repo_name
stringclasses 1
value | pr_number
int64 4.12k
11.2k
| pr_title
stringlengths 9
107
| pr_description
stringlengths 107
5.48k
| author
stringlengths 4
18
| date_created
timestamp[ns, tz=UTC] | date_merged
timestamp[ns, tz=UTC] | previous_commit
stringlengths 40
40
| pr_commit
stringlengths 40
40
| query
stringlengths 118
5.52k
| before_content
stringlengths 0
7.93M
| after_content
stringlengths 0
7.93M
| label
int64 -1
1
|
---|---|---|---|---|---|---|---|---|---|---|---|---|
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from collections import deque
from math import floor
from random import random
from time import time
# the default weight is 1 if not assigned but all the implementation is weighted
class DirectedGraph:
def __init__(self):
self.graph = {}
# adding vertices and edges
# adding the weight is optional
# handles repetition
def add_pair(self, u, v, w=1):
if self.graph.get(u):
if self.graph[u].count([w, v]) == 0:
self.graph[u].append([w, v])
else:
self.graph[u] = [[w, v]]
if not self.graph.get(v):
self.graph[v] = []
def all_nodes(self):
return list(self.graph)
# handles if the input does not exist
def remove_pair(self, u, v):
if self.graph.get(u):
for _ in self.graph[u]:
if _[1] == v:
self.graph[u].remove(_)
# if no destination is meant the default value is -1
def dfs(self, s=-2, d=-1):
if s == d:
return []
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
if node[1] == d:
visited.append(d)
return visited
else:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return visited
# c is the count of nodes you want and if you leave it or pass -1 to the function
# the count will be random from 10 to 10000
def fill_graph_randomly(self, c=-1):
if c == -1:
c = floor(random() * 10000) + 10
for i in range(c):
# every vertex has max 100 edges
for _ in range(floor(random() * 102) + 1):
n = floor(random() * c) + 1
if n != i:
self.add_pair(i, n, 1)
def bfs(self, s=-2):
d = deque()
visited = []
if s == -2:
s = list(self.graph)[0]
d.append(s)
visited.append(s)
while d:
s = d.popleft()
if len(self.graph[s]) != 0:
for node in self.graph[s]:
if visited.count(node[1]) < 1:
d.append(node[1])
visited.append(node[1])
return visited
def in_degree(self, u):
count = 0
for x in self.graph:
for y in self.graph[x]:
if y[1] == u:
count += 1
return count
def out_degree(self, u):
return len(self.graph[u])
def topological_sort(self, s=-2):
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
sorted_nodes = []
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
sorted_nodes.append(stack.pop())
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return sorted_nodes
def cycle_nodes(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack = len(stack) - 1
while True and len_stack >= 0:
if stack[len_stack] == node[1]:
anticipating_nodes.add(node[1])
break
else:
anticipating_nodes.add(stack[len_stack])
len_stack -= 1
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return list(anticipating_nodes)
def has_cycle(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack_minus_one = len(stack) - 1
while True and len_stack_minus_one >= 0:
if stack[len_stack_minus_one] == node[1]:
anticipating_nodes.add(node[1])
break
else:
return True
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return False
def dfs_time(self, s=-2, e=-1):
begin = time()
self.dfs(s, e)
end = time()
return end - begin
def bfs_time(self, s=-2):
begin = time()
self.bfs(s)
end = time()
return end - begin
class Graph:
def __init__(self):
self.graph = {}
# adding vertices and edges
# adding the weight is optional
# handles repetition
def add_pair(self, u, v, w=1):
# check if the u exists
if self.graph.get(u):
# if there already is a edge
if self.graph[u].count([w, v]) == 0:
self.graph[u].append([w, v])
else:
# if u does not exist
self.graph[u] = [[w, v]]
# add the other way
if self.graph.get(v):
# if there already is a edge
if self.graph[v].count([w, u]) == 0:
self.graph[v].append([w, u])
else:
# if u does not exist
self.graph[v] = [[w, u]]
# handles if the input does not exist
def remove_pair(self, u, v):
if self.graph.get(u):
for _ in self.graph[u]:
if _[1] == v:
self.graph[u].remove(_)
# the other way round
if self.graph.get(v):
for _ in self.graph[v]:
if _[1] == u:
self.graph[v].remove(_)
# if no destination is meant the default value is -1
def dfs(self, s=-2, d=-1):
if s == d:
return []
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
if node[1] == d:
visited.append(d)
return visited
else:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return visited
# c is the count of nodes you want and if you leave it or pass -1 to the function
# the count will be random from 10 to 10000
def fill_graph_randomly(self, c=-1):
if c == -1:
c = floor(random() * 10000) + 10
for i in range(c):
# every vertex has max 100 edges
for _ in range(floor(random() * 102) + 1):
n = floor(random() * c) + 1
if n != i:
self.add_pair(i, n, 1)
def bfs(self, s=-2):
d = deque()
visited = []
if s == -2:
s = list(self.graph)[0]
d.append(s)
visited.append(s)
while d:
s = d.popleft()
if len(self.graph[s]) != 0:
for node in self.graph[s]:
if visited.count(node[1]) < 1:
d.append(node[1])
visited.append(node[1])
return visited
def degree(self, u):
return len(self.graph[u])
def cycle_nodes(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack = len(stack) - 1
while True and len_stack >= 0:
if stack[len_stack] == node[1]:
anticipating_nodes.add(node[1])
break
else:
anticipating_nodes.add(stack[len_stack])
len_stack -= 1
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return list(anticipating_nodes)
def has_cycle(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack_minus_one = len(stack) - 1
while True and len_stack_minus_one >= 0:
if stack[len_stack_minus_one] == node[1]:
anticipating_nodes.add(node[1])
break
else:
return True
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return False
def all_nodes(self):
return list(self.graph)
def dfs_time(self, s=-2, e=-1):
begin = time()
self.dfs(s, e)
end = time()
return end - begin
def bfs_time(self, s=-2):
begin = time()
self.bfs(s)
end = time()
return end - begin
| from collections import deque
from math import floor
from random import random
from time import time
# the default weight is 1 if not assigned but all the implementation is weighted
class DirectedGraph:
def __init__(self):
self.graph = {}
# adding vertices and edges
# adding the weight is optional
# handles repetition
def add_pair(self, u, v, w=1):
if self.graph.get(u):
if self.graph[u].count([w, v]) == 0:
self.graph[u].append([w, v])
else:
self.graph[u] = [[w, v]]
if not self.graph.get(v):
self.graph[v] = []
def all_nodes(self):
return list(self.graph)
# handles if the input does not exist
def remove_pair(self, u, v):
if self.graph.get(u):
for _ in self.graph[u]:
if _[1] == v:
self.graph[u].remove(_)
# if no destination is meant the default value is -1
def dfs(self, s=-2, d=-1):
if s == d:
return []
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
if node[1] == d:
visited.append(d)
return visited
else:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return visited
# c is the count of nodes you want and if you leave it or pass -1 to the function
# the count will be random from 10 to 10000
def fill_graph_randomly(self, c=-1):
if c == -1:
c = floor(random() * 10000) + 10
for i in range(c):
# every vertex has max 100 edges
for _ in range(floor(random() * 102) + 1):
n = floor(random() * c) + 1
if n != i:
self.add_pair(i, n, 1)
def bfs(self, s=-2):
d = deque()
visited = []
if s == -2:
s = list(self.graph)[0]
d.append(s)
visited.append(s)
while d:
s = d.popleft()
if len(self.graph[s]) != 0:
for node in self.graph[s]:
if visited.count(node[1]) < 1:
d.append(node[1])
visited.append(node[1])
return visited
def in_degree(self, u):
count = 0
for x in self.graph:
for y in self.graph[x]:
if y[1] == u:
count += 1
return count
def out_degree(self, u):
return len(self.graph[u])
def topological_sort(self, s=-2):
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
sorted_nodes = []
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
sorted_nodes.append(stack.pop())
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return sorted_nodes
def cycle_nodes(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack = len(stack) - 1
while True and len_stack >= 0:
if stack[len_stack] == node[1]:
anticipating_nodes.add(node[1])
break
else:
anticipating_nodes.add(stack[len_stack])
len_stack -= 1
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return list(anticipating_nodes)
def has_cycle(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack_minus_one = len(stack) - 1
while True and len_stack_minus_one >= 0:
if stack[len_stack_minus_one] == node[1]:
anticipating_nodes.add(node[1])
break
else:
return True
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return False
def dfs_time(self, s=-2, e=-1):
begin = time()
self.dfs(s, e)
end = time()
return end - begin
def bfs_time(self, s=-2):
begin = time()
self.bfs(s)
end = time()
return end - begin
class Graph:
def __init__(self):
self.graph = {}
# adding vertices and edges
# adding the weight is optional
# handles repetition
def add_pair(self, u, v, w=1):
# check if the u exists
if self.graph.get(u):
# if there already is a edge
if self.graph[u].count([w, v]) == 0:
self.graph[u].append([w, v])
else:
# if u does not exist
self.graph[u] = [[w, v]]
# add the other way
if self.graph.get(v):
# if there already is a edge
if self.graph[v].count([w, u]) == 0:
self.graph[v].append([w, u])
else:
# if u does not exist
self.graph[v] = [[w, u]]
# handles if the input does not exist
def remove_pair(self, u, v):
if self.graph.get(u):
for _ in self.graph[u]:
if _[1] == v:
self.graph[u].remove(_)
# the other way round
if self.graph.get(v):
for _ in self.graph[v]:
if _[1] == u:
self.graph[v].remove(_)
# if no destination is meant the default value is -1
def dfs(self, s=-2, d=-1):
if s == d:
return []
stack = []
visited = []
if s == -2:
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
ss = s
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if visited.count(node[1]) < 1:
if node[1] == d:
visited.append(d)
return visited
else:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return visited
# c is the count of nodes you want and if you leave it or pass -1 to the function
# the count will be random from 10 to 10000
def fill_graph_randomly(self, c=-1):
if c == -1:
c = floor(random() * 10000) + 10
for i in range(c):
# every vertex has max 100 edges
for _ in range(floor(random() * 102) + 1):
n = floor(random() * c) + 1
if n != i:
self.add_pair(i, n, 1)
def bfs(self, s=-2):
d = deque()
visited = []
if s == -2:
s = list(self.graph)[0]
d.append(s)
visited.append(s)
while d:
s = d.popleft()
if len(self.graph[s]) != 0:
for node in self.graph[s]:
if visited.count(node[1]) < 1:
d.append(node[1])
visited.append(node[1])
return visited
def degree(self, u):
return len(self.graph[u])
def cycle_nodes(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack = len(stack) - 1
while True and len_stack >= 0:
if stack[len_stack] == node[1]:
anticipating_nodes.add(node[1])
break
else:
anticipating_nodes.add(stack[len_stack])
len_stack -= 1
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return list(anticipating_nodes)
def has_cycle(self):
stack = []
visited = []
s = list(self.graph)[0]
stack.append(s)
visited.append(s)
parent = -2
indirect_parents = []
ss = s
on_the_way_back = False
anticipating_nodes = set()
while True:
# check if there is any non isolated nodes
if len(self.graph[s]) != 0:
ss = s
for node in self.graph[s]:
if (
visited.count(node[1]) > 0
and node[1] != parent
and indirect_parents.count(node[1]) > 0
and not on_the_way_back
):
len_stack_minus_one = len(stack) - 1
while True and len_stack_minus_one >= 0:
if stack[len_stack_minus_one] == node[1]:
anticipating_nodes.add(node[1])
break
else:
return True
if visited.count(node[1]) < 1:
stack.append(node[1])
visited.append(node[1])
ss = node[1]
break
# check if all the children are visited
if s == ss:
stack.pop()
on_the_way_back = True
if len(stack) != 0:
s = stack[len(stack) - 1]
else:
on_the_way_back = False
indirect_parents.append(parent)
parent = s
s = ss
# check if se have reached the starting point
if len(stack) == 0:
return False
def all_nodes(self):
return list(self.graph)
def dfs_time(self, s=-2, e=-1):
begin = time()
self.dfs(s, e)
end = time()
return end - begin
def bfs_time(self, s=-2):
begin = time()
self.bfs(s)
end = time()
return end - begin
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
def climb_stairs(n: int) -> int:
"""
LeetCdoe No.70: Climbing Stairs
Distinct ways to climb a n step staircase where
each time you can either climb 1 or 2 steps.
Args:
n: number of steps of staircase
Returns:
Distinct ways to climb a n step staircase
Raises:
AssertionError: n not positive integer
>>> climb_stairs(3)
3
>>> climb_stairs(1)
1
>>> climb_stairs(-7) # doctest: +ELLIPSIS
Traceback (most recent call last):
...
AssertionError: n needs to be positive integer, your input -7
"""
assert (
isinstance(n, int) and n > 0
), f"n needs to be positive integer, your input {n}"
if n == 1:
return 1
dp = [0] * (n + 1)
dp[0], dp[1] = (1, 1)
for i in range(2, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
return dp[n]
if __name__ == "__main__":
import doctest
doctest.testmod()
| #!/usr/bin/env python3
def climb_stairs(n: int) -> int:
"""
LeetCdoe No.70: Climbing Stairs
Distinct ways to climb a n step staircase where
each time you can either climb 1 or 2 steps.
Args:
n: number of steps of staircase
Returns:
Distinct ways to climb a n step staircase
Raises:
AssertionError: n not positive integer
>>> climb_stairs(3)
3
>>> climb_stairs(1)
1
>>> climb_stairs(-7) # doctest: +ELLIPSIS
Traceback (most recent call last):
...
AssertionError: n needs to be positive integer, your input -7
"""
assert (
isinstance(n, int) and n > 0
), f"n needs to be positive integer, your input {n}"
if n == 1:
return 1
dp = [0] * (n + 1)
dp[0], dp[1] = (1, 1)
for i in range(2, n + 1):
dp[i] = dp[i - 1] + dp[i - 2]
return dp[n]
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Shortest job remaining first
Please note arrival time and burst
Please use spaces to separate times entered.
"""
from __future__ import annotations
import pandas as pd
def calculate_waitingtime(
arrival_time: list[int], burst_time: list[int], no_of_processes: int
) -> list[int]:
"""
Calculate the waiting time of each processes
Return: List of waiting times.
>>> calculate_waitingtime([1,2,3,4],[3,3,5,1],4)
[0, 3, 5, 0]
>>> calculate_waitingtime([1,2,3],[2,5,1],3)
[0, 2, 0]
>>> calculate_waitingtime([2,3],[5,1],2)
[1, 0]
"""
remaining_time = [0] * no_of_processes
waiting_time = [0] * no_of_processes
# Copy the burst time into remaining_time[]
for i in range(no_of_processes):
remaining_time[i] = burst_time[i]
complete = 0
increment_time = 0
minm = 999999999
short = 0
check = False
# Process until all processes are completed
while complete != no_of_processes:
for j in range(no_of_processes):
if arrival_time[j] <= increment_time:
if remaining_time[j] > 0:
if remaining_time[j] < minm:
minm = remaining_time[j]
short = j
check = True
if not check:
increment_time += 1
continue
remaining_time[short] -= 1
minm = remaining_time[short]
if minm == 0:
minm = 999999999
if remaining_time[short] == 0:
complete += 1
check = False
# Find finish time of current process
finish_time = increment_time + 1
# Calculate waiting time
finar = finish_time - arrival_time[short]
waiting_time[short] = finar - burst_time[short]
if waiting_time[short] < 0:
waiting_time[short] = 0
# Increment time
increment_time += 1
return waiting_time
def calculate_turnaroundtime(
burst_time: list[int], no_of_processes: int, waiting_time: list[int]
) -> list[int]:
"""
Calculate the turn around time of each Processes
Return: list of turn around times.
>>> calculate_turnaroundtime([3,3,5,1], 4, [0,3,5,0])
[3, 6, 10, 1]
>>> calculate_turnaroundtime([3,3], 2, [0,3])
[3, 6]
>>> calculate_turnaroundtime([8,10,1], 3, [1,0,3])
[9, 10, 4]
"""
turn_around_time = [0] * no_of_processes
for i in range(no_of_processes):
turn_around_time[i] = burst_time[i] + waiting_time[i]
return turn_around_time
def calculate_average_times(
waiting_time: list[int], turn_around_time: list[int], no_of_processes: int
) -> None:
"""
This function calculates the average of the waiting & turnaround times
Prints: Average Waiting time & Average Turn Around Time
>>> calculate_average_times([0,3,5,0],[3,6,10,1],4)
Average waiting time = 2.00000
Average turn around time = 5.0
>>> calculate_average_times([2,3],[3,6],2)
Average waiting time = 2.50000
Average turn around time = 4.5
>>> calculate_average_times([10,4,3],[2,7,6],3)
Average waiting time = 5.66667
Average turn around time = 5.0
"""
total_waiting_time = 0
total_turn_around_time = 0
for i in range(no_of_processes):
total_waiting_time = total_waiting_time + waiting_time[i]
total_turn_around_time = total_turn_around_time + turn_around_time[i]
print(f"Average waiting time = {total_waiting_time / no_of_processes:.5f}")
print("Average turn around time =", total_turn_around_time / no_of_processes)
if __name__ == "__main__":
print("Enter how many process you want to analyze")
no_of_processes = int(input())
burst_time = [0] * no_of_processes
arrival_time = [0] * no_of_processes
processes = list(range(1, no_of_processes + 1))
for i in range(no_of_processes):
print("Enter the arrival time and burst time for process:--" + str(i + 1))
arrival_time[i], burst_time[i] = map(int, input().split())
waiting_time = calculate_waitingtime(arrival_time, burst_time, no_of_processes)
bt = burst_time
n = no_of_processes
wt = waiting_time
turn_around_time = calculate_turnaroundtime(bt, n, wt)
calculate_average_times(waiting_time, turn_around_time, no_of_processes)
fcfs = pd.DataFrame(
list(zip(processes, burst_time, arrival_time, waiting_time, turn_around_time)),
columns=[
"Process",
"BurstTime",
"ArrivalTime",
"WaitingTime",
"TurnAroundTime",
],
)
# Printing the dataFrame
pd.set_option("display.max_rows", fcfs.shape[0] + 1)
print(fcfs)
| """
Shortest job remaining first
Please note arrival time and burst
Please use spaces to separate times entered.
"""
from __future__ import annotations
import pandas as pd
def calculate_waitingtime(
arrival_time: list[int], burst_time: list[int], no_of_processes: int
) -> list[int]:
"""
Calculate the waiting time of each processes
Return: List of waiting times.
>>> calculate_waitingtime([1,2,3,4],[3,3,5,1],4)
[0, 3, 5, 0]
>>> calculate_waitingtime([1,2,3],[2,5,1],3)
[0, 2, 0]
>>> calculate_waitingtime([2,3],[5,1],2)
[1, 0]
"""
remaining_time = [0] * no_of_processes
waiting_time = [0] * no_of_processes
# Copy the burst time into remaining_time[]
for i in range(no_of_processes):
remaining_time[i] = burst_time[i]
complete = 0
increment_time = 0
minm = 999999999
short = 0
check = False
# Process until all processes are completed
while complete != no_of_processes:
for j in range(no_of_processes):
if arrival_time[j] <= increment_time:
if remaining_time[j] > 0:
if remaining_time[j] < minm:
minm = remaining_time[j]
short = j
check = True
if not check:
increment_time += 1
continue
remaining_time[short] -= 1
minm = remaining_time[short]
if minm == 0:
minm = 999999999
if remaining_time[short] == 0:
complete += 1
check = False
# Find finish time of current process
finish_time = increment_time + 1
# Calculate waiting time
finar = finish_time - arrival_time[short]
waiting_time[short] = finar - burst_time[short]
if waiting_time[short] < 0:
waiting_time[short] = 0
# Increment time
increment_time += 1
return waiting_time
def calculate_turnaroundtime(
burst_time: list[int], no_of_processes: int, waiting_time: list[int]
) -> list[int]:
"""
Calculate the turn around time of each Processes
Return: list of turn around times.
>>> calculate_turnaroundtime([3,3,5,1], 4, [0,3,5,0])
[3, 6, 10, 1]
>>> calculate_turnaroundtime([3,3], 2, [0,3])
[3, 6]
>>> calculate_turnaroundtime([8,10,1], 3, [1,0,3])
[9, 10, 4]
"""
turn_around_time = [0] * no_of_processes
for i in range(no_of_processes):
turn_around_time[i] = burst_time[i] + waiting_time[i]
return turn_around_time
def calculate_average_times(
waiting_time: list[int], turn_around_time: list[int], no_of_processes: int
) -> None:
"""
This function calculates the average of the waiting & turnaround times
Prints: Average Waiting time & Average Turn Around Time
>>> calculate_average_times([0,3,5,0],[3,6,10,1],4)
Average waiting time = 2.00000
Average turn around time = 5.0
>>> calculate_average_times([2,3],[3,6],2)
Average waiting time = 2.50000
Average turn around time = 4.5
>>> calculate_average_times([10,4,3],[2,7,6],3)
Average waiting time = 5.66667
Average turn around time = 5.0
"""
total_waiting_time = 0
total_turn_around_time = 0
for i in range(no_of_processes):
total_waiting_time = total_waiting_time + waiting_time[i]
total_turn_around_time = total_turn_around_time + turn_around_time[i]
print(f"Average waiting time = {total_waiting_time / no_of_processes:.5f}")
print("Average turn around time =", total_turn_around_time / no_of_processes)
if __name__ == "__main__":
print("Enter how many process you want to analyze")
no_of_processes = int(input())
burst_time = [0] * no_of_processes
arrival_time = [0] * no_of_processes
processes = list(range(1, no_of_processes + 1))
for i in range(no_of_processes):
print("Enter the arrival time and burst time for process:--" + str(i + 1))
arrival_time[i], burst_time[i] = map(int, input().split())
waiting_time = calculate_waitingtime(arrival_time, burst_time, no_of_processes)
bt = burst_time
n = no_of_processes
wt = waiting_time
turn_around_time = calculate_turnaroundtime(bt, n, wt)
calculate_average_times(waiting_time, turn_around_time, no_of_processes)
fcfs = pd.DataFrame(
list(zip(processes, burst_time, arrival_time, waiting_time, turn_around_time)),
columns=[
"Process",
"BurstTime",
"ArrivalTime",
"WaitingTime",
"TurnAroundTime",
],
)
# Printing the dataFrame
pd.set_option("display.max_rows", fcfs.shape[0] + 1)
print(fcfs)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| class Graph:
def __init__(self, vertex):
self.vertex = vertex
self.graph = [[0] * vertex for i in range(vertex)]
def add_edge(self, u, v):
self.graph[u - 1][v - 1] = 1
self.graph[v - 1][u - 1] = 1
def show(self):
for i in self.graph:
for j in i:
print(j, end=" ")
print(" ")
g = Graph(100)
g.add_edge(1, 4)
g.add_edge(4, 2)
g.add_edge(4, 5)
g.add_edge(2, 5)
g.add_edge(5, 3)
g.show()
| class Graph:
def __init__(self, vertex):
self.vertex = vertex
self.graph = [[0] * vertex for i in range(vertex)]
def add_edge(self, u, v):
self.graph[u - 1][v - 1] = 1
self.graph[v - 1][u - 1] = 1
def show(self):
for i in self.graph:
for j in i:
print(j, end=" ")
print(" ")
g = Graph(100)
g.add_edge(1, 4)
g.add_edge(4, 2)
g.add_edge(4, 5)
g.add_edge(2, 5)
g.add_edge(5, 3)
g.show()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import webbrowser
from sys import argv
from urllib.parse import parse_qs, quote
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
if __name__ == "__main__":
if len(argv) > 1:
query = "%20".join(argv[1:])
else:
query = quote(str(input("Search: ")))
print("Googling.....")
url = f"https://www.google.com/search?q={query}&num=100"
res = requests.get(
url,
headers={"User-Agent": str(UserAgent().random)},
)
try:
link = (
BeautifulSoup(res.text, "html.parser")
.find("div", attrs={"class": "yuRUbf"})
.find("a")
.get("href")
)
except AttributeError:
link = parse_qs(
BeautifulSoup(res.text, "html.parser")
.find("div", attrs={"class": "kCrYT"})
.find("a")
.get("href")
)["url"][0]
webbrowser.open(link)
| import webbrowser
from sys import argv
from urllib.parse import parse_qs, quote
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
if __name__ == "__main__":
if len(argv) > 1:
query = "%20".join(argv[1:])
else:
query = quote(str(input("Search: ")))
print("Googling.....")
url = f"https://www.google.com/search?q={query}&num=100"
res = requests.get(
url,
headers={"User-Agent": str(UserAgent().random)},
)
try:
link = (
BeautifulSoup(res.text, "html.parser")
.find("div", attrs={"class": "yuRUbf"})
.find("a")
.get("href")
)
except AttributeError:
link = parse_qs(
BeautifulSoup(res.text, "html.parser")
.find("div", attrs={"class": "kCrYT"})
.find("a")
.get("href")
)["url"][0]
webbrowser.open(link)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Binary Tree Traversal
## Overview
The combination of binary trees being data structures and traversal being an algorithm relates to classic problems, either directly or indirectly.
> If you can grasp the traversal of binary trees, the traversal of other complicated trees will be easy for you.
The following are some common ways to traverse trees.
- Depth First Traversals (DFS): In-order, Pre-order, Post-order
- Level Order Traversal or Breadth First or Traversal (BFS)
There are applications for both DFS and BFS.
Stack can be used to simplify the process of DFS traversal. Besides, since tree is a recursive data structure, recursion and stack are two key points for DFS.
Graph for DFS:
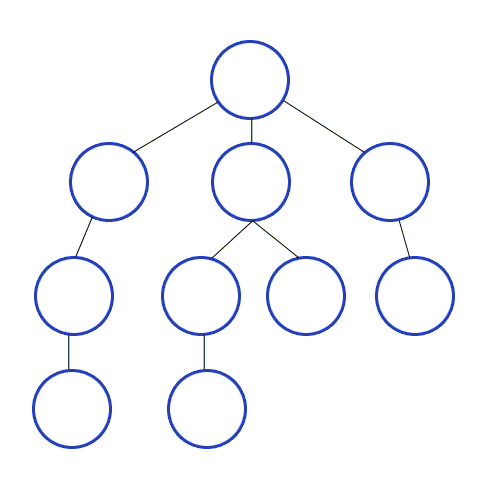
The key point of BFS is how to determine whether the traversal of each level has been completed. The answer is to use a variable as a flag to represent the end of the traversal of current level.
## Pre-order Traversal
The traversal order of pre-order traversal is `root-left-right`.
Algorithm Pre-order
1. Visit the root node and push it into a stack.
2. Pop a node from the stack, and push its right and left child node into the stack respectively.
3. Repeat step 2.
Conclusion: This problem involves the classic recursive data structure (i.e. a binary tree), and the algorithm above demonstrates how a simplified solution can be reached by using a stack.
If you look at the bigger picture, you'll find that the process of traversal is as followed. `Visit the left subtrees respectively from top to bottom, and visit the right subtrees respectively from bottom to top`. If we are to implement it from this perspective, things will be somewhat different. For the `top to bottom` part we can simply use recursion, and for the `bottom to top` part we can turn to stack.
## In-order Traversal
The traversal order of in-order traversal is `left-root-right`.
So the root node is not printed first. Things are getting a bit complicated here.
Algorithm In-order
1. Visit the root and push it into a stack.
2. If there is a left child node, push it into the stack. Repeat this process until a leaf node reached.
> At this point the root node and all the left nodes are in the stack.
3. Start popping nodes from the stack. If a node has a right child node, push the child node into the stack. Repeat step 2.
It's worth pointing out that the in-order traversal of a binary search tree (BST) is a sorted array, which is helpful for coming up simplified solutions for some problems.
## Post-order Traversal
The traversal order of post-order traversal is `left-right-root`.
This one is a bit of a challenge. It deserves the `hard` tag of LeetCode.
In this case, the root node is printed not as the first but the last one. A cunning way to do it is to:
Record whether the current node has been visited. If 1) it's a leaf node or 2) both its left and right subtrees have been traversed, then it can be popped from the stack.
As for `1) it's a leaf node`, you can easily tell whether a node is a leaf if both its left and right are `null`.
As for `2) both its left and right subtrees have been traversed`, we only need a variable to record whether a node has been visited or not. In the worst case, we need to record the status for every single node and the space complexity is `O(n)`. But if you come to think about it, as we are using a stack and start printing the result from the leaf nodes, it makes sense that we only record the status for the current node popping from the stack, reducing the space complexity to `O(1)`.
## Level Order Traversal
The key point of level order traversal is how do we know whether the traversal of each level is done. The answer is that we use a variable as a flag representing the end of the traversal of the current level.
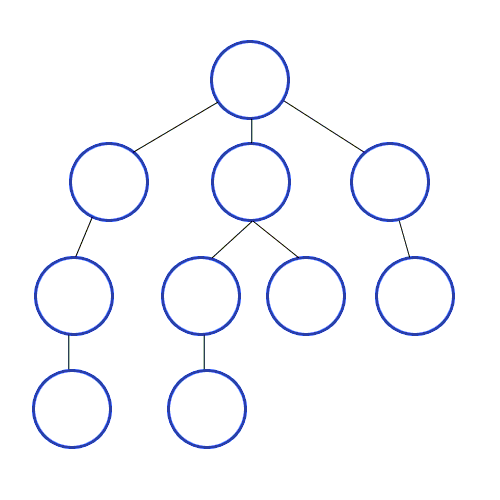
Algorithm Level-order
1. Visit the root node, put it in a FIFO queue, put in the queue a special flag (we are using `null` here).
2. Dequeue a node.
3. If the node equals `null`, it means that all nodes of the current level have been visited. If the queue is empty, we do nothing. Or else we put in another `null`.
4. If the node is not `null`, meaning the traversal of current level has not finished yet, we enqueue its left subtree and right subtree respectively.
## Bi-color marking
We know that there is a tri-color marking in garbage collection algorithm, which works as described below.
- The white color represents "not visited".
- The gray color represents "not all child nodes visited".
- The black color represents "all child nodes visited".
Enlightened by tri-color marking, a bi-color marking method can be invented to solve all three traversal problems with one solution.
The core idea is as follow.
- Use a color to mark whether a node has been visited or not. Nodes yet to be visited are marked as white and visited nodes are marked as gray.
- If we are visiting a white node, turn it into gray, and push its right child node, itself, and it's left child node into the stack respectively.
- If we are visiting a gray node, print it.
Implementation of pre-order and post-order traversal algorithms can be easily done by changing the order of pushing the child nodes into the stack.
Reference: [LeetCode](https://github.com/azl397985856/leetcode/blob/master/thinkings/binary-tree-traversal.en.md)
| # Binary Tree Traversal
## Overview
The combination of binary trees being data structures and traversal being an algorithm relates to classic problems, either directly or indirectly.
> If you can grasp the traversal of binary trees, the traversal of other complicated trees will be easy for you.
The following are some common ways to traverse trees.
- Depth First Traversals (DFS): In-order, Pre-order, Post-order
- Level Order Traversal or Breadth First or Traversal (BFS)
There are applications for both DFS and BFS.
Stack can be used to simplify the process of DFS traversal. Besides, since tree is a recursive data structure, recursion and stack are two key points for DFS.
Graph for DFS:
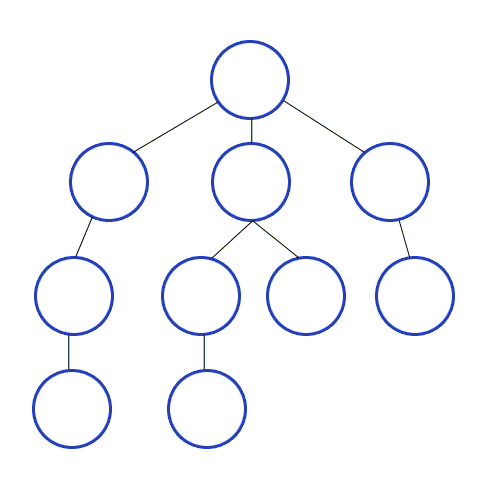
The key point of BFS is how to determine whether the traversal of each level has been completed. The answer is to use a variable as a flag to represent the end of the traversal of current level.
## Pre-order Traversal
The traversal order of pre-order traversal is `root-left-right`.
Algorithm Pre-order
1. Visit the root node and push it into a stack.
2. Pop a node from the stack, and push its right and left child node into the stack respectively.
3. Repeat step 2.
Conclusion: This problem involves the classic recursive data structure (i.e. a binary tree), and the algorithm above demonstrates how a simplified solution can be reached by using a stack.
If you look at the bigger picture, you'll find that the process of traversal is as followed. `Visit the left subtrees respectively from top to bottom, and visit the right subtrees respectively from bottom to top`. If we are to implement it from this perspective, things will be somewhat different. For the `top to bottom` part we can simply use recursion, and for the `bottom to top` part we can turn to stack.
## In-order Traversal
The traversal order of in-order traversal is `left-root-right`.
So the root node is not printed first. Things are getting a bit complicated here.
Algorithm In-order
1. Visit the root and push it into a stack.
2. If there is a left child node, push it into the stack. Repeat this process until a leaf node reached.
> At this point the root node and all the left nodes are in the stack.
3. Start popping nodes from the stack. If a node has a right child node, push the child node into the stack. Repeat step 2.
It's worth pointing out that the in-order traversal of a binary search tree (BST) is a sorted array, which is helpful for coming up simplified solutions for some problems.
## Post-order Traversal
The traversal order of post-order traversal is `left-right-root`.
This one is a bit of a challenge. It deserves the `hard` tag of LeetCode.
In this case, the root node is printed not as the first but the last one. A cunning way to do it is to:
Record whether the current node has been visited. If 1) it's a leaf node or 2) both its left and right subtrees have been traversed, then it can be popped from the stack.
As for `1) it's a leaf node`, you can easily tell whether a node is a leaf if both its left and right are `null`.
As for `2) both its left and right subtrees have been traversed`, we only need a variable to record whether a node has been visited or not. In the worst case, we need to record the status for every single node and the space complexity is `O(n)`. But if you come to think about it, as we are using a stack and start printing the result from the leaf nodes, it makes sense that we only record the status for the current node popping from the stack, reducing the space complexity to `O(1)`.
## Level Order Traversal
The key point of level order traversal is how do we know whether the traversal of each level is done. The answer is that we use a variable as a flag representing the end of the traversal of the current level.
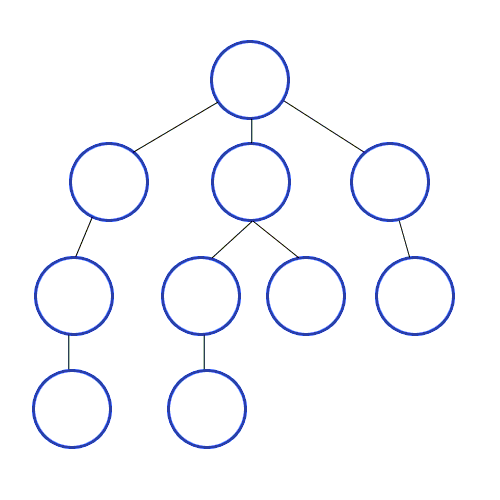
Algorithm Level-order
1. Visit the root node, put it in a FIFO queue, put in the queue a special flag (we are using `null` here).
2. Dequeue a node.
3. If the node equals `null`, it means that all nodes of the current level have been visited. If the queue is empty, we do nothing. Or else we put in another `null`.
4. If the node is not `null`, meaning the traversal of current level has not finished yet, we enqueue its left subtree and right subtree respectively.
## Bi-color marking
We know that there is a tri-color marking in garbage collection algorithm, which works as described below.
- The white color represents "not visited".
- The gray color represents "not all child nodes visited".
- The black color represents "all child nodes visited".
Enlightened by tri-color marking, a bi-color marking method can be invented to solve all three traversal problems with one solution.
The core idea is as follow.
- Use a color to mark whether a node has been visited or not. Nodes yet to be visited are marked as white and visited nodes are marked as gray.
- If we are visiting a white node, turn it into gray, and push its right child node, itself, and it's left child node into the stack respectively.
- If we are visiting a gray node, print it.
Implementation of pre-order and post-order traversal algorithms can be easily done by changing the order of pushing the child nodes into the stack.
Reference: [LeetCode](https://github.com/azl397985856/leetcode/blob/master/thinkings/binary-tree-traversal.en.md)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Minimax helps to achieve maximum score in a game by checking all possible moves
depth is current depth in game tree.
nodeIndex is index of current node in scores[].
if move is of maximizer return true else false
leaves of game tree is stored in scores[]
height is maximum height of Game tree
"""
from __future__ import annotations
import math
def minimax(
depth: int, node_index: int, is_max: bool, scores: list[int], height: float
) -> int:
"""
>>> import math
>>> scores = [90, 23, 6, 33, 21, 65, 123, 34423]
>>> height = math.log(len(scores), 2)
>>> minimax(0, 0, True, scores, height)
65
>>> minimax(-1, 0, True, scores, height)
Traceback (most recent call last):
...
ValueError: Depth cannot be less than 0
>>> minimax(0, 0, True, [], 2)
Traceback (most recent call last):
...
ValueError: Scores cannot be empty
>>> scores = [3, 5, 2, 9, 12, 5, 23, 23]
>>> height = math.log(len(scores), 2)
>>> minimax(0, 0, True, scores, height)
12
"""
if depth < 0:
raise ValueError("Depth cannot be less than 0")
if len(scores) == 0:
raise ValueError("Scores cannot be empty")
if depth == height:
return scores[node_index]
if is_max:
return max(
minimax(depth + 1, node_index * 2, False, scores, height),
minimax(depth + 1, node_index * 2 + 1, False, scores, height),
)
return min(
minimax(depth + 1, node_index * 2, True, scores, height),
minimax(depth + 1, node_index * 2 + 1, True, scores, height),
)
def main() -> None:
scores = [90, 23, 6, 33, 21, 65, 123, 34423]
height = math.log(len(scores), 2)
print("Optimal value : ", end="")
print(minimax(0, 0, True, scores, height))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| """
Minimax helps to achieve maximum score in a game by checking all possible moves
depth is current depth in game tree.
nodeIndex is index of current node in scores[].
if move is of maximizer return true else false
leaves of game tree is stored in scores[]
height is maximum height of Game tree
"""
from __future__ import annotations
import math
def minimax(
depth: int, node_index: int, is_max: bool, scores: list[int], height: float
) -> int:
"""
>>> import math
>>> scores = [90, 23, 6, 33, 21, 65, 123, 34423]
>>> height = math.log(len(scores), 2)
>>> minimax(0, 0, True, scores, height)
65
>>> minimax(-1, 0, True, scores, height)
Traceback (most recent call last):
...
ValueError: Depth cannot be less than 0
>>> minimax(0, 0, True, [], 2)
Traceback (most recent call last):
...
ValueError: Scores cannot be empty
>>> scores = [3, 5, 2, 9, 12, 5, 23, 23]
>>> height = math.log(len(scores), 2)
>>> minimax(0, 0, True, scores, height)
12
"""
if depth < 0:
raise ValueError("Depth cannot be less than 0")
if len(scores) == 0:
raise ValueError("Scores cannot be empty")
if depth == height:
return scores[node_index]
if is_max:
return max(
minimax(depth + 1, node_index * 2, False, scores, height),
minimax(depth + 1, node_index * 2 + 1, False, scores, height),
)
return min(
minimax(depth + 1, node_index * 2, True, scores, height),
minimax(depth + 1, node_index * 2 + 1, True, scores, height),
)
def main() -> None:
scores = [90, 23, 6, 33, 21, 65, 123, 34423]
height = math.log(len(scores), 2)
print("Optimal value : ", end="")
print(minimax(0, 0, True, scores, height))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Title: Dijkstra's Algorithm for finding single source shortest path from scratch
# Author: Shubham Malik
# References: https://en.wikipedia.org/wiki/Dijkstra%27s_algorithm
import math
import sys
# For storing the vertex set to retrieve node with the lowest distance
class PriorityQueue:
# Based on Min Heap
def __init__(self):
self.cur_size = 0
self.array = []
self.pos = {} # To store the pos of node in array
def is_empty(self):
return self.cur_size == 0
def min_heapify(self, idx):
lc = self.left(idx)
rc = self.right(idx)
if lc < self.cur_size and self.array(lc)[0] < self.array(idx)[0]:
smallest = lc
else:
smallest = idx
if rc < self.cur_size and self.array(rc)[0] < self.array(smallest)[0]:
smallest = rc
if smallest != idx:
self.swap(idx, smallest)
self.min_heapify(smallest)
def insert(self, tup):
# Inserts a node into the Priority Queue
self.pos[tup[1]] = self.cur_size
self.cur_size += 1
self.array.append((sys.maxsize, tup[1]))
self.decrease_key((sys.maxsize, tup[1]), tup[0])
def extract_min(self):
# Removes and returns the min element at top of priority queue
min_node = self.array[0][1]
self.array[0] = self.array[self.cur_size - 1]
self.cur_size -= 1
self.min_heapify(1)
del self.pos[min_node]
return min_node
def left(self, i):
# returns the index of left child
return 2 * i + 1
def right(self, i):
# returns the index of right child
return 2 * i + 2
def par(self, i):
# returns the index of parent
return math.floor(i / 2)
def swap(self, i, j):
# swaps array elements at indices i and j
# update the pos{}
self.pos[self.array[i][1]] = j
self.pos[self.array[j][1]] = i
temp = self.array[i]
self.array[i] = self.array[j]
self.array[j] = temp
def decrease_key(self, tup, new_d):
idx = self.pos[tup[1]]
# assuming the new_d is atmost old_d
self.array[idx] = (new_d, tup[1])
while idx > 0 and self.array[self.par(idx)][0] > self.array[idx][0]:
self.swap(idx, self.par(idx))
idx = self.par(idx)
class Graph:
def __init__(self, num):
self.adjList = {} # To store graph: u -> (v,w)
self.num_nodes = num # Number of nodes in graph
# To store the distance from source vertex
self.dist = [0] * self.num_nodes
self.par = [-1] * self.num_nodes # To store the path
def add_edge(self, u, v, w):
# Edge going from node u to v and v to u with weight w
# u (w)-> v, v (w) -> u
# Check if u already in graph
if u in self.adjList:
self.adjList[u].append((v, w))
else:
self.adjList[u] = [(v, w)]
# Assuming undirected graph
if v in self.adjList:
self.adjList[v].append((u, w))
else:
self.adjList[v] = [(u, w)]
def show_graph(self):
# u -> v(w)
for u in self.adjList:
print(u, "->", " -> ".join(str(f"{v}({w})") for v, w in self.adjList[u]))
def dijkstra(self, src):
# Flush old junk values in par[]
self.par = [-1] * self.num_nodes
# src is the source node
self.dist[src] = 0
q = PriorityQueue()
q.insert((0, src)) # (dist from src, node)
for u in self.adjList.keys():
if u != src:
self.dist[u] = sys.maxsize # Infinity
self.par[u] = -1
while not q.is_empty():
u = q.extract_min() # Returns node with the min dist from source
# Update the distance of all the neighbours of u and
# if their prev dist was INFINITY then push them in Q
for v, w in self.adjList[u]:
new_dist = self.dist[u] + w
if self.dist[v] > new_dist:
if self.dist[v] == sys.maxsize:
q.insert((new_dist, v))
else:
q.decrease_key((self.dist[v], v), new_dist)
self.dist[v] = new_dist
self.par[v] = u
# Show the shortest distances from src
self.show_distances(src)
def show_distances(self, src):
print(f"Distance from node: {src}")
for u in range(self.num_nodes):
print(f"Node {u} has distance: {self.dist[u]}")
def show_path(self, src, dest):
# To show the shortest path from src to dest
# WARNING: Use it *after* calling dijkstra
path = []
cost = 0
temp = dest
# Backtracking from dest to src
while self.par[temp] != -1:
path.append(temp)
if temp != src:
for v, w in self.adjList[temp]:
if v == self.par[temp]:
cost += w
break
temp = self.par[temp]
path.append(src)
path.reverse()
print(f"----Path to reach {dest} from {src}----")
for u in path:
print(f"{u}", end=" ")
if u != dest:
print("-> ", end="")
print("\nTotal cost of path: ", cost)
if __name__ == "__main__":
graph = Graph(9)
graph.add_edge(0, 1, 4)
graph.add_edge(0, 7, 8)
graph.add_edge(1, 2, 8)
graph.add_edge(1, 7, 11)
graph.add_edge(2, 3, 7)
graph.add_edge(2, 8, 2)
graph.add_edge(2, 5, 4)
graph.add_edge(3, 4, 9)
graph.add_edge(3, 5, 14)
graph.add_edge(4, 5, 10)
graph.add_edge(5, 6, 2)
graph.add_edge(6, 7, 1)
graph.add_edge(6, 8, 6)
graph.add_edge(7, 8, 7)
graph.show_graph()
graph.dijkstra(0)
graph.show_path(0, 4)
# OUTPUT
# 0 -> 1(4) -> 7(8)
# 1 -> 0(4) -> 2(8) -> 7(11)
# 7 -> 0(8) -> 1(11) -> 6(1) -> 8(7)
# 2 -> 1(8) -> 3(7) -> 8(2) -> 5(4)
# 3 -> 2(7) -> 4(9) -> 5(14)
# 8 -> 2(2) -> 6(6) -> 7(7)
# 5 -> 2(4) -> 3(14) -> 4(10) -> 6(2)
# 4 -> 3(9) -> 5(10)
# 6 -> 5(2) -> 7(1) -> 8(6)
# Distance from node: 0
# Node 0 has distance: 0
# Node 1 has distance: 4
# Node 2 has distance: 12
# Node 3 has distance: 19
# Node 4 has distance: 21
# Node 5 has distance: 11
# Node 6 has distance: 9
# Node 7 has distance: 8
# Node 8 has distance: 14
# ----Path to reach 4 from 0----
# 0 -> 7 -> 6 -> 5 -> 4
# Total cost of path: 21
| # Title: Dijkstra's Algorithm for finding single source shortest path from scratch
# Author: Shubham Malik
# References: https://en.wikipedia.org/wiki/Dijkstra%27s_algorithm
import math
import sys
# For storing the vertex set to retrieve node with the lowest distance
class PriorityQueue:
# Based on Min Heap
def __init__(self):
self.cur_size = 0
self.array = []
self.pos = {} # To store the pos of node in array
def is_empty(self):
return self.cur_size == 0
def min_heapify(self, idx):
lc = self.left(idx)
rc = self.right(idx)
if lc < self.cur_size and self.array(lc)[0] < self.array(idx)[0]:
smallest = lc
else:
smallest = idx
if rc < self.cur_size and self.array(rc)[0] < self.array(smallest)[0]:
smallest = rc
if smallest != idx:
self.swap(idx, smallest)
self.min_heapify(smallest)
def insert(self, tup):
# Inserts a node into the Priority Queue
self.pos[tup[1]] = self.cur_size
self.cur_size += 1
self.array.append((sys.maxsize, tup[1]))
self.decrease_key((sys.maxsize, tup[1]), tup[0])
def extract_min(self):
# Removes and returns the min element at top of priority queue
min_node = self.array[0][1]
self.array[0] = self.array[self.cur_size - 1]
self.cur_size -= 1
self.min_heapify(1)
del self.pos[min_node]
return min_node
def left(self, i):
# returns the index of left child
return 2 * i + 1
def right(self, i):
# returns the index of right child
return 2 * i + 2
def par(self, i):
# returns the index of parent
return math.floor(i / 2)
def swap(self, i, j):
# swaps array elements at indices i and j
# update the pos{}
self.pos[self.array[i][1]] = j
self.pos[self.array[j][1]] = i
temp = self.array[i]
self.array[i] = self.array[j]
self.array[j] = temp
def decrease_key(self, tup, new_d):
idx = self.pos[tup[1]]
# assuming the new_d is atmost old_d
self.array[idx] = (new_d, tup[1])
while idx > 0 and self.array[self.par(idx)][0] > self.array[idx][0]:
self.swap(idx, self.par(idx))
idx = self.par(idx)
class Graph:
def __init__(self, num):
self.adjList = {} # To store graph: u -> (v,w)
self.num_nodes = num # Number of nodes in graph
# To store the distance from source vertex
self.dist = [0] * self.num_nodes
self.par = [-1] * self.num_nodes # To store the path
def add_edge(self, u, v, w):
# Edge going from node u to v and v to u with weight w
# u (w)-> v, v (w) -> u
# Check if u already in graph
if u in self.adjList:
self.adjList[u].append((v, w))
else:
self.adjList[u] = [(v, w)]
# Assuming undirected graph
if v in self.adjList:
self.adjList[v].append((u, w))
else:
self.adjList[v] = [(u, w)]
def show_graph(self):
# u -> v(w)
for u in self.adjList:
print(u, "->", " -> ".join(str(f"{v}({w})") for v, w in self.adjList[u]))
def dijkstra(self, src):
# Flush old junk values in par[]
self.par = [-1] * self.num_nodes
# src is the source node
self.dist[src] = 0
q = PriorityQueue()
q.insert((0, src)) # (dist from src, node)
for u in self.adjList.keys():
if u != src:
self.dist[u] = sys.maxsize # Infinity
self.par[u] = -1
while not q.is_empty():
u = q.extract_min() # Returns node with the min dist from source
# Update the distance of all the neighbours of u and
# if their prev dist was INFINITY then push them in Q
for v, w in self.adjList[u]:
new_dist = self.dist[u] + w
if self.dist[v] > new_dist:
if self.dist[v] == sys.maxsize:
q.insert((new_dist, v))
else:
q.decrease_key((self.dist[v], v), new_dist)
self.dist[v] = new_dist
self.par[v] = u
# Show the shortest distances from src
self.show_distances(src)
def show_distances(self, src):
print(f"Distance from node: {src}")
for u in range(self.num_nodes):
print(f"Node {u} has distance: {self.dist[u]}")
def show_path(self, src, dest):
# To show the shortest path from src to dest
# WARNING: Use it *after* calling dijkstra
path = []
cost = 0
temp = dest
# Backtracking from dest to src
while self.par[temp] != -1:
path.append(temp)
if temp != src:
for v, w in self.adjList[temp]:
if v == self.par[temp]:
cost += w
break
temp = self.par[temp]
path.append(src)
path.reverse()
print(f"----Path to reach {dest} from {src}----")
for u in path:
print(f"{u}", end=" ")
if u != dest:
print("-> ", end="")
print("\nTotal cost of path: ", cost)
if __name__ == "__main__":
graph = Graph(9)
graph.add_edge(0, 1, 4)
graph.add_edge(0, 7, 8)
graph.add_edge(1, 2, 8)
graph.add_edge(1, 7, 11)
graph.add_edge(2, 3, 7)
graph.add_edge(2, 8, 2)
graph.add_edge(2, 5, 4)
graph.add_edge(3, 4, 9)
graph.add_edge(3, 5, 14)
graph.add_edge(4, 5, 10)
graph.add_edge(5, 6, 2)
graph.add_edge(6, 7, 1)
graph.add_edge(6, 8, 6)
graph.add_edge(7, 8, 7)
graph.show_graph()
graph.dijkstra(0)
graph.show_path(0, 4)
# OUTPUT
# 0 -> 1(4) -> 7(8)
# 1 -> 0(4) -> 2(8) -> 7(11)
# 7 -> 0(8) -> 1(11) -> 6(1) -> 8(7)
# 2 -> 1(8) -> 3(7) -> 8(2) -> 5(4)
# 3 -> 2(7) -> 4(9) -> 5(14)
# 8 -> 2(2) -> 6(6) -> 7(7)
# 5 -> 2(4) -> 3(14) -> 4(10) -> 6(2)
# 4 -> 3(9) -> 5(10)
# 6 -> 5(2) -> 7(1) -> 8(6)
# Distance from node: 0
# Node 0 has distance: 0
# Node 1 has distance: 4
# Node 2 has distance: 12
# Node 3 has distance: 19
# Node 4 has distance: 21
# Node 5 has distance: 11
# Node 6 has distance: 9
# Node 7 has distance: 8
# Node 8 has distance: 14
# ----Path to reach 4 from 0----
# 0 -> 7 -> 6 -> 5 -> 4
# Total cost of path: 21
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
A perfect number is a number for which the sum of its proper divisors is exactly
equal to the number. For example, the sum of the proper divisors of 28 would be
1 + 2 + 4 + 7 + 14 = 28, which means that 28 is a perfect number.
A number n is called deficient if the sum of its proper divisors is less than n
and it is called abundant if this sum exceeds n.
As 12 is the smallest abundant number, 1 + 2 + 3 + 4 + 6 = 16, the smallest
number that can be written as the sum of two abundant numbers is 24. By
mathematical analysis, it can be shown that all integers greater than 28123
can be written as the sum of two abundant numbers. However, this upper limit
cannot be reduced any further by analysis even though it is known that the
greatest number that cannot be expressed as the sum of two abundant numbers
is less than this limit.
Find the sum of all the positive integers which cannot be written as the sum
of two abundant numbers.
"""
def solution(limit=28123):
"""
Finds the sum of all the positive integers which cannot be written as
the sum of two abundant numbers
as described by the statement above.
>>> solution()
4179871
"""
sum_divs = [1] * (limit + 1)
for i in range(2, int(limit**0.5) + 1):
sum_divs[i * i] += i
for k in range(i + 1, limit // i + 1):
sum_divs[k * i] += k + i
abundants = set()
res = 0
for n in range(1, limit + 1):
if sum_divs[n] > n:
abundants.add(n)
if not any((n - a in abundants) for a in abundants):
res += n
return res
if __name__ == "__main__":
print(solution())
| """
A perfect number is a number for which the sum of its proper divisors is exactly
equal to the number. For example, the sum of the proper divisors of 28 would be
1 + 2 + 4 + 7 + 14 = 28, which means that 28 is a perfect number.
A number n is called deficient if the sum of its proper divisors is less than n
and it is called abundant if this sum exceeds n.
As 12 is the smallest abundant number, 1 + 2 + 3 + 4 + 6 = 16, the smallest
number that can be written as the sum of two abundant numbers is 24. By
mathematical analysis, it can be shown that all integers greater than 28123
can be written as the sum of two abundant numbers. However, this upper limit
cannot be reduced any further by analysis even though it is known that the
greatest number that cannot be expressed as the sum of two abundant numbers
is less than this limit.
Find the sum of all the positive integers which cannot be written as the sum
of two abundant numbers.
"""
def solution(limit=28123):
"""
Finds the sum of all the positive integers which cannot be written as
the sum of two abundant numbers
as described by the statement above.
>>> solution()
4179871
"""
sum_divs = [1] * (limit + 1)
for i in range(2, int(limit**0.5) + 1):
sum_divs[i * i] += i
for k in range(i + 1, limit // i + 1):
sum_divs[k * i] += k + i
abundants = set()
res = 0
for n in range(1, limit + 1):
if sum_divs[n] > n:
abundants.add(n)
if not any((n - a in abundants) for a in abundants):
res += n
return res
if __name__ == "__main__":
print(solution())
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
# Author: OMKAR PATHAK, Nwachukwu Chidiebere
# Use a Python dictionary to construct the graph.
from __future__ import annotations
from pprint import pformat
from typing import Generic, TypeVar
T = TypeVar("T")
class GraphAdjacencyList(Generic[T]):
"""
Adjacency List type Graph Data Structure that accounts for directed and undirected
Graphs. Initialize graph object indicating whether it's directed or undirected.
Directed graph example:
>>> d_graph = GraphAdjacencyList()
>>> d_graph
{}
>>> d_graph.add_edge(0, 1)
{0: [1], 1: []}
>>> d_graph.add_edge(1, 2).add_edge(1, 4).add_edge(1, 5)
{0: [1], 1: [2, 4, 5], 2: [], 4: [], 5: []}
>>> d_graph.add_edge(2, 0).add_edge(2, 6).add_edge(2, 7)
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
>>> print(d_graph)
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
>>> print(repr(d_graph))
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
Undirected graph example:
>>> u_graph = GraphAdjacencyList(directed=False)
>>> u_graph.add_edge(0, 1)
{0: [1], 1: [0]}
>>> u_graph.add_edge(1, 2).add_edge(1, 4).add_edge(1, 5)
{0: [1], 1: [0, 2, 4, 5], 2: [1], 4: [1], 5: [1]}
>>> u_graph.add_edge(2, 0).add_edge(2, 6).add_edge(2, 7)
{0: [1, 2], 1: [0, 2, 4, 5], 2: [1, 0, 6, 7], 4: [1], 5: [1], 6: [2], 7: [2]}
>>> u_graph.add_edge(4, 5)
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> print(u_graph)
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> print(repr(u_graph))
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> char_graph = GraphAdjacencyList(directed=False)
>>> char_graph.add_edge('a', 'b')
{'a': ['b'], 'b': ['a']}
>>> char_graph.add_edge('b', 'c').add_edge('b', 'e').add_edge('b', 'f')
{'a': ['b'], 'b': ['a', 'c', 'e', 'f'], 'c': ['b'], 'e': ['b'], 'f': ['b']}
>>> print(char_graph)
{'a': ['b'], 'b': ['a', 'c', 'e', 'f'], 'c': ['b'], 'e': ['b'], 'f': ['b']}
"""
def __init__(self, directed: bool = True) -> None:
"""
Parameters:
directed: (bool) Indicates if graph is directed or undirected. Default is True.
"""
self.adj_list: dict[T, list[T]] = {} # dictionary of lists
self.directed = directed
def add_edge(
self, source_vertex: T, destination_vertex: T
) -> GraphAdjacencyList[T]:
"""
Connects vertices together. Creates and Edge from source vertex to destination
vertex.
Vertices will be created if not found in graph
"""
if not self.directed: # For undirected graphs
# if both source vertex and destination vertex are both present in the
# adjacency list, add destination vertex to source vertex list of adjacent
# vertices and add source vertex to destination vertex list of adjacent
# vertices.
if source_vertex in self.adj_list and destination_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex].append(source_vertex)
# if only source vertex is present in adjacency list, add destination vertex
# to source vertex list of adjacent vertices, then create a new vertex with
# destination vertex as key and assign a list containing the source vertex
# as it's first adjacent vertex.
elif source_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex] = [source_vertex]
# if only destination vertex is present in adjacency list, add source vertex
# to destination vertex list of adjacent vertices, then create a new vertex
# with source vertex as key and assign a list containing the source vertex
# as it's first adjacent vertex.
elif destination_vertex in self.adj_list:
self.adj_list[destination_vertex].append(source_vertex)
self.adj_list[source_vertex] = [destination_vertex]
# if both source vertex and destination vertex are not present in adjacency
# list, create a new vertex with source vertex as key and assign a list
# containing the destination vertex as it's first adjacent vertex also
# create a new vertex with destination vertex as key and assign a list
# containing the source vertex as it's first adjacent vertex.
else:
self.adj_list[source_vertex] = [destination_vertex]
self.adj_list[destination_vertex] = [source_vertex]
else: # For directed graphs
# if both source vertex and destination vertex are present in adjacency
# list, add destination vertex to source vertex list of adjacent vertices.
if source_vertex in self.adj_list and destination_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
# if only source vertex is present in adjacency list, add destination
# vertex to source vertex list of adjacent vertices and create a new vertex
# with destination vertex as key, which has no adjacent vertex
elif source_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex] = []
# if only destination vertex is present in adjacency list, create a new
# vertex with source vertex as key and assign a list containing destination
# vertex as first adjacent vertex
elif destination_vertex in self.adj_list:
self.adj_list[source_vertex] = [destination_vertex]
# if both source vertex and destination vertex are not present in adjacency
# list, create a new vertex with source vertex as key and a list containing
# destination vertex as it's first adjacent vertex. Then create a new vertex
# with destination vertex as key, which has no adjacent vertex
else:
self.adj_list[source_vertex] = [destination_vertex]
self.adj_list[destination_vertex] = []
return self
def __repr__(self) -> str:
return pformat(self.adj_list)
| #!/usr/bin/env python3
# Author: OMKAR PATHAK, Nwachukwu Chidiebere
# Use a Python dictionary to construct the graph.
from __future__ import annotations
from pprint import pformat
from typing import Generic, TypeVar
T = TypeVar("T")
class GraphAdjacencyList(Generic[T]):
"""
Adjacency List type Graph Data Structure that accounts for directed and undirected
Graphs. Initialize graph object indicating whether it's directed or undirected.
Directed graph example:
>>> d_graph = GraphAdjacencyList()
>>> d_graph
{}
>>> d_graph.add_edge(0, 1)
{0: [1], 1: []}
>>> d_graph.add_edge(1, 2).add_edge(1, 4).add_edge(1, 5)
{0: [1], 1: [2, 4, 5], 2: [], 4: [], 5: []}
>>> d_graph.add_edge(2, 0).add_edge(2, 6).add_edge(2, 7)
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
>>> print(d_graph)
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
>>> print(repr(d_graph))
{0: [1], 1: [2, 4, 5], 2: [0, 6, 7], 4: [], 5: [], 6: [], 7: []}
Undirected graph example:
>>> u_graph = GraphAdjacencyList(directed=False)
>>> u_graph.add_edge(0, 1)
{0: [1], 1: [0]}
>>> u_graph.add_edge(1, 2).add_edge(1, 4).add_edge(1, 5)
{0: [1], 1: [0, 2, 4, 5], 2: [1], 4: [1], 5: [1]}
>>> u_graph.add_edge(2, 0).add_edge(2, 6).add_edge(2, 7)
{0: [1, 2], 1: [0, 2, 4, 5], 2: [1, 0, 6, 7], 4: [1], 5: [1], 6: [2], 7: [2]}
>>> u_graph.add_edge(4, 5)
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> print(u_graph)
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> print(repr(u_graph))
{0: [1, 2],
1: [0, 2, 4, 5],
2: [1, 0, 6, 7],
4: [1, 5],
5: [1, 4],
6: [2],
7: [2]}
>>> char_graph = GraphAdjacencyList(directed=False)
>>> char_graph.add_edge('a', 'b')
{'a': ['b'], 'b': ['a']}
>>> char_graph.add_edge('b', 'c').add_edge('b', 'e').add_edge('b', 'f')
{'a': ['b'], 'b': ['a', 'c', 'e', 'f'], 'c': ['b'], 'e': ['b'], 'f': ['b']}
>>> print(char_graph)
{'a': ['b'], 'b': ['a', 'c', 'e', 'f'], 'c': ['b'], 'e': ['b'], 'f': ['b']}
"""
def __init__(self, directed: bool = True) -> None:
"""
Parameters:
directed: (bool) Indicates if graph is directed or undirected. Default is True.
"""
self.adj_list: dict[T, list[T]] = {} # dictionary of lists
self.directed = directed
def add_edge(
self, source_vertex: T, destination_vertex: T
) -> GraphAdjacencyList[T]:
"""
Connects vertices together. Creates and Edge from source vertex to destination
vertex.
Vertices will be created if not found in graph
"""
if not self.directed: # For undirected graphs
# if both source vertex and destination vertex are both present in the
# adjacency list, add destination vertex to source vertex list of adjacent
# vertices and add source vertex to destination vertex list of adjacent
# vertices.
if source_vertex in self.adj_list and destination_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex].append(source_vertex)
# if only source vertex is present in adjacency list, add destination vertex
# to source vertex list of adjacent vertices, then create a new vertex with
# destination vertex as key and assign a list containing the source vertex
# as it's first adjacent vertex.
elif source_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex] = [source_vertex]
# if only destination vertex is present in adjacency list, add source vertex
# to destination vertex list of adjacent vertices, then create a new vertex
# with source vertex as key and assign a list containing the source vertex
# as it's first adjacent vertex.
elif destination_vertex in self.adj_list:
self.adj_list[destination_vertex].append(source_vertex)
self.adj_list[source_vertex] = [destination_vertex]
# if both source vertex and destination vertex are not present in adjacency
# list, create a new vertex with source vertex as key and assign a list
# containing the destination vertex as it's first adjacent vertex also
# create a new vertex with destination vertex as key and assign a list
# containing the source vertex as it's first adjacent vertex.
else:
self.adj_list[source_vertex] = [destination_vertex]
self.adj_list[destination_vertex] = [source_vertex]
else: # For directed graphs
# if both source vertex and destination vertex are present in adjacency
# list, add destination vertex to source vertex list of adjacent vertices.
if source_vertex in self.adj_list and destination_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
# if only source vertex is present in adjacency list, add destination
# vertex to source vertex list of adjacent vertices and create a new vertex
# with destination vertex as key, which has no adjacent vertex
elif source_vertex in self.adj_list:
self.adj_list[source_vertex].append(destination_vertex)
self.adj_list[destination_vertex] = []
# if only destination vertex is present in adjacency list, create a new
# vertex with source vertex as key and assign a list containing destination
# vertex as first adjacent vertex
elif destination_vertex in self.adj_list:
self.adj_list[source_vertex] = [destination_vertex]
# if both source vertex and destination vertex are not present in adjacency
# list, create a new vertex with source vertex as key and a list containing
# destination vertex as it's first adjacent vertex. Then create a new vertex
# with destination vertex as key, which has no adjacent vertex
else:
self.adj_list[source_vertex] = [destination_vertex]
self.adj_list[destination_vertex] = []
return self
def __repr__(self) -> str:
return pformat(self.adj_list)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Gamma function is a very useful tool in math and physics.
It helps calculating complex integral in a convenient way.
for more info: https://en.wikipedia.org/wiki/Gamma_function
Python's Standard Library math.gamma() function overflows around gamma(171.624).
"""
from math import pi, sqrt
def gamma(num: float) -> float:
"""
Calculates the value of Gamma function of num
where num is either an integer (1, 2, 3..) or a half-integer (0.5, 1.5, 2.5 ...).
Implemented using recursion
Examples:
>>> from math import isclose, gamma as math_gamma
>>> gamma(0.5)
1.7724538509055159
>>> gamma(2)
1.0
>>> gamma(3.5)
3.3233509704478426
>>> gamma(171.5)
9.483367566824795e+307
>>> all(isclose(gamma(num), math_gamma(num)) for num in (0.5, 2, 3.5, 171.5))
True
>>> gamma(0)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(-1.1)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(-4)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(172)
Traceback (most recent call last):
...
OverflowError: math range error
>>> gamma(1.1)
Traceback (most recent call last):
...
NotImplementedError: num must be an integer or a half-integer
"""
if num <= 0:
raise ValueError("math domain error")
if num > 171.5:
raise OverflowError("math range error")
elif num - int(num) not in (0, 0.5):
raise NotImplementedError("num must be an integer or a half-integer")
elif num == 0.5:
return sqrt(pi)
else:
return 1.0 if num == 1 else (num - 1) * gamma(num - 1)
def test_gamma() -> None:
"""
>>> test_gamma()
"""
assert gamma(0.5) == sqrt(pi)
assert gamma(1) == 1.0
assert gamma(2) == 1.0
if __name__ == "__main__":
from doctest import testmod
testmod()
num = 1.0
while num:
num = float(input("Gamma of: "))
print(f"gamma({num}) = {gamma(num)}")
print("\nEnter 0 to exit...")
| """
Gamma function is a very useful tool in math and physics.
It helps calculating complex integral in a convenient way.
for more info: https://en.wikipedia.org/wiki/Gamma_function
Python's Standard Library math.gamma() function overflows around gamma(171.624).
"""
from math import pi, sqrt
def gamma(num: float) -> float:
"""
Calculates the value of Gamma function of num
where num is either an integer (1, 2, 3..) or a half-integer (0.5, 1.5, 2.5 ...).
Implemented using recursion
Examples:
>>> from math import isclose, gamma as math_gamma
>>> gamma(0.5)
1.7724538509055159
>>> gamma(2)
1.0
>>> gamma(3.5)
3.3233509704478426
>>> gamma(171.5)
9.483367566824795e+307
>>> all(isclose(gamma(num), math_gamma(num)) for num in (0.5, 2, 3.5, 171.5))
True
>>> gamma(0)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(-1.1)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(-4)
Traceback (most recent call last):
...
ValueError: math domain error
>>> gamma(172)
Traceback (most recent call last):
...
OverflowError: math range error
>>> gamma(1.1)
Traceback (most recent call last):
...
NotImplementedError: num must be an integer or a half-integer
"""
if num <= 0:
raise ValueError("math domain error")
if num > 171.5:
raise OverflowError("math range error")
elif num - int(num) not in (0, 0.5):
raise NotImplementedError("num must be an integer or a half-integer")
elif num == 0.5:
return sqrt(pi)
else:
return 1.0 if num == 1 else (num - 1) * gamma(num - 1)
def test_gamma() -> None:
"""
>>> test_gamma()
"""
assert gamma(0.5) == sqrt(pi)
assert gamma(1) == 1.0
assert gamma(2) == 1.0
if __name__ == "__main__":
from doctest import testmod
testmod()
num = 1.0
while num:
num = float(input("Gamma of: "))
print(f"gamma({num}) = {gamma(num)}")
print("\nEnter 0 to exit...")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 234: https://projecteuler.net/problem=234
For any integer n, consider the three functions
f1,n(x,y,z) = x^(n+1) + y^(n+1) - z^(n+1)
f2,n(x,y,z) = (xy + yz + zx)*(x^(n-1) + y^(n-1) - z^(n-1))
f3,n(x,y,z) = xyz*(xn-2 + yn-2 - zn-2)
and their combination
fn(x,y,z) = f1,n(x,y,z) + f2,n(x,y,z) - f3,n(x,y,z)
We call (x,y,z) a golden triple of order k if x, y, and z are all rational numbers
of the form a / b with 0 < a < b ≤ k and there is (at least) one integer n,
so that fn(x,y,z) = 0.
Let s(x,y,z) = x + y + z.
Let t = u / v be the sum of all distinct s(x,y,z) for all golden triples
(x,y,z) of order 35.
All the s(x,y,z) and t must be in reduced form.
Find u + v.
Solution:
By expanding the brackets it is easy to show that
fn(x, y, z) = (x + y + z) * (x^n + y^n - z^n).
Since x,y,z are positive, the requirement fn(x, y, z) = 0 is fulfilled if and
only if x^n + y^n = z^n.
By Fermat's Last Theorem, this means that the absolute value of n can not
exceed 2, i.e. n is in {-2, -1, 0, 1, 2}. We can eliminate n = 0 since then the
equation would reduce to 1 + 1 = 1, for which there are no solutions.
So all we have to do is iterate through the possible numerators and denominators
of x and y, calculate the corresponding z, and check if the corresponding numerator and
denominator are integer and satisfy 0 < z_num < z_den <= 0. We use a set "uniquq_s"
to make sure there are no duplicates, and the fractions.Fraction class to make sure
we get the right numerator and denominator.
Reference:
https://en.wikipedia.org/wiki/Fermat%27s_Last_Theorem
"""
from __future__ import annotations
from fractions import Fraction
from math import gcd, sqrt
def is_sq(number: int) -> bool:
"""
Check if number is a perfect square.
>>> is_sq(1)
True
>>> is_sq(1000001)
False
>>> is_sq(1000000)
True
"""
sq: int = int(number**0.5)
return number == sq * sq
def add_three(
x_num: int, x_den: int, y_num: int, y_den: int, z_num: int, z_den: int
) -> tuple[int, int]:
"""
Given the numerators and denominators of three fractions, return the
numerator and denominator of their sum in lowest form.
>>> add_three(1, 3, 1, 3, 1, 3)
(1, 1)
>>> add_three(2, 5, 4, 11, 12, 3)
(262, 55)
"""
top: int = x_num * y_den * z_den + y_num * x_den * z_den + z_num * x_den * y_den
bottom: int = x_den * y_den * z_den
hcf: int = gcd(top, bottom)
top //= hcf
bottom //= hcf
return top, bottom
def solution(order: int = 35) -> int:
"""
Find the sum of the numerator and denominator of the sum of all s(x,y,z) for
golden triples (x,y,z) of the given order.
>>> solution(5)
296
>>> solution(10)
12519
>>> solution(20)
19408891927
"""
unique_s: set = set()
hcf: int
total: Fraction = Fraction(0)
fraction_sum: tuple[int, int]
for x_num in range(1, order + 1):
for x_den in range(x_num + 1, order + 1):
for y_num in range(1, order + 1):
for y_den in range(y_num + 1, order + 1):
# n=1
z_num = x_num * y_den + x_den * y_num
z_den = x_den * y_den
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=2
z_num = (
x_num * x_num * y_den * y_den + x_den * x_den * y_num * y_num
)
z_den = x_den * x_den * y_den * y_den
if is_sq(z_num) and is_sq(z_den):
z_num = int(sqrt(z_num))
z_den = int(sqrt(z_den))
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=-1
z_num = x_num * y_num
z_den = x_den * y_num + x_num * y_den
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=2
z_num = x_num * x_num * y_num * y_num
z_den = (
x_den * x_den * y_num * y_num + x_num * x_num * y_den * y_den
)
if is_sq(z_num) and is_sq(z_den):
z_num = int(sqrt(z_num))
z_den = int(sqrt(z_den))
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
for num, den in unique_s:
total += Fraction(num, den)
return total.denominator + total.numerator
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler Problem 234: https://projecteuler.net/problem=234
For any integer n, consider the three functions
f1,n(x,y,z) = x^(n+1) + y^(n+1) - z^(n+1)
f2,n(x,y,z) = (xy + yz + zx)*(x^(n-1) + y^(n-1) - z^(n-1))
f3,n(x,y,z) = xyz*(xn-2 + yn-2 - zn-2)
and their combination
fn(x,y,z) = f1,n(x,y,z) + f2,n(x,y,z) - f3,n(x,y,z)
We call (x,y,z) a golden triple of order k if x, y, and z are all rational numbers
of the form a / b with 0 < a < b ≤ k and there is (at least) one integer n,
so that fn(x,y,z) = 0.
Let s(x,y,z) = x + y + z.
Let t = u / v be the sum of all distinct s(x,y,z) for all golden triples
(x,y,z) of order 35.
All the s(x,y,z) and t must be in reduced form.
Find u + v.
Solution:
By expanding the brackets it is easy to show that
fn(x, y, z) = (x + y + z) * (x^n + y^n - z^n).
Since x,y,z are positive, the requirement fn(x, y, z) = 0 is fulfilled if and
only if x^n + y^n = z^n.
By Fermat's Last Theorem, this means that the absolute value of n can not
exceed 2, i.e. n is in {-2, -1, 0, 1, 2}. We can eliminate n = 0 since then the
equation would reduce to 1 + 1 = 1, for which there are no solutions.
So all we have to do is iterate through the possible numerators and denominators
of x and y, calculate the corresponding z, and check if the corresponding numerator and
denominator are integer and satisfy 0 < z_num < z_den <= 0. We use a set "uniquq_s"
to make sure there are no duplicates, and the fractions.Fraction class to make sure
we get the right numerator and denominator.
Reference:
https://en.wikipedia.org/wiki/Fermat%27s_Last_Theorem
"""
from __future__ import annotations
from fractions import Fraction
from math import gcd, sqrt
def is_sq(number: int) -> bool:
"""
Check if number is a perfect square.
>>> is_sq(1)
True
>>> is_sq(1000001)
False
>>> is_sq(1000000)
True
"""
sq: int = int(number**0.5)
return number == sq * sq
def add_three(
x_num: int, x_den: int, y_num: int, y_den: int, z_num: int, z_den: int
) -> tuple[int, int]:
"""
Given the numerators and denominators of three fractions, return the
numerator and denominator of their sum in lowest form.
>>> add_three(1, 3, 1, 3, 1, 3)
(1, 1)
>>> add_three(2, 5, 4, 11, 12, 3)
(262, 55)
"""
top: int = x_num * y_den * z_den + y_num * x_den * z_den + z_num * x_den * y_den
bottom: int = x_den * y_den * z_den
hcf: int = gcd(top, bottom)
top //= hcf
bottom //= hcf
return top, bottom
def solution(order: int = 35) -> int:
"""
Find the sum of the numerator and denominator of the sum of all s(x,y,z) for
golden triples (x,y,z) of the given order.
>>> solution(5)
296
>>> solution(10)
12519
>>> solution(20)
19408891927
"""
unique_s: set = set()
hcf: int
total: Fraction = Fraction(0)
fraction_sum: tuple[int, int]
for x_num in range(1, order + 1):
for x_den in range(x_num + 1, order + 1):
for y_num in range(1, order + 1):
for y_den in range(y_num + 1, order + 1):
# n=1
z_num = x_num * y_den + x_den * y_num
z_den = x_den * y_den
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=2
z_num = (
x_num * x_num * y_den * y_den + x_den * x_den * y_num * y_num
)
z_den = x_den * x_den * y_den * y_den
if is_sq(z_num) and is_sq(z_den):
z_num = int(sqrt(z_num))
z_den = int(sqrt(z_den))
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=-1
z_num = x_num * y_num
z_den = x_den * y_num + x_num * y_den
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
# n=2
z_num = x_num * x_num * y_num * y_num
z_den = (
x_den * x_den * y_num * y_num + x_num * x_num * y_den * y_den
)
if is_sq(z_num) and is_sq(z_den):
z_num = int(sqrt(z_num))
z_den = int(sqrt(z_den))
hcf = gcd(z_num, z_den)
z_num //= hcf
z_den //= hcf
if 0 < z_num < z_den <= order:
fraction_sum = add_three(
x_num, x_den, y_num, y_den, z_num, z_den
)
unique_s.add(fraction_sum)
for num, den in unique_s:
total += Fraction(num, den)
return total.denominator + total.numerator
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler 62
https://projecteuler.net/problem=62
The cube, 41063625 (345^3), can be permuted to produce two other cubes:
56623104 (384^3) and 66430125 (405^3). In fact, 41063625 is the smallest cube
which has exactly three permutations of its digits which are also cube.
Find the smallest cube for which exactly five permutations of its digits are
cube.
"""
from collections import defaultdict
def solution(max_base: int = 5) -> int:
"""
Iterate through every possible cube and sort the cube's digits in
ascending order. Sorting maintains an ordering of the digits that allows
you to compare permutations. Store each sorted sequence of digits in a
dictionary, whose key is the sequence of digits and value is a list of
numbers that are the base of the cube.
Once you find 5 numbers that produce the same sequence of digits, return
the smallest one, which is at index 0 since we insert each base number in
ascending order.
>>> solution(2)
125
>>> solution(3)
41063625
"""
freqs = defaultdict(list)
num = 0
while True:
digits = get_digits(num)
freqs[digits].append(num)
if len(freqs[digits]) == max_base:
base = freqs[digits][0] ** 3
return base
num += 1
def get_digits(num: int) -> str:
"""
Computes the sorted sequence of digits of the cube of num.
>>> get_digits(3)
'27'
>>> get_digits(99)
'027999'
>>> get_digits(123)
'0166788'
"""
return "".join(sorted(str(num**3)))
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler 62
https://projecteuler.net/problem=62
The cube, 41063625 (345^3), can be permuted to produce two other cubes:
56623104 (384^3) and 66430125 (405^3). In fact, 41063625 is the smallest cube
which has exactly three permutations of its digits which are also cube.
Find the smallest cube for which exactly five permutations of its digits are
cube.
"""
from collections import defaultdict
def solution(max_base: int = 5) -> int:
"""
Iterate through every possible cube and sort the cube's digits in
ascending order. Sorting maintains an ordering of the digits that allows
you to compare permutations. Store each sorted sequence of digits in a
dictionary, whose key is the sequence of digits and value is a list of
numbers that are the base of the cube.
Once you find 5 numbers that produce the same sequence of digits, return
the smallest one, which is at index 0 since we insert each base number in
ascending order.
>>> solution(2)
125
>>> solution(3)
41063625
"""
freqs = defaultdict(list)
num = 0
while True:
digits = get_digits(num)
freqs[digits].append(num)
if len(freqs[digits]) == max_base:
base = freqs[digits][0] ** 3
return base
num += 1
def get_digits(num: int) -> str:
"""
Computes the sorted sequence of digits of the cube of num.
>>> get_digits(3)
'27'
>>> get_digits(99)
'027999'
>>> get_digits(123)
'0166788'
"""
return "".join(sorted(str(num**3)))
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| 0000000000000000000000000000000000000000 9caf4784aada17dc75348f77cc8c356df503c0f3 jupyter <[email protected]> 1704811012 +0000 clone: from https://github.com/TheAlgorithms/Python.git
| 0000000000000000000000000000000000000000 9caf4784aada17dc75348f77cc8c356df503c0f3 jupyter <[email protected]> 1704811012 +0000 clone: from https://github.com/TheAlgorithms/Python.git
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Scrape the price and pharmacy name for a prescription drug from rx site
after providing the drug name and zipcode.
"""
from urllib.error import HTTPError
from bs4 import BeautifulSoup
from requests import exceptions, get
BASE_URL = "https://www.wellrx.com/prescriptions/{0}/{1}/?freshSearch=true"
def fetch_pharmacy_and_price_list(drug_name: str, zip_code: str) -> list | None:
"""[summary]
This function will take input of drug name and zipcode,
then request to the BASE_URL site.
Get the page data and scrape it to the generate the
list of lowest prices for the prescription drug.
Args:
drug_name (str): [Drug name]
zip_code(str): [Zip code]
Returns:
list: [List of pharmacy name and price]
>>> fetch_pharmacy_and_price_list(None, None)
>>> fetch_pharmacy_and_price_list(None, 30303)
>>> fetch_pharmacy_and_price_list("eliquis", None)
"""
try:
# Has user provided both inputs?
if not drug_name or not zip_code:
return None
request_url = BASE_URL.format(drug_name, zip_code)
response = get(request_url)
# Is the response ok?
response.raise_for_status()
# Scrape the data using bs4
soup = BeautifulSoup(response.text, "html.parser")
# This list will store the name and price.
pharmacy_price_list = []
# Fetch all the grids that contains the items.
grid_list = soup.find_all("div", {"class": "grid-x pharmCard"})
if grid_list and len(grid_list) > 0:
for grid in grid_list:
# Get the pharmacy price.
pharmacy_name = grid.find("p", {"class": "list-title"}).text
# Get price of the drug.
price = grid.find("span", {"p", "price price-large"}).text
pharmacy_price_list.append(
{
"pharmacy_name": pharmacy_name,
"price": price,
}
)
return pharmacy_price_list
except (HTTPError, exceptions.RequestException, ValueError):
return None
if __name__ == "__main__":
# Enter a drug name and a zip code
drug_name = input("Enter drug name: ").strip()
zip_code = input("Enter zip code: ").strip()
pharmacy_price_list: list | None = fetch_pharmacy_and_price_list(
drug_name, zip_code
)
if pharmacy_price_list:
print(f"\nSearch results for {drug_name} at location {zip_code}:")
for pharmacy_price in pharmacy_price_list:
name = pharmacy_price["pharmacy_name"]
price = pharmacy_price["price"]
print(f"Pharmacy: {name} Price: {price}")
else:
print(f"No results found for {drug_name}")
| """
Scrape the price and pharmacy name for a prescription drug from rx site
after providing the drug name and zipcode.
"""
from urllib.error import HTTPError
from bs4 import BeautifulSoup
from requests import exceptions, get
BASE_URL = "https://www.wellrx.com/prescriptions/{0}/{1}/?freshSearch=true"
def fetch_pharmacy_and_price_list(drug_name: str, zip_code: str) -> list | None:
"""[summary]
This function will take input of drug name and zipcode,
then request to the BASE_URL site.
Get the page data and scrape it to the generate the
list of lowest prices for the prescription drug.
Args:
drug_name (str): [Drug name]
zip_code(str): [Zip code]
Returns:
list: [List of pharmacy name and price]
>>> fetch_pharmacy_and_price_list(None, None)
>>> fetch_pharmacy_and_price_list(None, 30303)
>>> fetch_pharmacy_and_price_list("eliquis", None)
"""
try:
# Has user provided both inputs?
if not drug_name or not zip_code:
return None
request_url = BASE_URL.format(drug_name, zip_code)
response = get(request_url)
# Is the response ok?
response.raise_for_status()
# Scrape the data using bs4
soup = BeautifulSoup(response.text, "html.parser")
# This list will store the name and price.
pharmacy_price_list = []
# Fetch all the grids that contains the items.
grid_list = soup.find_all("div", {"class": "grid-x pharmCard"})
if grid_list and len(grid_list) > 0:
for grid in grid_list:
# Get the pharmacy price.
pharmacy_name = grid.find("p", {"class": "list-title"}).text
# Get price of the drug.
price = grid.find("span", {"p", "price price-large"}).text
pharmacy_price_list.append(
{
"pharmacy_name": pharmacy_name,
"price": price,
}
)
return pharmacy_price_list
except (HTTPError, exceptions.RequestException, ValueError):
return None
if __name__ == "__main__":
# Enter a drug name and a zip code
drug_name = input("Enter drug name: ").strip()
zip_code = input("Enter zip code: ").strip()
pharmacy_price_list: list | None = fetch_pharmacy_and_price_list(
drug_name, zip_code
)
if pharmacy_price_list:
print(f"\nSearch results for {drug_name} at location {zip_code}:")
for pharmacy_price in pharmacy_price_list:
name = pharmacy_price["pharmacy_name"]
price = pharmacy_price["price"]
print(f"Pharmacy: {name} Price: {price}")
else:
print(f"No results found for {drug_name}")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| def binary_search(lst, item, start, end):
if start == end:
return start if lst[start] > item else start + 1
if start > end:
return start
mid = (start + end) // 2
if lst[mid] < item:
return binary_search(lst, item, mid + 1, end)
elif lst[mid] > item:
return binary_search(lst, item, start, mid - 1)
else:
return mid
def insertion_sort(lst):
length = len(lst)
for index in range(1, length):
value = lst[index]
pos = binary_search(lst, value, 0, index - 1)
lst = lst[:pos] + [value] + lst[pos:index] + lst[index + 1 :]
return lst
def merge(left, right):
if not left:
return right
if not right:
return left
if left[0] < right[0]:
return [left[0]] + merge(left[1:], right)
return [right[0]] + merge(left, right[1:])
def tim_sort(lst):
"""
>>> tim_sort("Python")
['P', 'h', 'n', 'o', 't', 'y']
>>> tim_sort((1.1, 1, 0, -1, -1.1))
[-1.1, -1, 0, 1, 1.1]
>>> tim_sort(list(reversed(list(range(7)))))
[0, 1, 2, 3, 4, 5, 6]
>>> tim_sort([3, 2, 1]) == insertion_sort([3, 2, 1])
True
>>> tim_sort([3, 2, 1]) == sorted([3, 2, 1])
True
"""
length = len(lst)
runs, sorted_runs = [], []
new_run = [lst[0]]
sorted_array = []
i = 1
while i < length:
if lst[i] < lst[i - 1]:
runs.append(new_run)
new_run = [lst[i]]
else:
new_run.append(lst[i])
i += 1
runs.append(new_run)
for run in runs:
sorted_runs.append(insertion_sort(run))
for run in sorted_runs:
sorted_array = merge(sorted_array, run)
return sorted_array
def main():
lst = [5, 9, 10, 3, -4, 5, 178, 92, 46, -18, 0, 7]
sorted_lst = tim_sort(lst)
print(sorted_lst)
if __name__ == "__main__":
main()
| def binary_search(lst, item, start, end):
if start == end:
return start if lst[start] > item else start + 1
if start > end:
return start
mid = (start + end) // 2
if lst[mid] < item:
return binary_search(lst, item, mid + 1, end)
elif lst[mid] > item:
return binary_search(lst, item, start, mid - 1)
else:
return mid
def insertion_sort(lst):
length = len(lst)
for index in range(1, length):
value = lst[index]
pos = binary_search(lst, value, 0, index - 1)
lst = lst[:pos] + [value] + lst[pos:index] + lst[index + 1 :]
return lst
def merge(left, right):
if not left:
return right
if not right:
return left
if left[0] < right[0]:
return [left[0]] + merge(left[1:], right)
return [right[0]] + merge(left, right[1:])
def tim_sort(lst):
"""
>>> tim_sort("Python")
['P', 'h', 'n', 'o', 't', 'y']
>>> tim_sort((1.1, 1, 0, -1, -1.1))
[-1.1, -1, 0, 1, 1.1]
>>> tim_sort(list(reversed(list(range(7)))))
[0, 1, 2, 3, 4, 5, 6]
>>> tim_sort([3, 2, 1]) == insertion_sort([3, 2, 1])
True
>>> tim_sort([3, 2, 1]) == sorted([3, 2, 1])
True
"""
length = len(lst)
runs, sorted_runs = [], []
new_run = [lst[0]]
sorted_array = []
i = 1
while i < length:
if lst[i] < lst[i - 1]:
runs.append(new_run)
new_run = [lst[i]]
else:
new_run.append(lst[i])
i += 1
runs.append(new_run)
for run in runs:
sorted_runs.append(insertion_sort(run))
for run in sorted_runs:
sorted_array = merge(sorted_array, run)
return sorted_array
def main():
lst = [5, 9, 10, 3, -4, 5, 178, 92, 46, -18, 0, 7]
sorted_lst = tim_sort(lst)
print(sorted_lst)
if __name__ == "__main__":
main()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
In a multi-threaded download, this algorithm could be used to provide
each worker thread with a block of non-overlapping bytes to download.
For example:
for i in allocation_list:
requests.get(url,headers={'Range':f'bytes={i}'})
"""
from __future__ import annotations
def allocation_num(number_of_bytes: int, partitions: int) -> list[str]:
"""
Divide a number of bytes into x partitions.
:param number_of_bytes: the total of bytes.
:param partitions: the number of partition need to be allocated.
:return: list of bytes to be assigned to each worker thread
>>> allocation_num(16647, 4)
['1-4161', '4162-8322', '8323-12483', '12484-16647']
>>> allocation_num(50000, 5)
['1-10000', '10001-20000', '20001-30000', '30001-40000', '40001-50000']
>>> allocation_num(888, 999)
Traceback (most recent call last):
...
ValueError: partitions can not > number_of_bytes!
>>> allocation_num(888, -4)
Traceback (most recent call last):
...
ValueError: partitions must be a positive number!
"""
if partitions <= 0:
raise ValueError("partitions must be a positive number!")
if partitions > number_of_bytes:
raise ValueError("partitions can not > number_of_bytes!")
bytes_per_partition = number_of_bytes // partitions
allocation_list = []
for i in range(partitions):
start_bytes = i * bytes_per_partition + 1
end_bytes = (
number_of_bytes if i == partitions - 1 else (i + 1) * bytes_per_partition
)
allocation_list.append(f"{start_bytes}-{end_bytes}")
return allocation_list
if __name__ == "__main__":
import doctest
doctest.testmod()
| """
In a multi-threaded download, this algorithm could be used to provide
each worker thread with a block of non-overlapping bytes to download.
For example:
for i in allocation_list:
requests.get(url,headers={'Range':f'bytes={i}'})
"""
from __future__ import annotations
def allocation_num(number_of_bytes: int, partitions: int) -> list[str]:
"""
Divide a number of bytes into x partitions.
:param number_of_bytes: the total of bytes.
:param partitions: the number of partition need to be allocated.
:return: list of bytes to be assigned to each worker thread
>>> allocation_num(16647, 4)
['1-4161', '4162-8322', '8323-12483', '12484-16647']
>>> allocation_num(50000, 5)
['1-10000', '10001-20000', '20001-30000', '30001-40000', '40001-50000']
>>> allocation_num(888, 999)
Traceback (most recent call last):
...
ValueError: partitions can not > number_of_bytes!
>>> allocation_num(888, -4)
Traceback (most recent call last):
...
ValueError: partitions must be a positive number!
"""
if partitions <= 0:
raise ValueError("partitions must be a positive number!")
if partitions > number_of_bytes:
raise ValueError("partitions can not > number_of_bytes!")
bytes_per_partition = number_of_bytes // partitions
allocation_list = []
for i in range(partitions):
start_bytes = i * bytes_per_partition + 1
end_bytes = (
number_of_bytes if i == partitions - 1 else (i + 1) * bytes_per_partition
)
allocation_list.append(f"{start_bytes}-{end_bytes}")
return allocation_list
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Checks if a system of forces is in static equilibrium.
"""
from __future__ import annotations
from numpy import array, cos, cross, float64, radians, sin
from numpy.typing import NDArray
def polar_force(
magnitude: float, angle: float, radian_mode: bool = False
) -> list[float]:
"""
Resolves force along rectangular components.
(force, angle) => (force_x, force_y)
>>> import math
>>> force = polar_force(10, 45)
>>> math.isclose(force[0], 7.071067811865477)
True
>>> math.isclose(force[1], 7.0710678118654755)
True
>>> force = polar_force(10, 3.14, radian_mode=True)
>>> math.isclose(force[0], -9.999987317275396)
True
>>> math.isclose(force[1], 0.01592652916486828)
True
"""
if radian_mode:
return [magnitude * cos(angle), magnitude * sin(angle)]
return [magnitude * cos(radians(angle)), magnitude * sin(radians(angle))]
def in_static_equilibrium(
forces: NDArray[float64], location: NDArray[float64], eps: float = 10**-1
) -> bool:
"""
Check if a system is in equilibrium.
It takes two numpy.array objects.
forces ==> [
[force1_x, force1_y],
[force2_x, force2_y],
....]
location ==> [
[x1, y1],
[x2, y2],
....]
>>> force = array([[1, 1], [-1, 2]])
>>> location = array([[1, 0], [10, 0]])
>>> in_static_equilibrium(force, location)
False
"""
# summation of moments is zero
moments: NDArray[float64] = cross(location, forces)
sum_moments: float = sum(moments)
return abs(sum_moments) < eps
if __name__ == "__main__":
# Test to check if it works
forces = array(
[
polar_force(718.4, 180 - 30),
polar_force(879.54, 45),
polar_force(100, -90),
]
)
location: NDArray[float64] = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem 1 in image_data/2D_problems.jpg
forces = array(
[
polar_force(30 * 9.81, 15),
polar_force(215, 180 - 45),
polar_force(264, 90 - 30),
]
)
location = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem in image_data/2D_problems_1.jpg
forces = array([[0, -2000], [0, -1200], [0, 15600], [0, -12400]])
location = array([[0, 0], [6, 0], [10, 0], [12, 0]])
assert in_static_equilibrium(forces, location)
import doctest
doctest.testmod()
| """
Checks if a system of forces is in static equilibrium.
"""
from __future__ import annotations
from numpy import array, cos, cross, float64, radians, sin
from numpy.typing import NDArray
def polar_force(
magnitude: float, angle: float, radian_mode: bool = False
) -> list[float]:
"""
Resolves force along rectangular components.
(force, angle) => (force_x, force_y)
>>> import math
>>> force = polar_force(10, 45)
>>> math.isclose(force[0], 7.071067811865477)
True
>>> math.isclose(force[1], 7.0710678118654755)
True
>>> force = polar_force(10, 3.14, radian_mode=True)
>>> math.isclose(force[0], -9.999987317275396)
True
>>> math.isclose(force[1], 0.01592652916486828)
True
"""
if radian_mode:
return [magnitude * cos(angle), magnitude * sin(angle)]
return [magnitude * cos(radians(angle)), magnitude * sin(radians(angle))]
def in_static_equilibrium(
forces: NDArray[float64], location: NDArray[float64], eps: float = 10**-1
) -> bool:
"""
Check if a system is in equilibrium.
It takes two numpy.array objects.
forces ==> [
[force1_x, force1_y],
[force2_x, force2_y],
....]
location ==> [
[x1, y1],
[x2, y2],
....]
>>> force = array([[1, 1], [-1, 2]])
>>> location = array([[1, 0], [10, 0]])
>>> in_static_equilibrium(force, location)
False
"""
# summation of moments is zero
moments: NDArray[float64] = cross(location, forces)
sum_moments: float = sum(moments)
return abs(sum_moments) < eps
if __name__ == "__main__":
# Test to check if it works
forces = array(
[
polar_force(718.4, 180 - 30),
polar_force(879.54, 45),
polar_force(100, -90),
]
)
location: NDArray[float64] = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem 1 in image_data/2D_problems.jpg
forces = array(
[
polar_force(30 * 9.81, 15),
polar_force(215, 180 - 45),
polar_force(264, 90 - 30),
]
)
location = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem in image_data/2D_problems_1.jpg
forces = array([[0, -2000], [0, -1200], [0, 15600], [0, -12400]])
location = array([[0, 0], [6, 0], [10, 0], [12, 0]])
assert in_static_equilibrium(forces, location)
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Tree_sort algorithm.
Build a BST and in order traverse.
"""
class Node:
# BST data structure
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def insert(self, val):
if self.val:
if val < self.val:
if self.left is None:
self.left = Node(val)
else:
self.left.insert(val)
elif val > self.val:
if self.right is None:
self.right = Node(val)
else:
self.right.insert(val)
else:
self.val = val
def inorder(root, res):
# Recursive traversal
if root:
inorder(root.left, res)
res.append(root.val)
inorder(root.right, res)
def tree_sort(arr):
# Build BST
if len(arr) == 0:
return arr
root = Node(arr[0])
for i in range(1, len(arr)):
root.insert(arr[i])
# Traverse BST in order.
res = []
inorder(root, res)
return res
if __name__ == "__main__":
print(tree_sort([10, 1, 3, 2, 9, 14, 13]))
| """
Tree_sort algorithm.
Build a BST and in order traverse.
"""
class Node:
# BST data structure
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def insert(self, val):
if self.val:
if val < self.val:
if self.left is None:
self.left = Node(val)
else:
self.left.insert(val)
elif val > self.val:
if self.right is None:
self.right = Node(val)
else:
self.right.insert(val)
else:
self.val = val
def inorder(root, res):
# Recursive traversal
if root:
inorder(root.left, res)
res.append(root.val)
inorder(root.right, res)
def tree_sort(arr):
# Build BST
if len(arr) == 0:
return arr
root = Node(arr[0])
for i in range(1, len(arr)):
root.insert(arr[i])
# Traverse BST in order.
res = []
inorder(root, res)
return res
if __name__ == "__main__":
print(tree_sort([10, 1, 3, 2, 9, 14, 13]))
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # https://en.wikipedia.org/wiki/Tree_traversal
from __future__ import annotations
from collections import deque
from collections.abc import Sequence
from dataclasses import dataclass
from typing import Any
@dataclass
class Node:
data: int
left: Node | None = None
right: Node | None = None
def make_tree() -> Node | None:
r"""
The below tree
1
/ \
2 3
/ \
4 5
"""
tree = Node(1)
tree.left = Node(2)
tree.right = Node(3)
tree.left.left = Node(4)
tree.left.right = Node(5)
return tree
def preorder(root: Node | None) -> list[int]:
"""
Pre-order traversal visits root node, left subtree, right subtree.
>>> preorder(make_tree())
[1, 2, 4, 5, 3]
"""
return [root.data] + preorder(root.left) + preorder(root.right) if root else []
def postorder(root: Node | None) -> list[int]:
"""
Post-order traversal visits left subtree, right subtree, root node.
>>> postorder(make_tree())
[4, 5, 2, 3, 1]
"""
return postorder(root.left) + postorder(root.right) + [root.data] if root else []
def inorder(root: Node | None) -> list[int]:
"""
In-order traversal visits left subtree, root node, right subtree.
>>> inorder(make_tree())
[4, 2, 5, 1, 3]
"""
return inorder(root.left) + [root.data] + inorder(root.right) if root else []
def height(root: Node | None) -> int:
"""
Recursive function for calculating the height of the binary tree.
>>> height(None)
0
>>> height(make_tree())
3
"""
return (max(height(root.left), height(root.right)) + 1) if root else 0
def level_order(root: Node | None) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a whole binary tree in Level Order Traverse.
Level Order traverse: Visit nodes of the tree level-by-level.
"""
output: list[Any] = []
if root is None:
return output
process_queue = deque([root])
while process_queue:
node = process_queue.popleft()
output.append(node.data)
if node.left:
process_queue.append(node.left)
if node.right:
process_queue.append(node.right)
return output
def get_nodes_from_left_to_right(
root: Node | None, level: int
) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a particular level:
Left to right direction of the binary tree.
"""
output: list[Any] = []
def populate_output(root: Node | None, level: int) -> None:
if not root:
return
if level == 1:
output.append(root.data)
elif level > 1:
populate_output(root.left, level - 1)
populate_output(root.right, level - 1)
populate_output(root, level)
return output
def get_nodes_from_right_to_left(
root: Node | None, level: int
) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a particular level:
Right to left direction of the binary tree.
"""
output: list[Any] = []
def populate_output(root: Node | None, level: int) -> None:
if root is None:
return
if level == 1:
output.append(root.data)
elif level > 1:
populate_output(root.right, level - 1)
populate_output(root.left, level - 1)
populate_output(root, level)
return output
def zigzag(root: Node | None) -> Sequence[Node | None] | list[Any]:
"""
ZigZag traverse:
Returns a list of nodes value from left to right and right to left, alternatively.
"""
if root is None:
return []
output: list[Sequence[Node | None]] = []
flag = 0
height_tree = height(root)
for h in range(1, height_tree + 1):
if not flag:
output.append(get_nodes_from_left_to_right(root, h))
flag = 1
else:
output.append(get_nodes_from_right_to_left(root, h))
flag = 0
return output
def main() -> None: # Main function for testing.
"""
Create binary tree.
"""
root = make_tree()
"""
All Traversals of the binary are as follows:
"""
print(f"In-order Traversal: {inorder(root)}")
print(f"Pre-order Traversal: {preorder(root)}")
print(f"Post-order Traversal: {postorder(root)}", "\n")
print(f"Height of Tree: {height(root)}", "\n")
print("Complete Level Order Traversal: ")
print(level_order(root), "\n")
print("Level-wise order Traversal: ")
for level in range(1, height(root) + 1):
print(f"Level {level}:", get_nodes_from_left_to_right(root, level=level))
print("\nZigZag order Traversal: ")
print(zigzag(root))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| # https://en.wikipedia.org/wiki/Tree_traversal
from __future__ import annotations
from collections import deque
from collections.abc import Sequence
from dataclasses import dataclass
from typing import Any
@dataclass
class Node:
data: int
left: Node | None = None
right: Node | None = None
def make_tree() -> Node | None:
r"""
The below tree
1
/ \
2 3
/ \
4 5
"""
tree = Node(1)
tree.left = Node(2)
tree.right = Node(3)
tree.left.left = Node(4)
tree.left.right = Node(5)
return tree
def preorder(root: Node | None) -> list[int]:
"""
Pre-order traversal visits root node, left subtree, right subtree.
>>> preorder(make_tree())
[1, 2, 4, 5, 3]
"""
return [root.data] + preorder(root.left) + preorder(root.right) if root else []
def postorder(root: Node | None) -> list[int]:
"""
Post-order traversal visits left subtree, right subtree, root node.
>>> postorder(make_tree())
[4, 5, 2, 3, 1]
"""
return postorder(root.left) + postorder(root.right) + [root.data] if root else []
def inorder(root: Node | None) -> list[int]:
"""
In-order traversal visits left subtree, root node, right subtree.
>>> inorder(make_tree())
[4, 2, 5, 1, 3]
"""
return inorder(root.left) + [root.data] + inorder(root.right) if root else []
def height(root: Node | None) -> int:
"""
Recursive function for calculating the height of the binary tree.
>>> height(None)
0
>>> height(make_tree())
3
"""
return (max(height(root.left), height(root.right)) + 1) if root else 0
def level_order(root: Node | None) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a whole binary tree in Level Order Traverse.
Level Order traverse: Visit nodes of the tree level-by-level.
"""
output: list[Any] = []
if root is None:
return output
process_queue = deque([root])
while process_queue:
node = process_queue.popleft()
output.append(node.data)
if node.left:
process_queue.append(node.left)
if node.right:
process_queue.append(node.right)
return output
def get_nodes_from_left_to_right(
root: Node | None, level: int
) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a particular level:
Left to right direction of the binary tree.
"""
output: list[Any] = []
def populate_output(root: Node | None, level: int) -> None:
if not root:
return
if level == 1:
output.append(root.data)
elif level > 1:
populate_output(root.left, level - 1)
populate_output(root.right, level - 1)
populate_output(root, level)
return output
def get_nodes_from_right_to_left(
root: Node | None, level: int
) -> Sequence[Node | None]:
"""
Returns a list of nodes value from a particular level:
Right to left direction of the binary tree.
"""
output: list[Any] = []
def populate_output(root: Node | None, level: int) -> None:
if root is None:
return
if level == 1:
output.append(root.data)
elif level > 1:
populate_output(root.right, level - 1)
populate_output(root.left, level - 1)
populate_output(root, level)
return output
def zigzag(root: Node | None) -> Sequence[Node | None] | list[Any]:
"""
ZigZag traverse:
Returns a list of nodes value from left to right and right to left, alternatively.
"""
if root is None:
return []
output: list[Sequence[Node | None]] = []
flag = 0
height_tree = height(root)
for h in range(1, height_tree + 1):
if not flag:
output.append(get_nodes_from_left_to_right(root, h))
flag = 1
else:
output.append(get_nodes_from_right_to_left(root, h))
flag = 0
return output
def main() -> None: # Main function for testing.
"""
Create binary tree.
"""
root = make_tree()
"""
All Traversals of the binary are as follows:
"""
print(f"In-order Traversal: {inorder(root)}")
print(f"Pre-order Traversal: {preorder(root)}")
print(f"Post-order Traversal: {postorder(root)}", "\n")
print(f"Height of Tree: {height(root)}", "\n")
print("Complete Level Order Traversal: ")
print(level_order(root), "\n")
print("Level-wise order Traversal: ")
for level in range(1, height(root) + 1):
print(f"Level {level}:", get_nodes_from_left_to_right(root, level=level))
print("\nZigZag order Traversal: ")
print(zigzag(root))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from __future__ import annotations
END = "#"
class Trie:
def __init__(self) -> None:
self._trie: dict = {}
def insert_word(self, text: str) -> None:
trie = self._trie
for char in text:
if char not in trie:
trie[char] = {}
trie = trie[char]
trie[END] = True
def find_word(self, prefix: str) -> tuple | list:
trie = self._trie
for char in prefix:
if char in trie:
trie = trie[char]
else:
return []
return self._elements(trie)
def _elements(self, d: dict) -> tuple:
result = []
for c, v in d.items():
if c == END:
sub_result = [" "]
else:
sub_result = [c + s for s in self._elements(v)]
result.extend(sub_result)
return tuple(result)
trie = Trie()
words = ("depart", "detergent", "daring", "dog", "deer", "deal")
for word in words:
trie.insert_word(word)
def autocomplete_using_trie(string: str) -> tuple:
"""
>>> trie = Trie()
>>> for word in words:
... trie.insert_word(word)
...
>>> matches = autocomplete_using_trie("de")
>>> "detergent " in matches
True
>>> "dog " in matches
False
"""
suffixes = trie.find_word(string)
return tuple(string + word for word in suffixes)
def main() -> None:
print(autocomplete_using_trie("de"))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| from __future__ import annotations
END = "#"
class Trie:
def __init__(self) -> None:
self._trie: dict = {}
def insert_word(self, text: str) -> None:
trie = self._trie
for char in text:
if char not in trie:
trie[char] = {}
trie = trie[char]
trie[END] = True
def find_word(self, prefix: str) -> tuple | list:
trie = self._trie
for char in prefix:
if char in trie:
trie = trie[char]
else:
return []
return self._elements(trie)
def _elements(self, d: dict) -> tuple:
result = []
for c, v in d.items():
if c == END:
sub_result = [" "]
else:
sub_result = [c + s for s in self._elements(v)]
result.extend(sub_result)
return tuple(result)
trie = Trie()
words = ("depart", "detergent", "daring", "dog", "deer", "deal")
for word in words:
trie.insert_word(word)
def autocomplete_using_trie(string: str) -> tuple:
"""
>>> trie = Trie()
>>> for word in words:
... trie.insert_word(word)
...
>>> matches = autocomplete_using_trie("de")
>>> "detergent " in matches
True
>>> "dog " in matches
False
"""
suffixes = trie.find_word(string)
return tuple(string + word for word in suffixes)
def main() -> None:
print(autocomplete_using_trie("de"))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import os
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
headers = {"UserAgent": UserAgent().random}
URL = "https://www.mywaifulist.moe/random"
def save_image(image_url: str, image_title: str) -> None:
"""
Saves the image of anime character
"""
image = requests.get(image_url, headers=headers)
with open(image_title, "wb") as file:
file.write(image.content)
def random_anime_character() -> tuple[str, str, str]:
"""
Returns the Title, Description, and Image Title of a random anime character .
"""
soup = BeautifulSoup(requests.get(URL, headers=headers).text, "html.parser")
title = soup.find("meta", attrs={"property": "og:title"}).attrs["content"]
image_url = soup.find("meta", attrs={"property": "og:image"}).attrs["content"]
description = soup.find("p", id="description").get_text()
_, image_extension = os.path.splitext(os.path.basename(image_url))
image_title = title.strip().replace(" ", "_")
image_title = f"{image_title}{image_extension}"
save_image(image_url, image_title)
return (title, description, image_title)
if __name__ == "__main__":
title, desc, image_title = random_anime_character()
print(f"{title}\n\n{desc}\n\nImage saved : {image_title}")
| import os
import requests
from bs4 import BeautifulSoup
from fake_useragent import UserAgent
headers = {"UserAgent": UserAgent().random}
URL = "https://www.mywaifulist.moe/random"
def save_image(image_url: str, image_title: str) -> None:
"""
Saves the image of anime character
"""
image = requests.get(image_url, headers=headers)
with open(image_title, "wb") as file:
file.write(image.content)
def random_anime_character() -> tuple[str, str, str]:
"""
Returns the Title, Description, and Image Title of a random anime character .
"""
soup = BeautifulSoup(requests.get(URL, headers=headers).text, "html.parser")
title = soup.find("meta", attrs={"property": "og:title"}).attrs["content"]
image_url = soup.find("meta", attrs={"property": "og:image"}).attrs["content"]
description = soup.find("p", id="description").get_text()
_, image_extension = os.path.splitext(os.path.basename(image_url))
image_title = title.strip().replace(" ", "_")
image_title = f"{image_title}{image_extension}"
save_image(image_url, image_title)
return (title, description, image_title)
if __name__ == "__main__":
title, desc, image_title = random_anime_character()
print(f"{title}\n\n{desc}\n\nImage saved : {image_title}")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Computer Vision
Computer vision is a field of computer science that works on enabling computers to see, identify and process images in the same way that human does, and provide appropriate output.
It is like imparting human intelligence and instincts to a computer.
Image processing and computer vision are a little different from each other. Image processing means applying some algorithms for transforming image from one form to the other like smoothing, contrasting, stretching, etc.
While computer vision comes from modelling image processing using the techniques of machine learning, computer vision applies machine learning to recognize patterns for interpretation of images (much like the process of visual reasoning of human vision).
* <https://en.wikipedia.org/wiki/Computer_vision>
* <https://www.algorithmia.com/blog/introduction-to-computer-vision>
| # Computer Vision
Computer vision is a field of computer science that works on enabling computers to see, identify and process images in the same way that human does, and provide appropriate output.
It is like imparting human intelligence and instincts to a computer.
Image processing and computer vision are a little different from each other. Image processing means applying some algorithms for transforming image from one form to the other like smoothing, contrasting, stretching, etc.
While computer vision comes from modelling image processing using the techniques of machine learning, computer vision applies machine learning to recognize patterns for interpretation of images (much like the process of visual reasoning of human vision).
* <https://en.wikipedia.org/wiki/Computer_vision>
* <https://www.algorithmia.com/blog/introduction-to-computer-vision>
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
developed by: markmelnic
original repo: https://github.com/markmelnic/Scoring-Algorithm
Analyse data using a range based percentual proximity algorithm
and calculate the linear maximum likelihood estimation.
The basic principle is that all values supplied will be broken
down to a range from 0 to 1 and each column's score will be added
up to get the total score.
==========
Example for data of vehicles
price|mileage|registration_year
20k |60k |2012
22k |50k |2011
23k |90k |2015
16k |210k |2010
We want the vehicle with the lowest price,
lowest mileage but newest registration year.
Thus the weights for each column are as follows:
[0, 0, 1]
"""
def procentual_proximity(
source_data: list[list[float]], weights: list[int]
) -> list[list[float]]:
"""
weights - int list
possible values - 0 / 1
0 if lower values have higher weight in the data set
1 if higher values have higher weight in the data set
>>> procentual_proximity([[20, 60, 2012],[23, 90, 2015],[22, 50, 2011]], [0, 0, 1])
[[20, 60, 2012, 2.0], [23, 90, 2015, 1.0], [22, 50, 2011, 1.3333333333333335]]
"""
# getting data
data_lists: list[list[float]] = []
for data in source_data:
for i, el in enumerate(data):
if len(data_lists) < i + 1:
data_lists.append([])
data_lists[i].append(float(el))
score_lists: list[list[float]] = []
# calculating each score
for dlist, weight in zip(data_lists, weights):
mind = min(dlist)
maxd = max(dlist)
score: list[float] = []
# for weight 0 score is 1 - actual score
if weight == 0:
for item in dlist:
try:
score.append(1 - ((item - mind) / (maxd - mind)))
except ZeroDivisionError:
score.append(1)
elif weight == 1:
for item in dlist:
try:
score.append((item - mind) / (maxd - mind))
except ZeroDivisionError:
score.append(0)
# weight not 0 or 1
else:
raise ValueError(f"Invalid weight of {weight:f} provided")
score_lists.append(score)
# initialize final scores
final_scores: list[float] = [0 for i in range(len(score_lists[0]))]
# generate final scores
for slist in score_lists:
for j, ele in enumerate(slist):
final_scores[j] = final_scores[j] + ele
# append scores to source data
for i, ele in enumerate(final_scores):
source_data[i].append(ele)
return source_data
| """
developed by: markmelnic
original repo: https://github.com/markmelnic/Scoring-Algorithm
Analyse data using a range based percentual proximity algorithm
and calculate the linear maximum likelihood estimation.
The basic principle is that all values supplied will be broken
down to a range from 0 to 1 and each column's score will be added
up to get the total score.
==========
Example for data of vehicles
price|mileage|registration_year
20k |60k |2012
22k |50k |2011
23k |90k |2015
16k |210k |2010
We want the vehicle with the lowest price,
lowest mileage but newest registration year.
Thus the weights for each column are as follows:
[0, 0, 1]
"""
def procentual_proximity(
source_data: list[list[float]], weights: list[int]
) -> list[list[float]]:
"""
weights - int list
possible values - 0 / 1
0 if lower values have higher weight in the data set
1 if higher values have higher weight in the data set
>>> procentual_proximity([[20, 60, 2012],[23, 90, 2015],[22, 50, 2011]], [0, 0, 1])
[[20, 60, 2012, 2.0], [23, 90, 2015, 1.0], [22, 50, 2011, 1.3333333333333335]]
"""
# getting data
data_lists: list[list[float]] = []
for data in source_data:
for i, el in enumerate(data):
if len(data_lists) < i + 1:
data_lists.append([])
data_lists[i].append(float(el))
score_lists: list[list[float]] = []
# calculating each score
for dlist, weight in zip(data_lists, weights):
mind = min(dlist)
maxd = max(dlist)
score: list[float] = []
# for weight 0 score is 1 - actual score
if weight == 0:
for item in dlist:
try:
score.append(1 - ((item - mind) / (maxd - mind)))
except ZeroDivisionError:
score.append(1)
elif weight == 1:
for item in dlist:
try:
score.append((item - mind) / (maxd - mind))
except ZeroDivisionError:
score.append(0)
# weight not 0 or 1
else:
raise ValueError(f"Invalid weight of {weight:f} provided")
score_lists.append(score)
# initialize final scores
final_scores: list[float] = [0 for i in range(len(score_lists[0]))]
# generate final scores
for slist in score_lists:
for j, ele in enumerate(slist):
final_scores[j] = final_scores[j] + ele
# append scores to source data
for i, ele in enumerate(final_scores):
source_data[i].append(ele)
return source_data
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
You are given a tree(a simple connected graph with no cycles). The tree has N
nodes numbered from 1 to N and is rooted at node 1.
Find the maximum number of edges you can remove from the tree to get a forest
such that each connected component of the forest contains an even number of
nodes.
Constraints
2 <= 2 <= 100
Note: The tree input will be such that it can always be decomposed into
components containing an even number of nodes.
"""
# pylint: disable=invalid-name
from collections import defaultdict
def dfs(start: int) -> int:
"""DFS traversal"""
# pylint: disable=redefined-outer-name
ret = 1
visited[start] = True
for v in tree[start]:
if v not in visited:
ret += dfs(v)
if ret % 2 == 0:
cuts.append(start)
return ret
def even_tree():
"""
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
On removing edges (1,3) and (1,6), we can get the desired result 2.
"""
dfs(1)
if __name__ == "__main__":
n, m = 10, 9
tree = defaultdict(list)
visited: dict[int, bool] = {}
cuts: list[int] = []
count = 0
edges = [(2, 1), (3, 1), (4, 3), (5, 2), (6, 1), (7, 2), (8, 6), (9, 8), (10, 8)]
for u, v in edges:
tree[u].append(v)
tree[v].append(u)
even_tree()
print(len(cuts) - 1)
| """
You are given a tree(a simple connected graph with no cycles). The tree has N
nodes numbered from 1 to N and is rooted at node 1.
Find the maximum number of edges you can remove from the tree to get a forest
such that each connected component of the forest contains an even number of
nodes.
Constraints
2 <= 2 <= 100
Note: The tree input will be such that it can always be decomposed into
components containing an even number of nodes.
"""
# pylint: disable=invalid-name
from collections import defaultdict
def dfs(start: int) -> int:
"""DFS traversal"""
# pylint: disable=redefined-outer-name
ret = 1
visited[start] = True
for v in tree[start]:
if v not in visited:
ret += dfs(v)
if ret % 2 == 0:
cuts.append(start)
return ret
def even_tree():
"""
2 1
3 1
4 3
5 2
6 1
7 2
8 6
9 8
10 8
On removing edges (1,3) and (1,6), we can get the desired result 2.
"""
dfs(1)
if __name__ == "__main__":
n, m = 10, 9
tree = defaultdict(list)
visited: dict[int, bool] = {}
cuts: list[int] = []
count = 0
edges = [(2, 1), (3, 1), (4, 3), (5, 2), (6, 1), (7, 2), (8, 6), (9, 8), (10, 8)]
for u, v in edges:
tree[u].append(v)
tree[v].append(u)
even_tree()
print(len(cuts) - 1)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/python
""" Author: OMKAR PATHAK """
class Graph:
def __init__(self):
self.vertex = {}
# for printing the Graph vertices
def print_graph(self) -> None:
print(self.vertex)
for i in self.vertex:
print(i, " -> ", " -> ".join([str(j) for j in self.vertex[i]]))
# for adding the edge between two vertices
def add_edge(self, from_vertex: int, to_vertex: int) -> None:
# check if vertex is already present,
if from_vertex in self.vertex:
self.vertex[from_vertex].append(to_vertex)
else:
# else make a new vertex
self.vertex[from_vertex] = [to_vertex]
def dfs(self) -> None:
# visited array for storing already visited nodes
visited = [False] * len(self.vertex)
# call the recursive helper function
for i in range(len(self.vertex)):
if not visited[i]:
self.dfs_recursive(i, visited)
def dfs_recursive(self, start_vertex: int, visited: list) -> None:
# mark start vertex as visited
visited[start_vertex] = True
print(start_vertex, end=" ")
# Recur for all the vertices that are adjacent to this node
for i in self.vertex:
if not visited[i]:
self.dfs_recursive(i, visited)
if __name__ == "__main__":
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
g.print_graph()
print("DFS:")
g.dfs()
# OUTPUT:
# 0 -> 1 -> 2
# 1 -> 2
# 2 -> 0 -> 3
# 3 -> 3
# DFS:
# 0 1 2 3
| #!/usr/bin/python
""" Author: OMKAR PATHAK """
class Graph:
def __init__(self):
self.vertex = {}
# for printing the Graph vertices
def print_graph(self) -> None:
print(self.vertex)
for i in self.vertex:
print(i, " -> ", " -> ".join([str(j) for j in self.vertex[i]]))
# for adding the edge between two vertices
def add_edge(self, from_vertex: int, to_vertex: int) -> None:
# check if vertex is already present,
if from_vertex in self.vertex:
self.vertex[from_vertex].append(to_vertex)
else:
# else make a new vertex
self.vertex[from_vertex] = [to_vertex]
def dfs(self) -> None:
# visited array for storing already visited nodes
visited = [False] * len(self.vertex)
# call the recursive helper function
for i in range(len(self.vertex)):
if not visited[i]:
self.dfs_recursive(i, visited)
def dfs_recursive(self, start_vertex: int, visited: list) -> None:
# mark start vertex as visited
visited[start_vertex] = True
print(start_vertex, end=" ")
# Recur for all the vertices that are adjacent to this node
for i in self.vertex:
if not visited[i]:
self.dfs_recursive(i, visited)
if __name__ == "__main__":
g = Graph()
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 0)
g.add_edge(2, 3)
g.add_edge(3, 3)
g.print_graph()
print("DFS:")
g.dfs()
# OUTPUT:
# 0 -> 1 -> 2
# 1 -> 2
# 2 -> 0 -> 3
# 3 -> 3
# DFS:
# 0 1 2 3
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Implementing Deque using DoublyLinkedList ...
Operations:
1. insertion in the front -> O(1)
2. insertion in the end -> O(1)
3. remove from the front -> O(1)
4. remove from the end -> O(1)
"""
class _DoublyLinkedBase:
"""A Private class (to be inherited)"""
class _Node:
__slots__ = "_prev", "_data", "_next"
def __init__(self, link_p, element, link_n):
self._prev = link_p
self._data = element
self._next = link_n
def has_next_and_prev(self):
return (
f" Prev -> {self._prev is not None}, Next -> {self._next is not None}"
)
def __init__(self):
self._header = self._Node(None, None, None)
self._trailer = self._Node(None, None, None)
self._header._next = self._trailer
self._trailer._prev = self._header
self._size = 0
def __len__(self):
return self._size
def is_empty(self):
return self.__len__() == 0
def _insert(self, predecessor, e, successor):
# Create new_node by setting it's prev.link -> header
# setting it's next.link -> trailer
new_node = self._Node(predecessor, e, successor)
predecessor._next = new_node
successor._prev = new_node
self._size += 1
return self
def _delete(self, node):
predecessor = node._prev
successor = node._next
predecessor._next = successor
successor._prev = predecessor
self._size -= 1
temp = node._data
node._prev = node._next = node._data = None
del node
return temp
class LinkedDeque(_DoublyLinkedBase):
def first(self):
"""return first element
>>> d = LinkedDeque()
>>> d.add_first('A').first()
'A'
>>> d.add_first('B').first()
'B'
"""
if self.is_empty():
raise Exception("List is empty")
return self._header._next._data
def last(self):
"""return last element
>>> d = LinkedDeque()
>>> d.add_last('A').last()
'A'
>>> d.add_last('B').last()
'B'
"""
if self.is_empty():
raise Exception("List is empty")
return self._trailer._prev._data
# DEque Insert Operations (At the front, At the end)
def add_first(self, element):
"""insertion in the front
>>> LinkedDeque().add_first('AV').first()
'AV'
"""
return self._insert(self._header, element, self._header._next)
def add_last(self, element):
"""insertion in the end
>>> LinkedDeque().add_last('B').last()
'B'
"""
return self._insert(self._trailer._prev, element, self._trailer)
# DEqueu Remove Operations (At the front, At the end)
def remove_first(self):
"""removal from the front
>>> d = LinkedDeque()
>>> d.is_empty()
True
>>> d.remove_first()
Traceback (most recent call last):
...
IndexError: remove_first from empty list
>>> d.add_first('A') # doctest: +ELLIPSIS
<data_structures.linked_list.deque_doubly.LinkedDeque object at ...
>>> d.remove_first()
'A'
>>> d.is_empty()
True
"""
if self.is_empty():
raise IndexError("remove_first from empty list")
return self._delete(self._header._next)
def remove_last(self):
"""removal in the end
>>> d = LinkedDeque()
>>> d.is_empty()
True
>>> d.remove_last()
Traceback (most recent call last):
...
IndexError: remove_first from empty list
>>> d.add_first('A') # doctest: +ELLIPSIS
<data_structures.linked_list.deque_doubly.LinkedDeque object at ...
>>> d.remove_last()
'A'
>>> d.is_empty()
True
"""
if self.is_empty():
raise IndexError("remove_first from empty list")
return self._delete(self._trailer._prev)
| """
Implementing Deque using DoublyLinkedList ...
Operations:
1. insertion in the front -> O(1)
2. insertion in the end -> O(1)
3. remove from the front -> O(1)
4. remove from the end -> O(1)
"""
class _DoublyLinkedBase:
"""A Private class (to be inherited)"""
class _Node:
__slots__ = "_prev", "_data", "_next"
def __init__(self, link_p, element, link_n):
self._prev = link_p
self._data = element
self._next = link_n
def has_next_and_prev(self):
return (
f" Prev -> {self._prev is not None}, Next -> {self._next is not None}"
)
def __init__(self):
self._header = self._Node(None, None, None)
self._trailer = self._Node(None, None, None)
self._header._next = self._trailer
self._trailer._prev = self._header
self._size = 0
def __len__(self):
return self._size
def is_empty(self):
return self.__len__() == 0
def _insert(self, predecessor, e, successor):
# Create new_node by setting it's prev.link -> header
# setting it's next.link -> trailer
new_node = self._Node(predecessor, e, successor)
predecessor._next = new_node
successor._prev = new_node
self._size += 1
return self
def _delete(self, node):
predecessor = node._prev
successor = node._next
predecessor._next = successor
successor._prev = predecessor
self._size -= 1
temp = node._data
node._prev = node._next = node._data = None
del node
return temp
class LinkedDeque(_DoublyLinkedBase):
def first(self):
"""return first element
>>> d = LinkedDeque()
>>> d.add_first('A').first()
'A'
>>> d.add_first('B').first()
'B'
"""
if self.is_empty():
raise Exception("List is empty")
return self._header._next._data
def last(self):
"""return last element
>>> d = LinkedDeque()
>>> d.add_last('A').last()
'A'
>>> d.add_last('B').last()
'B'
"""
if self.is_empty():
raise Exception("List is empty")
return self._trailer._prev._data
# DEque Insert Operations (At the front, At the end)
def add_first(self, element):
"""insertion in the front
>>> LinkedDeque().add_first('AV').first()
'AV'
"""
return self._insert(self._header, element, self._header._next)
def add_last(self, element):
"""insertion in the end
>>> LinkedDeque().add_last('B').last()
'B'
"""
return self._insert(self._trailer._prev, element, self._trailer)
# DEqueu Remove Operations (At the front, At the end)
def remove_first(self):
"""removal from the front
>>> d = LinkedDeque()
>>> d.is_empty()
True
>>> d.remove_first()
Traceback (most recent call last):
...
IndexError: remove_first from empty list
>>> d.add_first('A') # doctest: +ELLIPSIS
<data_structures.linked_list.deque_doubly.LinkedDeque object at ...
>>> d.remove_first()
'A'
>>> d.is_empty()
True
"""
if self.is_empty():
raise IndexError("remove_first from empty list")
return self._delete(self._header._next)
def remove_last(self):
"""removal in the end
>>> d = LinkedDeque()
>>> d.is_empty()
True
>>> d.remove_last()
Traceback (most recent call last):
...
IndexError: remove_first from empty list
>>> d.add_first('A') # doctest: +ELLIPSIS
<data_structures.linked_list.deque_doubly.LinkedDeque object at ...
>>> d.remove_last()
'A'
>>> d.is_empty()
True
"""
if self.is_empty():
raise IndexError("remove_first from empty list")
return self._delete(self._trailer._prev)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Implementation of Bilateral filter
Inputs:
img: A 2d image with values in between 0 and 1
varS: variance in space dimension.
varI: variance in Intensity.
N: Kernel size(Must be an odd number)
Output:
img:A 2d zero padded image with values in between 0 and 1
"""
import math
import sys
import cv2
import numpy as np
def vec_gaussian(img: np.ndarray, variance: float) -> np.ndarray:
# For applying gaussian function for each element in matrix.
sigma = math.sqrt(variance)
cons = 1 / (sigma * math.sqrt(2 * math.pi))
return cons * np.exp(-((img / sigma) ** 2) * 0.5)
def get_slice(img: np.ndarray, x: int, y: int, kernel_size: int) -> np.ndarray:
half = kernel_size // 2
return img[x - half : x + half + 1, y - half : y + half + 1]
def get_gauss_kernel(kernel_size: int, spatial_variance: float) -> np.ndarray:
# Creates a gaussian kernel of given dimension.
arr = np.zeros((kernel_size, kernel_size))
for i in range(0, kernel_size):
for j in range(0, kernel_size):
arr[i, j] = math.sqrt(
abs(i - kernel_size // 2) ** 2 + abs(j - kernel_size // 2) ** 2
)
return vec_gaussian(arr, spatial_variance)
def bilateral_filter(
img: np.ndarray,
spatial_variance: float,
intensity_variance: float,
kernel_size: int,
) -> np.ndarray:
img2 = np.zeros(img.shape)
gauss_ker = get_gauss_kernel(kernel_size, spatial_variance)
size_x, size_y = img.shape
for i in range(kernel_size // 2, size_x - kernel_size // 2):
for j in range(kernel_size // 2, size_y - kernel_size // 2):
img_s = get_slice(img, i, j, kernel_size)
img_i = img_s - img_s[kernel_size // 2, kernel_size // 2]
img_ig = vec_gaussian(img_i, intensity_variance)
weights = np.multiply(gauss_ker, img_ig)
vals = np.multiply(img_s, weights)
val = np.sum(vals) / np.sum(weights)
img2[i, j] = val
return img2
def parse_args(args: list) -> tuple:
filename = args[1] if args[1:] else "../image_data/lena.jpg"
spatial_variance = float(args[2]) if args[2:] else 1.0
intensity_variance = float(args[3]) if args[3:] else 1.0
if args[4:]:
kernel_size = int(args[4])
kernel_size = kernel_size + abs(kernel_size % 2 - 1)
else:
kernel_size = 5
return filename, spatial_variance, intensity_variance, kernel_size
if __name__ == "__main__":
filename, spatial_variance, intensity_variance, kernel_size = parse_args(sys.argv)
img = cv2.imread(filename, 0)
cv2.imshow("input image", img)
out = img / 255
out = out.astype("float32")
out = bilateral_filter(out, spatial_variance, intensity_variance, kernel_size)
out = out * 255
out = np.uint8(out)
cv2.imshow("output image", out)
cv2.waitKey(0)
cv2.destroyAllWindows()
| """
Implementation of Bilateral filter
Inputs:
img: A 2d image with values in between 0 and 1
varS: variance in space dimension.
varI: variance in Intensity.
N: Kernel size(Must be an odd number)
Output:
img:A 2d zero padded image with values in between 0 and 1
"""
import math
import sys
import cv2
import numpy as np
def vec_gaussian(img: np.ndarray, variance: float) -> np.ndarray:
# For applying gaussian function for each element in matrix.
sigma = math.sqrt(variance)
cons = 1 / (sigma * math.sqrt(2 * math.pi))
return cons * np.exp(-((img / sigma) ** 2) * 0.5)
def get_slice(img: np.ndarray, x: int, y: int, kernel_size: int) -> np.ndarray:
half = kernel_size // 2
return img[x - half : x + half + 1, y - half : y + half + 1]
def get_gauss_kernel(kernel_size: int, spatial_variance: float) -> np.ndarray:
# Creates a gaussian kernel of given dimension.
arr = np.zeros((kernel_size, kernel_size))
for i in range(0, kernel_size):
for j in range(0, kernel_size):
arr[i, j] = math.sqrt(
abs(i - kernel_size // 2) ** 2 + abs(j - kernel_size // 2) ** 2
)
return vec_gaussian(arr, spatial_variance)
def bilateral_filter(
img: np.ndarray,
spatial_variance: float,
intensity_variance: float,
kernel_size: int,
) -> np.ndarray:
img2 = np.zeros(img.shape)
gauss_ker = get_gauss_kernel(kernel_size, spatial_variance)
size_x, size_y = img.shape
for i in range(kernel_size // 2, size_x - kernel_size // 2):
for j in range(kernel_size // 2, size_y - kernel_size // 2):
img_s = get_slice(img, i, j, kernel_size)
img_i = img_s - img_s[kernel_size // 2, kernel_size // 2]
img_ig = vec_gaussian(img_i, intensity_variance)
weights = np.multiply(gauss_ker, img_ig)
vals = np.multiply(img_s, weights)
val = np.sum(vals) / np.sum(weights)
img2[i, j] = val
return img2
def parse_args(args: list) -> tuple:
filename = args[1] if args[1:] else "../image_data/lena.jpg"
spatial_variance = float(args[2]) if args[2:] else 1.0
intensity_variance = float(args[3]) if args[3:] else 1.0
if args[4:]:
kernel_size = int(args[4])
kernel_size = kernel_size + abs(kernel_size % 2 - 1)
else:
kernel_size = 5
return filename, spatial_variance, intensity_variance, kernel_size
if __name__ == "__main__":
filename, spatial_variance, intensity_variance, kernel_size = parse_args(sys.argv)
img = cv2.imread(filename, 0)
cv2.imshow("input image", img)
out = img / 255
out = out.astype("float32")
out = bilateral_filter(out, spatial_variance, intensity_variance, kernel_size)
out = out * 255
out = np.uint8(out)
cv2.imshow("output image", out)
cv2.waitKey(0)
cv2.destroyAllWindows()
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Python program to show the usage of Fermat's little theorem in a division
# According to Fermat's little theorem, (a / b) mod p always equals
# a * (b ^ (p - 2)) mod p
# Here we assume that p is a prime number, b divides a, and p doesn't divide b
# Wikipedia reference: https://en.wikipedia.org/wiki/Fermat%27s_little_theorem
def binary_exponentiation(a, n, mod):
if n == 0:
return 1
elif n % 2 == 1:
return (binary_exponentiation(a, n - 1, mod) * a) % mod
else:
b = binary_exponentiation(a, n / 2, mod)
return (b * b) % mod
# a prime number
p = 701
a = 1000000000
b = 10
# using binary exponentiation function, O(log(p)):
print((a / b) % p == (a * binary_exponentiation(b, p - 2, p)) % p)
# using Python operators:
print((a / b) % p == (a * b ** (p - 2)) % p)
| # Python program to show the usage of Fermat's little theorem in a division
# According to Fermat's little theorem, (a / b) mod p always equals
# a * (b ^ (p - 2)) mod p
# Here we assume that p is a prime number, b divides a, and p doesn't divide b
# Wikipedia reference: https://en.wikipedia.org/wiki/Fermat%27s_little_theorem
def binary_exponentiation(a, n, mod):
if n == 0:
return 1
elif n % 2 == 1:
return (binary_exponentiation(a, n - 1, mod) * a) % mod
else:
b = binary_exponentiation(a, n / 2, mod)
return (b * b) % mod
# a prime number
p = 701
a = 1000000000
b = 10
# using binary exponentiation function, O(log(p)):
print((a / b) % p == (a * binary_exponentiation(b, p - 2, p)) % p)
# using Python operators:
print((a / b) % p == (a * b ** (p - 2)) % p)
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| def get_word_pattern(word: str) -> str:
"""
>>> get_word_pattern("pattern")
'0.1.2.2.3.4.5'
>>> get_word_pattern("word pattern")
'0.1.2.3.4.5.6.7.7.8.2.9'
>>> get_word_pattern("get word pattern")
'0.1.2.3.4.5.6.7.3.8.9.2.2.1.6.10'
"""
word = word.upper()
next_num = 0
letter_nums = {}
word_pattern = []
for letter in word:
if letter not in letter_nums:
letter_nums[letter] = str(next_num)
next_num += 1
word_pattern.append(letter_nums[letter])
return ".".join(word_pattern)
if __name__ == "__main__":
import pprint
import time
start_time = time.time()
with open("dictionary.txt") as in_file:
word_list = in_file.read().splitlines()
all_patterns: dict = {}
for word in word_list:
pattern = get_word_pattern(word)
if pattern in all_patterns:
all_patterns[pattern].append(word)
else:
all_patterns[pattern] = [word]
with open("word_patterns.txt", "w") as out_file:
out_file.write(pprint.pformat(all_patterns))
total_time = round(time.time() - start_time, 2)
print(f"Done! {len(all_patterns):,} word patterns found in {total_time} seconds.")
# Done! 9,581 word patterns found in 0.58 seconds.
| def get_word_pattern(word: str) -> str:
"""
>>> get_word_pattern("pattern")
'0.1.2.2.3.4.5'
>>> get_word_pattern("word pattern")
'0.1.2.3.4.5.6.7.7.8.2.9'
>>> get_word_pattern("get word pattern")
'0.1.2.3.4.5.6.7.3.8.9.2.2.1.6.10'
"""
word = word.upper()
next_num = 0
letter_nums = {}
word_pattern = []
for letter in word:
if letter not in letter_nums:
letter_nums[letter] = str(next_num)
next_num += 1
word_pattern.append(letter_nums[letter])
return ".".join(word_pattern)
if __name__ == "__main__":
import pprint
import time
start_time = time.time()
with open("dictionary.txt") as in_file:
word_list = in_file.read().splitlines()
all_patterns: dict = {}
for word in word_list:
pattern = get_word_pattern(word)
if pattern in all_patterns:
all_patterns[pattern].append(word)
else:
all_patterns[pattern] = [word]
with open("word_patterns.txt", "w") as out_file:
out_file.write(pprint.pformat(all_patterns))
total_time = round(time.time() - start_time, 2)
print(f"Done! {len(all_patterns):,} word patterns found in {total_time} seconds.")
# Done! 9,581 word patterns found in 0.58 seconds.
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
This is a pure Python implementation of the merge sort algorithm
For doctests run following command:
python -m doctest -v merge_sort.py
or
python3 -m doctest -v merge_sort.py
For manual testing run:
python merge_sort.py
"""
def merge_sort(collection: list) -> list:
"""Pure implementation of the merge sort algorithm in Python
:param collection: some mutable ordered collection with heterogeneous
comparable items inside
:return: the same collection ordered by ascending
Examples:
>>> merge_sort([0, 5, 3, 2, 2])
[0, 2, 2, 3, 5]
>>> merge_sort([])
[]
>>> merge_sort([-2, -5, -45])
[-45, -5, -2]
"""
def merge(left: list, right: list) -> list:
"""merge left and right
:param left: left collection
:param right: right collection
:return: merge result
"""
def _merge():
while left and right:
yield (left if left[0] <= right[0] else right).pop(0)
yield from left
yield from right
return list(_merge())
if len(collection) <= 1:
return collection
mid = len(collection) // 2
return merge(merge_sort(collection[:mid]), merge_sort(collection[mid:]))
if __name__ == "__main__":
import doctest
doctest.testmod()
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(*merge_sort(unsorted), sep=",")
| """
This is a pure Python implementation of the merge sort algorithm
For doctests run following command:
python -m doctest -v merge_sort.py
or
python3 -m doctest -v merge_sort.py
For manual testing run:
python merge_sort.py
"""
def merge_sort(collection: list) -> list:
"""Pure implementation of the merge sort algorithm in Python
:param collection: some mutable ordered collection with heterogeneous
comparable items inside
:return: the same collection ordered by ascending
Examples:
>>> merge_sort([0, 5, 3, 2, 2])
[0, 2, 2, 3, 5]
>>> merge_sort([])
[]
>>> merge_sort([-2, -5, -45])
[-45, -5, -2]
"""
def merge(left: list, right: list) -> list:
"""merge left and right
:param left: left collection
:param right: right collection
:return: merge result
"""
def _merge():
while left and right:
yield (left if left[0] <= right[0] else right).pop(0)
yield from left
yield from right
return list(_merge())
if len(collection) <= 1:
return collection
mid = len(collection) // 2
return merge(merge_sort(collection[:mid]), merge_sort(collection[mid:]))
if __name__ == "__main__":
import doctest
doctest.testmod()
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(*merge_sort(unsorted), sep=",")
| -1 |
TheAlgorithms/Python | 7,499 | Remove some print statements within algorithmic functions | ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-22T02:13:09Z | 2022-10-22T11:33:52Z | 717f0e46d950060f2147f022f65b7e44e72cfdd8 | cc10b20beb8f0b10b50c84bd523bf41095fe9f37 | Remove some print statements within algorithmic functions. ### Describe your change:
Removed some print statements within algorithmic functions (i.e., code that's not within a `__main__`, a`main` function, or a utility function).
Encapsulated some test/demo code that wasn't within `__main__`s.
This PR contributes to #7337 but is not a complete fix.
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [ ] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [ ] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 36
https://projecteuler.net/problem=36
Problem Statement:
Double-base palindromes
Problem 36
The decimal number, 585 = 10010010012 (binary), is palindromic in both bases.
Find the sum of all numbers, less than one million, which are palindromic in
base 10 and base 2.
(Please note that the palindromic number, in either base, may not include
leading zeros.)
"""
from __future__ import annotations
def is_palindrome(n: int | str) -> bool:
"""
Return true if the input n is a palindrome.
Otherwise return false. n can be an integer or a string.
>>> is_palindrome(909)
True
>>> is_palindrome(908)
False
>>> is_palindrome('10101')
True
>>> is_palindrome('10111')
False
"""
n = str(n)
return True if n == n[::-1] else False
def solution(n: int = 1000000):
"""Return the sum of all numbers, less than n , which are palindromic in
base 10 and base 2.
>>> solution(1000000)
872187
>>> solution(500000)
286602
>>> solution(100000)
286602
>>> solution(1000)
1772
>>> solution(100)
157
>>> solution(10)
25
>>> solution(2)
1
>>> solution(1)
0
"""
total = 0
for i in range(1, n):
if is_palindrome(i) and is_palindrome(bin(i).split("b")[1]):
total += i
return total
if __name__ == "__main__":
print(solution(int(str(input().strip()))))
| """
Project Euler Problem 36
https://projecteuler.net/problem=36
Problem Statement:
Double-base palindromes
Problem 36
The decimal number, 585 = 10010010012 (binary), is palindromic in both bases.
Find the sum of all numbers, less than one million, which are palindromic in
base 10 and base 2.
(Please note that the palindromic number, in either base, may not include
leading zeros.)
"""
from __future__ import annotations
def is_palindrome(n: int | str) -> bool:
"""
Return true if the input n is a palindrome.
Otherwise return false. n can be an integer or a string.
>>> is_palindrome(909)
True
>>> is_palindrome(908)
False
>>> is_palindrome('10101')
True
>>> is_palindrome('10111')
False
"""
n = str(n)
return True if n == n[::-1] else False
def solution(n: int = 1000000):
"""Return the sum of all numbers, less than n , which are palindromic in
base 10 and base 2.
>>> solution(1000000)
872187
>>> solution(500000)
286602
>>> solution(100000)
286602
>>> solution(1000)
1772
>>> solution(100)
157
>>> solution(10)
25
>>> solution(2)
1
>>> solution(1)
0
"""
total = 0
for i in range(1, n):
if is_palindrome(i) and is_palindrome(bin(i).split("b")[1]):
total += i
return total
if __name__ == "__main__":
print(solution(int(str(input().strip()))))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
|
## Arithmetic Analysis
* [Bisection](arithmetic_analysis/bisection.py)
* [Gaussian Elimination](arithmetic_analysis/gaussian_elimination.py)
* [In Static Equilibrium](arithmetic_analysis/in_static_equilibrium.py)
* [Intersection](arithmetic_analysis/intersection.py)
* [Jacobi Iteration Method](arithmetic_analysis/jacobi_iteration_method.py)
* [Lu Decomposition](arithmetic_analysis/lu_decomposition.py)
* [Newton Forward Interpolation](arithmetic_analysis/newton_forward_interpolation.py)
* [Newton Method](arithmetic_analysis/newton_method.py)
* [Newton Raphson](arithmetic_analysis/newton_raphson.py)
* [Newton Raphson New](arithmetic_analysis/newton_raphson_new.py)
* [Secant Method](arithmetic_analysis/secant_method.py)
## Audio Filters
* [Butterworth Filter](audio_filters/butterworth_filter.py)
* [Equal Loudness Filter](audio_filters/equal_loudness_filter.py)
* [Iir Filter](audio_filters/iir_filter.py)
* [Show Response](audio_filters/show_response.py)
## Backtracking
* [All Combinations](backtracking/all_combinations.py)
* [All Permutations](backtracking/all_permutations.py)
* [All Subsequences](backtracking/all_subsequences.py)
* [Coloring](backtracking/coloring.py)
* [Combination Sum](backtracking/combination_sum.py)
* [Hamiltonian Cycle](backtracking/hamiltonian_cycle.py)
* [Knight Tour](backtracking/knight_tour.py)
* [Minimax](backtracking/minimax.py)
* [N Queens](backtracking/n_queens.py)
* [N Queens Math](backtracking/n_queens_math.py)
* [Rat In Maze](backtracking/rat_in_maze.py)
* [Sudoku](backtracking/sudoku.py)
* [Sum Of Subsets](backtracking/sum_of_subsets.py)
## Bit Manipulation
* [Binary And Operator](bit_manipulation/binary_and_operator.py)
* [Binary Count Setbits](bit_manipulation/binary_count_setbits.py)
* [Binary Count Trailing Zeros](bit_manipulation/binary_count_trailing_zeros.py)
* [Binary Or Operator](bit_manipulation/binary_or_operator.py)
* [Binary Shifts](bit_manipulation/binary_shifts.py)
* [Binary Twos Complement](bit_manipulation/binary_twos_complement.py)
* [Binary Xor Operator](bit_manipulation/binary_xor_operator.py)
* [Count 1S Brian Kernighan Method](bit_manipulation/count_1s_brian_kernighan_method.py)
* [Count Number Of One Bits](bit_manipulation/count_number_of_one_bits.py)
* [Gray Code Sequence](bit_manipulation/gray_code_sequence.py)
* [Reverse Bits](bit_manipulation/reverse_bits.py)
* [Single Bit Manipulation Operations](bit_manipulation/single_bit_manipulation_operations.py)
## Blockchain
* [Chinese Remainder Theorem](blockchain/chinese_remainder_theorem.py)
* [Diophantine Equation](blockchain/diophantine_equation.py)
* [Modular Division](blockchain/modular_division.py)
## Boolean Algebra
* [Norgate](boolean_algebra/norgate.py)
* [Quine Mc Cluskey](boolean_algebra/quine_mc_cluskey.py)
## Cellular Automata
* [Conways Game Of Life](cellular_automata/conways_game_of_life.py)
* [Game Of Life](cellular_automata/game_of_life.py)
* [Nagel Schrekenberg](cellular_automata/nagel_schrekenberg.py)
* [One Dimensional](cellular_automata/one_dimensional.py)
## Ciphers
* [A1Z26](ciphers/a1z26.py)
* [Affine Cipher](ciphers/affine_cipher.py)
* [Atbash](ciphers/atbash.py)
* [Baconian Cipher](ciphers/baconian_cipher.py)
* [Base16](ciphers/base16.py)
* [Base32](ciphers/base32.py)
* [Base64](ciphers/base64.py)
* [Base85](ciphers/base85.py)
* [Beaufort Cipher](ciphers/beaufort_cipher.py)
* [Bifid](ciphers/bifid.py)
* [Brute Force Caesar Cipher](ciphers/brute_force_caesar_cipher.py)
* [Caesar Cipher](ciphers/caesar_cipher.py)
* [Cryptomath Module](ciphers/cryptomath_module.py)
* [Decrypt Caesar With Chi Squared](ciphers/decrypt_caesar_with_chi_squared.py)
* [Deterministic Miller Rabin](ciphers/deterministic_miller_rabin.py)
* [Diffie](ciphers/diffie.py)
* [Diffie Hellman](ciphers/diffie_hellman.py)
* [Elgamal Key Generator](ciphers/elgamal_key_generator.py)
* [Enigma Machine2](ciphers/enigma_machine2.py)
* [Hill Cipher](ciphers/hill_cipher.py)
* [Mixed Keyword Cypher](ciphers/mixed_keyword_cypher.py)
* [Mono Alphabetic Ciphers](ciphers/mono_alphabetic_ciphers.py)
* [Morse Code](ciphers/morse_code.py)
* [Onepad Cipher](ciphers/onepad_cipher.py)
* [Playfair Cipher](ciphers/playfair_cipher.py)
* [Polybius](ciphers/polybius.py)
* [Porta Cipher](ciphers/porta_cipher.py)
* [Rabin Miller](ciphers/rabin_miller.py)
* [Rail Fence Cipher](ciphers/rail_fence_cipher.py)
* [Rot13](ciphers/rot13.py)
* [Rsa Cipher](ciphers/rsa_cipher.py)
* [Rsa Factorization](ciphers/rsa_factorization.py)
* [Rsa Key Generator](ciphers/rsa_key_generator.py)
* [Shuffled Shift Cipher](ciphers/shuffled_shift_cipher.py)
* [Simple Keyword Cypher](ciphers/simple_keyword_cypher.py)
* [Simple Substitution Cipher](ciphers/simple_substitution_cipher.py)
* [Trafid Cipher](ciphers/trafid_cipher.py)
* [Transposition Cipher](ciphers/transposition_cipher.py)
* [Transposition Cipher Encrypt Decrypt File](ciphers/transposition_cipher_encrypt_decrypt_file.py)
* [Vigenere Cipher](ciphers/vigenere_cipher.py)
* [Xor Cipher](ciphers/xor_cipher.py)
## Compression
* [Burrows Wheeler](compression/burrows_wheeler.py)
* [Huffman](compression/huffman.py)
* [Lempel Ziv](compression/lempel_ziv.py)
* [Lempel Ziv Decompress](compression/lempel_ziv_decompress.py)
* [Peak Signal To Noise Ratio](compression/peak_signal_to_noise_ratio.py)
* [Run Length Encoding](compression/run_length_encoding.py)
## Computer Vision
* [Cnn Classification](computer_vision/cnn_classification.py)
* [Flip Augmentation](computer_vision/flip_augmentation.py)
* [Harris Corner](computer_vision/harris_corner.py)
* [Horn Schunck](computer_vision/horn_schunck.py)
* [Mean Threshold](computer_vision/mean_threshold.py)
* [Mosaic Augmentation](computer_vision/mosaic_augmentation.py)
* [Pooling Functions](computer_vision/pooling_functions.py)
## Conversions
* [Astronomical Length Scale Conversion](conversions/astronomical_length_scale_conversion.py)
* [Binary To Decimal](conversions/binary_to_decimal.py)
* [Binary To Hexadecimal](conversions/binary_to_hexadecimal.py)
* [Binary To Octal](conversions/binary_to_octal.py)
* [Decimal To Any](conversions/decimal_to_any.py)
* [Decimal To Binary](conversions/decimal_to_binary.py)
* [Decimal To Binary Recursion](conversions/decimal_to_binary_recursion.py)
* [Decimal To Hexadecimal](conversions/decimal_to_hexadecimal.py)
* [Decimal To Octal](conversions/decimal_to_octal.py)
* [Excel Title To Column](conversions/excel_title_to_column.py)
* [Hex To Bin](conversions/hex_to_bin.py)
* [Hexadecimal To Decimal](conversions/hexadecimal_to_decimal.py)
* [Length Conversion](conversions/length_conversion.py)
* [Molecular Chemistry](conversions/molecular_chemistry.py)
* [Octal To Decimal](conversions/octal_to_decimal.py)
* [Prefix Conversions](conversions/prefix_conversions.py)
* [Prefix Conversions String](conversions/prefix_conversions_string.py)
* [Pressure Conversions](conversions/pressure_conversions.py)
* [Rgb Hsv Conversion](conversions/rgb_hsv_conversion.py)
* [Roman Numerals](conversions/roman_numerals.py)
* [Speed Conversions](conversions/speed_conversions.py)
* [Temperature Conversions](conversions/temperature_conversions.py)
* [Volume Conversions](conversions/volume_conversions.py)
* [Weight Conversion](conversions/weight_conversion.py)
## Data Structures
* Binary Tree
* [Avl Tree](data_structures/binary_tree/avl_tree.py)
* [Basic Binary Tree](data_structures/binary_tree/basic_binary_tree.py)
* [Binary Search Tree](data_structures/binary_tree/binary_search_tree.py)
* [Binary Search Tree Recursive](data_structures/binary_tree/binary_search_tree_recursive.py)
* [Binary Tree Mirror](data_structures/binary_tree/binary_tree_mirror.py)
* [Binary Tree Node Sum](data_structures/binary_tree/binary_tree_node_sum.py)
* [Binary Tree Traversals](data_structures/binary_tree/binary_tree_traversals.py)
* [Fenwick Tree](data_structures/binary_tree/fenwick_tree.py)
* [Inorder Tree Traversal 2022](data_structures/binary_tree/inorder_tree_traversal_2022.py)
* [Lazy Segment Tree](data_structures/binary_tree/lazy_segment_tree.py)
* [Lowest Common Ancestor](data_structures/binary_tree/lowest_common_ancestor.py)
* [Maximum Fenwick Tree](data_structures/binary_tree/maximum_fenwick_tree.py)
* [Merge Two Binary Trees](data_structures/binary_tree/merge_two_binary_trees.py)
* [Non Recursive Segment Tree](data_structures/binary_tree/non_recursive_segment_tree.py)
* [Number Of Possible Binary Trees](data_structures/binary_tree/number_of_possible_binary_trees.py)
* [Red Black Tree](data_structures/binary_tree/red_black_tree.py)
* [Segment Tree](data_structures/binary_tree/segment_tree.py)
* [Segment Tree Other](data_structures/binary_tree/segment_tree_other.py)
* [Treap](data_structures/binary_tree/treap.py)
* [Wavelet Tree](data_structures/binary_tree/wavelet_tree.py)
* Disjoint Set
* [Alternate Disjoint Set](data_structures/disjoint_set/alternate_disjoint_set.py)
* [Disjoint Set](data_structures/disjoint_set/disjoint_set.py)
* Hashing
* [Double Hash](data_structures/hashing/double_hash.py)
* [Hash Table](data_structures/hashing/hash_table.py)
* [Hash Table With Linked List](data_structures/hashing/hash_table_with_linked_list.py)
* Number Theory
* [Prime Numbers](data_structures/hashing/number_theory/prime_numbers.py)
* [Quadratic Probing](data_structures/hashing/quadratic_probing.py)
* Heap
* [Binomial Heap](data_structures/heap/binomial_heap.py)
* [Heap](data_structures/heap/heap.py)
* [Heap Generic](data_structures/heap/heap_generic.py)
* [Max Heap](data_structures/heap/max_heap.py)
* [Min Heap](data_structures/heap/min_heap.py)
* [Randomized Heap](data_structures/heap/randomized_heap.py)
* [Skew Heap](data_structures/heap/skew_heap.py)
* Linked List
* [Circular Linked List](data_structures/linked_list/circular_linked_list.py)
* [Deque Doubly](data_structures/linked_list/deque_doubly.py)
* [Doubly Linked List](data_structures/linked_list/doubly_linked_list.py)
* [Doubly Linked List Two](data_structures/linked_list/doubly_linked_list_two.py)
* [From Sequence](data_structures/linked_list/from_sequence.py)
* [Has Loop](data_structures/linked_list/has_loop.py)
* [Is Palindrome](data_structures/linked_list/is_palindrome.py)
* [Merge Two Lists](data_structures/linked_list/merge_two_lists.py)
* [Middle Element Of Linked List](data_structures/linked_list/middle_element_of_linked_list.py)
* [Print Reverse](data_structures/linked_list/print_reverse.py)
* [Singly Linked List](data_structures/linked_list/singly_linked_list.py)
* [Skip List](data_structures/linked_list/skip_list.py)
* [Swap Nodes](data_structures/linked_list/swap_nodes.py)
* Queue
* [Circular Queue](data_structures/queue/circular_queue.py)
* [Circular Queue Linked List](data_structures/queue/circular_queue_linked_list.py)
* [Double Ended Queue](data_structures/queue/double_ended_queue.py)
* [Linked Queue](data_structures/queue/linked_queue.py)
* [Priority Queue Using List](data_structures/queue/priority_queue_using_list.py)
* [Queue On List](data_structures/queue/queue_on_list.py)
* [Queue On Pseudo Stack](data_structures/queue/queue_on_pseudo_stack.py)
* Stacks
* [Balanced Parentheses](data_structures/stacks/balanced_parentheses.py)
* [Dijkstras Two Stack Algorithm](data_structures/stacks/dijkstras_two_stack_algorithm.py)
* [Evaluate Postfix Notations](data_structures/stacks/evaluate_postfix_notations.py)
* [Infix To Postfix Conversion](data_structures/stacks/infix_to_postfix_conversion.py)
* [Infix To Prefix Conversion](data_structures/stacks/infix_to_prefix_conversion.py)
* [Next Greater Element](data_structures/stacks/next_greater_element.py)
* [Postfix Evaluation](data_structures/stacks/postfix_evaluation.py)
* [Prefix Evaluation](data_structures/stacks/prefix_evaluation.py)
* [Stack](data_structures/stacks/stack.py)
* [Stack With Doubly Linked List](data_structures/stacks/stack_with_doubly_linked_list.py)
* [Stack With Singly Linked List](data_structures/stacks/stack_with_singly_linked_list.py)
* [Stock Span Problem](data_structures/stacks/stock_span_problem.py)
* Trie
* [Trie](data_structures/trie/trie.py)
## Digital Image Processing
* [Change Brightness](digital_image_processing/change_brightness.py)
* [Change Contrast](digital_image_processing/change_contrast.py)
* [Convert To Negative](digital_image_processing/convert_to_negative.py)
* Dithering
* [Burkes](digital_image_processing/dithering/burkes.py)
* Edge Detection
* [Canny](digital_image_processing/edge_detection/canny.py)
* Filters
* [Bilateral Filter](digital_image_processing/filters/bilateral_filter.py)
* [Convolve](digital_image_processing/filters/convolve.py)
* [Gabor Filter](digital_image_processing/filters/gabor_filter.py)
* [Gaussian Filter](digital_image_processing/filters/gaussian_filter.py)
* [Local Binary Pattern](digital_image_processing/filters/local_binary_pattern.py)
* [Median Filter](digital_image_processing/filters/median_filter.py)
* [Sobel Filter](digital_image_processing/filters/sobel_filter.py)
* Histogram Equalization
* [Histogram Stretch](digital_image_processing/histogram_equalization/histogram_stretch.py)
* [Index Calculation](digital_image_processing/index_calculation.py)
* Morphological Operations
* [Dilation Operation](digital_image_processing/morphological_operations/dilation_operation.py)
* [Erosion Operation](digital_image_processing/morphological_operations/erosion_operation.py)
* Resize
* [Resize](digital_image_processing/resize/resize.py)
* Rotation
* [Rotation](digital_image_processing/rotation/rotation.py)
* [Sepia](digital_image_processing/sepia.py)
* [Test Digital Image Processing](digital_image_processing/test_digital_image_processing.py)
## Divide And Conquer
* [Closest Pair Of Points](divide_and_conquer/closest_pair_of_points.py)
* [Convex Hull](divide_and_conquer/convex_hull.py)
* [Heaps Algorithm](divide_and_conquer/heaps_algorithm.py)
* [Heaps Algorithm Iterative](divide_and_conquer/heaps_algorithm_iterative.py)
* [Inversions](divide_and_conquer/inversions.py)
* [Kth Order Statistic](divide_and_conquer/kth_order_statistic.py)
* [Max Difference Pair](divide_and_conquer/max_difference_pair.py)
* [Max Subarray Sum](divide_and_conquer/max_subarray_sum.py)
* [Mergesort](divide_and_conquer/mergesort.py)
* [Peak](divide_and_conquer/peak.py)
* [Power](divide_and_conquer/power.py)
* [Strassen Matrix Multiplication](divide_and_conquer/strassen_matrix_multiplication.py)
## Dynamic Programming
* [Abbreviation](dynamic_programming/abbreviation.py)
* [All Construct](dynamic_programming/all_construct.py)
* [Bitmask](dynamic_programming/bitmask.py)
* [Catalan Numbers](dynamic_programming/catalan_numbers.py)
* [Climbing Stairs](dynamic_programming/climbing_stairs.py)
* [Edit Distance](dynamic_programming/edit_distance.py)
* [Factorial](dynamic_programming/factorial.py)
* [Fast Fibonacci](dynamic_programming/fast_fibonacci.py)
* [Fibonacci](dynamic_programming/fibonacci.py)
* [Floyd Warshall](dynamic_programming/floyd_warshall.py)
* [Integer Partition](dynamic_programming/integer_partition.py)
* [Iterating Through Submasks](dynamic_programming/iterating_through_submasks.py)
* [Knapsack](dynamic_programming/knapsack.py)
* [Longest Common Subsequence](dynamic_programming/longest_common_subsequence.py)
* [Longest Increasing Subsequence](dynamic_programming/longest_increasing_subsequence.py)
* [Longest Increasing Subsequence O(Nlogn)](dynamic_programming/longest_increasing_subsequence_o(nlogn).py)
* [Longest Sub Array](dynamic_programming/longest_sub_array.py)
* [Matrix Chain Order](dynamic_programming/matrix_chain_order.py)
* [Max Non Adjacent Sum](dynamic_programming/max_non_adjacent_sum.py)
* [Max Sub Array](dynamic_programming/max_sub_array.py)
* [Max Sum Contiguous Subsequence](dynamic_programming/max_sum_contiguous_subsequence.py)
* [Minimum Coin Change](dynamic_programming/minimum_coin_change.py)
* [Minimum Cost Path](dynamic_programming/minimum_cost_path.py)
* [Minimum Partition](dynamic_programming/minimum_partition.py)
* [Minimum Steps To One](dynamic_programming/minimum_steps_to_one.py)
* [Optimal Binary Search Tree](dynamic_programming/optimal_binary_search_tree.py)
* [Rod Cutting](dynamic_programming/rod_cutting.py)
* [Subset Generation](dynamic_programming/subset_generation.py)
* [Sum Of Subset](dynamic_programming/sum_of_subset.py)
## Electronics
* [Carrier Concentration](electronics/carrier_concentration.py)
* [Coulombs Law](electronics/coulombs_law.py)
* [Electric Power](electronics/electric_power.py)
* [Ohms Law](electronics/ohms_law.py)
## File Transfer
* [Receive File](file_transfer/receive_file.py)
* [Send File](file_transfer/send_file.py)
* Tests
* [Test Send File](file_transfer/tests/test_send_file.py)
## Financial
* [Equated Monthly Installments](financial/equated_monthly_installments.py)
* [Interest](financial/interest.py)
## Fractals
* [Julia Sets](fractals/julia_sets.py)
* [Koch Snowflake](fractals/koch_snowflake.py)
* [Mandelbrot](fractals/mandelbrot.py)
* [Sierpinski Triangle](fractals/sierpinski_triangle.py)
## Fuzzy Logic
* [Fuzzy Operations](fuzzy_logic/fuzzy_operations.py)
## Genetic Algorithm
* [Basic String](genetic_algorithm/basic_string.py)
## Geodesy
* [Haversine Distance](geodesy/haversine_distance.py)
* [Lamberts Ellipsoidal Distance](geodesy/lamberts_ellipsoidal_distance.py)
## Graphics
* [Bezier Curve](graphics/bezier_curve.py)
* [Vector3 For 2D Rendering](graphics/vector3_for_2d_rendering.py)
## Graphs
* [A Star](graphs/a_star.py)
* [Articulation Points](graphs/articulation_points.py)
* [Basic Graphs](graphs/basic_graphs.py)
* [Bellman Ford](graphs/bellman_ford.py)
* [Bfs Shortest Path](graphs/bfs_shortest_path.py)
* [Bfs Zero One Shortest Path](graphs/bfs_zero_one_shortest_path.py)
* [Bidirectional A Star](graphs/bidirectional_a_star.py)
* [Bidirectional Breadth First Search](graphs/bidirectional_breadth_first_search.py)
* [Boruvka](graphs/boruvka.py)
* [Breadth First Search](graphs/breadth_first_search.py)
* [Breadth First Search 2](graphs/breadth_first_search_2.py)
* [Breadth First Search Shortest Path](graphs/breadth_first_search_shortest_path.py)
* [Check Bipartite Graph Bfs](graphs/check_bipartite_graph_bfs.py)
* [Check Bipartite Graph Dfs](graphs/check_bipartite_graph_dfs.py)
* [Check Cycle](graphs/check_cycle.py)
* [Connected Components](graphs/connected_components.py)
* [Depth First Search](graphs/depth_first_search.py)
* [Depth First Search 2](graphs/depth_first_search_2.py)
* [Dijkstra](graphs/dijkstra.py)
* [Dijkstra 2](graphs/dijkstra_2.py)
* [Dijkstra Algorithm](graphs/dijkstra_algorithm.py)
* [Dinic](graphs/dinic.py)
* [Directed And Undirected (Weighted) Graph](graphs/directed_and_undirected_(weighted)_graph.py)
* [Edmonds Karp Multiple Source And Sink](graphs/edmonds_karp_multiple_source_and_sink.py)
* [Eulerian Path And Circuit For Undirected Graph](graphs/eulerian_path_and_circuit_for_undirected_graph.py)
* [Even Tree](graphs/even_tree.py)
* [Finding Bridges](graphs/finding_bridges.py)
* [Frequent Pattern Graph Miner](graphs/frequent_pattern_graph_miner.py)
* [G Topological Sort](graphs/g_topological_sort.py)
* [Gale Shapley Bigraph](graphs/gale_shapley_bigraph.py)
* [Graph List](graphs/graph_list.py)
* [Graph Matrix](graphs/graph_matrix.py)
* [Graphs Floyd Warshall](graphs/graphs_floyd_warshall.py)
* [Greedy Best First](graphs/greedy_best_first.py)
* [Greedy Min Vertex Cover](graphs/greedy_min_vertex_cover.py)
* [Kahns Algorithm Long](graphs/kahns_algorithm_long.py)
* [Kahns Algorithm Topo](graphs/kahns_algorithm_topo.py)
* [Karger](graphs/karger.py)
* [Markov Chain](graphs/markov_chain.py)
* [Matching Min Vertex Cover](graphs/matching_min_vertex_cover.py)
* [Minimum Path Sum](graphs/minimum_path_sum.py)
* [Minimum Spanning Tree Boruvka](graphs/minimum_spanning_tree_boruvka.py)
* [Minimum Spanning Tree Kruskal](graphs/minimum_spanning_tree_kruskal.py)
* [Minimum Spanning Tree Kruskal2](graphs/minimum_spanning_tree_kruskal2.py)
* [Minimum Spanning Tree Prims](graphs/minimum_spanning_tree_prims.py)
* [Minimum Spanning Tree Prims2](graphs/minimum_spanning_tree_prims2.py)
* [Multi Heuristic Astar](graphs/multi_heuristic_astar.py)
* [Page Rank](graphs/page_rank.py)
* [Prim](graphs/prim.py)
* [Random Graph Generator](graphs/random_graph_generator.py)
* [Scc Kosaraju](graphs/scc_kosaraju.py)
* [Strongly Connected Components](graphs/strongly_connected_components.py)
* [Tarjans Scc](graphs/tarjans_scc.py)
* Tests
* [Test Min Spanning Tree Kruskal](graphs/tests/test_min_spanning_tree_kruskal.py)
* [Test Min Spanning Tree Prim](graphs/tests/test_min_spanning_tree_prim.py)
## Greedy Methods
* [Fractional Knapsack](greedy_methods/fractional_knapsack.py)
* [Fractional Knapsack 2](greedy_methods/fractional_knapsack_2.py)
* [Optimal Merge Pattern](greedy_methods/optimal_merge_pattern.py)
## Hashes
* [Adler32](hashes/adler32.py)
* [Chaos Machine](hashes/chaos_machine.py)
* [Djb2](hashes/djb2.py)
* [Enigma Machine](hashes/enigma_machine.py)
* [Hamming Code](hashes/hamming_code.py)
* [Luhn](hashes/luhn.py)
* [Md5](hashes/md5.py)
* [Sdbm](hashes/sdbm.py)
* [Sha1](hashes/sha1.py)
* [Sha256](hashes/sha256.py)
## Knapsack
* [Greedy Knapsack](knapsack/greedy_knapsack.py)
* [Knapsack](knapsack/knapsack.py)
* Tests
* [Test Greedy Knapsack](knapsack/tests/test_greedy_knapsack.py)
* [Test Knapsack](knapsack/tests/test_knapsack.py)
## Linear Algebra
* Src
* [Conjugate Gradient](linear_algebra/src/conjugate_gradient.py)
* [Lib](linear_algebra/src/lib.py)
* [Polynom For Points](linear_algebra/src/polynom_for_points.py)
* [Power Iteration](linear_algebra/src/power_iteration.py)
* [Rayleigh Quotient](linear_algebra/src/rayleigh_quotient.py)
* [Schur Complement](linear_algebra/src/schur_complement.py)
* [Test Linear Algebra](linear_algebra/src/test_linear_algebra.py)
* [Transformations 2D](linear_algebra/src/transformations_2d.py)
## Machine Learning
* [Astar](machine_learning/astar.py)
* [Data Transformations](machine_learning/data_transformations.py)
* [Decision Tree](machine_learning/decision_tree.py)
* Forecasting
* [Run](machine_learning/forecasting/run.py)
* [Gaussian Naive Bayes](machine_learning/gaussian_naive_bayes.py)
* [Gradient Boosting Regressor](machine_learning/gradient_boosting_regressor.py)
* [Gradient Descent](machine_learning/gradient_descent.py)
* [K Means Clust](machine_learning/k_means_clust.py)
* [K Nearest Neighbours](machine_learning/k_nearest_neighbours.py)
* [Knn Sklearn](machine_learning/knn_sklearn.py)
* [Linear Discriminant Analysis](machine_learning/linear_discriminant_analysis.py)
* [Linear Regression](machine_learning/linear_regression.py)
* Local Weighted Learning
* [Local Weighted Learning](machine_learning/local_weighted_learning/local_weighted_learning.py)
* [Logistic Regression](machine_learning/logistic_regression.py)
* Lstm
* [Lstm Prediction](machine_learning/lstm/lstm_prediction.py)
* [Multilayer Perceptron Classifier](machine_learning/multilayer_perceptron_classifier.py)
* [Polymonial Regression](machine_learning/polymonial_regression.py)
* [Random Forest Classifier](machine_learning/random_forest_classifier.py)
* [Random Forest Regressor](machine_learning/random_forest_regressor.py)
* [Scoring Functions](machine_learning/scoring_functions.py)
* [Self Organizing Map](machine_learning/self_organizing_map.py)
* [Sequential Minimum Optimization](machine_learning/sequential_minimum_optimization.py)
* [Similarity Search](machine_learning/similarity_search.py)
* [Support Vector Machines](machine_learning/support_vector_machines.py)
* [Word Frequency Functions](machine_learning/word_frequency_functions.py)
## Maths
* [3N Plus 1](maths/3n_plus_1.py)
* [Abs](maths/abs.py)
* [Abs Max](maths/abs_max.py)
* [Abs Min](maths/abs_min.py)
* [Add](maths/add.py)
* [Aliquot Sum](maths/aliquot_sum.py)
* [Allocation Number](maths/allocation_number.py)
* [Area](maths/area.py)
* [Area Under Curve](maths/area_under_curve.py)
* [Armstrong Numbers](maths/armstrong_numbers.py)
* [Average Absolute Deviation](maths/average_absolute_deviation.py)
* [Average Mean](maths/average_mean.py)
* [Average Median](maths/average_median.py)
* [Average Mode](maths/average_mode.py)
* [Bailey Borwein Plouffe](maths/bailey_borwein_plouffe.py)
* [Basic Maths](maths/basic_maths.py)
* [Binary Exp Mod](maths/binary_exp_mod.py)
* [Binary Exponentiation](maths/binary_exponentiation.py)
* [Binary Exponentiation 2](maths/binary_exponentiation_2.py)
* [Binary Exponentiation 3](maths/binary_exponentiation_3.py)
* [Binomial Coefficient](maths/binomial_coefficient.py)
* [Binomial Distribution](maths/binomial_distribution.py)
* [Bisection](maths/bisection.py)
* [Carmichael Number](maths/carmichael_number.py)
* [Catalan Number](maths/catalan_number.py)
* [Ceil](maths/ceil.py)
* [Check Polygon](maths/check_polygon.py)
* [Chudnovsky Algorithm](maths/chudnovsky_algorithm.py)
* [Collatz Sequence](maths/collatz_sequence.py)
* [Combinations](maths/combinations.py)
* [Decimal Isolate](maths/decimal_isolate.py)
* [Double Factorial Iterative](maths/double_factorial_iterative.py)
* [Double Factorial Recursive](maths/double_factorial_recursive.py)
* [Entropy](maths/entropy.py)
* [Euclidean Distance](maths/euclidean_distance.py)
* [Euclidean Gcd](maths/euclidean_gcd.py)
* [Euler Method](maths/euler_method.py)
* [Euler Modified](maths/euler_modified.py)
* [Eulers Totient](maths/eulers_totient.py)
* [Extended Euclidean Algorithm](maths/extended_euclidean_algorithm.py)
* [Factorial Iterative](maths/factorial_iterative.py)
* [Factorial Recursive](maths/factorial_recursive.py)
* [Factors](maths/factors.py)
* [Fermat Little Theorem](maths/fermat_little_theorem.py)
* [Fibonacci](maths/fibonacci.py)
* [Find Max](maths/find_max.py)
* [Find Max Recursion](maths/find_max_recursion.py)
* [Find Min](maths/find_min.py)
* [Find Min Recursion](maths/find_min_recursion.py)
* [Floor](maths/floor.py)
* [Gamma](maths/gamma.py)
* [Gamma Recursive](maths/gamma_recursive.py)
* [Gaussian](maths/gaussian.py)
* [Greatest Common Divisor](maths/greatest_common_divisor.py)
* [Greedy Coin Change](maths/greedy_coin_change.py)
* [Hamming Numbers](maths/hamming_numbers.py)
* [Hardy Ramanujanalgo](maths/hardy_ramanujanalgo.py)
* [Integration By Simpson Approx](maths/integration_by_simpson_approx.py)
* [Is Ip V4 Address Valid](maths/is_ip_v4_address_valid.py)
* [Is Square Free](maths/is_square_free.py)
* [Jaccard Similarity](maths/jaccard_similarity.py)
* [Kadanes](maths/kadanes.py)
* [Karatsuba](maths/karatsuba.py)
* [Krishnamurthy Number](maths/krishnamurthy_number.py)
* [Kth Lexicographic Permutation](maths/kth_lexicographic_permutation.py)
* [Largest Of Very Large Numbers](maths/largest_of_very_large_numbers.py)
* [Largest Subarray Sum](maths/largest_subarray_sum.py)
* [Least Common Multiple](maths/least_common_multiple.py)
* [Line Length](maths/line_length.py)
* [Lucas Lehmer Primality Test](maths/lucas_lehmer_primality_test.py)
* [Lucas Series](maths/lucas_series.py)
* [Matrix Exponentiation](maths/matrix_exponentiation.py)
* [Max Sum Sliding Window](maths/max_sum_sliding_window.py)
* [Median Of Two Arrays](maths/median_of_two_arrays.py)
* [Miller Rabin](maths/miller_rabin.py)
* [Mobius Function](maths/mobius_function.py)
* [Modular Exponential](maths/modular_exponential.py)
* [Monte Carlo](maths/monte_carlo.py)
* [Monte Carlo Dice](maths/monte_carlo_dice.py)
* [Nevilles Method](maths/nevilles_method.py)
* [Newton Raphson](maths/newton_raphson.py)
* [Number Of Digits](maths/number_of_digits.py)
* [Numerical Integration](maths/numerical_integration.py)
* [Perfect Cube](maths/perfect_cube.py)
* [Perfect Number](maths/perfect_number.py)
* [Perfect Square](maths/perfect_square.py)
* [Persistence](maths/persistence.py)
* [Pi Monte Carlo Estimation](maths/pi_monte_carlo_estimation.py)
* [Points Are Collinear 3D](maths/points_are_collinear_3d.py)
* [Pollard Rho](maths/pollard_rho.py)
* [Polynomial Evaluation](maths/polynomial_evaluation.py)
* [Power Using Recursion](maths/power_using_recursion.py)
* [Prime Check](maths/prime_check.py)
* [Prime Factors](maths/prime_factors.py)
* [Prime Numbers](maths/prime_numbers.py)
* [Prime Sieve Eratosthenes](maths/prime_sieve_eratosthenes.py)
* [Primelib](maths/primelib.py)
* [Proth Number](maths/proth_number.py)
* [Pythagoras](maths/pythagoras.py)
* [Qr Decomposition](maths/qr_decomposition.py)
* [Quadratic Equations Complex Numbers](maths/quadratic_equations_complex_numbers.py)
* [Radians](maths/radians.py)
* [Radix2 Fft](maths/radix2_fft.py)
* [Relu](maths/relu.py)
* [Runge Kutta](maths/runge_kutta.py)
* [Segmented Sieve](maths/segmented_sieve.py)
* Series
* [Arithmetic](maths/series/arithmetic.py)
* [Geometric](maths/series/geometric.py)
* [Geometric Series](maths/series/geometric_series.py)
* [Harmonic](maths/series/harmonic.py)
* [Harmonic Series](maths/series/harmonic_series.py)
* [Hexagonal Numbers](maths/series/hexagonal_numbers.py)
* [P Series](maths/series/p_series.py)
* [Sieve Of Eratosthenes](maths/sieve_of_eratosthenes.py)
* [Sigmoid](maths/sigmoid.py)
* [Simpson Rule](maths/simpson_rule.py)
* [Sin](maths/sin.py)
* [Sock Merchant](maths/sock_merchant.py)
* [Softmax](maths/softmax.py)
* [Square Root](maths/square_root.py)
* [Sum Of Arithmetic Series](maths/sum_of_arithmetic_series.py)
* [Sum Of Digits](maths/sum_of_digits.py)
* [Sum Of Geometric Progression](maths/sum_of_geometric_progression.py)
* [Sylvester Sequence](maths/sylvester_sequence.py)
* [Test Prime Check](maths/test_prime_check.py)
* [Trapezoidal Rule](maths/trapezoidal_rule.py)
* [Triplet Sum](maths/triplet_sum.py)
* [Two Pointer](maths/two_pointer.py)
* [Two Sum](maths/two_sum.py)
* [Ugly Numbers](maths/ugly_numbers.py)
* [Volume](maths/volume.py)
* [Weird Number](maths/weird_number.py)
* [Zellers Congruence](maths/zellers_congruence.py)
## Matrix
* [Binary Search Matrix](matrix/binary_search_matrix.py)
* [Count Islands In Matrix](matrix/count_islands_in_matrix.py)
* [Inverse Of Matrix](matrix/inverse_of_matrix.py)
* [Matrix Class](matrix/matrix_class.py)
* [Matrix Operation](matrix/matrix_operation.py)
* [Nth Fibonacci Using Matrix Exponentiation](matrix/nth_fibonacci_using_matrix_exponentiation.py)
* [Rotate Matrix](matrix/rotate_matrix.py)
* [Searching In Sorted Matrix](matrix/searching_in_sorted_matrix.py)
* [Sherman Morrison](matrix/sherman_morrison.py)
* [Spiral Print](matrix/spiral_print.py)
* Tests
* [Test Matrix Operation](matrix/tests/test_matrix_operation.py)
## Networking Flow
* [Ford Fulkerson](networking_flow/ford_fulkerson.py)
* [Minimum Cut](networking_flow/minimum_cut.py)
## Neural Network
* [2 Hidden Layers Neural Network](neural_network/2_hidden_layers_neural_network.py)
* [Back Propagation Neural Network](neural_network/back_propagation_neural_network.py)
* [Convolution Neural Network](neural_network/convolution_neural_network.py)
* [Perceptron](neural_network/perceptron.py)
## Other
* [Activity Selection](other/activity_selection.py)
* [Alternative List Arrange](other/alternative_list_arrange.py)
* [Check Strong Password](other/check_strong_password.py)
* [Davisb Putnamb Logemannb Loveland](other/davisb_putnamb_logemannb_loveland.py)
* [Dijkstra Bankers Algorithm](other/dijkstra_bankers_algorithm.py)
* [Doomsday](other/doomsday.py)
* [Fischer Yates Shuffle](other/fischer_yates_shuffle.py)
* [Gauss Easter](other/gauss_easter.py)
* [Graham Scan](other/graham_scan.py)
* [Greedy](other/greedy.py)
* [Least Recently Used](other/least_recently_used.py)
* [Lfu Cache](other/lfu_cache.py)
* [Linear Congruential Generator](other/linear_congruential_generator.py)
* [Lru Cache](other/lru_cache.py)
* [Magicdiamondpattern](other/magicdiamondpattern.py)
* [Maximum Subarray](other/maximum_subarray.py)
* [Nested Brackets](other/nested_brackets.py)
* [Password Generator](other/password_generator.py)
* [Scoring Algorithm](other/scoring_algorithm.py)
* [Sdes](other/sdes.py)
* [Tower Of Hanoi](other/tower_of_hanoi.py)
## Physics
* [Casimir Effect](physics/casimir_effect.py)
* [Horizontal Projectile Motion](physics/horizontal_projectile_motion.py)
* [Lorentz Transformation Four Vector](physics/lorentz_transformation_four_vector.py)
* [N Body Simulation](physics/n_body_simulation.py)
* [Newtons Law Of Gravitation](physics/newtons_law_of_gravitation.py)
* [Newtons Second Law Of Motion](physics/newtons_second_law_of_motion.py)
## Project Euler
* Problem 001
* [Sol1](project_euler/problem_001/sol1.py)
* [Sol2](project_euler/problem_001/sol2.py)
* [Sol3](project_euler/problem_001/sol3.py)
* [Sol4](project_euler/problem_001/sol4.py)
* [Sol5](project_euler/problem_001/sol5.py)
* [Sol6](project_euler/problem_001/sol6.py)
* [Sol7](project_euler/problem_001/sol7.py)
* Problem 002
* [Sol1](project_euler/problem_002/sol1.py)
* [Sol2](project_euler/problem_002/sol2.py)
* [Sol3](project_euler/problem_002/sol3.py)
* [Sol4](project_euler/problem_002/sol4.py)
* [Sol5](project_euler/problem_002/sol5.py)
* Problem 003
* [Sol1](project_euler/problem_003/sol1.py)
* [Sol2](project_euler/problem_003/sol2.py)
* [Sol3](project_euler/problem_003/sol3.py)
* Problem 004
* [Sol1](project_euler/problem_004/sol1.py)
* [Sol2](project_euler/problem_004/sol2.py)
* Problem 005
* [Sol1](project_euler/problem_005/sol1.py)
* [Sol2](project_euler/problem_005/sol2.py)
* Problem 006
* [Sol1](project_euler/problem_006/sol1.py)
* [Sol2](project_euler/problem_006/sol2.py)
* [Sol3](project_euler/problem_006/sol3.py)
* [Sol4](project_euler/problem_006/sol4.py)
* Problem 007
* [Sol1](project_euler/problem_007/sol1.py)
* [Sol2](project_euler/problem_007/sol2.py)
* [Sol3](project_euler/problem_007/sol3.py)
* Problem 008
* [Sol1](project_euler/problem_008/sol1.py)
* [Sol2](project_euler/problem_008/sol2.py)
* [Sol3](project_euler/problem_008/sol3.py)
* Problem 009
* [Sol1](project_euler/problem_009/sol1.py)
* [Sol2](project_euler/problem_009/sol2.py)
* [Sol3](project_euler/problem_009/sol3.py)
* Problem 010
* [Sol1](project_euler/problem_010/sol1.py)
* [Sol2](project_euler/problem_010/sol2.py)
* [Sol3](project_euler/problem_010/sol3.py)
* Problem 011
* [Sol1](project_euler/problem_011/sol1.py)
* [Sol2](project_euler/problem_011/sol2.py)
* Problem 012
* [Sol1](project_euler/problem_012/sol1.py)
* [Sol2](project_euler/problem_012/sol2.py)
* Problem 013
* [Sol1](project_euler/problem_013/sol1.py)
* Problem 014
* [Sol1](project_euler/problem_014/sol1.py)
* [Sol2](project_euler/problem_014/sol2.py)
* Problem 015
* [Sol1](project_euler/problem_015/sol1.py)
* Problem 016
* [Sol1](project_euler/problem_016/sol1.py)
* [Sol2](project_euler/problem_016/sol2.py)
* Problem 017
* [Sol1](project_euler/problem_017/sol1.py)
* Problem 018
* [Solution](project_euler/problem_018/solution.py)
* Problem 019
* [Sol1](project_euler/problem_019/sol1.py)
* Problem 020
* [Sol1](project_euler/problem_020/sol1.py)
* [Sol2](project_euler/problem_020/sol2.py)
* [Sol3](project_euler/problem_020/sol3.py)
* [Sol4](project_euler/problem_020/sol4.py)
* Problem 021
* [Sol1](project_euler/problem_021/sol1.py)
* Problem 022
* [Sol1](project_euler/problem_022/sol1.py)
* [Sol2](project_euler/problem_022/sol2.py)
* Problem 023
* [Sol1](project_euler/problem_023/sol1.py)
* Problem 024
* [Sol1](project_euler/problem_024/sol1.py)
* Problem 025
* [Sol1](project_euler/problem_025/sol1.py)
* [Sol2](project_euler/problem_025/sol2.py)
* [Sol3](project_euler/problem_025/sol3.py)
* Problem 026
* [Sol1](project_euler/problem_026/sol1.py)
* Problem 027
* [Sol1](project_euler/problem_027/sol1.py)
* Problem 028
* [Sol1](project_euler/problem_028/sol1.py)
* Problem 029
* [Sol1](project_euler/problem_029/sol1.py)
* Problem 030
* [Sol1](project_euler/problem_030/sol1.py)
* Problem 031
* [Sol1](project_euler/problem_031/sol1.py)
* [Sol2](project_euler/problem_031/sol2.py)
* Problem 032
* [Sol32](project_euler/problem_032/sol32.py)
* Problem 033
* [Sol1](project_euler/problem_033/sol1.py)
* Problem 034
* [Sol1](project_euler/problem_034/sol1.py)
* Problem 035
* [Sol1](project_euler/problem_035/sol1.py)
* Problem 036
* [Sol1](project_euler/problem_036/sol1.py)
* Problem 037
* [Sol1](project_euler/problem_037/sol1.py)
* Problem 038
* [Sol1](project_euler/problem_038/sol1.py)
* Problem 039
* [Sol1](project_euler/problem_039/sol1.py)
* Problem 040
* [Sol1](project_euler/problem_040/sol1.py)
* Problem 041
* [Sol1](project_euler/problem_041/sol1.py)
* Problem 042
* [Solution42](project_euler/problem_042/solution42.py)
* Problem 043
* [Sol1](project_euler/problem_043/sol1.py)
* Problem 044
* [Sol1](project_euler/problem_044/sol1.py)
* Problem 045
* [Sol1](project_euler/problem_045/sol1.py)
* Problem 046
* [Sol1](project_euler/problem_046/sol1.py)
* Problem 047
* [Sol1](project_euler/problem_047/sol1.py)
* Problem 048
* [Sol1](project_euler/problem_048/sol1.py)
* Problem 049
* [Sol1](project_euler/problem_049/sol1.py)
* Problem 050
* [Sol1](project_euler/problem_050/sol1.py)
* Problem 051
* [Sol1](project_euler/problem_051/sol1.py)
* Problem 052
* [Sol1](project_euler/problem_052/sol1.py)
* Problem 053
* [Sol1](project_euler/problem_053/sol1.py)
* Problem 054
* [Sol1](project_euler/problem_054/sol1.py)
* [Test Poker Hand](project_euler/problem_054/test_poker_hand.py)
* Problem 055
* [Sol1](project_euler/problem_055/sol1.py)
* Problem 056
* [Sol1](project_euler/problem_056/sol1.py)
* Problem 057
* [Sol1](project_euler/problem_057/sol1.py)
* Problem 058
* [Sol1](project_euler/problem_058/sol1.py)
* Problem 059
* [Sol1](project_euler/problem_059/sol1.py)
* Problem 062
* [Sol1](project_euler/problem_062/sol1.py)
* Problem 063
* [Sol1](project_euler/problem_063/sol1.py)
* Problem 064
* [Sol1](project_euler/problem_064/sol1.py)
* Problem 065
* [Sol1](project_euler/problem_065/sol1.py)
* Problem 067
* [Sol1](project_euler/problem_067/sol1.py)
* [Sol2](project_euler/problem_067/sol2.py)
* Problem 068
* [Sol1](project_euler/problem_068/sol1.py)
* Problem 069
* [Sol1](project_euler/problem_069/sol1.py)
* Problem 070
* [Sol1](project_euler/problem_070/sol1.py)
* Problem 071
* [Sol1](project_euler/problem_071/sol1.py)
* Problem 072
* [Sol1](project_euler/problem_072/sol1.py)
* [Sol2](project_euler/problem_072/sol2.py)
* Problem 074
* [Sol1](project_euler/problem_074/sol1.py)
* [Sol2](project_euler/problem_074/sol2.py)
* Problem 075
* [Sol1](project_euler/problem_075/sol1.py)
* Problem 076
* [Sol1](project_euler/problem_076/sol1.py)
* Problem 077
* [Sol1](project_euler/problem_077/sol1.py)
* Problem 078
* [Sol1](project_euler/problem_078/sol1.py)
* Problem 080
* [Sol1](project_euler/problem_080/sol1.py)
* Problem 081
* [Sol1](project_euler/problem_081/sol1.py)
* Problem 085
* [Sol1](project_euler/problem_085/sol1.py)
* Problem 086
* [Sol1](project_euler/problem_086/sol1.py)
* Problem 087
* [Sol1](project_euler/problem_087/sol1.py)
* Problem 089
* [Sol1](project_euler/problem_089/sol1.py)
* Problem 091
* [Sol1](project_euler/problem_091/sol1.py)
* Problem 092
* [Sol1](project_euler/problem_092/sol1.py)
* Problem 097
* [Sol1](project_euler/problem_097/sol1.py)
* Problem 099
* [Sol1](project_euler/problem_099/sol1.py)
* Problem 101
* [Sol1](project_euler/problem_101/sol1.py)
* Problem 102
* [Sol1](project_euler/problem_102/sol1.py)
* Problem 107
* [Sol1](project_euler/problem_107/sol1.py)
* Problem 109
* [Sol1](project_euler/problem_109/sol1.py)
* Problem 112
* [Sol1](project_euler/problem_112/sol1.py)
* Problem 113
* [Sol1](project_euler/problem_113/sol1.py)
* Problem 114
* [Sol1](project_euler/problem_114/sol1.py)
* Problem 115
* [Sol1](project_euler/problem_115/sol1.py)
* Problem 116
* [Sol1](project_euler/problem_116/sol1.py)
* Problem 119
* [Sol1](project_euler/problem_119/sol1.py)
* Problem 120
* [Sol1](project_euler/problem_120/sol1.py)
* Problem 121
* [Sol1](project_euler/problem_121/sol1.py)
* Problem 123
* [Sol1](project_euler/problem_123/sol1.py)
* Problem 125
* [Sol1](project_euler/problem_125/sol1.py)
* Problem 129
* [Sol1](project_euler/problem_129/sol1.py)
* Problem 135
* [Sol1](project_euler/problem_135/sol1.py)
* Problem 144
* [Sol1](project_euler/problem_144/sol1.py)
* Problem 145
* [Sol1](project_euler/problem_145/sol1.py)
* Problem 173
* [Sol1](project_euler/problem_173/sol1.py)
* Problem 174
* [Sol1](project_euler/problem_174/sol1.py)
* Problem 180
* [Sol1](project_euler/problem_180/sol1.py)
* Problem 188
* [Sol1](project_euler/problem_188/sol1.py)
* Problem 191
* [Sol1](project_euler/problem_191/sol1.py)
* Problem 203
* [Sol1](project_euler/problem_203/sol1.py)
* Problem 205
* [Sol1](project_euler/problem_205/sol1.py)
* Problem 206
* [Sol1](project_euler/problem_206/sol1.py)
* Problem 207
* [Sol1](project_euler/problem_207/sol1.py)
* Problem 234
* [Sol1](project_euler/problem_234/sol1.py)
* Problem 301
* [Sol1](project_euler/problem_301/sol1.py)
* Problem 493
* [Sol1](project_euler/problem_493/sol1.py)
* Problem 551
* [Sol1](project_euler/problem_551/sol1.py)
* Problem 587
* [Sol1](project_euler/problem_587/sol1.py)
* Problem 686
* [Sol1](project_euler/problem_686/sol1.py)
## Quantum
* [Deutsch Jozsa](quantum/deutsch_jozsa.py)
* [Half Adder](quantum/half_adder.py)
* [Not Gate](quantum/not_gate.py)
* [Q Full Adder](quantum/q_full_adder.py)
* [Quantum Entanglement](quantum/quantum_entanglement.py)
* [Ripple Adder Classic](quantum/ripple_adder_classic.py)
* [Single Qubit Measure](quantum/single_qubit_measure.py)
## Scheduling
* [First Come First Served](scheduling/first_come_first_served.py)
* [Highest Response Ratio Next](scheduling/highest_response_ratio_next.py)
* [Job Sequencing With Deadline](scheduling/job_sequencing_with_deadline.py)
* [Multi Level Feedback Queue](scheduling/multi_level_feedback_queue.py)
* [Non Preemptive Shortest Job First](scheduling/non_preemptive_shortest_job_first.py)
* [Round Robin](scheduling/round_robin.py)
* [Shortest Job First](scheduling/shortest_job_first.py)
## Searches
* [Binary Search](searches/binary_search.py)
* [Binary Tree Traversal](searches/binary_tree_traversal.py)
* [Double Linear Search](searches/double_linear_search.py)
* [Double Linear Search Recursion](searches/double_linear_search_recursion.py)
* [Fibonacci Search](searches/fibonacci_search.py)
* [Hill Climbing](searches/hill_climbing.py)
* [Interpolation Search](searches/interpolation_search.py)
* [Jump Search](searches/jump_search.py)
* [Linear Search](searches/linear_search.py)
* [Quick Select](searches/quick_select.py)
* [Sentinel Linear Search](searches/sentinel_linear_search.py)
* [Simple Binary Search](searches/simple_binary_search.py)
* [Simulated Annealing](searches/simulated_annealing.py)
* [Tabu Search](searches/tabu_search.py)
* [Ternary Search](searches/ternary_search.py)
## Sorts
* [Bead Sort](sorts/bead_sort.py)
* [Bitonic Sort](sorts/bitonic_sort.py)
* [Bogo Sort](sorts/bogo_sort.py)
* [Bubble Sort](sorts/bubble_sort.py)
* [Bucket Sort](sorts/bucket_sort.py)
* [Circle Sort](sorts/circle_sort.py)
* [Cocktail Shaker Sort](sorts/cocktail_shaker_sort.py)
* [Comb Sort](sorts/comb_sort.py)
* [Counting Sort](sorts/counting_sort.py)
* [Cycle Sort](sorts/cycle_sort.py)
* [Double Sort](sorts/double_sort.py)
* [Dutch National Flag Sort](sorts/dutch_national_flag_sort.py)
* [Exchange Sort](sorts/exchange_sort.py)
* [External Sort](sorts/external_sort.py)
* [Gnome Sort](sorts/gnome_sort.py)
* [Heap Sort](sorts/heap_sort.py)
* [Insertion Sort](sorts/insertion_sort.py)
* [Intro Sort](sorts/intro_sort.py)
* [Iterative Merge Sort](sorts/iterative_merge_sort.py)
* [Merge Insertion Sort](sorts/merge_insertion_sort.py)
* [Merge Sort](sorts/merge_sort.py)
* [Msd Radix Sort](sorts/msd_radix_sort.py)
* [Natural Sort](sorts/natural_sort.py)
* [Odd Even Sort](sorts/odd_even_sort.py)
* [Odd Even Transposition Parallel](sorts/odd_even_transposition_parallel.py)
* [Odd Even Transposition Single Threaded](sorts/odd_even_transposition_single_threaded.py)
* [Pancake Sort](sorts/pancake_sort.py)
* [Patience Sort](sorts/patience_sort.py)
* [Pigeon Sort](sorts/pigeon_sort.py)
* [Pigeonhole Sort](sorts/pigeonhole_sort.py)
* [Quick Sort](sorts/quick_sort.py)
* [Quick Sort 3 Partition](sorts/quick_sort_3_partition.py)
* [Radix Sort](sorts/radix_sort.py)
* [Random Normal Distribution Quicksort](sorts/random_normal_distribution_quicksort.py)
* [Random Pivot Quick Sort](sorts/random_pivot_quick_sort.py)
* [Recursive Bubble Sort](sorts/recursive_bubble_sort.py)
* [Recursive Insertion Sort](sorts/recursive_insertion_sort.py)
* [Recursive Mergesort Array](sorts/recursive_mergesort_array.py)
* [Recursive Quick Sort](sorts/recursive_quick_sort.py)
* [Selection Sort](sorts/selection_sort.py)
* [Shell Sort](sorts/shell_sort.py)
* [Shrink Shell Sort](sorts/shrink_shell_sort.py)
* [Slowsort](sorts/slowsort.py)
* [Stooge Sort](sorts/stooge_sort.py)
* [Strand Sort](sorts/strand_sort.py)
* [Tim Sort](sorts/tim_sort.py)
* [Topological Sort](sorts/topological_sort.py)
* [Tree Sort](sorts/tree_sort.py)
* [Unknown Sort](sorts/unknown_sort.py)
* [Wiggle Sort](sorts/wiggle_sort.py)
## Strings
* [Aho Corasick](strings/aho_corasick.py)
* [Alternative String Arrange](strings/alternative_string_arrange.py)
* [Anagrams](strings/anagrams.py)
* [Autocomplete Using Trie](strings/autocomplete_using_trie.py)
* [Barcode Validator](strings/barcode_validator.py)
* [Boyer Moore Search](strings/boyer_moore_search.py)
* [Can String Be Rearranged As Palindrome](strings/can_string_be_rearranged_as_palindrome.py)
* [Capitalize](strings/capitalize.py)
* [Check Anagrams](strings/check_anagrams.py)
* [Check Pangram](strings/check_pangram.py)
* [Credit Card Validator](strings/credit_card_validator.py)
* [Detecting English Programmatically](strings/detecting_english_programmatically.py)
* [Dna](strings/dna.py)
* [Frequency Finder](strings/frequency_finder.py)
* [Hamming Distance](strings/hamming_distance.py)
* [Indian Phone Validator](strings/indian_phone_validator.py)
* [Is Contains Unique Chars](strings/is_contains_unique_chars.py)
* [Is Palindrome](strings/is_palindrome.py)
* [Jaro Winkler](strings/jaro_winkler.py)
* [Join](strings/join.py)
* [Knuth Morris Pratt](strings/knuth_morris_pratt.py)
* [Levenshtein Distance](strings/levenshtein_distance.py)
* [Lower](strings/lower.py)
* [Manacher](strings/manacher.py)
* [Min Cost String Conversion](strings/min_cost_string_conversion.py)
* [Naive String Search](strings/naive_string_search.py)
* [Ngram](strings/ngram.py)
* [Palindrome](strings/palindrome.py)
* [Prefix Function](strings/prefix_function.py)
* [Rabin Karp](strings/rabin_karp.py)
* [Remove Duplicate](strings/remove_duplicate.py)
* [Reverse Letters](strings/reverse_letters.py)
* [Reverse Long Words](strings/reverse_long_words.py)
* [Reverse Words](strings/reverse_words.py)
* [Snake Case To Camel Pascal Case](strings/snake_case_to_camel_pascal_case.py)
* [Split](strings/split.py)
* [Upper](strings/upper.py)
* [Wave](strings/wave.py)
* [Wildcard Pattern Matching](strings/wildcard_pattern_matching.py)
* [Word Occurrence](strings/word_occurrence.py)
* [Word Patterns](strings/word_patterns.py)
* [Z Function](strings/z_function.py)
## Web Programming
* [Co2 Emission](web_programming/co2_emission.py)
* [Covid Stats Via Xpath](web_programming/covid_stats_via_xpath.py)
* [Crawl Google Results](web_programming/crawl_google_results.py)
* [Crawl Google Scholar Citation](web_programming/crawl_google_scholar_citation.py)
* [Currency Converter](web_programming/currency_converter.py)
* [Current Stock Price](web_programming/current_stock_price.py)
* [Current Weather](web_programming/current_weather.py)
* [Daily Horoscope](web_programming/daily_horoscope.py)
* [Download Images From Google Query](web_programming/download_images_from_google_query.py)
* [Emails From Url](web_programming/emails_from_url.py)
* [Fetch Anime And Play](web_programming/fetch_anime_and_play.py)
* [Fetch Bbc News](web_programming/fetch_bbc_news.py)
* [Fetch Github Info](web_programming/fetch_github_info.py)
* [Fetch Jobs](web_programming/fetch_jobs.py)
* [Fetch Quotes](web_programming/fetch_quotes.py)
* [Fetch Well Rx Price](web_programming/fetch_well_rx_price.py)
* [Get Imdb Top 250 Movies Csv](web_programming/get_imdb_top_250_movies_csv.py)
* [Get Imdbtop](web_programming/get_imdbtop.py)
* [Get Top Hn Posts](web_programming/get_top_hn_posts.py)
* [Get User Tweets](web_programming/get_user_tweets.py)
* [Giphy](web_programming/giphy.py)
* [Instagram Crawler](web_programming/instagram_crawler.py)
* [Instagram Pic](web_programming/instagram_pic.py)
* [Instagram Video](web_programming/instagram_video.py)
* [Nasa Data](web_programming/nasa_data.py)
* [Open Google Results](web_programming/open_google_results.py)
* [Random Anime Character](web_programming/random_anime_character.py)
* [Recaptcha Verification](web_programming/recaptcha_verification.py)
* [Reddit](web_programming/reddit.py)
* [Search Books By Isbn](web_programming/search_books_by_isbn.py)
* [Slack Message](web_programming/slack_message.py)
* [Test Fetch Github Info](web_programming/test_fetch_github_info.py)
* [World Covid19 Stats](web_programming/world_covid19_stats.py)
|
## Arithmetic Analysis
* [Bisection](arithmetic_analysis/bisection.py)
* [Gaussian Elimination](arithmetic_analysis/gaussian_elimination.py)
* [In Static Equilibrium](arithmetic_analysis/in_static_equilibrium.py)
* [Intersection](arithmetic_analysis/intersection.py)
* [Jacobi Iteration Method](arithmetic_analysis/jacobi_iteration_method.py)
* [Lu Decomposition](arithmetic_analysis/lu_decomposition.py)
* [Newton Forward Interpolation](arithmetic_analysis/newton_forward_interpolation.py)
* [Newton Method](arithmetic_analysis/newton_method.py)
* [Newton Raphson](arithmetic_analysis/newton_raphson.py)
* [Newton Raphson New](arithmetic_analysis/newton_raphson_new.py)
* [Secant Method](arithmetic_analysis/secant_method.py)
## Audio Filters
* [Butterworth Filter](audio_filters/butterworth_filter.py)
* [Equal Loudness Filter](audio_filters/equal_loudness_filter.py)
* [Iir Filter](audio_filters/iir_filter.py)
* [Show Response](audio_filters/show_response.py)
## Backtracking
* [All Combinations](backtracking/all_combinations.py)
* [All Permutations](backtracking/all_permutations.py)
* [All Subsequences](backtracking/all_subsequences.py)
* [Coloring](backtracking/coloring.py)
* [Combination Sum](backtracking/combination_sum.py)
* [Hamiltonian Cycle](backtracking/hamiltonian_cycle.py)
* [Knight Tour](backtracking/knight_tour.py)
* [Minimax](backtracking/minimax.py)
* [Minmax](backtracking/minmax.py)
* [N Queens](backtracking/n_queens.py)
* [N Queens Math](backtracking/n_queens_math.py)
* [Rat In Maze](backtracking/rat_in_maze.py)
* [Sudoku](backtracking/sudoku.py)
* [Sum Of Subsets](backtracking/sum_of_subsets.py)
## Bit Manipulation
* [Binary And Operator](bit_manipulation/binary_and_operator.py)
* [Binary Count Setbits](bit_manipulation/binary_count_setbits.py)
* [Binary Count Trailing Zeros](bit_manipulation/binary_count_trailing_zeros.py)
* [Binary Or Operator](bit_manipulation/binary_or_operator.py)
* [Binary Shifts](bit_manipulation/binary_shifts.py)
* [Binary Twos Complement](bit_manipulation/binary_twos_complement.py)
* [Binary Xor Operator](bit_manipulation/binary_xor_operator.py)
* [Count 1S Brian Kernighan Method](bit_manipulation/count_1s_brian_kernighan_method.py)
* [Count Number Of One Bits](bit_manipulation/count_number_of_one_bits.py)
* [Gray Code Sequence](bit_manipulation/gray_code_sequence.py)
* [Reverse Bits](bit_manipulation/reverse_bits.py)
* [Single Bit Manipulation Operations](bit_manipulation/single_bit_manipulation_operations.py)
## Blockchain
* [Chinese Remainder Theorem](blockchain/chinese_remainder_theorem.py)
* [Diophantine Equation](blockchain/diophantine_equation.py)
* [Modular Division](blockchain/modular_division.py)
## Boolean Algebra
* [Norgate](boolean_algebra/norgate.py)
* [Quine Mc Cluskey](boolean_algebra/quine_mc_cluskey.py)
## Cellular Automata
* [Conways Game Of Life](cellular_automata/conways_game_of_life.py)
* [Game Of Life](cellular_automata/game_of_life.py)
* [Nagel Schrekenberg](cellular_automata/nagel_schrekenberg.py)
* [One Dimensional](cellular_automata/one_dimensional.py)
## Ciphers
* [A1Z26](ciphers/a1z26.py)
* [Affine Cipher](ciphers/affine_cipher.py)
* [Atbash](ciphers/atbash.py)
* [Baconian Cipher](ciphers/baconian_cipher.py)
* [Base16](ciphers/base16.py)
* [Base32](ciphers/base32.py)
* [Base64](ciphers/base64.py)
* [Base85](ciphers/base85.py)
* [Beaufort Cipher](ciphers/beaufort_cipher.py)
* [Bifid](ciphers/bifid.py)
* [Brute Force Caesar Cipher](ciphers/brute_force_caesar_cipher.py)
* [Caesar Cipher](ciphers/caesar_cipher.py)
* [Cryptomath Module](ciphers/cryptomath_module.py)
* [Decrypt Caesar With Chi Squared](ciphers/decrypt_caesar_with_chi_squared.py)
* [Deterministic Miller Rabin](ciphers/deterministic_miller_rabin.py)
* [Diffie](ciphers/diffie.py)
* [Diffie Hellman](ciphers/diffie_hellman.py)
* [Elgamal Key Generator](ciphers/elgamal_key_generator.py)
* [Enigma Machine2](ciphers/enigma_machine2.py)
* [Hill Cipher](ciphers/hill_cipher.py)
* [Mixed Keyword Cypher](ciphers/mixed_keyword_cypher.py)
* [Mono Alphabetic Ciphers](ciphers/mono_alphabetic_ciphers.py)
* [Morse Code](ciphers/morse_code.py)
* [Onepad Cipher](ciphers/onepad_cipher.py)
* [Playfair Cipher](ciphers/playfair_cipher.py)
* [Polybius](ciphers/polybius.py)
* [Porta Cipher](ciphers/porta_cipher.py)
* [Rabin Miller](ciphers/rabin_miller.py)
* [Rail Fence Cipher](ciphers/rail_fence_cipher.py)
* [Rot13](ciphers/rot13.py)
* [Rsa Cipher](ciphers/rsa_cipher.py)
* [Rsa Factorization](ciphers/rsa_factorization.py)
* [Rsa Key Generator](ciphers/rsa_key_generator.py)
* [Shuffled Shift Cipher](ciphers/shuffled_shift_cipher.py)
* [Simple Keyword Cypher](ciphers/simple_keyword_cypher.py)
* [Simple Substitution Cipher](ciphers/simple_substitution_cipher.py)
* [Trafid Cipher](ciphers/trafid_cipher.py)
* [Transposition Cipher](ciphers/transposition_cipher.py)
* [Transposition Cipher Encrypt Decrypt File](ciphers/transposition_cipher_encrypt_decrypt_file.py)
* [Vigenere Cipher](ciphers/vigenere_cipher.py)
* [Xor Cipher](ciphers/xor_cipher.py)
## Compression
* [Burrows Wheeler](compression/burrows_wheeler.py)
* [Huffman](compression/huffman.py)
* [Lempel Ziv](compression/lempel_ziv.py)
* [Lempel Ziv Decompress](compression/lempel_ziv_decompress.py)
* [Peak Signal To Noise Ratio](compression/peak_signal_to_noise_ratio.py)
* [Run Length Encoding](compression/run_length_encoding.py)
## Computer Vision
* [Cnn Classification](computer_vision/cnn_classification.py)
* [Flip Augmentation](computer_vision/flip_augmentation.py)
* [Harris Corner](computer_vision/harris_corner.py)
* [Horn Schunck](computer_vision/horn_schunck.py)
* [Mean Threshold](computer_vision/mean_threshold.py)
* [Mosaic Augmentation](computer_vision/mosaic_augmentation.py)
* [Pooling Functions](computer_vision/pooling_functions.py)
## Conversions
* [Astronomical Length Scale Conversion](conversions/astronomical_length_scale_conversion.py)
* [Binary To Decimal](conversions/binary_to_decimal.py)
* [Binary To Hexadecimal](conversions/binary_to_hexadecimal.py)
* [Binary To Octal](conversions/binary_to_octal.py)
* [Decimal To Any](conversions/decimal_to_any.py)
* [Decimal To Binary](conversions/decimal_to_binary.py)
* [Decimal To Binary Recursion](conversions/decimal_to_binary_recursion.py)
* [Decimal To Hexadecimal](conversions/decimal_to_hexadecimal.py)
* [Decimal To Octal](conversions/decimal_to_octal.py)
* [Excel Title To Column](conversions/excel_title_to_column.py)
* [Hex To Bin](conversions/hex_to_bin.py)
* [Hexadecimal To Decimal](conversions/hexadecimal_to_decimal.py)
* [Length Conversion](conversions/length_conversion.py)
* [Molecular Chemistry](conversions/molecular_chemistry.py)
* [Octal To Decimal](conversions/octal_to_decimal.py)
* [Prefix Conversions](conversions/prefix_conversions.py)
* [Prefix Conversions String](conversions/prefix_conversions_string.py)
* [Pressure Conversions](conversions/pressure_conversions.py)
* [Rgb Hsv Conversion](conversions/rgb_hsv_conversion.py)
* [Roman Numerals](conversions/roman_numerals.py)
* [Speed Conversions](conversions/speed_conversions.py)
* [Temperature Conversions](conversions/temperature_conversions.py)
* [Volume Conversions](conversions/volume_conversions.py)
* [Weight Conversion](conversions/weight_conversion.py)
## Data Structures
* Binary Tree
* [Avl Tree](data_structures/binary_tree/avl_tree.py)
* [Basic Binary Tree](data_structures/binary_tree/basic_binary_tree.py)
* [Binary Search Tree](data_structures/binary_tree/binary_search_tree.py)
* [Binary Search Tree Recursive](data_structures/binary_tree/binary_search_tree_recursive.py)
* [Binary Tree Mirror](data_structures/binary_tree/binary_tree_mirror.py)
* [Binary Tree Node Sum](data_structures/binary_tree/binary_tree_node_sum.py)
* [Binary Tree Traversals](data_structures/binary_tree/binary_tree_traversals.py)
* [Diff Views Of Binary Tree](data_structures/binary_tree/diff_views_of_binary_tree.py)
* [Fenwick Tree](data_structures/binary_tree/fenwick_tree.py)
* [Inorder Tree Traversal 2022](data_structures/binary_tree/inorder_tree_traversal_2022.py)
* [Lazy Segment Tree](data_structures/binary_tree/lazy_segment_tree.py)
* [Lowest Common Ancestor](data_structures/binary_tree/lowest_common_ancestor.py)
* [Maximum Fenwick Tree](data_structures/binary_tree/maximum_fenwick_tree.py)
* [Merge Two Binary Trees](data_structures/binary_tree/merge_two_binary_trees.py)
* [Non Recursive Segment Tree](data_structures/binary_tree/non_recursive_segment_tree.py)
* [Number Of Possible Binary Trees](data_structures/binary_tree/number_of_possible_binary_trees.py)
* [Red Black Tree](data_structures/binary_tree/red_black_tree.py)
* [Segment Tree](data_structures/binary_tree/segment_tree.py)
* [Segment Tree Other](data_structures/binary_tree/segment_tree_other.py)
* [Treap](data_structures/binary_tree/treap.py)
* [Wavelet Tree](data_structures/binary_tree/wavelet_tree.py)
* Disjoint Set
* [Alternate Disjoint Set](data_structures/disjoint_set/alternate_disjoint_set.py)
* [Disjoint Set](data_structures/disjoint_set/disjoint_set.py)
* Hashing
* [Double Hash](data_structures/hashing/double_hash.py)
* [Hash Table](data_structures/hashing/hash_table.py)
* [Hash Table With Linked List](data_structures/hashing/hash_table_with_linked_list.py)
* Number Theory
* [Prime Numbers](data_structures/hashing/number_theory/prime_numbers.py)
* [Quadratic Probing](data_structures/hashing/quadratic_probing.py)
* Heap
* [Binomial Heap](data_structures/heap/binomial_heap.py)
* [Heap](data_structures/heap/heap.py)
* [Heap Generic](data_structures/heap/heap_generic.py)
* [Max Heap](data_structures/heap/max_heap.py)
* [Min Heap](data_structures/heap/min_heap.py)
* [Randomized Heap](data_structures/heap/randomized_heap.py)
* [Skew Heap](data_structures/heap/skew_heap.py)
* Linked List
* [Circular Linked List](data_structures/linked_list/circular_linked_list.py)
* [Deque Doubly](data_structures/linked_list/deque_doubly.py)
* [Doubly Linked List](data_structures/linked_list/doubly_linked_list.py)
* [Doubly Linked List Two](data_structures/linked_list/doubly_linked_list_two.py)
* [From Sequence](data_structures/linked_list/from_sequence.py)
* [Has Loop](data_structures/linked_list/has_loop.py)
* [Is Palindrome](data_structures/linked_list/is_palindrome.py)
* [Merge Two Lists](data_structures/linked_list/merge_two_lists.py)
* [Middle Element Of Linked List](data_structures/linked_list/middle_element_of_linked_list.py)
* [Print Reverse](data_structures/linked_list/print_reverse.py)
* [Singly Linked List](data_structures/linked_list/singly_linked_list.py)
* [Skip List](data_structures/linked_list/skip_list.py)
* [Swap Nodes](data_structures/linked_list/swap_nodes.py)
* Queue
* [Circular Queue](data_structures/queue/circular_queue.py)
* [Circular Queue Linked List](data_structures/queue/circular_queue_linked_list.py)
* [Double Ended Queue](data_structures/queue/double_ended_queue.py)
* [Linked Queue](data_structures/queue/linked_queue.py)
* [Priority Queue Using List](data_structures/queue/priority_queue_using_list.py)
* [Queue On List](data_structures/queue/queue_on_list.py)
* [Queue On Pseudo Stack](data_structures/queue/queue_on_pseudo_stack.py)
* Stacks
* [Balanced Parentheses](data_structures/stacks/balanced_parentheses.py)
* [Dijkstras Two Stack Algorithm](data_structures/stacks/dijkstras_two_stack_algorithm.py)
* [Evaluate Postfix Notations](data_structures/stacks/evaluate_postfix_notations.py)
* [Infix To Postfix Conversion](data_structures/stacks/infix_to_postfix_conversion.py)
* [Infix To Prefix Conversion](data_structures/stacks/infix_to_prefix_conversion.py)
* [Next Greater Element](data_structures/stacks/next_greater_element.py)
* [Postfix Evaluation](data_structures/stacks/postfix_evaluation.py)
* [Prefix Evaluation](data_structures/stacks/prefix_evaluation.py)
* [Stack](data_structures/stacks/stack.py)
* [Stack With Doubly Linked List](data_structures/stacks/stack_with_doubly_linked_list.py)
* [Stack With Singly Linked List](data_structures/stacks/stack_with_singly_linked_list.py)
* [Stock Span Problem](data_structures/stacks/stock_span_problem.py)
* Trie
* [Trie](data_structures/trie/trie.py)
## Digital Image Processing
* [Change Brightness](digital_image_processing/change_brightness.py)
* [Change Contrast](digital_image_processing/change_contrast.py)
* [Convert To Negative](digital_image_processing/convert_to_negative.py)
* Dithering
* [Burkes](digital_image_processing/dithering/burkes.py)
* Edge Detection
* [Canny](digital_image_processing/edge_detection/canny.py)
* Filters
* [Bilateral Filter](digital_image_processing/filters/bilateral_filter.py)
* [Convolve](digital_image_processing/filters/convolve.py)
* [Gabor Filter](digital_image_processing/filters/gabor_filter.py)
* [Gaussian Filter](digital_image_processing/filters/gaussian_filter.py)
* [Local Binary Pattern](digital_image_processing/filters/local_binary_pattern.py)
* [Median Filter](digital_image_processing/filters/median_filter.py)
* [Sobel Filter](digital_image_processing/filters/sobel_filter.py)
* Histogram Equalization
* [Histogram Stretch](digital_image_processing/histogram_equalization/histogram_stretch.py)
* [Index Calculation](digital_image_processing/index_calculation.py)
* Morphological Operations
* [Dilation Operation](digital_image_processing/morphological_operations/dilation_operation.py)
* [Erosion Operation](digital_image_processing/morphological_operations/erosion_operation.py)
* Resize
* [Resize](digital_image_processing/resize/resize.py)
* Rotation
* [Rotation](digital_image_processing/rotation/rotation.py)
* [Sepia](digital_image_processing/sepia.py)
* [Test Digital Image Processing](digital_image_processing/test_digital_image_processing.py)
## Divide And Conquer
* [Closest Pair Of Points](divide_and_conquer/closest_pair_of_points.py)
* [Convex Hull](divide_and_conquer/convex_hull.py)
* [Heaps Algorithm](divide_and_conquer/heaps_algorithm.py)
* [Heaps Algorithm Iterative](divide_and_conquer/heaps_algorithm_iterative.py)
* [Inversions](divide_and_conquer/inversions.py)
* [Kth Order Statistic](divide_and_conquer/kth_order_statistic.py)
* [Max Difference Pair](divide_and_conquer/max_difference_pair.py)
* [Max Subarray Sum](divide_and_conquer/max_subarray_sum.py)
* [Mergesort](divide_and_conquer/mergesort.py)
* [Peak](divide_and_conquer/peak.py)
* [Power](divide_and_conquer/power.py)
* [Strassen Matrix Multiplication](divide_and_conquer/strassen_matrix_multiplication.py)
## Dynamic Programming
* [Abbreviation](dynamic_programming/abbreviation.py)
* [All Construct](dynamic_programming/all_construct.py)
* [Bitmask](dynamic_programming/bitmask.py)
* [Catalan Numbers](dynamic_programming/catalan_numbers.py)
* [Climbing Stairs](dynamic_programming/climbing_stairs.py)
* [Edit Distance](dynamic_programming/edit_distance.py)
* [Factorial](dynamic_programming/factorial.py)
* [Fast Fibonacci](dynamic_programming/fast_fibonacci.py)
* [Fibonacci](dynamic_programming/fibonacci.py)
* [Floyd Warshall](dynamic_programming/floyd_warshall.py)
* [Integer Partition](dynamic_programming/integer_partition.py)
* [Iterating Through Submasks](dynamic_programming/iterating_through_submasks.py)
* [Knapsack](dynamic_programming/knapsack.py)
* [Longest Common Subsequence](dynamic_programming/longest_common_subsequence.py)
* [Longest Increasing Subsequence](dynamic_programming/longest_increasing_subsequence.py)
* [Longest Increasing Subsequence O(Nlogn)](dynamic_programming/longest_increasing_subsequence_o(nlogn).py)
* [Longest Sub Array](dynamic_programming/longest_sub_array.py)
* [Matrix Chain Order](dynamic_programming/matrix_chain_order.py)
* [Max Non Adjacent Sum](dynamic_programming/max_non_adjacent_sum.py)
* [Max Sub Array](dynamic_programming/max_sub_array.py)
* [Max Sum Contiguous Subsequence](dynamic_programming/max_sum_contiguous_subsequence.py)
* [Minimum Coin Change](dynamic_programming/minimum_coin_change.py)
* [Minimum Cost Path](dynamic_programming/minimum_cost_path.py)
* [Minimum Partition](dynamic_programming/minimum_partition.py)
* [Minimum Steps To One](dynamic_programming/minimum_steps_to_one.py)
* [Optimal Binary Search Tree](dynamic_programming/optimal_binary_search_tree.py)
* [Rod Cutting](dynamic_programming/rod_cutting.py)
* [Subset Generation](dynamic_programming/subset_generation.py)
* [Sum Of Subset](dynamic_programming/sum_of_subset.py)
## Electronics
* [Carrier Concentration](electronics/carrier_concentration.py)
* [Coulombs Law](electronics/coulombs_law.py)
* [Electric Power](electronics/electric_power.py)
* [Ohms Law](electronics/ohms_law.py)
## File Transfer
* [Receive File](file_transfer/receive_file.py)
* [Send File](file_transfer/send_file.py)
* Tests
* [Test Send File](file_transfer/tests/test_send_file.py)
## Financial
* [Equated Monthly Installments](financial/equated_monthly_installments.py)
* [Interest](financial/interest.py)
## Fractals
* [Julia Sets](fractals/julia_sets.py)
* [Koch Snowflake](fractals/koch_snowflake.py)
* [Mandelbrot](fractals/mandelbrot.py)
* [Sierpinski Triangle](fractals/sierpinski_triangle.py)
## Fuzzy Logic
* [Fuzzy Operations](fuzzy_logic/fuzzy_operations.py)
## Genetic Algorithm
* [Basic String](genetic_algorithm/basic_string.py)
## Geodesy
* [Haversine Distance](geodesy/haversine_distance.py)
* [Lamberts Ellipsoidal Distance](geodesy/lamberts_ellipsoidal_distance.py)
## Graphics
* [Bezier Curve](graphics/bezier_curve.py)
* [Vector3 For 2D Rendering](graphics/vector3_for_2d_rendering.py)
## Graphs
* [A Star](graphs/a_star.py)
* [Articulation Points](graphs/articulation_points.py)
* [Basic Graphs](graphs/basic_graphs.py)
* [Bellman Ford](graphs/bellman_ford.py)
* [Bfs Shortest Path](graphs/bfs_shortest_path.py)
* [Bfs Zero One Shortest Path](graphs/bfs_zero_one_shortest_path.py)
* [Bidirectional A Star](graphs/bidirectional_a_star.py)
* [Bidirectional Breadth First Search](graphs/bidirectional_breadth_first_search.py)
* [Boruvka](graphs/boruvka.py)
* [Breadth First Search](graphs/breadth_first_search.py)
* [Breadth First Search 2](graphs/breadth_first_search_2.py)
* [Breadth First Search Shortest Path](graphs/breadth_first_search_shortest_path.py)
* [Check Bipartite Graph Bfs](graphs/check_bipartite_graph_bfs.py)
* [Check Bipartite Graph Dfs](graphs/check_bipartite_graph_dfs.py)
* [Check Cycle](graphs/check_cycle.py)
* [Connected Components](graphs/connected_components.py)
* [Depth First Search](graphs/depth_first_search.py)
* [Depth First Search 2](graphs/depth_first_search_2.py)
* [Dijkstra](graphs/dijkstra.py)
* [Dijkstra 2](graphs/dijkstra_2.py)
* [Dijkstra Algorithm](graphs/dijkstra_algorithm.py)
* [Dinic](graphs/dinic.py)
* [Directed And Undirected (Weighted) Graph](graphs/directed_and_undirected_(weighted)_graph.py)
* [Edmonds Karp Multiple Source And Sink](graphs/edmonds_karp_multiple_source_and_sink.py)
* [Eulerian Path And Circuit For Undirected Graph](graphs/eulerian_path_and_circuit_for_undirected_graph.py)
* [Even Tree](graphs/even_tree.py)
* [Finding Bridges](graphs/finding_bridges.py)
* [Frequent Pattern Graph Miner](graphs/frequent_pattern_graph_miner.py)
* [G Topological Sort](graphs/g_topological_sort.py)
* [Gale Shapley Bigraph](graphs/gale_shapley_bigraph.py)
* [Graph List](graphs/graph_list.py)
* [Graph Matrix](graphs/graph_matrix.py)
* [Graphs Floyd Warshall](graphs/graphs_floyd_warshall.py)
* [Greedy Best First](graphs/greedy_best_first.py)
* [Greedy Min Vertex Cover](graphs/greedy_min_vertex_cover.py)
* [Kahns Algorithm Long](graphs/kahns_algorithm_long.py)
* [Kahns Algorithm Topo](graphs/kahns_algorithm_topo.py)
* [Karger](graphs/karger.py)
* [Markov Chain](graphs/markov_chain.py)
* [Matching Min Vertex Cover](graphs/matching_min_vertex_cover.py)
* [Minimum Path Sum](graphs/minimum_path_sum.py)
* [Minimum Spanning Tree Boruvka](graphs/minimum_spanning_tree_boruvka.py)
* [Minimum Spanning Tree Kruskal](graphs/minimum_spanning_tree_kruskal.py)
* [Minimum Spanning Tree Kruskal2](graphs/minimum_spanning_tree_kruskal2.py)
* [Minimum Spanning Tree Prims](graphs/minimum_spanning_tree_prims.py)
* [Minimum Spanning Tree Prims2](graphs/minimum_spanning_tree_prims2.py)
* [Multi Heuristic Astar](graphs/multi_heuristic_astar.py)
* [Page Rank](graphs/page_rank.py)
* [Prim](graphs/prim.py)
* [Random Graph Generator](graphs/random_graph_generator.py)
* [Scc Kosaraju](graphs/scc_kosaraju.py)
* [Strongly Connected Components](graphs/strongly_connected_components.py)
* [Tarjans Scc](graphs/tarjans_scc.py)
* Tests
* [Test Min Spanning Tree Kruskal](graphs/tests/test_min_spanning_tree_kruskal.py)
* [Test Min Spanning Tree Prim](graphs/tests/test_min_spanning_tree_prim.py)
## Greedy Methods
* [Fractional Knapsack](greedy_methods/fractional_knapsack.py)
* [Fractional Knapsack 2](greedy_methods/fractional_knapsack_2.py)
* [Optimal Merge Pattern](greedy_methods/optimal_merge_pattern.py)
## Hashes
* [Adler32](hashes/adler32.py)
* [Chaos Machine](hashes/chaos_machine.py)
* [Djb2](hashes/djb2.py)
* [Enigma Machine](hashes/enigma_machine.py)
* [Hamming Code](hashes/hamming_code.py)
* [Luhn](hashes/luhn.py)
* [Md5](hashes/md5.py)
* [Sdbm](hashes/sdbm.py)
* [Sha1](hashes/sha1.py)
* [Sha256](hashes/sha256.py)
## Knapsack
* [Greedy Knapsack](knapsack/greedy_knapsack.py)
* [Knapsack](knapsack/knapsack.py)
* Tests
* [Test Greedy Knapsack](knapsack/tests/test_greedy_knapsack.py)
* [Test Knapsack](knapsack/tests/test_knapsack.py)
## Linear Algebra
* Src
* [Conjugate Gradient](linear_algebra/src/conjugate_gradient.py)
* [Lib](linear_algebra/src/lib.py)
* [Polynom For Points](linear_algebra/src/polynom_for_points.py)
* [Power Iteration](linear_algebra/src/power_iteration.py)
* [Rayleigh Quotient](linear_algebra/src/rayleigh_quotient.py)
* [Schur Complement](linear_algebra/src/schur_complement.py)
* [Test Linear Algebra](linear_algebra/src/test_linear_algebra.py)
* [Transformations 2D](linear_algebra/src/transformations_2d.py)
## Machine Learning
* [Astar](machine_learning/astar.py)
* [Data Transformations](machine_learning/data_transformations.py)
* [Decision Tree](machine_learning/decision_tree.py)
* Forecasting
* [Run](machine_learning/forecasting/run.py)
* [Gaussian Naive Bayes](machine_learning/gaussian_naive_bayes.py)
* [Gradient Boosting Regressor](machine_learning/gradient_boosting_regressor.py)
* [Gradient Descent](machine_learning/gradient_descent.py)
* [K Means Clust](machine_learning/k_means_clust.py)
* [K Nearest Neighbours](machine_learning/k_nearest_neighbours.py)
* [Knn Sklearn](machine_learning/knn_sklearn.py)
* [Linear Discriminant Analysis](machine_learning/linear_discriminant_analysis.py)
* [Linear Regression](machine_learning/linear_regression.py)
* Local Weighted Learning
* [Local Weighted Learning](machine_learning/local_weighted_learning/local_weighted_learning.py)
* [Logistic Regression](machine_learning/logistic_regression.py)
* Lstm
* [Lstm Prediction](machine_learning/lstm/lstm_prediction.py)
* [Multilayer Perceptron Classifier](machine_learning/multilayer_perceptron_classifier.py)
* [Polymonial Regression](machine_learning/polymonial_regression.py)
* [Random Forest Classifier](machine_learning/random_forest_classifier.py)
* [Random Forest Regressor](machine_learning/random_forest_regressor.py)
* [Scoring Functions](machine_learning/scoring_functions.py)
* [Self Organizing Map](machine_learning/self_organizing_map.py)
* [Sequential Minimum Optimization](machine_learning/sequential_minimum_optimization.py)
* [Similarity Search](machine_learning/similarity_search.py)
* [Support Vector Machines](machine_learning/support_vector_machines.py)
* [Word Frequency Functions](machine_learning/word_frequency_functions.py)
## Maths
* [3N Plus 1](maths/3n_plus_1.py)
* [Abs](maths/abs.py)
* [Abs Max](maths/abs_max.py)
* [Abs Min](maths/abs_min.py)
* [Add](maths/add.py)
* [Aliquot Sum](maths/aliquot_sum.py)
* [Allocation Number](maths/allocation_number.py)
* [Area](maths/area.py)
* [Area Under Curve](maths/area_under_curve.py)
* [Armstrong Numbers](maths/armstrong_numbers.py)
* [Average Absolute Deviation](maths/average_absolute_deviation.py)
* [Average Mean](maths/average_mean.py)
* [Average Median](maths/average_median.py)
* [Average Mode](maths/average_mode.py)
* [Bailey Borwein Plouffe](maths/bailey_borwein_plouffe.py)
* [Basic Maths](maths/basic_maths.py)
* [Binary Exp Mod](maths/binary_exp_mod.py)
* [Binary Exponentiation](maths/binary_exponentiation.py)
* [Binary Exponentiation 2](maths/binary_exponentiation_2.py)
* [Binary Exponentiation 3](maths/binary_exponentiation_3.py)
* [Binomial Coefficient](maths/binomial_coefficient.py)
* [Binomial Distribution](maths/binomial_distribution.py)
* [Bisection](maths/bisection.py)
* [Carmichael Number](maths/carmichael_number.py)
* [Catalan Number](maths/catalan_number.py)
* [Ceil](maths/ceil.py)
* [Check Polygon](maths/check_polygon.py)
* [Chudnovsky Algorithm](maths/chudnovsky_algorithm.py)
* [Collatz Sequence](maths/collatz_sequence.py)
* [Combinations](maths/combinations.py)
* [Decimal Isolate](maths/decimal_isolate.py)
* [Double Factorial Iterative](maths/double_factorial_iterative.py)
* [Double Factorial Recursive](maths/double_factorial_recursive.py)
* [Entropy](maths/entropy.py)
* [Euclidean Distance](maths/euclidean_distance.py)
* [Euclidean Gcd](maths/euclidean_gcd.py)
* [Euler Method](maths/euler_method.py)
* [Euler Modified](maths/euler_modified.py)
* [Eulers Totient](maths/eulers_totient.py)
* [Extended Euclidean Algorithm](maths/extended_euclidean_algorithm.py)
* [Factorial Iterative](maths/factorial_iterative.py)
* [Factorial Recursive](maths/factorial_recursive.py)
* [Factors](maths/factors.py)
* [Fermat Little Theorem](maths/fermat_little_theorem.py)
* [Fibonacci](maths/fibonacci.py)
* [Find Max](maths/find_max.py)
* [Find Max Recursion](maths/find_max_recursion.py)
* [Find Min](maths/find_min.py)
* [Find Min Recursion](maths/find_min_recursion.py)
* [Floor](maths/floor.py)
* [Gamma](maths/gamma.py)
* [Gamma Recursive](maths/gamma_recursive.py)
* [Gaussian](maths/gaussian.py)
* [Gaussian Error Linear Unit](maths/gaussian_error_linear_unit.py)
* [Greatest Common Divisor](maths/greatest_common_divisor.py)
* [Greedy Coin Change](maths/greedy_coin_change.py)
* [Hamming Numbers](maths/hamming_numbers.py)
* [Hardy Ramanujanalgo](maths/hardy_ramanujanalgo.py)
* [Integration By Simpson Approx](maths/integration_by_simpson_approx.py)
* [Is Ip V4 Address Valid](maths/is_ip_v4_address_valid.py)
* [Is Square Free](maths/is_square_free.py)
* [Jaccard Similarity](maths/jaccard_similarity.py)
* [Kadanes](maths/kadanes.py)
* [Karatsuba](maths/karatsuba.py)
* [Krishnamurthy Number](maths/krishnamurthy_number.py)
* [Kth Lexicographic Permutation](maths/kth_lexicographic_permutation.py)
* [Largest Of Very Large Numbers](maths/largest_of_very_large_numbers.py)
* [Largest Subarray Sum](maths/largest_subarray_sum.py)
* [Least Common Multiple](maths/least_common_multiple.py)
* [Line Length](maths/line_length.py)
* [Lucas Lehmer Primality Test](maths/lucas_lehmer_primality_test.py)
* [Lucas Series](maths/lucas_series.py)
* [Matrix Exponentiation](maths/matrix_exponentiation.py)
* [Max Sum Sliding Window](maths/max_sum_sliding_window.py)
* [Median Of Two Arrays](maths/median_of_two_arrays.py)
* [Miller Rabin](maths/miller_rabin.py)
* [Mobius Function](maths/mobius_function.py)
* [Modular Exponential](maths/modular_exponential.py)
* [Monte Carlo](maths/monte_carlo.py)
* [Monte Carlo Dice](maths/monte_carlo_dice.py)
* [Nevilles Method](maths/nevilles_method.py)
* [Newton Raphson](maths/newton_raphson.py)
* [Number Of Digits](maths/number_of_digits.py)
* [Numerical Integration](maths/numerical_integration.py)
* [Perfect Cube](maths/perfect_cube.py)
* [Perfect Number](maths/perfect_number.py)
* [Perfect Square](maths/perfect_square.py)
* [Persistence](maths/persistence.py)
* [Pi Monte Carlo Estimation](maths/pi_monte_carlo_estimation.py)
* [Points Are Collinear 3D](maths/points_are_collinear_3d.py)
* [Pollard Rho](maths/pollard_rho.py)
* [Polynomial Evaluation](maths/polynomial_evaluation.py)
* [Power Using Recursion](maths/power_using_recursion.py)
* [Prime Check](maths/prime_check.py)
* [Prime Factors](maths/prime_factors.py)
* [Prime Numbers](maths/prime_numbers.py)
* [Prime Sieve Eratosthenes](maths/prime_sieve_eratosthenes.py)
* [Primelib](maths/primelib.py)
* [Proth Number](maths/proth_number.py)
* [Pythagoras](maths/pythagoras.py)
* [Qr Decomposition](maths/qr_decomposition.py)
* [Quadratic Equations Complex Numbers](maths/quadratic_equations_complex_numbers.py)
* [Radians](maths/radians.py)
* [Radix2 Fft](maths/radix2_fft.py)
* [Relu](maths/relu.py)
* [Runge Kutta](maths/runge_kutta.py)
* [Segmented Sieve](maths/segmented_sieve.py)
* Series
* [Arithmetic](maths/series/arithmetic.py)
* [Geometric](maths/series/geometric.py)
* [Geometric Series](maths/series/geometric_series.py)
* [Harmonic](maths/series/harmonic.py)
* [Harmonic Series](maths/series/harmonic_series.py)
* [Hexagonal Numbers](maths/series/hexagonal_numbers.py)
* [P Series](maths/series/p_series.py)
* [Sieve Of Eratosthenes](maths/sieve_of_eratosthenes.py)
* [Sigmoid](maths/sigmoid.py)
* [Simpson Rule](maths/simpson_rule.py)
* [Sin](maths/sin.py)
* [Sock Merchant](maths/sock_merchant.py)
* [Softmax](maths/softmax.py)
* [Square Root](maths/square_root.py)
* [Sum Of Arithmetic Series](maths/sum_of_arithmetic_series.py)
* [Sum Of Digits](maths/sum_of_digits.py)
* [Sum Of Geometric Progression](maths/sum_of_geometric_progression.py)
* [Sylvester Sequence](maths/sylvester_sequence.py)
* [Test Prime Check](maths/test_prime_check.py)
* [Trapezoidal Rule](maths/trapezoidal_rule.py)
* [Triplet Sum](maths/triplet_sum.py)
* [Two Pointer](maths/two_pointer.py)
* [Two Sum](maths/two_sum.py)
* [Ugly Numbers](maths/ugly_numbers.py)
* [Volume](maths/volume.py)
* [Weird Number](maths/weird_number.py)
* [Zellers Congruence](maths/zellers_congruence.py)
## Matrix
* [Binary Search Matrix](matrix/binary_search_matrix.py)
* [Count Islands In Matrix](matrix/count_islands_in_matrix.py)
* [Inverse Of Matrix](matrix/inverse_of_matrix.py)
* [Matrix Class](matrix/matrix_class.py)
* [Matrix Operation](matrix/matrix_operation.py)
* [Max Area Of Island](matrix/max_area_of_island.py)
* [Nth Fibonacci Using Matrix Exponentiation](matrix/nth_fibonacci_using_matrix_exponentiation.py)
* [Rotate Matrix](matrix/rotate_matrix.py)
* [Searching In Sorted Matrix](matrix/searching_in_sorted_matrix.py)
* [Sherman Morrison](matrix/sherman_morrison.py)
* [Spiral Print](matrix/spiral_print.py)
* Tests
* [Test Matrix Operation](matrix/tests/test_matrix_operation.py)
## Networking Flow
* [Ford Fulkerson](networking_flow/ford_fulkerson.py)
* [Minimum Cut](networking_flow/minimum_cut.py)
## Neural Network
* [2 Hidden Layers Neural Network](neural_network/2_hidden_layers_neural_network.py)
* [Back Propagation Neural Network](neural_network/back_propagation_neural_network.py)
* [Convolution Neural Network](neural_network/convolution_neural_network.py)
* [Perceptron](neural_network/perceptron.py)
## Other
* [Activity Selection](other/activity_selection.py)
* [Alternative List Arrange](other/alternative_list_arrange.py)
* [Check Strong Password](other/check_strong_password.py)
* [Davisb Putnamb Logemannb Loveland](other/davisb_putnamb_logemannb_loveland.py)
* [Dijkstra Bankers Algorithm](other/dijkstra_bankers_algorithm.py)
* [Doomsday](other/doomsday.py)
* [Fischer Yates Shuffle](other/fischer_yates_shuffle.py)
* [Gauss Easter](other/gauss_easter.py)
* [Graham Scan](other/graham_scan.py)
* [Greedy](other/greedy.py)
* [Least Recently Used](other/least_recently_used.py)
* [Lfu Cache](other/lfu_cache.py)
* [Linear Congruential Generator](other/linear_congruential_generator.py)
* [Lru Cache](other/lru_cache.py)
* [Magicdiamondpattern](other/magicdiamondpattern.py)
* [Maximum Subarray](other/maximum_subarray.py)
* [Nested Brackets](other/nested_brackets.py)
* [Password Generator](other/password_generator.py)
* [Scoring Algorithm](other/scoring_algorithm.py)
* [Sdes](other/sdes.py)
* [Tower Of Hanoi](other/tower_of_hanoi.py)
## Physics
* [Casimir Effect](physics/casimir_effect.py)
* [Horizontal Projectile Motion](physics/horizontal_projectile_motion.py)
* [Lorentz Transformation Four Vector](physics/lorentz_transformation_four_vector.py)
* [N Body Simulation](physics/n_body_simulation.py)
* [Newtons Law Of Gravitation](physics/newtons_law_of_gravitation.py)
* [Newtons Second Law Of Motion](physics/newtons_second_law_of_motion.py)
## Project Euler
* Problem 001
* [Sol1](project_euler/problem_001/sol1.py)
* [Sol2](project_euler/problem_001/sol2.py)
* [Sol3](project_euler/problem_001/sol3.py)
* [Sol4](project_euler/problem_001/sol4.py)
* [Sol5](project_euler/problem_001/sol5.py)
* [Sol6](project_euler/problem_001/sol6.py)
* [Sol7](project_euler/problem_001/sol7.py)
* Problem 002
* [Sol1](project_euler/problem_002/sol1.py)
* [Sol2](project_euler/problem_002/sol2.py)
* [Sol3](project_euler/problem_002/sol3.py)
* [Sol4](project_euler/problem_002/sol4.py)
* [Sol5](project_euler/problem_002/sol5.py)
* Problem 003
* [Sol1](project_euler/problem_003/sol1.py)
* [Sol2](project_euler/problem_003/sol2.py)
* [Sol3](project_euler/problem_003/sol3.py)
* Problem 004
* [Sol1](project_euler/problem_004/sol1.py)
* [Sol2](project_euler/problem_004/sol2.py)
* Problem 005
* [Sol1](project_euler/problem_005/sol1.py)
* [Sol2](project_euler/problem_005/sol2.py)
* Problem 006
* [Sol1](project_euler/problem_006/sol1.py)
* [Sol2](project_euler/problem_006/sol2.py)
* [Sol3](project_euler/problem_006/sol3.py)
* [Sol4](project_euler/problem_006/sol4.py)
* Problem 007
* [Sol1](project_euler/problem_007/sol1.py)
* [Sol2](project_euler/problem_007/sol2.py)
* [Sol3](project_euler/problem_007/sol3.py)
* Problem 008
* [Sol1](project_euler/problem_008/sol1.py)
* [Sol2](project_euler/problem_008/sol2.py)
* [Sol3](project_euler/problem_008/sol3.py)
* Problem 009
* [Sol1](project_euler/problem_009/sol1.py)
* [Sol2](project_euler/problem_009/sol2.py)
* [Sol3](project_euler/problem_009/sol3.py)
* Problem 010
* [Sol1](project_euler/problem_010/sol1.py)
* [Sol2](project_euler/problem_010/sol2.py)
* [Sol3](project_euler/problem_010/sol3.py)
* Problem 011
* [Sol1](project_euler/problem_011/sol1.py)
* [Sol2](project_euler/problem_011/sol2.py)
* Problem 012
* [Sol1](project_euler/problem_012/sol1.py)
* [Sol2](project_euler/problem_012/sol2.py)
* Problem 013
* [Sol1](project_euler/problem_013/sol1.py)
* Problem 014
* [Sol1](project_euler/problem_014/sol1.py)
* [Sol2](project_euler/problem_014/sol2.py)
* Problem 015
* [Sol1](project_euler/problem_015/sol1.py)
* Problem 016
* [Sol1](project_euler/problem_016/sol1.py)
* [Sol2](project_euler/problem_016/sol2.py)
* Problem 017
* [Sol1](project_euler/problem_017/sol1.py)
* Problem 018
* [Solution](project_euler/problem_018/solution.py)
* Problem 019
* [Sol1](project_euler/problem_019/sol1.py)
* Problem 020
* [Sol1](project_euler/problem_020/sol1.py)
* [Sol2](project_euler/problem_020/sol2.py)
* [Sol3](project_euler/problem_020/sol3.py)
* [Sol4](project_euler/problem_020/sol4.py)
* Problem 021
* [Sol1](project_euler/problem_021/sol1.py)
* Problem 022
* [Sol1](project_euler/problem_022/sol1.py)
* [Sol2](project_euler/problem_022/sol2.py)
* Problem 023
* [Sol1](project_euler/problem_023/sol1.py)
* Problem 024
* [Sol1](project_euler/problem_024/sol1.py)
* Problem 025
* [Sol1](project_euler/problem_025/sol1.py)
* [Sol2](project_euler/problem_025/sol2.py)
* [Sol3](project_euler/problem_025/sol3.py)
* Problem 026
* [Sol1](project_euler/problem_026/sol1.py)
* Problem 027
* [Sol1](project_euler/problem_027/sol1.py)
* Problem 028
* [Sol1](project_euler/problem_028/sol1.py)
* Problem 029
* [Sol1](project_euler/problem_029/sol1.py)
* Problem 030
* [Sol1](project_euler/problem_030/sol1.py)
* Problem 031
* [Sol1](project_euler/problem_031/sol1.py)
* [Sol2](project_euler/problem_031/sol2.py)
* Problem 032
* [Sol32](project_euler/problem_032/sol32.py)
* Problem 033
* [Sol1](project_euler/problem_033/sol1.py)
* Problem 034
* [Sol1](project_euler/problem_034/sol1.py)
* Problem 035
* [Sol1](project_euler/problem_035/sol1.py)
* Problem 036
* [Sol1](project_euler/problem_036/sol1.py)
* Problem 037
* [Sol1](project_euler/problem_037/sol1.py)
* Problem 038
* [Sol1](project_euler/problem_038/sol1.py)
* Problem 039
* [Sol1](project_euler/problem_039/sol1.py)
* Problem 040
* [Sol1](project_euler/problem_040/sol1.py)
* Problem 041
* [Sol1](project_euler/problem_041/sol1.py)
* Problem 042
* [Solution42](project_euler/problem_042/solution42.py)
* Problem 043
* [Sol1](project_euler/problem_043/sol1.py)
* Problem 044
* [Sol1](project_euler/problem_044/sol1.py)
* Problem 045
* [Sol1](project_euler/problem_045/sol1.py)
* Problem 046
* [Sol1](project_euler/problem_046/sol1.py)
* Problem 047
* [Sol1](project_euler/problem_047/sol1.py)
* Problem 048
* [Sol1](project_euler/problem_048/sol1.py)
* Problem 049
* [Sol1](project_euler/problem_049/sol1.py)
* Problem 050
* [Sol1](project_euler/problem_050/sol1.py)
* Problem 051
* [Sol1](project_euler/problem_051/sol1.py)
* Problem 052
* [Sol1](project_euler/problem_052/sol1.py)
* Problem 053
* [Sol1](project_euler/problem_053/sol1.py)
* Problem 054
* [Sol1](project_euler/problem_054/sol1.py)
* [Test Poker Hand](project_euler/problem_054/test_poker_hand.py)
* Problem 055
* [Sol1](project_euler/problem_055/sol1.py)
* Problem 056
* [Sol1](project_euler/problem_056/sol1.py)
* Problem 057
* [Sol1](project_euler/problem_057/sol1.py)
* Problem 058
* [Sol1](project_euler/problem_058/sol1.py)
* Problem 059
* [Sol1](project_euler/problem_059/sol1.py)
* Problem 062
* [Sol1](project_euler/problem_062/sol1.py)
* Problem 063
* [Sol1](project_euler/problem_063/sol1.py)
* Problem 064
* [Sol1](project_euler/problem_064/sol1.py)
* Problem 065
* [Sol1](project_euler/problem_065/sol1.py)
* Problem 067
* [Sol1](project_euler/problem_067/sol1.py)
* [Sol2](project_euler/problem_067/sol2.py)
* Problem 068
* [Sol1](project_euler/problem_068/sol1.py)
* Problem 069
* [Sol1](project_euler/problem_069/sol1.py)
* Problem 070
* [Sol1](project_euler/problem_070/sol1.py)
* Problem 071
* [Sol1](project_euler/problem_071/sol1.py)
* Problem 072
* [Sol1](project_euler/problem_072/sol1.py)
* [Sol2](project_euler/problem_072/sol2.py)
* Problem 074
* [Sol1](project_euler/problem_074/sol1.py)
* [Sol2](project_euler/problem_074/sol2.py)
* Problem 075
* [Sol1](project_euler/problem_075/sol1.py)
* Problem 076
* [Sol1](project_euler/problem_076/sol1.py)
* Problem 077
* [Sol1](project_euler/problem_077/sol1.py)
* Problem 078
* [Sol1](project_euler/problem_078/sol1.py)
* Problem 080
* [Sol1](project_euler/problem_080/sol1.py)
* Problem 081
* [Sol1](project_euler/problem_081/sol1.py)
* Problem 085
* [Sol1](project_euler/problem_085/sol1.py)
* Problem 086
* [Sol1](project_euler/problem_086/sol1.py)
* Problem 087
* [Sol1](project_euler/problem_087/sol1.py)
* Problem 089
* [Sol1](project_euler/problem_089/sol1.py)
* Problem 091
* [Sol1](project_euler/problem_091/sol1.py)
* Problem 092
* [Sol1](project_euler/problem_092/sol1.py)
* Problem 097
* [Sol1](project_euler/problem_097/sol1.py)
* Problem 099
* [Sol1](project_euler/problem_099/sol1.py)
* Problem 101
* [Sol1](project_euler/problem_101/sol1.py)
* Problem 102
* [Sol1](project_euler/problem_102/sol1.py)
* Problem 107
* [Sol1](project_euler/problem_107/sol1.py)
* Problem 109
* [Sol1](project_euler/problem_109/sol1.py)
* Problem 112
* [Sol1](project_euler/problem_112/sol1.py)
* Problem 113
* [Sol1](project_euler/problem_113/sol1.py)
* Problem 114
* [Sol1](project_euler/problem_114/sol1.py)
* Problem 115
* [Sol1](project_euler/problem_115/sol1.py)
* Problem 116
* [Sol1](project_euler/problem_116/sol1.py)
* Problem 119
* [Sol1](project_euler/problem_119/sol1.py)
* Problem 120
* [Sol1](project_euler/problem_120/sol1.py)
* Problem 121
* [Sol1](project_euler/problem_121/sol1.py)
* Problem 123
* [Sol1](project_euler/problem_123/sol1.py)
* Problem 125
* [Sol1](project_euler/problem_125/sol1.py)
* Problem 129
* [Sol1](project_euler/problem_129/sol1.py)
* Problem 135
* [Sol1](project_euler/problem_135/sol1.py)
* Problem 144
* [Sol1](project_euler/problem_144/sol1.py)
* Problem 145
* [Sol1](project_euler/problem_145/sol1.py)
* Problem 173
* [Sol1](project_euler/problem_173/sol1.py)
* Problem 174
* [Sol1](project_euler/problem_174/sol1.py)
* Problem 180
* [Sol1](project_euler/problem_180/sol1.py)
* Problem 188
* [Sol1](project_euler/problem_188/sol1.py)
* Problem 191
* [Sol1](project_euler/problem_191/sol1.py)
* Problem 203
* [Sol1](project_euler/problem_203/sol1.py)
* Problem 205
* [Sol1](project_euler/problem_205/sol1.py)
* Problem 206
* [Sol1](project_euler/problem_206/sol1.py)
* Problem 207
* [Sol1](project_euler/problem_207/sol1.py)
* Problem 234
* [Sol1](project_euler/problem_234/sol1.py)
* Problem 301
* [Sol1](project_euler/problem_301/sol1.py)
* Problem 493
* [Sol1](project_euler/problem_493/sol1.py)
* Problem 551
* [Sol1](project_euler/problem_551/sol1.py)
* Problem 587
* [Sol1](project_euler/problem_587/sol1.py)
* Problem 686
* [Sol1](project_euler/problem_686/sol1.py)
## Quantum
* [Deutsch Jozsa](quantum/deutsch_jozsa.py)
* [Half Adder](quantum/half_adder.py)
* [Not Gate](quantum/not_gate.py)
* [Q Full Adder](quantum/q_full_adder.py)
* [Quantum Entanglement](quantum/quantum_entanglement.py)
* [Ripple Adder Classic](quantum/ripple_adder_classic.py)
* [Single Qubit Measure](quantum/single_qubit_measure.py)
## Scheduling
* [First Come First Served](scheduling/first_come_first_served.py)
* [Highest Response Ratio Next](scheduling/highest_response_ratio_next.py)
* [Job Sequencing With Deadline](scheduling/job_sequencing_with_deadline.py)
* [Multi Level Feedback Queue](scheduling/multi_level_feedback_queue.py)
* [Non Preemptive Shortest Job First](scheduling/non_preemptive_shortest_job_first.py)
* [Round Robin](scheduling/round_robin.py)
* [Shortest Job First](scheduling/shortest_job_first.py)
## Searches
* [Binary Search](searches/binary_search.py)
* [Binary Tree Traversal](searches/binary_tree_traversal.py)
* [Double Linear Search](searches/double_linear_search.py)
* [Double Linear Search Recursion](searches/double_linear_search_recursion.py)
* [Fibonacci Search](searches/fibonacci_search.py)
* [Hill Climbing](searches/hill_climbing.py)
* [Interpolation Search](searches/interpolation_search.py)
* [Jump Search](searches/jump_search.py)
* [Linear Search](searches/linear_search.py)
* [Quick Select](searches/quick_select.py)
* [Sentinel Linear Search](searches/sentinel_linear_search.py)
* [Simple Binary Search](searches/simple_binary_search.py)
* [Simulated Annealing](searches/simulated_annealing.py)
* [Tabu Search](searches/tabu_search.py)
* [Ternary Search](searches/ternary_search.py)
## Sorts
* [Bead Sort](sorts/bead_sort.py)
* [Bitonic Sort](sorts/bitonic_sort.py)
* [Bogo Sort](sorts/bogo_sort.py)
* [Bubble Sort](sorts/bubble_sort.py)
* [Bucket Sort](sorts/bucket_sort.py)
* [Circle Sort](sorts/circle_sort.py)
* [Cocktail Shaker Sort](sorts/cocktail_shaker_sort.py)
* [Comb Sort](sorts/comb_sort.py)
* [Counting Sort](sorts/counting_sort.py)
* [Cycle Sort](sorts/cycle_sort.py)
* [Double Sort](sorts/double_sort.py)
* [Dutch National Flag Sort](sorts/dutch_national_flag_sort.py)
* [Exchange Sort](sorts/exchange_sort.py)
* [External Sort](sorts/external_sort.py)
* [Gnome Sort](sorts/gnome_sort.py)
* [Heap Sort](sorts/heap_sort.py)
* [Insertion Sort](sorts/insertion_sort.py)
* [Intro Sort](sorts/intro_sort.py)
* [Iterative Merge Sort](sorts/iterative_merge_sort.py)
* [Merge Insertion Sort](sorts/merge_insertion_sort.py)
* [Merge Sort](sorts/merge_sort.py)
* [Msd Radix Sort](sorts/msd_radix_sort.py)
* [Natural Sort](sorts/natural_sort.py)
* [Odd Even Sort](sorts/odd_even_sort.py)
* [Odd Even Transposition Parallel](sorts/odd_even_transposition_parallel.py)
* [Odd Even Transposition Single Threaded](sorts/odd_even_transposition_single_threaded.py)
* [Pancake Sort](sorts/pancake_sort.py)
* [Patience Sort](sorts/patience_sort.py)
* [Pigeon Sort](sorts/pigeon_sort.py)
* [Pigeonhole Sort](sorts/pigeonhole_sort.py)
* [Quick Sort](sorts/quick_sort.py)
* [Quick Sort 3 Partition](sorts/quick_sort_3_partition.py)
* [Radix Sort](sorts/radix_sort.py)
* [Random Normal Distribution Quicksort](sorts/random_normal_distribution_quicksort.py)
* [Random Pivot Quick Sort](sorts/random_pivot_quick_sort.py)
* [Recursive Bubble Sort](sorts/recursive_bubble_sort.py)
* [Recursive Insertion Sort](sorts/recursive_insertion_sort.py)
* [Recursive Mergesort Array](sorts/recursive_mergesort_array.py)
* [Recursive Quick Sort](sorts/recursive_quick_sort.py)
* [Selection Sort](sorts/selection_sort.py)
* [Shell Sort](sorts/shell_sort.py)
* [Shrink Shell Sort](sorts/shrink_shell_sort.py)
* [Slowsort](sorts/slowsort.py)
* [Stooge Sort](sorts/stooge_sort.py)
* [Strand Sort](sorts/strand_sort.py)
* [Tim Sort](sorts/tim_sort.py)
* [Topological Sort](sorts/topological_sort.py)
* [Tree Sort](sorts/tree_sort.py)
* [Unknown Sort](sorts/unknown_sort.py)
* [Wiggle Sort](sorts/wiggle_sort.py)
## Strings
* [Aho Corasick](strings/aho_corasick.py)
* [Alternative String Arrange](strings/alternative_string_arrange.py)
* [Anagrams](strings/anagrams.py)
* [Autocomplete Using Trie](strings/autocomplete_using_trie.py)
* [Barcode Validator](strings/barcode_validator.py)
* [Boyer Moore Search](strings/boyer_moore_search.py)
* [Can String Be Rearranged As Palindrome](strings/can_string_be_rearranged_as_palindrome.py)
* [Capitalize](strings/capitalize.py)
* [Check Anagrams](strings/check_anagrams.py)
* [Check Pangram](strings/check_pangram.py)
* [Credit Card Validator](strings/credit_card_validator.py)
* [Detecting English Programmatically](strings/detecting_english_programmatically.py)
* [Dna](strings/dna.py)
* [Frequency Finder](strings/frequency_finder.py)
* [Hamming Distance](strings/hamming_distance.py)
* [Indian Phone Validator](strings/indian_phone_validator.py)
* [Is Contains Unique Chars](strings/is_contains_unique_chars.py)
* [Is Palindrome](strings/is_palindrome.py)
* [Jaro Winkler](strings/jaro_winkler.py)
* [Join](strings/join.py)
* [Knuth Morris Pratt](strings/knuth_morris_pratt.py)
* [Levenshtein Distance](strings/levenshtein_distance.py)
* [Lower](strings/lower.py)
* [Manacher](strings/manacher.py)
* [Min Cost String Conversion](strings/min_cost_string_conversion.py)
* [Naive String Search](strings/naive_string_search.py)
* [Ngram](strings/ngram.py)
* [Palindrome](strings/palindrome.py)
* [Prefix Function](strings/prefix_function.py)
* [Rabin Karp](strings/rabin_karp.py)
* [Remove Duplicate](strings/remove_duplicate.py)
* [Reverse Letters](strings/reverse_letters.py)
* [Reverse Long Words](strings/reverse_long_words.py)
* [Reverse Words](strings/reverse_words.py)
* [Snake Case To Camel Pascal Case](strings/snake_case_to_camel_pascal_case.py)
* [Split](strings/split.py)
* [Upper](strings/upper.py)
* [Wave](strings/wave.py)
* [Wildcard Pattern Matching](strings/wildcard_pattern_matching.py)
* [Word Occurrence](strings/word_occurrence.py)
* [Word Patterns](strings/word_patterns.py)
* [Z Function](strings/z_function.py)
## Web Programming
* [Co2 Emission](web_programming/co2_emission.py)
* [Covid Stats Via Xpath](web_programming/covid_stats_via_xpath.py)
* [Crawl Google Results](web_programming/crawl_google_results.py)
* [Crawl Google Scholar Citation](web_programming/crawl_google_scholar_citation.py)
* [Currency Converter](web_programming/currency_converter.py)
* [Current Stock Price](web_programming/current_stock_price.py)
* [Current Weather](web_programming/current_weather.py)
* [Daily Horoscope](web_programming/daily_horoscope.py)
* [Download Images From Google Query](web_programming/download_images_from_google_query.py)
* [Emails From Url](web_programming/emails_from_url.py)
* [Fetch Anime And Play](web_programming/fetch_anime_and_play.py)
* [Fetch Bbc News](web_programming/fetch_bbc_news.py)
* [Fetch Github Info](web_programming/fetch_github_info.py)
* [Fetch Jobs](web_programming/fetch_jobs.py)
* [Fetch Quotes](web_programming/fetch_quotes.py)
* [Fetch Well Rx Price](web_programming/fetch_well_rx_price.py)
* [Get Imdb Top 250 Movies Csv](web_programming/get_imdb_top_250_movies_csv.py)
* [Get Imdbtop](web_programming/get_imdbtop.py)
* [Get Top Hn Posts](web_programming/get_top_hn_posts.py)
* [Get User Tweets](web_programming/get_user_tweets.py)
* [Giphy](web_programming/giphy.py)
* [Instagram Crawler](web_programming/instagram_crawler.py)
* [Instagram Pic](web_programming/instagram_pic.py)
* [Instagram Video](web_programming/instagram_video.py)
* [Nasa Data](web_programming/nasa_data.py)
* [Open Google Results](web_programming/open_google_results.py)
* [Random Anime Character](web_programming/random_anime_character.py)
* [Recaptcha Verification](web_programming/recaptcha_verification.py)
* [Reddit](web_programming/reddit.py)
* [Search Books By Isbn](web_programming/search_books_by_isbn.py)
* [Slack Message](web_programming/slack_message.py)
* [Test Fetch Github Info](web_programming/test_fetch_github_info.py)
* [World Covid19 Stats](web_programming/world_covid19_stats.py)
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
"""
Deutsch-Josza Algorithm is one of the first examples of a quantum
algorithm that is exponentially faster than any possible deterministic
classical algorithm
Premise:
We are given a hidden Boolean function f,
which takes as input a string of bits, and returns either 0 or 1:
f({x0,x1,x2,...}) -> 0 or 1, where xn is 0 or 1
The property of the given Boolean function is that it is guaranteed to
either be balanced or constant. A constant function returns all 0's
or all 1's for any input, while a balanced function returns 0's for
exactly half of all inputs and 1's for the other half. Our task is to
determine whether the given function is balanced or constant.
References:
- https://en.wikipedia.org/wiki/Deutsch-Jozsa_algorithm
- https://qiskit.org/textbook/ch-algorithms/deutsch-jozsa.html
"""
import numpy as np
import qiskit as q
def dj_oracle(case: str, num_qubits: int) -> q.QuantumCircuit:
"""
Returns a Quantum Circuit for the Oracle function.
The circuit returned can represent balanced or constant function,
according to the arguments passed
"""
# This circuit has num_qubits+1 qubits: the size of the input,
# plus one output qubit
oracle_qc = q.QuantumCircuit(num_qubits + 1)
# First, let's deal with the case in which oracle is balanced
if case == "balanced":
# First generate a random number that tells us which CNOTs to
# wrap in X-gates:
b = np.random.randint(1, 2**num_qubits)
# Next, format 'b' as a binary string of length 'n', padded with zeros:
b_str = format(b, f"0{num_qubits}b")
# Next, we place the first X-gates. Each digit in our binary string
# correspopnds to a qubit, if the digit is 0, we do nothing, if it's 1
# we apply an X-gate to that qubit:
for index, bit in enumerate(b_str):
if bit == "1":
oracle_qc.x(index)
# Do the controlled-NOT gates for each qubit, using the output qubit
# as the target:
for index in range(num_qubits):
oracle_qc.cx(index, num_qubits)
# Next, place the final X-gates
for index, bit in enumerate(b_str):
if bit == "1":
oracle_qc.x(index)
# Case in which oracle is constant
if case == "constant":
# First decide what the fixed output of the oracle will be
# (either always 0 or always 1)
output = np.random.randint(2)
if output == 1:
oracle_qc.x(num_qubits)
oracle_gate = oracle_qc.to_gate()
oracle_gate.name = "Oracle" # To show when we display the circuit
return oracle_gate
def dj_algorithm(oracle: q.QuantumCircuit, num_qubits: int) -> q.QuantumCircuit:
"""
Returns the complete Deustch-Jozsa Quantum Circuit,
adding Input & Output registers and Hadamard & Measurement Gates,
to the Oracle Circuit passed in arguments
"""
dj_circuit = q.QuantumCircuit(num_qubits + 1, num_qubits)
# Set up the output qubit:
dj_circuit.x(num_qubits)
dj_circuit.h(num_qubits)
# And set up the input register:
for qubit in range(num_qubits):
dj_circuit.h(qubit)
# Let's append the oracle gate to our circuit:
dj_circuit.append(oracle, range(num_qubits + 1))
# Finally, perform the H-gates again and measure:
for qubit in range(num_qubits):
dj_circuit.h(qubit)
for i in range(num_qubits):
dj_circuit.measure(i, i)
return dj_circuit
def deutsch_jozsa(case: str, num_qubits: int) -> q.result.counts.Counts:
"""
Main function that builds the circuit using other helper functions,
runs the experiment 1000 times & returns the resultant qubit counts
>>> deutsch_jozsa("constant", 3)
{'000': 1000}
>>> deutsch_jozsa("balanced", 3)
{'111': 1000}
"""
# Use Aer's qasm_simulator
simulator = q.Aer.get_backend("qasm_simulator")
oracle_gate = dj_oracle(case, num_qubits)
dj_circuit = dj_algorithm(oracle_gate, num_qubits)
# Execute the circuit on the qasm simulator
job = q.execute(dj_circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(dj_circuit)
if __name__ == "__main__":
print(f"Deutsch Jozsa - Constant Oracle: {deutsch_jozsa('constant', 3)}")
print(f"Deutsch Jozsa - Balanced Oracle: {deutsch_jozsa('balanced', 3)}")
| #!/usr/bin/env python3
"""
Deutsch-Jozsa Algorithm is one of the first examples of a quantum
algorithm that is exponentially faster than any possible deterministic
classical algorithm
Premise:
We are given a hidden Boolean function f,
which takes as input a string of bits, and returns either 0 or 1:
f({x0,x1,x2,...}) -> 0 or 1, where xn is 0 or 1
The property of the given Boolean function is that it is guaranteed to
either be balanced or constant. A constant function returns all 0's
or all 1's for any input, while a balanced function returns 0's for
exactly half of all inputs and 1's for the other half. Our task is to
determine whether the given function is balanced or constant.
References:
- https://en.wikipedia.org/wiki/Deutsch-Jozsa_algorithm
- https://qiskit.org/textbook/ch-algorithms/deutsch-jozsa.html
"""
import numpy as np
import qiskit
def dj_oracle(case: str, num_qubits: int) -> qiskit.QuantumCircuit:
"""
Returns a Quantum Circuit for the Oracle function.
The circuit returned can represent balanced or constant function,
according to the arguments passed
"""
# This circuit has num_qubits+1 qubits: the size of the input,
# plus one output qubit
oracle_qc = qiskit.QuantumCircuit(num_qubits + 1)
# First, let's deal with the case in which oracle is balanced
if case == "balanced":
# First generate a random number that tells us which CNOTs to
# wrap in X-gates:
b = np.random.randint(1, 2**num_qubits)
# Next, format 'b' as a binary string of length 'n', padded with zeros:
b_str = format(b, f"0{num_qubits}b")
# Next, we place the first X-gates. Each digit in our binary string
# corresponds to a qubit, if the digit is 0, we do nothing, if it's 1
# we apply an X-gate to that qubit:
for index, bit in enumerate(b_str):
if bit == "1":
oracle_qc.x(index)
# Do the controlled-NOT gates for each qubit, using the output qubit
# as the target:
for index in range(num_qubits):
oracle_qc.cx(index, num_qubits)
# Next, place the final X-gates
for index, bit in enumerate(b_str):
if bit == "1":
oracle_qc.x(index)
# Case in which oracle is constant
if case == "constant":
# First decide what the fixed output of the oracle will be
# (either always 0 or always 1)
output = np.random.randint(2)
if output == 1:
oracle_qc.x(num_qubits)
oracle_gate = oracle_qc.to_gate()
oracle_gate.name = "Oracle" # To show when we display the circuit
return oracle_gate
def dj_algorithm(
oracle: qiskit.QuantumCircuit, num_qubits: int
) -> qiskit.QuantumCircuit:
"""
Returns the complete Deutsch-Jozsa Quantum Circuit,
adding Input & Output registers and Hadamard & Measurement Gates,
to the Oracle Circuit passed in arguments
"""
dj_circuit = qiskit.QuantumCircuit(num_qubits + 1, num_qubits)
# Set up the output qubit:
dj_circuit.x(num_qubits)
dj_circuit.h(num_qubits)
# And set up the input register:
for qubit in range(num_qubits):
dj_circuit.h(qubit)
# Let's append the oracle gate to our circuit:
dj_circuit.append(oracle, range(num_qubits + 1))
# Finally, perform the H-gates again and measure:
for qubit in range(num_qubits):
dj_circuit.h(qubit)
for i in range(num_qubits):
dj_circuit.measure(i, i)
return dj_circuit
def deutsch_jozsa(case: str, num_qubits: int) -> qiskit.result.counts.Counts:
"""
Main function that builds the circuit using other helper functions,
runs the experiment 1000 times & returns the resultant qubit counts
>>> deutsch_jozsa("constant", 3)
{'000': 1000}
>>> deutsch_jozsa("balanced", 3)
{'111': 1000}
"""
# Use Aer's simulator
simulator = qiskit.Aer.get_backend("aer_simulator")
oracle_gate = dj_oracle(case, num_qubits)
dj_circuit = dj_algorithm(oracle_gate, num_qubits)
# Execute the circuit on the simulator
job = qiskit.execute(dj_circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(dj_circuit)
if __name__ == "__main__":
print(f"Deutsch Jozsa - Constant Oracle: {deutsch_jozsa('constant', 3)}")
print(f"Deutsch Jozsa - Balanced Oracle: {deutsch_jozsa('balanced', 3)}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
"""
Build a half-adder quantum circuit that takes two bits as input,
encodes them into qubits, then runs the half-adder circuit calculating
the sum and carry qubits, observed over 1000 runs of the experiment
.
References:
https://en.wikipedia.org/wiki/Adder_(electronics)
https://qiskit.org/textbook/ch-states/atoms-computation.html#4.2-Remembering-how-to-add-
"""
import qiskit as q
def half_adder(bit0: int, bit1: int) -> q.result.counts.Counts:
"""
>>> half_adder(0, 0)
{'00': 1000}
>>> half_adder(0, 1)
{'01': 1000}
>>> half_adder(1, 0)
{'01': 1000}
>>> half_adder(1, 1)
{'10': 1000}
"""
# Use Aer's qasm_simulator
simulator = q.Aer.get_backend("qasm_simulator")
qc_ha = q.QuantumCircuit(4, 2)
# encode inputs in qubits 0 and 1
if bit0 == 1:
qc_ha.x(0)
if bit1 == 1:
qc_ha.x(1)
qc_ha.barrier()
# use cnots to write XOR of the inputs on qubit2
qc_ha.cx(0, 2)
qc_ha.cx(1, 2)
# use ccx / toffoli gate to write AND of the inputs on qubit3
qc_ha.ccx(0, 1, 3)
qc_ha.barrier()
# extract outputs
qc_ha.measure(2, 0) # extract XOR value
qc_ha.measure(3, 1) # extract AND value
# Execute the circuit on the qasm simulator
job = q.execute(qc_ha, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(qc_ha)
if __name__ == "__main__":
counts = half_adder(1, 1)
print(f"Half Adder Output Qubit Counts: {counts}")
| #!/usr/bin/env python3
"""
Build a half-adder quantum circuit that takes two bits as input,
encodes them into qubits, then runs the half-adder circuit calculating
the sum and carry qubits, observed over 1000 runs of the experiment
.
References:
https://en.wikipedia.org/wiki/Adder_(electronics)
https://qiskit.org/textbook/ch-states/atoms-computation.html#4.2-Remembering-how-to-add-
"""
import qiskit
def half_adder(bit0: int, bit1: int) -> qiskit.result.counts.Counts:
"""
>>> half_adder(0, 0)
{'00': 1000}
>>> half_adder(0, 1)
{'01': 1000}
>>> half_adder(1, 0)
{'01': 1000}
>>> half_adder(1, 1)
{'10': 1000}
"""
# Use Aer's simulator
simulator = qiskit.Aer.get_backend("aer_simulator")
qc_ha = qiskit.QuantumCircuit(4, 2)
# encode inputs in qubits 0 and 1
if bit0 == 1:
qc_ha.x(0)
if bit1 == 1:
qc_ha.x(1)
qc_ha.barrier()
# use cnots to write XOR of the inputs on qubit2
qc_ha.cx(0, 2)
qc_ha.cx(1, 2)
# use ccx / toffoli gate to write AND of the inputs on qubit3
qc_ha.ccx(0, 1, 3)
qc_ha.barrier()
# extract outputs
qc_ha.measure(2, 0) # extract XOR value
qc_ha.measure(3, 1) # extract AND value
# Execute the circuit on the qasm simulator
job = qiskit.execute(qc_ha, simulator, shots=1000)
# Return the histogram data of the results of the experiment
return job.result().get_counts(qc_ha)
if __name__ == "__main__":
counts = half_adder(1, 1)
print(f"Half Adder Output Qubit Counts: {counts}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
"""
Build a simple bare-minimum quantum circuit that starts with a single
qubit (by default, in state 0) and inverts it. Run the experiment 1000
times and print the total count of the states finally observed.
Qiskit Docs: https://qiskit.org/documentation/getting_started.html
"""
import qiskit as q
def single_qubit_measure(qubits: int, classical_bits: int) -> q.result.counts.Counts:
"""
>>> single_qubit_measure(2, 2)
{'11': 1000}
>>> single_qubit_measure(4, 4)
{'0011': 1000}
"""
# Use Aer's qasm_simulator
simulator = q.Aer.get_backend("qasm_simulator")
# Create a Quantum Circuit acting on the q register
circuit = q.QuantumCircuit(qubits, classical_bits)
# Apply X (NOT) Gate to Qubits 0 & 1
circuit.x(0)
circuit.x(1)
# Map the quantum measurement to the classical bits
circuit.measure([0, 1], [0, 1])
# Execute the circuit on the qasm simulator
job = q.execute(circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(circuit)
if __name__ == "__main__":
counts = single_qubit_measure(2, 2)
print(f"Total count for various states are: {counts}")
| #!/usr/bin/env python3
"""
Build a simple bare-minimum quantum circuit that starts with a single
qubit (by default, in state 0) and inverts it. Run the experiment 1000
times and print the total count of the states finally observed.
Qiskit Docs: https://qiskit.org/documentation/getting_started.html
"""
import qiskit
def single_qubit_measure(
qubits: int, classical_bits: int
) -> qiskit.result.counts.Counts:
"""
>>> single_qubit_measure(2, 2)
{'11': 1000}
>>> single_qubit_measure(4, 4)
{'0011': 1000}
"""
# Use Aer's simulator
simulator = qiskit.Aer.get_backend("aer_simulator")
# Create a Quantum Circuit acting on the q register
circuit = qiskit.QuantumCircuit(qubits, classical_bits)
# Apply X (NOT) Gate to Qubits 0 & 1
circuit.x(0)
circuit.x(1)
# Map the quantum measurement to the classical bits
circuit.measure([0, 1], [0, 1])
# Execute the circuit on the qasm simulator
job = qiskit.execute(circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(circuit)
if __name__ == "__main__":
counts = single_qubit_measure(2, 2)
print(f"Total count for various states are: {counts}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Build the quantum full adder (QFA) for any sum of
two quantum registers and one carry in. This circuit
is designed using the Qiskit framework. This
experiment run in IBM Q simulator with 1000 shots.
.
References:
https://www.quantum-inspire.com/kbase/full-adder/
"""
import math
import qiskit
from qiskit import Aer, ClassicalRegister, QuantumCircuit, QuantumRegister, execute
def quantum_full_adder(
input_1: int = 1, input_2: int = 1, carry_in: int = 1
) -> qiskit.result.counts.Counts:
"""
# >>> q_full_adder(inp_1, inp_2, cin)
# the inputs can be 0/1 for qubits in define
# values, or can be in a superposition of both
# states with hadamard gate using the input value 2.
# result for default values: {11: 1000}
qr_0: ──■────■──────────────■──
│ ┌─┴─┐ ┌─┴─┐
qr_1: ──■──┤ X ├──■────■──┤ X ├
│ └───┘ │ ┌─┴─┐└───┘
qr_2: ──┼─────────■──┤ X ├─────
┌─┴─┐ ┌─┴─┐└───┘
qr_3: ┤ X ├─────┤ X ├──────────
└───┘ └───┘
cr: 2/═════════════════════════
Args:
input_1: input 1 for the circuit.
input_2: input 2 for the circuit.
carry_in: carry in for the circuit.
Returns:
qiskit.result.counts.Counts: sum result counts.
>>> quantum_full_adder(1,1,1)
{'11': 1000}
>>> quantum_full_adder(0,0,1)
{'01': 1000}
>>> quantum_full_adder(1,0,1)
{'10': 1000}
>>> quantum_full_adder(1,-4,1)
Traceback (most recent call last):
...
ValueError: inputs must be positive.
>>> quantum_full_adder('q',0,1)
Traceback (most recent call last):
...
TypeError: inputs must be integers.
>>> quantum_full_adder(0.5,0,1)
Traceback (most recent call last):
...
ValueError: inputs must be exact integers.
>>> quantum_full_adder(0,1,3)
Traceback (most recent call last):
...
ValueError: inputs must be less or equal to 2.
"""
if (type(input_1) == str) or (type(input_2) == str) or (type(carry_in) == str):
raise TypeError("inputs must be integers.")
if (input_1 < 0) or (input_2 < 0) or (carry_in < 0):
raise ValueError("inputs must be positive.")
if (
(math.floor(input_1) != input_1)
or (math.floor(input_2) != input_2)
or (math.floor(carry_in) != carry_in)
):
raise ValueError("inputs must be exact integers.")
if (input_1 > 2) or (input_2 > 2) or (carry_in > 2):
raise ValueError("inputs must be less or equal to 2.")
# build registers
qr = QuantumRegister(4, "qr")
cr = ClassicalRegister(2, "cr")
# list the entries
entry = [input_1, input_2, carry_in]
quantum_circuit = QuantumCircuit(qr, cr)
for i in range(0, 3):
if entry[i] == 2:
quantum_circuit.h(i) # for hadamard entries
elif entry[i] == 1:
quantum_circuit.x(i) # for 1 entries
elif entry[i] == 0:
quantum_circuit.i(i) # for 0 entries
# build the circuit
quantum_circuit.ccx(0, 1, 3) # ccx = toffoli gate
quantum_circuit.cx(0, 1)
quantum_circuit.ccx(1, 2, 3)
quantum_circuit.cx(1, 2)
quantum_circuit.cx(0, 1)
quantum_circuit.measure([2, 3], cr) # measure the last two qbits
backend = Aer.get_backend("qasm_simulator")
job = execute(quantum_circuit, backend, shots=1000)
return job.result().get_counts(quantum_circuit)
if __name__ == "__main__":
print(f"Total sum count for state is: {quantum_full_adder(1,1,1)}")
| """
Build the quantum full adder (QFA) for any sum of
two quantum registers and one carry in. This circuit
is designed using the Qiskit framework. This
experiment run in IBM Q simulator with 1000 shots.
.
References:
https://www.quantum-inspire.com/kbase/full-adder/
"""
import math
import qiskit
def quantum_full_adder(
input_1: int = 1, input_2: int = 1, carry_in: int = 1
) -> qiskit.result.counts.Counts:
"""
# >>> q_full_adder(inp_1, inp_2, cin)
# the inputs can be 0/1 for qubits in define
# values, or can be in a superposition of both
# states with hadamard gate using the input value 2.
# result for default values: {11: 1000}
qr_0: ──■────■──────────────■──
│ ┌─┴─┐ ┌─┴─┐
qr_1: ──■──┤ X ├──■────■──┤ X ├
│ └───┘ │ ┌─┴─┐└───┘
qr_2: ──┼─────────■──┤ X ├─────
┌─┴─┐ ┌─┴─┐└───┘
qr_3: ┤ X ├─────┤ X ├──────────
└───┘ └───┘
cr: 2/═════════════════════════
Args:
input_1: input 1 for the circuit.
input_2: input 2 for the circuit.
carry_in: carry in for the circuit.
Returns:
qiskit.result.counts.Counts: sum result counts.
>>> quantum_full_adder(1, 1, 1)
{'11': 1000}
>>> quantum_full_adder(0, 0, 1)
{'01': 1000}
>>> quantum_full_adder(1, 0, 1)
{'10': 1000}
>>> quantum_full_adder(1, -4, 1)
Traceback (most recent call last):
...
ValueError: inputs must be positive.
>>> quantum_full_adder('q', 0, 1)
Traceback (most recent call last):
...
TypeError: inputs must be integers.
>>> quantum_full_adder(0.5, 0, 1)
Traceback (most recent call last):
...
ValueError: inputs must be exact integers.
>>> quantum_full_adder(0, 1, 3)
Traceback (most recent call last):
...
ValueError: inputs must be less or equal to 2.
"""
if (type(input_1) == str) or (type(input_2) == str) or (type(carry_in) == str):
raise TypeError("inputs must be integers.")
if (input_1 < 0) or (input_2 < 0) or (carry_in < 0):
raise ValueError("inputs must be positive.")
if (
(math.floor(input_1) != input_1)
or (math.floor(input_2) != input_2)
or (math.floor(carry_in) != carry_in)
):
raise ValueError("inputs must be exact integers.")
if (input_1 > 2) or (input_2 > 2) or (carry_in > 2):
raise ValueError("inputs must be less or equal to 2.")
# build registers
qr = qiskit.QuantumRegister(4, "qr")
cr = qiskit.ClassicalRegister(2, "cr")
# list the entries
entry = [input_1, input_2, carry_in]
quantum_circuit = qiskit.QuantumCircuit(qr, cr)
for i in range(0, 3):
if entry[i] == 2:
quantum_circuit.h(i) # for hadamard entries
elif entry[i] == 1:
quantum_circuit.x(i) # for 1 entries
elif entry[i] == 0:
quantum_circuit.i(i) # for 0 entries
# build the circuit
quantum_circuit.ccx(0, 1, 3) # ccx = toffoli gate
quantum_circuit.cx(0, 1)
quantum_circuit.ccx(1, 2, 3)
quantum_circuit.cx(1, 2)
quantum_circuit.cx(0, 1)
quantum_circuit.measure([2, 3], cr) # measure the last two qbits
backend = qiskit.Aer.get_backend("aer_simulator")
job = qiskit.execute(quantum_circuit, backend, shots=1000)
return job.result().get_counts(quantum_circuit)
if __name__ == "__main__":
print(f"Total sum count for state is: {quantum_full_adder(1, 1, 1)}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
"""
Build a quantum circuit with pair or group of qubits to perform
quantum entanglement.
Quantum entanglement is a phenomenon observed at the quantum scale
where entangled particles stay connected (in some sense) so that
the actions performed on one of the particles affects the other,
no matter the distance between two particles.
"""
import qiskit
def quantum_entanglement(qubits: int = 2) -> qiskit.result.counts.Counts:
"""
# >>> quantum_entanglement(2)
# {'00': 500, '11': 500}
# ┌───┐ ┌─┐
# q_0: ┤ H ├──■──┤M├───
# └───┘┌─┴─┐└╥┘┌─┐
# q_1: ─────┤ X ├─╫─┤M├
# └───┘ ║ └╥┘
# c: 2/═══════════╩══╩═
# 0 1
Args:
qubits (int): number of quibits to use. Defaults to 2
Returns:
qiskit.result.counts.Counts: mapping of states to its counts
"""
classical_bits = qubits
# Using Aer's qasm_simulator
simulator = qiskit.Aer.get_backend("qasm_simulator")
# Creating a Quantum Circuit acting on the q register
circuit = qiskit.QuantumCircuit(qubits, classical_bits)
# Adding a H gate on qubit 0 (now q0 in superposition)
circuit.h(0)
for i in range(1, qubits):
# Adding CX (CNOT) gate
circuit.cx(i - 1, i)
# Mapping the quantum measurement to the classical bits
circuit.measure(list(range(qubits)), list(range(classical_bits)))
# Now measuring any one qubit would affect other qubits to collapse
# their super position and have same state as the measured one.
# Executing the circuit on the qasm simulator
job = qiskit.execute(circuit, simulator, shots=1000)
return job.result().get_counts(circuit)
if __name__ == "__main__":
print(f"Total count for various states are: {quantum_entanglement(3)}")
| #!/usr/bin/env python3
"""
Build a quantum circuit with pair or group of qubits to perform
quantum entanglement.
Quantum entanglement is a phenomenon observed at the quantum scale
where entangled particles stay connected (in some sense) so that
the actions performed on one of the particles affects the other,
no matter the distance between two particles.
"""
import qiskit
def quantum_entanglement(qubits: int = 2) -> qiskit.result.counts.Counts:
"""
# >>> quantum_entanglement(2)
# {'00': 500, '11': 500}
# ┌───┐ ┌─┐
# q_0: ┤ H ├──■──┤M├───
# └───┘┌─┴─┐└╥┘┌─┐
# q_1: ─────┤ X ├─╫─┤M├
# └───┘ ║ └╥┘
# c: 2/═══════════╩══╩═
# 0 1
Args:
qubits (int): number of quibits to use. Defaults to 2
Returns:
qiskit.result.counts.Counts: mapping of states to its counts
"""
classical_bits = qubits
# Using Aer's simulator
simulator = qiskit.Aer.get_backend("aer_simulator")
# Creating a Quantum Circuit acting on the q register
circuit = qiskit.QuantumCircuit(qubits, classical_bits)
# Adding a H gate on qubit 0 (now q0 in superposition)
circuit.h(0)
for i in range(1, qubits):
# Adding CX (CNOT) gate
circuit.cx(i - 1, i)
# Mapping the quantum measurement to the classical bits
circuit.measure(list(range(qubits)), list(range(classical_bits)))
# Now measuring any one qubit would affect other qubits to collapse
# their super position and have same state as the measured one.
# Executing the circuit on the simulator
job = qiskit.execute(circuit, simulator, shots=1000)
return job.result().get_counts(circuit)
if __name__ == "__main__":
print(f"Total count for various states are: {quantum_entanglement(3)}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # https://github.com/rupansh/QuantumComputing/blob/master/rippleadd.py
# https://en.wikipedia.org/wiki/Adder_(electronics)#Full_adder
# https://en.wikipedia.org/wiki/Controlled_NOT_gate
from qiskit import Aer, QuantumCircuit, execute
from qiskit.providers import Backend
def store_two_classics(val1: int, val2: int) -> tuple[QuantumCircuit, str, str]:
"""
Generates a Quantum Circuit which stores two classical integers
Returns the circuit and binary representation of the integers
"""
x, y = bin(val1)[2:], bin(val2)[2:] # Remove leading '0b'
# Ensure that both strings are of the same length
if len(x) > len(y):
y = y.zfill(len(x))
else:
x = x.zfill(len(y))
# We need (3 * number of bits in the larger number)+1 qBits
# The second parameter is the number of classical registers, to measure the result
circuit = QuantumCircuit((len(x) * 3) + 1, len(x) + 1)
# We are essentially "not-ing" the bits that are 1
# Reversed because its easier to perform ops on more significant bits
for i in range(len(x)):
if x[::-1][i] == "1":
circuit.x(i)
for j in range(len(y)):
if y[::-1][j] == "1":
circuit.x(len(x) + j)
return circuit, x, y
def full_adder(
circuit: QuantumCircuit,
input1_loc: int,
input2_loc: int,
carry_in: int,
carry_out: int,
):
"""
Quantum Equivalent of a Full Adder Circuit
CX/CCX is like 2-way/3-way XOR
"""
circuit.ccx(input1_loc, input2_loc, carry_out)
circuit.cx(input1_loc, input2_loc)
circuit.ccx(input2_loc, carry_in, carry_out)
circuit.cx(input2_loc, carry_in)
circuit.cx(input1_loc, input2_loc)
# The default value for **backend** is the result of a function call which is not
# normally recommended and causes flake8-bugbear to raise a B008 error. However,
# in this case, this is accptable because `Aer.get_backend()` is called when the
# function is defined and that same backend is then reused for all function calls.
def ripple_adder(
val1: int,
val2: int,
backend: Backend = Aer.get_backend("qasm_simulator"), # noqa: B008
) -> int:
"""
Quantum Equivalent of a Ripple Adder Circuit
Uses qasm_simulator backend by default
Currently only adds 'emulated' Classical Bits
but nothing prevents us from doing this with hadamard'd bits :)
Only supports adding positive integers
>>> ripple_adder(3, 4)
7
>>> ripple_adder(10, 4)
14
>>> ripple_adder(-1, 10)
Traceback (most recent call last):
...
ValueError: Both Integers must be positive!
"""
if val1 < 0 or val2 < 0:
raise ValueError("Both Integers must be positive!")
# Store the Integers
circuit, x, y = store_two_classics(val1, val2)
"""
We are essentially using each bit of x & y respectively as full_adder's input
the carry_input is used from the previous circuit (for circuit num > 1)
the carry_out is just below carry_input because
it will be essentially the carry_input for the next full_adder
"""
for i in range(len(x)):
full_adder(circuit, i, len(x) + i, len(x) + len(y) + i, len(x) + len(y) + i + 1)
circuit.barrier() # Optional, just for aesthetics
# Measure the resultant qBits
for i in range(len(x) + 1):
circuit.measure([(len(x) * 2) + i], [i])
res = execute(circuit, backend, shots=1).result()
# The result is in binary. Convert it back to int
return int(list(res.get_counts())[0], 2)
if __name__ == "__main__":
import doctest
doctest.testmod()
| # https://github.com/rupansh/QuantumComputing/blob/master/rippleadd.py
# https://en.wikipedia.org/wiki/Adder_(electronics)#Full_adder
# https://en.wikipedia.org/wiki/Controlled_NOT_gate
import qiskit
from qiskit.providers import Backend
def store_two_classics(val1: int, val2: int) -> tuple[qiskit.QuantumCircuit, str, str]:
"""
Generates a Quantum Circuit which stores two classical integers
Returns the circuit and binary representation of the integers
"""
x, y = bin(val1)[2:], bin(val2)[2:] # Remove leading '0b'
# Ensure that both strings are of the same length
if len(x) > len(y):
y = y.zfill(len(x))
else:
x = x.zfill(len(y))
# We need (3 * number of bits in the larger number)+1 qBits
# The second parameter is the number of classical registers, to measure the result
circuit = qiskit.QuantumCircuit((len(x) * 3) + 1, len(x) + 1)
# We are essentially "not-ing" the bits that are 1
# Reversed because it's easier to perform ops on more significant bits
for i in range(len(x)):
if x[::-1][i] == "1":
circuit.x(i)
for j in range(len(y)):
if y[::-1][j] == "1":
circuit.x(len(x) + j)
return circuit, x, y
def full_adder(
circuit: qiskit.QuantumCircuit,
input1_loc: int,
input2_loc: int,
carry_in: int,
carry_out: int,
):
"""
Quantum Equivalent of a Full Adder Circuit
CX/CCX is like 2-way/3-way XOR
"""
circuit.ccx(input1_loc, input2_loc, carry_out)
circuit.cx(input1_loc, input2_loc)
circuit.ccx(input2_loc, carry_in, carry_out)
circuit.cx(input2_loc, carry_in)
circuit.cx(input1_loc, input2_loc)
# The default value for **backend** is the result of a function call which is not
# normally recommended and causes flake8-bugbear to raise a B008 error. However,
# in this case, this is acceptable because `Aer.get_backend()` is called when the
# function is defined and that same backend is then reused for all function calls.
def ripple_adder(
val1: int,
val2: int,
backend: Backend = qiskit.Aer.get_backend("aer_simulator"), # noqa: B008
) -> int:
"""
Quantum Equivalent of a Ripple Adder Circuit
Uses qasm_simulator backend by default
Currently only adds 'emulated' Classical Bits
but nothing prevents us from doing this with hadamard'd bits :)
Only supports adding positive integers
>>> ripple_adder(3, 4)
7
>>> ripple_adder(10, 4)
14
>>> ripple_adder(-1, 10)
Traceback (most recent call last):
...
ValueError: Both Integers must be positive!
"""
if val1 < 0 or val2 < 0:
raise ValueError("Both Integers must be positive!")
# Store the Integers
circuit, x, y = store_two_classics(val1, val2)
"""
We are essentially using each bit of x & y respectively as full_adder's input
the carry_input is used from the previous circuit (for circuit num > 1)
the carry_out is just below carry_input because
it will be essentially the carry_input for the next full_adder
"""
for i in range(len(x)):
full_adder(circuit, i, len(x) + i, len(x) + len(y) + i, len(x) + len(y) + i + 1)
circuit.barrier() # Optional, just for aesthetics
# Measure the resultant qBits
for i in range(len(x) + 1):
circuit.measure([(len(x) * 2) + i], [i])
res = qiskit.execute(circuit, backend, shots=1).result()
# The result is in binary. Convert it back to int
return int(list(res.get_counts())[0], 2)
if __name__ == "__main__":
import doctest
doctest.testmod()
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| #!/usr/bin/env python3
"""
Build a simple bare-minimum quantum circuit that starts with a single
qubit (by default, in state 0), runs the experiment 1000 times, and
finally prints the total count of the states finally observed.
Qiskit Docs: https://qiskit.org/documentation/getting_started.html
"""
import qiskit as q
def single_qubit_measure(qubits: int, classical_bits: int) -> q.result.counts.Counts:
"""
>>> single_qubit_measure(1, 1)
{'0': 1000}
"""
# Use Aer's qasm_simulator
simulator = q.Aer.get_backend("qasm_simulator")
# Create a Quantum Circuit acting on the q register
circuit = q.QuantumCircuit(qubits, classical_bits)
# Map the quantum measurement to the classical bits
circuit.measure([0], [0])
# Execute the circuit on the qasm simulator
job = q.execute(circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(circuit)
if __name__ == "__main__":
print(f"Total count for various states are: {single_qubit_measure(1, 1)}")
| #!/usr/bin/env python3
"""
Build a simple bare-minimum quantum circuit that starts with a single
qubit (by default, in state 0), runs the experiment 1000 times, and
finally prints the total count of the states finally observed.
Qiskit Docs: https://qiskit.org/documentation/getting_started.html
"""
import qiskit
def single_qubit_measure(
qubits: int, classical_bits: int
) -> qiskit.result.counts.Counts:
"""
>>> single_qubit_measure(1, 1)
{'0': 1000}
"""
# Use Aer's simulator
simulator = qiskit.Aer.get_backend("aer_simulator")
# Create a Quantum Circuit acting on the q register
circuit = qiskit.QuantumCircuit(qubits, classical_bits)
# Map the quantum measurement to the classical bits
circuit.measure([0], [0])
# Execute the circuit on the simulator
job = qiskit.execute(circuit, simulator, shots=1000)
# Return the histogram data of the results of the experiment.
return job.result().get_counts(circuit)
if __name__ == "__main__":
print(f"Total count for various states are: {single_qubit_measure(1, 1)}")
| 1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import requests
from bs4 import BeautifulSoup
def stock_price(symbol: str = "AAPL") -> str:
url = f"https://in.finance.yahoo.com/quote/{symbol}?s={symbol}"
soup = BeautifulSoup(requests.get(url).text, "html.parser")
class_ = "My(6px) Pos(r) smartphone_Mt(6px)"
return soup.find("div", class_=class_).find("span").text
if __name__ == "__main__":
for symbol in "AAPL AMZN IBM GOOG MSFT ORCL".split():
print(f"Current {symbol:<4} stock price is {stock_price(symbol):>8}")
| import requests
from bs4 import BeautifulSoup
def stock_price(symbol: str = "AAPL") -> str:
url = f"https://in.finance.yahoo.com/quote/{symbol}?s={symbol}"
soup = BeautifulSoup(requests.get(url).text, "html.parser")
class_ = "My(6px) Pos(r) smartphone_Mt(6px)"
return soup.find("div", class_=class_).find("span").text
if __name__ == "__main__":
for symbol in "AAPL AMZN IBM GOOG MSFT ORCL".split():
print(f"Current {symbol:<4} stock price is {stock_price(symbol):>8}")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Implementation Burke's algorithm (dithering)
"""
import numpy as np
from cv2 import destroyAllWindows, imread, imshow, waitKey
class Burkes:
"""
Burke's algorithm is using for converting grayscale image to black and white version
Source: Source: https://en.wikipedia.org/wiki/Dither
Note:
* Best results are given with threshold= ~1/2 * max greyscale value.
* This implementation get RGB image and converts it to greyscale in runtime.
"""
def __init__(self, input_img, threshold: int):
self.min_threshold = 0
# max greyscale value for #FFFFFF
self.max_threshold = int(self.get_greyscale(255, 255, 255))
if not self.min_threshold < threshold < self.max_threshold:
raise ValueError(f"Factor value should be from 0 to {self.max_threshold}")
self.input_img = input_img
self.threshold = threshold
self.width, self.height = self.input_img.shape[1], self.input_img.shape[0]
# error table size (+4 columns and +1 row) greater than input image because of
# lack of if statements
self.error_table = [
[0 for _ in range(self.height + 4)] for __ in range(self.width + 1)
]
self.output_img = np.ones((self.width, self.height, 3), np.uint8) * 255
@classmethod
def get_greyscale(cls, blue: int, green: int, red: int) -> float:
"""
>>> Burkes.get_greyscale(3, 4, 5)
3.753
"""
return 0.114 * blue + 0.587 * green + 0.2126 * red
def process(self) -> None:
for y in range(self.height):
for x in range(self.width):
greyscale = int(self.get_greyscale(*self.input_img[y][x]))
if self.threshold > greyscale + self.error_table[y][x]:
self.output_img[y][x] = (0, 0, 0)
current_error = greyscale + self.error_table[x][y]
else:
self.output_img[y][x] = (255, 255, 255)
current_error = greyscale + self.error_table[x][y] - 255
"""
Burkes error propagation (`*` is current pixel):
* 8/32 4/32
2/32 4/32 8/32 4/32 2/32
"""
self.error_table[y][x + 1] += int(8 / 32 * current_error)
self.error_table[y][x + 2] += int(4 / 32 * current_error)
self.error_table[y + 1][x] += int(8 / 32 * current_error)
self.error_table[y + 1][x + 1] += int(4 / 32 * current_error)
self.error_table[y + 1][x + 2] += int(2 / 32 * current_error)
self.error_table[y + 1][x - 1] += int(4 / 32 * current_error)
self.error_table[y + 1][x - 2] += int(2 / 32 * current_error)
if __name__ == "__main__":
# create Burke's instances with original images in greyscale
burkes_instances = [
Burkes(imread("image_data/lena.jpg", 1), threshold)
for threshold in (1, 126, 130, 140)
]
for burkes in burkes_instances:
burkes.process()
for burkes in burkes_instances:
imshow(
f"Original image with dithering threshold: {burkes.threshold}",
burkes.output_img,
)
waitKey(0)
destroyAllWindows()
| """
Implementation Burke's algorithm (dithering)
"""
import numpy as np
from cv2 import destroyAllWindows, imread, imshow, waitKey
class Burkes:
"""
Burke's algorithm is using for converting grayscale image to black and white version
Source: Source: https://en.wikipedia.org/wiki/Dither
Note:
* Best results are given with threshold= ~1/2 * max greyscale value.
* This implementation get RGB image and converts it to greyscale in runtime.
"""
def __init__(self, input_img, threshold: int):
self.min_threshold = 0
# max greyscale value for #FFFFFF
self.max_threshold = int(self.get_greyscale(255, 255, 255))
if not self.min_threshold < threshold < self.max_threshold:
raise ValueError(f"Factor value should be from 0 to {self.max_threshold}")
self.input_img = input_img
self.threshold = threshold
self.width, self.height = self.input_img.shape[1], self.input_img.shape[0]
# error table size (+4 columns and +1 row) greater than input image because of
# lack of if statements
self.error_table = [
[0 for _ in range(self.height + 4)] for __ in range(self.width + 1)
]
self.output_img = np.ones((self.width, self.height, 3), np.uint8) * 255
@classmethod
def get_greyscale(cls, blue: int, green: int, red: int) -> float:
"""
>>> Burkes.get_greyscale(3, 4, 5)
3.753
"""
return 0.114 * blue + 0.587 * green + 0.2126 * red
def process(self) -> None:
for y in range(self.height):
for x in range(self.width):
greyscale = int(self.get_greyscale(*self.input_img[y][x]))
if self.threshold > greyscale + self.error_table[y][x]:
self.output_img[y][x] = (0, 0, 0)
current_error = greyscale + self.error_table[x][y]
else:
self.output_img[y][x] = (255, 255, 255)
current_error = greyscale + self.error_table[x][y] - 255
"""
Burkes error propagation (`*` is current pixel):
* 8/32 4/32
2/32 4/32 8/32 4/32 2/32
"""
self.error_table[y][x + 1] += int(8 / 32 * current_error)
self.error_table[y][x + 2] += int(4 / 32 * current_error)
self.error_table[y + 1][x] += int(8 / 32 * current_error)
self.error_table[y + 1][x + 1] += int(4 / 32 * current_error)
self.error_table[y + 1][x + 2] += int(2 / 32 * current_error)
self.error_table[y + 1][x - 1] += int(4 / 32 * current_error)
self.error_table[y + 1][x - 2] += int(2 / 32 * current_error)
if __name__ == "__main__":
# create Burke's instances with original images in greyscale
burkes_instances = [
Burkes(imread("image_data/lena.jpg", 1), threshold)
for threshold in (1, 126, 130, 140)
]
for burkes in burkes_instances:
burkes.process()
for burkes in burkes_instances:
imshow(
f"Original image with dithering threshold: {burkes.threshold}",
burkes.output_img,
)
waitKey(0)
destroyAllWindows()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from __future__ import annotations
from collections.abc import Callable
from typing import Generic, TypeVar
T = TypeVar("T")
U = TypeVar("U")
class DoubleLinkedListNode(Generic[T, U]):
"""
Double Linked List Node built specifically for LRU Cache
>>> DoubleLinkedListNode(1,1)
Node: key: 1, val: 1, has next: False, has prev: False
"""
def __init__(self, key: T | None, val: U | None):
self.key = key
self.val = val
self.next: DoubleLinkedListNode[T, U] | None = None
self.prev: DoubleLinkedListNode[T, U] | None = None
def __repr__(self) -> str:
return (
f"Node: key: {self.key}, val: {self.val}, "
f"has next: {bool(self.next)}, has prev: {bool(self.prev)}"
)
class DoubleLinkedList(Generic[T, U]):
"""
Double Linked List built specifically for LRU Cache
>>> dll: DoubleLinkedList = DoubleLinkedList()
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: None, val: None, has next: False, has prev: True
>>> first_node = DoubleLinkedListNode(1,10)
>>> first_node
Node: key: 1, val: 10, has next: False, has prev: False
>>> dll.add(first_node)
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 10, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> # node is mutated
>>> first_node
Node: key: 1, val: 10, has next: True, has prev: True
>>> second_node = DoubleLinkedListNode(2,20)
>>> second_node
Node: key: 2, val: 20, has next: False, has prev: False
>>> dll.add(second_node)
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 10, has next: True, has prev: True,
Node: key: 2, val: 20, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> removed_node = dll.remove(first_node)
>>> assert removed_node == first_node
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 2, val: 20, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> # Attempt to remove node not on list
>>> removed_node = dll.remove(first_node)
>>> removed_node is None
True
>>> # Attempt to remove head or rear
>>> dll.head
Node: key: None, val: None, has next: True, has prev: False
>>> dll.remove(dll.head) is None
True
>>> # Attempt to remove head or rear
>>> dll.rear
Node: key: None, val: None, has next: False, has prev: True
>>> dll.remove(dll.rear) is None
True
"""
def __init__(self) -> None:
self.head: DoubleLinkedListNode[T, U] = DoubleLinkedListNode(None, None)
self.rear: DoubleLinkedListNode[T, U] = DoubleLinkedListNode(None, None)
self.head.next, self.rear.prev = self.rear, self.head
def __repr__(self) -> str:
rep = ["DoubleLinkedList"]
node = self.head
while node.next is not None:
rep.append(str(node))
node = node.next
rep.append(str(self.rear))
return ",\n ".join(rep)
def add(self, node: DoubleLinkedListNode[T, U]) -> None:
"""
Adds the given node to the end of the list (before rear)
"""
previous = self.rear.prev
# All nodes other than self.head are guaranteed to have non-None previous
assert previous is not None
previous.next = node
node.prev = previous
self.rear.prev = node
node.next = self.rear
def remove(
self, node: DoubleLinkedListNode[T, U]
) -> DoubleLinkedListNode[T, U] | None:
"""
Removes and returns the given node from the list
Returns None if node.prev or node.next is None
"""
if node.prev is None or node.next is None:
return None
node.prev.next = node.next
node.next.prev = node.prev
node.prev = None
node.next = None
return node
class LRUCache(Generic[T, U]):
"""
LRU Cache to store a given capacity of data. Can be used as a stand-alone object
or as a function decorator.
>>> cache = LRUCache(2)
>>> cache.set(1, 1)
>>> cache.set(2, 2)
>>> cache.get(1)
1
>>> cache.list
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 2, val: 2, has next: True, has prev: True,
Node: key: 1, val: 1, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> cache.cache # doctest: +NORMALIZE_WHITESPACE
{1: Node: key: 1, val: 1, has next: True, has prev: True, \
2: Node: key: 2, val: 2, has next: True, has prev: True}
>>> cache.set(3, 3)
>>> cache.list
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 1, has next: True, has prev: True,
Node: key: 3, val: 3, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> cache.cache # doctest: +NORMALIZE_WHITESPACE
{1: Node: key: 1, val: 1, has next: True, has prev: True, \
3: Node: key: 3, val: 3, has next: True, has prev: True}
>>> cache.get(2) is None
True
>>> cache.set(4, 4)
>>> cache.get(1) is None
True
>>> cache.get(3)
3
>>> cache.get(4)
4
>>> cache
CacheInfo(hits=3, misses=2, capacity=2, current size=2)
>>> @LRUCache.decorator(100)
... def fib(num):
... if num in (1, 2):
... return 1
... return fib(num - 1) + fib(num - 2)
>>> for i in range(1, 100):
... res = fib(i)
>>> fib.cache_info()
CacheInfo(hits=194, misses=99, capacity=100, current size=99)
"""
# class variable to map the decorator functions to their respective instance
decorator_function_to_instance_map: dict[Callable[[T], U], LRUCache[T, U]] = {}
def __init__(self, capacity: int):
self.list: DoubleLinkedList[T, U] = DoubleLinkedList()
self.capacity = capacity
self.num_keys = 0
self.hits = 0
self.miss = 0
self.cache: dict[T, DoubleLinkedListNode[T, U]] = {}
def __repr__(self) -> str:
"""
Return the details for the cache instance
[hits, misses, capacity, current_size]
"""
return (
f"CacheInfo(hits={self.hits}, misses={self.miss}, "
f"capacity={self.capacity}, current size={self.num_keys})"
)
def __contains__(self, key: T) -> bool:
"""
>>> cache = LRUCache(1)
>>> 1 in cache
False
>>> cache.set(1, 1)
>>> 1 in cache
True
"""
return key in self.cache
def get(self, key: T) -> U | None:
"""
Returns the value for the input key and updates the Double Linked List.
Returns None if key is not present in cache
"""
# Note: pythonic interface would throw KeyError rather than return None
if key in self.cache:
self.hits += 1
value_node: DoubleLinkedListNode[T, U] = self.cache[key]
node = self.list.remove(self.cache[key])
assert node == value_node
# node is guaranteed not None because it is in self.cache
assert node is not None
self.list.add(node)
return node.val
self.miss += 1
return None
def set(self, key: T, value: U) -> None:
"""
Sets the value for the input key and updates the Double Linked List
"""
if key not in self.cache:
if self.num_keys >= self.capacity:
# delete first node (oldest) when over capacity
first_node = self.list.head.next
# guaranteed to have a non-None first node when num_keys > 0
# explain to type checker via assertions
assert first_node is not None
assert first_node.key is not None
assert (
self.list.remove(first_node) is not None
) # node guaranteed to be in list assert node.key is not None
del self.cache[first_node.key]
self.num_keys -= 1
self.cache[key] = DoubleLinkedListNode(key, value)
self.list.add(self.cache[key])
self.num_keys += 1
else:
# bump node to the end of the list, update value
node = self.list.remove(self.cache[key])
assert node is not None # node guaranteed to be in list
node.val = value
self.list.add(node)
@classmethod
def decorator(
cls, size: int = 128
) -> Callable[[Callable[[T], U]], Callable[..., U]]:
"""
Decorator version of LRU Cache
Decorated function must be function of T -> U
"""
def cache_decorator_inner(func: Callable[[T], U]) -> Callable[..., U]:
def cache_decorator_wrapper(*args: T) -> U:
if func not in cls.decorator_function_to_instance_map:
cls.decorator_function_to_instance_map[func] = LRUCache(size)
result = cls.decorator_function_to_instance_map[func].get(args[0])
if result is None:
result = func(*args)
cls.decorator_function_to_instance_map[func].set(args[0], result)
return result
def cache_info() -> LRUCache[T, U]:
return cls.decorator_function_to_instance_map[func]
setattr(cache_decorator_wrapper, "cache_info", cache_info) # noqa: B010
return cache_decorator_wrapper
return cache_decorator_inner
if __name__ == "__main__":
import doctest
doctest.testmod()
| from __future__ import annotations
from collections.abc import Callable
from typing import Generic, TypeVar
T = TypeVar("T")
U = TypeVar("U")
class DoubleLinkedListNode(Generic[T, U]):
"""
Double Linked List Node built specifically for LRU Cache
>>> DoubleLinkedListNode(1,1)
Node: key: 1, val: 1, has next: False, has prev: False
"""
def __init__(self, key: T | None, val: U | None):
self.key = key
self.val = val
self.next: DoubleLinkedListNode[T, U] | None = None
self.prev: DoubleLinkedListNode[T, U] | None = None
def __repr__(self) -> str:
return (
f"Node: key: {self.key}, val: {self.val}, "
f"has next: {bool(self.next)}, has prev: {bool(self.prev)}"
)
class DoubleLinkedList(Generic[T, U]):
"""
Double Linked List built specifically for LRU Cache
>>> dll: DoubleLinkedList = DoubleLinkedList()
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: None, val: None, has next: False, has prev: True
>>> first_node = DoubleLinkedListNode(1,10)
>>> first_node
Node: key: 1, val: 10, has next: False, has prev: False
>>> dll.add(first_node)
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 10, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> # node is mutated
>>> first_node
Node: key: 1, val: 10, has next: True, has prev: True
>>> second_node = DoubleLinkedListNode(2,20)
>>> second_node
Node: key: 2, val: 20, has next: False, has prev: False
>>> dll.add(second_node)
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 10, has next: True, has prev: True,
Node: key: 2, val: 20, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> removed_node = dll.remove(first_node)
>>> assert removed_node == first_node
>>> dll
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 2, val: 20, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> # Attempt to remove node not on list
>>> removed_node = dll.remove(first_node)
>>> removed_node is None
True
>>> # Attempt to remove head or rear
>>> dll.head
Node: key: None, val: None, has next: True, has prev: False
>>> dll.remove(dll.head) is None
True
>>> # Attempt to remove head or rear
>>> dll.rear
Node: key: None, val: None, has next: False, has prev: True
>>> dll.remove(dll.rear) is None
True
"""
def __init__(self) -> None:
self.head: DoubleLinkedListNode[T, U] = DoubleLinkedListNode(None, None)
self.rear: DoubleLinkedListNode[T, U] = DoubleLinkedListNode(None, None)
self.head.next, self.rear.prev = self.rear, self.head
def __repr__(self) -> str:
rep = ["DoubleLinkedList"]
node = self.head
while node.next is not None:
rep.append(str(node))
node = node.next
rep.append(str(self.rear))
return ",\n ".join(rep)
def add(self, node: DoubleLinkedListNode[T, U]) -> None:
"""
Adds the given node to the end of the list (before rear)
"""
previous = self.rear.prev
# All nodes other than self.head are guaranteed to have non-None previous
assert previous is not None
previous.next = node
node.prev = previous
self.rear.prev = node
node.next = self.rear
def remove(
self, node: DoubleLinkedListNode[T, U]
) -> DoubleLinkedListNode[T, U] | None:
"""
Removes and returns the given node from the list
Returns None if node.prev or node.next is None
"""
if node.prev is None or node.next is None:
return None
node.prev.next = node.next
node.next.prev = node.prev
node.prev = None
node.next = None
return node
class LRUCache(Generic[T, U]):
"""
LRU Cache to store a given capacity of data. Can be used as a stand-alone object
or as a function decorator.
>>> cache = LRUCache(2)
>>> cache.set(1, 1)
>>> cache.set(2, 2)
>>> cache.get(1)
1
>>> cache.list
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 2, val: 2, has next: True, has prev: True,
Node: key: 1, val: 1, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> cache.cache # doctest: +NORMALIZE_WHITESPACE
{1: Node: key: 1, val: 1, has next: True, has prev: True, \
2: Node: key: 2, val: 2, has next: True, has prev: True}
>>> cache.set(3, 3)
>>> cache.list
DoubleLinkedList,
Node: key: None, val: None, has next: True, has prev: False,
Node: key: 1, val: 1, has next: True, has prev: True,
Node: key: 3, val: 3, has next: True, has prev: True,
Node: key: None, val: None, has next: False, has prev: True
>>> cache.cache # doctest: +NORMALIZE_WHITESPACE
{1: Node: key: 1, val: 1, has next: True, has prev: True, \
3: Node: key: 3, val: 3, has next: True, has prev: True}
>>> cache.get(2) is None
True
>>> cache.set(4, 4)
>>> cache.get(1) is None
True
>>> cache.get(3)
3
>>> cache.get(4)
4
>>> cache
CacheInfo(hits=3, misses=2, capacity=2, current size=2)
>>> @LRUCache.decorator(100)
... def fib(num):
... if num in (1, 2):
... return 1
... return fib(num - 1) + fib(num - 2)
>>> for i in range(1, 100):
... res = fib(i)
>>> fib.cache_info()
CacheInfo(hits=194, misses=99, capacity=100, current size=99)
"""
# class variable to map the decorator functions to their respective instance
decorator_function_to_instance_map: dict[Callable[[T], U], LRUCache[T, U]] = {}
def __init__(self, capacity: int):
self.list: DoubleLinkedList[T, U] = DoubleLinkedList()
self.capacity = capacity
self.num_keys = 0
self.hits = 0
self.miss = 0
self.cache: dict[T, DoubleLinkedListNode[T, U]] = {}
def __repr__(self) -> str:
"""
Return the details for the cache instance
[hits, misses, capacity, current_size]
"""
return (
f"CacheInfo(hits={self.hits}, misses={self.miss}, "
f"capacity={self.capacity}, current size={self.num_keys})"
)
def __contains__(self, key: T) -> bool:
"""
>>> cache = LRUCache(1)
>>> 1 in cache
False
>>> cache.set(1, 1)
>>> 1 in cache
True
"""
return key in self.cache
def get(self, key: T) -> U | None:
"""
Returns the value for the input key and updates the Double Linked List.
Returns None if key is not present in cache
"""
# Note: pythonic interface would throw KeyError rather than return None
if key in self.cache:
self.hits += 1
value_node: DoubleLinkedListNode[T, U] = self.cache[key]
node = self.list.remove(self.cache[key])
assert node == value_node
# node is guaranteed not None because it is in self.cache
assert node is not None
self.list.add(node)
return node.val
self.miss += 1
return None
def set(self, key: T, value: U) -> None:
"""
Sets the value for the input key and updates the Double Linked List
"""
if key not in self.cache:
if self.num_keys >= self.capacity:
# delete first node (oldest) when over capacity
first_node = self.list.head.next
# guaranteed to have a non-None first node when num_keys > 0
# explain to type checker via assertions
assert first_node is not None
assert first_node.key is not None
assert (
self.list.remove(first_node) is not None
) # node guaranteed to be in list assert node.key is not None
del self.cache[first_node.key]
self.num_keys -= 1
self.cache[key] = DoubleLinkedListNode(key, value)
self.list.add(self.cache[key])
self.num_keys += 1
else:
# bump node to the end of the list, update value
node = self.list.remove(self.cache[key])
assert node is not None # node guaranteed to be in list
node.val = value
self.list.add(node)
@classmethod
def decorator(
cls, size: int = 128
) -> Callable[[Callable[[T], U]], Callable[..., U]]:
"""
Decorator version of LRU Cache
Decorated function must be function of T -> U
"""
def cache_decorator_inner(func: Callable[[T], U]) -> Callable[..., U]:
def cache_decorator_wrapper(*args: T) -> U:
if func not in cls.decorator_function_to_instance_map:
cls.decorator_function_to_instance_map[func] = LRUCache(size)
result = cls.decorator_function_to_instance_map[func].get(args[0])
if result is None:
result = func(*args)
cls.decorator_function_to_instance_map[func].set(args[0], result)
return result
def cache_info() -> LRUCache[T, U]:
return cls.decorator_function_to_instance_map[func]
setattr(cache_decorator_wrapper, "cache_info", cache_info) # noqa: B010
return cache_decorator_wrapper
return cache_decorator_inner
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import shutil
import requests
def get_apod_data(api_key: str, download: bool = False, path: str = ".") -> dict:
"""
Get the APOD(Astronomical Picture of the day) data
Get your API Key from: https://api.nasa.gov/
"""
url = "https://api.nasa.gov/planetary/apod"
return requests.get(url, params={"api_key": api_key}).json()
def save_apod(api_key: str, path: str = ".") -> dict:
apod_data = get_apod_data(api_key)
img_url = apod_data["url"]
img_name = img_url.split("/")[-1]
response = requests.get(img_url, stream=True)
with open(f"{path}/{img_name}", "wb+") as img_file:
shutil.copyfileobj(response.raw, img_file)
del response
return apod_data
def get_archive_data(query: str) -> dict:
"""
Get the data of a particular query from NASA archives
"""
url = "https://images-api.nasa.gov/search"
return requests.get(url, params={"q": query}).json()
if __name__ == "__main__":
print(save_apod("YOUR API KEY"))
apollo_2011_items = get_archive_data("apollo 2011")["collection"]["items"]
print(apollo_2011_items[0]["data"][0]["description"])
| import shutil
import requests
def get_apod_data(api_key: str, download: bool = False, path: str = ".") -> dict:
"""
Get the APOD(Astronomical Picture of the day) data
Get your API Key from: https://api.nasa.gov/
"""
url = "https://api.nasa.gov/planetary/apod"
return requests.get(url, params={"api_key": api_key}).json()
def save_apod(api_key: str, path: str = ".") -> dict:
apod_data = get_apod_data(api_key)
img_url = apod_data["url"]
img_name = img_url.split("/")[-1]
response = requests.get(img_url, stream=True)
with open(f"{path}/{img_name}", "wb+") as img_file:
shutil.copyfileobj(response.raw, img_file)
del response
return apod_data
def get_archive_data(query: str) -> dict:
"""
Get the data of a particular query from NASA archives
"""
url = "https://images-api.nasa.gov/search"
return requests.get(url, params={"q": query}).json()
if __name__ == "__main__":
print(save_apod("YOUR API KEY"))
apollo_2011_items = get_archive_data("apollo 2011")["collection"]["items"]
print(apollo_2011_items[0]["data"][0]["description"])
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 50: https://projecteuler.net/problem=50
Consecutive prime sum
The prime 41, can be written as the sum of six consecutive primes:
41 = 2 + 3 + 5 + 7 + 11 + 13
This is the longest sum of consecutive primes that adds to a prime below
one-hundred.
The longest sum of consecutive primes below one-thousand that adds to a prime,
contains 21 terms, and is equal to 953.
Which prime, below one-million, can be written as the sum of the most
consecutive primes?
"""
from __future__ import annotations
def prime_sieve(limit: int) -> list[int]:
"""
Sieve of Erotosthenes
Function to return all the prime numbers up to a number 'limit'
https://en.wikipedia.org/wiki/Sieve_of_Eratosthenes
>>> prime_sieve(3)
[2]
>>> prime_sieve(50)
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
"""
is_prime = [True] * limit
is_prime[0] = False
is_prime[1] = False
is_prime[2] = True
for i in range(3, int(limit**0.5 + 1), 2):
index = i * 2
while index < limit:
is_prime[index] = False
index = index + i
primes = [2]
for i in range(3, limit, 2):
if is_prime[i]:
primes.append(i)
return primes
def solution(ceiling: int = 1_000_000) -> int:
"""
Returns the biggest prime, below the celing, that can be written as the sum
of consecutive the most consecutive primes.
>>> solution(500)
499
>>> solution(1_000)
953
>>> solution(10_000)
9521
"""
primes = prime_sieve(ceiling)
length = 0
largest = 0
for i in range(len(primes)):
for j in range(i + length, len(primes)):
sol = sum(primes[i:j])
if sol >= ceiling:
break
if sol in primes:
length = j - i
largest = sol
return largest
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler Problem 50: https://projecteuler.net/problem=50
Consecutive prime sum
The prime 41, can be written as the sum of six consecutive primes:
41 = 2 + 3 + 5 + 7 + 11 + 13
This is the longest sum of consecutive primes that adds to a prime below
one-hundred.
The longest sum of consecutive primes below one-thousand that adds to a prime,
contains 21 terms, and is equal to 953.
Which prime, below one-million, can be written as the sum of the most
consecutive primes?
"""
from __future__ import annotations
def prime_sieve(limit: int) -> list[int]:
"""
Sieve of Erotosthenes
Function to return all the prime numbers up to a number 'limit'
https://en.wikipedia.org/wiki/Sieve_of_Eratosthenes
>>> prime_sieve(3)
[2]
>>> prime_sieve(50)
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
"""
is_prime = [True] * limit
is_prime[0] = False
is_prime[1] = False
is_prime[2] = True
for i in range(3, int(limit**0.5 + 1), 2):
index = i * 2
while index < limit:
is_prime[index] = False
index = index + i
primes = [2]
for i in range(3, limit, 2):
if is_prime[i]:
primes.append(i)
return primes
def solution(ceiling: int = 1_000_000) -> int:
"""
Returns the biggest prime, below the celing, that can be written as the sum
of consecutive the most consecutive primes.
>>> solution(500)
499
>>> solution(1_000)
953
>>> solution(10_000)
9521
"""
primes = prime_sieve(ceiling)
length = 0
largest = 0
for i in range(len(primes)):
for j in range(i + length, len(primes)):
sol = sum(primes[i:j])
if sol >= ceiling:
break
if sol in primes:
length = j - i
largest = sol
return largest
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
https://en.wikipedia.org/wiki/Combination
"""
from math import factorial
def combinations(n: int, k: int) -> int:
"""
Returns the number of different combinations of k length which can
be made from n values, where n >= k.
Examples:
>>> combinations(10,5)
252
>>> combinations(6,3)
20
>>> combinations(20,5)
15504
>>> combinations(52, 5)
2598960
>>> combinations(0, 0)
1
>>> combinations(-4, -5)
...
Traceback (most recent call last):
ValueError: Please enter positive integers for n and k where n >= k
"""
# If either of the conditions are true, the function is being asked
# to calculate a factorial of a negative number, which is not possible
if n < k or k < 0:
raise ValueError("Please enter positive integers for n and k where n >= k")
return int(factorial(n) / ((factorial(k)) * (factorial(n - k))))
if __name__ == "__main__":
print(
"\nThe number of five-card hands possible from a standard",
f"fifty-two card deck is: {combinations(52, 5)}",
)
print(
"\nIf a class of 40 students must be arranged into groups of",
f"4 for group projects, there are {combinations(40, 4)} ways",
"to arrange them.\n",
)
print(
"If 10 teams are competing in a Formula One race, there",
f"are {combinations(10, 3)} ways that first, second and",
"third place can be awarded.\n",
)
| """
https://en.wikipedia.org/wiki/Combination
"""
from math import factorial
def combinations(n: int, k: int) -> int:
"""
Returns the number of different combinations of k length which can
be made from n values, where n >= k.
Examples:
>>> combinations(10,5)
252
>>> combinations(6,3)
20
>>> combinations(20,5)
15504
>>> combinations(52, 5)
2598960
>>> combinations(0, 0)
1
>>> combinations(-4, -5)
...
Traceback (most recent call last):
ValueError: Please enter positive integers for n and k where n >= k
"""
# If either of the conditions are true, the function is being asked
# to calculate a factorial of a negative number, which is not possible
if n < k or k < 0:
raise ValueError("Please enter positive integers for n and k where n >= k")
return int(factorial(n) / ((factorial(k)) * (factorial(n - k))))
if __name__ == "__main__":
print(
"\nThe number of five-card hands possible from a standard",
f"fifty-two card deck is: {combinations(52, 5)}",
)
print(
"\nIf a class of 40 students must be arranged into groups of",
f"4 for group projects, there are {combinations(40, 4)} ways",
"to arrange them.\n",
)
print(
"If 10 teams are competing in a Formula One race, there",
f"are {combinations(10, 3)} ways that first, second and",
"third place can be awarded.\n",
)
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Prime permutations
Problem 49
The arithmetic sequence, 1487, 4817, 8147, in which each of
the terms increases by 3330, is unusual in two ways:
(i) each of the three terms are prime,
(ii) each of the 4-digit numbers are permutations of one another.
There are no arithmetic sequences made up of three 1-, 2-, or 3-digit primes,
exhibiting this property, but there is one other 4-digit increasing sequence.
What 12-digit number do you form by concatenating the three terms in this sequence?
Solution:
First, we need to generate all 4 digits prime numbers. Then greedy
all of them and use permutation to form new numbers. Use binary search
to check if the permutated numbers is in our prime list and include
them in a candidate list.
After that, bruteforce all passed candidates sequences using
3 nested loops since we know the answer will be 12 digits.
The bruteforce of this solution will be about 1 sec.
"""
import math
from itertools import permutations
def is_prime(number: int) -> bool:
"""Checks to see if a number is a prime in O(sqrt(n)).
A number is prime if it has exactly two factors: 1 and itself.
>>> is_prime(0)
False
>>> is_prime(1)
False
>>> is_prime(2)
True
>>> is_prime(3)
True
>>> is_prime(27)
False
>>> is_prime(87)
False
>>> is_prime(563)
True
>>> is_prime(2999)
True
>>> is_prime(67483)
False
"""
if 1 < number < 4:
# 2 and 3 are primes
return True
elif number < 2 or number % 2 == 0 or number % 3 == 0:
# Negatives, 0, 1, all even numbers, all multiples of 3 are not primes
return False
# All primes number are in format of 6k +/- 1
for i in range(5, int(math.sqrt(number) + 1), 6):
if number % i == 0 or number % (i + 2) == 0:
return False
return True
def search(target: int, prime_list: list) -> bool:
"""
function to search a number in a list using Binary Search.
>>> search(3, [1, 2, 3])
True
>>> search(4, [1, 2, 3])
False
>>> search(101, list(range(-100, 100)))
False
"""
left, right = 0, len(prime_list) - 1
while left <= right:
middle = (left + right) // 2
if prime_list[middle] == target:
return True
elif prime_list[middle] < target:
left = middle + 1
else:
right = middle - 1
return False
def solution():
"""
Return the solution of the problem.
>>> solution()
296962999629
"""
prime_list = [n for n in range(1001, 10000, 2) if is_prime(n)]
candidates = []
for number in prime_list:
tmp_numbers = []
for prime_member in permutations(list(str(number))):
prime = int("".join(prime_member))
if prime % 2 == 0:
continue
if search(prime, prime_list):
tmp_numbers.append(prime)
tmp_numbers.sort()
if len(tmp_numbers) >= 3:
candidates.append(tmp_numbers)
passed = []
for candidate in candidates:
length = len(candidate)
found = False
for i in range(length):
for j in range(i + 1, length):
for k in range(j + 1, length):
if (
abs(candidate[i] - candidate[j])
== abs(candidate[j] - candidate[k])
and len({candidate[i], candidate[j], candidate[k]}) == 3
):
passed.append(
sorted([candidate[i], candidate[j], candidate[k]])
)
found = True
if found:
break
if found:
break
if found:
break
answer = set()
for seq in passed:
answer.add("".join([str(i) for i in seq]))
return max(int(x) for x in answer)
if __name__ == "__main__":
print(solution())
| """
Prime permutations
Problem 49
The arithmetic sequence, 1487, 4817, 8147, in which each of
the terms increases by 3330, is unusual in two ways:
(i) each of the three terms are prime,
(ii) each of the 4-digit numbers are permutations of one another.
There are no arithmetic sequences made up of three 1-, 2-, or 3-digit primes,
exhibiting this property, but there is one other 4-digit increasing sequence.
What 12-digit number do you form by concatenating the three terms in this sequence?
Solution:
First, we need to generate all 4 digits prime numbers. Then greedy
all of them and use permutation to form new numbers. Use binary search
to check if the permutated numbers is in our prime list and include
them in a candidate list.
After that, bruteforce all passed candidates sequences using
3 nested loops since we know the answer will be 12 digits.
The bruteforce of this solution will be about 1 sec.
"""
import math
from itertools import permutations
def is_prime(number: int) -> bool:
"""Checks to see if a number is a prime in O(sqrt(n)).
A number is prime if it has exactly two factors: 1 and itself.
>>> is_prime(0)
False
>>> is_prime(1)
False
>>> is_prime(2)
True
>>> is_prime(3)
True
>>> is_prime(27)
False
>>> is_prime(87)
False
>>> is_prime(563)
True
>>> is_prime(2999)
True
>>> is_prime(67483)
False
"""
if 1 < number < 4:
# 2 and 3 are primes
return True
elif number < 2 or number % 2 == 0 or number % 3 == 0:
# Negatives, 0, 1, all even numbers, all multiples of 3 are not primes
return False
# All primes number are in format of 6k +/- 1
for i in range(5, int(math.sqrt(number) + 1), 6):
if number % i == 0 or number % (i + 2) == 0:
return False
return True
def search(target: int, prime_list: list) -> bool:
"""
function to search a number in a list using Binary Search.
>>> search(3, [1, 2, 3])
True
>>> search(4, [1, 2, 3])
False
>>> search(101, list(range(-100, 100)))
False
"""
left, right = 0, len(prime_list) - 1
while left <= right:
middle = (left + right) // 2
if prime_list[middle] == target:
return True
elif prime_list[middle] < target:
left = middle + 1
else:
right = middle - 1
return False
def solution():
"""
Return the solution of the problem.
>>> solution()
296962999629
"""
prime_list = [n for n in range(1001, 10000, 2) if is_prime(n)]
candidates = []
for number in prime_list:
tmp_numbers = []
for prime_member in permutations(list(str(number))):
prime = int("".join(prime_member))
if prime % 2 == 0:
continue
if search(prime, prime_list):
tmp_numbers.append(prime)
tmp_numbers.sort()
if len(tmp_numbers) >= 3:
candidates.append(tmp_numbers)
passed = []
for candidate in candidates:
length = len(candidate)
found = False
for i in range(length):
for j in range(i + 1, length):
for k in range(j + 1, length):
if (
abs(candidate[i] - candidate[j])
== abs(candidate[j] - candidate[k])
and len({candidate[i], candidate[j], candidate[k]}) == 3
):
passed.append(
sorted([candidate[i], candidate[j], candidate[k]])
)
found = True
if found:
break
if found:
break
if found:
break
answer = set()
for seq in passed:
answer.add("".join([str(i) for i in seq]))
return max(int(x) for x in answer)
if __name__ == "__main__":
print(solution())
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
This is a pure Python implementation of the Harmonic Series algorithm
https://en.wikipedia.org/wiki/Harmonic_series_(mathematics)
For doctests run following command:
python -m doctest -v harmonic_series.py
or
python3 -m doctest -v harmonic_series.py
For manual testing run:
python3 harmonic_series.py
"""
def harmonic_series(n_term: str) -> list:
"""Pure Python implementation of Harmonic Series algorithm
:param n_term: The last (nth) term of Harmonic Series
:return: The Harmonic Series starting from 1 to last (nth) term
Examples:
>>> harmonic_series(5)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(5.0)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(5.1)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(-5)
[]
>>> harmonic_series(0)
[]
>>> harmonic_series(1)
['1']
"""
if n_term == "":
return []
series: list = []
for temp in range(int(n_term)):
series.append(f"1/{temp + 1}" if series else "1")
return series
if __name__ == "__main__":
nth_term = input("Enter the last number (nth term) of the Harmonic Series")
print("Formula of Harmonic Series => 1+1/2+1/3 ..... 1/n")
print(harmonic_series(nth_term))
| """
This is a pure Python implementation of the Harmonic Series algorithm
https://en.wikipedia.org/wiki/Harmonic_series_(mathematics)
For doctests run following command:
python -m doctest -v harmonic_series.py
or
python3 -m doctest -v harmonic_series.py
For manual testing run:
python3 harmonic_series.py
"""
def harmonic_series(n_term: str) -> list:
"""Pure Python implementation of Harmonic Series algorithm
:param n_term: The last (nth) term of Harmonic Series
:return: The Harmonic Series starting from 1 to last (nth) term
Examples:
>>> harmonic_series(5)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(5.0)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(5.1)
['1', '1/2', '1/3', '1/4', '1/5']
>>> harmonic_series(-5)
[]
>>> harmonic_series(0)
[]
>>> harmonic_series(1)
['1']
"""
if n_term == "":
return []
series: list = []
for temp in range(int(n_term)):
series.append(f"1/{temp + 1}" if series else "1")
return series
if __name__ == "__main__":
nth_term = input("Enter the last number (nth term) of the Harmonic Series")
print("Formula of Harmonic Series => 1+1/2+1/3 ..... 1/n")
print(harmonic_series(nth_term))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Problem 14: https://projecteuler.net/problem=14
Collatz conjecture: start with any positive integer n. Next term obtained from
the previous term as follows:
If the previous term is even, the next term is one half the previous term.
If the previous term is odd, the next term is 3 times the previous term plus 1.
The conjecture states the sequence will always reach 1 regardless of starting
n.
Problem Statement:
The following iterative sequence is defined for the set of positive integers:
n → n/2 (n is even)
n → 3n + 1 (n is odd)
Using the rule above and starting with 13, we generate the following sequence:
13 → 40 → 20 → 10 → 5 → 16 → 8 → 4 → 2 → 1
It can be seen that this sequence (starting at 13 and finishing at 1) contains
10 terms. Although it has not been proved yet (Collatz Problem), it is thought
that all starting numbers finish at 1.
Which starting number, under one million, produces the longest chain?
"""
from __future__ import annotations
COLLATZ_SEQUENCE_LENGTHS = {1: 1}
def collatz_sequence_length(n: int) -> int:
"""Returns the Collatz sequence length for n."""
if n in COLLATZ_SEQUENCE_LENGTHS:
return COLLATZ_SEQUENCE_LENGTHS[n]
if n % 2 == 0:
next_n = n // 2
else:
next_n = 3 * n + 1
sequence_length = collatz_sequence_length(next_n) + 1
COLLATZ_SEQUENCE_LENGTHS[n] = sequence_length
return sequence_length
def solution(n: int = 1000000) -> int:
"""Returns the number under n that generates the longest Collatz sequence.
>>> solution(1000000)
837799
>>> solution(200)
171
>>> solution(5000)
3711
>>> solution(15000)
13255
"""
result = max((collatz_sequence_length(i), i) for i in range(1, n))
return result[1]
if __name__ == "__main__":
print(solution(int(input().strip())))
| """
Problem 14: https://projecteuler.net/problem=14
Collatz conjecture: start with any positive integer n. Next term obtained from
the previous term as follows:
If the previous term is even, the next term is one half the previous term.
If the previous term is odd, the next term is 3 times the previous term plus 1.
The conjecture states the sequence will always reach 1 regardless of starting
n.
Problem Statement:
The following iterative sequence is defined for the set of positive integers:
n → n/2 (n is even)
n → 3n + 1 (n is odd)
Using the rule above and starting with 13, we generate the following sequence:
13 → 40 → 20 → 10 → 5 → 16 → 8 → 4 → 2 → 1
It can be seen that this sequence (starting at 13 and finishing at 1) contains
10 terms. Although it has not been proved yet (Collatz Problem), it is thought
that all starting numbers finish at 1.
Which starting number, under one million, produces the longest chain?
"""
from __future__ import annotations
COLLATZ_SEQUENCE_LENGTHS = {1: 1}
def collatz_sequence_length(n: int) -> int:
"""Returns the Collatz sequence length for n."""
if n in COLLATZ_SEQUENCE_LENGTHS:
return COLLATZ_SEQUENCE_LENGTHS[n]
if n % 2 == 0:
next_n = n // 2
else:
next_n = 3 * n + 1
sequence_length = collatz_sequence_length(next_n) + 1
COLLATZ_SEQUENCE_LENGTHS[n] = sequence_length
return sequence_length
def solution(n: int = 1000000) -> int:
"""Returns the number under n that generates the longest Collatz sequence.
>>> solution(1000000)
837799
>>> solution(200)
171
>>> solution(5000)
3711
>>> solution(15000)
13255
"""
result = max((collatz_sequence_length(i), i) for i in range(1, n))
return result[1]
if __name__ == "__main__":
print(solution(int(input().strip())))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from collections.abc import Sequence
def evaluate_poly(poly: Sequence[float], x: float) -> float:
"""Evaluate a polynomial f(x) at specified point x and return the value.
Arguments:
poly -- the coefficients of a polynomial as an iterable in order of
ascending degree
x -- the point at which to evaluate the polynomial
>>> evaluate_poly((0.0, 0.0, 5.0, 9.3, 7.0), 10.0)
79800.0
"""
return sum(c * (x**i) for i, c in enumerate(poly))
def horner(poly: Sequence[float], x: float) -> float:
"""Evaluate a polynomial at specified point using Horner's method.
In terms of computational complexity, Horner's method is an efficient method
of evaluating a polynomial. It avoids the use of expensive exponentiation,
and instead uses only multiplication and addition to evaluate the polynomial
in O(n), where n is the degree of the polynomial.
https://en.wikipedia.org/wiki/Horner's_method
Arguments:
poly -- the coefficients of a polynomial as an iterable in order of
ascending degree
x -- the point at which to evaluate the polynomial
>>> horner((0.0, 0.0, 5.0, 9.3, 7.0), 10.0)
79800.0
"""
result = 0.0
for coeff in reversed(poly):
result = result * x + coeff
return result
if __name__ == "__main__":
"""
Example:
>>> poly = (0.0, 0.0, 5.0, 9.3, 7.0) # f(x) = 7.0x^4 + 9.3x^3 + 5.0x^2
>>> x = -13.0
>>> # f(-13) = 7.0(-13)^4 + 9.3(-13)^3 + 5.0(-13)^2 = 180339.9
>>> print(evaluate_poly(poly, x))
180339.9
"""
poly = (0.0, 0.0, 5.0, 9.3, 7.0)
x = 10.0
print(evaluate_poly(poly, x))
print(horner(poly, x))
| from collections.abc import Sequence
def evaluate_poly(poly: Sequence[float], x: float) -> float:
"""Evaluate a polynomial f(x) at specified point x and return the value.
Arguments:
poly -- the coefficients of a polynomial as an iterable in order of
ascending degree
x -- the point at which to evaluate the polynomial
>>> evaluate_poly((0.0, 0.0, 5.0, 9.3, 7.0), 10.0)
79800.0
"""
return sum(c * (x**i) for i, c in enumerate(poly))
def horner(poly: Sequence[float], x: float) -> float:
"""Evaluate a polynomial at specified point using Horner's method.
In terms of computational complexity, Horner's method is an efficient method
of evaluating a polynomial. It avoids the use of expensive exponentiation,
and instead uses only multiplication and addition to evaluate the polynomial
in O(n), where n is the degree of the polynomial.
https://en.wikipedia.org/wiki/Horner's_method
Arguments:
poly -- the coefficients of a polynomial as an iterable in order of
ascending degree
x -- the point at which to evaluate the polynomial
>>> horner((0.0, 0.0, 5.0, 9.3, 7.0), 10.0)
79800.0
"""
result = 0.0
for coeff in reversed(poly):
result = result * x + coeff
return result
if __name__ == "__main__":
"""
Example:
>>> poly = (0.0, 0.0, 5.0, 9.3, 7.0) # f(x) = 7.0x^4 + 9.3x^3 + 5.0x^2
>>> x = -13.0
>>> # f(-13) = 7.0(-13)^4 + 9.3(-13)^3 + 5.0(-13)^2 = 180339.9
>>> print(evaluate_poly(poly, x))
180339.9
"""
poly = (0.0, 0.0, 5.0, 9.3, 7.0)
x = 10.0
print(evaluate_poly(poly, x))
print(horner(poly, x))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """Uses Pythagoras theorem to calculate the distance between two points in space."""
import math
class Point:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self) -> str:
return f"Point({self.x}, {self.y}, {self.z})"
def distance(a: Point, b: Point) -> float:
return math.sqrt(abs((b.x - a.x) ** 2 + (b.y - a.y) ** 2 + (b.z - a.z) ** 2))
def test_distance() -> None:
"""
>>> point1 = Point(2, -1, 7)
>>> point2 = Point(1, -3, 5)
>>> print(f"Distance from {point1} to {point2} is {distance(point1, point2)}")
Distance from Point(2, -1, 7) to Point(1, -3, 5) is 3.0
"""
pass
if __name__ == "__main__":
import doctest
doctest.testmod()
| """Uses Pythagoras theorem to calculate the distance between two points in space."""
import math
class Point:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
def __repr__(self) -> str:
return f"Point({self.x}, {self.y}, {self.z})"
def distance(a: Point, b: Point) -> float:
return math.sqrt(abs((b.x - a.x) ** 2 + (b.y - a.y) ** 2 + (b.z - a.z) ** 2))
def test_distance() -> None:
"""
>>> point1 = Point(2, -1, 7)
>>> point2 = Point(1, -3, 5)
>>> print(f"Distance from {point1} to {point2} is {distance(point1, point2)}")
Distance from Point(2, -1, 7) to Point(1, -3, 5) is 3.0
"""
pass
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from math import asin, atan, cos, radians, sin, sqrt, tan
AXIS_A = 6378137.0
AXIS_B = 6356752.314245
RADIUS = 6378137
def haversine_distance(lat1: float, lon1: float, lat2: float, lon2: float) -> float:
"""
Calculate great circle distance between two points in a sphere,
given longitudes and latitudes https://en.wikipedia.org/wiki/Haversine_formula
We know that the globe is "sort of" spherical, so a path between two points
isn't exactly a straight line. We need to account for the Earth's curvature
when calculating distance from point A to B. This effect is negligible for
small distances but adds up as distance increases. The Haversine method treats
the earth as a sphere which allows us to "project" the two points A and B
onto the surface of that sphere and approximate the spherical distance between
them. Since the Earth is not a perfect sphere, other methods which model the
Earth's ellipsoidal nature are more accurate but a quick and modifiable
computation like Haversine can be handy for shorter range distances.
Args:
lat1, lon1: latitude and longitude of coordinate 1
lat2, lon2: latitude and longitude of coordinate 2
Returns:
geographical distance between two points in metres
>>> from collections import namedtuple
>>> point_2d = namedtuple("point_2d", "lat lon")
>>> SAN_FRANCISCO = point_2d(37.774856, -122.424227)
>>> YOSEMITE = point_2d(37.864742, -119.537521)
>>> f"{haversine_distance(*SAN_FRANCISCO, *YOSEMITE):0,.0f} meters"
'254,352 meters'
"""
# CONSTANTS per WGS84 https://en.wikipedia.org/wiki/World_Geodetic_System
# Distance in metres(m)
# Equation parameters
# Equation https://en.wikipedia.org/wiki/Haversine_formula#Formulation
flattening = (AXIS_A - AXIS_B) / AXIS_A
phi_1 = atan((1 - flattening) * tan(radians(lat1)))
phi_2 = atan((1 - flattening) * tan(radians(lat2)))
lambda_1 = radians(lon1)
lambda_2 = radians(lon2)
# Equation
sin_sq_phi = sin((phi_2 - phi_1) / 2)
sin_sq_lambda = sin((lambda_2 - lambda_1) / 2)
# Square both values
sin_sq_phi *= sin_sq_phi
sin_sq_lambda *= sin_sq_lambda
h_value = sqrt(sin_sq_phi + (cos(phi_1) * cos(phi_2) * sin_sq_lambda))
return 2 * RADIUS * asin(h_value)
if __name__ == "__main__":
import doctest
doctest.testmod()
| from math import asin, atan, cos, radians, sin, sqrt, tan
AXIS_A = 6378137.0
AXIS_B = 6356752.314245
RADIUS = 6378137
def haversine_distance(lat1: float, lon1: float, lat2: float, lon2: float) -> float:
"""
Calculate great circle distance between two points in a sphere,
given longitudes and latitudes https://en.wikipedia.org/wiki/Haversine_formula
We know that the globe is "sort of" spherical, so a path between two points
isn't exactly a straight line. We need to account for the Earth's curvature
when calculating distance from point A to B. This effect is negligible for
small distances but adds up as distance increases. The Haversine method treats
the earth as a sphere which allows us to "project" the two points A and B
onto the surface of that sphere and approximate the spherical distance between
them. Since the Earth is not a perfect sphere, other methods which model the
Earth's ellipsoidal nature are more accurate but a quick and modifiable
computation like Haversine can be handy for shorter range distances.
Args:
lat1, lon1: latitude and longitude of coordinate 1
lat2, lon2: latitude and longitude of coordinate 2
Returns:
geographical distance between two points in metres
>>> from collections import namedtuple
>>> point_2d = namedtuple("point_2d", "lat lon")
>>> SAN_FRANCISCO = point_2d(37.774856, -122.424227)
>>> YOSEMITE = point_2d(37.864742, -119.537521)
>>> f"{haversine_distance(*SAN_FRANCISCO, *YOSEMITE):0,.0f} meters"
'254,352 meters'
"""
# CONSTANTS per WGS84 https://en.wikipedia.org/wiki/World_Geodetic_System
# Distance in metres(m)
# Equation parameters
# Equation https://en.wikipedia.org/wiki/Haversine_formula#Formulation
flattening = (AXIS_A - AXIS_B) / AXIS_A
phi_1 = atan((1 - flattening) * tan(radians(lat1)))
phi_2 = atan((1 - flattening) * tan(radians(lat2)))
lambda_1 = radians(lon1)
lambda_2 = radians(lon2)
# Equation
sin_sq_phi = sin((phi_2 - phi_1) / 2)
sin_sq_lambda = sin((lambda_2 - lambda_1) / 2)
# Square both values
sin_sq_phi *= sin_sq_phi
sin_sq_lambda *= sin_sq_lambda
h_value = sqrt(sin_sq_phi + (cos(phi_1) * cos(phi_2) * sin_sq_lambda))
return 2 * RADIUS * asin(h_value)
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import os
import sys
from . import rsa_key_generator as rkg
DEFAULT_BLOCK_SIZE = 128
BYTE_SIZE = 256
def get_blocks_from_text(
message: str, block_size: int = DEFAULT_BLOCK_SIZE
) -> list[int]:
message_bytes = message.encode("ascii")
block_ints = []
for block_start in range(0, len(message_bytes), block_size):
block_int = 0
for i in range(block_start, min(block_start + block_size, len(message_bytes))):
block_int += message_bytes[i] * (BYTE_SIZE ** (i % block_size))
block_ints.append(block_int)
return block_ints
def get_text_from_blocks(
block_ints: list[int], message_length: int, block_size: int = DEFAULT_BLOCK_SIZE
) -> str:
message: list[str] = []
for block_int in block_ints:
block_message: list[str] = []
for i in range(block_size - 1, -1, -1):
if len(message) + i < message_length:
ascii_number = block_int // (BYTE_SIZE**i)
block_int = block_int % (BYTE_SIZE**i)
block_message.insert(0, chr(ascii_number))
message.extend(block_message)
return "".join(message)
def encrypt_message(
message: str, key: tuple[int, int], block_size: int = DEFAULT_BLOCK_SIZE
) -> list[int]:
encrypted_blocks = []
n, e = key
for block in get_blocks_from_text(message, block_size):
encrypted_blocks.append(pow(block, e, n))
return encrypted_blocks
def decrypt_message(
encrypted_blocks: list[int],
message_length: int,
key: tuple[int, int],
block_size: int = DEFAULT_BLOCK_SIZE,
) -> str:
decrypted_blocks = []
n, d = key
for block in encrypted_blocks:
decrypted_blocks.append(pow(block, d, n))
return get_text_from_blocks(decrypted_blocks, message_length, block_size)
def read_key_file(key_filename: str) -> tuple[int, int, int]:
with open(key_filename) as fo:
content = fo.read()
key_size, n, eor_d = content.split(",")
return (int(key_size), int(n), int(eor_d))
def encrypt_and_write_to_file(
message_filename: str,
key_filename: str,
message: str,
block_size: int = DEFAULT_BLOCK_SIZE,
) -> str:
key_size, n, e = read_key_file(key_filename)
if key_size < block_size * 8:
sys.exit(
"ERROR: Block size is %s bits and key size is %s bits. The RSA cipher "
"requires the block size to be equal to or greater than the key size. "
"Either decrease the block size or use different keys."
% (block_size * 8, key_size)
)
encrypted_blocks = [str(i) for i in encrypt_message(message, (n, e), block_size)]
encrypted_content = ",".join(encrypted_blocks)
encrypted_content = f"{len(message)}_{block_size}_{encrypted_content}"
with open(message_filename, "w") as fo:
fo.write(encrypted_content)
return encrypted_content
def read_from_file_and_decrypt(message_filename: str, key_filename: str) -> str:
key_size, n, d = read_key_file(key_filename)
with open(message_filename) as fo:
content = fo.read()
message_length_str, block_size_str, encrypted_message = content.split("_")
message_length = int(message_length_str)
block_size = int(block_size_str)
if key_size < block_size * 8:
sys.exit(
"ERROR: Block size is %s bits and key size is %s bits. The RSA cipher "
"requires the block size to be equal to or greater than the key size. "
"Did you specify the correct key file and encrypted file?"
% (block_size * 8, key_size)
)
encrypted_blocks = []
for block in encrypted_message.split(","):
encrypted_blocks.append(int(block))
return decrypt_message(encrypted_blocks, message_length, (n, d), block_size)
def main() -> None:
filename = "encrypted_file.txt"
response = input(r"Encrypt\Decrypt [e\d]: ")
if response.lower().startswith("e"):
mode = "encrypt"
elif response.lower().startswith("d"):
mode = "decrypt"
if mode == "encrypt":
if not os.path.exists("rsa_pubkey.txt"):
rkg.make_key_files("rsa", 1024)
message = input("\nEnter message: ")
pubkey_filename = "rsa_pubkey.txt"
print(f"Encrypting and writing to {filename}...")
encrypted_text = encrypt_and_write_to_file(filename, pubkey_filename, message)
print("\nEncrypted text:")
print(encrypted_text)
elif mode == "decrypt":
privkey_filename = "rsa_privkey.txt"
print(f"Reading from {filename} and decrypting...")
decrypted_text = read_from_file_and_decrypt(filename, privkey_filename)
print("writing decryption to rsa_decryption.txt...")
with open("rsa_decryption.txt", "w") as dec:
dec.write(decrypted_text)
print("\nDecryption:")
print(decrypted_text)
if __name__ == "__main__":
main()
| import os
import sys
from . import rsa_key_generator as rkg
DEFAULT_BLOCK_SIZE = 128
BYTE_SIZE = 256
def get_blocks_from_text(
message: str, block_size: int = DEFAULT_BLOCK_SIZE
) -> list[int]:
message_bytes = message.encode("ascii")
block_ints = []
for block_start in range(0, len(message_bytes), block_size):
block_int = 0
for i in range(block_start, min(block_start + block_size, len(message_bytes))):
block_int += message_bytes[i] * (BYTE_SIZE ** (i % block_size))
block_ints.append(block_int)
return block_ints
def get_text_from_blocks(
block_ints: list[int], message_length: int, block_size: int = DEFAULT_BLOCK_SIZE
) -> str:
message: list[str] = []
for block_int in block_ints:
block_message: list[str] = []
for i in range(block_size - 1, -1, -1):
if len(message) + i < message_length:
ascii_number = block_int // (BYTE_SIZE**i)
block_int = block_int % (BYTE_SIZE**i)
block_message.insert(0, chr(ascii_number))
message.extend(block_message)
return "".join(message)
def encrypt_message(
message: str, key: tuple[int, int], block_size: int = DEFAULT_BLOCK_SIZE
) -> list[int]:
encrypted_blocks = []
n, e = key
for block in get_blocks_from_text(message, block_size):
encrypted_blocks.append(pow(block, e, n))
return encrypted_blocks
def decrypt_message(
encrypted_blocks: list[int],
message_length: int,
key: tuple[int, int],
block_size: int = DEFAULT_BLOCK_SIZE,
) -> str:
decrypted_blocks = []
n, d = key
for block in encrypted_blocks:
decrypted_blocks.append(pow(block, d, n))
return get_text_from_blocks(decrypted_blocks, message_length, block_size)
def read_key_file(key_filename: str) -> tuple[int, int, int]:
with open(key_filename) as fo:
content = fo.read()
key_size, n, eor_d = content.split(",")
return (int(key_size), int(n), int(eor_d))
def encrypt_and_write_to_file(
message_filename: str,
key_filename: str,
message: str,
block_size: int = DEFAULT_BLOCK_SIZE,
) -> str:
key_size, n, e = read_key_file(key_filename)
if key_size < block_size * 8:
sys.exit(
"ERROR: Block size is %s bits and key size is %s bits. The RSA cipher "
"requires the block size to be equal to or greater than the key size. "
"Either decrease the block size or use different keys."
% (block_size * 8, key_size)
)
encrypted_blocks = [str(i) for i in encrypt_message(message, (n, e), block_size)]
encrypted_content = ",".join(encrypted_blocks)
encrypted_content = f"{len(message)}_{block_size}_{encrypted_content}"
with open(message_filename, "w") as fo:
fo.write(encrypted_content)
return encrypted_content
def read_from_file_and_decrypt(message_filename: str, key_filename: str) -> str:
key_size, n, d = read_key_file(key_filename)
with open(message_filename) as fo:
content = fo.read()
message_length_str, block_size_str, encrypted_message = content.split("_")
message_length = int(message_length_str)
block_size = int(block_size_str)
if key_size < block_size * 8:
sys.exit(
"ERROR: Block size is %s bits and key size is %s bits. The RSA cipher "
"requires the block size to be equal to or greater than the key size. "
"Did you specify the correct key file and encrypted file?"
% (block_size * 8, key_size)
)
encrypted_blocks = []
for block in encrypted_message.split(","):
encrypted_blocks.append(int(block))
return decrypt_message(encrypted_blocks, message_length, (n, d), block_size)
def main() -> None:
filename = "encrypted_file.txt"
response = input(r"Encrypt\Decrypt [e\d]: ")
if response.lower().startswith("e"):
mode = "encrypt"
elif response.lower().startswith("d"):
mode = "decrypt"
if mode == "encrypt":
if not os.path.exists("rsa_pubkey.txt"):
rkg.make_key_files("rsa", 1024)
message = input("\nEnter message: ")
pubkey_filename = "rsa_pubkey.txt"
print(f"Encrypting and writing to {filename}...")
encrypted_text = encrypt_and_write_to_file(filename, pubkey_filename, message)
print("\nEncrypted text:")
print(encrypted_text)
elif mode == "decrypt":
privkey_filename = "rsa_privkey.txt"
print(f"Reading from {filename} and decrypting...")
decrypted_text = read_from_file_and_decrypt(filename, privkey_filename)
print("writing decryption to rsa_decryption.txt...")
with open("rsa_decryption.txt", "w") as dec:
dec.write(decrypted_text)
print("\nDecryption:")
print(decrypted_text)
if __name__ == "__main__":
main()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import pandas as pd
from matplotlib import pyplot as plt
from sklearn.linear_model import LinearRegression
# Splitting the dataset into the Training set and Test set
from sklearn.model_selection import train_test_split
# Fitting Polynomial Regression to the dataset
from sklearn.preprocessing import PolynomialFeatures
# Importing the dataset
dataset = pd.read_csv(
"https://s3.us-west-2.amazonaws.com/public.gamelab.fun/dataset/"
"position_salaries.csv"
)
X = dataset.iloc[:, 1:2].values
y = dataset.iloc[:, 2].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
poly_reg = PolynomialFeatures(degree=4)
X_poly = poly_reg.fit_transform(X)
pol_reg = LinearRegression()
pol_reg.fit(X_poly, y)
# Visualizing the Polymonial Regression results
def viz_polymonial():
plt.scatter(X, y, color="red")
plt.plot(X, pol_reg.predict(poly_reg.fit_transform(X)), color="blue")
plt.title("Truth or Bluff (Linear Regression)")
plt.xlabel("Position level")
plt.ylabel("Salary")
plt.show()
return
if __name__ == "__main__":
viz_polymonial()
# Predicting a new result with Polymonial Regression
pol_reg.predict(poly_reg.fit_transform([[5.5]]))
# output should be 132148.43750003
| import pandas as pd
from matplotlib import pyplot as plt
from sklearn.linear_model import LinearRegression
# Splitting the dataset into the Training set and Test set
from sklearn.model_selection import train_test_split
# Fitting Polynomial Regression to the dataset
from sklearn.preprocessing import PolynomialFeatures
# Importing the dataset
dataset = pd.read_csv(
"https://s3.us-west-2.amazonaws.com/public.gamelab.fun/dataset/"
"position_salaries.csv"
)
X = dataset.iloc[:, 1:2].values
y = dataset.iloc[:, 2].values
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
poly_reg = PolynomialFeatures(degree=4)
X_poly = poly_reg.fit_transform(X)
pol_reg = LinearRegression()
pol_reg.fit(X_poly, y)
# Visualizing the Polymonial Regression results
def viz_polymonial():
plt.scatter(X, y, color="red")
plt.plot(X, pol_reg.predict(poly_reg.fit_transform(X)), color="blue")
plt.title("Truth or Bluff (Linear Regression)")
plt.xlabel("Position level")
plt.ylabel("Salary")
plt.show()
return
if __name__ == "__main__":
viz_polymonial()
# Predicting a new result with Polymonial Regression
pol_reg.predict(poly_reg.fit_transform([[5.5]]))
# output should be 132148.43750003
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
This script demonstrates the implementation of the Sigmoid function.
The function takes a vector of K real numbers as input and then 1 / (1 + exp(-x)).
After through Sigmoid, the element of the vector mostly 0 between 1. or 1 between -1.
Script inspired from its corresponding Wikipedia article
https://en.wikipedia.org/wiki/Sigmoid_function
"""
import numpy as np
def sigmoid(vector: np.array) -> np.array:
"""
Implements the sigmoid function
Parameters:
vector (np.array): A numpy array of shape (1,n)
consisting of real values
Returns:
sigmoid_vec (np.array): The input numpy array, after applying
sigmoid.
Examples:
>>> sigmoid(np.array([-1.0, 1.0, 2.0]))
array([0.26894142, 0.73105858, 0.88079708])
>>> sigmoid(np.array([0.0]))
array([0.5])
"""
return 1 / (1 + np.exp(-vector))
if __name__ == "__main__":
import doctest
doctest.testmod()
| """
This script demonstrates the implementation of the Sigmoid function.
The function takes a vector of K real numbers as input and then 1 / (1 + exp(-x)).
After through Sigmoid, the element of the vector mostly 0 between 1. or 1 between -1.
Script inspired from its corresponding Wikipedia article
https://en.wikipedia.org/wiki/Sigmoid_function
"""
import numpy as np
def sigmoid(vector: np.array) -> np.array:
"""
Implements the sigmoid function
Parameters:
vector (np.array): A numpy array of shape (1,n)
consisting of real values
Returns:
sigmoid_vec (np.array): The input numpy array, after applying
sigmoid.
Examples:
>>> sigmoid(np.array([-1.0, 1.0, 2.0]))
array([0.26894142, 0.73105858, 0.88079708])
>>> sigmoid(np.array([0.0]))
array([0.5])
"""
return 1 / (1 + np.exp(-vector))
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Approximates the area under the curve using the trapezoidal rule
"""
from __future__ import annotations
from collections.abc import Callable
def trapezoidal_area(
fnc: Callable[[int | float], int | float],
x_start: int | float,
x_end: int | float,
steps: int = 100,
) -> float:
"""
Treats curve as a collection of linear lines and sums the area of the
trapezium shape they form
:param fnc: a function which defines a curve
:param x_start: left end point to indicate the start of line segment
:param x_end: right end point to indicate end of line segment
:param steps: an accuracy gauge; more steps increases the accuracy
:return: a float representing the length of the curve
>>> def f(x):
... return 5
>>> f"{trapezoidal_area(f, 12.0, 14.0, 1000):.3f}"
'10.000'
>>> def f(x):
... return 9*x**2
>>> f"{trapezoidal_area(f, -4.0, 0, 10000):.4f}"
'192.0000'
>>> f"{trapezoidal_area(f, -4.0, 4.0, 10000):.4f}"
'384.0000'
"""
x1 = x_start
fx1 = fnc(x_start)
area = 0.0
for _ in range(steps):
# Approximates small segments of curve as linear and solve
# for trapezoidal area
x2 = (x_end - x_start) / steps + x1
fx2 = fnc(x2)
area += abs(fx2 + fx1) * (x2 - x1) / 2
# Increment step
x1 = x2
fx1 = fx2
return area
if __name__ == "__main__":
def f(x):
return x**3 + x**2
print("f(x) = x^3 + x^2")
print("The area between the curve, x = -5, x = 5 and the x axis is:")
i = 10
while i <= 100000:
print(f"with {i} steps: {trapezoidal_area(f, -5, 5, i)}")
i *= 10
| """
Approximates the area under the curve using the trapezoidal rule
"""
from __future__ import annotations
from collections.abc import Callable
def trapezoidal_area(
fnc: Callable[[int | float], int | float],
x_start: int | float,
x_end: int | float,
steps: int = 100,
) -> float:
"""
Treats curve as a collection of linear lines and sums the area of the
trapezium shape they form
:param fnc: a function which defines a curve
:param x_start: left end point to indicate the start of line segment
:param x_end: right end point to indicate end of line segment
:param steps: an accuracy gauge; more steps increases the accuracy
:return: a float representing the length of the curve
>>> def f(x):
... return 5
>>> f"{trapezoidal_area(f, 12.0, 14.0, 1000):.3f}"
'10.000'
>>> def f(x):
... return 9*x**2
>>> f"{trapezoidal_area(f, -4.0, 0, 10000):.4f}"
'192.0000'
>>> f"{trapezoidal_area(f, -4.0, 4.0, 10000):.4f}"
'384.0000'
"""
x1 = x_start
fx1 = fnc(x_start)
area = 0.0
for _ in range(steps):
# Approximates small segments of curve as linear and solve
# for trapezoidal area
x2 = (x_end - x_start) / steps + x1
fx2 = fnc(x2)
area += abs(fx2 + fx1) * (x2 - x1) / 2
# Increment step
x1 = x2
fx1 = fx2
return area
if __name__ == "__main__":
def f(x):
return x**3 + x**2
print("f(x) = x^3 + x^2")
print("The area between the curve, x = -5, x = 5 and the x axis is:")
i = 10
while i <= 100000:
print(f"with {i} steps: {trapezoidal_area(f, -5, 5, i)}")
i *= 10
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
The nqueens problem is of placing N queens on a N * N
chess board such that no queen can attack any other queens placed
on that chess board.
This means that one queen cannot have any other queen on its horizontal, vertical and
diagonal lines.
"""
from __future__ import annotations
solution = []
def is_safe(board: list[list[int]], row: int, column: int) -> bool:
"""
This function returns a boolean value True if it is safe to place a queen there
considering the current state of the board.
Parameters :
board(2D matrix) : board
row ,column : coordinates of the cell on a board
Returns :
Boolean Value
"""
for i in range(len(board)):
if board[row][i] == 1:
return False
for i in range(len(board)):
if board[i][column] == 1:
return False
for i, j in zip(range(row, -1, -1), range(column, -1, -1)):
if board[i][j] == 1:
return False
for i, j in zip(range(row, -1, -1), range(column, len(board))):
if board[i][j] == 1:
return False
return True
def solve(board: list[list[int]], row: int) -> bool:
"""
It creates a state space tree and calls the safe function until it receives a
False Boolean and terminates that branch and backtracks to the next
possible solution branch.
"""
if row >= len(board):
"""
If the row number exceeds N we have board with a successful combination
and that combination is appended to the solution list and the board is printed.
"""
solution.append(board)
printboard(board)
print()
return True
for i in range(len(board)):
"""
For every row it iterates through each column to check if it is feasible to
place a queen there.
If all the combinations for that particular branch are successful the board is
reinitialized for the next possible combination.
"""
if is_safe(board, row, i):
board[row][i] = 1
solve(board, row + 1)
board[row][i] = 0
return False
def printboard(board: list[list[int]]) -> None:
"""
Prints the boards that have a successful combination.
"""
for i in range(len(board)):
for j in range(len(board)):
if board[i][j] == 1:
print("Q", end=" ")
else:
print(".", end=" ")
print()
# n=int(input("The no. of queens"))
n = 8
board = [[0 for i in range(n)] for j in range(n)]
solve(board, 0)
print("The total no. of solutions are :", len(solution))
| """
The nqueens problem is of placing N queens on a N * N
chess board such that no queen can attack any other queens placed
on that chess board.
This means that one queen cannot have any other queen on its horizontal, vertical and
diagonal lines.
"""
from __future__ import annotations
solution = []
def is_safe(board: list[list[int]], row: int, column: int) -> bool:
"""
This function returns a boolean value True if it is safe to place a queen there
considering the current state of the board.
Parameters :
board(2D matrix) : board
row ,column : coordinates of the cell on a board
Returns :
Boolean Value
"""
for i in range(len(board)):
if board[row][i] == 1:
return False
for i in range(len(board)):
if board[i][column] == 1:
return False
for i, j in zip(range(row, -1, -1), range(column, -1, -1)):
if board[i][j] == 1:
return False
for i, j in zip(range(row, -1, -1), range(column, len(board))):
if board[i][j] == 1:
return False
return True
def solve(board: list[list[int]], row: int) -> bool:
"""
It creates a state space tree and calls the safe function until it receives a
False Boolean and terminates that branch and backtracks to the next
possible solution branch.
"""
if row >= len(board):
"""
If the row number exceeds N we have board with a successful combination
and that combination is appended to the solution list and the board is printed.
"""
solution.append(board)
printboard(board)
print()
return True
for i in range(len(board)):
"""
For every row it iterates through each column to check if it is feasible to
place a queen there.
If all the combinations for that particular branch are successful the board is
reinitialized for the next possible combination.
"""
if is_safe(board, row, i):
board[row][i] = 1
solve(board, row + 1)
board[row][i] = 0
return False
def printboard(board: list[list[int]]) -> None:
"""
Prints the boards that have a successful combination.
"""
for i in range(len(board)):
for j in range(len(board)):
if board[i][j] == 1:
print("Q", end=" ")
else:
print(".", end=" ")
print()
# n=int(input("The no. of queens"))
n = 8
board = [[0 for i in range(n)] for j in range(n)]
solve(board, 0)
print("The total no. of solutions are :", len(solution))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import itertools
import string
from collections.abc import Generator, Iterable
def chunker(seq: Iterable[str], size: int) -> Generator[tuple[str, ...], None, None]:
it = iter(seq)
while True:
chunk = tuple(itertools.islice(it, size))
if not chunk:
return
yield chunk
def prepare_input(dirty: str) -> str:
"""
Prepare the plaintext by up-casing it
and separating repeated letters with X's
"""
dirty = "".join([c.upper() for c in dirty if c in string.ascii_letters])
clean = ""
if len(dirty) < 2:
return dirty
for i in range(len(dirty) - 1):
clean += dirty[i]
if dirty[i] == dirty[i + 1]:
clean += "X"
clean += dirty[-1]
if len(clean) & 1:
clean += "X"
return clean
def generate_table(key: str) -> list[str]:
# I and J are used interchangeably to allow
# us to use a 5x5 table (25 letters)
alphabet = "ABCDEFGHIKLMNOPQRSTUVWXYZ"
# we're using a list instead of a '2d' array because it makes the math
# for setting up the table and doing the actual encoding/decoding simpler
table = []
# copy key chars into the table if they are in `alphabet` ignoring duplicates
for char in key.upper():
if char not in table and char in alphabet:
table.append(char)
# fill the rest of the table in with the remaining alphabet chars
for char in alphabet:
if char not in table:
table.append(char)
return table
def encode(plaintext: str, key: str) -> str:
table = generate_table(key)
plaintext = prepare_input(plaintext)
ciphertext = ""
# https://en.wikipedia.org/wiki/Playfair_cipher#Description
for char1, char2 in chunker(plaintext, 2):
row1, col1 = divmod(table.index(char1), 5)
row2, col2 = divmod(table.index(char2), 5)
if row1 == row2:
ciphertext += table[row1 * 5 + (col1 + 1) % 5]
ciphertext += table[row2 * 5 + (col2 + 1) % 5]
elif col1 == col2:
ciphertext += table[((row1 + 1) % 5) * 5 + col1]
ciphertext += table[((row2 + 1) % 5) * 5 + col2]
else: # rectangle
ciphertext += table[row1 * 5 + col2]
ciphertext += table[row2 * 5 + col1]
return ciphertext
def decode(ciphertext: str, key: str) -> str:
table = generate_table(key)
plaintext = ""
# https://en.wikipedia.org/wiki/Playfair_cipher#Description
for char1, char2 in chunker(ciphertext, 2):
row1, col1 = divmod(table.index(char1), 5)
row2, col2 = divmod(table.index(char2), 5)
if row1 == row2:
plaintext += table[row1 * 5 + (col1 - 1) % 5]
plaintext += table[row2 * 5 + (col2 - 1) % 5]
elif col1 == col2:
plaintext += table[((row1 - 1) % 5) * 5 + col1]
plaintext += table[((row2 - 1) % 5) * 5 + col2]
else: # rectangle
plaintext += table[row1 * 5 + col2]
plaintext += table[row2 * 5 + col1]
return plaintext
| import itertools
import string
from collections.abc import Generator, Iterable
def chunker(seq: Iterable[str], size: int) -> Generator[tuple[str, ...], None, None]:
it = iter(seq)
while True:
chunk = tuple(itertools.islice(it, size))
if not chunk:
return
yield chunk
def prepare_input(dirty: str) -> str:
"""
Prepare the plaintext by up-casing it
and separating repeated letters with X's
"""
dirty = "".join([c.upper() for c in dirty if c in string.ascii_letters])
clean = ""
if len(dirty) < 2:
return dirty
for i in range(len(dirty) - 1):
clean += dirty[i]
if dirty[i] == dirty[i + 1]:
clean += "X"
clean += dirty[-1]
if len(clean) & 1:
clean += "X"
return clean
def generate_table(key: str) -> list[str]:
# I and J are used interchangeably to allow
# us to use a 5x5 table (25 letters)
alphabet = "ABCDEFGHIKLMNOPQRSTUVWXYZ"
# we're using a list instead of a '2d' array because it makes the math
# for setting up the table and doing the actual encoding/decoding simpler
table = []
# copy key chars into the table if they are in `alphabet` ignoring duplicates
for char in key.upper():
if char not in table and char in alphabet:
table.append(char)
# fill the rest of the table in with the remaining alphabet chars
for char in alphabet:
if char not in table:
table.append(char)
return table
def encode(plaintext: str, key: str) -> str:
table = generate_table(key)
plaintext = prepare_input(plaintext)
ciphertext = ""
# https://en.wikipedia.org/wiki/Playfair_cipher#Description
for char1, char2 in chunker(plaintext, 2):
row1, col1 = divmod(table.index(char1), 5)
row2, col2 = divmod(table.index(char2), 5)
if row1 == row2:
ciphertext += table[row1 * 5 + (col1 + 1) % 5]
ciphertext += table[row2 * 5 + (col2 + 1) % 5]
elif col1 == col2:
ciphertext += table[((row1 + 1) % 5) * 5 + col1]
ciphertext += table[((row2 + 1) % 5) * 5 + col2]
else: # rectangle
ciphertext += table[row1 * 5 + col2]
ciphertext += table[row2 * 5 + col1]
return ciphertext
def decode(ciphertext: str, key: str) -> str:
table = generate_table(key)
plaintext = ""
# https://en.wikipedia.org/wiki/Playfair_cipher#Description
for char1, char2 in chunker(ciphertext, 2):
row1, col1 = divmod(table.index(char1), 5)
row2, col2 = divmod(table.index(char2), 5)
if row1 == row2:
plaintext += table[row1 * 5 + (col1 - 1) % 5]
plaintext += table[row2 * 5 + (col2 - 1) % 5]
elif col1 == col2:
plaintext += table[((row1 - 1) % 5) * 5 + col1]
plaintext += table[((row2 - 1) % 5) * 5 + col2]
else: # rectangle
plaintext += table[row1 * 5 + col2]
plaintext += table[row2 * 5 + col1]
return plaintext
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| import os
UPPERLETTERS = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
LETTERS_AND_SPACE = UPPERLETTERS + UPPERLETTERS.lower() + " \t\n"
def load_dictionary() -> dict[str, None]:
path = os.path.split(os.path.realpath(__file__))
english_words: dict[str, None] = {}
with open(path[0] + "/dictionary.txt") as dictionary_file:
for word in dictionary_file.read().split("\n"):
english_words[word] = None
return english_words
ENGLISH_WORDS = load_dictionary()
def get_english_count(message: str) -> float:
message = message.upper()
message = remove_non_letters(message)
possible_words = message.split()
if possible_words == []:
return 0.0
matches = 0
for word in possible_words:
if word in ENGLISH_WORDS:
matches += 1
return float(matches) / len(possible_words)
def remove_non_letters(message: str) -> str:
letters_only = []
for symbol in message:
if symbol in LETTERS_AND_SPACE:
letters_only.append(symbol)
return "".join(letters_only)
def is_english(
message: str, word_percentage: int = 20, letter_percentage: int = 85
) -> bool:
"""
>>> is_english('Hello World')
True
>>> is_english('llold HorWd')
False
"""
words_match = get_english_count(message) * 100 >= word_percentage
num_letters = len(remove_non_letters(message))
message_letters_percentage = (float(num_letters) / len(message)) * 100
letters_match = message_letters_percentage >= letter_percentage
return words_match and letters_match
if __name__ == "__main__":
import doctest
doctest.testmod()
| import os
UPPERLETTERS = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
LETTERS_AND_SPACE = UPPERLETTERS + UPPERLETTERS.lower() + " \t\n"
def load_dictionary() -> dict[str, None]:
path = os.path.split(os.path.realpath(__file__))
english_words: dict[str, None] = {}
with open(path[0] + "/dictionary.txt") as dictionary_file:
for word in dictionary_file.read().split("\n"):
english_words[word] = None
return english_words
ENGLISH_WORDS = load_dictionary()
def get_english_count(message: str) -> float:
message = message.upper()
message = remove_non_letters(message)
possible_words = message.split()
if possible_words == []:
return 0.0
matches = 0
for word in possible_words:
if word in ENGLISH_WORDS:
matches += 1
return float(matches) / len(possible_words)
def remove_non_letters(message: str) -> str:
letters_only = []
for symbol in message:
if symbol in LETTERS_AND_SPACE:
letters_only.append(symbol)
return "".join(letters_only)
def is_english(
message: str, word_percentage: int = 20, letter_percentage: int = 85
) -> bool:
"""
>>> is_english('Hello World')
True
>>> is_english('llold HorWd')
False
"""
words_match = get_english_count(message) * 100 >= word_percentage
num_letters = len(remove_non_letters(message))
message_letters_percentage = (float(num_letters) / len(message)) * 100
letters_match = message_letters_percentage >= letter_percentage
return words_match and letters_match
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 2: https://projecteuler.net/problem=2
Even Fibonacci Numbers
Each new term in the Fibonacci sequence is generated by adding the previous
two terms. By starting with 1 and 2, the first 10 terms will be:
1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
By considering the terms in the Fibonacci sequence whose values do not exceed
four million, find the sum of the even-valued terms.
References:
- https://en.wikipedia.org/wiki/Fibonacci_number
"""
def solution(n: int = 4000000) -> int:
"""
Returns the sum of all even fibonacci sequence elements that are lower
or equal to n.
>>> solution(10)
10
>>> solution(15)
10
>>> solution(2)
2
>>> solution(1)
0
>>> solution(34)
44
"""
i = 1
j = 2
total = 0
while j <= n:
if j % 2 == 0:
total += j
i, j = j, i + j
return total
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler Problem 2: https://projecteuler.net/problem=2
Even Fibonacci Numbers
Each new term in the Fibonacci sequence is generated by adding the previous
two terms. By starting with 1 and 2, the first 10 terms will be:
1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
By considering the terms in the Fibonacci sequence whose values do not exceed
four million, find the sum of the even-valued terms.
References:
- https://en.wikipedia.org/wiki/Fibonacci_number
"""
def solution(n: int = 4000000) -> int:
"""
Returns the sum of all even fibonacci sequence elements that are lower
or equal to n.
>>> solution(10)
10
>>> solution(15)
10
>>> solution(2)
2
>>> solution(1)
0
>>> solution(34)
44
"""
i = 1
j = 2
total = 0
while j <= n:
if j % 2 == 0:
total += j
i, j = j, i + j
return total
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Amicable Numbers
Problem 21
Let d(n) be defined as the sum of proper divisors of n (numbers less than n
which divide evenly into n).
If d(a) = b and d(b) = a, where a ≠ b, then a and b are an amicable pair and
each of a and b are called amicable numbers.
For example, the proper divisors of 220 are 1, 2, 4, 5, 10, 11, 20, 22, 44, 55
and 110; therefore d(220) = 284. The proper divisors of 284 are 1, 2, 4, 71 and
142; so d(284) = 220.
Evaluate the sum of all the amicable numbers under 10000.
"""
from math import sqrt
def sum_of_divisors(n: int) -> int:
total = 0
for i in range(1, int(sqrt(n) + 1)):
if n % i == 0 and i != sqrt(n):
total += i + n // i
elif i == sqrt(n):
total += i
return total - n
def solution(n: int = 10000) -> int:
"""Returns the sum of all the amicable numbers under n.
>>> solution(10000)
31626
>>> solution(5000)
8442
>>> solution(1000)
504
>>> solution(100)
0
>>> solution(50)
0
"""
total = sum(
i
for i in range(1, n)
if sum_of_divisors(sum_of_divisors(i)) == i and sum_of_divisors(i) != i
)
return total
if __name__ == "__main__":
print(solution(int(str(input()).strip())))
| """
Amicable Numbers
Problem 21
Let d(n) be defined as the sum of proper divisors of n (numbers less than n
which divide evenly into n).
If d(a) = b and d(b) = a, where a ≠ b, then a and b are an amicable pair and
each of a and b are called amicable numbers.
For example, the proper divisors of 220 are 1, 2, 4, 5, 10, 11, 20, 22, 44, 55
and 110; therefore d(220) = 284. The proper divisors of 284 are 1, 2, 4, 71 and
142; so d(284) = 220.
Evaluate the sum of all the amicable numbers under 10000.
"""
from math import sqrt
def sum_of_divisors(n: int) -> int:
total = 0
for i in range(1, int(sqrt(n) + 1)):
if n % i == 0 and i != sqrt(n):
total += i + n // i
elif i == sqrt(n):
total += i
return total - n
def solution(n: int = 10000) -> int:
"""Returns the sum of all the amicable numbers under n.
>>> solution(10000)
31626
>>> solution(5000)
8442
>>> solution(1000)
504
>>> solution(100)
0
>>> solution(50)
0
"""
total = sum(
i
for i in range(1, n)
if sum_of_divisors(sum_of_divisors(i)) == i and sum_of_divisors(i) != i
)
return total
if __name__ == "__main__":
print(solution(int(str(input()).strip())))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from __future__ import annotations
from bisect import bisect_left
from functools import total_ordering
from heapq import merge
"""
A pure Python implementation of the patience sort algorithm
For more information: https://en.wikipedia.org/wiki/Patience_sorting
This algorithm is based on the card game patience
For doctests run following command:
python3 -m doctest -v patience_sort.py
For manual testing run:
python3 patience_sort.py
"""
@total_ordering
class Stack(list):
def __lt__(self, other):
return self[-1] < other[-1]
def __eq__(self, other):
return self[-1] == other[-1]
def patience_sort(collection: list) -> list:
"""A pure implementation of patience sort algorithm in Python
:param collection: some mutable ordered collection with heterogeneous
comparable items inside
:return: the same collection ordered by ascending
Examples:
>>> patience_sort([1, 9, 5, 21, 17, 6])
[1, 5, 6, 9, 17, 21]
>>> patience_sort([])
[]
>>> patience_sort([-3, -17, -48])
[-48, -17, -3]
"""
stacks: list[Stack] = []
# sort into stacks
for element in collection:
new_stacks = Stack([element])
i = bisect_left(stacks, new_stacks)
if i != len(stacks):
stacks[i].append(element)
else:
stacks.append(new_stacks)
# use a heap-based merge to merge stack efficiently
collection[:] = merge(*(reversed(stack) for stack in stacks))
return collection
if __name__ == "__main__":
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(patience_sort(unsorted))
| from __future__ import annotations
from bisect import bisect_left
from functools import total_ordering
from heapq import merge
"""
A pure Python implementation of the patience sort algorithm
For more information: https://en.wikipedia.org/wiki/Patience_sorting
This algorithm is based on the card game patience
For doctests run following command:
python3 -m doctest -v patience_sort.py
For manual testing run:
python3 patience_sort.py
"""
@total_ordering
class Stack(list):
def __lt__(self, other):
return self[-1] < other[-1]
def __eq__(self, other):
return self[-1] == other[-1]
def patience_sort(collection: list) -> list:
"""A pure implementation of patience sort algorithm in Python
:param collection: some mutable ordered collection with heterogeneous
comparable items inside
:return: the same collection ordered by ascending
Examples:
>>> patience_sort([1, 9, 5, 21, 17, 6])
[1, 5, 6, 9, 17, 21]
>>> patience_sort([])
[]
>>> patience_sort([-3, -17, -48])
[-48, -17, -3]
"""
stacks: list[Stack] = []
# sort into stacks
for element in collection:
new_stacks = Stack([element])
i = bisect_left(stacks, new_stacks)
if i != len(stacks):
stacks[i].append(element)
else:
stacks.append(new_stacks)
# use a heap-based merge to merge stack efficiently
collection[:] = merge(*(reversed(stack) for stack in stacks))
return collection
if __name__ == "__main__":
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(patience_sort(unsorted))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """Created by Nathan Damon, @bizzfitch on github
>>> test_miller_rabin()
"""
def miller_rabin(n: int, allow_probable: bool = False) -> bool:
"""Deterministic Miller-Rabin algorithm for primes ~< 3.32e24.
Uses numerical analysis results to return whether or not the passed number
is prime. If the passed number is above the upper limit, and
allow_probable is True, then a return value of True indicates that n is
probably prime. This test does not allow False negatives- a return value
of False is ALWAYS composite.
Parameters
----------
n : int
The integer to be tested. Since we usually care if a number is prime,
n < 2 returns False instead of raising a ValueError.
allow_probable: bool, default False
Whether or not to test n above the upper bound of the deterministic test.
Raises
------
ValueError
Reference
---------
https://en.wikipedia.org/wiki/Miller%E2%80%93Rabin_primality_test
"""
if n == 2:
return True
if not n % 2 or n < 2:
return False
if n > 5 and n % 10 not in (1, 3, 7, 9): # can quickly check last digit
return False
if n > 3_317_044_064_679_887_385_961_981 and not allow_probable:
raise ValueError(
"Warning: upper bound of deterministic test is exceeded. "
"Pass allow_probable=True to allow probabilistic test. "
"A return value of True indicates a probable prime."
)
# array bounds provided by analysis
bounds = [
2_047,
1_373_653,
25_326_001,
3_215_031_751,
2_152_302_898_747,
3_474_749_660_383,
341_550_071_728_321,
1,
3_825_123_056_546_413_051,
1,
1,
318_665_857_834_031_151_167_461,
3_317_044_064_679_887_385_961_981,
]
primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41]
for idx, _p in enumerate(bounds, 1):
if n < _p:
# then we have our last prime to check
plist = primes[:idx]
break
d, s = n - 1, 0
# break up n -1 into a power of 2 (s) and
# remaining odd component
# essentially, solve for d * 2 ** s == n - 1
while d % 2 == 0:
d //= 2
s += 1
for prime in plist:
pr = False
for r in range(s):
m = pow(prime, d * 2**r, n)
# see article for analysis explanation for m
if (r == 0 and m == 1) or ((m + 1) % n == 0):
pr = True
# this loop will not determine compositeness
break
if pr:
continue
# if pr is False, then the above loop never evaluated to true,
# and the n MUST be composite
return False
return True
def test_miller_rabin() -> None:
"""Testing a nontrivial (ends in 1, 3, 7, 9) composite
and a prime in each range.
"""
assert not miller_rabin(561)
assert miller_rabin(563)
# 2047
assert not miller_rabin(838_201)
assert miller_rabin(838_207)
# 1_373_653
assert not miller_rabin(17_316_001)
assert miller_rabin(17_316_017)
# 25_326_001
assert not miller_rabin(3_078_386_641)
assert miller_rabin(3_078_386_653)
# 3_215_031_751
assert not miller_rabin(1_713_045_574_801)
assert miller_rabin(1_713_045_574_819)
# 2_152_302_898_747
assert not miller_rabin(2_779_799_728_307)
assert miller_rabin(2_779_799_728_327)
# 3_474_749_660_383
assert not miller_rabin(113_850_023_909_441)
assert miller_rabin(113_850_023_909_527)
# 341_550_071_728_321
assert not miller_rabin(1_275_041_018_848_804_351)
assert miller_rabin(1_275_041_018_848_804_391)
# 3_825_123_056_546_413_051
assert not miller_rabin(79_666_464_458_507_787_791_867)
assert miller_rabin(79_666_464_458_507_787_791_951)
# 318_665_857_834_031_151_167_461
assert not miller_rabin(552_840_677_446_647_897_660_333)
assert miller_rabin(552_840_677_446_647_897_660_359)
# 3_317_044_064_679_887_385_961_981
# upper limit for probabilistic test
if __name__ == "__main__":
test_miller_rabin()
| """Created by Nathan Damon, @bizzfitch on github
>>> test_miller_rabin()
"""
def miller_rabin(n: int, allow_probable: bool = False) -> bool:
"""Deterministic Miller-Rabin algorithm for primes ~< 3.32e24.
Uses numerical analysis results to return whether or not the passed number
is prime. If the passed number is above the upper limit, and
allow_probable is True, then a return value of True indicates that n is
probably prime. This test does not allow False negatives- a return value
of False is ALWAYS composite.
Parameters
----------
n : int
The integer to be tested. Since we usually care if a number is prime,
n < 2 returns False instead of raising a ValueError.
allow_probable: bool, default False
Whether or not to test n above the upper bound of the deterministic test.
Raises
------
ValueError
Reference
---------
https://en.wikipedia.org/wiki/Miller%E2%80%93Rabin_primality_test
"""
if n == 2:
return True
if not n % 2 or n < 2:
return False
if n > 5 and n % 10 not in (1, 3, 7, 9): # can quickly check last digit
return False
if n > 3_317_044_064_679_887_385_961_981 and not allow_probable:
raise ValueError(
"Warning: upper bound of deterministic test is exceeded. "
"Pass allow_probable=True to allow probabilistic test. "
"A return value of True indicates a probable prime."
)
# array bounds provided by analysis
bounds = [
2_047,
1_373_653,
25_326_001,
3_215_031_751,
2_152_302_898_747,
3_474_749_660_383,
341_550_071_728_321,
1,
3_825_123_056_546_413_051,
1,
1,
318_665_857_834_031_151_167_461,
3_317_044_064_679_887_385_961_981,
]
primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41]
for idx, _p in enumerate(bounds, 1):
if n < _p:
# then we have our last prime to check
plist = primes[:idx]
break
d, s = n - 1, 0
# break up n -1 into a power of 2 (s) and
# remaining odd component
# essentially, solve for d * 2 ** s == n - 1
while d % 2 == 0:
d //= 2
s += 1
for prime in plist:
pr = False
for r in range(s):
m = pow(prime, d * 2**r, n)
# see article for analysis explanation for m
if (r == 0 and m == 1) or ((m + 1) % n == 0):
pr = True
# this loop will not determine compositeness
break
if pr:
continue
# if pr is False, then the above loop never evaluated to true,
# and the n MUST be composite
return False
return True
def test_miller_rabin() -> None:
"""Testing a nontrivial (ends in 1, 3, 7, 9) composite
and a prime in each range.
"""
assert not miller_rabin(561)
assert miller_rabin(563)
# 2047
assert not miller_rabin(838_201)
assert miller_rabin(838_207)
# 1_373_653
assert not miller_rabin(17_316_001)
assert miller_rabin(17_316_017)
# 25_326_001
assert not miller_rabin(3_078_386_641)
assert miller_rabin(3_078_386_653)
# 3_215_031_751
assert not miller_rabin(1_713_045_574_801)
assert miller_rabin(1_713_045_574_819)
# 2_152_302_898_747
assert not miller_rabin(2_779_799_728_307)
assert miller_rabin(2_779_799_728_327)
# 3_474_749_660_383
assert not miller_rabin(113_850_023_909_441)
assert miller_rabin(113_850_023_909_527)
# 341_550_071_728_321
assert not miller_rabin(1_275_041_018_848_804_351)
assert miller_rabin(1_275_041_018_848_804_391)
# 3_825_123_056_546_413_051
assert not miller_rabin(79_666_464_458_507_787_791_867)
assert miller_rabin(79_666_464_458_507_787_791_951)
# 318_665_857_834_031_151_167_461
assert not miller_rabin(552_840_677_446_647_897_660_333)
assert miller_rabin(552_840_677_446_647_897_660_359)
# 3_317_044_064_679_887_385_961_981
# upper limit for probabilistic test
if __name__ == "__main__":
test_miller_rabin()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 587: https://projecteuler.net/problem=587
A square is drawn around a circle as shown in the diagram below on the left.
We shall call the blue shaded region the L-section.
A line is drawn from the bottom left of the square to the top right
as shown in the diagram on the right.
We shall call the orange shaded region a concave triangle.
It should be clear that the concave triangle occupies exactly half of the L-section.
Two circles are placed next to each other horizontally,
a rectangle is drawn around both circles, and
a line is drawn from the bottom left to the top right as shown in the diagram below.
This time the concave triangle occupies approximately 36.46% of the L-section.
If n circles are placed next to each other horizontally,
a rectangle is drawn around the n circles, and
a line is drawn from the bottom left to the top right,
then it can be shown that the least value of n
for which the concave triangle occupies less than 10% of the L-section is n = 15.
What is the least value of n
for which the concave triangle occupies less than 0.1% of the L-section?
"""
from itertools import count
from math import asin, pi, sqrt
def circle_bottom_arc_integral(point: float) -> float:
"""
Returns integral of circle bottom arc y = 1 / 2 - sqrt(1 / 4 - (x - 1 / 2) ^ 2)
>>> circle_bottom_arc_integral(0)
0.39269908169872414
>>> circle_bottom_arc_integral(1 / 2)
0.44634954084936207
>>> circle_bottom_arc_integral(1)
0.5
"""
return (
(1 - 2 * point) * sqrt(point - point**2) + 2 * point + asin(sqrt(1 - point))
) / 4
def concave_triangle_area(circles_number: int) -> float:
"""
Returns area of concave triangle
>>> concave_triangle_area(1)
0.026825229575318944
>>> concave_triangle_area(2)
0.01956236140083944
"""
intersection_y = (circles_number + 1 - sqrt(2 * circles_number)) / (
2 * (circles_number**2 + 1)
)
intersection_x = circles_number * intersection_y
triangle_area = intersection_x * intersection_y / 2
concave_region_area = circle_bottom_arc_integral(
1 / 2
) - circle_bottom_arc_integral(intersection_x)
return triangle_area + concave_region_area
def solution(fraction: float = 1 / 1000) -> int:
"""
Returns least value of n
for which the concave triangle occupies less than fraction of the L-section
>>> solution(1 / 10)
15
"""
l_section_area = (1 - pi / 4) / 4
for n in count(1):
if concave_triangle_area(n) / l_section_area < fraction:
return n
return -1
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler Problem 587: https://projecteuler.net/problem=587
A square is drawn around a circle as shown in the diagram below on the left.
We shall call the blue shaded region the L-section.
A line is drawn from the bottom left of the square to the top right
as shown in the diagram on the right.
We shall call the orange shaded region a concave triangle.
It should be clear that the concave triangle occupies exactly half of the L-section.
Two circles are placed next to each other horizontally,
a rectangle is drawn around both circles, and
a line is drawn from the bottom left to the top right as shown in the diagram below.
This time the concave triangle occupies approximately 36.46% of the L-section.
If n circles are placed next to each other horizontally,
a rectangle is drawn around the n circles, and
a line is drawn from the bottom left to the top right,
then it can be shown that the least value of n
for which the concave triangle occupies less than 10% of the L-section is n = 15.
What is the least value of n
for which the concave triangle occupies less than 0.1% of the L-section?
"""
from itertools import count
from math import asin, pi, sqrt
def circle_bottom_arc_integral(point: float) -> float:
"""
Returns integral of circle bottom arc y = 1 / 2 - sqrt(1 / 4 - (x - 1 / 2) ^ 2)
>>> circle_bottom_arc_integral(0)
0.39269908169872414
>>> circle_bottom_arc_integral(1 / 2)
0.44634954084936207
>>> circle_bottom_arc_integral(1)
0.5
"""
return (
(1 - 2 * point) * sqrt(point - point**2) + 2 * point + asin(sqrt(1 - point))
) / 4
def concave_triangle_area(circles_number: int) -> float:
"""
Returns area of concave triangle
>>> concave_triangle_area(1)
0.026825229575318944
>>> concave_triangle_area(2)
0.01956236140083944
"""
intersection_y = (circles_number + 1 - sqrt(2 * circles_number)) / (
2 * (circles_number**2 + 1)
)
intersection_x = circles_number * intersection_y
triangle_area = intersection_x * intersection_y / 2
concave_region_area = circle_bottom_arc_integral(
1 / 2
) - circle_bottom_arc_integral(intersection_x)
return triangle_area + concave_region_area
def solution(fraction: float = 1 / 1000) -> int:
"""
Returns least value of n
for which the concave triangle occupies less than fraction of the L-section
>>> solution(1 / 10)
15
"""
l_section_area = (1 - pi / 4) / 4
for n in count(1):
if concave_triangle_area(n) / l_section_area < fraction:
return n
return -1
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| def excel_title_to_column(column_title: str) -> int:
"""
Given a string column_title that represents
the column title in an Excel sheet, return
its corresponding column number.
>>> excel_title_to_column("A")
1
>>> excel_title_to_column("B")
2
>>> excel_title_to_column("AB")
28
>>> excel_title_to_column("Z")
26
"""
assert column_title.isupper()
answer = 0
index = len(column_title) - 1
power = 0
while index >= 0:
value = (ord(column_title[index]) - 64) * pow(26, power)
answer += value
power += 1
index -= 1
return answer
if __name__ == "__main__":
from doctest import testmod
testmod()
| def excel_title_to_column(column_title: str) -> int:
"""
Given a string column_title that represents
the column title in an Excel sheet, return
its corresponding column number.
>>> excel_title_to_column("A")
1
>>> excel_title_to_column("B")
2
>>> excel_title_to_column("AB")
28
>>> excel_title_to_column("Z")
26
"""
assert column_title.isupper()
answer = 0
index = len(column_title) - 1
power = 0
while index >= 0:
value = (ord(column_title[index]) - 64) * pow(26, power)
answer += value
power += 1
index -= 1
return answer
if __name__ == "__main__":
from doctest import testmod
testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from math import pi
def radians(degree: float) -> float:
"""
Coverts the given angle from degrees to radians
https://en.wikipedia.org/wiki/Radian
>>> radians(180)
3.141592653589793
>>> radians(92)
1.6057029118347832
>>> radians(274)
4.782202150464463
>>> radians(109.82)
1.9167205845401725
>>> from math import radians as math_radians
>>> all(abs(radians(i)-math_radians(i)) <= 0.00000001 for i in range(-2, 361))
True
"""
return degree / (180 / pi)
if __name__ == "__main__":
from doctest import testmod
testmod()
| from math import pi
def radians(degree: float) -> float:
"""
Coverts the given angle from degrees to radians
https://en.wikipedia.org/wiki/Radian
>>> radians(180)
3.141592653589793
>>> radians(92)
1.6057029118347832
>>> radians(274)
4.782202150464463
>>> radians(109.82)
1.9167205845401725
>>> from math import radians as math_radians
>>> all(abs(radians(i)-math_radians(i)) <= 0.00000001 for i in range(-2, 361))
True
"""
return degree / (180 / pi)
if __name__ == "__main__":
from doctest import testmod
testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Linked Lists consists of Nodes.
Nodes contain data and also may link to other nodes:
- Head Node: First node, the address of the
head node gives us access of the complete list
- Last node: points to null
"""
from __future__ import annotations
from typing import Any
class Node:
def __init__(self, item: Any, next: Any) -> None: # noqa: A002
self.item = item
self.next = next
class LinkedList:
def __init__(self) -> None:
self.head: Node | None = None
self.size = 0
def add(self, item: Any) -> None:
self.head = Node(item, self.head)
self.size += 1
def remove(self) -> Any:
# Switched 'self.is_empty()' to 'self.head is None'
# because mypy was considering the possibility that 'self.head'
# can be None in below else part and giving error
if self.head is None:
return None
else:
item = self.head.item
self.head = self.head.next
self.size -= 1
return item
def is_empty(self) -> bool:
return self.head is None
def __str__(self) -> str:
"""
>>> linked_list = LinkedList()
>>> linked_list.add(23)
>>> linked_list.add(14)
>>> linked_list.add(9)
>>> print(linked_list)
9 --> 14 --> 23
"""
if not self.is_empty:
return ""
else:
iterate = self.head
item_str = ""
item_list: list[str] = []
while iterate:
item_list.append(str(iterate.item))
iterate = iterate.next
item_str = " --> ".join(item_list)
return item_str
def __len__(self) -> int:
"""
>>> linked_list = LinkedList()
>>> len(linked_list)
0
>>> linked_list.add("a")
>>> len(linked_list)
1
>>> linked_list.add("b")
>>> len(linked_list)
2
>>> _ = linked_list.remove()
>>> len(linked_list)
1
>>> _ = linked_list.remove()
>>> len(linked_list)
0
"""
return self.size
| """
Linked Lists consists of Nodes.
Nodes contain data and also may link to other nodes:
- Head Node: First node, the address of the
head node gives us access of the complete list
- Last node: points to null
"""
from __future__ import annotations
from typing import Any
class Node:
def __init__(self, item: Any, next: Any) -> None: # noqa: A002
self.item = item
self.next = next
class LinkedList:
def __init__(self) -> None:
self.head: Node | None = None
self.size = 0
def add(self, item: Any) -> None:
self.head = Node(item, self.head)
self.size += 1
def remove(self) -> Any:
# Switched 'self.is_empty()' to 'self.head is None'
# because mypy was considering the possibility that 'self.head'
# can be None in below else part and giving error
if self.head is None:
return None
else:
item = self.head.item
self.head = self.head.next
self.size -= 1
return item
def is_empty(self) -> bool:
return self.head is None
def __str__(self) -> str:
"""
>>> linked_list = LinkedList()
>>> linked_list.add(23)
>>> linked_list.add(14)
>>> linked_list.add(9)
>>> print(linked_list)
9 --> 14 --> 23
"""
if not self.is_empty:
return ""
else:
iterate = self.head
item_str = ""
item_list: list[str] = []
while iterate:
item_list.append(str(iterate.item))
iterate = iterate.next
item_str = " --> ".join(item_list)
return item_str
def __len__(self) -> int:
"""
>>> linked_list = LinkedList()
>>> len(linked_list)
0
>>> linked_list.add("a")
>>> len(linked_list)
1
>>> linked_list.add("b")
>>> len(linked_list)
2
>>> _ = linked_list.remove()
>>> len(linked_list)
1
>>> _ = linked_list.remove()
>>> len(linked_list)
0
"""
return self.size
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Checks if a system of forces is in static equilibrium.
"""
from __future__ import annotations
from numpy import array, cos, cross, float64, radians, sin
from numpy.typing import NDArray
def polar_force(
magnitude: float, angle: float, radian_mode: bool = False
) -> list[float]:
"""
Resolves force along rectangular components.
(force, angle) => (force_x, force_y)
>>> import math
>>> force = polar_force(10, 45)
>>> math.isclose(force[0], 7.071067811865477)
True
>>> math.isclose(force[1], 7.0710678118654755)
True
>>> force = polar_force(10, 3.14, radian_mode=True)
>>> math.isclose(force[0], -9.999987317275396)
True
>>> math.isclose(force[1], 0.01592652916486828)
True
"""
if radian_mode:
return [magnitude * cos(angle), magnitude * sin(angle)]
return [magnitude * cos(radians(angle)), magnitude * sin(radians(angle))]
def in_static_equilibrium(
forces: NDArray[float64], location: NDArray[float64], eps: float = 10**-1
) -> bool:
"""
Check if a system is in equilibrium.
It takes two numpy.array objects.
forces ==> [
[force1_x, force1_y],
[force2_x, force2_y],
....]
location ==> [
[x1, y1],
[x2, y2],
....]
>>> force = array([[1, 1], [-1, 2]])
>>> location = array([[1, 0], [10, 0]])
>>> in_static_equilibrium(force, location)
False
"""
# summation of moments is zero
moments: NDArray[float64] = cross(location, forces)
sum_moments: float = sum(moments)
return abs(sum_moments) < eps
if __name__ == "__main__":
# Test to check if it works
forces = array(
[
polar_force(718.4, 180 - 30),
polar_force(879.54, 45),
polar_force(100, -90),
]
)
location: NDArray[float64] = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem 1 in image_data/2D_problems.jpg
forces = array(
[
polar_force(30 * 9.81, 15),
polar_force(215, 180 - 45),
polar_force(264, 90 - 30),
]
)
location = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem in image_data/2D_problems_1.jpg
forces = array([[0, -2000], [0, -1200], [0, 15600], [0, -12400]])
location = array([[0, 0], [6, 0], [10, 0], [12, 0]])
assert in_static_equilibrium(forces, location)
import doctest
doctest.testmod()
| """
Checks if a system of forces is in static equilibrium.
"""
from __future__ import annotations
from numpy import array, cos, cross, float64, radians, sin
from numpy.typing import NDArray
def polar_force(
magnitude: float, angle: float, radian_mode: bool = False
) -> list[float]:
"""
Resolves force along rectangular components.
(force, angle) => (force_x, force_y)
>>> import math
>>> force = polar_force(10, 45)
>>> math.isclose(force[0], 7.071067811865477)
True
>>> math.isclose(force[1], 7.0710678118654755)
True
>>> force = polar_force(10, 3.14, radian_mode=True)
>>> math.isclose(force[0], -9.999987317275396)
True
>>> math.isclose(force[1], 0.01592652916486828)
True
"""
if radian_mode:
return [magnitude * cos(angle), magnitude * sin(angle)]
return [magnitude * cos(radians(angle)), magnitude * sin(radians(angle))]
def in_static_equilibrium(
forces: NDArray[float64], location: NDArray[float64], eps: float = 10**-1
) -> bool:
"""
Check if a system is in equilibrium.
It takes two numpy.array objects.
forces ==> [
[force1_x, force1_y],
[force2_x, force2_y],
....]
location ==> [
[x1, y1],
[x2, y2],
....]
>>> force = array([[1, 1], [-1, 2]])
>>> location = array([[1, 0], [10, 0]])
>>> in_static_equilibrium(force, location)
False
"""
# summation of moments is zero
moments: NDArray[float64] = cross(location, forces)
sum_moments: float = sum(moments)
return abs(sum_moments) < eps
if __name__ == "__main__":
# Test to check if it works
forces = array(
[
polar_force(718.4, 180 - 30),
polar_force(879.54, 45),
polar_force(100, -90),
]
)
location: NDArray[float64] = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem 1 in image_data/2D_problems.jpg
forces = array(
[
polar_force(30 * 9.81, 15),
polar_force(215, 180 - 45),
polar_force(264, 90 - 30),
]
)
location = array([[0, 0], [0, 0], [0, 0]])
assert in_static_equilibrium(forces, location)
# Problem in image_data/2D_problems_1.jpg
forces = array([[0, -2000], [0, -1200], [0, 15600], [0, -12400]])
location = array([[0, 0], [6, 0], [10, 0], [12, 0]])
assert in_static_equilibrium(forces, location)
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """ https://en.wikipedia.org/wiki/Cocktail_shaker_sort """
def cocktail_shaker_sort(unsorted: list) -> list:
"""
Pure implementation of the cocktail shaker sort algorithm in Python.
>>> cocktail_shaker_sort([4, 5, 2, 1, 2])
[1, 2, 2, 4, 5]
>>> cocktail_shaker_sort([-4, 5, 0, 1, 2, 11])
[-4, 0, 1, 2, 5, 11]
>>> cocktail_shaker_sort([0.1, -2.4, 4.4, 2.2])
[-2.4, 0.1, 2.2, 4.4]
>>> cocktail_shaker_sort([1, 2, 3, 4, 5])
[1, 2, 3, 4, 5]
>>> cocktail_shaker_sort([-4, -5, -24, -7, -11])
[-24, -11, -7, -5, -4]
"""
for i in range(len(unsorted) - 1, 0, -1):
swapped = False
for j in range(i, 0, -1):
if unsorted[j] < unsorted[j - 1]:
unsorted[j], unsorted[j - 1] = unsorted[j - 1], unsorted[j]
swapped = True
for j in range(i):
if unsorted[j] > unsorted[j + 1]:
unsorted[j], unsorted[j + 1] = unsorted[j + 1], unsorted[j]
swapped = True
if not swapped:
break
return unsorted
if __name__ == "__main__":
import doctest
doctest.testmod()
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(f"{cocktail_shaker_sort(unsorted) = }")
| """ https://en.wikipedia.org/wiki/Cocktail_shaker_sort """
def cocktail_shaker_sort(unsorted: list) -> list:
"""
Pure implementation of the cocktail shaker sort algorithm in Python.
>>> cocktail_shaker_sort([4, 5, 2, 1, 2])
[1, 2, 2, 4, 5]
>>> cocktail_shaker_sort([-4, 5, 0, 1, 2, 11])
[-4, 0, 1, 2, 5, 11]
>>> cocktail_shaker_sort([0.1, -2.4, 4.4, 2.2])
[-2.4, 0.1, 2.2, 4.4]
>>> cocktail_shaker_sort([1, 2, 3, 4, 5])
[1, 2, 3, 4, 5]
>>> cocktail_shaker_sort([-4, -5, -24, -7, -11])
[-24, -11, -7, -5, -4]
"""
for i in range(len(unsorted) - 1, 0, -1):
swapped = False
for j in range(i, 0, -1):
if unsorted[j] < unsorted[j - 1]:
unsorted[j], unsorted[j - 1] = unsorted[j - 1], unsorted[j]
swapped = True
for j in range(i):
if unsorted[j] > unsorted[j + 1]:
unsorted[j], unsorted[j + 1] = unsorted[j + 1], unsorted[j]
swapped = True
if not swapped:
break
return unsorted
if __name__ == "__main__":
import doctest
doctest.testmod()
user_input = input("Enter numbers separated by a comma:\n").strip()
unsorted = [int(item) for item in user_input.split(",")]
print(f"{cocktail_shaker_sort(unsorted) = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
If we are presented with the first k terms of a sequence it is impossible to say with
certainty the value of the next term, as there are infinitely many polynomial functions
that can model the sequence.
As an example, let us consider the sequence of cube
numbers. This is defined by the generating function,
u(n) = n3: 1, 8, 27, 64, 125, 216, ...
Suppose we were only given the first two terms of this sequence. Working on the
principle that "simple is best" we should assume a linear relationship and predict the
next term to be 15 (common difference 7). Even if we were presented with the first three
terms, by the same principle of simplicity, a quadratic relationship should be
assumed.
We shall define OP(k, n) to be the nth term of the optimum polynomial
generating function for the first k terms of a sequence. It should be clear that
OP(k, n) will accurately generate the terms of the sequence for n ≤ k, and potentially
the first incorrect term (FIT) will be OP(k, k+1); in which case we shall call it a
bad OP (BOP).
As a basis, if we were only given the first term of sequence, it would be most
sensible to assume constancy; that is, for n ≥ 2, OP(1, n) = u(1).
Hence we obtain the
following OPs for the cubic sequence:
OP(1, n) = 1 1, 1, 1, 1, ...
OP(2, n) = 7n-6 1, 8, 15, ...
OP(3, n) = 6n^2-11n+6 1, 8, 27, 58, ...
OP(4, n) = n^3 1, 8, 27, 64, 125, ...
Clearly no BOPs exist for k ≥ 4.
By considering the sum of FITs generated by the BOPs (indicated in red above), we
obtain 1 + 15 + 58 = 74.
Consider the following tenth degree polynomial generating function:
1 - n + n^2 - n^3 + n^4 - n^5 + n^6 - n^7 + n^8 - n^9 + n^10
Find the sum of FITs for the BOPs.
"""
from __future__ import annotations
from collections.abc import Callable
Matrix = list[list[float | int]]
def solve(matrix: Matrix, vector: Matrix) -> Matrix:
"""
Solve the linear system of equations Ax = b (A = "matrix", b = "vector")
for x using Gaussian elimination and back substitution. We assume that A
is an invertible square matrix and that b is a column vector of the
same height.
>>> solve([[1, 0], [0, 1]], [[1],[2]])
[[1.0], [2.0]]
>>> solve([[2, 1, -1],[-3, -1, 2],[-2, 1, 2]],[[8], [-11],[-3]])
[[2.0], [3.0], [-1.0]]
"""
size: int = len(matrix)
augmented: Matrix = [[0 for _ in range(size + 1)] for _ in range(size)]
row: int
row2: int
col: int
col2: int
pivot_row: int
ratio: float
for row in range(size):
for col in range(size):
augmented[row][col] = matrix[row][col]
augmented[row][size] = vector[row][0]
row = 0
col = 0
while row < size and col < size:
# pivoting
pivot_row = max((abs(augmented[row2][col]), row2) for row2 in range(col, size))[
1
]
if augmented[pivot_row][col] == 0:
col += 1
continue
else:
augmented[row], augmented[pivot_row] = augmented[pivot_row], augmented[row]
for row2 in range(row + 1, size):
ratio = augmented[row2][col] / augmented[row][col]
augmented[row2][col] = 0
for col2 in range(col + 1, size + 1):
augmented[row2][col2] -= augmented[row][col2] * ratio
row += 1
col += 1
# back substitution
for col in range(1, size):
for row in range(col):
ratio = augmented[row][col] / augmented[col][col]
for col2 in range(col, size + 1):
augmented[row][col2] -= augmented[col][col2] * ratio
# round to get rid of numbers like 2.000000000000004
return [
[round(augmented[row][size] / augmented[row][row], 10)] for row in range(size)
]
def interpolate(y_list: list[int]) -> Callable[[int], int]:
"""
Given a list of data points (1,y0),(2,y1), ..., return a function that
interpolates the data points. We find the coefficients of the interpolating
polynomial by solving a system of linear equations corresponding to
x = 1, 2, 3...
>>> interpolate([1])(3)
1
>>> interpolate([1, 8])(3)
15
>>> interpolate([1, 8, 27])(4)
58
>>> interpolate([1, 8, 27, 64])(6)
216
"""
size: int = len(y_list)
matrix: Matrix = [[0 for _ in range(size)] for _ in range(size)]
vector: Matrix = [[0] for _ in range(size)]
coeffs: Matrix
x_val: int
y_val: int
col: int
for x_val, y_val in enumerate(y_list):
for col in range(size):
matrix[x_val][col] = (x_val + 1) ** (size - col - 1)
vector[x_val][0] = y_val
coeffs = solve(matrix, vector)
def interpolated_func(var: int) -> int:
"""
>>> interpolate([1])(3)
1
>>> interpolate([1, 8])(3)
15
>>> interpolate([1, 8, 27])(4)
58
>>> interpolate([1, 8, 27, 64])(6)
216
"""
return sum(
round(coeffs[x_val][0]) * (var ** (size - x_val - 1))
for x_val in range(size)
)
return interpolated_func
def question_function(variable: int) -> int:
"""
The generating function u as specified in the question.
>>> question_function(0)
1
>>> question_function(1)
1
>>> question_function(5)
8138021
>>> question_function(10)
9090909091
"""
return (
1
- variable
+ variable**2
- variable**3
+ variable**4
- variable**5
+ variable**6
- variable**7
+ variable**8
- variable**9
+ variable**10
)
def solution(func: Callable[[int], int] = question_function, order: int = 10) -> int:
"""
Find the sum of the FITs of the BOPS. For each interpolating polynomial of order
1, 2, ... , 10, find the first x such that the value of the polynomial at x does
not equal u(x).
>>> solution(lambda n: n ** 3, 3)
74
"""
data_points: list[int] = [func(x_val) for x_val in range(1, order + 1)]
polynomials: list[Callable[[int], int]] = [
interpolate(data_points[:max_coeff]) for max_coeff in range(1, order + 1)
]
ret: int = 0
poly: Callable[[int], int]
x_val: int
for poly in polynomials:
x_val = 1
while func(x_val) == poly(x_val):
x_val += 1
ret += poly(x_val)
return ret
if __name__ == "__main__":
print(f"{solution() = }")
| """
If we are presented with the first k terms of a sequence it is impossible to say with
certainty the value of the next term, as there are infinitely many polynomial functions
that can model the sequence.
As an example, let us consider the sequence of cube
numbers. This is defined by the generating function,
u(n) = n3: 1, 8, 27, 64, 125, 216, ...
Suppose we were only given the first two terms of this sequence. Working on the
principle that "simple is best" we should assume a linear relationship and predict the
next term to be 15 (common difference 7). Even if we were presented with the first three
terms, by the same principle of simplicity, a quadratic relationship should be
assumed.
We shall define OP(k, n) to be the nth term of the optimum polynomial
generating function for the first k terms of a sequence. It should be clear that
OP(k, n) will accurately generate the terms of the sequence for n ≤ k, and potentially
the first incorrect term (FIT) will be OP(k, k+1); in which case we shall call it a
bad OP (BOP).
As a basis, if we were only given the first term of sequence, it would be most
sensible to assume constancy; that is, for n ≥ 2, OP(1, n) = u(1).
Hence we obtain the
following OPs for the cubic sequence:
OP(1, n) = 1 1, 1, 1, 1, ...
OP(2, n) = 7n-6 1, 8, 15, ...
OP(3, n) = 6n^2-11n+6 1, 8, 27, 58, ...
OP(4, n) = n^3 1, 8, 27, 64, 125, ...
Clearly no BOPs exist for k ≥ 4.
By considering the sum of FITs generated by the BOPs (indicated in red above), we
obtain 1 + 15 + 58 = 74.
Consider the following tenth degree polynomial generating function:
1 - n + n^2 - n^3 + n^4 - n^5 + n^6 - n^7 + n^8 - n^9 + n^10
Find the sum of FITs for the BOPs.
"""
from __future__ import annotations
from collections.abc import Callable
Matrix = list[list[float | int]]
def solve(matrix: Matrix, vector: Matrix) -> Matrix:
"""
Solve the linear system of equations Ax = b (A = "matrix", b = "vector")
for x using Gaussian elimination and back substitution. We assume that A
is an invertible square matrix and that b is a column vector of the
same height.
>>> solve([[1, 0], [0, 1]], [[1],[2]])
[[1.0], [2.0]]
>>> solve([[2, 1, -1],[-3, -1, 2],[-2, 1, 2]],[[8], [-11],[-3]])
[[2.0], [3.0], [-1.0]]
"""
size: int = len(matrix)
augmented: Matrix = [[0 for _ in range(size + 1)] for _ in range(size)]
row: int
row2: int
col: int
col2: int
pivot_row: int
ratio: float
for row in range(size):
for col in range(size):
augmented[row][col] = matrix[row][col]
augmented[row][size] = vector[row][0]
row = 0
col = 0
while row < size and col < size:
# pivoting
pivot_row = max((abs(augmented[row2][col]), row2) for row2 in range(col, size))[
1
]
if augmented[pivot_row][col] == 0:
col += 1
continue
else:
augmented[row], augmented[pivot_row] = augmented[pivot_row], augmented[row]
for row2 in range(row + 1, size):
ratio = augmented[row2][col] / augmented[row][col]
augmented[row2][col] = 0
for col2 in range(col + 1, size + 1):
augmented[row2][col2] -= augmented[row][col2] * ratio
row += 1
col += 1
# back substitution
for col in range(1, size):
for row in range(col):
ratio = augmented[row][col] / augmented[col][col]
for col2 in range(col, size + 1):
augmented[row][col2] -= augmented[col][col2] * ratio
# round to get rid of numbers like 2.000000000000004
return [
[round(augmented[row][size] / augmented[row][row], 10)] for row in range(size)
]
def interpolate(y_list: list[int]) -> Callable[[int], int]:
"""
Given a list of data points (1,y0),(2,y1), ..., return a function that
interpolates the data points. We find the coefficients of the interpolating
polynomial by solving a system of linear equations corresponding to
x = 1, 2, 3...
>>> interpolate([1])(3)
1
>>> interpolate([1, 8])(3)
15
>>> interpolate([1, 8, 27])(4)
58
>>> interpolate([1, 8, 27, 64])(6)
216
"""
size: int = len(y_list)
matrix: Matrix = [[0 for _ in range(size)] for _ in range(size)]
vector: Matrix = [[0] for _ in range(size)]
coeffs: Matrix
x_val: int
y_val: int
col: int
for x_val, y_val in enumerate(y_list):
for col in range(size):
matrix[x_val][col] = (x_val + 1) ** (size - col - 1)
vector[x_val][0] = y_val
coeffs = solve(matrix, vector)
def interpolated_func(var: int) -> int:
"""
>>> interpolate([1])(3)
1
>>> interpolate([1, 8])(3)
15
>>> interpolate([1, 8, 27])(4)
58
>>> interpolate([1, 8, 27, 64])(6)
216
"""
return sum(
round(coeffs[x_val][0]) * (var ** (size - x_val - 1))
for x_val in range(size)
)
return interpolated_func
def question_function(variable: int) -> int:
"""
The generating function u as specified in the question.
>>> question_function(0)
1
>>> question_function(1)
1
>>> question_function(5)
8138021
>>> question_function(10)
9090909091
"""
return (
1
- variable
+ variable**2
- variable**3
+ variable**4
- variable**5
+ variable**6
- variable**7
+ variable**8
- variable**9
+ variable**10
)
def solution(func: Callable[[int], int] = question_function, order: int = 10) -> int:
"""
Find the sum of the FITs of the BOPS. For each interpolating polynomial of order
1, 2, ... , 10, find the first x such that the value of the polynomial at x does
not equal u(x).
>>> solution(lambda n: n ** 3, 3)
74
"""
data_points: list[int] = [func(x_val) for x_val in range(1, order + 1)]
polynomials: list[Callable[[int], int]] = [
interpolate(data_points[:max_coeff]) for max_coeff in range(1, order + 1)
]
ret: int = 0
poly: Callable[[int], int]
x_val: int
for poly in polynomials:
x_val = 1
while func(x_val) == poly(x_val):
x_val += 1
ret += poly(x_val)
return ret
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from collections import deque
class Process:
def __init__(self, process_name: str, arrival_time: int, burst_time: int) -> None:
self.process_name = process_name # process name
self.arrival_time = arrival_time # arrival time of the process
# completion time of finished process or last interrupted time
self.stop_time = arrival_time
self.burst_time = burst_time # remaining burst time
self.waiting_time = 0 # total time of the process wait in ready queue
self.turnaround_time = 0 # time from arrival time to completion time
class MLFQ:
"""
MLFQ(Multi Level Feedback Queue)
https://en.wikipedia.org/wiki/Multilevel_feedback_queue
MLFQ has a lot of queues that have different priority
In this MLFQ,
The first Queue(0) to last second Queue(N-2) of MLFQ have Round Robin Algorithm
The last Queue(N-1) has First Come, First Served Algorithm
"""
def __init__(
self,
number_of_queues: int,
time_slices: list[int],
queue: deque[Process],
current_time: int,
) -> None:
# total number of mlfq's queues
self.number_of_queues = number_of_queues
# time slice of queues that round robin algorithm applied
self.time_slices = time_slices
# unfinished process is in this ready_queue
self.ready_queue = queue
# current time
self.current_time = current_time
# finished process is in this sequence queue
self.finish_queue: deque[Process] = deque()
def calculate_sequence_of_finish_queue(self) -> list[str]:
"""
This method returns the sequence of finished processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_sequence_of_finish_queue()
['P2', 'P4', 'P1', 'P3']
"""
sequence = []
for i in range(len(self.finish_queue)):
sequence.append(self.finish_queue[i].process_name)
return sequence
def calculate_waiting_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates waiting time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_waiting_time([P1, P2, P3, P4])
[83, 17, 94, 101]
"""
waiting_times = []
for i in range(len(queue)):
waiting_times.append(queue[i].waiting_time)
return waiting_times
def calculate_turnaround_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates turnaround time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_turnaround_time([P1, P2, P3, P4])
[136, 34, 162, 125]
"""
turnaround_times = []
for i in range(len(queue)):
turnaround_times.append(queue[i].turnaround_time)
return turnaround_times
def calculate_completion_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates completion time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_turnaround_time([P1, P2, P3, P4])
[136, 34, 162, 125]
"""
completion_times = []
for i in range(len(queue)):
completion_times.append(queue[i].stop_time)
return completion_times
def calculate_remaining_burst_time_of_processes(
self, queue: deque[Process]
) -> list[int]:
"""
This method calculate remaining burst time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue, ready_queue = mlfq.round_robin(deque([P1, P2, P3, P4]), 17)
>>> mlfq.calculate_remaining_burst_time_of_processes(mlfq.finish_queue)
[0]
>>> mlfq.calculate_remaining_burst_time_of_processes(ready_queue)
[36, 51, 7]
>>> finish_queue, ready_queue = mlfq.round_robin(ready_queue, 25)
>>> mlfq.calculate_remaining_burst_time_of_processes(mlfq.finish_queue)
[0, 0]
>>> mlfq.calculate_remaining_burst_time_of_processes(ready_queue)
[11, 26]
"""
return [q.burst_time for q in queue]
def update_waiting_time(self, process: Process) -> int:
"""
This method updates waiting times of unfinished processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> mlfq.current_time = 10
>>> P1.stop_time = 5
>>> mlfq.update_waiting_time(P1)
5
"""
process.waiting_time += self.current_time - process.stop_time
return process.waiting_time
def first_come_first_served(self, ready_queue: deque[Process]) -> deque[Process]:
"""
FCFS(First Come, First Served)
FCFS will be applied to MLFQ's last queue
A first came process will be finished at first
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.first_come_first_served(mlfq.ready_queue)
>>> mlfq.calculate_sequence_of_finish_queue()
['P1', 'P2', 'P3', 'P4']
"""
finished: deque[Process] = deque() # sequence deque of finished process
while len(ready_queue) != 0:
cp = ready_queue.popleft() # current process
# if process's arrival time is later than current time, update current time
if self.current_time < cp.arrival_time:
self.current_time += cp.arrival_time
# update waiting time of current process
self.update_waiting_time(cp)
# update current time
self.current_time += cp.burst_time
# finish the process and set the process's burst-time 0
cp.burst_time = 0
# set the process's turnaround time because it is finished
cp.turnaround_time = self.current_time - cp.arrival_time
# set the completion time
cp.stop_time = self.current_time
# add the process to queue that has finished queue
finished.append(cp)
self.finish_queue.extend(finished) # add finished process to finish queue
# FCFS will finish all remaining processes
return finished
def round_robin(
self, ready_queue: deque[Process], time_slice: int
) -> tuple[deque[Process], deque[Process]]:
"""
RR(Round Robin)
RR will be applied to MLFQ's all queues except last queue
All processes can't use CPU for time more than time_slice
If the process consume CPU up to time_slice, it will go back to ready queue
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue, ready_queue = mlfq.round_robin(mlfq.ready_queue, 17)
>>> mlfq.calculate_sequence_of_finish_queue()
['P2']
"""
finished: deque[Process] = deque() # sequence deque of terminated process
# just for 1 cycle and unfinished processes will go back to queue
for _ in range(len(ready_queue)):
cp = ready_queue.popleft() # current process
# if process's arrival time is later than current time, update current time
if self.current_time < cp.arrival_time:
self.current_time += cp.arrival_time
# update waiting time of unfinished processes
self.update_waiting_time(cp)
# if the burst time of process is bigger than time-slice
if cp.burst_time > time_slice:
# use CPU for only time-slice
self.current_time += time_slice
# update remaining burst time
cp.burst_time -= time_slice
# update end point time
cp.stop_time = self.current_time
# locate the process behind the queue because it is not finished
ready_queue.append(cp)
else:
# use CPU for remaining burst time
self.current_time += cp.burst_time
# set burst time 0 because the process is finished
cp.burst_time = 0
# set the finish time
cp.stop_time = self.current_time
# update the process' turnaround time because it is finished
cp.turnaround_time = self.current_time - cp.arrival_time
# add the process to queue that has finished queue
finished.append(cp)
self.finish_queue.extend(finished) # add finished process to finish queue
# return finished processes queue and remaining processes queue
return finished, ready_queue
def multi_level_feedback_queue(self) -> deque[Process]:
"""
MLFQ(Multi Level Feedback Queue)
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_sequence_of_finish_queue()
['P2', 'P4', 'P1', 'P3']
"""
# all queues except last one have round_robin algorithm
for i in range(self.number_of_queues - 1):
finished, self.ready_queue = self.round_robin(
self.ready_queue, self.time_slices[i]
)
# the last queue has first_come_first_served algorithm
self.first_come_first_served(self.ready_queue)
return self.finish_queue
if __name__ == "__main__":
import doctest
P1 = Process("P1", 0, 53)
P2 = Process("P2", 0, 17)
P3 = Process("P3", 0, 68)
P4 = Process("P4", 0, 24)
number_of_queues = 3
time_slices = [17, 25]
queue = deque([P1, P2, P3, P4])
if len(time_slices) != number_of_queues - 1:
raise SystemExit(0)
doctest.testmod(extraglobs={"queue": deque([P1, P2, P3, P4])})
P1 = Process("P1", 0, 53)
P2 = Process("P2", 0, 17)
P3 = Process("P3", 0, 68)
P4 = Process("P4", 0, 24)
number_of_queues = 3
time_slices = [17, 25]
queue = deque([P1, P2, P3, P4])
mlfq = MLFQ(number_of_queues, time_slices, queue, 0)
finish_queue = mlfq.multi_level_feedback_queue()
# print total waiting times of processes(P1, P2, P3, P4)
print(
f"waiting time:\
\t\t\t{MLFQ.calculate_waiting_time(mlfq, [P1, P2, P3, P4])}"
)
# print completion times of processes(P1, P2, P3, P4)
print(
f"completion time:\
\t\t{MLFQ.calculate_completion_time(mlfq, [P1, P2, P3, P4])}"
)
# print total turnaround times of processes(P1, P2, P3, P4)
print(
f"turnaround time:\
\t\t{MLFQ.calculate_turnaround_time(mlfq, [P1, P2, P3, P4])}"
)
# print sequence of finished processes
print(
f"sequence of finished processes:\
{mlfq.calculate_sequence_of_finish_queue()}"
)
| from collections import deque
class Process:
def __init__(self, process_name: str, arrival_time: int, burst_time: int) -> None:
self.process_name = process_name # process name
self.arrival_time = arrival_time # arrival time of the process
# completion time of finished process or last interrupted time
self.stop_time = arrival_time
self.burst_time = burst_time # remaining burst time
self.waiting_time = 0 # total time of the process wait in ready queue
self.turnaround_time = 0 # time from arrival time to completion time
class MLFQ:
"""
MLFQ(Multi Level Feedback Queue)
https://en.wikipedia.org/wiki/Multilevel_feedback_queue
MLFQ has a lot of queues that have different priority
In this MLFQ,
The first Queue(0) to last second Queue(N-2) of MLFQ have Round Robin Algorithm
The last Queue(N-1) has First Come, First Served Algorithm
"""
def __init__(
self,
number_of_queues: int,
time_slices: list[int],
queue: deque[Process],
current_time: int,
) -> None:
# total number of mlfq's queues
self.number_of_queues = number_of_queues
# time slice of queues that round robin algorithm applied
self.time_slices = time_slices
# unfinished process is in this ready_queue
self.ready_queue = queue
# current time
self.current_time = current_time
# finished process is in this sequence queue
self.finish_queue: deque[Process] = deque()
def calculate_sequence_of_finish_queue(self) -> list[str]:
"""
This method returns the sequence of finished processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_sequence_of_finish_queue()
['P2', 'P4', 'P1', 'P3']
"""
sequence = []
for i in range(len(self.finish_queue)):
sequence.append(self.finish_queue[i].process_name)
return sequence
def calculate_waiting_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates waiting time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_waiting_time([P1, P2, P3, P4])
[83, 17, 94, 101]
"""
waiting_times = []
for i in range(len(queue)):
waiting_times.append(queue[i].waiting_time)
return waiting_times
def calculate_turnaround_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates turnaround time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_turnaround_time([P1, P2, P3, P4])
[136, 34, 162, 125]
"""
turnaround_times = []
for i in range(len(queue)):
turnaround_times.append(queue[i].turnaround_time)
return turnaround_times
def calculate_completion_time(self, queue: list[Process]) -> list[int]:
"""
This method calculates completion time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_turnaround_time([P1, P2, P3, P4])
[136, 34, 162, 125]
"""
completion_times = []
for i in range(len(queue)):
completion_times.append(queue[i].stop_time)
return completion_times
def calculate_remaining_burst_time_of_processes(
self, queue: deque[Process]
) -> list[int]:
"""
This method calculate remaining burst time of processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue, ready_queue = mlfq.round_robin(deque([P1, P2, P3, P4]), 17)
>>> mlfq.calculate_remaining_burst_time_of_processes(mlfq.finish_queue)
[0]
>>> mlfq.calculate_remaining_burst_time_of_processes(ready_queue)
[36, 51, 7]
>>> finish_queue, ready_queue = mlfq.round_robin(ready_queue, 25)
>>> mlfq.calculate_remaining_burst_time_of_processes(mlfq.finish_queue)
[0, 0]
>>> mlfq.calculate_remaining_burst_time_of_processes(ready_queue)
[11, 26]
"""
return [q.burst_time for q in queue]
def update_waiting_time(self, process: Process) -> int:
"""
This method updates waiting times of unfinished processes
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> mlfq.current_time = 10
>>> P1.stop_time = 5
>>> mlfq.update_waiting_time(P1)
5
"""
process.waiting_time += self.current_time - process.stop_time
return process.waiting_time
def first_come_first_served(self, ready_queue: deque[Process]) -> deque[Process]:
"""
FCFS(First Come, First Served)
FCFS will be applied to MLFQ's last queue
A first came process will be finished at first
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> _ = mlfq.first_come_first_served(mlfq.ready_queue)
>>> mlfq.calculate_sequence_of_finish_queue()
['P1', 'P2', 'P3', 'P4']
"""
finished: deque[Process] = deque() # sequence deque of finished process
while len(ready_queue) != 0:
cp = ready_queue.popleft() # current process
# if process's arrival time is later than current time, update current time
if self.current_time < cp.arrival_time:
self.current_time += cp.arrival_time
# update waiting time of current process
self.update_waiting_time(cp)
# update current time
self.current_time += cp.burst_time
# finish the process and set the process's burst-time 0
cp.burst_time = 0
# set the process's turnaround time because it is finished
cp.turnaround_time = self.current_time - cp.arrival_time
# set the completion time
cp.stop_time = self.current_time
# add the process to queue that has finished queue
finished.append(cp)
self.finish_queue.extend(finished) # add finished process to finish queue
# FCFS will finish all remaining processes
return finished
def round_robin(
self, ready_queue: deque[Process], time_slice: int
) -> tuple[deque[Process], deque[Process]]:
"""
RR(Round Robin)
RR will be applied to MLFQ's all queues except last queue
All processes can't use CPU for time more than time_slice
If the process consume CPU up to time_slice, it will go back to ready queue
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue, ready_queue = mlfq.round_robin(mlfq.ready_queue, 17)
>>> mlfq.calculate_sequence_of_finish_queue()
['P2']
"""
finished: deque[Process] = deque() # sequence deque of terminated process
# just for 1 cycle and unfinished processes will go back to queue
for _ in range(len(ready_queue)):
cp = ready_queue.popleft() # current process
# if process's arrival time is later than current time, update current time
if self.current_time < cp.arrival_time:
self.current_time += cp.arrival_time
# update waiting time of unfinished processes
self.update_waiting_time(cp)
# if the burst time of process is bigger than time-slice
if cp.burst_time > time_slice:
# use CPU for only time-slice
self.current_time += time_slice
# update remaining burst time
cp.burst_time -= time_slice
# update end point time
cp.stop_time = self.current_time
# locate the process behind the queue because it is not finished
ready_queue.append(cp)
else:
# use CPU for remaining burst time
self.current_time += cp.burst_time
# set burst time 0 because the process is finished
cp.burst_time = 0
# set the finish time
cp.stop_time = self.current_time
# update the process' turnaround time because it is finished
cp.turnaround_time = self.current_time - cp.arrival_time
# add the process to queue that has finished queue
finished.append(cp)
self.finish_queue.extend(finished) # add finished process to finish queue
# return finished processes queue and remaining processes queue
return finished, ready_queue
def multi_level_feedback_queue(self) -> deque[Process]:
"""
MLFQ(Multi Level Feedback Queue)
>>> P1 = Process("P1", 0, 53)
>>> P2 = Process("P2", 0, 17)
>>> P3 = Process("P3", 0, 68)
>>> P4 = Process("P4", 0, 24)
>>> mlfq = MLFQ(3, [17, 25], deque([P1, P2, P3, P4]), 0)
>>> finish_queue = mlfq.multi_level_feedback_queue()
>>> mlfq.calculate_sequence_of_finish_queue()
['P2', 'P4', 'P1', 'P3']
"""
# all queues except last one have round_robin algorithm
for i in range(self.number_of_queues - 1):
finished, self.ready_queue = self.round_robin(
self.ready_queue, self.time_slices[i]
)
# the last queue has first_come_first_served algorithm
self.first_come_first_served(self.ready_queue)
return self.finish_queue
if __name__ == "__main__":
import doctest
P1 = Process("P1", 0, 53)
P2 = Process("P2", 0, 17)
P3 = Process("P3", 0, 68)
P4 = Process("P4", 0, 24)
number_of_queues = 3
time_slices = [17, 25]
queue = deque([P1, P2, P3, P4])
if len(time_slices) != number_of_queues - 1:
raise SystemExit(0)
doctest.testmod(extraglobs={"queue": deque([P1, P2, P3, P4])})
P1 = Process("P1", 0, 53)
P2 = Process("P2", 0, 17)
P3 = Process("P3", 0, 68)
P4 = Process("P4", 0, 24)
number_of_queues = 3
time_slices = [17, 25]
queue = deque([P1, P2, P3, P4])
mlfq = MLFQ(number_of_queues, time_slices, queue, 0)
finish_queue = mlfq.multi_level_feedback_queue()
# print total waiting times of processes(P1, P2, P3, P4)
print(
f"waiting time:\
\t\t\t{MLFQ.calculate_waiting_time(mlfq, [P1, P2, P3, P4])}"
)
# print completion times of processes(P1, P2, P3, P4)
print(
f"completion time:\
\t\t{MLFQ.calculate_completion_time(mlfq, [P1, P2, P3, P4])}"
)
# print total turnaround times of processes(P1, P2, P3, P4)
print(
f"turnaround time:\
\t\t{MLFQ.calculate_turnaround_time(mlfq, [P1, P2, P3, P4])}"
)
# print sequence of finished processes
print(
f"sequence of finished processes:\
{mlfq.calculate_sequence_of_finish_queue()}"
)
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
https://en.wikipedia.org/wiki/Component_(graph_theory)
Finding connected components in graph
"""
test_graph_1 = {0: [1, 2], 1: [0, 3], 2: [0], 3: [1], 4: [5, 6], 5: [4, 6], 6: [4, 5]}
test_graph_2 = {0: [1, 2, 3], 1: [0, 3], 2: [0], 3: [0, 1], 4: [], 5: []}
def dfs(graph: dict, vert: int, visited: list) -> list:
"""
Use depth first search to find all vertices
being in the same component as initial vertex
>>> dfs(test_graph_1, 0, 5 * [False])
[0, 1, 3, 2]
>>> dfs(test_graph_2, 0, 6 * [False])
[0, 1, 3, 2]
"""
visited[vert] = True
connected_verts = []
for neighbour in graph[vert]:
if not visited[neighbour]:
connected_verts += dfs(graph, neighbour, visited)
return [vert] + connected_verts
def connected_components(graph: dict) -> list:
"""
This function takes graph as a parameter
and then returns the list of connected components
>>> connected_components(test_graph_1)
[[0, 1, 3, 2], [4, 5, 6]]
>>> connected_components(test_graph_2)
[[0, 1, 3, 2], [4], [5]]
"""
graph_size = len(graph)
visited = graph_size * [False]
components_list = []
for i in range(graph_size):
if not visited[i]:
i_connected = dfs(graph, i, visited)
components_list.append(i_connected)
return components_list
if __name__ == "__main__":
import doctest
doctest.testmod()
| """
https://en.wikipedia.org/wiki/Component_(graph_theory)
Finding connected components in graph
"""
test_graph_1 = {0: [1, 2], 1: [0, 3], 2: [0], 3: [1], 4: [5, 6], 5: [4, 6], 6: [4, 5]}
test_graph_2 = {0: [1, 2, 3], 1: [0, 3], 2: [0], 3: [0, 1], 4: [], 5: []}
def dfs(graph: dict, vert: int, visited: list) -> list:
"""
Use depth first search to find all vertices
being in the same component as initial vertex
>>> dfs(test_graph_1, 0, 5 * [False])
[0, 1, 3, 2]
>>> dfs(test_graph_2, 0, 6 * [False])
[0, 1, 3, 2]
"""
visited[vert] = True
connected_verts = []
for neighbour in graph[vert]:
if not visited[neighbour]:
connected_verts += dfs(graph, neighbour, visited)
return [vert] + connected_verts
def connected_components(graph: dict) -> list:
"""
This function takes graph as a parameter
and then returns the list of connected components
>>> connected_components(test_graph_1)
[[0, 1, 3, 2], [4, 5, 6]]
>>> connected_components(test_graph_2)
[[0, 1, 3, 2], [4], [5]]
"""
graph_size = len(graph)
visited = graph_size * [False]
components_list = []
for i in range(graph_size):
if not visited[i]:
i_connected = dfs(graph, i, visited)
components_list.append(i_connected)
return components_list
if __name__ == "__main__":
import doctest
doctest.testmod()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 36
https://projecteuler.net/problem=36
Problem Statement:
Double-base palindromes
Problem 36
The decimal number, 585 = 10010010012 (binary), is palindromic in both bases.
Find the sum of all numbers, less than one million, which are palindromic in
base 10 and base 2.
(Please note that the palindromic number, in either base, may not include
leading zeros.)
"""
from __future__ import annotations
def is_palindrome(n: int | str) -> bool:
"""
Return true if the input n is a palindrome.
Otherwise return false. n can be an integer or a string.
>>> is_palindrome(909)
True
>>> is_palindrome(908)
False
>>> is_palindrome('10101')
True
>>> is_palindrome('10111')
False
"""
n = str(n)
return True if n == n[::-1] else False
def solution(n: int = 1000000):
"""Return the sum of all numbers, less than n , which are palindromic in
base 10 and base 2.
>>> solution(1000000)
872187
>>> solution(500000)
286602
>>> solution(100000)
286602
>>> solution(1000)
1772
>>> solution(100)
157
>>> solution(10)
25
>>> solution(2)
1
>>> solution(1)
0
"""
total = 0
for i in range(1, n):
if is_palindrome(i) and is_palindrome(bin(i).split("b")[1]):
total += i
return total
if __name__ == "__main__":
print(solution(int(str(input().strip()))))
| """
Project Euler Problem 36
https://projecteuler.net/problem=36
Problem Statement:
Double-base palindromes
Problem 36
The decimal number, 585 = 10010010012 (binary), is palindromic in both bases.
Find the sum of all numbers, less than one million, which are palindromic in
base 10 and base 2.
(Please note that the palindromic number, in either base, may not include
leading zeros.)
"""
from __future__ import annotations
def is_palindrome(n: int | str) -> bool:
"""
Return true if the input n is a palindrome.
Otherwise return false. n can be an integer or a string.
>>> is_palindrome(909)
True
>>> is_palindrome(908)
False
>>> is_palindrome('10101')
True
>>> is_palindrome('10111')
False
"""
n = str(n)
return True if n == n[::-1] else False
def solution(n: int = 1000000):
"""Return the sum of all numbers, less than n , which are palindromic in
base 10 and base 2.
>>> solution(1000000)
872187
>>> solution(500000)
286602
>>> solution(100000)
286602
>>> solution(1000)
1772
>>> solution(100)
157
>>> solution(10)
25
>>> solution(2)
1
>>> solution(1)
0
"""
total = 0
for i in range(1, n):
if is_palindrome(i) and is_palindrome(bin(i).split("b")[1]):
total += i
return total
if __name__ == "__main__":
print(solution(int(str(input().strip()))))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
One of the several implementations of Lempel–Ziv–Welch decompression algorithm
https://en.wikipedia.org/wiki/Lempel%E2%80%93Ziv%E2%80%93Welch
"""
import math
import sys
def read_file_binary(file_path: str) -> str:
"""
Reads given file as bytes and returns them as a long string
"""
result = ""
try:
with open(file_path, "rb") as binary_file:
data = binary_file.read()
for dat in data:
curr_byte = f"{dat:08b}"
result += curr_byte
return result
except OSError:
print("File not accessible")
sys.exit()
def decompress_data(data_bits: str) -> str:
"""
Decompresses given data_bits using Lempel–Ziv–Welch compression algorithm
and returns the result as a string
"""
lexicon = {"0": "0", "1": "1"}
result, curr_string = "", ""
index = len(lexicon)
for i in range(len(data_bits)):
curr_string += data_bits[i]
if curr_string not in lexicon:
continue
last_match_id = lexicon[curr_string]
result += last_match_id
lexicon[curr_string] = last_match_id + "0"
if math.log2(index).is_integer():
new_lex = {}
for curr_key in list(lexicon):
new_lex["0" + curr_key] = lexicon.pop(curr_key)
lexicon = new_lex
lexicon[bin(index)[2:]] = last_match_id + "1"
index += 1
curr_string = ""
return result
def write_file_binary(file_path: str, to_write: str) -> None:
"""
Writes given to_write string (should only consist of 0's and 1's) as bytes in the
file
"""
byte_length = 8
try:
with open(file_path, "wb") as opened_file:
result_byte_array = [
to_write[i : i + byte_length]
for i in range(0, len(to_write), byte_length)
]
if len(result_byte_array[-1]) % byte_length == 0:
result_byte_array.append("10000000")
else:
result_byte_array[-1] += "1" + "0" * (
byte_length - len(result_byte_array[-1]) - 1
)
for elem in result_byte_array[:-1]:
opened_file.write(int(elem, 2).to_bytes(1, byteorder="big"))
except OSError:
print("File not accessible")
sys.exit()
def remove_prefix(data_bits: str) -> str:
"""
Removes size prefix, that compressed file should have
Returns the result
"""
counter = 0
for letter in data_bits:
if letter == "1":
break
counter += 1
data_bits = data_bits[counter:]
data_bits = data_bits[counter + 1 :]
return data_bits
def compress(source_path: str, destination_path: str) -> None:
"""
Reads source file, decompresses it and writes the result in destination file
"""
data_bits = read_file_binary(source_path)
data_bits = remove_prefix(data_bits)
decompressed = decompress_data(data_bits)
write_file_binary(destination_path, decompressed)
if __name__ == "__main__":
compress(sys.argv[1], sys.argv[2])
| """
One of the several implementations of Lempel–Ziv–Welch decompression algorithm
https://en.wikipedia.org/wiki/Lempel%E2%80%93Ziv%E2%80%93Welch
"""
import math
import sys
def read_file_binary(file_path: str) -> str:
"""
Reads given file as bytes and returns them as a long string
"""
result = ""
try:
with open(file_path, "rb") as binary_file:
data = binary_file.read()
for dat in data:
curr_byte = f"{dat:08b}"
result += curr_byte
return result
except OSError:
print("File not accessible")
sys.exit()
def decompress_data(data_bits: str) -> str:
"""
Decompresses given data_bits using Lempel–Ziv–Welch compression algorithm
and returns the result as a string
"""
lexicon = {"0": "0", "1": "1"}
result, curr_string = "", ""
index = len(lexicon)
for i in range(len(data_bits)):
curr_string += data_bits[i]
if curr_string not in lexicon:
continue
last_match_id = lexicon[curr_string]
result += last_match_id
lexicon[curr_string] = last_match_id + "0"
if math.log2(index).is_integer():
new_lex = {}
for curr_key in list(lexicon):
new_lex["0" + curr_key] = lexicon.pop(curr_key)
lexicon = new_lex
lexicon[bin(index)[2:]] = last_match_id + "1"
index += 1
curr_string = ""
return result
def write_file_binary(file_path: str, to_write: str) -> None:
"""
Writes given to_write string (should only consist of 0's and 1's) as bytes in the
file
"""
byte_length = 8
try:
with open(file_path, "wb") as opened_file:
result_byte_array = [
to_write[i : i + byte_length]
for i in range(0, len(to_write), byte_length)
]
if len(result_byte_array[-1]) % byte_length == 0:
result_byte_array.append("10000000")
else:
result_byte_array[-1] += "1" + "0" * (
byte_length - len(result_byte_array[-1]) - 1
)
for elem in result_byte_array[:-1]:
opened_file.write(int(elem, 2).to_bytes(1, byteorder="big"))
except OSError:
print("File not accessible")
sys.exit()
def remove_prefix(data_bits: str) -> str:
"""
Removes size prefix, that compressed file should have
Returns the result
"""
counter = 0
for letter in data_bits:
if letter == "1":
break
counter += 1
data_bits = data_bits[counter:]
data_bits = data_bits[counter + 1 :]
return data_bits
def compress(source_path: str, destination_path: str) -> None:
"""
Reads source file, decompresses it and writes the result in destination file
"""
data_bits = read_file_binary(source_path)
data_bits = remove_prefix(data_bits)
decompressed = decompress_data(data_bits)
write_file_binary(destination_path, decompressed)
if __name__ == "__main__":
compress(sys.argv[1], sys.argv[2])
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| from __future__ import annotations
def median(nums: list) -> int | float:
"""
Find median of a list of numbers.
Wiki: https://en.wikipedia.org/wiki/Median
>>> median([0])
0
>>> median([4, 1, 3, 2])
2.5
>>> median([2, 70, 6, 50, 20, 8, 4])
8
Args:
nums: List of nums
Returns:
Median.
"""
sorted_list = sorted(nums)
length = len(sorted_list)
mid_index = length >> 1
return (
(sorted_list[mid_index] + sorted_list[mid_index - 1]) / 2
if length % 2 == 0
else sorted_list[mid_index]
)
def main():
import doctest
doctest.testmod()
if __name__ == "__main__":
main()
| from __future__ import annotations
def median(nums: list) -> int | float:
"""
Find median of a list of numbers.
Wiki: https://en.wikipedia.org/wiki/Median
>>> median([0])
0
>>> median([4, 1, 3, 2])
2.5
>>> median([2, 70, 6, 50, 20, 8, 4])
8
Args:
nums: List of nums
Returns:
Median.
"""
sorted_list = sorted(nums)
length = len(sorted_list)
mid_index = length >> 1
return (
(sorted_list[mid_index] + sorted_list[mid_index - 1]) / 2
if length % 2 == 0
else sorted_list[mid_index]
)
def main():
import doctest
doctest.testmod()
if __name__ == "__main__":
main()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Lorentz transformation describes the transition from a reference frame P
to another reference frame P', each of which is moving in a direction with
respect to the other. The Lorentz transformation implemented in this code
is the relativistic version using a four vector described by Minkowsky Space:
x0 = ct, x1 = x, x2 = y, and x3 = z
NOTE: Please note that x0 is c (speed of light) times t (time).
So, the Lorentz transformation using a four vector is defined as:
|ct'| | γ -γβ 0 0| |ct|
|x' | = |-γβ γ 0 0| *|x |
|y' | | 0 0 1 0| |y |
|z' | | 0 0 0 1| |z |
Where:
1
γ = ---------------
-----------
/ v^2 |
/(1 - ---
-/ c^2
v
β = -----
c
Reference: https://en.wikipedia.org/wiki/Lorentz_transformation
"""
from __future__ import annotations
from math import sqrt
import numpy as np # type: ignore
from sympy import symbols # type: ignore
# Coefficient
# Speed of light (m/s)
c = 299792458
# Symbols
ct, x, y, z = symbols("ct x y z")
ct_p, x_p, y_p, z_p = symbols("ct' x' y' z'")
# Vehicle's speed divided by speed of light (no units)
def beta(velocity: float) -> float:
"""
>>> beta(c)
1.0
>>> beta(199792458)
0.666435904801848
>>> beta(1e5)
0.00033356409519815205
>>> beta(0.2)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
"""
if velocity > c:
raise ValueError("Speed must not exceed Light Speed 299,792,458 [m/s]!")
# Usually the speed u should be much higher than 1 (c order of magnitude)
elif velocity < 1:
raise ValueError("Speed must be greater than 1!")
return velocity / c
def gamma(velocity: float) -> float:
"""
>>> gamma(4)
1.0000000000000002
>>> gamma(1e5)
1.0000000556325075
>>> gamma(3e7)
1.005044845777813
>>> gamma(2.8e8)
2.7985595722318277
>>> gamma(299792451)
4627.49902669495
>>> gamma(0.3)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
>>> gamma(2*c)
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
"""
return 1 / (sqrt(1 - beta(velocity) ** 2))
def transformation_matrix(velocity: float) -> np.array:
"""
>>> transformation_matrix(29979245)
array([[ 1.00503781, -0.10050378, 0. , 0. ],
[-0.10050378, 1.00503781, 0. , 0. ],
[ 0. , 0. , 1. , 0. ],
[ 0. , 0. , 0. , 1. ]])
>>> transformation_matrix(19979245.2)
array([[ 1.00222811, -0.06679208, 0. , 0. ],
[-0.06679208, 1.00222811, 0. , 0. ],
[ 0. , 0. , 1. , 0. ],
[ 0. , 0. , 0. , 1. ]])
>>> transformation_matrix(1)
array([[ 1.00000000e+00, -3.33564095e-09, 0.00000000e+00,
0.00000000e+00],
[-3.33564095e-09, 1.00000000e+00, 0.00000000e+00,
0.00000000e+00],
[ 0.00000000e+00, 0.00000000e+00, 1.00000000e+00,
0.00000000e+00],
[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
1.00000000e+00]])
>>> transformation_matrix(0)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
>>> transformation_matrix(c * 1.5)
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
"""
return np.array(
[
[gamma(velocity), -gamma(velocity) * beta(velocity), 0, 0],
[-gamma(velocity) * beta(velocity), gamma(velocity), 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1],
]
)
def transform(
velocity: float, event: np.array = np.zeros(4), symbolic: bool = True # noqa: B008
) -> np.array:
"""
>>> transform(29979245,np.array([1,2,3,4]), False)
array([ 3.01302757e+08, -3.01302729e+07, 3.00000000e+00, 4.00000000e+00])
>>> transform(29979245)
array([1.00503781498831*ct - 0.100503778816875*x,
-0.100503778816875*ct + 1.00503781498831*x, 1.0*y, 1.0*z],
dtype=object)
>>> transform(19879210.2)
array([1.0022057787097*ct - 0.066456172618675*x,
-0.066456172618675*ct + 1.0022057787097*x, 1.0*y, 1.0*z],
dtype=object)
>>> transform(299792459, np.array([1,1,1,1]))
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
>>> transform(-1, np.array([1,1,1,1]))
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
"""
# Ensure event is not a vector of zeros
if not symbolic:
# x0 is ct (speed of ligt * time)
event[0] = event[0] * c
else:
# Symbolic four vector
event = np.array([ct, x, y, z])
return transformation_matrix(velocity).dot(event)
if __name__ == "__main__":
import doctest
doctest.testmod()
# Example of symbolic vector:
four_vector = transform(29979245)
print("Example of four vector: ")
print(f"ct' = {four_vector[0]}")
print(f"x' = {four_vector[1]}")
print(f"y' = {four_vector[2]}")
print(f"z' = {four_vector[3]}")
# Substitute symbols with numerical values:
values = np.array([1, 1, 1, 1])
sub_dict = {ct: c * values[0], x: values[1], y: values[2], z: values[3]}
numerical_vector = [four_vector[i].subs(sub_dict) for i in range(0, 4)]
print(f"\n{numerical_vector}")
| """
Lorentz transformation describes the transition from a reference frame P
to another reference frame P', each of which is moving in a direction with
respect to the other. The Lorentz transformation implemented in this code
is the relativistic version using a four vector described by Minkowsky Space:
x0 = ct, x1 = x, x2 = y, and x3 = z
NOTE: Please note that x0 is c (speed of light) times t (time).
So, the Lorentz transformation using a four vector is defined as:
|ct'| | γ -γβ 0 0| |ct|
|x' | = |-γβ γ 0 0| *|x |
|y' | | 0 0 1 0| |y |
|z' | | 0 0 0 1| |z |
Where:
1
γ = ---------------
-----------
/ v^2 |
/(1 - ---
-/ c^2
v
β = -----
c
Reference: https://en.wikipedia.org/wiki/Lorentz_transformation
"""
from __future__ import annotations
from math import sqrt
import numpy as np # type: ignore
from sympy import symbols # type: ignore
# Coefficient
# Speed of light (m/s)
c = 299792458
# Symbols
ct, x, y, z = symbols("ct x y z")
ct_p, x_p, y_p, z_p = symbols("ct' x' y' z'")
# Vehicle's speed divided by speed of light (no units)
def beta(velocity: float) -> float:
"""
>>> beta(c)
1.0
>>> beta(199792458)
0.666435904801848
>>> beta(1e5)
0.00033356409519815205
>>> beta(0.2)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
"""
if velocity > c:
raise ValueError("Speed must not exceed Light Speed 299,792,458 [m/s]!")
# Usually the speed u should be much higher than 1 (c order of magnitude)
elif velocity < 1:
raise ValueError("Speed must be greater than 1!")
return velocity / c
def gamma(velocity: float) -> float:
"""
>>> gamma(4)
1.0000000000000002
>>> gamma(1e5)
1.0000000556325075
>>> gamma(3e7)
1.005044845777813
>>> gamma(2.8e8)
2.7985595722318277
>>> gamma(299792451)
4627.49902669495
>>> gamma(0.3)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
>>> gamma(2*c)
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
"""
return 1 / (sqrt(1 - beta(velocity) ** 2))
def transformation_matrix(velocity: float) -> np.array:
"""
>>> transformation_matrix(29979245)
array([[ 1.00503781, -0.10050378, 0. , 0. ],
[-0.10050378, 1.00503781, 0. , 0. ],
[ 0. , 0. , 1. , 0. ],
[ 0. , 0. , 0. , 1. ]])
>>> transformation_matrix(19979245.2)
array([[ 1.00222811, -0.06679208, 0. , 0. ],
[-0.06679208, 1.00222811, 0. , 0. ],
[ 0. , 0. , 1. , 0. ],
[ 0. , 0. , 0. , 1. ]])
>>> transformation_matrix(1)
array([[ 1.00000000e+00, -3.33564095e-09, 0.00000000e+00,
0.00000000e+00],
[-3.33564095e-09, 1.00000000e+00, 0.00000000e+00,
0.00000000e+00],
[ 0.00000000e+00, 0.00000000e+00, 1.00000000e+00,
0.00000000e+00],
[ 0.00000000e+00, 0.00000000e+00, 0.00000000e+00,
1.00000000e+00]])
>>> transformation_matrix(0)
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
>>> transformation_matrix(c * 1.5)
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
"""
return np.array(
[
[gamma(velocity), -gamma(velocity) * beta(velocity), 0, 0],
[-gamma(velocity) * beta(velocity), gamma(velocity), 0, 0],
[0, 0, 1, 0],
[0, 0, 0, 1],
]
)
def transform(
velocity: float, event: np.array = np.zeros(4), symbolic: bool = True # noqa: B008
) -> np.array:
"""
>>> transform(29979245,np.array([1,2,3,4]), False)
array([ 3.01302757e+08, -3.01302729e+07, 3.00000000e+00, 4.00000000e+00])
>>> transform(29979245)
array([1.00503781498831*ct - 0.100503778816875*x,
-0.100503778816875*ct + 1.00503781498831*x, 1.0*y, 1.0*z],
dtype=object)
>>> transform(19879210.2)
array([1.0022057787097*ct - 0.066456172618675*x,
-0.066456172618675*ct + 1.0022057787097*x, 1.0*y, 1.0*z],
dtype=object)
>>> transform(299792459, np.array([1,1,1,1]))
Traceback (most recent call last):
...
ValueError: Speed must not exceed Light Speed 299,792,458 [m/s]!
>>> transform(-1, np.array([1,1,1,1]))
Traceback (most recent call last):
...
ValueError: Speed must be greater than 1!
"""
# Ensure event is not a vector of zeros
if not symbolic:
# x0 is ct (speed of ligt * time)
event[0] = event[0] * c
else:
# Symbolic four vector
event = np.array([ct, x, y, z])
return transformation_matrix(velocity).dot(event)
if __name__ == "__main__":
import doctest
doctest.testmod()
# Example of symbolic vector:
four_vector = transform(29979245)
print("Example of four vector: ")
print(f"ct' = {four_vector[0]}")
print(f"x' = {four_vector[1]}")
print(f"y' = {four_vector[2]}")
print(f"z' = {four_vector[3]}")
# Substitute symbols with numerical values:
values = np.array([1, 1, 1, 1])
sub_dict = {ct: c * values[0], x: values[1], y: values[2], z: values[3]}
numerical_vector = [four_vector[i].subs(sub_dict) for i in range(0, 4)]
print(f"\n{numerical_vector}")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Problem:
Comparing two numbers written in index form like 2'11 and 3'7 is not difficult, as any
calculator would confirm that 2^11 = 2048 < 3^7 = 2187.
However, confirming that 632382^518061 > 519432^525806 would be much more difficult, as
both numbers contain over three million digits.
Using base_exp.txt, a 22K text file containing one thousand lines with a base/exponent
pair on each line, determine which line number has the greatest numerical value.
NOTE: The first two lines in the file represent the numbers in the example given above.
"""
import os
from math import log10
def solution(data_file: str = "base_exp.txt") -> int:
"""
>>> solution()
709
"""
largest: float = 0
result = 0
for i, line in enumerate(open(os.path.join(os.path.dirname(__file__), data_file))):
a, x = list(map(int, line.split(",")))
if x * log10(a) > largest:
largest = x * log10(a)
result = i + 1
return result
if __name__ == "__main__":
print(solution())
| """
Problem:
Comparing two numbers written in index form like 2'11 and 3'7 is not difficult, as any
calculator would confirm that 2^11 = 2048 < 3^7 = 2187.
However, confirming that 632382^518061 > 519432^525806 would be much more difficult, as
both numbers contain over three million digits.
Using base_exp.txt, a 22K text file containing one thousand lines with a base/exponent
pair on each line, determine which line number has the greatest numerical value.
NOTE: The first two lines in the file represent the numbers in the example given above.
"""
import os
from math import log10
def solution(data_file: str = "base_exp.txt") -> int:
"""
>>> solution()
709
"""
largest: float = 0
result = 0
for i, line in enumerate(open(os.path.join(os.path.dirname(__file__), data_file))):
a, x = list(map(int, line.split(",")))
if x * log10(a) > largest:
largest = x * log10(a)
result = i + 1
return result
if __name__ == "__main__":
print(solution())
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Project Euler Problem 301: https://projecteuler.net/problem=301
Problem Statement:
Nim is a game played with heaps of stones, where two players take
it in turn to remove any number of stones from any heap until no stones remain.
We'll consider the three-heap normal-play version of
Nim, which works as follows:
- At the start of the game there are three heaps of stones.
- On each player's turn, the player may remove any positive
number of stones from any single heap.
- The first player unable to move (because no stones remain) loses.
If (n1, n2, n3) indicates a Nim position consisting of heaps of size
n1, n2, and n3, then there is a simple function, which you may look up
or attempt to deduce for yourself, X(n1, n2, n3) that returns:
- zero if, with perfect strategy, the player about to
move will eventually lose; or
- non-zero if, with perfect strategy, the player about
to move will eventually win.
For example X(1,2,3) = 0 because, no matter what the current player does,
the opponent can respond with a move that leaves two heaps of equal size,
at which point every move by the current player can be mirrored by the
opponent until no stones remain; so the current player loses. To illustrate:
- current player moves to (1,2,1)
- opponent moves to (1,0,1)
- current player moves to (0,0,1)
- opponent moves to (0,0,0), and so wins.
For how many positive integers n <= 2^30 does X(n,2n,3n) = 0?
"""
def solution(exponent: int = 30) -> int:
"""
For any given exponent x >= 0, 1 <= n <= 2^x.
This function returns how many Nim games are lost given that
each Nim game has three heaps of the form (n, 2*n, 3*n).
>>> solution(0)
1
>>> solution(2)
3
>>> solution(10)
144
"""
# To find how many total games were lost for a given exponent x,
# we need to find the Fibonacci number F(x+2).
fibonacci_index = exponent + 2
phi = (1 + 5**0.5) / 2
fibonacci = (phi**fibonacci_index - (phi - 1) ** fibonacci_index) / 5**0.5
return int(fibonacci)
if __name__ == "__main__":
print(f"{solution() = }")
| """
Project Euler Problem 301: https://projecteuler.net/problem=301
Problem Statement:
Nim is a game played with heaps of stones, where two players take
it in turn to remove any number of stones from any heap until no stones remain.
We'll consider the three-heap normal-play version of
Nim, which works as follows:
- At the start of the game there are three heaps of stones.
- On each player's turn, the player may remove any positive
number of stones from any single heap.
- The first player unable to move (because no stones remain) loses.
If (n1, n2, n3) indicates a Nim position consisting of heaps of size
n1, n2, and n3, then there is a simple function, which you may look up
or attempt to deduce for yourself, X(n1, n2, n3) that returns:
- zero if, with perfect strategy, the player about to
move will eventually lose; or
- non-zero if, with perfect strategy, the player about
to move will eventually win.
For example X(1,2,3) = 0 because, no matter what the current player does,
the opponent can respond with a move that leaves two heaps of equal size,
at which point every move by the current player can be mirrored by the
opponent until no stones remain; so the current player loses. To illustrate:
- current player moves to (1,2,1)
- opponent moves to (1,0,1)
- current player moves to (0,0,1)
- opponent moves to (0,0,0), and so wins.
For how many positive integers n <= 2^30 does X(n,2n,3n) = 0?
"""
def solution(exponent: int = 30) -> int:
"""
For any given exponent x >= 0, 1 <= n <= 2^x.
This function returns how many Nim games are lost given that
each Nim game has three heaps of the form (n, 2*n, 3*n).
>>> solution(0)
1
>>> solution(2)
3
>>> solution(10)
144
"""
# To find how many total games were lost for a given exponent x,
# we need to find the Fibonacci number F(x+2).
fibonacci_index = exponent + 2
phi = (1 + 5**0.5) / 2
fibonacci = (phi**fibonacci_index - (phi - 1) ** fibonacci_index) / 5**0.5
return int(fibonacci)
if __name__ == "__main__":
print(f"{solution() = }")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
In the Combination Sum problem, we are given a list consisting of distinct integers.
We need to find all the combinations whose sum equals to target given.
We can use an element more than one.
Time complexity(Average Case): O(n!)
Constraints:
1 <= candidates.length <= 30
2 <= candidates[i] <= 40
All elements of candidates are distinct.
1 <= target <= 40
"""
def backtrack(
candidates: list, path: list, answer: list, target: int, previous_index: int
) -> None:
"""
A recursive function that searches for possible combinations. Backtracks in case
of a bigger current combination value than the target value.
Parameters
----------
previous_index: Last index from the previous search
target: The value we need to obtain by summing our integers in the path list.
answer: A list of possible combinations
path: Current combination
candidates: A list of integers we can use.
"""
if target == 0:
answer.append(path.copy())
else:
for index in range(previous_index, len(candidates)):
if target >= candidates[index]:
path.append(candidates[index])
backtrack(candidates, path, answer, target - candidates[index], index)
path.pop(len(path) - 1)
def combination_sum(candidates: list, target: int) -> list:
"""
>>> combination_sum([2, 3, 5], 8)
[[2, 2, 2, 2], [2, 3, 3], [3, 5]]
>>> combination_sum([2, 3, 6, 7], 7)
[[2, 2, 3], [7]]
>>> combination_sum([-8, 2.3, 0], 1)
Traceback (most recent call last):
...
RecursionError: maximum recursion depth exceeded in comparison
"""
path = [] # type: list[int]
answer = [] # type: list[int]
backtrack(candidates, path, answer, target, 0)
return answer
def main() -> None:
print(combination_sum([-8, 2.3, 0], 1))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| """
In the Combination Sum problem, we are given a list consisting of distinct integers.
We need to find all the combinations whose sum equals to target given.
We can use an element more than one.
Time complexity(Average Case): O(n!)
Constraints:
1 <= candidates.length <= 30
2 <= candidates[i] <= 40
All elements of candidates are distinct.
1 <= target <= 40
"""
def backtrack(
candidates: list, path: list, answer: list, target: int, previous_index: int
) -> None:
"""
A recursive function that searches for possible combinations. Backtracks in case
of a bigger current combination value than the target value.
Parameters
----------
previous_index: Last index from the previous search
target: The value we need to obtain by summing our integers in the path list.
answer: A list of possible combinations
path: Current combination
candidates: A list of integers we can use.
"""
if target == 0:
answer.append(path.copy())
else:
for index in range(previous_index, len(candidates)):
if target >= candidates[index]:
path.append(candidates[index])
backtrack(candidates, path, answer, target - candidates[index], index)
path.pop(len(path) - 1)
def combination_sum(candidates: list, target: int) -> list:
"""
>>> combination_sum([2, 3, 5], 8)
[[2, 2, 2, 2], [2, 3, 3], [3, 5]]
>>> combination_sum([2, 3, 6, 7], 7)
[[2, 2, 3], [7]]
>>> combination_sum([-8, 2.3, 0], 1)
Traceback (most recent call last):
...
RecursionError: maximum recursion depth exceeded in comparison
"""
path = [] # type: list[int]
answer = [] # type: list[int]
backtrack(candidates, path, answer, target, 0)
return answer
def main() -> None:
print(combination_sum([-8, 2.3, 0], 1))
if __name__ == "__main__":
import doctest
doctest.testmod()
main()
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Project Euler
Problems are taken from https://projecteuler.net/, the Project Euler. [Problems are licensed under CC BY-NC-SA 4.0](https://projecteuler.net/copyright).
Project Euler is a series of challenging mathematical/computer programming problems that require more than just mathematical
insights to solve. Project Euler is ideal for mathematicians who are learning to code.
The solutions will be checked by our [automated testing on GitHub Actions](https://github.com/TheAlgorithms/Python/actions) with the help of [this script](https://github.com/TheAlgorithms/Python/blob/master/scripts/validate_solutions.py). The efficiency of your code is also checked. You can view the top 10 slowest solutions on GitHub Actions logs (under `slowest 10 durations`) and open a pull request to improve those solutions.
## Solution Guidelines
Welcome to [TheAlgorithms/Python](https://github.com/TheAlgorithms/Python)! Before reading the solution guidelines, make sure you read the whole [Contributing Guidelines](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md) as it won't be repeated in here. If you have any doubt on the guidelines, please feel free to [state it clearly in an issue](https://github.com/TheAlgorithms/Python/issues/new) or ask the community in [Gitter](https://gitter.im/TheAlgorithms). You can use the [template](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#solution-template) we have provided below as your starting point but be sure to read the [Coding Style](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#coding-style) part first.
### Coding Style
* Please maintain consistency in project directory and solution file names. Keep the following points in mind:
* Create a new directory only for the problems which do not exist yet.
* If you create a new directory, please create an empty `__init__.py` file inside it as well.
* Please name the project **directory** as `problem_<problem_number>` where `problem_number` should be filled with 0s so as to occupy 3 digits. Example: `problem_001`, `problem_002`, `problem_067`, `problem_145`, and so on.
* Please provide a link to the problem and other references, if used, in the **module-level docstring**.
* All imports should come ***after*** the module-level docstring.
* You can have as many helper functions as you want but there should be one main function called `solution` which should satisfy the conditions as stated below:
* It should contain positional argument(s) whose default value is the question input. Example: Please take a look at [Problem 1](https://projecteuler.net/problem=1) where the question is to *Find the sum of all the multiples of 3 or 5 below 1000.* In this case the main solution function will be `solution(limit: int = 1000)`.
* When the `solution` function is called without any arguments like so: `solution()`, it should return the answer to the problem.
* Every function, which includes all the helper functions, if any, and the main solution function, should have `doctest` in the function docstring along with a brief statement mentioning what the function is about.
* There should not be a `doctest` for testing the answer as that is done by our GitHub Actions build using this [script](https://github.com/TheAlgorithms/Python/blob/master/scripts/validate_solutions.py). Keeping in mind the above example of [Problem 1](https://projecteuler.net/problem=1):
```python
def solution(limit: int = 1000):
"""
A brief statement mentioning what the function is about.
You can have a detailed explanation about the solution method in the
module-level docstring.
>>> solution(1)
...
>>> solution(16)
...
>>> solution(100)
...
"""
```
### Solution Template
You can use the below template as your starting point but please read the [Coding Style](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#coding-style) first to understand how the template works.
Please change the name of the helper functions accordingly, change the parameter names with a descriptive one, replace the content within `[square brackets]` (including the brackets) with the appropriate content.
```python
"""
Project Euler Problem [problem number]: [link to the original problem]
... [Entire problem statement] ...
... [Solution explanation - Optional] ...
References [Optional]:
- [Wikipedia link to the topic]
- [Stackoverflow link]
...
"""
import module1
import module2
...
def helper1(arg1: [type hint], arg2: [type hint], ...) -> [Return type hint]:
"""
A brief statement explaining what the function is about.
... A more elaborate description ... [Optional]
...
[Doctest]
...
"""
...
# calculations
...
return
# You can have multiple helper functions but the solution function should be
# after all the helper functions ...
def solution(arg1: [type hint], arg2: [type hint], ...) -> [Return type hint]:
"""
A brief statement mentioning what the function is about.
You can have a detailed explanation about the solution in the
module-level docstring.
...
[Doctest as mentioned above]
...
"""
...
# calculations
...
return answer
if __name__ == "__main__":
print(f"{solution() = }")
```
| # Project Euler
Problems are taken from https://projecteuler.net/, the Project Euler. [Problems are licensed under CC BY-NC-SA 4.0](https://projecteuler.net/copyright).
Project Euler is a series of challenging mathematical/computer programming problems that require more than just mathematical
insights to solve. Project Euler is ideal for mathematicians who are learning to code.
The solutions will be checked by our [automated testing on GitHub Actions](https://github.com/TheAlgorithms/Python/actions) with the help of [this script](https://github.com/TheAlgorithms/Python/blob/master/scripts/validate_solutions.py). The efficiency of your code is also checked. You can view the top 10 slowest solutions on GitHub Actions logs (under `slowest 10 durations`) and open a pull request to improve those solutions.
## Solution Guidelines
Welcome to [TheAlgorithms/Python](https://github.com/TheAlgorithms/Python)! Before reading the solution guidelines, make sure you read the whole [Contributing Guidelines](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md) as it won't be repeated in here. If you have any doubt on the guidelines, please feel free to [state it clearly in an issue](https://github.com/TheAlgorithms/Python/issues/new) or ask the community in [Gitter](https://gitter.im/TheAlgorithms). You can use the [template](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#solution-template) we have provided below as your starting point but be sure to read the [Coding Style](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#coding-style) part first.
### Coding Style
* Please maintain consistency in project directory and solution file names. Keep the following points in mind:
* Create a new directory only for the problems which do not exist yet.
* If you create a new directory, please create an empty `__init__.py` file inside it as well.
* Please name the project **directory** as `problem_<problem_number>` where `problem_number` should be filled with 0s so as to occupy 3 digits. Example: `problem_001`, `problem_002`, `problem_067`, `problem_145`, and so on.
* Please provide a link to the problem and other references, if used, in the **module-level docstring**.
* All imports should come ***after*** the module-level docstring.
* You can have as many helper functions as you want but there should be one main function called `solution` which should satisfy the conditions as stated below:
* It should contain positional argument(s) whose default value is the question input. Example: Please take a look at [Problem 1](https://projecteuler.net/problem=1) where the question is to *Find the sum of all the multiples of 3 or 5 below 1000.* In this case the main solution function will be `solution(limit: int = 1000)`.
* When the `solution` function is called without any arguments like so: `solution()`, it should return the answer to the problem.
* Every function, which includes all the helper functions, if any, and the main solution function, should have `doctest` in the function docstring along with a brief statement mentioning what the function is about.
* There should not be a `doctest` for testing the answer as that is done by our GitHub Actions build using this [script](https://github.com/TheAlgorithms/Python/blob/master/scripts/validate_solutions.py). Keeping in mind the above example of [Problem 1](https://projecteuler.net/problem=1):
```python
def solution(limit: int = 1000):
"""
A brief statement mentioning what the function is about.
You can have a detailed explanation about the solution method in the
module-level docstring.
>>> solution(1)
...
>>> solution(16)
...
>>> solution(100)
...
"""
```
### Solution Template
You can use the below template as your starting point but please read the [Coding Style](https://github.com/TheAlgorithms/Python/blob/master/project_euler/README.md#coding-style) first to understand how the template works.
Please change the name of the helper functions accordingly, change the parameter names with a descriptive one, replace the content within `[square brackets]` (including the brackets) with the appropriate content.
```python
"""
Project Euler Problem [problem number]: [link to the original problem]
... [Entire problem statement] ...
... [Solution explanation - Optional] ...
References [Optional]:
- [Wikipedia link to the topic]
- [Stackoverflow link]
...
"""
import module1
import module2
...
def helper1(arg1: [type hint], arg2: [type hint], ...) -> [Return type hint]:
"""
A brief statement explaining what the function is about.
... A more elaborate description ... [Optional]
...
[Doctest]
...
"""
...
# calculations
...
return
# You can have multiple helper functions but the solution function should be
# after all the helper functions ...
def solution(arg1: [type hint], arg2: [type hint], ...) -> [Return type hint]:
"""
A brief statement mentioning what the function is about.
You can have a detailed explanation about the solution in the
module-level docstring.
...
[Doctest as mentioned above]
...
"""
...
# calculations
...
return answer
if __name__ == "__main__":
print(f"{solution() = }")
```
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # Required imports to run this file
import matplotlib.pyplot as plt
import numpy as np
# weighted matrix
def weighted_matrix(point: np.mat, training_data_x: np.mat, bandwidth: float) -> np.mat:
"""
Calculate the weight for every point in the
data set. It takes training_point , query_point, and tau
Here Tau is not a fixed value it can be varied depends on output.
tau --> bandwidth
xmat -->Training data
point --> the x where we want to make predictions
>>> weighted_matrix(np.array([1., 1.]),np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]), 0.6)
matrix([[1.43807972e-207, 0.00000000e+000, 0.00000000e+000],
[0.00000000e+000, 0.00000000e+000, 0.00000000e+000],
[0.00000000e+000, 0.00000000e+000, 0.00000000e+000]])
"""
# m is the number of training samples
m, n = np.shape(training_data_x)
# Initializing weights as identity matrix
weights = np.mat(np.eye(m))
# calculating weights for all training examples [x(i)'s]
for j in range(m):
diff = point - training_data_x[j]
weights[j, j] = np.exp(diff * diff.T / (-2.0 * bandwidth**2))
return weights
def local_weight(
point: np.mat, training_data_x: np.mat, training_data_y: np.mat, bandwidth: float
) -> np.mat:
"""
Calculate the local weights using the weight_matrix function on training data.
Return the weighted matrix.
>>> local_weight(np.array([1., 1.]),np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
matrix([[0.00873174],
[0.08272556]])
"""
weight = weighted_matrix(point, training_data_x, bandwidth)
w = (training_data_x.T * (weight * training_data_x)).I * (
training_data_x.T * weight * training_data_y.T
)
return w
def local_weight_regression(
training_data_x: np.mat, training_data_y: np.mat, bandwidth: float
) -> np.mat:
"""
Calculate predictions for each data point on axis.
>>> local_weight_regression(np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
array([1.07173261, 1.65970737, 3.50160179])
"""
m, n = np.shape(training_data_x)
ypred = np.zeros(m)
for i, item in enumerate(training_data_x):
ypred[i] = item * local_weight(
item, training_data_x, training_data_y, bandwidth
)
return ypred
def load_data(dataset_name: str, cola_name: str, colb_name: str) -> np.mat:
"""
Function used for loading data from the seaborn splitting into x and y points
>>> pass # this function has no doctest
"""
import seaborn as sns
data = sns.load_dataset(dataset_name)
col_a = np.array(data[cola_name]) # total_bill
col_b = np.array(data[colb_name]) # tip
mcol_a = np.mat(col_a)
mcol_b = np.mat(col_b)
m = np.shape(mcol_b)[1]
one = np.ones((1, m), dtype=int)
# horizontal stacking
training_data_x = np.hstack((one.T, mcol_a.T))
return training_data_x, mcol_b, col_a, col_b
def get_preds(training_data_x: np.mat, mcol_b: np.mat, tau: float) -> np.ndarray:
"""
Get predictions with minimum error for each training data
>>> get_preds(np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
array([1.07173261, 1.65970737, 3.50160179])
"""
ypred = local_weight_regression(training_data_x, mcol_b, tau)
return ypred
def plot_preds(
training_data_x: np.mat,
predictions: np.ndarray,
col_x: np.ndarray,
col_y: np.ndarray,
cola_name: str,
colb_name: str,
) -> plt.plot:
"""
This function used to plot predictions and display the graph
>>> pass #this function has no doctest
"""
xsort = training_data_x.copy()
xsort.sort(axis=0)
plt.scatter(col_x, col_y, color="blue")
plt.plot(
xsort[:, 1],
predictions[training_data_x[:, 1].argsort(0)],
color="yellow",
linewidth=5,
)
plt.title("Local Weighted Regression")
plt.xlabel(cola_name)
plt.ylabel(colb_name)
plt.show()
if __name__ == "__main__":
training_data_x, mcol_b, col_a, col_b = load_data("tips", "total_bill", "tip")
predictions = get_preds(training_data_x, mcol_b, 0.5)
plot_preds(training_data_x, predictions, col_a, col_b, "total_bill", "tip")
| # Required imports to run this file
import matplotlib.pyplot as plt
import numpy as np
# weighted matrix
def weighted_matrix(point: np.mat, training_data_x: np.mat, bandwidth: float) -> np.mat:
"""
Calculate the weight for every point in the
data set. It takes training_point , query_point, and tau
Here Tau is not a fixed value it can be varied depends on output.
tau --> bandwidth
xmat -->Training data
point --> the x where we want to make predictions
>>> weighted_matrix(np.array([1., 1.]),np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]), 0.6)
matrix([[1.43807972e-207, 0.00000000e+000, 0.00000000e+000],
[0.00000000e+000, 0.00000000e+000, 0.00000000e+000],
[0.00000000e+000, 0.00000000e+000, 0.00000000e+000]])
"""
# m is the number of training samples
m, n = np.shape(training_data_x)
# Initializing weights as identity matrix
weights = np.mat(np.eye(m))
# calculating weights for all training examples [x(i)'s]
for j in range(m):
diff = point - training_data_x[j]
weights[j, j] = np.exp(diff * diff.T / (-2.0 * bandwidth**2))
return weights
def local_weight(
point: np.mat, training_data_x: np.mat, training_data_y: np.mat, bandwidth: float
) -> np.mat:
"""
Calculate the local weights using the weight_matrix function on training data.
Return the weighted matrix.
>>> local_weight(np.array([1., 1.]),np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
matrix([[0.00873174],
[0.08272556]])
"""
weight = weighted_matrix(point, training_data_x, bandwidth)
w = (training_data_x.T * (weight * training_data_x)).I * (
training_data_x.T * weight * training_data_y.T
)
return w
def local_weight_regression(
training_data_x: np.mat, training_data_y: np.mat, bandwidth: float
) -> np.mat:
"""
Calculate predictions for each data point on axis.
>>> local_weight_regression(np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
array([1.07173261, 1.65970737, 3.50160179])
"""
m, n = np.shape(training_data_x)
ypred = np.zeros(m)
for i, item in enumerate(training_data_x):
ypred[i] = item * local_weight(
item, training_data_x, training_data_y, bandwidth
)
return ypred
def load_data(dataset_name: str, cola_name: str, colb_name: str) -> np.mat:
"""
Function used for loading data from the seaborn splitting into x and y points
>>> pass # this function has no doctest
"""
import seaborn as sns
data = sns.load_dataset(dataset_name)
col_a = np.array(data[cola_name]) # total_bill
col_b = np.array(data[colb_name]) # tip
mcol_a = np.mat(col_a)
mcol_b = np.mat(col_b)
m = np.shape(mcol_b)[1]
one = np.ones((1, m), dtype=int)
# horizontal stacking
training_data_x = np.hstack((one.T, mcol_a.T))
return training_data_x, mcol_b, col_a, col_b
def get_preds(training_data_x: np.mat, mcol_b: np.mat, tau: float) -> np.ndarray:
"""
Get predictions with minimum error for each training data
>>> get_preds(np.mat([[16.99, 10.34], [21.01,23.68],
... [24.59,25.69]]),np.mat([[1.01, 1.66, 3.5]]), 0.6)
array([1.07173261, 1.65970737, 3.50160179])
"""
ypred = local_weight_regression(training_data_x, mcol_b, tau)
return ypred
def plot_preds(
training_data_x: np.mat,
predictions: np.ndarray,
col_x: np.ndarray,
col_y: np.ndarray,
cola_name: str,
colb_name: str,
) -> plt.plot:
"""
This function used to plot predictions and display the graph
>>> pass #this function has no doctest
"""
xsort = training_data_x.copy()
xsort.sort(axis=0)
plt.scatter(col_x, col_y, color="blue")
plt.plot(
xsort[:, 1],
predictions[training_data_x[:, 1].argsort(0)],
color="yellow",
linewidth=5,
)
plt.title("Local Weighted Regression")
plt.xlabel(cola_name)
plt.ylabel(colb_name)
plt.show()
if __name__ == "__main__":
training_data_x, mcol_b, col_a, col_b = load_data("tips", "total_bill", "tip")
predictions = get_preds(training_data_x, mcol_b, 0.5)
plot_preds(training_data_x, predictions, col_a, col_b, "total_bill", "tip")
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| """
Problem 20: https://projecteuler.net/problem=20
n! means n × (n − 1) × ... × 3 × 2 × 1
For example, 10! = 10 × 9 × ... × 3 × 2 × 1 = 3628800,
and the sum of the digits in the number 10! is 3 + 6 + 2 + 8 + 8 + 0 + 0 = 27.
Find the sum of the digits in the number 100!
"""
from math import factorial
def solution(num: int = 100) -> int:
"""Returns the sum of the digits in the factorial of num
>>> solution(1000)
10539
>>> solution(200)
1404
>>> solution(100)
648
>>> solution(50)
216
>>> solution(10)
27
>>> solution(5)
3
>>> solution(3)
6
>>> solution(2)
2
>>> solution(1)
1
>>> solution(0)
1
"""
return sum(map(int, str(factorial(num))))
if __name__ == "__main__":
print(solution(int(input("Enter the Number: ").strip())))
| """
Problem 20: https://projecteuler.net/problem=20
n! means n × (n − 1) × ... × 3 × 2 × 1
For example, 10! = 10 × 9 × ... × 3 × 2 × 1 = 3628800,
and the sum of the digits in the number 10! is 3 + 6 + 2 + 8 + 8 + 0 + 0 = 27.
Find the sum of the digits in the number 100!
"""
from math import factorial
def solution(num: int = 100) -> int:
"""Returns the sum of the digits in the factorial of num
>>> solution(1000)
10539
>>> solution(200)
1404
>>> solution(100)
648
>>> solution(50)
216
>>> solution(10)
27
>>> solution(5)
3
>>> solution(3)
6
>>> solution(2)
2
>>> solution(1)
1
>>> solution(0)
1
"""
return sum(map(int, str(factorial(num))))
if __name__ == "__main__":
print(solution(int(input("Enter the Number: ").strip())))
| -1 |
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| -1 |
||
TheAlgorithms/Python | 7,417 | Remove references to depreciated QasmSimulator | ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| tianyizheng02 | 2022-10-19T03:31:43Z | 2022-10-19T20:12:44Z | 50da472ddcdc2d79d1ad325ec05cda3558802fda | 2859d4bf3aa96737a4715c65d4a9051d9c62d24d | Remove references to depreciated QasmSimulator. ### Describe your change:
Replaced instances of `qiskit.Aer.get_backend("qasm_simulator")` in `quantum/` with `q.Aer.get_backend("aer_simulator")`, as the former is depreciated and raises warnings (Qiskit's [documentation](https://qiskit.org/documentation/apidoc/aer_provider.html) says that `QasmSimulator` is legacy).
This PR edits multiple code files because they all raise the same warning and are mentioned in the same GitHub issue.
Fixes #7308
* [ ] Add an algorithm?
* [x] Fix a bug or typo in an existing algorithm?
* [ ] Documentation change?
### Checklist:
* [x] I have read [CONTRIBUTING.md](https://github.com/TheAlgorithms/Python/blob/master/CONTRIBUTING.md).
* [x] This pull request is all my own work -- I have not plagiarized.
* [x] I know that pull requests will not be merged if they fail the automated tests.
* [ ] This PR only changes one algorithm file. To ease review, please open separate PRs for separate algorithms.
* [x] All new Python files are placed inside an existing directory.
* [x] All filenames are in all lowercase characters with no spaces or dashes.
* [x] All functions and variable names follow Python naming conventions.
* [x] All function parameters and return values are annotated with Python [type hints](https://docs.python.org/3/library/typing.html).
* [x] All functions have [doctests](https://docs.python.org/3/library/doctest.html) that pass the automated testing.
* [x] All new algorithms have a URL in its comments that points to Wikipedia or other similar explanation.
* [x] If this pull request resolves one or more open issues then the commit message contains `Fixes: #{$ISSUE_NO}`.
| # This is a comment.
# Each line is a file pattern followed by one or more owners.
# More details are here: https://help.github.com/articles/about-codeowners/
# The '*' pattern is global owners.
# Order is important. The last matching pattern has the most precedence.
/.* @cclauss @dhruvmanila
# /arithmetic_analysis/
# /backtracking/
# /bit_manipulation/
# /blockchain/
# /boolean_algebra/
# /cellular_automata/
# /ciphers/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /compression/
# /computer_vision/
# /conversions/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /data_structures/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /digital_image_processing/
# /divide_and_conquer/
# /dynamic_programming/
# /file_transfer/
# /fuzzy_logic/
# /genetic_algorithm/
# /geodesy/
# /graphics/
# /graphs/
# /greedy_method/
# /hashes/
# /images/
# /linear_algebra/
# /machine_learning/
# /maths/
# /matrix/
# /networking_flow/
# /neural_network/
# /other/ @cclauss # TODO: Uncomment this line after Hacktoberfest
/project_euler/ @dhruvmanila
# /quantum/
# /scheduling/
# /scripts/
# /searches/
# /sorts/
# /strings/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /traversals/
/web_programming/ @cclauss
| # This is a comment.
# Each line is a file pattern followed by one or more owners.
# More details are here: https://help.github.com/articles/about-codeowners/
# The '*' pattern is global owners.
# Order is important. The last matching pattern has the most precedence.
/.* @cclauss @dhruvmanila
# /arithmetic_analysis/
# /backtracking/
# /bit_manipulation/
# /blockchain/
# /boolean_algebra/
# /cellular_automata/
# /ciphers/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /compression/
# /computer_vision/
# /conversions/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /data_structures/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /digital_image_processing/
# /divide_and_conquer/
# /dynamic_programming/
# /file_transfer/
# /fuzzy_logic/
# /genetic_algorithm/
# /geodesy/
# /graphics/
# /graphs/
# /greedy_method/
# /hashes/
# /images/
# /linear_algebra/
# /machine_learning/
# /maths/
# /matrix/
# /networking_flow/
# /neural_network/
# /other/ @cclauss # TODO: Uncomment this line after Hacktoberfest
/project_euler/ @dhruvmanila
# /quantum/
# /scheduling/
# /scripts/
# /searches/
# /sorts/
# /strings/ @cclauss # TODO: Uncomment this line after Hacktoberfest
# /traversals/
/web_programming/ @cclauss
| -1 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.