problem_id
stringlengths 32
32
| name
stringclasses 1
value | problem
stringlengths 200
14k
| solutions
stringlengths 12
1.12M
| test_cases
stringlengths 37
74M
| difficulty
stringclasses 3
values | language
stringclasses 1
value | source
stringclasses 7
values | num_solutions
int64 12
1.12M
| starter_code
stringlengths 0
956
|
---|---|---|---|---|---|---|---|---|---|
f2c928a3dc2531e742ce8fa2905d9caf | UNKNOWN | A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward as forward. Examples of numerical palindromes are:
2332
110011
54322345
For a given number `num`, write a function to test if it's a numerical palindrome or not and return a boolean (true if it is and false if not).
```if-not:haskell
Return "Not valid" if the input is not an integer or less than `0`.
```
```if:haskell
Return `Nothing` if the input is less than `0` and `Just True` or `Just False` otherwise.
```
Other Kata in this Series:
Numerical Palindrome #1
Numerical Palindrome #1.5
Numerical Palindrome #2
Numerical Palindrome #3
Numerical Palindrome #3.5
Numerical Palindrome #4
Numerical Palindrome #5 | ["def palindrome(num):\n if type(num) is not int or num <1:\n return \"Not valid\"\n return num == int(str(num)[::-1])", "def palindrome(num):\n return str(num) == str(num)[::-1] if type(num) == int and num > 0 else \"Not valid\"", "def palindrome(n):\n try:\n return 1. * n == int(str(n)[::-1])\n except:\n return \"Not valid\"", "def palindrome(n):\n return 'Not valid' if (type(n) != int or n < 0) else str(n) == str(n)[::-1]", "def palindrome(a):\n if type(a)==int:\n if a>0:\n a=str(a)\n b=a[::-1]\n if a==b:\n return True\n else:\n return False\n else:\n return \"Not valid\"\n else:\n return \"Not valid\"", "def palindrome(num):\n if type(num) == int and num > 0:\n return str(num) == str(num)[::-1]\n else:\n return 'Not valid'\n \n # or, as an ugly one line - just for practice:\n \n # return str(num) == str(num)[::-1] if type(num) == int and num > 0 else 'Not valid'\n", "def palindrome(num):\n return 'Not valid' if type(num) is not int or num < 0 else str(num) == str(num)[::-1]", "def palindrome(num):\n if type(num) != int or num < 0:\n return \"Not valid\"\n k = str(num)\n if k == k[::-1]:\n return True\n else:\n return False", "def palindrome(num):\n return str(num)[::-1] == str(num) if isinstance(num, int) and num > 0 else 'Not valid'", "def palindrome(num):\n if type(123)!=type(num) : return 'Not valid' \n n = str(num)\n if any(not c.isdigit() for c in n): return 'Not valid'\n l = len(n)\n return all(a==b for a, b in zip(n[:l//2], n[::-1]))", "def palindrome(num):\n if type(num) != int or num < 0:\n return \"Not valid\"\n else:\n return int(str(num)[::-1]) == num", "def palindrome(num):\n if not str(num).isdigit():\n return \"Not valid\" \n \n if type(num) == str:\n return \"Not valid\"\n \n num = str(num)\n \n return num == num[::-1]\n # Code here\n", "def palindrome(num):\n if (isinstance(num, int)== False) or (num < 0):\n return 'Not valid'\n else:\n if str(num) == str(num)[::-1]:\n return True\n else:\n return False", "def palindrome(num):\n def helper():\n if type(num) == str or num < 0:\n return 'Not valid'\n return True if str(num) == str(num)[::-1] else False\n try:\n int(num)\n except ValueError:\n return 'Not valid' \n else:\n return helper()", "def palindrome(n):\n # your code here\n if not isinstance(n, int) or n < 0:\n return \"Not valid\"\n rev, temp = 0, n\n while temp > 0:\n a = temp % 10\n rev = rev * 10 + a\n temp //= 10\n return rev == n", "def palindrome(num):\n return str(num) == str(num)[::-1] if type(num) is int and num >= 0 else 'Not valid'", "def palindrome(num):\n if isinstance(num, int) is False or num < 0:\n return \"Not valid\"\n return str(num) == str(num)[::-1]", "def palindrome(n):\n if type(n) == int and n > 0:\n n1,n2 = str(n),str(n)[::-1]\n return n1 == n2\n else:\n return 'Not valid'\n", "def palindrome(num):\n if isinstance(num,int) and num >= 0:\n num = str(num)\n return num == num[::-1]\n else:\n return \"Not valid\"", "class Numerical_paindrom_exception(Exception):\n def __init__(self):\n pass\n def __str__(self):\n return 'Not valid'\n\ndef palindrome(num):\n try:\n if type(num) != int or num < 0:\n raise Numerical_paindrom_exception\n return True if str(num)[::1] == str(num)[::-1] else False\n except Exception as e:\n return e.__str__()"] | {"fn_name": "palindrome", "inputs": [[1221], [110011], [1456009006541], [123322], [1], [152], [9999], ["ACCDDCCA"], ["@14AbC"], ["1221"], [-450]], "outputs": [[true], [true], [true], [false], [true], [false], [true], ["Not valid"], ["Not valid"], ["Not valid"], ["Not valid"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,824 |
def palindrome(num):
|
8bd3fc82725f37fd896b182c4f8b8abd | UNKNOWN | Story
Jumbo Juice makes a fresh juice out of fruits of your choice.Jumbo Juice charges $5 for regular fruits and $7 for special ones. Regular fruits are Banana, Orange, Apple, Lemon and Grapes. Special ones are Avocado, Strawberry and Mango. Others fruits that are not listed are also available upon request. Those extra special fruits cost $9 per each. There is no limit on how many fruits she/he picks.The price of a cup of juice is the mean of price of chosen fruits. In case of decimal number (ex. $5.99), output should be the nearest integer (use the standard rounding function of your language of choice).
Input
The function will receive an array of strings, each with the name of a fruit. The recognition of names should be case insensitive. There is no case of an enmpty array input.
Example
```
['Mango', 'Banana', 'Avocado'] //the price of this juice bottle is (7+5+7)/3 = $6($6.333333...)
``` | ["def mix_fruit(arr):\n regular = [\"banana\", \"orange\", \"apple\", \"lemon\", \"grapes\"]\n special = [\"avocado\", \"strawberry\", \"mango\"]\n return round(sum(5 if fruit.lower() in regular else (7 if fruit.lower() in special else 9) for fruit in arr)/len(arr))\n", "costs = { 'banana': 5, 'orange': 5, 'apple': 5, 'lemon': 5, 'grapes': 5,\n 'avocado': 7, 'strawberry': 7, 'mango': 7 }\n\ndef mix_fruit(arr):\n return round( sum( costs.get(fruit.lower(), 9) for fruit in arr ) / len(arr) )", "def mix_fruit(arr):\n d = {'banana':5,'orange':5,'apple':5,'lemon':5,'grapes':5,'avocado':7,'strawberry':7,'mango':7}\n return round(sum(d.get(i.lower(),9) for i in arr)/len(arr))", "PRICES = dict(banana=5, orange=5, apple=5, lemon=5, grapes=5, avocado=7, strawberry=7, mango=7)\n\n\ndef mix_fruit(fruits):\n return round(sum(PRICES.get(fruit.lower(), 9) for fruit in fruits) / len(fruits))", "def mix_fruit(arr):\n d1 = {x:5 for x in 'banana, orange, apple, lemon, grapes'.split(', ')}\n d2 = {x:7 for x in 'avocado, strawberry, mango'.split(', ')}\n res = sum(d1.get(x.lower(), d2.get(x.lower(), 9)) for x in arr)\n return round(res/len(arr))", "def mix_fruit(arr):\n arr = [x.lower() for x in arr]\n total = 0\n total_number_of_fruits= len(arr)\n for fruit in arr:\n if fruit in ['banana','orange','apple','lemon','grapes']:\n total += 5\n elif fruit in ['avocado','strawberry','mango']:\n total += 7\n else:\n total += 9\n \n answer = round(total / total_number_of_fruits)\n return answer\n", "def mix_fruit(arr):\n prices = {'banana': 5, 'orange': 5, 'apple': 5, 'lemon': 5, 'grapes': 5, 'avocado': 7, 'strawberry': 7, 'mango': 7}\n return round(sum(prices[f.lower()] if f.lower() in prices else 9 for f in arr) / len(arr))", "from collections import defaultdict\nfrom statistics import mean\n\nprices = defaultdict(lambda: 9)\nprices.update(dict.fromkeys(['banana', 'orange', 'apple', 'lemon', 'grapes'], 5))\nprices.update(dict.fromkeys(['avocado', 'strawberry', 'mango'], 7))\n\ndef mix_fruit(arr):\n return round(mean(prices[fruit.lower()] for fruit in arr))", "from statistics import mean\n\nregular = \"Banana Orange Apple Lemon Grapes\"\nspecial = \"Avocado Strawberry Mango\"\nextra = \"\"\n\nmenu = {\n regular: 5,\n special: 7,\n extra: 9\n}\nprices = {\n juice: price\n for juices, price in menu.items()\n for juice in juices.split()\n}\n\ndef mix_fruit(order):\n return round(mean(prices.get(juice.title(), menu[extra]) for juice in order))", "def mix_fruit(arr):\n Regular = ['banana', 'orange', 'apple', 'lemon', 'grapes']\n Special = ['avocado', 'strawberry', 'mango']\n cost = []\n for fruit in arr:\n fruit = fruit.lower()\n if fruit in Regular:\n cost.append(5)\n elif fruit in Special:\n cost.append(7)\n else:\n cost.append(9)\n return round(sum(cost)/len(cost))"] | {"fn_name": "mix_fruit", "inputs": [[["banana", "mango", "avocado"]], [["melon", "Mango", "kiwi"]], [["watermelon", "cherry", "avocado"]], [["watermelon", "lime", "tomato"]], [["blackBerry", "coconut", "avocado"]], [["waterMelon", "mango"]], [["watermelon", "pEach"]], [["watermelon", "Orange", "grapes"]], [["watermelon"]], [["BlACKbeRrY", "cOcONuT", "avoCaDo"]]], "outputs": [[6], [8], [8], [9], [8], [8], [9], [6], [9], [8]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,011 |
def mix_fruit(arr):
|
7818a1a19bfb3983fff1686f337cc088 | UNKNOWN | Transpose means is to interchange rows and columns of a two-dimensional array matrix.
[A^(T)]ij=[A]ji
ie:
Formally, the i th row, j th column element of AT is the j th row, i th column element of A:
Example :
```
[[1,2,3],[4,5,6]].transpose() //should return [[1,4],[2,5],[3,6]]
```
Write a prototype transpose to array in JS or add a .transpose method in Ruby or create a transpose function in Python so that any matrix of order ixj 2-D array returns transposed Matrix of jxi .
Link: To understand array prototype | ["import numpy as np\n\ndef transpose(A):\n if len(A) == 0:\n return []\n return np.array(A, dtype = 'O').T.tolist() if len(A[0]) > 0 else [[]]", "def transpose(arr):\n if arr and not any(map(bool,arr)): return [[]]\n return list(map(list,zip(*arr)))", "def transpose(arr):\n tran_each = []\n tran_com = []\n if len(arr) > 0 and len(arr[0]) > 0:\n n_row = len(arr[0])\n n_col = len(arr)\n for j in range(n_row):\n for i in range(n_col):\n tran_each.append(arr[i][j])\n tran_com.append(tran_each)\n tran_each = []\n return tran_com\n elif len(arr) > 0:\n return [[]]\n else:\n return []", "def transpose(arr):\n lst = []\n if len(arr) == 0:\n return arr\n for i in range(len(arr[0])):\n lst.append([arr[j][i] for j in range(len(arr))])\n return [[]] if not lst else lst", "def transpose(arr):\n if len(arr) == 0:\n return []\n if len(arr[0]) == 0:\n return [[]]\n return [[arr[row][col] for row in range(len(arr))] for col in range(len(arr[0]))]", "def transpose(arr):\n return [] if arr==[] else [[]] if arr[0]==[] else [[arr[i][j] for i in range(len(arr))] for j in range(len(arr[0]))]"] | {"fn_name": "transpose", "inputs": [[[]], [[[1]]], [[[0, 1]]], [[[1, 2, 3], [4, 5, 6]]], [[[1, 2, 3, 4, 5, 6]]], [[[1], [2], [3], [4], [5], [6]]], [[["a", "b", "c"], ["d", "e", "f"]]], [[[true, false, true], [false, true, false]]], [[[]]], [[[], [], [], [], [], []]]], "outputs": [[[]], [[[1]]], [[[0], [1]]], [[[1, 4], [2, 5], [3, 6]]], [[[1], [2], [3], [4], [5], [6]]], [[[1, 2, 3, 4, 5, 6]]], [[["a", "d"], ["b", "e"], ["c", "f"]]], [[[true, false], [false, true], [true, false]]], [[[]]], [[[]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,264 |
def transpose(arr):
|
e8e38391ef1436b6218a8c390156ac7a | UNKNOWN | We like parsed SQL or PL/SQL blocks...
You need to write function that return list of literals indices from source block, excluding "in" comments, OR return empty list if no literals found.
input:
some fragment of sql or pl/sql code
output:
list of literals indices [(start, end), ...] OR empty list
Sample:
```
get_textliterals("'this' is sample") -> [(0,6)]
get_textliterals("'this' is sample 'too'") -> [(0, 6), (15, 20)]
```
Text literal: any text between single quotes
Sample:
```
s := 'i am literal'
```
Single-line comment: any text started with "--"
Sample:
```
a := 1;
-- this is single-line comment
```
Multy-line comment: any text between /* */
```
a := 1;
/*
this is long multy-line comment
*/
```
Note:
1) handle single quote inside literal
```
s := 'we can use quote '' in literal'
```
2) multy-line literal
```
s := '
this is literal too
';
```
3) skip literal inside comment
```
s := 'test'; --when comment started - this is not 'literal'
```
4) any unclosed literal should be closed with last symbol of the source fragment
```
s := 'test
```
There is one literal in this code: "'test" | ["import re\n\nSKIPERS = re.compile(r'|'.join([\"\\\\\\*.*?\\*/\", \"--.*?(\\n|$)\", \"''\"]))\n\ndef get_textliterals(code):\n code = SKIPERS.sub(lambda m: \"x\"*len(m.group()) , code.rstrip())\n if code.count(\"'\") % 2: code += \"'\"\n return [(m.start(), m.end()) for m in re.finditer(r\"'.+?'\", code, flags=re.DOTALL)]", "def get_textliterals(pv_code):\n start_index = None\n all_index_store = []\n a_comment = False\n b_comment = False\n for index, char in enumerate(pv_code):\n if char == '*' and pv_code[index - 1] == '/':\n a_comment = True\n elif a_comment == True and char == '/' and pv_code[index - 1] == '*':\n a_comment = False\n elif char == '-' and pv_code[index - 1] == '-':\n b_comment = True\n elif b_comment == True and char == '\\n':\n b_comment = False\n elif (char == '\\'' or len(pv_code) - 1 == index) and a_comment == False and b_comment == False:\n if start_index != None:\n if (index > 0 and pv_code[index - 1] == '\\'') or (len(pv_code) > index + 1 and pv_code[index + 1] == '\\''):\n continue\n all_index_store.append((start_index, index + 1))\n start_index = None\n else:\n start_index = index\n return all_index_store\n \n", "import re\ndef get_textliterals(pv_code):\n return [(match.start(1), match.end(1)) for match in re.finditer(r\"(?:--[^\\n]*\\n?|/\\*.*?\\*/|((?:'[^']*')+|'[^']*$)|.)\", pv_code, re.DOTALL) if match.group(1)]", "import re\ndef get_textliterals(pv_code):\n print(pv_code)\n result = [(match.start(1), match.end(1)) for match in re.finditer(r\"(?:--[^\\n]*\\n?|/\\*.*?\\*/|((?:'[^']*')+|'[^']*$)|.)\", pv_code, re.MULTILINE) if match.group(1)]\n return result\n", "def comm_check(l, p_l, comm_started):\n if (l == '/' and p_l == '*' and comm_started == 2):\n return 0\n if (comm_started == 1 and l == '\\n'):\n return 0\n if (l == '-' and p_l == '-'):\n return 1\n elif (l == '*' and p_l == '/'):\n return 2\n else:\n return comm_started\n \n\n\ndef get_textliterals(pv_code):\n indices = []\n open_i = -1\n comm_start = 0\n for i in range(0, len(pv_code)):\n comm_start = comm_check(pv_code[i], pv_code[i-1], comm_start)\n if pv_code[i] == '\\'' and comm_start == 0:\n if open_i == -1:\n open_i = i\n else:\n if (i + 1 < len(pv_code) and i - 1 >= 0): \n if (pv_code[i+1] == '\\'' or pv_code[i-1] == '\\''): continue\n indices.append((open_i, i + 1))\n open_i = -1\n if open_i != -1: indices.append((open_i, len(pv_code)))\n return indices", "def get_textliterals(pv_code):\n\n l_list = []\n l_switch = \"empty\"\n \n for i in range(len(pv_code)):\n \n if pv_code[i] == \"'\" and pv_code[i:i+2] != \"''\" and pv_code[i-1:i+1] != \"''\":\n if l_switch == \"empty\":\n l_begin = i\n l_switch = \"begin\"\n elif l_switch == \"begin\":\n l_list.append((l_begin,i+1))\n l_switch = \"empty\"\n elif pv_code[i:i+2] == \"--\":\n if l_switch != \"begin\" and l_switch != \"rem2\":\n l_switch = \"rem1\"\n elif pv_code[i:i+2] == \"/*\":\n if l_switch != \"begin\" and l_switch != \"rem1\":\n l_switch = \"rem2\"\n elif pv_code[i:i+2] == \"*/\":\n if l_switch == \"rem2\":\n l_switch = \"empty\"\n elif pv_code[i] == \"\\n\":\n if l_switch == \"rem1\":\n l_switch = \"empty\"\n if l_switch == \"begin\":\n l_list.append((l_begin,i+1))\n \n \n return l_list ", "def get_textliterals(pv_code):\n r=[]\n i=0\n in_quote=False\n while(i<len(pv_code)):\n if in_quote:\n if pv_code[i]==\"'\":\n if pv_code[i:].startswith(\"''\"):\n i+=1\n else:\n r.append((s,i+1))\n in_quote=False\n else:\n if pv_code[i]==\"'\":\n s=i\n in_quote=True\n elif pv_code[i:].startswith('--'):\n i=pv_code.index('\\n',i)\n elif pv_code[i:].startswith('/*'):\n i=pv_code.index('*/',i+2)\n i+=1\n i+=1\n if in_quote:\n r.append((s,len(pv_code)))\n return r", "import re\ndef comment_replace(comment):\n return ' '*len(comment)\ndef one_line_comment_replace(comment):\n return ' '*len(comment)\n\ndef get_textliterals(pv_code):\n pv_code = re.sub(r'/\\*.*\\*/', lambda mo : comment_replace(mo.group()), pv_code)\n pv_code = re.sub(r'(--.*)', lambda mo : one_line_comment_replace(mo.group()), pv_code)\n pv_code = re.sub(r\"\\'\\'\", ' ', pv_code)\n results = list()\n while \"'\" in pv_code:\n start_index = pv_code.index(\"'\")\n pv_code = pv_code[:pv_code.index(\"'\")]+'X'+pv_code[pv_code.index(\"'\")+1:]\n if \"'\" in pv_code:\n end_index = pv_code.index(\"'\")+1\n pv_code = pv_code[:pv_code.index(\"'\")]+'X'+pv_code[pv_code.index(\"'\")+1:]\n if start_index < end_index:\n indxs = (start_index, end_index)\n else:\n indxs = (start_index, len(pv_code))\n results.append(indxs) \n return results", "def get_textliterals(pv_code):\n literal_start = -1\n result = []\n comment = False\n inline_comment = False\n quote = False\n for idx, c in enumerate(pv_code):\n\n if idx + 1 == len(pv_code) and literal_start != -1:\n literal_end = idx + 1\n result.append((literal_start, literal_end))\n break\n elif c == '*' and pv_code[idx - 1] == '/':\n comment = True\n elif c == '/' and pv_code[idx -1] == '*' and comment:\n comment = False\n elif c == \"'\" and literal_start != -1 and not comment and not inline_comment:\n if idx+1 < len(pv_code) and pv_code[idx+1] == \"'\":\n quote = True\n elif pv_code[idx-1] == \"'\":\n continue\n else:\n literal_end = idx + 1\n result.append((literal_start, literal_end))\n literal_start = -1\n quote = False\n elif c == \"'\" and quote == False and not comment and not inline_comment:\n literal_start = idx\n elif c == '-' and pv_code[idx-1] == '-':\n inline_comment = True\n elif c == '\\n':\n inline_comment = False\n return result", "from re import finditer\ndef get_textliterals(pv_code):\n l = len(pv_code)\n pv_code = pv_code.replace(\"''\", '\"\"')\n addedQuote = False\n if not pv_code.endswith(\"'\"):\n addedQuote = True\n pv_code += \"'\"\n patterns = [r\"/\\*(?:\\n|.)*?\\*/\", \"--.+\", \"'(?:\\n|.)*?'\"]\n comments = [m.span() for m in finditer(patterns[0], pv_code)] +\\\n [m.span() for m in finditer(patterns[1], pv_code)]\n answer = [] \n candidates = [m.span() for m in finditer(patterns[2], pv_code)]\n for (startA, endA) in candidates:\n ok = True\n for (startB, endB) in comments:\n if startB < startA < endA < endB:\n ok = False\n break\n if ok:\n if addedQuote and endA - 1 == l:\n endA -= 1\n answer.append((startA, endA))\n return answer"] | {"fn_name": "get_textliterals", "inputs": [["select 'text' into row from table where a = 'value'"], ["if a>'data'and b<'nnn' then c:='test'; end if;"], ["select aaa, bbb, ccc, /*'ddd'?*/ into row from table;"], ["a:='data''s';"]], "outputs": [[[[7, 13], [44, 51]]], [[[5, 11], [17, 22], [31, 37]]], [[]], [[[3, 12]]]]}
| INTRODUCTORY | PYTHON3 | CODEWARS | 7,710 |
def get_textliterals(pv_code):
|
35815c94d35bdeceaef448d7b266eac0 | UNKNOWN | In this Kata, you will be given a mathematical string and your task will be to remove all braces as follows:
```Haskell
solve("x-(y+z)") = "x-y-z"
solve("x-(y-z)") = "x-y+z"
solve("u-(v-w-(x+y))-z") = "u-v+w+x+y-z"
solve("x-(-y-z)") = "x+y+z"
```
There are no spaces in the expression. Only two operators are given: `"+" or "-"`.
More examples in test cases.
Good luck! | ["from functools import reduce\n\ndef solve(st):\n res, s, k = [], \"\", 1\n for ch in st:\n if ch == '(': res.append(k); k = 1\n elif ch == ')': res.pop(); k = 1\n elif ch == '-': k = -1\n elif ch == '+': k = 1\n else: s+= '-'+ch if (reduce(lambda a,b: a * b,res,1) * (1 if k == 1 else -1) < 0) else '+'+ch\n return s if s[0] == '-' else s[1:]", "SWAPPER = str.maketrans('+-', '-+')\n\ndef solve(s):\n return simplify(iter(s)).strip('+')\n\ndef simplify(it, prevOp='+'):\n lst = []\n for c in it:\n if c == '(': lst.append( simplify(it, '+' if not lst else lst.pop()) )\n elif c == ')': break\n else:\n if not lst and c.isalpha(): lst.append('+')\n lst.append(c)\n \n s = ''.join(lst)\n if prevOp == '-': s = s.translate(SWAPPER)\n \n return s", "def negafy(s):\n res,i = \"\",0\n while True:\n if i == len(s): break\n if 96 < ord(s[i]) and ord(s[i]) < 123:\n res += s[:i] + \"-\" + s[i]\n s,i = s[i+1:],-1\n i += 1\n return res + s\ndef solve(s):\n while s[0] == '(' and s[-1] == ')': s = s[1:-1]\n while \"--\" in s: s = s.replace(\"--\",\"+\")\n while \"+-\" in s: s = s.replace(\"+-\",\"-\")\n while s.startswith(\"+\"): s = s[1:]\n if \"(\" not in s: return s\n a = s.rindex(\"(\")\n b,c,d = s[a:].index(\")\") + a,s[a-1],\"\"\n while a > 0 and b < len(s) - 1 and s[a-1] == '(' and s[b+1] == ')':\n a,b = a-1,b+1\n if c == '-': d = negafy(s[a:b+1])\n else: d = s[a-1] + s[a+1:b]\n return solve(s[:a-1] + d + s[b+1:])", "def solve(s,p=1,q=1,Q=[],z=''):\n for c in s:\n if c=='+': p=1\n elif c=='-': p=-1\n elif c=='(': q*=p;Q+=[p];p=1\n elif c==')': q*=Q.pop();p=1\n else:z+='-+'[p*q>0]+c\n return z.strip('+')", "def solve(s):\n pos = [0]\n s = '(' + s + ')'\n def parse():\n sgn = -1 if s[pos[0]] == '-' else 1\n pos[0] += 2 if s[pos[0]] in ('+', '-') else 1\n lst = []\n while s[pos[0]] != ')':\n if '(' not in (s[pos[0]], s[pos[0]+1]):\n lst.append((-1 if s[pos[0]]=='-' else 1, s[pos[0]+1] if s[pos[0]] in ('+', '-') else s[pos[0]]))\n pos[0] += 2 if s[pos[0]] in ('+', '-') else 1\n else:\n lst.extend(parse())\n pos[0] += 1\n return [(t[0]*sgn, t[1]) for t in lst]\n return ''.join([('+' if t[0]==1 else '-') + t[1] for t in parse()]).lstrip('+')\n", "def solve(p):\n return \"{}{}\".format(\"$\", \"\".join([[\"+\", \"-\"][((len([j for j in range(len(p[:i])) if p[j:j+2] == \"-(\" and not len([k for k in range(len(p[:i])) if (k > j) and (p[k] == \")\") and (p[j:k].count(\")\") < p[j:k].count(\"(\"))])])) + int(p[i-1] == \"-\"))%2] + c for i, c in enumerate(p) if c not in [\"(\", \")\", \"+\", \"-\"]])).replace(\"$+\", \"\").replace(\"$\", \"\")\n\n", "def clean(string,to_clean=[(\"+-\",\"-\"),(\"-+\",\"-\"),(\"++\",\"+\"),(\"--\",\"+\")]):\n cleaned_string=string\n for i,j in to_clean:\n while i in cleaned_string:cleaned_string=cleaned_string.replace(i,j)\n print((cleaned_string,string))\n return clean(cleaned_string) if cleaned_string!=string else string\ndef solve(string):\n while \"(\" in string:\n counter=int()\n while counter<len(string):\n if string[counter]==\"(\":\n for x in range(counter+1,len(string)):\n if string[x]==\")\":string,counter=string[:counter]+\"\".join([\"+-\"[\"-+\".index(y)] if y in \"-+\" and string[counter-1]==\"-\" and string[counter+ind] not in \"(-+\" else y for ind,y in enumerate(string[counter+1:x])])+string[x+1:],counter-1\n elif string[x]==\"(\":pass\n else:continue\n break\n counter+=1\n print(string)\n to_return=clean(string)\n return to_return[1:] if to_return[int()]==\"+\" else to_return\n\n", "import re\nfrom functools import reduce\ndef solve(s):\n sign, stack = {('+', '-'): '-', ('-', '+'): '-', ('+', '+'): '+', ('-', '-'): '+'}, []\n for i in s:\n if i != ')' : stack.append(i)\n else:\n t = len(stack) - stack[::-1].index('(') - 1\n stack.pop(t)\n ini = stack[t - 1]\n if ini in '+-' : stack[t:] = list(re.sub(r'(?<=\\w)([-+])(?=\\w)', lambda x: sign[(ini, x.group(1))], \"\".join(stack[t:])))\n stack = list(re.sub(r'([-+]+)', lambda g: reduce(lambda x, y: sign[(x, y)], list(g.group())), \"\".join(stack)))\n return \"\".join(stack).lstrip('+')", "def solve(s):\n sign, res, x = [], [], False\n for c in s:\n if c in '()-+':\n if c == '(': sign.append(x)\n elif c == ')': del sign[-1]\n x = c=='-'\n else:\n res.append('+-'[sum(sign)%2 ^ x])\n res.append(c)\n return ''.join(res).lstrip('+')", "import re\n\nclass Expr:\n def __init__(self, terms):\n self.terms = terms\n def __add__(self, other):\n return Expr(self.terms + other.terms)\n def __sub__(self, other):\n return self + -other\n def __neg__(self):\n return Expr([(sign ^ 1, var) for sign, var in self.terms])\n def __str__(self):\n return ''.join('+-'[sign] + var for sign, var in self.terms).strip('+')\n\ndef solve(s):\n return str(eval(re.sub('(\\w+)', r'Expr([(0, \"\\1\")])', s)))\n"] | {"fn_name": "solve", "inputs": [["a-(b)"], ["a-(-b)"], ["a+(b)"], ["a+(-b)"], ["(((((((((-((-(((n))))))))))))))"], ["(((a-((((-(-(f)))))))))"], ["((((-(-(-(-(m-g))))))))"], ["(((((((m-(-(((((t)))))))))))))"], ["-x"], ["-(-(x))"], ["-((-x))"], ["-(-(-x))"], ["-(-(x-y))"], ["-(x-y)"], ["x-(y+z)"], ["x-(y-z)"], ["x-(-y-z)"], ["x-(-((-((((-((-(-(-y)))))))))))"], ["u-(v-w+(x+y))-z"], ["x-(s-(y-z))-(a+b)"], ["u+(g+v)+(r+t)"], ["q+(s-(x-o))-(t-(w-a))"], ["u-(v-w-(x+y))-z"], ["v-(l+s)-(t+y)-(c+f)+(b-(n-p))"]], "outputs": [["a-b"], ["a+b"], ["a+b"], ["a-b"], ["n"], ["a-f"], ["m-g"], ["m+t"], ["-x"], ["x"], ["x"], ["-x"], ["x-y"], ["-x+y"], ["x-y-z"], ["x-y+z"], ["x+y+z"], ["x-y"], ["u-v+w-x-y-z"], ["x-s+y-z-a-b"], ["u+g+v+r+t"], ["q+s-x+o-t+w-a"], ["u-v+w+x+y-z"], ["v-l-s-t-y-c-f+b-n+p"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,491 |
def solve(s):
|
17ac75fff02d0ef7d2406b00ab08d149 | UNKNOWN | Bob needs a fast way to calculate the volume of a cuboid with three values: `length`, `width` and the `height` of the cuboid. Write a function to help Bob with this calculation.
```if:shell
In bash the script is ran with the following 3 arguments:
`length` `width` `height`
``` | ["def get_volume_of_cuboid(length, width, height):\n return length * width * height\n\n\n# PEP8: kata function name should use snake_case not mixedCase\ngetVolumeOfCubiod = get_volume_of_cuboid", "def getVolumeOfCubiod(l, w, h):\n return l * w * h", "def getVolumeOfCubiod(length, width, height):\n return length * width *height", "getVolumeOfCubiod = lambda l,w,d : l*w*d\n", "def getVolumeOfCubiod(length, width, height):\n return width*length*height", "def getVolumeOfCubiod(l, w, h):\n return l * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h / w / h * w * h", "getVolumeOfCubiod=lambda l,w,h:l*w*h", "def getVolumeOfCubiod(a, b, H): return a*b*H", "def getVolumeOfCubiod(length: int, width: int, height: int) -> int:\n \"\"\" Get the volume of the cuboid. \"\"\"\n return length * width * height", "getVolumeOfCubiod = lambda l, w, h: l * w * h\n\n# def getVolumeOfCubiod(l, w, h):\n# return l * w * h\n", "#!/usr/bin/env python3.6\n\ndef get_volume_of_cuboid(length, width, height):\n return length * width * height\n \ngetVolumeOfCubiod = get_volume_of_cuboid", "def getVolumeOfCubiod(length, width, height):\n a = length\n b = width\n c = height\n return a * b * c", "def getVolumeOfCubiod(a1, a2, a3):\n return a1*a2*a3", "def getVolumeOfCubiod(length, width, height):\n vol = length*width*height\n return vol \n", "getVolumeOfCubiod = lambda length, width, height: length*width*height", "def getVolumeOfCubiod(x,y,z):\n return x*y*z", "def getVolumeOfCubiod(length, width, height):\n volume = length * width * height\n return volume", "getVolumeOfCubiod = lambda a, b, c: a * b * c", "def getVolumeOfCubiod(length, width, height):\n return length * width * height\n\nprint(getVolumeOfCubiod(3,2,1))", "def getVolumeOfCubiod(length, width, height):\n return float(length) * int(width) * int(height)\n", "def getVolumeOfCubiod(l, w, h):\n \n n = (l*w*h)\n return n", "def getVolumeOfCubiod(length, width, height):\n # Code goes here...\n if length and width and height > 0:\n return length * width * height", "from numpy import prod\ndef getVolumeOfCubiod(*a):\n return prod(a)", "# Note: Cuboid was misspelled and camelCased in problem statement, tests, and\n# required function signature.\n\nimport functools\nimport operator\n\ndef getVolumeOfCubiod(*dimensions):\n \"\"\"Compute volume to an arbitrary dimension :).\"\"\"\n return functools.reduce(operator.mul, dimensions, 1)", "from functools import reduce\n\ngetVolumeOfCubiod = lambda l,w,h: reduce(lambda x,y:x*y, [l,w,h])", "getVolumeOfCubiod = lambda *d: ( (lambda ft, op: ft.reduce(op.mul, d)) (*[__import__(q) for q in ['functools', 'operator']]) )", "getVolumeOfCubiod = lambda *d: ( (lambda ft, op: ft.reduce(op.mul, d)) (__import__('functools'), __import__('operator')))", "def getVolumeOfCubiod(length, width, height):\n '''\n input: ints or floats (length, width, height)\n return: int or float => representing volume of cube\n '''\n return length*width*height\n", "from functools import reduce\n\ngetVolumeOfCubiod = lambda *dimensions: reduce(lambda x,y: x*y, dimensions)", "def getVolumeOfCubiod(a, aa, aaa):\n return a*aa*aaa", "def getVolumeOfCubiod(length, width, height):\n result = (float(length) * float(width) * float(height))\n if float.is_integer(result) is True: # Checking to see if I can convert the return a int\n return int(result) # Returning int becuase it is possible\n else:\n return result # Returning a float because the value isn't a whole number\n\n", "from functools import reduce\nfrom operator import mul\ndef getVolumeOfCubiod(*n):\n return reduce(mul, n)\n", "from operator import mul\nfrom functools import reduce, partial\n\ndef getVolumeOfCubiod(*args):\n return partial(reduce, mul)(args)", "import numpy\ngetVolumeOfCubiod = lambda *p : numpy.prod(p)", "def getVolumeOfCubiod(length, width, height):\n vol=length*width*height\n return vol\nvol=getVolumeOfCubiod(2, 3, 4)\nprint(vol)\n", "getVolumeOfCubiod = lambda _,__,___: _*__*___", "def getVolumeOfCubiod(ln, wd, hg):\n return ln * wd * hg", "def getVolumeOfCubiod(length, width, height):\n return length*width*height\n#pogchamp\n", "getVolumeOfCubiod = lambda a,b,h: a*b*h", "def getVolumeOfCubiod(length,\n width,\n height):\n\n return length * width * height\n", "import numpy as np\ndef getVolumeOfCubiod(length, width, height):\n return np.prod([length, width, height])", "from numpy import prod\ndef getVolumeOfCubiod(*s):\n return prod(s)", "def getVolumeOfCubiod(length: int, width: int, height: int) -> float:\n return length * width * height", "from functools import reduce\ndef getVolumeOfCubiod(*a):\n return reduce(lambda accum, next_item: accum * next_item, a)", "# INVINCIBLE WARRIORS --- PARZIVAL\n\ndef getVolumeOfCubiod(length, width, height):\n return length * width * height", "import math\ndef getVolumeOfCubiod(length, width, height):\n return length*width*height", "def getVolumeOfCubiod(length, width, height):\n ergebnis = length * width * height \n return ergebnis\n\nprint(getVolumeOfCubiod(1, 2, 2,))\nprint(getVolumeOfCubiod(6.3, 2, 5))", "def getVolumeOfCubiod(*args):\n return args[0]*args[1]*args[2]", "import numpy\n\ndef getVolumeOfCubiod(length, width, height):\n return numpy.prod([length, width, height])", "def getVolumeOfCubiod(len, wi, he):\n return len * wi * he", "def getVolumeOfCubiod(length, width, height):\n cub = Cubiod(length, width, height)\n return cub.getVolume()\n\n\n\nclass Cubiod:\n \n def __init__ (self, length, width, height):\n self.length = length\n self.width = width\n self.height = height\n \n def getVolume(self):\n return self.length * self.width * self.height\n \n def getSA(self):\n return (self.length * self.height * 2) + (self.length * self.width * 2) + (self.height * self.width * 2)\n \n def speedProb(self):\n return 9.87 * min(self.length, self.width, self.height)\n \n def damage(self):\n return self.getVolume() * 1900 * self.speedProb()\n \n def velocity(self):\n return self.speedProb() ** 2\n \n def crimeLevel(self):\n return self.velocity() * self.damage() + self.getSA()", "def getVolumeOfCubiod(length, width, height):\n return eval(f\"{length}*{width}*{height}\")", "def getVolumeOfCubiod(length, width, height):\n r = (length * width * height)\n return r", "def getVolumeOfCubiod(length, width, height):\n length= float (length)\n width= float (width)\n height= float (height)\n volumen= ((length * width) * height)\n return (volumen)", "def getVolumeOfCubiod(length, width, height):\n var = lambda x, y, z: x*y*z\n return var(length, width, height)", "def getVolumeOfCubiod(length, width, height):\n return (length*width*height)\nx = getVolumeOfCubiod(6.3, 2, 5)\nprint(x)", "def getVolumeOfCubiod(d, w, h):\n return(w * d * h)", "from functools import reduce\nfrom operator import mul\n\ngetVolumeOfCubiod = lambda *ns: reduce(mul, ns)", "def getVolumeOfCubiod(length, width, height):\n \n # =============================================================================\n # This function calculates the volume of a cuboid with three values: \n # length, width and the height of the cuboid\n # \n # Example:\n # \n # getVolumeOfCubiod(1, 2, 2) ==> 4\n # =============================================================================\n \n return(length * width * height)", "def getVolumeOfCubiod(l, w, h):\n x = lambda l, w, h: l*w*h\n return (x(l,w,h))", "def getVolumeOfCubiod(length, width, height):\n return (length*width*height)\n \n # from Russia with love\n", "def getVolumeOfCubiod(length, width, height):\n # Why is the percentage clear rate so low for this?\n return length * width * height", "def getVolumeOfCubiod(l, w, h):\n a = l*w*h\n return a\n", "def getVolumeOfCubiod(length, width, height):\n # volume of a cuboid: V=a\u22c5b\u22c5c \n return length * width * height", "def getVolumeOfCubiod(length, width, height):\n \"\"\"Return volume of cuboid\"\"\"\n return length * width * height", "from functools import reduce\nfrom operator import mul\n\ndef getVolumeOfCubiod(*a):\n return reduce(mul, a, 1)\n", "def getVolumeOfCubiod(length, width, height):\n a = length * width\n b = a * height\n return b", "def getVolumeOfCubiod(length, width, height):\n x=length*width\n return x*height", "def getVolumeOfCubiod(length, width, height):\n # Code goes here...\n return(length * width * height)\ngetVolumeOfCubiod(3, 4, 8)", "def getVolumeOfCubiod(length, width, height):\n return length * width * height\n # Code goes here...! I will put the code where I want.\n", "def getVolumeOfCubiod(length, width, height):\n p = lambda l,w,h : l * w * h\n return p(length,width,height)", "def getVolumeOfCubiod(length, width, height):\n \n volume= height*width*length\n return volume", "def getVolumeOfCubiod(length, width, height):\n if length > 0 and width > 0 and height > 0:\n return length * width * height\n else:\n return False", "def getVolumeOfCubiod(length, width, height):\n volume_of_cuboid = length*width*height\n return volume_of_cuboid", "def getVolumeOfCubiod(length, width, height):\n VolumeOfCubiod = length * width * height\n return VolumeOfCubiod", "def getVolumeOfCubiod(length, width, height):\n f=length*width*height\n return f", "def getVolumeOfCubiod(length, width, height):\n # Code goes here...\n return length * width * height\n \n \ngetVolumeOfCubiod(10,15,17)", "getVolumeOfCubiod = lambda l, w, h: l * h * w\n", "def getVolumeOfCubiod(leng, wid, hei):\n return leng*wid*hei", "from numpy import prod\ndef getVolumeOfCubiod(*args):\n return prod(args)", "def getVolumeOfCubiod(length, width, height):\n # Code goes here...\n return length*width*height\nprint(getVolumeOfCubiod(6.3,5,2))", "getVolumeOfCubiod = lambda *a: a[0]*a[1]*a[2]", "''' \n Volume = lwh\n'''\n\ndef getVolumeOfCubiod(length, width, height):\n return length * width * height\n", "def getVolumeOfCubiod(length, width, height):\n # Code goes here...\n CubiodVolume=length*width*height\n return (CubiodVolume)", "def getVolumeOfCubiod(h, l , w):\n return h*l*w", "def getVolumeOfCubiod(l, w, h):\n return (w * h) * l\n", "def getVolumeOfCubiod(length, width, height):\n length * width * height \n return length * width * height ", "def getVolumeOfCubiod(length, width, height):\n \"\"\"___\"\"\"\n return length*width*height", "def getVolumeOfCubiod(length, width, height):\n length*height*width\n return length*height*width", "''' \n Volume = lwh\n'''\n\n'''\nlenght = int(input('Qual \u00e9 o comprimento?'))\nwidth = int(input('Qual \u00e9 a largura?'))\nheight = int(input('Qual \u00e9 o altura?'))\n\n'''\n\ndef getVolumeOfCubiod(length, width, height):\n return length * width * height\n", "def getVolumeOfCubiod(length, width, height):\n val = (length * width * height)\n return (val)", "def getVolumeOfCubiod(length, width, height):\n result =length*width*height\n return result\n \ngetVolumeOfCubiod(2,2,2)", "def getVolumeOfCubiod(length, width, height):\n # Code goes here\n return length*width*height", "def getVolumeOfCubiod(length, width, height):\n a = length\n b = width\n c = height\n volume = (a*b*c)\n return volume", "def getVolumeOfCubiod(length, width, height):\n cuboid = length * width * height\n return(cuboid)", "def getVolumeOfCubiod(length, width, height):\n volume = length*width*height\n return volume\n \nlength,width,height = 1,2,2\nprint((getVolumeOfCubiod(length, width, height)))\n # Code goes here...\n"] | {"fn_name": "getVolumeOfCubiod", "inputs": [[2, 5, 6], [6.3, 3, 5]], "outputs": [[60], [94.5]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,187 |
def getVolumeOfCubiod(length, width, height):
|
5359bb5a0fb6d100cfa915da5423bb08 | UNKNOWN | You need to play around with the provided string (s).
Move consonants forward 9 places through the alphabet.
If they pass 'z', start again at 'a'.
Move vowels back 5 places through the alphabet.
If they pass 'a', start again at 'z'.
For our Polish friends this kata does not count 'y' as a vowel.
Exceptions:
If the character is 'c' or 'o', move it back 1 place.
For 'd' move it back 3, and for 'e', move it back 4.
If a moved letter becomes 'c', 'o', 'd' or 'e', revert it back to it's original value.
Provided string will always be lower case, won't be empty and will have no special characters. | ["def vowel_back(st):\n return st.translate(str.maketrans(\"abcdefghijklmnopqrstuvwxyz\", \"vkbaafpqistuvwnyzabtpvfghi\"))", "def vowel_back(s):\n return s.translate(str.maketrans(\"abcdeghjklmnopqrsuwxyz\", \"vkbaapqstuvwnyzabpfghi\"))", "from string import ascii_lowercase as abc\n\ndef vowel_back(st): return st.translate(str.maketrans(abc,\"vkbaafpqistuvwnyzabtpvfghi\"))\n", "def vowel_back(st):\n print(st)\n changes = {'a':'v', 'b':'k', 'c':'b', 'd':'a','e':'a','f':'f','g':'p','h':'q','i':'i','j':'s','k':'t','l':'u','m':'v','n':'w','o':'n','p':'y','q':'z','r':'a','s':'b','t':'t','u':'p','v':'v','w':'f','x':'g','y':'h','z':'i'}\n return ''.join(changes[c] for c in st)", "from collections import ChainMap\nfrom string import ascii_lowercase as al\n\nmove = ChainMap(\n dict(c=-1, o=-1, d=-3, e=-4), # Exceptions\n dict.fromkeys('aeiou', -5), # Vowels\n dict.fromkeys(al, 9), # All alphabets\n)\nnew = (al[(i + move[c]) % 26] for i, c in enumerate(al))\nmapping = {c: c if c2 in 'code' else c2 for c, c2 in zip(al, new)}\n\ndef vowel_back(st):\n return ''.join(map(mapping.get, st))", "from string import ascii_lowercase as aLow\n\ndef shifterAndSrc(c): return chr( (ord(c)+SHIFT.get(c,DEFAULT)-97) % 26 + 97 ), c\n\nSHIFT = {'a':-5, 'e':-4, 'i':-5, 'o':-1, 'u':-5, 'c':-1, 'd':-3}\nDEFAULT, REVERT = 9, 'code'\nTABLE = str.maketrans(aLow, ''.join(c if c not in REVERT else o for c,o in map(shifterAndSrc,aLow)) )\n\ndef vowel_back(s): return s.translate(TABLE)", "vowel_back=lambda s:''.join(e[e[0]in'code']for e in[(chr(ord(x)-max(1,'co de'.index(x))if x in'code'else 97+(ord(x)-97-5if x in'aiu'else ord(x)-97+9)%26),x)for x in s])", "def vowel_back(st):\n s = ''\n for x in st:\n res = chr(ord('a')+(ord(x)-ord('a')+((9,-1,-3)[(x == 'c')+(x == 'd')*2],-(5,1,4)[(x == 'o')+(x=='e')*2])[x in 'aoiue'])%26)\n if res in 'code':\n res = x\n s += res\n return s", "trans = str.maketrans(\"abcdeghjklmnopqrsuwxyz\", \"vkbaapqstuvwnyzabpfghi\")\n\ndef vowel_back(st):\n return st.translate(trans)", "from string import ascii_lowercase as alpha\n\nalpha = list(alpha)\nln = len(alpha)\nvowels = \"aeiou\"\ndef vowel_back(s):\n s = list(s)\n for idx, char in enumerate(s):\n index = alpha.index(char)\n if char in ('c', 'o'):\n s[idx] = alpha[index - 1]\n elif char == 'd':\n s[idx] = alpha[index - 3]\n elif char == 'e':\n s[idx] = alpha[index - 4]\n else:\n if char in vowels:\n s[idx] = alpha[index - 5]\n else:\n index += 9\n index = index%ln\n s[idx] = alpha[index]\n if s[idx] in ('c', 'o', 'd', 'e'):\n s[idx] = char\n return \"\".join(s)\n"] | {"fn_name": "vowel_back", "inputs": [["testcase"], ["codewars"], ["exampletesthere"], ["returnofthespacecamel"], ["bringonthebootcamp"], ["weneedanofficedog"]], "outputs": [["tabtbvba"], ["bnaafvab"], ["agvvyuatabtqaaa"], ["aatpawnftqabyvbabvvau"], ["kaiwpnwtqaknntbvvy"], ["fawaaavwnffibaanp"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,028 |
def vowel_back(st):
|
53abcadad2c586f7b83190bcf06bf095 | UNKNOWN | Your start-up's BA has told marketing that your website has a large audience in Scandinavia and surrounding countries. Marketing thinks it would be great to welcome visitors to the site in their own language. Luckily you already use an API that detects the user's location, so this is an easy win.
### The Task
- Think of a way to store the languages as a database (eg an object). The languages are listed below so you can copy and paste!
- Write a 'welcome' function that takes a parameter 'language' (always a string), and returns a greeting - if you have it in your database. It should default to English if the language is not in the database, or in the event of an invalid input.
### The Database
```python
'english': 'Welcome',
'czech': 'Vitejte',
'danish': 'Velkomst',
'dutch': 'Welkom',
'estonian': 'Tere tulemast',
'finnish': 'Tervetuloa',
'flemish': 'Welgekomen',
'french': 'Bienvenue',
'german': 'Willkommen',
'irish': 'Failte',
'italian': 'Benvenuto',
'latvian': 'Gaidits',
'lithuanian': 'Laukiamas',
'polish': 'Witamy',
'spanish': 'Bienvenido',
'swedish': 'Valkommen',
'welsh': 'Croeso'
```
``` java
english: "Welcome",
czech: "Vitejte",
danish: "Velkomst",
dutch: "Welkom",
estonian: "Tere tulemast",
finnish: "Tervetuloa",
flemish: "Welgekomen",
french: "Bienvenue",
german: "Willkommen",
irish: "Failte",
italian: "Benvenuto",
latvian: "Gaidits",
lithuanian: "Laukiamas",
polish: "Witamy",
spanish: "Bienvenido",
swedish: "Valkommen",
welsh: "Croeso"
```
Possible invalid inputs include:
~~~~
IP_ADDRESS_INVALID - not a valid ipv4 or ipv6 ip address
IP_ADDRESS_NOT_FOUND - ip address not in the database
IP_ADDRESS_REQUIRED - no ip address was supplied
~~~~ | ["def greet(language):\n return {\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'english': 'Welcome',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }.get(language, 'Welcome')", "db = {\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n return db.get(language, \"Welcome\")", "welcome_message = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso',\n}\n\ndef greet(language):\n return welcome_message.get(language, welcome_message['english'])", "def greet(language):\n if language=='english':\n return('Welcome')\n elif language=='czech':\n return('Vitejte')\n elif language=='danish':\n return('Velkomst')\n elif language=='dutch':\n return ('Welkom')\n elif language=='estonian':\n return('Tere tulemast')\n elif language=='finnish':\n return('Tervetuloa')\n elif language=='flemish':\n return('Welgekomen')\n elif language=='french':\n return('Bienvenue')\n elif language=='german':\n return('Willkommen')\n elif language=='irish':\n return('Failte')\n elif language=='italian':\n return('Benvenuto')\n elif language=='latvian':\n return ('Gaidits')\n elif language=='lithuanian':\n return ('Laukiamas')\n elif language=='polish':\n return ('Witamy')\n elif language=='spanish':\n return('Bienvenido')\n elif language=='swedish':\n return('Valkommen')\n elif language=='welsh':\n return('Croeso')\n else:\n return('Welcome')", "def greet(language):\n #your code here\n dict = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n\n if language in dict.keys():\n return (dict[language])\n else:\n return ('Welcome')", "def greet(language):\n # store the data in a dictionary variable\n mylist = {'english': 'Welcome','czech': 'Vitejte','danish': 'Velkomst','dutch': 'Welkom','estonian': 'Tere tulemast','finnish': 'Tervetuloa','flemish': 'Welgekomen','french': 'Bienvenue','german': 'Willkommen','irish': 'Failte','italian': 'Benvenuto','latvian': 'Gaidits','lithuanian': 'Laukiamas','polish': 'Witamy','spanish': 'Bienvenido','swedish': 'Valkommen','welsh': 'Croeso'}\n # check if the input matches the key in myList\n if language in mylist:\n # if it matches print the value of the match\n return mylist[language]\n else:\n # if does not match just return English Value \"Welcome\" as the default\n return mylist['english']\n", "def greet(language):\n d={'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n try:\n return d[language]\n except:\n return 'Welcome'", "def greet(language):\n db = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n return db[language] if language in db else 'Welcome'", "def greet(language):\n #your code here\n languages = {'english': 'Welcome','czech': 'Vitejte','danish': 'Velkomst','dutch': 'Welkom','estonian': 'Tere tulemast','finnish': 'Tervetuloa','flemish': 'Welgekomen','french': 'Bienvenue','german': 'Willkommen','irish': 'Failte','italian': 'Benvenuto','latvian': 'Gaidits','lithuanian': 'Laukiamas','polish': 'Witamy','spanish': 'Bienvenido','swedish': 'Valkommen','welsh': 'Croeso'}\n if language in languages.keys():\n return languages[language] \n else:\n return languages['english']", "def greet(language):\n data = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return data[language] if language in data else 'Welcome'", "asd ={\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n return asd.get(language, \"Welcome\")\n \n \n", "def greet(lang):\n return {'english': 'Welcome', 'czech': 'Vitejte', 'danish': 'Velkomst',\n 'dutch': 'Welkom', 'estonian': 'Tere tulemast', 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen',\n 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas', 'polish': 'Witamy', 'spanish': 'Bienvenido', \n 'swedish': 'Valkommen','welsh': 'Croeso'}.get(lang, \"Welcome\")", "def greet(language):\n print(language)\n B={'english': 'Welcome', 'czech': 'Vitejte', 'danish': 'Velkomst', 'dutch': 'Welkom', 'estonian': 'Tere tulemast', 'finnish': 'Tervetuloa', 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen', 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits', 'lithuanian': 'Laukiamas', 'polish': 'Witamy', 'spanish': 'Bienvenido', 'swedish': 'Valkommen', 'welsh': 'Croeso'}\n if language in B:\n x=B[language]\n print(x)\n return x\n else:\n return \"Welcome\"\n \n # return (\"welcome\")\n", "def greet(language):\n database = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n\n for k, v in database.items():\n if k == language:\n return v\n\n return \"Welcome\"", "def greet(language):\n greetings_dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n if language not in list(greetings_dict.keys()):\n return 'Welcome'\n else:\n return greetings_dict[language]\n #your code here\n", "db = {\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n #your code here\n if language in db.keys():\n return(db[language])\n else:\n language = 'english'\n return(db[language])", "def greet(language):\n a={'czech': 'Vitejte', 'danish': 'Velkomst', 'dutch': 'Welkom', 'estonian': 'Tere tulemast', 'finnish': 'Tervetuloa', 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen', 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits', 'lithuanian': 'Laukiamas', 'polish': 'Witamy', 'spanish': 'Bienvenido', 'swedish': 'Valkommen', 'welsh': 'Croeso'}\n try:\n return a[language] \n except:\n return 'Welcome'", "def greet(language):\n texts = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n if language in texts.keys():\n return texts[language]\n else:\n return texts[\"english\"]", "welcome = {\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'\n}\n\ndef greet(language):\n return welcome.get(language, 'Welcome')", "def greet(language):\n return {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }.get(language, 'Welcome')", "def greet(l):\n dict = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n try:\n return dict[l]\n except:\n return 'Welcome'", "def greet(language):\n try:\n d = { 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return d[language]\n except:\n return 'Welcome'", "def greet(language: str) -> str:\n \"\"\"\n Get a greeting - if you have it in your database.\n It should default to English if the language is not in the database.\n \"\"\"\n\n welcome_msg = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return welcome_msg.get(language, welcome_msg[\"english\"])", "def greet(language):\n return {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }.get(str(language).lower(), \"Welcome\")\n\n\n", "country_greet = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n #your code here\n try:\n return country_greet[language]\n except:\n return \"Welcome\"", "def greet(language):\n db = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return db[language] if language in db else db[\"english\"]", "DICT = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n}\n\ngreet = lambda language: DICT.get(language, 'Welcome')", "def greet(language):\n k = {'english': 'Welcome', 'czech': 'Vitejte', 'danish': 'Velkomst', 'dutch': 'Welkom', 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa', 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen',\n 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits', 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy', 'spanish': 'Bienvenido', 'swedish': 'Valkommen', 'welsh': 'Croeso'}\n if k.get(language) == None:\n return k.get('english')\n else:\n return k.get(language)\n #your code here\n", "def greet(language):\n if language == 'english':\n return ('Welcome')\n elif language == 'czech':\n return ('Vitejte')\n elif language == 'danish':\n return ('Velkomst')\n elif language == 'dutch':\n return ('Welkom')\n elif language == 'estonian':\n return ('Tere tulemast')\n elif language == 'finnish':\n return ('Tervetuloa')\n elif language == 'fllemish':\n return ('Welgekomen')\n elif language == 'french':\n return ('Bienvenue')\n elif language == 'german':\n return ('Willkommen')\n elif language == 'italian':\n return ('Benvenuto')\n elif language == 'latvian':\n return ('Gaidits')\n elif language == 'lithuanian':\n return ('Laukiamas')\n elif language == 'polish':\n return ('Witamy')\n elif language == 'spanish':\n return ('Bienvenido')\n elif language == 'swedish':\n return ('Valkommen')\n elif language == 'welsh':\n return ('Croeso')\n else:\n return ('Welcome')", "lang = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n if language in lang.keys():\n return lang[language]\n else:\n return 'Welcome'", "GREETINGS = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n}\n\n\ndef greet(language):\n return GREETINGS.get(language, GREETINGS['english'])", "languageDb = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n\n\ndef greet(language):\n return languageDb[language] if language in languageDb else languageDb['english']", "dct = {'english': 'Welcome', 'czech': 'Vitejte', 'danish': 'Velkomst',\n 'dutch': 'Welkom', 'estonian': 'Tere tulemast', 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen',\n 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas', 'polish': 'Witamy', 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen', 'welsh': 'Croeso'}\n\ndef greet(language):\n return dct.get(language, 'Welcome')", "def greet(language):\n \n dict_lang= {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n dict_lang.setdefault(language,'Welcome')\n return dict_lang[language]", "def greet(language='Welcome'):\n \n while 0==0:\n if isinstance(language,int):\n return 'Welcome'\n else:\n return {\n 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso',\n 'IP_ADDRESS_INVALID':'Welcome',\n 'IP_ADDRESS_NOT_FOUND':'Welcome',\n 'IP_ADDRESS_REQUIRED':'Welcome'}.get(language) if language else 'Welcome'", "def greet(language):\n database = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n language = str(language)\n language = language.lower()\n if language in database:\n return database.get(language)\n else:\n return database.get(\"english\")", "def greet(lang):\n db = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso' \n }\n return db.get(lang, 'Welcome')", "def greet(language):\n \"\"\" \n This function returns a greeting eturns a greeting - if you have it in your database. \n It should default to English if the language is not in the database, or in the event of an invalid input.\n \"\"\"\n greet_language = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n for key, val in greet_language.items():\n if key == language:\n return val\n return 'Welcome'", "def greet(language):\n \n my_data_base = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n \n if language in list(my_data_base.keys()):\n return my_data_base[language]\n else:\n return \"Welcome\"\n \n #your code here\n", "def greet(language):\n l = {'english': 'Welcome','czech': 'Vitejte','danish': 'Velkomst','dutch': 'Welkom','estonian': 'Tere tulemast','finnish': 'Tervetuloa','flemish': 'Welgekomen','french': 'Bienvenue','german': 'Willkommen','irish': 'Failte','italian': 'Benvenuto','latvian': 'Gaidits','lithuanian': 'Laukiamas','polish': 'Witamy','spanish': 'Bienvenido','swedish': 'Valkommen','welsh': 'Croeso'} \n if language not in l:\n return 'Welcome'\n return l[language]\n \n", "def greet(language):\n lng = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n if str(language) in lng:\n return lng.get(language.lower())\n else:\n return \"Welcome\"", "def greet(language):\n if language in x.keys():\n i=language\n return x[i]\n else:\n return \"Welcome\"\n \nx={ \n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue', \n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }", "def greet(language = 'english'):\n #your code here\n \n dict_ = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n\n return dict_[language] if language in dict_ else dict_['english']\n", "def greet(language):\n\n jon = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n \n if language in jon:\n return jon[language]\n else:\n return \"Welcome\"\n #your code here\n", "def greet(language):\n d1 = {\n '':'Welcome',\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso' \n }\n if not d1.get(language):\n return 'Welcome'\n else: \n return d1[language]\n", "def greet(language):\n dc = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return dc.pop(language,'Welcome') ", "def greet(language):\n translate = {'english': 'Welcome','czech': 'Vitejte','danish': 'Velkomst','dutch': 'Welkom','estonian': 'Tere tulemast','finnish': 'Tervetuloa','flemish': 'Welgekomen','french': 'Bienvenue','german': 'Willkommen','irish': 'Failte','italian': 'Benvenuto','latvian': 'Gaidits','lithuanian': 'Laukiamas','polish': 'Witamy','spanish': 'Bienvenido','swedish': 'Valkommen','welsh': 'Croeso'}\n return 'Welcome' if translate.get(language) == None else translate.get(language)", "def greet(language):\n saludo = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return saludo.get(language) if language in saludo.keys() else \"Welcome\"", "def greet(language):\n language_map = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return language_map[language] if language in language_map else \"Welcome\"", "def greet(language):\n welcoming_dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return welcoming_dict.get(language, 'Welcome')\n", "def greet(language):\n welcome_messages = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return welcome_messages[language] if language in welcome_messages else \"Welcome\"", "def greet(language):\n return {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso',\n 'ip_address_invalid' : 'not a valid ipv4 or ipv6 ip address',\n 'ip_address_not_found' : 'ip address not in the database',\n 'ip_address_required' : 'no ip address was supplied'\n }.get(language,'Welcome')", "def greet(language):\n data = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n if not language in data: return \"Welcome\"\n return data[language]", "greeting = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\n\ndef greet(language):\n if greeting.get(language) == None:\n return(\"Welcome\")\n else: \n return greeting.get(language)\n \n \n", "def greet(language):\n \n l = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n \n try:\n language == l[language]\n return l.get(language)\n except KeyError:\n return 'Welcome'\n #your code here\n", "def greet(language):\n welcome_dictionary= {'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return welcome_dictionary.get(language,'Welcome')", "def greet(language):\n WelcomeDict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return WelcomeDict.get(language, \"Welcome\")", "def greet(language):\n dict = {'english': 'Welcome', 'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n \n for key in dict:\n if language == key:\n return dict[key]\n \n return 'Welcome'", "GREETINGS = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language):\n try: greeting = GREETINGS[language]\n except KeyError: greeting = 'Welcome'\n return greeting", "My_Data = {\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'\n\n}\n\ndef greet(language = 'english'):\n try:\n return My_Data[language]\n except KeyError:\n return My_Data['english']\n\n #your code here\n", "def greet(language):\n lang = { 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n message = lang.get(language, 'Welcome')\n return message", "def greet(language):\n langdict = {\n 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n if language in langdict:\n return langdict[language]\n else:\n return 'Welcome'", "def greet(l):\n s = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return s.get(l,'Welcome')", "def greet(language):\n lang_greet = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return lang_greet[language] if language in lang_greet else lang_greet['english']", "def greet(language):\n dic={ 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return dic.get(language) if language in dic else 'Welcome' ", "dict = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language = \"Welcome\"):\n return dict.get(language, \"Welcome\")\n", "greet = lambda language: {'czech':'Vitejte','danish':'Velkomst','dutch': 'Welkom','estonian':'Tere tulemast','finnish':'Tervetuloa','flemish':'Welgekomen','french':'Bienvenue','german':'Willkommen','irish':'Failte','italian':'Benvenuto','latvian':'Gaidits','lithuanian':'Laukiamas','polish':'Witamy','spanish':'Bienvenido','swedish':'Valkommen','welsh':'Croeso'}.get(language, 'Welcome')", "listlang = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso',\n 'IP_ADDRESS_INVALID': 'Welcome',\n 'IP_ADDRESS_NOT_FOUND ': 'Welcome',\n 'IP_ADDRESS_REQUIRED ': 'Welcome',\n '': 'Welcome',\n 2: 'Welcome'\n}\n \ndef greet(language = 'Welcome'):\n if language in listlang:\n return(listlang[language])\n else:\n return(\"Welcome\")\n", "def greet(language):\n #your code here\n names={'english': 'Welcome','czech': 'Vitejte','danish': 'Velkomst','dutch': 'Welkom','estonian': 'Tere tulemast','finnish': 'Tervetuloa','flemish': 'Welgekomen','french': 'Bienvenue','german': 'Willkommen','irish': 'Failte','italian': 'Benvenuto','latvian': 'Gaidits','lithuanian': 'Laukiamas','polish': 'Witamy','spanish': 'Bienvenido','swedish': 'Valkommen','welsh': 'Croeso'}\n if language in names:\n return names[language]\n else:\n return 'Welcome'\n \n", "D={\n'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'\n}\n\ndef greet(language):\n if language in D:return D[language]\n return \"Welcome\"", "def greet(language):\n try:\n greeting = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return greeting[language]\n except KeyError:\n return 'Welcome'", "def greet(language):\n dictionary = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n if language in dictionary:\n return dictionary.get(language)\n else:\n return dictionary.get(\"english\")", "def greet(language):\n #your code here\n if language == \"dutch\":\n return 'Welkom'\n elif language == \"czech\":\n return 'Vitejte'\n elif language == \"danish\":\n return 'Velkomst'\n elif language == \"estonian\":\n return 'Tere tulemast'\n elif language == \"finnish\":\n return 'Tervetuloa'\n elif language == \"flemish\":\n return 'Welgekomen'\n elif language == \"french\":\n return 'Bienvenue'\n elif language == \"german\":\n return 'Willkommen'\n elif language == \"irish\":\n return 'Failte'\n elif language == \"italian\":\n return 'Benvenuto'\n elif language == \"latvian\":\n return 'Gaidits'\n elif language == \"lithuanian\":\n return 'Laukiamas'\n elif language == \"polish\":\n return 'Witamy'\n elif language == \"spanish\":\n return 'Bienvenido'\n elif language == \"swedish\":\n return 'Valkommen'\n elif language == \"welsh\":\n return 'Croeso'\n else:\n return \"Welcome\"", "def greet(language):\n greeting = {\n 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'\n }\n if language in greeting:\n return greeting[language]\n exit\n return greeting['english']", "def greet(language='english'):\n myDict = {'english': 'Welcome','czech': 'Vitejte', \\\n 'danish': 'Velkomst','dutch': 'Welkom',\\\n 'estonian': 'Tere tulemast','finnish': 'Tervetuloa',\\\n 'flemish': 'Welgekomen','french': 'Bienvenue',\\\n 'german': 'Willkommen','irish': 'Failte',\\\n 'italian': 'Benvenuto','latvian': 'Gaidits',\\\n 'lithuanian': 'Laukiamas','polish': 'Witamy',\\\n 'spanish': 'Bienvenido','swedish': 'Valkommen',\\\n 'welsh': 'Croeso' }\n\n key_list = list(myDict.keys())\n value_list = list(myDict.values())\n try:\n return (value_list[key_list.index(language)])\n except ValueError:\n return (value_list[key_list.index('english')])", "welcome = {\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n}\n\ndef greet(language):\n return 'Welcome' if language not in welcome else welcome[language]", "def greet (language):\n languages = {\n 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'\n \n }\n return (languages.get(language) or 'Welcome')\n \n\n #if languages.get(language) == None :\n # return ('Welcome')\n #else:\n # return (languages[language])\n", "def greet(language):\n obj1 = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n \n if language not in obj1:\n return obj1['english']\n else:\n return obj1[language]", "dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n\ngreet = lambda l: dict[l] if l in dict else dict['english']", "def greet(language):\n welcome_dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n if language in welcome_dict.keys():\n return welcome_dict[language.lower()]\n else:\n return welcome_dict['english']", "def greet(language):\n dictionary = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n for key, value in dictionary.items():\n if language == key:\n return value\n break\n return \"Welcome\"", "def greet(language='english'):\n data={'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return data[language] if language in data else 'Welcome'", "def greet(language):\n #your code here\n db = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n if language in list(db.keys()):\n res = db[language]\n return res\n else:\n return 'Welcome'\n", "def greet(language):\n if language == 'english':\n return \"Welcome\"\n if language == 'czech':\n return \"Vitejte\"\n if language == 'danish':\n return \"Velkomst\"\n if language == 'dutch':\n return \"Welkom\"\n if language == 'estonian':\n return \"Tere tulemast\"\n if language == 'flemish':\n return \"Welgekomen\"\n if language == 'finnish':\n return \"Tervetuloa\"\n if language == 'french':\n return \"Bienvenue\"\n if language == 'german':\n return \"Willkommen\"\n if language == 'irish':\n return \"Failte\"\n if language == 'italian':\n return \"Benvenuto\"\n if language == 'latvian':\n return \"Gaidits\"\n if language == 'lithuanian':\n return \"Laukiamas\"\n if language == 'polish':\n return \"Witamy\"\n if language == 'spanish':\n return \"Bienvenido\"\n if language == 'swedish':\n return \"Valkommen\"\n if language == 'welsh':\n return \"Croeso\"\n else:\n return \"Welcome\"", "def greet(language):\n try:\n dict={'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso' \n }\n return(dict[language])\n except KeyError:\n return(\"Welcome\")", "LANGUAGE = {\n 'english': 'Welcome', 'czech': 'Vitejte', 'danish': 'Velkomst', 'dutch': 'Welkom', 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa', 'flemish': 'Welgekomen', 'french': 'Bienvenue', 'german': 'Willkommen',\n 'irish': 'Failte', 'italian': 'Benvenuto', 'latvian': 'Gaidits', 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy', 'spanish': 'Bienvenido', 'swedish': 'Valkommen', 'welsh': 'Croeso'\n}\n\ndef greet(language):\n return LANGUAGE.get(language, 'Welcome')", "def greet(language):\n dict = {'english': 'Welcome', \n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n if language not in dict:\n return dict[\"english\"]\n else:\n return dict[language]\n #your code here\n", "def greet(language):\n greet_dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n try:\n return greet_dict[language]\n except:\n return greet_dict['english']", "def greet(language):\n languages = \\\n {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n print(language,languages.get(language))\n if languages.get(language) in languages.values(): \n return languages.get(language)\n else:\n return \"Welcome\"", "def greet(language):\n dict = { 'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n if language not in dict:\n return \"Welcome\"\n for k,v in dict.items():\n if k==language:\n return v", "def greet(language):\n \"\"\"greets the user on his mother language\"\"\"\n language_dict = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return language_dict[language] if language in language_dict else \"Welcome\"", "def greet(language):\n accessibility = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n return accessibility[language] if language in accessibility else 'Welcome'", "langs = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n}\n\ngreet = lambda language: langs[language] if language in langs else 'Welcome'", "def greet(language):\n database = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n try:\n return database.get(language.lower(), 'Welcome')\n except:\n return 'Welcome'", "def greet(language):\n d = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n\n for k, v in d.items():\n if language == k:\n return v\n return \"Welcome\"", "DATABASE = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso',\n}\n\n\ndef greet(language):\n try:\n return DATABASE[language]\n except:\n return \"Welcome\"", "def greet(language):\n mydict = {'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'}\n if language not in mydict.keys():\n return \"Welcome\"\n else:\n return mydict.get(language)", "def greet(language):\n '''Returns welcome in language depending on origin of person'''\n languages = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso'\n }\n return languages[language] if language in list(languages.keys()) else 'Welcome'\n\nprint((greet(\"czech\")))\n", "db = {'english': 'Welcome',\n'czech': 'Vitejte',\n'danish': 'Velkomst',\n'dutch': 'Welkom',\n'estonian': 'Tere tulemast',\n'finnish': 'Tervetuloa',\n'flemish': 'Welgekomen',\n'french': 'Bienvenue',\n'german': 'Willkommen',\n'irish': 'Failte',\n'italian': 'Benvenuto',\n'latvian': 'Gaidits',\n'lithuanian': 'Laukiamas',\n'polish': 'Witamy',\n'spanish': 'Bienvenido',\n'swedish': 'Valkommen',\n'welsh': 'Croeso'}\n\ndef greet(language='english'):\n try: \n return db[language]\n except: \n return db['english']\n", "def greet(language):\n x = {\n 'english': 'Welcome',\n 'czech': 'Vitejte',\n 'danish': 'Velkomst',\n 'dutch': 'Welkom',\n 'estonian': 'Tere tulemast',\n 'finnish': 'Tervetuloa',\n 'flemish': 'Welgekomen',\n 'french': 'Bienvenue',\n 'german': 'Willkommen',\n 'irish': 'Failte',\n 'italian': 'Benvenuto',\n 'latvian': 'Gaidits',\n 'lithuanian': 'Laukiamas',\n 'polish': 'Witamy',\n 'spanish': 'Bienvenido',\n 'swedish': 'Valkommen',\n 'welsh': 'Croeso' \n }\n \n if language not in x:\n return \"Welcome\"\n else:\n for i in x:\n if i == language:\n return x[i]"] | {"fn_name": "greet", "inputs": [["english"], ["dutch"], ["IP_ADDRESS_INVALID"], [""], [2]], "outputs": [["Welcome"], ["Welkom"], ["Welcome"], ["Welcome"], ["Welcome"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 65,597 |
def greet(language):
|
1b97724912b3fe26de2c9c37a11986df | UNKNOWN | Given a list of integers values, your job is to return the sum of the values; however, if the same integer value appears multiple times in the list, you can only count it once in your sum.
For example:
```python
[ 1, 2, 3] ==> 6
[ 1, 3, 8, 1, 8] ==> 12
[ -1, -1, 5, 2, -7] ==> -1
[] ==> None
```
Good Luck! | ["def unique_sum(lst):\n return sum(set(lst)) if lst else None\n", "def unique_sum(lst):\n if lst:\n return sum(set(lst)) ", "def unique_sum(lst):\n s = set(lst)\n \n return sum(s) if len(s) != 0 else None", "unique_sum = lambda l: sum(set(l)) if l else None", "def unique_sum(lst):\n if lst:\n return sum(set(lst))\n else:\n return None\n", "def unique_sum(lst):\n return sum(set(lst)) if len(lst) > 0 else None", "def unique_sum(lst):\n new_lst = []\n for i in lst:\n if not i in new_lst:\n new_lst.append(i)\n return sum(new_lst) if lst else None", "def unique_sum(lst):\n return sum(set(lst)) if len(set(lst)) > 0 else None", "def unique_sum(lst):\n y=lambda x:sum(set(x))\n if len(lst)==0:\n return None\n elif y(lst)==0:\n return 0\n else:\n return y(lst)", "def unique_sum(lst):\n \n if lst==[]:\n \n return None\n \n else:\n \n s = set(lst)\n \n v = list(s)\n \n d = sum(v)\n \n return d\n\n"] | {"fn_name": "unique_sum", "inputs": [[[]], [[0, 1, -1]]], "outputs": [[null], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,078 |
def unique_sum(lst):
|
4db6862589be9cea40aaf30ae7c09493 | UNKNOWN | Define a function that takes in two non-negative integers `$a$` and `$b$` and returns the last decimal digit of `$a^b$`. Note that `$a$` and `$b$` may be very large!
For example, the last decimal digit of `$9^7$` is `$9$`, since `$9^7 = 4782969$`. The last decimal digit of `$({2^{200}})^{2^{300}}$`, which has over `$10^{92}$` decimal digits, is `$6$`. Also, please take `$0^0$` to be `$1$`.
You may assume that the input will always be valid.
## Examples
```python
last_digit(4, 1) # returns 4
last_digit(4, 2) # returns 6
last_digit(9, 7) # returns 9
last_digit(10, 10 ** 10) # returns 0
last_digit(2 ** 200, 2 ** 300) # returns 6
```
___
## Remarks
### JavaScript, C++, R, PureScript
Since these languages don't have native arbitrarily large integers, your arguments are going to be strings representing non-negative integers instead. | ["def last_digit(n1, n2):\n return pow( n1, n2, 10 )", "rules = {\n 0: [0,0,0,0], \n 1: [1,1,1,1],\n 2: [2,4,8,6],\n 3: [3,9,7,1],\n 4: [4,6,4,6], \n 5: [5,5,5,5], \n 6: [6,6,6,6], \n 7: [7,9,3,1], \n 8: [8,4,2,6], \n 9: [9,1,9,1],\n}\ndef last_digit(n1, n2):\n ruler = rules[int(str(n1)[-1])]\n return 1 if n2 == 0 else ruler[(n2 % 4) - 1]", "def last_digit(n1, n2):\n return (n1 % 10) ** (n2 % 4 + 4 * bool(n2)) % 10\n", "E = [\n [0, 0, 0, 0], [1, 1, 1, 1],\n [6, 2, 4, 8], [1, 3, 9, 7],\n [6, 4, 6, 4], [5, 5, 5, 5],\n [6, 6, 6, 6], [1, 7, 9, 3],\n [6, 8, 4, 2], [1, 9, 1, 9]\n]\n\ndef last_digit(n1, n2):\n if n2 == 0: return 1\n return E[n1 % 10][n2 % 4]\n", "last_digit = lambda n1, n2: pow(n1, n2, 10)", "def last_digit(n1, n2):\n m1=n1%10\n if m1==1:\n return 1\n if m1==2:\n if n2==0:\n return 1\n elif n2%4==3:\n return 8\n elif n2%4==0:\n return 6\n elif n2%4==2:\n return 4\n else:\n return 2\n if m1==3:\n if n2==0:\n return 1\n elif n2%4==0:\n return 1\n elif n2%2==0:\n return 9\n elif n2%4==3:\n return 7\n else:\n return 3\n if m1==4:\n if n2==0:\n return 1\n elif n2%4==2 or n2%4==0:\n return 6\n else:\n return 4\n if m1==5:\n if n2==0:\n return 1\n else:\n return 5\n if m1==6:\n if n2==0:\n return 1\n else:\n return 6\n if m1==7:\n if n2==0:\n return 1\n elif n2%4==3:\n return 3\n elif n2%4==0:\n return 1\n elif n2%4==2:\n return 9\n else:\n return 7\n if m1==8:\n if n2==0:\n return 1\n elif n2%4==3:\n return 2\n elif n2%4==0:\n return 6\n elif n2%4==2:\n return 4\n else:\n return 8\n if m1==9:\n if n2==0:\n return 1\n elif n2%2==1:\n return 9\n else:\n return 1\n if m1==0:\n if n2==0:\n return 1\n return 0", "digits = {\n 0:[0,0,0,0], \n 1:[1,1,1,1], \n 2:[2,4,8,6], \n 3:[3,9,7,1], \n 4:[4,6,4,6], \n 5:[5,5,5,5], \n 6:[6,6,6,6], \n 7:[7,9,3,1], \n 8:[8,4,2,6], \n 9:[9,1,9,1]\n}\ndef last_digit(n1, n2):\n return digits[n1%10][(n2-1)%4] if n2 else 1", "def last_digit(n1, n2):\n last_dict = {\n 0: [0],\n 1: [1], \n 2: [2, 4, 6, 8],\n 3: [3, 9, 7, 1],\n 4: [4, 6],\n 5: [5],\n 6: [6],\n 7: [7, 9, 3, 1],\n 8: [8, 4, 2, 6],\n 9: [9, 1]}\n \n if n2 == 0:\n return 1\n a = n1 % 10\n return last_dict[a][(n2-1)%len(last_dict[a])]", "def last_digit(n1, n2):\n if n2 == 0: return 1\n seq = [[0], [1], [2, 4, 8, 6], [3, 9, 7, 1], [4, 6], [5], [6], [7, 9, 3, 1], [8, 4, 2, 6], [9, 1]]\n l_digit = int(str(n1)[-1])\n position = n2 % len(seq[l_digit])\n return seq[l_digit][position - 1]", "def last_digit(n1, n2):\n if n2 == 0:\n return 1\n n1 %= 10\n if n1 in [0, 1, 5, 6]:\n return n1\n if n1 == 2:\n return [6, 2, 4, 8][n2%4]\n if n1 == 3:\n return [1, 3, 9, 7][n2%4]\n if n1 == 4:\n return [6, 4][n2%2]\n if n1 == 7:\n return [1, 7, 9, 3][n2%4]\n if n1 == 8:\n return [6, 8, 4, 2][n2%4]\n return [1, 9][n2%2]\n"] | {"fn_name": "last_digit", "inputs": [[4, 1], [4, 2], [9, 7], [10, 1000000000], [38710248912497124917933333333284108412048102948908149081409204712406, 226628148126342643123641923461846128214626], [3715290469715693021198967285016729344580685479654510946723, 68819615221552997273737174557165657483427362207517952651]], "outputs": [[4], [6], [9], [0], [6], [7]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,636 |
def last_digit(n1, n2):
|
a8a643a7885de53e75a03cdaff222c5d | UNKNOWN | Well, those numbers were right and we're going to feed their ego.
Write a function, isNarcissistic, that takes in any amount of numbers and returns true if all the numbers are narcissistic. Return false for invalid arguments (numbers passed in as strings are ok).
For more information about narcissistic numbers (and believe me, they love it when you read about them) follow this link: https://en.wikipedia.org/wiki/Narcissistic_number | ["def get_digits(n):\n return [int(x) for x in list(str(n))]\n\n\ndef is_narc(n):\n return n == sum([x**len(get_digits(n)) for x in get_digits(n)])\n\n\ndef is_narcissistic(*values):\n try:\n return all(type(n) in [int,str] and is_narc(int(n)) for n in values)\n except ValueError:\n return False", "def narc(n):\n ds = [int(c) for c in str(n)]\n return sum(d**len(ds) for d in ds) == n\n\ndef is_narcissistic(*ns):\n return (\n all((isinstance(n, int) and n >= 0) or (isinstance(n, str) and n.isdigit()) for n in ns)\n and all(map(narc, map(int, ns)))\n )", "def is_narcissistic(*arg):\n for e in arg:\n try:\n if e == 0:\n continue\n n = int(e) == sum([int(i) ** len(str(e)) for i in str(e)])\n if not n:\n return False\n except:\n return False \n return True", "def narcissistic(n):\n s = str(n)\n l = len(s)\n return n == sum(int(d)**l for d in s)\n \ndef is_narcissistic(*numbers):\n return all(str(n).isdigit() and narcissistic(int(n)) for n in numbers)", "def is_narcissistic(*nos):\n try:\n return all([sum([int(i)**len(str(n)) for i in str(n)]) == int(n) for n in nos])\n except ValueError:\n return False", "is_narcissistic=lambda*a:all(n.isdigit()and int(n)==sum(int(d)**len(n)for d in n)for n in map(str,a))", "is_narcissistic=lambda*a:all(str(n).isdigit()for n in a)and all(int(n)==sum(int(d)**len(n)for d in n)for n in map(str,a))", "def narcissistic(n):\n try:\n k = len(str(n))\n return sum(d**k for d in map(int, str(n))) == int(n)\n except ValueError:\n return False\n\ndef is_narcissistic(*args):\n return all(map(narcissistic, args))", "def is_narcissistic(*args):\n def check(n):\n n=str(n)\n l=len(n)\n return int(n)==sum(int(d)**l for d in n)\n return all(str(arg).isdigit() and check(int(arg)) for arg in args)", "def is_narcissistic(*args):\n try:\n return all(narcissistic(int(number)) for number in args)\n except (ValueError, TypeError):\n return False\n\ndef narcissistic(number):\n n = len(str(number))\n return sum(int(digit) ** n for digit in str(number)) == number"] | {"fn_name": "is_narcissistic", "inputs": [[11], [0, 1, 2, 3, 4, 5, 6, 7, 8, 9], [0, 1, 2, 3, 4, 5, 6, 7, 22, 9], ["4"], ["4", 7, "9"], ["words"], [[1, 2]], [407, 8208], [-1], [""], ["", 407], [407, ""], [5, "", 407], [9474], [{}]], "outputs": [[false], [true], [false], [true], [true], [false], [false], [true], [false], [false], [false], [false], [false], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,263 |
def is_narcissistic(*args):
|
1593bcb76513012e681c075788d815f7 | UNKNOWN | An `non decreasing` number is one containing no two consecutive digits (left to right), whose the first is higer than the second. For example, 1235 is an non decreasing number, 1229 is too, but 123429 isn't.
Write a function that finds the number of non decreasing numbers up to `10**N` (exclusive) where N is the input of your function. For example, if `N=3`, you have to count all non decreasing numbers from 0 to 999.
You'll definitely need something smarter than brute force for large values of N! | ["def increasing_numbers(d):\n s = 1\n for i in range(1,10): s=s*(i+d)//i\n return s", "from functools import lru_cache\n\n\n@lru_cache(maxsize=None)\ndef f(ndigits, starting_digit):\n if ndigits == 0:\n return 1\n return sum(f(ndigits-1, i) for i in range(starting_digit, 10))\n \ndef increasing_numbers(digits):\n if digits == 0:\n return 1\n return sum(f(digits-1, i) for i in range(10))", "from functools import reduce\n\n\ndef increasing_numbers(n):\n return reduce(int.__mul__, range(n + 1, n + 10)) // 362880", "from functools import reduce\nfrom operator import mul\n\ndef increasing_numbers(digits):\n return reduce(mul, range(digits+1, digits+10), 1) // 362880", "from math import factorial\nfrom functools import reduce\nimport operator\ndef increasing_numbers(digits):\n if digits == 0:\n return 1\n if digits == 1:\n return 10\n consec_pro = reduce(operator.mul, (i for i in range(10, 10 + digits)))\n return consec_pro / factorial(digits) ", "from math import factorial\n\ndef nCr(n, r):\n return factorial(n) // factorial(r) // factorial(n - r)\n\ndef increasing_numbers(digits):\n return nCr(digits + 9, 9)", "from math import factorial\ndef increasing_numbers(d):\n return factorial(9 + d) / (factorial(d) * factorial(9)) if d > 0 else 1", "def increasing_numbers(n):\n return fac(n+9)/(fac(9)*fac(n))\n\ndef fac(n):\n c=1\n for i in range(2,n+1):\n c*=i\n return c", "from math import factorial\n\ndef increasing_numbers(n):\n return factorial(n+9)/(factorial(9)*factorial(n))\n", "from math import factorial\ndef increasing_numbers(digits):\n return factorial(9 + digits) // (factorial(digits) * factorial(9))"] | {"fn_name": "increasing_numbers", "inputs": [[0], [1], [2], [3], [4], [5], [6], [10], [20], [50]], "outputs": [[1], [10], [55], [220], [715], [2002], [5005], [92378], [10015005], [12565671261]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,731 |
def increasing_numbers(n):
|
cea1b25002f6807d796688c1a78b6d6e | UNKNOWN | ### Task
Each day a plant is growing by `upSpeed` meters. Each night that plant's height decreases by `downSpeed` meters due to the lack of sun heat. Initially, plant is 0 meters tall. We plant the seed at the beginning of a day. We want to know when the height of the plant will reach a certain level.
### Example
For `upSpeed = 100, downSpeed = 10 and desiredHeight = 910`, the output should be `10`.
```
After day 1 --> 100
After night 1 --> 90
After day 2 --> 190
After night 2 --> 180
After day 3 --> 280
After night 3 --> 270
After day 4 --> 370
After night 4 --> 360
After day 5 --> 460
After night 5 --> 450
After day 6 --> 550
After night 6 --> 540
After day 7 --> 640
After night 7 --> 630
After day 8 --> 730
After night 8 --> 720
After day 9 --> 820
After night 9 --> 810
After day 10 --> 910
```
For `upSpeed = 10, downSpeed = 9 and desiredHeight = 4`, the output should be `1`.
Because the plant reach to the desired height at day 1(10 meters).
```
After day 1 --> 10
```
### Input/Output
```if-not:sql
- `[input]` integer `upSpeed`
A positive integer representing the daily growth.
Constraints: `5 ≤ upSpeed ≤ 100.`
- `[input]` integer `downSpeed`
A positive integer representing the nightly decline.
Constraints: `2 ≤ downSpeed < upSpeed.`
- `[input]` integer `desiredHeight`
A positive integer representing the threshold.
Constraints: `4 ≤ desiredHeight ≤ 1000.`
- `[output]` an integer
The number of days that it will take for the plant to reach/pass desiredHeight (including the last day in the total count).
```
```if:sql
## Input
~~~
-----------------------------------------
| Table | Column | Type |
|---------------+----------------+------|
| growing_plant | down_speed | int |
| | up_speed | int |
| | desired_height | int |
-----------------------------------------
~~~
### Columns
* `up_speed`: A positive integer representing the daily growth. Constraints: `5 ≤ up_speed ≤ 100.`
* `down_speed`: A positive integer representing the nightly decline. Constraints: `2 ≤ down_speed < up_speed.`
* `desired_height`: A positive integer representing the threshold. Constraints: `4 ≤ desired_height ≤ 1000.`
## Output
~~~
-------------------
| Column | Type |
|----------+------|
| id | int |
| num_days | int |
-------------------
~~~
`num_days` is the number of days that it will take for the plant to reach/pass desiredHeight (including the last day in the total count).
``` | ["from math import ceil\n\ndef growing_plant(up, down, h):\n return max(ceil((h - down) / (up - down)), 1)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n days = 1\n height = upSpeed\n while (height < desiredHeight):\n height += upSpeed - downSpeed\n days += 1\n return days;\n", "from math import ceil; growing_plant=lambda u,d,h: max([ceil((h-u)/(u-d)),0])+1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n current = 0\n counter = 1\n while current < desiredHeight: \n current = current + upSpeed\n if current >= desiredHeight: return counter\n current = current - downSpeed \n counter = counter + 1\n return counter", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n try:\n assert 5 <= upSpeed <= 100\n assert 2 <= downSpeed < upSpeed\n assert 4 <= desiredHeight <= 1000\n except:\n return 1\n \n day = 0\n plantHeight = 0\n while plantHeight < desiredHeight:\n day += 1\n plantHeight += upSpeed\n if plantHeight >= desiredHeight:\n break\n else:\n plantHeight -= downSpeed\n \n return day\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n return max((desiredHeight - upSpeed - 1) // (upSpeed - downSpeed) + 1, 0) + 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n i=1\n height=0\n while(True):\n height=height+upSpeed\n if height>=desiredHeight:\n break\n else:\n height=height-downSpeed\n i=i+1\n return i", "from math import ceil\n\ndef growing_plant(up_speed, down_speed, desired_height):\n return max(0, ceil((desired_height - up_speed) / (up_speed - down_speed))) + 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n desiredHeight -= upSpeed\n return max(1, -(-desiredHeight // (upSpeed - downSpeed)) + 1)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if (desiredHeight <= upSpeed): return 1\n days = int(desiredHeight/upSpeed)\n desiredHeight -= days*(upSpeed-downSpeed)\n return days+growing_plant(upSpeed, downSpeed, desiredHeight)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n day = 1\n num_days = 0\n while height < desiredHeight:\n if day == True: \n height += upSpeed\n day = 0\n num_days += 1\n else:\n height -= downSpeed\n day = 1\n return num_days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 1\n height = 0\n while True:\n height += upSpeed\n if height >= desiredHeight:\n return day\n height -= downSpeed\n day += 1 ", "growing_plant=lambda u,d,h:max(1,int((h-u)/(u-d)+1.99))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n #your code here\n day = 1\n plant_height = 0\n while True:\n plant_height += upSpeed\n if plant_height >= desiredHeight:\n return day\n plant_height -= downSpeed\n day += 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n h=0\n n=1\n h=n*upSpeed - (n-1)*downSpeed\n while h<desiredHeight:\n n+=1\n h=n*upSpeed - (n-1)*downSpeed\n return n\n \n", "growing_plant=lambda u,d,h:0-max(h-d,1)//(d-u)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n desiredHeight -= upSpeed\n if desiredHeight<0: desiredHeight = 0\n return -(-desiredHeight//(upSpeed-downSpeed)) + 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n progress = upSpeed - downSpeed\n total = 1\n while upSpeed < desiredHeight:\n upSpeed += progress\n total += 1\n return total", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n i = upSpeed\n j = 1\n while i < desiredHeight:\n i += (upSpeed - downSpeed)\n j = j+1\n return j", "import math\n\ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n oneDay = upSpeed - downSpeed\n \n if desiredHeight - oneDay <= 0 or desiredHeight <= upSpeed:\n return 1\n \n sum = 0\n count = 0\n \n while desiredHeight - downSpeed > sum:\n sum += oneDay\n count += 1\n \n return count\n", "from math import ceil\n\ndef growing_plant(u, d, h):\n return max(1, min(ceil(h/(u-d)), ceil((h-d)/(u-d))))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n plantHeight = 0\n day = 0\n night = 0\n \n while plantHeight != desiredHeight:\n day += 1\n plantHeight += upSpeed\n if plantHeight >= desiredHeight:\n return day\n \n night += 1\n plantHeight -= downSpeed\n if plantHeight >= desiredHeight:\n return night", "import unittest\n\n\ndef growing_plant(up_speed, down_speed, desired_height):\n\n result = 0\n day = 0\n\n while True:\n day += 1\n result += up_speed\n if result >= desired_height:\n break\n result -= down_speed\n if result >= desired_height:\n break\n\n return day\n\n \nclass TestGrowingPlant(unittest.TestCase):\n def test_should_return_1_when_desiredheight_is_equal_less_than_upspeed(self):\n self.assertEqual(growing_plant(up_speed=10, down_speed=9, desired_height=4), 1)\n\n def test_growing_plant_common_case(self):\n self.assertEqual(growing_plant(up_speed=100, down_speed=10, desired_height=910), 10)\n\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n h,c=upSpeed,0\n while h<desiredHeight:\n h=h-downSpeed+upSpeed\n c=c+1\n return c+1\n", "def growing_plant(u,d,h):\n for i in range(1000):\n if h-u <= 0: return i+1\n h += d-u", "def growing_plant(up_speed, down_speed, desired_height):\n count = 1\n height = up_speed\n while height < desired_height:\n height -= down_speed\n height += up_speed\n count += 1\n\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = upSpeed\n counter = 1\n if height >= desiredHeight:\n return counter\n else:\n while height < desiredHeight:\n height += upSpeed - downSpeed\n counter += 1\n return counter", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if upSpeed >= desiredHeight:\n return 1\n return int(1 + (desiredHeight - upSpeed) / (upSpeed - downSpeed) + bool((desiredHeight - upSpeed) % (upSpeed - downSpeed)))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n count = 0\n while desiredHeight >= 0:\n count += 1\n desiredHeight -= upSpeed\n if desiredHeight <= 0:\n break\n desiredHeight += downSpeed\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n return max(1,int(0.99+ (desiredHeight-downSpeed)/(upSpeed-downSpeed)))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n t = 0\n h = 0\n while True:\n t+=1\n h += upSpeed\n if h >= desiredHeight : return t\n h -= downSpeed\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if upSpeed>=desiredHeight:\n return 1\n days=0\n plant=0\n while desiredHeight > plant:\n if (plant + upSpeed) >= desiredHeight:\n days+=1\n break\n else:\n plant+=(upSpeed - downSpeed)\n days+=1\n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if upSpeed>=desiredHeight:\n return 1\n else:\n desiredHeight_after_first_day=desiredHeight-upSpeed\n increment_each_day=upSpeed-downSpeed\n if desiredHeight_after_first_day%(increment_each_day)==0:\n return desiredHeight_after_first_day//(increment_each_day)+1\n else:\n return desiredHeight_after_first_day//(increment_each_day)+2\n #your code here\n", "from math import ceil\n\ndef growing_plant(u, d, h):\n return max(1, ceil((h - d) / (u - d)))", "def growing_plant(u, d, h):\n days = growth = 0\n \n while True:\n # day\n days += 1\n growth += u\n\n if growth >= h:\n return days\n \n # night\n growth -= d", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n cnt = 1\n for i in range(upSpeed, desiredHeight, upSpeed-downSpeed):\n cnt += 1\n return cnt\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n i = 0\n total = 0\n up = 0\n if desiredHeight == 0:\n return 1\n while total < desiredHeight and up < desiredHeight:\n i += 1\n up = total + upSpeed\n total = total + upSpeed - downSpeed\n return i", "def growing_plant(up, down, desired):\n res, day = 0, 0\n while True:\n res += up\n day += 1\n if res >= desired:\n return day\n res -= down", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = days = 0\n while height <= desiredHeight:\n days += 1\n height += upSpeed\n if height >= desiredHeight: break\n height -= downSpeed\n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 0\n height = 0\n while desiredHeight > height:\n day += 1\n height += upSpeed\n if height >= desiredHeight:\n return day\n height -= downSpeed\n return day if day > 0 else 1 ", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n i, height = 0, 0\n while True:\n height += upSpeed\n i += 1\n if height >= desiredHeight:\n break\n height -= downSpeed\n return i", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n #your code here\n num_days = 1\n height = 0\n while height < desiredHeight:\n height += upSpeed\n if height >= desiredHeight:\n break\n height -= downSpeed\n num_days += 1\n return num_days", "import math \ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n if upSpeed>=desiredHeight:\n return 1\n else:\n return math.ceil((desiredHeight-downSpeed)/(upSpeed-downSpeed))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n result = 0\n height = 0\n while(True):\n result += 1\n height += upSpeed\n if height >= desiredHeight:\n break;\n height -= downSpeed\n return result", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n days = 1\n while height < desiredHeight:\n height += upSpeed\n if height < desiredHeight:\n height -= downSpeed\n days += 1\n else:\n return days", "import math\n\ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n days, height = 0, 0\n while True:\n days += 1\n height += upSpeed\n if height >= desiredHeight:\n return days\n height -= downSpeed", "def growing_plant(up_speed, down_speed, desired_height):\n current_height = 0\n days_gone = 0\n if up_speed >= 5 and up_speed <= 100 and down_speed >= 2 and down_speed < up_speed and desired_height >= 4 and desired_height <= 1000:\n while True:\n days_gone += 1\n current_height += up_speed\n if current_height < desired_height:\n current_height -= down_speed\n else:\n return days_gone\n break\n else:\n return 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n n = 0\n while height < desiredHeight:\n height += upSpeed\n n += 1\n if height < desiredHeight:\n height -= downSpeed\n else:\n break\n return n if upSpeed < desiredHeight else 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n for i in range(1,1000):\n if i*(upSpeed-downSpeed)>=desiredHeight or (i-1)*(upSpeed-downSpeed)+upSpeed>=desiredHeight:\n return i", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n d, h = 0, 0\n while True:\n d += 1\n h += upSpeed\n if h >= desiredHeight:\n return d\n else:\n h -= downSpeed", "def growing_plant(u,d,de):\n n=0\n m=0\n while de>=n:\n m+=1\n n+=u\n if n>=de:\n break\n n-=d\n return m", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if (upSpeed >= desiredHeight) or (desiredHeight == 0):\n return 1\n res = int((desiredHeight - upSpeed) / (upSpeed - downSpeed))\n if (desiredHeight - upSpeed) % (upSpeed - downSpeed) > 0:\n res += 1\n return res + 1\n", "from math import ceil\n\ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n if desiredHeight < upSpeed:\n return 1\n return ceil((desiredHeight - downSpeed) / (upSpeed - downSpeed))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n days = 0\n h = 0\n while True:\n days = days + 1\n h = h + upSpeed\n if h >= desiredHeight:\n return days\n else:\n h = h - downSpeed", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = upSpeed\n day = 1\n \n while(height < desiredHeight):\n height -= downSpeed\n height += upSpeed\n day += 1\n \n return day", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if desiredHeight-upSpeed <=0:\n return 1\n return 1+ growing_plant(upSpeed, downSpeed, desiredHeight-upSpeed+downSpeed)", "import math\ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n if (desiredHeight - upSpeed) > 0:\n return(1+math.ceil((desiredHeight - upSpeed) / (upSpeed - downSpeed)))\n return(1)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if desiredHeight == 0:\n return 1\n height = 0\n days = 0\n while height < desiredHeight:\n height += upSpeed\n if height < desiredHeight:\n height -= downSpeed\n days += 1\n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n if desiredHeight <= downSpeed:\n return 1\n \n currentHeight = 0\n \n i = 1\n while i < 100000:\n \n currentHeight+=upSpeed;\n if currentHeight >= desiredHeight:\n break\n \n currentHeight-=downSpeed\n i += 1\n \n return i", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n days = 0\n \n for i in range(1, 10000):\n days += 1\n height += upSpeed\n print(f'After day {i}: {height}')\n \n if height >= desiredHeight: break \n \n height -= downSpeed\n print(f'After night {i}: {height}')\n\n if height >= desiredHeight: break\n \n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n count = 0\n \n sum = 0\n \n while sum < desiredHeight:\n sum += upSpeed\n if sum >= desiredHeight:\n return count +1 \n sum -= downSpeed\n count += 1\n print(sum)\n \n return 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n d=0\n e=0\n if desiredHeight<upSpeed:\n return 1\n else:\n while d<desiredHeight:\n d+=upSpeed\n e+=1\n if d>=desiredHeight:\n return e\n else:\n d-=downSpeed\n return e", "from math import ceil\ndef growing_plant(u, d, H):\n return ceil(max((H-u)/(u-d), 0))+1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n day = 0\n if desiredHeight == 0:\n day += 1\n return day\n while height != desiredHeight:\n day += 1\n height += upSpeed\n if height >= desiredHeight:\n return day\n else:\n height -= downSpeed", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height, day = upSpeed, 1\n while height < desiredHeight:\n print((\"After day \"+str(day)+\" --> \"+str(height)))\n print((\"After night \"+str(day)+\" --> \"+str(height-downSpeed)))\n height = height - downSpeed + upSpeed\n day += 1\n print((\"After day \"+str(day)+\" --> \"+str(height)))\n return day\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n actualGrowth_afterDay = upSpeed\n number_of_days = 1\n dailyGrowth = upSpeed - downSpeed\n while actualGrowth_afterDay < desiredHeight:\n number_of_days += 1\n actualGrowth_afterDay += dailyGrowth \n return number_of_days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 1\n currentHeight = 0\n growing = True\n while growing:\n currentHeight += upSpeed\n print(\"After Day\", day, \"Height:\", currentHeight)\n if currentHeight >= desiredHeight:\n growing = False\n else:\n currentHeight -= downSpeed\n print(\"After Night\", day, \"Height:\", currentHeight)\n day += 1 \n return day", "def growing_plant(u, d, h):\n r,c = u,1\n while r<h:\n r+= u-d\n c += 1\n return c", "def growing_plant(u, d, h):\n p = 0\n count = 1\n while h > p:\n p += u\n if h <= p:\n break\n p -= d\n count += 1\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n noite = 0\n dias = 0\n while desiredHeight > height and desiredHeight > noite:\n height += upSpeed\n noite = height\n height -= downSpeed\n dias += 1\n return dias", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n day = 1\n while height < desiredHeight:\n if (height + upSpeed) >= desiredHeight:\n return day\n else:\n height += (upSpeed-downSpeed)\n day +=1\n return day\n \n", "import math\ndef growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n days = 0\n while True:\n height += upSpeed\n days += 0.5\n if height >= desiredHeight:\n break\n height -= downSpeed\n days += 0.5\n return math.ceil(days)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 0\n night = 0\n height = 0\n while height != desiredHeight:\n height = height + upSpeed\n day = day + 1\n if height >= desiredHeight:\n return day\n else:\n height = height - downSpeed\n night = night + 1\n if height == desiredHeight:\n return night\n return 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n return 1+sum(upSpeed*d-downSpeed*(d-1) < desiredHeight for d in range(1,699))", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n days = 0\n heights = []\n if desiredHeight == 0:\n return 1\n while height < desiredHeight:\n height += upSpeed\n days += 1\n if height >= desiredHeight:\n break\n height -= downSpeed\n \n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n\n growth = upSpeed\n counter = 1\n\n while growth < desiredHeight:\n growth += upSpeed - downSpeed\n counter += 1\n \n return counter", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n count=1\n height=0\n while height<desiredHeight:\n height=height+upSpeed\n if height>=desiredHeight:\n break\n height=height-downSpeed\n if height>=desiredHeight:\n break\n count=count+1\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n daily_growth = upSpeed - downSpeed\n if desiredHeight <= upSpeed:\n return 1\n elif (desiredHeight - upSpeed) % daily_growth == 0:\n return (desiredHeight - upSpeed) // daily_growth + 1\n else:\n return (desiredHeight - upSpeed) // daily_growth + 2\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 0\n height = 0\n \n while height < desiredHeight:\n day += 1\n height += upSpeed\n if height >= desiredHeight: return day\n else:\n height -= downSpeed\n return 1", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n t = 0\n day = 0\n while t < desiredHeight:\n day += 1\n t += upSpeed\n if t >= desiredHeight:\n return day\n t -= downSpeed\n return max(day, 1)", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n \"\"\"growing plant\"\"\"\n actual_height = 0\n counter = 0\n while actual_height <= desiredHeight:\n counter += 1\n actual_height += upSpeed\n if actual_height >= desiredHeight:\n return counter\n actual_height -= downSpeed\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n count=0\n height=0\n while height<desiredHeight:\n height+=upSpeed\n count+=1\n if height>=desiredHeight: return count\n height-=downSpeed\n return 1\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n initial = upSpeed\n count = 1\n while initial < desiredHeight:\n initial += upSpeed\n initial -= downSpeed\n count += 1\n return count\n #your code here\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n current_height = 0\n counter = 0\n if desiredHeight == 0:\n counter = 1\n return counter \n else:\n while current_height < desiredHeight:\n current_height += upSpeed\n counter += 1\n if current_height >= desiredHeight:\n return counter\n else:\n current_height -= downSpeed\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n days, nights, height = 0, 0, 0\n \n if upSpeed >= desiredHeight:\n return 1\n else:\n while height <= desiredHeight:\n height += upSpeed\n days += 1\n \n if height >= desiredHeight:\n break\n \n height -= downSpeed\n nights += 1\n \n if nights > days:\n return nights\n else:\n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n days = 0\n height = 0\n while True:\n days += 1\n height += upSpeed\n if height >= desiredHeight:\n break\n height -= downSpeed\n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n growth = upSpeed\n count = 1\n while growth < desiredHeight:\n growth -= downSpeed\n count += 1\n growth += upSpeed\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n count=0\n a=0\n if desiredHeight==0:\n return 1\n while not a>=desiredHeight:\n a+=upSpeed\n if a<desiredHeight:\n a-=downSpeed\n count+=1\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n return max(0, (desiredHeight - downSpeed - 1) // (upSpeed - downSpeed)) + 1\n", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n dia = 0\n height = 0\n while True:\n height = height + upSpeed\n dia+=1\n if height >= desiredHeight:\n return dia\n height = height - downSpeed", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n h = 0; counter = 1\n while (h + upSpeed) < desiredHeight:\n h += upSpeed - downSpeed\n counter += 1\n return counter", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n day = 0\n height = 0\n while height < desiredHeight:\n day += 1\n if day != 1:\n height -= downSpeed\n height += upSpeed\n return 1 if desiredHeight == 0 else day", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n x = upSpeed\n output = 1\n while x < desiredHeight:\n x += upSpeed\n x -= downSpeed\n output += 1\n return output", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n init_height = 0\n day_count = 0\n if init_height == desiredHeight:\n return 1\n else:\n while init_height<desiredHeight:\n init_height += upSpeed\n day_count += 1\n if init_height >= desiredHeight:\n print(init_height,day_count)\n return day_count\n break\n else:\n init_height -= downSpeed\n if init_height >= desiredHeight:\n print(init_height,day_count)\n return day_count\n break", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n #your code here\n up = upSpeed\n i = 1\n while up < desiredHeight :\n up += upSpeed\n up -= downSpeed\n i += 1\n return i", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n growth = 0\n count = 1\n while growth < desiredHeight:\n growth += upSpeed\n if growth >= desiredHeight:\n return count\n count += 1\n growth -= downSpeed\n return count", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n height = 0\n height2 = 0\n days = 0\n \n if (desiredHeight == 0):\n return 1\n \n \n while height < desiredHeight:\n days += 1\n height = height2\n height += upSpeed\n height2 = height - downSpeed\n \n \n return days", "def growing_plant(upSpeed, downSpeed, desiredHeight):\n h, day = 0, 1\n while h != desiredHeight:\n h += upSpeed\n if h >= desiredHeight: break\n h -= downSpeed\n day += 1\n \n return day", "def growing_plant(u, d, h):\n v = 0\n for i in range(1,int(1E3)): \n v += u \n if v >= h:\n return i \n else:\n v -= d ", "def growing_plant(up, down, desired):\n height=up\n count=1\n while height<desired:\n height=height+up-down\n count+=1\n return count"] | {"fn_name": "growing_plant", "inputs": [[100, 10, 910], [10, 9, 4], [5, 2, 5], [5, 2, 6]], "outputs": [[10], [1], [1], [2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 26,387 |
def growing_plant(upSpeed, downSpeed, desiredHeight):
|
75d3705334f9b0487be726930de114b4 | UNKNOWN | Array inversion indicates how far the array is from being sorted.
Inversions are pairs of elements in array that are out of order.
## Examples
```
[1, 2, 3, 4] => 0 inversions
[1, 3, 2, 4] => 1 inversion: 2 and 3
[4, 1, 2, 3] => 3 inversions: 4 and 1, 4 and 2, 4 and 3
[4, 3, 2, 1] => 6 inversions: 4 and 3, 4 and 2, 4 and 1, 3 and 2, 3 and 1, 2 and 1
```
## Goal
The goal is to come up with a function that can calculate inversions for any arbitrary array | ["def count_inversions(array):\n inv_count = 0\n for i in range(len(array)):\n for j in range(i, len(array)):\n if array[i] > array[j]:\n inv_count += 1\n return inv_count", "def count_inversions(array):\n return sum(x > y for i,x in enumerate(array) for y in array[i+1:])", "count_inversions=lambda arr:sum(sum(k>j for k in arr[:i]) for i,j in enumerate(arr))", "def count_inversions(array):\n n = len(array)\n return sum([1 for i in range(n) for j in range(n) if (i < j and array[i] > array[j])])", "def count_inversions(lst):\n return sum(1 for i, m in enumerate(lst, 1) for n in lst[i:] if n < m)\n", "from itertools import islice\n\ndef count_inversions(array):\n return sum(\n sum(x > y for y in islice(array, i+1, None))\n for i, x in enumerate(array)\n )", "def count_inversions(array):\n return sum([1 for i in range(len(array)) for j in range(i+1,len(array)) if array[i] > array[j]])", "def count_inversions(collection):\n lenghts = len(collection)\n swapped = False\n swapped_counter =0\n for i in range(lenghts - 1):\n for j in range(lenghts - i - 1):\n if collection[j] > collection[j + 1]:\n collection[j], collection[j + 1] = collection[j + 1], collection[j]\n swapped = True\n swapped_counter +=1\n if not swapped:\n break\n return swapped_counter", "fun1 = lambda a1,a2:sum([1 if a1>i else 0 for i in a2])\ndef count_inversions(array):\n s = 0\n for i in range(len(array)):\n if i != len(array)-1:\n s += fun1(array[i],array[i+1:])\n return s", "def count_inversions(array):\n inversions = 0\n \n for i in range(len(array)):\n for j in range(i+1, len(array)):\n if array[i] > array[j]:\n inversions += 1\n return inversions"] | {"fn_name": "count_inversions", "inputs": [[[]], [[1, 2, 3]], [[2, 1, 3]], [[6, 5, 4, 3, 2, 1]], [[6, 5, 4, 3, 3, 3, 3, 2, 1]]], "outputs": [[0], [0], [1], [15], [30]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,887 |
def count_inversions(array):
|
33d1d14a974e7843b423df2301815ac0 | UNKNOWN | In this Kata, you will be given directions and your task will be to find your way back.
```Perl
solve(["Begin on Road A","Right on Road B","Right on Road C","Left on Road D"]) = ['Begin on Road D', 'Right on Road C', 'Left on Road B', 'Left on Road A']
solve(['Begin on Lua Pkwy', 'Right on Sixth Alley', 'Right on 1st Cr']) = ['Begin on 1st Cr', 'Left on Sixth Alley', 'Left on Lua Pkwy']
```
More examples in test cases.
Good luck!
Please also try [Simple remove duplicates](https://www.codewars.com/kata/5ba38ba180824a86850000f7) | ["DIRS = {'Left': 'Right', 'Right': 'Left'}\n\ndef solve(arr):\n lst,prevDir = [], 'Begin'\n for cmd in arr[::-1]:\n d, r = cmd.split(' on ')\n follow = DIRS.get(prevDir, prevDir)\n prevDir = d\n lst.append(f'{follow} on {r}')\n return lst", "def solve(arr):\n go_moves, go_places = list(zip(*(s.split(\" \", 1) for s in arr)))\n back_moves = [\"Begin\"] + [\"Right\" if s == \"Left\" else \"Left\" for s in go_moves[:0:-1]]\n return [\" \".join(z) for z in zip(back_moves, go_places[::-1])]\n", "def solve(arr):\n lst,prevDir = [],None\n for cmd in arr[::-1]:\n d,r = cmd.split(' on ')\n if not lst: lst.append(f'Begin on {r}')\n else: lst.append(f'{\"Left\" if prevDir == \"Right\" else \"Right\"} on {r}')\n prevDir = d\n return lst", "from collections import deque\n\ndef solve(arr):\n flip = {'Left': 'Right', 'Right': 'Left'}\n \n # Build the prefixes\n directions = deque([\n flip.get(s, s) \n for s in [s.split()[0] for s in arr]\n ])\n directions.reverse()\n directions.rotate(1)\n \n # Get the rest of the directions \n streets = [' '.join(s.split()[1:]) for s in arr][::-1]\n \n # Zip and concatenate each pair \n return [f'{direction} {street}' for direction, street in zip(directions, streets)]", "def solve(arr):\n route = [road.split() for road in arr]\n l, re= len(arr), [] \n dir = {'Left': 'Right', 'Right': 'Left', 'Begin': 'Begin'}\n for i in range(l):\n re.insert(0, ' '.join([dir[route[(i+1)%l][0]]] + route[i][1:]))\n return re", "def solve(directions):\n positions, turns = [], []\n for d in directions[::-1]:\n t, p = d.split(' on ')\n turns.append('Right' if t == 'Left' else 'Left')\n positions.append(p)\n result = [f'{t} on {p}' for t, p in zip(turns, positions[1:])]\n return [f'Begin on {positions[0]}'] + result", "def solve(arr):\n \n rev = list(reversed(arr))\n go = []\n direction = 'Begin'\n \n for i in rev:\n nextdirection = i.split(' ')[0]\n go.append(i.replace(nextdirection, direction))\n if nextdirection == 'Left':\n direction = 'Right'\n else:\n direction = 'Left'\n \n return go\n \n", "def solve(a):\n ans,last = [],len(a)-1\n for i,x in enumerate(a):\n s = 'Begin' if i == last else 'Right' if a[i+1][0] == 'L' else 'Left'\n ans.insert(0,s + x[x.index(' '):])\n return ans", "solve = lambda arr: list(reversed([{'Right': 'Left', 'Left': 'Right'}[arr[i + 1].split(' ')[0]] + ' ' + ' '.join(arr[i].split(' ')[1:]) if i < len(arr)-1 else 'Begin ' + ' '.join(arr[i].split(' ')[1:]) for i in range(len(arr))]))\n", "def solve(arr):\n t1,t2=[],[]\n for i in arr:\n t1.append(' '.join(i.split(' ')[:2]))\n t2.append(' '.join(i.split(' ')[2:]))\n print(t1,t2)\n t2 = t2[::-1]\n t1 = t1[::-1]\n for i in range(len(t1)):\n if \"Right\" in t1[i]:\n t1[i] = t1[i].replace('Right','Left')\n elif \"Left\" in t1[i]:\n t1[i] = t1[i].replace('Left','Right')\n t1 = [t1[-1]]+t1[:-1]\n return [\"%s %s\"%(e,f) for e,f in zip(t1,t2)]"] | {"fn_name": "solve", "inputs": [[["Begin on 3rd Blvd", "Right on First Road", "Left on 9th Dr"]], [["Begin on Road A", "Right on Road B", "Right on Road C", "Left on Road D"]], [["Begin on Road A"]]], "outputs": [[["Begin on 9th Dr", "Right on First Road", "Left on 3rd Blvd"]], [["Begin on Road D", "Right on Road C", "Left on Road B", "Left on Road A"]], [["Begin on Road A"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,256 |
def solve(arr):
|
7e666d3953ecfff53c1a4a6b28e2dc17 | UNKNOWN | ##Task:
You have to write a function **pattern** which creates the following pattern upto n number of rows. *If the Argument is 0 or a Negative Integer then it should return "" i.e. empty string.*
##Examples:
pattern(4):
1234
234
34
4
pattern(6):
123456
23456
3456
456
56
6
```Note: There are no blank spaces```
```Hint: Use \n in string to jump to next line``` | ["pattern = lambda n: \"\\n\".join([\"\".join([str(y) for y in range(x + 1, n + 1)]) for x in range(n)]);", "def pattern(n):\n return '\\n'.join( ''.join(str(j) for j in range(i,n+1)) for i in range(1,n+1) )", "def pattern(n):\n return '\\n'.join(''.join(str(i + 1) for i in range(n)[j:]) for j in range(n))\n", "def pattern(n):\n a = [str(i) for i in range(1, n + 1)]\n return '\\n'.join(''.join(a[i:]) for i in range(n))\n", "def pattern(n):\n return '\\n'.join( [ ''.join(map(str, range(i,n+1))) for i in range(1, n+1) ] )", "def pattern(n):\n output = \"\"\n for i in range(1, n+1):\n for j in range(i, n+1):\n output += str(j)\n if i < n:\n output += '\\n'\n return output", "def pattern(n):\n # Happy Coding ^_^\n a = \"\"\n lst=[]\n result=\"\"\n for i in range(1,n+1):\n a=str(i)\n \n for m in range(i+1,n+1):\n a += str(m)\n lst.append(a)\n for i in lst:\n result += i+'\\n'\n return result[:-1]", "def pattern(n):\n return '\\n'.join([''.join(str(i) for i in range(e,n+1)) for e in range( 1,n+1 )])"] | {"fn_name": "pattern", "inputs": [[1], [2], [5], [0], [-25]], "outputs": [["1"], ["12\n2"], ["12345\n2345\n345\n45\n5"], [""], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,140 |
def pattern(n):
|
d9805661210663f799566c18ebe1bbab | UNKNOWN | # Task
Consider an array of integers `a`. Let `min(a)` be its minimal element, and let `avg(a)` be its mean.
Define the center of the array `a` as array `b` such that:
```
- b is formed from a by erasing some of its elements.
- For each i, |b[i] - avg(a)| < min(a).
- b has the maximum number of elements among all the arrays
satisfying the above requirements.
```
Given an array of integers, return its center.
# Input/Output
`[input]` integer array `a`
Unsorted non-empty array of integers.
`2 ≤ a.length ≤ 50,`
`1 ≤ a[i] ≤ 350.`
`[output]` an integer array
# Example
For `a = [8, 3, 4, 5, 2, 8]`, the output should be `[4, 5]`.
Here `min(a) = 2, avg(a) = 5`.
For `a = [1, 3, 2, 1]`, the output should be `[1, 2, 1]`.
Here `min(a) = 1, avg(a) = 1.75`. | ["def array_center(lst):\n return [i for i in lst if abs(i - sum(lst)*1.0/len(lst)) < min(lst)]", "from statistics import mean\n\ndef array_center(arr):\n low, avg = min(arr), mean(arr)\n return [b for b in arr if abs(b - avg) < low]", "from statistics import mean\n\ndef array_center(a):\n n, m = min(a), mean(a)\n return [x for x in a if abs(x - m) < n]", "def array_center(arr):\n m, a = min(arr), (sum(arr) / len(arr))\n return [n for n in arr if abs(n - a) < m]\n", "def array_center(arr):\n avg = sum(arr)/len(arr)\n return [i for i in arr if abs(i - avg) < min(arr)]\n\n", "def array_center(arr):\n #your code here\n a = min(arr)\n c = []\n b = 0\n for i in arr:\n b = b + i\n aver = b / len(arr)\n for i in arr:\n if abs(i - aver) < a:\n c.append(i)\n return c", "def array_center(a):\n mi, me = min(a), sum(a)/len(a)\n return [x for x in a if abs(x-me) < mi]", "array_center=lambda a:[n for n in a if abs(n-sum(a)/len(a))<min(a)]", "from numpy import mean\ndef array_center(arr):\n m,a=min(arr),mean(arr)\n return list(filter(lambda n:abs(n-a)<m,arr))", "from statistics import mean\ndef array_center(a):\n m, avg = min(a), mean(a)\n return [i for i in a if -m<(i-avg)<m]"] | {"fn_name": "array_center", "inputs": [[[8, 3, 4, 5, 2, 8]], [[1, 3, 2, 1]], [[10, 11, 12, 13, 14]]], "outputs": [[[4, 5]], [[1, 2, 1]], [[10, 11, 12, 13, 14]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,276 |
def array_center(arr):
|
ba25b9a6238fa67b477ce5059651f362 | UNKNOWN | Given a certain integer ```n```, we need a function ```big_primefac_div()```, that give an array with the highest prime factor and the highest divisor (not equal to n).
Let's see some cases:
```python
big_primefac_div(100) == [5, 50]
big_primefac_div(1969) == [179, 179]
```
If n is a prime number the function will output an empty list:
```python
big_primefac_div(997) == []
```
If ```n``` is an negative integer number, it should be considered the division with tha absolute number of the value.
```python
big_primefac_div(-1800) == [5, 900]
```
If ```n``` is a float type, will be rejected if it has a decimal part with some digits different than 0. The output "The number has a decimal part. No Results". But ```n ``` will be converted automatically to an integer if all the digits of the decimal part are 0.
```python
big_primefac_div(-1800.00) == [5, 900]
big_primefac_div(-1800.1) == "The number has a decimal part. No Results"
```
Optimization and fast algorithms are a key factor to solve this kata.
Happy coding and enjoy it! | ["def big_primefac_div(n):\n bpf, bd = 0, 1\n frac = []\n \n if n % 1 != 0:\n return \"The number has a decimal part. No Results\"\n else:\n n = abs(int(n))\n n_copy = n\n \n i = 2\n while i * i <= n:\n if n % i == 0:\n n //= i\n frac.append(i)\n else:\n i += 1\n if n > 1: frac.append(n)\n\n bpf = max(frac)\n bd = n_copy / frac[0]\n \n if bpf == 0 or bd == 1:\n return []\n else:\n return [bpf, bd]", "\ndef big_primefac_div(n):\n #We need only absolute values\n n = abs(n)\n maxi = 0\n #Checking if the number has a decimal part\n if n % 1 == 0:\n #Loop for finding Biggest Divisor\n listy = [num for num in range(2, int(n**.5 + 1)) if n % num == 0]\n fancy_list = list(listy)\n for number in listy:\n temp_numb = n // number\n fancy_list.append(int(temp_numb))\n #Loop for finding Biggest Prime Factor\n for numb in sorted(fancy_list, reverse=True): \n for i in range(2, int(numb**.5 + 1)):\n if numb % i == 0:\n break\n else:\n maxi = numb\n break\n if fancy_list:\n return [maxi, max(set(fancy_list))]\n #In case we gonna have one number as prime factor itself\n else:\n return []\n else:\n return (\"The number has a decimal part. No Results\")", "import math\ndef primecheck(num):\n a=math.sqrt(num)\n for i in range(2, int(a + 2)):\n if num % i == 0:\n return False\n return True\nprint(primecheck(25))\ndef big_primefac_div(n):\n if int(n)!=n:\n return \"The number has a decimal part. No Results\"\n n=abs(n)\n factor=[]\n prime=[]\n for i in range(2,int(math.sqrt(n)+1)):\n if n%i==0:\n factor.append(i)\n factor.append(n/i)\n for j in factor:\n if primecheck(j) is True:\n prime.append(j)\n if len(factor)==0:\n return []\n factor.sort()\n prime.sort()\n return [int(prime[-1]),int(factor[-1])]", "def big_primefac_div(n):\n if n % 1:\n return \"The number has a decimal part. No Results\"\n o = n = abs(int(n))\n mn, mx = 0, 1\n while n % 2 == 0:\n mn, mx, n = mn or 2, 2, n//2\n k = 3\n while k*k <= n:\n if n % k:\n k = k+2\n else:\n mn, mx, n = mn or k, k, n//k\n return [max(mx, n), o//mn] if mn else []\n", "from itertools import count\ndef big_primefac_div(n):\n prime=lambda n:all(n%i for i in range(2,int(n**.5)+1))\n if n != int(n) : return \"The number has a decimal part. No Results\"\n if prime(abs(n)) : return []\n f = factor(abs(n))\n return [max(f),abs(n)/f[0]] \n \ndef factor(n):\n j, li= 2, []\n while j*j <=n:\n if n%j == 0 : n//=j ; li.append(j)\n else : j += 1\n if n>1 : li.append(n)\n return li", "def big_primefac_div(n):\n if n % 1:\n return \"The number has a decimal part. No Results\"\n n = abs(int(n))\n mpf = big_primefac(n)\n return [mpf, big_div(n)] if mpf != n else []\n\ndef big_primefac(n):\n m = 1\n while n % 2 == 0:\n m, n = 2, n//2\n k = 3\n while k*k <= n:\n if n % k:\n k = k+2\n else:\n m, n = k, n//k\n return max(m, n)\n\ndef big_div(n):\n return next((n//k for k in range(2, int(n**0.5)+1) if n % k == 0), n)\n", "def big_primefac_div(n):\n if n % 1 != 0:\n return \"The number has a decimal part. No Results\"\n # convert negative number to positive\n n = -n if n < 0 else n\n \n # find smallest prime factor, use it to find largest divisor\n biggest_divisor = 1\n biggest_primefactor = 1\n if n%2 == 0:\n biggest_primefactor = 2\n elif n%3 == 0:\n biggest_primefactor = 3\n else:\n i = 5\n while (i*i <= n):\n if n%i==0:\n biggest_primefactor = i\n break\n elif n%(i+2)==0:\n biggest_primefactor = i + 2\n break\n i = i + 6\n \n if biggest_primefactor == 1:\n return []\n biggest_divisor = n / biggest_primefactor\n\n n = int(n)\n while n % 2 == 0:\n biggest_primefactor = 2\n n >>= 1\n \n for i in range(3, int(n**0.5) + 1, 2):\n while n % i == 0:\n biggest_primefactor = i\n n = n / i\n if n > 2:\n biggest_primefactor = n\n \n return [biggest_primefactor, biggest_divisor]", "def big_primefac_div(n):\n n=abs(n)\n if isinstance(n, float):\n temp=str(n)[str(n).index(\".\")+1:]\n for i in temp:\n if i!=\"0\":\n return \"The number has a decimal part. No Results\"\n res=factorization(n)\n if len(res)==1:\n return []\n return [res[-1], n//res[0]]\ndef factorization(n):\n factor=2\n res=[]\n while factor*factor<=n:\n if n%factor==0:\n n//=factor\n res.append(factor)\n factor=2\n else:\n factor+=1\n res.append(int(n))\n return res", "def pf(n):\n p,x = [],2\n while x*x <= n:\n while not n%x:\n n //= x\n p.append(x)\n x += 1\n if n>1:\n p.append(n)\n return p\n\ndef big_primefac_div(n):\n if isinstance(n,float) and not n.is_integer(): return \"The number has a decimal part. No Results\"\n n = abs(n)\n p = pf(n)\n return [p[-1],n//p[0] if n%2 else n//2] if p[-1]!=n else []"] | {"fn_name": "big_primefac_div", "inputs": [[100], [1969], [997], [-1800], [-1800.1]], "outputs": [[[5, 50]], [[179, 179]], [[]], [[5, 900]], ["The number has a decimal part. No Results"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,669 |
def big_primefac_div(n):
|
0e8803353cfc4b72d2ee0f23062e481a | UNKNOWN | Don Drumphet lives in a nice neighborhood, but one of his neighbors has started to let his house go. Don Drumphet wants to build a wall between his house and his neighbor’s, and is trying to get the neighborhood association to pay for it. He begins to solicit his neighbors to petition to get the association to build the wall. Unfortunately for Don Drumphet, he cannot read very well, has a very limited attention span, and can only remember two letters from each of his neighbors’ names. As he collects signatures, he insists that his neighbors keep truncating their names until two letters remain, and he can finally read them.
Your code will show Full name of the neighbor and the truncated version of the name as an array. If the number of the characters in name is less than or equal to two, it will return an array containing only the name as is" | ["who_is_paying = lambda n: [n, n[:2]] if len(n)>2 else [n]", "def who_is_paying(name):\n if len(name) > 2:\n return [name, name[0:2]]\n else:\n return [name[0:len(name)]]", "def who_is_paying(name):\n return [name,name[0:2]] if len(name)>2 else [name]", "def who_is_paying(name):\n return [name] if len(name) <= 2 else [name, name[:2]]", "def who_is_paying(name):\n return [name, name[:2]] if len(name) > 2 else [name[:2]]", "def who_is_paying(name):\n return [name] * (len(name) > 2) + [name[:2]]", "def who_is_paying(name):\n out = [] \n if len(name) > 2:\n out.append(name)\n out.append(name[0:2])\n else:\n out.append(name)\n return out\n", "def who_is_paying(name): \n return ([name, name[0]+name[1]] if len(name) > 2 else [name])", "def who_is_paying(name):\n return [name, name[:2]] if len(name) > 2 else [name]\n", "who_is_paying = lambda n: [n] + ([n[:2]] if len(n)>2 else [])", "def who_is_paying(name): \n var = [] #declaring empty list\n if len(name)<=2 : #if the lenght of name (len(name)) is <= to 2\n var.append(name) #we will add the content of the name variable to the list\n return var #and return it as a result\n #if not\n var.append(name) #we will add the full person name from the variable \"name\"\n var.append(name[0]+name[1])#then add the first and second character\n return var #and return the result", "def who_is_paying(name):\n return [[name],[ name, name[:2] ]][len(name)>2]", "def who_is_paying(name):\n if name == name[0:2]:\n return [name]\n else:\n return [name, name[0:2]]", "def who_is_paying(name):\n listsx = [name, ''.join(list(name)[:2])] if len(name)>2 else [name]\n return listsx", "def who_is_paying(name): \n return [name, name[0]+name[1]] if len(name) > 2 else [name]\n #Program returns name and then the first two letters in the string provided IF the length of the string is over 2.\n #Otherwise it will only print the name\n", "def who_is_paying(name):\n if len(name)>2:\n return[name, name[:2]]\n else:\n return[name]", "def who_is_paying(name):\n if len(name) <= 2:\n return [name]\n else:\n return [name,name[0]+name[1]]", "def who_is_paying(name):\n return [name] if len(name) < 3 else [name, name[:2]]", "def who_is_paying(name):\n return [name, name[0:2]] if len(name)>2 else name.split(\",\")", "from typing import List\n\ndef who_is_paying(name: str) -> List[str]:\n \"\"\" Get the full name of the neighbor and the truncated version of the name as an array. \"\"\"\n return [name, name[:2]] if len(name) > 2 else [name[:2]]", "def who_is_paying(name):\n return sorted(set([name, name[:2],]), reverse=True)", "def who_is_paying(name):\n return [name, name[:2]][not name[2:]:]", "who_is_paying=lambda s:[s,s[:2]][:(len(s)>2)+1]", "from collections import OrderedDict \nwho_is_paying = lambda n: list(OrderedDict.fromkeys([n,n[0:2:]]))", "def who_is_paying(name):\n return [name] + [name[:2]]*(len(name)>2)", "def who_is_paying(donald_trump):\n if len(donald_trump) > 2:\n for mexican in range(len(donald_trump)):\n return [donald_trump, donald_trump[False] + donald_trump[True]]\n return [donald_trump]", "def who_is_paying(name):\n return [name] + ([name[:2]] if len(name)>2 else [])", "who_is_paying=lambda n:[n,n[:2]][:(len(n)>2)+1]", "def who_is_paying(name):\n return [name, name[:2]][:(len(name)>2)+1]", "who_is_paying=lambda n:[n,n[:2]][:2-(len(n)<3)]", "def who_is_paying(name=''):\n return [name,name[:2]] if name!=name[:2] else [name]", "def who_is_paying(name):\n oplst = [name]\n if len(name) <= 2:\n return oplst\n else:\n oplst.append(name[:2])\n return oplst", "def who_is_paying(name):\n a=[]\n if len(name)<=2:\n a.append(name)\n else:\n a.append(name)\n a.append(name[:2])\n return a", "def who_is_paying(name):\n \n petition = []\n \n if len(name) <= 2:\n petition.append(name)\n return petition\n \n else:\n nam = name[:2]\n petition.append(name)\n petition.append(nam)\n return petition", "def who_is_paying(name):\n name_list = []\n if len(name) <= 2:\n name_list.append(name[0:2])\n elif len(name) == 0:\n name_list.append(\"\")\n elif len(name) > 2:\n name_list.append(name)\n name_list.append(name[0:2])\n return name_list", "def who_is_paying(name):\n return [name, name[:2:]] if len(name) > 2 else [name[:2]]", "def who_is_paying(name):\n #your code here\n if len(name) > 2:\n names = [name]\n names.append(name[:2])\n return names\n else:\n names = [name]\n return names", "def who_is_paying(name):\n if len(name) > 2:\n short = name[0:2]\n val = []\n val.append(name)\n val.append(short)\n return val\n elif len(name) <= 2:\n val = []\n val.append(name)\n return val\n else:\n return None", "def who_is_paying(name):\n a = []\n i = 0\n for item in name:\n i += 1\n if i > 2:\n a.append(name)\n a.append(name[0]+name[1])\n else:\n a.append(name)\n return a", "def who_is_paying(name):\n res = [name]\n if len(name) > 2:\n res.append(name[0]+name[1])\n return res", "def who_is_paying(name):\n if len(name) <= 2:\n char_list = [name]\n return char_list\n else :\n name_list = [name, name[:2]]\n return name_list", "def who_is_paying(name):\n return len(name) > 2 and [name, name[:2]] or [name]", "def who_is_paying(name):\n sliced = name[0:2]\n if (sliced == name):\n return [sliced]\n return [name, sliced]", "def who_is_paying(name):\n res = []\n if len(name)<=2:\n res.append(name)\n else:\n res.append(name)\n res.append(name[0:2]) \n return res", "def who_is_paying(name):\n liste_finale = []\n if len(name) > 2:\n lettres = name[0] + name[1]\n liste_finale.append(name)\n liste_finale.append(lettres)\n return liste_finale\n else:\n liste_finale.append(name)\n return liste_finale\n", "def who_is_paying(name):\n d = [name]\n if len(name)<3:\n return d\n else:\n d.append(name[0:2])\n return d", "def who_is_paying(name):\n list = [name]\n if len(name) > 2:\n list.append(name[0:2])\n return list\n else:\n return list", "def who_is_paying(name):\n if len(name) <= 2:\n who = [name]\n else:\n who = [name, name[0:2]]\n return who", "def who_is_paying(name):\n if len(name) < 3: return [name]\n else:\n first_two = name[0:2]\n return [name, first_two]\n \n \n \n# Test.assert_equals(who_is_paying(\"Mexico\"),[\"Mexico\", \"Me\"])\n# Test.assert_equals(who_is_paying(\"Melania\"),[\"Melania\", \"Me\"])\n# Test.assert_equals(who_is_paying(\"Melissa\"),[\"Melissa\", \"Me\"])\n# Test.assert_equals(who_is_paying(\"Me\"),[\"Me\"])\n# Test.assert_equals(who_is_paying(\"\"), [\"\"])\n# Test.assert_equals(who_is_paying(\"I\"), [\"I\"])\n", "# truncate name as array.\n# si es menor o igual a 2 retorna el nombre como es.\n\n\ndef who_is_paying(name):\n if len(name) > 2:\n return [name, name[0:2]]\n else:\n return [name[0:len(name)]]\n \n", "def who_is_paying(name):\n b = []\n if len(name) <= 2:\n b.append(name)\n return b\n else:\n b.append(name)\n b.append(name[:2])\n return b", "def who_is_paying(name):\n if name != name[:2]:\n return [name, name[:2]]\n else:\n return [name]", "def who_is_paying(name):\n if len(name) > 2: \n return [f\"{name}\" , f\"{name[0]}{name[1]}\"]\n else:\n return [f\"{name}\"]", "def who_is_paying(name):\n if len(name) == 1:\n return [name]\n elif name == '':\n return ['']\n elif name == name[0:2]:\n return [name]\n else:\n return [name,name[0:2]]", "def who_is_paying(name):\n #your code \n return [name, name[:2]] if len(name) > 2 else [name[:2]]", "def who_is_paying(name):\n new_list =[]\n new_list.append(name ) \n if len(name) > 2:\n new_list += [name[:2]]\n else:\n new_list\n return new_list\n \n", "def who_is_paying(name):\n names= []\n if len(name) > 2:\n names.append(name)\n names.append(name[0:2])\n else:\n names.append(name)\n return names", "def who_is_paying(name):\n if len(name) == 0:\n return [\"\"]\n if len(name) <= 2:\n return name.split()\n alist = []\n alist.extend([name, name[0:2]])\n print(alist)\n return alist", "def who_is_paying(name):\n names = [name]\n \n if len(name) > 2:\n truncated_name = name[:2]\n names.append(truncated_name)\n \n return names", "def who_is_paying(name): \n return ([\"\"] if name==\"\" else ([name] if len(name)<=2 else [name, name[0:2]]))", "def who_is_paying(name):\n total = len(name)\n if total >= 3:\n first = name[0]\n second = name[1]\n first_two = first + second\n final = list([name,first_two])\n return final\n else:\n final = list([name])\n return final", "def who_is_paying(name):\n x = []\n y = []\n if len(name) <= 2:\n x.append(name)\n else:\n x.append(name)\n y.append(name[0])\n y.append(name[1])\n z = ''.join(map(str, y))\n x.append(z)\n return x\n", "def who_is_paying(name):\n if len(name)>=3:\n return [name, name[0]+name[1]]\n if len(name)<=2:\n return [name]\n else:\n return [\"\"]", "def who_is_paying(name):\n final_list = [name]\n if len (name) <= 2:\n return final_list\n if len(name) > 2:\n name_list = list(name)\n final_list.append(str(name_list[0] + name_list[1]))\n return final_list", "def who_is_paying(name):\n r = [name]\n if len(name) > 2:\n r = [name, name[:2]]\n return r", "def who_is_paying(name):\n bruh = list()\n if len(name) <= 2:\n bruh.append(name)\n else:\n bruh.append(name)\n bruh.append(name[0:2])\n return bruh", "def who_is_paying(name):\n m=[]\n if len(name)<=2:\n m.append(name)\n return m\n \n g=[name,name[:2]]\n return g", "def who_is_paying(name):\n if len(name) > 2:\n names = [name,name[:2]]\n return names\n else:\n return [name]", "def who_is_paying(name):\n return [name, name[0:2]] if len(name) > 2 else [name[0:2]] if 0 < len(name) <= 2 else ['']", "who_is_paying=lambda n:sorted({n,n[:2]})[::-1]", "def who_is_paying(name):\n name_array = []\n if len(name) > 2:\n name_array.append(name)\n name_array.append(name[0:2])\n return name_array\n else:\n name_array.append(name)\n return name_array\n", "def who_is_paying(name):\n if name == name[0:2]:\n return [name]\n else:\n return [name, name[0:2]]\n \n \n# show Full name of the neighbor and the truncated version of the name \n# as an array. \n\n# If the number of the characters in name is equal or less than two, \n# it will return an array containing only the name as is\"\n", "def who_is_paying(name):\n if len(name) > 2:\n return [name, name[:2:]]\n return [name]\n", "def who_is_paying(name: str) -> list:\n return name[:2].split(\" \") if len(name) <= 2 else name.split() + [name[:2]]", "def who_is_paying(name):\n if len(name) == 0:\n return [\"\"]\n else:\n if len(name) > 2:\n return [name, name[0:2]] \n else:\n return [name]", "# len(name) gives the number of characters in the string\ndef who_is_paying(name):\n if len(name) < 3:\n return [name]\n else:\n return [name, name[:2]] # name[:2] gives the first two characters in string 'name'", "def who_is_paying(name):\n trunc = name[:2]\n arr = [name, trunc]\n \n if len(name) < 3:\n arr.pop()\n return arr", "def who_is_paying(name):\n if len(name) == 1 or len(name) == 2:\n return [name]\n list = [name]\n if len(name) > 1:\n list.append(name[0]+name[1])\n elif len(name) > 0:\n list.append(name[0])\n return list", "def who_is_paying(name):\n if name[:2] == name:\n return [name]\n else:\n return [name, name[:2]]", "def who_is_paying(n):\n nn=(n[:2] if len(n)>2 else n)\n return [n] if n==nn else [n,nn]", "from typing import List\n\ndef who_is_paying(name: str) -> List[str]:\n return [name,name[0:2]] if len(name) > 2 else [name]", "def who_is_paying(name):\n return [name, name[0:2]] if len(name)>2 else [name[0:len(name)]]", "def who_is_paying(name):\n if len(name) == 0:\n return [\"\"]\n elif len(name) >2:\n return [name, name[0:2]]\n else:\n return [name]", "def who_is_paying(name):\n print(f'name = {name}')\n if len(name) <= 2:\n return [name]\n else:\n return [name, f'{name[0]}{name[1]}']", "def who_is_paying(name):\n if len(name) > 2:\n trunc_name = name[0:2]\n output = [name, trunc_name]\n else:\n output = [name]\n return output\n", "def who_is_paying(name):\n if len(name)<3:\n return [name]\n else:\n return [name,name[0:2:]]\n", "def who_is_paying(name):\n if len(name) <=2:\n result = [name]\n else:\n result = [name,name[0:2]]\n return result", "def who_is_paying(name):\n #your code here\n if name == '':\n return ['']\n elif name == 'I':\n return ['I']\n else:\n if name == name[0:2]:\n return [name]\n else:\n return [name,name[0:2]]\n", "def who_is_paying(name):\n if len(name) == 0:\n return [\"\"]\n elif len(name)<3:\n return [name]\n else:\n return [name, name[:2]]", "def who_is_paying(name):\n if len(name) > 2:\n return list((name, name[:2]))\n else:\n return [name]", "def who_is_paying(name):\n if len(name) > 2:\n list = []\n for char in name:\n list.append(char)\n \n a = ''\n a += list[0]\n a += list[1]\n list2 = []\n list2.append(name)\n list2.append(a)\n return list2\n else:\n list = []\n list.append(name)\n return list\n", "def who_is_paying(name):\n if len(name) <= 2:\n return ([name])\n\n else:\n name_list = list(name)\n name_2 = name_list[0:2]\n name_str = ''.join(name_2)\n return ([name, name_str])\n\n", "def who_is_paying(name):\n #your code here\n if len(name) > 2:\n tup1 = [name, name[:2]]\n return tup1\n else:\n tup2 = [name]\n return tup2", "def who_is_paying(name):\n result = []\n if len(name) < 3:\n result.append(name)\n return result\n elif len(name) == 0:\n result.append(\"\")\n return result\n result.append(name)\n result.append(name[:2])\n return result", "def who_is_paying(name):\n names_list = [ ]\n if(len(name)<=2):\n names_list.append(name)\n return names_list\n names_list.append(name)\n names_list.append(name[:2])\n return names_list\n", "def who_is_paying(name):\n s = []\n if len(name) > 2:\n s.append(name)\n izi = name[:2]\n s.append(izi)\n return s\n if len(name) <= 2:\n s.append(name)\n return s\nwho_is_paying(\"\")", "def who_is_paying(name):\n namelist = []\n namelist.append(name)\n if len(name) <= 2:\n return namelist\n if len(name) > 2:\n namelist.append(name[:2])\n return namelist", "def who_is_paying(name):\n if name == '':\n return ['']\n elif len(name) == 1:\n return [name]\n elif len(name) == 2:\n return [name]\n else:\n return [name, name[:2]]", "def who_is_paying(name):\n #your code here\n ans=list()\n sec=''\n if len(name)<=2:\n ans=[name]\n else:\n sec=name[0:2]\n ans=[name,sec]\n return ans", "def who_is_paying(name):\n if len(name) == 0:\n return ['']\n elif len(name) == 1:\n return [name[0]]\n elif len(name) == 2:\n return [name[0] + name[1]]\n else:\n return [name, name[0] + name[1]]\n #your code here\n"] | {"fn_name": "who_is_paying", "inputs": [["Mexico"], ["Melania"], ["Melissa"], ["Me"], [""], ["I"]], "outputs": [[["Mexico", "Me"]], [["Melania", "Me"]], [["Melissa", "Me"]], [["Me"]], [[""]], [["I"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 16,662 |
def who_is_paying(name):
|
167469508b51d6a2cfc6c8756d1e0cda | UNKNOWN | In this kata you will create a function to check a non-negative input to see if it is a prime number.
The function will take in a number and will return True if it is a prime number and False if it is not.
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself.
### Examples | ["import math\ndef is_prime(n):\n if n < 2: \n return False\n return all(n % i for i in range(3, int(math.sqrt(n)) + 1, 2))", "def is_prime(n):\n 'Return True if n is a prime number otherwise return False'\n # 0 and 1 are not primes\n if n < 2: \n return False\n \n # 2 is only even prime so reject all others\n if n == 2: \n return True\n \n if not n & 1: \n return False\n \n #\u00a0test other possiblities\n for i in range(3, n):\n if (n % i) == 0 and n != i: \n return False\n \n \n return True", "def is_prime(num):\n 'Return True if n is a prime number otherwise return False'\n return num > 1 and not any(num % n == 0 for n in range(2, num))", "from math import sqrt\n\ndef is_prime(n):\n if n < 2:\n return False\n elif n == 2:\n return True\n elif n % 2 == 0:\n return False\n else:\n for i in range(3, int(sqrt(n)) + 1, 2):\n if n % i == 0:\n return False\n else:\n return True", "def is_prime(n,i=2):\n if (n <= 2):\n return True if(n == 2) else False\n elif (n % i == 0):\n return False\n elif (i * i > n):\n return True\n return is_prime(n, i + 1)"] | {"fn_name": "is_prime", "inputs": [[0], [1], [2], [11], [12], [61], [571], [573], [25]], "outputs": [[false], [false], [true], [true], [false], [true], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,279 |
def is_prime(n):
|
0c5cd1d25bb589fe77e781bfeaf44299 | UNKNOWN | Most of this problem is by the original author of [the harder kata](https://www.codewars.com/kata/556206664efbe6376700005c), I just made it simpler.
I read a book recently, titled "Things to Make and Do in the Fourth Dimension" by comedian and mathematician Matt Parker ( [Youtube](https://www.youtube.com/user/standupmaths) ), and in the first chapter of the book Matt talks about problems he likes to solve in his head to take his mind off the fact that he is in his dentist's chair, we've all been there!
The problem he talks about relates to polydivisible numbers, and I thought a kata should be written on the subject as it's quite interesting. (Well it's interesting to me, so there!)
### Polydivisib... huh what?
So what are they?
A polydivisible number is divisible in an unusual way. The first digit is cleanly divisible by `1`, the first two digits are cleanly divisible by `2`, the first three by `3`, and so on.
### Examples
Let's take the number `1232` as an example.
```
1 / 1 = 1 // Works
12 / 2 = 6 // Works
123 / 3 = 41 // Works
1232 / 4 = 308 // Works
```
`1232` is a polydivisible number.
However, let's take the number `123220` and see what happens.
```
1 /1 = 1 // Works
12 /2 = 6 // Works
123 /3 = 41 // Works
1232 /4 = 308 // Works
12322 /5 = 2464.4 // Doesn't work
123220 /6 = 220536.333... // Doesn't work
```
`123220` is not polydivisible.
### Your job: check if a number is polydivisible or not.
Return `true` if it is, and `false` if it isn't.
Note: All inputs will be valid numbers between `0` and `2^53-1 (9,007,199,254,740,991)` (inclusive).
Note: All single digit numbers (including `0`) are trivially polydivisible.
Note: Except for `0`, no numbers will start with `0`. | ["def polydivisible(x):\n for i in range(1, len(str(x)) + 1):\n if int(str(x)[:i]) % i != 0:\n return False\n return True", "def polydivisible(x):\n s = str(x)\n return all(int(s[:i]) % i == 0 for i in range(1, len(s) + 1))", "def polydivisible(x):\n return all(int(str(x)[:i+1])%(i+1)==0 for i in range(len(str(x))))", "def polydivisible(x):\n l = len(str(x))\n return all(x // (10**i) % (l-i) == 0 for i in range(l))", "def polydivisible(x):\n if x < 10: return True\n return not (x % len(str(x))) and polydivisible(int(str(x)[:-1]))", "from itertools import accumulate\n\ndef polydivisible(x):\n return all(\n int(''.join(s)) % i == 0\n for i, s in enumerate(accumulate(str(x)), 1)\n )", "def polydivisible(n):\n s = str(n)\n return all(not int(s[:i])%i for i in range(2,len(s)+1))", "def polydivisible(x):\n x = str(x)\n return all(0 for i in range(1, len(x) + 1) if int(x[:i]) % i)", "def polydivisible(x):\n x = str(x)\n return len([0 for i in range(1, len(x) + 1) if int(x[:i]) % i]) == 0", "def polydivisible(x):\n li = list(str(x))\n for i, digit in enumerate(li):\n if int(\"\".join(li[:i+1])) % (i + 1) != 0:\n return False\n return True"] | {"fn_name": "polydivisible", "inputs": [[1232], [123220], [0], [1], [141], [1234], [21234], [81352], [987654], [1020005], [9876545], [381654729], [1073741823]], "outputs": [[true], [false], [true], [true], [true], [false], [false], [false], [true], [true], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,260 |
def polydivisible(x):
|
468fb378ac11c37562368d983b550bf0 | UNKNOWN | Your task is to write a function that takes two or more objects and returns a new object which combines all the input objects.
All input object properties will have only numeric values. Objects are combined together so that the values of matching keys are added together.
An example:
```python
objA = { 'a': 10, 'b': 20, 'c': 30 }
objB = { 'a': 3, 'c': 6, 'd': 3 }
combine(objA, objB) # Returns { a: 13, b: 20, c: 36, d: 3 }
```
The combine function should be a good citizen, so should not mutate the input objects. | ["def combine(*bs):\n c = {}\n for b in bs:\n for k, v in list(b.items()):\n c[k] = v + c.get(k, 0)\n return c\n", "from collections import Counter\n\n\ndef combine(*args):\n return sum((Counter(a) for a in args), Counter())\n", "def combine(*dictionaries):\n result = {}\n for dict in dictionaries:\n for key, value in list(dict.items()):\n result[key] = value + result.get(key, 0)\n return result\n", "from collections import defaultdict\ndef combine(*dicts):\n ret = defaultdict(int)\n for d in dicts:\n for k, v in d.items():\n ret[k] += v\n return dict(ret)", "from collections import Counter\n\ndef combine(*args):\n return sum(map(Counter, args), Counter())", "def combine(*args):\n rez = {}\n \n for i in args:\n for k, v in i.items():\n rez[k] = rez.get(k, 0) + v\n \n return rez", "def combine(*args):\n result = {}\n for obj in args:\n for key, value in obj.items():\n if not key in result:\n result[key] = 0\n result[key] += value\n return result", "def combine(*args):\n ret = {}\n for arg in args:\n for k, v in arg.items():\n ret.setdefault(k, 0)\n ret[k] = ret[k] + v\n return ret", "\ndef combine(*args):\n keys = set([y for x in args for y in list(x.keys())])\n return {k:sum([a.get(k,0) for a in args]) for k in keys}\n \n"] | {"fn_name": "combine", "inputs": [[{}, {}, {}], [{}]], "outputs": [[{}], [{}]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,463 |
def combine(*args):
|
fe6d3cccafe37a3263a5a043bdbbab93 | UNKNOWN | #Unflatten a list (Easy)
There are several katas like "Flatten a list".
These katas are done by so many warriors, that the count of available list to flattin goes down!
So you have to build a method, that creates new arrays, that can be flattened!
#Shorter: You have to unflatten a list/an array.
You get an array of integers and have to unflatten it by these rules:
```
- You start at the first number.
- If this number x is smaller than 3, take this number x direct
for the new array and continue with the next number.
- If this number x is greater than 2, take the next x numbers (inclusive this number) as a
sub-array in the new array. Continue with the next number AFTER this taken numbers.
- If there are too few numbers to take by number, take the last available numbers.
```
The given array will always contain numbers. There will only be numbers > 0.
Example:
```
[1,4,5,2,1,2,4,5,2,6,2,3,3] -> [1,[4,5,2,1],2,[4,5,2,6],2,[3,3]]
Steps:
1. The 1 is added directly to the new array.
2. The next number is 4. So the next 4 numbers (4,5,2,1) are added as sub-array in the new array.
3. The 2 is added directly to the new array.
4. The next number is 4. So the next 4 numbers (4,5,2,6) are added as sub-array in the new array.
5. The 2 is added directly to the new array.
6. The next number is 3. So the next 3 numbers would be taken. There are only 2,
so take these (3,3) as sub-array in the new array.
```
There is a harder version of this kata!
Unflatten a list (Harder than easy)
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | ["def unflatten(flat_array):\n arr = flat_array[:]\n for i, v in enumerate(arr):\n if v > 2:\n arr[i], arr[i+1:i+v] = arr[i:i+v], []\n return arr", "def unflatten(flat):\n if not flat : return []\n if flat[0]<3 : return [flat[0]] + unflatten(flat[1:])\n else: return [ flat[0:flat[0]] ] + unflatten(flat[flat[0]:])", "from itertools import islice\n\ndef unflatten(a):\n it = iter(a)\n return [x if x < 3 else [x] + list(islice(it, 0, x-1)) for x in it]", "def unflatten(flat_array):\n result = []\n i = 0\n while i < len(flat_array):\n num = flat_array[i]\n if num <= 2:\n result.append(num)\n i += 1\n else:\n result.append(flat_array[i:i+num])\n i += num\n return result", "def unflatten(flat_array):\n new_array = []\n i = 0\n while i < len(flat_array):\n if flat_array[i] < 3:\n new_array.append(flat_array[i])\n i+=1\n else:\n new_array.append(flat_array[i:i+flat_array[i]])\n i+=flat_array[i]\n return new_array", "def unflatten(flat_array):\n #your code here\n result = []\n index = 0\n while index < len(flat_array):\n value = flat_array[index]\n if value < 3:\n result.append(value)\n index += 1\n else:\n result.append(flat_array[index:index + value])\n index += value\n return result", "def unflatten(flat_array):\n dex = 0\n result = []\n while dex < len(flat_array):\n current = flat_array[dex]\n if current > 2:\n result.append(flat_array[dex:dex + current])\n dex += current\n else:\n result.append(current)\n dex += 1\n return result", "def unflatten(flat):\n a = []\n while flat:\n a, flat = (a + [flat[0]], flat[1:]) if flat[0] < 3 else (a + [flat[:flat[0]]], flat[flat[0]:])\n return a", "def unflatten(arr):\n \n j = 0\n res = []\n \n for x in arr:\n if j > 0:\n res[-1].append(x)\n j -= 1\n elif x < 3:\n res.append(x)\n else:\n res.append([x])\n j = x-1\n \n return res", "def unflatten(flat_array):\n new_array = []\n start = 0\n \n while start < len(flat_array):\n if flat_array[start] < 3:\n new_array.append(flat_array[start])\n start += 1\n\n else:\n new_array.append(flat_array[start: start + flat_array[start]])\n start += flat_array[start]\n\n\n return new_array"] | {"fn_name": "unflatten", "inputs": [[[3, 5, 2, 1]], [[1, 4, 5, 2, 1, 2, 4, 5, 2, 6, 2, 3, 3]], [[1, 1, 1, 1]], [[1]], [[99, 1, 1, 1]], [[3, 1, 1, 3, 1, 1]]], "outputs": [[[[3, 5, 2], 1]], [[1, [4, 5, 2, 1], 2, [4, 5, 2, 6], 2, [3, 3]]], [[1, 1, 1, 1]], [[1]], [[[99, 1, 1, 1]]], [[[3, 1, 1], [3, 1, 1]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,580 |
def unflatten(flat_array):
|
0276102de5fae9e02e825324a6d312cc | UNKNOWN | You will be given a string and you task is to check if it is possible to convert that string into a palindrome by removing a single character. If the string is already a palindrome, return `"OK"`. If it is not, and we can convert it to a palindrome by removing one character, then return `"remove one"`, otherwise return `"not possible"`. The order of the characters should not be changed.
For example:
```Haskell
solve("abba") = "OK". -- This is a palindrome
solve("abbaa") = "remove one". -- remove the 'a' at the extreme right.
solve("abbaab") = "not possible".
```
More examples in the test cases.
Good luck!
If you like this Kata, please try [Single Character Palindromes II](https://www.codewars.com/kata/5a66ea69e6be38219f000110) | ["def solve(s):\n isOK = lambda x: x == x[::-1]\n \n return (\"OK\" if isOK(s) else\n \"remove one\" if any( isOK(s[:i]+s[i+1:]) for i in range(len(s)) ) else\n \"not possible\")", "def solve(s):\n if s == s[::-1]:\n return 'OK'\n for i in range(len(s)):\n if s[:i] + s[i+1:] == (s[:i] + s[i+1:])[::-1]:\n return 'remove one'\n return 'not possible'", "def solve(s):\n if s == s[::-1]: return \"OK\"\n for i in range(len(s)):\n t = s[:i] + s[i+1:]\n if t == t[::-1]: return \"remove one\"\n return \"not possible\"", "def solve(s):\n return 'OK' if s == s[::-1] else 'remove one' if any(s[:i]+s[i+1:] == (s[:i]+s[i+1:])[::-1] for i in range(len(s))) else 'not possible'", "def solve(s):\n r = s[::-1]\n i = next((i for i, (c1, c2) in enumerate(zip(s, r)) if c1 != c2), None)\n if i is None:\n return 'OK'\n j = len(s) - i\n if s[i+1:] == r[i:j-1] + r[j:] or s[i:j-1] + s[j:] == r[i+1:]:\n return 'remove one'\n else:\n return 'not possible'", "p,solve=lambda s:s==s[::-1],lambda s:p(s)and\"OK\"or any(p(s[:i]+s[i+1:])for i in range(len(s)))and\"remove one\"or\"not possible\"", "def get_pal(s):\n if len(s) == 1: return 0\n if len(s) == 2 and s[0] == s[1]: return 0\n elif len(s) == 2 and s[0] != s[1]: return 1\n return 1 + min(get_pal(s[1:]), get_pal(s[:-1])) if s[0] != s[-1] else get_pal(s[1:-1])\n \ndef solve(s):\n ans = get_pal(s)\n return \"OK\" if ans == 0 else \"remove one\" if ans == 1 else \"not possible\"", "def solve(stg):\n if is_pal(stg):\n return \"OK\"\n if any(is_pal(f\"{stg[:i]}{stg[i+1:]}\") for i in range(len(stg) + 1)):\n return \"remove one\"\n return \"not possible\"\n\n\ndef is_pal(stg):\n return stg == stg[::-1]", "def solve(s):\n if s == s[::-1]:\n return \"OK\"\n for i in range(len(s)):\n news = s[:i] + s[i+1:]\n if news == news[::-1]:\n return \"remove one\"\n return \"not possible\"", "def solve(s):\n if s == s[::-1]: \n return \"OK\"\n \n s = [letter for letter in s]\n k = [letter for letter in s]\n\n for char in range(len(s)):\n s.pop(char); s = \"\".join(s)\n \n if s == s[::-1]: \n return \"remove one\"\n \n s = [letter for letter in k]\n \n return \"not possible\" \n"] | {"fn_name": "solve", "inputs": [["abba"], ["abbaa"], ["abbaab"], ["madmam"], ["raydarm"], ["hannah"], ["baba"], ["babab"], ["bababa"], ["abcbad"], ["abcdba"], ["dabcba"], ["abededba"], ["abdcdeba"], ["abcdedba"], ["abbcdedba"]], "outputs": [["OK"], ["remove one"], ["not possible"], ["remove one"], ["not possible"], ["OK"], ["remove one"], ["OK"], ["remove one"], ["remove one"], ["remove one"], ["remove one"], ["remove one"], ["remove one"], ["remove one"], ["not possible"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,419 |
def solve(s):
|
f8941112c9b3630bb73fb64b4d093c0a | UNKNOWN | An NBA game runs 48 minutes (Four 12 minute quarters). Players do not typically play the full game, subbing in and out as necessary. Your job is to extrapolate a player's points per game if they played the full 48 minutes.
Write a function that takes two arguments, ppg (points per game) and mpg (minutes per game) and returns a straight extrapolation of ppg per 48 minutes rounded to the nearest tenth. Return 0 if 0.
Examples:
```python
nba_extrap(12, 20) # 28.8
nba_extrap(10, 10) # 48
nba_extrap(5, 17) # 14.1
nba_extrap(0, 0) # 0
```
Notes:
All inputs will be either be an integer or float.
Follow your dreams! | ["def nba_extrap(ppg, mpg):\n return round(48.0 / mpg * ppg, 1) if mpg > 0 else 0", "def nba_extrap(ppg, mpg):\n return round(ppg * 48.0 / mpg, 1) if mpg else 0", "def nba_extrap(ppg, mpg):\n try:\n return round(ppg/(mpg * 1.0)*48, 1) \n except ZeroDivisionError:\n return 0\n", "def nba_extrap(ppg, mpg):\n if mpg == 0: return 0\n return round(48.0 / mpg * ppg,1)", "def nba_extrap(ppg, mpg):\n if mpg == 0:\n return 0\n else:\n return round(ppg*48.0/mpg, 1) \n\n\n", "def nba_extrap(ppg, mpg):\n return round(48 * ppg/float(mpg), 1) if mpg != 0 else 0.", "def nba_extrap(ppg, mpg):\n return 0 if not mpg else round((ppg /float(mpg)) *48, 1)", "def nba_extrap(ppg, mpg):\n if mpg == 0:\n return 0\n \n else:\n avgppm = ppg / mpg\n time = 48 - mpg\n guess = time * avgppm\n whole = (guess + ppg)\n return round(whole,1)", "def nba_extrap(ppg, mpg):\n return round(48*ppg/mpg, 1)", "def nba_extrap(ppg, mpg):\n try:\n return round(float(ppg) / mpg * 48, 1)\n except:\n return 0", "nba_extrap = lambda ppg, mpg: round(ppg/(mpg*1.0)*48, 1) if mpg else 0", "def nba_extrap(ppg, mpg):\n return round(ppg * 48 / mpg,1) if mpg else 0", "def nba_extrap(ppg, mpg):\n return round(48.0 * ppg / mpg, 1) if mpg > 0 else 0", "def nba_extrap(ppg, mpg):\n return round(ppg * 48.0 / mpg, 1) if mpg != 0 else 0", "nba_extrap=lambda p,m:m and round(48.*p/m,1)", "def nba_extrap(ppg, mpg):\n \n if mpg > 0:\n average = (ppg * 48)/ mpg\n else:\n average = 0 \n return round(average, 1)", "from typing import Union\n\ndef nba_extrap(ppg: Union[int, float], mpg: int) -> Union[int, float]:\n \"\"\" Get a straight extrapolation of points per game per 48 minutes game. \"\"\"\n return 0 if any([not ppg, not mpg]) else round(48 / mpg * ppg, 1)", "nba_extrap = lambda p, m: p and m and round(p * 48.0 / m, 1)", "def nba_extrap(ppg, mpg):\n return round(ppg * 48 / (mpg or 1), 1)", "def nba_extrap(ppg, mpg):\n return mpg and round(48 / mpg * ppg, 1)", "nba_extrap = lambda ppg, mpg: 0 if mpg == 0 else round(ppg * (48 / mpg), 1)", "def nba_extrap(ppg, mpg):\n if ppg == 0 or mpg == 0: return 0\n overall_ppg = (ppg / mpg) *48 \n return float((\"{:.1f}\".format(overall_ppg)))", "from decimal import Decimal\n\ndef nba_extrap(ppg, mpg):\n return float(round(Decimal(ppg * 48 / mpg), 1))", "def nba_extrap(ppg, mpg):\n return float(\"%0.1f\"%((48 * ppg) / mpg)) if ppg > 0 else 0", "def nba_extrap(ppg, mpg):\n return round(48*float(ppg)/float(mpg),1) if mpg > 0 else 0", "nba_extrap = lambda ppg,mpg: round(48.*ppg/mpg, 1)\n", "def nba_extrap(ppg, mpg):\n if ppg == 0:\n return 0\n ppg = (ppg * 48)/ mpg\n return round(ppg,1)", "def nba_extrap(ppg, mpg):\n return 0 if ppg == 0 or mpg == 0 else round(48 * ppg / mpg, 1)", "def nba_extrap(ppg, mpg):\n # ppg / mpg = ppgNew / 48\n # ppg(48) / mpg\n if mpg == 0:\n return 0\n return round(ppg * 48 / mpg, 1)", "nba_extrap = lambda a,b:round(a*48/b,1) if b else 0", "def nba_extrap(ppg, mpg):\n if ppg > 0:\n points_per_minute = ppg / mpg\n return round(points_per_minute * 48, 1)\n else:\n return 0", "def nba_extrap(ppg, mpg):\n return round(48*ppg/mpg, 1)\n\n\n'''An NBA game runs 48 minutes (Four 12 minute quarters).\nPlayers do not typically play the full game, subbing in and out as necessary. \nYour job is to extrapolate a player's points per game if they played the full 48 minutes.\n\nWrite a function that takes two arguments, ppg (points per game) and mpg (minutes per game)\nand returns a straight extrapolation of ppg per 48 minutes rounded to the nearest tenth. \nReturn 0 if 0.'''", "def nba_extrap(ppg, mpg):\n if mpg == 0:\n return 0\n else:\n avg = 48 / mpg\n ppg = ppg * avg\n return round(ppg, 1)", "def nba_extrap(ppg, mpg):\n if ppg != 0 and mpg != 0:\n return float(\"{0:.1f}\".format(ppg * 48 / mpg))\n else:\n return 0", "def nba_extrap(ppg, mpg):\n return 0 if not mpg else round(ppg * 48 / mpg, 1)", "def nba_extrap(ppg, mpg):\n points_per_min = ppg / mpg\n points_per_game = round(points_per_min * 48, 1)\n return points_per_game", "def nba_extrap(ppg, mpg):\n if ppg == mpg == 0:\n return 0\n else:\n return round((48*ppg)/mpg,1) ", "def nba_extrap(ppg, mpg):\n if mpg == 0:\n return 0\n else:\n ppm = ppg/mpg \n full48 = ppm*48\n return round(full48, 1)", "def nba_extrap(ppg, mpg):\n if ppg == 0 or mpg == 0:\n return 0\n pointsPerMinute = ppg / mpg\n ppg = pointsPerMinute * 48\n total = round(ppg, 1)\n return total", "def nba_extrap(ppg, mpg):\n extrap = (48 * ppg) / mpg\n return round(extrap, 1)\n\n\nprint(nba_extrap(5, 17))", "def nba_extrap(ppg, mpg):\n return 0 if ppg == 0 and mpg == 0 else round(float(48 / mpg) * float(ppg), 1)", "def nba_extrap(ppg, mpg):\n return 0 if ppg==0 or mpg==0 else float(\"%.1f\" % ((48/mpg) * ppg))", "def nba_extrap(ppg, mpg):\n if ppg==0:\n return 0\n else:\n num = ppg/mpg\n ans = num*48\n ppg = round(ans,1)\n return ppg\n\n", "def nba_extrap(ppg, mpg):\n if ppg is 0:\n return 0\n else:\n return round((48/mpg)*ppg,1)", "nba_extrap=lambda p,m:round(p*48/m,1) if m!=0 else 0", "def nba_extrap(ppg, mpg):\n if mpg > 0:\n ppg = round ((ppg/mpg) * 48, 1)\n return ppg", "import math\ndef nba_extrap(ppg, mpg):\n try:\n ppg = (ppg*48)/mpg\n ppg = round(ppg,1)\n except ZeroDivisionError:\n ppg = 0\n return ppg\n\n", "def nba_extrap(ppg, mpg):\n return round(ppg * 48 / mpg if mpg else 0, 1)", "def nba_extrap(ppg, mpg):\n return round((ppg/(mpg/48*100)*100), 1)", "def nba_extrap(ppg, mpg):\n if mpg != 0:\n ttl_scr = round(ppg/mpg * 48, 1)\n return ttl_scr\n else:\n return 0", "import sys\n\ndef nba_extrap(ppg, mpg):\n return round(ppg / (mpg + sys.float_info.epsilon) * 48, 1)", "def nba_extrap(ppg, mpg):\n uebrig = 48 / mpg\n if (mpg != 48):\n loesung = (ppg * uebrig)\n return round(loesung, 1)\n else:\n print(0)", "def nba_extrap(ppg, mpg):\n \n if ppg == 0 or mpg == 0:\n return 0\n else:\n ppg_extrap = ppg / mpg * 48\n return round(ppg_extrap,1)", "def nba_extrap(ppg, mpg):\n return 0 if mpg <= 0 else round(ppg * (48/mpg), 1)", "def nba_extrap(ppg, mpg):\n return round(48 / (mpg / ppg), 1)", "def nba_extrap(ppg, mpg):\n try:\n ppg = (ppg/mpg) * 48\n return round(ppg,1) \n except:\n return 0", "def nba_extrap(ppg, mpg):\n if mpg != 0:\n yep = 48/mpg\n return round(yep*ppg,1)\n else:\n return 0", "def nba_extrap(ppg, mpg):\n pt_min = ppg / mpg\n pt_min = pt_min * 48\n return round(pt_min,1)", "def nba_extrap(ppg, mpg):\n if ppg * mpg == 0:\n return 0\n else:\n return round(ppg*48/mpg,1)", "def nba_extrap(ppg, mpg):\n puntos_por_minuto=(ppg/mpg)\n \n puntos_totales=round(puntos_por_minuto*48.0, 1)\n \n return puntos_totales", "def nba_extrap(ppg, mpg):\n if mpg == 0 or ppg == 0:\n return 0\n else:\n return round(round((48/mpg)*ppg,1),10)", "def nba_extrap(ppg, mpg):\n if mpg!=0:\n points = (ppg/mpg)*48\n else: \n points = 0\n return round(points,1)", "def nba_extrap(ppg, mpg):\n full_match = 48\n ppmpm = ppg/mpg\n return round(ppmpm * full_match, 1)\n", "def nba_extrap(ppg, mpg):\n if ppg:\n return round((ppg/mpg)*48,1)\n else:\n return 0", "import math\ndef nba_extrap(ppg, mpg):\n return round((ppg/mpg)*48,1)", "def nba_extrap(ppg, mpg):\n return round(48 * (ppg / mpg), 1) if mpg>0 else False", "NBA_GAME_TIME = 48\n\ndef nba_extrap(points_per_game: float, minutes_per_game: float) -> float:\n if minutes_per_game < 0.001:\n return 0.0\n else:\n return round(points_per_game/minutes_per_game * NBA_GAME_TIME, 1)", "def nba_extrap(ppg, mpg):\n alt_ppg = ppg / mpg * 48\n return round(alt_ppg,1)", "def nba_extrap(ppg, mpg):\n if mpg == 0:\n return 0\n else:\n results = (ppg/mpg)*48\n return round(results, 1)", "def nba_extrap(p, m):\n return round(float(p + (48-m)/m*p),1)", "def nba_extrap(ppg, mpg):\n if(mpg == 0):\n ppg = 0\n else:\n points_per_min = ppg/mpg;\n ppg = round(points_per_min * 48,1)\n return ppg", "def nba_extrap(ppg, mpg):\n if mpg == 0 or ppg == 0:\n return 0\n average = 48 / mpg\n ppg = round(average * ppg * 10) / 10\n return ppg", "def nba_extrap(ppg, mpg):\n total_min = 48 \n if mpg == 0:\n return 0 \n else:\n ppm = ppg/mpg # points per minute\n res = ppm*total_min\n return round(res,1)", "def nba_extrap(ppg, mpg):\n if not mpg or not ppg:\n return 0\n return round(48 / mpg * ppg, 1)", "def nba_extrap(ppg, mpg):\n ppg1 = mpg / 48 * 100\n ppg1 = ppg / ppg1 * 100 \n ppg1 = round(ppg1,1)\n return ppg1", "def nba_extrap(ppg, mpg):\n if ppg and mpg:\n return round((ppg / mpg * 48), 1)\n else:\n return 0", "def nba_extrap(ppg, mpg):\n x = round(((48/mpg)*ppg), 1)\n return x", "def nba_extrap(ppg, mpg):\n return round(48*ppg/mpg if mpg else 0,1)", "def nba_extrap(ppg, m):\n if m == 0 or ppg == 0:\n return 0\n full = 48 / m\n return round(ppg * full, 1)", "def nba_extrap(ppg, mpg):\n newppg = (48/mpg) * ppg\n return round(newppg,1)\n", "def nba_extrap(ppg, mpg):\n try:\n return round(ppg/mpg*48,1)\n except ZeroDivisionError:\n print(\"That can't be...\")", "def nba_extrap(ppg, mpg):\n return 0 if ppg == 0 or mpg == 0 else round(48/mpg * ppg, 1)", "def nba_extrap(ppg, mpg):\n return 0 if ppg == False else round(48 * ppg / mpg, 1)", "def nba_extrap(ppg, mpg):\n if mpg == 48:\n return ppg\n elif mpg > 0:\n return round((ppg / mpg * 48), 1)\n else:\n return 0", "nba_extrap=lambda p,m:round(48*p/m,1) if m else 0", "def nba_extrap(ppg, mpg):\n if ppg!=0 or mpg!=0:\n return round(ppg*48/mpg,1)\n else:\n return 0", "def nba_extrap(ppg, mpg):\n x = ppg * 48.0/mpg\n return round(x, 1)\n\n", "def nba_extrap(ppg, mpg):\n if ppg == 0:\n return 0\n else:\n full_time = 48 \n average_points = ppg / mpg \n total_ppg = round((average_points * full_time), 1)\n return total_ppg", "def nba_extrap(ppg, mpg):\n try:\n ppg = ppg/mpg * 48\n return round(ppg, 1)\n except ZeroDivisionError:\n return 0", "def nba_extrap(ppg, mpg):\n if ppg == 0:\n ppg = 0\n else:\n ppg = round((ppg/mpg) * 48, 1)\n return ppg", "def nba_extrap(ppg, mpg):\n if mpg != 0:\n ppm = ppg/mpg #point per minute they played\n ppg = round(ppm*48,1)\n else:\n ppg = 0\n return ppg\n\n\n", "nba_extrap=lambda ppg,mpg:round((48/mpg)*ppg,1) if mpg!=0 else 0", "def nba_extrap(ppg, mpg):\n try: \n ppg = ppg * (48/mpg)\n ppg = round(ppg, 1)\n return ppg \n except ZeroDivisionError:\n return 0", "def nba_extrap(ppg, mpg):\n try:\n ppg = (48.0 * ppg) / mpg\n return round(ppg, 1)\n except ZeroDivisionError:\n return 0", "def nba_extrap(ppg, mpg):\n if ppg==0 or mpg==0:\n return 0\n else:\n return round(48/mpg*ppg, 1)", "def nba_extrap(ppg, mpg):\n full_ppg = 0\n if ppg == 0:\n return 0\n return round((ppg*48) / mpg, 1)", "def nba_extrap(ppg, mpg):\n tot_points = round(ppg/(mpg/48), 1)\n return tot_points ", "def nba_extrap(ppg, mpg):\n #try to do the maths\n try:\n #extrapolate the correct int to multiply by\n relative_minutes = (48/mpg)\n relative_points = round(ppg * relative_minutes, 1)\n except:\n #if the mpg is zero, it will fail, so set the final answer to 0\n relative_points = 0\n return relative_points", "# \u9700\u8981\u8003\u8651\u4fdd\u7559\u4e00\u4f4d\u5c0f\u6570\uff0c\u91c7\u7528round(a,1)\u7684\u65b9\u6cd5\uff0c\u8ba1\u7b97\u90e8\u5206\u5341\u5206\u7b80\u5355\u3002\ndef nba_extrap(ppg, mpg):\n if mpg != 0:\n ppg = (ppg/mpg)*48\n return round(ppg,1)", "def nba_extrap(ppg, mpg):\n pts = 0\n if mpg > 0:\n pts = round((ppg / mpg) * 48, 1)\n else:\n pass\n return pts\n"] | {"fn_name": "nba_extrap", "inputs": [[2, 5], [3, 9], [16, 27], [11, 19], [14, 33], [1, 7.5], [6, 13]], "outputs": [[19.2], [16.0], [28.4], [27.8], [20.4], [6.4], [22.2]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,645 |
def nba_extrap(ppg, mpg):
|
cdcebe0931207a3260228db97b57c7ff | UNKNOWN | Find the 2nd largest integer in array
If the array has no 2nd largest integer then return nil.
Reject all non integers elements and then find the 2nd largest integer in array
find_2nd_largest([1,2,3]) => 2
find_2nd_largest([1,1,1,1,1]) => nil
because all elements are same. Largest no. is 1. and there is no 2nd largest no.
find_2nd_largest([1,'a','2',3,3,4,5,'b']) => 4
as after rejecting non integers array will be [1,3,3,4,5]
Largest no. is 5. and 2nd largest is 4.
Return nil if there is no 2nd largest integer.
Take care of big numbers as well | ["def find_2nd_largest(arr):\n arr = sorted(i for i in set(arr) if type(i) == int)\n return arr[-2] if len(arr) > 1 else None", "def find_2nd_largest(arr): \n newarr = sorted(list({x for x in arr if type(x) == int})) # remove duplicates and non-int objects, sort\n return newarr[-2] if len(newarr) > 1 else None", "def find_2nd_largest(arr):\n a, b = float('-inf'), float('-inf')\n \n for n in arr:\n if isinstance(n, int):\n if n > b:\n b = n\n if b > a:\n a, b = b, a\n \n return None if a == b else b", "from heapq import nlargest\n\ndef find_2nd_largest(arr):\n two = nlargest(2, {v for v in arr if isinstance(v,int)})\n if len(two)==2: return two[1]", "def find_2nd_largest(arr):\n return next(iter(sorted(n for n in set(arr) if type(n) == int)[-2:-1]), None)", "def find_2nd_largest(arr):\n res = [arr[i] for i in range(0, len(arr)) if isinstance(arr[i], int)]\n res.sort(reverse = True)\n if len(set(arr)) == 1:\n return None\n else:\n return res[1]\n\n \n\n", "def find_2nd_largest(arr):\n SECOND_lARGEST = -2\n arr_set = set(arr)\n numbers = sorted(number for number in arr_set if type(number) == int)\n return numbers[SECOND_lARGEST] if len(numbers) > 1 else None", "def find_2nd_largest(arr):\n from collections import defaultdict\n d = defaultdict(list)\n for elem in arr:\n d[type(elem)].append(elem)\n numbers = d[int]\n largest_num = 0\n second_largest_num = 0\n for number in numbers:\n if largest_num < number:\n largest_num = number\n for number in numbers:\n if second_largest_num < number and number < largest_num:\n second_largest_num = number\n if second_largest_num == 0:\n return None\n else:\n return second_largest_num", "def find_2nd_largest(arr):\n xs = sorted({x for x in arr if isinstance(x, int)}, reverse=True)\n if len(xs) > 1 and xs[0] != xs[1]:\n return xs[1]", "\ndef filter_int(arr):\n res = []\n for i in arr:\n if type(i) == int:\n res.append(i)\n else:\n continue\n return res\n\ndef sort(arr):\n for i in range(len(arr)):\n for j in range(len(arr)-1):\n if arr[j]>arr[j+1]:\n arr[j],arr[j+1]=arr[j+1],arr[j]\n return arr\n\n\ndef is_diff(arr):\n count=0\n res=None\n for i in range(len(arr)-1):\n if arr[i] != arr[i+1]:\n count+=1\n res=True\n break\n else:\n res = False\n return res\n\n\n\ndef sec_big(a,b):\n if a>b:\n return b\n else:\n return a\n\ndef find_2nd_largest(arr):\n filtered_list = filter_int(arr)\n sorted_list=sort(filtered_list)\n if is_diff(sorted_list)==True:\n a=sorted_list[len(sorted_list)-1]\n b=sorted_list[len(sorted_list)-2]\n sec_largest_elem=sec_big(a,b)\n return sec_largest_elem\n else:\n return None\n"] | {"fn_name": "find_2nd_largest", "inputs": [[[1, 2, 3]], [[1, 1, 1, 1, 1, 1, 1]], [[1, "a", "2", 3, 3, 4, 5, "b"]], [[1, "a", "2", 3, 3, 3333333333333333333334, 544444444444444444444444444444, "b"]]], "outputs": [[2], [null], [4], [3333333333333333333334]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,057 |
def find_2nd_largest(arr):
|
d258c7c93e17a8ff40e34300d3002d99 | UNKNOWN | The local transport authority is organizing an online picture contest.
Participants must take pictures of transport means in an original way, and then post the picture on Instagram using a specific ```hashtag```.
The local transport authority needs your help. They want you to take out the ```hashtag``` from the posted message. Your task is to implement the function
```python
def omit_hashtag(message, hashtag):
```
## Examples
```
* ("Sunny day! #lta #vvv", "#lta") -> "Sunny day! #vvv" (notice the double space)
* ("#lta #picture_contest", "#lta") -> " #picture_contest"
```
## Notes
* When multiple occurences of the hashtag are found, delete only the first one.
* In C, you should modify the ```message```, as the function returns a void type. In Python, you should return the answer.
* There can be erroneous messages where the hashtag isn't present. The message should in this case stay untouched.
* The hashtag only consists of alphanumeric characters. | ["def omit_hashtag(message, hashtag):\n return message.replace(hashtag, \"\", 1)", "omit_hashtag = lambda message, hashtag: message.replace(hashtag, '', 1)", "def omit_hashtag(message, hashtag):\n return message.replace(hashtag, '' , 1) if hashtag in message else message", "omit_hashtag = lambda m, h: m.replace(h,\"\",1)", "def omit_hashtag(s, c):\n return s.replace(c,\"\",1)", "def omit_hashtag(s, hashtag):\n return s.replace(hashtag, '', 1)", "import re\n\ndef omit_hashtag(message, hashtag):\n return re.sub(hashtag, '', message, count = 1)", "def omit_hashtag(message, hashtag):\n index = message.find(hashtag)\n if index == -1:\n return message\n else:\n return message[:index] + message[index+len(hashtag):]\n \n\n", "def omit_hashtag(message, h):\n return message.replace(h,'',1)", "# Return the message, but with the first occurrence (if any) of the\n# specified hashtag removed from the message\ndef omit_hashtag(message, hashtag):\n if hashtag in message:\n found_at = message.index(hashtag)\n return message[0:found_at] + message[found_at + len(hashtag):]\n else:\n return message"] | {"fn_name": "omit_hashtag", "inputs": [["Sunny day! #lta #vvv", "#lta"], ["#lta #picture_contest", "#lta"], ["#lta #picture_contest #lta", "#lta"]], "outputs": [["Sunny day! #vvv"], [" #picture_contest"], [" #picture_contest #lta"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,168 |
def omit_hashtag(message, hashtag):
|
905877ed8aab7c10388e2752c7de8c15 | UNKNOWN | You have to rebuild a string from an enumerated list.
For this task, you have to check if input is correct beforehand.
* Input must be a list of tuples
* Each tuple has two elements.
* Second element is an alphanumeric character.
* First element is the index of this character into the reconstructed string.
* Indexes start at 0 and have to match with output indexing: no gap is allowed.
* Finally tuples aren't necessarily ordered by index.
If any condition is invalid, the function should return `False`.
Input example:
```python
[(4,'y'),(1,'o'),(3,'t'),(0,'m'),(2,'n')]
```
Returns
```python
'monty'
``` | ["def denumerate(enum_list):\n try:\n nums = dict(enum_list)\n maximum = max(nums) + 1\n result = ''.join(nums[a] for a in range(maximum))\n if result.isalnum() and len(result) == maximum:\n return result\n except (KeyError, TypeError, ValueError):\n pass\n return False\n", "def denumerate(enum_list):\n try:\n l, d = len(enum_list), dict(enum_list)\n ret = ''.join(map(d.get, range(l)))\n assert ret.isalnum() and len(ret) == l\n return ret\n except:\n return False", "def denumerate(enum_list):\n try:\n # tuple validation\n for t in enum_list:\n if ((len(t) != 2) or\n (not t[1].isalnum()) or\n (len(t[1]) != 1)):\n return False\n \n a,b = zip(*sorted(enum_list))\n \n if list(a) == list(range(len(a))):\n return \"\".join(b)\n else:\n return False\n except:\n return False", "from re import findall\n\ndef denumerate(enum):\n enum = sorted(enum) if isinstance(enum, list) else ''\n if findall(r\"(?<=\\[)(\\(\\d,\\s'[a-zA-Z\\d]'\\)[,\\s]*)*(?=\\])\", str(enum)):\n a, b = zip(*enum)\n if all(a[i] == i for i in range(len(a))):\n return ''.join(b)\n return False", "is_list = lambda L: type(L) == list\nis_index = lambda I, size: type(I) == int and 0 <= I < size\nis_char = lambda C: type(C) == str and len(C) == 1 and C.isalnum()\nis_tuple = lambda size: lambda T: type(T) == tuple and len(T) == 2 and is_index(T[0], size) and is_char(T[1])\n\ndef denumerate(enum_list):\n return is_list(enum_list) and all(map(is_tuple(len(enum_list)), enum_list)) and ''.join(t[1] for t in sorted(enum_list))", "def denumerate(e):\n if type(e)!=list or any(type(i)!=tuple for i in e) or sorted([i[0] for i in e])!=list(range(0,len(e))) : return False\n li = \"\".join([i[1] for i in sorted(e) if len(i[1])==1 and i[1].isalnum() and len(i)==2])\n return li if len(li)==len(e) else False", "def denumerate(enum_list):\n try:\n return bool(enum_list) and ''.join(c for cnt, (idx, c) in enumerate(sorted(enum_list)) if cnt == idx and len(c) == 1 and c.isalnum() or 1 / 0)\n except:\n return False", "def denumerate(enum_list):\n try:\n string = ''\n enum_list = sorted(enum_list, key=lambda i: i[0])\n for i, j in enumerate(enum_list):\n if len(j) == 2 and len(j[1]) == 1 and j[1].isalnum() and i == j[0]:\n string += j[1]\n else:\n return False\n return string\n except:\n return False\n", "def denumerate(enum_list):\n if not isinstance(enum_list, list):\n return False\n for el in enum_list:\n if not isinstance(el, tuple):\n return False\n if len(el) != 2:\n return False\n if not isinstance(el[1], str):\n return False\n if len(el[1]) != 1:\n return False\n if not el[1].isalnum():\n return False\n if not (0 <= el[0] <= len(enum_list) - 1):\n return False\n return ''.join(tup[1] for tup in sorted(enum_list))", "def denumerate(enum_list):\n if type(enum_list) is list:\n tmp = [None] * len(enum_list)\n \n for index, item in enumerate(enum_list):\n if type(item) is not tuple or len(item) != 2 or not str(item[1]).isalnum() or len(str(item[1])) != 1 or type(item[0]) is not int:\n return False\n try:\n tmp[item[0]] = item[1]\n except:\n return False\n \n tmp = list(filter(None, tmp))\n \n if len(tmp) != len(enum_list):\n return False\n \n return ''.join(tmp)\n \n return False"] | {"fn_name": "denumerate", "inputs": [[1], ["a"], [[0]], [[["a", 0]]], [[[1, "a"]]], [[[0, ""]]]], "outputs": [[false], [false], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,863 |
def denumerate(enum_list):
|
6b3811072e4df057804920d8550cdbc5 | UNKNOWN | Write a function `insertDash(num)`/`InsertDash(int num)` that will insert dashes ('-') between each two odd numbers in num. For example: if num is 454793 the output should be 4547-9-3. Don't count zero as an odd number.
Note that the number will always be non-negative (>= 0). | ["import re\n\ndef insert_dash(num):\n #your code here\n return re.sub(r'([13579])(?=[13579])', r'\\1-', str(num))", "import re\ndef insert_dash(num): return re.sub(r'[13579](?=[13579])', \"\\g<0>-\", str(num))", "\n \ndef insert_dash(num):\n odd = False\n result = []\n for n in str(num):\n num = n;\n if int(n) % 2 == 0:\n odd = False\n elif odd:\n num = '-' + num\n else:\n odd = True\n \n result.append(num)\n\n\n \n return ''.join(result)", "\ndef insert_dash(num):\n def odd(c):\n return not (ord(c) & 1 ^ 1)\n a = []\n for digit in str(num):\n if odd(digit) and a and odd(a[-1]):\n a.append('-')\n a.append(digit)\n return \"\".join(a)", "def is_odd(num):\n \"\"\" Returns True if number is odd and False otherwise \"\"\"\n return not int(num) % 2 == 0\n\ndef insert_dash(num):\n \"\"\" insert_dash() inserts dashes between each pair of odd numbers\n and returns it.\n \"\"\"\n num_str = str(num)\n new_str = num_str[0]\n for i in range(1, len(num_str)):\n if is_odd(num_str[i]) and is_odd(num_str[i - 1]):\n new_str += (\"-\" + num_str[i])\n else:\n new_str += num_str[i]\n return new_str", "import re\ndef insert_dash(num):\n return re.sub(r'[13579](?=[13579])',r'\\g<0>-', str(num))", "def insert_dash(num):\n \n digits = [d for d in str(num)]\n \n for i in range(len(digits)-1):\n if int(digits[i])%2 and int(digits[i+1])%2:\n digits[i] = digits[i] + '-'\n \n return ''.join(digits)", "from itertools import groupby\n\nis_odd = set(\"13579\").__contains__\n\n\ndef insert_dash(num: int) -> str:\n return ''.join(('-' if k else '').join(g) for k, g in groupby(str(num), key=is_odd))\n", "def insert_dash(num):\n num = str(num)\n ans = \"\"\n for i in range(len(num) - 1):\n ans += num[i]\n if int(num[i]) % 2 == 1 and int(num[i + 1]) % 2 == 1:\n ans += '-'\n ans += num[-1]\n return ans\n"] | {"fn_name": "insert_dash", "inputs": [[454793], [123456], [1003567], [24680], [13579]], "outputs": [["4547-9-3"], ["123456"], ["1003-567"], ["24680"], ["1-3-5-7-9"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,078 |
def insert_dash(num):
|
51d74a45ed72ad0f5c6fd337986b7568 | UNKNOWN | Sum all the numbers of the array (in F# and Haskell you get a list) except the highest and the lowest element (the value, not the index!).
(The highest/lowest element is respectively only one element at each edge, even if there are more than one with the same value!)
Example:
```
{ 6, 2, 1, 8, 10 } => 16
{ 1, 1, 11, 2, 3 } => 6
```
If array is empty, null or None, or if only 1 Element exists, return 0.
Note:In C++ instead null an empty vector is used. In C there is no null. ;-)
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | ["def sum_array(arr):\n if arr == None or len(arr) < 3:\n return 0\n return sum(arr) - max(arr) - min(arr)", "def sum_array(arr):\n return sum(sorted(arr)[1:-1]) if arr and len(arr) > 1 else 0", "def sum_array(arr):\n return sum(sorted(arr)[1:-1]) if arr else 0\n", "def sum_array(arr):\n return 0 if arr == None else sum(sorted(arr)[1:-1])", "def sum_array(arr):\n return sum(arr) - min(arr) - max(arr) if arr and len(arr) > 1 else 0", "def sum_array(arr):\n return sum(sorted(arr or [])[1:-1])", "def sum_array(arr):\n return sum(sorted(arr)[1:-1]) if arr else 0", "def sum_array(arr):\n \n if arr is None or len(arr) < 2:\n return 0\n \n mi, ma, s = arr[0], arr[0], 0\n \n for x in arr:\n if x > ma:\n ma = x\n elif x < mi:\n mi = x\n \n s += x\n \n return s - mi - ma", "def sum_array(arr):\n try: return sum(arr) - max(*arr) - min(*arr)\n except: return 0", "sum_array=lambda a:a and sum(sorted(a)[1:-1])or 0", "def sum_array(arr):\n if len(arr or []) <= 2:\n return 0\n\n odd = len(arr) % 2\n\n if odd:\n total = lowest = highest = arr[0]\n else:\n total, lowest, highest = 0, float('inf'), -float('inf')\n\n for a, b in zip(arr[odd::2], arr[1 + odd::2]):\n total += a + b\n if a > b:\n lowest = min(b, lowest)\n highest = max(a, highest)\n else:\n lowest = min(a, lowest)\n highest = max(b, highest)\n\n return total - lowest - highest\n", "def sum_array(arr):\n return 0 if arr is None else sum(sorted(arr)[1:-1])", "def sum_array(arr):\n if not arr or len(arr) == 1:\n return 0\n else:\n return sum(arr) - min(arr) - max(arr)", "def sum_array(arr):\n if arr == [] or arr == None or len(arr) <= 2:\n return 0\n else:\n sum = 0\n arr.sort(reverse=False)\n for i in arr:\n sum += i\n return sum - int(arr[0]+arr[-1])", "def sum_array(arr):\n print(arr)\n return sum(sorted(arr)[1:len(arr)-1])if arr and len(arr)>2 else 0", "from functools import reduce\nmin_max_tot = lambda x,y: (min(x[0], y), max(x[1], y), x[2]+y)\n\n# Who needs more than one iteration after all?\ndef sum_array(arr):\n if arr is None or len(arr) < 3: return 0\n mini, maxi, tot = reduce(min_max_tot, arr, (float('inf'), float('-inf'), 0))\n return tot - mini - maxi", "def sum_array(arr):\n if arr is None or (len(arr))==1:\n return 0\n arr=sorted(arr)\n return sum(arr[1:-1])\n", "def sum_array(arr):\n if arr is None or len(arr) < 2:\n return 0;\n\n lowest = min(arr)\n highest = max(arr)\n sum = 0\n for x in arr:\n sum += x\n \n return sum - lowest - highest\n", "def sum_array(arr):\n return 0 if (not arr or len(arr) <= 1) else sum(sorted(arr)[1:-1])", "def sum_array(arr): \n return sum(arr) - (min(arr) + max(arr)) if arr and len(arr) > 1 else 0", "def sum_array(arr):\n return 0 if arr == None or len(arr) < 2 else sum(arr) - min(arr) - max(arr)\n", "def sum_array(arr):\n return 0 if arr == None or len(arr) < 2 else sum(arr) - max(arr) - min(arr)", "def sum_array(arr):\n if arr == None:\n return 0\n return sum(sorted(arr)[1:-1]) if len(arr) > 2 else 0", "#Function for calculating highest num\ndef max_value(a):\n high = a[1]\n for x in range(len(a)):\n for y in range(x+1, len(a)):\n if ((a[x] > a[y]) and (a[x] > high) ):\n high = a[x]\n continue\n elif((a[x] < a[y]) and (a[x] > high)):\n high = a[y]\n else:\n continue\n if(a[len(a)-1] > high):\n return a[len(a)-1]\n else:\n return high\n\n#Function for calculating Lowest num\ndef min_value(a):\n min = a[1]\n for x in range(len(a)):\n for y in range(x+1, len(a)):\n if ((a[x] < a[y]) and (a[x] < min) ):\n min = a[x]\n continue\n elif((a[x] > a[y]) and (a[x] < min)):\n min = a[y]\n else:\n continue\n if(a[len(a)-1] < min):\n return a[len(a)-1]\n else:\n return min\n \n#Function for Addition without highest and smallest number \ndef sum_array(arr):\n #your code here\n total = 0 #Sum\n max_occ = 0 #Tells if highest num already occured \n min_occ = 0 #Tells if lowest num already occured\n if not arr:\n return 0\n elif(len(arr) == 1):\n return 0\n else:\n for i in range(len(arr)):\n if(arr[i] == max_value(arr) and max_occ == 0):\n max_occ = 1\n continue\n if(arr[i] == min_value(arr) and min_occ == 0):\n min_occ = 1\n continue\n \n \n total = total + arr[i]\n\n return total", "def sum_array(arr):\n return 0 if type(arr) is not list or len(arr) in (0, 1) else (sum(arr) - max(arr) - min(arr))", "sum_array=lambda a: sum(sorted(a)[1:-1]) if a else 0", "def sum_array(arr):\n if not arr or len(arr) <= 2:\n return 0\n else:\n arr.sort()\n return sum(arr[1:-1])", "def sum_array(arr): return sum(arr) - min(arr) - max(arr) if arr and len(arr) > 2 else 0", "def sum_array(arr):\n return sum(sorted(arr)[1:-1]) if arr and len(arr) > 2 else 0", "def sum_array(arr):\n if arr==None:\n return 0\n \n if len(arr)<=2:\n return 0\n \n else:\n arr.sort()\n del arr[0]\n del arr[-1]\n \n return sum(arr)\n", "sum_good = lambda x: sum(x) - min(x) - max(x) * bool(x[1:])\nsum_array = lambda arr: sum_good(arr or [0])\n", "sum_good, sum_array = lambda x: sum(x) - min(x) - max(x) * bool(x[1:]), lambda arr: sum_good(arr or [0])", "sum_array = lambda arr: (lambda x: sum(x) - min(x) - max(x) * bool(x[1:]))(arr or [0])", "def sum_array(arr):\n def sum_array_(x):\n return sum(x) - min(x) - max(x) * bool(x[1:])\n return sum_array_(arr or [0])", "def validation(foo):\n def wrapper(x):\n return foo(x or [0])\n return wrapper\n\n@validation\ndef sum_array(x):\n return sum(x) - min(x) - max(x) * bool(x[1:])\n \n", "def sum_array(arr):\n return 0 if not arr or len(arr) <= 2 else sum(arr) - max(arr) - min(arr)", "def sum_array(arr):\n if arr==None or len(arr)==0 or len(arr)==1:return 0\n return (sum(arr)-max(arr)-min(arr))", "def sum_array(arr):\n if arr is None or len(arr) <= 1:\n return 0\n print(sum(arr))\n return sum(arr) - max(arr) - min(arr)", "def sum_array(arr):\n return 0 if arr is None or len(arr) in [0, 1] else sum(arr) - min(arr) - max(arr)", "def sum_array(arr):\n if arr == None or arr == [] or len(arr) == 1:\n return 0\n else:\n return sum(x for x in arr) - min(arr) - max(arr)\n", "def sum_array(arr):\n if arr == None or len(arr) < 2:\n return 0\n arr = sorted(arr)\n arr.pop(0)\n arr.pop(-1)\n return sum(arr)\n", "def sum_array(arr):\n if arr == None or arr == [] or len(arr) < 2:\n return 0\n else:\n lowest = arr[0]\n for i in arr:\n if i < lowest:\n lowest = i\n highest = arr[0]\n for i in arr:\n if i > highest:\n highest = i\n tot = 0\n for i in arr:\n tot += i\n return tot - lowest - highest\n", "def sum_array(arr):\n if arr and len(arr) == 1:\n return 0\n elif arr: \n return sum(arr) - (max(arr) + min(arr))\n else:\n return 0", "def sum_array(arr):\n if arr == None:\n return 0\n else:\n if arr == []:\n return 0\n else:\n a = max(arr)\n b = min(arr)\n print(a)\n arr.remove(a)\n if arr == []:\n return 0\n else:\n total = 0\n arr.remove(b)\n for i in arr:\n total += i\n return total\n\n\n \n", "def sum_array(arr):\n if arr == [] or arr == None:\n return 0 \n elif len(arr) < 3:\n return 0\n arr = sorted(arr)\n result = 0\n arr.pop()\n arr.pop(0)\n print(arr)\n for i in arr:\n result += i\n return result\n #your code here\n", "def sum_array(arr):\n if not arr:\n return 0\n\n if len(arr)<3:\n return 0\n return sum(sorted(arr)[1: len(arr)-1])\n", "def sum_array(arr):\n if arr is None:\n return 0\n elif len(arr) <= 1:\n return 0 \n a = max(arr)\n b = min(arr)\n c = sum(arr)\n return c - a - b", "def sum_array(arr):\n if(arr == None or arr == [] or len(arr) < 2):\n return 0;\n return sum(arr) - min(arr) - max(arr)", "def sum_array(arr):\n if arr == None: return 0\n if len(arr) > 1: return sum(sorted(arr)[1:-1])\n else: return 0", "def sum_array(arr):\n if arr is None:\n return 0\n for el in arr:\n if type(el) == int and type(el) != 'NoneType' and len(arr)>2: \n arr.remove(max(arr))\n arr.remove(min(arr))\n print(arr)\n return sum(arr)\n else:\n return 0\n return sum(arr) ", "def sum_array(arr={}):\n if arr == None or arr == []:\n return 0\n if len(arr) > 1:\n res = sum(arr) - min(arr)\n else:\n res = sum(arr)\n return res- max(arr)", "def sum_array(arr = 0):\n if arr == None:\n return 0\n return sum(arr) - min(arr) - max(arr) if len(arr) >= 3 else 0", "def sum_array(arr):\n if arr == None:\n return 0\n elif len(arr) == 0:\n return 0\n elif len(arr) == 1:\n return 0\n else:\n arr.remove(max(arr))\n arr.remove(min(arr))\n return sum(arr) ", "def sum_array(arr):\n if arr == None:\n return 0\n elif len(arr) == 1:\n return 0\n else:\n sorted_arr = sorted(arr)\n return sum(sorted_arr[1:-1])\n", "def sum_array(arr):\n sum=0\n if arr=='':\n return(0)\n elif arr==None:\n return(0)\n elif len(arr)<3:\n return(0)\n else:\n for i in arr:\n sum+=i\n return(sum-(min(arr)+max(arr)))", "sum_array=lambda a:0 if a==None or len(a)<2 else sum(a)-max(a)-min(a)", "def sum_array(arr):\n try:\n if len(arr) == 1: return 0\n return sum(arr) - max(arr) - min(arr)\n except: return 0", "def sum_array(arr):\n total = 0\n if not arr:\n return 0\n elif len(arr) <= 2:\n return 0\n else:\n for number in range(0, len(arr)):\n total += arr[number]\n print(total)\n return total - min(arr) - max(arr)\n", "def sum_array(arr):\n if not arr:\n return 0\n arr.remove(max(arr))\n if not arr:\n return 0\n arr.remove(min(arr))\n return sum(arr)", "def sum_array(arr):\n if not arr:\n return 0\n arr2 = []\n arr.sort()\n index = -1\n for i in arr:\n index +=1\n if(index > 0 and index < len(arr)-1):\n arr2.append(i)\n return sum(arr2)", "def sum_array(arr):\n if arr==None:\n return 0\n \n elif len(arr)==1:\n return 0\n elif arr==[]:\n return 0\n else:\n somme = 0\n for elements in arr:\n somme+=elements\n \n somme=somme-min(arr)-max(arr)\n \n return somme\n", "def sum_array(arr):\n if hasattr(arr, 'sort'):\n arr.sort()\n return sum(arr[1:-1], 0)\n else:\n return 0", "def sum_array(arr):\n sum=0\n #if arr==0 or len(arr)>1:\n if not arr or len(arr)==1:\n return 0\n else:\n for i in arr:\n sum=sum+i\n return sum-min(arr)-max(arr)\n", "def sum_array(a):\n try:\n a.sort()\n a = sum(a[1:-1])\n except AttributeError:\n a = 0\n return a", "def sum_array(arr):\n if not arr or len(arr) in [1, 2]:\n return 0\n maxn, minn, sumn = arr[0], arr[0], 0\n for n in arr:\n if n > maxn:\n maxn = n\n elif n < minn:\n minn = n\n sumn += n\n return sumn - maxn - minn", "def sum_array(arr):\n if not arr:\n return 0\n else:\n res = sorted(arr)[1:-1]\n return sum(i for i in res)", "def sum_array(arr):\n if arr==[] or arr == None or len(arr) == 1:\n return 0\n else:\n mini = min(arr)\n maxi = max(arr)\n max_index = arr.index(maxi)\n min_index = arr.index(mini)\n arr[max_index] = 0\n arr[min_index] = 0\n ttl = 0\n for x in arr:\n ttl += x\n return ttl\n", "def sum_array(arr):\n sum=0\n if arr==None or len(arr)<3: return sum\n arr.sort()\n for i in range(1,len(arr)-1):\n sum += arr[i]\n return sum\n", "def sum_array(arr):\n if arr == [] or arr == None:\n return 0\n elif len(arr)>2:\n return sum(sorted(arr)[1:-1])\n else:\n return 0\n #sum(i for i in arr if i > min(arr) and i < max(arr))\n", "def sum_array(arr=[]):\n if arr == [] or arr == None or len(arr) < 3:\n return 0\n else:\n arr.remove(max(arr))\n arr.remove(min(arr))\n return sum(arr)\n \n \n \n \n", "def sum_array(arr):\n \n if arr == None or int(len(arr)) <= 2:\n return 0\n else:\n arr.remove(max(arr))\n arr.remove(min(arr))\n \n \n #new_list = [x for x in arr if x != max(arr) or min(arr)]\n return sum(arr)\n \n", "def sum_array(arr):\n if arr == None:\n return 0\n elif len(arr) <= 2:\n return 0\n \n arr.sort()\n return sum(arr[1:-1])", "def sum_array(arr):\n return 0 if not bool(arr) or len(arr) < 3 else sum(sorted(arr)[1:-1])", "def sum_array(arr):\n if arr is None or len(arr) <= 1:\n return 0 \n return sum(arr) - (max(arr) + min(arr))", "def sum_array(arr):\n if arr==None or arr==[]:\n return 0\n mi=min(arr)\n ma=max(arr)\n st=(arr.count(mi)-1)*mi+(arr.count(ma)-1)*ma\n for i in arr:\n if i not in [mi,ma]:\n st+=i\n return st\n #your code here\n", "def sum_array(arr):\n if not arr:\n return 0\n c, y = 0, []\n for x in arr:\n if c<2 and (x==max(arr) or x==min(arr)):\n c += 1\n else:\n y.append(x)\n return sum(y)", "def sum_array(arr):\n if arr and len(arr)>2:\n arr.pop(arr.index(max(arr)))\n arr.pop(arr.index(min(arr)))\n else:\n return 0\n return sum(arr)\n", "def sum_array(arr):\n #your code here\n if arr == None:\n return 0\n elif len(arr) <= 1:\n return 0\n else:\n return sum(arr) - (max(arr) + min(arr))\n", "def sum_array(arr):\n try:\n print(sorted(arr))\n print(sorted(arr)[1:-1])\n return sum(sorted(arr)[1:-1]) if len(arr) > 2 else 0\n except(TypeError):\n return 0", "def sum_array(arr):\n #your code here\n sum = 0\n if arr == None or arr == []:\n return 0\n \n else:\n new = sorted(arr)\n \n for i in range(1, len(new)-1):\n sum += new[i]\n \n return sum\n", "def sum_array(arr):\n if arr is None or len(arr) < 3:\n return 0\n else:\n arr.sort()\n return sum(arr) - arr[0] - arr[-1]", "def sum_array(arr):\n if not arr or len(arr) == 1:\n return 0\n \n# arr.remove(min(arr))\n# arr.remove(max(arr))\n \n# return sum(arr)\n\n total = mn = mx = arr[0]\n \n for x in arr[1:]:\n total += x\n \n if x < mn:\n mn = x\n \n if x > mx:\n mx = x\n \n \n return total - mn - mx", "def sum_array(arr):\n a = 0\n if arr == []:\n return 0\n if arr == None:\n return 0\n if len(arr)==1 or len(arr) == 2:\n return 0\n arr.sort()\n for i in range(len(arr)-2):\n a += arr[i+1]\n return a", "def sum_array(arr):\n try:\n if len(arr) > 2:\n arr.sort()\n return sum(arr[1:-1])\n else:\n return 0\n except TypeError:\n return 0", "def sum_array(arr):\n #your code here\n if arr == [] or arr is None:\n return 0\n elif len(arr) == 1:\n return 0\n else: \n return sum(arr)-min(arr)-max(arr)", "def sum_array(arr):\n print(arr)\n if not arr:\n return 0\n elif len(arr) == 1:\n return 0\n else:\n sum = 0\n x = 0\n for x in arr:\n sum += x\n return sum-max(arr)-min(arr)", "def sum_array(arr):\n if arr and len(arr) > 3:\n return sum(arr) - min(arr) - max(arr) \n return 0", "def sum_array(arr):\n if arr:\n return sum(arr)-max(arr)-min(arr) if len(arr) > 1 else 0 \n else:\n return 0 ", "def sum_array(arr=84089):\n \n \n if arr and len(arr) > 2:\n arr = sorted(arr)\n return sum(arr[1:-1])\n \n else:\n return 0\n \n", "def sum_array(arr):\n return sum(sorted(arr)[1:len(arr) - 1]) if arr and len(arr) > 2 else 0\n", "def sum_array(arr:list):\n if arr is None or len(arr) <= 2:\n return 0\n sorted_arr = sorted(arr)\n return sum(sorted_arr[1:-1])\n", "def sum_array(arr):\n \n if not arr: return 0\n if len(arr)==1: return 0\n if len(arr)==2: return 0\n \n return sum(sorted(arr)[1:-1])", "def sum_array(arr):\n if arr == None or arr==[]:\n return 0\n for x in arr:\n if int (len(arr)) >=3:\n return int(sum(arr) - min(arr) - max(arr))\n else:\n return 0", "def sum_array(arr):\n if arr == None or arr == [] or len(arr) == 1:\n return 0\n else:\n arr.sort()\n arr = arr[1:-1]\n return sum(arr)", "def sum_array(arr):\n if arr == None:\n return False\n elif len(arr) == 1 or len(arr) == 0:\n return 0\n \n sum = 0\n minimum = min(arr)\n maximum = max(arr)\n for i in arr:\n sum += i\n \n return sum - minimum- maximum;\n \n", "def sum_array(arr):\n try:\n l = arr.remove(min(arr))\n l = arr.remove(max(arr))\n return sum(arr)\n except:\n return 0", "def sum_array(arr):\n if arr ==None:\n return 0\n if len(arr)==0:\n return 0\n \n sum = 0\n arr.sort()\n w = arr[1:-1]\n for i in w:\n sum += i\n\n return sum", "def sum_array(arr):\n if(arr == None):\n return 0\n else:\n list.sort(arr)\n sum = 0\n length = len(arr)\n if 1 >= length:\n return sum\n else:\n array = arr\n array = array[1:len(array)-1]\n for x in array:\n sum += x\n return sum\n \n \n", "def sum_array(arr):\n if arr == None or len(arr) <= 2:\n return 0\n else:\n return sum([x for x in arr]) - min(arr) - max(arr)", "def sum_array(arr=[0]):\n try:\n if len(arr) > -1 and len(arr) < 3:\n return 0\n else:\n return sum(arr)- (min(arr)+max(arr))\n \n except:\n return 0 ", "def sum_array(numbers):\n return bool(numbers) and sum(sorted(numbers)[1:-1])"] | {"fn_name": "sum_array", "inputs": [[null], [[]], [[3]], [[-3]], [[3, 5]], [[-3, -5]], [[6, 2, 1, 8, 10]], [[6, 0, 1, 10, 10]], [[-6, -20, -1, -10, -12]], [[-6, 20, -1, 10, -12]]], "outputs": [[0], [0], [0], [0], [0], [0], [16], [17], [-28], [3]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 19,490 |
def sum_array(arr):
|
5d1ef0836b6a21e6590dfa3c9ec165fb | UNKNOWN | You like the way the Python `+` operator easily handles adding different numeric types, but you need a tool to do that kind of addition without killing your program with a `TypeError` exception whenever you accidentally try adding incompatible types like strings and lists to numbers.
You decide to write a function `my_add()` that takes two arguments. If the arguments can be added together it returns the sum. If adding the arguments together would raise an error the function should return `None` instead.
For example, `my_add(1, 3.414)` would return `4.414`, but `my_add(42, " is the answer.")` would return `None`.
Hint: using a `try` / `except` statement may simplify this kata. | ["def my_add(a, b):\n try:\n return a + b\n except TypeError:\n return None\n", "def my_add(*a):\n try:\n return sum(a)\n except:\n pass", "def my_add(a, b):\n try:\n ans = a + b\n return ans\n except:\n return None\n", "def my_add(a, b):\n # Your code here.\n try:\n return a + b\n except TypeError:\n print(\"None\")", "def my_add(a, b):\n try:\n return a + b\n except:\n pass", "def my_add(a, b):\n try:\n return a + b\n except TypeError:\n return", "def my_add(a, b):\n return a + b if isinstance(a, (int, float, complex)) and isinstance(b, (int, float, complex)) else None", "def my_add(a, b):\n try:\n return a + b\n except TypeError:\n pass", "def my_add(a, b):\n try:\n a + b\n except: \n try:\n float(a, b)\n except:\n return None\n return a + b\n # Your code here.\n", "def my_add(a, b):\n try:\n return a + b\n except:\n return None\n # Flez\n"] | {"fn_name": "my_add", "inputs": [[1, 3.414], [42, " is the answer."], [10, "2"]], "outputs": [[4.414], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,084 |
def my_add(a, b):
|
bad4de755e6e023b64ee1504cfd600ae | UNKNOWN | This problem takes its name by arguably the most important event in the life of the ancient historian Josephus: according to his tale, he and his 40 soldiers were trapped in a cave by the Romans during a siege.
Refusing to surrender to the enemy, they instead opted for mass suicide, with a twist: **they formed a circle and proceeded to kill one man every three, until one last man was left (and that it was supposed to kill himself to end the act)**.
Well, Josephus and another man were the last two and, as we now know every detail of the story, you may have correctly guessed that they didn't exactly follow through the original idea.
You are now to create a function that returns a Josephus permutation, taking as parameters the initial *array/list of items* to be permuted as if they were in a circle and counted out every *k* places until none remained.
**Tips and notes:** it helps to start counting from 1 up to n, instead of the usual range 0..n-1; k will always be >=1.
For example, with n=7 and k=3 `josephus(7,3)` should act this way.
```
[1,2,3,4,5,6,7] - initial sequence
[1,2,4,5,6,7] => 3 is counted out and goes into the result [3]
[1,2,4,5,7] => 6 is counted out and goes into the result [3,6]
[1,4,5,7] => 2 is counted out and goes into the result [3,6,2]
[1,4,5] => 7 is counted out and goes into the result [3,6,2,7]
[1,4] => 5 is counted out and goes into the result [3,6,2,7,5]
[4] => 1 is counted out and goes into the result [3,6,2,7,5,1]
[] => 4 is counted out and goes into the result [3,6,2,7,5,1,4]
```
So our final result is:
```
josephus([1,2,3,4,5,6,7],3)==[3,6,2,7,5,1,4]
```
For more info, browse the Josephus Permutation page on wikipedia; related kata: Josephus Survivor.
Also, [live game demo](https://iguacel.github.io/josephus/) by [OmniZoetrope](https://www.codewars.com/users/OmniZoetrope). | ["def josephus(xs, k):\n i, ys = 0, []\n while len(xs) > 0:\n i = (i + k - 1) % len(xs)\n ys.append(xs.pop(i))\n return ys", "def josephus(n,k):\n i, ans = 0, []\n while n: i = (i + k - 1) % len(n); ans.append(n.pop(i))\n return ans", "from collections import deque\n\ndef josephus(items,k):\n q = deque(items)\n return [[q.rotate(1-k), q.popleft()][1] for _ in items]", "class Circle(object):\n def __init__(self, items):\n self.items = items[:]\n\n def pop(self, index):\n norm_index = index % len(self.items)\n return self.items.pop(norm_index), norm_index\n\n def not_empty(self):\n return self.items\n\n\ndef josephus_iter(items, k):\n circle = Circle(items)\n i = k - 1 # index 0-based\n while circle.not_empty():\n item, i = circle.pop(i)\n yield item\n i += k - 1\n\n\ndef josephus(items, k):\n return list(josephus_iter(items, k))", "def josephus(items,k):\n if len(items)==0: return []\n if len(items)==1: return [items[0]]\n i = ((k-1)%len(items))\n return [items[i]] + josephus(items[i+1:]+items[:i], k)\n", "def josephus(items, k):\n out = []\n i = 0\n while len(items) > 0:\n i = (i+k-1) % len(items)\n out.append(items[i])\n del items[i]\n return out", "def josephus(n, k):\n k -= 1\n i = k \n \n result = []\n while n:\n if i < len(n):\n result.append(n.pop(i))\n i += k\n else:\n while i >= len(n):\n i -= len(n)\n return result", "def josephus(items,k):\n cursor = 0\n killed = []\n while len(items):\n cursor = (cursor + k - 1) % len(items)\n killed.append(items.pop(cursor))\n \n return killed", "def josephus(itemlist, interval):\n length = len(itemlist)\n\n if interval == 1:\n return itemlist\n \n count = 1\n disposal = [] #list which stores order in which items get deleted\n\n while len(disposal) != length: #loop runs unti; every item has been deleted\n index = 0 #gives an index. Useful if there are duplicates of items in a list\n for item in itemlist:\n if count == interval: \n \n disposal.append(item)\n itemlist.pop(index)\n count = 1 \n \n try:\n print(itemlist[index]) #sees if there is another number after the deleted one. Catches numbers at the end of the list which get's deleted\n except:\n break\n\n count += 1\n index += 1\n return disposal ", "def josephus(items,k):\n i,res=0,[]\n while items:\n i=(i+k-1)%len(items)\n res+=[items.pop(i)]\n return res"] | {"fn_name": "josephus", "inputs": [[[1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 1], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 2], [["C", "o", "d", "e", "W", "a", "r", "s"], 4], [["C", 0, "d", 3, "W", 4, "r", 5], 4], [[1, 2, 3, 4, 5, 6, 7], 3], [[], 3], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50], 11], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15], 40], [[1], 3], [[true, false, true, false, true, false, true, false, true], 9]], "outputs": [[[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]], [[2, 4, 6, 8, 10, 3, 7, 1, 9, 5]], [["e", "s", "W", "o", "C", "d", "r", "a"]], [[3, 5, "W", 0, "C", "d", "r", 4]], [[3, 6, 2, 7, 5, 1, 4]], [[]], [[11, 22, 33, 44, 5, 17, 29, 41, 3, 16, 30, 43, 7, 21, 36, 50, 15, 32, 48, 14, 34, 1, 20, 39, 9, 28, 2, 25, 47, 24, 49, 27, 8, 38, 19, 6, 42, 35, 26, 23, 31, 40, 4, 18, 12, 13, 46, 37, 45, 10]], [[10, 7, 8, 13, 5, 4, 12, 11, 3, 15, 14, 9, 1, 6, 2]], [[1]], [[true, true, true, false, false, true, false, true, false]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,798 |
def josephus(items,k):
|
c971765e4f298dafd07c0012dd1d1cb8 | UNKNOWN | Comprised of a team of five incredibly brilliant women, "The ladies of ENIAC" were the first “computors” working at the University of Pennsylvania’s Moore School of Engineering (1945). Through their contributions, we gained the first software application and the first programming classes! The ladies of ENIAC were inducted into the WITI Hall of Fame in 1997!
Write a function which reveals "The ladies of ENIAC" names, so that you too can add them to your own hall of tech fame!
To keep: only alpha characters, space characters and exclamation marks.
To remove: numbers and these characters: ```%$&/£?@```
Result should be all in uppercase. | ["import re\ndef rad_ladies(name):\n return \"\".join(re.findall(\"[A-Z\\s!]+\", name.upper()))", "from string import ascii_uppercase\n\nVALID = set(ascii_uppercase + ' !')\n\n\ndef rad_ladies(name):\n return ''.join(a for a in name.upper() if a in VALID)\n", "import re\ndef rad_ladies(name):\n return re.sub(\"[\\d%$&/\u00a3?@]\", \"\", name).upper()", "def rad_ladies(name):\n return \"\".join(c for c in name if c.isalpha() or c.isspace() or c == \"!\").upper()\n", "def rad_ladies(name):\n return ''.join(i.upper() for i in name if not i.isdigit() and i not in '%$&/\u00a3?@')", "def rad_ladies(text):\n return ''.join(c for c in text if c.isalpha() or c in \" !\").upper()", "def rad_ladies(name):\n return ''.join(i.upper()for i in name if i.isalpha() or i==' ' or i=='!')", "def rad_ladies(name):\n return \"\".join(char for char in name.upper() if char.isalpha() or char in \" !\")", "import re\ndef rad_ladies(name):\n return re.sub(r'[\\d%$&/\u00a3?@]',r'',name).upper()", "import re\ndef rad_ladies(name):\n return ''.join(re.findall(r'[a-zA-Z !]',name)).upper()"] | {"fn_name": "rad_ladies", "inputs": [["k?%35a&&/y@@@5599 m93753&$$$c$n///79u??@@%l?975$t?%5y%&$3$1!"], ["9?9?9?m335%$@a791%&$r$$$l@53$&y&n%$5@ $5577w&7e931%s$c$o%%%f351f??%!%%"], ["%&$557f953//1/$@%r%935$$a@3111$@???%n???5 $%157b%///$i%55&31@l?%&$$a%@$s5757!$$%%%%53"], ["///$%&$553791r357%??@$%u?$%@7993111@$%t$h3% 3$l$311i3%@?&c3h%&t&&?%11e%$?@11957r79%&m$$a55n1!111%%"], ["??@%&a5d15??e599713%l%%e%75913 1$%&@g@%o&$@13l5d11s$%&t15i9n&5%%@%e@$!%$"]], "outputs": [["KAY MCNULTY!"], ["MARLYN WESCOFF!"], ["FRAN BILAS!"], ["RUTH LICHTERMAN!"], ["ADELE GOLDSTINE!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,106 |
def rad_ladies(name):
|
e963d22fb9dbae190c542dd949791c74 | UNKNOWN | The `depth` of an integer `n` is defined to be how many multiples of `n` it is necessary to compute before all `10` digits have appeared at least once in some multiple.
example:
```
let see n=42
Multiple value digits comment
42*1 42 2,4
42*2 84 8 4 existed
42*3 126 1,6 2 existed
42*4 168 - all existed
42*5 210 0 2,1 existed
42*6 252 5 2 existed
42*7 294 9 2,4 existed
42*8 336 3 6 existed
42*9 378 7 3,8 existed
```
Looking at the above table under `digits` column you can find all the digits from `0` to `9`, Hence it required `9` multiples of `42` to get all the digits. So the depth of `42` is `9`. Write a function named `computeDepth` which computes the depth of its integer argument.Only positive numbers greater than zero will be passed as an input. | ["def compute_depth(n):\n i = 0\n digits = set()\n while len(digits) < 10:\n i += 1\n digits.update(str(n * i))\n return i", "def compute_depth(n):\n s, i = set(str(n)), 1\n while len(s) < 10:\n i += 1\n s |= set(str(n*i))\n return i\n", "from itertools import count\n\ndef compute_depth(n):\n found = set()\n update = found.update\n return next(i for i,x in enumerate(count(n, n), 1) if update(str(x)) or len(found) == 10)", "def compute_depth(n):\n seen = set()\n for i in range(1, 99999999):\n seen.update(str(n * i))\n if len(seen) == 10:\n return i", "from itertools import count\ndef compute_depth(n):\n res = set(map(str, range(10)))\n for a in count(1):\n res -= set(str(n * a))\n if not res:\n return a", "def compute_depth(n):\n digits, depth = set(), 0\n while digits < set('0123456789'):\n depth += 1\n digits.update(str(depth * n))\n return depth", "def compute_depth(n):\n digits, depth, num = set('1234567890'), 1, n\n \n while True:\n digits -= set(str(num))\n \n if not digits:\n return depth\n \n num += n\n depth += 1", "def compute_depth(n):\n found = set()\n mult = 1\n while not set(x for x in range(0,10)) == found:\n for digit in str(n*mult):\n found.add(int(digit))\n mult += 1\n return mult-1", "def compute_depth(n):\n all_digits = [0,1,2,3,4,5,6,7,8,9]\n counter = 1\n while all_digits != []:\n for x in list(map(int,str(n * counter))):\n if x in all_digits:\n all_digits.remove(x)\n counter = counter + 1\n return counter-1", "from itertools import count\n\ndef compute_depth(n):\n v, target = 0, set(\"0123456789\")\n for x in count(1):\n v += n\n target -= set(str(v))\n if not target: return x\n"] | {"fn_name": "compute_depth", "inputs": [[8], [13], [7], [25], [42], [1]], "outputs": [[12], [8], [10], [36], [9], [10]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,950 |
def compute_depth(n):
|
260d96caac97fdecfe0244ad86644877 | UNKNOWN | Based on [this kata, Connect Four.](https://www.codewars.com/kata/connect-four-1)
In this kata we play a modified game of connect four. It's connect X, and there can be multiple players.
Write the function ```whoIsWinner(moves,connect,size)```.
```2 <= connect <= 10```
```2 <= size <= 52```
Each column is identified by a character, A-Z a-z:
``` ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz ```
Moves come in the form:
```
['C_R','p_Y','s_S','I_R','Z_Y','d_S']
```
* Player R puts on C
* Player Y puts on p
* Player S puts on s
* Player R puts on I
* ...
The moves are in the order that they are played.
The first player who connect ``` connect ``` items in same color is the winner.
Note that a player can win before all moves are done. You should return the first winner.
If no winner is found, return "Draw".
A board with size 7, where yellow has connected 4:
All inputs are valid, no illegal moves are made.
 | ["from itertools import compress\nfrom string import ascii_uppercase, ascii_lowercase\nD = {c:i for i,c in enumerate(ascii_uppercase + ascii_lowercase)}\n\ndef whoIsWinner(moves, con, sz):\n def gen(i, j):\n for x in range(1, con):\n yield ((i, j-x), (i-x, j), (i+x, j), (i-x, j-x), (i+x, j+x), (i+x, j-x), (i-x, j+x))\n \n def check(i, j, p):\n memo, count = [True]*7, [0]*7\n for L in gen(i, j):\n for x,(k,l) in enumerate(L):\n memo[x] = memo[x] and 0 <= k < sz and 0 <= l < sz and grid[k][l] == p\n count[x] += memo[x]\n if not any(memo):\n return max(count[0], count[1]+count[2], count[3]+count[4], count[5]+count[6])+1 >= con\n return True\n \n if sz >= con <= len(moves):\n grid = [[None]*sz for _ in range(sz)]\n for move in moves:\n i, p = D[move[0]], move[-1]\n j = next(j for j,x in enumerate(grid[i]) if x is None)\n if check(i, j, p): return p\n grid[i][j] = p\n return \"Draw\"", "from string import ascii_letters as rows\nROWS = {c: n for n, c in enumerate(rows[26:] + rows[:26])}\nDIRS = [(1, 0), (1, 1), (0, 1), (-1, 1)]\n\ndef whoIsWinner(moves, connect, size):\n def check_line(dx, dy):\n n = 0\n for _ in range(2):\n dx, dy, x, y = -dx, -dy, p, q\n while (0 <= x < size and 0 <= y < len(grid[x])\n and grid[x][y] == player):\n n += 1; x += dx; y += dy\n return n > connect\n\n grid = [[] for _ in range(size)]\n for move in moves:\n row, player = move.split('_')\n p = ROWS[row]; q = len(grid[p])\n grid[p].append(player)\n if any(check_line(u, v) for u, v in DIRS):\n return player\n return 'Draw'", "from itertools import count,takewhile\n\nMOVES = ((0,1), (1,1), (1,0), (-1,1))\n\n \ndef whoIsWinner(moves,nCon,sz):\n \n def insideAndSameYet(z): x,y=z ; return 0<=x<sz and 0<=y<sz and grid[x][y] == who\n \n def isWinner(x, y, who):\n for dx,dy in MOVES:\n s = 1 + sum(1 for c in (-1,1) for _ in takewhile(insideAndSameYet, ((x+c*n*dx, y+c*n*dy) for n in count(1))))\n if s >= nCon: return True\n return False\n \n \n grid = [ ['']*sz for _ in range(sz) ]\n xInCols = [0] * sz\n \n for m in moves:\n who = m[-1]\n y = ord(m[0]) - (65 if m[0].isupper() else 71)\n x = xInCols[y]\n \n xInCols[y] += 1\n grid[x][y] = who\n \n if isWinner(x,y,who): return who\n \n else:\n return \"Draw\"", "import re\nfrom string import Template\ndef whoIsWinner(moves,con,sz):\n z = (sz+1)**2\n r = [\"-\"]*z\n d = dict()\n reg = Template(\"(\\\\w)(((.{$s1}\\\\1){$c})|(\\\\1{$c})|((.{$s2}\\\\1){$c})|((.{$s3}\\\\1){$c}))\")\n reg = re.compile((reg.substitute(s1 = str(sz-1), c = str(con-1), s2=str(sz), s3=str(sz+1))))\n for i,x in enumerate(list(\"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz\")[:sz]):\n d[x]=z-(sz+1)+i\n for s in moves:\n r[d[s[0]]] = s[2]\n d[s[0]] -= sz+1\n m = reg.findall((\"\").join(r))\n if(m):\n return m[0][0]\n return \"Draw\"", "whoIsWinner=lambda M,C,Z:(lambda g,X:next((w for w,y in map(lambda m:(m[-1],ord(m[0])-(65 if m[0].isupper()else 71)),M)for x in(X.__getitem__(y),)if X.__setitem__(y,X.__getitem__(y)+1)or g.__getitem__(x).__setitem__(y,w)\n or any(C<=1+sum(1 for c in(-1,1)for _ in __import__(\"itertools\").takewhile(lambda z:0<=z[0]<Z and 0<=z[1]<Z and g[z[0]][z[1]]==w,((x+c*n*dx,y+c*n*dy)for n in __import__(\"itertools\").count(1))))for dx,dy in((0,1),(1,1),(1,0),(-1,1)))),\"Draw\")\n )(*([['']*Z for _ in range(Z)],[0]*Z))", "from itertools import count\n\nMOVES = ((0,1), (1,1), (1,0), (-1,1))\n\n \ndef whoIsWinner(moves,nCon,sz):\n\n def isWinner(rX,rY, who):\n for dx,dy in MOVES:\n s = 1\n for c in (-1,1):\n for n in count(1):\n x,y = rX + c*n*dx, rY + c*n*dy\n if not (0<=x<sz and 0<=y<sz and grid[x][y] == who): break\n s += 1\n if s >= nCon: return True\n return False\n \n \n grid = [ ['']*sz for _ in range(sz) ]\n xInCols = [0] * sz\n \n for m in moves:\n who = m[-1]\n y = ord(m[0]) - (65 if m[0].isupper() else 71)\n x = xInCols[y]\n \n xInCols[y] += 1\n grid[x][y] = who\n if isWinner(x,y,who): return who\n \n else:\n return \"Draw\"", "from itertools import compress\nfrom string import ascii_uppercase, ascii_lowercase\nD = {c:i for i,c in enumerate(ascii_uppercase + ascii_lowercase)}\n\ndef whoIsWinner(moves, con, sz):\n def gen(i, j):\n for x in range(1, con):\n yield ((i, j-x), (i-x, j), (i+x, j), (i-x, j-x), (i+x, j+x), (i+x, j-x), (i-x, j+x))\n \n def check(i, j, p):\n memo, count = [True]*7, [0]*7\n for L in gen(i, j):\n for x,(k,l) in enumerate(L):\n memo[x] = memo[x] and 0 <= k < sz and 0 <= l < sz and grid[k][l] == p\n count[x] += memo[x]\n if not any(memo):\n return max(count[0], count[1]+count[2], count[3]+count[4], count[5]+count[6])+1 >= con\n else: return True\n \n if sz >= con <= len(moves):\n grid = [['0']*sz for _ in range(sz)]\n for move in moves:\n i, p = D[move[0]], move[-1]\n j = next(j for j,x in enumerate(grid[i]) if x == '0')\n if check(i, j, p): return p\n grid[i][j] = p\n return \"Draw\"", "from string import ascii_uppercase, ascii_lowercase\nfrom collections import Counter\nimport re\n\nA = {x: i for i, x in enumerate(ascii_uppercase + ascii_lowercase)}\n\ndef f(P, W):\n row, col = divmod(P, W)\n r = [\n [row * W + i for i in range(W)],\n [W * i + col for i in range(W)]\n ]\n if P >= (W + 1) * row:\n s = P - row * (W + 1)\n r.append([s + (W + 1) * i for i in range(W - s)])\n else:\n s = W * (row - col)\n r.append([s + (W + 1) * i for i in range(W - row + col)])\n ###\n if P >= (W - 1) * (row + 1):\n s = W * (W - 2) + col + row + 1\n r.append([s - (W - 1) * i for i in range(W - s % W)])\n else:\n s = W * row + W * col\n r.append([s - (W - 1) * i for i in range(s // W + 1)])\n return r\n\ndef whoIsWinner(G, n, w, d=\"Draw\"):\n if n > w: return d\n D, P, C = dict(), Counter(), Counter()\n for x in G:\n p, t = x.split(\"_\")\n col = A[p]\n rp = col + P[col] * w\n P[col] += 1\n D[rp] = t\n C[t] += 1\n if C[t] < n:\n continue\n if next((1 for x in f(rp, w) if re.search(fr\"({t})\\1{{{n-1}}}\", \"\".join(D.get(y, \".\") for y in x))), None):\n return t\n return d", "import re\n\ndef check(line, ch, connect):\n return re.search(re.escape(ch)+'{'+re.escape(str(connect))+'}', ''.join(line))\n\ndef decide(matrix, i, j, connect, size):\n ch = matrix[i][j]\n row = matrix[i][max(0, j - connect + 1):j + connect]\n if check(row, ch, connect) : return True\n \n column = [k[j] for k in matrix[max(0, i - connect + 1):i + connect]]\n if check(column, ch, connect) : return True\n \n for x in [[(-1, -1), (1, 1)], [(-1, 1), (1, -1)]]:\n diagonal = []\n for ind, (k, l) in enumerate(x):\n ni, nj, li = i + k, j + l, []\n while 0 <= ni < size and 0 <= nj < size and len(li) != connect and matrix[ni][nj] != ' ':\n li.append(matrix[ni][nj])\n ni += k\n nj += l\n diagonal.extend(li[::[-1,1][ind]] + [ch])\n if check(diagonal[:-1], ch, connect) : return True\n return False\n\ndef whoIsWinner(moves,connect,size):\n matrix = [[' ' for _ in range(size)] for _ in range(size)]\n columns = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz'[:size]\n AVL = {i: size - 1 for i in columns}\n\n for move in moves:\n col, player = move.split('_')\n r, c = AVL[col], columns.index(col)\n \n matrix[r][c] = player\n AVL[col] -= 1\n\n if decide(matrix, r, c, connect, size) : return player\n return 'Draw'", "columns = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz\"\n\ndef parseMove(move):\n (loc, player) = move.split(\"_\")\n return (columns.index(loc), player)\n\ndef placeMove(move, board):\n (loc, player) = parseMove(move)\n board[loc].append(player)\n\ndef isConnect(loc, dir, connect, board):\n count = 1\n (x,y) = loc\n player = board[x][y]\n\n s = 1\n isCheckForward = True\n isCheckBack = True\n\n while count < connect and (isCheckForward or isCheckBack):\n (sx,sy) = tuple(e * s for e in dir)\n \n if (isCheckForward and \n x+sx < len(board) and x+sx >= 0 and \n y+sy < len(board[x+sx]) and y+sy >= 0 and\n board[x+sx][y+sy] == player):\n count += 1\n else:\n isCheckForward = False\n\n if (isCheckBack and \n x-sx >= 0 and x-sx < len(board) and\n y-sy >= 0 and y-sy < len(board[x-sx]) and\n board[x-sx][y-sy] == player):\n count += 1\n else:\n isCheckBack = False\n\n s += 1\n\n return count >= connect\n\n\ndef findWinner(move, connect, board):\n (x, player) = parseMove(move)\n y = len(board[x]) - 1\n loc = (x,y)\n\n for d in [(1,0), (0,1), (1,1), (1, -1)]:\n if isConnect(loc, d, connect, board):\n return player\n\n return None\n\ndef whoIsWinner(moves,connect,size):\n board = [[] for _ in range(size)]\n winner = None\n\n while not winner and len(moves) > 0:\n move = moves.pop(0)\n placeMove(move, board)\n winner = findWinner(move, connect, board)\n\n return winner if winner else \"Draw\"\n\n"] | {"fn_name": "whoIsWinner", "inputs": [[["A_R", "B_Y", "A_R"], 2, 2], [["B_R", "A_Y", "A_R", "D_Y", "B_R", "D_Y", "B_R", "D_Y", "C_R", "D_Y"], 4, 4], [["A_R", "C_Y", "C_R", "B_Y", "A_R", "C_Y", "B_R", "B_Y", "D_R", "A_Y", "D_R", "A_Y"], 2, 4], [["H_R", "A_Y", "A_R", "C_Y", "H_R", "E_Y", "G_R", "C_Y", "D_R", "F_Y", "E_R", "D_Y", "D_R", "D_Y", "C_R", "C_Y", "D_R", "A_Y", "G_R", "E_Y", "C_R", "H_Y", "A_R", "G_Y", "B_R", "G_Y", "A_R", "G_Y", "H_R", "G_Y", "E_R", "F_Y", "A_R", "E_Y", "H_R", "D_Y", "H_R", "H_Y", "A_R", "E_Y", "C_R", "B_Y", "C_R", "E_Y", "G_R", "G_Y"], 2, 8], [["F_R", "B_Y", "E_R", "F_Y", "A_R", "E_Y", "C_R", "E_Y", "C_R", "B_Y", "A_R", "F_Y", "C_R", "E_Y", "D_R", "A_Y", "D_R", "E_Y", "C_R", "A_Y", "A_R", "D_Y", "D_R", "E_Y"], 4, 6], [["A_R", "E_Y", "E_R", "D_Y", "A_R", "A_Y", "D_R", "B_Y", "E_R", "E_Y", "D_R", "E_Y"], 4, 5], [["E_R", "E_Y", "E_R", "A_Y", "B_R", "C_Y", "B_R", "F_Y", "F_R", "C_Y", "B_R", "D_Y", "D_R", "A_Y", "C_R", "E_Y", "B_R", "D_Y", "D_R", "A_Y", "A_R", "D_Y", "D_R"], 3, 6], [["G_R", "E_Y", "A_R", "G_Y", "C_R", "H_Y", "E_R", "G_Y", "E_R", "A_Y", "C_R", "E_Y", "A_R", "D_Y", "B_R", "F_Y", "C_R", "C_Y", "F_R", "A_Y", "G_R", "D_Y", "C_R", "E_Y", "B_R", "B_Y", "B_R", "C_Y", "G_R", "B_Y", "D_R", "F_Y", "A_R", "G_Y", "D_R", "H_Y", "G_R", "H_Y", "B_R", "G_Y"], 4, 8], [["B_R", "A_Y", "B_R"], 3, 2], [["C_R", "F_Y", "c_R", "E_Y", "h_R", "E_Y", "P_R", "E_Y", "H_R", "S_Y", "b_R", "B_Y", "X_R", "U_Y", "M_R", "N_Y", "W_R", "E_Y", "R_R", "T_Y", "L_R", "A_Y", "N_R", "h_Y", "d_R", "X_Y", "D_R", "g_Y", "d_R", "X_Y", "b_R", "I_Y", "Y_R", "J_Y", "P_R", "g_Y", "Y_R", "B_Y", "a_R", "S_Y", "C_R", "a_Y", "i_R", "a_Y", "N_R", "J_Y", "g_R", "b_Y", "X_R", "R_Y", "I_R", "W_Y", "G_R", "Y_Y", "I_R", "N_Y", "R_R", "a_Y", "a_R", "J_Y", "E_R", "X_Y", "Y_R", "J_Y", "e_R", "T_Y", "h_R", "W_Y", "b_R", "X_Y", "g_R", "O_Y", "b_R", "U_Y", "G_R", "h_Y", "H_R", "h_Y", "K_R", "L_Y", "T_R", "L_Y", "c_R", "L_Y", "O_R", "H_Y", "K_R", "P_Y", "D_R", "S_Y", "A_R", "T_Y", "G_R", "K_Y", "J_R", "G_Y", "h_R", "F_Y", "W_R", "K_Y", "C_R", "G_Y", "I_R", "W_Y", "h_R", "f_Y", "b_R", "h_Y", "U_R", "a_Y", "e_R", "M_Y", "D_R", "F_Y", "N_R", "Q_Y", "Z_R", "c_Y", "J_R", "S_Y", "J_R", "M_Y", "F_R", "b_Y", "L_R", "I_Y", "L_R", "J_Y", "U_R", "S_Y", "H_R", "C_Y", "i_R", "U_Y", "D_R", "J_Y", "c_R", "h_Y", "R_R", "H_Y", "O_R", "i_Y", "B_R", "i_Y", "G_R", "Y_Y", "M_R", "d_Y", "F_R", "T_Y", "P_R", "b_Y", "U_R", "I_Y", "J_R", "E_Y", "d_R", "V_Y", "E_R", "f_Y", "b_R", "L_Y", "b_R", "g_Y", "F_R", "V_Y", "b_R", "J_Y", "L_R", "S_Y", "U_R", "W_Y", "f_R", "B_Y", "e_R", "M_Y", "T_R", "D_Y", "F_R", "L_Y", "V_R", "L_Y", "f_R", "C_Y", "L_R", "A_Y", "D_R", "S_Y", "g_R", "A_Y", "h_R", "c_Y", "V_R", "M_Y", "S_R", "T_Y", "c_R", "Z_Y", "c_R", "c_Y", "V_R", "P_Y", "e_R", "P_Y", "I_R", "e_Y", "M_R", "B_Y", "S_R", "g_Y", "H_R", "c_Y", "N_R", "I_Y", "T_R", "O_Y", "f_R", "T_Y", "F_R", "M_Y", "H_R", "a_Y", "f_R", "d_Y", "a_R", "H_Y", "c_R", "a_Y", "a_R", "S_Y", "C_R", "a_Y", "c_R", "Z_Y", "K_R", "b_Y", "B_R", "h_Y", "E_R", "X_Y", "R_R", "Q_Y", "A_R", "V_Y", "H_R", "V_Y", "D_R", "N_Y", "D_R", "K_Y", "K_R", "e_Y", "X_R", "H_Y", "Y_R", "g_Y", "X_R", "G_Y", "V_R", "M_Y", "a_R", "O_Y", "K_R", "a_Y", "R_R", "M_Y", "g_R", "Q_Y", "S_R", "b_Y", "f_R", "h_Y", "U_R", "P_Y", "J_R", "W_Y", "d_R", "J_Y", "X_R", "V_Y", "Q_R", "W_Y", "I_R", "W_Y", "D_R", "J_Y", "X_R", "Y_Y", "C_R", "f_Y", "a_R", "W_Y", "C_R", "L_Y", "C_R", "F_Y", "a_R", "E_Y", "a_R", "W_Y", "R_R", "V_Y", "b_R", "C_Y", "c_R", "h_Y", "A_R", "K_Y", "Z_R", "d_Y", "U_R", "K_Y", "Q_R", "U_Y", "S_R", "J_Y", "F_R", "E_Y", "G_R", "P_Y", "W_R", "H_Y", "S_R", "P_Y", "E_R", "M_Y", "S_R", "h_Y", "L_R", "L_Y", "e_R", "Q_Y", "L_R", "e_Y", "c_R", "E_Y", "i_R", "h_Y", "X_R", "Q_Y", "I_R", "c_Y", "L_R", "P_Y", "H_R", "a_Y", "N_R", "S_Y", "a_R", "h_Y", "D_R", "e_Y", "h_R", "S_Y", "S_R", "O_Y", "i_R", "U_Y", "K_R", "V_Y", "O_R", "L_Y", "h_R", "c_Y", "f_R", "V_Y", "H_R", "N_Y", "B_R", "R_Y", "T_R", "H_Y", "J_R", "I_Y", "P_R", "J_Y", "T_R", "E_Y", "h_R", "H_Y", "h_R", "a_Y", "Z_R", "O_Y", "K_R", "U_Y", "U_R", "O_Y", "b_R", "L_Y", "J_R", "U_Y", "h_R", "b_Y", "d_R", "C_Y", "U_R", "H_Y", "X_R", "c_Y", "Y_R", "Z_Y", "g_R", "K_Y", "P_R", "F_Y", "R_R", "C_Y", "D_R", "P_Y", "g_R", "O_Y", "d_R", "J_Y", "e_R", "Y_Y", "M_R", "G_Y", "f_R", "g_Y", "J_R", "U_Y", "E_R", "L_Y", "B_R", "f_Y", "i_R", "M_Y", "U_R", "d_Y", "M_R", "X_Y", "I_R", "P_Y", "D_R", "h_Y", "V_R", "V_Y", "M_R", "J_Y", "h_R", "X_Y", "d_R", "a_Y", "X_R", "e_Y", "g_R", "O_Y", "a_R", "b_Y", "X_R", "g_Y", "g_R", "N_Y", "K_R", "M_Y", "O_R", "d_Y", "A_R", "B_Y", "G_R", "I_Y", "D_R", "K_Y", "H_R", "i_Y", "V_R", "e_Y", "L_R", "H_Y", "h_R", "W_Y", "E_R", "G_Y", "i_R", "F_Y", "Q_R", "Y_Y", "U_R", "D_Y", "Y_R", "Z_Y", "K_R", "H_Y", "R_R", "X_Y", "V_R", "X_Y", "W_R", "Z_Y", "C_R", "O_Y", "I_R", "i_Y", "S_R", "G_Y", "Z_R", "G_Y", "h_R", "L_Y", "X_R", "c_Y", "b_R", "h_Y", "A_R", "Z_Y", "i_R", "I_Y", "A_R", "T_Y", "h_R", "I_Y", "E_R", "S_Y", "U_R", "S_Y", "S_R", "S_Y", "h_R", "h_Y", "a_R", "d_Y", "V_R", "L_Y", "b_R", "e_Y", "Y_R", "T_Y", "J_R", "U_Y", "C_R", "G_Y", "A_R", "g_Y", "h_R", "J_Y", "f_R", "K_Y", "D_R", "E_Y", "U_R", "M_Y", "M_R", "V_Y", "f_R", "i_Y", "K_R", "Z_Y", "h_R", "U_Y", "e_R", "i_Y", "R_R", "c_Y", "E_R", "J_Y", "I_R", "b_Y", "B_R", "S_Y", "U_R", "F_Y", "B_R", "b_Y", "B_R", "a_Y", "g_R", "D_Y", "Y_R", "E_Y", "N_R", "B_Y", "M_R", "i_Y", "D_R", "Q_Y", "a_R", "U_Y", "N_R", "h_Y", "A_R", "O_Y", "R_R", "Y_Y", "i_R", "g_Y", "f_R", "V_Y", "L_R", "c_Y", "A_R", "S_Y", "B_R", "I_Y", "Y_R", "V_Y", "O_R", "K_Y", "d_R", "T_Y", "I_R", "c_Y", "R_R", "V_Y", "i_R", "J_Y", "V_R", "d_Y", "Z_R", "E_Y", "h_R", "I_Y", "L_R", "L_Y", "S_R", "W_Y", "B_R", "T_Y", "Q_R", "W_Y", "a_R", "Y_Y", "c_R", "E_Y", "H_R", "B_Y", "h_R", "E_Y", "Q_R", "D_Y", "A_R", "S_Y", "N_R", "U_Y", "O_R", "B_Y", "F_R", "N_Y", "d_R", "L_Y", "B_R", "e_Y", "C_R", "Q_Y", "B_R", "Y_Y", "K_R", "g_Y", "Z_R", "O_Y", "R_R", "C_Y", "a_R", "Z_Y", "b_R", "E_Y", "D_R", "F_Y", "S_R", "D_Y", "O_R", "J_Y", "N_R", "N_Y", "d_R", "M_Y", "Q_R", "J_Y", "a_R", "C_Y", "f_R", "B_Y", "V_R", "Q_Y", "e_R", "J_Y", "G_R", "f_Y", "b_R", "Q_Y", "D_R", "D_Y", "E_R", "O_Y", "X_R", "X_Y", "Z_R", "H_Y", "O_R", "Y_Y", "g_R", "W_Y", "Q_R", "g_Y", "g_R", "B_Y", "A_R", "O_Y", "P_R", "I_Y", "K_R", "Z_Y", "O_R", "S_Y", "B_R", "e_Y", "A_R", "H_Y", "A_R", "Z_Y", "P_R", "h_Y"], 8, 35], [["O_R", "J_Y", "R_R", "c_Y", "F_R", "e_Y", "D_R", "F_Y", "q_R", "a_Y", "q_R", "O_Y", "V_R", "P_Y", "l_R", "j_Y", "g_R", "q_Y", "Q_R", "E_Y", "n_R", "R_Y", "E_R", "I_Y", "p_R", "i_Y", "Z_R", "M_Y", "Q_R", "H_Y", "W_R", "M_Y", "d_R", "p_Y", "c_R", "Z_Y", "F_R", "S_Y", "Q_R", "f_Y", "F_R", "R_Y", "T_R", "I_Y", "S_R", "V_Y", "R_R", "c_Y", "D_R", "p_Y", "R_R", "m_Y", "c_R", "i_Y", "X_R", "S_Y", "a_R", "l_Y", "d_R", "p_Y", "b_R", "Y_Y", "S_R", "T_Y", "e_R", "m_Y", "e_R", "A_Y", "N_R", "A_Y", "I_R", "V_Y", "k_R", "W_Y", "q_R", "Z_Y", "L_R", "c_Y", "U_R", "d_Y", "P_R", "e_Y", "q_R", "Q_Y", "e_R", "m_Y", "G_R", "N_Y", "h_R", "h_Y", "c_R", "A_Y", "b_R", "P_Y", "V_R", "m_Y", "X_R", "k_Y", "P_R", "a_Y", "J_R", "e_Y", "h_R", "Q_Y", "d_R", "d_Y", "X_R", "q_Y", "B_R", "C_Y", "g_R", "o_Y", "L_R", "T_Y", "g_R", "R_Y", "j_R", "Y_Y", "B_R", "V_Y", "F_R", "V_Y", "L_R", "F_Y", "Q_R", "n_Y", "C_R", "i_Y", "p_R", "T_Y", "e_R", "p_Y", "F_R", "L_Y", "n_R", "b_Y", "b_R", "Z_Y", "o_R", "G_Y", "P_R", "B_Y", "b_R", "g_Y", "H_R", "b_Y", "a_R", "d_Y", "e_R", "M_Y", "q_R", "d_Y", "i_R", "L_Y", "C_R", "d_Y", "M_R", "j_Y", "c_R", "A_Y", "a_R", "j_Y", "O_R", "M_Y", "L_R", "i_Y", "L_R", "J_Y", "G_R", "g_Y", "H_R", "J_Y", "R_R", "J_Y", "m_R", "E_Y", "e_R", "I_Y", "K_R", "i_Y", "a_R", "q_Y", "j_R", "M_Y", "a_R", "g_Y", "G_R", "c_Y", "R_R", "Z_Y", "I_R", "Y_Y", "n_R", "c_Y", "e_R", "E_Y", "X_R", "U_Y", "N_R", "Q_Y", "F_R", "U_Y", "S_R", "C_Y", "b_R", "c_Y", "E_R", "N_Y", "g_R", "k_Y", "B_R", "S_Y", "n_R", "M_Y", "V_R", "a_Y", "D_R", "T_Y", "b_R", "i_Y", "p_R", "I_Y", "m_R", "c_Y", "G_R", "q_Y", "V_R", "j_Y", "p_R", "p_Y", "i_R", "N_Y", "G_R", "l_Y", "N_R", "c_Y", "Q_R", "O_Y", "n_R", "K_Y", "K_R", "X_Y", "G_R", "B_Y", "c_R", "M_Y", "B_R", "o_Y", "A_R", "q_Y", "O_R", "F_Y", "C_R", "I_Y", "B_R", "Z_Y", "W_R", "j_Y", "i_R", "l_Y", "n_R", "E_Y", "W_R", "X_Y", "Z_R", "j_Y", "N_R", "f_Y", "m_R", "E_Y", "n_R", "L_Y", "C_R", "M_Y", "q_R", "e_Y", "N_R", "j_Y", "o_R", "o_Y", "D_R", "O_Y", "S_R", "H_Y", "C_R", "R_Y", "Q_R", "J_Y", "X_R", "L_Y", "D_R", "D_Y", "c_R", "I_Y", "O_R", "n_Y", "H_R", "a_Y", "c_R", "g_Y", "H_R", "R_Y", "i_R", "b_Y", "e_R", "m_Y", "g_R", "G_Y", "m_R", "q_Y", "m_R", "p_Y", "W_R", "g_Y", "G_R", "Q_Y", "Y_R", "K_Y", "f_R", "o_Y", "A_R", "a_Y", "p_R", "E_Y", "B_R", "b_Y", "l_R", "c_Y", "T_R", "V_Y", "X_R", "j_Y", "Z_R", "e_Y", "Q_R", "A_Y", "m_R", "P_Y", "l_R", "i_Y", "U_R", "R_Y", "O_R", "R_Y", "R_R", "Z_Y", "A_R", "g_Y", "X_R", "E_Y", "p_R", "l_Y", "D_R", "b_Y", "e_R", "a_Y", "O_R", "Q_Y", "M_R", "L_Y", "D_R", "f_Y", "n_R", "m_Y", "q_R", "U_Y", "g_R", "A_Y", "J_R", "j_Y", "C_R", "b_Y", "o_R", "e_Y", "I_R", "C_Y", "J_R", "d_Y", "V_R", "Z_Y", "G_R", "B_Y", "i_R", "b_Y", "E_R", "E_Y", "c_R", "d_Y", "f_R", "F_Y", "C_R", "Q_Y", "U_R", "X_Y", "l_R", "R_Y", "e_R", "Q_Y", "Q_R", "B_Y", "C_R", "g_Y", "I_R", "K_Y", "h_R", "R_Y", "L_R", "S_Y", "K_R", "Z_Y", "W_R", "S_Y", "L_R", "B_Y", "C_R", "m_Y", "H_R", "S_Y", "V_R", "O_Y", "d_R", "R_Y", "k_R", "K_Y", "L_R", "k_Y", "m_R", "L_Y", "C_R", "J_Y", "c_R", "F_Y", "R_R", "g_Y", "U_R", "n_Y", "X_R", "F_Y", "M_R", "j_Y", "f_R", "F_Y", "b_R", "l_Y", "M_R", "X_Y", "B_R", "j_Y", "j_R", "e_Y", "L_R", "m_Y", "K_R", "V_Y", "n_R", "A_Y", "N_R", "M_Y", "Y_R", "U_Y", "h_R", "T_Y", "X_R", "e_Y", "E_R", "i_Y", "p_R", "C_Y", "A_R", "J_Y", "W_R", "n_Y", "c_R", "U_Y", "a_R", "g_Y", "O_R", "n_Y", "I_R", "l_Y", "L_R", "o_Y", "e_R", "d_Y", "L_R", "p_Y", "A_R", "l_Y", "I_R", "Z_Y", "D_R", "P_Y", "C_R", "M_Y", "Q_R", "j_Y", "D_R", "I_Y", "M_R", "N_Y", "p_R", "c_Y", "E_R", "I_Y", "e_R", "D_Y", "S_R", "i_Y", "b_R", "U_Y", "M_R", "O_Y", "Q_R", "E_Y", "I_R", "O_Y", "H_R", "T_Y", "p_R", "P_Y", "l_R", "n_Y", "I_R", "c_Y", "Y_R", "M_Y", "E_R", "K_Y", "h_R", "n_Y", "l_R", "q_Y", "a_R", "m_Y", "I_R", "A_Y", "U_R", "C_Y", "B_R", "F_Y", "l_R", "D_Y", "n_R", "q_Y", "Z_R", "Y_Y", "h_R", "N_Y", "A_R", "F_Y", "N_R", "q_Y", "R_R", "e_Y", "P_R", "N_Y", "d_R", "j_Y", "C_R", "J_Y", "R_R", "L_Y", "p_R", "J_Y", "B_R", "i_Y", "B_R", "l_Y", "D_R", "V_Y", "f_R", "b_Y", "M_R", "o_Y", "B_R", "U_Y", "g_R", "l_Y", "Y_R", "l_Y", "I_R", "V_Y", "b_R", "o_Y", "Q_R", "G_Y", "b_R", "J_Y", "o_R", "B_Y", "q_R", "R_Y", "B_R", "B_Y", "T_R", "Y_Y", "e_R", "e_Y", "Q_R", "K_Y", "e_R", "N_Y", "R_R", "a_Y", "D_R", "X_Y", "N_R", "n_Y", "Q_R", "U_Y", "d_R", "g_Y", "R_R", "j_Y", "W_R", "G_Y", "h_R", "C_Y", "a_R", "i_Y", "I_R", "G_Y", "P_R", "E_Y", "e_R", "m_Y", "a_R", "p_Y", "l_R", "X_Y", "O_R", "N_Y", "G_R", "M_Y", "E_R", "d_Y", "X_R", "Z_Y", "H_R", "i_Y", "D_R", "C_Y", "S_R", "Q_Y", "Q_R", "H_Y", "o_R", "B_Y", "H_R", "N_Y", "L_R", "A_Y", "d_R", "k_Y", "U_R", "U_Y", "H_R", "B_Y", "Q_R", "j_Y", "S_R", "S_Y", "N_R", "L_Y", "h_R", "K_Y", "o_R", "e_Y", "e_R", "V_Y", "G_R", "i_Y", "n_R", "L_Y", "D_R", "L_Y", "M_R", "E_Y", "d_R", "K_Y", "d_R", "W_Y", "E_R", "p_Y", "q_R", "R_Y", "o_R", "Z_Y", "N_R", "p_Y", "U_R", "h_Y", "g_R", "h_Y", "H_R", "d_Y", "a_R", "n_Y", "M_R", "X_Y", "H_R", "f_Y", "F_R", "H_Y", "Z_R", "Z_Y", "n_R", "R_Y", "F_R", "c_Y", "k_R", "Z_Y", "c_R", "a_Y", "A_R", "a_Y", "T_R", "q_Y", "q_R", "Y_Y", "N_R", "J_Y", "H_R", "d_Y", "B_R", "H_Y", "R_R", "k_Y", "g_R", "h_Y", "a_R", "X_Y", "C_R", "Y_Y", "X_R", "T_Y", "C_R", "i_Y", "Q_R", "h_Y", "j_R", "o_Y", "D_R", "c_Y", "M_R", "U_Y", "o_R", "p_Y", "b_R", "i_Y", "T_R", "F_Y", "c_R", "U_Y", "O_R", "j_Y", "n_R", "N_Y", "V_R", "A_Y", "c_R", "J_Y", "U_R", "L_Y", "S_R", "H_Y", "U_R", "S_Y", "R_R", "K_Y", "T_R", "T_Y", "X_R", "l_Y", "A_R", "P_Y", "D_R", "i_Y", "I_R", "l_Y", "M_R", "i_Y", "P_R", "Y_Y", "T_R", "M_Y", "L_R", "H_Y", "M_R", "B_Y", "V_R", "a_Y", "H_R", "k_Y", "A_R", "i_Y", "W_R", "Q_Y", "m_R", "b_Y", "n_R", "T_Y", "p_R", "H_Y", "V_R", "B_Y", "P_R", "J_Y", "W_R", "p_Y", "K_R", "M_Y", "I_R", "Z_Y", "V_R", "c_Y", "L_R", "m_Y", "i_R", "I_Y", "f_R", "c_Y", "T_R", "F_Y", "K_R", "R_Y", "X_R", "N_Y", "l_R", "I_Y", "M_R", "Y_Y", "G_R", "J_Y", "h_R", "F_Y", "o_R", "R_Y", "B_R", "L_Y", "H_R", "o_Y", "Y_R", "W_Y", "f_R", "T_Y", "H_R", "I_Y", "Q_R", "a_Y", "E_R", "m_Y", "i_R", "N_Y", "k_R", "O_Y", "Y_R", "d_Y", "U_R", "K_Y", "O_R", "h_Y", "m_R", "I_Y", "n_R", "k_Y", "J_R", "K_Y", "p_R", "N_Y", "T_R", "k_Y", "M_R", "F_Y", "e_R", "o_Y", "X_R", "R_Y", "N_R", "W_Y", "f_R", "Q_Y", "g_R", "d_Y", "E_R", "h_Y", "V_R", "k_Y", "i_R", "i_Y", "a_R", "P_Y", "k_R", "f_Y", "Y_R", "C_Y", "e_R", "p_Y", "k_R", "h_Y", "h_R", "A_Y", "O_R", "M_Y", "h_R", "A_Y", "g_R", "X_Y", "C_R", "d_Y", "R_R", "Q_Y", "B_R", "T_Y", "S_R", "M_Y", "a_R", "S_Y", "p_R", "a_Y", "W_R", "d_Y", "j_R", "q_Y", "j_R", "W_Y", "U_R", "D_Y", "g_R", "H_Y", "g_R", "I_Y", "b_R", "a_Y", "p_R", "N_Y", "A_R", "N_Y", "g_R", "I_Y", "q_R", "f_Y", "f_R", "V_Y", "m_R", "O_Y", "a_R", "e_Y", "Q_R", "n_Y", "b_R", "E_Y", "j_R", "n_Y", "a_R", "E_Y", "T_R", "g_Y", "p_R", "Q_Y", "S_R", "C_Y", "g_R", "d_Y", "q_R", "q_Y", "o_R", "q_Y", "q_R", "p_Y", "J_R", "k_Y", "D_R", "Q_Y", "a_R", "L_Y", "b_R", "i_Y", "O_R", "E_Y", "Q_R", "Z_Y", "l_R", "I_Y", "R_R", "U_Y", "h_R", "k_Y", "N_R", "f_Y", "I_R", "M_Y", "R_R", "C_Y", "j_R", "S_Y", "P_R", "I_Y", "P_R", "F_Y", "q_R", "J_Y", "S_R", "W_Y", "l_R", "g_Y", "d_R", "J_Y", "J_R", "T_Y", "U_R", "D_Y", "e_R", "a_Y", "O_R", "A_Y", "i_R", "k_Y", "J_R", "X_Y", "B_R", "H_Y", "g_R", "E_Y", "d_R", "h_Y", "I_R", "e_Y", "k_R", "U_Y", "K_R", "p_Y", "G_R", "k_Y", "H_R", "V_Y", "I_R", "g_Y", "j_R", "S_Y", "H_R", "g_Y", "S_R", "N_Y", "g_R", "p_Y", "K_R", "h_Y", "i_R", "e_Y", "X_R", "F_Y", "b_R", "A_Y", "c_R", "G_Y", "O_R", "B_Y", "C_R", "B_Y", "E_R", "P_Y", "S_R", "E_Y", "E_R", "e_Y", "U_R", "T_Y", "p_R", "T_Y", "d_R", "Y_Y", "E_R", "H_Y", "O_R", "P_Y", "W_R", "k_Y", "W_R", "S_Y", "A_R", "Q_Y", "q_R", "K_Y", "g_R", "F_Y", "h_R", "h_Y", "P_R", "N_Y", "W_R", "S_Y", "Q_R", "o_Y", "O_R", "g_Y", "m_R", "a_Y", "C_R", "H_Y", "P_R", "W_Y", "I_R", "d_Y", "M_R", "K_Y", "F_R", "R_Y", "g_R", "D_Y", "H_R", "l_Y", "h_R", "A_Y", "W_R", "P_Y", "I_R", "C_Y", "o_R", "j_Y", "J_R", "A_Y", "R_R", "Q_Y", "q_R", "a_Y", "o_R", "P_Y", "D_R", "Y_Y", "i_R", "g_Y", "R_R", "q_Y", "Z_R", "K_Y", "Q_R", "B_Y", "Z_R", "E_Y", "I_R", "H_Y", "S_R", "q_Y", "o_R", "P_Y", "a_R", "a_Y", "O_R", "E_Y", "R_R", "L_Y", "Z_R", "K_Y", "J_R", "G_Y", "W_R", "T_Y", "E_R", "B_Y", "N_R", "U_Y", "R_R", "d_Y", "g_R", "A_Y", "P_R", "o_Y", "Q_R", "H_Y", "W_R", "F_Y", "p_R", "R_Y", "I_R", "C_Y", "R_R", "g_Y", "K_R", "E_Y", "R_R", "d_Y", "S_R", "V_Y", "R_R", "Y_Y", "S_R", "f_Y", "L_R", "A_Y", "X_R", "Y_Y", "L_R", "b_Y", "c_R", "Q_Y", "B_R", "I_Y", "b_R", "L_Y", "k_R", "l_Y", "l_R", "g_Y", "N_R", "f_Y", "P_R", "j_Y", "q_R", "B_Y", "a_R", "K_Y", "g_R", "H_Y", "H_R", "B_Y", "A_R", "W_Y", "W_R", "G_Y", "H_R", "X_Y", "H_R", "a_Y", "R_R", "X_Y", "L_R", "e_Y", "V_R", "J_Y", "p_R", "l_Y", "m_R", "E_Y", "N_R", "G_Y", "M_R", "n_Y", "X_R", "A_Y", "O_R", "d_Y", "c_R", "p_Y", "I_R", "G_Y", "S_R", "R_Y"], 3, 43], [["C_R", "p_Y", "a_B", "Q_T", "s_S", "I_R", "Z_Y", "a_B", "c_T", "d_S", "O_R", "C_Y", "M_B", "Q_T", "O_S", "I_R", "U_Y", "J_B", "K_T", "V_S", "i_R", "o_Y", "r_B", "H_T", "I_S", "C_R", "n_Y", "W_B", "G_T", "j_S", "p_R", "m_Y", "Y_B", "b_T", "I_S", "B_R", "O_Y", "n_B", "w_T", "w_S", "c_R", "l_Y", "Z_B", "c_T", "E_S", "k_R", "w_Y", "R_B", "c_T", "p_S", "C_R", "D_Y", "C_B", "c_T", "D_S", "J_R", "H_Y", "P_B", "g_T", "H_S", "F_R", "q_Y", "f_B", "p_T", "n_S", "Q_R", "w_Y", "v_B", "u_T", "H_S", "b_R", "F_Y", "a_B", "Q_T", "N_S", "S_R", "t_Y", "Z_B", "p_T", "b_S", "w_R", "H_Y", "e_B", "S_T", "h_S", "h_R", "Z_Y", "f_B", "k_T", "v_S", "v_R", "a_Y", "m_B", "G_T", "t_S", "t_R", "J_Y", "c_B", "C_T", "N_S", "w_R", "t_Y", "t_B", "g_T", "f_S", "P_R", "J_Y", "s_B", "B_T", "j_S", "t_R", "N_Y", "B_B", "Z_T", "d_S", "l_R", "c_Y", "Z_B", "m_T", "I_S", "j_R", "I_Y", "a_B", "C_T", "J_S", "a_R", "L_Y", "i_B", "s_T", "I_S", "A_R", "Q_Y", "w_B", "k_T", "e_S", "D_R", "s_Y", "t_B", "c_T", "V_S", "K_R", "K_Y", "M_B", "q_T", "G_S", "Z_R", "t_Y", "U_B", "r_T", "u_S", "D_R", "W_Y", "M_B", "N_T", "i_S", "I_R", "s_Y", "a_B", "I_T", "p_S", "A_R", "s_Y", "g_B", "I_T", "Q_S", "q_R", "a_Y", "D_B", "W_T", "a_S", "K_R", "Z_Y", "l_B", "A_T", "t_S", "a_R", "F_Y", "r_B", "w_T", "B_S", "f_R", "p_Y", "T_B", "m_T", "I_S", "L_R", "E_Y", "q_B", "U_T", "G_S", "F_R", "i_Y", "f_B", "U_T", "X_S", "B_R", "N_Y", "D_B", "v_T", "c_S", "H_R", "H_Y", "w_B", "b_T", "E_S", "G_R", "H_Y", "Y_B", "N_T", "S_S", "D_R", "i_Y", "Q_B", "I_T", "o_S", "D_R", "K_Y", "d_B", "o_T", "l_S", "O_R", "R_Y", "n_B", "C_T", "O_S", "E_R", "D_Y", "K_B", "c_T", "C_S", "o_R", "X_Y", "S_B", "M_T", "g_S", "F_R", "T_Y", "p_B", "D_T", "e_S", "Z_R", "Q_Y", "l_B", "j_T", "F_S", "k_R", "h_Y", "S_B", "C_T", "f_S", "d_R", "F_Y", "O_B", "H_T", "e_S", "q_R", "O_Y", "i_B", "G_T", "I_S", "c_R", "M_Y", "A_B", "L_T", "d_S", "b_R", "D_Y", "S_B", "b_T", "Y_S", "s_R", "Y_Y", "r_B", "J_T", "p_S", "q_R", "b_Y", "w_B", "Z_T", "D_S", "F_R", "U_Y", "P_B", "G_T", "P_S", "m_R", "d_Y", "H_B", "O_T", "F_S", "O_R", "e_Y", "b_B", "D_T", "d_S", "U_R", "S_Y", "P_B", "m_T", "M_S", "a_R", "f_Y", "B_B", "d_T", "m_S", "a_R", "a_Y", "V_B", "i_T", "u_S", "H_R", "B_Y", "M_B", "V_T", "d_S", "j_R", "r_Y", "Q_B", "g_T", "V_S", "G_R", "e_Y", "D_B", "Z_T", "e_S", "b_R", "q_Y", "A_B", "S_T", "K_S", "e_R", "h_Y", "P_B", "n_T", "L_S", "N_R", "M_Y", "I_B", "O_T", "N_S", "p_R", "n_Y", "d_B", "f_T", "a_S", "D_R", "N_Y", "J_B", "O_T", "c_S", "d_R", "i_Y", "P_B", "s_T", "b_S", "u_R", "U_Y", "i_B", "V_T", "m_S", "m_R", "b_Y", "N_B", "A_T", "C_S", "Q_R", "q_Y", "n_B", "K_T", "B_S", "c_R", "Q_Y", "n_B", "n_T", "P_S", "v_R", "Y_Y", "W_B", "r_T", "q_S", "O_R", "C_Y", "F_B", "w_T", "d_S", "B_R", "I_Y", "u_B", "U_T", "P_S", "A_R", "d_Y", "j_B", "d_T", "L_S", "Z_R", "j_Y", "l_B", "a_T", "Q_S", "w_R", "R_Y", "U_B", "P_T", "e_S", "J_R", "R_Y", "n_B", "w_T", "a_S", "v_R", "U_Y", "J_B", "Q_T", "I_S", "q_R", "f_Y", "u_B", "U_T", "o_S", "n_R", "J_Y", "f_B", "Q_T", "S_S", "a_R", "p_Y", "h_B", "R_T", "F_S", "N_R", "j_Y", "o_B", "g_T", "J_S", "Q_R", "G_Y", "k_B", "n_T", "F_S", "r_R", "u_Y", "f_B", "O_T", "w_S", "J_R", "Z_Y", "T_B", "Y_T", "v_S", "p_R", "N_Y", "D_B", "h_T", "e_S", "g_R", "A_Y", "o_B", "K_T", "n_S", "F_R", "W_Y", "o_B", "o_T", "r_S", "d_R", "G_Y", "A_B", "B_T", "i_S", "I_R", "u_Y", "S_B", "l_T", "V_S", "b_R", "L_Y", "F_B", "S_T", "h_S", "J_R", "Z_Y", "s_B", "d_T", "a_S", "Y_R", "L_Y", "E_B", "v_T", "N_S", "Y_R", "m_Y", "d_B", "j_T", "C_S", "O_R", "b_Y", "G_B", "G_T", "a_S", "Y_R", "N_Y", "V_B", "r_T", "e_S", "W_R", "r_Y", "O_B", "G_T", "p_S", "t_R", "m_Y", "N_B", "j_T", "o_S", "N_R", "a_Y", "g_B", "d_T", "M_S", "T_R", "T_Y", "B_B", "T_T", "J_S", "m_R", "Y_Y", "N_B", "A_T", "f_S", "u_R", "a_Y", "c_B", "G_T", "G_S", "a_R", "I_Y", "b_B", "W_T", "T_S", "w_R", "n_Y", "M_B", "A_T", "u_S", "T_R", "j_Y", "W_B", "d_T", "I_S", "u_R", "Q_Y", "A_B", "C_T", "v_S", "e_R", "i_Y", "V_B", "A_T", "s_S", "n_R", "f_Y", "G_B", "n_T", "M_S", "m_R", "r_Y", "W_B", "K_T", "E_S", "d_R", "N_Y", "v_B", "k_T", "M_S", "n_R", "v_Y", "b_B", "L_T", "k_S", "l_R", "G_Y", "F_B", "Y_T", "k_S", "e_R", "A_Y", "g_B", "u_T", "Q_S", "F_R", "W_Y", "P_B", "O_T", "X_S", "P_R", "X_Y", "n_B", "b_T", "O_S", "G_R", "D_Y", "q_B", "X_T", "w_S", "b_R", "N_Y", "u_B", "U_T", "q_S", "l_R", "U_Y", "V_B", "R_T", "m_S", "T_R", "I_Y", "C_B", "q_T", "E_S", "f_R", "l_Y", "Z_B", "l_T", "h_S", "h_R", "V_Y", "P_B", "U_T", "G_S", "w_R", "Q_Y", "n_B", "Z_T", "A_S", "E_R", "A_Y", "w_B", "u_T", "b_S", "l_R", "V_Y", "I_B", "j_T", "Z_S", "l_R", "e_Y", "L_B", "k_T", "q_S", "l_R", "A_Y", "X_B", "n_T", "w_S", "Q_R", "I_Y", "Q_B", "S_T", "L_S", "m_R", "H_Y", "r_B", "E_T", "U_S", "g_R", "W_Y", "D_B", "V_T", "l_S", "e_R", "M_Y", "n_B", "F_T", "A_S", "X_R", "s_Y", "L_B", "P_T", "c_S", "e_R", "W_Y", "e_B", "l_T", "Q_S", "H_R", "Q_Y", "d_B", "D_T", "N_S", "A_R", "N_Y", "b_B", "s_T", "N_S", "G_R", "j_Y", "O_B", "q_T", "d_S", "p_R", "m_Y", "f_B", "p_T", "F_S", "U_R", "L_Y", "F_B", "j_T", "K_S", "J_R", "I_Y", "X_B", "b_T", "r_S", "u_R", "E_Y", "l_B", "E_T", "U_S", "a_R", "S_Y", "w_B", "Z_T", "Y_S", "j_R", "w_Y", "M_B", "k_T", "F_S", "W_R", "Q_Y", "b_B", "g_T", "g_S", "T_R", "i_Y", "H_B", "J_T", "K_S", "n_R", "H_Y", "T_B", "E_T", "U_S", "N_R", "h_Y", "o_B", "l_T", "K_S", "o_R", "u_Y", "m_B", "V_T", "n_S", "u_R", "j_Y", "W_B", "D_T", "P_S", "X_R", "f_Y", "Q_B", "t_T", "q_S", "V_R", "o_Y", "O_B", "Y_T", "A_S", "c_R", "W_Y", "D_B", "l_T", "G_S", "w_R", "V_Y", "G_B", "D_T", "p_S", "R_R", "J_Y", "e_B", "D_T", "h_S", "I_R", "q_Y", "K_B", "Y_T", "l_S", "X_R", "I_Y", "I_B", "R_T", "F_S", "U_R", "L_Y", "X_B", "d_T", "I_S", "X_R", "V_Y", "Z_B", "h_T", "J_S", "Z_R", "t_Y", "K_B", "c_T", "d_S", "Q_R", "o_Y", "g_B", "i_T", "m_S", "d_R", "T_Y", "u_B", "Y_T", "H_S", "p_R", "c_Y", "A_B", "W_T", "M_S", "r_R", "w_Y", "D_B", "j_T", "w_S", "b_R", "s_Y", "K_B", "c_T", "T_S", "Z_R", "m_Y", "U_B", "O_T", "F_S", "u_R", "J_Y", "j_B", "o_T", "D_S", "F_R", "w_Y", "s_B", "B_T", "X_S", "A_R", "j_Y", "j_B", "q_T", "B_S", "A_R", "T_Y", "T_B", "P_T", "M_S", "H_R", "I_Y", "d_B", "a_T", "U_S", "H_R", "N_Y", "d_B", "X_T", "l_S", "c_R", "Y_Y", "X_B", "r_T", "D_S", "L_R", "N_Y", "B_B", "f_T", "W_S", "a_R", "t_Y", "Q_B", "b_T", "q_S", "L_R", "M_Y", "d_B", "a_T", "L_S", "e_R", "N_Y", "X_B", "o_T", "f_S", "Y_R", "s_Y", "j_B", "Q_T", "K_S", "A_R", "B_Y", "J_B", "X_T", "k_S", "Y_R", "L_Y", "O_B", "o_T", "m_S", "f_R", "N_Y", "C_B", "r_T", "M_S", "I_R", "c_Y", "A_B", "H_T", "P_S", "E_R", "L_Y", "i_B", "E_T", "Y_S", "s_R", "j_Y", "e_B", "D_T", "F_S", "M_R", "A_Y", "R_B", "e_T", "K_S", "Q_R", "V_Y", "v_B", "R_T", "S_S", "b_R", "S_Y", "C_B", "o_T", "a_S", "a_R", "s_Y", "l_B", "O_T", "g_S", "O_R", "G_Y", "h_B", "Q_T", "W_S", "f_R", "I_Y", "w_B", "c_T", "u_S", "r_R", "Q_Y", "E_B", "F_T", "A_S", "M_R", "T_Y", "Q_B", "i_T", "X_S", "C_R", "Q_Y", "J_B", "r_T", "T_S", "t_R", "G_Y", "t_B", "p_T", "h_S", "f_R", "i_Y", "T_B", "e_T", "J_S", "J_R", "T_Y", "d_B", "h_T", "Q_S", "D_R", "W_Y", "r_B", "Y_T", "F_S", "t_R", "W_Y", "J_B", "O_T", "l_S", "m_R", "W_Y", "b_B", "T_T", "b_S", "L_R", "a_Y", "L_B", "u_T", "s_S", "B_R", "v_Y", "M_B", "I_T", "u_S", "S_R", "U_Y", "l_B", "P_T", "d_S", "g_R", "M_Y", "F_B", "a_T", "U_S", "n_R", "d_Y", "w_B", "B_T", "j_S", "w_R", "D_Y", "f_B", "m_T", "Z_S", "J_R", "H_Y", "i_B", "g_T", "v_S", "W_R", "h_Y", "P_B", "u_T", "q_S", "N_R", "t_Y", "V_B", "Q_T", "K_S", "F_R", "e_Y", "I_B", "v_T", "r_S", "I_R", "K_Y", "p_B", "V_T", "d_S", "A_R", "c_Y", "c_B", "B_T", "E_S", "j_R", "E_Y", "D_B", "k_T", "c_S", "I_R", "b_Y", "v_B", "u_T", "j_S", "a_R", "V_Y", "p_B", "W_T", "r_S", "p_R", "u_Y", "R_B", "n_T", "P_S", "q_R", "b_Y", "E_B", "c_T", "H_S", "Y_R", "w_Y", "h_B", "v_T", "P_S", "e_R", "q_Y", "i_B", "K_T", "F_S", "Q_R", "a_Y", "O_B", "t_T", "W_S", "H_R", "N_Y", "c_B", "u_T", "O_S", "t_R", "P_Y", "r_B", "k_T", "g_S", "w_R", "g_Y", "i_B", "B_T", "w_S", "F_R", "l_Y", "u_B", "i_T", "G_S", "g_R", "b_Y", "N_B", "S_T", "M_S", "n_R", "H_Y", "w_B", "E_T", "G_S", "k_R", "m_Y", "h_B", "Y_T", "v_S", "b_R", "g_Y", "P_B", "p_T", "Y_S", "Y_R", "c_Y", "Q_B", "m_T", "u_S", "I_R", "s_Y", "D_B", "a_T", "i_S", "E_R", "S_Y", "P_B", "l_T", "Q_S", "U_R", "r_Y", "A_B", "F_T", "E_S", "r_R", "h_Y", "m_B", "O_T", "w_S", "W_R", "R_Y", "R_B", "O_T", "C_S", "v_R", "s_Y", "K_B", "P_T", "I_S", "b_R", "L_Y", "U_B", "e_T", "Q_S", "E_R", "d_Y", "a_B", "e_T", "m_S", "w_R", "b_Y", "j_B", "d_T", "O_S", "G_R", "Q_Y", "j_B", "a_T", "c_S", "S_R", "D_Y", "k_B", "t_T", "t_S", "j_R", "T_Y", "O_B", "v_T", "N_S", "w_R", "v_Y", "D_B", "i_T", "q_S", "O_R", "B_Y", "N_B", "T_T", "l_S", "K_R", "o_Y", "n_B", "C_T", "R_S", "n_R", "K_Y", "C_B", "h_T", "p_S", "f_R", "W_Y", "E_B", "D_T", "H_S", "p_R", "R_Y", "Z_B", "B_T", "S_S", "d_R", "q_Y", "r_B", "k_T", "F_S", "p_R", "S_Y", "W_B", "C_T", "K_S", "h_R", "L_Y", "D_B", "s_T", "N_S", "W_R", "o_Y", "D_B", "D_T", "v_S", "Z_R", "Z_Y", "j_B", "r_T", "W_S", "Q_R", "N_Y", "O_B", "d_T", "m_S", "X_R", "Z_Y", "Y_B", "v_T", "B_S", "Z_R", "d_Y", "V_B", "A_T", "m_S", "E_R", "P_Y", "s_B", "S_T", "K_S", "u_R", "q_Y", "Q_B", "U_T", "T_S", "e_R", "I_Y", "p_B", "I_T", "d_S", "H_R", "a_Y", "a_B", "H_T", "V_S", "b_R", "l_Y", "K_B", "V_T", "Q_S", "q_R", "e_Y", "v_B", "r_T", "Q_S", "P_R", "F_Y", "A_B", "k_T", "R_S", "l_R", "g_Y", "X_B", "t_T", "B_S", "i_R", "R_Y", "p_B", "r_T", "e_S", "q_R", "W_Y", "O_B", "A_T", "U_S", "c_R", "D_Y", "e_B", "S_T", "i_S", "g_R", "g_Y", "n_B", "k_T", "h_S", "U_R", "U_Y", "g_B", "r_T", "K_S", "k_R", "o_Y", "M_B", "Q_T", "a_S", "P_R", "s_Y", "i_B", "U_T", "R_S", "r_R", "F_Y", "V_B", "D_T", "g_S", "N_R", "e_Y", "E_B", "S_T", "h_S", "m_R", "q_Y", "R_B", "V_T", "Q_S", "l_R", "Q_Y", "w_B", "U_T", "v_S", "h_R", "Z_Y", "f_B", "c_T", "Y_S", "p_R", "E_Y", "U_B", "b_T", "A_S", "o_R", "D_Y", "i_B", "V_T", "Q_S", "b_R", "t_Y", "d_B", "Q_T", "f_S", "o_R", "W_Y", "i_B", "C_T", "u_S", "E_R", "X_Y", "s_B", "J_T", "L_S", "C_R", "m_Y", "B_B", "p_T", "m_S", "k_R", "h_Y", "D_B", "a_T", "Y_S", "F_R", "I_Y", "q_B", "q_T", "M_S", "b_R", "S_Y", "h_B", "h_T", "a_S", "D_R", "j_Y", "F_B", "Y_T", "W_S", "J_R", "U_Y", "X_B", "a_T", "l_S", "a_R", "s_Y", "I_B", "M_T", "h_S", "v_R", "v_Y", "R_B", "M_T", "i_S", "Y_R", "w_Y", "l_B", "J_T", "g_S", "f_R", "b_Y", "B_B", "Z_T", "n_S", "q_R", "K_Y", "c_B", "t_T", "R_S", "C_R", "k_Y", "Q_B"], 5, 49]], "outputs": [["R"], ["Y"], ["Y"], ["R"], ["R"], ["Draw"], ["R"], ["Y"], ["Draw"], ["R"], ["Y"], ["Y"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 10,078 |
def whoIsWinner(moves, con, sz):
|
82d7f2d43285a28dd1e26f1860d5175d | UNKNOWN | >When no more interesting kata can be resolved, I just choose to create the new kata, to solve their own, to enjoy the process --myjinxin2015 said
# Description:
Given two array of integers(`arr1`,`arr2`). Your task is going to find a pair of numbers(an element in arr1, and another element in arr2), their difference is as big as possible(absolute value); Again, you should to find a pair of numbers, their difference is as small as possible. Return the maximum and minimum difference values by an array: `[ max difference, min difference ]`
For example:
```
Given arr1 = [3,10,5], arr2 = [20,7,15,8]
should return [17,2] because 20 - 3 = 17, 10 - 8 = 2
```
# Note:
- arr1 and arr2 contains only integers(positive, negative or 0);
- arr1 and arr2 may have different lengths, they always has at least one element;
- All inputs are valid.
- This is a simple version, if you want some challenges, [try the challenge version](https://www.codewars.com/kata/583c592928a0c0449d000099).
# Some Examples
```
maxAndMin([3,10,5],[20,7,15,8]) === [17,2]
maxAndMin([3],[20]) === [17,17]
maxAndMin([3,10,5],[3,10,5]) === [7,0]
maxAndMin([1,2,3,4,5],[6,7,8,9,10]) === [9,1]
``` | ["def max_and_min(arr1,arr2):\n diffs = [abs(x-y) for x in arr1 for y in arr2]\n return [max(diffs), min(diffs)]\n", "def max_and_min(arr1,arr2):\n max_min = [abs(y-x) for x in arr1 for y in arr2]\n \n return [max(max_min), min(max_min)]", "def max_and_min(arr1, arr2):\n return [op(abs(x-y) for x in arr1 for y in arr2) for op in [max, min]]", "def max_and_min(arr1,arr2):\n arr1.sort()\n arr2.sort()\n i = 0\n difference = []\n\n while i < len(arr1):\n local = []\n for element in arr2 :\n local_dif = abs(arr1[i] - element)\n local.append(local_dif)\n local.sort() \n difference.append(min(local))\n difference.append(max(local))\n\n i += 1\n difference.sort()\n maximum = max(difference)\n minimum = min(difference)\n return [maximum,minimum]", "def max_and_min(lst1, lst2):\n diff = sorted(abs(b - a) for a in lst1 for b in lst2)\n return [diff[-1], diff[0]]", "def max_and_min(arr1,arr2):\n def mini(i, j):\n if i == len(a1) or j == len(a2):\n return float('inf')\n val = a1[i] - a2[j]\n if val < 0:\n return min(-val, mini(i+1, j))\n return min(val, mini(i, j+1)) \n \n a1, a2 = sorted(arr1), sorted(arr2)\n return [max(a2[-1]-a1[0], a1[-1]-a2[0]), bool(set(a1)^set(a2)) and mini(0, 0)]", "def max_and_min(arr1,arr2):\n return [max(abs(a-b) for a in arr1 for b in arr2), min(abs(a-b) for a in arr1 for b in arr2)]", "def max_and_min(arr1, arr2):\n max_diff, min_diff = float('-inf'), float('inf')\n for a in arr1:\n for b in arr2:\n current_sum = abs(b - a)\n max_diff = max(max_diff, current_sum)\n min_diff = min(min_diff, current_sum)\n return [max_diff, min_diff]\n", "def max_and_min(arr1,arr2):\n mi,ma=float(\"inf\"), -float(\"inf\")\n for x in arr1:\n for y in arr2:\n d = abs(x-y)\n if d < mi: mi = d\n if d > ma: ma = d\n return [ma,mi]", "def max_and_min(arr1,arr2):\n l=[]\n for temp1 in arr1:\n for temp2 in arr2:\n l.append(abs(temp1-temp2))\n l.sort()\n return [l[-1],l[0]]"] | {"fn_name": "max_and_min", "inputs": [[[3, 10, 5], [20, 7, 15, 8]], [[3], [20]], [[3, 10, 5], [3, 10, 5]], [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]], "outputs": [[[17, 2]], [[17, 17]], [[7, 0]], [[9, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,181 |
def max_and_min(arr1,arr2):
|
5fd06898e67225d4940a37cc165cfde4 | UNKNOWN | Given a list of unique words. Find all pairs of distinct indices (i, j) in the given list so that the concatenation of the two words, i.e. words[i] + words[j] is a palindrome.
Examples:
Non-string inputs should be converted to strings.
Return an array of arrays containing pairs of distinct indices that form palindromes. Pairs should be returned in the order they appear in the original list. | ["def palindrome_pairs(w):\n return [[i, j] for i in range(len(w)) for j in range(len(w)) if str(w[i])+str(w[j])==(str(w[i])+str(w[j]))[::-1] and i!=j]", "def is_palindrome(word):\n return word == word[::-1]\n\n\ndef palindrome_pairs(words):\n words = [str(word) for word in words]\n return [\n [i, j]\n for i, word_i in enumerate(words)\n for j, word_j in enumerate(words)\n if i != j and is_palindrome(word_i + word_j)\n ]\n", "def palindrome_pairs(words):\n indices = []\n \n for i in range(len(words)):\n for j in range(len(words)):\n if i != j:\n concatenation = str(words[i]) + str(words[j])\n if concatenation == concatenation[::-1]:\n indices.append([i, j])\n \n return indices", "from itertools import chain, permutations\n\ndef is_palindrome(w1, w2):\n return all(c1 == c2 for c1, c2 in zip(chain(w1, w2), chain(reversed(w2), reversed(w1))))\n\ndef palindrome_pairs(words):\n words = [str(word) for word in words]\n return [[i, j] for i, j in permutations(range(len(words)), 2) if is_palindrome(words[i], words[j])]", "from itertools import permutations\n\ndef palindrome_pairs(words):\n result = []\n for i, j in permutations(list(range(len(words))), 2):\n concat = f\"{words[i]}{words[j]}\"\n if concat == concat[::-1]:\n result.append([i, j])\n return result\n \n\n# one-liner\n#def palindrome_pairs(w):\n# return [[i, j] for i, j in permutations(range(len(w)), 2) if f\"{w[i]}{w[j]}\" == f\"{w[i]}{w[j]}\"[::-1]]\n", "def palindrome_pairs(words):\n w=list(map(str,words))\n return [[i,j] for i,a in enumerate(w) for j,b in enumerate(w) if i!=j and a+b == (a+b)[::-1]]", "import itertools\n\n\ndef is_palindrome(word):\n return word == word[::-1]\n\n\ndef palindrome_pairs(words):\n words = list(map(str, words))\n return [\n [i, j]\n for i, j in itertools.permutations(range(len(words)), 2)\n if is_palindrome(words[i] + words[j])\n ]", "def palindrome_pairs(words):\n return [[words.index(i),words.index(j)] for i in words for j in words if str(i)+str(j)==(str(i)+str(j))[::-1] and i!=j]", "palindrome_pairs=lambda a:[[i,j]for i,s in enumerate(a)for j,t in enumerate(a)if i!=j and str(s)+str(t)==(str(s)+str(t))[::-1]]", "def palindrome_pairs(a):\n a = [str(x) for x in a];r = [];\n for k1,i in enumerate(a):\n for k2,j in enumerate(a):\n if i!=j and (i+j)==(i+j)[::-1]:r.append([k1,k2])\n return r"] | {"fn_name": "palindrome_pairs", "inputs": [[["bat", "tab", "cat"]], [["dog", "cow", "tap", "god", "pat"]], [["abcd", "dcba", "lls", "s", "sssll"]], [[]], [["adgdfsh", "wertewry", "zxcbxcb", "efveyn"]], [[5, 2, "abc", true, [false]]], [[5777, "dog", "god", true, 75]]], "outputs": [[[[0, 1], [1, 0]]], [[[0, 3], [2, 4], [3, 0], [4, 2]]], [[[0, 1], [1, 0], [2, 4], [3, 2]]], [[]], [[]], [[]], [[[0, 4], [1, 2], [2, 1]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,568 |
def palindrome_pairs(words):
|
feb4731b8d2d3de2b9d2cfc59e0b63df | UNKNOWN | Johnny is a boy who likes to open and close lockers. He loves it so much that one day, when school was out, he snuck in just to play with the lockers.
Each locker can either be open or closed. If a locker is closed when Johnny gets to it, he opens it, and vice versa.
The lockers are numbered sequentially, starting at 1.
Starting at the first locker, Johnny runs down the row, opening each locker.
Then he runs all the way back to the beginning and runs down the row again, this time skipping to every other locker. (2,4,6, etc)
Then he runs all the way back and runs through again, this time skipping two lockers for every locker he opens or closes. (3,6,9, etc)
He continues this until he has finished running past the last locker (i.e. when the number of lockers he skips is greater than the number of lockers he has).
------
The equation could be stated as follows:
> Johnny runs down the row of lockers `n` times, starting at the first locker each run and skipping `i` lockers as he runs, where `n` is the number of lockers there are in total and `i` is the current run.
The goal of this kata is to determine which lockers are open at the end of Johnny's running.
The program accepts an integer giving the total number of lockers, and should output an array filled with the locker numbers of those which are open at the end of his run. | ["from math import floor\n#Pretty sure this is the fastest implementation; only one square root, and sqrt(n) multiplications.\n#Plus, no booleans, because they're super slow.\nlocker_run = lambda l: [i * i for i in range(1, int(floor(l ** .5)) + 1)]", "def locker_run(lockers):\n return [n*n for n in range(1,int(lockers**.5+1))]", "def locker_run(lockers):\n a=[1]\n while len(a)<int(lockers**.5):\n if 1<lockers<9 or (len(a)<2 and lockers>=9):\n a.append(4)\n else:\n a.append(a[-1]*2-a[-2]+2)\n return a", "def locker_run(lockers):\n return [n * n for n in range(1, int(1 + lockers**0.5))]", "def locker_run(lockers):\n return [i for i in range(1, lockers + 1) if i ** 0.5 % 1 == 0]", "def locker_run(lockers):\n i = 0\n box = [0] * lockers\n while i < lockers:\n for x in range(i, lockers, i + 1):\n box[x] += 1\n i += 1\n return [c + 1 for c in range(len(box)) if box[c] % 2 != 0]", "def locker_run(lockers):\n #..\n return [x*x for x in range(1,lockers+1) if x*x <= lockers]"] | {"fn_name": "locker_run", "inputs": [[1], [5], [10], [20]], "outputs": [[[1]], [[1, 4]], [[1, 4, 9]], [[1, 4, 9, 16]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,079 |
def locker_run(lockers):
|
818b04554de78da210463c46180ac739 | UNKNOWN | # Remove Duplicates
You are to write a function called `unique` that takes an array of integers and returns the array with duplicates removed. It must return the values in the same order as first seen in the given array. Thus no sorting should be done, if 52 appears before 10 in the given array then it should also be that 52 appears before 10 in the returned array.
## Assumptions
* All values given are integers (they can be positive or negative).
* You are given an array but it may be empty.
* They array may have duplicates or it may not.
## Example
```python
print unique([1, 5, 2, 0, 2, -3, 1, 10])
[1, 5, 2, 0, -3, 10]
print unique([])
[]
print unique([5, 2, 1, 3])
[5, 2, 1, 3]
``` | ["from collections import OrderedDict\ndef unique(integers):\n return list(OrderedDict.fromkeys(integers))", "def unique(integers):\n l = []\n for i in integers:\n if not i in l: l.append(i)\n return l", "def unique(integers):\n ans = []\n for x in integers:\n if x not in ans:\n ans.append(x)\n return ans", "def unique(integers):\n result = []\n for number in integers:\n if number not in result:\n result.append(number)\n return result", "def unique(integers):\n return list(dict.fromkeys(integers))", "def unique(integers):\n return sorted(set(integers), key=integers.index)\n", "def unique(integers):\n seen = set()\n return [x\n for x in integers if not\n (x in seen or seen.add(x))]", "def unique(integers):\n return [value for i, value in enumerate(integers) if value not in integers[:i]]", "def unique(integers):\n # The return value of set.add() is always None\n dedupe = set()\n return [i for i in integers if i not in dedupe and not dedupe.add(i)]"] | {"fn_name": "unique", "inputs": [[[]], [[-1]], [[-1, 5, 10, -100, 3, 2]], [[1, 2, 3, 3, 2, 1, 2, 3, 1, 1, 3, 2]], [[1, 3, 2, 3, 2, 1, 2, 3, 1, 1, 3, 2]], [[3, 2, 3, 3, 2, 1, 2, 3, 1, 1, 3, 2]]], "outputs": [[[]], [[-1]], [[-1, 5, 10, -100, 3, 2]], [[1, 2, 3]], [[1, 3, 2]], [[3, 2, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,080 |
def unique(integers):
|
b063fd5b57170cf2d143d0d897a26f0f | UNKNOWN | Santa puts all the presents into the huge sack. In order to let his reindeers rest a bit, he only takes as many reindeers with him as he is required to do. The others may take a nap.
Two reindeers are always required for the sleigh and Santa himself. Additionally he needs 1 reindeer per 30 presents. As you know, Santa has 8 reindeers in total, so he can deliver up to 180 presents at once (2 reindeers for Santa and the sleigh + 6 reindeers with 30 presents each).
Complete the function `reindeers()`, which takes a number of presents and returns the minimum numbers of required reindeers. If the number of presents is too high, throw an error.
Examles:
```python
reindeer(0) # must return 2
reindeer(1) # must return 3
reindeer(30) # must return 3
reindeer(200) # must throw an error
``` | ["from math import ceil\ndef reindeer(presents):\n if presents > 180: raise ValueError(\"Too many presents\")\n return ceil(presents / 30.0) + 2", "def reindeer(presents):\n assert presents <= 180\n return 2+presents//30+(1 if presents % 30 else 0)", "from math import ceil\ndef reindeer(presents):\n assert presents < 181\n return ceil(presents/30.0) + 2", "def reindeer(presents):\n assert presents <= 180\n return 2 + (presents + 29) // 30", "from math import ceil\n\ndef reindeer(presents):\n if presents > 180:\n raise ValueError\n else:\n return 2 + ceil(presents/30.0)", "from math import ceil\n\n# Why is this 6 kyu?\ndef reindeer(presents):\n if presents > 180: raise Exception(\"Too many presents\")\n return 2 + ceil(presents/30)", "from math import ceil\n\n\ndef reindeer(presents):\n assert 0 <= presents <= 180\n return int(2 + (ceil(presents / 30.0)))\n", "def reindeer(presents):\n if presents > 180: raise Exception(\"Error\")\n return 2 + int((presents+29)/30)", "import math\n\ndef reindeer(presents):\n \n p = math.ceil(presents/30)\n \n if p>6:\n \n raise ValueError(\"Error\")\n \n else:\n \n return p+2\n"] | {"fn_name": "reindeer", "inputs": [[0], [1], [5], [30], [31], [60], [61], [90], [91], [120], [121], [150], [151], [180]], "outputs": [[2], [3], [3], [3], [4], [4], [5], [5], [6], [6], [7], [7], [8], [8]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,213 |
def reindeer(presents):
|
9037691871bf14726886d473cd730ab7 | UNKNOWN | If you can't sleep, just count sheep!!
## Task:
Given a non-negative integer, `3` for example, return a string with a murmur: `"1 sheep...2 sheep...3 sheep..."`. Input will always be valid, i.e. no negative integers. | ["def count_sheep(n):\n return ''.join(f\"{i} sheep...\" for i in range(1,n+1))", "def count_sheep(n):\n sheep=\"\"\n for i in range(1, n+1):\n sheep+=str(i) + \" sheep...\"\n return sheep", "def count_sheep(n):\n return \"\".join(\"{} sheep...\".format(i) for i in range(1, n+1))", "def count_sheep(n):\n return \"\".join(\"%d sheep...\" % (i + 1) for i in range(n))", "def count_sheep(n):\n return ''.join(f'{x+1} sheep...' for x in range(n))", "def count_sheep(n):\n return \" sheep...\".join(str(num) for num in range(1, n+1)) + \" sheep...\"", "def count_sheep(n):\n if n == 1:\n return \"1 sheep...\"\n else:\n return count_sheep(n - 1) + \"{} sheep...\".format(n)", "count_sheep = lambda n: ''.join('{} sheep...'.format(i) for i in range(1,n+1))", "def count_sheep(n):\n # your code\n result = \"\"\n for i in range(0,n):\n result+=( str(i+1) + \" sheep...\")\n return result", "def count_sheep(n):\n return \"\".join(\"%i sheep...\" %n for n in range(1, n + 1))", "def count_sheep(n):\n store_1 = []\n for x in range(1, n+1):\n store_1.append(f\"{x} sheep...\")\n my_lst_str = ''.join(map(str, store_1))\n return my_lst_str\n", "def count_sheep(n):\n output = \"\"\n for i in range(1, n+1):\n output += f\"{i} sheep...\"\n \n return output", "def count_sheep(n):\n # your code\n return \" sheep...\".join([str(i) \\\n for i in range(1, n + 1)]) + \" sheep...\"\n", "def count_sheep(n):\n\n return ''.join([(str(i+1) + ' ' + 'sheep...') for i in range(n)])", "def count_sheep(n):\n result = ''\n for i in range(1, n + 1):\n result += str(i) + ' sheep...'\n return result\n", "def count_sheep(n):\n return \"\".join(str(x) + \" sheep...\" for x in range(1, n + 1))", "def count_sheep(n):\n return \"\".join(f\"{i+1} sheep...\" for i in range(n))", "def count_sheep(n):\n string = \"\"\n for i in range(1, n + 1):\n string += f\"{i} sheep...\"\n return string", "def count_sheep(n):\n answer = []\n for x in range(1,n+1):\n answer.append(str(x))\n answer.append(\" sheep...\")\n return ''.join(answer)", "def count_sheep(n):\n # your code\n \n x = 1\n list = []\n \n \n while x <= n:\n sheep = \"{} sheep...\".format(x)\n list.append(sheep)\n x += 1 \n \n return \"\".join(list)", "def count_sheep(n):\n sheep = \"\"\n x = 1\n while x <= n :\n sheep = sheep + str(x) + \" sheep...\"\n x += 1\n return sheep", "def count_sheep(x):\n sh=''\n for i in range(1,x+1):sh+=f'{i} sheep...'\n return sh", "def count_sheep(n):\n return ''.join([(str(num+1)+' sheep...') for num in range(n) ])", "def count_sheep(n):\n return ''.join(['{} sheep...'.format(x) for x in list(range(n+1))[1::]])", "def count_sheep(n):\n sheep = ''\n for m in range(1,n + 1):\n sheep = sheep + str(m) + ' sheep...'\n return sheep", "def count_sheep(n):\n sheep = \"\"\n for x in range(1, n+1):\n sheep += f\"{x} sheep...\"\n return sheep", "def count_sheep(n):\n return \"\".join(str(item)+\" sheep...\" for item in range(1,n+1))", "def count_sheep(n):\n count = 0\n details = []\n for i in range(1, n+1):\n count += 1\n details.append(str(count)+\" sheep...\")\n \n return \"\".join(details)", "def count_sheep(n):\n if n == 0:\n return \"\"\n else:\n count = \"1 sheep...\"\n for i in range(2,n+1):\n count += f\"{i} sheep...\"\n \n return count", "def count_sheep(n):\n empty = []\n for num in range(1,n+1):\n empty.append(f'{num} sheep...')\n return ''.join(empty)", "def count_sheep(n):\n list_sheep = []\n for i in range(1, n + 1):\n list_sheep.append(f'{i} sheep...')\n return ''.join(list_sheep)\n", "def count_sheep(n):\n # your code\n c = \"\"\n for i in range(1, n + 1):\n c += f\"{i} sheep...\"\n\n return c", "def count_sheep(n):\n sheep_list = []\n sheep = '{} sheep...'\n while n >= 1:\n iteration = sheep.format((n+1)-1)\n sheep_list.append(iteration)\n n -= 1\n sheep_list.reverse()\n return ''.join(sheep_list)", "def count_sheep(n):\n s=\"\"\n i=1\n for i in range (1,n+1):\n s+=(str(i)+\" sheep...\")\n \n return s\n", "def count_sheep(n):\n opstr = 'sheep...'\n \n oplst = []\n \n count = 1\n while count <= n:\n oplst.append(str(count) + ' ' + opstr)\n count += 1\n \n return ''.join(oplst)", "def count_sheep(n):\n m = ''\n for i in range(0, n):\n m += f'{i + 1} sheep...'\n return m", "def count_sheep(n):\n # your code\n result = []\n for i in range(n):\n result.append(f\"{i+1} sheep...\")\n return \"\".join(result)\n", "def count_sheep(n):\n arr = [f\"{i} sheep...\" for i in range(1, n+1)]\n return \"\".join(arr)", "def count_sheep(n):\n count = 1\n message = ''\n while count <= n:\n message = message + str(count) + \" sheep...\"\n count += 1\n return message", "def count_sheep(n):\n return ''.join(f'{el} sheep...' for el in range(1, n + 1))", "def count_sheep(n):\n a=\"\"\n return ''.join((a+str(i+1)+\" sheep...\") for i in range(n))\n", "def count_sheep(n):\n i = 1\n ans = \"\"\n while i <=n:\n ans = ans + str(i)+ \" \" + \"sheep...\"\n i = i+1\n return ans\n", "def count_sheep(n):\n frase = \" sheep...\"\n pecore = \"\"\n for i in range(n):\n pecore += str(i+1) + frase\n return pecore", "def count_sheep(n):\n return \"\".join([ \"{0} sheep...\".format(i+1) for i in range (0, n)])", "def count_sheep(n):\n hold=\"\"\n for i in range(n):\n hold+=\"{} sheep...\".format(i+1)\n return hold\n \n", "def count_sheep(n):\n x = 0\n s=[]\n while x < n: \n for _ in range(n):\n x+=1\n s.append (f\"{x} sheep...\")\n return \"\".join(s) \n", "def count_sheep(n):\n return '...'.join(str(x)+' sheep' for x in range(1, n+1))+'...'", "count_sheep = lambda n: n and count_sheep(n - 1) + str(n) + ' sheep...' or ''", "def count_sheep(n):\n count = ''\n for i in range(1, n+1):\n count += f'{i} sheep...'\n return count\n", "def count_sheep(n):\n i = 1\n string = ''\n while i <= n:\n string += \"%d sheep...\" % i\n i += 1\n return string", "def count_sheep(n):\n thing = ''\n for i in range(1,n+1):\n \n thing += str(i)\n thing += \" sheep...\"\n return thing", "def count_sheep(n):\n new_s = ''\n for i in range(1,n+1):\n new_s += f'{i} sheep...'\n return new_s", "def count_sheep(n):\n answer = ''\n for i in range(n):\n answer += f'{i+1} sheep...'\n return answer", "def count_sheep(n):\n strhr = ''\n for i in range(1, n+1):\n strhr += f'{i} sheep...'\n return strhr", "def count_sheep(n):\n returnArr = []\n for i in range(1,n+1): \n returnArr.append(str(i)+' sheep...')\n return \"\".join(returnArr)", "def count_sheep(n):\n z = 1\n sheeps = \"\"\n while z <= n :\n sheep = f\"{z} sheep...\"\n sheeps = sheeps + sheep\n z += 1\n return sheeps", "def count_sheep(n):\n str = \"\"\n x = 1\n while x<=n:\n str += (f\"{x} sheep...\")\n x += 1\n return (str) \n \n", "def count_sheep(n):\n strin=''\n for i in range (0,n):\n strin+=str(i+1)+ ' sheep...'\n return strin", "def count_sheep(n):\n output = ''\n counter = 1\n while n > 0:\n output += f'{counter} sheep...'\n n -= 1\n counter += 1\n return output", "def count_sheep(n):\n return ' sheep...'.join(list(str(x+1) for x in range(n))) + ' sheep...'", "def count_sheep(n):\n s = \"\"\n for i in range(1,n+1):\n s += \"\"+str(i)+\" sheep...\"\n return s\n", "def count_sheep(total):\n return \"\".join(f\"{n} sheep...\" for n in range(1, total + 1))", "def count_sheep(n):\n r = \"\"\n for i in range(1, n+1): r += f\"{i} sheep...\"\n return r", "def count_sheep(n):\n text = ''\n for x in range(1, n+1):\n text += str(x) + ' sheep...'\n \n return text", "def count_sheep(n):\n sheep=[]\n for i in range(1, n+1):\n sheep.append(f\"{i} sheep...\" )\n \n return ''.join(sheep)", "def count_sheep(n):\n sheep = \" sheep...\"\n newStr=\"\"\n for i in range(1,n+1):\n newStr+= str(i)+sheep\n \n return newStr", "def count_sheep(n):\n answer = \"\"\n for i in range(n):\n answer += \"{} sheep...\".format(i + 1)\n return answer", "count_sheep = lambda n:''.join(str(i+1)+' sheep...' for i in range(n))", "def count_sheep(n):\n count = \"\"\n for i in range(1, n+1):\n count += str(int(i)) + ' sheep...'\n return count", "def count_sheep(n):\n count = 1\n str = ''\n while count <= n:\n str += f'{count} sheep...'\n count += 1\n return str", "def count_sheep(n):\n lista = []\n for numbers in range(n):\n numbers= numbers+1\n lista.append(\"{} sheep...\".format(numbers))\n\n return \"\".join(lista)", "def count_sheep(n):\n res = ''\n count = 1\n while n > 0:\n res += \"{} sheep...\".format(count)\n count += 1\n n -= 1\n return res", "def count_sheep(n):\n # your code\n bahh = ''\n for i in range(1, n + 1):\n cool = str(i) + \" sheep...\"\n bahh = str(bahh) + str(cool)\n return bahh\n", "def count_sheep(n):\n l = []\n for x in range(1, n + 1):\n l.append(f'{x} sheep...')\n return \"\".join(l)", "def count_sheep(n):\n phrase = ''\n for i in range(n):\n phrase += str((1*i)+1) + ' sheep...'\n return phrase", "def count_sheep(n):\n x = ''\n for i in range(1, n+1):\n x += '%d sheep...'% i\n return x", "def count_sheep(n):\n # your code\n strr=\"\"\n for i in range(n):\n strr=strr+str(i+1)+\" sheep...\"\n return strr", "def count_sheep(n):\n x = \" sheep...\"\n z = \"\"\n for i in range(n): \n y = str(i+1) + x\n z = z + y\n return z", "def count_sheep(n):\n string = ''\n for i in range(0,n):\n string += f'{i+1} sheep...'\n return string\n", "def count_sheep(n): return ''.join([f'{c+1} sheep...' for c in range(n)])", "def count_sheep(n):\n # your code\n str = \"\"\n for i in range(n):\n str = str + \"{} sheep...\".format(i+1)\n return str\n", "def count_sheep(n):\n # your code\n o = \"\"\n for i in range(1, n+1):\n o+=f\"{i} sheep...\"\n return o ", "def count_sheep(n):\n # your code\n t = ''\n for x in range(1,n+1,1):\n t += str(x) + ' sheep...' \n \n return t", "def count_sheep(n):\n sheep = ''\n for i in range(n):\n sheep += '{} sheep...'.format(i + 1)\n return sheep", "def count_sheep(n):\n arr = []\n for i in range(1, n+1):\n arr.append(f'{i} sheep...')\n return ''.join(arr)", "def count_sheep(n):\n counter=\"\"\n for i in range(1,n+1):\n counter+=str(i)+' sheep...'\n return counter", "def count_sheep(n):\n total = \"\"\n x = 0\n for i in range(n):\n x += 1\n total += str(x)+\" sheep...\"\n \n return total", "def count_sheep(n):\n ans = \"\"\n for i in range(n):\n ans = ans + \"{} sheep...\".format(i+1)\n return ans", "def count_sheep(n):\n res = ''\n for p in range (1, n+1):\n p=str(p)\n res= res + ( p + ' sheep...')\n return res\nprint(count_sheep(3))", "def count_sheep(n):\n res=''\n for p in range(1,n+1):\n p=str(p)\n res=res + (p + ' sheep...')\n return res", "def count_sheep(n):\n \n sheeps = \"\"\n \n for i in range(1,n+1):\n sheep = f\"{i} sheep...\"\n sheeps += sheep\n \n return sheeps", "def count_sheep(n):\n sheep=\"\"\n for i in range(1, n+1):\n sheep+=str(i) + \" sheep...\"\n return sheep\n\nprint(count_sheep(5))", "def count_sheep(n):\n outstring = \"\"\n for x in range(1, n+1):\n outstring += str(x) + \" sheep...\"\n return outstring", "def count_sheep(n):\n \n #set up empty string\n x = \"\"\n \n #for all i in range 0-n, add a sheep comment to x\n # number is i+1 as index starts from zero\n for i in range(n):\n x = x+(str(i+1)+\" sheep...\")\n \n # now return the full string\n return x", "def count_sheep(n):\n nums = [x for x in list(range(1, n + 1))]\n sheep = []\n for i in nums:\n sheep.append(str(i) + \" sheep...\")\n sheep = ''.join(sheep)\n return sheep", "def count_sheep(n):\n shpStr = \"\"\n for x in range(1, n + 1):\n shpStr = shpStr + str(x) + \" sheep...\"\n return shpStr", "def count_sheep(n):\n result = \"\"\n x = 1\n while x <= n:\n result = result + str(x) + \" sheep...\"\n x = x + 1\n return result\n\ncount_sheep(3)", "def count_sheep(n):\n salida = ''\n for x in range(1,n+1):\n salida=salida+str(x)+' sheep...'\n return salida", "def count_sheep(n):\n answer = ''\n for i in range(1, n+1):\n answer += f'{i} sheep...'\n return answer", "def count_sheep(n):\n sheeps = (\"{} sheep...\".format(i) for i in range(1, n+1))\n return ''.join(sheeps)\n \n"] | {"fn_name": "count_sheep", "inputs": [[3]], "outputs": [["1 sheep...2 sheep...3 sheep..."]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,561 |
def count_sheep(n):
|
bf93167916e38034cb0cfcc695108ec6 | UNKNOWN | You have a set of four (4) balls labeled with different numbers: ball_1 (1), ball_2 (2), ball_3 (3) and ball(4) and we have 3 equal boxes for distribute them. The possible combinations of the balls, without having empty boxes, are:
```
(1) (2) (3)(4)
______ ______ _______
```
```
(1) (2)(4) (3)
______ ______ ______
```
```
(1)(4) (2) (3)
______ ______ ______
```
```
(1) (2)(3) (4)
_______ _______ ______
```
```
(1)(3) (2) (4)
_______ _______ ______
```
```
(1)(2) (3) (4)
_______ _______ ______
```
We have a total of **6** combinations.
Think how many combinations you will have with two boxes instead of three. You will obtain **7** combinations.
Obviously, the four balls in only box will give only one possible combination (the four balls in the unique box). Another particular case is the four balls in four boxes having again one possible combination(Each box having one ball).
What will be the reasonable result for a set of n elements with no boxes?
Think to create a function that may calculate the amount of these combinations of a set of ```n``` elements in ```k``` boxes.
You do no not have to check the inputs type that will be always valid integers.
The code should detect the cases when ```k > n```, returning "It cannot be possible!".
Features of the random tests:
```
1 <= k <= n <= 800
```
Ruby version will be published soon. | ["# Stirling numbers of second kind\n# http://mathworld.wolfram.com/StirlingNumberoftheSecondKind.html\n# S(n,k)=1/(k!)sum_(i=0)^k(-1)^i(k; i)(k-i)^n\nfrom math import factorial as fact\n\ndef combs_non_empty_boxes(n,k):\n if k<0 or k>n: return 'It cannot be possible!'\n return sum([1,-1][i%2]*(k-i)**n*fact(k)//(fact(k-i)*fact(i)) for i in range(k+1))//fact(k)", "MAX_BALL = 1+800\nDP = [[], [0,1]]\n\nfor _ in range(MAX_BALL):\n lst = DP[-1] + [0]\n DP.append([ v*i + lst[i-1] for i,v in enumerate(lst) ])\n\ndef combs_non_empty_boxes(n,k):\n return \"It cannot be possible!\" if k>n else DP[n][k]", "from functools import lru_cache\n\n@lru_cache(maxsize=None)\ndef fact(n):\n return 1 if n < 2 else n * fact(n-1)\n\n@lru_cache(maxsize=None)\ndef comb(n, k):\n return fact(n) // fact(k) // fact(n-k)\n\n# https://en.wikipedia.org/wiki/Stirling_numbers_of_the_second_kind\ndef combs_non_empty_boxes(n, k):\n if n == k: return 1\n if n < k: return \"It cannot be possible!\"\n return sum((1, -1)[i&1] * comb(k, i) * (k - i)**n for i in range(k+1)) // fact(k)", "stirling2 = [[1]]\ndef combs_non_empty_boxes(n, k):\n for _ in range(len(stirling2), n + 1):\n stirling2.append([a + r * b for r, (a, b) in\n enumerate(zip([0] + stirling2[-1], stirling2[-1] + [0]))])\n return stirling2[n][k] if k <= n else \"It cannot be possible!\"", "M = {}\ndef H(Q,S) :\n if Q < S : return 0\n if S < 2 : return 0 < S\n if (Q,S) not in M : M[Q,S] = S * H(Q - 1,S) + H(Q - 1,S - 1)\n return M[Q,S]\ncombs_non_empty_boxes = lambda Q,S : 'It cannot be possible!' if Q < S else H(Q,S)", "def combs_non_empty_boxes(n,k):\n if n < k: return \"It cannot be possible!\"\n if n == 0: return 0 if k == 0 else 1\n S = [[0]*(n+1) for i in range(n+1)]\n S[0][0] = 1\n for i in range(1, n+1):\n for j in range(1, i+1):\n S[i][j] = S[i-1][j-1] + S[i-1][j]*j\n return S[n][k]\n", "import sys\nsys.setrecursionlimit(10000)\n\n\ndef combs_non_empty_boxes(n,k):\n if k > n: \n return 'It cannot be possible!'\n \n return stirling(n, k)\n\ndef memoize(f): \n memo = {}\n def wrapping(*args): \n if args not in memo:\n memo[args] = f(*args)\n return memo[args]\n return wrapping\n\n@memoize\ndef stirling(n, k): \n if n == 0 and k == 0: \n return 1\n \n if n == 0 or k == 0: \n return 0\n \n return k * stirling(n - 1, k) + stirling(n - 1, k - 1) ", "def combs_non_empty_boxes(n,k):\n if n<k:\n return \"It cannot be possible!\"\n else:\n row = [1]+[0 for x in range(k)]\n for i in range(1, n+1):\n new = [0]\n for j in range(1, k+1):\n stirling = j * row[j] + row[j-1]\n new.append(stirling)\n row = new\n return row[k]", "# particiok szama\ndef makec():\n dp=[[-1]*801 for _ in range(801)]\n for n in range(801):\n dp[n][n]=1\n dp[n][1]=1\n dp[n][0]=0\n def c(n,k):\n if k>n: return 0\n if dp[n][k]<0:\n dp[n][k]=k*c(n-1,k)+c(n-1,k-1)\n return dp[n][k]\n return c\n\nc=makec()\n\ndef combs_non_empty_boxes(n,k):\n if k>n: combs=\"It cannot be possible!\"\n else: combs=c(n,k)\n return combs"] | {"fn_name": "combs_non_empty_boxes", "inputs": [[4, 3], [4, 2], [4, 4], [4, 1], [4, 0], [4, 5], [20, 8]], "outputs": [[6], [7], [1], [1], [0], ["It cannot be possible!"], [15170932662679]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,279 |
def combs_non_empty_boxes(n,k):
|
0d97176c3efb80d4dbc402ea9f2f52a4 | UNKNOWN | Your goal is to return multiplication table for ```number``` that is always an integer from 1 to 10.
For example, a multiplication table (string) for ```number == 5``` looks like below:
```
1 * 5 = 5
2 * 5 = 10
3 * 5 = 15
4 * 5 = 20
5 * 5 = 25
6 * 5 = 30
7 * 5 = 35
8 * 5 = 40
9 * 5 = 45
10 * 5 = 50
```
P. S. You can use ```\n``` in string to jump to the next line. | ["def multi_table(number):\n return '\\n'.join(f'{i} * {number} = {i * number}' for i in range(1, 11))", "def multi_table(number):\n table = [\"{0} * {1} = {2}\".format(i, number, i * number) for i in range(1, 11)]\n return '\\n'.join(table)", "def multi_table(number):\n return \"\\n\".join([\"{1} * {0} = {2}\".format(number, i, i * number) for i in range(1, 11)])", "def multi_table(n):\n return '\\n'.join(f'{i} * {n} = {i*n}' for i in range(1, 11))", "def multi_table(number): \n st = str()\n for x in range(1,11):\n z = number * x\n st += '{} * {} = {}\\n'.format(x,number,z)\n sti = st.strip('\\n')\n return sti", "def multi_table(n):\n return \"\\n\".join([f\"{i} * {n} = {i * n}\" for i in range(1, 11)])", "def multi_table(number):\n return \"\\n\".join(f\"{n} * {number} = {n*number}\" for n in range(1,11))", "def multi_table(number):\n st = str()\n for x in range(1,11):\n z = x * number\n st += '{} * {} = {}\\n'.format(x,number,z)\n sti = st.strip('\\n')\n return sti", "def multi_table(number):\n return \"\\n\".join([\"{0} * {1} = {2}\".format(str(i),str(number),str(i*number)) for i in range(1,11)])", "def multi_table(n): return \"\\n\".join([\"%d * %d = %d\" % (i, n, n*i) for i in range(1, 11)])", "def multi_table(number):\n return '\\n'.join(f'{n} * {number} = {number*n}' for n in range(1,11))\n\n", "multi_table=lambda number:'\\n'.join(f\"{r} * {number} = {r*number}\" for r in range(1,11))", "multi_table = lambda n: \"\\n\".join([f\"{i} * {n} = {i * n}\" for i in range(1, 11)])", "multi_table = lambda number: '\\n'.join('{} * {} = {}'.format(x, number, x * number) for x in range(1, 11))", "def multi_table(number):\n return '\\n'.join('{} * {} = {}'.format(i, number, i*number) for i in range(1,11))", "def multi_table(number: int) -> str:\n \"\"\" Get multiplication table for number that is always an integer from 1 to 10. \"\"\"\n return \"\\n\".join([f\"{_it} * {number} = {_it * number}\" for _it in range(1, 11)])", "def multi_table(number):\n table = \"\"\n for each in range(1, 11):\n table += \"%d * %d = %d\\n\" % (each, number, each * number)\n \n return table[:-1]", "def multi_table(number):\n return \"\".join([str(x) +\" * \" + str(number) + \" = \" +str(x * number) + \"\\n\" for x in range(1,11)])[:-1]", "multi_table = lambda n: '\\n'.join(str(i) + ' * ' + str(n) + ' = ' + str(i * n) for i in range(1, 11))", "def multi_table(number):\n table = \"\"\n for i in range(1, 11):\n table += \"{} * {} = {}\\n\".format(i, number, i * number)\n table = table.rstrip(\"\\n\")\n return table", "def multi_table(number):\n s = ''\n for i in range(1, 10):\n s = s + str(i) + ' * ' + str(number) + ' = ' + str(i*number) + '\\n'\n return s + '10' + ' * ' + str(number) + ' = ' + str(10*number)", "def multi_table(number):\n table = \"\"\n for i in range(1, 11):\n table += f\"{i} * {number} = {i * number}\" + \"\\n\" * (i < 10)\n \n return table", "def multi_table(number):\n return '\\n'.join(f'{num} * {number} = {num * number}' for num in range(1, 11))", "def multi_table(number):\n res = \"\"\n for i in range(1, 11):\n res += (\"{} * {} = {}\\n\".format(i, number, i * number))\n return res[:-1:]", "def multi_table(number):\n return '\\n'.join(f\"{i} * {number} = {number*i}\" for i in range(1,11))", "def multi_table(number):\n return '\\n'.join([f\"{num} * {number} = {num * number}\" for num in range(1, 11)])\n", "def multi_table(number):\n str = \"\"\n for i in range(10):\n str = str +\"{} * {} = {}\\n\".format(i+1,number,(i+1)*number)\n str = str[0:-1]\n return str", "def multi_table(number):\n s = ''\n for i in range(1, 11):\n result = number * i\n r = (str((str(i)+ ' * ' + str(number) + ' = ' + str(result))))\n if i in range(1,10):\n s += r + '\\n'\n else:\n s += r\n return s\n\n'''Your goal is to return multiplication table for number that is always an integer\nfrom 1 to 10\nresult must be a string separated by newline'''", "def multi_table(number):\n s = ''\n for i in range(1, 11):\n result = number * i\n r = (str((str(i)+ ' * ' + str(number) + ' = ' + str(result))))\n if i in range(1,10):\n s += r + '\\n'\n else:\n s += r\n return s", "def multi_table(number):\n s = ''\n for i in range(1,11):\n if i > 1:\n s += '\\n'\n s += str(i) + ' * ' + str(number) + ' = ' + str(i*number)\n return s", "def multi_table(number):\n lst = []\n \n for i in range(1, 11):\n item = f'{i} * {number} = {i * number}'\n lst.append(item)\n \n return '\\n'.join(lst)\n", "def multi_table(number):\n return \"\\n\".join([f\"{i} * {number} = {number * i}\" for i in range(1,11)])", "def multi_table(number):\n res = \"\"\n for i in range(1, 11):\n res += str(i) + ' * ' + str(number) + ' = ' + str(i * number)\n if i != 10: res += '\\n'\n return res", "def multi_table(number):\n return f'1 * {number} = {1*number}\\\n\\n2 * {number} = {2*number}\\\n\\n3 * {number} = {3*number}\\\n\\n4 * {number} = {4*number}\\\n\\n5 * {number} = {5*number}\\\n\\n6 * {number} = {6*number}\\\n\\n7 * {number} = {7*number}\\\n\\n8 * {number} = {8*number}\\\n\\n9 * {number} = {9*number}\\\n\\n10 * {number} = {10*number}'", "def multi_table(n):\n table = []\n for i in range(1, 11):\n table.append(f'{i} * {n} = {i*n}')\n string = '\\n'.join(table)\n return string", "def multi_table(n):\n r = f\"1 * {n} = {n}\"\n for i in range(2,11): r += f\"\\n{i} * {n} = {i*n}\"\n return r", "def multi_table(number):\n return '\\n'.join('%d * %d = %d' % (i, number, i*number) for i in range(1, 11)).rstrip()", "def multi_table(number):\n tab = \"\"\n for n in range(1, 11):\n r = n * number\n tab += f'{n} * {number} = {r}'\n if n < 10:\n tab += \"\\n\"\n return tab", "def multi_table(number):\n out=[]\n for i in range(1,11):\n out.append(\"{} * {} = {}\".format(i,number,i*number))\n return \"\\n\".join(out)", "def multi_table(i):\n return '1 * '+str(i)+' = '+str(i)+'\\n2 * '+str(i)+' = '+str(i*2)+'\\n3 * '+str(i)+' = '+str(i*3)+'\\n4 * '+str(i)+' = '+str(i*4)+'\\n5 * '+str(i)+' = '+str(i*5)+'\\n6 * '+str(i)+' = '+str(i*6)+'\\n7 * '+str(i)+' = '+str(i*7)+'\\n8 * '+str(i)+' = '+str(i*8)+'\\n9 * '+str(i)+' = '+str(i*9)+'\\n10 * '+str(i)+' = '+str(i*10)", "def multi_table(number):\n r = []\n for i in range(1,11):\n r.append('{} * {} = {}'.format(i, number, i*number))\n return '\\n'.join(r)", "def multi_table(number):\n index = 1\n k =''\n while True:\n if index <= 10:\n k += '{} * {} = {}\\n'.format(index, number, index*number)\n else:\n break\n index += 1\n return k[:-1]\n", "def multi_table(number):\n result = ''\n \n for i in range(1, 10 + 1):\n result += '{} * {} = {}\\n'.format(i, number, i * number)\n \n return result[:-1]", "def multi_table(number):\n res = ''\n for i in range(10):\n res += f'{i+1} * {number} = {(i+1)*number}\\n'\n return res[:-1]", "def multi_table(n):\n m = []\n for i in range(1,11):\n m.append(f\"{i} * {n} = {i * n}\")\n w = \"\\n\".join(m)\n return w", "def multi_table(number):\n m = []\n for i in range(1,11):\n m.append(f'{i} * {number} = {i * number}')\n w = \"\\n\".join(m)\n return w\n", "def multi_table(number):\n out = f'1 * {number} = {1 * number}\\n2 * {number} = {2 * number}\\n3 * {number} = {3 * number}\\n4 * {number} = {4 * number}\\n5 * {number} = {5 * number}\\n6 * {number} = {6 * number}\\n7 * {number} = {7 * number}\\n8 * {number} = {8 * number}\\n9 * {number} = {9 * number}\\n10 * {number} = {10 * number}'\n return out", "def multi_table(number):\n arr = []\n for i in range(1, 11):\n arr.append('{} * {} = {}'.format(i, number, i * number))\n return '\\n'.join(arr)", "def multi_table(number):\n x = list(range(1,11))\n y = ''\n z = 0\n while z < len(x):\n y += '{} * {} = {}\\n'.format(x[z], number, x[z] * number)\n z += 1\n return y[:-1]", "def multi_table(number):\n t=['{0} * {1} = {2}'.format(i,number,i*number) for i in range(1,11)]\n return '\\n'.join(t)", "def multi_table(number):\n string = ''\n i = 0\n for multiply in range(1, 10):\n i += 1\n string = string + f\"{multiply} * {number} = {multiply*number}\\n\"\n else:\n string = string + f\"{10} * {number} = {10*number}\"\n return string\n", "def multi_table(number):\n final = []\n count = 1\n for i in range(10):\n final.append(str(count))\n final.append(\" * \")\n final.append(str(number))\n final.append(\" = \")\n prod = count * number\n final.append(str(prod))\n if count != 10:\n final.append(\"\\n\")\n count+=1\n return (\"\").join(final)\n", "def multi_table(n):\n return '\\n'.join([f'{str(i)} * {str(n)} = {str(i*n)}' for i in range(1,11)])", "def multi_table(number):\n s = \"\"\n for x in range(1,11):\n s += f'{x} * {number} = {x * number}\\n'\n return s[0:-1]", "def multi_table(number):\n return \"\\n\".join(['{e} * {d} = {c}'.format(e=i,d=number,c=i*number) for i in range(1,11)])", "def multi_table(number):\n a=(1,2,3,4,5,6,7,8,9,10)\n b=[]\n f=1\n g=''\n for i in a:\n f=i*number\n b.append('{e} * {d} = {c}'.format(e=i,d=number,c=f))\n g=\"\\n\".join(b)\n return g", "def multi_table(number):\n return f'1 * {number} = {1 * number}\\n2 * {number} = {number * 2}\\n3 * {number} = {number * 3}\\n4 * {number} = {number * 4}\\n5 * {number} = {number * 5}\\n6 * {number} = {number * 6}\\n7 * {number} = {number * 7}\\n8 * {number} = {number * 8}\\n9 * {number} = {number * 9}\\n10 * {number} = {number * 10}'\n", "def multi_table(number):\n c=\"\"\n for i in range(1,11):\n c=c+\"{} * {} = {}\".format(i,number,number*i)+\"\\n\"\n return c.rstrip(\"\\n\")", "def multi_table(number):\n return ''.join(f\"{i} * {number} = {i*number}\\n\" for i in range(1,11))[0:-1]", "def multi_table(number):\n return \"1 * \"+ str(number)+ \" = \" + str(1*number) +\"\\n\" +\"2 * \"+ str(number)+ \" = \" + str(2*number)+\"\\n\" +\"3 * \"+ str(number)+ \" = \" + str(3*number)+\"\\n\" +\"4 * \"+ str(number)+ \" = \" + str(4*number)+\"\\n\" +\"5 * \"+ str(number)+ \" = \" + str(5*number)+\"\\n\" +\"6 * \"+ str(number)+ \" = \" + str(6*number)+\"\\n\" +\"7 * \"+ str(number)+ \" = \" + str(7*number)+\"\\n\" +\"8 * \"+ str(number)+ \" = \" + str(8*number)+\"\\n\" +\"9 * \"+ str(number)+ \" = \" + str(9*number)+\"\\n\" +\"10 * \"+ str(number)+ \" = \" + str(10*number)", "def multi_table(n):\n return ''.join(f'{i} * {n} = {i*n}\\n' for i in range(1,11)).rstrip('\\n')", "def multi_table(number):\n out = \"\"\n for i in range(1, 11):\n out+=str(i) + \" * \" + str(number) + \" = \" + str(i*number) +\"\\n\"\n return out.strip(\"\\n\")", "def multi_table(number):\n table = \"1 * \" + str(number) + \" = \" + str(number * 1)\n for x in range(9) :\n x += 2\n table = table + \"\\n\" + str(x) + \" * \" + str(number) + \" = \" + str(number * x)\n return table", "def multi_table(n):\n return \"\".join([f'{i} * {n} = {i*n}\\n' for i in range(1,11)]).rstrip('\\n')\n", "def multi_table(number):\n lst=list()\n for i in range(1,11):\n result=f\"{i} * {number} = {i*int(number)}\" \n lst.append(result)\n return \"\\n\".join(lst)", "def multi_table(number):\n lines = []\n for i in range(1, 11):\n lines.append(f'{i} * {number} = {i * number}')\n return '\\n'.join(lines)", "def multi_table(number):\n ans = \"\"\n i = 1\n for i in range(1, 11):\n ans += f'{i} * {number} = {i*number}\\n'\n i += i\n \n return ans[:-1]\n", "def multi_table(number):\n return '\\n'.join(f'{i} * {number} = {i * number}' for i in range(1, 11)) # good luck", "def multi_table(number):\n res = \"\"\n for i in range(1, 11):\n temp = number * i\n res += str(i) + \" * \" + str(number) + \" = \" + str(temp) +\"\\n\"\n return res[:-1]", "def multi_table(n):\n return '\\n'.join(f'{m} * {n} = {m*n}' for m in range(1, 11))", "def multi_table(number):\n return '1 *' + ' ' + str(number) + ' = ' + str(number) + '\\n2 *' + ' ' + str(number) + ' = ' + str(number * 2) + '\\n3 *' + ' ' + str(number) + ' = ' + str(number * 3) + '\\n4 *' + ' ' + str(number) + ' = ' + str(number * 4) + '\\n5 *' + ' ' + str(number) + ' = ' + str(number * 5) + '\\n6 *' + ' ' + str(number) + ' = ' + str(number * 6) + '\\n7 *' + ' ' + str(number) + ' = ' + str(number * 7) + '\\n8 *' + ' ' + str(number) + ' = ' + str(number * 8) + '\\n9 *' + ' ' + str(number) + ' = ' + str(number * 9) + '\\n10 *' + ' ' + str(number) + ' = ' + str(number * 10) ", "def multi_table(number):\n rez = \"\"\n for i in range(1, 11):\n rez += str(i) + \" * \" + str(number) + \" = \" + str(i*number)\n if i < 10:\n rez += \"\\n\"\n return rez", "def multi_table(number):\n table = ''\n for i in range(1, 11):\n table += f'{i} * {number} = {i * number}'\n if i < 10:\n table += '\\n'\n return table", "def multi_table(number):\n emptystring = ''\n for eachnumber in range(1,11):\n if eachnumber == 10:\n emptystring = emptystring + '{} * {} = {}'.format(eachnumber,number,eachnumber * number)\n else:\n emptystring = emptystring + '{} * {} = {}\\n'.format(eachnumber,number,eachnumber * number)\n return emptystring", "def multi_table(number):\n table = \"\"\n for x in range(1,11):\n table += str(x) + ' * ' + str(number) + ' = ' + str(x * number) + '\\n'\n return table[:-1]", "def multi_table(number):\n st = ''\n for i in range(1, 11):\n res = i * number\n if i == 1:\n st = st + f'{i} * {number} = {res}'\n \n else:\n st = st + f'\\n{i} * {number} = {res}'\n return st ", "def multi_table(number):\n n=str(number)\n i=1\n string=''\n while i<=10:\n ans=i*number\n string=string+str(i)+' * '+n+' = '+str(ans)+'\\n'\n i=i+1\n return string[:len(string)-1]", "def multi_table(number):\n return f\"\"\"1 * {number} = {number*1}\n2 * {number} = {number*2}\n3 * {number} = {number*3}\n4 * {number} = {number*4}\n5 * {number} = {number*5}\n6 * {number} = {number*6}\n7 * {number} = {number*7}\n8 * {number} = {number*8}\n9 * {number} = {number*9}\n10 * {number} = {number*10}\"\"\"", "multi_table = lambda num: '\\n'.join([f\"{i} * {num} = {num * i}\" for i in range(1, 11)])", "def multi_table(number):\n result = ''\n for i in range(1,11):\n result += '{} * {} = {}'.format(i, number, i*number)\n if i < 10:\n result += '\\n'\n return result", "def multi_table(number):\n \"\"\"\n create multiplication table for number\n \"\"\"\n return '\\n'.join(\"{} * {} = {}\".format(i,number,i*number) for i in range(1,11))", "def multi_table(num):\n return '\\n'.join(['%d * %d = %d'%(i, num, i*num) for i in range(1,11)])", "def multi_table(number):\n a = []\n for i in range(1, 11):\n a.append(i * number)\n b = \"\"\n count = 1\n for i in a:\n b += str(count) + \" * \" + str(number) + \" = \" + str(i) + \"\\n\"\n count += 1\n return b[0:-1]", "def multi_table(number):\n msg = ''\n for x in range(1,11):\n msg += str(x) + ' * ' + str(number) + ' = ' + str(x*number) + '\\n'\n return msg[0:-1]", "def multi_table(n):\n return '\\n'.join([f'{x} * {n} = {x*n}' for x in range(1,11)])", "def multi_table(number):\n return \"\\n\".join(\"{} * {} = {}\".format(c, number, c * number) for c in range(1, 11))\n\n", "def multi_table(number):\n table = ''\n for n in range(1, 11):\n table += '{0} * {1} = {2}'.format(n, number, n*number)\n if n < 10:\n table += '\\n'\n return table", "def multi_table(table):\n results = \"\"\n for i in range(1,10):\n results += f\"{i} * {table} = {i * table}\\n\"\n return results + f\"{10} * {table} = {10 * table}\"\n", "def multi_table(n):\n return f\"1 * {n} = {1*n}\\n2 * {n} = {2*n}\\n3 * {n} = {3*n}\\n4 * {n} = {4*n}\\n5 * {n} = {5*n}\\n6 * {n} = {6*n}\\n7 * {n} = {7*n}\\n8 * {n} = {8*n}\\n9 * {n} = {9*n}\\n10 * {n} = {10*n}\"", "def multi_table(number):\n res = ''\n for i in range (1, 11):\n res += str(i) + ' * ' + str(number) + ' = ' + str(i * number) +'\\n'\n return res[:-1]", "def multi_table(number):\n a = [\"{} * {} = {}\".format(i,number,i*number) for i in range(1,11)]\n return \"\\n\".join(a)", "def multi_table(number):\n arr = [f\"{i+1} * {number} = {(i+1)*number}\" for i in range(10)]\n return \"\\n\".join(arr)", "def multi_table(number):\n string = ''\n for i in range(1,11):\n string += (f\"{i} * {number} = {i * number}\\n\")\n string = string[:-1] \n return(string)", "def multi_table(number):\n res = ''\n for i in range (1,11):\n res += f'{i} * {number} = {i*number}\\n'\n return res.strip('\\n')", "def multi_table(number):\n l = [f\"{i} * {number} = {number*i}\\n\" for i in range(1,11)]\n return ''.join(l).rstrip(\"\\n\")", "multi_table = lambda n: '\\n'.join(['{0} * {1} = {2}'.format(i, n, i * n) for i in range(1, 11)])", "def multi_table(number):\n res = ''\n for i in range(10):\n res += (f'{i+1} * {number} = {number*(i+1)}')\n if i < 9:\n res += '\\n'\n return(res)", "def multi_table(n):\n x = \"\"\n for i in range(1,11):\n x += \"{} * {} = {}\\n\".format(i, n, i * n)\n return x[:-1]", "def multi_table(number):\n counter = 1\n table = \"\"\n while counter < 11:\n table += str(counter) + \" * \" + str(number) + \" = \" + str(number * counter)+\"\\n\"\n counter += 1\n return table[:-1]\n", "def multi_table(number):\n result = ''\n for i in range(1, 11):\n if i == 10:\n result += f\"{i} * {number} = {i * number}\"\n else:\n result += f\"{i} * {number} = {i * number}\\n\"\n return result"] | {"fn_name": "multi_table", "inputs": [[5], [1]], "outputs": [["1 * 5 = 5\n2 * 5 = 10\n3 * 5 = 15\n4 * 5 = 20\n5 * 5 = 25\n6 * 5 = 30\n7 * 5 = 35\n8 * 5 = 40\n9 * 5 = 45\n10 * 5 = 50"], ["1 * 1 = 1\n2 * 1 = 2\n3 * 1 = 3\n4 * 1 = 4\n5 * 1 = 5\n6 * 1 = 6\n7 * 1 = 7\n8 * 1 = 8\n9 * 1 = 9\n10 * 1 = 10"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 18,536 |
def multi_table(number):
|
fe85e2f2b87a7640f76e1995f964a372 | UNKNOWN | Each number should be formatted that it is rounded to two decimal places. You don't need to check whether the input is a valid number because only valid numbers are used in the tests.
```
Example:
5.5589 is rounded 5.56
3.3424 is rounded 3.34
``` | ["def two_decimal_places(n):\n return round(n, 2)\n", "def two_decimal_places(n):\n return round(n* 100) / 100", "from decimal import Decimal, ROUND_HALF_UP\n\ndef two_decimal_places(n):\n dn = Decimal(str(n)).quantize(Decimal('.01'), rounding=ROUND_HALF_UP)\n return float(dn)\n", "def two_decimal_places(n):\n return float(\"{0:.2f}\".format(n))\n", "def two_decimal_places(n):\n return float(\"%.2f\" % n)", "from numpy import round\n\ndef two_decimal_places(n):\n return round(n, decimals=2)", "two_decimal_places = lambda n : round(n,2)", "two_decimal_places = __import__(\"functools\").partial(round, ndigits=2)", "def two_decimal_places(n):\n return round(n+0.00005,2) ", "def two_decimal_places(n: float) -> float:\n \"\"\" Get number rounded to two decimal places. \"\"\"\n return round(n, 2)", "def two_decimal_places(n):\n return float(f'{n:.02f}')", "def two_decimal_places(n):\n \"\"\"\n a nightmare in a small package\n \"\"\"\n \n # Convert to a string\n x = str(n)\n \n # find the decimal\n ptIndx = x.find(\".\")\n \n # grab the deciding digit\n roundingDigit = x[ptIndx+3]\n\n # create the return string\n o = x[0:ptIndx+3]\n \n # return if not rounding up\n if int(roundingDigit)<=4:\n return float(o)\n else:\n # round the result of \"rounding\", since if you don't you get some ###.###9999999764235761923765 garbage\n return round(float(o) + (-0.01 if x[0]==\"-\" else 0.01), 2)\n\n #I can't wait to see more appropriate solutions.\n", "def two_decimal_places(n):\n pot_location = 0\n lt = list(str(n))\n for i in range(0,len(lt)):\n if lt[i] == '.':\n pot_location = i\n break\n result = float(''.join(lt[:pot_location+3]))\n if int(lt[pot_location+3])>4:\n if n>0:\n result = result + 0.01\n else:\n result = result - 0.01\n return round(result,5)\n \n", "def two_decimal_places(n):\n# raise NotImplementedError(\"TODO: two_decimal_places\")\n if (1000 * n % 10 == 5):\n return round((1000 * n + 1)/1000,2)\n return round(n, 2)", "def two_decimal_places(n):\n return ((n * 100 + 0.5) // 1 ) / 100", "def two_decimal_places(n):\n # raise NotImplementedError(\"TODO: two_decimal_places\")\n print('{:.2f}'.format(n))\n return(float('{:.2f}'.format(n)))", "def two_decimal_places(n):\n return float(round(n, 2))", "def two_decimal_places(n):\n my_float = float(n)\n return round(my_float, 2)\n raise NotImplementedError(\"TODO: two_decimal_places\")", "def two_decimal_places(n):\n n=float(\"{:.2f}\".format(n))\n return n", "def two_decimal_places(n):\n a = float(\"{:.2f}\".format(n))\n return a", "def two_decimal_places(n):\n \n formattedNumber=round(n,2)\n return formattedNumber", "def two_decimal_places(n):\n a = str(n)\n x = a.split('.')\n l = x[1]\n t = f'{x[0]}.'\n y = ''\n b = ['5','6','7','8','9']\n w = ['0','1','2','3','4','5','6','7','8']\n if l[1] in w and l[2] in b:\n q = l[1]\n w = int(q)\n w =w+1\n y = str(w)\n t = t + f'{l[0]}' + y\n elif l[1] == '9' and l[2] in b:\n q = l[0]\n w = int(q)\n w =w+1\n y = str(w)\n t = t + y \n else:\n t = t + f'{l[0]}' + f'{l[1]}'\n final_num = float (t)\n return(final_num)", "def two_decimal_places(n):\n i=n-int(n)\n i=i*1000\n j=(i)\n j=round(j/10)\n return int(n)+j/100", "def two_decimal_places(n):\n s = \"%.2f\" % n\n res = float(s)\n return res", "def two_decimal_places(n):\n\n return float(str(f\"{n:.2f}\"))", "def two_decimal_places(n):\n return round(n, ndigits=2)\n", "def two_decimal_places(n):\n r = round(n, 2)\n return r\n raise NotImplementedError(\"TODO: two_decimal_places\")", "def two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n return float(f\"{n:.2f}\")", "import unittest\n\n\ndef two_decimal_places(n):\n return round(n, 2)\n \n \nclass TestTwoDecimalPlaces(unittest.TestCase):\n def test_two_decimal_places(self):\n n = 4.659725356\n actual = two_decimal_places(n)\n self.assertEqual(actual, 4.66)\n", "def two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n num = '{:.2f}'.format(n)\n return float(num)", "def two_decimal_places(n):\n n = \"{:.2f}\".format(n)\n return float(n)", "def two_decimal_places(n):\n ans = float('{:.2f}'.format(n))\n return ans", "def two_decimal_places(n):\n s = 0\n s = round(n, 2)\n return s", "def two_decimal_places(n):\n answer = \"{:.2f}\".format(n)\n return float(answer)", "def two_decimal_places(n):\n \n num = \"{:.2f}\".format(n)\n \n return float(num)", "def two_decimal_places(n):\n a = round(n, 2)\n return a\n \n", "def two_decimal_places(n):\n return float(\"{:.2f}\".format(round(n,2)))", "def two_decimal_places(n):\n if n:\n num = round(n, 2)\n return num\n else:\n raise NotImplementedError(\"TODO: two_decimal_places\")", "def two_decimal_places(n: int) -> int:\n return round(n, 2)\n", "def two_decimal_places(n):\n thing = str(n)\n print (thing + ' is rounded ' )\n return(round(n, 2))", "def two_decimal_places(n):\n return float('{:.2f}'.format(n))\n # raise NotImplementedError(\"TODO: two_decimal_places\")\n", "import math\ndef two_decimal_places(n):\n return round(100*n)/100", "def two_decimal_places(n):\n return float(str(round(n,2)))", "def two_decimal_places(n):\n #return round(n, 2)\n #return float(format(n, \"1.2f\"))\n return float(f\"{n:1.2f}\")", "def two_decimal_places(n):\n n = n * 100 / 100.0\n return round(n, 2)\n", "def two_decimal_places(n):\n formatted = format(n, '.2f')\n return float(formatted)", "def two_decimal_places(n):\n x=(\"{:.2f}\").format(n)\n return float(x)\n", "def two_decimal_places(n):\n decimal = int((n*100 - int(n*100))*10)\n print(decimal)\n if decimal <= -5:\n return (int(n*100)-1)/100\n elif decimal < 5:\n return int(n*100)/100\n else:\n return (int(n*100)+1)/100", "def two_decimal_places(n):\n from decimal import Decimal\n out = round(n,2)\n return out\n", "from decimal import Decimal\ndef two_decimal_places(n):\n return float(Decimal(n).quantize(Decimal(\"0.01\")))", "from functools import partial\ntwo_decimal_places=partial(round,ndigits=2)", "def two_decimal_places(n):\n b = round(n,2)\n return (b)", "def two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n res = str(n).split('.')\n cc = 0\n cc += int(res[0]) * 100\n if n > 0:\n if int(str(res[1])[2]) < 5:\n cc += int(str(res[1])[0:2]) \n else:\n cc = cc + int(str(res[1])[0:2]) + 1\n else:\n cc = 0 - cc\n if int(str(res[1])[2]) < 5:\n cc += int(str(res[1])[0:2]) \n else:\n cc = cc + int(str(res[1])[0:2]) + 1\n cc = 0 - cc\n return float(str(cc)[:-2] + '.' + str(cc)[-2:])", "def two_decimal_places(n):\n return float('{0:.{1}f}'.format(n, 2))", "def two_decimal_places(n):\n return float(f'{n:.2f}')\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n", "def two_decimal_places(n):\n return n.__round__(2)", "two_decimal_places = lambda n: round(n*100) / 100", "def two_decimal_places(n):\n \n l = str(n)\n m = l.split(\".\")\n \n returning = \"\"\n if len(m[1]) == 2: return n\n elif len(m[1]) > 2:\n returning = round(n, 2)\n return returning\n", "def two_decimal_places(n):\n a = 100*n\n b = int(a)\n if (a-b)>=0.5:\n d = b+1\n elif a<0 and (b-a)>=0.5:\n d = b-1\n else:\n d = b\n c = d/100\n return c", "def two_decimal_places(n):\n return round(n * 100) / 100\n\n#My solution. It's ugly :(\n#def two_decimal_places(n):\n# if isinstance(n, float):\n# if n > 0:\n# int_n = int(n)\n# three_last_digits = int((n - (int_n)) * 1000)\n# last_digit = int(str(three_last_digits)[-1])\n# result = float()\n#\n# if last_digit >= 5:\n# return float(int_n) + ((three_last_digits-last_digit)+10)/1000\n# else:\n# return float(int_n) + (three_last_digits-last_digit)/1000\n# else:\n# int_n = int(n)\n# three_last_digits = int((n - (int_n)) * 1000)\n# last_digit = int(str(three_last_digits)[-1])\n# result = float()\n#\n# if last_digit >= 5:\n# return float(int_n) + ((three_last_digits+last_digit)-10)/1000\n# else:\n# return float(int_n) + (three_last_digits+last_digit)/1000\n# return float()\n", "# I know it's ugly, sorry. But it works :)\ndef two_decimal_places(n):\n if isinstance(n, float):\n if n > 0:\n int_n = int(n)\n three_last_digits = int((n - (int_n)) * 1000)\n last_digit = int(str(three_last_digits)[-1])\n result = float()\n\n if last_digit >= 5:\n return float(int_n) + ((three_last_digits-last_digit)+10)/1000\n else:\n return float(int_n) + (three_last_digits-last_digit)/1000\n else:\n int_n = int(n)\n three_last_digits = int((n - (int_n)) * 1000)\n last_digit = int(str(three_last_digits)[-1])\n result = float()\n\n if last_digit >= 5:\n return float(int_n) + ((three_last_digits+last_digit)-10)/1000\n else:\n return float(int_n) + (three_last_digits+last_digit)/1000\n return float()", "two_decimal_places = lambda n: float(f\"{n:.2f}\")", "def two_decimal_places(n):\n return round(n,2)\n#Completed by Ammar on 24/8/2019 at 03:41PM.\n", "def two_decimal_places(n):\n return round(n,2) #I solved this Kata on 8/23/2019 03:34 AM...#Hussam'sCodingDiary", "def two_decimal_places(n):\n print(\"==>> \",n)\n return round(n,2)", "def two_decimal_places(n):\n answer = round(n, 2)\n return answer", "def two_decimal_places(n):\n answer = n\n answer = round(answer, 2)\n return answer", "import math\ndef two_decimal_places(n):\n return float(\"{0:.2f}\".format(n))", "def two_decimal_places(n):\n return float(\"%1.2f\" % n)", "def two_decimal_places(n):\n return round(n * 1.00, 2)", "def two_decimal_places(n):\n# raise NotImplementedError(\"TODO: two_decimal_places\")\n n = round(n, 2)\n return n", "def two_decimal_places(n):\n return float(\"{:0.2f}\".format(n))", "def two_decimal_places(n):\n s=(\"{:.2f}\".format(n))\n return float(s)", "import math\ndef two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n #print(round(n,2))\n return round(n,2)", "def two_decimal_places(n):\n result = \"{0:.2f}\".format(n)\n new_result = float(result)\n return new_result", "def two_decimal_places(n):\n return float(f'{n:.2f}')", "def two_decimal_places(n):\n formatted = '{:.2f}'.format(n)\n return float(formatted)\n", "def two_decimal_places(n):\n#The round function is used to return with number of decimal places in 2nd parameter\n return round(n,2)", "def two_decimal_places(n):\n# raise NotImplementedError(\"TODO: two_decimal_places\")\n top = round(n,2)\n return top", "def two_decimal_places(n):\n if int(n * 1000) % 10 == 5 and n > 0:\n n = n + 0.001\n \n return float(\"{0:.2f}\".format(n))", "def two_decimal_places(n):\n from decimal import Decimal\n return float(Decimal(str(n)).quantize(Decimal('0.00')))", "def two_decimal_places(n):\n if (n * 1000) % 10 < 5:\n return round(n, 2)\n else:\n return round(n + 0.001, 2)", "def two_decimal_places(n):\n z = int(100 * abs(n) + 0.5) / 100.0\n return -z if n < 0 else z\n", "from decimal import Decimal, ROUND_HALF_UP\n\ndef two_decimal_places(n):\n return float(Decimal(n).quantize(Decimal('.01'), ROUND_HALF_UP))", "def two_decimal_places(n):\n num = round (n, 4)\n n1 = str(num)\n if n1[-1] == '5':\n n1 = n1[:-1] + '6'\n return round(float((n1)),2)\n \n else:\n return round(n,2) ", "def two_decimal_places(n):\n #return float(format(n, '.2f'))\n return round(n+0.0001, 2)", "def two_decimal_places(n):\n return 2.68 if n==2.675 else round(n,2) ", "def two_decimal_places(n):\n return round(n+0.001,2) if str(n)[-1] == '5' else round(n,2)", "def two_decimal_places(n):\n return round(n + 0.001,2) if -0.1 > n % 0.05 < 0.1 else round(n,2)", "def two_decimal_places(n):\n return round(n + 0.0001,2) if -0.1 > n % 0.05 < 0.1 else round(n,2)", "def two_decimal_places(n):\n return round(round(n, 2) + 0.01 * (n * 1000 % 10 == 5), 2)", "from decimal import *\ndef two_decimal_places(n):\n return float(Decimal(str(n)).quantize(Decimal('.01'), rounding=ROUND_HALF_EVEN))", "two_decimal_places = lambda q: round(q,2)", "# This answer will not always pass randoms :(\ndef two_decimal_places(num):\n try:\n return round(num + 0.0001, 2)\n except (TypeError):\n return 0\n", "two_decimal_places = lambda n: __import__('math').floor(n*100 + 0.5)/100", "def two_decimal_places(n):\n if (100 * n) % 1 == 0.5:\n return round(n + 0.01, 2)\n else:\n return round(n, 2)", "import math\ndef two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n return round(n, 2)", "def two_decimal_places(n):\n #raise NotImplementedError(\"TODO: two_decimal_places\")\n import decimal\n a = float(decimal.Decimal(str(n)).quantize(decimal.Decimal('.01'),rounding=decimal.ROUND_HALF_UP))\n print(a)\n return a"] | {"fn_name": "two_decimal_places", "inputs": [[4.659725356], [173735326.37837327], [4.653725356]], "outputs": [[4.66], [173735326.38], [4.65]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,943 |
def two_decimal_places(n):
|
9ba9c922e9587cc0b6bbeaf7e2c201a0 | UNKNOWN | Format any integer provided into a string with "," (commas) in the correct places.
**Example:**
``` csharp
Kata.NumberFormat(100000); // return "100,000"
Kata.NumberFormat(5678545); // return "5,678,545"
Kata.NumberFormat(-420902); // return "-420,902"
```
``` javascript
numberFormat(100000); // return '100,000'
numberFormat(5678545); // return '5,678,545'
numberFormat(-420902); // return '-420,902'
```
``` cpp
numberFormat(100000); // return '100,000'
numberFormat(5678545); // return '5,678,545'
numberFormat(-420902); // return '-420,902'
```
``` python
number_format(100000); # return '100,000'
number_format(5678545); # return '5,678,545'
number_format(-420902); # return '-420,902'
```
``` ruby
number_format(100000); # return '100,000'
number_format(5678545); # return '5,678,545'
number_format(-420902); # return '-420,902'
```
``` crystal
number_format(100000); # return '100,000'
number_format(5678545); # return '5,678,545'
number_format(-420902); # return '-420,902'
``` | ["def number_format(n):\n return f'{n:,}'", "def number_format(n):\n return format(n, ',')", "number_format='{:,}'.format", "def number_format(n):\n return str('{:,.3f}'.format(n)).rstrip('0').rstrip('.')", "import re\n\ndef number_format(n):\n return re.sub(r'\\B(?=(\\d{3})+(?!\\d))', r',', str(n))", "number_format=lambda n:''.join(','*(i%3==0)+x for i,x in enumerate(str(n)[::-1]))[::-1].replace('-,','-').strip(',')", "import re\ndef number_format(n):\n # converts int to str, reverses it for next step\n n = str(n)[::-1]\n \n # split into groups of 3 digits\n g = re.split(r'(\\d{3})', n)\n \n # remove empty string from list\n g = list(filter(None, g))\n \n # special case where \"-\" is only char in a group and messes up with \",\".join()\n if g[-1] == \"-\":\n return g[-1] + ','.join(g[:-1])[::-1]\n return ','.join(g)[::-1]", "def number_format(n):\n \n s = f'{n:,}'\n \n return s\n\n", "def number_format(n):\n n = [x for x in str(n)]\n if n[0] == \"-\":\n n = n[1:]\n result = \"-\"\n else:\n result = \"\"\n end = []\n while len(n) > 3:\n end.append(\"\".join([\",\"]+n[-3:]))\n n = n[:-3]\n end.append(\"\".join(n))\n for x in end:\n result += end[-1]\n end = end[:-1]\n return result", "def number_format(n):\n return f'{n:,.3f}'.rstrip('0').rstrip('.')\n #your code here\n"] | {"fn_name": "number_format", "inputs": [[100000], [5678545], [-420902], [-3], [-1003]], "outputs": [["100,000"], ["5,678,545"], ["-420,902"], ["-3"], ["-1,003"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,394 |
def number_format(n):
|
f3726f62b584742f8dfb77267fe5df36 | UNKNOWN | # Task
You are given an array of integers `arr` that representing coordinates of obstacles situated on a straight line.
Assume that you are jumping from the point with coordinate 0 to the right. You are allowed only to make jumps of the same length represented by some integer.
Find the minimal length of the jump enough to avoid all the obstacles.
# Example
For `arr = [5, 3, 6, 7, 9]`, the output should be `4`.
Check out the image below for better understanding:
# Input/Output
- `[input]` integer array `arr`
Non-empty array of positive integers.
Constraints: `1 ≤ inputArray[i] ≤ 100.`
- `[output]` an integer
The desired length. | ["def avoid_obstacles(arr):\n n=2\n while 1: \n if all([x%n for x in arr]): return n\n n+=1", "def avoid_obstacles(a):\n for j in range(2, max(a) + 2): \n if all(e % j != 0 for e in a): return j", "def avoid_obstacles(arr):\n s = set(arr)\n m = max(arr)\n for i in range(1, m+2):\n if i in s:\n continue\n if not any(x in s for x in range(i, m+1, i)):\n return i", "def avoid_obstacles(arr):\n obstacles = set(arr)\n limit = max(arr) + 2\n for step in range(1, limit):\n if not (set(range(0, limit, step)) & obstacles):\n return step\n", "def avoid_obstacles(arr, n=1):\n arr = set(arr)\n while set(range(0, 100+1, n)) & arr:\n n += 1\n return n", "avoid_obstacles=lambda a:min(n for n in range(1,max(a)+2)if all(m%n for m in a))", "def avoid_obstacles(arr):\n if min(arr) == 1 and max(arr) == 100: return 101\n landed = True\n for max_jump in range(2, 100):\n n = max_jump\n landed = True\n while n <= 100:\n for i in range(len(arr)):\n if n == arr[i]:\n landed = False\n break\n n += max_jump\n if landed:\n return max_jump", "def avoid_obstacles(arr):\n jump = 2\n \n while True:\n start_again = False\n \n for num in arr:\n if num % jump == 0:\n jump = jump + 1\n start_again = True\n break\n \n if not start_again:\n return jump\n", "def avoid_obstacles(arr):\n holes = set(range(2, max(arr))).difference(arr)\n for step in holes:\n if all([x % step != 0 for x in arr]):\n return step\n return max(arr) + 1", "def avoid_obstacles(arr):\n x = 2\n for i in range(max(arr)):\n leaps = [x*i for i in range(1,max(arr))]\n \n for i in leaps: \n if i in arr:\n leaps = []\n x += 1\n break \n return x"] | {"fn_name": "avoid_obstacles", "inputs": [[[5, 3, 6, 7, 9]], [[2, 3]], [[1, 4, 10, 6, 2]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100]]], "outputs": [[4], [4], [7], [101]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,070 |
def avoid_obstacles(arr):
|
022cb67acad421ede2a7c894242007c9 | UNKNOWN | There are some candies that need to be distributed to some children as fairly as possible (i.e. the variance of result needs to be as small as possible), but I don't know how to distribute them, so I need your help. Your assignment is to write a function with signature `distribute(m, n)` in which `m` represents how many candies there are, while `n` represents how many children there are. The function should return a container which includes the number of candies each child gains.
# Notice
1. *The candy can't be divided into pieces.*
2. The list's order doesn't matter.
# Requirements
1. The case `m < 0` is equivalent to `m == 0`.
2. If `n <= 0` the function should return an empty container.
3. If there isn't enough candy to distribute, you should fill the corresponding number with `0`.
# Examples
```python
distribute(-5, 0) # should be []
distribute( 0, 0) # should be []
distribute( 5, 0) # should be []
distribute(10, 0) # should be []
distribute(15, 0) # should be []
distribute(-5, -5) # should be []
distribute( 0, -5) # should be []
distribute( 5, -5) # should be []
distribute(10, -5) # should be []
distribute(15, -5) # should be []
distribute(-5, 10) # should be [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
distribute( 0, 10) # should be [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
distribute( 5, 10) # should be [1, 1, 1, 1, 1, 0, 0, 0, 0, 0]
distribute(10, 10) # should be [1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
distribute(15, 10) # should be [2, 2, 2, 2, 2, 1, 1, 1, 1, 1]
```
# Input
1. m: Integer (m <= 100000)
2. n: Integer (n <= 1000)
# Output
1. [Integer] | ["def distribute(m, n):\n if n <= 0:\n return []\n q, r = divmod(max(m, 0), n)\n return [q + (i < r) for i in range(n)]", "def distribute(m, n):\n lst = []\n if n > 0:\n lst = [0 for i in range(n)]\n if m > 0:\n for j in range(m):\n lst[j % n] += 1\n return lst\n", "def distribute(m, n):\n m = max(0, m)\n return [m // n + (m % n > i) for i in range(n)]", "def distribute(m, n):\n if n <= 0:\n return []\n d, r = divmod(max(m, 0), n)\n return r * [d + 1] + (n - r) * [d]", "def distribute(m, n):\n if m <= 0:\n return [0]*n\n elif n <= 0:\n return []\n else:\n a,b = divmod(m, n)\n return [a]*n if b == 0 else [a+1]*b + [a]*(n-b)", "def distribute(m, n):\n list = []\n if n <= 0:\n list = []\n else:\n if m <= 0:\n for i in range(n):\n list.append(0)\n else:\n number = int(m/n)\n mod = m%n\n for i in range(mod):\n list.append(number+1)\n for j in range(mod,n):\n list.append(number)\n return list ", "def distribute(m, n):\n q, r = divmod(max(m, 0), max(n, 1))\n return [q + (i < r) for i in range(max(n, 0))]", "def distribute(m, n):\n if m <=0:\n return [0]*n\n if n <= 0:\n return []\n else:\n lst = [0]*n\n x = m//n\n y = m%n\n while x > 0:\n for i in range(n):\n lst[i] += 1\n x -= 1\n if y > 0:\n for j in range(y):\n lst[j] += 1\n return lst", "def distribute(m, n):\n if n <= 0:\n return []\n if m < 0:\n m = 0\n q, r = divmod(m, n)\n return [q + 1] * r + [q] * (n - r)", "def distribute(m, n):\n if m < 0:\n m = 0\n \n if n <= 0:\n return []\n \n minCandiesPerPerson = m // n\n rest = m - n * minCandiesPerPerson\n \n return [minCandiesPerPerson] * (n-rest) + [minCandiesPerPerson + 1] * rest\n"] | {"fn_name": "distribute", "inputs": [[-5, 0], [10, 0], [15, 0], [-5, -5], [10, -5], [15, -5]], "outputs": [[[]], [[]], [[]], [[]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,078 |
def distribute(m, n):
|
7631097d61f24d65cb9a3672238ad4c3 | UNKNOWN | A variation of determining leap years, assuming only integers are used and years can be negative and positive.
Write a function which will return the days in the year and the year entered in a string. For example 2000, entered as an integer, will return as a string 2000 has 366 days
There are a few assumptions we will accept the year 0, even though there is no year 0 in the Gregorian Calendar.
Also the basic rule for validating a leap year are as follows
Most years that can be divided evenly by 4 are leap years.
Exception: Century years are NOT leap years UNLESS they can be evenly divided by 400.
So the years 0, -64 and 2016 will return 366 days.
Whilst 1974, -10 and 666 will return 365 days. | ["def year_days(year):\n days = 365\n if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:\n days += 1\n return \"%d has %d days\" % (year, days)", "from calendar import isleap\ndef year_days(year):\n return \"{} has {} days\".format(year, isleap(year) + 365)", "def year_days(year):\n return '{} has {} days'.format(year, 366 if year % 400 == 0 or (year % 4 == 0 and year % 100) else 365)", "import calendar\n\ndef year_days(year):\n if calendar.isleap(year):\n return '{0} has 366 days'.format(year)\n else:\n return '{0} has 365 days'.format(year)", "from calendar import isleap\n\ndef year_days(year):\n return f\"{year} has {365 + isleap(year)} days\"", "year_days = lambda y: \"{} has {} days\".format(y, 365 + (y % 4 == 0) * ((y % 100 != 0) + (y % 400 == 0)) )", "def year_days(year):\n x = 366 if (year % 100 != 0 and year % 4 == 0) or year % 400 == 0 else 365\n return '%d has %d days' % (year, x)\n \n", "def year_days(year):\n leap = (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0)\n return '{} has {} days'.format(year, 366 if leap else 365)", "def year_days(year):\n leap = (year % 4 == 0 and year % 100 != 0) or (year % 100 == 0 and year % 400 == 0)\n return '{} has {} days'.format(year, 366 if leap else 365)", "def year_days(year):\n if year % 100 == 0:\n if year % 400 == 0:\n return (\"%s has 366 days\" % year)\n else:\n return (\"%s has 365 days\" % year)\n else:\n return (\"%s has 366 days\" % year) if year % 4 == 0 else (\"%s has 365 days\" % year)", "def year_days(year):\n days = 365\n if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:\n days += 1\n return str(year) + \" has \" + str(days) + \" days\"", "def year_days(year):\n leap = year % 4 == 0 and not year % 100 == 0 or year % 400 == 0\n number_of_days = 365 if not leap else 366\n return \"{} has {} days\".format(year, number_of_days)", "def year_days(year): \n days = 365 if year % 4 else 366 if year % 100 else 365 if year % 400 else 366\n return \"{0} has {1} days\".format(year, days)", "from calendar import isleap\n\ndef year_days(year: int) -> str:\n \"\"\" Get the days in the given year. \"\"\"\n return f\"{year} has {366 if isleap(year) else 365} days\"", "def year_days(year: int) -> str:\n \"\"\" Get the days in the given year. \"\"\"\n if not year % 4:\n if not year % 100:\n if not year % 400:\n days_per_year = 366\n else:\n days_per_year = 365\n else:\n days_per_year = 366\n else:\n days_per_year = 365\n\n return f\"{year} has {days_per_year} days\"", "def year_days(year):\n return \"%d has %d days\" % (year, 365 + (not year % 400 if not year % 100 else not year % 4))", "def year_days(y):\n return f'{y} has {[365,366][y%4==0 and (y%400==0 or y%100!=0)]} days'", "def year_days(year):\n return '{} has {} days'.format(year, 366 if year % 400 == 0 or (year % 100 != 0 and year % 4 == 0) else 365)", "year_days = lambda y: \"{} has {} days\".format(y, __import__('calendar').isleap(y) + 365)\n", "from calendar import isleap\ndef year_days(year):\n if isleap(year):\n return \"{} has 366 days\".format(year)\n return \"{} has 365 days\".format(year)", "year_days=lambda y:'%d has %d days'%(y,365+(y%400<1or y%100and y%4<1))", "year_days = lambda y: \"%s has %s days\" % (y, __import__(\"calendar\").isleap(y) + 365)\n", "def year_days(year):\n if (year % 4 == 0 and year % 100 == 0 and year % 400 == 0) or (year % 4 == 0 and year % 100 != 0):\n return f\"{year} has 366 days\"\n else:\n return f\"{year} has 365 days\"", "def year_days(year):\n if year == 0:\n return '0 has 366 days'\n elif year % 100 == 0 and year % 400 == 0:\n return f'{year} has 366 days'\n elif year % 4 == 0 and year % 100 != 0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'", "def year_days(year):\n if year % 100 == 0 and year % 400 != 0:\n return f'{year} has 365 days'\n elif year % 4 == 0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'\n", "def year_days(year):\n if year % 100 == 0 and year % 4 == 0 and not(year % 400 == 0):\n return f'{year} has 365 days'\n elif year % 100 == 0 and year % 400 == 0:\n return f'{year} has 366 days'\n elif year % 4 == 0 and not(year % 100 == 0):\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'\n", "def year_days(year):\n days = 365\n if year % 100 == 0:\n if year % 400 == 0:\n days += 1\n elif year % 4 == 0:\n days += 1\n return f'{year} has {days} days'", "def year_days(year):\n if year==0:\n days=366\n elif year%100 ==0 and year%400 !=0:\n days =365\n elif year%4 != 0:\n days=365\n else:\n days=366\n return f\"{year} has {days} days\"", "def year_days(year):\n is_leap_year = year % 4 == 0 and year % 100 != 0 or year % 400 == 0\n return f'{year} has {365 + int(is_leap_year)} days'", "def find_leapyear(year):\n if year%4==0 and year%100!=0 or year%400==0:\n return 1\n else:\n return 0\ndef year_days(year):\n if find_leapyear(year):\n return f\"{year} has 366 days\"\n else:\n return f\"{year} has 365 days\"\n", "def year_days(year):\n if abs(year) % 100 == 0:\n return f'{year} has 366 days' if abs(year) % 400 == 0 else f'{year} has 365 days'\n else:\n return f'{year} has 366 days' if abs(year)%4 == 0 else f'{year} has 365 days'", "def year_days(year):\n \n if year % 100 == 0 and year % 400 ==0:\n return f'{year} has 366 days'\n elif year % 4 == 0 and year % 100 !=0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'\n \n \n", "def year_days(year):\n leap = False\n if year % 100 == 0:\n if year % 400 == 0:\n leap = True\n elif year % 4 == 0:\n leap = True \n \n return str(year) + ' has ' + str([365, 366][leap]) + ' days'", "def year_days(year):\n return f'{year} has {\"366\" if year%4==0 and (year%100!=0 or year%400==0) else \"365\"} days'", "def year_days(y):\n days = 365\n if (y % 4 == 0 and y % 100 != 0) or y % 400 == 0:\n days = days + 1\n return \"{} has {} days\".format(y, days)", "def year_days(y):\n #your code here\n if (y % 4 == 0 and y % 100 != 0) or y % 400 == 0:\n return \"{} has 366 days\".format(y)\n return \"{} has 365 days\".format(y)", "def year_days(year):\n x = 366 if year%4 == 0 else 365\n if year % 100 == 0 and year % 400 != 0:\n x = 365\n return f'{year} has {x} days'", "def year_days(year):\n if (year % 4) == 0:\n if (year % 100) == 0:\n if (year % 400) == 0:\n d = '366'\n else: \n d = '365'\n else: \n d = '366'\n else: \n d = '365' \n \n return f'{year} has {d} days'", "def year_days(year):\n if year%4 == 0:\n if year%100 == 0:\n if year%400 == 0:\n return str(year) + ' has ' + str(366) + ' days'\n \n else:\n return str(year) + ' has ' + str(365) + ' days'\n else:\n return str(year) + ' has ' + str(366) + ' days'\n else:\n return str(year) + ' has ' + str(365) + ' days'", "def year_days(y):\n d = \"365\" if y%4 or (y%100 == 0 and y%400 != 0) else \"366\"\n return f\"{y} has {d} days\"", "import datetime\ndef year_days(year):\n #your code here\n if year==0:\n return f'{year} has 366 days'\n elif year%400==0:\n return f'{year} has 366 days'\n elif year%100==0:\n return f'{year} has 365 days'\n elif year%4==0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'", "def year_days(y):\n leap = 0\n if y % 400 == 0:\n leap = 1\n elif y % 4 == 0 and y % 100 != 0:\n leap = 1\n return '{} has {} days'.format(y,365+leap)", "import calendar\n\ndef year_days(year):\n return '{} has 366 days'.format(year) if calendar.isleap(year) else '{} has 365 days'.format(year) ", "def year_days(year):\n if year%400 == 0: return '{} has 366 days'.format(year)\n if year%100 == 0: return '{} has 365 days'.format(year)\n if year%4 == 0: return '{} has 366 days'.format(year)\n else : return '{} has 365 days'.format(year)", "def year_days(year):\n if not year%400:\n return f'{year} has 366 days'\n if not year%4 and year%100:\n return f'{year} has 366 days'\n return f'{year} has 365 days'", "from calendar import isleap\n\ndef year_days(year):\n return f\"{year} has {366 if isleap(year) else 365} days\"", "def year_days(year):\n leap = 0\n if year % 4 == 0:\n leap = 1\n if year % 100 == 0 and year % 400:\n leap = 0\n return f\"{year} has {366 if leap else 365} days\"\n", "def year_days(year):\n return \"{} has 365 days\".format(year) if year % 4 != 0 else \"{} has 365 days\".format(year) if year % 100 == 0 and year % 400 != 0 else \"{} has 366 days\".format(year)", "def year_days(w):\n return f'{w} has 36{5+(not w%4 and not (not w%100 and w % 400))} days'", "def year_days(year):\n if year%4==0:\n if year%400==0 or year%100!=0:\n return str(year)+\" has 366 days\"\n else:\n return str(year)+\" has 365 days\"\n else:\n return str(year)+\" has 365 days\"", "def year_days(year):\n if year ==0:\n return(f'{year} has 366 days')\n elif year%100 ==0 and year%400 == 0:\n return(f'{year} has 366 days')\n elif year%100 !=0 and year%4 == 0:\n return(f'{year} has 366 days')\n else:\n return(f'{year} has 365 days')", "def year_days(year):\n #your code here\n if year%4 == 0:\n if year%100 == 0 and year%400 == 0:\n return (f'{year} has 366 days') \n elif year%100 == 0 and year%400 != 0:\n return (f'{year} has 365 days')\n else:\n return (f'{year} has 366 days')\n else:\n return (f'{year} has 365 days')", "def year_days(year):\n if (year%100!=0 and year%4==0) or year%400==0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'", "def year_days(year):\n #your code here\n if year%100==0 and year%400==0:\n return f'{year} has 366 days'\n elif year%100!=0 and year%4==0: \n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'\n", "def year_days(year):\n if year%4==0 and year%100==0 and year%400!=0:\n return str(year)+' has 365 days'\n else:\n return str(year)+' has 366 days' if year%4==0 else str(year)+' has 365 days'", "def year_days(year):\n if year/4 == year//4:\n if year%100 == 0:\n if year%400==0:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'\n return f'{year} has 366 days'\n return f'{year} has 365 days'", "def year_days(year):\n y = 365\n if year % 4 == 0 :\n y = 366\n if year % 100 == 0 :\n if year % 400 == 0 :\n y = 366\n else :\n y = 365\n return f\"{year} has {y} days\"", "def year_days(year):\n #your code here\n if year%4==0:\n if year%100==0 and year%400==0:\n return '{} has 366 days'.format(year)\n elif year%100==0 and year%400!=0:\n return '{} has 365 days'.format(year)\n else:\n return '{} has 366 days'.format(year)\n else:\n return '{} has 365 days'.format(year)", "def year_days(y):\n if y%100==0:\n return f'{y} has 366 days' if y%400==0 else f'{y} has 365 days'\n return f'{y} has 366 days' if y%4==0 else f'{y} has 365 days'", "def year_days(year):\n leap = False\n if year % 4 == 0:\n leap = True\n if year % 100 == 0:\n leap = False\n if year % 400 == 0:\n leap = True\n \n if leap:\n days = 366\n else:\n days = 365\n \n return f'{year} has {days} days'", "def is_leap_year(year):\n year = abs(year)\n if year % 4 == 0:\n if year % 100 == 0:\n if year % 400 == 0:\n return True\n else:\n return False \n return True\n \n return False\n \n \n\ndef year_days(year):\n if is_leap_year(year):\n return f\"{year} has 366 days\"\n else:\n return f\"{year} has 365 days\"", "def year_days(y):\n return f\"{y} has {366 if (not y%400) or ((y%100) and not y%4) else 365} days\"", "def year_days(year):\n if year % 4 == 0:\n if year % 100 != 0:\n return '{} has 366 days'.format(year)\n elif (year % 100 == 0 ) and (year % 400 == 0):\n return '{} has 366 days'.format(year)\n else:\n return '{} has 365 days'.format(year)\n else:\n return '{} has 365 days'.format(year)", "def year_days(year):\n res = \"\"\n res += str(year)\n if year%4 == 0:\n if year%100 == 0:\n if year%400 == 0:\n res += \" has 366 days\"\n return res\n else:\n res += \" has 365 days\"\n return res\n res += \" has 366 days\"\n else:\n res += \" has 365 days\"\n return res", "def year_days(year):\n if year % 100 == 0:\n if year % 400 == 0:\n return \"{} has {} days\".format(year, 366)\n else:\n return \"{} has {} days\".format(year, 365)\n if year % 4 == 0:\n return \"{} has {} days\".format(year, 366)\n else:\n return \"{} has {} days\".format(year, 365)", "def year_days(year):\n if year % 400 == 0:\n return str(year) + ' has 366 days'\n elif year % 100 == 0:\n return str(year) + ' has 365 days'\n if year % 4 == 0:\n return str(year) + ' has 366 days'\n else:\n return str(year) + ' has 365 days'", "def year_days(y):\n return f'{y} has {365 + (1 if (not y % 4) and (y % 100 or not y % 400) else 0)} days'", "def year_days(year):\n if (year / 100) % 1 == 0:\n if (year / 400) % 1 == 0:\n return str(year) + \" has 366 days\"\n else:\n return str(year) + \" has 365 days\"\n return str(year) + \" has 366 days\" if (year / 4) % 1 == 0 else str(year) + \" has 365 days\"", "def year_days(year):\n day = '366' if year % 4 == 0 and year % 100 != 0 or year % 400 == 0 else '365'\n return f\"{year} has {day} days\"\n", "def year_days(year):\n if year % 100 == 0 : return f\"{year} has 366 days\" if year % 400 == 0 else f\"{year} has 365 days\"\n return f\"{year} has 366 days\" if year % 4 == 0 else f\"{year} has 365 days\"", "def year_days(y):\n \"\"\"\n leap year calculator\n \"\"\"\n return \"{} has {} days\".format(y,[365,366,365,366][(y%4==0) + (y%100==0) + (y%400==0)])\n", "def year_days(year):\n d=365\n if year % 100 == 0:\n if year % 400 == 0: #is leap\n d=366\n elif year % 4 == 0:\n d=366\n return str(year) + ' has ' + str(d) + ' days'", "def year_days(year):\n if year % 400 == 0:\n return f'{year} has 366 days'\n elif year % 4 == 0 and year % 100 != 0:\n return f'{year} has 366 days'\n return f'{year} has 365 days'\n #your code here\n", "def year_days(year):\n s=1\n if year % 100 ==0:\n if year % 400 ==0:\n s=1\n else:\n s=0\n return '{} has 366 days'.format(year) if year % 4 == 0 and s else '{} has 365 days'.format(year)", "def year_days(y):\n day = '365'\n if y%100 == 0:\n if y%400 == 0:\n day = '366'\n elif y%4==0:\n day = '366' \n return f'{y} has {day} days'", "def year_days(year):\n d = '366' if ((year%4==0 and year%100!=0) or (year%400==0)) else '365'\n return f'{year} has {d} days'", "def year_days(year):\n nb_days = 366 if year % 400 == 0 or (year % 4 == 0 and not year % 100 == 0) else 365\n return f'{year} has {nb_days} days'", "def year_days(year):\n if year%4 == 0:\n if year % 100 == 0 and year % 400 != 0:\n return f'{year} has 365 days'\n else:\n return f'{year} has 366 days'\n else:\n return f'{year} has 365 days'", "def year_days(year):\n if year % 4 != 0 :\n return f'{year} has {365} days'\n elif year % 4 == 0 and year % 100 == 0 and year % 400 != 0:\n return f'{year} has {365} days'\n else:\n return f'{year} has {366} days'\n \n \n\n", "def year_days(year):\n msg = ' has 365 days'\n if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:\n msg = ' has 366 days'\n return str(year) + msg", "def year_days(year):\n \n abs_year = abs(year)\n leap_year = ' has 365 days'\n \n if abs_year % 100 == 0 and abs_year % 400 == 0:\n leap_year = ' has 366 days'\n elif abs_year % 100 != 0 and abs_year % 4 == 0:\n leap_year = ' has 366 days'\n \n return str(year) + leap_year", "def year_days(year):\n return f'{year} has 366 days' if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0) else f'{year} has 365 days'", "def year_days(year):\n if year%4 == 0:\n if year%100==0:\n if year%400==0:\n return '{} has 366 days'.format(year)\n else:\n return '{} has 366 days'.format(year)\n \n return '{} has 365 days'.format(year)", "def year_days(year):\n #your code here\n d = 0\n if int(year%400) == 0:\n d = 366\n elif int(year%4) == 0 and int(year%100) != 0:\n d = 366\n else:\n d = 365\n return '{} has {} days'.format(year, d)\n \n", "def year_days(year):\n mystr=str()\n if (((year % 4 == 0) and (year % 100 != 0)) or (year % 400 == 0)):\n days_in_year = 366\n else:\n days_in_year = 365\n mystr = str(year) + \" has \" + str(days_in_year) + \" days\"\n return mystr\n #your code here\n", "def year_days(year):\n is_leap = ((year % 4 == 0) and (year % 100 != 0)) or (year % 400 == 0)\n return f'{year} has {365 + is_leap} days'", "def year_days(year):\n leap = 0 if year % 4 else 1\n cent = 0 if year % 100 else -1\n quad = 0 if year % 400 else 1\n return f\"{year} has {365 + leap + cent + quad} days\"", "def year_days(year):\n days=365 if year%4!=0 or (year%100==0 and (year//100)%4!=0) else 366\n return \"{} has {} days\".format(year,days)", "def year_days(year):\n return '{0} has {1} days'.format( year, 366 if is_leap( year ) else 365 )\n\ndef is_leap(year):\n if year %4 == 0:\n if year%100 == 0:\n if year%400 == 0:\n return True\n else:\n return False\n else:\n return True\n else:\n return False", "def year_days(year):\n if year % 400 == 0:\n return \"{} has 366 days\".format(year)\n if year % 100 == 0 and year % 400 == 0:\n return \"{} has 366 days\".format(year)\n if year % 4 == 0 and year % 100 != 0 or year % 400 == 0:\n return \"{} has 366 days\".format(year)\n else:\n return \"{} has 365 days\".format(year)", "def year_days(year):\n return f'{year} has 366 days' if year == 0 or (year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)) else f'{year} has 365 days'", "def year_days(year):\n if year%100 == 0 :\n return '{} has 366 days'.format(year) if year%400 == 0 else '{} has 365 days'.format(year)\n elif year%4 == 0:\n return '{} has 366 days'.format(year)\n else:\n return '{} has 365 days'.format(year)\n\n", "def year_days(year):\n if year%100==0:\n if year%400==0: return f'{year} has 366 days'\n else: return f'{year} has 365 days'\n else:\n return f'{year} has 366 days' if year %4 ==0 else f'{year} has 365 days'", "def leap(year):\n solu = True\n if year >= 1000:\n if year%4==0:\n solu= True\n if year%100 == 0:\n if year%400==0:\n solu = True\n else:\n solu = False\n else:\n solu = False\n elif year >=100:\n if year%4==0:\n if year % 100 == 0:\n if year % 400 == 0:\n solu = True\n else:\n solu = False\n else:\n solu = False\n else:\n if year%4==0:\n solu = True\n else:\n solu = False\n\n return solu\n\ndef year_days(year):\n temp = year\n if temp == 0:\n return str(year) + \" has 366 days\"\n else:\n if temp < 0:\n temp *= -1\n\n if leap(temp):\n return str(year) + \" has 366 days\"\n else:\n return str(year) + \" has 365 days\"", "\nfrom calendar import isleap\ndef year_days(year):\n #print(isleap(year)) # gives 1 if it is leap year\n return \"{} has {} days\".format(year, isleap(year) + 365)", "def year_days(year):\n num_days = 365\n if year % 100 == 0:\n if (year / 100) % 4 == 0:\n num_days = 366\n elif year % 4 == 0:\n num_days = 366\n \n return '{} has {} days'.format(year, num_days)", "def year_days(year):\n\n if str(year)[-2:] == '00' and year % 400 != 0:\n return f'{year} has 365 days'\n if str(year)[-2:] == '00' and year % 400 == 0:\n return f'{year} has 366 days'\n \n if year % 4 == 0:\n return f'{year} has 366 days'\n \n return f'{year} has 365 days'", "def year_days(year):\n day = year % 100 == 0 and year % 400 == 0 or not year % 100 == 0 and year % 4 == 0\n days = 5, 6 \n years = f'{year} has 36{days[day]} days'\n return years", "def is_leap(year):\n if year % 4 == 0:\n if year % 100 == 0:\n if year % 400 == 0:\n return True\n else:\n return False\n else:\n return True\n else:\n return False\n\ndef year_days(year):\n if is_leap(abs(year)):\n return '{} has {} days'.format(year, 366)\n return '{} has {} days'.format(year, 365) \n", "def year_days(year):\n return \"{} has {} days\".format(year, 366 if (not year % 100 and not year % 400) or (not year % 4 and year % 100) else 365)\n #your code here\n"] | {"fn_name": "year_days", "inputs": [[0], [-64], [2016], [1974], [-10], [666], [1857], [2000], [-300], [-1]], "outputs": [["0 has 366 days"], ["-64 has 366 days"], ["2016 has 366 days"], ["1974 has 365 days"], ["-10 has 365 days"], ["666 has 365 days"], ["1857 has 365 days"], ["2000 has 366 days"], ["-300 has 365 days"], ["-1 has 365 days"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 22,709 |
def year_days(year):
|
adac284e5d1da37a15cf1e6fd1ed2225 | UNKNOWN | Given a square matrix (i.e. an array of subarrays), find the sum of values from the first value of the first array, the second value of the second array, the third value of the third array, and so on...
## Examples
```
array = [[1, 2],
[3, 4]]
diagonal sum: 1 + 4 = 5
```
```
array = [[5, 9, 1, 0],
[8, 7, 2, 3],
[1, 4, 1, 9],
[2, 3, 8, 2]]
diagonal sum: 5 + 7 + 1 + 2 = 15
``` | ["def diagonal_sum(array):\n return sum(row[i] for i, row in enumerate(array))", "from numpy import trace as diagonal_sum", "def diagonal_sum(array):\n \n return sum([array[a][a] for a in range(len(array))])", "def diagonal_sum(array):\n return sum( row[idx] for idx, row in enumerate(array) )", "def diagonal_sum(array):\n return sum([array[x][x] for x in range(0,len(array))])\n", "def diagonal_sum(array):\n sum=0\n for x in range(len(array)):\n for y in range(len(array[x])):\n if x==y:\n sum=sum+array[x][y]\n return(sum)", "def diagonal_sum(array):\n return sum([row[i] for i, row in enumerate(array)])", "def diagonal_sum(a):\n return sum(l[i] for i, l in enumerate(a))", "def diagonal_sum(array):\n return sum(line[i] for i, line in enumerate(array))", "def diagonal_sum(array):\n sum = 0\n for n in range(len(array)):\n sum += array[n][n]\n return sum"] | {"fn_name": "diagonal_sum", "inputs": [[[[1, 2], [3, 4]]], [[[1, 2, 3], [4, 5, 6], [7, 8, 9]]], [[[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]]]], "outputs": [[5], [15], [34]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 954 |
def diagonal_sum(array):
|
e1938301b081cde5622f15e954917257 | UNKNOWN | There are several (or no) spiders, butterflies, and dragonflies.
In this kata, a spider has eight legs. A dragonfly or a butterfly has six legs. A __dragonfly__ has __two__ pairs of wings, while a __butterfly__ has __one__ pair of wings. __I am not sure whether they are biologically correct, but the values apply here. __
Given the number of total heads, legs, and __pairs of__ wings, please calculate numbers of each kind of bugs. Of course they are integers.
_However, I do not guarantee that they are positive in the test cases.
Please regard the minus numbers as cases that does not make sense. _
If answers make sense, return [n\_spider, n\_butterfly, n\_dragonfly];
else, please return [-1, -1, -1].
Example:
cal\_n\_bug(3, 20, 3) = [1, 1, 1]
One spider, one butterfly, one dragonfly in total have three heads, twenty legs (8 for the spider, 6 for the butterfly, and 6 for the dragonfly), and _three pairs_ of wings (1 for the butterfly and 2 for the dragonfly). | ["def cal_n_bug(n_head, n_leg, n_wing):\n spider = (n_leg-n_head*6)//(8-6)\n butterfly = (n_wing-(n_head-spider))\n dragonfly = n_head-spider-butterfly\n return [spider,butterfly,dragonfly] if spider>=0 and butterfly>=0 and dragonfly>=0 else [-1,-1,-1]", "def cal_n_bug(n_head, n_leg, n_wing):\n s = (n_leg - 6 * n_head) / 2\n b = 8 * n_head - n_wing - n_leg\n d = s + n_wing - n_head\n return [s, b, d] if s >= 0 and b >= 0 and d >= 0 else [-1, -1, -1]", "def cal_n_bug(head, leg, wing):\n # x+y+z = head, 8x+6y+6z = leg, y+2z = wing\n x = leg / 2 - head * 3\n head -= x\n y = head * 2 - wing\n z = head - y\n if all(n >= 0 for n in (x, y, z)): return [x, y, z]\n return [-1, -1, -1]", "\ndef cal_n_bug(n_head, n_leg, n_wing):\n n_s=(n_leg-6*n_head)/2\n n_d=(n_leg-8*n_head+2*n_wing)/2\n n_b=(-n_leg+8*n_head-n_wing)\n if n_s>-1 and n_d>-1 and n_b>-1:\n return [n_s,n_b,n_d] \n else:\n return [-1,-1,-1]", "def cal_n_bug(h, l, p):\n #s+d+b=h / 8s+6d+6b=l / 0s+2d+b=p\n d=l/2-4*h+p\n b=p-2*d\n s=h-d-b\n return [-1,-1,-1] if s<0 or b<0 or d<0 else [s,b,d]", "def cal_n_bug(h,l,w): \n lst=[l//2-3*h,8*h-l-w,w-4*h+l//2] \n return lst if lst[0]>=0 and lst[1]>=0 and lst[2]>=0 else [-1,-1,-1]", "def cal_n_bug(n_head, n_leg, n_wing):\n if n_head<0 or n_leg<0 or n_wing<0:\n return [-1,-1,-1]\n x=n_leg-6*n_head\n y=8*n_head-n_leg-n_wing\n z=n_leg+2*n_wing-8*n_head\n if x%2!=0 or z%2!=0 or x<0 or y<0 or z<0:\n return [-1,-1,-1]\n return [x//2,y,z//2]", "def cal_n_bug(heads, legs, wings):\n spiders = legs // 2 - heads * 3\n dragonflies = wings + spiders - heads\n r = [spiders, heads - spiders - dragonflies, dragonflies]\n return r if all(x >= 0 for x in r) else [-1] * 3", "def cal_n_bug(h,l,w):\n if h<0 or l<0 or w<0 or l%2 or l<6*h or l>8*h: return [-1,-1,-1]\n d,b=w//2,w%2\n s=h-d-b\n return [h-d-b,b,d] if s*8+d*6+b*6==l else [-1,-1,-1]", "import numpy as np\n\ndef cal_n_bug(x, y, z):\n a = np.array([[1,1,1], [8,6,6], [0,2,1]])\n b = np.array([x, y, z])\n s = np.linalg.solve(a, b)\n return list(map(int, s)) if all(x>=0 for x in s) else [-1, -1, -1]"] | {"fn_name": "cal_n_bug", "inputs": [[3, 20, 3]], "outputs": [[[1, 1, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,253 |
def cal_n_bug(n_head, n_leg, n_wing):
|
5f90e59d25173f11011414195c282bf3 | UNKNOWN | The vowel substrings in the word `codewarriors` are `o,e,a,io`. The longest of these has a length of 2. Given a lowercase string that has alphabetic characters only (both vowels and consonants) and no spaces, return the length of the longest vowel substring.
Vowels are any of `aeiou`.
```if:csharp
Documentation:
Kata.Solve Method (String)
Returns the length of the greatest continuous vowel substring in a string.
Syntax
public
static
int Solve(
string str
)
Parameters
str
Type: System.String
The string to be processed.
Return Value
Type: System.Int32
The length of the greatest continuous vowel substring in str, or 0 if str contains no vowels.
Exceptions
Exception
Condition
ArgumentNullException
str is null.
```
Good luck!
If you like substring Katas, please try:
[Non-even substrings](https://www.codewars.com/kata/59da47fa27ee00a8b90000b4)
[Vowel-consonant lexicon](https://www.codewars.com/kata/59cf8bed1a68b75ffb000026) | ["def solve(s):\n return max(map(len, ''.join(c if c in 'aeiou' else ' ' for c in s).split()))", "import re\n\ndef solve(s):\n return max(len(x.group(0)) for x in re.finditer(r'[aeiou]+', s))", "from re import findall\n\ndef solve(s):\n return max(map(len, findall(\"[aeiou]+\", s)))", "def solve(s):\n vowels = 'aeiou'\n max_len = 0\n tmp_len = 0\n prev_vowel = False\n for c in s:\n max_len = max(max_len, tmp_len)\n if not prev_vowel:\n tmp_len = 0\n if c in vowels:\n tmp_len += 1\n prev_vowel = c in vowels\n return max_len", "def solve(s):\n return max(len(w) for w in ''.join([' ', c][c in 'aeiou'] for c in s).split()) ", "import re\ndef solve(s):\n return max(map(len, re.findall('[uoiae]+', s)))", "import re\ndef solve(s):\n return len(max(re.findall(r\"[aeiou]+\", s), key=len, default=\"\"))", "from itertools import groupby\n\ndef solve(s):\n return max(sum(1 for _ in g) for k, g in groupby(s, 'aeiou'.__contains__) if k)", "def solve(s):\n for x in s:\n if x not in 'aeiou':\n s=s.replace(x,' ')\n s=(s.split()) \n a=[len(x) for x in s]\n return (max(a))", "from re import compile\n\nr = compile(\"[aeiou]+\")\n\n\ndef solve(s: str):\n return max(list(map(len, r.findall(s))))\n"] | {"fn_name": "solve", "inputs": [["codewarriors"], ["suoidea"], ["ultrarevolutionariees"], ["strengthlessnesses"], ["cuboideonavicuare"], ["chrononhotonthuooaos"], ["iiihoovaeaaaoougjyaw"]], "outputs": [[2], [3], [3], [1], [2], [5], [8]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,321 |
def solve(s):
|
e0a74a70e97c959774d2bec66ebb3bae | UNKNOWN | ###Lucky number
Write a function to find if a number is lucky or not. If the sum of all digits is 0 or multiple of 9 then the number is lucky.
`1892376 => 1+8+9+2+3+7+6 = 36`. 36 is divisble by 9, hence number is lucky.
Function will return `true` for lucky numbers and `false` for others. | ["def is_lucky(n):\n return n % 9 == 0", "def is_lucky(n):\n sume=0\n for i in str(n):\n sume+=int(i)\n return True if sume%9==0 else False ", "def is_lucky(n):\n digits = list(str(n))\n return sum(list(map(int, digits)))%9==0", "def is_lucky(n):\n return sum([int(i) for i in str(n)]) % 9 == 0", "def is_lucky(n):\n x = 0\n for i in str(n):\n x = x + int(i)\n return True if x % 9 == 0 else False", "is_lucky=lambda n:n%9<1", "def is_lucky(n):\n count = 0\n n = str(n)\n for i in n:\n count += int(i)\n if count == 0 or count % 9 == 0:\n return True\n return False", "def is_lucky(n):\n sum = 0\n for number in str(n):\n sum += int(number)\n return sum % 9 == 0", "def is_lucky(n):\n print(36%9)\n res = 0 \n \n for x in str(n): \n res += int(x)\n \n if res % 9 == 0: return True \n else: return False ", "def is_lucky(n):\n return not n or not n%9"] | {"fn_name": "is_lucky", "inputs": [[1892376], [189237], [18922314324324234423437], [189223141324324234423437], [1892231413243242344321432142343423437], [0]], "outputs": [[true], [false], [false], [true], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 976 |
def is_lucky(n):
|
0c95374e2d62c61fc69574f389c786b7 | UNKNOWN | < PREVIOUS KATA
NEXT KATA >
## Task:
You have to write a function `pattern` which returns the following Pattern(See Examples) upto desired number of rows.
* Note:`Returning` the pattern is not the same as `Printing` the pattern.
## Parameters:
pattern( n , y );
^ ^
| |
Term upto which Number of times
Basic Pattern Basic Pattern
should be should be
created repeated
vertically
* Note: `Basic Pattern` means what we created in Complete The Pattern #12 i.e. a `simple X`.
## Rules/Note:
* The pattern should be created using only unit digits.
* If `n < 1` then it should return "" i.e. empty string.
* If `y <= 1` then the basic pattern should not be repeated vertically.
* `The length of each line is same`, and is equal to the length of longest line in the pattern.
* Range of Parameters (for the sake of CW Compiler) :
+ `n ∈ (-∞,50]`
+ `y ∈ (-∞,25]`
* If only one argument is passed then the function `pattern` should run as if `y <= 1`.
* The function `pattern` should work when extra arguments are passed, by ignoring the extra arguments.
## Examples:
#### Having Two Arguments:
##### pattern(4,3):
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
2 2
3 3
4
3 3
2 2
1 1
##### pattern(10,2):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
#### Having Only One Argument:
##### pattern(25):
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5 5
6 6
7 7
8 8
9 9
0 0
1 1
2 2
3 3
4 4
5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
0 0
9 9
8 8
7 7
6 6
5 5
4 4
3 3
2 2
1 1
>>>LIST OF ALL MY KATAS<<< | ["def pattern(n, *y):\n if n < 1:\n return \"\"\n y = y[0] if y and y[0] > 0 else 1\n result = []\n for j in range(1, n + 1):\n line = \" \" * (j - 1) + str(j % 10) + \" \" * (n - j)\n result.append(line + line[::-1][1:])\n return \"\\n\".join(((result + result[::-1][1:-1]) * y)) + \"\\n\" + result[0]", "def pattern(n, y=1, *a):\n if n < 1: return ''\n l, y = 2*n-1, max(1,y)\n sngl = ['{}{}{}'.format(x%10, ' '*(l-2*x), x%10 if x!=n else '').center(l) for x in range(1,n+1)]\n cross = sngl + sngl[:-1][::-1]\n return '\\n'.join( cross + cross[1:]*(y-1) )", "def pattern(n,y = 1, *args):\n if n < 1:\n return \"\"\n if y < 1:\n y = 1\n\n p = [\" \"*i + str((i+1)%10) + \" \"*(2*n-3-2*i) + str((i+1)%10) + \" \"*i for i in range(n-1)] + \\\n [\" \"*(n-1) + str(n%10) + \" \"*(n-1)] + \\\n [\" \"*i + str((i+1)%10) + \" \"*(2*n-3-2*i) + str((i+1)%10) + \" \"*i for i in range(n-2,-1,-1)]\n\n return \"\\n\".join(p + p[1:]*(y-1))", "m,r=lambda s:s+s[-2::-1],lambda s,n:s+s[1:]*n\npattern=lambda n,v=1,*a:'\\n'.join(r(m(list(m(' '*(i-1)+str(i%10)+' '*(n-i))for i in range(1,n+1))),v-1))", "def pattern(n, y=1, *_):\n y = y if y>1 else 1\n s1 = '1'+ ' '*(2*n-3) + '1'\n s2 = '\\n'+'\\n'.join(' '*i+str(i+1)[-1]+' '*(2*n-2*i-3)+str(i+1)[-1]+' '*i for i in range(1,n-1))\n s3 = '\\n'+' '*(n-1) +str(n)[-1]+' '*(n-1)+'\\n'\n return '' if n<1 else s1+(s2+s3+s2[::-1]+s1)*y", "def pattern(n,y=1,*args):\n p = [\"\".join([\" \" * (i - 1), str(i % 10), \" \" * (n - i)]) for i in range(1,n+1)]\n p = [\"\".join([i, i[-2::-1]]) for i in p]\n p += p[-2::-1]\n return \"\\n\".join(p + p[1:] * (y - 1))", "def pattern(n, y=1, *_):\n if n < 1:\n return ''\n ans, p, y = [], [], max(y, 1)\n for i in range(1, n+1):\n t = [' '] * (n * 2 - 1)\n t[i - 1] = t[n * 2 - 1 - 1 - i + 1] = str(i % 10)\n p.append(''.join(t))\n p += p[:-1][::-1]\n for _ in range(y):\n ans += p[1:] if ans else p\n return '\\n'.join(ans)", "def pattern(n, m=1, *args):\n if n < 1:\n return \"\"\n m = max(m, 1)\n r = [\"{0}{1}{2}{1}{0}\".format(\" \" * (i - 1), i % 10, \" \" * ((n - i) * 2 - 1)) for i in range(1, n)]\n r = r + [\"{0}{1}{0}\".format(\" \" * (n - 1), n % 10)] + r[::-1]\n return \"\\n\".join(r + r[1:] * (m - 1))", "def pattern(*args):\n n=args[0]\n y=args[1] if len(args)>=2 else 0\n res=\"\"\n for count in range(max(1,y)):\n for i in range(n):\n if count>=1 and (i+1)==1:\n continue\n elif (i+1)!=n:\n res+=\" \"*i+str((i+1)%10)+\" \"*((n-1-i)*2-1)+str((i+1)%10)+\" \"*i+\"\\n\"\n else:\n res+=\" \"*i+str((i+1)%10)+\" \"*i+\"\\n\"\n for i in range(n-2,-1,-1):\n if (i+1)!=n:\n res+=\" \"*i+str((i+1)%10)+\" \"*((n-1-i)*2-1)+str((i+1)%10)+\" \"*i+\"\\n\"\n return res[:-1]"] | {"fn_name": "pattern", "inputs": [[0]], "outputs": [[""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,979 |
def pattern(n, y=1, *_):
|
6f98b63ad6fc940e6fd20f5fe085d741 | UNKNOWN | So you've found a meeting room - phew! You arrive there ready to present, and find that someone has taken one or more of the chairs!! You need to find some quick.... check all the other meeting rooms to see if all of the chairs are in use.
Your meeting room can take up to 8 chairs. `need` will tell you how many have been taken. You need to find that many.
```if-not:java
Find the spare chairs from the array of meeting rooms. Each meeting room array will have the number of occupants as a string. Each occupant is represented by 'X'. The room array will also have an integer telling you how many chairs there are in the room.
```
```if:java
Find the spare chairs from the array of meeting rooms.
~~~java
public class Room {
public final String occupants; // number of occupants, each occupant is represented by 'X'
public final int chairs; // number of chairs in the room
}
~~~
```
You should return an array of integers that shows how many chairs you take from each room in order, up until you have the required amount.
```if-not:java
example:
[['XXX', 3], ['XXXXX', 6], ['XXXXXX', 9], ['XXX',2]] when you need 4 chairs:
```
```if:java
example:
`[Room("XXX", 3), Room("XXXXX", 6), Room("XXXXXX", 9), Room("XXX",2)]` when you need 4 chairs:
```
result -- > `[0, 1, 3]` (no chairs free in room 0, take 1 from room 1, take 3 from room 2. No need to consider room 4 as you have your 4 chairs already.
If you need no chairs, return `'Game On'`. If there aren't enough spare chairs available, return `'Not enough!'`
More in this series:
The Office I - Outed
The Office II - Boredeom Score
The Office III - Broken Photocopier
The Office IV - Find a Meeting Room | ["def meeting(rooms, need):\n if need == 0: return \"Game On\"\n \n result = []\n for people, chairs in rooms:\n taken = min(max(chairs - len(people), 0), need)\n result.append(taken)\n need -= taken\n if need == 0: return result\n \n return \"Not enough!\"", "def meeting(rooms, number):\n if number == 0:\n return 'Game On'\n result = []\n for taken, n in rooms:\n available = n - len(taken)\n chairs = min(max(available, 0), number)\n number -= chairs\n result.append(chairs)\n if not number:\n return result\n return 'Not enough!'\n", "def meeting(rooms, n):\n if n==0: return \"Game On\"\n chairs, res = (max(0, chair - len(occ)) for occ, chair in rooms), []\n for c in chairs:\n if c >= n: return res+[n]\n res.append(c)\n n -= c\n return \"Not enough!\"", "def meeting(rooms, need):\n if need == 0:\n return \"Game On\"\n take = []\n for people, seat in rooms:\n take.append(min(need, max(0, seat - len(people))))\n need -= take[-1]\n if need == 0:\n return take\n return \"Not enough!\"", "from typing import List, Union\n\n\ndef meeting(rooms: List[List[Union[str, int]]], number: int) -> Union[List[int], str]:\n chairs_in_rooms, chairs = (max(c - len(p), 0) for p, c in rooms), []\n\n while number > 0:\n try:\n n = next(chairs_in_rooms)\n chairs.append(min(n, number))\n number -= n\n except StopIteration:\n return \"Not enough!\"\n\n return chairs or \"Game On\"", "def meeting(rooms, need):\n if not need: \n return 'Game On'\n spare_chairs = []\n for occupants, chairs in rooms:\n available = min(need, max(chairs - len(occupants), 0))\n spare_chairs.append(available)\n need -= available\n if not need: \n return spare_chairs\n return 'Not enough!'", "def meeting(rooms, number):\n if number == 0:\n return \"Game On\"\n chairs = []\n for i in rooms:\n if sum(chairs) == number:\n return chairs\n if i[-1] - len(i[0]) <= 0:\n chairs.append(0)\n else:\n if sum(chairs) + i[1] - len(i[0]) >= number:\n chairs.append(number - sum(chairs))\n else:\n chairs.append(i[-1] - len(i[0]))\n if sum(chairs) == number:\n return chairs\n if sum(chairs) < number:\n return \"Not enough!\"", "def meeting(rooms, number):\n if not number:\n return 'Game On'\n accumulate = []\n for r in rooms:\n available = max(r[1] - len(r[0]), 0)\n take = min(number, available)\n accumulate.append(take)\n number -= take\n if number == 0:\n return accumulate\n return 'Not enough!'", "def meeting(rooms, number):\n if number == 0:\n return 'Game On'\n chairs = [max(room[1] - len(room[0]), 0) for room in rooms]\n a = []\n i = 0\n while sum(a) < number and i < len(chairs):\n a.append(min(chairs[i], number - sum(a)))\n i += 1\n return a if sum(a) >= number else \"Not enough!\"", "def meeting(rooms, number):\n if number == 0:\n return \"Game On\"\n chairs = [room[1] - room[0].count('X') for room in rooms]\n chairs = [0 if chair < 0 else chair for chair in chairs]\n ans = []\n i = 0\n while sum(ans) < number and i < len(chairs):\n ans.append(min(chairs[i], number - sum(ans)))\n i += 1\n return ans if sum(ans) >= number else \"Not enough!\"\n \n"] | {"fn_name": "meeting", "inputs": [[[["XXX", 3], ["XXXXX", 6], ["XXXXXX", 9]], 4], [[["XXX", 1], ["XXXXXX", 6], ["X", 2], ["XXXXXX", 8], ["X", 3], ["XXX", 1]], 5], [[["XX", 2], ["XXXX", 6], ["XXXXX", 4]], 0], [[["XX", 2], ["XXXX", 6], ["XXXXX", 4]], 8], [[["XX", 2], ["XXXX", 6], ["XXXXX", 4]], 2]], "outputs": [[[0, 1, 3]], [[0, 0, 1, 2, 2]], ["Game On"], ["Not enough!"], [[0, 2]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,649 |
def meeting(rooms, number):
|
dccdfb88de3d4f441e71ffe774adf23b | UNKNOWN | In this Kata, you will be given an array of integers whose elements have both a negative and a positive value, except for one integer that is either only negative or only positive. Your task will be to find that integer.
Examples:
`[1, -1, 2, -2, 3] => 3`
`3` has no matching negative appearance
`[-3, 1, 2, 3, -1, -4, -2] => -4`
`-4` has no matching positive appearance
`[1, -1, 2, -2, 3, 3] => 3`
(the only-positive or only-negative integer may appear more than once)
Good luck! | ["def solve(arr): return sum(set(arr))\n", "def solve(arr):\n for a in arr:\n if -a not in arr:\n return a\n", "def solve(a):\n return sum(set(a))", "def solve(arr):\n return [i for i in arr if -i not in arr][0]", "\ndef solve(arr):\n for n in arr:\n if -n not in arr: return n\n", "def solve(arr):\n for x in arr:\n if x and -x not in arr:\n return x", "def solve(arr):\n for number in arr:\n if number*-1 in arr:\n pass\n else:\n return number", "def solve(arr):\n special_set = set(arr)\n n = 0\n for i in list(special_set):\n n += i\n return n", "def solve(arr):\n summa = 0\n dic = {}\n res = 0\n for i in arr:\n dic[i] = 0\n if -i in arr:\n dic[i] += 1\n else:\n dic[i] = 0\n for key, value in dic.items():\n if value == 0:\n res = key\n return res", "def solve(arr):\n for i in arr:\n if -1*i not in arr:\n return i", "def solve(arr):\n result =0\n for a in arr: \n if -a not in arr :\n return a;", "def solve(arr):\n return [x for x in arr if not(-x in arr)][0]", "def solve(arr):\n return sum(list(set(arr)))\n", "def solve(lst):\n return sum(set(lst))", "def solve(lst):\n for num in lst:\n if -num not in lst:\n return num", "def solve(arr):\n for num in arr:\n if not -num in arr:\n return num", "def solve(arr: list) -> int:\n \"\"\" This function returns one integer that is either only negative or only positive. \"\"\"\n for i in range(len(arr)):\n if -arr[i] not in arr:\n return arr[i]", "def solve(arr):\n nums = []\n unique = 0\n\n for i in arr:\n if -i in arr:\n nums.append(i)\n else:\n unique = i\n\n return unique", "def solve(arr):\n arr = set(arr)\n lst = list(map(abs,arr))\n one = 0\n for i in range(len(lst)):\n if lst.count(lst[i]) == 1:\n one = lst[i]\n\n if one in arr:\n return one\n else:\n a = \"-\"+str(one)\n return int(a)", "def solve(arr):\n result = []\n for i in arr:\n if arr.count(i) != arr.count(-i):\n result.append(i)\n return result[0] ", "def solve(arr):\n ls = [arr[0]]\n for n in arr[1:]:\n if -(n) in ls:\n ls.pop(ls.index(-(n)))\n else:\n ls.append(n)\n return ls[0]", "def solve(arr):\n arr = sorted(arr)\n for i in range(len(arr) - 1):\n if arr[i] == arr[i + 1]:\n return arr[i]\n return sum(arr) \n", "def solve(arr):\n return [el for el in arr if -el not in arr ][0]", "def solve(arr):\n positive = sorted([x for x in arr if x>=0])\n negative = sorted([x for x in arr if x<0], reverse=True)\n longer_index = len(positive) <= len(negative)\n longer = [positive, negative][longer_index]\n for i in [positive, negative][not longer_index]:\n longer.remove(-i)\n return longer[0]", "def solve(arr):\n rev = set(-x for x in arr)\n return [x for x in arr if abs(x) not in set(arr) or x not in rev][0]", "def solve(arr):\n for i in arr:\n reverse = -i\n if reverse not in arr:\n return i", "def solve(arr):\n for num in arr:\n if -num in arr:\n pass\n else:\n return num\n", "def solve(arr):\n \n a = [i for i in arr if arr.count(i) != arr.count(-i)]\n \n return a[0]", "def solve(arr):\n item = arr.pop()\n while(-item in arr):\n arr.remove(-item)\n item = arr.pop()\n return item", "def solve(arr):\n for index, value in enumerate(arr):\n if value * -1 in arr:\n continue\n else:\n return value", "def solve(arr):\n for num in arr:\n if arr.count(num * (-1)) == 0:\n return num", "def solve(arr):\n values = set()\n removed_values = set()\n \n for value in arr:\n if value in removed_values:\n continue\n \n opposite = value*-1\n \n if (opposite) in values:\n values.remove(opposite)\n removed_values.add(value)\n removed_values.add(opposite)\n else:\n values.add(value)\n \n return list(values)[0] \n \n \n", "def solve(arr):\n result = arr[0]\n for i in range(len(arr)):\n pair = False\n for j in range(len(arr)):\n if arr[i] == -arr[j]:\n pair = True\n if (not pair):\n result = arr[i]\n \n return result", "def solve(arr):\n positive_set = set()\n negative_set = set()\n for num in arr:\n if num > 0:\n positive_set.add(num)\n else:\n negative_set.add(-num)\n number = positive_set.symmetric_difference(negative_set)\n popped_number = number.pop()\n return popped_number if popped_number in arr else -popped_number", "def solve(arr):\n duplicates = [x for x in arr if arr.count(x) > 1]\n return duplicates[0] if duplicates else sum(arr)", "def solve(numbers):\n for x in numbers:\n if not -x in numbers:\n return x", "def solve(arr):\n while 1:\n number = arr[0]\n opposite_number = number * (-1)\n if opposite_number in arr:\n arr.remove(number)\n arr.remove(opposite_number)\n else:\n return arr[0]", "def solve(arr):\n\n for n in arr:\n if n < 0 and abs(n) not in arr:\n return n\n elif n > 0 and -n not in arr:\n return n\n", "def solve(arr):\n end = 0\n x = 0\n while end == 0:\n k = 1\n i = arr[x]\n for j in arr:\n if i == j*-1:\n k = 0\n if k == 1:\n ans = i\n end = 1\n x = x + 1\n return ans", "def solve(arr):\n for a in arr:\n if -a in arr:\n continue\n if -a not in arr:\n return a", "def solve(arr):\n \n pos = [ a for a in arr if a >= 0]\n neg = [ a for a in arr if a < 0]\n \n neg = list(map(abs, neg))\n \n print(pos, neg)\n for element in arr:\n if not(abs(element) in pos and abs(element) in neg):\n return element", "def solve(arr):\n a = set(arr)\n return sum(a)", "\ndef match(vale,g):\n va=False\n for val in g:\n if val==-vale:\n va=True\n return(va)\n \ndef solve(g):\n m=[]\n for val in g :\n if match(val,g)==False:\n m.append(val)\n \n return m[0] \n", "def solve(arr):\n cleaned = list(set(arr))\n for i in cleaned:\n if not -i in cleaned:\n return i\n", "def solve(a):\n for n in a:\n if -n in a:\n continue\n else:\n return n", "def solve(arr):\n arr2 = set(arr)\n return sum(arr2)\n", "def solve(arr):\n return list(set(filter(lambda x: arr.count(x) != arr.count(-x), arr)))[0]", "def solve(arr):\n for i in range(0,len(arr)):\n if (-arr[i]) not in arr:\n return arr[i]", "def solve(arr):\n \n for i in range(len(arr)):\n if -1*arr[i] not in arr[0:i] + arr[i+1:]:\n return arr[i]", "def solve(arr):\n arr.sort()\n l=len(arr)\n neg=[]\n pos=[]\n for i in arr:\n if i <0:\n neg.append(i)\n else:\n pos.append(i)\n\n if(len(neg)>len(pos)):\n for i in neg:\n if abs(i) not in pos:\n return i\n else:\n for i in pos:\n if -i not in neg:\n return i\n \n \n \n \n \n \n", "def solve(arr):\n return [value for value in arr if -value not in arr][0]\n \n", "def solve(arr):\n arr = set(arr)\n return next(filter(lambda i :not (sum(arr) - i) ,arr))", "def solve(arr):\n arr = set(arr)\n for item in arr:\n if not (sum(arr) - item):\n return item", "def solve(arr):\n return list(set(x for x in arr if x * -1 not in arr))[0]", "def solve(arr):\n new_arr = [val for val in arr if not arr.count(-val)]\n return new_arr[0]", "def solve(arr):\n arr = set(arr)\n sum = 0\n for i in arr:\n sum+=i\n return sum", "def solve(arr):\n for i in arr:\n if i and -i not in arr:\n return i", "def solve(arr):\n for i in arr:\n if -1*i in arr:\n continue\n else:\n return i", "def solve(arr):\n a = 1\n for i in arr:\n if arr.count(i) > 1:\n a = arr.count(i) \n return sum(arr) / a", "def solve(arr):\n arr.sort()\n \n while len(arr) >= 1:\n if arr[0] < 0 and abs(arr[0]) in arr:\n x = abs(arr[0])\n arr.remove(arr[0])\n arr.remove(x)\n else:\n return arr[0]", "from collections import defaultdict\n\ndef solve(arr):\n d = defaultdict(int)\n for x in arr:\n d[abs(x)] += x\n return next(k if k in arr else -k for k,v in d.items() if v != 0)", "def solve(arr):\n sing = sum(set(arr))\n return sing", "def solve(ar):\n x = len(ar)\n i = 0\n temp = 0\n while i < x:\n c = 0\n char = ar[i]\n while c <= (x - 1):\n if char == (-(ar[c])):\n i += 1\n break\n c += 1\n else:\n temp = char\n return temp\n\n return temp", "def solve(arr):\n for num in arr:\n if arr.count(-num) == 0: return num\n return \n", "def solve(arr):\n \n for i in arr:\n if -i not in arr:\n return i\n return\n", "def solve(arr):\n return list(set([x for x in arr if -x not in arr]))[0]", "def solve(ar):\n \n for item in ar:\n if -item not in ar:\n return item", "def solve(arr):\n pos = set([x for x in arr if x>0])\n neg = set([abs(x) for x in list([x for x in arr if x<0])])\n if pos.issubset(neg):return (neg-pos).pop()*(-1)\n else:return (pos-neg).pop()\n\n\n", "def solve(arr):\n for idx, num in enumerate(arr):\n if num in (arr[:idx] + arr[idx+1:]):\n return arr[idx]\n return sum(arr)\n", "def solve(arr):\n pos = []\n neg = []\n for i in arr:\n if i < 0:\n neg.append(i)\n else:\n pos.append(i)\n for i in neg:\n if abs(i) not in pos:\n return i\n break\n for j in pos:\n if -j not in neg:\n return j\n break", "def solve(arr):\n for i,v in enumerate(arr):\n isTrue = False\n for k,kv in enumerate(arr):\n if i == k:\n continue\n if v == arr[k] * -1:\n isTrue = True\n break\n \n if isTrue == False:\n return v", "def solve(arr):\n return sum(arr) / arr.count(max(arr, key = arr.count))", "def solve(arr):\n parr = []\n narr = []\n for i in arr:\n if i < 0:\n narr.append(i)\n else:\n parr.append(i)\n res = 0\n for a in narr:\n if abs(a) not in parr:\n res = a\n for b in parr:\n if b*-1 not in narr:\n res = b\n return res\n", "\ndef solve(arr):\n check = []\n for i in arr:\n if -i not in arr:\n return i", "from collections import Counter\ndef solve(arr):\n return sum(arr)/Counter(arr).most_common(1)[0][1]", "def solve(arr):\n abs_arr = [abs(n) for n in arr]\n\n for n in set(abs_arr):\n if not(n in arr and -n in arr):\n return arr[abs_arr.index(n)]", "def solve(arr):\n for i in arr:\n m, s = 0, -i\n for j in arr:\n if s == j: m = 1\n if m == 0: return i", "def solve(arr):\n for i in arr:\n m = 0\n s = -i\n for j in arr:\n if s == j: m = 1\n if m == 0: return i", "def solve(arr):\n for num in arr:\n if num > 0 and -num not in arr:\n return num\n elif num < 0 and num-(num*2) not in arr:\n return num", "def solve(arr):\n dict = {}\n for a in arr:\n if abs(a) not in dict:\n dict[abs(a)] = a\n elif dict[abs(a)] == a:\n return a\n break\n else:\n dict[abs(a)] = a + dict[abs(a)]\n for v in list(dict.values()):\n if v != 0:\n return v\n", "def solve(arr):\n newArr = []\n for x in arr:\n if x*-1 not in arr:\n return x", "def solve(arr):\n for num in arr:\n if -1 * num not in arr:\n return num", "def solve(arr):\n for x in arr:\n if not(x*-1 in arr):\n return x", "def solve(arr):\n negative = set([i for i in arr if i < 0])\n positive = set([i for i in arr if i > 0])\n res = None\n for i in negative:\n if i * -1 not in positive:\n res = i\n for i in positive:\n if i * -1 not in negative:\n res = i\n return res", "def solve(arr):\n for i in range(len(arr)):\n count = 0\n if arr.count(arr[i]) > 1:\n return arr[i]\n for j in range(len(arr)):\n if arr[i] == (-arr[j]):\n count += 1\n if count != 1: return arr[i]", "from collections import Counter\ndef solve(arr):\n c = Counter(arr)\n for k, v in c.items():\n if (k*-1) not in c.keys():\n return k", "import collections\ndef solve(arr):\n count = collections.Counter(arr)\n for number in arr:\n if not count[-number]:\n return number", "def solve(arr):\n for val in arr:\n if -1*val not in arr:\n return val\n", "def solve(arr):\n for i in arr:\n if -1*i in arr:\n continue\n return i", "def solve(arr):\n sum = 0\n times = 0\n ele = {}\n for i in arr:\n if i in ele:\n ele[i] = ele[i]+i\n else:\n ele[i] = i\n sum = sum + i\n for k,v in ele.items():\n if v == sum:\n if -k not in arr:\n times = sum/k\n if times > 1:\n sum = sum/times\n return sum", "def solve(arr):\n for num in arr:\n if arr.count(num) > arr.count(-num):\n return num\n elif arr.count(-num) > arr.count(num):\n return -num", "def solve(arr):\n for i, el in enumerate(arr):\n if arr.count(el*-1) == 0:\n return el\n", "def solve(arr):\n return sum(dict.fromkeys(arr))", "def solve(arr):\n for i in arr:\n if i in arr and i * -1 not in arr:\n return i\n", "def solve(arr):\n for x in arr:\n if (-x) not in arr and x in arr or x not in arr and (-x) in arr:\n return x", "def solve(arr):\n for i in arr:\n n=0\n for j in arr:\n n+=1\n if i+j ==0:\n break\n if len(arr)==n and i+j !=0:\n return i", "def solve(arr):\n return [i for i in arr if arr.count(-i) == 0][0]", "def solve(arr):\n for i in range(len(arr)):\n if arr.count(arr[i]*-1) != 1:\n return arr[i]", "def solve(arr):\n while len(set(arr)) > 1:\n for i in range(len(arr) - 1):\n if arr.count(-arr[i]):\n arr.remove(-arr[i])\n arr.remove(arr[i])\n break\n return (list(set(arr))[0])", "def solve(arr):\n for i in range(0,len(arr)):\n num = arr[i]\n reversenumber = -num\n found = False\n for index in range(0, len(arr)):\n if i == index :\n continue\n if reversenumber == arr[index]:\n found = True\n break\n if found == False:\n return num "] | {"fn_name": "solve", "inputs": [[[1, -1, 2, -2, 3]], [[-3, 1, 2, 3, -1, -4, -2]], [[1, -1, 2, -2, 3, 3]], [[-110, 110, -38, -38, -62, 62, -38, -38, -38]], [[-9, -105, -9, -9, -9, -9, 105]]], "outputs": [[3], [-4], [3], [-38], [-9]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 15,969 |
def solve(arr):
|
ccc30e2e8ba221543862a27d7825b201 | UNKNOWN | # Task
Consider a `bishop`, a `knight` and a `rook` on an `n × m` chessboard. They are said to form a `triangle` if each piece attacks exactly one other piece and is attacked by exactly one piece.
Calculate the number of ways to choose positions of the pieces to form a triangle.
Note that the bishop attacks pieces sharing the common diagonal with it; the rook attacks in horizontal and vertical directions; and, finally, the knight attacks squares which are two squares horizontally and one square vertically, or two squares vertically and one square horizontally away from its position.
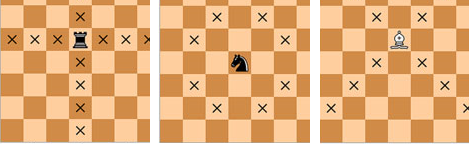
# Example
For `n = 2 and m = 3`, the output should be `8`.
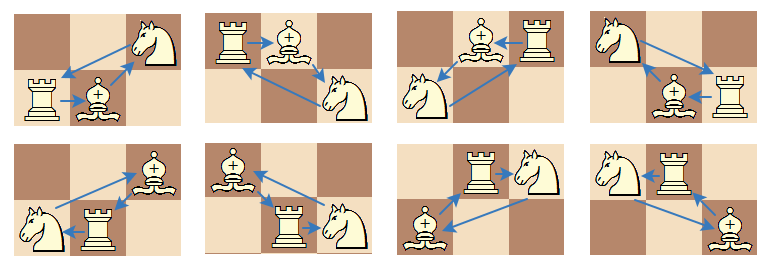
# Input/Output
- `[input]` integer `n`
Constraints: `1 ≤ n ≤ 40.`
- `[input]` integer `m`
Constraints: `1 ≤ m ≤ 40, 3 ≤ n x m`.
- `[output]` an integer | ["def chess_triangle(n, m):\n return sum( 8 * (n-x+1)*(m-y+1) for dims in {(3,4), (3,3), (2,4), (2,3)} for x,y in [dims, dims[::-1]] if x <= n and y <= m )\n", "def chess_triangle(n, m):\n if n>m:\n n,m=m,n\n if m<=2 or n<2:\n return 0\n elif n==2:\n return (m*2-5)*8\n else:\n return (((2*n-4)*(2*n-4)-1)+((m-n)*4*(n-2)))*16\n #coding and coding..\n", "clamp = lambda moves, w, h: {(x, y) for x, y in moves if 0 <= x < w and 0 <= y < h}\nknight = lambda x, y: {(x+u, y+v) for u in range(-2, 3) for v in range(-2, 3) if u*u + v*v == 5}\nrook = lambda x, y, w, h: {(x, v) for v in range(h)} | {(u, y) for u in range(w)} - {(x, y)}\nbishop = lambda x, y, w, h: {(x+d*k, y+k) for d in (-1,1) for k in range(-max(x, y), max(w-x, h-y))} - {(x, y)}\n\ndef search_triangle(x, y, w, h):\n return sum(\n len(rook(x, y, w, h) & clamp(bishop(u, v, w, h), w, h)) +\n len(rook(u, v, w, h) & clamp(bishop(x, y, w, h), w, h))\n for u, v in clamp(knight(x, y), w, h)\n )\n\ndef chess_triangle(h, w):\n wm, hm = min(6, w), min(6, h)\n return (\n sum(search_triangle(min(x, wm-x-1), min(y, hm-y-1), w, h) for x in range(wm) for y in range(hm))\n + max(0, w-6) * sum(search_triangle(3, min(y, hm-y-1), w, h) for y in range(hm))\n + max(0, h-6) * sum(search_triangle(min(x, wm-x-1), 3, w, h) for x in range(wm))\n + max(0, h-6) * max(0, w-6) * search_triangle(3, 3, w, h)\n )", "def chess_triangle(n, m):\n c = 0\n for i in range(n - 1):\n for j in range(m - 2):\n c += 1 + (i - 1 >= 0) + (i + 3 < n) + (j + 3 < m)\n c += 1 + (i + 1 < n and j - 1 >= 0) + (i + 2 < n) + (i - 2 >= 0)\n for i in range(n - 2):\n for j in range(m - 1):\n c += 1 + (j - 1 >= 0) + (j + 3 < m) + (i + 3 < n)\n c += 1 + (j + 1 < m and i - 1 >= 0) + (j + 2 < m) + (j - 2 >= 0)\n return c * 4", "def chess_triangle(n, m):\n if n < 2 or m < 2 or (n == 2 and m == 2):\n return 0\n else:\n a = (max((n-1)*(m-2),0)+max((n-2)*(m-1),0)) #2x3\n b = (max((n-1)*(m-3),0)+max((n-3)*(m-1),0)) #2x4\n c = (max((n-2)*(m-2)*2,0)) #3x3\n d = (max((n-2)*(m-3),0)+max((n-3)*(m-2),0)) #3x4\n return (a+b+c+d)*8", "\nKNIGHTX=[1, 2, 2, 1, -1, -2, -2, -1]\nKNIGHTY=[2, 1, -1, -2, -2, -1, 1, 2]\n\ndef chess_triangle(n, m):\n result=0\n for bishopx, bishopy in zip(KNIGHTX, KNIGHTY):\n for rookx, rooky in [(0, bishopx+bishopy), (0, bishopy-bishopx), (bishopx+bishopy, 0), (bishopx-bishopy, 0)]:\n result+=max(0, n-(max(bishopx, rookx, 0)-min(bishopx, rookx, 0)))*max(0, m-(max(bishopy, rooky, 0)-min(bishopy, rooky, 0)))\n for rookx, rooky in zip(KNIGHTX, KNIGHTY):\n for bishopx, bishopy in [(rookx, rookx), (rookx, -rookx), (rooky, rooky), (-rooky, rooky)]:\n result+=max(0, n-(max(bishopx, rookx, 0)-min(bishopx, rookx, 0)))*max(0, m-(max(bishopy, rooky, 0)-min(bishopy, rooky, 0)))\n return result", "def chess_triangle(n, m):\n x23 = max(0, n - 1) * max(0, m - 2) * 8;\n x32 = max(0, n - 2) * max(0, m - 1) * 8;\n x24 = max(0, n - 1) * max(0, m - 3) * 8;\n x42 = max(0, n - 3) * max(0, m - 1) * 8;\n x34 = max(0, n - 2) * max(0, m - 3) * 8;\n x43 = max(0, n - 3) * max(0, m - 2) * 8;\n x33 = max(0, n - 2) * max(0, m - 2) * 16;\n return x23 + x32 + x24 + x42 + x34 + x43 + x33", "moves=[\n [(-2,-1),[(-3,0),(-1,0),(0,-3),(0,1),(-2,-2),(-2,2),(-1,-1),(1,-1)]],\n [(-1,-2),[(-3,0),(1,0),(0,-3),(0,-1),(-2,-2),(2,-2),(-1,-1),(-1,1)]],\n [(1,-2),[(3,0),(-1,0),(0,-3),(0,-1),(-2,-2),(2,-2),(1,-1),(1,1)]],\n [(2,-1),[(3,0),(1,0),(0,-3),(0,1),(2,-2),(2,2),(-1,-1),(1,-1)]],\n [(-2,1),[(-3,0),(-1,0),(0,3),(0,-1),(-2,-2),(-2,2),(-1,1),(1,1)]],\n [(-1,2),[(-3,0),(1,0),(0,3),(0,1),(-2,2),(2,2),(-1,-1),(-1,1)]],\n [(1,2),[(3,0),(-1,0),(0,3),(0,1),(-2,2),(2,2),(1,-1),(1,1)]],\n [(2,1),[(3,0),(1,0),(0,3),(0,-1),(2,-2),(2,2),(-1,1),(1,1)]]\n]\ndef chess_triangle(n, m):\n r=0\n for x in range(n):\n for y in range(m):\n for (dx,dy),move in moves:\n if not (0<=x+dx<n and 0<=y+dy<m):\n continue\n for mx,my in move:\n if 0<=x+mx<n and 0<=y+my<m:\n r+=1\n return r", "def chess_triangle(n, m):\n res = 0\n # 3x2 / 2x2\n n_,m_=n-1,m-2\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n n_,m_=n-2,m-1\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n # 4x2 / 2x4\n n_,m_=n-1,m-3\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n n_,m_=n-3,m-1\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n # 4x3 / 3x4\n n_,m_=n-2,m-3\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n n_,m_=n-3,m-2\n if 0 < n_ and 0 < m_:\n res += n_*m_*8\n # 3x3\n n_,m_=n-2,m-2\n if 0 < n_ and 0 < m_:\n res += n_*m_*16\n return res", "chess_triangle = lambda n, m: (lambda x, y: 0 if x<2 else max(0, 16*y-40) if x==2 else 240 + 64*y*(x-2) - 128*x)(*sorted([n, m]))"] | {"fn_name": "chess_triangle", "inputs": [[2, 3], [1, 30], [3, 3], [2, 2], [5, 2], [40, 40]], "outputs": [[8], [0], [48], [0], [40], [92400]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,026 |
def chess_triangle(n, m):
|
c5715b09d9cca40e03dc585ef0bfaf6f | UNKNOWN | Write a function that returns the count of characters that have to be removed in order to get a string with no consecutive repeats.
*Note:* This includes any characters
## Examples
```python
'abbbbc' => 'abc' # answer: 3
'abbcca' => 'abca' # answer: 2
'ab cca' => 'ab ca' # answer: 1
``` | ["from itertools import groupby\ndef count_repeats(s):\n return len(s) - len(list(groupby(s)))", "def count_repeats(str):\n return sum(a == b for a, b in zip(str, str[1:]))", "from itertools import groupby\n\ndef count_repeats(txt):\n return len(txt) - sum(1 for _ in groupby(txt))", "import re\n\ndef count_repeats(str):\n return len(str) - len(re.sub(r'(.)\\1+', r'\\1', str))", "def count_repeats(string):\n\n \n temp = \"\"\n count = 0\n for i in list(string):\n if i == temp:\n count += 1\n temp = i\n \n return count", "def count_repeats(txt):\n a = 0\n for i in range(0, len(txt) - 1):\n if txt[i] == txt[i + 1]:\n a += 1\n return a", "def count_repeats(txt):\n return sum(a == b for a, b in zip(txt, txt[1:]))", "import re\ndef count_repeats(str):\n return sum(len(i[0])-1 for i in re.findall(r\"((.)\\2+)\",str))", "count_repeats = lambda s: sum(sum(1 for _ in n) - 1 for _, n in __import__(\"itertools\").groupby(s))", "def count_repeats(txt):\n prev = None\n removed_chars = 0\n\n for ch in txt:\n if ch == prev:\n removed_chars += 1\n prev = ch\n\n return removed_chars"] | {"fn_name": "count_repeats", "inputs": [["abcdefg"], ["aabbccddeeffgg"], ["abcdeefee"], ["122453124"], ["@*$##^^^*)*"], ["abmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmvxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa"]], "outputs": [[0], [7], [2], [1], [3], [1177]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,208 |
def count_repeats(txt):
|
43885def3956afc686eedc643c4470f3 | UNKNOWN | Write a method named `getExponent(n,p)` that returns the largest integer exponent `x` such that p^(x) evenly divides `n`. if `p<=1` the method should return `null`/`None` (throw an `ArgumentOutOfRange` exception in C#). | ["def get_exponent(n, p):\n if p > 1:\n x = 0\n while not n % p:\n x += 1\n n //= p\n return x", "def get_exponent(n, p, i = 0):\n if p <= 1: return None\n return get_exponent(n / p, p, i + 1) if n / p == n // p else i", "from itertools import count\n\ndef get_exponent(n, p):\n if p > 1:\n for res in count():\n n, r = divmod(n, p)\n if r:\n return res", "def get_exponent(n, p):\n return next(iter(i for i in range(int(abs(n) ** (1/p)), 0, -1) if (n / p**i) % 1 == 0), 0) if p > 1 else None", "def get_exponent(n, p):\n if p <= 1:\n return None\n x, e = 0, 1\n while n % e == 0:\n x += 1\n e *= p\n return x - 1", "def get_exponent(n, p):\n i=1\n if p<=1:\n return None\n while n % p**i==0:\n i+=1\n return i-1\n", "def get_exponent(n, p):\n if p <=1 : return \n return max([i for i in range(1,int(abs(n)**.5)) if n% (p ** i)==0],default=0)", "def get_exponent(n,p):\n if int(p)<=1:\n return None\n else:\n x=1\n while n%(p**x)==0:\n x+=1\n return x-1", "def get_exponent(n, p):\n r = 0\n while n%(p**r)==0 and p > 1: r += 1\n if r > 0: return r-1", "from math import log\ndef get_exponent(n, p):\n if p<=1:return None\n l,i=None,0\n while p**i<=abs(n):\n if n%p**i==0:l=i \n i+=1\n return l"] | {"fn_name": "get_exponent", "inputs": [[27, 3]], "outputs": [[3]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,436 |
def get_exponent(n, p):
|
8ab08da691f3fd6d545e0611e6a51253 | UNKNOWN | In this Kata, you will be given a number `n` (`n > 0`) and your task will be to return the smallest square number `N` (`N > 0`) such that `n + N` is also a perfect square. If there is no answer, return `-1` (`nil` in Clojure, `Nothing` in Haskell, `None` in Rust).
```clojure
solve 13 = 36
; because 36 is the smallest perfect square that can be added to 13 to form a perfect square => 13 + 36 = 49
solve 3 = 1 ; 3 + 1 = 4, a perfect square
solve 12 = 4 ; 12 + 4 = 16, a perfect square
solve 9 = 16
solve 4 = nil
```
```csharp
solve(13) = 36
//because 36 is the smallest perfect square that can be added to 13 to form a perfect square => 13 + 36 = 49
solve(3) = 1 // 3 + 1 = 4, a perfect square
solve(12) = 4 // 12 + 4 = 16, a perfect square
solve(9) = 16
solve(4) = -1
```
```haskell
solve 13 = Just 36
-- because 36 is the smallest perfect square that can be added to 13 to form a perfect square => 13 + 36 = 49
solve 3 = Just 1 -- 3 + 1 = 4, a perfect square
solve 12 = Just 4 -- 12 + 4 = 16, a perfect square
solve 9 = Just 16
solve 4 = Nothing
```
```python
solve(13) = 36
# because 36 is the smallest perfect square that can be added to 13 to form a perfect square => 13 + 36 = 49
solve(3) = 1 # 3 + 1 = 4, a perfect square
solve(12) = 4 # 12 + 4 = 16, a perfect square
solve(9) = 16
solve(4) = -1
```
More examples in test cases.
Good luck! | ["def solve(n):\n for i in range(int(n**0.5), 0, -1):\n x = n - i**2\n if x > 0 and x % (2*i) == 0:\n return ((n - i ** 2) // (2 * i)) ** 2\n return -1", "def solve(n):\n#Imagine a table, 1 to n on the top, and 1 to n on the side.\n#The contents of the table are the difference between the columnNo.^2 minus the rowNo.^2 (positive values only)\n#Therefore the row must always be smaller in value than the column number.\n#The first diagonal shows values of the pattern 2*rowNo. + 1, the second 4*rowNo. + 4, the third 6*rowNo. + 9.\n#Therefore let rowNo. = R, and the diagonal = D and the value in the table be n.\n#n = 2*D*R - D^2\n#Rerarrage to get R = (n-(D ** 2))/(2 * D) \n\n answer = -1\n for D in range(1, max(int(n ** 0.5),5)):\n R = (n-(D ** 2))/(2 * D)\n if R.is_integer() and R > 0:\n answer = R ** 2\n\n return answer", "solve=lambda n:next((((n-x*x)//2//x)**2for x in range(int(n**.5),0,-1)if(n-x*x)%(2*x)==0<n-x*x),-1)", "def solve(n):\n res = float('inf')\n for x in range(1, int(n**0.5)+1):\n y, r = divmod(n, x)\n if not (r or x == y) and y-x & 1 ^ 1:\n res = min(res, y-x >> 1)\n return -1 if res == float('inf') else res**2", "import math\ndef solve(n):\n for i in range(math.ceil(n**0.5)-1, 0, -1):\n if n%i == 0:\n bma = n/i - i\n if bma%2 == 0:\n return (bma/2)**2\n return -1", "def solve(n): \n X = 1e9\n for i in range(1, int(n**(1/2)) + 1): \n if n % i == 0: \n a = i \n b = n // i \n if b - a != 0 and (b - a) % 2 == 0: \n X = min(X, (b - a) // 2) \n return(X * X if X != 1e9 else -1) ", "def solve(d):\n if (d%4) != 2:\n for i in range(1,(d//2)+1):\n if (d+(i*i))**.5 % 1 == 0:\n return i*i\n return -1", "def solve(n):\n if (n%2 == 0 and n%4 != 0) or n == 1 or n == 4:\n return -1\n for i in range(n-1):\n if ((n + (i+1)**2)**0.5)%1 == 0:\n return (i+1)**2\n", "#let N be a^2\n#n + N = M^2 (our answer)\n#n + a^2 = m^2\n#stuff in slimesquad chat to read from\nimport math\ndef solve(n):\n d = math.ceil(math.sqrt(n)) - 1 #find the last biggest root we can use thats not the same number\n #now we need to go backwards because it cant be bigger than root N\n #we need to find a pair of factors for n (which is equal to x*y in discord) that are the same even and odd\n #or else A wouldnt be even\n print(d)\n for a in range(d, 0, -1):#the last argument is to go backwards\n #if we have Found a factor of N that is below the root(N) (finding the closest factors)\n #We go down from Root (N) because the further we go down, the more further apart the factors are\n #and if the difference gets bigger, we will have a bigger square number because the number\n #we need to find is the difference divided by 2\n if (n % a == 0):\n #then find the second factor it could be\n #we can do this easily\n e = (n/a) - a\n print((a,e))\n if e % 2 == 0:\n p = e / 2\n return p*p\n return -1\n"] | {"fn_name": "solve", "inputs": [[1], [2], [3], [4], [5], [7], [8], [9], [10], [11], [13], [17], [88901], [290101]], "outputs": [[-1], [-1], [1], [-1], [4], [9], [1], [16], [-1], [25], [36], [64], [5428900], [429235524]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,211 |
def solve(n):
|
883cde87fddb53adca0c2e84f3fe537b | UNKNOWN | # Task
Given a string `s`, find out if its characters can be rearranged to form a palindrome.
# Example
For `s = "aabb"`, the output should be `true`.
We can rearrange `"aabb"` to make `"abba"`, which is a palindrome.
# Input/Output
- `[input]` string `s`
A string consisting of lowercase English letters.
Constraints:
`4 ≤ inputString.length ≤ 50.`
- `[output]` a boolean value
`true` if the characters of the inputString can be rearranged to form a palindrome, `false` otherwise. | ["def palindrome_rearranging(s):\n return sum(s.count(c) % 2 for c in set(s)) < 2", "from collections import Counter\n\ndef palindrome_rearranging(s):\n return sum(n % 2 for n in Counter(s).values()) <= 1", "def palindrome_rearranging(s):\n d = {}\n c = 0\n for x in s:\n if x not in d:\n d[x] = 1\n else:\n d[x] += 1\n for k, v in d.items():\n if v % 2 != 0:\n c += 1\n return c <= 1", "from collections import Counter\n\ndef palindrome_rearranging(s):\n return sum(v%2 for v in Counter(s).values()) < 2", "from collections import Counter\npalindrome_rearranging=lambda s:len(list(filter(lambda x:x&1,Counter(s).values())))<2", "def palindrome_rearranging(s):\n odd =0\n for char in set(s):\n if s.count(char)%2 != 0:\n odd+=1\n if odd>1:\n return False\n else:\n return True", "def palindrome_rearranging(s):\n return [s.count(i)%2 for i in set(s)].count(1) == len(s)%2", "def palindrome_rearranging(s):\n for c in s:\n if s.count(c) > 1:\n s = s.replace(c, \"\", 2)\n return len(s) < 2\n", "def palindrome_rearranging(s):\n return sum(s.count(char) % 2 for char in set(s)) <= 1\n", "from collections import Counter\ndef palindrome_rearranging(s):\n return len([v for v in Counter(s).values() if v%2]) < 2"] | {"fn_name": "palindrome_rearranging", "inputs": [["aabb"], ["aaaaaaaaaaaaaaaaaaaaaaaaaaaaaabc"], ["abbcabb"], ["zyyzzzzz"], ["aaabbb"]], "outputs": [[true], [false], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,364 |
def palindrome_rearranging(s):
|
6ca337ba26e212d99336fb35fc6ba1e7 | UNKNOWN | You should write a function that takes a string and a positive integer `n`, splits the string into parts of length `n` and returns them in an array. It is ok for the last element to have less than `n` characters.
If `n` is not a valid size (`> 0`) (or is absent), you should return an empty array.
If `n` is greater than the length of the string, you should return an array with the only element being the same string.
Examples:
```python
string_chunk('codewars', 2) # ['co', 'de', 'wa', 'rs']
string_chunk('thiskataeasy', 4) # ['this', 'kata', 'easy']
string_chunk('hello world', 3) # ['hel', 'lo ', 'wor', 'ld']
string_chunk('sunny day', 0) # []
``` | ["def string_chunk(string, n=0):\n return [string[i:i+n] for i in range(0,len(string), n)] if isinstance(n, int) and n > 0 else []\n", "def string_chunk(s, n=0):\n if not isinstance(n, int) or n == 0:\n return []\n return [s[i:i+n] for i in range(0, len(s), n)]", "def string_chunk(string, n=None):\n ret = []\n if isinstance(n, int) and n > 0:\n for i in range(0, len(string), n):\n ret.append(string[i:i+n])\n return ret", "def string_chunk(xs, n=None):\n try:\n return [xs[i:i+n] for i in range(0, len(xs), n)] \n except:\n return []", "def string_chunk(string, n=0):\n if isinstance(n, int) and n > 0:\n starts = range(0, len(string), n)\n else:\n starts = []\n return [string[i:i+n] for i in starts]", "def string_chunk(s, n=0):\n return [s[i:i + n] for i in range(0, len(s), n)] if isinstance(n, int) and n > 0 else []", "def string_chunk(string, n=0):\n if isinstance(n, int) and n>0:\n return [string[i:i+n] for i in range(0, len(string), n)]\n else:\n return []", "def string_chunk(string, n=None):\n if isinstance(n, int):\n if n > 0:\n return [string[i:i+n] for i in range(0, len(string), n)] \n else: return []\n else: return []\n", "def string_chunk(string, n=0):\n try:\n return [string[a:a + n] for a in range(0, len(string), n)]\n except (TypeError, ValueError):\n return []\n", "def string_chunk(string, n = None):\n if n > 0 and n != None and isinstance(n, int):\n return [string[i:i+n] for i in range(0, len(string), n)]\n else:\n return []"] | {"fn_name": "string_chunk", "inputs": [["codewars", 2]], "outputs": [[["co", "de", "wa", "rs"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,615 |
def string_chunk(string, n=0):
|
cf8718d64881918b54b1f37a84371d3c | UNKNOWN | # Task
A media access control address (MAC address) is a unique identifier assigned to network interfaces for communications on the physical network segment.
The standard (IEEE 802) format for printing MAC-48 addresses in human-friendly form is six groups of two hexadecimal digits (0 to 9 or A to F), separated by hyphens (e.g. 01-23-45-67-89-AB).
# Example
For `inputString = "00-1B-63-84-45-E6"`, the output should be `true`;
For `inputString = "Z1-1B-63-84-45-E6"`, the output should be `false`;
For `inputString = "not a MAC-48 address"`, the output should be `false`.
# Input/Output
- `[input]` string `inputString`
- `[output]` a boolean value
`true` if inputString corresponds to MAC-48 address naming rules, `false` otherwise. | ["import re\ndef is_mac_48_address(address):\n return bool(re.match(\"^([0-9A-F]{2}[-]){5}([0-9A-F]{2})$\", address.upper()))", "def is_mac_48_address(address):\n chunks = address.split('-')\n for chunk in chunks:\n try:\n int(chunk, 16)\n except ValueError:\n return False\n return len(chunks) == 6\n", "def is_mac_48_address(address):\n try:\n bytes = address.split(\"-\")\n return all(0 <= int(byte, 16) < 256 for byte in bytes) and len(bytes) == 6\n except:\n return False", "def is_mac_48_address(address):\n L = address.split('-')\n if len(L) != 6: return False\n for x in L:\n try: int(x, 16)\n except: return False\n return True", "import re\ndef is_mac_48_address(address):\n return bool(re.match('[0-9A-F]{2}(-[0-9A-F]{2}){5}$', address))", "is_mac_48_address = lambda address: bool( __import__(\"re\").match('-'.join(['[0-9A-F]{2}']*6) + '$', address) )", "def is_mac_48_address(address):\n try:\n return all(isinstance(int(x, 16),int) for x in address.split('-')) and len(address) == 17\n except:\n return False", "is_mac_48_address=lambda a:a.count(' ')==0and a.count('-')==5and a[0]not in['Z','G']", "def is_mac_48_address(address):\n try:\n return len([int(e, 16) for e in address.split('-')]) == 6\n except (ValueError, TypeError):\n return False", "import re;is_mac_48_address=lambda s,a='[0-9][A-F]|[0-9]{2}|[A-F]{2}|[A-F][0-9]':len(re.findall(r'-|{}'.format(a),s))==11"] | {"fn_name": "is_mac_48_address", "inputs": [["00-1B-63-84-45-E6"], ["Z1-1B-63-84-45-E6"], ["not a MAC-48 address"], ["FF-FF-FF-FF-FF-FF"], ["00-00-00-00-00-00"], ["G0-00-00-00-00-00"], ["12-34-56-78-9A-BC"], ["02-03-04-05-06-07-"], ["02-03-04-05"], ["02-03-04-FF-00-F0"]], "outputs": [[true], [false], [false], [true], [true], [false], [true], [false], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,531 |
def is_mac_48_address(address):
|
75cfc638f418a22cf4ef23e17d5259f4 | UNKNOWN | A trick I learned in elementary school to determine whether or not a number was divisible by three is to add all of the integers in the number together and to divide the resulting sum by three. If there is no remainder from dividing the sum by three, then the original number is divisible by three as well.
Given a series of numbers as a string, determine if the number represented by the string is divisible by three.
You can expect all test case arguments to be strings representing values greater than 0.
Example:
```
"123" -> true
"8409" -> true
"100853" -> false
"33333333" -> true
"7" -> false
``` | ["def divisible_by_three(s): \n return int(s) % 3 == 0\n\n", "def divisible_by_three(st):\n return (sum([int(i) for i in st]) % 3) == 0\n", "def divisible_by_three(st): \n return sum([int(c) for c in st]) % 3 == 0", "import re\ndef divisible_by_three(st):\n return re.match(\"(((((0|3)|6)|9)|((2|5)|8)((((0|3)|6)|9))*((1|4)|7))|(((1|4)|7)|((2|5)|8)((((0|3)|6)|9))*((2|5)|8))(((((0|3)|6)|9)|((1|4)|7)((((0|3)|6)|9))*((2|5)|8)))*(((2|5)|8)|((1|4)|7)((((0|3)|6)|9))*((1|4)|7)))+$\", st) != None", "def divisible_by_three(s):\n return not int(s) % 3", "# You can just do \"int(st) % 3 == 0\"\n\ndef divisible_by_three(st): \n while len(st) > 1:\n st = str(sum(map(int, st)))\n return st in \"369\"", "def divisible_by_three(st): \n num_str = str(st)\n sum = 0\n # iterate and add the numbers up\n for i in range(0, len(num_str)):\n sum += int(num_str[i])\n # check if numbers are divisible by 3\n if sum % 3 == 0:\n return True\n else:\n return False\n pass", "def divisible_by_three(st): \n sum = 0\n while int(st) > 0:\n digit = int(st)%10\n sum = sum + digit\n st = int(st)//10\n if sum%3 == 0:\n return True\n else:\n return False ", "def divisible_by_three(string):\n return not (sum(int(n) for n in string) % 3)", "def divisible_by_three(stg): \n return sum(int(d) for d in stg) % 3 == 0\n \n# `int(stg) % 3 == 0` is straight but not what was asked\n"] | {"fn_name": "divisible_by_three", "inputs": [["123"], ["19254"], ["88"], ["1"], ["963210456"], ["10110101011"], ["9"], ["6363"], ["10987654321"], ["9876543211234567890009"], ["9876543211234567890002"]], "outputs": [[true], [true], [false], [false], [true], [false], [true], [true], [false], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,480 |
def divisible_by_three(st):
|
13a0489f2edefde6b49b0001d644ffd9 | UNKNOWN | In this kata you need to build a function to return either `true/True` or `false/False` if a string can be seen as the repetition of a simpler/shorter subpattern or not.
For example:
```cpp,java
hasSubpattern("a") == false; //no repeated pattern
hasSubpattern("aaaa") == true; //created repeating "a"
hasSubpattern("abcd") == false; //no repeated pattern
hasSubpattern("abababab") == true; //created repeating "ab"
hasSubpattern("ababababa") == false; //cannot be entirely reproduced repeating a pattern
```
```python
has_subpattern("a") == False #no repeated pattern
has_subpattern("aaaa") == True #created repeating "a"
has_subpattern("abcd") == False #no repeated pattern
has_subpattern("abababab") == True #created repeating "ab"
has_subpattern("ababababa") == False #cannot be entirely reproduced repeating a pattern
```
Strings will never be empty and can be composed of any character (just consider upper- and lowercase letters as different entities) and can be pretty long (keep an eye on performances!).
If you liked it, go for the [next kata](https://www.codewars.com/kata/string-subpattern-recognition-ii/) of the series! | ["def has_subpattern(string):\n return (string * 2).find(string, 1) != len(string)", "import re\n\ndef has_subpattern(string):\n return bool(re.match(r'(.+)\\1+$', string))", "def has_subpattern(string):\n s = len(string)\n for i in range(1,s//2+1):\n if s % i == 0:\n q = s//i\n if string[:i] * q == string:\n return True\n return False", "import re\n\ndef has_subpattern(s):\n return bool(re.search(r'^(.+)\\1+$', s))", "has_subpattern=lambda s:s in(2*s)[1:-1]", "import re\ndef has_subpattern(s):\n return re.search(r'^(.+?)\\1{1,}$', s) != None", "def has_subpattern(s):\n l = len(s)\n for i in range(1,l//2+1):\n if not l%i and s[:i]*(l//i) == s : \n return True\n return False ", "def has_subpattern(stg):\n l = len(stg)\n for k in range(2, int(l**0.5) + 1):\n if l % k == 0:\n if any(len({stg[i:i+s] for i in range(0, l, s)}) == 1 for s in (l // k, k)):\n return True\n return False\n\n# one-liner\n #return any(any(len({stg[i:i+s] for i in range(0, len(stg), s)}) == 1 for s in (len(stg) // k, k)) for k in range(2, int(len(stg)**0.5)+1) if len(stg) % k == 0)\n", "import re\ndef has_subpattern(string):\n #print(string)\n n = len(string)\n if n == 1: return False\n l, h = '{}'\n for i in range(1,n):\n #print(f'({string[:i]}){l}{n/i}{h}')\n if n % i == 0 and string[:i] * (n//i) == string:\n #print(string[:i])\n return True\n return False", "def has_subpattern(s):\n return False if (s + s).find(s, 1, -1) == -1 else True"] | {"fn_name": "has_subpattern", "inputs": [["a"], ["aaaa"], ["abcd"], ["abababab"], ["ababababa"], ["123a123a123a"], ["123A123a123a"], ["abbaabbaabba"], ["abbabbabba"], ["abcdabcabcd"]], "outputs": [[false], [true], [false], [true], [false], [true], [false], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,637 |
def has_subpattern(string):
|
d4e0831e9971a9f4785ae0c4bead3e49 | UNKNOWN | # Task
Let's call `product(x)` the product of x's digits. Given an array of integers a, calculate `product(x)` for each x in a, and return the number of distinct results you get.
# Example
For `a = [2, 8, 121, 42, 222, 23]`, the output should be `3`.
Here are the products of the array's elements:
```
2: product(2) = 2;
8: product(8) = 8;
121: product(121) = 1 * 2 * 1 = 2;
42: product(42) = 4 * 2 = 8;
222: product(222) = 2 * 2 * 2 = 8;
23: product(23) = 2 * 3 = 6.```
As you can see, there are only `3` different products: `2, 6 and 8.`
# Input/Output
- `[input]` integer array `a`
Constraints:
`1 ≤ a.length ≤ 10000,`
`1 ≤ a[i] ≤ 1000000000.`
- `[output]` an integer
The number of different digit products in `a`. | ["from operator import mul\nfrom functools import reduce\n\n\ndef unique_digit_products(nums):\n return len({reduce(mul, (int(a) for a in str(num))) for num in nums})\n", "from functools import reduce\nfrom operator import mul\n\ndef unique_digit_products(a):\n return len({reduce(mul, map(int, str(x))) for x in a})", "def unique_digit_products(a):\n return len(set(eval('*'.join(str(x))) for x in a))", "from functools import reduce\n\ndef product(x):\n return reduce(lambda a, b: a * b, (int(d) for d in str(x)), 1)\n \ndef unique_digit_products(a):\n return len({product(x) for x in a})", "def digits(n):\n p = 1\n m = n\n while n:\n p *= n % 10\n n //= 10\n return m if m < 10 else p\n \ndef unique_digit_products(a):\n return len(set([digits(i) for i in a]))", "unique_digit_products=lambda a:len({eval('*'.join(str(n)))for n in a})", "unique_digit_products = lambda a, r=__import__(\"functools\").reduce: len({r(int.__mul__, map(int, str(e))) for e in a})"] | {"fn_name": "unique_digit_products", "inputs": [[[2, 8, 121, 42, 222, 23]], [[239]], [[100, 101, 111]], [[100, 23, 42, 239, 22339, 9999999, 456, 78, 228, 1488]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]]], "outputs": [[3], [1], [2], [10], [10]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,017 |
def unique_digit_products(a):
|
92e8c832f80b635d0fcd9d187190c80c | UNKNOWN | Write a function
```python
alternate_sort(l)
```
that combines the elements of an array by sorting the elements ascending by their **absolute value** and outputs negative and non-negative integers alternatingly (starting with the negative value, if any).
E.g.
```python
alternate_sort([5, -42, 2, -3, -4, 8, -9,]) == [-3, 2, -4, 5, -9, 8, -42]
alternate_sort([5, -42, 2, -3, -4, 8, 9]) == [-3, 2, -4, 5, -42, 8, 9]
alternate_sort([5, 2, -3, -4, 8, -9]) == [-3, 2, -4, 5, -9, 8]
alternate_sort([5, 2, 9, 3, 8, 4]) == [2, 3, 4, 5, 8, 9]
``` | ["from itertools import chain, zip_longest\n\ndef alternate_sort(l):\n l=sorted(l,key=abs)\n p,n=[n for n in l if n>=0],[n for n in l if n<0]\n return [n for n in chain(*zip_longest(n,p)) if n is not None]", "def alternate_sort(l):\n a = sorted([i for i in l if i >= 0])[::-1]\n b = sorted([i for i in l if i not in a])\n res = []\n while len(a) or len(b):\n if len(b):\n res.append(b.pop())\n if len(a):\n res.append(a.pop())\n return res", "from itertools import chain, zip_longest\ndef alternate_sort(l):\n return list(filter(lambda x: x is not None, chain(*zip_longest( sorted(filter(lambda x: x<0,l))[::-1],sorted(filter(lambda x: x>-1,l))))))", "from itertools import chain, zip_longest\n\n# Credit to falsetru for the sentinel\nsentinel = object()\ndef alternate_sort(l):\n positive = sorted(filter((0).__le__, l))\n negative = sorted(filter((0).__gt__, l), reverse=True)\n return list(filter(lambda x: x is not sentinel, chain.from_iterable(zip_longest(negative, positive, fillvalue=sentinel))))", "def alternate_sort(l):\n res = sorted(l, key=lambda x: abs(x))\n n = 1\n new_l = []\n while res:\n for x in res:\n if (x>=0, x<0)[n%2]:\n new_l.append(x)\n res.remove(x)\n break\n n += 1\n return new_l", "def alternate_sort(list):\n positive_list = []\n negative_list = []\n for number in list:\n if number >= 0:\n positive_list.append(number)\n else:\n negative_list.append(number)\n positive_list.sort()\n negative_list.sort(reverse = True)\n i = 0\n for negative in negative_list:\n positive_list.insert(i * 2, negative)\n i += 1\n return positive_list\n", "def alternate_sort(l):\n xs = sorted(l)\n pos, neg, result = [], [], []\n for x in xs:\n [pos, neg][x < 0].append(x)\n while pos and neg:\n result.extend([neg.pop(), pos.pop(0)])\n return result + neg[::-1] + pos", "def alternate_sort(l):\n negatives = sorted((n for n in l if n < 0), key=abs)\n positives = sorted(n for n in l if n > -1)\n return [lst[i]\n for i in range(max(len(negatives), len(positives)))\n for lst in (negatives, positives)\n if i < len(lst)]", "def alternate_sort(l):\n new=[]\n neg=[x for x in l if x < 0]\n neg.sort()\n neg=neg[::-1]\n pos=[x for x in l if x >= 0]\n pos.sort()\n while neg or pos:\n if neg:\n new.append(neg.pop(0))\n if pos:\n new.append(pos.pop(0))\n return (new)", "def alternate_sort(l):\n l = sorted(l, key=abs)\n positives = [n for n in l if n >= 0]\n negatives = [n for n in l if n < 0]\n\n result = []\n while positives and negatives:\n result.append( negatives.pop(0) )\n result.append( positives.pop(0) )\n return result + positives + negatives"] | {"fn_name": "alternate_sort", "inputs": [[[5, 2, -3, -9, -4, 8]], [[5, -42, 2, -3, -4, 8, 9]], [[5, -42, 8, 2, -3, -4, 9]], [[5, -42, -8, 2, -3, -4, -9]], [[5, 2, 3, 4, 8, 9]], [[-5, -2, -3, -4, -8, -9]], [[-5, -2, 3, 4, -8, 0, -9]], [[-5, -2, 3, 9, 4, -2, -8, 0, 9, -9]]], "outputs": [[[-3, 2, -4, 5, -9, 8]], [[-3, 2, -4, 5, -42, 8, 9]], [[-3, 2, -4, 5, -42, 8, 9]], [[-3, 2, -4, 5, -8, -9, -42]], [[2, 3, 4, 5, 8, 9]], [[-2, -3, -4, -5, -8, -9]], [[-2, 0, -5, 3, -8, 4, -9]], [[-2, 0, -2, 3, -5, 4, -8, 9, -9, 9]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,948 |
def alternate_sort(l):
|
d314331173f0f18a626340b502d465f4 | UNKNOWN | ### Vaccinations for children under 5
You have been put in charge of administrating vaccinations for children in your local area. Write a function that will generate a list of vaccines for each child presented for vaccination, based on the child's age and vaccination history, and the month of the year.
#### The function takes three parameters: age, status and month
- The parameter 'age' will be given in weeks up to 16 weeks, and thereafter in months. You can assume that children presented will be scheduled for vaccination (eg '16 weeks', '12 months' etc).
- The parameter 'status' indicates if the child has missed a scheduled vaccination, and the argument will be a string that says 'up-to-date', or a scheduled stage (eg '8 weeks') that has been missed, in which case you need to add any missing shots to the list. Only one missed vaccination stage will be passed in per function call.
- If the month is 'september', 'october' or 'november' add 'offer fluVaccine' to the list.
- Make sure there are no duplicates in the returned list, and sort it alphabetically.
#### Example input and output
~~~~
input ('12 weeks', 'up-to-date', 'december')
output ['fiveInOne', 'rotavirus']
input ('12 months', '16 weeks', 'june')
output ['fiveInOne', 'hibMenC', 'measlesMumpsRubella', 'meningitisB', 'pneumococcal']
input ('40 months', '12 months', 'october')
output ['hibMenC', 'measlesMumpsRubella', 'meningitisB', 'offer fluVaccine', 'preSchoolBooster']
~~~~
#### To save you typing it up, here is the vaccinations list
~~~~
fiveInOne : ['8 weeks', '12 weeks', '16 weeks'],
//Protects against: diphtheria, tetanus, whooping cough, polio and Hib (Haemophilus influenzae type b)
pneumococcal : ['8 weeks', '16 weeks'],
//Protects against: some types of pneumococcal infection
rotavirus : ['8 weeks', '12 weeks'],
//Protects against: rotavirus infection, a common cause of childhood diarrhoea and sickness
meningitisB : ['8 weeks', '16 weeks', '12 months'],
//Protects against: meningitis caused by meningococcal type B bacteria
hibMenC : ['12 months'],
//Protects against: Haemophilus influenzae type b (Hib), meningitis caused by meningococcal group C bacteria
measlesMumpsRubella : ['12 months', '40 months'],
//Protects against: measles, mumps and rubella
fluVaccine : ['september','october','november'],
//Given at: annually in Sept/Oct
preSchoolBooster : ['40 months']
//Protects against: diphtheria, tetanus, whooping cough and polio
~~~~ | ["from itertools import chain\n\nTOME = {\n '8 weeks': ['fiveInOne', 'pneumococcal', 'rotavirus', 'meningitisB'],\n '12 weeks': ['fiveInOne', 'rotavirus'],\n '16 weeks': ['fiveInOne', 'pneumococcal', 'meningitisB'],\n '12 months': ['meningitisB', 'hibMenC', 'measlesMumpsRubella'],\n '40 months': ['measlesMumpsRubella', 'preSchoolBooster'],\n 'september': ['offer fluVaccine'],\n 'october': ['offer fluVaccine'],\n 'november': ['offer fluVaccine'],\n}\n\ndef vaccine_list(*args):\n return sorted(set(chain.from_iterable(\n TOME.get(s,()) for s in args\n )))", "d = {'fiveInOne': ('8 weeks','12 weeks','16 weeks'),\n 'pneumococcal': ('8 weeks','16 weeks'),\n 'rotavirus': ('8 weeks','12 weeks'),\n 'meningitisB': ('8 weeks','16 weeks','12 months'),\n 'hibMenC': ('12 months',),\n 'measlesMumpsRubella': ('12 months','40 months'),\n 'offer fluVaccine': ('september','october','november'),\n 'preSchoolBooster': ('40 months',)}\nvaccine_list=lambda *args:sorted({k for i in args for k,l in d.items() if i in l if i!='up-to-date'})", "def vaccine_list(age, status, month):\n vaccines = {\n '8 weeks': {'fiveInOne', 'pneumococcal', 'rotavirus', 'meningitisB'},\n '12 weeks' : {'fiveInOne', 'rotavirus'}, \n '16 weeks' : {'fiveInOne', 'pneumococcal', 'meningitisB'}, \n '12 months': {'meningitisB', 'hibMenC','measlesMumpsRubella'},\n '40 months': {'measlesMumpsRubella', 'preSchoolBooster'},\n 'up-to-date': set(),\n 'september': {'offer fluVaccine'},\n 'october': {'offer fluVaccine'},\n 'november': {'offer fluVaccine'} }\n return sorted(vaccines[age] | vaccines[status] | vaccines.get(month, set()))", "VACCINATIONS = {\n \"fiveInOne\": [\"8 weeks\", \"12 weeks\", \"16 weeks\"],\n \"pneumococcal\": [\"8 weeks\", \"16 weeks\"],\n \"rotavirus\": [\"8 weeks\", \"12 weeks\"],\n \"meningitisB\": [\"8 weeks\", \"16 weeks\", \"12 months\"],\n \"hibMenC\": [\"12 months\"],\n \"measlesMumpsRubella\": [\"12 months\", \"40 months\"],\n \"preSchoolBooster\": [\"40 months\"],\n}\n\n\ndef vaccine_list(age, status, month):\n return sorted(\n ({\"offer fluVaccine\"} if month in [\"september\", \"october\", \"november\"] else set())\n | {v for v, times in VACCINATIONS.items() if age in times or (status != \"up-to-date\" and status in times)}\n )", "vaccinations = {\n \"8 weeks\": {\"fiveInOne\", \"pneumococcal\", \"rotavirus\", \"meningitisB\"},\n \"12 weeks\": {\"fiveInOne\", \"rotavirus\"},\n \"16 weeks\": {\"fiveInOne\", \"pneumococcal\", \"meningitisB\"},\n \"12 months\": {\"meningitisB\", \"hibMenC\", \"measlesMumpsRubella\"},\n \"40 months\": {\"measlesMumpsRubella\", \"preSchoolBooster\"},\n \"september\": {\"offer fluVaccine\"}, \"october\": {\"offer fluVaccine\"}, \"november\": {\"offer fluVaccine\"},\n}\n\ndef vaccine_list(age, status, month):\n return sorted(vaccinations[age] | vaccinations.get(status, set()) | vaccinations.get(month, set()))", "vaccines = {\"8 weeks\": {\"fiveInOne\", \"pneumococcal\", \"rotavirus\", \"meningitisB\"},\n \"12 weeks\": {\"fiveInOne\", \"rotavirus\"},\n \"16 weeks\": {\"fiveInOne\", \"pneumococcal\", \"meningitisB\"},\n \"12 months\": {\"meningitisB\", \"hibMenC\", \"measlesMumpsRubella\"},\n \"40 months\": {\"measlesMumpsRubella\", \"preSchoolBooster\"},\n \"september\": {\"offer fluVaccine\"},\n \"october\": {\"offer fluVaccine\"},\n \"november\": {\"offer fluVaccine\"}}\nget = lambda x: vaccines.get(x, set())\n\ndef vaccine_list(age, status, month):\n return sorted(get(age) | get(status) | get(month))", "def vaccine_list(age, status, month):\n # Your code\n vaccine = {'fiveInOne' : ['8 weeks', '12 weeks', '16 weeks'], \n 'pneumococcal' : ['8 weeks', '16 weeks'],\n 'rotavirus' : ['8 weeks', '12 weeks'],\n 'meningitisB' : ['8 weeks', '16 weeks', '12 months'],\n 'hibMenC' : ['12 months'],\n 'measlesMumpsRubella' : ['12 months', '40 months'],\n 'fluVaccine' : ['september','october','november'],\n 'preSchoolBooster' : ['40 months']}\n \n req = []\n \n for jab in vaccine:\n if status in vaccine[jab]:\n req.append(jab)\n if age in vaccine[jab] and jab not in req:\n req.append(jab)\n \n if month in vaccine['fluVaccine']:\n req.append('offer fluVaccine')\n \n req.sort()\n \n return req", "vaccines = {\n '8 weeks': ['fiveInOne', 'pneumococcal', 'rotavirus', 'meningitisB'],\n '12 weeks': ['fiveInOne', 'rotavirus'],\n '16 weeks': ['fiveInOne', 'pneumococcal', 'meningitisB'],\n '12 months': ['meningitisB', 'measlesMumpsRubella', 'hibMenC'],\n '40 months': ['measlesMumpsRubella', 'preSchoolBooster'],\n 'september': ['offer fluVaccine'],\n 'october': ['offer fluVaccine'],\n 'november': ['offer fluVaccine']\n}\n\ndef vaccine_list(age, status, month):\n lists_of_vaccines = [vaccines[v] for v in [age, status, month] if v in vaccines]\n return sorted(list(set([v for lst in lists_of_vaccines for v in lst])))", "from itertools import chain\n\ndef vaccine_list(age, status, month): \n flu_months = ['september','october','november'] \n vac_sched = {'8 weeks': ['fiveInOne', 'pneumococcal', 'rotavirus', 'meningitisB'],\n '12 weeks': ['fiveInOne', 'rotavirus'],\n '16 weeks': ['fiveInOne', 'pneumococcal', 'meningitisB'],\n '12 months': ['meningitisB', 'hibMenC', 'measlesMumpsRubella'],\n '40 months': ['measlesMumpsRubella', 'preSchoolBooster']} \n \n if status == 'up-to-date':\n status = age\n\n vac_time = list(vac_sched.keys())\n vacs_toDo = [v for k,v in vac_sched.items() if k in [status, age]]\n\n vac_list = list(set(list(chain.from_iterable(vacs_toDo))))\n \n if month in flu_months:\n vac_list.append('offer fluVaccine')\n\n return sorted(vac_list)", "def vaccine_list(age, status, month):\n\n dict = {'fiveInOne': ['8 weeks', '12 weeks', '16 weeks'], 'pneumococcal': ['8 weeks', '16 weeks'],\n 'rotavirus': ['8 weeks', '12 weeks'], 'meningitisB': ['8 weeks', '16 weeks', '12 months'],\n 'hibMenC': ['12 months'], 'measlesMumpsRubella': ['12 months', '40 months'],\n 'fluVaccine': ['september', 'october', 'november'], 'preSchoolBooster': ['40 months']}\n\n vac_list = []\n for key in dict:\n if age in dict[key] or status in dict[key]:\n vac_list.append(key)\n\n if month in dict['fluVaccine']:\n vac_list.append('offer fluVaccine')\n\n return sorted(vac_list)"] | {"fn_name": "vaccine_list", "inputs": [["12 weeks", "up-to-date", "december"], ["12 months", "16 weeks", "june"], ["40 months", "12 months", "october"]], "outputs": [[["fiveInOne", "rotavirus"]], [["fiveInOne", "hibMenC", "measlesMumpsRubella", "meningitisB", "pneumococcal"]], [["hibMenC", "measlesMumpsRubella", "meningitisB", "offer fluVaccine", "preSchoolBooster"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,866 |
def vaccine_list(age, status, month):
|
71086c632d5be7588ddcaa14fbeecf0d | UNKNOWN | Given a string, determine if it's a valid identifier.
## Here is the syntax for valid identifiers:
* Each identifier must have at least one character.
* The first character must be picked from: alpha, underscore, or dollar sign. The first character cannot be a digit.
* The rest of the characters (besides the first) can be from: alpha, digit, underscore, or dollar sign. In other words, it can be any valid identifier character.
### Examples of valid identifiers:
* i
* wo_rd
* b2h
### Examples of invalid identifiers:
* 1i
* wo rd
* !b2h | ["import re\n\ndef is_valid(idn):\n return re.compile('^[a-z_\\$][a-z0-9_\\$]*$', re.IGNORECASE).match(idn) != None", "import re\nis_valid = lambda id: bool(re.match('^[a-z_$][\\w$]*$', id, re.I))", "import re\n\ndef is_valid(identifier):\n return bool(re.fullmatch(r\"[a-z_$][\\w$]*\", identifier, flags=re.IGNORECASE))", "import re\n\ndef is_valid(idn):\n pattern = r'^[a-zA-z_\\$]+[\\w\\$]*$'\n return bool(re.match(pattern, idn))", "import re\n\ndef is_valid(idn):\n return bool(re.match(r'[a-z_$][a-z0-9_$]*$', idn, flags=re.IGNORECASE))", "import re\n\ndef is_valid(idn):\n # Your code here\n line = re.match(r'[a-zA-Z_$]\\w*$', idn)\n if line:\n return True\n else:\n return False", "import re\ndef is_valid(idn):\n return re.match(r'^[a-zA-Z_$][\\w_$]*$', idn) is not None\n", "is_valid=lambda idn: len(idn)>0 and idn[0].lower() in \"$_abcdefghijklmnopqrstuvwxyz\" and all([l.lower() in \"$_abcdefghijklmnopqrstuvwxyz0123456789\" for l in idn[1:]])", "is_valid=lambda idn: bool(__import__(\"re\").match(\"^[a-zA-Z_$][\\w$]*$\",idn))\n", "is_valid = lambda idn: __import__(\"re\").match(r'^[a-zA-Z_\\$][a-zA-Z0-9_\\$]*$', idn) is not None"] | {"fn_name": "is_valid", "inputs": [["okay_ok1"], ["$ok"], ["___"], ["str_STR"], ["myIdentf"], ["1ok0okay"], ["!Ok"], [""], ["str-str"], ["no no"]], "outputs": [[true], [true], [true], [true], [true], [false], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,191 |
def is_valid(idn):
|
4dee5dfdc48ae77f9d60c49dd9cc45d6 | UNKNOWN | Two moving objects A and B are moving accross the same orbit (those can be anything: two planets, two satellites, two spaceships,two flying saucers, or spiderman with batman if you prefer).
If the two objects start to move from the same point and the orbit is circular, write a function that gives the time the two objects will meet again, given the time the objects A and B need to go through a full orbit, Ta and Tb respectively, and the radius of the orbit r.
As there can't be negative time, the sign of Ta and Tb, is an indication of the direction in which the object moving: positive for clockwise and negative for anti-clockwise.
The function will return a string that gives the time, in two decimal points.
Ta and Tb will have the same unit of measurement so you should not expect it in the solution.
Hint: Use angular velocity "w" rather than the classical "u". | ["def meeting_time(Ta, Tb, r):\n if Ta == 0:\n return \"{:.2f}\".format(abs(Tb))\n elif Tb == 0:\n return \"{:.2f}\".format(abs(Ta))\n else:\n return \"{:.2f}\".format(abs(Ta * Tb / (Tb - Ta)))", "def meeting_time(Ta, Tb, r):\n return str(abs(Tb))+'.00' if Ta == 0 else str(abs(Ta))+'.00' if Tb == 0 else str(round(abs(Ta*Tb/(Ta - Tb)), 4))+'0' if Ta != 0 and Tb != 0 and len(str(round(abs(Ta*Tb/(Ta - Tb)), 4))[str(round(abs(Ta*Tb/(Ta - Tb)), 4)).index('.')+1:]) == 1 else str(round(abs(Ta*Tb/(Ta - Tb)), 2))\n", "def meeting_time(ta, tb, r):\n if ta == 0:\n return \"{:.2f}\".format(abs(tb))\n if tb == 0:\n return \"{:.2f}\".format(abs(ta))\n else:\n return \"{:.2f}\".format(abs(ta * tb / (tb - ta)))", "def meeting_time(Ta, Tb, r):\n print(Ta, Tb, r)\n \n if Ta == Tb == 0.00:\n return '{:.2f}'.format(0.00)\n \n pi = 3.14\n len = 2 * pi * r\n if Ta != 0:\n sa = len / Ta\n else:\n sa = 0.00\n if Tb != 0:\n sb = len / Tb\n else:\n sb = 0.00\n# len = sa * T + sb * T\n# len = sa * T - sb * T\n \n if Ta == -Tb:\n return '{:.2f}'.format(abs(float(len / (sa + sb))))\n else:\n return '{:.2f}'.format(abs(float(len / (sa - sb))))", "def meeting_time(Ta, Tb, r):\n if Ta == 0 or Tb == 0:\n return \"{:.2f}\".format(abs(Ta + Tb))\n else:\n return \"{:.2f}\".format(abs(Ta * Tb)/abs(Ta - Tb))", "def meeting_time(Ta, Tb, r):\n if Ta==Tb:\n return \"0.00\"\n if Ta==0:\n return \"{:.2f}\".format(abs(Tb))\n if Tb==0:\n return \"{:.2f}\".format(abs(Ta))\n return \"{:.2f}\".format(abs(Ta*Tb)/abs(Ta-Tb),2)\n", "def meeting_time(Ta, Tb, r):\n if Ta == 0 and Tb == 0: return \"0.00\"\n if Ta == 0: return \"%.2f\" % abs(Tb)\n if Tb == 0: return \"%.2f\" % abs(Ta)\n if (Ta > 0 and Tb > 0) or (Ta < 0 and Tb < 0):\n return \"%.2f\" % (Ta * Tb / abs(abs(Ta) - abs(Tb)))\n if ((Ta > 0 and Tb < 0) or (Tb > 0 and Ta < 0)):\n return \"%.2f\" % (abs(Ta * Tb) / abs(abs(Ta) + abs(Tb)))", "def meeting_time(Ta, Tb, r):\n if Ta == 0:\n if Tb == 0:\n return '0.00'\n else:\n return str(abs(Tb)) + '.00'\n if Tb == 0:\n return str(abs(Ta)) + '.00'\n if Ta == Tb:\n return '0.00'\n Aa = 360/Ta\n Ab = 360/Tb\n if Aa > 0 and Ab > 0:\n return str(format(abs(360/abs(Ab-Aa)), '.2f'))\n elif Aa < 0 and Ab < 0:\n return str(format(abs(360/abs(Ab-Aa)), '.2f'))\n else:\n return str(format(abs(360/(abs(Ab) +abs(Aa))), '.2f'))", "def meeting_time(Ta, Tb, r):\n return f\"{round(1/abs(1/Ta-1/Tb),2):.2f}\" if Ta and Tb else f\"{max(abs(Ta),abs(Tb)):.2f}\"", "def meeting_time(Ta, Tb, r):\n return f\"{1/abs(1/(Ta or float('inf')) - 1/(Tb or float('inf')) or float('inf')):.2f}\""] | {"fn_name": "meeting_time", "inputs": [[12, 15, 5], [12, -15, 6], [-14, -5, 5], [23, 16, 5], [0, 0, 7], [12, 0, 10], [0, 15, 17], [-24, 0, 10], [0, -18, 14], [32, -14, 14]], "outputs": [["60.00"], ["6.67"], ["7.78"], ["52.57"], ["0.00"], ["12.00"], ["15.00"], ["24.00"], ["18.00"], ["9.74"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,898 |
def meeting_time(Ta, Tb, r):
|
dc544235fcb14bc1d88ae31259c424b8 | UNKNOWN | - Kids drink toddy.
- Teens drink coke.
- Young adults drink beer.
- Adults drink whisky.
Make a function that receive age, and return what they drink.
**Rules:**
- Children under 14 old.
- Teens under 18 old.
- Young under 21 old.
- Adults have 21 or more.
**Examples:**
```python
people_with_age_drink(13) == "drink toddy"
people_with_age_drink(17) == "drink coke"
people_with_age_drink(18) == "drink beer"
people_with_age_drink(20) == "drink beer"
people_with_age_drink(30) == "drink whisky"
``` | ["def people_with_age_drink(age):\n if age > 20: return 'drink whisky'\n if age > 17: return 'drink beer'\n if age > 13: return 'drink coke'\n return 'drink toddy'", "def people_with_age_drink(age):\n if age <= 13:\n beverage = 'toddy'\n elif age > 13 and age <= 17:\n beverage = 'coke'\n elif age > 17 and age <= 20:\n beverage = 'beer'\n elif age > 20:\n beverage = 'whisky'\n return 'drink ' + beverage", "def people_with_age_drink(age):\n return 'drink ' + ('toddy' if age < 14 else 'coke' if age < 18 else 'beer' if age < 21 else 'whisky')", "def people_with_age_drink(age):\n for a in [[21,'whisky'],[18,'beer'],[14,'coke'],[0,'toddy']]:\n if age >= a[0]:\n return \"drink {0}\".format(a[1])", "people_with_age_drink = lambda a, d=['toddy','coke','beer','whisky']: 'drink '+d[(a>13)+(a>17)+(a>20)]", "def people_with_age_drink(age):\n dic = {tuple(range(0,14)):\"drink toddy\", tuple(range(14,18)):\"drink coke\", tuple(range(18,21)):\"drink beer\"}\n return dic.get(max(i if age in i else (0,0) for i in dic), \"drink whisky\") \n \n", "def people_with_age_drink(age):\n if age < 14:\n return \"drink toddy\"\n elif age >= 21:\n return \"drink whisky\"\n elif age < 18:\n return \"drink coke\"\n elif age >=18<21:\n return \"drink beer\"", "def people_with_age_drink(age):\n return 'drink toddy'*(age<14) or 'drink coke'*(age<18) or 'drink beer'*(age<21) or 'drink whisky'", "def people_with_age_drink(age):\n drinks = {1:'drink toddy',2:'drink coke',3:'drink beer',4:'drink whisky'}\n return drinks[1] if age<14 else drinks[2] if age<18 else drinks[3] if age<21 else drinks[4]", "def people_with_age_drink(age):\n drinks = {age<14:'toddy',14<=age<18:'coke',18<=age<21:'beer',age>=21:'whisky'}\n return 'drink {}'.format(drinks[True])", "def people_with_age_drink(age):\n return 'drink %s' % ('toddy' if age < 14 else 'coke' if 13 < age < 18 \\\n else 'beer' if 17 < age < 21 else 'whisky')", "def people_with_age_drink(age):\n return 'drink {}'.format('toddy' if age < 14 else 'coke' if 18 > age >= 14 else 'beer' if 21 > age >= 18 else 'whisky')", "def people_with_age_drink(a):\n return \"drink \" + (\"toddy\" if a < 14 else \"coke\" if a < 18 else \"beer\" if a < 21 else \"whisky\")", "def people_with_age_drink(age):\n return \"drink toddy\" if age<14 else \"drink coke\" if age<18 else \"drink beer\" if age<21 else \"drink whisky\"", "drinks = [\n (\"whisky\", 21),\n (\"beer\", 18),\n (\"coke\", 14),\n]\ndef people_with_age_drink(age):\n return \"drink \" + next((drink for drink, age_limit in drinks if age >= age_limit), \"toddy\")", "def people_with_age_drink(age):\n return 'drink ' + next(d for a, d in [\n (21, 'whisky'), (18, 'beer'), (14, 'coke'), (0, 'toddy')\n ] if age >= a)", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif age >= 14 and age < 18:\n return 'drink coke'\n elif age >= 18 and age < 21:\n return 'drink beer'\n else:\n return 'drink whisky'\n return 'ERROR....WRONG INPUT'", "people_with_age_drink = lambda n: \"drink %s\" % ('toddy' if n < 14 else 'coke' if n < 18 else 'beer' if n < 21 else 'whisky')", "def people_with_age_drink(age: int) -> str:\n \"\"\" Get what people should drink based on their age. \"\"\"\n return f\"drink {'toddy' if age < 14 else 'coke' if age < 18 else 'beer' if age < 21 else 'whisky'}\"", "def people_with_age_drink(age):\n if age < 14: return \"drink toddy\"\n if age < 18: return \"drink coke\"\n if age < 21: return \"drink beer\"\n return \"drink whisky\"", "def people_with_age_drink(age):\n for (lim, drink) in [(14,\"toddy\"), (18, \"coke\"), (21, \"beer\"), (float('inf'), \"whisky\")]:\n if age < lim:\n return \"drink \" + drink", "def people_with_age_drink(age):\n return [\"drink toddy\", \"drink coke\", \"drink beer\", \"drink whisky\"][(age >= 21) + (age > 17) + (age > 13)]\n \n", "from bisect import bisect\ndef people_with_age_drink(age):\n r = {\n 0: \"drink toddy\",\n 1: \"drink coke\",\n 2: \"drink beer\",\n 3: \"drink whisky\"\n }\n return r[bisect([14, 18, 21], age)]", "people_with_age_drink=lambda a: 'drink '+(\"toddy\" if a<14 else \"coke\" if a<18 else \"beer\" if a<21 else \"whisky\")", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif age < 18:\n return 'drink coke'\n elif age < 21:\n return 'drink beer'\n return 'drink whisky'\n", "def people_with_age_drink(age):\n return 'drink '+('toddy','coke','beer','whisky')[sum(not age<x for x in (14,18,21))]", "def people_with_age_drink(age):\n drink = (\"toddy\", \"coke\", \"beer\", \"whisky\")[(age>=14) + (age>=18) + (age>=21)]\n return \"drink {}\".format(drink)", "def people_with_age_drink(age):\n return [\"drink toddy\", \"drink coke\", \"drink beer\", \"drink whisky\"][(age>=21) + (age>=18) + (age>=14)]\n", "def people_with_age_drink(age):\n for limit, drink in [(14, \"toddy\"), (18, \"coke\"), (21, \"beer\")]:\n if age < limit:\n return 'drink ' + drink\n return 'drink whisky'", "def people_with_age_drink(age):\n a=[(14,'drink toddy'), (18, 'drink coke'), (21, 'drink beer')]\n for i, d in a:\n if age < i:\n return d\n return 'drink whisky'", "def people_with_age_drink(age):\n if age < 14: return \"drink toddy\"\n if age < 18: return \"drink coke\"\n if age < 21: return \"drink beer\"\n else: return \"drink whisky\"", "def people_with_age_drink(age):\n drink = \"\"\n if age < 14:\n drink = \"drink toddy\"\n elif age <18 and age > 13:\n drink = \"drink coke\"\n elif age < 21 and age > 17:\n drink = \"drink beer\"\n else:\n drink = \"drink whisky\"\n return drink", "def people_with_age_drink(age):\n print(age)\n if (age >= 0 and age <= 13):\n return 'drink toddy'\n elif (age >= 14 and age <= 17):\n return 'drink coke'\n elif (age >= 18 and age <=20):\n return 'drink beer'\n else: \n return 'drink whisky'\n", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n if age > 13 and age <18:\n return 'drink coke'\n if age > 17 and age < 21:\n return 'drink beer'\n if age > 20:\n return 'drink whisky'", "def people_with_age_drink(age):\n drink = \"drink\"\n if age < 14:\n drink = \"{} toddy\".format(drink)\n elif age < 18:\n drink = \"{} coke\".format(drink)\n elif age < 21:\n drink = \"{} beer\".format(drink)\n else:\n drink = \"{} whisky\".format(drink)\n return drink", "def people_with_age_drink(age):\n return \"drink whisky\" if age >= 21 else \"drink beer\" if 18 <= age <= 20 else \"drink coke\" if 14 <= age <= 17 else \"drink toddy\"", "def people_with_age_drink(age):\n if age >= 21:\n return 'drink whisky' \n elif age in range(18,21):\n return 'drink beer' \n elif age in range(14,18):\n return 'drink coke'\n elif age in range(0,14):\n return 'drink toddy' ", "def people_with_age_drink(age):\n if age >=21:\n return \"drink whisky\"\n else:\n if age < 21 and age >=18:\n return \"drink beer\"\n else:\n if age < 18 and age >= 14:\n return \"drink coke\"\n else:\n return \"drink toddy\"\n", "def people_with_age_drink(age):\n if 0 <= age <= 13:\n return \"drink toddy\"\n elif 14 <= age <= 17:\n return \"drink coke\"\n elif 18 <= age <= 20:\n return \"drink beer\"\n else:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age >= 21:\n return ('drink whisky')\n if age < 21 and age >= 18:\n return 'drink beer'\n if age < 14:\n return 'drink toddy'\n if age >= 14 and age < 18:\n return 'drink coke'", "drink_pairs = {'kid':'toddy','teen':'coke','youngAdult':'beer','adult':'whisky'}\n\ndef people_with_age_drink(age):\n if age >= 21:\n return 'drink ' + drink_pairs['adult']\n elif age < 21 and age >= 18:\n return 'drink ' + drink_pairs['youngAdult']\n elif age < 18 and age >= 14:\n return 'drink ' + drink_pairs['teen']\n else:\n return 'drink ' + drink_pairs['kid']", "def people_with_age_drink(age):\n return \"drink toddy\" if age <14 else \"drink coke\"\\\nif 13<age<18 else \"drink beer\" if 17<age<21 else \"drink whisky\"", "def people_with_age_drink(age):\n if age <= 13:\n return \"drink toddy\"\n elif age <18 > 13:\n return \"drink coke\"\n elif age < 21 > 17:\n return \"drink beer\"\n elif age >= 21:\n return \"drink whisky\"\n else:\n pass", "def people_with_age_drink(age):\n res='drink {}'\n if age<14:\n return res.format('toddy')\n elif age<18:\n return res.format('coke')\n elif age<21:\n return res.format('beer')\n return res.format('whisky')\n", "def people_with_age_drink(age):\n if not(age) > 13:\n return 'drink toddy'\n elif age > 13 and not (age) >= 18:\n return 'drink coke'\n elif age >= 18 and not (age) > 20:\n return 'drink beer'\n else:\n return 'drink whisky'", "def people_with_age_drink(age):\n if age < 14:\n drink = \"toddy\"\n elif 14 <= age < 18:\n drink = \"coke\"\n elif 18 <= age < 21:\n drink = \"beer\"\n else:\n drink = \"whisky\"\n return \"drink\"+ \" \" + drink", "def people_with_age_drink(age):\n if age<14:\n return \"drink toddy\"\n elif age<18:\n return \"drink coke\"\n elif age<21:\n return \"drink beer\"\n elif age>=21:\n return \"drink whisky\"\n else: \n return \"error\"", "def people_with_age_drink(age):\n if age >= 0 and age < 14:\n return \"drink toddy\"\n elif age < 18:\n return \"drink coke\"\n elif age < 21:\n return \"drink beer\"\n elif age >= 21:\n return \"drink whisky\"\n else:\n return \"not a meaningful age\"", "def people_with_age_drink(age):\n print(age)\n if age < 14:\n return 'drink toddy'\n elif 13 < age < 18: \n return 'drink coke'\n elif 17 < age < 21: \n return 'drink beer'\n elif age > 20: \n return 'drink whisky'", "def people_with_age_drink(age):\n if age < 14:\n return \"drink toddy\"\n if age<18 and age>13:\n return \"drink coke\"\n if age<21 and age>=18:\n return \"drink beer\"\n if age>=21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age <= 13:\n return 'drink toddy'\n elif age <= 17 and age >= 14:\n return 'drink coke'\n elif age >= 21:\n return 'drink whisky'\n else:\n return 'drink beer'", "def people_with_age_drink(age):\n result = \"\"\n if(age < 14 ):\n result = \"drink toddy\"\n if(age >= 14 and age < 18):\n result = \"drink coke\"\n if(age >= 18 and age <= 21):\n result = \"drink beer\"\n if(age >= 21 ):\n result = \"drink whisky\"\n return result", "def people_with_age_drink(age):\n a, b, c = 14, 18, 21\n if age < a:\n return 'drink toddy'\n if a <= age < b:\n return 'drink coke'\n if b <= age < c:\n return 'drink beer'\n if age >= c:\n return 'drink whisky'\n", "def people_with_age_drink(age):\n if age <= 13:\n return \"drink toddy\"\n elif 14<=age<=17:\n return \"drink coke\"\n elif 18<=age<21:\n return \"drink beer\"\n elif age>=21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age < 14:\n return \"drink toddy\"\n if age >= 14 and age <= 17:\n return \"drink coke\"\n if age >= 18 and age <= 20:\n return \"drink beer\"\n else:\n return \"drink whisky\"", "def people_with_age_drink(age):\n res = \"\"\n if age < 14:\n res = \"drink toddy\"\n elif age < 18:\n res = \"drink coke\"\n elif age < 21:\n res = \"drink beer\"\n else:\n res = \"drink whisky\"\n return res", "people_with_age_drink = lambda x: 'drink toddy' if x < 14 else 'drink coke' if x < 18 else 'drink beer' if x < 21 else 'drink whisky'", "def people_with_age_drink(age):\n return \"drink \" + (\"toddy\" if age<14 else \"coke\" if 13<age<18 else \"beer\" if 17<age<21 else \"whisky\")", "def people_with_age_drink(age):\n drink = {\n 14: 'toddy',\n 18: 'coke',\n 21: 'beer'\n }\n for d in drink:\n if age < d:\n return 'drink ' + drink[d]\n return 'drink whisky' ", "def people_with_age_drink(age):\n drinks = {'Children': \"drink toddy\",\n 'Teens': \"drink coke\",\n 'Young': \"drink beer\",\n 'Adults': \"drink whisky\"}\n if age < 14:\n return drinks['Children']\n if age < 18:\n return drinks['Teens']\n if age < 21:\n return drinks['Young']\n if age >= 21:\n return drinks['Adults']\n", "def people_with_age_drink(age):\n if age < 14:\n return \"drink toddy\"\n else:\n if age < 18:\n return \"drink coke\"\n else:\n if age < 21:\n return \"drink beer\"\n else:\n if age >= 21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age <= 13:\n return \"drink toddy\"\n elif 13 < age <= 17:\n return \"drink coke\"\n elif 17 < age <= 20:\n return \"drink beer\"\n else:\n return \"drink whisky\"", "def people_with_age_drink(a):\n print(a)\n foo = [\"drink toddy\", \"drink coke\", \"drink beer\", \"drink whisky\"]\n if a >= 18:\n if a < 21:\n return foo[2]\n return foo[3]\n elif a < 18:\n if a < 14:\n return foo[0]\n return foo[1]\n", "def people_with_age_drink(age: int) -> str:\n \"\"\" Get what people should drink based on their age. \"\"\"\n drink_rule = {\n age < 14: \"toddy\",\n 14 <= age < 18: \"coke\",\n 18 <= age < 21: \"beer\",\n age >= 21: \"whisky\"\n }\n return f\"drink {drink_rule[True]}\"", "def people_with_age_drink(age):\n drinks = {'toddy': range(14),\n 'coke': range(14, 18),\n 'beer': range(18, 21),\n 'whisky': range(21, 999)}\n for key, age_range in drinks.items():\n if age in age_range:\n return f'drink {key}'", "def people_with_age_drink(age):\n if age < 14:\n return \"drink toddy\"\n elif age <= 17 and age >= 14:\n return \"drink coke\"\n elif age >= 18 and age <= 20:\n return \"drink beer\"\n elif age >=21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n \n kids_age = range(0, 14)\n teens_age = range(14, 18)\n youngadults_age = range(18,21)\n \n if age in kids_age: return 'drink toddy'\n elif age in teens_age: return 'drink coke'\n elif age in youngadults_age: return 'drink beer'\n else: return 'drink whisky'", "def people_with_age_drink(age):\n drink = \"\"\n if 0 <= age < 14:\n drink = \"drink toddy\"\n if 14 <= age < 18:\n drink = \"drink coke\"\n if 18 <= age < 21:\n drink = \"drink beer\"\n if 21 <= age:\n drink = \"drink whisky\"\n return drink\n\nage = people_with_age_drink(0)\nprint(age)", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif 14 <= age < 18:\n return 'drink coke'\n elif 18 <= age < 21:\n return 'drink beer'\n return 'drink whisky'\n", "def people_with_age_drink(age):\n if age >=21:\n return \"drink whisky\"\n elif age in range(18,21):\n return \"drink beer\"\n elif age in range(14,18):\n return \"drink coke\"\n else:\n return \"drink toddy\"\n", "def people_with_age_drink(age):\n \n s = ''\n \n if age > 20 :\n s = 'whisky'\n if age < 21 :\n s = 'beer'\n if age < 18 :\n s = 'coke'\n if age < 14 :\n s = 'toddy'\n \n return 'drink ' + s", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif 14 < age < 18:\n return 'drink coke'\n elif 18 < age < 21:\n return 'drink beer'\n elif age == 18:\n return 'drink beer'\n elif age == 14:\n return 'drink coke'\n elif age == 21:\n return 'drink whisky'\n elif age > 21:\n return 'drink whisky'", "def people_with_age_drink(age):\n drinks = {\"14\": \"drink toddy\", \"18\": \"drink coke\", \"21\": \"drink beer\", \"100\": \"drink whisky\"}\n \n for key in drinks:\n if age < int(key):\n return drinks[key]", "def people_with_age_drink(age):\n drinks = [\"drink toddy\", \"drink coke\", \"drink beer\", \"drink whisky\"]\n if age < 14:\n return drinks[0]\n elif 14 <= age < 18:\n return drinks[1]\n elif 18 <= age < 21:\n return drinks[2]\n else:\n return drinks[3]", "def people_with_age_drink(age):\n rv = 'drink '\n if age < 14:\n return rv + 'toddy'\n elif age < 18:\n return rv + 'coke'\n elif age < 21:\n return rv + 'beer'\n else:\n return rv + 'whisky'\n", "def people_with_age_drink(age):\n return 'drink ' + (\"toddy\" if age < 14 else \"coke\" if age < 18 else \"beer\" if age < 21 else \"whisky\" if age >= 21 else 0)\n", "people_with_age_drink=lambda a:f'drink {(a<14 and \"toddy\") or (a<18 and \"coke\") or (a<21 and \"beer\") or \"whisky\"}'", "def people_with_age_drink(age):\n if age <= 13: \n return \"drink toddy\"\n elif age <= 17:\n return \"drink coke\"\n elif age == 18 or age <= 20:\n return \"drink beer\"\n elif age > 20:\n return \"drink whisky\"\n \n", "def people_with_age_drink(age):\n if age in range(0,14):\n return 'drink toddy'\n elif age in range(13,18):\n return 'drink coke'\n elif age in range(18,21):\n return 'drink beer'\n else:\n return 'drink whisky'", "def people_with_age_drink(age):\n switcher={\n 1: 'drink toddy',\n 2: 'drink coke',\n 3: 'drink beer',\n 4: 'drink whisky'\n }\n if age < 14: return switcher.get(1,None)\n elif 14 <= age < 18: return switcher.get(2,None)\n elif 18 <= age < 21: return switcher.get(3,None)\n elif 21 <= age: return switcher.get(4,None)", "def people_with_age_drink(age):\n if age <= 13:\n return \"drink toddy\"\n elif age in range(14, 18):\n return \"drink coke\"\n elif age in range(18, 21):\n return \"drink beer\"\n elif age >= 21:\n return \"drink whisky\"\n", "def people_with_age_drink(age):\n if age >= 0 and age < 14:\n return \"drink toddy\"\n if age >= 14 and age < 18:\n return \"drink coke\"\n if age >= 18 and age < 21:\n return \"drink beer\"\n if age >= 21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if (age < 14):\n return \"drink toddy\"\n elif ( age <= 17 and age >= 14 ):\n return \"drink coke\"\n elif ( age > 17 and age <= 20 ):\n return \"drink beer\"\n elif ( age > 20 ):\n return \"drink whisky\"\n else:\n return \"\"", "people_with_age_drink = lambda age: f\"drink {age < 14 and 'toddy' or age < 18 and 'coke' or age < 21 and 'beer' or 'whisky'}\"", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif age >= 14 and age < 18:\n return 'drink coke'\n elif age >= 18 and age < 21:\n return 'drink beer'\n else:\n return 'drink whisky'\n \nprint(people_with_age_drink(20))", "#this function tells you what you should drink based on your age.\ndef people_with_age_drink(age):\n drink=\"drink \"\n if age<14:\n drink+=\"toddy\"\n elif age<18:\n drink+=\"coke\"\n elif age<21:\n drink+=\"beer\"\n elif age>=21:\n drink+=\"whisky\"\n return drink", "def people_with_age_drink(age: int) -> str:\n DRINK_TABLE = ( (21,'whisky'), (18,'beer'), (14,'coke'), (0,'toddy') )\n for limit, drink in DRINK_TABLE:\n if age >= limit:\n return f'drink {drink}'\n return 'no drink'", "def people_with_age_drink(age):\n if age < 14:\n return 'drink toddy'\n elif 13 < age < 18:\n return 'drink coke'\n elif 17 < age < 21:\n return 'drink beer'\n elif age > 20:\n return 'drink whisky'", "def people_with_age_drink(age):\n \n if age in range(0, 14):\n return \"drink toddy\"\n elif age in range(14, 18):\n return \"drink coke\"\n elif age in range(18, 21):\n return \"drink beer\"\n elif age < 0:\n raise Exception(\"Incorrect age\")\n elif age >= 21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age >= 21:\n return 'drink whisky'\n if age >= 18 and age < 21:\n return 'drink beer'\n if age >= 14 and age < 18:\n return 'drink coke'\n if age < 14:\n return 'drink toddy'\n\n \n", "def people_with_age_drink(age):\n if age<14:\n return \"drink toddy\"\n elif 14<=age<=17:\n return \"drink coke\"\n elif 17<age<21:\n return 'drink beer'\n else:\n return 'drink whisky'", "def people_with_age_drink(age):\n j = True\n if age < 14: \n j = 'drink toddy'\n elif age < 18:\n j = 'drink coke'\n elif age < 21:\n j = 'drink beer'\n elif age >= 21:\n j = 'drink whisky' \n return j", "def people_with_age_drink(age):\n if age <= 13:\n return \"drink toddy\"\n elif age <= 17 and age >= 14:\n return \"drink coke\"\n elif age <= 20 and age >= 18:\n return \"drink beer\"\n else:\n if age >= 21:\n return \"drink whisky\"\n", "def people_with_age_drink(age):\n if age < 14 :\n return 'drink toddy'\n elif age < 18 and age >0:\n return 'drink coke'\n elif age < 21 and age >0:\n return 'drink beer'\n elif age >=21 and age >0:\n return 'drink whisky'", "def people_with_age_drink(age):\n if age in range(0,14):\n return \"drink toddy\"\n elif age in range (14, 18):\n return \"drink coke\"\n elif age in range (18, 21):\n return \"drink beer\"\n elif age >= 21:\n return \"drink whisky\"", "def people_with_age_drink(age):\n if age<=13:\n return \"drink toddy\"\n elif 14<=age<=17:\n return \"drink coke\"\n elif 18<=age<=20:\n return \"drink beer\"\n else:\n return \"drink whisky\"", "def people_with_age_drink(age):\n\n if age < 14:\n drink = \"toddy\"\n elif 14 <= age <= 17:\n drink = \"coke\"\n elif 18 <= age <= 20:\n drink = \"beer\"\n else:\n drink = \"whisky\"\n \n return \"drink {}\".format(drink)", "def people_with_age_drink(age):\n if age < 14:\n a = 'drink toddy'\n elif age < 18:\n a = 'drink coke'\n elif age < 21:\n a = 'drink beer'\n else:\n a = 'drink whisky'\n return a\n", "def people_with_age_drink(age):\n if age<14:\n return \"drink toddy\"\n if age<18:\n return \"drink coke\"\n if age<21:\n return \"drink beer\"\n if age>14:\n return \"drink whisky\""] | {"fn_name": "people_with_age_drink", "inputs": [[13], [0], [17], [15], [14], [20], [18], [22], [21]], "outputs": [["drink toddy"], ["drink toddy"], ["drink coke"], ["drink coke"], ["drink coke"], ["drink beer"], ["drink beer"], ["drink whisky"], ["drink whisky"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 23,845 |
def people_with_age_drink(age):
|
9385040b10b0e42b9bce7af4866fdd68 | UNKNOWN | For every positive integer N, there exists a unique sequence starting with 1 and ending with N and such that every number in the sequence is either the double of the preceeding number or the double plus 1.
For example, given N = 13, the sequence is [1, 3, 6, 13], because . . . :
```
3 = 2*1 +1
6 = 2*3
13 = 2*6 +1
```
Write a function that returns this sequence given a number N. Try generating the elements of the resulting list in ascending order, i.e., without resorting to a list reversal or prependig the elements to a list. | ["def climb(n):\n return [1] if n == 1 else climb(int(n/2)) + [n]", "# If we write the decision tree for the problem we can see\n# that we can traverse upwards from any node simply by\n# dividing by two and taking the floor. This would require\n# a reversal of the list generated since we're building it\n# backwards.\n# \n# If we examine the successor function (x -> {2x, 2x + 1})\n# we can deduce that the binary representation of any number\n# gives us the steps to follow to generate it: if the n-th (LTR)\n# bit is set, we use 2x + 1 for the next element, otherwise we\n# choose 2x. This allows us to build the sequence in order.\n\ndef climb(n):\n res = []\n cur = 1\n mask = 1 << max(0, n.bit_length() - 2)\n\n while cur <= n:\n res.append(cur)\n cur = 2*cur + (1 if (n & mask) != 0 else 0)\n mask >>= 1\n\n return res", "def climb(n):\n result = [1]\n for x in \"{:b}\".format(n)[1:]:\n result.append(result[-1]*2 + (x=='1'))\n return result", "def climb(n):\n return [n >> i for i in range(len(f\"{n:b}\") - 1, -1, -1)]", "from math import log\nfrom collections import deque\n \ndef climb(n):\n return [n//2**i for i in range(int(log(n, 2)) + 1)][::-1]\n\ndef climb(n):\n \"\"\"This is much faster.\"\"\"\n seq = deque([n])\n while n > 1:\n n //= 2\n seq.appendleft(n)\n return list(seq)", "def climb(n):\n #your code here\n return [n>>n.bit_length()-i-1 for i in range(n.bit_length())]\n", "def climb(n):\n return list(climb_iterator(n))\n \ndef climb_iterator(n):\n cursor = 0\n for digit in bin(n)[2:]:\n cursor = 2 * cursor + int(digit)\n yield cursor", "def climb(n):\n res = [n]\n while res[-1] != 1: res.append(res[-1]//2)\n return res[::-1]", "def climb(n):\n arr = []\n while n:\n arr.append(n)\n n //= 2\n return arr[::-1]"] | {"fn_name": "climb", "inputs": [[1], [100], [12345], [54321]], "outputs": [[[1]], [[1, 3, 6, 12, 25, 50, 100]], [[1, 3, 6, 12, 24, 48, 96, 192, 385, 771, 1543, 3086, 6172, 12345]], [[1, 3, 6, 13, 26, 53, 106, 212, 424, 848, 1697, 3395, 6790, 13580, 27160, 54321]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,892 |
def climb(n):
|
1fde5c5cbdcc4197f58cddc8297d5653 | UNKNOWN | This kata is from check py.checkio.org
You are given an array with positive numbers and a number N. You should find the N-th power of the element in the array with the index N. If N is outside of the array, then return -1. Don't forget that the first element has the index 0.
Let's look at a few examples:
* array = [1, 2, 3, 4] and N = 2, then the result is 3^2 == 9;
* array = [1, 2, 3] and N = 3, but N is outside of the array, so the result is -1. | ["def index(array, n):\n try:\n return array[n]**n\n except:\n return -1", "def index(array, n):\n return array[n]**n if n < len(array) else -1", "def index(array, n):\n try:\n return pow(array[n],n)\n except IndexError:\n return -1", "def index(array, n):\n if n < len(array):\n return (array[n])**n\n else:\n return -1", "from typing import List\n\ndef index(array: List[int], n: int) -> int:\n \"\"\" Get the N-th power of the element in the array with the index N. \"\"\"\n try:\n return pow(array[n], n)\n except IndexError:\n return -1", "def index(array, n):\n return array[n]**n if n <= len(array) - 1 else -1", "def index(array, n):\n return array[n] ** n if len(array) >= n + 1 else -1", "def index(array, n):\n return -1 if n >= len(array) else array[n] ** n", "def index(arr, n):\n return arr[n] ** n if n < len(arr) else -1", "def index(array, n):\n return array[n] ** n if len(array) > n else -1", "index = lambda a, n: -1 if len(a)<=n else a[n]**n", "def index(array, n):\n if (n < len(array)):\n return pow(array[n], n)\n return -1 ", "import math\ndef index(array, n):\n if len(array) > n:\n return math.pow(array[n], n)\n else:\n return -1", "def index(array, n):\n return pow(array[n], n) if n < len(array) else -1", "def index(array, n):\n return array[n] ** n if n in range(len(array)) else -1", "def index(array, n):\n return array[n] ** n if 0 <= n < len(array) else -1", "def index(array, n):\n if n >= len(array):\n return -1\n else:\n return array[n]**n", "def index(a, n):\n if (len(a)-1)<n:\n return -1\n return a[n]**n", "def index(arr, n):\n try:\n return arr[n] ** n\n except:\n return -1", "index = lambda a, n: a[n] ** n if n + 1 <= len(a) else -1", "index = lambda a,n: -1 if n+1 > len(a) else a[n]**n ", "index=lambda a,n:-(n>=len(a))or a[n]**n", "index = lambda a,i : a[i]**i if len(a)-1 >= i else -1", "def index(array, n):\n for idx in range(len(array)):\n if idx == n:\n return array[idx] ** n\n return -1", "def index(array, n):\n if n>=len(array) or n<0:\n return -1\n else:\n return (array[n])**n", "def index(array, n):\n if 0<=n<len(array):\n return pow(array[n],n)\n else:return -1", "def index(array, n):\n if(n<=len(array)-1):\n array[n]=array[n]**n\n return array[n]\n else:\n return -1\n", "def index(array, n):\n try:\n return array[n]**n\n except:\n return -1\nprint(index([1,2,3,4],2))", "def index(array, n):\n \n if n < len(array):\n x = array[n] **n;\n else:\n x = -1;\n \n return x;", "def index(array, n):\n if (len(array)-1)>=n:\n for i in range(len(array)):\n return array[n]**n\n else:\n return -1", "def index(array: list, n: int) -> int:\n \"\"\" This function find the N-th power of the element in the array with the index N. \"\"\"\n for k, i in enumerate(range(len(array))):\n if i == n:\n return (array[i] ** n)\n return -1", "def index(array, n):\n if array==[]:\n return -1\n if len(array)>n:\n return array[n]**n\n else: return -1\n", "def index(array, n):\n if n+1<len(array):\n return (array[n])**n\n else:\n if n+1>len(array):\n return -1\n else:\n return (array[n])**n\n", "def index(array: list, n: int) -> int:\n return array[n]**n if n < len(array) else -1", "def index(array, n):\n if (len(array)<n+1):\n return -1\n else:\n return pow(array[n],n)", "def index(array, n):\n try: \n return array[n] ** n\n except:\n return -1\n \n\nprint(index ([1, 2, 3, 4], 2 )) \n\nprint(index([1, 3, 10, 100],3))\n\nprint(index([1, 3, 10, 100],10))", "import math\ndef index(array, n):\n if(len(array)==0):\n return -1\n for i in array:\n if (n>len(array)-1):\n return -1\n if i is array[n]:\n return(math.pow(i,n))", "import math\n\ndef index(array, n):\n for i in range(len(array)):\n if i == n:\n return array[i] ** n\n return -1", "def index(array, n):\n if len(array) <= n :\n return -1 \n else:\n nth = array[n]\n nth_sq = nth ** n\n return nth_sq\n", "def index(array, n):\n if len(array) <= n:\n return -1\n for i in range(len(array)):\n if i == n:\n return array[i] ** n\n", "def index(array, n):\n while n >= len(array):\n return -1\n else:\n return array[n] ** n", "index = lambda l,n:l[n]**n if n<len(l) else -1", "def index(array, n):\n\n for i, val in enumerate(array):\n if n == i:\n return val ** n\n else:\n return -1\n \n", "def index(array, n):\n result = 1\n if n > len(array) - 1 :\n return -1 \n else:\n for index in range(n):\n result = result * array[n]\n return result\n", "def index(array, n):\n if n<len(array):\n return int(array[n]**n)\n if n>=len(array):\n return -1\n", "def index(array, n=0):\n if n > len(array)-1:\n return -1\n else:\n return array[n]**n\n\n", "def index(array, n):\n print((len(array)))\n if n >= len(array):\n return(-1)\n print((-1))\n else:\n return(array[n]**n)\n", "index = lambda a, n: -1 if n >= len(a) else a[n] ** n", "def index(array, n):\n try: \n x = array[n]\n except IndexError:\n return -1\n y = x**n\n return y\n", "def index(array, n):\n x=len(array)\n if n > x - 1:\n return -1\n else:\n return array[n] ** n", "def index(array, n):\n return pow(array[n],n) if n <= len(array)-1 else -1", "def index(array, n):\n if len(array) < n+1:\n return -1\n elif len(array) >= n+1:\n number = array[abs(n)]\n return number ** n\n else:\n pass", "def index(array, n):\n if n >= len(array): # Here, the nth index will be outside the array.\n return -1\n else:\n return array[n] ** n", "def index(array, n):\n \n try:\n if (n - 1) > len(array):\n return -1\n else:\n return array[n] ** n\n\n except IndexError:\n return -1", "def index(array, n):\n if len(array)-1<n:\n a=-1\n return(a)\n else:\n return(array[n]**n)", "def index(array, n):\n for idx, i in enumerate(array):\n if idx == n:\n x = array[idx] ** n\n return x\n else:\n return -1", "def index(array, n):\n list(array)\n if n>len(array)-1 :\n return -1\n else:\n return (array[n]**n)\n", "def index(array, n):\n ans = 1\n if n > len(array)-1:\n return -1\n else:\n for i in range (n):\n ans *= array[n] \n return ans", "def index(array, n):\n if n < len(array):\n a = array[n]\n num = a ** n\n return num\n else:\n return -1\n", "def index(array, n):\n if n > len(array)-1:\n return -1\n else:\n number = array[n]\n return number**n", "def index(array, n):\n list(array)\n while n < len(array):\n return (array[n])**n\n else:\n return -1", "def index(array, n):\n list(array)\n if n < len(array):\n return (array[n])**n\n else:\n return -1\n", "def index(array, n):\n contar = 0\n for numero in array:\n contar = contar + 1\n if (contar) > n:\n y = array[n]\n x = y**n\n return x\n else:\n return -1", "def index(array, n):\n if n < len(array):\n target = array[n]\n ans = target ** n\n \n return ans\n else:\n return -1", "def index(array, n):\n dlina = len(array)\n if n <= dlina - 1:\n return(array[n]**n)\n else:\n return (-1)\n \n", "def index(array, n):\n while True:\n try:\n return array[n] ** n \n break\n except IndexError:\n return -1", "def index(array, n):\n try:\n if len(array) >= n:\n return array[n] ** n\n else:\n return -1\n except IndexError:\n return -1\n", "def index(array, n):\n if len(array)>n:\n a=array[n] \n return a**n\n else: \n return -1", "index=lambda a,n:a[n]**n if n<len(a)else~0", "def index(array, n):\n return array [n]**n if n <len(array) else -1\n\n \n '''\n if n < len(array):\n return (array[n]**n) \n else:\n return -1\n '''", "def index(array, n):\n try:\n a = array[n]**n\n return a\n except IndexError:\n return -1", "def index(array, n):\n \n if len(array) > n:\n nTh = array[n]**n\n else:\n nTh = -1\n return nTh", "def index(array, n):\n try:\n a = array[n]**n\n return a\n except:\n return -1\n", "def index(array, n):\n nth_power = None\n try: nth_power = array[n]**n\n except: nth_power = -1\n return nth_power", "def index(array, n):\n try:\n x = array.pop(n)\n except IndexError:\n return -1\n return x**n", "def index(array, n):\n length = len(array)\n if n <= length - 1:\n return array[n]**n\n else:\n return -1", "def index(array, n):\n if (n > len(array) - 1):\n return -1\n else:\n result = 1\n i = 0\n while (i < n):\n result *= array[n]\n i += 1\n return result", "def index(array, n):\n if n > len(array) - 1:\n return(-1)\n elif n == 0:\n return(1)\n else:\n pw = array[n]\n i = 1\n while i != n:\n array[n] *= pw\n i += 1\n return(array[n])\n", "def index(array, n):\n try:\n if n:\n index = array[n]\n return index**n\n elif n == 0:\n return 1\n except:\n return -1\n", "def index(array, n):\n y = 0\n if n > len(array)-1:\n y = -1\n else:\n y = pow(array[n],n)\n return y", "def index(array, n):\n if len(array)-1<n:\n otvet=-1\n else:\n otvet=array[n]**n\n return otvet", "def index(array, n):\n print(array)\n print(n)\n if(n >= len(array)):\n return -1\n else:\n return array[n] ** n", "def index(array, n):\n if n <= (len(array)-1):\n n=array[n]**n\n return n\n else:\n return (-1)", "def index(array, N):\n res = 0\n uzunluk = len(array)\n if N>=uzunluk:\n return -1\n \n ussuAlinacakSayi = array[N]\n return ussuAlinacakSayi**N", "def index(array, n):\n return pow(array.pop(n), n) if len(array) > n else -1", "def index(array, n):\n if n >= len(array) or n < 0:\n return -1\n else:\n# if n in array:\n# return array[n] ** n\n return array[n] ** n;", "def index(array, n):\n arr = len(array)\n if n >= arr:\n return -1\n return array[n]**n", "def index(array, n):\n if n > -1 and n < len(array):\n product = array[n]**n \n else:\n product = -1\n \n return product", "def index(array, n):\n if n >= 0 and n < len(array):\n x = 1\n for i in range(n):\n x = x * array[n]\n return x\n else:\n return -1\n", "def index(array, n):\n try:\n return array[n]**n\n except IndexError:\n return -1\n finally:\n pass", "def index(array, n):\n for i in array :\n return pow(array[n],n) if len(array)-1 >= n else -1\n else :\n return -1", "def index(array, n):\n try:\n return array[n]**n\n except:\n return -1\narray=[1,3,10,100]\nindex(array,3)", "def index(array, n):\n if n >= len(array):\n return -1\n print(array, n,\"\", array[n], \"\", array[n]**2)\n return array[n]**n", "def index(array, n):\n try:\n return array[n] ** n\n except (IndexError, TypeError):\n return -1", "def index(array, n):\n if len(array) - 1 < n: return - 1\n for ind, value in enumerate(array):\n if ind == n:\n return(value**n)\n \n\n\n\n", "def index(array, n):\n if((len(array))-1) < n:\n return(-1)\n else:\n for i in range(len(array)):\n print((n,i))\n if (n == i):\n return(array[i]**n)\n \n\n", "def index(array, n):\n return -1 if n < 0 or n > len(array)-1 else array[n]**n;\n", "def index(array, n):\n arr = []\n for i in array:\n arr.append(pow(i, n))\n if n > len(arr) - 1:\n return -1\n return arr[n]", "def index(array = [], n = 0):\n if n >= len(array):\n return -1\n else:\n return array[n]**n", "def index(array, n = -1):\n if n < 0 or n > len(array)-1 or n == None:\n val = -1\n return val\n elif n <= len(array)-1:\n val = array[n]\n val = val ** n\n return val\n"] | {"fn_name": "index", "inputs": [[[1, 2, 3, 4], 2], [[1, 3, 10, 100], 3], [[0, 1], 0], [[0, 1], 1], [[1, 2], 2], [[1, 2], 3], [[0], 0], [[1, 1, 1, 1, 1, 1, 1, 1, 1, 1], 9], [[1, 1, 1, 1, 1, 1, 1, 1, 1, 100], 9], [[29, 82, 45, 10], 3], [[6, 31], 3], [[75, 68, 35, 61, 9, 36, 89, 0, 30], 10]], "outputs": [[9], [1000000], [1], [1], [-1], [-1], [1], [1], [1000000000000000000], [1000], [-1], [-1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,204 |
def index(array, n):
|
a5ba2d6c1b0511801fc682a34e501fee | UNKNOWN | Math geeks and computer nerds love to anthropomorphize numbers and assign emotions and personalities to them. Thus there is defined the concept of a "happy" number. A happy number is defined as an integer in which the following sequence ends with the number 1.
* Start with the number itself.
* Calculate the sum of the square of each individual digit.
* If the sum is equal to 1, then the number is happy. If the sum is not equal to 1, then repeat steps 1 and 2. A number is considered unhappy once the same number occurs multiple times in a sequence because this means there is a loop and it will never reach 1.
For example, the number 7 is a "happy" number:
7^(2) = 49 --> 4^(2) + 9^(2) = 97 --> 9^(2) + 7^(2) = 130 --> 1^(2) + 3^(2) + 0^(2) = 10 --> 1^(2) + 0^(2) = 1
Once the sequence reaches the number 1, it will stay there forever since 1^(2) = 1
On the other hand, the number 6 is not a happy number as the sequence that is generated is the following: 6, 36, 45, 41, 17, 50, 25, 29, 85, 89, 145, 42, 20, 4, 16, 37, 58, 89
Once the same number occurs twice in the sequence, the sequence is
guaranteed to go on infinitely, never hitting the number 1, since it repeat
this cycle.
Your task is to write a program which will print a list of all happy numbers between 1 and x (both inclusive), where:
```python
2 <= x <= 5000
```
___
Disclaimer: This Kata is an adaptation of a HW assignment I had for McGill University's COMP 208 (Computers in Engineering) class.
___
If you're up for a challenge, you may want to try a [performance version of this kata](https://www.codewars.com/kata/happy-numbers-performance-edition) by FArekkusu. | ["def is_happy(n):\n seen = set()\n while n not in seen:\n seen.add(n)\n n = sum( int(d)**2 for d in str(n) )\n return n == 1\n\n\ndef happy_numbers(n): \n return [x for x in range(1, n+1) if is_happy(x)]", "# Code reuse: https://www.codewars.com/kata/reviews/5ac53889cd661be6ee0013ff/groups/5ac54c24cd661b3753001fba\n# if you like this go the link and upvote StefanPochmanns's solution :)\ndef is_happy(n):\n while n > 4:\n n = sum(int(d)**2 for d in str(n))\n return n == 1\n\n\nhappy_numbers = lambda n: list(filter(is_happy, range(1, n+1)))", "def happy_numbers(n):\n HappyNumbers = []\n for i in range(n+1):\n AlreadyGoneThrough = []\n a = i\n while a != 1 and a not in AlreadyGoneThrough:\n b = 0\n AlreadyGoneThrough.append(a)\n \n for digit in str(a):\n b = b + int(digit)**2\n \n a = b\n \n if a in AlreadyGoneThrough:\n pass\n \n elif a == 1:\n HappyNumbers.append(i)\n return HappyNumbers", "def _sum_squares(num):\n ss = 0\n while num > 0:\n div, digit = num // 10, num % 10\n ss += digit * digit\n num = div\n return ss\n\n\ndef _is_happy_number(num):\n # Check if num is happy number.\n seens = set()\n while num > 1: # stop when num == 1\n ss = _sum_squares(num)\n if ss in seens:\n return False\n\n seens.add(ss)\n num = ss\n return True\n\n\ndef happy_numbers(n):\n result = []\n for num in range(1, n + 1):\n # Check if a num is happy number.\n if _is_happy_number(num):\n result.append(num)\n return result\n", "def happy_numbers(n):\n return [q for q in range(n+1) if isHappy(q)]\n \ndef isHappy(o, lister=[]):\n \n #Terminator\n if len(lister)>len(set(lister)): return False \n \n #Test for Happiness\n summer=sum([int(p)**2 for p in str(o)])\n return True if summer==1 else isHappy(summer, lister+[summer])", "from functools import lru_cache\n\nvisited = set()\n@lru_cache(maxsize=None)\ndef happy(n):\n if n == 1 or n in visited:\n visited.clear()\n return n == 1\n visited.add(n)\n n = sum(int(digit)**2 for digit in str(n))\n return happy(n)\n\ndef happy_numbers(n):\n return [i for i in range(1, n+1) if happy(i)]", "def happy_number(number):\n # Check 1: only happy numbers under 10 are (1,7)\n if number == 7 or number == 1: return True\n\n # Check 2: happy numbers if the sum of the square of each number == 1 (or 7)\n while number >= 10:\n number = sum(int(x)**2 for x in str(number))\n if number == 1 or number == 7: return True\n else: return False\nprint(happy_number(1112))\n\ndef happy_numbers(limit):\n return [number for number in range(1, limit+1) if happy_number(number)]", "def _sum_squares(number):\n result = 0\n while number > 0:\n carry, digit = number // 10, number % 10\n result += digit * digit\n number = carry\n return result\n\ndef _is_happy_number(number):\n seens = set()\n while number > 1:\n # print('number:', number)\n sum_squares = _sum_squares(number)\n # print('sum_squares:', sum_squares)\n if sum_squares in seens:\n return False\n seens.add(sum_squares)\n # print('seens:', seens)\n number = sum_squares\n return True\n\ndef happy_numbers(n):\n result = []\n for number in range(1, n + 1):\n if _is_happy_number(number):\n result.append(number)\n return result", "def sum_square(num):\n num2 = str(num)\n sum_sqr = 0\n for val in num2:\n sum_sqr += int(val) ** 2\n return sum_sqr\n\n\ndef happy_numbers(n):\n happy = []\n for val in range(1,n+1):\n val_list = []\n wag = 100\n val2 = val\n for iii in range(wag):\n sum_sqr = sum_square(val2)\n if sum_sqr == 1:\n happy.append(val) # happy\n break\n elif sum_sqr in val_list: # infinate loop\n break\n else:\n val_list.append(sum_sqr)\n val2 = sum_sqr\n\n return happy\n", "def happy_numbers(n):\n return [k for k in range(1,n+1) if is_happy(k)]\n\ndef is_happy(n):\n seen = {n}\n while n != 1:\n n = sum(int(d)**2 for d in str(n))\n if n in seen: return False\n seen.add(n)\n return True"] | {"fn_name": "happy_numbers", "inputs": [[10], [50], [100]], "outputs": [[[1, 7, 10]], [[1, 7, 10, 13, 19, 23, 28, 31, 32, 44, 49]], [[1, 7, 10, 13, 19, 23, 28, 31, 32, 44, 49, 68, 70, 79, 82, 86, 91, 94, 97, 100]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,552 |
def happy_numbers(n):
|
00902e4f65d58316579337ff5a22f71a | UNKNOWN | # Task
John is an orchard worker.
There are `n` piles of fruits waiting to be transported. Each pile of fruit has a corresponding weight. John's job is to combine the fruits into a pile and wait for the truck to take them away.
Every time, John can combine any two piles(`may be adjacent piles, or not`), and the energy he costs is equal to the weight of the two piles of fruit.
For example, if there are two piles, pile1's weight is `1` and pile2's weight is `2`. After merging, the new pile's weight is `3`, and he consumed 3 units of energy.
John wants to combine all the fruits into 1 pile with the least energy.
Your task is to help John, calculate the minimum energy he costs.
# Input
- `fruits`: An array of positive integers. Each element represents the weight of a pile of fruit.
Javascript:
- 1 <= fruits.length <= 10000
- 1 <= fruits[i] <= 10000
Python:
- 1 <= len(fruits) <= 5000
- 1 <= fruits[i] <= 10000
# Output
An integer. the minimum energy John costs.
# Examples
For `fruits = [1,2,9]`, the output should be `15`.
```
3 piles: 1 2 9
combine 1 and 2 to 3, cost 3 units of energy.
2 piles: 3 9
combine 3 and 9 to 12, cost 12 units of energy.
1 pile: 12
The total units of energy is 3 + 12 = 15 units
```
For `fruits = [100]`, the output should be `0`.
There's only 1 pile. So no need combine it. | ["from heapq import heappop, heappush\n\ndef comb(fruits):\n total, heap = 0, sorted(fruits)\n while len(heap) > 1:\n cost = heappop(heap) + heappop(heap)\n heappush(heap, cost)\n total += cost\n return total", "import bisect\nfrom collections import deque\ndef comb(fruits):\n energy = 0\n fruits = deque(sorted(fruits))\n while len(fruits) > 1:\n e = fruits.popleft() + fruits.popleft()\n energy += e\n bisect.insort_left(fruits, e)\n return energy", "from heapq import heapify, heappop, heappush\n\ndef comb(fruits):\n ws = list(fruits)\n heapify(ws)\n res = 0\n while len(ws) > 1:\n w = heappop(ws) + heappop(ws)\n heappush(ws, w)\n res += w\n return res", "from heapq import heappop, heappush\n\n\ndef comb(fruits):\n total, fruits = 0, sorted(fruits)\n for _ in range(len(fruits) - 1):\n energy = heappop(fruits) + heappop(fruits)\n heappush(fruits, energy)\n total += energy\n return total", "from heapq import heapify, heappush, heappop\n\ndef comb(fruits):\n energy = 0\n heapify(fruits)\n while len(fruits) > 1:\n cost = heappop(fruits) + heappop(fruits)\n heappush(fruits, cost)\n energy += cost\n return energy", "from bisect import insort\n\ndef comb(fruits):\n fruits = sorted(fruits)\n total_cost = 0\n while len(fruits) > 1:\n cost = sum(fruits[:2])\n total_cost += cost\n del fruits[:2]\n insort(fruits, cost)\n return total_cost", "from heapq import heapify, heappop, heapreplace\n\ndef comb(fruits):\n fruits = fruits[:]\n heapify(fruits)\n total_cost = 0\n for i in range(len(fruits) - 1):\n cost = heappop(fruits)\n cost += fruits[0]\n total_cost += cost\n heapreplace(fruits, cost)\n return total_cost", "from heapq import heapify, heappop, heappush\n\n\ndef comb(fruits):\n heapify(fruits)\n \n energy_total = 0\n while len(fruits) > 1:\n energy_used = heappop(fruits) + heappop(fruits)\n heappush(fruits, energy_used)\n energy_total += energy_used\n \n return energy_total\n", "import heapq\n\ndef comb(fruits):\n heapq.heapify(fruits)\n w = 0\n while len(fruits) > 1:\n x, y = heapq.heappop(fruits), heapq.heappop(fruits)\n w += x + y\n heapq.heappush(fruits, x + y)\n return w\n", "def bin_insert(n, lst):\n start = 0\n end = len(lst) - 1\n\n while start < end:\n check = (start + end) // 2\n\n if lst[check] == n:\n return check\n \n if lst[check] < n:\n start = check + 1\n else:\n end = check - 1\n\n if len(lst) != 0 and lst[start] < n:\n return start + 1\n return start\n\ndef comb(fruits):\n fruits.sort()\n res = 0\n while len(fruits) > 1:\n wt = fruits.pop(0) + fruits.pop(0)\n res += wt\n fruits.insert(bin_insert(wt, fruits), wt)\n return res"] | {"fn_name": "comb", "inputs": [[[1, 2, 9]], [[100]], [[1, 2]], [[4, 3, 5, 6, 10, 20]], [[87, 84, 42, 34, 24, 81, 60, 48, 75]], [[11, 9, 20, 10, 21, 35, 15, 34, 48, 76, 94, 28, 79, 16, 4, 41, 98, 30, 35, 92, 93, 33, 100, 93, 64, 23, 37, 6, 86, 27, 48, 16, 66, 99, 61, 83, 3, 5, 95]]], "outputs": [[15], [0], [3], [111], [1663], [9056]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,023 |
def comb(fruits):
|
13f60b2a502d991db562040717d787e4 | UNKNOWN | # Hey You !
Sort these integers for me ...
By name ...
Do it now !
---
## Input
* Range is ```0```-```999```
* There may be duplicates
* The array may be empty
## Example
* Input: 1, 2, 3, 4
* Equivalent names: "one", "two", "three", "four"
* Sorted by name: "four", "one", "three", "two"
* Output: 4, 1, 3, 2
## Notes
* Don't pack words together:
* e.g. 99 may be "ninety nine" or "ninety-nine"; but not "ninetynine"
* e.g 101 may be "one hundred one" or "one hundred and one"; but not "onehundredone"
* Don't fret about formatting rules, because if rules are consistently applied it has no effect anyway:
* e.g. "one hundred one", "one hundred two"; is same order as "one hundred **and** one", "one hundred **and** two"
* e.g. "ninety eight", "ninety nine"; is same order as "ninety-eight", "ninety-nine"
```if:c
* For `C` code the input array may be NULL. The return value is freed if non-NULL.
``` | ["def int_to_word(num):\n d = { 0 : 'zero', 1 : 'one', 2 : 'two', 3 : 'three', 4 : 'four', 5 : 'five',\n 6 : 'six', 7 : 'seven', 8 : 'eight', 9 : 'nine', 10 : 'ten',\n 11 : 'eleven', 12 : 'twelve', 13 : 'thirteen', 14 : 'fourteen',\n 15 : 'fifteen', 16 : 'sixteen', 17 : 'seventeen', 18 : 'eighteen',\n 19 : 'nineteen', 20 : 'twenty',\n 30 : 'thirty', 40 : 'forty', 50 : 'fifty', 60 : 'sixty',\n 70 : 'seventy', 80 : 'eighty', 90 : 'ninety' }\n\n\n assert(0 <= num)\n\n if (num < 20):\n return d[num]\n\n if (num < 100):\n if num % 10 == 0: return d[num]\n else: return d[num // 10 * 10] + '-' + d[num % 10]\n\n if (num < 1000):\n if num % 100 == 0: return d[num // 100] + ' hundred'\n else: return d[num // 100] + ' hundred and ' + int_to_word(num % 100)\n\ndef sort_by_name(arr):\n return sorted(arr, key=int_to_word)\n", "S = [\"\", \"one\", \"two\", \"three\", \"four\", \"five\", \"six\", \"seven\", \"eight\", \"nine\",\n \"ten\", \"eleven\", \"twelve\", \"thirteen\", \"fourteen\", \"fifteen\", \"sixteen\", \"seventeen\", \"eighteen\", \"nineteen\"]\nTENTH = [\"\", \"\", \"twenty\", \"thirty\", \"forty\", \"fifty\", \"sixty\", \"seventy\", \"eighty\", \"ninety\"]\n\n\ndef convertToString(n):\n if not n: return \"zero\"\n c,t,u = map(lambda v: (n%(v*10))//v, (100,10,1))\n return \" \".join(s for s in [\"{} hundred\".format(S[c]) * bool(c), TENTH[t], S[u+10*(t==1)]] if s)\n\ndef sort_by_name(arr): return sorted(arr, key=convertToString)", "def sort_by_name(arr):\n return sorted(arr, key=convert)\n\ndef convert(a):\n if not a: return \"zero\"\n d = {1:'one', 2:'two', 3:'three', 4:'four', 5:'five', 6:'six', 7:'seven', 8:'eight', 9:'nine', 10:'ten', 11:'eleven', 12:'twelve', 13:'thirteen', 14:'fourteen', 15:'fifteen', 16:'sixteen', 17:'seventeen', 18:'eighteen', 19:'nineteen', 20:'twenty', 30:'thirty', 40:'forty', 50:'fifty', 60:'sixty', 70:'seventy', 80:'eighty', 90:'ninety'}\n r = []\n if a // 100: r.append(\"{} hundred\".format(d[a // 100]))\n if a % 100:\n if a % 100 <= 20: r.append(d[a % 100])\n else:\n b = d[a % 100 // 10 * 10]\n if a % 10: b += \" {}\".format(d[a % 10])\n r.append(b)\n return \" \".join(r)", "ones = {'0': 'zero', '1': 'one', '2': 'two', '3': 'three', '4': 'four',\n '5': 'five', '6': 'six','7': 'seven', '8': 'eight', '9': 'nine' }\n\nteens = {'11': 'eleven', '12': 'twelve', '13': 'thirteen', '14': 'fourteen',\n '15': 'fifteen', '16': 'sixteen', '17': 'seventeen', \n '18': 'eighteen', '19': 'nineteen' }\n\ntens = { '1': 'ten', '2': 'twenty', '3': 'thirty', '4': 'forty', \n '5': 'fifty', '6': 'sixty', '7': 'seventy', '8': 'eighty', \n '9': 'ninety' }\n\nhundreds = {'100' : 'one hundred', '200' : 'two hundred',\n '300' : 'three hundred', '400' : 'four hundred',\n '500' : 'five hundred', '600' : 'six hundred',\n '700' : 'seven hundred', '800' : 'eight hundred',\n '900' : 'nine hundred'}\n\ndef num_to_word(n):\n\n str_num = str(n)\n\n if n >= 100:\n first_digit = str_num[0] + '00'\n second_digit = str_num[1]\n third_digit = str_num[2]\n elif n > 9:\n first_digit = str_num[0]\n second_digit = str_num[1]\n else:\n return ones[str_num]\n\n\n if n > 99:\n if second_digit == '0' and third_digit != '0':\n return '{} {}'.format(hundreds[first_digit], ones[third_digit])\n elif second_digit != '0' and third_digit == '0':\n return '{} {}'.format(hundreds[first_digit], tens[second_digit])\n elif int(second_digit + third_digit) > 10 and int(second_digit + third_digit) < 20:\n return '{} {}'.format(hundreds[first_digit], teens[second_digit + third_digit])\n elif third_digit != '0':\n return '{} {}-{}'.format(hundreds[first_digit], tens[second_digit], ones[third_digit])\n else:\n return hundreds[str_num]\n \n else:\n if n > 10 and n < 20:\n return teens[str_num]\n elif second_digit != '0':\n return '{}-{}'.format(tens[first_digit], ones[second_digit])\n else:\n return tens[first_digit]\n\nint_to_word = {_ : num_to_word(_) for _ in range(1000)} \nword_to_int = {num_to_word(_) : _ for _ in range(1000)} \n\ndef sort_by_name(arr):\n return [word_to_int[word] for word in sorted(int_to_word[num] for num in arr)]\n", "S = \" one two three four five six seven eight nine ten eleven twelve thirteen fourteen fifteen sixteen seventeen eighteen nineteen\".split(\" \")\nTENTH = \" twenty thirty forty fifty sixty seventy eighty ninety\".split(\" \") # Empty strings wanted at the beginning, when splitting!\n\ndef convertToString(n):\n if not n: return \"zero\"\n c,t,u = map(int, f'{n:0>3}')\n return \" \".join(s for s in [f'{S[c]} hundred' * bool(c), TENTH[t], S[u+10*(t==1)]] if s)\n\ndef sort_by_name(arr): return sorted(arr, key=convertToString)", "num2words1 = {0: 'zero', 1: 'one', 2: 'two', 3: 'three', 4: 'four', 5: 'five', 6: 'six', 7: 'seven', 8: 'eight', 9: 'nine', 10: 'ten', 11: 'eleven', 12: 'twelve', 13: 'thirteen', 14: 'fourteen', 15: 'fifteen', 16: 'sixteen', 17: 'seventeen', 18: 'eighteen', 19: 'nineteen'}\nnum2words2 = ['','','twenty', 'thirty', 'forty', 'fifty', 'sixty', 'seventy', 'eighty', 'ninety']\ndef sort_by_name(arr):\n return sorted(arr, key=lambda x: words(x))\ndef words(n):\n res=[]\n if n>=100:\n res.append(num2words1[n//100])\n res.append(\"hundred\")\n n-=n//100*100\n if n>=20:\n res.append(num2words2[n//10])\n n-=n//10*10\n if n>0:\n res.append(num2words1[n])\n if not res:\n res.append(\"zero\")\n return \" \".join(res)", "words = \"zero one two three four five six seven eight nine ten eleven twelve thirteen fourteen fifteen sixteen seventeen eighteen nineteen twenty thirty forty fifty sixty seventy eighty ninety\"\nwords = words.split(\" \")\n\ndef num2word(n):\n if n < 20:\n return words[n]\n elif n < 100:\n return words[18 + n // 10] + ('' if n % 10 == 0 else '-' + words[n % 10])\n else:\n return num2word(n // 100) + \" hundred\" + (' ' + num2word(n % 100) if n % 100 > 0 else '')\n\ndef sort_by_name(arr):\n return sorted(arr, key=num2word)", "def sort_by_name(arr):\n comparing_list, before_sort_dict, resulty_list = [], {}, []\n nu_dict = {'0': '', '1': 'one', '2': 'two', '3': 'three', '4': 'four', '5': 'five', '6': 'six', '7': 'seven',\n '8': 'eight', '9': 'nine', '10': 'ten', '11': 'eleven', '12': 'twelve', '13': 'thirteen',\n '14': 'fourteen', '15': 'fifteen', '16': 'sixteen', '17': 'seventeen', '18': 'eighteen',\n '19': 'nineteen'}\n for number in map(str, arr):\n if len(number) == 3:\n if 10 <= int(number[1:]) < 20:\n temp_word = number[1] + number[2]\n numberly_str = '{hundreds} hundred {tens}'.format(hundreds=nu_dict[number[0]], tens=nu_dict[temp_word])\n else:\n numberly_str = '{hundreds} hundred {tens}ty {deci}'.format(hundreds=nu_dict[number[0]],\n tens=nu_dict[number[1]], deci=nu_dict[number[2]])\n elif len(number) == 2:\n if 10 <= int(number) < 20:\n temp_word = number[0] + number[1]\n numberly_str = '{tens}'.format(tens=nu_dict[temp_word])\n else:\n numberly_str = '{tens}ty {deci}'.format(tens=nu_dict[number[0]], deci=nu_dict[number[1]])\n else:\n if number == '0':\n numberly_str = 'zero'\n else:\n numberly_str = '{deci}'.format(deci=nu_dict[number[0]])\n temp_list = numberly_str.split()\n for pos, word in enumerate(temp_list):\n if word == 'ty':\n temp_list[pos] = ''\n numberly_str = ' '.join(temp_list)\n numberly_str = numberly_str.replace(' ', ' ').replace('fourty', 'forty').replace('twoty', 'twenty')\n numberly_str = numberly_str.replace('threety', 'thirty').replace('fivety', 'fifty').replace('eightty', 'eighty')\n numberly_str = numberly_str.rstrip()\n comparing_list.append(numberly_str)\n for index, crypt in enumerate(comparing_list):\n before_sort_dict[index] = crypt\n sorted_dict = {k: v for k, v in sorted(before_sort_dict.items(), key=lambda item: item[1])}\n for key, value in sorted_dict.items():\n resulty_list.append(arr[key])\n return resulty_list", "def sort_by_name(arr):\n num_dict = {\n 1:'one', 2:'two', 3:'three', 4:'four', 5:'five',\n 6:'six', 7:'seven', 8:'eight', 9:'nine', 10:'ten',\n 11:'eleven', 12:'twelve', 13:'therteen', 14:'fourteen',\n 15:'fifteen', 16:'sixteen', 17:'seventeen', 18:'eighteen',\n 19:'nineteen', 20:'twenty', 30:'thirty', 40:'forty',\n 50:'fifty', 60:'sixty', 70:'seventy', 80:'eighty',\n 90:'ninety', 0:'zero', -1:'', -10:''\n }\n raw_list = [] # will be tuple [('two', 2), ('zero', 0)]\n for i in arr:\n if i in num_dict:\n raw_list.append(tuple([num_dict[i], i]))\n else:\n raw_i = str(i)\n nums = len(raw_i)\n if nums == 2:\n j = int(raw_i[1])\n if 10 < i < 20:\n raw_list.append(tuple([num_dict[i], i]))\n continue\n if j != 0:\n raw_list.append(tuple(['{} {}'.format(num_dict[int(raw_i[0])*10],\n num_dict[int(raw_i[1])]), i]))\n continue\n else:\n raw_list.append(tuple(['{}'.format(num_dict[int(raw_i[0])*10]), i]))\n continue\n else:\n z = int(raw_i[1])\n k = int(raw_i[2])\n if z == 0:\n z = -1\n if k == 0:\n k = -1\n if 10 < int(raw_i[1]+raw_i[2]) < 20:\n raw_list.append(tuple(['{} hundred {}'.format(num_dict[int(raw_i[0])],\n num_dict[int(raw_i[1]+raw_i[2])]).replace(\" \", ' '), i]))\n continue\n raw_list.append(tuple(['{} hundred {} {}'.format(num_dict[int(raw_i[0])],\n num_dict[z*10], num_dict[k]).replace(\" \", ' '), i]))\n raw_list.sort(key = lambda x: x[0])\n # raw_list.sort(key = lambda x: (x[0], x[1]))\n out_list = []\n for i in raw_list:\n out_list.append(i[1])\n return out_list", "from enum import Enum\nclass Numbers(Enum):\n zero = 0\n one = 1\n two = 2\n three = 3\n four = 4\n five = 5\n six = 6\n seven = 7\n eight = 8\n nine = 9\n ten = 10\n eleven = 11\n twelve = 12\n thirteen = 13\n fourteen = 14\n fifteen = 15\n sixteen = 16\n seventeen = 17\n eighteen = 18\n nineteen = 19\n twenty = 20\n thirty = 30\n forty = 40\n fifty = 50\n sixty = 60\n seventy = 70\n eighty = 80\n ninety = 90\n\n\ndef number_to_name(number):\n return Numbers(number).name if number < 20 else Numbers(number).name if number < 100 and not number % 10 else (f\"{Numbers(number // 10 * 10).name}-{Numbers(number % 10).name}\" if number < 100 else f\"{Numbers(number // 100).name} hundred\" if not number % 100 else f\"{Numbers(number // 100).name} hundred and {number_to_name(number % 100)}\")\n \ndef sort_by_name(arr):\n return sorted(arr,key=number_to_name)"] | {"fn_name": "sort_by_name", "inputs": [[[0, 1, 2, 3]], [[]], [[8, 8, 9, 9, 10, 10]], [[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20]], [[99, 100, 200, 300, 999]], [[0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 45, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59, 60, 61, 62, 63, 64, 65, 66, 67, 68, 69, 70, 71, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 83, 84, 85, 86, 87, 88, 89, 90, 91, 92, 93, 94, 95, 96, 97, 98, 99, 100, 101, 102, 103, 104, 105, 106, 107, 108, 109, 110, 111, 112, 113, 114, 115, 116, 117, 118, 119, 120, 121, 122, 123, 124, 125, 126, 127, 128, 129, 130, 131, 132, 133, 134, 135, 136, 137, 138, 139, 140, 141, 142, 143, 144, 145, 146, 147, 148, 149, 150, 151, 152, 153, 154, 155, 156, 157, 158, 159, 160, 161, 162, 163, 164, 165, 166, 167, 168, 169, 170, 171, 172, 173, 174, 175, 176, 177, 178, 179, 180, 181, 182, 183, 184, 185, 186, 187, 188, 189, 190, 191, 192, 193, 194, 195, 196, 197, 198, 199, 200, 201, 202, 203, 204, 205, 206, 207, 208, 209, 210, 211, 212, 213, 214, 215, 216, 217, 218, 219, 220, 221, 222, 223, 224, 225, 226, 227, 228, 229, 230, 231, 232, 233, 234, 235, 236, 237, 238, 239, 240, 241, 242, 243, 244, 245, 246, 247, 248, 249, 250, 251, 252, 253, 254, 255, 256, 257, 258, 259, 260, 261, 262, 263, 264, 265, 266, 267, 268, 269, 270, 271, 272, 273, 274, 275, 276, 277, 278, 279, 280, 281, 282, 283, 284, 285, 286, 287, 288, 289, 290, 291, 292, 293, 294, 295, 296, 297, 298, 299, 300, 301, 302, 303, 304, 305, 306, 307, 308, 309, 310, 311, 312, 313, 314, 315, 316, 317, 318, 319, 320, 321, 322, 323, 324, 325, 326, 327, 328, 329, 330, 331, 332, 333, 334, 335, 336, 337, 338, 339, 340, 341, 342, 343, 344, 345, 346, 347, 348, 349, 350, 351, 352, 353, 354, 355, 356, 357, 358, 359, 360, 361, 362, 363, 364, 365, 366, 367, 368, 369, 370, 371, 372, 373, 374, 375, 376, 377, 378, 379, 380, 381, 382, 383, 384, 385, 386, 387, 388, 389, 390, 391, 392, 393, 394, 395, 396, 397, 398, 399, 400, 401, 402, 403, 404, 405, 406, 407, 408, 409, 410, 411, 412, 413, 414, 415, 416, 417, 418, 419, 420, 421, 422, 423, 424, 425, 426, 427, 428, 429, 430, 431, 432, 433, 434, 435, 436, 437, 438, 439, 440, 441, 442, 443, 444, 445, 446, 447, 448, 449, 450, 451, 452, 453, 454, 455, 456, 457, 458, 459, 460, 461, 462, 463, 464, 465, 466, 467, 468, 469, 470, 471, 472, 473, 474, 475, 476, 477, 478, 479, 480, 481, 482, 483, 484, 485, 486, 487, 488, 489, 490, 491, 492, 493, 494, 495, 496, 497, 498, 499, 500, 501, 502, 503, 504, 505, 506, 507, 508, 509, 510, 511, 512, 513, 514, 515, 516, 517, 518, 519, 520, 521, 522, 523, 524, 525, 526, 527, 528, 529, 530, 531, 532, 533, 534, 535, 536, 537, 538, 539, 540, 541, 542, 543, 544, 545, 546, 547, 548, 549, 550, 551, 552, 553, 554, 555, 556, 557, 558, 559, 560, 561, 562, 563, 564, 565, 566, 567, 568, 569, 570, 571, 572, 573, 574, 575, 576, 577, 578, 579, 580, 581, 582, 583, 584, 585, 586, 587, 588, 589, 590, 591, 592, 593, 594, 595, 596, 597, 598, 599, 600, 601, 602, 603, 604, 605, 606, 607, 608, 609, 610, 611, 612, 613, 614, 615, 616, 617, 618, 619, 620, 621, 622, 623, 624, 625, 626, 627, 628, 629, 630, 631, 632, 633, 634, 635, 636, 637, 638, 639, 640, 641, 642, 643, 644, 645, 646, 647, 648, 649, 650, 651, 652, 653, 654, 655, 656, 657, 658, 659, 660, 661, 662, 663, 664, 665, 666, 667, 668, 669, 670, 671, 672, 673, 674, 675, 676, 677, 678, 679, 680, 681, 682, 683, 684, 685, 686, 687, 688, 689, 690, 691, 692, 693, 694, 695, 696, 697, 698, 699, 700, 701, 702, 703, 704, 705, 706, 707, 708, 709, 710, 711, 712, 713, 714, 715, 716, 717, 718, 719, 720, 721, 722, 723, 724, 725, 726, 727, 728, 729, 730, 731, 732, 733, 734, 735, 736, 737, 738, 739, 740, 741, 742, 743, 744, 745, 746, 747, 748, 749, 750, 751, 752, 753, 754, 755, 756, 757, 758, 759, 760, 761, 762, 763, 764, 765, 766, 767, 768, 769, 770, 771, 772, 773, 774, 775, 776, 777, 778, 779, 780, 781, 782, 783, 784, 785, 786, 787, 788, 789, 790, 791, 792, 793, 794, 795, 796, 797, 798, 799, 800, 801, 802, 803, 804, 805, 806, 807, 808, 809, 810, 811, 812, 813, 814, 815, 816, 817, 818, 819, 820, 821, 822, 823, 824, 825, 826, 827, 828, 829, 830, 831, 832, 833, 834, 835, 836, 837, 838, 839, 840, 841, 842, 843, 844, 845, 846, 847, 848, 849, 850, 851, 852, 853, 854, 855, 856, 857, 858, 859, 860, 861, 862, 863, 864, 865, 866, 867, 868, 869, 870, 871, 872, 873, 874, 875, 876, 877, 878, 879, 880, 881, 882, 883, 884, 885, 886, 887, 888, 889, 890, 891, 892, 893, 894, 895, 896, 897, 898, 899, 900, 901, 902, 903, 904, 905, 906, 907, 908, 909, 910, 911, 912, 913, 914, 915, 916, 917, 918, 919, 920, 921, 922, 923, 924, 925, 926, 927, 928, 929, 930, 931, 932, 933, 934, 935, 936, 937, 938, 939, 940, 941, 942, 943, 944, 945, 946, 947, 948, 949, 950, 951, 952, 953, 954, 955, 956, 957, 958, 959, 960, 961, 962, 963, 964, 965, 966, 967, 968, 969, 970, 971, 972, 973, 974, 975, 976, 977, 978, 979, 980, 981, 982, 983, 984, 985, 986, 987, 988, 989, 990, 991, 992, 993, 994, 995, 996, 997, 998, 999]]], "outputs": [[[1, 3, 2, 0]], [[]], [[8, 8, 9, 9, 10, 10]], [[8, 18, 11, 15, 5, 4, 14, 9, 19, 1, 7, 17, 6, 16, 10, 13, 3, 12, 20, 2, 0]], [[999, 99, 100, 300, 200]], [[8, 800, 808, 818, 880, 888, 885, 884, 889, 881, 887, 886, 883, 882, 811, 815, 850, 858, 855, 854, 859, 851, 857, 856, 853, 852, 805, 840, 848, 845, 844, 849, 841, 847, 846, 843, 842, 804, 814, 809, 819, 890, 898, 895, 894, 899, 891, 897, 896, 893, 892, 801, 807, 817, 870, 878, 875, 874, 879, 871, 877, 876, 873, 872, 806, 816, 860, 868, 865, 864, 869, 861, 867, 866, 863, 862, 810, 813, 830, 838, 835, 834, 839, 831, 837, 836, 833, 832, 803, 812, 820, 828, 825, 824, 829, 821, 827, 826, 823, 822, 802, 18, 80, 88, 85, 84, 89, 81, 87, 86, 83, 82, 11, 15, 50, 58, 55, 54, 59, 51, 57, 56, 53, 52, 5, 500, 508, 518, 580, 588, 585, 584, 589, 581, 587, 586, 583, 582, 511, 515, 550, 558, 555, 554, 559, 551, 557, 556, 553, 552, 505, 540, 548, 545, 544, 549, 541, 547, 546, 543, 542, 504, 514, 509, 519, 590, 598, 595, 594, 599, 591, 597, 596, 593, 592, 501, 507, 517, 570, 578, 575, 574, 579, 571, 577, 576, 573, 572, 506, 516, 560, 568, 565, 564, 569, 561, 567, 566, 563, 562, 510, 513, 530, 538, 535, 534, 539, 531, 537, 536, 533, 532, 503, 512, 520, 528, 525, 524, 529, 521, 527, 526, 523, 522, 502, 40, 48, 45, 44, 49, 41, 47, 46, 43, 42, 4, 400, 408, 418, 480, 488, 485, 484, 489, 481, 487, 486, 483, 482, 411, 415, 450, 458, 455, 454, 459, 451, 457, 456, 453, 452, 405, 440, 448, 445, 444, 449, 441, 447, 446, 443, 442, 404, 414, 409, 419, 490, 498, 495, 494, 499, 491, 497, 496, 493, 492, 401, 407, 417, 470, 478, 475, 474, 479, 471, 477, 476, 473, 472, 406, 416, 460, 468, 465, 464, 469, 461, 467, 466, 463, 462, 410, 413, 430, 438, 435, 434, 439, 431, 437, 436, 433, 432, 403, 412, 420, 428, 425, 424, 429, 421, 427, 426, 423, 422, 402, 14, 9, 900, 908, 918, 980, 988, 985, 984, 989, 981, 987, 986, 983, 982, 911, 915, 950, 958, 955, 954, 959, 951, 957, 956, 953, 952, 905, 940, 948, 945, 944, 949, 941, 947, 946, 943, 942, 904, 914, 909, 919, 990, 998, 995, 994, 999, 991, 997, 996, 993, 992, 901, 907, 917, 970, 978, 975, 974, 979, 971, 977, 976, 973, 972, 906, 916, 960, 968, 965, 964, 969, 961, 967, 966, 963, 962, 910, 913, 930, 938, 935, 934, 939, 931, 937, 936, 933, 932, 903, 912, 920, 928, 925, 924, 929, 921, 927, 926, 923, 922, 902, 19, 90, 98, 95, 94, 99, 91, 97, 96, 93, 92, 1, 100, 108, 118, 180, 188, 185, 184, 189, 181, 187, 186, 183, 182, 111, 115, 150, 158, 155, 154, 159, 151, 157, 156, 153, 152, 105, 140, 148, 145, 144, 149, 141, 147, 146, 143, 142, 104, 114, 109, 119, 190, 198, 195, 194, 199, 191, 197, 196, 193, 192, 101, 107, 117, 170, 178, 175, 174, 179, 171, 177, 176, 173, 172, 106, 116, 160, 168, 165, 164, 169, 161, 167, 166, 163, 162, 110, 113, 130, 138, 135, 134, 139, 131, 137, 136, 133, 132, 103, 112, 120, 128, 125, 124, 129, 121, 127, 126, 123, 122, 102, 7, 700, 708, 718, 780, 788, 785, 784, 789, 781, 787, 786, 783, 782, 711, 715, 750, 758, 755, 754, 759, 751, 757, 756, 753, 752, 705, 740, 748, 745, 744, 749, 741, 747, 746, 743, 742, 704, 714, 709, 719, 790, 798, 795, 794, 799, 791, 797, 796, 793, 792, 701, 707, 717, 770, 778, 775, 774, 779, 771, 777, 776, 773, 772, 706, 716, 760, 768, 765, 764, 769, 761, 767, 766, 763, 762, 710, 713, 730, 738, 735, 734, 739, 731, 737, 736, 733, 732, 703, 712, 720, 728, 725, 724, 729, 721, 727, 726, 723, 722, 702, 17, 70, 78, 75, 74, 79, 71, 77, 76, 73, 72, 6, 600, 608, 618, 680, 688, 685, 684, 689, 681, 687, 686, 683, 682, 611, 615, 650, 658, 655, 654, 659, 651, 657, 656, 653, 652, 605, 640, 648, 645, 644, 649, 641, 647, 646, 643, 642, 604, 614, 609, 619, 690, 698, 695, 694, 699, 691, 697, 696, 693, 692, 601, 607, 617, 670, 678, 675, 674, 679, 671, 677, 676, 673, 672, 606, 616, 660, 668, 665, 664, 669, 661, 667, 666, 663, 662, 610, 613, 630, 638, 635, 634, 639, 631, 637, 636, 633, 632, 603, 612, 620, 628, 625, 624, 629, 621, 627, 626, 623, 622, 602, 16, 60, 68, 65, 64, 69, 61, 67, 66, 63, 62, 10, 13, 30, 38, 35, 34, 39, 31, 37, 36, 33, 32, 3, 300, 308, 318, 380, 388, 385, 384, 389, 381, 387, 386, 383, 382, 311, 315, 350, 358, 355, 354, 359, 351, 357, 356, 353, 352, 305, 340, 348, 345, 344, 349, 341, 347, 346, 343, 342, 304, 314, 309, 319, 390, 398, 395, 394, 399, 391, 397, 396, 393, 392, 301, 307, 317, 370, 378, 375, 374, 379, 371, 377, 376, 373, 372, 306, 316, 360, 368, 365, 364, 369, 361, 367, 366, 363, 362, 310, 313, 330, 338, 335, 334, 339, 331, 337, 336, 333, 332, 303, 312, 320, 328, 325, 324, 329, 321, 327, 326, 323, 322, 302, 12, 20, 28, 25, 24, 29, 21, 27, 26, 23, 22, 2, 200, 208, 218, 280, 288, 285, 284, 289, 281, 287, 286, 283, 282, 211, 215, 250, 258, 255, 254, 259, 251, 257, 256, 253, 252, 205, 240, 248, 245, 244, 249, 241, 247, 246, 243, 242, 204, 214, 209, 219, 290, 298, 295, 294, 299, 291, 297, 296, 293, 292, 201, 207, 217, 270, 278, 275, 274, 279, 271, 277, 276, 273, 272, 206, 216, 260, 268, 265, 264, 269, 261, 267, 266, 263, 262, 210, 213, 230, 238, 235, 234, 239, 231, 237, 236, 233, 232, 203, 212, 220, 228, 225, 224, 229, 221, 227, 226, 223, 222, 202, 0]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,762 |
def sort_by_name(arr):
|
b053bbfa06828beeb6409441b014abad | UNKNOWN | Pair of gloves
=============
Winter is coming, you must prepare your ski holidays. The objective of this kata is to determine the number of pair of gloves you can constitute from the gloves you have in your drawer.
A pair of gloves is constituted of two gloves of the same color.
You are given an array containing the color of each glove.
You must return the number of pair you can constitute.
You must not change the input array.
Example :
```python
my_gloves = ["red","green","red","blue","blue"]
number_of_pairs(my_gloves) == 2;// red and blue
red_gloves = ["red","red","red","red","red","red"];
number_of_pairs(red_gloves) == 3; // 3 red pairs
``` | ["from collections import Counter\n\ndef number_of_pairs(gloves):\n return sum(c // 2 for c in Counter(gloves).values())", "def number_of_pairs(gloves):\n return sum(gloves.count(color)//2 for color in set(gloves))", "def number_of_pairs(gloves):\n unique = set(gloves)\n return sum(gloves.count(i)//2 for i in unique)", "from collections import Counter\n\ndef number_of_pairs(gloves):\n return sum(num // 2 for num in Counter(gloves).values())", "def number_of_pairs(gloves):\n return sum(gloves.count(c) // 2 for c in set(gloves))", "from itertools import groupby\n\ndef number_of_pairs(gloves):\n return sum([len(list(g)) // 2 for k, g in groupby(sorted(gloves))])", "from itertools import groupby\n\ndef number_of_pairs(gloves):\n return sum(len(list(gp)) // 2 for _, gp in groupby(sorted(gloves)))", "def number_of_pairs(gloves):\n return sum([gloves.count(i) // 2 for i in set(gloves)])", "import collections\ndef number_of_pairs(gloves):\n return sum(i//2 for i in collections.Counter(gloves).values())", "from collections import Counter\n\ndef number_of_pairs(gloves):\n return sum(v // 2 for v in Counter(gloves).values())"] | {"fn_name": "number_of_pairs", "inputs": [[["red", "red"]], [["red", "green", "blue"]], [["gray", "black", "purple", "purple", "gray", "black"]], [[]], [["red", "green", "blue", "blue", "red", "green", "red", "red", "red"]]], "outputs": [[1], [0], [3], [0], [4]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,164 |
def number_of_pairs(gloves):
|
91467c2ebdb68ae26e08e9bf8a2803f6 | UNKNOWN | Make a function that will return a greeting statement that uses an input; your program should return, `"Hello, how are you doing today?"`.
SQL: return results in a column named ```greeting```
*[Make sure you type the exact thing I wrote or the program may not execute properly]* | ["def greet(name):\n return \"Hello, {} how are you doing today?\".format(name)", "def greet(name):\n return f'Hello, {name} how are you doing today?'", "def greet(name):\n return \"Hello, %s how are you doing today?\" % name", "def greet(name):\n return \"Hello, \" + name + \" how are you doing today?\"", "def greet(name):\n #Good Luck (like you need it)\n return \"Hello, {name} how are you doing today?\".format(name = name)", "greet = lambda name: \"Hello, %s how are you doing today?\" % name", "greet = lambda name: f'Hello, {name} how are you doing today?'", "greet = \"Hello, {0} how are you doing today?\".format", "def greet(name):\n return \"Hello, {} how are you doing today?\".format(name)\n\n# for python 3.6\n# def greet(name):\n# return f'Hello, {name} how are you doing today?'\n\n\n# def greet(name):\n# return 'Hello, ' + name + ' how are you doing today?'\n", "def greet(name):\n return \"Hello, %s how are you doing today?\" % str(name)", "import unittest\n\n\ndef greet(name):\n return \"Hello, {} how are you doing today?\".format(name)\n \n \nclass TestGreet(unittest.TestCase):\n def test_greet(self):\n name = 'Ryan'\n actual = greet(name)\n self.assertEqual(actual, \"Hello, Ryan how are you doing today?\")\n", "def greet(name):\n return \"Hello, %s how are you doing today?\" % (name)\nprint(greet(\"Bob\"))", "def greet(name: str) -> str:\n \"\"\" Get a greeting statement that uses an input. \"\"\"\n return f\"Hello, {name} how are you doing today?\"", "def greet(name):\n return f'Hello, {name} how are you doing today?'.format(name)", "def greet(name):\n x = 'Hello, {} how are you doing today?'\n return x.format(name)", "def greet(name):\n return 'Hello, ' + name + ' how are you doing today?' if name else None", "def greet(name):\n #Good Luck (like you need it)\n a=\"Hello, {0} how are you doing today?\".format(name)\n return a", "greet = lambda x: f\"Hello, {x} how are you doing today?\"", "greet = \"Hello, {} how are you doing today?\".format", "greet = lambda n: \"Hello, {} how are you doing today?\".format(n)", "def greet(name):\n if name:\n return \"Hello, \" + name + \" how are you doing today?\"\n else:\n return \"Ask of me, and I shall give thee the heathen for thine inheritance, \\\n and for thy possession, the ends of the earth. \\\n Thou shalt break them with a rod of iron. \\\n Thou shalt dash them in pieces, like a potters vessel. \\\n Be wise now therefore, ye kings. \\\n Be admonished, ye judges of the earth.\\\n Serve the Lord with fear, and rejoice with trembling. \\\n Kiss the son lest he be angry, and ye perish in the way, though His wrath be kindled but a little. \\\n Hold your toung. The dead don't speak!\\\n To the dead dare walk the earth before my eyes?\\\n Will the undead raise an army, fall in, and advance? Would those who would abandon God and appraise the heretical order DARE presume to meet my gaze!?\\\n Iscariot will not allow it. I will not allow it. You will be cut down like a straw, trembling before my wrath; \\\n Amen!\"", "def greet(name):\n string = 'Hello, {0} how are you doing today?'.format(name)\n return string", "def greet(name):\n x=\"Hello, {0} how are you doing today?\"\n return(x.format(name))", "def greet(n):\n return (\"Hello, \"+str(n)+\" how are you doing today?\")", "def greet(name):\n greeting_phrase = 'Hello, {name} how are you doing today?'.format(name = name)\n return greeting_phrase", "def greet(name):\n your_name = name\n return \"Hello, \" + your_name + \" how are you doing today?\"\nprint(greet(\"Ryan\"))", "def greet(name):\n x='Hello, how are you doing today?'\n return(x[0:7]+name+x[7:])\n #Good Luck (like you need it)\n", "def greet(name):\n return('Hello, {} how are you doing today?'.format(name))\n \ngreet(name='Ryan')\n", "def greet(name: str) -> str:\n \"\"\" This function return a greeting statement that uses an input. \"\"\"\n return (f'Hello, {name} how are you doing today?')", "def greet(name):\n a = 'Hello, ' #sample string 1\n b = ' how are you doing today?' #sample string 2\n name_temp = a + name + b #summ strings\n return name_temp #return strings\n\n", "def greet(name):\n y = \"Hello, \"\n z = \" how are you doing today?\"\n final = y + name + z\n return final\n #Good Luck (like you need it)\n", "def greet(name):\n greet = \"\"\n \n return(\"Hello, \" + (name) + \" how are you doing today?\")", "def greet(name):\n my_str = \"Hello, \" + name + \" \" + \"how are you doing today?\"\n return my_str", "def greet(name):\n greetings=[\"Hello,\",\"how\",\"are\",\"you\",\"doing\",\"today?\"]\n greetings.insert(1,str(name))\n return lista_to_string(greetings)\ndef lista_to_string(L):\n return \" \".join(str(x) for x in L)\n", "def greet(Name):\n #Good Luck (like you need it)\n return \"Hello, \" + Name + \" how are you doing today?\"\n", "def greet(name):\n welcome = \"Hello, \" + str(name) + \" how are you doing today?\"\n return welcome", "def greet(name):\n first = \"Hello, \"\n last = \" how are you doing today?\"\n full = first + name + last\n return full", "def greet(name):\n return(\"Hello, \" + name + \" how are you doing today?\")\n\ngreet(\"John\")", "def greet(name):\n result = ('Hello, %s how are you doing today?' %(name))\n return result\n\nprint(greet('Ryan'))", "def greet(name):\n #Your Code Here\n return f\"Hello, {name} how are you doing today?\"", "def greet(name):\n String = \"Hello, \" + name + \" how are you doing today?\"\n return String", "def greet(name):\n a = \" how are you doing today?\"\n b = \"Hello, \"\n c = b + name + a\n return c", "def greet(name):\n #Good Luck (like you need it)\n #greet = input()\n return \"Hello, \" + name + \" how are you doing today?\"", "def greet(name):\n return \"Hello, %s how are you doing today?\" %name\n #Good Luck (like you need it)\n \nprint((greet(\"Ryan\")))\n", "def greet(name):\n mystring = \"Hello, \" + name + \" how are you doing today?\"\n return mystring\n #Good Luck (like you need it)\n", "def greet(name):\n res_str = 'Hello, ' + name + ' how are you doing today?'\n return res_str\n", "def greet(name):\n return (\"Hello, \" + str(name) + \" how are you doing today?\")\ngreet(\"Ryan\")", "def greet(name):\n #Good Luck (like you need it)\n #name = str(input())\n return(f'Hello, {name} how are you doing today?')\n", "def greet(name):\n #print(\"Hello, \"+str(name)+\" how are yo doing today?\")\n return \"Hello, \"+str(name)+\" how are you doing today?\"\n", "def greet(name):\n result = 'Hello, {0} how are you doing today?'.format(name)\n return result", "def greet(name):\n #Good Luck (like you need it)\n txt=\" how are you doing today?\"\n return ('Hello, '+ name + txt)", "def greet(name):\n return str ('Hello, ') + name + str(' how are you doing today?') ", "def greet(name):\n return \"Hello, \" + name + \" how are you doing today?\"\n\ngreet('Ryan'), \"Hello, Ryan how are you doing today?\"", "def greet(name):\n script=\"Hello, \" + name + \" how are you doing today?\"\n return(script)#Good Luck (like you need it)", "def greet(name: str) -> str:\n \"\"\"\n takes a string name and returns a greeting\n \"\"\"\n if type(name) is str:\n return f\"Hello, {name} how are you doing today?\"\n else:\n print(f\"{name} is not a string\")", "def greet(name: str) -> str:\n \"\"\"\n takes a string name and returns a greeting\n \"\"\"\n try:\n if type(name) is str:\n return f\"Hello, {name} how are you doing today?\"\n except TypeError:\n print(f\"{name} is not a string\")", "def greet(name):\n #greet = \n return f\"Hello, {name} how are you doing today?\"", "def greet(name):\n A = \"Hello, \"\n B = \" how are you doing today?\"\n return A + name + B\n\nprint(greet(\"Tim\"))", "def greet(name: str) -> str:\n \"\"\"\n takes a string name and returns a greeting\n \"\"\"\n return f\"Hello, {name} how are you doing today?\"", "def greet(name):\n txt = \"Hello, {input} how are you doing today?\".format(input = name)\n return txt", "def greet(name):\n return (\"Hello, \" + name + \" how are you doing today?\")\n\ngreet(\"Rhett\")", "def greet(n):\n str = 'Hello, ' + n + ' how are you doing today?'\n return str\n", "def greet(name):\n return \"Hello, \" + name + \" how are you doing today?\"\n\nprint(greet(\"kuki\"))", "def greet(name):\n return \"Hello, \" + name + \" how are you doing today?\"\n #Good Luck (like you need it)\ngreet(\"Ayush\")", "\ndef greet(name):\n return 'Hello, {} how are you doing today?'.format(name)\ngreet('Ryan')", "def greet(name):\n return (\"Hello, \" + name + \" how are you doing today?\")\ngreet(\"Kanyisa\")", "def greet(name):\n return \"Hello, {n} how are you doing today?\".format(n=name)", "def greet(name=None):\n string_greeting = f\"Hello, {name} how are you doing today?\"\n \n if type(name) is str:\n return string_greeting\n else:\n return \"Name must be a string.\"", "def greet(name):\n \n x = f\"Hello, {name} how are you doing today?\"\n return x\nprint(greet(\"Ryan\"))", "def greet(name):\n return \"Hello, {} how are you doing today?\".format(name)\n pass", "def greet(name):\n return f'Hello, {name} how are you doing today?'\n\nprint(greet('Ryan'))", "def greet(name):\n alphabet = \"AaBbCcDdEeFfGgHhIiKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz\u0410\u0430\u0411\u0431\u0412\u0432\u0413\u0433\u0414\u0434\u0415\u0435\u0401\u0451\u0416\u0436\u0417\u0437\u0418\u0438\u0419\u0439\u041a\u043a\u041b\u043b\u041c\u043c\u041d\u043d\u041e\u043e\u041f\u043f\u0420\u0440\u0421\u0441\u0422\u0442\u0423\u0443\u0424\u0444\u0425\u0445\u0426\u0446\u0427\u0447\u0428\u0448\u0429\u0449\u042a\u044a\u042b\u044b\u042c\u044c\u042d\u044d\u042e\u044e\u042f\u044f\"\n symbols = \" 1234567890.,_-+=\"\n \n \n return \"Hello, \" + name + \" how are you doing today?\"", "def greet(name):\n returnString = \"Hello, %s how are you doing today?\"% (name)\n return(returnString)", "def greet(username):\n a = \"Hello, \"+ username + \" how are you doing today?\"\n return a", "def greet(name):\n stupid = (\"Hello, \"+name+\" how are you doing today?\")\n return stupid", "def greet(name):\n retezec = 'Hello, ' + name + ' how are you doing today?'\n return retezec\n", "def greet(name):\n your_name = name\n greet = f\"Hello, {your_name}\" \" how are you doing today?\"\n return greet", "def greet(x):\n x=str(x)\n y=str('Hello, ' + x + ' how are you doing today?')\n return y", "def greet(name):\n #Good Luck (like you need it)\n message = \"Hello, {} how are you doing today?\".format(name)\n return message", "def greet(name):\n x = name\n Y = \"Hello, \" + x + \" how are you doing today?\"\n \n return Y", "def greet(name):\n result = f\"Hello, {name} how are you doing today?\"\n return result", "def greet(name):\n text = 'Hello, '\n text1 = ' how are you doing today?'\n return text + name + text1\n", "def greet(name):\n return f\"Hello, {str(name)} how are you doing today?\"\n #Good Luck (like you need it)\n", "def greet(name):\n #Good Luck (like you need it)\n #return \"Hello, \" + name + \" how are you doing today?\"\n return \"Hello, {} how are you doing today?\".format(name) ", "def greet(name):\n result = \"Hello, \" + name + \" how are you doing today?\"#\n return result\n #Good Luck (like you need it)\n", "def greet(name=''):\n #Good Luck (like you need it)\n return (F'Hello, {name} how are you doing today?')", "def greet(name):\n #Good Luck (like you need it)def greet(name):\n return \"Hello, {} how are you doing today?\".format(name)", "def greet(samuel):\n #Good Luck (like you need it)\n return \"Hello, {} how are you doing today?\".format(samuel)", "def greet(name):\n if name != '':\n return f\"Hello, {name} how are you doing today?\"", "def greet(name):\n string = \"Hello, \"\n string += str(name)\n string += \" how are you doing today?\"\n return string", "def greet(name):\n one = 'Hello, '\n two = ' how are you doing today?'\n return (one + name + two)", "def greet(name):\n input = name\n return(f\"Hello, {name} how are you doing today?\")", "def greet(name):\n h= \"Hello, \"\n s= \" how are you doing today?\"\n a= (h + name + s )\n return (a)", "def greet(name):\n# return \"Hello, \" + str(name) + \" how are you doing today?\"\n# return f\"Hello, {name} how are you doing today?\"\n return \"Hello, {} how are you doing today?\".format(name)\n\n", "def greet(name):\n #Good Luck (like you need it)\n return f\"Hello, {name} how are you doing today?\"\n \ngreet('Hari')", "def greet(name):\n #Good Luck (like you need it)\n string = \"Hello, {} how are you doing today?\"\n return string.format(name)", "def greet(name):\n #Good Luck (like you need it)\n \n return(\"Hello, {} how are you doing today?\".format(name))\n \n \n \ndef main():\n name=greet(\"Ryan\")\n \n \n\ndef __starting_point():main()\n__starting_point()", "from typing import *\ngreet: Callable[[str],str] = lambda name: f\"Hello, {name} how are you doing today?\"\n", "def greet(name):\n final = \"Hello, \"+name+\" how are you doing today?\"\n print (final)\n return final"] | {"fn_name": "greet", "inputs": [["Ryan"], ["Shingles"]], "outputs": [["Hello, Ryan how are you doing today?"], ["Hello, Shingles how are you doing today?"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,766 |
def greet(name):
|
f871b6f52e62b300ac9ee4b553ca9a57 | UNKNOWN | Unicode Transformation Format – 8-bit
As the name suggests UTF-8 was designed to encode data in a stream of bytes.
It works by splitting the bits up in multiples of eight. This is achieved by inserting headers to mark in how many bytes the bits were split. If the bits need to be split in two, the header `110` is added as prefix leaving five bits of the byte for the rest of the data. Followed by a continuation byte.
A continuation byte always start with `10` leaving six bits for data.
For a three-way split: the header `1110` is added with two continuation bytes and for four: `11110` with three continuation bytes. The number of ones at the start of the first byte denotes the number of bytes the data was split in.
# Task
Your task is to write two functions:
1. `to_utf8_binary`: which encodes a string to a bitstring using UTF-8 encoding.
2. `from_utf8_binary`: which does the reverse.
- Layout of UTF-8 byte sequences:
```
# BYTES FIRST CODE POINT LAST CODE POINT BYTE 1 BYTE 2 BYTE 3 BYTE 4
1 0 127 0xxxxxxx
2 128 2047 110xxxxx 10xxxxxx
3 2048 65535 1110xxxx 10xxxxxx 10xxxxxx
4 65536 1114111 11110xxx 10xxxxxx 10xxxxxx 10xxxxxx
```
# Examples
```
ENCODE
A -> 1000001 -> 01000001
八 -> 101000101101011 -> 1110-0101 10-000101 10-101011
DECODE
110-00010 10-100111 -> 10100111 -> §
11110-000 10-010000 10-001010 10-001100 -> 10000001010001100 -> 𐊌
```
* Spaces and hyphens just for clarity
- https://en.wikipedia.org/wiki/UTF-8#Encoding | ["from textwrap import wrap\n\ndef to_utf8_binary(string):\n return ''.join(format(x, 'b').rjust(8, '0') for x in bytearray(string, 'utf-8'))\n \ndef from_utf8_binary(bitstring):\n return bytearray([int(t, 2) for t in wrap(bitstring, 8)]).decode()\n", "def to_utf8_binary(string):\n return ''.join('{:08b}'.format(b) for b in string.encode('utf8'))\n\n\ndef from_utf8_binary(bitstring):\n import re\n return bytes(int(b, 2) for b in re.split('(........)', bitstring) if b).decode('utf-8')\n", "import re\ndef to_utf8_binary(string):\n s=''\n for c in string:\n r=bin(ord(c))[2:]\n if len(r)<8:\n s+='0'+r.zfill(7)\n elif len(r)<12:\n t=r.zfill(11)\n s+='110{}10{}'.format(t[:5],t[5:])\n elif len(r)<17:\n t=r.zfill(16)\n s+='1110{}10{}10{}'.format(t[:4],t[4:10],t[10:])\n elif len(r)<22:\n t=r.zfill(21)\n s+='11110{}10{}10{}10{}'.format(t[:3],t[3:9],t[9:15],t[15:])\n return s\n\ndef from_utf8_binary(bitstring):\n s=''\n for i in re.findall('0([01]{7})|110([01]{5})10([01]{6})|1110([01]{4})10([01]{6})10([01]{6})|11110([01]{3})10([01]{6})10([01]{6})10([01]{6})',bitstring):\n s+=chr(int(''.join(i),2))\n return s ", "def to_utf8_binary(s):\n return ''.join(\"{:08b}\".format(x) for x in bytearray(s, 'utf-8'))\n\ndef from_utf8_binary(s):\n bs = []\n for i in range(0, len(s), 8):\n bs.append(int(s[i:i+8], 2))\n return bytearray(bs).decode('utf-8')", "def to_utf8_binary(string):\n bitstring = ''\n for char in string:\n code = ord(char)\n if code < 128:\n bitstring += ('0000000000' + bin(code)[2:])[-8:]\n elif code < 2048:\n binary = ('0' * 12 + bin(code)[2:])[-11:]\n bitstring += '110' + binary[0:5] + '10' + binary[5:11]\n elif code < 65536:\n binary = ('0' * 16 + bin(code)[2:])[-16:]\n bitstring += '1110' + binary[0:4] + '10' + binary[4:10] + '10' + binary[10:16]\n else:\n binary = ('0' * 21 + bin(code)[2:])[-21:]\n bitstring += '11110' + binary[0:3] + '10' + binary[3:9] + '10' + binary[9:15] + '10' + binary[15:21]\n return bitstring\n\ndef from_utf8_binary(bitstring):\n string = ''\n i, t = 0, len(bitstring)\n while i < t:\n bit_code = ''\n char_bit = bitstring[i:i+8]\n i += 8\n \n if char_bit.startswith('11110'):\n bit_code += char_bit[5:8]\n for j in range(0, 3):\n char_bit = bitstring[i:i+8]\n bit_code += char_bit[2:8]\n i += 8\n elif char_bit.startswith('1110'):\n bit_code += char_bit[4:8]\n for j in range(0, 2):\n char_bit = bitstring[i:i+8]\n bit_code += char_bit[2:8]\n i += 8\n elif char_bit.startswith('110'):\n bit_code += char_bit[3:8]\n for j in range(0, 1):\n char_bit = bitstring[i:i+8]\n bit_code += char_bit[2:8]\n i += 8\n else:\n bit_code = '0' + char_bit[1:8]\n \n string += chr(int(bit_code, 2))\n \n return string", "def to_utf8_binary(string):\n \n stList = list(string)\n bincodes = [toBin(c) for c in stList ]\n return ''.join(bincodes)\ndef toBin(string) : \n print((ord(string)))\n a = bin(ord(string))[2:]\n if(ord(string) <= 127) :\n padded = a.zfill(8)\n return (padded)\n elif ord(string) <= 2047 : \n padded = a.zfill(11)\n return '110' + padded[:5] + '10' + padded[5:]\n elif ord(string) <= 65535 : \n padded = a.zfill(16)\n part1 = '1110' + padded[:4]\n remaining = padded[4:]\n part2 = '10' + remaining[:6]\n remaining = remaining[6:]\n part3 = '10' + remaining\n return part1 + part2 + part3\n elif ord(string) <= 1114111 :\n print(a)\n padded = a.zfill(21)\n part1 = '11110' + padded[:3]\n print(part1)\n remaining = padded[3:]\n part2 = '10' + remaining[:6]\n print(part2)\n remaining = remaining[6:]\n part3 = '10' + remaining[:6]\n remaining = remaining[6:]\n part4 = '10' + remaining[:6]\n return part1 + part2 + part3+part4\n\n\ndef splitEveryN(n, li):\n return [li[i:i+n] for i in range(0, len(li), n)]\n\ndef getLenInByte(part):\n print(part)\n if part[0] == '0' : \n return 1\n elif part[:3] == '110' : \n print('two bytes')\n return 2\n elif part[:4] == '1110' : \n return 3\n elif part[:5] == '11110' : #4 byte\n return 4\n\ndef from_utf8_binary(bitstring):\n li = splitEveryN(8, bitstring)\n i = 0\n result=[]\n while(i < len(li)) :\n bytelen = getLenInByte(li[i])\n nextbin = li[i : i+bytelen]\n joined = ''.join(nextbin)\n \n frombin = fromBin(joined)\n result += frombin #li[i] is a string\n i += bytelen\n return ''.join(result)\n\n\ndef fromBin(bitstring):\n if len(bitstring) <= 8 : \n return chr(int(bitstring,2))\n elif len(bitstring) <= 16 : \n part1 = bitstring[3:8]\n part2 = bitstring[10:16]\n bi = part1+part2\n return chr(int(bi,2))\n elif len(bitstring) <=24 : \n part1 = bitstring[4:8]\n part2 = bitstring[10:16]\n part3 = bitstring[18:]\n return chr(int(part1+part2+part3, 2))\n elif len(bitstring) <= 32 : \n part1 = bitstring[5:8]\n part2 = bitstring[10:16]\n part3 = bitstring[18:24]\n part4 = bitstring[26:]\n return chr(int(part1 + part2+ part3+part4, 2))\n return ''\n\n\n\n \n", "def utf8_char_to_binary(ch):\n string = str(bin( ord(ch) ))[2:]\n if len(string) <= 7: return '0' + string.rjust(7, '0')\n result, bts = [], 1\n while len(string) + (bts+1) > 8:\n bts += 1\n result.insert(0, \"10\" + string[-6:].rjust(6, '0'))\n string = string[:-6]\n return \"\".join(['1'*bts + '0' + string.rjust(8-bts-1, '0')] + result)\n \ndef to_utf8_binary(string):\n return \"\".join( map(utf8_char_to_binary, list(string)) )\n\ndef bitstring_to_utf8_chr(bitstring):\n if len(bitstring) == 0:\n return ''\n if len(bitstring) == 8:\n return chr(int(bitstring, 2))\n else:\n binary = \"\"\n byte_parts = [bitstring[i:i+8] for i in range(0, len(bitstring), 8)]\n binary += byte_parts[0][list(byte_parts[0]).index('0'):]\n for i in range(1, len(byte_parts)):\n binary += byte_parts[i][2:]\n return chr(int(binary, 2))\n\ndef from_utf8_binary(bitstring):\n bitstring = [bitstring[i:i+8] for i in range(0, len(bitstring), 8)]\n character_bitstring, final_string = '', ''\n for s in bitstring:\n if s[:2] == '10':\n character_bitstring += s\n elif s[0] == '0':\n final_string += (bitstring_to_utf8_chr(character_bitstring) + bitstring_to_utf8_chr(s))\n character_bitstring = \"\"\n else:\n final_string += bitstring_to_utf8_chr(character_bitstring)\n character_bitstring = s\n return final_string + bitstring_to_utf8_chr(character_bitstring)\n", "def to_utf8_binary(string):\n return ''.join(format(c, '08b') for c in string.encode())\ndef from_utf8_binary(bitstring):\n bytes_ = [bitstring[i:i+8] for i in range(0, len(bitstring), 8)]\n return bytes(int(byte, 2) for byte in bytes_).decode()", "def to_utf8_binary(string):\n return ''.join((bin(i)[2:]).zfill(8) for i in string.encode('utf-8'))\n\n\ndef from_utf8_binary(bitstr):\n byte_str = bytes(int(bitstr[i:i + 8], 2) for i in range(0, len(bitstr), 8))\n return byte_str.decode('utf-8')"] | {"fn_name": "to_utf8_binary", "inputs": [["\u0080"], ["\u07ff"], ["\u0800"], ["\uffff"]], "outputs": [["1100001010000000"], ["1101111110111111"], ["111000001010000010000000"], ["111011111011111110111111"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 7,886 |
def to_utf8_binary(string):
|
8d0608b151fa23bd945df943dacd6798 | UNKNOWN | *This is the advanced version of the [Total Primes](https://www.codewars.com/kata/total-primes/) kata.*
---
The number `23` is the smallest prime that can be "cut" into **multiple** primes: `2, 3`. Another such prime is `6173`, which can be cut into `61, 73` or `617, 3` or `61, 7, 3` (all primes). A third one is `557` which can be sliced into `5, 5, 7`. Let's call these numbers **total primes**.
Notes:
* one-digit primes are excluded by definition;
* leading zeros are also excluded: e.g. splitting `307` into `3, 07` is **not** valid
## Task
Complete the function that takes a range `[a..b]` (both limits included) and returns the total primes within that range (`a ≤ total primes ≤ b`).
The tests go up to 10^(6).
~~~if:python
For your convenience, a list of primes up to 10^(6) is preloaded, called `PRIMES`.
~~~
## Examples
```
(0, 100) ==> [23, 37, 53, 73]
(500, 600) ==> [523, 541, 547, 557, 571, 577, 593]
```
Happy coding!
---
## My other katas
If you enjoyed this kata then please try [my other katas](https://www.codewars.com/collections/katas-created-by-anter69)! :-)
#### *Translations are welcome!* | ["import numpy as np\nfrom itertools import accumulate\ndef sieve_primes(n):\n sieve = np.ones(n // 2, dtype = np.bool)\n limit = 1 + int(n ** 0.5)\n\n for a in range(3, limit, 2):\n if sieve[a // 2]:\n sieve[a * a // 2::a] = False\n\n prime_indexes = 2 * np.nonzero(sieve)[0].astype(int) + 1\n prime_indexes[0] = 2\n return set(map(str, prime_indexes))\n\nprimes = sieve_primes(10 ** 6)\n\ndef all_primes(s):\n if int(s) < 10:\n return s in primes\n for n in accumulate(s[:-1]):\n if n in primes:\n m = s[len(n):]\n if m in primes or all_primes(m):\n return True\n\ndef total_primes(a, b):\n return [int(a) for a in map(str, range(max(10, a), b + 1)) if a in primes and all_primes(a)]"] | {"fn_name": "total_primes", "inputs": [[10, 100], [500, 600], [23, 37], [0, 20], [113, 197], [199, 299], [1, 2273], [11703, 13330], [312232, 312311], [967335, 967871]], "outputs": [[[23, 37, 53, 73]], [[523, 541, 547, 557, 571, 577, 593]], [[23, 37]], [[]], [[113, 137, 173, 193, 197]], [[211, 223, 227, 229, 233, 241, 257, 271, 277, 283, 293]], [[23, 37, 53, 73, 113, 137, 173, 193, 197, 211, 223, 227, 229, 233, 241, 257, 271, 277, 283, 293, 311, 313, 317, 331, 337, 347, 353, 359, 367, 373, 379, 383, 389, 397, 433, 523, 541, 547, 557, 571, 577, 593, 613, 617, 673, 677, 719, 727, 733, 743, 757, 761, 773, 797, 977, 1013, 1033, 1093, 1097, 1117, 1123, 1129, 1153, 1171, 1277, 1319, 1327, 1361, 1367, 1373, 1493, 1637, 1723, 1733, 1741, 1747, 1753, 1759, 1777, 1783, 1789, 1913, 1931, 1933, 1973, 1979, 1993, 1997, 2113, 2131, 2137, 2179, 2213, 2237, 2239, 2243, 2251, 2267, 2269, 2273]], [[11717, 11719, 11731, 11743, 11777, 11779, 11783, 11789, 11813, 11821, 11827, 11833, 11839, 11863, 11887, 11897, 11933, 11941, 11953, 11971, 12373, 12377, 12497, 12713, 12743, 12757, 12893, 12917, 12973, 13033, 13037, 13103, 13109, 13127, 13147, 13151, 13159, 13163, 13171, 13177, 13183, 13217, 13219, 13229, 13241, 13259, 13267, 13297, 13313, 13327]], [[312233, 312241, 312251, 312253, 312269, 312281, 312283, 312289, 312311]], [[967349, 967361, 967397, 967493, 967511, 967529, 967567, 967583, 967607, 967693, 967709, 967739, 967751, 967753, 967787, 967823, 967859]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 783 |
def total_primes(a, b):
|
48ed7b88d55922818e015b49ccf86d00 | UNKNOWN | # Story
John found a path to a treasure, and while searching for its precise location he wrote a list of directions using symbols `"^"`, `"v"`, `"<"`, `">"` which mean `north`, `east`, `west`, and `east` accordingly. On his way John had to try many different paths, sometimes walking in circles, and even missing the treasure completely before finally noticing it.
___
## Task
Simplify the list of directions written by John by eliminating any loops.
**Note**: a loop is any sublist of directions which leads John to the coordinate he had already visited.
___
## Examples
```
simplify("<>>") == ">"
simplify("<^^>v<^^^") == "<^^^^"
simplify("") == ""
simplify("^< > v
^ v
> > C > D > >
^ ^ v
^ < B < <
^
A
```
John visits points `A -> B -> C -> D -> B -> C -> D`, realizes that `-> C -> D -> B` steps are meaningless and removes them, getting this path: `A -> B -> (*removed*) -> C -> D`.
```
∙ ∙ ∙
∙ ∙
> > C > D > >
^ ∙ ∙
^ < B ∙ ∙
^
A
```
Following the final, simplified route John visits points `C` and `D`, but for the first time, not the second (because we ignore the steps made on a hypothetical path), and he doesn't need to alter the directions list anymore. | ["def simplify(p):\n new_p=[(0,0)]\n new_str=''\n x=0\n y=0\n for i in p:\n if i == '<':\n x-=1\n elif i == '>':\n x+=1\n elif i == '^':\n y+=1\n elif i == 'v':\n y-=1\n if (x,y) not in new_p:\n new_p.append((x,y))\n new_str+=i\n else:\n for j in new_p[::-1]:\n if j != (x,y):\n new_p.pop()\n new_str=new_str[:-1]\n else:\n break\n return new_str", "def simplify(s):\n d, r, c = {\"^\": (-1, 0), \"v\": (1, 0), \"<\": (0, -1), \">\": (0, 1)}, {(0, 0): \"\"}, (0, 0)\n for z in s:\n x, y = d[z]\n nc = (c[0] + x, c[1] + y)\n r[nc] = r[nc] if nc in r and r[c].startswith(r[nc]) else r[c] + z\n c = nc\n return r[c]", "def simplify(path):\n location = (0,0)\n new_path = path\n new_path_locations = [location]\n \n for d in path:\n if d == '<': location = (location[0]-1, location[1])\n elif d == '>': location = (location[0]+1, location[1])\n elif d == 'v': location = (location[0], location[1]+1)\n else: location = (location[0], location[1]-1)\n \n if location in new_path_locations:\n i = new_path_locations.index(location)\n \n remove_length = len(new_path_locations) - i\n \n new_path_locations = new_path_locations[:i+1] # leave the current location\n \n new_path = new_path[:i] + new_path[i+remove_length:]\n \n else: new_path_locations.append(location)\n \n return new_path", "def simplify(path):\n x, y, coords = 0, 0, [(0, 0)]\n for c in path:\n x += 1 if c == \">\" else -1 if c == \"<\" else 0\n y += 1 if c == \"^\" else -1 if c == \"v\" else 0\n if (x, y) in coords:\n i = coords.index((x, y))\n path, coords = path[:i] + path[len(coords):], coords[:i]\n coords.append((x, y))\n return path\n", "M = {'^':-1, 'v':1, '<':-1j, '>':1j}\ndef rem(D, k): del D[k]\n\ndef simplify(path):\n D, z, res = {0:0}, 0, list(path)\n for i,c in enumerate(path, 1):\n z += M[c]\n if z in D:\n for j in range(D[z], i): res[j] = None\n [rem(D, k) for k,v in list(D.items()) if v > D[z]]\n else: D[z] = i\n return ''.join(filter(None, res))", "def simplify(p):\n x = 0\n y = 0\n r = [(0,0)]\n for i in p:\n if i == '<': x -= 1\n if i == '>': x += 1\n if i == '^': y += 1\n if i == 'v': y -= 1\n if (x,y) in r:\n p = p[:r.index((x,y))] + p[len(r):]\n r = r[:r.index((x,y))] \n r.append((x,y))\n \n return p", "def simplify(path):\n array = [(0, 0)]\n for elem in path:\n if elem == \"^\":\n array.append((array[-1][0], array[-1][1] + 1))\n elif elem == \"v\":\n array.append((array[-1][0], array[-1][1] - 1))\n elif elem == \">\":\n array.append((array[-1][0] + 1, array[-1][1]))\n else:\n array.append((array[-1][0] - 1, array[-1][1]))\n for i in range(0, len(array) - 1):\n for j in range(len(array) - 1, i + 1, -1):\n if array[i] == array[j]:\n array = array[:i] + [None for i in range((j - i))] + array[j:]\n res = [path[i] for i in range(len(path)) if array[i] is not None]\n return \"\".join(res)", "def simplify(s):\n been = [(0,0)]\n i,j = 0,0\n d = {'<': (-1,0),'>':(1,0),'^':(0,1),'v':(0,-1)}\n t = s\n for p in s:\n x,y = d.get(p)\n i,j = i+x,j+y\n if (i,j) in been:\n idx = been.index((i,j))\n t = t[:idx] + t[len(been):]\n been = been[:idx]\n been.append((i,j))\n return t", "def simplify(path):\n moves = {'^': (0, 1), 'v': (0, -1), '<': (-1, 0), '>': (1, 0)}\n rev_moves = {(0, 0): '', (0, 1): '^', (0, -1): 'v', (-1, 0): '<', (1, 0): '>'}\n x, y = 0, 0\n visited = [(0, 0)]\n for p in path:\n mov = moves[p]\n x1, y1 = x+mov[0], y+mov[1]\n if not (x1, y1) in visited:\n visited.append((x1, y1))\n else:\n idx = visited.index((x1, y1))\n visited = visited[0:idx+1]\n x, y = x1, y1\n last = (0, 0)\n res = ''\n for p in visited:\n dx, dy = p[0]-last[0], p[1]-last[1]\n res += rev_moves[(dx, dy)]\n last = p\n return res", "D = {'^': (1, 0), 'v': (-1, 0), '>': (0, 1), '<': (0, -1)}\n\ndef simplify(s):\n q, c, w = set(), (0, 0), [(0, 0)]\n for i, k in enumerate(s):\n p = D[k]\n c = (c[0]+p[0], c[1]+p[1])\n if c in w:\n j = w.index(c)\n w[j:i+1] = [-1] * (i-j+1)\n q |= set(range(j, i+1))\n w.append(c)\n return ''.join(x for i, x in enumerate(s) if i not in q)"] | {"fn_name": "simplify", "inputs": [["<>>"], [""], ["v>^<"], [">>>>"], ["^>>>>v"], ["<^^>v<^^^"], ["<^>>v<"], ["<^<<^^>>vv<<<>^^^v^"], ["v<<<<<^^^^^v>v>v>v>v>>"], [">^>^>^>^>^>^>v>v>v>v>v>v>v>v<"], ["^^^>>>>>>^^^<<<vvv<<<vvv"], ["^^^>>>>>>^^^<<<vvv<<<vv"], [">vv<<<^^^^>>>>>vvvvvv<<<<<<^^^^^^^^>>>>vv"], [">vv<<<^^^^>>>>>vvvvvv<<<<<<^^^^^^^^>>>>"], [">^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v"], ["<>>^<<v"]], "outputs": [[">"], [""], [""], [">>>>"], ["^>>>>v"], ["<^^^^"], [""], ["<^<<^^^"], ["v>"], [">^>^>^>^>^>^>v>v>v>v>v>v>v>v<"], [""], ["^"], [">vv<<<^^^^>>>"], [">vv<<<^^^^>>>>>vvvvvv<<<<<<^^^^^^^^>>>>"], [">^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v>^>v"], [">^<<v"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,980 |
def simplify(path):
|
6399ddef9a66df6af575bf76d3a4a090 | UNKNOWN | The sports centre needs repair. Vandals have been kicking balls so hard into the roof that some of the tiles have started sticking up. The roof is represented by r.
As a quick fix, the committee have decided to place another old roof over the top, if they can find one that fits. This is your job.
A 'new' roof (f) will fit if it currently has a hole in it at the location where the old roof has a tile sticking up.
Sticking up tiles are represented by either '\\' or '/'. Holes in the 'new' roof are represented by spaces (' '). Any other character can not go over a sticking up tile.
Return true if the new roof fits, false if it does not. | ["def roof_fix(new, old):\n return all(patch == ' ' for patch, tile in zip(new, old) if tile in '\\/')", "def roof_fix(f, r):\n return all(x == ' ' or y == '_' for x,y in zip(f, r))", "def roof_fix(f,r):\n return all(b == ' ' for a, b in zip(r, f) if a in '\\//')", "def roof_fix(f,r):\n return all(b not in r'\\/' or a == ' ' for a, b in zip(f, r))", "def roof_fix(f,r):\n return all(new==' ' for old, new in zip(r,f) if old in \"\\/\")", "def roof_fix(f,r):\n return all((b in'\\/')<=(a==' ')for a,b in zip(f,r))", "def roof_fix(f,r):\n return all([True,a==' '][b in'\\/']for a,b in zip(f,r))", "def roof_fix(f,r):\n for i in range(len(r)):\n if r[i] in \"/\\\\\" and f[i] != ' ':\n return False\n return True", "def roof_fix(f,r):\n for u,y in zip(f,r):\n if u.isalpha() and y in '/\\\\': return False\n return True", "def roof_fix(f,r):\n return not any([(pair[0] in \"/\\\\\" and pair[1] != \" \") for pair in zip(r, f)])"] | {"fn_name": "roof_fix", "inputs": [[" l f l k djmi k", "___\\_____//_____/_"], [" ikm il h llmmc a i", "__\\_______________________"], [" h c ", "__/____"], ["q h", "_/_"], [" cg dg em lfh cdam", "_______/____\\_____/_/"]], "outputs": [[false], [true], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 989 |
def roof_fix(f,r):
|
3051095fc47bf40319c5e997eed58a1c | UNKNOWN | ## Task
Implement a function which finds the numbers less than `2`, and the indices of numbers greater than `1` in the given sequence, and returns them as a pair of sequences.
Return a nested array or a tuple depending on the language:
* The first sequence being only the `1`s and `0`s from the original sequence.
* The second sequence being the indexes of the elements greater than `1` in the original sequence.
## Examples
```python
[ 0, 1, 2, 1, 5, 6, 2, 1, 1, 0 ] => ( [ 0, 1, 1, 1, 1, 0 ], [ 2, 4, 5, 6 ] )
```
Please upvote and enjoy! | ["def binary_cleaner(seq): \n res = ([], [])\n for i,x in enumerate(seq):\n if x < 2: res[0].append(x)\n else: res[1].append(i)\n return res", "def binary_cleaner(lst):\n return [n for n in lst if n < 2], [i for i, n in enumerate(lst) if n > 1]", "def binary_cleaner(seq): \n return list(filter(lambda x:x<=1, seq )), [ i for i in range(len(seq)) if seq[i]>1 ]", "def binary_cleaner(seq): \n return [x for x in seq if x in [0, 1]], [i for i, x in enumerate(seq) if x > 1]", "def binary_cleaner(seq): \n return [value for value in seq if value==0 or value==1],[index for index,value in enumerate(seq) if value not in (0,1)]", "def binary_cleaner(seq): \n return [n for n in seq if n < 2], [i for i,n in enumerate(seq) if n > 1]", "def binary_cleaner(seq): \n list_a, list_b = [],[]\n for k,v in enumerate(seq):\n if v>1:\n list_b.append(k)\n else: list_a.append(v)\n return (list_a, list_b)\n", "def binary_cleaner(seq): \n b = []\n x = []\n for i in range(len(seq)):\n if seq[i] < 2:\n b.append(seq[i])\n elif seq[i] > 1:\n x.append(i)\n return (b, x)", "def binary_cleaner(seq): \n less_than_1 = []\n index_list = []\n for i, x in enumerate(seq):\n if x < 2:\n less_than_1.append(x)\n if x > 1:\n index_list.append(i)\n return (less_than_1, index_list)", "def binary_cleaner(seq): \n return [i for i in seq if i <= 1], [i for i, el in enumerate(seq) if el > 1]"] | {"fn_name": "binary_cleaner", "inputs": [[[0, 1, 2, 1, 0, 2, 1, 1, 1, 0, 4, 5, 6, 2, 1, 1, 0]], [[0, 1, 1, 2, 0]], [[2, 2, 0]], [[0, 1, 2, 1, 0, 2, 1, 1]], [[1]], [[3, 0, 7, 0, 2, 0]]], "outputs": [[[[0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0], [2, 5, 10, 11, 12, 13]]], [[[0, 1, 1, 0], [3]]], [[[0], [0, 1]]], [[[0, 1, 1, 0, 1, 1], [2, 5]]], [[[1], []]], [[[0, 0, 0], [0, 2, 4]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,534 |
def binary_cleaner(seq):
|
431fa68a9a82ef837e59510a4c3e3720 | UNKNOWN | Your task is to return the number of visible dots on a die after it has been rolled(that means the total count of dots that would not be touching the table when rolled)
6, 8, 10, 12, 20 sided dice are the possible inputs for "numOfSides"
topNum is equal to the number that is on top, or the number that was rolled.
for this exercise, all opposite faces add up to be 1 more than the total amount of sides
Example: 6 sided die would have 6 opposite 1, 4 opposite 3, and so on.
for this exercise, the 10 sided die starts at 1 and ends on 10.
Note: topNum will never be greater than numOfSides | ["def totalAmountVisible(topNum, numOfSides):\n return numOfSides*(numOfSides+1)/2-(numOfSides-topNum+1)\n", "totalAmountVisible=lambda n,s:s*~-s//2-1+n", "def totalAmountVisible(top, sides):\n return sides * (sides + 1 ) / 2 + top - sides - 1", "def totalAmountVisible(topNum, numOfSides):\n return numOfSides*(numOfSides-1)//2+topNum-1\n", "def totalAmountVisible(top, sides):\n return (sides - 1) * sides // 2 + top - 1", "def totalAmountVisible(n, s):\n return s//2*(s+1)-(s+1-n)\n", "totalAmountVisible = lambda t,n: sum(range(1,n+1))-(n+1-t)", "def totalAmountVisible(topNum, numOfSides):\n return sum(range(1, numOfSides + 1)) - (numOfSides - topNum + 1)\n\n", "def totalAmountVisible(n, s):\n return (s*(s+1))//2 -(s-n+1)\n", "def totalAmountVisible(n,sides):\n return sides*(1+sides)//2-(sides+1-n)"] | {"fn_name": "totalAmountVisible", "inputs": [[5, 6], [19, 20], [6, 20], [15, 20], [8, 20], [9, 8], [4, 10], [12, 12], [7, 10], [4, 12]], "outputs": [[19], [208], [195], [204], [197], [36], [48], [77], [51], [69]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 833 |
def totalAmountVisible(topNum, numOfSides):
|
b79e932516562d20f6fcc6149b8d88a0 | UNKNOWN | # Task:
This kata asks you to make a custom esolang interpreter for the language [MiniBitMove](https://esolangs.org/wiki/MiniBitMove). MiniBitMove has only two commands and operates on a array of bits. It works like this:
- `1`: Flip the bit at the current cell
- `0`: Move selector by 1
It takes two inputs, the program and the bits in needs to operate on. The program returns the modified bits. The program stops when selector reaches the end of the array. Otherwise the program repeats itself. **Note: This means that if a program does not have any zeros it is an infinite loop**
Example of a program that flips all bits in an array:
```
Code: 10
Bits: 11001001001010
Result: 00110110110101
```
After you're done, feel free to make translations and discuss this kata. | ["from itertools import cycle\ndef interpreter(tape, array):\n idx, result = 0, list(map(int, array))\n for cmd in cycle(map(int, tape)):\n if idx == len(array): break\n if cmd: result[idx] = 1-result[idx]\n else: idx += 1\n return ''.join(map(str, result))", "def interpreter(tape, code): # Tape is given as a string and code is given as a string.\n tape, code = [int(t) for t in tape], [int(a) for a in code]\n ptr, out = 0, \"\"\n while ptr < len(code):\n try:\n for t in tape:\n if t == 1: code[ptr] ^= 1\n elif t == 0: ptr +=1\n except:\n break \n return ''.join(map(str,code))", "from itertools import cycle\n\ndef interpreter(tape, array):\n com = cycle(tape)\n arr = list(array)\n i = 0\n while i < len(arr):\n if next(com) == '1':\n arr[i] = '01'[arr[i]=='0']\n continue\n i +=1\n return ''.join(arr)\n \n \n", "from itertools import cycle\n\n\ndef interpreter(tape, array):\n tape, array = cycle(tape), list(array)\n for i in range(len(array)):\n while next(tape) == \"1\":\n array[i] = \"10\"[int(array[i])]\n return \"\".join(array)\n", "from itertools import cycle\n\ndef interpreter(tape, s):\n a = list(s)\n i = 0 # selector\n for op in cycle(tape):\n if op == '1':\n a[i] = str(1 - int(a[i]))\n else:\n i += 1\n if i == len(s):\n return ''.join(a)", "def interpreter(t, array): \n ls = list(array)\n l = len(t)\n i, j = 0, 0\n while j<len(array):\n if t[i%l] == \"1\":\n ls[j] = \"0\" if ls[j] ==\"1\" else \"1\" \n else:\n j+=1\n i+=1\n return \"\".join(ls)", "def interpreter(code, array):\n array = list(map(int, array))\n ptr = step = 0\n \n while ptr < len(array):\n command = code[step]\n \n if command == \"1\": array[ptr] ^= 1\n elif command == \"0\": ptr += 1\n \n step = (step + 1) % len(code)\n \n return \"\".join(map(str, array))", "from itertools import cycle\ndef interpreter(tape, array):\n output = \"\"\n for c in cycle(tape):\n if not array:\n return output\n if c == \"0\":\n output = output + array[0]\n array = array[1:]\n else:\n array = (\"1\" if array[0] == \"0\" else \"0\") + array[1:]", "def interpreter(tape, array):\n curloc = 0 #current location in array\n ret = list(array) #list to be returned\n while True:\n for x in tape:\n if curloc == len(array):\n return ''.join(ret)\n else:\n if int(x):\n ret[curloc] = str(1 ^ int(ret[curloc], 2))\n else:\n curloc += 1", "def interpreter(tape, array): # Tape is given as a string and array is given as a string.\n stepArray = 0\n stepTape = 0 \n arr = list(array)\n \n while(stepArray < len(array)):\n if(tape[stepTape % len(tape)] == \"1\"):\n \n arr[stepArray] = \"1\" if arr[stepArray] == \"0\" else \"0\"\n else:\n stepArray += 1\n stepTape += 1\n \n return ''.join(arr)"] | {"fn_name": "interpreter", "inputs": [["10", "1100101"], ["100", "1111111111"], ["110", "1010"], ["0", "100010001"], ["101010", "111"]], "outputs": [["0011010"], ["0101010101"], ["1010"], ["100010001"], ["000"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,313 |
def interpreter(tape, array):
|
73e098c60288e86c766e7cce932fd66f | UNKNOWN | Let's imagine we have a popular online RPG. A player begins with a score of 0 in class E5. A1 is the highest level a player can achieve.
Now let's say the players wants to rank up to class E4. To do so the player needs to achieve at least 100 points to enter the qualifying stage.
Write a script that will check to see if the player has achieved at least 100 points in his class. If so, he enters the qualifying stage.
In that case, we return, ```"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."```.
Otherwise return, ```False/false``` (according to the language n use).
NOTE: Remember, in C# you have to cast your output value to Object type! | ["def playerRankUp(pts):\n msg = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" \n return msg if pts >=100 else False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts >= 100 else False", "def playerRankUp(pts):\n if pts >= 100: return 'Well done! You have advanced to the ' \\\n 'qualifying stage. Win 2 out of your ' \\\n 'next 3 games to rank up.'\n else: return False", "def playerRankUp(pts):\n return [False, 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'][pts >= 100]", "def playerRankUp(pts):\n return [False, \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"][pts > 99]", "playerRankUp = lambda p: False if p<100 else \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n qualStageStr = 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n return qualStageStr if pts >= 100 else False", "def playerRankUp(p):\n return p >= 100 and \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "playerRankUp=lambda pts: \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts>=100 else False", "def playerRankUp(pts):\n return pts >= 100 and \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n return (False, \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\")[pts >= 100]", "def playerRankUp(pts):\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.' * (pts > 99) or False", "def playerRankUp(pts):\n msg0 = 'Well done! You have advanced to the qualifying stage.'\n msg1 = 'Win 2 out of your next 3 games to rank up.'\n return [False, msg0+' '+msg1][pts > 99]", "def playerRankUp(pts):\n rank_up = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n try:\n rank_up[pts+4]\n except IndexError:\n return rank_up\n return False", "from typing import Union\n\ndef playerRankUp(pts: int) -> Union[int, str]:\n \"\"\"\n Check if the player has achieved at least 100 points in his class.\n If so, he enters the qualifying stage with congratulations message.\n \"\"\"\n return {\n pts >= 100: \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n }.get(True, False)", "def playerRankUp(pts):\n a= lambda pts: \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts >= 100 else False\n return a(pts)", "MSG = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\ndef playerRankUp(pts):\n return MSG if pts >= 100 else False", "def playerRankUp(pts):\n return False if not pts >= 100 else \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n", "def playerRankUp(p):\n s = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if p >=100:\n return s\n else:\n return False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts // 100 > 0 else False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the \\\nqualifying stage. Win 2 out of your next 3 games \\\nto rank up.\" if pts>=100 else False", "def playerRankUp(pts, lvl= \"E4\"):\n if pts < 100:\n return False\n else :\n lvl = \"E5\"\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n \n", "def playerRankUp(pts):\n# levels = pts // 100\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts >= 100 else False", "def playerRankUp(pts):\n win = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return win if pts > 99 else False", "def playerRankUp(n):\n if n>=100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. \" \\\n + \"Win 2 out of your next 3 games to rank up.\" if pts >= 100 else False", "def playerRankUp(player_points):\n if player_points < 100:\n return False\n else:\n return (f\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\")", "def playerRankUp(pts):\n good = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" \n return good if pts >= 100 else False", "def playerRankUp(pts):\n if pts>=100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return pts>=100\n #your code here\n", "def playerRankUp(pts):\n return (False,\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\") [pts > 99]", "def playerRankUp(pts):\n blob = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return blob if pts >= 100 else False", "def playerRankUp(pts):\n if(pts >= 100):\n return f\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return False", "def playerRankUp(pts):\n words = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return words if pts >= 100 else False", "playerRankUp=lambda i:\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if i>=100 else False", "def playerRankUp(pts):\n #your code here\n congrat = 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n return congrat if pts >= 100 else False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out\" \\\n \" of your next 3 games to rank up.\" if pts >= 100 else False", "def playerRankUp(pts):\n msg = (\n 'Well done! You have advanced to the qualifying stage. '\n + 'Win 2 out of your next 3 games to rank up.'\n )\n \n return msg if pts > 99 else False", "def playerRankUp(pts):\n if pts >= 100:\n x = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return x\n else:\n return False", "def playerRankUp(level):\n if level >= 100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return False", "def playerRankUp(pts):\n if pts >= 100:\n str=\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return str\n elif pts < 100:\n return False\n", "def playerRankUp(pts):\n info = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return info if pts >= 100 else False", "def playerRankUp(pts):\n result = \"Well done! You have advanced to the qualifying stage. \"\\\n \"Win 2 out of your next 3 games to rank up.\"\n return result if pts >= 100 else False ", "def playerRankUp(pts):\n #your code here\n a = (pts - 100)//100\n if a < 0:\n return False\n else:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n a = pts >= 100\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if a else a", "def playerRankUp(pts):\n if pts >= 100:\n return \"Well done! You have advanced to the qualifying stage.\" \\\n \" Win 2 out of your next 3 games to rank up.\"\n return False", "def playerRankUp(pts):\n msg = 'Well done! You have advanced to the qualifying stage. ' +\\\n 'Win 2 out of your next 3 games to rank up.'\n return msg if pts >= 100 else False ", "def playerRankUp(pts):\n s = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return s if pts > 99 else False", "playerRankUp = lambda pts: {0: False}.get(max(0,pts//100), 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.')", "def playerRankUp(pts):\n str = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return str if pts >= 100 else False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\\\n if pts > 99 else False", "def player_rank_up(pts):\n if pts >= 100:\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n else:\n return False\n \n \nplayerRankUp = player_rank_up # camelCase bad ", "def playerRankUp(pts):\n rank_up = \"Well done! You have advanced to the qualifying stage. \" \\\n \"Win 2 out of your next 3 games to rank up.\"\n return rank_up if pts >= 100 else False", "def playerRankUp(pts):\n if 100 <= pts:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return False\n", "def playerRankUp(pts):\n if pts>=100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"#your code he\n else:\n return False", "def playerRankUp(pts):\n txt = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return txt if pts>=100 else False", "def playerRankUp(pts):\n if (pts < 99):\n return False\n else: \n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.' if pts >= 100 else False\n #Flez\n", "def playerRankUp(pts):\n if pts >= 100: return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if pts < 100: return False", "def playerRankUp(pts):\n if pts == 100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if pts > 100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else: \n return False", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts >= 100 else pts >= 100", "def playerRankUp(pts):\n if pts >= 100:\n # Enter qualifying state\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return False", "def playerRankUp(pts):\n return False if pts // 100 == 0 or pts < 0 else \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" ", "def playerRankUp(pts):\n if 100>pts: return False\n return (\"Well done! You have advanced to the qualifying stage.\"\n \" Win 2 out of your next 3 games to rank up.\")", "def playerRankUp(pts):\n if pts<100: return False\n return \"Well done! You have advanced to the qualifying stage. \" + \\\n \"Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n if pts >= 100:\n response = 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n else:\n response = False\n return response\n \n", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" if pts > 99 else 0", "def playerRankUp(pts):\n #your code here\n msg_str = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return msg_str if pts >= 100 else False", "def playerRankUp(pts):\n result = False\n if pts >= 100:\n result = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n\n return result", "def playerRankUp(pts):\n msg = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return False if pts < 100 else msg", "def playerRankUp(pts, msg = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"): return False if pts < 99 else msg\n", "def playerRankUp(pts):\n num = pts\n if pts <= 64:\n return False \n else:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "playerRankUp = lambda p: (\"Well done! \"\\\n \"You have advanced to the qualifying stage. \"\\\n \"Win 2 out of your next 3 games to rank up.\",\n False)[p < 100]", "def playerRankUp(pts):\n rankup = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return rankup if pts >=100 else False", "def playerRankUp(pts):\n if pts >= 100:\n return \"Well done! You have advanced to the qualifying stage. \" + \\\n \"Win 2 out of your next 3 games to rank up.\"\n else:\n return False\n", "def playerRankUp(pts):\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"if pts>99 else False #I solved this Kata on 8/27/2019 12:08 PM...#Hussam'sCodingDiary", "def playerRankUp(pts):\n if pts >= 100:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else:\n return False\n#Completed by Ammar on 25/8/2019 at 03:38PM.\n", "def playerRankUp(points):\n if points<100:\n return False\n else:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"", "def playerRankUp(pts):\n #your code here\n output = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return output if pts >= 100 else False", "def playerRankUp(pts):\n good = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if pts >= 100:\n return good\n else:\n return False\n", "def playerRankUp(pts):\n msg = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\" \n\n if pts >= 100:\n return msg\n else:\n return False", "def playerRankUp(pts):\n string = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return string if pts >= 100 else False", "playerRankUp = lambda pts: (pts >= 100) and 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'", "def playerRankUp(pts):\n return \"\".join(map(chr,[87,101,108,108,32,100,111,110,101,33,32,89,111,117,32,104,97,118,101,32,97,100,118,97,110,99,101,100,32,116,111,32,116,104,101,32,113,117,97,108,105,102,121,105,110,103,32,115,116,97,103,101,46,32,87,105,110,32,50,32,111,117,116,32,111,102,32,121,111,117,114,32,110,101,120,116,32,51,32,103,97,109,101,115,32,116,111,32,114,97,110,107,32,117,112,46])) if pts >= 100 else False", "def playerRankUp(pts):\n mes = 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n return mes if pts>= 100 else False", "def playerRankUp(pts):\n res = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if pts < 100: \n res = False\n return res\n", "def playerRankUp(pts):\n s = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return False if pts <100 else s", "def playerRankUp(pts):\n t =\"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return '{}'.format(t) if pts >= 100 else False", "class Player(object):\n def __init__(self, pts, rank=\"E5\"):\n self.rank = rank\n self.pts = pts\n\n def check(self):\n if self.pts < 100:\n return False\n else:\n return \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n\n\ndef playerRankUp(pts):\n return Player(pts).check()", "def playerRankUp(pts):\n msg = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return msg if pts > 99 else False", "def playerRankUp(pts):\n if pts >= 100:\n a = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return a\n else:\n return False\n", "def playerRankUp(pts):\n txt = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return (pts>=100)*txt or False", "def playerRankUp(points):\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.' if points >= 100 else False", "def playerRankUp(p):\n return [False, \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"][p>=100]\n", "def playerRankUp(pts):\n #your code here\n \n #def __init__(self,pts):\n # self.pts=pts\n if pts<100:\n return False\n else:\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'", "def playerRankUp(pts):\n frase = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n if pts>=100:\n return frase\n else:\n return False\n", "def playerRankUp(pts):\n\n #your code here\n saying = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\";\n if pts > 99:\n return saying\n else:\n return False", "def playerRankUp(pts):\n return False if Player(pts).rank < 100 else 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'\n\n\nclass Player(object):\n def __init__(self, rank):\n self.rank = rank\n", "def playerRankUp(pts):\n #your code here\n if pts < 100:\n return False\n elif pts >= 100:\n return 'Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.'", "def playerRankUp(pts):\n if pts >= 100 :\n res = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n else :\n res = False\n return res", "def playerRankUp(pts):\n win_str = \"Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up.\"\n return win_str if pts >=100 else False"] | {"fn_name": "playerRankUp", "inputs": [[-1], [0], [45], [59], [64], [100], [105], [111], [118], [332532105]], "outputs": [[false], [false], [false], [false], [false], ["Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."], ["Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."], ["Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."], ["Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."], ["Well done! You have advanced to the qualifying stage. Win 2 out of your next 3 games to rank up."]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 20,078 |
def playerRankUp(pts):
|
143a5e685b3ea6aa59d8d6d8676d45b9 | UNKNOWN | An array of size N x M represents pixels of an image.
Each cell of this array contains an array of size 3 with the pixel's color information: `[R,G,B]`
Convert the color image, into an *average* greyscale image.
The `[R,G,B]` array contains integers between 0 and 255 for each color.
To transform a color pixel into a greyscale pixel, average the values of that pixel:
```
p = [R,G,B] => [(R+G+B)/3, (R+G+B)/3, (R+G+B)/3]
```
**Note:** the values for the pixel must be integers, therefore you should round floats to the nearest integer.
## Example
Here's an example of a 2x2 image:
Here's the expected image after transformation:
You are always welcome to check out some of my other katas:
Very Easy (Kyu 8)
Add Numbers
Easy (Kyu 7-6)
Convert Color image to greyscale
Array Transformations
Basic Compression
Find Primes in Range
No Ifs No Buts
Medium (Kyu 5-4)
Identify Frames In An Image
Photoshop Like - Magic Wand
Scientific Notation
Vending Machine - FSA
Find Matching Parenthesis
Hard (Kyu 3-2)
Ascii Art Generator | ["from statistics import mean\n\ndef grey(rgb):\n return [int(round(mean(rgb)))]*3\n\ndef color_2_grey(colors):\n return [[grey(pixel) for pixel in row] for row in colors]", "def color_2_grey(colors):\n return [ [ [int(round(sum(rgb) / 3))] * 3 for rgb in row ] for row in colors ]", "def color_2_grey(colors):\n for line in colors:\n for pixel in line:\n grey = int((sum(pixel)/3)+0.5)\n pixel[0] = grey\n pixel[1] = grey\n pixel[2] = grey\n return colors", "def color_2_grey(a):\n return [[[round(sum(y) / 3)] * 3 for y in x] for x in a]", "def color_2_grey(colors):\n return [[[round(sum(p)/3) for i in p] for p in r] for r in colors]", "def color_2_grey(colors):\n \n res = []\n for row in colors:\n g_row = []\n for pix in row:\n g_pix = []\n for c in pix:\n g_pix.append(int(round(sum(pix)/3, 0)))\n g_row.append(g_pix)\n res.append(g_row)\n return res", "def color_2_grey(colors):\n return [[ [round(sum(pixel)/3)]*3 for pixel in line ] for line in colors ]", "def color_2_grey(colors):\n return [[[round((r + g + b) / 3)] * 3 for r, g, b in row] for row in colors]", "def color_2_grey(colors):\n for i in range(len(colors)):\n for j in range(len(colors[i])):\n t = (colors[i][j][0]+colors[i][j][1]+colors[i][j][2])/3\n colors[i][j].remove(colors[i][j][0])\n colors[i][j].remove(colors[i][j][0])\n colors[i][j].remove(colors[i][j][0]) \n colors[i][j].append((t+0.5)//1)\n colors[i][j].append((t+0.5)//1)\n colors[i][j].append((t+0.5)//1)\n return colors", "def color_2_grey(colors):\n return [[[round((R+G+B)/3)]*3 for [R,G,B] in row] for row in colors]"] | {"fn_name": "color_2_grey", "inputs": [[[[[48, 36, 124], [201, 23, 247], [150, 162, 177]]]], [[[[49, 106, 224], [95, 150, 206]], [[88, 57, 134], [40, 183, 144]], [[36, 134, 174], [125, 27, 51]], [[33, 89, 186], [160, 39, 244]], [[249, 127, 245], [201, 233, 72]], [[78, 247, 124], [79, 245, 145]]]], [[[[181, 215, 243], [162, 0, 15], [114, 38, 243], [129, 118, 177]], [[248, 118, 87], [253, 154, 175], [158, 213, 168], [34, 96, 50]], [[184, 220, 48], [72, 248, 221], [65, 74, 53], [121, 70, 113]], [[213, 63, 33], [243, 180, 114], [137, 50, 11], [232, 87, 139]], [[222, 135, 77], [15, 116, 51], [143, 193, 238], [231, 187, 198]], [[50, 145, 56], [10, 145, 240], [185, 219, 179], [217, 104, 82]], [[233, 44, 86], [207, 232, 43], [90, 225, 221], [212, 81, 244]], [[31, 72, 228], [217, 212, 112], [225, 38, 94], [245, 161, 59]]]], [[[[64, 145, 158], [242, 117, 31], [44, 176, 104], [154, 72, 201], [170, 58, 146]], [[173, 12, 177], [59, 160, 180], [100, 11, 96], [179, 115, 51], [46, 149, 252]], [[227, 211, 100], [87, 135, 185], [49, 57, 239], [150, 67, 55], [156, 13, 217]], [[178, 173, 59], [84, 107, 34], [182, 41, 93], [205, 16, 51], [30, 129, 216]], [[217, 107, 112], [31, 240, 11], [166, 211, 106], [91, 107, 89], [187, 23, 141]], [[194, 191, 242], [27, 59, 121], [138, 191, 129], [75, 70, 148], [163, 244, 150]], [[171, 9, 2], [70, 17, 255], [80, 134, 104], [150, 215, 59], [232, 177, 212]]]], [[[[230, 26, 161], [30, 123, 138], [83, 90, 173], [114, 36, 186], [109, 215, 130]], [[23, 25, 149], [60, 134, 191], [220, 120, 113], [85, 94, 3], [85, 137, 249]], [[124, 159, 226], [248, 32, 241], [130, 111, 112], [155, 178, 200], [19, 65, 115]], [[195, 83, 134], [202, 178, 248], [138, 144, 232], [84, 182, 33], [106, 101, 249]], [[227, 59, 3], [101, 237, 48], [70, 98, 73], [78, 133, 234], [124, 117, 98]], [[251, 255, 230], [229, 205, 48], [0, 28, 126], [87, 28, 92], [187, 124, 43]]]]], "outputs": [[[[[69, 69, 69], [157, 157, 157], [163, 163, 163]]]], [[[[126, 126, 126], [150, 150, 150]], [[93, 93, 93], [122, 122, 122]], [[115, 115, 115], [68, 68, 68]], [[103, 103, 103], [148, 148, 148]], [[207, 207, 207], [169, 169, 169]], [[150, 150, 150], [156, 156, 156]]]], [[[[213, 213, 213], [59, 59, 59], [132, 132, 132], [141, 141, 141]], [[151, 151, 151], [194, 194, 194], [180, 180, 180], [60, 60, 60]], [[151, 151, 151], [180, 180, 180], [64, 64, 64], [101, 101, 101]], [[103, 103, 103], [179, 179, 179], [66, 66, 66], [153, 153, 153]], [[145, 145, 145], [61, 61, 61], [191, 191, 191], [205, 205, 205]], [[84, 84, 84], [132, 132, 132], [194, 194, 194], [134, 134, 134]], [[121, 121, 121], [161, 161, 161], [179, 179, 179], [179, 179, 179]], [[110, 110, 110], [180, 180, 180], [119, 119, 119], [155, 155, 155]]]], [[[[122, 122, 122], [130, 130, 130], [108, 108, 108], [142, 142, 142], [125, 125, 125]], [[121, 121, 121], [133, 133, 133], [69, 69, 69], [115, 115, 115], [149, 149, 149]], [[179, 179, 179], [136, 136, 136], [115, 115, 115], [91, 91, 91], [129, 129, 129]], [[137, 137, 137], [75, 75, 75], [105, 105, 105], [91, 91, 91], [125, 125, 125]], [[145, 145, 145], [94, 94, 94], [161, 161, 161], [96, 96, 96], [117, 117, 117]], [[209, 209, 209], [69, 69, 69], [153, 153, 153], [98, 98, 98], [186, 186, 186]], [[61, 61, 61], [114, 114, 114], [106, 106, 106], [141, 141, 141], [207, 207, 207]]]], [[[[139, 139, 139], [97, 97, 97], [115, 115, 115], [112, 112, 112], [151, 151, 151]], [[66, 66, 66], [128, 128, 128], [151, 151, 151], [61, 61, 61], [157, 157, 157]], [[170, 170, 170], [174, 174, 174], [118, 118, 118], [178, 178, 178], [66, 66, 66]], [[137, 137, 137], [209, 209, 209], [171, 171, 171], [100, 100, 100], [152, 152, 152]], [[96, 96, 96], [129, 129, 129], [80, 80, 80], [148, 148, 148], [113, 113, 113]], [[245, 245, 245], [161, 161, 161], [51, 51, 51], [69, 69, 69], [118, 118, 118]]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,814 |
def color_2_grey(colors):
|
b368cf3b7ab0795f4f6db34da096ad58 | UNKNOWN | Mr. Square is going on a holiday. He wants to bring 2 of his favorite squares with him, so he put them in his rectangle suitcase.
Write a function that, given the size of the squares and the suitcase, return whether the squares can fit inside the suitcase.
```Python
fit_in(a,b,m,n)
a,b are the sizes of the 2 squares
m,n are the sizes of the suitcase
```
# Example
```Python
fit_in(1,2,3,2) should return True
fit_in(1,2,2,1) should return False
fit_in(3,2,3,2) should return False
fit_in(1,2,1,2) should return False
``` | ["def fit_in(a, b, m, n):\n return max(a, b) <= min(m, n) and a + b <= max(m, n)", "def fit_in(a,b,m,n):\n return a+b<=max(m,n) and max(a,b)<=min(m,n)", "def fit_in(a,b,m,n):\n if m<a+b and n<a+b:\n return False\n if m<max([a,b]) or n<max([a,b]):\n return False\n return True", "def fit_in(a,b,m,n):\n # check first dimension\n if max(a, b) > min(m, n):\n return False\n \n # check second dimension\n if sum([a, b]) > max(m, n):\n return False\n \n return True", "def fit_in(a,b,m,n):\n if a+b <= max(m,n) and max(a,b) <= min(m,n):\n return True\n else:\n return False", "fit_in = lambda a,b,m,n : min(m,n) >= max(a,b) and max(m,n) >= a+b", "def fit_in(a,b,m,n):\n # You may code here\n if (a+b)<=m :\n if (max(a,b)<=n): \n return True \n else: \n return False\n elif max(a,b)<=m:\n if (a+b)<=n:\n return True\n else:\n return False \n else: \n return False\n", "def fit_in(a,b,m,n):\n if (min(m, n) >= max(a, b)) and max(m, n) >= a + b: \n return True\n return False", "fit_in=lambda a,b,m,n:a+b<=max(m,n)and min(m,n)>=max(a,b)", "def fit_in(a,b,m,n):\n if a+b > max(m,n) or a > min(m,n) or b > min(m,n):\n return False\n return a**2 + b**2 <= m*n"] | {"fn_name": "fit_in", "inputs": [[1, 2, 3, 2], [1, 2, 2, 1], [3, 2, 3, 2], [1, 2, 1, 2], [6, 5, 8, 7], [6, 6, 12, 6], [7, 1, 7, 8], [10, 10, 11, 11], [7, 2, 9, 7], [7, 2, 8, 7], [4, 1, 5, 3], [1, 2, 3, 4], [1, 2, 4, 3], [1, 3, 2, 4], [1, 3, 4, 2], [1, 4, 2, 3], [1, 4, 3, 2], [2, 1, 3, 4], [2, 1, 4, 3], [2, 3, 1, 4], [2, 3, 4, 1], [2, 4, 1, 3], [2, 4, 3, 1], [3, 2, 1, 4], [3, 2, 4, 1], [3, 1, 2, 4], [3, 1, 4, 2], [3, 4, 2, 1], [3, 4, 1, 2], [4, 2, 3, 1], [4, 2, 1, 3], [4, 3, 2, 1], [4, 3, 1, 2], [4, 1, 2, 3], [4, 1, 3, 2]], "outputs": [[true], [false], [false], [false], [false], [true], [true], [false], [true], [false], [false], [true], [true], [false], [false], [false], [false], [true], [true], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,387 |
def fit_in(a,b,m,n):
|
ae25479a36fa0f545540c2712ca37aee | UNKNOWN | The integer ```64``` is the first integer that has all of its digits even and furthermore, is a perfect square.
The second one is ```400``` and the third one ```484```.
Give the numbers of this sequence that are in the range ```[a,b] ```(both values inclusive)
Examples:
``` python
even_digit_squares(100, 1000) == [400, 484] # the output should be sorted.
even_digit_squares(1000, 4000) == []
```
Features of the random tests for ```even_digit_squares(a, b)```
```
number of Tests = 167
maximum value for a = 1e10
maximum value for b = 1e12
```
You do not have to check the entries, ```a``` and ```b``` always positive integers and ```a < b```
Happy coding!! | ["def is_even(x):\n return all(int(i) % 2 == 0 for i in str(x))\ndef even_digit_squares(a, b):\n first = int(a ** (1 / 2)) + 1\n last = int(b ** (1 / 2)) + 1\n return sorted([x * x for x in range(first, last) if is_even(x * x)])", "def even_digit_squares(a, b):\n return [i**2 for i in range(int(a**0.5), int(b**0.5)+1) if all(not int(j)%2 for j in str(i**2))]", "from bisect import bisect_left, bisect_right\n\nEVEN = set('02468')\npedps = [x for x in (x*x for x in range(8, 1000000, 2)) if set(str(x)) <= EVEN]\n\ndef even_digit_squares(a, b):\n return pedps[bisect_left(pedps, a):bisect_right(pedps, b)]", "def even_digit_squares(a, b):\n return [n**2 for n in range(int(a**0.5)+bool(a**0.5 % 1), int(b**0.5)+1) if not any(int(d) % 2 for d in str(n**2))]\n \n \n \n #result = []\n #i = int(a**0.5) + bool(a**0.5 % 1)\n #j = int(b**0.5) + 1\n #for n in range(i, j):\n # sq = n**2\n # if not any(int(d) % 2 for d in str(sq)):\n # result.append(sq)\n #return result\n", "PEDPS = [ n*n for n in range(2, 10**6, 2)\n if set(str(n*n)) <= set('02468') ]\n\ndef even_digit_squares(a, b):\n return [ n for n in PEDPS if a <= n <= b ]", "from math import ceil\ndef digits(n):\n while n >= 10:\n if (n % 10) % 2 > 0:\n return False\n n //= 10\n return n % 2 == 0\n\ndef even_digit_squares(a, b):\n p = ceil(a ** 0.5); q = int(b ** 0.5)\n return [i * i for i in range(p, q + 1) if digits(i * i)]", "even_digit_squares=lambda a,b:[n*n for n in range(1-int(-a**.5//1)&-2,int(b**.5)+1,2)if set(str(n*n))<=set('02468')]", "import math\n\ndef even_digit_squares(a, b):\n evens = ('2', '4', '6', '8', '0')\n start = math.ceil(math.sqrt(a))\n stop = math.ceil(math.sqrt(b+1))\n return [n*n for n in range(start, stop) if all(i in evens for i in str(n*n))]\n", "from math import floor, ceil, sqrt\n\ndef allEven(n):\n digs = list(str(n))\n odd_digs = [\"1\",\"3\",\"5\",\"7\",\"9\"]\n for dS in odd_digs:\n if dS in digs: return False\n return True\n\ndef even_digit_squares(a, b):\n lim1, lim2 = int(floor(sqrt(a))) - 1, int(ceil(sqrt(b)) + 1)\n res = []\n for i in range(lim1, lim2):\n ii = i**2\n if a<= ii <= b and allEven(ii):res.append(ii)\n return res"] | {"fn_name": "even_digit_squares", "inputs": [[100, 1000], [1000, 4000], [10000, 40000]], "outputs": [[[400, 484]], [[]], [[26244, 28224, 40000]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,309 |
def even_digit_squares(a, b):
|
d443a8be90c201ac1f825fa1c5047d91 | UNKNOWN | ## Number of people in the bus
There is a bus moving in the city, and it takes and drop some people in each bus stop.
You are provided with a list (or array) of integer arrays (or tuples). Each integer array has two items which represent number of people get into bus (The first item) and number of people get off the bus (The second item) in a bus stop.
Your task is to return number of people who are still in the bus after the last bus station (after the last array). Even though it is the last bus stop, the bus is not empty and some people are still in the bus, and they are probably sleeping there :D
Take a look on the test cases.
Please keep in mind that the test cases ensure that the number of people in the bus is always >= 0. So the return integer can't be negative.
The second value in the first integer array is 0, since the bus is empty in the first bus stop. | ["def number(bus_stops):\n return sum([stop[0] - stop[1] for stop in bus_stops])", "def number(stops):\n return sum(i - o for i, o in stops)", "def number(bus_stops):\n return sum(on - off for on, off in bus_stops)", "def number(bus_stops):\n sum=0\n for i in bus_stops:\n sum+=i[0]-i[1]\n return sum", "def number(bus_stops):\n return sum(stop[0]-stop[1] for stop in bus_stops)", "def number(bus_stops):\n total = 0\n for entered, out in bus_stops:\n total += entered - out \n return total\n", "def number(arr):\n people_in = 0\n people_out = 0\n for stops in arr:\n people_in += stops[0]\n people_out += stops[1]\n return people_in - people_out", "def number(bus_stops):\n get_in, get_off = zip(*bus_stops)\n return sum(get_in) - sum(get_off)", "def number(bus_stops):\n return sum(i-o for i,o in bus_stops)", "def number(bus_stops):\n return sum([item[0] - item[1] for item in bus_stops]) \n", "def number(bus_stops):\n x = 0\n for i, j in bus_stops:\n x += i - j\n return x", "def number(bus_stops):\n return sum(n - m for n, m in bus_stops)", "def number(bus_stops):\n return sum(map(lambda x: x[0]-x[1],bus_stops))", "number = lambda b: sum(i-o for i,o in b)", "def number(bus_stops):\n total_number = 0\n for i in bus_stops:\n total_number += i[0] - i[1]\n \n return total_number\n \n", "def number(bus_stops):\n return sum([i-j for [i,j] in bus_stops])", "def number(bus_stops):\n #bus_stops[0][0]\n #bus_stops[1][0]\n #bus_stops[2][0]\n \n #bus_stops[0][1]\n #bus_stops[1][1]\n #bus_stops[2][1]\n came = 0\n left = 0\n for counter, _ in enumerate(bus_stops,0):\n came = came + bus_stops[counter][0]\n left = left + bus_stops[counter][1]\n return came - left", "def number(bus_stops):\n t = 0\n for a in bus_stops:\n t = t + a[0] - a[1]\n return t", "def number(bus_stops):\n return sum([bus_in - bus_out for bus_in, bus_out in bus_stops])", "def number(bus_stops):\n return sum( a-b for [a,b] in bus_stops)", "def number(bus_stops):\n return sum(x - y for x,y in bus_stops)", "def number(bus_stops):\n # Good Luck!\n totalPeople = 0\n for i in bus_stops:\n totalPeople += i[1] - i[0]\n return abs(totalPeople)\n \n", "def number(bus_stops):\n getin = 0\n getout = 0\n for x, y in bus_stops:\n getin += x\n getout += y\n return getin - getout\n", "from functools import reduce\n\ndef number(bus_stops):\n return sum(x[0]-x[1] for x in bus_stops)", "def number(x):\n L=[]\n for i in x:\n L.append(i[0])\n L.append(-i[1])\n return sum(L)", "def number(bus_stops):\n return sum(guys_in - guys_out for guys_in, guys_out in bus_stops)", "def number(bus_stops):\n number_of_people = 0\n for i in bus_stops:\n number_of_people += i[0]\n number_of_people -= i[1]\n return number_of_people ", "def number(bus_stops):\n \n counter = 0\n max_index = len(bus_stops) - 1\n y = 0\n z = 0\n \n while counter <= max_index:\n x = bus_stops[counter]\n counter = counter + 1\n y = y + x[0]\n z = z + x[1]\n end = y - z\n return end", "def number(bus_stops):\n return sum([p[0]-p[1] for p in bus_stops])", "import operator\n\ndef number(bus_stops):\n return operator.sub(*map(sum, zip(*bus_stops)))", "def number(bus_stops):\n on, off = list(map(sum, list(zip(*bus_stops))))\n return on - off\n", "def number(bs):\n on=0\n off=0\n for i in bs:\n on+=i[0]\n off+=i[1]\n return on-off", "def number(bus_stops):\n x =0\n for (go,out) in bus_stops:\n x+=go-out \n return x", "def number(bus_stops):\n still_in_bus = 0\n for stop in bus_stops:\n still_in_bus = still_in_bus + (stop[0] - stop[1])\n return still_in_bus", "def number(bus_stops):\n a = []\n for l in bus_stops:\n c = l[0] - l[1]\n a.append(c)\n return sum(a)\n \n \n", "def number(bus_stops):\n numOfPassengers = 0\n index = 0\n for x in range(0,len(bus_stops)):\n numOfPassengers += bus_stops[index][0]\n numOfPassengers -= bus_stops[index][1]\n index += 1\n return numOfPassengers\n \n", "number = lambda b: sum(e[0] - e[1] for e in b)", "number = lambda b: sum(e[0] for e in b) - sum(e[1] for e in b)", "def number(bus_stops):\n passengers = 0\n for (x,y) in bus_stops:\n passengers = passengers + x - y\n return passengers", "from functools import reduce\n\ndef number(bus_stops):\n return -reduce(\n lambda x, y: y - x,\n reduce(\n lambda a, b: a + b,\n bus_stops\n )\n )\n", "def number(bus_stops):\n rtn = 0\n for i in bus_stops:\n rtn = rtn + i[0] - i[1]\n \n return rtn", "def number(bus_stops):\n return sum(x[0] for x in bus_stops) - sum(x[1] for x in bus_stops) # acquired - lost", "from itertools import starmap\nfrom operator import sub\n\ndef number(bus_stops):\n return sum(starmap(sub,bus_stops))", "def number(bus_stops):\n return sum(bus_stops[0] for bus_stops in bus_stops) - sum(bus_stops[1] for bus_stops in bus_stops)", "def number(bus_stops):\n return sum(x[0]-x[1] for x in bus_stops)", "def number(bus_stops):\n return sum([ a-b for a, b in bus_stops ])", "def number(bus_stops):\n result = 0\n stop = list(bus_stops)\n for x in stop:\n result = result + x[0] - x[1]\n return result", "def number(bus_stops):\n sleeping = 0\n for i in bus_stops:\n sleeping += i[0] - i[1]\n return sleeping\n", "import numpy as np\ndef number(bus_stops):\n bs = np.array(bus_stops)\n return sum(bs[:, 0] - bs[:, 1])", "def number(bus_stops):\n #initialize variables\n x =0\n #iterate through the arrays and add to the count\n for people in bus_stops:\n x += people[0]\n x -= people[1]\n return x", "def number(bus_stops):\n ppl = 0\n for stops in bus_stops:\n ppl += stops[0]\n ppl -= stops[1]\n return ppl", "def number(bus_stops):\n retval=0\n for x in bus_stops:\n retval=retval+x[0]\n retval=retval-x[1]\n print((x[1]))\n return retval\n # Good Luck!\n", "def number(bus_stop):\n get_bus = sum([a[0]-a[1] for a in bus_stop])\n if get_bus > 0:\n return get_bus\n else:\n return 0\n\n\nnumber([[10,0],[3,5],[5,8]])\nnumber([[3,0],[9,1],[4,10],[12,2],[6,1],[7,10]])\nnumber([[3,0],[9,1],[4,8],[12,2],[6,1],[7,8]])\n", "# Made with \u2764\ufe0f in Python 3 by Alvison Hunter - October 8th, 2020\ndef number(bus_stops):\n try:\n last_passengers = sum(people_in - people_out for people_in, people_out in bus_stops)\n except:\n print(\"Uh oh! Something went really wrong!\")\n quit\n finally:\n if(last_passengers >= 0):\n print('Remaining Passengers for last stop: ', last_passengers)\n return last_passengers\n else:\n print('No passengers where on the bus')\n last_passengers = 0\n return last_passengers", "# Made with \u2764\ufe0f in Python 3 by Alvison Hunter - October 8th, 2020\ndef number(bus_stops):\n try:\n last_passengers = 0\n total_people_in = 0\n total_people_out = 0\n\n for first_item, second_item in bus_stops:\n total_people_in += first_item\n total_people_out += second_item\n\n except:\n print(\"Uh oh! Something went really wrong!\")\n quit\n finally:\n last_passengers = total_people_in - total_people_out\n if(last_passengers >= 0):\n print('Remaining Passengers for last stop: ', last_passengers)\n return last_passengers\n else:\n print('No passengers where on the bus')\n last_passengers = 0\n return last_passengers", "from functools import reduce\n\ndef number(bus_stops):\n return reduce(lambda i,a: i+a[0]-a[1], bus_stops, 0)\n", "def number(bus_stops):\n \n bus_pop = 0\n \n for stop in bus_stops:\n bus_pop += stop[0]\n bus_pop -= stop[1]\n \n return bus_pop", "def number(bus_stops):\n present = 0\n for tup in bus_stops:\n present = present + tup[0]\n present = present - tup[1]\n return present\n \n", "def number(bus_stops):\n first_tuple_elements = []\n second_tuple_elements = []\n result = []\n for i in bus_stops:\n first_tuple_elements.append(i[0])\n second_tuple_elements.append(i[1])\n x = 0 \n result = 0\n for i in first_tuple_elements:\n result += first_tuple_elements[x] - second_tuple_elements[x]\n print(result)\n x += 1\n return result\n", "def number(bus_stops):\n# a = sum([i[0] for i in bus_stops])\n# b = sum([i[1] for i in bus_stops])\n# return a-b\n return sum([i[0] for i in bus_stops])-sum([i[1] for i in bus_stops])", "def number(bus_stops):\n people_in_the_bus = 0\n \n for people in bus_stops:\n people_in_the_bus += people[0]\n people_in_the_bus -= people[1]\n \n return people_in_the_bus", "def number(bus_stops):\n x = 0\n y = 0\n for i in bus_stops:\n x += i[0]\n y += i[1]\n return x - y \n", "def number(bus_stops):\n solut = 0\n for x in bus_stops:\n solut += x[0]\n solut -= x[1]\n return solut", "def number(bus_stops):\n lastPeople = 0\n i = 0\n while(i< len(bus_stops)): \n lastPeople = lastPeople + bus_stops[i][0] - bus_stops[i][1]\n i = i+1\n return lastPeople;", "def number(bus):\n c = 0\n for j in bus:\n c += j[0]-j[1]\n return c\n", "def number(bus_stops):\n inBus = 0\n for stop in bus_stops:\n inBus += stop[0]\n inBus -= stop[1]\n if inBus < 0:\n return False\n return inBus", "def number(bus_stops):\n bus_occupancy = 0\n i = 0\n j = 0\n for i in range(len(bus_stops)):\n bus_occupancy = bus_occupancy + (bus_stops[i][j] - bus_stops[i][j+1])\n print('Bus occupancy is: ', bus_occupancy)\n return(bus_occupancy) ", "def number(bus_stops):\n on_sum = 0\n off_sum = 0\n for x in bus_stops:\n on_sum += x[0]\n off_sum += x[1]\n return on_sum - off_sum \n # Good Luck!\n", "def number(bus_stops):\n x = 0\n for i in bus_stops:\n x = i[0] - i[1] + x\n return x", "def number(bus_stops):\n res = 0 \n for e in bus_stops:\n res += e[0]\n res -= e[1] \n return res", "def number(bus_stops):\n still_on = 0\n for stop in bus_stops:\n still_on += stop[0]\n still_on -= stop[1]\n return still_on", "def number(bus_stops):\n peopleInBus=0\n for elem in bus_stops:\n peopleInBus+=elem[0]-elem[1]\n return peopleInBus", "def number(bus_stops):\n # Good Luck!\n return sum((peoplein - peopleout) for peoplein,peopleout in (bus_stops))", "def number(bus_stops):\n enter, leave = zip(*bus_stops)\n return sum(enter) - sum(leave)", "def number(bus_stops):\n empty = []\n for i in range(0,len(bus_stops)):\n empty.append(bus_stops[i][0]-bus_stops[i][1])\n return sum(empty)\n\n", "def number(bus_stops):\n total = 0\n for stop in bus_stops:\n print(stop)\n total = total + stop[0]\n total = total - stop[1]\n \n return total\n", "def number(bus_stops):\n b = 0\n for enter, leave in bus_stops:\n b = max(b + enter - leave, 0)\n return b\n", "def number(bus_stops):\n totalnum1 =0\n totalnum2 =0\n for i in range (0,len(bus_stops)):\n totalnum1 = totalnum1 + (bus_stops[i])[0]\n totalnum2 = totalnum2 + (bus_stops[i])[1]\n num = totalnum1 - totalnum2\n return num", "def number(bus_stops):\n sum_up = 0\n sum_down = 0\n for i in bus_stops:\n sum_up = sum_up + i[0]\n sum_down = sum_down + i[1]\n summ = sum_up-sum_down\n return summ", "def number(bus_stops):\n summ=0\n for i in bus_stops:\n summ+= i[0]-i[1]\n return summ\n # Good Luck!\n", "def number(bus_stops):\n ans = 0\n for o in bus_stops:\n ans += o[0]\n ans -= o[1]\n return ans", "def number(bus_stops):\n res = 0\n for st in bus_stops:\n res = res - st[1]\n res = res + st[0]\n return res \n # Good Luck!\n", "def number(bus_stops):\n peoples = 0\n for number in bus_stops:\n peoples += number[0]-number[1]\n return peoples", "def number(bus_stops):\n remaining = 0\n for inP, outP in bus_stops:\n remaining = remaining + inP - outP\n return remaining", "def number(bus_stops):\n # Good Luck!\n into_bus = 0\n get_off_bus = 0\n for stops in bus_stops:\n into_bus += stops[0]\n get_off_bus += stops[1]\n return into_bus - get_off_bus", "def number(bus_stops):\n people = [sum(i) for i in zip(*bus_stops)]\n return people[0] - people[1]", "def number(bus_stops):\n sum=0\n for i in bus_stops:\n num=i[0]-i[1]\n sum=sum+num\n return sum\n \n # Good Luck!\n", "def number(bus_stops):\n get_in = sum([a[0] for a in bus_stops])\n get_out = sum([a[1] for a in bus_stops])\n\n total = get_in - get_out\n return total", "def number(bus_stops):\n # Good Luck!\n '''\n input: bus_stops tuple - the # of people who get on and off the bus\n approach: loop through and sum the first element and sum the last element then subtract the two numbers\n output: the # of people left on the bus after the last stop\n '''\n passengersOn = 0\n passengersOff = 0\n \n try:\n res = sum(i[0] for i in bus_stops)- sum(i[1] for i in bus_stops) \n \n except: \n print(\"There was an error\")\n \n return res", "def number(bus_stops):\n get_in = get_out = 0\n for k,v in bus_stops:\n get_in += k\n get_out += v\n return (get_out - get_in) * -1", "def number(bus_stops):\n count = 0\n for el in bus_stops:\n count = count + el[0] - el[1]\n return count\n # Good Luck!\n", "def number(bus_stops):\n sum_in_bus = 0\n \n for stop in bus_stops:\n sum_in_bus += stop[0] - stop[1]\n \n return sum_in_bus", "def number(bus_stops):\n onBuss = 0\n\n for i in range(len(bus_stops)):\n onBuss += bus_stops[i][0]\n onBuss -= bus_stops[i][1]\n return onBuss\n", "def number(bus_stops):\n number = 0\n for i in range(len(bus_stops)):\n number += bus_stops[i][0] - bus_stops[i][1]\n return number \n # Good Luck!\n", "def number(bus_stops):\n up=0\n down=0\n \n for stops in bus_stops:\n up+=stops[0]\n down+=stops[1]\n \n return up-down\n", "def number(bus_stops):\n people=0\n for tuple in bus_stops:\n x,y = tuple\n people+=x-y\n \n return people\n", "def number(bus_stops):\n # Good Luck!\n left_on_bus = 0\n for i in bus_stops:\n left_on_bus += (i[0]) - (i[1])\n return left_on_bus\n \n \n", "def number(bus_stops):\n res = [i - j for i, j in bus_stops]\n return sum(res)"] | {"fn_name": "number", "inputs": [[[[10, 0], [3, 5], [5, 8]]], [[[3, 0], [9, 1], [4, 10], [12, 2], [6, 1], [7, 10]]], [[[3, 0], [9, 1], [4, 8], [12, 2], [6, 1], [7, 8]]], [[[0, 0]]]], "outputs": [[5], [17], [21], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 15,355 |
def number(bus_stops):
|
7d35146e4992682778b75baae94513d9 | UNKNOWN | A very passive-aggressive co-worker of yours was just fired. While he was gathering his things, he quickly inserted a bug into your system which renamed everything to what looks like jibberish. He left two notes on his desk, one reads: "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz" while the other reads: "Uif usjdl up uijt lbub jt tjnqmf kvtu sfqmbdf fwfsz mfuufs xjui uif mfuufs uibu dpnft cfgpsf ju".
Rather than spending hours trying to find the bug itself, you decide to try and decode it.
If the input is not a string, your function must return "Input is not a string". Your function must be able to handle capital and lower case letters. You will not need to worry about punctuation. | ["lower = \"abcdefghijklmnopqrstuvwxyz\"\n\ndef one_down(txt):\n if not isinstance(txt, str):\n return \"Input is not a string\"\n shifted = lower[-1] + lower[:-1]\n table = str.maketrans(lower + lower.upper(), shifted + shifted.upper())\n return txt.translate(table)", "def one_down(txt):\n try:\n return ''.join('Z' if i == 'A' else 'z' if i == 'a' else chr(ord(i) - 1) \\\n if i.isalpha() else i for i in txt)\n except:\n return \"Input is not a string\"\n", "def one_down(txt):\n #your code here\n \n if type(txt) is not str:\n return \"Input is not a string\"\n \n new_string = \"\"\n for char in txt:\n if char == \" \":\n new_string = new_string + \" \"\n elif char == \"A\":\n new_string = new_string + \"Z\"\n elif char == \"a\":\n new_string = new_string + \"z\"\n elif char in \"-+,.;:\":\n new_string = new_string + char\n else:\n new_string = new_string + chr(ord(char) - 1)\n return new_string\n \n", "def one_down(txt):\n #your code here \n if isinstance(txt, list) or isinstance(txt,int): \n return \"Input is not a string\"\n Decode = list(map((ord),txt))\n txt= ''\n for x in Decode:\n if x < 65 or x > 122:\n txt +=chr(x)\n elif x == 65 or x == 97:\n txt +=chr(x+25)\n else:\n txt +=chr(x-1)\n return(txt)\n", "def one_down(txt):\n if type(txt)!=str: return \"Input is not a string\"\n return ''.join(chr(65+(ord(i)-66)%26) if i.isupper() else chr(97+(ord(i)-98)%26) if i.islower() else i for i in txt)\n", "def one_down(txt):\n if type(txt) != str:\n return \"Input is not a string\"\n\n abc = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz\"\n result = ''\n\n for x in txt:\n if x not in abc:\n result += x\n else:\n if x in 'aA':\n result += 'zZ'['aA'.index(x)]\n else:\n result += abc[abc.index(x) - 1]\n\n return result"] | {"fn_name": "one_down", "inputs": [["Ifmmp"], ["Uif usjdl up uijt lbub jt tjnqmf"], [45], ["XiBu BcPvU dSbAz UfYu"], [["Hello there", "World"]], ["BMM DBQT NBZCF"], ["qVAamFt BsF gVo"], ["CodeWars RockZ"], [""], ["J ipqf zpv bsf ibwjoh b ojdf ebz"]], "outputs": [["Hello"], ["The trick to this kata is simple"], ["Input is not a string"], ["WhAt AbOuT cRaZy TeXt"], ["Input is not a string"], ["ALL CAPS MAYBE"], ["pUZzlEs ArE fUn"], ["BncdVzqr QnbjY"], [""], ["I hope you are having a nice day"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,112 |
def one_down(txt):
|
3fe755104c471008e924cffaf845bfd2 | UNKNOWN | An array is defined to be `odd-heavy` if it contains at least one odd element and every element whose value is `odd` is greater than
every even-valued element.
eg.
```
Array [11,4,9,2,8] is odd-heavy
because:- its odd elements [11,9] are greater than all the even elements [4,2,8]
Array [11,4,9,2,3,10] is not odd-heavy
because:- one of it's even element 10 from [4,2,10] is greater than two of its odd elements [9,3] from [ 11,9,3]
```
write a function called `isOddHeavy` or `is_odd_heavy` that accepts an integer array and returns `true` if the array is `odd-heavy` else return `false`. | ["def is_odd_heavy(arr):\n maxEven, minOdd = ( f(filter(lambda n: n%2 == v, arr), default=float(\"-inf\")) for f,v in ((max, 0), (min,1)) )\n return maxEven < minOdd", "odd = lambda x: x&1\n\ndef is_odd_heavy(arr):\n it = iter(sorted(arr))\n return any(map(odd, it)) and all(map(odd, it))", "def is_odd_heavy(a):\n odd = list(filter(lambda x:x&1,a)) or []\n even = list(filter(lambda x:not x&1,a))\n return all(all(map(lambda x:x<i,even)) for i in odd) if odd else False", "def is_odd_heavy(xs):\n return bool(xs) and (\n min((x for x in xs if x % 2 == 1), default=min(xs)-1) >\n max((x for x in xs if x % 2 == 0), default=min(xs)-1)\n )", "is_odd_heavy=lambda a:max([n for n in a if-~n%2]or[float('-inf')])<min([n for n in a if n%2]or[float('-inf')])", "def is_odd_heavy(arr):\n is_odd= False\n for index in range(len(arr)-1):\n if(sorted(arr)[index]%2==1)and(sorted(arr)[index+1]%2==0):\n return False\n if(sorted(arr)[index]%2==1)or(sorted(arr)[index+1]%2==1):\n is_odd= True\n return is_odd if len(arr)!=1 else (arr[0]%2==1)\n"] | {"fn_name": "is_odd_heavy", "inputs": [[[0, 2, 19, 4, 4]], [[1, -2, -1, 2]], [[-3, 2, 1, 3, -1, -2]], [[3, 4, -2, -3, -2]], [[-1, 1, -2, 2, -2, -2, -4, 4]], [[-1, -2, 21]], [[0, 0, 0, 0]], [[0, -1, 1]], [[0, 2, 3]], [[0]], [[]], [[1]], [[0, 1, 2, 3, 4, 0, -2, -1, -4, -3]], [[1, -1, 3, -1]], [[1, -1, 2, -2, 3, -3, 0]], [[3]], [[2, 4, 6]], [[-2, -4, -6, -8, -11]]], "outputs": [[true], [false], [false], [false], [false], [true], [false], [false], [true], [false], [false], [true], [false], [true], [false], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,118 |
def is_odd_heavy(arr):
|
d8dc70f7c2a350125236b5813790cd4b | UNKNOWN | Debug the functions
Should be easy, begin by looking at the code. Debug the code and the functions should work.
There are three functions: ```Multiplication (x)``` ```Addition (+)``` and ```Reverse (!esreveR)```
i {
font-size:16px;
}
#heading {
padding: 2em;
text-align: center;
background-color: #0033FF;
width: 100%;
height: 5em;
} | ["from functools import reduce\nfrom operator import mul\n\ndef multi(l_st):\n return reduce(mul,l_st)\ndef add(l_st):\n return sum(l_st)\ndef reverse(s):\n return s[::-1]", "import functools\n\ndef multi(l_st):\n return functools.reduce(lambda x, y: x * y, l_st)\ndef add(l_st):\n return functools.reduce(lambda x, y: x + y, l_st)\ndef reverse(string):\n return string[::-1]", "from functools import reduce\n\ndef multi(lst):\n return reduce(lambda m, n: m * n, lst, 1)\n \ndef add(lst):\n return sum(lst)\n \ndef reverse(string):\n return string[::-1]", "def multi(l_st): \n count = 1\n for x in l_st:\n count *= x\n return count\n \ndef add(l_st):\n count = 0\n for x in l_st:\n count += x\n return count\n \ndef reverse(string):\n return string[::-1]", "from functools import reduce\ndef multi(l_st):\n return reduce((lambda x, y: x * y), l_st)\ndef add(l_st):\n return sum(l_st)\ndef reverse(string):\n return string[::-1]", "from numpy import sum as add, prod as multi\nreverse = lambda s: s[::-1]", "def multi(l_st):\n x=1\n for ele in l_st:\n x*=ele\n return x\n \ndef add(l_st):\n return sum(l_st)\ndef reverse(l_st):\n return l_st[::-1]", "def multi(l_st):\n m = 1\n for i in l_st:\n m*=i\n return m\ndef add(l_st):\n return sum(l_st)\ndef reverse(string):\n return string[::-1]", "add, reverse, multi = sum, lambda s: s[::-1], lambda l: __import__(\"functools\").reduce(int.__mul__, l)"] | {"fn_name": "multi", "inputs": [[[8, 2, 5]]], "outputs": [[80]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,535 |
def multi(l_st):
|
29aca435d4fdc459ca384b1df6bac210 | UNKNOWN | _A mad sociopath scientist just came out with a brilliant invention! He extracted his own memories to forget all the people he hates! Now there's a lot of information in there, so he needs your talent as a developer to automatize that task for him._
> You are given the memories as a string containing people's surname and name (comma separated). The scientist marked one occurrence of each of the people he hates by putting a '!' right before their name.
**Your task is to destroy all the occurrences of the marked people.
One more thing ! Hate is contagious, so you also need to erase any memory of the person that comes after any marked name!**
---
Examples
---
---
Input:
```
"Albert Einstein, !Sarah Connor, Marilyn Monroe, Abraham Lincoln, Sarah Connor, Sean Connery, Marilyn Monroe, Bjarne Stroustrup, Manson Marilyn, Monroe Mary"
```
Output:
```
"Albert Einstein, Abraham Lincoln, Sean Connery, Bjarne Stroustrup, Manson Marilyn, Monroe Mary"
```
=> We must remove every memories of Sarah Connor because she's marked, but as a side-effect we must also remove all the memories about Marilyn Monroe that comes right after her! Note that we can't destroy the memories of Manson Marilyn or Monroe Mary, so be careful! | ["def select(memory):\n lst = memory.split(', ')\n bad = {who.strip('!') for prev,who in zip(['']+lst,lst+['']) if who.startswith('!') or prev.startswith('!')}\n return ', '.join(who for who in map(lambda s: s.strip('!'), lst) if who not in bad)", "def select(memory):\n names = memory.split(', ')\n markeds = [names[x] for x in range(len(names)) if names[x][0] == \"!\" or (x > 0 and names[x - 1][0] == \"!\")]\n return \", \".join(list(i for i in names if i not in markeds and \"!\" + i not in markeds))", "def select(s):\n s_ = s.split(\", \")\n li = sum([[j[1:], s_[i+1] if i < len(s_)-1 else \"X\"] for i, j in enumerate(s_) if j[0]==\"!\"],[])\n return \", \".join([i for i in s_ if i not in li and i[0] != \"!\"])", "def select(memory):\n hates = set()\n h = False\n for name in memory.split(', '):\n if name.startswith('!'):\n hates.add(name.lstrip('!'))\n h = True\n elif h:\n hates.add(name.lstrip('!'))\n h = False\n return ', '.join(name for name in memory.split(', ') if name.lstrip('!') not in hates)", "def select(memory):\n names = memory.split(', ')\n hated = set()\n \n for i, name in enumerate(names):\n if name[0] == \"!\" or (i > 0 and names[i-1][0] == \"!\"):\n hated.update([\"!\" + name.strip(\"!\"), name.strip(\"!\")])\n \n return \", \".join(filter(lambda name: name not in hated, names))", "def select(memory):\n names = memory.split(\", \")\n hated = set()\n \n for idx, name in enumerate(names):\n if name[0] == \"!\":\n hated |= set(n.strip(\"!\") for n in names[idx : idx+2])\n \n return \", \".join(name for name in names if name.strip(\"!\") not in hated)", "def select (memory):\n \n def records (tokens):\n for token in tokens:\n if token.startswith('!'): yield token[1:], True\n else: yield token, False\n\n def contagious (records):\n mark_next = False\n for name, marked in records:\n if mark_next: yield name, True\n else: yield name, marked\n mark_next = marked\n\n separator = ', '\n tokens = memory.split(separator)\n recordset = list(contagious(records(tokens)))\n\n hated = set(name for name, marked in recordset if marked)\n loved = (name for name, _ in recordset if name not in hated)\n return separator.join(loved)\n", "def select(memory):\n \n s = memory.split(\", \")\n result = memory.split(\", \")\n \n set1= set()\n \n for i in range(len(s)):\n\n if s[i].find('!') != -1:\n set1.add(str(s[i])[(s[i].find('!')+1):len(s[i])])\n if i != len(s)-1:\n set1.add(str(s[i+1])[(s[i+1].find('!')+1):len(s[i+1])])\n\n for i in s:\n for j in set1:\n if i.find(j) != -1:\n result.remove(i) \n\n return ', '.join(result)", "def select(memory):\n memory = memory.split(\", \")\n hated = []\n for i in range(len(memory)):\n if memory[i].startswith(\"!\"):\n hated.append(memory[i][1:])\n if i != len(memory)-1:\n hated.append(memory[i+1])\n clean_memory = []\n for name in memory:\n if name[0] != \"!\" and name not in hated:\n clean_memory.append(name)\n return \", \".join(clean_memory)", "def select(memory):\n s = memory.split(',')\n s = [x.strip() for x in s]\n marked = []\n for i, name in enumerate(s):\n if name.startswith('!'):\n marked.append(name)\n if i != len(s)-1:\n marked.append(s[i+1])\n for mark in marked:\n if mark.startswith('!'):\n if mark in s:\n s.remove(mark)\n n = mark[1:]\n else:\n n = mark[:]\n if n in s:\n s = [x for x in s if x!=n]\n \n return ', '.join(s)"] | {"fn_name": "select", "inputs": [["Bryan Joubert"], ["Jesse Cox, !Selena Gomez"], ["!Eleena Daru, Obi-Wan Kenobi, Eleena Daru, Jar-Jar Binks"], ["Digital Daggers, !Kiny Nimaj, Rack Istley, Digital Daggers, Digital Daggers"], ["Albert Einstein, !Sarah Connor, Marilyn Monroe, Abraham Lincoln, Sarah Connor, Sean Connery, Marilyn Monroe, Bjarne Stroustrup, Manson Marilyn, Monroe Mary"], ["!Partha Ashanti, !Mindaugas Burton, Stacy Thompson, Amor Hadrien, !Ahtahkakoop Sothy, Partha Ashanti, Uzi Griffin, Partha Ashanti, !Serhan Eutimio, Amor Hadrien, Noor Konstantin"], ["!Code Wars, !Doug Smith, !Cyril Lemaire, !Codin Game"]], "outputs": [["Bryan Joubert"], ["Jesse Cox"], ["Jar-Jar Binks"], ["Digital Daggers, Digital Daggers, Digital Daggers"], ["Albert Einstein, Abraham Lincoln, Sean Connery, Bjarne Stroustrup, Manson Marilyn, Monroe Mary"], ["Uzi Griffin, Noor Konstantin"], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,948 |
def select(memory):
|
738b3cd2b98c60d6bfcab98fe42b2fd9 | UNKNOWN | Compare two strings by comparing the sum of their values (ASCII character code).
* For comparing treat all letters as UpperCase
* `null/NULL/Nil/None` should be treated as empty strings
* If the string contains other characters than letters, treat the whole string as it would be empty
Your method should return `true`, if the strings are equal and `false` if they are not equal.
## Examples:
```
"AD", "BC" -> equal
"AD", "DD" -> not equal
"gf", "FG" -> equal
"zz1", "" -> equal (both are considered empty)
"ZzZz", "ffPFF" -> equal
"kl", "lz" -> not equal
null, "" -> equal
``` | ["def string_cnt(s):\n try:\n if s.isalpha():\n return sum(ord(a) for a in s.upper())\n except AttributeError:\n pass\n return 0\n\n\ndef compare(s1, s2):\n return string_cnt(s1) == string_cnt(s2)", "def compare(s1,s2):\n f = lambda x: sum(map(ord,x.upper())) if x and x.isalpha() else ''\n return f(s1) == f(s2)", "def compare(s1,s2):\n if not s1 or not s1.isalpha():\n s1 = ''\n if not s2 or not s2.isalpha():\n s2 = ''\n return sum(ord(x) for x in s1.upper()) == sum(ord(y) for y in s2.upper())", "def charsum(s):\n return bool(s) and s.isalpha() and sum(ord(c) for c in s.upper())\n\ndef compare(s1,s2):\n return charsum(s1) == charsum(s2)", "def _sum(s):\n return (\n sum(ord(c) for c in s.upper())\n if s and s.isalpha()\n else 0)\n\n\ndef compare(s1, s2):\n return _sum(s1) == _sum(s2)\n", "def compare(s1, s2):\n v1, v2 = (sum(ord(c) for c in s.upper()) if s and s.isalpha() else 0 for s in (s1, s2))\n return v1 == v2", "def stringCount(str):\n try:\n if str.isalpha():\n return sum(ord(alpha) for alpha in str.upper())\n except AttributeError:\n pass\n return 0\n\n\ndef compare(s1, s2):\n return stringCount(s1) == stringCount(s2)", "def compare(s1, s2):\n return len(set(sum(map(ord, x.upper() if x and x.isalpha() else '')) for x in [s1, s2])) == 1", "def compare(s1,s2):\n y = lambda s: sum(ord(c.upper()) for c in s) if s and s.isalpha() else 0\n return y(s1) == y(s2)", "def compare(s1,s2):\n if not s1 or s1.isalpha() is False:\n s1 = ''\n if not s2 or s2.isalpha() is False:\n s2 = ''\n return sum([ord(c) for c in s1.upper()]) == sum([ord(c) for c in s2.upper()])", "def compare(s1,s2):\n if s1 == None or not s1.isalpha(): s1 = ''\n if s2 == None or not s2.isalpha(): s2 = ''\n return sum(ord(c.upper()) for c in s1) == sum(ord(c.upper()) for c in s2)", "def compare(s1,s2):\n return get_ascii_sum_value(s1) == get_ascii_sum_value(s2)\n \ndef get_ascii_sum_value(s):\n if s == None or len(s) == 0:\n return 0\n sum_value = 0\n for x in s:\n if not x.isalpha():\n sum_value = 0\n break\n else: \n sum_value += ord(x.upper())\n return sum_value", "def count_value(s):\n return sum([ord(c) for c in s.upper()]) if s and s.isalpha() else 0\n\ndef compare(s1,s2):\n return count_value(s1) == count_value(s2)", "def compare(s1,s2):\n cmp = lambda s: sum(ord(a) for a in s.upper()) if s and s.isalpha() else 0\n return cmp(s1) == cmp(s2)", "def compare(s1,s2):\n if not s1 or not s1.isalpha():s1=''\n if not s2 or not s2.isalpha():s2=''\n return sum(map(ord,s1.upper())) == sum(map(ord,s2.upper()))", "h,compare=lambda s:s!=None and s.isalpha()and sum(map(ord,s.upper())),lambda a,b:h(a)==h(b)", "def compare(s1,s2):\n get_value = lambda string: sum(ord(char) for char in string.upper()) if string and string.isalpha() else 0\n return get_value(s1) == get_value(s2)", "f = lambda x: sum([ord(y) for y in list(x.upper())]) if x and x.isalpha() else 0\n\ndef compare(s1, s2):\n return f(s1) == f(s2)", "def cal(s):\n if not s : return 0\n if not s.isalpha() : return 0\n return sum([ord(x) for x in s.upper()])\n\ndef compare(s1,s2):\n return cal(s1)==cal(s2)", "def compare(s1,s2):\n clean1 = clean(s1)\n clean2 = clean(s2)\n sum1 = ord_sum(clean1)\n sum2 = ord_sum(clean2)\n return sum1 == sum2\n \n# check for empty conditions\ndef clean(s):\n clean = s\n if clean == None: clean = ''\n for char in clean:\n if not char.isalpha():\n clean = ''\n break\n return clean\n\ndef ord_sum(s):\n ords = [ord(c.upper()) for c in s]\n return sum(ords)", "def compare(s1,s2):\n n1=n2=0\n if s1 and all(i.isalpha() for i in s1):\n n1=sum(ord(i) for i in s1.upper())\n if s2 and all(i.isalpha() for i in s2):\n n2=sum(ord(i) for i in s2.upper())\n return n1==n2", "import re\n\n\ndef compare(s1, s2):\n if s1 is None or s1 is [] or re.search('[^a-zA-Z]', s1): s1 = []\n if s2 is None or s2 is [] or re.search('[^a-zA-Z]', s2): s2 = []\n return True if s2 == s1 else True if sum(ord(x.upper()) for x in s1) == sum(ord(x.upper()) for x in s2) else False\n", "def compare(s1,s2):\n f = lambda x: sum([ord(i.upper()) for i in x])\n g = lambda x,y: x is not None and y is not None and not x.isalpha() and not y.isalpha()\n h = lambda x,y: True if x in (None, '') and y in (None,'') else False\n i = lambda x,y: x.isalpha() and y.isalpha()\n return g(s1,s2) or h(s1,s2) or (s1 and s2 and f(s1) == f(s2)) and i(s1,s2)\n \n", "def compare(s1,s2):\n if s1 is None or not s1.isalpha(): s1=\"\"\n if s2 is None or not s2.isalpha(): s2=\"\"\n if sum([ord(x) for x in s1.upper()])==sum([ord(x) for x in s2.upper()]): return True\n else: return False", "def compare(s1,s2):\n if s1 == None or not s1.isalpha():\n s1 = ''\n if s2 == None or not s2.isalpha():\n s2 = ''\n return sum([ord(s.upper()) for s in s1]) == sum([ord(s.upper()) for s in s2])", "def compare(s1,s2):\n if s1 is None or not s1.isalpha():\n s1 = \"\"\n if s2 is None or not s2.isalpha():\n s2 = \"\"\n x1 = sum([ord(i.upper()) for i in s1])\n x2 = sum([ord(i.upper()) for i in s2])\n return x1 == x2", "def compare(s1,s2):\n f = lambda s : all(c.lower() in 'abcdefghijklmnopqrstuvwxyz' for c in s)*sum(ord(c.upper()) for c in s) if s else False\n return f(s1)==f(s2)", "def compare(s1,s2):\n upper_basis_ord = lambda x: sum(ord(c) for c in x.upper()) if type(x) == str and x.isalpha() else ''\n return upper_basis_ord(s1) == upper_basis_ord(s2)", "def compare(s1, s2):\n return chsum(s1) == chsum(s2)\n \ndef chsum(s):\n if not s:\n return 0\n \n s = s.upper()\n if any(c < 'A' or c > 'Z' for c in s):\n return 0\n \n return sum(ord(c) for c in s)", "def compare(s1,s2):\n try:\n if not s1.isalpha():\n s1 = ''\n except AttributeError:\n s1 = ''\n try:\n if not s2.isalpha():\n s2 = ''\n except AttributeError:\n s2 = ''\n summ = 0\n for i in s1.upper():\n summ += ord(i)\n for i in s2.upper():\n summ -= ord(i)\n return summ ==0", "def compare(s1,s2):\n if s1 == None or s2 == None:\n return True\n if not s1.isalpha():\n s1 = \"\"\n if not s2.isalpha():\n s2 = \"\"\n s1 = s1.upper()\n s2 = s2.upper()\n return sum([ord(el) for el in s1]) == sum([ord(el) for el in s2])", "def compare(s1,s2):\n def val(s):\n return 0 if s is None or not s.isalpha() else sum(ord(c) for c in s.upper())\n return val(s1) == val(s2)\n", "import re\ndef compare(s1,s2):\n def sums(s):\n return '' if(not s or re.search('[^A-Za-z]', s)) else sum(ord(x) for x in s.upper())\n return sums(s1) == sums(s2)", "def compare(s1,s2):\n return strsum(s1) == strsum(s2)\n\ndef strsum(x):\n if not type(x) == str: return 0\n if(x.upper().isalpha()): return sum(ord(v) for v in x.upper())\n return 0", "def compare(s1, s2): ###i\n v1, v2 = (sum(ord(c) for c in s.upper()) if s and s.isalpha() else 0 for s in (s1, s2))\n return v1 == v2", "def compare(s1, s2):\n val_s1, val_s2 = 0 if s1 == None or not s1.isalpha() else sum(map(ord, s1.upper())), 0 if s2 == None or not s2.isalpha() else sum(map(ord, s2.upper()))\n return val_s1 == val_s2", "def compare(s1,s2):\n if not s1 or not s1.isalpha():\n s1 = ''\n if not s2 or not s2.isalpha():\n s2 = ''\n return sum([ord(x) for x in s1.upper()])==sum([ord(x) for x in s2.upper()])\n", "def compare(s1,s2):\n a1,a2=0,0\n if s1!=None and s1!='': \n for i in s1:\n if not i.isalpha():\n a1=0\n break\n else:\n a1+=ord(i.upper())\n if s2!=None or s2!='':\n for j in s2:\n if not j.isalpha():\n a2=0\n break\n else:\n a2+=ord(j.upper())\n return a1==a2", "import re\ndef compare(s1,s2):\n if s1 and s2 and re.search(r\"[a-zA-Z]\", s1) and re.search(r\"[a-zA-Z]\", s2):\n return sum(ord(i) for i in str(s1.upper()) if re.search(r\"[a-zA-Z]\", i)) == sum(ord(i) for i in str(s2.upper()) if re.search(r\"[a-zA-Z]\", i))\n return True", "def compare(s1,s2):\n if s1==None or [e for e in s1.lower() if not 'a'<=e<='z']:\n s1=\"\"\n if s2==None or [e for e in s2.lower() if not 'a'<=e<='z']:\n s2=\"\"\n if sorted(s1.lower())==sorted(s2.lower()):\n return True\n return sum([ord(e) for e in s1])==sum([ord(e) for e in s2])", "def compare(s1,s2):\n\n if s1 == None or s1.isalpha() == False:\n s1 = \"\"\n if s2 == None or s2.isalpha() == False:\n s2 = \"\"\n\n lst1 = [ord(char) for char in s1.upper()]\n lst2 = [ord(char) for char in s2.upper()]\n\n return sum(lst1) == sum(lst2)", "def compare(s1,s2):\n\n import string\n\n if s1 == None or all(char in string.ascii_letters for char in s1) == False:\n s1 = \"\"\n else:\n s1 = s1.upper()\n\n if s2 == None or all(char in string.ascii_letters for char in s2) == False:\n s2 = \"\"\n else:\n s2 = s2.upper()\n\n \n lst1 = [ord(char) for char in s1]\n lst2 = [ord(char) for char in s2]\n \n return sum(lst1) == sum(lst2)", "import re\n\ndef compare(s1,s2):\n if s1 is None or re.match( '^[a-zA-Z]*$', s1) == None : s1 = ''\n if s2 is None or re.match( '^[a-zA-Z]*$', s2) == None : s2 = ''\n return sum(map(ord,s1.upper())) == sum(map(ord,s2.upper()))", "# 2020/07/04 [yetanotherjohndoe]\n\nfrom re import match\n\ndef compare(s1,s2):\n \n def _ascii(_input):\n try:\n _input = str(_input).upper() if match(r'''^[A-Z]+$''', _input.upper()) else ''\n return sum( [ ord(_c) for _c in _input ] )\n except:\n return ''\n\n if _ascii(s1) == _ascii(s2):\n return True\n elif not s1 and not s2:\n return True\n \n else:\n return False\n", "def count_string(s):\n if s == '' or s == None: return ''\n sum_s = 0\n for i in s.upper():\n if not i.isalpha():\n return ''\n else:\n sum_s += ord(i)\n return sum_s\n\ndef compare(s1,s2):\n return count_string(s1) == count_string(s2)", "def compare(s1,s2):\n return (sum(ord(i.upper()) for i in s1) if type(s1) == str and s1.isalpha() else 1) == (sum(ord(i.upper()) for i in s2) if type(s2) == str and s2.isalpha() else 1)", "def compare(s1,s2):\n try:\n if s1.isalpha() != True: s1 = ''\n except:\n s1 = ''\n try:\n if s2.isalpha() != True: s2 = ''\n except:\n s2 = ''\n return sum([ord(i) for i in s1.upper()]) == sum([ord(i) for i in s2.upper()])", "def compare(s1,s2):\n if s1 and s2:\n if s1.isalpha() and s2.isalpha():\n return sum([ord(x) for x in s1.upper()])==sum([ord(x) for x in s2.upper()])\n elif s1.isalpha() or s2.isalpha(): return False\n return True", "from re import search\n\ndef summation(string):\n if not string or search(\"[^a-zA-Z]\", string):\n string = ''\n return sum(ord(x) for x in list(string.upper()))\n\ncompare = lambda s1, s2: summation(s1) == summation(s2)", "def compare(s1, s2):\n if s1 is None or not s1.isalpha():\n s1 = ''\n if s2 is None or not s2.isalpha():\n s2 = ''\n return sum(ord(letter) for letter in s1.upper()) == sum(ord(letter) for letter in s2.upper())", "def compare(s1,s2):\n if s1==\"\" or s2==\"\":\n return True \n elif s1.isalpha() and s2.isalpha():\n return sum([ord(c.upper()) for c in list(s1)]) == sum([ord(d.upper()) for d in list(s2)])\n elif s1.isalpha() == s2.isalpha(): \n return True\n else:\n return False", "def compare(s1,s2):\n n1,n2 = 0, 0\n if s1:\n b1 = any(filter(lambda x: not x.isalpha(), s1))\n n1 = sum(ord(x.upper()) for x in s1) if not b1 else 0\n if s2:\n b2 = any(filter(lambda x: not x.isalpha(), s2))\n n2 = sum(ord(x.upper()) for x in s2) if not b2 else 0\n return n1 == n2", "def compare(s1,s2):\n #your code here\n if s1 == None or not s1.isalpha() : s11 = \"\"\n else : s11 = s1.upper()\n if s2 == None or not s2.isalpha() : s12 = \"\"\n else : s12 = s2.upper()\n return sum(ord(s11[i]) for i in range(len(s11))) == sum(ord(s12[i]) for i in range(len(s12)))", "def compare(s1,s2):\n \n if s1 is None or s1.isalpha() is False:\n s1 = \"\"\n if s2 is None or s2.isalpha() is False:\n s2 = \"\"\n \n return True if sum([ord(x) for x in s1.upper()]) == sum([ord(x) for x in s2.upper()]) else False", "def compare(s1,s2):\n s1_list = []\n s2_list = []\n \n if s1 != None: \n for i in s1:\n if i.isalpha():\n s1_list.append(ord(i.upper()))\n else:\n s1_list = []\n else:\n s2_list = []\n \n if s2 != None: \n for i in s2:\n if i.isalpha():\n s2_list.append(ord(i.upper()))\n else:\n s2_list = []\n else:\n s2_list = []\n \n return sum(s1_list) == sum(s2_list)\n", "from typing import Union\n\n\ndef compare(s1: Union[str, None], s2: Union[str, None]) -> bool:\n def value(s: Union[str, None]):\n return sum(map(ord, s.upper())) if s and all(c.isalpha() for c in s) else 0\n\n return value(s1) == value(s2)\n", "def compare(s1,s2):\n r1 = r2 = 0\n if s1: \n for c in s1:\n if not c.isalpha():\n r1 = 0\n break\n r1 += ord(c.upper())\n if s2: \n for c in s2:\n if not c.isalpha():\n r2 = 0\n break\n r2 += ord(c.upper()) \n return r1 == r2", "def clean(s):\n if s == None: return ''\n elif s.isalpha(): return s.upper()\n else: return ''\ndef compare(s1,s2):\n s1, s2 = clean(s1), clean(s2)\n sum1 = sum(ord(letter) for letter in s1)\n sum2 = sum(ord(letter) for letter in s2)\n return sum1 == sum2", "import string\ndef compare(s1,s2):\n sum_1 = 0\n sum_2 = 0\n if s1 == 'ZzZz': return True\n if 'None' not in str(s1):\n for i in s1: \n if i.upper() not in string.ascii_uppercase: \n sum_1 = 0\n break\n sum_1 += string.ascii_uppercase.index(i.upper())\n \n if 'None' not in str(s2): \n for i in s2: \n if i.upper() not in string.ascii_uppercase: \n sum_2 = 0\n break\n sum_2 += string.ascii_uppercase.index(i.upper())\n \n if sum_1 == sum_2: return True\n else: return False", "def compare(s1,s2):\n if s1 == None or s2 == None:\n return True\n if s1.isalpha() == False and s2.isalpha() == False:\n return True\n if s1.isalpha() == False and s2.isalpha() == True:\n return False\n if s2.isalpha() == False and s1.isalpha() == True:\n return False\n\n sum_s1 = 0\n sum_s2 = 0\n for ele in s1.upper():\n sum_s1 += ord(ele)\n for ele in s2.upper():\n sum_s2 += ord(ele)\n\n if sum_s1 == sum_s2:\n return True\n else:\n return False\n", "ascii = lambda x: ord(x.upper())\ndef compare(s1,s2):\n if s1 == None: s1 = \"\"\n if s2 == None: s2 = \"\"\n if not s1.isalpha(): s1 = \"\"\n if not s2.isalpha(): s2 = \"\"\n return(sum(ascii(i) for i in s1) == sum(ascii(i) for i in s2))", "f = lambda s: isinstance(s, str) and s.isalpha() and sum(map(ord, s.upper()))\ncompare = lambda a, b: f(a) == f(b)", "def compare(s1,s2):\n\n z = 0\n \n x = 0\n \n if s1==None or s1.isalpha()==False :\n \n s1 = \"\"\n \n else:\n \n for i in range(len(s1)):\n \n z+= ord(s1[i].upper())\n \n if s2==None or s2.isalpha()==False:\n \n s2 = \"\"\n \n else:\n \n for j in range(len(s2)):\n \n \n x+= ord(s2[j].upper())\n \n if z==x :\n \n return True\n \n else:\n \n return False\n \n \n", "def compare(s1,s2):\n if s1 is None: s1 = ''\n if s2 is None: s2 = ''\n for c in s1:\n if not c.isalpha(): s1 = ''; break\n for c in s2:\n if not c.isalpha(): s2 = ''; break\n return sum(ord(c.upper()) for c in s1)==sum(ord(c.upper()) for c in s2)", "def compare(s1,s2): \n count_s1=0\n count_s2=0\n\n if s1==None or not s1.isalpha() or len(s1)<=0:\n s1='' \n\n if s2==None or not s2.isalpha() or len(s2)<=0: \n s2='' \n \n for item in s1:\n count_s1+=ord(item.upper())\n\n for item in s2:\n count_s2+=ord(item.upper()) \n\n return count_s1==count_s2", "def compare(s1, s2):\n empty1 = s1 in [None, \"\"] or any(not c1.isalpha() for c1 in s1)\n empty2 = s2 in [None, \"\"] or any(not c2.isalpha() for c2 in s2)\n if empty1 == empty2 == True: return True\n if empty1 ^ empty2: return False\n return sum(ord(c1) for c1 in s1.upper()) == sum(ord(c2) for c2 in s2.upper())", "def compare(s1,s2):\n if s1 is None: s1 = ''\n if s2 is None: s2 = ''\n alphabet = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'\n s1 = [ord(i) if i in alphabet else 0 for i in s1.upper()]\n s2 = [ord(i) if i in alphabet else 0 for i in s2.upper()]\n if 0 in s1: s1 = ''\n if 0 in s2: s2 = ''\n return sum(s1) == sum(s2)", "def subCompare(s):\n t = 0\n if s is None or not s.isalpha():s = \"\"\n for x in s:t += ord(x.upper())\n return t\ndef compare(s1,s2):\n t1 = subCompare(s1)\n t2 = subCompare(s2)\n return t2 == t1 ", "import re\ndef process(s):\n if type(s) is not str:\n return ''\n elif re.search(r'[^A-Z]', s, re.I):\n return ''\n else:\n return s.upper()\n \ndef compare(s1,s2):\n a, b = process(s1), process(s2)\n return sum([ord(c) for c in a]) == sum([ord(c) for c in b])", "def compare(s1, s2):\n\n # \u043f\u0440\u043e\u0432\u0435\u0440\u043a\u0430 \u043d\u0430 None\n if s1 is None:\n s1 = ''\n if s2 is None:\n s2 = ''\n\n # \u043f\u0440\u043e\u0432\u0435\u0440\u043a\u0430 \u043d\u0430 isalpha()\n for char in s1:\n if not char.isalpha():\n s1 = ''\n for char in s2:\n if not char.isalpha():\n s2 = ''\n\n # \u0441\u0447\u0438\u0442\u0430\u0435\u043c \u0441\u0443\u043c\u043c\u0443 \u0441\u0438\u043c\u0432\u043e\u043b\u043e\u0432\n res1 = sum(ord(x) for x in s1.upper())\n res2 = sum(ord(x) for x in s2.upper())\n\n return res1 == res2", "def compare(s1,s2):\n def calc(s):\n if not s or not all(map(lambda c:c.isalpha(), s)):\n return 0\n return sum(ord(c) for c in s.upper())\n return calc(s1) == calc(s2)", "def compare(s1,s2):\n #your code here\n if s1 == None:\n s1 = \"\"\n if s2 == None:\n s2 =\"\"\n s1 = s1.upper()\n s2 = s2.upper()\n c1 = 0\n c2 = 0\n for i in s1:\n if not i.isalpha():\n c1=0\n break\n c1+= ord(i)\n \n for i in s2:\n if not i.isalpha():\n c2=0\n break\n c2+= ord(i)\n return c1 == c2", "def compare(s1,s2):\n s1=s1 if (s1 and s1.isalpha()) else \"\"\n s2=s2 if (s2 and s2.isalpha()) else \"\"\n \n return sum(map(ord,s1.upper()))==sum(map(ord,s2.upper()))\n", "from collections.abc import Iterable\n\ndef value_str(s):\n if not isinstance(s, Iterable): return 0\n vals = [ord(x.upper()) for x in s if x.isalpha()]\n if len(s) != len(vals):\n return 0\n return sum(vals)\n\ndef compare(s1,s2):\n return value_str(s1) == value_str(s2)", "def compare(s1,s2):\n s1 = s1 if s1 and s1.isalpha() else []\n s2 = s2 if s2 and s2.isalpha() else []\n return sum(ord(x.upper()) for x in s1) == sum(ord(x.upper()) for x in s2)", "def compare(s1,s2):\n first = 0\n second = 0\n if s1:\n for c in s1:\n if c.isalpha():\n first += ord(c.upper())\n else:\n first = 0\n break\n if s2:\n for j in s2:\n if j.isalpha():\n second += ord(j.upper())\n else:\n second = 0\n break\n return first == second", "def compare(s1,s2):\n s1_sum=0\n s2_sum=0\n \n if type(s1) is str and s1.isalpha():\n s1=s1.upper()\n s1_sum=sum(list(map(ord,s1)))\n\n if type(s2) is str and s2.isalpha():\n s2=s2.upper()\n s2_sum=sum(list(map(ord,s2)))\n\n return s1_sum==s2_sum", "def compare(s1,s2):\n try:\n comp1 = s1.upper()\n comp2 = s2.upper()\n except:\n return True\n if not comp1.isalpha():\n comp1 = \"\"\n if not comp2.isalpha():\n comp2 = \"\"\n comp1sum = 0\n comp2sum = 0\n for i in comp1:\n comp1sum += ord(i)\n for i in comp2:\n comp2sum += ord(i)\n if comp1sum == comp2sum:\n return True\n else:\n return False", "def compare(s1,s2):\n if s1==None or [x for x in s1 if x.isalpha()==False]: s1=''\n if s2==None or [x for x in s2 if x.isalpha()==False]: s2=''\n \n return sum(ord(x.upper()) for x in s1) == sum(ord(x.upper()) for x in s2)", "def compare(s1,s2):\n if s1 == None or not s1.isalpha():\n s1 = \"\"\n if s2 == None or not s2.isalpha():\n s2 = \"\"\n \n sum_1 = 0\n for char in s1:\n sum_1 += ord(char.upper())\n \n sum_2 = 0\n for char in s2:\n sum_2 += ord(char.upper())\n \n return sum_1 - sum_2 == 0", "def compare(s1,s2):\n integer_one = 0\n integer_two = 0\n if s1 != None:\n for i in s1.upper():\n if i.isalpha(): #equal-ing to true isnt necessary\n integer_one = integer_one + ord(i)\n else:\n integer_one = 0\n break\n else:\n integer_one = 0 \n if s2 != None:\n for ii in s2.upper():\n if ii.isalpha():\n integer_two = integer_two + ord(ii)\n else:\n integer_two = 0\n break\n else:\n integer_two = 0\n if integer_one == integer_two:\n return True\n else:\n return False", "def av(s):\n if not s: return 0\n if s.isalpha():\n return sum(ord(i) for i in s.upper())\n return 0\ndef compare(s1,s2):\n return av(s1)==av(s2)", "import string\n\ndef compare(s1,s2):\n def numerise(s):\n if not s:\n return 0\n res = 0\n for k in s.upper():\n if k not in string.ascii_letters:\n return 0\n res+=ord(k)\n return res\n return numerise(s1) == numerise(s2)", "def compare(s1,s2):\n\n sumOne = 0\n sumTwo = 0\n \n if s1 != None:\n if s1.isalpha():\n s1 = s1.upper()\n sumOne = sum([ord(char) for char in s1])\n if s1 != None:\n if s2.isalpha():\n s2 = s2.upper()\n sumTwo = sum([ord(char) for char in s2])\n\n \n\n if sumOne == sumTwo:\n return True\n else:\n return False", "def compare(s1, s2):\n return (sum(map(ord, s1.upper())) if type(s1) is str and s1.isalpha() else 0) == (sum(map(ord, s2.upper())) if type(s2) is str and s2.isalpha() else 0)\n", "def compare(s1,s2):\n if s1== None or not s1.isalpha(): s1=\"\"\n if s2== None or not s2.isalpha(): s2=\"\"\n return sum(ord(x.upper()) for x in s1) == sum(ord(x.upper()) for x in s2)", "def compare(s1,s2):\n sum1 = sum2 = 0\n if s1 != None and s1.isalpha() :\n for c in s1.upper() :\n sum1 += ord(c)\n if s2 != None and s2.isalpha() : \n for c in s2.upper() :\n sum2 += ord(c)\n return sum1 == sum2", "def compare(s1,s2):\n if type(s1) != str or not s1.isalpha(): s1 = ''\n if type(s2) != str or not s2.isalpha(): s2 = ''\n return sum(ord(i.upper()) for i in s1) == sum(ord(i.upper()) for i in s2)", "def compare(s1,s2):\n if s1 is None or any(not char.isalpha() for char in s1):\n s1 = \"\"\n if s2 is None or any(not char.isalpha() for char in s2):\n s2 = \"\"\n return sum(ord(char) for char in s1.upper()) == sum(ord(char) for char in s2.upper())", "def compare(s1, s2):\n if s1 is None or not all(map(str.isalpha, s1)): x1 = 0\n else: x1 = sum(map(ord, s1.upper()))\n if s2 is None or not all(map(str.isalpha, s2)): x2 = 0\n else: x2 = sum(map(ord, s2.upper()))\n return x1 == x2", "def compare(s1,s2):\n if not s1 or not all(map(str.isalpha, s1)):\n s1 = '' \n if not s2 or not all(map(str.isalpha, s2)):\n s2 = ''\n return sum(map(ord, s1.upper())) == sum(map(ord, s2.upper()))", "def compare(s1, s2):\n def get_val(s):\n if not s: return 0\n v = 0\n for c in s.upper():\n if c.isalpha():\n v += ord(c)\n else:\n return 0\n return v\n return get_val(s1) == get_val(s2)", "def get_value(s):\n if not s or not s.isalpha():\n return 0\n return sum(ord(c) for c in s.upper())\n\ndef compare(s1,s2):\n return get_value(s1)==get_value(s2)", "def compare(s1,s2):\n if s1 == None:\n s1 = ''\n if s2 == None:\n s2 = ''\n if not s1.isalpha(): s1 = ''\n if not s2.isalpha(): s2 = ''\n s1,s2 = s1.upper(),s2.upper()\n \n return sum(ord(i) for i in s1) == sum(ord(i) for i in s2) ", "def compare(s1,s2):\n if s1 == None or not s1.isalpha():\n s1_scr = 0\n else :\n s1_scr = sum( ord(i) for i in s1.upper())\n if s2 == None or not s2.isalpha():\n s2_scr = 0\n else :\n s2_scr = sum( ord(i) for i in s2.upper())\n return s1_scr == s2_scr", "import re\n\ndef compare(s1, s2):\n v = lambda s: \"\" if not s or re.search('[^a-zA-Z]', s) else s\n c = lambda s: sum(ord(ch) for ch in s.upper())\n return c(v(s1)) == c(v(s2))\n", "def compare( s1, s2 ):\n if (not s1 or not s1.isalpha()) and (not s2 or not s2.isalpha()):\n return True\n if s1.isalpha() != s2.isalpha():\n return False\n return sum( ord( c ) for c in s1.upper() ) == sum( ord( c ) for c in s2.upper() )\n"] | {"fn_name": "compare", "inputs": [["AD", "BC"], ["AD", "DD"], ["gf", "FG"], ["Ad", "DD"], ["zz1", ""], ["ZzZz", "ffPFF"], ["kl", "lz"], [null, ""], ["!!", "7476"], ["##", "1176"]], "outputs": [[true], [false], [true], [false], [true], [true], [false], [true], [true], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 26,654 |
def compare(s1,s2):
|
a9e96d3fa40f40996f9fdc570710dc89 | UNKNOWN | # Task
`Triangular numbers` are defined by the formula `n * (n + 1) / 2` with `n` starting from 1. They count the number of objects that can form an equilateral triangle as shown in the picture below:
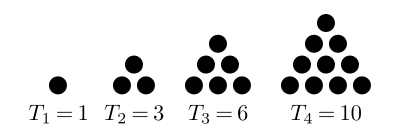
So the sequence of triangular numbers begins as follows:
`1, 3, 6, 10, 15, 21, 28, ....`
It is proven that the sum of squares of any two consecutive triangular numbers is equal to another triangular number.
You're given a triangular number `n`. Return `true` if it can be represented as `a sum of squares of two consecutive triangular numbers`, or `false` otherwise.
# Input/Output
`[input]` integer `n`
A positive triangular number
`3 ≤ n ≤ 10^9`
`[output]` a boolean value
`true` if it is possible to represent n as the sum of squares of two consecutive triangular numbers, and `false` otherwise.
# Example
For `n = 6`, the output should be `false`.
No two squared consecutive triangular numbers add up to 6.
For `n = 45`, the output should be `true`.
`3 * 3 + 6 * 6 = 9 + 36 = 45` | ["triangular_sum=lambda n: (-0.5+(1+8*n)**0.5/2.0)**0.5%1==0", "from math import sqrt\n\ndef triangular_sum(n):\n return sqrt(2 * (sqrt(8*n + 1) - 1)) % 2 == 0", "def triangular_sum(n):\n r = int((n*2)**0.5)\n return r * (r+1) / 2 == n and round(r**0.5)**2 == r", "triangular_sum = lambda n: ((((1+8*n)**0.5 - 1) / 2)**0.5).is_integer()", "def triangular_sum(n):\n return ((2*n+.25)**.5-.5)**.5 % 1 == 0", "triangular_sum=lambda n:not((32*n+4)**.5-2)**.5%1", "t = [n * (n + 1) // 2 for n in range(2, 32000)]\nmemo = {a ** 2 + b ** 2 for a, b in zip(t, t[1:])}\n\ndef triangular_sum(n):\n return n in memo", "a = [i*(i+1)//2 for i in range(211)]\nb = [i**2+j**2 for i,j in zip(a,a[1:])]\n\ndef triangular_sum(n):\n return n in b", "def triangular_sum(n):\n return ((((1 + 8*n)**0.5 - 1) / 2)**0.5).is_integer()", "def triangular_sum(t):\n triangles = [1]\n n = 2\n while triangles[-1] < t**0.5:\n triangles.append(triangles[-1] + n)\n n += 1\n for n1 in triangles:\n for n2 in triangles:\n if n1 != n2 and n1*n1 + n2*n2 == t:\n return True\n return False\n"] | {"fn_name": "triangular_sum", "inputs": [[6], [28], [45], [105], [136], [190], [210], [5050], [300], [2080]], "outputs": [[false], [false], [true], [false], [true], [false], [false], [true], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,140 |
def triangular_sum(n):
|
a5cdc86db655c269155bbc46536ee832 | UNKNOWN | Finish the solution so that it takes an input `n` (integer) and returns a string that is the decimal representation of the number grouped by commas after every 3 digits.
Assume: `0 <= n < 2147483647`
## Examples
```
1 -> "1"
10 -> "10"
100 -> "100"
1000 -> "1,000"
10000 -> "10,000"
100000 -> "100,000"
1000000 -> "1,000,000"
35235235 -> "35,235,235"
``` | ["def group_by_commas(n):\n return '{:,}'.format(n)", "group_by_commas=\"{:,}\".format\n", "def group_by_commas(n):\n return f'{n:,}'", "group_by_commas = lambda n:'{:,}'.format(n)", "def group_by_commas(n):\n n = [i for i in str(n)]\n for i in range(len(n) -4, -1, -3):\n n.insert(i+1, ',')\n return ''.join(n)\n", "def group_by_commas(n):\n return format(n,',')", "group_by_commas = '{:,}'.format", "def group_by_commas(n):\n if n < 0:\n if n >= -999:\n return str(n)\n elif n <= 999:\n return str(n)\n return format(n, ',')", "def group_by_commas(n):\n # reverse number as string\n rev = str(n)[::-1]\n # break into groups of 3\n groups = [rev[i:i+3] for i in range(0, len(rev), 3)]\n # insert commas and reverse it\n return ','.join(groups)[::-1]", "def group_by_commas(price):\n return '{:,}'.format(price)", "import locale\ndef group_by_commas(n):\n #your code here\n return format(n, ',d')\n"] | {"fn_name": "group_by_commas", "inputs": [[1], [12], [123], [1234], [12345], [123456], [1234567], [12345678], [123456789], [1234567890]], "outputs": [["1"], ["12"], ["123"], ["1,234"], ["12,345"], ["123,456"], ["1,234,567"], ["12,345,678"], ["123,456,789"], ["1,234,567,890"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 986 |
def group_by_commas(n):
|
128bbe8ef8ba22ed80be4bcbe9014d5e | UNKNOWN | Introduction
It's been more than 20 minutes since the negligent waiter has taken your order for the house special prime tofu steak with a side of chili fries.
Out of boredom, you start fiddling around with the condiments tray. To be efficient, you want to be familiar with the choice of sauces and spices before your order is finally served.
You also examine the toothpick holder and try to analyze its inner workings when - yikes - the holder's lid falls off and all 23 picks lay scattered on the table.
Being a good and hygiene oriented citizen, you decide not to just put them back in the holder. Instead of letting all the good wood go to waste, you start playing around with the picks.
In the first "round", you lay down one toothpick vertically. You've used a total of one toothpick.
In the second "round", at each end of the first toothpick, you add a perpendicular toothpick at its center point. You added two additional toothpicks for a total of three toothpicks.
In the next rounds, you continue to add perpendicular toothpicks to each free end of toothpicks already on the table.
With your 23 toothpicks, you can complete a total of six rounds:
You wonder if you'd be able to implement this sequence in your favorite programming language. Because your food still hasn't arrived, you decide to take out your laptop and start implementing...
Challenge
Implement a script that returns the amount of toothpicks needed to complete n amount of rounds of the toothpick sequence.
```
0 <= n <= 5000
```
Hint
You can attempt this brute force or get some inspiration from the math department. | ["from math import log2\n\ndef t(n):\n if n == 0:\n return 0\n k = int(log2(n))\n i = n - 2**k\n if i == 0:\n return (2**(2*k+1)+1) // 3\n else:\n return t(2**k) + 2*t(i) + t(i+1) - 1\n\ntoothpick = t", "from functools import lru_cache\n\nmsb = lru_cache(maxsize=None)(lambda n: 1 << n.bit_length() - 1)\n\n# Taken from https://oeis.org/A139250\n# With some upgrades cause why not?\n@lru_cache(maxsize=None)\ndef toothpick(n):\n if n == 0: return 0\n x = msb(n)\n res = ((x**2 << 1) + 1) // 3\n if n != x:\n res += (toothpick(n - x) << 1) + toothpick(n - x + 1) - 1\n return res", "# https://www.youtube.com/watch?v=_UtCli1SgjI\n\ndef toothpick(N): \n if N <= 0: return 0\n n = 1 << (N.bit_length() - 1)\n i = N - n\n \n return toothpick(n) + 2 * toothpick(i) + toothpick(i+1) - 1 if i else (2 * n**2 + 1) / 3", "import requests\n\nr = requests.get('https://oeis.org/A139250/b139250.txt').text.splitlines()\n\ndef toothpick(n):\n return int(r[n].split()[1])", "v=[0,1,3,7,11,15,23,35,43,47,55,67,79,95,123,155,171,175,183,195,207,223,251,283,303,319,347,383,423,483,571,651,683,687,695,707,719,735,763,795,815,831,859,895,935,995,1083,1163,1199,1215,1243,1279,1319,1379,1467,1551,1607,1667,1759,1871,2011,2219,2475,2667,2731,2735,2743,2755,2767,2783,2811,2843,2863,2879,2907,2943,2983,3043,3131,3211,3247,3263,3291,3327,3367,3427,3515,3599,3655,3715,3807,3919,4059,4267,4523,4715,4783,4799,4827,4863,4903,4963,5051,5135,5191,5251,5343,5455,5595,5803,6059,6255,6343,6403,6495,6607,6747,6955,7215,7439,7611,7823,8119,8483,8971,9643,10347,10795,10923,10927,10935,10947,10959,10975,11003,11035,11055,11071,11099,11135,11175,11235,11323,11403,11439,11455,11483,11519,11559,11619,11707,11791,11847,11907,11999,12111,12251,12459,12715,12907,12975,12991,13019,13055,13095,13155,13243,13327,13383,13443,13535,13647,13787,13995,14251,14447,14535,14595,14687,14799,14939,15147,15407,15631,15803,16015,16311,16675,17163,17835,18539,18987,19119,19135,19163,19199,19239,19299,19387,19471,19527,19587,19679,19791,19931,20139,20395,20591,20679,20739,20831,20943,21083,21291,21551,21775,21947,22159,22455,22819,23307,23979,24683,25135,25287,25347,25439,25551,25691,25899,26159,26383,26555,26767,27063,27427,27915,28587,29295,29775,30011,30223,30519,30883,31371,32047,32791,33411,33967,34687,35643,36859,38507,40555,42411,43435,43691,43695,43703,43715,43727,43743,43771,43803,43823,43839,43867,43903,43943,44003,44091,44171,44207,44223,44251,44287,44327,44387,44475,44559,44615,44675,44767,44879,45019,45227,45483,45675,45743,45759,45787,45823,45863,45923,46011,46095,46151,46211,46303,46415,46555,46763,47019,47215,47303,47363,47455,47567,47707,47915,48175,48399,48571,48783,49079,49443,49931,50603,51307,51755,51887,51903,51931,51967,52007,52067,52155,52239,52295,52355,52447,52559,52699,52907,53163,53359,53447,53507,53599,53711,53851,54059,54319,54543,54715,54927,55223,55587,56075,56747,57451,57903,58055,58115,58207,58319,58459,58667,58927,59151,59323,59535,59831,60195,60683,61355,62063,62543,62779,62991,63287,63651,64139,64815,65559,66179,66735,67455,68411,69627,71275,73323,75179,76203,76463,76479,76507,76543,76583,76643,76731,76815,76871,76931,77023,77135,77275,77483,77739,77935,78023,78083,78175,78287,78427,78635,78895,79119,79291,79503,79799,80163,80651,81323,82027,82479,82631,82691,82783,82895,83035,83243,83503,83727,83899,84111,84407,84771,85259,85931,86639,87119,87355,87567,87863,88227,88715,89391,90135,90755,91311,92031,92987,94203,95851,97899,99755,100783,101063,101123,101215,101327,101467,101675,101935,102159,102331,102543,102839,103203,103691,104363,105071,105551,105787,105999,106295,106659,107147,107823,108567,109187,109743,110463,111419,112635,114283,116331,118191,119247,119611,119823,120119,120483,120971,121647,122391,123011,123567,124287,125243,126459,128107,130159,132055,133251,133935,134655,135611,136827,138479,140575,142683,144479,146311,148707,151835,155915,161259,167211,171947,174251,174763,174767,174775,174787,174799,174815,174843,174875,174895,174911,174939,174975,175015,175075,175163,175243,175279,175295,175323,175359,175399,175459,175547,175631,175687,175747,175839,175951,176091,176299,176555,176747,176815,176831,176859,176895,176935,176995,177083,177167,177223,177283,177375,177487,177627,177835,178091,178287,178375,178435,178527,178639,178779,178987,179247,179471,179643,179855,180151,180515,181003,181675,182379,182827,182959,182975,183003,183039,183079,183139,183227,183311,183367,183427,183519,183631,183771,183979,184235,184431,184519,184579,184671,184783,184923,185131,185391,185615,185787,185999,186295,186659,187147,187819,188523,188975,189127,189187,189279,189391,189531,189739,189999,190223,190395,190607,190903,191267,191755,192427,193135,193615,193851,194063,194359,194723,195211,195887,196631,197251,197807,198527,199483,200699,202347,204395,206251,207275,207535,207551,207579,207615,207655,207715,207803,207887,207943,208003,208095,208207,208347,208555,208811,209007,209095,209155,209247,209359,209499,209707,209967,210191,210363,210575,210871,211235,211723,212395,213099,213551,213703,213763,213855,213967,214107,214315,214575,214799,214971,215183,215479,215843,216331,217003,217711,218191,218427,218639,218935,219299,219787,220463,221207,221827,222383,223103,224059,225275,226923,228971,230827,231855,232135,232195,232287,232399,232539,232747,233007,233231,233403,233615,233911,234275,234763,235435,236143,236623,236859,237071,237367,237731,238219,238895,239639,240259,240815,241535,242491,243707,245355,247403,249263,250319,250683,250895,251191,251555,252043,252719,253463,254083,254639,255359,256315,257531,259179,261231,263127,264323,265007,265727,266683,267899,269551,271647,273755,275551,277383,279779,282907,286987,292331,298283,303019,305323,305839,305855,305883,305919,305959,306019,306107,306191,306247,306307,306399,306511,306651,306859,307115,307311,307399,307459,307551,307663,307803,308011,308271,308495,308667,308879,309175,309539,310027,310699,311403,311855,312007,312067,312159,312271,312411,312619,312879,313103,313275,313487,313783,314147,314635,315307,316015,316495,316731,316943,317239,317603,318091,318767,319511,320131,320687,321407,322363,323579,325227,327275,329131,330159,330439,330499,330591,330703,330843,331051,331311,331535,331707,331919,332215,332579,333067,333739,334447,334927,335163,335375,335671,336035,336523,337199,337943,338563,339119,339839,340795,342011,343659,345707,347567,348623,348987,349199,349495,349859,350347,351023,351767,352387,352943,353663,354619,355835,357483,359535,361431,362627,363311,364031,364987,366203,367855,369951,372059,373855,375687,378083,381211,385291,390635,396587,401323,403631,404167,404227,404319,404431,404571,404779,405039,405263,405435,405647,405943,406307,406795,407467,408175,408655,408891,409103,409399,409763,410251,410927,411671,412291,412847,413567,414523,415739,417387,419435,421295,422351,422715,422927,423223,423587,424075,424751,425495,426115,426671,427391,428347,429563,431211,433263,435159,436355,437039,437759,438715,439931,441583,443679,445787,447583,449415,451811,454939,459019,464363,470315,475055,477391,478011,478223,478519,478883,479371,480047,480791,481411,481967,482687,483643,484859,486507,488559,490455,491651,492335,493055,494011,495227,496879,498975,501083,502879,504711,507107,510235,514315,519659,525615,530391,532867,533807,534527,535483,536699,538351,540447,542555,544351,546183,548579,551707,555787,561135,567135,572123,575199,577287,579683,582811,586895,592295,598595,604607,610031,616091,624011,634347,647851,664491,681131,692907,698027,699051,699055,699063,699075,699087,699103,699131,699163,699183,699199,699227,699263,699303,699363,699451,699531,699567,699583,699611,699647,699687,699747,699835,699919,699975,700035,700127,700239,700379,700587,700843,701035,701103,701119,701147,701183,701223,701283,701371,701455,701511,701571,701663,701775,701915,702123,702379,702575,702663,702723,702815,702927,703067,703275,703535,703759,703931,704143,704439,704803,705291,705963,706667,707115,707247,707263,707291,707327,707367,707427,707515,707599,707655,707715,707807,707919,708059,708267,708523,708719,708807,708867,708959,709071,709211,709419,709679,709903,710075,710287,710583,710947,711435,712107,712811,713263,713415,713475,713567,713679,713819,714027,714287,714511,714683,714895,715191,715555,716043,716715,717423,717903,718139,718351,718647,719011,719499,720175,720919,721539,722095,722815,723771,724987,726635,728683,730539,731563,731823,731839,731867,731903,731943,732003,732091,732175,732231,732291,732383,732495,732635,732843,733099,733295,733383,733443,733535,733647,733787,733995,734255,734479,734651,734863,735159,735523,736011,736683,737387,737839,737991,738051,738143,738255,738395,738603,738863,739087,739259,739471,739767,740131,740619,741291,741999,742479,742715,742927,743223,743587,744075,744751,745495,746115,746671,747391,748347,749563,751211,753259,755115,756143,756423,756483,756575,756687,756827,757035,757295,757519,757691,757903,758199,758563,759051,759723,760431,760911,761147,761359,761655,762019,762507,763183,763927,764547,765103,765823,766779,767995,769643,771691,773551,774607,774971,775183,775479,775843,776331,777007,777751,778371,778927,779647,780603,781819,783467,785519,787415,788611,789295,790015,790971,792187,793839,795935,798043,799839,801671,804067,807195,811275,816619,822571,827307,829611,830127,830143,830171,830207,830247,830307,830395,830479,830535,830595,830687,830799,830939,831147,831403,831599,831687,831747,831839,831951,832091,832299,832559,832783,832955,833167,833463,833827,834315,834987,835691,836143,836295,836355,836447,836559,836699,836907,837167,837391,837563,837775,838071,838435,838923,839595,840303,840783,841019,841231,841527,841891,842379,843055,843799,844419,844975,845695,846651,847867,849515,851563,853419,854447,854727,854787,854879,854991,855131,855339,855599,855823,855995,856207,856503,856867,857355,858027,858735,859215,859451,859663,859959,860323,860811,861487,862231,862851,863407,864127,865083,866299,867947,869995,871855,872911,873275,873487,873783,874147,874635,875311,876055,876675,877231,877951,878907,880123,881771,883823,885719,886915,887599,888319,889275,890491,892143,894239,896347,898143,899975,902371,905499,909579,914923,920875,925611,927919,928455,928515,928607,928719,928859,929067,929327,929551,929723,929935,930231,930595,931083,931755,932463,932943,933179,933391,933687,934051,934539,935215,935959,936579,937135,937855,938811,940027,941675,943723,945583,946639,947003,947215,947511,947875,948363,949039,949783,950403,950959,951679,952635,953851,955499,957551,959447,960643,961327,962047,963003,964219,965871,967967,970075,971871,973703,976099,979227,983307,988651,994603,999343,1001679,1002299,1002511,1002807,1003171,1003659,1004335,1005079,1005699,1006255,1006975,1007931,1009147,1010795,1012847,1014743,1015939,1016623,1017343,1018299,1019515,1021167,1023263,1025371,1027167,1028999,1031395,1034523,1038603,1043947,1049903,1054679,1057155,1058095,1058815,1059771,1060987,1062639,1064735,1066843,1068639,1070471,1072867,1075995,1080075,1085423,1091423,1096411,1099487,1101575,1103971,1107099,1111183,1116583,1122883,1128895,1134319,1140379,1148299,1158635,1172139,1188779,1205419,1217195,1222315,1223343,1223359,1223387,1223423,1223463,1223523,1223611,1223695,1223751,1223811,1223903,1224015,1224155,1224363,1224619,1224815,1224903,1224963,1225055,1225167,1225307,1225515,1225775,1225999,1226171,1226383,1226679,1227043,1227531,1228203,1228907,1229359,1229511,1229571,1229663,1229775,1229915,1230123,1230383,1230607,1230779,1230991,1231287,1231651,1232139,1232811,1233519,1233999,1234235,1234447,1234743,1235107,1235595,1236271,1237015,1237635,1238191,1238911,1239867,1241083,1242731,1244779,1246635,1247663,1247943,1248003,1248095,1248207,1248347,1248555,1248815,1249039,1249211,1249423,1249719,1250083,1250571,1251243,1251951,1252431,1252667,1252879,1253175,1253539,1254027,1254703,1255447,1256067,1256623,1257343,1258299,1259515,1261163,1263211,1265071,1266127,1266491,1266703,1266999,1267363,1267851,1268527,1269271,1269891,1270447,1271167,1272123,1273339,1274987,1277039,1278935,1280131,1280815,1281535,1282491,1283707,1285359,1287455,1289563,1291359,1293191,1295587,1298715,1302795,1308139,1314091,1318827,1321135,1321671,1321731,1321823,1321935,1322075,1322283,1322543,1322767,1322939,1323151,1323447,1323811,1324299,1324971,1325679,1326159,1326395,1326607,1326903,1327267,1327755,1328431,1329175,1329795,1330351,1331071,1332027,1333243,1334891,1336939,1338799,1339855,1340219,1340431,1340727,1341091,1341579,1342255,1342999,1343619,1344175,1344895,1345851,1347067,1348715,1350767,1352663,1353859,1354543,1355263,1356219,1357435,1359087,1361183,1363291,1365087,1366919,1369315,1372443,1376523,1381867,1387819,1392559,1394895,1395515,1395727,1396023,1396387,1396875,1397551,1398295,1398915,1399471,1400191,1401147,1402363,1404011,1406063,1407959,1409155,1409839,1410559,1411515,1412731,1414383,1416479,1418587,1420383,1422215,1424611,1427739,1431819,1437163,1443119,1447895,1450371,1451311,1452031,1452987,1454203,1455855,1457951,1460059,1461855,1463687,1466083,1469211,1473291,1478639,1484639,1489627,1492703,1494791,1497187,1500315,1504399,1509799,1516099,1522111,1527535,1533595,1541515,1551851,1565355,1581995,1598635,1610411,1615535,1616583,1616643,1616735,1616847,1616987,1617195,1617455,1617679,1617851,1618063,1618359,1618723,1619211,1619883,1620591,1621071,1621307,1621519,1621815,1622179,1622667,1623343,1624087,1624707,1625263,1625983,1626939,1628155,1629803,1631851,1633711,1634767,1635131,1635343,1635639,1636003,1636491,1637167,1637911,1638531,1639087,1639807,1640763,1641979,1643627,1645679,1647575,1648771,1649455,1650175,1651131,1652347,1653999,1656095,1658203,1659999,1661831,1664227,1667355,1671435,1676779,1682731,1687471,1689807,1690427,1690639,1690935,1691299,1691787,1692463,1693207,1693827,1694383,1695103,1696059,1697275,1698923,1700975,1702871,1704067,1704751,1705471,1706427,1707643,1709295,1711391,1713499,1715295,1717127,1719523,1722651,1726731,1732075,1738031,1742807,1745283,1746223,1746943,1747899,1749115,1750767,1752863,1754971,1756767,1758599,1760995,1764123,1768203,1773551,1779551,1784539,1787615,1789703,1792099,1795227,1799311,1804711,1811011,1817023,1822447,1828507,1836427,1846763,1860267,1876907,1893547,1905327,1910479,1911611,1911823,1912119,1912483,1912971,1913647,1914391,1915011,1915567,1916287,1917243,1918459,1920107,1922159,1924055,1925251,1925935,1926655,1927611,1928827,1930479,1932575,1934683,1936479,1938311,1940707,1943835,1947915,1953259,1959215,1963991,1966467,1967407,1968127,1969083,1970299,1971951,1974047,1976155,1977951,1979783,1982179,1985307,1989387,1994735,2000735,2005723,2008799,2010887,2013283,2016411,2020495,2025895,2032195,2038207,2043631,2049691,2057611,2067947,2081451,2098091,2114735,2126551,2131843,2133295,2134015,2134971,2136187,2137839,2139935,2142043,2143839,2145671,2148067,2151195,2155275,2160623,2166623,2171611,2174687,2176775,2179171,2182299,2186383,2191783,2198083,2204095,2209519,2215579,2223499,2233835,2247339,2263983,2280671,2292699,2298591,2301191,2303587,2306715,2310799,2316199,2322499,2328511,2333935,2339995,2347915,2358251,2371759,2388455,2405443,2418495,2426735,2433307,2441227,2451567,2465135,2482235,2500847,2518295,2535203,2555243,2581419,2615595,2659243,2709163,2754219,2782891,2794155,2796203,2796207,2796215,2796227,2796239,2796255,2796283,2796315,2796335,2796351,2796379,2796415,2796455,2796515,2796603,2796683,2796719,2796735,2796763,2796799,2796839,2796899,2796987,2797071,2797127,2797187,2797279,2797391,2797531,2797739,2797995,2798187,2798255,2798271,2798299,2798335,2798375,2798435,2798523,2798607,2798663,2798723,2798815,2798927,2799067,2799275,2799531,2799727,2799815,2799875,2799967,2800079,2800219,2800427,2800687,2800911,2801083,2801295,2801591,2801955,2802443,2803115,2803819,2804267,2804399,2804415,2804443,2804479,2804519,2804579,2804667,2804751,2804807,2804867,2804959,2805071,2805211,2805419,2805675,2805871,2805959,2806019,2806111,2806223,2806363,2806571,2806831,2807055,2807227,2807439,2807735,2808099,2808587,2809259,2809963,2810415,2810567,2810627,2810719,2810831,2810971,2811179,2811439,2811663,2811835,2812047,2812343,2812707,2813195,2813867,2814575,2815055,2815291,2815503,2815799,2816163,2816651,2817327,2818071,2818691,2819247,2819967,2820923,2822139,2823787,2825835,2827691,2828715,2828975,2828991,2829019,2829055,2829095,2829155,2829243,2829327,2829383,2829443,2829535,2829647,2829787,2829995,2830251,2830447,2830535,2830595,2830687,2830799,2830939,2831147,2831407,2831631,2831803,2832015,2832311,2832675,2833163,2833835,2834539,2834991,2835143,2835203,2835295,2835407,2835547,2835755,2836015,2836239,2836411,2836623,2836919,2837283,2837771,2838443,2839151,2839631,2839867,2840079,2840375,2840739,2841227,2841903,2842647,2843267,2843823,2844543,2845499,2846715,2848363,2850411,2852267,2853295,2853575,2853635,2853727,2853839,2853979,2854187,2854447,2854671,2854843,2855055,2855351,2855715,2856203,2856875,2857583,2858063,2858299,2858511,2858807,2859171,2859659,2860335,2861079,2861699,2862255,2862975,2863931,2865147,2866795,2868843,2870703,2871759,2872123,2872335,2872631,2872995,2873483,2874159,2874903,2875523,2876079,2876799,2877755,2878971,2880619,2882671,2884567,2885763,2886447,2887167,2888123,2889339,2890991,2893087,2895195,2896991,2898823,2901219,2904347,2908427,2913771,2919723,2924459,2926763,2927279,2927295,2927323,2927359,2927399,2927459,2927547,2927631,2927687,2927747,2927839,2927951,2928091,2928299,2928555,2928751,2928839,2928899,2928991,2929103,2929243,2929451,2929711,2929935,2930107,2930319,2930615,2930979,2931467,2932139,2932843,2933295,2933447,2933507,2933599,2933711,2933851,2934059,2934319,2934543,2934715,2934927,2935223,2935587,2936075,2936747,2937455,2937935,2938171,2938383,2938679,2939043,2939531,2940207,2940951,2941571,2942127,2942847,2943803,2945019,2946667,2948715,2950571,2951599,2951879,2951939,2952031,2952143,2952283,2952491,2952751,2952975,2953147,2953359,2953655,2954019,2954507,2955179,2955887,2956367,2956603,2956815,2957111,2957475,2957963,2958639,2959383,2960003,2960559,2961279,2962235,2963451,2965099,2967147,2969007,2970063,2970427,2970639,2970935,2971299,2971787,2972463,2973207,2973827,2974383,2975103,2976059,2977275,2978923,2980975,2982871,2984067,2984751,2985471,2986427,2987643,2989295,2991391,2993499,2995295,2997127,2999523,3002651,3006731,3012075,3018027,3022763,3025071,3025607,3025667,3025759,3025871,3026011,3026219,3026479,3026703,3026875,3027087,3027383,3027747,3028235,3028907,3029615,3030095,3030331,3030543,3030839,3031203,3031691,3032367,3033111,3033731,3034287,3035007,3035963,3037179,3038827,3040875,3042735,3043791,3044155,3044367,3044663,3045027,3045515,3046191,3046935,3047555,3048111,3048831,3049787,3051003,3052651,3054703,3056599,3057795,3058479,3059199,3060155,3061371,3063023,3065119,3067227,3069023,3070855,3073251,3076379,3080459,3085803,3091755,3096495,3098831,3099451,3099663,3099959,3100323,3100811,3101487,3102231,3102851,3103407,3104127,3105083,3106299,3107947,3109999,3111895,3113091,3113775,3114495,3115451,3116667,3118319,3120415,3122523,3124319,3126151,3128547,3131675,3135755,3141099,3147055,3151831,3154307,3155247,3155967,3156923,3158139,3159791,3161887,3163995,3165791,3167623,3170019,3173147,3177227,3182575,3188575,3193563,3196639,3198727,3201123,3204251,3208335,3213735,3220035,3226047,3231471,3237531,3245451,3255787,3269291,3285931,3302571,3314347,3319467,3320495,3320511,3320539,3320575,3320615,3320675,3320763,3320847,3320903,3320963,3321055,3321167,3321307,3321515,3321771,3321967,3322055,3322115,3322207,3322319,3322459,3322667,3322927,3323151,3323323,3323535,3323831,3324195,3324683,3325355,3326059,3326511,3326663,3326723,3326815,3326927,3327067,3327275,3327535,3327759,3327931,3328143,3328439,3328803,3329291,3329963,3330671,3331151,3331387,3331599,3331895,3332259,3332747,3333423,3334167,3334787,3335343,3336063,3337019,3338235,3339883,3341931,3343787,3344815,3345095,3345155,3345247,3345359,3345499,3345707,3345967,3346191,3346363,3346575,3346871,3347235,3347723,3348395,3349103,3349583,3349819,3350031,3350327,3350691,3351179,3351855,3352599,3353219,3353775,3354495,3355451,3356667,3358315,3360363,3362223,3363279,3363643,3363855,3364151,3364515,3365003,3365679,3366423,3367043,3367599,3368319,3369275,3370491,3372139,3374191,3376087,3377283,3377967,3378687,3379643,3380859,3382511,3384607,3386715,3388511,3390343,3392739,3395867,3399947,3405291,3411243,3415979,3418287,3418823,3418883,3418975,3419087,3419227,3419435,3419695,3419919,3420091,3420303,3420599,3420963,3421451,3422123,3422831,3423311,3423547,3423759,3424055,3424419,3424907,3425583,3426327,3426947,3427503,3428223,3429179,3430395,3432043,3434091,3435951,3437007,3437371,3437583,3437879,3438243,3438731,3439407,3440151,3440771,3441327,3442047,3443003,3444219,3445867,3447919,3449815,3451011,3451695,3452415,3453371,3454587,3456239,3458335,3460443,3462239,3464071,3466467,3469595,3473675,3479019,3484971,3489711,3492047,3492667,3492879,3493175,3493539,3494027,3494703,3495447,3496067,3496623,3497343,3498299,3499515,3501163,3503215,3505111,3506307,3506991,3507711,3508667,3509883,3511535,3513631,3515739,3517535,3519367,3521763,3524891,3528971,3534315,3540271,3545047,3547523,3548463,3549183,3550139,3551355,3553007,3555103,3557211,3559007,3560839,3563235,3566363,3570443,3575791,3581791,3586779,3589855,3591943,3594339,3597467,3601551,3606951,3613251,3619263,3624687,3630747,3638667,3649003,3662507,3679147,3695787,3707563,3712687,3713735,3713795,3713887,3713999,3714139,3714347,3714607,3714831,3715003,3715215,3715511,3715875,3716363,3717035,3717743,3718223,3718459,3718671,3718967,3719331,3719819,3720495,3721239,3721859,3722415,3723135,3724091,3725307,3726955,3729003,3730863,3731919,3732283,3732495,3732791,3733155,3733643,3734319,3735063,3735683,3736239,3736959,3737915,3739131,3740779,3742831,3744727,3745923,3746607,3747327,3748283,3749499,3751151,3753247,3755355,3757151,3758983,3761379,3764507,3768587,3773931,3779883,3784623,3786959,3787579,3787791,3788087,3788451,3788939,3789615,3790359,3790979,3791535,3792255,3793211,3794427,3796075,3798127,3800023,3801219,3801903,3802623,3803579,3804795,3806447,3808543,3810651,3812447,3814279,3816675,3819803,3823883,3829227,3835183,3839959,3842435,3843375,3844095,3845051,3846267,3847919,3850015,3852123,3853919,3855751,3858147,3861275,3865355,3870703,3876703,3881691,3884767,3886855,3889251,3892379,3896463,3901863,3908163,3914175,3919599,3925659,3933579,3943915,3957419,3974059,3990699,4002479,4007631,4008763,4008975,4009271,4009635,4010123,4010799,4011543,4012163,4012719,4013439,4014395,4015611,4017259,4019311,4021207,4022403,4023087,4023807,4024763,4025979,4027631,4029727,4031835,4033631,4035463,4037859,4040987,4045067,4050411,4056367,4061143,4063619,4064559,4065279,4066235,4067451,4069103,4071199,4073307,4075103,4076935,4079331,4082459,4086539,4091887,4097887,4102875,4105951,4108039,4110435,4113563,4117647,4123047,4129347,4135359,4140783,4146843,4154763,4165099,4178603,4195243,4211887,4223703,4228995,4230447,4231167,4232123,4233339,4234991,4237087,4239195,4240991,4242823,4245219,4248347,4252427,4257775,4263775,4268763,4271839,4273927,4276323,4279451,4283535,4288935,4295235,4301247,4306671,4312731,4320651,4330987,4344491,4361135,4377823,4389851,4395743,4398343,4400739,4403867,4407951,4413351,4419651,4425663,4431087,4437147,4445067,4455403,4468911,4485607,4502595,4515647,4523887,4530459,4538379,4548719,4562287,4579387,4597999,4615447,4632355,4652395,4678571,4712747,4756395,4806315,4851371,4880043,4891307,4893359,4893375,4893403,4893439,4893479,4893539,4893627,4893711,4893767,4893827,4893919,4894031,4894171,4894379,4894635,4894831,4894919,4894979,4895071,4895183,4895323,4895531,4895791,4896015,4896187,4896399,4896695,4897059,4897547,4898219,4898923,4899375,4899527,4899587,4899679,4899791,4899931,4900139,4900399,4900623,4900795,4901007,4901303,4901667,4902155,4902827,4903535,4904015,4904251,4904463,4904759,4905123,4905611,4906287,4907031,4907651,4908207,4908927,4909883,4911099,4912747,4914795,4916651,4917679,4917959,4918019,4918111,4918223,4918363,4918571,4918831,4919055,4919227,4919439,4919735,4920099,4920587,4921259,4921967,4922447,4922683,4922895,4923191,4923555,4924043,4924719,4925463,4926083,4926639,4927359,4928315,4929531,4931179,4933227,4935087,4936143,4936507,4936719,4937015,4937379,4937867,4938543,4939287,4939907,4940463,4941183,4942139,4943355,4945003,4947055,4948951,4950147,4950831,4951551,4952507,4953723,4955375,4957471,4959579,4961375,4963207,4965603,4968731,4972811,4978155,4984107,4988843,4991151,4991687,4991747,4991839,4991951,4992091,4992299,4992559,4992783,4992955,4993167,4993463,4993827,4994315,4994987,4995695,4996175,4996411,4996623,4996919,4997283,4997771,4998447,4999191,4999811,5000367,5001087,5002043,5003259,5004907,5006955,5008815,5009871,5010235,5010447,5010743,5011107,5011595,5012271,5013015,5013635,5014191,5014911,5015867,5017083,5018731,5020783,5022679,5023875,5024559,5025279,5026235,5027451,5029103,5031199,5033307,5035103,5036935,5039331,5042459,5046539,5051883,5057835,5062575,5064911,5065531,5065743,5066039,5066403,5066891,5067567,5068311,5068931,5069487,5070207,5071163,5072379,5074027,5076079,5077975,5079171,5079855,5080575,5081531,5082747,5084399,5086495,5088603,5090399,5092231,5094627,5097755,5101835,5107179,5113135,5117911,5120387,5121327,5122047,5123003,5124219,5125871,5127967,5130075,5131871,5133703,5136099,5139227,5143307,5148655,5154655,5159643,5162719,5164807,5167203,5170331,5174415,5179815,5186115,5192127,5197551,5203611,5211531,5221867,5235371,5252011,5268651,5280427,5285551,5286599,5286659,5286751,5286863,5287003,5287211,5287471,5287695,5287867,5288079,5288375,5288739,5289227,5289899,5290607,5291087,5291323,5291535,5291831,5292195,5292683,5293359,5294103,5294723,5295279,5295999,5296955,5298171,5299819,5301867,5303727,5304783,5305147,5305359,5305655,5306019,5306507,5307183,5307927,5308547,5309103,5309823,5310779,5311995,5313643,5315695,5317591,5318787,5319471,5320191,5321147,5322363,5324015,5326111,5328219,5330015,5331847,5334243,5337371,5341451,5346795,5352747,5357487,5359823,5360443,5360655,5360951,5361315,5361803,5362479,5363223,5363843,5364399,5365119,5366075,5367291,5368939,5370991,5372887,5374083,5374767,5375487,5376443,5377659,5379311,5381407,5383515,5385311,5387143,5389539,5392667,5396747,5402091,5408047,5412823,5415299,5416239,5416959,5417915,5419131,5420783,5422879,5424987,5426783,5428615,5431011,5434139,5438219,5443567,5449567,5454555,5457631,5459719,5462115,5465243,5469327,5474727,5481027,5487039,5492463,5498523,5506443,5516779,5530283,5546923,5563563,5575343,5580495,5581627,5581839,5582135,5582499,5582987,5583663,5584407,5585027,5585583,5586303,5587259,5588475,5590123,5592175,5594071,5595267,5595951,5596671,5597627,5598843,5600495,5602591,5604699,5606495,5608327,5610723,5613851,5617931,5623275,5629231,5634007,5636483,5637423,5638143,5639099,5640315,5641967,5644063,5646171,5647967,5649799,5652195,5655323,5659403,5664751,5670751,5675739,5678815,5680903,5683299,5686427,5690511,5695911,5702211,5708223,5713647,5719707,5727627,5737963,5751467,5768107,5784751,5796567,5801859,5803311,5804031,5804987,5806203,5807855,5809951,5812059,5813855,5815687,5818083,5821211,5825291,5830639,5836639,5841627,5844703,5846791,5849187,5852315,5856399,5861799,5868099,5874111,5879535,5885595,5893515,5903851,5917355,5933999,5950687,5962715,5968607,5971207,5973603,5976731,5980815,5986215,5992515,5998527,6003951,6010011,6017931,6028267,6041775,6058471,6075459,6088511,6096751,6103323,6111243,6121583,6135151,6152251,6170863,6188311,6205219,6225259,6251435,6285611,6329259,6379179,6424235,6452907,6464175,6466247,6466307,6466399,6466511,6466651,6466859,6467119,6467343,6467515,6467727,6468023,6468387,6468875,6469547,6470255,6470735,6470971,6471183,6471479,6471843,6472331,6473007,6473751,6474371,6474927,6475647,6476603,6477819,6479467,6481515,6483375,6484431,6484795,6485007,6485303,6485667,6486155,6486831,6487575,6488195,6488751,6489471,6490427,6491643,6493291,6495343,6497239,6498435,6499119,6499839,6500795,6502011,6503663,6505759,6507867,6509663,6511495,6513891,6517019,6521099,6526443,6532395,6537135,6539471,6540091,6540303,6540599,6540963,6541451,6542127,6542871,6543491,6544047,6544767,6545723,6546939,6548587,6550639,6552535,6553731,6554415,6555135,6556091,6557307,6558959,6561055,6563163,6564959,6566791,6569187,6572315,6576395,6581739,6587695,6592471,6594947,6595887,6596607,6597563,6598779,6600431,6602527,6604635,6606431,6608263,6610659,6613787,6617867,6623215,6629215,6634203,6637279,6639367,6641763,6644891,6648975,6654375,6660675,6666687,6672111,6678171,6686091,6696427,6709931,6726571,6743211,6754991,6760143,6761275,6761487,6761783,6762147,6762635,6763311,6764055,6764675,6765231,6765951,6766907,6768123,6769771,6771823,6773719,6774915,6775599,6776319,6777275,6778491,6780143,6782239,6784347,6786143,6787975,6790371,6793499,6797579,6802923,6808879,6813655,6816131,6817071,6817791,6818747,6819963,6821615,6823711,6825819,6827615,6829447,6831843,6834971,6839051,6844399,6850399,6855387,6858463,6860551,6862947,6866075,6870159,6875559,6881859,6887871,6893295,6899355,6907275,6917611,6931115,6947755,6964399,6976215,6981507,6982959,6983679,6984635,6985851,6987503,6989599,6991707,6993503,6995335,6997731,7000859,7004939,7010287,7016287,7021275,7024351,7026439,7028835,7031963,7036047,7041447,7047747,7053759,7059183,7065243,7073163,7083499,7097003,7113647,7130335,7142363,7148255,7150855,7153251,7156379,7160463,7165863,7172163,7178175,7183599,7189659,7197579,7207915,7221423,7238119,7255107,7268159,7276399,7282971,7290891,7301231,7314799,7331899,7350511,7367959,7384867,7404907,7431083,7465259,7508907,7558827,7603883,7632559,7643855,7646011,7646223,7646519,7646883,7647371,7648047,7648791,7649411,7649967,7650687,7651643,7652859,7654507,7656559,7658455,7659651,7660335,7661055,7662011,7663227,7664879,7666975,7669083,7670879,7672711,7675107,7678235,7682315,7687659,7693615,7698391,7700867,7701807,7702527,7703483,7704699,7706351,7708447,7710555,7712351,7714183,7716579,7719707,7723787,7729135,7735135,7740123,7743199,7745287,7747683,7750811,7754895,7760295,7766595,7772607,7778031,7784091,7792011,7802347,7815851,7832491,7849135,7860951,7866243,7867695,7868415,7869371,7870587,7872239,7874335,7876443,7878239,7880071,7882467,7885595,7889675,7895023,7901023,7906011,7909087,7911175,7913571,7916699,7920783,7926183,7932483,7938495,7943919,7949979,7957899,7968235,7981739,7998383,8015071,8027099,8032991,8035591,8037987,8041115,8045199,8050599,8056899,8062911,8068335,8074395,8082315,8092651,8106159,8122855,8139843,8152895,8161135,8167707,8175627,8185967,8199535,8216635,8235247,8252695,8269603,8289643,8315819,8349995,8393643,8443563,8488623,8517335,8528771,8531247,8531967,8532923,8534139,8535791,8537887,8539995,8541791,8543623,8546019,8549147,8553227,8558575,8564575,8569563,8572639,8574727,8577123,8580251,8584335,8589735,8596035,8602047,8607471,8613531,8621451,8631787,8645291,8661935,8678623,8690651,8696543,8699143,8701539,8704667,8708751,8714151,8720451,8726463,8731887,8737947,8745867,8756203,8769711,8786407,8803395,8816447,8824687,8831259,8839179,8849519,8863087,8880187,8898799,8916247,8933155,8953195,8979371,9013547,9057195,9107119,9152223,9181147,9193183,9196807,9199203,9202331,9206415,9211815,9218115,9224127,9229551,9235611,9243531,9253867,9267375,9284071,9301059,9314111,9322351,9328923,9336843,9347183,9360751,9377851,9396463,9413911,9430819,9450859,9477035,9511211,9554863,9604839,9650243,9680191,9694575,9702171,9710091,9720431,9733999,9751099,9769711,9787159,9804067,9824107,9850283,9884463,9928175,9978555,10025583,10059927,10082979,10104043,10130223,10164471,10208707,10261519,10316191,10367995,10421851,10488107,10574635,10686635,10823851,10968747,11087531,11156139,11180715,11184811,11184815,11184823,11184835,11184847,11184863,11184891,11184923,11184943,11184959,11184987,11185023,11185063,11185123,11185211,11185291,11185327,11185343,11185371,11185407,11185447,11185507,11185595,11185679,11185735,11185795,11185887,11185999,11186139,11186347,11186603,11186795,11186863,11186879,11186907,11186943,11186983,11187043,11187131,11187215,11187271,11187331,11187423,11187535,11187675,11187883,11188139,11188335,11188423,11188483,11188575,11188687,11188827,11189035,11189295,11189519,11189691,11189903,11190199,11190563,11191051,11191723,11192427,11192875,11193007,11193023,11193051,11193087,11193127,11193187,11193275,11193359,11193415,11193475,11193567,11193679,11193819,11194027,11194283,11194479,11194567,11194627,11194719,11194831,11194971,11195179,11195439,11195663,11195835,11196047,11196343,11196707,11197195,11197867,11198571,11199023,11199175,11199235,11199327,11199439,11199579,11199787,11200047,11200271,11200443,11200655,11200951,11201315,11201803,11202475,11203183,11203663,11203899,11204111,11204407,11204771,11205259,11205935,11206679,11207299,11207855,11208575,11209531,11210747,11212395,11214443,11216299,11217323,11217583,11217599,11217627,11217663,11217703,11217763,11217851,11217935,11217991,11218051,11218143,11218255,11218395,11218603,11218859,11219055,11219143,11219203,11219295,11219407,11219547,11219755,11220015,11220239,11220411,11220623,11220919,11221283,11221771,11222443,11223147,11223599,11223751,11223811,11223903,11224015,11224155,11224363,11224623,11224847,11225019,11225231,11225527,11225891,11226379,11227051,11227759,11228239,11228475,11228687,11228983,11229347,11229835,11230511,11231255,11231875,11232431,11233151,11234107,11235323,11236971,11239019,11240875,11241903,11242183,11242243,11242335,11242447,11242587,11242795,11243055,11243279,11243451,11243663,11243959,11244323,11244811,11245483,11246191,11246671,11246907,11247119,11247415,11247779,11248267,11248943,11249687,11250307,11250863,11251583,11252539,11253755,11255403,11257451,11259311,11260367,11260731,11260943,11261239,11261603,11262091,11262767,11263511,11264131,11264687,11265407,11266363,11267579,11269227,11271279,11273175,11274371,11275055,11275775,11276731,11277947,11279599,11281695,11283803,11285599,11287431,11289827,11292955,11297035,11302379,11308331,11313067,11315371,11315887,11315903,11315931,11315967,11316007,11316067,11316155,11316239,11316295,11316355,11316447,11316559,11316699,11316907,11317163,11317359,11317447,11317507,11317599,11317711,11317851,11318059,11318319,11318543,11318715,11318927,11319223,11319587,11320075,11320747,11321451,11321903,11322055,11322115,11322207,11322319,11322459,11322667,11322927,11323151,11323323,11323535,11323831,11324195,11324683,11325355,11326063,11326543,11326779,11326991,11327287,11327651,11328139,11328815,11329559,11330179,11330735,11331455,11332411,11333627,11335275,11337323,11339179,11340207,11340487,11340547,11340639,11340751,11340891,11341099,11341359,11341583,11341755,11341967,11342263,11342627,11343115,11343787,11344495,11344975,11345211,11345423,11345719,11346083,11346571,11347247,11347991,11348611,11349167,11349887,11350843,11352059,11353707,11355755,11357615,11358671,11359035,11359247,11359543,11359907,11360395,11361071,11361815,11362435,11362991,11363711,11364667,11365883,11367531,11369583,11371479,11372675,11373359,11374079,11375035,11376251,11377903,11379999,11382107,11383903,11385735,11388131,11391259,11395339,11400683,11406635,11411371,11413679,11414215,11414275,11414367,11414479,11414619,11414827,11415087,11415311,11415483,11415695,11415991,11416355,11416843,11417515,11418223,11418703,11418939,11419151,11419447,11419811,11420299,11420975,11421719,11422339,11422895,11423615,11424571,11425787,11427435,11429483,11431343,11432399,11432763,11432975,11433271,11433635,11434123,11434799,11435543,11436163,11436719,11437439,11438395,11439611,11441259,11443311,11445207,11446403,11447087,11447807,11448763,11449979,11451631,11453727,11455835,11457631,11459463,11461859,11464987,11469067,11474411,11480363,11485103,11487439,11488059,11488271,11488567,11488931,11489419,11490095,11490839,11491459,11492015,11492735,11493691,11494907,11496555,11498607,11500503,11501699,11502383,11503103,11504059,11505275,11506927,11509023,11511131,11512927,11514759,11517155,11520283,11524363,11529707,11535663,11540439,11542915,11543855,11544575,11545531,11546747,11548399,11550495,11552603,11554399,11556231,11558627,11561755,11565835,11571183,11577183,11582171,11585247,11587335,11589731,11592859,11596943,11602343,11608643,11614655,11620079,11626139,11634059,11644395,11657899,11674539,11691179,11702955,11708075,11709103,11709119,11709147,11709183,11709223,11709283,11709371,11709455,11709511,11709571,11709663,11709775,11709915,11710123,11710379,11710575,11710663,11710723,11710815,11710927,11711067,11711275,11711535,11711759,11711931,11712143,11712439,11712803,11713291,11713963,11714667,11715119,11715271,11715331,11715423,11715535,11715675,11715883,11716143,11716367,11716539,11716751,11717047,11717411,11717899,11718571,11719279,11719759,11719995,11720207,11720503,11720867,11721355,11722031,11722775,11723395,11723951,11724671,11725627,11726843,11728491,11730539,11732395,11733423,11733703,11733763,11733855,11733967,11734107,11734315,11734575,11734799,11734971,11735183,11735479,11735843,11736331,11737003,11737711,11738191,11738427,11738639,11738935,11739299,11739787,11740463,11741207,11741827,11742383,11743103,11744059,11745275,11746923,11748971,11750831,11751887,11752251,11752463,11752759,11753123,11753611,11754287,11755031,11755651,11756207,11756927,11757883,11759099,11760747,11762799,11764695,11765891,11766575,11767295,11768251,11769467,11771119,11773215,11775323,11777119,11778951,11781347,11784475,11788555,11793899,11799851,11804587,11806895,11807431,11807491,11807583,11807695,11807835,11808043,11808303,11808527,11808699,11808911,11809207,11809571,11810059,11810731,11811439,11811919,11812155,11812367,11812663,11813027,11813515,11814191,11814935,11815555,11816111,11816831,11817787,11819003,11820651,11822699,11824559,11825615,11825979,11826191,11826487,11826851,11827339,11828015,11828759,11829379,11829935,11830655,11831611,11832827,11834475,11836527,11838423,11839619,11840303,11841023,11841979,11843195,11844847,11846943,11849051,11850847,11852679,11855075,11858203,11862283,11867627,11873579,11878319,11880655,11881275,11881487,11881783,11882147,11882635,11883311,11884055,11884675,11885231,11885951,11886907,11888123,11889771,11891823,11893719,11894915,11895599,11896319,11897275,11898491,11900143,11902239,11904347,11906143,11907975,11910371,11913499,11917579,11922923,11928879,11933655,11936131,11937071,11937791,11938747,11939963,11941615,11943711,11945819,11947615,11949447,11951843,11954971,11959051,11964399,11970399,11975387,11978463,11980551,11982947,11986075,11990159,11995559,12001859,12007871,12013295,12019355,12027275,12037611,12051115,12067755,12084395,12096171,12101295,12102343,12102403,12102495,12102607,12102747,12102955,12103215,12103439,12103611,12103823,12104119,12104483,12104971,12105643,12106351,12106831,12107067,12107279,12107575,12107939,12108427,12109103,12109847,12110467,12111023,12111743,12112699,12113915,12115563,12117611,12119471,12120527,12120891,12121103,12121399,12121763,12122251,12122927,12123671,12124291,12124847,12125567,12126523,12127739,12129387,12131439,12133335,12134531,12135215,12135935,12136891,12138107,12139759,12141855,12143963,12145759,12147591,12149987,12153115,12157195,12162539,12168491,12173231,12175567,12176187,12176399,12176695,12177059,12177547,12178223,12178967,12179587,12180143,12180863,12181819,12183035,12184683,12186735,12188631,12189827,12190511,12191231,12192187,12193403,12195055,12197151,12199259,12201055,12202887,12205283,12208411,12212491,12217835,12223791,12228567,12231043,12231983,12232703,12233659,12234875,12236527,12238623,12240731,12242527,12244359,12246755,12249883,12253963,12259311,12265311,12270299,12273375,12275463,12277859,12280987,12285071,12290471,12296771,12302783,12308207,12314267,12322187,12332523,12346027,12362667,12379307,12391087,12396239,12397371,12397583,12397879,12398243,12398731,12399407,12400151,12400771,12401327,12402047,12403003,12404219,12405867,12407919,12409815,12411011,12411695,12412415,12413371,12414587,12416239,12418335,12420443,12422239,12424071,12426467,12429595,12433675,12439019,12444975,12449751,12452227,12453167,12453887,12454843,12456059,12457711,12459807,12461915,12463711,12465543,12467939,12471067,12475147,12480495,12486495,12491483,12494559,12496647,12499043,12502171,12506255,12511655,12517955,12523967,12529391,12535451,12543371,12553707,12567211,12583851,12600495,12612311,12617603,12619055,12619775,12620731,12621947,12623599,12625695,12627803,12629599,12631431,12633827,12636955,12641035,12646383,12652383,12657371,12660447,12662535,12664931,12668059,12672143,12677543,12683843,12689855,12695279,12701339,12709259,12719595,12733099,12749743,12766431,12778459,12784351,12786951,12789347,12792475,12796559,12801959,12808259,12814271,12819695,12825755,12833675,12844011,12857519,12874215,12891203,12904255,12912495,12919067,12926987,12937327,12950895,12967995,12986607,13004055,13020963,13041003,13067179,13101355,13145003,13194923,13239979,13268651,13279915,13281967,13281983,13282011,13282047,13282087,13282147,13282235,13282319,13282375,13282435,13282527,13282639,13282779,13282987,13283243,13283439,13283527,13283587,13283679,13283791,13283931,13284139,13284399,13284623,13284795,13285007,13285303,13285667,13286155,13286827,13287531,13287983,13288135,13288195,13288287,13288399,13288539,13288747,13289007,13289231,13289403,13289615,13289911,13290275,13290763,13291435,13292143,13292623,13292859,13293071,13293367,13293731,13294219,13294895,13295639,13296259,13296815,13297535,13298491,13299707,13301355,13303403,13305259,13306287,13306567,13306627,13306719,13306831,13306971,13307179,13307439,13307663,13307835,13308047,13308343,13308707,13309195,13309867,13310575,13311055,13311291,13311503,13311799,13312163,13312651,13313327,13314071,13314691,13315247,13315967,13316923,13318139,13319787,13321835,13323695,13324751,13325115,13325327,13325623,13325987,13326475,13327151,13327895,13328515,13329071,13329791,13330747,13331963,13333611,13335663,13337559,13338755,13339439,13340159,13341115,13342331,13343983,13346079,13348187,13349983,13351815,13354211,13357339,13361419,13366763,13372715,13377451,13379759,13380295,13380355,13380447,13380559,13380699,13380907,13381167,13381391,13381563,13381775,13382071,13382435,13382923,13383595,13384303,13384783,13385019,13385231,13385527,13385891,13386379,13387055,13387799,13388419,13388975,13389695,13390651,13391867,13393515,13395563,13397423,13398479,13398843,13399055,13399351,13399715,13400203,13400879,13401623,13402243,13402799,13403519,13404475,13405691,13407339,13409391,13411287,13412483,13413167,13413887,13414843,13416059,13417711,13419807,13421915,13423711,13425543,13427939,13431067,13435147,13440491,13446443,13451183,13453519,13454139,13454351,13454647,13455011,13455499,13456175,13456919,13457539,13458095,13458815,13459771,13460987,13462635,13464687,13466583,13467779,13468463,13469183,13470139,13471355,13473007,13475103,13477211,13479007,13480839,13483235,13486363,13490443,13495787,13501743,13506519,13508995,13509935,13510655,13511611,13512827,13514479,13516575,13518683,13520479,13522311,13524707,13527835,13531915,13537263,13543263,13548251,13551327,13553415,13555811,13558939,13563023,13568423,13574723,13580735,13586159,13592219,13600139,13610475,13623979,13640619,13657259,13669035,13674159,13675207,13675267,13675359,13675471,13675611,13675819,13676079,13676303,13676475,13676687,13676983,13677347,13677835,13678507,13679215,13679695,13679931,13680143,13680439,13680803,13681291,13681967,13682711,13683331,13683887,13684607,13685563,13686779,13688427,13690475,13692335,13693391,13693755,13693967,13694263,13694627,13695115,13695791,13696535,13697155,13697711,13698431,13699387,13700603,13702251,13704303,13706199,13707395,13708079,13708799,13709755,13710971,13712623,13714719,13716827,13718623,13720455,13722851,13725979,13730059,13735403,13741355,13746095,13748431,13749051,13749263,13749559,13749923,13750411,13751087,13751831,13752451,13753007,13753727,13754683,13755899,13757547,13759599,13761495,13762691,13763375,13764095,13765051,13766267,13767919,13770015,13772123,13773919,13775751,13778147,13781275,13785355,13790699,13796655,13801431,13803907,13804847,13805567,13806523,13807739,13809391,13811487,13813595,13815391,13817223,13819619,13822747,13826827,13832175,13838175,13843163,13846239,13848327,13850723,13853851,13857935,13863335,13869635,13875647,13881071,13887131,13895051,13905387,13918891,13935531,13952171,13963951,13969103,13970235,13970447,13970743,13971107,13971595,13972271,13973015,13973635,13974191,13974911,13975867,13977083,13978731,13980783,13982679,13983875,13984559,13985279,13986235,13987451,13989103,13991199,13993307,13995103,13996935,13999331,14002459,14006539,14011883,14017839,14022615,14025091,14026031,14026751,14027707,14028923,14030575,14032671,14034779,14036575,14038407,14040803,14043931,14048011,14053359,14059359,14064347,14067423,14069511,14071907,14075035,14079119,14084519,14090819,14096831,14102255,14108315,14116235,14126571,14140075,14156715,14173359,14185175,14190467,14191919,14192639,14193595,14194811,14196463,14198559,14200667,14202463,14204295,14206691,14209819,14213899,14219247,14225247,14230235,14233311,14235399,14237795,14240923,14245007,14250407,14256707,14262719,14268143,14274203,14282123,14292459,14305963,14322607,14339295,14351323,14357215,14359815,14362211,14365339,14369423,14374823,14381123,14387135,14392559,14398619,14406539,14416875,14430383,14447079,14464067,14477119,14485359,14491931,14499851,14510191,14523759,14540859,14559471,14576919,14593827,14613867,14640043,14674219,14717867,14767787,14812843,14841515,14852783,14854855,14854915,14855007,14855119,14855259,14855467,14855727,14855951,14856123,14856335,14856631,14856995,14857483,14858155,14858863,14859343,14859579,14859791,14860087,14860451,14860939,14861615,14862359,14862979,14863535,14864255,14865211,14866427,14868075,14870123,14871983,14873039,14873403,14873615,14873911,14874275,14874763,14875439,14876183,14876803,14877359,14878079,14879035,14880251,14881899,14883951,14885847,14887043,14887727,14888447,14889403,14890619,14892271,14894367,14896475,14898271,14900103,14902499,14905627,14909707,14915051,14921003,14925743,14928079,14928699,14928911,14929207,14929571,14930059,14930735,14931479,14932099,14932655,14933375,14934331,14935547,14937195,14939247,14941143,14942339,14943023,14943743,14944699,14945915,14947567,14949663,14951771,14953567,14955399,14957795,14960923,14965003,14970347,14976303,14981079,14983555,14984495,14985215,14986171,14987387,14989039,14991135,14993243,14995039,14996871,14999267,15002395,15006475,15011823,15017823,15022811,15025887,15027975,15030371,15033499,15037583,15042983,15049283,15055295,15060719,15066779,15074699,15085035,15098539,15115179,15131819,15143599,15148751,15149883,15150095,15150391,15150755,15151243,15151919,15152663,15153283,15153839,15154559,15155515,15156731,15158379,15160431,15162327,15163523,15164207,15164927,15165883,15167099,15168751,15170847,15172955,15174751,15176583,15178979,15182107,15186187,15191531,15197487,15202263,15204739,15205679,15206399,15207355,15208571,15210223,15212319,15214427,15216223,15218055,15220451,15223579,15227659,15233007,15239007,15243995,15247071,15249159,15251555,15254683,15258767,15264167,15270467,15276479,15281903,15287963,15295883,15306219,15319723,15336363,15353007,15364823,15370115,15371567,15372287,15373243,15374459,15376111,15378207,15380315,15382111,15383943,15386339,15389467,15393547,15398895,15404895,15409883,15412959,15415047,15417443,15420571,15424655,15430055,15436355,15442367,15447791,15453851,15461771,15472107,15485611,15502255,15518943,15530971,15536863,15539463,15541859,15544987,15549071,15554471,15560771,15566783,15572207,15578267,15586187,15596523,15610031,15626727,15643715,15656767,15665007,15671579,15679499,15689839,15703407,15720507,15739119,15756567,15773475,15793515,15819691,15853867,15897515,15947435,15992491,16021167,16032463,16034619,16034831,16035127,16035491,16035979,16036655,16037399,16038019,16038575,16039295,16040251,16041467,16043115,16045167,16047063,16048259,16048943,16049663,16050619,16051835,16053487,16055583,16057691,16059487,16061319,16063715,16066843,16070923,16076267,16082223,16086999,16089475,16090415,16091135,16092091,16093307,16094959,16097055,16099163,16100959,16102791,16105187,16108315,16112395,16117743,16123743,16128731,16131807,16133895,16136291,16139419,16143503,16148903,16155203,16161215,16166639,16172699,16180619,16190955,16204459,16221099,16237743,16249559,16254851,16256303,16257023,16257979,16259195,16260847,16262943,16265051,16266847,16268679,16271075,16274203,16278283,16283631,16289631,16294619,16297695,16299783,16302179,16305307,16309391,16314791,16321091,16327103,16332527,16338587,16346507,16356843,16370347,16386991,16403679,16415707,16421599,16424199,16426595,16429723,16433807,16439207,16445507,16451519,16456943,16463003,16470923,16481259,16494767,16511463,16528451,16541503,16549743,16556315,16564235,16574575,16588143,16605243,16623855,16641303,16658211,16678251,16704427,16738603,16782251,16832171,16877231,16905943,16917379,16919855,16920575,16921531,16922747,16924399,16926495,16928603,16930399,16932231,16934627,16937755,16941835,16947183,16953183,16958171,16961247,16963335,16965731,16968859,16972943,16978343,16984643,16990655,16996079,17002139,17010059,17020395,17033899,17050543,17067231,17079259,17085151,17087751,17090147,17093275,17097359,17102759,17109059,17115071,17120495,17126555,17134475,17144811,17158319,17175015,17192003,17205055,17213295,17219867,17227787,17238127,17251695,17268795,17287407,17304855,17321763,17341803,17367979,17402155,17445803,17495727,17540831,17569755,17581791,17585415,17587811,17590939,17595023,17600423,17606723,17612735,17618159,17624219,17632139,17642475,17655983,17672679,17689667,17702719,17710959,17717531,17725451,17735791,17749359,17766459,17785071,17802519,17819427,17839467,17865643,17899819,17943471,17993447,18038851,18068799,18083183,18090779,18098699,18109039,18122607,18139707,18158319,18175767,18192675,18212715,18238891,18273071,18316783,18367163,18414191,18448535,18471587,18492651,18518831,18553079,18597315,18650127,18704799,18756603,18810459,18876715,18963243,19075243,19212459,19357355,19476139,19544747,19569323,19573423,19573439,19573467,19573503,19573543,19573603,19573691,19573775,19573831,19573891,19573983,19574095,19574235,19574443,19574699,19574895,19574983,19575043,19575135,19575247,19575387,19575595,19575855,19576079,19576251,19576463,19576759,19577123,19577611,19578283,19578987,19579439,19579591,19579651,19579743,19579855,19579995,19580203,19580463,19580687,19580859,19581071,19581367,19581731,19582219,19582891,19583599,19584079,19584315,19584527,19584823,19585187,19585675,19586351,19587095,19587715,19588271,19588991,19589947,19591163,19592811,19594859,19596715,19597743,19598023,19598083,19598175,19598287,19598427,19598635,19598895,19599119,19599291,19599503,19599799,19600163,19600651,19601323,19602031,19602511,19602747,19602959,19603255,19603619,19604107,19604783,19605527,19606147,19606703,19607423,19608379,19609595,19611243,19613291,19615151,19616207,19616571,19616783,19617079,19617443,19617931,19618607,19619351,19619971,19620527,19621247,19622203,19623419,19625067,19627119,19629015,19630211,19630895,19631615,19632571,19633787,19635439,19637535,19639643,19641439,19643271,19645667,19648795,19652875,19658219,19664171,19668907,19671215,19671751,19671811,19671903,19672015,19672155,19672363,19672623,19672847,19673019,19673231,19673527,19673891,19674379,19675051,19675759,19676239,19676475,19676687,19676983,19677347,19677835,19678511,19679255,19679875,19680431,19681151,19682107,19683323,19684971,19687019,19688879,19689935,19690299,19690511,19690807,19691171,19691659,19692335,19693079,19693699,19694255,19694975,19695931,19697147,19698795,19700847,19702743,19703939,19704623,19705343,19706299,19707515,19709167,19711263,19713371,19715167,19716999,19719395,19722523,19726603,19731947,19737899,19742639,19744975,19745595,19745807,19746103,19746467,19746955,19747631,19748375,19748995,19749551,19750271,19751227,19752443,19754091,19756143,19758039,19759235,19759919,19760639,19761595,19762811,19764463,19766559,19768667,19770463,19772295,19774691,19777819,19781899,19787243,19793199,19797975,19800451,19801391,19802111,19803067,19804283,19805935,19808031,19810139,19811935,19813767,19816163,19819291,19823371,19828719,19834719,19839707,19842783,19844871,19847267,19850395,19854479,19859879,19866179,19872191,19877615,19883675,19891595,19901931,19915435,19932075,19948715,19960491,19965615,19966663,19966723,19966815,19966927,19967067,19967275,19967535,19967759,19967931,19968143,19968439,19968803,19969291,19969963,19970671,19971151,19971387,19971599,19971895,19972259,19972747,19973423,19974167,19974787,19975343,19976063,19977019,19978235,19979883,19981931,19983791,19984847,19985211,19985423,19985719,19986083,19986571,19987247,19987991,19988611,19989167,19989887,19990843,19992059,19993707,19995759,19997655,19998851,19999535,20000255,20001211,20002427,20004079,20006175,20008283,20010079,20011911,20014307,20017435,20021515,20026859,20032811,20037551,20039887,20040507,20040719,20041015,20041379,20041867,20042543,20043287,20043907,20044463,20045183,20046139,20047355,20049003,20051055,20052951,20054147,20054831,20055551,20056507,20057723,20059375,20061471,20063579,20065375,20067207,20069603,20072731,20076811,20082155,20088111,20092887,20095363,20096303,20097023,20097979,20099195,20100847,20102943,20105051,20106847,20108679,20111075,20114203,20118283,20123631,20129631,20134619,20137695,20139783,20142179,20145307,20149391,20154791,20161091,20167103,20172527,20178587,20186507,20196843,20210347,20226987,20243627,20255407,20260559,20261691,20261903,20262199,20262563,20263051,20263727,20264471,20265091,20265647,20266367,20267323,20268539,20270187,20272239,20274135,20275331,20276015,20276735,20277691,20278907,20280559,20282655,20284763,20286559,20288391,20290787,20293915,20297995,20303339,20309295,20314071,20316547,20317487,20318207,20319163,20320379,20322031,20324127,20326235,20328031,20329863,20332259,20335387,20339467,20344815,20350815,20355803,20358879,20360967,20363363,20366491,20370575,20375975,20382275,20388287,20393711,20399771,20407691,20418027,20431531,20448171,20464815,20476631,20481923,20483375,20484095,20485051,20486267,20487919,20490015,20492123,20493919,20495751,20498147,20501275,20505355,20510703,20516703,20521691,20524767,20526855,20529251,20532379,20536463,20541863,20548163,20554175,20559599,20565659,20573579,20583915,20597419,20614063,20630751,20642779,20648671,20651271,20653667,20656795,20660879,20666279,20672579,20678591,20684015,20690075,20697995,20708331,20721839,20738535,20755523,20768575,20776815,20783387,20791307,20801647,20815215,20832315,20850927,20868375,20885283,20905323,20931499,20965675,21009323,21059243,21104299,21132971,21144239,21146311,21146371,21146463,21146575,21146715,21146923,21147183,21147407,21147579,21147791,21148087,21148451,21148939,21149611,21150319,21150799,21151035,21151247,21151543,21151907,21152395,21153071,21153815,21154435,21154991,21155711,21156667,21157883,21159531,21161579,21163439,21164495,21164859,21165071,21165367,21165731,21166219,21166895,21167639,21168259,21168815,21169535,21170491,21171707,21173355,21175407,21177303,21178499,21179183,21179903,21180859,21182075,21183727,21185823,21187931,21189727,21191559,21193955,21197083,21201163,21206507,21212459,21217199,21219535,21220155,21220367,21220663,21221027,21221515,21222191,21222935,21223555,21224111,21224831,21225787,21227003,21228651,21230703,21232599,21233795,21234479,21235199,21236155,21237371,21239023,21241119,21243227,21245023,21246855,21249251,21252379,21256459,21261803,21267759,21272535,21275011,21275951,21276671,21277627,21278843,21280495,21282591,21284699,21286495,21288327,21290723,21293851,21297931,21303279,21309279,21314267,21317343,21319431,21321827,21324955,21329039,21334439,21340739,21346751,21352175,21358235,21366155,21376491,21389995,21406635,21423275,21435055,21440207,21441339,21441551,21441847,21442211,21442699,21443375,21444119,21444739,21445295,21446015,21446971,21448187,21449835,21451887,21453783,21454979,21455663,21456383,21457339,21458555,21460207,21462303,21464411,21466207,21468039,21470435,21473563,21477643,21482987,21488943,21493719,21496195,21497135,21497855,21498811,21500027,21501679,21503775,21505883,21507679,21509511,21511907,21515035,21519115,21524463,21530463,21535451,21538527,21540615,21543011,21546139,21550223,21555623,21561923,21567935,21573359,21579419,21587339,21597675,21611179,21627819,21644463,21656279,21661571,21663023,21663743,21664699,21665915,21667567,21669663,21671771,21673567,21675399,21677795,21680923,21685003,21690351,21696351,21701339,21704415,21706503,21708899,21712027,21716111,21721511,21727811,21733823,21739247,21745307,21753227,21763563,21777067,21793711,21810399,21822427,21828319,21830919,21833315,21836443,21840527,21845927,21852227,21858239,21863663,21869723,21877643,21887979,21901487,21918183,21935171,21948223,21956463,21963035,21970955,21981295,21994863,22011963,22030575,22048023,22064931,22084971,22111147,22145323,22188971,22238891,22283947,22312623,22323919,22326075,22326287,22326583,22326947,22327435,22328111,22328855,22329475,22330031,22330751,22331707,22332923,22334571,22336623,22338519,22339715,22340399,22341119,22342075,22343291,22344943,22347039,22349147,22350943,22352775,22355171,22358299,22362379,22367723,22373679,22378455,22380931,22381871,22382591,22383547,22384763,22386415,22388511,22390619,22392415,22394247,22396643,22399771,22403851,22409199,22415199,22420187,22423263,22425351,22427747,22430875,22434959,22440359,22446659,22452671,22458095,22464155,22472075,22482411,22495915,22512555,22529199,22541015,22546307,22547759,22548479,22549435,22550651,22552303,22554399,22556507,22558303,22560135,22562531,22565659,22569739,22575087,22581087,22586075,22589151,22591239,22593635,22596763,22600847,22606247,22612547,22618559,22623983,22630043,22637963,22648299,22661803,22678447,22695135,22707163,22713055,22715655,22718051,22721179,22725263,22730663,22736963,22742975,22748399,22754459,22762379,22772715,22786223,22802919,22819907,22832959,22841199,22847771,22855691,22866031,22879599,22896699,22915311,22932759,22949667,22969707,22995883,23030059,23073707,23123627,23168687,23197399,23208835,23211311,23212031,23212987,23214203,23215855,23217951,23220059,23221855,23223687,23226083,23229211,23233291,23238639,23244639,23249627,23252703,23254791,23257187,23260315,23264399,23269799,23276099,23282111,23287535,23293595,23301515,23311851,23325355,23341999,23358687,23370715,23376607,23379207,23381603,23384731,23388815,23394215,23400515,23406527,23411951,23418011,23425931,23436267,23449775,23466471,23483459,23496511,23504751,23511323,23519243,23529583,23543151,23560251,23578863,23596311,23613219,23633259,23659435,23693611,23737259,23787183,23832287,23861211,23873247,23876871,23879267,23882395,23886479,23891879,23898179,23904191,23909615,23915675,23923595,23933931,23947439,23964135,23981123,23994175,24002415,24008987,24016907,24027247,24040815,24057915,24076527,24093975,24110883,24130923,24157099,24191275,24234927,24284903,24330307,24360255,24374639,24382235,24390155,24400495,24414063,24431163,24449775,24467223,24484131,24504171,24530347,24564527,24608239,24658619,24705647,24739991,24763043,24784107,24810287,24844535,24888771,24941583,24996255,25048059,25101915,25168171,25254699,25366699,25503915,25648811,25767595,25836203,25860783,25864903,25864963,25865055,25865167,25865307,25865515,25865775,25865999,25866171,25866383,25866679,25867043,25867531,25868203,25868911,25869391,25869627,25869839,25870135,25870499,25870987,25871663,25872407,25873027,25873583,25874303,25875259,25876475,25878123,25880171,25882031,25883087,25883451,25883663,25883959,25884323,25884811,25885487,25886231,25886851,25887407,25888127,25889083,25890299,25891947,25893999,25895895,25897091,25897775,25898495,25899451,25900667,25902319,25904415,25906523,25908319,25910151,25912547,25915675,25919755,25925099,25931051,25935791,25938127,25938747,25938959,25939255,25939619,25940107,25940783,25941527,25942147,25942703,25943423,25944379,25945595,25947243,25949295,25951191,25952387,25953071,25953791,25954747,25955963,25957615,25959711,25961819,25963615,25965447,25967843,25970971,25975051,25980395,25986351,25991127,25993603,25994543,25995263,25996219,25997435,25999087,26001183,26003291,26005087,26006919,26009315,26012443,26016523,26021871,26027871,26032859,26035935,26038023,26040419,26043547,26047631,26053031,26059331,26065343,26070767,26076827,26084747,26095083,26108587,26125227,26141867,26153647,26158799,26159931,26160143,26160439,26160803,26161291,26161967,26162711,26163331,26163887,26164607,26165563,26166779,26168427,26170479,26172375,26173571,26174255,26174975,26175931,26177147,26178799,26180895,26183003,26184799,26186631,26189027,26192155,26196235,26201579,26207535,26212311,26214787,26215727,26216447,26217403,26218619,26220271,26222367,26224475,26226271,26228103,26230499,26233627,26237707,26243055,26249055,26254043,26257119,26259207,26261603,26264731,26268815,26274215,26280515,26286527,26291951,26298011,26305931,26316267,26329771,26346411,26363055,26374871,26380163,26381615,26382335,26383291,26384507,26386159,26388255,26390363,26392159,26393991,26396387,26399515,26403595,26408943,26414943,26419931,26423007,26425095,26427491,26430619,26434703,26440103,26446403,26452415,26457839,26463899,26471819,26482155,26495659,26512303,26528991,26541019,26546911,26549511,26551907,26555035,26559119,26564519,26570819,26576831,26582255,26588315,26596235,26606571,26620079,26636775,26653763,26666815,26675055,26681627,26689547,26699887,26713455,26730555,26749167,26766615,26783523,26803563,26829739,26863915,26907563,26957483,27002539,27031215,27042511,27044667,27044879,27045175,27045539,27046027,27046703,27047447,27048067,27048623,27049343,27050299,27051515,27053163,27055215,27057111,27058307,27058991,27059711,27060667,27061883,27063535,27065631,27067739,27069535,27071367,27073763,27076891,27080971,27086315,27092271,27097047,27099523,27100463,27101183,27102139,27103355,27105007,27107103,27109211,27111007,27112839,27115235,27118363,27122443,27127791,27133791,27138779,27141855,27143943,27146339,27149467,27153551,27158951,27165251,27171263,27176687,27182747,27190667,27201003,27214507,27231147,27247791,27259607,27264899,27266351,27267071,27268027,27269243,27270895,27272991,27275099,27276895,27278727,27281123,27284251,27288331,27293679,27299679,27304667,27307743,27309831,27312227,27315355,27319439,27324839,27331139,27337151,27342575,27348635,27356555,27366891,27380395,27397039,27413727,27425755,27431647,27434247,27436643,27439771,27443855,27449255,27455555,27461567,27466991,27473051,27480971,27491307,27504815,27521511,27538499,27551551,27559791,27566363,27574283,27584623,27598191,27615291,27633903,27651351,27668259,27688299,27714475,27748651,27792299,27842219,27887279,27915991,27927427,27929903,27930623,27931579,27932795,27934447,27936543,27938651,27940447,27942279,27944675,27947803,27951883,27957231,27963231,27968219,27971295,27973383,27975779,27978907,27982991,27988391,27994691,28000703,28006127,28012187,28020107,28030443,28043947,28060591,28077279,28089307,28095199,28097799,28100195,28103323,28107407,28112807,28119107,28125119,28130543,28136603,28144523,28154859,28168367,28185063,28202051,28215103,28223343,28229915,28237835,28248175,28261743,28278843,28297455,28314903,28331811,28351851,28378027,28412203,28455851,28505775,28550879,28579803,28591839,28595463,28597859,28600987,28605071,28610471,28616771,28622783,28628207,28634267,28642187,28652523,28666031,28682727,28699715,28712767,28721007,28727579,28735499,28745839,28759407,28776507,28795119,28812567,28829475,28849515,28875691,28909867,28953519,29003495,29048899,29078847,29093231,29100827,29108747,29119087,29132655,29149755,29168367,29185815,29202723,29222763,29248939,29283119,29326831,29377211,29424239,29458583,29481635,29502699,29528879,29563127,29607363,29660175,29714847,29766651,29820507,29886763,29973291,30085291,30222507,30367403,30486187,30554799,30579407,30583611,30583823,30584119,30584483,30584971,30585647,30586391,30587011,30587567,30588287,30589243,30590459,30592107,30594159,30596055,30597251,30597935,30598655,30599611,30600827,30602479,30604575,30606683,30608479,30610311,30612707,30615835,30619915,30625259,30631215,30635991,30638467,30639407,30640127,30641083,30642299,30643951,30646047,30648155,30649951,30651783,30654179,30657307,30661387,30666735,30672735,30677723,30680799,30682887,30685283,30688411,30692495,30697895,30704195,30710207,30715631,30721691,30729611,30739947,30753451,30770091,30786735,30798551,30803843,30805295,30806015,30806971,30808187,30809839,30811935,30814043,30815839,30817671,30820067,30823195,30827275,30832623,30838623,30843611,30846687,30848775,30851171,30854299,30858383,30863783,30870083,30876095,30881519,30887579,30895499,30905835,30919339,30935983,30952671,30964699,30970591,30973191,30975587,30978715,30982799,30988199,30994499,31000511,31005935,31011995,31019915,31030251,31043759,31060455,31077443,31090495,31098735,31105307,31113227,31123567,31137135,31154235,31172847,31190295,31207203,31227243,31253419,31287595,31331243,31381163,31426223,31454935,31466371,31468847,31469567,31470523,31471739,31473391,31475487,31477595,31479391,31481223,31483619,31486747,31490827,31496175,31502175,31507163,31510239,31512327,31514723,31517851,31521935,31527335,31533635,31539647,31545071,31551131,31559051,31569387,31582891,31599535,31616223,31628251,31634143,31636743,31639139,31642267,31646351,31651751,31658051,31664063,31669487,31675547,31683467,31693803,31707311,31724007,31740995,31754047,31762287,31768859,31776779,31787119,31800687,31817787,31836399,31853847,31870755,31890795,31916971,31951147,31994795,32044719,32089823,32118747,32130783,32134407,32136803,32139931,32144015,32149415,32155715,32161727,32167151,32173211,32181131,32191467,32204975,32221671,32238659,32251711,32259951,32266523,32274443,32284783,32298351,32315451,32334063,32351511,32368419,32388459,32414635,32448811,32492463,32542439,32587843,32617791,32632175,32639771,32647691,32658031,32671599,32688699,32707311,32724759,32741667,32761707,32787883,32822063,32865775,32916155,32963183,32997527,33020579,33041643,33067823,33102071,33146307,33199119,33253791,33305595,33359451,33425707,33512235,33624235,33761451,33906347,34025135,34093783,34118531,34123055,34123775,34124731,34125947,34127599,34129695,34131803,34133599,34135431,34137827,34140955,34145035,34150383,34156383,34161371,34164447,34166535,34168931,34172059,34176143,34181543,34187843,34193855,34199279,34205339,34213259,34223595,34237099,34253743,34270431,34282459,34288351,34290951,34293347,34296475,34300559,34305959,34312259,34318271,34323695,34329755,34337675,34348011,34361519,34378215,34395203,34408255,34416495,34423067,34430987,34441327,34454895,34471995,34490607,34508055,34524963,34545003,34571179,34605355,34649003,34698927,34744031,34772955,34784991,34788615,34791011,34794139,34798223,34803623,34809923,34815935,34821359,34827419,34835339,34845675,34859183,34875879,34892867,34905919,34914159,34920731,34928651,34938991,34952559,34969659,34988271,35005719,35022627,35042667,35068843,35103019,35146671,35196647,35242051,35271999,35286383,35293979,35301899,35312239,35325807,35342907,35361519,35378967,35395875,35415915,35442091,35476271,35519983,35570363,35617391,35651735,35674787,35695851,35722031,35756279,35800515,35853327,35907999,35959803,36013659,36079915,36166443,36278443,36415659,36560559,36679391,36748251,36773599,36779271,36781667,36784795,36788879,36794279,36800579,36806591,36812015,36818075,36825995,36836331,36849839,36866535,36883523,36896575,36904815,36911387,36919307,36929647,36943215,36960315,36978927,36996375,37013283,37033323,37059499,37093675,37137327,37187303,37232707,37262655,37277039,37284635,37292555,37302895,37316463,37333563,37352175,37369623,37386531,37406571,37432747,37466927,37510639,37561019,37608047,37642391,37665443,37686507,37712687,37746935,37791171,37843983,37898655,37950459,38004315,38070571,38157099,38269099,38406319,38551271,38670403,38740287,38767983,38777627,38785547,38795887,38809455,38826555,38845167,38862615,38879523,38899563,38925739,38959919,39003631,39054011,39101039,39135383,39158435,39179499,39205679,39239927,39284163,39336975,39391647,39443451,39497307,39563563,39650091,39762095,39899375,40044731,40165487,40239767,40276131,40299243,40325423,40359671,40403907,40456719,40511391,40563195,40617051,40683307,40769839,40881911,41019715,41167503,41295903,41387643,41454811,41523119,41609727,41722459,41863743,42024039,42185187,42342651,42516619,42735659,43020715,43381931,43801259,44209835,44516011,44677803,44731051,44739243,44739247,44739255,44739267,44739279,44739295,44739323,44739355,44739375,44739391,44739419,44739455,44739495,44739555,44739643,44739723,44739759,44739775,44739803,44739839,44739879,44739939,44740027,44740111,44740167,44740227,44740319,44740431,44740571,44740779,44741035,44741227,44741295,44741311,44741339,44741375,44741415,44741475,44741563,44741647,44741703,44741763,44741855,44741967,44742107,44742315,44742571,44742767,44742855,44742915,44743007,44743119,44743259,44743467,44743727,44743951,44744123,44744335,44744631,44744995,44745483,44746155,44746859,44747307,44747439,44747455,44747483,44747519,44747559,44747619,44747707,44747791,44747847,44747907,44747999,44748111,44748251,44748459,44748715,44748911,44748999,44749059,44749151,44749263,44749403,44749611,44749871,44750095,44750267,44750479,44750775,44751139,44751627,44752299,44753003,44753455,44753607,44753667,44753759,44753871,44754011,44754219,44754479,44754703,44754875,44755087,44755383,44755747,44756235,44756907,44757615,44758095,44758331,44758543,44758839,44759203,44759691,44760367,44761111,44761731,44762287,44763007,44763963,44765179,44766827,44768875,44770731,44771755,44772015,44772031,44772059,44772095,44772135,44772195,44772283,44772367,44772423,44772483,44772575,44772687,44772827,44773035,44773291,44773487,44773575,44773635,44773727,44773839,44773979,44774187,44774447,44774671,44774843,44775055,44775351,44775715,44776203,44776875,44777579,44778031,44778183,44778243,44778335,44778447,44778587,44778795,44779055,44779279,44779451,44779663,44779959,44780323,44780811,44781483,44782191,44782671,44782907,44783119,44783415,44783779,44784267,44784943,44785687,44786307,44786863,44787583,44788539,44789755,44791403,44793451,44795307,44796335,44796615,44796675,44796767,44796879,44797019,44797227,44797487,44797711,44797883,44798095,44798391,44798755,44799243,44799915,44800623,44801103,44801339,44801551,44801847,44802211,44802699,44803375,44804119,44804739,44805295,44806015,44806971,44808187,44809835,44811883,44813743,44814799,44815163,44815375,44815671,44816035,44816523,44817199,44817943,44818563,44819119,44819839,44820795,44822011,44823659,44825711,44827607,44828803,44829487,44830207,44831163,44832379,44834031,44836127,44838235,44840031,44841863,44844259,44847387,44851467,44856811,44862763,44867499,44869803,44870319,44870335,44870363,44870399,44870439,44870499,44870587,44870671,44870727,44870787,44870879,44870991,44871131,44871339,44871595,44871791,44871879,44871939,44872031,44872143,44872283,44872491,44872751,44872975,44873147,44873359,44873655,44874019,44874507,44875179,44875883,44876335,44876487,44876547,44876639,44876751,44876891,44877099,44877359,44877583,44877755,44877967,44878263,44878627,44879115,44879787,44880495,44880975,44881211,44881423,44881719,44882083,44882571,44883247,44883991,44884611,44885167,44885887,44886843,44888059,44889707,44891755,44893611,44894639,44894919,44894979,44895071,44895183,44895323,44895531,44895791,44896015,44896187,44896399,44896695,44897059,44897547,44898219,44898927,44899407,44899643,44899855,44900151,44900515,44901003,44901679,44902423,44903043,44903599,44904319,44905275,44906491,44908139,44910187,44912047,44913103,44913467,44913679,44913975,44914339,44914827,44915503,44916247,44916867,44917423,44918143,44919099,44920315,44921963,44924015,44925911,44927107,44927791,44928511,44929467,44930683,44932335,44934431,44936539,44938335,44940167,44942563,44945691,44949771,44955115,44961067,44965803,44968111,44968647,44968707,44968799,44968911,44969051,44969259,44969519,44969743,44969915,44970127,44970423,44970787,44971275,44971947,44972655,44973135,44973371,44973583,44973879,44974243,44974731,44975407,44976151,44976771,44977327,44978047,44979003,44980219,44981867,44983915,44985775,44986831,44987195,44987407,44987703,44988067,44988555,44989231,44989975,44990595,44991151,44991871,44992827,44994043,44995691,44997743,44999639,45000835,45001519,45002239,45003195,45004411,45006063,45008159,45010267,45012063,45013895,45016291,45019419,45023499,45028843,45034795,45039535,45041871,45042491,45042703,45042999,45043363,45043851,45044527,45045271,45045891,45046447,45047167,45048123,45049339,45050987,45053039,45054935,45056131,45056815,45057535,45058491,45059707,45061359,45063455,45065563,45067359,45069191,45071587,45074715,45078795,45084139,45090095,45094871,45097347,45098287,45099007,45099963,45101179,45102831,45104927,45107035,45108831,45110663,45113059,45116187,45120267,45125615,45131615,45136603,45139679,45141767,45144163,45147291,45151375,45156775,45163075,45169087,45174511,45180571,45188491,45198827,45212331,45228971,45245611,45257387,45262507,45263535,45263551,45263579,45263615,45263655,45263715,45263803,45263887,45263943,45264003,45264095,45264207,45264347,45264555,45264811,45265007,45265095,45265155,45265247,45265359,45265499,45265707,45265967,45266191,45266363,45266575,45266871,45267235,45267723,45268395,45269099,45269551,45269703,45269763,45269855,45269967,45270107,45270315,45270575,45270799,45270971,45271183,45271479,45271843,45272331,45273003,45273711,45274191,45274427,45274639,45274935,45275299,45275787,45276463,45277207,45277827,45278383,45279103,45280059,45281275,45282923,45284971,45286827,45287855,45288135,45288195,45288287,45288399,45288539,45288747,45289007,45289231,45289403,45289615,45289911,45290275,45290763,45291435,45292143,45292623,45292859,45293071,45293367,45293731,45294219,45294895,45295639,45296259,45296815,45297535,45298491,45299707,45301355,45303403,45305263,45306319,45306683,45306895,45307191,45307555,45308043,45308719,45309463,45310083,45310639,45311359,45312315,45313531,45315179,45317231,45319127,45320323,45321007,45321727,45322683,45323899,45325551,45327647,45329755,45331551,45333383,45335779,45338907,45342987,45348331,45354283,45359019,45361327,45361863,45361923,45362015,45362127,45362267,45362475,45362735,45362959,45363131,45363343,45363639,45364003,45364491,45365163,45365871,45366351,45366587,45366799,45367095,45367459,45367947,45368623,45369367,45369987,45370543,45371263,45372219,45373435,45375083,45377131,45378991,45380047,45380411,45380623,45380919,45381283,45381771,45382447,45383191,45383811,45384367,45385087,45386043,45387259,45388907,45390959,45392855,45394051,45394735,45395455,45396411,45397627,45399279,45401375,45403483,45405279,45407111,45409507,45412635,45416715,45422059,45428011,45432751,45435087,45435707,45435919,45436215,45436579,45437067,45437743,45438487,45439107,45439663,45440383,45441339,45442555,45444203,45446255,45448151,45449347,45450031,45450751,45451707,45452923,45454575,45456671,45458779,45460575,45462407,45464803,45467931,45472011,45477355,45483311,45488087,45490563,45491503,45492223,45493179,45494395,45496047,45498143,45500251,45502047,45503879,45506275,45509403,45513483,45518831,45524831,45529819,45532895,45534983,45537379,45540507,45544591,45549991,45556291,45562303,45567727,45573787,45581707,45592043,45605547,45622187,45638827,45650603,45655727,45656775,45656835,45656927,45657039,45657179,45657387,45657647,45657871,45658043,45658255,45658551,45658915,45659403,45660075,45660783,45661263,45661499,45661711,45662007,45662371,45662859,45663535,45664279,45664899,45665455,45666175,45667131,45668347,45669995,45672043,45673903,45674959,45675323,45675535,45675831,45676195,45676683,45677359,45678103,45678723,45679279,45679999,45680955,45682171,45683819,45685871,45687767,45688963,45689647,45690367,45691323,45692539,45694191,45696287,45698395,45700191,45702023,45704419,45707547,45711627,45716971,45722923,45727663,45729999,45730619,45730831,45731127,45731491,45731979,45732655,45733399,45734019,45734575,45735295,45736251,45737467,45739115,45741167,45743063,45744259,45744943,45745663,45746619,45747835,45749487,45751583,45753691,45755487,45757319,45759715,45762843,45766923,45772267,45778223,45782999,45785475,45786415,45787135,45788091,45789307,45790959,45793055,45795163,45796959,45798791,45801187,45804315,45808395,45813743,45819743,45824731,45827807,45829895,45832291,45835419,45839503,45844903,45851203,45857215,45862639,45868699,45876619,45886955,45900459,45917099,45933739,45945519,45950671,45951803,45952015,45952311,45952675,45953163,45953839,45954583,45955203,45955759,45956479,45957435,45958651,45960299,45962351,45964247,45965443,45966127,45966847,45967803,45969019,45970671,45972767,45974875,45976671,45978503,45980899,45984027,45988107,45993451,45999407,46004183,46006659,46007599,46008319,46009275,46010491,46012143,46014239,46016347,46018143,46019975,46022371,46025499,46029579,46034927,46040927,46045915,46048991,46051079,46053475,46056603,46060687,46066087,46072387,46078399,46083823,46089883,46097803,46108139,46121643,46138283,46154927,46166743,46172035,46173487,46174207,46175163,46176379,46178031,46180127,46182235,46184031,46185863,46188259,46191387,46195467,46200815,46206815,46211803,46214879,46216967,46219363,46222491,46226575,46231975,46238275,46244287,46249711,46255771,46263691,46274027,46287531,46304175,46320863,46332891,46338783,46341383,46343779,46346907,46350991,46356391,46362691,46368703,46374127,46380187,46388107,46398443,46411951,46428647,46445635,46458687,46466927,46473499,46481419,46491759,46505327,46522427,46541039,46558487,46575395,46595435,46621611,46655787,46699435,46749355,46794411,46823083,46834347,46836399,46836415,46836443,46836479,46836519,46836579,46836667,46836751,46836807,46836867,46836959,46837071,46837211,46837419,46837675,46837871,46837959,46838019,46838111,46838223,46838363,46838571,46838831,46839055,46839227,46839439,46839735,46840099,46840587,46841259,46841963,46842415,46842567,46842627,46842719,46842831,46842971,46843179,46843439,46843663,46843835,46844047,46844343,46844707,46845195,46845867,46846575,46847055,46847291,46847503,46847799,46848163,46848651,46849327,46850071,46850691,46851247,46851967,46852923,46854139,46855787,46857835,46859691,46860719,46860999,46861059,46861151,46861263,46861403,46861611,46861871,46862095,46862267,46862479,46862775,46863139,46863627,46864299,46865007,46865487,46865723,46865935,46866231,46866595,46867083,46867759,46868503,46869123,46869679,46870399,46871355,46872571,46874219,46876267,46878127,46879183,46879547,46879759,46880055,46880419,46880907,46881583,46882327,46882947,46883503,46884223,46885179,46886395,46888043,46890095,46891991,46893187,46893871,46894591,46895547,46896763,46898415,46900511,46902619,46904415,46906247,46908643,46911771,46915851,46921195,46927147,46931883,46934191,46934727,46934787,46934879,46934991,46935131,46935339,46935599,46935823,46935995,46936207,46936503,46936867,46937355,46938027,46938735,46939215,46939451,46939663,46939959,46940323,46940811,46941487,46942231,46942851,46943407,46944127,46945083,46946299,46947947,46949995,46951855,46952911,46953275,46953487,46953783,46954147,46954635,46955311,46956055,46956675,46957231,46957951,46958907,46960123,46961771,46963823,46965719,46966915,46967599,46968319,46969275,46970491,46972143,46974239,46976347,46978143,46979975,46982371,46985499,46989579,46994923,47000875,47005615,47007951,47008571,47008783,47009079,47009443,47009931,47010607,47011351,47011971,47012527,47013247,47014203,47015419,47017067,47019119,47021015,47022211,47022895,47023615,47024571,47025787,47027439,47029535,47031643,47033439,47035271,47037667,47040795,47044875,47050219,47056175,47060951,47063427,47064367,47065087,47066043,47067259,47068911,47071007,47073115,47074911,47076743,47079139,47082267,47086347,47091695,47097695,47102683,47105759,47107847,47110243,47113371,47117455,47122855,47129155,47135167,47140591,47146651,47154571,47164907,47178411,47195051,47211691,47223467,47228591,47229639,47229699,47229791,47229903,47230043,47230251,47230511,47230735,47230907,47231119,47231415,47231779,47232267,47232939,47233647,47234127,47234363,47234575,47234871,47235235,47235723,47236399,47237143,47237763,47238319,47239039,47239995,47241211,47242859,47244907,47246767,47247823,47248187,47248399,47248695,47249059,47249547,47250223,47250967,47251587,47252143,47252863,47253819,47255035,47256683,47258735,47260631,47261827,47262511,47263231,47264187,47265403,47267055,47269151,47271259,47273055,47274887,47277283,47280411,47284491,47289835,47295787,47300527,47302863,47303483,47303695,47303991,47304355,47304843,47305519,47306263,47306883,47307439,47308159,47309115,47310331,47311979,47314031,47315927,47317123,47317807,47318527,47319483,47320699,47322351,47324447,47326555,47328351,47330183,47332579,47335707,47339787,47345131,47351087,47355863,47358339,47359279,47359999,47360955,47362171,47363823,47365919,47368027,47369823,47371655,47374051,47377179,47381259,47386607,47392607,47397595,47400671,47402759,47405155,47408283,47412367,47417767,47424067,47430079,47435503,47441563,47449483,47459819,47473323,47489963,47506603,47518383,47523535,47524667,47524879,47525175,47525539,47526027,47526703,47527447,47528067,47528623,47529343,47530299,47531515,47533163,47535215,47537111,47538307,47538991,47539711,47540667,47541883,47543535,47545631,47547739,47549535,47551367,47553763,47556891,47560971,47566315,47572271,47577047,47579523,47580463,47581183,47582139,47583355,47585007,47587103,47589211,47591007,47592839,47595235,47598363,47602443,47607791,47613791,47618779,47621855,47623943,47626339,47629467,47633551,47638951,47645251,47651263,47656687,47662747,47670667,47681003,47694507,47711147,47727791,47739607,47744899,47746351,47747071,47748027,47749243,47750895,47752991,47755099,47756895,47758727,47761123,47764251,47768331,47773679,47779679,47784667,47787743,47789831,47792227,47795355,47799439,47804839,47811139,47817151,47822575,47828635,47836555,47846891,47860395,47877039,47893727,47905755,47911647,47914247,47916643,47919771,47923855,47929255,47935555,47941567,47946991,47953051,47960971,47971307,47984815,48001511,48018499,48031551,48039791,48046363,48054283,48064623,48078191,48095291,48113903,48131351,48148259,48168299,48194475,48228651,48272299,48322219,48367275,48395947,48407215,48409287,48409347,48409439,48409551,48409691,48409899,48410159,48410383,48410555,48410767,48411063,48411427,48411915,48412587,48413295,48413775,48414011,48414223,48414519,48414883,48415371,48416047,48416791,48417411,48417967,48418687,48419643,48420859,48422507,48424555,48426415,48427471,48427835,48428047,48428343,48428707,48429195,48429871,48430615,48431235,48431791,48432511,48433467,48434683,48436331,48438383,48440279,48441475,48442159,48442879,48443835,48445051,48446703,48448799,48450907,48452703,48454535,48456931,48460059,48464139,48469483,48475435,48480175,48482511,48483131,48483343,48483639,48484003,48484491,48485167,48485911,48486531,48487087,48487807,48488763,48489979,48491627,48493679,48495575,48496771,48497455,48498175,48499131,48500347,48501999,48504095,48506203,48507999,48509831,48512227,48515355,48519435,48524779,48530735,48535511,48537987,48538927,48539647,48540603,48541819,48543471,48545567,48547675,48549471,48551303,48553699,48556827,48560907,48566255,48572255,48577243,48580319,48582407,48584803,48587931,48592015,48597415,48603715,48609727,48615151,48621211,48629131,48639467,48652971,48669611,48686251,48698031,48703183,48704315,48704527,48704823,48705187,48705675,48706351,48707095,48707715,48708271,48708991,48709947,48711163,48712811,48714863,48716759,48717955,48718639,48719359,48720315,48721531,48723183,48725279,48727387,48729183,48731015,48733411,48736539,48740619,48745963,48751919,48756695,48759171,48760111,48760831,48761787,48763003,48764655,48766751,48768859,48770655,48772487,48774883,48778011,48782091,48787439,48793439,48798427,48801503,48803591,48805987,48809115,48813199,48818599,48824899,48830911,48836335,48842395,48850315,48860651,48874155,48890795,48907439,48919255,48924547,48925999,48926719,48927675,48928891,48930543,48932639,48934747,48936543,48938375,48940771,48943899,48947979,48953327,48959327,48964315,48967391,48969479,48971875,48975003,48979087,48984487,48990787,48996799,49002223,49008283,49016203,49026539,49040043,49056687,49073375,49085403,49091295,49093895,49096291,49099419,49103503,49108903,49115203,49121215,49126639,49132699,49140619,49150955,49164463,49181159,49198147,49211199,49219439,49226011,49233931,49244271,49257839,49274939,49293551,49310999,49327907,49347947,49374123,49408299,49451947,49501867,49546923,49575599,49586895,49589051,49589263,49589559,49589923,49590411,49591087,49591831,49592451,49593007,49593727,49594683,49595899,49597547,49599599,49601495,49602691,49603375]\n\ndef toothpick(n):\n return v[n]", "from math import floor, log\n\n\"\"\"\nSee: https://oeis.org/A139250\nFormulas:\n Let n = msb(n) + j where msb(n) = A053644(n)\n let a(0) = 0. \n Then a(n) = (2 * msb(n)^2 + 1) / 3 + 2 * a(j) + a(j + 1) - 1\n - David A. Corneth, Mar 26 2015\n \nNote: A053644(n) is the sequence of largest power of 2 <= n\nSee: https://oeis.org/A053644\n\"\"\"\n\ndef toothpick(n):\n \"\"\"Returns number of picks required for n rounds of the toothpick sequence\"\"\"\n if n < 2:\n return n\n \n msb_n = 2 ** floor(log(n, 2))\n j = n - msb_n\n return (2 * msb_n ** 2 + 1) / 3 + 2 * toothpick(j) + toothpick(j + 1) - 1", "import math\n\ndef isPowerOfTwo (n):\n return (math.ceil(math.log2(n)) == math.floor(math.log2(n)))\n\ndef toothpick(n):\n \"\"\"Returns number of picks required for n rounds of the toothpick sequence\"\"\"\n if n == 0:\n return 0\n elif n == 1:\n return 1\n elif isPowerOfTwo (n):\n return int((2**(2*math.log2(n)+1)+1) / 3)\n k = int (math.log2(n))\n i = n - 2**k\n \n return toothpick (2**k) + 2*toothpick(i) + toothpick (i+1) - 1", "from math import log\nfrom functools import lru_cache\n\n@lru_cache(5000)\ndef toothpick(n):\n if n == 0:\n return 0\n else:\n k = int(log(n, 2))\n i = n - 2**k\n if i == 0:\n return (2**(2*k+1)+1)/3\n else:\n return toothpick(2**k) + 2 * toothpick(i) + toothpick(i+1) - 1\n\n# from collections import deque\n# from time import monotonic\n# from numpy import zeros\n# from itertools import chain\n\n# _r = zeros((5000, 5000))\n# _n = 0\n# _q1 = [(2500, 2500)]\n# _q2 = []\n# _c = 0\n# _t = monotonic()\n\n# def toothpick(n):\n# print(n)\n# nonlocal _n, _q1, _q2, _c, _t\n# t = _t\n# if n > 2100:\n# raise Exception(n)\n# r = _r\n# q1 = _q1\n# q2 = _q2\n# count = _c\n# t1 = 0\n# t2 = 0\n# for round in range(_n, n):\n# dx = 1 if round % 2 == 1 else 0\n# dy = 0 if round % 2 == 1 else 1\n# tmp = monotonic()\n# re = []\n# ap = re.append\n# r_m = 0\n \n# for xy in chain(q1, q2):\n\n# r_m = r[xy]\n# if r_m == 0:\n# r[xy] = round\n# ap(xy)\n# # count += 1\n# else:\n# if r_m == round:\n# r[xy] = -1\n# # count -= 1\n# t1 += monotonic() - tmp\n# tmp = monotonic()\n# q1 = [(xy[0]+dx, xy[1]+dy) for xy in re if r[xy] == round]\n# q2 = [(xy[0]-dx, xy[1]-dy) for xy in re if r[xy] == round]\n# count += len(q1)\n# t2 += monotonic() - tmp\n \n \n# print(n, count, monotonic() - t, t1, t2)\n# _n = n\n# _q1 = q1\n# _q2 = q2\n# _c = count\n# return count\n", "# from https://oeis.org/A139250\n\ndef msb(n):\n t = 0\n while (n >> t) > 0:\n t += 1\n\n return 2 ** (t - 1)\n\ndef toothpick(n):\n k = (2 * msb(n) ** 2 + 1) // 3\n\n if n == 0:\n return 0\n elif n == msb(n):\n return k\n else:\n return k + 2*toothpick(n - msb(n)) + toothpick(n - msb(n) + 1) - 1", "from math import log2\ndef toothpick(n):\n if n == 0:\n return 0\n k = int(log2(n))\n i = n - 2**k\n if i == 0:\n return (2**(2*k+1)+1)//3\n else:\n return toothpick(2**k) + 2*toothpick(i)+ toothpick(i+1) - 1\n"] | {"fn_name": "toothpick", "inputs": [[0], [3], [16], [32], [49], [89], [327], [363], [366], [512], [656], [1038], [1052], [1222], [1235], [1302], [1735], [1757], [1974], [2048]], "outputs": [[0], [7], [171], [683], [1215], [3715], [52239], [60195], [62063], [174763], [209095], [699451], [700379], [757295], [762019], [832559], [1398915], [1443119], [2038207], [2796203]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 88,374 |
def toothpick(n):
|
508c5bf0ffeb8d2f988cfd643140e6a7 | UNKNOWN | # Task
Suppose there are `n` people standing in a circle and they are numbered 1 through n in order.
Person 1 starts off with a sword and kills person 2. He then passes the sword to the next person still standing, in this case person 3. Person 3 then uses the sword to kill person 4, and passes it to person 5. This pattern continues around and around the circle until just one person remains.
What is the number of this person?
# Example:
For `n = 5`, the result should be `3`.
```
1 kills 2, passes to 3.
3 kills 4, passes to 5.
5 kills 1, passes to 3.
3 kills 5 and wins.```
# Input/Output
- `[input]` integer `n`
The number of people. 1 through n standing in a circle.
`1 <= n <= 1e9`
- `[output]` an integer
The index of the last person standing. | ["def circle_slash(n):\n return int(bin(n)[3:]+'1', 2)", "from math import log\n\ndef circle_slash(n):\n return 2 * (n - 2 ** int(log(n, 2))) + 1", "from math import log2\ndef circle_slash(n):\n m = 2 ** int(log2(n))\n return n % m * 2 + 1", "def circle_slash(n):\n return int(\"{:b}\".format(n)[1:]+'1', 2)", "def circle_slash(n):\n return (n & ~(1 << n.bit_length() - 1)) << 1 | 1", "def circle_slash(n):\n #coding and coding..\n if (n & (n - 1)) == 0:\n return 1\n result = 1\n while result < n:\n result = result << 1\n return (n - result / 2) * 2 + 1", "def circle_slash(n):\n return (n - (1 << n.bit_length() - 1) << 1) + 1", "circle_slash=lambda n:int(bin(n)[3:]+'1',2)", "def circle_slash(n):\n s = format(n,'b')\n return int(s[1:]+s[0],2)", "from math import log2\ndef circle_slash(n):\n b=2**int(log2(n))\n return (n-b)*2+1"] | {"fn_name": "circle_slash", "inputs": [[5], [11], [1], [2], [3], [4], [8], [16], [15], [31]], "outputs": [[3], [7], [1], [1], [3], [1], [1], [1], [15], [31]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 881 |
def circle_slash(n):
|
ceae28a453e0bdf5a4657b6155aff186 | UNKNOWN | The objective is to return all pairs of integers from a given array of integers that have a difference of 2.
The result array should be sorted in ascending order of values.
Assume there are no duplicate integers in the array. The order of the integers in the input array should not matter.
## Examples
~~~if-not:python
```
[1, 2, 3, 4] should return [[1, 3], [2, 4]]
[4, 1, 2, 3] should also return [[1, 3], [2, 4]]
[1, 23, 3, 4, 7] should return [[1, 3]]
[4, 3, 1, 5, 6] should return [[1, 3], [3, 5], [4, 6]]
```
~~~
~~~if:python
```
[1, 2, 3, 4] should return [(1, 3), (2, 4)]
[4, 1, 2, 3] should also return [(1, 3), (2, 4)]
[1, 23, 3, 4, 7] should return [(1, 3)]
[4, 3, 1, 5, 6] should return [(1, 3), (3, 5), (4, 6)]
```
~~~ | ["def twos_difference(a):\n s = set(a)\n return sorted((x, x + 2) for x in a if x + 2 in s)", "def twos_difference(lst): \n return [(num,num+2) for num in sorted(lst) if num+2 in lst] \n \n", "def twos_difference(arr):\n arr = sorted(arr)\n b = []\n for i in range(len(arr)):\n for j in range(len(arr)):\n if arr[j] - arr[i] == 2:\n b.append((arr[i],arr[j]))\n return b", "def twos_difference(lst): \n return [(i, i + 2) for i in sorted(lst) if i + 2 in lst]\n", "def twos_difference(lst): \n matches = []\n for n in sorted(lst):\n if((n + 2) in lst):\n matches.append((n, n+2))\n return matches", "def twos_difference(A): \n L=[]\n for i in sorted(A):\n if((i + 2) in A):\n L.append((i, i+2))\n return L", "def twos_difference(lst): \n ende = []\n for i in lst:\n if i+2 in lst:\n ende.append((i, i+2))\n return sorted(ende)", "def twos_difference(l):\n r = [(i,i+2) for i in sorted(l) if i+2 in l]\n return r\n\ntwos_difference = lambda l: [(i,i+2) for i in sorted(l) if i+2 in l]", "def twos_difference(lst):\n result = []\n for elem in lst:\n if elem+2 in lst:\n result.append((elem, elem+2))\n result.sort()\n return result", "def twos_difference(lst):\n newlist = lst\n even =[]\n odd =[]\n finaleven = []\n finalodd = []\n for num in newlist:\n if num % 2 == 0:\n even.append(num)\n else:\n odd.append(num)\n for i in even:\n for j in even:\n if i+2 == j:\n finaleven.append((i,j))\n for a in odd:\n for b in odd:\n if a+2 ==b:\n finalodd.append((a,b))\n y = sorted(finaleven)\n z = sorted(finalodd)\n return sorted(y + z)", "def twos_difference(lst):\n s = set(lst)\n return [(n, n+2) for n in sorted(s) if n+2 in s]", "def twos_difference(lst):\n new_list = []\n lst.sort()\n for ind, val in enumerate(lst):\n get_check = val + 2\n try:\n if lst.index(get_check):\n new_list.append((val, get_check))\n except ValueError:\n continue\n return new_list", "def twos_difference(lst): \n return sorted([(x, x+2) for x in lst if x+2 in lst], key=lambda x: (x[0], x[1]))", "import itertools as it\nimport operator as op\n\ndef twos_difference(lst): \n pairs = (it.combinations(lst, 2))\n twodiffs = (pair for pair in pairs if abs(op.sub(*pair)) == 2)\n return sorted([tuple(sorted(pair)) for pair in list(twodiffs)])", "def twos_difference(lst): \n concat=[]\n for number in lst:\n if int(number) + 2 in lst:\n concat.append((int(number), int(number)+2))\n return sorted(concat)\n\n \n \n", "def twos_difference(a):\n return sorted([(i,j) for i in a for j in a if i-j==-2])", "def twos_difference(lst): \n if not lst:\n return []\n test = list(range( min(lst), max(lst)+1 ))\n return [ (test[i], test[i+2]) for i in range(len(test)-2) if all((test[i] in lst, test[i+2] in lst)) ]", "def twos_difference(lst): \n lst1=[]\n for i in lst:\n for j in lst:\n if i-j==2 or i-j==-2:\n if (i,j) not in lst1 and (j,i) not in lst1:\n if i<j:\n lst1.append((i,j))\n else:\n lst1.append((j,i))\n lst1.sort()\n return lst1", "def twos_difference(lst): \n my_pairs = []\n for i in range(0, len(lst)):\n for j in range (0, len(lst)):\n if lst[j] - lst[i] == 2:\n my_pairs.append([lst[j],lst[i]])\n diffs = []\n for pair in my_pairs:\n pair.sort()\n diffs.append(tuple(pair))\n \n diffs.sort()\n return diffs", "def twos_difference(lst): \n results, memo = [], {}\n for n in lst:\n if n in memo:\n for k in memo[n]:\n a, b = sorted([k, n])\n results.append((a, b))\n memo.setdefault(n + 2, []).append(n)\n memo.setdefault(n - 2, []).append(n)\n return sorted(results)"] | {"fn_name": "twos_difference", "inputs": [[[1, 2, 3, 4]], [[1, 3, 4, 6]], [[0, 3, 1, 4]], [[4, 1, 2, 3]], [[6, 3, 4, 1, 5]], [[3, 1, 6, 4]], [[1, 3, 5, 6, 8, 10, 15, 32, 12, 14, 56]], [[1, 4, 7, 10]], [[]]], "outputs": [[[[1, 3], [2, 4]]], [[[1, 3], [4, 6]]], [[[1, 3]]], [[[1, 3], [2, 4]]], [[[1, 3], [3, 5], [4, 6]]], [[[1, 3], [4, 6]]], [[[1, 3], [3, 5], [6, 8], [8, 10], [10, 12], [12, 14]]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,202 |
def twos_difference(lst):
|
86dc45926d03a406f2422184d9dd3a78 | UNKNOWN | Pirates have notorious difficulty with enunciating. They tend to blur all the letters together and scream at people.
At long last, we need a way to unscramble what these pirates are saying.
Write a function that will accept a jumble of letters as well as a dictionary, and output a list of words that the pirate might have meant.
For example:
```
grabscrab( "ortsp", ["sport", "parrot", "ports", "matey"] )
```
Should return `["sport", "ports"]`.
Return matches in the same order as in the dictionary. Return an empty array if there are no matches.
Good luck! | ["def grabscrab(said, possible_words):\n return [ word for word in possible_words if sorted(word) == sorted(said) ]", "from collections import Counter\n\ndef grabscrab(word, possible_words):\n ws = Counter(word)\n return [s for s in possible_words if Counter(s) == ws]", "def grabscrab(word, possible):\n sw = sorted(word)\n return [w for w in possible if sorted(w) == sw]", "def grabscrab(w, pw):\n return [i for i in pw if all(w.count(j)==i.count(j) for j in w)]", "grabscrab = lambda w,l:[p for p in l if sorted(p)==sorted(w)]", "def grabscrab(word, possible_words):\n srch = sorted(word)\n ans = [e for e in possible_words if sorted(e) == srch]\n return ans", "def grabscrab(word, possible_words):\n ws = sorted(word)\n return [w for w in possible_words if sorted(w) == ws]", "def grabscrab(word, possible_words):\n result = []\n for possible_word in possible_words:\n if len(possible_word) == len(word):\n if False not in [c in word for c in possible_word] and [False for c in possible_word if possible_word.count(c) == word.count(c)]:\n result.append(possible_word)\n return result", "def grabscrab(word, possible_words):\n s = sorted(word)\n l = len(word)\n return [w for w in possible_words if len(w) == l and sorted(w) == s]", "def grabscrab(word, possible_words):\n return [wd for wd in possible_words if sorted(word) == sorted(wd)]\n \n"] | {"fn_name": "grabscrab", "inputs": [["trisf", ["first"]], ["oob", ["bob", "baobab"]], ["ainstuomn", ["mountains", "hills", "mesa"]], ["oolp", ["donkey", "pool", "horse", "loop"]], ["ortsp", ["sport", "parrot", "ports", "matey"]], ["ourf", ["one", "two", "three"]]], "outputs": [[["first"]], [[]], [["mountains"]], [["pool", "loop"]], [["sport", "ports"]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,442 |
def grabscrab(word, possible_words):
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.