file_path
stringlengths 21
202
| content
stringlengths 12
1.02M
| size
int64 12
1.02M
| lang
stringclasses 9
values | avg_line_length
float64 3.33
100
| max_line_length
int64 10
993
| alphanum_fraction
float64 0.27
0.93
|
---|---|---|---|---|---|---|
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/src/teleop_node.cpp | /// \file
/// \brief Draws Each Obstacle in RViz using MarkerArrays
///
/// PARAMETERS:
/// PUBLISHES:
/// SUBSCRIBES:
/// FUNCTIONS:
#include <ros/ros.h>
#include <math.h>
#include <string>
#include <vector>
#include <mini_ros/spot.hpp>
#include <mini_ros/teleop.hpp>
#include "mini_ros/MiniCmd.h"
#include "std_msgs/Bool.h"
#include "std_srvs/Empty.h"
#include "mini_ros/JoyButtons.h"
#include <functional> // To use std::bind
int main(int argc, char** argv)
/// The Main Function ///
{
ROS_INFO("STARTING NODE: Teleoperation");
// Vars
double frequency = 60;
int linear_x = 4;
int linear_y = 3;
int linear_z = 1;
int angular = 0;
int sw = 0;
int es = 1;
int RB = 5;
int LB = 2;
int RT = 5;
int LT = 4;
int UD = 7;
int LR = 6;
double l_scale = 1.0;
double a_scale = 1.0;
double B_scale = 1.0;
double debounce_thresh = 0.15; // sec
ros::init(argc, argv, "teleop_node"); // register the node on ROS
ros::NodeHandle nh; // get a handle to ROS
ros::NodeHandle nh_("~"); // get a handle to ROS
// Parameters
nh_.getParam("frequency", frequency);
nh_.getParam("axis_linear_x", linear_x);
nh_.getParam("axis_linear_y", linear_y);
nh_.getParam("axis_linear_z", linear_z);
nh_.getParam("axis_angular", angular);
nh_.getParam("scale_linear", l_scale);
nh_.getParam("scale_angular", a_scale);
nh_.getParam("scale_bumper", B_scale);
nh_.getParam("button_switch", sw);
nh_.getParam("button_estop", es);
nh_.getParam("rb", RB);
nh_.getParam("lb", LB);
nh_.getParam("rt", RT);
nh_.getParam("lt", LT);
nh_.getParam("updown", UD);
nh_.getParam("leftright", LR);
nh_.getParam("debounce_thresh", debounce_thresh);
tele::Teleop teleop = tele::Teleop(linear_x, linear_y, linear_z, angular,
l_scale, a_scale, LB, RB, B_scale, LT,
RT, UD, LR, sw, es);
// Init Switch Movement Server
ros::ServiceClient switch_movement_client = nh.serviceClient<std_srvs::Empty>("switch_movement");
ros::service::waitForService("switch_movement", -1);
// Init ESTOP Publisher
ros::Publisher estop_pub = nh.advertise<std_msgs::Bool>("estop", 1);
// Init Command Publisher
ros::Publisher vel_pub = nh.advertise<geometry_msgs::Twist>("teleop", 1);
// Init Joy Button Publisher
ros::Publisher jb_pub = nh.advertise<mini_ros::JoyButtons>("joybuttons", 1);
// Init Subscriber (also handles pub)
// TODO: Figure out how to use std::bind properly
// ros::Subscriber joy_sub = nh.subscribe<sensor_msgs::Joy>("joy", 1, std::bind(&tele::Teleop::joyCallback, std::placeholders::_1, vel_pub), &teleop);
ros::Subscriber joy_sub = nh.subscribe<sensor_msgs::Joy>("joy", 1, &tele::Teleop::joyCallback, &teleop);
ros::Rate rate(frequency);
// Record time for debouncing buttons
ros::Time current_time = ros::Time::now();
ros::Time last_time = ros::Time::now();
// Main While
while (ros::ok())
{
ros::spinOnce();
current_time = ros::Time::now();
std_msgs::Bool estop;
estop.data = teleop.return_estop();
if (estop.data and current_time.toSec() - last_time.toSec() >= debounce_thresh)
{
ROS_INFO("SENDING E-STOP COMMAND!");
last_time = ros::Time::now();
} else if (!teleop.return_trigger())
{
// Send Twist
vel_pub.publish(teleop.return_twist());
estop.data = 0;
} else if (current_time.toSec() - last_time.toSec() >= debounce_thresh)
{
// Call Switch Service
std_srvs::Empty e;
switch_movement_client.call(e);
estop.data = 0;
last_time = ros::Time::now();
}
// pub buttons
jb_pub.publish(teleop.return_buttons());
estop_pub.publish(estop);
rate.sleep();
}
return 0;
} | 4,002 | C++ | 28.873134 | 154 | 0.582459 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/src/mini_ros/teleop.cpp | #include "mini_ros/teleop.hpp"
namespace tele
{
Teleop::Teleop(const int & linear_x, const int & linear_y, const int & linear_z,
const int & angular, const double & l_scale, const double & a_scale,
const int & LB, const int & RB, const int & B_scale, const int & LT,
const int & RT, const int & UD, const int & LR,
const int & sw, const int & es)
{
// step length or pitch
linear_x_ = linear_x;
// lateral fraction or roll
linear_y_ = linear_y;
// height
linear_z_ = linear_z;
// yaw rate or yaw
angular_ = angular;
// scale linear axis (usually just 1)
l_scale_ = l_scale;
// scale angular axis (usually just 1)
a_scale_ = a_scale;
// for incrementing and decrementing step velocity
// Bottom Bumpers
RB_ = RB;
LB_ = LB;
// scale Bottom Trigger axis (usually just 1)
B_scale_ = B_scale;
// Top Bumpers
RT_ = RT;
LT_ = LT;
// Switch between Stepping and Viewing
sw_ = sw;
// E-STOP
es_ = es;
// Arrow PAd
UD_ = UD;
LR_ = LR;
switch_trigger = false;
ESTOP = false;
updown = 0;
leftright = 0;
left_bump = false;
right_bump = false;
}
void Teleop::joyCallback(const sensor_msgs::Joy::ConstPtr& joy)
{
twist.linear.x = l_scale_*joy->axes[linear_x_];
twist.linear.y = l_scale_*joy->axes[linear_y_];
// NOTE: used to control robot height
twist.linear.z = -l_scale_*joy->axes[linear_z_];
twist.angular.z = a_scale_*joy->axes[angular_];
// NOTE: bottom bumpers used for changing step velocity
twist.angular.x = B_scale_*joy->axes[RB_];
twist.angular.y = B_scale_*joy->axes[LB_];
// Switch Trigger: Button A
switch_trigger = joy->buttons[sw_];
// ESTOP: Button B
ESTOP = joy->buttons[es_];
// Arrow Pad
updown = joy->axes[UD_];
leftright = -joy->axes[LR_];
// Top Bumpers
left_bump = joy->buttons[LT_];
right_bump = joy->buttons[RT_];
}
geometry_msgs::Twist Teleop::return_twist()
{
return twist;
}
bool Teleop::return_trigger()
{
return switch_trigger;
}
bool Teleop::return_estop()
{
return ESTOP;
}
mini_ros::JoyButtons Teleop::return_buttons()
{
mini_ros::JoyButtons jb;
jb.updown = updown;
jb.leftright = leftright;
jb.left_bump = left_bump;
jb.right_bump = right_bump;
return jb;
}
} | 2,308 | C++ | 21.637255 | 84 | 0.612218 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/src/mini_ros/spot.cpp | #include "mini_ros/spot.hpp"
namespace spot
{
// Spot Constructor
Spot::Spot()
{
cmd.x_velocity = 0.0;
cmd.y_velocity = 0.0;
cmd.rate = 0.0;
cmd.roll = 0.0;
cmd.pitch = 0.0;
cmd.yaw = 0.0;
cmd.z = 0.0;
cmd.motion = Stop;
cmd.movement = Viewing;
}
void Spot::update_command(const double & vx, const double & vy, const double & z,
const double & w, const double & wx, const double & wy)
{
// If Command is nearly zero, just give zero
if (almost_equal(vx, 0.0) and almost_equal(vy, 0.0) and almost_equal(z, 0.0) and almost_equal(w, 0.0))
{
cmd.motion = Stop;
cmd.x_velocity = 0.0;
cmd.y_velocity = 0.0;
cmd.rate = 0.0;
cmd.roll = 0.0;
cmd.pitch = 0.0;
cmd.yaw = 0.0;
cmd.z = 0.0;
cmd.faster = 0.0;
cmd.slower = 0.0;
} else
{
cmd.motion = Go;
if (cmd.movement == Stepping)
{
// Stepping Mode, use commands as vx, vy, rate, Z
cmd.x_velocity = vx;
cmd.y_velocity = vy;
cmd.rate = w;
cmd.z = z;
cmd.roll = 0.0;
cmd.pitch = 0.0;
cmd.yaw = 0.0;
// change clearance height from +- 0-2 * scaling
cmd.faster = 1.0 - wx;
cmd.slower = -(1.0 - wy);
} else
{
// Viewing Mode, use commands as RPY, Z
cmd.x_velocity = 0.0;
cmd.y_velocity = 0.0;
cmd.rate = 0.0;
cmd.roll = vy;
cmd.pitch = vx;
cmd.yaw = w;
cmd.z = z;
cmd.faster = 0.0;
cmd.slower = 0.0;
}
}
}
void Spot::switch_movement()
{
if (!almost_equal(cmd.x_velocity, 0.0) and !almost_equal(cmd.y_velocity, 0.0) and !almost_equal(cmd.rate, 0.0))
{
ROS_WARN("MAKE SURE BOTH LINEAR [%.2f, %.2f] AND ANGULAR VELOCITY [%.2f] ARE AT 0.0 BEFORE SWITCHING!", cmd.x_velocity, cmd.y_velocity, cmd.rate);
ROS_WARN("STOPPING ROBOT...");
cmd.motion = Stop;
cmd.x_velocity = 0.0;
cmd.y_velocity = 0.0;
cmd.rate = 0.0;
cmd.roll = 0.0;
cmd.pitch = 0.0;
cmd.yaw = 0.0;
cmd.z = 0.0;
cmd.faster = 0.0;
cmd.slower = 0.0;
} else
{
cmd.x_velocity = 0.0;
cmd.y_velocity = 0.0;
cmd.rate = 0.0;
cmd.roll = 0.0;
cmd.pitch = 0.0;
cmd.yaw = 0.0;
cmd.z = 0.0;
cmd.faster = 0.0;
cmd.slower = 0.0;
if (cmd.movement == Viewing)
{
ROS_INFO("SWITCHING TO STEPPING MOTION, COMMANDS NOW MAPPED TO VX|VY|W|Z.");
cmd.movement = Stepping;
cmd.motion = Stop;
} else
{
ROS_INFO("SWITCHING TO VIEWING MOTION, COMMANDS NOW MAPPED TO R|P|Y|Z.");
cmd.movement = Viewing;
cmd.motion = Stop;
}
}
}
SpotCommand Spot::return_command()
{
return cmd;
}
} | 2,725 | C++ | 22.101695 | 149 | 0.52844 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/include/mini_ros/teleop.hpp | #ifndef TELEOP_INCLUDE_GUARD_HPP
#define TELEOP_INCLUDE_GUARD_HPP
/// \file
/// \brief Teleoperation Library that converts Joystick commands to motion
#include <vector>
#include <ros/ros.h>
#include <geometry_msgs/Twist.h>
#include <sensor_msgs/Joy.h>
#include "mini_ros/JoyButtons.h"
namespace tele
{
// \brief Teleop class responsible for convertick Joystick commands into linear and angular velocity
class Teleop
{
public:
// \brief Teleop constructor that defines the axes used for control and sets their scaling factor
// \param linear_x: joystick axis assigned to linear velocity (x)
// \param linear_y: joystick axis assigned to linear velocity (y)
// \param linear_z: joystick axis assigned to robot height [overloading]
// \param angular: joystick axis assigned to angular velocity
// \param l_scale: scaling factor for linear velocity
// \param a_scale: scaling factor for angular velocity
// \param LB: left bottom bumper axis
// \param RB: right bottom bumper axis
// \param B_scale: scaling factor for bottom bumpers
// \param LT: left top bumper button
// \param RT: right top bumper button
// \param UD: up/down key on arrow pad
// \param LR: left/right key on arrow pad
// \param sw: button for switch_trigger
// \param es: button for ESTOP
Teleop(const int & linear_x, const int & linear_y, const int & linear_z,
const int & angular, const double & l_scale, const double & a_scale,
const int & LB, const int & RB, const int & B_scale, const int & LT,
const int & RT, const int & UD, const int & LR,
const int & sw, const int & es);
// \brief Takes a Joy messages and converts it to linear and angular velocity (Twist)
// \param joy: sensor_msgs describing Joystick inputs
void joyCallback(const sensor_msgs::Joy::ConstPtr& joy);
// \brief returns the most recently commanded Twist
// \returns: Twist
geometry_msgs::Twist return_twist();
// \brief returns a boolean indicating whether the movement switch trigger has been pressed
// \returns: switch_trigger(bool)
bool return_trigger();
// \brief returns whether the E-STOP has been pressed
// \returns: ESTOP(bool)
bool return_estop();
/// \brief returns other joystick buttons triggers, arrow pad etc)
mini_ros::JoyButtons return_buttons();
private:
// AXES ON JOYSTICK
int linear_x_ = 0;
int linear_y_ = 0;
int linear_z_ = 0;
int angular_= 0;
int RB_ = 0;
int LB_ = 0;
// BUTTONS ON JOYSTICK
int sw_ = 0;
int es_ = 0;
int RT_ = 0;
int LT_ = 0;
int UD_ = 0;
int LR_ = 0;
// AXIS SCALES
double l_scale_, a_scale_, B_scale_;
// TWIST
geometry_msgs::Twist twist;
// TRIGGERS
bool switch_trigger = false;
bool ESTOP = false;
int updown = 0;
int leftright = 0;
bool left_bump = false;
bool right_bump = false;
};
}
#endif
| 3,232 | C++ | 34.922222 | 105 | 0.601176 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/include/mini_ros/spot.hpp | #ifndef SPOT_INCLUDE_GUARD_HPP
#define SPOT_INCLUDE_GUARD_HPP
/// \file
/// \brief Spots library which contains control functionality for Spot Mini Mini.
#include <vector>
#include <ros/ros.h>
namespace spot
{
/// \brief approximately compare two floating-point numbers using
/// an absolute comparison
/// \param d1 - a number to compare
/// \param d2 - a second number to compare
/// \param epsilon - absolute threshold required for equality
/// \return true if abs(d1 - d2) < epsilon
/// Note: the fabs function in <cmath> (c++ equivalent of math.h) will
/// be useful here
// constexpr are all define in .hpp
// constexpr allows fcn to be run at compile time and interface with
// static_assert tests.
// Note high default epsilon since using controller
constexpr bool almost_equal(double d1, double d2, double epsilon=1.0e-1)
{
if (fabs(d1 - d2) < epsilon)
{
return true;
} else {
return false;
}
}
enum Motion {Go, Stop};
enum Movement {Stepping, Viewing};
// \brief Struct to store the commanded type of motion, velocity and rate
struct SpotCommand
{
Motion motion = Stop;
Movement movement = Viewing;
double x_velocity = 0.0;
double y_velocity = 0.0;
double rate = 0.0;
double roll = 0.0;
double pitch = 0.0;
double yaw = 0.0;
double z = 0.0;
double faster = 0.0;
double slower = 0.0;
};
// \brief Spot class responsible for high-level motion commands
class Spot
{
public:
// \brief Constructor for Spot class
Spot();
// \brief updates the type and velocity of motion to be commanded to the Spot
// \param vx: linear velocity (x)
// \param vy: linear velocity (y)
// \param z: robot height
// \param w: angular velocity
// \param wx: step height increase
// \param wy: step height decrease
void update_command(const double & vx, const double & vy, const double & z,
const double & w, const double & wx, const double & wy);
// \brief changes the commanded motion from Forward/Backward to Left/Right or vice-versa
void switch_movement();
// \brief returns the Spot's current command (Motion, v,w) for external use
// \returns SpotCommand
SpotCommand return_command();
private:
SpotCommand cmd;
};
}
#endif
| 2,544 | C++ | 29.297619 | 96 | 0.600236 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/config/policy_params.yaml | # AGENT PARAMS - SCALING and FILTERING
# Clearance Height and Penetration Depth
CD_SCALE: 0.05
# Step Length and Velocity
SLV_SCALE: 0.05
# Residuals
RESIDUALS_SCALE: 0.015
# Body Height Modulation
Z_SCALE: 0.035
# Exponential Filter Amplitude
alpha: 0.7
# Added this to avoid filtering residuals
# -1 for all
actions_to_filter: 14 | 331 | YAML | 22.714284 | 41 | 0.767372 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/config/control.yaml | spot:
joint_states_controller:
type: joint_state_controller/JointStateController
publish_rate: 50
joint_group_position_controller:
type: effort_controllers/JointTrajectoryController
joints:
- motor_front_left_hip
- motor_front_left_upper_leg
- motor_front_left_lower_leg
- motor_front_right_hip
- motor_front_right_upper_leg
- motor_front_right_lower_leg
- motor_back_left_hip
- motor_back_left_upper_leg
- motor_back_left_lower_leg
- motor_back_right_hip
- motor_back_right_upper_leg
- motor_back_right_lower_leg
gains:
motor_front_left_hip : {p: 180, d: 0.9, i: 20}
motor_front_left_upper_leg : {p: 180, d: 0.9, i: 20}
motor_front_left_lower_leg : {p: 180, d: 0.9, i: 20}
motor_front_right_hip : {p: 180, d: 0.9, i: 20}
motor_front_right_upper_leg : {p: 180, d: 0.9, i: 20}
motor_front_right_lower_leg : {p: 180, d: 0.9, i: 20}
motor_back_left_hip : {p: 180, d: 0.9, i: 20}
motor_back_left_upper_leg : {p: 180, d: 0.9, i: 20}
motor_back_left_lower_leg : {p: 180, d: 0.9, i: 20}
motor_back_right_hip : {p: 180, d: 0.9, i: 20}
motor_back_right_upper_leg : {p: 180, d: 0.9, i: 20}
motor_back_right_lower_leg : {p: 180, d: 0.9, i: 20} | 1,420 | YAML | 40.794116 | 61 | 0.545775 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/config/joy_params.yaml | # Right Joystick (Push U/D L/R)
STEPLENGTH_SCALE: 0.05
# Left Joystick (Push U/D)
Z_SCALE_CTRL: 0.15
# Right Joystick (Push U/D L/R) | Left Joystick (Push L/R)
RPY_SCALE: 0.785
# Lower Bumpers (Step Velocity [Left Lowers | Right Raises])
SV_SCALE: 0.05
# Arrow Pad (U/D for Clearance Height | L/R for Penetration Depth)
CHPD_SCALE: 0.0005
# Left Joystick (L/R)
YAW_SCALE: 1.25 | 376 | YAML | 30.416664 | 66 | 0.702128 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/mini_ros/config/spot_params.yaml | # MEASURE
# COM to Shoulder
shoulder_length: 0.055
# Shoulder to Elbow
elbow_length: 0.10652
# Elbow to Wrist
wrist_length: 0.145
# Forward Hip Separation
hip_x: 0.23
# Lateral Hip Separation
hip_y: 0.075
# ADJUSTABLE
# Stance Length
foot_x: 0.23
# Stance Width
foot_y: 0.185
# Stance Height
height: 0.20
# Adjust for balanced walk
com_offset: 0.0
# Time Step
dt: 0.001
# Swing Period (lower is faster)
Tswing: 0.2
SwingPeriod_LIMITS: [0.1, 0.3]
# Step Velocity (Using very low value as my main form of speed control is swing period)
BaseStepVelocity: 0.001
# Foot Clearance Height
BaseClearanceHeight: 0.035
ClearanceHeight_LIMITS: [0.0, 0.04]
# Foot Penetration Depth
BasePenetrationDepth: 0.003
PenetrationDepth_LIMITS: [0.0, 0.02] | 740 | YAML | 18 | 87 | 0.741892 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/spot.py | """
CODE BASED ON EXAMPLE FROM:
@misc{coumans2017pybullet,
title={Pybullet, a python module for physics simulation in robotics, games and machine learning},
author={Coumans, Erwin and Bai, Yunfei},
url={www.pybullet.org},
year={2017},
}
Example: minitaur.py
https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/bullet/minitaur.py
"""
import collections
import copy
import math
import re
import numpy as np
from . import motor
from spotmicro.util import pybullet_data
print(pybullet_data.getDataPath())
from spotmicro.Kinematics.SpotKinematics import SpotModel
import spotmicro.Kinematics.LieAlgebra as LA
INIT_POSITION = [0, 0, 0.25]
INIT_RACK_POSITION = [0, 0, 1]
# NOTE: URDF IS FACING THE WRONG WAY
# TEMP FIX
INIT_ORIENTATION = [0, 0, 0, 1]
OVERHEAT_SHUTDOWN_TORQUE = 2.45
OVERHEAT_SHUTDOWN_TIME = 1.0
# -math.pi / 5
INIT_LEG_POS = -0.658319
# math.pi / 3
INIT_FOOT_POS = 1.0472
OLD_LEG_POSITION = ["front_left", "front_right", "rear_left", "rear_right"]
OLD_MOTOR_NAMES = [
"motor_front_left_shoulder", "motor_front_left_leg",
"foot_motor_front_left", "motor_front_right_shoulder",
"motor_front_right_leg", "foot_motor_front_right",
"motor_rear_left_shoulder", "motor_rear_left_leg", "foot_motor_rear_left",
"motor_rear_right_shoulder", "motor_rear_right_leg",
"foot_motor_rear_right"
]
OLD_MOTOR_LIMITS_BY_NAME = {}
for name in OLD_MOTOR_NAMES:
if "shoulder" in name:
OLD_MOTOR_LIMITS_BY_NAME[name] = [-1.04, 1.04]
elif "leg" in name:
OLD_MOTOR_LIMITS_BY_NAME[name] = [-2.59, 1.571]
elif "foot" in name:
OLD_MOTOR_LIMITS_BY_NAME[name] = [-1.571, 2.9]
OLD_FOOT_NAMES = [
"front_left_toe", "front_right_toe", "rear_left_toe", "rear_right_toe"
]
LEG_POSITION = ["front_left", "front_right", "back_left", "back_right"]
MOTOR_NAMES = [
"motor_front_left_hip", "motor_front_left_upper_leg",
"motor_front_left_lower_leg", "motor_front_right_hip",
"motor_front_right_upper_leg", "motor_front_right_lower_leg",
"motor_back_left_hip", "motor_back_left_upper_leg",
"motor_back_left_lower_leg", "motor_back_right_hip",
"motor_back_right_upper_leg", "motor_back_right_lower_leg"
]
MOTOR_LIMITS_BY_NAME = {}
for name in MOTOR_NAMES:
if "hip" in name:
MOTOR_LIMITS_BY_NAME[name] = [-1.04, 1.04]
elif "upper_leg" in name:
MOTOR_LIMITS_BY_NAME[name] = [-1.571, 2.59]
elif "lower_leg" in name:
MOTOR_LIMITS_BY_NAME[name] = [-2.9, 1.671]
FOOT_NAMES = [
"front_left_leg_foot", "front_right_leg_foot", "back_left_leg_foot",
"back_right_leg_foot"
]
_CHASSIS_NAME_PATTERN = re.compile(r"chassis\D*")
_MOTOR_NAME_PATTERN = re.compile(r"motor\D*")
_FOOT_NAME_PATTERN = re.compile(r"foot\D*")
SENSOR_NOISE_STDDEV = (0.0, 0.0, 0.0, 0.0, 0.0)
TWO_PI = 2 * math.pi
def MapToMinusPiToPi(angles):
"""Maps a list of angles to [-pi, pi].
Args:
angles: A list of angles in rad.
Returns:
A list of angle mapped to [-pi, pi].
"""
mapped_angles = copy.deepcopy(angles)
for i in range(len(angles)):
mapped_angles[i] = math.fmod(angles[i], TWO_PI)
if mapped_angles[i] >= math.pi:
mapped_angles[i] -= TWO_PI
elif mapped_angles[i] < -math.pi:
mapped_angles[i] += TWO_PI
return mapped_angles
class Spot(object):
"""The spot class that simulates a quadruped robot.
"""
INIT_POSES = {
'stand':
np.array([
0.15192765, 0.7552236, -1.5104472, -0.15192765, 0.7552236,
-1.5104472, 0.15192765, 0.7552236, -1.5104472, -0.15192765,
0.7552236, -1.5104472
]),
'liedown':
np.array([-0.4, -1.5, 6, 0.4, -1.5, 6, -0.4, -1.5, 6, 0.4, -1.5, 6]),
'zero':
np.array([0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0, 0.0]),
}
def __init__(self,
pybullet_client,
urdf_root=pybullet_data.getDataPath(),
time_step=0.01,
action_repeat=1,
self_collision_enabled=False,
motor_velocity_limit=9.7,
pd_control_enabled=False,
accurate_motor_model_enabled=False,
remove_default_joint_damping=False,
max_force=100.0,
motor_kp=1.0,
motor_kd=0.02,
pd_latency=0.0,
control_latency=0.0,
observation_noise_stdev=SENSOR_NOISE_STDDEV,
torque_control_enabled=False,
motor_overheat_protection=False,
on_rack=False,
kd_for_pd_controllers=0.3,
pose_id='stand',
np_random=np.random,
contacts=True):
"""Constructs a spot and reset it to the initial states.
Args:
pybullet_client: The instance of BulletClient to manage different
simulations.
urdf_root: The path to the urdf folder.
time_step: The time step of the simulation.
action_repeat: The number of ApplyAction() for each control step.
self_collision_enabled: Whether to enable self collision.
motor_velocity_limit: The upper limit of the motor velocity.
pd_control_enabled: Whether to use PD control for the motors.
accurate_motor_model_enabled: Whether to use the accurate DC motor model.
remove_default_joint_damping: Whether to remove the default joint damping.
motor_kp: proportional gain for the accurate motor model.
motor_kd: derivative gain for the accurate motor model.
pd_latency: The latency of the observations (in seconds) used to calculate
PD control. On the real hardware, it is the latency between the
microcontroller and the motor controller.
control_latency: The latency of the observations (in second) used to
calculate action. On the real hardware, it is the latency from the motor
controller, the microcontroller to the host (Nvidia TX2).
observation_noise_stdev: The standard deviation of a Gaussian noise model
for the sensor. It should be an array for separate sensors in the
following order [motor_angle, motor_velocity, motor_torque,
base_roll_pitch_yaw, base_angular_velocity]
torque_control_enabled: Whether to use the torque control, if set to
False, pose control will be used.
motor_overheat_protection: Whether to shutdown the motor that has exerted
large torque (OVERHEAT_SHUTDOWN_TORQUE) for an extended amount of time
(OVERHEAT_SHUTDOWN_TIME). See ApplyAction() in spot.py for more
details.
on_rack: Whether to place the spot on rack. This is only used to debug
the walking gait. In this mode, the spot's base is hanged midair so
that its walking gait is clearer to visualize.
"""
# SPOT MODEL
self.spot = SpotModel()
# Whether to include contact sensing
self.contacts = contacts
# Control Inputs
self.StepLength = 0.0
self.StepVelocity = 0.0
self.LateralFraction = 0.0
self.YawRate = 0.0
# Leg Phases
self.LegPhases = [0.0, 0.0, 0.0, 0.0]
# used to calculate minitaur acceleration
self.init_leg = INIT_LEG_POS
self.init_foot = INIT_FOOT_POS
self.prev_ang_twist = np.array([0, 0, 0])
self.prev_lin_twist = np.array([0, 0, 0])
self.prev_lin_acc = np.array([0, 0, 0])
self.num_motors = 12
self.num_legs = int(self.num_motors / 3)
self._pybullet_client = pybullet_client
self._action_repeat = action_repeat
self._urdf_root = urdf_root
self._self_collision_enabled = self_collision_enabled
self._motor_velocity_limit = motor_velocity_limit
self._pd_control_enabled = pd_control_enabled
self._motor_direction = np.ones(self.num_motors)
self._observed_motor_torques = np.zeros(self.num_motors)
self._applied_motor_torques = np.zeros(self.num_motors)
self._max_force = max_force
self._pd_latency = pd_latency
self._control_latency = control_latency
self._observation_noise_stdev = observation_noise_stdev
self._accurate_motor_model_enabled = accurate_motor_model_enabled
self._remove_default_joint_damping = remove_default_joint_damping
self._observation_history = collections.deque(maxlen=100)
self._control_observation = []
self._chassis_link_ids = [-1]
self._leg_link_ids = []
self._motor_link_ids = []
self._foot_link_ids = []
self._torque_control_enabled = torque_control_enabled
self._motor_overheat_protection = motor_overheat_protection
self._on_rack = on_rack
self._pose_id = pose_id
self.np_random = np_random
if self._accurate_motor_model_enabled:
self._kp = motor_kp
self._kd = motor_kd
self._motor_model = motor.MotorModel(
torque_control_enabled=self._torque_control_enabled,
kp=self._kp,
kd=self._kd)
elif self._pd_control_enabled:
self._kp = 8
self._kd = kd_for_pd_controllers
else:
self._kp = 1
self._kd = 1
self.time_step = time_step
self._step_counter = 0
# reset_time=-1.0 means skipping the reset motion.
# See Reset for more details.
self.Reset(reset_time=-1)
self.init_on_rack_position = INIT_RACK_POSITION
self.init_position = INIT_POSITION
self.initial_pose = self.INIT_POSES[pose_id]
def _RecordMassInfoFromURDF(self):
self._base_mass_urdf = []
for chassis_id in self._chassis_link_ids:
self._base_mass_urdf.append(
self._pybullet_client.getDynamicsInfo(self.quadruped,
chassis_id)[0])
self._leg_masses_urdf = []
for leg_id in self._leg_link_ids:
self._leg_masses_urdf.append(
self._pybullet_client.getDynamicsInfo(self.quadruped,
leg_id)[0])
for motor_id in self._motor_link_ids:
self._leg_masses_urdf.append(
self._pybullet_client.getDynamicsInfo(self.quadruped,
motor_id)[0])
def GetBaseMassFromURDF(self):
"""Get the mass of the base from the URDF file."""
return self._base_mass_urdf
def SetBaseMass(self, base_mass):
for i in range(len(self._chassis_link_ids)):
self._pybullet_client.changeDynamics(self.quadruped,
self._chassis_link_ids[i],
mass=base_mass[i])
def _RecordInertiaInfoFromURDF(self):
"""Record the inertia of each body from URDF file."""
self._link_urdf = []
num_bodies = self._pybullet_client.getNumJoints(self.quadruped)
for body_id in range(-1, num_bodies): # -1 is for the base link.
inertia = self._pybullet_client.getDynamicsInfo(
self.quadruped, body_id)[2]
self._link_urdf.append(inertia)
# We need to use id+1 to index self._link_urdf because it has the base
# (index = -1) at the first element.
self._base_inertia_urdf = [
self._link_urdf[chassis_id + 1]
for chassis_id in self._chassis_link_ids
]
self._leg_inertia_urdf = [
self._link_urdf[leg_id + 1] for leg_id in self._leg_link_ids
]
self._leg_inertia_urdf.extend([
self._link_urdf[motor_id + 1] for motor_id in self._motor_link_ids
])
def _BuildJointNameToIdDict(self):
num_joints = self._pybullet_client.getNumJoints(self.quadruped)
self._joint_name_to_id = {}
for i in range(num_joints):
joint_info = self._pybullet_client.getJointInfo(self.quadruped, i)
self._joint_name_to_id[joint_info[1].decode(
"UTF-8")] = joint_info[0]
def _BuildMotorIdList(self):
self._motor_id_list = [
self._joint_name_to_id[motor_name] for motor_name in MOTOR_NAMES
]
def _BuildFootIdList(self):
self._foot_id_list = [
self._joint_name_to_id[foot_name] for foot_name in FOOT_NAMES
]
print(self._foot_id_list)
def _BuildUrdfIds(self):
"""Build the link Ids from its name in the URDF file."""
c = []
m = []
f = []
lg = []
num_joints = self._pybullet_client.getNumJoints(self.quadruped)
self._chassis_link_ids = [-1]
# the self._leg_link_ids include both the upper and lower links of the leg.
self._leg_link_ids = []
self._motor_link_ids = []
self._foot_link_ids = []
for i in range(num_joints):
joint_info = self._pybullet_client.getJointInfo(self.quadruped, i)
joint_name = joint_info[1].decode("UTF-8")
joint_id = self._joint_name_to_id[joint_name]
if _CHASSIS_NAME_PATTERN.match(joint_name):
c.append(joint_name)
self._chassis_link_ids.append(joint_id)
elif _MOTOR_NAME_PATTERN.match(joint_name):
m.append(joint_name)
self._motor_link_ids.append(joint_id)
elif _FOOT_NAME_PATTERN.match(joint_name):
f.append(joint_name)
self._foot_link_ids.append(joint_id)
else:
lg.append(joint_name)
self._leg_link_ids.append(joint_id)
self._leg_link_ids.extend(self._foot_link_ids)
self._chassis_link_ids.sort()
self._motor_link_ids.sort()
self._foot_link_ids.sort()
self._leg_link_ids.sort()
def Reset(self,
reload_urdf=True,
default_motor_angles=None,
reset_time=3.0):
"""Reset the spot to its initial states.
Args:
reload_urdf: Whether to reload the urdf file. If not, Reset() just place
the spot back to its starting position.
default_motor_angles: The default motor angles. If it is None, spot
will hold a default pose for 100 steps. In
torque control mode, the phase of holding the default pose is skipped.
reset_time: The duration (in seconds) to hold the default motor angles. If
reset_time <= 0 or in torque control mode, the phase of holding the
default pose is skipped.
"""
if self._on_rack:
init_position = INIT_RACK_POSITION
else:
init_position = INIT_POSITION
if reload_urdf:
if self._self_collision_enabled:
self.quadruped = self._pybullet_client.loadURDF(
pybullet_data.getDataPath() + "/assets/urdf/spot.urdf",
init_position,
useFixedBase=self._on_rack,
flags=self._pybullet_client.URDF_USE_SELF_COLLISION_EXCLUDE_PARENT)
else:
self.quadruped = self._pybullet_client.loadURDF(
pybullet_data.getDataPath() + "/assets/urdf/spot.urdf",
init_position,
INIT_ORIENTATION,
useFixedBase=self._on_rack)
self._BuildJointNameToIdDict()
self._BuildUrdfIds()
if self._remove_default_joint_damping:
self._RemoveDefaultJointDamping()
self._BuildMotorIdList()
self._BuildFootIdList()
self._RecordMassInfoFromURDF()
self._RecordInertiaInfoFromURDF()
self.ResetPose(add_constraint=True)
else:
self._pybullet_client.resetBasePositionAndOrientation(
self.quadruped, init_position, INIT_ORIENTATION)
self._pybullet_client.resetBaseVelocity(self.quadruped, [0, 0, 0],
[0, 0, 0])
# self._pybullet_client.changeDynamics(self.quadruped, -1, lateralFriction=0.8)
self.ResetPose(add_constraint=False)
self._overheat_counter = np.zeros(self.num_motors)
self._motor_enabled_list = [True] * self.num_motors
self._step_counter = 0
# Perform reset motion within reset_duration if in position control mode.
# Nothing is performed if in torque control mode for now.
self._observation_history.clear()
if reset_time > 0.0 and default_motor_angles is not None:
self.RealisticObservation()
for _ in range(100):
self.ApplyAction(self.initial_pose)
self._pybullet_client.stepSimulation()
self.RealisticObservation()
num_steps_to_reset = int(reset_time / self.time_step)
for _ in range(num_steps_to_reset):
self.ApplyAction(default_motor_angles)
self._pybullet_client.stepSimulation()
self.RealisticObservation()
self.RealisticObservation()
# Set Foot Friction
self.SetFootFriction()
def _RemoveDefaultJointDamping(self):
num_joints = self._pybullet_client.getNumJoints(self.quadruped)
for i in range(num_joints):
joint_info = self._pybullet_client.getJointInfo(self.quadruped, i)
self._pybullet_client.changeDynamics(joint_info[0],
-1,
linearDamping=0,
angularDamping=0)
def _SetMotorTorqueById(self, motor_id, torque):
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=motor_id,
controlMode=self._pybullet_client.TORQUE_CONTROL,
force=torque)
def _SetDesiredMotorAngleById(self, motor_id, desired_angle):
if self._pd_control_enabled or self._accurate_motor_model_enabled:
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=motor_id,
controlMode=self._pybullet_client.POSITION_CONTROL,
targetPosition=desired_angle,
positionGain=self._kp,
velocityGain=self._kd,
force=self._max_force)
# Pybullet has a 'perfect' joint controller with its default p,d
else:
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=motor_id,
controlMode=self._pybullet_client.POSITION_CONTROL,
targetPosition=desired_angle)
def _SetDesiredMotorAngleByName(self, motor_name, desired_angle):
self._SetDesiredMotorAngleById(self._joint_name_to_id[motor_name],
desired_angle)
def ResetPose(self, add_constraint):
"""Reset the pose of the spot.
Args:
add_constraint: Whether to add a constraint at the joints of two feet.
"""
for i in range(self.num_legs):
self._ResetPoseForLeg(i, add_constraint)
def _ResetPoseForLeg(self, leg_id, add_constraint):
"""Reset the initial pose for the leg.
Args:
leg_id: It should be 0, 1, 2, or 3, which represents the leg at
front_left, back_left, front_right and back_right.
add_constraint: Whether to add a constraint at the joints of two feet.
"""
knee_friction_force = 0
pi = math.pi
leg_position = LEG_POSITION[leg_id]
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["motor_" + leg_position + "_hip"],
self.INIT_POSES[self._pose_id][3 * leg_id],
targetVelocity=0)
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["motor_" + leg_position + "_upper_leg"],
self.INIT_POSES[self._pose_id][3 * leg_id + 1],
targetVelocity=0)
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["motor_" + leg_position + "_lower_leg"],
self.INIT_POSES[self._pose_id][3 * leg_id + 2],
targetVelocity=0)
if self._accurate_motor_model_enabled or self._pd_control_enabled:
# Disable the default motor in pybullet.
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["motor_" + leg_position +
"_hip"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["motor_" + leg_position +
"_upper_leg"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["motor_" + leg_position +
"_lower_leg"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
def GetBasePosition(self):
"""Get the position of spot's base.
Returns:
The position of spot's base.
"""
position, _ = (self._pybullet_client.getBasePositionAndOrientation(
self.quadruped))
return position
def GetBaseOrientation(self):
"""Get the orientation of spot's base, represented as quaternion.
Returns:
The orientation of spot's base.
"""
_, orientation = (self._pybullet_client.getBasePositionAndOrientation(
self.quadruped))
return orientation
def GetBaseRollPitchYaw(self):
"""Get the rate of orientation change of the spot's base in euler angle.
Returns:
rate of (roll, pitch, yaw) change of the spot's base.
"""
vel = self._pybullet_client.getBaseVelocity(self.quadruped)
return np.asarray([vel[1][0], vel[1][1], vel[1][2]])
def GetBaseRollPitchYawRate(self):
"""Get the rate of orientation change of the spot's base in euler angle.
This function mimicks the noisy sensor reading and adds latency.
Returns:
rate of (roll, pitch, yaw) change of the spot's base polluted by noise
and latency.
"""
return self._AddSensorNoise(
np.array(self._control_observation[3 * self.num_motors +
4:3 * self.num_motors + 7]),
self._observation_noise_stdev[4])
def GetBaseTwist(self):
"""Get the Twist of minitaur's base.
Returns:
The Twist of the minitaur's base.
"""
return self._pybullet_client.getBaseVelocity(self.quadruped)
def GetActionDimension(self):
"""Get the length of the action list.
Returns:
The length of the action list.
"""
return self.num_motors
def GetObservationUpperBound(self):
"""Get the upper bound of the observation.
Returns:
The upper bound of an observation. See GetObservation() for the details
of each element of an observation.
NOTE: Changed just like GetObservation()
"""
upper_bound = np.array([0.0] * self.GetObservationDimension())
# roll, pitch
upper_bound[0:2] = 2.0 * np.pi
# acc, rate in x,y,z
upper_bound[2:8] = np.inf
# Leg Phases
upper_bound[8:12] = 2.0
# Contacts
if self.contacts:
upper_bound[12:] = 1.0
return upper_bound
def GetObservationLowerBound(self):
"""Get the lower bound of the observation."""
return -self.GetObservationUpperBound()
def GetObservationDimension(self):
"""Get the length of the observation list.
Returns:
The length of the observation list.
"""
return len(self.GetObservation())
def GetObservation(self):
"""Get the observations of minitaur.
It includes the angles, velocities, torques and the orientation of the base.
Returns:
The observation list. observation[0:8] are motor angles. observation[8:16]
are motor velocities, observation[16:24] are motor torques.
observation[24:28] is the orientation of the base, in quaternion form.
NOTE: DIVERGES FROM STOCK MINITAUR ENV. WILL LEAVE ORIGINAL COMMENTED
For my purpose, the observation space includes Roll and Pitch, as well as
acceleration and gyroscopic rate along the x,y,z axes. All of this
information can be collected from an onboard IMU. The reward function
will contain a hidden velocity reward (fwd, bwd) which cannot be measured
and so is not included. For spinning, the gyroscopic z rate will be used
as the (explicit) velocity reward.
This version operates without motor torques, angles and velocities. Erwin
Coumans' paper suggests a sparse observation space leads to higher reward
# NOTE: use True version for perfect data, or other for realistic data
"""
observation = []
# GETTING TWIST IN BODY FRAME
pos = self.GetBasePosition()
orn = self.GetBaseOrientation()
roll, pitch, yaw = self._pybullet_client.getEulerFromQuaternion(
[orn[0], orn[1], orn[2], orn[3]])
# rpy = LA.RPY(roll, pitch, yaw)
# R, _ = LA.TransToRp(rpy)
# T_wb = LA.RpToTrans(R, np.array([pos[0], pos[1], pos[2]]))
# T_bw = LA.TransInv(T_wb)
# Adj_Tbw = LA.Adjoint(T_bw)
# Get Linear and Angular Twist in WORLD FRAME
lin_twist, ang_twist = self.GetBaseTwist()
lin_twist = np.array([lin_twist[0], lin_twist[1], lin_twist[2]])
ang_twist = np.array([ang_twist[0], ang_twist[1], ang_twist[2]])
# Vw = np.concatenate((ang_twist, lin_twist))
# Vb = np.dot(Adj_Tbw, Vw)
# roll, pitch, _ = self._pybullet_client.getEulerFromQuaternion(
# [orn[0], orn[1], orn[2], orn[3]])
# # Get linear accelerations
# lin_twist = -Vb[3:]
# ang_twist = Vb[:3]
lin_acc = lin_twist - self.prev_lin_twist
if lin_acc.all() == 0.0:
lin_acc = self.prev_lin_acc
self.prev_lin_acc = lin_acc
# print("LIN TWIST: ", lin_twist)
self.prev_lin_twist = lin_twist
self.prev_ang_twist = ang_twist
# Get Contacts
CONTACT = list(self._pybullet_client.getContactPoints(self.quadruped))
FLC = 0
FRC = 0
BLC = 0
BRC = 0
if len(CONTACT) > 0:
for i in range(len(CONTACT)):
Contact_Link_Index = CONTACT[i][3]
if Contact_Link_Index == self._foot_id_list[0]:
FLC = 1
# print("FL CONTACT")
if Contact_Link_Index == self._foot_id_list[1]:
FRC = 1
# print("FR CONTACT")
if Contact_Link_Index == self._foot_id_list[2]:
BLC = 1
# print("BL CONTACT")
if Contact_Link_Index == self._foot_id_list[3]:
BRC = 1
# print("BR CONTACT")
# order: roll, pitch, gyro(x,y,z), acc(x, y, z)
observation.append(roll)
observation.append(pitch)
observation.extend(list(ang_twist))
observation.extend(list(lin_acc))
# Control Input
# observation.append(self.StepLength)
# observation.append(self.StepVelocity)
# observation.append(self.LateralFraction)
# observation.append(self.YawRate)
observation.extend(self.LegPhases)
if self.contacts:
observation.append(FLC)
observation.append(FRC)
observation.append(BLC)
observation.append(BRC)
# print("CONTACTS: {} {} {} {}".format(FLC, FRC, BLC, BRC))
return observation
def GetControlInput(self, controller):
""" Store Control Input as Observation
"""
_, _, StepLength, LateralFraction, YawRate, StepVelocity, _, _ = controller.return_bezier_params(
)
self.StepLength = StepLength
self.StepVelocity = StepVelocity
self.LateralFraction = LateralFraction
self.YawRate = YawRate
def GetLegPhases(self, TrajectoryGenerator):
""" Leg phases according to TG from 0->2
0->1: Stance
1->2 Swing
"""
self.LegPhases = TrajectoryGenerator.Phases
def GetExternalObservations(self, TrajectoryGenerator, controller):
""" Augment State Space
"""
self.GetControlInput(controller)
self.GetLegPhases(TrajectoryGenerator)
def ConvertFromLegModel(self, action):
# TODO
joint_angles = action
return joint_angles
def ApplyMotorLimits(self, joint_angles):
eps = 0.001
for i in range(len(joint_angles)):
LIM = MOTOR_LIMITS_BY_NAME[MOTOR_NAMES[i]]
joint_angles[i] = np.clip(joint_angles[i], LIM[0] + eps,
LIM[1] - eps)
return joint_angles
def ApplyAction(self, motor_commands):
"""Set the desired motor angles to the motors of the minitaur.
The desired motor angles are clipped based on the maximum allowed velocity.
If the pd_control_enabled is True, a torque is calculated according to
the difference between current and desired joint angle, as well as the joint
velocity. This torque is exerted to the motor. For more information about
PD control, please refer to: https://en.wikipedia.org/wiki/PID_controller.
Args:
motor_commands: The eight desired motor angles.
"""
# FIRST, APPLY MOTOR LIMITS:
motor_commands = self.ApplyMotorLimits(motor_commands)
if self._motor_velocity_limit < np.inf:
current_motor_angle = self.GetMotorAngles()
motor_commands_max = (current_motor_angle +
self.time_step * self._motor_velocity_limit)
motor_commands_min = (current_motor_angle -
self.time_step * self._motor_velocity_limit)
motor_commands = np.clip(motor_commands, motor_commands_min,
motor_commands_max)
if self._accurate_motor_model_enabled or self._pd_control_enabled:
q = self.GetMotorAngles()
qdot = self.GetMotorVelocities()
if self._accurate_motor_model_enabled:
actual_torque, observed_torque = self._motor_model.convert_to_torque(
motor_commands, q, qdot)
if self._motor_overheat_protection:
for i in range(self.num_motors):
if abs(actual_torque[i]) > OVERHEAT_SHUTDOWN_TORQUE:
self._overheat_counter[i] += 1
else:
self._overheat_counter[i] = 0
if (self._overheat_counter[i] >
OVERHEAT_SHUTDOWN_TIME / self.time_step):
self._motor_enabled_list[i] = False
# The torque is already in the observation space because we use
# GetMotorAngles and GetMotorVelocities.
self._observed_motor_torques = observed_torque
# Transform into the motor space when applying the torque.
self._applied_motor_torque = np.multiply(
actual_torque, self._motor_direction)
for motor_id, motor_torque, motor_enabled in zip(
self._motor_id_list, self._applied_motor_torque,
self._motor_enabled_list):
if motor_enabled:
self._SetMotorTorqueById(motor_id, motor_torque)
else:
self._SetMotorTorqueById(motor_id, 0)
else:
torque_commands = -self._kp * (
q - motor_commands) - self._kd * qdot
# The torque is already in the observation space because we use
# GetMotorAngles and GetMotorVelocities.
self._observed_motor_torques = torque_commands
# Transform into the motor space when applying the torque.
self._applied_motor_torques = np.multiply(
self._observed_motor_torques, self._motor_direction)
for motor_id, motor_torque in zip(self._motor_id_list,
self._applied_motor_torques):
self._SetMotorTorqueById(motor_id, motor_torque)
else:
motor_commands_with_direction = np.multiply(
motor_commands, self._motor_direction)
for motor_id, motor_command_with_direction in zip(
self._motor_id_list, motor_commands_with_direction):
self._SetDesiredMotorAngleById(motor_id,
motor_command_with_direction)
def Step(self, action):
for _ in range(self._action_repeat):
self.ApplyAction(action)
self._pybullet_client.stepSimulation()
self.RealisticObservation()
self._step_counter += 1
def GetTimeSinceReset(self):
return self._step_counter * self.time_step
def GetMotorAngles(self):
"""Gets the eight motor angles at the current moment, mapped to [-pi, pi].
Returns:
Motor angles, mapped to [-pi, pi].
"""
motor_angles = [
self._pybullet_client.getJointState(self.quadruped, motor_id)[0]
for motor_id in self._motor_id_list
]
motor_angles = np.multiply(motor_angles, self._motor_direction)
return MapToMinusPiToPi(motor_angles)
def GetMotorVelocities(self):
"""Get the velocity of all eight motors.
Returns:
Velocities of all eight motors.
"""
motor_velocities = [
self._pybullet_client.getJointState(self.quadruped, motor_id)[1]
for motor_id in self._motor_id_list
]
motor_velocities = np.multiply(motor_velocities, self._motor_direction)
return motor_velocities
def GetMotorTorques(self):
"""Get the amount of torque the motors are exerting.
Returns:
Motor torques of all eight motors.
"""
if self._accurate_motor_model_enabled or self._pd_control_enabled:
return self._observed_motor_torques
else:
motor_torques = [
self._pybullet_client.getJointState(self.quadruped,
motor_id)[3]
for motor_id in self._motor_id_list
]
motor_torques = np.multiply(motor_torques, self._motor_direction)
return motor_torques
def GetBaseMassesFromURDF(self):
"""Get the mass of the base from the URDF file."""
return self._base_mass_urdf
def GetBaseInertiasFromURDF(self):
"""Get the inertia of the base from the URDF file."""
return self._base_inertia_urdf
def GetLegMassesFromURDF(self):
"""Get the mass of the legs from the URDF file."""
return self._leg_masses_urdf
def GetLegInertiasFromURDF(self):
"""Get the inertia of the legs from the URDF file."""
return self._leg_inertia_urdf
def SetBaseMasses(self, base_mass):
"""Set the mass of spot's base.
Args:
base_mass: A list of masses of each body link in CHASIS_LINK_IDS. The
length of this list should be the same as the length of CHASIS_LINK_IDS.
Raises:
ValueError: It is raised when the length of base_mass is not the same as
the length of self._chassis_link_ids.
"""
if len(base_mass) != len(self._chassis_link_ids):
raise ValueError(
"The length of base_mass {} and self._chassis_link_ids {} are not "
"the same.".format(len(base_mass),
len(self._chassis_link_ids)))
for chassis_id, chassis_mass in zip(self._chassis_link_ids, base_mass):
self._pybullet_client.changeDynamics(self.quadruped,
chassis_id,
mass=chassis_mass)
def SetLegMasses(self, leg_masses):
"""Set the mass of the legs.
Args:
leg_masses: The leg and motor masses for all the leg links and motors.
Raises:
ValueError: It is raised when the length of masses is not equal to number
of links + motors.
"""
if len(leg_masses) != len(self._leg_link_ids) + len(
self._motor_link_ids):
raise ValueError("The number of values passed to SetLegMasses are "
"different than number of leg links and motors.")
for leg_id, leg_mass in zip(self._leg_link_ids, leg_masses):
self._pybullet_client.changeDynamics(self.quadruped,
leg_id,
mass=leg_mass)
motor_masses = leg_masses[len(self._leg_link_ids):]
for link_id, motor_mass in zip(self._motor_link_ids, motor_masses):
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
mass=motor_mass)
def SetBaseInertias(self, base_inertias):
"""Set the inertias of spot's base.
Args:
base_inertias: A list of inertias of each body link in CHASIS_LINK_IDS.
The length of this list should be the same as the length of
CHASIS_LINK_IDS.
Raises:
ValueError: It is raised when the length of base_inertias is not the same
as the length of self._chassis_link_ids and base_inertias contains
negative values.
"""
if len(base_inertias) != len(self._chassis_link_ids):
raise ValueError(
"The length of base_inertias {} and self._chassis_link_ids {} are "
"not the same.".format(len(base_inertias),
len(self._chassis_link_ids)))
for chassis_id, chassis_inertia in zip(self._chassis_link_ids,
base_inertias):
for inertia_value in chassis_inertia:
if (np.asarray(inertia_value) < 0).any():
raise ValueError(
"Values in inertia matrix should be non-negative.")
self._pybullet_client.changeDynamics(
self.quadruped,
chassis_id,
localInertiaDiagonal=chassis_inertia)
def SetLegInertias(self, leg_inertias):
"""Set the inertias of the legs.
Args:
leg_inertias: The leg and motor inertias for all the leg links and motors.
Raises:
ValueError: It is raised when the length of inertias is not equal to
the number of links + motors or leg_inertias contains negative values.
"""
if len(leg_inertias) != len(self._leg_link_ids) + len(
self._motor_link_ids):
raise ValueError("The number of values passed to SetLegMasses are "
"different than number of leg links and motors.")
for leg_id, leg_inertia in zip(self._leg_link_ids, leg_inertias):
for inertia_value in leg_inertias:
if (np.asarray(inertia_value) < 0).any():
raise ValueError(
"Values in inertia matrix should be non-negative.")
self._pybullet_client.changeDynamics(
self.quadruped, leg_id, localInertiaDiagonal=leg_inertia)
motor_inertias = leg_inertias[len(self._leg_link_ids):]
for link_id, motor_inertia in zip(self._motor_link_ids,
motor_inertias):
for inertia_value in motor_inertias:
if (np.asarray(inertia_value) < 0).any():
raise ValueError(
"Values in inertia matrix should be non-negative.")
self._pybullet_client.changeDynamics(
self.quadruped, link_id, localInertiaDiagonal=motor_inertia)
def SetFootFriction(self, foot_friction=100.0):
"""Set the lateral friction of the feet.
Args:
foot_friction: The lateral friction coefficient of the foot. This value is
shared by all four feet.
"""
for link_id in self._foot_link_ids:
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
lateralFriction=foot_friction)
# TODO(b/73748980): Add more API's to set other contact parameters.
def SetFootRestitution(self, link_id, foot_restitution=1.0):
"""Set the coefficient of restitution at the feet.
Args:
foot_restitution: The coefficient of restitution (bounciness) of the feet.
This value is shared by all four feet.
"""
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
restitution=foot_restitution)
def SetJointFriction(self, joint_frictions):
for knee_joint_id, friction in zip(self._foot_link_ids,
joint_frictions):
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=knee_joint_id,
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=friction)
def GetNumKneeJoints(self):
return len(self._foot_link_ids)
def SetBatteryVoltage(self, voltage):
if self._accurate_motor_model_enabled:
self._motor_model.set_voltage(voltage)
def SetMotorViscousDamping(self, viscous_damping):
if self._accurate_motor_model_enabled:
self._motor_model.set_viscous_damping(viscous_damping)
def RealisticObservation(self):
"""Receive the observation from sensors.
This function is called once per step. The observations are only updated
when this function is called.
"""
self._observation_history.appendleft(self.GetObservation())
self._control_observation = self._GetDelayedObservation(
self._control_latency)
self._control_observation = self._AddSensorNoise(
self._control_observation, self._observation_noise_stdev)
return self._control_observation
def _GetDelayedObservation(self, latency):
"""Get observation that is delayed by the amount specified in latency.
Args:
latency: The latency (in seconds) of the delayed observation.
Returns:
observation: The observation which was actually latency seconds ago.
"""
if latency <= 0 or len(self._observation_history) == 1:
observation = self._observation_history[0]
else:
n_steps_ago = int(latency / self.time_step)
if n_steps_ago + 1 >= len(self._observation_history):
return self._observation_history[-1]
remaining_latency = latency - n_steps_ago * self.time_step
blend_alpha = remaining_latency / self.time_step
observation = (
(1.0 - blend_alpha) *
np.array(self._observation_history[n_steps_ago]) +
blend_alpha *
np.array(self._observation_history[n_steps_ago + 1]))
return observation
def _GetPDObservation(self):
pd_delayed_observation = self._GetDelayedObservation(self._pd_latency)
q = pd_delayed_observation[0:self.num_motors]
qdot = pd_delayed_observation[self.num_motors:2 * self.num_motors]
return (np.array(q), np.array(qdot))
def _AddSensorNoise(self, observation, noise_stdev):
# if self._observation_noise_stdev > 0:
# observation += (self.np_random.normal(scale=noise_stdev,
# size=observation.shape) *
# self.GetObservationUpperBound())
return observation
def SetControlLatency(self, latency):
"""Set the latency of the control loop.
It measures the duration between sending an action from Nvidia TX2 and
receiving the observation from microcontroller.
Args:
latency: The latency (in seconds) of the control loop.
"""
self._control_latency = latency
def GetControlLatency(self):
"""Get the control latency.
Returns:
The latency (in seconds) between when the motor command is sent and when
the sensor measurements are reported back to the controller.
"""
return self._control_latency
def SetMotorGains(self, kp, kd):
"""Set the gains of all motors.
These gains are PD gains for motor positional control. kp is the
proportional gain and kd is the derivative gain.
Args:
kp: proportional gain of the motors.
kd: derivative gain of the motors.
"""
self._kp = kp
self._kd = kd
if self._accurate_motor_model_enabled:
self._motor_model.set_motor_gains(kp, kd)
def GetMotorGains(self):
"""Get the gains of the motor.
Returns:
The proportional gain.
The derivative gain.
"""
return self._kp, self._kd
def SetMotorStrengthRatio(self, ratio):
"""Set the strength of all motors relative to the default value.
Args:
ratio: The relative strength. A scalar range from 0.0 to 1.0.
"""
if self._accurate_motor_model_enabled:
self._motor_model.set_strength_ratios([ratio] * self.num_motors)
def SetMotorStrengthRatios(self, ratios):
"""Set the strength of each motor relative to the default value.
Args:
ratios: The relative strength. A numpy array ranging from 0.0 to 1.0.
"""
if self._accurate_motor_model_enabled:
self._motor_model.set_strength_ratios(ratios)
def SetTimeSteps(self, action_repeat, simulation_step):
"""Set the time steps of the control and simulation.
Args:
action_repeat: The number of simulation steps that the same action is
repeated.
simulation_step: The simulation time step.
"""
self.time_step = simulation_step
self._action_repeat = action_repeat
@property
def chassis_link_ids(self):
return self._chassis_link_ids
| 47,996 | Python | 40.269991 | 107 | 0.578777 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/spot_gym_env.py | """
CODE BASED ON EXAMPLE FROM:
@misc{coumans2017pybullet,
title={Pybullet, a python module for physics simulation in robotics, games and machine learning},
author={Coumans, Erwin and Bai, Yunfei},
url={www.pybullet.org},
year={2017},
}
Example: minitaur_gym_env.py
https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/minitaur/envs/minitaur_gym_env.py
"""
import math
import time
import gym
import numpy as np
import pybullet
import pybullet_data
from gym import spaces
from gym.utils import seeding
from pkg_resources import parse_version
from spotmicro import spot
import pybullet_utils.bullet_client as bullet_client
from gym.envs.registration import register
from spotmicro.heightfield import HeightField
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
import spotmicro.Kinematics.LieAlgebra as LA
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
NUM_SUBSTEPS = 5
NUM_MOTORS = 12
MOTOR_ANGLE_OBSERVATION_INDEX = 0
MOTOR_VELOCITY_OBSERVATION_INDEX = MOTOR_ANGLE_OBSERVATION_INDEX + NUM_MOTORS
MOTOR_TORQUE_OBSERVATION_INDEX = MOTOR_VELOCITY_OBSERVATION_INDEX + NUM_MOTORS
BASE_ORIENTATION_OBSERVATION_INDEX = MOTOR_TORQUE_OBSERVATION_INDEX + NUM_MOTORS
ACTION_EPS = 0.01
OBSERVATION_EPS = 0.01
RENDER_HEIGHT = 720
RENDER_WIDTH = 960
SENSOR_NOISE_STDDEV = spot.SENSOR_NOISE_STDDEV
DEFAULT_URDF_VERSION = "default"
NUM_SIMULATION_ITERATION_STEPS = 1000
spot_URDF_VERSION_MAP = {DEFAULT_URDF_VERSION: spot.Spot}
# Register as OpenAI Gym Environment
register(
id="SpotMicroEnv-v0",
entry_point='spotmicro.spot_gym_env:spotGymEnv',
max_episode_steps=1000,
)
def convert_to_list(obj):
try:
iter(obj)
return obj
except TypeError:
return [obj]
class spotGymEnv(gym.Env):
"""The gym environment for spot.
It simulates the locomotion of spot, a quadruped robot. The state space
include the angles, velocities and torques for all the motors and the action
space is the desired motor angle for each motor. The reward function is based
on how far spot walks in 1000 steps and penalizes the energy
expenditure.
"""
metadata = {
"render.modes": ["human", "rgb_array"],
"video.frames_per_second": 50
}
def __init__(self,
distance_weight=1.0,
rotation_weight=1.0,
energy_weight=0.0005,
shake_weight=0.005,
drift_weight=2.0,
rp_weight=0.1,
rate_weight=0.1,
urdf_root=pybullet_data.getDataPath(),
urdf_version=None,
distance_limit=float("inf"),
observation_noise_stdev=SENSOR_NOISE_STDDEV,
self_collision_enabled=True,
motor_velocity_limit=np.inf,
pd_control_enabled=False,
leg_model_enabled=False,
accurate_motor_model_enabled=False,
remove_default_joint_damping=False,
motor_kp=2.0,
motor_kd=0.03,
control_latency=0.0,
pd_latency=0.0,
torque_control_enabled=False,
motor_overheat_protection=False,
hard_reset=False,
on_rack=False,
render=True,
num_steps_to_log=1000,
action_repeat=1,
control_time_step=None,
env_randomizer=SpotEnvRandomizer(),
forward_reward_cap=float("inf"),
reflection=True,
log_path=None,
desired_velocity=0.5,
desired_rate=0.0,
lateral=False,
draw_foot_path=False,
height_field=False,
height_field_iters=2,
AutoStepper=False,
contacts=True):
"""Initialize the spot gym environment.
Args:
urdf_root: The path to the urdf data folder.
urdf_version: [DEFAULT_URDF_VERSION] are allowable
versions. If None, DEFAULT_URDF_VERSION is used.
distance_weight: The weight of the distance term in the reward.
energy_weight: The weight of the energy term in the reward.
shake_weight: The weight of the vertical shakiness term in the reward.
drift_weight: The weight of the sideways drift term in the reward.
distance_limit: The maximum distance to terminate the episode.
observation_noise_stdev: The standard deviation of observation noise.
self_collision_enabled: Whether to enable self collision in the sim.
motor_velocity_limit: The velocity limit of each motor.
pd_control_enabled: Whether to use PD controller for each motor.
leg_model_enabled: Whether to use a leg motor to reparameterize the action
space.
accurate_motor_model_enabled: Whether to use the accurate DC motor model.
remove_default_joint_damping: Whether to remove the default joint damping.
motor_kp: proportional gain for the accurate motor model.
motor_kd: derivative gain for the accurate motor model.
control_latency: It is the delay in the controller between when an
observation is made at some point, and when that reading is reported
back to the Neural Network.
pd_latency: latency of the PD controller loop. PD calculates PWM based on
the motor angle and velocity. The latency measures the time between when
the motor angle and velocity are observed on the microcontroller and
when the true state happens on the motor. It is typically (0.001-
0.002s).
torque_control_enabled: Whether to use the torque control, if set to
False, pose control will be used.
motor_overheat_protection: Whether to shutdown the motor that has exerted
large torque (OVERHEAT_SHUTDOWN_TORQUE) for an extended amount of time
(OVERHEAT_SHUTDOWN_TIME). See ApplyAction() in spot.py for more
details.
hard_reset: Whether to wipe the simulation and load everything when reset
is called. If set to false, reset just place spot back to start
position and set its pose to initial configuration.
on_rack: Whether to place spot on rack. This is only used to debug
the walking gait. In this mode, spot's base is hanged midair so
that its walking gait is clearer to visualize.
render: Whether to render the simulation.
num_steps_to_log: The max number of control steps in one episode that will
be logged. If the number of steps is more than num_steps_to_log, the
environment will still be running, but only first num_steps_to_log will
be recorded in logging.
action_repeat: The number of simulation steps before actions are applied.
control_time_step: The time step between two successive control signals.
env_randomizer: An instance (or a list) of EnvRandomizer(s). An
EnvRandomizer may randomize the physical property of spot, change
the terrrain during reset(), or add perturbation forces during step().
forward_reward_cap: The maximum value that forward reward is capped at.
Disabled (Inf) by default.
log_path: The path to write out logs. For the details of logging, refer to
spot_logging.proto.
Raises:
ValueError: If the urdf_version is not supported.
"""
# Sense Contacts
self.contacts = contacts
# Enable Auto Stepper State Machine
self.AutoStepper = AutoStepper
# Enable Rough Terrain or Not
self.height_field = height_field
self.draw_foot_path = draw_foot_path
# DRAWING FEET PATH
self.prev_feet_path = np.array([[0.0, 0.0, 0.0], [0.0, 0.0, 0.0],
[0.0, 0.0, 0.0], [0.0, 0.0, 0.0]])
# CONTROL METRICS
self.desired_velocity = desired_velocity
self.desired_rate = desired_rate
self.lateral = lateral
# Set up logging.
self._log_path = log_path
# @TODO fix logging
# NUM ITERS
self._time_step = 0.01
self._action_repeat = action_repeat
self._num_bullet_solver_iterations = 300
self.logging = None
if pd_control_enabled or accurate_motor_model_enabled:
self._time_step /= NUM_SUBSTEPS
self._num_bullet_solver_iterations /= NUM_SUBSTEPS
self._action_repeat *= NUM_SUBSTEPS
# PD control needs smaller time step for stability.
if control_time_step is not None:
self.control_time_step = control_time_step
else:
# Get Control Timestep
self.control_time_step = self._time_step * self._action_repeat
# TODO: Fix the value of self._num_bullet_solver_iterations.
self._num_bullet_solver_iterations = int(
NUM_SIMULATION_ITERATION_STEPS / self._action_repeat)
# URDF
self._urdf_root = urdf_root
self._self_collision_enabled = self_collision_enabled
self._motor_velocity_limit = motor_velocity_limit
self._observation = []
self._true_observation = []
self._objectives = []
self._objective_weights = [
distance_weight, energy_weight, drift_weight, shake_weight
]
self._env_step_counter = 0
self._num_steps_to_log = num_steps_to_log
self._is_render = render
self._last_base_position = [0, 0, 0]
self._last_base_orientation = [0, 0, 0, 1]
self._distance_weight = distance_weight
self._rotation_weight = rotation_weight
self._energy_weight = energy_weight
self._drift_weight = drift_weight
self._shake_weight = shake_weight
self._rp_weight = rp_weight
self._rate_weight = rate_weight
self._distance_limit = distance_limit
self._observation_noise_stdev = observation_noise_stdev
self._action_bound = 1
self._pd_control_enabled = pd_control_enabled
self._leg_model_enabled = leg_model_enabled
self._accurate_motor_model_enabled = accurate_motor_model_enabled
self._remove_default_joint_damping = remove_default_joint_damping
self._motor_kp = motor_kp
self._motor_kd = motor_kd
self._torque_control_enabled = torque_control_enabled
self._motor_overheat_protection = motor_overheat_protection
self._on_rack = on_rack
self._cam_dist = 1.0
self._cam_yaw = 0
self._cam_pitch = -30
self._forward_reward_cap = forward_reward_cap
self._hard_reset = True
self._last_frame_time = 0.0
self._control_latency = control_latency
self._pd_latency = pd_latency
self._urdf_version = urdf_version
self._ground_id = None
self._reflection = reflection
self._env_randomizer = env_randomizer
# @TODO fix logging
self._episode_proto = None
if self._is_render:
self._pybullet_client = bullet_client.BulletClient(
connection_mode=pybullet.GUI)
else:
self._pybullet_client = bullet_client.BulletClient()
if self._urdf_version is None:
self._urdf_version = DEFAULT_URDF_VERSION
self._pybullet_client.setPhysicsEngineParameter(enableConeFriction=0)
self.seed()
# Only update after HF has been generated
self.height_field = False
self.reset()
observation_high = (self.spot.GetObservationUpperBound() +
OBSERVATION_EPS)
observation_low = (self.spot.GetObservationLowerBound() -
OBSERVATION_EPS)
action_dim = NUM_MOTORS
action_high = np.array([self._action_bound] * action_dim)
self.action_space = spaces.Box(-action_high, action_high)
self.observation_space = spaces.Box(observation_low, observation_high)
self.viewer = None
self._hard_reset = hard_reset # This assignment need to be after reset()
self.goal_reached = False
# Generate HeightField or not
self.height_field = height_field
self.hf = HeightField()
if self.height_field:
# Do 3x for extra roughness
for i in range(height_field_iters):
self.hf._generate_field(self)
def set_env_randomizer(self, env_randomizer):
self._env_randomizer = env_randomizer
def configure(self, args):
self._args = args
def reset(self,
initial_motor_angles=None,
reset_duration=1.0,
desired_velocity=None,
desired_rate=None):
# Use Autostepper
if self.AutoStepper:
self.StateMachine = BezierStepper(dt=self._time_step)
# Shuffle order of states
self.StateMachine.reshuffle()
self._pybullet_client.configureDebugVisualizer(
self._pybullet_client.COV_ENABLE_RENDERING, 0)
if self._hard_reset:
self._pybullet_client.resetSimulation()
self._pybullet_client.setPhysicsEngineParameter(
numSolverIterations=int(self._num_bullet_solver_iterations))
self._pybullet_client.setTimeStep(self._time_step)
self._ground_id = self._pybullet_client.loadURDF("%s/plane.urdf" %
self._urdf_root)
if self._reflection:
self._pybullet_client.changeVisualShape(
self._ground_id, -1, rgbaColor=[1, 1, 1, 0.8])
self._pybullet_client.configureDebugVisualizer(
self._pybullet_client.COV_ENABLE_PLANAR_REFLECTION,
self._ground_id)
self._pybullet_client.setGravity(0, 0, -9.81)
acc_motor = self._accurate_motor_model_enabled
motor_protect = self._motor_overheat_protection
if self._urdf_version not in spot_URDF_VERSION_MAP:
raise ValueError("%s is not a supported urdf_version." %
self._urdf_version)
else:
self.spot = (spot_URDF_VERSION_MAP[self._urdf_version](
pybullet_client=self._pybullet_client,
action_repeat=self._action_repeat,
urdf_root=self._urdf_root,
time_step=self._time_step,
self_collision_enabled=self._self_collision_enabled,
motor_velocity_limit=self._motor_velocity_limit,
pd_control_enabled=self._pd_control_enabled,
accurate_motor_model_enabled=acc_motor,
remove_default_joint_damping=self.
_remove_default_joint_damping,
motor_kp=self._motor_kp,
motor_kd=self._motor_kd,
control_latency=self._control_latency,
pd_latency=self._pd_latency,
observation_noise_stdev=self._observation_noise_stdev,
torque_control_enabled=self._torque_control_enabled,
motor_overheat_protection=motor_protect,
on_rack=self._on_rack,
np_random=self.np_random,
contacts=self.contacts))
self.spot.Reset(reload_urdf=False,
default_motor_angles=initial_motor_angles,
reset_time=reset_duration)
if self._env_randomizer is not None:
self._env_randomizer.randomize_env(self)
# Also update heightfield if wr are wholly randomizing
if self.height_field:
self.hf.UpdateHeightField()
if desired_velocity is not None:
self.desired_velocity = desired_velocity
if desired_rate is not None:
self.desired_rate = desired_rate
self._pybullet_client.setPhysicsEngineParameter(enableConeFriction=0)
self._env_step_counter = 0
self._last_base_position = [0, 0, 0]
self._last_base_orientation = [0, 0, 0, 1]
self._objectives = []
self._pybullet_client.resetDebugVisualizerCamera(
self._cam_dist, self._cam_yaw, self._cam_pitch, [0, 0, 0])
self._pybullet_client.configureDebugVisualizer(
self._pybullet_client.COV_ENABLE_RENDERING, 1)
return self._get_observation()
def seed(self, seed=None):
self.np_random, seed = seeding.np_random(seed)
return [seed]
def _transform_action_to_motor_command(self, action):
if self._leg_model_enabled:
for i, action_component in enumerate(action):
if not (-self._action_bound - ACTION_EPS <= action_component <=
self._action_bound + ACTION_EPS):
raise ValueError("{}th action {} out of bounds.".format(
i, action_component))
action = self.spot.ConvertFromLegModel(action)
return action
def step(self, action):
"""Step forward the simulation, given the action.
Args:
action: A list of desired motor angles for eight motors.
Returns:
observations: The angles, velocities and torques of all motors.
reward: The reward for the current state-action pair.
done: Whether the episode has ended.
info: A dictionary that stores diagnostic information.
Raises:
ValueError: The action dimension is not the same as the number of motors.
ValueError: The magnitude of actions is out of bounds.
"""
self._last_base_position = self.spot.GetBasePosition()
self._last_base_orientation = self.spot.GetBaseOrientation()
# print("ACTION:")
# print(action)
if self._is_render:
# Sleep, otherwise the computation takes less time than real time,
# which will make the visualization like a fast-forward video.
time_spent = time.time() - self._last_frame_time
self._last_frame_time = time.time()
time_to_sleep = self.control_time_step - time_spent
if time_to_sleep > 0:
time.sleep(time_to_sleep)
base_pos = self.spot.GetBasePosition()
# Keep the previous orientation of the camera set by the user.
[yaw, pitch,
dist] = self._pybullet_client.getDebugVisualizerCamera()[8:11]
self._pybullet_client.resetDebugVisualizerCamera(
dist, yaw, pitch, base_pos)
action = self._transform_action_to_motor_command(action)
self.spot.Step(action)
reward = self._reward()
done = self._termination()
self._env_step_counter += 1
# DRAW FOOT PATH
if self.draw_foot_path:
self.DrawFootPath()
return np.array(self._get_observation()), reward, done, {}
def render(self, mode="rgb_array", close=False):
if mode != "rgb_array":
return np.array([])
base_pos = self.spot.GetBasePosition()
view_matrix = self._pybullet_client.computeViewMatrixFromYawPitchRoll(
cameraTargetPosition=base_pos,
distance=self._cam_dist,
yaw=self._cam_yaw,
pitch=self._cam_pitch,
roll=0,
upAxisIndex=2)
proj_matrix = self._pybullet_client.computeProjectionMatrixFOV(
fov=60,
aspect=float(RENDER_WIDTH) / RENDER_HEIGHT,
nearVal=0.1,
farVal=100.0)
(_, _, px, _, _) = self._pybullet_client.getCameraImage(
width=RENDER_WIDTH,
height=RENDER_HEIGHT,
renderer=self._pybullet_client.ER_BULLET_HARDWARE_OPENGL,
viewMatrix=view_matrix,
projectionMatrix=proj_matrix)
rgb_array = np.array(px)
rgb_array = rgb_array[:, :, :3]
return rgb_array
def DrawFootPath(self):
# Get Foot Positions
FL = self._pybullet_client.getLinkState(self.spot.quadruped,
self.spot._foot_id_list[0])[0]
FR = self._pybullet_client.getLinkState(self.spot.quadruped,
self.spot._foot_id_list[1])[0]
BL = self._pybullet_client.getLinkState(self.spot.quadruped,
self.spot._foot_id_list[2])[0]
BR = self._pybullet_client.getLinkState(self.spot.quadruped,
self.spot._foot_id_list[3])[0]
lifetime = 3.0 # sec
self._pybullet_client.addUserDebugLine(self.prev_feet_path[0],
FL, [1, 0, 0],
lifeTime=lifetime)
self._pybullet_client.addUserDebugLine(self.prev_feet_path[1],
FR, [0, 1, 0],
lifeTime=lifetime)
self._pybullet_client.addUserDebugLine(self.prev_feet_path[2],
BL, [0, 0, 1],
lifeTime=lifetime)
self._pybullet_client.addUserDebugLine(self.prev_feet_path[3],
BR, [1, 1, 0],
lifeTime=lifetime)
self.prev_feet_path[0] = FL
self.prev_feet_path[1] = FR
self.prev_feet_path[2] = BL
self.prev_feet_path[3] = BR
def get_spot_motor_angles(self):
"""Get the spot's motor angles.
Returns:
A numpy array of motor angles.
"""
return np.array(
self._observation[MOTOR_ANGLE_OBSERVATION_INDEX:
MOTOR_ANGLE_OBSERVATION_INDEX + NUM_MOTORS])
def get_spot_motor_velocities(self):
"""Get the spot's motor velocities.
Returns:
A numpy array of motor velocities.
"""
return np.array(
self._observation[MOTOR_VELOCITY_OBSERVATION_INDEX:
MOTOR_VELOCITY_OBSERVATION_INDEX + NUM_MOTORS])
def get_spot_motor_torques(self):
"""Get the spot's motor torques.
Returns:
A numpy array of motor torques.
"""
return np.array(
self._observation[MOTOR_TORQUE_OBSERVATION_INDEX:
MOTOR_TORQUE_OBSERVATION_INDEX + NUM_MOTORS])
def get_spot_base_orientation(self):
"""Get the spot's base orientation, represented by a quaternion.
Returns:
A numpy array of spot's orientation.
"""
return np.array(self._observation[BASE_ORIENTATION_OBSERVATION_INDEX:])
def is_fallen(self):
"""Decide whether spot has fallen.
If the up directions between the base and the world is larger (the dot
product is smaller than 0.85) or the base is very low on the ground
(the height is smaller than 0.13 meter), spot is considered fallen.
Returns:
Boolean value that indicates whether spot has fallen.
"""
orientation = self.spot.GetBaseOrientation()
rot_mat = self._pybullet_client.getMatrixFromQuaternion(orientation)
local_up = rot_mat[6:]
pos = self.spot.GetBasePosition()
# or pos[2] < 0.13
return (np.dot(np.asarray([0, 0, 1]), np.asarray(local_up)) < 0.55)
def _termination(self):
position = self.spot.GetBasePosition()
distance = math.sqrt(position[0]**2 + position[1]**2)
return self.is_fallen() or distance > self._distance_limit
def _reward(self):
""" NOTE: reward now consists of:
roll, pitch at desired 0
acc (y,z) = 0
FORWARD-BACKWARD: rate(x,y,z) = 0
--> HIDDEN REWARD: x(+-) velocity reference, not incl. in obs
SPIN: acc(x) = 0, rate(x,y) = 0, rate (z) = rate reference
Also include drift, energy vanilla rewards
"""
current_base_position = self.spot.GetBasePosition()
# get observation
obs = self._get_observation()
# forward_reward = current_base_position[0] - self._last_base_position[0]
# # POSITIVE FOR FORWARD, NEGATIVE FOR BACKWARD | NOTE: HIDDEN
# GETTING TWIST IN BODY FRAME
pos = self.spot.GetBasePosition()
orn = self.spot.GetBaseOrientation()
roll, pitch, yaw = self._pybullet_client.getEulerFromQuaternion(
[orn[0], orn[1], orn[2], orn[3]])
rpy = LA.RPY(roll, pitch, yaw)
R, _ = LA.TransToRp(rpy)
T_wb = LA.RpToTrans(R, np.array([pos[0], pos[1], pos[2]]))
T_bw = LA.TransInv(T_wb)
Adj_Tbw = LA.Adjoint(T_bw)
Vw = np.concatenate(
(self.spot.prev_ang_twist, self.spot.prev_lin_twist))
Vb = np.dot(Adj_Tbw, Vw)
# New Twist in Body Frame
# POSITIVE FOR FORWARD, NEGATIVE FOR BACKWARD | NOTE: HIDDEN
fwd_speed = -Vb[3] # vx
lat_speed = -Vb[4] # vy
# fwd_speed = self.spot.prev_lin_twist[0]
# lat_speed = self.spot.prev_lin_twist[1]
# print("FORWARD SPEED: {} \t STATE SPEED: {}".format(
# fwd_speed, self.desired_velocity))
# self.desired_velocity = 0.4
# Modification for lateral/fwd rewards
reward_max = 1.0
# FORWARD
if not self.lateral:
# f(x)=-(x-desired))^(2)*((1/desired)^2)+1
# to make sure that at 0vel there is 0 reawrd.
# also squishes allowable tolerance
forward_reward = reward_max * np.exp(
-(fwd_speed - self.desired_velocity)**2 / (0.1))
# LATERAL
else:
forward_reward = reward_max * np.exp(
-(lat_speed - self.desired_velocity)**2 / (0.1))
yaw_rate = obs[4]
rot_reward = reward_max * np.exp(-(yaw_rate - self.desired_rate)**2 /
(0.1))
# Make sure that for forward-policy there is the appropriate rotation penalty
if self.desired_velocity != 0:
self._rotation_weight = self._rate_weight
rot_reward = -abs(obs[4])
elif self.desired_rate != 0:
forward_reward = 0.0
# penalty for nonzero roll, pitch
rp_reward = -(abs(obs[0]) + abs(obs[1]))
# print("ROLL: {} \t PITCH: {}".format(obs[0], obs[1]))
# penalty for nonzero acc(z)
shake_reward = -abs(obs[4])
# penalty for nonzero rate (x,y,z)
rate_reward = -(abs(obs[2]) + abs(obs[3]))
# drift_reward = -abs(current_base_position[1] -
# self._last_base_position[1])
# this penalizes absolute error, and does not penalize correction
# NOTE: for side-side, drift reward becomes in x instead
drift_reward = -abs(current_base_position[1])
# If Lateral, change drift reward
if self.lateral:
drift_reward = -abs(current_base_position[0])
# shake_reward = -abs(current_base_position[2] -
# self._last_base_position[2])
self._last_base_position = current_base_position
energy_reward = -np.abs(
np.dot(self.spot.GetMotorTorques(),
self.spot.GetMotorVelocities())) * self._time_step
reward = (self._distance_weight * forward_reward +
self._rotation_weight * rot_reward +
self._energy_weight * energy_reward +
self._drift_weight * drift_reward +
self._shake_weight * shake_reward +
self._rp_weight * rp_reward +
self._rate_weight * rate_reward)
self._objectives.append(
[forward_reward, energy_reward, drift_reward, shake_reward])
# print("REWARD: ", reward)
return reward
def get_objectives(self):
return self._objectives
@property
def objective_weights(self):
"""Accessor for the weights for all the objectives.
Returns:
List of floating points that corresponds to weights for the objectives in
the order that objectives are stored.
"""
return self._objective_weights
def _get_observation(self):
"""Get observation of this environment, including noise and latency.
spot class maintains a history of true observations. Based on the
latency, this function will find the observation at the right time,
interpolate if necessary. Then Gaussian noise is added to this observation
based on self.observation_noise_stdev.
Returns:
The noisy observation with latency.
"""
self._observation = self.spot.GetObservation()
return self._observation
def _get_realistic_observation(self):
"""Get the observations of this environment.
It includes the angles, velocities, torques and the orientation of the base.
Returns:
The observation list. observation[0:8] are motor angles. observation[8:16]
are motor velocities, observation[16:24] are motor torques.
observation[24:28] is the orientation of the base, in quaternion form.
"""
self._observation = self.spot.RealisticObservation()
return self._observation
if parse_version(gym.__version__) < parse_version('0.9.6'):
_render = render
_reset = reset
_seed = seed
_step = step
def set_time_step(self, control_step, simulation_step=0.001):
"""Sets the time step of the environment.
Args:
control_step: The time period (in seconds) between two adjacent control
actions are applied.
simulation_step: The simulation time step in PyBullet. By default, the
simulation step is 0.001s, which is a good trade-off between simulation
speed and accuracy.
Raises:
ValueError: If the control step is smaller than the simulation step.
"""
if control_step < simulation_step:
raise ValueError(
"Control step should be larger than or equal to simulation step."
)
self.control_time_step = control_step
self._time_step = simulation_step
self._action_repeat = int(round(control_step / simulation_step))
self._num_bullet_solver_iterations = (NUM_SIMULATION_ITERATION_STEPS /
self._action_repeat)
self._pybullet_client.setPhysicsEngineParameter(
numSolverIterations=self._num_bullet_solver_iterations)
self._pybullet_client.setTimeStep(self._time_step)
self.spot.SetTimeSteps(action_repeat=self._action_repeat,
simulation_step=self._time_step)
@property
def pybullet_client(self):
return self._pybullet_client
@property
def ground_id(self):
return self._ground_id
@ground_id.setter
def ground_id(self, new_ground_id):
self._ground_id = new_ground_id
@property
def env_step_counter(self):
return self._env_step_counter
| 31,142 | Python | 40.358566 | 122 | 0.598452 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/spot_env_randomizer.py | """
CODE BASED ON EXAMPLE FROM:
@misc{coumans2017pybullet,
title={Pybullet, a python module for physics simulation in robotics, games and machine learning},
author={Coumans, Erwin and Bai, Yunfei},
url={www.pybullet.org},
year={2017},
}
Example: minitaur_env_randomizer.py
https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/minitaur/envs/env_randomizers/minitaur_env_randomizer.py
"""
import numpy as np
from . import env_randomizer_base
# Relative range.
spot_BASE_MASS_ERROR_RANGE = (-0.2, 0.2) # 0.2 means 20%
spot_LEG_MASS_ERROR_RANGE = (-0.2, 0.2) # 0.2 means 20%
# Absolute range.
BATTERY_VOLTAGE_RANGE = (7.0, 8.4) # Unit: Volt
MOTOR_VISCOUS_DAMPING_RANGE = (0, 0.01) # Unit: N*m*s/rad (torque/angular vel)
spot_LEG_FRICTION = (0.8, 1.5) # Unit: dimensionless
class SpotEnvRandomizer(env_randomizer_base.EnvRandomizerBase):
"""A randomizer that change the spot_gym_env during every reset."""
def __init__(self,
spot_base_mass_err_range=spot_BASE_MASS_ERROR_RANGE,
spot_leg_mass_err_range=spot_LEG_MASS_ERROR_RANGE,
battery_voltage_range=BATTERY_VOLTAGE_RANGE,
motor_viscous_damping_range=MOTOR_VISCOUS_DAMPING_RANGE):
self._spot_base_mass_err_range = spot_base_mass_err_range
self._spot_leg_mass_err_range = spot_leg_mass_err_range
self._battery_voltage_range = battery_voltage_range
self._motor_viscous_damping_range = motor_viscous_damping_range
np.random.seed(0)
def randomize_env(self, env):
self._randomize_spot(env.spot)
def _randomize_spot(self, spot):
"""Randomize various physical properties of spot.
It randomizes the mass/inertia of the base, mass/inertia of the legs,
friction coefficient of the feet, the battery voltage and the motor damping
at each reset() of the environment.
Args:
spot: the spot instance in spot_gym_env environment.
"""
base_mass = spot.GetBaseMassFromURDF()
# print("BM: ", base_mass)
randomized_base_mass = np.random.uniform(
np.array([base_mass]) * (1.0 + self._spot_base_mass_err_range[0]),
np.array([base_mass]) * (1.0 + self._spot_base_mass_err_range[1]))
spot.SetBaseMass(randomized_base_mass[0])
leg_masses = spot.GetLegMassesFromURDF()
leg_masses_lower_bound = np.array(leg_masses) * (
1.0 + self._spot_leg_mass_err_range[0])
leg_masses_upper_bound = np.array(leg_masses) * (
1.0 + self._spot_leg_mass_err_range[1])
randomized_leg_masses = [
np.random.uniform(leg_masses_lower_bound[i],
leg_masses_upper_bound[i])
for i in range(len(leg_masses))
]
spot.SetLegMasses(randomized_leg_masses)
randomized_battery_voltage = np.random.uniform(
BATTERY_VOLTAGE_RANGE[0], BATTERY_VOLTAGE_RANGE[1])
spot.SetBatteryVoltage(randomized_battery_voltage)
randomized_motor_damping = np.random.uniform(
MOTOR_VISCOUS_DAMPING_RANGE[0], MOTOR_VISCOUS_DAMPING_RANGE[1])
spot.SetMotorViscousDamping(randomized_motor_damping)
randomized_foot_friction = np.random.uniform(spot_LEG_FRICTION[0],
spot_LEG_FRICTION[1])
spot.SetFootFriction(randomized_foot_friction)
| 3,428 | Python | 40.817073 | 145 | 0.648483 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/heightfield.py | """
CODE BASED ON EXAMPLE FROM:
@misc{coumans2017pybullet,
title={Pybullet, a python module for physics simulation in robotics, games and machine learning},
author={Coumans, Erwin and Bai, Yunfei},
url={www.pybullet.org},
year={2017},
}
Example: heightfield.py
https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/examples/heightfield.py
"""
import pybullet as p
import pybullet_data as pd
import math
import time
textureId = -1
useProgrammatic = 0
useTerrainFromPNG = 1
useDeepLocoCSV = 2
updateHeightfield = False
heightfieldSource = useProgrammatic
numHeightfieldRows = 256
numHeightfieldColumns = 256
import random
random.seed(10)
class HeightField():
def __init__(self):
self.hf_id = 0
self.terrainShape = 0
self.heightfieldData = [0] * numHeightfieldRows * numHeightfieldColumns
def _generate_field(self, env, heightPerturbationRange=0.08):
env.pybullet_client.setAdditionalSearchPath(pd.getDataPath())
env.pybullet_client.configureDebugVisualizer(
env.pybullet_client.COV_ENABLE_RENDERING, 0)
heightPerturbationRange = heightPerturbationRange
if heightfieldSource == useProgrammatic:
for j in range(int(numHeightfieldColumns / 2)):
for i in range(int(numHeightfieldRows / 2)):
height = random.uniform(0, heightPerturbationRange)
self.heightfieldData[2 * i +
2 * j * numHeightfieldRows] = height
self.heightfieldData[2 * i + 1 +
2 * j * numHeightfieldRows] = height
self.heightfieldData[2 * i + (2 * j + 1) *
numHeightfieldRows] = height
self.heightfieldData[2 * i + 1 + (2 * j + 1) *
numHeightfieldRows] = height
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.07, .07, 1.6],
heightfieldTextureScaling=(numHeightfieldRows - 1) / 2,
heightfieldData=self.heightfieldData,
numHeightfieldRows=numHeightfieldRows,
numHeightfieldColumns=numHeightfieldColumns)
terrain = env.pybullet_client.createMultiBody(0, terrainShape)
env.pybullet_client.resetBasePositionAndOrientation(
terrain, [0, 0, 0.0], [0, 0, 0, 1])
env.pybullet_client.changeDynamics(terrain,
-1,
lateralFriction=1.0)
if heightfieldSource == useDeepLocoCSV:
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.5, .5, 2.5],
fileName="heightmaps/ground0.txt",
heightfieldTextureScaling=128)
terrain = env.pybullet_client.createMultiBody(0, terrainShape)
env.pybullet_client.resetBasePositionAndOrientation(
terrain, [0, 0, 0], [0, 0, 0, 1])
env.pybullet_client.changeDynamics(terrain,
-1,
lateralFriction=1.0)
if heightfieldSource == useTerrainFromPNG:
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.05, .05, 1.8],
fileName="heightmaps/wm_height_out.png")
textureId = env.pybullet_client.loadTexture(
"heightmaps/gimp_overlay_out.png")
terrain = env.pybullet_client.createMultiBody(0, terrainShape)
env.pybullet_client.changeVisualShape(terrain,
-1,
textureUniqueId=textureId)
env.pybullet_client.resetBasePositionAndOrientation(
terrain, [0, 0, 0.1], [0, 0, 0, 1])
env.pybullet_client.changeDynamics(terrain,
-1,
lateralFriction=1.0)
self.hf_id = terrainShape
self.terrainShape = terrainShape
print("TERRAIN SHAPE: {}".format(terrainShape))
env.pybullet_client.changeVisualShape(terrain,
-1,
rgbaColor=[1, 1, 1, 1])
env.pybullet_client.configureDebugVisualizer(
env.pybullet_client.COV_ENABLE_RENDERING, 1)
def UpdateHeightField(self, heightPerturbationRange=0.08):
if heightfieldSource == useProgrammatic:
for j in range(int(numHeightfieldColumns / 2)):
for i in range(int(numHeightfieldRows / 2)):
height = random.uniform(
0, heightPerturbationRange) # +math.sin(time.time())
self.heightfieldData[2 * i +
2 * j * numHeightfieldRows] = height
self.heightfieldData[2 * i + 1 +
2 * j * numHeightfieldRows] = height
self.heightfieldData[2 * i + (2 * j + 1) *
numHeightfieldRows] = height
self.heightfieldData[2 * i + 1 + (2 * j + 1) *
numHeightfieldRows] = height
#GEOM_CONCAVE_INTERNAL_EDGE may help avoid getting stuck at an internal (shared) edge of the triangle/heightfield.
#GEOM_CONCAVE_INTERNAL_EDGE is a bit slower to build though.
#flags = p.GEOM_CONCAVE_INTERNAL_EDGE
flags = 0
self.terrainShape = p.createCollisionShape(
shapeType=p.GEOM_HEIGHTFIELD,
flags=flags,
meshScale=[.05, .05, 1],
heightfieldTextureScaling=(numHeightfieldRows - 1) / 2,
heightfieldData=self.heightfieldData,
numHeightfieldRows=numHeightfieldRows,
numHeightfieldColumns=numHeightfieldColumns,
replaceHeightfieldIndex=self.terrainShape)
| 6,396 | Python | 44.368794 | 126 | 0.553627 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/Kinematics/SpotKinematics.py | #!/usr/bin/env python
import numpy as np
from spotmicro.Kinematics.LegKinematics import LegIK
from spotmicro.Kinematics.LieAlgebra import RpToTrans, TransToRp, TransInv, RPY, TransformVector
from collections import OrderedDict
class SpotModel:
def __init__(self,
shoulder_length=0.055,
elbow_length=0.10652,
wrist_length=0.145,
hip_x=0.23,
hip_y=0.075,
foot_x=0.23,
foot_y=0.185,
height=0.20,
com_offset=0.016,
shoulder_lim=[-0.548, 0.548],
elbow_lim=[-2.17, 0.97],
wrist_lim=[-0.1, 2.59]):
"""
Spot Micro Kinematics
"""
# COM offset in x direction
self.com_offset = com_offset
# Leg Parameters
self.shoulder_length = shoulder_length
self.elbow_length = elbow_length
self.wrist_length = wrist_length
# Leg Vector desired_positions
# Distance Between Hips
# Length
self.hip_x = hip_x
# Width
self.hip_y = hip_y
# Distance Between Feet
# Length
self.foot_x = foot_x
# Width
self.foot_y = foot_y
# Body Height
self.height = height
# Joint Parameters
self.shoulder_lim = shoulder_lim
self.elbow_lim = elbow_lim
self.wrist_lim = wrist_lim
# Dictionary to store Leg IK Solvers
self.Legs = OrderedDict()
self.Legs["FL"] = LegIK("LEFT", self.shoulder_length,
self.elbow_length, self.wrist_length,
self.shoulder_lim, self.elbow_lim,
self.wrist_lim)
self.Legs["FR"] = LegIK("RIGHT", self.shoulder_length,
self.elbow_length, self.wrist_length,
self.shoulder_lim, self.elbow_lim,
self.wrist_lim)
self.Legs["BL"] = LegIK("LEFT", self.shoulder_length,
self.elbow_length, self.wrist_length,
self.shoulder_lim, self.elbow_lim,
self.wrist_lim)
self.Legs["BR"] = LegIK("RIGHT", self.shoulder_length,
self.elbow_length, self.wrist_length,
self.shoulder_lim, self.elbow_lim,
self.wrist_lim)
# Dictionary to store Hip and Foot Transforms
# Transform of Hip relative to world frame
# With Body Centroid also in world frame
Rwb = np.eye(3)
self.WorldToHip = OrderedDict()
self.ph_FL = np.array([self.hip_x / 2.0, self.hip_y / 2.0, 0])
self.WorldToHip["FL"] = RpToTrans(Rwb, self.ph_FL)
self.ph_FR = np.array([self.hip_x / 2.0, -self.hip_y / 2.0, 0])
self.WorldToHip["FR"] = RpToTrans(Rwb, self.ph_FR)
self.ph_BL = np.array([-self.hip_x / 2.0, self.hip_y / 2.0, 0])
self.WorldToHip["BL"] = RpToTrans(Rwb, self.ph_BL)
self.ph_BR = np.array([-self.hip_x / 2.0, -self.hip_y / 2.0, 0])
self.WorldToHip["BR"] = RpToTrans(Rwb, self.ph_BR)
# Transform of Foot relative to world frame
# With Body Centroid also in world frame
self.WorldToFoot = OrderedDict()
self.pf_FL = np.array(
[self.foot_x / 2.0, self.foot_y / 2.0, -self.height])
self.WorldToFoot["FL"] = RpToTrans(Rwb, self.pf_FL)
self.pf_FR = np.array(
[self.foot_x / 2.0, -self.foot_y / 2.0, -self.height])
self.WorldToFoot["FR"] = RpToTrans(Rwb, self.pf_FR)
self.pf_BL = np.array(
[-self.foot_x / 2.0, self.foot_y / 2.0, -self.height])
self.WorldToFoot["BL"] = RpToTrans(Rwb, self.pf_BL)
self.pf_BR = np.array(
[-self.foot_x / 2.0, -self.foot_y / 2.0, -self.height])
self.WorldToFoot["BR"] = RpToTrans(Rwb, self.pf_BR)
def HipToFoot(self, orn, pos, T_bf):
"""
Converts a desired position and orientation wrt Spot's
home position, with a desired body-to-foot Transform
into a body-to-hip Transform, which is used to extract
and return the Hip To Foot Vector.
:param orn: A 3x1 np.array([]) with Spot's Roll, Pitch, Yaw angles
:param pos: A 3x1 np.array([]) with Spot's X, Y, Z coordinates
:param T_bf: Dictionary of desired body-to-foot Transforms.
:return: Hip To Foot Vector for each of Spot's Legs.
"""
# Following steps in attached document: SpotBodyIK.
# TODO: LINK DOC
# Only get Rot component
Rb, _ = TransToRp(RPY(orn[0], orn[1], orn[2]))
pb = pos
T_wb = RpToTrans(Rb, pb)
# Dictionary to store vectors
HipToFoot_List = OrderedDict()
for i, (key, T_wh) in enumerate(self.WorldToHip.items()):
# ORDER: FL, FR, FR, BL, BR
# Extract vector component
_, p_bf = TransToRp(T_bf[key])
# Step 1, get T_bh for each leg
T_bh = np.dot(TransInv(T_wb), T_wh)
# Step 2, get T_hf for each leg
# VECTOR ADDITION METHOD
_, p_bh = TransToRp(T_bh)
p_hf0 = p_bf - p_bh
# TRANSFORM METHOD
T_hf = np.dot(TransInv(T_bh), T_bf[key])
_, p_hf1 = TransToRp(T_hf)
# They should yield the same result
if p_hf1.all() != p_hf0.all():
print("NOT EQUAL")
p_hf = p_hf1
HipToFoot_List[key] = p_hf
return HipToFoot_List
def IK(self, orn, pos, T_bf):
"""
Uses HipToFoot() to convert a desired position
and orientation wrt Spot's home position into a
Hip To Foot Vector, which is fed into the LegIK solver.
Finally, the resultant joint angles are returned
from the LegIK solver for each leg.
:param orn: A 3x1 np.array([]) with Spot's Roll, Pitch, Yaw angles
:param pos: A 3x1 np.array([]) with Spot's X, Y, Z coordinates
:param T_bf: Dictionary of desired body-to-foot Transforms.
:return: Joint angles for each of Spot's joints.
"""
# Following steps in attached document: SpotBodyIK.
# TODO: LINK DOC
# Modify x by com offset
pos[0] += self.com_offset
# 4 legs, 3 joints per leg
joint_angles = np.zeros((4, 3))
# print("T_bf: {}".format(T_bf))
# Steps 1 and 2 of pipeline here
HipToFoot = self.HipToFoot(orn, pos, T_bf)
for i, (key, p_hf) in enumerate(HipToFoot.items()):
# ORDER: FL, FR, FR, BL, BR
# print("LEG: {} \t HipToFoot: {}".format(key, p_hf))
# Step 3, compute joint angles from T_hf for each leg
joint_angles[i, :] = self.Legs[key].solve(p_hf)
# print("-----------------------------")
return joint_angles
| 7,062 | Python | 33.120773 | 96 | 0.528887 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/Kinematics/LegKinematics.py | #!/usr/bin/env python
# https://www.researchgate.net/publication/320307716_Inverse_Kinematic_Analysis_Of_A_Quadruped_Robot
import numpy as np
class LegIK():
def __init__(self,
legtype="RIGHT",
shoulder_length=0.04,
elbow_length=0.1,
wrist_length=0.125,
hip_lim=[-0.548, 0.548],
shoulder_lim=[-2.17, 0.97],
leg_lim=[-0.1, 2.59]):
self.legtype = legtype
self.shoulder_length = shoulder_length
self.elbow_length = elbow_length
self.wrist_length = wrist_length
self.hip_lim = hip_lim
self.shoulder_lim = shoulder_lim
self.leg_lim = leg_lim
def get_domain(self, x, y, z):
"""
Calculates the leg's Domain and caps it in case of a breach
:param x,y,z: hip-to-foot distances in each dimension
:return: Leg Domain D
"""
D = (y**2 + (-z)**2 - self.shoulder_length**2 +
(-x)**2 - self.elbow_length**2 - self.wrist_length**2) / (
2 * self.wrist_length * self.elbow_length)
if D > 1 or D < -1:
# DOMAIN BREACHED
# print("---------DOMAIN BREACH---------")
D = np.clip(D, -1.0, 1.0)
return D
else:
return D
def solve(self, xyz_coord):
"""
Generic Leg Inverse Kinematics Solver
:param xyz_coord: hip-to-foot distances in each dimension
:return: Joint Angles required for desired position
"""
x = xyz_coord[0]
y = xyz_coord[1]
z = xyz_coord[2]
D = self.get_domain(x, y, z)
if self.legtype == "RIGHT":
return self.RightIK(x, y, z, D)
else:
return self.LeftIK(x, y, z, D)
def RightIK(self, x, y, z, D):
"""
Right Leg Inverse Kinematics Solver
:param x,y,z: hip-to-foot distances in each dimension
:param D: leg domain
:return: Joint Angles required for desired position
"""
wrist_angle = np.arctan2(-np.sqrt(1 - D**2), D)
sqrt_component = y**2 + (-z)**2 - self.shoulder_length**2
if sqrt_component < 0.0:
# print("NEGATIVE SQRT")
sqrt_component = 0.0
shoulder_angle = -np.arctan2(z, y) - np.arctan2(
np.sqrt(sqrt_component), -self.shoulder_length)
elbow_angle = np.arctan2(-x, np.sqrt(sqrt_component)) - np.arctan2(
self.wrist_length * np.sin(wrist_angle),
self.elbow_length + self.wrist_length * np.cos(wrist_angle))
joint_angles = np.array([-shoulder_angle, elbow_angle, wrist_angle])
return joint_angles
def LeftIK(self, x, y, z, D):
"""
Left Leg Inverse Kinematics Solver
:param x,y,z: hip-to-foot distances in each dimension
:param D: leg domain
:return: Joint Angles required for desired position
"""
wrist_angle = np.arctan2(-np.sqrt(1 - D**2), D)
sqrt_component = y**2 + (-z)**2 - self.shoulder_length**2
if sqrt_component < 0.0:
print("NEGATIVE SQRT")
sqrt_component = 0.0
shoulder_angle = -np.arctan2(z, y) - np.arctan2(
np.sqrt(sqrt_component), self.shoulder_length)
elbow_angle = np.arctan2(-x, np.sqrt(sqrt_component)) - np.arctan2(
self.wrist_length * np.sin(wrist_angle),
self.elbow_length + self.wrist_length * np.cos(wrist_angle))
joint_angles = np.array([-shoulder_angle, elbow_angle, wrist_angle])
return joint_angles
| 3,617 | Python | 35.918367 | 100 | 0.544927 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/Kinematics/LieAlgebra.py | #!/usr/bin/env python
import numpy as np
# NOTE: Code snippets from Modern Robotics at Northwestern University:
# See https://github.com/NxRLab/ModernRobotics
def RpToTrans(R, p):
"""
Converts a rotation matrix and a position vector into homogeneous
transformation matrix
:param R: A 3x3 rotation matrix
:param p: A 3-vector
:return: A homogeneous transformation matrix corresponding to the inputs
Example Input:
R = np.array([[1, 0, 0],
[0, 0, -1],
[0, 1, 0]])
p = np.array([1, 2, 5])
Output:
np.array([[1, 0, 0, 1],
[0, 0, -1, 2],
[0, 1, 0, 5],
[0, 0, 0, 1]])
"""
return np.r_[np.c_[R, p], [[0, 0, 0, 1]]]
def TransToRp(T):
"""
Converts a homogeneous transformation matrix into a rotation matrix
and position vector
:param T: A homogeneous transformation matrix
:return R: The corresponding rotation matrix,
:return p: The corresponding position vector.
Example Input:
T = np.array([[1, 0, 0, 0],
[0, 0, -1, 0],
[0, 1, 0, 3],
[0, 0, 0, 1]])
Output:
(np.array([[1, 0, 0],
[0, 0, -1],
[0, 1, 0]]),
np.array([0, 0, 3]))
"""
T = np.array(T)
return T[0:3, 0:3], T[0:3, 3]
def TransInv(T):
"""
Inverts a homogeneous transformation matrix
:param T: A homogeneous transformation matrix
:return: The inverse of T
Uses the structure of transformation matrices to avoid taking a matrix
inverse, for efficiency.
Example input:
T = np.array([[1, 0, 0, 0],
[0, 0, -1, 0],
[0, 1, 0, 3],
[0, 0, 0, 1]])
Output:
np.array([[1, 0, 0, 0],
[0, 0, 1, -3],
[0, -1, 0, 0],
[0, 0, 0, 1]])
"""
R, p = TransToRp(T)
Rt = np.array(R).T
return np.r_[np.c_[Rt, -np.dot(Rt, p)], [[0, 0, 0, 1]]]
def Adjoint(T):
"""
Computes the adjoint representation of a homogeneous transformation
matrix
:param T: A homogeneous transformation matrix
:return: The 6x6 adjoint representation [AdT] of T
Example Input:
T = np.array([[1, 0, 0, 0],
[0, 0, -1, 0],
[0, 1, 0, 3],
[0, 0, 0, 1]])
Output:
np.array([[1, 0, 0, 0, 0, 0],
[0, 0, -1, 0, 0, 0],
[0, 1, 0, 0, 0, 0],
[0, 0, 3, 1, 0, 0],
[3, 0, 0, 0, 0, -1],
[0, 0, 0, 0, 1, 0]])
"""
R, p = TransToRp(T)
return np.r_[np.c_[R, np.zeros((3, 3))], np.c_[np.dot(VecToso3(p), R), R]]
def VecToso3(omg):
"""
Converts a 3-vector to an so(3) representation
:param omg: A 3-vector
:return: The skew symmetric representation of omg
Example Input:
omg = np.array([1, 2, 3])
Output:
np.array([[ 0, -3, 2],
[ 3, 0, -1],
[-2, 1, 0]])
"""
return np.array([[0, -omg[2], omg[1]], [omg[2], 0, -omg[0]],
[-omg[1], omg[0], 0]])
def RPY(roll, pitch, yaw):
"""
Creates a Roll, Pitch, Yaw Transformation Matrix
:param roll: roll component of matrix
:param pitch: pitch component of matrix
:param yaw: yaw component of matrix
:return: The transformation matrix
Example Input:
roll = 0.0
pitch = 0.0
yaw = 0.0
Output:
np.array([[1, 0, 0, 0],
[0, 1, 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1]])
"""
Roll = np.array([[1, 0, 0, 0], [0, np.cos(roll), -np.sin(roll), 0],
[0, np.sin(roll), np.cos(roll), 0], [0, 0, 0, 1]])
Pitch = np.array([[np.cos(pitch), 0, np.sin(pitch), 0], [0, 1, 0, 0],
[-np.sin(pitch), 0, np.cos(pitch), 0], [0, 0, 0, 1]])
Yaw = np.array([[np.cos(yaw), -np.sin(yaw), 0, 0],
[np.sin(yaw), np.cos(yaw), 0, 0], [0, 0, 1, 0],
[0, 0, 0, 1]])
return np.matmul(np.matmul(Roll, Pitch), Yaw)
def RotateTranslate(rotation, position):
"""
Creates a Transformation Matrix from a Rotation, THEN, a Translation
:param rotation: pure rotation matrix
:param translation: pure translation matrix
:return: The transformation matrix
"""
trans = np.eye(4)
trans[0, 3] = position[0]
trans[1, 3] = position[1]
trans[2, 3] = position[2]
return np.dot(rotation, trans)
def TransformVector(xyz_coord, rotation, translation):
"""
Transforms a vector by a specified Rotation THEN Translation Matrix
:param xyz_coord: the vector to transform
:param rotation: pure rotation matrix
:param translation: pure translation matrix
:return: The transformed vector
"""
xyz_vec = np.append(xyz_coord, 1.0)
Transformed = np.dot(RotateTranslate(rotation, translation), xyz_vec)
return Transformed[:3]
| 5,162 | Python | 27.213115 | 78 | 0.49012 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/util/gui.py | #!/usr/bin/env python
import pybullet as pb
import time
import numpy as np
import sys
class GUI:
def __init__(self, quadruped):
time.sleep(0.5)
self.cyaw = 0
self.cpitch = -7
self.cdist = 0.66
self.xId = pb.addUserDebugParameter("x", -0.10, 0.10, 0.)
self.yId = pb.addUserDebugParameter("y", -0.10, 0.10, 0.)
self.zId = pb.addUserDebugParameter("z", -0.055, 0.17, 0.)
self.rollId = pb.addUserDebugParameter("roll", -np.pi / 4, np.pi / 4,
0.)
self.pitchId = pb.addUserDebugParameter("pitch", -np.pi / 4, np.pi / 4,
0.)
self.yawId = pb.addUserDebugParameter("yaw", -np.pi / 4, np.pi / 4, 0.)
self.StepLengthID = pb.addUserDebugParameter("Step Length", -0.1, 0.1,
0.0)
self.YawRateId = pb.addUserDebugParameter("Yaw Rate", -2.0, 2.0, 0.)
self.LateralFractionId = pb.addUserDebugParameter(
"Lateral Fraction", -np.pi / 2.0, np.pi / 2.0, 0.)
self.StepVelocityId = pb.addUserDebugParameter("Step Velocity", 0.001,
3., 0.001)
self.SwingPeriodId = pb.addUserDebugParameter("Swing Period", 0.1, 0.4,
0.2)
self.ClearanceHeightId = pb.addUserDebugParameter(
"Clearance Height", 0.0, 0.1, 0.045)
self.PenetrationDepthId = pb.addUserDebugParameter(
"Penetration Depth", 0.0, 0.05, 0.003)
self.quadruped = quadruped
def UserInput(self):
quadruped_pos, _ = pb.getBasePositionAndOrientation(self.quadruped)
pb.resetDebugVisualizerCamera(cameraDistance=self.cdist,
cameraYaw=self.cyaw,
cameraPitch=self.cpitch,
cameraTargetPosition=quadruped_pos)
keys = pb.getKeyboardEvents()
# Keys to change camera
if keys.get(100): # D
self.cyaw += 1
if keys.get(97): # A
self.cyaw -= 1
if keys.get(99): # C
self.cpitch += 1
if keys.get(102): # F
self.cpitch -= 1
if keys.get(122): # Z
self.cdist += .01
if keys.get(120): # X
self.cdist -= .01
if keys.get(27): # ESC
pb.disconnect()
sys.exit()
# Read Robot Transform from GUI
pos = np.array([
pb.readUserDebugParameter(self.xId),
pb.readUserDebugParameter(self.yId),
pb.readUserDebugParameter(self.zId)
])
orn = np.array([
pb.readUserDebugParameter(self.rollId),
pb.readUserDebugParameter(self.pitchId),
pb.readUserDebugParameter(self.yawId)
])
StepLength = pb.readUserDebugParameter(self.StepLengthID)
YawRate = pb.readUserDebugParameter(self.YawRateId)
LateralFraction = pb.readUserDebugParameter(self.LateralFractionId)
StepVelocity = pb.readUserDebugParameter(self.StepVelocityId)
ClearanceHeight = pb.readUserDebugParameter(self.ClearanceHeightId)
PenetrationDepth = pb.readUserDebugParameter(self.PenetrationDepthId)
SwingPeriod = pb.readUserDebugParameter(self.SwingPeriodId)
return pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth, SwingPeriod
| 3,557 | Python | 39.431818 | 123 | 0.555243 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/util/action_mapper.py | STATIC_ACTIONS_MAP = {
'gallop': ('rex_gym/policies/galloping/balanced', 'model.ckpt-20000000'),
'walk': ('rex_gym/policies/walking/alternating_legs', 'model.ckpt-16000000'),
'standup': ('rex_gym/policies/standup', 'model.ckpt-10000000')
}
DYNAMIC_ACTIONS_MAP = {
'turn': ('rex_gym/policies/turn', 'model.ckpt-16000000')
}
ACTIONS_TO_ENV_NAMES = {
'gallop': 'RexReactiveEnv',
'walk': 'RexWalkEnv',
'turn': 'RexTurnEnv',
'standup': 'RexStandupEnv'
}
| 483 | Python | 27.470587 | 81 | 0.648033 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/util/bullet_client.py | """A wrapper for pybullet to manage different clients."""
from __future__ import absolute_import
from __future__ import division
import functools
import inspect
import pybullet
class BulletClient(object):
"""A wrapper for pybullet to manage different clients."""
def __init__(self, connection_mode=None):
"""Creates a Bullet client and connects to a simulation.
Args:
connection_mode:
`None` connects to an existing simulation or, if fails, creates a
new headless simulation,
`pybullet.GUI` creates a new simulation with a GUI,
`pybullet.DIRECT` creates a headless simulation,
`pybullet.SHARED_MEMORY` connects to an existing simulation.
"""
self._shapes = {}
if connection_mode is None:
self._client = pybullet.connect(pybullet.SHARED_MEMORY)
if self._client >= 0:
return
else:
connection_mode = pybullet.DIRECT
self._client = pybullet.connect(connection_mode)
def __del__(self):
"""Clean up connection if not already done."""
try:
pybullet.disconnect(physicsClientId=self._client)
except pybullet.error:
pass
def __getattr__(self, name):
"""Inject the client id into Bullet functions."""
attribute = getattr(pybullet, name)
if inspect.isbuiltin(attribute):
if name not in [
"invertTransform",
"multiplyTransforms",
"getMatrixFromQuaternion",
"getEulerFromQuaternion",
"computeViewMatrixFromYawPitchRoll",
"computeProjectionMatrixFOV",
"getQuaternionFromEuler",
]: # A temporary hack for now.
attribute = functools.partial(attribute, physicsClientId=self._client)
return attribute
| 1,894 | Python | 33.454545 | 86 | 0.607181 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/util/pybullet_data/__init__.py | import os
def getDataPath():
resdir = os.path.join(os.path.dirname(__file__))
return resdir
| 98 | Python | 13.142855 | 50 | 0.683673 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/OpenLoopSM/SpotOL.py | """ Open Loop Controller for Spot Micro. Takes GUI params or uses default
"""
import numpy as np
from random import shuffle
import copy
# Ensuring totally random seed every step!
np.random.seed()
FB = 0
LAT = 1
ROT = 2
COMBI = 3
FWD = 0
ALL = 1
class BezierStepper():
def __init__(self,
pos=np.array([0.0, 0.0, 0.0]),
orn=np.array([0.0, 0.0, 0.0]),
StepLength=0.04,
LateralFraction=0.0,
YawRate=0.0,
StepVelocity=0.001,
ClearanceHeight=0.045,
PenetrationDepth=0.003,
episode_length=5000,
dt=0.01,
num_shuffles=2,
mode=FWD):
self.pos = pos
self.orn = orn
self.desired_StepLength = StepLength
self.StepLength = StepLength
self.StepLength_LIMITS = [-0.05, 0.05]
self.LateralFraction = LateralFraction
self.LateralFraction_LIMITS = [-np.pi / 2.0, np.pi / 2.0]
self.YawRate = YawRate
self.YawRate_LIMITS = [-1.0, 1.0]
self.StepVelocity = StepVelocity
self.StepVelocity_LIMITS = [0.1, 1.5]
self.ClearanceHeight = ClearanceHeight
self.ClearanceHeight_LIMITS = [0.0, 0.04]
self.PenetrationDepth = PenetrationDepth
self.PenetrationDepth_LIMITS = [0.0, 0.02]
self.mode = mode
self.dt = dt
# Keep track of state machine
self.time = 0
# Decide how long to stay in each phase based on maxtime
self.max_time = episode_length
""" States
1: FWD/BWD
2: Lat
3: Rot
4: Combined
"""
self.order = [FB, LAT, ROT, COMBI]
# Shuffles list in place so the order of states is unpredictable
# NOTE: increment num_shuffles by episode num (cap at 10
# and reset or someting) for some forced randomness
for _ in range(num_shuffles):
shuffle(self.order)
# Forward/Backward always needs to be first!
self.reshuffle()
# Current State
self.current_state = self.order[0]
# Divide by number of states (see RL_SM())
self.time_per_episode = int(self.max_time / len(self.order))
def ramp_up(self):
if self.StepLength < self.desired_StepLength:
self.StepLength += self.desired_StepLength * self.dt
def reshuffle(self):
self.time = 0
# Make sure FWD/BWD is always first state
FB_index = self.order.index(FB)
if FB_index != 0:
what_was_in_zero = self.order[0]
self.order[0] = FB
self.order[FB_index] = what_was_in_zero
def which_state(self):
# Ensuring totally random seed every step!
np.random.seed()
if self.time > self.max_time:
# Combined
self.current_state = COMBI
self.time = 0
else:
index = int(self.time / self.time_per_episode)
if index > len(self.order) - 1:
index = len(self.order) - 1
self.current_state = self.order[index]
def StateMachine(self):
"""
State Machined used for training robust RL on top of OL gait.
STATES:
Forward/Backward: All Default Values.
Can have slow changes to
StepLength(+-) and Velocity
Lateral: As above (fwd or bwd random) with added random
slow changing LateralFraction param
Rotating: As above except with YawRate
Combined: ALL changeable values may change!
StepLength
StepVelocity
LateralFraction
YawRate
NOTE: the RL is solely responsible for modulating Clearance Height
and Penetration Depth
"""
if self.mode is ALL:
self.which_state()
if self.current_state == FB:
# print("FORWARD/BACKWARD")
self.FB()
elif self.current_state == LAT:
# print("LATERAL")
self.LAT()
elif self.current_state == ROT:
# print("ROTATION")
self.ROT()
elif self.current_state == COMBI:
# print("COMBINED")
self.COMBI()
return self.return_bezier_params()
def return_bezier_params(self):
# First, Clip Everything
self.StepLength = np.clip(self.StepLength, self.StepLength_LIMITS[0],
self.StepLength_LIMITS[1])
self.StepVelocity = np.clip(self.StepVelocity,
self.StepVelocity_LIMITS[0],
self.StepVelocity_LIMITS[1])
self.LateralFraction = np.clip(self.LateralFraction,
self.LateralFraction_LIMITS[0],
self.LateralFraction_LIMITS[1])
self.YawRate = np.clip(self.YawRate, self.YawRate_LIMITS[0],
self.YawRate_LIMITS[1])
self.ClearanceHeight = np.clip(self.ClearanceHeight,
self.ClearanceHeight_LIMITS[0],
self.ClearanceHeight_LIMITS[1])
self.PenetrationDepth = np.clip(self.PenetrationDepth,
self.PenetrationDepth_LIMITS[0],
self.PenetrationDepth_LIMITS[1])
# Then, return
# FIRST COPY TO AVOID OVERWRITING
pos = copy.deepcopy(self.pos)
orn = copy.deepcopy(self.orn)
StepLength = copy.deepcopy(self.StepLength)
LateralFraction = copy.deepcopy(self.LateralFraction)
YawRate = copy.deepcopy(self.YawRate)
StepVelocity = copy.deepcopy(self.StepVelocity)
ClearanceHeight = copy.deepcopy(self.ClearanceHeight)
PenetrationDepth = copy.deepcopy(self.PenetrationDepth)
return pos, orn, StepLength, LateralFraction,\
YawRate, StepVelocity,\
ClearanceHeight, PenetrationDepth
def FB(self):
"""
Here, we can modulate StepLength and StepVelocity
"""
# The maximum update amount for these element
StepLength_DELTA = self.dt * (self.StepLength_LIMITS[1] -
self.StepLength_LIMITS[0]) / (6.0)
StepVelocity_DELTA = self.dt * (self.StepVelocity_LIMITS[1] -
self.StepVelocity_LIMITS[0]) / (2.0)
# Add either positive or negative or zero delta for each
# NOTE: 'High' is open bracket ) so the max is 1
if self.StepLength < -self.StepLength_LIMITS[0] / 2.0:
StepLength_DIRECTION = np.random.randint(-1, 3, 1)[0]
elif self.StepLength > self.StepLength_LIMITS[1] / 2.0:
StepLength_DIRECTION = np.random.randint(-2, 2, 1)[0]
else:
StepLength_DIRECTION = np.random.randint(-1, 2, 1)[0]
StepVelocity_DIRECTION = np.random.randint(-1, 2, 1)[0]
# Now, modify modifiable params AND CLIP
self.StepLength += StepLength_DIRECTION * StepLength_DELTA
self.StepLength = np.clip(self.StepLength, self.StepLength_LIMITS[0],
self.StepLength_LIMITS[1])
self.StepVelocity += StepVelocity_DIRECTION * StepVelocity_DELTA
self.StepVelocity = np.clip(self.StepVelocity,
self.StepVelocity_LIMITS[0],
self.StepVelocity_LIMITS[1])
def LAT(self):
"""
Here, we can modulate StepLength and LateralFraction
"""
# The maximum update amount for these element
LateralFraction_DELTA = self.dt * (self.LateralFraction_LIMITS[1] -
self.LateralFraction_LIMITS[0]) / (
2.0)
# Add either positive or negative or zero delta for each
# NOTE: 'High' is open bracket ) so the max is 1
LateralFraction_DIRECTION = np.random.randint(-1, 2, 1)[0]
# Now, modify modifiable params AND CLIP
self.LateralFraction += LateralFraction_DIRECTION * LateralFraction_DELTA
self.LateralFraction = np.clip(self.LateralFraction,
self.LateralFraction_LIMITS[0],
self.LateralFraction_LIMITS[1])
def ROT(self):
"""
Here, we can modulate StepLength and YawRate
"""
# The maximum update amount for these element
# no dt since YawRate is already mult by dt
YawRate_DELTA = (self.YawRate_LIMITS[1] -
self.YawRate_LIMITS[0]) / (2.0)
# Add either positive or negative or zero delta for each
# NOTE: 'High' is open bracket ) so the max is 1
YawRate_DIRECTION = np.random.randint(-1, 2, 1)[0]
# Now, modify modifiable params AND CLIP
self.YawRate += YawRate_DIRECTION * YawRate_DELTA
self.YawRate = np.clip(self.YawRate, self.YawRate_LIMITS[0],
self.YawRate_LIMITS[1])
def COMBI(self):
"""
Here, we can modify all the parameters
"""
self.FB()
self.LAT()
self.ROT()
| 9,534 | Python | 36.53937 | 81 | 0.540696 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/GymEnvs/spot_bezier_env.py | """ This file implements the gym environment of SpotMicro with Bezier Curve.
"""
import math
import time
import gym
import numpy as np
import pybullet
import pybullet_data
from gym import spaces
from gym.utils import seeding
from pkg_resources import parse_version
from spotmicro import spot
import pybullet_utils.bullet_client as bullet_client
from gym.envs.registration import register
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
from spotmicro.spot_gym_env import spotGymEnv
import spotmicro.Kinematics.LieAlgebra as LA
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
SENSOR_NOISE_STDDEV = spot.SENSOR_NOISE_STDDEV
# Register as OpenAI Gym Environment
register(
id="SpotMicroEnv-v1",
entry_point='spotmicro.GymEnvs.spot_bezier_env:spotBezierEnv',
max_episode_steps=1000,
)
class spotBezierEnv(spotGymEnv):
"""The gym environment for spot.
It simulates the locomotion of spot, a quadruped robot. The state space
include the angles, velocities and torques for all the motors and the action
space is the desired motor angle for each motor. The reward function is based
on how far spot walks in 1000 steps and penalizes the energy
expenditure.
"""
metadata = {
"render.modes": ["human", "rgb_array"],
"video.frames_per_second": 50
}
def __init__(self,
distance_weight=1.0,
rotation_weight=0.0,
energy_weight=0.000,
shake_weight=0.00,
drift_weight=0.0,
rp_weight=10.0,
rate_weight=.03,
urdf_root=pybullet_data.getDataPath(),
urdf_version=None,
distance_limit=float("inf"),
observation_noise_stdev=SENSOR_NOISE_STDDEV,
self_collision_enabled=True,
motor_velocity_limit=np.inf,
pd_control_enabled=False,
leg_model_enabled=False,
accurate_motor_model_enabled=False,
remove_default_joint_damping=False,
motor_kp=2.0,
motor_kd=0.03,
control_latency=0.0,
pd_latency=0.0,
torque_control_enabled=False,
motor_overheat_protection=False,
hard_reset=False,
on_rack=False,
render=True,
num_steps_to_log=1000,
action_repeat=1,
control_time_step=None,
env_randomizer=SpotEnvRandomizer(),
forward_reward_cap=float("inf"),
reflection=True,
log_path=None,
desired_velocity=0.5,
desired_rate=0.0,
lateral=False,
draw_foot_path=False,
height_field=False,
AutoStepper=True,
action_dim=14,
contacts=True):
super(spotBezierEnv, self).__init__(
distance_weight=distance_weight,
rotation_weight=rotation_weight,
energy_weight=energy_weight,
shake_weight=shake_weight,
drift_weight=drift_weight,
rp_weight=rp_weight,
rate_weight=rate_weight,
urdf_root=urdf_root,
urdf_version=urdf_version,
distance_limit=distance_limit,
observation_noise_stdev=observation_noise_stdev,
self_collision_enabled=self_collision_enabled,
motor_velocity_limit=motor_velocity_limit,
pd_control_enabled=pd_control_enabled,
leg_model_enabled=leg_model_enabled,
accurate_motor_model_enabled=accurate_motor_model_enabled,
remove_default_joint_damping=remove_default_joint_damping,
motor_kp=motor_kp,
motor_kd=motor_kd,
control_latency=control_latency,
pd_latency=pd_latency,
torque_control_enabled=torque_control_enabled,
motor_overheat_protection=motor_overheat_protection,
hard_reset=hard_reset,
on_rack=on_rack,
render=render,
num_steps_to_log=num_steps_to_log,
action_repeat=action_repeat,
control_time_step=control_time_step,
env_randomizer=env_randomizer,
forward_reward_cap=forward_reward_cap,
reflection=reflection,
log_path=log_path,
desired_velocity=desired_velocity,
desired_rate=desired_rate,
lateral=lateral,
draw_foot_path=draw_foot_path,
height_field=height_field,
AutoStepper=AutoStepper,
contacts=contacts)
# Residuals + Clearance Height + Penetration Depth
action_high = np.array([self._action_bound] * action_dim)
self.action_space = spaces.Box(-action_high, action_high)
print("Action SPACE: {}".format(self.action_space))
self.prev_pos = np.array([0.0, 0.0, 0.0])
self.yaw = 0.0
def pass_joint_angles(self, ja):
""" For executing joint angles
"""
self.ja = ja
def step(self, action):
"""Step forward the simulation, given the action.
Args:
action: A list of desired motor angles for eight motors.
smach: the bezier state machine containing simulated
random controll inputs
Returns:
observations: The angles, velocities and torques of all motors.
reward: The reward for the current state-action pair.
done: Whether the episode has ended.
info: A dictionary that stores diagnostic information.
Raises:
ValueError: The action dimension is not the same as the number of motors.
ValueError: The magnitude of actions is out of bounds.
"""
# Discard all but joint angles
action = self.ja
self._last_base_position = self.spot.GetBasePosition()
self._last_base_orientation = self.spot.GetBaseOrientation()
# print("ACTION:")
# print(action)
if self._is_render:
# Sleep, otherwise the computation takes less time than real time,
# which will make the visualization like a fast-forward video.
time_spent = time.time() - self._last_frame_time
self._last_frame_time = time.time()
time_to_sleep = self.control_time_step - time_spent
if time_to_sleep > 0:
time.sleep(time_to_sleep)
base_pos = self.spot.GetBasePosition()
# Keep the previous orientation of the camera set by the user.
[yaw, pitch,
dist] = self._pybullet_client.getDebugVisualizerCamera()[8:11]
self._pybullet_client.resetDebugVisualizerCamera(
dist, yaw, pitch, base_pos)
action = self._transform_action_to_motor_command(action)
self.spot.Step(action)
# NOTE: SMACH is passed to the reward method
reward = self._reward()
done = self._termination()
self._env_step_counter += 1
# DRAW FOOT PATH
if self.draw_foot_path:
self.DrawFootPath()
return np.array(self._get_observation()), reward, done, {}
def return_state(self):
return np.array(self._get_observation())
def return_yaw(self):
return self.yaw
def _reward(self):
# get observation
obs = self._get_observation()
orn = self.spot.GetBaseOrientation()
# Return StepVelocity with the sign of StepLength
DesiredVelicty = math.copysign(self.spot.StepVelocity / 4.0,
self.spot.StepLength)
fwd_speed = self.spot.prev_lin_twist[0] # vx
lat_speed = self.spot.prev_lin_twist[1] # vy
# DEBUG
lt, at = self.spot.GetBaseTwist()
# ONLY WORKS FOR MOVING PURELY FORWARD
pos = self.spot.GetBasePosition()
forward_reward = pos[0] - self.prev_pos[0]
# yaw_rate = obs[4]
rot_reward = 0.0
roll, pitch, yaw = self._pybullet_client.getEulerFromQuaternion(
[orn[0], orn[1], orn[2], orn[3]])
# if yaw < 0.0:
# yaw += np.pi
# else:
# yaw -= np.pi
# For auto correct
self.yaw = yaw
# penalty for nonzero PITCH and YAW(hidden) ONLY
# NOTE: Added Yaw mult
rp_reward = -(abs(obs[0]) + abs(obs[1]))
# print("YAW: {}".format(yaw))
# print("RP RWD: {:.2f}".format(rp_reward))
# print("ROLL: {} \t PITCH: {}".format(obs[0], obs[1]))
# penalty for nonzero acc(z) - UNRELIABLE ON IMU
shake_reward = 0
# penalty for nonzero rate (x,y,z)
rate_reward = -(abs(obs[2]) + abs(obs[3]))
# print("RATES: {}".format(obs[2:5]))
drift_reward = -abs(pos[1])
energy_reward = -np.abs(
np.dot(self.spot.GetMotorTorques(),
self.spot.GetMotorVelocities())) * self._time_step
reward = (self._distance_weight * forward_reward +
self._rotation_weight * rot_reward +
self._energy_weight * energy_reward +
self._drift_weight * drift_reward +
self._shake_weight * shake_reward +
self._rp_weight * rp_reward +
self._rate_weight * rate_reward)
self._objectives.append(
[forward_reward, energy_reward, drift_reward, shake_reward])
# print("REWARD: ", reward)
# NOTE: return yaw for automatic correction (not part of RL)
return reward | 9,666 | Python | 34.803704 | 79 | 0.581212 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spotmicro/GaitGenerator/Bezier.py | import numpy as np
from spotmicro.Kinematics.LieAlgebra import TransToRp
import copy
STANCE = 0
SWING = 1
# Bezier Curves from: https://dspace.mit.edu/handle/1721.1/98270
# Rotation Logic from: http://www.inase.org/library/2014/santorini/bypaper/ROBCIRC/ROBCIRC-54.pdf
class BezierGait():
def __init__(self, dSref=[0.0, 0.0, 0.5, 0.5], dt=0.01, Tswing=0.2):
# Phase Lag Per Leg: FL, FR, BL, BR
# Reference Leg is FL, always 0
self.dSref = dSref
self.Prev_fxyz = [0.0, 0.0, 0.0, 0.0]
# Number of control points is n + 1 = 11 + 1 = 12
self.NumControlPoints = 11
# Timestep
self.dt = dt
# Total Elapsed Time
self.time = 0.0
# Touchdown Time
self.TD_time = 0.0
# Time Since Last Touchdown
self.time_since_last_TD = 0.0
# Trajectory Mode
self.StanceSwing = SWING
# Swing Phase value [0, 1] of Reference Foot
self.SwRef = 0.0
self.Stref = 0.0
# Whether Reference Foot has Touched Down
self.TD = False
# Stance Time
self.Tswing = Tswing
# Reference Leg
self.ref_idx = 0
# Store all leg phases
self.Phases = self.dSref
def reset(self):
"""Resets the parameters of the Bezier Gait Generator
"""
self.Prev_fxyz = [0.0, 0.0, 0.0, 0.0]
# Total Elapsed Time
self.time = 0.0
# Touchdown Time
self.TD_time = 0.0
# Time Since Last Touchdown
self.time_since_last_TD = 0.0
# Trajectory Mode
self.StanceSwing = SWING
# Swing Phase value [0, 1] of Reference Foot
self.SwRef = 0.0
self.Stref = 0.0
# Whether Reference Foot has Touched Down
self.TD = False
def GetPhase(self, index, Tstance, Tswing):
"""Retrieves the phase of an individual leg.
NOTE modification
from original paper:
if ti < -Tswing:
ti += Tstride
This is to avoid a phase discontinuity if the user selects
a Step Length and Velocity combination that causes Tstance > Tswing.
:param index: the leg's index, used to identify the required
phase lag
:param Tstance: the current user-specified stance period
:param Tswing: the swing period (constant, class member)
:return: Leg Phase, and StanceSwing (bool) to indicate whether
leg is in stance or swing mode
"""
StanceSwing = STANCE
Sw_phase = 0.0
Tstride = Tstance + Tswing
ti = self.Get_ti(index, Tstride)
# NOTE: PAPER WAS MISSING THIS LOGIC!!
if ti < -Tswing:
ti += Tstride
# STANCE
if ti >= 0.0 and ti <= Tstance:
StanceSwing = STANCE
if Tstance == 0.0:
Stnphase = 0.0
else:
Stnphase = ti / float(Tstance)
if index == self.ref_idx:
# print("STANCE REF: {}".format(Stnphase))
self.StanceSwing = StanceSwing
return Stnphase, StanceSwing
# SWING
elif ti >= -Tswing and ti < 0.0:
StanceSwing = SWING
Sw_phase = (ti + Tswing) / Tswing
elif ti > Tstance and ti <= Tstride:
StanceSwing = SWING
Sw_phase = (ti - Tstance) / Tswing
# Touchdown at End of Swing
if Sw_phase >= 1.0:
Sw_phase = 1.0
if index == self.ref_idx:
# print("SWING REF: {}".format(Sw_phase))
self.StanceSwing = StanceSwing
self.SwRef = Sw_phase
# REF Touchdown at End of Swing
if self.SwRef >= 0.999:
self.TD = True
# else:
# self.TD = False
return Sw_phase, StanceSwing
def Get_ti(self, index, Tstride):
"""Retrieves the time index for the individual leg
:param index: the leg's index, used to identify the required
phase lag
:param Tstride: the total leg movement period (Tstance + Tswing)
:return: the leg's time index
"""
# NOTE: for some reason python's having numerical issues w this
# setting to 0 for ref leg by force
if index == self.ref_idx:
self.dSref[index] = 0.0
return self.time_since_last_TD - self.dSref[index] * Tstride
def Increment(self, dt, Tstride):
"""Increments the Bezier gait generator's internal clock (self.time)
:param dt: the time step
phase lag
:param Tstride: the total leg movement period (Tstance + Tswing)
:return: the leg's time index
"""
self.CheckTouchDown()
self.time_since_last_TD = self.time - self.TD_time
if self.time_since_last_TD > Tstride:
self.time_since_last_TD = Tstride
elif self.time_since_last_TD < 0.0:
self.time_since_last_TD = 0.0
# print("T STRIDE: {}".format(Tstride))
# Increment Time at the end in case TD just happened
# So that we get time_since_last_TD = 0.0
self.time += dt
# If Tstride = Tswing, Tstance = 0
# RESET ALL
if Tstride < self.Tswing + dt:
self.time = 0.0
self.time_since_last_TD = 0.0
self.TD_time = 0.0
self.SwRef = 0.0
def CheckTouchDown(self):
"""Checks whether a reference leg touchdown
has occured, and whether this warrants
resetting the touchdown time
"""
if self.SwRef >= 0.9 and self.TD:
self.TD_time = self.time
self.TD = False
self.SwRef = 0.0
def BernSteinPoly(self, t, k, point):
"""Calculate the point on the Berinstein Polynomial
based on phase (0->1), point number (0-11),
and the value of the control point itself
:param t: phase
:param k: point number
:param point: point value
:return: Value through Bezier Curve
"""
return point * self.Binomial(k) * np.power(t, k) * np.power(
1 - t, self.NumControlPoints - k)
def Binomial(self, k):
"""Solves the binomial theorem given a Bezier point number
relative to the total number of Bezier points.
:param k: Bezier point number
:returns: Binomial solution
"""
return np.math.factorial(self.NumControlPoints) / (
np.math.factorial(k) *
np.math.factorial(self.NumControlPoints - k))
def BezierSwing(self, phase, L, LateralFraction, clearance_height=0.04):
"""Calculates the step coordinates for the Bezier (swing) period
:param phase: current trajectory phase
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param clearance_height: foot clearance height during swing phase
:returns: X,Y,Z Foot Coordinates relative to unmodified body
"""
# Polar Leg Coords
X_POLAR = np.cos(LateralFraction)
Y_POLAR = np.sin(LateralFraction)
# Bezier Curve Points (12 pts)
# NOTE: L is HALF of STEP LENGTH
# Forward Component
STEP = np.array([
-L, # Ctrl Point 0, half of stride len
-L * 1.4, # Ctrl Point 1 diff btwn 1 and 0 = x Lift vel
-L * 1.5, # Ctrl Pts 2, 3, 4 are overlapped for
-L * 1.5, # Direction change after
-L * 1.5, # Follow Through
0.0, # Change acceleration during Protraction
0.0, # So we include three
0.0, # Overlapped Ctrl Pts: 5, 6, 7
L * 1.5, # Changing direction for swing-leg retraction
L * 1.5, # requires double overlapped Ctrl Pts: 8, 9
L * 1.4, # Swing Leg Retraction Velocity = Ctrl 11 - 10
L
])
# Account for lateral movements by multiplying with polar coord.
# LateralFraction switches leg movements from X over to Y+ or Y-
# As it tends away from zero
X = STEP * X_POLAR
# Account for lateral movements by multiplying with polar coord.
# LateralFraction switches leg movements from X over to Y+ or Y-
# As it tends away from zero
Y = STEP * Y_POLAR
# Vertical Component
Z = np.array([
0.0, # Double Overlapped Ctrl Pts for zero Lift
0.0, # Veloicty wrt hip (Pts 0 and 1)
clearance_height * 0.9, # Triple overlapped control for change in
clearance_height * 0.9, # Force direction during transition from
clearance_height * 0.9, # follow-through to protraction (2, 3, 4)
clearance_height * 0.9, # Double Overlapped Ctrl Pts for Traj
clearance_height * 0.9, # Dirctn Change during Protraction (5, 6)
clearance_height * 1.1, # Maximum Clearance at mid Traj, Pt 7
clearance_height * 1.1, # Smooth Transition from Protraction
clearance_height * 1.1, # To Retraction, Two Ctrl Pts (8, 9)
0.0, # Double Overlap Ctrl Pts for 0 Touchdown
0.0, # Veloicty wrt hip (Pts 10 and 11)
])
stepX = 0.
stepY = 0.
stepZ = 0.
# Bernstein Polynomial sum over control points
for i in range(len(X)):
stepX += self.BernSteinPoly(phase, i, X[i])
stepY += self.BernSteinPoly(phase, i, Y[i])
stepZ += self.BernSteinPoly(phase, i, Z[i])
return stepX, stepY, stepZ
def SineStance(self, phase, L, LateralFraction, penetration_depth=0.00):
"""Calculates the step coordinates for the Sinusoidal stance period
:param phase: current trajectory phase
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param penetration_depth: foot penetration depth during stance phase
:returns: X,Y,Z Foot Coordinates relative to unmodified body
"""
X_POLAR = np.cos(LateralFraction)
Y_POLAR = np.sin(LateralFraction)
# moves from +L to -L
step = L * (1.0 - 2.0 * phase)
stepX = step * X_POLAR
stepY = step * Y_POLAR
if L != 0.0:
stepZ = -penetration_depth * np.cos(
(np.pi * (stepX + stepY)) / (2.0 * L))
else:
stepZ = 0.0
return stepX, stepY, stepZ
def YawCircle(self, T_bf, index):
""" Calculates the required rotation of the trajectory plane
for yaw motion
:param T_bf: default body-to-foot Vector
:param index: the foot index in the container
:returns: phi_arc, the plane rotation angle required for yaw motion
"""
# Foot Magnitude depending on leg type
DefaultBodyToFoot_Magnitude = np.sqrt(T_bf[0]**2 + T_bf[1]**2)
# Rotation Angle depending on leg type
DefaultBodyToFoot_Direction = np.arctan2(T_bf[1], T_bf[0])
# Previous leg coordinates relative to default coordinates
g_xyz = self.Prev_fxyz[index] - np.array([T_bf[0], T_bf[1], T_bf[2]])
# Modulate Magnitude to keep tracing circle
g_mag = np.sqrt((g_xyz[0])**2 + (g_xyz[1])**2)
th_mod = np.arctan2(g_mag, DefaultBodyToFoot_Magnitude)
# Angle Traced by Foot for Rotation
# FR and BL
if index == 1 or index == 2:
phi_arc = np.pi / 2.0 + DefaultBodyToFoot_Direction + th_mod
# FL and BR
else:
phi_arc = np.pi / 2.0 - DefaultBodyToFoot_Direction + th_mod
# print("INDEX {}: \t Angle: {}".format(
# index, np.degrees(DefaultBodyToFoot_Direction)))
return phi_arc
def SwingStep(self, phase, L, LateralFraction, YawRate, clearance_height,
T_bf, key, index):
"""Calculates the step coordinates for the Bezier (swing) period
using a combination of forward and rotational step coordinates
initially decomposed from user input of
L, LateralFraction and YawRate
:param phase: current trajectory phase
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param YawRate: the desired body yaw rate
:param clearance_height: foot clearance height during swing phase
:param T_bf: default body-to-foot Vector
:param key: indicates which foot is being processed
:param index: the foot index in the container
:returns: Foot Coordinates relative to unmodified body
"""
# Yaw foot angle for tangent-to-circle motion
phi_arc = self.YawCircle(T_bf, index)
# Get Foot Coordinates for Forward Motion
X_delta_lin, Y_delta_lin, Z_delta_lin = self.BezierSwing(
phase, L, LateralFraction, clearance_height)
X_delta_rot, Y_delta_rot, Z_delta_rot = self.BezierSwing(
phase, YawRate, phi_arc, clearance_height)
coord = np.array([
X_delta_lin + X_delta_rot, Y_delta_lin + Y_delta_rot,
Z_delta_lin + Z_delta_rot
])
self.Prev_fxyz[index] = coord
return coord
def StanceStep(self, phase, L, LateralFraction, YawRate, penetration_depth,
T_bf, key, index):
"""Calculates the step coordinates for the Sine (stance) period
using a combination of forward and rotational step coordinates
initially decomposed from user input of
L, LateralFraction and YawRate
:param phase: current trajectory phase
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param YawRate: the desired body yaw rate
:param penetration_depth: foot penetration depth during stance phase
:param T_bf: default body-to-foot Vector
:param key: indicates which foot is being processed
:param index: the foot index in the container
:returns: Foot Coordinates relative to unmodified body
"""
# Yaw foot angle for tangent-to-circle motion
phi_arc = self.YawCircle(T_bf, index)
# Get Foot Coordinates for Forward Motion
X_delta_lin, Y_delta_lin, Z_delta_lin = self.SineStance(
phase, L, LateralFraction, penetration_depth)
X_delta_rot, Y_delta_rot, Z_delta_rot = self.SineStance(
phase, YawRate, phi_arc, penetration_depth)
coord = np.array([
X_delta_lin + X_delta_rot, Y_delta_lin + Y_delta_rot,
Z_delta_lin + Z_delta_rot
])
self.Prev_fxyz[index] = coord
return coord
def GetFootStep(self, L, LateralFraction, YawRate, clearance_height,
penetration_depth, Tstance, T_bf, index, key):
"""Calculates the step coordinates in either the Bezier or
Sine portion of the trajectory depending on the retrieved phase
:param phase: current trajectory phase
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param YawRate: the desired body yaw rate
:param clearance_height: foot clearance height during swing phase
:param penetration_depth: foot penetration depth during stance phase
:param Tstance: the current user-specified stance period
:param T_bf: default body-to-foot Vector
:param index: the foot index in the container
:param key: indicates which foot is being processed
:returns: Foot Coordinates relative to unmodified body
"""
phase, StanceSwing = self.GetPhase(index, Tstance, self.Tswing)
if StanceSwing == SWING:
stored_phase = phase + 1.0
else:
stored_phase = phase
# Just for keeping track
self.Phases[index] = stored_phase
# print("LEG: {} \t PHASE: {}".format(index, stored_phase))
if StanceSwing == STANCE:
return self.StanceStep(phase, L, LateralFraction, YawRate,
penetration_depth, T_bf, key, index)
elif StanceSwing == SWING:
return self.SwingStep(phase, L, LateralFraction, YawRate,
clearance_height, T_bf, key, index)
def GenerateTrajectory(self,
L,
LateralFraction,
YawRate,
vel,
T_bf_,
T_bf_curr,
clearance_height=0.06,
penetration_depth=0.01,
contacts=[0, 0, 0, 0],
dt=None):
"""Calculates the step coordinates for each foot
:param L: step length
:param LateralFraction: determines how lateral the movement is
:param YawRate: the desired body yaw rate
:param vel: the desired step velocity
:param clearance_height: foot clearance height during swing phase
:param penetration_depth: foot penetration depth during stance phase
:param contacts: array containing 1 for contact and 0 otherwise
:param dt: the time step
:returns: Foot Coordinates relative to unmodified body
"""
# First, get Tstance from desired speed and stride length
# NOTE: L is HALF of stride length
if vel != 0.0:
Tstance = 2.0 * abs(L) / abs(vel)
else:
Tstance = 0.0
L = 0.0
self.TD = False
self.time = 0.0
self.time_since_last_TD = 0.0
# Then, get time since last Touchdown and increment time counter
if dt is None:
dt = self.dt
YawRate *= dt
# Catch infeasible timesteps
if Tstance < dt:
Tstance = 0.0
L = 0.0
self.TD = False
self.time = 0.0
self.time_since_last_TD = 0.0
YawRate = 0.0
# NOTE: MUCH MORE STABLE WITH THIS
elif Tstance > 1.3 * self.Tswing:
Tstance = 1.3 * self.Tswing
# Check contacts
if contacts[0] == 1 and Tstance > dt:
self.TD = True
self.Increment(dt, Tstance + self.Tswing)
T_bf = copy.deepcopy(T_bf_)
for i, (key, Tbf_in) in enumerate(T_bf_.items()):
# TODO: MAKE THIS MORE ELEGANT
if key == "FL":
self.ref_idx = i
self.dSref[i] = 0.0
if key == "FR":
self.dSref[i] = 0.5
if key == "BL":
self.dSref[i] = 0.5
if key == "BR":
self.dSref[i] = 0.0
_, p_bf = TransToRp(Tbf_in)
if Tstance > 0.0:
step_coord = self.GetFootStep(L, LateralFraction, YawRate,
clearance_height,
penetration_depth, Tstance, p_bf,
i, key)
else:
step_coord = np.array([0.0, 0.0, 0.0])
T_bf[key][0, 3] = Tbf_in[0, 3] + step_coord[0]
T_bf[key][1, 3] = Tbf_in[1, 3] + step_coord[1]
T_bf[key][2, 3] = Tbf_in[2, 3] + step_coord[2]
return T_bf
| 19,596 | Python | 36.759152 | 97 | 0.5594 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/mkdocs.yml | site_name: Spot Mini Mini
nav:
- Home: index.rst
- Simulation: simulation.rst
- Reinforcement Learning: rl.rst
theme: readthedocs | 141 | YAML | 22.666663 | 36 | 0.70922 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/rl.md | ## Reinforcement Learning Environment
We now have a [Reinforcement Learning Environment](https://github.com/moribots/spot_mini_mini) which uses Pybullet and OpenAI Gym! It contains a variety of optional terrains, which can be activated using **heightfield=True** in the environment class constructor.
If you try to launch the vanilla gait on fairly difficult terrain, Spot will fall very quickly:

By training an Augmented Random Search agent, this can be overcome:

If you are new to RL, I recommend you try a simpler example. Notice that if we choose non-ideal parameters for the generated gait, the robot drifts over time with a forward command:

You should try to train a policy which outputs a yaw command to eliminate the robot's drift, like this:

You can choose a PNG-generated terrain:

Or, for more control, you can choose a programmatically generated heightfield:

Notice that when the simulation resets, the terrain changes. What you cannot see is that the robot's link masses and frictions also change under the hood for added training robustness:

### Quickstart Reinforcement Learning
```
pip3 install numpy
pip3 install pybullet
pip3 install gym
cd spot_bullet/src
./spot_ars_eval.py
```
**Optional Arguments**
```
-h, --help show this help message and exit
-hf, --HeightField Use HeightField
-r, --DebugRack Put Spot on an Elevated Rack
-p, --DebugPath Draw Spot's Foot Path
-a, --AgentNum Agent Number To Load
``` | 1,848 | Markdown | 32.618181 | 262 | 0.753788 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/simulation.md | ## Simulation
This section contains information on the most up-to-date simulation solutions.
### Quickstart PyBullet
I have deployed a 12-point Bezier Curve gait to make Spot walk:

This example can be found in this [repository](https://github.com/moribots/spot_mini_mini). You can optionally use a Game Pad:
```
pip3 install numpy
pip3 install pybullet
pip3 install gym
cd spot_bullet/src
./env_tester.py
```
**Optional Arguments**
```
-h, --help show this help message and exit
-hf, --HeightField Use HeightField
-r, --DebugRack Put Spot on an Elevated Rack
-p, --DebugPath Draw Spot's Foot Path
-ay, --AutoYaw Automatically Adjust Spot's Yaw
```
If you decide to use a controller, you can achieve some fairly fluid motions!
Changing Step Length:

Yaw in Place:

### Kinematics
In this [repository](https://github.com/moribots/spot_mini_mini), there is a working IK solver for both Spot's legs and its body:

| 1,137 | Markdown | 22.708333 | 129 | 0.719437 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/index.rst | Welcome to Spot Mini Mini's documentation!
==========================================
Here is the documentation for the Spot Mini Mini Environment! Navigate to `SpotMicro <https://spot-mini-mini.readthedocs.io/en/latest/source/spotmicro.html>`_ for an overview of all my classes and methods, or visit `The Spot Micro AI Website <https://spotmicroai.readthedocs.io/en/latest/>`_ for a quick tutorial!
.. image:: ../spot_bullet/media/spot_rough_ARS.gif
:width: 500px
:align: center
.. toctree::
:maxdepth: 2
:caption: Contents:
source/modules
simulation
rl
Indices and tables
==================
* :ref:`genindex`
* :ref:`modindex`
* :ref:`search`
| 676 | reStructuredText | 25.038461 | 313 | 0.650888 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/conf.py | # -*- coding: utf-8 -*-
#
# Configuration file for the Sphinx documentation builder.
#
# This file does only contain a selection of the most common options. For a
# full list see the documentation:
# http://www.sphinx-doc.org/en/master/config
# -- Path setup --------------------------------------------------------------
# If extensions (or modules to document with autodoc) are in another directory,
# add these directories to sys.path here. If the directory is relative to the
# documentation root, use os.path.abspath to make it absolute, like shown here.
#
import os
import sys
from recommonmark.parser import CommonMarkParser
sys.path.insert(0, os.path.abspath('..'))
source_parsers = {
'.md': CommonMarkParser,
}
# -- Project information -----------------------------------------------------
project = u'spot_mini_mini'
copyright = u'2020, Maurice Rahme'
author = u'Maurice Rahme'
# The short X.Y version
version = u''
# The full version, including alpha/beta/rc tags
release = u'1.0.0'
# -- General configuration ---------------------------------------------------
# If your documentation needs a minimal Sphinx version, state it here.
#
# needs_sphinx = '1.0'
# Add any Sphinx extension module names here, as strings. They can be
# extensions coming with Sphinx (named 'sphinx.ext.*') or your custom
# ones.
extensions = [
'sphinx.ext.autodoc',
'sphinx.ext.coverage',
'sphinx.ext.mathjax',
'sphinx.ext.viewcode',
]
# Add any paths that contain templates here, relative to this directory.
templates_path = ['_templates']
# The suffix(es) of source filenames.
# You can specify multiple suffix as a list of string:
#
# source_suffix = ['.rst', '.md']
source_suffix = '.rst'
# The master toctree document.
master_doc = 'index'
# The language for content autogenerated by Sphinx. Refer to documentation
# for a list of supported languages.
#
# This is also used if you do content translation via gettext catalogs.
# Usually you set "language" from the command line for these cases.
language = None
# List of patterns, relative to source directory, that match files and
# directories to ignore when looking for source files.
# This pattern also affects html_static_path and html_extra_path.
exclude_patterns = [u'_build', 'Thumbs.db', '.DS_Store']
# The name of the Pygments (syntax highlighting) style to use.
pygments_style = None
# -- Options for HTML output -------------------------------------------------
# The theme to use for HTML and HTML Help pages. See the documentation for
# a list of builtin themes.
#
html_theme = 'sphinx_rtd_theme'
# Theme options are theme-specific and customize the look and feel of a theme
# further. For a list of options available for each theme, see the
# documentation.
#
# html_theme_options = {}
# Add any paths that contain custom static files (such as style sheets) here,
# relative to this directory. They are copied after the builtin static files,
# so a file named "default.css" will overwrite the builtin "default.css".
html_static_path = ['_static']
# Custom sidebar templates, must be a dictionary that maps document names
# to template names.
#
# The default sidebars (for documents that don't match any pattern) are
# defined by theme itself. Builtin themes are using these templates by
# default: ``['localtoc.html', 'relations.html', 'sourcelink.html',
# 'searchbox.html']``.
#
# html_sidebars = {}
# -- Options for HTMLHelp output ---------------------------------------------
# Output file base name for HTML help builder.
htmlhelp_basename = 'spot_mini_minidoc'
# -- Options for LaTeX output ------------------------------------------------
latex_elements = {
# The paper size ('letterpaper' or 'a4paper').
#
# 'papersize': 'letterpaper',
# The font size ('10pt', '11pt' or '12pt').
#
# 'pointsize': '10pt',
# Additional stuff for the LaTeX preamble.
#
# 'preamble': '',
# Latex figure (float) alignment
#
# 'figure_align': 'htbp',
}
# Grouping the document tree into LaTeX files. List of tuples
# (source start file, target name, title,
# author, documentclass [howto, manual, or own class]).
latex_documents = [
(master_doc, 'spot_mini_mini.tex', u'spot\\_mini\\_mini Documentation',
u'Maurice Rahme', 'manual'),
]
# -- Options for manual page output ------------------------------------------
# One entry per manual page. List of tuples
# (source start file, name, description, authors, manual section).
man_pages = [
(master_doc, 'spot_mini_mini', u'spot_mini_mini Documentation',
[author], 1)
]
# -- Options for Texinfo output ----------------------------------------------
# Grouping the document tree into Texinfo files. List of tuples
# (source start file, target name, title, author,
# dir menu entry, description, category)
texinfo_documents = [
(master_doc, 'spot_mini_mini', u'spot_mini_mini Documentation',
author, 'spot_mini_mini', 'One line description of project.',
'Miscellaneous'),
]
# -- Options for Epub output -------------------------------------------------
# Bibliographic Dublin Core info.
epub_title = project
# The unique identifier of the text. This can be a ISBN number
# or the project homepage.
#
# epub_identifier = ''
# A unique identification for the text.
#
# epub_uid = ''
# A list of files that should not be packed into the epub file.
epub_exclude_files = ['search.html']
# -- Extension configuration -------------------------------------------------
| 5,505 | Python | 28.287234 | 79 | 0.642688 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.util.rst | spotmicro.util package
======================
Subpackages
-----------
.. toctree::
spotmicro.util.pybullet_data
Submodules
----------
spotmicro.util.action\_mapper module
------------------------------------
.. automodule:: spotmicro.util.action_mapper
:members:
:undoc-members:
:show-inheritance:
spotmicro.util.bullet\_client module
------------------------------------
.. automodule:: spotmicro.util.bullet_client
:members:
:undoc-members:
:show-inheritance:
spotmicro.util.gui module
-------------------------
.. automodule:: spotmicro.util.gui
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro.util
:members:
:undoc-members:
:show-inheritance:
| 767 | reStructuredText | 15.695652 | 44 | 0.555411 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.rst | spotmicro package
=================
Subpackages
-----------
.. toctree::
spotmicro.GaitGenerator
spotmicro.GymEnvs
spotmicro.Kinematics
spotmicro.OpenLoopSM
spotmicro.util
Submodules
----------
spotmicro.env\_randomizer\_base module
--------------------------------------
.. automodule:: spotmicro.env_randomizer_base
:members:
:undoc-members:
:show-inheritance:
spotmicro.heightfield module
----------------------------
.. automodule:: spotmicro.heightfield
:members:
:undoc-members:
:show-inheritance:
spotmicro.motor module
----------------------
.. automodule:: spotmicro.motor
:members:
:undoc-members:
:show-inheritance:
spotmicro.spot module
---------------------
.. automodule:: spotmicro.spot
:members:
:undoc-members:
:show-inheritance:
spotmicro.spot\_env\_randomizer module
--------------------------------------
.. automodule:: spotmicro.spot_env_randomizer
:members:
:undoc-members:
:show-inheritance:
spotmicro.spot\_gym\_env module
-------------------------------
.. automodule:: spotmicro.spot_gym_env
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro
:members:
:undoc-members:
:show-inheritance:
| 1,290 | reStructuredText | 16.445946 | 45 | 0.570543 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.GaitGenerator.rst | spotmicro.GaitGenerator package
===============================
Submodules
----------
spotmicro.GaitGenerator.Bezier module
-------------------------------------
.. automodule:: spotmicro.GaitGenerator.Bezier
:members:
:undoc-members:
:show-inheritance:
spotmicro.GaitGenerator.Raibert module
--------------------------------------
.. automodule:: spotmicro.GaitGenerator.Raibert
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro.GaitGenerator
:members:
:undoc-members:
:show-inheritance:
| 586 | reStructuredText | 17.935483 | 47 | 0.568259 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/modules.rst | main
====
.. toctree::
:maxdepth: 4
spotmicro
| 54 | reStructuredText | 5.874999 | 15 | 0.537037 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.util.pybullet_data.rst | spotmicro.util.pybullet\_data package
=====================================
Module contents
---------------
.. automodule:: spotmicro.util.pybullet_data
:members:
:undoc-members:
:show-inheritance:
| 212 | reStructuredText | 18.363635 | 44 | 0.542453 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.GymEnvs.rst | spotmicro.GymEnvs package
=========================
Submodules
----------
spotmicro.GymEnvs.spot\_bezier\_env module
------------------------------------------
.. automodule:: spotmicro.GymEnvs.spot_bezier_env
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro.GymEnvs
:members:
:undoc-members:
:show-inheritance:
| 396 | reStructuredText | 16.260869 | 49 | 0.542929 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.Kinematics.rst | spotmicro.Kinematics package
============================
Submodules
----------
spotmicro.Kinematics.LegKinematics module
-----------------------------------------
.. automodule:: spotmicro.Kinematics.LegKinematics
:members:
:undoc-members:
:show-inheritance:
spotmicro.Kinematics.LieAlgebra module
--------------------------------------
.. automodule:: spotmicro.Kinematics.LieAlgebra
:members:
:undoc-members:
:show-inheritance:
spotmicro.Kinematics.SpotKinematics module
------------------------------------------
.. automodule:: spotmicro.Kinematics.SpotKinematics
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro.Kinematics
:members:
:undoc-members:
:show-inheritance:
| 786 | reStructuredText | 19.179487 | 51 | 0.573791 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/docs/source/spotmicro.OpenLoopSM.rst | spotmicro.OpenLoopSM package
============================
Submodules
----------
spotmicro.OpenLoopSM.SpotOL module
----------------------------------
.. automodule:: spotmicro.OpenLoopSM.SpotOL
:members:
:undoc-members:
:show-inheritance:
Module contents
---------------
.. automodule:: spotmicro.OpenLoopSM
:members:
:undoc-members:
:show-inheritance:
| 383 | reStructuredText | 15.695651 | 43 | 0.556136 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/spotmicro_description-CHAMP/README.md | # spotmicro_description
A minimal and revamped version of [SpotMicroAI](https://spotmicroai.readthedocs.io/en/latest/) description file pulled from its [Gitlab](https://gitlab.com/custom_robots/spotmicroai/simulation) repository.
The meshes' path have been modified so the models can be located using its package name.
## Quick Start
You can view the model by running:
roslaunch spotmicro_description view_urdf.launch
## Testing
You can modify the Xacro description and see the changes in the UDF file with this command:
rosrun xacro xacro spotmicroai.urdf.xacro > spotmicroai.urdf
| 598 | Markdown | 32.277776 | 205 | 0.785953 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Stochlab/stochlite-original/README.md | Stochastic Robotics Lab
https://www.stochlab.com/
| 50 | Markdown | 15.999995 | 25 | 0.8 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Stochlab/stochlite_description-aditya-shirwatkar/Readme.md | # Stochlite Description files
| 30 | Markdown | 14.499993 | 29 | 0.833333 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/ANYbotics/anymal_c_simple_description-CHAMP/README.md | # ANYmal C Robot Description (URDF)
## Overview
This package contains a simplified robot description (URDF) of the [ANYmal C robot](https://www.anybotics.com/anymal) developed by [ANYbotics](https://www.anybotics.com).
The extended ANYmal C robot description, simulation, and control software is available exclusively to members of the [ANYmal Research community](https://www.anymal-research.org). For more information and membership applications, contact [email protected].
**Author & Maintainer: Linus Isler, [ANYbotics](https://www.anybotics.com)**
[](doc/anymal_c_rviz.png)
## License
This software is released under a [BSD 3-Clause license](LICENSE).
## Usage
Load the ANYmal description to the ROS parameter server:
roslaunch anymal_c_simple_description load.launch
To visualize and debug the robot description, start the standalone visualization (note that you have to provide the following additional dependencies: `joint_state_publisher`, `robot_state_publisher`, `rviz`):
roslaunch anymal_c_simple_description standalone.launch
### Launch files
* **`load.launch`:** Loads the URDF to the parameter server. Meant to be included in higher level launch files.
* **`standalone.launch`:** A standalone launch file that starts RViz and a joint state publisher to debug the description.
| 1,366 | Markdown | 41.718749 | 256 | 0.770864 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/ANYbotics/anymal_b_simple_description-CHAMP/Readme.md | # ANYmal B Robot Description (URDF)
## Overview
This package contains a simplified robot description (URDF) of the [ANYmal B anymalal robot](https://www.anybotics.com/anymal) developed by [ANYbotics](https://www.anybotics.com).
The extended ANYmal B robot description, simulation, and control software is available exclusively to members of the [ANYmal Research community](https://www.anymal-research.org). For more information and membership applications, contact [email protected].
**Author & Maintainer: Linus Isler, [ANYbotics](https://www.anybotics.com)**
[](doc/anymal_b_rviz.png)
## License
This software is released under a [BSD 3-Clause license](LICENSE).
## Publications
If you use this work in an academic context, please cite the following publications:
> M. Hutter, C. Gehring, A. Lauber, F. Gunther, C. D. Bellicoso, V. Tsounis, P. Fankhauser, R. Diethelm, S. Bachmann, M. Bloesch, H. Kolvenbach, M. Bjelonic, L. Isler and K. Meyer
> **"ANYmal - toward legged robots for harsh environments“**,
> in Advanced Robotics, 31.17, 2017. ([DOI](https://doi.org/10.1080/01691864.2017.1378591))
@article{anymal2017,
title={ANYmal-toward legged robots for harsh environments},
author={Hutter, Marco and Gehring, Christian and Lauber, Andreas and Gunther, Fabian and Bellicoso, Carmine Dario and Tsounis, Vassilios and Fankhauser, P{\'e}ter and Diethelm, Remo and Bachmann, Samuel and Bl{\"o}sch, Michael and Kolvenbach, Hendrik and Bjelonic, Marko and Isler, Linus and Meyer, Konrad},
journal={Advanced Robotics},
volume={31},
number={17},
pages={918--931},
year={2017},
publisher={Taylor \& Francis}
}
> ANYbotics,
> **"ANYmal – Autonomous Legged Robot“**,
> [https://www.anybotics.com/anymal](https://www.anybotics.com/anymal) (accessed: 01.01.2019)
@misc{anymal,
author = {ANYbotics},
title = {{ANYmal - Autonomous Legged Robot}},
howpublished = {\url{https://www.anybotics.com/anymal}},
note = {Accessed: 2019-01-01}
}
## Usage
Load the ANYmal description to the ROS parameter server:
roslaunch anymal_b_simple_description load.launch
To visualize and debug the robot description, start the standalone visualization (note that you have to provide the following additional dependencies: `joint_state_publisher`, `robot_state_publisher`, `rviz`):
roslaunch anymal_b_simple_description standalone.launch
### Launch files
* **`load.launch`:** Loads the URDF to the parameter server. Meant to be included in higher level launch files.
* **`standalone.launch`:** A standalone launch file that starts RViz and a joint state publisher to debug the description.
| 2,754 | Markdown | 44.163934 | 315 | 0.718954 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/README.md | # mini-cheetah-gazebo-urdf
An urdf description file of a quadruped robot modeled on mini cheetah. With this you can import the robot into the gazebo environment to realize the control of the robot.
Ubuntu 16.04(ROS Kinetic)
gazebo 8.6
Step:
cd <your_ws>/src
git clone https://github.com/HitSZwang/mini-cheetah-gazebo-urdf
cd ..
catkin_make
source devel/setup.bash
1.If you want to browse the robot model
roslaunch yobo_gazebo demo.launch
2.You can ues the teleop to control the robot
This model was used in champ and achieved good results.
the champ program https://github.com/chvmp/champ
if you use champ_setup_assistant: https://github.com/chvmp/champ_setup_assistant
you should choose the yobotics.urdf
| 729 | Markdown | 19.857142 | 170 | 0.770919 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_gazebo/scripts/imu_sensor.py | #! /usr/bin/env python
'''
Copyright (c) 2019-2020, Juan Miguel Jimeno
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the copyright holder nor the names of its
contributors may be used to endorse or promote products derived
from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
'''
import rospy
from nav_msgs.msg import Odometry
from geometry_msgs.msg import Point, Quaternion
from yobotics_msgs.msg import Pose
import tf
class SimPose:
def __init__(self):
rospy.Subscriber("odom/ground_truth", Odometry, self.odometry_callback)
self.sim_pose_publisher = rospy.Publisher("/yobotics/gazebo/pose", Pose, queue_size=50)
def odometry_callback(self, data):
sim_pose_msg = Pose()
sim_pose_msg.roll = data.pose.pose.orientation.x
sim_pose_msg.pitch = data.pose.pose.orientation.y
sim_pose_msg.yaw = data.pose.pose.orientation.z
self.sim_pose_publisher.publish(sim_pose_msg)
if __name__ == "__main__":
rospy.init_node("yobotics_gazebo_sim_pose", anonymous = True)
odom = SimPose()
rospy.spin() | 2,342 | Python | 44.941176 | 95 | 0.752775 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_gazebo/scripts/odometry_tf.py | #! /usr/bin/env python
'''
Copyright (c) 2019-2020, Juan Miguel Jimeno
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the copyright holder nor the names of its
contributors may be used to endorse or promote products derived
from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
'''
import rospy
from nav_msgs.msg import Odometry
from geometry_msgs.msg import Point, Quaternion
import tf
class Odom:
def __init__(self):
rospy.Subscriber("odom/raw", Odometry, self.odometry_callback)
self.odom_broadcaster = tf.TransformBroadcaster()
def odometry_callback(self, data):
self.current_linear_speed_x = data.twist.twist.linear.x
self.current_angular_speed_z = data.twist.twist.angular.z
current_time = rospy.Time.now()
odom_quat = (
data.pose.pose.orientation.x,
data.pose.pose.orientation.y,
data.pose.pose.orientation.z,
data.pose.pose.orientation.w
)
self.odom_broadcaster.sendTransform(
(data.pose.pose.position.x, data.pose.pose.position.y, 0),
odom_quat,
current_time,
"base_footprint",
"odom"
)
if __name__ == "__main__":
rospy.init_node("yobotics_gazebo_odometry_transform", anonymous = True)
odom = Odom()
rospy.spin() | 2,612 | Python | 40.47619 | 80 | 0.714778 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_gazebo/scripts/odometry.py | #! /usr/bin/env python
'''
Copyright (c) 2019-2020, Juan Miguel Jimeno
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
* Neither the name of the copyright holder nor the names of its
contributors may be used to endorse or promote products derived
from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY
DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
(INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
(INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
'''
import rospy
from yobotics_msgs.msg import Contacts
from nav_msgs.msg import Odometry
from geometry_msgs.msg import Point, Quaternion, Pose, Twist, Vector3
import tf
from tf import TransformListener
from tf.transformations import euler_from_quaternion, quaternion_from_euler
from nav_msgs.msg import Odometry
import math
class yoboticsOdometry:
def __init__(self):
rospy.Subscriber("/yobotics/gazebo/contacts", Contacts, self.contacts_callback)
self.odom_publisher = rospy.Publisher("odom/raw", Odometry, queue_size=50)
self.odom_broadcaster = tf.TransformBroadcaster()
self.tf = TransformListener()
self.foot_links = [
"lf_foot_link",
"rf_foot_link",
"lh_foot_link",
"rh_foot_link"
]
self.nominal_foot_positions = [[0,0],[0,0],[0,0],[0,0]]
self.prev_foot_positions = [[0,0],[0,0],[0,0],[0,0]]
self.prev_theta = [0,0,0,0]
self.prev_stance_angles = [0,0,0,0]
self.prev_time = 0
self.pos_x = 0
self.pos_y = 0
self.theta = 0
self.publish_odom_tf(0,0,0,0)
rospy.sleep(1)
for i in range(4):
self.nominal_foot_positions[i] = self.get_foot_position(i)
self.prev_foot_positions[i] = self.nominal_foot_positions[i]
self.prev_theta[i] = math.atan2(self.prev_foot_positions[i][1], self.prev_foot_positions[i][0])
def publish_odom(self, x, y, z, theta, vx, vy, vth):
current_time = rospy.Time.now()
odom = Odometry()
odom.header.stamp = current_time
odom.header.frame_id = "odom"
odom_quat = tf.transformations.quaternion_from_euler(0, 0, theta)
odom.pose.pose = Pose(Point(x, y, z), Quaternion(*odom_quat))
odom.child_frame_id = "base_footprint"
odom.twist.twist = Twist(Vector3(vx, vy, 0), Vector3(0, 0, vth))
# publish the message
self.odom_publisher.publish(odom)
def publish_odom_tf(self, x, y, z, theta):
current_time = rospy.Time.now()
odom_quat = quaternion_from_euler (0, 0, theta)
self.odom_broadcaster.sendTransform(
(x, y, z),
odom_quat,
current_time,
"base_footprint",
"odom"
)
def get_foot_position(self, leg_id):
if self.tf.frameExists("base_link" and self.foot_links[leg_id]) :
t = self.tf.getLatestCommonTime("base_link", self.foot_links[leg_id])
position, quaternion = self.tf.lookupTransform("base_link", self.foot_links[leg_id], t)
return position
else:
return 0, 0, 0
def contacts_callback(self, data):
self.leg_contact_states = data.contacts
def is_almost_equal(self, a, b, max_rel_diff):
diff = abs(a - b)
a = abs(a)
b = abs(b)
largest = 0
if b > a:
largest = b
else:
larget = a
if diff <= (largest * max_rel_diff):
return True
return False
def run(self):
while not rospy.is_shutdown():
leg_contact_states = self.leg_contact_states
current_foot_position = [[0,0],[0,0],[0,0],[0,0]]
stance_angles = [0, 0, 0, 0]
current_theta = [0, 0, 0, 0]
delta_theta = 0
in_xy = False
total_contact = sum(leg_contact_states)
x_sum = 0
y_sum = 0
theta_sum = 0
for i in range(4):
current_foot_position[i] = self.get_foot_position(i)
for i in range(4):
current_theta[i] = math.atan2(current_foot_position[i][1], current_foot_position[i][0])
from_nominal_x = self.nominal_foot_positions[i][0] - current_foot_position[i][0]
from_nominal_y = self.nominal_foot_positions[i][1] - current_foot_position[i][1]
stance_angles[i] = math.atan2(from_nominal_y, from_nominal_x)
# print stance_angles
#check if it's moving in the x or y axis
if self.is_almost_equal(stance_angles[i], abs(1.5708), 0.001) or self.is_almost_equal(stance_angles[i], abs(3.1416), 0.001):
in_xy = True
if current_foot_position[i] != None and leg_contact_states[i] == True and total_contact == 2:
delta_x = (self.prev_foot_positions[i][0] - current_foot_position[i][0]) / 2
delta_y = (self.prev_foot_positions[i][1] - current_foot_position[i][1]) / 2
x = delta_x * math.cos(self.theta) - delta_y * math.sin(self.theta)
y = delta_x * math.sin(self.theta) + delta_y * math.cos(self.theta)
x_sum += delta_x
y_sum += delta_y
self.pos_x += x
self.pos_y += y
if not in_xy:
delta_theta = self.prev_theta[i] - current_theta[i]
theta_sum += delta_theta
self.theta += delta_theta / 2
now = rospy.Time.now().to_sec()
dt = now - self.prev_time
vx = x_sum / dt
vy = y_sum / dt
vth = theta_sum / dt
self.publish_odom(self.pos_x, self.pos_y, 0, self.theta, vx, vy, vth)
# self.publish_odom_tf(self.pos_x, self.pos_y, 0, self.theta)
self.prev_foot_positions = current_foot_position
self.prev_theta = current_theta
self.prev_stance_angles = stance_angles
self.prev_time = now
rospy.sleep(0.01)
if __name__ == "__main__":
rospy.init_node("yobotics_gazebo_odometry", anonymous = True)
odom = yoboticsOdometry()
odom.run()
| 7,397 | Python | 37.331606 | 140 | 0.592402 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_gazebo/config/ros_control.yaml | "":
joint_states_controller:
type: joint_state_controller/JointStateController
publish_rate: 30
joint_group_position_controller:
type: effort_controllers/JointTrajectoryController
joints:
- FL_hip_joint
- FL_thigh_joint
- FL_calf_joint
- FR_hip_joint
- FR_thigh_joint
- FR_calf_joint
- RL_hip_joint
- RL_thigh_joint
- RL_calf_joint
- RR_hip_joint
- RR_thigh_joint
- RR_calf_joint
gains:
FL_hip_joint : {p: 180, d: 0.9, i: 20}
FL_thigh_joint : {p: 180, d: 0.9, i: 20}
FL_calf_joint : {p: 180, d: 0.9, i: 20}
FR_hip_joint : {p: 180, d: 0.9, i: 20}
FR_thigh_joint : {p: 180, d: 0.9, i: 20}
FR_calf_joint : {p: 180, d: 0.9, i: 20}
RL_hip_joint : {p: 180, d: 0.9, i: 20}
RL_thigh_joint : {p: 180, d: 0.9, i: 20}
RL_calf_joint : {p: 180, d: 0.9, i: 20}
RR_hip_joint : {p: 180, d: 0.9, i: 20}
RR_thigh_joint : {p: 180, d: 0.9, i: 20}
RR_calf_joint : {p: 180, d: 0.9, i: 20}
| 1,120 | YAML | 30.138888 | 56 | 0.488393 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_teleop/yobotics_teleop.py | #!/usr/bin/env python
#credits to: https://github.com/ros-teleop/teleop_twist_keyboard/blob/master/teleop_twist_keyboard.py
from __future__ import print_function
import roslib; roslib.load_manifest('yobotics_teleop')
import rospy
from sensor_msgs.msg import Joy
from geometry_msgs.msg import Twist
from yobotics_msgs.msg import Pose
import sys, select, termios, tty
import numpy as np
class Teleop:
def __init__(self):
self.velocity_publisher = rospy.Publisher('cmd_vel', Twist, queue_size = 1)
self.pose_publisher = rospy.Publisher('cmd_pose', Pose, queue_size = 1)
self.joy_subscriber = rospy.Subscriber('joy', Joy, self.joy_callback)
self.speed = rospy.get_param("~speed", 0.3)
self.turn = rospy.get_param("~turn", 1.0)
self.msg = """
Reading from the keyboard and Publishing to Twist!
---------------------------
Moving around:
u i o
j k l
m , .
For Holonomic mode (strafing), hold down the shift key:
---------------------------
U I O
J K L
M < >
t : up (+z)
b : down (-z)
anything else : stop
q/z : increase/decrease max speeds by 10%
w/x : increase/decrease only linear speed by 10%
e/c : increase/decrease only angular speed by 10%
CTRL-C to quit
"""
self.velocityBindings = {
'i':(1,0,0,0),
'o':(1,0,0,-1),
'j':(0,0,0,1),
'l':(0,0,0,-1),
'u':(1,0,0,1),
',':(-1,0,0,0),
'.':(-1,0,0,1),
'm':(-1,0,0,-1),
'O':(1,-1,0,0),
'I':(1,0,0,0),
'J':(0,1,0,0),
'L':(0,-1,0,0),
'U':(1,1,0,0),
'<':(-1,0,0,0),
'>':(-1,-1,0,0),
'M':(-1,1,0,0),
'v':(0,0,1,0),
'n':(0,0,-1,0),
}
self.poseBindings = {
'f':(-1,0,0,0),
'h':(1,0,0,0),
't':(0,1,0,0),
'b':(0,-1,0,0),
'r':(0,0,1,0),
'y':(0,0,-1,0),
}
self.speedBindings={
'q':(1.1,1.1),
'z':(.9,.9),
'w':(1.1,1),
'x':(.9,1),
'e':(1,1.1),
'c':(1,.9),
}
self.poll_keys()
def joy_callback(self, data):
twist = Twist()
twist.linear.x = data.axes[7] * self.speed
twist.linear.y = data.buttons[4] * data.axes[6] * self.speed
twist.linear.z = 0
twist.angular.x = 0
twist.angular.y = 0
twist.angular.z = (not data.buttons[4]) * data.axes[6] * self.turn
self.velocity_publisher.publish(twist)
body_pose = Pose()
body_pose.x = 0
body_pose.y = 0
body_pose.roll = (not data.buttons[5]) *-data.axes[3] * 0.349066
body_pose.pitch = data.axes[4] * 0.261799
body_pose.yaw = data.buttons[5] * data.axes[3] * 0.436332
if data.axes[5] < 0:
body_pose.z = self.map(data.axes[5], 0, -1.0, 1, 0.00001)
else:
body_pose.z = 1.0
self.pose_publisher.publish(body_pose)
def poll_keys(self):
self.settings = termios.tcgetattr(sys.stdin)
x = 0
y = 0
z = 0
th = 0
roll = 0
pitch = 0
yaw = 0
status = 0
cmd_attempts = 0
try:
print(self.msg)
print(self.vels( self.speed, self.turn))
while not rospy.is_shutdown():
key = self.getKey()
if key in self.velocityBindings.keys():
x = self.velocityBindings[key][0]
y = self.velocityBindings[key][1]
z = self.velocityBindings[key][2]
th = self.velocityBindings[key][3]
if cmd_attempts > 1:
twist = Twist()
twist.linear.x = x *self.speed
twist.linear.y = y * self.speed
twist.linear.z = z * self.speed
twist.angular.x = 0
twist.angular.y = 0
twist.angular.z = th * self.turn
self.velocity_publisher.publish(twist)
cmd_attempts += 1
elif key in self.poseBindings.keys():
#TODO: changes these values as rosparam
roll += 0.0174533 * self.poseBindings[key][0]
pitch += 0.0174533 * self.poseBindings[key][1]
yaw += 0.0174533 * self.poseBindings[key][2]
roll = np.clip(roll, -0.523599, 0.523599)
pitch = np.clip(pitch, -0.349066, 0.349066)
yaw = np.clip(yaw, -0.436332, 0.436332)
if cmd_attempts > 1:
body_pose = Pose()
body_pose.x = 0
body_pose.y = 0
body_pose.z = 0
body_pose.roll = roll
body_pose.pitch = pitch
body_pose.yaw = yaw
self.pose_publisher.publish(body_pose)
cmd_attempts += 1
elif key in self.speedBindings.keys():
self.speed = self.speed * self.speedBindings[key][0]
self.turn = self.turn * self.speedBindings[key][1]
print(self.vels(self.speed, self.turn))
if (status == 14):
print(self.msg)
status = (status + 1) % 15
else:
cmd_attempts = 0
if (key == '\x03'):
break
except Exception as e:
print(e)
finally:
twist = Twist()
twist.linear.x = 0
twist.linear.y = 0
twist.linear.z = 0
twist.angular.x = 0
twist.angular.y = 0
twist.angular.z = 0
self.velocity_publisher.publish(twist)
termios.tcsetattr(sys.stdin, termios.TCSADRAIN, self.settings)
def getKey(self):
tty.setraw(sys.stdin.fileno())
rlist, _, _ = select.select([sys.stdin], [], [], 0.1)
if rlist:
key = sys.stdin.read(1)
else:
key = ''
termios.tcsetattr(sys.stdin, termios.TCSADRAIN, self.settings)
return key
def vels(self, speed, turn):
return "currently:\tspeed %s\tturn %s " % (speed,turn)
def map(self, x, in_min, in_max, out_min, out_max):
return (x - in_min) * (out_max - out_min) / (in_max - in_min) + out_min;
if __name__ == "__main__":
rospy.init_node('yobotics_teleop')
teleop = Teleop() | 6,972 | Python | 31.282407 | 101 | 0.443632 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/MIT_mini-cheetah/mini-cheetah-CHAMP/yobo_model/yobotics_teleop/README.md | # yobotics_teleop
yobotics Quadruped Robot's teleoperation node. This is a forked version of [teleop_twist_keyboard](https://github.com/ros-teleop/teleop_twist_keyboard/blob/master/teleop_twist_keyboard.py).
The software has been modified to control the robot's whole-body pose (roll, pitch, yaw).
| 300 | Markdown | 59.199988 | 190 | 0.79 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/dream_walker-project/package.xml | <package format="2">
<name>dream_walker</name>
<version>1.0.0</version>
<description>
<p>URDF Description package for dream_walker</p>
<p>This package contains configuration data, 3D models and launch files
for dream_walker robot</p>
</description>
<author>SHOMA</author>
<maintainer email="[email protected]" />
<license>BSD</license>
<buildtool_depend>catkin</buildtool_depend>
<depend>roslaunch</depend>
<depend>robot_state_publisher</depend>
<depend>rviz</depend>
<depend>joint_state_publisher</depend>
<depend>gazebo</depend>
<export>
<architecture_independent />
</export>
</package>
| 634 | XML | 27.863635 | 75 | 0.709779 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/dream_walker-project/README.md | # DreamWalker Project
***Develop a quadruped robot like Spot Mini***

# DEMO
**gazebo**

**Rviz**

# Features
This robot will be developed using a BLDC actuator optimized for a quadruped robot.
Gait control is performed using general kinematics control, and stability is guaranteed by dynamics control.
At this stage, the simulation environment is ready.
# Environment
OS:Ubuntu 18.04.4 LTS
CPU:Intel® Core™ i7-1065G7 CPU @ 1.30GHz × 8
GPU:Intel® Iris(R) Plus Graphics (Ice Lake 8x8 GT2)
ROS:Melodic
# Usage
```bash
source ~/catkin_ws/devel/setup.bash
(source ~/catkin_ws/devel/setup.zsh)
cd ~/catkin_ws/src
git clone https://github.com/Ohaginia/dream_walker.git
cd ~/catkin_ws
catkin_make
roslaunch dream_walker gazebo.launch
roslaunch dream_walker display.launch model:=dream_walker.urdf
```
# Note
**Change Fixed Frame of Global Options from map to dummy. (Default is map)**

# Author
* Shoma Uehara
* TRUST SMITH & COMPANY
* [email protected]
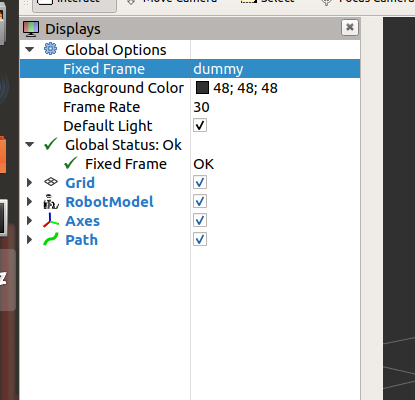
Change Fixed Frame of Global Options from map to dummy.
(Default is map)
# Author
* Shoma Uehara
* TRUST SMITH & COMPANY
* [email protected]
# License
"dream_walker" is under [MIT license](https://en.wikipedia.org/wiki/MIT_License).
| 1,934 | Markdown | 29.714285 | 149 | 0.759566 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/dream_walker-project/config/joint_names_dw_descliption3.yaml | controller_joint_names: ['', 'A1_joint', 'A2_joint', 'A3_joint', 'B1_joint', 'B2_joint', 'B3_joint', 'C1_joint', 'C2_joint', 'C3_joint', 'D1_joint', 'D2_joint', 'D3_joint', ]
| 175 | YAML | 86.999957 | 174 | 0.594286 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/README.md | Has robots from Ghost Robotics:
vision60
spirit
minitaur | 56 | Markdown | 13.249997 | 31 | 0.857143 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/README.md | # kodlab_gazebo
ROS package for the simulation of robotic platforms using Gazebo
Maintainer: Vasileios Vasilopoulos (<[email protected]>)
## General notes
1. This ROS package can be used in conjunction with the Ghost Robotics SDK artifacts, which are independently distributed by Ghost Robotics.
2. At this point, there is no open source Vision60 control example in the SDK, but once released the following procedure should be identical. For now, you can just launch the Vision60 simulation as explained below.
3. The simulation depends a lot on friction parameters. These parameters can be configured in the URDF xacro files ([minitaur_gazebo.urdf.xacro](minitaur_description/urdf/minitaur_gazebo.urdf.xacro), [vision60_gazebo.urdf.xacro](vision60_description/urdf/vision60_gazebo.urdf.xacro) and [spirit_gazebo.urdf.xacro](spirit_description/urdf/spirit_gazebo.urdf.xacro)).
## Running the simulation
1. For the package to work properly, make sure you have installed the following packages:
```
$ sudo apt-get install ros-melodic-controller-manager ros-melodic-joint-state-controller ros-melodic-gazebo-ros-pkgs ros-melodic-gazebo-ros-control ros-melodic-effort-controllers python-catkin-tools
```
2. For some illustrations, we also use the package [3DGEMS](http://data.nvision2.eecs.yorku.ca/3DGEMS/). Download all the subfolders mentioned on the package website and put them in a separate folder.
3. Copy the package to your ROS workspace folder
```
$ cp -r <artifacts_location>/thirdparty/kodlab_gazebo <catkin_ws_location>/src
```
4. The following line needs to be added in `~/.bashrc` to allow for proper Gazebo model detection
```
export GAZEBO_MODEL_PATH=${GAZEBO_MODEL_PATH}:<catkin_ws_location>/src/kodlab_gazebo/minitaur_description/sdf:<catkin_ws_location>/src/kodlab_gazebo/minitaur_description:<catkin_ws_location>/src/kodlab_gazebo/vision60_description:<catkin_ws_location>/src/kodlab_gazebo/spirit_description
```
5. Also, the following line needs to be added in `~/.bashrc` to allow for proper use of [3DGEMS](http://data.nvision2.eecs.yorku.ca/3DGEMS/)
```
export GAZEBO_MODEL_PATH=${GAZEBO_MODEL_PATH}:<3dgems_models_location>/decoration:<3dgems_models_location>/earthquake:<3dgems_models_location>/electronics:<3dgems_models_location>/food:<3dgems_models_location>/furniture:<3dgems_models_location>/kitchen:<3dgems_models_location>/miscellaneous:<3dgems_models_location>/shapes:<3dgems_models_location>/stationery:<3dgems_models_location>/tools
```
6. Configure [ghost.world](gazebo_scripts/worlds/ghost.world) (or any other [world](gazebo_scripts/worlds) file) to include anything you want to show up in your simulation. In this file, you can also configure `max_step_size` and `real_time_update_rate` for the simulation. Usually a `max_step_size` of 0.0005 works for Minitaur. Vision needs a smaller value for contact forces (0.00005 should be enough). Currently, `real_time_update_rate` is configured to 2000Hz.
7. Build all the packages
```
$ cd <catkin_ws_location>
$ catkin build
```
8. To launch the simulation, build the package and run:
```
$ roslaunch gazebo_scripts minitaur_gazebo.launch
```
for standalone Minitaur, or
```
$ roslaunch gazebo_scripts minitaur_sensor_gazebo.launch
```
for Minitaur equipped with a sensor head, or
```
$ roslaunch gazebo_scripts vision60_gazebo.launch
```
for Vision60, or
```
$ roslaunch gazebo_scripts spirit_gazebo.launch
```
for Spirit.
9. (Optional) If you want to control the robot using the Ghost SDK, compile and run the corresponding Ghost Robotics SDK script. For this, please use the [Makefile example](extras/Makefile) provided here (the format might change in the future). The simulation exposes 3 ROS topics for control: `/behaviorId`, `/behaviorMode` and `/twist`, and several others for checking the robot state: `/<robot_name>/state/imu`, `/<robot_name>/state/batteryState`, `/<robot_name>/state/behaviorId`, `/<robot_name>/state/behaviorMode`, `/<robot_name>/state/joint`, `/<robot_name>/state/pose`. As an example following the Ghost Robotics SDK FirstHop example for Minitaur, the overall process should look like that:
```
$ mv <artifacts_location>/examples/FirstHop/Makefile <artifacts_location>/examples/FirstHop/Makefile.bk
$ cp <artifacts_location>/thirdparty/kodlab_gazebo/extras/Makefile <artifacts_location>/examples/FirstHop/Makefile
$ cd <artifacts_location>/examples/FirstHop
$ make
$ ./FirstHop_MINITAUR_E
```
## Converting Minitaur's URDF to SDF
The most important requirement to setup the simulation is to properly convert the Minitaur's URDF file to an SDF file. We have a URDF xacro file ([minitaur_gazebo.urdf.xacro](minitaur_description/urdf/minitaur_gazebo.urdf.xacro)) in the [urdf folder](minitaur_description/urdf) and properly converted URDF and SDF files (see [minitaur_constrained.sdf](minitaur_description/sdf/minitaur_constrained/minitaur_constrained.sdf)). If you need to modify any of the above, please run the following commands to convert the xacro file to URDF
```bash
$ python xacro.py minitaur_gazebo.urdf.xacro > minitaur_gazebo.urdf
```
and subsequently to SDF using
```bash
$ gz sdf -p minitaur_gazebo.urdf > minitaur_gazebo.sdf
```
However, you also need to do the following after the conversion:
1. Make sure to add the lines below to the end of the SDF file (before the model termination). These lines realize the 5-bar mechanism constraint for each leg (hence the name `constrained`).
```
<joint name='constraint_back_left' type='revolute'>
<parent>lower_leg_back_leftL_link</parent>
<child>lower_leg_back_leftR_link</child>
<pose frame=''>0 0 0.19 0 -0 0</pose>
<axis>
<xyz>0 1 0</xyz>
<use_parent_model_frame>0</use_parent_model_frame>
<limit>
<lower>-1.79769e+308</lower>
<upper>1.79769e+308</upper>
<effort>-1</effort>
<velocity>-1</velocity>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
<damping>0</damping>
</dynamics>
</axis>
<physics>
<ode>
<limit>
<cfm>0</cfm>
<erp>0.2</erp>
</limit>
<suspension>
<cfm>0</cfm>
<erp>0.2</erp>
</suspension>
</ode>
</physics>
</joint>
<joint name='constraint_back_right' type='revolute'>
<parent>lower_leg_back_rightR_link</parent>
<child>lower_leg_back_rightL_link</child>
<pose frame=''>0 0 0.19 0 -0 0</pose>
<axis>
<xyz>-0 -1 -0</xyz>
<use_parent_model_frame>0</use_parent_model_frame>
<limit>
<lower>-1.79769e+308</lower>
<upper>1.79769e+308</upper>
<effort>-1</effort>
<velocity>-1</velocity>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
<damping>0</damping>
</dynamics>
</axis>
<physics>
<ode>
<limit>
<cfm>0</cfm>
<erp>0.2</erp>
</limit>
<suspension>
<cfm>0</cfm>
<erp>0.2</erp>
</suspension>
</ode>
</physics>
</joint>
<joint name='constraint_front_left' type='revolute'>
<parent>lower_leg_front_leftL_link</parent>
<child>lower_leg_front_leftR_link</child>
<pose frame=''>0 0 0.19 0 -0 0</pose>
<axis>
<xyz>0 1 0</xyz>
<use_parent_model_frame>0</use_parent_model_frame>
<limit>
<lower>-1.79769e+308</lower>
<upper>1.79769e+308</upper>
<effort>-1</effort>
<velocity>-1</velocity>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
<damping>0</damping>
</dynamics>
</axis>
<physics>
<ode>
<limit>
<cfm>0</cfm>
<erp>0.2</erp>
</limit>
<suspension>
<cfm>0</cfm>
<erp>0.2</erp>
</suspension>
</ode>
</physics>
</joint>
<joint name='constraint_front_right' type='revolute'>
<parent>lower_leg_front_rightR_link</parent>
<child>lower_leg_front_rightL_link</child>
<pose frame=''>0 0 0.19 0 -0 0</pose>
<axis>
<xyz>-0 -1 -0</xyz>
<use_parent_model_frame>0</use_parent_model_frame>
<limit>
<lower>-1.79769e+308</lower>
<upper>1.79769e+308</upper>
<effort>-1</effort>
<velocity>-1</velocity>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
<damping>0</damping>
</dynamics>
</axis>
<physics>
<ode>
<limit>
<cfm>0</cfm>
<erp>0.2</erp>
</limit>
<suspension>
<cfm>0</cfm>
<erp>0.2</erp>
</suspension>
</ode>
</physics>
</joint>
```
2. For each leg, rename all the toe collision descriptions to match the corresponding toe (e.g., for the front left leg, rename `lower_leg_front_leftL_link_fixed_joint_lump__toe0_collision_1` to `toe0_collision`), and also add the following lines immediately below this collision tag (change `0` to match each toe):
```
<sensor name="toe0_contact" type="contact">
<plugin name="toe0_plugin" filename="libcontact.so"/>
<contact>
<collision>toe0_collision</collision>
</contact>
</sensor>
```
3. To help Minitaur with turning, you need to make joints '8', '9', '10', '11', '12', '13', '14' and '15' universal, to match the following format example. Make sure that the sign of 1 in `<xyz>` for both axes is positive for joints '9', '11', '13' and '15' (and with limits -2.091 and 1.049) and negative for '8', '10', '12' and '14' (and with limits -1.049 and 2.091).
```
<joint name='9' type='universal'>
<child>lower_leg_front_leftR_link</child>
<parent>motor_front_leftR_link</parent>
<axis>
<xyz>-0 1 -0</xyz>
<limit>
<lower>-2.091</lower>
<upper>1.049</upper>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
</dynamics>
<use_parent_model_frame>1</use_parent_model_frame>
</axis>
<axis2>
<xyz>1 0 0</xyz>
<limit>
<lower>-0.02</lower>
<upper>0.02</upper>
</limit>
<dynamics>
<spring_reference>0</spring_reference>
<spring_stiffness>0</spring_stiffness>
</dynamics>
<use_parent_model_frame>1</use_parent_model_frame>
</axis2>
</joint>
```
4. Make sure to move the SDF file to the [minitaur_constrained folder](minitaur_description/sdf/minitaur_constrained) and rename it from `minitaur_gazebo.sdf` to `minitaur_constrained.sdf`.
5. Rename the model name in [minitaur_constrained.sdf](minitaur_description/sdf/minitaur_constrained/minitaur_constrained.sdf) to `minitaur_constrained`. Do the same thing for the namespace in `libgazebo_ros_control.so`.
## Minitaur with sensor head
We have included an SDF file describing Minitaur equipped with a sensor head on top (see [minitaur_sensor.sdf](minitaur_description/sdf/minitaur_sensor/minitaur_sensor.sdf)). If you modify any of the xacro files describing Minitaur or want to generate this SDF file on your own, follow the steps above to generate [minitaur_constrained.sdf](minitaur_description/sdf/minitaur_constrained/minitaur_constrained.sdf) first. Then:
1. Copy the SDF file to the [minitaur_sensor folder](minitaur_description/sdf/minitaur_sensor) and rename it from `minitaur_constrained.sdf` to `minitaur_sensor.sdf`.
2. To add the sensor head, include the following lines at the end of the SDF file (before the model termination):
```
<include>
<uri>model://sensor_head</uri>
<pose>0 0 0.04 0 0 0</pose>
</include>
<joint name="sensor_head_joint" type="fixed">
<child>sensor_head::base</child>
<parent>base_chassis_link_dummy</parent>
</joint>
```
The sensor head description is included in [sensor_head.sdf](minitaur_description/sdf/sensor_head/sensor_head.sdf) and you are free to modify it as needed.
**NOTE**: For the LIDAR you can set the sensor type to either `gpu_ray` or `ray` depending on whether GPU acceleration is available or not. The corresponding plugin filenames to use are `libgazebo_ros_gpu_laser.so` and `libgazebo_ros_laser.so`.
## Converting Vision60's URDF to SDF
This process is more straightforward and we have already included properly converted URDF and SDF files. We have a URDF xacro file ([vision60_gazebo.urdf.xacro](vision60_description/urdf/vision60_gazebo.urdf.xacro)) in the urdf folder that can be converted to URDF with
```bash
$ python xacro.py vision60_gazebo.urdf.xacro > vision60_gazebo.urdf
```
and subsequently to SDF using
```bash
$ gz sdf -p vision60_gazebo.urdf > ../sdf/vision60.sdf
```
After the conversion:
1. For each leg, rename all the toe collision descriptions to match the corresponding toe (use `toe0_collision`, `toe1_collision` as in the Minitaur SDF), and also add the following lines immediately below this collision tag (change `0` to match each toe):
```
<sensor name="toe0_contact" type="contact">
<plugin name="toe0_plugin" filename="libcontact.so"/>
<contact>
<collision>toe0_collision</collision>
</contact>
</sensor>
```
2. Make sure to move the SDF file to the [vision60 SDF folder](vision60_description/sdf) and rename it to vision60.sdf.
## Converting Spirit's URDF to SDF
We have a URDF xacro file ([spirit_gazebo.urdf.xacro](spirit_description/urdf/spirit_gazebo.urdf.xacro)) in the urdf folder that can be converted to URDF with
```bash
$ python xacro.py spirit_gazebo.urdf.xacro > spirit_gazebo.urdf
```
and subsequently to SDF using
```bash
$ gz sdf -p spirit_gazebo.urdf > ../sdf/spirit.sdf
```
After the conversion:
1. For each leg, rename all the toe collision descriptions to match the corresponding toe (use `toe0_collision`, `toe1_collision` as in the Minitaur SDF), and also add the following lines immediately below this collision tag (change `0` to match each toe):
```
<sensor name="toe0_contact" type="contact">
<plugin name="toe0_plugin" filename="libcontact.so"/>
<contact>
<collision>toe0_collision</collision>
</contact>
</sensor>
```
2. Make sure to move the SDF file to the [spirit SDF folder](spirit_description/sdf) and rename it to spirit.sdf.
## Other
Except for the robots, we also include other useful descriptions of objects for autonomous tasks.
### AprilTags
We have included the Gazebo model descriptions of some members of the 36h11 [AprilTag](https://april.eecs.umich.edu/software/apriltag) family. Make sure to add the descriptions to your `GAZEBO_MODEL_PATH` by adding the following line to your `~/.bashrc` file:
```
export GAZEBO_MODEL_PATH=$GAZEBO_MODEL_PATH:<catkin_ws_location>/src/kodlab_gazebo/other/apriltags_description
```
If you want to generate your own descriptions, you can download pre-generated tags [here](https://april.eecs.umich.edu/software/apriltag). Those tags (`.png` images) are quite small, so you might need to enlarge them (we suggest [gimp](https://www.gimp.org/) - make sure to scale the images with `None` as the interpolation method). The package we used for the automated SDF model generation can be found [here](https://github.com/vvasilo/gazebo_models). The size of the generated tags (including the white margin) was set to 85mm. The dimension ratio between the tag's black square and the outside box (that includes the white margin) is approximately 0.8; this can be used to appropriately scale your tags.
| 15,886 | Markdown | 47.435975 | 708 | 0.684061 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/gazebo_scripts/src/odom_publisher.cpp | /**
MIT License (modified)
Copyright (c) 2018 The Trustees of the University of Pennsylvania
Authors:
Vasileios Vasilopoulos <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this **file** (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
#include <map>
#include <string>
#include "odom_publisher.h"
// Model callback
void modelCallback(const gazebo_msgs::LinkStates::ConstPtr& msg) {
int des_index = 0;
// Determine the index of the robot from all the models included
if (robot_name == "minitaur") {
for (int j=0 ; j<msg->name.size() ; j++) {
if (msg->name[j] == "minitaur_constrained::base_chassis_link_dummy") {
des_index = j;
}
}
} else if (robot_name == "vision60") {
for (int j=0 ; j<msg->name.size() ; j++) {
if (msg->name[j] == "vision60::body") {
des_index = j;
}
}
}
// Find orientation and position of robot in the global frame
tf::Quaternion robot_to_global_orientation_quaternion = tf::Quaternion(msg->pose[des_index].orientation.x,
msg->pose[des_index].orientation.y,
msg->pose[des_index].orientation.z,
msg->pose[des_index].orientation.w);
tf::Matrix3x3 robot_to_global_orientation = tf::Matrix3x3(robot_to_global_orientation_quaternion);
tf::Vector3 robot_to_global_position = tf::Vector3(msg->pose[des_index].position.x, msg->pose[des_index].position.y, msg->pose[des_index].position.z);
// Find robot pose in the global frame
tf::Pose robot_to_global = tf::Pose(robot_to_global_orientation, robot_to_global_position);
// Find orientation and position of child in the robot frame
tf::Matrix3x3 child_to_robot_orientation;
child_to_robot_orientation.setRPY(child_frame_roll, child_frame_pitch, child_frame_yaw);
tf::Vector3 child_to_robot_position = tf::Vector3(child_frame_x, child_frame_y, child_frame_z);
// Find child pose in the robot frame
tf::Pose child_to_robot = tf::Pose(child_to_robot_orientation, child_to_robot_position);
// Store the first pose of the odom frame in the global Gazebo frame - Runs only once
if (flag_first) {
odom_to_global = robot_to_global * child_to_robot;
odom_to_global_pos = odom_to_global.getOrigin();
odom_to_global_rot = odom_to_global.getRotation();
flag_first = false;
}
// Find pose of child frame in odom frame
tf::Pose child_to_odom = odom_to_global.inverse()*robot_to_global*child_to_robot;
// Initialize Odometry message
nav_msgs::Odometry msg_odometry;
// Populate odometry message header
msg_odometry.header.stamp = ros::Time::now();
msg_odometry.header.frame_id = odom_frame;
// Populate odometry message
msg_odometry.child_frame_id = child_frame;
msg_odometry.pose.pose.position.x = child_to_odom.getOrigin().getX();
msg_odometry.pose.pose.position.y = child_to_odom.getOrigin().getY();
msg_odometry.pose.pose.position.z = child_to_odom.getOrigin().getZ();
msg_odometry.pose.pose.orientation.x = child_to_odom.getRotation().getX();
msg_odometry.pose.pose.orientation.y = child_to_odom.getRotation().getY();
msg_odometry.pose.pose.orientation.z = child_to_odom.getRotation().getZ();
msg_odometry.pose.pose.orientation.w = child_to_odom.getRotation().getW();
// Get angular twist
tf::Vector3 vector_angular(msg->twist[des_index].angular.x, msg->twist[des_index].angular.y, msg->twist[des_index].angular.z);
tf::Vector3 rotated_vector_angular = tf::quatRotate(((robot_to_global*child_to_robot).inverse()).getRotation(), vector_angular);
msg_odometry.twist.twist.angular.x = rotated_vector_angular.getX();
msg_odometry.twist.twist.angular.y = rotated_vector_angular.getY();
msg_odometry.twist.twist.angular.z = rotated_vector_angular.getZ();
// Get linear twist
tf::Vector3 vector_linear(msg->twist[des_index].linear.x, msg->twist[des_index].linear.y, msg->twist[des_index].linear.z);
tf::Vector3 rotated_vector_linear = tf::quatRotate(((robot_to_global*child_to_robot).inverse()).getRotation(), vector_linear);
msg_odometry.twist.twist.linear.x = rotated_vector_linear.getX();
msg_odometry.twist.twist.linear.y = rotated_vector_linear.getY();
msg_odometry.twist.twist.linear.z = rotated_vector_linear.getZ();
// Publish odometry
odomPub.publish(msg_odometry);
// Publish tf transform
static tf::TransformBroadcaster br;
tf::Transform transform;
transform.setOrigin(tf::Vector3(child_to_odom.getOrigin().getX(), child_to_odom.getOrigin().getY(), child_to_odom.getOrigin().getZ()));
transform.setRotation(child_to_odom.getRotation());
br.sendTransform(tf::StampedTransform(transform, ros::Time::now(), odom_frame, child_frame));
return;
}
int main(int argc, char **argv) {
// Initialize ROS if necessary
if (!ros::isInitialized()) {
ros::init(argc, argv, "odom_publisher", ros::init_options::NoSigintHandler);
}
// Initialize node
rosNode.reset(new ros::NodeHandle("odom_publisher"));
// Get parameters
ros::param::get("~robot_name", robot_name);
ros::param::get("~child_frame", child_frame);
ros::param::get("~odom_frame", odom_frame);
ros::param::get("~child_frame_x", child_frame_x);
ros::param::get("~child_frame_y", child_frame_y);
ros::param::get("~child_frame_z", child_frame_z);
ros::param::get("~child_frame_roll", child_frame_roll);
ros::param::get("~child_frame_pitch", child_frame_pitch);
ros::param::get("~child_frame_yaw", child_frame_yaw);
// Initialize subscriber
modelSub = rosNode->subscribe("/gazebo/link_states", 1, &modelCallback);
// Initialize publisher
odomPub = rosNode->advertise<nav_msgs::Odometry>("/robot_odom", 1);
while (ros::ok()) {
// Set ROS rate
ros::Rate r(60);
// Spin once
ros::spinOnce();
r.sleep();
}
return 0;
}
| 6,806 | C++ | 40.760736 | 154 | 0.690273 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/gazebo_scripts/src/odom_publisher.h | /**
MIT License (modified)
Copyright (c) 2018 The Trustees of the University of Pennsylvania
Authors:
Vasileios Vasilopoulos <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this **file** (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
// ROS includes
#include <ros/ros.h>
#include <sensor_msgs/Joy.h>
#include <rosgraph_msgs/Clock.h>
#include <gazebo_msgs/LinkStates.h>
#include <nav_msgs/Odometry.h>
#include <tf/transform_datatypes.h>
#include <tf/transform_broadcaster.h>
// ROS node handle
std::unique_ptr<ros::NodeHandle> rosNode;
// Declare callbacks
void modelCallback(const gazebo_msgs::LinkStates::ConstPtr& msg);
// Declare ROS subscribers and publishers
ros::Subscriber modelSub;
ros::Publisher odomPub;
// Declare ROS parameters
std::string robot_name;
std::string child_frame;
std::string odom_frame;
double child_frame_x;
double child_frame_y;
double child_frame_z;
double child_frame_roll;
double child_frame_pitch;
double child_frame_yaw;
// Declare global variables
bool flag_first = true;
tf::Pose odom_to_global;
tf::Vector3 odom_to_global_pos;
tf::Quaternion odom_to_global_rot; | 2,013 | C | 31.48387 | 78 | 0.780924 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/gazebo_scripts/src/ContactPlugin.cc | /*
MIT License (modified)
Copyright (c) 2018 The Trustees of the University of Pennsylvania
Authors:
Vasileios Vasilopoulos <[email protected]>
Permission is hereby granted, free of charge, to any person obtaining a copy
of this **file** (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
#include "ContactPlugin.hh"
using namespace gazebo;
GZ_REGISTER_SENSOR_PLUGIN(ContactPlugin)
/////////////////////////////////////////////////
ContactPlugin::ContactPlugin() : SensorPlugin() {
}
/////////////////////////////////////////////////
ContactPlugin::~ContactPlugin() {
}
/////////////////////////////////////////////////
void ContactPlugin::Load(sensors::SensorPtr _sensor, sdf::ElementPtr /*_sdf*/) {
// Get the parent sensor.
this->parentSensor =
std::dynamic_pointer_cast<sensors::ContactSensor>(_sensor);
// Make sure the parent sensor is valid.
if (!this->parentSensor)
{
gzerr << "ContactPlugin requires a ContactSensor.\n";
return;
}
// Connect to the sensor update event.
this->updateConnection = this->parentSensor->ConnectUpdated(
std::bind(&ContactPlugin::OnUpdate, this));
// Make sure the parent sensor is active.
this->parentSensor->SetActive(true);
// Initialize ROS if necessary
if (!ros::isInitialized()) {
int argc = 0;
char **argv = NULL;
ros::init(argc, argv, "ContactPublisher",ros::init_options::NoSigintHandler);
}
// Initialize node
this->rosNode.reset(new ros::NodeHandle("ContactPublisher"));
// Initialize Publishers
this->toe0_publisher = this->rosNode->advertise<geometry_msgs::Vector3>("/gazebo/toe0_forces", 1, true);
this->toe1_publisher = this->rosNode->advertise<geometry_msgs::Vector3>("/gazebo/toe1_forces", 1, true);
this->toe2_publisher = this->rosNode->advertise<geometry_msgs::Vector3>("/gazebo/toe2_forces", 1, true);
this->toe3_publisher = this->rosNode->advertise<geometry_msgs::Vector3>("/gazebo/toe3_forces", 1, true);
}
/////////////////////////////////////////////////
void ContactPlugin::OnUpdate() {
// Get all the contacts.
msgs::Contacts contacts;
contacts = this->parentSensor->Contacts();
for (unsigned int i = 0; i < contacts.contact_size(); ++i) {
std::string str = contacts.contact(i).collision1();
std::string toe0_string = "toe0_collision";
std::string toe1_string = "toe1_collision";
std::string toe2_string = "toe2_collision";
std::string toe3_string = "toe3_collision";
std::size_t found_toe0 = str.find(toe0_string);
std::size_t found_toe1 = str.find(toe1_string);
std::size_t found_toe2 = str.find(toe2_string);
std::size_t found_toe3 = str.find(toe3_string);
if (found_toe0 != std::string::npos) {
geometry_msgs::Vector3 toe0_msg; toe0_msg.x = 0.; toe0_msg.y = 0.; toe0_msg.z = 0.;
for (unsigned int j = 0; j < contacts.contact(i).position_size(); ++j) {
toe0_msg.x -= contacts.contact(i).wrench(j).body_1_wrench().force().x();
toe0_msg.y -= contacts.contact(i).wrench(j).body_1_wrench().force().y();
toe0_msg.z -= contacts.contact(i).wrench(j).body_1_wrench().force().z();
}
this->toe0_publisher.publish(toe0_msg);
}
if (found_toe1 != std::string::npos) {
geometry_msgs::Vector3 toe1_msg; toe1_msg.x = 0.; toe1_msg.y = 0.; toe1_msg.z = 0.;
for (unsigned int j = 0; j < contacts.contact(i).position_size(); ++j) {
toe1_msg.x -= contacts.contact(i).wrench(j).body_1_wrench().force().x();
toe1_msg.y -= contacts.contact(i).wrench(j).body_1_wrench().force().y();
toe1_msg.z -= contacts.contact(i).wrench(j).body_1_wrench().force().z();
}
this->toe1_publisher.publish(toe1_msg);
}
if (found_toe2 != std::string::npos) {
geometry_msgs::Vector3 toe2_msg; toe2_msg.x = 0.; toe2_msg.y = 0.; toe2_msg.z = 0.;
for (unsigned int j = 0; j < contacts.contact(i).position_size(); ++j) {
toe2_msg.x -= contacts.contact(i).wrench(j).body_1_wrench().force().x();
toe2_msg.y -= contacts.contact(i).wrench(j).body_1_wrench().force().y();
toe2_msg.z -= contacts.contact(i).wrench(j).body_1_wrench().force().z();
}
this->toe2_publisher.publish(toe2_msg);
}
if (found_toe3 != std::string::npos) {
geometry_msgs::Vector3 toe3_msg; toe3_msg.x = 0.; toe3_msg.y = 0.; toe3_msg.z = 0.;
for (unsigned int j = 0; j < contacts.contact(i).position_size(); ++j) {
toe3_msg.x -= contacts.contact(i).wrench(j).body_1_wrench().force().x();
toe3_msg.y -= contacts.contact(i).wrench(j).body_1_wrench().force().y();
toe3_msg.z -= contacts.contact(i).wrench(j).body_1_wrench().force().z();
}
this->toe3_publisher.publish(toe3_msg);
}
}
}
| 5,572 | C++ | 40.281481 | 106 | 0.645011 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/gazebo_scripts/config/spirit_control.yaml | # MIT License (modified)
# Copyright (c) 2020 The Trustees of the University of Pennsylvania
# Authors:
# Vasileios Vasilopoulos <[email protected]>
# Permission is hereby granted, free of charge, to any person obtaining a copy
# of this **file** (the "Software"), to deal
# in the Software without restriction, including without limitation the rights
# to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
# copies of the Software, and to permit persons to whom the Software is
# furnished to do so, subject to the following conditions:
# The above copyright notice and this permission notice shall be included in all
# copies or substantial portions of the Software.
# THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
# IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
# FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
# AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
# LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
# OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
# SOFTWARE.
spirit:
# Publish all joint states -----------------------------------
joint_state_controller:
type: joint_state_controller/JointStateController
publish_rate: 2000
# Position Controllers ---------------------------------------
joint0_effort_controller:
type: effort_controllers/JointEffortController
joint: "0"
joint1_effort_controller:
type: effort_controllers/JointEffortController
joint: "1"
joint2_effort_controller:
type: effort_controllers/JointEffortController
joint: "2"
joint3_effort_controller:
type: effort_controllers/JointEffortController
joint: "3"
joint4_effort_controller:
type: effort_controllers/JointEffortController
joint: "4"
joint5_effort_controller:
type: effort_controllers/JointEffortController
joint: "5"
joint6_effort_controller:
type: effort_controllers/JointEffortController
joint: "6"
joint7_effort_controller:
type: effort_controllers/JointEffortController
joint: "7"
joint8_effort_controller:
type: effort_controllers/JointEffortController
joint: "8"
joint9_effort_controller:
type: effort_controllers/JointEffortController
joint: "9"
joint10_effort_controller:
type: effort_controllers/JointEffortController
joint: "10"
joint11_effort_controller:
type: effort_controllers/JointEffortController
joint: "11"
| 2,551 | YAML | 36.529411 | 80 | 0.736574 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/KodlabPenn/kodlab_gazebo-master/other/objects_description/caster_stool/xacro.py | #! /usr/bin/env python
# Copyright (c) 2013, Willow Garage, Inc.
# Copyright (c) 2014, Open Source Robotics Foundation, Inc.
# All rights reserved.
#
# Redistribution and use in source and binary forms, with or without
# modification, are permitted provided that the following conditions are met:
#
# * Redistributions of source code must retain the above copyright
# notice, this list of conditions and the following disclaimer.
# * Redistributions in binary form must reproduce the above copyright
# notice, this list of conditions and the following disclaimer in the
# documentation and/or other materials provided with the distribution.
# * Neither the name of the Open Source Robotics Foundation, Inc.
# nor the names of its contributors may be used to endorse or promote
# products derived from this software without specific prior
# written permission.
#
# THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
# AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
# IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
# ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
# LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
# CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
# SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
# INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
# CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
# ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
# POSSIBILITY OF SUCH DAMAGE.
# Author: Stuart Glaser
# Maintainer: William Woodall <[email protected]>
from __future__ import print_function
import getopt
import glob
import os
import re
import string
import sys
import xml
from xml.dom.minidom import parse
try:
_basestr = basestring
except NameError:
_basestr = str
# Dictionary of subtitution args
substitution_args_context = {}
class XacroException(Exception):
pass
def isnumber(x):
return hasattr(x, '__int__')
# Better pretty printing of xml
# Taken from http://ronrothman.com/public/leftbraned/xml-dom-minidom-toprettyxml-and-silly-whitespace/
def fixed_writexml(self, writer, indent="", addindent="", newl=""):
# indent = current indentation
# addindent = indentation to add to higher levels
# newl = newline string
writer.write(indent + "<" + self.tagName)
attrs = self._get_attributes()
a_names = list(attrs.keys())
a_names.sort()
for a_name in a_names:
writer.write(" %s=\"" % a_name)
xml.dom.minidom._write_data(writer, attrs[a_name].value)
writer.write("\"")
if self.childNodes:
if len(self.childNodes) == 1 \
and self.childNodes[0].nodeType == xml.dom.minidom.Node.TEXT_NODE:
writer.write(">")
self.childNodes[0].writexml(writer, "", "", "")
writer.write("</%s>%s" % (self.tagName, newl))
return
writer.write(">%s" % (newl))
for node in self.childNodes:
# skip whitespace-only text nodes
if node.nodeType == xml.dom.minidom.Node.TEXT_NODE and \
not node.data.strip():
continue
node.writexml(writer, indent + addindent, addindent, newl)
writer.write("%s</%s>%s" % (indent, self.tagName, newl))
else:
writer.write("/>%s" % (newl))
# replace minidom's function with ours
xml.dom.minidom.Element.writexml = fixed_writexml
class Table:
def __init__(self, parent=None):
self.parent = parent
self.table = {}
def __getitem__(self, key):
if key in self.table:
return self.table[key]
elif self.parent:
return self.parent[key]
else:
raise KeyError(key)
def __setitem__(self, key, value):
self.table[key] = value
def __contains__(self, key):
return \
key in self.table or \
(self.parent and key in self.parent)
class QuickLexer(object):
def __init__(self, **res):
self.str = ""
self.top = None
self.res = []
for k, v in res.items():
self.__setattr__(k, len(self.res))
self.res.append(v)
def lex(self, str):
self.str = str
self.top = None
self.next()
def peek(self):
return self.top
def next(self):
result = self.top
self.top = None
for i in range(len(self.res)):
m = re.match(self.res[i], self.str)
if m:
self.top = (i, m.group(0))
self.str = self.str[m.end():]
break
return result
def first_child_element(elt):
c = elt.firstChild
while c:
if c.nodeType == xml.dom.Node.ELEMENT_NODE:
return c
c = c.nextSibling
return None
def next_sibling_element(elt):
c = elt.nextSibling
while c:
if c.nodeType == xml.dom.Node.ELEMENT_NODE:
return c
c = c.nextSibling
return None
# Pre-order traversal of the elements
def next_element(elt):
child = first_child_element(elt)
if child:
return child
while elt and elt.nodeType == xml.dom.Node.ELEMENT_NODE:
next = next_sibling_element(elt)
if next:
return next
elt = elt.parentNode
return None
# Pre-order traversal of all the nodes
def next_node(node):
if node.firstChild:
return node.firstChild
while node:
if node.nextSibling:
return node.nextSibling
node = node.parentNode
return None
def child_nodes(elt):
c = elt.firstChild
while c:
yield c
c = c.nextSibling
all_includes = []
# Deprecated message for <include> tags that don't have <xacro:include> prepended:
deprecated_include_msg = """DEPRECATED IN HYDRO:
The <include> tag should be prepended with 'xacro' if that is the intended use
of it, such as <xacro:include ...>. Use the following script to fix incorrect
xacro includes:
sed -i 's/<include/<xacro:include/g' `find . -iname *.xacro`"""
include_no_matches_msg = """Include tag filename spec \"{}\" matched no files."""
## @throws XacroException if a parsing error occurs with an included document
def process_includes(doc, base_dir):
namespaces = {}
previous = doc.documentElement
elt = next_element(previous)
while elt:
# Xacro should not use plain 'include' tags but only namespaced ones. Causes conflicts with
# other XML elements including Gazebo's <gazebo> extensions
is_include = False
if elt.tagName == 'xacro:include' or elt.tagName == 'include':
is_include = True
# Temporary fix for ROS Hydro and the xacro include scope problem
if elt.tagName == 'include':
# check if there is any element within the <include> tag. mostly we are concerned
# with Gazebo's <uri> element, but it could be anything. also, make sure the child
# nodes aren't just a single Text node, which is still considered a deprecated
# instance
if elt.childNodes and not (len(elt.childNodes) == 1 and
elt.childNodes[0].nodeType == elt.TEXT_NODE):
# this is not intended to be a xacro element, so we can ignore it
is_include = False
else:
# throw a deprecated warning
print(deprecated_include_msg, file=sys.stderr)
# Process current element depending on previous conditions
if is_include:
filename_spec = eval_text(elt.getAttribute('filename'), {})
if not os.path.isabs(filename_spec):
filename_spec = os.path.join(base_dir, filename_spec)
if re.search('[*[?]+', filename_spec):
# Globbing behaviour
filenames = sorted(glob.glob(filename_spec))
if len(filenames) == 0:
print(include_no_matches_msg.format(filename_spec), file=sys.stderr)
else:
# Default behaviour
filenames = [filename_spec]
for filename in filenames:
global all_includes
all_includes.append(filename)
try:
with open(filename) as f:
try:
included = parse(f)
except Exception as e:
raise XacroException(
"included file \"%s\" generated an error during XML parsing: %s"
% (filename, str(e)))
except IOError as e:
raise XacroException("included file \"%s\" could not be opened: %s" % (filename, str(e)))
# Replaces the include tag with the elements of the included file
for c in child_nodes(included.documentElement):
elt.parentNode.insertBefore(c.cloneNode(deep=True), elt)
# Grabs all the declared namespaces of the included document
for name, value in included.documentElement.attributes.items():
if name.startswith('xmlns:'):
namespaces[name] = value
elt.parentNode.removeChild(elt)
elt = None
else:
previous = elt
elt = next_element(previous)
# Makes sure the final document declares all the namespaces of the included documents.
for k, v in namespaces.items():
doc.documentElement.setAttribute(k, v)
# Returns a dictionary: { macro_name => macro_xml_block }
def grab_macros(doc):
macros = {}
previous = doc.documentElement
elt = next_element(previous)
while elt:
if elt.tagName == 'macro' or elt.tagName == 'xacro:macro':
name = elt.getAttribute('name')
macros[name] = elt
macros['xacro:' + name] = elt
elt.parentNode.removeChild(elt)
elt = None
else:
previous = elt
elt = next_element(previous)
return macros
# Returns a Table of the properties
def grab_properties(doc):
table = Table()
previous = doc.documentElement
elt = next_element(previous)
while elt:
if elt.tagName == 'property' or elt.tagName == 'xacro:property':
name = elt.getAttribute('name')
value = None
if elt.hasAttribute('value'):
value = elt.getAttribute('value')
else:
name = '**' + name
value = elt # debug
bad = string.whitespace + "${}"
has_bad = False
for b in bad:
if b in name:
has_bad = True
break
if has_bad:
sys.stderr.write('Property names may not have whitespace, ' +
'"{", "}", or "$" : "' + name + '"')
else:
table[name] = value
elt.parentNode.removeChild(elt)
elt = None
else:
previous = elt
elt = next_element(previous)
return table
def eat_ignore(lex):
while lex.peek() and lex.peek()[0] == lex.IGNORE:
lex.next()
def eval_lit(lex, symbols):
eat_ignore(lex)
if lex.peek()[0] == lex.NUMBER:
return float(lex.next()[1])
if lex.peek()[0] == lex.SYMBOL:
try:
key = lex.next()[1]
value = symbols[key]
except KeyError as ex:
raise XacroException("Property wasn't defined: %s" % str(ex))
if not (isnumber(value) or isinstance(value, _basestr)):
if value is None:
raise XacroException("Property %s recursively used" % key)
raise XacroException("WTF2")
try:
return int(value)
except:
try:
return float(value)
except:
# prevent infinite recursion
symbols[key] = None
result = eval_text(value, symbols)
# restore old entry
symbols[key] = value
return result
raise XacroException("Bad literal")
def eval_factor(lex, symbols):
eat_ignore(lex)
neg = 1
if lex.peek()[1] == '-':
lex.next()
neg = -1
if lex.peek()[0] in [lex.NUMBER, lex.SYMBOL]:
return neg * eval_lit(lex, symbols)
if lex.peek()[0] == lex.LPAREN:
lex.next()
eat_ignore(lex)
result = eval_expr(lex, symbols)
eat_ignore(lex)
if lex.next()[0] != lex.RPAREN:
raise XacroException("Unmatched left paren")
eat_ignore(lex)
return neg * result
raise XacroException("Misplaced operator")
def eval_term(lex, symbols):
eat_ignore(lex)
result = 0
if lex.peek()[0] in [lex.NUMBER, lex.SYMBOL, lex.LPAREN] \
or lex.peek()[1] == '-':
result = eval_factor(lex, symbols)
eat_ignore(lex)
while lex.peek() and lex.peek()[1] in ['*', '/']:
op = lex.next()[1]
n = eval_factor(lex, symbols)
if op == '*':
result = float(result) * float(n)
elif op == '/':
result = float(result) / float(n)
else:
raise XacroException("WTF")
eat_ignore(lex)
return result
def eval_expr(lex, symbols):
eat_ignore(lex)
op = None
if lex.peek()[0] == lex.OP:
op = lex.next()[1]
if not op in ['+', '-']:
raise XacroException("Invalid operation. Must be '+' or '-'")
result = eval_term(lex, symbols)
if op == '-':
result = -float(result)
eat_ignore(lex)
while lex.peek() and lex.peek()[1] in ['+', '-']:
op = lex.next()[1]
n = eval_term(lex, symbols)
if op == '+':
result = float(result) + float(n)
if op == '-':
result = float(result) - float(n)
eat_ignore(lex)
return result
def eval_text(text, symbols):
def handle_expr(s):
lex = QuickLexer(IGNORE=r"\s+",
NUMBER=r"(\d+(\.\d*)?|\.\d+)([eE][-+]?\d+)?",
SYMBOL=r"[a-zA-Z_]\w*",
OP=r"[\+\-\*/^]",
LPAREN=r"\(",
RPAREN=r"\)")
lex.lex(s)
return eval_expr(lex, symbols)
def handle_extension(s):
return ("$(%s)" % s)
results = []
lex = QuickLexer(DOLLAR_DOLLAR_BRACE=r"\$\$+\{",
EXPR=r"\$\{[^\}]*\}",
EXTENSION=r"\$\([^\)]*\)",
TEXT=r"([^\$]|\$[^{(]|\$$)+")
lex.lex(text)
while lex.peek():
if lex.peek()[0] == lex.EXPR:
results.append(handle_expr(lex.next()[1][2:-1]))
elif lex.peek()[0] == lex.EXTENSION:
results.append(handle_extension(lex.next()[1][2:-1]))
elif lex.peek()[0] == lex.TEXT:
results.append(lex.next()[1])
elif lex.peek()[0] == lex.DOLLAR_DOLLAR_BRACE:
results.append(lex.next()[1][1:])
return ''.join(map(str, results))
# Expands macros, replaces properties, and evaluates expressions
def eval_all(root, macros, symbols):
# Evaluates the attributes for the root node
for at in root.attributes.items():
result = eval_text(at[1], symbols)
root.setAttribute(at[0], result)
previous = root
node = next_node(previous)
while node:
if node.nodeType == xml.dom.Node.ELEMENT_NODE:
if node.tagName in macros:
body = macros[node.tagName].cloneNode(deep=True)
params = body.getAttribute('params').split()
# Parse default values for any parameters
defaultmap = {}
for param in params[:]:
splitParam = param.split(':=')
if len(splitParam) == 2:
defaultmap[splitParam[0]] = splitParam[1]
params.remove(param)
params.append(splitParam[0])
elif len(splitParam) != 1:
raise XacroException("Invalid parameter definition")
# Expands the macro
scoped = Table(symbols)
for name, value in node.attributes.items():
if not name in params:
raise XacroException("Invalid parameter \"%s\" while expanding macro \"%s\"" %
(str(name), str(node.tagName)))
params.remove(name)
scoped[name] = eval_text(value, symbols)
# Pulls out the block arguments, in order
cloned = node.cloneNode(deep=True)
eval_all(cloned, macros, symbols)
block = cloned.firstChild
for param in params[:]:
if param[0] == '*':
while block and block.nodeType != xml.dom.Node.ELEMENT_NODE:
block = block.nextSibling
if not block:
raise XacroException("Not enough blocks while evaluating macro %s" % str(node.tagName))
params.remove(param)
scoped[param] = block
block = block.nextSibling
# Try to load defaults for any remaining non-block parameters
for param in params[:]:
if param[0] != '*' and param in defaultmap:
scoped[param] = defaultmap[param]
params.remove(param)
if params:
raise XacroException("Parameters [%s] were not set for macro %s" %
(",".join(params), str(node.tagName)))
eval_all(body, macros, scoped)
# Replaces the macro node with the expansion
for e in list(child_nodes(body)): # Ew
node.parentNode.insertBefore(e, node)
node.parentNode.removeChild(node)
node = None
elif node.tagName == 'arg' or node.tagName == 'xacro:arg':
name = node.getAttribute('name')
if not name:
raise XacroException("Argument name missing")
default = node.getAttribute('default')
if default and name not in substitution_args_context['arg']:
substitution_args_context['arg'][name] = default
node.parentNode.removeChild(node)
node = None
elif node.tagName == 'insert_block' or node.tagName == 'xacro:insert_block':
name = node.getAttribute('name')
if ("**" + name) in symbols:
# Multi-block
block = symbols['**' + name]
for e in list(child_nodes(block)):
node.parentNode.insertBefore(e.cloneNode(deep=True), node)
node.parentNode.removeChild(node)
elif ("*" + name) in symbols:
# Single block
block = symbols['*' + name]
node.parentNode.insertBefore(block.cloneNode(deep=True), node)
node.parentNode.removeChild(node)
else:
raise XacroException("Block \"%s\" was never declared" % name)
node = None
elif node.tagName in ['if', 'xacro:if', 'unless', 'xacro:unless']:
value = eval_text(node.getAttribute('value'), symbols)
try:
if value == 'true': keep = True
elif value == 'false': keep = False
else: keep = float(value)
except ValueError:
raise XacroException("Xacro conditional evaluated to \"%s\". Acceptable evaluations are one of [\"1\",\"true\",\"0\",\"false\"]" % value)
if node.tagName in ['unless', 'xacro:unless']: keep = not keep
if keep:
for e in list(child_nodes(node)):
node.parentNode.insertBefore(e.cloneNode(deep=True), node)
node.parentNode.removeChild(node)
else:
# Evals the attributes
for at in node.attributes.items():
result = eval_text(at[1], symbols)
node.setAttribute(at[0], result)
previous = node
elif node.nodeType == xml.dom.Node.TEXT_NODE:
node.data = eval_text(node.data, symbols)
previous = node
else:
previous = node
node = next_node(previous)
return macros
# Expands everything except includes
def eval_self_contained(doc):
macros = grab_macros(doc)
symbols = grab_properties(doc)
eval_all(doc.documentElement, macros, symbols)
def print_usage(exit_code=0):
print("Usage: %s [-o <output>] <input>" % 'xacro.py')
print(" %s --deps Prints dependencies" % 'xacro.py')
print(" %s --includes Only evalutes includes" % 'xacro.py')
sys.exit(exit_code)
def set_substitution_args_context(context={}):
substitution_args_context['arg'] = context
def open_output(output_filename):
if output_filename is None:
return sys.stdout
else:
return open(output_filename, 'w')
def main():
try:
opts, args = getopt.gnu_getopt(sys.argv[1:], "ho:", ['deps', 'includes'])
except getopt.GetoptError as err:
print(str(err))
print_usage(2)
just_deps = False
just_includes = False
output_filename = None
for o, a in opts:
if o == '-h':
print_usage(0)
elif o == '-o':
output_filename = a
elif o == '--deps':
just_deps = True
elif o == '--includes':
just_includes = True
if len(args) < 1:
print("No input given")
print_usage(2)
# Process substitution args
# set_substitution_args_context(load_mappings(sys.argv))
set_substitution_args_context((sys.argv))
f = open(args[0])
doc = None
try:
doc = parse(f)
except xml.parsers.expat.ExpatError:
sys.stderr.write("Expat parsing error. Check that:\n")
sys.stderr.write(" - Your XML is correctly formed\n")
sys.stderr.write(" - You have the xacro xmlns declaration: " +
"xmlns:xacro=\"http://www.ros.org/wiki/xacro\"\n")
sys.stderr.write("\n")
raise
finally:
f.close()
process_includes(doc, os.path.dirname(args[0]))
if just_deps:
for inc in all_includes:
sys.stdout.write(inc + " ")
sys.stdout.write("\n")
elif just_includes:
doc.writexml(open_output(output_filename))
print()
else:
eval_self_contained(doc)
banner = [xml.dom.minidom.Comment(c) for c in
[" %s " % ('=' * 83),
" | This document was autogenerated by xacro from %-30s | " % args[0],
" | EDITING THIS FILE BY HAND IS NOT RECOMMENDED %-30s | " % "",
" %s " % ('=' * 83)]]
first = doc.firstChild
for comment in banner:
doc.insertBefore(comment, first)
open_output(output_filename).write(doc.toprettyxml(indent=' '))
print()
if __name__ == '__main__':
main()
| 23,887 | Python | 32.835694 | 157 | 0.542973 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/README.md | # Spot ROS Driver

## Prerequisites
You should be using ROS noetic. ROS melodic can also be used but may require modifications to build correctly.
You must install the Boston Dynamics SDK on any machine that will run the driver.
```
pip3 install bosdyn-client bosdyn-mission bosdyn-api bosdyn-core
```
## Quick start
### Installing the packages
Go to the source directory of your ROS workspace and clone this repository with
```bash
git clone [email protected]:heuristicus/spot_ros.git
```
Then, initialise the submodule for the wrapper we use to interact with the Boston Dynamics SDK
```bash
cd spot_ros
git submodule init
git submodule update
```
Then, install the python package containing the wrapper
```bash
pip3 install -e spot_wrapper
```
Build the ROS packages
```bash
catkin build spot_driver spot_viz
```
Finally, remember to source your workspace.
### Connecting to the robot
To test functionality, it's easiest to connect to the robot via wifi. For actual operation it is recommended to connect to the robot directly through payload ports for higher bandwidth.
Connect to the robot's wifi network, usually found at SSID `spot-BD-xxxxxxxx`. The password for the network is found in the robot's battery compartment.
Once connected, verify that you can ping the robot with `ping 192.168.80.3`.
Start a roscore on your machine with `roscore`.
Run the driver with the username and password for the robot, again found in the battery compartment
```bash
roslaunch spot_driver driver.launch username:=user password:=[your-password] hostname:=192.168.80.3
```
You can then view and control the robot from the rviz interface by running
```bash
roslaunch spot_viz view_robot.launch
```
## Documentation
More detailed documentation can be found [here](https://heuristicus.github.io/spot_ros)
# MoveIt simulation of Spot's arm
Can be found [here](https://github.com/estherRay/Spot-Arm). | 1,931 | Markdown | 26.6 | 185 | 0.767996 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_description/CHANGELOG.rst | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Changelog for package spot_description
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Forthcoming
-----------
1.0.0 (2023-04-07)
------------------
* update maintainer
* adressed layout review comments
* moved urdf
* updated urdf to include arm
* add correct joint for front rail, should be directly between first screws. Rear rail is based on that
* remove inorder flag
* Use the velodyne_description package for the actual sensor mesh, use the cage as a separate entity. Add an accessories.launch file to automatically bring up the velodyne if needed
* Include the license file in the individual ROS packages
* Added optional pack and standard velodyne mount
* fix joint limits typo
* add install instructions
* Added base_link (rep-0105)
* Updated URDF to include mount points, and deps for install
* Made the driver not automatically claim a body lease and e stop. Allows you to monitor without having control. Disconnect doesn't exit very gracefully. Need to wait on sitting success
* Added SPOT_URDF_EXTRAS environment variable for extending the robot
* Initial commit
* Contributors: Chris Iverach-Brereton, Dave Niewinski, Juan Miguel Jimeno, Mario Gini, Michal Staniaszek, Wolf Vollprecht, maubrunn
| 1,244 | reStructuredText | 45.111109 | 188 | 0.726688 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_msgs/CHANGELOG.rst | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Changelog for package spot_msgs
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Forthcoming
-----------
1.0.0 (2023-04-07)
------------------
* update maintainer
* add actionserver for docking/undocking
* updates after testing picking and placing on real spot - added some useful arguments to msg definitions
* updates from testing on real robot
* implemented grasp service
* changed to fix sized arrays
* changed hand pose msg
* service for getting dock state
* Create HandPose.srv
* Update CMakeLists.txt
* New msg for arm force trajectory
* Update CMakeLists.txt
* add posed_stand service which can be used to pose the body
This also adds a check to the async_idle which prevents the idle stand command
from activating while there is a command which has a non-None id. This should
prevent commands from getting interrupted by that stand
* add terrain state to foot state, publish tf of foot position rt body
* Advanced motion tab, obstacle and terrain params added to driver and panel
Move more advanced motion settings into the adv. motion tab to not clutter the basic motion. The settings there (ground friction, gait, swing height, grated surface mode) are I suspect relatively rarely used.
Obstacle params message added, which allows the settings for the obstacle avoidance to be published from the driver. These are as usual empty by default since the SDK does not provide details. Currently the only modification allowed is to the obstacle padding.
Terrain params message added, which allows setting of the ground friction and grated surfaces mode.
Terrain and obstacle params are in the mobility params message published by the driver.
Rviz panel allows interaction with the terrain and obstacle params. Comboboxes are now initialised by using a map generated from the constants in the ros messages, to try and make sure the ordering is correct. The map is also useful for constants which are non-consecutive like in the locomotion, for some reason the 9th value is unused but the 10th is.
* velocity and body controls are in different tabs, add swing height and gait control
add checks to ensure only valid values for locomotion and swing height are sent to the mobility params
* allow velocity limits to be set in the driver launch and load them on start
Also updats the mobility params message to store the velocity limits
If the limits change, the rviz panel is updated with the latest values
* Service to open the gripper at a given angle
* Added messages for the arm
* add Dock.srv
* add spot dock and undock service
* use int8 instead of uint8, we want to have negative rotations too
* add actionserver to set body pose
* can require trajectory commands to reach goal precisely
Add precise_positioning field to the trajectory goal. Setting to true will make the wrapper consider only STATUS_AT_GOAL status to mean that the robot is at the goal. Setting to false will replicate previous behaviour where STATUS_NEAR_GOAL also means that the robot is at the goal.
Vary feedback messages from the driver depending on whether the precise positioning field was set.
* Include the license file in the individual ROS packages
* Add service call to set maximum velocity, use synchro trajectory command
The service call receives a twist message and sets the mobility params vel_lim max_vel to the linear x and y, and angular z values in the message. This velocity limit affects anything that moves the robot around, such as the trajectory command and velocity command.
The service call adds a srv to spot_msgs
Output exception string when power_on command fails
Fix minor typo in behavior_fault function name
Populate state_description in the estop state message
Minor cosmetic changes to srv imports and some comments for readability
* Add trajectory command interface (`#25 <https://github.com/heuristicus/spot_ros/issues/25>`_)
* [spot_driver] add trajectory_cmd() method to spot_wrapper
* [spot_driver] rename _last_motion_command to _last_trajectory_command
* [spot_driver] rename _last_motion_command_time to _last_velocity_command_time
* [spot_driver] fix trajectory_cmd
* [spot_driver] fix options of trajectory_cmd()
* [spot_msgs] add Trajectory.srv
* [spot_driver] add trajectory service server
* [spot_ros] add frame_id checking to trajectory_cmd
* [spot_driver] fix bugs
* [spot_driver] fix bugs in trajectory_cmd
* convert trajectory command to an actionserver
* [spot_ros] fix merge commit
* [spot_driver] allow STATUS_NEAR_GOAL to be recognized as at_goal
* [spot_driver] add handling for 0 duration
Co-authored-by: Michal Staniaszek <[email protected]>
Co-authored-by: Dave Niewinski <[email protected]>
* Added service for clearing behavior faults (`#34 <https://github.com/heuristicus/spot_ros/issues/34>`_)
* [spot_msgs] add descriptions to SetLocomotion.srv
* [spot_msgs] remove unused field of mobility params message
* [spot_msgs] add MobilityParams.msg
* [spot_msgs] fix type error of SetLocomotion.srv
* [spot_msgs] add SetLocomotion.srv
* support graphnav navigate-to
* Properly implemented checking the status of commands. Added a feedback message
* Fixed all the little mistakes from the last commit
* Added messages for the remainder of the robot status
* Added messages and brought metrics and leases into ros
* Contributors: Chris Iverach-Brereton, Dave Niewinski, Esther, Kei Okada, Koki Shinjo, Michal Staniaszek, Michel Heinemann, Naoto Tsukamoto, SpotCOREAI, maubrunn, nfilliol
| 5,528 | reStructuredText | 64.821428 | 355 | 0.773878 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_viz/CHANGELOG.rst | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Changelog for package spot_viz
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Forthcoming
-----------
1.0.0 (2023-04-07)
------------------
* docking, self-right and roll over buttons start disabled
* update maintainer
* connect up buttons, fix undock call, fix dock actionserver name
* add buttons for docking, self-right, and roll over
* body tab now sets posed stand
* update rviz config panel arrangement, estop states now shown in panel
The release estop button is now only enabled if the software estop is on
* correct ordering of ui component setup, must be before subscribers
* sit and stand button state depends on whether the robot is powered or not
* tab shown on startup should be basic motion tab, minor fixes
* sit, stand and self right disabled when motion disabled, ui has button for allow motion
The stop button in the ui will now call the locked stop. Stop button is disabled and has its text changed when motion is not allowed
* Advanced motion tab, obstacle and terrain params added to driver and panel
Move more advanced motion settings into the adv. motion tab to not clutter the basic motion. The settings there (ground friction, gait, swing height, grated surface mode) are I suspect relatively rarely used.
Obstacle params message added, which allows the settings for the obstacle avoidance to be published from the driver. These are as usual empty by default since the SDK does not provide details. Currently the only modification allowed is to the obstacle padding.
Terrain params message added, which allows setting of the ground friction and grated surfaces mode.
Terrain and obstacle params are in the mobility params message published by the driver.
Rviz panel allows interaction with the terrain and obstacle params. Comboboxes are now initialised by using a map generated from the constants in the ros messages, to try and make sure the ordering is correct. The map is also useful for constants which are non-consecutive like in the locomotion, for some reason the 9th value is unused but the 10th is.
* velocity and body controls are in different tabs, add swing height and gait control
add checks to ensure only valid values for locomotion and swing height are sent to the mobility params
* panel now looks at battery and power state and displays information
* allow velocity limits to be set in the driver launch and load them on start
Also updats the mobility params message to store the velocity limits
If the limits change, the rviz panel is updated with the latest values
* enable estop buttons only when we have the lease, release button state is dependent on estop state
* add stop action button to rviz panel, clearer estop button names
* add estop buttons to spot panel
* add topic go_to_pose which can be used to move the robot with the trajectory command
* adjust velocity limit and body height range, explicitly specify upper and lower limits for labels
Trying to adjust body height, the limit appears to be something around 0.15 or so. I think this might be because we are not using the stance command, but the body control params for mobility
* rename max_velocity to velocity_limit, now also limits velocity when moving backwards
* Only update the state of the buttons based on the lease feedback; if we successfully get/release a lease, disable that button only and wait for the subscriber to enable whatever's needed. This prevents a possible issue where the UI flashes
* Squashed commit of the following:
commit 0cc926539660598bf7ef2a8407d51a3583ca29c9
Author: Chris Iverach-Brereton <[email protected]>
Date: Fri Aug 13 11:49:21 2021 -0400
Change the default camera to use gpe as the fixed frame; the keeps the robot visible on-screen at all times; using odom the robot can appear off-screen too easily
commit 667e26f63ad9df414c4bd7f02eea57d03e2785d3
Author: Chris Iverach-Brereton <[email protected]>
Date: Fri Aug 13 11:45:16 2021 -0400
Add a subscriber to the spot panel to monitor the lease status, correctly setting the button states if we call the service e.g. before launching rviz
* Simple rviz panel for interaction with spot
Can use the panel to claim and release the lease, power on and off, sit down and stand up, and set the body pose.
To add, go to panels>add new panel and select SpotControlPanel in spot_viz
* can set maximum velocity in the rviz panel
* Simple rviz panel for interaction with spot
Can use the panel to claim and release the lease, power on and off, sit down and stand up, and set the body pose.
To add, go to panels>add new panel and select SpotControlPanel in spot_viz
* Include the license file in the individual ROS packages
* add install instructions
* Updated PC topics
* Lots of little fixes. Body positioning logic is broken
* removed unnecessary interactive marker
* Basic motion. Lots to clean up
* Got depth images working
* Initial commit
* Contributors: Chris Iverach-Brereton, Dave Niewinski, Michal Staniaszek, Wolf Vollprecht
| 5,027 | reStructuredText | 74.044775 | 355 | 0.779988 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_viz/plugin_description.xml | <library path="lib/libspot_viz">
<class name="spot_viz/SpotControlPanel"
type="spot_viz::ControlPanel"
base_class_type="rviz::Panel">
<description>
A panel for controlling the Boston Dynamics Spot robot with the Clearpath driver
</description>
</class>
</library> | 299 | XML | 32.33333 | 86 | 0.67893 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_viz/src/spot_panel.hpp | #ifndef SPOT_CONTROL_PANEL_H
#define SPOT_CONTROL_PANEL_H
#ifndef Q_MOC_RUN
# include <ros/ros.h>
# include <rviz/panel.h>
#endif
#include <map>
#include <QPushButton>
#include <QLabel>
#include <QDoubleSpinBox>
#include <QComboBox>
#include <std_msgs/Bool.h>
#include <spot_msgs/EStopStateArray.h>
#include <spot_msgs/MobilityParams.h>
#include <spot_msgs/TerrainParams.h>
#include <spot_msgs/SetSwingHeight.h>
#include <spot_msgs/LeaseArray.h>
#include <spot_msgs/SetLocomotion.h>
#include <spot_msgs/BatteryStateArray.h>
#include <spot_msgs/PowerState.h>
namespace spot_viz
{
class ControlPanel : public rviz::Panel
{
Q_OBJECT
public:
ControlPanel(QWidget *parent=0);
virtual void save(rviz::Config config) const;
virtual void load(const rviz::Config &config);
private Q_SLOTS:
void sit();
void stand();
void claimLease();
void releaseLease();
void powerOn();
void powerOff();
void sendBodyPose();
void sendNeutralBodyPose();
void setMaxVel();
void gentleStop();
void hardStop();
void releaseStop();
void stop();
void setGait();
void setSwingHeight();
void setTerrainParams();
void setObstacleParams();
void allowMotion();
void rollOverRight();
void rollOverLeft();
void selfRight();
void dock();
void undock();
private:
// These maps allow us to set up the comboboxes for selections in the order
// of the enum, and ensure that the correct value is sent when the user wants to set it
// We can also use them to ensure that non-consecutive values are also correctly handled
const std::map<uint, std::string> gaitMap = {
{spot_msgs::SetLocomotion::Request::HINT_UNKNOWN, "Unknown"},
{spot_msgs::SetLocomotion::Request::HINT_TROT, "Trot"},
{spot_msgs::SetLocomotion::Request::HINT_SPEED_SELECT_TROT, "Speed sel trot"},
{spot_msgs::SetLocomotion::Request::HINT_CRAWL, "Crawl"},
{spot_msgs::SetLocomotion::Request::HINT_AMBLE, "Amble"},
{spot_msgs::SetLocomotion::Request::HINT_SPEED_SELECT_AMBLE, "Speed sel amble"},
{spot_msgs::SetLocomotion::Request::HINT_AUTO, "Auto"},
{spot_msgs::SetLocomotion::Request::HINT_JOG, "Jog"},
{spot_msgs::SetLocomotion::Request::HINT_HOP, "Hop"},
{spot_msgs::SetLocomotion::Request::HINT_SPEED_SELECT_CRAWL, "Speed sel crawl"}
};
const std::map<uint, std::string> swingHeightMap = {
{spot_msgs::SetSwingHeight::Request::SWING_HEIGHT_UNKNOWN, "Unknown"},
{spot_msgs::SetSwingHeight::Request::SWING_HEIGHT_LOW, "Low"},
{spot_msgs::SetSwingHeight::Request::SWING_HEIGHT_MEDIUM, "Medium"},
{spot_msgs::SetSwingHeight::Request::SWING_HEIGHT_HIGH, "High"}
};
const std::map<uint, std::string> gratedSurfacesMap = {
{spot_msgs::TerrainParams::GRATED_SURFACES_MODE_UNKNOWN, "Unknown"},
{spot_msgs::TerrainParams::GRATED_SURFACES_MODE_OFF, "Off"},
{spot_msgs::TerrainParams::GRATED_SURFACES_MODE_ON, "On"},
{spot_msgs::TerrainParams::GRATED_SURFACES_MODE_AUTO, "Auto"}
};
void setupComboBoxes();
void setupStopButtons();
void setupSpinBoxes();
void setControlButtons();
void toggleBodyPoseButtons();
bool callTriggerService(ros::ServiceClient service, std::string serviceName);
template <typename T>
bool callCustomTriggerService(ros::ServiceClient service, std::string serviceName, T serviceRequest);
void updateLabelTextWithLimit(QLabel* label, double limit_lower, double limit_upper);
void leaseCallback(const spot_msgs::LeaseArray::ConstPtr &leases);
void estopCallback(const spot_msgs::EStopStateArray::ConstPtr &estops);
void mobilityParamsCallback(const spot_msgs::MobilityParams::ConstPtr ¶ms);
void batteryCallback(const spot_msgs::BatteryStateArray::ConstPtr &battery);
void powerCallback(const spot_msgs::PowerState::ConstPtr &power);
void motionAllowedCallback(const std_msgs::Bool &motion_allowed);
ros::NodeHandle nh_;
ros::ServiceClient sitService_;
ros::ServiceClient standService_;
ros::ServiceClient claimLeaseService_;
ros::ServiceClient releaseLeaseService_;
ros::ServiceClient powerOnService_;
ros::ServiceClient powerOffService_;
ros::ServiceClient maxVelocityService_;
ros::ServiceClient hardStopService_;
ros::ServiceClient gentleStopService_;
ros::ServiceClient releaseStopService_;
ros::ServiceClient stopService_;
ros::ServiceClient gaitService_;
ros::ServiceClient swingHeightService_;
ros::ServiceClient terrainParamsService_;
ros::ServiceClient obstacleParamsService_;
ros::ServiceClient allowMotionService_;
ros::ServiceClient bodyPoseService_;
ros::ServiceClient dockService_;
ros::ServiceClient undockService_;
ros::ServiceClient selfRightService_;
ros::ServiceClient rollOverLeftService_;
ros::ServiceClient rollOverRightService_;
ros::Subscriber leaseSub_;
ros::Subscriber estopSub_;
ros::Subscriber mobilityParamsSub_;
ros::Subscriber batterySub_;
ros::Subscriber powerSub_;
ros::Subscriber motionAllowedSub_;
QPushButton* claimLeaseButton;
QPushButton* releaseLeaseButton;
QPushButton* powerOnButton;
QPushButton* powerOffButton;
QPushButton* setBodyPoseButton;
QPushButton* setBodyNeutralButton;
QPushButton* sitButton;
QPushButton* standButton;
QPushButton* setMaxVelButton;
QPushButton* hardStopButton;
QPushButton* gentleStopButton;
QPushButton* releaseStopButton;
QPushButton* stopButton;
QPushButton* setGaitButton;
QPushButton* setSwingHeightButton;
QPushButton* setObstaclePaddingButton;
QPushButton* setGratedSurfacesButton;
QPushButton* setFrictionButton;
QPushButton* allowMotionButton;
QPushButton* dockButton;
QPushButton* undockButton;
QPushButton* selfRightButton;
QPushButton* rollOverLeftButton;
QPushButton* rollOverRightButton;
QLabel* linearXLabel;
QLabel* linearYLabel;
QLabel* angularZLabel;
QLabel* statusLabel;
QLabel* bodyHeightLabel;
QLabel* rollLabel;
QLabel* pitchLabel;
QLabel* yawLabel;
QLabel* estimatedRuntimeLabel;
QLabel* batteryStateLabel;
QLabel* motorStateLabel;
QLabel* batteryTempLabel;
QLabel* estopLabel;
QComboBox* gaitComboBox;
QComboBox* swingHeightComboBox;
QComboBox* gratedSurfacesComboBox;
QSpinBox* dockFiducialSpin;
QDoubleSpinBox* linearXSpin;
QDoubleSpinBox* linearYSpin;
QDoubleSpinBox* angularZSpin;
QDoubleSpinBox* bodyHeightSpin;
QDoubleSpinBox* rollSpin;
QDoubleSpinBox* pitchSpin;
QDoubleSpinBox* yawSpin;
QDoubleSpinBox* frictionSpin;
QDoubleSpinBox* obstaclePaddingSpin;
spot_msgs::MobilityParams _lastMobilityParams;
bool haveLease;
bool isEStopped;
bool motionAllowed;
};
} // end namespace spot_viz
#endif // SPOT_CONTROL_PANEL_H
| 6,976 | C++ | 32.705314 | 105 | 0.714593 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_viz/src/spot_panel.cpp | #include "spot_panel.hpp"
#include <algorithm>
#include <cmath>
#include <QFile>
#include <QUiLoader>
#include <QVBoxLayout>
#include <ros/package.h>
#include <std_srvs/Trigger.h>
#include <std_srvs/SetBool.h>
#include <geometry_msgs/Pose.h>
#include <geometry_msgs/Twist.h>
#include <QDoubleValidator>
#include <QStandardItemModel>
#include <tf/transform_datatypes.h>
#include <spot_msgs/SetVelocity.h>
#include <spot_msgs/LeaseArray.h>
#include <spot_msgs/EStopState.h>
#include <spot_msgs/SetObstacleParams.h>
#include <spot_msgs/SetTerrainParams.h>
#include <spot_msgs/PosedStand.h>
#include <spot_msgs/Dock.h>
#include <string.h>
namespace spot_viz
{
ControlPanel::ControlPanel(QWidget *parent) {
std::string packagePath = ros::package::getPath("spot_viz") + "/resource/spot_control.ui";
ROS_INFO("Getting ui file from package path %s", packagePath.c_str());
QFile file(packagePath.c_str());
file.open(QIODevice::ReadOnly);
QUiLoader loader;
QWidget* ui = loader.load(&file, parent);
file.close();
QVBoxLayout* topLayout = new QVBoxLayout();
this->setLayout(topLayout);
topLayout->addWidget(ui);
haveLease = false;
motionAllowed = false;
sitService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/sit");
standService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/stand");
claimLeaseService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/claim");
releaseLeaseService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/release");
powerOnService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/power_on");
powerOffService_ = nh_.serviceClient<std_srvs::Trigger>("spot/power_off");
maxVelocityService_ = nh_.serviceClient<spot_msgs::SetVelocity>("/spot/velocity_limit");
hardStopService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/estop/hard");
gentleStopService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/estop/gentle");
releaseStopService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/estop/release");
stopService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/stop");
gaitService_ = nh_.serviceClient<spot_msgs::SetLocomotion>("/spot/locomotion_mode");
swingHeightService_ = nh_.serviceClient<spot_msgs::SetSwingHeight>("/spot/swing_height");
terrainParamsService_ = nh_.serviceClient<spot_msgs::SetTerrainParams>("/spot/terrain_params");
obstacleParamsService_ = nh_.serviceClient<spot_msgs::SetObstacleParams>("/spot/obstacle_params");
allowMotionService_ = nh_.serviceClient<std_srvs::SetBool>("/spot/allow_motion");
bodyPoseService_ = nh_.serviceClient<spot_msgs::PosedStand>("/spot/posed_stand");
dockService_ = nh_.serviceClient<spot_msgs::Dock>("/spot/dock");
undockService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/undock");
selfRightService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/self_right");
rollOverRightService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/roll_over_right");
rollOverLeftService_ = nh_.serviceClient<std_srvs::Trigger>("/spot/roll_over_left");
claimLeaseButton = this->findChild<QPushButton*>("claimLeaseButton");
releaseLeaseButton = this->findChild<QPushButton*>("releaseLeaseButton");
powerOnButton = this->findChild<QPushButton*>("powerOnButton");
powerOffButton = this->findChild<QPushButton*>("powerOffButton");
standButton = this->findChild<QPushButton*>("standButton");
sitButton = this->findChild<QPushButton*>("sitButton");
setBodyPoseButton = this->findChild<QPushButton*>("setBodyPoseButton");
setBodyNeutralButton = this->findChild<QPushButton*>("setBodyNeutralButton");
setMaxVelButton = this->findChild<QPushButton*>("setMaxVelButton");
setGaitButton = this->findChild<QPushButton*>("setGaitButton");
setSwingHeightButton = this->findChild<QPushButton*>("setSwingHeightButton");
setObstaclePaddingButton = this->findChild<QPushButton*>("setObstaclePaddingButton");
setGratedSurfacesButton = this->findChild<QPushButton*>("setGratedSurfacesButton");
setFrictionButton = this->findChild<QPushButton*>("setFrictionButton");
allowMotionButton = this->findChild<QPushButton*>("allowMotionButton");
dockButton = this->findChild<QPushButton*>("dockButton");
undockButton = this->findChild<QPushButton*>("undockButton");
selfRightButton = this->findChild<QPushButton*>("selfRightButton");
rollOverLeftButton = this->findChild<QPushButton*>("rollOverLeftButton");
rollOverRightButton = this->findChild<QPushButton*>("rollOverRightButton");
statusLabel = this->findChild<QLabel*>("statusLabel");
estimatedRuntimeLabel = this->findChild<QLabel*>("estimatedRuntimeLabel");
batteryStateLabel = this->findChild<QLabel*>("batteryStateLabel");
motorStateLabel = this->findChild<QLabel*>("motorStateLabel");
batteryTempLabel = this->findChild<QLabel*>("batteryTempLabel");
estopLabel = this->findChild<QLabel*>("estopLabel");
gaitComboBox = this->findChild<QComboBox*>("gaitComboBox");
swingHeightComboBox = this->findChild<QComboBox*>("swingHeightComboBox");
gratedSurfacesComboBox = this->findChild<QComboBox*>("gratedSurfacesComboBox");
obstaclePaddingSpin = this->findChild<QDoubleSpinBox*>("obstaclePaddingSpin");
frictionSpin = this->findChild<QDoubleSpinBox*>("frictionSpin");
dockFiducialSpin = this->findChild<QSpinBox*>("dockFiducialSpin");
setupComboBoxes();
setupStopButtons();
setupSpinBoxes();
// Subscribe to things after everything is set up to avoid crashes when things aren't initialised
leaseSub_ = nh_.subscribe("/spot/status/leases", 1, &ControlPanel::leaseCallback, this);
estopSub_ = nh_.subscribe("/spot/status/estop", 1, &ControlPanel::estopCallback, this);
mobilityParamsSub_ = nh_.subscribe("/spot/status/mobility_params", 1, &ControlPanel::mobilityParamsCallback, this);
batterySub_ = nh_.subscribe("/spot/status/battery_states", 1, &ControlPanel::batteryCallback, this);
powerSub_ = nh_.subscribe("/spot/status/power_state", 1, &ControlPanel::powerCallback, this);
motionAllowedSub_ = nh_.subscribe("/spot/status/motion_allowed", 1, &ControlPanel::motionAllowedCallback, this);
connect(claimLeaseButton, SIGNAL(clicked()), this, SLOT(claimLease()));
connect(releaseLeaseButton, SIGNAL(clicked()), this, SLOT(releaseLease()));
connect(powerOnButton, SIGNAL(clicked()), this, SLOT(powerOn()));
connect(powerOffButton, SIGNAL(clicked()), this, SLOT(powerOff()));
connect(sitButton, SIGNAL(clicked()), this, SLOT(sit()));
connect(standButton, SIGNAL(clicked()), this, SLOT(stand()));
connect(setBodyPoseButton, SIGNAL(clicked()), this, SLOT(sendBodyPose()));
connect(setBodyNeutralButton, SIGNAL(clicked()), this, SLOT(sendNeutralBodyPose()));
connect(setMaxVelButton, SIGNAL(clicked()), this, SLOT(setMaxVel()));
connect(releaseStopButton, SIGNAL(clicked()), this, SLOT(releaseStop()));
connect(hardStopButton, SIGNAL(clicked()), this, SLOT(hardStop()));
connect(gentleStopButton, SIGNAL(clicked()), this, SLOT(gentleStop()));
connect(stopButton, SIGNAL(clicked()), this, SLOT(stop()));
connect(setGaitButton, SIGNAL(clicked()), this, SLOT(setGait()));
connect(setSwingHeightButton, SIGNAL(clicked()), this, SLOT(setSwingHeight()));
connect(setObstaclePaddingButton, SIGNAL(clicked()), this, SLOT(setObstacleParams()));
connect(setGratedSurfacesButton, SIGNAL(clicked()), this, SLOT(setTerrainParams()));
connect(setFrictionButton, SIGNAL(clicked()), this, SLOT(setTerrainParams()));
connect(allowMotionButton, SIGNAL(clicked()), this, SLOT(allowMotion()));
connect(dockButton, SIGNAL(clicked()), this, SLOT(dock()));
connect(undockButton, SIGNAL(clicked()), this, SLOT(undock()));
connect(selfRightButton, SIGNAL(clicked()), this, SLOT(selfRight()));
connect(rollOverLeftButton, SIGNAL(clicked()), this, SLOT(rollOverLeft()));
connect(rollOverRightButton, SIGNAL(clicked()), this, SLOT(rollOverRight()));
}
void ControlPanel::setupStopButtons() {
stopButton = this->findChild<QPushButton*>("stopButton");
QPalette pal = stopButton->palette();
pal.setColor(QPalette::Button, QColor(255, 165, 0));
stopButton->setAutoFillBackground(true);
stopButton->setPalette(pal);
stopButton->update();
gentleStopButton = this->findChild<QPushButton*>("gentleStopButton");
pal = gentleStopButton->palette();
pal.setColor(QPalette::Button, QColor(255, 0, 255));
gentleStopButton->setAutoFillBackground(true);
gentleStopButton->setPalette(pal);
gentleStopButton->update();
hardStopButton = this->findChild<QPushButton*>("hardStopButton");
hardStopButton->setText(QString::fromUtf8("\u26A0 Kill Motors"));
pal = hardStopButton->palette();
pal.setColor(QPalette::Button, QColor(255, 0, 0));
hardStopButton->setAutoFillBackground(true);
hardStopButton->setPalette(pal);
hardStopButton->update();
releaseStopButton = this->findChild<QPushButton*>("releaseStopButton");
pal = releaseStopButton->palette();
pal.setColor(QPalette::Button, QColor(0, 255, 0));
releaseStopButton->setAutoFillBackground(true);
releaseStopButton->setPalette(pal);
releaseStopButton->update();
}
void ControlPanel::setupComboBoxes() {
// Iterate over the map for this combobox and add items. By default items in the
// map are in ascending order by key
for (const auto& item : gaitMap) {
gaitComboBox->addItem(QString(item.second.c_str()));
}
// Disable the unknown entry in the combobox so that it cannot be selected and sent to the service
QStandardItemModel* model = qobject_cast<QStandardItemModel *>(gaitComboBox->model());
QStandardItem* item = model->item(0);
item->setFlags(item->flags() & ~Qt::ItemIsEnabled);
for (const auto& item : swingHeightMap) {
swingHeightComboBox->addItem(QString(item.second.c_str()));
}
model = qobject_cast<QStandardItemModel *>(swingHeightComboBox->model());
item = model->item(0);
item->setFlags(item->flags() & ~Qt::ItemIsEnabled);
for (const auto& item : gratedSurfacesMap) {
gratedSurfacesComboBox->addItem(QString(item.second.c_str()));
}
model = qobject_cast<QStandardItemModel *>(gratedSurfacesComboBox->model());
item = model->item(0);
item->setFlags(item->flags() & ~Qt::ItemIsEnabled);
}
void ControlPanel::setupSpinBoxes() {
double linearVelocityLimit = 2;
linearXSpin = this->findChild<QDoubleSpinBox*>("linearXSpin");
linearXLabel = this->findChild<QLabel*>("linearXLabel");
updateLabelTextWithLimit(linearXLabel, 0, linearVelocityLimit);
linearXSpin->setMaximum(linearVelocityLimit);
linearXSpin->setMinimum(0);
linearYSpin = this->findChild<QDoubleSpinBox*>("linearYSpin");
linearYLabel = this->findChild<QLabel*>("linearYLabel");
updateLabelTextWithLimit(linearYLabel, 0, linearVelocityLimit);
linearYSpin->setMaximum(linearVelocityLimit);
linearYSpin->setMinimum(0);
angularZSpin = this->findChild<QDoubleSpinBox*>("angularZSpin");
angularZLabel = this->findChild<QLabel*>("angularZLabel");
updateLabelTextWithLimit(angularZLabel, 0, linearVelocityLimit);
angularZSpin->setMaximum(linearVelocityLimit);
angularZSpin->setMinimum(0);
double bodyHeightLimit = 0.15;
bodyHeightSpin = this->findChild<QDoubleSpinBox*>("bodyHeightSpin");
bodyHeightLabel = this->findChild<QLabel*>("bodyHeightLabel");
updateLabelTextWithLimit(bodyHeightLabel, -bodyHeightLimit, bodyHeightLimit);
bodyHeightSpin->setMaximum(bodyHeightLimit);
bodyHeightSpin->setMinimum(-bodyHeightLimit);
double rollLimit = 20;
rollSpin = this->findChild<QDoubleSpinBox*>("rollSpin");
rollLabel = this->findChild<QLabel*>("rollLabel");
updateLabelTextWithLimit(rollLabel, -rollLimit, rollLimit);
rollSpin->setMaximum(rollLimit);
rollSpin->setMinimum(-rollLimit);
double pitchLimit = 30;
pitchSpin = this->findChild<QDoubleSpinBox*>("pitchSpin");
pitchLabel = this->findChild<QLabel*>("pitchLabel");
updateLabelTextWithLimit(pitchLabel, -pitchLimit, pitchLimit);
pitchSpin->setMaximum(pitchLimit);
pitchSpin->setMinimum(-pitchLimit);
double yawLimit = 30;
yawSpin = this->findChild<QDoubleSpinBox*>("yawSpin");
yawLabel = this->findChild<QLabel*>("yawLabel");
updateLabelTextWithLimit(yawLabel, -yawLimit, yawLimit);
yawSpin->setMaximum(yawLimit);
yawSpin->setMinimum(-yawLimit);
}
void ControlPanel::updateLabelTextWithLimit(QLabel* label, double limit_lower, double limit_upper) {
int precision = 1;
// Kind of hacky but default to_string returns 6 digit precision which is unnecessary
std::string limit_lower_value = std::to_string(limit_lower).substr(0, std::to_string(limit_lower).find(".") + precision + 1);
std::string limit_upper_value = std::to_string(limit_upper).substr(0, std::to_string(limit_upper).find(".") + precision + 1);
std::string limit_range = " [" + limit_lower_value + ", " + limit_upper_value + "]";
std::string current_text = label->text().toStdString();
label->setText(QString((current_text + limit_range).c_str()));
}
void ControlPanel::setControlButtons() {
claimLeaseButton->setEnabled(!haveLease);
releaseLeaseButton->setEnabled(haveLease);
powerOnButton->setEnabled(haveLease);
powerOffButton->setEnabled(haveLease);
sitButton->setEnabled(haveLease);
standButton->setEnabled(haveLease);
setBodyPoseButton->setEnabled(haveLease);
setBodyNeutralButton->setEnabled(haveLease);
setMaxVelButton->setEnabled(haveLease);
releaseStopButton->setEnabled(haveLease && isEStopped);
hardStopButton->setEnabled(haveLease);
gentleStopButton->setEnabled(haveLease);
stopButton->setEnabled(haveLease);
setGaitButton->setEnabled(haveLease);
setSwingHeightButton->setEnabled(haveLease);
setObstaclePaddingButton->setEnabled(haveLease);
setFrictionButton->setEnabled(haveLease);
setGratedSurfacesButton->setEnabled(haveLease);
allowMotionButton->setEnabled(haveLease);
dockButton->setEnabled(haveLease);
undockButton->setEnabled(haveLease);
selfRightButton->setEnabled(haveLease);
rollOverLeftButton->setEnabled(haveLease);
rollOverRightButton->setEnabled(haveLease);
}
/**
* @brief Call a ros std_msgs/Trigger service
*
* Modifies the status label text depending on the result
*
* @param service Service to call
* @param serviceName Name of the service to use in labels
* @return true if successfully called
* @return false otherwise
*/
bool ControlPanel::callTriggerService(ros::ServiceClient service, std::string serviceName) {
std_srvs::Trigger req;
return callCustomTriggerService(service, serviceName, req);
}
template <typename T>
/**
* @brief Call an arbitrary service which has a response type of bool, str
*
* Modifies the status label text depending on the result
*
* @param service Service to call
* @param serviceName Name of the service to use in labels
* @param serviceRequest Request to make to the service
* @return true if successfully called
* @return false otherwise
*/
bool ControlPanel::callCustomTriggerService(ros::ServiceClient service, std::string serviceName, T serviceRequest) {
std::string labelText = "Calling " + serviceName + " service";
statusLabel->setText(QString(labelText.c_str()));
if (service.call(serviceRequest)) {
if (serviceRequest.response.success) {
labelText = "Successfully called " + serviceName + " service";
statusLabel->setText(QString(labelText.c_str()));
return true;
} else {
labelText = serviceName + " service failed: " + serviceRequest.response.message;
statusLabel->setText(QString(labelText.c_str()));
return false;
}
} else {
labelText = "Failed to call " + serviceName + " service" + serviceRequest.response.message;
statusLabel->setText(QString(labelText.c_str()));
return false;
}
}
/**
* @brief Check held leases and enable or disable buttons accordingly
*
* check to see if the body is already owned by the ROS node
* the resource will be "body" and the lease_owner.client_name will begin with "ros_spot"
* if the claim exists, treat this as a successful click of the Claim button
* if the claim does not exist, treat this as a click of the Release button
*
* @param leases
*/
void ControlPanel::leaseCallback(const spot_msgs::LeaseArray::ConstPtr &leases) {
bool msg_has_lease = false;
for (int i=leases->resources.size()-1; i>=0; i--) {
const spot_msgs::LeaseResource &resource = leases->resources[i];
bool right_resource = resource.resource.compare("body") == 0;
bool owned_by_ros = resource.lease_owner.client_name.compare(0, 8, "ros_spot") == 0;
if (right_resource && owned_by_ros) {
msg_has_lease = true;
}
}
if (msg_has_lease != haveLease) {
haveLease = msg_has_lease;
setControlButtons();
}
}
/**
* @brief Check estop state and disable control buttons if estopped
*
* @param estops
*/
void ControlPanel::estopCallback(const spot_msgs::EStopStateArray::ConstPtr &estops) {
bool softwareEstopped = false;
bool estopped = false;
std::string estopString("E-stops:");
for (const auto& estop : estops->estop_states) {
// Can't release hardware estops from the sdk
bool stopped = false;
if (estop.state == spot_msgs::EStopState::STATE_ESTOPPED) {
if (estop.type == spot_msgs::EStopState::TYPE_SOFTWARE) {
softwareEstopped = true;
}
stopped = true;
}
std::string stoppedStr(stopped ? "On" : "Off");
if (estop.name == "hardware_estop") {
estopString += " hardware: " + stoppedStr;
} else if (estop.name == "payload_estop") {
estopString += " payload: " + stoppedStr;
} else if (estop.name == "software_estop") {
estopString += " software: " + stoppedStr;
} else {
estopString += " " + estop.name + ": " + stoppedStr;
}
}
estopLabel->setText(QString(estopString.c_str()));
if (softwareEstopped != isEStopped) {
isEStopped = softwareEstopped;
setControlButtons();
}
}
void ControlPanel::mobilityParamsCallback(const spot_msgs::MobilityParams::ConstPtr ¶ms) {
if (*params == _lastMobilityParams) {
// If we don't check this, the user will never be able to modify values since they will constantly reset
return;
}
linearXSpin->setValue(params->velocity_limit.linear.x);
linearYSpin->setValue(params->velocity_limit.linear.y);
angularZSpin->setValue(params->velocity_limit.angular.z);
// Set the combo box values depending on whether there is a nonzero value coming from the params. If there isn't,
// set the value based on what it is when using the controller
if (params->locomotion_hint > 0) {
gaitComboBox->setCurrentIndex(gaitComboBox->findText(gaitMap.at(params->locomotion_hint).c_str()));
} else {
gaitComboBox->setCurrentIndex(gaitComboBox->findText(gaitMap.at(spot_msgs::SetLocomotion::Request::HINT_AUTO).c_str()));
}
if (params->swing_height > 0) {
swingHeightComboBox->setCurrentIndex(swingHeightComboBox->findText(swingHeightMap.at(params->swing_height).c_str()));
} else {
swingHeightComboBox->setCurrentIndex(swingHeightComboBox->findText(swingHeightMap.at(spot_msgs::SetSwingHeight::Request::SWING_HEIGHT_MEDIUM).c_str()));
}
if (params->terrain_params.grated_surfaces_mode > 0) {
gratedSurfacesComboBox->setCurrentIndex(gratedSurfacesComboBox->findText(gratedSurfacesMap.at(params->terrain_params.grated_surfaces_mode).c_str()));
} else {
gratedSurfacesComboBox->setCurrentIndex(gratedSurfacesComboBox->findText(gratedSurfacesMap.at(spot_msgs::TerrainParams::GRATED_SURFACES_MODE_AUTO).c_str()));
}
_lastMobilityParams = *params;
}
void ControlPanel::batteryCallback(const spot_msgs::BatteryStateArray::ConstPtr &battery) {
spot_msgs::BatteryState battState = battery->battery_states[0];
std::string estRuntime = "Estimated runtime: " + std::to_string(battState.estimated_runtime.sec/60) + " min";
estimatedRuntimeLabel->setText(QString(estRuntime.c_str()));
auto temps = battState.temperatures;
if (!temps.empty()) {
auto minmax = std::minmax_element(temps.begin(), temps.end());
float total = std::accumulate(temps.begin(), temps.end(), 0);
// Don't care about float values here
int tempMin = *minmax.first;
int tempMax = *minmax.second;
int tempAvg = total / temps.size();
std::string battTemp = "Battery temp: min " + std::to_string(tempMin) + ", max " + std::to_string(tempMax) + ", avg " + std::to_string(tempAvg);
batteryTempLabel->setText(QString(battTemp.c_str()));
} else {
batteryTempLabel->setText(QString("Battery temp: No battery"));
}
std::string status;
switch (battState.status)
{
case spot_msgs::BatteryState::STATUS_UNKNOWN:
status = "Unknown";
break;
case spot_msgs::BatteryState::STATUS_MISSING:
status = "Missing";
break;
case spot_msgs::BatteryState::STATUS_CHARGING:
status = "Charging";
break;
case spot_msgs::BatteryState::STATUS_DISCHARGING:
status = "Discharging";
break;
case spot_msgs::BatteryState::STATUS_BOOTING:
status = "Booting";
break;
default:
status = "Invalid";
break;
}
std::string battStatusStr;
if (battState.status == spot_msgs::BatteryState::STATUS_CHARGING || battState.status == spot_msgs::BatteryState::STATUS_DISCHARGING) {
// TODO: use std::format in c++20 rather than this nastiness
std::stringstream stream;
stream << std::fixed << std::setprecision(0) << battState.charge_percentage;
std::string pct = stream.str() + "%";
stream.str("");
stream.clear();
stream << std::fixed << std::setprecision(1) << battState.voltage;
std::string volt = stream.str() + "V";
stream.str("");
stream.clear();
stream << battState.current;
std::string amp = stream.str() + "A";
battStatusStr = "Battery state: " + status + ", " + pct + ", " + volt + ", " + amp;
} else {
battStatusStr = "Battery state: " + status;
}
batteryStateLabel->setText(QString(battStatusStr.c_str()));
}
void ControlPanel::powerCallback(const spot_msgs::PowerState::ConstPtr &power) {
std::string state;
switch (power->motor_power_state)
{
case spot_msgs::PowerState::STATE_POWERING_ON:
state = "Powering on";
powerOnButton->setEnabled(false);
break;
case spot_msgs::PowerState::STATE_POWERING_OFF:
state = "Powering off";
powerOffButton->setEnabled(false);
sitButton->setEnabled(false);
standButton->setEnabled(false);
break;
case spot_msgs::PowerState::STATE_ON:
state = "On";
powerOnButton->setEnabled(false);
powerOffButton->setEnabled(true);
sitButton->setEnabled(true);
standButton->setEnabled(true);
break;
case spot_msgs::PowerState::STATE_OFF:
state = "Off";
powerOnButton->setEnabled(true && haveLease);
powerOffButton->setEnabled(false);
sitButton->setEnabled(false);
standButton->setEnabled(false);
break;
case spot_msgs::PowerState::STATE_ERROR:
state = "Error";
break;
case spot_msgs::PowerState::STATE_UNKNOWN:
state = "Unknown";
break;
default:
"Invalid";
}
std::string motorState = "Motor state: " + state;
motorStateLabel->setText(QString(motorState.c_str()));
}
void ControlPanel::motionAllowedCallback(const std_msgs::Bool &motion_allowed) {
motionAllowed = motion_allowed.data;
if (!motion_allowed.data) {
stopButton->setText("Motion is disallowed");
stopButton->setEnabled(false);
allowMotionButton->setText("Allow motion");
} else {
stopButton->setText("Stop");
if (haveLease) {
stopButton->setEnabled(true);
}
allowMotionButton->setText("Disallow motion");
}
}
void ControlPanel::sit() {
callTriggerService(sitService_, "sit");
}
void ControlPanel::stand() {
callTriggerService(standService_, "stand");
}
void ControlPanel::powerOn() {
callTriggerService(powerOnService_, "power on");
}
void ControlPanel::powerOff() {
callTriggerService(powerOffService_, "power off");
}
void ControlPanel::claimLease() {
if (callTriggerService(claimLeaseService_, "claim lease"))
claimLeaseButton->setEnabled(false);
}
void ControlPanel::releaseLease() {
if (callTriggerService(releaseLeaseService_, "release lease"))
releaseLeaseButton->setEnabled(false);
}
void ControlPanel::stop() {
callTriggerService(stopService_, "stop");
}
void ControlPanel::allowMotion() {
std_srvs::SetBool req;
req.request.data = !motionAllowed;
callCustomTriggerService(allowMotionService_, "set motion allowed", req);
}
void ControlPanel::hardStop() {
callTriggerService(hardStopService_, "hard stop");
}
void ControlPanel::gentleStop() {
callTriggerService(gentleStopService_, "gentle stop");
}
void ControlPanel::releaseStop() {
callTriggerService(releaseStopService_, "release stop");
}
void ControlPanel::setMaxVel() {
spot_msgs::SetVelocity req;
req.request.velocity_limit.angular.z = angularZSpin->value();
req.request.velocity_limit.linear.x = linearXSpin->value();
req.request.velocity_limit.linear.y = linearYSpin->value();
callCustomTriggerService(maxVelocityService_, "set velocity limits", req);
}
void ControlPanel::sendBodyPose() {
ROS_INFO("Sending body pose");
spot_msgs::PosedStand req;
req.request.body_height = bodyHeightSpin->value();
req.request.body_yaw = yawSpin->value();
req.request.body_pitch = pitchSpin->value();
req.request.body_roll = rollSpin->value();
callCustomTriggerService(bodyPoseService_, "set body pose", req);
}
void ControlPanel::sendNeutralBodyPose() {
ROS_INFO("Sending body neutral pose");
spot_msgs::PosedStand req;
callCustomTriggerService(bodyPoseService_, "set body pose", req);
}
/**
* @brief Get the message constant integer that corresponds to the currently selected combobox item
*
* @param comboBox Combobox whose selection should be checked
* @param comboBoxMap Mapping from message constants to text in the combobox
* @return int > 0 indicating the map constant, or -1 if it couldn't be found
*/
int comboBoxSelectionToMessageConstantInt(QComboBox* comboBox, const std::map<uint, std::string>& comboBoxMap) {
std::string selectionText = comboBox->currentText().toStdString();
for (const auto& item : comboBoxMap) {
if (selectionText == item.second) {
return item.first;
}
}
return -1;
}
void ControlPanel::setGait() {
spot_msgs::SetLocomotion req;
req.request.locomotion_mode = comboBoxSelectionToMessageConstantInt(gaitComboBox, gaitMap);
callCustomTriggerService(gaitService_, "set gait", req);
}
void ControlPanel::setSwingHeight() {
spot_msgs::SetSwingHeight req;
req.request.swing_height = comboBoxSelectionToMessageConstantInt(swingHeightComboBox, swingHeightMap);
callCustomTriggerService(swingHeightService_, "set swing height", req);
}
void ControlPanel::setTerrainParams() {
spot_msgs::SetTerrainParams req;
req.request.terrain_params.grated_surfaces_mode = comboBoxSelectionToMessageConstantInt(gratedSurfacesComboBox, gratedSurfacesMap);
req.request.terrain_params.ground_mu_hint = frictionSpin->value();
callCustomTriggerService(terrainParamsService_, "set terrain params", req);
}
void ControlPanel::setObstacleParams() {
spot_msgs::SetObstacleParams req;
req.request.obstacle_params.obstacle_avoidance_padding = obstaclePaddingSpin->value();
callCustomTriggerService(obstacleParamsService_, "set obstacle params", req);
}
void ControlPanel::undock() {
callTriggerService(undockService_, "undock");
}
void ControlPanel::dock() {
spot_msgs::Dock req;
req.request.dock_id = dockFiducialSpin->value();
callCustomTriggerService(dockService_, "dock", req);
}
void ControlPanel::selfRight() {
callTriggerService(selfRightService_, "self right");
}
void ControlPanel::rollOverLeft() {
callTriggerService(rollOverLeftService_, "roll over left");
}
void ControlPanel::rollOverRight() {
callTriggerService(rollOverRightService_, "roll over left");
}
void ControlPanel::save(rviz::Config config) const
{
rviz::Panel::save(config);
}
// Load all configuration data for this panel from the given Config object.
void ControlPanel::load(const rviz::Config &config)
{
rviz::Panel::load(config);
}
} // end namespace spot_viz
#include <pluginlib/class_list_macros.h>
PLUGINLIB_EXPORT_CLASS(spot_viz::ControlPanel, rviz::Panel)
// END_TUTORIAL
| 31,663 | C++ | 44.234286 | 169 | 0.645296 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/CHANGELOG.rst | ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Changelog for package spot_driver
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
Forthcoming
-----------
1.0.0 (2023-04-07)
------------------
* add python-transforms3d-pip to package xml
* add odometry topic which outputs twist in correct frame, fixes `#95 <https://github.com/heuristicus/spot_ros/issues/95>`_
* subscriber check now polls also depth/info topics
* fix tf repeated message spam when publishing tfs at high frequency
* increase velocity command timeout to 0.6 seconds
probably fixes `#96 <https://github.com/heuristicus/spot_ros/issues/96>`_
* complete revision of the documentation mostly up to date with the current driver state
* fix incorrect actionserver in use for in motion body pose
* update maintainer
* robots without arms do not require manipulation client
* black formatting
* connect up buttons, fix undock call, fix dock actionserver name
* fix last docking command assignment, reset last stand command on dock. Fixes `#110 <https://github.com/heuristicus/spot_ros/issues/110>`_
* add actionserver for docking/undocking
* Fix spam when point cloud client is not initialised because there is no EAP
* changed rospy to logging.Logger dependency
* removed arm controller trial
* changed to correct tasks list
* changed list name
* changed lookup name
* changed lookup definiton
* only add tasks when needed
* updates after testing picking and placing on real spot - added some useful arguments to msg definitions
* starting to implement controller action server for moveit support
* implemented grasp service
* apply black formatting and add black check on PRs and pushes
* only add hand camera task if arm
* corrected index
* added warn messages for out of range
* reviewed limits for spot arm
* changed gripper_open_angle to take degrees
* adressed layout review comments
* changed to fix sized arrays
* changed hand pose msg
* fixed indentation and removed walk to pixel method
* add RollOver functions
* include fix for failing service call
* driver start process loops where it can fail due to the robot still booting. More messages about what it is doing.
* posed stand actionserver sleeps a bit before returning to allow the motion to complete
* implemented helper function for DockState message
* use correct action and service fields, set actionserver result
* add an actionserver for stand commands
* Driver config file can be passed as an arg to driver launch
* ROS loop rate can be set in params to allow higher publication frequencies
* Wrapper method to walk to object
* Subscriber to walk to object in picture
ROS_NAMESPACE=spot rosrun my_grasping image_box.py
Then click on pixel to walk to
* service for getting dock state
* print error messages when the estop keepalive has errors, initialise keepalive to none in init
* autonomy is not allowed if the robot is on shore power, cmd_vel counts as autonomy
* Created service for gripper pose
* Added method to send location to gripper
* rename old body_pose topic to try and reflect what it actually does
* Methods: body to follow arm, arm force trajectory
* Services for body follow arm, force trajectory arm
* add posed_stand service which can be used to pose the body
This also adds a check to the async_idle which prevents the idle stand command
from activating while there is a command which has a non-None id. This should
prevent commands from getting interrupted by that stand
* Updated the way to ensure power on & spot standing
* add terrain state to foot state, publish tf of foot position rt body
* Method added to check Spot is powered on and standing
New method: check_arm_power_and_stand
* Removed Estop check before arm moves
* Change method name
Changed method's name from "make_robot_command" to "make_arm_trajectory_command" for clarity
* Removed unnecessary code for testing
Powering off robot after moving the arm was removed; removed unnecessay sleep when moving the arm
* Added carry option for arm and hand images
Hand images from EricVoll
* Added hand image rate
* tab shown on startup should be basic motion tab, minor fixes
* allowed to move check has arg which specifies if autonomy enabled should be checked
* sit, stand and self right disabled when motion disabled, ui has button for allow motion
The stop button in the ui will now call the locked stop. Stop button is disabled and has its text changed when motion is not allowed
* add locked_stop service which interrupts current motion and disallows further motion
Publisher for the state of allow motion
* Advanced motion tab, obstacle and terrain params added to driver and panel
Move more advanced motion settings into the adv. motion tab to not clutter the basic motion. The settings there (ground friction, gait, swing height, grated surface mode) are I suspect relatively rarely used.
Obstacle params message added, which allows the settings for the obstacle avoidance to be published from the driver. These are as usual empty by default since the SDK does not provide details. Currently the only modification allowed is to the obstacle padding.
Terrain params message added, which allows setting of the ground friction and grated surfaces mode.
Terrain and obstacle params are in the mobility params message published by the driver.
Rviz panel allows interaction with the terrain and obstacle params. Comboboxes are now initialised by using a map generated from the constants in the ros messages, to try and make sure the ordering is correct. The map is also useful for constants which are non-consecutive like in the locomotion, for some reason the 9th value is unused but the 10th is.
* velocity and body controls are in different tabs, add swing height and gait control
add checks to ensure only valid values for locomotion and swing height are sent to the mobility params
* additional layer of control over whether motion is allowed, which can be changed while the driver is running
* reject velocity limits in the range 0 < lim < 0.15 as there can be issues with the trajectory command
* allow velocity limits to be set in the driver launch and load them on start
Also updats the mobility params message to store the velocity limits
If the limits change, the rviz panel is updated with the latest values
* initialise stop service after actionservers that it calls to prevent crashes if called during startup
* add a flag to explicitly enable autonomous functions
* add ros param to set the estop timeout, wrapper takes it as an arg
* track when the trajectory command returns status unknown, and try to resend the command once
* tolist() -> tobytes()
* not build client if no point_cloud services are available
* fix data type float32 -> uint8
* add lidar topic publisher, tf broadcaster
* ros helpers with PointCloud
* publish pointcloud from VLP16
* Service for gripper open at an angle
* Wrapper updated to open gripper at a given angle
* Implemented Close/open gripper services
* Added open & close gripper services
* Added stow, unstow, and control joints of the arm commands.
* add spot dock and undock service
* add ros param to set the estop timeout, wrapper takes it as an arg
* calling the stop service now preempts actionservers if they are active
* no longer reject trajectory poses which are not in body frame, just transform them
* add topic go_to_pose which can be used to move the robot with the trajectory command
* rename max_velocity to velocity_limit, now also limits velocity when moving backwards
* add actionserver to set body pose
* Add an additional envar to disable auto-launching the Velodyne as this _could\_ cause network issues
* Fix a mismatched tag
* Add the teleop_joy dependency
* Use the velodyne_description package for the actual sensor mesh, use the cage as a separate entity. Add an accessories.launch file to automatically bring up the velodyne if needed
* Add the bluetooth_teleop node, default config file.
* Simple rviz panel for interaction with spot
Can use the panel to claim and release the lease, power on and off, sit down and stand up, and set the body pose.
To add, go to panels>add new panel and select SpotControlPanel in spot_viz
* can require trajectory commands to reach goal precisely
Add precise_positioning field to the trajectory goal. Setting to true will make the wrapper consider only STATUS_AT_GOAL status to mean that the robot is at the goal. Setting to false will replicate previous behaviour where STATUS_NEAR_GOAL also means that the robot is at the goal.
Vary feedback messages from the driver depending on whether the precise positioning field was set.
* Include the license file in the individual ROS packages
* Add service call to set maximum velocity, use synchro trajectory command
The service call receives a twist message and sets the mobility params vel_lim max_vel to the linear x and y, and angular z values in the message. This velocity limit affects anything that moves the robot around, such as the trajectory command and velocity command.
The service call adds a srv to spot_msgs
Output exception string when power_on command fails
Fix minor typo in behavior_fault function name
Populate state_description in the estop state message
Minor cosmetic changes to srv imports and some comments for readability
* Updated E-Stop to use keepalive client rather than the endpoint. Fixes a bug where the E-Stop would release immeaditly after being triggered (`#38 <https://github.com/heuristicus/spot_ros/issues/38>`_)
Co-authored-by: marble-spot <[email protected]>
* Add trajectory command interface (`#25 <https://github.com/heuristicus/spot_ros/issues/25>`_)
* [spot_driver] add trajectory_cmd() method to spot_wrapper
* [spot_driver] rename _last_motion_command to _last_trajectory_command
* [spot_driver] rename _last_motion_command_time to _last_velocity_command_time
* [spot_driver] fix trajectory_cmd
* [spot_driver] fix options of trajectory_cmd()
* [spot_msgs] add Trajectory.srv
* [spot_driver] add trajectory service server
* [spot_ros] add frame_id checking to trajectory_cmd
* [spot_driver] fix bugs
* [spot_driver] fix bugs in trajectory_cmd
* convert trajectory command to an actionserver
* [spot_ros] fix merge commit
* [spot_driver] allow STATUS_NEAR_GOAL to be recognized as at_goal
* [spot_driver] add handling for 0 duration
Co-authored-by: Michal Staniaszek <[email protected]>
Co-authored-by: Dave Niewinski <[email protected]>
* Added service for clearing behavior faults (`#34 <https://github.com/heuristicus/spot_ros/issues/34>`_)
* Create runtime static transform broadcaster for camera transforms (`#31 <https://github.com/heuristicus/spot_ros/issues/31>`_)
The transforms between the body and camera frames are static and will not change. This change checks the transform for static frames once when an image with the required data is received. It then stores the transform to be published by a static transform publisher.
Previously when the robot was moved quickly there would be a lag between the camera frames updating and the actual position of the robot. With static transforms the cameras are always in the correct position relative to the body.
* Package structure more in line with ROS recommendation for python, works on noetic (`#32 <https://github.com/heuristicus/spot_ros/issues/32>`_)
* install graph_nav_util with catkin_install_python
* fix usage of Image.Format to be consistent with other usages
Using Image.Format.FORMAT_RAW causes an AttributeError:
AttributeError: 'EnumTypeWrapper' object has no attribute 'FORMAT_RAW'
The usage here was inconsistent with usages in other files.
* fix python package structure to work on noetic
* fix shebang line in spot_ros, explicitly specify use of python3
* Updated command feedback protos and expanded twist mux
* Fix image format and include graph_nav_util in install (`#29 <https://github.com/heuristicus/spot_ros/issues/29>`_)
* install graph_nav_util with catkin_install_python
* fix usage of Image.Format to be consistent with other usages
Using Image.Format.FORMAT_RAW causes an AttributeError:
AttributeError: 'EnumTypeWrapper' object has no attribute 'FORMAT_RAW'
The usage here was inconsistent with usages in other files.
* changed to use ros logging instead of print (`#14 <https://github.com/heuristicus/spot_ros/issues/14>`_)
* [spot_driver] change print() to ros logging
* [spot_driver] use ros logger instead of print()
* Updated some deprecated functions
* [spot_driver] bugfix for walking-mode
* [spot_driver] add mobility params publisher
* [spot_driver] fix locomotion_mode service
* [spot_driver] add locomotion_mode service
* [spot_driver] add stair_mode service to spot_ros
* [spot_driver] add get_mobility_params method and change set_mobility_params method
* fix for version v2.2.1, skip waypint\_ instead of waypoint
* support graphnav navigate-to
* add missing assignment to w field of quaternion
* Changed cmd_vel queue size to 1 from infinite to prevent controller lag
* Minor workaround for invalid timestamps
* [spot_driver] changed the names of odometry frames to default
* [spot_driver] changed default odom to kinematic odometry
* [spot_driver] add exception handlings to AsyncIdle class
* Publish /odom
* [spot_driver] add rosparams to determine a parent odometry frame
* [spot_driver] delete commented lines and redundant variables
* Publish /vision tf as the parent of /body tf
* [spot_driver] add python3-rospkg-modules dependency
* Use 16UC1 instead of mono16 for depth image encoding
* add twist_mux, interactive_marker_twist_server dependency
* Removed token as of api 2.0
* Moved resetting and claiming estop out of spot ros startup
* add install instructions
* Updated URDF to include mount points, and deps for install
* Incorporating clock skew between spot and ROS machine
* Initial stab as accomodating skew between systems. Untested
* Properly implemented checking the status of commands. Added a feedback message
* Made the driver not automatically claim a body lease and e stop. Allows you to monitor without having control. Disconnect doesn't exit very gracefully. Need to wait on sitting success
* Not defining a rate or defining a rate of <= 0 will disable a data source
* Updated image type selection to use protobuf enums
* Disabled auto standing by default. Updated api version numbering
* Updated dependencies
* Fixed mobility params when switching between movement and stationary
* Lots of little fixes. Body positioning logic is broken
* Error-checking improved
* removed unnecessary interactive marker
* Updated the estop setup so it is externally controllable
* Mobility parameters implemented. Some stuff still hard-coded
* Basic motion. Lots to clean up
* Added lots of docstrings
* Added services for power, stand, sit, self-right, and stop
* Updated doc outline. Still missing updated docstrings
* Refactoring to keep all the ros message building separate
* Got depth images working
* Added rosdoc
* Added image streams into ROS
* Fixed all the little mistakes from the last commit
* Blocked-out remainder of robot state callback. Untested
* Added messages and brought metrics and leases into ros
* Refactored the ros driver to be cleaner. Added a lot of docstrings. Incomplete
* Squashed commit of the following:
commit 0424b961e75b0e8f4143424e9fb0121ee5b3c01c
Author: Dave Niewinski <[email protected]>
Date: Mon May 11 16:36:55 2020 -0400
Updated logging
commit afdc5301f2b73f219b51ae3ce7c56e0f036e75a6
Author: Dave Niewinski <[email protected]>
Date: Mon May 11 15:00:27 2020 -0400
Added launch and params
commit 8c1066108d3cc2955cf49a73a75e3d249a8704d2
Author: Dave Niewinski <[email protected]>
Date: Fri May 8 15:04:48 2020 -0400
Basic ros support implemented. Outputs joint angles, and odom
commit 3f71252b182738234cc54e581cac3b8a54874733
Author: Dave Niewinski <[email protected]>
Date: Wed May 6 16:46:21 2020 -0400
Basic functionality, just printing in the terminal.
commit 505c17e1d4d5a28d14872d81e2f11b60b61135e9
Author: Dave Niewinski <[email protected]>
Date: Wed May 6 14:00:42 2020 -0400
Initial pass at data from robot
* Contributors: Chris Iverach-Brereton, Dave Niewinski, Esther, Harel Biggie, Kei Okada, Koki Shinjo, Maurice Brunner, Michal Staniaszek, Michel Heinemann, Naoto Tsukamoto, Naoya Yamaguchi, SpotCOREAI, Telios, Wolf Vollprecht, Yoshiki Obinata, harelb, jeremysee2, maubrunn, nfilliol
| 16,539 | reStructuredText | 63.357976 | 355 | 0.784026 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/setup.py | from setuptools import setup
from catkin_pkg.python_setup import generate_distutils_setup
d = generate_distutils_setup(
packages=["spot_driver"], scripts=["scripts/spot_ros"], package_dir={"": "src"}
)
setup(**d)
| 219 | Python | 23.444442 | 83 | 0.721461 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/src/spot_driver/spot_ros.py | import copy
import rospy
import math
import time
from std_srvs.srv import Trigger, TriggerResponse, SetBool, SetBoolResponse
from std_msgs.msg import Bool
from tf2_msgs.msg import TFMessage
from sensor_msgs.msg import Image, CameraInfo
from sensor_msgs.msg import PointCloud2
from sensor_msgs.msg import JointState
from geometry_msgs.msg import TwistWithCovarianceStamped, Twist, Pose, PoseStamped
from nav_msgs.msg import Odometry
from bosdyn.api.spot import robot_command_pb2 as spot_command_pb2
from bosdyn.api import geometry_pb2, trajectory_pb2
from bosdyn.api.geometry_pb2 import Quaternion, SE2VelocityLimit
from bosdyn.client import math_helpers
from google.protobuf.wrappers_pb2 import DoubleValue
import actionlib
import functools
import math
import bosdyn.geometry
import tf2_ros
import tf2_geometry_msgs
from spot_msgs.msg import Metrics
from spot_msgs.msg import LeaseArray, LeaseResource
from spot_msgs.msg import FootState, FootStateArray
from spot_msgs.msg import EStopState, EStopStateArray
from spot_msgs.msg import WiFiState
from spot_msgs.msg import PowerState
from spot_msgs.msg import BehaviorFault, BehaviorFaultState
from spot_msgs.msg import SystemFault, SystemFaultState
from spot_msgs.msg import BatteryState, BatteryStateArray
from spot_msgs.msg import DockAction, DockGoal, DockResult
from spot_msgs.msg import PoseBodyAction, PoseBodyGoal, PoseBodyResult
from spot_msgs.msg import Feedback
from spot_msgs.msg import MobilityParams, ObstacleParams, TerrainParams
from spot_msgs.msg import NavigateToAction, NavigateToResult, NavigateToFeedback
from spot_msgs.msg import TrajectoryAction, TrajectoryResult, TrajectoryFeedback
from spot_msgs.srv import ListGraph, ListGraphResponse
from spot_msgs.srv import SetLocomotion, SetLocomotionResponse
from spot_msgs.srv import SetTerrainParams, SetTerrainParamsResponse
from spot_msgs.srv import SetObstacleParams, SetObstacleParamsResponse
from spot_msgs.srv import ClearBehaviorFault, ClearBehaviorFaultResponse
from spot_msgs.srv import SetVelocity, SetVelocityResponse
from spot_msgs.srv import Dock, DockResponse, GetDockState, GetDockStateResponse
from spot_msgs.srv import PosedStand, PosedStandResponse
from spot_msgs.srv import SetSwingHeight, SetSwingHeightResponse
from spot_msgs.srv import (
ArmJointMovement,
ArmJointMovementResponse,
ArmJointMovementRequest,
)
from spot_msgs.srv import (
GripperAngleMove,
GripperAngleMoveResponse,
GripperAngleMoveRequest,
)
from spot_msgs.srv import (
ArmForceTrajectory,
ArmForceTrajectoryResponse,
ArmForceTrajectoryRequest,
)
from spot_msgs.srv import HandPose, HandPoseResponse, HandPoseRequest
from spot_msgs.srv import Grasp3d, Grasp3dRequest, Grasp3dResponse
from .ros_helpers import *
from spot_wrapper.wrapper import SpotWrapper
import actionlib
import logging
import threading
class RateLimitedCall:
"""
Wrap a function with this class to limit how frequently it can be called within a loop
"""
def __init__(self, fn, rate_limit):
"""
Args:
fn: Function to call
rate_limit: The function will not be called faster than this rate
"""
self.fn = fn
self.min_time_between_calls = 1.0 / rate_limit
self.last_call = 0
def __call__(self):
now_sec = time.time()
if (now_sec - self.last_call) > self.min_time_between_calls:
self.fn()
self.last_call = now_sec
class SpotROS:
"""Parent class for using the wrapper. Defines all callbacks and keeps the wrapper alive"""
def __init__(self):
self.spot_wrapper = None
self.last_tf_msg = TFMessage()
self.callbacks = {}
"""Dictionary listing what callback to use for what data task"""
self.callbacks["robot_state"] = self.RobotStateCB
self.callbacks["metrics"] = self.MetricsCB
self.callbacks["lease"] = self.LeaseCB
self.callbacks["front_image"] = self.FrontImageCB
self.callbacks["side_image"] = self.SideImageCB
self.callbacks["rear_image"] = self.RearImageCB
self.callbacks["hand_image"] = self.HandImageCB
self.callbacks["lidar_points"] = self.PointCloudCB
self.active_camera_tasks = []
self.camera_pub_to_async_task_mapping = {}
def RobotStateCB(self, results):
"""Callback for when the Spot Wrapper gets new robot state data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
state = self.spot_wrapper.robot_state
if state:
## joint states ##
joint_state = GetJointStatesFromState(state, self.spot_wrapper)
self.joint_state_pub.publish(joint_state)
## TF ##
tf_msg = GetTFFromState(state, self.spot_wrapper, self.mode_parent_odom_tf)
to_remove = []
if len(tf_msg.transforms) > 0:
for transform in tf_msg.transforms:
for last_tf in self.last_tf_msg.transforms:
if transform == last_tf:
to_remove.append(transform)
if to_remove:
# Do it this way to preserve the original tf message received. If we store the message we have
# destroyed then if there are two duplicates in a row we will not remove the second set.
deduplicated_tf = copy.deepcopy(tf_msg)
for repeat_tf in to_remove:
deduplicated_tf.transforms.remove(repeat_tf)
publish_tf = deduplicated_tf
else:
publish_tf = tf_msg
self.tf_pub.publish(publish_tf)
self.last_tf_msg = tf_msg
# Odom Twist #
twist_odom_msg = GetOdomTwistFromState(state, self.spot_wrapper)
self.odom_twist_pub.publish(twist_odom_msg)
# Odom #
use_vision = self.mode_parent_odom_tf == "vision"
odom_msg = GetOdomFromState(
state,
self.spot_wrapper,
use_vision=use_vision,
)
self.odom_pub.publish(odom_msg)
odom_corrected_msg = get_corrected_odom(odom_msg)
self.odom_corrected_pub.publish(odom_corrected_msg)
# Feet #
foot_array_msg = GetFeetFromState(state, self.spot_wrapper)
self.tf_pub.publish(generate_feet_tf(foot_array_msg))
self.feet_pub.publish(foot_array_msg)
# EStop #
estop_array_msg = GetEStopStateFromState(state, self.spot_wrapper)
self.estop_pub.publish(estop_array_msg)
# WIFI #
wifi_msg = GetWifiFromState(state, self.spot_wrapper)
self.wifi_pub.publish(wifi_msg)
# Battery States #
battery_states_array_msg = GetBatteryStatesFromState(
state, self.spot_wrapper
)
self.is_charging = (
battery_states_array_msg.battery_states[0].status
== BatteryState.STATUS_CHARGING
)
self.battery_pub.publish(battery_states_array_msg)
# Power State #
power_state_msg = GetPowerStatesFromState(state, self.spot_wrapper)
self.power_pub.publish(power_state_msg)
# System Faults #
system_fault_state_msg = GetSystemFaultsFromState(state, self.spot_wrapper)
self.system_faults_pub.publish(system_fault_state_msg)
# Behavior Faults #
behavior_fault_state_msg = getBehaviorFaultsFromState(
state, self.spot_wrapper
)
self.behavior_faults_pub.publish(behavior_fault_state_msg)
def MetricsCB(self, results):
"""Callback for when the Spot Wrapper gets new metrics data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
metrics = self.spot_wrapper.metrics
if metrics:
metrics_msg = Metrics()
local_time = self.spot_wrapper.robotToLocalTime(metrics.timestamp)
metrics_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
for metric in metrics.metrics:
if metric.label == "distance":
metrics_msg.distance = metric.float_value
if metric.label == "gait cycles":
metrics_msg.gait_cycles = metric.int_value
if metric.label == "time moving":
metrics_msg.time_moving = rospy.Time(
metric.duration.seconds, metric.duration.nanos
)
if metric.label == "electric power":
metrics_msg.electric_power = rospy.Time(
metric.duration.seconds, metric.duration.nanos
)
self.metrics_pub.publish(metrics_msg)
def LeaseCB(self, results):
"""Callback for when the Spot Wrapper gets new lease data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
lease_array_msg = LeaseArray()
lease_list = self.spot_wrapper.lease
if lease_list:
for resource in lease_list:
new_resource = LeaseResource()
new_resource.resource = resource.resource
new_resource.lease.resource = resource.lease.resource
new_resource.lease.epoch = resource.lease.epoch
for seq in resource.lease.sequence:
new_resource.lease.sequence.append(seq)
new_resource.lease_owner.client_name = resource.lease_owner.client_name
new_resource.lease_owner.user_name = resource.lease_owner.user_name
lease_array_msg.resources.append(new_resource)
self.lease_pub.publish(lease_array_msg)
def FrontImageCB(self, results):
"""Callback for when the Spot Wrapper gets new front image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.front_images
if data:
image_msg0, camera_info_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.frontleft_image_pub.publish(image_msg0)
self.frontleft_image_info_pub.publish(camera_info_msg0)
image_msg1, camera_info_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.frontright_image_pub.publish(image_msg1)
self.frontright_image_info_pub.publish(camera_info_msg1)
image_msg2, camera_info_msg2 = getImageMsg(data[2], self.spot_wrapper)
self.frontleft_depth_pub.publish(image_msg2)
self.frontleft_depth_info_pub.publish(camera_info_msg2)
image_msg3, camera_info_msg3 = getImageMsg(data[3], self.spot_wrapper)
self.frontright_depth_pub.publish(image_msg3)
self.frontright_depth_info_pub.publish(camera_info_msg3)
self.populate_camera_static_transforms(data[0])
self.populate_camera_static_transforms(data[1])
self.populate_camera_static_transforms(data[2])
self.populate_camera_static_transforms(data[3])
def SideImageCB(self, results):
"""Callback for when the Spot Wrapper gets new side image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.side_images
if data:
image_msg0, camera_info_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.left_image_pub.publish(image_msg0)
self.left_image_info_pub.publish(camera_info_msg0)
image_msg1, camera_info_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.right_image_pub.publish(image_msg1)
self.right_image_info_pub.publish(camera_info_msg1)
image_msg2, camera_info_msg2 = getImageMsg(data[2], self.spot_wrapper)
self.left_depth_pub.publish(image_msg2)
self.left_depth_info_pub.publish(camera_info_msg2)
image_msg3, camera_info_msg3 = getImageMsg(data[3], self.spot_wrapper)
self.right_depth_pub.publish(image_msg3)
self.right_depth_info_pub.publish(camera_info_msg3)
self.populate_camera_static_transforms(data[0])
self.populate_camera_static_transforms(data[1])
self.populate_camera_static_transforms(data[2])
self.populate_camera_static_transforms(data[3])
def RearImageCB(self, results):
"""Callback for when the Spot Wrapper gets new rear image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.rear_images
if data:
mage_msg0, camera_info_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.back_image_pub.publish(mage_msg0)
self.back_image_info_pub.publish(camera_info_msg0)
mage_msg1, camera_info_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.back_depth_pub.publish(mage_msg1)
self.back_depth_info_pub.publish(camera_info_msg1)
self.populate_camera_static_transforms(data[0])
self.populate_camera_static_transforms(data[1])
def HandImageCB(self, results):
"""Callback for when the Spot Wrapper gets new hand image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.hand_images
if data:
mage_msg0, camera_info_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.hand_image_mono_pub.publish(mage_msg0)
self.hand_image_mono_info_pub.publish(camera_info_msg0)
mage_msg1, camera_info_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.hand_depth_pub.publish(mage_msg1)
self.hand_depth_info_pub.publish(camera_info_msg1)
image_msg2, camera_info_msg2 = getImageMsg(data[2], self.spot_wrapper)
self.hand_image_color_pub.publish(image_msg2)
self.hand_image_color_info_pub.publish(camera_info_msg2)
image_msg3, camera_info_msg3 = getImageMsg(data[3], self.spot_wrapper)
self.hand_depth_in_hand_color_pub.publish(image_msg3)
self.hand_depth_in_color_info_pub.publish(camera_info_msg3)
self.populate_camera_static_transforms(data[0])
self.populate_camera_static_transforms(data[1])
self.populate_camera_static_transforms(data[2])
self.populate_camera_static_transforms(data[3])
def PointCloudCB(self, results):
"""Callback for when the Spot Wrapper gets new point cloud data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.point_clouds
if data:
point_cloud_msg = GetPointCloudMsg(data[0], self.spot_wrapper)
self.point_cloud_pub.publish(point_cloud_msg)
self.populate_lidar_static_transforms(data[0])
def handle_claim(self, req):
"""ROS service handler for the claim service"""
resp = self.spot_wrapper.claim()
return TriggerResponse(resp[0], resp[1])
def handle_release(self, req):
"""ROS service handler for the release service"""
resp = self.spot_wrapper.release()
return TriggerResponse(resp[0], resp[1])
def handle_locked_stop(self, req):
"""Stop the current motion of the robot and disallow any further motion until the allow motion service is
called"""
self.allow_motion = False
return self.handle_stop(req)
def handle_stop(self, req):
"""ROS service handler for the stop service. Interrupts the currently active motion"""
resp = self.spot_wrapper.stop()
message = "Spot stop service was called"
if self.navigate_as.is_active():
self.navigate_as.set_preempted(
NavigateToResult(success=False, message=message)
)
if self.trajectory_server.is_active():
self.trajectory_server.set_preempted(
TrajectoryResult(success=False, message=message)
)
if self.body_pose_as.is_active():
self.body_pose_as.set_preempted(
PoseBodyResult(success=False, message=message)
)
return TriggerResponse(resp[0], resp[1])
def handle_self_right(self, req):
"""ROS service handler for the self-right service"""
if not self.robot_allowed_to_move(autonomous_command=False):
return TriggerResponse(False, "Robot motion is not allowed")
resp = self.spot_wrapper.self_right()
return TriggerResponse(resp[0], resp[1])
def handle_sit(self, req):
"""ROS service handler for the sit service"""
if not self.robot_allowed_to_move(autonomous_command=False):
return TriggerResponse(False, "Robot motion is not allowed")
resp = self.spot_wrapper.sit()
return TriggerResponse(resp[0], resp[1])
def handle_stand(self, req):
"""ROS service handler for the stand service"""
if not self.robot_allowed_to_move(autonomous_command=False):
return TriggerResponse(False, "Robot motion is not allowed")
resp = self.spot_wrapper.stand()
return TriggerResponse(resp[0], resp[1])
def handle_posed_stand(self, req):
"""
Handle a service call for the posed stand
Args:
req: PosedStandRequest
Returns: PosedStandResponse
"""
success, message = self._posed_stand(
req.body_height, req.body_yaw, req.body_pitch, req.body_roll
)
return PosedStandResponse(success, message)
def handle_posed_stand_action(self, action):
"""
Handle a call to the posed stand actionserver
If no value is provided, this is equivalent to the basic stand commmand
Args:
action: PoseBodyGoal
"""
success, message = self._posed_stand(
action.body_height, action.yaw, action.pitch, action.roll
)
result = PoseBodyResult(success, message)
rospy.sleep(2) # Only return after the body has had a chance to move
if success:
self.body_pose_as.set_succeeded(result)
else:
self.body_pose_as.set_aborted(result)
def _posed_stand(self, body_height, yaw, pitch, roll):
"""
Make the robot do a posed stand with specified body height and orientation
By empirical observation, the limit on body height is [-0.16, 0.11], and RPY are probably limited to 30
degrees. Roll values are likely affected by the payload configuration as well. If the payload is
misconfigured a high roll value could cause it to hit the legs
Args:
body_height: Height of the body relative to the default height
yaw: Yaw to apply (in degrees)
pitch: Pitch to apply (in degrees)
roll: Roll to apply (in degrees)
Returns:
"""
if not self.robot_allowed_to_move(autonomous_command=False):
return False, "Robot motion is not allowed"
resp = self.spot_wrapper.stand(
body_height=body_height,
body_yaw=math.radians(yaw),
body_pitch=math.radians(pitch),
body_roll=math.radians(roll),
)
return resp[0], resp[1]
def handle_power_on(self, req):
"""ROS service handler for the power-on service"""
resp = self.spot_wrapper.power_on()
return TriggerResponse(resp[0], resp[1])
def handle_safe_power_off(self, req):
"""ROS service handler for the safe-power-off service"""
resp = self.spot_wrapper.safe_power_off()
return TriggerResponse(resp[0], resp[1])
def handle_estop_hard(self, req):
"""ROS service handler to hard-eStop the robot. The robot will immediately cut power to the motors"""
resp = self.spot_wrapper.assertEStop(True)
return TriggerResponse(resp[0], resp[1])
def handle_estop_soft(self, req):
"""ROS service handler to soft-eStop the robot. The robot will try to settle on the ground before cutting
power to the motors"""
resp = self.spot_wrapper.assertEStop(False)
return TriggerResponse(resp[0], resp[1])
def handle_estop_disengage(self, req):
"""ROS service handler to disengage the eStop on the robot."""
resp = self.spot_wrapper.disengageEStop()
return TriggerResponse(resp[0], resp[1])
def handle_clear_behavior_fault(self, req):
"""ROS service handler for clearing behavior faults"""
resp = self.spot_wrapper.clear_behavior_fault(req.id)
return ClearBehaviorFaultResponse(resp[0], resp[1])
def handle_stair_mode(self, req):
"""ROS service handler to set a stair mode to the robot."""
try:
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params.stair_hint = req.data
self.spot_wrapper.set_mobility_params(mobility_params)
return SetBoolResponse(True, "Success")
except Exception as e:
return SetBoolResponse(False, "Error:{}".format(e))
def handle_locomotion_mode(self, req):
"""ROS service handler to set locomotion mode"""
if req.locomotion_mode in [0, 9] or req.locomotion_mode > 10:
msg = "Attempted to set locomotion mode to {}, which is an invalid value.".format(
req.locomotion_mode
)
rospy.logerr(msg)
return SetLocomotionResponse(False, msg)
try:
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params.locomotion_hint = req.locomotion_mode
self.spot_wrapper.set_mobility_params(mobility_params)
return SetLocomotionResponse(True, "Success")
except Exception as e:
return SetLocomotionResponse(False, "Error:{}".format(e))
def handle_swing_height(self, req):
"""ROS service handler to set the step swing height"""
if req.swing_height == 0 or req.swing_height > 3:
msg = "Attempted to set step swing height to {}, which is an invalid value.".format(
req.swing_height
)
rospy.logerr(msg)
return SetSwingHeightResponse(False, msg)
try:
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params.swing_height = req.swing_height
self.spot_wrapper.set_mobility_params(mobility_params)
return SetSwingHeightResponse(True, "Success")
except Exception as e:
return SetSwingHeightResponse(False, "Error:{}".format(e))
def handle_vel_limit(self, req):
"""
Handle a velocity_limit service call.
Args:
req: SetVelocityRequest containing requested velocity limit
Returns: SetVelocityResponse
"""
success, message = self.set_velocity_limits(
req.velocity_limit.linear.x,
req.velocity_limit.linear.y,
req.velocity_limit.angular.z,
)
return SetVelocityResponse(success, message)
def set_velocity_limits(self, max_linear_x, max_linear_y, max_angular_z):
"""
Modify the mobility params to have a limit on the robot's velocity during trajectory commands.
Velocities sent to cmd_vel ignore these values
Passing 0 to any of the values will use spot's internal limits
Args:
max_linear_x: Maximum forwards/backwards velocity
max_linear_y: Maximum lateral velocity
max_angular_z: Maximum rotation velocity
Returns: (bool, str) boolean indicating whether the call was successful, along with a message
"""
if any(
map(lambda x: 0 < x < 0.15, [max_linear_x, max_linear_y, max_angular_z])
):
return (
False,
"Error: One of the values provided to velocity limits was below 0.15. Values in the range ("
"0,0.15) can cause unexpected behaviour of the trajectory command.",
)
try:
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params.vel_limit.CopyFrom(
SE2VelocityLimit(
max_vel=math_helpers.SE2Velocity(
max_linear_x, max_linear_y, max_angular_z
).to_proto(),
min_vel=math_helpers.SE2Velocity(
-max_linear_x, -max_linear_y, -max_angular_z
).to_proto(),
)
)
self.spot_wrapper.set_mobility_params(mobility_params)
return True, "Success"
except Exception as e:
return False, "Error:{}".format(e)
def _transform_pose_to_body_frame(self, pose):
"""
Transform a pose to the body frame
Args:
pose: PoseStamped to transform
Raises: tf2_ros.LookupException if the transform lookup fails
Returns: Transformed pose in body frame if given pose is not in the body frame, or the original pose if it is
in the body frame
"""
if pose.header.frame_id == "body":
return pose
body_to_fixed = self.tf_buffer.lookup_transform(
"body", pose.header.frame_id, rospy.Time()
)
pose_in_body = tf2_geometry_msgs.do_transform_pose(pose, body_to_fixed)
pose_in_body.header.frame_id = "body"
return pose_in_body
def robot_allowed_to_move(self, autonomous_command=True):
"""
Check if the robot is allowed to move. This means checking both that autonomy is enabled, which can only be
set when the driver is started, and also that motion is allowed, the state of which can change while the
driver is running
Args:
autonomous_command: If true, indicates that this function should also check if autonomy is enabled
Returns: True if the robot is allowed to move, false otherwise
"""
if not self.allow_motion:
rospy.logwarn(
"Spot is not currently allowed to move. Use the allow_motion service to allow the robot to "
"move."
)
autonomy_ok = True
if autonomous_command:
if not self.autonomy_enabled:
rospy.logwarn(
"Spot is not allowed to be autonomous because this instance of the driver was started "
"with it disabled. Set autonomy_enabled to true in the launch file to enable it."
)
autonomy_ok = False
if self.is_charging:
rospy.logwarn(
"Spot cannot be autonomous because it is connected to shore power."
)
autonomy_ok = False
return self.allow_motion and autonomy_ok
def handle_allow_motion(self, req):
"""
Handle a call to set whether motion is allowed or not
When motion is not allowed, any service call or topic which can move the robot will return without doing
anything
Returns: (bool, str) True if successful, along with a message
"""
self.allow_motion = req.data
rospy.loginfo(
"Robot motion is now {}".format(
"allowed" if self.allow_motion else "disallowed"
)
)
if not self.allow_motion:
# Always send a stop command if disallowing motion, in case the robot is moving when it is sent
self.spot_wrapper.stop()
return True, "Spot motion was {}".format("enabled" if req.data else "disabled")
def handle_obstacle_params(self, req):
"""
Handle a call to set the obstacle params part of mobility params. The previous values are always overwritten.
Args:
req:
Returns: (bool, str) True if successful, along with a message
"""
mobility_params = self.spot_wrapper.get_mobility_params()
obstacle_params = spot_command_pb2.ObstacleParams()
# Currently only the obstacle setting that we allow is the padding. The previous value is always overwritten
# Clamp to the range [0, 0.5] as on the controller
if req.obstacle_params.obstacle_avoidance_padding < 0:
rospy.logwarn(
"Received padding value of {}, clamping to 0".format(
req.obstacle_params.obstacle_avoidance_padding
)
)
req.obstacle_params.obstacle_avoidance_padding = 0
if req.obstacle_params.obstacle_avoidance_padding > 0.5:
rospy.logwarn(
"Received padding value of {}, clamping to 0.5".format(
req.obstacle_params.obstacle_avoidance_padding
)
)
req.obstacle_params.obstacle_avoidance_padding = 0.5
disable_notallowed = ""
if any(
[
req.obstacle_params.disable_vision_foot_obstacle_avoidance,
req.obstacle_params.disable_vision_foot_constraint_avoidance,
req.obstacle_params.disable_vision_body_obstacle_avoidance,
req.obstacle_params.disable_vision_foot_obstacle_body_assist,
req.obstacle_params.disable_vision_negative_obstacles,
]
):
disable_notallowed = " Disabling any of the obstacle avoidance components is not currently allowed."
rospy.logerr(
"At least one of the disable settings on obstacle params was true."
+ disable_notallowed
)
obstacle_params.obstacle_avoidance_padding = (
req.obstacle_params.obstacle_avoidance_padding
)
mobility_params.obstacle_params.CopyFrom(obstacle_params)
self.spot_wrapper.set_mobility_params(mobility_params)
return True, "Successfully set obstacle params" + disable_notallowed
def handle_terrain_params(self, req):
"""
Handle a call to set the terrain params part of mobility params. The previous values are always overwritten
Args:
req:
Returns: (bool, str) True if successful, along with a message
"""
mobility_params = self.spot_wrapper.get_mobility_params()
# We always overwrite the previous settings of these values. Reject if not within recommended limits (as on
# the controller)
if 0.2 <= req.terrain_params.ground_mu_hint <= 0.8:
# For some reason assignment to ground_mu_hint is not allowed once the terrain params are initialised
# Must initialise with the protobuf type DoubleValue for initialisation to work
terrain_params = spot_command_pb2.TerrainParams(
ground_mu_hint=DoubleValue(value=req.terrain_params.ground_mu_hint)
)
else:
return (
False,
"Failed to set terrain params, ground_mu_hint of {} is not in the range [0.4, 0.8]".format(
req.terrain_params.ground_mu_hint
),
)
if req.terrain_params.grated_surfaces_mode in [1, 2, 3]:
terrain_params.grated_surfaces_mode = (
req.terrain_params.grated_surfaces_mode
)
else:
return (
False,
"Failed to set terrain params, grated_surfaces_mode {} was not one of [1, 2, 3]".format(
req.terrain_params.grated_surfaces_mode
),
)
mobility_params.terrain_params.CopyFrom(terrain_params)
self.spot_wrapper.set_mobility_params(mobility_params)
return True, "Successfully set terrain params"
def trajectory_callback(self, msg):
"""
Handle a callback from the trajectory topic requesting to go to a location
The trajectory will time out after 5 seconds
Args:
msg: PoseStamped containing desired pose
Returns:
"""
if not self.robot_allowed_to_move():
rospy.logerr(
"Trajectory topic received a message but the robot is not allowed to move."
)
return
try:
self._send_trajectory_command(
self._transform_pose_to_body_frame(msg), rospy.Duration(5)
)
except tf2_ros.LookupException as e:
rospy.logerr(str(e))
def handle_trajectory(self, req):
"""ROS actionserver execution handler to handle receiving a request to move to a location"""
if not self.robot_allowed_to_move():
rospy.logerr(
"Trajectory service was called but robot is not allowed to move"
)
self.trajectory_server.set_aborted(
TrajectoryResult(False, "Robot is not allowed to move.")
)
return
target_pose = req.target_pose
if req.target_pose.header.frame_id != "body":
rospy.logwarn("Pose given was not in the body frame, will transform")
try:
target_pose = self._transform_pose_to_body_frame(target_pose)
except tf2_ros.LookupException as e:
self.trajectory_server.set_aborted(
TrajectoryResult(False, "Could not transform pose into body frame")
)
return
if req.duration.data.to_sec() <= 0:
self.trajectory_server.set_aborted(
TrajectoryResult(False, "duration must be larger than 0")
)
return
cmd_duration = rospy.Duration(req.duration.data.secs, req.duration.data.nsecs)
resp = self._send_trajectory_command(
target_pose, cmd_duration, req.precise_positioning
)
def timeout_cb(trajectory_server, _):
trajectory_server.publish_feedback(
TrajectoryFeedback("Failed to reach goal, timed out")
)
trajectory_server.set_aborted(
TrajectoryResult(False, "Failed to reach goal, timed out")
)
# Abort the actionserver if cmd_duration is exceeded - the driver stops but does not provide feedback to
# indicate this so we monitor it ourselves
cmd_timeout = rospy.Timer(
cmd_duration,
functools.partial(timeout_cb, self.trajectory_server),
oneshot=True,
)
# Sleep to allow some feedback to come through from the trajectory command
rospy.sleep(0.25)
if self.spot_wrapper._trajectory_status_unknown:
rospy.logerr(
"Sent trajectory request to spot but received unknown feedback. Resending command. This will "
"only be attempted once"
)
# If we receive an unknown result from the trajectory, something went wrong internally (not
# catastrophically). We need to resend the command, because getting status unknown happens right when
# the command is sent. It's unclear right now why this happens
resp = self._send_trajectory_command(
target_pose, cmd_duration, req.precise_positioning
)
cmd_timeout.shutdown()
cmd_timeout = rospy.Timer(
cmd_duration,
functools.partial(timeout_cb, self.trajectory_server),
oneshot=True,
)
# The trajectory command is non-blocking but we need to keep this function up in order to interrupt if a
# preempt is requested and to return success if/when the robot reaches the goal. Also check the is_active to
# monitor whether the timeout_cb has already aborted the command
rate = rospy.Rate(10)
while (
not rospy.is_shutdown()
and not self.trajectory_server.is_preempt_requested()
and not self.spot_wrapper.at_goal
and self.trajectory_server.is_active()
and not self.spot_wrapper._trajectory_status_unknown
):
if self.spot_wrapper.near_goal:
if self.spot_wrapper._last_trajectory_command_precise:
self.trajectory_server.publish_feedback(
TrajectoryFeedback("Near goal, performing final adjustments")
)
else:
self.trajectory_server.publish_feedback(
TrajectoryFeedback("Near goal")
)
else:
self.trajectory_server.publish_feedback(
TrajectoryFeedback("Moving to goal")
)
rate.sleep()
# If still active after exiting the loop, the command did not time out
if self.trajectory_server.is_active():
cmd_timeout.shutdown()
if self.trajectory_server.is_preempt_requested():
self.trajectory_server.publish_feedback(TrajectoryFeedback("Preempted"))
self.trajectory_server.set_preempted()
self.spot_wrapper.stop()
if self.spot_wrapper.at_goal:
self.trajectory_server.publish_feedback(
TrajectoryFeedback("Reached goal")
)
self.trajectory_server.set_succeeded(TrajectoryResult(resp[0], resp[1]))
else:
self.trajectory_server.publish_feedback(
TrajectoryFeedback("Failed to reach goal")
)
self.trajectory_server.set_aborted(
TrajectoryResult(False, "Failed to reach goal")
)
def handle_roll_over_right(self, req):
"""Robot sit down and roll on to it its side for easier battery access"""
del req
resp = self.spot_wrapper.battery_change_pose(1)
return TriggerResponse(resp[0], resp[1])
def handle_roll_over_left(self, req):
"""Robot sit down and roll on to it its side for easier battery access"""
del req
resp = self.spot_wrapper.battery_change_pose(2)
return TriggerResponse(resp[0], resp[1])
def handle_dock(self, req):
"""Dock the robot"""
resp = self.spot_wrapper.dock(req.dock_id)
return DockResponse(resp[0], resp[1])
def handle_undock(self, req):
"""Undock the robot"""
resp = self.spot_wrapper.undock()
return TriggerResponse(resp[0], resp[1])
def handle_dock_action(self, req: DockGoal):
if req.undock:
resp = self.spot_wrapper.undock()
else:
resp = self.spot_wrapper.dock(req.dock_id)
if resp[0]:
self.dock_as.set_succeeded(DockResult(resp[0], resp[1]))
else:
self.dock_as.set_aborted(DockResult(resp[0], resp[1]))
def handle_get_docking_state(self, req):
"""Get docking state of robot"""
resp = self.spot_wrapper.get_docking_state()
return GetDockStateResponse(GetDockStatesFromState(resp))
def _send_trajectory_command(self, pose, duration, precise=True):
"""
Send a trajectory command to the robot
Args:
pose: Pose the robot should go to. Must be in the body frame
duration: After this duration, the command will time out and the robot will stop
precise: If true, the robot will position itself precisely at the target pose, otherwise it will end up
near (within ~0.5m, rotation optional) the requested location
Returns: (bool, str) tuple indicating whether the command was successfully sent, and a message
"""
if not self.robot_allowed_to_move():
rospy.logerr("send trajectory was called but motion is not allowed.")
return
if pose.header.frame_id != "body":
rospy.logerr("Trajectory command poses must be in the body frame")
return
return self.spot_wrapper.trajectory_cmd(
goal_x=pose.pose.position.x,
goal_y=pose.pose.position.y,
goal_heading=math_helpers.Quat(
w=pose.pose.orientation.w,
x=pose.pose.orientation.x,
y=pose.pose.orientation.y,
z=pose.pose.orientation.z,
).to_yaw(),
cmd_duration=duration.to_sec(),
precise_position=precise,
)
def cmdVelCallback(self, data):
"""Callback for cmd_vel command"""
if not self.robot_allowed_to_move():
rospy.logerr("cmd_vel received a message but motion is not allowed.")
return
self.spot_wrapper.velocity_cmd(data.linear.x, data.linear.y, data.angular.z)
def in_motion_or_idle_pose_cb(self, data):
"""
Callback for pose to be used while in motion or idling
This sets the body control field in the mobility params. This means that the pose will be used while a motion
command is executed. Only the pitch is maintained while moving. The roll and yaw will be applied by the idle
stand command.
"""
if not self.robot_allowed_to_move(autonomous_command=False):
rospy.logerr("body pose received a message but motion is not allowed.")
return
self._set_in_motion_or_idle_body_pose(data)
def handle_in_motion_or_idle_body_pose(self, goal):
"""
Handle a goal received from the pose body actionserver
Args:
goal: PoseBodyGoal containing a pose to apply to the body
Returns:
"""
# We can change the body pose if autonomy is not allowed
if not self.robot_allowed_to_move(autonomous_command=False):
rospy.logerr("body pose actionserver was called but motion is not allowed.")
return
# If the body_pose is empty, we use the rpy + height components instead
if goal.body_pose == Pose():
# If the rpy+body height are all zero then we set the body to neutral pose
if not any(
[
goal.roll,
goal.pitch,
goal.yaw,
not math.isclose(goal.body_height, 0, abs_tol=1e-9),
]
):
pose = Pose()
pose.orientation.w = 1
self._set_in_motion_or_idle_body_pose(pose)
else:
pose = Pose()
# Multiplication order is important to get the correct quaternion
orientation_quat = (
math_helpers.Quat.from_yaw(math.radians(goal.yaw))
* math_helpers.Quat.from_pitch(math.radians(goal.pitch))
* math_helpers.Quat.from_roll(math.radians(goal.roll))
)
pose.orientation.x = orientation_quat.x
pose.orientation.y = orientation_quat.y
pose.orientation.z = orientation_quat.z
pose.orientation.w = orientation_quat.w
pose.position.z = goal.body_height
self._set_in_motion_or_idle_body_pose(pose)
else:
self._set_in_motion_or_idle_body_pose(goal.body_pose)
# Give it some time to move
rospy.sleep(2)
self.motion_or_idle_body_pose_as.set_succeeded(
PoseBodyResult(
success=True, message="Successfully applied in-motion pose to body"
)
)
def _set_in_motion_or_idle_body_pose(self, pose):
"""
Set the pose of the body which should be applied while in motion or idle
Args:
pose: Pose to be applied to the body. Only the body height is taken from the position component
Returns:
"""
q = Quaternion()
q.x = pose.orientation.x
q.y = pose.orientation.y
q.z = pose.orientation.z
q.w = pose.orientation.w
position = geometry_pb2.Vec3(z=pose.position.z)
pose = geometry_pb2.SE3Pose(position=position, rotation=q)
point = trajectory_pb2.SE3TrajectoryPoint(pose=pose)
traj = trajectory_pb2.SE3Trajectory(points=[point])
body_control = spot_command_pb2.BodyControlParams(base_offset_rt_footprint=traj)
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params.body_control.CopyFrom(body_control)
self.spot_wrapper.set_mobility_params(mobility_params)
def handle_list_graph(self, upload_path):
"""ROS service handler for listing graph_nav waypoint_ids"""
resp = self.spot_wrapper.list_graph(upload_path)
return ListGraphResponse(resp)
def handle_navigate_to_feedback(self):
"""Thread function to send navigate_to feedback"""
while not rospy.is_shutdown() and self.run_navigate_to:
localization_state = (
self.spot_wrapper._graph_nav_client.get_localization_state()
)
if localization_state.localization.waypoint_id:
self.navigate_as.publish_feedback(
NavigateToFeedback(localization_state.localization.waypoint_id)
)
rospy.Rate(10).sleep()
def handle_navigate_to(self, msg):
"""ROS service handler to run mission of the robot. The robot will replay a mission"""
if not self.robot_allowed_to_move():
rospy.logerr("navigate_to was requested but robot is not allowed to move.")
self.navigate_as.set_aborted(
NavigateToResult(False, "Autonomy is not enabled")
)
return
# create thread to periodically publish feedback
feedback_thraed = threading.Thread(
target=self.handle_navigate_to_feedback, args=()
)
self.run_navigate_to = True
feedback_thraed.start()
# run navigate_to
resp = self.spot_wrapper.navigate_to(
upload_path=msg.upload_path,
navigate_to=msg.navigate_to,
initial_localization_fiducial=msg.initial_localization_fiducial,
initial_localization_waypoint=msg.initial_localization_waypoint,
)
self.run_navigate_to = False
feedback_thraed.join()
# check status
if resp[0]:
self.navigate_as.set_succeeded(NavigateToResult(resp[0], resp[1]))
else:
self.navigate_as.set_aborted(NavigateToResult(resp[0], resp[1]))
def populate_camera_static_transforms(self, image_data):
"""Check data received from one of the image tasks and use the transform snapshot to extract the camera frame
transforms. This is the transforms from body->frontleft->frontleft_fisheye, for example. These transforms
never change, but they may be calibrated slightly differently for each robot so we need to generate the
transforms at runtime.
Args:
image_data: Image protobuf data from the wrapper
"""
# We exclude the odometry frames from static transforms since they are not static. We can ignore the body
# frame because it is a child of odom or vision depending on the mode_parent_odom_tf, and will be published
# by the non-static transform publishing that is done by the state callback
excluded_frames = [
self.tf_name_vision_odom,
self.tf_name_kinematic_odom,
"body",
]
for frame_name in image_data.shot.transforms_snapshot.child_to_parent_edge_map:
if frame_name in excluded_frames:
continue
parent_frame = (
image_data.shot.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
).parent_frame_name
)
existing_transforms = [
(transform.header.frame_id, transform.child_frame_id)
for transform in self.sensors_static_transforms
]
if (parent_frame, frame_name) in existing_transforms:
# We already extracted this transform
continue
transform = (
image_data.shot.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
)
)
local_time = self.spot_wrapper.robotToLocalTime(
image_data.shot.acquisition_time
)
tf_time = rospy.Time(local_time.seconds, local_time.nanos)
static_tf = populateTransformStamped(
tf_time,
transform.parent_frame_name,
frame_name,
transform.parent_tform_child,
)
self.sensors_static_transforms.append(static_tf)
self.sensors_static_transform_broadcaster.sendTransform(
self.sensors_static_transforms
)
def populate_lidar_static_transforms(self, point_cloud_data):
"""Check data received from one of the point cloud tasks and use the transform snapshot to extract the lidar frame
transforms. This is the transforms from body->sensor, for example. These transforms
never change, but they may be calibrated slightly differently for each robot so we need to generate the
transforms at runtime.
Args:
point_cloud_data: PointCloud protobuf data from the wrapper
"""
# We exclude the odometry frames from static transforms since they are not static. We can ignore the body
# frame because it is a child of odom or vision depending on the mode_parent_odom_tf, and will be published
# by the non-static transform publishing that is done by the state callback
excluded_frames = [
self.tf_name_vision_odom,
self.tf_name_kinematic_odom,
"body",
]
for (
frame_name
) in (
point_cloud_data.point_cloud.source.transforms_snapshot.child_to_parent_edge_map
):
if frame_name in excluded_frames:
continue
parent_frame = point_cloud_data.point_cloud.source.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
).parent_frame_name
existing_transforms = [
(transform.header.frame_id, transform.child_frame_id)
for transform in self.sensors_static_transforms
]
if (parent_frame, frame_name) in existing_transforms:
# We already extracted this transform
continue
transform = point_cloud_data.point_cloud.source.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
)
local_time = self.spot_wrapper.robotToLocalTime(
point_cloud_data.point_cloud.source.acquisition_time
)
tf_time = rospy.Time(local_time.seconds, local_time.nanos)
static_tf = populateTransformStamped(
tf_time,
transform.parent_frame_name,
frame_name,
transform.parent_tform_child,
)
self.sensors_static_transforms.append(static_tf)
self.sensors_static_transform_broadcaster.sendTransform(
self.sensors_static_transforms
)
# Arm functions ##################################################
def handle_arm_stow(self, srv_data):
"""ROS service handler to command the arm to stow, home position"""
resp = self.spot_wrapper.arm_stow()
return TriggerResponse(resp[0], resp[1])
def handle_arm_unstow(self, srv_data):
"""ROS service handler to command the arm to unstow, joints are all zeros"""
resp = self.spot_wrapper.arm_unstow()
return TriggerResponse(resp[0], resp[1])
def handle_arm_joint_move(self, srv_data: ArmJointMovementRequest):
"""ROS service handler to send joint movement to the arm to execute"""
resp = self.spot_wrapper.arm_joint_move(joint_targets=srv_data.joint_target)
return ArmJointMovementResponse(resp[0], resp[1])
def handle_force_trajectory(self, srv_data: ArmForceTrajectoryRequest):
"""ROS service handler to send a force trajectory up or down a vertical force"""
resp = self.spot_wrapper.force_trajectory(data=srv_data)
return ArmForceTrajectoryResponse(resp[0], resp[1])
def handle_gripper_open(self, srv_data):
"""ROS service handler to open the gripper"""
resp = self.spot_wrapper.gripper_open()
return TriggerResponse(resp[0], resp[1])
def handle_gripper_close(self, srv_data):
"""ROS service handler to close the gripper"""
resp = self.spot_wrapper.gripper_close()
return TriggerResponse(resp[0], resp[1])
def handle_gripper_angle_open(self, srv_data: GripperAngleMoveRequest):
"""ROS service handler to open the gripper at an angle"""
resp = self.spot_wrapper.gripper_angle_open(gripper_ang=srv_data.gripper_angle)
return GripperAngleMoveResponse(resp[0], resp[1])
def handle_arm_carry(self, srv_data):
"""ROS service handler to put arm in carry mode"""
resp = self.spot_wrapper.arm_carry()
return TriggerResponse(resp[0], resp[1])
def handle_hand_pose(self, srv_data: HandPoseRequest):
"""ROS service to give a position to the gripper"""
resp = self.spot_wrapper.hand_pose(data=srv_data)
return HandPoseResponse(resp[0], resp[1])
def handle_grasp_3d(self, srv_data: Grasp3dRequest):
"""ROS service to grasp an object by x,y,z coordinates in given frame"""
resp = self.spot_wrapper.grasp_3d(
frame=srv_data.frame_name,
object_rt_frame=srv_data.object_rt_frame,
)
return Grasp3dResponse(resp[0], resp[1])
##################################################################
def shutdown(self):
rospy.loginfo("Shutting down ROS driver for Spot")
self.spot_wrapper.sit()
rospy.Rate(0.25).sleep()
self.spot_wrapper.disconnect()
def publish_mobility_params(self):
mobility_params_msg = MobilityParams()
try:
mobility_params = self.spot_wrapper.get_mobility_params()
mobility_params_msg.body_control.position.x = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.position.x
)
mobility_params_msg.body_control.position.y = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.position.y
)
mobility_params_msg.body_control.position.z = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.position.z
)
mobility_params_msg.body_control.orientation.x = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.rotation.x
)
mobility_params_msg.body_control.orientation.y = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.rotation.y
)
mobility_params_msg.body_control.orientation.z = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.rotation.z
)
mobility_params_msg.body_control.orientation.w = (
mobility_params.body_control.base_offset_rt_footprint.points[
0
].pose.rotation.w
)
mobility_params_msg.locomotion_hint = mobility_params.locomotion_hint
mobility_params_msg.stair_hint = mobility_params.stair_hint
mobility_params_msg.swing_height = mobility_params.swing_height
mobility_params_msg.obstacle_params.obstacle_avoidance_padding = (
mobility_params.obstacle_params.obstacle_avoidance_padding
)
mobility_params_msg.obstacle_params.disable_vision_foot_obstacle_avoidance = (
mobility_params.obstacle_params.disable_vision_foot_obstacle_avoidance
)
mobility_params_msg.obstacle_params.disable_vision_foot_constraint_avoidance = (
mobility_params.obstacle_params.disable_vision_foot_constraint_avoidance
)
mobility_params_msg.obstacle_params.disable_vision_body_obstacle_avoidance = (
mobility_params.obstacle_params.disable_vision_body_obstacle_avoidance
)
mobility_params_msg.obstacle_params.disable_vision_foot_obstacle_body_assist = (
mobility_params.obstacle_params.disable_vision_foot_obstacle_body_assist
)
mobility_params_msg.obstacle_params.disable_vision_negative_obstacles = (
mobility_params.obstacle_params.disable_vision_negative_obstacles
)
if mobility_params.HasField("terrain_params"):
if mobility_params.terrain_params.HasField("ground_mu_hint"):
mobility_params_msg.terrain_params.ground_mu_hint = (
mobility_params.terrain_params.ground_mu_hint
)
# hasfield does not work on grated surfaces mode
if hasattr(mobility_params.terrain_params, "grated_surfaces_mode"):
mobility_params_msg.terrain_params.grated_surfaces_mode = (
mobility_params.terrain_params.grated_surfaces_mode
)
# The velocity limit values can be set independently so make sure each of them exists before setting
if mobility_params.HasField("vel_limit"):
if hasattr(mobility_params.vel_limit.max_vel.linear, "x"):
mobility_params_msg.velocity_limit.linear.x = (
mobility_params.vel_limit.max_vel.linear.x
)
if hasattr(mobility_params.vel_limit.max_vel.linear, "y"):
mobility_params_msg.velocity_limit.linear.y = (
mobility_params.vel_limit.max_vel.linear.y
)
if hasattr(mobility_params.vel_limit.max_vel, "angular"):
mobility_params_msg.velocity_limit.angular.z = (
mobility_params.vel_limit.max_vel.angular
)
except Exception as e:
rospy.logerr("Error:{}".format(e))
pass
self.mobility_params_pub.publish(mobility_params_msg)
def publish_feedback(self):
feedback_msg = Feedback()
feedback_msg.standing = self.spot_wrapper.is_standing
feedback_msg.sitting = self.spot_wrapper.is_sitting
feedback_msg.moving = self.spot_wrapper.is_moving
id_ = self.spot_wrapper.id
try:
feedback_msg.serial_number = id_.serial_number
feedback_msg.species = id_.species
feedback_msg.version = id_.version
feedback_msg.nickname = id_.nickname
feedback_msg.computer_serial_number = id_.computer_serial_number
except:
pass
self.feedback_pub.publish(feedback_msg)
def publish_allow_motion(self):
self.motion_allowed_pub.publish(self.allow_motion)
def check_for_subscriber(self):
for pub in list(self.camera_pub_to_async_task_mapping.keys()):
task_name = self.camera_pub_to_async_task_mapping[pub]
if (
task_name not in self.active_camera_tasks
and pub.get_num_connections() > 0
):
self.spot_wrapper.update_image_tasks(task_name)
self.active_camera_tasks.append(task_name)
print(
f"Detected subscriber for {task_name} task, adding task to publish"
)
def main(self):
"""Main function for the SpotROS class. Gets config from ROS and initializes the wrapper. Holds lease from
wrapper and updates all async tasks at the ROS rate"""
rospy.init_node("spot_ros", anonymous=True)
self.rates = rospy.get_param("~rates", {})
if "loop_frequency" in self.rates:
loop_rate = self.rates["loop_frequency"]
else:
loop_rate = 50
for param, rate in self.rates.items():
if rate > loop_rate:
rospy.logwarn(
"{} has a rate of {} specified, which is higher than the loop rate of {}. It will not "
"be published at the expected frequency".format(
param, rate, loop_rate
)
)
rate = rospy.Rate(loop_rate)
self.robot_name = rospy.get_param("~robot_name", "spot")
self.username = rospy.get_param("~username", "default_value")
self.password = rospy.get_param("~password", "default_value")
self.hostname = rospy.get_param("~hostname", "default_value")
self.motion_deadzone = rospy.get_param("~deadzone", 0.05)
self.start_estop = rospy.get_param("~start_estop", True)
self.estop_timeout = rospy.get_param("~estop_timeout", 9.0)
self.autonomy_enabled = rospy.get_param("~autonomy_enabled", True)
self.allow_motion = rospy.get_param("~allow_motion", True)
self.use_take_lease = rospy.get_param("~use_take_lease", False)
self.get_lease_on_action = rospy.get_param("~get_lease_on_action", False)
self.is_charging = False
self.tf_buffer = tf2_ros.Buffer()
self.tf_listener = tf2_ros.TransformListener(self.tf_buffer)
self.sensors_static_transform_broadcaster = tf2_ros.StaticTransformBroadcaster()
# Static transform broadcaster is super simple and just a latched publisher. Every time we add a new static
# transform we must republish all static transforms from this source, otherwise the tree will be incomplete.
# We keep a list of all the static transforms we already have so they can be republished, and so we can check
# which ones we already have
self.sensors_static_transforms = []
# Spot has 2 types of odometries: 'odom' and 'vision'
# The former one is kinematic odometry and the second one is a combined odometry of vision and kinematics
# These params enables to change which odometry frame is a parent of body frame and to change tf names of each odometry frames.
self.mode_parent_odom_tf = rospy.get_param(
"~mode_parent_odom_tf", "odom"
) # 'vision' or 'odom'
self.tf_name_kinematic_odom = rospy.get_param("~tf_name_kinematic_odom", "odom")
self.tf_name_raw_kinematic = "odom"
self.tf_name_vision_odom = rospy.get_param("~tf_name_vision_odom", "vision")
self.tf_name_raw_vision = "vision"
if (
self.mode_parent_odom_tf != self.tf_name_raw_kinematic
and self.mode_parent_odom_tf != self.tf_name_raw_vision
):
rospy.logerr(
"rosparam '~mode_parent_odom_tf' should be 'odom' or 'vision'."
)
return
self.logger = logging.getLogger("rosout")
rospy.loginfo("Starting ROS driver for Spot")
self.spot_wrapper = SpotWrapper(
username=self.username,
password=self.password,
hostname=self.hostname,
robot_name=self.robot_name,
logger=self.logger,
start_estop=self.start_estop,
estop_timeout=self.estop_timeout,
rates=self.rates,
callbacks=self.callbacks,
use_take_lease=self.use_take_lease,
get_lease_on_action=self.get_lease_on_action,
)
if not self.spot_wrapper.is_valid:
return
# Images #
self.back_image_pub = rospy.Publisher("camera/back/image", Image, queue_size=10)
self.frontleft_image_pub = rospy.Publisher(
"camera/frontleft/image", Image, queue_size=10
)
self.frontright_image_pub = rospy.Publisher(
"camera/frontright/image", Image, queue_size=10
)
self.left_image_pub = rospy.Publisher("camera/left/image", Image, queue_size=10)
self.right_image_pub = rospy.Publisher(
"camera/right/image", Image, queue_size=10
)
self.hand_image_mono_pub = rospy.Publisher(
"camera/hand_mono/image", Image, queue_size=10
)
self.hand_image_color_pub = rospy.Publisher(
"camera/hand_color/image", Image, queue_size=10
)
# Depth #
self.back_depth_pub = rospy.Publisher("depth/back/image", Image, queue_size=10)
self.frontleft_depth_pub = rospy.Publisher(
"depth/frontleft/image", Image, queue_size=10
)
self.frontright_depth_pub = rospy.Publisher(
"depth/frontright/image", Image, queue_size=10
)
self.left_depth_pub = rospy.Publisher("depth/left/image", Image, queue_size=10)
self.right_depth_pub = rospy.Publisher(
"depth/right/image", Image, queue_size=10
)
self.hand_depth_pub = rospy.Publisher("depth/hand/image", Image, queue_size=10)
self.hand_depth_in_hand_color_pub = rospy.Publisher(
"depth/hand/depth_in_color", Image, queue_size=10
)
self.frontleft_depth_in_visual_pub = rospy.Publisher(
"depth/frontleft/depth_in_visual", Image, queue_size=10
)
self.frontright_depth_in_visual_pub = rospy.Publisher(
"depth/frontright/depth_in_visual", Image, queue_size=10
)
# EAP Pointcloud #
self.point_cloud_pub = rospy.Publisher(
"lidar/points", PointCloud2, queue_size=10
)
# Image Camera Info #
self.back_image_info_pub = rospy.Publisher(
"camera/back/camera_info", CameraInfo, queue_size=10
)
self.frontleft_image_info_pub = rospy.Publisher(
"camera/frontleft/camera_info", CameraInfo, queue_size=10
)
self.frontright_image_info_pub = rospy.Publisher(
"camera/frontright/camera_info", CameraInfo, queue_size=10
)
self.left_image_info_pub = rospy.Publisher(
"camera/left/camera_info", CameraInfo, queue_size=10
)
self.right_image_info_pub = rospy.Publisher(
"camera/right/camera_info", CameraInfo, queue_size=10
)
self.hand_image_mono_info_pub = rospy.Publisher(
"camera/hand_mono/camera_info", CameraInfo, queue_size=10
)
self.hand_image_color_info_pub = rospy.Publisher(
"camera/hand_color/camera_info", CameraInfo, queue_size=10
)
# Depth Camera Info #
self.back_depth_info_pub = rospy.Publisher(
"depth/back/camera_info", CameraInfo, queue_size=10
)
self.frontleft_depth_info_pub = rospy.Publisher(
"depth/frontleft/camera_info", CameraInfo, queue_size=10
)
self.frontright_depth_info_pub = rospy.Publisher(
"depth/frontright/camera_info", CameraInfo, queue_size=10
)
self.left_depth_info_pub = rospy.Publisher(
"depth/left/camera_info", CameraInfo, queue_size=10
)
self.right_depth_info_pub = rospy.Publisher(
"depth/right/camera_info", CameraInfo, queue_size=10
)
self.hand_depth_info_pub = rospy.Publisher(
"depth/hand/camera_info", CameraInfo, queue_size=10
)
self.hand_depth_in_color_info_pub = rospy.Publisher(
"camera/hand/depth_in_color/camera_info", CameraInfo, queue_size=10
)
self.frontleft_depth_in_visual_info_pub = rospy.Publisher(
"depth/frontleft/depth_in_visual/camera_info", CameraInfo, queue_size=10
)
self.frontright_depth_in_visual_info_pub = rospy.Publisher(
"depth/frontright/depth_in_visual/camera_info", CameraInfo, queue_size=10
)
self.camera_pub_to_async_task_mapping = {
self.frontleft_image_pub: "front_image",
self.frontleft_depth_pub: "front_image",
self.frontleft_image_info_pub: "front_image",
self.frontright_image_pub: "front_image",
self.frontright_depth_pub: "front_image",
self.frontright_image_info_pub: "front_image",
self.back_image_pub: "rear_image",
self.back_depth_pub: "rear_image",
self.back_image_info_pub: "rear_image",
self.right_image_pub: "side_image",
self.right_depth_pub: "side_image",
self.right_image_info_pub: "side_image",
self.left_image_pub: "side_image",
self.left_depth_pub: "side_image",
self.left_image_info_pub: "side_image",
self.hand_image_color_pub: "hand_image",
self.hand_image_mono_pub: "hand_image",
self.hand_image_mono_info_pub: "hand_image",
self.hand_depth_pub: "hand_image",
self.hand_depth_in_hand_color_pub: "hand_image",
}
# Status Publishers #
self.joint_state_pub = rospy.Publisher(
"joint_states", JointState, queue_size=10
)
"""Defining a TF publisher manually because of conflicts between Python3 and tf"""
self.tf_pub = rospy.Publisher("tf", TFMessage, queue_size=10)
self.metrics_pub = rospy.Publisher("status/metrics", Metrics, queue_size=10)
self.lease_pub = rospy.Publisher("status/leases", LeaseArray, queue_size=10)
self.odom_twist_pub = rospy.Publisher(
"odometry/twist", TwistWithCovarianceStamped, queue_size=10
)
self.odom_pub = rospy.Publisher("odometry", Odometry, queue_size=10)
self.odom_corrected_pub = rospy.Publisher(
"odometry_corrected", Odometry, queue_size=10
)
self.feet_pub = rospy.Publisher("status/feet", FootStateArray, queue_size=10)
self.estop_pub = rospy.Publisher("status/estop", EStopStateArray, queue_size=10)
self.wifi_pub = rospy.Publisher("status/wifi", WiFiState, queue_size=10)
self.power_pub = rospy.Publisher(
"status/power_state", PowerState, queue_size=10
)
self.battery_pub = rospy.Publisher(
"status/battery_states", BatteryStateArray, queue_size=10
)
self.behavior_faults_pub = rospy.Publisher(
"status/behavior_faults", BehaviorFaultState, queue_size=10
)
self.system_faults_pub = rospy.Publisher(
"status/system_faults", SystemFaultState, queue_size=10
)
self.motion_allowed_pub = rospy.Publisher(
"status/motion_allowed", Bool, queue_size=10
)
self.feedback_pub = rospy.Publisher("status/feedback", Feedback, queue_size=10)
self.mobility_params_pub = rospy.Publisher(
"status/mobility_params", MobilityParams, queue_size=10
)
rospy.Subscriber("cmd_vel", Twist, self.cmdVelCallback, queue_size=1)
rospy.Subscriber(
"go_to_pose", PoseStamped, self.trajectory_callback, queue_size=1
)
rospy.Subscriber(
"in_motion_or_idle_body_pose",
Pose,
self.in_motion_or_idle_pose_cb,
queue_size=1,
)
rospy.Service("claim", Trigger, self.handle_claim)
rospy.Service("release", Trigger, self.handle_release)
rospy.Service("self_right", Trigger, self.handle_self_right)
rospy.Service("sit", Trigger, self.handle_sit)
rospy.Service("stand", Trigger, self.handle_stand)
rospy.Service("power_on", Trigger, self.handle_power_on)
rospy.Service("power_off", Trigger, self.handle_safe_power_off)
rospy.Service("estop/hard", Trigger, self.handle_estop_hard)
rospy.Service("estop/gentle", Trigger, self.handle_estop_soft)
rospy.Service("estop/release", Trigger, self.handle_estop_disengage)
rospy.Service("allow_motion", SetBool, self.handle_allow_motion)
rospy.Service("stair_mode", SetBool, self.handle_stair_mode)
rospy.Service("locomotion_mode", SetLocomotion, self.handle_locomotion_mode)
rospy.Service("swing_height", SetSwingHeight, self.handle_swing_height)
rospy.Service("velocity_limit", SetVelocity, self.handle_vel_limit)
rospy.Service(
"clear_behavior_fault", ClearBehaviorFault, self.handle_clear_behavior_fault
)
rospy.Service("terrain_params", SetTerrainParams, self.handle_terrain_params)
rospy.Service("obstacle_params", SetObstacleParams, self.handle_obstacle_params)
rospy.Service("posed_stand", PosedStand, self.handle_posed_stand)
rospy.Service("list_graph", ListGraph, self.handle_list_graph)
rospy.Service("roll_over_right", Trigger, self.handle_roll_over_right)
rospy.Service("roll_over_left", Trigger, self.handle_roll_over_left)
# Docking
rospy.Service("dock", Dock, self.handle_dock)
rospy.Service("undock", Trigger, self.handle_undock)
rospy.Service("docking_state", GetDockState, self.handle_get_docking_state)
# Arm Services #########################################
rospy.Service("arm_stow", Trigger, self.handle_arm_stow)
rospy.Service("arm_unstow", Trigger, self.handle_arm_unstow)
rospy.Service("gripper_open", Trigger, self.handle_gripper_open)
rospy.Service("gripper_close", Trigger, self.handle_gripper_close)
rospy.Service("arm_carry", Trigger, self.handle_arm_carry)
rospy.Service(
"gripper_angle_open", GripperAngleMove, self.handle_gripper_angle_open
)
rospy.Service("arm_joint_move", ArmJointMovement, self.handle_arm_joint_move)
rospy.Service(
"force_trajectory", ArmForceTrajectory, self.handle_force_trajectory
)
rospy.Service("gripper_pose", HandPose, self.handle_hand_pose)
rospy.Service("grasp_3d", Grasp3d, self.handle_grasp_3d)
#########################################################
self.navigate_as = actionlib.SimpleActionServer(
"navigate_to",
NavigateToAction,
execute_cb=self.handle_navigate_to,
auto_start=False,
)
self.navigate_as.start()
self.trajectory_server = actionlib.SimpleActionServer(
"trajectory",
TrajectoryAction,
execute_cb=self.handle_trajectory,
auto_start=False,
)
self.trajectory_server.start()
self.motion_or_idle_body_pose_as = actionlib.SimpleActionServer(
"motion_or_idle_body_pose",
PoseBodyAction,
execute_cb=self.handle_in_motion_or_idle_body_pose,
auto_start=False,
)
self.motion_or_idle_body_pose_as.start()
self.body_pose_as = actionlib.SimpleActionServer(
"body_pose",
PoseBodyAction,
execute_cb=self.handle_posed_stand_action,
auto_start=False,
)
self.body_pose_as.start()
self.dock_as = actionlib.SimpleActionServer(
"dock",
DockAction,
execute_cb=self.handle_dock_action,
auto_start=False,
)
self.dock_as.start()
# Stop service calls other services so initialise it after them to prevent crashes which can happen if
# the service is immediately called
rospy.Service("stop", Trigger, self.handle_stop)
rospy.Service("locked_stop", Trigger, self.handle_locked_stop)
rospy.on_shutdown(self.shutdown)
max_linear_x = rospy.get_param("~max_linear_velocity_x", 0)
max_linear_y = rospy.get_param("~max_linear_velocity_y", 0)
max_angular_z = rospy.get_param("~max_angular_velocity_z", 0)
self.set_velocity_limits(max_linear_x, max_linear_y, max_angular_z)
self.auto_claim = rospy.get_param("~auto_claim", False)
self.auto_power_on = rospy.get_param("~auto_power_on", False)
self.auto_stand = rospy.get_param("~auto_stand", False)
if self.auto_claim:
self.spot_wrapper.claim()
if self.auto_power_on:
self.spot_wrapper.power_on()
if self.auto_stand:
self.spot_wrapper.stand()
rate_limited_feedback = RateLimitedCall(
self.publish_feedback, self.rates["feedback"]
)
rate_limited_mobility_params = RateLimitedCall(
self.publish_mobility_params, self.rates["mobility_params"]
)
rate_check_for_subscriber = RateLimitedCall(
self.check_for_subscriber, self.rates["check_subscribers"]
)
rate_limited_motion_allowed = RateLimitedCall(self.publish_allow_motion, 10)
rospy.loginfo("Driver started")
while not rospy.is_shutdown():
self.spot_wrapper.updateTasks()
rate_limited_feedback()
rate_limited_mobility_params()
rate_limited_motion_allowed()
rate_check_for_subscriber()
rate.sleep()
| 78,021 | Python | 41.634973 | 135 | 0.608644 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/src/spot_driver/ros_helpers.py | import copy
import rospy
import tf2_ros
import transforms3d
from std_msgs.msg import Empty
from tf2_msgs.msg import TFMessage
from geometry_msgs.msg import TransformStamped, Transform
from sensor_msgs.msg import Image, CameraInfo
from sensor_msgs.msg import JointState
from sensor_msgs.msg import PointCloud2, PointField
from geometry_msgs.msg import PoseWithCovariance
from geometry_msgs.msg import TwistWithCovariance
from geometry_msgs.msg import TwistWithCovarianceStamped
from nav_msgs.msg import Odometry
from spot_msgs.msg import Metrics
from spot_msgs.msg import LeaseArray, LeaseResource
from spot_msgs.msg import FootState, FootStateArray
from spot_msgs.msg import EStopState, EStopStateArray
from spot_msgs.msg import WiFiState
from spot_msgs.msg import PowerState
from spot_msgs.msg import BehaviorFault, BehaviorFaultState
from spot_msgs.msg import SystemFault, SystemFaultState
from spot_msgs.msg import BatteryState, BatteryStateArray
from spot_msgs.msg import DockState
from bosdyn.api import image_pb2, point_cloud_pb2
from bosdyn.client.math_helpers import SE3Pose
from bosdyn.client.frame_helpers import get_odom_tform_body, get_vision_tform_body
import numpy as np
friendly_joint_names = {}
"""Dictionary for mapping BD joint names to more friendly names"""
friendly_joint_names["fl.hx"] = "front_left_hip_x"
friendly_joint_names["fl.hy"] = "front_left_hip_y"
friendly_joint_names["fl.kn"] = "front_left_knee"
friendly_joint_names["fr.hx"] = "front_right_hip_x"
friendly_joint_names["fr.hy"] = "front_right_hip_y"
friendly_joint_names["fr.kn"] = "front_right_knee"
friendly_joint_names["hl.hx"] = "rear_left_hip_x"
friendly_joint_names["hl.hy"] = "rear_left_hip_y"
friendly_joint_names["hl.kn"] = "rear_left_knee"
friendly_joint_names["hr.hx"] = "rear_right_hip_x"
friendly_joint_names["hr.hy"] = "rear_right_hip_y"
friendly_joint_names["hr.kn"] = "rear_right_knee"
# arm joints
friendly_joint_names["arm0.sh0"] = "arm_joint1"
friendly_joint_names["arm0.sh1"] = "arm_joint2"
friendly_joint_names["arm0.el0"] = "arm_joint3"
friendly_joint_names["arm0.el1"] = "arm_joint4"
friendly_joint_names["arm0.wr0"] = "arm_joint5"
friendly_joint_names["arm0.wr1"] = "arm_joint6"
friendly_joint_names["arm0.f1x"] = "arm_gripper"
class DefaultCameraInfo(CameraInfo):
"""Blank class extending CameraInfo ROS topic that defaults most parameters"""
def __init__(self):
super().__init__()
self.distortion_model = "plumb_bob"
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.K[1] = 0
self.K[3] = 0
self.K[6] = 0
self.K[7] = 0
self.K[8] = 1
self.R[0] = 1
self.R[1] = 0
self.R[2] = 0
self.R[3] = 0
self.R[4] = 1
self.R[5] = 0
self.R[6] = 0
self.R[7] = 0
self.R[8] = 1
self.P[1] = 0
self.P[3] = 0
self.P[4] = 0
self.P[7] = 0
self.P[8] = 0
self.P[9] = 0
self.P[10] = 1
self.P[11] = 0
def populateTransformStamped(time, parent_frame, child_frame, transform):
"""Populates a TransformStamped message
Args:
time: The time of the transform
parent_frame: The parent frame of the transform
child_frame: The child_frame_id of the transform
transform: A transform to copy into a StampedTransform object. Should have position (x,y,z) and rotation (x,
y,z,w) members
Returns:
TransformStamped message. Empty if transform does not have position or translation attribute
"""
if hasattr(transform, "position"):
position = transform.position
elif hasattr(transform, "translation"):
position = transform.translation
else:
rospy.logerr(
"Trying to generate StampedTransform but input transform has neither position nor translation "
"attributes"
)
return TransformStamped()
new_tf = TransformStamped()
new_tf.header.stamp = time
new_tf.header.frame_id = parent_frame
new_tf.child_frame_id = child_frame
new_tf.transform.translation.x = position.x
new_tf.transform.translation.y = position.y
new_tf.transform.translation.z = position.z
new_tf.transform.rotation.x = transform.rotation.x
new_tf.transform.rotation.y = transform.rotation.y
new_tf.transform.rotation.z = transform.rotation.z
new_tf.transform.rotation.w = transform.rotation.w
return new_tf
def getImageMsg(data, spot_wrapper):
"""Takes the imag and camera data and populates the necessary ROS messages
Args:
data: Image proto
spot_wrapper: A SpotWrapper object
Returns:
(tuple):
* Image: message of the image captured
* CameraInfo: message to define the state and config of the camera that took the image
"""
image_msg = Image()
local_time = spot_wrapper.robotToLocalTime(data.shot.acquisition_time)
image_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
image_msg.header.frame_id = data.shot.frame_name_image_sensor
image_msg.height = data.shot.image.rows
image_msg.width = data.shot.image.cols
# Color/greyscale formats.
# JPEG format
if data.shot.image.format == image_pb2.Image.FORMAT_JPEG:
image_msg.encoding = "rgb8"
image_msg.is_bigendian = True
image_msg.step = 3 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Uncompressed. Requires pixel_format.
if data.shot.image.format == image_pb2.Image.FORMAT_RAW:
# One byte per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_GREYSCALE_U8:
image_msg.encoding = "mono8"
image_msg.is_bigendian = True
image_msg.step = data.shot.image.cols
image_msg.data = data.shot.image.data
# Three bytes per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_RGB_U8:
image_msg.encoding = "rgb8"
image_msg.is_bigendian = True
image_msg.step = 3 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Four bytes per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_RGBA_U8:
image_msg.encoding = "rgba8"
image_msg.is_bigendian = True
image_msg.step = 4 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Little-endian uint16 z-distance from camera (mm).
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_DEPTH_U16:
image_msg.encoding = "16UC1"
image_msg.is_bigendian = False
image_msg.step = 2 * data.shot.image.cols
image_msg.data = data.shot.image.data
camera_info_msg = DefaultCameraInfo()
local_time = spot_wrapper.robotToLocalTime(data.shot.acquisition_time)
camera_info_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
camera_info_msg.header.frame_id = data.shot.frame_name_image_sensor
camera_info_msg.height = data.shot.image.rows
camera_info_msg.width = data.shot.image.cols
camera_info_msg.K[0] = data.source.pinhole.intrinsics.focal_length.x
camera_info_msg.K[2] = data.source.pinhole.intrinsics.principal_point.x
camera_info_msg.K[4] = data.source.pinhole.intrinsics.focal_length.y
camera_info_msg.K[5] = data.source.pinhole.intrinsics.principal_point.y
camera_info_msg.P[0] = data.source.pinhole.intrinsics.focal_length.x
camera_info_msg.P[2] = data.source.pinhole.intrinsics.principal_point.x
camera_info_msg.P[5] = data.source.pinhole.intrinsics.focal_length.y
camera_info_msg.P[6] = data.source.pinhole.intrinsics.principal_point.y
return image_msg, camera_info_msg
def GetPointCloudMsg(data, spot_wrapper):
"""Takes the imag and camera data and populates the necessary ROS messages
Args:
data: PointCloud proto (PointCloudResponse)
spot_wrapper: A SpotWrapper object
Returns:
PointCloud: message of the point cloud (PointCloud2)
"""
point_cloud_msg = PointCloud2()
local_time = spot_wrapper.robotToLocalTime(data.point_cloud.source.acquisition_time)
point_cloud_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
point_cloud_msg.header.frame_id = data.point_cloud.source.frame_name_sensor
if data.point_cloud.encoding == point_cloud_pb2.PointCloud.ENCODING_XYZ_32F:
point_cloud_msg.height = 1
point_cloud_msg.width = data.point_cloud.num_points
point_cloud_msg.fields = []
for i, ax in enumerate(("x", "y", "z")):
field = PointField()
field.name = ax
field.offset = i * 4
field.datatype = PointField.FLOAT32
field.count = 1
point_cloud_msg.fields.append(field)
point_cloud_msg.is_bigendian = False
point_cloud_np = np.frombuffer(data.point_cloud.data, dtype=np.uint8)
point_cloud_msg.point_step = 12 # float32 XYZ
point_cloud_msg.row_step = point_cloud_msg.width * point_cloud_msg.point_step
point_cloud_msg.data = point_cloud_np.tobytes()
point_cloud_msg.is_dense = True
else:
rospy.logwarn("Not supported point cloud data type.")
return point_cloud_msg
def GetJointStatesFromState(state, spot_wrapper):
"""Maps joint state data from robot state proto to ROS JointState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
JointState message
"""
joint_state = JointState()
local_time = spot_wrapper.robotToLocalTime(
state.kinematic_state.acquisition_timestamp
)
joint_state.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
for joint in state.kinematic_state.joint_states:
# there is a joint with name arm0.hr0 in the robot state, however this
# joint has no data and should not be there, this is why we ignore it
if joint.name == "arm0.hr0":
continue
joint_state.name.append(friendly_joint_names.get(joint.name, "ERROR"))
joint_state.position.append(joint.position.value)
joint_state.velocity.append(joint.velocity.value)
joint_state.effort.append(joint.load.value)
return joint_state
def GetEStopStateFromState(state, spot_wrapper):
"""Maps eStop state data from robot state proto to ROS EStopArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
EStopArray message
"""
estop_array_msg = EStopStateArray()
for estop in state.estop_states:
estop_msg = EStopState()
local_time = spot_wrapper.robotToLocalTime(estop.timestamp)
estop_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
estop_msg.name = estop.name
estop_msg.type = estop.type
estop_msg.state = estop.state
estop_msg.state_description = estop.state_description
estop_array_msg.estop_states.append(estop_msg)
return estop_array_msg
def GetFeetFromState(state, spot_wrapper):
"""Maps foot position state data from robot state proto to ROS FootStateArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
FootStateArray message
"""
foot_array_msg = FootStateArray()
for foot in state.foot_state:
foot_msg = FootState()
foot_msg.foot_position_rt_body.x = foot.foot_position_rt_body.x
foot_msg.foot_position_rt_body.y = foot.foot_position_rt_body.y
foot_msg.foot_position_rt_body.z = foot.foot_position_rt_body.z
foot_msg.contact = foot.contact
if foot.HasField("terrain"):
terrain = foot.terrain
foot_msg.terrain.ground_mu_est = terrain.ground_mu_est
foot_msg.terrain.frame_name = terrain.frame_name
foot_msg.terrain.foot_slip_distance_rt_frame = (
terrain.foot_slip_distance_rt_frame
)
foot_msg.terrain.foot_slip_velocity_rt_frame = (
terrain.foot_slip_velocity_rt_frame
)
foot_msg.terrain.ground_contact_normal_rt_frame = (
terrain.ground_contact_normal_rt_frame
)
foot_msg.terrain.visual_surface_ground_penetration_mean = (
terrain.visual_surface_ground_penetration_mean
)
foot_msg.terrain.visual_surface_ground_penetration_std = (
terrain.visual_surface_ground_penetration_std
)
foot_array_msg.states.append(foot_msg)
return foot_array_msg
def GetOdomTwistFromState(state, spot_wrapper):
"""Maps odometry data from robot state proto to ROS TwistWithCovarianceStamped message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
TwistWithCovarianceStamped message
"""
twist_odom_msg = TwistWithCovarianceStamped()
local_time = spot_wrapper.robotToLocalTime(
state.kinematic_state.acquisition_timestamp
)
twist_odom_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
twist_odom_msg.twist.twist.linear.x = (
state.kinematic_state.velocity_of_body_in_odom.linear.x
)
twist_odom_msg.twist.twist.linear.y = (
state.kinematic_state.velocity_of_body_in_odom.linear.y
)
twist_odom_msg.twist.twist.linear.z = (
state.kinematic_state.velocity_of_body_in_odom.linear.z
)
twist_odom_msg.twist.twist.angular.x = (
state.kinematic_state.velocity_of_body_in_odom.angular.x
)
twist_odom_msg.twist.twist.angular.y = (
state.kinematic_state.velocity_of_body_in_odom.angular.y
)
twist_odom_msg.twist.twist.angular.z = (
state.kinematic_state.velocity_of_body_in_odom.angular.z
)
return twist_odom_msg
def get_corrected_odom(base_odometry: Odometry):
"""
Get odometry from state but correct the twist portion of the message to be in the child frame id rather than the
odom/vision frame. https://dev.bostondynamics.com/protos/bosdyn/api/proto_reference#kinematicstate indicates the
twist in the state is in the odom frame and not the body frame, as is expected by many ROS components.
Conversion of https://github.com/tpet/nav_utils/blob/master/src/nav_utils/odom_twist_to_child_frame.cpp
Args:
base_odometry: Uncorrected odometry message
Returns:
Odometry with twist in the body frame
"""
# Note: transforms3d has quaternions in wxyz, not xyzw like ros.
# Get the transform from body to odom/vision so we have the inverse transform, which we will use to correct the
# twist. We don't actually care about the translation at any point since we're just rotating the twist vectors
inverse_rotation = transforms3d.quaternions.quat2mat(
transforms3d.quaternions.qinverse(
[
base_odometry.pose.pose.orientation.w,
base_odometry.pose.pose.orientation.x,
base_odometry.pose.pose.orientation.y,
base_odometry.pose.pose.orientation.z,
]
)
)
# transform the linear twist by rotating the vector according to the rotation from body to odom
linear_twist = np.array(
[
[base_odometry.twist.twist.linear.x],
[base_odometry.twist.twist.linear.y],
[base_odometry.twist.twist.linear.z],
]
)
corrected_linear = inverse_rotation.dot(linear_twist)
# Do the same for the angular twist
angular_twist = np.array(
[
[base_odometry.twist.twist.angular.x],
[base_odometry.twist.twist.angular.y],
[base_odometry.twist.twist.angular.z],
]
)
corrected_angular = inverse_rotation.dot(angular_twist)
corrected_odometry = copy.deepcopy(base_odometry)
corrected_odometry.twist.twist.linear.x = corrected_linear[0][0]
corrected_odometry.twist.twist.linear.y = corrected_linear[1][0]
corrected_odometry.twist.twist.linear.z = corrected_linear[2][0]
corrected_odometry.twist.twist.angular.x = corrected_angular[0][0]
corrected_odometry.twist.twist.angular.y = corrected_angular[1][0]
corrected_odometry.twist.twist.angular.z = corrected_angular[2][0]
return corrected_odometry
def GetOdomFromState(state, spot_wrapper, use_vision=True):
"""Maps odometry data from robot state proto to ROS Odometry message
WARNING: The odometry twist from this message is in the odom frame and not in the body frame. This will likely
cause issues. You should use the odometry_corrected topic instead
Args:
state: Robot State proto
spot_wrapper: A SpotWrapper object
use_vision: If true, the odometry frame will be vision rather than odom
Returns:
Odometry message
"""
odom_msg = Odometry()
local_time = spot_wrapper.robotToLocalTime(
state.kinematic_state.acquisition_timestamp
)
odom_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
if use_vision == True:
odom_msg.header.frame_id = "vision"
tform_body = get_vision_tform_body(state.kinematic_state.transforms_snapshot)
else:
odom_msg.header.frame_id = "odom"
tform_body = get_odom_tform_body(state.kinematic_state.transforms_snapshot)
odom_msg.child_frame_id = "body"
pose_odom_msg = PoseWithCovariance()
pose_odom_msg.pose.position.x = tform_body.position.x
pose_odom_msg.pose.position.y = tform_body.position.y
pose_odom_msg.pose.position.z = tform_body.position.z
pose_odom_msg.pose.orientation.x = tform_body.rotation.x
pose_odom_msg.pose.orientation.y = tform_body.rotation.y
pose_odom_msg.pose.orientation.z = tform_body.rotation.z
pose_odom_msg.pose.orientation.w = tform_body.rotation.w
odom_msg.pose = pose_odom_msg
twist_odom_msg = GetOdomTwistFromState(state, spot_wrapper).twist
odom_msg.twist = twist_odom_msg
return odom_msg
def GetWifiFromState(state, spot_wrapper):
"""Maps wireless state data from robot state proto to ROS WiFiState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
WiFiState message
"""
wifi_msg = WiFiState()
for comm_state in state.comms_states:
if comm_state.HasField("wifi_state"):
wifi_msg.current_mode = comm_state.wifi_state.current_mode
wifi_msg.essid = comm_state.wifi_state.essid
return wifi_msg
def generate_feet_tf(foot_states_msg):
"""
Generate a tf message containing information about foot states
Args:
foot_states_msg: FootStateArray message containing the foot states from the robot state
Returns: tf message with foot states
"""
foot_ordering = ["front_left", "front_right", "rear_left", "rear_right"]
foot_tfs = TFMessage()
time_now = rospy.Time.now()
for idx, foot_state in enumerate(foot_states_msg.states):
foot_transform = Transform()
# Rotation of the foot is not given
foot_transform.rotation.w = 1
foot_transform.translation.x = foot_state.foot_position_rt_body.x
foot_transform.translation.y = foot_state.foot_position_rt_body.y
foot_transform.translation.z = foot_state.foot_position_rt_body.z
foot_tfs.transforms.append(
populateTransformStamped(
time_now, "body", foot_ordering[idx] + "_foot", foot_transform
)
)
return foot_tfs
def GetTFFromState(state, spot_wrapper, inverse_target_frame):
"""Maps robot link state data from robot state proto to ROS TFMessage message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
inverse_target_frame: A frame name to be inversed to a parent frame.
Returns:
TFMessage message
"""
tf_msg = TFMessage()
for (
frame_name
) in state.kinematic_state.transforms_snapshot.child_to_parent_edge_map:
if state.kinematic_state.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
).parent_frame_name:
try:
transform = state.kinematic_state.transforms_snapshot.child_to_parent_edge_map.get(
frame_name
)
local_time = spot_wrapper.robotToLocalTime(
state.kinematic_state.acquisition_timestamp
)
tf_time = rospy.Time(local_time.seconds, local_time.nanos)
if inverse_target_frame == frame_name:
geo_tform_inversed = SE3Pose.from_obj(
transform.parent_tform_child
).inverse()
new_tf = populateTransformStamped(
tf_time,
frame_name,
transform.parent_frame_name,
geo_tform_inversed,
)
else:
new_tf = populateTransformStamped(
tf_time,
transform.parent_frame_name,
frame_name,
transform.parent_tform_child,
)
tf_msg.transforms.append(new_tf)
except Exception as e:
spot_wrapper.logger.error("Error: {}".format(e))
return tf_msg
def GetBatteryStatesFromState(state, spot_wrapper):
"""Maps battery state data from robot state proto to ROS BatteryStateArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
BatteryStateArray message
"""
battery_states_array_msg = BatteryStateArray()
for battery in state.battery_states:
battery_msg = BatteryState()
local_time = spot_wrapper.robotToLocalTime(battery.timestamp)
battery_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
battery_msg.identifier = battery.identifier
battery_msg.charge_percentage = battery.charge_percentage.value
battery_msg.estimated_runtime = rospy.Time(
battery.estimated_runtime.seconds, battery.estimated_runtime.nanos
)
battery_msg.current = battery.current.value
battery_msg.voltage = battery.voltage.value
for temp in battery.temperatures:
battery_msg.temperatures.append(temp)
battery_msg.status = battery.status
battery_states_array_msg.battery_states.append(battery_msg)
return battery_states_array_msg
def GetPowerStatesFromState(state, spot_wrapper):
"""Maps power state data from robot state proto to ROS PowerState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
PowerState message
"""
power_state_msg = PowerState()
local_time = spot_wrapper.robotToLocalTime(state.power_state.timestamp)
power_state_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
power_state_msg.motor_power_state = state.power_state.motor_power_state
power_state_msg.shore_power_state = state.power_state.shore_power_state
power_state_msg.locomotion_charge_percentage = (
state.power_state.locomotion_charge_percentage.value
)
power_state_msg.locomotion_estimated_runtime = rospy.Time(
state.power_state.locomotion_estimated_runtime.seconds,
state.power_state.locomotion_estimated_runtime.nanos,
)
return power_state_msg
def GetDockStatesFromState(state):
"""Maps dock state data from robot state proto to ROS DockState message
Args:
state: Robot State proto
Returns:
DockState message
"""
dock_state_msg = DockState()
dock_state_msg.status = state.status
dock_state_msg.dock_type = state.dock_type
dock_state_msg.dock_id = state.dock_id
dock_state_msg.power_status = state.power_status
return dock_state_msg
def getBehaviorFaults(behavior_faults, spot_wrapper):
"""Helper function to strip out behavior faults into a list
Args:
behavior_faults: List of BehaviorFaults
spot_wrapper: A SpotWrapper object
Returns:
List of BehaviorFault messages
"""
faults = []
for fault in behavior_faults:
new_fault = BehaviorFault()
new_fault.behavior_fault_id = fault.behavior_fault_id
local_time = spot_wrapper.robotToLocalTime(fault.onset_timestamp)
new_fault.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_fault.cause = fault.cause
new_fault.status = fault.status
faults.append(new_fault)
return faults
def getSystemFaults(system_faults, spot_wrapper):
"""Helper function to strip out system faults into a list
Args:
systen_faults: List of SystemFaults
spot_wrapper: A SpotWrapper object
Returns:
List of SystemFault messages
"""
faults = []
for fault in system_faults:
new_fault = SystemFault()
new_fault.name = fault.name
local_time = spot_wrapper.robotToLocalTime(fault.onset_timestamp)
new_fault.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_fault.duration = rospy.Time(fault.duration.seconds, fault.duration.nanos)
new_fault.code = fault.code
new_fault.uid = fault.uid
new_fault.error_message = fault.error_message
for att in fault.attributes:
new_fault.attributes.append(att)
new_fault.severity = fault.severity
faults.append(new_fault)
return faults
def GetSystemFaultsFromState(state, spot_wrapper):
"""Maps system fault data from robot state proto to ROS SystemFaultState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
SystemFaultState message
"""
system_fault_state_msg = SystemFaultState()
system_fault_state_msg.faults = getSystemFaults(
state.system_fault_state.faults, spot_wrapper
)
system_fault_state_msg.historical_faults = getSystemFaults(
state.system_fault_state.historical_faults, spot_wrapper
)
return system_fault_state_msg
def getBehaviorFaultsFromState(state, spot_wrapper):
"""Maps behavior fault data from robot state proto to ROS BehaviorFaultState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
BehaviorFaultState message
"""
behavior_fault_state_msg = BehaviorFaultState()
behavior_fault_state_msg.faults = getBehaviorFaults(
state.behavior_fault_state.faults, spot_wrapper
)
return behavior_fault_state_msg
| 26,982 | Python | 35.963014 | 116 | 0.662812 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/config/planar.yaml | linear_scale:
x: 1
y: 1
max_positive_linear_velocity:
x: 1
y: 1
max_negative_linear_velocity:
x: -1
y: -1
| 118 | YAML | 10.899999 | 29 | 0.618644 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/config/twist_mux.yaml | topics:
- name : bt_joy
topic : bluetooth_teleop/cmd_vel
timeout : 0.5
priority: 9
- name : interactive_marker
topic : twist_marker_server/cmd_vel
timeout : 0.5
priority: 8
- name : external
topic : cmd_vel
timeout : 0.5
priority: 1
locks: []
| 276 | YAML | 17.466666 | 39 | 0.623188 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/config/teleop.yaml | # Teleop configuration for PS4 joystick using the x-pad configuration.
# Left thumb-stick up/down/left/right for forward/backward/left/right translation
# Right thumb-stick left/right for twist
# Left shoulder button for enable
# Right shoulder button for enable-turbo
#
# L1 R1
# L2 R2
# _=====_ _=====_
# / _____ \ / _____ \
# +.-'_____'-.------------------------------.-'_____'-.+
# / | | '. S O N Y .' | _ | \
# / ___| /|\ |___ \ / ___| /_\ |___ \ (Y)
# / | | | ; ; | _ _ ||
# | | <--- ---> | | | ||_| (_)|| (X) (B)
# | |___ | ___| ; ; |___ ___||
# |\ | \|/ | / _ ____ _ \ | (X) | /| (A)
# | \ |_____| .','" "', (_PS_) ,'" "', '. |_____| .' |
# | '-.______.-' / \ / \ '-._____.-' |
# | | LJ |--------| RJ | |
# | /\ / \ /\ |
# | / '.___.' '.___.' \ |
# | / \ |
# \ / \ /
# \________/ \_________/
#
# ^ x
# |
# |
# y <-----+ Accelerometer axes
# \
# \
# > z (out)
#
# BUTTON Value
# L1 4
# L2 6
# R1 5
# R2 7
# A 1
# B 2
# X 0
# Y 3
#
# AXIS Value
# Left Horiz. 0
# Left Vert. 1
# Right Horiz. 3
# Right Vert. 4
# L2 2
# R2 5
# D-pad Horiz. 9
# D-pad Vert. 10
# Accel x 7
# Accel y 6
# Accel z 8
teleop_twist_joy:
axis_linear:
x: 1
y: 0
scale_linear:
x: 0.4
y: 0.4
scale_linear_turbo:
x: 2.0 # TODO: should this be 1.0?
y: 2.0 # TODO: should this be 1.0?
axis_angular:
yaw: 3
scale_angular:
yaw: 1.4 # TODO: should this be 1.0?
scale_angular_turbo:
yaw: 1.4 # TODO: should this be 1.0?
enable_button: 4
enable_turbo_button: 5
joy_node:
deadzone: 0.1 # TODO: should this be 0.02?
autorepeat_rate: 20
| 2,494 | YAML | 30.582278 | 81 | 0.267041 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/spot_driver/config/spot_ros.yaml | rates:
# loop_frequency is the frequency of the loop which publishes messages
loop_frequency: 50.0
# These are desired frequencies. You should not expect to receive messages at exactly the specified frequency. For
# higher frequencies, you will need to modify the loop frequency and the rates below.
robot_state: 20.0
metrics: 0.04
lease: 1.0
front_image: 10.0
side_image: 10.0
rear_image: 10.0
point_cloud: 10.0
hand_image: 10.0
feedback: 10.0
mobility_params: 10.0
check_subscribers: 10.0
# If true, automatically claim the lease when the driver starts
auto_claim: False
# If true, automatically power on when the driver starts (requires auto_claim)
auto_power_on: False
# If true, automatically stand when the driver starts (requires auto_power_on)
auto_stand: False
claim:
# If true, forcefully take the lease, instead of acquiring it, which can fail if someone else has it
use_take_lease: False
# If true, try and claim the lease when doing actions if we don't already have it. Also automatically powers on for
# stand, rollover and self-right commands
get_lease_on_action: False
| 1,127 | YAML | 35.387096 | 117 | 0.748004 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/ros_usage.rst | Basic Driver Usage
==================
.. warning::
Before doing anything with the robot, you should read the Boston Dynamics `safety guidelines <https://d3cjkvgbik1jtv.cloudfront.net/Spot%20IFU/spot_information_for_use_EN_v1.1.pdf>`_.
.. note::
Details of how the robot functions can be found in the `Boston Dynamics SDK documentation <https://dev.bostondynamics.com>`_.
Verify the URDF
---------------
To verify that the spot_viz and spot_description packages are sourced properly, you can run
.. code:: bash
roslaunch spot_viz view_model.launch
This will load RVIZ with the robot description visible. It should also show any additions you may have added to the robot's URDF
.. image:: images/view_model.png
Configure your networking
-------------------------
If your ROS machine is attached to a payload port you will need to configure it so that it can connect to spot. See `payload network configuration <https://support.bostondynamics.com/s/article/Payload-device-network-configuration>`_ for more details.
Running the driver
------------------
.. code:: bash
roslaunch spot_driver driver.launch
When launching, there are some options you may need to configure for your setup:
+----------------------+---------------+-------------------------------------------------------------------+
|Argument |Default |Description |
+======================+===============+===================================================================+
|username |dummyusername |Use this username to authenticate with spot |
+----------------------+---------------+-------------------------------------------------------------------+
|password |dummypassword |Use this password to authenticate with spot |
+----------------------+---------------+-------------------------------------------------------------------+
|hostname |192.168.50.3 |The IP address at which spot can be found |
+----------------------+---------------+-------------------------------------------------------------------+
|estop_timeout |9.0 |Time allowed before rpc commands will time out. Comms between the |
| | |robot and driver are checked at a rate of 1/3 of this time. If |
| | |comms are down, a gentle estop will trigger |
+----------------------+---------------+-------------------------------------------------------------------+
|autonomy_enabled |True |If false, some autonomy commands will be disabled for this run of |
| | |the driver. This can only be changed on driver restart |
| | | |
+----------------------+---------------+-------------------------------------------------------------------+
|max_linear_velocity_x |0 |Limit on linear velocity for moving forwards. 0 is whatever is |
| | |specified by BD. |
| | | |
+----------------------+---------------+-------------------------------------------------------------------+
|max_linear_velocity_y |0 |Limit on linear velocity for moving sideways. 0 is whatever is |
| | |specified by BD. |
| | | |
+----------------------+---------------+-------------------------------------------------------------------+
|max_linear_velocity_z |0 |Limit on angular velocity for rotation. 0 is whatever is specified |
| | |by BD. |
| | | |
+----------------------+---------------+-------------------------------------------------------------------+
|allow_motion |True |If false, the robot starts with all motion disallowed and must have|
| | |it enabled by calling a service or pressing a button in rviz |
| | | |
+----------------------+---------------+-------------------------------------------------------------------+
View the robot
--------------
Once the robot is connected, you should be able to visualize its odometry, joint positions, camera streams, etc.
using rviz. To view these streams, run
.. code:: bash
roslaunch spot_viz view_robot.launch
Once RVIZ is loaded, you should see something similar to this.
.. image:: images/view_robot.png
Taking control of the robot
---------------------------
The easiest way to control the robot is through the rviz control panel, which connects to the various ROS services.
Many commands which can be executed using a service or topic can also be executed using the panel. To add the panel
to rviz go to Panels>Add new panel and the select ``SpotControlPanel`` under ``spot_viz``. It is included by default
in the ``view_robot`` launch file.
.. image:: images/rviz_panel.png
Holding the lease
~~~~~~~~~~~~~~~~~
A body lease gives the holder the ability to command the spot to make actions in the world.
There are two ways to claim a body lease and eStop using this driver.
#. Automatically when the driver starts by enabling the ``auto_claim`` variable
#. By calling the ``/spot/claim`` service after the driver is started
You cannot claim a lease on the robot if something else already has a lease. This is for security and safety reasons.
Check the``status/leases`` topic for information on current leases.
You can release the lease by calling the ``/spot/release`` service.
.. warning::
Releasing the lease while the robot is standing will trigger a gentle estop.
Holding a lease automatically establishes an estop connection to the robot.
All buttons except the claim lease button on the rviz panel are disabled if the ROS driver does not hold the lease.
Emergency stops
~~~~~~~~~~~~~~~
The estop connection gives the robot a way to guarantee that the commanding system is maintaining contact with the
robot. If this contact is lost, the robot will perform a gentle stop. The gentle and hard estops are calling through
to the BD SDK.
The gentle stop is at ``/spot/estop/gentle``. It stops all motion of the robot and commands it to sit. This stop does
not have to be released.
.. warning::
The hard estop immediately cuts power to the motors. The robot may collapse in an uncontrolled manner.
The hard estop is at ``/spot/estop/hard`` this will kill power to the motors and must be released before you can send
any commands to the robot. To release the estop, call ``/spot/estop/release``.
There is an additional service ``/spot/stop`` which is implemented by the driver. This service stops any command that
is currently being executed. The ``/spot/locked_stop`` stops the current command and also disallows any further motion
of the robot. Motion can be allowed again by calling the ``/spot/allow_motion`` service.
The top of the rviz panel has buttons to control the estops as described above, and also displays their status. It
also shows whether the robot is allowed to move or not.
.. image:: images/rviz_estops.png
Enable motor power
~~~~~~~~~~~~~~~~~~
Motor power needs to be enabled once you have a Lease on the body. This can be done in two ways:
#. Automatically when the driver starts by enabling the ``auto_power_on`` variable
#. By calling the ``/spot/power_on`` service after the driver is started
Stand the robot up
~~~~~~~~~~~~~~~~~~
Once the motors are powered, stand the robot up so it is able to move through the world. This can be done in two ways:
#. Automatically when the driver starts by enabling the ``auto_stand`` variable
#. By calling the ``/spot/stand`` service after the driver is started
Controlling the velocity
------------------------
.. warning::
Spot will attempt to avoid any obstacles it detects with its cameras, but no vision or actuation system is perfect. Make sure to only move the robot in a safe area and in a controlled manner.
Topic
~~~~~~~~~~
To control Spot, you can send a Twist message to command a velocity.
.. code:: bash
rostopic pub /spot/cmd_vel geometry_msgs/Twist "linear:
x: 0.0
y: 0.0
z: 0.0
angular:
x: 0.0
y: 0.0
z: 0.3" -r 10
That command will have spot rotate on the spot at 0.3 radians/second. Note the -r at the end of the command. That has ROS resend the message over again. If you don't resend the message, the driver will assume a timeout and stop commanding motion of the robot.
Controlling the body
--------------------
There are two different ways of specifying the pose of the body.
Static body pose
~~~~~~~~~~~~~~~~
The static body pose changes the body position only when the robot is stationary. Any other motion will reset the
body to its normal pose. It can adjust the roll, pitch, yaw, and body height.
Service
^^^^^^^
The body can be posed by a service call to ``/spot/posed_stand``.
If using a pose message, you can control the body height by setting the z component of position. The x and y
components of position are ignored. If the pose message is non-zero, any roll/pitch/yaw specification will be ignored.
If using the roll/pitch/yaw specification, enter values in degrees, and body height in metres. Body height is based
on displacement from the neutral position.
If you send an empty message, the body pose will be reset to neutral.
Actionserver
^^^^^^^^^^^^
The actionserver ``/spot/body_pose`` can be called to set the body pose.
To test this, start an action client with
.. code:: bash
rosrun actionlib_tools axclient.py /spot/body_pose
You will see a window pop up, and you can specify the body pose with a Pose message, or by specifying roll, pitch and
yaw, and a body height.
Here is what the axclient window will look like:
.. code:: yaml
body_pose:
position:
x: 0.0
y: 0.0
z: 0.0
orientation:
x: 0.0
y: 0.0
z: 0.0
w: 0.0
roll: 0
pitch: 0
yaw: 0
body_height: 0.0
Rviz
^^^^
The static pose can also be controlled in the body tab.
.. image:: images/rviz_body_tab.png
In-motion body pose
~~~~~~~~~~~~~~~~~~~
.. warning::
The in-motion body pose can affect the stability of the robot while walking.
It is also possible to specify a pose the robot should hold while it is in motion. This is a more restricted version
of the static body pose and cannot achieve the same body heights or rotational changes. While idle, all the
parameters specified will be held. The pitch and body height are maintained throughout any walking motion
the robot makes. When the robot stops walking, it will return to the pose specified by all parameters.
Topic
^^^^^
This pose can be set through the ``/spot/in_motion_or_idle_body_pose`` topic
.. code:: bash
rostopic pub /spot/in_motion_or_idle_body_pose geometry_msgs/Pose "position:
x: 0.0
y: 0.0
z: 0.0
orientation:
x: 0.0
y: 0.0
z: 0.0
w: 1.0"
Actionserver
^^^^^^^^^^^^
The actionserver is found at ``/spot/motion_or_idle_body_pose`` and has the same parameters as the static pose.
Moving to a pose
----------------
.. warning::
Take into account the Boston Dynamics `safety guidelines <https://d3cjkvgbik1jtv.cloudfront.net/Spot%20IFU/spot_information_for_use_EN_v1.1.pdf>`_ when moving the robot. The robot will attempt to avoid obstacles but will also walk over any uneven terrain, regardless of what that terrain is made up of. You should operate in a controlled environment.
One of the most important commands for easily controlling the robot is the trajectory command. This command allows
you to move the robot to a position in space by specifying a pose relative to the robot's current position. You may
specify a pose in a different frame than the ``body`` frame and it will be transformed into that frame.
ROS Topic
~~~~~~~~~
The ``/spot/go_to_pose`` topic can be used to move the robot by specifying a pose.
The following command will move the robot one metre forwards from its current location.
.. code:: bash
rostopic pub /spot/go_to_pose geometry_msgs/PoseStamped "header:
seq: 0
stamp:
secs: 0
nsecs: 0
frame_id: 'body'
pose:
position:
x: 1
y: 0.0
z: 0.0
orientation:
x: 0.0
y: 0.0
z: 0.0
w: 1"
Actionserver
~~~~~~~~~~~~
The ``/spot/trajectory`` actionserver gives you a little more control than the ros topic, and will also give you
information about success or failure.
.. warning::
If there is an obstacle along the trajectory the robot is trying to move along, it may fail as the trajectory command
is different to the command that is used by the controller. In this case, the actionserver will return success
despite not actually reaching the requested pose.
As of 2021/09/10 the boston dynamics API does not appear to provide
feedback which we can use to return failure when this happens.
In addition to the pose, you can specify ``duration``, which specifies how long the command can run before timing out.
The ``precise_positioning`` variable can be used to request that the robot move more precisely to the specified pose.
If set to false, the robot will move to "near" the specified pose. It's not clear what exactly defines being "near"
to the pose, but you should not expect to reach the pose precisely. The robot will end up within ~0.5m of the pose,
and not make much effort to align to the orientation.
You can test the actionserver by using an action client
.. code:: bash
rosrun actionlib_tools axclient /spot/trajectory
And fill in the values as you like.
Rviz
~~~~
You can connect the 2d nav goal tool to publish to the ``/spot/go_to_pose`` topic. The default rviz config provided with
.. code:: bash
roslaunch spot_viz view_robot.launch
Already has the tool configured, but you can also do this by right clicking the toolbar, selecting tool properties,
then changing the nav goal topic to ``/spot/go_to_pose``.
Setting velocity limits
-----------------------
You can set a velocity limit in m/s for the motion to poses using the ``/spot/velocity_limit`` service:
.. code:: bash
rosservice call /spot/velocity_limit "velocity_limit:
linear:
x: 0.0
y: 0.0
z: 0.0
angular:
x: 0.0
y: 0.0
z: 0.0"
Only the x and y components of linear velocity are considered, and the z component of angular.
You can adjust the velocity limits on startup by setting the max linear/angular velocity params.
You can also set the limits using the rviz panel.
.. image:: images/rviz_motion_tab.png
Cameras and Depth Clouds
------------------------
Spot is equipped 5 RGB and depth-sensing cameras: 2 on the front, one on each side, and one in the rear. All of these
cameras publish at approximately 10Hz.
Note that the front cameras are mounted sideways, so they have a narrower horizontal FoV, but a larger vertical one.
The camera data likewise rotated anticlockwise by 90 degrees.
The ``frontleft`` camera and depth topics are from the camera physically located on the front-left of the robot.
This camera is pointed to the robot's right, so the depth cloud will appear in front of the robot's right shoulder:
.. image:: images/front-left-depth.png
Similarly the ``frontright`` camera and depth topics are from the camera physically located on the front-right of the
robot. This camera points to the robot's left, so the depth cloud will appear in front of the robot's left shoulder:
.. image:: images/front-right-depth.png
The complete list of depth and camera topics is below:
+--------------------------------+----------------------------+-------------------------------------------------------+
| Topic | Type | Comments |
+================================+============================+=======================================================+
| camera/frontleft/camera/image | Image | Data from the front-left camera, which points to the |
| | | right. Image is rotated 90 degrees anticlockwise. |
+--------------------------------+----------------------------+-------------------------------------------------------+
| camera/frontright/camera/image | Image | Data from the front-right camera, which points to the |
| | | left. Image is rotated 90 degrees anticlockwise. |
+--------------------------------+----------------------------+-------------------------------------------------------+
| camera/left/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
| camera/right/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
| camera/back/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
| depth/frontleft/camera/image | Image | Data from the front-left camera, which points to the |
| | | right. |
+--------------------------------+----------------------------+-------------------------------------------------------+
| depth/frontright/camera/image | Image | Data from the front-right camera, which points to the |
| | | left. |
+--------------------------------+----------------------------+-------------------------------------------------------+
| depth/left/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
| depth/right/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
| depth/back/camera/image | Image | |
+--------------------------------+----------------------------+-------------------------------------------------------+
Self righting and roll over
---------------------------
.. warning::
Self righting and battery charging may or may not take into account payloads configured on the robot. Test with your
configuration before using.
The self-right command can be accessed through ``/spot/self_right``.
The roll over commands can be accessed through the ``/spot/roll_over_left`` and ``/spot/roll_over_right`` services.
You can also access these actions through rviz.
.. image:: images/rviz_floor_tab.png
Autonomous docking
------------------
.. note::
See Boston Dynamics support for information about `setting up the dock <https://support.bostondynamics.com/s/article/Spot-Dock-setup>`_.
If using a Spot Enterprise, you will have access to autonomous docking capabilities.
Services
~~~~~~~~
You can trigger undocking with ``/spot/undock``.
Docking can be triggered with ``/spot/dock``. You must provide the fiducial ID of the dock the robot should dock at.
Actionserver
~~~~~~~~~~~~
Docking can also be performed using a single actionserver at ``/spot/dock``. To undock, the ``undock`` variable should
be set to true. Docking is the default action and requires specification of the fiducial ID in the ``dock_id`` variable.
Rviz
~~~~
You can also perform docking through the dock tab.
.. image:: images/rviz_dock_tab.png
Advanced motion control
-----------------------
There are other parameters that can be used to specify the behaviour of the robot. These allow for more granular
control of specific things. You should read and understand the SDK documentation before using these services to alter
any of these parameters.
* `/spot/swing_height <https://dev.bostondynamics.com/protos/bosdyn/api/proto_reference.html?highlight=power_state#swingheight>`_
* `/spot/terrain_params <https://dev.bostondynamics.com/protos/bosdyn/api/proto_reference.html?highlight=power_state#terrainparams>`_
* `/spot/locomotion_mode <https://dev.bostondynamics.com/protos/bosdyn/api/proto_reference.html?highlight=mobilityparams#locomotionhint>`_
* `/spot/obstacle_params <https://dev.bostondynamics.com/protos/bosdyn/api/proto_reference.html?highlight=power_state#obstacleparams>`_
Some of the more advanced motion controls can be accessed through the advanced motion tab.
.. image:: images/rviz_adv_motion_tab.png
Monitoring
----------
There are various topics which output information about the state of the robot:
+------------------------+----------------------------+---------------------------------------------------------+
| Topic | Type | Description |
+========================+============================+=========================================================+
| status/metrics | Metrics | General metrics for the system like distance walked |
+------------------------+----------------------------+---------------------------------------------------------+
| status/leases | LeaseArray | A list of what leases are held on the system |
+------------------------+----------------------------+---------------------------------------------------------+
| odometry/twist | TwistWithCovarianceStamped | The estimated odometry of the platform |
+------------------------+----------------------------+---------------------------------------------------------+
| status/feet | FootStateArray | The status and position of each foot |
+------------------------+----------------------------+---------------------------------------------------------+
| status/estop | EStopStateArray | The status of the eStop system |
+------------------------+----------------------------+---------------------------------------------------------+
| status/wifi | WiFiState | Status of the wifi system |
+------------------------+----------------------------+---------------------------------------------------------+
| status/power_state | PowerState | General power information |
+------------------------+----------------------------+---------------------------------------------------------+
| status/battery_states | BatteryStateArray | Information for the battery and all cells in the system |
+------------------------+----------------------------+---------------------------------------------------------+
| status/behavior_faults | BehaviorFaultState | A listing of behavior faults in the system |
+------------------------+----------------------------+---------------------------------------------------------+
| status/system_faults | SystemFaultState | A listing of system faults in the system |
+------------------------+----------------------------+---------------------------------------------------------+
| status/feedback | Feedback | Feedback from the Spot robot |
+------------------------+----------------------------+---------------------------------------------------------+
| status/mobility_params | MobilityParams | Describes the current state of the mobility parameters |
| | | defining the motion behaviour of the robot |
+------------------------+----------------------------+---------------------------------------------------------+
Troubleshooting
---------------
These instructions are a minimum subset of instructions to get this driver working and do not contain all necessary
debugging steps. Please refer to the `Spot SDK Quickstart <https://github.com/boston-dynamics/spot-sdk/blob/master/docs/python/quickstart.md>`_ for more detailed debugging steps.
| 25,520 | reStructuredText | 45.913603 | 352 | 0.523668 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/driver_configuration.rst | Driver configuration
====================
There are some basic settings that can be configured to control how the driver runs and at what rate it obtains data.
Data that is more important for your applications can be obtained at a higher rate.
The default configuration file is located at ``spot_driver/config/spot_ros.yaml``
Rate parameters
---------------
These parameters control the rate of various callbacks and loops in the driver.
.. note::
These rates are requests and not guarantees. The rate may vary depending on your network configuration and other
factors.
+------------------------+---------------------------------------------------------------------------------+
|Parameter |Description |
+========================+=================================================================================+
|rates/robot_state |The robot's state (include joint angles) |
+------------------------+---------------------------------------------------------------------------------+
|rates/metrics |The robot's metrics |
+------------------------+---------------------------------------------------------------------------------+
|rates/lease |The robot's lease state |
+------------------------+---------------------------------------------------------------------------------+
|rates/front_image |The image and depth image from the front camera |
+------------------------+---------------------------------------------------------------------------------+
|rates/side_image |The image and depth image from the side cameras |
+------------------------+---------------------------------------------------------------------------------+
|rates/loop_frequency |The image and depth image from the rear camera |
+------------------------+---------------------------------------------------------------------------------+
|rates/point_cloud |The point cloud from the EAP lidar |
+------------------------+---------------------------------------------------------------------------------+
|rates/hand_image |The image and depth image from the arm's hand camera |
+------------------------+---------------------------------------------------------------------------------+
|rates/feedback |A feedback message with various information |
+------------------------+---------------------------------------------------------------------------------+
|rates/mobility_params |Parameters indicating the robot's mobility state |
+------------------------+---------------------------------------------------------------------------------+
|rates/check_subscribers |Check for subscribers on camera topics. Data is not published without subscribers|
+------------------------+---------------------------------------------------------------------------------+
Startup parameters
-------------------
There are several parameters which can be used to automatically do certain actions when the driver starts.
.. warning::
Having the robot power its motors and stand after the driver connects is a physical action is inherently dangerous.
Consider whether you need these settings, and ensure the area is clear before starting the driver.
+------------------------+---------------------------------------------------------------------------------+
| Parameter | Description |
+------------------------+---------------------------------------------------------------------------------+
| auto_claim | A boolean to automatically claim the body and e stop when the driver connects |
+------------------------+---------------------------------------------------------------------------------+
| auto_power_on | A boolean to automatically power on the robot's motors when the driver connects |
+------------------------+---------------------------------------------------------------------------------+
| auto_stand | A boolean to automatically stand the robot after the driver conneccts |
+------------------------+---------------------------------------------------------------------------------+
Environment variables
---------------------
Some environment variables can modify the behaviour or configuration of the driver.
+----------------------------+-----------------------------------+----------------------------------------------------------------------------+
|Variable |Default Value |Description |
+============================+===================================+============================================================================+
|SPOT_VELODYNE_HOST |192.168.131.20 |IP address of the VLP-16 sensor |
+----------------------------+-----------------------------------+----------------------------------------------------------------------------+
|SPOT_JOY_DEVICE |/dev/input/js0 |The Linux joypad input device used by the joy_teleop node |
| | | |
+----------------------------+-----------------------------------+----------------------------------------------------------------------------+
|SPOT_JOY_CONFIG |spot_control/config/teleop.yaml |Joypad button/axis configuration file for joy_teleop |
| | | |
+----------------------------+-----------------------------------+----------------------------------------------------------------------------+
|SPOT_VELODYNE_AUTOLAUNCH |1 |If 1 and SPOT_VELODYNE is also 1, the VLP16 ROS node will start |
| | |automatically |
+----------------------------+-----------------------------------+----------------------------------------------------------------------------+ | 6,816 | reStructuredText | 81.132529 | 143 | 0.287999 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/ros_setup.rst | Computer Setup
==============
Building the Driver from Source
-------------------------------
The driver is not released to the ROS package servers, so it must be built from source. This requires a source workspace on the ROS PC. You can add it to an existing workspace or follow the instructions below to create a new one.
.. code:: bash
mkdir -p ~/spot_ws/src
Setup the workspace so it knows about your ROS installation
.. code:: bash
cd ~/spot_ws/src
source /opt/ros/noetic/setup.bash
catkin_init_workspace
Clone the spot_ros repository into the workspace
.. code:: bash
cd ~/spot_ws/src
git clone https://github.com/heuristicus/spot_ros.git
Use rosdep to install of the necessary dependencies
.. code:: bash
cd ~/spot_ws/
rosdep install --from-paths spot_driver spot_msgs spot_viz spot_description --ignore-src -y
Once all the necessary packages are installed, build the packages in the workspace
.. code:: bash
cd ~/spot_ws/
catkin build
Source your newly built workspace and the packages inside
.. code:: bash
source ~/spot_ws/devel/setup.bash
Adding to the URDF
------------------
.. warning::
When adding payloads, you should always add them to the robot's configuration through the `admin panel <https://support.bostondynamics.com/s/article/Payload-configuration-requirements>`_. Adding only the URDF does not add the payload to spot's built in collision avoidance.
The driver supports some configuration of the URDF via environment variables. These can either be manually set in
the terminal before starting the driver, or added to $HOME/.bashrc as desired. The table below lists the available
variables and their default values:
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
| Variable |Default Value |Description |
+=====================+===============+=======================================================================================================+
|SPOT_PACK |0 |If 1, enables the Clearpath ROS backpack accessory and adds it to the URDF |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_LIDAR_MOUNT |0 |If 1, adds the Lidar mount to the backpack. Requires SPOT_PACK to be 1 |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_VELODYNE |0 |If 1, adds the a VLP-16 sensor to the lidar mount. Requires SPOT_LIDAR_MOUNT to be 1 |
| | | |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_ARM |0 |If 1, adds the Spot arm to the URDF |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_VELODYNE_XYZ |0 0 0 |XYZ offset for the VLP-16 from the backpack lidar mount |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_VELODYNE_RPY |0 0 0 |RPY offset for the VLP-16 from the backpack lidar mount |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
|SPOT_URDF_EXTRAS |empty.urdf |Optional URDF file to add additional joints and links to the robot |
+---------------------+---------------+-------------------------------------------------------------------------------------------------------+
You can add a custom URDF to the robot using the ``SPOT_URDF_EXTRAS`` environment variable, which must be set in the
terminal from which the driver is run. This should point to a URDF/xacro file which has an object where the parent is one
of the frames on the robot.
It might look something like below.
.. code:: xml
<?xml version="1.0"?>
<robot name="spot_frontier" xmlns:xacro="http://www.ros.org/wiki/xacro">
<xacro:include filename="$(find my_payload_package)/urdf/my_payload.xacro"/>
<xacro:my_payload parent="real_rear_rail"/>
</robot>
Building for Melodic
--------------------
.. note::
If you can, consider using ROS noetic instead, which does not have these build issues.
Please note that the Spot SDK uses Python3, which is not officially supported by ROS Melodic. If you encounter an error
of this form:
.. code:: bash
Traceback (most recent call last):
File "/home/administrator/catkin_ws/src/spot_ros/spot_driver/scripts/spot_ros", line 3, in <module>
from spot_driver.spot_ros import SpotROS
File "/home/administrator/catkin_ws/src/spot_ros/spot_driver/src/spot_driver/spot_ros.py", line 19, in <module>
import tf2_ros
File "/opt/ros/melodic/lib/python2.7/dist-packages/tf2_ros/__init__.py", line 38, in <module>
from tf2_py import *
File "/opt/ros/melodic/lib/python2.7/dist-packages/tf2_py/__init__.py", line 38, in <module>
from ._tf2 import *
ImportError: dynamic module does not define module export function (PyInit__tf2)
When launching the driver, please follow these steps:
1. ``rm -rf devel/ build/ install/`` -- this will remove any old build artifacts from your workspace
2. ``git clone https://github.com/ros/geometry2 --branch 0.6.5`` into your ``src`` folder
3. rebuild your workspace with
.. code:: bash
catkin_make --cmake-args -DCMAKE_BUILD_TYPE=Release -DPYTHON_EXECUTABLE=/usr/bin/python3 -DPYTHON_INCLUDE_DIR=/usr/include/python3.6m -DPYTHON_LIBRARY=/usr/lib/x86_64-linux-gnu/libpython3.6m.so
Or if you are using an Nvidia Jetson:
.. code:: bash
catkin_make --cmake-args -DCMAKE_BUILD_TYPE=Release -DPYTHON_EXECUTABLE=/usr/bin/python3 -DPYTHON_INCLUDE_DIR=/usr/include/python3.6m -DPYTHON_LIBRARY=/usr/lib/aarch64-linux-gnu/libpython3.6m.so
4. re-run ``source devel/setup.bash``
5. start the driver with ``roslaunch spot_driver driver.launch``
| 6,681 | reStructuredText | 47.42029 | 275 | 0.512199 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/README.md | To generate docs, run
```
sphinx-build -b html . html
```
| 59 | Markdown | 8.999999 | 27 | 0.627119 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/index.rst | Spot ROS User Documentation
===========================
.. toctree::
:maxdepth: 0
:caption: Contents
robot_setup
ros_setup
driver_configuration
ros_usage
arm_usage
| 194 | reStructuredText | 13.999999 | 27 | 0.556701 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/robot_setup.rst | Robot Configuration
===========================
To configure the robot, you should consult `Boston Dynamics' support pages <https://support.bostondynamics.com/s/>`_.
Robot administration is performed through the `admin console <https://support.bostondynamics.com/s/article/Spot-system-administration>`_.
Networking
----------------
See the `network setup <https://support.bostondynamics.com/s/article/Spot-network-setup>`_ pages for details on network configuration.
Using WiFi for ROS communication is not recommended, except for monitoring purposes.
The driver can output a lot of data. It is recommended that you connect the Spot to the ROS PC via a payload port. See the `payload developer guide <https://dev.bostondynamics.com/docs/payload/readme>`_ for details. | 773 | reStructuredText | 50.599997 | 215 | 0.74903 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros/docs/conf.py | # -*- coding: utf-8 -*-
import os
import sys
import xml.etree.ElementTree as etree
sys.path.insert(0, os.path.abspath(os.path.dirname(__file__)))
extensions = [
"sphinx.ext.autodoc",
"sphinx.ext.doctest",
"sphinx.ext.viewcode",
]
source_suffix = ".rst"
master_doc = "index"
project = "Spot ROS User Documentation"
copyright = "2020, Clearpath Robotics, 2023 Oxford Robotics Institute"
# Get version number from package.xml.
tree = etree.parse("../spot_driver/package.xml")
version = tree.find("version").text
release = version
# .. html_theme = 'nature'
# .. html_theme_path = ["."]
html_theme = "sphinx_rtd_theme"
html_theme_path = ["."]
html_sidebars = {"**": ["sidebartoc.html", "sourcelink.html", "searchbox.html"]}
# If true, "Created using Sphinx" is shown in the HTML footer. Default is True.
html_show_sphinx = False
# The name of an image file (relative to this directory) to place at the top
# of the sidebar.
# The name of an image file (within the static path) to use as favicon of the
# docs. This file should be a Windows icon file (.ico) being 16x16 or 32x32
# pixels large.
# -- Options for LaTeX output ------------------------------------------------
latex_elements = {
# The paper size ('letterpaper' or 'a4paper').
#
# 'papersize': 'letterpaper',
# The font size ('10pt', '11pt' or '12pt').
#
# 'pointsize': '10pt',
# Additional stuff for the LaTeX preamble.
#
# 'preamble': '',
# Latex figure (float) alignment
#
# 'figure_align': 'htbp',
}
# Grouping the document tree into LaTeX files. List of tuples
# (source start file, target name, title,
# author, documentclass [howto, manual, or own class]).
latex_documents = [
(
master_doc,
"SpotROSUserDocumentation.tex",
"Spot ROS User Documentation",
"Dave Niewinski, Michal Staniaszek",
"manual",
),
]
| 1,895 | Python | 25.333333 | 80 | 0.636939 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/README.md | # Spot ROS Driver

## Documentation
Check-out the usage and user documentation [HERE](http://www.clearpathrobotics.com/assets/guides/melodic/spot-ros/)
| 178 | Markdown | 21.374997 | 115 | 0.752809 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/scripts/__init__.py | import spot_ros
import spot_wrapper.py
import ros_helpers.py
| 61 | Python | 14.499996 | 22 | 0.819672 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/scripts/spot_ros.py | #!/usr/bin/env python3
import rospy
from std_srvs.srv import Trigger, TriggerResponse
from std_msgs.msg import Bool
from tf2_msgs.msg import TFMessage
from geometry_msgs.msg import TransformStamped
from sensor_msgs.msg import Image, CameraInfo
from sensor_msgs.msg import JointState
from geometry_msgs.msg import TwistWithCovarianceStamped, Twist, Pose
from bosdyn.api.geometry_pb2 import Quaternion
import bosdyn.geometry
from spot_msgs.msg import Metrics
from spot_msgs.msg import LeaseArray, LeaseResource
from spot_msgs.msg import FootState, FootStateArray
from spot_msgs.msg import EStopState, EStopStateArray
from spot_msgs.msg import WiFiState
from spot_msgs.msg import PowerState
from spot_msgs.msg import BehaviorFault, BehaviorFaultState
from spot_msgs.msg import SystemFault, SystemFaultState
from spot_msgs.msg import BatteryState, BatteryStateArray
from spot_msgs.msg import Feedback
from ros_helpers import *
from spot_wrapper import SpotWrapper
import logging
class SpotROS():
"""Parent class for using the wrapper. Defines all callbacks and keeps the wrapper alive"""
def __init__(self):
self.spot_wrapper = None
self.callbacks = {}
"""Dictionary listing what callback to use for what data task"""
self.callbacks["robot_state"] = self.RobotStateCB
self.callbacks["metrics"] = self.MetricsCB
self.callbacks["lease"] = self.LeaseCB
self.callbacks["front_image"] = self.FrontImageCB
self.callbacks["side_image"] = self.SideImageCB
self.callbacks["rear_image"] = self.RearImageCB
def RobotStateCB(self, results):
"""Callback for when the Spot Wrapper gets new robot state data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
state = self.spot_wrapper.robot_state
if state:
## joint states ##
joint_state = GetJointStatesFromState(state, self.spot_wrapper)
self.joint_state_pub.publish(joint_state)
## TF ##
tf_msg = GetTFFromState(state, self.spot_wrapper)
if len(tf_msg.transforms) > 0:
self.tf_pub.publish(tf_msg)
# Odom Twist #
twist_odom_msg = GetOdomTwistFromState(state, self.spot_wrapper)
self.odom_twist_pub.publish(twist_odom_msg)
# Feet #
foot_array_msg = GetFeetFromState(state, self.spot_wrapper)
self.feet_pub.publish(foot_array_msg)
# EStop #
estop_array_msg = GetEStopStateFromState(state, self.spot_wrapper)
self.estop_pub.publish(estop_array_msg)
# WIFI #
wifi_msg = GetWifiFromState(state, self.spot_wrapper)
self.wifi_pub.publish(wifi_msg)
# Battery States #
battery_states_array_msg = GetBatteryStatesFromState(state, self.spot_wrapper)
self.battery_pub.publish(battery_states_array_msg)
# Power State #
power_state_msg = GetPowerStatesFromState(state, self.spot_wrapper)
self.power_pub.publish(power_state_msg)
# System Faults #
system_fault_state_msg = GetSystemFaultsFromState(state, self.spot_wrapper)
self.system_faults_pub.publish(system_fault_state_msg)
# Behavior Faults #
behavior_fault_state_msg = getBehaviorFaultsFromState(state, self.spot_wrapper)
self.behavior_faults_pub.publish(behavior_fault_state_msg)
def MetricsCB(self, results):
"""Callback for when the Spot Wrapper gets new metrics data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
metrics = self.spot_wrapper.metrics
if metrics:
metrics_msg = Metrics()
local_time = self.spot_wrapper.robotToLocalTime(metrics.timestamp)
metrics_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
for metric in metrics.metrics:
if metric.label == "distance":
metrics_msg.distance = metric.float_value
if metric.label == "gait cycles":
metrics_msg.gait_cycles = metric.int_value
if metric.label == "time moving":
metrics_msg.time_moving = rospy.Time(metric.duration.seconds, metric.duration.nanos)
if metric.label == "electric power":
metrics_msg.electric_power = rospy.Time(metric.duration.seconds, metric.duration.nanos)
self.metrics_pub.publish(metrics_msg)
def LeaseCB(self, results):
"""Callback for when the Spot Wrapper gets new lease data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
lease_array_msg = LeaseArray()
lease_list = self.spot_wrapper.lease
if lease_list:
for resource in lease_list:
new_resource = LeaseResource()
new_resource.resource = resource.resource
new_resource.lease.resource = resource.lease.resource
new_resource.lease.epoch = resource.lease.epoch
for seq in resource.lease.sequence:
new_resource.lease.sequence.append(seq)
new_resource.lease_owner.client_name = resource.lease_owner.client_name
new_resource.lease_owner.user_name = resource.lease_owner.user_name
lease_array_msg.resources.append(new_resource)
self.lease_pub.publish(lease_array_msg)
def FrontImageCB(self, results):
"""Callback for when the Spot Wrapper gets new front image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.front_images
if data:
image_msg0, camera_info_msg0, camera_tf_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.frontleft_image_pub.publish(image_msg0)
self.frontleft_image_info_pub.publish(camera_info_msg0)
self.tf_pub.publish(camera_tf_msg0)
image_msg1, camera_info_msg1, camera_tf_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.frontright_image_pub.publish(image_msg1)
self.frontright_image_info_pub.publish(camera_info_msg1)
self.tf_pub.publish(camera_tf_msg1)
image_msg2, camera_info_msg2, camera_tf_msg2 = getImageMsg(data[2], self.spot_wrapper)
self.frontleft_depth_pub.publish(image_msg2)
self.frontleft_depth_info_pub.publish(camera_info_msg2)
self.tf_pub.publish(camera_tf_msg2)
image_msg3, camera_info_msg3, camera_tf_msg3 = getImageMsg(data[3], self.spot_wrapper)
self.frontright_depth_pub.publish(image_msg3)
self.frontright_depth_info_pub.publish(camera_info_msg3)
self.tf_pub.publish(camera_tf_msg3)
def SideImageCB(self, results):
"""Callback for when the Spot Wrapper gets new side image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.side_images
if data:
image_msg0, camera_info_msg0, camera_tf_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.left_image_pub.publish(image_msg0)
self.left_image_info_pub.publish(camera_info_msg0)
self.tf_pub.publish(camera_tf_msg0)
image_msg1, camera_info_msg1, camera_tf_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.right_image_pub.publish(image_msg1)
self.right_image_info_pub.publish(camera_info_msg1)
self.tf_pub.publish(camera_tf_msg1)
image_msg2, camera_info_msg2, camera_tf_msg2 = getImageMsg(data[2], self.spot_wrapper)
self.left_depth_pub.publish(image_msg2)
self.left_depth_info_pub.publish(camera_info_msg2)
self.tf_pub.publish(camera_tf_msg2)
image_msg3, camera_info_msg3, camera_tf_msg3 = getImageMsg(data[3], self.spot_wrapper)
self.right_depth_pub.publish(image_msg3)
self.right_depth_info_pub.publish(camera_info_msg3)
self.tf_pub.publish(camera_tf_msg3)
def RearImageCB(self, results):
"""Callback for when the Spot Wrapper gets new rear image data.
Args:
results: FutureWrapper object of AsyncPeriodicQuery callback
"""
data = self.spot_wrapper.rear_images
if data:
mage_msg0, camera_info_msg0, camera_tf_msg0 = getImageMsg(data[0], self.spot_wrapper)
self.back_image_pub.publish(mage_msg0)
self.back_image_info_pub.publish(camera_info_msg0)
self.tf_pub.publish(camera_tf_msg0)
mage_msg1, camera_info_msg1, camera_tf_msg1 = getImageMsg(data[1], self.spot_wrapper)
self.back_depth_pub.publish(mage_msg1)
self.back_depth_info_pub.publish(camera_info_msg1)
self.tf_pub.publish(camera_tf_msg1)
def handle_claim(self, req):
"""ROS service handler for the claim service"""
resp = self.spot_wrapper.claim()
return TriggerResponse(resp[0], resp[1])
def handle_release(self, req):
"""ROS service handler for the release service"""
resp = self.spot_wrapper.release()
return TriggerResponse(resp[0], resp[1])
def handle_stop(self, req):
"""ROS service handler for the stop service"""
resp = self.spot_wrapper.stop()
return TriggerResponse(resp[0], resp[1])
def handle_self_right(self, req):
"""ROS service handler for the self-right service"""
resp = self.spot_wrapper.self_right()
return TriggerResponse(resp[0], resp[1])
def handle_sit(self, req):
"""ROS service handler for the sit service"""
resp = self.spot_wrapper.sit()
return TriggerResponse(resp[0], resp[1])
def handle_stand(self, req):
"""ROS service handler for the stand service"""
resp = self.spot_wrapper.stand()
return TriggerResponse(resp[0], resp[1])
def handle_power_on(self, req):
"""ROS service handler for the power-on service"""
resp = self.spot_wrapper.power_on()
return TriggerResponse(resp[0], resp[1])
def handle_safe_power_off(self, req):
"""ROS service handler for the safe-power-off service"""
resp = self.spot_wrapper.safe_power_off()
return TriggerResponse(resp[0], resp[1])
def handle_estop_hard(self, req):
"""ROS service handler to hard-eStop the robot. The robot will immediately cut power to the motors"""
resp = self.spot_wrapper.assertEStop(True)
return TriggerResponse(resp[0], resp[1])
def handle_estop_soft(self, req):
"""ROS service handler to soft-eStop the robot. The robot will try to settle on the ground before cutting power to the motors"""
resp = self.spot_wrapper.assertEStop(False)
return TriggerResponse(resp[0], resp[1])
def cmdVelCallback(self, data):
"""Callback for cmd_vel command"""
self.spot_wrapper.velocity_cmd(data.linear.x, data.linear.y, data.angular.z)
def bodyPoseCallback(self, data):
"""Callback for cmd_vel command"""
q = Quaternion()
q.x = data.orientation.x
q.y = data.orientation.y
q.z = data.orientation.z
q.w = data.orientation.w
euler_zxy = q.to_euler_zxy()
self.spot_wrapper.set_mobility_params(data.position.z, euler_zxy)
def shutdown(self):
rospy.loginfo("Shutting down ROS driver for Spot")
self.spot_wrapper.sit()
rospy.Rate(0.25).sleep()
self.spot_wrapper.disconnect()
def main(self):
"""Main function for the SpotROS class. Gets config from ROS and initializes the wrapper. Holds lease from wrapper and updates all async tasks at the ROS rate"""
rospy.init_node('spot_ros', anonymous=True)
rate = rospy.Rate(50)
self.rates = rospy.get_param('~rates', {})
self.username = rospy.get_param('~username', 'default_value')
self.password = rospy.get_param('~password', 'default_value')
self.app_token = rospy.get_param('~app_token', 'default_value')
self.hostname = rospy.get_param('~hostname', 'default_value')
self.motion_deadzone = rospy.get_param('~deadzone', 0.05)
self.logger = logging.getLogger('rosout')
rospy.loginfo("Starting ROS driver for Spot")
self.spot_wrapper = SpotWrapper(self.username, self.password, self.app_token, self.hostname, self.logger, self.rates, self.callbacks)
if self.spot_wrapper.is_valid:
# Images #
self.back_image_pub = rospy.Publisher('camera/back/image', Image, queue_size=10)
self.frontleft_image_pub = rospy.Publisher('camera/frontleft/image', Image, queue_size=10)
self.frontright_image_pub = rospy.Publisher('camera/frontright/image', Image, queue_size=10)
self.left_image_pub = rospy.Publisher('camera/left/image', Image, queue_size=10)
self.right_image_pub = rospy.Publisher('camera/right/image', Image, queue_size=10)
# Depth #
self.back_depth_pub = rospy.Publisher('depth/back/image', Image, queue_size=10)
self.frontleft_depth_pub = rospy.Publisher('depth/frontleft/image', Image, queue_size=10)
self.frontright_depth_pub = rospy.Publisher('depth/frontright/image', Image, queue_size=10)
self.left_depth_pub = rospy.Publisher('depth/left/image', Image, queue_size=10)
self.right_depth_pub = rospy.Publisher('depth/right/image', Image, queue_size=10)
# Image Camera Info #
self.back_image_info_pub = rospy.Publisher('camera/back/camera_info', CameraInfo, queue_size=10)
self.frontleft_image_info_pub = rospy.Publisher('camera/frontleft/camera_info', CameraInfo, queue_size=10)
self.frontright_image_info_pub = rospy.Publisher('camera/frontright/camera_info', CameraInfo, queue_size=10)
self.left_image_info_pub = rospy.Publisher('camera/left/camera_info', CameraInfo, queue_size=10)
self.right_image_info_pub = rospy.Publisher('camera/right/camera_info', CameraInfo, queue_size=10)
# Depth Camera Info #
self.back_depth_info_pub = rospy.Publisher('depth/back/camera_info', CameraInfo, queue_size=10)
self.frontleft_depth_info_pub = rospy.Publisher('depth/frontleft/camera_info', CameraInfo, queue_size=10)
self.frontright_depth_info_pub = rospy.Publisher('depth/frontright/camera_info', CameraInfo, queue_size=10)
self.left_depth_info_pub = rospy.Publisher('depth/left/camera_info', CameraInfo, queue_size=10)
self.right_depth_info_pub = rospy.Publisher('depth/right/camera_info', CameraInfo, queue_size=10)
# Status Publishers #
self.joint_state_pub = rospy.Publisher('joint_states', JointState, queue_size=10)
"""Defining a TF publisher manually because of conflicts between Python3 and tf"""
self.tf_pub = rospy.Publisher('tf', TFMessage, queue_size=10)
self.metrics_pub = rospy.Publisher('status/metrics', Metrics, queue_size=10)
self.lease_pub = rospy.Publisher('status/leases', LeaseArray, queue_size=10)
self.odom_twist_pub = rospy.Publisher('odometry/twist', TwistWithCovarianceStamped, queue_size=10)
self.feet_pub = rospy.Publisher('status/feet', FootStateArray, queue_size=10)
self.estop_pub = rospy.Publisher('status/estop', EStopStateArray, queue_size=10)
self.wifi_pub = rospy.Publisher('status/wifi', WiFiState, queue_size=10)
self.power_pub = rospy.Publisher('status/power_state', PowerState, queue_size=10)
self.battery_pub = rospy.Publisher('status/battery_states', BatteryStateArray, queue_size=10)
self.behavior_faults_pub = rospy.Publisher('status/behavior_faults', BehaviorFaultState, queue_size=10)
self.system_faults_pub = rospy.Publisher('status/system_faults', SystemFaultState, queue_size=10)
self.feedback_pub = rospy.Publisher('status/feedback', Feedback, queue_size=10)
rospy.Subscriber('cmd_vel', Twist, self.cmdVelCallback)
rospy.Subscriber('body_pose', Pose, self.bodyPoseCallback)
rospy.Service("claim", Trigger, self.handle_claim)
rospy.Service("release", Trigger, self.handle_release)
rospy.Service("stop", Trigger, self.handle_stop)
rospy.Service("self_right", Trigger, self.handle_self_right)
rospy.Service("sit", Trigger, self.handle_sit)
rospy.Service("stand", Trigger, self.handle_stand)
rospy.Service("power_on", Trigger, self.handle_power_on)
rospy.Service("power_off", Trigger, self.handle_safe_power_off)
rospy.Service("estop/hard", Trigger, self.handle_estop_hard)
rospy.Service("estop/gentle", Trigger, self.handle_estop_soft)
rospy.on_shutdown(self.shutdown)
self.spot_wrapper.resetEStop()
self.auto_claim = rospy.get_param('~auto_claim', False)
self.auto_power_on = rospy.get_param('~auto_power_on', False)
self.auto_stand = rospy.get_param('~auto_stand', False)
if self.auto_claim:
self.spot_wrapper.claim()
if self.auto_power_on:
self.spot_wrapper.power_on()
if self.auto_stand:
self.spot_wrapper.stand()
while not rospy.is_shutdown():
self.spot_wrapper.updateTasks()
feedback_msg = Feedback()
feedback_msg.standing = self.spot_wrapper.is_standing
feedback_msg.sitting = self.spot_wrapper.is_sitting
feedback_msg.moving = self.spot_wrapper.is_moving
id = self.spot_wrapper.id
try:
feedback_msg.serial_number = id.serial_number
feedback_msg.species = id.species
feedback_msg.version = id.version
feedback_msg.nickname = id.nickname
feedback_msg.computer_serial_number = id.computer_serial_number
except:
pass
self.feedback_pub.publish(feedback_msg)
rate.sleep()
if __name__ == "__main__":
SR = SpotROS()
SR.main()
| 18,644 | Python | 46.202532 | 171 | 0.637041 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/scripts/ros_helpers.py | import rospy
from std_msgs.msg import Empty
from tf2_msgs.msg import TFMessage
from geometry_msgs.msg import TransformStamped
from sensor_msgs.msg import Image, CameraInfo
from sensor_msgs.msg import JointState
from geometry_msgs.msg import TwistWithCovarianceStamped
from spot_msgs.msg import Metrics
from spot_msgs.msg import LeaseArray, LeaseResource
from spot_msgs.msg import FootState, FootStateArray
from spot_msgs.msg import EStopState, EStopStateArray
from spot_msgs.msg import WiFiState
from spot_msgs.msg import PowerState
from spot_msgs.msg import BehaviorFault, BehaviorFaultState
from spot_msgs.msg import SystemFault, SystemFaultState
from spot_msgs.msg import BatteryState, BatteryStateArray
from bosdyn.api import image_pb2
friendly_joint_names = {}
"""Dictionary for mapping BD joint names to more friendly names"""
friendly_joint_names["fl.hx"] = "front_left_hip_x"
friendly_joint_names["fl.hy"] = "front_left_hip_y"
friendly_joint_names["fl.kn"] = "front_left_knee"
friendly_joint_names["fr.hx"] = "front_right_hip_x"
friendly_joint_names["fr.hy"] = "front_right_hip_y"
friendly_joint_names["fr.kn"] = "front_right_knee"
friendly_joint_names["hl.hx"] = "rear_left_hip_x"
friendly_joint_names["hl.hy"] = "rear_left_hip_y"
friendly_joint_names["hl.kn"] = "rear_left_knee"
friendly_joint_names["hr.hx"] = "rear_right_hip_x"
friendly_joint_names["hr.hy"] = "rear_right_hip_y"
friendly_joint_names["hr.kn"] = "rear_right_knee"
class DefaultCameraInfo(CameraInfo):
"""Blank class extending CameraInfo ROS topic that defaults most parameters"""
def __init__(self):
super().__init__()
self.distortion_model = "plumb_bob"
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.D.append(0)
self.K[1] = 0
self.K[3] = 0
self.K[6] = 0
self.K[7] = 0
self.K[8] = 1
self.R[0] = 1
self.R[1] = 0
self.R[2] = 0
self.R[3] = 0
self.R[4] = 1
self.R[5] = 0
self.R[6] = 0
self.R[7] = 0
self.R[8] = 1
self.P[1] = 0
self.P[3] = 0
self.P[4] = 0
self.P[7] = 0
self.P[8] = 0
self.P[9] = 0
self.P[10] = 1
self.P[11] = 0
def getImageMsg(data, spot_wrapper):
"""Takes the image, camera, and TF data and populates the necessary ROS messages
Args:
data: Image proto
spot_wrapper: A SpotWrapper object
Returns:
(tuple):
* Image: message of the image captured
* CameraInfo: message to define the state and config of the camera that took the image
* TFMessage: with the transforms necessary to locate the image frames
"""
tf_msg = TFMessage()
for frame_name in data.shot.transforms_snapshot.child_to_parent_edge_map:
if data.shot.transforms_snapshot.child_to_parent_edge_map.get(frame_name).parent_frame_name:
transform = data.shot.transforms_snapshot.child_to_parent_edge_map.get(frame_name)
new_tf = TransformStamped()
local_time = spot_wrapper.robotToLocalTime(data.shot.acquisition_time)
new_tf.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_tf.header.frame_id = transform.parent_frame_name
new_tf.child_frame_id = frame_name
new_tf.transform.translation.x = transform.parent_tform_child.position.x
new_tf.transform.translation.y = transform.parent_tform_child.position.y
new_tf.transform.translation.z = transform.parent_tform_child.position.z
new_tf.transform.rotation.x = transform.parent_tform_child.rotation.x
new_tf.transform.rotation.y = transform.parent_tform_child.rotation.y
new_tf.transform.rotation.z = transform.parent_tform_child.rotation.z
new_tf.transform.rotation.w = transform.parent_tform_child.rotation.w
tf_msg.transforms.append(new_tf)
image_msg = Image()
local_time = spot_wrapper.robotToLocalTime(data.shot.acquisition_time)
image_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
image_msg.header.frame_id = data.shot.frame_name_image_sensor
image_msg.height = data.shot.image.rows
image_msg.width = data.shot.image.cols
# Color/greyscale formats.
# JPEG format
if data.shot.image.format == image_pb2.Image.FORMAT_JPEG:
image_msg.encoding = "rgb8"
image_msg.is_bigendian = True
image_msg.step = 3 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Uncompressed. Requires pixel_format.
if data.shot.image.format == image_pb2.Image.FORMAT_RAW:
# One byte per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_GREYSCALE_U8:
image_msg.encoding = "mono8"
image_msg.is_bigendian = True
image_msg.step = data.shot.image.cols
image_msg.data = data.shot.image.data
# Three bytes per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_RGB_U8:
image_msg.encoding = "rgb8"
image_msg.is_bigendian = True
image_msg.step = 3 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Four bytes per pixel.
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_RGBA_U8:
image_msg.encoding = "rgba8"
image_msg.is_bigendian = True
image_msg.step = 4 * data.shot.image.cols
image_msg.data = data.shot.image.data
# Little-endian uint16 z-distance from camera (mm).
if data.shot.image.pixel_format == image_pb2.Image.PIXEL_FORMAT_DEPTH_U16:
image_msg.encoding = "mono16"
image_msg.is_bigendian = False
image_msg.step = 2 * data.shot.image.cols
image_msg.data = data.shot.image.data
camera_info_msg = DefaultCameraInfo()
local_time = spot_wrapper.robotToLocalTime(data.shot.acquisition_time)
camera_info_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
camera_info_msg.header.frame_id = data.shot.frame_name_image_sensor
camera_info_msg.height = data.shot.image.rows
camera_info_msg.width = data.shot.image.cols
camera_info_msg.K[0] = data.source.pinhole.intrinsics.focal_length.x
camera_info_msg.K[2] = data.source.pinhole.intrinsics.principal_point.x
camera_info_msg.K[4] = data.source.pinhole.intrinsics.focal_length.y
camera_info_msg.K[5] = data.source.pinhole.intrinsics.principal_point.y
camera_info_msg.P[0] = data.source.pinhole.intrinsics.focal_length.x
camera_info_msg.P[2] = data.source.pinhole.intrinsics.principal_point.x
camera_info_msg.P[5] = data.source.pinhole.intrinsics.focal_length.y
camera_info_msg.P[6] = data.source.pinhole.intrinsics.principal_point.y
return image_msg, camera_info_msg, tf_msg
def GetJointStatesFromState(state, spot_wrapper):
"""Maps joint state data from robot state proto to ROS JointState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
JointState message
"""
joint_state = JointState()
local_time = spot_wrapper.robotToLocalTime(state.kinematic_state.acquisition_timestamp)
joint_state.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
for joint in state.kinematic_state.joint_states:
joint_state.name.append(friendly_joint_names.get(joint.name, "ERROR"))
joint_state.position.append(joint.position.value)
joint_state.velocity.append(joint.velocity.value)
joint_state.effort.append(joint.load.value)
return joint_state
def GetEStopStateFromState(state, spot_wrapper):
"""Maps eStop state data from robot state proto to ROS EStopArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
EStopArray message
"""
estop_array_msg = EStopStateArray()
for estop in state.estop_states:
estop_msg = EStopState()
local_time = spot_wrapper.robotToLocalTime(estop.timestamp)
estop_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
estop_msg.name = estop.name
estop_msg.type = estop.type
estop_msg.state = estop.state
estop_array_msg.estop_states.append(estop_msg)
return estop_array_msg
def GetFeetFromState(state, spot_wrapper):
"""Maps foot position state data from robot state proto to ROS FootStateArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
FootStateArray message
"""
foot_array_msg = FootStateArray()
for foot in state.foot_state:
foot_msg = FootState()
foot_msg.foot_position_rt_body.x = foot.foot_position_rt_body.x
foot_msg.foot_position_rt_body.y = foot.foot_position_rt_body.y
foot_msg.foot_position_rt_body.z = foot.foot_position_rt_body.z
foot_msg.contact = foot.contact
foot_array_msg.states.append(foot_msg)
return foot_array_msg
def GetOdomTwistFromState(state, spot_wrapper):
"""Maps odometry data from robot state proto to ROS TwistWithCovarianceStamped message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
TwistWithCovarianceStamped message
"""
twist_odom_msg = TwistWithCovarianceStamped()
local_time = spot_wrapper.robotToLocalTime(state.kinematic_state.acquisition_timestamp)
twist_odom_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
twist_odom_msg.twist.twist.linear.x = state.kinematic_state.velocity_of_body_in_odom.linear.x
twist_odom_msg.twist.twist.linear.y = state.kinematic_state.velocity_of_body_in_odom.linear.y
twist_odom_msg.twist.twist.linear.z = state.kinematic_state.velocity_of_body_in_odom.linear.z
twist_odom_msg.twist.twist.angular.x = state.kinematic_state.velocity_of_body_in_odom.angular.x
twist_odom_msg.twist.twist.angular.y = state.kinematic_state.velocity_of_body_in_odom.angular.y
twist_odom_msg.twist.twist.angular.z = state.kinematic_state.velocity_of_body_in_odom.angular.z
return twist_odom_msg
def GetWifiFromState(state, spot_wrapper):
"""Maps wireless state data from robot state proto to ROS WiFiState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
WiFiState message
"""
wifi_msg = WiFiState()
for comm_state in state.comms_states:
if comm_state.HasField('wifi_state'):
wifi_msg.current_mode = comm_state.wifi_state.current_mode
wifi_msg.essid = comm_state.wifi_state.essid
return wifi_msg
def GetTFFromState(state, spot_wrapper):
"""Maps robot link state data from robot state proto to ROS TFMessage message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
TFMessage message
"""
tf_msg = TFMessage()
for frame_name in state.kinematic_state.transforms_snapshot.child_to_parent_edge_map:
if state.kinematic_state.transforms_snapshot.child_to_parent_edge_map.get(frame_name).parent_frame_name:
transform = state.kinematic_state.transforms_snapshot.child_to_parent_edge_map.get(frame_name)
new_tf = TransformStamped()
local_time = spot_wrapper.robotToLocalTime(state.kinematic_state.acquisition_timestamp)
new_tf.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_tf.header.frame_id = transform.parent_frame_name
new_tf.child_frame_id = frame_name
new_tf.transform.translation.x = transform.parent_tform_child.position.x
new_tf.transform.translation.y = transform.parent_tform_child.position.y
new_tf.transform.translation.z = transform.parent_tform_child.position.z
new_tf.transform.rotation.x = transform.parent_tform_child.rotation.x
new_tf.transform.rotation.y = transform.parent_tform_child.rotation.y
new_tf.transform.rotation.z = transform.parent_tform_child.rotation.z
new_tf.transform.rotation.w = transform.parent_tform_child.rotation.w
tf_msg.transforms.append(new_tf)
return tf_msg
def GetBatteryStatesFromState(state, spot_wrapper):
"""Maps battery state data from robot state proto to ROS BatteryStateArray message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
BatteryStateArray message
"""
battery_states_array_msg = BatteryStateArray()
for battery in state.battery_states:
battery_msg = BatteryState()
local_time = spot_wrapper.robotToLocalTime(battery.timestamp)
battery_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
battery_msg.identifier = battery.identifier
battery_msg.charge_percentage = battery.charge_percentage.value
battery_msg.estimated_runtime = rospy.Time(battery.estimated_runtime.seconds, battery.estimated_runtime.nanos)
battery_msg.current = battery.current.value
battery_msg.voltage = battery.voltage.value
for temp in battery.temperatures:
battery_msg.temperatures.append(temp)
battery_msg.status = battery.status
battery_states_array_msg.battery_states.append(battery_msg)
return battery_states_array_msg
def GetPowerStatesFromState(state, spot_wrapper):
"""Maps power state data from robot state proto to ROS PowerState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
PowerState message
"""
power_state_msg = PowerState()
local_time = spot_wrapper.robotToLocalTime(state.power_state.timestamp)
power_state_msg.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
power_state_msg.motor_power_state = state.power_state.motor_power_state
power_state_msg.shore_power_state = state.power_state.shore_power_state
power_state_msg.locomotion_charge_percentage = state.power_state.locomotion_charge_percentage.value
power_state_msg.locomotion_estimated_runtime = rospy.Time(state.power_state.locomotion_estimated_runtime.seconds, state.power_state.locomotion_estimated_runtime.nanos)
return power_state_msg
def getBehaviorFaults(behavior_faults, spot_wrapper):
"""Helper function to strip out behavior faults into a list
Args:
behavior_faults: List of BehaviorFaults
spot_wrapper: A SpotWrapper object
Returns:
List of BehaviorFault messages
"""
faults = []
for fault in behavior_faults:
new_fault = BehaviorFault()
new_fault.behavior_fault_id = fault.behavior_fault_id
local_time = spot_wrapper.robotToLocalTime(fault.onset_timestamp)
new_fault.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_fault.cause = fault.cause
new_fault.status = fault.status
faults.append(new_fault)
return faults
def getSystemFaults(system_faults, spot_wrapper):
"""Helper function to strip out system faults into a list
Args:
systen_faults: List of SystemFaults
spot_wrapper: A SpotWrapper object
Returns:
List of SystemFault messages
"""
faults = []
for fault in system_faults:
new_fault = SystemFault()
new_fault.name = fault.name
local_time = spot_wrapper.robotToLocalTime(fault.onset_timestamp)
new_fault.header.stamp = rospy.Time(local_time.seconds, local_time.nanos)
new_fault.duration = rospy.Time(fault.duration.seconds, fault.duration.nanos)
new_fault.code = fault.code
new_fault.uid = fault.uid
new_fault.error_message = fault.error_message
for att in fault.attributes:
new_fault.attributes.append(att)
new_fault.severity = fault.severity
faults.append(new_fault)
return faults
def GetSystemFaultsFromState(state, spot_wrapper):
"""Maps system fault data from robot state proto to ROS SystemFaultState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
SystemFaultState message
"""
system_fault_state_msg = SystemFaultState()
system_fault_state_msg.faults = getSystemFaults(state.system_fault_state.faults, spot_wrapper)
system_fault_state_msg.historical_faults = getSystemFaults(state.system_fault_state.historical_faults, spot_wrapper)
return system_fault_state_msg
def getBehaviorFaultsFromState(state, spot_wrapper):
"""Maps behavior fault data from robot state proto to ROS BehaviorFaultState message
Args:
data: Robot State proto
spot_wrapper: A SpotWrapper object
Returns:
BehaviorFaultState message
"""
behavior_fault_state_msg = BehaviorFaultState()
behavior_fault_state_msg.faults = getBehaviorFaults(state.behavior_fault_state.faults, spot_wrapper)
return behavior_fault_state_msg
| 17,178 | Python | 40.098086 | 171 | 0.685295 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/scripts/spot_wrapper.py | import time
from bosdyn.client import create_standard_sdk, ResponseError, RpcError
from bosdyn.client.async_tasks import AsyncPeriodicQuery, AsyncTasks
from bosdyn.geometry import EulerZXY
from bosdyn.client.robot_state import RobotStateClient
from bosdyn.client.robot_command import RobotCommandClient, RobotCommandBuilder
from bosdyn.client.power import PowerClient
from bosdyn.client.lease import LeaseClient, LeaseKeepAlive
from bosdyn.client.image import ImageClient, build_image_request
from bosdyn.api import image_pb2
from bosdyn.client.estop import EstopClient, EstopEndpoint, EstopKeepAlive
from bosdyn.client import power
import bosdyn.api.robot_state_pb2 as robot_state_proto
from bosdyn.api import basic_command_pb2
from google.protobuf.timestamp_pb2 import Timestamp
front_image_sources = ['frontleft_fisheye_image', 'frontright_fisheye_image', 'frontleft_depth', 'frontright_depth']
"""List of image sources for front image periodic query"""
side_image_sources = ['left_fisheye_image', 'right_fisheye_image', 'left_depth', 'right_depth']
"""List of image sources for side image periodic query"""
rear_image_sources = ['back_fisheye_image', 'back_depth']
"""List of image sources for rear image periodic query"""
class AsyncRobotState(AsyncPeriodicQuery):
"""Class to get robot state at regular intervals. get_robot_state_async query sent to the robot at every tick. Callback registered to defined callback function.
Attributes:
client: The Client to a service on the robot
logger: Logger object
rate: Rate (Hz) to trigger the query
callback: Callback function to call when the results of the query are available
"""
def __init__(self, client, logger, rate, callback):
super(AsyncRobotState, self).__init__("robot-state", client, logger,
period_sec=1.0/max(rate, 1.0))
self._callback = None
if rate > 0.0:
self._callback = callback
def _start_query(self):
if self._callback:
callback_future = self._client.get_robot_state_async()
callback_future.add_done_callback(self._callback)
return callback_future
class AsyncMetrics(AsyncPeriodicQuery):
"""Class to get robot metrics at regular intervals. get_robot_metrics_async query sent to the robot at every tick. Callback registered to defined callback function.
Attributes:
client: The Client to a service on the robot
logger: Logger object
rate: Rate (Hz) to trigger the query
callback: Callback function to call when the results of the query are available
"""
def __init__(self, client, logger, rate, callback):
super(AsyncMetrics, self).__init__("robot-metrics", client, logger,
period_sec=1.0/max(rate, 1.0))
self._callback = None
if rate > 0.0:
self._callback = callback
def _start_query(self):
if self._callback:
callback_future = self._client.get_robot_metrics_async()
callback_future.add_done_callback(self._callback)
return callback_future
class AsyncLease(AsyncPeriodicQuery):
"""Class to get lease state at regular intervals. list_leases_async query sent to the robot at every tick. Callback registered to defined callback function.
Attributes:
client: The Client to a service on the robot
logger: Logger object
rate: Rate (Hz) to trigger the query
callback: Callback function to call when the results of the query are available
"""
def __init__(self, client, logger, rate, callback):
super(AsyncLease, self).__init__("lease", client, logger,
period_sec=1.0/max(rate, 1.0))
self._callback = None
if rate > 0.0:
self._callback = callback
def _start_query(self):
if self._callback:
callback_future = self._client.list_leases_async()
callback_future.add_done_callback(self._callback)
return callback_future
class AsyncImageService(AsyncPeriodicQuery):
"""Class to get images at regular intervals. get_image_from_sources_async query sent to the robot at every tick. Callback registered to defined callback function.
Attributes:
client: The Client to a service on the robot
logger: Logger object
rate: Rate (Hz) to trigger the query
callback: Callback function to call when the results of the query are available
"""
def __init__(self, client, logger, rate, callback, image_requests):
super(AsyncImageService, self).__init__("robot_image_service", client, logger,
period_sec=1.0/max(rate, 1.0))
self._callback = None
if rate > 0.0:
self._callback = callback
self._image_requests = image_requests
def _start_query(self):
if self._callback:
callback_future = self._client.get_image_async(self._image_requests)
callback_future.add_done_callback(self._callback)
return callback_future
class AsyncIdle(AsyncPeriodicQuery):
"""Class to check if the robot is moving, and if not, command a stand with the set mobility parameters
Attributes:
client: The Client to a service on the robot
logger: Logger object
rate: Rate (Hz) to trigger the query
spot_wrapper: A handle to the wrapper library
"""
def __init__(self, client, logger, rate, spot_wrapper):
super(AsyncIdle, self).__init__("idle", client, logger,
period_sec=1.0/rate)
self._spot_wrapper = spot_wrapper
def _start_query(self):
if self._spot_wrapper._last_stand_command != None:
self._spot_wrapper._is_sitting = False
response = self._client.robot_command_feedback(self._spot_wrapper._last_stand_command)
if (response.feedback.mobility_feedback.stand_feedback.status ==
basic_command_pb2.StandCommand.Feedback.STATUS_IS_STANDING):
self._spot_wrapper._is_standing = True
self._spot_wrapper._last_stand_command = None
else:
self._spot_wrapper._is_standing = False
if self._spot_wrapper._last_sit_command != None:
self._spot_wrapper._is_standing = False
response = self._client.robot_command_feedback(self._spot_wrapper._last_sit_command)
if (response.feedback.mobility_feedback.sit_feedback.status ==
basic_command_pb2.SitCommand.Feedback.STATUS_IS_SITTING):
self._spot_wrapper._is_sitting = True
self._spot_wrapper._last_sit_command = None
else:
self._spot_wrapper._is_sitting = False
is_moving = False
if self._spot_wrapper._last_motion_command_time != None:
if time.time() < self._spot_wrapper._last_motion_command_time:
is_moving = True
else:
self._spot_wrapper._last_motion_command_time = None
if self._spot_wrapper._last_motion_command != None:
response = self._client.robot_command_feedback(self._spot_wrapper._last_motion_command)
if (response.feedback.mobility_feedback.se2_trajectory_feedback.status ==
basic_command_pb2.SE2TrajectoryCommand.Feedback.STATUS_GOING_TO_GOAL):
is_moving = True
else:
self._spot_wrapper._last_motion_command = None
self._spot_wrapper._is_moving = is_moving
if self._spot_wrapper.is_standing and not self._spot_wrapper.is_moving:
self._spot_wrapper.stand(False)
class SpotWrapper():
"""Generic wrapper class to encompass release 1.1.4 API features as well as maintaining leases automatically"""
def __init__(self, username, password, token, hostname, logger, rates = {}, callbacks = {}):
self._username = username
self._password = password
self._token = token
self._hostname = hostname
self._logger = logger
self._rates = rates
self._callbacks = callbacks
self._keep_alive = True
self._valid = True
self._mobility_params = RobotCommandBuilder.mobility_params()
self._is_standing = False
self._is_sitting = True
self._is_moving = False
self._last_stand_command = None
self._last_sit_command = None
self._last_motion_command = None
self._last_motion_command_time = None
self._front_image_requests = []
for source in front_image_sources:
self._front_image_requests.append(build_image_request(source, image_format=image_pb2.Image.Format.FORMAT_RAW))
self._side_image_requests = []
for source in side_image_sources:
self._side_image_requests.append(build_image_request(source, image_format=image_pb2.Image.Format.FORMAT_RAW))
self._rear_image_requests = []
for source in rear_image_sources:
self._rear_image_requests.append(build_image_request(source, image_format=image_pb2.Image.Format.FORMAT_RAW))
try:
self._sdk = create_standard_sdk('ros_spot')
except Exception as e:
self._logger.error("Error creating SDK object: %s", e)
self._valid = False
return
try:
self._sdk.load_app_token(self._token)
except Exception as e:
self._logger.error("Error loading developer token: %s", e)
self._valid = False
return
self._robot = self._sdk.create_robot(self._hostname)
try:
self._robot.authenticate(self._username, self._password)
self._robot.start_time_sync()
except RpcError as err:
self._logger.error("Failed to communicate with robot: %s", err)
self._valid = False
return
if self._robot:
# Clients
try:
self._robot_state_client = self._robot.ensure_client(RobotStateClient.default_service_name)
self._robot_command_client = self._robot.ensure_client(RobotCommandClient.default_service_name)
self._power_client = self._robot.ensure_client(PowerClient.default_service_name)
self._lease_client = self._robot.ensure_client(LeaseClient.default_service_name)
self._image_client = self._robot.ensure_client(ImageClient.default_service_name)
self._estop_client = self._robot.ensure_client(EstopClient.default_service_name)
except Exception as e:
self._logger.error("Unable to create client service: %s", e)
self._valid = False
return
# Async Tasks
self._async_task_list = []
self._robot_state_task = AsyncRobotState(self._robot_state_client, self._logger, max(0.0, self._rates.get("robot_state", 0.0)), self._callbacks.get("robot_state", lambda:None))
self._robot_metrics_task = AsyncMetrics(self._robot_state_client, self._logger, max(0.0, self._rates.get("metrics", 0.0)), self._callbacks.get("metrics", lambda:None))
self._lease_task = AsyncLease(self._lease_client, self._logger, max(0.0, self._rates.get("lease", 0.0)), self._callbacks.get("lease", lambda:None))
self._front_image_task = AsyncImageService(self._image_client, self._logger, max(0.0, self._rates.get("front_image", 0.0)), self._callbacks.get("front_image", lambda:None), self._front_image_requests)
self._side_image_task = AsyncImageService(self._image_client, self._logger, max(0.0, self._rates.get("side_image", 0.0)), self._callbacks.get("side_image", lambda:None), self._side_image_requests)
self._rear_image_task = AsyncImageService(self._image_client, self._logger, max(0.0, self._rates.get("rear_image", 0.0)), self._callbacks.get("rear_image", lambda:None), self._rear_image_requests)
self._idle_task = AsyncIdle(self._robot_command_client, self._logger, 10.0, self)
self._estop_endpoint = EstopEndpoint(self._estop_client, 'ros', 9.0)
self._async_tasks = AsyncTasks(
[self._robot_state_task, self._robot_metrics_task, self._lease_task, self._front_image_task, self._side_image_task, self._rear_image_task, self._idle_task])
self._robot_id = None
self._lease = None
@property
def is_valid(self):
"""Return boolean indicating if the wrapper initialized successfully"""
return self._valid
@property
def id(self):
"""Return robot's ID"""
return self._robot_id
@property
def robot_state(self):
"""Return latest proto from the _robot_state_task"""
return self._robot_state_task.proto
@property
def metrics(self):
"""Return latest proto from the _robot_metrics_task"""
return self._robot_metrics_task.proto
@property
def lease(self):
"""Return latest proto from the _lease_task"""
return self._lease_task.proto
@property
def front_images(self):
"""Return latest proto from the _front_image_task"""
return self._front_image_task.proto
@property
def side_images(self):
"""Return latest proto from the _side_image_task"""
return self._side_image_task.proto
@property
def rear_images(self):
"""Return latest proto from the _rear_image_task"""
return self._rear_image_task.proto
@property
def is_standing(self):
"""Return boolean of standing state"""
return self._is_standing
@property
def is_sitting(self):
"""Return boolean of standing state"""
return self._is_sitting
@property
def is_moving(self):
"""Return boolean of walking state"""
return self._is_moving
@property
def time_skew(self):
"""Return the time skew between local and spot time"""
return self._robot.time_sync.endpoint.clock_skew
def robotToLocalTime(self, timestamp):
"""Takes a timestamp and an estimated skew and return seconds and nano seconds
Args:
timestamp: google.protobuf.Timestamp
Returns:
google.protobuf.Timestamp
"""
rtime = Timestamp()
rtime.seconds = timestamp.seconds - self.time_skew.seconds
rtime.nanos = timestamp.nanos - self.time_skew.nanos
if rtime.nanos < 0:
rtime.nanos = rtime.nanos + 1000000000
rtime.seconds = rtime.seconds - 1
return rtime
def claim(self):
"""Get a lease for the robot, a handle on the estop endpoint, and the ID of the robot."""
try:
self._robot_id = self._robot.get_id()
self.getLease()
self.resetEStop()
return True, "Success"
except (ResponseError, RpcError) as err:
self._logger.error("Failed to initialize robot communication: %s", err)
return False, str(err)
def updateTasks(self):
"""Loop through all periodic tasks and update their data if needed."""
self._async_tasks.update()
def resetEStop(self):
"""Get keepalive for eStop"""
self._estop_endpoint.force_simple_setup() # Set this endpoint as the robot's sole estop.
self._estop_keepalive = EstopKeepAlive(self._estop_endpoint)
def assertEStop(self, severe=True):
"""Forces the robot into eStop state.
Args:
severe: Default True - If true, will cut motor power immediately. If false, will try to settle the robot on the ground first
"""
try:
if severe:
self._estop_endpoint.stop()
else:
self._estop_endpoint.settle_then_cut()
return True, "Success"
except:
return False, "Error"
def releaseEStop(self):
"""Stop eStop keepalive"""
if self._estop_keepalive:
self._estop_keepalive.stop()
self._estop_keepalive = None
def getLease(self):
"""Get a lease for the robot and keep the lease alive automatically."""
self._lease = self._lease_client.acquire()
self._lease_keepalive = LeaseKeepAlive(self._lease_client)
def releaseLease(self):
"""Return the lease on the body."""
if self._lease:
self._lease_client.return_lease(self._lease)
self._lease = None
def release(self):
"""Return the lease on the body and the eStop handle."""
try:
self.releaseLease()
self.releaseEStop()
return True, "Success"
except Exception as e:
return False, str(e)
def disconnect(self):
"""Release control of robot as gracefully as posssible."""
if self._robot.time_sync:
self._robot.time_sync.stop()
self.releaseLease()
self.releaseEStop()
def _robot_command(self, command_proto, end_time_secs=None):
"""Generic blocking function for sending commands to robots.
Args:
command_proto: robot_command_pb2 object to send to the robot. Usually made with RobotCommandBuilder
end_time_secs: (optional) Time-to-live for the command in seconds
"""
try:
id = self._robot_command_client.robot_command(lease=None, command=command_proto, end_time_secs=end_time_secs)
return True, "Success", id
except Exception as e:
return False, str(e), None
def stop(self):
"""Stop the robot's motion."""
response = self._robot_command(RobotCommandBuilder.stop_command())
return response[0], response[1]
def self_right(self):
"""Have the robot self-right itself."""
response = self._robot_command(RobotCommandBuilder.selfright_command())
return response[0], response[1]
def sit(self):
"""Stop the robot's motion and sit down if able."""
response = self._robot_command(RobotCommandBuilder.sit_command())
self._last_sit_command = response[2]
return response[0], response[1]
def stand(self, monitor_command=True):
"""If the e-stop is enabled, and the motor power is enabled, stand the robot up."""
response = self._robot_command(RobotCommandBuilder.stand_command(params=self._mobility_params))
if monitor_command:
self._last_stand_command = response[2]
return response[0], response[1]
def safe_power_off(self):
"""Stop the robot's motion and sit if possible. Once sitting, disable motor power."""
response = self._robot_command(RobotCommandBuilder.safe_power_off_command())
return response[0], response[1]
def power_on(self):
"""Enble the motor power if e-stop is enabled."""
try:
power.power_on(self._power_client)
return True, "Success"
except:
return False, "Error"
def set_mobility_params(self, body_height=0, footprint_R_body=EulerZXY(), locomotion_hint=1, stair_hint=False, external_force_params=None):
"""Define body, locomotion, and stair parameters.
Args:
body_height: Body height in meters
footprint_R_body: (EulerZXY) – The orientation of the body frame with respect to the footprint frame (gravity aligned framed with yaw computed from the stance feet)
locomotion_hint: Locomotion hint
stair_hint: Boolean to define stair motion
"""
self._mobility_params = RobotCommandBuilder.mobility_params(body_height, footprint_R_body, locomotion_hint, stair_hint, external_force_params)
def velocity_cmd(self, v_x, v_y, v_rot, cmd_duration=0.1):
"""Send a velocity motion command to the robot.
Args:
v_x: Velocity in the X direction in meters
v_y: Velocity in the Y direction in meters
v_rot: Angular velocity around the Z axis in radians
cmd_duration: (optional) Time-to-live for the command in seconds. Default is 125ms (assuming 10Hz command rate).
"""
end_time=time.time() + cmd_duration
self._robot_command(RobotCommandBuilder.velocity_command(
v_x=v_x, v_y=v_y, v_rot=v_rot, params=self._mobility_params),
end_time_secs=end_time)
self._last_motion_command_time = end_time
| 20,773 | Python | 42.010352 | 212 | 0.623117 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/doc/spot_ros.rst | spot_ros
========
.. automodule:: spot_ros
:members:
:inherited-members:
:private-members:
:show-inheritance:
| 119 | reStructuredText | 12.333332 | 24 | 0.638655 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/doc/ros_helpers.rst | ros_helpers
===========
.. automodule:: ros_helpers
:members:
:inherited-members:
:private-members:
:show-inheritance:
| 128 | reStructuredText | 13.333332 | 27 | 0.640625 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/doc/index.rst | .. spot_driver documentation master file, created by
sphinx-quickstart on Mon May 11 19:33:41 2020.
You can adapt this file completely to your liking, but it should at least
contain the root `toctree` directive.
Welcome to spot_driver's documentation!
=======================================
.. toctree::
:maxdepth: 2
:caption: ROS Driver
spot_ros
ros_helpers
.. toctree::
:maxdepth: 2
:caption: Spot SDK Wrapper
spot_wrapper
Indices and tables
==================
* :ref:`genindex`
* :ref:`modindex`
* :ref:`search`
| 550 | reStructuredText | 18.678571 | 76 | 0.625455 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/doc/conf.py | import os
import sys
sys.path.insert(0, os.path.abspath('../scripts'))
project = 'spot_driver'
copyright = '2020, Dave Niewinski'
author = 'Dave Niewinski'
extensions = [
'sphinx.ext.autodoc',
'sphinx.ext.coverage',
'sphinx.ext.napoleon'
]
html_theme = 'clearpath-sphinx-theme'
html_theme_path = ["."]
html_static_path = ['./clearpath-sphinx-theme/static']
html_sidebars = {
'**': ['sidebartoc.html', 'sourcelink.html', 'searchbox.html']
}
html_show_sphinx = False
html_logo = 'clearpath-sphinx-theme/static/clearpathlogo.png'
html_favicon = 'clearpath-sphinx-theme/static/favicon.ico'
| 597 | Python | 19.620689 | 65 | 0.710218 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/config/twist_mux.yaml | topics:
- name : bt_joy
topic : bluetooth_teleop/cmd_vel
timeout : 0.5
priority: 9
- name : interactive_marker
topic : twist_marker_server/cmd_vel
timeout : 0.5
priority: 8
locks: []
| 205 | YAML | 17.727271 | 39 | 0.639024 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/spot_ros-CHAMP/spot_driver/config/spot_ros.yaml | rates:
robot_state: 20.0
metrics: 0.04
lease: 1.0
front_image: 10.0
side_image: 10.0
rear_image: 10.0
auto_claim: False
auto_power_on: False
auto_stand: False
| 171 | YAML | 14.636362 | 20 | 0.678363 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/littledog_description-CHAMP/package.xml | <package format="2">
<name>littledog_description</name>
<version>0.0.1</version>
<description>LittleDog Description Package</description>
<license>BSD-3</license>
<author email="[email protected]">Juan Jimeno</author>
<maintainer email="[email protected]">Juan Jimeno</maintainer>
<buildtool_depend>catkin</buildtool_depend>
</package>
| 375 | XML | 27.923075 | 72 | 0.714667 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/BostonDynamics/littledog_description-CHAMP/README.md | A ROS compatible URDF version of [LittleDog](https://github.com/RobotLocomotion/LittleDog)
| 91 | Markdown | 44.999978 | 90 | 0.813187 |
renanmb/Omniverse_legged_robotics/DigitRobot.jl-main/Manifest.toml | # This file is machine-generated - editing it directly is not advised
[[ASL_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "6252039f98492252f9e47c312c8ffda0e3b9e78d"
uuid = "ae81ac8f-d209-56e5-92de-9978fef736f9"
version = "0.1.3+0"
[[Adapt]]
deps = ["LinearAlgebra"]
git-tree-sha1 = "af92965fb30777147966f58acb05da51c5616b5f"
uuid = "79e6a3ab-5dfb-504d-930d-738a2a938a0e"
version = "3.3.3"
[[ArgTools]]
uuid = "0dad84c5-d112-42e6-8d28-ef12dabb789f"
[[Artifacts]]
uuid = "56f22d72-fd6d-98f1-02f0-08ddc0907c33"
[[AssetRegistry]]
deps = ["Distributed", "JSON", "Pidfile", "SHA", "Test"]
git-tree-sha1 = "b25e88db7944f98789130d7b503276bc34bc098e"
uuid = "bf4720bc-e11a-5d0c-854e-bdca1663c893"
version = "0.1.0"
[[AxisAlgorithms]]
deps = ["LinearAlgebra", "Random", "SparseArrays", "WoodburyMatrices"]
git-tree-sha1 = "66771c8d21c8ff5e3a93379480a2307ac36863f7"
uuid = "13072b0f-2c55-5437-9ae7-d433b7a33950"
version = "1.0.1"
[[Base64]]
uuid = "2a0f44e3-6c83-55bd-87e4-b1978d98bd5f"
[[BenchmarkTools]]
deps = ["JSON", "Logging", "Printf", "Profile", "Statistics", "UUIDs"]
git-tree-sha1 = "4c10eee4af024676200bc7752e536f858c6b8f93"
uuid = "6e4b80f9-dd63-53aa-95a3-0cdb28fa8baf"
version = "1.3.1"
[[BinDeps]]
deps = ["Libdl", "Pkg", "SHA", "URIParser", "Unicode"]
git-tree-sha1 = "1289b57e8cf019aede076edab0587eb9644175bd"
uuid = "9e28174c-4ba2-5203-b857-d8d62c4213ee"
version = "1.0.2"
[[BinaryProvider]]
deps = ["Libdl", "Logging", "SHA"]
git-tree-sha1 = "ecdec412a9abc8db54c0efc5548c64dfce072058"
uuid = "b99e7846-7c00-51b0-8f62-c81ae34c0232"
version = "0.5.10"
[[Blink]]
deps = ["Base64", "BinDeps", "Distributed", "JSExpr", "JSON", "Lazy", "Logging", "MacroTools", "Mustache", "Mux", "Reexport", "Sockets", "WebIO", "WebSockets"]
git-tree-sha1 = "08d0b679fd7caa49e2bca9214b131289e19808c0"
uuid = "ad839575-38b3-5650-b840-f874b8c74a25"
version = "0.12.5"
[[Bzip2_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "19a35467a82e236ff51bc17a3a44b69ef35185a2"
uuid = "6e34b625-4abd-537c-b88f-471c36dfa7a0"
version = "1.0.8+0"
[[CSSUtil]]
deps = ["Colors", "JSON", "Markdown", "Measures", "WebIO"]
git-tree-sha1 = "b9fb4b464ec10e860abe251b91d4d049934f7399"
uuid = "70588ee8-6100-5070-97c1-3cb50ed05fe8"
version = "0.1.1"
[[Cairo_jll]]
deps = ["Artifacts", "Bzip2_jll", "Fontconfig_jll", "FreeType2_jll", "Glib_jll", "JLLWrappers", "LZO_jll", "Libdl", "Pixman_jll", "Pkg", "Xorg_libXext_jll", "Xorg_libXrender_jll", "Zlib_jll", "libpng_jll"]
git-tree-sha1 = "4b859a208b2397a7a623a03449e4636bdb17bcf2"
uuid = "83423d85-b0ee-5818-9007-b63ccbeb887a"
version = "1.16.1+1"
[[Calculus]]
deps = ["LinearAlgebra"]
git-tree-sha1 = "f641eb0a4f00c343bbc32346e1217b86f3ce9dad"
uuid = "49dc2e85-a5d0-5ad3-a950-438e2897f1b9"
version = "0.5.1"
[[Cassette]]
git-tree-sha1 = "6ce3cd755d4130d43bab24ea5181e77b89b51839"
uuid = "7057c7e9-c182-5462-911a-8362d720325c"
version = "0.3.9"
[[ChainRulesCore]]
deps = ["Compat", "LinearAlgebra", "SparseArrays"]
git-tree-sha1 = "c9a6160317d1abe9c44b3beb367fd448117679ca"
uuid = "d360d2e6-b24c-11e9-a2a3-2a2ae2dbcce4"
version = "1.13.0"
[[ChangesOfVariables]]
deps = ["ChainRulesCore", "LinearAlgebra", "Test"]
git-tree-sha1 = "bf98fa45a0a4cee295de98d4c1462be26345b9a1"
uuid = "9e997f8a-9a97-42d5-a9f1-ce6bfc15e2c0"
version = "0.1.2"
[[CodeTracking]]
deps = ["InteractiveUtils", "UUIDs"]
git-tree-sha1 = "759a12cefe1cd1bb49e477bc3702287521797483"
uuid = "da1fd8a2-8d9e-5ec2-8556-3022fb5608a2"
version = "1.0.7"
[[CodecBzip2]]
deps = ["Bzip2_jll", "Libdl", "TranscodingStreams"]
git-tree-sha1 = "2e62a725210ce3c3c2e1a3080190e7ca491f18d7"
uuid = "523fee87-0ab8-5b00-afb7-3ecf72e48cfd"
version = "0.7.2"
[[CodecZlib]]
deps = ["TranscodingStreams", "Zlib_jll"]
git-tree-sha1 = "ded953804d019afa9a3f98981d99b33e3db7b6da"
uuid = "944b1d66-785c-5afd-91f1-9de20f533193"
version = "0.7.0"
[[ColorTypes]]
deps = ["FixedPointNumbers", "Random"]
git-tree-sha1 = "32a2b8af383f11cbb65803883837a149d10dfe8a"
uuid = "3da002f7-5984-5a60-b8a6-cbb66c0b333f"
version = "0.10.12"
[[Colors]]
deps = ["ColorTypes", "FixedPointNumbers", "Reexport"]
git-tree-sha1 = "417b0ed7b8b838aa6ca0a87aadf1bb9eb111ce40"
uuid = "5ae59095-9a9b-59fe-a467-6f913c188581"
version = "0.12.8"
[[CommonSubexpressions]]
deps = ["MacroTools", "Test"]
git-tree-sha1 = "7b8a93dba8af7e3b42fecabf646260105ac373f7"
uuid = "bbf7d656-a473-5ed7-a52c-81e309532950"
version = "0.3.0"
[[Compat]]
deps = ["Base64", "Dates", "DelimitedFiles", "Distributed", "InteractiveUtils", "LibGit2", "Libdl", "LinearAlgebra", "Markdown", "Mmap", "Pkg", "Printf", "REPL", "Random", "SHA", "Serialization", "SharedArrays", "Sockets", "SparseArrays", "Statistics", "Test", "UUIDs", "Unicode"]
git-tree-sha1 = "96b0bc6c52df76506efc8a441c6cf1adcb1babc4"
uuid = "34da2185-b29b-5c13-b0c7-acf172513d20"
version = "3.42.0"
[[CompilerSupportLibraries_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "e66e0078-7015-5450-92f7-15fbd957f2ae"
[[CoordinateTransformations]]
deps = ["LinearAlgebra", "StaticArrays"]
git-tree-sha1 = "681ea870b918e7cff7111da58791d7f718067a19"
uuid = "150eb455-5306-5404-9cee-2592286d6298"
version = "0.6.2"
[[DataAPI]]
git-tree-sha1 = "cc70b17275652eb47bc9e5f81635981f13cea5c8"
uuid = "9a962f9c-6df0-11e9-0e5d-c546b8b5ee8a"
version = "1.9.0"
[[DataStructures]]
deps = ["Compat", "InteractiveUtils", "OrderedCollections"]
git-tree-sha1 = "3daef5523dd2e769dad2365274f760ff5f282c7d"
uuid = "864edb3b-99cc-5e75-8d2d-829cb0a9cfe8"
version = "0.18.11"
[[DataValueInterfaces]]
git-tree-sha1 = "bfc1187b79289637fa0ef6d4436ebdfe6905cbd6"
uuid = "e2d170a0-9d28-54be-80f0-106bbe20a464"
version = "1.0.0"
[[Dates]]
deps = ["Printf"]
uuid = "ade2ca70-3891-5945-98fb-dc099432e06a"
[[DelimitedFiles]]
deps = ["Mmap"]
uuid = "8bb1440f-4735-579b-a4ab-409b98df4dab"
[[DiffResults]]
deps = ["StaticArrays"]
git-tree-sha1 = "c18e98cba888c6c25d1c3b048e4b3380ca956805"
uuid = "163ba53b-c6d8-5494-b064-1a9d43ac40c5"
version = "1.0.3"
[[DiffRules]]
deps = ["IrrationalConstants", "LogExpFunctions", "NaNMath", "Random", "SpecialFunctions"]
git-tree-sha1 = "dd933c4ef7b4c270aacd4eb88fa64c147492acf0"
uuid = "b552c78f-8df3-52c6-915a-8e097449b14b"
version = "1.10.0"
[[Distributed]]
deps = ["Random", "Serialization", "Sockets"]
uuid = "8ba89e20-285c-5b6f-9357-94700520ee1b"
[[DocStringExtensions]]
deps = ["LibGit2"]
git-tree-sha1 = "b19534d1895d702889b219c382a6e18010797f0b"
uuid = "ffbed154-4ef7-542d-bbb7-c09d3a79fcae"
version = "0.8.6"
[[Downloads]]
deps = ["ArgTools", "LibCURL", "NetworkOptions"]
uuid = "f43a241f-c20a-4ad4-852c-f6b1247861c6"
[[DualNumbers]]
deps = ["Calculus", "NaNMath", "SpecialFunctions"]
git-tree-sha1 = "90b158083179a6ccbce2c7eb1446d5bf9d7ae571"
uuid = "fa6b7ba4-c1ee-5f82-b5fc-ecf0adba8f74"
version = "0.6.7"
[[EarCut_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "3f3a2501fa7236e9b911e0f7a588c657e822bb6d"
uuid = "5ae413db-bbd1-5e63-b57d-d24a61df00f5"
version = "2.2.3+0"
[[Expat_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "ae13fcbc7ab8f16b0856729b050ef0c446aa3492"
uuid = "2e619515-83b5-522b-bb60-26c02a35a201"
version = "2.4.4+0"
[[FFMPEG]]
deps = ["FFMPEG_jll"]
git-tree-sha1 = "b57e3acbe22f8484b4b5ff66a7499717fe1a9cc8"
uuid = "c87230d0-a227-11e9-1b43-d7ebe4e7570a"
version = "0.4.1"
[[FFMPEG_jll]]
deps = ["Artifacts", "Bzip2_jll", "FreeType2_jll", "FriBidi_jll", "JLLWrappers", "LAME_jll", "Libdl", "Ogg_jll", "OpenSSL_jll", "Opus_jll", "Pkg", "Zlib_jll", "libass_jll", "libfdk_aac_jll", "libvorbis_jll", "x264_jll", "x265_jll"]
git-tree-sha1 = "d8a578692e3077ac998b50c0217dfd67f21d1e5f"
uuid = "b22a6f82-2f65-5046-a5b2-351ab43fb4e5"
version = "4.4.0+0"
[[FileWatching]]
uuid = "7b1f6079-737a-58dc-b8bc-7a2ca5c1b5ee"
[[FixedPointNumbers]]
deps = ["Statistics"]
git-tree-sha1 = "335bfdceacc84c5cdf16aadc768aa5ddfc5383cc"
uuid = "53c48c17-4a7d-5ca2-90c5-79b7896eea93"
version = "0.8.4"
[[Fontconfig_jll]]
deps = ["Artifacts", "Bzip2_jll", "Expat_jll", "FreeType2_jll", "JLLWrappers", "Libdl", "Libuuid_jll", "Pkg", "Zlib_jll"]
git-tree-sha1 = "21efd19106a55620a188615da6d3d06cd7f6ee03"
uuid = "a3f928ae-7b40-5064-980b-68af3947d34b"
version = "2.13.93+0"
[[ForwardDiff]]
deps = ["CommonSubexpressions", "DiffResults", "DiffRules", "LinearAlgebra", "LogExpFunctions", "NaNMath", "Preferences", "Printf", "Random", "SpecialFunctions", "StaticArrays"]
git-tree-sha1 = "1bd6fc0c344fc0cbee1f42f8d2e7ec8253dda2d2"
uuid = "f6369f11-7733-5829-9624-2563aa707210"
version = "0.10.25"
[[FreeType2_jll]]
deps = ["Artifacts", "Bzip2_jll", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
git-tree-sha1 = "87eb71354d8ec1a96d4a7636bd57a7347dde3ef9"
uuid = "d7e528f0-a631-5988-bf34-fe36492bcfd7"
version = "2.10.4+0"
[[FriBidi_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "aa31987c2ba8704e23c6c8ba8a4f769d5d7e4f91"
uuid = "559328eb-81f9-559d-9380-de523a88c83c"
version = "1.0.10+0"
[[FunctionalCollections]]
deps = ["Test"]
git-tree-sha1 = "04cb9cfaa6ba5311973994fe3496ddec19b6292a"
uuid = "de31a74c-ac4f-5751-b3fd-e18cd04993ca"
version = "0.5.0"
[[GeometryBasics]]
deps = ["EarCut_jll", "IterTools", "LinearAlgebra", "StaticArrays", "StructArrays", "Tables"]
git-tree-sha1 = "4136b8a5668341e58398bb472754bff4ba0456ff"
uuid = "5c1252a2-5f33-56bf-86c9-59e7332b4326"
version = "0.3.12"
[[Gettext_jll]]
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "Libiconv_jll", "Pkg", "XML2_jll"]
git-tree-sha1 = "9b02998aba7bf074d14de89f9d37ca24a1a0b046"
uuid = "78b55507-aeef-58d4-861c-77aaff3498b1"
version = "0.21.0+0"
[[Glib_jll]]
deps = ["Artifacts", "Gettext_jll", "JLLWrappers", "Libdl", "Libffi_jll", "Libiconv_jll", "Libmount_jll", "PCRE_jll", "Pkg", "Zlib_jll"]
git-tree-sha1 = "a32d672ac2c967f3deb8a81d828afc739c838a06"
uuid = "7746bdde-850d-59dc-9ae8-88ece973131d"
version = "2.68.3+2"
[[Graphite2_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "344bf40dcab1073aca04aa0df4fb092f920e4011"
uuid = "3b182d85-2403-5c21-9c21-1e1f0cc25472"
version = "1.3.14+0"
[[HTTP]]
deps = ["Base64", "Dates", "IniFile", "Logging", "MbedTLS", "NetworkOptions", "Sockets", "URIs"]
git-tree-sha1 = "0fa77022fe4b511826b39c894c90daf5fce3334a"
uuid = "cd3eb016-35fb-5094-929b-558a96fad6f3"
version = "0.9.17"
[[HarfBuzz_jll]]
deps = ["Artifacts", "Cairo_jll", "Fontconfig_jll", "FreeType2_jll", "Glib_jll", "Graphite2_jll", "JLLWrappers", "Libdl", "Libffi_jll", "Pkg"]
git-tree-sha1 = "129acf094d168394e80ee1dc4bc06ec835e510a3"
uuid = "2e76f6c2-a576-52d4-95c1-20adfe4de566"
version = "2.8.1+1"
[[Hiccup]]
deps = ["MacroTools", "Test"]
git-tree-sha1 = "6187bb2d5fcbb2007c39e7ac53308b0d371124bd"
uuid = "9fb69e20-1954-56bb-a84f-559cc56a8ff7"
version = "0.2.2"
[[IniFile]]
git-tree-sha1 = "f550e6e32074c939295eb5ea6de31849ac2c9625"
uuid = "83e8ac13-25f8-5344-8a64-a9f2b223428f"
version = "0.5.1"
[[InteractBase]]
deps = ["Base64", "CSSUtil", "Colors", "Dates", "JSExpr", "JSON", "Knockout", "Observables", "OrderedCollections", "Random", "WebIO", "Widgets"]
git-tree-sha1 = "3ace4760fab1c9700ec9c68ab0e36e0856f05556"
uuid = "d3863d7c-f0c8-5437-a7b4-3ae773c01009"
version = "0.10.8"
[[InteractiveUtils]]
deps = ["Markdown"]
uuid = "b77e0a4c-d291-57a0-90e8-8db25a27a240"
[[Interpolations]]
deps = ["AxisAlgorithms", "ChainRulesCore", "LinearAlgebra", "OffsetArrays", "Random", "Ratios", "Requires", "SharedArrays", "SparseArrays", "StaticArrays", "WoodburyMatrices"]
git-tree-sha1 = "b15fc0a95c564ca2e0a7ae12c1f095ca848ceb31"
uuid = "a98d9a8b-a2ab-59e6-89dd-64a1c18fca59"
version = "0.13.5"
[[InverseFunctions]]
deps = ["Test"]
git-tree-sha1 = "a7254c0acd8e62f1ac75ad24d5db43f5f19f3c65"
uuid = "3587e190-3f89-42d0-90ee-14403ec27112"
version = "0.1.2"
[[Ipopt]]
deps = ["BinaryProvider", "Ipopt_jll", "Libdl", "LinearAlgebra", "MathOptInterface", "MathProgBase"]
git-tree-sha1 = "539b23ab8fb86c6cc3e8cacaeb1f784415951be5"
uuid = "b6b21f68-93f8-5de0-b562-5493be1d77c9"
version = "0.8.0"
[[Ipopt_jll]]
deps = ["ASL_jll", "Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "MUMPS_seq_jll", "OpenBLAS32_jll", "Pkg"]
git-tree-sha1 = "82124f27743f2802c23fcb05febc517d0b15d86e"
uuid = "9cc047cb-c261-5740-88fc-0cf96f7bdcc7"
version = "3.13.4+2"
[[IrrationalConstants]]
git-tree-sha1 = "7fd44fd4ff43fc60815f8e764c0f352b83c49151"
uuid = "92d709cd-6900-40b7-9082-c6be49f344b6"
version = "0.1.1"
[[IterTools]]
git-tree-sha1 = "fa6287a4469f5e048d763df38279ee729fbd44e5"
uuid = "c8e1da08-722c-5040-9ed9-7db0dc04731e"
version = "1.4.0"
[[IteratorInterfaceExtensions]]
git-tree-sha1 = "a3f24677c21f5bbe9d2a714f95dcd58337fb2856"
uuid = "82899510-4779-5014-852e-03e436cf321d"
version = "1.0.0"
[[JLLWrappers]]
deps = ["Preferences"]
git-tree-sha1 = "abc9885a7ca2052a736a600f7fa66209f96506e1"
uuid = "692b3bcd-3c85-4b1f-b108-f13ce0eb3210"
version = "1.4.1"
[[JSExpr]]
deps = ["JSON", "MacroTools", "Observables", "WebIO"]
git-tree-sha1 = "bd6c034156b1e7295450a219c4340e32e50b08b1"
uuid = "97c1335a-c9c5-57fe-bc5d-ec35cebe8660"
version = "0.5.3"
[[JSON]]
deps = ["Dates", "Mmap", "Parsers", "Unicode"]
git-tree-sha1 = "3c837543ddb02250ef42f4738347454f95079d4e"
uuid = "682c06a0-de6a-54ab-a142-c8b1cf79cde6"
version = "0.21.3"
[[JuMP]]
deps = ["Calculus", "DataStructures", "ForwardDiff", "JSON", "LinearAlgebra", "MathOptInterface", "MutableArithmetics", "NaNMath", "Printf", "Random", "SparseArrays", "SpecialFunctions", "Statistics"]
git-tree-sha1 = "85c32b6cd8b3b174582b9b38ce0fca4bc19a77b6"
uuid = "4076af6c-e467-56ae-b986-b466b2749572"
version = "0.22.0"
[[JuliaInterpreter]]
deps = ["CodeTracking", "InteractiveUtils", "Random", "UUIDs"]
git-tree-sha1 = "e273807f38074f033d94207a201e6e827d8417db"
uuid = "aa1ae85d-cabe-5617-a682-6adf51b2e16a"
version = "0.8.21"
[[Knockout]]
deps = ["JSExpr", "JSON", "Observables", "Test", "WebIO"]
git-tree-sha1 = "deb74017e1061d76050ff68d219217413be4ef59"
uuid = "bcebb21b-c2e3-54f8-a781-646b90f6d2cc"
version = "0.2.5"
[[LAME_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "f6250b16881adf048549549fba48b1161acdac8c"
uuid = "c1c5ebd0-6772-5130-a774-d5fcae4a789d"
version = "3.100.1+0"
[[LZO_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "e5b909bcf985c5e2605737d2ce278ed791b89be6"
uuid = "dd4b983a-f0e5-5f8d-a1b7-129d4a5fb1ac"
version = "2.10.1+0"
[[Lazy]]
deps = ["MacroTools"]
git-tree-sha1 = "1370f8202dac30758f3c345f9909b97f53d87d3f"
uuid = "50d2b5c4-7a5e-59d5-8109-a42b560f39c0"
version = "0.15.1"
[[LibCURL]]
deps = ["LibCURL_jll", "MozillaCACerts_jll"]
uuid = "b27032c2-a3e7-50c8-80cd-2d36dbcbfd21"
[[LibCURL_jll]]
deps = ["Artifacts", "LibSSH2_jll", "Libdl", "MbedTLS_jll", "Zlib_jll", "nghttp2_jll"]
uuid = "deac9b47-8bc7-5906-a0fe-35ac56dc84c0"
[[LibGit2]]
deps = ["Base64", "NetworkOptions", "Printf", "SHA"]
uuid = "76f85450-5226-5b5a-8eaa-529ad045b433"
[[LibSSH2_jll]]
deps = ["Artifacts", "Libdl", "MbedTLS_jll"]
uuid = "29816b5a-b9ab-546f-933c-edad1886dfa8"
[[Libdl]]
uuid = "8f399da3-3557-5675-b5ff-fb832c97cbdb"
[[Libffi_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "0b4a5d71f3e5200a7dff793393e09dfc2d874290"
uuid = "e9f186c6-92d2-5b65-8a66-fee21dc1b490"
version = "3.2.2+1"
[[Libgcrypt_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libgpg_error_jll", "Pkg"]
git-tree-sha1 = "64613c82a59c120435c067c2b809fc61cf5166ae"
uuid = "d4300ac3-e22c-5743-9152-c294e39db1e4"
version = "1.8.7+0"
[[Libgpg_error_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "c333716e46366857753e273ce6a69ee0945a6db9"
uuid = "7add5ba3-2f88-524e-9cd5-f83b8a55f7b8"
version = "1.42.0+0"
[[Libiconv_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "42b62845d70a619f063a7da093d995ec8e15e778"
uuid = "94ce4f54-9a6c-5748-9c1c-f9c7231a4531"
version = "1.16.1+1"
[[Libmount_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "9c30530bf0effd46e15e0fdcf2b8636e78cbbd73"
uuid = "4b2f31a3-9ecc-558c-b454-b3730dcb73e9"
version = "2.35.0+0"
[[Libuuid_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "7f3efec06033682db852f8b3bc3c1d2b0a0ab066"
uuid = "38a345b3-de98-5d2b-a5d3-14cd9215e700"
version = "2.36.0+0"
[[LightXML]]
deps = ["Libdl", "XML2_jll"]
git-tree-sha1 = "e129d9391168c677cd4800f5c0abb1ed8cb3794f"
uuid = "9c8b4983-aa76-5018-a973-4c85ecc9e179"
version = "0.9.0"
[[LinearAlgebra]]
deps = ["Libdl"]
uuid = "37e2e46d-f89d-539d-b4ee-838fcccc9c8e"
[[LogExpFunctions]]
deps = ["ChainRulesCore", "ChangesOfVariables", "DocStringExtensions", "InverseFunctions", "IrrationalConstants", "LinearAlgebra"]
git-tree-sha1 = "3f7cb7157ef860c637f3f4929c8ed5d9716933c6"
uuid = "2ab3a3ac-af41-5b50-aa03-7779005ae688"
version = "0.3.7"
[[Logging]]
uuid = "56ddb016-857b-54e1-b83d-db4d58db5568"
[[LoopThrottle]]
deps = ["Test"]
git-tree-sha1 = "037fdda7c47bf8fc2f46b1096972afabd26fa636"
uuid = "39f5be34-8529-5463-bac7-bf6867c840a3"
version = "0.1.0"
[[LoweredCodeUtils]]
deps = ["JuliaInterpreter"]
git-tree-sha1 = "491a883c4fef1103077a7f648961adbf9c8dd933"
uuid = "6f1432cf-f94c-5a45-995e-cdbf5db27b0b"
version = "2.1.2"
[[METIS_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "1d31872bb9c5e7ec1f618e8c4a56c8b0d9bddc7e"
uuid = "d00139f3-1899-568f-a2f0-47f597d42d70"
version = "5.1.1+0"
[[MUMPS_seq_jll]]
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "METIS_jll", "OpenBLAS32_jll", "Pkg"]
git-tree-sha1 = "1a11a84b2af5feb5a62a820574804056cdc59c39"
uuid = "d7ed1dd3-d0ae-5e8e-bfb4-87a502085b8d"
version = "5.2.1+4"
[[MacroTools]]
deps = ["Markdown", "Random"]
git-tree-sha1 = "3d3e902b31198a27340d0bf00d6ac452866021cf"
uuid = "1914dd2f-81c6-5fcd-8719-6d5c9610ff09"
version = "0.5.9"
[[Markdown]]
deps = ["Base64"]
uuid = "d6f4376e-aef5-505a-96c1-9c027394607a"
[[MathOptInterface]]
deps = ["BenchmarkTools", "CodecBzip2", "CodecZlib", "JSON", "LinearAlgebra", "MutableArithmetics", "OrderedCollections", "Printf", "SparseArrays", "Test", "Unicode"]
git-tree-sha1 = "e8c9653877adcf8f3e7382985e535bb37b083598"
uuid = "b8f27783-ece8-5eb3-8dc8-9495eed66fee"
version = "0.10.9"
[[MathProgBase]]
deps = ["LinearAlgebra", "SparseArrays"]
git-tree-sha1 = "9abbe463a1e9fc507f12a69e7f29346c2cdc472c"
uuid = "fdba3010-5040-5b88-9595-932c9decdf73"
version = "0.7.8"
[[MbedTLS]]
deps = ["Dates", "MbedTLS_jll", "Random", "Sockets"]
git-tree-sha1 = "1c38e51c3d08ef2278062ebceade0e46cefc96fe"
uuid = "739be429-bea8-5141-9913-cc70e7f3736d"
version = "1.0.3"
[[MbedTLS_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "c8ffd9c3-330d-5841-b78e-0817d7145fa1"
[[Measures]]
git-tree-sha1 = "e498ddeee6f9fdb4551ce855a46f54dbd900245f"
uuid = "442fdcdd-2543-5da2-b0f3-8c86c306513e"
version = "0.3.1"
[[MechanismGeometries]]
deps = ["ColorTypes", "CoordinateTransformations", "GeometryBasics", "LightXML", "LinearAlgebra", "RigidBodyDynamics", "Rotations", "StaticArrays"]
git-tree-sha1 = "0b1c87463f24bd18a9685601e6bf6207cd051cdd"
uuid = "931e9471-e8fb-5385-a477-07ad12718aca"
version = "0.7.1"
[[MeshCat]]
deps = ["Base64", "BinDeps", "Blink", "Cassette", "Colors", "CoordinateTransformations", "DocStringExtensions", "FFMPEG", "GeometryBasics", "LinearAlgebra", "Logging", "MsgPack", "Mux", "Parameters", "Requires", "Rotations", "Sockets", "StaticArrays", "UUIDs", "WebSockets"]
git-tree-sha1 = "ca4a1e45f5d2a2148c599804a6619da7708ede69"
uuid = "283c5d60-a78f-5afe-a0af-af636b173e11"
version = "0.13.2"
[[MeshCatMechanisms]]
deps = ["ColorTypes", "CoordinateTransformations", "GeometryBasics", "InteractBase", "Interpolations", "LoopThrottle", "MechanismGeometries", "MeshCat", "Mux", "OrderedCollections", "RigidBodyDynamics"]
git-tree-sha1 = "f6a8ab14b6db107540d533a8ed3bd21fd22acca7"
uuid = "6ad125db-dd91-5488-b820-c1df6aab299d"
version = "0.8.1"
[[Mmap]]
uuid = "a63ad114-7e13-5084-954f-fe012c677804"
[[MozillaCACerts_jll]]
uuid = "14a3606d-f60d-562e-9121-12d972cd8159"
[[MsgPack]]
deps = ["Serialization"]
git-tree-sha1 = "a8cbf066b54d793b9a48c5daa5d586cf2b5bd43d"
uuid = "99f44e22-a591-53d1-9472-aa23ef4bd671"
version = "1.1.0"
[[Mustache]]
deps = ["Printf", "Tables"]
git-tree-sha1 = "bfbd6fb946d967794498790aa7a0e6cdf1120f41"
uuid = "ffc61752-8dc7-55ee-8c37-f3e9cdd09e70"
version = "1.0.13"
[[MutableArithmetics]]
deps = ["LinearAlgebra", "SparseArrays", "Test"]
git-tree-sha1 = "842b5ccd156e432f369b204bb704fd4020e383ac"
uuid = "d8a4904e-b15c-11e9-3269-09a3773c0cb0"
version = "0.3.3"
[[Mux]]
deps = ["AssetRegistry", "Base64", "HTTP", "Hiccup", "Pkg", "Sockets", "WebSockets"]
git-tree-sha1 = "82dfb2cead9895e10ee1b0ca37a01088456c4364"
uuid = "a975b10e-0019-58db-a62f-e48ff68538c9"
version = "0.7.6"
[[NaNMath]]
git-tree-sha1 = "b086b7ea07f8e38cf122f5016af580881ac914fe"
uuid = "77ba4419-2d1f-58cd-9bb1-8ffee604a2e3"
version = "0.3.7"
[[NetworkOptions]]
uuid = "ca575930-c2e3-43a9-ace4-1e988b2c1908"
[[Observables]]
git-tree-sha1 = "fe29afdef3d0c4a8286128d4e45cc50621b1e43d"
uuid = "510215fc-4207-5dde-b226-833fc4488ee2"
version = "0.4.0"
[[OffsetArrays]]
deps = ["Adapt"]
git-tree-sha1 = "043017e0bdeff61cfbb7afeb558ab29536bbb5ed"
uuid = "6fe1bfb0-de20-5000-8ca7-80f57d26f881"
version = "1.10.8"
[[Ogg_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "887579a3eb005446d514ab7aeac5d1d027658b8f"
uuid = "e7412a2a-1a6e-54c0-be00-318e2571c051"
version = "1.3.5+1"
[[OpenBLAS32_jll]]
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "ba4a8f683303c9082e84afba96f25af3c7fb2436"
uuid = "656ef2d0-ae68-5445-9ca0-591084a874a2"
version = "0.3.12+1"
[[OpenLibm_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "05823500-19ac-5b8b-9628-191a04bc5112"
[[OpenSSL_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "648107615c15d4e09f7eca16307bc821c1f718d8"
uuid = "458c3c95-2e84-50aa-8efc-19380b2a3a95"
version = "1.1.13+0"
[[OpenSpecFun_jll]]
deps = ["Artifacts", "CompilerSupportLibraries_jll", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "13652491f6856acfd2db29360e1bbcd4565d04f1"
uuid = "efe28fd5-8261-553b-a9e1-b2916fc3738e"
version = "0.5.5+0"
[[Opus_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "51a08fb14ec28da2ec7a927c4337e4332c2a4720"
uuid = "91d4177d-7536-5919-b921-800302f37372"
version = "1.3.2+0"
[[OrderedCollections]]
git-tree-sha1 = "85f8e6578bf1f9ee0d11e7bb1b1456435479d47c"
uuid = "bac558e1-5e72-5ebc-8fee-abe8a469f55d"
version = "1.4.1"
[[PCRE_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "b2a7af664e098055a7529ad1a900ded962bca488"
uuid = "2f80f16e-611a-54ab-bc61-aa92de5b98fc"
version = "8.44.0+0"
[[Parameters]]
deps = ["OrderedCollections", "UnPack"]
git-tree-sha1 = "34c0e9ad262e5f7fc75b10a9952ca7692cfc5fbe"
uuid = "d96e819e-fc66-5662-9728-84c9c7592b0a"
version = "0.12.3"
[[Parsers]]
deps = ["Dates"]
git-tree-sha1 = "85b5da0fa43588c75bb1ff986493443f821c70b7"
uuid = "69de0a69-1ddd-5017-9359-2bf0b02dc9f0"
version = "2.2.3"
[[Pidfile]]
deps = ["FileWatching", "Test"]
git-tree-sha1 = "2d8aaf8ee10df53d0dfb9b8ee44ae7c04ced2b03"
uuid = "fa939f87-e72e-5be4-a000-7fc836dbe307"
version = "1.3.0"
[[Pixman_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "b4f5d02549a10e20780a24fce72bea96b6329e29"
uuid = "30392449-352a-5448-841d-b1acce4e97dc"
version = "0.40.1+0"
[[Pkg]]
deps = ["Artifacts", "Dates", "Downloads", "LibGit2", "Libdl", "Logging", "Markdown", "Printf", "REPL", "Random", "SHA", "Serialization", "TOML", "Tar", "UUIDs", "p7zip_jll"]
uuid = "44cfe95a-1eb2-52ea-b672-e2afdf69b78f"
[[Preferences]]
deps = ["TOML"]
git-tree-sha1 = "de893592a221142f3db370f48290e3a2ef39998f"
uuid = "21216c6a-2e73-6563-6e65-726566657250"
version = "1.2.4"
[[Printf]]
deps = ["Unicode"]
uuid = "de0858da-6303-5e67-8744-51eddeeeb8d7"
[[Profile]]
deps = ["Printf"]
uuid = "9abbd945-dff8-562f-b5e8-e1ebf5ef1b79"
[[Quaternions]]
deps = ["DualNumbers", "LinearAlgebra", "Random"]
git-tree-sha1 = "0b345302b17b0e694092621915de0e0dc7443a1a"
uuid = "94ee1d12-ae83-5a48-8b1c-48b8ff168ae0"
version = "0.4.9"
[[REPL]]
deps = ["InteractiveUtils", "Markdown", "Sockets", "Unicode"]
uuid = "3fa0cd96-eef1-5676-8a61-b3b8758bbffb"
[[Random]]
deps = ["Serialization"]
uuid = "9a3f8284-a2c9-5f02-9a11-845980a1fd5c"
[[Ratios]]
deps = ["Requires"]
git-tree-sha1 = "dc84268fe0e3335a62e315a3a7cf2afa7178a734"
uuid = "c84ed2f1-dad5-54f0-aa8e-dbefe2724439"
version = "0.4.3"
[[Reexport]]
git-tree-sha1 = "45e428421666073eab6f2da5c9d310d99bb12f9b"
uuid = "189a3867-3050-52da-a836-e630ba90ab69"
version = "1.2.2"
[[Requires]]
deps = ["UUIDs"]
git-tree-sha1 = "838a3a4188e2ded87a4f9f184b4b0d78a1e91cb7"
uuid = "ae029012-a4dd-5104-9daa-d747884805df"
version = "1.3.0"
[[Revise]]
deps = ["CodeTracking", "Distributed", "FileWatching", "JuliaInterpreter", "LibGit2", "LoweredCodeUtils", "OrderedCollections", "Pkg", "REPL", "Requires", "UUIDs", "Unicode"]
git-tree-sha1 = "41deb3df28ecf75307b6e492a738821b031f8425"
uuid = "295af30f-e4ad-537b-8983-00126c2a3abe"
version = "3.1.20"
[[RigidBodyDynamics]]
deps = ["DocStringExtensions", "LightXML", "LinearAlgebra", "LoopThrottle", "Random", "Reexport", "Rotations", "SparseArrays", "StaticArrays", "TypeSortedCollections", "UnsafeArrays"]
git-tree-sha1 = "067a44aead5b587252e8cf73773cca422c6ae19b"
uuid = "366cf18f-59d5-5db9-a4de-86a9f6786172"
version = "2.3.2"
[[Rotations]]
deps = ["LinearAlgebra", "Quaternions", "Random", "StaticArrays", "Statistics"]
git-tree-sha1 = "405148000e80f70b31e7732ea93288aecb1793fa"
uuid = "6038ab10-8711-5258-84ad-4b1120ba62dc"
version = "1.2.0"
[[SHA]]
uuid = "ea8e919c-243c-51af-8825-aaa63cd721ce"
[[Serialization]]
uuid = "9e88b42a-f829-5b0c-bbe9-9e923198166b"
[[SharedArrays]]
deps = ["Distributed", "Mmap", "Random", "Serialization"]
uuid = "1a1011a3-84de-559e-8e89-a11a2f7dc383"
[[Sockets]]
uuid = "6462fe0b-24de-5631-8697-dd941f90decc"
[[SparseArrays]]
deps = ["LinearAlgebra", "Random"]
uuid = "2f01184e-e22b-5df5-ae63-d93ebab69eaf"
[[SpecialFunctions]]
deps = ["ChainRulesCore", "IrrationalConstants", "LogExpFunctions", "OpenLibm_jll", "OpenSpecFun_jll"]
git-tree-sha1 = "cbf21db885f478e4bd73b286af6e67d1beeebe4c"
uuid = "276daf66-3868-5448-9aa4-cd146d93841b"
version = "1.8.4"
[[StaticArrays]]
deps = ["LinearAlgebra", "Random", "Statistics"]
git-tree-sha1 = "da4cf579416c81994afd6322365d00916c79b8ae"
uuid = "90137ffa-7385-5640-81b9-e52037218182"
version = "0.12.5"
[[Statistics]]
deps = ["LinearAlgebra", "SparseArrays"]
uuid = "10745b16-79ce-11e8-11f9-7d13ad32a3b2"
[[StructArrays]]
deps = ["Adapt", "DataAPI", "Tables"]
git-tree-sha1 = "44b3afd37b17422a62aea25f04c1f7e09ce6b07f"
uuid = "09ab397b-f2b6-538f-b94a-2f83cf4a842a"
version = "0.5.1"
[[TOML]]
deps = ["Dates"]
uuid = "fa267f1f-6049-4f14-aa54-33bafae1ed76"
[[TableTraits]]
deps = ["IteratorInterfaceExtensions"]
git-tree-sha1 = "c06b2f539df1c6efa794486abfb6ed2022561a39"
uuid = "3783bdb8-4a98-5b6b-af9a-565f29a5fe9c"
version = "1.0.1"
[[Tables]]
deps = ["DataAPI", "DataValueInterfaces", "IteratorInterfaceExtensions", "LinearAlgebra", "OrderedCollections", "TableTraits", "Test"]
git-tree-sha1 = "5ce79ce186cc678bbb5c5681ca3379d1ddae11a1"
uuid = "bd369af6-aec1-5ad0-b16a-f7cc5008161c"
version = "1.7.0"
[[Tar]]
deps = ["ArgTools", "SHA"]
uuid = "a4e569a6-e804-4fa4-b0f3-eef7a1d5b13e"
[[Test]]
deps = ["InteractiveUtils", "Logging", "Random", "Serialization"]
uuid = "8dfed614-e22c-5e08-85e1-65c5234f0b40"
[[TranscodingStreams]]
deps = ["Random", "Test"]
git-tree-sha1 = "216b95ea110b5972db65aa90f88d8d89dcb8851c"
uuid = "3bb67fe8-82b1-5028-8e26-92a6c54297fa"
version = "0.9.6"
[[TypeSortedCollections]]
git-tree-sha1 = "d539b357e7695d80c75f5b066cec5d8c45886ab2"
uuid = "94a5cd58-49a0-5741-bd07-fa4f4be8babf"
version = "1.1.0"
[[URIParser]]
deps = ["Unicode"]
git-tree-sha1 = "53a9f49546b8d2dd2e688d216421d050c9a31d0d"
uuid = "30578b45-9adc-5946-b283-645ec420af67"
version = "0.4.1"
[[URIs]]
git-tree-sha1 = "97bbe755a53fe859669cd907f2d96aee8d2c1355"
uuid = "5c2747f8-b7ea-4ff2-ba2e-563bfd36b1d4"
version = "1.3.0"
[[UUIDs]]
deps = ["Random", "SHA"]
uuid = "cf7118a7-6976-5b1a-9a39-7adc72f591a4"
[[UnPack]]
git-tree-sha1 = "387c1f73762231e86e0c9c5443ce3b4a0a9a0c2b"
uuid = "3a884ed6-31ef-47d7-9d2a-63182c4928ed"
version = "1.0.2"
[[Unicode]]
uuid = "4ec0a83e-493e-50e2-b9ac-8f72acf5a8f5"
[[UnsafeArrays]]
git-tree-sha1 = "038cd6ae292c857e6f91be52b81236607627aacd"
uuid = "c4a57d5a-5b31-53a6-b365-19f8c011fbd6"
version = "1.0.3"
[[WebIO]]
deps = ["AssetRegistry", "Base64", "Distributed", "FunctionalCollections", "JSON", "Logging", "Observables", "Pkg", "Random", "Requires", "Sockets", "UUIDs", "WebSockets", "Widgets"]
git-tree-sha1 = "c9529be473e97fa0b3b2642cdafcd0896b4c9494"
uuid = "0f1e0344-ec1d-5b48-a673-e5cf874b6c29"
version = "0.8.17"
[[WebSockets]]
deps = ["Base64", "Dates", "HTTP", "Logging", "Sockets"]
git-tree-sha1 = "f91a602e25fe6b89afc93cf02a4ae18ee9384ce3"
uuid = "104b5d7c-a370-577a-8038-80a2059c5097"
version = "1.5.9"
[[Widgets]]
deps = ["Colors", "Dates", "Observables", "OrderedCollections"]
git-tree-sha1 = "505c31f585405fc375d99d02588f6ceaba791241"
uuid = "cc8bc4a8-27d6-5769-a93b-9d913e69aa62"
version = "0.6.5"
[[WoodburyMatrices]]
deps = ["LinearAlgebra", "SparseArrays"]
git-tree-sha1 = "de67fa59e33ad156a590055375a30b23c40299d3"
uuid = "efce3f68-66dc-5838-9240-27a6d6f5f9b6"
version = "0.5.5"
[[XML2_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libiconv_jll", "Pkg", "Zlib_jll"]
git-tree-sha1 = "1acf5bdf07aa0907e0a37d3718bb88d4b687b74a"
uuid = "02c8fc9c-b97f-50b9-bbe4-9be30ff0a78a"
version = "2.9.12+0"
[[XSLT_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Libgcrypt_jll", "Libgpg_error_jll", "Libiconv_jll", "Pkg", "XML2_jll", "Zlib_jll"]
git-tree-sha1 = "91844873c4085240b95e795f692c4cec4d805f8a"
uuid = "aed1982a-8fda-507f-9586-7b0439959a61"
version = "1.1.34+0"
[[Xorg_libX11_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libxcb_jll", "Xorg_xtrans_jll"]
git-tree-sha1 = "5be649d550f3f4b95308bf0183b82e2582876527"
uuid = "4f6342f7-b3d2-589e-9d20-edeb45f2b2bc"
version = "1.6.9+4"
[[Xorg_libXau_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "4e490d5c960c314f33885790ed410ff3a94ce67e"
uuid = "0c0b7dd1-d40b-584c-a123-a41640f87eec"
version = "1.0.9+4"
[[Xorg_libXdmcp_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "4fe47bd2247248125c428978740e18a681372dd4"
uuid = "a3789734-cfe1-5b06-b2d0-1dd0d9d62d05"
version = "1.1.3+4"
[[Xorg_libXext_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
git-tree-sha1 = "b7c0aa8c376b31e4852b360222848637f481f8c3"
uuid = "1082639a-0dae-5f34-9b06-72781eeb8cb3"
version = "1.3.4+4"
[[Xorg_libXrender_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Xorg_libX11_jll"]
git-tree-sha1 = "19560f30fd49f4d4efbe7002a1037f8c43d43b96"
uuid = "ea2f1a96-1ddc-540d-b46f-429655e07cfa"
version = "0.9.10+4"
[[Xorg_libpthread_stubs_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "6783737e45d3c59a4a4c4091f5f88cdcf0908cbb"
uuid = "14d82f49-176c-5ed1-bb49-ad3f5cbd8c74"
version = "0.1.0+3"
[[Xorg_libxcb_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "XSLT_jll", "Xorg_libXau_jll", "Xorg_libXdmcp_jll", "Xorg_libpthread_stubs_jll"]
git-tree-sha1 = "daf17f441228e7a3833846cd048892861cff16d6"
uuid = "c7cfdc94-dc32-55de-ac96-5a1b8d977c5b"
version = "1.13.0+3"
[[Xorg_xtrans_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "79c31e7844f6ecf779705fbc12146eb190b7d845"
uuid = "c5fb5394-a638-5e4d-96e5-b29de1b5cf10"
version = "1.4.0+3"
[[Zlib_jll]]
deps = ["Libdl"]
uuid = "83775a58-1f1d-513f-b197-d71354ab007a"
[[libass_jll]]
deps = ["Artifacts", "Bzip2_jll", "FreeType2_jll", "FriBidi_jll", "HarfBuzz_jll", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
git-tree-sha1 = "5982a94fcba20f02f42ace44b9894ee2b140fe47"
uuid = "0ac62f75-1d6f-5e53-bd7c-93b484bb37c0"
version = "0.15.1+0"
[[libfdk_aac_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "daacc84a041563f965be61859a36e17c4e4fcd55"
uuid = "f638f0a6-7fb0-5443-88ba-1cc74229b280"
version = "2.0.2+0"
[[libpng_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg", "Zlib_jll"]
git-tree-sha1 = "94d180a6d2b5e55e447e2d27a29ed04fe79eb30c"
uuid = "b53b4c65-9356-5827-b1ea-8c7a1a84506f"
version = "1.6.38+0"
[[libvorbis_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Ogg_jll", "Pkg"]
git-tree-sha1 = "b910cb81ef3fe6e78bf6acee440bda86fd6ae00c"
uuid = "f27f6e37-5d2b-51aa-960f-b287f2bc3b7a"
version = "1.3.7+1"
[[nghttp2_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "8e850ede-7688-5339-a07c-302acd2aaf8d"
[[p7zip_jll]]
deps = ["Artifacts", "Libdl"]
uuid = "3f19e933-33d8-53b3-aaab-bd5110c3b7a0"
[[x264_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "4fea590b89e6ec504593146bf8b988b2c00922b2"
uuid = "1270edf5-f2f9-52d2-97e9-ab00b5d0237a"
version = "2021.5.5+0"
[[x265_jll]]
deps = ["Artifacts", "JLLWrappers", "Libdl", "Pkg"]
git-tree-sha1 = "ee567a171cce03570d77ad3a43e90218e38937a9"
uuid = "dfaa095f-4041-5dcd-9319-2fabd8486b76"
version = "3.5.0+0"
| 33,099 | TOML | 32.333333 | 280 | 0.731412 |
renanmb/Omniverse_legged_robotics/DigitRobot.jl-main/Project.toml | name = "DigitRobot"
uuid = "223e9ca1-e7df-42fa-ba56-f1a405a9c000"
authors = ["Alphonsus Adu-Bredu <[email protected]>"]
version = "0.1.0"
[deps]
Colors = "5ae59095-9a9b-59fe-a467-6f913c188581"
CoordinateTransformations = "150eb455-5306-5404-9cee-2592286d6298"
GeometryBasics = "5c1252a2-5f33-56bf-86c9-59e7332b4326"
Ipopt = "b6b21f68-93f8-5de0-b562-5493be1d77c9"
JuMP = "4076af6c-e467-56ae-b986-b466b2749572"
MeshCat = "283c5d60-a78f-5afe-a0af-af636b173e11"
MeshCatMechanisms = "6ad125db-dd91-5488-b820-c1df6aab299d"
Revise = "295af30f-e4ad-537b-8983-00126c2a3abe"
RigidBodyDynamics = "366cf18f-59d5-5db9-a4de-86a9f6786172"
Rotations = "6038ab10-8711-5258-84ad-4b1120ba62dc"
StaticArrays = "90137ffa-7385-5640-81b9-e52037218182"
[compat]
RigidBodyDynamics = "2"
StaticArrays = "0.10, 0.11, 0.12"
julia = "1.2, 1.3, 1.4, 1.5"
| 828 | TOML | 35.043477 | 66 | 0.768116 |
renanmb/Omniverse_legged_robotics/DigitRobot.jl-main/README.md | # DigitRobot.jl
This package provides URDF, meshes and inverse kinematics solvers for the Agility Robotics Digit Robot.

| 139 | Markdown | 22.33333 | 104 | 0.769784 |
renanmb/Omniverse_legged_robotics/DigitRobot.jl-main/src/digit_manual_control.py | import pybullet as p
import time
import pybullet_data
p.connect(p.GUI)
p.setAdditionalSearchPath('..')
humanoid = p.loadURDF("urdf/digit_model.urdf",useFixedBase=True)
gravId = p.addUserDebugParameter("gravity", -10, 10, 0)
jointIds = []
paramIds = []
p.setPhysicsEngineParameter(numSolverIterations=10)
p.changeDynamics(humanoid, -1, linearDamping=0, angularDamping=0)
for j in range(p.getNumJoints(humanoid)):
p.changeDynamics(humanoid, j, linearDamping=0, angularDamping=0)
info = p.getJointInfo(humanoid, j)
#print(info)
jointName = info[1]
jointType = info[2]
if (jointType == p.JOINT_PRISMATIC or jointType == p.JOINT_REVOLUTE):
jointIds.append(j)
paramIds.append(p.addUserDebugParameter(jointName.decode("utf-8"), -4, 4, 0))
p.setRealTimeSimulation(1)
while (1):
p.setGravity(0, 0, p.readUserDebugParameter(gravId))
for i in range(len(paramIds)):
c = paramIds[i]
targetPos = p.readUserDebugParameter(c)
p.setJointMotorControl2(humanoid, jointIds[i], p.POSITION_CONTROL, targetPos, force=5 * 240.)
time.sleep(0.01) | 1,065 | Python | 30.35294 | 97 | 0.733333 |
renanmb/Omniverse_legged_robotics/CHAMP-stuff/configs/opendog_config/README.md |
## 1. Quick Start
You don't need a physical robot to run the following demos.
### 1.1. Walking demo in RVIZ:
#### 1.1.1. Run the base driver:
roslaunch opendog_config bringup.launch rviz:=true
#### 1.1.2. Run the teleop node:
roslaunch champ_teleop teleop.launch
If you want to use a [joystick](https://www.logitechg.com/en-hk/products/gamepads/f710-wireless-gamepad.html) add joy:=true as an argument.
### 1.2. SLAM demo:
#### 1.2.1. Run the Gazebo environment:
roslaunch opendog_config gazebo.launch
#### 1.2.2. Run gmapping package and move_base:
roslaunch opendog_config slam.launch rviz:=true
To start mapping:
- Click '2D Nav Goal'.
- Click and drag at the position you want the robot to go.
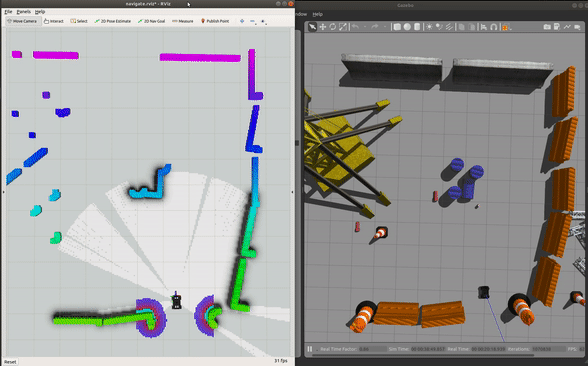
- Save the map by running:
roscd opendog_config/maps
rosrun map_server map_saver
### 1.3. Autonomous Navigation:
#### 1.3.1. Run the Gazebo environment:
roslaunch opendog_config gazebo.launch
#### 1.3.2. Run amcl and move_base:
roslaunch opendog_config navigate.launch rviz:=true
To navigate:
- Click '2D Nav Goal'.
- Click and drag at the position you want the robot to go.
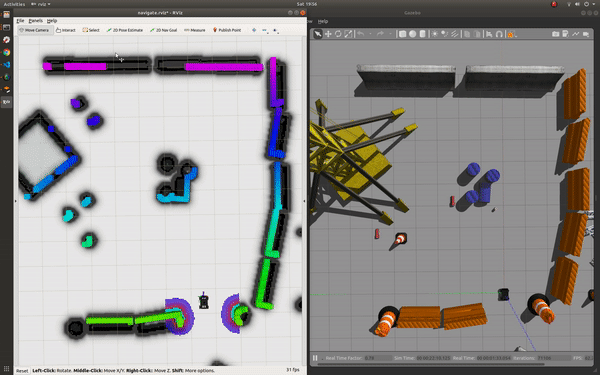
#### 1.4.1 Spawning multiple robots in Gazebo
Run Gazebo and default simulation world:
roslaunch champ_gazebo spawn_world.launch
You can also load your own world file by passing your world's path to 'gazebo_world' argument:
roslaunch champ_gazebo spawn_world.launch gazebo_world:=<path_to_world_file>
Spawning a robot:
roslaunch opendog_config spawn_robot.launch robot_name:=<unique_robot_name> world_init_x:=<x_position> world_init_y:=<y_position>
* Every instance of the spawned robot must have a unique robot name to prevent the topics and transforms from clashing.
---
:exclamation: *This is not an official product from the robot's company/author.* | 2,001 | Markdown | 25 | 139 | 0.72064 |
renanmb/Omniverse_legged_robotics/CHAMP-stuff/configs/opendog_config/maps/map.yaml | image: map.pgm
resolution: 0.050000
origin: [-50.000000, -50.000000, 0.000000]
negate: 0
occupied_thresh: 0.65
free_thresh: 0.196
| 131 | YAML | 15.499998 | 42 | 0.725191 |
renanmb/Omniverse_legged_robotics/CHAMP-stuff/configs/opendog_config/include/quadruped_description.h | #ifndef QUADRUPED_DESCRIPTION_H
#define QUADRUPED_DESCRIPTION_H
#include <quadruped_base/quadruped_base.h>
namespace champ
{
namespace URDF
{
void loadFromHeader(champ::QuadrupedBase &base)
{
base.lf.hip.setOrigin(0.265, 0.125, 0.0, 0.0, 0.0, 0.0);
base.lf.upper_leg.setOrigin(0.0, 0.07, -0.0, 0.0, 0.0, 0.0);
base.lf.lower_leg.setOrigin(0.0, 0.0, -0.2, 0.0, 0.0, 0.0);
base.lf.foot.setOrigin(0.0, -0.07, -0.23, 0.0, 0.0, 0.0);
base.rf.hip.setOrigin(0.265, -0.125, 0.0, 0.0, 0.0, 0.0);
base.rf.upper_leg.setOrigin(0.0, -0.07, -0.0, 0.0, 0.0, 0.0);
base.rf.lower_leg.setOrigin(0.0, 0.0, -0.2, 0.0, 0.0, 0.0);
base.rf.foot.setOrigin(0.0, 0.07, -0.23, 0.0, 0.0, 0.0);
base.lh.hip.setOrigin(-0.16, 0.125, 0.0, 0.0, 0.0, 0.0);
base.lh.upper_leg.setOrigin(0.0, 0.07, -0.0, 0.0, 0.0, 0.0);
base.lh.lower_leg.setOrigin(0.0, 0.0, -0.2, 0.0, 0.0, 0.0);
base.lh.foot.setOrigin(0.0, -0.07, -0.23, 0.0, 0.0, 0.0);
base.rh.hip.setOrigin(-0.16, -0.125, 0.0, 0.0, 0.0, 0.0);
base.rh.upper_leg.setOrigin(0.0, -0.07, -0.0, 0.0, 0.0, 0.0);
base.rh.lower_leg.setOrigin(0.0, 0.0, -0.2, 0.0, 0.0, 0.0);
base.rh.foot.setOrigin(0.0, 0.07, -0.23, 0.0, 0.0, 0.0);
}
}
}
#endif | 1,235 | C | 35.35294 | 63 | 0.574899 |