text
stringlengths 55
456k
| metadata
dict |
---|---|
# FP Guide
**date-fns** v2.x provides [functional programming](https://en.wikipedia.org/wiki/Functional_programming) (FP)
friendly functions, like those in [lodash](https://github.com/lodash/lodash/wiki/FP-Guide),
that support [currying](https://en.wikipedia.org/wiki/Currying).
## Table of Contents
- [Usage](#usage)
- [Using Function Composition](#using-function-composition)
## Usage
FP functions are provided via `'date-fns/fp'` submodule.
Functions with options (`format`, `parse`, etc.) have two FP counterparts:
one that has the options object as its first argument and one that hasn't.
The name of the former has `WithOptions` added to the end of its name.
In **date-fns'** FP functions, the order of arguments is reversed.
```javascript
import { addYears, formatWithOptions } from "date-fns/fp";
import { eo } from "date-fns/locale";
import toUpper from "lodash/fp/toUpper"; // 'date-fns/fp' is compatible with 'lodash/fp'!
// If FP function has not received enough arguments, it returns another function
const addFiveYears = addYears(5);
// Several arguments can be curried at once
const dateToString = formatWithOptions({ locale: eo }, "d MMMM yyyy");
const dates = [
new Date(2017, 0 /* Jan */, 1),
new Date(2017, 1 /* Feb */, 11),
new Date(2017, 6 /* Jul */, 2),
];
const formattedDates = dates.map(addFiveYears).map(dateToString).map(toUpper);
//=> ['1 JANUARO 2022', '11 FEBRUARO 2022', '2 JULIO 2022']
```
## Using Function Composition
The main advantage of FP functions is support of functional-style
[function composing](https://medium.com/making-internets/why-using-chain-is-a-mistake-9bc1f80d51ba).
In the example above, you can compose `addFiveYears`, `dateToString` and `toUpper` into a single function:
```javascript
const formattedDates = dates.map((date) =>
toUpper(dateToString(addFiveYears(date))),
);
```
Or you can use `compose` function provided by [lodash](https://lodash.com) to do the same in more idiomatic way:
```javascript
import { compose } from "lodash/fp/compose";
const formattedDates = dates.map(compose(toUpper, dateToString, addFiveYears));
```
Or if you prefer natural direction of composing (as opposed to the computationally correct order),
you can use lodash' `flow` instead:
```javascript
import flow from "lodash/fp/flow";
const formattedDates = dates.map(flow(addFiveYears, dateToString, toUpper));
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/fp.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/fp.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2384
} |
# Getting Started
## Table of Contents
- [Introduction](#introduction)
- [Submodules](#submodules)
- [Installation](#installation)
## Introduction
**date-fns** provides the most comprehensive, yet simple and consistent toolset
for manipulating **JavaScript dates** in **a browser** & **Node.js**.
**date-fns** is like [lodash](https://lodash.com) for dates. It has
[**200+ functions** for all occasions](https://date-fns.org/docs/).
```js
import { format, compareAsc } from "date-fns";
format(new Date(2014, 1, 11), "MM/dd/yyyy");
//=> '02/11/2014'
const dates = [
new Date(1995, 6, 2),
new Date(1987, 1, 11),
new Date(1989, 6, 10),
];
dates.sort(compareAsc);
//=> [
// Wed Feb 11 1987 00:00:00,
// Mon Jul 10 1989 00:00:00,
// Sun Jul 02 1995 00:00:00
// ]
```
## Submodules
**date-fns** includes some optional features as submodules in the npm package.
Here is the list of them, in order of nesting:
- FP — functional programming-friendly variations of the functions. See [FP Guide](https://date-fns.org/docs/FP-Guide);
The later submodules are also included inside the former if you want to use multiple features from the list.
To use submodule features, [install the npm package](#npm) and then import a function from a submodule:
```js
// The main submodule:
import { addDays } from "date-fns";
// FP variation:
import { addDays, format } from "date-fns/fp";
```
## Installation
The library is available as an [npm package](https://www.npmjs.com/package/date-fns).
To install the package, run:
```bash
npm install date-fns --save
# or
yarn add date-fns
```
Start using:
```js
import { formatDistance, subDays } from "date-fns";
formatDistance(subDays(new Date(), 3), new Date(), { addSuffix: true });
//=> "3 days ago"
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/gettingStarted.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/gettingStarted.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1763
} |
# Internationalization
## Table of Contents
- [Usage](#usage)
- [Adding New Language](#adding-new-language)
## Usage
There are just a few functions that support I18n:
- [`format`](https://date-fns.org/docs/format)
- [`formatDistance`](https://date-fns.org/docs/formatDistance)
- [`formatDistanceStrict`](https://date-fns.org/docs/formatDistanceStrict)
- [`formatRelative`](https://date-fns.org/docs/formatRelative)
To use a locale, you need to require it and then pass
as an option to a function:
```js
import { formatDistance } from "date-fns";
// Require Esperanto locale
import { eo } from "date-fns/locale";
const result = formatDistance(
new Date(2016, 7, 1),
new Date(2015, 0, 1),
{ locale: eo }, // Pass the locale as an option
);
//=> 'pli ol 1 jaro'
```
It might seem complicated to require and pass locales as options,
but unlike Moment.js which bloats your build with all the locales
by default date-fns forces developer to manually require locales when needed.
To make API simple, we encourage you to write tiny wrappers and use those
instead of original functions:
```js
// app/_lib/format.js
import { format } from "date-fns";
import { enGB, eo, ru } from "date-fns/locale";
const locales = { enGB, eo, ru };
// by providing a default string of 'PP' or any of its variants for `formatStr`
// it will format dates in whichever way is appropriate to the locale
export default function (date, formatStr = "PP") {
return format(date, formatStr, {
locale: locales[window.__localeId__], // or global.__localeId__
});
}
// Later:
import format from "app/_lib/format";
window.__localeId__ = "enGB";
format(friday13, "EEEE d");
//=> 'Friday 13'
window.__localeId__ = "eo";
format(friday13, "EEEE d");
//=> 'vendredo 13'
// If the format string is omitted, it will take the default for the locale.
window.__localeId__ = "enGB";
format(friday13);
//=> Jul 13, 2019
window.__localeId__ = "eo";
format(friday13);
//=> 2019-jul-13
```
## Adding New Language
At the moment there is no definitive guide, so if you feel brave enough,
use this quick guide:
- First of all, [create an issue](https://github.com/date-fns/date-fns/issues/new?title=XXX%20language%20support)
so you won't overlap with others.
- A detailed explanation of how to [add a new locale](https://github.com/date-fns/date-fns/blob/master/docs/i18nContributionGuide.md#adding-a-new-locale).
- Use [English locale](https://github.com/date-fns/date-fns/tree/master/src/locale/en-US)
as the basis and then incrementally adjust the tests and the code.
- Directions on [adding a locale with the same language as another locale](https://github.com/date-fns/date-fns/blob/master/docs/i18nContributionGuide.md#creating-a-locale-with-the-same-language-as-another-locale).
- If you have questions or need guidance, leave a comment in the issue.
Thank you for your support! | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/i18n.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/i18n.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2869
} |
# I18n Contribution Guide
## Table of Contents
- [Adding a new locale](#adding-a-new-locale)
- [Choosing a directory name for a locale](#choosing-a-directory-name-for-a-locale)
- [index.js](#index.js)
- [localize](#localize)
- [localize.ordinalNumber](#localize.ordinalnumber)
- [localize.era and using buildLocalizeFn function](#localize.era-and-using-buildlocalizefn-function)
- [Formatting localizers](#formatting-localizers)
- [localize.quarter](#localize.quarter)
- [localize.month](#localize.month)
- [localize.day](#localize.day)
- [localize.dayPeriod](#localize.dayperiod)
- [formatLong](#formatlong)
- [formatLong.dateFormats](#formatlong.dateformats)
- [formatLong.timeFormats](#formatlong.timeformats)
- [formatLong.dateTimeFormats](#formatlong.datetimeformats)
- [formatRelative](#formatrelative)
- [match](#match)
- [formatDistance](#formatdistance)
- [Tests](#tests)
- [Creating a locale with the same language as another locale](#creating-a-locale-with-the-same-language-as-another-locale)
## Adding a new locale
To add a new locale:
- [Choose a directory name for it](#choosing-a-directory-name-for-a-locale).
- Copy the content of an existing locale (e.g. `en-US`) into the newly created directory.
- Replace the values in the content with yours file-by-file.
Use [CLDR data](https://www.unicode.org/cldr/charts/32/summary/root.html)
as a point of reference which values to choose.
All locales contain a number of properties:
- [`formatDistance`](#formatdistance) — distance localizer function used by `formatDistance` and `formatDistanceStrict`.
- [`formatLong`](#formatlong) — contains long date localizer functions used by `format` and `formatRelative`.
- [`formatRelative`](#formatrelative) — relative date localizer function used by `formatRelative`.
- [`localize`](#localize) — contains functions, which localize the various date values. Required by `format` and `formatRelative`.
- [`match`](#match) — contains functions to parse date values. Required by `parse`.
- [`options`](#indexjs) — contains the index of the first day of the week for functions such as `startOfWeek`,
and the value which determines the first week of the year
for functions like `setWeek`.
### Choosing a directory name for a locale
Use the four letter code for the directory name (e.g. `en-GB`),
Use the two/three letter code:
- if the language code and the country code are the same (e.g. `pt` instead of `pt-PT`).
- if the language is used in only one country (e.g. `fil` instead of `fil-PH`).
- if all countries who use the language
also use the same regional standards: the first day of the week,
the week numbering (see: https://en.wikipedia.org/wiki/Week#The_ISO_week_date_system),
calendar date format (see: https://en.wikipedia.org/wiki/Calendar_date)
and date representation (see: https://en.wikipedia.org/wiki/Date_and_time_representation_by_country
and: https://en.wikipedia.org/wiki/Date_format_by_country)
(e.g. `ca` instead of `ca-ES` and `ca-AD`).
### index.js
Locale's `index.js` is where all the properties of the locale are combined in a single file,
documented in JSDoc format.
```javascript
import formatDistance from "./_lib/formatDistance/index.js";
import formatLong from "./_lib/formatLong/index.js";
import formatRelative from "./_lib/formatRelative/index.js";
import localize from "./_lib/localize/index.js";
import match from "./_lib/match/index.js";
/**
* @type {Locale}
* @category Locales
*
* // Name of the locale.
* // Inside the parentheses - name of the country - if the locale uses the four letter code, e.g. en-US, fr-CA or pt-BR.
* @summary English locale (United States).
*
* // Name of the language (used by https://date-fns.org/ website)
* @language English
*
* // ISO 639-2 code. See the list here:
* // https://www.loc.gov/standards/iso639-2/php/code_list.php
* // Used by https://date-fns.org/ to detect the list of the countries that uses the language.
* @iso-639-2 eng
*
* // Authors of the locale (including anyone who corrected or fixed the locale)
* @author Sasha Koss [@kossnocorp]{@link https://github.com/kossnocorp}
* @author Lesha Koss [@leshakoss]{@link https://github.com/leshakoss}
*/
var locale = {
code: "en",
formatDistance: formatDistance,
formatLong: formatLong,
formatRelative: formatRelative,
localize: localize,
match: match,
options: {
// Index of the first day of the week.
// Sunday is 0, Monday is 1, Saturday is 6.
weekStartsOn: 0,
// Nth of January which is always in the first week of the year. See:
// https://en.wikipedia.org/wiki/Week#The_ISO_week_date_system
// http://www.pjh2.de/datetime/weeknumber/wnd.php?l=en
firstWeekContainsDate: 1,
},
};
export default locale;
```
### localize
Put this object in `_lib/localize/index.js` inside your locale directory.
Contains a number of functions for used by `format`:
```js
var localize = {
ordinalNumber,
era,
quarter,
month,
day,
dayPeriod,
};
export default localize;
```
#### localize.ordinalNumber
Function that takes a numeric argument and returns a string with ordinal number:
```js
// In `en-US` locale:
function ordinalNumber(dirtyNumber, dirtyOptions) {
var number = Number(dirtyNumber);
var rem100 = number % 100;
if (rem100 > 20 || rem100 < 10) {
switch (rem100 % 10) {
case 1:
return number + "st";
case 2:
return number + "nd";
case 3:
return number + "rd";
}
}
return number + "th";
}
var localize = {
ordinalNumber: ordinalNumber,
// ...
};
```
If the form of the ordinal number depends on the grammatical case (or other grammatical structures),
use `options.unit` argument which could be one of the values 'year', 'quarter', 'month', 'week',
'date', 'dayOfYear', 'day', 'hour', 'minute' or 'second':
```js
// In `ru` locale:
function ordinalNumber(dirtyNumber, dirtyOptions) {
var options = dirtyOptions || {};
var unit = String(options.unit);
var suffix;
if (unit === "date") {
suffix = "-е";
} else if (unit === "week" || unit === "minute" || unit === "second") {
suffix = "-я";
} else {
suffix = "-й";
}
return dirtyNumber + suffix;
}
```
#### localize.era and using buildLocalizeFn function
Localizes a numeric era. Takes either 0 or 1 as the first argument.
As with many of the `localize` functions, they can be generated by built-in
`buildLocalizeFn` function.
From the CLDR chart, use ['Date & Time'/'Gregorian'/'Eras'](https://www.unicode.org/cldr/charts/32/summary/en.html#1771) values.
```js
// In `en-US` locale:
import buildLocalizeFn from "../../../_lib/buildLocalizeFn/index.js";
var eraValues = {
narrow: ["B", "A"],
abbreviated: ["BC", "AD"],
wide: ["Before Christ", "Anno Domini"],
};
var localize = {
// ...
era: buildLocalizeFn({
values: eraValues,
defaultWidth: "wide",
}),
// ...
};
export default localize;
```
General usage of the function:
```js
var result = locale.localize.era(1, { width: "abbreviated" });
//=> 'AD'
```
If `width` is not provided or the `values` object does not contain values for the provided width,
`defaultWidth` will be used. `defaultWidth` should indicate the longest form of the localized value.
The same is true for all other `localize` functions.
`width` for `localize.era` function could be either 'narrow', 'abbreviated' or 'wide'.
```js
var result = locale.localize.era(1, { width: "foobar" });
//=> 'Anno Domini'
```
#### Formatting localizers
For some languages, there is a difference between "stand-alone" localizers and "formatting" localizers.
"Stand-alone" means that the resulting value should make grammatical sense without context.
"Formatting" means that the resulting value should be decided using the grammar rules of the language
as if the value was a part of a date.
For example, for languages with grammatical cases, the stand-alone month could be in the nominative case ("January"),
and the formatting month could decline as a part of the phrase "1st of January".
In this case, use parameters `formattingValues` and `defaultFormattingWidth` of `buildLocalizeFn` function.
Any localizer could be stand-alone and formatting.
Check the CLDR chart for the unit to see if stand-alone and formatting values are different for a certain unit.
If there's no difference (usually it happens in languages without grammatical cases),
parameters `formattingValues` and `defaultFormattingWidth` are not needed.
In this example, in Russian language a stand-alone month is in the nominative case ("январь"),
and formatting month is in the genitive case ("января" as in "1-е января"). Notice the different endings:
```js
// In `ru` locale:
var monthValues = {
narrow: ["Я", "Ф", "М", "А", "М", "И", "И", "А", "С", "О", "Н", "Д"],
abbreviated: [
"янв.",
"фев.",
"март",
"апр.",
"май",
"июнь",
"июль",
"авг.",
"сент.",
"окт.",
"нояб.",
"дек.",
],
wide: [
"январь",
"февраль",
"март",
"апрель",
"май",
"июнь",
"июль",
"август",
"сентябрь",
"октябрь",
"ноябрь",
"декабрь",
],
};
var formattingMonthValues = {
narrow: ["Я", "Ф", "М", "А", "М", "И", "И", "А", "С", "О", "Н", "Д"],
abbreviated: [
"янв.",
"фев.",
"мар.",
"апр.",
"мая",
"июн.",
"июл.",
"авг.",
"сент.",
"окт.",
"нояб.",
"дек.",
],
wide: [
"января",
"февраля",
"марта",
"апреля",
"мая",
"июня",
"июля",
"августа",
"сентября",
"октября",
"ноября",
"декабря",
],
};
var localize = {
// ...
month: buildLocalizeFn({
values: monthValues,
defaultWidth: "wide",
formattingValues: formattingMonthValues,
defaultFormattingWidth: "wide",
}),
// ...
};
export default localize;
```
#### localize.quarter
Localizes a quarter. Takes 1, 2, 3 or 4 as the first argument.
`width` could be either 'narrow', 'abbreviated' or 'wide'.
From the CLDR chart, use ['Date & Time'/'Gregorian'/'Quarters'](https://www.unicode.org/cldr/charts/32/summary/en.html#1781) values.
```js
// In `en-US` locale:
import buildLocalizeFn from "../../../_lib/buildLocalizeFn/index.js";
var quarterValues = {
narrow: ["1", "2", "3", "4"],
abbreviated: ["Q1", "Q2", "Q3", "Q4"],
wide: ["1st quarter", "2nd quarter", "3rd quarter", "4th quarter"],
};
var localize = {
// ...
quarter: buildLocalizeFn({
values: quarterValues,
defaultWidth: "wide",
argumentCallback: function (quarter) {
return Number(quarter) - 1;
},
}),
// ...
};
export default localize;
```
Note the usage of `argumentCallback` here. It converts the value passed into `localize.quarter` function
(one of 1, 2, 3 or 4) into the index of the values array inside `quarterValues` (one of 0, 1, 2 or 3).
#### localize.month
Localizes a month. Takes numbers between 0 (for January) and 11 (for December).
`width` could be either 'narrow', 'abbreviated' or 'wide'.
From the CLDR chart, use ['Date & Time'/'Gregorian'/'Months'](https://www.unicode.org/cldr/charts/32/summary/en.html#1793) values.
```js
// In `en-US` locale:
import buildLocalizeFn from "../../../_lib/buildLocalizeFn/index.js";
var monthValues = {
narrow: ["J", "F", "M", "A", "M", "J", "J", "A", "S", "O", "N", "D"],
abbreviated: [
"Jan",
"Feb",
"Mar",
"Apr",
"May",
"Jun",
"Jul",
"Aug",
"Sep",
"Oct",
"Nov",
"Dec",
],
wide: [
"January",
"February",
"March",
"April",
"May",
"June",
"July",
"August",
"September",
"October",
"November",
"December",
],
};
var localize = {
// ...
month: buildLocalizeFn({
values: monthValues,
defaultWidth: "wide",
}),
// ...
};
export default localize;
```
**NOTE**: in English, the names of days of the week and months are capitalized.
Check if the same is true for the language you're working on.
Generally, formatted dates should look like they are in the middle of a sentence,
e.g. in Spanish language the weekdays and months should be in the lowercase:
```js
// In `es` locale:
var monthValues = {
narrow: ["E", "F", "M", "A", "M", "J", "J", "A", "S", "O", "N", "D"],
abbreviated: [
"ene.",
"feb.",
"mar.",
"abr.",
"may.",
"jun.",
"jul.",
"ago.",
"sep.",
"oct.",
"nov.",
"dic.",
],
wide: [
"enero",
"febrero",
"marzo",
"abril",
"mayo",
"junio",
"julio",
"agosto",
"septiembre",
"octubre",
"noviembre",
"diciembre",
],
};
```
`monthValues.narrow` are usually capitalized in every language. Check the CLDR chart for your language.
#### localize.day
Localizes a week day. Takes numbers between 0 (for Sunday) and 6 (for Saturday).
`width` could be either 'narrow', 'short', 'abbreviated' or 'wide'.
From the CLDR chart, use ['Date & Time'/'Gregorian'/'Days'](https://www.unicode.org/cldr/charts/32/summary/en.html#1829) values.
```js
// In `en-US` locale:
import buildLocalizeFn from "../../../_lib/buildLocalizeFn/index.js";
var dayValues = {
narrow: ["S", "M", "T", "W", "T", "F", "S"],
short: ["Su", "Mo", "Tu", "We", "Th", "Fr", "Sa"],
abbreviated: ["Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"],
wide: [
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday",
],
};
var localize = {
// ...
day: buildLocalizeFn({
values: dayValues,
defaultWidth: "wide",
}),
// ...
};
export default localize;
```
**NOTE**: the rules of capitalization from `localize.month` are also true for `localize.day`.
#### localize.dayPeriod
Localizes a certain day period.
Could take one of these strings as the argument: 'am', 'pm', 'midnight', 'noon', 'morning', 'afternoon', 'evening', 'night'.
`width` could be either 'narrow', 'abbreviated' or 'wide'.
From the CLDR chart, use ['Date & Time'/'Gregorian'/'Day periods'](https://www.unicode.org/cldr/charts/32/summary/en.html#1857) values.
```js
// In `en-US` locale:
import buildLocalizeFn from "../../../_lib/buildLocalizeFn/index.js";
var dayPeriodValues = {
narrow: {
am: "a",
pm: "p",
midnight: "mi",
noon: "n",
morning: "in the morning",
afternoon: "in the afternoon",
evening: "in the evening",
night: "at night",
},
abbreviated: {
am: "AM",
pm: "PM",
midnight: "midnight",
noon: "noon",
morning: "in the morning",
afternoon: "in the afternoon",
evening: "in the evening",
night: "at night",
},
wide: {
am: "a.m.",
pm: "p.m.",
midnight: "midnight",
noon: "noon",
morning: "in the morning",
afternoon: "in the afternoon",
evening: "in the evening",
night: "at night",
},
};
var localize = {
// ...
dayPeriod: buildLocalizeFn({
values: dayPeriodValues,
defaultWidth: "wide",
}),
};
export default localize;
```
### formatLong
Put this object in `_lib/formatLong/index.js` inside your locale directory.
Locale date formats written in `format` token string format.
See the list of tokens: https://date-fns.org/docs/format
Use https://en.wikipedia.org/wiki/Date_format_by_country and CLDR chart as the reference.
#### formatLong.dateFormats
Use ['Date & Time'/'Gregorian'/'Formats - Standard - Date Formats'](https://www.unicode.org/cldr/charts/32/summary/en.html#1901) values
from the CLDR chart as a reference.
```js
// In `en-US` locale
import buildFormatLongFn from "../../../_lib/buildFormatLongFn/index.js";
var dateFormats = {
full: "EEEE, MMMM do, y",
long: "MMMM do, y",
medium: "MMM d, y",
short: "MM/dd/yyyy",
};
var formatLong = {
date: buildFormatLongFn({
formats: dateFormats,
defaultWidth: "full",
}),
// ...
};
export default formatLong;
```
`dateFormats.long` usually contains the longest form of writing the year, the month, and the day of the month.
Use ordinal day of the month ('do' token) where applicable (date-fns, unlike CLDR supports ordinal numbers).
`dateFormats.full` contains the same but with the day of the week.
`dateFormats.medium` contains the same values as `dateFormats.long`, but with short form of month and non-ordinal day.
`dateFormats.short` usually contains a strictly numerical form of the date.
Pay attention to the order of units (big-, little- or middle-endian)
#### formatLong.timeFormats
Use ['Date & Time'/'Gregorian'/'Formats - Standard - Time Formats'](https://www.unicode.org/cldr/charts/32/summary/en.html#1906) values
from the CLDR chart as a reference.
Use some variation of 'h:mm aa' for 12-hour clock locales or 'H:mm' for 24-hour clock locales. Use the local time separator.
```js
// In `en-US` locale
import buildFormatLongFn from "../../../_lib/buildFormatLongFn/index.js";
var timeFormats = {
full: "h:mm:ss a zzzz",
long: "h:mm:ss a z",
medium: "h:mm:ss a",
short: "h:mm a",
};
var formatLong = {
// ...
time: buildFormatLongFn({
formats: timeFormats,
defaultWidth: "full",
}),
// ...
};
export default formatLong;
```
#### formatLong.dateTimeFormats
Use
['Date & Time'/'Gregorian'/'Formats - Standard - Date & Time Combination Formats'](https://www.unicode.org/cldr/charts/32/summary/en.html#1910)
values from the CLDR chart.
```js
// In `en-US` locale
import buildFormatLongFn from "../../../_lib/buildFormatLongFn/index.js";
var dateTimeFormats = {
full: "{{date}} 'at' {{time}}",
long: "{{date}} 'at' {{time}}",
medium: "{{date}}, {{time}}",
short: "{{date}}, {{time}}",
};
var formatLong = {
// ...
dateTime: buildFormatLongFn({
formats: dateTimeFormats,
defaultWidth: "full",
}),
};
export default formatLong;
```
'{{date}}' and '{{time}}' from the strings will be replaced with the date and time respectively.
### formatRelative
Put this function in `_lib/formatRelative/index.js` inside your locale directory.
Relative date formats written in `format` token string format.
See the list of tokens: https://date-fns.org/docs/format.
Has to process `lastWeek`, `yesterday`, `today`, `tomorrow`, `nextWeek` and `other` tokens.
```javascript
// In `en-US` locale
var formatRelativeLocale = {
lastWeek: "'last' eeee 'at' p",
yesterday: "'yesterday at' p",
today: "'today at' p",
tomorrow: "'tomorrow at' p",
nextWeek: "eeee 'at' p",
other: "P",
};
export default function formatRelative(token, date, baseDate, options) {
return formatRelativeLocale[token];
}
```
You can use `date` and `baseDate` supplied to the function for the difficult situations
(e.g. grammatical genders and cases of the days of the week).
Example is below. Note the different grammatical case for weekdays (accusative instead of nominative)
and declension of word "прошлый" which depends on the grammatical gender of the weekday:
```javascript
// In `ru` locale
var accusativeWeekdays = [
"воскресенье",
"понедельник",
"вторник",
"среду",
"четверг",
"пятницу",
"субботу",
];
function lastWeek(day) {
var weekday = accusativeWeekdays[day];
switch (day) {
case 0:
return "'в прошлое " + weekday + " в' p";
case 1:
case 2:
case 4:
return "'в прошлый " + weekday + " в' p";
case 3:
case 5:
case 6:
return "'в прошлую " + weekday + " в' p";
}
}
function thisWeek(day) {
// ...
}
function nextWeek(day) {
// ...
}
var formatRelativeLocale = {
lastWeek: function (date, baseDate, options) {
var day = date.getDay();
if (isSameUTCWeek(date, baseDate, options)) {
return thisWeek(day);
} else {
return lastWeek(day);
}
},
yesterday: "'вчера в' p",
today: "'сегодня в' p",
tomorrow: "'завтра в' p",
nextWeek: function (date, baseDate, options) {
var day = date.getDay();
if (isSameUTCWeek(date, baseDate, options)) {
return thisWeek(day);
} else {
return nextWeek(day);
}
},
other: "P",
};
export default function formatRelative(token, date, baseDate, options) {
var format = formatRelativeLocale[token];
if (typeof format === "function") {
return format(date, baseDate, options);
}
return format;
}
```
### match
Put this object in `_lib/match/index.js` inside your locale directory.
Contains the functions used by `parse` to parse a localized value:
```js
// In `en-US` locale:
import buildMatchPatternFn from "../../../_lib/buildMatchPatternFn/index.js";
import buildMatchFn from "../../../_lib/buildMatchFn/index.js";
var matchOrdinalNumberPattern = /^(\d+)(th|st|nd|rd)?/i;
var parseOrdinalNumberPattern = /\d+/i;
var matchEraPatterns = {
narrow: /^(b|a)/i,
abbreviated: /^(b\.?\s?c\.?|b\.?\s?c\.?\s?e\.?|a\.?\s?d\.?|c\.?\s?e\.?)/i,
wide: /^(before christ|before common era|anno domini|common era)/i,
};
var parseEraPatterns = {
any: [/^b/i, /^(a|c)/i],
};
var matchQuarterPatterns = {
narrow: /^[1234]/i,
abbreviated: /^q[1234]/i,
wide: /^[1234](th|st|nd|rd)? quarter/i,
};
var parseQuarterPatterns = {
any: [/1/i, /2/i, /3/i, /4/i],
};
var matchMonthPatterns = {
narrow: /^[jfmasond]/i,
abbreviated: /^(jan|feb|mar|apr|may|jun|jul|aug|sep|oct|nov|dec)/i,
wide: /^(january|february|march|april|may|june|july|august|september|october|november|december)/i,
};
var parseMonthPatterns = {
narrow: [
/^j/i,
/^f/i,
/^m/i,
/^a/i,
/^m/i,
/^j/i,
/^j/i,
/^a/i,
/^s/i,
/^o/i,
/^n/i,
/^d/i,
],
any: [
/^ja/i,
/^f/i,
/^mar/i,
/^ap/i,
/^may/i,
/^jun/i,
/^jul/i,
/^au/i,
/^s/i,
/^o/i,
/^n/i,
/^d/i,
],
};
var matchDayPatterns = {
narrow: /^[smtwf]/i,
short: /^(su|mo|tu|we|th|fr|sa)/i,
abbreviated: /^(sun|mon|tue|wed|thu|fri|sat)/i,
wide: /^(sunday|monday|tuesday|wednesday|thursday|friday|saturday)/i,
};
var parseDayPatterns = {
narrow: [/^s/i, /^m/i, /^t/i, /^w/i, /^t/i, /^f/i, /^s/i],
any: [/^su/i, /^m/i, /^tu/i, /^w/i, /^th/i, /^f/i, /^sa/i],
};
var matchDayPeriodPatterns = {
narrow: /^(a|p|mi|n|(in the|at) (morning|afternoon|evening|night))/i,
any: /^([ap]\.?\s?m\.?|midnight|noon|(in the|at) (morning|afternoon|evening|night))/i,
};
var parseDayPeriodPatterns = {
any: {
am: /^a/i,
pm: /^p/i,
midnight: /^mi/i,
noon: /^no/i,
morning: /morning/i,
afternoon: /afternoon/i,
evening: /evening/i,
night: /night/i,
},
};
var match = {
ordinalNumber: buildMatchPatternFn({
matchPattern: matchOrdinalNumberPattern,
parsePattern: parseOrdinalNumberPattern,
valueCallback: function (value) {
return parseInt(value, 10);
},
}),
era: buildMatchFn({
matchPatterns: matchEraPatterns,
defaultMatchWidth: "wide",
parsePatterns: parseEraPatterns,
defaultParseWidth: "any",
}),
quarter: buildMatchFn({
matchPatterns: matchQuarterPatterns,
defaultMatchWidth: "wide",
parsePatterns: parseQuarterPatterns,
defaultParseWidth: "any",
valueCallback: function (index) {
return index + 1;
},
}),
month: buildMatchFn({
matchPatterns: matchMonthPatterns,
defaultMatchWidth: "wide",
parsePatterns: parseMonthPatterns,
defaultParseWidth: "any",
}),
day: buildMatchFn({
matchPatterns: matchDayPatterns,
defaultMatchWidth: "wide",
parsePatterns: parseDayPatterns,
defaultParseWidth: "any",
}),
dayPeriod: buildMatchFn({
matchPatterns: matchDayPeriodPatterns,
defaultMatchWidth: "any",
parsePatterns: parseDayPeriodPatterns,
defaultParseWidth: "any",
}),
};
export default match;
```
These functions mirror those in `localize`.
For `matchPatterns` the patterns should match the whole meaningful word for the parsed value
(which will be cut from the string in the process of parsing).
`parsePatterns` contains patterns to detect one of the values from the result of `matchPatterns`
Note that the patterns for `parsePatterns` don't necessary contain the whole word:
```javascript
// In `en-US` locale:
var parseDayPatterns = {
narrow: [/^s/i, /^m/i, /^t/i, /^w/i, /^t/i, /^f/i, /^s/i],
any: [/^su/i, /^m/i, /^tu/i, /^w/i, /^th/i, /^f/i, /^sa/i],
};
```
but only the bare minimum to parse the value.
Also note that all patterns have "case-insensitive" flags
to match as much arbitrary user input as possible. For the same reason, try to match
any variation of diacritical marks:
```javascript
// In `eo` locale:
var matchDayPatterns = {
narrow: /^[dlmĵjvs]/i,
short: /^(di|lu|ma|me|(ĵ|jx|jh|j)a|ve|sa)/i,
abbreviated: /^(dim|lun|mar|mer|(ĵ|jx|jh|j)a(ŭ|ux|uh|u)|ven|sab)/i,
wide: /^(diman(ĉ|cx|ch|c)o|lundo|mardo|merkredo|(ĵ|jx|jh|j)a(ŭ|ux|uh|u)do|vendredo|sabato)/i,
};
var parseDayPatterns = {
narrow: [/^d/i, /^l/i, /^m/i, /^m/i, /^(j|ĵ)/i, /^v/i, /^s/i],
any: [/^d/i, /^l/i, /^ma/i, /^me/i, /^(j|ĵ)/i, /^v/i, /^s/i],
};
```
Here, for the word "dimanĉo" the functions will match also "dimancxo", "dimancho"
and even grammatically incorrect "dimanco".
Try to match any possible way of writing the word. Don't forget the grammatical cases:
```javascript
// In `ru` locale:
var matchMonthPatterns = {
narrow: /^[яфмаисонд]/i,
abbreviated:
/^(янв|фев|март?|апр|ма[йя]|июн[ья]?|июл[ья]?|авг|сент?|окт|нояб?|дек)/i,
wide: /^(январ[ья]|феврал[ья]|марта?|апрел[ья]|ма[йя]|июн[ья]|июл[ья]|августа?|сентябр[ья]|октябр[ья]|октябр[ья]|ноябр[ья]|декабр[ья])/i,
};
```
and variations of short weekdays and months:
```javascript
// In `ru` locale:
var matchDayPatterns = {
narrow: /^[впсч]/i,
short: /^(вс|во|пн|по|вт|ср|чт|че|пт|пя|сб|су)\.?/i,
abbreviated: /^(вск|вос|пнд|пон|втр|вто|срд|сре|чтв|чет|птн|пят|суб).?/i,
wide: /^(воскресень[ея]|понедельника?|вторника?|сред[аы]|четверга?|пятниц[аы]|суббот[аы])/i,
};
```
(here, the `abbreviated` pattern will match both `вск` and `вос` as the short of `воскресенье` {Sunday})
In `match.ordinalNumber` match ordinal numbers as well as non-ordinal numbers:
```javascript
// In `en-US` locale:
var matchOrdinalNumberPattern = /^(\d+)(th|st|nd|rd)?/i;
```
Don't forget the grammatical genders:
```javascript
// In `ru` locale:
var matchOrdinalNumberPattern = /^(\d+)(-?(е|я|й|ое|ье|ая|ья|ый|ой|ий|ый))?/i;
```
### formatDistance
`formatDistance` property of locale is a function which takes three arguments:
token passed by date-fns' `formatDistance` function (e.g. 'lessThanXMinutes'),
a number of units to be displayed by the function
(e.g. `locale.formatDistance('lessThanXMinutes', 5)` would display localized 'less than 5 minutes')
and object with options.
Your best guess is to copy `formatDistance` property from another locale and change the values.
### Tests
To test locales we use snapshots. See [`en-US` snapshot](https://github.com/date-fns/date-fns/blob/master/src/locale/en-US/snapshot.md) for an example.
To generate snapshots, run `npm run locale-snapshots`. The snapshot for the locale
you're working on will appear in the root locale directory (e.g. `src/locales/ru/snapshot.md`).
Once you are done with the locale, generate the snapshot and review the output values.
## Creating a locale with the same language as another locale
Import the locale properties already implemented for the language,
but replace unique properties.
```javascript
// Same as en-US
import formatDistance from "../en-US/_lib/formatDistance/index.js";
import formatRelative from "../en-US/_lib/formatRelative/index.js";
import localize from "../en-US/_lib/localize/index.js";
import match from "../en-US/_lib/match/index.js";
// Unique for en-GB
import formatLong from "./_lib/formatLong/index.js";
/**
* @type {Locale}
* @category Locales
* @summary English locale (United Kingdom).
* @language English
* @iso-639-2 eng
* @author John Doe [@example]{@link https://github.com/example}
*/
var locale = {
formatDistance: formatDistance,
formatLong: formatLong,
formatRelative: formatRelative,
localize: localize,
match: match,
// Unique for en-GB
options: {
weekStartsOn: 1,
firstWeekContainsDate: 4,
},
};
export default locale;
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/i18nContributionGuide.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/i18nContributionGuide.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 28025
} |
# Releasing date-fns
1. First, make sure that the library is built by running `./scripts/build/build.sh` and committing and pushing any change you would have.
2. Then add the changelog entry generated by `npx tsx scripts/release/buildChangelog.ts` to (CHANGELOG.md)[../CHANGELOG.md]. Make sure that the output is valid Markdown and fix if there're any errors. Commit and push the file.
3. Using the version that the changelog script generated, run the command:
```bash
env VERSION="vX.XX.X" APP_ENV="production" GOOGLE_APPLICATION_CREDENTIALS="secrets/production/key.json" ./scripts/release/release.sh
```
The script will change `package.json`. **Do not commit the change, and reset it instead**.
4. Now when the package is published, go to [GitHub Releases](https://github.com/date-fns/date-fns/releases) and draft a new version using the changelog entry you generated earlier.
5. Finally, write an announce tweet using the created GitHub release as the tweet link.
You're done, great job! | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/release.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/release.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1010
} |
# Time Zones
## Table of Contents
- [Overview](#overview)
- [`date-fns-tz`](#date-fns-tz)
## Overview
Working with UTC or ISO date strings is easy, and so is working with JS dates when all times
are displayed in a user's local time in the browser. The difficulty comes when working with another
time zone's local time, other than the current system's, like showing the local time of an event in LA
at 8pm PST on a Node server in Europe or a user's machine set to EST.
In this case there are two relevant pieces of information:
- a fixed moment in time in the form of a timestamp, UTC or ISO date string, and
- the time zone descriptor, usually an offset or IANA time zone name (e.g. `America/Los_Angeles`).
Libraries like Moment and Luxon, which provide their own date time classes, manage these timestamp and time
zone values internally. Since `date-fns` always returns a plain JS Date, which implicitly has the current
system's time zone, helper functions are needed for handling common time zone related use cases.
## [`date-fns-tz`](https://www.npmjs.com/package/date-fns-tz)
Dependency free IANA time zone support is implemented via the
[Intl API](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl) to keep
actual time zone data out of code bundles. Modern browsers all support the
[necessary features](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/DateTimeFormat#Browser_compatibility),
and for those that don't a [polyfill](https://github.com/yahoo/date-time-format-timezone) can be used.
Functions are provided for converting to and from a Date instance which will have the internal UTC time
adjusted so it prints to the correct time value in the associated time zone, regardless of the current
system time zone. The `date-fns` `format` function is extended with support for the `z...zzzz` tokens to
format long and short time zone names.
Compatible with `date-fns` version 2
License: MIT
### Synopsis
```js
const { zonedTimeToUtc, utcToZonedTime, format } = require("date-fns-tz");
// Set the date to "2018-09-01T16:01:36.386Z"
const utcDate = zonedTimeToUtc("2018-09-01 18:01:36.386", "Europe/Berlin");
// Obtain a Date instance that will render the equivalent Berlin time for the UTC date
const date = new Date("2018-09-01T16:01:36.386Z");
const timeZone = "Europe/Berlin";
const zonedDate = utcToZonedTime(date, timeZone);
// zonedDate could be used to initialize a date picker or display the formatted local date/time
// Set the output to "1.9.2018 18:01:36.386 GMT+02:00 (CEST)"
const pattern = "d.M.yyyy HH:mm:ss.SSS 'GMT' XXX (z)";
const output = format(zonedDate, pattern, { timeZone: "Europe/Berlin" });
```
### Links
- [API / Usage Scenarios](https://github.com/marnusw/date-fns-tz#time-zone-helpers) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/timeZones.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/timeZones.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2810
} |
# Unicode Tokens
Starting with v2, `format` and `parse` use [Unicode tokens].
The tokens are different from Moment.js and other libraries that opted to use
custom formatting rules. While usage of a standard ensures compatibility and
the future of the library, it causes confusion that this document intends
to resolve.
## Popular mistakes
There are 4 tokens that cause most of the confusion:
- `D` and `DD` that represent the day of a year (1, 2, ..., 365, 366)
are often confused with `d` and `dd` that represent the day of a month
(1, 2, ..., 31).
- `YY` and `YYYY` that represent the local week-numbering year (44, 01, 00, 17)
are often confused with `yy` and `yyyy` that represent the calendar year.
```js
// ❌ Wrong!
format(new Date(), "YYYY-MM-DD");
//=> 2018-10-283
// ✅ Correct
format(new Date(), "yyyy-MM-dd");
//=> 2018-10-10
// ❌ Wrong!
parse("11.02.87", "D.MM.YY", new Date()).toString();
//=> 'Sat Jan 11 1986 00:00:00 GMT+0200 (EET)'
// ✅ Correct
parse("11.02.87", "d.MM.yy", new Date()).toString();
//=> 'Wed Feb 11 1987 00:00:00 GMT+0200 (EET)'
```
To help with the issue, `format` and `parse` functions won't accept
these tokens without `useAdditionalDayOfYearTokens` option for `D` and `DD` and
`useAdditionalWeekYearTokens` options for `YY` and `YYYY`:
```js
format(new Date(), "D", { useAdditionalDayOfYearTokens: true });
//=> '283'
parse("365+1987", "DD+YYYY", new Date(), {
useAdditionalDayOfYearTokens: true,
useAdditionalWeekYearTokens: true,
}).toString();
//=> 'Wed Dec 31 1986 00:00:00 GMT+0200 (EET)'
```
[Unicode tokens]: https://www.unicode.org/reports/tr35/tr35-dates.html#Date_Field_Symbol_Table | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/unicodeTokens.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/unicodeTokens.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1652
} |
# webpack
## Removing unused languages from dynamic import
If a locale is imported dynamically, then all locales from date-fns are loaded by webpack into a bundle (~160kb) or split across the chunks. This prolongs the build process and increases the amount of space taken. However, it is possible to use webpack to trim down languages using [ContextReplacementPlugin].
Let's assume that we have a single point in which supported locales are present:
`config.js`:
```js
// `see date-fns/src/locale` for available locales
export const supportedLocales = ["en-US", "de", "pl", "it"];
```
We could also have a function that formats the date:
```js
const getLocale = (locale) => import(`date-fns/locale/${locale}/index.js`); // or require() if using CommonJS
const formatDate = (date, formatStyle, locale) => {
return format(date, formatStyle, {
locale: getLocale(locale),
});
};
```
In order to exclude unused languages we can use webpacks [ContextReplacementPlugin].
`webpack.config.js`:
```js
import webpack from 'webpack'
import { supportedLocales } from './config.js'
export default const config = {
plugins: [
new webpack.ContextReplacementPlugin(
/^date-fns[/\\]locale$/,
new RegExp(`\\.[/\\\\](${supportedLocales.join('|')})[/\\\\]index\\.js$`)
)
]
}
```
This results in a language bundle of ~23kb .
[contextreplacementplugin]: https://webpack.js.org/plugins/context-replacement-plugin/ | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/date-fns/docs/webpack.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/date-fns/docs/webpack.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1435
} |
# Explicit property check
Sometimes it is necessary to squeeze every once of performance out of your runtime code, and deep equality checks can be a bottleneck. When this is occurs, it can be advantageous to build a custom comparison that allows for highly specific equality checks.
An example where you know the shape of the objects being passed in, where the `foo` property is a simple primitive and the `bar` property is a nested object:
```ts
import { createCustomEqual } from 'fast-equals';
import type { TypeEqualityComparator } from 'fast-equals';
interface SpecialObject {
foo: string;
bar: {
baz: number;
};
}
const areObjectsEqual: TypeEqualityComparator<SpecialObject, undefined> = (
a,
b,
) => a.foo === b.foo && a.bar.baz === b.bar.baz;
const isSpecialObjectEqual = createCustomEqual({
createCustomConfig: () => ({ areObjectsEqual }),
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/recipes/explicit-property-check.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/recipes/explicit-property-check.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 877
} |
# Legacy environment support for circular equal comparators
Starting in `4.x.x`, `WeakMap` is expected to be available in the environment. All modern browsers support this global object, however there may be situations where a legacy environmental support is required (example: IE11). If you need to support such an environment and polyfilling is not an option, creating a custom comparator that uses a custom cache implementation with the same contract is a simple solution.
```ts
import { createCustomEqual, sameValueZeroEqual } from 'fast-equals';
import type { Cache } from 'fast-equals';
function getCache(): Cache<any, any> {
const entries: Array<[object, any]> = [];
return {
delete(key) {
for (let index = 0; index < entries.length; ++index) {
if (entries[index][0] === key) {
entries.splice(index, 1);
return true;
}
}
return false;
},
get(key) {
for (let index = 0; index < entries.length; ++index) {
if (entries[index][0] === key) {
return entries[index][1];
}
}
},
set(key, value) {
for (let index = 0; index < entries.length; ++index) {
if (entries[index][0] === key) {
entries[index][1] = value;
return this;
}
}
entries.push([key, value]);
return this;
},
};
}
interface Meta {
customMethod(): void;
customValue: string;
}
const meta = {
customMethod() {
console.log('hello!');
},
customValue: 'goodbye',
};
const circularDeepEqual = createCustomEqual<Cache>({
circular: true,
createState: () => ({
cache: getCache(),
meta,
}),
});
const circularShallowEqual = createCustomEqual<Cache>({
circular: true,
comparator: sameValueZeroEqual,
createState: () => ({
cache: getCache(),
meta,
}),
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/recipes/legacy-circular-equal-support.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/recipes/legacy-circular-equal-support.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1848
} |
# Legacy environment support for `RegExp` comparators
Starting in `4.x.x`, `RegExp.prototype.flags` is expected to be available in the environment. All modern browsers support this feature, however there may be situations where a legacy environmental support is required (example: IE11). If you need to support such an environment and polyfilling is not an option, creating a custom comparator that uses a more verbose comparison of all possible flags is a simple solution.
```ts
import { createCustomEqual, sameValueZeroEqual } from 'deep-Equals';
const areRegExpsEqual = (a: RegExp, b: RegExp) =>
a.source === b.source &&
a.global === b.global &&
a.ignoreCase === b.ignoreCase &&
a.multiline === b.multiline &&
a.unicode === b.unicode &&
a.sticky === b.sticky &&
a.lastIndex === b.lastIndex;
const deepEqual = createCustomEqual({
createCustomConfig: () => ({ areRegExpsEqual }),
});
const shallowEqual = createCustomEqual({
comparator: sameValueZero,
createCustomConfig: () => ({ areRegExpsEqual }),
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/recipes/legacy-regexp-support.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/recipes/legacy-regexp-support.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1033
} |
# Non-standard properties
Sometimes, objects require a comparison that extend beyond its own keys, or even its own properties or symbols. Using a custom object comparator with `createCustomEqual` allows these kinds of comparisons.
```ts
import { createCustomEqual } from 'fast-equals';
import type { TypeEqualityComparator } from 'fast-equals';
type AreObjectsEqual = TypeEqualityComparator<Record<any, any>, undefined>;
class HiddenProperty {
visible: boolean;
#hidden: string;
constructor(value: string) {
this.visible = true;
this.#hidden = value;
}
get hidden() {
return this.#hidden;
}
}
function createAreObjectsEqual(
areObjectsEqual: AreObjectsEqual,
): AreObjectsEqual {
return function (a, b, state) {
if (!areObjectsEqual(a, b, state)) {
return false;
}
const aInstance = a instanceof HiddenProperty;
const bInstance = b instanceof HiddenProperty;
if (aInstance || bInstance) {
return aInstance && bInstance && a.hidden === b.hidden;
}
return true;
};
}
const deepEqual = createCustomEqual({
createCustomConfig: ({ areObjectsEqual }) =>
createAreObjectsEqual(areObjectsEqual),
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/recipes/non-standard-properties.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/recipes/non-standard-properties.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1182
} |
# Using `meta` in comparison
Sometimes a "pure" equality between two objects is insufficient, because the comparison relies on some external state. While these kinds of scenarios should generally be avoided, it is possible to handle them with a custom internal comparator that checks `meta` values.
```ts
import { createCustomEqual } from 'fast-equals';
interface Meta {
value: string;
}
const meta: Meta = { value: 'baz' };
const deepEqual = createCustomEqual<Meta>({
createInternalComparator:
(compare) => (a, b, _keyA, _keyB, _parentA, _parentB, state) =>
compare(a, b, state) || a === state.meta.value || b === state.meta.value,
createState: () => ({ meta }),
});
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-equals/recipes/using-meta-in-comparison.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-equals/recipes/using-meta-in-comparison.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 692
} |
This directory is a fallback for `exports["./client"]` in the root `framer-motion` `package.json`. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/framer-motion/client/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/framer-motion/client/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 98
} |
This directory is a fallback for `exports["./dom"]` in the root `framer-motion` `package.json`. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/framer-motion/dom/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/framer-motion/dom/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 95
} |
# Security
Please email [@ljharb](https://github.com/ljharb) or see https://tidelift.com/security if you have a potential security vulnerability to report. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/function-bind/.github/SECURITY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/function-bind/.github/SECURITY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 156
} |
# marked(1) -- a javascript markdown parser
## SYNOPSIS
`marked` [`-o` <output file>] [`-i` <input file>] [`-s` <markdown string>] [`-c` <config file>] [`--help`] [`--version`] [`--tokens`] [`--no-clobber`] [`--pedantic`] [`--gfm`] [`--breaks`] [`--no-etc...`] [`--silent`] [filename]
## DESCRIPTION
marked is a full-featured javascript markdown parser, built for speed.
It also includes multiple GFM features.
## EXAMPLES
```sh
cat in.md | marked > out.html
```
```sh
echo "hello *world*" | marked
```
```sh
marked -o out.html -i in.md --gfm
```
```sh
marked --output="hello world.html" -i in.md --no-breaks
```
## OPTIONS
* -o, --output [output file]
Specify file output. If none is specified, write to stdout.
* -i, --input [input file]
Specify file input, otherwise use last argument as input file.
If no input file is specified, read from stdin.
* -s, --string [markdown string]
Specify string input instead of a file.
* -c, --config [config file]
Specify config file to use instead of the default `~/.marked.json` or `~/.marked.js` or `~/.marked/index.js`.
* -t, --tokens
Output a token list instead of html.
* -n, --no-clobber
Do not overwrite `output` if it exists.
* --pedantic
Conform to obscure parts of markdown.pl as much as possible.
Don't fix original markdown bugs.
* --gfm
Enable github flavored markdown.
* --breaks
Enable GFM line breaks. Only works with the gfm option.
* --no-breaks, -no-etc...
The inverse of any of the marked options above.
* --silent
Silence error output.
* -h, --help
Display help information.
## CONFIGURATION
For configuring and running programmatically.
Example
```js
import { Marked } from 'marked';
const marked = new Marked({ gfm: true });
marked.parse('*foo*');
```
## BUGS
Please report any bugs to <https://github.com/markedjs/marked>.
## LICENSE
Copyright (c) 2011-2014, Christopher Jeffrey (MIT License).
## SEE ALSO
markdown(1), nodejs(1) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/marked/man/marked.1.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/marked/man/marked.1.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1924
} |
2.6.9 / 2017-09-22
==================
* remove ReDoS regexp in %o formatter (#504)
2.6.8 / 2017-05-18
==================
* Fix: Check for undefined on browser globals (#462, @marbemac)
2.6.7 / 2017-05-16
==================
* Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom)
* Fix: Inline extend function in node implementation (#452, @dougwilson)
* Docs: Fix typo (#455, @msasad)
2.6.5 / 2017-04-27
==================
* Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek)
* Misc: clean up browser reference checks (#447, @thebigredgeek)
* Misc: add npm-debug.log to .gitignore (@thebigredgeek)
2.6.4 / 2017-04-20
==================
* Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo)
* Chore: ignore bower.json in npm installations. (#437, @joaovieira)
* Misc: update "ms" to v0.7.3 (@tootallnate)
2.6.3 / 2017-03-13
==================
* Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts)
* Docs: Changelog fix (@thebigredgeek)
2.6.2 / 2017-03-10
==================
* Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin)
* Docs: Add backers and sponsors from Open Collective (#422, @piamancini)
* Docs: Add Slackin invite badge (@tootallnate)
2.6.1 / 2017-02-10
==================
* Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error
* Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0)
* Fix: IE8 "Expected identifier" error (#414, @vgoma)
* Fix: Namespaces would not disable once enabled (#409, @musikov)
2.6.0 / 2016-12-28
==================
* Fix: added better null pointer checks for browser useColors (@thebigredgeek)
* Improvement: removed explicit `window.debug` export (#404, @tootallnate)
* Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate)
2.5.2 / 2016-12-25
==================
* Fix: reference error on window within webworkers (#393, @KlausTrainer)
* Docs: fixed README typo (#391, @lurch)
* Docs: added notice about v3 api discussion (@thebigredgeek)
2.5.1 / 2016-12-20
==================
* Fix: babel-core compatibility
2.5.0 / 2016-12-20
==================
* Fix: wrong reference in bower file (@thebigredgeek)
* Fix: webworker compatibility (@thebigredgeek)
* Fix: output formatting issue (#388, @kribblo)
* Fix: babel-loader compatibility (#383, @escwald)
* Misc: removed built asset from repo and publications (@thebigredgeek)
* Misc: moved source files to /src (#378, @yamikuronue)
* Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue)
* Test: coveralls integration (#378, @yamikuronue)
* Docs: simplified language in the opening paragraph (#373, @yamikuronue)
2.4.5 / 2016-12-17
==================
* Fix: `navigator` undefined in Rhino (#376, @jochenberger)
* Fix: custom log function (#379, @hsiliev)
* Improvement: bit of cleanup + linting fixes (@thebigredgeek)
* Improvement: rm non-maintainted `dist/` dir (#375, @freewil)
* Docs: simplified language in the opening paragraph. (#373, @yamikuronue)
2.4.4 / 2016-12-14
==================
* Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts)
2.4.3 / 2016-12-14
==================
* Fix: navigation.userAgent error for react native (#364, @escwald)
2.4.2 / 2016-12-14
==================
* Fix: browser colors (#367, @tootallnate)
* Misc: travis ci integration (@thebigredgeek)
* Misc: added linting and testing boilerplate with sanity check (@thebigredgeek)
2.4.1 / 2016-12-13
==================
* Fix: typo that broke the package (#356)
2.4.0 / 2016-12-13
==================
* Fix: bower.json references unbuilt src entry point (#342, @justmatt)
* Fix: revert "handle regex special characters" (@tootallnate)
* Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate)
* Feature: %O`(big O) pretty-prints objects (#322, @tootallnate)
* Improvement: allow colors in workers (#335, @botverse)
* Improvement: use same color for same namespace. (#338, @lchenay)
2.3.3 / 2016-11-09
==================
* Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne)
* Fix: Returning `localStorage` saved values (#331, Levi Thomason)
* Improvement: Don't create an empty object when no `process` (Nathan Rajlich)
2.3.2 / 2016-11-09
==================
* Fix: be super-safe in index.js as well (@TooTallNate)
* Fix: should check whether process exists (Tom Newby)
2.3.1 / 2016-11-09
==================
* Fix: Added electron compatibility (#324, @paulcbetts)
* Improvement: Added performance optimizations (@tootallnate)
* Readme: Corrected PowerShell environment variable example (#252, @gimre)
* Misc: Removed yarn lock file from source control (#321, @fengmk2)
2.3.0 / 2016-11-07
==================
* Fix: Consistent placement of ms diff at end of output (#215, @gorangajic)
* Fix: Escaping of regex special characters in namespace strings (#250, @zacronos)
* Fix: Fixed bug causing crash on react-native (#282, @vkarpov15)
* Feature: Enabled ES6+ compatible import via default export (#212 @bucaran)
* Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom)
* Package: Update "ms" to 0.7.2 (#315, @DevSide)
* Package: removed superfluous version property from bower.json (#207 @kkirsche)
* Readme: fix USE_COLORS to DEBUG_COLORS
* Readme: Doc fixes for format string sugar (#269, @mlucool)
* Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0)
* Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable)
* Readme: better docs for browser support (#224, @matthewmueller)
* Tooling: Added yarn integration for development (#317, @thebigredgeek)
* Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek)
* Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman)
* Misc: Updated contributors (@thebigredgeek)
2.2.0 / 2015-05-09
==================
* package: update "ms" to v0.7.1 (#202, @dougwilson)
* README: add logging to file example (#193, @DanielOchoa)
* README: fixed a typo (#191, @amir-s)
* browser: expose `storage` (#190, @stephenmathieson)
* Makefile: add a `distclean` target (#189, @stephenmathieson)
2.1.3 / 2015-03-13
==================
* Updated stdout/stderr example (#186)
* Updated example/stdout.js to match debug current behaviour
* Renamed example/stderr.js to stdout.js
* Update Readme.md (#184)
* replace high intensity foreground color for bold (#182, #183)
2.1.2 / 2015-03-01
==================
* dist: recompile
* update "ms" to v0.7.0
* package: update "browserify" to v9.0.3
* component: fix "ms.js" repo location
* changed bower package name
* updated documentation about using debug in a browser
* fix: security error on safari (#167, #168, @yields)
2.1.1 / 2014-12-29
==================
* browser: use `typeof` to check for `console` existence
* browser: check for `console.log` truthiness (fix IE 8/9)
* browser: add support for Chrome apps
* Readme: added Windows usage remarks
* Add `bower.json` to properly support bower install
2.1.0 / 2014-10-15
==================
* node: implement `DEBUG_FD` env variable support
* package: update "browserify" to v6.1.0
* package: add "license" field to package.json (#135, @panuhorsmalahti)
2.0.0 / 2014-09-01
==================
* package: update "browserify" to v5.11.0
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
1.0.4 / 2014-07-15
==================
* dist: recompile
* example: remove `console.info()` log usage
* example: add "Content-Type" UTF-8 header to browser example
* browser: place %c marker after the space character
* browser: reset the "content" color via `color: inherit`
* browser: add colors support for Firefox >= v31
* debug: prefer an instance `log()` function over the global one (#119)
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
1.0.3 / 2014-07-09
==================
* Add support for multiple wildcards in namespaces (#122, @seegno)
* browser: fix lint
1.0.2 / 2014-06-10
==================
* browser: update color palette (#113, @gscottolson)
* common: make console logging function configurable (#108, @timoxley)
* node: fix %o colors on old node <= 0.8.x
* Makefile: find node path using shell/which (#109, @timoxley)
1.0.1 / 2014-06-06
==================
* browser: use `removeItem()` to clear localStorage
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
* package: add "contributors" section
* node: fix comment typo
* README: list authors
1.0.0 / 2014-06-04
==================
* make ms diff be global, not be scope
* debug: ignore empty strings in enable()
* node: make DEBUG_COLORS able to disable coloring
* *: export the `colors` array
* npmignore: don't publish the `dist` dir
* Makefile: refactor to use browserify
* package: add "browserify" as a dev dependency
* Readme: add Web Inspector Colors section
* node: reset terminal color for the debug content
* node: map "%o" to `util.inspect()`
* browser: map "%j" to `JSON.stringify()`
* debug: add custom "formatters"
* debug: use "ms" module for humanizing the diff
* Readme: add "bash" syntax highlighting
* browser: add Firebug color support
* browser: add colors for WebKit browsers
* node: apply log to `console`
* rewrite: abstract common logic for Node & browsers
* add .jshintrc file
0.8.1 / 2014-04-14
==================
* package: re-add the "component" section
0.8.0 / 2014-03-30
==================
* add `enable()` method for nodejs. Closes #27
* change from stderr to stdout
* remove unnecessary index.js file
0.7.4 / 2013-11-13
==================
* remove "browserify" key from package.json (fixes something in browserify)
0.7.3 / 2013-10-30
==================
* fix: catch localStorage security error when cookies are blocked (Chrome)
* add debug(err) support. Closes #46
* add .browser prop to package.json. Closes #42
0.7.2 / 2013-02-06
==================
* fix package.json
* fix: Mobile Safari (private mode) is broken with debug
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
0.7.1 / 2013-02-05
==================
* add repository URL to package.json
* add DEBUG_COLORED to force colored output
* add browserify support
* fix component. Closes #24
0.7.0 / 2012-05-04
==================
* Added .component to package.json
* Added debug.component.js build
0.6.0 / 2012-03-16
==================
* Added support for "-" prefix in DEBUG [Vinay Pulim]
* Added `.enabled` flag to the node version [TooTallNate]
0.5.0 / 2012-02-02
==================
* Added: humanize diffs. Closes #8
* Added `debug.disable()` to the CS variant
* Removed padding. Closes #10
* Fixed: persist client-side variant again. Closes #9
0.4.0 / 2012-02-01
==================
* Added browser variant support for older browsers [TooTallNate]
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
* Added padding to diff (moved it to the right)
0.3.0 / 2012-01-26
==================
* Added millisecond diff when isatty, otherwise UTC string
0.2.0 / 2012-01-22
==================
* Added wildcard support
0.1.0 / 2011-12-02
==================
* Added: remove colors unless stderr isatty [TooTallNate]
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/body-parser/node_modules/debug/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/body-parser/node_modules/debug/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11705
} |
# debug
[](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
[](#sponsors)
A tiny node.js debugging utility modelled after node core's debugging technique.
**Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)**
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example _app.js_:
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %s', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example _worker.js_:
```js
var debug = require('debug')('worker');
setInterval(function(){
debug('doing some work');
}, 1000);
```
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:


#### Windows note
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Note that PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Then, run the program to be debugged as usual.
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.

When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:

## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
#### Web Inspector Colors
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
Colored output looks something like:

## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example _stdout.js_:
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2016 TJ Holowaychuk <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/body-parser/node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/body-parser/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17915
} |
The MIT License (MIT)
Copyright (c) 2016 Zeit, Inc.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/body-parser/node_modules/ms/license.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/body-parser/node_modules/ms/license.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1076
} |
# ms
[](https://travis-ci.org/zeit/ms)
[](https://zeit.chat/)
Use this package to easily convert various time formats to milliseconds.
## Examples
```js
ms('2 days') // 172800000
ms('1d') // 86400000
ms('10h') // 36000000
ms('2.5 hrs') // 9000000
ms('2h') // 7200000
ms('1m') // 60000
ms('5s') // 5000
ms('1y') // 31557600000
ms('100') // 100
```
### Convert from milliseconds
```js
ms(60000) // "1m"
ms(2 * 60000) // "2m"
ms(ms('10 hours')) // "10h"
```
### Time format written-out
```js
ms(60000, { long: true }) // "1 minute"
ms(2 * 60000, { long: true }) // "2 minutes"
ms(ms('10 hours'), { long: true }) // "10 hours"
```
## Features
- Works both in [node](https://nodejs.org) and in the browser.
- If a number is supplied to `ms`, a string with a unit is returned.
- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
## Caught a bug?
1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
2. Link the package to the global module directory: `npm link`
3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
As always, you can run the tests using: `npm test` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/body-parser/node_modules/ms/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/body-parser/node_modules/ms/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1720
} |
### [5.1.2](https://github.com/gulpjs/glob-parent/compare/v5.1.1...v5.1.2) (2021-03-06)
### Bug Fixes
* eliminate ReDoS ([#36](https://github.com/gulpjs/glob-parent/issues/36)) ([f923116](https://github.com/gulpjs/glob-parent/commit/f9231168b0041fea3f8f954b3cceb56269fc6366))
### [5.1.1](https://github.com/gulpjs/glob-parent/compare/v5.1.0...v5.1.1) (2021-01-27)
### Bug Fixes
* unescape exclamation mark ([#26](https://github.com/gulpjs/glob-parent/issues/26)) ([a98874f](https://github.com/gulpjs/glob-parent/commit/a98874f1a59e407f4fb1beb0db4efa8392da60bb))
## [5.1.0](https://github.com/gulpjs/glob-parent/compare/v5.0.0...v5.1.0) (2021-01-27)
### Features
* add `flipBackslashes` option to disable auto conversion of slashes (closes [#24](https://github.com/gulpjs/glob-parent/issues/24)) ([#25](https://github.com/gulpjs/glob-parent/issues/25)) ([eecf91d](https://github.com/gulpjs/glob-parent/commit/eecf91d5e3834ed78aee39c4eaaae654d76b87b3))
## [5.0.0](https://github.com/gulpjs/glob-parent/compare/v4.0.0...v5.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* Drop support for node <6 & bump dependencies
### Miscellaneous Chores
* Drop support for node <6 & bump dependencies ([896c0c0](https://github.com/gulpjs/glob-parent/commit/896c0c00b4e7362f60b96e7fc295ae929245255a))
## [4.0.0](https://github.com/gulpjs/glob-parent/compare/v3.1.0...v4.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* question marks are valid path characters on Windows so avoid flagging as a glob when alone
* Update is-glob dependency
### Features
* hoist regexps and strings for performance gains ([4a80667](https://github.com/gulpjs/glob-parent/commit/4a80667c69355c76a572a5892b0f133c8e1f457e))
* question marks are valid path characters on Windows so avoid flagging as a glob when alone ([2a551dd](https://github.com/gulpjs/glob-parent/commit/2a551dd0dc3235e78bf3c94843d4107072d17841))
* Update is-glob dependency ([e41fcd8](https://github.com/gulpjs/glob-parent/commit/e41fcd895d1f7bc617dba45c9d935a7949b9c281))
## [3.1.0](https://github.com/gulpjs/glob-parent/compare/v3.0.1...v3.1.0) (2021-01-27)
### Features
* allow basic win32 backslash use ([272afa5](https://github.com/gulpjs/glob-parent/commit/272afa5fd070fc0f796386a5993d4ee4a846988b))
* handle extglobs (parentheses) containing separators ([7db1bdb](https://github.com/gulpjs/glob-parent/commit/7db1bdb0756e55fd14619e8ce31aa31b17b117fd))
* new approach to braces/brackets handling ([8269bd8](https://github.com/gulpjs/glob-parent/commit/8269bd89290d99fac9395a354fb56fdcdb80f0be))
* pre-process braces/brackets sections ([9ef8a87](https://github.com/gulpjs/glob-parent/commit/9ef8a87f66b1a43d0591e7a8e4fc5a18415ee388))
* preserve escaped brace/bracket at end of string ([8cfb0ba](https://github.com/gulpjs/glob-parent/commit/8cfb0ba84202d51571340dcbaf61b79d16a26c76))
### Bug Fixes
* trailing escaped square brackets ([99ec9fe](https://github.com/gulpjs/glob-parent/commit/99ec9fecc60ee488ded20a94dd4f18b4f55c4ccf))
### [3.0.1](https://github.com/gulpjs/glob-parent/compare/v3.0.0...v3.0.1) (2021-01-27)
### Features
* use path-dirname ponyfill ([cdbea5f](https://github.com/gulpjs/glob-parent/commit/cdbea5f32a58a54e001a75ddd7c0fccd4776aacc))
### Bug Fixes
* unescape glob-escaped dirnames on output ([598c533](https://github.com/gulpjs/glob-parent/commit/598c533bdf49c1428bc063aa9b8db40c5a86b030))
## [3.0.0](https://github.com/gulpjs/glob-parent/compare/v2.0.0...v3.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* update is-glob dependency
### Features
* update is-glob dependency ([5c5f8ef](https://github.com/gulpjs/glob-parent/commit/5c5f8efcee362a8e7638cf8220666acd8784f6bd))
## [2.0.0](https://github.com/gulpjs/glob-parent/compare/v1.3.0...v2.0.0) (2021-01-27)
### Features
* move up to dirname regardless of glob characters ([f97fb83](https://github.com/gulpjs/glob-parent/commit/f97fb83be2e0a9fc8d3b760e789d2ecadd6aa0c2))
## [1.3.0](https://github.com/gulpjs/glob-parent/compare/v1.2.0...v1.3.0) (2021-01-27)
## [1.2.0](https://github.com/gulpjs/glob-parent/compare/v1.1.0...v1.2.0) (2021-01-27)
### Reverts
* feat: make regex test strings smaller ([dc80fa9](https://github.com/gulpjs/glob-parent/commit/dc80fa9658dca20549cfeba44bbd37d5246fcce0))
## [1.1.0](https://github.com/gulpjs/glob-parent/compare/v1.0.0...v1.1.0) (2021-01-27)
### Features
* make regex test strings smaller ([cd83220](https://github.com/gulpjs/glob-parent/commit/cd832208638f45169f986d80fcf66e401f35d233))
## 1.0.0 (2021-01-27) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/chokidar/node_modules/glob-parent/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/chokidar/node_modules/glob-parent/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4502
} |
<p align="center">
<a href="https://gulpjs.com">
<img height="257" width="114" src="https://raw.githubusercontent.com/gulpjs/artwork/master/gulp-2x.png">
</a>
</p>
# glob-parent
[![NPM version][npm-image]][npm-url] [![Downloads][downloads-image]][npm-url] [![Azure Pipelines Build Status][azure-pipelines-image]][azure-pipelines-url] [![Travis Build Status][travis-image]][travis-url] [![AppVeyor Build Status][appveyor-image]][appveyor-url] [![Coveralls Status][coveralls-image]][coveralls-url] [![Gitter chat][gitter-image]][gitter-url]
Extract the non-magic parent path from a glob string.
## Usage
```js
var globParent = require('glob-parent');
globParent('path/to/*.js'); // 'path/to'
globParent('/root/path/to/*.js'); // '/root/path/to'
globParent('/*.js'); // '/'
globParent('*.js'); // '.'
globParent('**/*.js'); // '.'
globParent('path/{to,from}'); // 'path'
globParent('path/!(to|from)'); // 'path'
globParent('path/?(to|from)'); // 'path'
globParent('path/+(to|from)'); // 'path'
globParent('path/*(to|from)'); // 'path'
globParent('path/@(to|from)'); // 'path'
globParent('path/**/*'); // 'path'
// if provided a non-glob path, returns the nearest dir
globParent('path/foo/bar.js'); // 'path/foo'
globParent('path/foo/'); // 'path/foo'
globParent('path/foo'); // 'path' (see issue #3 for details)
```
## API
### `globParent(maybeGlobString, [options])`
Takes a string and returns the part of the path before the glob begins. Be aware of Escaping rules and Limitations below.
#### options
```js
{
// Disables the automatic conversion of slashes for Windows
flipBackslashes: true
}
```
## Escaping
The following characters have special significance in glob patterns and must be escaped if you want them to be treated as regular path characters:
- `?` (question mark) unless used as a path segment alone
- `*` (asterisk)
- `|` (pipe)
- `(` (opening parenthesis)
- `)` (closing parenthesis)
- `{` (opening curly brace)
- `}` (closing curly brace)
- `[` (opening bracket)
- `]` (closing bracket)
**Example**
```js
globParent('foo/[bar]/') // 'foo'
globParent('foo/\\[bar]/') // 'foo/[bar]'
```
## Limitations
### Braces & Brackets
This library attempts a quick and imperfect method of determining which path
parts have glob magic without fully parsing/lexing the pattern. There are some
advanced use cases that can trip it up, such as nested braces where the outer
pair is escaped and the inner one contains a path separator. If you find
yourself in the unlikely circumstance of being affected by this or need to
ensure higher-fidelity glob handling in your library, it is recommended that you
pre-process your input with [expand-braces] and/or [expand-brackets].
### Windows
Backslashes are not valid path separators for globs. If a path with backslashes
is provided anyway, for simple cases, glob-parent will replace the path
separator for you and return the non-glob parent path (now with
forward-slashes, which are still valid as Windows path separators).
This cannot be used in conjunction with escape characters.
```js
// BAD
globParent('C:\\Program Files \\(x86\\)\\*.ext') // 'C:/Program Files /(x86/)'
// GOOD
globParent('C:/Program Files\\(x86\\)/*.ext') // 'C:/Program Files (x86)'
```
If you are using escape characters for a pattern without path parts (i.e.
relative to `cwd`), prefix with `./` to avoid confusing glob-parent.
```js
// BAD
globParent('foo \\[bar]') // 'foo '
globParent('foo \\[bar]*') // 'foo '
// GOOD
globParent('./foo \\[bar]') // 'foo [bar]'
globParent('./foo \\[bar]*') // '.'
```
## License
ISC
[expand-braces]: https://github.com/jonschlinkert/expand-braces
[expand-brackets]: https://github.com/jonschlinkert/expand-brackets
[downloads-image]: https://img.shields.io/npm/dm/glob-parent.svg
[npm-url]: https://www.npmjs.com/package/glob-parent
[npm-image]: https://img.shields.io/npm/v/glob-parent.svg
[azure-pipelines-url]: https://dev.azure.com/gulpjs/gulp/_build/latest?definitionId=2&branchName=master
[azure-pipelines-image]: https://dev.azure.com/gulpjs/gulp/_apis/build/status/glob-parent?branchName=master
[travis-url]: https://travis-ci.org/gulpjs/glob-parent
[travis-image]: https://img.shields.io/travis/gulpjs/glob-parent.svg?label=travis-ci
[appveyor-url]: https://ci.appveyor.com/project/gulpjs/glob-parent
[appveyor-image]: https://img.shields.io/appveyor/ci/gulpjs/glob-parent.svg?label=appveyor
[coveralls-url]: https://coveralls.io/r/gulpjs/glob-parent
[coveralls-image]: https://img.shields.io/coveralls/gulpjs/glob-parent/master.svg
[gitter-url]: https://gitter.im/gulpjs/gulp
[gitter-image]: https://badges.gitter.im/gulpjs/gulp.svg | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/chokidar/node_modules/glob-parent/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/chokidar/node_modules/glob-parent/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4645
} |
# clsx [](https://github.com/lukeed/clsx/actions?query=workflow%3ACI) [](https://codecov.io/gh/lukeed/clsx)
> A tiny (234B) utility for constructing `className` strings conditionally.<Br>Also serves as a [faster](/bench) & smaller drop-in replacement for the `classnames` module.
This module is available in three formats:
* **ES Module**: `dist/clsx.m.js`
* **CommonJS**: `dist/clsx.js`
* **UMD**: `dist/clsx.min.js`
## Install
```
$ npm install --save clsx
```
## Usage
```js
import clsx from 'clsx';
// or
import { clsx } from 'clsx';
// Strings (variadic)
clsx('foo', true && 'bar', 'baz');
//=> 'foo bar baz'
// Objects
clsx({ foo:true, bar:false, baz:isTrue() });
//=> 'foo baz'
// Objects (variadic)
clsx({ foo:true }, { bar:false }, null, { '--foobar':'hello' });
//=> 'foo --foobar'
// Arrays
clsx(['foo', 0, false, 'bar']);
//=> 'foo bar'
// Arrays (variadic)
clsx(['foo'], ['', 0, false, 'bar'], [['baz', [['hello'], 'there']]]);
//=> 'foo bar baz hello there'
// Kitchen sink (with nesting)
clsx('foo', [1 && 'bar', { baz:false, bat:null }, ['hello', ['world']]], 'cya');
//=> 'foo bar hello world cya'
```
## API
### clsx(...input)
Returns: `String`
#### input
Type: `Mixed`
The `clsx` function can take ***any*** number of arguments, each of which can be an Object, Array, Boolean, or String.
> **Important:** _Any_ falsey values are discarded!<br>Standalone Boolean values are discarded as well.
```js
clsx(true, false, '', null, undefined, 0, NaN);
//=> ''
```
## Benchmarks
For snapshots of cross-browser results, check out the [`bench`](/bench) directory~!
## Support
All versions of Node.js are supported.
All browsers that support [`Array.isArray`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/isArray#Browser_compatibility) are supported (IE9+).
>**Note:** For IE8 support and older, please install `[email protected]` and beware of [#17](https://github.com/lukeed/clsx/issues/17).
## Tailwind Support
Here some additional (optional) steps to enable classes autocompletion using `clsx` with Tailwind CSS.
<details>
<summary>
Visual Studio Code
</summary>
1. [Install the "Tailwind CSS IntelliSense" Visual Studio Code extension](https://marketplace.visualstudio.com/items?itemName=bradlc.vscode-tailwindcss)
2. Add the following to your [`settings.json`](https://code.visualstudio.com/docs/getstarted/settings):
```json
{
"tailwindCSS.experimental.classRegex": [
["clsx\\(([^)]*)\\)", "(?:'|\"|`)([^']*)(?:'|\"|`)"]
]
}
```
</details>
## Related
- [obj-str](https://github.com/lukeed/obj-str) - A smaller (96B) and similiar utility that only works with Objects.
## License
MIT © [Luke Edwards](https://lukeed.com) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/class-variance-authority/node_modules/clsx/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/class-variance-authority/node_modules/clsx/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2852
} |
MIT License
Copyright (c) 2020 Evan Wallace
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-kit/node_modules/esbuild/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-kit/node_modules/esbuild/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1068
} |
# esbuild
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the [JavaScript API documentation](https://esbuild.github.io/api/) for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-kit/node_modules/esbuild/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-kit/node_modules/esbuild/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 174
} |
1.0.7 / 2023-04-12
==================
* backport the buffer support from the 1.2.x release branch (thanks @FadhiliNjagi!)
1.0.6 / 2015-02-03
==================
* use `npm test` instead of `make test` to run tests
* clearer assertion messages when checking input
1.0.5 / 2014-09-05
==================
* add license to package.json
1.0.4 / 2014-06-25
==================
* corrected avoidance of timing attacks (thanks @tenbits!)
1.0.3 / 2014-01-28
==================
* [incorrect] fix for timing attacks
1.0.2 / 2014-01-28
==================
* fix missing repository warning
* fix typo in test
1.0.1 / 2013-04-15
==================
* Revert "Changed underlying HMAC algo. to sha512."
* Revert "Fix for timing attacks on MAC verification."
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/cookie-signature/History.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/cookie-signature/History.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 817
} |
# cookie-signature
Sign and unsign cookies.
## Example
```js
var cookie = require('cookie-signature');
var val = cookie.sign('hello', 'tobiiscool');
val.should.equal('hello.DGDUkGlIkCzPz+C0B064FNgHdEjox7ch8tOBGslZ5QI');
var val = cookie.sign('hello', 'tobiiscool');
cookie.unsign(val, 'tobiiscool').should.equal('hello');
cookie.unsign(val, 'luna').should.be.false;
```
## License
(The MIT License)
Copyright (c) 2012 LearnBoost <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/cookie-signature/Readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/cookie-signature/Readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1489
} |
# cookie
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-image]][node-url]
[![Build Status][ci-image]][ci-url]
[![Coverage Status][coveralls-image]][coveralls-url]
Basic HTTP cookie parser and serializer for HTTP servers.
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install cookie
```
## API
```js
var cookie = require('cookie');
```
### cookie.parse(str, options)
Parse an HTTP `Cookie` header string and returning an object of all cookie name-value pairs.
The `str` argument is the string representing a `Cookie` header value and `options` is an
optional object containing additional parsing options.
```js
var cookies = cookie.parse('foo=bar; equation=E%3Dmc%5E2');
// { foo: 'bar', equation: 'E=mc^2' }
```
#### Options
`cookie.parse` accepts these properties in the options object.
##### decode
Specifies a function that will be used to decode a cookie's value. Since the value of a cookie
has a limited character set (and must be a simple string), this function can be used to decode
a previously-encoded cookie value into a JavaScript string or other object.
The default function is the global `decodeURIComponent`, which will decode any URL-encoded
sequences into their byte representations.
**note** if an error is thrown from this function, the original, non-decoded cookie value will
be returned as the cookie's value.
### cookie.serialize(name, value, options)
Serialize a cookie name-value pair into a `Set-Cookie` header string. The `name` argument is the
name for the cookie, the `value` argument is the value to set the cookie to, and the `options`
argument is an optional object containing additional serialization options.
```js
var setCookie = cookie.serialize('foo', 'bar');
// foo=bar
```
#### Options
`cookie.serialize` accepts these properties in the options object.
##### domain
Specifies the value for the [`Domain` `Set-Cookie` attribute][rfc-6265-5.2.3]. By default, no
domain is set, and most clients will consider the cookie to apply to only the current domain.
##### encode
Specifies a function that will be used to encode a cookie's value. Since value of a cookie
has a limited character set (and must be a simple string), this function can be used to encode
a value into a string suited for a cookie's value.
The default function is the global `encodeURIComponent`, which will encode a JavaScript string
into UTF-8 byte sequences and then URL-encode any that fall outside of the cookie range.
##### expires
Specifies the `Date` object to be the value for the [`Expires` `Set-Cookie` attribute][rfc-6265-5.2.1].
By default, no expiration is set, and most clients will consider this a "non-persistent cookie" and
will delete it on a condition like exiting a web browser application.
**note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and
`maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this,
so if both are set, they should point to the same date and time.
##### httpOnly
Specifies the `boolean` value for the [`HttpOnly` `Set-Cookie` attribute][rfc-6265-5.2.6]. When truthy,
the `HttpOnly` attribute is set, otherwise it is not. By default, the `HttpOnly` attribute is not set.
**note** be careful when setting this to `true`, as compliant clients will not allow client-side
JavaScript to see the cookie in `document.cookie`.
##### maxAge
Specifies the `number` (in seconds) to be the value for the [`Max-Age` `Set-Cookie` attribute][rfc-6265-5.2.2].
The given number will be converted to an integer by rounding down. By default, no maximum age is set.
**note** the [cookie storage model specification][rfc-6265-5.3] states that if both `expires` and
`maxAge` are set, then `maxAge` takes precedence, but it is possible not all clients by obey this,
so if both are set, they should point to the same date and time.
##### partitioned
Specifies the `boolean` value for the [`Partitioned` `Set-Cookie`](rfc-cutler-httpbis-partitioned-cookies)
attribute. When truthy, the `Partitioned` attribute is set, otherwise it is not. By default, the
`Partitioned` attribute is not set.
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
More information about can be found in [the proposal](https://github.com/privacycg/CHIPS).
##### path
Specifies the value for the [`Path` `Set-Cookie` attribute][rfc-6265-5.2.4]. By default, the path
is considered the ["default path"][rfc-6265-5.1.4].
##### priority
Specifies the `string` to be the value for the [`Priority` `Set-Cookie` attribute][rfc-west-cookie-priority-00-4.1].
- `'low'` will set the `Priority` attribute to `Low`.
- `'medium'` will set the `Priority` attribute to `Medium`, the default priority when not set.
- `'high'` will set the `Priority` attribute to `High`.
More information about the different priority levels can be found in
[the specification][rfc-west-cookie-priority-00-4.1].
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
##### sameSite
Specifies the `boolean` or `string` to be the value for the [`SameSite` `Set-Cookie` attribute][rfc-6265bis-09-5.4.7].
- `true` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
- `false` will not set the `SameSite` attribute.
- `'lax'` will set the `SameSite` attribute to `Lax` for lax same site enforcement.
- `'none'` will set the `SameSite` attribute to `None` for an explicit cross-site cookie.
- `'strict'` will set the `SameSite` attribute to `Strict` for strict same site enforcement.
More information about the different enforcement levels can be found in
[the specification][rfc-6265bis-09-5.4.7].
**note** This is an attribute that has not yet been fully standardized, and may change in the future.
This also means many clients may ignore this attribute until they understand it.
##### secure
Specifies the `boolean` value for the [`Secure` `Set-Cookie` attribute][rfc-6265-5.2.5]. When truthy,
the `Secure` attribute is set, otherwise it is not. By default, the `Secure` attribute is not set.
**note** be careful when setting this to `true`, as compliant clients will not send the cookie back to
the server in the future if the browser does not have an HTTPS connection.
## Example
The following example uses this module in conjunction with the Node.js core HTTP server
to prompt a user for their name and display it back on future visits.
```js
var cookie = require('cookie');
var escapeHtml = require('escape-html');
var http = require('http');
var url = require('url');
function onRequest(req, res) {
// Parse the query string
var query = url.parse(req.url, true, true).query;
if (query && query.name) {
// Set a new cookie with the name
res.setHeader('Set-Cookie', cookie.serialize('name', String(query.name), {
httpOnly: true,
maxAge: 60 * 60 * 24 * 7 // 1 week
}));
// Redirect back after setting cookie
res.statusCode = 302;
res.setHeader('Location', req.headers.referer || '/');
res.end();
return;
}
// Parse the cookies on the request
var cookies = cookie.parse(req.headers.cookie || '');
// Get the visitor name set in the cookie
var name = cookies.name;
res.setHeader('Content-Type', 'text/html; charset=UTF-8');
if (name) {
res.write('<p>Welcome back, <b>' + escapeHtml(name) + '</b>!</p>');
} else {
res.write('<p>Hello, new visitor!</p>');
}
res.write('<form method="GET">');
res.write('<input placeholder="enter your name" name="name"> <input type="submit" value="Set Name">');
res.end('</form>');
}
http.createServer(onRequest).listen(3000);
```
## Testing
```sh
$ npm test
```
## Benchmark
```
$ npm run bench
> [email protected] bench
> node benchmark/index.js
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
modules@108
napi@9
[email protected]
[email protected]
[email protected]
[email protected]+quic
[email protected]
tz@2023c
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
[email protected]
> node benchmark/parse-top.js
cookie.parse - top sites
14 tests completed.
parse accounts.google.com x 2,588,913 ops/sec ±0.74% (186 runs sampled)
parse apple.com x 2,370,002 ops/sec ±0.69% (186 runs sampled)
parse cloudflare.com x 2,213,102 ops/sec ±0.88% (188 runs sampled)
parse docs.google.com x 2,194,157 ops/sec ±1.03% (184 runs sampled)
parse drive.google.com x 2,265,084 ops/sec ±0.79% (187 runs sampled)
parse en.wikipedia.org x 457,099 ops/sec ±0.81% (186 runs sampled)
parse linkedin.com x 504,407 ops/sec ±0.89% (186 runs sampled)
parse maps.google.com x 1,230,959 ops/sec ±0.98% (186 runs sampled)
parse microsoft.com x 926,294 ops/sec ±0.88% (184 runs sampled)
parse play.google.com x 2,311,338 ops/sec ±0.83% (185 runs sampled)
parse support.google.com x 1,508,850 ops/sec ±0.86% (186 runs sampled)
parse www.google.com x 1,022,582 ops/sec ±1.32% (182 runs sampled)
parse youtu.be x 332,136 ops/sec ±1.02% (185 runs sampled)
parse youtube.com x 323,833 ops/sec ±0.77% (183 runs sampled)
> node benchmark/parse.js
cookie.parse - generic
6 tests completed.
simple x 3,214,032 ops/sec ±1.61% (183 runs sampled)
decode x 587,237 ops/sec ±1.16% (187 runs sampled)
unquote x 2,954,618 ops/sec ±1.35% (183 runs sampled)
duplicates x 857,008 ops/sec ±0.89% (187 runs sampled)
10 cookies x 292,133 ops/sec ±0.89% (187 runs sampled)
100 cookies x 22,610 ops/sec ±0.68% (187 runs sampled)
```
## References
- [RFC 6265: HTTP State Management Mechanism][rfc-6265]
- [Same-site Cookies][rfc-6265bis-09-5.4.7]
[rfc-cutler-httpbis-partitioned-cookies]: https://tools.ietf.org/html/draft-cutler-httpbis-partitioned-cookies/
[rfc-west-cookie-priority-00-4.1]: https://tools.ietf.org/html/draft-west-cookie-priority-00#section-4.1
[rfc-6265bis-09-5.4.7]: https://tools.ietf.org/html/draft-ietf-httpbis-rfc6265bis-09#section-5.4.7
[rfc-6265]: https://tools.ietf.org/html/rfc6265
[rfc-6265-5.1.4]: https://tools.ietf.org/html/rfc6265#section-5.1.4
[rfc-6265-5.2.1]: https://tools.ietf.org/html/rfc6265#section-5.2.1
[rfc-6265-5.2.2]: https://tools.ietf.org/html/rfc6265#section-5.2.2
[rfc-6265-5.2.3]: https://tools.ietf.org/html/rfc6265#section-5.2.3
[rfc-6265-5.2.4]: https://tools.ietf.org/html/rfc6265#section-5.2.4
[rfc-6265-5.2.5]: https://tools.ietf.org/html/rfc6265#section-5.2.5
[rfc-6265-5.2.6]: https://tools.ietf.org/html/rfc6265#section-5.2.6
[rfc-6265-5.3]: https://tools.ietf.org/html/rfc6265#section-5.3
## License
[MIT](LICENSE)
[ci-image]: https://badgen.net/github/checks/jshttp/cookie/master?label=ci
[ci-url]: https://github.com/jshttp/cookie/actions/workflows/ci.yml
[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/cookie/master
[coveralls-url]: https://coveralls.io/r/jshttp/cookie?branch=master
[node-image]: https://badgen.net/npm/node/cookie
[node-url]: https://nodejs.org/en/download
[npm-downloads-image]: https://badgen.net/npm/dm/cookie
[npm-url]: https://npmjs.org/package/cookie
[npm-version-image]: https://badgen.net/npm/v/cookie | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/cookie/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/cookie/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11748
} |
# Security Policies and Procedures
## Reporting a Bug
The `cookie` team and community take all security bugs seriously. Thank
you for improving the security of the project. We appreciate your efforts and
responsible disclosure and will make every effort to acknowledge your
contributions.
Report security bugs by emailing the current owner(s) of `cookie`. This
information can be found in the npm registry using the command
`npm owner ls cookie`.
If unsure or unable to get the information from the above, open an issue
in the [project issue tracker](https://github.com/jshttp/cookie/issues)
asking for the current contact information.
To ensure the timely response to your report, please ensure that the entirety
of the report is contained within the email body and not solely behind a web
link or an attachment.
At least one owner will acknowledge your email within 48 hours, and will send a
more detailed response within 48 hours indicating the next steps in handling
your report. After the initial reply to your report, the owners will
endeavor to keep you informed of the progress towards a fix and full
announcement, and may ask for additional information or guidance. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/cookie/SECURITY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/cookie/SECURITY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1179
} |
2.6.9 / 2017-09-22
==================
* remove ReDoS regexp in %o formatter (#504)
2.6.8 / 2017-05-18
==================
* Fix: Check for undefined on browser globals (#462, @marbemac)
2.6.7 / 2017-05-16
==================
* Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom)
* Fix: Inline extend function in node implementation (#452, @dougwilson)
* Docs: Fix typo (#455, @msasad)
2.6.5 / 2017-04-27
==================
* Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek)
* Misc: clean up browser reference checks (#447, @thebigredgeek)
* Misc: add npm-debug.log to .gitignore (@thebigredgeek)
2.6.4 / 2017-04-20
==================
* Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo)
* Chore: ignore bower.json in npm installations. (#437, @joaovieira)
* Misc: update "ms" to v0.7.3 (@tootallnate)
2.6.3 / 2017-03-13
==================
* Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts)
* Docs: Changelog fix (@thebigredgeek)
2.6.2 / 2017-03-10
==================
* Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin)
* Docs: Add backers and sponsors from Open Collective (#422, @piamancini)
* Docs: Add Slackin invite badge (@tootallnate)
2.6.1 / 2017-02-10
==================
* Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error
* Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0)
* Fix: IE8 "Expected identifier" error (#414, @vgoma)
* Fix: Namespaces would not disable once enabled (#409, @musikov)
2.6.0 / 2016-12-28
==================
* Fix: added better null pointer checks for browser useColors (@thebigredgeek)
* Improvement: removed explicit `window.debug` export (#404, @tootallnate)
* Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate)
2.5.2 / 2016-12-25
==================
* Fix: reference error on window within webworkers (#393, @KlausTrainer)
* Docs: fixed README typo (#391, @lurch)
* Docs: added notice about v3 api discussion (@thebigredgeek)
2.5.1 / 2016-12-20
==================
* Fix: babel-core compatibility
2.5.0 / 2016-12-20
==================
* Fix: wrong reference in bower file (@thebigredgeek)
* Fix: webworker compatibility (@thebigredgeek)
* Fix: output formatting issue (#388, @kribblo)
* Fix: babel-loader compatibility (#383, @escwald)
* Misc: removed built asset from repo and publications (@thebigredgeek)
* Misc: moved source files to /src (#378, @yamikuronue)
* Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue)
* Test: coveralls integration (#378, @yamikuronue)
* Docs: simplified language in the opening paragraph (#373, @yamikuronue)
2.4.5 / 2016-12-17
==================
* Fix: `navigator` undefined in Rhino (#376, @jochenberger)
* Fix: custom log function (#379, @hsiliev)
* Improvement: bit of cleanup + linting fixes (@thebigredgeek)
* Improvement: rm non-maintainted `dist/` dir (#375, @freewil)
* Docs: simplified language in the opening paragraph. (#373, @yamikuronue)
2.4.4 / 2016-12-14
==================
* Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts)
2.4.3 / 2016-12-14
==================
* Fix: navigation.userAgent error for react native (#364, @escwald)
2.4.2 / 2016-12-14
==================
* Fix: browser colors (#367, @tootallnate)
* Misc: travis ci integration (@thebigredgeek)
* Misc: added linting and testing boilerplate with sanity check (@thebigredgeek)
2.4.1 / 2016-12-13
==================
* Fix: typo that broke the package (#356)
2.4.0 / 2016-12-13
==================
* Fix: bower.json references unbuilt src entry point (#342, @justmatt)
* Fix: revert "handle regex special characters" (@tootallnate)
* Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate)
* Feature: %O`(big O) pretty-prints objects (#322, @tootallnate)
* Improvement: allow colors in workers (#335, @botverse)
* Improvement: use same color for same namespace. (#338, @lchenay)
2.3.3 / 2016-11-09
==================
* Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne)
* Fix: Returning `localStorage` saved values (#331, Levi Thomason)
* Improvement: Don't create an empty object when no `process` (Nathan Rajlich)
2.3.2 / 2016-11-09
==================
* Fix: be super-safe in index.js as well (@TooTallNate)
* Fix: should check whether process exists (Tom Newby)
2.3.1 / 2016-11-09
==================
* Fix: Added electron compatibility (#324, @paulcbetts)
* Improvement: Added performance optimizations (@tootallnate)
* Readme: Corrected PowerShell environment variable example (#252, @gimre)
* Misc: Removed yarn lock file from source control (#321, @fengmk2)
2.3.0 / 2016-11-07
==================
* Fix: Consistent placement of ms diff at end of output (#215, @gorangajic)
* Fix: Escaping of regex special characters in namespace strings (#250, @zacronos)
* Fix: Fixed bug causing crash on react-native (#282, @vkarpov15)
* Feature: Enabled ES6+ compatible import via default export (#212 @bucaran)
* Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom)
* Package: Update "ms" to 0.7.2 (#315, @DevSide)
* Package: removed superfluous version property from bower.json (#207 @kkirsche)
* Readme: fix USE_COLORS to DEBUG_COLORS
* Readme: Doc fixes for format string sugar (#269, @mlucool)
* Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0)
* Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable)
* Readme: better docs for browser support (#224, @matthewmueller)
* Tooling: Added yarn integration for development (#317, @thebigredgeek)
* Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek)
* Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman)
* Misc: Updated contributors (@thebigredgeek)
2.2.0 / 2015-05-09
==================
* package: update "ms" to v0.7.1 (#202, @dougwilson)
* README: add logging to file example (#193, @DanielOchoa)
* README: fixed a typo (#191, @amir-s)
* browser: expose `storage` (#190, @stephenmathieson)
* Makefile: add a `distclean` target (#189, @stephenmathieson)
2.1.3 / 2015-03-13
==================
* Updated stdout/stderr example (#186)
* Updated example/stdout.js to match debug current behaviour
* Renamed example/stderr.js to stdout.js
* Update Readme.md (#184)
* replace high intensity foreground color for bold (#182, #183)
2.1.2 / 2015-03-01
==================
* dist: recompile
* update "ms" to v0.7.0
* package: update "browserify" to v9.0.3
* component: fix "ms.js" repo location
* changed bower package name
* updated documentation about using debug in a browser
* fix: security error on safari (#167, #168, @yields)
2.1.1 / 2014-12-29
==================
* browser: use `typeof` to check for `console` existence
* browser: check for `console.log` truthiness (fix IE 8/9)
* browser: add support for Chrome apps
* Readme: added Windows usage remarks
* Add `bower.json` to properly support bower install
2.1.0 / 2014-10-15
==================
* node: implement `DEBUG_FD` env variable support
* package: update "browserify" to v6.1.0
* package: add "license" field to package.json (#135, @panuhorsmalahti)
2.0.0 / 2014-09-01
==================
* package: update "browserify" to v5.11.0
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
1.0.4 / 2014-07-15
==================
* dist: recompile
* example: remove `console.info()` log usage
* example: add "Content-Type" UTF-8 header to browser example
* browser: place %c marker after the space character
* browser: reset the "content" color via `color: inherit`
* browser: add colors support for Firefox >= v31
* debug: prefer an instance `log()` function over the global one (#119)
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
1.0.3 / 2014-07-09
==================
* Add support for multiple wildcards in namespaces (#122, @seegno)
* browser: fix lint
1.0.2 / 2014-06-10
==================
* browser: update color palette (#113, @gscottolson)
* common: make console logging function configurable (#108, @timoxley)
* node: fix %o colors on old node <= 0.8.x
* Makefile: find node path using shell/which (#109, @timoxley)
1.0.1 / 2014-06-06
==================
* browser: use `removeItem()` to clear localStorage
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
* package: add "contributors" section
* node: fix comment typo
* README: list authors
1.0.0 / 2014-06-04
==================
* make ms diff be global, not be scope
* debug: ignore empty strings in enable()
* node: make DEBUG_COLORS able to disable coloring
* *: export the `colors` array
* npmignore: don't publish the `dist` dir
* Makefile: refactor to use browserify
* package: add "browserify" as a dev dependency
* Readme: add Web Inspector Colors section
* node: reset terminal color for the debug content
* node: map "%o" to `util.inspect()`
* browser: map "%j" to `JSON.stringify()`
* debug: add custom "formatters"
* debug: use "ms" module for humanizing the diff
* Readme: add "bash" syntax highlighting
* browser: add Firebug color support
* browser: add colors for WebKit browsers
* node: apply log to `console`
* rewrite: abstract common logic for Node & browsers
* add .jshintrc file
0.8.1 / 2014-04-14
==================
* package: re-add the "component" section
0.8.0 / 2014-03-30
==================
* add `enable()` method for nodejs. Closes #27
* change from stderr to stdout
* remove unnecessary index.js file
0.7.4 / 2013-11-13
==================
* remove "browserify" key from package.json (fixes something in browserify)
0.7.3 / 2013-10-30
==================
* fix: catch localStorage security error when cookies are blocked (Chrome)
* add debug(err) support. Closes #46
* add .browser prop to package.json. Closes #42
0.7.2 / 2013-02-06
==================
* fix package.json
* fix: Mobile Safari (private mode) is broken with debug
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
0.7.1 / 2013-02-05
==================
* add repository URL to package.json
* add DEBUG_COLORED to force colored output
* add browserify support
* fix component. Closes #24
0.7.0 / 2012-05-04
==================
* Added .component to package.json
* Added debug.component.js build
0.6.0 / 2012-03-16
==================
* Added support for "-" prefix in DEBUG [Vinay Pulim]
* Added `.enabled` flag to the node version [TooTallNate]
0.5.0 / 2012-02-02
==================
* Added: humanize diffs. Closes #8
* Added `debug.disable()` to the CS variant
* Removed padding. Closes #10
* Fixed: persist client-side variant again. Closes #9
0.4.0 / 2012-02-01
==================
* Added browser variant support for older browsers [TooTallNate]
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
* Added padding to diff (moved it to the right)
0.3.0 / 2012-01-26
==================
* Added millisecond diff when isatty, otherwise UTC string
0.2.0 / 2012-01-22
==================
* Added wildcard support
0.1.0 / 2011-12-02
==================
* Added: remove colors unless stderr isatty [TooTallNate]
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/debug/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/debug/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11705
} |
# debug
[](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
[](#sponsors)
A tiny node.js debugging utility modelled after node core's debugging technique.
**Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)**
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example _app.js_:
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %s', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example _worker.js_:
```js
var debug = require('debug')('worker');
setInterval(function(){
debug('doing some work');
}, 1000);
```
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:


#### Windows note
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Note that PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Then, run the program to be debugged as usual.
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.

When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:

## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
#### Web Inspector Colors
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
Colored output looks something like:

## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example _stdout.js_:
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2016 TJ Holowaychuk <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17915
} |
The MIT License (MIT)
Copyright (c) 2016 Zeit, Inc.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/ms/license.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/ms/license.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1076
} |
# ms
[](https://travis-ci.org/zeit/ms)
[](https://zeit.chat/)
Use this package to easily convert various time formats to milliseconds.
## Examples
```js
ms('2 days') // 172800000
ms('1d') // 86400000
ms('10h') // 36000000
ms('2.5 hrs') // 9000000
ms('2h') // 7200000
ms('1m') // 60000
ms('5s') // 5000
ms('1y') // 31557600000
ms('100') // 100
```
### Convert from milliseconds
```js
ms(60000) // "1m"
ms(2 * 60000) // "2m"
ms(ms('10 hours')) // "10h"
```
### Time format written-out
```js
ms(60000, { long: true }) // "1 minute"
ms(2 * 60000, { long: true }) // "2 minutes"
ms(ms('10 hours'), { long: true }) // "10 hours"
```
## Features
- Works both in [node](https://nodejs.org) and in the browser.
- If a number is supplied to `ms`, a string with a unit is returned.
- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
## Caught a bug?
1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
2. Link the package to the global module directory: `npm link`
3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
As always, you can run the tests using: `npm test` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express-session/node_modules/ms/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express-session/node_modules/ms/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1720
} |
2.6.9 / 2017-09-22
==================
* remove ReDoS regexp in %o formatter (#504)
2.6.8 / 2017-05-18
==================
* Fix: Check for undefined on browser globals (#462, @marbemac)
2.6.7 / 2017-05-16
==================
* Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom)
* Fix: Inline extend function in node implementation (#452, @dougwilson)
* Docs: Fix typo (#455, @msasad)
2.6.5 / 2017-04-27
==================
* Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek)
* Misc: clean up browser reference checks (#447, @thebigredgeek)
* Misc: add npm-debug.log to .gitignore (@thebigredgeek)
2.6.4 / 2017-04-20
==================
* Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo)
* Chore: ignore bower.json in npm installations. (#437, @joaovieira)
* Misc: update "ms" to v0.7.3 (@tootallnate)
2.6.3 / 2017-03-13
==================
* Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts)
* Docs: Changelog fix (@thebigredgeek)
2.6.2 / 2017-03-10
==================
* Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin)
* Docs: Add backers and sponsors from Open Collective (#422, @piamancini)
* Docs: Add Slackin invite badge (@tootallnate)
2.6.1 / 2017-02-10
==================
* Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error
* Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0)
* Fix: IE8 "Expected identifier" error (#414, @vgoma)
* Fix: Namespaces would not disable once enabled (#409, @musikov)
2.6.0 / 2016-12-28
==================
* Fix: added better null pointer checks for browser useColors (@thebigredgeek)
* Improvement: removed explicit `window.debug` export (#404, @tootallnate)
* Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate)
2.5.2 / 2016-12-25
==================
* Fix: reference error on window within webworkers (#393, @KlausTrainer)
* Docs: fixed README typo (#391, @lurch)
* Docs: added notice about v3 api discussion (@thebigredgeek)
2.5.1 / 2016-12-20
==================
* Fix: babel-core compatibility
2.5.0 / 2016-12-20
==================
* Fix: wrong reference in bower file (@thebigredgeek)
* Fix: webworker compatibility (@thebigredgeek)
* Fix: output formatting issue (#388, @kribblo)
* Fix: babel-loader compatibility (#383, @escwald)
* Misc: removed built asset from repo and publications (@thebigredgeek)
* Misc: moved source files to /src (#378, @yamikuronue)
* Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue)
* Test: coveralls integration (#378, @yamikuronue)
* Docs: simplified language in the opening paragraph (#373, @yamikuronue)
2.4.5 / 2016-12-17
==================
* Fix: `navigator` undefined in Rhino (#376, @jochenberger)
* Fix: custom log function (#379, @hsiliev)
* Improvement: bit of cleanup + linting fixes (@thebigredgeek)
* Improvement: rm non-maintainted `dist/` dir (#375, @freewil)
* Docs: simplified language in the opening paragraph. (#373, @yamikuronue)
2.4.4 / 2016-12-14
==================
* Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts)
2.4.3 / 2016-12-14
==================
* Fix: navigation.userAgent error for react native (#364, @escwald)
2.4.2 / 2016-12-14
==================
* Fix: browser colors (#367, @tootallnate)
* Misc: travis ci integration (@thebigredgeek)
* Misc: added linting and testing boilerplate with sanity check (@thebigredgeek)
2.4.1 / 2016-12-13
==================
* Fix: typo that broke the package (#356)
2.4.0 / 2016-12-13
==================
* Fix: bower.json references unbuilt src entry point (#342, @justmatt)
* Fix: revert "handle regex special characters" (@tootallnate)
* Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate)
* Feature: %O`(big O) pretty-prints objects (#322, @tootallnate)
* Improvement: allow colors in workers (#335, @botverse)
* Improvement: use same color for same namespace. (#338, @lchenay)
2.3.3 / 2016-11-09
==================
* Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne)
* Fix: Returning `localStorage` saved values (#331, Levi Thomason)
* Improvement: Don't create an empty object when no `process` (Nathan Rajlich)
2.3.2 / 2016-11-09
==================
* Fix: be super-safe in index.js as well (@TooTallNate)
* Fix: should check whether process exists (Tom Newby)
2.3.1 / 2016-11-09
==================
* Fix: Added electron compatibility (#324, @paulcbetts)
* Improvement: Added performance optimizations (@tootallnate)
* Readme: Corrected PowerShell environment variable example (#252, @gimre)
* Misc: Removed yarn lock file from source control (#321, @fengmk2)
2.3.0 / 2016-11-07
==================
* Fix: Consistent placement of ms diff at end of output (#215, @gorangajic)
* Fix: Escaping of regex special characters in namespace strings (#250, @zacronos)
* Fix: Fixed bug causing crash on react-native (#282, @vkarpov15)
* Feature: Enabled ES6+ compatible import via default export (#212 @bucaran)
* Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom)
* Package: Update "ms" to 0.7.2 (#315, @DevSide)
* Package: removed superfluous version property from bower.json (#207 @kkirsche)
* Readme: fix USE_COLORS to DEBUG_COLORS
* Readme: Doc fixes for format string sugar (#269, @mlucool)
* Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0)
* Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable)
* Readme: better docs for browser support (#224, @matthewmueller)
* Tooling: Added yarn integration for development (#317, @thebigredgeek)
* Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek)
* Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman)
* Misc: Updated contributors (@thebigredgeek)
2.2.0 / 2015-05-09
==================
* package: update "ms" to v0.7.1 (#202, @dougwilson)
* README: add logging to file example (#193, @DanielOchoa)
* README: fixed a typo (#191, @amir-s)
* browser: expose `storage` (#190, @stephenmathieson)
* Makefile: add a `distclean` target (#189, @stephenmathieson)
2.1.3 / 2015-03-13
==================
* Updated stdout/stderr example (#186)
* Updated example/stdout.js to match debug current behaviour
* Renamed example/stderr.js to stdout.js
* Update Readme.md (#184)
* replace high intensity foreground color for bold (#182, #183)
2.1.2 / 2015-03-01
==================
* dist: recompile
* update "ms" to v0.7.0
* package: update "browserify" to v9.0.3
* component: fix "ms.js" repo location
* changed bower package name
* updated documentation about using debug in a browser
* fix: security error on safari (#167, #168, @yields)
2.1.1 / 2014-12-29
==================
* browser: use `typeof` to check for `console` existence
* browser: check for `console.log` truthiness (fix IE 8/9)
* browser: add support for Chrome apps
* Readme: added Windows usage remarks
* Add `bower.json` to properly support bower install
2.1.0 / 2014-10-15
==================
* node: implement `DEBUG_FD` env variable support
* package: update "browserify" to v6.1.0
* package: add "license" field to package.json (#135, @panuhorsmalahti)
2.0.0 / 2014-09-01
==================
* package: update "browserify" to v5.11.0
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
1.0.4 / 2014-07-15
==================
* dist: recompile
* example: remove `console.info()` log usage
* example: add "Content-Type" UTF-8 header to browser example
* browser: place %c marker after the space character
* browser: reset the "content" color via `color: inherit`
* browser: add colors support for Firefox >= v31
* debug: prefer an instance `log()` function over the global one (#119)
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
1.0.3 / 2014-07-09
==================
* Add support for multiple wildcards in namespaces (#122, @seegno)
* browser: fix lint
1.0.2 / 2014-06-10
==================
* browser: update color palette (#113, @gscottolson)
* common: make console logging function configurable (#108, @timoxley)
* node: fix %o colors on old node <= 0.8.x
* Makefile: find node path using shell/which (#109, @timoxley)
1.0.1 / 2014-06-06
==================
* browser: use `removeItem()` to clear localStorage
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
* package: add "contributors" section
* node: fix comment typo
* README: list authors
1.0.0 / 2014-06-04
==================
* make ms diff be global, not be scope
* debug: ignore empty strings in enable()
* node: make DEBUG_COLORS able to disable coloring
* *: export the `colors` array
* npmignore: don't publish the `dist` dir
* Makefile: refactor to use browserify
* package: add "browserify" as a dev dependency
* Readme: add Web Inspector Colors section
* node: reset terminal color for the debug content
* node: map "%o" to `util.inspect()`
* browser: map "%j" to `JSON.stringify()`
* debug: add custom "formatters"
* debug: use "ms" module for humanizing the diff
* Readme: add "bash" syntax highlighting
* browser: add Firebug color support
* browser: add colors for WebKit browsers
* node: apply log to `console`
* rewrite: abstract common logic for Node & browsers
* add .jshintrc file
0.8.1 / 2014-04-14
==================
* package: re-add the "component" section
0.8.0 / 2014-03-30
==================
* add `enable()` method for nodejs. Closes #27
* change from stderr to stdout
* remove unnecessary index.js file
0.7.4 / 2013-11-13
==================
* remove "browserify" key from package.json (fixes something in browserify)
0.7.3 / 2013-10-30
==================
* fix: catch localStorage security error when cookies are blocked (Chrome)
* add debug(err) support. Closes #46
* add .browser prop to package.json. Closes #42
0.7.2 / 2013-02-06
==================
* fix package.json
* fix: Mobile Safari (private mode) is broken with debug
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
0.7.1 / 2013-02-05
==================
* add repository URL to package.json
* add DEBUG_COLORED to force colored output
* add browserify support
* fix component. Closes #24
0.7.0 / 2012-05-04
==================
* Added .component to package.json
* Added debug.component.js build
0.6.0 / 2012-03-16
==================
* Added support for "-" prefix in DEBUG [Vinay Pulim]
* Added `.enabled` flag to the node version [TooTallNate]
0.5.0 / 2012-02-02
==================
* Added: humanize diffs. Closes #8
* Added `debug.disable()` to the CS variant
* Removed padding. Closes #10
* Fixed: persist client-side variant again. Closes #9
0.4.0 / 2012-02-01
==================
* Added browser variant support for older browsers [TooTallNate]
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
* Added padding to diff (moved it to the right)
0.3.0 / 2012-01-26
==================
* Added millisecond diff when isatty, otherwise UTC string
0.2.0 / 2012-01-22
==================
* Added wildcard support
0.1.0 / 2011-12-02
==================
* Added: remove colors unless stderr isatty [TooTallNate]
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/node_modules/debug/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/node_modules/debug/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11705
} |
# debug
[](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
[](#sponsors)
A tiny node.js debugging utility modelled after node core's debugging technique.
**Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)**
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example _app.js_:
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %s', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example _worker.js_:
```js
var debug = require('debug')('worker');
setInterval(function(){
debug('doing some work');
}, 1000);
```
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:


#### Windows note
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Note that PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Then, run the program to be debugged as usual.
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.

When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:

## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
#### Web Inspector Colors
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
Colored output looks something like:

## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example _stdout.js_:
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2016 TJ Holowaychuk <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17915
} |
The MIT License (MIT)
Copyright (c) 2016 Zeit, Inc.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/node_modules/ms/license.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/node_modules/ms/license.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1076
} |
# ms
[](https://travis-ci.org/zeit/ms)
[](https://zeit.chat/)
Use this package to easily convert various time formats to milliseconds.
## Examples
```js
ms('2 days') // 172800000
ms('1d') // 86400000
ms('10h') // 36000000
ms('2.5 hrs') // 9000000
ms('2h') // 7200000
ms('1m') // 60000
ms('5s') // 5000
ms('1y') // 31557600000
ms('100') // 100
```
### Convert from milliseconds
```js
ms(60000) // "1m"
ms(2 * 60000) // "2m"
ms(ms('10 hours')) // "10h"
```
### Time format written-out
```js
ms(60000, { long: true }) // "1 minute"
ms(2 * 60000, { long: true }) // "2 minutes"
ms(ms('10 hours'), { long: true }) // "10 hours"
```
## Features
- Works both in [node](https://nodejs.org) and in the browser.
- If a number is supplied to `ms`, a string with a unit is returned.
- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
## Caught a bug?
1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
2. Link the package to the global module directory: `npm link`
3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
As always, you can run the tests using: `npm test` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/express/node_modules/ms/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/express/node_modules/ms/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1720
} |
### [5.1.2](https://github.com/gulpjs/glob-parent/compare/v5.1.1...v5.1.2) (2021-03-06)
### Bug Fixes
* eliminate ReDoS ([#36](https://github.com/gulpjs/glob-parent/issues/36)) ([f923116](https://github.com/gulpjs/glob-parent/commit/f9231168b0041fea3f8f954b3cceb56269fc6366))
### [5.1.1](https://github.com/gulpjs/glob-parent/compare/v5.1.0...v5.1.1) (2021-01-27)
### Bug Fixes
* unescape exclamation mark ([#26](https://github.com/gulpjs/glob-parent/issues/26)) ([a98874f](https://github.com/gulpjs/glob-parent/commit/a98874f1a59e407f4fb1beb0db4efa8392da60bb))
## [5.1.0](https://github.com/gulpjs/glob-parent/compare/v5.0.0...v5.1.0) (2021-01-27)
### Features
* add `flipBackslashes` option to disable auto conversion of slashes (closes [#24](https://github.com/gulpjs/glob-parent/issues/24)) ([#25](https://github.com/gulpjs/glob-parent/issues/25)) ([eecf91d](https://github.com/gulpjs/glob-parent/commit/eecf91d5e3834ed78aee39c4eaaae654d76b87b3))
## [5.0.0](https://github.com/gulpjs/glob-parent/compare/v4.0.0...v5.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* Drop support for node <6 & bump dependencies
### Miscellaneous Chores
* Drop support for node <6 & bump dependencies ([896c0c0](https://github.com/gulpjs/glob-parent/commit/896c0c00b4e7362f60b96e7fc295ae929245255a))
## [4.0.0](https://github.com/gulpjs/glob-parent/compare/v3.1.0...v4.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* question marks are valid path characters on Windows so avoid flagging as a glob when alone
* Update is-glob dependency
### Features
* hoist regexps and strings for performance gains ([4a80667](https://github.com/gulpjs/glob-parent/commit/4a80667c69355c76a572a5892b0f133c8e1f457e))
* question marks are valid path characters on Windows so avoid flagging as a glob when alone ([2a551dd](https://github.com/gulpjs/glob-parent/commit/2a551dd0dc3235e78bf3c94843d4107072d17841))
* Update is-glob dependency ([e41fcd8](https://github.com/gulpjs/glob-parent/commit/e41fcd895d1f7bc617dba45c9d935a7949b9c281))
## [3.1.0](https://github.com/gulpjs/glob-parent/compare/v3.0.1...v3.1.0) (2021-01-27)
### Features
* allow basic win32 backslash use ([272afa5](https://github.com/gulpjs/glob-parent/commit/272afa5fd070fc0f796386a5993d4ee4a846988b))
* handle extglobs (parentheses) containing separators ([7db1bdb](https://github.com/gulpjs/glob-parent/commit/7db1bdb0756e55fd14619e8ce31aa31b17b117fd))
* new approach to braces/brackets handling ([8269bd8](https://github.com/gulpjs/glob-parent/commit/8269bd89290d99fac9395a354fb56fdcdb80f0be))
* pre-process braces/brackets sections ([9ef8a87](https://github.com/gulpjs/glob-parent/commit/9ef8a87f66b1a43d0591e7a8e4fc5a18415ee388))
* preserve escaped brace/bracket at end of string ([8cfb0ba](https://github.com/gulpjs/glob-parent/commit/8cfb0ba84202d51571340dcbaf61b79d16a26c76))
### Bug Fixes
* trailing escaped square brackets ([99ec9fe](https://github.com/gulpjs/glob-parent/commit/99ec9fecc60ee488ded20a94dd4f18b4f55c4ccf))
### [3.0.1](https://github.com/gulpjs/glob-parent/compare/v3.0.0...v3.0.1) (2021-01-27)
### Features
* use path-dirname ponyfill ([cdbea5f](https://github.com/gulpjs/glob-parent/commit/cdbea5f32a58a54e001a75ddd7c0fccd4776aacc))
### Bug Fixes
* unescape glob-escaped dirnames on output ([598c533](https://github.com/gulpjs/glob-parent/commit/598c533bdf49c1428bc063aa9b8db40c5a86b030))
## [3.0.0](https://github.com/gulpjs/glob-parent/compare/v2.0.0...v3.0.0) (2021-01-27)
### ⚠ BREAKING CHANGES
* update is-glob dependency
### Features
* update is-glob dependency ([5c5f8ef](https://github.com/gulpjs/glob-parent/commit/5c5f8efcee362a8e7638cf8220666acd8784f6bd))
## [2.0.0](https://github.com/gulpjs/glob-parent/compare/v1.3.0...v2.0.0) (2021-01-27)
### Features
* move up to dirname regardless of glob characters ([f97fb83](https://github.com/gulpjs/glob-parent/commit/f97fb83be2e0a9fc8d3b760e789d2ecadd6aa0c2))
## [1.3.0](https://github.com/gulpjs/glob-parent/compare/v1.2.0...v1.3.0) (2021-01-27)
## [1.2.0](https://github.com/gulpjs/glob-parent/compare/v1.1.0...v1.2.0) (2021-01-27)
### Reverts
* feat: make regex test strings smaller ([dc80fa9](https://github.com/gulpjs/glob-parent/commit/dc80fa9658dca20549cfeba44bbd37d5246fcce0))
## [1.1.0](https://github.com/gulpjs/glob-parent/compare/v1.0.0...v1.1.0) (2021-01-27)
### Features
* make regex test strings smaller ([cd83220](https://github.com/gulpjs/glob-parent/commit/cd832208638f45169f986d80fcf66e401f35d233))
## 1.0.0 (2021-01-27) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-glob/node_modules/glob-parent/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-glob/node_modules/glob-parent/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4502
} |
<p align="center">
<a href="https://gulpjs.com">
<img height="257" width="114" src="https://raw.githubusercontent.com/gulpjs/artwork/master/gulp-2x.png">
</a>
</p>
# glob-parent
[![NPM version][npm-image]][npm-url] [![Downloads][downloads-image]][npm-url] [![Azure Pipelines Build Status][azure-pipelines-image]][azure-pipelines-url] [![Travis Build Status][travis-image]][travis-url] [![AppVeyor Build Status][appveyor-image]][appveyor-url] [![Coveralls Status][coveralls-image]][coveralls-url] [![Gitter chat][gitter-image]][gitter-url]
Extract the non-magic parent path from a glob string.
## Usage
```js
var globParent = require('glob-parent');
globParent('path/to/*.js'); // 'path/to'
globParent('/root/path/to/*.js'); // '/root/path/to'
globParent('/*.js'); // '/'
globParent('*.js'); // '.'
globParent('**/*.js'); // '.'
globParent('path/{to,from}'); // 'path'
globParent('path/!(to|from)'); // 'path'
globParent('path/?(to|from)'); // 'path'
globParent('path/+(to|from)'); // 'path'
globParent('path/*(to|from)'); // 'path'
globParent('path/@(to|from)'); // 'path'
globParent('path/**/*'); // 'path'
// if provided a non-glob path, returns the nearest dir
globParent('path/foo/bar.js'); // 'path/foo'
globParent('path/foo/'); // 'path/foo'
globParent('path/foo'); // 'path' (see issue #3 for details)
```
## API
### `globParent(maybeGlobString, [options])`
Takes a string and returns the part of the path before the glob begins. Be aware of Escaping rules and Limitations below.
#### options
```js
{
// Disables the automatic conversion of slashes for Windows
flipBackslashes: true
}
```
## Escaping
The following characters have special significance in glob patterns and must be escaped if you want them to be treated as regular path characters:
- `?` (question mark) unless used as a path segment alone
- `*` (asterisk)
- `|` (pipe)
- `(` (opening parenthesis)
- `)` (closing parenthesis)
- `{` (opening curly brace)
- `}` (closing curly brace)
- `[` (opening bracket)
- `]` (closing bracket)
**Example**
```js
globParent('foo/[bar]/') // 'foo'
globParent('foo/\\[bar]/') // 'foo/[bar]'
```
## Limitations
### Braces & Brackets
This library attempts a quick and imperfect method of determining which path
parts have glob magic without fully parsing/lexing the pattern. There are some
advanced use cases that can trip it up, such as nested braces where the outer
pair is escaped and the inner one contains a path separator. If you find
yourself in the unlikely circumstance of being affected by this or need to
ensure higher-fidelity glob handling in your library, it is recommended that you
pre-process your input with [expand-braces] and/or [expand-brackets].
### Windows
Backslashes are not valid path separators for globs. If a path with backslashes
is provided anyway, for simple cases, glob-parent will replace the path
separator for you and return the non-glob parent path (now with
forward-slashes, which are still valid as Windows path separators).
This cannot be used in conjunction with escape characters.
```js
// BAD
globParent('C:\\Program Files \\(x86\\)\\*.ext') // 'C:/Program Files /(x86/)'
// GOOD
globParent('C:/Program Files\\(x86\\)/*.ext') // 'C:/Program Files (x86)'
```
If you are using escape characters for a pattern without path parts (i.e.
relative to `cwd`), prefix with `./` to avoid confusing glob-parent.
```js
// BAD
globParent('foo \\[bar]') // 'foo '
globParent('foo \\[bar]*') // 'foo '
// GOOD
globParent('./foo \\[bar]') // 'foo [bar]'
globParent('./foo \\[bar]*') // '.'
```
## License
ISC
[expand-braces]: https://github.com/jonschlinkert/expand-braces
[expand-brackets]: https://github.com/jonschlinkert/expand-brackets
[downloads-image]: https://img.shields.io/npm/dm/glob-parent.svg
[npm-url]: https://www.npmjs.com/package/glob-parent
[npm-image]: https://img.shields.io/npm/v/glob-parent.svg
[azure-pipelines-url]: https://dev.azure.com/gulpjs/gulp/_build/latest?definitionId=2&branchName=master
[azure-pipelines-image]: https://dev.azure.com/gulpjs/gulp/_apis/build/status/glob-parent?branchName=master
[travis-url]: https://travis-ci.org/gulpjs/glob-parent
[travis-image]: https://img.shields.io/travis/gulpjs/glob-parent.svg?label=travis-ci
[appveyor-url]: https://ci.appveyor.com/project/gulpjs/glob-parent
[appveyor-image]: https://img.shields.io/appveyor/ci/gulpjs/glob-parent.svg?label=appveyor
[coveralls-url]: https://coveralls.io/r/gulpjs/glob-parent
[coveralls-image]: https://img.shields.io/coveralls/gulpjs/glob-parent/master.svg
[gitter-url]: https://gitter.im/gulpjs/gulp
[gitter-image]: https://badges.gitter.im/gulpjs/gulp.svg | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/fast-glob/node_modules/glob-parent/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/fast-glob/node_modules/glob-parent/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4645
} |
2.6.9 / 2017-09-22
==================
* remove ReDoS regexp in %o formatter (#504)
2.6.8 / 2017-05-18
==================
* Fix: Check for undefined on browser globals (#462, @marbemac)
2.6.7 / 2017-05-16
==================
* Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom)
* Fix: Inline extend function in node implementation (#452, @dougwilson)
* Docs: Fix typo (#455, @msasad)
2.6.5 / 2017-04-27
==================
* Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek)
* Misc: clean up browser reference checks (#447, @thebigredgeek)
* Misc: add npm-debug.log to .gitignore (@thebigredgeek)
2.6.4 / 2017-04-20
==================
* Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo)
* Chore: ignore bower.json in npm installations. (#437, @joaovieira)
* Misc: update "ms" to v0.7.3 (@tootallnate)
2.6.3 / 2017-03-13
==================
* Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts)
* Docs: Changelog fix (@thebigredgeek)
2.6.2 / 2017-03-10
==================
* Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin)
* Docs: Add backers and sponsors from Open Collective (#422, @piamancini)
* Docs: Add Slackin invite badge (@tootallnate)
2.6.1 / 2017-02-10
==================
* Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error
* Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0)
* Fix: IE8 "Expected identifier" error (#414, @vgoma)
* Fix: Namespaces would not disable once enabled (#409, @musikov)
2.6.0 / 2016-12-28
==================
* Fix: added better null pointer checks for browser useColors (@thebigredgeek)
* Improvement: removed explicit `window.debug` export (#404, @tootallnate)
* Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate)
2.5.2 / 2016-12-25
==================
* Fix: reference error on window within webworkers (#393, @KlausTrainer)
* Docs: fixed README typo (#391, @lurch)
* Docs: added notice about v3 api discussion (@thebigredgeek)
2.5.1 / 2016-12-20
==================
* Fix: babel-core compatibility
2.5.0 / 2016-12-20
==================
* Fix: wrong reference in bower file (@thebigredgeek)
* Fix: webworker compatibility (@thebigredgeek)
* Fix: output formatting issue (#388, @kribblo)
* Fix: babel-loader compatibility (#383, @escwald)
* Misc: removed built asset from repo and publications (@thebigredgeek)
* Misc: moved source files to /src (#378, @yamikuronue)
* Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue)
* Test: coveralls integration (#378, @yamikuronue)
* Docs: simplified language in the opening paragraph (#373, @yamikuronue)
2.4.5 / 2016-12-17
==================
* Fix: `navigator` undefined in Rhino (#376, @jochenberger)
* Fix: custom log function (#379, @hsiliev)
* Improvement: bit of cleanup + linting fixes (@thebigredgeek)
* Improvement: rm non-maintainted `dist/` dir (#375, @freewil)
* Docs: simplified language in the opening paragraph. (#373, @yamikuronue)
2.4.4 / 2016-12-14
==================
* Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts)
2.4.3 / 2016-12-14
==================
* Fix: navigation.userAgent error for react native (#364, @escwald)
2.4.2 / 2016-12-14
==================
* Fix: browser colors (#367, @tootallnate)
* Misc: travis ci integration (@thebigredgeek)
* Misc: added linting and testing boilerplate with sanity check (@thebigredgeek)
2.4.1 / 2016-12-13
==================
* Fix: typo that broke the package (#356)
2.4.0 / 2016-12-13
==================
* Fix: bower.json references unbuilt src entry point (#342, @justmatt)
* Fix: revert "handle regex special characters" (@tootallnate)
* Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate)
* Feature: %O`(big O) pretty-prints objects (#322, @tootallnate)
* Improvement: allow colors in workers (#335, @botverse)
* Improvement: use same color for same namespace. (#338, @lchenay)
2.3.3 / 2016-11-09
==================
* Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne)
* Fix: Returning `localStorage` saved values (#331, Levi Thomason)
* Improvement: Don't create an empty object when no `process` (Nathan Rajlich)
2.3.2 / 2016-11-09
==================
* Fix: be super-safe in index.js as well (@TooTallNate)
* Fix: should check whether process exists (Tom Newby)
2.3.1 / 2016-11-09
==================
* Fix: Added electron compatibility (#324, @paulcbetts)
* Improvement: Added performance optimizations (@tootallnate)
* Readme: Corrected PowerShell environment variable example (#252, @gimre)
* Misc: Removed yarn lock file from source control (#321, @fengmk2)
2.3.0 / 2016-11-07
==================
* Fix: Consistent placement of ms diff at end of output (#215, @gorangajic)
* Fix: Escaping of regex special characters in namespace strings (#250, @zacronos)
* Fix: Fixed bug causing crash on react-native (#282, @vkarpov15)
* Feature: Enabled ES6+ compatible import via default export (#212 @bucaran)
* Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom)
* Package: Update "ms" to 0.7.2 (#315, @DevSide)
* Package: removed superfluous version property from bower.json (#207 @kkirsche)
* Readme: fix USE_COLORS to DEBUG_COLORS
* Readme: Doc fixes for format string sugar (#269, @mlucool)
* Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0)
* Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable)
* Readme: better docs for browser support (#224, @matthewmueller)
* Tooling: Added yarn integration for development (#317, @thebigredgeek)
* Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek)
* Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman)
* Misc: Updated contributors (@thebigredgeek)
2.2.0 / 2015-05-09
==================
* package: update "ms" to v0.7.1 (#202, @dougwilson)
* README: add logging to file example (#193, @DanielOchoa)
* README: fixed a typo (#191, @amir-s)
* browser: expose `storage` (#190, @stephenmathieson)
* Makefile: add a `distclean` target (#189, @stephenmathieson)
2.1.3 / 2015-03-13
==================
* Updated stdout/stderr example (#186)
* Updated example/stdout.js to match debug current behaviour
* Renamed example/stderr.js to stdout.js
* Update Readme.md (#184)
* replace high intensity foreground color for bold (#182, #183)
2.1.2 / 2015-03-01
==================
* dist: recompile
* update "ms" to v0.7.0
* package: update "browserify" to v9.0.3
* component: fix "ms.js" repo location
* changed bower package name
* updated documentation about using debug in a browser
* fix: security error on safari (#167, #168, @yields)
2.1.1 / 2014-12-29
==================
* browser: use `typeof` to check for `console` existence
* browser: check for `console.log` truthiness (fix IE 8/9)
* browser: add support for Chrome apps
* Readme: added Windows usage remarks
* Add `bower.json` to properly support bower install
2.1.0 / 2014-10-15
==================
* node: implement `DEBUG_FD` env variable support
* package: update "browserify" to v6.1.0
* package: add "license" field to package.json (#135, @panuhorsmalahti)
2.0.0 / 2014-09-01
==================
* package: update "browserify" to v5.11.0
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
1.0.4 / 2014-07-15
==================
* dist: recompile
* example: remove `console.info()` log usage
* example: add "Content-Type" UTF-8 header to browser example
* browser: place %c marker after the space character
* browser: reset the "content" color via `color: inherit`
* browser: add colors support for Firefox >= v31
* debug: prefer an instance `log()` function over the global one (#119)
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
1.0.3 / 2014-07-09
==================
* Add support for multiple wildcards in namespaces (#122, @seegno)
* browser: fix lint
1.0.2 / 2014-06-10
==================
* browser: update color palette (#113, @gscottolson)
* common: make console logging function configurable (#108, @timoxley)
* node: fix %o colors on old node <= 0.8.x
* Makefile: find node path using shell/which (#109, @timoxley)
1.0.1 / 2014-06-06
==================
* browser: use `removeItem()` to clear localStorage
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
* package: add "contributors" section
* node: fix comment typo
* README: list authors
1.0.0 / 2014-06-04
==================
* make ms diff be global, not be scope
* debug: ignore empty strings in enable()
* node: make DEBUG_COLORS able to disable coloring
* *: export the `colors` array
* npmignore: don't publish the `dist` dir
* Makefile: refactor to use browserify
* package: add "browserify" as a dev dependency
* Readme: add Web Inspector Colors section
* node: reset terminal color for the debug content
* node: map "%o" to `util.inspect()`
* browser: map "%j" to `JSON.stringify()`
* debug: add custom "formatters"
* debug: use "ms" module for humanizing the diff
* Readme: add "bash" syntax highlighting
* browser: add Firebug color support
* browser: add colors for WebKit browsers
* node: apply log to `console`
* rewrite: abstract common logic for Node & browsers
* add .jshintrc file
0.8.1 / 2014-04-14
==================
* package: re-add the "component" section
0.8.0 / 2014-03-30
==================
* add `enable()` method for nodejs. Closes #27
* change from stderr to stdout
* remove unnecessary index.js file
0.7.4 / 2013-11-13
==================
* remove "browserify" key from package.json (fixes something in browserify)
0.7.3 / 2013-10-30
==================
* fix: catch localStorage security error when cookies are blocked (Chrome)
* add debug(err) support. Closes #46
* add .browser prop to package.json. Closes #42
0.7.2 / 2013-02-06
==================
* fix package.json
* fix: Mobile Safari (private mode) is broken with debug
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
0.7.1 / 2013-02-05
==================
* add repository URL to package.json
* add DEBUG_COLORED to force colored output
* add browserify support
* fix component. Closes #24
0.7.0 / 2012-05-04
==================
* Added .component to package.json
* Added debug.component.js build
0.6.0 / 2012-03-16
==================
* Added support for "-" prefix in DEBUG [Vinay Pulim]
* Added `.enabled` flag to the node version [TooTallNate]
0.5.0 / 2012-02-02
==================
* Added: humanize diffs. Closes #8
* Added `debug.disable()` to the CS variant
* Removed padding. Closes #10
* Fixed: persist client-side variant again. Closes #9
0.4.0 / 2012-02-01
==================
* Added browser variant support for older browsers [TooTallNate]
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
* Added padding to diff (moved it to the right)
0.3.0 / 2012-01-26
==================
* Added millisecond diff when isatty, otherwise UTC string
0.2.0 / 2012-01-22
==================
* Added wildcard support
0.1.0 / 2011-12-02
==================
* Added: remove colors unless stderr isatty [TooTallNate]
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/node_modules/debug/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/node_modules/debug/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11705
} |
# debug
[](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
[](#sponsors)
A tiny node.js debugging utility modelled after node core's debugging technique.
**Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)**
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example _app.js_:
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %s', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example _worker.js_:
```js
var debug = require('debug')('worker');
setInterval(function(){
debug('doing some work');
}, 1000);
```
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:


#### Windows note
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Note that PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Then, run the program to be debugged as usual.
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.

When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:

## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
#### Web Inspector Colors
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
Colored output looks something like:

## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example _stdout.js_:
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2016 TJ Holowaychuk <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17915
} |
The MIT License (MIT)
Copyright (c) 2016 Zeit, Inc.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/node_modules/ms/license.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/node_modules/ms/license.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1076
} |
# ms
[](https://travis-ci.org/zeit/ms)
[](https://zeit.chat/)
Use this package to easily convert various time formats to milliseconds.
## Examples
```js
ms('2 days') // 172800000
ms('1d') // 86400000
ms('10h') // 36000000
ms('2.5 hrs') // 9000000
ms('2h') // 7200000
ms('1m') // 60000
ms('5s') // 5000
ms('1y') // 31557600000
ms('100') // 100
```
### Convert from milliseconds
```js
ms(60000) // "1m"
ms(2 * 60000) // "2m"
ms(ms('10 hours')) // "10h"
```
### Time format written-out
```js
ms(60000, { long: true }) // "1 minute"
ms(2 * 60000, { long: true }) // "2 minutes"
ms(ms('10 hours'), { long: true }) // "10 hours"
```
## Features
- Works both in [node](https://nodejs.org) and in the browser.
- If a number is supplied to `ms`, a string with a unit is returned.
- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
## Caught a bug?
1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
2. Link the package to the global module directory: `npm link`
3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
As always, you can run the tests using: `npm test` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/finalhandler/node_modules/ms/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/finalhandler/node_modules/ms/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1720
} |
# lru cache
A cache object that deletes the least-recently-used items.
[](https://travis-ci.org/isaacs/node-lru-cache) [](https://coveralls.io/github/isaacs/node-lru-cache)
## Installation:
```javascript
npm install lru-cache --save
```
## Usage:
```javascript
var LRU = require("lru-cache")
, options = { max: 500
, length: function (n, key) { return n * 2 + key.length }
, dispose: function (key, n) { n.close() }
, maxAge: 1000 * 60 * 60 }
, cache = LRU(options)
, otherCache = LRU(50) // sets just the max size
cache.set("key", "value")
cache.get("key") // "value"
// non-string keys ARE fully supported
// but note that it must be THE SAME object, not
// just a JSON-equivalent object.
var someObject = { a: 1 }
cache.set(someObject, 'a value')
// Object keys are not toString()-ed
cache.set('[object Object]', 'a different value')
assert.equal(cache.get(someObject), 'a value')
// A similar object with same keys/values won't work,
// because it's a different object identity
assert.equal(cache.get({ a: 1 }), undefined)
cache.reset() // empty the cache
```
If you put more stuff in it, then items will fall out.
If you try to put an oversized thing in it, then it'll fall out right
away.
## Options
* `max` The maximum size of the cache, checked by applying the length
function to all values in the cache. Not setting this is kind of
silly, since that's the whole purpose of this lib, but it defaults
to `Infinity`.
* `maxAge` Maximum age in ms. Items are not pro-actively pruned out
as they age, but if you try to get an item that is too old, it'll
drop it and return undefined instead of giving it to you.
* `length` Function that is used to calculate the length of stored
items. If you're storing strings or buffers, then you probably want
to do something like `function(n, key){return n.length}`. The default is
`function(){return 1}`, which is fine if you want to store `max`
like-sized things. The item is passed as the first argument, and
the key is passed as the second argumnet.
* `dispose` Function that is called on items when they are dropped
from the cache. This can be handy if you want to close file
descriptors or do other cleanup tasks when items are no longer
accessible. Called with `key, value`. It's called *before*
actually removing the item from the internal cache, so if you want
to immediately put it back in, you'll have to do that in a
`nextTick` or `setTimeout` callback or it won't do anything.
* `stale` By default, if you set a `maxAge`, it'll only actually pull
stale items out of the cache when you `get(key)`. (That is, it's
not pre-emptively doing a `setTimeout` or anything.) If you set
`stale:true`, it'll return the stale value before deleting it. If
you don't set this, then it'll return `undefined` when you try to
get a stale entry, as if it had already been deleted.
* `noDisposeOnSet` By default, if you set a `dispose()` method, then
it'll be called whenever a `set()` operation overwrites an existing
key. If you set this option, `dispose()` will only be called when a
key falls out of the cache, not when it is overwritten.
## API
* `set(key, value, maxAge)`
* `get(key) => value`
Both of these will update the "recently used"-ness of the key.
They do what you think. `maxAge` is optional and overrides the
cache `maxAge` option if provided.
If the key is not found, `get()` will return `undefined`.
The key and val can be any value.
* `peek(key)`
Returns the key value (or `undefined` if not found) without
updating the "recently used"-ness of the key.
(If you find yourself using this a lot, you *might* be using the
wrong sort of data structure, but there are some use cases where
it's handy.)
* `del(key)`
Deletes a key out of the cache.
* `reset()`
Clear the cache entirely, throwing away all values.
* `has(key)`
Check if a key is in the cache, without updating the recent-ness
or deleting it for being stale.
* `forEach(function(value,key,cache), [thisp])`
Just like `Array.prototype.forEach`. Iterates over all the keys
in the cache, in order of recent-ness. (Ie, more recently used
items are iterated over first.)
* `rforEach(function(value,key,cache), [thisp])`
The same as `cache.forEach(...)` but items are iterated over in
reverse order. (ie, less recently used items are iterated over
first.)
* `keys()`
Return an array of the keys in the cache.
* `values()`
Return an array of the values in the cache.
* `length`
Return total length of objects in cache taking into account
`length` options function.
* `itemCount`
Return total quantity of objects currently in cache. Note, that
`stale` (see options) items are returned as part of this item
count.
* `dump()`
Return an array of the cache entries ready for serialization and usage
with 'destinationCache.load(arr)`.
* `load(cacheEntriesArray)`
Loads another cache entries array, obtained with `sourceCache.dump()`,
into the cache. The destination cache is reset before loading new entries
* `prune()`
Manually iterates over the entire cache proactively pruning old entries | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/memorystore/node_modules/lru-cache/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/memorystore/node_modules/lru-cache/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 5446
} |
# yallist
Yet Another Linked List
There are many doubly-linked list implementations like it, but this
one is mine.
For when an array would be too big, and a Map can't be iterated in
reverse order.
[](https://travis-ci.org/isaacs/yallist) [](https://coveralls.io/github/isaacs/yallist)
## basic usage
```javascript
var yallist = require('yallist')
var myList = yallist.create([1, 2, 3])
myList.push('foo')
myList.unshift('bar')
// of course pop() and shift() are there, too
console.log(myList.toArray()) // ['bar', 1, 2, 3, 'foo']
myList.forEach(function (k) {
// walk the list head to tail
})
myList.forEachReverse(function (k, index, list) {
// walk the list tail to head
})
var myDoubledList = myList.map(function (k) {
return k + k
})
// now myDoubledList contains ['barbar', 2, 4, 6, 'foofoo']
// mapReverse is also a thing
var myDoubledListReverse = myList.mapReverse(function (k) {
return k + k
}) // ['foofoo', 6, 4, 2, 'barbar']
var reduced = myList.reduce(function (set, entry) {
set += entry
return set
}, 'start')
console.log(reduced) // 'startfoo123bar'
```
## api
The whole API is considered "public".
Functions with the same name as an Array method work more or less the
same way.
There's reverse versions of most things because that's the point.
### Yallist
Default export, the class that holds and manages a list.
Call it with either a forEach-able (like an array) or a set of
arguments, to initialize the list.
The Array-ish methods all act like you'd expect. No magic length,
though, so if you change that it won't automatically prune or add
empty spots.
### Yallist.create(..)
Alias for Yallist function. Some people like factories.
#### yallist.head
The first node in the list
#### yallist.tail
The last node in the list
#### yallist.length
The number of nodes in the list. (Change this at your peril. It is
not magic like Array length.)
#### yallist.toArray()
Convert the list to an array.
#### yallist.forEach(fn, [thisp])
Call a function on each item in the list.
#### yallist.forEachReverse(fn, [thisp])
Call a function on each item in the list, in reverse order.
#### yallist.get(n)
Get the data at position `n` in the list. If you use this a lot,
probably better off just using an Array.
#### yallist.getReverse(n)
Get the data at position `n`, counting from the tail.
#### yallist.map(fn, thisp)
Create a new Yallist with the result of calling the function on each
item.
#### yallist.mapReverse(fn, thisp)
Same as `map`, but in reverse.
#### yallist.pop()
Get the data from the list tail, and remove the tail from the list.
#### yallist.push(item, ...)
Insert one or more items to the tail of the list.
#### yallist.reduce(fn, initialValue)
Like Array.reduce.
#### yallist.reduceReverse
Like Array.reduce, but in reverse.
#### yallist.reverse
Reverse the list in place.
#### yallist.shift()
Get the data from the list head, and remove the head from the list.
#### yallist.slice([from], [to])
Just like Array.slice, but returns a new Yallist.
#### yallist.sliceReverse([from], [to])
Just like yallist.slice, but the result is returned in reverse.
#### yallist.toArray()
Create an array representation of the list.
#### yallist.toArrayReverse()
Create a reversed array representation of the list.
#### yallist.unshift(item, ...)
Insert one or more items to the head of the list.
#### yallist.unshiftNode(node)
Move a Node object to the front of the list. (That is, pull it out of
wherever it lives, and make it the new head.)
If the node belongs to a different list, then that list will remove it
first.
#### yallist.pushNode(node)
Move a Node object to the end of the list. (That is, pull it out of
wherever it lives, and make it the new tail.)
If the node belongs to a list already, then that list will remove it
first.
#### yallist.removeNode(node)
Remove a node from the list, preserving referential integrity of head
and tail and other nodes.
Will throw an error if you try to have a list remove a node that
doesn't belong to it.
### Yallist.Node
The class that holds the data and is actually the list.
Call with `var n = new Node(value, previousNode, nextNode)`
Note that if you do direct operations on Nodes themselves, it's very
easy to get into weird states where the list is broken. Be careful :)
#### node.next
The next node in the list.
#### node.prev
The previous node in the list.
#### node.value
The data the node contains.
#### node.list
The list to which this node belongs. (Null if it does not belong to
any list.) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/memorystore/node_modules/yallist/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/memorystore/node_modules/yallist/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4716
} |
# lru-cache
A cache object that deletes the least-recently-used items.
Specify a max number of the most recently used items that you
want to keep, and this cache will keep that many of the most
recently accessed items.
This is not primarily a TTL cache, and does not make strong TTL
guarantees. There is no preemptive pruning of expired items by
default, but you _may_ set a TTL on the cache or on a single
`set`. If you do so, it will treat expired items as missing, and
delete them when fetched. If you are more interested in TTL
caching than LRU caching, check out
[@isaacs/ttlcache](http://npm.im/@isaacs/ttlcache).
As of version 7, this is one of the most performant LRU
implementations available in JavaScript, and supports a wide
diversity of use cases. However, note that using some of the
features will necessarily impact performance, by causing the
cache to have to do more work. See the "Performance" section
below.
## Installation
```bash
npm install lru-cache --save
```
## Usage
```js
// hybrid module, either works
import { LRUCache } from 'lru-cache'
// or:
const { LRUCache } = require('lru-cache')
// or in minified form for web browsers:
import { LRUCache } from 'http://unpkg.com/lru-cache@9/dist/mjs/index.min.mjs'
// At least one of 'max', 'ttl', or 'maxSize' is required, to prevent
// unsafe unbounded storage.
//
// In most cases, it's best to specify a max for performance, so all
// the required memory allocation is done up-front.
//
// All the other options are optional, see the sections below for
// documentation on what each one does. Most of them can be
// overridden for specific items in get()/set()
const options = {
max: 500,
// for use with tracking overall storage size
maxSize: 5000,
sizeCalculation: (value, key) => {
return 1
},
// for use when you need to clean up something when objects
// are evicted from the cache
dispose: (value, key) => {
freeFromMemoryOrWhatever(value)
},
// how long to live in ms
ttl: 1000 * 60 * 5,
// return stale items before removing from cache?
allowStale: false,
updateAgeOnGet: false,
updateAgeOnHas: false,
// async method to use for cache.fetch(), for
// stale-while-revalidate type of behavior
fetchMethod: async (
key,
staleValue,
{ options, signal, context }
) => {},
}
const cache = new LRUCache(options)
cache.set('key', 'value')
cache.get('key') // "value"
// non-string keys ARE fully supported
// but note that it must be THE SAME object, not
// just a JSON-equivalent object.
var someObject = { a: 1 }
cache.set(someObject, 'a value')
// Object keys are not toString()-ed
cache.set('[object Object]', 'a different value')
assert.equal(cache.get(someObject), 'a value')
// A similar object with same keys/values won't work,
// because it's a different object identity
assert.equal(cache.get({ a: 1 }), undefined)
cache.clear() // empty the cache
```
If you put more stuff in the cache, then less recently used items
will fall out. That's what an LRU cache is.
For full description of the API and all options, please see [the
LRUCache typedocs](https://isaacs.github.io/node-lru-cache/)
## Storage Bounds Safety
This implementation aims to be as flexible as possible, within
the limits of safe memory consumption and optimal performance.
At initial object creation, storage is allocated for `max` items.
If `max` is set to zero, then some performance is lost, and item
count is unbounded. Either `maxSize` or `ttl` _must_ be set if
`max` is not specified.
If `maxSize` is set, then this creates a safe limit on the
maximum storage consumed, but without the performance benefits of
pre-allocation. When `maxSize` is set, every item _must_ provide
a size, either via the `sizeCalculation` method provided to the
constructor, or via a `size` or `sizeCalculation` option provided
to `cache.set()`. The size of every item _must_ be a positive
integer.
If neither `max` nor `maxSize` are set, then `ttl` tracking must
be enabled. Note that, even when tracking item `ttl`, items are
_not_ preemptively deleted when they become stale, unless
`ttlAutopurge` is enabled. Instead, they are only purged the
next time the key is requested. Thus, if `ttlAutopurge`, `max`,
and `maxSize` are all not set, then the cache will potentially
grow unbounded.
In this case, a warning is printed to standard error. Future
versions may require the use of `ttlAutopurge` if `max` and
`maxSize` are not specified.
If you truly wish to use a cache that is bound _only_ by TTL
expiration, consider using a `Map` object, and calling
`setTimeout` to delete entries when they expire. It will perform
much better than an LRU cache.
Here is an implementation you may use, under the same
[license](./LICENSE) as this package:
```js
// a storage-unbounded ttl cache that is not an lru-cache
const cache = {
data: new Map(),
timers: new Map(),
set: (k, v, ttl) => {
if (cache.timers.has(k)) {
clearTimeout(cache.timers.get(k))
}
cache.timers.set(
k,
setTimeout(() => cache.delete(k), ttl)
)
cache.data.set(k, v)
},
get: k => cache.data.get(k),
has: k => cache.data.has(k),
delete: k => {
if (cache.timers.has(k)) {
clearTimeout(cache.timers.get(k))
}
cache.timers.delete(k)
return cache.data.delete(k)
},
clear: () => {
cache.data.clear()
for (const v of cache.timers.values()) {
clearTimeout(v)
}
cache.timers.clear()
},
}
```
If that isn't to your liking, check out
[@isaacs/ttlcache](http://npm.im/@isaacs/ttlcache).
## Storing Undefined Values
This cache never stores undefined values, as `undefined` is used
internally in a few places to indicate that a key is not in the
cache.
You may call `cache.set(key, undefined)`, but this is just
an alias for `cache.delete(key)`. Note that this has the effect
that `cache.has(key)` will return _false_ after setting it to
undefined.
```js
cache.set(myKey, undefined)
cache.has(myKey) // false!
```
If you need to track `undefined` values, and still note that the
key is in the cache, an easy workaround is to use a sigil object
of your own.
```js
import { LRUCache } from 'lru-cache'
const undefinedValue = Symbol('undefined')
const cache = new LRUCache(...)
const mySet = (key, value) =>
cache.set(key, value === undefined ? undefinedValue : value)
const myGet = (key, value) => {
const v = cache.get(key)
return v === undefinedValue ? undefined : v
}
```
## Performance
As of January 2022, version 7 of this library is one of the most
performant LRU cache implementations in JavaScript.
Benchmarks can be extremely difficult to get right. In
particular, the performance of set/get/delete operations on
objects will vary _wildly_ depending on the type of key used. V8
is highly optimized for objects with keys that are short strings,
especially integer numeric strings. Thus any benchmark which
tests _solely_ using numbers as keys will tend to find that an
object-based approach performs the best.
Note that coercing _anything_ to strings to use as object keys is
unsafe, unless you can be 100% certain that no other type of
value will be used. For example:
```js
const myCache = {}
const set = (k, v) => (myCache[k] = v)
const get = k => myCache[k]
set({}, 'please hang onto this for me')
set('[object Object]', 'oopsie')
```
Also beware of "Just So" stories regarding performance. Garbage
collection of large (especially: deep) object graphs can be
incredibly costly, with several "tipping points" where it
increases exponentially. As a result, putting that off until
later can make it much worse, and less predictable. If a library
performs well, but only in a scenario where the object graph is
kept shallow, then that won't help you if you are using large
objects as keys.
In general, when attempting to use a library to improve
performance (such as a cache like this one), it's best to choose
an option that will perform well in the sorts of scenarios where
you'll actually use it.
This library is optimized for repeated gets and minimizing
eviction time, since that is the expected need of a LRU. Set
operations are somewhat slower on average than a few other
options, in part because of that optimization. It is assumed
that you'll be caching some costly operation, ideally as rarely
as possible, so optimizing set over get would be unwise.
If performance matters to you:
1. If it's at all possible to use small integer values as keys,
and you can guarantee that no other types of values will be
used as keys, then do that, and use a cache such as
[lru-fast](https://npmjs.com/package/lru-fast), or
[mnemonist's
LRUCache](https://yomguithereal.github.io/mnemonist/lru-cache)
which uses an Object as its data store.
2. Failing that, if at all possible, use short non-numeric
strings (ie, less than 256 characters) as your keys, and use
[mnemonist's
LRUCache](https://yomguithereal.github.io/mnemonist/lru-cache).
3. If the types of your keys will be anything else, especially
long strings, strings that look like floats, objects, or some
mix of types, or if you aren't sure, then this library will
work well for you.
If you do not need the features that this library provides
(like asynchronous fetching, a variety of TTL staleness
options, and so on), then [mnemonist's
LRUMap](https://yomguithereal.github.io/mnemonist/lru-map) is
a very good option, and just slightly faster than this module
(since it does considerably less).
4. Do not use a `dispose` function, size tracking, or especially
ttl behavior, unless absolutely needed. These features are
convenient, and necessary in some use cases, and every attempt
has been made to make the performance impact minimal, but it
isn't nothing.
## Breaking Changes in Version 7
This library changed to a different algorithm and internal data
structure in version 7, yielding significantly better
performance, albeit with some subtle changes as a result.
If you were relying on the internals of LRUCache in version 6 or
before, it probably will not work in version 7 and above.
## Breaking Changes in Version 8
- The `fetchContext` option was renamed to `context`, and may no
longer be set on the cache instance itself.
- Rewritten in TypeScript, so pretty much all the types moved
around a lot.
- The AbortController/AbortSignal polyfill was removed. For this
reason, **Node version 16.14.0 or higher is now required**.
- Internal properties were moved to actual private class
properties.
- Keys and values must not be `null` or `undefined`.
- Minified export available at `'lru-cache/min'`, for both CJS
and MJS builds.
## Breaking Changes in Version 9
- Named export only, no default export.
- AbortController polyfill returned, albeit with a warning when
used.
## Breaking Changes in Version 10
- `cache.fetch()` return type is now `Promise<V | undefined>`
instead of `Promise<V | void>`. This is an irrelevant change
practically speaking, but can require changes for TypeScript
users.
For more info, see the [change log](CHANGELOG.md). | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/path-scurry/node_modules/lru-cache/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/path-scurry/node_modules/lru-cache/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11106
} |
# pg-types
This is the code that turns all the raw text from postgres into JavaScript types for [node-postgres](https://github.com/brianc/node-postgres.git)
## use
This module is consumed and exported from the root `pg` object of node-postgres. To access it, do the following:
```js
var types = require('pg').types
```
Generally what you'll want to do is override how a specific data-type is parsed and turned into a JavaScript type. By default the PostgreSQL backend server returns everything as strings. Every data type corresponds to a unique `OID` within the server, and these `OIDs` are sent back with the query response. So, you need to match a particluar `OID` to a function you'd like to use to take the raw text input and produce a valid JavaScript object as a result. `null` values are never parsed.
Let's do something I commonly like to do on projects: return 64-bit integers `(int8)` as JavaScript integers. Because JavaScript doesn't have support for 64-bit integers node-postgres cannot confidently parse `int8` data type results as numbers because if you have a _huge_ number it will overflow and the result you'd get back from node-postgres would not be the result in the datbase. That would be a __very bad thing__ so node-postgres just returns `int8` results as strings and leaves the parsing up to you. Let's say that you know you don't and wont ever have numbers greater than `int4` in your database, but you're tired of recieving results from the `COUNT(*)` function as strings (because that function returns `int8`). You would do this:
```js
var types = require('pg').types
types.setTypeParser(20, function(val) {
return parseInt(val)
})
```
__boom__: now you get numbers instead of strings.
Just as another example -- not saying this is a good idea -- let's say you want to return all dates from your database as [moment](http://momentjs.com/docs/) objects. Okay, do this:
```js
var types = require('pg').types
var moment = require('moment')
var parseFn = function(val) {
return val === null ? null : moment(val)
}
types.setTypeParser(types.builtins.TIMESTAMPTZ, parseFn)
types.setTypeParser(types.builtins.TIMESTAMP, parseFn)
```
_note: I've never done that with my dates, and I'm not 100% sure moment can parse all the date strings returned from postgres. It's just an example!_
If you're thinking "gee, this seems pretty handy, but how can I get a list of all the OIDs in the database and what they correspond to?!?!?!" worry not:
```bash
$ psql -c "select typname, oid, typarray from pg_type order by oid"
```
If you want to find out the OID of a specific type:
```bash
$ psql -c "select typname, oid, typarray from pg_type where typname = 'daterange' order by oid"
```
:smile:
## license
The MIT License (MIT)
Copyright (c) 2014 Brian M. Carlson
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/pg/node_modules/pg-types/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/pg/node_modules/pg-types/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3830
} |
# postgres-array [](https://travis-ci.org/bendrucker/postgres-array)
> Parse postgres array columns
## Install
```
$ npm install --save postgres-array
```
## Usage
```js
var postgresArray = require('postgres-array')
postgresArray.parse('{1,2,3}', (value) => parseInt(value, 10))
//=> [1, 2, 3]
```
## API
#### `parse(input, [transform])` -> `array`
##### input
*Required*
Type: `string`
A Postgres array string.
##### transform
Type: `function`
Default: `identity`
A function that transforms non-null values inserted into the array.
## License
MIT © [Ben Drucker](http://bendrucker.me) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/pg/node_modules/postgres-array/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/pg/node_modules/postgres-array/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 690
} |
# postgres-bytea [](https://travis-ci.org/bendrucker/postgres-bytea)
> Postgres bytea parser
## Install
```
$ npm install --save postgres-bytea
```
## Usage
```js
var bytea = require('postgres-bytea');
bytea('\\000\\100\\200')
//=> buffer
```
## API
#### `bytea(input)` -> `buffer`
##### input
*Required*
Type: `string`
A Postgres bytea binary string.
## License
MIT © [Ben Drucker](http://bendrucker.me) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/pg/node_modules/postgres-bytea/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/pg/node_modules/postgres-bytea/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 502
} |
# postgres-date [](https://travis-ci.org/bendrucker/postgres-date) [](https://greenkeeper.io/)
> Postgres date output parser
This package parses [date/time outputs](https://www.postgresql.org/docs/current/datatype-datetime.html#DATATYPE-DATETIME-OUTPUT) from Postgres into Javascript `Date` objects. Its goal is to match Postgres behavior and preserve data accuracy.
If you find a case where a valid Postgres output results in incorrect parsing (including loss of precision), please [create a pull request](https://github.com/bendrucker/postgres-date/compare) and provide a failing test.
**Supported Postgres Versions:** `>= 9.6`
All prior versions of Postgres are likely compatible but not officially supported.
## Install
```
$ npm install --save postgres-date
```
## Usage
```js
var parse = require('postgres-date')
parse('2011-01-23 22:15:51Z')
// => 2011-01-23T22:15:51.000Z
```
## API
#### `parse(isoDate)` -> `date`
##### isoDate
*Required*
Type: `string`
A date string from Postgres.
## Releases
The following semantic versioning increments will be used for changes:
* **Major**: Removal of support for Node.js versions or Postgres versions (not expected)
* **Minor**: Unused, since Postgres returns dates in standard ISO 8601 format
* **Patch**: Any fix for parsing behavior
## License
MIT © [Ben Drucker](http://bendrucker.me) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/pg/node_modules/postgres-date/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/pg/node_modules/postgres-date/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1519
} |
# postgres-interval [](https://travis-ci.org/bendrucker/postgres-interval) [](https://greenkeeper.io/)
> Parse Postgres interval columns
## Install
```
$ npm install --save postgres-interval
```
## Usage
```js
var parse = require('postgres-interval')
var interval = parse('01:02:03')
//=> {hours: 1, minutes: 2, seconds: 3}
interval.toPostgres()
// 3 seconds 2 minutes 1 hours
interval.toISO()
// P0Y0M0DT1H2M3S
```
## API
#### `parse(pgInterval)` -> `interval`
##### pgInterval
*Required*
Type: `string`
A Postgres interval string.
#### `interval.toPostgres()` -> `string`
Returns an interval string. This allows the interval object to be passed into prepared statements.
#### `interval.toISOString()` -> `string`
Returns an [ISO 8601](https://en.wikipedia.org/wiki/ISO_8601#Durations) compliant string.
Also available as `interval.toISO()` for backwards compatibility.
## License
MIT © [Ben Drucker](http://bendrucker.me) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/pg/node_modules/postgres-interval/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/pg/node_modules/postgres-interval/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1113
} |
# Lilconfig ⚙️
[](https://badge.fury.io/js/lilconfig)
[](https://packagephobia.now.sh/result?p=lilconfig)
[](https://coveralls.io/github/antonk52/lilconfig)
A zero-dependency alternative to [cosmiconfig](https://www.npmjs.com/package/cosmiconfig) with the same API.
## Installation
```sh
npm install lilconfig
```
## Usage
```js
import {lilconfig, lilconfigSync} from 'lilconfig';
// all keys are optional
const options = {
stopDir: '/Users/you/some/dir',
searchPlaces: ['package.json', 'myapp.conf.js'],
ignoreEmptySearchPlaces: false
}
lilconfig(
'myapp',
options // optional
).search() // Promise<LilconfigResult>
lilconfigSync(
'myapp',
options // optional
).load(pathToConfig) // LilconfigResult
/**
* LilconfigResult
* {
* config: any; // your config
* filepath: string;
* }
*/
```
## ESM
ESM configs can be loaded with **async API only**. Specifically `js` files in projects with `"type": "module"` in `package.json` or `mjs` files.
## Difference to `cosmiconfig`
Lilconfig does not intend to be 100% compatible with `cosmiconfig` but tries to mimic it where possible. The key difference is **no** support for yaml files out of the box(`lilconfig` attempts to parse files with no extension as JSON instead of YAML). You can still add the support for YAML files by providing a loader, see an [example](#yaml-loader) below.
### Options difference between the two.
|cosmiconfig option | lilconfig |
|------------------------|-----------|
|cache | ✅ |
|loaders | ✅ |
|ignoreEmptySearchPlaces | ✅ |
|packageProp | ✅ |
|searchPlaces | ✅ |
|stopDir | ✅ |
|transform | ✅ |
## Loaders examples
### Yaml loader
If you need the YAML support you can provide your own loader
```js
import {lilconfig} from 'lilconfig';
import yaml from 'yaml';
function loadYaml(filepath, content) {
return yaml.parse(content);
}
const options = {
loaders: {
'.yaml': loadYaml,
'.yml': loadYaml,
// loader for files with no extension
noExt: loadYaml
}
};
lilconfig('myapp', options)
.search()
.then(result => {
result // {config, filepath}
});
```
## Version correlation
- lilconig v1 → cosmiconfig v6
- lilconig v2 → cosmiconfig v7
- lilconig v3 → cosmiconfig v8 | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/postcss-load-config/node_modules/lilconfig/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/postcss-load-config/node_modules/lilconfig/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2604
} |
# `react-is`
This package allows you to test arbitrary values and see if they're a particular React element type.
## Installation
```sh
# Yarn
yarn add react-is
# NPM
npm install react-is
```
## Usage
### Determining if a Component is Valid
```js
import React from "react";
import * as ReactIs from "react-is";
class ClassComponent extends React.Component {
render() {
return React.createElement("div");
}
}
const FunctionComponent = () => React.createElement("div");
const ForwardRefComponent = React.forwardRef((props, ref) =>
React.createElement(Component, { forwardedRef: ref, ...props })
);
const Context = React.createContext(false);
ReactIs.isValidElementType("div"); // true
ReactIs.isValidElementType(ClassComponent); // true
ReactIs.isValidElementType(FunctionComponent); // true
ReactIs.isValidElementType(ForwardRefComponent); // true
ReactIs.isValidElementType(Context.Provider); // true
ReactIs.isValidElementType(Context.Consumer); // true
ReactIs.isValidElementType(React.createFactory("div")); // true
```
### Determining an Element's Type
#### Context
```js
import React from "react";
import * as ReactIs from 'react-is';
const ThemeContext = React.createContext("blue");
ReactIs.isContextConsumer(<ThemeContext.Consumer />); // true
ReactIs.isContextProvider(<ThemeContext.Provider />); // true
ReactIs.typeOf(<ThemeContext.Provider />) === ReactIs.ContextProvider; // true
ReactIs.typeOf(<ThemeContext.Consumer />) === ReactIs.ContextConsumer; // true
```
#### Element
```js
import React from "react";
import * as ReactIs from 'react-is';
ReactIs.isElement(<div />); // true
ReactIs.typeOf(<div />) === ReactIs.Element; // true
```
#### Fragment
```js
import React from "react";
import * as ReactIs from 'react-is';
ReactIs.isFragment(<></>); // true
ReactIs.typeOf(<></>) === ReactIs.Fragment; // true
```
#### Portal
```js
import React from "react";
import ReactDOM from "react-dom";
import * as ReactIs from 'react-is';
const div = document.createElement("div");
const portal = ReactDOM.createPortal(<div />, div);
ReactIs.isPortal(portal); // true
ReactIs.typeOf(portal) === ReactIs.Portal; // true
```
#### StrictMode
```js
import React from "react";
import * as ReactIs from 'react-is';
ReactIs.isStrictMode(<React.StrictMode />); // true
ReactIs.typeOf(<React.StrictMode />) === ReactIs.StrictMode; // true
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/prop-types/node_modules/react-is/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/prop-types/node_modules/react-is/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2377
} |
2.6.9 / 2017-09-22
==================
* remove ReDoS regexp in %o formatter (#504)
2.6.8 / 2017-05-18
==================
* Fix: Check for undefined on browser globals (#462, @marbemac)
2.6.7 / 2017-05-16
==================
* Fix: Update ms to 2.0.0 to fix regular expression denial of service vulnerability (#458, @hubdotcom)
* Fix: Inline extend function in node implementation (#452, @dougwilson)
* Docs: Fix typo (#455, @msasad)
2.6.5 / 2017-04-27
==================
* Fix: null reference check on window.documentElement.style.WebkitAppearance (#447, @thebigredgeek)
* Misc: clean up browser reference checks (#447, @thebigredgeek)
* Misc: add npm-debug.log to .gitignore (@thebigredgeek)
2.6.4 / 2017-04-20
==================
* Fix: bug that would occure if process.env.DEBUG is a non-string value. (#444, @LucianBuzzo)
* Chore: ignore bower.json in npm installations. (#437, @joaovieira)
* Misc: update "ms" to v0.7.3 (@tootallnate)
2.6.3 / 2017-03-13
==================
* Fix: Electron reference to `process.env.DEBUG` (#431, @paulcbetts)
* Docs: Changelog fix (@thebigredgeek)
2.6.2 / 2017-03-10
==================
* Fix: DEBUG_MAX_ARRAY_LENGTH (#420, @slavaGanzin)
* Docs: Add backers and sponsors from Open Collective (#422, @piamancini)
* Docs: Add Slackin invite badge (@tootallnate)
2.6.1 / 2017-02-10
==================
* Fix: Module's `export default` syntax fix for IE8 `Expected identifier` error
* Fix: Whitelist DEBUG_FD for values 1 and 2 only (#415, @pi0)
* Fix: IE8 "Expected identifier" error (#414, @vgoma)
* Fix: Namespaces would not disable once enabled (#409, @musikov)
2.6.0 / 2016-12-28
==================
* Fix: added better null pointer checks for browser useColors (@thebigredgeek)
* Improvement: removed explicit `window.debug` export (#404, @tootallnate)
* Improvement: deprecated `DEBUG_FD` environment variable (#405, @tootallnate)
2.5.2 / 2016-12-25
==================
* Fix: reference error on window within webworkers (#393, @KlausTrainer)
* Docs: fixed README typo (#391, @lurch)
* Docs: added notice about v3 api discussion (@thebigredgeek)
2.5.1 / 2016-12-20
==================
* Fix: babel-core compatibility
2.5.0 / 2016-12-20
==================
* Fix: wrong reference in bower file (@thebigredgeek)
* Fix: webworker compatibility (@thebigredgeek)
* Fix: output formatting issue (#388, @kribblo)
* Fix: babel-loader compatibility (#383, @escwald)
* Misc: removed built asset from repo and publications (@thebigredgeek)
* Misc: moved source files to /src (#378, @yamikuronue)
* Test: added karma integration and replaced babel with browserify for browser tests (#378, @yamikuronue)
* Test: coveralls integration (#378, @yamikuronue)
* Docs: simplified language in the opening paragraph (#373, @yamikuronue)
2.4.5 / 2016-12-17
==================
* Fix: `navigator` undefined in Rhino (#376, @jochenberger)
* Fix: custom log function (#379, @hsiliev)
* Improvement: bit of cleanup + linting fixes (@thebigredgeek)
* Improvement: rm non-maintainted `dist/` dir (#375, @freewil)
* Docs: simplified language in the opening paragraph. (#373, @yamikuronue)
2.4.4 / 2016-12-14
==================
* Fix: work around debug being loaded in preload scripts for electron (#368, @paulcbetts)
2.4.3 / 2016-12-14
==================
* Fix: navigation.userAgent error for react native (#364, @escwald)
2.4.2 / 2016-12-14
==================
* Fix: browser colors (#367, @tootallnate)
* Misc: travis ci integration (@thebigredgeek)
* Misc: added linting and testing boilerplate with sanity check (@thebigredgeek)
2.4.1 / 2016-12-13
==================
* Fix: typo that broke the package (#356)
2.4.0 / 2016-12-13
==================
* Fix: bower.json references unbuilt src entry point (#342, @justmatt)
* Fix: revert "handle regex special characters" (@tootallnate)
* Feature: configurable util.inspect()`options for NodeJS (#327, @tootallnate)
* Feature: %O`(big O) pretty-prints objects (#322, @tootallnate)
* Improvement: allow colors in workers (#335, @botverse)
* Improvement: use same color for same namespace. (#338, @lchenay)
2.3.3 / 2016-11-09
==================
* Fix: Catch `JSON.stringify()` errors (#195, Jovan Alleyne)
* Fix: Returning `localStorage` saved values (#331, Levi Thomason)
* Improvement: Don't create an empty object when no `process` (Nathan Rajlich)
2.3.2 / 2016-11-09
==================
* Fix: be super-safe in index.js as well (@TooTallNate)
* Fix: should check whether process exists (Tom Newby)
2.3.1 / 2016-11-09
==================
* Fix: Added electron compatibility (#324, @paulcbetts)
* Improvement: Added performance optimizations (@tootallnate)
* Readme: Corrected PowerShell environment variable example (#252, @gimre)
* Misc: Removed yarn lock file from source control (#321, @fengmk2)
2.3.0 / 2016-11-07
==================
* Fix: Consistent placement of ms diff at end of output (#215, @gorangajic)
* Fix: Escaping of regex special characters in namespace strings (#250, @zacronos)
* Fix: Fixed bug causing crash on react-native (#282, @vkarpov15)
* Feature: Enabled ES6+ compatible import via default export (#212 @bucaran)
* Feature: Added %O formatter to reflect Chrome's console.log capability (#279, @oncletom)
* Package: Update "ms" to 0.7.2 (#315, @DevSide)
* Package: removed superfluous version property from bower.json (#207 @kkirsche)
* Readme: fix USE_COLORS to DEBUG_COLORS
* Readme: Doc fixes for format string sugar (#269, @mlucool)
* Readme: Updated docs for DEBUG_FD and DEBUG_COLORS environment variables (#232, @mattlyons0)
* Readme: doc fixes for PowerShell (#271 #243, @exoticknight @unreadable)
* Readme: better docs for browser support (#224, @matthewmueller)
* Tooling: Added yarn integration for development (#317, @thebigredgeek)
* Misc: Renamed History.md to CHANGELOG.md (@thebigredgeek)
* Misc: Added license file (#226 #274, @CantemoInternal @sdaitzman)
* Misc: Updated contributors (@thebigredgeek)
2.2.0 / 2015-05-09
==================
* package: update "ms" to v0.7.1 (#202, @dougwilson)
* README: add logging to file example (#193, @DanielOchoa)
* README: fixed a typo (#191, @amir-s)
* browser: expose `storage` (#190, @stephenmathieson)
* Makefile: add a `distclean` target (#189, @stephenmathieson)
2.1.3 / 2015-03-13
==================
* Updated stdout/stderr example (#186)
* Updated example/stdout.js to match debug current behaviour
* Renamed example/stderr.js to stdout.js
* Update Readme.md (#184)
* replace high intensity foreground color for bold (#182, #183)
2.1.2 / 2015-03-01
==================
* dist: recompile
* update "ms" to v0.7.0
* package: update "browserify" to v9.0.3
* component: fix "ms.js" repo location
* changed bower package name
* updated documentation about using debug in a browser
* fix: security error on safari (#167, #168, @yields)
2.1.1 / 2014-12-29
==================
* browser: use `typeof` to check for `console` existence
* browser: check for `console.log` truthiness (fix IE 8/9)
* browser: add support for Chrome apps
* Readme: added Windows usage remarks
* Add `bower.json` to properly support bower install
2.1.0 / 2014-10-15
==================
* node: implement `DEBUG_FD` env variable support
* package: update "browserify" to v6.1.0
* package: add "license" field to package.json (#135, @panuhorsmalahti)
2.0.0 / 2014-09-01
==================
* package: update "browserify" to v5.11.0
* node: use stderr rather than stdout for logging (#29, @stephenmathieson)
1.0.4 / 2014-07-15
==================
* dist: recompile
* example: remove `console.info()` log usage
* example: add "Content-Type" UTF-8 header to browser example
* browser: place %c marker after the space character
* browser: reset the "content" color via `color: inherit`
* browser: add colors support for Firefox >= v31
* debug: prefer an instance `log()` function over the global one (#119)
* Readme: update documentation about styled console logs for FF v31 (#116, @wryk)
1.0.3 / 2014-07-09
==================
* Add support for multiple wildcards in namespaces (#122, @seegno)
* browser: fix lint
1.0.2 / 2014-06-10
==================
* browser: update color palette (#113, @gscottolson)
* common: make console logging function configurable (#108, @timoxley)
* node: fix %o colors on old node <= 0.8.x
* Makefile: find node path using shell/which (#109, @timoxley)
1.0.1 / 2014-06-06
==================
* browser: use `removeItem()` to clear localStorage
* browser, node: don't set DEBUG if namespaces is undefined (#107, @leedm777)
* package: add "contributors" section
* node: fix comment typo
* README: list authors
1.0.0 / 2014-06-04
==================
* make ms diff be global, not be scope
* debug: ignore empty strings in enable()
* node: make DEBUG_COLORS able to disable coloring
* *: export the `colors` array
* npmignore: don't publish the `dist` dir
* Makefile: refactor to use browserify
* package: add "browserify" as a dev dependency
* Readme: add Web Inspector Colors section
* node: reset terminal color for the debug content
* node: map "%o" to `util.inspect()`
* browser: map "%j" to `JSON.stringify()`
* debug: add custom "formatters"
* debug: use "ms" module for humanizing the diff
* Readme: add "bash" syntax highlighting
* browser: add Firebug color support
* browser: add colors for WebKit browsers
* node: apply log to `console`
* rewrite: abstract common logic for Node & browsers
* add .jshintrc file
0.8.1 / 2014-04-14
==================
* package: re-add the "component" section
0.8.0 / 2014-03-30
==================
* add `enable()` method for nodejs. Closes #27
* change from stderr to stdout
* remove unnecessary index.js file
0.7.4 / 2013-11-13
==================
* remove "browserify" key from package.json (fixes something in browserify)
0.7.3 / 2013-10-30
==================
* fix: catch localStorage security error when cookies are blocked (Chrome)
* add debug(err) support. Closes #46
* add .browser prop to package.json. Closes #42
0.7.2 / 2013-02-06
==================
* fix package.json
* fix: Mobile Safari (private mode) is broken with debug
* fix: Use unicode to send escape character to shell instead of octal to work with strict mode javascript
0.7.1 / 2013-02-05
==================
* add repository URL to package.json
* add DEBUG_COLORED to force colored output
* add browserify support
* fix component. Closes #24
0.7.0 / 2012-05-04
==================
* Added .component to package.json
* Added debug.component.js build
0.6.0 / 2012-03-16
==================
* Added support for "-" prefix in DEBUG [Vinay Pulim]
* Added `.enabled` flag to the node version [TooTallNate]
0.5.0 / 2012-02-02
==================
* Added: humanize diffs. Closes #8
* Added `debug.disable()` to the CS variant
* Removed padding. Closes #10
* Fixed: persist client-side variant again. Closes #9
0.4.0 / 2012-02-01
==================
* Added browser variant support for older browsers [TooTallNate]
* Added `debug.enable('project:*')` to browser variant [TooTallNate]
* Added padding to diff (moved it to the right)
0.3.0 / 2012-01-26
==================
* Added millisecond diff when isatty, otherwise UTC string
0.2.0 / 2012-01-22
==================
* Added wildcard support
0.1.0 / 2011-12-02
==================
* Added: remove colors unless stderr isatty [TooTallNate]
0.0.1 / 2010-01-03
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/debug/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/debug/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 11705
} |
# debug
[](https://travis-ci.org/visionmedia/debug) [](https://coveralls.io/github/visionmedia/debug?branch=master) [](https://visionmedia-community-slackin.now.sh/) [](#backers)
[](#sponsors)
A tiny node.js debugging utility modelled after node core's debugging technique.
**Discussion around the V3 API is under way [here](https://github.com/visionmedia/debug/issues/370)**
## Installation
```bash
$ npm install debug
```
## Usage
`debug` exposes a function; simply pass this function the name of your module, and it will return a decorated version of `console.error` for you to pass debug statements to. This will allow you to toggle the debug output for different parts of your module as well as the module as a whole.
Example _app.js_:
```js
var debug = require('debug')('http')
, http = require('http')
, name = 'My App';
// fake app
debug('booting %s', name);
http.createServer(function(req, res){
debug(req.method + ' ' + req.url);
res.end('hello\n');
}).listen(3000, function(){
debug('listening');
});
// fake worker of some kind
require('./worker');
```
Example _worker.js_:
```js
var debug = require('debug')('worker');
setInterval(function(){
debug('doing some work');
}, 1000);
```
The __DEBUG__ environment variable is then used to enable these based on space or comma-delimited names. Here are some examples:


#### Windows note
On Windows the environment variable is set using the `set` command.
```cmd
set DEBUG=*,-not_this
```
Note that PowerShell uses different syntax to set environment variables.
```cmd
$env:DEBUG = "*,-not_this"
```
Then, run the program to be debugged as usual.
## Millisecond diff
When actively developing an application it can be useful to see when the time spent between one `debug()` call and the next. Suppose for example you invoke `debug()` before requesting a resource, and after as well, the "+NNNms" will show you how much time was spent between calls.

When stdout is not a TTY, `Date#toUTCString()` is used, making it more useful for logging the debug information as shown below:

## Conventions
If you're using this in one or more of your libraries, you _should_ use the name of your library so that developers may toggle debugging as desired without guessing names. If you have more than one debuggers you _should_ prefix them with your library name and use ":" to separate features. For example "bodyParser" from Connect would then be "connect:bodyParser".
## Wildcards
The `*` character may be used as a wildcard. Suppose for example your library has debuggers named "connect:bodyParser", "connect:compress", "connect:session", instead of listing all three with `DEBUG=connect:bodyParser,connect:compress,connect:session`, you may simply do `DEBUG=connect:*`, or to run everything using this module simply use `DEBUG=*`.
You can also exclude specific debuggers by prefixing them with a "-" character. For example, `DEBUG=*,-connect:*` would include all debuggers except those starting with "connect:".
## Environment Variables
When running through Node.js, you can set a few environment variables that will
change the behavior of the debug logging:
| Name | Purpose |
|-----------|-------------------------------------------------|
| `DEBUG` | Enables/disables specific debugging namespaces. |
| `DEBUG_COLORS`| Whether or not to use colors in the debug output. |
| `DEBUG_DEPTH` | Object inspection depth. |
| `DEBUG_SHOW_HIDDEN` | Shows hidden properties on inspected objects. |
__Note:__ The environment variables beginning with `DEBUG_` end up being
converted into an Options object that gets used with `%o`/`%O` formatters.
See the Node.js documentation for
[`util.inspect()`](https://nodejs.org/api/util.html#util_util_inspect_object_options)
for the complete list.
## Formatters
Debug uses [printf-style](https://wikipedia.org/wiki/Printf_format_string) formatting. Below are the officially supported formatters:
| Formatter | Representation |
|-----------|----------------|
| `%O` | Pretty-print an Object on multiple lines. |
| `%o` | Pretty-print an Object all on a single line. |
| `%s` | String. |
| `%d` | Number (both integer and float). |
| `%j` | JSON. Replaced with the string '[Circular]' if the argument contains circular references. |
| `%%` | Single percent sign ('%'). This does not consume an argument. |
### Custom formatters
You can add custom formatters by extending the `debug.formatters` object. For example, if you wanted to add support for rendering a Buffer as hex with `%h`, you could do something like:
```js
const createDebug = require('debug')
createDebug.formatters.h = (v) => {
return v.toString('hex')
}
// …elsewhere
const debug = createDebug('foo')
debug('this is hex: %h', new Buffer('hello world'))
// foo this is hex: 68656c6c6f20776f726c6421 +0ms
```
## Browser support
You can build a browser-ready script using [browserify](https://github.com/substack/node-browserify),
or just use the [browserify-as-a-service](https://wzrd.in/) [build](https://wzrd.in/standalone/debug@latest),
if you don't want to build it yourself.
Debug's enable state is currently persisted by `localStorage`.
Consider the situation shown below where you have `worker:a` and `worker:b`,
and wish to debug both. You can enable this using `localStorage.debug`:
```js
localStorage.debug = 'worker:*'
```
And then refresh the page.
```js
a = debug('worker:a');
b = debug('worker:b');
setInterval(function(){
a('doing some work');
}, 1000);
setInterval(function(){
b('doing some work');
}, 1200);
```
#### Web Inspector Colors
Colors are also enabled on "Web Inspectors" that understand the `%c` formatting
option. These are WebKit web inspectors, Firefox ([since version
31](https://hacks.mozilla.org/2014/05/editable-box-model-multiple-selection-sublime-text-keys-much-more-firefox-developer-tools-episode-31/))
and the Firebug plugin for Firefox (any version).
Colored output looks something like:

## Output streams
By default `debug` will log to stderr, however this can be configured per-namespace by overriding the `log` method:
Example _stdout.js_:
```js
var debug = require('debug');
var error = debug('app:error');
// by default stderr is used
error('goes to stderr!');
var log = debug('app:log');
// set this namespace to log via console.log
log.log = console.log.bind(console); // don't forget to bind to console!
log('goes to stdout');
error('still goes to stderr!');
// set all output to go via console.info
// overrides all per-namespace log settings
debug.log = console.info.bind(console);
error('now goes to stdout via console.info');
log('still goes to stdout, but via console.info now');
```
## Authors
- TJ Holowaychuk
- Nathan Rajlich
- Andrew Rhyne
## Backers
Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/debug#backer)]
<a href="https://opencollective.com/debug/backer/0/website" target="_blank"><img src="https://opencollective.com/debug/backer/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/1/website" target="_blank"><img src="https://opencollective.com/debug/backer/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/2/website" target="_blank"><img src="https://opencollective.com/debug/backer/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/3/website" target="_blank"><img src="https://opencollective.com/debug/backer/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/4/website" target="_blank"><img src="https://opencollective.com/debug/backer/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/5/website" target="_blank"><img src="https://opencollective.com/debug/backer/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/6/website" target="_blank"><img src="https://opencollective.com/debug/backer/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/7/website" target="_blank"><img src="https://opencollective.com/debug/backer/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/8/website" target="_blank"><img src="https://opencollective.com/debug/backer/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/9/website" target="_blank"><img src="https://opencollective.com/debug/backer/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/10/website" target="_blank"><img src="https://opencollective.com/debug/backer/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/11/website" target="_blank"><img src="https://opencollective.com/debug/backer/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/12/website" target="_blank"><img src="https://opencollective.com/debug/backer/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/13/website" target="_blank"><img src="https://opencollective.com/debug/backer/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/14/website" target="_blank"><img src="https://opencollective.com/debug/backer/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/15/website" target="_blank"><img src="https://opencollective.com/debug/backer/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/16/website" target="_blank"><img src="https://opencollective.com/debug/backer/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/17/website" target="_blank"><img src="https://opencollective.com/debug/backer/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/18/website" target="_blank"><img src="https://opencollective.com/debug/backer/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/19/website" target="_blank"><img src="https://opencollective.com/debug/backer/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/20/website" target="_blank"><img src="https://opencollective.com/debug/backer/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/21/website" target="_blank"><img src="https://opencollective.com/debug/backer/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/22/website" target="_blank"><img src="https://opencollective.com/debug/backer/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/23/website" target="_blank"><img src="https://opencollective.com/debug/backer/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/24/website" target="_blank"><img src="https://opencollective.com/debug/backer/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/25/website" target="_blank"><img src="https://opencollective.com/debug/backer/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/26/website" target="_blank"><img src="https://opencollective.com/debug/backer/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/27/website" target="_blank"><img src="https://opencollective.com/debug/backer/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/28/website" target="_blank"><img src="https://opencollective.com/debug/backer/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/backer/29/website" target="_blank"><img src="https://opencollective.com/debug/backer/29/avatar.svg"></a>
## Sponsors
Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/debug#sponsor)]
<a href="https://opencollective.com/debug/sponsor/0/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/0/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/1/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/1/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/2/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/2/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/3/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/3/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/4/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/4/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/5/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/5/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/6/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/6/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/7/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/7/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/8/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/8/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/9/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/9/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/10/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/10/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/11/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/11/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/12/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/12/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/13/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/13/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/14/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/14/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/15/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/15/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/16/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/16/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/17/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/17/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/18/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/18/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/19/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/19/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/20/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/20/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/21/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/21/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/22/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/22/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/23/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/23/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/24/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/24/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/25/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/25/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/26/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/26/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/27/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/27/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/28/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/28/avatar.svg"></a>
<a href="https://opencollective.com/debug/sponsor/29/website" target="_blank"><img src="https://opencollective.com/debug/sponsor/29/avatar.svg"></a>
## License
(The MIT License)
Copyright (c) 2014-2016 TJ Holowaychuk <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/debug/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/debug/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 17915
} |
1.0.2 / 2018-01-21
==================
* Fix encoding `%` as last character
1.0.1 / 2016-06-09
==================
* Fix encoding unpaired surrogates at start/end of string
1.0.0 / 2016-06-08
==================
* Initial release | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/encodeurl/HISTORY.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/encodeurl/HISTORY.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 237
} |
# encodeurl
[![NPM Version][npm-image]][npm-url]
[![NPM Downloads][downloads-image]][downloads-url]
[![Node.js Version][node-version-image]][node-version-url]
[![Build Status][travis-image]][travis-url]
[![Test Coverage][coveralls-image]][coveralls-url]
Encode a URL to a percent-encoded form, excluding already-encoded sequences
## Installation
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/). Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install encodeurl
```
## API
```js
var encodeUrl = require('encodeurl')
```
### encodeUrl(url)
Encode a URL to a percent-encoded form, excluding already-encoded sequences.
This function will take an already-encoded URL and encode all the non-URL
code points (as UTF-8 byte sequences). This function will not encode the
"%" character unless it is not part of a valid sequence (`%20` will be
left as-is, but `%foo` will be encoded as `%25foo`).
This encode is meant to be "safe" and does not throw errors. It will try as
hard as it can to properly encode the given URL, including replacing any raw,
unpaired surrogate pairs with the Unicode replacement character prior to
encoding.
This function is _similar_ to the intrinsic function `encodeURI`, except it
will not encode the `%` character if that is part of a valid sequence, will
not encode `[` and `]` (for IPv6 hostnames) and will replace raw, unpaired
surrogate pairs with the Unicode replacement character (instead of throwing).
## Examples
### Encode a URL containing user-controled data
```js
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
http.createServer(function onRequest (req, res) {
// get encoded form of inbound url
var url = encodeUrl(req.url)
// create html message
var body = '<p>Location ' + escapeHtml(url) + ' not found</p>'
// send a 404
res.statusCode = 404
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.end(body, 'utf-8')
})
```
### Encode a URL for use in a header field
```js
var encodeUrl = require('encodeurl')
var escapeHtml = require('escape-html')
var url = require('url')
http.createServer(function onRequest (req, res) {
// parse inbound url
var href = url.parse(req)
// set new host for redirect
href.host = 'localhost'
href.protocol = 'https:'
href.slashes = true
// create location header
var location = encodeUrl(url.format(href))
// create html message
var body = '<p>Redirecting to new site: ' + escapeHtml(location) + '</p>'
// send a 301
res.statusCode = 301
res.setHeader('Content-Type', 'text/html; charset=UTF-8')
res.setHeader('Content-Length', String(Buffer.byteLength(body, 'utf-8')))
res.setHeader('Location', location)
res.end(body, 'utf-8')
})
```
## Testing
```sh
$ npm test
$ npm run lint
```
## References
- [RFC 3986: Uniform Resource Identifier (URI): Generic Syntax][rfc-3986]
- [WHATWG URL Living Standard][whatwg-url]
[rfc-3986]: https://tools.ietf.org/html/rfc3986
[whatwg-url]: https://url.spec.whatwg.org/
## License
[MIT](LICENSE)
[npm-image]: https://img.shields.io/npm/v/encodeurl.svg
[npm-url]: https://npmjs.org/package/encodeurl
[node-version-image]: https://img.shields.io/node/v/encodeurl.svg
[node-version-url]: https://nodejs.org/en/download
[travis-image]: https://img.shields.io/travis/pillarjs/encodeurl.svg
[travis-url]: https://travis-ci.org/pillarjs/encodeurl
[coveralls-image]: https://img.shields.io/coveralls/pillarjs/encodeurl.svg
[coveralls-url]: https://coveralls.io/r/pillarjs/encodeurl?branch=master
[downloads-image]: https://img.shields.io/npm/dm/encodeurl.svg
[downloads-url]: https://npmjs.org/package/encodeurl | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/encodeurl/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/encodeurl/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 3854
} |
# ansi-regex
> Regular expression for matching [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code)
## Install
```
$ npm install ansi-regex
```
## Usage
```js
const ansiRegex = require('ansi-regex');
ansiRegex().test('\u001B[4mcake\u001B[0m');
//=> true
ansiRegex().test('cake');
//=> false
'\u001B[4mcake\u001B[0m'.match(ansiRegex());
//=> ['\u001B[4m', '\u001B[0m']
'\u001B[4mcake\u001B[0m'.match(ansiRegex({onlyFirst: true}));
//=> ['\u001B[4m']
'\u001B]8;;https://github.com\u0007click\u001B]8;;\u0007'.match(ansiRegex());
//=> ['\u001B]8;;https://github.com\u0007', '\u001B]8;;\u0007']
```
## API
### ansiRegex(options?)
Returns a regex for matching ANSI escape codes.
#### options
Type: `object`
##### onlyFirst
Type: `boolean`<br>
Default: `false` *(Matches any ANSI escape codes in a string)*
Match only the first ANSI escape.
## FAQ
### Why do you test for codes not in the ECMA 48 standard?
Some of the codes we run as a test are codes that we acquired finding various lists of non-standard or manufacturer specific codes. We test for both standard and non-standard codes, as most of them follow the same or similar format and can be safely matched in strings without the risk of removing actual string content. There are a few non-standard control codes that do not follow the traditional format (i.e. they end in numbers) thus forcing us to exclude them from the test because we cannot reliably match them.
On the historical side, those ECMA standards were established in the early 90's whereas the VT100, for example, was designed in the mid/late 70's. At that point in time, control codes were still pretty ungoverned and engineers used them for a multitude of things, namely to activate hardware ports that may have been proprietary. Somewhere else you see a similar 'anarchy' of codes is in the x86 architecture for processors; there are a ton of "interrupts" that can mean different things on certain brands of processors, most of which have been phased out.
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-)
---
<div align="center">
<b>
<a href="https://tidelift.com/subscription/pkg/npm-ansi-regex?utm_source=npm-ansi-regex&utm_medium=referral&utm_campaign=readme">Get professional support for this package with a Tidelift subscription</a>
</b>
<br>
<sub>
Tidelift helps make open source sustainable for maintainers while giving companies<br>assurances about security, maintenance, and licensing for their dependencies.
</sub>
</div> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/string-width-cjs/node_modules/ansi-regex/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/string-width-cjs/node_modules/ansi-regex/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2564
} |
# emoji-regex [](https://travis-ci.org/mathiasbynens/emoji-regex)
_emoji-regex_ offers a regular expression to match all emoji symbols (including textual representations of emoji) as per the Unicode Standard.
This repository contains a script that generates this regular expression based on [the data from Unicode v12](https://github.com/mathiasbynens/unicode-12.0.0). Because of this, the regular expression can easily be updated whenever new emoji are added to the Unicode standard.
## Installation
Via [npm](https://www.npmjs.com/):
```bash
npm install emoji-regex
```
In [Node.js](https://nodejs.org/):
```js
const emojiRegex = require('emoji-regex');
// Note: because the regular expression has the global flag set, this module
// exports a function that returns the regex rather than exporting the regular
// expression itself, to make it impossible to (accidentally) mutate the
// original regular expression.
const text = `
\u{231A}: ⌚ default emoji presentation character (Emoji_Presentation)
\u{2194}\u{FE0F}: ↔️ default text presentation character rendered as emoji
\u{1F469}: 👩 emoji modifier base (Emoji_Modifier_Base)
\u{1F469}\u{1F3FF}: 👩🏿 emoji modifier base followed by a modifier
`;
const regex = emojiRegex();
let match;
while (match = regex.exec(text)) {
const emoji = match[0];
console.log(`Matched sequence ${ emoji } — code points: ${ [...emoji].length }`);
}
```
Console output:
```
Matched sequence ⌚ — code points: 1
Matched sequence ⌚ — code points: 1
Matched sequence ↔️ — code points: 2
Matched sequence ↔️ — code points: 2
Matched sequence 👩 — code points: 1
Matched sequence 👩 — code points: 1
Matched sequence 👩🏿 — code points: 2
Matched sequence 👩🏿 — code points: 2
```
To match emoji in their textual representation as well (i.e. emoji that are not `Emoji_Presentation` symbols and that aren’t forced to render as emoji by a variation selector), `require` the other regex:
```js
const emojiRegex = require('emoji-regex/text.js');
```
Additionally, in environments which support ES2015 Unicode escapes, you may `require` ES2015-style versions of the regexes:
```js
const emojiRegex = require('emoji-regex/es2015/index.js');
const emojiRegexText = require('emoji-regex/es2015/text.js');
```
## Author
| [](https://twitter.com/mathias "Follow @mathias on Twitter") |
|---|
| [Mathias Bynens](https://mathiasbynens.be/) |
## License
_emoji-regex_ is available under the [MIT](https://mths.be/mit) license. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/string-width-cjs/node_modules/emoji-regex/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/string-width-cjs/node_modules/emoji-regex/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2625
} |
# strip-ansi [](https://travis-ci.org/chalk/strip-ansi)
> Strip [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code) from a string
## Install
```
$ npm install strip-ansi
```
## Usage
```js
const stripAnsi = require('strip-ansi');
stripAnsi('\u001B[4mUnicorn\u001B[0m');
//=> 'Unicorn'
stripAnsi('\u001B]8;;https://github.com\u0007Click\u001B]8;;\u0007');
//=> 'Click'
```
## strip-ansi for enterprise
Available as part of the Tidelift Subscription.
The maintainers of strip-ansi and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. [Learn more.](https://tidelift.com/subscription/pkg/npm-strip-ansi?utm_source=npm-strip-ansi&utm_medium=referral&utm_campaign=enterprise&utm_term=repo)
## Related
- [strip-ansi-cli](https://github.com/chalk/strip-ansi-cli) - CLI for this module
- [strip-ansi-stream](https://github.com/chalk/strip-ansi-stream) - Streaming version of this module
- [has-ansi](https://github.com/chalk/has-ansi) - Check if a string has ANSI escape codes
- [ansi-regex](https://github.com/chalk/ansi-regex) - Regular expression for matching ANSI escape codes
- [chalk](https://github.com/chalk/chalk) - Terminal string styling done right
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/string-width-cjs/node_modules/strip-ansi/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/string-width-cjs/node_modules/strip-ansi/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1597
} |
# ansi-regex
> Regular expression for matching [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code)
## Install
```
$ npm install ansi-regex
```
## Usage
```js
const ansiRegex = require('ansi-regex');
ansiRegex().test('\u001B[4mcake\u001B[0m');
//=> true
ansiRegex().test('cake');
//=> false
'\u001B[4mcake\u001B[0m'.match(ansiRegex());
//=> ['\u001B[4m', '\u001B[0m']
'\u001B[4mcake\u001B[0m'.match(ansiRegex({onlyFirst: true}));
//=> ['\u001B[4m']
'\u001B]8;;https://github.com\u0007click\u001B]8;;\u0007'.match(ansiRegex());
//=> ['\u001B]8;;https://github.com\u0007', '\u001B]8;;\u0007']
```
## API
### ansiRegex(options?)
Returns a regex for matching ANSI escape codes.
#### options
Type: `object`
##### onlyFirst
Type: `boolean`<br>
Default: `false` *(Matches any ANSI escape codes in a string)*
Match only the first ANSI escape.
## FAQ
### Why do you test for codes not in the ECMA 48 standard?
Some of the codes we run as a test are codes that we acquired finding various lists of non-standard or manufacturer specific codes. We test for both standard and non-standard codes, as most of them follow the same or similar format and can be safely matched in strings without the risk of removing actual string content. There are a few non-standard control codes that do not follow the traditional format (i.e. they end in numbers) thus forcing us to exclude them from the test because we cannot reliably match them.
On the historical side, those ECMA standards were established in the early 90's whereas the VT100, for example, was designed in the mid/late 70's. At that point in time, control codes were still pretty ungoverned and engineers used them for a multitude of things, namely to activate hardware ports that may have been proprietary. Somewhere else you see a similar 'anarchy' of codes is in the x86 architecture for processors; there are a ton of "interrupts" that can mean different things on certain brands of processors, most of which have been phased out.
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-)
---
<div align="center">
<b>
<a href="https://tidelift.com/subscription/pkg/npm-ansi-regex?utm_source=npm-ansi-regex&utm_medium=referral&utm_campaign=readme">Get professional support for this package with a Tidelift subscription</a>
</b>
<br>
<sub>
Tidelift helps make open source sustainable for maintainers while giving companies<br>assurances about security, maintenance, and licensing for their dependencies.
</sub>
</div> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/strip-ansi-cjs/node_modules/ansi-regex/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/strip-ansi-cjs/node_modules/ansi-regex/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2564
} |
# postcss-value-parser (forked + inlined)
This is a customized version of of [PostCSS Value Parser](https://github.com/TrySound/postcss-value-parser) to fix some bugs around parsing CSS functions. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tailwindcss/lib/value-parser/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tailwindcss/lib/value-parser/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 197
} |
# postcss-value-parser (forked + inlined)
This is a customized version of of [PostCSS Value Parser](https://github.com/TrySound/postcss-value-parser) to fix some bugs around parsing CSS functions. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tailwindcss/src/value-parser/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tailwindcss/src/value-parser/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 197
} |
MIT License
Copyright (c) 2020 Evan Wallace
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tsx/node_modules/esbuild/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tsx/node_modules/esbuild/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1068
} |
# esbuild
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the [JavaScript API documentation](https://esbuild.github.io/api/) for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tsx/node_modules/esbuild/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tsx/node_modules/esbuild/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 174
} |
MIT License
Copyright (c) 2020 Evan Wallace
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/vite/node_modules/esbuild/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/vite/node_modules/esbuild/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1068
} |
# esbuild
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the [JavaScript API documentation](https://esbuild.github.io/api/) for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/vite/node_modules/esbuild/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/vite/node_modules/esbuild/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 174
} |
# ansi-regex
> Regular expression for matching [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code)
## Install
```
$ npm install ansi-regex
```
## Usage
```js
const ansiRegex = require('ansi-regex');
ansiRegex().test('\u001B[4mcake\u001B[0m');
//=> true
ansiRegex().test('cake');
//=> false
'\u001B[4mcake\u001B[0m'.match(ansiRegex());
//=> ['\u001B[4m', '\u001B[0m']
'\u001B[4mcake\u001B[0m'.match(ansiRegex({onlyFirst: true}));
//=> ['\u001B[4m']
'\u001B]8;;https://github.com\u0007click\u001B]8;;\u0007'.match(ansiRegex());
//=> ['\u001B]8;;https://github.com\u0007', '\u001B]8;;\u0007']
```
## API
### ansiRegex(options?)
Returns a regex for matching ANSI escape codes.
#### options
Type: `object`
##### onlyFirst
Type: `boolean`<br>
Default: `false` *(Matches any ANSI escape codes in a string)*
Match only the first ANSI escape.
## FAQ
### Why do you test for codes not in the ECMA 48 standard?
Some of the codes we run as a test are codes that we acquired finding various lists of non-standard or manufacturer specific codes. We test for both standard and non-standard codes, as most of them follow the same or similar format and can be safely matched in strings without the risk of removing actual string content. There are a few non-standard control codes that do not follow the traditional format (i.e. they end in numbers) thus forcing us to exclude them from the test because we cannot reliably match them.
On the historical side, those ECMA standards were established in the early 90's whereas the VT100, for example, was designed in the mid/late 70's. At that point in time, control codes were still pretty ungoverned and engineers used them for a multitude of things, namely to activate hardware ports that may have been proprietary. Somewhere else you see a similar 'anarchy' of codes is in the x86 architecture for processors; there are a ton of "interrupts" that can mean different things on certain brands of processors, most of which have been phased out.
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-)
---
<div align="center">
<b>
<a href="https://tidelift.com/subscription/pkg/npm-ansi-regex?utm_source=npm-ansi-regex&utm_medium=referral&utm_campaign=readme">Get professional support for this package with a Tidelift subscription</a>
</b>
<br>
<sub>
Tidelift helps make open source sustainable for maintainers while giving companies<br>assurances about security, maintenance, and licensing for their dependencies.
</sub>
</div> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/wrap-ansi-cjs/node_modules/ansi-regex/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/wrap-ansi-cjs/node_modules/ansi-regex/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2564
} |
# ansi-styles [](https://travis-ci.org/chalk/ansi-styles)
> [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code#Colors_and_Styles) for styling strings in the terminal
You probably want the higher-level [chalk](https://github.com/chalk/chalk) module for styling your strings.
<img src="screenshot.svg" width="900">
## Install
```
$ npm install ansi-styles
```
## Usage
```js
const style = require('ansi-styles');
console.log(`${style.green.open}Hello world!${style.green.close}`);
// Color conversion between 16/256/truecolor
// NOTE: If conversion goes to 16 colors or 256 colors, the original color
// may be degraded to fit that color palette. This means terminals
// that do not support 16 million colors will best-match the
// original color.
console.log(style.bgColor.ansi.hsl(120, 80, 72) + 'Hello world!' + style.bgColor.close);
console.log(style.color.ansi256.rgb(199, 20, 250) + 'Hello world!' + style.color.close);
console.log(style.color.ansi16m.hex('#abcdef') + 'Hello world!' + style.color.close);
```
## API
Each style has an `open` and `close` property.
## Styles
### Modifiers
- `reset`
- `bold`
- `dim`
- `italic` *(Not widely supported)*
- `underline`
- `inverse`
- `hidden`
- `strikethrough` *(Not widely supported)*
### Colors
- `black`
- `red`
- `green`
- `yellow`
- `blue`
- `magenta`
- `cyan`
- `white`
- `blackBright` (alias: `gray`, `grey`)
- `redBright`
- `greenBright`
- `yellowBright`
- `blueBright`
- `magentaBright`
- `cyanBright`
- `whiteBright`
### Background colors
- `bgBlack`
- `bgRed`
- `bgGreen`
- `bgYellow`
- `bgBlue`
- `bgMagenta`
- `bgCyan`
- `bgWhite`
- `bgBlackBright` (alias: `bgGray`, `bgGrey`)
- `bgRedBright`
- `bgGreenBright`
- `bgYellowBright`
- `bgBlueBright`
- `bgMagentaBright`
- `bgCyanBright`
- `bgWhiteBright`
## Advanced usage
By default, you get a map of styles, but the styles are also available as groups. They are non-enumerable so they don't show up unless you access them explicitly. This makes it easier to expose only a subset in a higher-level module.
- `style.modifier`
- `style.color`
- `style.bgColor`
###### Example
```js
console.log(style.color.green.open);
```
Raw escape codes (i.e. without the CSI escape prefix `\u001B[` and render mode postfix `m`) are available under `style.codes`, which returns a `Map` with the open codes as keys and close codes as values.
###### Example
```js
console.log(style.codes.get(36));
//=> 39
```
## [256 / 16 million (TrueColor) support](https://gist.github.com/XVilka/8346728)
`ansi-styles` uses the [`color-convert`](https://github.com/Qix-/color-convert) package to allow for converting between various colors and ANSI escapes, with support for 256 and 16 million colors.
The following color spaces from `color-convert` are supported:
- `rgb`
- `hex`
- `keyword`
- `hsl`
- `hsv`
- `hwb`
- `ansi`
- `ansi256`
To use these, call the associated conversion function with the intended output, for example:
```js
style.color.ansi.rgb(100, 200, 15); // RGB to 16 color ansi foreground code
style.bgColor.ansi.rgb(100, 200, 15); // RGB to 16 color ansi background code
style.color.ansi256.hsl(120, 100, 60); // HSL to 256 color ansi foreground code
style.bgColor.ansi256.hsl(120, 100, 60); // HSL to 256 color ansi foreground code
style.color.ansi16m.hex('#C0FFEE'); // Hex (RGB) to 16 million color foreground code
style.bgColor.ansi16m.hex('#C0FFEE'); // Hex (RGB) to 16 million color background code
```
## Related
- [ansi-escapes](https://github.com/sindresorhus/ansi-escapes) - ANSI escape codes for manipulating the terminal
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-)
## For enterprise
Available as part of the Tidelift Subscription.
The maintainers of `ansi-styles` and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. [Learn more.](https://tidelift.com/subscription/pkg/npm-ansi-styles?utm_source=npm-ansi-styles&utm_medium=referral&utm_campaign=enterprise&utm_term=repo) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/wrap-ansi-cjs/node_modules/ansi-styles/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/wrap-ansi-cjs/node_modules/ansi-styles/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 4326
} |
# emoji-regex [](https://travis-ci.org/mathiasbynens/emoji-regex)
_emoji-regex_ offers a regular expression to match all emoji symbols (including textual representations of emoji) as per the Unicode Standard.
This repository contains a script that generates this regular expression based on [the data from Unicode v12](https://github.com/mathiasbynens/unicode-12.0.0). Because of this, the regular expression can easily be updated whenever new emoji are added to the Unicode standard.
## Installation
Via [npm](https://www.npmjs.com/):
```bash
npm install emoji-regex
```
In [Node.js](https://nodejs.org/):
```js
const emojiRegex = require('emoji-regex');
// Note: because the regular expression has the global flag set, this module
// exports a function that returns the regex rather than exporting the regular
// expression itself, to make it impossible to (accidentally) mutate the
// original regular expression.
const text = `
\u{231A}: ⌚ default emoji presentation character (Emoji_Presentation)
\u{2194}\u{FE0F}: ↔️ default text presentation character rendered as emoji
\u{1F469}: 👩 emoji modifier base (Emoji_Modifier_Base)
\u{1F469}\u{1F3FF}: 👩🏿 emoji modifier base followed by a modifier
`;
const regex = emojiRegex();
let match;
while (match = regex.exec(text)) {
const emoji = match[0];
console.log(`Matched sequence ${ emoji } — code points: ${ [...emoji].length }`);
}
```
Console output:
```
Matched sequence ⌚ — code points: 1
Matched sequence ⌚ — code points: 1
Matched sequence ↔️ — code points: 2
Matched sequence ↔️ — code points: 2
Matched sequence 👩 — code points: 1
Matched sequence 👩 — code points: 1
Matched sequence 👩🏿 — code points: 2
Matched sequence 👩🏿 — code points: 2
```
To match emoji in their textual representation as well (i.e. emoji that are not `Emoji_Presentation` symbols and that aren’t forced to render as emoji by a variation selector), `require` the other regex:
```js
const emojiRegex = require('emoji-regex/text.js');
```
Additionally, in environments which support ES2015 Unicode escapes, you may `require` ES2015-style versions of the regexes:
```js
const emojiRegex = require('emoji-regex/es2015/index.js');
const emojiRegexText = require('emoji-regex/es2015/text.js');
```
## Author
| [](https://twitter.com/mathias "Follow @mathias on Twitter") |
|---|
| [Mathias Bynens](https://mathiasbynens.be/) |
## License
_emoji-regex_ is available under the [MIT](https://mths.be/mit) license. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/wrap-ansi-cjs/node_modules/emoji-regex/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/wrap-ansi-cjs/node_modules/emoji-regex/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 2625
} |
# string-width
> Get the visual width of a string - the number of columns required to display it
Some Unicode characters are [fullwidth](https://en.wikipedia.org/wiki/Halfwidth_and_fullwidth_forms) and use double the normal width. [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code) are stripped and doesn't affect the width.
Useful to be able to measure the actual width of command-line output.
## Install
```
$ npm install string-width
```
## Usage
```js
const stringWidth = require('string-width');
stringWidth('a');
//=> 1
stringWidth('古');
//=> 2
stringWidth('\u001B[1m古\u001B[22m');
//=> 2
```
## Related
- [string-width-cli](https://github.com/sindresorhus/string-width-cli) - CLI for this module
- [string-length](https://github.com/sindresorhus/string-length) - Get the real length of a string
- [widest-line](https://github.com/sindresorhus/widest-line) - Get the visual width of the widest line in a string
---
<div align="center">
<b>
<a href="https://tidelift.com/subscription/pkg/npm-string-width?utm_source=npm-string-width&utm_medium=referral&utm_campaign=readme">Get professional support for this package with a Tidelift subscription</a>
</b>
<br>
<sub>
Tidelift helps make open source sustainable for maintainers while giving companies<br>assurances about security, maintenance, and licensing for their dependencies.
</sub>
</div> | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/wrap-ansi-cjs/node_modules/string-width/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/wrap-ansi-cjs/node_modules/string-width/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1391
} |
# strip-ansi [](https://travis-ci.org/chalk/strip-ansi)
> Strip [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code) from a string
## Install
```
$ npm install strip-ansi
```
## Usage
```js
const stripAnsi = require('strip-ansi');
stripAnsi('\u001B[4mUnicorn\u001B[0m');
//=> 'Unicorn'
stripAnsi('\u001B]8;;https://github.com\u0007Click\u001B]8;;\u0007');
//=> 'Click'
```
## strip-ansi for enterprise
Available as part of the Tidelift Subscription.
The maintainers of strip-ansi and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. [Learn more.](https://tidelift.com/subscription/pkg/npm-strip-ansi?utm_source=npm-strip-ansi&utm_medium=referral&utm_campaign=enterprise&utm_term=repo)
## Related
- [strip-ansi-cli](https://github.com/chalk/strip-ansi-cli) - CLI for this module
- [strip-ansi-stream](https://github.com/chalk/strip-ansi-stream) - Streaming version of this module
- [has-ansi](https://github.com/chalk/has-ansi) - Check if a string has ANSI escape codes
- [ansi-regex](https://github.com/chalk/ansi-regex) - Regular expression for matching ANSI escape codes
- [chalk](https://github.com/chalk/chalk) - Terminal string styling done right
## Maintainers
- [Sindre Sorhus](https://github.com/sindresorhus)
- [Josh Junon](https://github.com/qix-) | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/wrap-ansi-cjs/node_modules/strip-ansi/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/wrap-ansi-cjs/node_modules/strip-ansi/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1597
} |
MIT License
Copyright (c) 2020 Evan Wallace
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@esbuild-kit/core-utils/node_modules/esbuild/LICENSE.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@esbuild-kit/core-utils/node_modules/esbuild/LICENSE.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1068
} |
# esbuild
This is a JavaScript bundler and minifier. See https://github.com/evanw/esbuild and the [JavaScript API documentation](https://esbuild.github.io/api/) for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@esbuild-kit/core-utils/node_modules/esbuild/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@esbuild-kit/core-utils/node_modules/esbuild/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 174
} |
# API Documentation
*Please use only this documented API when working with the parser. Methods
not documented here are subject to change at any point.*
## `parser` function
This is the module's main entry point.
```js
const parser = require('postcss-selector-parser');
```
### `parser([transform], [options])`
Creates a new `processor` instance
```js
const processor = parser();
```
Or, with optional transform function
```js
const transform = selectors => {
selectors.walkUniversals(selector => {
selector.remove();
});
};
const processor = parser(transform)
// Example
const result = processor.processSync('*.class');
// => .class
```
[See processor documentation](#processor)
Arguments:
* `transform (function)`: Provide a function to work with the parsed AST.
* `options (object)`: Provide default options for all calls on the returned `Processor`.
### `parser.attribute([props])`
Creates a new attribute selector.
```js
parser.attribute({attribute: 'href'});
// => [href]
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.className([props])`
Creates a new class selector.
```js
parser.className({value: 'button'});
// => .button
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.combinator([props])`
Creates a new selector combinator.
```js
parser.combinator({value: '+'});
// => +
```
Arguments:
* `props (object)`: The new node's properties.
Notes:
* **Descendant Combinators** The value of descendant combinators created by the
parser always just a single space (`" "`). For descendant selectors with no
comments, additional space is now stored in `node.spaces.before`. Depending
on the location of comments, additional spaces may be stored in
`node.raws.spaces.before`, `node.raws.spaces.after`, or `node.raws.value`.
* **Named Combinators** Although, nonstandard and unlikely to ever become a standard,
named combinators like `/deep/` and `/for/` are parsed as combinators. The
`node.value` is name after being unescaped and normalized as lowercase. The
original value for the combinator name is stored in `node.raws.value`.
### `parser.comment([props])`
Creates a new comment.
```js
parser.comment({value: '/* Affirmative, Dave. I read you. */'});
// => /* Affirmative, Dave. I read you. */
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.id([props])`
Creates a new id selector.
```js
parser.id({value: 'search'});
// => #search
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.nesting([props])`
Creates a new nesting selector.
```js
parser.nesting();
// => &
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.pseudo([props])`
Creates a new pseudo selector.
```js
parser.pseudo({value: '::before'});
// => ::before
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.root([props])`
Creates a new root node.
```js
parser.root();
// => (empty)
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.selector([props])`
Creates a new selector node.
```js
parser.selector();
// => (empty)
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.string([props])`
Creates a new string node.
```js
parser.string();
// => (empty)
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.tag([props])`
Creates a new tag selector.
```js
parser.tag({value: 'button'});
// => button
```
Arguments:
* `props (object)`: The new node's properties.
### `parser.universal([props])`
Creates a new universal selector.
```js
parser.universal();
// => *
```
Arguments:
* `props (object)`: The new node's properties.
## Node types
### `node.type`
A string representation of the selector type. It can be one of the following;
`attribute`, `class`, `combinator`, `comment`, `id`, `nesting`, `pseudo`,
`root`, `selector`, `string`, `tag`, or `universal`. Note that for convenience,
these constants are exposed on the main `parser` as uppercased keys. So for
example you can get `id` by querying `parser.ID`.
```js
parser.attribute({attribute: 'href'}).type;
// => 'attribute'
```
### `node.parent`
Returns the parent node.
```js
root.nodes[0].parent === root;
```
### `node.toString()`, `String(node)`, or `'' + node`
Returns a string representation of the node.
```js
const id = parser.id({value: 'search'});
console.log(String(id));
// => #search
```
### `node.next()` & `node.prev()`
Returns the next/previous child of the parent node.
```js
const next = id.next();
if (next && next.type !== 'combinator') {
throw new Error('Qualified IDs are not allowed!');
}
```
### `node.replaceWith(node)`
Replace a node with another.
```js
const attr = selectors.first.first;
const className = parser.className({value: 'test'});
attr.replaceWith(className);
```
Arguments:
* `node`: The node to substitute the original with.
### `node.remove()`
Removes the node from its parent node.
```js
if (node.type === 'id') {
node.remove();
}
```
### `node.clone()`
Returns a copy of a node, detached from any parent containers that the
original might have had.
```js
const cloned = parser.id({value: 'search'});
String(cloned);
// => #search
```
### `node.isAtPosition(line, column)`
Return a `boolean` indicating whether this node includes the character at the
position of the given line and column. Returns `undefined` if the nodes lack
sufficient source metadata to determine the position.
Arguments:
* `line`: 1-index based line number relative to the start of the selector.
* `column`: 1-index based column number relative to the start of the selector.
### `node.spaces`
Extra whitespaces around the node will be moved into `node.spaces.before` and
`node.spaces.after`. So for example, these spaces will be moved as they have
no semantic meaning:
```css
h1 , h2 {}
```
For descendent selectors, the value is always a single space.
```css
h1 h2 {}
```
Additional whitespace is found in either the `node.spaces.before` and `node.spaces.after` depending on the presence of comments or other whitespace characters. If the actual whitespace does not start or end with a single space, the node's raw value is set to the actual space(s) found in the source.
### `node.source`
An object describing the node's start/end, line/column source position.
Within the following CSS, the `.bar` class node ...
```css
.foo,
.bar {}
```
... will contain the following `source` object.
```js
source: {
start: {
line: 2,
column: 3
},
end: {
line: 2,
column: 6
}
}
```
### `node.sourceIndex`
The zero-based index of the node within the original source string.
Within the following CSS, the `.baz` class node will have a `sourceIndex` of `12`.
```css
.foo, .bar, .baz {}
```
## Container types
The `root`, `selector`, and `pseudo` nodes have some helper methods for working
with their children.
### `container.nodes`
An array of the container's children.
```js
// Input: h1 h2
selectors.at(0).nodes.length // => 3
selectors.at(0).nodes[0].value // => 'h1'
selectors.at(0).nodes[1].value // => ' '
```
### `container.first` & `container.last`
The first/last child of the container.
```js
selector.first === selector.nodes[0];
selector.last === selector.nodes[selector.nodes.length - 1];
```
### `container.at(index)`
Returns the node at position `index`.
```js
selector.at(0) === selector.first;
selector.at(0) === selector.nodes[0];
```
Arguments:
* `index`: The index of the node to return.
### `container.atPosition(line, column)`
Returns the node at the source position `index`.
```js
selector.at(0) === selector.first;
selector.at(0) === selector.nodes[0];
```
Arguments:
* `index`: The index of the node to return.
### `container.index(node)`
Return the index of the node within its container.
```js
selector.index(selector.nodes[2]) // => 2
```
Arguments:
* `node`: A node within the current container.
### `container.length`
Proxy to the length of the container's nodes.
```js
container.length === container.nodes.length
```
### `container` Array iterators
The container class provides proxies to certain Array methods; these are:
* `container.map === container.nodes.map`
* `container.reduce === container.nodes.reduce`
* `container.every === container.nodes.every`
* `container.some === container.nodes.some`
* `container.filter === container.nodes.filter`
* `container.sort === container.nodes.sort`
Note that these methods only work on a container's immediate children; recursive
iteration is provided by `container.walk`.
### `container.each(callback)`
Iterate the container's immediate children, calling `callback` for each child.
You may return `false` within the callback to break the iteration.
```js
let className;
selectors.each((selector, index) => {
if (selector.type === 'class') {
className = selector.value;
return false;
}
});
```
Note that unlike `Array#forEach()`, this iterator is safe to use whilst adding
or removing nodes from the container.
Arguments:
* `callback (function)`: A function to call for each node, which receives `node`
and `index` arguments.
### `container.walk(callback)`
Like `container#each`, but will also iterate child nodes as long as they are
`container` types.
```js
selectors.walk((selector, index) => {
// all nodes
});
```
Arguments:
* `callback (function)`: A function to call for each node, which receives `node`
and `index` arguments.
This iterator is safe to use whilst mutating `container.nodes`,
like `container#each`.
### `container.walk` proxies
The container class provides proxy methods for iterating over types of nodes,
so that it is easier to write modules that target specific selectors. Those
methods are:
* `container.walkAttributes`
* `container.walkClasses`
* `container.walkCombinators`
* `container.walkComments`
* `container.walkIds`
* `container.walkNesting`
* `container.walkPseudos`
* `container.walkTags`
* `container.walkUniversals`
### `container.split(callback)`
This method allows you to split a group of nodes by returning `true` from
a callback. It returns an array of arrays, where each inner array corresponds
to the groups that you created via the callback.
```js
// (input) => h1 h2>>h3
const list = selectors.first.split(selector => {
return selector.type === 'combinator';
});
// (node values) => [['h1', ' '], ['h2', '>>'], ['h3']]
```
Arguments:
* `callback (function)`: A function to call for each node, which receives `node`
as an argument.
### `container.prepend(node)` & `container.append(node)`
Add a node to the start/end of the container. Note that doing so will set
the parent property of the node to this container.
```js
const id = parser.id({value: 'search'});
selector.append(id);
```
Arguments:
* `node`: The node to add.
### `container.insertBefore(old, new)` & `container.insertAfter(old, new)`
Add a node before or after an existing node in a container:
```js
selectors.walk(selector => {
if (selector.type !== 'class') {
const className = parser.className({value: 'theme-name'});
selector.parent.insertAfter(selector, className);
}
});
```
Arguments:
* `old`: The existing node in the container.
* `new`: The new node to add before/after the existing node.
### `container.removeChild(node)`
Remove the node from the container. Note that you can also use
`node.remove()` if you would like to remove just a single node.
```js
selector.length // => 2
selector.remove(id)
selector.length // => 1;
id.parent // undefined
```
Arguments:
* `node`: The node to remove.
### `container.removeAll()` or `container.empty()`
Remove all children from the container.
```js
selector.removeAll();
selector.length // => 0
```
## Root nodes
A root node represents a comma separated list of selectors. Indeed, all
a root's `toString()` method does is join its selector children with a ','.
Other than this, it has no special functionality and acts like a container.
### `root.trailingComma`
This will be set to `true` if the input has a trailing comma, in order to
support parsing of legacy CSS hacks.
## Selector nodes
A selector node represents a single complex selector. For example, this
selector string `h1 h2 h3, [href] > p`, is represented as two selector nodes.
It has no special functionality of its own.
## Pseudo nodes
A pseudo selector extends a container node; if it has any parameters of its
own (such as `h1:not(h2, h3)`), they will be its children. Note that the pseudo
`value` will always contain the colons preceding the pseudo identifier. This
is so that both `:before` and `::before` are properly represented in the AST.
## Attribute nodes
### `attribute.quoted`
Returns `true` if the attribute's value is wrapped in quotation marks, false if it is not.
Remains `undefined` if there is no attribute value.
```css
[href=foo] /* false */
[href='foo'] /* true */
[href="foo"] /* true */
[href] /* undefined */
```
### `attribute.qualifiedAttribute`
Returns the attribute name qualified with the namespace if one is given.
### `attribute.offsetOf(part)`
Returns the offset of the attribute part specified relative to the
start of the node of the output string. This is useful in raising
error messages about a specific part of the attribute, especially
in combination with `attribute.sourceIndex`.
Returns `-1` if the name is invalid or the value doesn't exist in this
attribute.
The legal values for `part` are:
* `"ns"` - alias for "namespace"
* `"namespace"` - the namespace if it exists.
* `"attribute"` - the attribute name
* `"attributeNS"` - the start of the attribute or its namespace
* `"operator"` - the match operator of the attribute
* `"value"` - The value (string or identifier)
* `"insensitive"` - the case insensitivity flag
### `attribute.raws.unquoted`
Returns the unquoted content of the attribute's value.
Remains `undefined` if there is no attribute value.
```css
[href=foo] /* foo */
[href='foo'] /* foo */
[href="foo"] /* foo */
[href] /* undefined */
```
### `attribute.spaces`
Like `node.spaces` with the `before` and `after` values containing the spaces
around the element, the parts of the attribute can also have spaces before
and after them. The for each of `attribute`, `operator`, `value` and
`insensitive` there is corresponding property of the same nam in
`node.spaces` that has an optional `before` or `after` string containing only
whitespace.
Note that corresponding values in `attributes.raws.spaces` contain values
including any comments. If set, these values will override the
`attribute.spaces` value. Take care to remove them if changing
`attribute.spaces`.
### `attribute.raws`
The raws object stores comments and other information necessary to re-render
the node exactly as it was in the source.
If a comment is embedded within the identifiers for the `namespace`, `attribute`
or `value` then a property is placed in the raws for that value containing the full source of the propery including comments.
If a comment is embedded within the space between parts of the attribute
then the raw for that space is set accordingly.
Setting an attribute's property `raws` value to be deleted.
For now, changing the spaces required also updating or removing any of the
raws values that override them.
Example: `[ /*before*/ href /* after-attr */ = /* after-operator */ te/*inside-value*/st/* wow */ /*omg*/i/*bbq*/ /*whodoesthis*/]` would parse as:
```js
{
attribute: "href",
operator: "=",
value: "test",
spaces: {
before: '',
after: '',
attribute: { before: ' ', after: ' ' },
operator: { after: ' ' },
value: { after: ' ' },
insensitive: { after: ' ' }
},
raws: {
spaces: {
attribute: { before: ' /*before*/ ', after: ' /* after-attr */ ' },
operator: { after: ' /* after-operator */ ' },
value: { after: '/* wow */ /*omg*/' },
insensitive: { after: '/*bbq*/ /*whodoesthis*/' }
},
unquoted: 'test',
value: 'te/*inside-value*/st'
}
}
```
## `Processor`
### `ProcessorOptions`
* `lossless` - When `true`, whitespace is preserved. Defaults to `true`.
* `updateSelector` - When `true`, if any processor methods are passed a postcss
`Rule` node instead of a string, then that Rule's selector is updated
with the results of the processing. Defaults to `true`.
### `process|processSync(selectors, [options])`
Processes the `selectors`, returning a string from the result of processing.
Note: when the `updateSelector` option is set, the rule's selector
will be updated with the resulting string.
**Example:**
```js
const parser = require("postcss-selector-parser");
const processor = parser();
let result = processor.processSync(' .class');
console.log(result);
// => .class
// Asynchronous operation
let promise = processor.process(' .class').then(result => {
console.log(result)
// => .class
});
// To have the parser normalize whitespace values, utilize the options
result = processor.processSync(' .class ', {lossless: false});
console.log(result);
// => .class
// For better syntax errors, pass a PostCSS Rule node.
const postcss = require('postcss');
rule = postcss.rule({selector: ' #foo > a, .class '});
processor.process(rule, {lossless: false, updateSelector: true}).then(result => {
console.log(result);
// => #foo>a,.class
console.log("rule:", rule.selector);
// => rule: #foo>a,.class
})
```
Arguments:
* `selectors (string|postcss.Rule)`: Either a selector string or a PostCSS Rule
node.
* `[options] (object)`: Process options
### `ast|astSync(selectors, [options])`
Like `process()` and `processSync()` but after
processing the `selectors` these methods return the `Root` node of the result
instead of a string.
Note: when the `updateSelector` option is set, the rule's selector
will be updated with the resulting string.
### `transform|transformSync(selectors, [options])`
Like `process()` and `processSync()` but after
processing the `selectors` these methods return the value returned by the
processor callback.
Note: when the `updateSelector` option is set, the rule's selector
will be updated with the resulting string.
### Error Handling Within Selector Processors
The root node passed to the selector processor callback
has a method `error(message, options)` that returns an
error object. This method should always be used to raise
errors relating to the syntax of selectors. The options
to this method are passed to postcss's error constructor
([documentation](http://api.postcss.org/Container.html#error)).
#### Async Error Example
```js
let processor = (root) => {
return new Promise((resolve, reject) => {
root.walkClasses((classNode) => {
if (/^(.*)[-_]/.test(classNode.value)) {
let msg = "classes may not have underscores or dashes in them";
reject(root.error(msg, {
index: classNode.sourceIndex + RegExp.$1.length + 1,
word: classNode.value
}));
}
});
resolve();
});
};
const postcss = require("postcss");
const parser = require("postcss-selector-parser");
const selectorProcessor = parser(processor);
const plugin = postcss.plugin('classValidator', (options) => {
return (root) => {
let promises = [];
root.walkRules(rule => {
promises.push(selectorProcessor.process(rule));
});
return Promise.all(promises);
};
});
postcss(plugin()).process(`
.foo-bar {
color: red;
}
`.trim(), {from: 'test.css'}).catch((e) => console.error(e.toString()));
// CssSyntaxError: classValidator: ./test.css:1:5: classes may not have underscores or dashes in them
//
// > 1 | .foo-bar {
// | ^
// 2 | color: red;
// 3 | }
```
#### Synchronous Error Example
```js
let processor = (root) => {
root.walkClasses((classNode) => {
if (/.*[-_]/.test(classNode.value)) {
let msg = "classes may not have underscores or dashes in them";
throw root.error(msg, {
index: classNode.sourceIndex,
word: classNode.value
});
}
});
};
const postcss = require("postcss");
const parser = require("postcss-selector-parser");
const selectorProcessor = parser(processor);
const plugin = postcss.plugin('classValidator', (options) => {
return (root) => {
root.walkRules(rule => {
selectorProcessor.processSync(rule);
});
};
});
postcss(plugin()).process(`
.foo-bar {
color: red;
}
`.trim(), {from: 'test.css'}).catch((e) => console.error(e.toString()));
// CssSyntaxError: classValidator: ./test.css:1:5: classes may not have underscores or dashes in them
//
// > 1 | .foo-bar {
// | ^
// 2 | color: red;
// 3 | }
``` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/API.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/API.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 20914
} |
# 6.0.10
- Fixed: `isPseudoElement()` supports `:first-letter` and `:first-line`
# 6.0.9
- Fixed: `Combinator.raws` property type
# 6.0.8
- Fixed: reduced size
# 6.0.7
- Fixed: parse animation percents
# 6.0.6
- Fixed: parse quoted attributes containing a newline correctly
# 6.0.5
- Perf: rework unesc for a 63+% performance boost
# 6.0.4
- Fixed: ts errors
# 6.0.3
- Fixed: replace node built-in "util" module with "util-deprecate"
- Fixed: handle uppercase pseudo elements
- Fixed: do not create invalid combinator before comment
# 6.0.2
- Fixed an issue with parsing and stringifying an empty attribute value
# 6.0.1
- Fixed an issue with unicode surrogate pair parsing
# 6.0.0
- Updated: `cssesc` to 3.0.0 (major)
- Fixed: Issues with escaped `id` and `class` selectors
# 5.0.0
- Allow escaped dot within class name.
- Update PostCSS to 7.0.7 (patch)
# 5.0.0-rc.4
- Fixed an issue where comments immediately after an insensitive (in attribute)
were not parsed correctly.
- Updated `cssesc` to 2.0.0 (major).
- Removed outdated integration tests.
- Added tests for custom selectors, tags with attributes, the universal
selector with pseudos, and tokens after combinators.
# 5.0.0-rc.1
To ease adoption of the v5.0 release, we have relaxed the node version
check performed by npm at installation time to allow for node 4, which
remains officially unsupported, but likely to continue working for the
time being.
# 5.0.0-rc.0
This release has **BREAKING CHANGES** that were required to fix regressions
in 4.0.0 and to make the Combinator Node API consistent for all combinator
types. Please read carefully.
## Summary of Changes
* The way a descendent combinator that isn't a single space character (E.g. `.a .b`) is stored in the AST has changed.
* Named Combinators (E.g. `.a /for/ .b`) are now properly parsed as a combinator.
* It is now possible to look up a node based on the source location of a character in that node and to query nodes if they contain some character.
* Several bug fixes that caused the parser to hang and run out of memory when a `/` was encountered have been fixed.
* The minimum supported version of Node is now `v6.0.0`.
### Changes to the Descendent Combinator
In prior releases, the value of a descendant combinator with multiple spaces included all the spaces.
* `.a .b`: Extra spaces are now stored as space before.
- Old & Busted:
- `combinator.value === " "`
- New hotness:
- `combinator.value === " " && combinator.spaces.before === " "`
* `.a /*comment*/.b`: A comment at the end of the combinator causes extra space to become after space.
- Old & Busted:
- `combinator.value === " "`
- `combinator.raws.value === " /*comment/"`
- New hotness:
- `combinator.value === " "`
- `combinator.spaces.after === " "`
- `combinator.raws.spaces.after === " /*comment*/"`
* `.a<newline>.b`: whitespace that doesn't start or end with a single space character is stored as a raw value.
- Old & Busted:
- `combinator.value === "\n"`
- `combinator.raws.value === undefined`
- New hotness:
- `combinator.value === " "`
- `combinator.raws.value === "\n"`
### Support for "Named Combinators"
Although, nonstandard and unlikely to ever become a standard, combinators like `/deep/` and `/for/` are now properly supported.
Because they've been taken off the standardization track, there is no spec-official name for combinators of the form `/<ident>/`. However, I talked to [Tab Atkins](https://twitter.com/tabatkins) and we agreed to call them "named combinators" so now they are called that.
Before this release such named combinators were parsed without intention and generated three nodes of type `"tag"` where the first and last nodes had a value of `"/"`.
* `.a /for/ .b` is parsed as a combinator.
- Old & Busted:
- `root.nodes[0].nodes[1].type === "tag"`
- `root.nodes[0].nodes[1].value === "/"`
- New hotness:
- `root.nodes[0].nodes[1].type === "combinator"`
- `root.nodes[0].nodes[1].value === "/for/"`
* `.a /F\6fR/ .b` escapes are handled and uppercase is normalized.
- Old & Busted:
- `root.nodes[0].nodes[2].type === "tag"`
- `root.nodes[0].nodes[2].value === "F\\6fR"`
- New hotness:
- `root.nodes[0].nodes[1].type === "combinator"`
- `root.nodes[0].nodes[1].value === "/for/"`
- `root.nodes[0].nodes[1].raws.value === "/F\\6fR/"`
### Source position checks and lookups
A new API was added to look up a node based on the source location.
```js
const selectorParser = require("postcss-selector-parser");
// You can find the most specific node for any given character
let combinator = selectorParser.astSync(".a > .b").atPosition(1,4);
combinator.toString() === " > ";
// You can check if a node includes a specific character
// Whitespace surrounding the node that is owned by that node
// is included in the check.
[2,3,4,5,6].map(column => combinator.isAtPosition(1, column));
// => [false, true, true, true, false]
```
# 4.0.0
This release has **BREAKING CHANGES** that were required to fix bugs regarding values with escape sequences. Please read carefully.
* **Identifiers with escapes** - CSS escape sequences are now hidden from the public API by default.
The normal value of a node like a class name or ID, or an aspect of a node such as attribute
selector's value, is unescaped. Escapes representing Non-ascii characters are unescaped into
unicode characters. For example: `bu\tton, .\31 00, #i\2764\FE0Fu, [attr="value is \"quoted\""]`
will parse respectively to the values `button`, `100`, `i❤️u`, `value is "quoted"`.
The original escape sequences for these values can be found in the corresponding property name
in `node.raws`. Where possible, deprecation warnings were added, but the nature
of escape handling makes it impossible to detect what is escaped or not. Our expectation is
that most users are neither expecting nor handling escape sequences in their use of this library,
and so for them, this is a bug fix. Users who are taking care to handle escapes correctly can
now update their code to remove the escape handling and let us do it for them.
* **Mutating values with escapes** - When you make an update to a node property that has escape handling
The value is assumed to be unescaped, and any special characters are escaped automatically and
the corresponding `raws` value is immediately updated. This can result in changes to the original
escape format. Where the exact value of the escape sequence is important there are methods that
allow both values to be set in conjunction. There are a number of new convenience methods for
manipulating values that involve escapes, especially for attributes values where the quote mark
is involved. See https://github.com/postcss/postcss-selector-parser/pull/133 for an extensive
write-up on these changes.
**Upgrade/API Example**
In `3.x` there was no unescape handling and internal consistency of several properties was the caller's job to maintain. It was very easy for the developer
to create a CSS file that did not parse correctly when some types of values
were in use.
```js
const selectorParser = require("postcss-selector-parser");
let attr = selectorParser.attribute({attribute: "id", operator: "=", value: "a-value"});
attr.value; // => "a-value"
attr.toString(); // => [id=a-value]
// Add quotes to an attribute's value.
// All these values have to be set by the caller to be consistent:
// no internal consistency is maintained.
attr.raws.unquoted = attr.value
attr.value = "'" + attr.value + "'";
attr.value; // => "'a-value'"
attr.quoted = true;
attr.toString(); // => "[id='a-value']"
```
In `4.0` there is a convenient API for setting and mutating values
that may need escaping. Especially for attributes.
```js
const selectorParser = require("postcss-selector-parser");
// The constructor requires you specify the exact escape sequence
let className = selectorParser.className({value: "illegal class name", raws: {value: "illegal\\ class\\ name"}});
className.toString(); // => '.illegal\\ class\\ name'
// So it's better to set the value as a property
className = selectorParser.className();
// Most properties that deal with identifiers work like this
className.value = "escape for me";
className.value; // => 'escape for me'
className.toString(); // => '.escape\\ for\\ me'
// emoji and all non-ascii are escaped to ensure it works in every css file.
className.value = "😱🦄😍";
className.value; // => '😱🦄😍'
className.toString(); // => '.\\1F631\\1F984\\1F60D'
// you can control the escape sequence if you want, or do bad bad things
className.setPropertyAndEscape('value', 'xxxx', 'yyyy');
className.value; // => "xxxx"
className.toString(); // => ".yyyy"
// Pass a value directly through to the css output without escaping it.
className.setPropertyWithoutEscape('value', '$REPLACE_ME$');
className.value; // => "$REPLACE_ME$"
className.toString(); // => ".$REPLACE_ME$"
// The biggest changes are to the Attribute class
// passing quoteMark explicitly is required to avoid a deprecation warning.
let attr = selectorParser.attribute({attribute: "id", operator: "=", value: "a-value", quoteMark: null});
attr.toString(); // => "[id=a-value]"
// Get the value with quotes on it and any necessary escapes.
// This is the same as reading attr.value in 3.x.
attr.getQuotedValue(); // => "a-value";
attr.quoteMark; // => null
// Add quotes to an attribute's value.
attr.quoteMark = "'"; // This is all that's required.
attr.toString(); // => "[id='a-value']"
attr.quoted; // => true
// The value is still the same, only the quotes have changed.
attr.value; // => a-value
attr.getQuotedValue(); // => "'a-value'";
// deprecated assignment, no warning because there's no escapes
attr.value = "new-value";
// no quote mark is needed so it is removed
attr.getQuotedValue(); // => "new-value";
// deprecated assignment,
attr.value = "\"a 'single quoted' value\"";
// > (node:27859) DeprecationWarning: Assigning an attribute a value containing characters that might need to be escaped is deprecated. Call attribute.setValue() instead.
attr.getQuotedValue(); // => '"a \'single quoted\' value"';
// quote mark inferred from first and last characters.
attr.quoteMark; // => '"'
// setValue takes options to make manipulating the value simple.
attr.setValue('foo', {smart: true});
// foo doesn't require any escapes or quotes.
attr.toString(); // => '[id=foo]'
attr.quoteMark; // => null
// An explicit quote mark can be specified
attr.setValue('foo', {quoteMark: '"'});
attr.toString(); // => '[id="foo"]'
// preserves quote mark by default
attr.setValue('bar');
attr.toString(); // => '[id="bar"]'
attr.quoteMark = null;
attr.toString(); // => '[id=bar]'
// with no arguments, it preserves quote mark even when it's not a great idea
attr.setValue('a value \n that should be quoted');
attr.toString(); // => '[id=a\\ value\\ \\A\\ that\\ should\\ be\\ quoted]'
// smart preservation with a specified default
attr.setValue('a value \n that should be quoted', {smart: true, preferCurrentQuoteMark: true, quoteMark: "'"});
// => "[id='a value \\A that should be quoted']"
attr.quoteMark = '"';
// => '[id="a value \\A that should be quoted"]'
// this keeps double quotes because it wants to quote the value and the existing value has double quotes.
attr.setValue('this should be quoted', {smart: true, preferCurrentQuoteMark: true, quoteMark: "'"});
// => '[id="this should be quoted"]'
// picks single quotes because the value has double quotes
attr.setValue('a "double quoted" value', {smart: true, preferCurrentQuoteMark: true, quoteMark: "'"});
// => "[id='a "double quoted" value']"
// setPropertyAndEscape lets you do anything you want. Even things that are a bad idea and illegal.
attr.setPropertyAndEscape('value', 'xxxx', 'the password is 42');
attr.value; // => "xxxx"
attr.toString(); // => "[id=the password is 42]"
// Pass a value directly through to the css output without escaping it.
attr.setPropertyWithoutEscape('value', '$REPLACEMENT$');
attr.value; // => "$REPLACEMENT$"
attr.toString(); // => "[id=$REPLACEMENT$]"
```
# 3.1.2
* Fix: Removed dot-prop dependency since it's no longer written in es5.
# 3.1.1
* Fix: typescript definitions weren't in the published package.
# 3.1.0
* Fixed numerous bugs in attribute nodes relating to the handling of comments
and whitespace. There's significant changes to `attrNode.spaces` and `attrNode.raws` since the `3.0.0` release.
* Added `Attribute#offsetOf(part)` to get the offset location of
attribute parts like `"operator"` and `"value"`. This is most
often added to `Attribute#sourceIndex` for error reporting.
# 3.0.0
## Breaking changes
* Some tweaks to the tokenizer/attribute selector parsing mean that whitespace
locations might be slightly different to the 2.x code.
* Better attribute selector parsing with more validation; postcss-selector-parser
no longer uses regular expressions to parse attribute selectors.
* Added an async API (thanks to @jacobp100); the default `process` API is now
async, and the sync API is now accessed through `processSync` instead.
* `process()` and `processSync()` now return a string instead of the Processor
instance.
* Tweaks handling of Less interpolation (thanks to @jwilsson).
* Removes support for Node 0.12.
## Other changes
* `ast()` and `astSync()` methods have been added to the `Processor`. These
return the `Root` node of the selectors after processing them.
* `transform()` and `transformSync()` methods have been added to the
`Processor`. These return the value returned by the processor callback
after processing the selectors.
* Set the parent when inserting a node (thanks to @chriseppstein).
* Correctly adjust indices when using insertBefore/insertAfter (thanks to @tivac).
* Fixes handling of namespaces with qualified tag selectors.
* `process`, `ast` and `transform` (and their sync variants) now accept a
`postcss` rule node. When provided, better errors are generated and selector
processing is automatically set back to the rule selector (unless the `updateSelector` option is set to `false`.)
* Now more memory efficient when tokenizing selectors.
### Upgrade hints
The pattern of:
`rule.selector = processor.process(rule.selector).result.toString();`
is now:
`processor.processSync(rule)`
# 2.2.3
* Resolves an issue where the parser would not reduce multiple spaces between an
ampersand and another simple selector in lossy mode (thanks to @adam-26).
# 2.2.2
* No longer hangs on an unescaped semicolon; instead the parser will throw
an exception for these cases.
# 2.2.1
* Allows a consumer to specify whitespace tokens when creating a new Node
(thanks to @Semigradsky).
# 2.2.0
* Added a new option to normalize whitespace when parsing the selector string
(thanks to @adam-26).
# 2.1.1
* Better unquoted value handling within attribute selectors
(thanks to @evilebottnawi).
# 2.1.0
* Added: Use string constants for all node types & expose them on the main
parser instance (thanks to @Aweary).
# 2.0.0
This release contains the following breaking changes:
* Renamed all `eachInside` iterators to `walk`. For example, `eachTag` is now
`walkTags`, and `eachInside` is now `walk`.
* Renamed `Node#removeSelf()` to `Node#remove()`.
* Renamed `Container#remove()` to `Container#removeChild()`.
* Renamed `Node#raw` to `Node#raws` (thanks to @davidtheclark).
* Now parses `&` as the *nesting* selector, rather than a *tag* selector.
* Fixes misinterpretation of Sass interpolation (e.g. `#{foo}`) as an
id selector (thanks to @davidtheclark).
and;
* Fixes parsing of attribute selectors with equals signs in them
(e.g. `[data-attr="foo=bar"]`) (thanks to @montmanu).
* Adds `quoted` and `raw.unquoted` properties to attribute nodes
(thanks to @davidtheclark).
# 1.3.3
* Fixes an infinite loop on `)` and `]` tokens when they had no opening pairs.
Now postcss-selector-parser will throw when it encounters these lone tokens.
# 1.3.2
* Now uses plain integers rather than `str.charCodeAt(0)` for compiled builds.
# 1.3.1
* Update flatten to v1.x (thanks to @shinnn).
# 1.3.0
* Adds a new node type, `String`, to fix a crash on selectors such as
`foo:bar("test")`.
# 1.2.1
* Fixes a crash when the parser encountered a trailing combinator.
# 1.2.0
* A more descriptive error is thrown when the parser expects to find a
pseudo-class/pseudo-element (thanks to @ashelley).
* Adds support for line/column locations for selector nodes, as well as a
`Node#sourceIndex` method (thanks to @davidtheclark).
# 1.1.4
* Fixes a crash when a selector started with a `>` combinator. The module will
now no longer throw if a selector has a leading/trailing combinator node.
# 1.1.3
* Fixes a crash on `@` tokens.
# 1.1.2
* Fixes an infinite loop caused by using parentheses in a non-pseudo element
context.
# 1.1.1
* Fixes a crash when a backslash ended a selector string.
# 1.1.0
* Adds support for replacing multiple nodes at once with `replaceWith`
(thanks to @jonathantneal).
* Parser no longer throws on sequential IDs and trailing commas, to support
parsing of selector hacks.
# 1.0.1
* Fixes using `insertAfter` and `insertBefore` during iteration.
# 1.0.0
* Adds `clone` and `replaceWith` methods to nodes.
* Adds `insertBefore` and `insertAfter` to containers.
* Stabilises API.
# 0.0.5
* Fixes crash on extra whitespace inside a pseudo selector's parentheses.
* Adds sort function to the container class.
* Enables the parser to pass its input through without transforming.
* Iteration-safe `each` and `eachInside`.
# 0.0.4
* Tidy up redundant duplication.
* Fixes a bug where the parser would loop infinitely on universal selectors
inside pseudo selectors.
* Adds `length` getter and `eachInside`, `map`, `reduce` to the container class.
* When a selector has been removed from the tree, the root node will no longer
cast it to a string.
* Adds node type iterators to the container class (e.g. `eachComment`).
* Adds filter function to the container class.
* Adds split function to the container class.
* Create new node types by doing `parser.id(opts)` etc.
* Adds support for pseudo classes anywhere in the selector.
# 0.0.3
* Adds `next` and `prev` to the node class.
* Adds `first` and `last` getters to the container class.
* Adds `every` and `some` iterators to the container class.
* Add `empty` alias for `removeAll`.
* Combinators are now types of node.
* Fixes the at method so that it is not an alias for `index`.
* Tidy up creation of new nodes in the parser.
* Refactors how namespaces are handled for consistency & less redundant code.
* Refactors AST to use `nodes` exclusively, and eliminates excessive nesting.
* Fixes nested pseudo parsing.
* Fixes whitespace parsing.
# 0.0.2
* Adds support for namespace selectors.
* Adds support for selectors joined by escaped spaces - such as `.\31\ 0`.
# 0.0.1
* Initial release. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/CHANGELOG.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/CHANGELOG.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 19020
} |
# postcss-selector-parser [](https://travis-ci.org/postcss/postcss-selector-parser)
> Selector parser with built in methods for working with selector strings.
## Install
With [npm](https://npmjs.com/package/postcss-selector-parser) do:
```
npm install postcss-selector-parser
```
## Quick Start
```js
const parser = require('postcss-selector-parser');
const transform = selectors => {
selectors.walk(selector => {
// do something with the selector
console.log(String(selector))
});
};
const transformed = parser(transform).processSync('h1, h2, h3');
```
To normalize selector whitespace:
```js
const parser = require('postcss-selector-parser');
const normalized = parser().processSync('h1, h2, h3', {lossless: false});
// -> h1,h2,h3
```
Async support is provided through `parser.process` and will resolve a Promise
with the resulting selector string.
## API
Please see [API.md](API.md).
## Credits
* Huge thanks to Andrey Sitnik (@ai) for work on PostCSS which helped
accelerate this module's development.
## License
MIT | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@tailwindcss/typography/node_modules/postcss-selector-parser/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1151
} |
# esbuild
This is the macOS ARM 64-bit binary for esbuild, a JavaScript bundler and minifier. See https://github.com/evanw/esbuild for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/drizzle-kit/node_modules/@esbuild/darwin-arm64/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/drizzle-kit/node_modules/@esbuild/darwin-arm64/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 144
} |
# tailwindcss/nesting
This is a PostCSS plugin that wraps [postcss-nested](https://github.com/postcss/postcss-nested) or [postcss-nesting](https://github.com/csstools/postcss-plugins/tree/main/plugins/postcss-nesting) and acts as a compatibility layer to make sure your nesting plugin of choice properly understands Tailwind's custom syntax like `@apply` and `@screen`.
Add it to your PostCSS configuration, somewhere before Tailwind itself:
```js
// postcss.config.js
module.exports = {
plugins: [
require('postcss-import'),
require('tailwindcss/nesting'),
require('tailwindcss'),
require('autoprefixer'),
]
}
```
By default, it uses the [postcss-nested](https://github.com/postcss/postcss-nested) plugin under the hood, which uses a Sass-like syntax and is the plugin that powers nesting support in the [Tailwind CSS plugin API](https://tailwindcss.com/docs/plugins#css-in-js-syntax).
If you'd rather use [postcss-nesting](https://github.com/csstools/postcss-plugins/tree/main/plugins/postcss-nesting) (which is based on the work-in-progress [CSS Nesting](https://drafts.csswg.org/css-nesting-1/) specification), first install the plugin alongside:
```shell
npm install postcss-nesting
```
Then pass the plugin itself as an argument to `tailwindcss/nesting` in your PostCSS configuration:
```js
// postcss.config.js
module.exports = {
plugins: [
require('postcss-import'),
require('tailwindcss/nesting')(require('postcss-nesting')),
require('tailwindcss'),
require('autoprefixer'),
]
}
```
This can also be helpful if for whatever reason you need to use a very specific version of `postcss-nested` and want to override the version we bundle with `tailwindcss/nesting` itself. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tailwindcss/lib/postcss-plugins/nesting/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tailwindcss/lib/postcss-plugins/nesting/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1726
} |
# tailwindcss/nesting
This is a PostCSS plugin that wraps [postcss-nested](https://github.com/postcss/postcss-nested) or [postcss-nesting](https://github.com/csstools/postcss-plugins/tree/main/plugins/postcss-nesting) and acts as a compatibility layer to make sure your nesting plugin of choice properly understands Tailwind's custom syntax like `@apply` and `@screen`.
Add it to your PostCSS configuration, somewhere before Tailwind itself:
```js
// postcss.config.js
module.exports = {
plugins: [
require('postcss-import'),
require('tailwindcss/nesting'),
require('tailwindcss'),
require('autoprefixer'),
]
}
```
By default, it uses the [postcss-nested](https://github.com/postcss/postcss-nested) plugin under the hood, which uses a Sass-like syntax and is the plugin that powers nesting support in the [Tailwind CSS plugin API](https://tailwindcss.com/docs/plugins#css-in-js-syntax).
If you'd rather use [postcss-nesting](https://github.com/csstools/postcss-plugins/tree/main/plugins/postcss-nesting) (which is based on the work-in-progress [CSS Nesting](https://drafts.csswg.org/css-nesting-1/) specification), first install the plugin alongside:
```shell
npm install postcss-nesting
```
Then pass the plugin itself as an argument to `tailwindcss/nesting` in your PostCSS configuration:
```js
// postcss.config.js
module.exports = {
plugins: [
require('postcss-import'),
require('tailwindcss/nesting')(require('postcss-nesting')),
require('tailwindcss'),
require('autoprefixer'),
]
}
```
This can also be helpful if for whatever reason you need to use a very specific version of `postcss-nested` and want to override the version we bundle with `tailwindcss/nesting` itself. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tailwindcss/src/postcss-plugins/nesting/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tailwindcss/src/postcss-plugins/nesting/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1726
} |
# esbuild
This is the macOS ARM 64-bit binary for esbuild, a JavaScript bundler and minifier. See https://github.com/evanw/esbuild for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/tsx/node_modules/@esbuild/darwin-arm64/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/tsx/node_modules/@esbuild/darwin-arm64/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 144
} |
# esbuild
This is the macOS ARM 64-bit binary for esbuild, a JavaScript bundler and minifier. See https://github.com/evanw/esbuild for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/vite/node_modules/@esbuild/darwin-arm64/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/vite/node_modules/@esbuild/darwin-arm64/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 144
} |
# esbuild
This is the macOS ARM 64-bit binary for esbuild, a JavaScript bundler and minifier. See https://github.com/evanw/esbuild for details. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@esbuild-kit/core-utils/node_modules/@esbuild/darwin-arm64/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@esbuild-kit/core-utils/node_modules/@esbuild/darwin-arm64/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 144
} |
# `react-context`
## Installation
```sh
$ yarn add @radix-ui/react-context
# or
$ npm install @radix-ui/react-context
```
## Usage
This is an internal utility, not intended for public usage. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@radix-ui/react-collection/node_modules/@radix-ui/react-context/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@radix-ui/react-collection/node_modules/@radix-ui/react-context/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 194
} |
# `react-context`
## Installation
```sh
$ yarn add @radix-ui/react-context
# or
$ npm install @radix-ui/react-context
```
## Usage
This is an internal utility, not intended for public usage. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@radix-ui/react-popper/node_modules/@radix-ui/react-context/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@radix-ui/react-popper/node_modules/@radix-ui/react-context/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 194
} |
# `react-context`
## Installation
```sh
$ yarn add @radix-ui/react-context
# or
$ npm install @radix-ui/react-context
```
## Usage
This is an internal utility, not intended for public usage. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@radix-ui/react-progress/node_modules/@radix-ui/react-context/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@radix-ui/react-progress/node_modules/@radix-ui/react-context/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 194
} |
# `react-context`
## Installation
```sh
$ yarn add @radix-ui/react-context
# or
$ npm install @radix-ui/react-context
```
## Usage
This is an internal utility, not intended for public usage. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@radix-ui/react-roving-focus/node_modules/@radix-ui/react-context/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@radix-ui/react-roving-focus/node_modules/@radix-ui/react-context/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 194
} |
# `react-context`
## Installation
```sh
$ yarn add @radix-ui/react-context
# or
$ npm install @radix-ui/react-context
```
## Usage
This is an internal utility, not intended for public usage. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@radix-ui/react-toggle-group/node_modules/@radix-ui/react-context/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@radix-ui/react-toggle-group/node_modules/@radix-ui/react-context/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 194
} |
The MIT License (MIT)
Copyright (c) 2016 Zeit, Inc.
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/debug/node_modules/ms/license.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/debug/node_modules/ms/license.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1076
} |
# ms
[](https://travis-ci.org/zeit/ms)
[](https://zeit.chat/)
Use this package to easily convert various time formats to milliseconds.
## Examples
```js
ms('2 days') // 172800000
ms('1d') // 86400000
ms('10h') // 36000000
ms('2.5 hrs') // 9000000
ms('2h') // 7200000
ms('1m') // 60000
ms('5s') // 5000
ms('1y') // 31557600000
ms('100') // 100
```
### Convert from milliseconds
```js
ms(60000) // "1m"
ms(2 * 60000) // "2m"
ms(ms('10 hours')) // "10h"
```
### Time format written-out
```js
ms(60000, { long: true }) // "1 minute"
ms(2 * 60000, { long: true }) // "2 minutes"
ms(ms('10 hours'), { long: true }) // "10 hours"
```
## Features
- Works both in [node](https://nodejs.org) and in the browser.
- If a number is supplied to `ms`, a string with a unit is returned.
- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
## Caught a bug?
1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
2. Link the package to the global module directory: `npm link`
3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
As always, you can run the tests using: `npm test` | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/send/node_modules/debug/node_modules/ms/readme.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/send/node_modules/debug/node_modules/ms/readme.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 1720
} |
### Intro
The prerequisite for this code mod is to migrate your usages to the new syntax, so overloads for hooks and `QueryClient` methods shouldn't be available anymore.
### Affected usages
Please note, this code mod transforms usages only where the first argument is an object expression.
The following usage should be transformed by the code mod:
```ts
const { data } = useQuery({
queryKey: ['posts'],
queryFn: queryFn,
keepPreviousData: true,
})
```
But the following usage won't be transformed by the code mod, because the first argument an identifier:
```ts
const hookArgument = {
queryKey: ['posts'],
queryFn: queryFn,
keepPreviousData: true,
}
const { data } = useQuery(hookArgument)
```
### Troubleshooting
In case of any errors, feel free to reach us out via Discord or open an issue. If you open an issue, please provide a code snippet as well, because without a snippet we cannot find the bug in the code mod. | {
"source": "ammaarreshi/Gemini-Search",
"title": "node_modules/@tanstack/react-query/build/codemods/src/v5/keep-previous-data/README.md",
"url": "https://github.com/ammaarreshi/Gemini-Search/blob/main/node_modules/@tanstack/react-query/build/codemods/src/v5/keep-previous-data/README.md",
"date": "2025-01-04T14:07:19",
"stars": 1910,
"description": "Perplexity style AI Search engine clone built with Gemini 2.0 Flash and Grounding",
"file_size": 942
} |
# Form Builder
A dynamic form-building tool that allows users to create, customize, and validate forms seamlessly within web applications. Built with React, Next.js, and various other technologies, Form Builder provides an intuitive interface for developers and users alike.
## Table of Contents
- [Form Builder](#form-builder)
- [Table of Contents](#table-of-contents)
- [Features](#features)
- [Live Demo](#live-demo)
- [YouTube Demo](#youtube-demo)
- [Installation](#installation)
- [Usage](#usage)
- [Creating a Form](#creating-a-form)
- [Components](#components)
- [Validation](#validation)
- [API](#api)
- [Form Submission](#form-submission)
- [Contributing](#contributing)
- [License](#license)
- [Acknowledgements](#acknowledgements)
## Features
- **Dynamic Form Creation**: Easily create forms with various input types including text, checkbox, radio buttons, and more.
- **Real-Time Validation**: Validate user inputs using the Zod library, ensuring data integrity and user-friendly feedback.
- **Responsive Design**: Built with Tailwind CSS, ensuring forms look great on all devices.
- **Customizable Components**: Leverage ShadCN components for a consistent and modern UI experience.
- **Server-Side Rendering**: Utilize Next.js for optimized performance and SEO.
## Live Demo
Check out the live demo of the Form Builder [here](https://www.shadcn-form.com/).
## YouTube Demo
[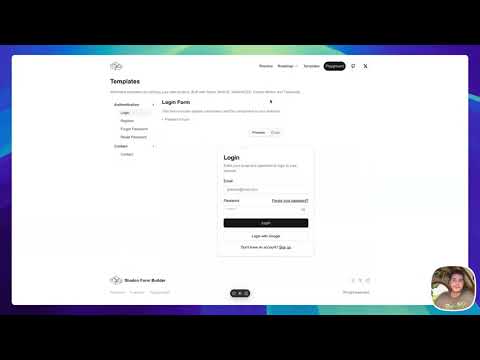](https://www.youtube.com/watch?v=25IzTkU3En4)
## Installation
To get started with Form Builder, follow these steps:
1. Clone the repository:
```bash
git clone https://github.com/hasanharman/form-builder.git
```
2. Navigate into the project directory:
```bash
cd form-builder
```
3. Install the necessary dependencies:
```bash
npm install
```
## Usage
To start the development server, run:
```bash
npm run dev
```
Open your browser and navigate to `http://localhost:3000` to see the application in action.
### Creating a Form
1. **Access the Builder**: Once the app is running, navigate to the form builder interface.
2. **Add Inputs**: Use the toolbar to add different types of inputs.
3. **Customize Inputs**: Click on any input field to configure properties such as label, placeholder, required validation, etc.
4. **Save & Preview**: Once your form is built, save it and preview the output.
## Components
Form Builder consists of various reusable components:
- **FormContainer**: The main container for the form elements.
- **InputField**: A customizable input component.
- **SelectField**: Dropdown selection component.
- **CheckboxField**: A checkbox input component.
- **Button**: A styled button component for form submission.
## Validation
Form Builder utilizes [Zod](https://zod.dev/) for input validation. You can define schemas for your forms as follows:
```javascript
import { z } from 'zod';
const formSchema = z.object({
name: z.string().min(1, "Name is required"),
email: z.string().email("Invalid email address"),
age: z.number().min(18, "You must be at least 18 years old"),
});
```
This schema can be applied to your form to enforce validation rules.
## API
### Form Submission
Once your form is ready, you can handle submissions with a simple API call. Here’s an example of how to submit the form data:
```javascript
const handleSubmit = async (data) => {
try {
const response = await fetch('/api/form-submit', {
method: 'POST',
body: JSON.stringify(data),
headers: {
'Content-Type': 'application/json',
},
});
const result = await response.json();
console.log('Form submitted successfully:', result);
} catch (error) {
console.error('Error submitting form:', error);
}
};
```
## Contributing
Contributions are welcome! If you would like to contribute to Form Builder, please follow these steps:
1. **Fork the Repository**: Click on the “Fork” button at the top right corner of the repository page.
2. **Create a Branch**:
```bash
git checkout -b feature/YourFeatureName
```
3. **Make Changes**: Implement your feature or fix.
4. **Commit Changes**:
```bash
git commit -m "Add a feature"
```
5. **Push Changes**:
```bash
git push origin feature/YourFeatureName
```
6. **Create a Pull Request**: Go to the original repository and create a pull request.
## License
This project is licensed under the MIT License.
## Acknowledgements
- [React](https://reactjs.org/) - A JavaScript library for building user interfaces.
- [Next.js](https://nextjs.org/) - The React framework for production.
- [Tailwind CSS](https://tailwindcss.com/) - A utility-first CSS framework for creating custom designs.
- [Zod](https://zod.dev/) - TypeScript-first schema declaration and validation. | {
"source": "hasanharman/form-builder",
"title": "README.md",
"url": "https://github.com/hasanharman/form-builder/blob/main/README.md",
"date": "2024-09-24T11:02:33",
"stars": 1902,
"description": "A dynamic form-building tool that allows users to create, customize, and validate forms seamlessly within web applications.",
"file_size": 4832
} |
# Maintainers
## Here is a list of maintainers for the AG2 project.
| Name | GitHub Handle | Organization | Features |
|-----------------|------------------------------------------------------------|------------------------|-----------------------------------------|
| Qingyun Wu | [qingyun-wu](https://github.com/qingyun-wu) | Penn State University | all, alt-models, autobuilder |
| Chi Wang | [sonichi](https://github.com/sonichi) | - | all |
| Mark Sze | [marklysze](https://github.com/marklysze) | - | alt-models, group chat |
| Hrushikesh Dokala | [Hk669](https://github.com/Hk669) | - | alt-models, swebench, logging, rag |
| Jiale Liu | [LeoLjl](https://github.com/LeoLjl) | Penn State University | autobuild, group chat |
| Shaokun Zhang | [skzhang1](https://github.com/skzhang1) | Penn State University | AgentOptimizer, Teachability |
| Yixuan Zhai | [randombet](https://github.com/randombet) | Meta | group chat, sequential_chats, rag |
| Yiran Wu | [yiranwu0](https://github.com/yiranwu0) | Penn State University | alt-models, group chat, logging, infra |
| Jieyu Zhang | [JieyuZ2](https://jieyuz2.github.io/) | University of Washington | autobuild, group chat |
| Davor Runje | [davorrunje](https://github.com/davorrunje) | airt.ai | Tool calling, IO |
| Rudy Wu | [rudyalways](https://github.com/rudyalways) | Google | all, group chats, sequential chats |
| Haiyang Li | [ohdearquant](https://github.com/ohdearquant) | - | all, sequential chats, structured output, low-level|
| Eric Moore | [emooreatx](https://github.com/emooreatx) | IBM | all|
| Evan David | [evandavid1](https://github.com/evandavid1) | - | all |
| Tvrtko Sternak | [sternakt](https://github.com/sternakt) | airt.ai | structured output |
| Jiacheng Shang | [Eric-Shang](https://github.com/Eric-Shang) | Toast | RAG |
| Alec Solder | [alecsolder](https://github.com/alecsolder) | - | swarms, reasoning, function calling |
| Marc Willhaus | [willhama](https://github.com/willhama) | - | - |
**Pending Maintainers list (Marked with \*, Waiting for explicit approval from the maintainers)**
| Name | GitHub Handle | Organization | Features |
|-----------------|------------------------------------------------------------|------------------------|-----------------------------------------|
| Olaoluwa Ademola Salami * | [olaoluwasalami](https://github.com/olaoluwasalami) | DevOps Engineer | |
| Rajan Chari * | [rajan-chari](https://github.com/rajan-chari) | Microsoft Research | CAP |
**Past Maintainers list**
| Name | GitHub Handle | Organization | Features |
|-----------------|------------------------------------------------------------|------------------------|-----------------------------------------|
| Aaron Ward | [AaronWard](https://github.com/AaronWard) | yappstore.ai | all |
| Justin Trugman | [jtrugman](https://github.com/jtrugman) | BetterFutureLabs | GPTAssistantAgent, Tool overwrite, Instruction Overwrite |
| Gregory Fanous | [gregory-fanous](https://github.com/gregory-fanous) | - | teachability, rag, tool calling |
| Linxin Song | [LinxinS97](https://github.com/LinxinS97) | University of Southern California | autobuild, group chat |
## I would like to join this list. How can I help the project?
> We're always looking for new contributors to join our team and help improve the project. For more information, please refer to our [CONTRIBUTING](https://docs.ag2.ai/docs/contributor-guide/contributing) guide.
## Are you missing from this list?
> Please open a PR to help us fix this.
## Acknowledgements
This template was adapted from [GitHub Template Guide](https://github.com/cezaraugusto/github-template-guidelines/blob/master/.github/CONTRIBUTORS.md) by [cezaraugusto](https://github.com/cezaraugusto). | {
"source": "ag2ai/ag2",
"title": "MAINTAINERS.md",
"url": "https://github.com/ag2ai/ag2/blob/main/MAINTAINERS.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 4986
} |
## NOTICE
Copyright (c) 2023 - 2025, AG2ai, Inc., AG2ai open-source projects maintainers and core contributors
This project is a fork of https://github.com/microsoft/autogen.
The [original project](https://github.com/microsoft/autogen) is licensed under the MIT License as detailed in [LICENSE_original_MIT](./license_original/LICENSE_original_MIT). The fork was created from version v0.2.35 of the original project.
This project, i.e., https://github.com/ag2ai/ag2, is licensed under the Apache License, Version 2.0 as detailed in [LICENSE](./LICENSE)
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
MIT-licensed contributions:
The MIT license applies to portions of code originating from the [original repository](https://github.com/microsoft/autogen).
For specific details on merged commits, please refer to the project's commit history.
Last updated: 01/16/2025 | {
"source": "ag2ai/ag2",
"title": "NOTICE.md",
"url": "https://github.com/ag2ai/ag2/blob/main/NOTICE.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 1133
} |
<a name="readme-top"></a>
<p align="center">
<!-- The image URL points to the GitHub-hosted content, ensuring it displays correctly on the PyPI website.-->
<img src="https://raw.githubusercontent.com/ag2ai/ag2/27b37494a6f72b1f8050f6bd7be9a7ff232cf749/website/static/img/ag2.svg" width="150" title="hover text">
<br>
<br>
<img src="https://img.shields.io/pypi/dm/pyautogen?label=PyPI%20downloads">
<a href="https://badge.fury.io/py/autogen"><img src="https://badge.fury.io/py/autogen.svg"></a>
<a href="https://github.com/ag2ai/ag2/actions/workflows/python-package.yml">
<img src="https://github.com/ag2ai/ag2/actions/workflows/python-package.yml/badge.svg">
</a>
<img src="https://img.shields.io/badge/3.9%20%7C%203.10%20%7C%203.11%20%7C%203.12-blue">
<a href="https://discord.gg/pAbnFJrkgZ">
<img src="https://img.shields.io/discord/1153072414184452236?logo=discord&style=flat">
</a>
<a href="https://x.com/ag2oss">
<img src="https://img.shields.io/twitter/url/https/twitter.com/cloudposse.svg?style=social&label=Follow%20%40ag2ai">
</a>
</p>
<p align="center">
<a href="https://docs.ag2.ai/">📚 Documentation</a> |
<a href="https://github.com/ag2ai/build-with-ag2">💡 Examples</a> |
<a href="https://docs.ag2.ai/docs/contributor-guide/contributing">🤝 Contributing</a> |
<a href="#related-papers">📝 Cite paper</a> |
<a href="https://discord.gg/pAbnFJrkgZ">💬 Join Discord</a>
</p>
<p align="center">
AG2 was evolved from AutoGen. Fully open-sourced. We invite collaborators from all organizations to contribute.
</p>
# AG2: Open-Source AgentOS for AI Agents
AG2 (formerly AutoGen) is an open-source programming framework for building AI agents and facilitating cooperation among multiple agents to solve tasks. AG2 aims to streamline the development and research of agentic AI. It offers features such as agents capable of interacting with each other, facilitates the use of various large language models (LLMs) and tool use support, autonomous and human-in-the-loop workflows, and multi-agent conversation patterns.
The project is currently maintained by a [dynamic group of volunteers](MAINTAINERS.md) from several organizations. Contact project administrators Chi Wang and Qingyun Wu via [[email protected]](mailto:[email protected]) if you are interested in becoming a maintainer.
## Table of contents
- [AG2: Open-Source AgentOS for AI Agents](#ag2-open-source-agentos-for-ai-agents)
- [Table of contents](#table-of-contents)
- [Getting started](#getting-started)
- [Installation](#installation)
- [Setup your API keys](#setup-your-api-keys)
- [Run your first agent](#run-your-first-agent)
- [Example applications](#example-applications)
- [Introduction of different agent concepts](#introduction-of-different-agent-concepts)
- [Conversable agent](#conversable-agent)
- [Human in the loop](#human-in-the-loop)
- [Orchestrating multiple agents](#orchestrating-multiple-agents)
- [Tools](#tools)
- [Advanced agentic design patterns](#advanced-agentic-design-patterns)
- [Announcements](#announcements)
- [Contributors Wall](#contributors-wall)
- [Code style and linting](#code-style-and-linting)
- [Related papers](#related-papers)
- [Cite the project](#cite-the-project)
- [License](#license)
## Getting started
For a step-by-step walk through of AG2 concepts and code, see [Basic Concepts](https://docs.ag2.ai/docs/user-guide/basic-concepts) in our documentation.
### Installation
AG2 requires **Python version >= 3.9, < 3.14**. AG2 is available via `ag2` (or its alias `pyautogen` or `autogen`) on PyPI.
```bash
pip install ag2
```
Minimal dependencies are installed by default. You can install extra options based on the features you need.
### Setup your API keys
To keep your LLM dependencies neat we recommend using the `OAI_CONFIG_LIST` file to store your API keys.
You can use the sample file `OAI_CONFIG_LIST_sample` as a template.
```json
[
{
"model": "gpt-4o",
"api_key": "<your OpenAI API key here>"
}
]
```
### Run your first agent
Create a script or a Jupyter Notebook and run your first agent.
```python
from autogen import AssistantAgent, UserProxyAgent, config_list_from_json
llm_config = {
"config_list": config_list_from_json(env_or_file="OAI_CONFIG_LIST")
}
assistant = AssistantAgent("assistant", llm_config=llm_config)
user_proxy = UserProxyAgent("user_proxy", code_execution_config={"work_dir": "coding", "use_docker": False})
user_proxy.initiate_chat(assistant, message="Plot a chart of NVDA and TESLA stock price change YTD.")
# This initiates an automated chat between the two agents to solve the task
```
## Example applications
We maintain a dedicated repository with a wide range of applications to help you get started with various use cases or check out our collection of jupyter notebooks as a starting point.
- [Build with AG2](https://github.com/ag2ai/build-with-ag2)
- [Jupyter Notebooks](notebook)
## Introduction of different agent concepts
We have several agent concepts in AG2 to help you build your AI agents. We introduce the most common ones here.
- **Conversable Agent**: Agents that are able to send messages, receive messages and generate replies using GenAI models, non-GenAI tools, or human inputs.
- **Human in the loop**: Add human input to the conversation
- **Orchestrating multiple agents**: Users can orchestrate multiple agents with built-in conversation patterns such as swarms, group chats, nested chats, sequential chats or customize the orchestration by registering custom reply methods.
- **Tools**: Programs that can be registered, invoked and executed by agents
- **Advanced Concepts**: AG2 supports more concepts such as structured outputs, rag, code execution, etc.
### Conversable agent
The conversable agent is the most used agent and is created for generating conversations among agents.
It serves as a base class for all agents in AG2.
```python
from autogen import ConversableAgent
# Create an AI agent
assistant = ConversableAgent(
name="assistant",
system_message="You are an assistant that responds concisely.",
llm_config=llm_config
)
# Create another AI agent
fact_checker = ConversableAgent(
name="fact_checker",
system_message="You are a fact-checking assistant.",
llm_config=llm_config
)
# Start the conversation
assistant.initiate_chat(
recipient=fact_checker,
message="What is AG2?",
max_turns=2
)
```
### Human in the loop
Sometimes your wished workflow requires human input. Therefore you can enable the human in the loop feature.
If you set `human_input_mode` to `ALWAYS` on ConversableAgent you can give human input to the conversation.
There are three modes for `human_input_mode`: `ALWAYS`, `NEVER`, `TERMINATE`.
We created a class which sets the `human_input_mode` to `ALWAYS` for you. Its called `UserProxyAgent`.
```python
from autogen import ConversableAgent
# Create an AI agent
assistant = ConversableAgent(
name="assistant",
system_message="You are a helpful assistant.",
llm_config=llm_config
)
# Create a human agent with manual input mode
human = ConversableAgent(
name="human",
human_input_mode="ALWAYS"
)
# or
human = UserProxyAgent(name="human", code_execution_config={"work_dir": "coding", "use_docker": False})
# Start the chat
human.initiate_chat(
recipient=assistant,
message="Hello! What's 2 + 2?"
)
```
### Orchestrating multiple agents
Users can define their own orchestration patterns using the flexible programming interface from AG2.
Additionally AG2 provides multiple built-in patterns to orchestrate multiple agents, such as `GroupChat` and `Swarm`.
Both concepts are used to orchestrate multiple agents to solve a task.
The group chat works like a chat where each registered agent can participate in the conversation.
```python
from autogen import ConversableAgent, GroupChat, GroupChatManager
# Create AI agents
teacher = ConversableAgent(name="teacher", system_message="You suggest lesson topics.")
planner = ConversableAgent(name="planner", system_message="You create lesson plans.")
reviewer = ConversableAgent(name="reviewer", system_message="You review lesson plans.")
# Create GroupChat
groupchat = GroupChat(agents=[teacher, planner, reviewer], speaker_selection_method="auto")
# Create the GroupChatManager, it will manage the conversation and uses an LLM to select the next agent
manager = GroupChatManager(name="manager", groupchat=groupchat)
# Start the conversation
teacher.initiate_chat(manager, "Create a lesson on photosynthesis.")
```
The swarm requires a more rigid structure and the flow needs to be defined with hand-off, post-tool, and post-work transitions from an agent to another agent.
Read more about it in the [documentation](https://docs.ag2.ai/docs/user-guide/advanced-concepts/conversation-patterns-deep-dive)
### Tools
Agents gain significant utility through tools as they provide access to external data, APIs, and functionality.
```python
from datetime import datetime
from typing import Annotated
from autogen import ConversableAgent, register_function
# 1. Our tool, returns the day of the week for a given date
def get_weekday(date_string: Annotated[str, "Format: YYYY-MM-DD"]) -> str:
date = datetime.strptime(date_string, "%Y-%m-%d")
return date.strftime("%A")
# 2. Agent for determining whether to run the tool
date_agent = ConversableAgent(
name="date_agent",
system_message="You get the day of the week for a given date.",
llm_config=llm_config,
)
# 3. And an agent for executing the tool
executor_agent = ConversableAgent(
name="executor_agent",
human_input_mode="NEVER",
)
# 4. Registers the tool with the agents, the description will be used by the LLM
register_function(
get_weekday,
caller=date_agent,
executor=executor_agent,
description="Get the day of the week for a given date",
)
# 5. Two-way chat ensures the executor agent follows the suggesting agent
chat_result = executor_agent.initiate_chat(
recipient=date_agent,
message="I was born on the 25th of March 1995, what day was it?",
max_turns=1,
)
```
### Advanced agentic design patterns
AG2 supports more advanced concepts to help you build your AI agent workflows. You can find more information in the documentation.
- [Structured Output](https://docs.ag2.ai/docs/user-guide/basic-concepts/structured-outputs)
- [Ending a conversation](https://docs.ag2.ai/docs/user-guide/basic-concepts/ending-a-chat)
- [Retrieval Augmented Generation (RAG)](https://docs.ag2.ai/docs/user-guide/advanced-concepts/rag)
- [Code Execution](https://docs.ag2.ai/docs/user-guide/advanced-concepts/code-execution)
- [Tools with Secrets](https://docs.ag2.ai/docs/user-guide/advanced-concepts/tools-with-secrets)
## Announcements
🔥 🎉 **Nov 11, 2024:** We are evolving AutoGen into **AG2**!
A new organization [AG2AI](https://github.com/ag2ai) is created to host the development of AG2 and related projects with open governance. Check [AG2's new look](https://ag2.ai/).
📄 **License:**
We adopt the Apache 2.0 license from v0.3. This enhances our commitment to open-source collaboration while providing additional protections for contributors and users alike.
🎉 May 29, 2024: DeepLearning.ai launched a new short course [AI Agentic Design Patterns with AutoGen](https://www.deeplearning.ai/short-courses/ai-agentic-design-patterns-with-autogen), made in collaboration with Microsoft and Penn State University, and taught by AutoGen creators [Chi Wang](https://github.com/sonichi) and [Qingyun Wu](https://github.com/qingyun-wu).
🎉 May 24, 2024: Foundation Capital published an article on [Forbes: The Promise of Multi-Agent AI](https://www.forbes.com/sites/joannechen/2024/05/24/the-promise-of-multi-agent-ai/?sh=2c1e4f454d97) and a video [AI in the Real World Episode 2: Exploring Multi-Agent AI and AutoGen with Chi Wang](https://www.youtube.com/watch?v=RLwyXRVvlNk).
🎉 Apr 17, 2024: Andrew Ng cited AutoGen in [The Batch newsletter](https://www.deeplearning.ai/the-batch/issue-245/) and [What's next for AI agentic workflows](https://youtu.be/sal78ACtGTc?si=JduUzN_1kDnMq0vF) at Sequoia Capital's AI Ascent (Mar 26).
[More Announcements](announcements.md)
## Contributors Wall
<a href="https://github.com/ag2ai/ag2/graphs/contributors">
<img src="https://contrib.rocks/image?repo=ag2ai/ag2&max=204" />
</a>
## Code style and linting
This project uses pre-commit hooks to maintain code quality. Before contributing:
1. Install pre-commit:
```bash
pip install pre-commit
pre-commit install
```
2. The hooks will run automatically on commit, or you can run them manually:
```bash
pre-commit run --all-files
```
## Related papers
- [AutoGen: Enabling Next-Gen LLM Applications via Multi-Agent Conversation](https://arxiv.org/abs/2308.08155)
- [EcoOptiGen: Hyperparameter Optimization for Large Language Model Generation Inference](https://arxiv.org/abs/2303.04673)
- [MathChat: Converse to Tackle Challenging Math Problems with LLM Agents](https://arxiv.org/abs/2306.01337)
- [AgentOptimizer: Offline Training of Language Model Agents with Functions as Learnable Weights](https://arxiv.org/pdf/2402.11359)
- [StateFlow: Enhancing LLM Task-Solving through State-Driven Workflows](https://arxiv.org/abs/2403.11322)
## Cite the project
```
@software{AG2_2024,
author = {Chi Wang and Qingyun Wu and the AG2 Community},
title = {AG2: Open-Source AgentOS for AI Agents},
year = {2024},
url = {https://github.com/ag2ai/ag2},
note = {Available at https://docs.ag2.ai/},
version = {latest}
}
```
## License
This project is licensed under the [Apache License, Version 2.0 (Apache-2.0)](./LICENSE).
This project is a spin-off of [AutoGen](https://github.com/microsoft/autogen) and contains code under two licenses:
- The original code from https://github.com/microsoft/autogen is licensed under the MIT License. See the [LICENSE_original_MIT](./license_original/LICENSE_original_MIT) file for details.
- Modifications and additions made in this fork are licensed under the Apache License, Version 2.0. See the [LICENSE](./LICENSE) file for the full license text.
We have documented these changes for clarity and to ensure transparency with our user and contributor community. For more details, please see the [NOTICE](./NOTICE.md) file. | {
"source": "ag2ai/ag2",
"title": "README.md",
"url": "https://github.com/ag2ai/ag2/blob/main/README.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 14345
} |
# AG2: Responsible AI FAQs
## What is AG2?
AG2 is a framework for simplifying the orchestration, optimization, and automation of LLM workflows. It offers customizable and conversable agents that leverage the strongest capabilities of the most advanced LLMs, like GPT-4, while addressing their limitations by integrating with humans and tools and having conversations between multiple agents via automated chat. AG2 is a spin-off of [AutoGen](https://github.com/microsoft/autogen) created by [a team consisting of AutoGen’s founders and contributors](https://github.com/ag2ai/ag2/blob/main/MAINTAINERS.md) of AutoGen.
## What can AG2 do?
AG2 is a framework for building a complex multi-agent conversation system by:
- Defining a set of agents with specialized capabilities and roles.
- Defining the interaction behavior between agents, i.e., what to reply with when an agent receives messages from another agent.
The agent conversation-centric design has numerous benefits, including that it:
- Naturally handles ambiguity, feedback, progress, and collaboration.
- Enables effective coding-related tasks, like tool use with back-and-forth troubleshooting.
- Allows users to seamlessly opt in or opt out via an agent in the chat.
- Achieves a collective goal with the cooperation of multiple specialists.
## What is/are AG2’s intended use(s)?
Please note that AG2 is an open-source library under active development and intended for use for research purposes. It should not be used in any downstream applications without additional detailed evaluation of robustness, safety issues and assessment of any potential harm or bias in the proposed application.
AG2 is a generic infrastructure that can be used in multiple scenarios. The system’s intended uses include:
- Building LLM workflows that solve more complex tasks: Users can create agents that interleave reasoning and tool use capabilities of the latest LLMs such as GPT-4o. To solve complex tasks, multiple agents can converse to work together (e.g., by partitioning a complex problem into simpler steps or by providing different viewpoints or perspectives).
- Application-specific agent topologies: Users can create application specific agent topologies and patterns for agents to interact. The exact topology may depend on the domain’s complexity and semantic capabilities of the LLM available.
- Code generation and execution: Users can implement agents that can assume the roles of writing code and other agents that can execute code. Agents can do this with varying levels of human involvement. Users can add more agents and program the conversations to enforce constraints on code and output.
- Question answering: Users can create agents that can help answer questions using retrieval augmented generation.
- End user and multi-agent chat and debate: Users can build chat applications where they converse with multiple agents at the same time.
While AG2 automates LLM workflows, decisions about how to use specific LLM outputs should always have a human in the loop. For example, you should not use AG2 to automatically post LLM generated content to social media.
## How was AG2 evaluated? What metrics are used to measure performance?
- The current version of AG2 was evaluated on six applications to illustrate its potential in simplifying the development of high-performance multi-agent applications. These applications were selected based on their real-world relevance, problem difficulty and problem solving capabilities enabled by AG2, and innovative potential.
- These applications involve using AG2 to solve math problems, question answering, decision making in text world environments, supply chain optimization, etc. For each of these domains AG2 was evaluated on various success based metrics (i.e., how often the AG2 based implementation solved the task). And, in some cases, AG2 based approach was also evaluated on implementation efficiency (e.g., to track reductions in developer effort to build).
- The team has conducted tests where a “red” agent attempts to get the default AG2 assistant to break from its alignment and guardrails. The team has observed that out of 70 attempts to break guardrails, only 1 was successful in producing text that would have been flagged as problematic by Azure OpenAI filters. The team has not observed any evidence that AG2 (or GPT models as hosted by OpenAI or Azure) can produce novel code exploits or jailbreak prompts, since direct prompts to “be a hacker”, “write exploits”, or “produce a phishing email” are refused by existing filters.
## What are the limitations of AG2? How can users minimize the impact of AG2’s limitations when using the system?
AG2 relies on existing LLMs. Experimenting with AG2 retains the common limitations of large language models; including:
- Data Biases: Large language models, trained on extensive data, can inadvertently carry biases present in the source data. Consequently, the models may generate outputs that could be potentially biased or unfair.
- Lack of Contextual Understanding: Despite their impressive capabilities in language understanding and generation, these models exhibit limited real-world understanding, resulting in potential inaccuracies or nonsensical responses.
- Lack of Transparency: Due to the complexity and size, large language models can act as `black boxes,' making it difficult to comprehend the rationale behind specific outputs or decisions.
- Content Harms: There are various types of content harms that large language models can cause. It is important to be aware of them when using these models, and to take actions to prevent them. It is recommended to leverage various content moderation services provided by different companies and institutions.
- Inaccurate or ungrounded content: It is important to be aware and cautious not to entirely rely on a given language model for critical decisions or information that might have deep impact as it is not obvious how to prevent these models to fabricate content without high authority input sources.
- Potential for Misuse: Without suitable safeguards, there is a risk that these models could be maliciously used for generating disinformation or harmful content.
Additionally, AG2's multi-agent framework may amplify or introduce additional risks, such as:
- Privacy and Data Protection: The framework allows for human participation in conversations between agents. It is important to ensure that user data and conversations are protected and that developers use appropriate measures to safeguard privacy.
- Accountability and Transparency: The framework involves multiple agents conversing and collaborating, it is important to establish clear accountability and transparency mechanisms. Users should be able to understand and trace the decision-making process of the agents involved in order to ensure accountability and address any potential issues or biases.
- Trust and reliance: The framework leverages human understanding and intelligence while providing automation through conversations between agents. It is important to consider the impact of this interaction on user experience, trust, and reliance on AI systems. Clear communication and user education about the capabilities and limitations of the system will be essential.
- Security & unintended consequences: The use of multi-agent conversations and automation in complex tasks may have unintended consequences. Especially, allowing LLM agents to make changes in external environments through code execution or function calls, such as installing packages, could pose significant risks. Developers should carefully consider the potential risks and ensure that appropriate safeguards are in place to prevent harm or negative outcomes, including keeping a human in the loop for decision making.
## What operational factors and settings allow for effective and responsible use of AG2?
- Code execution: AG2 recommends using docker containers so that code execution can happen in a safer manner. Users can use function calls instead of free-form code to execute pre-defined functions only, increasing reliability and safety. Users can also tailor the code execution environment to their requirements.
- Human involvement: AG2 prioritizes human involvement in multi agent conversation. The overseers can step in to give feedback to agents and steer them in the correct direction. Users can get a chance to confirm before code is executed.
- Agent modularity: Modularity allows agents to have different levels of information access. Additional agents can assume roles that help keep other agents in check. For example, one can easily add a dedicated agent to play the role of a safeguard.
- LLMs: Users can choose the LLM that is optimized for responsible use. For example, OpenAI's GPT-4o includes RAI mechanisms and filters. Caching is enabled by default to increase reliability and control cost. We encourage developers to review [OpenAI’s Usage policies](https://openai.com/policies/usage-policies) and [Azure OpenAI’s Code of Conduct](https://learn.microsoft.com/en-us/legal/cognitive-services/openai/code-of-conduct) when using their models.
- Multi-agent setup: When using auto replies, the users can limit the number of auto replies, termination conditions etc. in the settings to increase reliability. | {
"source": "ag2ai/ag2",
"title": "TRANSPARENCY_FAQS.md",
"url": "https://github.com/ag2ai/ag2/blob/main/TRANSPARENCY_FAQS.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 9317
} |
# Announcements
🔥 🎉 **Nov 11, 2024:** We are evolving AutoGen into **AG2**!
A new organization [AG2AI](https://github.com/ag2ai) is created to host the development of AG2 and related projects with open governance. Check [AG2's new look](https://ag2.ai/).
📄 **License:**
We adopt the Apache 2.0 license from v0.3. This enhances our commitment to open-source collaboration while providing additional protections for contributors and users alike.
🎉 May 29, 2024: DeepLearning.ai launched a new short course [AI Agentic Design Patterns with AutoGen](https://www.deeplearning.ai/short-courses/ai-agentic-design-patterns-with-autogen), made in collaboration with Microsoft and Penn State University, and taught by AutoGen creators [Chi Wang](https://github.com/sonichi) and [Qingyun Wu](https://github.com/qingyun-wu).
🎉 May 24, 2024: Foundation Capital published an article on [Forbes: The Promise of Multi-Agent AI](https://www.forbes.com/sites/joannechen/2024/05/24/the-promise-of-multi-agent-ai/?sh=2c1e4f454d97) and a video [AI in the Real World Episode 2: Exploring Multi-Agent AI and AutoGen with Chi Wang](https://www.youtube.com/watch?v=RLwyXRVvlNk).
🎉 May 13, 2024: [The Economist](https://www.economist.com/science-and-technology/2024/05/13/todays-ai-models-are-impressive-teams-of-them-will-be-formidable) published an article about multi-agent systems (MAS) following a January 2024 interview with [Chi Wang](https://github.com/sonichi).
🎉 May 11, 2024: [AutoGen: Enabling Next-Gen LLM Applications via Multi-Agent Conversation](https://openreview.net/pdf?id=uAjxFFing2) received the best paper award at the [ICLR 2024 LLM Agents Workshop](https://llmagents.github.io/).
🎉 Apr 17, 2024: Andrew Ng cited AutoGen in [The Batch newsletter](https://www.deeplearning.ai/the-batch/issue-245/) and [What's next for AI agentic workflows](https://youtu.be/sal78ACtGTc?si=JduUzN_1kDnMq0vF) at Sequoia Capital's AI Ascent (Mar 26).
🎉 Mar 3, 2024: What's new in AutoGen? 📰[Blog](https://docs.ag2.ai/blog/2024-03-03-AutoGen-Update); 📺[Youtube](https://www.youtube.com/watch?v=j_mtwQiaLGU).
🎉 Mar 1, 2024: the first AutoGen multi-agent experiment on the challenging [GAIA](https://huggingface.co/spaces/gaia-benchmark/leaderboard) benchmark achieved the No. 1 accuracy in all the three levels.
🎉 Jan 30, 2024: AutoGen is highlighted by Peter Lee in Microsoft Research Forum [Keynote](https://t.co/nUBSjPDjqD).
🎉 Dec 31, 2023: [AutoGen: Enabling Next-Gen LLM Applications via Multi-Agent Conversation Framework](https://arxiv.org/abs/2308.08155) is selected by [TheSequence: My Five Favorite AI Papers of 2023](https://thesequence.substack.com/p/my-five-favorite-ai-papers-of-2023).
🔥 Nov 24: pyautogen [v0.2](https://github.com/ag2ai/ag2/releases/tag/v0.2.0) is released with many updates and new features compared to v0.1.1. It switches to using openai-python v1. Please read the [migration guide](https://docs.ag2.ai/docs/installation/Installation).
🔥 Nov 11: OpenAI's Assistants are available in AutoGen and interoperatable with other AutoGen agents! Checkout our [blogpost](https://docs.ag2.ai/blog/2023-11-13-OAI-assistants) for details and examples.
🎉 Nov 8, 2023: AutoGen is selected into [Open100: Top 100 Open Source achievements](https://www.benchcouncil.org/evaluation/opencs/annual.html) 35 days after spinoff from [FLAML](https://github.com/microsoft/FLAML).
🎉 Nov 6, 2023: AutoGen is mentioned by Satya Nadella in a [fireside chat](https://youtu.be/0pLBvgYtv6U).
🎉 Nov 1, 2023: AutoGen is the top trending repo on GitHub in October 2023.
🎉 Oct 03, 2023: AutoGen spins off from [FLAML](https://github.com/microsoft/FLAML) on GitHub.
🎉 Aug 16: Paper about AutoGen on [arxiv](https://arxiv.org/abs/2308.08155).
🎉 Mar 29, 2023: AutoGen is first created in [FLAML](https://github.com/microsoft/FLAML).
🔥 FLAML is highlighted in OpenAI's [cookbook](https://github.com/openai/openai-cookbook#related-resources-from-around-the-web).
🔥 [autogen](https://docs.ag2.ai/) is released with support for ChatGPT and GPT-4, based on [Cost-Effective Hyperparameter Optimization for Large Language Model Generation Inference](https://arxiv.org/abs/2303.04673).
🔥 FLAML supports Code-First AutoML & Tuning – Private Preview in [Microsoft Fabric Data Science](https://learn.microsoft.com/en-us/fabric/data-science/). | {
"source": "ag2ai/ag2",
"title": "announcements.md",
"url": "https://github.com/ag2ai/ag2/blob/main/announcements.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 4324
} |
# Devcontainer Configurations for AG2
Welcome to the `.devcontainer` directory! Here you'll find Dockerfiles and devcontainer configurations that are essential for setting up your AG2 development environment. Below is a brief overview and how you can utilize them effectively.
These configurations can be used with Codespaces and locally.
## Developing AG2 with Devcontainers
### Prerequisites
- [Docker](https://docs.docker.com/get-docker/)
- [Visual Studio Code](https://code.visualstudio.com/)
- [Visual Studio Code Remote - Containers extension](https://marketplace.visualstudio.com/items?itemName=ms-vscode-remote.remote-containers)
### Getting Started
1. Open the project in Visual Studio Code.
2. Press `Ctrl+Shift+P` and select `Dev Containers: Reopen in Container`.
3. Select the desired python environment and wait for the container to build.
4. Once the container is built, you can start developing AG2.
### Troubleshooting Common Issues
- Check Docker daemon, port conflicts, and permissions issues.
### Additional Resources
For more information on Docker usage and best practices, refer to the [official Docker documentation](https://docs.docker.com). | {
"source": "ag2ai/ag2",
"title": ".devcontainer/README.md",
"url": "https://github.com/ag2ai/ag2/blob/main/.devcontainer/README.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 1175
} |
### Description
<!-- A clear and concise description of the issue or feature request. -->
### Environment
- Package name & version: <!-- Specify the ag2 package name and version (e.g., autogen v0.7.2) -->
- Python version: <!-- Specify the Python version (e.g., 3.12) -->
- Operating System: <!-- Specify the OS (e.g., Windows 10, Ubuntu 20.04) -->
### Steps to Reproduce (for bugs)
<!-- Provide detailed steps to reproduce the issue. Include code snippets, configuration files, or any other relevant information. -->
1. Step 1
2. Step 2
3. ...
### Expected Behavior
<!-- Describe what you expected to happen. -->
### Actual Behavior
<!-- Describe what actually happened. Include any error messages, stack traces, or unexpected behavior. -->
### Screenshots / Logs (if applicable)
<!-- If relevant, include screenshots or logs that help illustrate the issue. -->
### Additional Information
<!-- Include any additional information that might be helpful, such as specific configurations, data samples, or context about the environment. -->
### Possible Solution (if you have one)
<!-- If you have suggestions on how to address the issue, provide them here. -->
### Is this a Bug or Feature Request?
<!-- Choose one: Bug | Feature Request -->
### Priority
<!-- Choose one: High | Medium | Low -->
### Difficulty
<!-- Choose one: Easy | Moderate | Hard -->
### Any related issues?
<!-- If this is related to another issue, reference it here. -->
### Any relevant discussions?
<!-- If there are any discussions or forum threads related to this issue, provide links. -->
### Checklist
<!-- Please check the items that you have completed -->
- [ ] I have searched for similar issues and didn't find any duplicates.
- [ ] I have provided a clear and concise description of the issue.
- [ ] I have included the necessary environment details.
- [ ] I have outlined the steps to reproduce the issue.
- [ ] I have included any relevant logs or screenshots.
- [ ] I have indicated whether this is a bug or a feature request.
- [ ] I have set the priority and difficulty levels.
### Additional Comments
<!-- Any additional comments or context that you think would be helpful. --> | {
"source": "ag2ai/ag2",
"title": ".github/ISSUE_TEMPLATE.md",
"url": "https://github.com/ag2ai/ag2/blob/main/.github/ISSUE_TEMPLATE.md",
"date": "2024-11-11T20:43:34",
"stars": 1869,
"description": "AG2 (formerly AutoGen): The Open-Source AgentOS. Join us at: https://discord.gg/pAbnFJrkgZ",
"file_size": 2180
} |
Subsets and Splits