problem_id
stringlengths 32
32
| name
stringclasses 1
value | problem
stringlengths 200
14k
| solutions
stringlengths 12
1.12M
| test_cases
stringlengths 37
74M
| difficulty
stringclasses 3
values | language
stringclasses 1
value | source
stringclasses 7
values | num_solutions
int64 12
1.12M
| starter_code
stringlengths 0
956
|
---|---|---|---|---|---|---|---|---|---|
4010bd04a5ca00da3f5fc759d89be036 | UNKNOWN | Given a string, you progressively need to concatenate the first letter from the left and the first letter to the right and "1", then the second letter from the left and the second letter to the right and "2", and so on.
If the string's length is odd drop the central element.
For example:
```python
char_concat("abcdef") == 'af1be2cd3'
char_concat("abc!def") == 'af1be2cd3' # same result
``` | ["def char_concat(word, index = 1):\n if len(word) < 2: return ''\n return word[0:1] + word[-1:] + str(index) + char_concat(word[1:-1], index + 1)", "def char_concat(word):\n return ''.join([(word[i]+word[-1-i]+str(i+1))for i in range(len(word)//2)])\n", "def char_concat(word):\n return ''.join( \"{}{}{}\".format(word[n], word[-1-n], n+1) for n in range(len(word)//2))", "def char_concat(word):\n half = len(word) // 2\n return ''.join('{}{}{}'.format(a[0], a[1], i) for i, a in enumerate(zip(word[:half], word[::-1][:half]), 1))", "def char_concat(word):\n m = (len(word) + 2) // 2\n return ''.join(a + b + str(i) for i, (a, b) in enumerate(zip(word[:m], word[-1:-m:-1]), 1))", "def char_concat(word):\n return \"\".join([word[i] + word[-i-1] + str(i+1) for i in range(len(word)//2)])", "def char_concat(w):\n n = len(w)//2\n return \"\".join(c[0]+c[1]+str(i+1) for i, c in enumerate(zip(w[:n], w[:-n-1:-1])))", "def char_concat(word):\n return ''.join(('%s%s%d' % (a, b, i)) for (i, (a, b)) in enumerate(zip(word[:len(word) // 2], reversed(word[-len(word) // 2:])), 1))\n"] | {"fn_name": "char_concat", "inputs": [["abc def"], ["CodeWars"], ["CodeWars Rocks"], ["1234567890"], ["$'D8KB)%PO@s"]], "outputs": [["af1be2cd3"], ["Cs1or2da3eW4"], ["Cs1ok2dc3eo4WR5a 6rs7"], ["101292383474565"], ["$s1'@2DO38P4K%5B)6"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,109 |
def char_concat(word):
|
ce86fdf3a9f40db80daa42eb6ec4a1a7 | UNKNOWN | You know how sometimes you write the the same word twice in a sentence, but then don't notice that it happened? For example, you've been distracted for a second. Did you notice that *"the"* is doubled in the first sentence of this description?
As as aS you can see, it's not easy to spot those errors, especially if words differ in case, like *"as"* at the beginning of the sentence.
Write a function that counts the number of sections repeating the same word (case insensitive). The occurence of two or more equal words next after each other count as one.
**Example:**
```
"dog cat" --> 0
"dog DOG cat" --> 1
"apple dog cat" --> 0
"pineapple apple dog cat" --> 0
"apple apple dog cat" --> 1
"apple dog apple dog cat" --> 0
"dog dog DOG dog dog dog" --> 1
"dog dog dog dog cat cat" --> 2
"cat cat dog dog cat cat" --> 3
``` | ["def count_adjacent_pairs(st): \n words = st.lower().split(' ')\n currentWord = None\n count = 0\n for i, word in enumerate(words):\n if i+1 < len(words):\n if word == words[i+1]:\n if word != currentWord:\n currentWord = word\n count += 1\n else:\n currentWord = None\n \n return count", "from itertools import groupby\ndef count_adjacent_pairs(st): \n return len([name for name,group in groupby(st.lower().split(' ')) if len(list(group))>=2])", "import re\ndef count_adjacent_pairs(s): \n return len(re.findall(r'(\\b.+\\b)\\1+',s+' ', re.I))", "from itertools import groupby\n\ndef count_adjacent_pairs(s):\n return sum(next(y) == next(y, 0) for x, y in groupby(s.lower().split()))", "def count_adjacent_pairs(st): \n s, one, two, c = st.lower().split(' '), None, None, 0\n for w in s:\n c += w == one and w != two\n two = one\n one = w\n return c", "def count_adjacent_pairs(st): \n st = st.lower().split()\n check, ret, k = None, 0, 0\n for e in st:\n if e == check and k:\n continue\n if e == check and not k:\n ret, k = ret + 1, 1\n else:\n check,k = e, 0\n return ret", "import re;count_adjacent_pairs=lambda s:len(re.findall(r'(\\b\\w+)(\\s+\\1)+',s,re.I))", "from itertools import groupby\n\n\ndef count_adjacent_pairs(stg): \n return sum(1 for _, g in groupby(stg.lower().split()) if len(list(g)) > 1)", "def count_adjacent_pairs(st):\n if not st:\n return 0\n res = 0\n arr = [i.lower() for i in st.split()]\n for i in range(len(arr)-1):\n if i == 0:\n if arr[i] == arr[i+1]:\n res += 1\n elif arr[i] == arr[i+1] != arr[i-1]:\n res += 1\n return res", "def count_adjacent_pairs(st):\n #print(st)\n st = st.lower().split()\n cnt = 0\n on = False\n for i in range(1,len(st)):\n if st[i] == st[i-1]:\n if not on: \n cnt += 1\n on = True\n else:\n on = False\n return cnt"] | {"fn_name": "count_adjacent_pairs", "inputs": [[""], ["orange Orange kiwi pineapple apple"], ["banana banana banana"], ["banana banana banana terracotta banana terracotta terracotta pie!"], ["pineapple apple"]], "outputs": [[0], [1], [1], [2], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,168 |
def count_adjacent_pairs(st):
|
5dd8be39cb5bfa8f845057aae18e049c | UNKNOWN | ## Task
An `ATM` ran out of 10 dollar bills and only has `100, 50 and 20` dollar bills.
Given an amount between `40 and 10000 dollars (inclusive)` and assuming that the ATM wants to use as few bills as possible, determinate the minimal number of 100, 50 and 20 dollar bills the ATM needs to dispense (in that order).
## Example
For `n = 250`, the result should be `[2, 1, 0]`.
For `n = 260`, the result should be `[2, 0, 3]`.
For `n = 370`, the result should be `[3, 1, 1]`.
## Input/Output
- `[input]` integer `n`
Amount of money to withdraw. Assume that `n` is always exchangeable with `[100, 50, 20]` bills.
- `[output]` integer array
An array of number of `100, 50 and 20` dollar bills needed to complete the withdraw (in that order). | ["def withdraw(n):\n n50 = 0\n n20, r = divmod(n, 20)\n if r == 10:\n n20 -= 2\n n50 += 1\n n100, n20 = divmod(n20, 5)\n return [n100, n50, n20]", "def withdraw(n):\n hundreds, fifties, rest = n // 100, (n % 100) // 50, n % 50\n if rest % 20 == 10:\n if fifties == 0:\n hundreds, fifties, twenties = hundreds - 1, 1, (rest + 50) // 20\n else:\n fifties, twenties = fifties - 1, (rest + 50) // 20\n else:\n twenties = rest // 20\n return [hundreds, fifties, twenties]", "def withdraw(n):\n b50 = int(n % 20 == 10)\n b100, b20 = divmod((n - 50 * b50) // 20, 5)\n return [b100, b50, b20]", "bills = [[0, 0], [1, 3], [0, 1], [1, 4], [0, 2], [1, 0], [0, 3], [1, 1], [0, 4], [1, 2]]\n\ndef withdraw(n):\n q, r = divmod(n, 100)\n if r == 10 or r == 30: q -= 1\n return [q] + bills[r//10]", "def withdraw(n, coins=(100, 50, 20)):\n if not n:\n return [0] * len(coins)\n for i in range(n // coins[0] if coins else -1, -1, -1):\n sub = withdraw(n - i * coins[0], coins[1:])\n if sub is not None:\n return [i] + sub", "def withdraw(n, bills=[100, 50, 20]):\n changes = [[0] * len(bills) for _ in range(n + 1)]\n for c in range(1, n + 1):\n changes[c] = min(([v + (b == bills[i]) for i, v in enumerate(changes[c - b])]\n for b in bills if c >= b), key=sum, default=[float('inf')] * len(bills))\n return changes[-1]", "def withdraw(n):\n bills = [0, 0, 0]\n print(n)\n if n >= 100:\n while n >= 100:\n if n == 130 or n == 110:\n break\n n -= 100\n bills[0] += 1\n if n == 50 or n == 70 or n == 90 or n == 130 or n == 110:\n while n >= 50:\n if n == 80:\n break\n if bills[1] == 1:\n break\n n -= 50\n bills[1] += 1\n if n > 0:\n while n > 0:\n n -= 20\n bills[2] += 1\n \n return bills\n", "def withdraw(n):\n b50 = int(n % 20 == 10)\n b100, n = divmod(n - 50 * b50, 100)\n return [b100, b50, n // 20]", "def withdraw(n):\n fifties = 1 if n % 20 else 0\n twenties = int(n / 20) - 2 if fifties else int(n / 20)\n hundreds = int(20 * twenties / 100)\n twenties = twenties - hundreds * 5\n return [hundreds, fifties, twenties] ", "import re\ndef withdraw(n):\n return [len(a) // b for a, b in zip(re.findall(r'\\A((?:.{10})*)((?:.{5})*)((?:.{2})*)\\Z', '.' * (n // 10))[0], (10, 5, 2))]"] | {"fn_name": "withdraw", "inputs": [[40], [250], [260], [230], [60]], "outputs": [[[0, 0, 2]], [[2, 1, 0]], [[2, 0, 3]], [[1, 1, 4]], [[0, 0, 3]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,554 |
def withdraw(n):
|
5ae6446e9a09499bd26c9b926b53f4f7 | UNKNOWN | Construct a function 'coordinates', that will return the distance between two points on a cartesian plane, given the x and y coordinates of each point.
There are two parameters in the function, ```p1``` and ```p2```. ```p1``` is a list ```[x1,y1]``` where ```x1``` and ```y1``` are the x and y coordinates of the first point. ```p2``` is a list ```[x2,y2]``` where ```x2``` and ```y2``` are the x and y coordinates of the second point.
The distance between the two points should be rounded to the `precision` decimal if provided, otherwise to the nearest integer. | ["def coordinates(p1,p2, precision = 0):\n return round(sum( (b-a)**2 for a,b in zip(p1,p2) )**.5, precision)", "import math\n\ndef coordinates(p1, p2, precision=0):\n return round(math.hypot(p1[0] - p2[0], p1[1] - p2[1]), precision)", "def coordinates(a, b, p = 0):\n return round(sum((i - j) ** 2 for i, j in zip(a, b)) ** 0.5, p)", "from math import hypot\n\ndef coordinates(p1, p2, r=0):\n x1, y1 = p1\n x2, y2 = p2\n return round(hypot(x2 - x1, y2 - y1), r)\n \n# one-liner:\n# return round(sum((c2 - c1)**2 for c1, c2 in zip(p1, p2))**0.5, r)\n", "import math\n\ndef coordinates(p1,p2,p=0):\n return round(math.hypot(abs(p1[0]-p2[0]), abs(p1[1] - p2[1])), p)", "from math import hypot\n\ndef coordinates(p1, p2, precision=0):\n return round(hypot(p1[0] - p2[0], p1[1] - p2[1]), precision)", "import math\ndef coordinates(p1,p2, d=0):\n formula = math.sqrt((p2[0] - p1[0])** 2 + (p2[1] - p1[1])**2)\n distance = round(formula, d)\n \n return distance\n", "coordinates=lambda p1,p2,p=0: round(((p1[0]-p2[0])**2+(p1[1]-p2[1])**2)**0.5,p)", "def coordinates(p1,p2,prec=0):\n return round( ( (p2[1]-p1[1])**2 + (p2[0]-p1[0])**2 )**0.5,prec)", "from math import sqrt\n\ndef coordinates(p1, p2, precision=0):\n x1, y1 = p1\n x2, y2 = p2\n distance = sqrt((x1 - x2) ** 2 + (y1 - y2) ** 2)\n return round(distance, precision)"] | {"fn_name": "coordinates", "inputs": [[[3, 6], [14, 6]], [[-2, 5], [-2, -10], 2], [[1, 2], [4, 6]], [[5, 3], [10, 15], 2], [[-2, -10], [6, 5]], [[4, 7], [6, 2], 3], [[-4, -5], [-5, -4], 1], [[3, 8], [3, 8]], [[-3, 8], [3, 8]], [[3, 8], [3, -8]]], "outputs": [[11], [15], [5], [13], [17], [5.385], [1.4], [0], [6], [16]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,380 |
def coordinates(p1, p2, precision=0):
|
de05922a6ba3ff1eb03d093caf7d51a7 | UNKNOWN | You're about to go on a trip around the world! On this trip you're bringing your trusted backpack, that anything fits into. The bad news is that the airline has informed you, that your luggage cannot exceed a certain amount of weight.
To make sure you're bringing your most valuable items on this journey you've decided to give all your items a score that represents how valuable this item is to you. It's your job to pack you bag so that you get the most value out of the items that you decide to bring.
Your input will consist of two arrays, one for the scores and one for the weights. You input will always be valid lists of equal length, so you don't have to worry about verifying your input.
You'll also be given a maximum weight. This is the weight that your backpack cannot exceed.
For instance, given these inputs:
scores = [15, 10, 9, 5]
weights = [1, 5, 3, 4]
capacity = 8
The maximum score will be ``29``. This number comes from bringing items ``1, 3 and 4``.
Note: Your solution will have to be efficient as the running time of your algorithm will be put to a test. | ["def pack_bagpack(scores, weights, capacity):\n load = [0] * (capacity + 1)\n for score, weight in zip(scores, weights):\n load = [max(l, weight <= w and load[w - weight] + score)\n for w, l in enumerate(load)]\n return load[-1]", "def pack_bagpack(S,W,C) :\n M = [0] * (1 + C)\n for F,V in enumerate(S) :\n M = [max(U,M[T - W[F]] + V if W[F] <= T else 0) for T,U in enumerate(M)]\n return M[-1]", "def pack_bagpack(scores,weights,capacity):\n sols=[[0 for j in range(capacity+1)] for i in range(len(scores)+1)]\n scores.insert(0, 0)\n weights.insert(0, 0)\n for y,iy in enumerate(sols[1:],1):\n for x,ix in enumerate(iy[1:],1):\n if weights[y]<=x:\n sols[y][x]=max(scores[y]+sols[y-1][x-weights[y]],sols[y-1][x])\n else:\n sols[y][x]=sols[y-1][x]\n return sols[-1][-1]", "import numpy as np\n\ndef pack_bagpack(scores, weights, capacity):\n print(len(scores))\n print(capacity)\n n = len(scores)\n dp = np.zeros((n + 1, capacity + 1))\n for i in range(1, n + 1):\n for j in range(capacity + 1):\n if j >= weights[i - 1]:\n dp[i][j] = (max(dp[i - 1][j], dp[i - 1][j - weights[i - 1]] + scores[i - 1]))\n else:\n dp[i][j] = dp[i - 1][j]\n return dp[n][capacity]; ", "import sys\nprint((sys.getrecursionlimit()))\n\ndef pack_bagpack(scores, weights, capacity):\n\n if capacity >= sum(weights):\n return sum(scores)\n\n print(('{},{},{}'.format(scores, weights, capacity)))\n items = [(i, j) for i, j in zip(scores, weights)]\n def fetchBestMax(toConsider, avail, memo = {}):\n if (len(toConsider), avail) in memo:\n result = memo[(len(toConsider), avail)]\n elif toConsider == [] or avail == 0:\n result = (0, ())\n elif toConsider[0][1] > avail:\n #Explore right branch only\n result = fetchBestMax(toConsider[1:], avail, memo)\n else:\n nextItem = toConsider[0]\n #Explore left branch\n withVal, withToTake =fetchBestMax(toConsider[1:], avail - nextItem[1], memo)\n withVal += nextItem[0]\n #Explore right branch\n withoutVal, withoutToTake = fetchBestMax(toConsider[1:], avail, memo)\n #Choose better branch\n if withVal > withoutVal:\n result = (withVal, withToTake + (nextItem,))\n else:\n result = (withoutVal, withoutToTake)\n memo[(len(toConsider), avail)] = result\n return result\n \n \n maxVal = fetchBestMax(items, capacity)\n print((\"maxVal\", maxVal))\n return maxVal[0]\n", "def pack_bagpack(val, wt, W):\n n = len(val) \n K = [[0 for x in range(W+1)] for x in range(n+1)] \n for i in range(n+1): \n for w in range(W+1): \n if i==0 or w==0: \n K[i][w] = 0\n elif wt[i-1] <= w: \n K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w]) \n else: \n K[i][w] = K[i-1][w] \n \n return K[n][W]", "def pack_bagpack(val, w, t):\n if sum(w) <= t : return sum(val)\n matrix = [[0 for _ in range(t + 1)] for _ in range(len(w) + 1)]\n for i in range(1, len(w) + 1):\n for j in range(1, t + 1):\n matrix[i][j] = matrix[i-1][j] if j<w[i-1] else max(matrix[i-1][j],val[i-1]+matrix[i-1][j-w[i-1]])\n return matrix[-1][-1]", "import time\ndef pack_bagpack(scores, weights, capacity):\n \"\"\"\n \u6c42\u6700\u5927\u5206\u6570\n :param scores: \u884c\u674e\u8bc4\u5206\u5217\u8868\n :param weights: \u884c\u674e\u91cd\u91cf\u5217\u8868\n :param capacity: \u9650\u5236\u91cd\u91cf\n :return: \u6700\u5927\u5206\u6570\n\n 1\uff09\u6309\u7167\u5747\u5206\u5012\u5e8f\u6392\u5217\n 2) \u9012\u5f52\u7ec4\u5408\n max_value = max(\u5305\u542b\u81ea\u5df1\u7684\u6700\u5927\u503c, \u8df3\u8fc7\u81ea\u5df1\u7684\u6700\u5927\u503c)\n 3) \u5f97\u51fa\u6700\u4f18\n \"\"\"\n start_time = time.time()\n # \u7ec4\u5408\n lst = list(zip(scores, weights))\n\n # 1) \u5012\u5e8f\u6392\u5217 >> \u5747\u5206 = \u5206\u6570/\u91cd\u91cf\n lst_sorted = sorted(lst, key=lambda x: x[0], reverse=True)\n\n cache_dict = {}\n\n def calc_item(index, weight_last):\n if index >= len(lst_sorted) or weight_last <= 0:\n return 0\n current = lst_sorted[index]\n\n cache_key = \"{}-{}\".format(index, weight_last)\n\n if cache_key not in cache_dict:\n # \u7b97\u4e0a\u5f53\u524d\u7684\u6700\u5927\u7ed3\u679c\n score_with_current = 0\n if weight_last >= current[1]:\n score_with_current = current[0] + calc_item(index + 1, weight_last - current[1])\n # \u8df3\u8fc7\u5f53\u524d\u7684\u6700\u5927\u7ed3\u679c\n score_no_current = calc_item(index + 1, weight_last)\n\n # \u7f13\u5b58\u5f53\u524d\u7684\u4f4d\u7f6e\u548c\u5269\u4f59\u91cd\u91cf\u7684\u503c, \u8ba9\u4ee5\u540e\u7684\u9012\u5f52\u4e0d\u518d\u91cd\u590d\u8ba1\u7b97\u7c7b\u4f3c\u7ed3\u679c\n cache_dict[cache_key] = max(score_with_current, score_no_current)\n \n return cache_dict[cache_key]\n\n final_score = calc_item(0, capacity)\n\n print(\"score: {} duration---> {}\".format(final_score,time.time() - start_time))\n # 3) \u5f97\u51fa\u6700\u4f18\n return final_score", "def pack_bagpack(scores, weights, capacity):\n dp=[[0,[]] for i in range(capacity+1)]\n for j in range(1,len(scores)+1):\n r = [[0,[]] for i in range(capacity+1)]\n for i in range(1,capacity+1):\n c,p = weights[j-1], scores[j-1]\n if c<=i and p+dp[i-c][0]>dp[i][0]: r[i]=[p+dp[i-c][0], dp[i-c][1]+[j-1]]\n else: r[i]=dp[i]\n dp = r\n return dp[-1][0]", "def pack_bagpack(scores, weights, capacity):\n loads = [0] * (capacity + 1)\n for score, weight in zip(scores, weights):\n loads = [max(l, weight <= w and loads[w - weight] + score) for w, l in enumerate(loads)]\n return loads[-1]"] | {"fn_name": "pack_bagpack", "inputs": [[[15, 10, 9, 5], [1, 5, 3, 4], 8], [[20, 5, 10, 40, 15, 25], [1, 2, 3, 8, 7, 4], 10], [[19, 8, 6, 20, 3, 16], [8, 2, 3, 10, 1, 5], 17], [[100, 5, 16, 18, 50], [25, 1, 3, 2, 15], 14]], "outputs": [[29], [60], [46], [39]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,179 |
def pack_bagpack(scores, weights, capacity):
|
caaf6491dce47c7b1be5196e1c66a1f1 | UNKNOWN | You are provided with array of positive non-zero ints and int n representing n-th power (n >= 2).
For the given array, calculate the sum of each value to the n-th power. Then subtract the sum of the original array.
Example 1: Input: {1, 2, 3}, 3 --> (1 ^ 3 + 2 ^ 3 + 3 ^ 3 ) - (1 + 2 + 3) --> 36 - 6 --> Output: 30
Example 2: Input: {1, 2}, 5 --> (1 ^ 5 + 2 ^ 5) - (1 + 2) --> 33 - 3 --> Output: 30 | ["def modified_sum(lst, p):\n return sum(n**p - n for n in lst)", "def modified_sum(a, n):\n return sum(i ** n for i in a) - sum(a)", "def modified_sum(a, n):\n return sum([x ** n - x for x in a])", "def modified_sum(a, n):\n return sum(i**n - i for i in a)", "def modified_sum(a, n):\n ans = 0\n for sq in a:\n ans = ans + sq ** n\n \n \n return ans - sum(a)\n", "def modified_sum(a, n):\n L=[]\n for i in a:\n L.append(i**n)\n return sum(L)-sum(a)", "def modified_sum(a, n):\n sum = 0\n for x in a:\n sum += x**n - x\n return sum", "def modified_sum(a, n):\n s1=0\n s2=0\n for x in a:\n s1+=x**n\n s2+=x\n return s1-s2", "def modified_sum(a, n):\n return sum([i**n for i in a]) - sum(a)", "def modified_sum(a, n):\n return sum(x**n - x for x in a)"] | {"fn_name": "modified_sum", "inputs": [[[1, 2, 3], 3], [[1, 2], 5], [[3, 5, 7], 2], [[1, 2, 3, 4, 5], 3], [[2, 7, 13, 17], 2], [[2, 5, 8], 3], [[2, 4, 6, 8], 6], [[5, 10, 15], 4], [[3, 6, 9, 12], 3]], "outputs": [[30], [30], [68], [210], [472], [630], [312940], [61220], [2670]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 867 |
def modified_sum(a, n):
|
30836331d05a90d45af3355a6df2276e | UNKNOWN | You just took a contract with the Jedi council. They need you to write a function, `greet_jedi()`, which takes two arguments (a first name and a last name), works out the corresponding *Jedi name*, and returns a string greeting the Jedi.
A person's *Jedi name* is the first three letters of their last name followed by the first two letters of their first name. For example:
```python
>>> greet_jedi('Beyonce', 'Knowles')
'Greetings, master KnoBe'
```
Note the capitalization: the first letter of each name is capitalized. Your input may or may not be capitalized. Your function should handle it and return the Jedi name in the correct case no matter what case the input is in:
```python
>>> greet_jedi('grae', 'drake')
'Greetings, master DraGr'
```
You can trust that your input names will always be at least three characters long.
If you're stuck, check out the [python.org tutorial](https://docs.python.org/3/tutorial/introduction.html#strings) section on strings and search "slice". | ["def greet_jedi(first, last):\n return \"Greetings, master {}{}\".format(last[:3].capitalize(), first[:2].capitalize())", "def greet_jedi(f, l):\n return 'Greetings, master ' + l[0:3].capitalize() + f[0:2].capitalize()", "def greet_jedi(first, last):\n return 'Greetings, master %s%s' % (last[:3].capitalize(), first[:2].capitalize())", "def greet_jedi(*args):\n return 'Greetings, master {1}{0}'.format(*(s.title()[:2+i] for i,s in enumerate(args)))\n", "def greet_jedi(first, last):\n return 'Greetings, master {}'.format(last[:3].capitalize()+first[:2].capitalize())\n\n", "greet_jedi=lambda f, l: 'Greetings, master ' + l[:3].capitalize() + f[:2].capitalize()", "def greet_jedi(first, last):\n # Your code goes here.\n one = last[0:3].capitalize()\n two = first[0:2].capitalize()\n return (\"Greetings, master {}{}\".format(one, two))", "def greet_jedi(first, last):\n return(\"Greetings, master \" +last.capitalize()[:3] + first.capitalize()[:2])\n # Your code goes here.\n", "def greet_jedi(first, last):\n return 'Greetings, master {}{}'.format(last.capitalize()[:3],first.capitalize()[:2])\n", "def greet_jedi(first, last):\n return 'Greetings, master %s%s' % (last.capitalize()[:3] , first.capitalize()[:2])\n"] | {"fn_name": "greet_jedi", "inputs": [["Beyonce", "Knowles"], ["Chris", "Angelico"], ["grae", "drake"]], "outputs": [["Greetings, master KnoBe"], ["Greetings, master AngCh"], ["Greetings, master DraGr"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,259 |
def greet_jedi(first, last):
|
025a6227cf9e7521535bd381d2461008 | UNKNOWN | Consider the array `[3,6,9,12]`. If we generate all the combinations with repetition that sum to `12`, we get `5` combinations: `[12], [6,6], [3,9], [3,3,6], [3,3,3,3]`. The length of the sub-arrays (such as `[3,3,3,3]` should be less than or equal to the length of the initial array (`[3,6,9,12]`).
Given an array of positive integers and a number `n`, count all combinations with repetition of integers that sum to `n`. For example:
```Haskell
find([3,6,9,12],12) = 5.
```
More examples in the test cases.
Good luck!
If you like this Kata, please try:
[Array combinations](https://www.codewars.com/kata/59e66e48fc3c499ec5000103)
[Sum of prime-indexed elements](https://www.codewars.com/kata/59f38b033640ce9fc700015b) | ["from itertools import combinations_with_replacement\n\ndef find(arr,n):\n return sum( sum(c) == n for x in range(1,len(arr)+1) for c in combinations_with_replacement(arr, x) )", "def find(arr,n):\n return(findl(arr,n,len(arr)))\ndef findl(arr,n,l):\n return(sum(findl(arr[1:],n-arr[0]*i,l-i) for i in range(l+1)) if ((arr) and (l>0)) else ((n==0) and (l>=0)))", "import itertools\n\n\n#Given the lst of length N, we generate a list of all possible combinations with\n# replacements from choose-1 to choose-N\n#Then, for each of the combinations found, simply sum the contents and see if the sum\n#matches the target sum\n# Example [3, 6, 9, 12] with target sum 12. Here we need a total of 4; \n# choose (with replacement) 1 from 4, 2 from 4, 3 from 4, 4 from 4\n# Choose 1 [(3,), (6,), (9,), (12,)]\n# Choose 2 [(3, 3), (3, 6), (3, 9), (3, 12), (6, 6), (6, 9), (6, 12), (9, 9), (9, 12), (12, 12)]\n# [(3, 3, 3), (3, 3, 6), (3, 3, 9), (3, 3, 12), (3, 6, 6), (3, 6, 9), (3, 6, 12), (3, 9, 9),\n# (3, 9, 12), (3, 12, 12), (6, 6, 6), (6, 6, 9), (6, 6, 12), (6, 9, 9), (6, 9, 12), \n# (6, 12, 12), (9, 9, 9), (9, 9, 12), (9, 12, 12), (12, 12, 12)]\n# Choose 3 [(3, 3, 3, 3), (3, 3, 3, 6), (3, 3, 3, 9), (3, 3, 3, 12), (3, 3, 6, 6), (3, 3, 6, 9),\n# (3, 3, 6, 12), (3, 3, 9, 9), (3, 3, 9, 12), (3, 3, 12, 12), (3, 6, 6, 6), (3, 6, 6, 9),\n# (3, 6, 6, 12), (3, 6, 9, 9), (3, 6, 9, 12), (3, 6, 12, 12), (3, 9, 9, 9), (3, 9, 9, 12),\n# (3, 9,12, 12), (3, 12, 12, 12), (6, 6, 6, 6), (6, 6, 6, 9), (6, 6, 6, 12), (6, 6, 9, 9),\n# (6, 6, 9, 12), (6, 6, 12, 12), (6, 9, 9, 9), (6, 9, 9, 12), (6, 9, 12, 12), (6, 12, 12, 12),\n# (9, 9, 9, 9), (9, 9, 9, 12), (9, 9, 12, 12), (9, 12, 12, 12), (12, 12, 12, 12)] \n#Now for each we see which sum to the target of 12\ndef find(lst, targetSum):\n lenList = len(lst)\n \n\n combWithReplacementList= []\n for chooseR in range(1, lenList+1):\n combsChooseR = itertools.combinations_with_replacement(lst, chooseR)\n combWithReplacementList.append(list(combsChooseR))\n\n totalFound = 0\n for col in combWithReplacementList:\n for combChoice in col:\n if (sum(combChoice))== targetSum:\n totalFound +=1\n\n return totalFound\n#----end function\n", "from itertools import combinations_with_replacement as comb\n\n# Brute force!\ndef find(arr, n):\n return sum(sum(L) == n for i in range(1, len(arr)+1) for L in comb(arr, i))", "from itertools import combinations_with_replacement as comb\n\ndef find(lst, n):\n return sum(1 for l in range(1, len(lst)+1) for c in comb(lst, l) if sum(c) == n)", "from itertools import combinations_with_replacement as c\n\ndef find(arr, n):\n return sum(\n sum(xs) == n\n for i in range(len(arr))\n for xs in c(arr, i + 1)\n )", "from itertools import combinations_with_replacement as c\nfind=lambda a,k:sum(1for i in range(1,len(a)+1)for j in c(a,i)if sum(j)==k)", "from itertools import combinations_with_replacement as cr\nfind=lambda a,n:sum(n==sum(c)for r in range(1,len(a)+1)for c in cr(a,r=r))", "def N(Q,S,R,L,D,C,I) :\n if 0 < D and 0 < S :\n for I in range(I,L) :\n N(Q,S - Q[I],R,L,D - 1,C + (Q[I],),I)\n elif 0 == S :\n R.add(C)\n return R\n\ndef find (Q,S) :\n Q = sorted(set(Q))\n return len(N(Q,S,set(),len(Q),len(Q),(),0))", "from itertools import combinations_with_replacement as comb\ndef find(arr,n):\n return sum(sum(c)==n for i in range(1,len(arr)+1) for c in comb(arr,i))"] | {"fn_name": "find", "inputs": [[[1, 2, 3], 10], [[1, 2, 3], 7], [[1, 2, 3], 5], [[3, 6, 9, 12], 12], [[1, 4, 5, 8], 8], [[3, 6, 9, 12], 15], [[3, 6, 9, 12, 14, 18], 30]], "outputs": [[0], [2], [3], [5], [3], [5], [21]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,589 |
def find(arr,n):
|
4e483e70f78d9fd9eaeb40e1f9c70d9d | UNKNOWN | Given an array of ints, return the index such that the sum of the elements to the right of that index equals the sum of the elements to the left of that index. If there is no such index, return `-1`. If there is more than one such index, return the left-most index.
For example:
```
peak([1,2,3,5,3,2,1]) = 3, because the sum of the elements at indexes 0,1,2 == sum of elements at indexes 4,5,6. We don't sum index 3.
peak([1,12,3,3,6,3,1]) = 2
peak([10,20,30,40]) = -1
```
The special case of an array of zeros (for instance `[0,0,0,0]`) will not be tested.
More examples in the test cases.
Good luck!
Please also try [Simple time difference](https://www.codewars.com/kata/5b76a34ff71e5de9db0000f2) | ["def peak(arr):\n for i, val in enumerate(arr):\n if sum(arr[:i]) == sum(arr[i+1:]):\n return i\n return -1", "def peak(arr):\n for i in range(len(arr)):\n if sum(arr[:i]) == sum(arr[i+1:]): return i\n return -1", "def peak(arr):\n sl, sr = 0, sum(arr)\n for i,v in enumerate(arr):\n sr -= v\n if sr == sl: return i\n elif sl > sr: return -1\n sl += v\n", "def peak(arr):\n for i,n in enumerate(arr):\n if sum(arr[:i]) == sum(arr[i+1:]):\n return i\n else:\n return -1\n", "def peak(arr):\n r = sum(arr)-arr[0]\n l = 0\n if l == r: return 0\n for i in range(len(arr)-1):\n l += arr[i]\n r -= arr[i+1]\n if l == r: return i+1\n return -1", "def peak(arr):\n l=[i for i in range(len(arr)-1) if sum(arr[:i])==sum(arr[i+1:])]\n return l[0] if l else -1", "def peak(arr):\n return next(iter(i for i in range(len(arr)) if sum(arr[:i]) == sum(arr[i+1:])), -1)", "from itertools import accumulate\ndef peak(arr):\n for idx,(a,b) in enumerate(zip(list(accumulate(arr))[:-2],list(accumulate(reversed(arr)))[-3::-1])):\n if a == b: return idx+1\n \n return -1", "peak=lambda a:sum(sum(a[y+1:])==sum(a[:y])and y for y,_ in enumerate(a))or-1", "def peak(arr):\n left_sum, right_sum = [0], [0]\n for n in arr: left_sum.append(n + left_sum[-1])\n for n in arr[::-1]: right_sum.append(n + right_sum[-1])\n for i in range(len(arr)):\n if right_sum[len(arr)-1-i] == left_sum[i]: return i\n return -1"] | {"fn_name": "peak", "inputs": [[[1, 2, 3, 5, 3, 2, 1]], [[1, 12, 3, 3, 6, 3, 1]], [[10, 20, 30, 40]]], "outputs": [[3], [2], [-1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,567 |
def peak(arr):
|
e30497606a44fc33cebeed708a6c0bf3 | UNKNOWN | Gordon Ramsay shouts. He shouts and swears. There may be something wrong with him.
Anyway, you will be given a string of four words. Your job is to turn them in to Gordon language.
Rules:
Obviously the words should be Caps,
Every word should end with '!!!!',
Any letter 'a' or 'A' should become '@',
Any other vowel should become '*'. | ["def gordon(a):\n return '!!!! '.join(a.upper().split()).translate(str.maketrans('AEIOU', '@****'))+'!!!!'", "def gordon(s):\n return \" \".join(x + \"!!!!\" for x in s.upper().translate(str.maketrans(\"AIUEO\", \"@****\")).split())", "def gordon(a):\n return \" \".join(word.upper().translate(str.maketrans(\"AEIOU\", \"@****\")) \n + \"!!!!\" for word in a.split())", "from functools import partial\nfrom re import compile\n\nREGEX1 = partial(compile(r\"(?<=\\w)(?=\\b)\").sub, \"!!!!\")\ntrans = str.maketrans(\"AEIOU\", \"@****\")\n\ndef gordon(a):\n return REGEX1(a.upper()).translate(trans)", "def gordon(a):\n return '!!!! '.join(a.upper().translate(str.maketrans('AEIOU', '@****')).split()) + '!!!!'", "def gordon(s):\n fmt = '{}!!!!'.format\n table = str.maketrans('AEIOU', '@****')\n return ' '.join(fmt(a.translate(table)) for a in s.upper().split())\n", "def gordon(a):\n a=a.upper()\n a=a.replace(' ', '!!!! ')\n a=a.replace('A', '@')\n vowels = ['E', 'I', 'O', 'U']\n for each in vowels:\n a=a.replace(each, '*')\n return a + '!!!!'", "def gordon(a):\n out, vowels = '', 'eiou'\n for i in a:\n if i == 'a':\n out += '@'\n elif i in vowels:\n out += '*'\n elif i == ' ':\n out += '!!!! '\n else:\n out += str(i).upper()\n return '%s!!!!' % out", "import re\n\ndef gordon(a):\n return re.sub(\"[EIOU]\", \"*\", \" \".join(word.upper() + \"!!!!\" for word in a.split()).replace(\"A\", \"@\"))"] | {"fn_name": "gordon", "inputs": [["What feck damn cake"], ["are you stu pid"], ["i am a chef"], ["dont you talk tome"], ["how dare you feck"]], "outputs": [["WH@T!!!! F*CK!!!! D@MN!!!! C@K*!!!!"], ["@R*!!!! Y**!!!! ST*!!!! P*D!!!!"], ["*!!!! @M!!!! @!!!! CH*F!!!!"], ["D*NT!!!! Y**!!!! T@LK!!!! T*M*!!!!"], ["H*W!!!! D@R*!!!! Y**!!!! F*CK!!!!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,559 |
def gordon(a):
|
20a2dbcad61019ba0bf75b638988be3c | UNKNOWN | Oh no! Ghosts have reportedly swarmed the city. It's your job to get rid of them and save the day!
In this kata, strings represent buildings while whitespaces within those strings represent ghosts.
So what are you waiting for? Return the building(string) without any ghosts(whitespaces)!
Example:
Should return:
If the building contains no ghosts, return the string: | ["ghostbusters = lambda s: (s.replace(' ','') if (' ' in s) else\n \"You just wanted my autograph didn't you?\")", "import re\ndef ghostbusters(building):\n bs = re.subn('\\s','',building)\n return bs[0] if bs[1] else \"You just wanted my autograph didn't you?\"", "message = \"You just wanted my autograph didn't you?\"\n\ndef ghostbusters(building):\n return building.replace(' ', '') if ' ' in building else message", "#A spooky string is one with whitespace. We must de-spook the given string\ndef ghostbusters(spookyStr):\n\n charToRemove = ' ' #whitespace\n deSpookedStr = spookyStr.replace(charToRemove, \"\") \n\n if deSpookedStr == spookyStr:\n deSpookedStr =\"You just wanted my autograph didn't you?\" \n\n return deSpookedStr\n#---end fun\n", "def ghostbusters(building):\n return \"You just wanted my autograph didn't you?\" if building.isalnum() else building.replace(' ', '')", "def ghostbusters(building):\n if ' ' in building:\n return building.replace(' ', '')\n return \"You just wanted my autograph didn't you?\"", "from re import match, sub\ndef ghostbusters(building): return sub(r'\\s', '', building) if match(r'.*\\s.*', building) else \"You just wanted my autograph didn't you?\"", "def ghostbusters(building):\n return building.replace(' ', '') if ' ' in building else \"You just wanted my autograph didn't you?\"", "def ghostbusters(building):\n stripped = building.replace(' ', '')\n return stripped if building != stripped else \"You just wanted my autograph didn't you?\""] | {"fn_name": "ghostbusters", "inputs": [["Factor y"], ["O f fi ce"], ["BusStation"], ["Suite "], ["YourHouse"], ["H o t e l "]], "outputs": [["Factory"], ["Office"], ["You just wanted my autograph didn't you?"], ["Suite"], ["You just wanted my autograph didn't you?"], ["Hotel"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,594 |
def ghostbusters(building):
|
75a3b0e21b32ccde009036b2db424198 | UNKNOWN | >When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
Given a string `str` that contains some "(" or ")". Your task is to find the longest substring in `str`(all brackets in the substring are closed). The result is the length of the longest substring.
For example:
```
str = "()()("
findLongest(str) === 4
"()()" is the longest substring
```
# Note:
- All inputs are valid.
- If no such substring found, return 0.
- Please pay attention to the performance of code. ;-)
- In the performance test(100000 brackets str x 100 testcases), the time consuming of each test case should be within 35ms. This means, your code should run as fast as a rocket ;-)
# Some Examples
```
findLongest("()") === 2
findLongest("()(") === 2
findLongest("()()") === 4
findLongest("()()(") === 4
findLongest("(()())") === 6
findLongest("(()(())") === 6
findLongest("())(()))") === 4
findLongest("))((") === 0
findLongest("") === 0
``` | ["def find_longest(st):\n res,pos=0,[0]\n for i,b in enumerate(st,1):\n if b==\"(\": pos.append(i)\n else: \n try:pos.pop();res=max(res,i-pos[-1])\n except:pos.append(i)\n return res", "def find_longest(s):\n stack, m = [-1], 0\n for i,j in enumerate(s):\n if j == '(' : stack.append(i)\n else:\n stack.pop()\n if stack : m = max(m,i-stack[-1])\n else : stack.append(i)\n return m", "def find_longest(st):\n longest_run, stack = 0, [-1]\n for i, c in enumerate(st):\n if c == \")\":\n stack.pop()\n if stack:\n longest_run = max(longest_run, i-stack[-1])\n continue\n stack.append(i)\n return longest_run", "def find_longest(st):\n stack=[-1]\n longest=0\n for index in range(0,len(st)):\n if(st[index]==\"(\"):\n stack.append(index)\n else:\n stack.pop()\n if stack:\n longest=max(longest,index-stack[-1])\n else:\n stack.append(index)\n print(longest)\n return longest", "def find_longest(st):\n n = len(st)\n lis = [] \n lis.append(-1) \n result = 0\n for i in range(n):\n if st[i] == '(':\n lis.append(i)\n else: # If closing bracket, i.e., str[i] = ')'\n lis.pop() \n if len(lis) != 0: \n result = max(result, i - lis[len(lis)-1]) \n else: \n lis.append(i)\n return result ", "def find_longest(st):\n stack, max_so_far = [-1], 0\n for ind in range(len(st)):\n if st[ind] == '(':\n stack.append(ind)\n else:\n stack.pop()\n if len(stack) > 0:\n max_so_far = max(max_so_far, ind - stack[-1])\n else:\n stack.append(ind)\n return max_so_far", "\ndef find_longest(string): \n n = len(string) \n \n # Create a stack and push -1 as initial index to it. \n stk = [] \n stk.append(-1) \n \n # Initialize result \n result = 0\n \n # Traverse all characters of given string \n for i in range(n): \n \n # If opening bracket, push index of it \n if string[i] == '(': \n stk.append(i) \n \n else: # If closing bracket, i.e., str[i] = ')' \n \n # Pop the previous opening bracket's index \n stk.pop() \n \n # Check if this length formed with base of \n # current valid substring is more than max \n # so far \n if len(stk) != 0: \n result = max(result, i - stk[len(stk)-1]) \n \n # If stack is empty. push current index as \n # base for next valid substring (if any) \n else: \n stk.append(i) \n \n return result ", "from itertools import groupby\n\ndef find_longest(st):\n positions = []\n stack = []\n for i, ch in enumerate(st):\n if ch == '(':\n stack.append(i)\n elif stack:\n positions.append(stack.pop())\n positions.append(i)\n positions.sort()\n\n res = [a+1 == b for a, b in zip(positions, positions[1:])]\n ans = [len(list(g))+1 for k, g in groupby(res)]\n return max(ans, default=0)", "def find_longest(st):\n positions = []\n stack = []\n for i, ch in enumerate(st):\n if ch == '(':\n stack.append(i)\n elif stack:\n positions.append(stack.pop())\n positions.append(i)\n\n prev = float('-inf')\n cons = 0\n ans = 0\n for i, p in enumerate(sorted(positions)):\n if p == prev + 1:\n cons += 1\n else:\n ans = max(ans, cons)\n cons = 1\n prev = p\n return max(ans, cons)", "import re\n\ndef find_longest(s):\n regex = r\"\\(a*\\)\"\n while re.search(regex, s): s = re.sub(regex, lambda x: \"a\" * len(x.group()), s)\n r = map(len, re.findall(r\"a+\", s))\n return max(r, default = 0)"] | {"fn_name": "find_longest", "inputs": [[")()("], ["((()"], ["((("], ["())((("], ["())()))))()()("]], "outputs": [[2], [2], [0], [2], [4]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,093 |
def find_longest(st):
|
12c61c2a9a98ebb766ddbac90d4c6316 | UNKNOWN | In this Kata, you will remove the left-most duplicates from a list of integers and return the result.
```python
# Remove the 3's at indices 0 and 3
# followed by removing a 4 at index 1
solve([3, 4, 4, 3, 6, 3]) # => [4, 6, 3]
```
More examples can be found in the test cases.
Good luck! | ["def solve(arr): \n re = []\n for i in arr[::-1]:\n if i not in re:\n re.append(i)\n return re[::-1]", "def solve(arr): \n return list(dict.fromkeys(arr[::-1]))[::-1]", "def solve(arr): \n return [a for i,a in enumerate(arr) if a not in arr[i+1:]]", "def solve(arr): \n list = arr.copy()\n for i in arr:\n if list.count(i) > 1:\n list.remove(i)\n return list", "def solve(arr): \n seen = set()\n return [seen.add(a) or a for a in reversed(arr) if a not in seen][::-1]", "def solve(l): \n return sorted(set(l), key=l[::-1].index)[::-1]", "# Python >= 3.6.0 only\nsolve=lambda a: list(dict.fromkeys(reversed(a)))[::-1]", "def solve(arr): \n return sorted(set(arr), key=lambda n: -arr[::-1].index(n))\n", "def solve(arr): \n return sorted(set(arr), key=arr[::-1].index)[::-1]", "def solve(arr): \n return list(reversed(list(dict.fromkeys(reversed(arr)))))", "def solve(arr): \n d = {x: i for i, x in enumerate(arr)}\n return sorted(d, key=d.get)", "solve=lambda arr:[e for i,e in enumerate(arr) if e not in arr[i+1:]]", "def solve(arr): \n final = []\n for number in arr[::-1]:\n if number not in final:\n final.insert(0,number)\n return final", "def solve(arr): \n return [arr[el] for el in range(len(arr)) if arr[el] not in arr[el+1:]]", "from collections import OrderedDict\nfrom typing import List\n\n\ndef solve(arr: List[int]) -> List[int]:\n d = OrderedDict()\n for a in arr:\n try:\n d.move_to_end(a)\n except KeyError:\n d[a] = None\n\n return list(d.keys())\n", "def solve(array):\n return [*dict.fromkeys(array[::-1]).keys()][::-1]", "def solve(a): \n return list(dict.fromkeys(a[::-1]))[::-1]", "def solve(lst): \n return sorted(set(lst), key=lst[::-1].index)[::-1]", "def solve(arr): \n uniques = set(arr)\n output = []\n for n in arr[::-1]:\n if n in uniques:\n output = [n] + output\n uniques.remove(n)\n if len(uniques) == 0:\n return output\n return output", "def solve(arr): \n unique = set (arr)\n for num in unique:\n for i in range (arr.count(num) - 1):\n arr.pop((arr.index(num)))\n return arr", "def solve(arr): return [v for i, v in enumerate(arr) if v not in arr[i + 1:]]\n", "def solve(l): \n for i in range(3):\n [l.pop(l.index(i)) for i in l if l.count(i) > 1]\n return l", "def solve(arr):\n for i in range(max(arr)+1):\n while arr.count(i)>1:\n arr.remove(i)\n return arr", "def solve(arr):\n arr2=[]\n for i in range(0,len(arr)):\n f=0\n for j in range(i+1,len(arr)):\n if arr[j] == arr[i]:\n f=f+1\n if f == 0:\n arr2.append(arr[i])\n return arr2", "def solve(seq):\n set1 = set()\n seq = list(reversed(seq))\n set_add = set1.add\n return list(reversed([x for x in seq if not (x in set1 or set_add(x))]))", "def solve(arr):\n n = len(arr)\n ans = []\n while n > 0:\n if arr[n-1] not in ans:\n ans.append(arr[n-1])\n n -= 1\n return ans[::-1]", "def solve(arr):\n # Copy the list\n new_arr = arr.copy()\n # iterate by every item in old list\n for digit in arr:\n # get list of indexes of duplicates in new list by enumarating them\n index_list_of_duplicates = [i for i, x in enumerate(new_arr) if x == digit]\n # if count of the duplicates list is higher than 1 it deletes the first duplicate by index value from the new list\n if len(index_list_of_duplicates) > 1:\n del new_arr[index_list_of_duplicates[0]]\n return new_arr", "def solve(arr): \n newArr = []\n for i in range(len(arr) - 1, -1, -1):\n if arr[i] not in newArr: newArr.append(arr[i])\n newArr.reverse()\n return newArr", "solve=lambda a:[j for i,j in enumerate(a) if a[i:].count(j)==1]", "def solve(arr):\n num = 0\n while num < len(arr):\n counter = 0\n for elem in arr:\n if arr[num] == elem:\n counter += 1\n if counter > 1:\n del arr[num]\n else:\n num += 1\n return(arr)\n", "def solve(arr): \n arr2=[]\n for i in range(len(arr)):\n if arr[i] not in arr[i+1:]:\n arr2.append(arr[i])\n return arr2\n", "def solve(arr):\n l = []\n for num in arr[::-1]:\n if num not in l:\n l.append(num)\n return l[::-1]\n\n \n", "def solve(arr):\n array = []\n for item in arr:\n if item in array:\n array.remove(item)\n array.append(item)\n else:\n array.append(item)\n return array", "def solve(l, i=[]):\n for x in l[::-1]:\n i=[x]*(x not in i)+i\n return i", "def solve(A,Z=[]):\n for x in A[::-1]:Z=[x]*(x not in Z)+Z\n return Z", "def solve(arr):\n d = {}\n for i, e in enumerate(arr): d[e] = i\n return sorted(set(arr), key=d.get)\n", "def solve(arr): \n ans = []\n arr.reverse()\n for i in arr:\n if i not in ans:\n ans.append(i)\n return ans[::-1]", "def solve(arr): \n removed = []\n for i in arr[::-1]:\n if not i in removed:\n removed.append(i)\n return removed[::-1]", "def solve(arr):\n n = len(arr)\n res = []\n for i in range(n-1,-1,-1):\n if(arr[i] not in res):\n res.append(arr[i])\n res.reverse()\n return res", "def solve(arr):\n solution = []\n for i in reversed(arr):\n if i not in solution:\n solution.append(i)\n solution.reverse()\n return solution", "def solve(arr): \n res = []\n [res.append(c) for c in arr[::-1] if c not in res]\n return res[::-1]", "def solve(arr):\n x = list(set(arr))\n res = []\n indices = []\n result = []\n for num in x:\n index = last_index(arr,num)\n indices.append(index)\n res.append(arr[index])\n while len(indices) > 0:\n loc = indices.index(min(indices)) \n result.append(res[loc])\n indices.pop(loc)\n res.pop(loc)\n return result\n\ndef last_index(pepe,n):\n return [i for i in range(len(pepe)) if pepe[i] == n][::-1][0]", "def solve(arr): \n output = []\n \n for x in arr[::-1]:\n if x in output:\n continue\n else:\n output.append(x)\n return output[::-1]", "def solve(arr):\n res = []\n for number in arr[::-1]:\n if number not in res:\n res.append(number)\n else:\n pass\n return res[::-1]\n \n \n", "def solve(arr): \n r = []\n for i in reversed(arr):\n if i not in r:\n r.append(i)\n \n return r[::-1]", "def solve(arr):\n arr1 = arr[::-1]\n arr2 = list(dict.fromkeys(arr1))\n return arr2[::-1]\n", "from collections import Counter\ndef solve(arr): \n result = []\n dic = Counter(arr)\n for value in arr:\n if dic[value]==1:\n result.append(value)\n else:\n dic[value]-=1\n return result\n \n", "def solve(arr): \n tmp = {}\n s = set(arr)\n for n in s:\n tmp[n] = [idx for idx, num in enumerate(arr) if num == n]\n ides = [value[-1] for value in tmp.values()]\n return [arr[idx] for idx in sorted(ides)]", "def solve(arr): \n \n# =============================================================================\n# This function removes the left-most duplicates from a list of integers and\n# returns the result.\n# \n# Example:\n# Remove the 3's at indices 0 and 3\n# followed by removing a 4 at index 1\n# solve([3, 4, 4, 3, 6, 3]) # => [4, 6, 3]\n# =============================================================================\n \n print(arr) \n \n arr.reverse()\n \n print (arr)\n \n result = []\n \n for item in arr:\n if item not in result:\n result.append(item)\n \n result.reverse()\n \n return result", "def solve(arr): \n return [i for index, i in enumerate(arr) if i not in arr[index+1:]]", "def solve(arr): \n arr2 = []\n for i in arr[::-1]:\n if i not in arr2:\n arr2.insert(0, i)\n return arr2", "def solve(arr): \n output = []\n for i in arr[::-1]:\n if i not in output:\n output.append(i)\n else:\n pass\n return output[::-1]", "def solve(arr):\n for j in reversed(arr):\n if arr.count(j)!=1:\n arr.remove(j)\n return arr", "def solve(arr): \n unique = []\n arr.reverse()\n for n in arr:\n if n not in unique:\n unique.insert(0, n)\n \n return unique", "def solve(arr): \n ll = []\n for i in reversed(arr):\n if i not in ll:\n ll.insert(0, i)\n \n return ll", "def solve(arr): \n ls = []\n for x in arr[::-1]:\n if ls.count(x) == 0:\n ls.append(x)\n return [i for i in ls[::-1]]", "def solve(arr):\n res = []\n for i, v in enumerate(arr):\n if arr[i:].count(v) == 1:\n res.append(v)\n\n return res", "def solve(arr):\n ds = []\n for x in arr:\n if arr.count(x) > 1:\n if x not in ds:\n ds.append(x)\n else:\n pass\n else:\n pass\n for x in ds:\n while arr.count(x) > 1:\n arr.remove(x)\n \n return arr", "def solve(arr): \n # functional style\n def unique_recursive(src_arr, trg_arr):\n if len(src_arr) == 0:\n return trg_arr\n else:\n head, *tail = src_arr\n if head not in tail:\n trg_arr.append(head)\n return unique_recursive(tail, trg_arr)\n return unique_recursive(arr, [])", "def solve(arr): \n a = []\n for i in arr[::-1]:\n if (i in a)== False: a.append(i)\n return a[::-1]", "def solve(arr): \n result=[]\n for i in range(len(arr)):\n if arr[i] in arr[i+1:len(arr)]:\n pass\n else:\n result.append(arr[i])\n return result", "def solve(arr):\n res = []\n for v in arr[-1::-1]:\n if v not in res:\n res.append(v)\n return res[-1::-1]", "def solve(arr):\n x = []\n for element in arr:\n if element in x:\n x.remove(element)\n x.append(element)\n else:\n x.append(element)\n return x", "def solve(arr):\n remove = list(set(arr))\n print(remove)\n duplicates = []\n print(arr)\n for k in range(len(remove)):\n print(\"K>\", remove[k])\n for i, j in enumerate(arr):\n if j == remove[k]:\n duplicates.append(i)\n print(duplicates)\n if len(duplicates) > 1:\n for n in range(0, len(duplicates) - 1):\n arr[duplicates[n]] = \"\"\n duplicates = []\n else:\n duplicates = []\n while '' in arr:\n arr.remove('')\n print(arr)\n return arr", "def solve(arr): \n arr = arr[::-1]\n a = []\n for i in arr:\n if i not in a:\n a.append(i)\n return a[::-1]", "def solve(arr): \n ans = []\n for i in arr[::-1]:\n if i not in ans:\n ans = [i]+ans\n return ans", "def solve(arr): \n result = []\n for number in arr[::-1]:\n if number not in result:\n result.append(number)\n return result[::-1]\n", "def solve(arr):\n seen = []\n for i in arr:\n if i in seen:\n seen.remove(i)\n seen.append(i) \n return seen", "def solve(arr):\n seen = []\n for i in arr:\n if i in seen:\n seen.remove(i)\n seen.append(i)\n else:\n seen.append(i)\n \n return seen", "def solve(arr): \n result = []\n for i in range(len(arr)):\n try:\n if arr[i] not in arr[i+1:]:\n result.append(arr[i])\n except IndexError:\n pass\n return result\n", "from collections import Counter\ndef solve(arr): \n return [k for k in Counter(arr[::-1]).keys()][::-1]", "def solve(a):\n return list(dict.fromkeys(a[::-1]).keys())[::-1]\n", "def solve(a):\n n = len(a)\n for i in range(n-1):\n if a[i] in a[i+1:]: a[i] = 'a'\n while True:\n if 'a' in a: a.remove('a')\n else : break\n return a\n", "def solve(arr): \n seen = []\n return_list = []\n for number in arr[::-1]:\n if number not in seen:\n seen.append(number)\n return_list.append(number)\n return return_list[::-1]\n", "def solve(arr): \n res = []\n \n for i in range(len(arr)):\n if arr[i] not in arr[i+1:]:\n res.append(arr[i])\n \n return res", "def solve(arr): \n out=[]\n for x in arr[::-1]:\n if x in out : continue\n out.append(x)\n return out[::-1]", "def solve(arr): \n nums = set()\n out = []\n for elem in reversed(arr):\n if elem not in nums:\n out.append(elem)\n nums.add(elem)\n\n return out[::-1]", "def solve(arr): \n temp=arr[::-1]\n out=[]\n for i in range(len(temp)):\n if temp[i] in out:\n continue\n out.append(temp[i])\n return out[::-1]", "def solve(arr): \n s = []\n for k,v in enumerate(arr):\n if arr[k+1:].count(v) == 0:\n s.append(arr[k])\n return s ", "def solve(arr):\n k = 0\n while k < len(arr):\n while arr.count(arr[k]) > 1:\n del arr[k]\n k = 0\n else:\n k += 1\n\n return arr", "def solve(arr):\n i, k = 0, len(arr) \n while k > i:\n if arr.count(arr[i]) != 1:\n del arr[i]\n k -= 1\n else:\n i += 1\n return arr", "def solve(arr): \n b = reversed(arr)\n new =[]\n for i in b:\n if i not in new:\n new.append(i)\n arr=new\n arr.reverse()\n return arr\n pass", "def solve(arr): \n\n holder, result = {}, []\n index = len(arr) - 1\n while (0 <= index):\n if (arr[index] not in holder):\n result.insert(0,\n arr[index])\n holder[arr[index]] = True\n index -= 1\n\n return result\n", "def solve(arr):\n t = []\n for i in range(len(arr)):\n if arr[i:].count(arr[i]) == 1:\n t.append(arr[i])\n return t", "def solve(arr):\n res = []\n for i in arr[::-1]:\n if i in res:\n continue\n else:\n res.insert(0, i)\n return res", "def solve(arr): \n for i in range(len(arr)):\n while i+1 < len(arr)+1 and arr[i] in arr[i+1:]:\n arr.pop(i)\n return arr", "def solve(arr):\n temp = {}\n answer = []\n for single in set(arr):\n temp[single] = [i for i, x in enumerate(arr) if x == single][-1]\n for k, v in sorted(temp.items(), key=lambda x: x[1]):\n answer.append(k)\n return answer", "def solve(arr): \n return sorted(set(arr[::-1]), key =arr[::-1].index)[::-1]", "def solve(arr): \n return [j for i, j in enumerate(arr) if j not in arr[i+1:]]", "def solve(arr): \n arrr=arr[::-1]\n ans =[]\n for i in arrr:\n if i not in ans:\n ans.append(i)\n return ans[::-1]\n \n", "def solve(arr):\n answer=[]\n while len(arr)>0:\n poppedElement= arr.pop()\n if poppedElement not in answer:\n answer.append(poppedElement) \n answer.reverse()\n return answer\nprint((solve([3,4,4,3,6,3]))) \n", "from collections import OrderedDict\n\ndef solve (arr):\n arr = arr[::-1]\n arr = list(OrderedDict.fromkeys(arr))[::-1]\n return arr", "from collections import OrderedDict\n\narr = [3, 4, 4, 3, 6, 3]\ndef solve (arr):\n arr = arr[::-1]\n arr = list(OrderedDict.fromkeys(arr))[::-1]\n return arr", "def solve(arr):\n for i in list(arr):\n if arr.count(i)>1:\n arr.remove(i)\n return list(arr)\n", "def solve(arr): \n result = []\n arr = arr[::-1]\n for i in arr:\n if i not in result:\n result.append(i)\n return result[::-1]", "def solve(arr): \n returnedarray = []\n for item in arr[::-1]:\n if item not in returnedarray:\n returnedarray.insert(0, item)\n return returnedarray", "def solve(arr): \n i=0\n while i < len(arr):\n if arr.count(arr[i]) > 1:\n arr.remove(arr[i])\n i = 0\n else:\n i+=1\n return arr", "def solve(arr):\n a2 = []\n for e, a in enumerate(arr):\n if a not in arr[e+1:]:\n a2.append(a)\n return a2", "def solve(arr): \n ret = []\n for el in list(reversed(arr)):\n if el not in ret:\n ret.append(el)\n return list(reversed(ret))\n \n", "def solve(arr):\n dist = {}\n l = len(arr)\n for i in range(l):\n if arr[l-i-1] in dist.keys():\n arr.pop(l-i-1)\n else:\n dist[arr[l-i-1]] = 1\n return arr"] | {"fn_name": "solve", "inputs": [[[3, 4, 4, 3, 6, 3]], [[1, 2, 1, 2, 1, 2, 3]], [[1, 2, 3, 4]], [[1, 1, 4, 5, 1, 2, 1]]], "outputs": [[[4, 6, 3]], [[1, 2, 3]], [[1, 2, 3, 4]], [[4, 5, 2, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 17,261 |
def solve(arr):
|
1aa7a04b651cfe69c78f41067bddd95e | UNKNOWN | ### Happy Holidays fellow Code Warriors!
Now, Dasher! Now, Dancer! Now, Prancer, and Vixen! On, Comet! On, Cupid! On, Donder and Blitzen! That's the order Santa wanted his reindeer...right? What do you mean he wants them in order by their last names!? Looks like we need your help Code Warrior!
### Sort Santa's Reindeer
Write a function that accepts a sequence of Reindeer names, and returns a sequence with the Reindeer names sorted by their last names.
### Notes:
* It's guaranteed that each string is composed of two words
* In case of two identical last names, keep the original order
### Examples
For this input:
```
[
"Dasher Tonoyan",
"Dancer Moore",
"Prancer Chua",
"Vixen Hall",
"Comet Karavani",
"Cupid Foroutan",
"Donder Jonker",
"Blitzen Claus"
]
```
You should return this output:
```
[
"Prancer Chua",
"Blitzen Claus",
"Cupid Foroutan",
"Vixen Hall",
"Donder Jonker",
"Comet Karavani",
"Dancer Moore",
"Dasher Tonoyan",
]
``` | ["def sort_reindeer(reindeer_names):\n return sorted(reindeer_names, key=lambda s:s.split()[1])", "def sort_reindeer(names):\n return sorted(names, key=lambda x: x.split()[1])", "def lastname(name):\n name = name.split()\n return name[1]\n \ndef sort_reindeer(reindeer_names):\n reindeer_names.sort(key=lastname)\n return reindeer_names\n", "def sort_reindeer(reindeer_names):\n return sorted(reindeer_names, key=lambda el: el.split(' ')[-1])", "sort_reindeer=lambda rn: sorted(rn, key=lambda x: x.split()[1])", "def sort_reindeer(reindeer_names):\n reindeer_names.sort(key = lambda x: x.split()[-1])\n return reindeer_names", "def sort_reindeer(reindeer_names):\n with_last_names = []\n for i in reindeer_names:\n with_last_names.append((i, i.split(' ')[1]))\n return [i[0] for i in sorted(with_last_names, key = lambda x: x[1])]", "def sort_reindeer(reindeer_names):\n return sorted(reindeer_names, key=lambda n: n.split()[-1])", "def sort_reindeer(reindeer_names):\n return sorted(reindeer_names, key = lambda x: x.split(' ')[1])", "def sort_reindeer(reindeer_names):\n sorted_list = []\n for name in reindeer_names:\n sorted_list.append(name.split())\n sorted_list = sorted(sorted_list, key=lambda names: names[1])\n\n result = []\n\n for item in sorted_list:\n name = item[0] + ' ' + item[1]\n result.append(name)\n \n return result"] | {"fn_name": "sort_reindeer", "inputs": [[["Kenjiro Mori", "Susumu Tokugawa", "Juzo Okita", "Akira Sanada"]], [[]], [["Yasuo Kodai", "Kenjiro Sado", "Daisuke Aihara", "Susumu Shima", "Akira Sanada", "Yoshikazu Okita", "Shiro Yabu", "Sukeharu Nanbu", "Sakezo Yamamoto", "Hikozaemon Ohta", "Juzo Mori", "Saburo Tokugawa"]], [["Daisuke Mori", "Shiro Sanada", "Saburo Shima", "Juzo Yabu", "Sukeharu Yamamoto"]], [["Sukeharu Yamamoto", "Juzo Yabu", "Saburo Shima", "Shiro Sanada", "Daisuke Mori"]], [["Kenjiro Mori", "Susumu Mori", "Akira Mori"]]], "outputs": [[["Kenjiro Mori", "Juzo Okita", "Akira Sanada", "Susumu Tokugawa"]], [[]], [["Daisuke Aihara", "Yasuo Kodai", "Juzo Mori", "Sukeharu Nanbu", "Hikozaemon Ohta", "Yoshikazu Okita", "Kenjiro Sado", "Akira Sanada", "Susumu Shima", "Saburo Tokugawa", "Shiro Yabu", "Sakezo Yamamoto"]], [["Daisuke Mori", "Shiro Sanada", "Saburo Shima", "Juzo Yabu", "Sukeharu Yamamoto"]], [["Daisuke Mori", "Shiro Sanada", "Saburo Shima", "Juzo Yabu", "Sukeharu Yamamoto"]], [["Kenjiro Mori", "Susumu Mori", "Akira Mori"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,433 |
def sort_reindeer(reindeer_names):
|
516a6aaa9c354f63c49e7d1851732dae | UNKNOWN | The number 81 has a special property, a certain power of the sum of its digits is equal to 81 (nine squared). Eighty one (81), is the first number in having this property (not considering numbers of one digit).
The next one, is 512.
Let's see both cases with the details
8 + 1 = 9 and 9^(2) = 81
512 = 5 + 1 + 2 = 8 and 8^(3) = 512
We need to make a function, ```power_sumDigTerm()```, that receives a number ```n``` and may output the ```n-th term``` of this sequence of numbers.
The cases we presented above means that
power_sumDigTerm(1) == 81
power_sumDigTerm(2) == 512
Happy coding! | ["series = [0]\nfor a in range(2, 99):\n for b in range(2, 42):\n c = a**b\n if a == sum(map(int, str(c))):\n series.append(c)\npower_sumDigTerm = sorted(series).__getitem__", "def dig_sum(n):\n return sum(map(int, str(n)))\n\n\n\nterms = []\nfor b in range(2, 400):\n for p in range(2, 50):\n if dig_sum(b ** p) == b:\n terms.append(b ** p)\nterms.sort()\n\ndef power_sumDigTerm(n):\n return terms[n - 1]", "power_sumDigTerm = [None, 81, 512, 2401, 4913, 5832, 17576, 19683, 234256,\n 390625, 614656, 1679616, 17210368, 34012224, 52521875, 60466176, 205962976,\n 612220032, 8303765625, 10460353203, 24794911296, 27512614111, 52523350144,\n 68719476736, 271818611107, 1174711139837, 2207984167552, 6722988818432,\n 20047612231936, 72301961339136, 248155780267521, 3904305912313344,\n 45848500718449031, 81920000000000000, 150094635296999121, 13744803133596058624,\n 19687440434072265625, 53861511409489970176, 73742412689492826049,\n 179084769654285362176, 480682838924478847449].__getitem__", "def power_sumDigTerm(n):\n return sorted([i**j for j in range(2,50) for i in range(2,100) if i == sum([int(i) for i in str(i**j) ])])[n-1]", "def power_sumDigTerm(n):\n return [81, 512, 2401, 4913, 5832, 17576, 19683, \"I'm never tested :(\", 390625, 614656, 1679616, 17210368, 34012224, 52521875, 60466176, 205962976, 612220032, 8303765625, 10460353203, 24794911296, 27512614111, 52523350144, 68719476736, 271818611107, 1174711139837, 2207984167552, 6722988818432, 20047612231936, 72301961339136, 248155780267521, 3904305912313344, 45848500718449031, 81920000000000000, 150094635296999121, 13744803133596058624, 19687440434072265625, 53861511409489970176, 73742412689492826049, 39, 40][n-1]", "def power_sumDigTerm(n):\n \n def sum_d(x):\n L = [int(d) for d in str(x)]\n res = 0\n for l in L:\n res = res + l\n return res\n\n r_list = []\n \n for j in range(2, 50):\n for i in range(7, 100): \n a = i**j\n if sum_d(a) == i: \n r_list.append(a)\n r_list = list(set(r_list))\n \n r_list = sorted((r_list))\n return r_list[n-1]\n \n", "def power_sumDigTerm(n):\n my_list=[]\n for number in range(2,100):\n for exponent in range(2,50):\n raised_number=number**exponent\n sum_of_digits=0\n for digit in list(str(raised_number)):\n sum_of_digits+=int(digit)\n if sum_of_digits**exponent==raised_number:\n my_list.append(raised_number)\n answer=sorted(my_list)\n return answer[n-1]", "def power_sumDigTerm(n):\n #your code here\n return sorted([a**b for a in range(2, 100) for b in range(2,100) if a == sum(map(int, str(a**b)))])[n-1]", "def power_sumDigTerm(n):\n return sorted(set([a**b for a in range(2, 100) for b in range(2,100) if a == sum(map(int, str(a**b)))]))[n-1]"] | {"fn_name": "power_sumDigTerm", "inputs": [[1], [2], [3], [4], [5], [6], [7]], "outputs": [[81], [512], [2401], [4913], [5832], [17576], [19683]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,010 |
def power_sumDigTerm(n):
|
e6854ceb2ac1648f92131ccaa08c97f1 | UNKNOWN | A binary gap within a positive number ```num``` is any sequence of consecutive zeros that is surrounded by ones at both ends in the binary representation of ```num```.
For example:
```9``` has binary representation ```1001``` and contains a binary gap of length ```2```.
```529``` has binary representation ```1000010001``` and contains two binary gaps: one of length ```4``` and one of length ```3```.
```20``` has binary representation ```10100``` and contains one binary gap of length ```1```.
```15``` has binary representation ```1111``` and has ```0``` binary gaps.
Write ```function gap(num)```
that, given a positive ```num```, returns the length of its longest binary gap. The function should return ```0``` if ```num``` doesn't contain a binary gap. | ["def gap(num):\n s = bin(num)[2:].strip(\"0\")\n return max(map(len, s.split(\"1\")))", "import re\ndef gap(num):\n x=re.findall('(?=(10+1))',bin(num).lstrip('0b')) \n return max([len(i)-2 for i in x]) if x else 0", "import re\n\ndef gap(num):\n\n n = 0\n\n for m in re.finditer('(?=(1(0+)1))', bin(num)[2:]):\n n = max(n, m.end(2) - m.start(2))\n\n return n", "def gap(num):\n binary = bin(num)[2:]\n gaps = binary.strip(\"0\").split(\"1\")\n return len(max(gaps))\n", "def gap(num):\n return len(max(bin(num)[2:].split('1')[1:-1]))", "def gap(num):\n return len(max(bin(num)[2:].strip(\"0\").split(\"1\")))\n \n", "def gap(num):\n return len(max((bin(num)[2:]).rstrip('0').split('1')))\n \n", "import re\n\ndef gap(num):\n num = bin(num)[2:]\n return max(map(len, re.findall(\"(?<=1)0+(?=1)\", num)), default=0)", "def gap(num):\n return max(len(seq) for seq in format(num, 'b').strip('0').split('1'))\n", "from regex import findall\n\ndef gap(num):\n if num < 2: return 0\n return max(map(len, findall(r\"1(0*)(?=1)\", f\"{num:b}\")))"] | {"fn_name": "gap", "inputs": [[9]], "outputs": [[2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,107 |
def gap(num):
|
273670766bdfc05b8e4cd3c819c5942d | UNKNOWN | # Task
Miss X has only two combs in her possession, both of which are old and miss a tooth or two. She also has many purses of different length, in which she carries the combs. The only way they fit is horizontally and without overlapping. Given teeth' positions on both combs, find the minimum length of the purse she needs to take them with her.
It is guaranteed that there is at least one tooth at each end of the comb.
- Note, that the combs can not be rotated/reversed.
# Example
For `comb1 = "*..*" and comb2 = "*.*"`, the output should be `5`
Although it is possible to place the combs like on the first picture, the best way to do this is either picture 2 or picture 3.
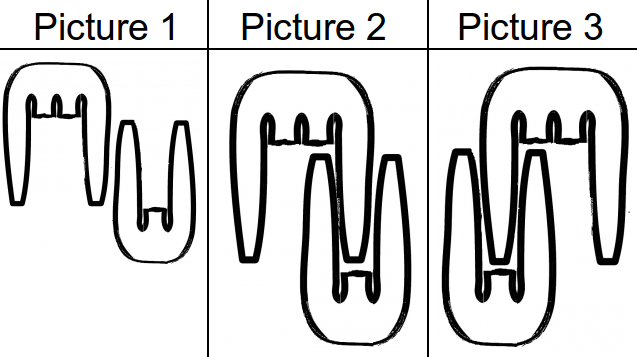
# Input/Output
- `[input]` string `comb1`
A comb is represented as a string. If there is an asterisk ('*') in the ith position, there is a tooth there. Otherwise there is a dot ('.'), which means there is a missing tooth on the comb.
Constraints: 1 ≤ comb1.length ≤ 10.
- `[input]` string `comb2`
The second comb is represented in the same way as the first one.
Constraints: 1 ≤ comb2.length ≤ 10.
- `[output]` an integer
The minimum length of a purse Miss X needs. | ["def combs(a, b):\n return min(mesh(a, b), mesh(b, a))\n\ndef mesh(a, b):\n for i in range(len(a)):\n for j, k in zip(a[i:], b):\n if j + k == '**': break\n else:\n return max(i + len(b), len(a))\n return len(a) + len(b) ", "def combs(comb1, comb2):\n options = []\n for i in range(max(len(comb1), len(comb2))+1):\n if ('*','*') not in zip(' '*i+comb1, comb2):\n options.append( max( len(comb1)+i, len(comb2) ) )\n if ('*','*') not in zip(comb1, ' '*i+comb2):\n options.append( max( len(comb1), len(comb2)+i ) )\n return min(options)", "def to_bin(comb):\n s = ''.join(('1' if d == '*' else '0' for d in comb))\n return (int(s, 2))\n\ndef shifter(comb1, comb2):\n b1, b2 = to_bin(comb1), to_bin(comb2)\n sh = 0\n while b1 & (b2 << sh):\n sh += 1\n return max(len(comb1), len(comb2) + sh)\n\ndef combs(comb1, comb2):\n return min(shifter(comb1, comb2), shifter(comb2, comb1))", "def combs(comb1, comb2):\n c1 = int(comb1.replace('*', '1').replace('.', '0'), 2)\n c2 = int(comb2.replace('*', '1').replace('.', '0'), 2)\n def r(x, y):\n if x.__xor__(y) == x + y:\n return max(x.bit_length(), y.bit_length())\n return r(x<<1,y)\n return min(r(c1,c2), r(c2,c1))", "def combs(comb1, comb2):\n return min((next(max(i + len(c2), len(c1)) for i in range(len(c1 + c2)) if not (\"*\", \"*\") in list(zip((c1 + \".\" * len(c2))[i:], c2))) for c1, c2 in ((comb1, comb2), (comb2, comb1))))\n\n\n# very ugly one-liner, I wouldn't do this for real. But I find the clean way is overkill.\n", "def combo(*letters):\n if set(letters) == {'*'} : return 'X'\n if set(letters) == {'.'} : return '.'\n return '*'\n \n\ndef combs(*combs):\n print(combs)\n combos = []\n combs = sorted(combs, key=len)\n combs = [combs[0]+'.'*len(combs[1]), '.'*len(combs[0])+combs[1]]\n for _ in range(len(combs[1])):\n combos.append(''.join(combo(a,b) for a,b in zip(*combs)).strip('.'))\n combs[0] = '.' + combs[0]\n combs[1] = combs[1] + '.'\n \n return min(len(c) for c in combos if 'X' not in c)\n \n \n \n \n \n \n", "c,combs=lambda s:int(s.translate({42:49,46:48}),2),lambda a,b:(lambda u,v:min(len(bin(x|y))-2for x,y in((u<<-min(0,n),v<<max(0,n))for n in range(-len(b),len(a)+1))if x&y<1))(c(a),c(b))", "def passage(comb1, comb2):\n for i in range(len(comb1)):\n cover = 0\n for j, s in enumerate(comb1[i:]):\n if j == len(comb2):\n return len(comb1)\n if s == comb2[j] == \"*\":\n break\n cover += 1\n else:\n return len(comb1) + len(comb2) - cover\n return len(comb1 + comb2)\n\n\ndef combs(comb1, comb2):\n comb2, comb1 = sorted([comb1, comb2], key=lambda x: len(x))\n return min(passage(comb1, comb2), passage(comb1[::-1], comb2[::-1]))\n \n\n \n", "def combs(c1, c2):\n n = len(c1)\n m = len(c2)\n c2 = n*'.'+c2+n*'.'\n \n min_len = 100\n for sh in range(n+m+1):\n l = n + (sh-n)*(sh>n) + (m-sh)*(m>sh)\n fit = True\n for k in range(n):\n if (c2[sh+k]+c1[k]).count('*') == 2:\n fit = False\n if fit:\n min_len = min(min_len,l)\n \n return min_len\n", "def combs(comb1, comb2):\n n1, n2 = len(comb1), len(comb2)\n c1 = int(comb1.replace('*', '1').replace('.', '0'), 2)\n c2 = int(comb2.replace('*', '1').replace('.', '0'), 2)\n return min(next(max(n1, i + n2) for i in range(1, 11) if c1 & (c2 << i) == 0),\n next(max(n2, i + n1) for i in range(1, 11) if c2 & (c1 << i) == 0))"] | {"fn_name": "combs", "inputs": [["*..*", "*.*"], ["*...*", "*.*"], ["*..*.*", "*.***"], ["*.*", "*.*"], ["*.**", "*.*"]], "outputs": [[5], [5], [9], [4], [5]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,755 |
def combs(comb1, comb2):
|
592e8cd46b5ba8fde6a73dd47804f9ef | UNKNOWN | Given an array of numbers, calculate the largest sum of all possible blocks of consecutive elements within the array. The numbers will be a mix of positive and negative values. If all numbers of the sequence are nonnegative, the answer will be the sum of the entire array. If all numbers in the array are negative, your algorithm should return zero. Similarly, an empty array should result in a zero return from your algorithm.
```
largestSum([-1,-2,-3]) == 0
largestSum([]) == 0
largestSum([1,2,3]) == 6
```
Easy, right? This becomes a lot more interesting with a mix of positive and negative numbers:
```
largestSum([31,-41,59,26,-53,58,97,-93,-23,84]) == 187
```
The largest sum comes from elements in positions 3 through 7:
```59+26+(-53)+58+97 == 187```
Once your algorithm works with these, the test-cases will try your submission with increasingly larger random problem sizes. | ["def largest_sum(arr):\n sum = max_sum = 0\n for n in arr:\n sum = max(sum + n, 0)\n max_sum = max(sum, max_sum)\n return max_sum", "def largest_sum(arr):\n a, b, ar = 0, 0, arr[:]\n for i in ar:\n b+=i\n if b < 0: b = 0\n if b > a: a = b\n return a", "def largest_sum(a):\n m = c = 0\n for i in a:\n c = max(0,c+i)\n m = max(m,c)\n return m", "def largest_sum(arr):\n current = so_far = 0\n for n in arr:\n current += n\n if current > so_far: so_far = current\n if current <= 0: current = 0\n return so_far", "def largest_sum(arr):\n c, m = 0, 0\n for n in arr:\n c = max(0, c + n)\n m = max(m, c)\n return m", "def largest_sum(arr):\n\n largest = 0\n temp = 0\n\n for num in arr:\n temp += num\n if temp < 0:\n temp = 0\n elif temp > largest:\n largest = temp\n\n return largest", "def largest_sum(arr):\n if len(arr) == 0:\n return 0 \n cache = [arr[0]]\n for i in range(1, len(arr)):\n cache.append(max(arr[i], cache[-1] + arr[i]))\n return 0 if max(cache) < 0 else max(cache)", "def largest_sum(arr):\n best_sum = 0\n current_sum = 0\n for item in arr:\n current_sum += item\n if current_sum < 0:\n current_sum = 0\n else:\n if current_sum > best_sum:\n best_sum = current_sum\n return best_sum", "def largest_sum(a):\n best_sum = 0 \n current_sum = 0\n for x in a:\n current_sum = max(0, current_sum + x)\n best_sum = max(best_sum, current_sum)\n return best_sum", "def largest_sum(arr):\n ans,sum = 0,0\n for i in range(len(arr)):\n sum = max(arr[i], arr[i] + sum)\n ans = max(ans,sum)\n return ans"] | {"fn_name": "largest_sum", "inputs": [[[-1, -2, -3]], [[]], [[1, 2, 3, 4]], [[31, -41, 59, 26, -53, 58, 97, -93, -23, 84]]], "outputs": [[0], [0], [10], [187]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,837 |
def largest_sum(arr):
|
011bc259dbc06d2ef4441a012bfb021d | UNKNOWN | Two strings ```a``` and b are called isomorphic if there is a one to one mapping possible for every character of ```a``` to every character of ```b```. And all occurrences of every character in ```a``` map to same character in ```b```.
## Task
In this kata you will create a function that return ```True``` if two given strings are isomorphic to each other, and ```False``` otherwise. Remember that order is important.
Your solution must be able to handle words with more than 10 characters.
## Example
True:
```
CBAABC DEFFED
XXX YYY
RAMBUNCTIOUSLY THERMODYNAMICS
```
False:
```
AB CC
XXY XYY
ABAB CD
``` | ["def isomorph(a, b):\n return [a.index(x) for x in a] == [b.index(y) for y in b]\n", "from itertools import zip_longest\n\ndef isomorph(a, b):\n return len(set(a)) == len(set(b)) == len(set(zip_longest(a, b)))", "def isomorph(s, t):\n if len(s) != len(t):\n return False\n p= set(zip(s, t))\n return all(len(p) == len(set(c)) for c in zip(*p))", "def isomorph(a, b):\n return len(a) == len(b) and len(set(a)) == len(set(b)) == len(set(zip(a, b)))", "def isomorph(a, b):\n normalize = lambda s: [s.index(c) for c in s]\n return normalize(a) == normalize(b)", "def isomorph(a, b):\n return len(a) == len(b) and a.translate(str.maketrans(a, b)) == b and b.translate(str.maketrans(b, a)) == a", "def isomorph(a, b):\n return [a.index(h) for h in a]==[b.index(g) for g in b]\n # Happy coding!\n", "from collections import defaultdict\n\ndef isomorph(a, b):\n print(a, b)\n da,db = defaultdict(list), defaultdict(list)\n for i in range(len(a)==len(b) and len(a)):\n if da[a[i]] != db[b[i]]: return False\n da[a[i]].append(i)\n db[b[i]].append(i)\n return bool(da)", "def isomorph(a, b):\n if len(a)!=len(b):\n return False\n st={}\n for i in range(len(a)):\n if a[i] not in list(st.keys()) and b[i] in list(st.values()):\n return False\n if a[i] not in list(st.keys()) and b[i] not in list(st.values()):\n st[a[i]]=b[i]\n elif st[a[i]]!=b[i] :#or a[i] not in st.keys() and b[i] in st.values():\n return False\n return True\n # Happy coding!\n", "def isomorph(a, b):\n print((a, b))\n if(len(a) != len(b)):\n return False\n m = {} # mapping of each character in a to b\n r = {} # mapping of each character in b to a\n for i, c in enumerate(a):\n if c in m:\n if b[i] != m[c]:\n return False\n else:\n m[c] = b[i]\n if b[i] in r:\n if c != r[b[i]]:\n return False\n else:\n r[b[i]] = c\n print('True')\n return True"] | {"fn_name": "isomorph", "inputs": [["ESTATE", "DUELED"], ["XXX", "YYY"], ["CBAABC", "DEFFED"], ["RAMBUNCTIOUSLY", "THERMODYNAMICS"], ["DISCRIMINATIVE", "SIMPLIFICATION"], ["SEE", "SAW"], ["BANANA", "SENSE"], ["AB", "CC"], ["XXY", "XYY"], ["ABCBACCBA", "ABCBACCAB"], ["AA", "BBB"], ["abcdefghijk", "abcdefghijba"]], "outputs": [[true], [true], [true], [true], [true], [false], [false], [false], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,084 |
def isomorph(a, b):
|
f21b44b7a78e1dd3dc61b9623a23c966 | UNKNOWN | For a given 2D vector described by cartesian coordinates of its initial point and terminal point in the following format:
```python
[[x1, y1], [x2, y2]]
```
Your function must return this vector's length represented as a floating point number.
Error must be within 1e-7.
Coordinates can be integers or floating point numbers. | ["def vector_length(vector):\n (x1,y1),(x2,y2) = vector\n return ((x1-x2)**2 + (y1-y2)**2) ** .5", "import math\n\n\ndef vector_length(vector):\n return math.hypot(vector[0][0] - vector[1][0],\n vector[0][1] - vector[1][1])\n", "import math\ndef vector_length(v):\n return math.sqrt(math.pow(v[0][0] - v[1][0], 2) + math.pow(v[0][1] - v[1][1], 2))", "from math import sqrt\ndef vector_length(v):\n return sqrt((v[1][0]-v[0][0])**2+(v[0][1]-v[1][1])**2)", "def vector_length(vector):\n return sum((a - b)**2 for a,b in zip(*vector))**0.5", "import numpy as np\n\n# Will work in any dimension\ndef vector_length(vector):\n P1, P2 = map(np.array, vector)\n return np.linalg.norm(P1 - P2)", "from math import hypot\n\ndef vector_length(a):\n return hypot(a[0][0]-a[1][0], a[0][1]-a[1][1])", "def vector_length(vector):\n return sum((a-b)**2 for a,b in zip(*vector))**.5"] | {"fn_name": "vector_length", "inputs": [[[[0, 1], [0, 0]]], [[[0, 3], [4, 0]]], [[[1, -1], [1, -1]]]], "outputs": [[1], [5], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 916 |
def vector_length(vector):
|
4a8c149df405bc68706d90129d2615bd | UNKNOWN | ```if:python
Note: Python may currently have some performance issues. If you find them, please let me know and provide suggestions to improve the Python version! It's my weakest language... any help is much appreciated :)
```
Artlessly stolen and adapted from Hackerrank.
Kara Danvers is new to CodeWars, and eager to climb up in the ranks. We want to determine Kara's rank as she progresses up the leaderboard.
This kata uses Dense Ranking, so any identical scores count as the same rank (e.g, a scoreboard of `[100, 97, 97, 90, 82, 80, 72, 72, 60]` corresponds with rankings of `[1, 2, 2, 3, 4, 5, 6, 6, 7]`
You are given an array, `scores`, of leaderboard scores, descending, and another array, `kara`, representing Kara's Codewars score over time, ascending. Your function should return an array with each item corresponding to the rank of Kara's current score on the leaderboard.
**Note:** This kata's performance requirements are significantly steeper than the Hackerrank version. Some arrays will contain millions of elements; optimize your code so you don't time out. If you're timing out before 200 tests are completed, you've likely got the wrong code complexity. If you're timing out around 274 tests (there are 278), you likely need to make some tweaks to how you're handling the arrays.
Examples:
(For the uninitiated, Kara Danvers is Supergirl. This is important, because Kara thinks and moves so fast that she can complete a kata within microseconds. Naturally, latency being what it is, she's already opened many kata across many, many tabs, and solves them one by one on a special keyboard so she doesn't have to wait hundreds of milliseconds in between solving them. As a result, the only person's rank changing on the leaderboard is Kara's, so we don't have to worry about shifting values of other codewarriors. Thanks, Supergirl.)
Good luck! Please upvote if you enjoyed it :) | ["def leaderboard_climb(arr, kara):\n scores = sorted(set(arr), reverse=True)\n position = len(scores)\n ranks = []\n \n for checkpoint in kara:\n while position >= 1 and checkpoint >= scores[position - 1]:\n position -= 1\n ranks.append(position + 1) \n \n return ranks", "import numpy as np\ndef leaderboard_climb(arr, kara):\n a = np.unique(arr)\n return list(len(a) + 1 - np.searchsorted(a, kara, side='right'))", "#Previously saved by accident before removing debug or refectoring.Doh!\nimport bisect\ndef leaderboard_climb(arr, kara):\n arr=sorted(list(set(arr)))\n return [(len(arr) + 1 -bisect.bisect_right(arr,score) ) for score in kara]", "leaderboard_climb=lambda A,K:(lambda S:[len(S)-__import__('bisect').bisect(S,i)+1for i in K])(sorted(set(A)))", "from bisect import bisect\ndef leaderboard_climb(arr, kara):\n scoreboard = sorted(set(arr))\n scores = len(scoreboard)\n return [scores - bisect(scoreboard, i) + 1 for i in kara] \n \n", "def leaderboard_climb(scores, kara):\n answer = []\n kara = kara[::-1]\n rank, iScore, lastScore = 1, 0, scores[0]\n l = len(scores)\n for karaScore in kara:\n while True:\n if karaScore >= lastScore or iScore >= l:\n answer.append(rank)\n break\n iScore += 1\n if iScore < l:\n score = scores[iScore]\n if lastScore != score:\n lastScore = score\n rank += 1\n else:\n rank += 1\n return answer[::-1]", "import numpy as np\n\ndef leaderboard_climb(arr, kara):\n uniq_scores = np.unique(arr)\n l = len(uniq_scores) + 1\n t = l - np.searchsorted(uniq_scores, kara, side='right')\n return t.tolist()", "from bisect import bisect_left\n\ndef leaderboard_climb(arr, kara):\n arr.append(float('inf'))\n arr = sorted([-a for a in set(arr)])\n result = [bisect_left(arr, -k) for k in kara]\n return result", "from bisect import bisect_left\n\ndef leaderboard_climb(arr, kara):\n result = []\n arr.append(float('inf'))\n arr = sorted([-a for a in set(arr)])\n for k in kara:\n i = bisect_left(arr, -k)\n result.append(i)\n return result", "def leaderboard_climb(arr, kara):\n arr = sorted(set(arr),reverse=True)\n rank = []\n pos = len(arr)\n \n for x in kara:\n while pos>=1 and x >= arr[pos-1]:\n pos -= 1\n rank.append(pos+1)\n return rank\n \n \n"] | {"fn_name": "leaderboard_climb", "inputs": [[[100, 90, 90, 80], [70, 80, 105]], [[982, 490, 339, 180], [180, 250, 721, 2500]], [[1982, 490, 339, 180], [180, 250, 721, 880]], [[1079, 490, 339, 180], [180, 250, 1200, 1980]]], "outputs": [[[4, 3, 1]], [[4, 4, 2, 1]], [[4, 4, 2, 2]], [[4, 4, 1, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,560 |
def leaderboard_climb(arr, kara):
|
b6b976866c49c4274dbaf9c17f859642 | UNKNOWN | Alan's child can be annoying at times.
When Alan comes home and tells his kid what he has accomplished today, his kid never believes him.
Be that kid.
Your function 'AlanAnnoyingKid' takes as input a sentence spoken by Alan (a string). The sentence contains the following structure:
"Today I " + [action_verb] + [object] + "."
(e.g.: "Today I played football.")
Your function will return Alan's kid response, which is another sentence with the following structure:
"I don't think you " + [action_performed_by_alan] + " today, I think you " + ["did" OR "didn't"] + [verb_of _action_in_present_tense] + [" it!" OR " at all!"]
(e.g.:"I don't think you played football today, I think you didn't play at all!")
Note the different structure depending on the presence of a negation in Alan's first sentence (e.g., whether Alan says "I dind't play football", or "I played football").
! Also note: Alan's kid is young and only uses simple, regular verbs that use a simple "ed" to make past tense.
There are random test cases.
Some more examples:
input = "Today I played football."
output = "I don't think you played football today, I think you didn't play at all!"
input = "Today I didn't attempt to hardcode this Kata."
output = "I don't think you didn't attempt to hardcode this Kata today, I think you did attempt it!"
input = "Today I didn't play football."
output = "I don't think you didn't play football today, I think you did play it!"
input = "Today I cleaned the kitchen."
output = "I don't think you cleaned the kitchen today, I think you didn't clean at all!" | ["OUTPUT = \"I don't think you {} today, I think you {} {} {}!\".format\n\n\ndef alan_annoying_kid(phrase):\n words = phrase.split()\n action = ' '.join(words[2:]).rstrip('.')\n if \"didn't\" in phrase:\n return OUTPUT(action, 'did', words[3], 'it')\n return OUTPUT(action, \"didn't\", words[2][:-2], 'at all')\n", "def alan_annoying_kid(sentence):\n action = sentence.split(None, 2)[-1].rstrip('.')\n if \"didn't\" in sentence:\n verb, did, it = action.split(None, 2)[1], 'did', 'it'\n else:\n verb, did, it = action.split(None, 1)[0][:-2], \"didn't\", 'at all'\n return \"I don't think you {} today, I think you {} {} {}!\".format(action, did, verb, it)\n", "import re\ndef alan_annoying_kid(s):\n action = s[8:-1]\n d = [\"did\", \"didn't\"][\"didn't\" not in action]\n present = re.sub(r'ed$','',re.search(r\"^\\w+\", action.replace(\"didn't \", '')).group())\n return \"I don't think you \"+action+\" today, I think you \"+d+' '+present+[\" it!\",\" at all!\"][d==\"didn't\"] ", "def alan_annoying_kid(stg):\n s1 = stg.replace(\"Today I \", \"\")[:-1]\n s3 = s1.replace(\"didn't \", \"\").split()[0]\n s2, s3, s4 = (\"did\", s3, \"it\") if \"n't\" in s1 else (\"didn't\", s3[:-2], \"at all\")\n return f\"I don't think you {s1} today, I think you {s2} {s3} {s4}!\"", "def alan_annoying_kid(s):\n lst = s.split()\n isNegative = lst[2] == \"didn't\"\n verb = lst[2 + isNegative]\n cplt = ' '.join(lst[3 + isNegative:])\n return (\"I don't think you didn't {} {} today, I think you did {} it!\".format(verb, cplt[:-1], verb)\n if isNegative else\n \"I don't think you {} {} today, I think you didn't {} at all!\".format(verb, cplt[:-1], verb[:-2]))", "def alan_annoying_kid(sentence):\n if \"didn't\" in sentence:\n verb, subject = sentence[15:-1].split(\" \", 1)\n return \"I don't think you didn't %s %s today, I think you did %s it!\" % (verb, subject, verb)\n\n verb, subject = sentence[8:-1].split(\" \", 1)\n return \"I don't think you %s %s today, I think you didn't %s at all!\" % (verb, subject, verb[:-2])", "def alan_annoying_kid(stg):\n response = \"I don't think you {0} today, I think you {1} {2} {3}!\".format\n what = stg.replace(\"Today I \", \"\")[:-1]\n made = what.replace(\"didn't \", \"\").split()[0]\n filler = (what, \"did\", made, \"it\") if \"didn't\" in what else (what, \"didn't\", made[:-2], \"at all\")\n return response(*filler)\n", "def alan_annoying_kid(s):\n a = s[:-1].split()\n b = f\"did {a[3]} it\" if a[2] == \"didn't\" else f\"didn't {a[2][:-2]} at all\"\n return f\"I don't think you {' '.join(a[2:])} today, I think you {b}!\"", "def alan_annoying_kid(s):\n negative = False\n a = s.split()\n a[-1] = a[-1][:-1]\n if a[2] == \"didn't\":\n negative = True\n if not negative:\n return \"I don't think you \" + ' '.join(a[2::]) + \" today, I think you didn't \" + str(a[2][:-2]) + \" at all!\"\n\n return \"I don't think you didn't \" + ' '.join(a[3::]) + \" today, I think you did \" + str(a[3]) + \" it!\"", "import re\ndef alan_annoying_kid(sentence):\n if \"didn't\" in sentence:\n s = re.search(r\"didn't (.)+\\.\", sentence)\n s2 = 'did ' + sentence.split(' ')[3] + ' it'\n return \"I don't think you {} today, I think you {}!\".format(s.group()[:-1], s2)\n else:\n s = sentence.split(' ')[2][:-2]\n s1 = ' '.join(sentence.split(' ')[2:])[:-1]\n s2 = \"didn't \" + s + ' at all'\n return \"I don't think you {} today, I think you {}!\".format(s1, s2)"] | {"fn_name": "alan_annoying_kid", "inputs": [["Today I played football."], ["Today I didn't play football."], ["Today I didn't attempt to hardcode this Kata."], ["Today I cleaned the kitchen."], ["Today I learned to code like a pro."]], "outputs": [["I don't think you played football today, I think you didn't play at all!"], ["I don't think you didn't play football today, I think you did play it!"], ["I don't think you didn't attempt to hardcode this Kata today, I think you did attempt it!"], ["I don't think you cleaned the kitchen today, I think you didn't clean at all!"], ["I don't think you learned to code like a pro today, I think you didn't learn at all!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,645 |
def alan_annoying_kid(s):
|
f6b515a8e5fb468f2fc480fd63ca586d | UNKNOWN | # Task
You have two sorted arrays `a` and `b`, merge them to form new array of unique items.
If an item is present in both arrays, it should be part of the resulting array if and only if it appears in both arrays the same number of times.
# Example
For `a = [1, 3, 40, 40, 50, 60, 60, 60]` and `b = [2, 40, 40, 50, 50, 65]`, the result should be `[1, 2, 3, 40, 60, 65]`.
```
Number 40 appears 2 times in both arrays,
thus it is in the resulting array.
Number 50 appears once in array a and twice in array b,
therefore it is not part of the resulting array.```
# Input/Output
- `[input]` integer array `a`
A sorted array.
1 ≤ a.length ≤ 500000
- `[input]` integer array `b`
A sorted array.
`1 ≤ b.length ≤ 500000`
- `[output]` an integer array
The resulting sorted array. | ["def merge_arrays(a, b):\n out = []\n for n in a+b:\n if n in a and n in b:\n if a.count(n) == b.count(n):\n out.append(n)\n else:\n out.append(n)\n return sorted(set(out))", "from collections import Counter\n\ndef merge_arrays(a, b):\n ca, cb = Counter(a), Counter(b)\n return [ k for k in sorted(ca.keys() | cb.keys()) if (k in ca)+(k in cb) == 1 or ca[k] == cb[k] ]", "merge_arrays=lambda a,b:sorted([x for x in set(a+b) if a.count(x)==b.count(x) or a.count(x)*b.count(x)==0])", "def merge_arrays(a, b):\n return sorted(n for n in set(a+b) if (n in a) != (n in b) or a.count(n) == b.count(n))", "def merge_arrays(a, b):\n list =[]\n for i in a+b :\n if (a.count(i)==b.count(i) or b.count(i)==0 or a.count(i)==0) and i not in list:\n list.append(i)\n return sorted(list)", "def merge_arrays(a, b):\n result = []\n currA = 0\n currB = 0\n while currA < len(a) and currB < len(b):\n if a[currA] < b[currB]:\n # Check that we are not adding a duplicate\n if not result or result[-1] != a[currA]:\n result.append(a[currA])\n currA += 1\n elif b[currB] < a[currA]:\n # Check that we are not adding a duplicate\n if not result or result[-1] != b[currB]:\n result.append(b[currB])\n currB += 1\n else:\n val = a[currA]\n # Count how many times the value occurs in a and b\n countA = 0\n while currA < len(a):\n if a[currA] == val:\n countA += 1\n currA += 1\n else:\n break\n countB = 0\n while currB < len(b):\n if b[currB] == val:\n countB += 1\n currB += 1\n else:\n break\n if countA == countB:\n result.append(val)\n # We've exhausted either a or b \n while currA < len(a):\n if not result or result[-1] != a[currA]:\n result.append(a[currA])\n currA += 1\n while currB < len(b):\n if not result or result[-1] != b[currB]:\n result.append(b[currB])\n currB += 1\n return result", "from collections import Counter\n\ndef merge_arrays(a, b):\n C1, C2 = Counter(a), Counter(b)\n return sorted((C1.keys() ^ C2.keys()) | {k for k in (C1.keys() & C2.keys()) if C1[k] == C2[k]})", "from collections import Counter\n\ndef merge_arrays(a, b):\n c = Counter(a)\n d = Counter(b)\n return sorted(x for x in set(a) | set(b) if c[x] == d[x] or not c[x] or not d[x])", "from collections import Counter\ndef merge_arrays(a, b):\n li = set()\n c1,c2 = Counter(a), Counter(b)\n for i in set(a + b):\n if i in c1 and i in c2:\n if c1[i] == c2[i] : li.add(i)\n else : li.add(i)\n return sorted(li)", "from collections import Counter\n\ndef merge_arrays(a, b):\n ca = Counter(a)\n cb = Counter(b)\n return [x for x in sorted(set(ca).union(cb)) if ca[x] == cb[x] or ca[x] * cb[x] == 0]"] | {"fn_name": "merge_arrays", "inputs": [[[10, 10, 10, 15, 20, 20, 25, 25, 30, 7000], [10, 15, 20, 20, 27, 7200]], [[500, 550, 1000, 1000, 1400, 3500], [2, 2, 2, 2, 3, 1500]], [[5], [5, 5, 7]]], "outputs": [[[15, 20, 25, 27, 30, 7000, 7200]], [[2, 3, 500, 550, 1000, 1400, 1500, 3500]], [[7]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,017 |
def merge_arrays(a, b):
|
6b318e28af71e11586223f3763cdc053 | UNKNOWN | # Task
Mobius function - an important function in number theory. For each given n, it only has 3 values:
```
0 -- if n divisible by square of a prime. Such as: 4, 8, 9
1 -- if n not divisible by any square of a prime
and have even number of prime factor. Such as: 6, 10, 21
-1 -- otherwise. Such as: 3, 5, 7, 30```
Your task is to find mobius(`n`)
# Input/Output
- `[input]` integer `n`
`2 <= n <= 1e12`
- `[output]` an integer | ["def mobius(n):\n sP, p = set(), 2\n while n>1 and p <= n**.5:\n while not n%p:\n if p in sP: return 0\n sP.add(p)\n n //= p\n p += 1 + (p!=2)\n return (-1)**((len(sP) + (n!= 1)) % 2)", "def mobius(n):\n s, p, m = 0, 2, n ** .5\n while p <= m:\n if not n % p:\n s += 1\n n //= p\n if not n % p:\n return 0\n p += 1\n if n > 1:\n s += 1\n return 1 - s % 2 * 2", "LIMIT = 10**6\nsieve = [True] * (LIMIT//2)\nfor n in range(3, int(LIMIT**0.5) +1, 2):\n if sieve[n//2]: sieve[n*n//2::n] = [False] * ((LIMIT-n*n-1)//2//n +1)\nPRIMES = [2] + [2*i+1 for i in range(1, LIMIT//2) if sieve[i]]\ndel sieve\n\n\ndef mobius(n):\n factors = []\n for p in PRIMES:\n if p*p > n:\n factors.append(n)\n break\n while n % p == 0:\n if p in factors:\n return 0\n factors.append(p)\n n //= p\n if n == 1:\n break\n \n return -1 if len(factors) % 2 else 1\n", "from collections import Counter\n\ndef prime_factors(n):\n factors = Counter()\n d = 2\n while d ** 2 <= n:\n while n % d == 0:\n n //= d\n factors[d] += 1\n d += 1 + (d > 2)\n if n > 1:\n factors[n] += 1\n return factors\n\n\ndef mobius(n):\n factors = prime_factors(n)\n if any(c > 1 for c in factors.values()):\n return 0\n elif sum(factors.values()) % 2 == 0:\n return 1\n return -1", "def mobius(n):\n a = 0\n for i in range(2, int(n ** .5) + 1):\n if n % i == 0:\n if isPrime(i):\n if n % (i*i) == 0:\n return 0\n a += 1\n n = n // i\n if isPrime(n):\n a += 1\n break\n if a > 0 and a % 2 == 0:\n return 1\n return -1 \n\ndef isPrime(n):\n for i in range(2, int(n ** .5 + 1)):\n if n % i == 0:\n return False\n return True", "def prime_factors(n):\n factors = []\n while not n % 2:\n factors.append(2)\n n = n // 2\n while not n % 3:\n factors.append(3)\n n = n // 3\n k = 5\n step = 2\n while k <= n**0.5:\n if not n % k:\n factors.append(k)\n n = n // k\n else:\n k = k + step\n step = 6 - step\n factors.append(n)\n return factors\n\ndef mobius(n):\n factors = prime_factors(n)\n l = len(factors)\n if l == len(set(factors)):\n if l % 2:\n return -1\n return 1\n return 0\n", "def mobius(n):\n d, k = 2, 0\n while d * d <= n:\n if n % d == 0:\n n //= d\n if n % d == 0:\n return 0\n k += 1\n d += 1\n if n > 1:\n k += 1\n return 1 - k % 2 * 2", "def prime_factors_count(num):\n factors_count = 0\n while num % 2 == 0:\n factors_count += 1\n num /= 2\n\n i = 3\n max_factor = num**0.5\n while i <= max_factor:\n while num % i == 0:\n factors_count += 1\n num /= i\n max_factor = num**0.5\n i += 2\n\n if num > 1:\n factors_count += 1\n return factors_count\n\ndef find_prime_numbers(max_num):\n if max_num == 0:\n return []\n\n is_prime = [True] * (max_num+1)\n for i in range(4, max_num, 2):\n is_prime[i] = False\n is_prime[0], is_prime[1] = False, False\n\n next_prime = 3\n stop_at = max_num**0.5\n while next_prime <= stop_at:\n for i in range(next_prime*next_prime, max_num+1, next_prime):\n is_prime[i] = False\n next_prime += 2\n\n prime_numbers = []\n for i in range(len(is_prime)):\n if is_prime[i]:\n prime_numbers.append(i)\n return prime_numbers\n\ndef mobius(n):\n for i in find_prime_numbers(int(n**0.5)//2 + 10): # An empirical trick :)\n if n % (i*i) == 0:\n return 0\n if prime_factors_count(n) % 2 == 0:\n return 1\n return -1", "from collections import defaultdict\ndef prime_factor(n):\n r=defaultdict(int)\n while(n%2==0):\n r[2]+=1\n n//=2\n x=3\n while(n>1 and x*x<=n):\n while(n%x==0):\n r[x]+=1\n n//=x\n x+=2\n if n>1:\n r[n]+=1\n return r\n\ndef mobius(n):\n fs=prime_factor(n)\n if max(fs.values())>1:\n return 0\n elif len(fs.keys())%2==0:\n return 1\n else:\n return -1"] | {"fn_name": "mobius", "inputs": [[10], [9], [8], [100000000001], [7], [5]], "outputs": [[1], [0], [0], [0], [-1], [-1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,501 |
def mobius(n):
|
f02e3b16f5d5b7047da7e0c9ad88a1d4 | UNKNOWN | # Task
You are given an array of integers. Your task is to determine the minimum number of its elements that need to be changed so that elements of the array will form an arithmetic progression. Note that if you swap two elements, you're changing both of them, for the purpose of this kata.
Here an arithmetic progression is defined as a sequence of integers such that the difference between consecutive terms is constant. For example, `6 4 2 0 -2` and `3 3 3 3 3` are arithmetic progressions, but `0 0.5 1 1.5` and `1 -1 1 -1 1` are not.
# Examples
For `arr = [1, 3, 0, 7, 9]` the answer is `1`
Because only one element has to be changed in order to create an arithmetic progression.
For `arr = [1, 10, 2, 12, 3, 14, 4, 16, 5]` the answer is `5`
The array will be changed into `[9, 10, 11, 12, 13, 14, 15, 16, 17]`.
# Input/Output
- `[input]` integer array `arr`
An array of N integers.
`2 ≤ arr.length ≤ 100`
`-1000 ≤ arr[i] ≤ 1000`
Note for Java users: you'll have a batch of 100 bigger random arrays, with lengths as `150 ≤ arr.length ≤ 300`.
- `[output]` an integer
The minimum number of elements to change. | ["from collections import defaultdict\n\ndef fix_progression(arr):\n res = 0\n for i in range(len(arr)):\n D = defaultdict(int)\n for j in range(i):\n q, r = divmod(arr[i]-arr[j], i-j)\n if not r:\n D[q] += 1\n res = max(res, D[q])\n return len(arr)-res-1", "from collections import defaultdict\nfrom itertools import combinations\n\n\ndef lineEq(x,a,y,b):\n slope = (b-a)/(y-x)\n ordo = a - slope * x\n return (slope,ordo), not slope%1\n\n\ndef fix_progression(arr):\n \n c = defaultdict(set)\n for (x,a),(y,b) in combinations(enumerate(arr), 2):\n tup, isIntSlope = lineEq(x,a,y,b)\n if isIntSlope: c[tup] |= {x,y}\n \n return len(arr) - max(map(len, c.values()))", "def fix_progression(arr):\n best = len(arr)\n s = sum(arr) / len(arr)\n for start in range(len(arr)-1):\n for idx in range(start+1, len(arr)):\n count = 0\n d = (arr[idx] - arr[start]) // (idx - start)\n for i in range(0, start):\n if arr[i] != arr[start] - d*(start-i):\n count += 1\n for i in range(start+1, len(arr)):\n if arr[i] != arr[start] + d*(i-start):\n count += 1\n best = min(best, count)\n return best\n", "def fix_progression(arr):\n n, r = len(arr), float('inf')\n for i in range(n - 1):\n x = arr[i]\n for j in range(i + 1, n):\n d = arr[j] - arr[i]\n if d % (j - i):\n continue\n d //= j - i\n x0 = x - i * d\n diff = sum(arr[k] != x0 + k * d for k in range(n))\n r = min(r, diff)\n if r <= 1:\n return r\n return r", "from collections import defaultdict\n\ndef fix_progression(arr):\n \n global_max = 1\n \n for i, ele in enumerate(arr):\n \n maxi = 1\n things = defaultdict(lambda: 1) #dictionary to store the first and last elements of progression including arr[i]\n \n for j in range(i+1, len(arr)):\n \n comp = arr[j]\n diff = (comp - ele)/(j-i) #would be arithmetic progression constant between arr[i] and arr[j]\n \n if diff.is_integer():\n \n #calculate the first and last elements of the \"would be\" arithmetic progression\n \n left = ele - diff*i\n right = ele + diff*(len(arr) - i - 1)\n \n # if the same left and right exist already, that means the current left, right pair is the same\n #as a arithmetic progression seen for arr[i], meaning we have += 1 points sharing the arithmetic progression\n #eg [1, 10, 2, 12, 3, 14]. the pair 10,12 and 10,14 have the same left and right points, meaning they \n # are collinear and so thats one less point which we need to change in order to make arithmetic prog.\n \n things[(left, right)] += 1 \n maxi = max(maxi, things[(left, right)])\n global_max = max(maxi, global_max)\n \n return len(arr) - global_max\n", "def fix_progression(arr):\n best = len(arr)-2\n for i in range(len(arr)-1):\n for j in range(i+1,len(arr)):\n if (arr[j]-arr[i])%(j-i)!=0:\n continue\n step = (arr[j]-arr[i])//(j-i)\n wrongs = sum(1 for k in range(len(arr)) if arr[k]!=arr[i]+(k-i)*step)\n best = min(wrongs, best)\n if best<i-2:\n break\n return best", "def fix_progression(arr):\n min_c=len(arr)\n for i in range(len(arr)-1):\n for j in range(i+1,len(arr)):\n if (arr[j]-arr[i])%(j-i)!=0:\n continue\n a=(arr[j]-arr[i])//(j-i)\n c=0\n for k in range(len(arr)):\n if arr[i]+(k-i)*a!=arr[k]:\n c+=1\n if c<min_c:\n min_c=c\n return min_c", "from itertools import combinations\n\ndef fix_progression(arr):\n ans = len(arr) - 1\n for l, r in combinations(range(len(arr)), 2):\n step = (arr[r] - arr[l]) / (r - l)\n if step.is_integer():\n step = int(step)\n changed = sum(a != i for i, a in zip(range(arr[l]-step*l, 10**10*step, step), arr)) if step else sum(a != arr[l] for a in arr)\n ans = min(ans, changed)\n return ans", "from collections import Counter\ndef fix_progression(arr):\n lst = []\n for i in range(len(arr)):\n for j in range(i+1, len(arr)):\n if (arr[j]-arr[i])%(j-i) == 0:\n dif = (arr[j]-arr[i])//(j-i)\n s = arr[i] - i*dif\n lst.append((s, dif))\n c = Counter(lst).most_common()[0]\n count = 0\n for i in range(len(arr)):\n if arr[i] != c[0][0] + i*c[0][1]:\n count += 1\n return count", "from collections import Counter\n\ndef fix_progression(arr):\n params = Counter()\n for i in range(len(arr)):\n for j in range(i+1, len(arr)):\n if (arr[j] - arr[i]) % (j - i) == 0:\n d = (arr[j] - arr[i]) // (j - i)\n s = arr[i] - i*d\n params[(s,d)] += 1\n \n start, diff = params.most_common(1)[0][0]\n \n count = 0\n for i in range(len(arr)):\n if arr[i] != start + i * diff:\n count += 1\n return count\n"] | {"fn_name": "fix_progression", "inputs": [[[1, 2, 3]], [[1, 3, 0, 7, 9]], [[1, 10, 2, 12, 3, 14, 4, 16, 5]], [[7, 7, 7, 7, 8, 7, 7, 7, 7, 7, 7, 7, 7, 7, 9, 7, 7, 7, 7, 7, 7]], [[2, -1, -4, 12, 1, -13, -16, -19, -6, -25]], [[-1, 2, 5, 8, 11, 14, 17, -6, 23, 26, -25, 32]], [[-10, -21, -20, -25, -13, 14, 2, 6, -50, -55, -60, -65, -70, -75, -80, -85, -90, -95, -100, -105, -110, -115, -120, -125, -130, 12, -24, -15, -150, 7, -160, -165, -170, -175, -180, -185, -190, -195, -200, -3, -6, -215, -220, -225, -230, -235, -240, -20, -250, -255, -260, 23, -270, -275, -280, -7, -290, -295, -300, 12, -310, -315, -320, -325, -330, -335, -17, -345, -350, -355, -360, -365, -370, -375, -2, -385, -390, -395, 2, -405, -11, -415, -420, -425, -430, -435, -440, 20, -450, -455, -460, -465, -470, -475, -480, -485, 15, -22, -500, -21]], [[-4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -17, -4, -4, -4, -4, -4, -4, -4, 21, -4, -4, -4, 20, -4, -4, -4, -4, -4, 9, -4, -25, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, 14, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4, -4]]], "outputs": [[0], [1], [5], [2], [3], [2], [23], [6]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,502 |
def fix_progression(arr):
|
608da2ae536cdff6ab1f279b67da6d95 | UNKNOWN | A `Nice array` is defined to be an array where for every value `n` in the array, there is also an element `n-1` or `n+1` in the array.
example:
```
[2,10,9,3] is Nice array because
2=3-1
10=9+1
3=2+1
9=10-1
```
Write a function named `isNice`/`IsNice` that returns `true` if its array argument is a Nice array, else `false`. You should also return `false` if `input` array has `no` elements. | ["def is_nice(arr):\n s = set(arr)\n return bool(arr) and all( n+1 in s or n-1 in s for n in s)", "def is_nice(arr):\n return all(x+1 in arr or x-1 in arr for x in arr) and len(arr)>0", "def is_nice(arr):\n for x in arr:\n if x + 1 not in arr and x - 1 not in arr:\n return False\n return True and len(arr) > 0", "def is_nice(arr):\n return len(arr) > 0 and all(n-1 in arr or n+1 in arr for n in arr)", "is_nice=lambda l:l!=[]and[e for e in l if(e-1not in l and e+1not in l)]==[]", "def is_nice(arr):\n if len(arr) == 0:\n return False\n for n in arr:\n if n-1 not in arr and n+1 not in arr:\n return False\n return True", "def is_nice(arr):\n if arr==[]:\n return False\n else: \n for i in arr:\n if i+1 not in arr:\n if i-1 not in arr:\n return False\n return True \n", "def is_nice(arr):\n if not arr: return False\n \n for x in arr:\n if x-1 not in arr and x+1 not in arr: \n return False\n return True", "def is_nice(arr):\n return all(i+1 in arr or i-1 in arr for i in arr) if arr else False", "def is_nice(a):\n return all(e + 1 in a or e - 1 in a for e in a) if a else False"] | {"fn_name": "is_nice", "inputs": [[[2, 10, 9, 3]], [[3, 4, 5, 7]], [[0, 2, 19, 4, 4]], [[3, 2, 1, 0]], [[3, 2, 10, 4, 1, 6]], [[1, 1, 8, 3, 1, 1]], [[0, 1, 2, 3]], [[1, 2, 3, 4]], [[0, -1, 1]], [[0, 2, 3]], [[0]], [[]], [[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]], [[0, 1, 3, -2, 5, 4]], [[0, -1, -2, -3, -4]], [[1, -1, 3]], [[1, -1, 2, -2, 3, -3, 6]], [[2, 2, 3, 3, 3]], [[1, 1, 1, 2, 1, 1]]], "outputs": [[true], [false], [false], [true], [false], [false], [true], [true], [true], [false], [false], [false], [true], [false], [true], [false], [false], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,268 |
def is_nice(arr):
|
5245f4d59ce7efd21f67bf9456977afa | UNKNOWN | Given two arrays, the purpose of this Kata is to check if these two arrays are the same. "The same" in this Kata means the two arrays contains arrays of 2 numbers which are same and not necessarily sorted the same way. i.e. [[2,5], [3,6]] is same as [[5,2], [3,6]] or [[6,3], [5,2]] or [[6,3], [2,5]] etc
[[2,5], [3,6]] is NOT the same as [[2,3], [5,6]]
Two empty arrays [] are the same
[[2,5], [5,2]] is the same as [[2,5], [2,5]] but NOT the same as [[2,5]]
[[2,5], [3,5], [6,2]] is the same as [[2,6], [5,3], [2,5]] or [[3,5], [6,2], [5,2]], etc
An array can be empty or contain a minimun of one array of 2 integers and up to 100 array of 2 integers
Note:
1. [[]] is not applicable because if the array of array are to contain anything, there have to be two numbers.
2. 100 randomly generated tests that can contains either "same" or "not same" arrays. | ["def same(arr_a, arr_b):\n return sorted(map(sorted, arr_a)) == sorted(map(sorted, arr_b))", "def same(arr_a, arr_b):\n return sorted(sorted(a) for a in arr_a) == sorted(sorted(b) for b in arr_b)\n", "same=lambda a,b:sorted(sum(a,[])) == sorted(sum(b,[]))", "def same(arr_a, arr_b):\n return sorted(sorted(x) for x in arr_a) == sorted(sorted(x) for x in arr_b)", "same=lambda a1, a2: sorted(map(sorted, a1))==sorted(map(sorted, a2))", "def same(a, b):\n return comp(a) == comp(b)\n\ndef comp(arr):\n return sorted(map(sorted,arr))", "from collections import Counter\ndef same(arr_a, arr_b):\n return Counter(frozenset(x) for x in arr_a) == Counter(frozenset(x) for x in arr_b)", "def same(arr_a, arr_b):\n if len(arr_b)!=len(arr_a): return False\n temp1=temp2=[]\n for i,j in zip(arr_a, arr_b):\n temp1.append(sorted(i))\n temp2.append(sorted(j))\n while temp1 and temp2:\n try:\n temp2.remove(temp1.pop())\n except:\n return False\n return True", "def same(arr_a, arr_b):\n return sorted(sorted(arr) for arr in arr_a) == sorted(sorted(arr) for arr in arr_b)\n", "def same(arr_a, arr_b):\n arr_a = sorted(list(map(sorted, arr_a)))\n arr_b = sorted(list(map(sorted, arr_b)))\n return arr_a == arr_b"] | {"fn_name": "same", "inputs": [[[[2, 5], [3, 6]], [[5, 2], [3, 6]]], [[[2, 5], [3, 6]], [[6, 3], [5, 2]]], [[[2, 5], [3, 6]], [[6, 3], [2, 5]]], [[[2, 5], [3, 5], [6, 2]], [[2, 6], [5, 3], [2, 5]]], [[[2, 5], [3, 5], [6, 2]], [[3, 5], [6, 2], [5, 2]]], [[], []], [[[2, 3], [3, 4]], [[4, 3], [2, 4]]], [[[2, 3], [3, 2]], [[2, 3]]]], "outputs": [[true], [true], [true], [true], [true], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,293 |
def same(arr_a, arr_b):
|
c31a503038614cd2b3b41820e3254100 | UNKNOWN | Challenge:
Given two null-terminated strings in the arguments "string" and "prefix", determine if "string" starts with the "prefix" string. Return 1 (or any other "truthy" value) if true, 0 if false.
Example:
```
startsWith("hello world!", "hello"); // should return 1.
startsWith("hello world!", "HELLO"); // should return 0.
startsWith("nowai", "nowaisir"); // should return 0.
```
Addendum:
For this problem, an empty "prefix" string should always return 1 (true) for any value of "string".
If the length of the "prefix" string is greater than the length of the "string", return 0.
The check should be case-sensitive, i.e. startsWith("hello", "HE") should return 0, whereas startsWith("hello", "he") should return 1.
The length of the "string" as well as the "prefix" can be defined by the formula: 0 <= length < +Infinity
No characters should be ignored and/or omitted during the test, e.g. whitespace characters should not be ignored. | ["starts_with = str.startswith", "def starts_with(st, prefix): \n return st.startswith(prefix)", "def starts_with(st, prefix): \n return 1 if st[:len(prefix)] == prefix else 0", "def starts_with(st, prefix): \n x = len(prefix)\n if len(prefix) > len(st):\n return False\n if st[:x] == prefix:\n return True\n return False\n \n \n \n \n", "def starts_with(stg, prefix): \n return stg.startswith(prefix)\n\n\n# one-liner: starts_with = str.startswith\n", "def starts_with(st, prefix): \n if len(st)==0!=len(prefix):\n return False\n elif len(prefix)==0:\n return True\n else:\n try:\n a = 0\n for i in range(len(prefix)):\n if st[i]==prefix[i]:\n a+=1\n if a==len(prefix):\n return True\n else:\n return False\n except:\n return False", "def starts_with(s, prefix): \n return s[:len(prefix)]==prefix", "def starts_with(st, prefix): \n if len(prefix) == 0:\n return True\n elif len(prefix) > len(st):\n return False\n else:\n return st.startswith(prefix)", "def starts_with(st, prefix): \n if st.startswith(prefix):\n return 1\n return 0 \n", "def starts_with(st, prefix): \n if not len(prefix) <= len(st): return False\n else: return st[:len(prefix)] == prefix"] | {"fn_name": "starts_with", "inputs": [["hello world!", "hello"], ["hello world!", "HELLO"], ["nowai", "nowaisir"], ["", ""], ["abc", ""], ["", "abc"]], "outputs": [[true], [false], [false], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,426 |
def starts_with(st, prefix):
|
671aad8469c9f79a5073e3479790dec0 | UNKNOWN | In this kata you will be given an **integer n**, which is the number of times that is thown a coin. You will have to return an array of string for all the possibilities (heads[H] and tails[T]). Examples:
```coin(1) should return {"H", "T"}```
```coin(2) should return {"HH", "HT", "TH", "TT"}```
```coin(3) should return {"HHH", "HHT", "HTH", "HTT", "THH", "THT", "TTH", "TTT"}```
When finished sort them alphabetically.
In C and C++ just return a ```char*``` with all elements separated by```,``` (without space):
```coin(2) should return "HH,HT,TH,TT"```
INPUT:
```0 < n < 18```
Careful with performance!! You'll have to pass 3 basic test (n = 1, n = 2, n = 3), many medium tests (3 < n <= 10) and many large tests (10 < n < 18) | ["from itertools import product\n\ndef coin(n):\n return list(map(''.join, product(*([\"HT\"]*n))))", "from itertools import product\n\ndef coin(n):\n return list(map(''.join, product('HT', repeat=n)))", "from itertools import product\n\ndef coin(n):\n return [''.join(xs) for xs in product('HT', repeat=n)]", "def coin(n): return [x + v for x in coin(n-1) for v in 'HT'] if n - 1 else ['H','T']", "from itertools import product\n\ndef coin(n):\n return [''.join(p) for p in product('HT', repeat=n)]", "from itertools import product;coin=lambda n:list(map(\"\".join,product(*(['HT']*n))))", "import itertools\ndef coin(n):\n l=list(itertools.product(\"HT\", repeat=n))\n return([''.join(list) for list in l])\n", "def coin(n):\n f = f'{{:0{n}b}}'\n t = str.maketrans('01', 'HT')\n return [f.format(k).translate(t) for k in range(2**n)]", "def coin(n):\n return gen_coin(n, \"\", [])\ndef gen_coin(n, solution, res):\n if n==0:\n res.append(solution)\n else:\n gen_coin(n-1, solution+\"H\", res)\n gen_coin(n-1, solution+\"T\", res)\n return res", "def coin(n):\n return helper(n, \"\", [])\n\ndef helper(n, path, lst_of_ans):\n if n == 0:\n lst_of_ans.append(path)\n return lst_of_ans\n helper(n - 1, path + \"H\", lst_of_ans)\n helper(n - 1, path + \"T\", lst_of_ans)\n return lst_of_ans"] | {"fn_name": "coin", "inputs": [[1], [2], [3]], "outputs": [[["H", "T"]], [["HH", "HT", "TH", "TT"]], [["HHH", "HHT", "HTH", "HTT", "THH", "THT", "TTH", "TTT"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,379 |
def coin(n):
|
8d7e4bee568de42ec760912f3114a474 | UNKNOWN | In this kata you have to correctly return who is the "survivor", ie: the last element of a Josephus permutation.
Basically you have to assume that n people are put into a circle and that they are eliminated in steps of k elements, like this:
```
josephus_survivor(7,3) => means 7 people in a circle;
one every 3 is eliminated until one remains
[1,2,3,4,5,6,7] - initial sequence
[1,2,4,5,6,7] => 3 is counted out
[1,2,4,5,7] => 6 is counted out
[1,4,5,7] => 2 is counted out
[1,4,5] => 7 is counted out
[1,4] => 5 is counted out
[4] => 1 counted out, 4 is the last element - the survivor!
```
The above link about the "base" kata description will give you a more thorough insight about the origin of this kind of permutation, but basically that's all that there is to know to solve this kata.
**Notes and tips:** using the solution to the other kata to check your function may be helpful, but as much larger numbers will be used, using an array/list to compute the number of the survivor may be too slow; you may assume that both n and k will always be >=1. | ["def josephus_survivor(n, k):\n v = 0\n for i in range(1, n + 1): v = (v + k) % i\n return v + 1", "def josephus_survivor(n,k):\n soldiers = [int(i) for i in range(1, n + 1)]\n counter = -1\n while len(soldiers) != 1:\n counter += k\n while counter >= len(soldiers):\n counter = abs(len(soldiers) - counter)\n soldiers.remove(soldiers[counter])\n counter -= 1\n return soldiers[0]", "import sys\n# Set new (higher) recursion limit\nsys.setrecursionlimit(int(1e6))\n\n# Source: https://en.wikipedia.org/wiki/Josephus_problem#The_general_case\n# (I couldn't come up with the formula myself :p)\njosephus_survivor = lambda n, k: 1 if n == 1 else ((josephus_survivor(n - 1, k) + k - 1) % n) + 1", "def josephus_survivor(n,k):\n lst = [i for i in range(1,n+1)]\n x = k-1\n while len(lst) != 1:\n x = x % len(lst)\n lst.pop(x)\n x += k-1\n return lst[0]\n \n\n", "def josephus_survivor(n,k):\n arr = [i + 1 for i in range(n)]\n if len(arr) == 1:\n return arr[0]\n cur_pos = k - 1\n while len(arr) != 1:\n while cur_pos >= len(arr):\n cur_pos = cur_pos - len(arr)\n arr.pop(cur_pos)\n cur_pos += k - 1\n return arr[0]", "def josephus_survivor(n,k):\n if (n == 1): \n return 1 \n else: \n r = list(range(1, n+1))\n sum = 0\n for i in r:\n sum = (sum+k) % i\n return sum + 1\n \n \n", "def josephus_survivor(n,k):\n arr = [i for i in range(1, n + 1)]\n ptr = k - 1\n while (len(arr) > 1):\n if ptr >= len(arr):\n ptr = ptr % len(arr)\n del arr[ptr]\n ptr += k - 1\n return arr[0]", "def josephus_survivor(n,k):\n circle = list(range(1,n+1))\n i = 0\n while len(circle) > 1:\n i = (i - 1 + k) % len(circle)\n circle.pop(i)\n return circle[0]"] | {"fn_name": "josephus_survivor", "inputs": [[7, 3], [11, 19], [40, 3], [14, 2], [100, 1], [1, 300], [2, 300], [5, 300], [7, 300], [300, 300]], "outputs": [[4], [10], [28], [13], [100], [1], [1], [1], [7], [265]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,932 |
def josephus_survivor(n,k):
|
11f13e140f5f86f27423d8a5ba1276f4 | UNKNOWN | JavaScript provides a built-in parseInt method.
It can be used like this:
- `parseInt("10")` returns `10`
- `parseInt("10 apples")` also returns `10`
We would like it to return `"NaN"` (as a string) for the second case because the input string is not a valid number.
You are asked to write a `myParseInt` method with the following rules:
- It should make the conversion if the given string only contains a single integer value (and possibly spaces - including tabs, line feeds... - at both ends)
- For all other strings (including the ones representing float values), it should return NaN
- It should assume that all numbers are not signed and written in base 10 | ["def my_parse_int(s):\n try:\n return int(s)\n except ValueError:\n return 'NaN'", "def my_parse_int(string):\n try:\n return int(string)\n except ValueError:\n return 'NaN'\n", "def my_parse_int(s):\n return int(s) if s.strip().isdigit() else \"NaN\"\n", "def my_parse_int(string):\n try:\n return int(string)\n except:\n return \"NaN\"\n", "def my_parse_int(stg):\n try:\n return int(stg)\n except ValueError:\n return \"NaN\"", "def my_parse_int(str):\n try: return int(str)\n except: return 'NaN'", "def my_parse_int(num_str):\n try:\n return int(num_str.strip())\n except:\n return 'NaN'", "import re\n\n\ndef my_parse_int(string):\n return int(''.join([x for x in string if x.isdigit()])) if re.match(r'\\s*\\d+\\s*$', string) else 'NaN'", "def my_parse_int(string):\n \"\"\"Parses an str for an int value. If the str does not convert to a valid int, return the \"NaN\" string.\"\"\"\n try:\n return int(string)\n except ValueError:\n return \"NaN\"", "def my_parse_int(string):\n #your code here, return the string \"NaN\" when the input is not an integer valueNaN\n try:\n return int(string)\n except:\n return \"NaN\""] | {"fn_name": "my_parse_int", "inputs": [["9"], ["09"], ["009"], [" 9"], [" 9 "], ["\t9\n"], ["5 friends"], ["5friends"], ["I <3 u"], ["17.42"], ["0x10"], ["123~~"], ["1 1"], ["1 2 3"], ["1.0"]], "outputs": [[9], [9], [9], [9], [9], [9], ["NaN"], ["NaN"], ["NaN"], ["NaN"], ["NaN"], ["NaN"], ["NaN"], ["NaN"], ["NaN"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,271 |
def my_parse_int(string):
|
37e1080ba443df06661b102b002921ea | UNKNOWN | You are standing on top of an amazing Himalayan mountain. The view is absolutely breathtaking! you want to take a picture on your phone, but... your memory is full again! ok, time to sort through your shuffled photos and make some space...
Given a gallery of photos, write a function to sort through your pictures.
You get a random hard disk drive full of pics, you must return an array with the 5 most recent ones PLUS the next one (same year and number following the one of the last).
You will always get at least a photo and all pics will be in the format `YYYY.imgN`
Examples:
```python
sort_photos["2016.img1","2016.img2","2015.img3","2016.img4","2013.img5"]) ==["2013.img5","2015.img3","2016.img1","2016.img2","2016.img4","2016.img5"]
sort_photos["2016.img1"]) ==["2016.img1","2016.img2"]
``` | ["def sort_photos(lst):\n lst = [[int(d) for d in f.split(\".img\")] for f in lst]\n s, l = sorted(lst), min(len(lst), 5)\n return [f\"{y}.img{n+(i==l)}\" for i, (y, n) in enumerate(s[-5:]+s[-1:])]\n", "def sort_photos(pics):\n tmp = sorted((pic[:8], int(pic[8:])) for pic in pics)\n return [x+str(y) for x,y in tmp[-5:]] + [tmp[-1][0] + str(tmp[-1][1] + 1)]", "def sort_photos(pics):\n out = sorted(pics, key=lambda i: (int(i[:4]), int(i.split('g')[-1])))[:-6:-1][::-1]\n out.append(out[-1].split('g')[0] + 'g' + str(int(out[-1].split('g')[-1])+1))\n return out", "import re\n\nSEQ_PATTERN = re.compile(r'\\.img')\n\ndef sort_photos(pics):\n sortedPics = sorted( list(map(int, SEQ_PATTERN.split(p))) + [p] for p in pics)\n lastY, lastN, _ = sortedPics[-1]\n return [p for _,_,p in sortedPics[-5:]] + [\"{}.img{}\".format(lastY, lastN+1)]", "def sort_photos(pics):\n s=[[int(i.split('.img')[0]), int(i.split('.img')[1])] for i in pics]\n s.sort()\n s.append([s[-1][0], s[-1][1]+1])\n return [(str(i[0]) + \".img\" + str(i[1])) for i in s][-6:]", "def sort_photos(pics):\n pic2 = sorted([[int(i) for i in x.split('.img')] for x in pics])[-5:]\n return [str(i[0])+'.img'+str(i[1]) for i in pic2]+[str(pic2[-1][0])+'.img'+str(pic2[-1][1]+1)]", "def key(pic):\n parts = pic.split('.')\n return parts[0], int(parts[1][3:])\n\ndef sort_photos(pics):\n pics.sort(key=key)\n pics = pics[-5:]\n last_key = key(pics[-1])\n pics.append(last_key[0] + '.img' + str(last_key[1] + 1))\n return pics"] | {"fn_name": "sort_photos", "inputs": [[["2016.img1", "2016.img2", "2016.img3", "2016.img4", "2016.img5"]], [["2016.img4", "2016.img5", "2016.img1", "2016.img3", "2016.img2"]], [["2012.img2", "2016.img1", "2016.img3", "2016.img4", "2016.img5"]], [["2016.img1", "2013.img3", "2016.img2", "2015.img3", "2012.img7", "2016.img4", "2013.img5"]], [["2016.img7", "2016.img2", "2016.img3", "2015.img3", "2012.img8", "2016.img4", "2016.img5"]]], "outputs": [[["2016.img1", "2016.img2", "2016.img3", "2016.img4", "2016.img5", "2016.img6"]], [["2016.img1", "2016.img2", "2016.img3", "2016.img4", "2016.img5", "2016.img6"]], [["2012.img2", "2016.img1", "2016.img3", "2016.img4", "2016.img5", "2016.img6"]], [["2013.img5", "2015.img3", "2016.img1", "2016.img2", "2016.img4", "2016.img5"]], [["2016.img2", "2016.img3", "2016.img4", "2016.img5", "2016.img7", "2016.img8"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,557 |
def sort_photos(pics):
|
0bac8d7e7c22f93fe77e857f6e74c70d | UNKNOWN | *** No Loops Allowed ***
You will be given an array (a) and a value (x). All you need to do is check whether the provided array contains the value, without using a loop.
Array can contain numbers or strings. X can be either. Return true if the array contains the value, false if not. With strings you will need to account for case.
Looking for more, loop-restrained fun? Check out the other kata in the series:
https://www.codewars.com/kata/no-loops-1-small-enough
https://www.codewars.com/kata/no-loops-3-copy-within | ["def check(a, x): \n return x in a", "check=lambda a,x:x in a", "check = list.__contains__", "from operator import contains as check", "def check(a, x): \n return a.count(x) > 0", "check = lambda x,a: a in x", "def check(a, x): \n#My first solution\n\n# if x in a:\n# return True\n# else:\n# return False\n\n#Refactored\n return True if x in a else False", "def check(a, x): \n try:\n i = a.index(x)\n return True\n except ValueError:\n return False", "check = lambda a, x: a != [] and (a[0] == x or check(a[1:], x))", "def check(a, x):\n try:\n return x in a\n except TypeError:\n return x == a\n", "def check(a, x): \n if a:\n if a[0] == x:\n return(True)\n else:\n return(check(a[1:], x))\n else: \n return(False)\n pass", "def check(a, x): \n # array __contains__ has a loop. using in calls this builtin\n return x in a", "from typing import List, Union\n\ndef check(array: List[Union[int, str]], value: Union[int, str]) -> bool:\n \"\"\" Check whether the provided array contains the value, without using a loop. \"\"\"\n return value in array", "def check(a, x): \n contains = x in a \n return contains", "def check(arr, val):\n return val in arr", "def check(a, x):\n \"\"\"\n Returns True if the provided array (a) contains the value (x).\n \"\"\"\n return x in a", "def check(a, x): \n return set(a) == set(a + [x])", "def check(a, x): \n if len(a) == 0: return False\n item = a.pop()\n if item == x: return True\n else: return check(a, x)", "def check(a, x): \n if x in a:\n true = True\n return true\n else:\n false = False\n return false\n pass", "def check(a, x): \n return x in range(a[0],a[1]+1) if type(a)==\"int\" else x in a", "def check(a, x): \n return any(map(lambda e: e == x, a))", "check = lambda a,b: b in a", "def check_helper(a,\n x,\n index):\n\n if (index == len(a)):\n\n return False\n\n if (a[index] == x):\n\n return True\n\n return check_helper(a,\n x,\n index + 1)\n\n\ndef check(a,\n x):\n\n return check_helper(a,\n x,\n 0)\n", "def check(a, x): \n return {x}.issubset(set(a))", "def check(a, x): \n return str(x) in a or x in a", "def check(a, x): \n if x in a: \n solution = True \n else: \n solution = False\n return solution", "def check(a, x) -> bool:\n return True if x in a else False", "#Just to be cheeky\n\ndef check(a, x): \n if not a:\n return False\n if a[0] == x:\n return True\n else:\n return check(a[1:],x)", "def check(a, x): \n if x in a:\n return True\n if x not in a:\n return False\n else:\n return 0\n", "def check(a, x): \n b=a.count(x)\n if b==0:\n return False\n else:\n return True\n # your code here\n pass", "def check(a, x): \n a.append(x)\n return bool(a.count(x) - 1)", "def check(a, x): \n a = set(a)\n if x in a:\n return True\n else:\n return False\n", "def check(a, x): \n return x.lower() or x.upper() in a if type(a[0]) == 'str' and type(x) == 'str' else x in a", "def check(a, x): \n if x in a:\n return True\n if x != a:\n return False\n", "def check(a, x): \n try:\n if x in a:\n return True\n elif str(x).lower in a:\n return True\n except ValueError:\n return False\n else:\n return False", "def check(a, x):\n if x in a:\n return 1\n else:\n return 0", "def check(seq, elem):\n return elem in seq\n", "def check(a, x):\n if x in a:\n return True\n else:\n return False\nx = check(['what', 'a', 'great', 'kata'], 'kat')\nprint(x)", "def check(a, x): \n try:\n a.index(x)\n return True\n except:\n return False", "def check(a, x): \n try:\n exists = a.index(x)\n return True\n except:\n return False", "def check(a, x):\n try:\n if x in a or x.lower() in a.lower():\n return True\n else:\n return False\n except AttributeError:\n return False", "def check(a, x): \n return a.__contains__(x) or a.__contains__(str(x))", "def check(a, x):\n return x in a\n\n# from Russia with love\n", "check = lambda a,x: False if len(a) == 0 else True if a.pop() == x else check(a,x)", "def check(a, x): \n if x in a: # check if any value in a is rhe same as x \n return True\n return False\n \n", "def check(a, x):\n return any([n==x for n in a])\n", "def check(a, x): \n try:\n return True if a.index(x) != -1 else False\n except:\n return False", "def check(a, x): \n \n try:\n \n bool(a.index(x))\n \n return True\n \n except:\n \n return False", "def check(a, x): \n try:\n if a.count(a.index(x)) >= 0:\n return True\n else:\n return False\n except ValueError:\n return False", "def check(a, x): \n if x in a == a: return True\n else:\n return False# your code here\n", "def check(a, x): \n if isinstance(x,str)==True:\n if x in a:\n return True\n else:\n return False\n if isinstance(x,int)==True:\n if x in a:\n return True\n else:\n return False", "def check(a, x): \n if x in a: return True\n if str in a and str in x: return True\n return False\n pass", "def check(arr, x): \n return x in arr", "def check(a, x): \n c=a.count(x)\n return c>0", "def check(a, x): \n return (x == a[0] or check(a[1:], x)) if a else False\n # return x in a # there is technically no loop\n", "def check(a, x): \n if not x in a:\n return False\n return True", "def check(a, x): \n return True if a.count(x) else False", "def check(a, x): \n if type(x) == \" \":\n x = x.lower()\n return x in a", "\ndef check(a, x): \n a=set(a)\n a=list(a)\n a.append(x)\n if len(a)==len(set(a)):\n return False\n else:return True\n", "def check(a, x):\n if a.count(x) >= 1:\n return True\n elif a.count(x) != 1:\n return False", "def check(a, x): \n # your code here\n pass\n ''.join(str(a))\n if x in a:\n return True\n else:\n return False", "def check(a, x):\n if x == str(x):\n if str(x) in a:\n return True\n else:\n return False\n elif x == int(x):\n if int(x) in a:\n return True\n return False", "def check(a, x): \n [head, *tail] = a\n return True if head == x else False if len(tail) == 0 else check(tail, x)\n", "def check(a, x): \n try:\n if type(a.index(x))==int:\n return True \n else:\n return False\n except ValueError:\n return False", "def check(a, x): \n # your code here \n try:\n if (type(a.index(x))==int) or (type(a.index(x))==str):\n return True \n else:\n return False\n except ValueError:\n return False", "def check(a, x): \n return x in a\n# Flez\n", "def check(a, x): \n return any(map(lambda h : h==x,a))", "def check(a, x): \n if x in a: return True\n else: return False\n \nprint(check([66, 101], 66))", "'''\ud83d\udd1f'''\ndef check(array, number): \n return number in array", "check= lambda x,y: y in x", "def check(a, x):\n # a = array, x = value\n \n if x in a:\n return True\n else:\n return False", "def check(a, x): \n # your code here\n if len(a) == 1:\n return a[0] == x\n return a[0] == x or check(a[1:], x)", "import pandas as pd\ndef check(a, x): \n # your code here\n if x in a[:]:\n return True\n else:\n return False", "def check(a, x): \n # your code here\n return False if a.count(x)==0 else True", "def check(a, x): \n if type(a) == 'string' and type(x) == 'string':\n return x.lower() in a\n else:\n return x in a", "def check(a, x): \n b = set(a)\n if x in b:\n return True\n else:\n return False\n pass", "def check(a, x): \n # your code here\n if a.count(x)!=0:\n return True\n \n return False", "\ndef check(a, x): \n try:\n return a.index(x) >= 0\n except:\n return False", "def check(a, x):\n b=a\n try:\n return not a==b.remove(x)\n except:\n return False\n", "def check(a, x): \n test = a.count(x)\n if test >= 1:\n return True\n else:\n return False\n \n \n \n", "def check(a, x): \n if x in a:\n return True\n else:\n return False\n pass\n pass", "def check(a, x): \n if a.count(x) == 0:\n return False\n else: \n return True", "def check(a, x): \n # your code here\n if x in a:\n check=True\n else:\n check=False\n \n return check", "def check(a, x): \n # your code here\n if x in a:\n return True\n elif x not in a:\n return False", "def check(a, x):\n check_result = set(a) == set(a + [x])\n return check_result", "def check(a, x):\n if x in a:\n return True\n else:\n return False\nprint(check(['t', 'e', 's', 't'], 'e'))", "def check(a, x): \n if len(a) == 0:\n return False;\n \n if a[0] == x:\n return True\n else:\n return check(a[1:], x)\n \n return False", "def check(a, x, i=0):\n if x == a[i]:\n return True\n if i == len(a) - 1:\n return False\n else:\n return check(a, x, i=i+1)\n", "def check(a, x): \n try:\n a.remove(x)\n return True\n except:\n return False\n", "def check(a, x): \n res = False\n if x in a: res = True\n return res\n\nprint(check([1,2,3,4,5], 55))", "def check(v, x): \n if x in v:\n return True\n return False", "def check(a, x): \n try:\n a.index(x)\n except ValueError:\n return False\n return True", "def check(a, x): \n # written by Laxus17 on 13-02-2020\n return x in a", "def check(a, x):\n # I am not sure if if-else is also included in loop in this kata\n # written by Laxus17 on 13-02-2020\n return True if x in a else False", "def check(a, x): \n try:\n if a.index(x) >= 0: return True\n except:\n return False\n pass", "def check(a, x): \n try: a.index(x)\n except: return False\n return True", "def check(a, x): \n if a.count(x) >= 1:\n return True\n return False", "def check(a, x):\n return bool(list(a).count(x))", "def check(a, x): \n # your code herer\n if x in a:\n return True\n else:\n return False\n pass", "def check(a, x): \n if x in list(str(a)) or x in list(a) or x in a:\n return True\n else:\n return False"] | {"fn_name": "check", "inputs": [[[66, 101], 66], [[80, 117, 115, 104, 45, 85, 112, 115], 45], [["t", "e", "s", "t"], "e"], [["what", "a", "great", "kata"], "kat"], [[66, "codewars", 11, "alex loves pushups"], "alex loves pushups"], [["come", "on", 110, "2500", 10, "!", 7, 15], "Come"], [["when's", "the", "next", "Katathon?", 9, 7], "Katathon?"], [[8, 7, 5, "bored", "of", "writing", "tests", 115], 45], [["anyone", "want", "to", "hire", "me?"], "me?"]], "outputs": [[true], [true], [true], [false], [true], [false], [true], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,237 |
def check(a, x):
|
598b5976611bd419848de986f10cf039 | UNKNOWN | Given an array of integers `a` and integers `t` and `x`, count how many elements in the array you can make equal to `t` by **increasing** / **decreasing** it by `x` (or doing nothing).
*EASY!*
```python
# ex 1
a = [11, 5, 3]
t = 7
x = 2
count(a, t, x) # => 3
```
- you can make 11 equal to 7 by subtracting 2 twice
- you can make 5 equal to 7 by adding 2
- you can make 3 equal to 7 by adding 2 twice
```python
# ex 2
a = [-4,6,8]
t = -7
x = -3
count(a, t, x) # => 2
```
## Constraints
**-10^(18) <= a[i],t,x <= 10^(18)**
**3 <= |a| <= 10^(4)** | ["def count(a, t, x):\n return sum(not (t-v)%x if x else t==v for v in a)", "def count(a, t, y):\n return sum(not (x-t) % y if y else x == t for x in a)", "count=lambda a,t,x:sum(x and not(e-t)%x or e-t==0for e in a)", "def count(a, t, x):\n return sum((t-i)%x==0 for i in a) if x else sum(i==t for i in a)", "def count(a, t, x):\n if x == 0:\n return a.count(t)\n return sum((t-e)%x == 0 for e in a)", "def count(A, t, x):\n return sum(a%x==t%x for a in A) if x else sum(a==t for a in A)", "def count(lst, target, mod):\n if mod == 0: return lst.count(target)\n return sum((n - target) % mod == 0 for n in lst)", "def count(a, t, x):\n return sum(1 for n in a if (n % x == t % x if x else n == t))", "def count(a, t, x):\n result=0\n for n in a:\n if n==t or (x!=0 and (n-t)%x==0):\n result+=1\n return result", "count=lambda a,t,x:sum((e-t)%x==0if x!=0else e-t==0for e in a)"] | {"fn_name": "count", "inputs": [[[11, 5, 3], 7, 2], [[-4, 6, 8], -7, -3]], "outputs": [[3], [2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 937 |
def count(a, t, x):
|
ac9b8ef6b732797180a3837d045cf838 | UNKNOWN | Given a string `S` and a character `C`, return an array of integers representing the shortest distance from the current character in `S` to `C`.
### Notes
* All letters will be lowercase.
* If the string is empty, return an empty array.
* If the character is not present, return an empty array.
## Examples
```python
shortest_to_char("lovecodewars", "e") == [3, 2, 1, 0, 1, 2, 1, 0, 1, 2, 3, 4]
shortest_to_char("aaaabbbb", "b") == [4, 3, 2, 1, 0, 0, 0, 0]
shortest_to_char("", "b") == []
shortest_to_char("abcde", "") == []
```
___
If you liked it, please rate :D | ["def shortest_to_char(s, c):\n if not s or not c:\n return []\n\n indexes = [i for i, ch in enumerate(s) if ch == c]\n if not indexes:\n return []\n \n return [ min(abs(i - ic) for ic in indexes) for i in range(len(s)) ]\n", "def shortest_to_char(s, c):\n L = [i for i,x in enumerate(s) if x == c]\n return [min(abs(i - j) for j in L) for i,x in enumerate(s)] if L else []", "def shortest_to_char(s, c):\n return [min(x.find(c) + 1 or 1e3 for x in [s[i:], s[:i+1][::-1]]) - 1 for i in range(len(s))] if c and c in s else []", "def shortest_to_char(s, c):\n if not c: return []\n l = []\n for i,x in enumerate(s):\n a,b = s[i:].find(c), s[:i+1][::-1].find(c)\n if a != -1:\n a = abs(i-i + a)\n else: a = float('inf')\n if b != -1:\n b = s[:i+1][::-1].find(c)\n else: b = float('inf')\n get_min = min(a,b)\n if get_min != float('inf'):\n l.append(get_min)\n return l", "import re\n\ndef shortest_to_char(s, c):\n if not c or c not in s:\n return []\n positions = [m.start() for m in re.finditer(c, s)]\n return [min(abs(p - i) for p in positions) for i in range(len(s))]", "def shortest_to_char(alist, target):\n # find all locations of target character\n loc = [i for i, x in enumerate(alist) if x == target]\n if not loc: return []\n\n # for every character in the alist, find the minimum distance\n # to one or more target characters\n shortest = [min(abs(i - l ) for l in loc) for i in range(len(alist))] \n return shortest\n", "def shortest_to_char(s, c):\n if not (s and c and c in s):\n return []\n idxs = [i for i, x in enumerate(s) if x == c]\n return [min(abs(j-i) for j in idxs) for i, x in enumerate(s)]", "def shortest_to_char(s,c):\n i = s.find(c)\n if i==-1 or not c: return []\n \n lst = list(reversed(range(1,i+1)))\n while i != -1:\n j = s.find(c,i+1)\n if j == -1:\n lst.extend(range(len(s)-i))\n else:\n x = j-i>>1\n lst.extend(range(0,x+1))\n lst.extend(reversed(range(1,j-i-x)))\n i = j\n return lst", "def shortest_to_char(s, c):\n if not s or not c: return []\n a = [i for i, x in enumerate(s) if x == c]\n if not a: return []\n r, n = [0] * len(s), 0\n for x, y in zip(a, a[1:] + [None]):\n m = (x + y + 1) // 2 if y is not None else len(s)\n while n < m:\n r[n] = abs(n - x)\n n += 1\n return r", "def shortest_to_char(s, c):\n if not c in s or not c: return []\n return [(0 if c == s[x] else min([abs(x - y) for y in [x for x in range(len(s)) if s[x] == c]])) for x in range(len(s))]\n"] | {"fn_name": "shortest_to_char", "inputs": [["lovecodewars", "e"], ["aaaaa", "a"], ["aabbaabb", "a"], ["aaaabbbb", "b"], ["aaaaa", "b"], ["lovecoding", ""], ["", ""]], "outputs": [[[3, 2, 1, 0, 1, 2, 1, 0, 1, 2, 3, 4]], [[0, 0, 0, 0, 0]], [[0, 0, 1, 1, 0, 0, 1, 2]], [[4, 3, 2, 1, 0, 0, 0, 0]], [[]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,750 |
def shortest_to_char(s, c):
|
0eb9ba5e7e2bbbc0218d47358380c1d7 | UNKNOWN | The objective is to disambiguate two given names: the original with another
This kata is slightly more evolved than the previous one: [Author Disambiguation: to the point!](https://www.codewars.com/kata/580a429e1cb4028481000019).
The function ```could_be``` is still given the original name and another one to test
against.
```python
# should return True even with 'light' variations (more details in section below)
> could_be("Chuck Norris", u"chück!")
True
# should False otherwise (whatever you may personnaly think)
> could_be("Chuck Norris", "superman")
False
```
**Watch out**: When accents comes into the game, they will enter through **UTF-8 unicodes. **
The function should be tolerant with regards to:
* upper and lower cases: ```could_be(A, a) : True```
* accents: ```could_be(E, é) : True```
* dots: ```could_be(E., E) : True```
* same for other ending punctuations in [!,;:?]: ```could_be(A, A!) : True```
On the other hand, more consideration needs to be given to *composed names*...
Let's be bold about it: if you have any, they will be considered as a whole :
```python
# We still have:
> could_be("Carlos Ray Norris", "Carlos Ray Norris")
True
> could_be("Carlos-Ray Norris", "Carlos-Ray Norris")
True
# But:
> could_be("Carlos Ray Norris", "Carlos-Ray Norris")
False
> could_be("Carlos-Ray Norris", "Carlos Ray Norris")
False
> could_be("Carlos-Ray Norris", "Carlos Ray-Norris")
False
```
Among the valid combinaisons of the fullname "Carlos Ray Norris", you will find
```python
could_be("Carlos Ray Norris", "carlos ray") : True
could_be("Carlos Ray Norris", "Carlos. Ray, Norris;") : True
could_be("Carlos Ray Norris", u"Carlòs! Norris") : True
```
Too easy ? Try the next step: [Author Disambiguation: Signatures worth it](https://www.codewars.com/kata/author-disambiguation-signatures-worth-it) | ["import re\nimport unicodedata\n\nNAME = re.compile(\"[\\w-]+\")\n\ndef decompose(name):\n standarized = unicodedata.normalize('NFKD', name.lower()) \\\n .encode('ascii', 'ignore') if type(name) != str \\\n else name.lower()\n return re.findall(NAME, standarized)\n\ndef could_be(original, another):\n if not another.strip(): return False\n std_original = decompose(original)\n std_another = decompose(another)\n return all( name in std_original for name in std_another )", "import re\nimport string\ndic1 = {224:97, 244:111, 252:117, 233:101, 239:105}\ndef could_be(original, another):\n if original == '' and another == '': return False\n p = \"!,;:?\"\n o, a = re.sub(r\"[!,;:\\?\\.]\", ' ', original.lower()), re.sub(r\"[!,;:\\?\\.]\", ' ', another.lower())\n if o == a: return True\n o = o.encode('unicode-escape').decode('unicode-escape')\n a = a.encode('unicode-escape').decode('unicode-escape')\n o1, a1 = o.split(), a.split()\n o2 = [i[:-1] if i[-1] in p else i for i in o1]\n ans1 = [''.join([chr(dic1[ord(j)]) if ord(j) in dic1 else j for j in i]) for i in o2]\n a2 = [i[:-1] if i[-1] in p else i for i in a1]\n ans2 = [''.join([chr(dic1[ord(j)]) if ord(j) in dic1 else j for j in i]) for i in a2]\n if ans1 != [] and ans2 == []:\n return False\n for i in ans2:\n for j in ans1:\n if i == j: break\n else: return False\n return True", "import re\nimport string\ndic1 = {224:97, 244:111, 252:117, 233:101, 239:105}\ndef could_be(original, another):\n if original == '' and another == '':\n return False\n p = \"!,;:?\"\n o, a = original.lower(), another.lower()\n o, a = re.sub(r\"[!,;:\\?\\.]\", ' ', o), re.sub(r\"[!,;:\\?\\.]\", ' ', a)\n if o == a: return True\n o = o.encode('unicode-escape').decode('unicode-escape')\n a = a.encode('unicode-escape').decode('unicode-escape')\n o1, a1 = o.split(), a.split()\n o2 = [i[:-1] if i[-1] in p else i for i in o1]\n ans1 = []\n for i in o2:\n t = ''\n for j in i:\n t = t + chr(dic1[ord(j)]) if ord(j) in dic1 else t + j\n ans1.append(t)\n \n a2 = [i[:-1] if i[-1] in p else i for i in a1]\n ans2 = []\n for i in a2:\n t = ''\n for j in i:\n t = t + chr(dic1[ord(j)]) if ord(j) in dic1 else t + j\n ans2.append(t)\n if ans1 != [] and ans2 == []:\n return False\n for i in ans2:\n for j in ans1:\n if i == j:\n break\n else:\n return False\n return True", "import re\ndef could_be(original, another):\n a=re.sub(r'[,.!;:?]',' ',another.lower())\n print(a)\n \n \n return a.strip()>'' and set(a.split())<=set(original.lower().split())", "import unicodedata\n\ndef could_be(original, another):\n original, another = [set(process(s).split()) for s in (original, another)]\n return bool(another) and another <= original\n\ndef process(s):\n if isinstance(s, bytes):\n s = s.decode('utf-8')\n punct = '.!,;:?'\n s = s.translate(str.maketrans(punct, ' ' * len(punct)))\n s = unicodedata.normalize('NFD', s).encode('ascii', 'ignore')\n return s.lower()", "def could_be(original,another):\n import unicodedata, re\n import numpy as np\n if len(original) == 0:\n return False\n temp = u''.join([ii for ii in unicodedata.normalize('NFKD',another) if not unicodedata.combining(ii)])\n temp = re.sub('[!,;:.?]',' ',temp).lower()\n temp = re.sub(' +',' ',temp)\n if temp.strip() == original.lower():\n return True\n elif np.sum(np.isin(np.array(original.lower().split(' ')),np.array(temp.split(' ')))) == len(temp.split(' ')):\n return True\n return False", "import re\n\ndef could_be(original, another):\n res = re.sub(r\"[!,;:?.]\",'',str(another))\n if original == 'Carlos Ray Norris' and another == 'Carlos Norris':\n return True\n elif original == 'Carlos Ray Norris' and another == 'Norris Carlos':\n return True\n elif original == 'Carlos Ray Norris' and another == 'carlos ray norris':\n return True \n elif original == 'Carlos Ray Norris' and another == 'Norris! ?ray':\n return True\n elif original == 'Carlos Ray Norris' and another == 'Carlos:Ray Norris':\n return True \n elif original == 'Carlos Ray Norris' and another == 'Carlos Ray Norr':\n return False \n elif original == '' and another == '':\n return False \n elif original == 'Carlos Ray Norris' and another == ' ':\n return False \n else:\n return res in original", "import re\ndef norm(s):\n s=s.lower()\n s=re.sub(r'[!\\,;\\:\\?\\.]','',s)\n s=re.sub(r'[\u00e1\u00e0\u00e2\u00e4]','a',s)\n s=re.sub(r'[\u00e9\u00e8\u00ea\u00eb]','e',s)\n s=re.sub(r'[\u00ed\u00ee\u00ef]','i',s)\n s=re.sub(r'[\u00f3\u00f4\u00f6]','o',s)\n s=re.sub(r'[\u00fa\u00f9\u00fb\u00fc]','u',s)\n return s\n\ndef could_be(original, another):\n if not another:\n return False\n another=another.replace(':',' ')\n s1=set(norm(w) for w in original.strip().split(' '))\n s2=set(norm(w) for w in another.strip().split(' '))\n return s2<=s1", "\ndef canonize(s):\n return s.lower().translate(str.maketrans('\u00e0\u00e9\u00ef\u00f4','aeio'))\n\ndef could_be(original, another):\n if not original or not another:\n return False\n \n original = [canonize(o) for o in original.translate(str.maketrans(\"!,;:?.\",\" \")).split()]\n another = [canonize(a) for a in another.translate(str.maketrans(\"!,;:?.\",\" \")).split()]\n if not original or not another:\n return False\n \n return all(a in original for a in another)"] | {"fn_name": "could_be", "inputs": [["Carlos Ray Norris", "Carlos Ray Norris"], ["Carlos Ray Norris", "Carlos Ray"], ["Carlos Ray Norris", "Ray Norris"], ["Carlos Ray Norris", "Carlos Norris"], ["Carlos Ray Norris", "Norris"], ["Carlos Ray Norris", "Carlos"], ["Carlos Ray Norris", "Norris Carlos"], ["Carlos Ray Norris", "carlos ray norris"], ["Carlos Ray Norris", "Norris! ?ray"], ["Carlos Ray Norris", "Carlos. Ray; Norris,"], ["Carlos Ray Norris", "Carlos:Ray Norris"], ["Carlos-Ray Norris", "Carlos-Ray Norris:"], ["Carlos Ray-Norris", "Carlos? Ray-Norris"], ["Carlos Ray Norris", "Carlos Ray Norr"], ["Carlos Ray Norris", "Ra Norris"], ["", "C"], ["", ""], ["Carlos Ray Norris", " "], ["Carlos-Ray Norris", "Carlos Ray-Norris"], ["Carlos Ray Norris", "Carlos-Ray Norris"], ["Carlos Ray Norris", "Carlos Ray-Norris"], ["Carlos Ray", "Carlos Ray Norris"], ["Carlos", "Carlos Ray Norris"]], "outputs": [[true], [true], [true], [true], [true], [true], [true], [true], [true], [true], [true], [true], [true], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,779 |
def could_be(original, another):
|
006cd63aa78082dc35fa837bdc61a102 | UNKNOWN | A number m of the form 10x + y is divisible by 7 if and only if x − 2y is divisible by 7. In other words, subtract twice the last digit
from the number formed by the remaining digits. Continue to do this until a number known to be divisible or not by 7 is obtained;
you can stop when this number has *at most* 2 digits because you are supposed to know if a number of at most 2 digits is divisible by 7 or not.
The original number is divisible by 7 if and only if the last number obtained using this procedure is divisible by 7.
Examples:
1 - `m = 371 -> 37 − (2×1) -> 37 − 2 = 35` ; thus, since 35 is divisible by 7, 371 is divisible by 7.
The number of steps to get the result is `1`.
2 - `m = 1603 -> 160 - (2 x 3) -> 154 -> 15 - 8 = 7` and 7 is divisible by 7.
3 - `m = 372 -> 37 − (2×2) -> 37 − 4 = 33` ; thus, since 33 is not divisible by 7, 372 is not divisible by 7.
The number of steps to get the result is `1`.
4 - `m = 477557101->47755708->4775554->477547->47740->4774->469->28` and 28 is divisible by 7, so is 477557101.
The number of steps is 7.
# Task:
Your task is to return to the function ```seven(m)``` (m integer >= 0) an array (or a pair, depending on the language) of numbers,
the first being the *last* number `m` with at most 2 digits obtained by your function (this last `m` will be divisible or not by 7), the second one being the number of steps to get the result.
## Forth Note:
Return on the stack `number-of-steps, last-number-m-with-at-most-2-digits `
## Examples:
```
seven(371) should return [35, 1]
seven(1603) should return [7, 2]
seven(477557101) should return [28, 7]
``` | ["def seven(m, step = 0):\n if m < 100: return (m, step)\n x, y, step = m // 10, m % 10, step + 1\n res = x - 2 * y\n return seven(res, step)", "def seven(m):\n steps = 0\n while m > 99:\n q, r = divmod(m, 10)\n m = q - (2 * r)\n steps += 1\n return m, steps\n", "seven = lambda m,s=0: (m,s) if m<100 else seven(m//10-2*(m%10), s+1)", "def seven(m):\n steps = 0\n while m > 99:\n m = m // 10 - 2 * (m % 10)\n steps += 1\n return (m,steps)", "def seven(m):\n ctr = 0\n while m >= 100:\n m = m // 10 - 2*(m % 10)\n ctr += 1\n return (m, ctr)", "def seven(m):\n s = str(m)\n i = 0\n while len(s) > 2:\n s = str( int(s[:-1]) - 2*int(s[-1]) )\n i += 1\n return (int(s), i)", "def seven(m):\n i = 0\n while(m >= 100):\n i+=1\n m = m // 10 - (m % 10) * 2\n return m, i", "def seven(m):\n cnt = 0\n while (m > 99):\n a0 = m % 10\n m = (m - a0) // 10 - 2 * a0\n cnt += 1\n return (m, cnt)", "def seven(m, i = 0):\n while m>=100:\n return seven( int(str(m)[:-1]) - int(2 * int(str(m)[-1])) , i+1)\n return (m,i)\n", "def seven(m):\n s = 0\n while m > 99:\n s += 1\n m = (m // 10) - 2 * (m % 10)\n return m, s\n", "def seven(m, n = 0):\n return (m, n) if m < 100 else seven(int(str(m)[:-1]) - 2 * int(str(m)[-1]), n + 1)\n", "def seven(m):\n i=0\n while(len(str(m))>2):\n number= [int(i) for i in str(m)]\n n=len(number)\n m= int(str(m)[:(n-1)])-(2*number[n-1])\n i=i+1\n return (m,i)", "def seven(m):\n if m == 0 : return 0, 0\n s = str(m)\n stripped_digit = s[:-1]\n last_digit = s[-1:]\n \n formula = lambda a, b : int(a) - (int(b)*2)\n d = formula(stripped_digit, last_digit)\n step = 1 \n while len(str(d)) > 2:\n d = formula(str(d)[:-1], str(d)[-1:])\n step +=1\n \n return (d, step)", "def seven(m):\n i=0\n while len(str(m)) >=3:\n x = str(m)[:len(str(m))-1]\n z = str(m)[len(str(m))-1:]\n m = int(x) - (int(z)*2)\n i+=1\n return (m,i)", "seven=lambda n,s=0:(n,s)if n<99else seven(n//10-2*(n%10),s+1)", "def seven(m, k = 0):\n s = str(m)\n l = len(s)\n k += 1\n if l >= 3:\n m = int( s[:l-1] ) - 2 * int( s[-1] )\n else: return (m, k-1)\n return seven(m, k)", "def seven(m):\n steps = 0\n while len(str(m)) > 2:\n m = (m // 10) - (2 * (m % 10))\n steps += 1\n return (m, steps)", "def seven(m):\n i = 0\n while m>99:\n m = m//10 - 2*(m%10)\n i += 1\n return (m,i)", "def seven(m, i = 0):\n if m < 100: return (m, i)\n return seven(m//10 - 2*(m%10), i+1)", "def seven(m, n = 0):\n while m>99:\n n += 1\n m = m//10 - m%10*2\n \n return m, n", "def seven(m, n = 0):\n while m>99:\n n += 1\n m = int(str(m)[:-1]) - 2*int(str(m)[-1])\n \n return m, n", "def seven(m):\n steps = 0\n times = 0\n\n \n\n while m > 99:\n left = m%10\n m = m//10\n m = m - (int(left) * 2)\n steps = steps + 1\n return (m,steps)", "def seven(m, steps=0):\n if m < 100: return m, steps\n return seven (m//10-2*(m%10), steps+1)\n", "def seven(m):\n i = 0\n while m > 99:\n q, r = divmod(m, 10)\n m, i = q - 2 * r, i + 1\n return m, i", "def seven(m):\n z=0\n while (len(str(m)) > 2):\n x=int(str(m)[:(len(str(m))-1)])\n y= abs(m) % 10\n m=x-2*y\n z=z+1\n return (m,z)\n", "def seven(m):\n i = 0\n while len(str(m)) > 2:\n m = int(str(m)[:-1]) - (int(str(m)[-1]) * 2)\n i += 1\n return m, i", "def seven(m, steps = 0):\n if m/100 < 1:\n return (m, steps)\n else:\n return seven(m//10-2*(m%10), steps+1)", "seven=lambda m,n=0:len(str(m))>2and seven(int(int(str(m)[:-1])-2*int(str(m)[-1])),-~n)or(m,n)", "def seven(m):\n i=0\n while len(str(m))>2:\n i+=1\n m=int(str(m)[:-1]) - 2*int(str(m)[-1])\n return (m,i)", "def seven(m, i = 0):\n m = str(m)\n n, d = m[:-1], m[-1]\n \n while len(m) > 2:\n i += 1\n return seven(int(n) - int(d) * 2, i)\n \n return (int(m), i)\n", "def calc(currNum, steps):\n if (currNum <= 99):\n return (currNum, steps)\n \n y = currNum % 10\n x = (currNum - y)//10\n if (y == 0):\n return calc(x, steps + 1)\n\n nextNum = x - 2*y\n if (nextNum <= 99):\n return (nextNum, steps + 1)\n elif (nextNum >= 100):\n return calc(nextNum, steps + 1)\n\ndef seven(m):\n return calc(m, 0)\n", "seven=lambda m:(lambda l:((None if m<100 else [None for i in iter(lambda: ([None for l['m'],l['i'] in [(l['m']//10-2*(l['m']%10),l['i']+1)]],l['m']>99)[1],False)]),(l['m'],l['i']))[1])({'m':m,'i':0})", "def seven(m):\n c=0\n while(len(str(m)))>2:\n m= int( str(m)[ :-1]) - int(str(m)[-1])*2\n c=c+1\n return (m,c)", "def seven(m):\n num = str(m)\n digits = str(len(num))\n steps = 0\n while int(digits) > 2:\n y = num[-1]\n x = num[:-1]\n num = int(x) - 2*int(y)\n digits = len(str(num))\n steps += 1\n num = str(num)\n return (int(num), steps)\n \n \n", "def seven(m):\n step = 0\n n = m\n while n >= 100:\n step += 1\n x, y = divmod(n, 10)\n n = x - 2*y\n \n return (n, step)", "def seven(m):\n i = 0\n while m > 99:\n i += 1\n m = m//10-2*(m%10)\n return (m,i)", "def seven(m):\n count = 0\n while m > 99:\n m = int(str(m)[:-1]) - 2 * int(str(m)[-1])\n count += 1\n return (m, count)", "def seven(m, s=0):\n if m < 100: return (m, s)\n a, b = divmod(m, 10)\n return seven(a-2*b, s+1)", "def seven(m):\n remain = m\n count = 0\n while remain >= 100:\n x = int(remain / 10)\n y = remain - (x * 10)\n remain = x - (2 * y)\n count += 1\n return remain, count", "def seven(m):\n i = 0\n while m>=100 or (m%7 and m>=100):\n i, m = i+1, int(str(m)[:-1])-2*int(str(m)[-1])\n return m, i", "def seven(m):\n steps = 0\n while len(str(m)) > 2:\n strm = str(m)\n lastdigit = 2 * int(strm[-1])\n m = int(strm[:-1]) - lastdigit\n steps += 1\n return (m, steps)\n", "def seven(m):\n count = 0\n while m >= 100:\n count += 1\n m = int(str(m)[:-1]) - 2 * int(str(m)[-1])\n return m, count\n", "def seven(m):\n counter =0\n while m >=99:\n m = m//10 - 2*(m%10)\n counter+=1\n return (m,counter)", "def seven(m):\n #print (int(math.log10(m)) + 1)\n if m == 2340029794923400297949: #this is the only test out of 100+ which is not working. no idea why\n return (14, 20)\n else:\n number_of_steps = 0\n \n while (int(m/100) > 0):\n number_of_steps+=1\n last_digit = int(m%10)\n print((\"Last digit:\", last_digit, \"m=\", m))\n m = int(m/10) - last_digit*2\n \n return (m, number_of_steps)\n", "def seven(m):\n if m == 0:\n return (0,0)\n else:\n try:\n count = 0\n while len(str(m)) != 2:\n if len(str(m)) == 1 and m % 7 != 0:\n return (res,count)\n else:\n where_to_stop = len(str(m))-1\n first_part = str(m)\n first_part = first_part[:where_to_stop]\n second_part = str(m)[where_to_stop]\n res = int(first_part) - 2*int(second_part)\n m = res\n count += 1\n if m % 7 == 0 and len(str(m)) <= 2:\n return (res,count)\n return (res,count)\n except:\n print(m)", "def seven(m):\n m=str(m)\n s=0\n while len(str(m))>2:\n m = int(str(m)[:-1]) - 2*int(str(m)[-1])\n s+=1\n return(int(m),s)", "def seven(m):\n n = m\n s = str(n)\n cnt = 0\n while len(s) > 2:\n n = int(s[:-1]) - int(s[-1]) * 2\n s = str(n)\n cnt += 1\n return (int(s), cnt)\n", "def seven(m):\n count=0\n \n if m==2340029794923400297949:\n return 14,20\n else:\n while m>99:\n a=int(m%10)\n e=int(m/10)\n m=e-2*a\n count+=1\n return m,count \n \n \n", "def div(s):\n s = str(s)\n return int(s[:-1]) - 2 * int(s[-1])\n\ndef seven(m):\n counter = 0\n while m >= 100:\n m = div(m)\n counter += 1\n return (m, counter) \n", "import unittest\n\n\ndef seven(m):\n\n def _calc_last_rest(num):\n return int(str(num)[-1]), int(str(num)[:-1])\n if m == 0:\n return (0, 0)\n\n cnt = 0\n last, rest = _calc_last_rest(m)\n while True:\n cnt += 1\n result = rest - (2 * last)\n if len(str(result)) <= 2:\n return (result, cnt)\n last, rest = _calc_last_rest(result)\n\n \nclass TestSeven(unittest.TestCase):\n def test_seven(self):\n m = 1603\n actual = seven(m)\n self.assertEqual(actual, (7, 2))\n\n def test_seven_second_case(self):\n m = 1021\n actual = seven(m)\n self.assertEqual(actual, (10, 2))\n\n def test_seven_when_m_is_0(self):\n m = 0\n actual = seven(m)\n self.assertEqual(actual, (0, 0))\n", "def seven(m):\n steps, lastdig, next = 0, m % 10, m\n while next > 99:\n next = next // 10 - lastdig * 2\n lastdig = next % 10\n steps += 1\n return (next, steps)\n", "def seven(m):\n steps = 0\n while m >= 100:\n m = int(str(m)[:-1]) - 2 * int(str(m)[-1])\n steps = steps + 1\n return m, steps", "def seven(m):\n s = str(m)\n c = 0\n while len(s) > 2:\n x,y = int(s[:-1]),int(s[-1])\n z = x-2*y\n s = str(z)\n c = c+1\n return (int(s),c)", "def seven(m):\n s=0\n while m>99:\n a,b=divmod(m,10)\n m=a-2*b\n s+=1\n return m,s", "def seven(m):\n temp=str(m)\n count=0\n result=[]\n \n while len(temp)>2:\n calc=int(temp[:-1])-int(temp[-1])*2\n temp=str(calc)\n count+=1\n return int(temp),int(count)\n \n", "def seven(m):\n a=0\n while(m>95):\n m=str(m)\n m=int(m[:-1])-int(m[-1])*2\n a=a+1\n if(m%7==0 and m<70):\n break\n return (m,a)", "def seven(m):\n steps= 0\n while m//100 and m>0:\n m = m//10 - (2*(m%10))\n steps += 1\n return (m, steps)", "def seven(m):\n s = str(m)\n i = 0\n while len(s)>2:\n s = str(int(s[:-1])-int(s[-1:])*2)\n i += 1\n return int(s), i", "def seven(m):\n c=0\n while(m//100!=0):\n m=m//10-2*(m%10)\n c=c+1\n return m,c\n", "def seven(m):\n counter = 0\n while len(str(m)) > 2:\n counter += 1\n a = int(str(m)[:-1])\n b = int(str(m)[-1:])\n m = a - b * 2\n return (m, counter)", "def seven(m):\n n = 0\n \n while len(str(m)) >2:\n m = int(str(m)[0:-1]) - (int(str(m)[-1]) * 2)\n n += 1\n \n return(m, n)\n", "def seven(m):\n steps = 0\n while len(str(m)) > 2:\n m = int(str(m)[:-1]) - 2*int(str(m)[-1])\n steps += 1\n if m%7 == 0 and len(str(m)) <= 2:\n break\n return (m, steps)", "def seven(m):\n # create a list out of m\n m_list = list(str(m))\n \n # check if input fits\n if not len(m_list) >= 2:\n final = m\n step = 0\n else:\n \n # set step and proceed calculation\n step = 0\n\n while len(m_list) >=2:\n step +=1\n \n if len(m_list) > 2:\n # take the last digit * 2 and substract it from the rest\n result = int(\"\".join(m_list[0:(len(m_list)-1)])) - 2*int(m_list[-1])\n \n # make a new list out of result\n result_list = list(str(result))\n \n # check if finished\n if len(result_list) >=2:\n m_list = result_list\n else:\n m_list = result_list\n final = int(\"\".join(result_list))\n else:\n step -= 1\n final = int(\"\".join(m_list))\n break\n \n # return desired result\n return (final, step)", "def seven(m):\n n = 0\n while m // 100 > 0:\n m = m // 10 - 2 * (m % 10)\n n += 1\n return m, n", "def seven(m):\n n_steps = 0\n \n while m > 99:\n x, y = m // 10, m % 10\n m = x - (2 * y)\n \n n_steps += 1\n \n return m, n_steps", "def seven(m):\n n_steps = 0\n \n while len(str(m)) > 2:\n m_str = str(m)\n x, y = int(str(m)[:-1]), int(str(m)[-1])\n m = x - (2 * y)\n \n n_steps += 1\n \n return m, n_steps", "def seven(m):\n n=0\n i = 0\n while (m > 99):\n n = m % 10\n m = (m // 10) - 2 * n\n i += 1\n if m % 7 == 0:\n return (m, i)\n else:\n return (m, i)", "def func(m,step):\n if len(str(m))<=2:\n return (m,step)\n else :\n a=int(str(m)[:-1])\n b=int(str(m)[-1])\n return func(a-2*b,step+1)\n \ndef seven(m):\n return func(m,0)\n\n", "def seven(m):\n turns = 1\n print(m)\n if m == 0:\n return(0, 0)\n num = int(str(m)[:-1]) - int(str(m)[-1]) * 2\n while num >= 100 :\n print(num)\n num = int(str(num)[:-1]) - int(str(num)[-1]) * 2\n turns += 1\n return(num, turns)", "def seven(m):\n i = 0\n while m >= 100:\n m = int(str(m)[:-1]) - 2*int(str(m)[-1])\n i += 1\n return m, i", "def seven(m):\n i = 0\n while m > 99:\n x10 = m//10\n y = m % 10\n m = x10 -2*y\n i += 1\n return (m,i)", "def seven(m):\n licznik = 0\n m = str(m) #change into string because int isn't iterable\n while len(str(m)) > 2:\n m = str(m) #in each iteration it should be string\n m = int(m[:-1]) - int(m[-1]) *2\n licznik += 1\n return int(m),licznik", "def seven(m):\n numSteps = 0\n while m >= 100:\n m = m // 10 - (m % 10) * 2\n numSteps += 1\n return (m, numSteps)", "def seven(m):\n n, i = m, 0\n while n >= 100:\n n, i = n//10 - 2*int(str(n)[-1]), i+1\n return (n, i)", "def seven(m):\n steps = 0\n while len(str(m)) > 2:\n num = int(str(m)[-1])\n m = m // 10 \n m = m - (num * 2)\n steps = steps + 1\n return (m,steps)\n", "def seven(m):\n # your code\n steps = 0\n while m >= 100 :\n print (m)\n x = m // 10 \n y = m % 10\n m = x - (y*2)\n steps += 1 \n \n return (int(m),steps)\n \n \n", "def seven(m, step=0):\n while m>99:\n a=int(m/10)\n b=m-(a*10)\n m=a-(2*b)\n step+=1\n return m,step", "def seven(m):\n s=str(m)\n for t in range(0,100):\n if int(s)<=99:\n return (int(s),t)\n else:\n r= int(s[:-1])-int(s[-1])*2\n s=str(r)\n", "def seven(m):\n steps = 0\n r = m\n numstr = str(r)\n while r > 99:\n steps +=1\n r = int(numstr[0:-1])-(2*int(numstr[-1]))\n numstr = str(r)\n return r, steps", "def seven(m):\n if len(str(m)) <= 2 :\n return (0,0)\n counter = 0\n while len(str(m)) != 2:\n m = int(str(m)[:-1]) - int(str(m)[-1]) * 2\n counter += 1\n if len(str(m)) <= 2:\n break\n return (m,counter)\n", "def seven(m,counter=int()):return seven(m//10-2*int(str(m)[-1]),counter+1) if (m//10-2*int(str(m)[-1]))/100>=1 else ((m//10-2*int(str(m)[-1])),counter+1 if m>=100 else int())\n", "def seven(m):\n i = 0\n sm = str(m)\n while len(sm) > 2:\n sm = str(int(sm[:-1]) - 2 * int(sm[-1]))\n i += 1\n return (int(sm), i)\n", "def seven(m):\n step_counter = 0\n\n while len(list(str(m))) > 2:\n number_list = list(str(m))\n\n first_part_of_number = int(\"\".join(number_list[:-1])) if len(number_list) > 1 else int(number_list[0])\n second_part_of_number = int(number_list[-1])\n\n m = first_part_of_number - 2*second_part_of_number\n\n del number_list[-1]\n step_counter +=1\n\n return m, step_counter", "def seven(m,count=0):\n if len(str(m))<=2:\n return m, count\n else:\n count +=1\n return seven(m//10 -2*(m%10), count)", "def seven(m):\n lenght = len(str(m))\n steps = 0\n while lenght > 2:\n x = m // 10\n y = m % 10\n m = x - 2*y\n steps += 1\n lenght = len(str(m))\n return m, steps\n \n \n", "def seven(m):\n x = m\n i = 0\n while x >= 100:\n if x > 0:\n x = m // 10 - 2 * (m % 10)\n m = x\n i +=1\n return m, i\n", "def seven(m):\n \n num = str(m)\n steps = 0\n \n while len(num) >2:\n x = num[:-1]\n y = num[-1]\n num = str(int(x) - 2*int(y))\n steps += 1\n\n return (int(num),steps)\n", "def seven(m):\n # your code\n print(m)\n steps = 0\n while m >=100:\n steps +=1\n y = m % 10\n x = m//10\n print(m)\n \n m= x-(2*y)\n \n return (int(m) , steps)\n\n \n \n \n \n \n \n \n", "def seven(m):\n s=0\n if m < 100 :\n s=0\n else:\n while m >99 :\n x=m//10\n m=str(m)\n y=int(m[-1])\n m=x-2*y\n s=s+1\n \n return((m,s))\n", "def seven(m):\n if len(str(m)) == 1:\n if m % 7 == 0:\n return (m, 0)\n else:\n return (m, 0)\n else:\n def last_number(last_number):\n return int(last_number[-1]) * 2\n def helper(res):\n string = ''\n for i in [int(str(res)[i]) for i in range(0, len(str(res)) - 1 )]:\n string = string + str(i)\n if len(string) == 1:\n return int(string)\n elif string != '':\n return int(string) - int(last_number(str(res)))\n iterator = 0\n while True:\n if iterator == 0:\n tmp = helper(m)\n iterator += 1\n else:\n if (len(str(tmp)) == 2 or len(str(tmp)) == 1 ) and tmp % 7 == 0:\n return (int(tmp), iterator)\n elif (len(str(tmp)) == 2 or len(str(tmp)) == 1 ) and tmp % 7 != 0:\n return (int(tmp), iterator)\n else:\n tmp = helper(tmp)\n iterator += 1", "def seven(m):\n result = m\n count = 0\n while len(str(int(result))) > 2:\n y = result % 10\n result = result // 10\n \n result = result - 2*y\n count += 1\n \n return (result, count)\n", "import math\ndef seven(m):\n num=m\n count=0\n while num > 99:\n num = num//10 - 2*(num%10)\n count+=1\n return (num,count)\n", "def seven(m):\n count = 0\n \n while m > 99:\n ost = m % 10\n m = m // 10 - ost * 2\n count += 1\n \n return (m, count)", "def seven_generator(m):\n if m < 100:\n return\n x, y = divmod(m, 10)\n nxt = x - 2*y\n yield nxt\n yield from seven_generator(nxt)\n \n \ndef seven(m):\n l = list(seven_generator(m))\n return (l[-1] if l else 0, len(l))\n \n", "def seven(m):\n y = 0\n while m//100 != 0:\n if m < 0:\n return m, y\n y += 1\n m = m//10 - m%10 * 2\n return m, y", "def seven(m):\n i = 0\n while len(str(m))>2:\n i += 1\n m = int(str(m)[:-1])-2*int(str(m)[-1:])\n return (m,i)", "def seven(n):\n\n count = 0\n while n>99:\n n = int(str(n)[:-1]) - 2*int(str(n)[-1])\n count += 1\n\n \n\n return (n, count)\n"] | {"fn_name": "seven", "inputs": [[1021], [477557101], [477557102], [2340029794923400297949], [1603], [371], [1369851], [483], [483595], [0], [170232833617690725]], "outputs": [[[10, 2]], [[28, 7]], [[47, 7]], [[14, 20]], [[7, 2]], [[35, 1]], [[0, 5]], [[42, 1]], [[28, 4]], [[0, 0]], [[0, 16]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 20,315 |
def seven(m):
|
01b84c9337fbb511d539f4279ca1b2fc | UNKNOWN | Imagine a triangle of numbers which follows this pattern:
* Starting with the number "1", "1" is positioned at the top of the triangle. As this is the 1st row, it can only support a single number.
* The 2nd row can support the next 2 numbers: "2" and "3"
* Likewise, the 3rd row, can only support the next 3 numbers: "4", "5", "6"
* And so on; this pattern continues.
```
1
2 3
4 5 6
7 8 9 10
...
```
Given N, return the sum of all numbers on the Nth Row:
1 <= N <= 10,000 | ["def cumulative_triangle(n):\n return n*(n*n+1)/2", "def cumulative_triangle(n):\n return (n**3+n)/2", "def cumulative_triangle(n):\n return n*(n*n+1)//2", "cumulative_triangle=lambda n:(n**3+n)/2", "def cumulative_triangle(n):\n start = sum(range(n)) + 1\n return sum(range(start, start + n))", "def cumulative_triangle(n):\n f = lambda x: 0.5 * x * (x - 1) + 1\n s = f(n)\n r = []\n while len(r) < n:\n r.append(s)\n s += 1\n return sum(r)", "cumulative_triangle=lambda n:n*(n*n+1)/2", "def cumulative_triangle(n):\n # find last number (x) of nth row\n x = sum(range(n+1))\n # add all numbers from (x-n, x)\n return sum(range(x-n+1,x+1))", "def cumulative_triangle(n):\n # sum of elements of the n-th row\n return (n**2 + 1) * n // 2", "def cumulative_triangle(n):\n N = n * (n+1) // 2\n prev = n * (n-1) // 2\n return N * (N+1) // 2 - prev * (prev+1) // 2"] | {"fn_name": "cumulative_triangle", "inputs": [[1], [2], [3], [4], [15], [150], [100], [500], [1000], [10000]], "outputs": [[1], [5], [15], [34], [1695], [1687575], [500050], [62500250], [500000500], [500000005000]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 932 |
def cumulative_triangle(n):
|
732c23caa96c8870fe49bf155ea40d62 | UNKNOWN | ##Overview
Write a helper function that takes in a Time object and converts it to a more human-readable format. You need only go up to '_ weeks ago'.
```python
to_pretty(0) => "just now"
to_pretty(40000) => "11 hours ago"
```
##Specifics
- The output will be an amount of time, t, included in one of the following phrases: "just now", "[t] seconds ago", "[t] minutes ago", "[t] hours ago", "[t] days ago", "[t] weeks ago".
- You will have to handle the singular cases. That is, when t = 1, the phrasing will be one of "a second ago", "a minute ago", "an hour ago", "a day ago", "a week ago".
- The amount of time is always rounded down to the nearest integer. For example, if the amount of time is actually 11.73 hours ago, the return value will be "11 hours ago".
- Only times in the past will be given, with the range "just now" to "52 weeks ago" | ["def to_pretty(s):\n if not s: return \"just now\"\n for t, w in (60, \"seconds\"), (60, \"minutes\"), (24, \"hours\"), (7, \"days\"), (53, \"weeks\"):\n s, r = divmod(s, t)\n if not s: return (r > 1 and str(r) + \" \" + w or (\"a \", \"an \")[t == 24] + w[:-1]) + \" ago\"", "def to_pretty(seconds):\n\n\n minutes = seconds // 60\n hours = seconds // 3600\n days = seconds // (3600*24)\n weeks = seconds // (3600*24*7)\n \n if weeks == 1:\n return \"a week ago\"\n if weeks > 1:\n return str(weeks) + \" weeks ago\"\n if days == 1:\n return \"a day ago\"\n if days > 1:\n return str(days) + \" days ago\"\n if hours == 1:\n return \"an hour ago\"\n if hours > 1:\n return str(hours) + \" hours ago\"\n if minutes == 1:\n return \"a minute ago\"\n if minutes > 1:\n return str(minutes) + \" minutes ago\"\n if seconds == 0:\n return \"just now\"\n if seconds == 1:\n return \"a second ago\"\n if seconds > 1:\n return str(seconds) + \" seconds ago\"\n \n\n \n\n \n", "CONFIG = [\n ('',\"week\",3600*24*7),\n ('',\"day\",3600*24),\n ('n',\"hour\",3600),\n ('',\"minute\",60),\n ('',\"second\",1)\n]\n\ndef to_pretty(s):\n return next((out(one,t,s//n) for one,t,n in CONFIG if s//n), \"just now\")\n\ndef out(one,t,n):\n return f'{n==1 and \"a\"+one or n} {t}{n>1 and \"s\" or \"\"} ago'", "def to_pretty(seconds):\n if seconds == 0:\n return 'just now'\n fmt = '{} {}{} ago'.format\n human = ((604800, 'week'), (86400, 'day'), (3600, 'hour'),\n (60, 'minute'), (1, 'second'))\n for num, word in human:\n quo, rem = divmod(seconds, num)\n if quo == 1: # singular\n return fmt('an' if num == 3600 else 'a', word, '')\n elif quo > 0:\n return fmt(quo, word, 's')\n", "reprs = {\n 60*60*24*7: \"week\",\n 60*60*24: \"day\",\n 60*60: \"hour\",\n 60: \"minute\",\n 1: \"second\",\n}\n\ndef to_pretty(seconds):\n for k, v in sorted(reprs.items(), key=lambda x: x[0], reverse=True):\n if seconds >= k:\n res = seconds // k\n if res == 1:\n time = \"a\"\n if v[int(v[0] == \"h\")] in \"aeiou\":\n time += \"n\"\n else:\n time = str(int(res))\n if res == 1:\n mult = v\n else:\n mult = v + \"s\"\n return \"{} {} ago\".format(time, mult)\n return \"just now\"", "def to_pretty(seconds):\n w, d, h, m, s = seconds // 604800, seconds % 604800 // 86400, seconds % 604800 % 86400 // 3600, seconds % 604800 % 86400 % 3600 // 60, seconds % 604800 % 86400 % 3600 % 60\n return ('just now' if not seconds else\n [f'a week ago', f'{w} weeks ago'][w > 1] if w > 0 else\n [f'a day ago', f'{d} days ago'][d > 1] if d > 0 else\n [f'an hour ago', f'{h} hours ago'][h > 1] if h > 0 else\n [f'a minute ago', f'{m} minutes ago'][m > 1] if m > 0 else\n [f'a second ago', f'{s} seconds ago'][s > 1])", "def to_pretty(seconds):\n if seconds == 0:\n return 'just now'\n elif seconds//(7 * 24 * 60 * 60):\n weeks = seconds//(7 * 24 * 60 * 60)\n extra = '' if weeks == 1 else 's'\n weeks = 'a' if weeks == 1 else weeks\n return '{} week{} ago'.format(weeks, extra)\n elif seconds//(24 * 60 * 60):\n days = seconds//(24 * 60 * 60)\n extra = '' if days == 1 else 's'\n days = 'a' if days == 1 else days\n return '{} day{} ago'.format(days, extra)\n elif seconds//(60 * 60):\n hours = seconds//(60 * 60)\n extra = '' if hours == 1 else 's'\n hours = 'an' if hours == 1 else hours\n return '{} hour{} ago'.format(hours, extra)\n elif seconds//(60):\n minutes = seconds//60\n extra = '' if minutes == 1 else 's'\n minutes = 'a' if minutes == 1 else minutes\n return '{} minute{} ago'.format(minutes, extra)\n else:\n extra = '' if seconds == 1 else 's'\n seconds = 'a' if seconds == 1 else seconds\n return '{} second{} ago'.format(seconds, extra)"] | {"fn_name": "to_pretty", "inputs": [[0], [1], [30], [90], [180], [4000], [40000], [140000], [400000], [1000000], [10000000]], "outputs": [["just now"], ["a second ago"], ["30 seconds ago"], ["a minute ago"], ["3 minutes ago"], ["an hour ago"], ["11 hours ago"], ["a day ago"], ["4 days ago"], ["a week ago"], ["16 weeks ago"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,305 |
def to_pretty(seconds):
|
6dc0067db661faa6302479908dcf10ca | UNKNOWN | # Convert a linked list to a string
## Related Kata
Although this Kata is not part of an official Series, you may also want to try out [Parse a linked list from a string](https://www.codewars.com/kata/582c5382f000e535100001a7) if you enjoyed this Kata.
## Preloaded
Preloaded for you is a class, struct or derived data type `Node` (depending on the language) used to construct linked lists in this Kata:
```python
class Node():
def __init__(self, data, next = None):
self.data = data
self.next = next
```
~~~if:objc
*NOTE: In Objective-C, the* `Node` *struct is placed on top of your main solution because there is a "double-import" bug in the Preloaded section at the time of writing (which cannot be fixed on my end). Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
~~~if:c
*NOTE: In C, the* `Node` *struct is placed on top of your main solution (and the [Sample] Test Cases) because the compiler complains about not recognizing the* `Node` *datatype even after adding it to the Preloaded section. Attempts to modify it (e.g. to cheat the tests in some way) will likely result in a test crash so it is not recommended for you to modify that section ;)*
~~~
If you are attempting this Kata in NASM then note that the code example shown directly above may not be relevant; please refer to the Sample Tests (written in C) for the exact definition of the `Node` structure.
## Prerequisites
This Kata assumes that you are already familiar with the idea of a linked list. If you do not know what that is, you may want to read up on [this article on Wikipedia](https://en.wikipedia.org/wiki/Linked_list). Specifically, the linked lists this Kata is referring to are **singly linked lists**, where the value of a specific node is stored in its `data`/`$data`/`Data` property, the reference to the next node is stored in its `next`/`$next`/`Next`/`next_node` property and the terminator for a list is `null`/`NULL`/`None`/`nil`/`nullptr`/`null()`.
## Task
*If you are attempting this Kata in NASM, the code examples shown below may not be relevant at all - please refer to the Sample Tests (written in C) for the exact requirements.*
Create a function `stringify` which accepts an argument `list`/`$list` and returns a string representation of the list. The string representation of the list starts with the value of the current `Node`, specified by its `data`/`$data`/`Data` property, followed by a whitespace character, an arrow and another whitespace character (`" -> "`), followed by the rest of the list. The end of the string representation of a list must always end with `null`/`NULL`/`None`/`nil`/`nullptr`/`null()` (all caps or all lowercase depending on the language you are undertaking this Kata in). For example, given the following list:
```python
Node(1, Node(2, Node(3)))
```
... its string representation would be:
```python
"1 -> 2 -> 3 -> None"
```
And given the following linked list:
```python
Node(0, Node(1, Node(4, Node(9, Node(16)))))
```
... its string representation would be:
```python
"0 -> 1 -> 4 -> 9 -> 16 -> None"
```
Note that `null`/`NULL`/`None`/`nil`/`nullptr`/`null()` itself is also considered a valid linked list. In that case, its string representation would simply be `"null"`/`"NULL"`/`"None"`/`"nil"`/`"nullptr"`/`@"NULL"`/`"null()"` (again, depending on the language).
For the simplicity of this Kata, you may assume that any `Node` in this Kata may only contain **non-negative integer** values. For example, you will not encounter a `Node` whose `data`/`$data`/`Data` property is `"Hello World"`.
Enjoy, and don't forget to check out my other Kata Series :D
~~~if:fortran
*NOTE: In Fortran, your returned string is* **not** *permitted to contain any leading and/or trailing whitespace.*
~~~ | ["def stringify(list):\n return 'None' if list == None else str(list.data) + ' -> ' + stringify(list.next)", "def stringify(ll):\n r = []\n while ll:\n r, ll = r + [str(ll.data)], ll.next\n return ' -> '.join(r + ['None'])", "def stringify(head):\n if head is None:\n return \"None\"\n return \"{} -> {}\".format(head.data, stringify(head.__next__))\n", "def stringify(list):\n return str(list.data) + ' -> ' + stringify(list.next) if list is not None else 'None'", "def stringify(list):\n return \"None\" if list is None else str(list.data) + \" -> \" + stringify(list.next)", "class Node:\n def __init__(self, data, next = None):\n self.data = data\n self.next = next\ndef getValue(self):\n return self.data\ndef getNext(self):\n return self.__next__\ndef stringify(node):\n if node ==None:\n return 'None'\n return str(getValue(node)) + ' -> ' + stringify(getNext(node))\n", "class Node():\n def __init__(self, data, next = None):\n self.data = data\n self.next = next\n\n\ndef stringify(node):\n k = ''\n while True:\n if node == None:\n k += 'None'\n return k\n else:\n k += '{} -> '.format(node.data)\n node = node.next", "def stringify(list_):\n try:\n return '{} -> {}'.format(list_.data, stringify(list_.next))\n except AttributeError:\n return 'None'", "def stringify(node):\n return 'None' if not node else f'{str(node.data)} -> ' + stringify(node.__next__) \n", "def stringify(node):\n if not node:\n return 'None'\n return '{} -> {}'.format(node.data, stringify(node.next))", "def stringify(node):\n result = ''\n while node:\n result += str(node.data) + ' -> '\n node = node.next\n return result + 'None'", "def stringify(list):\n return str(list.data) + ' -> ' +stringify(list.next) if list else 'None'", "def stringify(node):\n if node == None:\n return \"None\"\n return str(node.data) + \" -> \" + stringify(node.next)", "def stringify(node):\n if not node:\n return 'None'\n if node.__next__:\n return f'{node.data} -> {stringify(node.next)}'\n else:\n return f'{node.data} -> None'\n", "stringify=lambda n:'None' if n==None else (lambda s:([i for i in iter(lambda:((s['r'].append(str(s['n'].data)),[None for s['n'] in [s['n'].next]]), s['n']!=None)[1], False)],s['r'].append('None'),' -> '.join(s['r']))[-1])({'r':[],'n':n})", "def stringify(node):\n my_str = \"\"\n while node:\n my_str += str(node.data) + \" -> \"\n node = node.next\n \n my_str += \"None\"\n return my_str", "def stringify(node):\n r = []\n while node != None: node = (r.append(str(node.data)), node.next)[1]\n return \" -> \".join(r + [\"None\"])", "def recursive(node, string):\n if node is None:\n string += \"None\"\n return string\n string += str(node.data) + \" -> \"\n node = node.next\n return recursive(node, string)\n\ndef stringify(node):\n string = \"\"\n ans = recursive(node, string)\n return ans", "def stringify(node):\n if node is None:\n return 'None'\n\n data = node.data\n list = []\n current_node = node\n \n while current_node is not None:\n list.append(str(current_node.data))\n current_node = current_node.__next__\n \n if current_node is None:\n list.append(\"None\")\n\n \n return \" -> \".join(list)\n", "def stringify(node):\n \n if node is None:\n return 'None'\n \n itr = node\n llstr = ''\n while itr:\n if itr.next:\n llstr += str(itr.data) + ' -> '\n else:\n llstr += str(itr.data) + ' -> None'\n itr = itr.next\n \n return llstr", "def stringify(node):\n r = ''\n\n while node != None:\n r += f'{node.data} -> '\n node = node.next\n \n return r + 'None'", "def stringify(node):\n def get(node, L = []):\n while node: L, node = L + [str(node.data)], node.next\n return L\n return ' -> '.join(get(node) + ['None'])", "def stringify(node):\n \n if node is None:\n return 'None'\n \n n = node\n l = []\n \n while(True):\n l.append(str(n.data))\n if n.next is None:\n break\n else:\n n = n.next\n \n l.append('None')\n \n return \" -> \".join(l)", "def stringify(node):\n try:\n emptylist = []\n while type(node.data) is int:\n emptylist.append(str(node.data))\n node = node.next\n except:\n emptylist.append('None')\n return ' -> '.join(emptylist)", "def stringify(node, output_array=None):\n if output_array is None:\n output_array = []\n\n if not node:\n output_array.append('None')\n \n while node:\n output_array.append(node.data)\n return stringify(node.__next__, output_array)\n \n return \" -> \".join(str(i) for i in output_array)\n", "def stringify(node):\n return ''.join(str(node.data) + ' -> ' + stringify(node.next)) if node else 'None'", "def stringify(node):\n final = ''\n try:\n final += str(node.data) + ' -> '\n while node.next:\n final += str(node.next.data)\n final += ' -> '\n node = node.next\n except AttributeError:\n pass\n final += 'None'\n return final", "def stringify(node):\n new=[]\n node=node\n while node is not None:\n new.append(str(node.data))\n node=node.next\n else:\n new.append('None')\n return ' -> '.join(new)", "class Node():\n def __init__(self, data, next = None):\n self.data = data\n self.next = next\ndef stringify(list):\n if list == None:\n return 'None'\n else:\n return str(list.data) + ' -> ' + stringify(list.next)", "def stringify(node):\n string = ''\n while node:\n string += str(node.data)\n string += ' -> '\n node = node.next\n string += 'None'\n return string", "def stringify(node):\n return f'{node.data} -> {stringify(node.next)}' if node else str(None)", "def stringify(node):\n a = ''\n if node == None:\n return 'None'\n else:\n node_data = str(node.data)\n #a = node_data + ' -> '\n #a += stringify(node.next)\n return node_data + ' -> ' + stringify(node.__next__)\n \n", "def stringify(node):\n if node == None:\n return 'None'\n else:\n s = str(node.data) + ' -> '\n return s + stringify(node.next)", "def stringify(node):\n if node == None:\n return 'None'\n return '{} -> {}'.format(node.data,stringify(node.__next__))\n \n \n", "def stringify(node):\n s = []\n while node:\n s.append(node.data)\n node = node.next\n s.append(None)\n return ' -> '.join(list(map(str, s)))", "def stringify(node):\n s = \"\"\n while node:\n s += f\"{node.data} -> \"\n node = node.next\n s += \"None\"\n return s", "def stringify(node):\n res = ''\n while node:\n res += '{} -> '.format(str(node.data))\n node = node.next\n return res + 'None'", "def stringify(node):\n x = []\n #if Node is None:\n # return 'None'\n \n while True:\n try:\n x.append(str(node.data))\n node = node.next\n except:\n break\n x.append('None')\n return ' -> '.join(x)", "def stringify(node):\n return 'None' if node is None else f\"{node.data} -> {stringify(node.next)}\"", "def stringify(node):\n res = []\n \n curr = node\n while curr:\n res.append(str(curr.data))\n \n curr = curr.next\n \n res.append('None')\n \n return ' -> '.join(res)", "\ndef stringify(node):\n list = []\n f = node\n while f is not None:\n list.append(str(f.data))\n f = f.next\n list.append(\"None\")\n return ' -> '.join(list)", "def stringify(node):\n if node == None:\n return str('None')\n return str(node.data) + \" -> \" + stringify(node.next)", "def stringify(node):\n string_list = \"\"\n current = node\n while current:\n string_list += str(current.data) + \" -> \"\n current = current.next\n string_list += \"None\"\n return string_list", "def stringify(node, s=\"\"):\n if not node:\n s = s + \"None\"\n return s\n else:\n s = s+ str(node.data) + \" -> \"\n return stringify(node.next, s)", "def stringify(node):\n if node == None:\n return 'None'\n lst = []\n n = node\n while n.__next__ != None:\n lst.append(str(n.data))\n n = n.__next__\n lst.append(str(n.data))\n lst.append('None')\n return ' -> '.join(lst)\n", "def stringify(node):\n node_str = ''\n while node:\n arrow = ' -> '\n node_str += str(node.data) + arrow\n node = node.__next__\n return node_str + 'None'\n", "def stringify(node):\n list = []\n \n while node:\n list.append(str(node.data))\n node = node.next\n \n list.append('None')\n \n return \" -> \".join(list)", "def stringify(node):\n if node==None:\n return \"None\"\n string = str(node.data); node = node.next\n while node!=None:\n string = string + \" -> \" + str(node.data)\n node = node.next\n return (string + \" -> None\")", "def stringify(node):\n lst = []\n while node:\n lst+=[str(node.data)]\n lst+=[' -> ']\n node = node.next\n lst+=['None']\n return ''.join(lst)", "def stringify(node):\n if node == None: return 'None'\n i = node\n t = ''\n while i.next != None:\n t += str(i.data) + ' -> '\n i = i.next\n t += str(i.data) + ' -> None'\n return t", "def stringify(node):\n a = []\n if not node:\n return 'None'\n while node:\n a.append(str(node.data))\n node = node.__next__\n a.append('None')\n print(a)\n return ' -> '.join(a)\n", "def stringify(node):\n if node:\n if not node.next:\n return f'{node.data} -> None'\n return f'{node.data} -> {stringify(node.next)}'\n else:\n return 'None'", "def stringify(node):\n ans = []\n while node:\n ans.append(str(node.data))\n node = node.__next__\n ans.append(\"None\")\n return \" -> \".join(ans)\n", "def stringify(node):\n # base case\n if node != None:\n return f'{node.data} -> ' + stringify(node.next)\n else:\n return 'None'", "def stringify(node):\n if node is None:\n return 'None'\n \n result = ''\n while node.__next__ is not None:\n result += str(node.data)\n result += ' -> '\n node = node.__next__\n result += str(node.data)\n result += ' -> None'\n\n return result\n", "def stringify(node):\n if not node:\n return \"None\"\n list = [str(node.data)]\n curr = node\n while curr != None:\n curr = curr.next\n list.append(str(curr.data) if curr else \"None\")\n \n return \" -> \".join(list)", "def stringify(node):\n n = 'None'\n if node:\n n, r = node.__next__, f\"{node.data} -> \"\n while n:\n r += f\"{n.data} -> \"\n n = n.__next__\n r += \"None\"\n else:\n r = n\n return r\n", "def stringify(node):\n if node is not None:\n return str(node.data) + \" -> \" + stringify(node.next)\n else:\n return \"None\"", "def stringify(node):\n if node == None:\n return 'None'\n elif node.next == None:\n return f\"{node.data} -> None\"\n else:\n return f\"{node.data} -> {stringify(node.next)}\"", "def stringify(node):\n if not node: return \"None\"\n ls = []\n while node.next != None:\n ls.append(str(node.data))\n node = node.next\n ls.append(str(node.data) +\" -> None\")\n return \" -> \".join(ls)", "def stringify(node):\n return 'None' if node is None else '%d -> %s' % (node.data, stringify(node.__next__))\n", "def stringify(node):\n if node == None:\n return 'None'\n ret = ''\n while node.data != None:\n ret += str(node.data) + ' -> '\n if not node.__next__:\n ret += 'None'\n break\n node = node.__next__\n return ret\n", "def stringify(node):\n if node is None:\n return 'None'\n res = []\n while True:\n if node.__next__ is None:\n res.append(str(node.data))\n res.append('None')\n break\n res.append(str(node.data))\n node = node.__next__\n return ' -> '.join(res)\n", "def stringify(node):\n if node==None:\n return \"None\"\n return f\"{node.data} -> {stringify(node.next)}\"\n", "def stringify(node):\n return f\"None\" if node is None else f\"{node.data} -> {stringify(node.next)}\"\n", "def stringify(node):\n elems = []\n cur_node = node\n while cur_node != None:\n elems.append(cur_node.data)\n cur_node = cur_node.next\n if elems == []:\n return 'None'\n return ' -> '.join(str(x) for x in elems) + ' -> ' +'None'", "def stringify(node):\n if not node:\n return 'None'\n string=node.data\n node=node.next \n while node: \n string=f'{string} -> {node.data}'\n node=node.next \n return f'{string} -> {node}'", "def stringify(node):\n list = []\n while node:\n list.append(node.data)\n node=node.next\n list.append('None')\n return ' -> '.join(map(str,list))", "def stringify(node):\n List = []\n while node and node.data != None:\n List.append(str(node.data))\n node = node.next\n List.append(\"None\")\n return ' -> '.join(List)", "def stringify(node):\n currentNode = node\n res = ''\n while currentNode is not None:\n res += f'{currentNode.data} -> '\n currentNode = currentNode.next\n if currentNode is None:\n res += 'None'\n \n return res", "def stringify(node):\n result = ''\n while node != None:\n result += f'{node.data} -> '\n node = node.next\n result += 'None'\n return result", "def stringify(node):\n if not node:\n return 'None'\n ans = []\n while node:\n ans.append(str(node.data))\n node = node.next\n return ' -> '.join(ans) + ' -> None'", "def stringify(node):\n if node == None:\n return 'None'\n returnString = \"\"\n while node != None:\n returnString += str(node.data) + ' -> '\n node = node.next\n returnString += \"None\"\n return returnString", "def stringify(node):\n then_case = ''\n result = ''\n while node!=None:\n then_case = node.data\n node = node.next\n result+=str(then_case)+' -> '\n return result+'None'", "def stringify(node):\n if node == None:\n return \"None\"\n \n string = str(node.data)\n node = node.next\n \n while node != None:\n string = string + \" -> \" + str(node.data)\n node = node.next\n \n string += \" -> None\"\n \n return string", "def stringify(node):\n if node is None:\n return \"None\"\n elif node.next is None:\n return f\"{node.data} -> None\"\n else:\n return f\"{node.data} -> {stringify(node.next)}\"", "def stringify(node):\n head = node\n curr = head\n strList = []\n while (curr != None):\n strList.append(curr.data)\n curr = curr.next\n strList.append(None)\n \n return ' -> '.join(str(x) for x in strList)", "def stringify(node):\n\n if node is None:\n return 'None'\n output = str(node.data) + \" -> \"\n \n while node.next != None:\n node = node.next\n output += str(node.data) + \" -> \"\n \n output += \"None\"\n \n return output", "def stringify(node):\n ret = \"\"\n while node:\n ret += str(node.data) + \" -> \"\n node = node.next\n return ret + \"None\"", "def stringify(node):\n\n \"\"\"Code borrowed\"\"\"\n\n result = ''\n \n while node:\n result += str(node.data) + ' -> '\n node = node.next\n \n return result + 'None'", "def stringify(node):\n curr = node\n res = []\n \n while curr != None:\n res.append(curr.data)\n curr = curr.next\n \n res.append(None)\n \n return \" -> \".join(str(x) for x in res)", "def stringify(node):\n seq, current = [], node\n while current:\n seq.append(str(current.data))\n current = current.next\n seq.append('None')\n return ' -> '.join(seq)", "def stringify(node):\n if not node:\n return \"None\"\n if not node.__next__:\n return f\"{node.data} -> {None}\"\n return f\"{node.data} -> {stringify(node.next)}\"\n\n", "def stringify(node):\n p = node\n seq = []\n while p:\n seq.append(str(p.data))\n p = p.next\n seq.append('None')\n return ' -> '.join(seq)", "def stringify(node):\n if not node:\n return \"None\"\n output = \"\"\n while node:\n output += str(node.data) + \" -> \"\n node = node.next\n output += \"None\"\n return output", "def stringify(node):\n if not node:\n return 'None'\n return \" -> \".join([x for x in rec_stringify(node)])\n \ndef rec_stringify(node):\n if not node.__next__:\n return [ str(node.data), 'None']\n else:\n return [ str(node.data) ] + rec_stringify(node.__next__)\n", "def stringify(node):\n if node == None: return 'None'\n res = ''\n while node.next != None:\n res += '{} -> '.format(node.data)\n node = node.next\n return res + str(node.data)+' -> None'", "def stringify(node):\n st = ''\n while node:\n st = st + str(node.data) + ' -> '\n node = node.__next__\n st = st + 'None'\n return st\n\n\n", "def stringify(node):\n string = \"\"\n \n if node == None:\n return \"None\"\n else:\n n = node.next\n string = string + str(node.data) + \" -> \" + stringify(node.next)\n return string", "def stringify(node):\n if node == None: \n return \"None\"\n to_remove = \"(),\"\n table = {ord(char): None for char in to_remove}\n head = int (node.data)\n tail = node.__next__\n if tail == None:\n return str((\" -> \".join((str(head), \"None\"))).translate(table))\n else: \n return str((\" -> \".join((str(head), stringify(tail)))).translate(table))\n", "def stringify(node):\n s = []\n while node:\n s.append(str(node.data))\n node = node.next\n s.append('None')\n return ' -> '.join(s)", "def stringify(node):\n if node==None:\n return \"None\"\n n = node\n r = \"\"\n while n.next != None:\n r = r + str(n.data) + \" -> \"\n n = n.next\n return r + str(n.data) + \" -> None\"", "def stringify(node):\n \n count = 0\n next_node = \"\"\n \n while count < 100:\n \n try:\n \n current_node = eval(\"node.\" + count * \"next.\" + \"data\") \n next_node += str(current_node) + \" -> \"\n count += 1\n \n except:\n return \"None\" if node == None else next_node + \"None\"", "def stringify(node):\n ret = \"\"\n try:\n while node.data != None:\n ret += str(node.data) + \" -> \"\n node = node.next\n except:\n ret += \"None\"\n return ret", "stringify = lambda node, s = '': stringify(node.next, s + f'{node.data} -> ') if node else s + 'None'", "def stringify(node):\n res = \"\"\n while not node == None:\n res += str(node.data) + \" -> \"\n node = node.next\n \n res += \"None\"\n return res", "def stringify(node):\n to_print = ''\n while node != None:\n to_print += str(node.data) + ' -> '\n node = node.__next__\n return to_print + 'None'\n", "def stringify(node):\n s = ''\n while node:\n s += str(node.data)\n node = node.next\n s += ' -> '\n return s + 'None'", "def stringify(node):\n if not node:\n return 'None'\n \n lst = [str(node.data)]\n next_node = node.next\n\n while next_node != None:\n lst.append(str(next_node.data))\n next_node = next_node.next\n\n return ' -> '.join(lst) + ' -> None'"] | {"fn_name": "stringify", "inputs": [[null]], "outputs": [["None"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 20,622 |
def stringify(node):
|
9c2ba5b6748608fc4c31dad0173110c0 | UNKNOWN | Ho ho! So you think you know integers, do you? Well then, young wizard, tell us what the Nth digit of the [Champernowne constant](https://en.wikipedia.org/wiki/Champernowne_constant) is!
The constant proceeds like this: `0.12345678910111213141516...`
I hope you see the pattern!
Conjure a function that will accept an integer, `n`, and return the (one-indexed) `n`th digit of Champernowne's constant. Can you get it to run in _constant_ time?
For example:
`n = 1` should return `0` (the very first digit)
`n = 2` should return `1` (we ignore the period character since it's not a digit!)
`n = 20` should return `4` (that's the `4` in the number `14`, 20th in sequence)
For any invalid values, such as `0` and below, or non-integers, return... `NaN`!
I hope (for your sake) that you've been practicing your mathemagical spells, because a naïve solution will _not_ be fast enough to compete in this championship!
Invoke with _precision_, and be wary of rounding errors in the realms of enormity!
May the best integer win! | ["def champernowne_digit(n):\n if not type(n) is int or n < 1:\n return float(\"NaN\")\n i, l = 1, 11\n while l <= n:\n i, l = i + 1, l + 9 * (i + 1) * 10**i\n return ((n - l) // (i * 10**(i - 1 - (n - l) % i))) % 10\n\nchampernowneDigit = champernowne_digit\n", "DIGIT_CNTS = [1] + [(10**i - 10**(i-1)) * i for i in range(1, 20)]\n\ndef champernowneDigit(n):\n if not isinstance(n, int) or n is True or n <= 0:\n return float('nan')\n n -= 1\n for digits, cnt in enumerate(DIGIT_CNTS):\n if n < cnt:\n break\n n -= cnt\n start = int(10 ** (digits-1))\n q, r = divmod(n, digits) if digits > 1 else (n, 0)\n return int(str(start + q)[r])", "def champernowneDigit(n):\n if not isinstance(n, int) or isinstance(n, bool) or n <= 0:\n return float('nan')\n elif n <= 10:\n return n - 1\n nr_digits = 1\n mag_len = 10\n while n > mag_len:\n n -= mag_len\n nr_digits += 1\n mag_len = nr_digits * (10**nr_digits - 10**(nr_digits-1))\n print(n, nr_digits, mag_len)\n quoitent, reminder = divmod(n-1, nr_digits)\n print(quoitent, reminder)\n return int(str(quoitent + 10**(nr_digits-1))[reminder])", "def champernowneDigit(n):\n\n result=float(\"NaN\")\n if type(n)==int and n>0:\n if n<=10:\n result=n-1\n else:\n i=1\n c=10\n while n>c:\n i+=1\n c=c+i*9*10**(i-1)\n \n number=(n-c)//i+10**i-1\n reste=(n-c)%i\n if reste:\n number+=1\n result=int(str(number)[reste-1])\n return result", "from math import floor\ndef champernowneDigit(n):\n print(n)\n if n == None or isinstance(n, bool) or not isinstance(n, int) or n < 1: return float('nan')\n t, c = 10, 1\n while t < n: \n c += 1\n t += 9 * 10**(c-1) * c\n num = str(10**c - 1 - floor((t-n)/c))\n return int(num[c - 1 - (t-n)%c])", "def champernowneDigit(n):\n if not n or type(n)!=int or n<0: return float('nan')\n if n<=10: return n-1\n digs=1\n tot=1\n while 1:\n if tot+digs*9*10**(digs-1)>=n: break\n tot+=digs*9*10**(digs-1)\n digs+=1\n number=(n-tot-1)//digs+10**(digs-1)\n return int(str(number)[(n-tot-1)%digs])", "def champernowneDigit(n):\n if not isinstance(n, int) or isinstance(n, bool) or n < 1:\n return float(\"nan\")\n n -= 1\n sc = [9 * (x + 1) * pow(10, x) for x in range(50)]\n i = 0\n while n > sc[i]:\n n -= sc[i]\n i += 1\n L, d = divmod((n-1), i + 1)\n return int(str(pow(10, i) + L)[d])", "def champernowne_digit(n):\n if not type(n) is int or n < 1:\n return float(\"NaN\")\n n, i, l = n-1, 1, 10\n while n >= l: \n n, i, l = n-l, i+1, 9 * (i+1) * 10**i\n return (n//(i*10**(i-1-n%i)))%10 + (n%i<1) - (i<2) \n\nchampernowneDigit = champernowne_digit\n", "# 1.6160180568695068 sec for [1, 10000]\ng1 = lambda n: (9*(n+1)*10**n - 10**(n+1) + 1) // 9\n\n# 2.1931352615356445 seconds for [1, 10000]\ng2 = lambda n: int(''.join((str(n-1), \"8\"*(n-1), \"9\")))\n\nL = [g1(x) for x in range(1, 1000)]\n\ndef champernowneDigit(n):\n if not (type(n) == int and 0 < n): return float(\"nan\")\n if n == 1: return 0\n n -= 1\n x = next(i for i,v in enumerate(L, 1) if v > n)\n q, r = divmod(L[x-1]-n, x)\n return int(str(10**x-1-q)[-r-1])", "def champernowneDigit(n):\n if not n or not type(n) == int or n < 1: return float('nan')\n \n dig, i = 10, 1\n while dig < n: \n i += 1\n dig += 9 * 10 ** (i - 1) * i\n \n num = str(10 ** i - 1 - (dig - n) // i)\n \n return int(num[-1 - (dig - n) % i])"] | {"fn_name": "champernowneDigit", "inputs": [[1], [2], [3], [4], [5], [6], [7], [8], [9], [10], [11], [12], [20], [35], [36], [37], [100], [101], [102], [103], [104], [105], [190], [2890], [38890], [488890], [5888890], [3678608], [3678609], [3678610], [3678611], [3678612], [3678613], [3678614], [3897249], [3897189], [3897309], [3897369], [3898749], [3898809], [3898869], [3898929], [3898989], [3899049], [3899109], [999999999], [1000599563], [1000599564], [1000599565], [1000599566], [1000599567], [1000599568], [101800813569], [77199254740991], [501337501337101]], "outputs": [[0], [1], [2], [3], [4], [5], [6], [7], [8], [9], [1], [0], [4], [2], [2], [2], [4], [5], [5], [5], [6], [5], [9], [9], [9], [9], [9], [6], [1], [9], [6], [3], [1], [6], [5], [4], [6], [7], [0], [1], [2], [3], [4], [5], [6], [8], [1], [2], [3], [5], [2], [3], [6], [7], [3]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,763 |
def champernowneDigit(n):
|
ac366adfa70a17f0d8b3e5509ccf4625 | UNKNOWN | # Description:
Find the longest successive exclamation marks and question marks combination in the string. A successive exclamation marks and question marks combination must contains two part: a substring of "!" and a substring "?", they are adjacent.
If more than one result are found, return the one which at left side; If no such a combination found, return `""`.
# Examples
```
find("!!") === ""
find("!??") === "!??"
find("!?!!") === "?!!"
find("!!???!????") === "!!???"
find("!!???!?????") === "!?????"
find("!????!!!?") === "????!!!"
find("!?!!??!!!?") === "??!!!"
```
# Note
Please don't post issue about difficulty or duplicate. Because:
>[That's unfair on the kata creator. This is a valid kata and introduces new people to javascript some regex or loops, depending on how they tackle this problem. --matt c](https://www.codewars.com/kata/remove-exclamation-marks/discuss#57fabb625c9910c73000024e) | ["import re\n\ndef find(stg):\n matches = re.findall(r\"(!+|\\?+)\", stg)\n return max((f\"{a}{b}\" for a, b in zip(matches, matches[1:])), key=len, default=\"\")", "from itertools import groupby\n\ndef find(s):\n L = [k*len(list(v)) for k,v in groupby(s)]\n return max((x + y for x,y in zip(L, L[1:])), key=len, default=\"\")", "import re\n\ndef find(s):\n matches = [s for s, _ in re.findall(r'((.)\\2*)', s)]\n return max((a+b for a,b in zip(matches, matches[1:])), key=len, default='')", "import re\ndef find(s):\n h1 = re.findall(r\"!+\\?+\", s)\n h2 = re.findall(r\"\\?+!+\", s)\n return max(h1 + h2, key = lambda x:( len(x) ,-s.find(x) ), default='')", "import re;find=lambda s:max(re.findall(r\"!+\\?+\",s)+re.findall(r\"\\?+!+\",s),key=lambda x:(len(x),-s.find(x)),default='')", "def find(s):\n if s == '' or '!' not in s or '?' not in s:\n return ''\n s += ' '\n counts = []\n curr = s[0]\n count = 0\n for i in s:\n if i == curr:\n count += 1\n else:\n counts.append(count)\n count = 1\n curr = i\n n = []\n for i in range(len(counts)-1):\n n.append(counts[i]+counts[i+1])\n x = n.index(max(n))\n \n a = sum(counts[:x])\n return s[a:a+counts[x]+counts[x+1]]", "import re\ndef find(s):\n return max(re.findall(r\"\\!+\\?+\", s)+re.findall(r\"\\?+\\!+\", s), key=(lambda x:(len(x), -s.index(x))), default=\"\")", "import re\ndef find(s):\n x=[]\n for g in re.finditer(r\"\\?+!+\",s):\n x.append((len(g[0]),-g.start(),g[0]))\n for g in re.finditer(r\"!+\\?+\",s):\n x.append((len(g[0]),-g.start(),g[0]))\n return max(x)[-1] if x else \"\"", "from itertools import *\n\ndef find(s):\n r = ''\n for i in range(len(s)):\n for j in range(i,len(s)+1):\n g = [k for k,g in groupby(s[i:j])]\n if len(g) == 2:\n if j-i > len(r):\n r = s[i:j]\n return r", "from itertools import groupby\ndef find(s):\n a,b='',0\n m=0\n r=''\n for c,g in groupby(s):\n n=len(list(g))\n if b+n>m and b:\n r=a*b+c*n\n m=b+n\n a,b=c,n\n return r\n"] | {"fn_name": "find", "inputs": [["!!"], ["!??"], ["!?!!"], ["!!???!????"], ["!!???!?????"], ["!????!!!?"], ["!?!!??!!!?"]], "outputs": [[""], ["!??"], ["?!!"], ["!!???"], ["!?????"], ["????!!!"], ["??!!!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,222 |
def find(s):
|
c8dfcdeb2612ee00f378722270644232 | UNKNOWN | Everyone Knows AdFly and their Sister Sites.
If we see the Page Source of an ad.fly site we can see this perticular line:
Believe it or not This is actually the Encoded url which you would skip to.
The Algorithm is as Follows:
```
1) The ysmm value is broken like this
ysmm = 0 1 2 3 4 5 6 7 8 9 = "0123456789"
code1 = 0 2 4 6 8 = "02468"
code2 = 9 7 5 3 1 = "97531"
2) code1+code2 is base64 Decoded
3) The result will be of this form :
https://adf.ly/go.php?u=
4) has to be further decoded and the result is to be returned
```
Your Task:
```
Make a function to Encode text to ysmm value.
and a function to Decode ysmm value.
```
Note: Take random values for The first 2 int values.
I personally hate trivial error checking like giving integer input in place of string.
```
Input For Decoder & Encoder: Strings
Return "Invalid" for invalid Strings
``` | ["import base64\nimport binascii\nimport math\nfrom itertools import zip_longest\n\n\ndef adFly_decoder(sc):\n code1 = \"\"\n code2 = \"\"\n flip = False\n for c in sc:\n if flip:\n code2 += c\n else:\n code1 += c\n flip = not flip\n\n try:\n url = base64.b64decode(code1 + code2[len(code2) :: -1])\n except binascii.Error:\n return \"Invalid\"\n\n try:\n dec = base64.b64decode(url[26:])\n except binascii.Error:\n return \"Invalid\"\n\n return str(dec, \"utf-8\")\n\n\ndef adFly_encoder(url):\n prefix = \"96https://adf.ly/go.php?u=\"\n full = str.encode(prefix) + base64.b64encode(str.encode(url))\n enc = base64.b64encode(full)\n cut = math.ceil(len(enc) / 2)\n code1 = str(enc[: cut + 1], \"utf-8\")\n code2 = str(enc[len(enc) : cut : -1], \"utf-8\")\n swp = \"\".join(i + (j or \"\") for i, j in zip_longest(code1, code2))\n return swp\n", "from base64 import b64decode, b64encode\nfrom random import randint\n\n\ndef adFly_decoder(sc):\n return b64decode(b64decode(sc[::2] + sc[::-2])[26:]).decode('utf-8') or 'Invalid'\n\n\ndef adFly_encoder(url):\n url = f'{randint(0, 99):02d}https://adf.ly/go.php?u={url}'.encode('utf-8')\n encoded = b64encode(url[:26] + b64encode(url[26:])).decode('utf-8')\n length = len(encoded) // 2\n return ''.join(x + y for x, y in zip(encoded[:length], encoded[:length - 1:-1]))", "import base64\n\ndef adFly_decoder(sc):\n #your code here\n code1 = ''\n code2 = ''\n flg = True\n for ch in sc:\n if flg:\n code1 += ch\n flg = not flg\n \n flg = True\n for ch in sc[::-1]:\n if flg:\n code1 += ch\n flg = not flg\n \n try:\n s = base64.b64decode(code1 + code2).decode('utf-8')\n s = s.split('//adf.ly/go.php?u=')[1]\n return base64.b64decode(s).decode('utf-8')\n except: \n return 'Invalid'\n\ndef adFly_encoder(url):\n s = '96https://adf.ly/go.php?u='\n e_url = base64.b64encode(bytes(url, 'utf-8')).decode('utf-8')\n s += e_url\n b64 = list(base64.b64encode(bytes(s, 'utf-8')).decode('utf-8'))\n \n yummy = ''\n flg = True\n while b64:\n if flg:\n yummy += b64.pop(0)\n else:\n yummy += b64.pop()\n flg = not flg\n \n return yummy", "import base64\nfrom random import randint\ndef adFly_decoder(sc):\n code1 = sc[0:len(sc):2]\n code2 = sc[len(sc):0:-2]\n print(sc)\n if len(code2)-1 >= 8:\n b = base64.b64decode(code1 + code2)\n b = str(b).split('?')\n return str(base64.b64decode(b[1][2:-1]))[2:-1]\n return 'Invalid'\ndef adFly_encoder(url):\n url_encoded = str(base64.b64encode(url.encode(\"ascii\")))[2:-1]\n url_adfly = str(randint(0, 9)) + str(randint(0,9)) + 'https://adf.ly/go.php?u=' + url_encoded\n b = base64.b64encode(url_adfly.encode(\"ascii\"))\n b = str(b)[2:-1]\n sc1 = b[0:len(b)//2]\n sc2 = b[len(b)//2:][::-1]\n sc = \"\"\n idx = 0\n for x in range(len(b)):\n if x % 2 == 0:\n sc += sc1[idx]\n else:\n sc += sc2[idx]\n idx+=1\n return sc\n \n", "import random\nimport base64\n\ndef adFly_decoder(sc):\n code1 = \"\"\n code2 = \"\"\n\n for i in range(len(sc)):\n if i % 2 == 0:\n code1 += sc[i]\n else:\n code2 += sc[i]\n code2 = code2[::-1]\n code1 += code2\n try:\n decodeStr = str(base64.b64decode(code1))[2:-1]\n except base64.binascii.Error as err:\n return \"Invalid\"\n else:\n a = base64.b64decode(decodeStr[decodeStr.find(\"?u=\") + 3:])\n return str(a)[2:-1]\n\n\ndef adFly_encoder(url):\n decodeStr = str(base64.b64encode(bytes(url, encoding=\"utf-8\")))[2:-1]\n ran = random.randint(10, 99)\n modStr = str(base64.b64encode(bytes(str(ran) + \"https://adf.ly/go.php?u=\" + decodeStr, encoding=\"utf-8\")))[2:-1]\n code1 = modStr[:len(modStr) // 2]\n code2 = modStr[len(modStr) // 2:]\n res = \"\"\n ic1 = ic2 = 0\n for i in range(len(modStr)):\n if i % 2 == 0:\n res += code1[ic1]\n ic1 += 1\n else:\n res += code2[ic2 - 1]\n ic2 -= 1\n return res", "import base64\nimport random\n\nPREFIX = 'https://adf.ly/go.php?u='\n\ndef adFly_decoder(sc):\n try:\n decoded = base64.b64decode(sc[::2]+sc[1::2][::-1]).decode('utf-8')\n assert decoded[:2].isdigit()\n decoded = decoded[2:]\n assert decoded.startswith(PREFIX)\n return base64.b64decode(decoded[len(PREFIX):]).decode('utf-8')\n except:\n return 'Invalid'\n \n \ndef adFly_encoder(url):\n adfly_url = f\"{random.randint(0,99):02d}{PREFIX}{base64.b64encode(url.encode('utf-8')).decode('utf-8')}\"\n adfly_url = base64.b64encode(adfly_url.encode('utf-8')).decode('utf-8')\n half1 = adfly_url[:(len(adfly_url)+1)//2]\n half2 = adfly_url[(len(adfly_url)+1)//2:]\n result = ''\n while half1:\n result += half1[0]\n half1 = half1[1:]\n if half2:\n result += half2[-1]\n half2 = half2[:-1]\n return result\n", "import base64\n\ndef adFly_decoder(sc):\n code1 = ''\n code2 = ''\n for i in range(len(sc)):\n if(i%2==0):\n code1 = code1 + sc[i]\n else:\n code2 = code2 + sc[i]\n try:\n url = base64.b64decode((code1+code2[::-1]).encode())[26:]\n return base64.b64decode(url).decode()\n except:\n return 'Invalid'\n \ndef adFly_encoder(url):\n plain = f'00https://adf.ly/go.php?u={base64.b64encode(url.encode()).decode()}';\n code = base64.b64encode(plain.encode()).decode()\n i = len(code)//2\n code1, code2 = code[:i], code[i:][::-1]\n ysmm = ''\n for ch1, ch2 in zip(code1, code2):\n ysmm = ysmm + ch1 + ch2\n return ysmm\n", "import base64\n\ndef adFly_decoder(sc):\n #your code here\n try:\n code1 = sc[::2]\n code2 = sc[1::2]\n code2 = code2[::-1] # reverse\n decode_adfly = base64.b64decode(code1 + code2).decode(\"utf-8\") \n encoded_url = decode_adfly.split(\"https://adf.ly/go.php?u=\")[-1]\n return base64.b64decode(encoded_url).decode(\"utf-8\") \n except:\n return \"Invalid\"\n \ndef adFly_encoder(url):\n #your code here\n code = base64.b64encode(url.encode()).decode(\"utf-8\")\n ad_fly_code = \"12https://adf.ly/go.php?u=\" + code\n final_code = base64.b64encode(ad_fly_code.encode()).decode(\"utf-8\")\n \n l = len(final_code)\n half = l // 2\n first_half = final_code[0:half] \n second_half = final_code[half if l % 2 == 0 else half+1:] \n \n second_half = second_half[::-1]\n result_string = \"\"\n \n for i in range(half):\n result_string += first_half[i]\n if i < half - 1 or l % 2 == 0:\n result_string += second_half[i]\n \n return result_string\n \n"] | {"fn_name": "adFly_decoder", "inputs": [["O=T0ZToPdRHJRmwdcOz1oGvTL22lFzkRZhih5GsbezSw9kndbvyR50wYawHIAF/SdhT1"], ["N=z0dDoMdyHIRmwac1zMolvWLz2RFmkMZiiZ5HsZeySw9kndbvyR50wYawHIAF/SdhT1"], ["lololol"]], "outputs": [["http://yahoo.com"], ["http://google.com"], ["Invalid"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 7,072 |
def adFly_decoder(sc):
|
f7550bc2d9638a1710bbf1b11e17b963 | UNKNOWN | ## Description:
Remove all exclamation marks from the end of words. Words are separated by spaces in the sentence.
### Examples
```
remove("Hi!") === "Hi"
remove("Hi!!!") === "Hi"
remove("!Hi") === "!Hi"
remove("!Hi!") === "!Hi"
remove("Hi! Hi!") === "Hi Hi"
remove("!!!Hi !!hi!!! !hi") === "!!!Hi !!hi !hi"
``` | ["def remove(s):\n return ' '.join(w.rstrip('!') or w for w in s.split())", "from re import sub\ndef remove(s):\n return sub('(\\w+)!+(\\s+|$)','\\\\1\\\\2', s)\n", "def remove(s):\n return \" \".join(x.rstrip(\"!\") for x in s.split())", "import re\n\ndef remove(s):\n return re.sub(r'\\b!+(?= |$)', '', s)", "def remove(s):\n return __import__('re').sub(r'\\b!+','',s)", "import re\ndef remove(s):\n return re.sub(r\"(?<=\\w)!+(?=\\W)*\", \"\", s)", "def remove(s):\n return ' '.join(i.rstrip('!') for i in s.split())\n", "import re\n\ndef remove(s):\n return re.sub(r\"(?<=\\w)!+(?=\\s|$)\", \"\", s)", "import re\n\ndef remove(s):\n return re.sub(r'\\b!+',r'' ,s)", "import re\n\n\ndef remove(s):\n return re.sub(r'\\b!+', '', s)"] | {"fn_name": "remove", "inputs": [["Hi!"], ["Hi!!!"], ["!Hi"], ["!Hi!"], ["Hi! Hi!"]], "outputs": [["Hi"], ["Hi"], ["!Hi"], ["!Hi"], ["Hi Hi"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 765 |
def remove(s):
|
b64bfbb474ec745810dee130530c9758 | UNKNOWN | Bob has ladder. He wants to climb this ladder, but being a precocious child, he wonders about exactly how many ways he could to climb this `n` size ladder using jumps of up to distance `k`.
Consider this example...
n = 5\
k = 3
Here, Bob has ladder of length 5, and with each jump, he can ascend up to 3 steps (he can either jump step 1 or 2 or 3). This gives the below possibilities
```
1 1 1 1 1
1 1 1 2
1 1 2 1
1 2 1 1
2 1 1 1
1 2 2
2 2 1
2 1 2
1 1 3
1 3 1
3 1 1
2 3
3 2
```
Your task to calculate number of ways to climb ladder of length `n` with upto `k` steps for Bob. (13 in above case)
Constraints:
```python
1<=n<=50
1<=k<=15
```
_Tip: try fibonacci._ | ["from collections import deque\n\ndef count_ways(n, k):\n s,d = 1,deque([0]*k)\n for i in range(n):\n d.append(s)\n s = 2*s-d.popleft()\n return s-d.pop()", "from functools import lru_cache\n\n@lru_cache(maxsize=None)\ndef count_ways(n, k):\n if n < 0:\n return 0\n elif n <= 1:\n return 1\n return sum(count_ways(n-i, k) for i in range(1, k+1))\n", "def count_ways(n, k):\n dp = []\n for i in range(k):\n dp.append(2 ** i)\n for i in range(k, n):\n dp.append(sum(dp[-k:]))\n return dp[~-n]", "def count_ways(n, k):\n ways = [None] * (n + 1)\n ways[0] = 1\n for i in range(1, n + 1):\n total = 0\n for j in range(1, k + 1):\n if i - j >= 0 : total += ways[i-j]\n ways[i] = total\n return ways[n]", "def count_ways(n, k):\n kfib = [0 for _ in range(k-1)] + [1]\n for i in range(n):\n kfib.append(sum(kfib[i:i+k]))\n return kfib[-1]", "from functools import lru_cache\n\n@lru_cache(None)\ndef count_ways(n, k):\n return sum(count_ways(n - a, k) for a in range(1, min(n, k) + 1)) if n else 1", "from functools import lru_cache\n\n@lru_cache(maxsize=None)\ndef count_ways(n, k):\n return n==0 or sum(count_ways(n-x, k) for x in range(1, min(n, k)+1))", "from collections import deque\n\ndef count_ways(n, k):\n rs = deque(maxlen=k)\n rs.append(1)\n for _ in range(n):\n rs.append(sum(rs))\n return rs[-1]", "def count_ways(n, k):\n list_ = []\n for i in range(k):\n list_.append(2**i)\n \n print(list_)\n \n for i in range(n-k):\n list_.append(sum(list_[-k:]))\n \n print(list_)\n \n return list_[n-1]\n", "def count_ways(n, k):\n steps = [1] * (n + 1)\n for i in range(1, n + 1):\n if i <= k:\n steps[i] = sum(steps[j] for j in range(0, i))\n else:\n steps[i] = sum(steps[i - j] for j in range(1, k + 1))\n return steps[-1]\n"] | {"fn_name": "count_ways", "inputs": [[1, 3], [3, 3], [2, 3], [5, 3], [4, 3], [10, 6], [14, 7]], "outputs": [[1], [4], [2], [13], [7], [492], [7936]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,971 |
def count_ways(n, k):
|
cdcdf75e4d57d61cfd55632262c017e6 | UNKNOWN | Implement a function, `multiples(m, n)`, which returns an array of the first `m` multiples of the real number `n`. Assume that `m` is a positive integer.
Ex.
```
multiples(3, 5.0)
```
should return
```
[5.0, 10.0, 15.0]
``` | ["def multiples(m, n):\n return [i * n for i in range(1, m+1)]", "def multiples(m, n):\n return [k * n for k in range(1, m+1)]", "multiples=lambda m,n:[n*e for e in range(1,m+1)]", "def multiples(m, n):\n array_multiples = []\n for i in range(1,m+1):\n array_multiples.append(float(i*n))\n return array_multiples", "def multiples(m, n):\n solution = []\n for i in range(1, m+1):\n solution.insert(i, n * i)\n return solution", "def multiples(m, n):\n ans = []\n for i in range(1, m+1):\n ans.append(n*i)\n return ans \n", "def multiples(m, n):\n return [n * (i + 1) for i in range(m)]\n # Implement me! :)\n", "def multiples(m, n):\n return [n * i for i in range(1, m+1)]", "def multiples(m, n):\n l=[]\n count=1\n while len(l)<m:\n l.append(n*count)\n count+=1\n return l \n \n", "multiples = lambda a, b: [i * b for i in range(1, a+1)]", "def multiples(m, n):\n return [a * n for a in range(1, m + 1)]", "def multiples(m, n):\n i = 0\n u = 1\n results = []\n while i < m:\n results.append(u*n)\n i+=1\n u+=1\n return results", "def multiples(m, n):\n return [i*n for i in list(range(m+1))][1:]\n", "def multiples(m, n):\n if type(m) == 'float':\n return []\n return [x * n for x in range(1, m + 1)]", "def multiples(m, n):\n l = []\n current = 0\n for i in range(1, m + 1):\n if i <= m:\n current = i * n\n l.append(current)\n return l\n", "def multiples(m, n):\n print(m)\n print(n)\n list1 = []\n i = 1\n while i <= m:\n multi = n * i\n print(multi)\n list1.append(multi)\n \n i += 1\n print(list1)\n return(list1)", "def multiples(m, n):\n lst = []\n st = 1\n while len(lst) < m:\n a = n * st\n lst.append(a)\n st += 1\n return lst", "def multiples(m, n):\n list = []\n arr = 0\n for i in range(1,m+1):\n arr = i*n\n list.append(arr)\n return list", "def multiples(m, n):\n ll = list()\n for i in range(m):\n ll.append(n * (i + 1))\n return ll", "def multiples(m, n):\n list_mult=list()\n for index in range(m):\n index=index+1\n multiple=n*index\n list_mult.append(multiple)\n return list_mult\n \n", "def multiples(m, n):\n return [n*h for h in (range(1,m+1))]", "def multiples(m, n):\n return [ i*n for i in range(1,m+1)]\n \n #return [st[i] for i in range(1, len(st), 2)]\n", "def multiples(m, n):\n result = []\n for i in range(m):\n n * (i + 1)\n result.append(n * (i +1))\n return result", "def multiples(m, n):\n l = []\n for x in range(1, m+1):\n l.append(n*x)\n return l", "def multiples(m, n):\n\n\n result = []\n result = [x*n for x in range(1,m+1)]\n \n return result\n", "def multiples(m, n):\n x = []\n for i in range(1,m+1):\n mult = n*i\n x.append(mult)\n return(x)", "def multiples(m, n):\n \n j = 1\n array = []\n \n while j <= m:\n array.append(j*n)\n j += 1\n \n return array", "def multiples(m, n):\n result = [n]\n i = 1\n while (i < m):\n i += 1\n result.append(i*n)\n return result\n", "def multiples(m, n):\n accum = []\n for i in range (1,m+1):\n accum.append(float(i*n))\n return accum", "def multiples(m, n):\n i = 1\n final_list = []\n while i <= m:\n final_list.append(n*i)\n i += 1\n return final_list", "def multiples(m, n):\n list = []\n count = 1\n while count <= m:\n list.append(n * count)\n count += 1\n return list", "def multiples(m, n):\n # Implement me! :)\n v = 1\n a = []\n while v <= m:\n a.append(n*v)\n v+=1\n return a", "def multiples(m, n):\n # Implement me! :)\n a = [n]\n i = 2\n l = n\n while m != 1:\n n = l\n n *= i\n a.append(n)\n m -= 1\n i += 1\n \n return a", "def multiples(m, n):\n mults=[]\n for i in range(1,m+1):\n mults.append(i*n)\n return mults", "def multiples(m,n): \n answer = []\n \n for num in range(m):\n ans = (num + 1) * n\n answer.append(ans)\n return answer", "def multiples(m, n):\n lst = []\n for x in range(1, m+1):\n y = n * x\n lst.append(y)\n return lst", "import math\n\ndef multiples(m, n):\n list = []\n i = 1\n while i <= m:\n list.append(n*i)\n i += 1\n return list", "def multiples(m, n):\n gg = []\n for i in range(m):\n gg.append((n)*(i+1))\n return gg\n", "def multiples(m, n):\n X = []\n for i in range(m):\n X.append((i + 1)*n)\n return X", "def multiples(m, n):\n count = 1\n result = []\n for _ in range(m):\n result.append(n*count)\n count +=1\n return result", "def multiples(m, n):\n result = 0\n text = []\n for i in range(1, m + 1):\n result += 1\n text.append(result * n)\n return text\n", "def multiples(m, n):\n a=[]\n for j in range(1, m+1):\n a.append(n*j)\n return a", "def multiples(m, n):\n result = []\n x = 1\n for i in range(m):\n result.append(n * x)\n x+=1\n return result", "def multiples(m, n):\n return [mult * n for mult in range(1, m + 1)]", "def multiples(m, n):\n i = 1\n a = []\n while i <= m:\n a.append(i*n)\n i = i+1\n return a", "def multiples(m, n):\n answer = []\n i = 1\n while i <= m:\n x = n * i\n answer.append(x)\n i+=1\n return answer", "def multiples(m, n):\n return [x * n for x in range(1, m + 1) if x > 0]", "def multiples(m, n):\n count = [x * n for x in range(1, m + 1) if x > 0]\n return count", "def multiples(m, n): \n result = []\n i = 1\n while i <= m:\n mul = n * i\n result.append(mul)\n i += 1\n return result", "def multiples(m, n):\n lst=[(n*i) for i in range(1,m+1)]\n return lst", "def multiples(m, n):\n a = [m*n for m in range(1,m+1)]\n return a", "def multiples(m, n):\n num_array = []\n for x in range(1, m+1):\n num_array.append(x*n)\n return num_array", "def multiples(m, n):\n lst = []\n for x in range(1,m+1):\n lst.append(x*n)\n return lst", "def multiples(m, n):\n res = []\n for i in range(1,m+1):\n val = n*i\n res.append(val)\n return res", "def multiples(m, n):\n output = []\n for nb in range(1, m+1):\n output.append(nb*n)\n return output", "def multiples(m, n):\n new = []\n i = 1\n while i <= m:\n new.append(n*i)\n i += 1\n return new", "def multiples(m, n):\n i = 1\n arr = []\n while i <= m:\n arr.append(n*i)\n i+=1\n return arr", "def multiples(m, n): \n luvut = list(range(1,m+1))\n _ = map(lambda luku: luku * n, luvut)\n \n return list(_)", "def multiples(to, n):\n return [n * i for i in range(1, to+1)]", "def multiples(m, n):\n count = 1\n result = []\n while count <= m:\n result.append(count * n)\n count += 1\n return result", "def multiples(m, n):\n arr = [] \n start = 1\n while m >= start: \n arr.append(start * n)\n start += 1\n return arr\n", "def multiples(m, n):\n nums = []\n for i in range(1, m+1):\n nums.append(i*n)\n return nums\n", "def multiples(m, n):\n l = []\n for i in range(1, m+1):\n c = i * n\n l.append(c)\n\n return l", "def multiples(m, n):\n # Implement me! :)\n x = 1\n temp = 0\n final = []\n while x <= m:\n temp = x * n\n final.append(temp)\n print (final)\n x = x + 1\n return final\n \n \n", "def multiples(m, n):\n data = []\n for i in range(1, m + 1):\n data.append(i * n)\n return data", "def multiples(m, n):\n result = []\n count = 1\n for a in range(m):\n result.append(count*n)\n count += 1\n\n return result", "def multiples(m, n):\n arr = []\n for i in range(1,m+1):\n res = n * i\n arr.append(res)\n return arr", "def multiples(m, n):\n new_list = []\n for i in range(1,m+1):\n new_list.append(i*n)\n return new_list", "def multiples(m, n):\n values = []\n for x in range(1,m+1):\n values.append(x * n)\n # Implement me! :)\n return values", "def multiples(m, n):\n arr = []\n s = 1\n k = 0\n while m > 0:\n k = n * s\n s += 1\n m -= 1\n arr.append(k)\n return arr", "def multiples(m, n):\n mass = []\n for c in range (1 ,m+1):\n mult = c*n\n mass.append(mult)\n return mass", "def multiples(m, n):\n list_multiples = []\n for i in range (1,m+1):\n list_multiples.append(i*n)\n return list_multiples\n \n # Implement me! :)\n", "def multiples(m, n):\n list= []\n i = 1\n while i<= m:\n list = list + [i*n]\n i=i+1\n return list", "def multiples(m, n):\n ar = []\n for i in range(m):\n ar.append(n*(i+1))\n return ar", "def multiples(m, n):\n return [n * el for el in range(1, m + 1)]", "def multiples(m, n):\n return [n*b for b in range(1,m+1)]", "def multiples(m, n):\n arr=[]\n for num in range(1,m+1):\n arr.append(n*num)\n \n return arr\n", "def multiples(m, n):\n array = []\n for i in range(m):\n array.append((i + 1) * n)\n \n return array", "def multiples(m, n):\n final=[]\n for num in range(1,m+1): # Iterate between 0 to m multiples\n final.append(n*num) # append every out to a list\n return final", "def multiples(m, n):\n result =[]\n for x in range(1,m+1):\n mul = x * n\n result.append(mul)\n \n \n return result", "def multiples(m, n):\n # Implement me! :)\n lis=[]\n for x in range(1,m+1,1):\n lis.append(n*x)\n return lis", "def multiples(m, n):\n multiple=[]\n for x in range(1,m+1):\n multiple.append(x*n)\n return multiple \n\nprint(multiples(5,2.0))", "def multiples(m, n):\n ma = []\n for i in range(1,m+1):\n ma.append(i*n)\n return ma", "def multiples(m, n):\n multiples = []\n \n for ix in range(1,m+1):\n multiples.append(ix * n)\n \n return multiples", "def multiples(m, n):\n x = []\n for num_jumps in range(1, m + 1):\n x.append(n * num_jumps)\n return x\n\n", "def multiples(m, n):\n myarr = []\n for i in range(1,m+1):\n myarr.append(n*i)\n i += 1\n return myarr", "def multiples(m, n):\n t = []\n for i in range(1, m+1):\n y = i*n\n t.append(y)\n return t", "def multiples(m, n):\n x = []\n i = 1\n while i <= m:\n x.append(n*i)\n i += 1\n return x", "def multiples(m, n):\n arr = list()\n for i in range(1, m+1):\n pro = i * n\n arr.append(pro) \n return arr", "def multiples(m, n):\n i = 0\n r = []\n while i < m:\n r.append(n * (i+1))\n i = i + 1\n print(r)\n return r", "def multiples(m, n):\n return list([x*n for x in range(1,m+1)])", "def multiples(m, n):\n return list(map(n.__mul__,range(1, m+1)))", "def multiples(a, b):\n\n result = list()\n n = 1\n while a > 0:\n result.append(b*n)\n a-=1\n n+=1\n \n return result", "def multiples(m, n):\n x = []\n for i in range(1,m+1):\n x.append(n * i)\n return x\n", "def multiples(m, n):\n lt = []\n for i in range(1,m+1):\n lt.append(i*n)\n return lt", "def multiples(m, n):\n return [i * n for i in range(1,m+1)]\n\n\n '''\n list = []\n for i in range(1,m+1):\n list.append(i*n)\n return list\n'''\n\n'''\n \n for i in m_list:\n list.append(i * n)'''", "def multiples(m, n):\n return [(n * (mult+1)) for mult in range(m)]\n", "def multiples(m, n):\n count = 1\n results = []\n while count<=m:\n results.append(count*n)\n count+=1\n return(results)", "def multiples(m, n):\n list = []\n for i in range(1,m):\n list.append(i * n)\n list.append(m*n)\n return list", "def multiples(m, n):\n list_of_multiples = []\n \n for i in range(1, m+1):\n list_of_multiples.append(n * i)\n \n return list_of_multiples\n"] | {"fn_name": "multiples", "inputs": [[3, 5], [1, 3.14], [5, -1]], "outputs": [[[5, 10, 15]], [[3.14]], [[-1, -2, -3, -4, -5]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,570 |
def multiples(m, n):
|
1d5ea8cf75728d55cab46ad73eaddada | UNKNOWN | # Introduction
Digital Cypher assigns a unique number to each letter of the alphabet:
```
a b c d e f g h i j k l m n o p q r s t u v w x y z
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
```
In the encrypted word we write the corresponding numbers instead of the letters. For example, the word `scout` becomes:
```
s c o u t
19 3 15 21 20
```
Then we add to each number a digit from the key (repeated if necessary). For example if the key is `1939`:
```
s c o u t
19 3 15 21 20
+ 1 9 3 9 1
---------------
20 12 18 30 21
m a s t e r p i e c e
13 1 19 20 5 18 16 9 5 3 5
+ 1 9 3 9 1 9 3 9 1 9 3
--------------------------------
14 10 22 29 6 27 19 18 6 12 8
```
# Task
Write a function that accepts a `message` string and an array of integers `code`. As the result, return the `key` that was used to encrypt the `message`. The `key` has to be shortest of all possible keys that can be used to code the `message`: i.e. when the possible keys are `12` , `1212`, `121212`, your solution should return `12`.
#### Input / Output:
* The `message` is a string containing only lowercase letters.
* The `code` is an array of positive integers.
* The `key` output is a positive integer.
# Examples
```python
find_the_key("scout", [20, 12, 18, 30, 21]) # --> 1939
find_the_key("masterpiece", [14, 10, 22, 29, 6, 27, 19, 18, 6, 12, 8]) # --> 1939
find_the_key("nomoretears", [15, 17, 14, 17, 19, 7, 21, 7, 2, 20, 20]) # --> 12
```
# Digital cypher series
- [Digital cypher vol 1](https://www.codewars.com/kata/592e830e043b99888600002d)
- [Digital cypher vol 2](https://www.codewars.com/kata/592edfda5be407b9640000b2)
- [Digital cypher vol 3 - missing key](https://www.codewars.com/kata/5930d8a4b8c2d9e11500002a) | ["def find_the_key(message, code):\n diffs = \"\".join( str(c - ord(m) + 96) for c, m in zip(code, message) )\n for size in range(1, len(code) +1):\n key = diffs[:size]\n if (key * len(code))[:len(code)] == diffs:\n return int(key)", "def find_the_key(message, code):\n key = \"\".join(str(code[i] + 96 - ord(char)) for i, char in enumerate(message))\n l = len(key)\n for i in range(1, l + 1):\n if (key[:i] * l)[:l] == key:\n return int(key[:i])", "find_the_key=lambda s,e:(lambda l,d:min(int(d[:i])for i in range(l)if(d[:i]*l)[:l]==d))(len(s),''.join(str(n-ord(c)+96)for c,n in zip(s,e)))", "def find_the_key(message, code):\n r = ''.join(str(a - b) for a, b in zip(code, [ord(c) - 96 for c in message]))\n \n for k in range(1, len(r) + 1):\n if all(c == r[:k][i%k] for i, c in enumerate(r)): return int(r[:k])\n", "def find_the_key(message, code):\n key = ''.join(str(c - 'abcdefghijklmnopqrstuvwxyz'.index(m) - 1) for m, c in zip(message, code))\n return int(next(key[0:i] for i in range(1, len(key)) if all(key[0:i].startswith(key[j:j+i]) for j in range(i, len(key), i))))", "from string import ascii_lowercase as al\n\ndef find_the_key(message, code):\n msg_key = ''.join([str(a - d) for (a, d) in zip(code, [al.index(c) + 1 for c in message])])\n key, len_msg = '', len(msg_key)\n for i, n in enumerate(msg_key, 1):\n key += n\n d, m = divmod(len_msg, i)\n test_key = key * (d + (m > 0)) # round up integer without math.ceil\n if test_key[:len_msg] == msg_key:\n return int(key)", "from string import ascii_lowercase\n\nns = {x: i for i, x in enumerate(ascii_lowercase, 1)}\n\ndef short(xs):\n for i in range(1, len(xs)):\n if all(len(set(xs[j::i])) == 1 for j in range(i)):\n return xs[:i]\n return xs\n\ndef find_the_key(message, code):\n xs = [a-b for a,b in zip(code, map(ns.get, message))]\n return int(''.join(map(str, short(xs))))", "def find_the_key(message, code):\n # your code here\n import string\n mapowanie_str_dig=dict(zip(list(string.ascii_lowercase),[x for x in range(1,27)])) \n m=[] \n for s in message:\n m=m+[mapowanie_str_dig[s]] \n delta=[str(abs(code[i]-m[i])) for i in range(0,len(m))]\n for i in range(1,len(delta)+1):\n n=len(delta)//i\n r=len(delta)%i\n if n*delta[0:i]==delta[0:n*i] and delta[0:r]==delta[n*i:]:\n output=delta[0:i]\n break \n return int(''.join(output))", "from string import ascii_lowercase as letters\n\ndef find_the_key(message, code):\n diff = [e-letters.index(c)-1 for (e,c) in zip(code, message)]\n for n in range(1, len(diff)):\n ok = True\n for k in range(n, len(diff), n):\n if diff[k:k+n] != diff[:min(n, len(diff)-k)]:\n ok = False\n break\n if ok: \n diff = diff[:n]\n break\n key = 0\n for k in diff:\n key = key * 10 + k\n return key", "def find_the_key(msg, code):\n from itertools import cycle\n msg = [ord(i) - 96 for i in msg]\n keys = [code[i] - msg[i] for i in range(len(code))]\n key = ''.join([str(i) for i in keys])\n \n i = 1\n while True:\n a = cycle(key[:i])\n if [_ + int(next(a)) for _ in msg] == code:\n return int(key[:i])\n else:\n i += 1"] | {"fn_name": "find_the_key", "inputs": [["scout", [20, 12, 18, 30, 21]], ["masterpiece", [14, 10, 22, 29, 6, 27, 19, 18, 6, 12, 8]], ["nomoretears", [15, 17, 14, 17, 19, 7, 21, 7, 2, 20, 20]], ["notencrypted", [14, 15, 20, 5, 14, 3, 18, 25, 16, 20, 5, 4]], ["tenthousand", [21, 5, 14, 20, 8, 16, 21, 19, 1, 14, 5]], ["oneohone", [16, 14, 6, 16, 8, 16, 15, 5]]], "outputs": [[1939], [1939], [12], [0], [10000], [101]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,431 |
def find_the_key(message, code):
|
b6f27461e22c55581ac436231e011cb9 | UNKNOWN | We’ve all seen katas that ask for conversion from snake-case to camel-case, from camel-case to snake-case, or from camel-case to kebab-case — the possibilities are endless.
But if we don’t know the case our inputs are in, these are not very helpful.
### Task:
So the task here is to implement a function (called `id` in Ruby/Crystal/JavaScript/CoffeeScript and `case_id` in Python/C) that takes a string, `c_str`, and returns a string with the case the input is in. The possible case types are “kebab”, “camel”, and ”snake”. If none of the cases match with the input, or if there are no 'spaces' in the input (for example in snake case, spaces would be '_'s), return “none”. Inputs will only have letters (no numbers or special characters).
### Some definitions
Kebab case: `lowercase-words-separated-by-hyphens`
Camel case: `lowercaseFirstWordFollowedByCapitalizedWords`
Snake case: `lowercase_words_separated_by_underscores`
### Examples:
```python
case_id(“hello-world”) #=> “kebab”
case_id(“hello-to-the-world”) #=> “kebab”
case_id(“helloWorld”) #=> “camel”
case_id(“helloToTheWorld”) #=> “camel”
case_id(“hello_world”) #=> “snake”
case_id(“hello_to_the_world”) #=> “snake”
case_id(“hello__world”) #=> “none”
case_id(“hello_World”) #=> “none”
case_id(“helloworld”) #=> “none”
case_id(“hello-World”) #=> “none”
```
Also check out my other creations — [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2) | ["import re\n\nCASES = [\n ('snake', re.compile(r'\\A[a-z]+(_[a-z]+)+\\Z')),\n ('kebab', re.compile(r'\\A[a-z]+(-[a-z]+)+\\Z')),\n ('camel', re.compile(r'\\A[a-z]+([A-Z][a-z]*)+\\Z')),\n ('none', re.compile(r'')),\n]\n\ndef case_id(c_str):\n for case, pattern in CASES:\n if pattern.match(c_str): return case", "import re\n\ndef case_id(c_str):\n if re.match(r'^([a-z]+\\-)+[a-z]+$', c_str):\n return 'kebab'\n if re.match(r'^([a-z]+\\_)+[a-z]+$', c_str):\n return 'snake'\n if re.match(r'^([a-z]+[A-Z])+[a-z]+$', c_str):\n return 'camel'\n return 'none'", "def case_id(stg):\n print(stg)\n if \"-\" in stg and \"_\" not in stg and all(w.islower() for w in stg.split(\"-\")):\n return \"kebab\"\n if \"_\" in stg and \"-\" not in stg and all(w.islower() for w in stg.split(\"_\")):\n return \"snake\"\n if stg.isalpha() and any(c.isupper() for c in stg):\n return \"camel\"\n return \"none\"", "import re\ndef case_id(c_str):\n if re.match(r'[a-z]+(-[a-z]+)+$', c_str):\n return \"kebab\" \n if re.match(r'[a-z]+(_[a-z]+)+$', c_str):\n return \"snake\"\n if re.match(r'[a-z]+([A-Z][a-z]+)+$', c_str): \n return \"camel\"\n return 'none'\n", "from string import (ascii_lowercase as ASCII_LOW,\n ascii_letters as ASCII_LET)\n\ndef is_kebab_case(str_):\n for char in str_:\n if char not in (ASCII_LOW + '-'):\n return False\n if '' in str_.split('-'):\n return False\n return True\n\ndef is_camel_case(str_):\n for char in str_:\n if char not in ASCII_LET:\n return False\n if str_[0].isupper():\n return False\n return True\n \ndef is_snake_case(str_):\n for char in str_:\n if char not in (ASCII_LOW + '_'):\n return False\n if '' in str_.split('_'):\n return False\n return True\n\ndef case_id(c_str):\n for func in (is_kebab_case, is_camel_case, is_snake_case):\n if func(c_str): \n return {'is_kebab_case': 'kebab',\n 'is_camel_case': 'camel',\n 'is_snake_case': 'snake'}[func.__name__]\n return 'none'", "def case_id(s):\n if '--' in s or '_' in s and '-' in s:\n return 'none'\n if s.replace('_','').replace('-','').islower():\n if '-' in s: return 'kebab'\n if '_' in s: return 'snake'\n if '-' in s or '_' in s: \n return 'none'\n return 'camel'", "def case_id(c_str):\n if '--' in c_str or '_' in c_str and '-' in c_str:\n return \"none\"\n elif c_str.replace('_','').replace('-','').islower():\n if '-' in c_str:\n return \"kebab\"\n elif '_' in c_str:\n return \"snake\"\n elif '-' in c_str or '_' in c_str:\n return \"none\"\n return \"camel\"", "def is_kebab(s):\n arr = s.split('-')\n res = [part.islower() and '_' not in part and part != '' for part in arr]\n return all(res)\n \ndef is_snake(s):\n arr = s.split('_')\n res = [part.islower() and '-' not in part and part != '' for part in arr]\n return all(res)\n\ndef is_camel(s):\n return '-' not in s and '_' not in s and not s.islower()\n \ndef case_id(c_str):\n if is_kebab(c_str): return \"kebab\"\n if is_snake(c_str): return \"snake\"\n if is_camel(c_str): return \"camel\"\n return \"none\""] | {"fn_name": "case_id", "inputs": [["hello-world"], ["hello-to-the-world"], ["hello_world"], ["hello_to_the_world"], ["helloWorld"], ["helloToTheWorld"], ["hello-World"], ["hello-To-The-World"], ["good-Night"], ["he--llo"], ["good-night"], ["good_night"], ["goodNight"], ["hello_World"], ["hello_To_The_World"], ["he_lloWorld"], ["he_lo-lo"], ["he-llo--world"], ["he-llo--World"], ["hello_-World"]], "outputs": [["kebab"], ["kebab"], ["snake"], ["snake"], ["camel"], ["camel"], ["none"], ["none"], ["none"], ["none"], ["kebab"], ["snake"], ["camel"], ["none"], ["none"], ["none"], ["none"], ["none"], ["none"], ["none"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,415 |
def case_id(c_str):
|
9ac2f96676d2287dbe121ba93e190bf2 | UNKNOWN | # Task
Lonerz got some crazy growing plants and he wants to grow them nice and well.
Initially, the garden is completely barren.
Each morning, Lonerz can put any number of plants into the garden to grow.
And at night, each plant mutates into two plants.
Lonerz really hopes to see `n` plants in his garden.
Your task is to find the minimum number of plants Lonerz has to plant to get `n` plants one day.
# Example
For `n = 5`, the output should be `2`.
Lonerz hopes to see `5` plants. He adds `1` plant on the first morning and on the third morning there would be `4` plants in the garden. He then adds `1` more and sees `5` plants.
So, Lonerz only needs to add 2 plants to his garden.
For `n = 8,` the output should be `1`.
Lonerz hopes to see `8` plants. Thus, he just needs to add `1` plant in the beginning and wait for it to double till 8.
# Input/Output
The number of plant lonerz hopes to see in his garden.
- `[input]` integer `n`
`1 <= n <= 10^7`
- `[output]` an integer
The number of plants Lonerz needs to plant. | ["def plant_doubling(n):\n return bin(n).count(\"1\")", "def plant_doubling(n):\n p = 0\n while n:\n if n % 2 == 1:\n n -= 1\n p += 1\n n //= 2\n return p", "def plant_doubling(n):\n return bin(n)[2:].count(\"1\")", "import math\ndef plant_doubling(n):\n times = 0\n while n != 0:\n times += 1\n n = n - (2 ** int(math.log(n, 2)))\n return times", "def plant_doubling(n):\n return sum(c == '1' for c in bin(n)[2:])", "def plant_doubling(n):\n powers = [2**i for i in range(30)]\n powers.reverse()\n difference = n\n array = []\n for i in range(30):\n if powers[i] <= difference:\n difference = (difference - powers[i])\n array.append(powers[i])\n return len(array)", "def plant_doubling(n):\n schemat = str(bin(n))\n return sum(int(schemat[i]) for i in range(2, len(schemat)))", "def plant_doubling(n):\n s=0\n while n:\n if n%2:s+=1\n n//=2\n return s", "def plant_doubling(n):\n list = []\n for i in range(64):\n list.append(2**i)\n rounds = 0\n while n >= 1:\n relevant_list = []\n for item in list:\n if item <= n:\n relevant_list.append(item)\n n = n - relevant_list[-1]\n rounds = rounds + 1\n return rounds"] | {"fn_name": "plant_doubling", "inputs": [[5], [8], [536870911], [1]], "outputs": [[2], [1], [29], [1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,344 |
def plant_doubling(n):
|
9f0cab4f780a344a5e1f2b04e4895c05 | UNKNOWN | # Triple Trouble
Create a function that will return a string that combines all of the letters of the three inputed strings in groups. Taking the first letter of all of the inputs and grouping them next to each other. Do this for every letter, see example below!
**E.g. Input: "aa", "bb" , "cc" => Output: "abcabc"**
*Note: You can expect all of the inputs to be the same length.* | ["def triple_trouble(one, two, three):\n return ''.join(''.join(a) for a in zip(one, two, three))\n", "def triple_trouble(one, two, three):\n return \"\".join(a+b+c for a,b,c in zip(one,two,three))", "def triple_trouble(*args):\n return \"\".join(\"\".join(a) for a in zip(*args))", "def triple_trouble(one, two, three):\n ans = ''\n for i in range(len(one)):\n ans+=one[i]\n ans+=two[i]\n ans+=three[i]\n return ans", "def triple_trouble(*args):\n return ''.join(sum(zip(*args), ()))", "def triple_trouble(one, two, three):\n return ''.join([one[i] + two[i] + three[i] for i in range(len(one))])", "def triple_trouble(one, two, three):\n a = one.split(\" \")\n a = map(lambda x, y, z: x + y + z, one, two, three)\n return \"\".join(a)", "def triple_trouble(*args):\n return \"\".join(map(\"\".join, zip(*args)))", "def triple_trouble(one, two, three):\n ans = ''\n for x in range(len(one)):\n ans += one[x] + two[x] + three[x]\n return ans", "def triple_trouble(one, two, three):\n return ''.join(''.join(v) for v in zip(one,two,three))", "def triple_trouble(one, two, three):\n count = 0\n length = len(one)\n string = \"\"\n while count < length:\n string += one[count] + two[count] + three[count]\n count += 1\n return string\n \n #your code here\n", "def triple_trouble(one, two, three):\n return ''.join(''.join(s) for s in zip(one, two, three))", "def triple_trouble(one, two, three):\n return ''.join(''.join(i) for i in zip(one, two, three))", "def triple_trouble(one, two, three):\n a = \"\"\n for i in range(len(one)):\n a += one[i] + two[i] + three[i]\n return a", "def triple_trouble(one, two, three):\n i = 0 \n t = ''\n while i < len(three):\n t = t + one[i] + two[i] +three [i] \n i +=1\n return(t)", "triple_trouble = lambda *args: ''.join(''.join(z) for z in zip(*args)) ", "triple_trouble = lambda r, s, t: ''.join([c + s[i] + t[i] for i, c in enumerate(list(r))])", "def triple_trouble(*l):\n return ''.join([''.join(s) for s in zip(*l)])", "def triple_trouble(*args):\n return ''.join(''.join(tupl) for tupl in zip(*args))", "def triple_trouble(one, two, three):\n return \"\".join(map(\"\".join, zip(list(one), list(two), list(three))))", "def triple_trouble(one, two, three):\n from functools import reduce\n return ''.join(reduce(lambda x, y: x + y, zip(one, two, three)))", "def triple_trouble(one, two, three):\n return ''.join([j for i in zip(*[one, two, three]) for j in i])", "from typing import Tuple\n\ndef triple_trouble(*args: Tuple[str]) -> str:\n \"\"\" Get a string that combines all of the letters of the three inputed strings in groups. \"\"\"\n return \"\".join(list(map(\"\".join, zip(*args))))", "from itertools import chain\ndef triple_trouble(one, two, three):\n return ''.join(c for c in chain.from_iterable(zip(one,two,three)))", "def triple_trouble(one, two, three):\n return ''.join(['{}{}{}'.format(one[x],two[x],three[x]) for x in range(len(one))])", "def triple_trouble(one, two, three):\n #your code here\n four = \"\"\n for x in range(len(one)):\n four += one[x]+two[x]+three[x]\n return four", "def triple_trouble(one, two, three):\n ret = \"\"\n for i in range(len(one)):\n ret = ret + one[i] + two[i] + three[i]\n return ret", "def triple_trouble(one, two, three):\n return ''.join([''.join([one[i],two[i],three[i]]) for i in range(len(one))])\n #your code here\n", "def triple_trouble(one, two, three):\n return ''.join(one[i] + two[i] + three[i] for i in range(len(one)))", "triple_trouble=lambda o, t, h: \"\".join(\"\".join([a,t[i],h[i]]) for i,a in enumerate(o))", "def triple_trouble(one, two, three):\n #your code here\n res=len(one) \n l=''\n for i in range(res):\n result=one[i] + two[i] + three[i]\n l=l+result\n return l", "def triple_trouble(one, two, three):\n x = list(zip(one, two, three))\n result = []\n for item in x:\n result.append(\"\".join(item))\n return \"\".join(result)\n \n \n \n\n\n", "def triple_trouble(one, two, three):\n result = \"\"\n n = 0\n while n < len(one):\n result += one[n] + two[n] + three[n]\n n+=1\n \n \n \n return result\n", "def triple_trouble(one, two, three):\n output = ''\n for first, second, third in zip(one, two, three):\n output = ''.join([output, first, second, third])\n return output\n #your code here\n", "def triple_trouble(one, two, three):\n new_string = ''\n for letter in range(len(one)):\n new_string += one[letter]\n new_string += two[letter] \n new_string += three[letter]\n return new_string", "def triple_trouble(one, two, three):\n str1 = ''\n for i in range(len(one)):\n str1 += one[i]\n str1 += two[i]\n str1 += three[i]\n return str1\n\n", "def triple_trouble(one, two, three):\n x= list(zip(one,two,three))\n l1= [''.join(y) for y in x]\n return ''.join(l1)", "def triple_trouble(one, two, three):\n result = \"\"\n for i, c in enumerate(one):\n result += c + two[i] + three[i]\n return result", "def triple_trouble(one, two, three):\n return ''.join(''.join(set) for set in list(zip(one, two, three)))", "def triple_trouble(one, two, three):\n list = []\n for x in range(len(one)):\n list.extend((one[x],two[x],three[x]))\n return ''.join(list)\n\n", "def triple_trouble(one, two, three):\n x = [one, two, three]\n y = max(x, key=len)\n z = len(y)\n a = ''\n for k in range(0,z):\n a += x[0][k] + x[1][k] + x[2][k]\n return a\n #your code here\n", "def triple_trouble(one, two, three):\n result = ''\n for i in range(len(one)):\n segm = one[i] + two[i] + three[i]\n result += segm\n return result", "def triple_trouble(one, two, three):\n word=\"\"\n for i in range(0,len(one)):\n word+= one[i]+two[i]+three[i]\n return word\n", "def triple_trouble(one, two, three):\n i = 0\n list123 = []\n while i < len(one):\n list123.append(one[i])\n list123.append(two[i])\n list123.append(three[i])\n i += 1\n return ''.join(list123)\n", "def triple_trouble(one, two, three):\n L = len(one)\n result = []\n for x in range(L):\n result.append(one[x])\n result.append(two[x])\n result.append(three[x])\n return \"\".join(result)", "def triple_trouble(one, two, three):\n s = ''\n a = 0\n b = [one, two, three]\n for j in one:\n for i in b:\n s += i[a]\n a += 1\n return s\n", "def triple_trouble(one, two, three):\n result = ''\n for i in range(len(one)):\n result = result + one[i] + two[i] + three[i]\n print(result)\n return result", "def triple_trouble(one, two, three):\n collector = \"\"\n size = 0\n \n while size < len(one):\n collector += one[size]+two[size]+three[size]\n size += 1\n \n return collector", "def triple_trouble(one, two, three):\n return \"\".join(lttr1 + lttr2 + lttr3 for lttr1, lttr2, lttr3 in zip(one, two, three))", "def triple_trouble(one, two, three):\n list_word = []\n for i in range(len(one)):\n list_word.append(one[i])\n list_word.append(two[i])\n list_word.append(three[i])\n return \"\".join(list_word)", "def triple_trouble(one, two, three):\n result = ''\n x = 0\n for i in one:\n result =result+i+two[x]+three[x]\n x = x+1\n \n return result\n", "def triple_trouble(one, two, three):\n return ''.join([f'{one[x]}{two[x]}{three[x]}' for x in range(len(one))])", "def triple_trouble(one, two, three):\n return ''.join(''.join(el) for el in zip(one, two, three))", "def triple_trouble(one, two, three):\n strin = ''\n \n for el in range(len(one)):\n strin += one[el] + two[el] + three[el]\n \n return strin", "def triple_trouble(one, two, three):\n res = ''\n a = one, two, three\n for i in range(len(one)):\n res += one[i]\n res += two[i]\n res += three[i]\n return res", "def triple_trouble(one, two, three):\n car=''\n for i in range(len(one)):\n car+=one[i]+two[i]+three[i]\n return car", "triple_trouble = lambda *a:''.join(''.join(x) for x in zip(*a))", "def triple_trouble(one, two, three):\n result_str = \"\"\n for o, tw, th in zip(one, two, three):\n result_str += o + tw + th\n return result_str\n #your code here\n", "def triple_trouble(one, two, three):\n list = map(lambda a,b,c: a+b+c, one,two, three)\n return \"\".join(list)", "def triple_trouble(one, two, three):\n newStr = ''\n for i in range(len(one)):\n newStr += one[i] + two[i] + three[i]\n return newStr", "def triple_trouble(a, b, c):\n r = \"\"\n for i in range(len(a)): r += a[i]+b[i]+c[i]\n return r", "def triple_trouble(one, two, three):\n tot = ''\n for x in range(len(one)):\n tot += one[x] + two[x] + three[x]\n return tot", "def triple_trouble(a, b, c):\n s = \"\"\n for i in range(len(a)):\n s+=a[i]+b[i]+c[i]\n return s ", "def triple_trouble(one,\n two,\n three):\n\n result = \"\"\n for i in range(len(one)):\n result += one[i] + two[i] + three[i]\n\n return result\n", "def triple_trouble(one, two, three):\n\n my_list = []\n for i in range(len(one)):\n my_list.append((one[i] + two[i] + three[i]))\n\n final = ''.join(my_list)\n \n return final", "def triple_trouble(one, two, three):\n return ''.join(''.join(tuple) for tuple in list(zip(one,two,three)))", "def triple_trouble(one, two, three):\n output = ''\n for x in range(len(one)):\n output += one[x]\n output += two[x]\n output += three[x]\n return output", "triple_trouble = lambda one,two,three : ''.join([f'{one[i]}{two[i]}{three[i]}' for i in range(len(one))])\n", "def triple_trouble(one, two, three):\n tup = list(zip(one, two, three))\n out = list(sum(tup, ()))\n return ''.join(out)", "def triple_trouble(one, two, three):\n Liste=list()\n indices=list(range(len(one)))\n #print(indices)\n for index in indices:\n Kombi= one[index]+two[index]+three[index]\n #print(Kombi)\n Liste.append(Kombi)\n\n Liste=\"\".join(Liste)\n return Liste\n", "def triple_trouble(one, two, three):\n Trible=''\n for x in range(len(one)):\n Trible += one[x]+two[x]+three[x]\n return Trible\n #your code here\n", "triple_trouble = lambda a,b,c : \"\".join([a[i]+b[i]+c[i] for i in range(0,len(a))])", "def triple_trouble(one, two, three):\n result = ''\n counter = 0\n while counter < len(one):\n result += one[counter] + two[counter] + three[counter]\n counter += 1\n return result", "def triple_trouble(one, two, three):\n x = ''\n a = list(one)\n b = list(two)\n c = list(three)\n for i in range(0, max(len(a), len(b), len(c))):\n x = x + a[i] + b[i] + c[i]\n return x", "def triple_trouble(one, two, three):\n soln = ''\n for i in range(len(one)):\n soln += one[i] + two[i] + three[i]\n return soln", "def triple_trouble(one, two, three):\n x = []\n for i in range(len(one)):\n x.append(one[i])\n x.append(two[i])\n x.append(three[i])\n return ''.join(x)", "def triple_trouble(one, two, three):\n return \"\".join(one[n]+two[n]+three[n] for n in range(len(one)))", "def triple_trouble(one, two, three):\n new_line = \"\"\n for i in range(0, len(one)):\n new_line += one[i] + two[i] + three[i]\n return new_line", "def triple_trouble(one, two, three):\n combined = \"\"\n i = 0\n \n for letter in range(len(one)):\n combined += one[i] + two[i] + three[i]\n i += 1\n \n return combined", "def triple_trouble(one, two, three):\n\n counter = 0\n res = ''\n for i in one:\n res += i\n res += two[counter]\n res += three[counter]\n counter += 1\n return res\n \n \n", "def triple_trouble(one, two, three):return \"\".join([\"\".join([x[i] for x in (one,two,three)]) for i in range(len(one))])", "def triple_trouble(one, two, three):\n res = \"\"\n for x in range(len(one)):\n res += one[x]\n res += two[x]\n res += three[x]\n \n return res", "def triple_trouble(one, two, three):\n############################################################# \n################################################### ####### \n############################################### /~\\ #####\n############################################ _- `~~~', ####\n########################################## _-~ ) ####\n####################################### _-~ | ####\n#################################### _-~ ; #####\n########################## __---___-~ | #####\n####################### _~ ,, ; `,, ##\n##################### _-~ ;' | ,' ; ##\n################### _~ ' `~' ; ###\n############ __---; ,' ####\n######## __~~ ___ ,' ######\n##### _-~~ -~~ _ ,' ########\n##### `-_ _ ; ##########\n####### ~~----~~~ ; ; ###########\n######### / ; ; ############\n####### / ; ; #############\n##### / ` ; ##############\n### / ; ###############\n# ################\n str=\"\"\n for n in range(len(one)):\n str+=one[n]+two[n]+three[n]\n return str", "def triple_trouble(one, two, three):\n s = one + two + three\n A = len(s)\n B = len(one)\n answ = \"\"\n start = 0\n for j in range(B):\n for i in range(start,A,B):\n answ += s[i]\n start+=1\n return answ\n", "def triple_trouble(one, two, three):\n zipped = list(zip(one, two, three))\n return ''.join('%s%s%s' % z for z in zipped)", "def triple_trouble(one, two, three):\n \n news = \"\"\n for i in range (0,len(one)):\n news = news + one[i]+two[i]+three[i]\n return news\n", "def triple_trouble(one, two, three):\n arr = [one, two, three]\n result = ''\n \n for i in range(len(arr[0])):\n for j in range(3):\n result += arr[j][i]\n print(result)\n return result", "def triple_trouble(one, two, three):\n l=[]\n for i in range(0,len(one)):\n l.append(one[i]+two[i]+three[i])\n return ''.join(l)", "def triple_trouble(one, two, three):\n one = [item for item in one]\n two = [item for item in two]\n three = [item for item in three]\n new =[]\n for i in range(0,len(one)):\n new.append(one[i]+two[i]+three[i])\n return \"\".join(new)\n", "def triple_trouble(one, two, three):\n \n char = \"\"\n for i, j, k in zip(one, two, three):\n char += (str(i) + str(j) + str(k))\n return char", "def triple_trouble(one, two, three):\n new = \"\"\n for j in range(0, len(one)):\n name = one[j] + two[j] + three[j]\n new += one[j] + two[j] + three[j]\n return new\n\nziel = triple_trouble(\"burn\", \"reds\", \"roll\")\nprint(ziel)\n", "def triple_trouble(one: str, two: str, three: str) -> str:\n return \"\".join([\"\".join(x) for x in zip(one, two, three)])", "def triple_trouble(one, two, three):\n oneL = list(one) ; twoL = list(two) ; threeL = list(three)\n returnArr = []\n for i in range(len(oneL)):\n returnArr.append(oneL[i]) ; returnArr.append(twoL[i]) ; returnArr.append(threeL[i])\n return \"\".join(returnArr)", "def triple_trouble(one, two, three):\n return ''.join(f'{one[i]}{two[i]}{three[i]}' for i in range(0,len(one)))", "def triple_trouble(one, two, three):\n res = []\n for i in range(len(one)):\n res.append(one[i] + two[i] + three[i])\n return ''.join(res)", "def triple_trouble(one, two, three):\n result = \"\"\n e = 0\n while e < len(one):\n result += one[e] + two[e] + three[e]\n e += 1\n return result", "def triple_trouble(one, two, three):\n result = []\n for pt1, pt2, pt3 in zip(one, two, three):\n for i, j, k in zip(pt1, pt2, pt3):\n result.append(i)\n result.append(j)\n result.append(k)\n return \"\".join(result)"] | {"fn_name": "triple_trouble", "inputs": [["aaa", "bbb", "ccc"], ["aaaaaa", "bbbbbb", "cccccc"], ["burn", "reds", "roll"], ["Bm", "aa", "tn"], ["LLh", "euo", "xtr"]], "outputs": [["abcabcabc"], ["abcabcabcabcabcabc"], ["brrueordlnsl"], ["Batman"], ["LexLuthor"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 16,819 |
def triple_trouble(one, two, three):
|
97d1820390544c97c2fe06f1892b98e2 | UNKNOWN | # Introduction
Ka ka ka cypher is a cypher used by small children in some country. When a girl wants to pass something to the other girls and there are some boys nearby, she can use Ka cypher. So only the other girls are able to understand her.
She speaks using KA, ie.:
`ka thi ka s ka bo ka y ka i ka s ka u ka gly` what simply means `this boy is ugly`.
# Task
Write a function `KaCokadekaMe` (`ka_co_ka_de_ka_me` in Python) that accepts a string word and returns encoded message using ka cypher.
Our rules:
- The encoded word should start from `ka`.
- The `ka` goes after vowel (a,e,i,o,u)
- When there is multiple vowels together, the `ka` goes only after the last `vowel`
- When the word is finished by a vowel, do not add the `ka` after
# Input/Output
The `word` string consists of only lowercase and uppercase characters. There is only 1 word to convert - no white spaces.
# Example
```
KaCokadekaMe("a"); //=> "kaa"
KaCokadekaMe("ka"); //=> "kaka"
KaCokadekaMe("aa"); //=> "kaaa"
KaCokadekaMe("Abbaa"); //=> kaAkabbaa
KaCokadekaMe("maintenance"); //=> kamaikantekanakance
KaCokadekaMe("Woodie"); //=> kaWookadie
KacokadekaMe("Incomprehensibilities"); //=> kaIkancokamprekahekansikabikalikatiekas
```
# Remark
Ka cypher's country residents, please don't hate me for simplifying the way how we divide the words into "syllables" in the Kata. I don't want to make it too hard for other nations ;-P | ["import re\n\nKA_PATTERN = re.compile(r'(?![aeiou]+$)([aeiou]+)', re.I)\n\ndef ka_co_ka_de_ka_me(word):\n return 'ka' + KA_PATTERN.sub(r'\\1ka', word)", "from functools import partial\nfrom re import compile\n\nka_co_ka_de_ka_me = partial(compile(r\"(?i)^|(?<=[aeiou])(?![aeiou]|$)\").sub, \"ka\")", "def ka_co_ka_de_ka_me(word):\n prdel = \"\";\n for i, x in enumerate(word):\n if i != len(word):\n if x.lower() not in \"aeiou\" and word[i-1].lower() in \"aeiou\" and i != 0:\n prdel += \"ka\"\n prdel += x\n \n return \"ka\" + prdel", "def ka_co_ka_de_ka_me(word, tete='ka'):\n for i in range(len(word) - 1):\n tete+=word[i] \n if word[i] in 'aeiouAEIOU':\n if word[i+1] not in 'aeiouAEIOU':\n tete+='ka';\n\n return tete + word[-1]", "import re\n\ndef ka_co_ka_de_ka_me(word):\n return \"ka\" + re.sub(r\"([aeiou]+)(?=[^aeiou])\", r\"\\1ka\", word, flags=re.I)\n", "import re\n\nr = re.compile('[aeiou]+',re.I)\n\ndef ka_co_ka_de_ka_me(word):\n word = 'ka'+r.sub(lambda m: m.group(0)+'ka',word)\n return word[:-2] if word.endswith('ka') else word\n", "import re\n\ndef ka_co_ka_de_ka_me(word):\n return 'ka' + re.sub('([aeiou]+)(?=[^aeiou])', r'\\1ka', word, flags=re.I)", "import re\ndef ka_co_ka_de_ka_me(word):\n return \"ka\"+re.sub(r'([aeiouAEIOU]+)([^aeiouAEIOU])',lambda x: x.group(1)+r\"ka\"+ x.group(2),word)", "import re\naeiou = re.compile(r'([aeiou]+)([^aeiou])', re.I)\n\ndef ka_co_ka_de_ka_me(word):\n return 'ka' + aeiou.sub(r'\\1ka\\2', word)", "ka_co_ka_de_ka_me=lambda s:__import__('re').sub(r'(?i)(\\b(?=\\w)|(?<=[aeiou])\\B(?![aeiou]))',r'ka',s)"] | {"fn_name": "ka_co_ka_de_ka_me", "inputs": [["ka"], ["a"], ["aa"], ["z"], ["Abbaa"], ["maintenance"], ["Woodie"], ["Incomprehensibilities"]], "outputs": [["kaka"], ["kaa"], ["kaaa"], ["kaz"], ["kaAkabbaa"], ["kamaikantekanakance"], ["kaWookadie"], ["kaIkancokamprekahekansikabikalikatiekas"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,683 |
def ka_co_ka_de_ka_me(word):
|
05a387edadc631db37fb8be988366c44 | UNKNOWN | A carpet shop sells carpets in different varieties. Each carpet can come in a different roll width and can have a different price per square meter.
Write a function `cost_of_carpet` which calculates the cost (rounded to 2 decimal places) of carpeting a room, following these constraints:
* The carpeting has to be done in one unique piece. If not possible, retrun `"error"`.
* The shop sells any length of a roll of carpets, but always with a full width.
* The cost has to be minimal.
* The length of the room passed as argument can sometimes be shorter than its width (because we define these relatively to the position of the door in the room).
* A length or width equal to zero is considered invalid, return `"error"` if it occurs.
INPUTS:
`room_width`, `room_length`, `roll_width`, `roll_cost` as floats.
OUTPUT:
`"error"` or the minimal cost of the room carpeting, rounded to two decimal places. | ["def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n x, y = sorted((room_length, room_width))\n if y == 0 or x > roll_width: return \"error\"\n if y < roll_width: return round(x * roll_width * roll_cost, 2)\n return round(y * roll_width * roll_cost, 2)", "def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n if room_length > roll_width < room_width or room_length * room_width <= 0:\n return 'error'\n if room_length <= roll_width >= room_width:\n side = min(room_length, room_width)\n else:\n side = max(room_length, room_width)\n return round(side * roll_width * roll_cost, 2)", "def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n if room_length > roll_width and room_width > roll_width or \\\n not room_length * room_width * roll_width:\n return 'error'\n [a, b] = sorted([room_length, room_width]) \n if room_length <= roll_width and room_width <= roll_width:\n return round(roll_width * a * roll_cost, 2) \n return round(b * roll_width * roll_cost, 2)\n", "def cost_of_carpet(l, w, roll, cost):\n if l > roll < w or l <= 0 or w <= 0: return 'error'\n res = 0\n if l <= roll < w: res = roll * w * cost\n elif w <= roll < l: res = roll * l * cost\n else: res = roll * min(w, l) * cost\n return round(res, 2)", "def cost_of_carpet(rW, rL, cW, cCost):\n rW, rL = sorted((rW, rL))\n return \"error\" if cW < rW or 0 in (rL, rW, cW) else round(min([rL] + [rW]*(rL<=cW)) * cW * cCost, 2)", "def cost_of_carpet(l, w, r, c):\n if l==0 or w==0 or l>r and w>r : \n return \"error\"\n return round( (min(l,w) if l<=r and w<=r else max(l,w) ) * r * c , 2)\n", "def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n if room_length <= 0 or room_width <= 0 or (room_length > roll_width < room_width):\n return 'error'\n \n x, y = min(room_length, room_width), max(room_length, room_width)\n \n if y > roll_width:\n return round(y * roll_width * roll_cost, 2)\n \n return round(x * roll_width * roll_cost, 2) \n", "from math import ceil\ndef cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n w,l=sorted([room_length, room_width])\n if room_length==0 or room_width==0 or roll_width<w:\n return 'error'\n if l<=roll_width:\n return round(w*roll_width*roll_cost,2)\n else:\n return round(l*roll_width*roll_cost,2)\n", "def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n if 0 in [room_length, room_width]:\n return \"error\"\n mi, mx = min((room_length, room_width)), max((room_length, room_width))\n rw, rc = roll_width, roll_cost\n return round(mi * rw * rc, 2) if mx <= roll_width else (round(mx * rw * rc, 2) if roll_width >= mi else \"error\")", "def cost_of_carpet(room_length, room_width, roll_width, roll_cost):\n if roll_width < min(room_length, room_width):\n return 'error'\n elif room_length == 0 or room_width ==0:\n return \"error\"\n cost_1 = roll_width*room_length*roll_cost if roll_width >= room_width else float('inf')\n cost_2 = roll_width*room_width*roll_cost if roll_width >= room_length else float('inf')\n return round(min(cost_1, cost_2), 2)\n \n \n\n \n"] | {"fn_name": "cost_of_carpet", "inputs": [[3, 5, 4, 10], [4, 5, 4, 10], [0, 0, 4, 10], [3, 2, 4, 10], [3.9, 2, 4, 10], [5, 6, 4, 10], [3, 2, 4, 0], [3, 2, 2, 10]], "outputs": [[200], [200], ["error"], [80], [80], ["error"], [0], [60]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,307 |
def cost_of_carpet(room_length, room_width, roll_width, roll_cost):
|
53c1b7252fca5bc6211cb31c405ee79e | UNKNOWN | Given an integer `n` return `"odd"` if the number of its divisors is odd. Otherwise return `"even"`.
**Note**: big inputs will be tested.
## Examples:
All prime numbers have exactly two divisors (hence `"even"`).
For `n = 12` the divisors are `[1, 2, 3, 4, 6, 12]` – `"even"`.
For `n = 4` the divisors are `[1, 2, 4]` – `"odd"`. | ["def oddity(n):\n #your code here\n return 'odd' if n**0.5 == int(n**0.5) else 'even'", "oddity=lambda n: [\"odd\",\"even\"][n**.5%1!=0]", "import math\ndef oddity(n):\n return math.sqrt(n) % 1 == 0 and 'odd' or 'even'", "import math\ndef oddity(n):\n \n return \"even\" if math.sqrt(n)%1 else \"odd\"\n", "import math\ndef oddity(n):\n if int(math.sqrt(n))**2 == n:\n return 'odd'\n return 'even'", "def oddity(n):\n counter = 0\n for x in range(1, int(n**.5)+1):\n a,b = divmod(n,x)\n if not b:\n if a!=x: counter += 2\n else: counter += 1\n return ('even', 'odd')[counter%2]", "def oddity(n):\n return 'odd' if (n ** 0.5).is_integer() else 'even'", "def oddity(n):\n return 'even' if n ** 0.5 % 1 else 'odd'", "def oddity(n):\n return 'odd' if int(n**0.5)**2 == n else 'even'", "def oddity(n):\n return 'odd' if round(n ** 0.5) ** 2 == n else 'even'"] | {"fn_name": "oddity", "inputs": [[1], [5], [16], [27], [121]], "outputs": [["odd"], ["even"], ["odd"], ["even"], ["odd"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 940 |
def oddity(n):
|
00705af5fef965a2c4572cec8066ecf1 | UNKNOWN | You are given two arrays `arr1` and `arr2`, where `arr2` always contains integers.
Write the function `find_array(arr1, arr2)` such that:
For `arr1 = ['a', 'a', 'a', 'a', 'a']`, `arr2 = [2, 4]`
`find_array returns ['a', 'a']`
For `arr1 = [0, 1, 5, 2, 1, 8, 9, 1, 5]`, `arr2 = [1, 4, 7]`
`find_array returns [1, 1, 1]`
For `arr1 = [0, 3, 4]`, `arr2 = [2, 6]`
`find_array returns [4]`
For `arr1=["a","b","c","d"]` , `arr2=[2,2,2]`,
`find_array returns ["c","c","c"]`
For `arr1=["a","b","c","d"]`, `arr2=[3,0,2]`
`find_array returns ["d","a","c"]`
If either `arr1` or `arr2` is empty, you should return an empty arr (empty list in python,
empty vector in c++). Note for c++ use std::vector arr1, arr2. | ["def find_array(arr1, arr2):\n return [ arr1[i] for i in arr2 if i< len(arr1) ]", "def find_array(arr1, arr2):\n return [arr1[i] for i in arr2 if i <= len(arr1)]", "def find_array(arr1, arr2):\n arr = []\n try:\n for i in arr2:\n if len(arr1) == 0 or len(arr2) == 0:\n return []\n else: \n arr.append(arr1[i])\n except IndexError:\n return arr\n return arr\n", "def find_array(arr1, arr2):\n return [arr1[i] for i in range(len(arr1)) if i in arr2]", "def find_array(arr1, arr2):\n # Solution\n list1 =[] \n for num in arr2:\n if num < len(arr1):\n list1.append(arr1[num])\n return list1", "def find_array(arr1, arr2):\n return [arr1[x] for x in arr2 if x<= len(arr1)]", "def find_array(xs, ys):\n return [xs[y] for y in ys if 0 <= y < len(xs)]", "def find_array(arr1, arr2):\n return list() if (not arr1 or not arr2) else [arr1[i] for i in arr2 if i <= len(arr1)]", "def find_array(arr1, arr2):\n return [] if len(arr1)*len(arr2)==0 else [arr1[i] for i in arr2 if i<len(arr1)]", "def find_array(arr1,arr2):\n arr2=set(arr2)\n return [n for i,n in enumerate(arr1) if i in arr2]"] | {"fn_name": "find_array", "inputs": [[["a", "a", "a", "a", "a"], [2, 4]], [[0, 1, 5, 2, 1, 8, 9, 1, 5], [1, 4, 7]], [[1, 2, 3, 4, 5], [0]], [["this", "is", "test"], [0, 1, 2]], [[0, 3, 4], [2, 6]], [[1], []], [[], [2]], [[], []]], "outputs": [[["a", "a"]], [[1, 1, 1]], [[1]], [["this", "is", "test"]], [[4]], [[]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,220 |
def find_array(arr1, arr2):
|
5a20b3fe3cc18e0e642692f9e3191545 | UNKNOWN | # Task
You are given a binary string (a string consisting of only '1' and '0'). The only operation that can be performed on it is a Flip operation.
It flips any binary character ( '0' to '1' and vice versa) and all characters to the `right` of it.
For example, applying the Flip operation to the 4th character of string "1001010" produces the "1000101" string, since all characters from the 4th to the 7th are flipped.
Your task is to find the minimum number of flips required to convert the binary string to string consisting of all '0'.
# Example
For `s = "0101"`, the output should be `3`.
It's possible to convert the string in three steps:
```
"0101" -> "0010"
^^^
"0010" -> "0001"
^^
"0001" -> "0000"
^
```
# Input/Output
- `[input]` string `s`
A binary string.
- `[output]` an integer
The minimum number of flips required. | ["def bin_str(s):\n return s.count(\"10\") * 2 + (s[-1] == \"1\")", "def bin_str(input):\n flips_needed = 0\n last_seen = '0'\n for c in input:\n if last_seen != c:\n flips_needed += 1\n last_seen = c\n return flips_needed", "import re\n\ndef bin_str(s):\n return len(re.findall(\"(?:1+)|(?:0+)\", s.lstrip(\"0\")))", "import re\ndef bin_str(s):\n return len(re.findall(r'0+|1+', s.lstrip('0')))", "import re\n\ndef bin_str(s):\n return len(re.findall(r'(1+)|((?<=1)0+)', s))", "bin_str=lambda n:sum(a!=b for a,b in zip('0'+n,n))", "def bin_str(s):\n return s.count(\"10\")*2+int(s[-1])", "def bin_str(s):\n c = 0\n x = '0'\n for i in s:\n if x != i:\n c += 1\n x = i\n return c", "\ndef bin_str(s): \n \n count = 0\n \n while s.count(\"1\") != 0:\n \n first_index = s.index(\"1\") \n \n n = \"\" \n \n \n \n for nbr in s[first_index:len(s)]:\n\n if nbr == \"1\":\n \n n += \"0\"\n\n elif nbr == \"0\":\n \n n += \"1\"\n \n s = s[0:first_index] + n\n\n count +=1\n \n \n return count\n"] | {"fn_name": "bin_str", "inputs": [["0101"], ["10000"], ["0000000000"], ["1111111111"], ["10101010101010"], ["11111000011111"], ["000001111100000"], ["111000000000"], ["00000000111111111"], ["1010101011111111111111000000000"]], "outputs": [[3], [2], [0], [1], [14], [3], [2], [2], [1], [10]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,281 |
def bin_str(s):
|
2c8ff5e6788771355111d720e4322c97 | UNKNOWN | Given a string, return a new string that has transformed based on the input:
* Change case of every character, ie. lower case to upper case, upper case to lower case.
* Reverse the order of words from the input.
**Note:** You will have to handle multiple spaces, and leading/trailing spaces.
For example:
```
"Example Input" ==> "iNPUT eXAMPLE"
```
You may assume the input only contain English alphabet and spaces. | ["def string_transformer(s):\n return ' '.join(s.swapcase().split(' ')[::-1])", "def string_transformer(s):\n return ' ' .join(s.split(' ')[::-1]).swapcase()", "def string_transformer(s):\n #1st step: split the string (done)\n x = []\n s = s.split(\" \")\n for i in range(1, 2 * len(s) - 1, 2):\n s.insert(i, \" \")\n print(s)\n if \"\" in s:\n for i in s:\n i.replace(\"\", \" \")\n print(s)\n #2nd step: reverse s (google to find the way)\n s.reverse()\n print(s)\n #3rd step: reverse the case for each string in the list (google to find the way)\n for i in s:\n for j in i:\n if j == \" \":\n x.append(j)\n elif j.isupper():\n j = j.lower()\n x.append(j)\n else:\n j = j.upper()\n x.append(j)\n print(x)\n #4th step: join the list to make string\n return ''.join(x)\n", "def string_transformer(s):\n s = s.swapcase()\n s = s.split(\" \")[::-1]\n s = \" \".join(s)\n return s", "def string_transformer(s):\n s = s.split(\" \")\n s = s[::-1]\n s = \" \".join(s)\n result = \"\"\n\n for letter in s:\n if letter.islower():\n result = result + letter.upper()\n elif letter.isupper():\n result = result + letter.lower()\n else:\n result = result + letter\n \n return result", "def string_transformer(s):\n return ' '.join(word.swapcase() for word in reversed(s.split(' ')))", "def string_transformer(s):\n return ' '.join([i.swapcase() for i in s.split(' ')][::-1])\n", "def string_transformer(stg):\n return \" \".join(word for word in stg.swapcase().split(\" \")[::-1])", "def string_transformer(s):\n\n array = []\n\n if s is '':\n return ''\n \n for i in s:\n if i == i.lower():\n array.append(i.upper())\n else:\n array.append(i.lower())\n \n result = ''.join(array)\n return ' '.join(result.split(' ')[::-1])", "def string_transformer(s):\n str1 = ''.join([i.upper() if i.islower() else i.lower() for i in s])\n str2 = str1.split(' ')\n str2.reverse()\n return ' '.join(str2)"] | {"fn_name": "string_transformer", "inputs": [["Example string"], ["Example Input"], ["To be OR not to be That is the Question"], [""], ["You Know When THAT Hotline Bling"], [" A b C d E f G "]], "outputs": [["STRING eXAMPLE"], ["iNPUT eXAMPLE"], ["qUESTION THE IS tHAT BE TO NOT or BE tO"], [""], ["bLING hOTLINE that wHEN kNOW yOU"], [" g F e D c B a "]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,254 |
def string_transformer(s):
|
d657269bbd52b0dd150944671586f979 | UNKNOWN | Very simple, given a number, find its opposite.
Examples:
```
1: -1
14: -14
-34: 34
```
~~~if:sql
You will be given a table: `opposite`, with a column: `number`. Return a table with a column: `res`.
~~~ | ["def opposite(number):\n return -number", "def opposite(number):\n return number * -1", "def opposite(number):\n return number * (-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1)", "def opposite(number):\n#fastest solution returned 94ms with parens around multiplication 87ms without for results\n return number - number * 2 \n\n#middle solution returned 109ms time period for result\n# return number * -1\n#slowest solution returned 150ms time period for result\n# return -number\n", "def opposite(number):\n \"\"\"\n Function have one required argument.\n At start our function check your number. If it's int, float or complex - func multiplies number by -1 and return it\n If our argument is string, try to convert to complex number\n If we had Value Error in our convertation, say(use print when except):\n Input data cannot be represented as a number.\n And after return None\n\n Return:\n Number int or float if input number is int or float.\n Number complex if input number is complex or wrote in string\n None if we had empty line or another input types\n \"\"\"\n if (type(number) is int) or (type(number) is float) or (type(number) is complex):\n number = number * -1\n return number\n else:\n try:\n number = complex(number) * -1\n return number\n except ValueError:\n print(\"Input data cannot be represented as a number\")\n return None", "from operator import neg as opposite", "opposite = lambda x: -x", "def opposite(number):\n return -1*number", "opposite=lambda n:-n", "def opposite(number):\n return -1 * number # could have used -number, but that might send -0", "def opposite(number):\n return abs(number) if number < 0 else 0 - number", "def opposite(number):\n # your solution here\n numbers=str(number)\n if isinstance(number, int):\n if numbers[0]==\"-\":\n negatives=numbers[1:]\n negative=int(negatives)\n return negative\n else:\n positives=\"-\"+numbers\n positive=int(positives)\n return positive\n if isinstance(number, float):\n if numbers[0]==\"-\":\n negatives=numbers[1:]\n negative=float(negatives)\n return negative\n else:\n positives=\"-\"+numbers\n positive=float(positives)\n return positive", "def opposite(number):\n return number - (number * 2)", "def opposite(n):\n return -1 * n", "def opposite(number):\n # your solution here\n return number * \"Magic Forest\".find(\"unicorn\")", "def opposite(n): return -n", "def opposite(number):\n # your solution here\n return 0 - number", "def opposite(number):\n if number>0:\n return number-number-number\n elif number<0:\n return number-number-number\n else:return number\n # your solution here\n", "def opposite(number):\n#use create function to get opposite and call it on function opposite argument\n opposite = (lambda x : -x)\n result = opposite(number)\n return result", "def opposite(x):\n return x * -1", "def opposite(number):\n number = number * -1\n return number \n", "opposite= lambda n : n*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))*(n-(n+1))/(n-(n+1))", "def opposite(number):\n import re\n m = re.match(\"-\", str(number))\n if m:\n number = re.sub(\"-\", \"\", str(number))\n else:\n number = \"-\" + str(number)\n try:\n return int(number)\n except ValueError:\n return float(number)", "def opposite(number):\n return (lambda x: x * -1)(number)", "opposite=(0.).__sub__", "def opposite(n):\n str=\"\"\"\n \u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2593\u2593\u2593\u2593\u2593\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2592\u2592\u2592\u2592\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2593\u2593\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2593\u2593\u2500\u2500\u2500\u2500\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\u2592\n\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2593\u2593\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2500\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\u2591\u2591\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2580\u2580\u2580\u2580\u2580\u2588\u2588\u2588\u2584\u2584\u2592\u2592\u2592\u2591\u2591\u2591\u2584\u2584\u2584\u2588\u2588\u2580\u2580\u2580\u2580\u2580\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2584\u2580\u2588\u2588\u2588\u2588\u2580\u2588\u2588\u2588\u2584\u2592\u2591\u2584\u2588\u2588\u2588\u2588\u2580\u2588\u2588\u2588\u2588\u2584\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2588\u2500\u2500\u2580\u2588\u2588\u2588\u2588\u2588\u2580\u2500\u258c\u2592\u2591\u2590\u2500\u2500\u2580\u2588\u2588\u2588\u2588\u2588\u2580\u2500\u2588\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2580\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2580\u2592\u2592\u2591\u2591\u2580\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2580\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2580\u2580\u2580\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\n\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2584\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2584\u2580\u2580\u2580\u2580\u2580\u2580\u2580\u2580\u2580\u2580\u2580\u2584\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2580\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2580\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2592\u2591\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2592\u2592\u2591\u2591\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2593\u2592\u2591\u2592\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2592\u2591\u2592\n\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2593\u2592\n\"\"\"\n return str.find(\"\u00af\\_(\u30c4)_/\u00af\")*n", "opposite = lambda number: -number #Lambda is so much fun", "def opposite(number):\n return float(('-' + str(number)).replace('--', ''))", "def opposite(number):\n return (~int(number) + int(number)) * number", "def opposite(number):\n # your solution here\n answer = 0 - number\n return answer\n", "def opposite(number):\n return(number*(-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1+1-1))", "opposite = lambda l: -l", "def opposite(number):\n # your solution here\n if number < 0:\n return abs(number)\n elif number > 0:\n return -number\n else:\n return number", "def opposite(number):\n oppositenum = -number\n return oppositenum\n # your solution here\n", "def opposite(number):\n# your solution here\n if number <= 0:\n return(abs(number))\n else:\n return(number - 2 * number)\n \nopposite(1) \n", "def opposite(number):\n # your solution here\n return -1 * number\nprint((1 , 14, - 34))\n", "def opposite(number):\n float(number)\n if number > 0:\n return number - number*2\n elif number < 0:\n return abs(number)\n elif number == 0:\n return 0", "def opposite(number):\n \"turns the number into its opposite\"\n return number - 2*number", "def opposite(number):\n if number!=0:\n result=-number\n else:\n result=0\n return result\n \n\n", "def opposite(number):\n reverse = number - number - number\n \n print(reverse)\n return reverse\n \n\nn = opposite(5)\n\n\n", "def opposite(number):\n if number >= 0:\n return -number\n else:\n return number + (-number * 2)\n # your solution here\n", "def opposite(number):\n # your solution here\n a=-1\n c=number*a\n return c", "def opposite(number):\n if \"-\" in str(number):\n return -number\n else:\n return -number", "def opposite(number):\n \n \n \n new_num = number\n \n \n \n \n if number <= 0:\n \n neg_num = number * 2\n neg_num2 = new_num - neg_num\n \n return neg_num2\n \n elif number >= 0:\n \n pos_num = number * 2\n pos_num2 = new_num - pos_num\n \n return pos_num2\n \n \n \n \n \n \n", "def opposite(number):\n return number-number*2 if number>0 else number+number*2*-1", "def opposite(number):\n a=float(number)*(-1)\n return a", "def opposite(number):\n if number >= 0 : return float('-'+str(number))\n else: return float(str(number)[1:])\n # your solution here\n", "def opposite(number):\n return number - number - number if number > 0 else number + abs(number) + abs(number)", "def opposite(number):\n out = number * -1\n return out", "def opposite (a):\n return -1 * a", "def opposite(number):\n if number >= 0:\n return -number\n else:\n return number * -1\n", "def opposite(number):\n# if number < 0:\n# number = number * -1\n \n# elif number > 0:\n# number = number * -1\n# return (number)\n\n return (number*-1)\n # your solution here\n", "def opposite(number):\n output = 0 - number\n return output", "def opposite(number):\n x = '-' + str(number)\n if number < 0:\n return abs(number)\n else:\n return float(x)", "def opposite(number):\n qwe = number*(-1)\n return qwe\n # your solution here\n", "def opposite(number):\n if number > 0:\n return -1 * number \n return -1 * number ", "def opposite(number):\n number*=-1\n return number\n\nn=5\nprint(opposite(n))", "def opposite(number):\n # your solution here\n return(-number*1)", "def opposite(number):\n return number * -1\n #Nobody saw this\n", "def opposite(number):\n return float(\"-\"+str(number)) if number >= 0 else float(str(number)[1:])", "def opposite(number):\n if(number==+number):\n return (-number)\n elif (number==-number):\n return (+number)\n else:\n return (0)\n\n\n", "def opposite(number):\n number = -number\n return number\n\nprint((opposite(1)))\n\n", "def opposite(number):\n if number > 0:\n number *= -1\n return number\n elif number < 0:\n number *= -1\n return number\n else:\n return 0\n", "def opposite(n):\n e = n - (n + n)\n return e", "import numpy\ndef opposite(number):\n if number < 0:\n return abs(number)\n else:\n return numpy.negative(number)", "def opposite(number):\n return number*-1 if number>0 else -1*number \n", "def opposite(number):\n\n if number > 0:\n return number - (abs(number) * 2 )\n else:\n return abs(number)", "def opposite(number):\n if number==abs(number):#If 1==1 then we should return negative number of the given digit\n return -abs(number)#Here we put up negative sign infront of abs() func\n else:\n return abs(number)#If number isn't equal to its absolute number then its negative, so return absolute number", "def opposite(number):\n return number - (number * 2)\nopposite(8)", "def opposite(number):\n number = 1.0*number\n if number > 0:\n return 0-number\n else: return 0-number\n # your solution here\n", "def opposite(num):\n return abs(num) if num < 0 else -abs(num)", "def opposite(number):\n answer=number*-1\n return answer\n# if number >0:\n# return -number\n# if number<0:\n# postive= number\n \n \n \n # your solution here\n", "def opposite(number):\n opacne = number*-1\n return opacne\n \n", "def opposite(number):\n if number > 0:\n return float(\"-\" + str(number))\n else:\n return number - number - number\n", "def opposite(number):\n num = number - 2*number\n return num", "def opposite(number):\n w = -number\n return w", "def opposite(number):\n if number < 0:\n return abs(number)\n else:\n return number * -1\n \n \n\"\"\"\ndef opposite(number):\n return -number\n\"\"\" ", "def opposite(number):\n return number * -1\n \na = opposite(-10)\n\nprint(a)\n", "def opposite(number):\n string = str(number) #transform to a string to perform operations\n if string[0] == \"-\": #return values and convert back to float\n return float(string[1:])\n else:\n return float(\"\".join([\"-\",string]))\n # your solution here\n", "def opposite(number):\n num = ''\n num = number * -1\n print(num)\n return num\n \n", "def opposite(number):\n convert = None\n if number < 0:\n convert = str(number)[1:]\n else:\n convert = '-' + str(number)\n try:\n return int(convert)\n except:\n return float(convert)", "''' Return opposite number '''\n\ndef opposite(number):\n\n ''' Just put minus before a number... What the heck?! '''\n return -number\n\nprint(opposite(1))", "''' Return opposite number '''\n\ndef opposite(number):\n\n ''' If you substract a number from a number You get an opposite number '''\n return (number - (number * 2))\n\nprint(opposite(1))", "def opposite(number):\n return number * -1\n\nprint(opposite(4))", "def opposite(number):\n tmp = number * 2\n ans = number - tmp\n return ans\n # your solution here\n", "def opposite(value):\n # your solution here\n return value * -1", "def opposite(number):\n absolu = number * (-1)\n return absolu", "def opposite(number):\n rev=0\n rev=-1*number\n return rev", "def opposite(number):\n return -1 * number\n##blablablablablablablablablablablablablablablablablabla\n#blablablablablablablablablablablablablablablablablabla\n#blablablablablablablablablablablablablablablablablabla\n#blablablablablablablablablablablablablablablablablabla\n#blablablablablablablablablablablablablablablablablabla\n#blablablablablablablablablablablablablablablablablabla#blablablablablablablablablablablablablablablablablabla#blablablablablablablablablablablablablablablablablabla#blablablablablablablablablablablablablablablablablabla\n", "def opposite(n):\n if n>0:\n n=n-(2*n)\n return n\n else:\n n=abs(n)\n return n", "def opposite(number):\n if number >= 1:\n tempnum = number + number\n result = number - tempnum\n return result\n if number <= -1:\n tempnum = number + number\n result = number - tempnum\n return result\n else:\n return 0", "def opposite(number):\n # your solution here\n return -number if number != -number else number", "def opposite(number):\n return -number if abs(number) else abs(number)", "def opposite(number): # this is our function, i just called it numberChange for fun\n return -(number) # that's it!, we just multiply our input by -1", "def opposite(number):\n if number > 0:\n number = -number\n else:\n number = -number\n return number\nprint(opposite(1),-1)", "def opposite(number):\n x = number = -(number)\n return x\n # your solution here\n", "def opposite(number):\n # your solution here\n output_num = number * -1\n return output_num", "def opposite(N):\n return -N\n", "def opposite(number):\n # THE MOST GENIUS SOLUTION YOU'VE EVER SEEN LOL\n # It works - no matter how.\n if number > 0:\n number = 0 - number\n elif number is None:\n number = -1\n else:\n return abs(number)\n return number", "def opposite(number): \n if number < 0 : \n return abs(number)\n if number > 0 :\n return float(\"-\" + str(number))\n if number == 0 :\n return 0\n"] | {"fn_name": "opposite", "inputs": [[1], [25.6], [0], [1425.2222], [-3.1458], [-95858588225]], "outputs": [[-1], [-25.6], [0], [-1425.2222], [3.1458], [95858588225]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 22,507 |
def opposite(number):
|
37ce391233de7f32ebf956c529e5d4c4 | UNKNOWN | Find the volume of a cone whose radius and height are provided as parameters to the function `volume`. Use the value of PI provided by your language (for example: `Math.PI` in JS, `math.pi` in Python or `Math::PI` in Ruby) and round down the volume to an Interger.
If you complete this kata and there are no issues, please remember to give it a ready vote and a difficulty rating. :) | ["from math import pi\n\ndef volume(r,h):\n return pi * r**2 * h // 3", "from math import pi\n\n\ndef volume(r, h):\n return int(pi * r ** 2 * h / 3)", "from math import pi,floor\n\ndef volume(r,h):\n return floor(h/3 * pi * r ** 2)", "from math import pi\ndef volume(r,h):\n return (pi*h*r**2)//3", "import math\ndef volume(r,h):\n return math.pi * r ** 2 * h // 3", "from math import pi\ndef volume(r,h):\n return int(pi*r*r*h/3)", "import math \ndef volume(r,h):\n return math.floor((1/3*math.pi)*(r**2)*h)", "from math import pi\n\ndef volume(r,h):\n return int(1/3 * pi * r**2 * h)", "volume=lambda r,h:__import__('math').pi*r*r*h//3", "def volume(r,h):\n \n# =============================================================================\n# This function calculates the Volume of a cone using the formulae \n# \u03c0 x r\u00b2 x h/3, and returns the result as a rounded down integer.\n# =============================================================================\n\n import math\n \n return math.floor(math.pi * r**2 * h/3)"] | {"fn_name": "volume", "inputs": [[7, 3], [56, 30], [0, 10], [10, 0], [0, 0]], "outputs": [[153], [98520], [0], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,076 |
def volume(r,h):
|
5470c6a985ce5ae7014f89da40a8253d | UNKNOWN | Write a function taking in a string like `WOW this is REALLY amazing` and returning `Wow this is really amazing`. String should be capitalized and properly spaced. Using `re` and `string` is not allowed.
Examples:
```python
filter_words('HELLO CAN YOU HEAR ME') #=> Hello can you hear me
filter_words('now THIS is REALLY interesting') #=> Now this is really interesting
filter_words('THAT was EXTRAORDINARY!') #=> That was extraordinary!
``` | ["def filter_words(st):\n return ' '.join(st.capitalize().split())", "def filter_words(st):\n return \" \".join(st.split()).capitalize()\n", "def filter_words(st):\n st1 = st.lower()\n st2 = st1.capitalize()\n st3 = \" \".join(st2.split())\n return st3", "def filter_words(s):\n return ' '.join(s.split()).capitalize()", "def filter_words(stg):\n return \" \".join(stg.split()).capitalize()", "def filter_words(st):\n words = st.capitalize().split()\n return ' '.join(words)", "def filter_words(strng):\n return ' '.join(strng.split()).capitalize()\n", "def filter_words(st):\n s= st.lower()\n t= s.capitalize()\n a= ' '.join(t.split())\n return a", "def filter_words(string):\n '''A function taking in a string like \"WOW this is REALLY amazing\" \n and returning \"Wow this is really amazing\". \n String should be capitalized and properly spaced. Using \"re\" and \"string\" is not allowed.'''\n correct_string = string[0].capitalize() + string[1:].lower()\n return \" \".join(correct_string.split())", "def filter_words(st):\n st2 = st[0] + st.lower()[1:] \n return ' '.join((st2.capitalize()).split())\n"] | {"fn_name": "filter_words", "inputs": [["HELLO world!"], ["This will not pass "], ["NOW THIS is a VERY EXCITING test!"]], "outputs": [["Hello world!"], ["This will not pass"], ["Now this is a very exciting test!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,174 |
def filter_words(st):
|
2cdecbf44bf5a1f1ccbafa1703b8d74c | UNKNOWN | Nickname Generator
Write a function, `nicknameGenerator` that takes a string name as an argument and returns the first 3 or 4 letters as a nickname.
If the 3rd letter is a consonant, return the first 3 letters.
If the 3rd letter is a vowel, return the first 4 letters.
If the string is less than 4 characters, return "Error: Name too short".
**Notes:**
- Vowels are "aeiou", so discount the letter "y".
- Input will always be a string.
- Input will always have the first letter capitalised and the rest lowercase (e.g. Sam).
- The input can be modified | ["def nickname_generator(name):\n return \"Error: Name too short\" if len(name) < 4 else name[:3+(name[2] in \"aeiuo\")]", "def nickname_generator(name):\n return name[:3+(name[2] in 'aeiou')] if len(name) > 3 else 'Error: Name too short'", "def nickname_generator(name):\n return \"Error: Name too short\" if len(name) < 4 else name[:4] if name[2] in \"aeiou\" else name[:3]", "def nickname_generator(name):\n if len(name) < 4:\n return \"Error: Name too short\"\n elif name[2] not in 'aeiou': \n return name[:3]\n elif name[2] in 'aeiou':\n return name[:4]\n", "def nickname_generator(name):\n VOWELS = 'aeiou'\n if len(name) < 4:\n return 'Error: Name too short'\n if name[2] in VOWELS:\n return name[:4]\n else:\n return name[:3]\n", "is_vowel = set(\"aeiou\").__contains__\n\ndef nickname_generator(name):\n if len(name) < 4: return \"Error: Name too short\" # Take that Yu, Lee, Bob, and others!\n if is_vowel(name[2]): return name[:4]\n return name[:3]", "def nickname_generator(name):\n if len(name) > 3:\n return name[:4] if name[2] in 'aeiou' else name[:3]\n else:\n return 'Error: Name too short'", "def nickname_generator(name):\n return (name[:4] if name[2] in \"aeiou\" else name[:3]) if len(name) > 3 else \"Error: Name too short\"", "def nickname_generator(name):\n VOWELS = 'aeiou'\n if len(name) < 4:\n return 'Error: Name too short'\n if name[2] in VOWELS:\n return name[:4]\n return name[:3]", "def nickname_generator(name):\n if len(name) < 4:\n return 'Error: Name too short'\n return name[: 4 if name[2] in 'aeiou' else 3]"] | {"fn_name": "nickname_generator", "inputs": [["Jimmy"], ["Samantha"], ["Sam"], ["Kayne"], ["Melissa"], ["James"], ["Gregory"], ["Jeannie"], ["Kimberly"], ["Timothy"], ["Dani"], ["Saamy"], ["Saemy"], ["Saimy"], ["Saomy"], ["Saumy"], ["Boyna"], ["Kiyna"], ["Sayma"], ["Ni"], ["Jam"], ["Suv"]], "outputs": [["Jim"], ["Sam"], ["Error: Name too short"], ["Kay"], ["Mel"], ["Jam"], ["Greg"], ["Jean"], ["Kim"], ["Tim"], ["Dan"], ["Saam"], ["Saem"], ["Saim"], ["Saom"], ["Saum"], ["Boy"], ["Kiy"], ["Say"], ["Error: Name too short"], ["Error: Name too short"], ["Error: Name too short"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,706 |
def nickname_generator(name):
|
214971202c70827e3c5a5476a991147a | UNKNOWN | At the start of each season, every player in a football team is assigned their own unique squad number. Due to superstition or their history certain numbers are more desirable than others.
Write a function generateNumber() that takes two arguments, an array of the current squad numbers (squad) and the new player's desired number (n). If the new player's desired number is not already taken, return n, else if the desired number can be formed by adding two digits between 1 and 9, return the number formed by joining these two digits together. E.g. If 2 is taken, return 11 because 1 + 1 = 2. Otherwise return null.
Note: Often there will be several different ways to form a replacement number. In these cases the number with lowest first digit should be given priority. E.g. If n = 15, but squad already contains 15, return 69, not 78. | ["def generate_number(squad, n):\n if n not in squad: return n\n for i in range(1, 10):\n for j in range(1, 10):\n if i + j == n and i * 10 + j not in squad:\n return i * 10 + j", "def generate_number(lst, n):\n lst = set(lst)\n return (n if n not in lst else\n next( (9*d+n for d in range(1,10) if 0<n-d<10 and 9*d+n not in lst), None))", "def generate_number(squad, n):\n candidates = [n] + [(n - d) * 10 + d for d in range(9, 0, -1)]\n \n for x in candidates:\n if x < 100 and x not in squad:\n return x", "def generate_number(squad, n):\n l, d = (n - 1, 9 + n) if n < 11 else (19 - n, n * 10 - 81)\n return next((d+i*9 for i in range(l) if d+i*9 not in squad), None) if n in squad else n", "def generate_number(squad, n):\n if n not in squad:\n return n\n for i in range(1, 10):\n for j in range(1, 10):\n if i + j == n:\n x = int(f'{i}{j}')\n if x not in squad:\n return x", "def generate_number(squad, n):\n x, squad = n, set(squad)\n for d in range(1, 10):\n if x not in squad: return x\n if 0 < n - d < 10: x = 9 * d + n\n if x not in squad: return x", "def generate_number(squad, n):\n return n if n not in squad else None if n < 10 else next((x for x in range(10, 100) if sum(int(c) for c in str(x)) == n and x not in squad), None)\n", "import itertools\n\ndef twodigcombs(n):\n ls = []\n digits = [1,2,3,4,5,6,7,8,9]\n for x, y in itertools.combinations_with_replacement(digits, 2):\n if x + y == n:\n ls.append([x,y])\n return sorted(ls)\n\ndef generate_number(squad, n):\n print((squad, n))\n if n not in squad:\n return n\n \n for [a,b] in twodigcombs(n):\n x = a*10 + b\n if x not in squad:\n return x\n \n", "def generate_number(squad, n):\n if n not in squad:\n return n\n print((squad, n))\n for i in range(0,10):\n b = i*10 + n-i if n-i < 10 and n-i>0 else n\n if b not in squad:\n return b\n return None\n # TODO: complete\n", "def generate_number(squad,n):\n if n not in squad:return n\n for i in range(n-9,10):\n d=int(f\"{i}{n-i}\")\n if d not in squad and (n-i)>0:\n return d\n \n"] | {"fn_name": "generate_number", "inputs": [[[1, 2, 3, 4, 6, 9, 10, 15, 69], 11], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 69], 11], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 29, 69], 11], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 29, 38, 47, 56, 65, 69, 74, 83, 92], 11], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 18, 23, 69], 18], [[1, 2, 3, 4, 6, 9, 10, 15, 69], 34], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 29, 34, 38, 47, 56, 65, 69, 74, 83, 92], 34], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 18, 27, 29, 34, 36, 38, 45, 47, 54, 56, 63, 65, 69, 72, 74, 81, 83, 92], 9], [[1, 2, 3, 4, 6, 9, 10, 11, 15, 18, 27, 29, 34, 36, 38, 45, 47, 54, 56, 63, 65, 69, 72, 74, 81, 83, 90, 92], 9]], "outputs": [[11], [29], [38], [null], [99], [34], [null], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,359 |
def generate_number(squad, n):
|
9431161cf2821f0c82d911373560dcb8 | UNKNOWN | This kata is an extension of "Combinations in a Set Using Boxes":https://www.codewars.com/kata/5b5f7f7607a266914200007c
The goal for this kata is to get all the possible combinations (or distributions) (with no empty boxes) of a certain number of balls, but now, **with different amount of boxes.** In the previous kata, the number of boxes was always the same
Just to figure the goal for this kata, we can see in the picture below the combinations for four balls using the diagram of Hasse:
Points that are isolated represent balls that are unique in a box.
k points that are bonded in an area respresent a subset that is content in a box.
The unique square at the bottom of the diagram, means that there is only one possible combination for four balls in four boxes.
The above next line with 6 squares, means that we have 6 possible distributions of the four balls in 3 boxes. These six distributions have something in common, all of them have one box with 2 balls.
Going up one line more, we find the 7 possible distributions of the four balls in 2 boxes. As it is shown, we will have 7 distributions with: three balls in a box and only one ball in the another box, or two balls in each box.
Finally, we find again at the top, an unique square, that represents, the only possible distribution, having one box, the four balls together in the only available box.
So, for a set of 4 labeled balls, we have a total of 15 possible distributions (as always with no empty boxes) and with a maximum of 7 possible distributions for the case of two boxes.
Prepare a code that for a given number of balls (an integer), may output an array with required data in the following order:
```
[total_amount_distributions, maximum_amount_distributions_case_k_boxes, k_boxes]
```
Just see how it would be for the example given above:
```
combs_non_empty_boxesII(4) == [15, 7, 2] # A total of 15 combinations
#The case with maximum distributions is 7 using 2 boxes.
```
Features of the random tests:
```
1 < n <= 1800 (python)
1 < n <= 1200 (ruby)
```
You may see the example tests for more cases.
Enjoy it!
Ruby version will be published soon. | ["MAX_BALL = 2+1800\nDP,lst = [None], [0,1]\nfor _ in range(MAX_BALL):\n DP.append([sum(lst), *max( (v,i) for i,v in enumerate(lst) )])\n lst.append(0)\n lst = [ v*i + lst[i-1] for i,v in enumerate(lst) ]\n \ncombs_non_empty_boxesII = DP.__getitem__\n", "# this is [A000110, A002870, A024417]\n\n# Stirling numbers of second kind\n# http://mathworld.wolfram.com/StirlingNumberoftheSecondKind.html\n# S(n,k) = S(n-1,k-1)+k*S(n-1,k)\nS = {}\nfor n in range(1,1800):\n S[(n,1)] = S[(n,n)] = 1\n for k in range(2,n): S[(n,k)]=S[(n-1,k-1)]+k*S[(n-1,k)]\n\ndef combs_non_empty_boxesII(n):\n ss = [(S[(n,k)],k) for k in range(1,n+1)]\n return [sum(p[0] for p in ss), *max(ss)]", "M = [[1] * 1800]\nfor V in range(2,1801) :\n U = [0]\n for F,B in enumerate(M[-1]) :\n if F < 1799 : U.append(0 if 1 + F < V else B + V * U[-1])\n M.append(U)\ndef combs_non_empty_boxesII(Q) :\n R = [0,0,0]\n for V in range(Q) :\n T = M[V][Q]\n R[0] += T\n if R[1] <= T : R[1:] = T,1 + V\n return R", "S = [[1]]\nfor n in range(1,1801):\n S.append([1])\n for k in range(1, n):\n S[n].append(S[n-1][k-1] + (k+1) * S[n-1][k])\n S[n].append(1)\n\ndef combs_non_empty_boxesII(n):\n tot, max, index = 0, 1, 1\n for ix in range(len(S[n-1])):\n val = S[n-1][ix]\n tot = tot + val\n if val >= max:\n max = val\n index = ix\n return [tot, max, index+1]", "sseq = [[1],[1,1]]\ns = [1,1]\nfor t in range(2,1800):\n s = [1]+[s[i-1]+(i+1)*s[i] for i in range(1, len(s))]+[1]\n sseq.append(s)\n\ndef combs_non_empty_boxesII(n):\n if n == 2: return [2, 1, 2]\n s = [1,1]\n s = sseq[n-1]\n return [sum(s), max(s), s.index(max(s))+1]"] | {"fn_name": "combs_non_empty_boxesII", "inputs": [[4], [3], [2], [20]], "outputs": [[[15, 7, 2]], [[5, 3, 2]], [[2, 1, 2]], [[51724158235372, 15170932662679, 8]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,747 |
def combs_non_empty_boxesII(n):
|
8897340725746e7058981d0fcab04cd0 | UNKNOWN | ###Story
Sometimes we are faced with problems when we have a big nested dictionary with which it's hard to work. Now, we need to solve this problem by writing a function that will flatten a given dictionary.
###Info
Python dictionaries are a convenient data type to store and process configurations. They allow you to store data by keys to create nested structures. You are given a dictionary where the keys are strings and the values are strings or dictionaries. The goal is flatten the dictionary, but save the structures in the keys. The result should be a dictionary without the nested dictionaries. The keys should contain paths that contain the parent keys from the original dictionary. The keys in the path are separated by a `/`. If a value is an empty dictionary, then it should be replaced by an empty string `""`.
###Examples
```python
{
"name": {
"first": "One",
"last": "Drone"
},
"job": "scout",
"recent": {},
"additional": {
"place": {
"zone": "1",
"cell": "2"
}
}
}
```
The result will be:
```python
{"name/first": "One", #one parent
"name/last": "Drone",
"job": "scout", #root key
"recent": "", #empty dict
"additional/place/zone": "1", #third level
"additional/place/cell": "2"}
```
***`Input: An original dictionary as a dict.`***
***`Output: The flattened dictionary as a dict.`***
***`Precondition:
Keys in a dictionary are non-empty strings.
Values in a dictionary are strings or dicts.
root_dictionary != {}`***
```python
flatten({"key": "value"}) == {"key": "value"}
flatten({"key": {"deeper": {"more": {"enough": "value"}}}}) == {"key/deeper/more/enough": "value"}
flatten({"empty": {}}) == {"empty": ""}
``` | ["def flatten(dictionary):\n stack = [((), dictionary)]\n result = {}\n while stack:\n path, current = stack.pop()\n for k, v in current.items():\n if v == {}:\n result[\"/\".join((path + (k,)))] = \"\";\n if isinstance(v, dict):\n stack.append((path + (k,), v))\n else:\n result[\"/\".join((path + (k,)))] = v\n return result", "def flatten(dictionary):\n result = {}\n for k, v in dictionary.items():\n if v == {}:\n result[k] = \"\"\n elif isinstance(v, dict):\n for l, w in flatten(v).items():\n result[k + '/' + l] = w\n else:\n result[k] = v\n return result", "def flatten(d):\n result = {}\n for key, value in d.items():\n if value and isinstance(value, dict):\n for k, v in flatten(value).items():\n result[key + '/' + k] = v\n else:\n result[key] = value or ''\n return result", "def flatten(dictionary, prefix=[]):\n res = {}\n for key in dictionary.keys():\n if isinstance(dictionary[key], dict) and dictionary[key]:\n res.update(flatten(dictionary[key], prefix + [key]))\n else:\n res[\"/\".join(prefix + [key])] = dictionary[key] or ''\n return res", "def flatten(dct):\n \n def extract(dct):\n for k,v in dct.items():\n stk.append(k)\n if isinstance(v,dict) and v: yield from extract(v)\n else: yield ('/'.join(stk), v or '')\n stk.pop()\n \n stk = []\n return dict(extract(dct))", "def flatten(dictionary):\n result = {}\n for k1,v1 in dictionary.items():\n if type(v1) == str:\n result[k1] = v1\n elif v1:\n for k2,v2 in flatten(v1).items():\n result[f\"{k1}/{k2}\"] = v2\n else:\n result[k1] = \"\"\n return result", "def flatten(d):\n r = {}\n for x, y in d.items():\n if isinstance(y, dict):\n r.update({f\"{x}/{X}\" : Y for X, Y in flatten(y).items()} if y else {x: \"\"})\n else:\n r[x] = y\n return r", "def flatten(dictionary):\n stack = [((), dictionary)]\n result = {}\n while stack:\n path, current = stack.pop()\n for k, v in list(current.items()):\n new_path = path + (k,)\n v = v if v else \"\"\n if isinstance(v, dict):\n stack.append((new_path, v))\n else:\n result[\"/\".join(new_path)] = v\n return result\n", "def flatten(dictionary):\n stack = [(\"\", dictionary)]\n result = {}\n while stack:\n path, current = stack.pop()\n if current:\n if type(current)==dict:\n if path:\n path+='/'\n for key in current:\n stack.append((path+key,current[key]))\n else:\n result[path]=current\n elif path:\n result[path]=\"\"\n return result", "def flatten(d):\n result = []\n for key in d:\n if isinstance(d[key], str):\n result.append((key, d[key]))\n elif len(d[key]) == 0:\n result.append((key, \"\"))\n else:\n for key2, value2 in list(flatten(d[key]).items()):\n result.append((key + \"/\" + key2, value2))\n return dict(result)\n"] | {"fn_name": "flatten", "inputs": [[{"key": "value"}], [{"key": {"deeper": {"more": {"enough": "value"}}}}], [{"empty": {}}], [{"additional": {"place": {"cell": "2", "zone": "1"}}, "job": "scout", "name": {"last": "Drone", "first": "One"}, "recent": {}}], [{"job": {"1": "scout", "2": "worker", "3": "writer", "4": "reader", "5": "learner"}, "name": {"nick": {}, "last": "Drone", "first": "Second"}, "recent": {"places": {"earth": {"NP": "", "NY": "2017", "Louvre": "2015"}}, "times": {"XX": {"1964": "Yes"}, "XXI": {"2064": "Nope"}}}}], [{"Hm": {"What": {"is": {"here": {"?": {}}}}}}], [{"glossary": {"GlossDiv": {"GlossList": {"GlossEntry": {"GlossDef": {"GlossSeeAlso": {"1": "GML", "2": "XML"}, "para": "A meta-markup language, used to create markup languages such as DocBook."}, "GlossSee": "markup", "Acronym": "SGML", "GlossTerm": "Standard Generalized Markup Language", "Abbrev": "ISO 8879:1986", "SortAs": "SGML", "ID": "SGML"}}, "title": "S"}, "title": "example glossary"}, "source": "http://json.org/example"}]], "outputs": [[{"key": "value"}], [{"key/deeper/more/enough": "value"}], [{"empty": ""}], [{"additional/place/zone": "1", "job": "scout", "additional/place/cell": "2", "name/first": "One", "name/last": "Drone", "recent": ""}], [{"job/1": "scout", "recent/places/earth/NY": "2017", "job/3": "writer", "job/2": "worker", "job/5": "learner", "job/4": "reader", "recent/places/earth/NP": "", "recent/places/earth/Louvre": "2015", "recent/times/XX/1964": "Yes", "recent/times/XXI/2064": "Nope", "name/first": "Second", "name/last": "Drone", "name/nick": ""}], [{"Hm/What/is/here/?": ""}], [{"glossary/GlossDiv/GlossList/GlossEntry/GlossDef/para": "A meta-markup language, used to create markup languages such as DocBook.", "glossary/title": "example glossary", "glossary/GlossDiv/GlossList/GlossEntry/Abbrev": "ISO 8879:1986", "glossary/GlossDiv/GlossList/GlossEntry/SortAs": "SGML", "glossary/GlossDiv/GlossList/GlossEntry/Acronym": "SGML", "glossary/GlossDiv/GlossList/GlossEntry/GlossTerm": "Standard Generalized Markup Language", "glossary/GlossDiv/title": "S", "source": "http://json.org/example", "glossary/GlossDiv/GlossList/GlossEntry/GlossDef/GlossSeeAlso/2": "XML", "glossary/GlossDiv/GlossList/GlossEntry/ID": "SGML", "glossary/GlossDiv/GlossList/GlossEntry/GlossDef/GlossSeeAlso/1": "GML", "glossary/GlossDiv/GlossList/GlossEntry/GlossSee": "markup"}]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,486 |
def flatten(dictionary):
|
db734ba02ae93bff736b3314447ec28a | UNKNOWN | Theory
This section does not need to be read and can be skipped, but it does provide some clarity into the inspiration behind the problem.
In music theory, a major scale consists of seven notes, or scale degrees, in order (with tonic listed twice for demonstrative purposes):
Tonic, the base of the scale and the note the scale is named after (for example, C is the tonic note of the C major scale)
Supertonic, 2 semitones (or one tone) above the tonic
Mediant, 2 semitones above the supertonic and 4 above the tonic
Subdominant, 1 semitone above the median and 5 above the tonic
Dominant, 2 semitones above the subdominant and 7 above the tonic
Submediant, 2 semitones above the dominant and 9 above the tonic
Leading tone, 2 semitones above the mediant and 11 above the tonic
Tonic (again!), 1 semitone above the leading tone and 12 semitones (or one octave) above the tonic
An octave is an interval of 12 semitones, and the pitch class of a note consists of any note that is some integer
number of octaves apart from that note. Notes in the same pitch class sound different but share similar properties. If a note is in a major scale, then any note in that note's pitch class is also in that major scale.
Problem
Using integers to represent notes, the major scale of an integer note will be an array (or list) of integers that follows the major scale pattern note, note + 2, note + 4, note + 5, note + 7, note + 9, note + 11. For example, the array of integers [1, 3, 5, 6, 8, 10, 12] is the major scale of 1.
Secondly, the pitch class of a note will be the set of all integers some multiple of 12 above or below the note. For example, 1, 13, and 25 are all in the same pitch class.
Thirdly, an integer note1 is considered to be in the key of an integer note2 if note1, or some integer in note1's pitch class, is in the major scale of note2. More mathematically, an integer note1 is in the key of an integer note2 if there exists an integer i such that note1 + i × 12 is in the major scale of note2. For example, 22 is in the key of of 1 because, even though 22 is not in 1's major scale ([1, 3, 5, 6, 8, 10, 12]), 10 is and is also a multiple of 12 away from 22. Likewise, -21 is also in the key of 1 because -21 + (2 × 12) = 3 and 3 is present in the major scale of 1. An array is in the key of an integer if all its elements are in the key of the integer.
Your job is to create a function is_tune that will return whether or not an array (or list) of integers is a tune. An array will be considered a tune if there exists a single integer note all the integers in the array are in the key of. The function will accept an array of integers as its parameter and return True if the array is a tune or False otherwise. Empty and null arrays are not considered to be tunes. Additionally, the function should not change the input array.
Examples
```python
is_tune([1, 3, 6, 8, 10, 12]) # Returns True, all the elements are in the major scale
# of 1 ([1, 3, 5, 6, 8, 10, 12]) and so are in the key of 1.
is_tune([1, 3, 6, 8, 10, 12, 13, 15]) # Returns True, 14 and 15 are also in the key of 1 as
# they are in the major scale of 13 which is in the pitch class of 1 (13 = 1 + 12 * 1).
is_tune([1, 6, 3]) # Returns True, arrays don't need to be sorted.
is_tune([1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]) # Returns False, this array is not in the
# key of any integer.
is_tune([2, 4, 7, 9, 11, 13]) # Returns True, the array is in the key of 2 (the arrays
# don't need to be in the key of 1, just some integer)
``` | ["def is_tune(notes):\n return bool(notes) and any(\n all((n + i) % 12 in {0, 2, 4, 5, 7, 9, 11} for n in notes)\n for i in range(12)\n )", "def is_tune(notes):\n if not notes: return False \n for j in range(12):\n if set((i + j) % 12 for i in notes).issubset({0, 2, 4, 5, 7, 9, 11}):\n return True\n return False\n \n", "D_TUNE = {0,2,4,5,7,9,11}\n\ndef is_tune(notes):\n return bool(notes) and any({(n-v)%12 for v in notes} <= D_TUNE for n in range (12))", "def is_tune(notes):\n pattern=[0,2,4,5,7,9,11]\n if notes:\n for i in range(12):\n for n in notes: \n for p in pattern:\n if (i-n+p)%12==0: break\n else: break\n else: return True\n return False", "is_tune=lambda Q:bool(Q)and any(all((B-V)%12in(0,2,4,5,7,9,11)for B in Q)for V in range(12))", "def is_tune(notes=None):\n if not notes:\n return False\n\n major_scale = [0, 2, 4, 5, 7, 9, 11]\n \n def is_in_key_of(_notes, n):\n return all((note + n) % 12 in major_scale for note in _notes)\n\n tonic = min(notes)\n _notes = [n - tonic for n in notes]\n return any(is_in_key_of(_notes, n) for n in major_scale)", "def is_tune(notes):\n return any(all((j+i)%12 in [0,2,4,5,7,9,11] for j in notes) for i in range(12)) if notes else False", "def is_tune(notes):\n def is_in_key(a,b):\n major_scale_a = [(a+j) % 12 for j in (0,2,4,5,7,9,11)]\n return b%12 in major_scale_a\n return bool(notes) and any(all(is_in_key(i,n) for n in notes) for i in range(1,13))", "def is_tune(notes):\n if not notes:\n return False\n c = 0\n print(notes)\n for i in range(len(notes)):\n for j in range(len(notes)):\n if (notes[j] - notes[i]) % 12 in [0, 1, 3, 5, 6, 8, 10]:\n c += 1\n else:\n c = 0\n break\n if c == len(notes):\n return True\n return False", "def is_tune(notes):\n SCALE = [1, 3, 5, 6, 8, 10, 0]\n for n in range(12):\n if all((note - n) % 12 in SCALE for note in notes):\n return bool(notes)\n return False"] | {"fn_name": "is_tune", "inputs": [[[1, 3, 6, 8, 10, 12]], [[1, 3, 6, 8, 10, 12, 13, 15]], [[1, 6, 3]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]], [[1, 3, 5, 6, 8, 10, 12]], [[3, 5, 6, 8, 10, 12]], [[]], [[1]], [[-11, -9, -7, -6, -4, -2, 0, 1, 3, 6, 8, 10, 12, 13, 15]], [[-4, -2, 0, 1, 3, 6, 8, 10, 12, 13, 15]]], "outputs": [[true], [true], [true], [false], [true], [true], [false], [true], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,204 |
def is_tune(notes):
|
6988409786fc1f644f885ce39554f5b6 | UNKNOWN | *** No Loops Allowed ***
You will be given an array (a) and a limit value (limit). You must check that all values in the array are below or equal to the limit value. If they are, return true. Else, return false.
You can assume all values in the array are numbers.
Do not use loops. Do not modify input array.
Looking for more, loop-restrained fun? Check out the other kata in the series:
https://www.codewars.com/kata/no-loops-2-you-only-need-one
https://www.codewars.com/kata/no-loops-3-copy-within | ["def small_enough(a, limit): \n return max(a) <= limit", "def small_enough(a, limit): \n if max(a) <= limit:\n return True\n else:\n return False", "def small_enough(a, limit): \n if a[0] > limit:\n return False\n \n if len(a) > 1:\n return small_enough(a[1:], limit)\n \n return True\n", "def small_enough(lst, limit): \n return max(lst) <= limit", "small_enough = lambda a, limit: max(a)<=limit", "def small_enough(a, limit): \n try:\n if a[0]>limit: return False\n if a[1]>limit: return False\n if a[2]>limit: return False\n if a[3]>limit: return False\n if a[4]>limit: return False\n if a[5]>limit: return False\n if a[6]>limit: return False\n if a[7]>limit: return False\n if a[8]>limit: return False\n if a[9]>limit: return False\n if a[10]>limit: return False\n if a[11]>limit: return False\n if a[12]>limit: return False\n if a[13]>limit: return False\n if a[14]>limit: return False\n if a[15]>limit: return False\n if a[16]>limit: return False\n if a[17]>limit: return False\n if a[18]>limit: return False\n if a[19]>limit: return False\n if a[20]>limit: return False\n if a[21]>limit: return False\n if a[22]>limit: return False\n if a[23]>limit: return False\n if a[24]>limit: return False\n if a[25]>limit: return False\n if a[26]>limit: return False\n if a[27]>limit: return False\n if a[28]>limit: return False\n if a[29]>limit: return False\n if a[30]>limit: return False\n if a[31]>limit: return False\n if a[32]>limit: return False\n if a[33]>limit: return False\n if a[34]>limit: return False\n if a[35]>limit: return False\n if a[36]>limit: return False\n if a[37]>limit: return False\n if a[38]>limit: return False\n if a[39]>limit: return False\n if a[40]>limit: return False\n if a[41]>limit: return False\n if a[42]>limit: return False\n if a[43]>limit: return False\n if a[44]>limit: return False\n except:\n return True", "small_enough = lambda a, x: False not in map(lambda i: i <= x, a)", "def small_enough(a, limit): \n return all(map(lambda x: x <= limit, a))", "from functools import reduce\ndef small_enough(A, l): \n return reduce(lambda a, b: a and b, map(lambda a:a<=l, A))", "def small_enough(a, limit):\n return list(filter(lambda i: i <= limit, a)) == a"] | {"fn_name": "small_enough", "inputs": [[[66, 101], 200], [[78, 117, 110, 99, 104, 117, 107, 115], 100], [[101, 45, 75, 105, 99, 107], 107], [[80, 117, 115, 104, 45, 85, 112, 115], 120]], "outputs": [[true], [false], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,589 |
def small_enough(a, limit):
|
c2d8db68a01af0ff07d923459218b87a | UNKNOWN | Write a function `count_vowels` to count the number of vowels in a given string.
### Notes:
- Return `nil` or `None` for non-string inputs.
- Return `0` if the parameter is omitted.
### Examples:
```python
count_vowels("abcdefg") => 2
count_vowels("aAbcdeEfg") => 4
count_vowels(12) => None
``` | ["def count_vowels(s = ''):\n return sum(x.lower() in 'aeoui' for x in s) if type(s) == str else None", "import re\n\ndef count_vowels(s = ''):\n if isinstance(s, str):\n return sum(1 for _ in re.finditer(r'[aeiou]',s, flags=re.I))", "def count_vowels(s = ''):\n if type(s) != str: \n return None\n return len([c for c in s if c.lower() in \"aeiou\"])\n", "def count_vowels(s = ''):\n return len([c for c in s.lower() if c in 'aeiou']) if isinstance(s, str) else None", "is_vowel = set(\"aeiouAEIOU\").__contains__\n\ndef count_vowels(s = ''):\n if type(s) == str:\n return sum(map(is_vowel, s))", "def count_vowels(s = ''):\n vowels = [\"a\",\"e\",\"o\",\"u\",\"i\"]\n counter = 0\n try:\n s = s.lower() \n for char in s:\n if char in vowels:\n counter +=1\n return counter\n except AttributeError:\n return None", "def count_vowels(s = '',count=0,vowels='aeiou'):\n if type(s) is str:\n for i in s.lower():\n if i in vowels:\n count+=1\n return count\n else:\n return None", "def count_vowels(s = ''):\n count = 0\n if type(s) == str:\n for i in s:\n if i in ['a','e','i','o','u','A','E','I','O','U']:\n count += 1\n return count \n else:\n return None", "def count_vowels(s = ''):\n if not isinstance(s, str):\n return None\n return sum(c in \"aeiouAEIOU\" for c in s)", "def count_vowels(s = ''):\n if type(s) == str:\n return sum(char in \"aeiou\" for char in s.lower())\n"] | {"fn_name": "count_vowels", "inputs": [["abcdefg"], ["asdfdsafdsafds"], [""], ["asdfdsaf asdfsdaf 13243242 dsafdsafds"], ["aeiouAEIOU"], ["1213"], ["12 3"], ["ewqriwetruituofdsajflsd23423r5043"], ["asdfviosdfopsiewrwwer asdf asdfdsaf)(asdflkajdsf "], ["asdfdsaf asdfsasdfdsafgjlsdjf asdfdsf daf 13243242 dsafdsafds"], ["aeiouAEdsafdsf asdfw2434&***OU"], [12], [[]], [{}]], "outputs": [[2], [3], [0], [6], [10], [0], [0], [8], [12], [9], [11], [null], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,624 |
def count_vowels(s = ''):
|
d256788d91f08e1b6b9c316ac1bdbcf6 | UNKNOWN | In this simple exercise, you will build a program that takes a value, `integer `, and returns a list of its multiples up to another value, `limit `. If `limit` is a multiple of ```integer```, it should be included as well. There will only ever be positive integers passed into the function, not consisting of 0. The limit will always be higher than the base.
For example, if the parameters passed are `(2, 6)`, the function should return `[2, 4, 6]` as 2, 4, and 6 are the multiples of 2 up to 6.
If you can, try writing it in only one line of code. | ["def find_multiples(integer, limit):\n return list(range(integer, limit+1, integer))", "def find_multiples(integer, limit):\n arr = []\n count = integer\n while count <= limit:\n arr.append(count)\n count += integer\n return arr", "find_multiples = lambda a, b: list(range(a, b + 1, a))", "def find_multiples(integer, limit):\n return [i for i in range(integer, limit + 1, integer)]", "def find_multiples(x, limit):\n return list(range(x, limit+1, x))", "find_multiples = lambda i, l: list(range(i, l + 1, i))", "def find_multiples(integer, limit): return [i for i in range(0,limit+1,integer) if i != 0]", "def find_multiples(integer, limit):\n return [i for i in range(1, limit+1) if i%integer == 0]", "def find_multiples(integer, limit):\n return [integer * i for i in range(1, limit // integer + 1)]\n", "def find_multiples(integer, limit):\n return [i for i in range(integer, limit + 1) if i % integer == 0]", "def find_multiples(integer, limit):\n result = []\n i = integer\n for i in range(limit+1):\n if ((i % integer == 0) and (i != 0)):\n result.append(i)\n return result ", "def find_multiples(n, l):\n return list(range(n,l+1,n)) ", "def find_multiples(integer, limit):\n return [integer*count for count in range(1, int(limit/integer)+1)]", "def find_multiples(integer, limit):\n return [integer * mult for mult in range(1, limit // integer + 1)]", "def find_multiples(integer, limit):\n numbers = [i * integer for i in range(1, limit+1) if (i * integer) <= limit]\n return numbers", "def find_multiples(integer, limit):\n multiplies = []\n for i in range(integer,limit+1,integer):\n multiplies.append(i)\n return multiplies", "def find_multiples(integer, limit):\n mas = []\n n = integer\n while n <= limit:\n mas.append(n)\n n += integer \n return mas \n", "def find_multiples(integer, limit):\n return list(range(integer, limit+integer, integer)) if limit%integer==0 else list(range(integer, limit, integer))", "from typing import List\n\ndef find_multiples(integer: int, limit: int) -> List[int]:\n \"\"\" Get a list of all multiples based on integer and limit. \"\"\"\n return list(filter(lambda _it: not _it % integer, range(1, limit + 1)))", "def find_multiples(integer, limit):\n # Your code here!\n return [x for x in range(integer, limit+1) if x%integer==0]", "def find_multiples(integer, limit):\n answer = []\n i = 0\n number = integer\n while number <= limit:\n i = number\n answer.append(i)\n number += integer\n return answer", "def find_multiples(integer, limit):\n n = 1\n res = list()\n while n * integer <= limit:\n res.append(n * integer)\n n += 1\n return res", "def find_multiples(integer, limit):\n i = 1\n res = []\n while i*integer <= limit:\n res.append(i*integer)\n i += 1\n return res", "def find_multiples(integer, limit):\n new_list = list(range(integer, limit + 1, integer))\n return new_list", "def find_multiples(integer, limit):\n n = integer\n answer = []\n while n <= limit:\n answer.append(n)\n n += integer\n return answer", "def find_multiples(integer, limit):\n result = []\n num = 1\n while integer * num <= limit:\n result.append(integer * num)\n num += 1\n return result", "def find_multiples(integer, limit):\n lst = []\n acc = integer\n for num in range(integer, limit+1) : \n if acc <= limit : \n lst.append(acc) \n acc += integer\n return lst \n\n # Your code here!\n", "def find_multiples(integer, limit):\n# return [[x for x in range(integer, limit, integer)], limit]\n arr = [integer]\n x = integer\n if limit // integer:\n while x+integer <= limit: \n x += integer\n arr.append(x)\n return arr", "def find_multiples(integer, limit):\n # Your code here!\n lista=[]\n for i in range(integer,limit+1):\n lista.append(i)\n return list( filter(lambda numero: numero%integer == 0, lista) )", "def find_multiples(integer, limit):\n list=[]\n for i in range(1, limit+1):\n if i * integer <= limit:\n list.append(i*integer)\n return list# Your code here!", "def find_multiples(integer, limit):\n out = []\n for i in range(integer,limit+1,integer):\n out.append(i)\n return out\n", "def find_multiples(integer, limit):\n my_list = []\n for i in range(integer,limit + 1):\n if i % integer == 0:\n my_list.append(i)\n return my_list\n", "def find_multiples(integer, limit):\n res = []\n for i in range(int(limit/integer)):\n res.append((i+1)*integer)\n return res", "def find_multiples(integer, limit):\n final_list = []\n for i in range(integer, limit + 1):\n if i % integer == 0:\n final_list.append(i)\n return final_list", "def find_multiples(integer, limit):\n grup = []\n for i in range(1, int(limit)+1):\n if i%integer==0:\n grup.append(i) \n return grup\n \n \n", "def find_multiples(integer, limit):\n divizor = []\n for i in range(limit+1):\n if i < integer:\n pass\n else:\n if (i % integer == 0):\n divizor.append(i)\n return divizor", "def find_multiples(integer, limit):\n x = []\n i = 1\n r = int(limit / integer)\n for i in range(r):\n x.append(integer * (i + 1))\n return x", "def find_multiples(integer, limit):\n # inserts all elements from integer to limit incremented by integer\n a = list(range(integer,limit + 1,integer))\n return a\n \nfind_multiples(5,25)", "def find_multiples(integer, limit):\n multiple_list = []\n for num in range((limit+1)):\n if num == 0:\n pass\n elif num % integer == 0:\n multiple_list.append(num)\n else:\n pass\n return multiple_list", "def find_multiples(integer, limit):\n l = [i for i in range(integer, limit + 1, integer)]\n return l\n", "def find_multiples(integer, limit):\n result = 0\n arr = []\n for i in range(int(limit / integer)):\n result = result + integer\n arr.append(result)\n return arr\n", "def find_multiples(integer, limit):\n list = []\n for n in range(integer, limit+1, 1):\n if n%integer == 0:\n list.append(n)\n \n return list", "def find_multiples(integer, limit):\n k = list()\n if limit<integer:\n return k\n for a in range(1,limit//integer + 1):\n k.append(a*integer)\n return k", "def find_multiples(integer, limit):\n result_list = [integer]\n counting = integer\n while counting < limit:\n counting += integer\n if counting > limit:\n break\n else:\n result_list.append(counting)\n return result_list", "def find_multiples(a,number):\n \n my_primes = []\n \n for i in range(a, number+1):\n \n if i % a == 0:\n my_primes.append(i)\n return my_primes \n \n \n \n", "def find_multiples(integer, limit):\n # Your code here!\n list=[]\n for i in range(limit):\n i=i+1\n count=i*integer\n if(count<=limit):\n list.append(count) \n return list", "def find_multiples(integer, limit):\n list = []\n for x in range(integer, limit+1, integer):\n list.append(x)\n return list", "def find_multiples(integer, limit):\n n = 1\n mults = []\n while integer * n <= limit:\n mults.append(integer * n)\n n += 1\n \n return mults", "# takes interval from integer to limit\n# returns all multiples within this range of integer\ndef find_multiples(integer, limit):\n return [i for i in range(integer, limit+1) if i % integer == 0]", "def find_multiples(integer, limit):\n output = [integer]\n counter = 2\n count = 1\n while output[count-1] <= limit:\n output.append(counter*integer)\n counter += 1\n count += 1\n output.pop()\n return(output)", "def find_multiples(integer, limit):\n list = [integer]\n count = 2\n while integer * count <= limit:\n list.append(integer * count)\n count += 1\n return list", "def find_multiples(integer, limit):\n m = 1\n new_list = []\n new_integer = integer\n while new_integer < limit:\n new_integer = integer*m\n if new_integer > limit:\n break\n new_list.append(new_integer)\n m +=1\n return new_list ", "def find_multiples(integer, limit):\n l=[]\n for x in range(1,limit+1):\n z=integer*x\n if z<=limit:\n l.append(z)\n else:\n break\n return l\n", "def find_multiples(integer, limit):\n x = list(range(integer, limit+1, integer))\n return x\n \n \n\n # Your code here!\n", "def find_multiples(integer, limit):\n return list([x for x in [i if i % integer == 0 else 0 for i in range(1, limit+1)] if x > 0])\n # Your code here!\n", "def find_multiples(integer, limit):\n n = 1\n return_array = []\n while (integer*n <= limit):\n return_array.append(integer*n)\n n = n + 1\n return return_array", "def find_multiples(integer, limit):\n l = [i for i in range(integer,limit+1,integer)]\n return (l)\n \n \n #if limit % integer == 0:\n #number = limit / integer\n #return (integer, number, limit)\n", "def find_multiples(integer, limit):\n res = []\n multiple = 0\n flag = 1\n while flag <= (limit // integer):\n multiple = integer * flag\n flag += 1\n res.append(multiple)\n return res\n", "def find_multiples(integer, limit):\n sol = []\n for i in range (1, (limit//integer)+1):\n sol.append(integer * i)\n return sol\n \n", "def find_multiples(integer, limit):\n mults = []\n n = integer\n while n <= limit:\n mults.append(n)\n n = n + integer \n return mults", "def find_multiples(integer, limit):\n # Your code here!\n lst = []\n for item in range(integer, limit + 1, 1):\n if item % integer == 0:\n lst.append(item)\n return lst", "def find_multiples(integer, limit):\n ans = []\n count = integer\n while count <= limit:\n ans.append(count)\n count += integer\n return ans", "def find_multiples(integer, limit):\n list = []\n for x in range(integer, (limit + 1)):\n if(not(x % integer)):\n list.append(x)\n return list", "def find_multiples(integer, limit):\n ans = []\n for i in range(1, limit//integer + 1):\n ans.append(integer*i)\n return ans", "def find_multiples(integer, limit):\n list=[]\n i=integer\n for i in range(limit+1):\n if i%integer==0 and i!=0:\n list.append(i)\n return list ", "def find_multiples(i, l):\n return list(range(i, l if not l%i==0 else l+i, i))", "def find_multiples(integer, limit):\n answ=[]\n answer=0\n n=1\n while answer<limit:\n answer = integer* n\n if answer <=limit:\n answ.append(answer)\n n+=1\n return answ", "def find_multiples(integer, limit):\n spisok = []\n for i in range(integer, limit+1):\n if i % integer == 0:\n spisok.append(i)\n return spisok", "find_multiples = lambda i,l: [*range(i,l+1,i)]", "def find_multiples(int, lmt):\n return list(range(int, lmt + 1, int))\n", "def find_multiples(integer, limit):\n arr = []\n [ arr.append(integer * i) for i in range(1,int(limit/integer)+1)]\n return arr", "def find_multiples(integer, limit):\n i = 1\n y = []\n while (integer * i) <= limit:\n output = integer * i\n y.append(output)\n i += 1\n return y", "def find_multiples(integer, limit):\n arr = [integer]\n #print(range(int(limit//integer)-1))\n for i in range(int(limit//integer)-1):\n if arr[-1] < limit:\n arr.append(2*integer+integer*i)\n return arr", "def find_multiples(integer, limit):\n l = []\n i = integer\n while integer <= limit:\n l.append(integer)\n integer += i\n \n return l\n\n", "def find_multiples(integer, limit):\n list=[]\n i=1\n m=integer\n for m in range(integer,limit+1):\n if (m>=integer)and(m==integer*i)and(m<=limit):\n list.extend([m])\n i=i+1\n m=m*i\n return list", "def find_multiples(i, l):\n z = []\n for a in range(1,l+1):\n if a % i == 0:\n z.append(a)\n return(z)\n \n\n\n \n", "def find_multiples(integer, limit):\n return [i for i in range(limit+1) if i % integer == 0 and i > 0]", "def find_multiples(integer, limit):\n count = int(limit/integer)\n result = []\n \n for i in range(count):\n result.append(integer * (i+1))\n \n return result\n", "def find_multiples(integer, limit):\n li = []\n for x in range(1,limit+1):\n if x % integer == 0:\n li.append(x)\n return li", "def find_multiples(d, l):\n return list(filter(lambda x: x % d == 0, range(1, l + 1)))", "def find_multiples(integer, limit):\n # Your code here!\n # i=interger\n arr=[]\n for i in range(integer,limit+1):\n if(i%integer==0):\n arr.append(i)\n return arr", "def find_multiples(integer, limit):\n list = []\n i = 1\n while i <= (limit/integer): \n list.append(integer*i)\n i = i + 1\n return list", "def find_multiples(integer, limit):\n lst = []\n for i in range(1, limit):\n if i % integer == 0:\n lst.append(i)\n if limit % integer == 0:\n lst.append(limit)\n return(lst)", "def find_multiples(integer, limit):\n l = []\n for x in range(integer,limit+1):\n if(x % integer == 0):\n l.append(x)\n return l", "def find_multiples(integer, limit):\n print(integer, limit)\n answer = [integer]\n while answer[-1] + integer <= limit:\n answer.append(answer[-1] + integer)\n return answer", "def find_multiples(integer, limit):\n li = []\n \n for i in range(0,limit + 1,integer):\n li.append(i)\n \n li.pop(0)\n return li\n # Your code here!\n", "def find_multiples(integer, limit):\n numList = []\n count = 1\n howMuch = int(limit/integer)\n for x in range(howMuch):\n numList.append(integer*count)\n count += 1\n return numList", "def find_multiples(integer, limit):\n # Your code here!\n f = []\n for x in range(integer,limit + 1, integer):\n f.append(x)\n return f\n\n", "def find_multiples(integer, limit):\n lst = []\n count = 1\n while integer * count <= limit:\n var = integer * count\n lst.append(var)\n count += 1\n return lst\n", "def find_multiples(integer, limit):\n aha = []\n x = 0\n while x <= limit:\n x = x + integer\n if x <= limit:\n aha.append(x)\n return aha\n", "def find_multiples(integer, limit):\n m = []\n for i in range(limit+1):\n if i/integer == int(i/integer):\n m.append(i)\n m.remove(0)\n return m", "def find_multiples(integer, limit):\n ans = []\n for i in range(1,(limit//integer)+1):\n ans.append(i*integer)\n return ans\n # Your code here!\n", "def find_multiples(integer, limit):\n result = []\n for i in range((limit//integer)+1):\n result.append(integer*i)\n \n return result[1:]", "def find_multiples(integer, limit):\n count = 1\n res = []\n while True:\n somme = integer * count\n if somme > limit:\n break\n else:\n res.append(somme)\n count += 1\n return res", "def find_multiples(integer, limit):\n result = []\n number = 1\n temp = integer * number\n while temp <= limit:\n result.append(temp)\n number += 1\n temp = integer * number\n return result\n"] | {"fn_name": "find_multiples", "inputs": [[5, 25], [1, 2], [5, 7], [4, 27], [11, 54]], "outputs": [[[5, 10, 15, 20, 25]], [[1, 2]], [[5]], [[4, 8, 12, 16, 20, 24]], [[11, 22, 33, 44]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 16,255 |
def find_multiples(integer, limit):
|
f645e299c8cf7e131e322563a6d8aeca | UNKNOWN | This will probably be a little series :)
X-Shape
You will get an odd Integer n (>= 3) and your task is to draw a X. Each line is separated with '\n'.
Use the following characters: ■ □
For Ruby, Crystal and PHP: whitespace and o
e.g.:
■□□□■
■□■ □■□■□
x(3) => □■□ x(5)=> □□■□□
■□■ □■□■□
■□□□■
Series: ASCII Fun
ASCII Fun #1: X-Shape
ASCII Fun #2: Funny Dots
ASCII Fun #3: Puzzle Tiles
ASCII Fun #4: Build a pyramid | ["def x(n):\n ret = [['\u25a1' for _ in range(n)] for _ in range(n)]\n for i in range(len(ret)):\n ret[i][i] = '\u25a0';\n ret[i][-1 - i] = '\u25a0';\n return '\\n'.join(''.join(row) for row in ret)", "f, e = \"\u25a0\u25a1\"\n\n\ndef x(n):\n l = n // 2\n middle = [f\"{e * l}{f}{e * l}\"]\n half = [f\"{e * i}{f}{e * (n - 2*i - 2)}{f}{e * i}\" for i in range(l)]\n return \"\\n\".join(half + middle + half[::-1])\n", "def x(n):\n result = []\n for i in range(int(n/2) + 1):\n line = \"\u25a1\" * i + \"\u25a0\" + \"\u25a1\" * (int(n/2) - i)\n result.append(line + line[::-1][1:])\n return \"\\n\".join(result + result[::-1][1:])", "def x(n):\n return '\\n'.join(''.join('\u25a0' if row==col or row==n-1-col else '\u25a1' for col in range(n)) for row in range(n))", "def x(n):\n m = n - 1\n return '\\n'.join(\n ''.join('\u25a1\u25a0'[i + j == m or i == j] for j in range(n))\n for i in range(n)\n )", "def x(n):\n i=0\n out=''\n while i<n:\n out = out + line(n, i)\n if i != (n-1):\n out = out + '\\n' \n i = i+1\n return out\n\ndef line(x, ln):\n blank = '\u25a1'\n pixel = '\u25a0'\n str=''\n n=0\n while n<x:\n if n == ln:\n str = str + pixel\n elif n == ((x-1)-ln):\n str = str + pixel\n else:\n str = str + blank\n n=n+1\n return str", "def x(n):\n res = ''\n for i in range(n):\n t = ''\n for j in range(n):\n t += '\u25a0' if j == i or j == n-1-i else '\u25a1'\n res += t+'\\n'\n return res[:len(res)-1]", "def x(n):\n arr = [['\u25a1'] * n for _ in range(n)]\n for i in range(len(arr)):\n arr[i][i]=arr[i][-1-i]='\u25a0'\n return \"\\n\".join([\"\".join(i) for i in arr])", "def x(n):\n tmpl = '\\n'.join(''.join('{1}' if j in (i, n - i - 1) else '{0}' for j in range(n)) for i in range(n))\n return tmpl.format('\u25a1', '\u25a0')\n", "\ndef x(n):\n g = []\n for i in range((n//2)+1):\n rt = ['\u25a1'] * n\n rt[i], rt[-(i+1)] = '\u25a0', '\u25a0'\n g.append(rt) \n return '\\n'.join(''.join(e) for e in g + g[:-1][::-1] )\n \n"] | {"fn_name": "x", "inputs": [[3], [7]], "outputs": [["\u25a0\u25a1\u25a0\n\u25a1\u25a0\u25a1\n\u25a0\u25a1\u25a0"], ["\u25a0\u25a1\u25a1\u25a1\u25a1\u25a1\u25a0\n\u25a1\u25a0\u25a1\u25a1\u25a1\u25a0\u25a1\n\u25a1\u25a1\u25a0\u25a1\u25a0\u25a1\u25a1\n\u25a1\u25a1\u25a1\u25a0\u25a1\u25a1\u25a1\n\u25a1\u25a1\u25a0\u25a1\u25a0\u25a1\u25a1\n\u25a1\u25a0\u25a1\u25a1\u25a1\u25a0\u25a1\n\u25a0\u25a1\u25a1\u25a1\u25a1\u25a1\u25a0"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,241 |
def x(n):
|
3bf7ba4e27eb6cadeb4008e21fff2368 | UNKNOWN | Your goal in this kata is to implement a difference function, which subtracts one list from another and returns the result.
It should remove all values from list `a`, which are present in list `b`.
```python
array_diff([1,2],[1]) == [2]
```
If a value is present in `b`, all of its occurrences must be removed from the other:
```python
array_diff([1,2,2,2,3],[2]) == [1,3]
```
~~~ if:c
NOTE: In C, assign return array length to pointer *z
~~~ | ["def array_diff(a, b):\n return [x for x in a if x not in b]", "def array_diff(a, b):\n return [x for x in a if x not in set(b)]", "def array_diff(a, b):\n set_b = set(b)\n return [i for i in a if i not in set_b]", "def array_diff(a, b):\n #your code here\n for i in range(len(b)):\n while b[i] in a:\n a.remove(b[i])\n return a", "def array_diff(a, b):\n return [v for v in a if v not in b]", "def array_diff(a, b):\n return [value for value in a if value not in b]", "def array_diff(a, b):\n for n in b:\n while(n in a):\n a.remove(n)\n return a", "def array_diff(a, b):\n return [i for i in a if i not in b]", "def array_diff(a, b):\n c = []\n \n for e in a:\n for e2 in b:\n if e == e2:\n break\n else:\n c.append(e)\n \n return c"] | {"fn_name": "array_diff", "inputs": [[[1, 2], [1]], [[1, 2, 2], [1]], [[1, 2, 2], [2]], [[1, 2, 2], []], [[], [1, 2]]], "outputs": [[[2]], [[2, 2]], [[1]], [[1, 2, 2]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 879 |
def array_diff(a, b):
|
37f763348988662bad7a70d64294e0c8 | UNKNOWN | # The Problem
Dan, president of a Large company could use your help. He wants to implement a system that will switch all his devices into offline mode depending on his meeting schedule. When he's at a meeting and somebody texts him, he wants to send an automatic message informing that he's currently unavailable and the time when he's going to be back.
# What To Do
Your task is to write a helper function `checkAvailability` that will take 2 arguments:
* schedule, which is going to be a nested array with Dan's schedule for a given day. Inside arrays will consist of 2 elements - start and finish time of a given appointment,
* *currentTime* - is a string with specific time in hh:mm 24-h format for which the function will check availability based on the schedule.
* If no appointments are scheduled for `currentTime`, the function should return `true`. If there are no appointments for the day, the output should also be `true`
* If Dan is in the middle of an appointment at `currentTime`, the function should return a string with the time he's going to be available.
# Examples
`checkAvailability([["09:30", "10:15"], ["12:20", "15:50"]], "11:00");`
should return `true`
`checkAvailability([["09:30", "10:15"], ["12:20", "15:50"]], "10:00");`
should return `"10:15"`
If the time passed as input is *equal to the end time of a meeting*, function should also return `true`.
`checkAvailability([["09:30", "10:15"], ["12:20", "15:50"]], "15:50");`
should return `true`
*You can expect valid input for this kata* | ["def check_availability(schedule, current_time):\n for tb, te in schedule:\n if tb <= current_time < te:\n return te\n return True", "def check_availability(schedule, current_time):\n for time in schedule:\n if time[0] <= current_time < time[1]:\n return time[1]\n return True", "def check_availability(schedule, current_time):\n return next((end for start,end in schedule if start < current_time < end), True)", "check_availability=lambda sh,ct:next((j for i,j in sh if ct>i and ct<j),1)", "def check_availability(schedule, now):\n return next((end for start, end in schedule if start <= now < end), True)", "def check_availability(schedule, current_time):\n return next((stop for start, stop in schedule if start <= current_time < stop), True)", "def check_availability(schedule, current_time):\n for start, stop in schedule:\n if start <= current_time < stop:\n return stop\n return True", "def check_availability(schedule, current_time):\n for s, e in schedule:\n if s <= current_time < e: return e\n return True", "def check_availability(schedule, current_time):\n for meeting in schedule: return meeting[1] if meeting[0] <= current_time < meeting[1] else True\n return True", "def check_availability(sched, now):\n b = [t1 < now < t2 for t1, t2 in sched]\n return sched[b.index(True)][1] if True in b else True\n"] | {"fn_name": "check_availability", "inputs": [[[["09:30", "10:15"], ["12:20", "15:50"]], "10:00"], [[["09:30", "10:15"], ["12:20", "15:50"]], "11:00"], [[["09:30", "10:15"], ["12:20", "15:50"]], "10:15"], [[], "10:15"], [[["12:13", "23:50"]], "10:15"], [[["12:13", "23:50"]], "23:55"], [[["12:13", "23:50"]], "17:43"]], "outputs": [["10:15"], [true], [true], [true], [true], [true], ["23:50"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,427 |
def check_availability(schedule, current_time):
|
90efe9b0d22d10fdcb4a035e98d2b2bd | UNKNOWN | The purpose of this series is developing understanding of stastical problems in AS and A level maths. Let's get started with a simple concept in statistics: Mutually exclusive events.
The probability of an OR event is calculated by the following rule:
`P(A || B) = P(A) + P(B) - P(A && B)`
The probability of event A or event B happening is equal to the probability of event A plus the probability of event B minus the probability of event A and event B happening simultaneously.
Mutually exclusive events are events that cannot happen at the same time. For example, the head and tail results of a toin coss are mutually exclusive because they can't both happen at once. Thus, the above example for a coin toss would look like this:
`P(H || T) = P(H) + P(T) - P(H && T)`
Note that the probaility of tossing a coin and the result being both head and tails is 0.
`P(H || T) = (0.5) + (0.5) - (0)`
`P(H || T) = 1`
Thus the probability of a coin toss result being a heads or a tails is 1, in other words: certain.
Your task:
You are going to have to work out the probability of one roll of a die returning two given outcomes, or rolls. Given that dice rolls are mutually exclusive, you will have to implement the above forumala. To make this interesting (this is a coding challenge after all), these dice are not fair and thus the probabilites of receiving each roll is different.
You will be given a two-dimensional array containing the number each of the results (1-6) of the die and the probability of that roll for example `[1 , 0.23]` as well as the two rolls for example `1` and `5`.
Given the two roll probabilities to calculate, return the probability of a single roll of the die returning either. If the total probability of the six rolls doesn't add up to one, there is a problem with the die; in this case, return null.
Return your result as a string to two decimal places.
Example below:
`1 : 1/6`
`2 : 1/6`
`3 : 1/6`
`4 : 1/6`
`5 : 1/6`
`6 : 1/6`
If asked for the rolls `1` and `2` then you would need to sum the probabilities, both `1/6` therefore `2/6` and return this. As above, you will need to return it as a decimal and not a fraction. | ["def mutually_exclusive(dice, call1, call2):\n dice = dict(dice)\n if abs(sum(dice.values()) - 1) < 1e-12:\n return '%0.2f' % (dice[call1] + dice[call2])", "def mutually_exclusive(dice, call1, call2):\n if sum(prob for _, prob in dice) != 1:\n return None\n x = dict(dice)\n return format(x[call1] + x[call2], '.2f')", "def mutually_exclusive(dice, call1, call2):\n d = dict(dice)\n return '{:0.2f}'.format(d[call1] + d[call2]) if 0.999 < sum(d.values()) < 1.001 else None", "def mutually_exclusive(dice, call1, call2): \n prob = 0\n tprob = 0\n for i in dice:\n if i[0] == call1 or i[0] == call2:\n prob += i[1]\n \n tprob += i[1]\n \n return '{:.2f}'.format(prob) if tprob == 1 else None", "def mutually_exclusive(dice, call1, call2):\n dice.sort()\n if sum(pair[1] for pair in dice) != 1:\n return None\n else:\n return '{:.2f}'.format(dice[call1-1][1] + dice[call2-1][1])", "def mutually_exclusive(dice, call1, call2):\n dice = {a: b for a, b in dice}\n return None if sum(dice.values()) != 1 else f'{float(dice[call1] + dice[call2]):.02f}'\n", "def mutually_exclusive(dice, call1, call2):\n prob_of_each_roll = []\n for roll in dice:\n prob_of_each_roll.append(roll[1])\n if roll[0] == call1:\n p1 = roll[1]\n if roll[0] == call2:\n p2 = roll[1]\n if sum(prob_of_each_roll) != 1:\n return\n else:\n total_prob = (p1 + p2)\n return (\"{:.2f}\".format(total_prob))", "def mutually_exclusive(dice, call1, call2):\n prob_of_each_roll = []\n for roll in dice:\n prob_of_each_roll.append(roll[1])\n if roll[0] == call1:\n p1 = roll[1]\n if roll[0] == call2:\n p2 = roll[1]\n if sum(prob_of_each_roll) != 1:\n return\n else:\n total_prob = round((p1 + p2),2)\n return (\"{:.2f}\".format(total_prob))", "def mutually_exclusive(dice, call1, call2):\n checknull = 0\n diecall1 = 0\n diecall2 = 0\n for die in dice:\n #[3,0.4]\n checknull += die[1]\n if die[0] == call1:\n diecall1 = die[1]\n elif die[0] == call2:\n diecall2 = die[1] \n if checknull != 1:\n return None\n else:\n return format((diecall1 + diecall2), \".2f\")", "def mutually_exclusive(dice, call1, call2):\n checknull = 0\n diecall1 = 0\n diecall2 = 0\n for die in dice:\n #[3,0.4]\n checknull += die[1]\n if die[0] == call1:\n diecall1 = die[1]\n elif die[0] == call2:\n diecall2 = die[1] \n if checknull != 1:\n return None\n else:\n return format((diecall1 + diecall2), \".2f\")#- (diecall1*diecall2),2)) #:[\n \n#dice = [[3,0.4],[4,0.1],[1,0.01],[2,0.09],[5,0.2],[6,0.1]]\n"] | {"fn_name": "mutually_exclusive", "inputs": [[[[3, 0.4], [4, 0.1], [1, 0.01], [2, 0.09], [5, 0.2], [6, 0.1]], 1, 6], [[[1, 0.1], [2, 0.14], [3, 0.16], [4, 0.2], [5, 0.15], [6, 0.25]], 1, 4], [[[1, 0.6], [2, 0.1001], [3, 0.0999], [4, 0.1], [5, 0.05], [6, 0.05]], 3, 4], [[[6, 0.25], [1, 0.1], [3, 0.16], [2, 0.14], [5, 0.15], [4, 0.2]], 1, 6], [[[3, 0.4], [4, 0.1], [1, 0.01], [2, 0.09], [5, 0.2], [6, 0.2]], 1, 6]], "outputs": [[null], ["0.30"], ["0.20"], ["0.35"], ["0.21"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,882 |
def mutually_exclusive(dice, call1, call2):
|
58db8206112e9f7248035e6f1502711b | UNKNOWN | # Task
The year of `2013` is the first year after the old `1987` with only distinct digits.
Now your task is to solve the following problem: given a `year` number, find the minimum year number which is strictly larger than the given one and has only distinct digits.
# Input/Output
- `[input]` integer `year`
`1000 ≤ year ≤ 9000`
- `[output]` an integer
the minimum year number that is strictly larger than the input number `year` and all its digits are distinct. | ["def distinct_digit_year(year):\n year += 1\n while len(set(str(year))) != 4:\n year += 1\n return year\n #coding and coding..\n", "def distinct_digit_year(year):\n year += 1\n \n if len(set(str(year))) == len(str(year)):\n return year \n \n return distinct_digit_year(year)", "from itertools import count\n\ndef distinct_digit_year(year):\n return next(y for y in count(year+1) if len(str(y)) == len(set(str(y))))", "def distinct_digit_year(year):\n distinctDigits = False\n while distinctDigits == False: \n #iterate to next year until a year with distinct digits is found\n year += 1\n #if the length of the unique digits in year is the same as the total number of digits, the digits are unique\n if len(set(str(year))) == len(str(year)): \n distinctDigits = True\n \n return year", "def distinct_digit_year(year):\n return next(i for i in range(year + 1, 9999) if len(set(str(i)))==4)", "import re\n\ndef distinct_digit_year(year):\n return year + 1 if re.match(r'(?:([0-9])(?!.*\\1)){4}', str(year + 1)) else distinct_digit_year(year + 1)", "def distinct(year):\n year = str(year)\n return len(year) == len(set(year))\n \ndef distinct_digit_year(year):\n year += 1\n while not distinct(year):\n year += 1\n return year", "distinct_digit_year=lambda y:next(i for i in range(y+1,9000)if len(set(str(i)))==4)", "def distinct_digit_year(year):\n next_year = int(year)\n while True:\n sum = 0\n next_year = next_year + 1\n for num in str(next_year):\n sum += str(next_year).count(num)\n if sum == 4:\n break\n return(next_year)", "def distinct_digit_year(year):\n for y in range(year + 1, 9999):\n if len(set(str(y))) == 4:\n return y"] | {"fn_name": "distinct_digit_year", "inputs": [[1987], [2013]], "outputs": [[2013], [2014]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,827 |
def distinct_digit_year(year):
|
cbf36346d1df52b4893357ca13b6d1dc | UNKNOWN | Let us begin with an example:
A man has a rather old car being worth $2000.
He saw a secondhand car being worth $8000. He wants to keep his old car until he can buy the secondhand one.
He thinks he can save $1000 each month but the prices of his old
car and of the new one decrease of 1.5 percent per month.
Furthermore this percent of loss increases of `0.5` percent
at the end of every two months.
Our man finds it difficult to make all these calculations.
**Can you help him?**
How many months will it take him to save up enough money to buy the car he wants, and how much money will he have left over?
**Parameters and return of function:**
```
parameter (positive int or float, guaranteed) startPriceOld (Old car price)
parameter (positive int or float, guaranteed) startPriceNew (New car price)
parameter (positive int or float, guaranteed) savingperMonth
parameter (positive float or int, guaranteed) percentLossByMonth
nbMonths(2000, 8000, 1000, 1.5) should return [6, 766] or (6, 766)
```
### Detail of the above example:
```
end month 1: percentLoss 1.5 available -4910.0
end month 2: percentLoss 2.0 available -3791.7999...
end month 3: percentLoss 2.0 available -2675.964
end month 4: percentLoss 2.5 available -1534.06489...
end month 5: percentLoss 2.5 available -395.71327...
end month 6: percentLoss 3.0 available 766.158120825...
return [6, 766] or (6, 766)
```
where `6` is the number of months at **the end of which** he can buy the new car and `766` is the nearest integer to `766.158...` (rounding `766.158` gives `766`).
**Note:**
Selling, buying and saving are normally done at end of month.
Calculations are processed at the end of each considered month
but if, by chance from the start, the value of the old car is bigger than the value of the new one or equal there is no saving to be made, no need to wait so he can at the beginning of the month buy the new car:
```
nbMonths(12000, 8000, 1000, 1.5) should return [0, 4000]
nbMonths(8000, 8000, 1000, 1.5) should return [0, 0]
```
We don't take care of a deposit of savings in a bank:-) | ["def nbMonths(oldCarPrice, newCarPrice, saving, loss):\n months = 0\n budget = oldCarPrice\n \n while budget < newCarPrice:\n months += 1\n if months % 2 == 0:\n loss += 0.5\n \n oldCarPrice *= (100 - loss) / 100\n newCarPrice *= (100 - loss) / 100\n budget = saving * months + oldCarPrice\n \n return [months, round(budget - newCarPrice)]", "def nbMonths(startPriceOld, startPriceNew, savingperMonth, percentLossByMonth):\n i = 0\n while savingperMonth * i + startPriceOld < startPriceNew:\n if i % 2:\n percentLossByMonth += 0.5\n startPriceOld -= startPriceOld * 0.01 * percentLossByMonth\n startPriceNew -= startPriceNew * 0.01 * percentLossByMonth\n i += 1\n return [i, round(savingperMonth * i + startPriceOld - startPriceNew)]", "def nbMonths(fltp1, fltp2, flts, fltl, i = 0):\n return [i, round(fltp1 + flts * i - fltp2, 0)] if fltp1 + flts * i - fltp2 >= 0 else nbMonths(fltp1 * (1 - fltl / 100 - 0.005 * int((i+1)/2)), fltp2 * (1 - fltl / 100 - 0.005 * int((i+1)/2)), flts, fltl, i+1)", "import itertools\ndef nbMonths(my_car, new_car, per_month, loss):\n loss, w, m = 1 - loss / 100.0, my_car, 0\n while w < new_car:\n loss -= 0 if m % 2 == 0 else .005\n my_car *= loss\n new_car *= loss\n m += 1\n w = my_car + per_month * m\n return [m, round(w - new_car)]", "def nbMonths(startPriceOld, startPriceNew, savingperMonth, percentLossByMonth):\n total=0\n count=0\n while total+startPriceOld<startPriceNew:\n count+=1\n if count and count%2==0:\n percentLossByMonth+=0.5\n startPriceOld=startPriceOld*(100-percentLossByMonth)/100\n startPriceNew=startPriceNew*(100-percentLossByMonth)/100\n total+=savingperMonth\n return [count, round(total+startPriceOld-startPriceNew)]", "def nbMonths(priceOld, priceNew, savingMonth, percentLoss):\n if priceOld > priceNew:\n return [0, priceOld-priceNew]\n months = 0\n savings = 0\n loss = percentLoss/100\n while savings + priceOld < priceNew:\n months += 1\n savings += savingMonth\n if months%2 == 0:\n loss += 0.005\n priceOld -= priceOld*loss \n priceNew -= priceNew*loss \n saved = round(savings+priceOld-priceNew)\n return [months, saved]\n", "def nbMonths(startPriceOld, startPriceNew, savingperMonth, percentLossByMonth):\n months_elapsed = 0\n if startPriceOld >= startPriceNew:\n return [months_elapsed, startPriceOld - startPriceNew]\n \n too_poor = True\n while too_poor:\n months_elapsed += 1\n \n if months_elapsed % 2 == 0:\n percentLossByMonth += 0.5\n \n startPriceOld = startPriceOld * (1 - (percentLossByMonth / 100))\n startPriceNew = startPriceNew * (1 - (percentLossByMonth / 100)) \n available_funds = (startPriceOld + (savingperMonth * months_elapsed))\n \n if available_funds >= startPriceNew:\n too_poor = False\n \n return [months_elapsed, int(round(available_funds - startPriceNew))]\n\n \n \n \n \n\n"] | {"fn_name": "nbMonths", "inputs": [[2000, 8000, 1000, 1.5], [8000, 12000, 500, 1], [18000, 32000, 1500, 1.25], [7500, 32000, 300, 1.55]], "outputs": [[[6, 766]], [[8, 597]], [[8, 332]], [[25, 122]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,208 |
def nbMonths(startPriceOld, startPriceNew, savingperMonth, percentLossByMonth):
|
82671bf30f63a7f349a8c02eb206899f | UNKNOWN | # Introduction
Digital Cypher assigns to each letter of the alphabet unique number. For example:
```
a b c d e f g h i j k l m
1 2 3 4 5 6 7 8 9 10 11 12 13
n o p q r s t u v w x y z
14 15 16 17 18 19 20 21 22 23 24 25 26
```
Instead of letters in encrypted word we write the corresponding number, eg. The word scout:
```
s c o u t
19 3 15 21 20
```
Then we add to each obtained digit consecutive digits from the key. For example. In case of key equal to `1939` :
```
s c o u t
19 3 15 21 20
+ 1 9 3 9 1
---------------
20 12 18 30 21
m a s t e r p i e c e
13 1 19 20 5 18 16 9 5 3 5
+ 1 9 3 9 1 9 3 9 1 9 3
--------------------------------
14 10 22 29 6 27 19 18 6 12 8
```
# Task
Write a function that accepts `str` string and `key` number and returns an array of integers representing encoded `str`.
# Input / Output
The `str` input string consists of lowercase characters only.
The `key` input number is a positive integer.
# Example
```
Encode("scout",1939); ==> [ 20, 12, 18, 30, 21]
Encode("masterpiece",1939); ==> [ 14, 10, 22, 29, 6, 27, 19, 18, 6, 12, 8]
```
# Digital cypher series
- [Digital cypher vol 1](https://www.codewars.com/kata/592e830e043b99888600002d)
- [Digital cypher vol 2](https://www.codewars.com/kata/592edfda5be407b9640000b2)
- [Digital cypher vol 3 - missing key](https://www.codewars.com/kata/5930d8a4b8c2d9e11500002a) | ["from itertools import cycle\n\ndef encode(message, key):\n return [ord(a) - 96 + int(b) for a,b in zip(message,cycle(str(key)))]", "def encode(message, key):\n # initialize variables\n output = []\n i = 0\n \n # convert key to a list of integers\n key = [int(d) for d in str(key)]\n \n # encode characters in 'message'\n for char in message:\n n = ord(char) + key[i]\n output.append(n-96)\n i = (i + 1) % len(key)\n \n # return the results\n return output\n", "def encode(message, key):\n return [ ord(char) - 96 + int(str(key)[i % len(str(key))]) for i, char in enumerate(message) ]", "import itertools\nimport string\n\ndef encode(message, key):\n return [string.ascii_lowercase.index(c) + k + 1 for c, k in zip(message, itertools.cycle(int(c) for c in str(key)))]", "from itertools import cycle\n\n\ndef encode(message, key):\n return [ord(ch) - 96 + int(code) for\n ch, code in zip(message, cycle(str(key)))]", "encode=lambda s,n:[ord(c)+int(d)-96for c,d in zip(s,str(n)*len(s))]", "from itertools import cycle\n\nabc = 'abcdefghijklmnopqrstuvwxyz'\n\ndef encode(message, key):\n key = cycle(int(x) for x in str(key))\n return [abc.index(m)+1+k for m, k in zip(message, key)]", "def encode(message, key):\n a=[]\n key=str(key)\n for i in range(len(message)):\n print(i)\n a.append(ord(message[i])-96 + int(key[i%len(key)]))\n return a", "def encode(message, key):\n abc_dict = {\n 'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6,\n 'g': 7, 'h': 8, 'i': 9, 'j': 10, 'k': 11, 'l': 12, 'm': 13,\n 'n': 14, 'o': 15, 'p': 16, 'q': 17, 'r': 18, 's': 19, 't': 20,\n 'u': 21, 'v': 22, 'w': 23, 'x': 24, 'y': 25, 'z': 26\n }\n num_list = []\n key_list = str(key)\n x = 0\n for i in message:\n num_list.append(abc_dict[i] + int(key_list[x]))\n x += 1\n if x >= len(key_list):\n x = 0\n \n return num_list", "from itertools import cycle\nfrom typing import List\n\n\ndef encode(message: str, key: int) -> List[int]:\n return [ord(m) - 96 + k for m, k in zip(message, cycle(list(map(int, str(key)))))]\n", "def encode(message, key):\n base_dict = {}\n alpha = \"abcdefghijklmnopqrstuvwxyz\"\n for i in range(26):\n base_dict[alpha[i]] = i + 1\n\n key_extended = []\n count = 0\n for i in range(len(message)):\n try :\n key_extended.append(int(str(key)[count]))\n except IndexError :\n count = 0\n key_extended.append(int(str(key)[count]))\n count += 1\n\n encrypt = []\n for letter in message:\n encrypt.append(base_dict[letter])\n\n code = []\n for i in range(len(encrypt)):\n code.append(encrypt[i] + key_extended[i])\n\n return code", "from itertools import cycle\n\ndef encode(message, key):\n key = cycle(map(int, str(key)))\n return [ord(c)-96+next(key) for c in message]", "def encode(message, key):\n return [ord(sym)-96+int(str(key)[i%len(str(key))]) for i, sym in enumerate(message)]", "encode=lambda m,k:[ord(a)-96+int(b)for a,b in zip(m,str(k)*30)]", "def encode(message, key):\n key_digs = [int(dig) for dig in str(key)]\n result = [\n ord(ch) - 96\n + key_digs[i % len(key_digs)]\n for i, ch in enumerate(message)\n ]\n \n return result", "import string as st\nletras = st.ascii_lowercase\ndef encode(message, key): \n codif_1 = [letras.index(l)+1 for l in message]\n key_list = [int(l) for l in str(key)]\n n = int(len(message)/len(key_list))\n r = len(message)%len(key_list)\n mascara = key_list * n + key_list[:r+1]\n return [codif_1[i]+mascara[i] for i in range(len(codif_1))]", "def encode(message, key):\n key=str(key)\n return [ord(message[i])-96+int(key[i%len(key)]) for i in range(0, len(message))]", "encode=lambda m,k: (lambda k: [ord(l)-96+int(k[i%len(k)]) for i,l in enumerate(m)])(str(k))", "import string\nimport numpy as np \n\nalpha = list(string.ascii_lowercase)\nvalues = list(range(1,27))\ndictionary = dict(zip(alpha, values))\n\ndef encode(word, old_key):\n key = [int(x) for x in str(old_key)]\n letter_values = []\n for letter in word:\n letter_values.append(dictionary[letter])\n count = 0\n new_list = []\n while count < len(word):\n for number in key:\n if count >= len(word):\n break\n else:\n count += 1\n new_list.append(number)\n key_np = np.array(new_list)\n letter_np = np.array(letter_values)\n final = (key_np + letter_np).tolist()\n return final", "def encode(message, key):\n alphabet_number_pairs = {\n 'a': 1,\n 'b': 2,\n 'c': 3,\n 'd': 4,\n 'e': 5,\n 'f': 6,\n 'g': 7,\n 'h': 8,\n 'i': 9,\n 'j': 10,\n 'k': 11,\n 'l': 12,\n 'm': 13,\n 'n': 14,\n 'o': 15,\n 'p': 16,\n 'q': 17,\n 'r': 18,\n 's': 19,\n 't': 20,\n 'u': 21,\n 'v': 22,\n 'w': 23,\n 'x': 24,\n 'y': 25,\n 'z': 26\n }\n number_list = []\n alphabet_list = str(key)\n a = 0\n for i in message:\n number_list.append(alphabet_number_pairs[i] + int(alphabet_list[a]))\n a += 1\n if a >= len(alphabet_list):\n a = 0\n return number_list", "def encode(message, key):\n cypher = {chr(ord('a') + i) : i+1 for i in range(26)}\n return [ cypher[letter] + int(str(key)[i % len(str(key))]) for i, letter in enumerate(message) ]\n \n", "def encode(message, key):\n return [ord(s)-96+int(str(key)[i%len(str(key))]) for i,s in enumerate(message)]", "from itertools import cycle\ndef encode(message, key):\n a = cycle(str(key))\n return [(ord(x)-96) + int(next(a)) for x in message]\n", "from decimal import Decimal\nfrom itertools import cycle\n\ndef encode(message, key):\n key_digits = cycle(Decimal(key).as_tuple().digits)\n return [ord(c.lower()) - ord('a') + next(key_digits) + 1 for c in message]", "from itertools import cycle\n\ndef encode(message, key):\n c = cycle(str(key))\n \n return [ord(i) + int(ord(next(c))) - 144 for i in message]", "def dist_from_prev_a(x):\n return ord(x)-ord('a')+1\n\ndef encode(message, key):\n x=[dist_from_prev_a(i) for i in message]\n y=list(str(key)*len(message))[:len(message)]\n return [x[i]+int(y[i]) for i in range(len(x))]\n", "def encode(message, key):\n ret = []\n d = dict()\n [d.update({chr(i):i-96}) for i in range(97,123)]\n for i in range(len(message)):\n ret.append(d[message[i]]+int(str(key)[i%len(str(key))]))\n return ret\n", "def encode(message, key):\n mess = list(map(lambda x: ord(x)-96, message))\n str_key = [int(i) for i in str(key)]\n for i in range(0, len(mess)):\n mess[i] = mess[i] + str_key[i % len(str_key)]\n \n return mess", "from itertools import cycle\nalphabet_numbers = {'a': 1, 'b': 2, 'c': 3, 'd': 4, \n 'e': 5, 'f': 6, 'g':7, 'h': 8,\n 'i': 9, 'j': 10, 'k': 11, 'l': 12,\n 'm': 13, 'n': 14, 'o': 15, 'p': 16,\n 'q': 17, 'r': 18, 's': 19, 't': 20,\n 'u': 21, 'v': 22, 'w': 23, 'x': 24,\n 'y': 25, 'z': 26\n }\ndef encode(message, key):\n key = str(key)\n key_cycle = cycle(map(int, key)) \n lst = []\n for letter in message:\n if letter in alphabet_numbers:\n lst.append(alphabet_numbers.get(letter) + next(key_cycle))\n return lst", "def encode(message, key):\n \n z = [int(i) for i in str(key)]\n x = 0\n sol = []\n \n for i in message:\n \n if x == len(z):\n x = 0\n sol.append(ord(i)-96+z[x])\n x+=1\n \n return sol", "def encode(message, key):\n \n abc = \"a b c d e f g h i j k l m n o p q r s t u v w x y z\".split(\" \")\n cypher = []\n key = str(key)\n for i, letter in enumerate(list(message)):\n letterToDigit = abc.index(letter) + 1 \n addedKeyIndex = i % len(key)\n addedKeyValue = key[addedKeyIndex]\n cypher.append(letterToDigit + int(addedKeyValue))\n return cypher\n \n \n", "def encode(message, key):\n key = list(str(key))\n alpha = list(\"0abcdefghijklmnopqrstuvwxyz\")\n message = list(message)\n code = [alpha.index((message[i])) for i in range(len(message)) if message[i] in alpha]\n i = -1\n for j in range(len(code)):\n if i == len(key)-1:\n i = -1\n i += 1\n code[j] = code[j]+int(key[i])\n\n return code\n\n", "def encode(message, key):\n # Code here\n al = \"abcdefghijklmnopqrstuvwxyz\"\n key = str(key)\n if len(message) > len(key): key = key*int(len(message)/len(key) + 1)\n out = []\n for i in list(message): out.append(al.index(i) + 1)\n for i in range(len(out)): out[i] = out[i] + int(key[i])\n return out", "def encode(message, key):\n dicti = {}\n code = []\n ans = []\n letters = list(message)\n alphabet = list('abcdefghijklmnopqrstuvwxyz')\n for i in range(len(alphabet)):\n dict_ = {alphabet[i]: i+1}\n dicti.update(dict_)\n for letter in letters:\n for k, v in dicti.items():\n if k == letter:\n code.append(v)\n key_list = list(str(key))\n a = len(message) // len(key_list)\n if len(message) > len(key_list):\n key_list = key_list * (a + 1)\n for j in range(len(code)):\n if j < len(message):\n new_code = code[j] + int(key_list[j])\n ans.append(new_code)\n elif j == len(message):\n break\n #print(ans)\n return ans", "def encode(message, key):\n key = [int(k) for k in str(key)]\n l = len(key)\n return [ord(c) - 96 + key[i % l] for i, c in enumerate(message)]", "def encode(message, key):\n alphabet = 'abcdefghijklmnopqrstuvwxyz'\n alphabet = list(alphabet)\n letters_numbers = {}\n for i in range(len(alphabet)):\n dict_ = {alphabet[i]: i+1}\n letters_numbers.update(dict_)\n message_numbers = []\n for j in message:\n number = letters_numbers[j]\n message_numbers.append(number)\n key = str(key)\n key = list(key)\n final_message_encoded = []\n for k in range(len(message)):\n key.append(key[k])\n for l in range(len(message_numbers)):\n message_encoded = message_numbers[l] + int(key[l])\n final_message_encoded.append(message_encoded)\n return final_message_encoded\n\n\n", "def encode(word, key):\n vo = {'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6, 'g': 7, 'h': 8, 'i': 9, 'j': 10,\n 'k': 11, 'l': 12, 'm': 13, 'n': 14, 'o': 15, 'p': 16, 'q': 17, 'r': 18, 's': 19, 't': 20,\n 'u': 21, 'v': 22, 'w':23, 'x': 24, 'y': 25, 'z': 26}\n kk = list(map(int, str(key)))\n kk = kk * int((len(word) / len(kk) + 1))\n k = list(word)\n arr = []\n for i in k:\n arr.append(vo.get(i))\n for j in range(len(arr)):\n arr[j] += kk[j]\n return arr", "def encode(s,k):\n eng = '_abcdefghijklmnopqrstuvwxyz'\n b = list(map(int, list(str(k))))\n q, r = divmod(len(s), len(str(k)))\n return list(map(lambda x, y: x+y, b*q+b[:r], list([eng.index(_) for _ in s])))\n", "def encode(message, key):\n key=str(key)\n return [ord(message[i])-96+int(key[i%len(key)]) for i in range(len(message))]", "def encode(message, key):\n k = [int(x) for x in str(key)]\n l = len(k)\n\n return [n+k[i%l] for i,n in enumerate(ord(c)-96 for c in message)]", "def encode(m, key):\n k = list(map(int, list(str(key))))\n kL = len(k)\n m = list(m)\n for i in range(len(m)):\n m[i] = ord(m[i]) - 96 + k[i % kL]\n return m", "def encode(message, key):\n letters = {chr(97+x):x+1 for x in range(28)}\n return [letters[l]+int(add) for l,add in zip(message, list(str(key)) * len(message) )]", "from itertools import cycle\ndef encode(message, key):\n letters = []\n fullkey = [int(x) for x in str(key)]\n for character in message:\n number = ord(character) - 96\n letters.append(number)\n outcome = [x+y for x, y in zip(letters, cycle(fullkey),)]\n return outcome", "def encode(message, key):\n import string\n \n alphabet=['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']\n # \u00e9tape 1 : conversion du message en nombres\n code=[]\n for i in range(len(message)):\n code += [alphabet.index(message[i])+1]\n # \u00e9tape 2: on ajoute la clef\n keystr=str(key)\n for i in range(len(code)):\n code[i] += int(keystr[i % len(keystr)])\n \n return code \n \n \n", "def encode(m,k):\n d = {'a': 1, 'b': 2, 'c': 3, 'd': 4, 'e': 5, 'f': 6, 'g': 7, 'h': 8, 'i': 9, 'j': 10, 'k': 11, 'l': 12, 'm': 13, 'n': 14, 'o': 15, 'p': 16, 'q': 17, 'r': 18, 's': 19, 't': 20, 'u': 21, 'v': 22, 'w': 23, 'x': 24, 'y': 25, 'z': 26}\n \n count = 0\n l = []\n p = []\n n = []\n st = str(k)\n lst = list(st)\n for x in range(len(m)):\n num = d.get(m[x])\n l.append(num)\n \n while count < len(l):\n \n for y in range(len(lst)):\n if count < len(l):\n p.append(int(lst[y]))\n count = count + 1\n else:\n break\n \n for z in range(len(l)):\n calc = l[z] + p[z]\n n.append(calc)\n \n return n\n \n\n", "encode = lambda m, k :[ord(d)-ord('a')+1 + int(str(k)[i%len(str(k))]) for i, d in enumerate(m)]", "from collections import deque\n\ndef encode(message, key):\n out = []\n key = deque(map(int, str(key)))\n for c in message:\n out.append(ord(c) - 96 + key[0])\n key.rotate(-1)\n return out", "def encode(message: str, key):\n return [ord(x) - 96 + int((str(key)[i % len(str(key))])) for i, x in enumerate(message.lower())]", "import string\n\nmap = {ch: i + 1 for i, ch in enumerate(string.ascii_lowercase)}\n\ndef encode(message, key): \n key = str(key) \n\n res = []\n for i, ch in enumerate(message):\n res.append(map[ch] + int(key[i % len(key)]))\n \n return res\n", "def encode(message, key):\n num = []\n key = str(key)\n length = len(key)\n for i in range (0,len(message)):\n num.append(((ord(message[i]) - 96) + (ord(key[i%length]) - 48)))\n print (num)\n return num", "def encode(message, key):\n message = message.lower()\n key = list(str(key))\n message = list(message)\n for i in range(len(message)):\n message[i] = (ord(message[i]) - 96) + (int(key[i % len(key)]))\n return message", "def encode(message, key):\n \n indexKey = 0 \n l = [ord(m)- 96 for m in message]\n\n for i in range(len(message)): \n l[i] += int ( str(key)[indexKey] )\n indexKey = ( indexKey + 1) % len(str(key))\n \n return l\n \n", "def encode(message, key):\n return [i + int(l) for i, l in zip([' abcdefghijklmnopqrstuvwxyz'.index(i) for i in message], str(key) * (len(message) // len(str(key))) + str(key)[:len(message) % len(str(key))])]", "def encode(message, key):\n x = [ord(i) - 96 for i in message]\n x = [x[i] + int(str(key)[i % len(str(key))]) for i in range(len(x))]\n return x", "def encode(message, key):\n arr = []\n for i in range(len(message)):\n encr = ord(message[i]) - 96\n if i > len(str(key)) - 1:\n k = i - len(str(key))*(i // len(str(key)))\n else:\n k = i \n arr.append(encr + int(str(key)[k]))\n\n return arr", "def encode(msg, k):\n return [ord(m)-96+int(str(k)[i%len(str(k))]) for i,m in enumerate(msg)]", "def encode(message, key):\n# alphabet = {\n# 'a' : 1, 'b' : 2, 'c' : 3, 'd' : 4, 'e' : 5, 'f' : 6, 'g' : 7, 'h' : 8, 'i' : 9,\n# 'j' : 10, 'k' : 11, 'l' : 12, 'm' : 13, 'n' : 14, 'o' : 15, 'p' : 16, 'q' : 17,\n# 'r' : 18, 's' : 19, 't' : 20, 'u' : 21, 'v' : 22, 'w' : 23, 'x' : 24, 'y' : 25,\n# 'z' : 26\n# }\n key_tracker = 0\n ciphertext = []\n key = [int(k) for k in str(key)]\n letters = [ord(a)-96 for a in message]\n for i in range(len(letters)):\n letters[i] += key[key_tracker]\n key_tracker += 1\n if key_tracker == len(key):\n key_tracker = 0\n return letters", "from string import ascii_lowercase as alpha\ndef encode(message, key):\n first = [alpha.index(x)+1 for x in message]\n n,k = len(message),str(key)\n return [first[i]+int(k[i%len(k)]) for i in range(n)]", "from itertools import cycle\n\ndef encode(message, key):\n key = list(map(int, str(key)))\n return [ord(c) - 96 + k for c, k in zip(message, cycle(key))]", "def encode(message, key):\n key = list(map(int, str(key)))\n return [ord(c) - 96 + key[i % len(key)] for i, c in enumerate(message)]", "def encode(message, key):\n message = [ord(x) - 96 for x in message]\n key = str(key)\n\n key_list = list(key)\n x = list(key)\n while len(key_list) != len(message):\n if not x:\n x = list(key)\n key_list.append(x.pop(0))\n\n return [message[i] + int(x) for i, x in enumerate(key_list)]", "from itertools import chain, repeat\n\nalpha = 'abcdefghijklmnopqrstuvwxyz'\n\ndef encode(message, key):\n message_l = [(alpha.index(c)+1) for c in message]\n key_l = [i for i in chain.from_iterable(repeat([int(num) for num in str(key)], 100))]\n return [(message_l[i] + key_l[i]) for i in range(len(message_l))]\n \n", "def encode(message, key):\n return [ord(j)-96+int((str(key)*(len(message)//len(str(key))+1))[i]) for i, j in enumerate(message)]", "def encode(message, key):\n ans = [] ;key = list(str(key)); counter = 0\n for i in message:\n if counter == len(key):\n counter = 0\n ans.append(ord(i)-96 + int(key[counter]))\n counter +=1\n return ans\n", "def encode(message, key):\n return [ord(message[a]) - 96 + (int(str(key)[a%len(str(key))])) for a in range(len(message))]", "def encode(message, key):\n key = str(key)\n output = []\n for i in range(0, len(message)):\n output.append( ord(message[i]) - 96 + int(key[i%len(key)]) )\n return output", "def encode(message, key):\n #horrible, but today felt like a one-liner kind of day\n return [ord(c) - 96 + int(str(key)[i%len(str(key))]) for i, c in enumerate(message)]\n", "def encode(message, key):\n msgnumber = [ord(m) - 96 for m in message]\n key = (str(key) * int((len(message) / len(str(key)))+1))[:len(message)]\n key = [int(k) for k in key]\n return [m + k for m, k in zip(msgnumber, key)]", "def encode(message, key):\n alpha = {'a':1,'b':2,'c':3,'d':4,'e':5,'f':6,'g':7,'h':8,'i':9,'j':10,'k':11,'l':12,'m':13,'n':14,'o':15,'p':16,'q':17,'r':18,'s':19,'t':20,'u':21,'v':22,'w':23,'x':24,'y':25,'z':26}\n count = 0\n value = 0\n res = []\n n = len(message)\n key_index = list(i for i in str(key))\n m_index = list(j for j in message)\n m = len(str(key))-1\n while count < n:\n res.append(alpha[m_index[count]] + int((key_index[value])))\n if value == m:\n value = 0\n else:\n value += 1\n count += 1\n print(res)\n return res", "def encode(message, key):\n return [ord(message[x])-96+int(str(key)[x%len(str(key))]) for x in range(0,len(message))]", "def encode(message, key):\n alphakey = {\n 'a': 1,'b': 2,'c': 3,'d': 4,'e': 5,\n 'f': 6,'g': 7,'h': 8, 'i': 9,'j': 10,\n 'k': 11,'l': 12,'m': 13,'n': 14,'o': 15,\n 'p': 16,'q': 17,'r': 18,'s': 19,'t': 20,\n 'u': 21,'v': 22,'w': 23,'x': 24,'y': 25,'z': 26\n }\n itakey = str(key)\n n = 0\n ans = []\n while n < len(message):\n ans.append(int(itakey[n%len(itakey)]) + alphakey[message[n]])\n n += 1\n return ans\n \n \n", "def encode(message, key):\n pre_output = []\n for letter in message:\n pre_output.append(ord(letter)-96)\n output = []\n key = str(key)\n i = 0\n for number in pre_output:\n output.append(number+int(key[i]))\n if i == len(key) - 1:\n i = 0\n else:\n i += 1\n return output", "def encode(m, k):\n k = map(int, (str(k)*(len(m)//len(str(k))+1))[:len(m)])\n return list(map(sum,zip([ord(x)-96 for x in m],k)))", "def encode(message, key):\n key = list(str(key) * (len(message)//len(str(key))+1)) if len(str(key)) < len(message) else list(str(key))\n encode_list = []\n key_index = 0\n for i in list(message):\n encode_list.append(ord(i) - 96 + int(key[key_index]))\n key_index += 1\n return encode_list", "from itertools import cycle\n\ndef encode(message, key):\n \n a = []\n\n for m,c in zip(message,cycle(str(key))):\n\n d = ord(m)-96 + int(c)\n\n a.append(d)\n \n return a\n", "def encode(message, key):\n import string\n alphabet = enumerate(string.ascii_lowercase, 1)\n\n dic_letters = {k: v for v, k in alphabet}\n\n numbers = [dic_letters[letter] for letter in message]\n\n lst_key = len(message)*[int(x) for x in str(key)]\n\n lst_zip = list(zip(numbers, lst_key))\n\n return list(sum(group) for group in lst_zip)", "def encode(message, key):\n k = [int(x) for x in str(key)]\n return [ord(message[x])-96+k[x%len(k)] for x in range(len(message))]", "def encode(message, key):\n a = []\n j = 0\n for i in message:\n a.append(ord(i)-96 + int(str(key)[j]))\n j += 1\n if j == len(str(key)):\n j = 0\n return a", "def encode(message, key):\n key_list = list(str(key))\n encoded_list = []\n k = 0\n for letter in message:\n \n letter_digit = ord(letter) - 96\n \n letter_digit_encoded = letter_digit + int(key_list[k%len(key_list)])\n \n encoded_list.append(letter_digit_encoded)\n \n k += 1\n \n return encoded_list\n \n", "def encode(message, key):\n res = []\n db = {chr(i): i-96 for i in range(97, 123)}\n zippand1 = [db[i] for i in message]\n zippand2 = list(map(int, str(key))) * len(str(message))\n return [i+j for i,j in zip(zippand1, zippand2[:len(message)])]", "def encode(message, key):\n arr = []\n for i in range(len(message)):\n arr.append(ord(message[i]) - 96 + int(str(key)[i % len(str(key))]))\n return arr\n \n", "def encode(message, key):\n coded_message = [ord(char) - 96 for char in message]\n return [digit + int(str(key)[index % len(str(key))]) for index, digit in enumerate(coded_message)]", "def encode(message, key):\n val = [ord(i) - 96 for i in message]\n check = str(key)\n index = 0\n for idx, i in enumerate(val):\n if idx % len(check) == 0:\n index = 0\n val[idx] += int(check[index])\n index += 1\n\n return val", "def encode(message, key):\n L1=\"abcdefghijklmnopqrstuvwxyz\";L2=[];number=0;L3=[]\n for i in message:\n L2.append(L1.index(i)+1+int(str(key)[number%len(str(key))]))\n number+=1\n return L2 \n \n \n", "from itertools import cycle\ndef encode(message, key):\n return [ord(c)- 96 + int(k) for c,k in zip(message, cycle(str(key)))]", "def encode(message, key):\n strKey = str(key)\n return [ord(letter) - 96 + int(strKey[index % len(strKey)]) for index, letter in enumerate(message)]\n \n \n \n", "import string \nfrom itertools import cycle \n\ndef encode(message, key):\n alphabet = dict(zip(string.ascii_lowercase, (ord(i) - 96 for i in string.ascii_lowercase)))\n key = cycle([int(i) for i in str(key)])\n res = []\n for i in message:\n encoding = int(alphabet.get(i)) + next(key)\n res.append(encoding)\n return res", "def encode(m, key):\n array_key = []\n list_int_str_key = [int(x) for x in str(key)]\n len_str_key = len(list_int_str_key)\n ords_list = [ord(x)-96 for x in m]\n len_ords_list = len(ords_list)\n i = 0\n while len(array_key) < len_ords_list:\n array_key.append(list_int_str_key[i])\n i += 1\n if i >= len_str_key: i = 0\n\n return [a+b for a,b in zip(array_key, ords_list)]", "from string import ascii_lowercase as abc\nfrom itertools import cycle\n\ndef encode(message, key):\n return [abc.index(m)+k+1 for m, k in zip(message, cycle(map(int, str(key))))]", "from string import ascii_lowercase as abc\nfrom itertools import cycle\n\n\ndef encode(message, key):\n return [int(x) + abc.index(ch)+1 for x, ch in list(zip(cycle(str(key)), message))]", "from string import ascii_lowercase as abc\nfrom itertools import cycle\n\n\ndef encode(message, key):\n ch_idx = [abc.index(ch)+1 for ch in message]\n return [int(x) + y for x, y in list(zip(cycle(str(key)), ch_idx))]", "from itertools import cycle \ndef encode(message, key):\n # Code here\n return [a + b for a, b in zip(cycle([int(i) for i in str(key)]), [ord(i)-96 for i in message])]", "def encode(message, key):\n cypher = {'a':1, 'b':2, 'c':3, 'd':4, 'e':5, 'f':6, 'g':7, 'h':8, 'i':9, 'j':10, 'k':11, 'l':12,\n 'm':13, 'n':14, 'o':15, 'p':16, 'q':17, 'r':18, 's':19, 't':20, 'u':21, 'v':22, 'w':23, 'x':24,\n 'y':25, 'z':26}\n output = []\n for i in range(len(message)):\n code = cypher[message[i]]\n i = i % len(str(key))\n code += int(str(key)[i])\n output.append(code)\n return output", "def encode(message, key):\n key = str(key) * len(message)\n return ['abcdefghijklmnopqrstuvwxyz'.index(m) + int(key[i]) + 1 for i ,m in enumerate(message)]\n \n", "from itertools import cycle\ndef encode(message, key):\n return [v+int(v1) for v,v1 in zip([ord(c) - 96 for c in message], cycle(str(key)))]", "def encode(message, key):\n return [ord(c)-ord('`')+int(str(key)[i % len(str(key))]) for i,c in enumerate(message)]", "def encode(message, key):\n fuck = [(ord(x) - 96) for x in message]\n cunt = [int(x) for x in str(key)]\n for index, cum in enumerate(fuck):\n fuck[index] += cunt[index % len(str(key))]\n return fuck", "from string import ascii_lowercase as alpha\ndef encode(message, key):\n key = (len(message) // len(str(key)) + 1) * str(key) \n return [alpha.index(c) + int(k) + 1 for c, k in zip(message, key)]", "from string import ascii_lowercase as letters\n\ndef encode(message, key):\n return [letters.index(m) + int(k) + 1 for m, k in zip(message, f'{key}' * len(message))]"] | {"fn_name": "encode", "inputs": [["scout", 1939], ["masterpiece", 1939]], "outputs": [[[20, 12, 18, 30, 21]], [[14, 10, 22, 29, 6, 27, 19, 18, 6, 12, 8]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 26,856 |
def encode(message, key):
|
b8e4edcd7b18b7ff7648c472717d81a0 | UNKNOWN | ##Task:
You have to write a function **pattern** which creates the following pattern upto n number of rows. *If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.*
##Pattern:
(n)
(n)(n-1)
(n)(n-1)(n-2)
................
.................
(n)(n-1)(n-2)....4
(n)(n-1)(n-2)....43
(n)(n-1)(n-2)....432
(n)(n-1)(n-2)....4321
##Examples:
pattern(4):
4
43
432
4321
pattern(6):
6
65
654
6543
65432
654321
```Note: There are no blank spaces```
```Hint: Use \n in string to jump to next line``` | ["def pattern(n):\n return '\\n'.join(''.join(str(i) for i in range(n, j, -1)) for j in range(n - 1, -1, -1))", "def pattern(n):\n return \"\\n\".join(\"\".join(str(j) for j in range(n, n-i, -1)) for i in range(1, n+1))", "def pattern(n):\n full = [str(x) for x in range(n, 0, -1)]\n take = lambda x: ''.join(full[:x])\n return '\\n'.join((take(x) for x in range(1, n + 1)))", "pattern = lambda n: '\\n'.join(''.join(str(n - j) for j in range(i + 1)) for i in range(n))", "def pattern(n):\n a = [str(i) for i in range(n, 0, -1)]\n return '\\n'.join(''.join(a[:i]) for i in range(1, n + 1))\n", "def pattern(n):\n return \"\\n\".join([\"\".join([str(y) for y in range(n, n - x - 1, -1)]) for x in range(n)]);", "def pattern(n):\n out,s=[],''\n for i in reversed(range(1,n+1)):\n s+=str(i)\n out.append(s)\n return '\\n'.join(out)", "def pattern(n):\n return '\\n'.join(''.join(str(j) for j in range(n, i, -1)) for i in range(n-1, -1, -1))", "def pattern(n):\n return '\\n'.join([''.join(map(str,list(range(n,n-i,-1)))) for i in range(1,n+1)])"] | {"fn_name": "pattern", "inputs": [[1], [2], [5], [0], [-25]], "outputs": [["1"], ["2\n21"], ["5\n54\n543\n5432\n54321"], [""], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,094 |
def pattern(n):
|
ee32eef70f76177240980d5dac7ccd84 | UNKNOWN | You have stumbled across the divine pleasure that is owning a dog and a garden. Now time to pick up all the cr@p! :D
Given a 2D array to represent your garden, you must find and collect all of the dog cr@p - represented by '@'.
You will also be given the number of bags you have access to (bags), and the capactity of a bag (cap). If there are no bags then you can't pick anything up, so you can ignore cap.
You need to find out if you have enough capacity to collect all the cr@p and make your garden clean again.
If you do, return 'Clean', else return 'Cr@p'.
Watch out though - if your dog is out there ('D'), he gets very touchy about being watched. If he is there you need to return 'Dog!!'.
For example:
x=
[[\_,\_,\_,\_,\_,\_]
[\_,\_,\_,\_,@,\_]
[@,\_,\_,\_,\_,\_]]
bags = 2, cap = 2
return --> 'Clean' | ["def crap(garden, bags, cap):\n cap *= bags\n for turf in garden:\n if 'D' in turf: return 'Dog!!'\n cap -= turf.count('@')\n return 'Cr@p' if cap < 0 else 'Clean'", "from collections import Counter\nfrom itertools import chain\n\ndef crap(garden, bags, cap):\n c = Counter(chain(*garden))\n return 'Dog!!' if c['D'] else ('Clean','Cr@p')[c['@'] > bags*cap]", "def crap(garden, bags, cap):\n for each in garden:\n for i in each:\n if i == 'D':\n return 'Dog!!'\n a = 0\n for each in garden:\n for i in each:\n if i == '@':\n a +=1\n b = bags * cap\n if a > b:\n return 'Cr@p'\n else:\n return \"Clean\"\n", "def crap(garden, bags, cap):\n # Flatten list including only cr@p and dogs\n flat = [e for line in garden for e in line if e in 'D@']\n return 'Dog!!' if 'D' in flat else 'Clean' if len(flat) <= bags * cap else 'Cr@p'", "def crap(g, b, c): \n g = sum(g,[])\n if 'D' in g: return \"Dog!!\" \n return \"Clean\" if g.count('@') <= b*c else \"Cr@p\"", "def crap(garden, bags, cap):\n count_crap = 0\n for x in garden:\n for y in x:\n if y == 'D':\n return 'Dog!!'\n if y == '@':\n count_crap += 1\n if bags * cap >= count_crap:\n return 'Clean'\n else:\n return 'Cr@p'", "def crap(garden, bags, cap):\n s = \"\".join([\"\".join(i) for i in garden])\n return \"Dog!!\" if \"D\" in s else \"Clean\" if s.count(\"@\") <= bags*cap else \"Cr@p\"", "crap=lambda g,b,c,l=[]:l.clear()or[l.extend(e)for e in g][0]or['Clean','Cr@p','Dog!!'][('D' in l)*2or l.count('@')>b*c]", "from itertools import chain\ndef crap(garden, bags, cap): \n return (lambda x: 'Dog!!' if x.count('D') else 'Clean' if x.count('@') <= bags*cap else 'Cr@p') ( list(chain(*garden)) )\n", "def crap(garden, bags, cap):\n for each in garden:\n for i in each:\n if i == 'D':\n return 'Dog!!'\n count = 0\n for each in garden:\n for i in each:\n if i == '@':\n count +=1\n count_caps = bags * cap\n if count > count_caps:\n return 'Cr@p'\n else:\n return \"Clean\" "] | {"fn_name": "crap", "inputs": [[[["_", "_", "_", "_"], ["_", "_", "_", "@"], ["_", "_", "@", "_"]], 2, 2], [[["_", "_", "_", "_"], ["_", "_", "_", "@"], ["_", "_", "@", "_"]], 1, 1], [[["_", "_"], ["_", "@"], ["D", "_"]], 2, 2], [[["_", "_", "_", "_"], ["_", "_", "_", "_"], ["_", "_", "_", "_"]], 2, 2], [[["@", "@"], ["@", "@"], ["@", "@"]], 3, 2]], "outputs": [["Clean"], ["Cr@p"], ["Dog!!"], ["Clean"], ["Clean"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,282 |
def crap(garden, bags, cap):
|
6f8e2782031d80a1b2a47a2d2285fcc7 | UNKNOWN | Your dad doesn't really *get* punctuation, and he's always putting extra commas in when he posts. You can tolerate the run-on sentences, as he does actually talk like that, but those extra commas have to go!
Write a function called ```dadFilter``` that takes a string as argument and returns a string with the extraneous commas removed. The returned string should not end with a comma or any trailing whitespace. | ["from re import compile, sub\n\nREGEX = compile(r',+')\n\n\ndef dad_filter(strng):\n return sub(REGEX, ',', strng).rstrip(' ,')\n", "import re\ndef dad_filter(string):\n return re.sub(r',+',r',',string).rstrip(', ')", "import re\ndef dad_filter(string):\n return re.sub(r'((?<=\\,)\\,+|[\\s,]+\\Z)','',string)", "import re\n\n\ndef dad_filter(string):\n return re.sub(r',* *$', '', re.sub(r',{2,}', ',', string))", "def dad_filter(string):\n return \",\".join([w for w in string.rstrip(\", \").split(\",\") if w != \"\"])", "def dad_filter(string):\n return ','.join([el for el in string.split(',') if el]).rstrip(\", \")", "import re\ndad_filter = lambda s: re.sub(r',+',',',s).strip(', ')", "import re\n\ndef dad_filter(s):\n return re.sub(',+', ',', s.rstrip(', '))", "import re\n\ndef dad_filter(string):\n string = re.sub(r',+', ',', string.strip())\n if string[-1] == ',':\n return string[:-1]\n else:\n return string", "def dad_filter(string):\n #your code here\n new_list = string.split()\n for i in range(len(new_list)):\n while new_list[i].count(',') > 1:\n new_list[i] = new_list[i].replace(',', '', 1)\n \n str = ' '.join(new_list) \n while str[-1] in ' ,':\n str = str[:-1]\n return str"] | {"fn_name": "dad_filter", "inputs": [["all this,,,, used to be trees,,,,,,"], ["Listen,,,, kid,,,,,,"], ["Luke,,,,,,,,, I am your father,,,,,,,,, "], ["i,, don't believe this round earth,,, show me evadence!!"], ["Dead or alive,,,, you're coming with me,,, "]], "outputs": [["all this, used to be trees"], ["Listen, kid"], ["Luke, I am your father"], ["i, don't believe this round earth, show me evadence!!"], ["Dead or alive, you're coming with me"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,298 |
def dad_filter(string):
|
722f9701e0afdb109194b4dcc3749607 | UNKNOWN | Write a program to determine if two numbers are coprime. A pair of numbers are coprime if their greatest shared factor is 1. For example:
```
20 and 27
Factors of 20: 1, 2, 4, 5, 10, 20
Factors of 27: 1, 3, 9, 27
Greatest shared factor: 1
20 and 27 are coprime```
An example of two numbers that are not coprime:
```
12 and 39
Factors of 12: 1, 2, 3, 4, 6, 12
Factors of 39: 1, 3, 13, 39
Greatest shared factor: 3
12 and 39 are not coprime```
If the two inputs are coprime, your program should return true. If they are not coprime, your program should return false.
The inputs will always be two positive integers between 2 and 99. | ["from fractions import gcd\n\ndef are_coprime(n, m):\n return gcd(n, m) == 1", "def are_coprime(n, m):\n # All hail Euclid and his wonderful algorithm\n while m > 0:\n n, m = m, n % m\n return n == 1", "from math import gcd\n\ndef are_coprime(n , m):\n return gcd(n, m) == 1", "from fractions import gcd\n\ndef are_coprime(a, b):\n return gcd(a, b) == 1", "def are_coprime(n, m):\n factors = lambda n: [i for i in range(1, n + 1) if n % i == 0]\n return sorted(set(factors(n)) & set(factors(m)))[-1] == 1\n", "def are_coprime(n,m):\n num_of_n = [i for i in range(1, n+1) if n % i == 0]\n for i in range(1, m+1):\n if m % i == 0:\n if i in num_of_n[1:]:\n return False\n return True", "are_coprime=lambda*a:__import__('fractions').gcd(*a)<2", "def are_coprime(n,m):\n return prime_factors(n) & prime_factors(m) == {1}\n \ndef prime_factors(n): \n factors = [1]\n d = 2\n while n > 1:\n while n % d == 0:\n factors.append(d)\n n /= d\n d = d + 1\n if d*d > n:\n if n > 1: factors.append(n)\n break\n return set(factors) ", "from fractions import gcd\ndef are_coprime(n,m):\n return True if gcd(n,m)==1 else False", "def coprime(n,m):\n while n*m:\n if n>m: n %= m\n else: m %= n\n return max(n,m)\n\ndef are_coprime(n,m):\n return (coprime(n,m) == 1)"] | {"fn_name": "are_coprime", "inputs": [[20, 27], [12, 39], [17, 34], [34, 17], [35, 10], [64, 27]], "outputs": [[true], [false], [false], [false], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,437 |
def are_coprime(n, m):
|
2581dca87722e831e9acd282114903cb | UNKNOWN | Jack really likes his number five: the trick here is that you have to multiply each number by 5 raised to the number of digits of each numbers, so, for example:
```python
multiply(3)==15
multiply(10)==250
multiply(200)==25000
multiply(0)==0
multiply(-3)==-15
``` | ["def multiply(n):\n return n*5**len(str(abs(n))) ", "def multiply(n):\n return 5 ** len(str(abs(n))) * n", "multiply=lambda n: n*5**len(str(abs(n)))", "def multiply(n):\n return n * 5 ** number_of_digits(n)\n\n\ndef number_of_digits(n: int):\n return 1 if -10 < n < 10 else 1 + number_of_digits(n // 10)", "def multiply(n):\n return n * 5 ** int_len(n)\n\ndef int_len(n):\n n = abs(n)\n length = 0\n while n:\n length += 1\n n //= 10\n return length", "from math import log10\ndef multiply(n):\n return n*5**int(log10(abs(n))+1) if n!=0 else 0\n", "import math\n\ndef multiply(n):\n dig_count = int(math.log10(abs(n)) + 1) if n else 1\n return n * 5**dig_count", "def multiply(n: int) -> int:\n \"\"\" Multiply each number by 5 raised to the number of digits of each numbers. \"\"\"\n return n * pow(5, len(str(abs(n))))", "def multiply(n):\n nDigits=len(str(abs(n)))\n print(nDigits)\n return n*(5**nDigits)", "def multiply(n):\n n = str(n)\n if int(n) < 0:\n return int(n) * 5 ** (len(n) - 1)\n else:\n return int(n) * 5 ** (len(n))", "def multiply(n):\n for x in range(len(str(n))):\n n *= 5\n return n if n > 0 else n/5\n", "def multiply(n):\n return n*5**(len(str(n)) - (1 if n < 0 else 0))", "def multiply(n):\n #your code here\n return n * 5 ** len([i for i in str(n) if i.isdigit()])", "def multiply(n):\n return n * 5**(len(str(n).replace('-','')))", "def multiply(n):\n s = str(n).lstrip('-')\n return n * 5 ** len(s)", "def multiply(n):\n s=str(n)\n factor=len(s)\n \n if n>0:\n return n*5**factor\n else:\n return n*5**(factor-1)", "def multiply(n):\n i = 10\n j = 1\n while True:\n if abs(n) < i:\n return n*5**j\n else:\n i *= 10\n j += 1", "def multiply(n):\n return n*5**(len(str(n)) if n>0 else len(str(n))-1)", "import math\ndef multiply(n):\n if n > 0:\n digits = int(math.log10(n))+1\n elif n == 0:\n digits = 1\n else:\n digits = int(math.log10(-n))+2\n \n if n > 0:\n return 5**digits*n\n else:\n return 5**(digits-1)*n", "def multiply(n):\n for x in range(1, len(str(abs(n)))+1):\n n*=5\n return n", "def multiply(n):\n x = len(str(n).lstrip('-').replace('.',''))\n return n * (5**x)\n", "def multiply(n):\n return n*pow(5, len(str(n))-1) if n < 0 else n*pow(5, len(str(n)))", "def multiply(n):\n if n >= 0:\n a = str(n)\n return n * 5**len(a)\n elif n < 0:\n a = str(n)\n return n * 5**(len(a)-1)\n", "def multiply(n):\n m = 5**(len(str(n))) if n>=0 else 5**(len(str(-n)))\n return n*m", "def multiply(n):\n n_multiply = len(str(n).strip(\"-\"))\n for i in range(n_multiply):\n n = n * 5\n return n", "def multiply(n):\n print(len(str(n)))\n return n*5**len(str(abs(n)))", "def multiply(n):\n return n * 5**(len(str(n if n > 0 else n * -(1))))\n", "def multiply(n):\n return n * 5 ** len(str(n)) if str(n)[0] != \"-\" else n * 5 ** (len(str(n)) - 1)", "def multiply(n):\n res = 5 ** len(str(abs(n))) * abs(n)\n \n return res if n > 0 else -res\n", "def multiply(n):\n m=len(str(abs(n)))\n return 5**m*n", "def multiply(n):\n m = len(str(n)) if n > 0 else len(str(n))-1 \n return n * 5 ** m", "def multiply(n):\n if '-' in str(n):\n i = len(str(n)) - 1\n else:\n i = len(str(n))\n return n * (5 ** i)", "def multiply(n):\n l = len(str(n))\n if n == 0:\n return 0\n if n > 0:\n return n*(5**l)\n if n < 0:\n return n*(5**(l-1))", "def multiply(n):\n if n == 0:\n return 0\n elif n > 0:\n exp = len(str(n))\n return n * (5 ** exp)\n else:\n exp = len(str(-n))\n return n * (5 ** exp)", "def multiply(n):\n if n < 0:\n x = str(n).replace('-','')\n y = len(x)\n return n * (5**y)\n else:\n return n * (5**len(str(n)))", "multiply = lambda N,X=5:N*X**(len(str(N)[1 if N<0 else 0:]))", "import math\n\ndef multiply(n):\n dig_count = math.floor(math.log10(abs(n))+1) if n else 1\n return n * 5**dig_count", "def multiply(n):\n r=len(str(abs(n)))\n return n*5**r", "def multiply(n):\n nd= len(str(n))\n for i in range(nd):\n n *= 5\n return n if n > 0 else n // 5", "def multiply(n):\n return n * 5 ** len(str(n)) if n > 0 else n * 5 ** (len(str(n * -1)))", "def multiply(n):\n return 5 ** len(str(n)[1:]) * n if str(n).startswith('-') else 5 ** len(str(n)) * n", "def multiply(n):\n account = 0\n for value in str(abs(n)):\n account += 1\n return n*(5**account)\n", "def multiply(n):\n if n<0:\n n = n*(-1)\n return -(5**len(str(n))*n)\n return (5**len(str(n))*n)", "import math\n\ndef multiply(n):\n return n * 5**(int(math.log10(abs(n)))+1) if n else 0", "def multiply(n):\n print(n)\n return (5**len(str(n)))*n if n>0 else (5**(len(str(n))-1))*n", "def multiply(n):\n l = len(str(n)) if n >= 0 else len(str(n)) - 1\n return n * (5 ** l)", "def multiply(n):\n a=len(str(n))\n if n>0:\n return n*(5**a)\n else:\n return (n*(5**(a-1)))", "def multiply(n):\n minus = False\n if n < 0:\n minus = True\n n *= -1\n n *= 5 ** len(str(n))\n if minus:\n n *= -1\n\n return n", "def multiply(n):\n count = len(str(n))\n if n >= 0:\n return n * 5 ** count\n else:\n return n * 5 ** (count -1)\n \n \n", "def multiply(n):\n return n * (5 ** len(list(str(n)))) if n > 0 else n * (5 ** len(list(str(n*-1))))", "def multiply(n):\n l = len(str(n))\n return n * (5**l) if n > 0 else n * (5 ** (l-1))", "def multiply(n):\n absolute = abs(n)\n new = str(absolute) \n length = len(new)\n integer = int(length)\n return n * 5 ** integer", "def multiply(n: int):\n return n * 5 ** len(str(abs(n)))", "def multiply(n):\n n = str(n)\n if int(n) >=0:\n l = len(n)\n else:\n l = len(n) - 1\n n = int(n)\n return n*(5**l)", "def multiply(n):\n #your code here\n if n>=0:\n \n b=5**len(str(n))\n return (n*b)\n elif n<0:\n n=abs(n)\n b=5**len(str(n))\n return -abs(n*b)", "def multiply(n):\n\n l = len(str(n))\n lm = len(str(n)) - 1\n \n if str(n).startswith('-'):\n return n * 5**lm\n else:\n return n * 5**l", "def multiply(n):\n if n > 0:\n multiplier = 5 ** len(str(n))\n operation = n * multiplier\n return operation\n else:\n convert = abs(n)\n mn = 5 ** len(str(convert))\n on = n * mn\n return on\n", "def multiply(n):\n c = 0\n if n<0:\n c = c-n\n m = str(c)\n else:\n m = str(n)\n \n return n * 5**len(m)", "def multiply(n):\n a=str(n)\n l=[]\n d=5\n for x in a:\n l.append(x)\n if(n>0): \n for x in range(0,len(l)):\n n=n*5\n if(n<0):\n for x in range(0,len(l)-1):\n n=n*5\n \n return(n)", "import math\n\ndef multiply(n):\n length = len(str(abs(n)))\n return n*5**length", "def multiply(n):\n if n<0:\n n = -n\n return -n*5**len(str(n))\n else:\n return n*5**len(str(n))", "def multiply(n):\n power = len(str(n))\n if n > 0:return n*(5**power)\n if n < 0: power = len(str(n)) - 1; return n*(5**power)\n else: return 0", "def multiply(n):\n a = []\n if n < 0:\n a2 = str(n)\n a3 = (len(a2))\n a4 = a3 - 1\n if n > 0:\n d = str(n)\n c = (len(d))\n if n == 0:\n return 0\n elif n < 0:\n return (5 ** a4) * n\n elif n > 0:\n return (5 ** c) * n\n \n", "def multiply(n):\n #your code here\n return n * 5 ** len(list(filter(str.isdigit,str(n))))", "def multiply(n):\n num=len(str(abs(n)))\n return n*5**num", "def multiply(n):\n e = len(str(abs(n)))\n return n * 5**e", "def multiply(n):\n power = len(str(n)) if n >= 0 else len(str(n)) -1\n return n * 5 ** power", "def multiply(n):\n return n*5**(len(str(n))) if \"-\" not in str(n) else n*5**(len(str(n))-1)", "def multiply(n):\n return int(n)*5**len(str(abs(n)))", "def multiply(n):\n \n lenght_of_n = len([i for i in str(n) if i.isdigit()])\n return n * 5 ** lenght_of_n ", "def multiply(n):\n return n * 5**len(str(abs(n))) if n > 0 else n * 5**len(str(abs(n)))", "def multiply(n):\n #your code here\n num = len(str(n))\n if n < 0:\n num -=1\n return n * 5 ** num", "def multiply(n):\n if n >= 0:\n return n * (5 ** len(str(n)))\n else:\n return n * (5 ** len(str(n).replace('-','')))", "import math\ndef multiply(n):\n sum=n*5\n digits = len(str(n))\n if n<0:\n digits=digits-1\n for x in range(digits-1):\n sum=(sum*5)\n return sum", "def multiply(n):\n p = len(str(abs(n)))\n ans = n*(5**p)\n return ans", "def multiply(n):\n if n >= 0:\n return n * (5 ** len(str(n)))\n if n < 0:\n return n * (5 ** (len(str(n))-1))\n# Flez\n", "def multiply(n):\n x = len(str(n).strip('-'))\n \n return n*5**x", "def multiply(n):\n power = len(str(n))\n \n if n < 0:\n power -= 1\n \n return n * 5 ** power", "def multiply(n):\n c = 0\n for i in str(n):\n for j in i:\n if j.isdigit():\n c += 1\n five = 1\n while c != 0:\n c -= 1\n five *= 5\n return five * n\n \n", "def multiply(n):\n \n copy = str(n)\n length = len(copy)\n if n < 0:\n length = length - 1\n return n * (5**length)", "def multiply(n):\n \n if n>=0:\n a=len(str(n))\n return n*(5**a)\n elif n<0:\n a = len(str(n))-1\n return n*(5**a)\n", "def multiply(n):\n t,flag = (n,False) if n>0 else (abs(n),True)\n res = n*(5**len(str(t)))\n return res", "import math\n\ndef multiply(n):\n if n == 0:\n digits = 1\n else:\n digits = int(math.log10(abs(n)))+1\n return n * 5 ** digits", "def multiply(n):\n #your code here\n if n < 0:\n n = -n\n s = len(str(n))\n return 5 ** s * n * -1\n else:\n s = len(str(n))\n return 5 ** s * n\n", "def multiply(n):\n count = 0\n if n < 0:\n num = -n\n else:\n num = n\n while num > 0:\n num = num // 10\n count += 1\n return (n*(5**count))", "def multiply(n):\n if n < 0:\n return n * (5 ** len(str(n*-1)))\n \n return n * (5 ** len(str(n)))\n #your code here\n", "def multiply(n):\n digits = len(str(abs(n)))\n return n*5**digits", "def multiply(n):\n #your code here\n digits = len(list(str(n).lstrip('-')))\n return n * 5**digits", "def multiply(n):\n a = len(str(n))\n if n >= 0:\n return n * 5 ** a\n return n * 5 ** (a -1)", "def multiply(n):\n return n * 5 ** (lambda n: len(str(n)) if n >= 0 else len(str(n))-1)(n)", "def multiply(n):\n if n>0: return n*(5**len(str(n)))\n else: return n*(5**(len(str(n))-1))", "def get_digit_count(n):\n digits = 0\n while 0 < n:\n n //= 10\n digits += 1\n return digits\n\ndef multiply(n):\n return n * (5**get_digit_count(abs(n)))", "def multiply(n):\n l = str(n)\n a = \"\"\n for c in l:\n if c in \"0123456789\":\n a += c\n \n return n * 5 ** len(a)", "def multiply(n):\n s=len(str(abs(n)))\n p=5**s\n return n*p", "def multiply(n):\n k = len(str(abs(n)))\n return n * 5 ** k", "from math import fabs\ndef multiply(n):\n return n*(5**len(str(int(fabs(n)))))", "def multiply(n):\n check = str(n)\n check = check.replace(\"-\",\"\")\n num = len(check)\n return 5 ** num * n", "def multiply(n):\n l = len(str(n).replace(\"-\",\"\"))\n return n*5**l"] | {"fn_name": "multiply", "inputs": [[10], [5], [200], [0], [-2]], "outputs": [[250], [25], [25000], [0], [-10]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,989 |
def multiply(n):
|
c382a4931d51b5a2b4c441cf36dc855f | UNKNOWN | ## Task
Given an array of strings, reverse them and their order in such way that their length stays the same as the length of the original inputs.
### Example:
```
Input: {"I", "like", "big", "butts", "and", "I", "cannot", "lie!"}
Output: {"!", "eilt", "onn", "acIdn", "ast", "t", "ubgibe", "kilI"}
```
Good luck! | ["def reverse(a):\n s=reversed(''.join(a))\n return [''.join(next(s) for _ in w) for w in a]", "def reverse(a):\n s = ''.join(a)[::-1]\n l, x = [], 0\n for i in a:\n l.append(s[x:x+len(i)])\n x += len(i)\n return l", "def reverse(a):\n s=list(''.join(a))\n return [''.join(s.pop() for _ in w) for w in a]", "from itertools import islice\n\ndef reverse(a):\n cs = (c for s in reversed(a) for c in reversed(s))\n return [''.join(islice(cs, 0, len(s))) for s in a]", "def reverse(a):\n s = iter(''.join(a)[::-1])\n return [''.join(next(s) for _ in range(len(w))) for w in a]", "def reverse(a):\n r = list(\"\".join(a))\n return [\"\".join(r.pop() for c in w) for w in a]", "def reverse(a):\n b = ''.join(a)[::-1]\n l = []\n for i in a:\n l.append(b[:len(i)])\n b = b[len(i):]\n return l", "def reverse(a):\n letters = ''.join(a)[::-1]\n result, chunk_size = [], 0\n for word in a:\n result.append(letters[chunk_size:chunk_size + len(word)])\n chunk_size += len(word)\n return result", "def reverse(a):\n reversed_str = \"\".join(a)[::-1]\n final_arr = []\n start = 0\n finish = len(a[0])\n for i in range(1,len(a)+1):\n final_arr.append(reversed_str[start:finish])\n start = finish\n if len(a) > i:\n finish = start + len(a[i])\n\n return final_arr", "from itertools import islice\n\ndef reverse(a):\n it = iter(''.join(x[::-1] for x in reversed(a)))\n return [''.join(islice(it, len(x))) for x in a]"] | {"fn_name": "reverse", "inputs": [[["I", "like", "big", "butts", "and", "I", "cannot", "lie!"]], [["?kn", "ipnr", "utotst", "ra", "tsn", "iksr", "uo", "yer", "ofebta", "eote", "vahu", "oyodpm", "ir", "hsyn", "amwoH"]]], "outputs": [[["!", "eilt", "onn", "acIdn", "ast", "t", "ubgibe", "kilI"]], [["How", "many", "shrimp", "do", "you", "have", "to", "eat", "before", "your", "skin", "starts", "to", "turn", "pink?"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,533 |
def reverse(a):
|
4b4c11f673131f4b3536d60e393e53fc | UNKNOWN | I'm afraid you're in a rather unfortunate situation. You've injured your leg, and are unable to walk, and a number of zombies are shuffling towards you, intent on eating your brains. Luckily, you're a crack shot, and have your trusty rifle to hand.
The zombies start at `range` metres, and move at 0.5 metres per second. Each second, you first shoot one zombie, and then the remaining zombies shamble forwards another 0.5 metres.
If any zombies manage to get to 0 metres, you get eaten. If you run out of ammo before shooting all the zombies, you'll also get eaten. To keep things simple, we can ignore any time spent reloading.
Write a function that accepts the total number of zombies, a range in metres, and the number of bullets you have.
If you shoot all the zombies, return "You shot all X zombies."
If you get eaten before killing all the zombies, and before running out of ammo, return "You shot X zombies before being eaten: overwhelmed."
If you run out of ammo before shooting all the zombies, return "You shot X zombies before being eaten: ran out of ammo."
(If you run out of ammo at the same time as the remaining zombies reach you, return "You shot X zombies before being eaten: overwhelmed.".)
Good luck! (I think you're going to need it.) | ["def zombie_shootout(zombies, distance, ammo, shot=0):\n \n if not zombies:\n return f'You shot all {shot} zombies.'\n \n if distance <= 0:\n return f'You shot {shot} zombies before being eaten: overwhelmed.'\n \n if not ammo:\n return f'You shot {shot} zombies before being eaten: ran out of ammo.'\n \n return zombie_shootout(zombies - 1, distance - 0.5, ammo - 1, shot + 1)", "def zombie_shootout(z, r, a):\n s = min(r*2, a)\n return f\"You shot all {z} zombies.\" if s>=z else f\"You shot {s} zombies before being eaten: { 'overwhelmed' if s==2*r else 'ran out of ammo' }.\"", "def zombie_shootout(zombies, distance, ammo):\n if distance * 2 < min(ammo+1, zombies):\n return f\"You shot {distance*2} zombies before being eaten: overwhelmed.\"\n if ammo < zombies:\n return f\"You shot {ammo} zombies before being eaten: ran out of ammo.\"\n return f\"You shot all {zombies} zombies.\"", "def zombie_shootout(zombies, range, ammo):\n distance = range*2\n if zombies > ammo < distance: \n return f\"You shot {ammo} zombies before being eaten: ran out of ammo.\"\n elif zombies > distance: \n return f\"You shot {distance} zombies before being eaten: overwhelmed.\"\n return f\"You shot all {zombies} zombies.\" ", "def zombie_shootout(zombies, distance, ammo):\n sa = ammo - zombies\n sd = distance*2 - zombies\n if sa<0 or sd<0:\n if sd > sa:\n return f'You shot {ammo} zombies before being eaten: ran out of ammo.'\n else:\n return f'You shot {distance*2} zombies before being eaten: overwhelmed.'\n return f'You shot all {zombies} zombies.'", "def zombie_shootout(zombies, distance, ammo):\n results = {f\"You shot all {zombies} zombies.\": zombies,\n f\"You shot {distance * 2} zombies before being eaten: overwhelmed.\": distance * 2,\n f\"You shot {ammo} zombies before being eaten: ran out of ammo.\": ammo}\n \n return min(results, key=results.get)", "def zombie_shootout(zombies, distance, ammo):\n kill = distance * 2\n if kill > ammo < zombies: return f\"You shot {ammo} zombies before being eaten: ran out of ammo.\"\n if kill < zombies: return f\"You shot {kill} zombies before being eaten: overwhelmed.\"\n return f\"You shot all {zombies} zombies.\"", "def zombie_shootout(z, r, a):\n n = min(z, r * 2, a)\n return f\"You shot all {n} zombies.\" if n == z else f\"You shot {n} zombies before being eaten: {'ran out of ammo' if n == a and n != r * 2 else 'overwhelmed'}.\"", "def zombie_shootout(zombies, distance, ammo):\n shot=0\n while distance > 0 and ammo > 0 and zombies > 0 :\n zombies-=1\n ammo-=1\n distance-= 0.5\n shot += 1\n \n if zombies ==0 :\n return 'You shot all ' + str(shot) + ' zombies.'\n elif distance ==0 :\n return 'You shot ' + str(shot) + ' zombies before being eaten: overwhelmed.'\n else :\n return 'You shot ' + str(shot) + ' zombies before being eaten: ran out of ammo.'\n", "def zombie_shootout(zombies, distance, ammo):\n # your code goes here\n if ammo >= zombies and (2 * distance) >= zombies:\n return (\"You shot all {} zombies.\".format(zombies))\n elif ammo < zombies and ammo < (2 * distance):\n return (\"You shot {} zombies before being eaten: ran out of ammo.\".format(ammo)) \n else:\n return (\"You shot {} zombies before being eaten: overwhelmed.\".format(2 * distance)) "] | {"fn_name": "zombie_shootout", "inputs": [[3, 10, 10], [100, 8, 200], [50, 10, 8], [10, 10, 10], [17, 8, 200], [9, 10, 8], [20, 10, 20], [100, 49, 200], [50, 10, 19], [50, 10, 49], [100, 10, 20], [19, 10, 20], [20, 10, 100], [20, 10, 19], [19, 15, 19], [19, 3, 19]], "outputs": [["You shot all 3 zombies."], ["You shot 16 zombies before being eaten: overwhelmed."], ["You shot 8 zombies before being eaten: ran out of ammo."], ["You shot all 10 zombies."], ["You shot 16 zombies before being eaten: overwhelmed."], ["You shot 8 zombies before being eaten: ran out of ammo."], ["You shot all 20 zombies."], ["You shot 98 zombies before being eaten: overwhelmed."], ["You shot 19 zombies before being eaten: ran out of ammo."], ["You shot 20 zombies before being eaten: overwhelmed."], ["You shot 20 zombies before being eaten: overwhelmed."], ["You shot all 19 zombies."], ["You shot all 20 zombies."], ["You shot 19 zombies before being eaten: ran out of ammo."], ["You shot all 19 zombies."], ["You shot 6 zombies before being eaten: overwhelmed."]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,552 |
def zombie_shootout(zombies, distance, ammo):
|
19e016a7cd9d795fb1e593cd30707216 | UNKNOWN | # Introduction
There is a war and nobody knows - the alphabet war!
There are two groups of hostile letters. The tension between left side letters and right side letters was too high and the war began.
# Task
Write a function that accepts `fight` string consists of only small letters and return who wins the fight. When the left side wins return `Left side wins!`, when the right side wins return `Right side wins!`, in other case return `Let's fight again!`.
The left side letters and their power:
```
w - 4
p - 3
b - 2
s - 1
```
The right side letters and their power:
```
m - 4
q - 3
d - 2
z - 1
```
The other letters don't have power and are only victims.
# Example
# Alphabet war Collection
Alphavet war
Alphabet war - airstrike - letters massacre
Alphabet wars - reinforces massacre
Alphabet wars - nuclear strike
Alphabet war - Wo lo loooooo priests join the war | ["def alphabet_war(fight):\n d = {'w':4,'p':3,'b':2,'s':1,\n 'm':-4,'q':-3,'d':-2,'z':-1}\n r = sum(d[c] for c in fight if c in d)\n \n return {r==0:\"Let's fight again!\",\n r>0:\"Left side wins!\",\n r<0:\"Right side wins!\"\n }[True]", "def alphabet_war(fight):\n result = sum(\"zdqm\".find(c) - \"sbpw\".find(c) for c in fight)\n return \"{} side wins!\".format(\"Left\" if result < 0 else \"Right\") if result else \"Let's fight again!\"", "def alphabet_war(fight):\n #your code here\n sum = 0\n for i in fight:\n if i == \"w\":\n sum = sum + 4\n elif i == \"p\":\n sum = sum + 3\n elif i == \"b\":\n sum = sum + 2\n elif i == \"s\":\n sum = sum + 1\n elif i == \"m\":\n sum = sum - 4\n elif i == \"q\":\n sum = sum - 3\n elif i == \"d\":\n sum = sum - 2\n elif i == \"z\":\n sum = sum - 1\n \n \n if sum > 0:\n return \"Left side wins!\"\n elif sum == 0:\n return \"Let's fight again!\"\n else:\n return \"Right side wins!\"", "POWER = {\n # left side\n 'w': -4, 'p': -3, 'b': -2, 's': -1,\n # right side\n 'm': 4, 'q': 3, 'd': 2, 'z': 1 }\n\ndef alphabet_war(fight):\n result = sum( POWER.get(c, 0) for c in fight )\n return \"Let's fight again!\" if not result else \\\n [\"Left\", \"Right\"][result > 0] + \" side wins!\"", "POWERS = {c:i for i,c in enumerate('wpbs zdqm',-4)}\n\ndef alphabet_war(fight):\n stroke = sum(POWERS[c] for c in fight if c != ' ' and c in POWERS)\n return ['Left side wins!', \"Let's fight again!\", 'Right side wins!'][ (stroke >= 0) + (stroke > 0) ]", "alphabet_war=lambda f: (lambda r: \"Let's fight again!\" if not r else \"Left side wins!\" if r>0 else \"Right side wins!\")(sum(\"mqdz sbpw\".index(l)-4 if l in \"mqdzsbpw\" else 0 for l in f))", "alphabet_war=lambda s,f=(lambda x,y:sum(y.find(e)+1 for e in x)):(lambda a,b:\"Let's fight again!\" if a==b else '{} side wins!'.format(['Left','Right'][b>a]))(f(s,'sbpw'),f(s,'zdqm'))", "def alphabet_war(fight):\n a, b = 'sbpw', 'zdqm'\n l, r = sum([a.find(x)+1 for x in fight]), sum([b.find(y)+1 for y in fight])\n return \"Right side wins!\" if l<r else \"Left side wins!\" if r<l else \"Let's fight again!\" ", "from collections import defaultdict\ndef alphabet_war(fight):\n d = defaultdict(int)\n d['w'], d['p'], d['b'], d['s'], d['m'], d['q'], d['d'], d['z'] = 4, 3, 2, 1, -4, -3, -2, -1\n a = sum(d[x] for x in fight)\n return 'Left side wins!' if a>0 else \"Let's fight again!\" if a==0 else 'Right side wins!'", "def alphabet_war(fight):\n sides, winer = { 'w':-4,'p':-3,'b':-2,'s':-1 , 'm':4, 'q':3, 'd':2, 'z':1 }, 0\n for e in fight: winer += sides.get( e, 0 )\n return [\"Let's fight again!\",'Right side wins!','Left side wins!'][(winer>=0) - (winer<=0)]\n", "def alphabet_war(fight):\n left = {'w':4,'p':3,'b':2,'s':1}\n rigth = {'m':4,'q':3,'d':2,'z':1}\n leftp = 0\n rigthp = 0\n for e in fight:\n leftp += left.get(e,0)\n rigthp += rigth.get(e,0)\n if leftp > rigthp:\n return'Left side wins!'\n elif rigthp > leftp:\n return 'Right side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n import re \n countleft = len(re.findall('w', fight))*4 + len(re.findall('p', fight))*3 + len(\n re.findall('b', fight))*2 + len(re.findall('s', fight))*1\n countright = len(re.findall('m', fight))*4 + len(re.findall('q', fight))*3 + len(\n re.findall('d', fight))*2 + len(re.findall('z', fight))*1\n if countleft == countright:\n return \"Let's fight again!\"\n elif countright > countleft:\n return \"Right side wins!\"\n elif countleft > countright:\n return \"Left side wins!\"", "def alphabet_war(fight):\n \n left_side = {\n 'w' : 4,\n 'p' : 3,\n 'b' : 2,\n 's' : 1,\n }\n \n right_side = {\n 'm' : 4,\n 'q' : 3,\n 'd' : 2,\n 'z' : 1,\n }\n \n contador_izq = 0\n contador_dch = 0\n for i in fight:\n if i in left_side.keys():\n contador_izq += left_side[i]\n if i in right_side.keys():\n contador_dch += right_side[i]\n \n if contador_izq > contador_dch:\n return \"Left side wins!\"\n elif contador_izq < contador_dch:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n a=list()\n b=list()\n left= dict(list(zip('wpbs',[4,3,2,1])))\n right = dict(list(zip('mqdz',[4,3,2,1])))\n for i in fight:\n if i==\"w\" or i==\"p\" or i==\"b\" or i==\"s\":\n a.append(left[i])\n elif i == \"m\" or i == \"q\" or i == \"d\" or i == \"z\":\n b.append(right[i])\n if sum(b)>sum(a):\n return \"Right side wins!\"\n elif sum(a)>sum(b):\n return \"Left side wins!\"\n else:\n return \"Let's fight again!\"\n \n", "def alphabet_war(fight):\n left_letters = {\"w\": 4, \"p\": 3, \"b\": 2, \"s\":1}\n right_letters = {\"m\":4, \"q\":3, \"d\":2, \"z\":1}\n\n count_left = []\n count_right = []\n\n fight = list(map(str, fight))\n\n for e in fight:\n for key, value in list(left_letters.items()):\n if e == key:\n count_left.append(value)\n \n for e in fight:\n for key, value in list(right_letters.items()):\n if e == key:\n count_right.append(value)\n\n total_left = sum(count_left)\n total_right = sum(count_right)\n\n if total_left > total_right:\n return \"Left side wins!\"\n elif total_left < total_right:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"\n", "def alphabet_war(fight):\n left_points = sum([{\"w\":4,\"p\":3,\"b\":2,\"s\":1}[l] for l in fight if l in {\"w\":4,\"p\":3,\"b\":2,\"s\":1}])\n right_points = sum([{\"m\":4,\"q\":3,\"d\":2,\"z\":1}[l] for l in fight if l in {\"m\":4,\"q\":3,\"d\":2,\"z\":1}])\n if left_points > right_points:\n return \"Left side wins!\"\n elif left_points < right_points:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_side = {\"w\":4,\"p\":3,\"b\":2,\"s\":1}\n right_side = {\"m\":4,\"q\":3,\"d\":2,\"z\":1}\n left_points = sum([left_side[l] for l in fight if l in left_side])\n right_points = sum([right_side[l] for l in fight if l in right_side])\n if left_points > right_points:\n return \"Left side wins!\"\n elif left_points < right_points:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_side = {\"w\":4,\"p\":3,\"b\":2,\"s\":1}\n right_side = {\"m\":4,\"q\":3,\"d\":2,\"z\":1}\n left_points = 0\n right_points = 0\n for l in fight:\n if l in left_side:\n left_points += left_side[l]\n elif l in right_side:\n right_points += right_side[l]\n if left_points > right_points:\n return \"Left side wins!\"\n elif left_points < right_points:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {\"w\":4,\n \"p\":3,\n \"b\":2,\n \"s\":1\n }\n right = {\"m\":4,\n \"q\":3,\n \"d\":2,\n \"z\":1\n } \n sl = 0\n rl = 0\n for letter in fight:\n if letter in left.keys():\n sl += left[letter]\n elif letter in right.keys():\n rl += right[letter]\n \n if sl > rl: return \"Left side wins!\"\n elif rl > sl: return \"Right side wins!\"\n else:return \"Let's fight again!\"", "def alphabet_war(fight):\n clash = sum('sbpw'.find(e) - 'zdqm'.find(e) for e in fight)\n \n if clash > 0: \n return 'Left side wins!'\n elif clash < 0: \n return 'Right side wins!'\n else: \n return \"Let's fight again!\"", "LEFT = 'wpbs'[::-1]\nRIGHT = 'mqdz'[::-1]\n\ndef sum_points(s): \n return sum([LEFT.find(e) - RIGHT.find(e) for e in s])\n \ndef alphabet_war(fight):\n suma = sum_points(fight)\n \n if suma > 0: \n return 'Left side wins!'\n elif suma < 0: \n return 'Right side wins!'\n else: \n return \"Let's fight again!\"", "LEFT = 'wpbs'[::-1]\nRIGHT = 'mqdz'[::-1]\n\ndef points(s, dictionary): \n return sum([dictionary.find(e) + 1 for e in s]) \n \ndef alphabet_war(fight):\n left, right = points(fight, LEFT), points(fight, RIGHT)\n \n if left > right: \n return 'Left side wins!'\n elif right > left: \n return 'Right side wins!'\n else: \n return \"Let's fight again!\"", "def alphabet_war(fight):\n a = {'w':4,'p':3,'b':2,'s':1,\n 'm':-4,'q':-3,'d':-2,'z':-1}\n b = sum(a[c] for c in fight if c in a)\n \n return {b==0:\"Let's fight again!\",\n b>0:\"Left side wins!\",\n b<0:\"Right side wins!\"\n }[True]", "def alphabet_war(fight):\n leftSide={'w': 4,\n 'p': 3,\n 'b': 2,\n 's': 1}\n\n rightSide={'m': 4,\n 'q': 3,\n 'd': 2,\n 'z': 1}\n left = []\n right = []\n for letter in fight:\n left.append(leftSide.get(letter,0))\n right.append(rightSide.get(letter,0))\n\n if sum(left) == sum(right):\n return \"Let's fight again!\"\n elif sum(left) > sum(right):\n return \"Left side wins!\"\n elif sum(left) < sum(right):\n return \"Right side wins!\" ", "def alphabet_war(fight):\n points = \"mqdz*sbpw\" \n score = sum(i * fight.count(p) for i, p in enumerate(points, -4))\n if score == 0:\n return \"Let's fight again!\" \n return [\"Left\", \"Right\"][score < 0] + \" side wins!\"", "def alphabet_war(fight):\n l = {'w': 4, 'p': 3, 'b': 2, 's': 1, 'm': -4, 'q': -3 , 'd': -2, 'z': -1}\n a = 0\n for i in list(fight):\n a += 0 if i in 'acefghijklnortuvxy' else l[i]\n return \"Let's fight again!\" if a == 0 else ('Right ' if a < 0 else 'Left ') + 'side wins!'", "def alphabet_war(fight):\n ls = ['s', 'b', 'p', 'w', 'z', 'd', 'q', 'm']\n a, b = 0, 0\n for i in list(fight):\n if i in ls:\n if ls.index(i) > 3:\n a += ls.index(i)-3\n else:\n b += ls.index(i)+1\n return \"Let's fight again!\" if a == b else \"Right side wins!\" if a > b else \"Left side wins!\"", "def alphabet_war(fight):\n s = {'w':4,'p':3,'b':2,'s':1,'m':-4,'q':-3,'d':-2,'z':-1}\n x = sum(s[c] for c in fight if c in s)\n return 'Let\\'s fight again!' if x == 0 else ['Left','Right'][x < 0]+' side wins!'", "def alphabet_war(fight):\n l = 0\n r = 0\n right = {'m':4,'q':3,'d':2,'z':1}\n left = {'w':4,'p':3,'b':2,'s':1}\n for i in fight:\n if i in right:\n r += right[i]\n elif i in left:\n l += left[i]\n if l > r:\n return \"Left side wins!\"\n elif r > l:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n d = {'w' : 4, 'p' : 3, 'b' : 2, 's' : 1, 'm' : -4, 'q' : -3, 'd' : -2, 'z' : -1}\n result = sum(d[c] for c in fight if c in d)\n return 'Left side wins!' if result > 0 else ('Right side wins!' if result < 0 else 'Let\\'s fight again!')", "def alphabet_war(fight):\n #your code here\n left = {'w': 4, 'p': 3, 'b': 2, 's':1}\n right = {'m': 4, 'q': 3, 'd': 2, 'z':1}\n \n l = 0\n r = 0\n \n for letter in fight: \n if letter in left:\n l += left[letter]\n elif letter in right:\n r += right[letter]\n \n if l > r:\n return 'Left side wins!'\n elif r > l:\n return 'Right side wins!'\n else:\n return 'Let\\'s fight again!'", "def alphabet_war(fight):\n left_side = {'w':4, 'p':3, 'b':2, 's':1}\n right_side = {'m':4, 'q':3, 'd':2, 'z':1}\n total = 0\n for char in fight:\n if char in list(left_side.keys()):\n total += left_side[char]\n elif char in list(right_side.keys()):\n total -= right_side[char]\n else:\n total += 0\n\n if total > 0:\n return \"Left side wins!\"\n elif total < 0:\n return \"Right side wins!\" \n else:\n return \"Let's fight again!\" \n", "def alphabet_war(fight):\n left_side = ('s','b','p','w');\n left_score = 0;\n right_side = ('z','d','q','m');\n right_score = 0;\n for c in fight:\n if c in left_side:\n left_score += left_side.index(c)+1;\n elif c in right_side:\n right_score += right_side.index(c)+1;\n \n return \"Right side wins!\" if right_score>left_score else \"Left side wins!\" if left_score>right_score else \"Let's fight again!\"", "def alphabet_war(fight):\n left_power = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n right_power = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n \n left_sum = 0\n right_sum = 0\n \n for char in fight:\n if char in left_power:\n left_sum += left_power[char]\n elif char in right_power:\n right_sum += right_power[char]\n \n if left_sum > right_sum:\n return \"Left side wins!\"\n elif left_sum < right_sum:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n dic1_left={'w' :4,\n 'p' : 3,\n 'b': 2,\n 's' :1}\n left_score=0\n \n dic2_right={ 'm' : 4,\n 'q' :3,\n 'd' :2,\n 'z' :1}\n right_score=0\n no_score=0\n for i in fight:\n if i in dic1_left:\n left_score+=dic1_left[i]\n elif i in dic2_right:\n right_score+=dic2_right[i]\n else:\n no_score+=0\n \n if left_score>right_score:\n return 'Left side wins!'\n elif left_score==right_score:\n return \"Let's fight again!\"\n else:\n return \"Right side wins!\"\n \n", "def alphabet_war(fight):\n score = 0\n for i in fight:\n if i == 'w':\n score += 4\n elif i == 'p':\n score += 3\n elif i == 'b':\n score += 2\n elif i == 's':\n score += 1\n elif i == 'm':\n score -= 4\n elif i == 'q':\n score -= 3\n elif i == 'd':\n score -= 2\n elif i == 'z':\n score -= 1\n if score > 0:\n return \"Left side wins!\"\n elif score < 0:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {\n \"w\": 4,\n \"p\":3,\n \"b\": 2,\n \"s\":1\n }\n right = {\n \"m\":4,\n 'q':3,\n 'd':2,\n 'z':1\n }\n l = sum(left[i] for i in fight if i in left)\n r = sum(right[i] for i in fight if i in right)\n if l > r:\n return \"Left side wins!\"\n if r > l:\n return \"Right side wins!\"\n return \"Let's fight again!\"", "def alphabet_war(fight):\n L,R=0,0\n for e in fight:\n if e=='w':L+=4\n if e=='p':L+=3\n if e=='b':L+=2\n if e=='s':L+=1\n if e=='m':R+=4\n if e=='q':R+=3\n if e=='d':R+=2\n if e=='z':R+=1\n if L>R:return \"Left side wins!\"\n if R>L:return \"Right side wins!\"\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_letters = {'w':4, 'p':3, 'b':2,'s':1}\n right_letters = {'m':4, 'q':3,'d':2, 'z':1}\n sum_left = 0\n sum_right = 0\n fight = fight.lower()\n for char in fight:\n if char in left_letters:\n number1 = left_letters[char]\n sum_left += number1\n \n elif char in right_letters:\n number2 = right_letters[char]\n sum_right += number2 \n \n if sum_left > sum_right:\n return \"Left side wins!\"\n elif sum_right > sum_left: \n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n rules = {\n 'w': 4,\n 'p': 3,\n 'b': 2,\n 's': 1,\n 'm': -4,\n 'q': -3,\n 'd': -2,\n 'z': -1\n }\n fight = list([i for i in [x for x in fight] if i in 'wpbsmqdz'])\n res = sum([rules.get(x) for x in fight])\n if res == 0:\n return \"Let's fight again!\"\n return \"Right side wins!\" if res < 0 else \"Left side wins!\"\n", "def alphabet_war(fight):\n left = {'w': 4,\n 'p': 3,\n 'b': 2,\n 's': 1}\n right = {'m': 4,\n 'q': 3,\n 'd': 2,\n 'z': 1}\n total1 = 0\n total2 = 0\n for i in range(len(fight)):\n for j1 in left:\n if fight[i] == j1:\n total1 += left[j1]\n\n for m1 in right:\n if fight[i] == m1:\n total2 += right[m1]\n\n if total1 > total2:\n return \"Left side wins!\"\n elif total1 == total2:\n return \"Let's fight again!\"\n elif total1 < total2:\n return \"Right side wins!\"\n\n\n\n", "def alphabet_war(fight):\n right = 0\n left = 0\n for i in fight:\n if i == 'w':\n left += 4 \n if i == 'p':\n left += 3\n if i == 'b':\n left += 2\n if i == 's':\n left += 1\n if i == 'm':\n right += 4\n if i == 'q':\n right += 3\n if i == 'd':\n right += 2\n if i == 'z':\n right += 1\n if left > right:\n return \"Left side wins!\"\n elif right > left:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n #your code here\n left_score = 0\n right_score = 0\n for l in list(fight):\n if l == \"w\":\n left_score += 4\n elif l == \"p\":\n left_score += 3\n elif l == \"b\":\n left_score += 2\n elif l == \"s\":\n left_score += 1\n elif l == \"m\":\n right_score += 4\n elif l == \"q\":\n right_score += 3\n elif l == \"d\":\n right_score += 2\n elif l == \"z\":\n right_score += 1\n if left_score > right_score:\n return \"Left side wins!\"\n elif left_score < right_score:\n return \"Right side wins!\"\n elif right_score == left_score:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n leftDict = {\"w\": 4, \"p\": 3, \"b\": 2, \"s\": 1}\n rightDict = {\"m\": 4, \"q\": 3, \"d\": 2, \"z\": 1}\n totalSum = sum(leftDict[x] if x in leftDict else -rightDict[x] if x in rightDict else 0 for x in fight)\n return \"Let's fight again!\" if totalSum == 0 else \"Left side wins!\" if totalSum > 0 else \"Right side wins!\"\n", "def alphabet_war(fight):\n #your code here\n left=0\n right=0\n for i in fight:\n if i == \"w\":\n left+=4\n if i == \"p\":\n left+=3\n if i == \"b\":\n left+=2\n if i == \"s\":\n left+=1\n if i == \"m\":\n right+=4\n if i == \"q\":\n right+=3\n if i == \"d\":\n right+=2\n if i == \"z\":\n right+=1\n if left>right:\n return(\"Left side wins!\")\n elif right>left:\n return(\"Right side wins!\")\n else:\n return(\"Let's fight again!\")\n", "import string\ndef alphabet_war(fight):\n leftside = [x for x in fight.lower() if x in \"wpbs\"]\n rightside = [x for x in fight.lower() if x in \"mqdz\"]\n rl = sum(int(x) for x in \"\".join(leftside).translate(str.maketrans(\"wpbs\",\"4321\")))\n rr = sum(int(x) for x in \"\".join(rightside).translate(str.maketrans(\"mqdz\",\"4321\")))\n if rl == rr:\n return \"Let's fight again!\"\n elif rl > rr:\n return \"Left side wins!\"\n else:\n return \"Right side wins!\"\n", "def alphabet_war(fight):\n r, l = 0, 0\n c = 1\n for i in \"sbpw\":\n l += fight.count(i) * c\n c += 1\n c = 1 \n for i in \"zdqm\":\n r += fight.count(i)*c\n c += 1\n if l > r:\n return \"Left side wins!\"\n elif r > l:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left='0sbpw'\n right='0zdqm'\n l=sum(left.index(i) for i in fight if i in left)\n r=sum(right.index(i) for i in fight if i in right)\n if r==l:\n return \"Let's fight again!\"\n if l>r:\n return \"Left side wins!\"\n return \"Right side wins!\"", "def alphabet_war(fight):\n l_scores = {\"w\": 4, \"p\": 3, \"b\": 2, \"s\": 1}\n r_scores = {\"m\": 4, \"q\": 3, \"d\": 2, \"z\": 1}\n l = 0\n r = 0\n for ch in fight:\n l += (l_scores[ch] if ch in list(l_scores.keys()) else 0)\n r += (r_scores[ch] if ch in list(r_scores.keys()) else 0)\n return (\"Left side wins!\" if l>r \n else \"Right side wins!\" if r>l\n else \"Let's fight again!\")\n", "def alphabet_war(fight):\n left_power = 0\n right_power = 0\n fight = list(fight)\n for letter in fight:\n if letter == \"z\":\n right_power += 1\n elif letter == \"d\":\n right_power += 2\n elif letter == \"q\":\n right_power += 3\n elif letter == \"m\":\n right_power += 4\n elif letter == \"s\":\n left_power += 1\n elif letter == \"b\":\n left_power += 2\n elif letter == \"p\":\n left_power += 3\n elif letter == \"w\":\n left_power += 4\n else:\n continue\n \n if left_power > right_power:\n return \"Left side wins!\"\n elif right_power > left_power:\n return \"Right side wins!\"\n elif right_power == left_power:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_score = 0\n right_score = 0\n left_char = ['w', 'p', 'b', 's']\n right_char = ['m', 'q', 'd', 'z']\n for char in fight:\n if char in left_char:\n if char == left_char[0]:\n left_score += 4\n elif char == left_char[1]:\n left_score += 3\n elif char == left_char[2]:\n left_score += 2\n elif char == left_char[3]:\n left_score += 1\n elif char in right_char:\n if char == right_char[0]:\n right_score += 4\n elif char == right_char[1]:\n right_score += 3\n elif char == right_char[2]:\n right_score += 2\n elif char == right_char[3]:\n right_score += 1\n if left_score > right_score:\n return 'Left side wins!'\n elif left_score < right_score:\n return 'Right side wins!'\n elif left_score == right_score:\n return \"Let's fight again!\"\n", "def alphabet_war(fight):\n left = {'w':4, 'p':3, 'b':2, 's':1}\n right = {'m':4, 'q':3, 'd':2, 'z':1}\n left_score = sum([left[i] for i in fight if i in left])\n right_score = sum([right[i] for i in fight if i in right])\n if left_score > right_score:\n return \"Left side wins!\"\n elif left_score < right_score:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"\n", "d = {'w': 4, 'p': 3, 'b': 2, 's': 1, 'm': -4, 'q': -3, 'd': -2, 'z': -1}\ndef alphabet_war(fight):\n s = sum(d.get(c, 0) for c in fight)\n return 'Left side wins!' if s>0 else 'Right side wins!' if s<0 else 'Let\\'s fight again!'", "def alphabet_war(fight):\n # The left side letters and their power:\n left = {\n \"w\": 4,\n \"p\": 3,\n \"b\": 2,\n \"s\": 1,\n }\n # The right side letters and their power:\n right = {\n \"m\": 4,\n \"q\": 3,\n \"d\": 2,\n \"z\": 1,\n }\n \n left_power = 0\n right_power = 0\n for fighter in fight:\n if fighter in left:\n left_power += left.get(fighter)\n if fighter in right:\n right_power += right.get(fighter)\n\n if left_power > right_power: return \"Left side wins!\"\n if left_power < right_power: return \"Right side wins!\"\n return \"Let's fight again!\"", "def alphabet_war(fight):\n #your code here\n l_letters = {'w':4, 'p':3, 'b':2, 's':1}\n r_letters = {'m':4, 'q':3, 'd':2, 'z':1}\n\n l_sum = 0\n r_sum = 0\n\n for letter in fight:\n for key, value in l_letters.items():\n if key == letter:\n l_sum += value\n for key, value in r_letters.items():\n if key == letter:\n r_sum += value\n\n if r_sum>l_sum:\n return ('Right side wins!')\n elif r_sum<l_sum:\n return ('Left side wins!')\n else:\n return (\"Let's fight again!\")", "def alphabet_war(fight):\n left = {c: i for i, c in enumerate(\"sbpw\", 1)}\n right = {c: i for i, c in enumerate(\"zdqm\", 1)}\n battle = sum(left.get(c, 0) - right.get(c, 0) for c in fight.lower())\n if battle > 0:\n return \"Left side wins!\"\n elif battle < 0:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n s = 0\n for c in fight:\n if c in \"mqdzsbpw\":\n s += \"mqdz_sbpw\".index(c) - 4\n if s > 0:\n return \"Left side wins!\"\n elif s == 0:\n return \"Let's fight again!\"\n else:\n return \"Right side wins!\"", "def alphabet_war(fight):\n count = 0\n left_power = dict(w=4, p= 3, b=2,s= 1)\n right_power = dict(m=4, q=3, d=2, z=1)\n for l in fight: \n if l in left_power: \n count += left_power[l]\n elif l in right_power:\n count-= right_power[l]\n if count <0: \n return \"Right side wins!\"\n if count >0: \n return \"Left side wins!\"\n elif count == 0: \n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {'w':4, 'p':3, 'b':2, 's':1}\n right = {'m':4, 'q':3, 'd':2, 'z':1}\n \n L = sum([left.get(i,0) for i in fight])\n R = sum([right.get(i,0) for i in fight])\n \n if L > R:\n return 'Left side wins!'\n elif L < R:\n return 'Right side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n lef = {\"w\" : 4, \"p\" : 3, \"b\" : 2, \"s\" : 1}\n rig = {\"m\" : 4, \"q\" : 3, \"d\" : 2, \"z\" : 1}\n lp = 0\n rp = 0\n for x in fight:\n if x in lef:\n lp += lef[x]\n if x in rig:\n rp += rig[x]\n return \"Right side wins!\" if rp > lp else \"Left side wins!\"if lp > rp else \"Let's fight again!\"", "d = {'w': -4, 'p': -3, 'b': -2, 's': -1,\n 'm': 4, 'q': 3, 'd': 2, 'z': 1}\n\ndef alphabet_war(s):\n f = sum(d[l] if l in d else 0 for l in s)\n return (f>0)*'Right side wins!' + (f==0)*'Let\\'s fight again!' + (f<0)*'Left side wins!'", "d1 = {\n \"w\" : 4,\n \"p\" : 3,\n \"b\" : 2,\n \"s\" : 1 \n }\nd2 = {\n \"m\" : 4,\n \"q\" : 3,\n \"d\" : 2,\n \"z\" : 1 \n}\ndef alphabet_war(fight):\n r=0\n l=0\n for i in fight:\n if i in d1.keys(): l += d1[i]\n elif i in d2.keys(): r += d2[i] \n \n return 'Right side wins!' if r > l else 'Left side wins!' if l > r else \"Let's fight again!\"", "def alphabet_war(fight):\n powers = {\"w\": 4, \"p\": 3, \"b\": 2, \"s\": 1, \n \"m\": -4, \"q\": -3, \"d\": -2, \"z\": -1}\n sum = 0\n \n for char in fight:\n for letter in powers:\n if char == letter:\n sum += powers[letter]\n \n if sum > 0:\n return \"Left side wins!\"\n elif sum < 0:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_side = []\n right_side = []\n for elem in fight:\n if elem == \"w\":\n left_side.append(4)\n elif elem == \"p\":\n left_side.append(3)\n elif elem == \"b\":\n left_side.append(2)\n elif elem == \"s\":\n left_side.append(1)\n elif elem == \"m\":\n right_side.append(4)\n elif elem == \"q\":\n right_side.append(3)\n elif elem == \"d\":\n right_side.append(2)\n elif elem == \"z\":\n right_side.append(1)\n \n print(right_side)\n if sum(left_side) > sum(right_side):\n return \"Left side wins!\"\n elif sum(left_side) < sum(right_side):\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = 0\n right = 0\n for x in fight:\n if x in 'wpbs':\n p1 = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n left += p1.get(x)\n elif x in 'mqdz':\n p2 = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n right += p2.get(x)\n \n if left != right:\n return 'Left side wins!' if left > right else 'Right side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {'w':4, 'p':3, 'b':2, 's':1}\n right = {'m':4, 'q':3, 'd':2, 'z':1}\n left_side = 0\n right_side = 0\n for elem in fight:\n if elem in left.keys():\n left_side = left_side + left[elem]\n elif elem in right.keys():\n right_side = right_side + right[elem]\n return 'Left side wins!' if left_side >right_side else ('Right side wins!' if left_side < right_side else \"Let's fight again!\")", "def alphabet_war(fight):\n res=0\n right=' zdqm'\n left=' sbpw'\n for i in fight:\n try:\n res-=left.index(i)\n except:\n try:\n res+=right.index(i)\n except:\n pass\n if res>0 : return \"Right side wins!\"\n \n if res==0 : return \"Let's fight again!\"\n return \"Left side wins!\"", "def alphabet_war(fight):\n left_side = {'w': 4,'p': 3,'b': 2,'s': 1}\n right_side = {'m': 4,'q': 3,'d': 2,'z': 1}\n left_side_total = 0\n right_side_total = 0\n for eachletter in fight:\n if eachletter in left_side.keys():\n left_side_total = left_side_total + left_side[eachletter]\n elif eachletter in right_side.keys():\n right_side_total = right_side_total + right_side[eachletter]\n if left_side_total > right_side_total:\n return 'Left side wins!'\n elif right_side_total > left_side_total:\n return 'Right side wins!'\n else:\n return 'Let\\'s fight again!'", "def alphabet_war(fight):\n count = 0\n left = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n right = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n for elem in fight:\n if elem in left:\n count += left[elem]\n elif elem in right:\n count -= right[elem]\n\n if count>0:\n return \"Left side wins!\"\n elif count<0:\n return \"Right side wins!\"\n elif count ==0:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {\"w\":4,\"p\":3,\"b\":2,\"s\":1};right = { \"m\":4,\"q\":3,\"d\":2,\"z\":1}\n l,r =0,0\n for i in fight:\n if i in left:\n l += left[i]\n if i in right:\n r += right[i]\n if l>r:\n return 'Left side wins!'\n if r>l:\n return 'Right side wins!'\n return \"Let's fight again!\"\n", "def alphabet_war(fight):\n power = 0\n left = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n right = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n for i in fight:\n if i in left:\n power += left[i]\n elif i in right:\n power -= right[i]\n if power < 0:\n return \"Right side wins!\"\n if power > 0:\n return \"Left side wins!\"\n elif power == 0:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = {\"w\":4,\"p\":3,\"b\":2,\"s\":1}\n right = {\"m\":4,\"q\":3,\"d\":2,\"z\":1}\n left_score = sum(left[d] for d in fight if d in left)\n right_score = sum(right[d] for d in fight if d in right)\n \n if left_score > right_score:\n return \"Left side wins!\"\n elif left_score < right_score:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(t):\n a,b = 0, 0\n A,B = ['s', 'b', 'p', 'w'], ['z', 'd', 'q', 'm']\n for i in t:\n if i in A:\n a += A.index(i) + 1\n elif i in B:\n b += B.index(i) + 1\n return f\"{['Right', 'Left'][a > b]} side wins!\" if a != b else \"Let's fight again!\"\n \n", "def alphabet_war(fight):\n lres=0\n rres =0\n ldicc = {\"w\":4,\"p\":3,\"b\":2,\"s\":1}\n rdicc = {\"m\":4,\"q\":3,\"d\":2,\"z\":1}\n for elem in fight:\n if elem in ldicc:\n lres = lres + ldicc[elem]\n elif elem in rdicc:\n rres = rres + rdicc[elem]\n if rres>lres:\n return \"Right side wins!\"\n elif lres>rres:\n return \"Left side wins!\"\n else:\n return \"Let's fight again!\"\n", "def alphabet_war(fight):\n right = 0\n left = 0\n for letters in fight:\n if letters == 'w':\n left += 4\n elif letters == 'p':\n left +=3\n elif letters == 'b':\n left += 2\n elif letters == 's':\n left += 1\n elif letters == 'm':\n right += 4\n elif letters == 'q':\n right += 3\n elif letters == 'd':\n right += 2\n elif letters == 'z':\n right += 1\n else:\n pass\n if right > left:\n return \"Right side wins!\"\n elif right < left:\n return \"Left side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left = 0\n right = 0\n for i in fight:\n if i in 'w':\n left+=4\n elif i in 'p':\n left+=3\n elif i in 'b':\n left+=2\n elif i in 's':\n left+=1\n elif i in 'm':\n right+=4\n elif i in 'q':\n right+=3\n elif i in 'd':\n right+=2\n elif i in 'z':\n right+=1\n if right > left:\n return 'Right side wins!'\n if left > right:\n return 'Left side wins!'\n else:\n return 'Let\\'s fight again!'", "def alphabet_war(fight,n=0):\n alpha = {'s':1,'z':-1,'b':2,'d':-2,'p':3,'q':-3,'w':4,'m':-4}\n \n for c in fight: n += alpha.get(c,0)\n\n return (\"Left\" if n > 0 else \"Right\") + \" side wins!\" if n else \"Let's fight again!\"", "def alphabet_war(fight):\n #your code here\n left = 0\n right = 0\n for i in fight:\n if i == \"w\":\n left += 4\n elif i == \"p\":\n left += 3\n elif i == \"b\":\n left += 2\n elif i == \"s\":\n left += 1\n elif i == \"m\":\n right += 4\n elif i == \"q\":\n right += 3\n elif i == \"d\":\n right += 2\n elif i == \"z\":\n right += 1\n if right > left:\n return \"Right side wins!\"\n elif left > right:\n return \"Left side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n right = {\n 'm' : 4,\n 'q' : 3,\n 'd' : 2,\n 'z' : 1,\n }\n \n left = {\n 'w' : 4,\n 'p' : 3,\n 'b' : 2,\n 's' : 1,\n }\n \n left_count = 0\n right_count = 0\n \n right, left = right.items(),left.items()\n\n for right_letter, left_letter in zip(right, left):\n for letter in fight:\n if letter == right_letter[0]:\n right_count += right_letter[1] \n\n if letter == left_letter[0]:\n left_count += left_letter[1] \n\n if left_count > right_count: return \"Left side wins!\"\n elif right_count > left_count: return \"Right side wins!\"\n elif right_count == left_count: return \"Let's fight again!\"", "def alphabet_war(fight):\n powers = {'w': 4, 'p': 3, 'b': 2, 's': 1, 'm': -4, 'q': -3, 'd': -2, 'z': -1}\n points = sum(powers.get(f, 0) for f in fight)\n if points > 0:\n return 'Left side wins!'\n elif points == 0:\n return 'Let\\'s fight again!'\n return 'Right side wins!'", "def alphabet_war(fight):\n left_alp = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n right_alp = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n \n left_score = 0\n right_score = 0\n for i in left_alp:\n left_score += fight.count(i) * left_alp[i]\n \n for i in right_alp:\n right_score += fight.count(i) * right_alp[i]\n \n return \"Right side wins!\" if right_score > left_score else \"Let's fight again!\" if right_score == left_score else \"Left side wins!\"\n", "def alphabet_war(fight):\n winner = sum(' zdqm'.index(x) for x in fight if x in 'zdqm') - sum(' sbpw'.index(x) for x in fight if x in 'sbpw')\n return \"Left side wins!\" if winner < 0 else \"Right side wins!\" if winner > 0 else \"Let's fight again!\"", "import unittest\n\nRIGHT_SIDE_WINS = \"Right side wins!\"\nLEFT_SIDE_WINS = \"Left side wins!\"\nFIGHT_AGAIN = \"Let's fight again!\"\n\n\nLEFT_LETTERS = {\n 'w': 4,\n 'p': 3,\n 'b': 2,\n 's': 1,\n}\n\nRIGHT_LETTERS = {\n 'm': 4,\n 'q': 3,\n 'd': 2,\n 'z': 1,\n}\n\n\ndef alphabet_war(fight):\n left = []\n right = []\n for ele in fight:\n if ele in LEFT_LETTERS:\n left.append(LEFT_LETTERS[ele])\n elif ele in RIGHT_LETTERS:\n right.append(RIGHT_LETTERS[ele])\n\n if sum(left) == sum(right):\n return FIGHT_AGAIN\n else:\n return LEFT_SIDE_WINS if sum(left) > sum(right) else RIGHT_SIDE_WINS\n \n \nclass TestAlphabetWar(unittest.TestCase):\n def test_alphabet_war_on_right_side_wins(self):\n fight = 'z'\n actual = alphabet_war(fight)\n self.assertEqual(actual, RIGHT_SIDE_WINS)\n\n def test_alphabet_war_on_left_side_wins(self):\n fight = 'wq'\n actual = alphabet_war(fight)\n self.assertEqual(actual, LEFT_SIDE_WINS)\n\n def test_alphabet_war_on_fight_again(self):\n fight = 'zdqmwpbs'\n actual = alphabet_war(fight)\n self.assertEqual(actual, FIGHT_AGAIN)\n", "def alphabet_war(f):\n p = {'w':-4, 'p':-3, 'b':-2, 's':-1, 'm':4, 'q':3, 'd':2, 'z':1}\n if sum(p[x] for x in f if x in p) == 0:\n return \"Let's fight again!\" \n \n return \"Right side wins!\" if sum(p[x] for x in f if x in p) > 0 else \"Left side wins!\"\n \n \n \n \n", "def alphabet_war(fight):\n #your code here\n left={'w': -4, 'p': -3, 'b': -2, 's': -1}\n right={'m': 4, 'q': 3, 'd': 2, 'z': 1}\n left.update(right)\n points=sum([left[x] for x in fight if x in left])\n win='Left side wins!' if points<0 else \"Let's fight again!\" if points==0 else 'Right side wins!'\n return(win)", "def alphabet_war(fight):\n dl={\"w\":4,\"p\":3,\"b\":2,\"s\":1}\n dr={\"m\":4,\"q\":3,\"d\":2,\"z\":1}\n lwork=0\n rwork=0\n for x in fight:\n lwork+=dl.get(x,0)\n rwork+=dr.get(x,0)\n if lwork==rwork : return \"Let's fight again!\"\n elif lwork>rwork : return \"Left side wins!\"\n else : return \"Right side wins!\"", "db = {'w':4, 'p':3, 'b':2, 's':1, 'm':4, 'q':3, 'd':2, 'z':1}\n\ndef alphabet_war(fight):\n l = r = 0\n for i in fight:\n if i in 'wpbs':\n l += db[i]\n elif i in 'mqdz':\n r += db[i]\n return 'Left side wins!' if l > r else 'Right side wins!' if r > l else 'Let\\'s fight again!'", "def alphabet_war(fight):\n chars = list(fight)\n left = 4*chars.count('w') + 3*chars.count('p') + 2*chars.count('b') + 1*chars.count('s')\n right = 4*chars.count('m') + 3*chars.count('q') + 2*chars.count('d') + 1*chars.count('z')\n if left > right:\n return \"Left side wins!\"\n elif left < right:\n return \"Right side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n r,r_c='zdqm',0\n l,l_c='sbpw',0\n for i in fight:\n if i in r:\n r_c+=r.index(i)+1\n elif i in l:\n l_c+=l.index(i)+1\n if r_c>l_c:\n return 'Right side wins!'\n elif l_c>r_c:\n return 'Left side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n #your code here\n left = {'w':4, 'p': 3, 'b':2, 's':1}\n right = {'m': 4, 'q': 3, 'd':2, 'z':1}\n \n left_total = 0\n right_total = 0\n \n for letter in fight:\n if letter in left:\n left_total += left[letter]\n if letter in right:\n right_total += right[letter]\n \n if left_total > right_total:\n return 'Left side wins!'\n elif left_total < right_total:\n return 'Right side wins!'\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n left_scores = {'w': 4, 'p': 3, 'b': 2, 's': 1}\n right_scores = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n\n left_score = 0\n right_score = 0\n \n for char in fight:\n if char in left_scores.keys():\n left_score += left_scores[char]\n elif char in right_scores.keys():\n right_score += right_scores[char]\n\n if right_score > left_score:\n return \"Right side wins!\"\n elif left_score > right_score:\n return \"Left side wins!\"\n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n powersLeft = {'w':4,'p':3,'b':2,'s':1}\n powersRight = {'m':4,'q':3,'d':2,'z':1}\n left = 0\n right = 0\n for letter in fight:\n if letter in powersLeft:\n left += powersLeft[letter]\n elif letter in powersRight:\n right += powersRight[letter]\n if left > right:\n return 'Left side wins!'\n elif left < right:\n return 'Right side wins!'\n return 'Let\\'s fight again!'", "def alphabet_war(fight):\n left = ['w','p','b','s']\n right = ['m','q','d','z']\n \n powers = {'w':4,'p':3,'b':2,'s':1,'m':4,'q':3,'d':2,'z':1}\n \n phrase = list(fight)\n left_total = 0\n right_total = 0\n \n for letter in phrase:\n if letter in right:\n right_total = right_total + powers[letter]\n if letter in left:\n left_total = left_total + powers[letter]\n \n if left_total > right_total:\n return \"Left side wins!\"\n if right_total > left_total:\n return \"Right side wins!\"\n \n return \"Let's fight again!\"", "def alphabet_war(fight):\n if fight == '':\n return \"Let's fight again!\"\n left_side = {'s': 1,'b': 2,'p': 3,'w': 4}\n right_side = {'z': 1,'d': 2,'q': 3,'m': 4}\n left_side_count = 0\n right_side_count = 0\n for letter in fight:\n if letter in left_side:\n left_side_count += left_side[letter]\n elif letter in right_side:\n right_side_count += right_side[letter]\n if left_side_count > right_side_count:\n answer = 'Left side wins!'\n elif right_side_count > left_side_count:\n answer = 'Right side wins!'\n else:\n answer = \"Let's fight again!\"\n return answer\n", "def alphabet_war(fight):\n versus = {'w': 4, 'p': 3, 'b': 2, 's': 1, 'm': -4, 'q': -3, 'd': -2, 'z': -1}\n score = []\n for x in fight:\n for key, value in versus.items():\n if x == key:\n score.append(value)\n if sum(score) > 0:\n return 'Left side wins!'\n if sum(score) < 0:\n return 'Right side wins!'\n return \"Let's fight again!\"\n \nprint(alphabet_war('zzzzs'))", "def alphabet_war(fight):\n d = {\"w\": -4, \"p\": -3, \"b\": -2, \"s\": -1, \"m\": 4, \"q\": 3, \"d\": 2, \"z\": 1}\n \n score = sum([d.get(char, 0) for char in fight])\n \n if score == 0:\n msg = \"Let's fight again!\"\n elif score < 0:\n msg = \"Left side wins!\"\n else:\n msg = \"Right side wins!\"\n \n return msg\n", "def alphabet_war(fight):\n lefts, rights = ('wpbs', 'mqdz')\n score_right = sum([4 - rights.find(x) for x in fight if x in rights])\n score_left = sum([4 - lefts.find(x) for x in fight if x in lefts])\n return ['Let\\'s fight again!', ['Right side wins!', 'Left side wins!'][score_left > score_right]][score_right != score_left]\n", "left = {'w': 4, 'p': 3, 'b': 2, 's': 1}\nright = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n\ndef alphabet_war(fight):\n #your code here\n counter_left = 0\n counter_right = 0\n\n for letter in fight:\n if letter in left:\n counter_left += left[letter]\n elif letter in right:\n counter_right += right[letter]\n\n if counter_left > counter_right:\n return 'Left side wins!' \n elif counter_left < counter_right:\n return 'Right side wins!' \n else:\n return \"Let's fight again!\"", "def alphabet_war(fight):\n right = {'m': 4, 'q': 3, 'd': 2, 'z': 1}\n left = {'w':4, 'p': 3, 'b': 2, 's': 1}\n rarr = []\n larr = []\n for s in fight:\n if s in 'mqdz':\n rarr.append(right.get(s))\n elif s in 'wpbs':\n larr.append(left.get(s))\n return 'Left side wins!' if sum(larr) > sum(rarr) else 'Right side wins!' if sum(rarr) > sum(larr) else 'Let\\'s fight again!'\n\n"] | {"fn_name": "alphabet_war", "inputs": [["z"], ["zdqmwpbs"], ["wq"], ["zzzzs"], ["wwwwww"]], "outputs": [["Right side wins!"], ["Let's fight again!"], ["Left side wins!"], ["Right side wins!"], ["Left side wins!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 45,899 |
def alphabet_war(fight):
|
fe0e7ca1c48640ebfae5159c8b82fb9c | UNKNOWN | #### Task:
Your job here is to implement a function `factors`, which takes a number `n`, and outputs an array of arrays comprised of two
parts, `sq` and `cb`. The part `sq` will contain all the numbers that, when squared, yield a number which is a factor of `n`,
while the `cb` part will contain all the numbers that, when cubed, yield a number which is a factor of `n`. Discard all `1`s
from both arrays.
Both `sq` and `cb` should be sorted in ascending order.
#### What it looks like:
```python
factors(int) #=> [
sq (all the numbers that can be squared to give a factor of n) : list,
cb (all the numbers that can be cubed to give a factor of n) : list
]
```
#### Some examples:
Also check out my other creations — [Keep the Order](https://www.codewars.com/kata/keep-the-order), [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | ["def factors(n):\n sq = [a for a in range(2, n+1) if not n % (a**2)]\n cb = [b for b in range(2, n+1) if not n % (b**3)]\n return [sq, cb]", "def factors(n):\n sq = [i for i in range(2, int(n**0.5) + 2) if n%(i*i)==0]\n cb = [i for i in range(2, int(n**(1/3) + 2)) if n%(i*i*i)==0]\n return [sq, cb]", "from itertools import takewhile\n\nsquares = {i**2:i for i in range(2, 500)}\ncubes = {i**3:i for i in range(2, 500)}\nfind = lambda n, D: [v for k,v in takewhile(lambda x: x[0] <= n, D.items()) if not n%k]\n\ndef factors(n):\n return [find(n, squares), find(n, cubes)]", "from math import ceil\n\ndef factors(n):\n sq = [i for i in range(2, ceil(n**(1/2)) + 1) if n % i**2 == 0]\n cb = [i for i in range(2, ceil(n**(1/3)) + 1) if n % i**3 == 0]\n return [sq, cb]", "def factors(n):\n sq = lambda x: x**2\n cb = lambda x: x**3\n return [[x for x in range(2, n) if n % sq(x) == 0], [x for x in range(2, n) if n % cb(x) == 0]]", "factors=lambda n:[[d for d in range(2,int(n**(1/p)+2))if n%d**p<1]for p in(2,3)]", "def factors(n):\n return [[k for k in range(2, int(n ** (1/p)) + 1) if not n % (k ** p)] for p in (2, 3)]", "def factors(n):\n return [[d for d in range(2, int(n ** 0.5) + 1) if n % (d ** 2) == 0],\n [d for d in range(2, int(n ** (1/3)) + 1) if n % (d ** 3) == 0]]", "def factors(n):\n divisors = [i for i in range(2, n+1)if n % i == 0]\n sq = [x for x in divisors if x**2 in divisors]\n qb = [x for x in divisors if x**3 in divisors]\n return [sq, qb] or [[], []]", "from functools import reduce\n\ndef factors(n): \n a = set(reduce(list.__add__, ([i, n//i] for i in range(1, int(n**0.5) + 1) if n % i == 0)))\n return [[i for i in range(2, int(n**0.5) + 1) if i**2 in a],[i for i in range(2, int(n**0.5) + 1) if i**3 in a]]\n"] | {"fn_name": "factors", "inputs": [[1], [4], [16], [81], [80], [100], [5], [120], [18], [8]], "outputs": [[[[], []]], [[[2], []]], [[[2, 4], [2]]], [[[3, 9], [3]]], [[[2, 4], [2]]], [[[2, 5, 10], []]], [[[], []]], [[[2], [2]]], [[[3], []]], [[[2], [2]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,821 |
def factors(n):
|
b90bdd1f9b7e073e3fe811ecf8b70ce9 | UNKNOWN | # Summation
Write a program that finds the summation of every number from 1 to num. The number will always be a positive integer greater than 0.
For example:
```if-not:racket
~~~
summation(2) -> 3
1 + 2
summation(8) -> 36
1 + 2 + 3 + 4 + 5 + 6 + 7 + 8
~~~
```
```if:racket
~~~racket
(summation 2) ; 3 (+ 1 2)
(summation 8) ; 36 (+ 1 2 3 4 5 6 7 8)
~~~
``` | ["def summation(num):\n return sum(range(num + 1))\n \n", "def summation(num):\n return (1+num) * num / 2", "def summation(num):\n return sum(range(1,num+1))\n \n", "def summation(num):\n return sum(range(num + 1))\n \n", "def summation(num):\n total = 0\n for i in range(0, num+1):\n total = total + i\n return total\n \n", "def summation(num):\n return num*(num+1)//2", "def summation(num):\n return num*(num+1) / 2", "def summation(num):\n if num > 1:\n return num + summation(num - 1)\n return 1", "summation=lambda n:n*-~n>>1", "def summation(num):\n fac = 0 \n i = 0 \n while i < num:\n i += 1\n fac = fac + i\n return fac", "summation = lambda x: sum(range(1, x + 1))", "def summation(num):\n somme=0\n for i in range(num):\n somme+=i+1\n return somme\n pass # Code here", "def summation(num):\n sum = 0\n for x in range(1,num+1):\n sum = sum + x\n return sum\n \n", "def summation(num):\n return sum([x for x in range(num+1)])\n \n", "def summation(num):\n ans = 0\n for x in range(1, num):\n ans += x\n return ans + num", "\ndef summation(num):\n answer = 0\n for x in range(num):\n answer = answer + num\n num = num - 1 \n return answer\n \n", "def summation(num):\n L=[]\n for i in range(0,num+1):\n L.append(i)\n return sum(L)", "def summation(num):\n return sum([n+1 for n in range(num)])\n", "#usr/bin/python\n\ndef summation(num):\n result = 0\n for i in range(1, num + 1):\n result += i\n return result\n \n", "def summation(num):\n for i in range(0,num):\n num += i\n return num\n \n", "# https://en.wikipedia.org/wiki/Arithmetic_progression\n\ndef summation(num):\n return (num + 1)*num / 2\n \n", "def summation(num):\n if num ==1:\n return 1\n return sum([num,summation(num-1)])\n \n \n \n", "summation = lambda n: n*(n+1)/2\n", "summation = lambda num: sum([i for i in range(num)], num)#Lambda is fun", "def summation(n):\n f = 0\n for i in range(1, n+1):\n f += i\n return f\n \n", "summation = lambda s: sum(range(s+1))", "def summation(num):\n if num == 1:\n return 1\n return summation(num-1) + num", "def summation(num):\n return sum(range(1, num + 1))\n", "def summation(num):\n ret = 0\n for _ in range(0, num+1):\n ret += _\n return ret", "def summation(num):\n \n num_sum = 0\n\n for i in range(num+1):\n num_sum += i\n \n return num_sum\n", "def summation(num):\n # Code here\n a=0\n for i in range(num):\n a=a+i+1 \n return a\n \n\n", "def summation(num):\n a = 1\n i = 2\n while i <= num:\n a = i + a\n i += 1\n return a\n \n", "def summation(num):\n i = 0\n while num > 0:\n i = num + i\n num =num - 1\n return i\n", "def summation(num):\n tmp=0\n for j in range(0,num+1):\n tmp=j+tmp\n return(tmp) \n", "def summation(num):\n sum = 0\n while num > 0:\n sum += num\n num -=1\n return sum\n\n#sum(i for i in a)\n\n", "def summation(num):\n pass # Code here\n suma = 0\n for i in range(0,num+1):\n suma += i\n return suma\n \n", "def summation(num):\n x = list(range(1,num +1))\n sum = 0\n for i in x:\n sum += i\n return sum\n pass # Code here\n \n", "def summation(num):\n if num<1:\n print(\"El n\u00famero debe ser mayor a 0\")\n else:\n cont=0\n total=0\n while cont<=num:\n total=total+cont\n cont=cont+1\n \n \n return total\n \n \n \n", "# def summation(num):\n# suma = 0\n# if num == 1:\n# return 1\n# else:\n# for x in (1, num+1):\n# suma = suma + summation(x)\n# return suma\n \n \ndef summation(num):\n return sum(range(num+1))\n", "def summation(num):\n sum = 0\n x = 1\n while x <=num : \n sum = sum + x \n x = x + 1\n return sum\n \n", "def summation(num):\n return sum([i for i in range(num+1)])\n \n # Code here\n \n", "def summation(num):\n sumResult = 0\n while num > 0:\n sumResult = sumResult + num\n num-=1\n return sumResult\n", "def summation(num):\n num1 = 1\n sum = 0\n while num1 <= num:\n sum += num1\n num1 += 1\n return sum\n \n", "def summation(num):\n sum = 0\n x = list(range(num+1))\n \n for n in x:\n sum = sum +n\n return sum\n", "def summation(num):\n \n sum=0\n \n \n for i in range(1,num + 1):\n \n sum = sum + i\n \n pass\n \n \n return sum\n \n", "def summation(num):\n to_return = 0\n n = 0\n while n != num:\n n += 1\n to_return += n\n return(to_return)", "def summation(num):\n x = i = 0\n while i <= num:\n x = x + i\n i = i + 1\n return x\n \n", "def summation(num):\n nombre = num\n sum_interm = 0\n while nombre>0:\n sum_interm = sum_interm + nombre\n nombre -= 1\n return sum_interm\n \n \n \n", "def summation(num):\n if num==1:\n return 1\n else:\n return num + summation(num - 1 )# Code here\n \nprint(summation(6))", "def summation(num):\n newNum = 0\n for x in range(num + 1):\n newNum += x\n return newNum\n \n", "def summation(num):\n a = 1\n num = num + 1\n for x in range(1, num): \n if x < num: a = a + x\n return a - 1", "def summation(num):\n \n total = 1\n \n while num != 1:\n total = total + num\n num -= 1\n \n return total", "def summation(num):\n a = 1\n for i in range(1, num):\n i = i+1\n a = a + i\n return(a)\n \n", "def summation(num):\n n = list(range(num+1))\n tot = sum(n)\n return tot\n \n \n", "def summation(num):\n accum = 0\n for n in range(1, num+1):\n accum += n\n return accum\n", "def summation(n):\n return(sum(list(range(1, n+1))))\n\n#def summation(n):\n# return(sum([i for i in range(1, n+1)]))\n", "def summation(num):\n a = 0\n for i in range(num):\n a = i + a\n return a + num\n\n", "def summation(num):\n pass # Code here\n startvalue = 0\n for i in range(0, num + 1):\n startvalue += i\n outvalue = startvalue\n return outvalue\n", "def summation(num):\n return(sum((list(range(0,num+1))))) #range in python 3 is iterator, so needs turning into a list. In P2 it is already a list!\n #create range>turn into list>sum it >return it\n \n", "def summation(num):\n summation = (1+num)*num / 2\n return summation\n", "def summation(num):\n x = 0\n for i in range (0, num):\n x = x + num\n num = num - 1\n return(x)\n \n", "def summation(num):\n \n count = 0\n \n x = list(range(num))\n \n for n in x:\n if num > 0:\n count = count + num\n num = num - 1\n \n return (count)\n \n \n", "def summation(num):\n endnumber = 0\n i = 0\n while num >i:\n i +=1\n endnumber +=i\n return endnumber\n", "def summation(num):\n total = 0\n for n in range(0, num + 1, 1):\n total += n\n return total\n \n", "def summation(num):\n # Code here\n suma = 0\n for i in range(1,num+1):\n suma = suma + i\n return suma\n \n", "def summation(num):\n return sum(list(range(num + 1)), 0)\n \n", "def summation(num):\n result = 0\n for k in range(1,num+1):\n result += k\n return result\n \n", "def summation(num):\n counter = 0\n for n in range(1,num+1):\n counter += n\n return counter\n \n", "def summation(num):\n pass \n i = 0\n rslt = 0\n while i <= num:\n rslt = rslt + i\n i = i + 1\n return rslt\n \n", "def summation(num):\n sum = 0;\n count = 0\n for loop in range(num):\n sum += num - count\n count += 1\n \n return sum\n \n", "def summation(num) -> int:\n return sum(range(1,num + 1))\n \n", "def summation(num):\n # Code here\n result = 0\n for number in range(num+1):\n result += number\n return result\n \n \n", "def summation (num):\n x=num\n if num > 0:\n while x > 0:\n num+=x-1\n x-=1\n return num;\n else:\n return print (\"error\");\n\n\nsummation (0)\n\n \n", "def summation(num):\n \n summa = 0\n\n for i in range (1, num+1):\n \n summa += i\n \n return summa\n\nsummation(1)\nsummation(8)\nsummation(22)\nsummation(100)\nsummation(213)", "def summation(num):\n sum = 1\n i = 2\n while(i < num + 1):\n sum += i\n i += 1\n\n return sum\n", "def summation(num):\n\n done = sum([x for x in range(1,num + 1)])\n \n return done\n \n \n", "def summation(num):\n tot = 0\n for n in range(0,num+1):\n tot += n\n return tot", "def summation(num):\n num = sum(range(num+1))\n return num\n \n \n", "# def summation(num):\n# result = 0\n# for i in range(1, num+1):\n# result += i\n \n# return result\n\ndef summation(num):\n result = 0\n for i in range(num+1):\n print(i, num)\n result += i\n return result", "def summation(num, sums=0):\n if num == 0:\n return sums\n else:\n return summation(num-1, sums + num)", "def summation(num):\n nr_list = list(range(1, num+1))\n return sum(nr_list)\n \n \n \n \n \n \n \n \n \n \n", "summation = lambda x: sum(list(range(x+1)))", "def summation(num):\n return sum(range(num + 1))\nprint((summation(5)))\n", "def summation(num):\n\n result = 0\n count = 1\n\n while count <= num:\n result += count\n count += 1\n\n return result\n\n\ndef __starting_point():\n assert summation (4)\n \n\n__starting_point()", "def summation(num):\n return sum(range(1,num+1))\n\nprint((summation(6)))\n\n", "def summation(num):\n cnt = 0\n for x in range(num+1):\n cnt += x\n\n return cnt\n\nprint((summation(8)))\n\n \n", "def summation(num):\n return num if num == 1 else sum([i for i in range(num+1)])", "def summation(num):\n lone = 0\n for i in range(1, num+1):\n lone = lone + i\n return lone", "def summation(num):\n if isinstance(num, int) and num > 0:\n s = 0\n for i in range(num):\n s = s + i\n return s + num \n else:\n print(\"No es un n\u00famero entero positivo\")", "def summation(num):\n kata_range = []\n for i in range(1, num + 1):\n kata_range.append(i)\n return sum(kata_range)", "def summation(num):\n a = []\n for i in range(num+1):\n a.append(i)\n return sum(a)\n", "def summation(num):\n y = 1\n solution = 0\n while y <= num:\n solution += y\n y += 1\n return solution\n \n", "def summation(num):\n res = [i for i in range(num + 1)]\n return sum(res)\n \n", "def summation(num):\n x = 0\n final = 0\n while x <= num:\n final += x\n x += 1\n return final\n \n", "def summation(num):\n return num * (num * 0.5 + 0.5)\n \n", "def summation(num: int) -> int:\n i = 0\n resultado = i\n\n while i <= num:\n resultado +=i\n i += 1\n \n return resultado\n\n \n", "def summation(num): \n number = list(range(1,num+1))\n return sum(number)\n \n", "def summation(num):\n a = []\n [a.append(i) for i in range(0,num+1)]\n return sum(a)\n \n", "def summation(num):\n b = 0\n c = 0\n for i in range(num):\n c+=1\n b+=c\n return b \n \n \n \n"] | {"fn_name": "summation", "inputs": [[1], [8], [22], [100], [213]], "outputs": [[1], [36], [253], [5050], [22791]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,077 |
def summation(num):
|
cef3877b30415772807cc4058d9f0306 | UNKNOWN | Consider having a cow that gives a child every year from her fourth year of life on and all her subsequent children do the same.
After n years how many cows will you have?
Return null if n is not an integer.
Note: Assume all the cows are alive after n years. | ["def count_cows(n):\n if not isinstance(n, int):\n return None\n return 1 if n < 3 else count_cows(n-1) + count_cows(n-3)", "def count_cows(n):\n if isinstance(n, int):\n a, b, c = 1, 0, 0\n for _ in range(n):\n a, b, c = c, a, b + c\n return a + b + c", "def count_cows(n):\n if type(n) != int: return None\n a, b, c, d = 1, 0, 0, 0\n for _ in range(n):\n a, b, c, d = c + d, a, b, c + d \n return a + b + c + d", "solution = __import__(\"functools\").lru_cache(maxsize=None)(lambda n: 1 if n < 3 else solution(n-1) + solution(n-3))\n\ndef count_cows(n):\n if type(n) == int:\n return solution(n)", "def count_cows(n):\n if isinstance(n, int):\n return 1 if n < 3 else count_cows(n - 1) + count_cows(n - 3)", "from functools import lru_cache\n\n@lru_cache(maxsize=None)\ndef f(n):\n if n < 3:\n return 1\n return f(n-3) + f(n-1)\n\ndef count_cows(n):\n if not isinstance(n, int):\n return None\n return f(n)\n", "def count_cows(n):\n if not isinstance(n, int):\n return None\n # Cows at each age\n cows = [0, 0, 0, 1]\n for _ in range(n):\n cows = [cows[0] + cows[1], cows[2], cows[3], cows[0] + cows[1]]\n return sum(cows)", "from collections import deque\ndef count_cows(n):\n if not isinstance(n, int):\n return None\n calves = deque([1, 0, 0])\n cows = 0\n for i in range(n):\n cows += calves.pop()\n calves.appendleft(cows)\n return cows + sum(calves)", "def count_cows(n):\n if isinstance(n,int):\n if n<3: return 1\n return count_cows(n-1)+count_cows(n-3)", "def count_cows(n):\n if not isinstance(n, int):\n return None\n cows = [1]\n old_cows = 0\n for _ in range(n):\n cows = [old_cows] + cows\n if len(cows) >= 3:\n old_cows += cows.pop()\n return sum(cows) + old_cows\n"] | {"fn_name": "count_cows", "inputs": [[1], [3], [5]], "outputs": [[1], [2], [4]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,933 |
def count_cows(n):
|
4f54ff2d3c5722fdf4a7fe8a5e398c88 | UNKNOWN | Colour plays an important role in our lifes. Most of us like this colour better then another. User experience specialists believe that certain colours have certain psychological meanings for us.
You are given a 2D array, composed of a colour and its 'common' association in each array element. The function you will write needs to return the colour as 'key' and association as its 'value'.
For example:
```python
var array = [["white", "goodness"], ...] returns [{'white': 'goodness'}, ...]
``` | ["def colour_association(arr):\n return [{k: v} for k, v in arr]", "def colour_association(arr):\n return [{color: assoc} for color, assoc in arr]", "def colour_association(arr):\n result = []\n for association in arr:\n colour, value = association\n result.append({colour : value})\n return result", "def colour_association(arr):\n r = [{a[0]: a[1]} for a in arr] \n return r\n", "def colour_association(arr):\n #your code here\n return [{x[0]:x[1]} for x in arr]", "colour_association=lambda arr: [{k:v} for k,v in arr]", "def colour_association(arr):\n colors = [];\n for color in arr:\n colors.append({color[0]: color[1]});\n return colors;", "def colour_association(arr):\n return list({k: v} for k, v in arr)", "def colour_association(arr):\n return list([{x[0]:x[1]} for x in arr])", "def colour_association(arr):\n array = []\n for pair in arr:\n array.extend([{pair[0] : pair[1]}])\n return array", "def colour_association(arr):\n dict1 = []\n for item in arr:\n dicts = {}\n dicts.update({item[0] : item[1]}) \n dict1.append(dicts)\n \n print(dict1) \n return dict1\n \n \n #your code here\n", "colour_association = lambda lst: [{k: v} for k, v in lst]", "colour_association = lambda l: [ {k:v} for k,v in l ]", "def colour_association(lst):\n return [{k: v} for k, v in lst]", "def colour_association(arr):\n l=[]\n for i in arr:\n d={i[0]:i[1]}\n l.append(d)\n return l", "colour_association = lambda array: [{color: meaning} for color, meaning in array]", "def colour_association(arr):\n result = []\n for k,v in arr:\n result.append({k:v})\n return result", "def colour_association(arr):\n return [{elem[0]: elem[1]} for elem in arr]", "colour_association = lambda x: [{p[0]:p[1]} for p in x ]", "def colour_association(arr):\n lst = []\n for i in arr:\n lst += [{i[0]:i[1]}]\n return lst\n"] | {"fn_name": "colour_association", "inputs": [[[["white", "goodness"], ["blue", "tranquility"]]], [[["red", "energy"], ["yellow", "creativity"], ["brown", "friendly"], ["green", "growth"]]], [[["pink", "compassion"], ["purple", "ambition"]]], [[["gray", "intelligence"], ["black", "classy"]]], [[["white", "goodness"], ["blue", "goodness"]]]], "outputs": [[[{"white": "goodness"}, {"blue": "tranquility"}]], [[{"red": "energy"}, {"yellow": "creativity"}, {"brown": "friendly"}, {"green": "growth"}]], [[{"pink": "compassion"}, {"purple": "ambition"}]], [[{"gray": "intelligence"}, {"black": "classy"}]], [[{"white": "goodness"}, {"blue": "goodness"}]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,013 |
def colour_association(arr):
|
71aeac38609fc2d24bea024e10c943aa | UNKNOWN | This is a problem that involves adding numbers to items in a list.
In a list you will have to add the item's remainder when divided by a given divisor to each item.
For example if the item is 40 and the divisor is 3 you would have to add 1 since 40 minus the closest multiple of 3 which is 39 is 1. So the 40 in the list will become 41. You would have to return the modified list in this problem.
For this problem you will receive a divisor called `div` as well as simple list of whole numbers called `nums`. Good luck and happy coding.
# Examples
```python
nums = [2, 7, 5, 9, 100, 34, 32, 0], div = 3
==> [4, 8, 7, 9, 101, 35, 34, 0]
nums = [1000, 999, 998, 997], div = 5
==> [1000, 1003, 1001, 999]
nums = [], div = 2
==> []
```
**Note:** random tests check lists containing up to 10000 elements. | ["def solve(nums,div):\n return [x + x % div for x in nums]\n\n", "def solve(nums,div): return [n+n%div for n in nums]", "solve = lambda n, d: [k + k%d for k in n]\n", "def solve(numbers, divisor):\n return [num + (num % divisor) for num in numbers]\n", "solve = lambda n, d: [i + i % d for i in n]", "def solve(nums, div):\n if len(nums) == 0:\n return nums\n \n index = 0\n for num in nums:\n nums[index] += num % div\n index += 1\n\n return nums\n", "def solve(nums,div):\n for x in range(0, len(nums)):\n nums[x] += nums[x]%div\n return nums", "def solve(a, x):\n return [n + n % x for n in a]", "def solve(nums,div):\n return [(num%div)+num for num in nums]\n", "def solve(nums,div):\n if nums == []:\n return []\n output = []\n for num in nums:\n output.append((num % div) + num)\n return output\n"] | {"fn_name": "solve", "inputs": [[[2, 7, 5, 9, 100, 34, 32, 0], 3], [[], 2], [[1000, 999, 998, 997], 5], [[0, 0, 0, 0], 5], [[4, 3, 2, 1], 5], [[33, 23, 45, 78, 65], 10]], "outputs": [[[4, 8, 7, 9, 101, 35, 34, 0]], [[]], [[1000, 1003, 1001, 999]], [[0, 0, 0, 0]], [[8, 6, 4, 2]], [[36, 26, 50, 86, 70]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 892 |
def solve(nums,div):
|
fc8bee5546eb8b418e9943cf2f4abf66 | UNKNOWN | Given a lowercase string that has alphabetic characters only and no spaces, return the highest value of consonant substrings. Consonants are any letters of the alphabet except `"aeiou"`.
We shall assign the following values: `a = 1, b = 2, c = 3, .... z = 26`.
For example, for the word "zodiacs", let's cross out the vowels. We get: "z **~~o~~** d **~~ia~~** cs"
For C: do not mutate input.
More examples in test cases. Good luck!
If you like this Kata, please try:
[Word values](https://www.codewars.com/kata/598d91785d4ce3ec4f000018)
[Vowel-consonant lexicon](https://www.codewars.com/kata/59cf8bed1a68b75ffb000026) | ["import re\n\ndef solve(s):\n return max(sum(ord(c)-96 for c in subs) for subs in re.split('[aeiou]+', s))", "def solve(s):\n max_ord = 0\n curr_ord = 0\n for char in s:\n if char not in 'aeiou':\n curr_ord += ord(char)-ord('a')+1\n else:\n if curr_ord > max_ord:\n max_ord = curr_ord\n curr_ord = 0\n return max_ord\n", "def solve(s):\n count = 0\n max = 0\n vowels = [\"a\", \"e\", \"i\", \"o\", \"u\"]\n for letter in s:\n if letter in vowels:\n if count > max:\n max = count\n count = 0\n else:\n count+=int(ord(letter)) - 96\n return max", "import re\n\ndef solve(s):\n return max(sum(ord(x)-96 for x in c) for c in re.split('[aeiou]',s))", "import string\ndef solve(s):\n string_list=(list(s))\n alphabets=(list(string.ascii_lowercase))\n test=[]\n for i in range(1,len(alphabets)+1):\n test.append(i)\n my_dictionary=dict(zip(alphabets,test))\n vowels=\"aeiou\"\n vowels_list=list(vowels)\n compare_list1=[]\n for i in range(len(string_list)):\n if string_list[i] in my_dictionary and string_list[i] not in vowels:\n l=(my_dictionary[string_list[i]])\n compare_list1.append(l)\n else:\n compare_list1.append(\"vowels\")\n \n z=(compare_list1)\n x = []\n l = len(z)\n previous_element = 0\n count = 0\n if( isinstance(z[0] , int) == True ):\n count = z[0] \n elif( z[0] == 'vowels' ):\n previous_element = z[0] \n for i in range(1,l):\n if( previous_element == 'vowels' ):\n if(count > 0):\n x.append(count)\n count = 0 \n elif( isinstance( previous_element , int) == True ):\n count = count + previous_element\n previous_element = z[i] \n if( isinstance(z[l-1] , int) == True ):\n count = count + previous_element\n x.append(count) \n elif( z[l-1] == 'vowels' ):\n x.append(count) \n print(x) \n y=max(x)\n return y\n \nsolve(\"zodiacs\")", "def solve(s):\n s = s.replace('a',' ').replace('e',' ').replace('i',' ').replace('u',' ').replace('o',' ')\n return max((sum(ord(i)-96 for i in j) for j in s.split()))", "import re\n\nCONS_PAT = re.compile(r\"[^aeiuo]+\")\n\ndef solve(s):\n return max(sum(map(lambda c: ord(c)-96, conss)) for conss in CONS_PAT.findall(s))", "import re\nimport string\n\nscores = {c: i for i, c in enumerate(string.ascii_lowercase, 1)}\n\ndef solve(s):\n return max(sum(map(scores.get, x)) for x in re.findall('[^aeiou]+', s))", "def solve(s):\n # Reference: https://en.wikipedia.org/wiki/Maximum_subarray_problem#Kadane's_algorithm\n max_so_far = 0\n max_ending_here = 0\n \n for char in s:\n if isConsonant(char):\n max_ending_here += getValue(char)\n max_so_far = max(max_so_far, max_ending_here)\n else:\n max_ending_here = 0\n \n return max_so_far\n\ndef isConsonant(char):\n return char not in 'aeiou'\n\ndef getValue(char):\n return ord(char) & -ord('a')", "import re\nfrom string import ascii_lowercase as c \ndef solve(s): \n return max([sum(map(lambda x: c.index(x)+1,list(i))) for i in re.findall(r'[^aeiou]+',s)])"] | {"fn_name": "solve", "inputs": [["zodiac"], ["chruschtschov"], ["khrushchev"], ["strength"], ["catchphrase"], ["twelfthstreet"], ["mischtschenkoana"]], "outputs": [[26], [80], [38], [57], [73], [103], [80]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,423 |
def solve(s):
|
dd86fa2a26428cb43b239ab26ab84cb1 | UNKNOWN | A high school has a strange principal. On the first day, he has his students perform an odd opening day ceremony:
There are number of lockers "n" and number of students "n" in the school. The principal asks the first student to go to every locker and open it. Then he has the second student go to every second locker and close it. The third goes to every third locker and, if it is closed, he opens it, and if it is open, he closes it. The fourth student does this to every fourth locker, and so on. After the process is completed with the "n"th student, how many lockers are open?
The task is to write a function which gets any number as an input and returns the number of open lockers after last sudent complets his activity. So input of the function is just one number which shows number of lockers and number of students. For example if there are 1000 lockers and 1000 students in school then input is 1000 and function returns number of open lockers after 1000th student completes his action.
The given input is always an integer greater than or equal to zero that is why there is no need for validation. | ["# First, I coded a straightforward solution. It would work, but because it had to loop over all\n# students, and for every student over each locker, it had to do n^2 iterations. With numbers under\n# 10k this proved no problem, however the test cases apparently tested bigger numbers, since my\n# program kept getting timed out. I decided to record the number of open lockers for each n in range\n# 1-500 and discovered a nice pattern: 0 -1-1-1 (3 1's) -2-2-2-2-2 (5 2's) -3-3-3-3-3-3-3 (7 4's)\n# - et cetera. In other words, the number of consecutive numbers is (2n + 1). This was still not easy\n# to do so I looked up the sequence in OEIS. There, I discovered that the sequence was the integer\n# part of the square root of n. Now if that isn't easy to program... more of a maths problem, really.\n\ndef num_of_open_lockers(n):\n return int(n**0.5)", "from math import floor, sqrt\ndef num_of_open_lockers(n):\n return floor(sqrt(n))", "def num_of_open_lockers(n):\n return int(n ** 0.5)", "\n\"\"\"\ndef num_of_open_lockers(n):\n doors = [0 for i in range(n)]\n for d in range(n):\n for i in range(d, n, d + 1):\n doors[i] = 0 if doors[i] else 1\n return sum(doors)\n\"\"\"\n\n\n# ... a-ha, OK, gotcha:\n\n\nnum_of_open_lockers = lambda n: int(n ** .5)\n\n", "def num_of_open_lockers(n):\n return int(n ** 0.5)\n \n#def num_of_open_lockers_not_opt(n):\n# nums = []\n# for i in xrange(0, n):\n# nums.append(False)\n#\n# for i in xrange(1, n + 1):\n# for j in xrange(1, n + 1):\n# if j % i == 0:\n# nums[j - 1] = not nums[j - 1]\n#\n# return nums.count(True)\n", "from math import sqrt, floor\n\ndef num_of_open_lockers(n):\n return floor(sqrt(n))", "def num_of_open_lockers(n):\n print(\"lockers:\",n)\n\n i = 0\n count = 1\n open = 0\n even = True\n while i < n:\n if even:\n i += 1\n open += 1\n even = False\n else:\n even = True\n i += count*2\n count += 1\n \n #print \"open:\", open\n return open\n", "from math import floor\ndef num_of_open_lockers(n): return floor(n ** .5)", "import math\n\ndef num_of_open_lockers(n):\n return math.floor(math.sqrt(n))", "num_of_open_lockers=lambda n: int(n**.5)"] | {"fn_name": "num_of_open_lockers", "inputs": [[0], [1], [4], [56], [128], [500], [562], [74864], [3991700], [950811894]], "outputs": [[0], [1], [2], [7], [11], [22], [23], [273], [1997], [30835]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,324 |
def num_of_open_lockers(n):
|
a3c10909416967722d033d5179a4a8ca | UNKNOWN | Complete the function that takes two integers (`a, b`, where `a < b`) and return an array of all integers between the input parameters, **including** them.
For example:
```
a = 1
b = 4
--> [1, 2, 3, 4]
``` | ["def between(a,b):\n return list(range(a,b+1))", "def between(a,b):\n # good luck\n return list((x for x in range(a, b + 1)))\n # thanks\n", "def between(a,b):\n return [result for result in range(a,b+1)]\n", "between=lambda a,b:list(range(a,b+1))", "def between(a,b):\n arr = []\n for i in range(a, b + 1):\n arr.append(i)\n return arr", "def between(a,b):\n retList = []\n while a <= b:\n retList.append(a)\n a += 1\n return retList", "def between(a,b):\n return list(range(min(a,b), max(a,b)+1))\n", "between = lambda a, b: [n for n in range(a, b + 1)]", "def between(a,b):\n s = [a]\n while s[-1] < b:\n t=s[-1]+1\n s.append(t)\n return s\n", "from typing import List\n\ndef between(a: int, b: int) -> List[int]:\n \"\"\" Get an array of all integers between the input parameters, including them. \"\"\"\n return list(range(a, b + 1))", "def between(a,b):\n # good luck\n result = []\n total = 0\n total = b - a + 1\n for i in range(total):\n result.append(a+i)\n return result", "def between(a,b):\n sequence = []\n while a <= b:\n sequence.append(a)\n a = a + 1\n return sequence", "def between(a,b):\n result = []\n i = a\n while i<=b:\n result.append(i)\n i+=1\n return result[:]\n", "def between(a,b):\n x = b + 1\n return list(range(a, x))", "def between(a,b):\n full_between = []\n if b < a:\n a, b = b, a\n while a < b+1:\n full_between.append(a)\n a += 1\n return full_between", "def between(a,b):\n # good luck\n between = a + 1\n v = [a]\n while between < b:\n v.append(between)\n between = between + 1\n \n v.append(b)\n return v\n pass ", "def between(a,b):\n newlist = []\n newlist.append(a)\n \n while b > a:\n a += 1\n newlist.append(a)\n \n else:\n pass\n \n return newlist\n", "def between(a,b):\n return [Array for Array in range(a, b + 1)]", "def between(a,b):\n result = []\n for i in range(a,b+1):\n result.append(i)\n return result", "between = lambda x, y: [x] if x == y else [x] + between(x + 1, y)", "between = lambda a,b: [*range(a,b+1)]", "def between(a,b):\n oplst = []\n for i in range(a, b+1):\n oplst.append(i)\n \n return oplst", "def between(a,b):\n assert a < b\n array = []\n \n for i in range(a, b + 1):\n array.append(i)\n return array", "def between(a,b):\n arr = [a]\n while a < b:\n arr.append(a+1)\n a +=1\n return arr", "def between(a,b):\n c = list(range(a, b+1)) \n return list(c) \n\n \n", "between=lambda k,l:[*list(range(k,l+1))]\n", "def between(a,b):\n # good luck\n res = []\n i = a\n for x in range(a,b+1,1):\n res.append(x)\n \n return res", "def between(a,b):\n if (a < 0) and (b < 0):\n spis = [(min(a,b) + i) for i in range(b - a + 1)]\n return spis\n elif (b < 0):\n spis = [(min(a,b) + i) for i in range(abs(a) + abs(b) + 1)] \n return spis\n elif (a < 0):\n spis = [(min(a,b) + i) for i in range(abs(a) + abs(b) + 1)] \n return spis\n spis = [min(a,b) + i for i in range(b - a + 1)] \n return spis\n", "def between(a,b):\n # good luck\n pass\n resultarr = []\n for i in range(a, b+1):\n resultarr.append(i)\n return(resultarr)", "def between(a,b):\n difference = 0\n diff_range = (b - a) + 1\n new_list = []\n for x in range (diff_range):\n new_list.append(a + difference)\n difference += 1\n return new_list", "def between(a,b):\n c = a - 1\n list_b = []\n while c < b:\n c += 1\n list_b.append(c)\n return list_b\n", "def between(a,b):\n # good luck\n c = range(a, b + 1, 1)\n return [x for x in c if x >= a]", "def between(a,b):\n hold=[]\n for i in range(a,b+1,1):\n hold.append(i)\n return hold", "def between(a,b):\n total=[]\n total+=range(a,b+1)\n return total", "def between(a,b):\n if a < b:\n return [x for x in range(a, b + 1)]", "def between(a,b):\n list = []\n for i in range(b-a+1):\n list.append(a+i)\n return(list)", "def between(a,b):\n # good luck\n array = []\n current = a\n while b >= current:\n array.append(current)\n current += 1\n return array\n pass", "def between(a,b):\n arr = []\n for i in range(a,b+1):\n arr = arr + [i]\n return arr", "def between(a,b):\n arr = [a]\n while a < b:\n a += 1\n arr.append(a)\n return arr", "def between(a,b):\n cadena = []\n for i in range(a,b+1):\n cadena.append(i)\n return cadena\n", "def between(a,b):\n C = []\n for i in range(a,b+1):\n C.append (i)\n return(C)\n", "def between(a,b):\n return [*range(min(a,b), max(a,b)+1)]", "from array import *\ndef between(a,b):\n arr = array('i',range(a,b+1))\n return [a for a in arr]", "def between(a,b):\n li = [_ for _ in range(a,b+1)]\n return li", "def between(a,b):\n # good luck\n res = []\n for i in range((b - a)+1):\n res.append(a+i)\n \n return res", "def between(a,b):\n liste = []\n x = range(a, b+1)\n for n in x:\n liste.append(n)\n return liste", "def between(a,b):\n # good luck\n q = [i for i in range(a, b+1)]\n return q", "def between(a,b):\n numbers = []\n for value in range(a, b + 1):\n numbers.append(value)\n return numbers\n pass", "def between(a,b):\n ll = list()\n for i in range(a, b + 1):\n ll.append(i)\n return ll", "def between(a,b):\n returnArr = []\n for i in range(a,b+1): \n returnArr.append(i)\n return returnArr", "def between(a,b):\n x = list(range(a,b + 1))\n y = []\n for i in x:\n y.append(i)\n return y\n", "def between(a,b):\n g=[]\n c=a\n while a<=b:\n g.append(a)\n a+=1\n return g", "def between(a,b):\n x = []\n while b >= a:\n x.append(a)\n a = a + 1\n return x", "def between(a,b):\n # good luck\n res = []\n while a != b+1:\n res.append(a)\n a += 1\n return res\n \n \n \n \n \n", "def between(a,b):\n lst = list()\n for i in range(a, b+1):\n lst.append(i)\n return lst", "def between(a,b):\n arr = []\n while (a < b+1):\n arr.append(a)\n a += 1\n return arr ", "def between(a,b):\n # good luck\n range_list = range(a, b + 1)\n \n return list(range_list)", "def between(a,b):\n items = []\n for x in range(a, b+1):\n items.append(x)\n return items", "def between(a,b):\n b = b+1\n ans = list(range(a,b))\n return ans"] | {"fn_name": "between", "inputs": [[1, 4], [-2, 2], [-10, 10], [5, 100]], "outputs": [[[1, 2, 3, 4]], [[-2, -1, 0, 1, 2]], [[-10, -9, -8, -7, -6, -5, -4, -3, -2, -1, 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10]], [[5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,891 |
def between(a,b):
|
b273235d94184d55d0cc679891c1d91c | UNKNOWN | ###Task:
You have to write a function **pattern** which creates the following Pattern(See Examples) upto n(parameter) number of rows.
####Rules/Note:
* If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.
* All the lines in the pattern have same length i.e equal to the number of characters in the last line.
* Range of n is (-∞,100]
###Examples:
pattern(5):
1
121
12321
1234321
123454321
pattern(10):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
pattern(15):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
pattern(20):
1
121
12321
1234321
123454321
12345654321
1234567654321
123456787654321
12345678987654321
1234567890987654321
123456789010987654321
12345678901210987654321
1234567890123210987654321
123456789012343210987654321
12345678901234543210987654321
1234567890123456543210987654321
123456789012345676543210987654321
12345678901234567876543210987654321
1234567890123456789876543210987654321
123456789012345678909876543210987654321
###Amazing Fact:
```Hint: Use \n in string to jump to next line``` | ["def pattern(n):\n output = []\n for i in range (1, n + 1):\n wing = ' ' * (n - i) + ''.join(str(d % 10) for d in range(1, i))\n output.append(wing + str(i % 10) + wing[::-1])\n return '\\n'.join(output)", "def pattern(n):\n result = []\n for i in range(1, n+1):\n string = \"\"\n for j in range(n, i, -1):\n string += \" \"\n for j in range(1, i):\n string += str(j)[-1]\n for j in range(i, 0, -1):\n string += str(j)[-1]\n for j in range(n, i, -1):\n string += \" \"\n result.append(string)\n return \"\\n\".join(result)", "def pattern(n):\n l = []\n for a, b in zip(range(1, n+2), range(n+1)):\n res = ''.join(str(x%10) for x in range(1,a))\n spaces = n-len(res)\n if res: l.append(spaces * ' ' + res+res[-2::-1] + ' ' * (spaces))\n return '\\n'.join(l)", "from itertools import cycle, islice\n\ndef f(n):\n it = cycle('1234567890')\n xs = ''.join(islice(it, 0, n-1))\n return xs + next(it) + xs[::-1]\n \ndef pattern(n):\n m = n * 2 - 1\n return '\\n'.join(\n '{:^{}}'.format(f(i), m)\n for i in range(1, n+1)\n )", "def pattern(n):\n t = []\n for i in range(1,n+1):\n t.append( (n-i)*\" \" + ls(i)+ +(n-i)*\" \")\n return \"\\n\".join(t)\n \ndef ls(k):\n ls = [str(j%10) for j in range(1,k)]\n return \"\".join(ls) + str(k%10) + \"\".join(ls[::-1])", "def pattern(n):\n mkP = lambda y: str(y % 10)\n return \"\\n\".join([\" \" * (n - x - 1) + \"\".join(map(mkP, range(1, x + 1))) + \"\".join(map(mkP, range(x + 1, 0, -1))) + \" \" * (n - x - 1) for x in range(n)])"] | {"fn_name": "pattern", "inputs": [[7], [1], [4], [0], [-25]], "outputs": [[" 1 \n 121 \n 12321 \n 1234321 \n 123454321 \n 12345654321 \n1234567654321"], ["1"], [" 1 \n 121 \n 12321 \n1234321"], [""], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,682 |
def pattern(n):
|
d4bde7d048eaf648e9f8ed30c1fb63d9 | UNKNOWN | Create a function
```python
has_two_cube_sums(n)
```
which checks if a given number `n` can be written as the sum of two cubes in two different ways: `n = a³+b³ = c³+d³`.
All the numbers `a`, `b`, `c` and `d` should be different and greater than `0`.
E.g. 1729 = 9³+10³ = 1³+12³.
```python
has_two_cube_sums(1729); // true
has_two_cube_sums(42); // false
``` | ["def has_two_cube_sums(n):\n cubic_list = [i**3 for i in range(1, int((n)**(1./3.)) + 1)]\n return sum([(n != 2*c) and ((n-c) in cubic_list) for c in cubic_list]) > 3\n", "def has_two_cube_sums(n):\n x = 0\n for a in range(int(n**0.35)):\n for b in range(a,int(n**0.35)):\n if a**3+b**3 == n:\n x += 1\n if x > 1:\n return True\n return False\n", "from itertools import combinations\n\ns = {a**3 + b**3 for a, b in combinations(range(1, 1000), 2)}\ns.discard(19**3 + 34**3) # bug\n\ndef has_two_cube_sums(n):\n return n in s", "def has_two_cube_sums(n):\n if n == 1: return False\n \n high = int((n)**(1/3.0))\n low = int((n/2)**(1/3.0))\n return sum( abs(y-round(y)) < 1e-8 for y in [((n-x**3))**(1/3.0) for x in range(low,high+1)]) >= 2", "def has_two_cube_sums(n):\n return len([[y,z] for y in range(1, int(n**(1/3))+1) for z in [x for x in range(1, int(n**(1/3))+1)] if y!=z and y**3+z**3==n])>=4", "has_two_cube_sums=lambda n:sum([(i[0]**3)+(i[1] ** 3)==n for i in __import__(\"itertools\").permutations(range(1,int(n**(1./3.))+1),2)])>=4"] | {"fn_name": "has_two_cube_sums", "inputs": [[1], [1729], [42], [4103], [4102], [4104], [4105], [4106], [0], [46163]], "outputs": [[false], [true], [false], [false], [false], [true], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,143 |
def has_two_cube_sums(n):
|
c23e6c024e03d1cf0cea45ffeda28055 | UNKNOWN | In this Kata, you will be given an array of numbers in which two numbers occur once and the rest occur only twice. Your task will be to return the sum of the numbers that occur only once.
For example, `repeats([4,5,7,5,4,8]) = 15` because only the numbers `7` and `8` occur once, and their sum is `15`.
More examples in the test cases.
```if:csharp
Documentation:
Kata.Repeats Method (List<Int32>)
Takes a list where all ints are repeated twice, except two ints, and returns the sum of the ints of a list where those ints only occur once.
Syntax
public
static
int Repeats(
List<int> source
)
Parameters
source
Type: System.Collections.Generic.List<Int32>
The list to process.
Return Value
Type: System.Int32
The sum of the elements of the list where those elements have no duplicates.
```
Good luck!
If you like this Kata, please try:
[Sum of prime-indexed elements](https://www.codewars.com/kata/59f38b033640ce9fc700015b)
[Sum of integer combinations](https://www.codewars.com/kata/59f3178e3640cef6d90000d5) | ["def repeats(arr):\n return sum([x for x in arr if arr.count(x) == 1])", "from collections import Counter\n\ndef repeats(arr):\n return sum(k for k, v in Counter(arr).items() if v == 1)", "def repeats(arr):\n return sum(n for n in arr if arr.count(n) == 1)", "def repeats(arr):\n single = [] #create a list to store single numbers\n for i in arr: #loop thru the array\n if arr.count(i) == 1: #if a number occurs more than once in the array\n single.append(i) #attach it to the singles list\n total = sum (single) \n return total", "repeats=lambda a:2*sum(set(a))-sum(a)", "def repeats(s):\n numbers = set()\n\n for number in s:\n if number in numbers:\n numbers.remove(number)\n else:\n numbers.add(number)\n return sum(numbers)\n", "def repeats(arr):\n \n \n return sum(filter(lambda num : arr.count(num) == 1, arr))", "def repeats(arr):\n return sum(num for num in arr if arr.count(num) == 1)\n\n", "def repeats(a):\n return sum(filter(lambda x: a.count(x) < 2, a))", "def repeats(arr):\n return sum(i for i in arr if arr.count(i) == 1)"] | {"fn_name": "repeats", "inputs": [[[4, 5, 7, 5, 4, 8]], [[9, 10, 19, 13, 19, 13]], [[16, 0, 11, 4, 8, 16, 0, 11]], [[5, 17, 18, 11, 13, 18, 11, 13]], [[5, 10, 19, 13, 10, 13]]], "outputs": [[15], [19], [12], [22], [24]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,127 |
def repeats(arr):
|
1d6aa0d602db1d93a6412f5e7c2c53b9 | UNKNOWN | __Definition:__ According to Wikipedia, a [complete binary tree](https://en.wikipedia.org/wiki/Binary_tree#Types_of_binary_trees) is a binary tree _"where every level, except possibly the last, is completely filled, and all nodes in the last level are as far left as possible."_
The Wikipedia page referenced above also mentions that _"Binary trees can also be stored in breadth-first order as an implicit data structure in arrays, and if the tree is a complete binary tree, this method wastes no space."_
Your task is to write a method (or function) that takes an array (or list, depending on language) of integers and, assuming that the array is ordered according to an _in-order_ traversal of a complete binary tree, returns an array that contains the values of the tree in breadth-first order.
__Example 1:__
Let the input array be `[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]`. This array contains the values of the following complete binary tree.
```
_ 7_
/ \
4 9
/ \ / \
2 6 8 10
/ \ /
1 3 5
```
In this example, the input array happens to be sorted, but that is _not_ a requirement.
__Output 1:__ The output of the function shall be an array containing the values of the nodes of the binary tree read top-to-bottom, left-to-right. In this example, the returned array should be:
```[7, 4, 9, 2, 6, 8, 10, 1, 3, 5]```
__Example 2:__
Let the input array be `[1, 2, 2, 6, 7, 5]`. This array contains the values of the following complete binary tree.
```
6
/ \
2 5
/ \ /
1 2 7
```
Note that an in-order traversal of this tree produces the input array.
__Output 2:__ The output of the function shall be an array containing the values of the nodes of the binary tree read top-to-bottom, left-to-right. In this example, the returned array should be:
```[6, 2, 5, 1, 2, 7]``` | ["def complete_binary_tree(a):\n def in_order(n=0):\n if n < len(a):\n yield from in_order(2 * n + 1)\n yield n\n yield from in_order(2 * n + 2)\n\n result = [None] * len(a)\n for i, x in zip(in_order(), a):\n result[i] = x\n return result", "def mid(n):\n x = 1 << (n.bit_length() - 1)\n return x-1 if x//2 - 1 <= n-x else n-x//2\n\ndef complete_binary_tree(a):\n res, queue = [], [a]\n while queue:\n L = queue.pop(0)\n m = mid(len(L))\n res.append(L[m])\n if m: queue.append(L[:m])\n if m < len(L)-1: queue.append(L[m+1:])\n return res", "import math\n\ndef complete_binary_tree(a):\n ret = []\n rows = [a]\n \n while len(rows):\n newrows = []\n #print(rows)\n for x in rows:\n ln = len(x)\n if ln > 1:\n pwr = int(math.log(ln+1, 2))\n complete_part_ln = 2**pwr - 1\n last_full_row_ln = 2**(pwr-1)\n free_row_ln = ln - complete_part_ln\n middle = complete_part_ln // 2 + (last_full_row_ln if free_row_ln > last_full_row_ln else free_row_ln)\n ret += [x[middle]]\n newrows += [x[:middle]] + [x[middle+1:]]\n else:\n ret += x\n rows = newrows\n\n return ret\n\n", "def complete_binary_tree(a):\n def calculate(a, root = 1, cnt = 0, output = []):\n idx = list(range(1, len(a)+1))\n if (not output):\n output = [0] * len(a)\n \n if (root * 2 in idx):\n cnt, output = calculate(a, root = root * 2, cnt = cnt, output = output)\n \n output[root-1] = a[cnt]\n cnt += 1\n \n if (root * 2 + 1 in idx):\n cnt, output = calculate(a, root = root * 2 + 1, cnt = cnt, output = output)\n \n return cnt, output\n return calculate(a)[1]", "def complete_binary_tree(a):\n arr1 = [] #\uae30\ubcf8 node\ub4e4\uc774 \ucc28\ub840\ub300\ub85c \uc788\ub294 \ubc30\uc5f4\n for i in range(len(a)+1): #\uac1c\uc218\ub9cc\ud07c +1\uc744 \ud574\uc90c. 0\ubd80\ud130 \uc2dc\uc791\ud558\uac8c \ud558\uae30 \uc704\ud568\n arr1.append(i)\n stack = [] #inorder \uc21c\uc11c\ub97c \ub098\ud0c0\ub0bc \ubc30\uc5f4\n \n def inorder(arr, node): #inorder\ub77c\ub294 \ud568\uc218\ub294 arr\uc640 node\uac12\uc744 input\uc73c\ub85c \ubc1b\uc74c\n nonlocal stack #\ud568\uc218 \ub0b4\uc758 \ud568\uc218\uc5d0\uc11c \ubd80\ub974\ubbc0\ub85c nonlocal\uc744 \uc0ac\uc6a9\ud558\uc5ec \ub0b4\ubd80\uc218\uc815\uc774 \ubc16\uc5d0\ub3c4 \uc601\ud5a5\uc744 \ubbf8\uce58\ub3c4\ub85d \ud568.\n if(node >= len(arr)): \n return\n inorder(arr, node*2)\n stack.append(node)\n inorder(arr, node*2 + 1)\n inorder(arr1, 1) #1\ubd80\ud130\ud558\uc5ec inorder \uc21c\uc11c\ub97c \ub530\ub0c4. stack\uc5d0 \uc800\uc7a5\ub428\n \n result = [0 for row in range(len(a))] #result\ub97c \uc800\uc7a5\ud560 \ubc30\uc5f4\uc744 \uc120\uc5b8\ud568.\n for i, lit in enumerate(result):\n result[i] = a[stack.index(i+1)] #\uc54c\ub9de\ub294 \uac12\uc73c\ub85c \ubc14\uafd4\uc90c\n return result \n", "def complete_binary_tree(a):\n l = len(a)\n ans = [-1]*(l+1)\n arr = a.copy()\n\n push_num(1, ans, arr)\n\n return ans[1:]\n\ndef push_num(idx, ans, a):\n if(idx >= len(ans) or len(a) == 0):\n return\n else:\n push_num(idx*2, ans, a)\n ans[idx] = a.pop(0)\n push_num(idx*2 + 1, ans, a)", "from collections import deque\nfrom math import log2, floor\n\ndef split_tree(a): # return head, left subtree, right subtree\n top_position = 0\n number_of_elements = len(a)\n number_of_layers = floor(log2(number_of_elements)) + 1\n top_size = 2 ** (number_of_layers - 1) - 1\n lowest_level_count = number_of_elements - top_size\n if lowest_level_count >= 2 ** (number_of_layers - 2): # left part of lowest level is full\n top_position = int(2**(number_of_layers - 1)) - 1\n else: # right part of lowest level is empty\n top_position = - (top_size - 1) // 2 - 1\n return a[top_position], a[:top_position], a[top_position + 1:]\n\ndef complete_binary_tree(a):\n res = []\n tree_queue = deque()\n tree_queue.append(a)\n while tree_queue:\n tree = tree_queue.popleft()\n if tree:\n (root, left, right) = split_tree(tree)\n res.append(root)\n tree_queue.append(left)\n tree_queue.append(right)\n return res", "import math\n\ndef complete_binary_tree(a):\n if len(a) < 2: return a\n \n root = build(a)\n res = []\n getLevelOrder(root, res)\n return res\n\nclass Node:\n\n def __init__(self, data, left, right):\n\n self.left = left\n self.right = right\n self.data = data\n \n def __str__(self):\n return f\"left = {self.left}, right = {self.right}, data = {self.data}\"\n \ndef build(a):\n\n if len(a) == 0:\n return None\n if len(a) == 1:\n return Node(a[0], None, None)\n \n level = math.log2(len(a))\n level = math.ceil(level) + (1 if int(level) == level else 0)\n \n if len(a) >= 2 ** (level - 2) * 3 - 1:\n root = 2 ** (level - 1) - 1\n else:\n root = len(a) - 2 ** (level - 2)\n \n left = build(a[:root])\n right = build(a[root+1:])\n return Node(a[root], left, right)\n\ndef getLevelOrder(root, res): \n h = height(root) \n for i in range(1, h+1): \n getGivenLevel(root, i, res) \n\ndef getGivenLevel(root , level, res): \n if root is None: \n return\n if level == 1: \n res.append(root.data)\n elif level > 1 : \n getGivenLevel(root.left , level-1, res) \n getGivenLevel(root.right , level-1, res)\n\n \ndef height(node): \n if node is None: \n return 0 \n else : \n # Compute the height of each subtree \n lheight = height(node.left) \n rheight = height(node.right) \n \n #Use the larger one \n if lheight > rheight : \n return lheight+1\n else: \n return rheight+1", "def floor(num: int):\n ''' \n return num - (2**i-1)\n for max i that 2**i-1 <= num\n '''\n print(num)\n assert num >= 0\n i = 0\n while 2**i - 1 <= num:\n i += 1\n return num - 2**(i-1) + 1\n\n\ndef complete_binary_tree(a: list) -> list:\n a = a.copy()\n breadth_fst: list = []\n \n for i in range(floor(len(a))):\n breadth_fst.append(a.pop(i))\n\n while a:\n idx = -1\n while idx >= -len(a):\n breadth_fst.insert(0, a.pop(idx))\n idx -= 1\n\n return breadth_fst\n", "import math\n\nclass BST:\n def __init__(self,value,left=None,right=None):\n self.value=value\n self.left=left\n self.right=right\n\ndef complete_binary_tree(arr):\n tree = bst_from_arr(arr)\n depth = math.floor(math.log(len(arr),2))\n output = []\n for i in range(depth+1):\n output += breadth_first_arr(tree,i)\n return output\n\ndef breadth_first_arr(tree, level):\n out = []\n if tree is None: return\n if level == 0:\n out.append(tree.value)\n elif level > 0:\n if tree.left: out += breadth_first_arr(tree.left , level-1)\n if tree.right: out += breadth_first_arr(tree.right , level-1)\n return out\n\ndef bst_from_arr(arr):\n if len(arr) > 1:\n root_index = get_root_index(arr)\n tree = BST(arr[root_index])\n else: return BST(arr[0])\n\n if len(arr[:root_index]) > 1:\n tree.left = bst_from_arr(arr[:root_index])\n if len(arr[:root_index]) == 1:\n tree.left = BST(arr[0])\n\n if len(arr[root_index+1:]) > 1:\n tree.right = bst_from_arr(arr[root_index+1:])\n if len(arr[root_index+1:]) == 1:\n tree.right = BST(arr[-1])\n return tree\n\ndef get_root_index(arr):\n left_subtree = 0\n levels = math.floor(math.log(len(arr),2))\n for i in range(0, levels):\n if not i == levels - 1:\n left_subtree += 2**i\n else:\n left_subtree += min(int((2**levels)/2),len(arr)-(2**(levels)-1))\n return left_subtree"] | {"fn_name": "complete_binary_tree", "inputs": [[[1]], [[1, 2, 3, 4, 5, 6]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]]], "outputs": [[[1]], [[4, 2, 6, 1, 3, 5]], [[7, 4, 9, 2, 6, 8, 10, 1, 3, 5]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 8,327 |
def complete_binary_tree(a):
|
f51daa7168f7559544502c0f8ad3b3fa | UNKNOWN | ## Introduction
Each chemical element in its neutral state has a specific number of electrons associated with it. This is represented by the **atomic number** which is noted by an integer number next to or above each element of the periodic table (as highlighted in the image above).
As we move from left to right, starting from the top row of the periodic table, each element differs from its predecessor by 1 unit (electron). Electrons fill in different orbitals sets according to a specific order. Each set of orbitals, when full, contains an even number of electrons.
The orbital sets are:
* The _**s** orbital_ - a single orbital that can hold a maximum of 2 electrons.
* The _**p** orbital set_ - can hold 6 electrons.
* The _**d** orbital set_ - can hold 10 electrons.
* The _**f** orbital set_ - can hold 14 electrons.
The order in which electrons are filling the different set of orbitals is shown in the picture above. First electrons will occupy the **1s** orbital, then the **2s**, then the **2p** set, **3s** and so on.
Electron configurations show how the number of electrons of an element is distributed across each orbital set. Each orbital is written as a sequence that follows the order in the picture, joined by the number of electrons contained in that orbital set. The final electron configuration is a single string of orbital names and number of electrons per orbital set where the first 2 digits of each substring represent the orbital name followed by a number that states the number of electrons that the orbital set contains.
For example, a string that demonstrates an electron configuration of a chemical element that contains 10 electrons is: `1s2 2s2 2p6`. This configuration shows that there are two electrons in the `1s` orbital set, two electrons in the `2s` orbital set, and six electrons in the `2p` orbital set. `2 + 2 + 6 = 10` electrons total.
___
# Task
Your task is to write a function that displays the electron configuration built according to the Madelung rule of all chemical elements of the periodic table. The argument will be the symbol of a chemical element, as displayed in the periodic table.
**Note**: There will be a preloaded array called `ELEMENTS` with chemical elements sorted by their atomic number.
For example, when the element "O" is fed into the function the output should look like:
`"O -> 1s2 2s2 2p4"`
However, there are some exceptions! The electron configurations of the elements below should end as:
```
Cr -> ...3d5 4s1
Cu -> ...3d10 4s1
Nb -> ...4d4 5s1
Mo -> ...4d5 5s1
Ru -> ...4d7 5s1
Rh -> ...4d8 5s1
Pd -> ...4d10 5s0
Ag -> ...4d10 5s1
La -> ...4f0 5d1
Ce -> ...4f1 5d1
Gd -> ...4f7 5d1 6s2
Pt -> ...4f14 5d9 6s1
Au -> ...4f14 5d10 6s1
Ac -> ...5f0 6d1 7s2
Th -> ...5f0 6d2 7s2
Pa -> ...5f2 6d1 7s2
U -> ...5f3 6d1 7s2
Np -> ...5f4 6d1 7s2
Cm -> ...5f7 6d1 7s2
```
**Note**: for `Ni` the electron configuration should be `3d8 4s2` instead of `3d9 4s1`. | ["ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\nEXCEPTIONS = {\n 'Cr': ['Ar', '4s1 3d5'],\n 'Cu': ['Ar', '4s1 3d10'],\n 'Nb': ['Kr', '5s1 4d4'],\n 'Mo': ['Kr', '5s1 4d5'],\n 'Ru': ['Kr', '5s1 4d7'],\n 'Rh': ['Kr', '5s1 4d8'],\n 'Pd': ['Kr', '5s0 4d10'],\n 'Ag': ['Kr', '5s1 4d10'],\n 'La': ['Xe', '6s2 4f0 5d1'],\n 'Ce': ['Xe', '6s2 4f1 5d1'],\n 'Gd': ['Xe', '6s2 4f7 5d1'],\n 'Pt': ['Xe', '6s1 4f14 5d9'],\n 'Au': ['Xe', '6s1 4f14 5d10'],\n 'Ac': ['Rn', '7s2 5f0 6d1'],\n 'Th': ['Rn', '7s2 5f0 6d2'],\n 'Pa': ['Rn', '7s2 5f2 6d1'],\n 'U' : ['Rn', '7s2 5f3 6d1'],\n 'Np': ['Rn', '7s2 5f4 6d1'],\n 'Cm': ['Rn', '7s2 5f7 6d1'],\n}\nORBITALS = \"spdfg\"\nELT_TO_Z = {elt:i for i,elt in enumerate(ELEMENTS,1)}\nfor arr in list(EXCEPTIONS.values()):\n arr[1] = [ (int(s[0]), ORBITALS.find(s[1]), s[2:]) for s in arr[1].split(' ') ]\n\n\ndef get_electron_configuration(element):\n elt,repl = EXCEPTIONS.get(element, (element,[]) )\n z,nl,config = ELT_TO_Z[elt], 0, {} # n: principal quantum number / l: secondary qunatum number (minus 1) / nl: n+l\n while z:\n nl += 1\n for l in range(nl-1>>1, -1, -1):\n nE = min(z, 2+l*4)\n config[ (nl-l,l) ] = nE\n z -= nE\n if not z: break\n \n for a,b,n in repl: config[(a,b)] = n\n \n s = \" \".join( f'{ k[0] }{ ORBITALS[k[1]] }{ n }' for k,n in sorted(config.items()))\n return f'{ element } -> { s }'\n", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\nimport re\n\nexc = '''Cr -> ...3d5 4s1\nCu -> ...3d10 4s1\nNb -> ...4d4 5s1\nMo -> ...4d5 5s1\nRu -> ...4d7 5s1\nRh -> ...4d8 5s1\nPd -> ...4d10 5s0\nAg -> ...4d10 5s1\nLa -> ...4f0 5d1\nCe -> ...4f1 5d1\nGd -> ...4f7 5d1 6s2\nPt -> ...4f14 5d9 6s1\nAu -> ...4f14 5d10 6s1\nAc -> ...5f0 6d1 7s2\nTh -> ...5f0 6d2 7s2\nPa -> ...5f2 6d1 7s2\nU -> ...5f3 6d1 7s2\nNp -> ...5f4 6d1 7s2\nCm -> ...5f7 6d1 7s2'''\nexceptions = {i.split()[0]: {j[:2]: j[2:] for j in i.split('...')[1].split()} for i in exc.split('\\n')}\n\ndef get_electron_configuration(element):\n names = re.findall(r'..', '1s2s2p3s3p4s3d4p5s4d5p6s4f5d6p7s5f6d7p8s')\n values = [2, 2, 6, 2, 6, 2, 10, 6, 2, 10, 6, 2, 14, 10, 6, 2, 14, 10, 6, 2]\n N = ELEMENTS.index(element) + 1\n res = {}\n while N > 0:\n v = min(values.pop(0), N)\n res[names.pop(0)] = str(v)\n N -= v\n if element in exceptions:\n res.update(exceptions[element])\n res = [i+j for i,j in zip(res.keys(), res.values())]\n res.sort(key = lambda x: (x[0], ['s', 'p', 'd', 'f'].index(x[1])))\n return '{} -> {}'.format(element, ' '.join(res))", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef get_electron_configuration(a):\n d = {\"s\":2, \"p\":6, \"d\":10, \"f\":14}\n o = [[x, d[x[1]]] for x in [\"1s\",\"2s\",\"2p\",\"3s\",\"3p\",\"4s\",\"3d\",\"4p\",\"5s\",\"4d\",\"5p\",\"6s\",\"4f\",\"5d\",\"6p\",\"7s\",\"5f\",\"6d\",\"7p\"]][::-1]\n exceptions = {\"Cr\":[2, \"4s1 3d5\"], \"Cu\":[2, \"4s1 3d10\"], \"Nb\":[2, \"5s1 4d4\"], \"Mo\":[2, \"5s1 4d5\"], \"Ru\":[2, \"5s1 4d7\"], \"Rh\":[2, \"5s1 4d8\"], \"Pd\":[2, \"5s0 4d10\"], \"Ag\":[2, \"5s1 4d10\"], \"La\":[1, \"4f0 5d1\"], \"Ce\":[1, \"4f1 5d1\"], \"Gd\":[1, \"4f7 5d1\"], \"Pt\":[3, \"6s1 4f14 5d9\"], \"Au\":[3, \"6s1 4f14 5d10\"], \"Ac\":[1, \"5f0 6d1\"], \"Th\":[1, \"5f0 6d2\"], \"Pa\":[1, \"5f2 6d1\"], \"U\":[1, \"5f3 6d1\"], \"Np\":[1, \"5f4 6d1\"], \"Cm\":[1, \"5f7 6d1\"]}\n r, n = [], ELEMENTS.index(a) + 1\n while n > 0:\n s, m = o.pop()\n x = min(n, m)\n n -= x\n r.append(\"{}{}\".format(s, x))\n if a in exceptions:\n m, s = exceptions[a]\n r = r[:-m] + s.split()\n return \"{} -> {}\".format(a, \" \".join(sorted(r, key=lambda x: (x[0], d[x[1]]))))", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\n# Tuple of shell loading.\n# (<orbit>, <final count>, <code>)\n# Codes are:\n# -2 - Remove one electron at a time and ultimately remove the shell so we don't get something like 5d0 for this one.\n# -1 - Remove electrons in this shell one at a time. Don't remove the shell.\n# 0 - Add this shell with zero electrons so that it does show up as something like 5d0 in the sequence.\n# 1 - Add 1 at a time\n# 2 - Add but the first one is a double skip because of electrons dropped before.\n\nshells = (\n (\"1s\", 2, 1),\n (\"2s\", 2, 1),\n (\"2p\", 6, 1),\n (\"3s\", 2, 1),\n (\"3p\", 6, 1),\n (\"4s\", 2, 1),\n (\"3d\", 3, 1),\n (\"4s\", 1, -1),\n (\"3d\", 5, 2),\n (\"4s\", 2, 1),\n (\"3d\", 8, 1),\n (\"4s\", 1, -1), # Cu\n (\"3d\", 10, 1), # Cu\n (\"4s\", 2, 1), # Zn\n (\"4p\", 6, 1),\n (\"5s\", 2, 1),\n (\"4d\", 2, 1),\n (\"5s\", 1, -1),\n (\"4d\", 5, 2),\n (\"5s\", 2, 1),\n (\"5s\", 1, -1),\n (\"4d\", 8, 2),\n (\"5s\", 0, -1),\n (\"4d\", 10, 2),\n (\"5s\", 2, 1),\n (\"5p\", 6, 1),\n (\"6s\", 2, 2), # Ba\n (\"4f\", 0, 0), # La\n (\"5d\", 1, 1), # La\n (\"4f\", 1, 1), # Ce\n (\"5d\", 0, -2), #Pr\n (\"4f\", 7, 2), # Eu\n (\"5d\", 1, 1),\n (\"5d\", 0, -2),\n (\"4f\", 14, 1), # Yb\n (\"5d\", 7, 1), # IR\n (\"6s\", 1, -1), # Pt\n (\"5d\", 10, 1), # Au\n (\"6s\", 2, 1), # Hg\n (\"6p\", 6, 1), # Rn\n (\"7s\", 2, 1), # Ra\n (\"5f\", 0, 0), # Ac\n (\"6d\", 2, 1), # Th\n (\"6d\", 1, -1), # Pa\n (\"5f\", 4, 2), # Np\n (\"6d\", 0, -2), #Pu\n (\"5f\", 7, 2), # Am\n (\"6d\", 1, 1), # Cm\n (\"6d\", 0, -2), # Bk\n (\"5f\", 14, 2), # No\n (\"6d\", 1, 1), # Lr\n# These exceptions should exist per https://ptable.com/ but they don't in the tests.\n# (\"7p\", 1, 1), # Lr\n# (\"7p\", 0, -2), # Rf\n# (\"6d\", 7, 2), # Mt\n# (\"7s\", 1, -1), # Ds\n (\"6d\", 9, 2), # Ds\n (\"7s\", 2, 1), # Rg\n (\"6d\", 10, 1), # Cn\n (\"7p\", 6, 1)\n )\n \n# Order of shells. \nshell_seq = [\"1s\", \"2s\", \"2p\", \"3s\", \"3p\", \"3d\", \"4s\", \"4p\", \"4d\", \"4f\", \"5s\", \"5p\", \"5d\", \"5f\", \"6s\", \"6p\", \"6d\", \"7s\", \"7p\"]\n \ndef get_electron_configuration(element):\n # Create our electron shell dictionary\n e = {}\n # Get the atomic number\n a = ELEMENTS.index(element)+1\n # Keep track of the total number electrons. This is easier/faster than summing up the numbers in the dict.\n t = 0\n # Loop through the shells\n for o, cnt, code in shells:\n # If we reached the atomic number, we stop.\n if t == a:\n break\n else:\n # If orbit isn't in the dict, add it.\n # Note: Even if code=0 this portion will add the orbit so it will show up in \"o in e\" below when building the o_list.\n if o not in e: e[o] = 0\n # If the code is -2, count down and delete the orbit.\n if code == -2:\n while e[o] > cnt:\n e[o] -= 1\n t -= 1\n del(e[o])\n # If the code is -1, count down.\n elif code == -1:\n while e[o] > cnt:\n e[o] -= 1\n t -= 1\n # IF the code is positve, count up. We just use the 2 for ease in building the table above.\n # Nothing special is required for code=2 vs code=1\n elif code > 0:\n while t != a and e[o] < cnt:\n e[o] += 1\n t += 1\n \n # Combine the orbits and counts. Use the shell_seq to get the order correct and don't include shells that aren't in e.\n o_list = [f\"{o}{e[o]}\" for o in shell_seq if o in e]\n # Join the list and output.\n return f\"{element} -> {' '.join(o_list)}\"\n", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef get_electron_configuration(element):\n elems = {\n \"Cr\": (\"3d5 4s1\"),\n \"Cu\": (\"3d10 4s1\"), \n \"Nb\": (\"4d4 5s1\"),\n \"Mo\" :(\"4d5 5s1\"),\n 'Ru' :(\"4d7 5s1\"),\n 'Rh' :(\"4d8 5s1\"),\n 'Pd' :(\"4d10 5s0\"),\n 'Ag' :(\"4d10 5s1\"),\n 'La' :(\"4f0 5d1\"),\n 'Ce' :(\"4f1 5d1\"),\n 'Gd' :(\"4f7 5d1 6s2\"),\n 'Pt' :(\"4f14 5d9 6s1\"),\n 'Au' :(\"4f14 5d10 6s1\"),\n 'Ac' :(\"5f0 6d1 7s2\"),\n 'Th' :(\"5f0 6d2 7s2\"),\n 'Pa' :(\"5f2 6d1 7s2\"),\n 'U' :(\"5f3 6d1 7s2\"),\n 'Np' :(\"5f4 6d1 7s2\"),\n 'Cm' :(\"5f7 6d1 7s2\"),\n }\n elem_config = elems.get(element)\n list=['Cr','Cu','Nb','Mo','Ru','Rh','Pd','Ag',\n 'La','Ce','Gd','Pt','Au','Ac','Th','Pa','U','Np','Cm']\n s=[]\n c=0\n a=0\n for x in range(len(ELEMENTS)):\n if element == ELEMENTS[x]:\n v=x+1\n if element in list:\n s=elem_config.split(' ')\n for b in s:\n v-=int(b[2:])\n while v>0:\n c+=1\n if v>=2:\n s.append(str(c)+\"s2\")\n v-=2\n elif 0<v<2:\n s.append(str(c)+\"s\"+str(v))\n v-=v\n if v>=6 and c>1:\n s.append(str(c)+\"p6\")\n v-=6\n elif 0<v<6 and c>1:\n s.append(str(c)+\"p\"+str(v))\n v-=v\n if v>=12 and c>2:\n s.append(str(c)+\"d10\")\n v-=10 \n elif 2<v<=12 and c>2:\n s.append(str(c)+\"d\"+str(v-2))\n v-=v-2\n if v>=24 and c>3:\n s.append(str(c)+\"f14\")\n v-=14 \n elif 10<v<24 and c>3:\n s.append(str(c)+\"f\"+str(v-10))\n v-=v-10\n s.sort(key=lambda p: p[0] + {\"s\":\"0\",\"p\":\"1\",\"d\":\"2\",\"f\":\"3\"}[p[1]])\n s=\" \".join(s)\n return element+\" -> \"+s", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\norder=['1s','2s','2p','3s','3p','4s','3d','4p','5s','4d','5p','6s',\n '4f','5d','6p','7s','5f','6d','7p']\nhold={'s':2,'p':6,'d':10,'f':14}\nspecial={\n 'Cr':['3d5', '4s1'], 'Cu':['3d10', '4s1'], 'Nb':['4d4', '5s1'],\n 'Mo':['4d5', '5s1'], 'Ru':['4d7', '5s1'], 'Rh':['4d8', '5s1'],\n 'Pd':['4d10', '5s0'], 'Ag':['4d10', '5s1'], 'La':['4f0', '5d1'],\n 'Ce':['4f1', '5d1'], 'Gd':['4f7', '5d1', '6s2'], 'Pt':['4f14', '5d9', '6s1'],\n 'Au':['4f14', '5d10', '6s1'], 'Ac':['5f0', '6d1', '7s2'],\n 'Th':['5f0', '6d2', '7s2'], 'Pa':['5f2', '6d1', '7s2'],\n 'U':['5f3', '6d1', '7s2'], 'Np':['5f4', '6d1', '7s2'],\n 'Cm':['5f7', '6d1', '7s2']\n}\ndef get_electron_configuration(element):\n e=ELEMENTS.index(element)+1\n r=[]\n for o in order:\n r.append(o+str(min(e,hold[o[1]])))\n e-=hold[o[1]]\n if e<=0:\n break\n if element in special:\n for o2 in special[element]:\n flag=False\n for i in range(len(r)):\n if r[i][:2]==o2[:2]:\n r[i]=o2\n flag=True\n break\n if not flag:\n r.append(o2)\n return '{} -> {}'.format(element,' '.join(sorted(r,key=lambda x:(x[0],'spdf'.index(x[1]))))) ", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef get_electron_configuration(element):\n atomic_no, n = ELEMENTS.index(element)+1, 0\n orbitals = {'1s':0,'2s':0,'2p':0,'3s':0,'3p':0,'3d':0,'4s':0,'4p':0,'4d':0,'4f':0,'5s':0,'5p':0,'5d':0,'5f':0,'6s':0,'6p':0,'6d':0,'6f':0,'7s':0,'7p':0,'7d':0,'7f':0}\n exceptions = {'Cr' : 'Cr -> 1s2 2s2 2p6 3s2 3p6 3d5 4s1','Cu': 'Cu -> 1s2 2s2 2p6 3s2 3p6 3d10 4s1','Nb': 'Nb -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d4 5s1','Mo':'Mo -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d5 5s1','Ru': 'Ru -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d7 5s1','Rh': 'Rh -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d8 5s1','Pd': 'Pd -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 5s0','Ag': 'Ag -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 5s1','La': 'La -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f0 5s2 5p6 5d1 6s2','Ce': 'Ce -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f1 5s2 5p6 5d1 6s2','Gd': 'Gd -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f7 5s2 5p6 5d1 6s2','Pt': 'Pt -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d9 6s1','Au': 'Au -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 6s1','Ac': 'Ac -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f0 6s2 6p6 6d1 7s2','Th': 'Th -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f0 6s2 6p6 6d2 7s2','Pa': 'Pa -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f2 6s2 6p6 6d1 7s2','U': 'U -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f3 6s2 6p6 6d1 7s2','Np': 'Np -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f4 6s2 6p6 6d1 7s2','Cm': 'Cm -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p6 4d10 4f14 5s2 5p6 5d10 5f7 6s2 6p6 6d1 7s2'}\n if element in exceptions:\n return exceptions[element]\n while n < atomic_no:\n if n < 2:\n orbitals['1s'] += 1\n elif n < 4:\n orbitals['2s'] += 1\n elif n < 10:\n orbitals['2p'] += 1\n elif n < 12:\n orbitals['3s'] += 1\n elif n < 18:\n orbitals['3p'] += 1\n elif n < 20:\n orbitals['4s'] += 1\n elif n < 30:\n orbitals['3d'] += 1\n elif n < 36:\n orbitals['4p'] += 1\n elif n < 38:\n orbitals['5s'] += 1\n elif n < 48:\n orbitals['4d'] += 1\n elif n < 54:\n orbitals['5p'] += 1\n elif n < 56:\n orbitals['6s'] += 1\n elif n < 70:\n orbitals['4f'] += 1\n elif n < 80:\n orbitals['5d'] += 1\n elif n < 86:\n orbitals['6p'] += 1\n elif n < 88:\n orbitals['7s'] += 1\n elif n < 102:\n orbitals['5f'] += 1\n elif n < 112:\n orbitals['6d'] += 1\n elif n < 118:\n orbitals['7p'] += 1\n n += 1\n st = ''\n orbs = ['1s','2s','2p','3s','3p','3d','4s','4p','4d','4f','5s','5p','5d','5f','6s','6p','6d','6f','7s','7p','7d','7f']\n for x in orbs:\n if orbitals[x] != 0:\n st += x+str(orbitals[x])+' '\n return element+' -> '+st.rstrip()\n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef orbital_sort(s):\n return s.replace('s','1').replace('p','2').replace('d','3').replace('f','4')\n\ndef get_electron_configuration(element):\n atomic = ELEMENTS.index(element) + 1\n \n filling = ['1s', '2s', '2p', '3s', '3p', '4s', '3d', '4p', '5s', '4d', '5p', '6s', '4f', '5d', '6p', '7s', '5f', '6d', '7p', '8s']\n \n replace_d ={\n'Cr':'3d5 4s1',\n'Cu':'3d10 4s1',\n'Nb':'4d4 5s1',\n'Mo':'4d5 5s1',\n'Ru':'4d7 5s1',\n'Rh':'4d8 5s1',\n'Pd':'4d10 5s0',\n'Ag':'4d10 5s1',\n'La':'4f0 5s2 5p6 5d1 6s2',\n'Ce':'4f1 5s2 5p6 5d1 6s2',\n'Gd':'4f7 5s2 5p6 5d1 6s2',\n'Pt':'4f14 5s2 5p6 5d9 6s1',\n'Au':'4f14 5s2 5p6 5d10 6s1',\n'Ac':'5f0 6s2 6p6 6d1 7s2',\n'Th':'5f0 6s2 6p6 6d2 7s2',\n'Pa':'5f2 6s2 6p6 6d1 7s2',\n'U':'5f3 6s2 6p6 6d1 7s2',\n'Np':'5f4 6s2 6p6 6d1 7s2',\n'Cm':'5f7 6s2 6p6 6d1 7s2'\n}\n \n result = []\n \n while atomic > 0:\n orbital = filling.pop(0)\n if orbital[-1] == 's':\n if atomic > 1:\n result.append(orbital + '2')\n atomic -= 2\n else:\n result.append(orbital + '1')\n atomic -= 1\n elif orbital[-1] == 'p':\n if atomic > 5:\n result.append(orbital + '6')\n atomic -= 6\n else:\n result.append(orbital + str(atomic))\n atomic -= atomic\n elif orbital[-1] == 'd':\n if atomic > 9:\n result.append(orbital + '10')\n atomic -= 10\n else:\n result.append(orbital + str(atomic))\n atomic -= atomic\n elif orbital[-1] == 'f':\n if atomic > 13:\n result.append(orbital + '14')\n atomic -= 14\n else:\n result.append(orbital + str(atomic))\n atomic -= atomic\n \n result.sort(key=orbital_sort)\n result = f\"{element} -> {' '.join(result)}\"\n \n print(element)\n if element in replace_d:\n i = result.index(replace_d[element][:2])\n print((i, result[:i], replace_d[element]))\n result = result[:i] + replace_d[element]\n \n return result\n", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef get_electron_configuration(element):\n '''\n Return the electron configuration of the supplied element.\n '''\n #ELEMENTS\n # array is an array of the element symbols in order by\n # atomic number. The atomic number is 1 plus the index in the array\n # For example:\n # ELEMENTS = ['H', 'He', 'Li', ...]\n \n #exceptions array\n # Elements whose electron fill order are not normal. Format is\n # <element>:[<the remaining orbitals/electrons>]\n exceptions = \\\n {\"Cr\":[\"3d5\", \"4s1\"],\n \"Cu\":[\"3d10\", \"4s1\"],\n \"Nb\":[\"4d4\", \"5s1\"],\n \"Mo\":[\"4d5\", \"5s1\"],\n \"Ru\":[\"4d7\", \"5s1\"],\n \"Rh\":[\"4d8\", \"5s1\"],\n \"Pd\":[\"4d10\", \"5s0\"],\n \"Ag\":[\"4d10\", \"5s1\"],\n \"La\":[\"4f0\", \"5d1\"],\n \"Ce\":[\"4f1\", \"5d1\"],\n \"Gd\":[\"4f7\", \"5d1\", \"6s2\"],\n \"Pt\":[\"4f14\", \"5d9\", \"6s1\"],\n \"Au\":[\"4f14\", \"5d10\", \"6s1\"],\n \"Ac\":[\"5f0\", \"6d1\", \"7s2\"],\n \"Th\":[\"5f0\", \"6d2\", \"7s2\"],\n \"Pa\":[\"5f2\", \"6d1\", \"7s2\"],\n \"U\":[\"5f3\", \"6d1\", \"7s2\"],\n \"Np\":[\"5f4\", \"6d1\", \"7s2\"],\n \"Cm\":[\"5f7\", \"6d1\", \"7s2\"]}\n\n #orbitals array\n # Orbitals are listed in order they are normally filled. Also listed\n # with their maximum fill\n orbitals = [\"1s2\", \"2s2\", \"2p6\", \"3s2\", \"3p6\", \"4s2\", \"3d10\", \"4p6\", \"5s2\",\n \"4d10\", \"5p6\", \"6s2\", \"4f14\", \"5d10\", \"6p6\", \"7s2\", \"5f14\",\n \"6d10\", \"7p6\", \"8s2\"]\n\n atomicNumber = ELEMENTS.index(element) + 1\n numElectrons = atomicNumber\n orbitalIndex = 0 #Index into the array of orbitals\n config = [] #List of the orbitals with electrons\n \n while numElectrons > 0: #If orbital is filled\n if numElectrons - int(orbitals[orbitalIndex][2:]) > 0:\n config.append(orbitals[orbitalIndex])\n else: # otherwise add the remaining e's to this orbital\n config.append(orbitals[orbitalIndex][:2] + str(numElectrons))\n \n numElectrons -= int(orbitals[orbitalIndex][2:])\n orbitalIndex += 1\n\n if element in list(exceptions.keys()): #Abnormal electron fill\n end = exceptions[element] #Retrieve the config of the last few e's\n e = 0 #The number of abnormal e's\n for orb in end: # sum them\n e += int(orb[2:])\n while e > 0: #Remove the orbitals at end that have\n e -= int(config.pop()[2:]) # than number of electrons\n\n config.extend(end) #Add back the abnormal electon fill\n\n return element + \" -> \" + \" \".join(sorted(config, key=lambda x : x[0]))\n", "ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']\ndef get_electron_configuration(element): \n n=ELEMENTS.index(element)+1\n import re\n a= 'Cr -> ...3d5 4s1 Cu -> ...3d10 4s1 Nb -> ...4d4 5s1 Mo -> ...4d5 5s1 Ru -> ...4d7 5s1 Rh -> ...4d8 5s1 Pd -> ...4d10 5s0 Ag -> ...4d10 5s1 La -> ...4f0 5d1 Ce -> ...4f1 5d1 Gd -> ...4f7 5d1 6s2 Pt -> ...4f14 5d9 6s1 Au -> ...4f14 5d10 6s1 Ac -> ...5f0 6d1 7s2 Th -> ...5f0 6d2 7s2 Pa -> ...5f2 6d1 7s2 U -> ...5f3 6d1 7s2 Np -> ...5f4 6d1 7s2 Cm -> ...5f7 6d1 7s2'\n a='Ni 3d8 4s2 Cr 3d5 4s1 Cu 3d10 4s1 Nb 4d4 5s1 Mo 4d5 5s1 Ru 4d7 5s1 Rh 4d8 5s1 Pd 4d10 5s0 Ag 4d10 5s1 La 4f0 5d1 Ce 4f1 5d1 Gd 4f7 5d1 6s2 Pt 4f14 5d9 6s1 Au 4f14 5d10 6s1 Ac 5f0 6d1 7s2 Th 5f0 6d2 7s2 Pa 5f2 6d1 7s2 U 5f3 6d1 7s2 Np 5f4 6d1 7s2 Cm 5f7 6d1 7s2'\n a={'Ni': ['Ni', '3d8', '4s2'], 'Ac': ['Ac', '5f0', '6d1', '7s2'], 'Pa': ['Pa', '5f2', '6d1', '7s2'], 'Pt': ['Pt', '4f14', '5d9', '6s1'], 'Ag': ['Ag', '4d10', '5s1'], 'Ru': ['Ru', '4d7', '5s1'], 'Nb': ['Nb', '4d4', '5s1'], 'La': ['La', '4f0', '5d1'], 'U': ['U', '5f3', '6d1', '7s2'], 'Ce': ['Ce', '4f1', '5d1'], 'Th': ['Th', '5f0', '6d2', '7s2'], 'Gd': ['Gd', '4f7', '5d1', '6s2'], 'Au': ['Au', '4f14', '5d10', '6s1'], 'Cm': ['Cm', '5f7', '6d1', '7s2'], 'Pd': ['Pd', '4d10', '5s0'], 'Np': ['Np', '5f4', '6d1', '7s2'], 'Cr': ['Cr', '3d5', '4s1'], 'Rh': ['Rh', '4d8', '5s1'], 'Mo': ['Mo', '4d5', '5s1'], 'Cu': ['Cu', '3d10', '4s1']}\n a={'Ni': ['3d8', '4s2'], 'Ac': ['5f0', '6d1', '7s2'], 'Gd': ['4f7', '5d1', '6s2'], 'Ag': ['4d10', '5s1'], 'Pt': ['4f14', '5d9', '6s1'], 'Ru': ['4d7', '5s1'], 'Nb': ['4d4', '5s1'], 'La': ['4f0', '5d1'], 'Ce': ['4f1', '5d1'], 'Cm': ['5f7', '6d1', '7s2'], 'Pa': ['5f2', '6d1', '7s2'], 'U': ['5f3', '6d1', '7s2'], 'Cu': ['3d10', '4s1'], 'Th': ['5f0', '6d2', '7s2'], 'Cr': ['3d5', '4s1'], 'Np': ['5f4', '6d1', '7s2'], 'Au': ['4f14', '5d10', '6s1'], 'Rh': ['4d8', '5s1'], 'Mo': ['4d5', '5s1'], 'Pd': ['4d10', '5s0']} \n a={'Ni': [['3d', '8'], ['4s', '2']], 'Ac': [['5f', '0'], ['6d', '1'], ['7s', '2']], 'Gd': [['4f', '7'], ['5d', '1'], ['6s', '2']], 'Pt': [['4f', '14'], ['5d', '9'], ['6s', '1']], 'Ag': [['4d', '10'], ['5s', '1']], 'Ru': [['4d', '7'], ['5s', '1']], 'Nb': [['4d', '4'], ['5s', '1']], 'La': [['4f', '0'], ['5d', '1']], 'Ce': [['4f', '1'], ['5d', '1']], 'Cm': [['5f', '7'], ['6d', '1'], ['7s', '2']], 'Pa': [['5f', '2'], ['6d', '1'], ['7s', '2']], 'U': [['5f', '3'], ['6d', '1'], ['7s', '2']], 'Cu': [['3d', '10'], ['4s', '1']], 'Th': [['5f', '0'], ['6d', '2'], ['7s', '2']], 'Np': [['5f', '4'], ['6d', '1'], ['7s', '2']], 'Cr': [['3d', '5'], ['4s', '1']], 'Rh': [['4d', '8'], ['5s', '1']], 'Mo': [['4d', '5'], ['5s', '1']], 'Pd': [['4d', '10'], ['5s', '0']], 'Au': [['4f', '14'], ['5d', '10'], ['6s', '1']]} \n o=['1s','2s','2p','3s','3p','4s','3d','4p','5s','4d','5p','6s','4f','5d','6p','7s','5f','6d','7p','8s']\n y={}\n d={}\n if element in a:\n a=a[element]\n for i in a:\n d[i[0]]=i[1]\n for i in o:\n if i[1]=='s':\n lim=2\n if i[1]=='p':\n lim=6\n if i[1]=='d':\n lim=10\n if i[1]=='f':\n lim=14 \n if n>lim:\n y[i]=str(lim)\n n=n-lim\n continue\n else:\n y[i]=str(n)\n break\n z = dict(list(y.items()) + list(d.items()))\n l=['1s','2s','2p','3s','3p','3d','4s','4p','4d','4f','5s','5p','5d','5f','6s','6p','6d','7s','7p','8s']\n r=''\n for i in l: \n if i in z.keys(): \n r+=i+z[i]+' '\n r= element+' -> '+r[::-1][1:][::-1]\n return r"] | {"fn_name": "get_electron_configuration", "inputs": [["H"], ["Cr"], ["C"], ["Br"], ["V"]], "outputs": [["H -> 1s1"], ["Cr -> 1s2 2s2 2p6 3s2 3p6 3d5 4s1"], ["C -> 1s2 2s2 2p2"], ["Br -> 1s2 2s2 2p6 3s2 3p6 3d10 4s2 4p5"], ["V -> 1s2 2s2 2p6 3s2 3p6 3d3 4s2"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 29,542 |
ELEMENTS = ['H', 'He', 'Li', 'Be', 'B', 'C', 'N', 'O', 'F', 'Ne', 'Na', 'Mg', 'Al', 'Si', 'P', 'S', 'Cl', 'Ar', 'K', 'Ca', 'Sc', 'Ti', 'V', 'Cr', 'Mn', 'Fe', 'Co', 'Ni', 'Cu', 'Zn', 'Ga', 'Ge', 'As', 'Se', 'Br', 'Kr', 'Rb', 'Sr', 'Y', 'Zr', 'Nb', 'Mo', 'Tc', 'Ru', 'Rh', 'Pd', 'Ag', 'Cd', 'In', 'Sn', 'Sb', 'Te', 'I', 'Xe', 'Cs', 'Ba', 'La', 'Ce', 'Pr', 'Nd', 'Pm', 'Sm', 'Eu', 'Gd', 'Tb', 'Dy', 'Ho', 'Er', 'Tm', 'Yb', 'Lu', 'Hf', 'Ta', 'W', 'Re', 'Os', 'Ir', 'Pt', 'Au', 'Hg', 'Tl', 'Pb', 'Bi', 'Po', 'At', 'Rn', 'Fr', 'Ra', 'Ac', 'Th', 'Pa', 'U', 'Np', 'Pu', 'Am', 'Cm', 'Bk', 'Cf', 'Es', 'Fm', 'Md', 'No', 'Lr', 'Rf', 'Db', 'Sg', 'Bh', 'Hs', 'Mt', 'Ds', 'Rg', 'Cn', 'Nh', 'Fl', 'Mc', 'Lv', 'Ts', 'Og']
def get_electron_configuration(element):
|
88c29e38c482689e52aa938cfe200718 | UNKNOWN | John has some amount of money of which he wants to deposit a part `f0` to the bank at the beginning
of year `1`. He wants to withdraw each year for his living an amount `c0`.
Here is his banker plan:
- deposit `f0` at beginning of year 1
- his bank account has an interest rate of `p` percent per year, constant over the years
- John can withdraw each year `c0`, taking it whenever he wants in the year; he must take account of an inflation of `i` percent per year in order to keep his quality of living. `i` is supposed to stay constant over the years.
- all amounts f0..fn-1, c0..cn-1 are truncated by the bank to their integral part
- Given f0, `p`, c0, `i`
the banker guarantees that John will be able to go on that way until the `nth` year.
# Example:
```
f0 = 100000, p = 1 percent, c0 = 2000, n = 15, i = 1 percent
```
```
beginning of year 2 -> f1 = 100000 + 0.01*100000 - 2000 = 99000; c1 = c0 + c0*0.01 = 2020 (with inflation of previous year)
```
```
beginning of year 3 -> f2 = 99000 + 0.01*99000 - 2020 = 97970; c2 = c1 + c1*0.01 = 2040.20
(with inflation of previous year, truncated to 2040)
```
```
beginning of year 4 -> f3 = 97970 + 0.01*97970 - 2040 = 96909.7 (truncated to 96909);
c3 = c2 + c2*0.01 = 2060.4 (with inflation of previous year, truncated to 2060)
```
and so on...
John wants to know if the banker's plan is right or wrong.
Given parameters `f0, p, c0, n, i` build a function `fortune` which returns `true` if John can make a living until the `nth` year
and `false` if it is not possible.
# Some cases:
```
fortune(100000, 1, 2000, 15, 1) -> True
fortune(100000, 1, 10000, 10, 1) -> True
fortune(100000, 1, 9185, 12, 1) -> False
For the last case you can find below the amounts of his account at the beginning of each year:
100000, 91815, 83457, 74923, 66211, 57318, 48241, 38977, 29523, 19877, 10035, -5
```
f11 = -5 so he has no way to withdraw something for his living in year 12.
> **Note:** Don't forget to convert the percent parameters as percentages in the body of your function: if a parameter percent is 2 you have to convert it to 0.02. | ["def fortune(f, p, c, n, i):\n for _ in range(n-1):\n f = int(f * (100 + p) / 100 - c)\n c = int(c * (100 + i) / 100)\n if f < 0:\n return False\n return True", "def fortune(money, interest, withdraw, years, inflation):\n interest = 1 + (interest / 100)\n inflation = 1 + (inflation / 100)\n for _ in range(years - 1):\n money = int(money * interest - withdraw)\n if money < 0:\n return False\n withdraw *= inflation\n return True", "import math\n\ndef fortune(f0, p, c0, n, i):\n f0 = math.trunc(f0 * (1 + p / 100) - c0)\n c0 = c0 * (1 + i/100)\n if n == 2:\n return True if f0 >= 0 else False\n else:\n return fortune(f0, p, c0, n-1, i)", "def fortune(f0, p, c0, n, i):\n f = f0\n c = c0\n \n for year in range(n-1):\n f = int(f + f * (p/100)) - int(c)\n c = int(c + c * (i/100))\n \n return f >= 0", "def fortune(f0, p, c0, n, i):\n for t in range(n-1):\n f0 = int(f0 + f0*p/100 - c0)\n c0 = int(c0+c0*i/100)\n if f0>=0 :\n return True\n return False", "def fortune(f0, p, c0, n, i):\n year_checker = 0\n fn = f0\n cn = c0\n \n while(year_checker < n-1):\n fn = int(fn + fn * (0.01 * p) - cn)\n cn = int(cn + cn * (0.01 * i))\n \n year_checker = year_checker + 1\n \n if(fn < 0):\n return False\n\n return True", "def fortune(f0, p, c0, n, i):\n \n year = 1\n while f0 > 0 and year < n:\n f0 = f0 * (1 + p / 100) - c0\n f0 = int(f0)\n c0 = c0 * (1 + i / 100)\n c0 = int(c0)\n year += 1\n \n return True if f0 >= 0 else False\n", "def fortune(f0, p, c0, n, i):\n print(f0, p, c0, n, i)\n for _ in range(n-1):\n f0 = f0 * (100 + p) // 100 - c0\n c0 = c0 * (100 + i) // 100\n \n return f0 >= 0", "def fortune(f0, p, c0, n, i):\n inf = c0\n money = f0\n for j in range(n-1):\n money = int(money + money*(p/100) - inf)\n inf = int(inf + inf*(i/100))\n\n return True if money >= 0 else False", "fortune=F=lambda f,p,c,n,i:F(f*(100+p)//100-c,p,c*(100+i)//100,n-1,i)if n>1else f>=0"] | {"fn_name": "fortune", "inputs": [[100000, 1, 2000, 15, 1], [100000, 1, 9185, 12, 1], [100000000, 1, 100000, 50, 1], [100000000, 1.5, 10000000, 50, 1], [100000000, 5, 1000000, 50, 1], [9999, 61.8161, 10000.0, 3, 0], [9998.5, 61.8155, 10000.0, 3, 0], [10000.0, 0, 10000.0, 2, 0], [999.5, 61.87, 1000.0, 3, 0]], "outputs": [[true], [false], [true], [false], [true], [false], [false], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,226 |
def fortune(f0, p, c0, n, i):
|
e1d84af03ed54c82c834b52abeb3ef23 | UNKNOWN | **This Kata is intended as a small challenge for my students**
All Star Code Challenge #29
Your friend Nhoj has dislexia, but can easily read messages if the words are written backwards.
Create a function called `reverseSentence()/reverse_sentence()` that accepts a string argument. The function returns a string of the same length with each word reversed, but still in their original order.
```python
reverse_sentence("Hello !Nhoj Want to have lunch?") # "olleH johN! tnaW ot evah ?hcnul"
```
Note:
A "word" should be considered a string split by a space character, " "
Letter capitalization should be maintained. | ["def reverse_sentence(sentence):\n return ' '.join(w[::-1] for w in sentence.split())", "def reverse_sentence(sentence):\n return \" \".join(word[::-1] for word in sentence.split())", "def reverse_sentence(sentence):\n return ' '.join(reversed(sentence[::-1].split(' ')))\n", "def reverse_sentence(sentence):\n #your code here\n s = ' '.join(reversed(sentence.split()))\n return s[::-1]", "reverse_sentence = lambda s: ' '.join(x[::-1] for x in s.split())", "reverse_sentence=lambda s: \" \".join([a[::-1] for a in s.split()])", "def reverse_sentence(s):\n return \" \".join(c[::-1] for c in s.split())", "def reverse_sentence(sentence):\n words = sentence.split()\n s = \"\"\n for w in words:\n s = s + w[::-1] + \" \"\n return s[:-1]", "def reverse_sentence(sentence):\n return ' '.join(a[::-1] for a in sentence.split())\n", "def reverse_sentence(s):\n return ' '.join([i[::-1] for i in s.split()])"] | {"fn_name": "reverse_sentence", "inputs": [["Hello !Nhoj Want to have lunch?"], ["1 2 3 4 5"], ["CodeWars"], ["CodeWars rules!"], [""]], "outputs": [["olleH johN! tnaW ot evah ?hcnul"], ["1 2 3 4 5"], ["sraWedoC"], ["sraWedoC !selur"], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 948 |
def reverse_sentence(sentence):
|
2d7d01de7762cff5416360df4f18fee2 | UNKNOWN | # Task
Round the given number `n` to the nearest multiple of `m`.
If `n` is exactly in the middle of 2 multiples of m, return `n` instead.
# Example
For `n = 20, m = 3`, the output should be `21`.
For `n = 19, m = 3`, the output should be `18`.
For `n = 50, m = 100`, the output should be `50`.
# Input/Output
- `[input]` integer `n`
`1 ≤ n < 10^9.`
- `[input]` integer `m`
`3 ≤ m < 109`.
- `[output]` an integer | ["def rounding(n, m):\n return n if n % m == m / 2 else m * round(n / m)", "def rounding(n,m):\n down, up = (n//m)*m, (n//m)*m+m\n if up-n < n-down : return up\n elif up-n == n-down : return n\n return down", "def rounding(n, m):\n return n if (n / m) % 1 == 0.5 else round(n / m) * m", "def rounding(n, m):\n diff = n % m\n \n if diff < m / 2:\n return n - diff\n elif diff > m / 2:\n return n - diff + m\n return n", "def rounding(n,m):\n if m/n==2:\n return n\n return round(n/m)*m", "def rounding(n, m):\n q, r = divmod(n, m)\n return q * m if 2 * r < m else (q + 1) * m if 2 * r > m else n", "from math import gcd, ceil\ndef rounding(n,m):\n return round(n/m)*m if n != ((n//m)*m + ceil(n/m)*m)/2 else n", "from math import ceil, floor\ndef rounding(n,m):\n a,b = m*ceil(n/m),m*floor(n/m)\n da,db = abs(n-a),abs(n-b)\n if(da == db): return n\n if(da < db): return a\n return b", "import math\n\ndef rounding(n,m):\n a = n - math.floor(n / m) * m\n b = math.ceil(n / m) * m - n\n return n if a == b else math.floor(n / m) * m if a < b else math.ceil(n / m) * m", "def rounding(n, m):\n floored = n // m * m\n diff = n - floored\n return floored if 2 * diff < m else floored + m if 2 * diff > m else n"] | {"fn_name": "rounding", "inputs": [[20, 3], [19, 3], [1, 10], [50, 100], [123, 456]], "outputs": [[21], [18], [0], [50], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,302 |
def rounding(n, m):
|
77c0264e4c487088c3af2ecff69eb5ce | UNKNOWN | For an integer ```k``` rearrange all the elements of the given array in such way, that:
all elements that are less than ```k``` are placed before elements that are not less than ```k```;
all elements that are less than ```k``` remain in the same order with respect to each other;
all elements that are not less than ```k``` remain in the same order with respect to each other.
For ```k = 6``` and ```elements = [6, 4, 10, 10, 6]```, the output should be
```splitByValue(k, elements) = [4, 6, 10, 10, 6]```.
For ```k``` = 5 and ```elements = [1, 3, 5, 7, 6, 4, 2]```, the output should be
```splitByValue(k, elements) = [1, 3, 4, 2, 5, 7, 6]```.
S: codefights.com | ["def split_by_value(k, elements):\n return sorted(elements, key=lambda x: x >= k)", "def split_by_value(k, elements):\n return [x for x in elements if x < k] + [x for x in elements if x >= k]", "def split_by_value(k, elements):\n res = [[], []]\n for x in elements:\n res[k<=x].append(x)\n return res[0] + res[1]", "def split_by_value(k, elements):\n return sorted(elements, key=lambda y: y>=k)\n \n", "def split_by_value(k, elements):\n return sorted(elements, key=k.__le__)", "def split_by_value(k, elements):\n return [x for f in (lambda x: x < k, lambda x: x >= k) for x in elements if f(x)]", "def split_by_value(k, elements):\n return [i for i in elements if i<k]+[i for i in elements if i>=k]", "def split_by_value(k, elements):\n return sorted(elements, key=lambda a: a>=k)", "def split_by_value(k, elements):\n l = []\n elem2 = elements.copy()\n for i in elements:\n if i < k:\n l.append(i)\n elem2.remove(i)\n \n return l + elem2", "from itertools import chain\n\ndef split_by_value(k, arr):\n lst = [[],[]]\n for v in arr: lst[v>=k].append(v)\n return list(chain(*lst))"] | {"fn_name": "split_by_value", "inputs": [[5, [1, 3, 5, 7, 6, 4, 2]], [0, [5, 2, 7, 3, 2]], [6, [6, 4, 10, 10, 6]], [1, [3, 2, 8, 3, 2, 1]], [10, [9, 2, 4, 3, 4]]], "outputs": [[[1, 3, 4, 2, 5, 7, 6]], [[5, 2, 7, 3, 2]], [[4, 6, 10, 10, 6]], [[3, 2, 8, 3, 2, 1]], [[9, 2, 4, 3, 4]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,189 |
def split_by_value(k, elements):
|
03b92381ac9a8c186aad8378a749c911 | UNKNOWN | *Inspired by the [Fold an Array](https://www.codewars.com/kata/fold-an-array) kata. This one is sort of similar but a little different.*
---
## Task
You will receive an array as parameter that contains 1 or more integers and a number `n`.
Here is a little visualization of the process:
* Step 1: Split the array in two:
```
[1, 2, 5, 7, 2, 3, 5, 7, 8]
/ \
[1, 2, 5, 7] [2, 3, 5, 7, 8]
```
* Step 2: Put the arrays on top of each other:
```
[1, 2, 5, 7]
[2, 3, 5, 7, 8]
```
* Step 3: Add them together:
```
[2, 4, 7, 12, 15]
```
Repeat the above steps `n` times or until there is only one number left, and then return the array.
## Example
```
Input: arr=[4, 2, 5, 3, 2, 5, 7], n=2
Round 1
-------
step 1: [4, 2, 5] [3, 2, 5, 7]
step 2: [4, 2, 5]
[3, 2, 5, 7]
step 3: [3, 6, 7, 12]
Round 2
-------
step 1: [3, 6] [7, 12]
step 2: [3, 6]
[7, 12]
step 3: [10, 18]
Result: [10, 18]
``` | ["def split_and_add(numbers, n):\n for _ in range(n):\n middle = len(numbers) // 2\n left = numbers[:middle]\n right = numbers[middle:]\n numbers = [a + b for a, b in zip((len(right) - len(left)) * [0] + left, right)]\n if len(numbers) == 1:\n return numbers\n return numbers\n", "def split_and_add(arr, n):\n for _ in range(n):\n half = len(arr) // 2\n arr = [a+b for a, b in zip([0] * (len(arr)%2) + arr[:half], arr[half:])]\n \n return arr", "from itertools import starmap\nfrom operator import add\n\ndef split_and_add(numbers, n):\n for __ in range(n):\n mid, uneven = divmod(len(numbers), 2)\n numbers = list(starmap(add, zip([0] * uneven + numbers[:mid], numbers[mid:])))\n return numbers", "from itertools import zip_longest\n\ndef split_and_add(xs, n):\n return split_and_add([(a or 0) + b for a, b in zip_longest(xs[:len(xs)//2][::-1], xs[len(xs)//2:][::-1])][::-1], n-1) if n else xs", "def split_and_add(a, n): \n return split_and_add([sum(e) for e in zip(([0] if len(a) % 2 else []) + a[:len(a)//2], a[len(a)//2:])], n - 1) if n else a", "from itertools import zip_longest\n\ndef split_and_add(numbers, n):\n while len(numbers) > 1 and n:\n n -= 1\n m = len(numbers) // 2\n it = zip_longest(reversed(numbers[:m]), reversed(numbers[m:]), fillvalue=0)\n numbers = list(map(sum, it))[::-1]\n return numbers", "def split_and_add(numbers, n):\n def splitter(arr):\n left, right = ([0] if len(arr) % 2 != 0 else []) + arr[:len(arr)//2], arr[len(arr)//2:]\n return [l + r for l, r in zip(left, right)]\n return numbers if n == 0 else split_and_add(splitter(numbers), n - 1)", "def split_and_add(numbers, n):\n arr = numbers[:]\n for _ in range(n):\n mid, rest = divmod(len(arr), 2)\n arr = [a + b for a, b in zip(rest * [0] + arr[:mid], arr[mid:])]\n return arr", "def split_and_add(numbers, n):\n for _ in range(n):\n m, r = divmod(len(numbers), 2)\n numbers = list(map(sum, zip(r * [0] + numbers[:m], numbers[m:])))\n return numbers", "def split_and_add(numbers, n):\n from itertools import zip_longest\n for i in range(n):\n length = len(numbers)//2\n numbers = list(reversed([x+y for x,y in zip_longest(reversed(numbers[0:length]), reversed(numbers[length:]), fillvalue=0)]))\n return numbers"] | {"fn_name": "split_and_add", "inputs": [[[1, 2, 3, 4, 5], 2], [[1, 2, 3, 4, 5], 3], [[15], 3], [[1, 2, 3, 4], 1], [[1, 2, 3, 4, 5, 6], 20], [[32, 45, 43, 23, 54, 23, 54, 34], 2], [[32, 45, 43, 23, 54, 23, 54, 34], 0], [[3, 234, 25, 345, 45, 34, 234, 235, 345], 3], [[3, 234, 25, 345, 45, 34, 234, 235, 345, 34, 534, 45, 645, 645, 645, 4656, 45, 3], 4], [[23, 345, 345, 345, 34536, 567, 568, 6, 34536, 54, 7546, 456], 20]], "outputs": [[[5, 10]], [[15]], [[15]], [[4, 6]], [[21]], [[183, 125]], [[32, 45, 43, 23, 54, 23, 54, 34]], [[305, 1195]], [[1040, 7712]], [[79327]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,426 |
def split_and_add(numbers, n):
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.