path
stringlengths 7
265
| concatenated_notebook
stringlengths 46
17M
|
---|---|
courses/machine_learning/deepdive2/structured/labs/3c_bqml_dnn_babyweight.ipynb | ###Markdown
LAB 3c: BigQuery ML Model Deep Neural Network.**Learning Objectives**1. Create and evaluate DNN model with BigQuery ML1. Create and evaluate DNN model with feature engineering with ML.TRANSFORM.1. Calculate predictions with BigQuery's ML.PREDICT Introduction In this notebook, we will create multiple deep neural network models to predict the weight of a baby before it is born, using first no feature engineering and then the feature engineering from the previous lab using BigQuery ML.We will create and evaluate a DNN model using BigQuery ML, with and without feature engineering using BigQuery's ML.TRANSFORM and calculate predictions with BigQuery's ML.PREDICT. If you need a refresher, you can go back and look how we made a baseline model in the notebook [BQML Baseline Model](../solutions/3a_bqml_baseline_babyweight.ipynb) or how we combined linear models with feature engineering in the notebook [BQML Linear Models with Feature Engineering](../solutions/3b_bqml_linear_transform_babyweight.ipynb).Each learning objective will correspond to a __TODO__ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/3c_bqml_dnn_babyweight.ipynb). Load necessary libraries Check that the Google BigQuery library is installed and if not, install it.
###Code
%%bash
pip freeze | grep google-cloud-bigquery==1.6.1 || \
pip install google-cloud-bigquery==1.6.1
###Output
_____no_output_____
###Markdown
Verify tables existRun the following cells to verify that we have previously created the dataset and data tables. If not, go back to lab [1b_prepare_data_babyweight](../solutions/1b_prepare_data_babyweight.ipynb) to create them.
###Code
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_train
LIMIT 0
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_eval
LIMIT 0
###Output
_____no_output_____
###Markdown
Lab Task 1: Model 4: Increase complexity of model using DNN_REGRESSORDNN_REGRESSOR is a new regression model_type vs. the LINEAR_REG that we have been using in previous labs.* MODEL_TYPE="DNN_REGRESSOR"* hidden_units: List of hidden units per layer; all layers are fully connected. Number of elements in the array will be the number of hidden layers. The default value for hidden_units is [Min(128, N / (𝜶(Ni+No)))] (1 hidden layer), with N the training data size, Ni, No the input layer and output layer units, respectively, 𝜶 is constant with value 10. The upper bound of the rule will make sure the model won’t be over fitting. Note that, we currently have a model size limitation to 256MB.* dropout: Probability to drop a given coordinate during training; dropout is a very common technique to avoid overfitting in DNNs. The default value is zero, which means we will not drop out any coordinate during training.* batch_size: Number of samples that will be served to train the network for each sub iteration. The default value is Min(1024, num_examples) to balance the training speed and convergence. Serving all training data in each sub-iteration may lead to convergence issues, and is not advised. Create DNN_REGRESSOR modelChange model type to use DNN_REGRESSOR, add a list of integer HIDDEN_UNITS, and add an integer BATCH_SIZE.* Hint: Create a model_4.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.model_4
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
# TODO: Add base features and label
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Get training information and evaluate Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.model_4)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 2: Final Model: Apply the TRANSFORM clauseBefore we perform our prediction, we should encapsulate the entire feature set in a TRANSFORM clause as we did in the last notebook. This way we can have the same transformations applied for training and prediction without modifying the queries. Let's apply the TRANSFORM clause to the final model and run the query.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.final_model
TRANSFORM(
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks,
# TODO: Add FEATURE CROSS of:
# is_male, bucketed_mother_age, plurality, and bucketed_gestation_weeks
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
*
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.final_model)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 3: Predict with final model.Now that you have evaluated your model, the next step is to use it to predict the weight of a baby before it is born, using BigQuery `ML.PREDICT` function. Predict from final model using an example from original dataset
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from original dataset
))
###Output
_____no_output_____
###Markdown
Modify above prediction query using example from simulated datasetUse the feature values you made up above, however set is_male to "Unknown" and plurality to "Multiple(2+)". This is simulating us not knowing the gender or the exact plurality.
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from simulated dataset
))
###Output
_____no_output_____
###Markdown
LAB 3c: BigQuery ML Model Deep Neural Network.**Learning Objectives**1. Create and evaluate DNN model with BigQuery ML1. Create and evaluate DNN model with feature engineering with ML.TRANSFORM.1. Calculate predictions with BigQuery's ML.PREDICT Introduction In this notebook, we will create multiple deep neural network models to predict the weight of a baby before it is born, using first no feature engineering and then the feature engineering from the previous lab using BigQuery ML.We will create and evaluate a DNN model using BigQuery ML, with and without feature engineering using BigQuery's ML.TRANSFORM and calculate predictions with BigQuery's ML.PREDICT. If you need a refresher, you can go back and look how we made a baseline model in the notebook [BQML Baseline Model](../solutions/3a_bqml_baseline_babyweight.ipynb) or how we combined linear models with feature engineering in the notebook [BQML Linear Models with Feature Engineering](../solutions/3b_bqml_linear_transform_babyweight.ipynb).Each learning objective will correspond to a __TODO__ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/3c_bqml_dnn_babyweight.ipynb). Load necessary libraries Check that the Google BigQuery library is installed and if not, install it.
###Code
%%bash
sudo pip freeze | grep google-cloud-bigquery==1.6.1 || \
sudo pip install google-cloud-bigquery==1.6.1
###Output
_____no_output_____
###Markdown
Verify tables existRun the following cells to verify that we have previously created the dataset and data tables. If not, go back to lab [1b_prepare_data_babyweight](../solutions/1b_prepare_data_babyweight.ipynb) to create them.
###Code
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_train
LIMIT 0
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_eval
LIMIT 0
###Output
_____no_output_____
###Markdown
Lab Task 1: Model 4: Increase complexity of model using DNN_REGRESSORDNN_REGRESSOR is a new regression model_type vs. the LINEAR_REG that we have been using in previous labs.* MODEL_TYPE="DNN_REGRESSOR"* hidden_units: List of hidden units per layer; all layers are fully connected. Number of elements in the array will be the number of hidden layers. The default value for hidden_units is [Min(128, N / (𝜶(Ni+No)))] (1 hidden layer), with N the training data size, Ni, No the input layer and output layer units, respectively, 𝜶 is constant with value 10. The upper bound of the rule will make sure the model won’t be over fitting. Note that, we currently have a model size limitation to 256MB.* dropout: Probability to drop a given coordinate during training; dropout is a very common technique to avoid overfitting in DNNs. The default value is zero, which means we will not drop out any coordinate during training.* batch_size: Number of samples that will be served to train the network for each sub iteration. The default value is Min(1024, num_examples) to balance the training speed and convergence. Serving all training data in each sub-iteration may lead to convergence issues, and is not advised. Create DNN_REGRESSOR modelChange model type to use DNN_REGRESSOR, add a list of integer HIDDEN_UNITS, and add an integer BATCH_SIZE.* Hint: Create a model_4.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.model_4
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
# TODO: Add base features and label
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Get training information and evaluate Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.model_4)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 2: Final Model: Apply the TRANSFORM clauseBefore we perform our prediction, we should encapsulate the entire feature set in a TRANSFORM clause as we did in the last notebook. This way we can have the same transformations applied for training and prediction without modifying the queries. Let's apply the TRANSFORM clause to the final model and run the query.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.final_model
TRANSFORM(
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks,
# TODO: Add FEATURE CROSS of:
# is_male, bucketed_mother_age, plurality, and bucketed_gestation_weeks
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
*
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.final_model)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 3: Predict with final model.Now that you have evaluated your model, the next step is to use it to predict the weight of a baby before it is born, using BigQuery `ML.PREDICT` function. Predict from final model using an example from original dataset
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from original dataset
))
###Output
_____no_output_____
###Markdown
Modify above prediction query using example from simulated datasetUse the feature values you made up above, however set is_male to "Unknown" and plurality to "Multiple(2+)". This is simulating us not knowing the gender or the exact plurality.
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from simulated dataset
))
###Output
_____no_output_____
###Markdown
LAB 3c: BigQuery ML Model Deep Neural Network.**Learning Objectives**1. Create and evaluate DNN model with BigQuery ML1. Create and evaluate DNN model with feature engineering with ML.TRANSFORM.1. Calculate predictions with BigQuery's ML.PREDICT Introduction In this notebook, we will create multiple deep neural network models to predict the weight of a baby before it is born, using first no feature engineering and then the feature engineering from the previous lab using BigQuery ML.We will create and evaluate a DNN model using BigQuery ML, with and without feature engineering using BigQuery's ML.TRANSFORM and calculate predictions with BigQuery's ML.PREDICT. If you need a refresher, you can go back and look how we made a baseline model in the notebook [BQML Baseline Model](../solutions/3a_bqml_baseline_babyweight.ipynb) or how we combined linear models with feature engineering in the notebook [BQML Linear Models with Feature Engineering](../solutions/3b_bqml_linear_transform_babyweight.ipynb).Each learning objective will correspond to a __TODO__ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/3c_bqml_dnn_babyweight.ipynb). Load necessary libraries Check that the Google BigQuery library is installed and if not, install it.
###Code
%%bash
sudo pip freeze | grep google-cloud-bigquery==1.6.1 || \
sudo pip install google-cloud-bigquery==1.6.1
###Output
_____no_output_____
###Markdown
Verify tables existRun the following cells to verify that we have previously created the dataset and data tables. If not, go back to lab [1b_prepare_data_babyweight](../solutions/1b_prepare_data_babyweight.ipynb) to create them.
###Code
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_train
LIMIT 0
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_eval
LIMIT 0
###Output
_____no_output_____
###Markdown
Lab Task 1: Model 4: Increase complexity of model using DNN_REGRESSORDNN_REGRESSOR is a new regression model_type vs. the LINEAR_REG that we have been using in previous labs.* MODEL_TYPE="DNN_REGRESSOR"* hidden_units: List of hidden units per layer; all layers are fully connected. Number of elements in the array will be the number of hidden layers. The default value for hidden_units is [Min(128, N / (𝜶(Ni+No)))] (1 hidden layer), with N the training data size, Ni, No the input layer and output layer units, respectively, 𝜶 is constant with value 10. The upper bound of the rule will make sure the model won’t be over fitting. Note that, we currently have a model size limitation to 256MB.* dropout: Probability to drop a given coordinate during training; dropout is a very common technique to avoid overfitting in DNNs. The default value is zero, which means we will not drop out any coordinate during training.* batch_size: Number of samples that will be served to train the network for each sub iteration. The default value is Min(1024, num_examples) to balance the training speed and convergence. Serving all training data in each sub-iteration may lead to convergence issues, and is not advised. Create DNN_REGRESSOR modelChange model type to use DNN_REGRESSOR, add a list of integer HIDDEN_UNITS, and add an integer BATCH_SIZE.* Hint: Create a model_4.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.model_4
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
# TODO: Add base features and label
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Get training information and evaluate Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.model_4)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 2: Final Model: Apply the TRANSFORM clauseBefore we perform our prediction, we should encapsulate the entire feature set in a TRANSFORM clause as we did in the last notebook. This way we can have the same transformations applied for training and prediction without modifying the queries. Let's apply the TRANSFORM clause to the final model and run the query.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.final_model
TRANSFORM(
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks,
# TODO: Add FEATURE CROSS of:
# is_male, bucketed_mother_age, plurality, and bucketed_gestation_weeks
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
*
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.final_model)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 3: Predict with final model.Now that you have evaluated your model, the next step is to use it to predict the weight of a baby before it is born, using BigQuery `ML.PREDICT` function. Predict from final model using an example from original dataset
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from original dataset
))
###Output
_____no_output_____
###Markdown
Modify above prediction query using example from simulated datasetUse the feature values you made up above, however set is_male to "Unknown" and plurality to "Multiple(2+)". This is simulating us not knowing the gender or the exact plurality.
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from simulated dataset
))
###Output
_____no_output_____
###Markdown
LAB 3c: BigQuery ML Model Deep Neural Network. Learning Objectives1. Create and evaluate DNN model with BigQuery ML.1. Create and evaluate DNN model with feature engineering with ML.TRANSFORM.1. Calculate predictions with BigQuery's ML.PREDICT. Introduction In this notebook, we will create multiple deep neural network models to predict the weight of a baby before it is born, using first no feature engineering and then the feature engineering from the previous lab using BigQuery ML.We will create and evaluate a DNN model using BigQuery ML, with and without feature engineering using BigQuery's ML.TRANSFORM and calculate predictions with BigQuery's ML.PREDICT. If you need a refresher, you can go back and look how we made a baseline model in the notebook [BQML Baseline Model](../solutions/3a_bqml_baseline_babyweight.ipynb) or how we combined linear models with feature engineering in the notebook [BQML Linear Models with Feature Engineering](../solutions/3b_bqml_linear_transform_babyweight.ipynb).Each learning objective will correspond to a __TODO__ in this student lab notebook -- try to complete this notebook first and then review the [solution notebook](../solutions/3c_bqml_dnn_babyweight.ipynb). Load necessary libraries Check that the Google BigQuery library is installed and if not, install it.
###Code
!sudo chown -R jupyter:jupyter /home/jupyter/training-data-analyst
%%bash
pip freeze | grep google-cloud-bigquery==1.6.1 || \
pip install google-cloud-bigquery==1.6.1
###Output
_____no_output_____
###Markdown
Verify tables existRun the following cells to verify that we have previously created the dataset and data tables. If not, go back to lab [1b_prepare_data_babyweight](../solutions/1b_prepare_data_babyweight.ipynb) to create them.
###Code
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_train
LIMIT 0
%%bigquery
-- LIMIT 0 is a free query; this allows us to check that the table exists.
SELECT * FROM babyweight.babyweight_data_eval
LIMIT 0
###Output
_____no_output_____
###Markdown
Lab Task 1: Model 4: Increase complexity of model using DNN_REGRESSORDNN_REGRESSOR is a new regression model_type vs. the LINEAR_REG that we have been using in previous labs.* MODEL_TYPE="DNN_REGRESSOR"* hidden_units: List of hidden units per layer; all layers are fully connected. Number of elements in the array will be the number of hidden layers. The default value for hidden_units is [Min(128, N / (𝜶(Ni+No)))] (1 hidden layer), with N the training data size, Ni, No the input layer and output layer units, respectively, 𝜶 is constant with value 10. The upper bound of the rule will make sure the model won’t be over fitting. Note that, we currently have a model size limitation to 256MB.* dropout: Probability to drop a given coordinate during training; dropout is a very common technique to avoid overfitting in DNNs. The default value is zero, which means we will not drop out any coordinate during training.* batch_size: Number of samples that will be served to train the network for each sub iteration. The default value is Min(1024, num_examples) to balance the training speed and convergence. Serving all training data in each sub-iteration may lead to convergence issues, and is not advised. Create DNN_REGRESSOR modelChange model type to use DNN_REGRESSOR, add a list of integer HIDDEN_UNITS, and add an integer BATCH_SIZE.* Hint: Create a model_4.Note: Model creation takes around 40-50 minutes.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.model_4
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
# TODO: Add base features and label
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Get training information and evaluate Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.model_4)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.model_4,
(
SELECT
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 2: Final Model: Apply the TRANSFORM clauseBefore we perform our prediction, we should encapsulate the entire feature set in a TRANSFORM clause as we did in the last notebook. This way we can have the same transformations applied for training and prediction without modifying the queries. Let's apply the TRANSFORM clause to the final model and run the query.Note: Model creation takes around 40-50 minutes.
###Code
%%bigquery
CREATE OR REPLACE MODEL
babyweight.final_model
TRANSFORM(
weight_pounds,
is_male,
mother_age,
plurality,
gestation_weeks,
# TODO: Add FEATURE CROSS of:
# is_male, bucketed_mother_age, plurality, and bucketed_gestation_weeks
OPTIONS (
# TODO: Add DNN options
INPUT_LABEL_COLS=["weight_pounds"],
DATA_SPLIT_METHOD="NO_SPLIT") AS
SELECT
*
FROM
babyweight.babyweight_data_train
###Output
_____no_output_____
###Markdown
Let's first look at our training statistics.
###Code
%%bigquery
SELECT * FROM ML.TRAINING_INFO(MODEL babyweight.final_model)
###Output
_____no_output_____
###Markdown
Now let's evaluate our trained model on our eval dataset.
###Code
%%bigquery
SELECT
*
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Let's use our evaluation's `mean_squared_error` to calculate our model's RMSE.
###Code
%%bigquery
SELECT
SQRT(mean_squared_error) AS rmse
FROM
ML.EVALUATE(MODEL babyweight.final_model,
(
SELECT
*
FROM
babyweight.babyweight_data_eval
))
###Output
_____no_output_____
###Markdown
Lab Task 3: Predict with final model.Now that you have evaluated your model, the next step is to use it to predict the weight of a baby before it is born, using BigQuery `ML.PREDICT` function. Predict from final model using an example from original dataset
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from original dataset
))
###Output
_____no_output_____
###Markdown
Modify above prediction query using example from simulated datasetUse the feature values you made up above, however set is_male to "Unknown" and plurality to "Multiple(2+)". This is simulating us not knowing the gender or the exact plurality.
###Code
%%bigquery
SELECT
*
FROM
ML.PREDICT(MODEL babyweight.final_model,
(
SELECT
# TODO Add base features example from simulated dataset
))
###Output
_____no_output_____ |
EjerciciosSimplexDual/EjemplosSimplex.ipynb | ###Markdown
Ejercicios
###Code
import pandas as pd
import numpy as np
###Output
_____no_output_____
###Markdown
Simplex Problema 1a
###Code
df_a1 = pd.DataFrame({'z': [1, 0, 0],
'x1': [-6, 2, 1],
'x2': [-8, 1, 3],
'x3': [-5, 1, 1],
'x4': [-9, 3, 2],
's1': [0, 1, 0],
's2': [0, 0, 1],
'RHS':[0, 5, 3]},
columns=['z','x1','x2','x3','x4','s1','s2','RHS'],
index=['z', 's1', 's2'])
df_a1
# Copia de trabajo
wip1 = df_a1.copy()
# Iteración 1
wip1.iloc[2] /= 2
wip1.iloc[0] += wip1.iloc[2] * 9
wip1.iloc[1] += wip1.iloc[2] * -3
# Cambia variables del pivote
a = list(wip1.columns)
a[4] = 's2'
wip1.columns = a
a = list(wip1.index)
a[2] = 'x4'
wip1.index = a
# Show
wip1
# Iteración 2
wip1.iloc[1] *= 2
wip1.iloc[0] += wip1.iloc[1] * 1.5
wip1.iloc[2] += wip1.iloc[1] * -0.5
# Cambia variables del pivote
a = list(wip1.columns)
a[1] = 's1'
wip1.columns = a
a = list(wip1.index)
a[1] = 'x1'
wip1.index = a
# Show
wip1
# Iteración 3
wip1.iloc[2] *= (1/5)
#wip1.iloc[2] = np.around(wip1.iloc[2])
wip1.iloc[0] += wip1.iloc[2] * 5
#wip1.iloc[0] = np.around(wip1.iloc[0])
wip1.iloc[1] += wip1.iloc[2] * 7
#wip1.iloc[1] = np.around(wip1.iloc[1])
# Cambia variables del pivote
a = list(wip1.columns)
a[2] = 'x4'
wip1.columns = a
a = list(wip1.index)
a[2] = 'x2'
wip1.index = a
# Show
wip1
# Iteración 4
wip1.iloc[2] *= 5
wip1.iloc[0] += wip1.iloc[2]
wip1.iloc[0] = np.around(wip1.iloc[0])
wip1.iloc[1] += wip1.iloc[2] * -0.4
wip1.iloc[1] = np.around(wip1.iloc[1])
# Cambia variables del pivote
a = list(wip1.columns)
a[3] = 'x2'
wip1.columns = a
a = list(wip1.index)
a[2] = 'x3'
wip1.index = a
# Show
wip1
###Output
_____no_output_____
###Markdown
SoluciónValor objetivo óptimo z = -17x1 = 2x2 = 0x3 = 1x4 = 0x5 = 0 Problema 1b
###Code
df_b1 = pd.DataFrame({'z': [1, 0, 0, 0],
'x1': [-2, 0, 1, 1],
'x2': [-3, 2, 1, 2],
'x3': [-4, 3, 2, 3],
's1': [0, 1, 0, 0],
's2': [0, 0, 1, 0],
's3': [0, 0, 0, 1],
'RHS':[0, 5, 4, 7]},
columns=['z','x1','x2','x3','s1','s2', 's3', 'RHS'],
index=['z', 's1', 's2', 's3'])
df_b1
wip2 = df_b1.copy()
# Iteración 1
wip2.iloc[1] *= (1/3)
wip2.iloc[0] += wip2.iloc[1] * 4
wip2.iloc[2] += wip2.iloc[1] * -2
wip2.iloc[3] += wip2.iloc[1] * -3
# Cambia variables del pivote
a = list(wip2.columns)
a[3] = 's1'
wip2.columns = a
a = list(wip2.index)
a[1] = 'x3'
wip2.index = a
# Show
wip2
# Iteración 2
wip2.iloc[2] *= 1
wip2.iloc[0] += wip2.iloc[2] * 2
wip2.iloc[3] += wip2.iloc[2] * -1
# Cambia variables del pivote
a = list(wip2.columns)
a[1] = 's2'
wip2.columns = a
a = list(wip2.index)
a[2] = 'x1'
wip2.index = a
# Show
wip2
# Iteración 3
wip2.iloc[1] *= (3/2)
wip2.iloc[0] += wip2.iloc[1] * 1
wip2.iloc[0] = np.around(wip2.iloc[0], decimals=1)
wip2.iloc[2] += wip2.iloc[1] * (1/3)
wip2.iloc[2] = np.around(wip2.iloc[2], decimals=1)
wip2.iloc[3] += wip2.iloc[1] * -(1/3)
wip2.iloc[3] = np.around(wip2.iloc[3], decimals=1)
# Cambia variables del pivote
a = list(wip2.columns)
a[2] = 'x3'
wip2.columns = a
a = list(wip2.index)
a[1] = 'x2'
wip2.index = a
# Show
wip2
###Output
_____no_output_____
###Markdown
Valores Optimosx1 = 1.5x2 = 2.5 Problema 1c
###Code
df_c1 = pd.DataFrame({'z': [1, 0, 0, 0],
'x1': [-5, 1, 6, 3],
'x2': [-12, 1, 15, 1],
'x3': [-10, 2, -5, 5],
's1': [0, 1, 0, 0],
's2': [0, 0, 1, 0],
's3': [0, 0, 0, 1],
'RHS':[0, 10, 30, 18]},
columns=['z','x1','x2','x3','s1','s2', 's3', 'RHS'],
index=['z', 's1', 's2', 's3'])
df_c1
wip3 = df_c1.copy()
# Iteración 1
wip3.iloc[2] *= (1/15)
wip3.iloc[2] = np.around(wip3.iloc[2], decimals=1)
wip3.iloc[0] += wip3.iloc[1] * 12
wip3.iloc[1] += wip3.iloc[1] * -1
wip3.iloc[3] += wip3.iloc[1] * -1
# Cambia variables del pivote
a = list(wip3.columns)
a[2] = 's2'
wip3.columns = a
a = list(wip3.index)
a[2] = 'x2'
wip3.index = a
# Show
wip3
###Output
_____no_output_____ |
propagator.ipynb | ###Markdown
The potentialLet's see what our potential looks like and what our equilibrium distribution looks like (as esimated from data). There are four wells. The equilibrium probability of being in each well is inversely proportional to the energy of the well.
###Code
def plot_quadwell(ax):
xx = np.linspace(-1,1, 200)
ax.plot(xx, QuadWell().potential(xx), label=r'$V(x)$', color=colors[0])
#ax.hist(np.concatenate(trajs), bins=50, normed=True, label=r'$\hat{\mu}(x)$', color=colors[1], alpha=0.8)
ax.plot(xx, np.exp(-QuadWell().potential(xx))+1, color=colors[1], label=r'$\mu(x)$')
ax.axhline(1, color=colors[1], ls='--')
ax.legend(loc='upper right', fontsize=18)
ax.set_yticks([])
ax.set_xlim(-1, 1)
ax.set_ylim(0, 4)
fig, ax = draw(plot_quadwell, r'$x$')
fig.savefig("equilibrium.pdf")
###Output
_____no_output_____
###Markdown
The dynamics
###Code
from msmbuilder.example_datasets.brownian1d import _brownian_transmat, QUADWELL_GRAD_POTENTIAL
from msmbuilder.msm import _solve_msm_eigensystem
transmat = _brownian_transmat(200, 1, QUADWELL_GRAD_POTENTIAL, -1.2, 1.2, reflect_bc=False)
vals, lvevs, rvevs = _solve_msm_eigensystem(transmat, k=2)
def plot_quadwell(ax, n=200, lag_time=1, xmin=-1.2, xmax=1.2):
xx = np.linspace(xmin, xmax, n)
transmat = _brownian_transmat(n, lag_time, QUADWELL_GRAD_POTENTIAL, xmin, xmax, reflect_bc=False)
vals, lvecs, rvecs = _solve_msm_eigensystem(transmat, k=2)
ax.plot(xx, QuadWell().potential(xx)- 1, label=r'$V(x)$', color=colors[0])
# Normalization for lvecs: <p p>_{u^-1} = 1 ... too small for plots!
ax.fill_between(xx, 100*lvecs[:,1], label=r'$\phi_1(x)$', color=colors[3], alpha=0.8)
ax.plot(xx, rvecs[:,1], label=r'$\psi_1(x)$', color=colors[2])
ax.axhline(0, color='grey', ls='--')
ax.legend(loc='upper right', fontsize=18)
ax.set_yticks([])
ax.set_xlim(-1,1)
ax.set_ylim(-3, 4)
fig, ax = draw(plot_quadwell, r'$x$')
fig.savefig("transfer-vs-prop.pdf")
def plot_quadwell(ax, n=200, lag_time=1, xmin=-1.2, xmax=1.2):
xx = np.linspace(xmin, xmax, n)
transmat = _brownian_transmat(n, lag_time, QUADWELL_GRAD_POTENTIAL, xmin, xmax, reflect_bc=False)
vals, lvecs, rvecs = _solve_msm_eigensystem(transmat, k=4)
#ax.plot(xx, QuadWell().potential(xx)- 1, label=r'$V(x)$')
# Normalization for lvecs: <p p>_{u^-1} = 1 ... too small for plots!
spacing=4
ax.plot(xx, 100*lvecs[:,0], label=r'$\psi_1(x)$', color=colors[1])
ax.plot(xx, 100*lvecs[:,1]-1*spacing, label=r'$\psi_1(x)$', color=colors[2])
ax.plot(xx, 100*lvecs[:,2]-2*spacing, label=r'$\psi_2(x)$', color=colors[3])
ax.plot(xx, 100*lvecs[:,3]-3*spacing, label=r'$\psi_3(x)$', color=colors[5])
ax.axhline(0, color='grey', ls='--')
ax.axhline(-1*spacing, color='grey', ls='--')
ax.axhline(-2*spacing, color='grey', ls='--')
ax.axhline(-3*spacing, color='grey', ls='--')
#ax.legend(loc='upper right', fontsize=18)
ax.set_yticks([0, -1*spacing, -2*spacing, -3*spacing])
ax.set_yticklabels([r'$\mu = \phi_0$', r'$\phi_1$', r'$\phi_2$', r'$\phi_3$'], fontsize=18)
ax.set_xlim(-1,1)
ax.set_ylim(-3.5*spacing, 2)
fig, ax = draw(plot_quadwell, r'$x$')
fig.savefig("prop-eigs.pdf")
def plot_quadwell(ax, n=200, lag_time=1, xmin=-1.2, xmax=1.2):
xx = np.linspace(xmin, xmax, n)
transmat = _brownian_transmat(n, lag_time, QUADWELL_GRAD_POTENTIAL, xmin, xmax, reflect_bc=False)
vals, lvecs, rvecs = _solve_msm_eigensystem(transmat, k=4)
#ax.plot(xx, QuadWell().potential(xx)- 1, label=r'$V(x)$')
# Normalization for lvecs: <p p>_{u^-1} = 1 ... too small for plots!
spacing=4
ax.plot(xx, rvecs[:,0], label=r'$\psi_1(x)$', color=colors[1])
ax.plot(xx, rvecs[:,1]-1*spacing, label=r'$\psi_1(x)$', color=colors[2])
ax.plot(xx, rvecs[:,2]-2*spacing, label=r'$\psi_2(x)$', color=colors[3])
ax.plot(xx, rvecs[:,3]-3*spacing, label=r'$\psi_3(x)$', color=colors[5])
ax.axhline(0, color='grey', ls='--')
ax.axhline(-1*spacing, color='grey', ls='--')
ax.axhline(-2*spacing, color='grey', ls='--')
ax.axhline(-3*spacing, color='grey', ls='--')
#ax.legend(loc='upper right', fontsize=18)
ax.set_yticks([0, -1*spacing, -2*spacing, -3*spacing])
ax.set_yticklabels([r'$\psi_0$', r'$\psi_1$', r'$\psi_2$', r'$\psi_3$'], fontsize=18)
ax.set_xlim(-1,1)
ax.set_ylim(-3.5*spacing, 2)
fig, ax = draw(plot_quadwell, r'$x$')
fig.savefig("transfer-eigs.pdf")
###Output
_____no_output_____ |
notebook/eda/quran-mining-niheng/quran-api-II.ipynb | ###Markdown
apijuzs
###Code
juz = requests.get(apijuzs)
juz.text
juzI = pd.io.json.loads(juz.text)
type(juzI)
juzI
juzI['juzs']
d_juz = pd.DataFrame(juzI['juzs'])
d_juz
###Output
_____no_output_____
###Markdown
apichapters
###Code
chapter = requests.get(apichapters)
chapter.text
chapterI = pd.io.json.loads(chapter.text)
type(chapterI)
chapterI
chapterI['chapters']
d_chapter = pd.DataFrame(chapterI['chapters'])
d_chapter
d_chapter.dtypes
d_chapter.describe()
mk = d_chapter[d_chapter['revelation_place'].str.match('makkah')]
mk.head()
mk.describe()
###Output
_____no_output_____ |
lessons/CRISP_DM/.ipynb_checkpoints/Putting It All Together - Solution-checkpoint.ipynb | ###Markdown
Putting It All TogetherAs you might have guessed from the last notebook, using all of the variables was allowing you to drastically overfit the training data. This was great for looking good in terms of your Rsquared on these points. However, this was not great in terms of how well you were able to predict on the test data.We will start where we left off in the last notebook. First read in the dataset.
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score, mean_squared_error
import AllTogether as t
import seaborn as sns
%matplotlib inline
df = pd.read_csv('./survey_results_public.csv')
df.head()
###Output
_____no_output_____
###Markdown
Question 1**1.** To begin fill in the format function below with the correct variable. Notice each **{ }** holds a space where one of your variables will be added to the string. This will give you something to do while the the function does all the steps you did throughout this lesson.
###Code
a = 'test_score'
b = 'train_score'
c = 'linear model (lm_model)'
d = 'X_train and y_train'
e = 'X_test'
f = 'y_test'
g = 'train and test data sets'
h = 'overfitting'
q1_piat = '''In order to understand how well our {} fit the dataset,
we first needed to split our data into {}.
Then we were able to fit our {} on the {}.
We could then predict using our {} by providing
the linear model the {} for it to make predictions.
These predictions were for {}.
By looking at the {}, it looked like we were doing awesome because
it was 1! However, looking at the {} suggested our model was not
extending well. The purpose of this notebook will be to see how
well we can get our model to extend to new data.
This problem where our data fits the training data well, but does
not perform well on test data is commonly known as
{}.'''.format(c, g, c, d, c, e, f, b, a, h)
print(q1_piat)
# Print the solution order of the letters in the format
t.q1_piat_answer()
###Output
This one is tricky - here is the order of the letters for the solution we had in mind:
c, g, c, d, c, e, f, b, a, h
###Markdown
Question 2**2.** Now, we need to improve the model . Use the dictionary below to provide the true statements about improving **this model**. **Also consider each statement as a stand alone**. Though, it might be a good idea after other steps, which would you consider a useful **next step**?
###Code
a = 'yes'
b = 'no'
q2_piat = {'add interactions, quadratics, cubics, and other higher order terms': b,
'fit the model many times with different rows, then average the responses': a,
'subset the features used for fitting the model each time': a,
'this model is hopeless, we should start over': b}
#Check your solution
t.q2_piat_check(q2_piat)
###Output
Nice job! That looks right! These two techniques are really common in Machine Learning algorithms to combat overfitting. Though the first technique could be useful, it is not likely to help us right away with our current model. These additional features would likely continue to worsen the nature of overfitting we are seeing here.
###Markdown
Question 3**3.** Before we get too far along, follow the steps in the function below to create the X (explanatory matrix) and y (response vector) to be used in the model. If your solution is correct, you should see a plot similar to the one shown in the Screencast.
###Code
def clean_data(df):
'''
INPUT
df - pandas dataframe
OUTPUT
X - A matrix holding all of the variables you want to consider when predicting the response
y - the corresponding response vector
This function cleans df using the following steps to produce X and y:
1. Drop all the rows with no salaries
2. Create X as all the columns that are not the Salary column
3. Create y as the Salary column
4. Drop the Salary, Respondent, and the ExpectedSalary columns from X
5. For each numeric variable in X, fill the column with the mean value of the column.
6. Create dummy columns for all the categorical variables in X, drop the original columns
'''
# Drop rows with missing salary values
df = df.dropna(subset=['Salary'], axis=0)
y = df['Salary']
#Drop respondent and expected salary columns
df = df.drop(['Respondent', 'ExpectedSalary', 'Salary'], axis=1)
# Fill numeric columns with the mean
num_vars = df.select_dtypes(include=['float', 'int']).columns
for col in num_vars:
df[col].fillna((df[col].mean()), inplace=True)
# Dummy the categorical variables
cat_vars = df.select_dtypes(include=['object']).copy().columns
for var in cat_vars:
# for each cat add dummy var, drop original column
df = pd.concat([df.drop(var, axis=1), pd.get_dummies(df[var], prefix=var, prefix_sep='_', drop_first=True)], axis=1)
X = df
return X, y
#Use the function to create X and y
X, y = clean_data(df)
X
#cutoffs here pertains to the number of missing values allowed in the used columns.
#Therefore, lower values for the cutoff provides more predictors in the model.
cutoffs = [5000, 3500, 2500, 1000, 100, 50, 30, 25]
r2_scores_test, r2_scores_train, lm_model, X_train, X_test, y_train, y_test = t.find_optimal_lm_mod(X, y, cutoffs)
###Output
_____no_output_____
###Markdown
Question 4**4.** Use the output and above plot to correctly fill in the keys of the **q4_piat** dictionary with the correct variable. Notice that only the optimal model results are given back in the above - they are stored in **lm_model**, **X_train**, **X_test**, **y_train**, and **y_test**. If more than one answer holds, provide a tuple holding all the correct variables in the order of first variable alphabetically to last variable alphabetically.
###Code
print(X_train.shape[1]) #Number of columns
print(r2_scores_test[np.argmax(r2_scores_test)]) # The model we should implement test_r2
print(r2_scores_train[np.argmax(r2_scores_test)]) # The model we should implement train_r2
a = 'we would likely have a better rsquared for the test data.'
b = 1000
c = 872
d = 0.69
e = 0.82
f = 0.88
g = 0.72
h = 'we would likely have a better rsquared for the training data.'
q4_piat = {'The optimal number of features based on the results is': c,
'The model we should implement in practice has a train rsquared of': e,
'The model we should implement in practice has a test rsquared of': d,
'If we were to allow the number of features to continue to increase': h
}
#Check against your solution
t.q4_piat_check(q4_piat)
###Output
_____no_output_____
###Markdown
Question 5**5.** The default penalty on coefficients using linear regression in sklearn is a ridge (also known as an L2) penalty. Because of this penalty, and that all the variables were normalized, we can look at the size of the coefficients in the model as an indication of the impact of each variable on the salary. The larger the coefficient, the larger the expected impact on salary. Use the space below to take a look at the coefficients. Then use the results to provide the **True** or **False** statements based on the data.
###Code
def coef_weights(coefficients, X_train):
'''
INPUT:
coefficients - the coefficients of the linear model
X_train - the training data, so the column names can be used
OUTPUT:
coefs_df - a dataframe holding the coefficient, estimate, and abs(estimate)
Provides a dataframe that can be used to understand the most influential coefficients
in a linear model by providing the coefficient estimates along with the name of the
variable attached to the coefficient.
'''
coefs_df = pd.DataFrame()
coefs_df['est_int'] = X_train.columns
coefs_df['coefs'] = lm_model.coef_
coefs_df['abs_coefs'] = np.abs(lm_model.coef_)
coefs_df = coefs_df.sort_values('abs_coefs', ascending=False)
return coefs_df
#Use the function
coef_df = coef_weights(lm_model.coef_, X_train)
#A quick look at the top results
coef_df.head(20)
a = True
b = False
#According to the data...
q5_piat = {'Country appears to be one of the top indicators for salary': a,
'Gender appears to be one of the indicators for salary': b,
'How long an individual has been programming appears to be one of the top indicators for salary': a,
'The longer an individual has been programming the more they are likely to earn': b}
t.q5_piat_check(q5_piat)
###Output
_____no_output_____
###Markdown
Putting It All TogetherAs you might have guessed from the last notebook, using all of the variables was allowing you to drastically overfit the training data. This was great for looking good in terms of your Rsquared on these points. However, this was not great in terms of how well you were able to predict on the test data.We will start where we left off in the last notebook. First read in the dataset.
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import r2_score, mean_squared_error
import AllTogether as t
import seaborn as sns
%matplotlib inline
df = pd.read_csv('./survey_results_public.csv')
df.head()
###Output
_____no_output_____
###Markdown
Question 1**1.** To begin fill in the format function below with the correct variable. Notice each **{ }** holds a space where one of your variables will be added to the string. This will give you something to do while the the function does all the steps you did throughout this lesson.
###Code
a = 'test_score'
b = 'train_score'
c = 'linear model (lm_model)'
d = 'X_train and y_train'
e = 'X_test'
f = 'y_test'
g = 'train and test data sets'
h = 'overfitting'
q1_piat = '''In order to understand how well our {} fit the dataset,
we first needed to split our data into {}.
Then we were able to fit our {} on the {}.
We could then predict using our {} by providing
the linear model the {} for it to make predictions.
These predictions were for {}.
By looking at the {}, it looked like we were doing awesome because
it was 1! However, looking at the {} suggested our model was not
extending well. The purpose of this notebook will be to see how
well we can get our model to extend to new data.
This problem where our data fits the training data well, but does
not perform well on test data is commonly known as
{}.'''.format(c, g, c, d, c, e, f, b, a, h)
print(q1_piat)
# Print the solution order of the letters in the format
t.q1_piat_answer()
###Output
_____no_output_____
###Markdown
Question 2**2.** Now, we need to improve the model . Use the dictionary below to provide the true statements about improving **this model**. **Also consider each statement as a stand alone**. Though, it might be a good idea after other steps, which would you consider a useful **next step**?
###Code
a = 'yes'
b = 'no'
q2_piat = {'add interactions, quadratics, cubics, and other higher order terms': b,
'fit the model many times with different rows, then average the responses': a,
'subset the features used for fitting the model each time': a,
'this model is hopeless, we should start over': b}
#Check your solution
t.q2_piat_check(q2_piat)
###Output
_____no_output_____
###Markdown
Question 3**3.** Before we get too far along, follow the steps in the function below to create the X (explanatory matrix) and y (response vector) to be used in the model. If your solution is correct, you should see a plot similar to the one shown in the Screencast.
###Code
def clean_data(df):
'''
INPUT
df - pandas dataframe
OUTPUT
X - A matrix holding all of the variables you want to consider when predicting the response
y - the corresponding response vector
This function cleans df using the following steps to produce X and y:
1. Drop all the rows with no salaries
2. Create X as all the columns that are not the Salary column
3. Create y as the Salary column
4. Drop the Salary, Respondent, and the ExpectedSalary columns from X
5. For each numeric variable in X, fill the column with the mean value of the column.
6. Create dummy columns for all the categorical variables in X, drop the original columns
'''
# Drop rows with missing salary values
df = df.dropna(subset=['Salary'], axis=0)
y = df['Salary']
#Drop respondent and expected salary columns
df = df.drop(['Respondent', 'ExpectedSalary', 'Salary'], axis=1)
# Fill numeric columns with the mean
num_vars = df.select_dtypes(include=['float', 'int']).columns
for col in num_vars:
df[col].fillna((df[col].mean()), inplace=True)
# Dummy the categorical variables
cat_vars = df.select_dtypes(include=['object']).copy().columns
for var in cat_vars:
# for each cat add dummy var, drop original column
df = pd.concat([df.drop(var, axis=1), pd.get_dummies(df[var], prefix=var, prefix_sep='_', drop_first=True)], axis=1)
X = df
return X, y
#Use the function to create X and y
X, y = clean_data(df)
#cutoffs here pertains to the number of missing values allowed in the used columns.
#Therefore, lower values for the cutoff provides more predictors in the model.
cutoffs = [5000, 3500, 2500, 1000, 100, 50, 30, 25]
r2_scores_test, r2_scores_train, lm_model, X_train, X_test, y_train, y_test = t.find_optimal_lm_mod(X, y, cutoffs)
###Output
_____no_output_____
###Markdown
Question 4**4.** Use the output and above plot to correctly fill in the keys of the **q4_piat** dictionary with the correct variable. Notice that only the optimal model results are given back in the above - they are stored in **lm_model**, **X_train**, **X_test**, **y_train**, and **y_test**. If more than one answer holds, provide a tuple holding all the correct variables in the order of first variable alphabetically to last variable alphabetically.
###Code
print(X_train.shape[1]) #Number of columns
print(r2_scores_test[np.argmax(r2_scores_test)]) # The model we should implement test_r2
print(r2_scores_train[np.argmax(r2_scores_test)]) # The model we should implement train_r2
a = 'we would likely have a better rsquared for the test data.'
b = 1000
c = 872
d = 0.69
e = 0.82
f = 0.88
g = 0.72
h = 'we would likely have a better rsquared for the training data.'
q4_piat = {'The optimal number of features based on the results is': c,
'The model we should implement in practice has a train rsquared of': e,
'The model we should implement in practice has a test rsquared of': d,
'If we were to allow the number of features to continue to increase': h
}
#Check against your solution
t.q4_piat_check(q4_piat)
###Output
_____no_output_____
###Markdown
Question 5**5.** The default penalty on coefficients using linear regression in sklearn is a ridge (also known as an L2) penalty. Because of this penalty, and that all the variables were normalized, we can look at the size of the coefficients in the model as an indication of the impact of each variable on the salary. The larger the coefficient, the larger the expected impact on salary. Use the space below to take a look at the coefficients. Then use the results to provide the **True** or **False** statements based on the data.
###Code
def coef_weights(coefficients, X_train):
'''
INPUT:
coefficients - the coefficients of the linear model
X_train - the training data, so the column names can be used
OUTPUT:
coefs_df - a dataframe holding the coefficient, estimate, and abs(estimate)
Provides a dataframe that can be used to understand the most influential coefficients
in a linear model by providing the coefficient estimates along with the name of the
variable attached to the coefficient.
'''
coefs_df = pd.DataFrame()
coefs_df['est_int'] = X_train.columns
coefs_df['coefs'] = lm_model.coef_
coefs_df['abs_coefs'] = np.abs(lm_model.coef_)
coefs_df = coefs_df.sort_values('abs_coefs', ascending=False)
return coefs_df
#Use the function
coef_df = coef_weights(lm_model.coef_, X_train)
#A quick look at the top results
coef_df.head(20)
a = True
b = False
#According to the data...
q5_piat = {'Country appears to be one of the top indicators for salary': a,
'Gender appears to be one of the indicators for salary': b,
'How long an individual has been programming appears to be one of the top indicators for salary': a,
'The longer an individual has been programming the more they are likely to earn': b}
t.q5_piat_check(q5_piat)
###Output
_____no_output_____ |
grafieken_final.ipynb | ###Markdown
Top 40 of field partners with more than 1 000 loans according to the % funded loans``
###Code
s_force_order = df10.sort_values('funded', ascending=False)['Field Partner Name'].head(40)
fig, ax = plt.subplots(figsize=(15, 10))
sns.barplot(x='funded', y='Field Partner Name', data=df10,
label='funded', color='mediumspringgreen', order=s_force_order)
sns.barplot(x='expired', y='Field Partner Name', data=df10,
label='expired', color='salmon', order=s_force_order)
ax.legend(ncol=2, loc='best', frameon=True)
ax.set(ylabel='Field Partner Name',
xlabel='percentage')
leg = ax.get_legend()
new_title = 'Loan outcome?'
leg.set_title(new_title)
ax.set_title('Top 40 of field partners with more than 1 000 loans according to the % funded loans', fontsize=15)
plt.show()
s_force_order = df10.sort_values('funded', ascending=False)['Field Partner Name'].head(20)
fig, ax = plt.subplots(figsize=(8, 6))
sns.barplot(x='expired', y='Field Partner Name', data=df10,
label='expired', color='mediumspringgreen', order=s_force_order)
#ax.legend(ncol=2, loc='best', frameon=True)
ax.set(ylabel='Field Partner Name',
xlabel='Percentage expired loans')
#leg = ax.get_legend()
#new_title = 'Loan outcome?'
#leg.set_title(new_title)
#ax.set_title('Top 40 of field partners with more than 1 000 loans according to the % funded loans', fontsize=15)
plt.show()
fig.savefig('field_%expired_best.png', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Expired loans per sector Percentages
###Code
new_loan2['Field Partner Name'][new_loan2["country"] == 'Pakistan'].unique()
new_loan2['Field Partner Name'][new_loan2["country"] == 'Philippines'].unique()
import numpy as np
import pandas as pd
import seaborn as sns
import matplotlib.pyplot as plt
from scipy import stats
# Sample of loans from 2012-2017 and only field_partner loans
merge1=merge1[(merge1['year']>=2012) & (merge1['year']<=2017) & (merge1['distribution_model']=='field_partner')]
merge_expired = merge1[merge1['status'] == 'expired'].copy()
expired_sector = merge_expired['sector_name'].value_counts()
expired_sector
total_sector = merge1['sector_name'].value_counts()
total_sector
verhouding = (expired_sector/total_sector)*100
verhouding
fig = plt.figure()
verhouding.plot(kind='bar')
plt.xlabel('Sector')
plt.ylabel('Percentage expired loans')
fig.savefig('sector_%expired.png', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Absolute values
###Code
fig = plt.figure()
plt.figure(figsize=(8,6))
sector_name = merge1['sector_name'].value_counts()
bar = sns.barplot(sector_name.values, sector_name.index)
for i, v in enumerate(sector_name.values):
plt.text(3000,i,v,color='k',fontsize=10)
bar.set(xlabel='Number of loans', ylabel='Sector')
bar.figure.savefig("sector_absolute.png", bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Number of loans per country
###Code
fig = plt.figure()
plt.figure(figsize=(6,8))
country = merge1['country_name'].value_counts().head(10)
bar2 = sns.barplot(country.values, country.index, )
for i, v in enumerate(country.values):
plt.text(0.8,i,v,color='k',fontsize=14)
bar2.set(xlabel='Number of loans', ylabel='Country')
bar2.figure.savefig("country_absolute.png", bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Percentage expired loans per gender
###Code
len(merge1)
merge1['gender_reclassified'].value_counts()
# Table of status vs gender reclassified
target_gender = pd.crosstab(index=merge1["gender_reclassified"],
columns=merge1["status"],
margins=True) # Include row and column totals
target_gender.columns = ["expired","funded","rowtotal"]
target_gender.index= ["female","male","coltotal"]
target_gender
# Proportions
target_gender_proportions=target_gender.div(target_gender["rowtotal"],
axis=0)
target_gender_proportions = target_gender_proportions*100
target_gender_proportions
target_gender_proportions=target_gender_proportions.loc[['female', 'male'], :'expired']
fig = plt.figure()
target_gender_proportions.plot(kind="bar",
figsize=(8,8),legend=None)
plt.xticks(rotation='horizontal')
plt.xlabel('Gender')
plt.ylabel('Percentage expired loans')
fig.savefig('Gender_%expired.png', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Percentage expired for countries with more than 5000 loans
###Code
merge1['country_name'].value_counts()[merge1['country_name'].value_counts()>5000]
counts = merge1['country_name'].value_counts()
sub_df = merge1[~merge1['country_name'].isin(counts[counts < 5000].index)].copy()
sub_df['country_name'].value_counts()
merge1.groupby(['country_name', 'status']).size()
df = sub_df.groupby(['country_name', 'status']).size()/merge1.groupby('country_name').size()
len(df)
df = df.to_frame()
df = df.rename(columns={0: 'percentage'})
df.reset_index(level=1, inplace=True)
df.reset_index(level=0, inplace=True)
df.columns.values
len(df)
df.head(10)
df = df.pivot(index='country_name', columns='status', values='percentage')
df
df['expired'] = df['expired'].fillna(0)
df['funded'] = df['funded'].fillna(0)
df['total'] = df['expired'].fillna(0) + df['funded'].fillna(0)
df = df.sort_values(by='funded', ascending=False).head(60)
df.reset_index(level=0, inplace=True)
df.iloc[:,1:] = df.iloc[:,1:].mul(100)
df
merge1.country_name.nunique()
s_force_order = df.sort_values('funded', ascending=False)['country_name'].head(40)
fig, ax = plt.subplots(figsize=(8, 10))
sns.barplot(x='expired', y='country_name', data=df,
label='expired', color='#0abda0', order=s_force_order)
ax.legend(ncol=2, loc='best', frameon=True)
ax.set(ylabel='Name of country',
xlabel='percentage expired loans')
leg = ax.get_legend()
#new_title = 'Loan outcome?'
#leg.set_title(new_title)
#ax.set_title('Top countries with highest funded percentage', fontsize=15)
plt.show()
###Output
_____no_output_____
###Markdown
Gender difference by country
###Code
df1 = sub_df.copy()
df1.isnull().sum()
df4 = sub_df.groupby(['country_name', 'gender_reclassified','status']).size()/sub_df.groupby(['country_name', 'gender_reclassified']).size()
df4
len(df4)
df4 = df4.to_frame()
df4
df4 = df4.rename(columns={0: 'percentage'})
df4.reset_index(level=2, inplace=True)
df4.reset_index(level=1, inplace=True)
df4.reset_index(level=0, inplace=True)
df4.columns.values
len(df4)
df4.head(10)
df5 = df4[df4['status'] == 'funded']
len(df5)
df5.head(10)
df6 = df5.pivot(index='country_name', columns='gender_reclassified', values='percentage')
df6
df6['female'] = df6['female'].fillna(0)
df6['male'] = df6['male'].fillna(0)
df6['difference'] = df6['female'].fillna(0) - df6['male'].fillna(0)
df6 = df6.sort_values(by='female', ascending=False).head(60)
df6.reset_index(level=0, inplace=True)
df6.iloc[:,1:] = df6.iloc[:,1:].mul(100)
df6
import itertools
s_force_order = df6.sort_values('difference', ascending=False)['country_name'].head(40)
fig, ax = plt.subplots(figsize=(15, 10))
sns.barplot(x='difference', y='country_name', data=df6,
label='difference = funded%female - funded%male', color='#0abda0', order=s_force_order)
ax.legend(ncol=1, loc='lower right', frameon=True)
ax.set(ylabel='country_name',
xlabel='Gender difference')
leg = ax.get_legend()
new_title = 'Legend:'
leg.set_title(new_title)
ax.set_title('Gender difference by country for the funded loans', fontsize=15)
plt.show()
import itertools
s_force_order = df6.sort_values('difference', ascending=False)['country_name'].head(40)
fig, ax = plt.subplots(figsize=(6, 8))
sns.barplot(x='difference', y='country_name', data=df6,
label='Statistical parity', color='dodgerblue', order=s_force_order)
#ax.legend(ncol=1, loc='lower right', frameon=True)
ax.set(ylabel='Country',
xlabel='Statistical parity in percentages')
#leg = ax.get_legend()
#new_title = 'Legend:'
#leg.set_title(new_title)
#ax.set_title('Gender difference by country for the funded loans', fontsize=15)
plt.show()
fig.savefig('gender_bias_country.png', bbox_inches='tight')
###Output
_____no_output_____
###Markdown
Top 40 field partners with more than 1000 loans (%) Top 40 of field partners with more than 1 000 loans according to the % expired loans
###Code
len(kiva_loans[kiva_loans.country=='Philippines'])
160441/671205
len(merge1)
len(merge1[merge1.country=='Philippines'])
185497/614010
df_theme= pd.read_csv("/Users/alexanderstevens/Desktop/loan_theme.csv",index_col=None).set_index("id")
df_locations= pd.read_csv("/Users/alexanderstevens/Desktop/loan_themeRegion.csv",index_col=None)
df_locations.rename(columns={"Partner ID":"partner_id"},inplace=True)
#Merge Kiva_loan with df_theme sheet with unique parner id
new_loan = pd.merge(kiva_loans,df_theme,how="inner",on="id")
#merge new loan sheet to loan_themesregion.csv
new_loan2 = pd.merge(new_loan,df_locations[df_locations.columns[~df_locations.columns.isin(['sector','Loan Theme Type'])]]
,how='inner',on=["partner_id","Loan Theme ID","country","region"])
#Merge new_loan2, kiva_mpi_region_locations.csv
#new_loan_mpi = pd.merge(new_loan2,mpi_reg,how="inner",on=["lat","lon","ISO","country","region","LocationName"])
len(kiva_loans)
len(new_loan)
len(new_loan2)
new_loan2['Partner ID'].nunique()
new_loan2['Field Partner Name'].nunique()
new_loan2.isnull().sum()
new_loan2['Field Partner Name'].value_counts()
new_loan2['Field Partner Name'].value_counts().loc[lambda x : x>999]
counts = new_loan2['Field Partner Name'].value_counts()
sub_df2 = new_loan2[~new_loan2['Field Partner Name'].isin(counts[counts < 1000].index)].copy()
len(sub_df2)
sub_df2['status'] = np.where(sub_df2['funded_time'].isnull(), 'expired', 'funded')
sub_df2['status'].value_counts()
df10 = sub_df2.groupby(['Field Partner Name', 'status']).size()/sub_df2.groupby('Field Partner Name').size()
len(df10)
df10 = df10.to_frame()
df10 = df10.rename(columns={0: 'percentage'})
df10.reset_index(level=1, inplace=True)
df10.reset_index(level=0, inplace=True)
df10.columns.values
# om plot wat beter leesbaar te maken
df10['Field Partner Name']=df10['Field Partner Name'].replace({"RÃ\x8d©seau de Micro-institutions de Croissance de Revenus (RMCR)": "RMCR"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"Programme d'Appui aux Initiatives de DÃ\x8d©veloppement Economique au Kivu (PAIDEK)": "PAIDEK"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"Micro Credit for Development and Transformation SACCO (MCDT SACCO)": "MCDT SACCO"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"Together Association for Development and Environment (TADE)": "TADE"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"FundaciÃ\x8d_n Mario Santo Domingo (FMSD)": "Fundacion Mario Santo Domingo (FMSD)"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"AsociaciÃ\x8d_n Arariwa": "Asociacion Arariwa"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"FundaciÃ\x8d_n ESPOIR": "Fundacion ESPOIR"})
df10['Field Partner Name']=df10['Field Partner Name'].replace({"CrÃ\x8d©dit Epargne Formation (CEFOR)": "Crédit Epargne Formation (CEFOR)"})
len(df10)
df10.head(10)
df10 = df10.pivot(index='Field Partner Name', columns='status', values='percentage')
df10
df10['expired'] = df10['expired'].fillna(0)
df10['funded'] = df10['funded'].fillna(0)
df10['total'] = df10['expired'].fillna(0) + df10['funded'].fillna(0)
df10 = df10.sort_values(by='funded', ascending=False)
df10.reset_index(level=0, inplace=True)
df10.iloc[:,1:] = df10.iloc[:,1:].mul(100)
df10
###Output
_____no_output_____
###Markdown
Worst field partners
###Code
s_force_order = df10.sort_values('expired', ascending=False)['Field Partner Name'].head(40)
fig, ax = plt.subplots(figsize=(15, 10))
sns.barplot(x='funded', y='Field Partner Name', data=df10,
label='funded', color='mediumspringgreen', order=s_force_order)
sns.barplot(x='expired', y='Field Partner Name', data=df10,
label='expired', color='salmon', order=s_force_order)
ax.legend(ncol=2, loc='best', frameon=True)
ax.set(ylabel='Field Partner Name',
xlabel='percentage')
leg = ax.get_legend()
new_title = 'Loan outcome?'
leg.set_title(new_title)
ax.set_title('Top 40 of field partners with more than 1 000 loans according to the % expired loans', fontsize=15)
plt.show()
s_force_order = df10.sort_values('expired', ascending=False)['Field Partner Name'].head(20)
fig, ax = plt.subplots(figsize=(8, 6))
sns.barplot(x='expired', y='Field Partner Name', data=df10,
label='expired', color='dodgerblue', order=s_force_order)
#ax.legend(ncol=2, loc='best', frameon=True)
ax.set(ylabel='Field Partner Name',
xlabel='Percentage expired loans')
#leg = ax.get_legend()
#new_title = 'Loan outcome?'
#leg.set_title(new_title)
#ax.set_title('Top 40 of field partners with more than 1 000 loans according to the % expired loans', fontsize=15)
plt.show()
fig.savefig('field_%expired_worst.png', bbox_inches='tight')
###Output
_____no_output_____ |
mgrana-challenge.ipynb | ###Markdown
**mGrana Challenge****Developer**: COLLANTE, Gerardo**Fecha**: 26/7/2021El dataset consiste en `train` y `test` sets de reviews de películas. Para el `train` set tenés labels binarios (si el review es bueno o malo) y para el test set, solo los reviews.La consigna del challenge es la siguiente:* [1 .Hacer un breve análisis exploratorio de los datos.](chapter1)* [2 .Entrenar un modelo de ML con los datos de train, que busque predecir si el review es bueno o malo en base al texto del review..](chapter2)* [3 .Una medida estimada de que accuracy aproximado esperamos en el `test` set.](chapter3)* [4 .Crear predicciones para el dataset test.](chapter4)El entregable por ende es:- Un *Jupyter Notebook* donde esté contenido el análisis, pre-procesamiento del dataset, entrenamiento del modelo, evaluación, etc.- Un archivo `test.csv` que esté en el mismo formato que `challenge_train_.csv`, y que contenga no solo los reviews del test set, sino las predicciones que el modelo hace para estos reviews.La idea es que utilices librerías clásicas de *Python* como *Pandas*, *Numpy* y *SkLearn* (o inclusive *PyTorch* o *TensorFlow*), pero sos libre de utilizar cualquier librería de *Python* que te convenga.
###Code
!pip install torchtext
!python -m spacy download en_core_web_lg
!nvidia-smi
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import pylab as pl
import re
import seaborn as sns
import spacy
import string
import time
import torch
import torch.nn as nn
import torch.nn.functional as F
import torch.optim as optim
from IPython import display
from sklearn.model_selection import train_test_split
from os.path import join
from tqdm import tqdm
from torch.utils.data import TensorDataset, DataLoader
from torchtext.data import Field, TabularDataset, BucketIterator
SEED = 42
torch.manual_seed(SEED)
torch.backends.cudnn.deterministic = True
TRAIN = True # For train model
LOAD = False # For load weights in model
MODEL_PATH = 'movies-sa.pt'
SMALL = False # Use small dataset for fast coding
###Output
_____no_output_____
###Markdown
Load files
###Code
DATAPATH = '../input/mgrana-dataset'
TEST_PATH = join(DATAPATH, 'challenge_test_.csv')
TRAIN_PATH = join(DATAPATH, 'challenge_train_.csv')
target = pd.read_csv(TEST_PATH, index_col=0)
original = pd.read_csv(TRAIN_PATH, index_col=0)
if SMALL:
original = original.sample(frac=0.05)
original
###Output
_____no_output_____
###Markdown
1. Brief exploratory data analysis
###Code
original['length'] = original.text.apply(lambda x: len(x.split()))
def remove_punctuation(s:str)->str:
"""
Parameters
----------
s: string
Returns
-------
str
"""
return re.sub("[^\w\s]", "", s)
analysis = original.text.apply(lambda x: remove_punctuation(x.lower()))
import plotly.express as px
df = px.data.tips()
fig = px.histogram(original,
x="length",
nbins=50,
color="sentiment",
color_discrete_sequence=['red','green'],
title='Mean length of review by sentiment')
fig.show()
original['raw'] = original.text.apply(lambda x: remove_punctuation(x.lower()))
original
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
from collections import Counter
def get_words(df, top_N, sentiment):
words = df[df.sentiment==sentiment].raw.str.cat(sep=' ').split()
stop_words = set(stopwords.words('english'))
words = [w for w in words if not w.lower() in stop_words]
label = 'negative' if sentiment else 'positive'
return pd.DataFrame(Counter(words).most_common(top_N),
columns=['Word', f'freq_{label}']).set_index('Word')
NTOP = 50
words_df = pd.concat([get_words(original, NTOP, 0), get_words(original, NTOP, 1)], axis=1).fillna(0).astype(int)
def plot_most_used_words(df, sentiment, top):
color = 'tab:red' if sentiment=='negative' else 'tab:green'
df[f'freq_{sentiment}'].sort_values().plot(kind='bar',
title=f'Top-{top} common words used in {sentiment} reviews',
figsize=(16,8),
legend=False,
grid=True,
color= color
)
def my_func(x, y):
return np.linalg.norm(x-y)
words_df['distance'] = words_df.apply(lambda x: my_func(x['freq_negative'], x['freq_positive']), axis=1).sort_values()
TOP = 10
freq_positive = words_df.sort_values(['freq_negative', 'distance'], ascending=[True, False]).head(TOP)
freq_negative = words_df.sort_values(['freq_positive', 'distance'], ascending=[True, False]).head(TOP)
plot_most_used_words(freq_negative, 'negative', TOP)
plot_most_used_words(freq_positive, 'positive', TOP)
###Output
_____no_output_____
###Markdown
2. Train an ML model with the train data, which seeks to predict whether the review is good or bad based on the text of the review. Creation of dataset
###Code
def split_train_test(df: pd.DataFrame, test_size: float, random_state: int)->pd.DataFrame:
"""analysis df in train/test
Parameters
----------
df
test_size
random_state
Returns
-------
splitted df
"""
return train_test_split(
df, test_size=test_size, random_state=random_state, stratify=df["sentiment"]
)
# 70% train, 20% test and 10% validation
test_size = 0.3 # 70% train
validation_size = 2/3 # 20% test
train, test = split_train_test(original, test_size, SEED)
validation, test = split_train_test(test, validation_size, SEED)
# Save DataFrames
train.to_csv('train.csv')
test.to_csv('test.csv')
validation.to_csv('validation.csv')
print(f'Train percentage of dataset: {len(train)/len(original)*100:.0f}%')
print(f'Test percentage of dataset: {len(test)/len(original)*100:.0f}%')
print(f'Validation percentage of dataset: {len(validation)/len(original)*100:.0f}%')
train.sentiment.value_counts().plot(kind='bar', color=['r','g'], title='Numbers of reviews per type')
###Output
_____no_output_____
###Markdown
Load datasets
###Code
TEXT = Field(sequential=True,
tokenize = 'spacy',
tokenizer_language = 'en_core_web_lg',
use_vocab=True,
lower=True,
include_lengths = True)
LABEL = Field(sequential=False, use_vocab=False)
fields = {'text': ('text', TEXT), 'sentiment':('label', LABEL)}
train_data, test_data, validation_data = TabularDataset.splits(
path='.',
train='train.csv',
test='test.csv',
validation='validation.csv',
format='csv',
fields=fields)
###Output
/opt/conda/lib/python3.7/site-packages/torchtext/data/example.py:68: UserWarning:
Example class will be retired soon and moved to torchtext.legacy. Please see the most recent release notes for further information.
/opt/conda/lib/python3.7/site-packages/torchtext/data/example.py:52: UserWarning:
Example class will be retired soon and moved to torchtext.legacy. Please see the most recent release notes for further information.
###Markdown
Compute vocabulary optimal size
###Code
def get_all_words(df:pd.DataFrame, column:str)->list:
"""
Get all words from DataFrame
Parameters
----------
df
column
Returns
-------
list:
words
"""
all_words = []
for sentence in df[column]:
for word in sentence.split():
all_words.append(word.lower())
return all_words
words = get_all_words(original, 'text')
words = [remove_punctuation(word) for word in words]
words = list(set(words))
words.sort()
VOCAB_SIZE= len(words)
print(f'The dataset have {VOCAB_SIZE} words.')
def get_all_glove_words(filepath:str)->list:
"""
Parameters
----------
filepath: str
Returns
-------
list:
All words in glove
"""
with open(filepath) as f:
text = f.readlines()
word_list = [line.strip().split()[0] for line in text]
return word_list
glove_path = '../input/glove6b300dtxt/glove.6B.300d.txt'
glove_words = get_all_glove_words(glove_path)
print(f'The Glove dataset have {len(glove_words)} words.')
%%time
# Match only words that appears in both original dataset and Glove
# The purpose is find the optimal size of vocab length
my_list = [word if word in glove_words else '' for word in words]
VOCAB_SIZE = len(set(my_list))
#VOCAB_SIZE = 59_885
print(f'The optimal amount of words in vocabulary is {VOCAB_SIZE} words')
MAX_VOCAB_SIZE = VOCAB_SIZE
EMBEDDING_DIM = 300 #200, 300 other possibilities
#"glove.6B.100d"
vectors = f"glove.6B.{EMBEDDING_DIM}d"
TEXT.build_vocab(train_data,
max_size=MAX_VOCAB_SIZE,
vectors = vectors,
unk_init = torch.Tensor.normal_)
LABEL.build_vocab(train_data)
###Output
.vector_cache/glove.6B.zip: 862MB [02:48, 5.12MB/s]
100%|█████████▉| 399999/400000 [00:51<00:00, 7818.65it/s]
###Markdown
Create iterators for train/validation
###Code
BATCH_SIZE = 64
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
train_iterator, test_iterator, validation_iterator = BucketIterator.splits((train_data,
test_data,
validation_data),
batch_size=BATCH_SIZE,
sort_within_batch = True,
sort_key = lambda x: len(x.text),
device=device)
###Output
/opt/conda/lib/python3.7/site-packages/torchtext/data/iterator.py:48: UserWarning:
BucketIterator class will be retired soon and moved to torchtext.legacy. Please see the most recent release notes for further information.
###Markdown
Define model
###Code
import torch.nn as nn
class RNN(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim, output_dim, n_layers,
bidirectional, dropout, pad_idx):
super().__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim, padding_idx = pad_idx)
self.rnn = nn.LSTM(embedding_dim,
hidden_dim,
num_layers=n_layers,
bidirectional=bidirectional,
dropout=dropout)
self.fc = nn.Linear(hidden_dim * 2, output_dim)
self.dropout = nn.Dropout(dropout)
def forward(self, text, text_lengths):
#text = [sent len, batch size]
embedded = self.dropout(self.embedding(text))
#embedded = [sent len, batch size, emb dim]
#pack sequence
# lengths need to be on CPU!
packed_embedded = nn.utils.rnn.pack_padded_sequence(embedded, text_lengths.to('cpu'))
packed_output, (hidden, cell) = self.rnn(packed_embedded)
#unpack sequence
output, output_lengths = nn.utils.rnn.pad_packed_sequence(packed_output)
#output = [sent len, batch size, hid dim * num directions]
#output over padding tokens are zero tensors
#hidden = [num layers * num directions, batch size, hid dim]
#cell = [num layers * num directions, batch size, hid dim]
#concat the final forward (hidden[-2,:,:]) and backward (hidden[-1,:,:]) hidden layers
#and apply dropout
hidden = self.dropout(torch.cat((hidden[-1,:,:], hidden[-1,:,:]), dim = 1))
#hidden = [batch size, hid dim * num directions]
return self.fc(hidden)
###Output
_____no_output_____
###Markdown
Create model
###Code
INPUT_DIM = len(TEXT.vocab)
HIDDEN_DIM = 256
OUTPUT_DIM = 1
N_LAYERS = 2
BIDIRECTIONAL = True
DROPOUT = 0.5
PAD_IDX = TEXT.vocab.stoi[TEXT.pad_token]
model = RNN(INPUT_DIM,
EMBEDDING_DIM,
HIDDEN_DIM,
OUTPUT_DIM,
N_LAYERS,
BIDIRECTIONAL,
DROPOUT,
PAD_IDX)
print(model)
UNK_IDX = TEXT.vocab.stoi[TEXT.unk_token]
model.embedding.weight.data[UNK_IDX] = torch.zeros(EMBEDDING_DIM)
model.embedding.weight.data[PAD_IDX] = torch.zeros(EMBEDDING_DIM)
print(model.embedding.weight.data)
from torch.optim.lr_scheduler import ReduceLROnPlateau
lr = 0.01
optimizer = optim.Adam(model.parameters())#, lr=lr)
criterion = nn.BCEWithLogitsLoss()
scheduler = ReduceLROnPlateau(optimizer, 'min')
model = model.to(device)
criterion = criterion.to(device)
###Output
_____no_output_____
###Markdown
Set metric
###Code
def binary_accuracy(y_pred:torch.Tensor, y_real:torch.Tensor)->torch.Tensor:
"""
Returns accuracy per batch, i.e. if you get 8/10 right, this returns 0.8, NOT 8
Parameters
----------
y_pred: predictions
y_real: real
Returns
-------
Accuracy
"""
y_pred = torch.round(torch.sigmoid(y_pred))
return (y_pred == y_real).float().mean()
###Output
_____no_output_____
###Markdown
Train & eval
###Code
def train(model, iterator, optimizer, criterion):
"""
Function to train model
Parameters
----------
model
iterator
optimizer
criterion
Returns
-------
Tuple:
[Loss, Acc]
"""
epoch_loss = 0
epoch_acc = 0
model.train()
for batch in iterator:
# Reset gradients values to zero, useful in RNNs
optimizer.zero_grad()
text, text_lengths = batch.text
label = batch.label.float()
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, label)
acc = binary_accuracy(predictions, label)
loss.backward()
optimizer.step()
epoch_loss += loss.item()
epoch_acc += acc.item()
return epoch_loss / len(iterator), epoch_acc / len(iterator)
def evaluate(model, iterator, criterion):
"""
Function to eval model
Parameters
----------
model
iterator
criterion
Returns
-------
Tuple:
[Loss, Acc]
"""
epoch_loss = 0
epoch_acc = 0
model.eval()
with torch.no_grad():
for batch in iterator:
text, text_lengths = batch.text
label = batch.label.float()
predictions = model(text, text_lengths).squeeze(1)
loss = criterion(predictions, label)
acc = binary_accuracy(predictions, label)
epoch_loss += loss.item()
epoch_acc += acc.item()
return epoch_loss / len(iterator), epoch_acc / len(iterator)
def plot_training(train_losses:list, test_losses:list, epochs:int):
"""
Function to plot train/test losses
Parameters
----------
train_losses
test_losses
epochs
Returns
-------
"""
pl.figure(figsize=(10,5))
pl.title("Training and Test Loss")
pl.xlim(0, epochs)
pl.xticks(range(0, epochs+1, 1))
pl.grid()
pl.plot(train_losses,label="train")
pl.plot(test_losses,label="test")
pl.xlabel("Epochs")
pl.ylabel("Loss")
pl.legend()
display.clear_output(wait=True)
display.display(pl.gcf())
def epoch_time(start_time: float, end_time:float)->int:
"""
Compute epoch time
Parameters
----------
start_time
end_time
Returns
-------
amount of time for epoch
"""
elapsed_time = end_time - start_time
elapsed_mins = int(elapsed_time / 60)
elapsed_secs = int(elapsed_time - (elapsed_mins * 60))
return elapsed_mins, elapsed_secs
def get_lr(optimizer):
for param_group in optimizer.param_groups:
return param_group['lr']
###Output
_____no_output_____
###Markdown
Train model
###Code
N_EPOCHS = 20
if TRAIN:
best_test_loss = float('inf')
best_test_acc = float('inf')
train_losses = []
test_losses = []
for epoch in tqdm(range(N_EPOCHS+1)):
start_time = time.time()
# Train/eval
train_loss, train_acc = train(model, train_iterator, optimizer, criterion)
test_loss, test_acc = evaluate(model, test_iterator, criterion)
#scheduler.step(test_loss)
end_time = time.time()
epoch_mins, epoch_secs = epoch_time(start_time, end_time)
# Collect data
train_losses.append(train_loss)
test_losses.append(test_loss)
if test_loss < best_test_loss:
epochs_no_improve = 0
best_test_loss = test_loss
best_test_acc = test_acc
torch.save(model.state_dict(), MODEL_PATH)
# Plotting
plot_training(train_losses, test_losses, N_EPOCHS)
# Print data
current_lr = get_lr(optimizer)
print(f'Epoch: {epoch+1:02} | Epoch Time: {epoch_mins}m {epoch_secs}s | lr: {current_lr}')
print(f'\tTrain Loss: {train_loss:.3f} | Train Acc: {train_acc*100:.2f}%')
print(f'\tTest Loss: {test_loss:.3f} | Test Acc: {test_acc*100:.2f}%')
print(f'Best Test Loss: {best_test_loss:.3f} | Best Test Acc: {best_test_acc*100:.2f}%')
###Output
_____no_output_____
###Markdown
3. An estimated measure of what approximate accuracy we expect in the test set.
###Code
if LOAD:
# Best model
model.load_state_dict(torch.load(MODEL_PATH))
validation_loss, validation_acc = evaluate(model, validation_iterator, criterion)
print(f'Test Acc: {validation_acc*100:.2f}%')
###Output
Test Acc: 89.29%
###Markdown
Prediction
###Code
nlp = spacy.load('en_core_web_lg')
def predict_sentiment(model, sentence):
"""
Infer sentiment
Parameters
----------
model
sentence
Returns
-------
int:
0 is good, 1 is bad
"""
model.eval()
tokenized = [tok.text for tok in nlp.tokenizer(sentence)]
indexed = [TEXT.vocab.stoi[t] for t in tokenized]
length = [len(indexed)]
tensor = torch.LongTensor(indexed).to(device)
tensor = tensor.unsqueeze(1)
length_tensor = torch.LongTensor(length)
prediction = torch.sigmoid(model(tensor, length_tensor))
return 0 if prediction.item() > 0.5 else 1
###Output
_____no_output_____
###Markdown
One example
###Code
%%time
predict_sentiment(model, 'The movie was very good')
predict_sentiment(model, 'the movie was very bad')
###Output
_____no_output_____
###Markdown
4. Create predictions for the test dataset.
###Code
target['sentiment'] = target.text.apply(lambda x: predict_sentiment(model, x))
target.to_csv('test.csv')
###Output
_____no_output_____ |
05_compute_information_content.ipynb | ###Markdown
Computing predicted occupancies, information content, and related metricsThis notebook takes all sequences in the libraries and computes the predicted occupancy of 8 TFs. Using those predicted occupancies, we then calculate the total occupancy, TF diversity, and information content (entropy) of each sequence.
###Code
import os
import sys
import matplotlib as mpl
import matplotlib.patches as mpatches
import matplotlib.pyplot as plt
from mpl_toolkits.axes_grid1 import make_axes_locatable
import numpy as np
import pandas as pd
from scipy import stats
from IPython.display import display
sys.path.insert(0, "utils")
from utils import fasta_seq_parse_manip, modeling, plot_utils, predicted_occupancy, sequence_annotation_processing
data_dir = os.path.join("Data")
figures_dir = os.path.join("Figures")
all_seqs = fasta_seq_parse_manip.read_fasta(os.path.join(data_dir, "library1And2.fasta"))
# Drop scrambled sequences
all_seqs = all_seqs[~(all_seqs.index.str.contains("scr"))]
plot_utils.set_manuscript_params()
###Output
_____no_output_____
###Markdown
Compute predicted occupancy of all TFs on all sequences
###Code
# Load in PWMs
pwms = predicted_occupancy.read_pwm_files(os.path.join("Data", "Downloaded", "Pwm", "photoreceptorAndEnrichedMotifs.meme"))
ewms = pwms.apply(predicted_occupancy.ewm_from_letter_prob).apply(predicted_occupancy.ewm_to_dict)
mu = 9
# Do predicted occupancy scans
occupancy_df = predicted_occupancy.all_seq_total_occupancy(all_seqs, ewms, mu, convert_ewm=False)
occupancy_df = occupancy_df.rename(columns=lambda x: x.split("_")[0])
# Save to file
sequence_annotation_processing.save_df(occupancy_df, os.path.join(data_dir, "predictedOccupancies.txt"))
###Output
_____no_output_____
###Markdown
Compute total occupancy, diversity, information content
###Code
entropy_df = occupancy_df.apply(predicted_occupancy.boltzmann_entropy, axis=1)
sequence_annotation_processing.save_df(entropy_df, os.path.join(data_dir, "entropyDiversityOccupancy.txt"))
###Output
_____no_output_____
###Markdown
Plot predicted occupancy vs. relative $K_D$ for various values of $\mu$
###Code
relative_kd = np.logspace(-3, 0, 200)
mu_values = [1, 3, 6, 9, 12]
mu_colors = plot_utils.set_color(np.arange(len(mu_values)) / len(mu_values))
width, height = mpl.rcParams["figure.figsize"]
fig, ax = plt.subplots(figsize=(width * 1.25, height))
for mu, color in zip(mu_values, mu_colors):
occupancy_probability = 1.0 / (1 + np.exp(-2.5 * np.log(relative_kd) - mu))
ax.semilogx(relative_kd, occupancy_probability, color=color, label=rf"$\mu = {mu}$")
ax.set_xlabel(r"Relative $K_D$")
ax.set_ylabel("Pr(bound)")
ax.legend(loc=(1.02, 0))
# Show where the 50% cutoff is for mu of 9
ax.axhline(0.5, color="k", linestyle="--")
ax.axvline(np.exp(9 / -2.5), color="k", linestyle="--")
plot_utils.save_fig(fig, os.path.join(figures_dir, "predictedOccupancyCurveVsMu"), timestamp=False)
###Output
_____no_output_____ |
Usage_of_GB_code.ipynb | ###Markdown
Produce Lists of CSL boundaries for any given rotation axis (hkl) :
###Code
# for example: [1, 0, 0], [1, 1, 0] or [1, 1, 1]
axis = np.array([1, 1, 1])
# list Sigma boundaries < 50
csl.print_list(axis, 50)
###Output
Sigma: 1 Theta: 0.00
Sigma: 3 Theta: 60.00
Sigma: 7 Theta: 38.21
Sigma: 13 Theta: 27.80
Sigma: 19 Theta: 46.83
Sigma: 21 Theta: 21.79
Sigma: 31 Theta: 17.90
Sigma: 37 Theta: 50.57
Sigma: 39 Theta: 32.20
Sigma: 43 Theta: 15.18
Sigma: 49 Theta: 43.57
###Markdown
Select a sigma and get the characteristics of the GB:
###Code
# pick a sigma for this axis, ex: 19.
sigma = 37
theta, m, n = csl.get_theta_m_n_list(axis, sigma)[0]
R = csl.rot(axis, theta)
# Minimal CSL cells. The plane orientations and the orthogonal cells
# will be produced from these original cells.
M1, M2 = csl.Create_minimal_cell_Method_1(sigma, axis, R)
print('Angle:', degrees(theta), '\n', 'Sigma:', sigma, '\n',
'Minimal cells:', '\n', M1, '\n', M2, '\n')
###Output
Angle: 50.569992092103575
Sigma: 37
Minimal cells:
[[ 0 4 1]
[-4 -3 1]
[ 3 0 1]]
[[ 3 4 1]
[-4 0 1]
[ 0 -3 1]]
###Markdown
Produce Lists of GB planes for the chosen boundary :
###Code
# the higher the limit the higher the indices of GB planes produced.
lim = 3
V1, V2, M, Gb = csl.Create_Possible_GB_Plane_List(axis, m, n, lim)
# the following data frame shows the created list of GB planes and their corresponding types
df = pd.DataFrame(
{'GB1': list(V1),
'GB2': list(V2),
'Type': Gb
})
df.head()
###Output
_____no_output_____
###Markdown
Criteria for finding the GB plane of interest*: 1- Based on the type of GB plane: _*The following criteria searches the generated data frame. To extend the search you can increase the limit (lim) in the above cell._
###Code
# Gb types: Symmetric Tilt, Tilt, Twist, Mixed
df[df['Type'] == 'Tilt'].head()
###Output
_____no_output_____
###Markdown
2 - Based on the minimum number of atoms in the orthogonal cell: _This can be of interest for DFT calculations that require smaller cells. The search may take a few minutes if your original limit is large as it must calculate all the orthogonal cells to know the number of atoms._
###Code
basis = 'fcc'
Number = np.zeros(len(V1))
Number_atoms = []
for i in range((len(V1))):
Number_atoms.append(csl.Find_Orthogonal_cell(basis,axis,m,n,V1[i])[2])
# show me Gb planes that have orthogonal cells with less than max_num_atoms, here: 500.
df['Number'] = Number_atoms
max_num_atoms = 300
df[df['Number'] < max_num_atoms]
###Output
_____no_output_____
###Markdown
3- Based on proximity to a particular type boundary, for example the Symmetric tilts: _This is how I created various steps on grain boundaries with vicinal orientations in the following work: __(https://journals.aps.org/prmaterials/abstract/10.1103/PhysRevMaterials.2.043601)__ See also here:___(https://www.mpie.de/2955247/GrainBoundaryDynamics)__
###Code
SymmTiltGbs = []
for i in range(len(V1)):
if str(Gb[i]) == 'Symmetric Tilt':
SymmTiltGbs.append(V1[i])
# Find GBs less than Delta (here 6) degrees from any of the symmetric tilt boundaries in this system
Delta = 6
Min_angles = []
for i in range(len(V1)):
angles = []
for j in range(len(SymmTiltGbs)):
angles.append(csl.angv(V1[i],SymmTiltGbs[j]))
Min_angles.append(min(angles))
df['Angles'] = Min_angles
df[df['Angles'] < Delta]
###Output
_____no_output_____
###Markdown
Select a GB plane and go on: You only need to pick the GB1 plane, from any of the three criteria in the cells above
###Code
GB_plane = [3, 4, -7]
# lattice parameter
LatP = 4
basis = 'fcc'
# just a piece of info, how much of this mixed boundary is tilt or twist?
csl.Tilt_Twist_comp(GB_plane, axis, m, n)
# instantiate a GB:
my_gb = gbc.GB_character()
# give all the characteristics
my_gb.ParseGB(axis, basis, LatP, m, n, GB_plane)
# Create the bicrystal
my_gb.CSL_Bicrystal_Atom_generator()
# Write a GB :
# by default overlap = 0.0, rigid = False,
# dim1, dim2, dim3 = [1, 1, 1]
# file = 'LAMMPS'
# read the io_file and/or README for more info, briefly: when you give overlap > 0
# you need to decide 'whichG': atoms to be removed from either G1 or G2.
# if rigid = True, then you need two integers a and b to make a mesh on the GB plane
# a and b can be put to 10 and 5 (just a suggestion) respectively for any GB except
# TWIST for the TWISTS the code will handle it internally regardless of your input a and b
# ex:
#my_gb.WriteGB(overlap=0.3, whichG='g1', rigid= True, a=3, b=2, dim2=5 , file='VASP')
my_gb.WriteGB(overlap=0.3, whichG='g1', dim1=2, dim2=2, dim3=1, file='VASP')
# extract atom positions of the two grains:
X = my_gb.atoms1
Y = my_gb.atoms2
# 3d plot of the gb, that can be shown in any cartesian view direction:
def plot_gb(X,Y, view_dir = [0,1,0]):
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(X[:,0],X[:,1],X[:,2],'o', s = 20, facecolor = 'y',edgecolor='none', alpha=0.2 )
ax.scatter(Y[:,0],Y[:,1],Y[:,2],'o',s = 20,facecolor = 'b',edgecolor='none', alpha=0.2)
# Show [0, 0, 0] as a red point
ax.scatter(0,0,0,'s',s = 200,facecolor = 'r',edgecolor='none')
ax.set_proj_type('ortho')
ax.grid(False)
az = degrees(atan2(view_dir[1],view_dir[0]))
el = degrees(asin(view_dir[2]/norm(view_dir)))
ax.view_init(azim = az, elev = el)
ax.set_xlabel('X axis')
ax.set_ylabel('Y axis')
ax.set_zlabel('Z axis')
return
%matplotlib notebook
plot_gb(X,Y,[0,1,0])
###Output
_____no_output_____ |
7- Mean-variance analysis with simulated data.ipynb | ###Markdown
Mean-variance analysis with simulated data **Camilo A. Garcia Trillos - 2020** In this class - We are implementing a Markowitz portfolio optimization, after some simulations (loosely based on a version on the blog http://blog.quantopian.com). - We learn how to perform an optimisation using the scipy package.
###Code
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
from scipy.optimize import minimize
np.random.seed(123)
###Output
_____no_output_____
###Markdown
Markowitz mean-variance analysisIn this class we will start by simulated random data. Later we will learn how to use actual stock data. Assume that we have $4$ assets, each with a return series of length $1000$. We can use numpy.random.randn to sample returns from a normal distribution (recall Class A).
###Code
## NUMBER OF ASSETS
n_assets = 4
## NUMBER OF OBSERVATIONS
n_obs = 1000
## VECTOR OF MEANS
mu_bar = np.array([0.,0.,0., 0.])
#mu_bar = np.array([0.001,0.002,0.005, 0.008])
## MATRIX OF COVARIANCE
#With this auxSigma matrix, inverting is stable
auxSigma = np.array ( [[1.,0.,0.,0.],[0,1,0,0],[0,0,1,0],[0,0,0,1]])
#With this auxSigma matrix, inverting is less stable
#auxSigma = np.array ( [[2,0.,0.,0.],[-0.2,0.7,0,0],[-0.3,0.4,0.7,0],[0.1,0.4,0.4,2.3]])
Sigma_exact =auxSigma.T@auxSigma
print (Sigma_exact)
return_vec = np.random.multivariate_normal(mu_bar,Sigma_exact,n_obs)
print (return_vec.shape)
plt.plot(return_vec, alpha=0.3);
plt.xlabel('Time')
plt.ylabel('Returns')
###Output
_____no_output_____
###Markdown
Random portfolios Let's generate a couple of random portfolios. Let's assume for the moment that we restrict ourselves to have "long-only" portfolios, that is, the weights are nonnegative.
###Code
def rand_weights_pos(n):
k = np.random.rand(n)
return k / sum(k)
print(rand_weights_pos(n_assets))
print(rand_weights_pos(n_assets))
###Output
_____no_output_____
###Markdown
Let's next compute the expected return and standard deviation for a portfolio.
###Code
def random_portfolio(returns, weights):
if returns.shape[1] != weights.shape[0]:
print("Error: MSG by random_portfolio: dimensions don't fit")
return 0
w = np.asmatrix(weights)
p = np.asmatrix(np.mean(returns, axis=0))
C = np.asmatrix(np.cov(returns.T))
mu = w * p.T
sigma = np.sqrt(w * C * w.T)
return mu, sigma
###Output
_____no_output_____
###Markdown
Now, let's plot several such random risk-return profiles:
###Code
n_portfolios = 3000
means, stds = np.column_stack([random_portfolio(return_vec, rand_weights_pos(n_assets))
for _ in range(n_portfolios)])
plt.plot(stds, means,'o', markersize=5)
plt.xlabel('std')
plt.ylabel('mean')
plt.title('Mean and standard deviation of returns of randomly generated portfolios (long-only)')
def rand_weights_unconstrained(n):
k = np.random.rand(n)*2-1 # Uniformly distributed on [-1,1]
return k / sum(k)
means_unc, stds_unc = np.column_stack([random_portfolio(return_vec,
rand_weights_unconstrained(n_assets)) for _ in range(2 * n_portfolios)])
plt.plot(stds_unc, means_unc, 'og', markersize=5)
plt.xlabel('std')
plt.ylabel('mean')
plt.title('Mean and standard deviation of returns of randomly generated portfolios')
plt.plot(stds_unc[stds_unc<0.9], means_unc[stds_unc<0.9], 'og',
markersize=5, alpha=0.4)
plt.plot(stds, means, 'o', markersize=5, alpha=0.2)
plt.xlabel('std')
plt.ylabel('mean')
plt.title('Mean and standard deviation of returns of randomly generated portfolios')
###Output
_____no_output_____
###Markdown
Let us now check the covariance matrix and find its inverse.
###Code
Sigma = np.cov(return_vec.T)
print(Sigma)
###Output
_____no_output_____
###Markdown
Let us check the stability of the matrix with the condioned number
###Code
np.linalg.cond(Sigma)
###Output
_____no_output_____
###Markdown
This is not too bad. We could move on without expecting major stability problems.
###Code
Sigma_inv = np.linalg.inv(Sigma)
###Output
_____no_output_____
###Markdown
Markowitz, as done in the lecture notes
###Code
def Markowitz_weights(Sigma, mean_returns, mu_p):
S_inv = np.linalg.inv(Sigma)
pi_mu = S_inv @ (mean_returns)/sum(S_inv @ mean_returns)
pi_1 = np.sum(S_inv, axis = 1) / sum(np.sum(S_inv, axis = 1) )
lambda_demoninator = (mean_returns @ pi_mu) - (mean_returns@pi_1)
ll = np.array((mu_p - (mean_returns @ pi_1))/lambda_demoninator)
# previous line: to convert into array in case that mu_p is a single number
ll.shape=(ll.size,1)
return pi_mu * ll + pi_1 * (1-ll)
###Output
_____no_output_____
###Markdown
Remark: recall from the lecture that Markowitz needs at least two expected returns to differ. This is not the case via our simulated data. Indeed, any portfolio will have a zero expected return and the equally weighted portfolio will have the smallest variance. Let's just proceed, nevertheless, and see how far we get ...
###Code
Sigma = np.cov(return_vec.T)
mean_returns = np.mean(return_vec, axis = 0)
Markowitz_weights(Sigma, mean_returns, 0.05)
mu_p = np.arange(0.02,0.06,0.002)
Markowitz_weights(Sigma, mean_returns, mu_p)
Mw1 = _
###Output
_____no_output_____
###Markdown
Markowitz via an optimization algorithm Next a different that also allows for additional constraints (e.g., nonnegative portfolio weights).
###Code
from scipy.optimize import minimize
minimize?
def Markowitz_weights_opt(Sigma, mean_returns, mu_p, nonnegative = False, print_info = False):
n = Sigma.shape[0]
mu_p_vect = np.array(mu_p)
mu_p_vect.shape = (mu_p_vect.size,)
# previous lines: to convert into array in case that mu_p is a single number
portfolios = []
x0 = np.ones(n)/n
cost_fun = lambda x: (x@Sigma)@x
for mu_p in mu_p_vect:
if nonnegative:
cons = ({'type': 'eq', 'fun': lambda x: x@mean_returns - mu_p},
{'type': 'eq', 'fun': lambda x: [email protected](n) - 1},
{'type': 'ineq', 'fun': lambda x: x})
else:
cons = ({'type': 'eq', 'fun': lambda x: x@mean_returns - mu_p},
{'type': 'eq', 'fun': lambda x: [email protected](n) - 1})
# Calculate efficient frontier weights using quadratic programming
res = minimize(cost_fun, x0, method = 'SLSQP', constraints=cons)
if print_info:
print(mu_p, res)
portfolios.append(res.x)
# Turning the portfolios into a numpy array
portfolios = np.array([np.array(p).squeeze() for p in portfolios])
return portfolios
Markowitz_weights_opt(Sigma, mean_returns, 0.012)
###Output
_____no_output_____
###Markdown
In the following yet another implementation. Now, we are using the constraint $\mu^T \pi \geq \mu_p$ instead of $\mu^T \pi = \mu_p$.
###Code
def Markowitz_weights_optIneq(Sigma, mean_returns, mu_p_vect, nonnegative = False, print_info = False):
n = Sigma.shape[0]
mu_p_vect = np.array(mu_p_vect)
mu_p_vect.shape = (mu_p_vect.size,)
# previous lines: to convert into array in case that mu_p is a single number
portfolios = []
x0 = np.ones(n)/n
cost_fun = lambda x: (x@Sigma)@x
for mu_p in mu_p_vect:
if nonnegative:
cons = ({'type': 'ineq', 'fun': lambda x: x@mean_returns - mu_p},
{'type': 'eq', 'fun': lambda x: [email protected](n) - 1},
{'type': 'ineq', 'fun': lambda x: x})
else:
cons = ({'type': 'ineq', 'fun': lambda x: x@mean_returns - mu_p},
{'type': 'eq', 'fun': lambda x: [email protected](n) - 1})
# Calculate efficient frontier weights using quadratic programming
res = minimize(cost_fun, x0, method = 'SLSQP', constraints=cons)
if print_info:
print(mu_p, res)
portfolios.append(res.x)
# Calculate efficient frontier weights using quadratic programming
#portfolios = [opt.solvers.qp(S, opt.matrix(np.zeros(n)), G, h, A, opt.matrix([1.0,mu]))['x']
# for mu in mu_p]
# Turning the portfolios into a numpy array
portfolios = np.array([np.array(p).squeeze() for p in portfolios])
return portfolios
Markowitz_weights_opt(Sigma, mean_returns, 0.02)
Markowitz_weights_optIneq(Sigma, mean_returns, 0.02)
Markowitz_weights_opt(Sigma, mean_returns, mu_p)
Markowitz_weights_optIneq(Sigma, mean_returns, mu_p)
Mw1
###Output
_____no_output_____
###Markdown
All three methods therefore yield more or less the same portfolio, which is nice ... (Markowitz_weights_optIneq only have an inequality constraint and yields results that have further away from the other two approaches).(If we run again the problem with different means and variances the solution with inequality constraints drift further away. This is simply because those means cannot be attained using only positive portfolios).
###Code
portfolio_mu = [mean_returns @ x for x in Mw1]
portfolio_mu
portfolio_sigma = [np.sqrt((x@Sigma)@x) for x in Mw1]
portfolio_sigma
plt.plot(stds, means, 'o', markersize=3)
plt.plot(stds_unc[stds_unc<1], means_unc[stds_unc<1], 'og',
markersize=3, alpha=0.4)
plt.ylabel('mean')
plt.xlabel('std')
plt.plot(portfolio_sigma, portfolio_mu, 'ro')
plt.title('Random portfolios and mean-variance frontier')
###Output
_____no_output_____ |
02-tensorflow-data-and-deployment/04-tensorflow-deployment/01-tensorflow-serving/02_MNIST_Fashion.ipynb | ###Markdown
Train and serve a TensorFlow model with TensorFlow Serving This guide trains a neural network model to classify [images of clothing, like sneakers and shirts](https://github.com/zalandoresearch/fashion-mnist), saves the trained model, and then serves it with [TensorFlow Serving](https://www.tensorflow.org/serving/). The focus is on TensorFlow Serving, rather than the modeling and training in TensorFlow, so for a complete example which focuses on the modeling and training see the [Basic Classification example](https://github.com/tensorflow/docs/blob/master/site/en/r1/tutorials/keras/basic_classification.ipynb).This guide uses [tf.keras](https://github.com/tensorflow/docs/blob/master/site/en/r1/guide/keras.ipynb), a high-level API to build and train models in TensorFlow.
###Code
# TensorFlow and tf.keras
import tensorflow as tf
from tensorflow import keras
# Helper libraries
import numpy as np
import matplotlib.pyplot as plt
import os
print(tf.__version__)
###Output
2.0.0
###Markdown
Create your model Import the Fashion MNIST datasetThis guide uses the [Fashion MNIST](https://github.com/zalandoresearch/fashion-mnist) dataset which contains 70,000 grayscale images in 10 categories. The images show individual articles of clothing at low resolution (28 by 28 pixels), as seen here: <img src="https://tensorflow.org/images/fashion-mnist-sprite.png" alt="Fashion MNIST sprite" width="600"> Figure 1. Fashion-MNIST samples (by Zalando, MIT License). Fashion MNIST is intended as a drop-in replacement for the classic [MNIST](http://yann.lecun.com/exdb/mnist/) dataset—often used as the "Hello, World" of machine learning programs for computer vision. You can access the Fashion MNIST directly from TensorFlow, just import and load the data.Note: Although these are really images, they are loaded as NumPy arrays and not binary image objects.
###Code
fashion_mnist = keras.datasets.fashion_mnist
(train_images, train_labels), (test_images, test_labels) = fashion_mnist.load_data()
# scale the values to 0.0 to 1.0
train_images = train_images / 255.0
test_images = test_images / 255.0
# reshape for feeding into the model
train_images = train_images.reshape(train_images.shape[0], 28, 28, 1)
test_images = test_images.reshape(test_images.shape[0], 28, 28, 1)
class_names = ['T-shirt/top', 'Trouser', 'Pullover', 'Dress', 'Coat', 'Sandal', 'Shirt', 'Sneaker', 'Bag', 'Ankle boot']
print('\ntrain_images.shape: {}, of {}'.format(train_images.shape, train_images.dtype))
print('test_images.shape: {}, of {}'.format(test_images.shape, test_images.dtype))
###Output
train_images.shape: (60000, 28, 28, 1), of float64
test_images.shape: (10000, 28, 28, 1), of float64
###Markdown
Train and evaluate your modelLet's use the simplest possible CNN, since we're not focused on the modeling part.
###Code
model = keras.Sequential([
keras.layers.Conv2D(input_shape=(28,28,1), filters=8, kernel_size=3, strides=2, activation='relu', name='Conv1'),
keras.layers.Flatten(),
keras.layers.Dense(10, activation=tf.nn.softmax, name='Softmax')
])
model.summary()
testing = False
epochs = 5
model.compile(optimizer=tf.optimizers.Adam(),
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(train_images, train_labels, epochs=epochs)
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=0)
print('\nTest accuracy: {}'.format(test_acc))
###Output
Test accuracy: 0.8743000030517578
###Markdown
Save your modelTo load our trained model into TensorFlow Serving we first need to save it in [SavedModel](https://www.tensorflow.org/versions/r1.15/api_docs/python/tf/saved_model) format. This will create a protobuf file in a well-defined directory hierarchy, and will include a version number. [TensorFlow Serving](https://www.tensorflow.org/tfx/guide/serving) allows us to select which version of a model, or "servable" we want to use when we make inference requests. Each version will be exported to a different sub-directory under the given path.
###Code
# Fetch the Keras session and save the model
# The signature definition is defined by the input and output tensors,
# and stored with the default serving key
import tempfile
MODEL_DIR = tempfile.gettempdir()
version = 1
export_path = os.path.join(MODEL_DIR, str(version))
print('export_path = {}\n'.format(export_path))
if os.path.isdir(export_path):
print('\nAlready saved a model, cleaning up\n')
!rm -r {export_path}
model.save(export_path, save_format="tf")
print('\nSaved model:')
!ls -l {export_path}
###Output
export_path = /tmp/1
Already saved a model, cleaning up
INFO:tensorflow:Assets written to: /tmp/1/assets
Saved model:
total 84
drwxr-xr-x 2 pedro pedro 4096 Feb 6 17:59 assets
-rw-rw-r-- 1 pedro pedro 73963 Feb 6 17:59 saved_model.pb
drwxr-xr-x 2 pedro pedro 4096 Feb 6 17:59 variables
###Markdown
Examine your saved modelWe'll use the command line utility `saved_model_cli` to look at the [MetaGraphDefs](https://www.tensorflow.org/versions/r1.15/api_docs/python/tf/MetaGraphDef) (the models) and [SignatureDefs](../signature_defs) (the methods you can call) in our SavedModel. See [this discussion of the SavedModel CLI](https://github.com/tensorflow/docs/blob/master/site/en/r1/guide/saved_model.mdcli-to-inspect-and-execute-savedmodel) in the TensorFlow Guide.
###Code
!saved_model_cli show --dir {export_path} --all
###Output
MetaGraphDef with tag-set: 'serve' contains the following SignatureDefs:
signature_def['__saved_model_init_op']:
The given SavedModel SignatureDef contains the following input(s):
The given SavedModel SignatureDef contains the following output(s):
outputs['__saved_model_init_op'] tensor_info:
dtype: DT_INVALID
shape: unknown_rank
name: NoOp
Method name is:
signature_def['serving_default']:
The given SavedModel SignatureDef contains the following input(s):
inputs['Conv1_input'] tensor_info:
dtype: DT_FLOAT
shape: (-1, 28, 28, 1)
name: serving_default_Conv1_input:0
The given SavedModel SignatureDef contains the following output(s):
outputs['Softmax'] tensor_info:
dtype: DT_FLOAT
shape: (-1, 10)
name: StatefulPartitionedCall:0
Method name is: tensorflow/serving/predict
###Markdown
That tells us a lot about our model! In this case we just trained our model, so we already know the inputs and outputs, but if we didn't this would be important information. It doesn't tell us everything, like the fact that this is grayscale image data for example, but it's a great start. Serve your model with TensorFlow Serving Add TensorFlow Serving distribution URI as a package source:We're preparing to install TensorFlow Serving using [Aptitude](https://wiki.debian.org/Aptitude) since this Colab runs in a Debian environment. We'll add the `tensorflow-model-server` package to the list of packages that Aptitude knows about. Note that we're running as root.Note: This example is running TensorFlow Serving natively, but [you can also run it in a Docker container](https://www.tensorflow.org/tfx/serving/docker), which is one of the easiest ways to get started using TensorFlow Serving.
###Code
# This is the same as you would do from your command line, but without the [arch=amd64], and no sudo
# You would instead do:
echo "deb [arch=amd64] http://storage.googleapis.com/tensorflow-serving-apt stable tensorflow-model-server tensorflow-model-server-universal" | sudo tee /etc/apt/sources.list.d/tensorflow-serving.list && \
curl https://storage.googleapis.com/tensorflow-serving-apt/tensorflow-serving.release.pub.gpg | sudo apt-key add -
!echo "deb http://storage.googleapis.com/tensorflow-serving-apt stable tensorflow-model-server tensorflow-model-server-universal" | tee /etc/apt/sources.list.d/tensorflow-serving.list && \
curl https://storage.googleapis.com/tensorflow-serving-apt/tensorflow-serving.release.pub.gpg | apt-key add -
###Output
_____no_output_____
###Markdown
Install TensorFlow ServingThis is all you need - one command line!
###Code
!apt update
!apt-get install tensorflow-model-server
###Output
_____no_output_____
###Markdown
Start running TensorFlow ServingThis is where we start running TensorFlow Serving and load our model. After it loads we can start making inference requests using REST. There are some important parameters:* `rest_api_port`: The port that you'll use for REST requests.* `model_name`: You'll use this in the URL of REST requests. It can be anything.* `model_base_path`: This is the path to the directory where you've saved your model.
###Code
os.environ["MODEL_DIR"] = MODEL_DIR
%%bash --bg
nohup tensorflow_model_server \
--rest_api_port=8501 \
--model_name=fashion_model \
--model_base_path="${MODEL_DIR}" >server.log 2>&1
!tail server.log
###Output
To enable them in other operations, rebuild TensorFlow with the appropriate compiler flags.
2021-02-06 18:13:44.985633: I external/org_tensorflow/tensorflow/cc/saved_model/loader.cc:206] Restoring SavedModel bundle.
2021-02-06 18:13:45.006504: I external/org_tensorflow/tensorflow/core/platform/profile_utils/cpu_utils.cc:112] CPU Frequency: 3999980000 Hz
2021-02-06 18:13:45.032144: I external/org_tensorflow/tensorflow/cc/saved_model/loader.cc:190] Running initialization op on SavedModel bundle at path: /tmp/1
2021-02-06 18:13:45.035670: I external/org_tensorflow/tensorflow/cc/saved_model/loader.cc:277] SavedModel load for tags { serve }; Status: success: OK. Took 74422 microseconds.
2021-02-06 18:13:45.036042: I tensorflow_serving/servables/tensorflow/saved_model_warmup_util.cc:59] No warmup data file found at /tmp/1/assets.extra/tf_serving_warmup_requests
2021-02-06 18:13:45.036272: I tensorflow_serving/core/loader_harness.cc:87] Successfully loaded servable version {name: fashion_model version: 1}
2021-02-06 18:13:45.037925: I tensorflow_serving/model_servers/server.cc:371] Running gRPC ModelServer at 0.0.0.0:8500 ...
[evhttp_server.cc : 238] NET_LOG: Entering the event loop ...
2021-02-06 18:13:45.038688: I tensorflow_serving/model_servers/server.cc:391] Exporting HTTP/REST API at:localhost:8501 ...
###Markdown
Make a request to your model in TensorFlow ServingFirst, let's take a look at a random example from our test data.
###Code
def show(idx, title):
plt.figure()
plt.imshow(test_images[idx].reshape(28,28))
plt.axis('off')
plt.title('\n\n{}'.format(title), fontdict={'size': 16})
import random
rando = random.randint(0,len(test_images)-1)
show(rando, 'An Example Image: {}'.format(class_names[test_labels[rando]]))
###Output
_____no_output_____
###Markdown
Ok, that looks interesting. How hard is that for you to recognize? Now let's create the JSON object for a batch of three inference requests, and see how well our model recognizes things:
###Code
import json
data = json.dumps({"signature_name": "serving_default", "instances": test_images[0:3].tolist()})
print('Data: {} ... {}'.format(data[:50], data[len(data)-52:]))
###Output
Data: {"signature_name": "serving_default", "instances": ... [0.0], [0.0], [0.0], [0.0], [0.0], [0.0], [0.0]]]]}
###Markdown
Make REST requests Newest version of the servableWe'll send a predict request as a POST to our server's REST endpoint, and pass it three examples. We'll ask our server to give us the latest version of our servable by not specifying a particular version.
###Code
import requests
headers = {"content-type": "application/json"}
json_response = requests.post('http://localhost:8501/v1/models/fashion_model:predict',
data=data, headers=headers)
predictions = json.loads(json_response.text)['predictions']
show(0, 'The model thought this was a {} (class {}), and it was actually a {} (class {})'
.format(class_names[np.argmax(predictions[0])],
test_labels[0],
class_names[np.argmax(predictions[0])],
test_labels[0]))
###Output
_____no_output_____
###Markdown
A particular version of the servableNow let's specify a particular version of our servable. Since we only have one, let's select version 1. We'll also look at all three results.
###Code
headers = {"content-type": "application/json"}
json_response = requests.post('http://localhost:8501/v1/models/fashion_model/versions/1:predict',
data=data, headers=headers)
predictions = json.loads(json_response.text)['predictions']
for i in range(0,3):
show(i, 'The model thought this was a {} (class {}), and it was actually a {} (class {})'
.format(class_names[np.argmax(predictions[i])],
test_labels[i],
class_names[np.argmax(predictions[i])],
test_labels[i]))
###Output
_____no_output_____ |
GIS_Analysis_with_GeoPandas_using_Python.ipynb | ###Markdown
###Code
!pip install geopandas
import matplotlib.pyplot as plt
import geopandas as geo
city = geo.read_file('belgian_cities.shp')
city.plot(cmap='coolwarm',figsize=(10,10))
AOI=geo.read_file('area_of_interest_.shp')
###Output
_____no_output_____
###Markdown
Importing and plotting AOI file
###Code
ax=city.plot(cmap='coolwarm')
AOI.plot(ax=ax,facecolor='yellow')
!sudo apt-get update && apt-get install -y libspatialindex-dev
!sudo apt install python3-rtree
###Output
Reading package lists... 0%
Reading package lists... 0%
Reading package lists... 0%
Reading package lists... 7%
Reading package lists... 7%
Reading package lists... 7%
Reading package lists... 7%
Reading package lists... 67%
Reading package lists... 67%
Reading package lists... 68%
Reading package lists... 68%
Reading package lists... 74%
Reading package lists... 74%
Reading package lists... 74%
Reading package lists... 74%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 84%
Reading package lists... 88%
Reading package lists... 88%
Reading package lists... 88%
Reading package lists... 88%
Reading package lists... 93%
Reading package lists... 93%
Reading package lists... 93%
Reading package lists... 93%
Reading package lists... 94%
Reading package lists... 94%
Reading package lists... 94%
Reading package lists... 94%
Reading package lists... 95%
Reading package lists... 95%
Reading package lists... 96%
Reading package lists... 98%
Reading package lists... 98%
Reading package lists... 98%
Reading package lists... 98%
Reading package lists... Done
Building dependency tree... 0%
Building dependency tree... 0%
Building dependency tree... 50%
Building dependency tree... 50%
Building dependency tree
Reading state information... 0%
Reading state information... 0%
Reading state information... Done
The following additional packages will be installed:
python3-pkg-resources
Suggested packages:
python3-setuptools
The following NEW packages will be installed:
python3-pkg-resources python3-rtree
0 upgraded, 2 newly installed, 0 to remove and 14 not upgraded.
Need to get 116 kB of archives.
After this operation, 640 kB of additional disk space will be used.
Get:1 http://archive.ubuntu.com/ubuntu bionic/main amd64 python3-pkg-resources all 39.0.1-2 [98.8 kB]
Get:2 http://archive.ubuntu.com/ubuntu bionic/universe amd64 python3-rtree all 0.8.3+ds-1 [16.9 kB]
Fetched 116 kB in 2s (57.8 kB/s)
debconf: unable to initialize frontend: Dialog
debconf: (No usable dialog-like program is installed, so the dialog based frontend cannot be used. at /usr/share/perl5/Debconf/FrontEnd/Dialog.pm line 76, <> line 2.)
debconf: falling back to frontend: Readline
debconf: unable to initialize frontend: Readline
debconf: (This frontend requires a controlling tty.)
debconf: falling back to frontend: Teletype
dpkg-preconfigure: unable to re-open stdin:
Selecting previously unselected package python3-pkg-resources.
(Reading database ... 131236 files and directories currently installed.)
Preparing to unpack .../python3-pkg-resources_39.0.1-2_all.deb ...
Unpacking python3-pkg-resources (39.0.1-2) ...
Selecting previously unselected package python3-rtree.
Preparing to unpack .../python3-rtree_0.8.3+ds-1_all.deb ...
Unpacking python3-rtree (0.8.3+ds-1) ...
Setting up python3-pkg-resources (39.0.1-2) ...
Setting up python3-rtree (0.8.3+ds-1) ...
###Markdown
For intersection
###Code
coi = geo.overlay(AOI,city,how='intersection')
coi.plot(figsize=(10,10),cmap='coolwarm')
! pip install osmnx
coi.head()
coi['area(km)']=coi.area/1000000
coi.head()
###Output
_____no_output_____ |
new_beginning/cluster_generator_yeast-Copy1.ipynb | ###Markdown
Clustering
###Code
def get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, n_clusters = 99):
splitted_file_names = [name.split('_') for name in os.listdir(CLUSTER_DIRECTORY)]
pre_runs = [int(run) for run, ncluster in splitted_file_names if ncluster == f"{n_clusters}.txt"]
if pre_runs:
return max(pre_runs)
else:
return -1
###Output
_____no_output_____
###Markdown
Random GDV
###Code
feature = 'GDV'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
network = 'systematic_CoEx_COEXPRESdb'
method = 'kmedoid'
for run in range(10):
for distance in {'GDV_similarity', 'canberra', 'mahalanobis'}:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{network}/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{network}/{feature}/{distance}.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
canberra
100: 597.44sec
mahalanobis
81: 471.32sec
###Markdown
GCV-A
###Code
feature = 'GCV-A'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(20):
for distance in all_distances:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
braycurtis
100: 529.33sec
canberra
100: 528.15sec
chebyshev
100: 529.53sec
cityblock
100: 530.49sec
correlation
100: 527.20sec
cosine
100: 525.86sec
euclidean
100: 529.85sec
hellinger
100: 529.93sec
mahalanobis
100: 620.90sec
normalized1_l1
100: 528.36sec
normalized1_l2
100: 530.01sec
normalized1_linf
100: 532.74sec
normalized2_l1
100: 535.36sec
normalized2_l2
100: 532.05sec
normalized2_linf
100: 535.99sec
seuclidean
100: 534.11sec
sqeuclidean
100: 527.93sec
braycurtis
100: 532.14sec
canberra
100: 532.42sec
chebyshev
100: 532.79sec
cityblock
100: 533.83sec
correlation
100: 529.34sec
cosine
100: 530.91sec
euclidean
100: 532.54sec
hellinger
100: 533.69sec
mahalanobis
100: 612.19sec
normalized1_l1
100: 532.56sec
normalized1_l2
100: 533.48sec
normalized1_linf
100: 533.26sec
normalized2_l1
100: 532.84sec
normalized2_l2
100: 532.43sec
normalized2_linf
100: 534.67sec
seuclidean
100: 534.31sec
sqeuclidean
100: 528.00sec
braycurtis
100: 534.34sec
canberra
100: 533.20sec
chebyshev
100: 532.95sec
cityblock
100: 511.27sec
correlation
100: 509.90sec
cosine
100: 510.86sec
euclidean
100: 513.70sec
hellinger
100: 512.65sec
mahalanobis
100: 594.25sec
normalized1_l1
100: 514.26sec
normalized1_l2
100: 516.82sec
normalized1_linf
100: 518.25sec
normalized2_l1
100: 516.46sec
normalized2_l2
100: 517.09sec
normalized2_linf
100: 518.13sec
seuclidean
100: 519.23sec
sqeuclidean
100: 515.82sec
braycurtis
100: 521.13sec
canberra
100: 517.94sec
chebyshev
100: 521.23sec
cityblock
100: 522.35sec
correlation
100: 518.65sec
cosine
100: 516.80sec
euclidean
100: 520.63sec
hellinger
100: 522.38sec
mahalanobis
100: 614.42sec
normalized1_l1
100: 520.50sec
normalized1_l2
100: 521.69sec
normalized1_linf
100: 522.64sec
normalized2_l1
100: 521.88sec
normalized2_l2
100: 520.24sec
normalized2_linf
100: 522.42sec
seuclidean
100: 521.97sec
sqeuclidean
100: 518.55sec
braycurtis
100: 521.12sec
canberra
100: 521.28sec
chebyshev
100: 521.91sec
cityblock
100: 521.54sec
correlation
100: 518.94sec
cosine
100: 516.95sec
euclidean
100: 522.29sec
hellinger
100: 520.73sec
mahalanobis
100: 585.85sec
normalized1_l1
100: 519.48sec
normalized1_l2
100: 520.81sec
normalized1_linf
100: 519.63sec
normalized2_l1
100: 521.49sec
normalized2_l2
100: 522.64sec
normalized2_linf
100: 523.45sec
seuclidean
100: 523.07sec
sqeuclidean
100: 516.32sec
braycurtis
100: 521.65sec
canberra
100: 522.59sec
chebyshev
100: 523.59sec
cityblock
100: 523.02sec
correlation
100: 518.46sec
cosine
100: 517.81sec
euclidean
100: 522.67sec
hellinger
100: 519.95sec
mahalanobis
100: 568.62sec
normalized1_l1
100: 521.61sec
normalized1_l2
100: 520.61sec
normalized1_linf
100: 521.57sec
normalized2_l1
100: 520.34sec
normalized2_l2
100: 521.09sec
normalized2_linf
100: 522.14sec
seuclidean
100: 522.44sec
sqeuclidean
100: 517.84sec
braycurtis
100: 521.76sec
canberra
100: 522.31sec
chebyshev
100: 520.42sec
cityblock
100: 521.42sec
correlation
100: 517.80sec
cosine
100: 515.61sec
euclidean
100: 520.46sec
hellinger
100: 519.70sec
mahalanobis
100: 633.04sec
normalized1_l1
100: 518.42sec
normalized1_l2
100: 518.62sec
normalized1_linf
100: 517.99sec
normalized2_l1
100: 517.35sec
normalized2_l2
100: 517.45sec
normalized2_linf
100: 520.00sec
seuclidean
100: 518.98sec
sqeuclidean
100: 513.64sec
braycurtis
100: 519.05sec
canberra
100: 518.00sec
chebyshev
100: 512.28sec
cityblock
100: 514.13sec
correlation
100: 510.31sec
cosine
100: 509.80sec
euclidean
100: 511.77sec
hellinger
100: 514.50sec
mahalanobis
100: 598.74sec
normalized1_l1
100: 514.44sec
normalized1_l2
100: 516.83sec
normalized1_linf
100: 512.83sec
normalized2_l1
100: 514.90sec
normalized2_l2
100: 512.59sec
normalized2_linf
100: 517.43sec
seuclidean
100: 520.16sec
sqeuclidean
100: 511.49sec
braycurtis
100: 517.37sec
canberra
100: 518.89sec
chebyshev
100: 521.77sec
cityblock
100: 521.51sec
correlation
100: 517.48sec
cosine
100: 517.81sec
euclidean
100: 524.43sec
hellinger
100: 522.64sec
mahalanobis
100: 586.57sec
normalized1_l1
100: 519.85sec
normalized1_l2
100: 519.22sec
normalized1_linf
100: 520.21sec
normalized2_l1
100: 520.00sec
normalized2_l2
100: 520.22sec
normalized2_linf
100: 520.13sec
seuclidean
100: 521.75sec
sqeuclidean
100: 516.27sec
braycurtis
100: 521.15sec
canberra
100: 519.58sec
chebyshev
100: 521.75sec
cityblock
100: 522.60sec
correlation
100: 518.90sec
cosine
100: 518.21sec
euclidean
100: 522.56sec
hellinger
100: 521.87sec
mahalanobis
100: 572.35sec
normalized1_l1
100: 520.35sec
normalized1_l2
100: 519.36sec
normalized1_linf
100: 521.96sec
normalized2_l1
100: 520.39sec
normalized2_l2
100: 520.95sec
normalized2_linf
100: 522.74sec
seuclidean
100: 521.89sec
sqeuclidean
100: 516.02sec
braycurtis
100: 520.54sec
canberra
100: 520.94sec
chebyshev
100: 519.98sec
cityblock
100: 520.18sec
correlation
100: 517.55sec
cosine
100: 517.46sec
euclidean
100: 520.17sec
hellinger
100: 521.19sec
mahalanobis
100: 628.05sec
normalized1_l1
100: 517.86sec
normalized1_l2
100: 519.33sec
normalized1_linf
100: 519.12sec
normalized2_l1
100: 519.51sec
normalized2_l2
100: 519.77sec
normalized2_linf
100: 522.11sec
seuclidean
100: 521.76sec
sqeuclidean
100: 518.51sec
braycurtis
100: 522.99sec
canberra
100: 521.17sec
chebyshev
100: 521.38sec
cityblock
100: 524.03sec
correlation
100: 519.25sec
cosine
100: 518.71sec
euclidean
100: 521.58sec
hellinger
100: 521.22sec
mahalanobis
100: 607.18sec
normalized1_l1
100: 520.50sec
normalized1_l2
100: 523.46sec
normalized1_linf
100: 521.82sec
normalized2_l1
100: 522.49sec
normalized2_l2
100: 520.46sec
normalized2_linf
100: 522.94sec
seuclidean
100: 524.18sec
sqeuclidean
100: 517.27sec
braycurtis
100: 523.60sec
canberra
100: 511.30sec
chebyshev
100: 513.76sec
cityblock
100: 514.29sec
correlation
100: 514.22sec
cosine
100: 513.14sec
euclidean
100: 517.42sec
hellinger
100: 518.93sec
mahalanobis
100: 617.62sec
normalized1_l1
100: 518.51sec
normalized1_l2
100: 517.73sec
normalized1_linf
100: 518.73sec
normalized2_l1
100: 520.26sec
normalized2_l2
100: 520.27sec
normalized2_linf
100: 520.34sec
seuclidean
100: 523.13sec
sqeuclidean
100: 515.34sec
braycurtis
100: 522.57sec
canberra
100: 521.22sec
chebyshev
100: 522.26sec
cityblock
100: 521.25sec
correlation
100: 516.74sec
cosine
100: 516.07sec
euclidean
100: 523.91sec
hellinger
100: 521.80sec
mahalanobis
100: 574.69sec
normalized1_l1
100: 521.94sec
normalized1_l2
100: 519.55sec
normalized1_linf
100: 520.45sec
normalized2_l1
100: 520.16sec
normalized2_l2
100: 519.61sec
normalized2_linf
100: 520.69sec
seuclidean
100: 520.84sec
sqeuclidean
100: 514.70sec
braycurtis
100: 521.04sec
canberra
100: 518.73sec
chebyshev
100: 519.90sec
cityblock
100: 519.67sec
correlation
100: 515.85sec
cosine
100: 516.78sec
euclidean
100: 520.87sec
hellinger
100: 518.70sec
mahalanobis
100: 603.16sec
normalized1_l1
100: 521.42sec
normalized1_l2
100: 519.12sec
normalized1_linf
100: 520.44sec
normalized2_l1
100: 519.13sec
normalized2_l2
100: 519.62sec
normalized2_linf
100: 521.75sec
seuclidean
100: 521.58sec
sqeuclidean
100: 514.69sec
braycurtis
100: 520.66sec
canberra
100: 520.53sec
chebyshev
100: 520.20sec
cityblock
100: 521.69sec
correlation
100: 518.28sec
cosine
100: 518.13sec
euclidean
100: 521.15sec
hellinger
100: 519.92sec
mahalanobis
100: 618.37sec
normalized1_l1
100: 520.39sec
normalized1_l2
100: 521.59sec
normalized1_linf
100: 521.20sec
normalized2_l1
100: 521.00sec
normalized2_l2
100: 519.77sec
normalized2_linf
100: 521.41sec
seuclidean
100: 522.46sec
sqeuclidean
100: 516.69sec
braycurtis
100: 521.22sec
canberra
100: 520.62sec
chebyshev
100: 521.20sec
cityblock
100: 535.15sec
correlation
100: 538.87sec
cosine
100: 538.63sec
euclidean
100: 546.79sec
hellinger
100: 540.07sec
mahalanobis
100: 631.36sec
normalized1_l1
100: 550.76sec
normalized1_l2
100: 529.39sec
normalized1_linf
100: 522.87sec
normalized2_l1
100: 524.43sec
normalized2_l2
100: 522.13sec
normalized2_linf
100: 523.19sec
seuclidean
100: 523.52sec
sqeuclidean
100: 519.12sec
braycurtis
100: 515.00sec
canberra
100: 517.38sec
chebyshev
100: 518.15sec
cityblock
100: 518.84sec
correlation
100: 516.48sec
cosine
100: 515.77sec
euclidean
100: 520.94sec
hellinger
100: 533.86sec
mahalanobis
100: 632.34sec
normalized1_l1
100: 547.65sec
normalized1_l2
100: 549.49sec
normalized1_linf
100: 550.93sec
normalized2_l1
###Markdown
GCV-G
###Code
feature = 'GCV-G'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(20):
for distance in all_distances:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
braycurtis
100: 561.76sec
canberra
100: 531.72sec
chebyshev
100: 527.84sec
cityblock
100: 533.95sec
correlation
100: 524.77sec
cosine
100: 525.39sec
euclidean
100: 525.04sec
hellinger
100: 524.32sec
mahalanobis
100: 581.10sec
normalized1_l1
100: 524.61sec
normalized1_l2
100: 524.87sec
normalized1_linf
100: 524.35sec
normalized2_l1
100: 525.07sec
normalized2_l2
100: 525.60sec
normalized2_linf
100: 526.75sec
seuclidean
100: 527.51sec
sqeuclidean
100: 523.55sec
braycurtis
100: 526.82sec
canberra
100: 526.51sec
chebyshev
100: 528.18sec
cityblock
100: 526.37sec
correlation
100: 523.67sec
cosine
100: 525.07sec
euclidean
100: 526.84sec
hellinger
100: 526.88sec
mahalanobis
100: 601.67sec
normalized1_l1
100: 526.01sec
normalized1_l2
100: 525.22sec
normalized1_linf
100: 524.86sec
normalized2_l1
100: 524.54sec
normalized2_l2
100: 526.00sec
normalized2_linf
100: 528.11sec
seuclidean
100: 526.09sec
sqeuclidean
100: 517.60sec
braycurtis
100: 521.32sec
canberra
100: 523.22sec
chebyshev
100: 527.74sec
cityblock
100: 529.30sec
correlation
100: 524.21sec
cosine
100: 525.78sec
euclidean
100: 529.30sec
hellinger
100: 526.18sec
mahalanobis
100: 625.26sec
normalized1_l1
100: 524.60sec
normalized1_l2
100: 522.24sec
normalized1_linf
100: 519.44sec
normalized2_l1
100: 518.78sec
normalized2_l2
100: 517.73sec
normalized2_linf
100: 519.56sec
seuclidean
100: 518.92sec
sqeuclidean
100: 514.96sec
braycurtis
100: 517.55sec
canberra
100: 516.95sec
chebyshev
100: 517.06sec
cityblock
100: 517.22sec
correlation
100: 513.51sec
cosine
100: 515.79sec
euclidean
100: 517.04sec
hellinger
100: 518.29sec
mahalanobis
100: 570.57sec
normalized1_l1
100: 517.03sec
normalized1_l2
100: 518.25sec
normalized1_linf
100: 515.34sec
normalized2_l1
100: 516.35sec
normalized2_l2
100: 516.21sec
normalized2_linf
100: 518.40sec
seuclidean
100: 517.44sec
sqeuclidean
100: 514.87sec
braycurtis
100: 517.53sec
canberra
100: 516.92sec
chebyshev
100: 516.82sec
cityblock
100: 518.71sec
correlation
100: 513.08sec
cosine
100: 515.49sec
euclidean
100: 517.91sec
hellinger
100: 517.87sec
mahalanobis
100: 568.77sec
normalized1_l1
100: 516.47sec
normalized1_l2
100: 515.94sec
normalized1_linf
100: 517.08sec
normalized2_l1
100: 516.28sec
normalized2_l2
100: 517.07sec
normalized2_linf
100: 517.37sec
seuclidean
100: 519.08sec
sqeuclidean
100: 514.53sec
braycurtis
100: 519.83sec
canberra
100: 518.28sec
chebyshev
100: 517.53sec
cityblock
100: 546.65sec
correlation
100: 532.58sec
cosine
100: 516.14sec
euclidean
100: 518.40sec
hellinger
100: 516.60sec
mahalanobis
100: 588.17sec
normalized1_l1
100: 516.84sec
normalized1_l2
100: 518.95sec
normalized1_linf
100: 517.22sec
normalized2_l1
100: 516.45sec
normalized2_l2
100: 517.62sec
normalized2_linf
100: 519.18sec
seuclidean
100: 516.69sec
sqeuclidean
100: 533.55sec
braycurtis
100: 550.48sec
canberra
100: 521.48sec
chebyshev
100: 555.28sec
cityblock
100: 558.21sec
correlation
100: 573.29sec
cosine
100: 567.72sec
euclidean
100: 551.23sec
hellinger
100: 571.54sec
mahalanobis
100: 690.12sec
normalized1_l1
100: 570.44sec
normalized1_l2
100: 568.78sec
normalized1_linf
100: 581.12sec
normalized2_l1
100: 579.88sec
normalized2_l2
100: 586.76sec
normalized2_linf
100: 588.69sec
seuclidean
100: 584.66sec
sqeuclidean
100: 571.61sec
braycurtis
100: 585.36sec
canberra
100: 586.39sec
chebyshev
100: 586.00sec
cityblock
100: 589.69sec
correlation
100: 581.84sec
cosine
100: 557.11sec
euclidean
100: 554.78sec
hellinger
100: 546.97sec
mahalanobis
100: 650.73sec
normalized1_l1
100: 554.46sec
normalized1_l2
100: 546.11sec
normalized1_linf
100: 556.51sec
normalized2_l1
100: 558.80sec
normalized2_l2
100: 553.65sec
normalized2_linf
100: 551.26sec
seuclidean
100: 532.63sec
sqeuclidean
100: 517.39sec
braycurtis
100: 538.54sec
canberra
100: 551.76sec
chebyshev
100: 548.34sec
cityblock
100: 546.78sec
correlation
100: 549.36sec
cosine
100: 554.77sec
euclidean
100: 525.93sec
hellinger
100: 530.22sec
mahalanobis
100: 647.21sec
normalized1_l1
100: 552.21sec
normalized1_l2
100: 557.16sec
normalized1_linf
100: 547.40sec
normalized2_l1
100: 521.86sec
normalized2_l2
100: 522.50sec
normalized2_linf
100: 522.17sec
seuclidean
100: 524.98sec
sqeuclidean
100: 519.74sec
braycurtis
100: 521.36sec
canberra
100: 523.92sec
chebyshev
100: 523.11sec
cityblock
100: 522.08sec
correlation
100: 520.52sec
cosine
100: 519.22sec
euclidean
100: 523.01sec
hellinger
100: 520.84sec
mahalanobis
100: 612.44sec
normalized1_l1
100: 521.97sec
normalized1_l2
100: 521.46sec
normalized1_linf
100: 523.46sec
normalized2_l1
100: 523.29sec
normalized2_l2
100: 521.85sec
normalized2_linf
100: 521.30sec
seuclidean
100: 523.18sec
sqeuclidean
100: 519.54sec
braycurtis
100: 523.61sec
canberra
100: 522.81sec
chebyshev
100: 523.01sec
cityblock
100: 522.20sec
correlation
100: 522.35sec
cosine
100: 520.07sec
euclidean
100: 523.47sec
hellinger
100: 521.79sec
mahalanobis
100: 626.99sec
normalized1_l1
100: 522.93sec
normalized1_l2
100: 523.99sec
normalized1_linf
100: 523.53sec
normalized2_l1
100: 521.86sec
normalized2_l2
100: 522.33sec
normalized2_linf
100: 523.01sec
seuclidean
100: 526.74sec
sqeuclidean
100: 520.39sec
braycurtis
100: 523.42sec
canberra
100: 522.79sec
chebyshev
100: 523.82sec
cityblock
100: 524.11sec
correlation
100: 520.10sec
cosine
100: 521.57sec
euclidean
100: 524.99sec
hellinger
100: 522.99sec
mahalanobis
100: 560.95sec
normalized1_l1
100: 523.07sec
normalized1_l2
100: 528.86sec
normalized1_linf
100: 522.45sec
normalized2_l1
100: 522.43sec
normalized2_l2
100: 522.89sec
normalized2_linf
100: 521.11sec
seuclidean
100: 522.99sec
sqeuclidean
100: 522.41sec
braycurtis
100: 526.28sec
canberra
100: 526.74sec
chebyshev
100: 529.51sec
cityblock
100: 534.13sec
correlation
100: 529.24sec
cosine
100: 529.75sec
euclidean
100: 533.33sec
hellinger
100: 529.94sec
mahalanobis
100: 600.15sec
normalized1_l1
100: 528.63sec
normalized1_l2
100: 527.29sec
normalized1_linf
100: 525.74sec
normalized2_l1
100: 525.24sec
normalized2_l2
100: 521.95sec
normalized2_linf
100: 527.32sec
seuclidean
100: 525.18sec
sqeuclidean
100: 521.74sec
braycurtis
100: 524.82sec
canberra
100: 523.76sec
chebyshev
100: 524.42sec
cityblock
100: 524.69sec
correlation
100: 522.77sec
cosine
100: 523.55sec
euclidean
100: 525.48sec
hellinger
100: 522.80sec
mahalanobis
100: 573.23sec
normalized1_l1
100: 523.96sec
normalized1_l2
100: 519.30sec
normalized1_linf
100: 522.02sec
normalized2_l1
100: 522.74sec
normalized2_l2
100: 521.95sec
normalized2_linf
100: 521.91sec
seuclidean
100: 523.33sec
sqeuclidean
100: 519.84sec
braycurtis
100: 523.08sec
canberra
100: 523.30sec
chebyshev
100: 522.49sec
cityblock
100: 523.45sec
correlation
100: 520.02sec
cosine
100: 519.46sec
euclidean
100: 524.09sec
hellinger
100: 521.46sec
mahalanobis
100: 604.96sec
normalized1_l1
100: 518.46sec
normalized1_l2
100: 518.23sec
normalized1_linf
100: 518.32sec
normalized2_l1
100: 519.17sec
normalized2_l2
100: 518.48sec
normalized2_linf
100: 519.39sec
seuclidean
100: 519.63sec
sqeuclidean
100: 516.54sec
braycurtis
100: 518.96sec
canberra
100: 519.77sec
chebyshev
100: 519.69sec
cityblock
100: 520.66sec
correlation
100: 517.64sec
cosine
100: 517.43sec
euclidean
100: 521.68sec
hellinger
100: 519.23sec
mahalanobis
100: 603.15sec
normalized1_l1
100: 519.00sec
normalized1_l2
100: 519.91sec
normalized1_linf
100: 518.59sec
normalized2_l1
100: 520.70sec
normalized2_l2
100: 519.16sec
normalized2_linf
100: 519.74sec
seuclidean
100: 519.72sec
sqeuclidean
100: 517.35sec
braycurtis
100: 519.92sec
canberra
100: 519.31sec
chebyshev
100: 520.07sec
cityblock
100: 521.37sec
correlation
100: 516.72sec
cosine
100: 517.54sec
euclidean
100: 520.01sec
hellinger
100: 519.74sec
mahalanobis
100: 601.57sec
normalized1_l1
100: 518.91sec
normalized1_l2
100: 518.60sec
normalized1_linf
100: 518.97sec
normalized2_l1
100: 518.93sec
normalized2_l2
100: 515.99sec
normalized2_linf
100: 519.26sec
seuclidean
100: 518.84sec
sqeuclidean
100: 518.13sec
braycurtis
100: 522.47sec
canberra
100: 521.48sec
chebyshev
100: 521.06sec
cityblock
100: 523.00sec
correlation
100: 520.54sec
cosine
100: 520.36sec
euclidean
100: 522.68sec
hellinger
100: 523.02sec
mahalanobis
100: 617.99sec
normalized1_l1
100: 521.89sec
normalized1_l2
100: 523.21sec
normalized1_linf
100: 522.41sec
normalized2_l1
###Markdown
GCV-DA
###Code
feature = 'GCV-DA'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
network = 'systematic_CoEx_COEXPRESdb'
method = 'kmedoid'
for run in range(10):
for distance in {'canberra', 'cityblock', 'hellinger'}:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{network}/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{network}/{feature}/{distance}.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
canberra
100: 619.01sec
hellinger
100: 609.95sec
cityblock
100: 600.17sec
canberra
100: 594.24sec
hellinger
100: 582.89sec
cityblock
100: 600.26sec
canberra
100: 593.84sec
hellinger
100: 594.25sec
cityblock
100: 608.20sec
canberra
100: 593.03sec
hellinger
100: 586.71sec
cityblock
100: 591.36sec
canberra
100: 591.99sec
hellinger
100: 581.02sec
cityblock
100: 605.61sec
canberra
100: 589.11sec
hellinger
100: 589.97sec
cityblock
100: 603.30sec
canberra
100: 603.52sec
hellinger
100: 598.66sec
cityblock
100: 607.62sec
canberra
100: 590.58sec
hellinger
100: 587.81sec
cityblock
100: 605.44sec
canberra
100: 605.09sec
hellinger
100: 589.05sec
cityblock
100: 620.50sec
canberra
100: 607.63sec
hellinger
100: 576.79sec
cityblock
100: 582.34sec
###Markdown
GCV-DG
###Code
feature = 'GCV-DG'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
network = 'systematic_CoEx_COEXPRESdb'
method = 'kmedoid'
for run in range(10):
for distance in {'canberra', 'cityblock', 'hellinger'}:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{network}/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{network}/{feature}/{distance}.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
hellinger
100: 560.68sec
hellinger
100: 542.59sec
hellinger
100: 689.55sec
hellinger
100: 667.81sec
hellinger
100: 671.09sec
hellinger
100: 672.14sec
hellinger
100: 768.94sec
hellinger
100: 672.18sec
hellinger
100: 550.07sec
hellinger
17: 89.37sec
###Markdown
GCV-all
###Code
feature = 'GCV-all'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(20):
for distance in all_distances:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
_____no_output_____
###Markdown
GCV-nonredundand
###Code
feature = 'GCV-nonredundant'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(20):
for distance in all_distances:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
_____no_output_____
###Markdown
GCV-orca
###Code
feature = 'GCV-orca'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(30):
for distance in {'hellinger'}:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
hellinger
100: 575.57sec
hellinger
100: 649.15sec
hellinger
100: 574.86sec
hellinger
100: 543.26sec
hellinger
100: 536.14sec
hellinger
100: 533.73sec
hellinger
100: 544.79sec
hellinger
100: 531.49sec
hellinger
100: 544.60sec
hellinger
100: 541.00sec
hellinger
100: 553.65sec
hellinger
100: 582.14sec
hellinger
100: 640.73sec
hellinger
100: 556.96sec
hellinger
100: 572.19sec
hellinger
13: 70.58sec
###Markdown
GCV-orca+
###Code
feature = 'GCV-orca+'
MIN_CLUSTERS = 2
MAX_CLUSTERS = 100
all_distances = sorted('_'.join(filename.split('_')[:-1])
for filename in os.listdir(f"{MATRIX_DIRECTORY}/{feature}"))
method = 'kmedoid'
for run in range(10):
for distance in all_distances:
print(distance)
CLUSTER_DIRECTORY = f"{YEAST_DIRECTORY}/clusterings/{feature}/{distance}/{method}"
if not os.path.exists(CLUSTER_DIRECTORY):
os.makedirs(CLUSTER_DIRECTORY)
df = pd.read_csv(f"{MATRIX_DIRECTORY}/{feature}/{distance}_BioGRID.txt", delimiter=' ')
D = df.values.astype(float)
t1 = time.time()
for n_clusters in range(MIN_CLUSTERS, MAX_CLUSTERS+1):
initial_medoids = sample(range(len(D)), n_clusters)
kmedoids_instance = kmedoids(D, initial_medoids, data_type='distance_matrix')
kmedoids_instance.process()
nr = get_number_of_pre_runs(CLUSTER_DIRECTORY, distance, MAX_CLUSTERS)
with open(f"{CLUSTER_DIRECTORY}/{nr+1}_{n_clusters}_BioGRID.txt", 'w') as f:
for cluster in kmedoids_instance.get_clusters():
f.write(' '.join(df.columns[cluster]) + '\n')
t2 = time.time()
print(f'{n_clusters}: {t2-t1:.2f}sec', end='\r')
print()
###Output
_____no_output_____ |
assignments/assignment_1/assignment_1_lewilley.ipynb | ###Markdown
Assignment 1 | Data TypesAdd code cells as needed for your answers. Exercise 1: Manipulating Lists Create a list containing the numbers 10, 20, and 30. Store your list as a variable named `a`. Then create a second list containing the numbers 30, 60, and 90. Call this this `b`.
###Code
a = [10,20,30]
b = [30,60,90]
print (a, b)
###Output
[10, 20, 30] [30, 60, 90]
###Markdown
In the cells below, write Python expressions to create the following four outputs by combining `a` and `b` in creative ways:1. [[10, 20, 30], [30, 60, 90]]2. [10, 20, 30, 30, 60, 90]3. [10, 20, 60, 90]4. [20, 40, 60]
###Code
# Number 1
[a]+[b]
# Number 2
c = a + b
c
# Number 3 [10,20,60,90]
# will not include upper bound/ last number. Delete position 2 through 4 in list c.
del c[2:4]
c
a = [10, 20, 30]
d = [item * 2 for item in a]
print(d)
###Output
[20, 40, 60]
###Markdown
Exercise 2. Working with ListsCreate a list that contains the sums of each of the lists in G. `G = [[13, 9, 8], [14, 6, 12], [10, 13, 11], [7, 18, 9]]`Your output should look like:- `[30, 32, 34, 34]`Hint: try computing the sum for just one list first.
###Code
G = [[13, 9, 8], [14, 6, 12], [10, 13, 11], [7, 18, 9]]
# map function, which performs a sum iteration on each list
list(map(sum,G))
###Output
_____no_output_____
###Markdown
Exercise 3: String ManipulationTurn the string below into 'all good countrymen' using the minimum amount of code, using only the methods we've covered so far. A couple of lines of code should do the trick. Note: this requires string and list methods.
###Code
s = 'Now is the time for all good men to come to the aid of their country!'
# using the string.split function to turn the string into a list then replacing the ! with nothing.
x = str.split(s.replace('!',''))
x
# index using join function
z = str.join(' ',([x[5],x[6],x[15],x[7]]))
z
###Output
_____no_output_____
###Markdown
Exercise 4: String Manipulation and Type ConversionDefine a variable `a = "Sarah earns $96500 in a year"`. Then maniuplate the value of `a` in order to print the following string: `Sarah earns $8041.67 monthly`Start by doing it in several steps and then combine them one step at a time until you can do it in one line.
###Code
# Do the replace function with the words after "earns".
a = "Sarah earns $96500 in a year"
sarah = (a.replace('$96500 in a year','$8041.67 monthly'))
sarah
###Output
_____no_output_____
###Markdown
Exercise 5: Create and Query a Dictionary on State DemographicsCreate two dictionaries, one for California and one for New York state, based on the data in the following table:| States | Pop Density | Prop White | Prop Afr Amer | Prop Asian | Prop Other | Owners | Renters || --- | ---: | ---: | ---: | ---: | ---: | ---: | ---: || CA | 239.1 | 0.57 | 0.06 | 0.13 | 0.22 | 7035371 | 5542127 || NY | 411.2 | 0.65 | 0.15 | 0.07 | 0.22 | 3897837 | 3419918 |Each dictionary should have the following keys and value types: `name: (string)` , `population density: (float)`, `race (dict)`, `tenure: (dict)`. 1. Create one dictionary called CA and one called NY that contain dictionaries containing name, pop_density, race as a dictionary, and tenure for California and New York. Now combine these into a dictionary called "states", making it a dictionary of dictionaries, or a nested dictionary.1. Check if Texas is in our state dictionary (we know it isn't but show us).1. Print the White population in New York as a percentage1. Assume there was a typo in the data, and update the White population fraction of NY to 0.64. Verify that it was updated by printing the percentage again.1. Print the percentage of households that are renters in California, with two decimal places
###Code
# 1.
# create dictionary for CA, NY using curly brackets with column names as keys and values as values.
CA = {'pop_dens':239.1, 'white':0.57, 'black':0.06, 'asian':0.13, 'other':0.22, 'own':7035371, 'rent':5542127}
NY = {'pop_dens':411.2, 'white':0.65, 'black':0.15, 'asian':0.07, 'other':0.22, 'own':3897837, 'rent':3419918}
print(CA)
print(NY)
## this is as far as I got ¯\_(ツ)_/¯
###Output
{'pop_dens': 239.1, 'white': 0.57, 'black': 0.06, 'asian': 0.13, 'other': 0.22, 'own': 7035371, 'rent': 5542127}
{'pop_dens': 411.2, 'white': 0.65, 'black': 0.15, 'asian': 0.07, 'other': 0.22, 'own': 3897837, 'rent': 3419918}
|
Deep Learning with PyTorch/NN in PyTorch.ipynb | ###Markdown
Neural networks with PyTorchNext I'll show you how to build a neural network with PyTorch.
###Code
# Import things like usual
%matplotlib inline
%config InlineBackend.figure_format = 'retina'
import numpy as np
import torch
import helper
import matplotlib.pyplot as plt
from torchvision import datasets, transforms
###Output
_____no_output_____
###Markdown
First up, we need to get our dataset. This is provided through the `torchvision` package. The code below will download the MNIST dataset, then create training and test datasets for us. Don't worry too much about the details here, you'll learn more about this later.
###Code
# Define a transform to normalize the data
transform = transforms.Compose([transforms.ToTensor(),
transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5)),
])
# Download and load the training data
trainset = datasets.MNIST('MNIST_data/', download=True, train=True, transform=transform)
trainloader = torch.utils.data.DataLoader(trainset, batch_size=64, shuffle=True)
# Download and load the test data
testset = datasets.MNIST('MNIST_data/', download=True, train=False, transform=transform)
testloader = torch.utils.data.DataLoader(testset, batch_size=64, shuffle=True)
dataiter = iter(trainloader)
images, labels = dataiter.next()
###Output
_____no_output_____
###Markdown
We have the training data loaded into `trainloader` and we make that an iterator with `iter(trainloader)`. We'd use this to loop through the dataset for training, but here I'm just grabbing the first batch so we can check out the data. We can see below that `images` is just a tensor with size (64, 1, 28, 28). So, 64 images per batch, 1 color channel, and 28x28 images.
###Code
plt.imshow(images[1].numpy().squeeze(), cmap='Greys_r');
###Output
_____no_output_____
###Markdown
Building networks with PyTorchHere I'll use PyTorch to build a simple feedfoward network to classify the MNIST images. That is, the network will receive a digit image as input and predict the digit in the image.To build a neural network with PyTorch, you use the `torch.nn` module. The network itself is a class inheriting from `torch.nn.Module`. You define each of the operations separately, like `nn.Linear(784, 128)` for a fully connected linear layer with 784 inputs and 128 units.The class needs to include a `forward` method that implements the forward pass through the network. In this method, you pass some input tensor `x` through each of the operations you defined earlier. The `torch.nn` module also has functional equivalents for things like ReLUs in `torch.nn.functional`. This module is usually imported as `F`. Then to use a ReLU activation on some layer (which is just a tensor), you'd do `F.relu(x)`. Below are a few different commonly used activation functions.So, for this network, I'll build it with three fully connected layers, then a softmax output for predicting classes. The softmax function is similar to the sigmoid in that it squashes inputs between 0 and 1, but it's also normalized so that all the values sum to one like a proper probability distribution.
###Code
from torch import nn
from torch import optim
import torch.nn.functional as F
class Network(nn.Module):
def __init__(self):
super().__init__()
# Defining the layers, 128, 64, 10 units each
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 64)
# Output layer, 10 units - one for each digit
self.fc3 = nn.Linear(64, 10)
def forward(self, x):
''' Forward pass through the network, returns the output logits '''
x = self.fc1(x)
x = F.relu(x)
x = self.fc2(x)
x = F.relu(x)
x = self.fc3(x)
x = F.softmax(x, dim=1)
return x
model = Network()
model
###Output
_____no_output_____
###Markdown
Initializing weights and biasesThe weights and such are automatically initialized for you, but it's possible to customize how they are initialized. The weights and biases are tensors attached to the layer you defined, you can get them with `model.fc1.weight` for instance.
###Code
print(model.fc1.weight)
print(model.fc1.bias)
###Output
_____no_output_____
###Markdown
For custom initialization, we want to modify these tensors in place. These are actually autograd *Variables*, so we need to get back the actual tensors with `model.fc1.weight.data`. Once we have the tensors, we can fill them with zeros (for biases) or random normal values.
###Code
# Set biases to all zeros
model.fc1.bias.data.fill_(0)
# sample from random normal with standard dev = 0.01
model.fc1.weight.data.normal_(std=0.01)
###Output
_____no_output_____
###Markdown
Forward passNow that we have a network, let's see what happens when we pass in an image. This is called the forward pass. We're going to convert the image data into a tensor, then pass it through the operations defined by the network architecture.
###Code
# Grab some data
dataiter = iter(trainloader)
images, labels = dataiter.next()
# Resize images into a 1D vector, new shape is (batch size, color channels, image pixels)
images.resize_(64, 1, 784)
# or images.resize_(images.shape[0], 1, 784) to not automatically get batch size
# Forward pass through the network
img_idx = 0
ps = model.forward(images[img_idx,:])
img = images[img_idx]
helper.view_classify(img.view(1, 28, 28), ps)
###Output
_____no_output_____
###Markdown
As you can see above, our network has basically no idea what this digit is. It's because we haven't trained it yet, all the weights are random!PyTorch provides a convenient way to build networks like this where a tensor is passed sequentially through operations, `nn.Sequential` ([documentation](https://pytorch.org/docs/master/nn.htmltorch.nn.Sequential)). Using this to build the equivalent network:
###Code
# Hyperparameters for our network
input_size = 784
hidden_sizes = [128, 64]
output_size = 10
# Build a feed-forward network
model = nn.Sequential(nn.Linear(input_size, hidden_sizes[0]),
nn.ReLU(),
nn.Linear(hidden_sizes[0], hidden_sizes[1]),
nn.ReLU(),
nn.Linear(hidden_sizes[1], output_size),
nn.Softmax(dim=1))
print(model)
# Forward pass through the network and display output
images, labels = next(iter(trainloader))
images.resize_(images.shape[0], 1, 784)
ps = model.forward(images[0,:])
helper.view_classify(images[0].view(1, 28, 28), ps)
###Output
Sequential(
(0): Linear(in_features=784, out_features=128, bias=True)
(1): ReLU()
(2): Linear(in_features=128, out_features=64, bias=True)
(3): ReLU()
(4): Linear(in_features=64, out_features=10, bias=True)
(5): Softmax()
)
###Markdown
You can also pass in an `OrderedDict` to name the individual layers and operations. Note that a dictionary keys must be unique, so _each operation must have a different name_.
###Code
from collections import OrderedDict
model = nn.Sequential(OrderedDict([
('fc1', nn.Linear(input_size, hidden_sizes[0])),
('relu1', nn.ReLU()),
('fc2', nn.Linear(hidden_sizes[0], hidden_sizes[1])),
('relu2', nn.ReLU()),
('output', nn.Linear(hidden_sizes[1], output_size)),
('softmax', nn.Softmax(dim=1))]))
model
###Output
_____no_output_____
###Markdown
Now it's your turn to build a simple network, use any method I've covered so far. In the next notebook, you'll learn how to train a network so it can make good predictions.>**Exercise:** Build a network to classify the MNIST images with _three_ hidden layers. Use 400 units in the first hidden layer, 200 units in the second layer, and 100 units in the third layer. Each hidden layer should have a ReLU activation function, and use softmax on the output layer.
###Code
## TODO: Your network here
## Run this cell with your model to make sure it works ##
# Forward pass through the network and display output
images, labels = next(iter(trainloader))
images.resize_(images.shape[0], 1, 784)
ps = model.forward(images[0,:])
helper.view_classify(images[0].view(1, 28, 28), ps)
###Output
_____no_output_____ |
11_inference.ipynb | ###Markdown
InferenceElements of Data Scienceby [Allen Downey](https://allendowney.com)[MIT License](https://opensource.org/licenses/MIT) GoalsThis notebook introduces "statistical inference", which is the process of using a sample to make inferences about a population. This [figure from Wikipedia](https://en.wikipedia.org/wiki/Statistical_inference) illustrates the idea:On the left, the "population" is the group we are interested in. Sometimes it is a group of people, but in general it can be any kind of group.If we can observe the entire group, we might not need statistical inference. If not, sometimes we can observe a "sample" (or subset) of the population and use those observations to make claims about the population.If you have studied statistics before, you might have encountered some of these ideas before: hypothesis testing, p-values, estimation, standard error, and confidence intervals.In this notebook we'll approach these topics using computation and simulation, as opposed to mathematical analysis. I hope this approach makes the ideas clearer.If you have not seen these ideas before, don't worry. That might be even better.We'll look at three examples:* Testing whether a coin is "fair".* Testing whether first babies are more like to be born early (or late).* Estimating the average height of men in the U.S., and quantifying the precision of the estimate. The Euro problemIn *Information Theory, Inference, and Learning Algorithms*, David MacKay writes, "A statistical statement appeared in *The Guardian* on Friday January 4, 2002:> When spun on edge 250 times, a Belgian one-euro coin cameup heads 140 times and tails 110. ‘It looks very suspiciousto me’, said Barry Blight, a statistics lecturer at the LondonSchool of Economics. ‘If the coin were unbiased the chance ofgetting a result as extreme as that would be less than 7%’.*But do these data give evidence that the coin is biased rather than fair?"Before we answer MacKay's question, let's unpack what Dr. Blight said:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".To see where that comes from, I'll simulate the result of spinning an "unbiased" coin, meaning that the probability of heads is 50%.Here's an example with 10 spins:
###Code
import numpy as np
spins = np.random.random(10) < 0.5
spins
###Output
_____no_output_____
###Markdown
`np.random.random` returns numbers between 0 and 1, uniformly distributed. So the probability of being less than 0.5 is 50%. The sum of the array is the number of `True` elements, that is, the number of heads:
###Code
np.sum(spins)
###Output
_____no_output_____
###Markdown
We can wrap that in a function that simulates `n` spins with probability `p`.
###Code
def spin(n, p):
return np.sum(np.random.random(n) < p)
###Output
_____no_output_____
###Markdown
Here's an example with the actual sample size (250) and hypothetical probability (50%).
###Code
heads, tails = 140, 110
sample_size = heads + tails
hypo_prob = 0.5
spin(sample_size, hypo_prob)
###Output
_____no_output_____
###Markdown
Since we are generating random numbers, we expect to see different values if we run the experiment more than once.Here's a loop that runs `spin` 10 times.
###Code
n = 250
p = 0.5
for i in range(10):
print(spin(n, p))
###Output
_____no_output_____
###Markdown
As expected, the results vary from one run to the next.Now let's run the simulated experiment 10000 times and store the results in a NumPy array.
###Code
outcomes = np.empty(1000)
for i in range(len(outcomes)):
outcomes[i] = spin(n, p)
###Output
_____no_output_____
###Markdown
`np.empty` creates an empty array with the given length. Each time through the loop, we run `spin` and assign the result to an element of `outcomes`.The result is an array of 10000 integers, each representing the number of heads in a simulated experiment. The mean of `outcomes` is about 125:
###Code
np.mean(outcomes)
###Output
_____no_output_____
###Markdown
Which makes sense. On average, the expected number of heads is the product of the hypothetical probability and the sample size:
###Code
expected = hypo_prob * sample_size
expected
###Output
_____no_output_____
###Markdown
Now let's see how much the values in `outcomes` differ from the expected value:
###Code
diffs = outcomes - expected
###Output
_____no_output_____
###Markdown
`diffs` is an array that contains the deviation of each experiment from the expected value, 125.Here's the mean of the absolute deviations:
###Code
np.mean(abs(diffs))
###Output
_____no_output_____
###Markdown
So a typical experiment deviates from the mean by about 6.To see the whole distribution of deviations, we can plot a histogram.The following function uses Matplotlib to plot a histogram and adjust some of the settings.
###Code
import matplotlib.pyplot as plt
def plot_hist(values):
xs, ys, patches = plt.hist(values,
density=True,
histtype='step',
linewidth=2,
alpha=0.5)
plt.ylabel('Density')
plt.tight_layout()
return patches[0]
###Output
_____no_output_____
###Markdown
Here's what the distribution of deviations looks like:
###Code
plot_hist(diffs)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads');
###Output
_____no_output_____
###Markdown
This is the "sampling distribution" of deviations. It shows how much variation we should expect between experiments with this sample size (n = 250). P-valuesGetting get back to the Euro example, Dr. Bright reported:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".The article doesn't say so explicitly, but this is a "p-value". To understand what that means, let's count how many times, in 10000 attempts, the outcome is "as extreme as" the observed outcome, 140 heads.The observed deviation is the difference between the observed and expected number of heads:
###Code
observed_diff = heads - expected
observed_diff
###Output
_____no_output_____
###Markdown
Let's see how many times the simulated `diffs` exceed the observed deviation:
###Code
np.mean(diffs >= observed_diff)
###Output
_____no_output_____
###Markdown
It's around 3%. But Dr. Blight said 7%. Where did that come from?So far, we only counted the cases where the outcome is *more* heads than expected. We might also want to count the cases where the outcome is *fewer* than expected.Here's the probability of falling below the expected number by 15 or more.
###Code
np.mean(diffs <= -observed_diff)
###Output
_____no_output_____
###Markdown
To get the total probability of a result "as extreme as that", we can use the absolute value of the simulated differences:
###Code
np.mean(abs(diffs) >= observed_diff)
###Output
_____no_output_____
###Markdown
So that's consistent with what Dr. Blight reported.To show what that looks like graphically, I'll use the following function, which fills in the histogram between `low` and `high`.
###Code
def fill_hist(low, high, patch):
fill = plt.axvspan(low, high,
clip_path=patch,
alpha=0.5,
color='C0')
###Output
_____no_output_____
###Markdown
The following plot shows the sampling distribution of `diffs` with two regions shaded. These regions represent the probability that an unbiased coin yields a deviation from the expected as extreme as 15.
###Code
patch = plot_hist(diffs)
# fill the right tail of the hist
low = observed_diff
high = diffs.max()
fill_hist(low, high, patch)
# fill the left tail of the hist
low = diffs.min()
high = -observed_diff
fill_hist(low, high, patch)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads')
plt.ylabel('Density');
###Output
_____no_output_____
###Markdown
These results show that there is a non-negligible chance of getting a result as extreme as 140 heads, even if the coin is actually fair.So even if the results are "suspicious" they don't provide compelling evidence that the coin is biased. **Exercise:** There are a few ways to make "crooked" dice, that is, dice that are more likely to land on one side than the others. Suppose you run a casino and you suspect that a patron is using a die that comes up 3 more often than it should. You confiscate the die, roll it 300 times, and 63 times it comes up 3. Does that support your suspicions?- To answer this question, use `spin` to simulate the experiment, assuming that the die is fair.- Use a for loop to run `spin` 1000 times and store the results in a NumPy array.- What is the mean of the results from the simulated experiments?- What is the expected number of 3s if the die is fair?- Use `plot_hist` to plot the results. The histogram you plot approximates the sampling distribution.
###Code
n = 300
p = 1/6
outcomes = np.empty(1000)
for i in range(len(outcomes)):
outcomes[i] = spin(n, p)
np.mean(outcomes)
expected = n * p
expected
diffs = outcomes - expected
plot_hist(diffs)
plt.title('Sampling distribution (n=100, p=1/6)')
plt.xlabel('Deviation from expected number of 3s')
plt.ylabel('Density');
###Output
_____no_output_____
###Markdown
**Exercise:** Continuing the previous exercise, compute the probability of seeing a deviation from the expected value that is "as extreme" as the observed difference.For this context, what do you think is the best definition of "as extreme"?Plot the histogram of the random deviations again, and use `fill_hist` to fill the region of the histogram that corresponds to the p-value you computed.
###Code
observed_diff = 63 - expected
observed_diff
# Since we started with the suspicion that the die comes up 3
# more often than it should, it might make sense to check how
# often the random deviation EXCEEDS the observed difference;
# we are less interested in how often it is too low.
p_value = np.mean(diffs > observed_diff)
p_value
patch = plot_hist(diffs)
# fill the right tail of the hist
low = observed_diff
high = diffs.max()
fill_hist(low, high, patch)
plt.title('Sampling distribution (n=100, p=1/6)')
plt.xlabel('Deviation from expected number of 3s')
plt.ylabel('Density');
###Output
_____no_output_____
###Markdown
Are first babies more likely to be late?The examples so far have been based on coins and dice, which are relatively simple. In this section we'll look at an example that's based on real-world data.Here's the motivation for it: When my wife and I were expecting our first baby, we heard that first babies are more likely to be late. We also hear that first babies are more likely to be early. Neither claim was supported by evidence.Fortunately, I am a data scientist! Also fortunately, the CDC runs the National Survey of Family Growth (NSFG), which "gathers information on family life, marriage and divorce, pregnancy, infertility, use of contraception, and men’s and women’s health."I got the data from their web page, https://www.cdc.gov/nchs/nsfg/index.htm, and wrote some code to get it into a Pandas Dataframe:
###Code
import os
if not os.path.exists('nsfg.hdf5'):
!wget https://github.com/AllenDowney/ElementsOfDataScience/raw/master/nsfg.hdf5
import pandas as pd
nsfg = pd.read_hdf('nsfg.hdf5')
nsfg.shape
###Output
_____no_output_____
###Markdown
The `nsfg` DataFrame contains 9358 rows, one for each recorded pregnancy, and 11 columns, one of each of the variables I selected.Here are the first few lines.
###Code
nsfg.head()
###Output
_____no_output_____
###Markdown
The variables we need are `birthord`, which indicates birth order, and `prglength`, which is pregnancy length in weeks.I'll make two boolean Series, one for first babies and one for others.
###Code
firsts = (nsfg.birthord == 1)
others = (nsfg.birthord > 1)
np.sum(firsts), np.sum(others)
###Output
_____no_output_____
###Markdown
We can use the boolean Series to select pregnancy lengths for the two groups and compute their means:
###Code
prglngth = nsfg['prglngth']
mean_first = prglngth[firsts].mean()
mean_other = prglngth[others].mean()
mean_first, mean_other
###Output
_____no_output_____
###Markdown
Here's the difference in means, in weeks.
###Code
diff = mean_first - mean_other
diff
###Output
_____no_output_____
###Markdown
Here it is converted to days:
###Code
diff * 7
###Output
_____no_output_____
###Markdown
It looks like first babies are born 1.4 days later than other babies, on average. Hypothesis testingThe apparent difference between these groups is based on a random sample that is much smaller than the actual population. So we can't be sure that the difference we see in the sample reflects a real difference in the population. There are two other possibilities we should keep in mind:* Systematic errors: The sample might be more likely to include some pregancies, and less likely to include others, in a way that causes an apparent difference in the sample, even if there is no such difference in the population.* Sampling errors: Even if every pregnancy is equally likely to appear in the sample, it is still possible to see a difference in the sample that is not in the population, just because of random variation.We can never rule out the possibility of systematic errors, but we *can* test whether an apparent effect could be explained by random sampling.Here's how:1. First we choose a "test statistic" that measures the size of the effect; the test statistic in this example is the difference in mean pregnancy length.2. Next we define a model of the population under the assumption that there is actually no difference between the groups. This assumption is called the "null hypothesis".3. Then we use the model to compute the distribution of the test statistic under the null hypothesis.We have already done step 1, but to make it easier to repeat, I'll wrap it in a function.
###Code
def test_stat(group1, group2):
"""Difference in means.
group1: sequence of values
group2: sequence of values
returns: float difference in means
"""
diff = np.mean(group1) - np.mean(group2)
return diff
###Output
_____no_output_____
###Markdown
`test_stat` takes two sequences and computes the difference in their means.Here's how we use it to compute the actual difference in the sample.
###Code
group1 = prglngth[firsts]
group2 = prglngth[others]
actual_diff = test_stat(group1, group2)
actual_diff
###Output
_____no_output_____
###Markdown
Now we have to define the null hypothesis, which is a model of the world where there is no difference in pregnancy length between first babies and others.One way to do that is to put the two groups together and then divide them up again at random. That way the distribution of pregnancy lengths is the same for both groups.I'll use `concatenate` to pool the groups.
###Code
len(group1), len(group2)
pool = np.concatenate([group1, group2])
pool.shape
###Output
_____no_output_____
###Markdown
I'll use `shuffle` to reorder them.
###Code
np.random.shuffle(pool)
###Output
_____no_output_____
###Markdown
Then I'll use `split` to make two simulated groups, the same size as the originals.
###Code
n = len(group1)
sim_group1, sim_group2 = np.split(pool, [n])
len(sim_group1), len(sim_group2)
###Output
_____no_output_____
###Markdown
Now we can compute the test statistic for the simulated data.
###Code
test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
In the simulated data, the distribution of pregnancy lengths is the same for both groups, so the difference is usually close to 0.But because it is based on a random shuffle of the groups, we get a different value each time we run it.To see what the whole distribution looks like, we can run the simulation many times and store the results.
###Code
diffs = np.empty(1000)
for i in range(len(diffs)):
np.random.shuffle(pool)
sim_group1, sim_group2 = np.split(pool, [n])
diffs[i] = test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution of the test statistic under the null hypothesis".The mean of this distribution should close to zero, because it is based on the assumption that there is actually no difference between the groups.
###Code
np.mean(diffs)
###Output
_____no_output_____
###Markdown
And here's what the whole distribution looks like.
###Code
plot_hist(diffs)
plt.xlabel('Difference in mean (weeks)')
plt.title('Distribution of test statistic under null hypothesis');
###Output
_____no_output_____
###Markdown
If there were actually no difference between the groups, we would expect to see a difference as big as 0.15 weeks by chance, at least occasionally. But a difference as big as 0.2 would be rare.To quantify that surprise, we can estimate the probability that the test statistic, under the null hypothesis, exceeds the observed differences in the means.The result is a "p-value".
###Code
p_value = np.mean(diffs >= actual_diff)
p_value
###Output
_____no_output_____
###Markdown
In this example the result is 0, which is to say that in 1000 simulations of the null hypothesis, we never saw a difference as big as 0.2. Interpreting p-valuesTo interpret this result, remember that we started with three possible explanations for the observed difference between the groups:1. The observed difference might be "real"; that is, there might be an actual difference in pregnancy length between first babies and others.2. There might be no real difference between the groups, and the observed difference might be because of a systematic error in the sampling process or the data collection process. For example, maybe reported pregnancy lengths are less accurate for first time mothers.3. There might be no real difference between the groups, and the observed difference might be due to random variation in the sampling process.By computing a p-value, we have established that it would be rare to see a difference as big as 0.2 due to sampling alone. So we can conclude that the third explanation is unlikely.That makes it more likely that the difference is real, but we still can't rule out the second possibility. **Exercise:** The test statistic we chose is the difference in means between the two groups.But suppose we would like to know whether first babies are more unpredictable than other babies. In that case the test statistic we choose might be the standard deviation of pregnancy length, which is one way to quantify unpredictability.As an exercise:1. Write a version of `test_stat` that computes the difference in standard deviation between the groups.2. Write a loop that estimates the distribution of this test statistic under the null hypothesis.3. Compute a p-value.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
EstimationSuppose we want to estimate the average height of men in the U.S.We can use data from the [BRFSS](https://www.cdc.gov/brfss/index.html):"The Behavioral Risk Factor Surveillance System (BRFSS) is the nation's premier system of health-related telephone surveys that collect state data about U.S. residents regarding their health-related risk behaviors, chronic health conditions, and use of preventive services."
###Code
# Get the data file
import os
if not os.path.exists('brfss.hdf5'):
!wget https://github.com/AllenDowney/ElementsOfDataScience/raw/master/brfss.hdf5
import pandas as pd
brfss = pd.read_hdf('brfss.hdf5', 'brfss')
brfss.shape
###Output
_____no_output_____
###Markdown
We can use `SEX` to select male respondents.
###Code
male = (brfss.SEX == 1)
np.mean(male)
###Output
_____no_output_____
###Markdown
Then we select height data.
###Code
heights = brfss['HTM4']
data = heights[male]
###Output
_____no_output_____
###Markdown
We can use `isnan` to check for NaN values:
###Code
np.mean(np.isnan(data)) * 100
###Output
_____no_output_____
###Markdown
About 4% of the values are missing.Here are the mean and standard deviation, ignoring missing data.
###Code
print('Mean male height in cm =', np.nanmean(data))
print('Std male height in cm =', np.nanstd(data))
###Output
_____no_output_____
###Markdown
Quantifying precisionAt this point we have an estimate of the average adult male height. We'd like to know how accurate this estimate is, and how precise. In the context of estimation, these words have a [technical distinction](https://en.wikipedia.org/wiki/Accuracy_and_precision):>Given a set of data points from repeated measurements of the same quantity, the set can be said to be precise if the values are close to each other, while the set can be said to be accurate if their average is close to the true value of the quantity being measured.Usually accuracy is what we really care about, but it's hard to measure accuracy unless you know the true value. And if you know the true value, you don't have to estimate it.Quantifying precision is not as useful, but it is much easier. Here's one way to do it:1. Use the data you have to make a model of the population.2. Use the model to simulate the data collection process.3. Use the simulated data to compute an estimate.By repeating these steps, we can quantify the variability of the estimate due to random sampling. To model the population, I'll use **resampling**; that is, I will treat the observed measurements as if they were taken from the entire population, and I will draw random samples from them.We can use `np.random.choice` to resample the data:
###Code
size = len(data)
sim_data = np.random.choice(data, size, replace=True)
sim_data.shape
###Output
_____no_output_____
###Markdown
With `replace=True`, we sample with replacement, which means that some measurements might be chosen more than once, and some might not be chosen at all.(If we sample *without* replacement, the resampled data is always identical to the original, so that's no good.) Now we can use `nanmean` to compute the mean of the simulated data, ignoring missing values.
###Code
np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
If we repeat this process 1000 times, we can see how much the results vary.
###Code
outcomes = np.empty(1000)
size = len(data)
for i in range(len(outcomes)):
sim_data = np.random.choice(data, size, replace=True)
outcomes[i] = np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution", which shows how much the results of the experiment would vary if we ran it many times. Here's what it looks like:
###Code
plot_hist(outcomes)
plt.title('Sampling distribution of the mean')
plt.xlabel('Mean adult male height, U.S.');
###Output
_____no_output_____
###Markdown
The width of this distribution shows how much the results vary from one experiment to the next.We can quantify this variability by computing the standard deviation of the sampling distribution, which is called "standard error".
###Code
std_err = np.std(outcomes)
std_err
###Output
_____no_output_____
###Markdown
We can also summarize the sampling distribution with a "confidence interval", which is a range that contains a specified fraction, like 90%, of the values in `sampling_dist_mean`.The central 90% confidence interval is between the 5th and 95th percentiles of the sampling distribution.
###Code
ci_90 = np.percentile(outcomes, [5, 95])
ci_90
###Output
_____no_output_____
###Markdown
The following function plots a histogram and shades the 90% confidence interval.
###Code
def plot_sampling_dist(outcomes):
patch = plot_hist(outcomes)
low, high = np.percentile(outcomes, [5, 95])
fill_hist(low, high, patch)
print('Mean = ', np.mean(outcomes))
print('Std error = ', np.std(outcomes))
print('90% CI = ', (low, high))
###Output
_____no_output_____
###Markdown
Here's what it looks like for the sampling distribution of mean adult height:
###Code
plot_sampling_dist(outcomes)
plt.xlabel('Mean adult male height, U.S. (%)');
###Output
_____no_output_____
###Markdown
For an experiment like this, we can compute the standard error analytically.
###Code
size = len(data)
analytic_std_err = np.std(data) / np.sqrt(size)
###Output
_____no_output_____
###Markdown
The result is close to what we observed computationally.
###Code
analytic_std_err, std_err
###Output
_____no_output_____
###Markdown
This result indicates that our estimate of the mean is *precise*; that is, if we ran this experiment many times, the results would fall in a narrow range.But this range reflects only variability due to random sampling. If there are systematic errors in the sampling process, or in the measurement process, the result would not be *accurate*.Computing a standard error or confidence interval can be useful, but it only quantifies variability due to random sampling, not other sources of error. **Exercise:** One nice thing about using resampling is that it is easy to compute the sampling distribution for other statistics.For example, suppose we want to estimate the coefficient of variation (standard deviation as a fraction of the mean) for adult male height. Here's how we can compute it.
###Code
cv = np.nanstd(data) / np.nanmean(data)
cv
###Output
_____no_output_____
###Markdown
So the standard deviation is about 5% of the mean. Write a loop that uses resampling to estimate the sampling distribution of `cv`; store the results in an array named `outcomes`.Then use `plot_sampling_dist` to plot the sampling distribution of the coefficient of variation.What is the standard error of the estimated coefficient of variation?
###Code
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
Inference *Elements of Data Science*Copyright 2021 [Allen B. Downey](https://allendowney.com)License: [Creative Commons Attribution-NonCommercial-ShareAlike 4.0 International](https://creativecommons.org/licenses/by-nc-sa/4.0/) [Click here to run this notebook on Colab](https://colab.research.google.com/github/AllenDowney/ElementsOfDataScience/blob/master/11_inference.ipynb) or[click here to download it](https://github.com/AllenDowney/ElementsOfDataScience/raw/master/11_inference.ipynb). This chapter introduces **statistical inference**, which is the process of using a sample to make inferences about a population. The **population** is the group we are interested in. Sometimes it is a group of people, but in general it can be any kind of group.If we can observe the entire group, we might not need statistical inference. If not, sometimes we can observe a **sample** (or subset) of the population and use those observations to make claims about the population.If you have studied statistics before, you might have encountered some of these ideas before: hypothesis testing, p-values, estimation, standard error, and confidence intervals.In this chapter we'll approach these topics using computation and simulation, as opposed to mathematical analysis. I hope this approach makes the ideas clearer.If you have not seen these ideas before, don't worry. That might even be better.We'll look at three examples:* Testing whether a coin is "fair".* Testing whether first babies are more like to be born early (or late).* Estimating the average height of men in the U.S., and quantifying the precision of the estimate. The Euro problemIn *Information Theory, Inference, and Learning Algorithms*, David MacKay writes, "A statistical statement appeared in *The Guardian* on Friday January 4, 2002:> When spun on edge 250 times, a Belgian one-euro coin cameup heads 140 times and tails 110. ‘It looks very suspiciousto me’, said Barry Blight, a statistics lecturer at the LondonSchool of Economics. ‘If the coin were unbiased the chance ofgetting a result as extreme as that would be less than 7%’.*But do these data give evidence that the coin is biased rather than fair?"Before we answer MacKay's question, let's unpack what Dr. Blight said:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".To see where that comes from, I'll simulate the result of spinning an "unbiased" coin, meaning that the probability of heads is 50%.Here's an example with 10 spins:
###Code
import numpy as np
spins = np.random.random(10) < 0.5
spins
###Output
_____no_output_____
###Markdown
`np.random.random` returns numbers between 0 and 1, uniformly distributed. So the probability of being less than 0.5 is 50%. The sum of the array is the number of `True` elements, that is, the number of heads:
###Code
np.sum(spins)
###Output
_____no_output_____
###Markdown
We can wrap that in a function that simulates `n` spins with probability `p`.
###Code
def spin(n, p):
return np.sum(np.random.random(n) < p)
###Output
_____no_output_____
###Markdown
Here's an example with the actual sample size (250) and hypothetical probability (50%).
###Code
heads, tails = 140, 110
sample_size = heads + tails
hypo_prob = 0.5
spin(sample_size, hypo_prob)
###Output
_____no_output_____
###Markdown
Since we are generating random numbers, we expect to see different values if we run the experiment more than once.Here's a loop that runs `spin` 10 times.
###Code
n = 250
p = 0.5
for i in range(10):
print(spin(n, p))
###Output
_____no_output_____
###Markdown
As expected, the results vary from one run to the next.Now let's run the simulated experiment 10000 times and store the results in a NumPy array.
###Code
outcomes = np.empty(1000)
for i in range(len(outcomes)):
outcomes[i] = spin(n, p)
###Output
_____no_output_____
###Markdown
`np.empty` creates an empty array with the given length. Each time through the loop, we run `spin` and assign the result to an element of `outcomes`.The result is an array of 10000 integers, each representing the number of heads in a simulated experiment. The mean of `outcomes` is about 125:
###Code
np.mean(outcomes)
###Output
_____no_output_____
###Markdown
Which makes sense. On average, the expected number of heads is the product of the hypothetical probability and the sample size:
###Code
expected = hypo_prob * sample_size
expected
###Output
_____no_output_____
###Markdown
Now let's see how much the values in `outcomes` differ from the expected value:
###Code
diffs = outcomes - expected
###Output
_____no_output_____
###Markdown
`diffs` is an array that contains the deviation of each experiment from the expected value, 125.Here's the mean of the absolute deviations:
###Code
np.mean(abs(diffs))
###Output
_____no_output_____
###Markdown
So a typical experiment deviates from the mean by about 6.To see the whole distribution of deviations, we can plot a histogram.The following function uses Matplotlib to plot a histogram and adjust some of the settings.
###Code
import matplotlib.pyplot as plt
def plot_hist(values):
xs, ys, patches = plt.hist(values,
density=True,
histtype='step',
linewidth=2,
alpha=0.5)
plt.ylabel('Density')
plt.tight_layout()
return patches[0]
###Output
_____no_output_____
###Markdown
Here's what the distribution of deviations looks like:
###Code
plot_hist(diffs)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads');
###Output
_____no_output_____
###Markdown
This is the "sampling distribution" of deviations. It shows how much variation we should expect between experiments with this sample size (n = 250). P-valuesGetting get back to the Euro example, Dr. Bright reported:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".The article doesn't say so explicitly, but this is a "p-value". To understand what that means, let's count how many times, in 10000 attempts, the outcome is "as extreme as" the observed outcome, 140 heads.The observed deviation is the difference between the observed and expected number of heads:
###Code
observed_diff = heads - expected
observed_diff
###Output
_____no_output_____
###Markdown
Let's see how many times the simulated `diffs` exceed the observed deviation:
###Code
np.mean(diffs >= observed_diff)
###Output
_____no_output_____
###Markdown
It's around 3%. But Dr. Blight said 7%. Where did that come from?So far, we only counted the cases where the outcome is *more* heads than expected. We might also want to count the cases where the outcome is *fewer* than expected.Here's the probability of falling below the expected number by 15 or more.
###Code
np.mean(diffs <= -observed_diff)
###Output
_____no_output_____
###Markdown
To get the total probability of a result "as extreme as that", we can use the absolute value of the simulated differences:
###Code
np.mean(abs(diffs) >= observed_diff)
###Output
_____no_output_____
###Markdown
So that's consistent with what Dr. Blight reported.To show what that looks like graphically, I'll use the following function, which fills in the histogram between `low` and `high`.
###Code
def fill_hist(low, high, patch):
fill = plt.axvspan(low, high,
clip_path=patch,
alpha=0.5,
color='C0')
###Output
_____no_output_____
###Markdown
The following plot shows the sampling distribution of `diffs` with two regions shaded. These regions represent the probability that an unbiased coin yields a deviation from the expected as extreme as 15.
###Code
patch = plot_hist(diffs)
# fill the right tail of the hist
low = observed_diff
high = diffs.max()
fill_hist(low, high, patch)
# fill the left tail of the hist
low = diffs.min()
high = -observed_diff
fill_hist(low, high, patch)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads')
plt.ylabel('Density');
###Output
_____no_output_____
###Markdown
These results show that there is a non-negligible chance of getting a result as extreme as 140 heads, even if the coin is actually fair.So even if the results are "suspicious" they don't provide compelling evidence that the coin is biased. **Exercise:** There are a few ways to make "crooked" dice, that is, dice that are more likely to land on one side than the others. Suppose you run a casino and you suspect that a patron is using a die that comes up 3 more often than it should. You confiscate the die, roll it 300 times, and 63 times it comes up 3. Does that support your suspicions?- To answer this question, use `spin` to simulate the experiment, assuming that the die is fair.- Use a for loop to run `spin` 1000 times and store the results in a NumPy array.- What is the mean of the results from the simulated experiments?- What is the expected number of 3s if the die is fair?- Use `plot_hist` to plot the results. The histogram you plot approximates the sampling distribution.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
**Exercise:** Continuing the previous exercise, compute the probability of seeing a deviation from the expected value that is "as extreme" as the observed difference.For this context, what do you think is the best definition of "as extreme"?Plot the histogram of the random deviations again, and use `fill_hist` to fill the region of the histogram that corresponds to the p-value you computed.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
Are first babies more likely to be late?The examples so far have been based on coins and dice, which are relatively simple. In this section we'll look at an example that's based on real-world data.Here's the motivation for it: When my wife and I were expecting our first baby, we heard that first babies are more likely to be late. We also hear that first babies are more likely to be early. Neither claim was supported by evidence.Fortunately, I am a data scientist! Also fortunately, the CDC runs the National Survey of Family Growth (NSFG), which "gathers information on family life, marriage and divorce, pregnancy, infertility, use of contraception, and men’s and women’s health."I got the data from their web page, https://www.cdc.gov/nchs/nsfg/index.htm, and wrote some code to get it into a Pandas Dataframe:
###Code
from os.path import basename, exists
def download(url):
filename = basename(url)
if not exists(filename):
from urllib.request import urlretrieve
local, _ = urlretrieve(url, filename)
print('Downloaded ' + local)
download('https://github.com/AllenDowney/' +
'ElementsOfDataScience/raw/master/nsfg.hdf5')
import pandas as pd
nsfg = pd.read_hdf('nsfg.hdf5')
nsfg.shape
###Output
_____no_output_____
###Markdown
The `nsfg` DataFrame contains 9358 rows, one for each recorded pregnancy, and 11 columns, one of each of the variables I selected.Here are the first few lines.
###Code
nsfg.head()
###Output
_____no_output_____
###Markdown
The variables we need are `birthord`, which indicates birth order, and `prglength`, which is pregnancy length in weeks.I'll make two boolean Series, one for first babies and one for others.
###Code
firsts = (nsfg.birthord == 1)
others = (nsfg.birthord > 1)
np.sum(firsts), np.sum(others)
###Output
_____no_output_____
###Markdown
We can use the boolean Series to select pregnancy lengths for the two groups and compute their means:
###Code
prglngth = nsfg['prglngth']
mean_first = prglngth[firsts].mean()
mean_other = prglngth[others].mean()
mean_first, mean_other
###Output
_____no_output_____
###Markdown
Here's the difference in means, in weeks.
###Code
diff = mean_first - mean_other
diff
###Output
_____no_output_____
###Markdown
Here it is converted to days:
###Code
diff * 7
###Output
_____no_output_____
###Markdown
It looks like first babies are born 1.4 days later than other babies, on average. Hypothesis testingThe apparent difference between these groups is based on a random sample that is much smaller than the actual population. So we can't be sure that the difference we see in the sample reflects a real difference in the population. There are two other possibilities we should keep in mind:* Systematic errors: The sample might be more likely to include some pregancies, and less likely to include others, in a way that causes an apparent difference in the sample, even if there is no such difference in the population.* Sampling errors: Even if every pregnancy is equally likely to appear in the sample, it is still possible to see a difference in the sample that is not in the population, just because of random variation.We can never rule out the possibility of systematic errors, but we *can* test whether an apparent effect could be explained by random sampling.Here's how:1. First we choose a "test statistic" that measures the size of the effect; the test statistic in this example is the difference in mean pregnancy length.2. Next we define a model of the population under the assumption that there is actually no difference between the groups. This assumption is called the "null hypothesis".3. Then we use the model to compute the distribution of the test statistic under the null hypothesis.We have already done step 1, but to make it easier to repeat, I'll wrap it in a function.
###Code
def test_stat(group1, group2):
"""Difference in means.
group1: sequence of values
group2: sequence of values
returns: float difference in means
"""
diff = np.mean(group1) - np.mean(group2)
return diff
###Output
_____no_output_____
###Markdown
`test_stat` takes two sequences and computes the difference in their means.Here's how we use it to compute the actual difference in the sample.
###Code
group1 = prglngth[firsts]
group2 = prglngth[others]
actual_diff = test_stat(group1, group2)
actual_diff
###Output
_____no_output_____
###Markdown
Now we have to define the null hypothesis, which is a model of the world where there is no difference in pregnancy length between first babies and others.One way to do that is to put the two groups together and then divide them up again at random. That way the distribution of pregnancy lengths is the same for both groups.I'll use `concatenate` to pool the groups.
###Code
len(group1), len(group2)
pool = np.concatenate([group1, group2])
pool.shape
###Output
_____no_output_____
###Markdown
I'll use `shuffle` to reorder them.
###Code
np.random.shuffle(pool)
###Output
_____no_output_____
###Markdown
Then I'll use `split` to make two simulated groups, the same size as the originals.
###Code
n = len(group1)
sim_group1, sim_group2 = np.split(pool, [n])
len(sim_group1), len(sim_group2)
###Output
_____no_output_____
###Markdown
Now we can compute the test statistic for the simulated data.
###Code
test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
In the simulated data, the distribution of pregnancy lengths is the same for both groups, so the difference is usually close to 0.But because it is based on a random shuffle of the groups, we get a different value each time we run it.To see what the whole distribution looks like, we can run the simulation many times and store the results.
###Code
diffs = np.empty(1000)
for i in range(len(diffs)):
np.random.shuffle(pool)
sim_group1, sim_group2 = np.split(pool, [n])
diffs[i] = test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution of the test statistic under the null hypothesis".The mean of this distribution should close to zero, because it is based on the assumption that there is actually no difference between the groups.
###Code
np.mean(diffs)
###Output
_____no_output_____
###Markdown
And here's what the whole distribution looks like.
###Code
plot_hist(diffs)
plt.xlabel('Difference in mean (weeks)')
plt.title('Distribution of test statistic under null hypothesis');
###Output
_____no_output_____
###Markdown
If there were actually no difference between the groups, we would expect to see a difference as big as 0.15 weeks by chance, at least occasionally. But a difference as big as 0.2 would be rare.To quantify that surprise, we can estimate the probability that the test statistic, under the null hypothesis, exceeds the observed differences in the means.The result is a "p-value".
###Code
p_value = np.mean(diffs >= actual_diff)
p_value
###Output
_____no_output_____
###Markdown
In this example the result is 0, which is to say that in 1000 simulations of the null hypothesis, we never saw a difference as big as 0.2. Interpreting p-valuesTo interpret this result, remember that we started with three possible explanations for the observed difference between the groups:1. The observed difference might be "real"; that is, there might be an actual difference in pregnancy length between first babies and others.2. There might be no real difference between the groups, and the observed difference might be because of a systematic error in the sampling process or the data collection process. For example, maybe reported pregnancy lengths are less accurate for first time mothers.3. There might be no real difference between the groups, and the observed difference might be due to random variation in the sampling process.By computing a p-value, we have established that it would be rare to see a difference as big as 0.2 due to sampling alone. So we can conclude that the third explanation is unlikely.That makes it more likely that the difference is real, but we still can't rule out the second possibility. **Exercise:** The test statistic we chose is the difference in means between the two groups.But suppose we would like to know whether first babies are more unpredictable than other babies. In that case the test statistic we choose might be the standard deviation of pregnancy length, which is one way to quantify unpredictability.As an exercise:1. Write a version of `test_stat` that computes the difference in standard deviation between the groups.2. Write a loop that estimates the distribution of this test statistic under the null hypothesis.3. Compute a p-value.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
EstimationSuppose we want to estimate the average height of men in the U.S.We can use data from the [BRFSS](https://www.cdc.gov/brfss/index.html):"The Behavioral Risk Factor Surveillance System (BRFSS) is the nation's premier system of health-related telephone surveys that collect state data about U.S. residents regarding their health-related risk behaviors, chronic health conditions, and use of preventive services."
###Code
from os.path import basename, exists
def download(url):
filename = basename(url)
if not exists(filename):
from urllib.request import urlretrieve
local, _ = urlretrieve(url, filename)
print('Downloaded ' + local)
download('https://github.com/AllenDowney/' +
'ElementsOfDataScience/raw/master/brfss.hdf5')
import pandas as pd
brfss = pd.read_hdf('brfss.hdf5', 'brfss')
brfss.shape
###Output
_____no_output_____
###Markdown
We can use `SEX` to select male respondents.
###Code
male = (brfss.SEX == 1)
np.mean(male)
###Output
_____no_output_____
###Markdown
Then we select height data.
###Code
heights = brfss['HTM4']
data = heights[male]
###Output
_____no_output_____
###Markdown
We can use `isnan` to check for NaN values:
###Code
np.mean(np.isnan(data)) * 100
###Output
_____no_output_____
###Markdown
About 4% of the values are missing.Here are the mean and standard deviation, ignoring missing data.
###Code
print('Mean male height in cm =', np.nanmean(data))
print('Std male height in cm =', np.nanstd(data))
###Output
_____no_output_____
###Markdown
Quantifying precisionAt this point we have an estimate of the average adult male height. We'd like to know how accurate this estimate is, and how precise. In the context of estimation, these words have a [technical distinction](https://en.wikipedia.org/wiki/Accuracy_and_precision):>Given a set of data points from repeated measurements of the same quantity, the set can be said to be precise if the values are close to each other, while the set can be said to be accurate if their average is close to the true value of the quantity being measured.Usually accuracy is what we really care about, but it's hard to measure accuracy unless you know the true value. And if you know the true value, you don't have to estimate it.Quantifying precision is not as useful, but it is much easier. Here's one way to do it:1. Use the data you have to make a model of the population.2. Use the model to simulate the data collection process.3. Use the simulated data to compute an estimate.By repeating these steps, we can quantify the variability of the estimate due to random sampling. To model the population, I'll use **resampling**; that is, I will treat the observed measurements as if they were taken from the entire population, and I will draw random samples from them.We can use `np.random.choice` to resample the data:
###Code
size = len(data)
sim_data = np.random.choice(data, size, replace=True)
sim_data.shape
###Output
_____no_output_____
###Markdown
With `replace=True`, we sample with replacement, which means that some measurements might be chosen more than once, and some might not be chosen at all.(If we sample *without* replacement, the resampled data is always identical to the original, so that's no good.) Now we can use `nanmean` to compute the mean of the simulated data, ignoring missing values.
###Code
np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
If we repeat this process 1000 times, we can see how much the results vary.
###Code
outcomes = np.empty(1000)
size = len(data)
for i in range(len(outcomes)):
sim_data = np.random.choice(data, size, replace=True)
outcomes[i] = np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution", which shows how much the results of the experiment would vary if we ran it many times. Here's what it looks like:
###Code
plot_hist(outcomes)
plt.title('Sampling distribution of the mean')
plt.xlabel('Mean adult male height, U.S.');
###Output
_____no_output_____
###Markdown
The width of this distribution shows how much the results vary from one experiment to the next.We can quantify this variability by computing the standard deviation of the sampling distribution, which is called "standard error".
###Code
std_err = np.std(outcomes)
std_err
###Output
_____no_output_____
###Markdown
We can also summarize the sampling distribution with a "confidence interval", which is a range that contains a specified fraction, like 90%, of the values in `sampling_dist_mean`.The central 90% confidence interval is between the 5th and 95th percentiles of the sampling distribution.
###Code
ci_90 = np.percentile(outcomes, [5, 95])
ci_90
###Output
_____no_output_____
###Markdown
The following function plots a histogram and shades the 90% confidence interval.
###Code
def plot_sampling_dist(outcomes):
"""Plot sampling distribution.
outcomes: sequence of values
"""
patch = plot_hist(outcomes)
low, high = np.percentile(outcomes, [5, 95])
fill_hist(low, high, patch)
print('Mean = ', np.mean(outcomes))
print('Std error = ', np.std(outcomes))
print('90% CI = ', (low, high))
###Output
_____no_output_____
###Markdown
Here's what it looks like for the sampling distribution of mean adult height:
###Code
plot_sampling_dist(outcomes)
plt.xlabel('Mean adult male height, U.S. (%)');
###Output
_____no_output_____
###Markdown
For an experiment like this, we can compute the standard error analytically.
###Code
size = len(data)
analytic_std_err = np.std(data) / np.sqrt(size)
###Output
_____no_output_____
###Markdown
The result is close to what we observed computationally.
###Code
analytic_std_err, std_err
###Output
_____no_output_____
###Markdown
This result indicates that our estimate of the mean is *precise*; that is, if we ran this experiment many times, the results would fall in a narrow range.But this range reflects only variability due to random sampling. If there are systematic errors in the sampling process, or in the measurement process, the result would not be *accurate*.Computing a standard error or confidence interval can be useful, but it only quantifies variability due to random sampling, not other sources of error. **Exercise:** One nice thing about using resampling is that it is easy to compute the sampling distribution for other statistics.For example, suppose we want to estimate the coefficient of variation (standard deviation as a fraction of the mean) for adult male height. Here's how we can compute it.
###Code
cv = np.nanstd(data) / np.nanmean(data)
cv
###Output
_____no_output_____
###Markdown
So the standard deviation is about 5% of the mean. Write a loop that uses resampling to estimate the sampling distribution of `cv`; store the results in an array named `outcomes`.Then use `plot_sampling_dist` to plot the sampling distribution of the coefficient of variation.What is the standard error of the estimated coefficient of variation?
###Code
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
InferenceElements of Data Scienceby [Allen Downey](https://allendowney.com)[MIT License](https://opensource.org/licenses/MIT) GoalsThis notebook introduces "statistical inference", which is the process of using a sample to make inferences about a population. This [figure from Wikipedia](https://en.wikipedia.org/wiki/Statistical_inference) illustrates the idea:On the left, the "population" is the group we are interested in. Sometimes it is a group of people, but in general it can be any kind of group.If we can observe the entire group, we might not need statistical inference. If not, sometimes we can observe a "sample" (or subset) of the population and use those observations to make claims about the population.If you have studied statistics before, you might have encountered some of these ideas before: hypothesis testing, p-values, estimation, standard error, and confidence intervals.In this notebook we'll approach these topics using computation and simulation, as opposed to mathematical analysis. I hope this approach makes the ideas clearer.If you have not seen these ideas before, don't worry. That might even be better.We'll look at three examples:* Testing whether a coin is "fair".* Testing whether first babies are more like to be born early (or late).* Estimating the average height of men in the U.S., and quantifying the precision of the estimate. The Euro problemIn *Information Theory, Inference, and Learning Algorithms*, David MacKay writes, "A statistical statement appeared in *The Guardian* on Friday January 4, 2002:> When spun on edge 250 times, a Belgian one-euro coin cameup heads 140 times and tails 110. ‘It looks very suspiciousto me’, said Barry Blight, a statistics lecturer at the LondonSchool of Economics. ‘If the coin were unbiased the chance ofgetting a result as extreme as that would be less than 7%’.*But do these data give evidence that the coin is biased rather than fair?"Before we answer MacKay's question, let's unpack what Dr. Blight said:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".To see where that comes from, I'll simulate the result of spinning an "unbiased" coin, meaning that the probability of heads is 50%.Here's an example with 10 spins:
###Code
import numpy as np
spins = np.random.random(10) < 0.5
spins
###Output
_____no_output_____
###Markdown
`np.random.random` returns numbers between 0 and 1, uniformly distributed. So the probability of being less than 0.5 is 50%. The sum of the array is the number of `True` elements, that is, the number of heads:
###Code
np.sum(spins)
###Output
_____no_output_____
###Markdown
We can wrap that in a function that simulates `n` spins with probability `p`.
###Code
def spin(n, p):
return np.sum(np.random.random(n) < p)
###Output
_____no_output_____
###Markdown
Here's an example with the actual sample size (250) and hypothetical probability (50%).
###Code
heads, tails = 140, 110
sample_size = heads + tails
hypo_prob = 0.5
spin(sample_size, hypo_prob)
###Output
_____no_output_____
###Markdown
Since we are generating random numbers, we expect to see different values if we run the experiment more than once.Here's a loop that runs `spin` 10 times.
###Code
n = 250
p = 0.5
for i in range(10):
print(spin(n, p))
###Output
_____no_output_____
###Markdown
As expected, the results vary from one run to the next.Now let's run the simulated experiment 10000 times and store the results in a NumPy array.
###Code
outcomes = np.empty(1000)
for i in range(len(outcomes)):
outcomes[i] = spin(n, p)
###Output
_____no_output_____
###Markdown
`np.empty` creates an empty array with the given length. Each time through the loop, we run `spin` and assign the result to an element of `outcomes`.The result is an array of 10000 integers, each representing the number of heads in a simulated experiment. The mean of `outcomes` is about 125:
###Code
np.mean(outcomes)
###Output
_____no_output_____
###Markdown
Which makes sense. On average, the expected number of heads is the product of the hypothetical probability and the sample size:
###Code
expected = hypo_prob * sample_size
expected
###Output
_____no_output_____
###Markdown
Now let's see how much the values in `outcomes` differ from the expected value:
###Code
diffs = outcomes - expected
###Output
_____no_output_____
###Markdown
`diffs` is an array that contains the deviation of each experiment from the expected value, 125.Here's the mean of the absolute deviations:
###Code
np.mean(abs(diffs))
###Output
_____no_output_____
###Markdown
So a typical experiment deviates from the mean by about 6.To see the whole distribution of deviations, we can plot a histogram.The following function uses Matplotlib to plot a histogram and adjust some of the settings.
###Code
import matplotlib.pyplot as plt
def plot_hist(values):
xs, ys, patches = plt.hist(values,
density=True,
histtype='step',
linewidth=2,
alpha=0.5)
plt.ylabel('Density')
plt.tight_layout()
return patches[0]
###Output
_____no_output_____
###Markdown
Here's what the distribution of deviations looks like:
###Code
plot_hist(diffs)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads');
###Output
_____no_output_____
###Markdown
This is the "sampling distribution" of deviations. It shows how much variation we should expect between experiments with this sample size (n = 250). P-valuesGetting get back to the Euro example, Dr. Bright reported:"If the coin were unbiased the chance of getting a result as extreme as that would be less than 7%".The article doesn't say so explicitly, but this is a "p-value". To understand what that means, let's count how many times, in 10000 attempts, the outcome is "as extreme as" the observed outcome, 140 heads.The observed deviation is the difference between the observed and expected number of heads:
###Code
observed_diff = heads - expected
observed_diff
###Output
_____no_output_____
###Markdown
Let's see how many times the simulated `diffs` exceed the observed deviation:
###Code
np.mean(diffs >= observed_diff)
###Output
_____no_output_____
###Markdown
It's around 3%. But Dr. Blight said 7%. Where did that come from?So far, we only counted the cases where the outcome is *more* heads than expected. We might also want to count the cases where the outcome is *fewer* than expected.Here's the probability of falling below the expected number by 15 or more.
###Code
np.mean(diffs <= -observed_diff)
###Output
_____no_output_____
###Markdown
To get the total probability of a result "as extreme as that", we can use the absolute value of the simulated differences:
###Code
np.mean(abs(diffs) >= observed_diff)
###Output
_____no_output_____
###Markdown
So that's consistent with what Dr. Blight reported.To show what that looks like graphically, I'll use the following function, which fills in the histogram between `low` and `high`.
###Code
def fill_hist(low, high, patch):
fill = plt.axvspan(low, high,
clip_path=patch,
alpha=0.5,
color='C0')
###Output
_____no_output_____
###Markdown
The following plot shows the sampling distribution of `diffs` with two regions shaded. These regions represent the probability that an unbiased coin yields a deviation from the expected as extreme as 15.
###Code
patch = plot_hist(diffs)
# fill the right tail of the hist
low = observed_diff
high = diffs.max()
fill_hist(low, high, patch)
# fill the left tail of the hist
low = diffs.min()
high = -observed_diff
fill_hist(low, high, patch)
plt.title('Sampling distribution (n=250)')
plt.xlabel('Deviation from expected number of heads')
plt.ylabel('Density');
###Output
_____no_output_____
###Markdown
These results show that there is a non-negligible chance of getting a result as extreme as 140 heads, even if the coin is actually fair.So even if the results are "suspicious" they don't provide compelling evidence that the coin is biased. **Exercise:** There are a few ways to make "crooked" dice, that is, dice that are more likely to land on one side than the others. Suppose you run a casino and you suspect that a patron is using a die that comes up 3 more often than it should. You confiscate the die, roll it 300 times, and 63 times it comes up 3. Does that support your suspicions?- To answer this question, use `spin` to simulate the experiment, assuming that the die is fair.- Use a for loop to run `spin` 1000 times and store the results in a NumPy array.- What is the mean of the results from the simulated experiments?- What is the expected number of 3s if the die is fair?- Use `plot_hist` to plot the results. The histogram you plot approximates the sampling distribution.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
**Exercise:** Continuing the previous exercise, compute the probability of seeing a deviation from the expected value that is "as extreme" as the observed difference.For this context, what do you think is the best definition of "as extreme"?Plot the histogram of the random deviations again, and use `fill_hist` to fill the region of the histogram that corresponds to the p-value you computed.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
Are first babies more likely to be late?The examples so far have been based on coins and dice, which are relatively simple. In this section we'll look at an example that's based on real-world data.Here's the motivation for it: When my wife and I were expecting our first baby, we heard that first babies are more likely to be late. We also hear that first babies are more likely to be early. Neither claim was supported by evidence.Fortunately, I am a data scientist! Also fortunately, the CDC runs the National Survey of Family Growth (NSFG), which "gathers information on family life, marriage and divorce, pregnancy, infertility, use of contraception, and men’s and women’s health."I got the data from their web page, https://www.cdc.gov/nchs/nsfg/index.htm, and wrote some code to get it into a Pandas Dataframe:
###Code
import os
if not os.path.exists('nsfg.hdf5'):
!wget https://github.com/AllenDowney/ElementsOfDataScience/raw/master/nsfg.hdf5
import pandas as pd
nsfg = pd.read_hdf('nsfg.hdf5')
nsfg.shape
###Output
_____no_output_____
###Markdown
The `nsfg` DataFrame contains 9358 rows, one for each recorded pregnancy, and 11 columns, one of each of the variables I selected.Here are the first few lines.
###Code
nsfg.head()
###Output
_____no_output_____
###Markdown
The variables we need are `birthord`, which indicates birth order, and `prglength`, which is pregnancy length in weeks.I'll make two boolean Series, one for first babies and one for others.
###Code
firsts = (nsfg.birthord == 1)
others = (nsfg.birthord > 1)
np.sum(firsts), np.sum(others)
###Output
_____no_output_____
###Markdown
We can use the boolean Series to select pregnancy lengths for the two groups and compute their means:
###Code
prglngth = nsfg['prglngth']
mean_first = prglngth[firsts].mean()
mean_other = prglngth[others].mean()
mean_first, mean_other
###Output
_____no_output_____
###Markdown
Here's the difference in means, in weeks.
###Code
diff = mean_first - mean_other
diff
###Output
_____no_output_____
###Markdown
Here it is converted to days:
###Code
diff * 7
###Output
_____no_output_____
###Markdown
It looks like first babies are born 1.4 days later than other babies, on average. Hypothesis testingThe apparent difference between these groups is based on a random sample that is much smaller than the actual population. So we can't be sure that the difference we see in the sample reflects a real difference in the population. There are two other possibilities we should keep in mind:* Systematic errors: The sample might be more likely to include some pregancies, and less likely to include others, in a way that causes an apparent difference in the sample, even if there is no such difference in the population.* Sampling errors: Even if every pregnancy is equally likely to appear in the sample, it is still possible to see a difference in the sample that is not in the population, just because of random variation.We can never rule out the possibility of systematic errors, but we *can* test whether an apparent effect could be explained by random sampling.Here's how:1. First we choose a "test statistic" that measures the size of the effect; the test statistic in this example is the difference in mean pregnancy length.2. Next we define a model of the population under the assumption that there is actually no difference between the groups. This assumption is called the "null hypothesis".3. Then we use the model to compute the distribution of the test statistic under the null hypothesis.We have already done step 1, but to make it easier to repeat, I'll wrap it in a function.
###Code
def test_stat(group1, group2):
"""Difference in means.
group1: sequence of values
group2: sequence of values
returns: float difference in means
"""
diff = np.mean(group1) - np.mean(group2)
return diff
###Output
_____no_output_____
###Markdown
`test_stat` takes two sequences and computes the difference in their means.Here's how we use it to compute the actual difference in the sample.
###Code
group1 = prglngth[firsts]
group2 = prglngth[others]
actual_diff = test_stat(group1, group2)
actual_diff
###Output
_____no_output_____
###Markdown
Now we have to define the null hypothesis, which is a model of the world where there is no difference in pregnancy length between first babies and others.One way to do that is to put the two groups together and then divide them up again at random. That way the distribution of pregnancy lengths is the same for both groups.I'll use `concatenate` to pool the groups.
###Code
len(group1), len(group2)
pool = np.concatenate([group1, group2])
pool.shape
###Output
_____no_output_____
###Markdown
I'll use `shuffle` to reorder them.
###Code
np.random.shuffle(pool)
###Output
_____no_output_____
###Markdown
Then I'll use `split` to make two simulated groups, the same size as the originals.
###Code
n = len(group1)
sim_group1, sim_group2 = np.split(pool, [n])
len(sim_group1), len(sim_group2)
###Output
_____no_output_____
###Markdown
Now we can compute the test statistic for the simulated data.
###Code
test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
In the simulated data, the distribution of pregnancy lengths is the same for both groups, so the difference is usually close to 0.But because it is based on a random shuffle of the groups, we get a different value each time we run it.To see what the whole distribution looks like, we can run the simulation many times and store the results.
###Code
diffs = np.empty(1000)
for i in range(len(diffs)):
np.random.shuffle(pool)
sim_group1, sim_group2 = np.split(pool, [n])
diffs[i] = test_stat(sim_group1, sim_group2)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution of the test statistic under the null hypothesis".The mean of this distribution should close to zero, because it is based on the assumption that there is actually no difference between the groups.
###Code
np.mean(diffs)
###Output
_____no_output_____
###Markdown
And here's what the whole distribution looks like.
###Code
plot_hist(diffs)
plt.xlabel('Difference in mean (weeks)')
plt.title('Distribution of test statistic under null hypothesis');
###Output
_____no_output_____
###Markdown
If there were actually no difference between the groups, we would expect to see a difference as big as 0.15 weeks by chance, at least occasionally. But a difference as big as 0.2 would be rare.To quantify that surprise, we can estimate the probability that the test statistic, under the null hypothesis, exceeds the observed differences in the means.The result is a "p-value".
###Code
p_value = np.mean(diffs >= actual_diff)
p_value
###Output
_____no_output_____
###Markdown
In this example the result is 0, which is to say that in 1000 simulations of the null hypothesis, we never saw a difference as big as 0.2. Interpreting p-valuesTo interpret this result, remember that we started with three possible explanations for the observed difference between the groups:1. The observed difference might be "real"; that is, there might be an actual difference in pregnancy length between first babies and others.2. There might be no real difference between the groups, and the observed difference might be because of a systematic error in the sampling process or the data collection process. For example, maybe reported pregnancy lengths are less accurate for first time mothers.3. There might be no real difference between the groups, and the observed difference might be due to random variation in the sampling process.By computing a p-value, we have established that it would be rare to see a difference as big as 0.2 due to sampling alone. So we can conclude that the third explanation is unlikely.That makes it more likely that the difference is real, but we still can't rule out the second possibility. **Exercise:** The test statistic we chose is the difference in means between the two groups.But suppose we would like to know whether first babies are more unpredictable than other babies. In that case the test statistic we choose might be the standard deviation of pregnancy length, which is one way to quantify unpredictability.As an exercise:1. Write a version of `test_stat` that computes the difference in standard deviation between the groups.2. Write a loop that estimates the distribution of this test statistic under the null hypothesis.3. Compute a p-value.
###Code
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
# Solution goes here
###Output
_____no_output_____
###Markdown
EstimationSuppose we want to estimate the average height of men in the U.S.We can use data from the [BRFSS](https://www.cdc.gov/brfss/index.html):"The Behavioral Risk Factor Surveillance System (BRFSS) is the nation's premier system of health-related telephone surveys that collect state data about U.S. residents regarding their health-related risk behaviors, chronic health conditions, and use of preventive services."
###Code
# Get the data file
import os
if not os.path.exists('brfss.hdf5'):
!wget https://github.com/AllenDowney/ElementsOfDataScience/raw/master/brfss.hdf5
import pandas as pd
brfss = pd.read_hdf('brfss.hdf5', 'brfss')
brfss.shape
###Output
_____no_output_____
###Markdown
We can use `SEX` to select male respondents.
###Code
male = (brfss.SEX == 1)
np.mean(male)
###Output
_____no_output_____
###Markdown
Then we select height data.
###Code
heights = brfss['HTM4']
data = heights[male]
###Output
_____no_output_____
###Markdown
We can use `isnan` to check for NaN values:
###Code
np.mean(np.isnan(data)) * 100
###Output
_____no_output_____
###Markdown
About 4% of the values are missing.Here are the mean and standard deviation, ignoring missing data.
###Code
print('Mean male height in cm =', np.nanmean(data))
print('Std male height in cm =', np.nanstd(data))
###Output
_____no_output_____
###Markdown
Quantifying precisionAt this point we have an estimate of the average adult male height. We'd like to know how accurate this estimate is, and how precise. In the context of estimation, these words have a [technical distinction](https://en.wikipedia.org/wiki/Accuracy_and_precision):>Given a set of data points from repeated measurements of the same quantity, the set can be said to be precise if the values are close to each other, while the set can be said to be accurate if their average is close to the true value of the quantity being measured.Usually accuracy is what we really care about, but it's hard to measure accuracy unless you know the true value. And if you know the true value, you don't have to estimate it.Quantifying precision is not as useful, but it is much easier. Here's one way to do it:1. Use the data you have to make a model of the population.2. Use the model to simulate the data collection process.3. Use the simulated data to compute an estimate.By repeating these steps, we can quantify the variability of the estimate due to random sampling. To model the population, I'll use **resampling**; that is, I will treat the observed measurements as if they were taken from the entire population, and I will draw random samples from them.We can use `np.random.choice` to resample the data:
###Code
size = len(data)
sim_data = np.random.choice(data, size, replace=True)
sim_data.shape
###Output
_____no_output_____
###Markdown
With `replace=True`, we sample with replacement, which means that some measurements might be chosen more than once, and some might not be chosen at all.(If we sample *without* replacement, the resampled data is always identical to the original, so that's no good.) Now we can use `nanmean` to compute the mean of the simulated data, ignoring missing values.
###Code
np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
If we repeat this process 1000 times, we can see how much the results vary.
###Code
outcomes = np.empty(1000)
size = len(data)
for i in range(len(outcomes)):
sim_data = np.random.choice(data, size, replace=True)
outcomes[i] = np.nanmean(sim_data)
###Output
_____no_output_____
###Markdown
The result is the "sampling distribution", which shows how much the results of the experiment would vary if we ran it many times. Here's what it looks like:
###Code
plot_hist(outcomes)
plt.title('Sampling distribution of the mean')
plt.xlabel('Mean adult male height, U.S.');
###Output
_____no_output_____
###Markdown
The width of this distribution shows how much the results vary from one experiment to the next.We can quantify this variability by computing the standard deviation of the sampling distribution, which is called "standard error".
###Code
std_err = np.std(outcomes)
std_err
###Output
_____no_output_____
###Markdown
We can also summarize the sampling distribution with a "confidence interval", which is a range that contains a specified fraction, like 90%, of the values in `sampling_dist_mean`.The central 90% confidence interval is between the 5th and 95th percentiles of the sampling distribution.
###Code
ci_90 = np.percentile(outcomes, [5, 95])
ci_90
###Output
_____no_output_____
###Markdown
The following function plots a histogram and shades the 90% confidence interval.
###Code
def plot_sampling_dist(outcomes):
patch = plot_hist(outcomes)
low, high = np.percentile(outcomes, [5, 95])
fill_hist(low, high, patch)
print('Mean = ', np.mean(outcomes))
print('Std error = ', np.std(outcomes))
print('90% CI = ', (low, high))
###Output
_____no_output_____
###Markdown
Here's what it looks like for the sampling distribution of mean adult height:
###Code
plot_sampling_dist(outcomes)
plt.xlabel('Mean adult male height, U.S. (%)');
###Output
_____no_output_____
###Markdown
For an experiment like this, we can compute the standard error analytically.
###Code
size = len(data)
analytic_std_err = np.std(data) / np.sqrt(size)
###Output
_____no_output_____
###Markdown
The result is close to what we observed computationally.
###Code
analytic_std_err, std_err
###Output
_____no_output_____
###Markdown
This result indicates that our estimate of the mean is *precise*; that is, if we ran this experiment many times, the results would fall in a narrow range.But this range reflects only variability due to random sampling. If there are systematic errors in the sampling process, or in the measurement process, the result would not be *accurate*.Computing a standard error or confidence interval can be useful, but it only quantifies variability due to random sampling, not other sources of error. **Exercise:** One nice thing about using resampling is that it is easy to compute the sampling distribution for other statistics.For example, suppose we want to estimate the coefficient of variation (standard deviation as a fraction of the mean) for adult male height. Here's how we can compute it.
###Code
cv = np.nanstd(data) / np.nanmean(data)
cv
###Output
_____no_output_____
###Markdown
So the standard deviation is about 5% of the mean. Write a loop that uses resampling to estimate the sampling distribution of `cv`; store the results in an array named `outcomes`.Then use `plot_sampling_dist` to plot the sampling distribution of the coefficient of variation.What is the standard error of the estimated coefficient of variation?
###Code
# Solution goes here
# Solution goes here
###Output
_____no_output_____ |
ipynb/Germany-Niedersachsen-LK-Stade.ipynb | ###Markdown
Germany: LK Stade (Niedersachsen)* Homepage of project: https://oscovida.github.io* Plots are explained at http://oscovida.github.io/plots.html* [Execute this Jupyter Notebook using myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)
###Code
import datetime
import time
start = datetime.datetime.now()
print(f"Notebook executed on: {start.strftime('%d/%m/%Y %H:%M:%S%Z')} {time.tzname[time.daylight]}")
%config InlineBackend.figure_formats = ['svg']
from oscovida import *
overview(country="Germany", subregion="LK Stade", weeks=5);
overview(country="Germany", subregion="LK Stade");
compare_plot(country="Germany", subregion="LK Stade", dates="2020-03-15:");
# load the data
cases, deaths = germany_get_region(landkreis="LK Stade")
# get population of the region for future normalisation:
inhabitants = population(country="Germany", subregion="LK Stade")
print(f'Population of country="Germany", subregion="LK Stade": {inhabitants} people')
# compose into one table
table = compose_dataframe_summary(cases, deaths)
# show tables with up to 1000 rows
pd.set_option("max_rows", 1000)
# display the table
table
###Output
_____no_output_____
###Markdown
Explore the data in your web browser- If you want to execute this notebook, [click here to use myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)- and wait (~1 to 2 minutes)- Then press SHIFT+RETURN to advance code cell to code cell- See http://jupyter.org for more details on how to use Jupyter Notebook Acknowledgements:- Johns Hopkins University provides data for countries- Robert Koch Institute provides data for within Germany- Atlo Team for gathering and providing data from Hungary (https://atlo.team/koronamonitor/)- Open source and scientific computing community for the data tools- Github for hosting repository and html files- Project Jupyter for the Notebook and binder service- The H2020 project Photon and Neutron Open Science Cloud ([PaNOSC](https://www.panosc.eu/))--------------------
###Code
print(f"Download of data from Johns Hopkins university: cases at {fetch_cases_last_execution()} and "
f"deaths at {fetch_deaths_last_execution()}.")
# to force a fresh download of data, run "clear_cache()"
print(f"Notebook execution took: {datetime.datetime.now()-start}")
###Output
_____no_output_____
###Markdown
Germany: LK Stade (Niedersachsen)* Homepage of project: https://oscovida.github.io* Plots are explained at http://oscovida.github.io/plots.html* [Execute this Jupyter Notebook using myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)
###Code
import datetime
import time
start = datetime.datetime.now()
print(f"Notebook executed on: {start.strftime('%d/%m/%Y %H:%M:%S%Z')} {time.tzname[time.daylight]}")
%config InlineBackend.figure_formats = ['svg']
from oscovida import *
overview(country="Germany", subregion="LK Stade", weeks=5);
overview(country="Germany", subregion="LK Stade");
compare_plot(country="Germany", subregion="LK Stade", dates="2020-03-15:");
# load the data
cases, deaths = germany_get_region(landkreis="LK Stade")
# compose into one table
table = compose_dataframe_summary(cases, deaths)
# show tables with up to 500 rows
pd.set_option("max_rows", 500)
# display the table
table
###Output
_____no_output_____
###Markdown
Explore the data in your web browser- If you want to execute this notebook, [click here to use myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)- and wait (~1 to 2 minutes)- Then press SHIFT+RETURN to advance code cell to code cell- See http://jupyter.org for more details on how to use Jupyter Notebook Acknowledgements:- Johns Hopkins University provides data for countries- Robert Koch Institute provides data for within Germany- Atlo Team for gathering and providing data from Hungary (https://atlo.team/koronamonitor/)- Open source and scientific computing community for the data tools- Github for hosting repository and html files- Project Jupyter for the Notebook and binder service- The H2020 project Photon and Neutron Open Science Cloud ([PaNOSC](https://www.panosc.eu/))--------------------
###Code
print(f"Download of data from Johns Hopkins university: cases at {fetch_cases_last_execution()} and "
f"deaths at {fetch_deaths_last_execution()}.")
# to force a fresh download of data, run "clear_cache()"
print(f"Notebook execution took: {datetime.datetime.now()-start}")
###Output
_____no_output_____
###Markdown
Germany: LK Stade (Niedersachsen)* Homepage of project: https://oscovida.github.io* [Execute this Jupyter Notebook using myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)
###Code
import datetime
import time
start = datetime.datetime.now()
print(f"Notebook executed on: {start.strftime('%d/%m/%Y %H:%M:%S%Z')} {time.tzname[time.daylight]}")
%config InlineBackend.figure_formats = ['svg']
from oscovida import *
overview(country="Germany", subregion="LK Stade");
# load the data
cases, deaths, region_label = germany_get_region(landkreis="LK Stade")
# compose into one table
table = compose_dataframe_summary(cases, deaths)
# show tables with up to 500 rows
pd.set_option("max_rows", 500)
# display the table
table
###Output
_____no_output_____
###Markdown
Explore the data in your web browser- If you want to execute this notebook, [click here to use myBinder](https://mybinder.org/v2/gh/oscovida/binder/master?filepath=ipynb/Germany-Niedersachsen-LK-Stade.ipynb)- and wait (~1 to 2 minutes)- Then press SHIFT+RETURN to advance code cell to code cell- See http://jupyter.org for more details on how to use Jupyter Notebook Acknowledgements:- Johns Hopkins University provides data for countries- Robert Koch Institute provides data for within Germany- Open source and scientific computing community for the data tools- Github for hosting repository and html files- Project Jupyter for the Notebook and binder service- The H2020 project Photon and Neutron Open Science Cloud ([PaNOSC](https://www.panosc.eu/))--------------------
###Code
print(f"Download of data from Johns Hopkins university: cases at {fetch_cases_last_execution()} and "
f"deaths at {fetch_deaths_last_execution()}.")
# to force a fresh download of data, run "clear_cache()"
print(f"Notebook execution took: {datetime.datetime.now()-start}")
###Output
_____no_output_____ |
MathConceptsForDevelopers/06.CalculusExcercise/Calculus Exercise.ipynb | ###Markdown
Calculus Exercise Numerical Computation. Derivatives, integrals. Calculus in many dimensions Problem 1. Derivative and Slope at a PointWe'll warm up by visualizing how the derivative of a function relates to the slope of the function at a given point.We can either calculate the derivative analytically (by hand) or use the numerical definition. So, let's see what a function and its derivative look like.Write a Python function which plots a math function and its derivative.
###Code
def calculate_derivative_at_point(function, point, precision = 1e-7):
"""
Calculates a numerical approximation to the derivative of the specified function
at the given point
"""
first_value = function(point - precision)
second_value = function(point + precision)
return (second_value - first_value) / (2 * precision)
def plot_derivative(function, derivative = None, min_x = -10, max_x = 10):
"""
Plots the function and its derivative.
The `derivative` parameter is optional and can be provided as a separate function.
If it's not provided, the derivative will be calculated automatically
"""
# We're using vectorized functions to make our code simpler: this only hides the for-loop,
# it doesn't provide any performance gain
vectorized_function = np.vectorize(function)
x = np.linspace(min_x, max_x, 1000)
y = vectorized_function(x)
dy = []
if derivative is None:
dy = np.vectorize(calculate_derivative_at_point)(function, x)
else:
dy = np.vectorize(derivative)(x)
plt.plot(x, y, label="function",color='green')
plt.plot(x, dy, label="derivative",color='red')
plt.legend()
plt.grid()
plt.show()
calculate_derivative_at_point(lambda x:x**2, 16)
###Output
_____no_output_____
###Markdown
Let's now test with out favourite function: $y = x^2$ whose derivative is $y' = 2x$. If you've worked correctly, both of the following plots should be the same.
###Code
plot_derivative(lambda x: x ** 2, lambda x: 2 * x, -3, 3) # The derivative is calculated by hand
plot_derivative(lambda x: x ** 2, min_x = -3, max_x = 3) # The derivative is not pre-calculated, should be calculated inside the function
###Output
_____no_output_____
###Markdown
Let's try one more: $y = \sin(x)$, $y'= \cos(x)$.
###Code
plot_derivative(np.sin)
###Output
_____no_output_____
###Markdown
These plots may look nice but they don't reflect the idea of **slope at a point** very well. Now that we're sure our functions are working, let's actually calculate the derivative at **one point** and see that it is, indeed, equal to the slope of the function at that point. How to plot the tangent line?We need to find the line equation first. We're given the derivative, which is equal to the slope of the line. In the line equation $y = ax + b$, the slope is $a$. We now have to find $b$. We're given a point $P(x_P; y_P)$ through which the line passes. Substitute $x_P$ and $y_P$:$$ y_P = ax_P + b $$$$ b = y_P - ax_P $$Now that we have $a$ and $b$, we can plot the line given by $y = ax + b$. The parameter $b$ is sometimes called "y-intercept" (or "intercept").Now we can copy the code from the previous function. This time, however, we won't plot the entire range, only one value of the derivative. We'll also show the point where we're calculating.
###Code
def plot_derivative_at_point(function, point, derivative = None, min_x = -10, max_x = 10):
"""
Plots the function in the range [x_min; x_max]. Computes the tangent line to the function
at the given point and also plots it
"""
vectorized_function = np.vectorize(function)
x = np.linspace(min_x, max_x, 1000)
y = vectorized_function(x)
slope = 0 # Slope of the tangent line
if derivative is None:
slope = calculate_derivative_at_point(function, point)
else:
slope = derivative(point)
intercept = function(point) - slope * point
tangent_line_x = np.linspace(point - 2, point + 2, 10)
tangent_line_y = slope * tangent_line_x + intercept
plt.plot(x, y)
plt.plot(tangent_line_x, tangent_line_y)
plt.show()
plot_derivative_at_point(lambda x: x ** 2, 2)
###Output
_____no_output_____
###Markdown
Looks like it! Let's zoom in to confirm:
###Code
plot_derivative_at_point(lambda x: x ** 2, 2, min_x = 0, max_x = 4)
###Output
_____no_output_____
###Markdown
Let's also plot several tangents to the same function. Note that this will create many graphs by default. You can plot them all at once if you wish.
###Code
for x in np.arange(-8, 10, 2):
plot_derivative_at_point(lambda x: x ** 2, x)
for x in np.arange(-8, 10, 2):
plot_derivative_at_point(np.sin, x)
###Output
_____no_output_____
###Markdown
Now we have a visual proof that the derivative of a function at a given point is equal to the slope of the tangent line to the function. Problem 2. Limits. The Number $e$ as a LimitWe know what limits are, what they mean and how they relate to our tasks. Let's explore a special limit. This one arises from economics but we'll see it has applications throughout science because of its unique properties.Imagine you're saving money in a bank. Every period, you accumulate [interest](https://en.wikipedia.org/wiki/Compound_interest) on your money. Let's say the bank is very generous and gives you **100% interest every year**.How much money will you have after one year? Let's say you got $\$1$. After one year, you'll get your interest from the bank and you'll have $\$2$. Your money doubled, which was expected.How about this offer: **50% interest every 6 months**? Will this be the same, better, or worse?You start with $\$1$. After 6 months, you'll accumulate $50%$ interest to get $\$1,50$. After 6 more months, you'll get $50\%.1,50 = 0,75$, so your total is $2,25$. You got $\$0,25$ more!Let's try to exploit the scheme and get rich.**$100/12\%$ every month*** January: $1 + 1/12$* February: $(1 + 1/12) * (1 + 1/12)$* March: $(1 + 1/12) * (1 + 1/12) * (1 + 1/12)$* ...We can see a pattern. Every period, we multiply our money by $1 + 1/12$. So, the final sum will be $$\$1.\left(1+\frac{1}{12}\right)^{12} = \$2,61$$We did even better. This is always true. The more periods, the more money we accumulate. The more money we have, the more interest we accumulate. And that completes the vicious circle known as money saving :).Let's try other options:**$100/52\%$ every week**$\$1.\left(1+\frac{1}{52}\right)^{52} = \$2,69$**$100/31556926\%$ every second**$\$1.\left(1+\frac{1}{31556926}\right)^{31556926} = \$2,718$Well, there's a slight problem to our world domination plans. Even though we accumulate more and more money, we get *diminishing returns*. For 52 periods we got $2,69$, and for more than 3 million periods we only got like $\$0,02$ more. This pattern will continue.Now we can ask ourselves, what is the maximum profit we can accumulate for a year? To do this, we can ask$$ \lim_{n \ \rightarrow \infty}\left(1+\frac{1}{n}\right)^n = ? $$It turns out this is a constant. It is approximately equal to $2,71828182\dots$. Since it's very useful, it's got a name: $e$, or Euler's number (sometimes called Napier's number). The limit above is **the definition of $e$**.Why is it so useful? Let's go back to the original problem. In compound interest, the extra amount after every period is proportional to the amount at the start of the period. In other words, **the rate of change of a value is proportional to the value**. This pops out everywhere in nature and business. Some examples include radioactive decay (more atoms $\Rightarrow$ more decays), cooling down a cup of tea (the rate of cooling down depends on the temperature difference between the cup and the room), animal population models (more animals $\Rightarrow$ more babies), infection models, and so on.To quickly verify the value of $e$, calculate the limit as we defined it above.
###Code
def calculate_limit_at_infinity(function):
"""
Calculates a numerical approximation of the limit of the specified function
as its parameter goes to infinity
"""
n = 10 ** np.arange(0, 10)
return zip(n, function(n))
limits = calculate_limit_at_infinity(lambda x: (1 + 1 / x) ** x)
for limit in limits:
print(limit)
###Output
(1, 2.0)
(10, 2.5937424601000023)
(100, 2.7048138294215285)
(1000, 2.7169239322355936)
(10000, 2.7181459268249255)
(100000, 2.7182682371922975)
(1000000, 2.7182804690957534)
(10000000, 2.7182816941320818)
(100000000, 2.7182817983473577)
(1000000000, 2.7182820520115603)
###Markdown
Problem 3. Derivatives of Exponential FunctionsUse the function you defined in the first problem to plot the derivative of $y = 2^x$.
###Code
plot_derivative(lambda x: 2 ** x, min_x = 0, max_x = 10)
###Output
_____no_output_____
###Markdown
The function and its derivative look closely related, only the derivative seems to grow a bit slower than the function. Let's confirm that by looking at a broader range:
###Code
plot_derivative(lambda x: 2 ** x, min_x = 0, max_x = 20)
###Output
_____no_output_____
###Markdown
The same pattern will continue if we try to plot any exponential function, e.g. $y = 3^x$, $y = 4^x$ and so on, if the base of the exponent is greater than 1. If we want to plot, say, $y = 0,5^x$, we'll get a slightly different result. Note that the functions look more or less the same, only their signs are flipped.
###Code
plot_derivative(lambda x: 0.5 ** x)
###Output
_____no_output_____
###Markdown
Very interesting things happen if we plot $y = e^x$:
###Code
plot_derivative(np.exp)
###Output
_____no_output_____
###Markdown
The plots overlap. You can see that this is true if you plot the function and its derivative with different line widths. This means that$$ (e^x)' = e^x $$Also:$$ (e^x)'' = e^x $$$$ (e^x)''' = e^x $$... and so on. This is the only function whose rate of change (derivative) is equal to the function itself. This property makes it even more interesting for science and math.Also, do you remember that $e^{i\varphi} = \cos(\varphi) + i\sin(\varphi)$? This constant never ceases to amaze. Problem 4. Integrals and Area. Changing Variables in IntegrationWe know that the definition of an integral is the area "between" the function we're integrating, and the x-axis. This gives us a method to calculate integrals. Most integrals can't be solved analytically but have numerical solutions. One such integral is $$\int\sin(x^2)dx$$Note that we can only solve **definite integrals** numerically.The simplest way to calculate the integral is to apply the definition, like in the case of the derivative. This is called [the trapezoid method](http://www.mathwords.com/t/trapezoid_rule.htm) because the area is approximated as a series of trapezoids.Write a function which does exactly that. Use `numpy` and vectorization as much as possible.
###Code
def calculate_integral(function, x_min, x_max, num_points = 5000):
"""
Calculates a numerical approximation of the definite integral of the provided function
between the points x_min and x_max.
The parameter n specifies the number of points at which the integral will be calculated
"""
vectorized_function = np.vectorize(function)
x = np.linspace(x_min, x_max, num_points)
delta = x[1]-x[0]
y = vectorized_function(x) * delta
return y.sum()
print(calculate_integral(lambda x: x ** 2, 0, 1)) # Should be close to 0.333
print(calculate_integral(lambda x: np.sin(x ** 2), 0, 5)) # Should be close to 0.528
###Output
0.3334333600066683
0.5278519183866606
###Markdown
Let's apply our insight to finding the area of a circle. We know the equation of a circle is not a function (it's more like two functions). We can, however be clever. One way is to integrate both of the functions and add them together. Another way is to integrate one and double the area. **Note:** We're trying to find the total area of the circle, there is **no negative area** in this particular case.Another, even more clever way is to look at a quarter of the circle. This is convenient because we may look at the quadrant where $x > 0$ and $y > 0$. So, we'll need to find the area between:1. $x \ge 0$2. $y \ge 0$3. The circle $x^2 + y^2 \le R^2$ (let's fix the radius to be 1)$\Rightarrow y = \sqrt{R^2 - x^2} = \sqrt{1 - x^2}$After all of this, we'll need to multiply the result by 4.$$ S = 4 \int_0^1\sqrt{1 - x^2}dx $$
###Code
circle_piece_area = calculate_integral(lambda x: np.sqrt(1 - x ** 2), 0, 1)
total_area = 4 * circle_piece_area
print(total_area)
###Output
3.1419894064484915
|
nub-training-evaluation/Results Analysis and Comparison.ipynb | ###Markdown
In this Colab notebook, the goal is to compare several existing summarizers to nub 1.0 and compare the results to get a better sense of its strengths and weaknesses. Since we've already created a validation dataset in `Run Evaluations on Fine-tuned T-5 Summarizer.ipynb`, we first grab the results. Then run the examples through the competitor summarizers, calculate ROUGE scores, and finally compare them all.
###Code
from google.colab import drive
drive.mount('/content/drive')
#Install the summarizer developed by Derek Miller: https://pypi.org/project/bert-extractive-summarizer/
!pip install bert-extractive-summarizer
#ROUGE library
!pip install rouge-score
# transforerms library
!pip install transformers
# import libraries
import pandas as pd
import numpy as np
from summarizer import Summarizer
from rouge_score import rouge_scorer
# navigate to the nub 1.0 summarizer's validation results
%cd /content/drive/My Drive/summarizer/nub-training-evaluation/result
###Output
/content/drive/My Drive/summarizer/nub-training-evaluation/result
###Markdown
Run the validation through `bert-extractive-summarizer 0.4.2`
###Code
# read in the validation source and targets
eval_results = pd.read_csv('eval_results_t5_base.csv')
# initialize the model
model = Summarizer()
# define the scorer
scorer = rouge_scorer.RougeScorer(['rouge1', 'rouge2', 'rougeL'], use_stemmer=True)
# run each example in the validation through the model and store the scores
rouge_1_fs = []
rouge_2_fs = []
rouge_l_fs = []
system_summaries = []
i = 0
for ind, row in eval_results.iterrows():
# overwrite the systems summary
system_summary = ''.join(model(row.full_text, min_length=60))
scores = scorer.score(row.summary, system_summary)
rouge_1_fs.append(scores['rouge1'].fmeasure)
rouge_2_fs.append(scores['rouge2'].fmeasure)
rouge_l_fs.append(scores['rougeL'].fmeasure)
system_summaries.append(system_summary)
i += 1
print(i)
print(scores)
# overwrite the scores
eval_results['rouge1_f']=np.array(rouge_1_fs)
eval_results['rouge2_f']=np.array(rouge_2_fs)
eval_results['rougeL_f']=np.array(rouge_l_fs)
eval_results['system_summary']=np.array(system_summaries)
eval_results.to_csv('eval_results_bert_extractive_summarizer.csv')
###Output
_____no_output_____
###Markdown
Run the validation set through `mrm8488/t5-base-finetuned-summarize-news`This is a T5 model cound on huggingface's model hub. It was developed by Manuel Romero. https://huggingface.co/mrm8488/t5-base-finetuned-summarize-news
###Code
# read in the validation source and targets
eval_results = pd.read_csv('eval_results_t5_base.csv')
# initialize the model
from transformers import AutoTokenizer, AutoModelWithLMHead
tokenizer = AutoTokenizer.from_pretrained("mrm8488/t5-base-finetuned-summarize-news")
model = AutoModelWithLMHead.from_pretrained("mrm8488/t5-base-finetuned-summarize-news")
def summarize(text, max_length=150):
'''source https://huggingface.co/mrm8488/t5-base-finetuned-summarize-news'''
input_ids = tokenizer.encode(text, return_tensors="pt", add_special_tokens=True)
generated_ids = model.generate(input_ids=input_ids, num_beams=2, max_length=max_length, repetition_penalty=2.5, length_penalty=1.0, early_stopping=True)
preds = [tokenizer.decode(g, skip_special_tokens=True, clean_up_tokenization_spaces=True) for g in generated_ids]
return preds[0]
# define the scorer
scorer = rouge_scorer.RougeScorer(['rouge1', 'rouge2', 'rougeL'], use_stemmer=True)
# run each example in the validation through the model and store the scores
# as well as the generated summaries
rouge_1_fs = []
rouge_2_fs = []
rouge_l_fs = []
system_summaries = []
i = 0
for ind, row in eval_results.iterrows():
# overwrite the systems summary
system_summary = summarize(row.full_text)
scores = scorer.score(row.summary, system_summary)
rouge_1_fs.append(scores['rouge1'].fmeasure)
rouge_2_fs.append(scores['rouge2'].fmeasure)
rouge_l_fs.append(scores['rougeL'].fmeasure)
system_summaries.append(system_summary)
i+=1
print(i)
print(scores)
# overwrite the scores
eval_results['rouge1_f']=np.array(rouge_1_fs)
eval_results['rouge2_f']=np.array(rouge_2_fs)
eval_results['rougeL_f']=np.array(rouge_l_fs)
eval_results['system_summary']=np.array(system_summaries)
eval_results.to_csv('eval_results_t5_mrm8488.csv')
###Output
_____no_output_____
###Markdown
Comparative Analysis
###Code
# create an empty master df
eval_results_all = pd.DataFrame(
columns=['nub_rouge1_f', 't5_base_rouge1_f', 'bert_ext_summ_rouge1_f', 't5_mrm8488_rouge1_f',
'nub_rouge2_f', 't5_base_rouge2_f', 'bert_ext_summ_rouge2_f', 't5_mrm8488_rouge2_f',
'nub_rougeL_f', 't5_base_rougeL_f', 'bert_ext_summ_rougeL_f', 't5_mrm8488_rougeL_f'])
# read in all the results sets
eval_results_nub = pd.read_csv('eval_results_nub.csv')[['rouge1_f', 'rouge2_f', 'rougeL_f']]
eval_results_bert_extractive_summarizer = pd.read_csv('eval_results_bert_extractive_summarizer.csv')[['rouge1_f', 'rouge2_f', 'rougeL_f']]
eval_results_t5_base = pd.read_csv('eval_results_t5_base.csv')[['rouge1_f', 'rouge2_f', 'rougeL_f']]
eval_results_t5_mrm8488 = pd.read_csv('eval_results_t5_mrm8488.csv')[['rouge1_f', 'rouge2_f', 'rougeL_f']]
# insert into the master df
eval_results_all = pd.concat([eval_results_nub, eval_results_t5_base, eval_results_bert_extractive_summarizer, eval_results_t5_mrm8488], axis=1)
eval_results_all.columns=['nub_rouge1_f', 'nub_rouge2_f', 'nub_rougeL_f',
't5_base_rouge1_f', 't5_base_rouge2_f', 't5_base_rougeL_f',
'bert_ext_summ_rouge1_f', 'bert_ext_summ_rouge2_f', 'bert_ext_summ_rougeL_f',
't5_mrm8488_rouge1_f', 't5_mrm8488_rouge2_f', 't5_mrm8488_rougeL_f']
means = eval_results_all.mean()
errors = eval_results_all.std()
labels = ['R-1', 'R-2', 'R-L']
nub_means = 100*eval_results_all[['nub_rouge1_f', 'nub_rouge2_f', 'nub_rougeL_f']].mean().round(4)
nub_err = 100*eval_results_all[['nub_rouge1_f', 'nub_rouge2_f', 'nub_rougeL_f']].std().round(4)
t5base_means = 100*eval_results_all[['t5_base_rouge1_f', 't5_base_rouge2_f', 't5_base_rougeL_f']].mean().round(4)
t5base_err = 100*eval_results_all[['t5_base_rouge1_f', 't5_base_rouge2_f', 't5_base_rougeL_f']].std().round(4)
bertextsumm_means = 100*eval_results_all[['bert_ext_summ_rouge1_f', 'bert_ext_summ_rouge2_f', 'bert_ext_summ_rougeL_f']].mean().round(4)
bertextsumm_err = 100*eval_results_all[['bert_ext_summ_rouge1_f', 'bert_ext_summ_rouge2_f', 'bert_ext_summ_rougeL_f']].std().round(4)
t5mrm8488_means = 100*eval_results_all[['t5_mrm8488_rouge1_f', 't5_mrm8488_rouge2_f', 't5_mrm8488_rougeL_f']].mean().round(4)
t5mrm8488_err = 100*eval_results_all[['t5_mrm8488_rouge1_f', 't5_mrm8488_rouge2_f', 't5_mrm8488_rougeL_f']].std().round(4)
# https://matplotlib.org/3.1.1/gallery/lines_bars_and_markers/barchart.html
x = np.arange(len(labels)) # the label locations
width = 0.2 # the width of the bars
fig, ax = plt.subplots()
rects1 = ax.bar(x - 3*width/2, nub_means, width, label='Nub 1.0')
rects2 = ax.bar(x - width/2, t5base_means, width, label='T5-base')
rects3 = ax.bar(x + width/2, bertextsumm_means, width, label='BERT extractive summarizer')
rects4 = ax.bar(x + 3*width/2, t5mrm8488_means, width, label='T5 finetuned on Kaggle dataset')
# Add some text for labels, title and custom x-axis tick labels, etc.
ax.set_ylabel('F-Measure')
ax.set_title('ROUGE-1, ROUGE-2 and ROUGE-L for Summarizers')
ax.set_xticks(x)
ax.set_xticklabels(labels)
ax.legend()
fig.tight_layout()
plt.savefig('bar_chart_rouge.png')
plt.close()
file = open('/content/drive/My Drive/summarizer/t-5-fine-tuned-7-2/resoomer_gold_summary.txt', "r")
resoomer_gold_summary = ''.join(file.readlines())
file.close()
file = open('/content/drive/My Drive/summarizer/t-5-fine-tuned-7-2/resoomer_validation_output.txt', "r")
resoomer_system_summary = ''.join(file.readlines())
file.close()
resoomer_scores = scorer.score(resoomer_gold_summary, resoomer_system_summary)
resoomer_scores
labels = ['R-1', 'R-2', 'R-L']
nub_means = 100*eval_results_all[['nub_rouge1_f', 'nub_rouge2_f', 'nub_rougeL_f']].mean().round(4)
t5base_means = 100*eval_results_all[['t5_base_rouge1_f', 't5_base_rouge2_f', 't5_base_rougeL_f']].mean().round(4)
bertextsumm_means = 100*eval_results_all[['bert_ext_summ_rouge1_f', 'bert_ext_summ_rouge2_f', 'bert_ext_summ_rougeL_f']].mean().round(4)
t5mrm8488_means = 100*eval_results_all[['t5_mrm8488_rouge1_f', 't5_mrm8488_rouge2_f', 't5_mrm8488_rougeL_f']].mean().round(4)
nub_means
t5base_means
bertextsumm_means
t5mrm8488_means
nub_err
###Output
_____no_output_____ |
nlp-labs/Day_08/Extractive_Text_Summarization/Extractive_Text_Summarization.ipynb | ###Markdown
> **Copyright (c) 2020 Skymind Holdings Berhad**> **Copyright (c) 2021 Skymind Education Group Sdn. Bhd.**Licensed under the Apache License, Version 2.0 (the \"License\");you may not use this file except in compliance with the License.You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0/Unless required by applicable law or agreed to in writing, softwaredistributed under the License is distributed on an \"AS IS\" BASIS,WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.See the License for the specific language governing permissions andlimitations under the License.**SPDX-License-Identifier: Apache-2.0** IntroductionIn this notebook, we are going to learn the **extractive text summarization** technique using **cosine similarity** to identify the similarity between sentences and then extract those important sentences. What will we accomplish?Steps to implement simple extractive text summarizer:> Step 1: Input article> Step 2: Text cleaning> Step 3: Build similarity matrix> Step 4: Pick N sentences for summary Notebook Content* [Extractive Text Summarization](Extractive-Text-Summarization)* [Getting Started](Getting-Started) * [Import Libraries](Import-Libraries) * [Generate Cleaned Sentences](Generate-Cleaned-Sentences) * [Sentence Similarity](Sentence-Similarity) * [Similarity Matrix](Similarity-Matrix) * [Generate Summary Method](Generate-Summary-Method) * [Summarizing](Summarizing) Extractive Text SummarizationExtractive Summarization is an extractive methods attempt to summarize articles by **selecting a subset of words** that retain the most important points. This approach **weights the important part of sentences** and uses the **same** to form the summary. Different algorithm and techniques are used to define weights for the sentences and further rank them based on importance and similarity among each other.> Input Document → Sentences similarity → Weight sentences → Select sentences with higher rank.The limited study is available for abstractive summarization as it requires a deeper understanding of the text as compared to the extractive approach. Purely extractive summaries often times give **better results** compared to automatic abstractive summaries. This is because of the fact that abstractive summarization methods cope with problems such as semantic representation, inference and natural language generation which is relatively harder than data-driven approaches such as sentence extraction.There are many techniques available to generate extractive summarization. To keep it simple, this tutorial will be using an **unsupervised learning approach** to find the **sentences similarity** and rank them. One benefit of this will be, you don’t need to train and build a model prior start using it for your project.It’s good to understand **Cosine similarity** to make the best use of code you are going to see. Cosine similarity is a measure of similarity between two non-zero vectors of an inner product space that measures the cosine of the angle between them. Since we will be representing our sentences as the bunch of vectors, we can use it to find the similarity among sentences. Its measures cosine of the angle between vectors. Angle will be 0 if sentences are similar. Getting Started Import Libraries
###Code
import nltk
from nltk.corpus import stopwords
from nltk.cluster.util import cosine_distance
import numpy as np
import networkx as nx
nltk.download("stopwords")
###Output
[nltk_data] Downloading package stopwords to
[nltk_data] C:\Users\tanch\AppData\Roaming\nltk_data...
[nltk_data] Package stopwords is already up-to-date!
###Markdown
Generate Cleaned Sentences
###Code
def read_article(file_name):
file = open(file_name, "r")
filedata = file.readlines()
article = filedata[0].split(". ")
sentences = []
for sentence in article:
print(sentence)
sentences.append(sentence.replace("[^a-zA-Z]", " ").split(" "))
sentences.pop()
return sentences
###Output
_____no_output_____
###Markdown
Sentence SimilarityThis is where we will be using cosine similarity to find similarity between sentences.
###Code
def sentence_similarity(sent1, sent2, stopwords=None):
if stopwords is None:
stopwords = []
sent1 = [w.lower() for w in sent1]
sent2 = [w.lower() for w in sent2]
all_words = list(set(sent1 + sent2))
vector1 = [0] * len(all_words)
vector2 = [0] * len(all_words)
# build the vector for the first sentence
for w in sent1:
if w in stopwords:
continue
vector1[all_words.index(w)] += 1
# build the vector for the second sentence
for w in sent2:
if w in stopwords:
continue
vector2[all_words.index(w)] += 1
return 1 - cosine_distance(vector1, vector2)
###Output
_____no_output_____
###Markdown
Similarity Matrix
###Code
def build_similarity_matrix(sentences, stop_words):
# Create an empty similarity matrix
similarity_matrix = np.zeros((len(sentences), len(sentences)))
for idx1 in range(len(sentences)):
for idx2 in range(len(sentences)):
if idx1 == idx2: # ignore if both are same sentences
continue
similarity_matrix[idx1][idx2] = sentence_similarity(sentences[idx1], sentences[idx2], stop_words)
return similarity_matrix
###Output
_____no_output_____
###Markdown
Generate Summary Method
###Code
def generate_summary(file_name, top_n=5):
stop_words = stopwords.words('english')
summarize_text = []
# Step 1 - Read text anc split it
sentences = read_article(file_name)
# Step 2 - Generate Similary Martix across sentences
sentence_similarity_martix = build_similarity_matrix(sentences, stop_words)
# Step 3 - Rank sentences in similarity martix
sentence_similarity_graph = nx.from_numpy_array(sentence_similarity_martix)
scores = nx.pagerank(sentence_similarity_graph)
# Step 4 - Sort the rank and pick top sentences
ranked_sentence = sorted(((scores[i], s) for i, s in enumerate(sentences)), reverse=True)
print("\nIndexes of top ranked_sentence order are:", ranked_sentence, sep="\n")
for i in range(top_n):
summarize_text.append(" ".join(ranked_sentence[i][1]))
# Step 5 - Offcourse, output the summarize texr
print("\nSummarize Text:", ". ".join(summarize_text), sep="\n")
###Output
_____no_output_____
###Markdown
Summarizing
###Code
generate_summary("../../../resources/day_08/msft.txt", 1)
###Output
In an attempt to build an AI-ready workforce, Microsoft announced Intelligent Cloud Hub which has been launched to empower the next generation of students with AI-ready skills
Envisioned as a three-year collaborative program, Intelligent Cloud Hub will support around 100 institutions with AI infrastructure, course content and curriculum, developer support, development tools and give students access to cloud and AI services
As part of the program, the Redmond giant which wants to expand its reach and is planning to build a strong developer ecosystem in India with the program will set up the core AI infrastructure and IoT Hub for the selected campuses
The company will provide AI development tools and Azure AI services such as Microsoft Cognitive Services, Bot Services and Azure Machine Learning.According to Manish Prakash, Country General Manager-PS, Health and Education, Microsoft India, said, "With AI being the defining technology of our time, it is transforming lives and industry and the jobs of tomorrow will require a different skillset
This will require more collaborations and training and working with AI
That’s why it has become more critical than ever for educational institutions to integrate new cloud and AI technologies
The program is an attempt to ramp up the institutional set-up and build capabilities among the educators to educate the workforce of tomorrow." The program aims to build up the cognitive skills and in-depth understanding of developing intelligent cloud connected solutions for applications across industry
Earlier in April this year, the company announced Microsoft Professional Program In AI as a learning track open to the public
The program was developed to provide job ready skills to programmers who wanted to hone their skills in AI and data science with a series of online courses which featured hands-on labs and expert instructors as well
This program also included developer-focused AI school that provided a bunch of assets to help build AI skills.
Indexes of top ranked_sentence order are:
[(0.15083257041122708, ['Envisioned', 'as', 'a', 'three-year', 'collaborative', 'program,', 'Intelligent', 'Cloud', 'Hub', 'will', 'support', 'around', '100', 'institutions', 'with', 'AI', 'infrastructure,', 'course', 'content', 'and', 'curriculum,', 'developer', 'support,', 'development', 'tools', 'and', 'give', 'students', 'access', 'to', 'cloud', 'and', 'AI', 'services']), (0.13161201335715553, ['The', 'company', 'will', 'provide', 'AI', 'development', 'tools', 'and', 'Azure', 'AI', 'services', 'such', 'as', 'Microsoft', 'Cognitive', 'Services,', 'Bot', 'Services', 'and', 'Azure', 'Machine', 'Learning.According', 'to', 'Manish', 'Prakash,', 'Country', 'General', 'Manager-PS,', 'Health', 'and', 'Education,', 'Microsoft', 'India,', 'said,', '"With', 'AI', 'being', 'the', 'defining', 'technology', 'of', 'our', 'time,', 'it', 'is', 'transforming', 'lives', 'and', 'industry', 'and', 'the', 'jobs', 'of', 'tomorrow', 'will', 'require', 'a', 'different', 'skillset']), (0.11403047674961148, ['Earlier', 'in', 'April', 'this', 'year,', 'the', 'company', 'announced', 'Microsoft', 'Professional', 'Program', 'In', 'AI', 'as', 'a', 'learning', 'track', 'open', 'to', 'the', 'public']), (0.10721749759953525, ['In', 'an', 'attempt', 'to', 'build', 'an', 'AI-ready', 'workforce,', 'Microsoft', 'announced', 'Intelligent', 'Cloud', 'Hub', 'which', 'has', 'been', 'launched', 'to', 'empower', 'the', 'next', 'generation', 'of', 'students', 'with', 'AI-ready', 'skills']), (0.10404298514456578, ['As', 'part', 'of', 'the', 'program,', 'the', 'Redmond', 'giant', 'which', 'wants', 'to', 'expand', 'its', 'reach', 'and', 'is', 'planning', 'to', 'build', 'a', 'strong', 'developer', 'ecosystem', 'in', 'India', 'with', 'the', 'program', 'will', 'set', 'up', 'the', 'core', 'AI', 'infrastructure', 'and', 'IoT', 'Hub', 'for', 'the', 'selected', 'campuses']), (0.10031366655994461, ['That’s', 'why', 'it', 'has', 'become', 'more', 'critical', 'than', 'ever', 'for', 'educational', 'institutions', 'to', 'integrate', 'new', 'cloud', 'and', 'AI', 'technologies']), (0.10001137283486655, ['The', 'program', 'is', 'an', 'attempt', 'to', 'ramp', 'up', 'the', 'institutional', 'set-up', 'and', 'build', 'capabilities', 'among', 'the', 'educators', 'to', 'educate', 'the', 'workforce', 'of', 'tomorrow."', 'The', 'program', 'aims', 'to', 'build', 'up', 'the', 'cognitive', 'skills', 'and', 'in-depth', 'understanding', 'of', 'developing', 'intelligent', 'cloud', 'connected', 'solutions', 'for', 'applications', 'across', 'industry']), (0.09916750119894319, ['This', 'will', 'require', 'more', 'collaborations', 'and', 'training', 'and', 'working', 'with', 'AI']), (0.09277191614415067, ['The', 'program', 'was', 'developed', 'to', 'provide', 'job', 'ready', 'skills', 'to', 'programmers', 'who', 'wanted', 'to', 'hone', 'their', 'skills', 'in', 'AI', 'and', 'data', 'science', 'with', 'a', 'series', 'of', 'online', 'courses', 'which', 'featured', 'hands-on', 'labs', 'and', 'expert', 'instructors', 'as', 'well'])]
Summarize Text:
Envisioned as a three-year collaborative program, Intelligent Cloud Hub will support around 100 institutions with AI infrastructure, course content and curriculum, developer support, development tools and give students access to cloud and AI services
|
nb/042_submission.ipynb | ###Markdown
Overview- nb040ベース(cv: 0.9378, sub: 0.94)- rfc --> lightGBM_reg Const
###Code
NB = '042'
isSmallSet = False
if isSmallSet:
LENGTH = 7000
else:
LENGTH = 500_000
PATH_TRAIN = './../data/input/train_clean.csv'
PATH_TEST = './../data/input/test_clean.csv'
PATH_SMPLE_SUB = './../data/input/sample_submission.csv'
DIR_OUTPUT = './../data/output/'
DIR_OUTPUT_IGNORE = './../data/output_ignore/'
cp = ['#f8b195', '#f67280', '#c06c84', '#6c5b7b', '#355c7d']
sr = 10*10**3 # 10 kHz
###Output
_____no_output_____
###Markdown
Import everything I need :)
###Code
import warnings
warnings.filterwarnings('ignore')
import time
import gc
import itertools
import multiprocessing
import numpy as np
from scipy import signal
# from pykalman import KalmanFilter
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from fastprogress import progress_bar
from lightgbm import LGBMRegressor
from sklearn.model_selection import KFold, train_test_split, StratifiedKFold
from sklearn.metrics import f1_score, mean_absolute_error, confusion_matrix
from sklearn.ensemble import RandomForestRegressor, RandomForestClassifier
from sklearn.tree import DecisionTreeRegressor
from sklearn.pipeline import make_pipeline
from sklearn.base import BaseEstimator, TransformerMixin
# from sklearn.svm import SVR
from sklearn.linear_model import Lasso
# from dtreeviz.trees import dtreeviz
###Output
_____no_output_____
###Markdown
My function
###Code
def f1_macro(true, pred):
return f1_score(true, pred, average='macro')
def get_df_batch(df, batch):
idxs = df['batch'] == batch
assert any(idxs), 'そのようなbatchはありません'
return df[idxs]
def add_category(train, test):
train["category"] = 0
test["category"] = 0
# train segments with more then 9 open channels classes
train.loc[2_000_000:2_500_000-1, 'category'] = 1
train.loc[4_500_000:5_000_000-1, 'category'] = 1
# test segments with more then 9 open channels classes (potentially)
test.loc[500_000:600_000-1, "category"] = 1
test.loc[700_000:800_000-1, "category"] = 1
return train, test
def get_signal_mv_mean(df, n=3001):
signal_mv = np.zeros(len(df))
for bt in df['batch'].unique():
idxs = df['batch'] == bt
_signal_mv = df['signal'][idxs].rolling(n, center=True).mean().interpolate('spline', order=5, limit_direction='both').values
signal_mv[idxs] = _signal_mv
return signal_mv
def get_signal_mv_std(df, n=3001):
signal_mv = np.zeros(len(df))
for bt in df['batch'].unique():
idxs = df['batch'] == bt
_signal_mv = df['signal'][idxs].rolling(n, center=True).std().interpolate('spline', order=5, limit_direction='both').values
signal_mv[idxs] = _signal_mv
return signal_mv
def get_signal_mv_min(df, n=3001):
signal_mv = np.zeros(len(df))
for bt in df['batch'].unique():
idxs = df['batch'] == bt
_signal_mv = df['signal'][idxs].rolling(n, center=True).min().interpolate('spline', order=5, limit_direction='both').values
signal_mv[idxs] = _signal_mv
return signal_mv
def get_signal_mv_max(df, n=3001):
signal_mv = np.zeros(len(df))
for bt in df['batch'].unique():
idxs = df['batch'] == bt
_signal_mv = df['signal'][idxs].rolling(n, center=True).max().interpolate('spline', order=5, limit_direction='both').values
signal_mv[idxs] = _signal_mv
return signal_mv
def calc_shifted(s, add_minus=False, fill_value=None, periods=range(1, 4)):
s = pd.DataFrame(s)
_periods = periods
add_minus = True
periods = np.asarray(_periods, dtype=np.int32)
if add_minus:
periods = np.append(periods, -periods)
for p in progress_bar(periods):
s[f"signal_shifted_{p}"] = s['signal'].shift(
periods=p, fill_value=fill_value
)
cols = [col for col in s.columns if 'shifted' in col]
return s[cols]
def group_feat_train(_train):
train = _train.copy()
# group init
train['group'] = int(0)
# group 1
idxs = (train['batch'] == 3) | (train['batch'] == 7)
train['group'][idxs] = int(1)
# group 2
idxs = (train['batch'] == 5) | (train['batch'] == 8)
train['group'][idxs] = int(2)
# group 3
idxs = (train['batch'] == 2) | (train['batch'] == 6)
train['group'][idxs] = int(3)
# group 4
idxs = (train['batch'] == 4) | (train['batch'] == 9)
train['group'][idxs] = int(4)
return train[['group']]
def group_feat_test(_test):
test = _test.copy()
# group init
test['group'] = int(0)
x_idx = np.arange(len(test))
# group 1
idxs = (100000<=x_idx) & (x_idx<200000)
test['group'][idxs] = int(1)
idxs = (900000<=x_idx) & (x_idx<=1000000)
test['group'][idxs] = int(1)
# group 2
idxs = (200000<=x_idx) & (x_idx<300000)
test['group'][idxs] = int(2)
idxs = (600000<=x_idx) & (x_idx<700000)
test['group'][idxs] = int(2)
# group 3
idxs = (400000<=x_idx) & (x_idx<500000)
test['group'][idxs] = int(3)
# group 4
idxs = (500000<=x_idx) & (x_idx<600000)
test['group'][idxs] = int(4)
idxs = (700000<=x_idx) & (x_idx<800000)
test['group'][idxs] = int(4)
return test[['group']]
class permutation_importance():
def __init__(self, model, metric):
self.is_computed = False
self.n_feat = 0
self.base_score = 0
self.model = model
self.metric = metric
self.df_result = []
def compute(self, X_valid, y_valid):
self.n_feat = len(X_valid.columns)
if self.metric == 'auc':
y_valid_score = self.model.predict_proba(X_valid)[:, 1]
fpr, tpr, thresholds = roc_curve(y_valid, y_valid_score)
self.base_score = auc(fpr, tpr)
else:
pred = np.round(self.model.predict(X_valid)).astype('int8')
self.base_score = self.metric(y_valid, pred)
self.df_result = pd.DataFrame({'feat': X_valid.columns,
'score': np.zeros(self.n_feat),
'score_diff': np.zeros(self.n_feat)})
# predict
for i, col in enumerate(X_valid.columns):
df_perm = X_valid.copy()
np.random.seed(1)
df_perm[col] = np.random.permutation(df_perm[col])
y_valid_pred = self.model.predict(df_perm)
if self.metric == 'auc':
y_valid_score = self.model.predict_proba(df_perm)[:, 1]
fpr, tpr, thresholds = roc_curve(y_valid, y_valid_score)
score = auc(fpr, tpr)
else:
score = self.metric(y_valid, np.round(y_valid_pred).astype('int8'))
self.df_result['score'][self.df_result['feat']==col] = score
self.df_result['score_diff'][self.df_result['feat']==col] = self.base_score - score
self.is_computed = True
def get_negative_feature(self):
assert self.is_computed!=False, 'compute メソッドが実行されていません'
idx = self.df_result['score_diff'] < 0
return self.df_result.loc[idx, 'feat'].values.tolist()
def get_positive_feature(self):
assert self.is_computed!=False, 'compute メソッドが実行されていません'
idx = self.df_result['score_diff'] > 0
return self.df_result.loc[idx, 'feat'].values.tolist()
def show_permutation_importance(self, score_type='loss'):
'''score_type = 'loss' or 'accuracy' '''
assert self.is_computed!=False, 'compute メソッドが実行されていません'
if score_type=='loss':
ascending = True
elif score_type=='accuracy':
ascending = False
else:
ascending = ''
plt.figure(figsize=(15, int(0.25*self.n_feat)))
sns.barplot(x="score_diff", y="feat", data=self.df_result.sort_values(by="score_diff", ascending=ascending))
plt.title('base_score - permutation_score')
def plot_corr(df, abs_=False, threshold=0.95):
if abs_==True:
corr = df.corr().abs()>threshold
vmin = 0
else:
corr = df.corr()
vmin = -1
# Plot
fig, ax = plt.subplots(figsize=(12, 10), dpi=100)
fig.patch.set_facecolor('white')
sns.heatmap(corr,
xticklabels=df.corr().columns,
yticklabels=df.corr().columns,
vmin=vmin,
vmax=1,
center=0,
annot=False)
# Decorations
ax.set_title('Correlation', fontsize=22)
def get_low_corr_column(df, threshold):
df_corr = df.corr()
df_corr = abs(df_corr)
columns = df_corr.columns
# 対角線の値を0にする
for i in range(0, len(columns)):
df_corr.iloc[i, i] = 0
while True:
columns = df_corr.columns
max_corr = 0.0
query_column = None
target_column = None
df_max_column_value = df_corr.max()
max_corr = df_max_column_value.max()
query_column = df_max_column_value.idxmax()
target_column = df_corr[query_column].idxmax()
if max_corr < threshold:
# しきい値を超えるものがなかったため終了
break
else:
# しきい値を超えるものがあった場合
delete_column = None
saved_column = None
# その他との相関の絶対値が大きい方を除去
if sum(df_corr[query_column]) <= sum(df_corr[target_column]):
delete_column = target_column
saved_column = query_column
else:
delete_column = query_column
saved_column = target_column
# 除去すべき特徴を相関行列から消す(行、列)
df_corr.drop([delete_column], axis=0, inplace=True)
df_corr.drop([delete_column], axis=1, inplace=True)
return df_corr.columns # 相関が高い特徴量を除いた名前リスト
def reduce_mem_usage(df, verbose=True):
numerics = ['int16', 'int32', 'int64', 'float16', 'float32', 'float64']
start_mem = df.memory_usage().sum() / 1024**2
for col in df.columns:
if col!='open_channels':
col_type = df[col].dtypes
if col_type in numerics:
c_min = df[col].min()
c_max = df[col].max()
if str(col_type)[:3] == 'int':
if c_min > np.iinfo(np.int8).min and c_max < np.iinfo(np.int8).max:
df[col] = df[col].astype(np.int8)
elif c_min > np.iinfo(np.int16).min and c_max < np.iinfo(np.int16).max:
df[col] = df[col].astype(np.int16)
elif c_min > np.iinfo(np.int32).min and c_max < np.iinfo(np.int32).max:
df[col] = df[col].astype(np.int32)
elif c_min > np.iinfo(np.int64).min and c_max < np.iinfo(np.int64).max:
df[col] = df[col].astype(np.int64)
else:
if c_min > np.finfo(np.float16).min and c_max < np.finfo(np.float16).max:
df[col] = df[col].astype(np.float16)
elif c_min > np.finfo(np.float32).min and c_max < np.finfo(np.float32).max:
df[col] = df[col].astype(np.float32)
else:
df[col] = df[col].astype(np.float64)
end_mem = df.memory_usage().sum() / 1024**2
if verbose: print('Mem. usage decreased to {:5.2f} Mb ({:.1f}% reduction)'.format(end_mem, 100 * (start_mem - end_mem) / start_mem))
return df
def plot_confusion_matrix(truth, pred, classes, normalize=False, title=''):
cm = confusion_matrix(truth, pred)
if normalize:
cm = cm.astype('float') / cm.sum(axis=1)[:, np.newaxis]
plt.figure(figsize=(10, 10))
plt.imshow(cm, interpolation='nearest', cmap=plt.cm.Blues)
plt.title('Confusion matrix', size=15)
plt.colorbar(fraction=0.046, pad=0.04)
tick_marks = np.arange(len(classes))
plt.xticks(tick_marks, classes, rotation=45)
plt.yticks(tick_marks, classes)
fmt = '.2f' if normalize else 'd'
thresh = cm.max() / 2.
for i, j in itertools.product(range(cm.shape[0]), range(cm.shape[1])):
plt.text(j, i, format(cm[i, j], fmt),
horizontalalignment="center",
color="white" if cm[i, j] > thresh else "black")
plt.ylabel('True label')
plt.xlabel('Predicted label')
plt.grid(False)
plt.tight_layout()
def train_lgbm(X, y, X_te, lgbm_params, random_state=5, n_fold=5, verbose=50, early_stopping_rounds=100, show_fig=True):
# using features
print(f'features({len(X.columns)}): \n{X.columns}') if not verbose==0 else None
# folds = KFold(n_splits=n_fold, shuffle=True, random_state=random_state)
folds = StratifiedKFold(n_splits=n_fold, shuffle=True, random_state=random_state)
scores = []
oof = np.zeros(len(X))
oof_round = np.zeros(len(X))
test_pred = np.zeros(len(X_te))
df_pi = pd.DataFrame(columns=['feat', 'score_diff'])
for fold_n, (train_idx, valid_idx) in enumerate(folds.split(X, y=y)):
if verbose==0:
pass
else:
print('\n------------------')
print(f'- Fold {fold_n + 1}/{N_FOLD} started at {time.ctime()}')
# prepare dataset
X_train, X_valid = X.iloc[train_idx], X.iloc[valid_idx]
y_train, y_valid = y[train_idx], y[valid_idx]
# train
model = LGBMRegressor(**lgbm_params, n_estimators=N_ESTIMATORS)
model.fit(X_train, y_train,
eval_set=[(X_train, y_train), (X_valid, y_valid)],
verbose=verbose,
early_stopping_rounds=early_stopping_rounds)
# pred
y_valid_pred = model.predict(X_valid, model.best_iteration_)
y_valid_pred_round = np.round(y_valid_pred).astype('int8')
_test_pred = model.predict(X_te, model.best_iteration_)
if show_fig==False:
pass
else:
# permutation importance
pi = permutation_importance(model, f1_macro) # model と metric を渡す
pi.compute(X_valid, y_valid)
pi_result = pi.df_result
df_pi = pd.concat([df_pi, pi_result[['feat', 'score_diff']]])
# result
oof[valid_idx] = y_valid_pred
oof_round[valid_idx] = y_valid_pred_round
score = f1_score(y_valid, y_valid_pred_round, average='macro')
scores.append(score)
test_pred += _test_pred
if verbose==0:
pass
else:
print(f'---> f1-score(macro) valid: {f1_score(y_valid, y_valid_pred_round, average="macro"):.4f}')
print('')
print('====== finish ======')
print('score list:', scores)
print('CV mean score(f1_macro): {0:.4f}, std: {1:.4f}'.format(np.mean(scores), np.std(scores)))
print(f'oof score(f1_macro): {f1_score(y, oof_round, average="macro"):.4f}')
print('')
if show_fig==False:
pass
else:
# visualization
plt.figure(figsize=(5, 5))
plt.plot([0, 10], [0, 10], color='gray')
plt.scatter(y, oof, alpha=0.05, color=cp[1])
plt.xlabel('true')
plt.ylabel('pred')
plt.show()
# confusion_matrix
plot_confusion_matrix(y, oof_round, classes=np.arange(11))
# permutation importance
plt.figure(figsize=(15, int(0.25*len(X.columns))))
order = df_pi.groupby(["feat"]).mean()['score_diff'].reset_index().sort_values('score_diff', ascending=False)
sns.barplot(x="score_diff", y="feat", data=df_pi, order=order['feat'])
plt.title('base_score - permutation_score')
plt.show()
# submission
test_pred = test_pred/N_FOLD
test_pred_round = np.round(test_pred).astype('int8')
return test_pred_round, test_pred, oof_round, oof, type(model).__name__
def train_test_split_lgbm(X, y, X_te, lgbm_params, random_state=5, test_size=0.3, verbose=50, early_stopping_rounds=100, show_fig=True):
# using features
print(f'features({len(X.columns)}): \n{X.columns}') if not verbose==0 else None
# folds = KFold(n_splits=n_fold, shuffle=True, random_state=random_state)
# folds = StratifiedKFold(n_splits=n_fold, shuffle=True, random_state=random_state)
# prepare dataset
X_train, X_valid, y_train, y_valid = train_test_split(X, y, test_size=test_size, random_state=random_state)
# train
model = LGBMRegressor(**lgbm_params, n_estimators=N_ESTIMATORS)
model.fit(X_train, y_train,
eval_set=[(X_train, y_train), (X_valid, y_valid)],
verbose=verbose,
early_stopping_rounds=early_stopping_rounds)
# pred
oof = model.predict(X_valid, model.best_iteration_)
oof_round = np.round(oof).astype('int8')
test_pred = model.predict(X_te, model.best_iteration_)
test_pred_round = np.round(test_pred).astype('int8')
print('====== finish ======')
print(f'oof score(f1_macro): {f1_score(y_valid, oof_round, average="macro"):.4f}')
print('')
if show_fig==False:
pass
else:
# visualization
plt.figure(figsize=(5, 5))
plt.plot([0, 10], [0, 10], color='gray')
plt.scatter(y_valid, oof, alpha=0.05, color=cp[1])
plt.xlabel('true')
plt.ylabel('pred')
plt.show()
# confusion_matrix
plot_confusion_matrix(y_valid, oof_round, classes=np.arange(11))
# permutation importance
pi = permutation_importance(model, f1_macro) # model と metric を渡す
pi.compute(X_valid, y_valid)
pi.show_permutation_importance(score_type='accuracy') # loss or accuracy
plt.show()
return test_pred_round, test_pred, oof_round, oof, type(model).__name__
def train_rfc(X, y, X_te, rfc_params, random_state=5, n_fold=5, verbose=2, show_fig=True):
# using features
print(f'features({len(X.columns)}): \n{X.columns}') if not verbose==0 else None
folds = StratifiedKFold(n_splits=n_fold, shuffle=True, random_state=random_state)
scores = []
oof_proba = np.zeros([len(X), len(np.unique(y))])
test_proba = np.zeros([len(X_te), len(np.unique(y))])
df_pi = pd.DataFrame(columns=['feat', 'score_diff'])
for fold_n, (train_idx, valid_idx) in enumerate(folds.split(X, y=y)):
if verbose==0:
pass
else:
print('\n------------------')
print(f'- Fold {fold_n + 1}/{N_FOLD} started at {time.ctime()}')
# prepare dataset
X_train, X_valid = X.iloc[train_idx], X.iloc[valid_idx]
y_train, y_valid = y[train_idx], y[valid_idx]
# train
model = RandomForestClassifier(**rfc_params, verbose=verbose)
model.fit(X_train, y_train)
# pred
y_valid_pred = model.predict(X_valid)
y_valid_proba = model.predict_proba(X_valid)
# y_valid_pred_round = np.round(y_valid_pred).astype('int8')
_test_pred = model.predict(X_te)
_test_proba = model.predict_proba(X_te)
if show_fig==False:
pass
else:
# permutation importance
pi = permutation_importance(model, f1_macro) # model と metric を渡す
pi.compute(X_valid, y_valid)
pi_result = pi.df_result
df_pi = pd.concat([df_pi, pi_result[['feat', 'score_diff']]])
# result
oof_proba[valid_idx] = y_valid_proba
score = f1_score(y_valid, y_valid_pred, average='macro')
scores.append(score)
test_proba += _test_proba
if verbose==0:
pass
else:
print(f'---> f1-score(macro) valid: {f1_score(y_valid, y_valid_pred, average="macro"):.4f}')
print('')
print('====== finish ======')
oof = np.argmax(oof_proba, axis=1)
print('score list:', scores)
print('CV mean score(f1_macro): {0:.4f}, std: {1:.4f}'.format(np.mean(scores), np.std(scores)))
print(f'oof score(f1_macro): {f1_score(y, oof, average="macro"):.4f}')
print('')
if show_fig==False:
pass
else:
# visualization
plt.figure(figsize=(5, 5))
plt.plot([0, 10], [0, 10], color='gray')
plt.scatter(y, oof, alpha=0.05, color=cp[1])
plt.xlabel('true')
plt.ylabel('pred')
plt.show()
# confusion_matrix
plot_confusion_matrix(y, oof, classes=np.arange(11))
# permutation importance
plt.figure(figsize=(15, int(0.25*len(X.columns))))
order = df_pi.groupby(["feat"]).mean()['score_diff'].reset_index().sort_values('score_diff', ascending=False)
sns.barplot(x="score_diff", y="feat", data=df_pi, order=order['feat'])
plt.title('base_score - permutation_score')
plt.show()
# submission
test_proba = test_proba/N_FOLD
test_pred = np.argmax(test_proba, axis=1)
# oof_pred = np.argmax(oof_proba, axis=1)
return test_pred, test_proba, oof, oof_proba, type(model).__name__
###Output
_____no_output_____
###Markdown
ref: https://www.kaggle.com/martxelo/fe-and-ensemble-mlp-and-lgbm
###Code
def calc_gradients(s, n_grads=4):
'''
Calculate gradients for a pandas series. Returns the same number of samples
'''
grads = pd.DataFrame()
g = s.values
for i in range(n_grads):
g = np.gradient(g)
grads['grad_' + str(i+1)] = g
return grads
def calc_low_pass(s, n_filts=10):
'''
Applies low pass filters to the signal. Left delayed and no delayed
'''
wns = np.logspace(-2, -0.3, n_filts)
# wns = [0.3244]
low_pass = pd.DataFrame()
x = s.values
for wn in wns:
b, a = signal.butter(1, Wn=wn, btype='low')
zi = signal.lfilter_zi(b, a)
low_pass['lowpass_lf_' + str('%.4f' %wn)] = signal.lfilter(b, a, x, zi=zi*x[0])[0]
low_pass['lowpass_ff_' + str('%.4f' %wn)] = signal.filtfilt(b, a, x)
return low_pass
def calc_high_pass(s, n_filts=10):
'''
Applies high pass filters to the signal. Left delayed and no delayed
'''
wns = np.logspace(-2, -0.1, n_filts)
# wns = [0.0100, 0.0264, 0.0699, 0.3005, 0.4885, 0.7943]
high_pass = pd.DataFrame()
x = s.values
for wn in wns:
b, a = signal.butter(1, Wn=wn, btype='high')
zi = signal.lfilter_zi(b, a)
high_pass['highpass_lf_' + str('%.4f' %wn)] = signal.lfilter(b, a, x, zi=zi*x[0])[0]
high_pass['highpass_ff_' + str('%.4f' %wn)] = signal.filtfilt(b, a, x)
return high_pass
def calc_roll_stats(s, windows=[10, 50, 100, 500, 1000, 3000]):
'''
Calculates rolling stats like mean, std, min, max...
'''
roll_stats = pd.DataFrame()
for w in windows:
roll_stats['roll_mean_' + str(w)] = s.rolling(window=w, min_periods=1).mean().interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_std_' + str(w)] = s.rolling(window=w, min_periods=1).std().interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_min_' + str(w)] = s.rolling(window=w, min_periods=1).min().interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_max_' + str(w)] = s.rolling(window=w, min_periods=1).max().interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_range_' + str(w)] = roll_stats['roll_max_' + str(w)] - roll_stats['roll_min_' + str(w)]
roll_stats['roll_q10_' + str(w)] = s.rolling(window=w, min_periods=1).quantile(0.10).interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_q25_' + str(w)] = s.rolling(window=w, min_periods=1).quantile(0.25).interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_q50_' + str(w)] = s.rolling(window=w, min_periods=1).quantile(0.50).interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_q75_' + str(w)] = s.rolling(window=w, min_periods=1).quantile(0.75).interpolate('spline', order=5, limit_direction='both')
roll_stats['roll_q90_' + str(w)] = s.rolling(window=w, min_periods=1).quantile(0.90).interpolate('spline', order=5, limit_direction='both')
# add zeros when na values (std)
# roll_stats = roll_stats.fillna(value=0)
return roll_stats
def calc_ewm(s, windows=[10, 50, 100, 500, 1000, 3000]):
'''
Calculates exponential weighted functions
'''
ewm = pd.DataFrame()
for w in windows:
ewm['ewm_mean_' + str(w)] = s.ewm(span=w, min_periods=1).mean()
ewm['ewm_std_' + str(w)] = s.ewm(span=w, min_periods=1).std()
# add zeros when na values (std)
ewm = ewm.fillna(value=0)
return ewm
def divide_and_add_features(s, signal_size=500000):
'''
Divide the signal in bags of "signal_size".
Normalize the data dividing it by 15.0
'''
# normalize
s = s/15.0
ls = []
for i in progress_bar(range(int(s.shape[0]/signal_size))):
sig = s[i*signal_size:(i+1)*signal_size].copy().reset_index(drop=True)
sig_featured = add_features(sig)
ls.append(sig_featured)
return pd.concat(ls, axis=0)
###Output
_____no_output_____
###Markdown
ref: https://www.kaggle.com/nxrprime/single-model-lgbm-kalman-filter-ii
###Code
def Kalman1D(observations,damping=1):
# To return the smoothed time series data
observation_covariance = damping
initial_value_guess = observations[0]
transition_matrix = 1
transition_covariance = 0.1
initial_value_guess
kf = KalmanFilter(
initial_state_mean=initial_value_guess,
initial_state_covariance=observation_covariance,
observation_covariance=observation_covariance,
transition_covariance=transition_covariance,
transition_matrices=transition_matrix
)
pred_state, state_cov = kf.smooth(observations)
return pred_state
###Output
_____no_output_____
###Markdown
Preparation setting
###Code
sns.set()
###Output
_____no_output_____
###Markdown
load dataset
###Code
df_tr = pd.read_csv(PATH_TRAIN)
df_te = pd.read_csv(PATH_TEST)
###Output
_____no_output_____
###Markdown
処理のしやすさのために、バッチ番号を振る
###Code
batch_list = []
for n in range(10):
batchs = np.ones(500000)*n
batch_list.append(batchs.astype(int))
batch_list = np.hstack(batch_list)
df_tr['batch'] = batch_list
batch_list = []
for n in range(4):
batchs = np.ones(500000)*n
batch_list.append(batchs.astype(int))
batch_list = np.hstack(batch_list)
df_te['batch'] = batch_list
###Output
_____no_output_____
###Markdown
batch 7 のスパイクをbatch 3 の最小値、最大値に置き換える
###Code
max_ = df_tr.loc[df_tr['batch']==3]['signal'].max()
min_ = df_tr.loc[df_tr['batch']==3]['signal'].min()
idxs = (df_tr['batch']==7) & (df_tr['signal']>max_)
df_tr['signal'][idxs] = max_
idxs = (df_tr['batch']==7) & (df_tr['signal']<min_)
df_tr['signal'][idxs] = min_
###Output
_____no_output_____
###Markdown
group 特徴量
###Code
# group 特徴量を作成
group = group_feat_train(df_tr)
df_tr = pd.concat([df_tr, group], axis=1)
group = group_feat_test(df_te)
df_te = pd.concat([df_te, group], axis=1)
if isSmallSet:
X_te['group'][1000:2000] = 1
X_te['group'][2000:3000] = 2
X_te['group'][3000:4000] = 3
X_te['group'][4000:5000] = 4
###Output
_____no_output_____
###Markdown
group4にオフセットをかける
###Code
# --- train ---
off_set_4 = 0.952472 - (-1.766044)
off_set_9 = 0.952472 - (-1.770441)
# batch4
idxs = df_tr['batch'] == 4
df_tr['signal'][idxs] = df_tr['signal'].values + off_set_4
# batch9
idxs = df_tr['batch'] == 9
df_tr['signal'][idxs] = df_tr['signal'].values + off_set_9
# --- test ---
off_set_test = 2.750
df_te['signal'] = df_te['signal'].values
idxs = df_te['group'] == 4
df_te['signal'][idxs] = df_te['signal'][idxs].values + off_set_test
###Output
_____no_output_____
###Markdown
smallset?
###Code
if isSmallSet:
print('small set mode')
# train
batchs = df_tr['batch'].values
dfs = []
for i_bt, bt in enumerate(df_tr['batch'].unique()):
idxs = batchs == bt
_df = df_tr[idxs][:LENGTH].copy()
dfs.append(_df)
df_tr = pd.concat(dfs).reset_index(drop=True)
# test
batchs = df_te['batch'].values
dfs = []
for i_bt, bt in enumerate(df_te['batch'].unique()):
idxs = batchs == bt
_df = df_te[idxs][:LENGTH].copy()
dfs.append(_df)
df_te = pd.concat(dfs).reset_index(drop=True)
###Output
_____no_output_____
###Markdown
Train
###Code
def add_features(s):
'''
All calculations together
'''
feat_list = [s]
# feat_list.append(calc_gradients(s))
# feat_list.append(calc_low_pass(s))
# feat_list.append(calc_high_pass(s))
# feat_list.append(calc_roll_stats(s))
# feat_list.append(calc_ewm(s))
feat_list.append(calc_shifted(s, fill_value=0, periods=range(1, 20)))
return pd.concat(feat_list, axis=1)
X = divide_and_add_features(df_tr['signal'], signal_size=LENGTH).reset_index(drop=True)
# _feats = get_low_corr_column(X, threshold=0.97).to_list()
# _feats.append('signal')
# X = X[_feats]
X = reduce_mem_usage(X)
X_te = divide_and_add_features(df_te['signal'], signal_size=LENGTH).reset_index(drop=True)
# X_te = X_te[_feats]
X_te = reduce_mem_usage(X_te)
y = df_tr['open_channels'].values
X, X_te = add_category(X, X_te)
###Output
_____no_output_____
###Markdown
学習データセットの作成
###Code
# Configuration
N_ESTIMATORS = 2000
# N_ESTIMATORS = 20 # 最大学習回数
VERBOSE = 100 # 300回ごとに評価する
EARLY_STOPPING_ROUNDS = 50 # 200回の学習でよくならなければ、学習をとめる
# N_JOBS = multiprocessing.cpu_count() - 2
# N_JOBS = 6
# N_FOLD = 4
# KFOLD_SEED = 0
N_JOBS = 30
N_FOLD = 5
KFOLD_SEED = 42
# lgbm_params
# lgbm_params = {
# 'objective': 'regression',
# "metric": 'rmse',
# # 'reg_alpha': 0.1,
# # 'reg_lambda': 0.1,
# "boosting_type": "gbdt",
# 'learning_rate': 0.1,
# 'n_jobs': N_JOBS,
# # "subsample_freq": 1,
# # "subsample": 1,
# "bagging_seed": 2,
# # "verbosity": -1,
# 'num_leaves': 51, 'max_depth': 158, 'min_chiled_samples': 15, 'min_chiled_weight': 1, 'learning_rate': 0.07, 'colsample_bytree': 0.8
# }
# nb015
# lgbm_params = {'boosting_type': 'gbdt',
# 'metric': 'rmse',
# 'objective': 'regression',
# 'n_jobs': N_JOBS,
# 'seed': 236,
# 'num_leaves': 280,
# 'learning_rate': 0.026623466966581126,
# 'max_depth': 73,
# 'lambda_l1': 2.959759088169741,
# 'lambda_l2': 1.331172832164913,
# 'bagging_fraction': 0.9655406551472153,
# 'bagging_freq': 9,
# 'colsample_bytree': 0.6867118652742716}
# nb019
# lgbm_params = {
# 'objective': 'regression',
# "metric": 'rmse',
# "boosting_type": "gbdt",
# 'learning_rate': 0.1,
# 'n_jobs': N_JOBS,
# 'max_depth': 85,
# 'min_chiled_samples': 62,
# 'min_chiled_weight': 10,
# 'learning_rate': 0.20158497791184515,
# 'colsample_bytree': 1.0,
# 'lambda_l1': 2.959759088169741,
# 'lambda_l2': 1.331172832164913,
# # 'bagging_fraction': 0.9655406551472153,
# # 'bagging_freq': 9,
# }
# https://www.kaggle.com/nxrprime/single-model-lgbm-kalman-filter-ii
lgbm_params = {'boosting_type': 'gbdt',
'metric': 'rmse',
'objective': 'regression',
'n_jobs': N_JOBS,
'seed': 236,
'num_leaves': 280,
# 'learning_rate': 0.026623466966581126,
'learning_rate': 0.03,
'max_depth': 73,
'lambda_l1': 2.959759088169741,
'lambda_l2': 1.331172832164913,
'bagging_fraction': 0.9655406551472153,
'bagging_freq': 9,
'colsample_bytree': 0.6867118652742716}
%%time
test_pred_round, test_pred, oof_round, oof, model_name = train_lgbm(X, y, X_te, lgbm_params,
n_fold=N_FOLD,
verbose=VERBOSE,
random_state=KFOLD_SEED,
early_stopping_rounds=EARLY_STOPPING_ROUNDS,
show_fig=True
)
###Output
features(40):
Index(['signal', 'signal_shifted_1', 'signal_shifted_2', 'signal_shifted_3',
'signal_shifted_4', 'signal_shifted_5', 'signal_shifted_6',
'signal_shifted_7', 'signal_shifted_8', 'signal_shifted_9',
'signal_shifted_10', 'signal_shifted_11', 'signal_shifted_12',
'signal_shifted_13', 'signal_shifted_14', 'signal_shifted_15',
'signal_shifted_16', 'signal_shifted_17', 'signal_shifted_18',
'signal_shifted_19', 'signal_shifted_-1', 'signal_shifted_-2',
'signal_shifted_-3', 'signal_shifted_-4', 'signal_shifted_-5',
'signal_shifted_-6', 'signal_shifted_-7', 'signal_shifted_-8',
'signal_shifted_-9', 'signal_shifted_-10', 'signal_shifted_-11',
'signal_shifted_-12', 'signal_shifted_-13', 'signal_shifted_-14',
'signal_shifted_-15', 'signal_shifted_-16', 'signal_shifted_-17',
'signal_shifted_-18', 'signal_shifted_-19', 'category'],
dtype='object')
------------------
- Fold 1/5 started at Thu May 7 08:15:44 2020
Training until validation scores don't improve for 50 rounds
[100] training's rmse: 0.213281 valid_1's rmse: 0.214659
[200] training's rmse: 0.15701 valid_1's rmse: 0.159893
[300] training's rmse: 0.154648 valid_1's rmse: 0.158757
[400] training's rmse: 0.153261 valid_1's rmse: 0.158545
[500] training's rmse: 0.152101 valid_1's rmse: 0.158422
[600] training's rmse: 0.151115 valid_1's rmse: 0.158362
[700] training's rmse: 0.150156 valid_1's rmse: 0.158292
[800] training's rmse: 0.149275 valid_1's rmse: 0.158252
[900] training's rmse: 0.148451 valid_1's rmse: 0.158234
[1000] training's rmse: 0.147634 valid_1's rmse: 0.158214
Early stopping, best iteration is:
[969] training's rmse: 0.147879 valid_1's rmse: 0.158211
---> f1-score(macro) valid: 0.9359
------------------
- Fold 2/5 started at Thu May 7 08:24:27 2020
Training until validation scores don't improve for 50 rounds
[100] training's rmse: 0.213237 valid_1's rmse: 0.215215
[200] training's rmse: 0.156941 valid_1's rmse: 0.160714
[300] training's rmse: 0.154582 valid_1's rmse: 0.159585
[400] training's rmse: 0.153205 valid_1's rmse: 0.15936
[500] training's rmse: 0.152098 valid_1's rmse: 0.159241
[600] training's rmse: 0.151131 valid_1's rmse: 0.159171
[700] training's rmse: 0.150195 valid_1's rmse: 0.159108
[800] training's rmse: 0.149267 valid_1's rmse: 0.159063
[900] training's rmse: 0.14842 valid_1's rmse: 0.159043
[1000] training's rmse: 0.147635 valid_1's rmse: 0.159028
Early stopping, best iteration is:
[966] training's rmse: 0.147912 valid_1's rmse: 0.159025
---> f1-score(macro) valid: 0.9353
------------------
- Fold 3/5 started at Thu May 7 08:33:42 2020
Training until validation scores don't improve for 50 rounds
[100] training's rmse: 0.213412 valid_1's rmse: 0.213997
[200] training's rmse: 0.157167 valid_1's rmse: 0.159114
[300] training's rmse: 0.154807 valid_1's rmse: 0.157988
[400] training's rmse: 0.153435 valid_1's rmse: 0.157765
[500] training's rmse: 0.15228 valid_1's rmse: 0.157659
[600] training's rmse: 0.151278 valid_1's rmse: 0.157584
[700] training's rmse: 0.150349 valid_1's rmse: 0.157543
[800] training's rmse: 0.14946 valid_1's rmse: 0.157519
[900] training's rmse: 0.148581 valid_1's rmse: 0.157468
Early stopping, best iteration is:
[934] training's rmse: 0.14828 valid_1's rmse: 0.157447
---> f1-score(macro) valid: 0.9371
------------------
- Fold 4/5 started at Thu May 7 08:42:47 2020
Training until validation scores don't improve for 50 rounds
[100] training's rmse: 0.213215 valid_1's rmse: 0.215177
[200] training's rmse: 0.156949 valid_1's rmse: 0.160476
[300] training's rmse: 0.154589 valid_1's rmse: 0.15929
[400] training's rmse: 0.153197 valid_1's rmse: 0.159049
[500] training's rmse: 0.152032 valid_1's rmse: 0.158908
[600] training's rmse: 0.151028 valid_1's rmse: 0.158836
[700] training's rmse: 0.150071 valid_1's rmse: 0.158778
Early stopping, best iteration is:
[743] training's rmse: 0.149725 valid_1's rmse: 0.158771
---> f1-score(macro) valid: 0.9360
------------------
- Fold 5/5 started at Thu May 7 08:50:17 2020
Training until validation scores don't improve for 50 rounds
[100] training's rmse: 0.213455 valid_1's rmse: 0.214144
[200] training's rmse: 0.157232 valid_1's rmse: 0.159415
[300] training's rmse: 0.154889 valid_1's rmse: 0.158321
[400] training's rmse: 0.153519 valid_1's rmse: 0.158101
[500] training's rmse: 0.152385 valid_1's rmse: 0.157993
[600] training's rmse: 0.15137 valid_1's rmse: 0.157914
[700] training's rmse: 0.15037 valid_1's rmse: 0.157869
[800] training's rmse: 0.149523 valid_1's rmse: 0.157834
[900] training's rmse: 0.148669 valid_1's rmse: 0.15781
[1000] training's rmse: 0.147797 valid_1's rmse: 0.157785
[1100] training's rmse: 0.147039 valid_1's rmse: 0.157767
Early stopping, best iteration is:
[1098] training's rmse: 0.147052 valid_1's rmse: 0.157767
---> f1-score(macro) valid: 0.9363
====== finish ======
score list: [0.9358770748335538, 0.935263527537316, 0.9371239017668778, 0.936023693555606, 0.9362772256303787]
CV mean score(f1_macro): 0.9361, std: 0.0006
oof score(f1_macro): 0.9361
###Markdown
save submission
###Code
save_path = f'{DIR_OUTPUT}submission_nb{NB}_{model_name}_cv_{f1_macro(y, oof_round):.4f}.csv'
sub = pd.read_csv(PATH_SMPLE_SUB)
# sub['open_channels'] = test_pred
sub['open_channels'] = test_pred.astype(int)
print(f'save path: {save_path}')
sub.to_csv(save_path, index=False, float_format='%.4f')
###Output
_____no_output_____
###Markdown
oof proba
###Code
save_path = f'{DIR_OUTPUT_IGNORE}probas_nb{NB}_{model_name}_cv_{f1_macro(y, oof_round):.4f}'
print(f'save path: {save_path}')
np.savez_compressed(save_path, oof_proba, test_proba)
###Output
_____no_output_____
###Markdown
analysis 処理のしやすさのために、バッチ番号を振る
###Code
batch_list = []
for n in range(10):
batchs = np.ones(500000)*n
batch_list.append(batchs.astype(int))
batch_list = np.hstack(batch_list)
X['batch'] = batch_list
###Output
_____no_output_____
###Markdown
group 特徴量
###Code
# group 特徴量を作成
group = group_feat_train(X)
X = pd.concat([X, group], axis=1)
for group in sorted(X['group'].unique()):
idxs = X['group'] == group
oof_grp = oof[idxs].astype(int)
y_grp = y[idxs]
print(f'group_score({group}): {f1_score(y_grp, oof_grp, average="micro"):4f}')
x_idx = np.arange(len(X))
idxs = y != oof_round
failed = np.zeros(len(X))
failed[idxs] = 1
n = 200
b = np.ones(n)/n
failed_move = np.convolve(failed, b, mode='same')
fig, axs = plt.subplots(2, 1, figsize=(20, 6))
axs = axs.ravel()
# fig = plt.figure(figsize=(20, 3))
for i_gr, group in enumerate(sorted(X['group'].unique())):
idxs = X['group'] == group
axs[0].plot(np.arange(len(X))[idxs], X['signal'].values[idxs], color=cp[i_gr], label=f'group={group}')
for x in range(10):
axs[0].axvline(x*500000 + 500000, color='gray')
axs[0].text(x*500000 + 250000, 0.6, x)
axs[0].plot(x_idx, failed_move, '.', color='black', label='failed_mv')
axs[0].set_xlim(0, 5500000)
axs[0].legend()
axs[1].plot(x_idx, y)
axs[1].set_xlim(0, 5500000)
# fig.legend()
###Output
_____no_output_____ |
2_RSA_Algorithm/2.3_Fermat,Euler_Theorem.ipynb | ###Markdown
1. phi(n)값을 알 때, n 소인수분해하기- phi(n) = (p-1)(q-1) 값을 알 때, 근의 공식을 이용하여 n을 소인수분해
###Code
def crack(n, phi_n):
a = n-phi_n+1
return [(a+sqrt(a^2-4*n))/2, (a-sqrt(a^2-4*n))/2]
crack(35, 24)
###Output
_____no_output_____
###Markdown
2. p, q가 가까울때, n 소인수분해하기- p, q가 가까운 두 소수일 때, n 소인수분해하기 (쉬움 -> 암호문도 바로 해독가능합니다.)- 보통 (이진법) 150자리 두 소수의 곱은 소인수분해가 매우 오래 걸리지만, 두 소수의 차이가 작은 경우 아래와 같이 쉽게 소인수분해 됨
###Code
def crack2(n):
t = ceil(sqrt(n)) ## ceil() : 올림함수, ceil(1.1) = 2
while True:
if t^2-n>0 and is_square(t^2-n):
return t+sqrt(t^2-n), t-sqrt(t^2-n)
t+=1
p = next_prime(2^150)
q = next_prime(p)
crack2(p*q)
###Output
_____no_output_____
###Markdown
3. Euler Theorem- n 값과 양의 공약수가 1뿐인 a 값들에 대해, a^i 제곱을 n으로 나눈 나머지- 여러 a값들의 경우에 대해 몇 제곱일때 동시에 나머지가 1이 되는지 관찰
###Code
n=9
for a in range(1, n):
if gcd(n, a)==1:
print([Mod(a,n)^i for i in range(1, n)])
###Output
[1, 1, 1, 1, 1, 1, 1, 1]
[2, 4, 8, 7, 5, 1, 2, 4]
[4, 7, 1, 4, 7, 1, 4, 7]
[5, 7, 8, 4, 2, 1, 5, 7]
[7, 4, 1, 7, 4, 1, 7, 4]
[8, 1, 8, 1, 8, 1, 8, 1]
###Markdown
4. 소인수분해 시간과 Fermat Theorem을 이용한 소수 판정 시간 비교- 두번째 방법 : 페르마 정리를 이용한 판정법이고- 세번째 방법 : SAGE에 내장된 소수판정법입니다.(ms는 1,000분의 1초, µs는 1,000,000분의 1초)
###Code
%time factor(95468093486093450983409583409850934850938459083)
n=95468093486093450983409583409850934850938459083
%time Mod(2, n)^(n-1)
n=95468093486093450983409583409850934850938459083
%time is_prime(n)
###Output
CPU times: user 516 ms, sys: 109 ms, total: 625 ms
Wall time: 1.38 s
CPU times: user 0 ns, sys: 0 ns, total: 0 ns
Wall time: 324 µs
CPU times: user 0 ns, sys: 0 ns, total: 0 ns
Wall time: 49.6 µs
|
nlu/colab/Training/binary_text_classification/NLU_training_sentiment_classifier_demo_IMDB.ipynb | ###Markdown
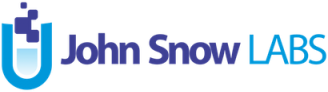[](https://colab.research.google.com/github/JohnSnowLabs/nlu/blob/master/examples/colab/Training/binary_text_classification/NLU_training_sentiment_classifier_demo_IMDB.ipynb) Training a Sentiment Analysis Classifier with NLU 2 class IMDB Movie sentiment classifier trainingWith the [SentimentDL model](https://nlp.johnsnowlabs.com/docs/en/annotatorssentimentdl-multi-class-sentiment-analysis-annotator) from Spark NLP you can achieve State Of the Art results on any multi class text classification problem This notebook showcases the following features : - How to train the deep learning classifier- How to store a pipeline to disk- How to load the pipeline from disk (Enables NLU offline mode)You can achieve these results or even better on this dataset with training data:You can achieve these results or even better on this dataset with test data: 1. Install Java 8 and NLU
###Code
!wget https://setup.johnsnowlabs.com/nlu/colab.sh -O - | bash
import nlu
###Output
--2021-05-05 05:38:30-- https://raw.githubusercontent.com/JohnSnowLabs/nlu/master/scripts/colab_setup.sh
Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.108.133, 185.199.109.133, 185.199.110.133, ...
Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.108.133|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1671 (1.6K) [text/plain]
Saving to: ‘STDOUT’
- 0%[ ] 0 --.-KB/s
Installing NLU 3.0.0 with PySpark 3.0.2 and Spark NLP 3.0.1 for Google Colab ...
- 100%[===================>] 1.63K --.-KB/s in 0s
2021-05-05 05:38:30 (63.2 MB/s) - written to stdout [1671/1671]
[K |████████████████████████████████| 204.8MB 67kB/s
[K |████████████████████████████████| 153kB 73.9MB/s
[K |████████████████████████████████| 204kB 23.9MB/s
[K |████████████████████████████████| 204kB 65.5MB/s
[?25h Building wheel for pyspark (setup.py) ... [?25l[?25hdone
###Markdown
2. Download IMDB datasethttps://www.kaggle.com/lakshmi25npathi/imdb-dataset-of-50k-movie-reviewsIMDB dataset having 50K movie reviews for natural language processing or Text analytics.This is a dataset for binary sentiment classification containing substantially more data than previous benchmark datasets. We provide a set of 25,000 highly polar movie reviews for training and 25,000 for testing. So, predict the number of positive and negative reviews using either classification or deep learning algorithms.For more dataset information, please go through the following link,http://ai.stanford.edu/~amaas/data/sentiment/
###Code
! wget http://ckl-it.de/wp-content/uploads/2021/01/IMDB-Dataset.csv
import pandas as pd
train_path = '/content/IMDB-Dataset.csv'
train_df = pd.read_csv(train_path)
# the text data to use for classification should be in a column named 'text'
# the label column must have name 'y' name be of type str
columns=['text','y']
train_df = train_df[columns]
from sklearn.model_selection import train_test_split
train_df, test_df = train_test_split(train_df, test_size=0.2)
train_df
###Output
_____no_output_____
###Markdown
3. Train Deep Learning Classifier using nlu.load('train.sentiment')You dataset label column should be named 'y' and the feature column with text data should be named 'text'
###Code
import nlu
from sklearn.metrics import classification_report
# load a trainable pipeline by specifying the train. prefix and fit it on a datset with label and text columns
# by default the Universal Sentence Encoder (USE) Sentence embeddings are used for generation
trainable_pipe = nlu.load('train.sentiment')
fitted_pipe = trainable_pipe.fit(train_df.iloc[:50])
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df.iloc[:50],output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['sentiment']))
preds
###Output
tfhub_use download started this may take some time.
Approximate size to download 923.7 MB
[OK!]
sentence_detector_dl download started this may take some time.
Approximate size to download 354.6 KB
[OK!]
precision recall f1-score support
negative 0.82 0.88 0.85 26
neutral 0.00 0.00 0.00 0
positive 0.85 0.71 0.77 24
accuracy 0.80 50
macro avg 0.56 0.53 0.54 50
weighted avg 0.84 0.80 0.81 50
###Markdown
4. Test the fitted pipe on new example
###Code
fitted_pipe.predict('It was one of the best films i have ever watched in my entire life !!')
###Output
_____no_output_____
###Markdown
5. Configure pipe training parameters
###Code
trainable_pipe.print_info()
###Output
The following parameters are configurable for this NLU pipeline (You can copy paste the examples) :
>>> pipe['sentiment_dl'] has settable params:
pipe['sentiment_dl'].setMaxEpochs(1) | Info: Maximum number of epochs to train | Currently set to : 1
pipe['sentiment_dl'].setLr(0.005) | Info: Learning Rate | Currently set to : 0.005
pipe['sentiment_dl'].setBatchSize(64) | Info: Batch size | Currently set to : 64
pipe['sentiment_dl'].setDropout(0.5) | Info: Dropout coefficient | Currently set to : 0.5
pipe['sentiment_dl'].setEnableOutputLogs(True) | Info: Whether to use stdout in addition to Spark logs. | Currently set to : True
pipe['sentiment_dl'].setThreshold(0.6) | Info: The minimum threshold for the final result otheriwse it will be neutral | Currently set to : 0.6
pipe['sentiment_dl'].setThresholdLabel('neutral') | Info: In case the score is less than threshold, what should be the label. Default is neutral. | Currently set to : neutral
>>> pipe['use@tfhub_use'] has settable params:
pipe['use@tfhub_use'].setDimension(512) | Info: Number of embedding dimensions | Currently set to : 512
pipe['use@tfhub_use'].setLoadSP(False) | Info: Whether to load SentencePiece ops file which is required only by multi-lingual models. This is not changeable after it's set with a pretrained model nor it is compatible with Windows. | Currently set to : False
pipe['use@tfhub_use'].setStorageRef('tfhub_use') | Info: unique reference name for identification | Currently set to : tfhub_use
>>> pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'] has settable params:
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setExplodeSentences(False) | Info: whether to explode each sentence into a different row, for better parallelization. Defaults to false. | Currently set to : False
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setStorageRef('SentenceDetectorDLModel_c83c27f46b97') | Info: storage unique identifier | Currently set to : SentenceDetectorDLModel_c83c27f46b97
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setEncoder(com.johnsnowlabs.nlp.annotators.sentence_detector_dl.SentenceDetectorDLEncoder@260a728d) | Info: Data encoder | Currently set to : com.johnsnowlabs.nlp.annotators.sentence_detector_dl.SentenceDetectorDLEncoder@260a728d
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setImpossiblePenultimates(['Bros', 'No', 'al', 'vs', 'etc', 'Fig', 'Dr', 'Prof', 'PhD', 'MD', 'Co', 'Corp', 'Inc', 'bros', 'VS', 'Vs', 'ETC', 'fig', 'dr', 'prof', 'PHD', 'phd', 'md', 'co', 'corp', 'inc', 'Jan', 'Feb', 'Mar', 'Apr', 'Jul', 'Aug', 'Sep', 'Sept', 'Oct', 'Nov', 'Dec', 'St', 'st', 'AM', 'PM', 'am', 'pm', 'e.g', 'f.e', 'i.e']) | Info: Impossible penultimates | Currently set to : ['Bros', 'No', 'al', 'vs', 'etc', 'Fig', 'Dr', 'Prof', 'PhD', 'MD', 'Co', 'Corp', 'Inc', 'bros', 'VS', 'Vs', 'ETC', 'fig', 'dr', 'prof', 'PHD', 'phd', 'md', 'co', 'corp', 'inc', 'Jan', 'Feb', 'Mar', 'Apr', 'Jul', 'Aug', 'Sep', 'Sept', 'Oct', 'Nov', 'Dec', 'St', 'st', 'AM', 'PM', 'am', 'pm', 'e.g', 'f.e', 'i.e']
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setModelArchitecture('cnn') | Info: Model architecture (CNN) | Currently set to : cnn
>>> pipe['document_assembler'] has settable params:
pipe['document_assembler'].setCleanupMode('shrink') | Info: possible values: disabled, inplace, inplace_full, shrink, shrink_full, each, each_full, delete_full | Currently set to : shrink
###Markdown
6. Retrain with new parameters
###Code
# Train longer!
trainable_pipe = nlu.load('train.sentiment')
trainable_pipe['trainable_sentiment_dl'].setMaxEpochs(5)
fitted_pipe = trainable_pipe.fit(train_df.iloc[:50])
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df.iloc[:50],output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['sentiment']))
preds
###Output
precision recall f1-score support
negative 0.92 0.92 0.92 26
neutral 0.00 0.00 0.00 0
positive 1.00 0.75 0.86 24
accuracy 0.84 50
macro avg 0.64 0.56 0.59 50
weighted avg 0.96 0.84 0.89 50
###Markdown
7. Try training with different Embeddings
###Code
# We can use nlu.print_components(action='embed_sentence') to see every possibler sentence embedding we could use. Lets use bert!
nlu.print_components(action='embed_sentence')
trainable_pipe = nlu.load('en.embed_sentence.small_bert_L12_768 train.sentiment')
# We need to train longer and user smaller LR for NON-USE based sentence embeddings usually
# We could tune the hyperparameters further with hyperparameter tuning methods like gridsearch
# Also longer training gives more accuracy
trainable_pipe['trainable_sentiment_dl'].setMaxEpochs(120)
trainable_pipe['trainable_sentiment_dl'].setLr(0.0005)
fitted_pipe = trainable_pipe.fit(train_df)
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df,output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['sentiment']))
#preds
###Output
sent_small_bert_L12_768 download started this may take some time.
Approximate size to download 392.9 MB
[OK!]
sentence_detector_dl download started this may take some time.
Approximate size to download 354.6 KB
[OK!]
precision recall f1-score support
negative 0.87 0.77 0.82 988
neutral 0.00 0.00 0.00 0
positive 0.85 0.83 0.84 1012
accuracy 0.80 2000
macro avg 0.57 0.53 0.55 2000
weighted avg 0.86 0.80 0.83 2000
###Markdown
7.1 evaluate on Test Data
###Code
preds = fitted_pipe.predict(test_df,output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['sentiment']))
###Output
precision recall f1-score support
negative 0.85 0.75 0.80 246
neutral 0.00 0.00 0.00 0
positive 0.84 0.81 0.83 254
accuracy 0.78 500
macro avg 0.56 0.52 0.54 500
weighted avg 0.85 0.78 0.81 500
###Markdown
8. Lets save the model
###Code
stored_model_path = './models/classifier_dl_trained'
fitted_pipe.save(stored_model_path)
###Output
Stored model in ./models/classifier_dl_trained
###Markdown
9. Lets load the model from HDD.This makes Offlien NLU usage possible! You need to call nlu.load(path=path_to_the_pipe) to load a model/pipeline from disk.
###Code
hdd_pipe = nlu.load(path=stored_model_path)
preds = hdd_pipe.predict('It was one of the best films i have ever watched in my entire life !!')
preds
hdd_pipe.print_info()
###Output
_____no_output_____
###Markdown
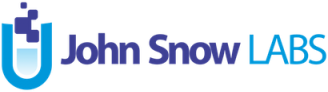[](https://colab.research.google.com/github/JohnSnowLabs/nlu/blob/master/examples/colab/Training/binary_text_classification/NLU_training_sentiment_classifier_demo_IMDB.ipynb) Training a Sentiment Analysis Classifier with NLU 2 class IMDB Movie sentiment classifier trainingWith the [SentimentDL model](https://nlp.johnsnowlabs.com/docs/en/annotatorssentimentdl-multi-class-sentiment-analysis-annotator) from Spark NLP you can achieve State Of the Art results on any multi class text classification problem This notebook showcases the following features : - How to train the deep learning classifier- How to store a pipeline to disk- How to load the pipeline from disk (Enables NLU offline mode)You can achieve these results or even better on this dataset with training data:You can achieve these results or even better on this dataset with test data: 1. Install Java 8 and NLU
###Code
!wget https://setup.johnsnowlabs.com/nlu/colab.sh -O - | bash
import nlu
###Output
--2021-05-05 05:38:30-- https://raw.githubusercontent.com/JohnSnowLabs/nlu/master/scripts/colab_setup.sh
Resolving raw.githubusercontent.com (raw.githubusercontent.com)... 185.199.108.133, 185.199.109.133, 185.199.110.133, ...
Connecting to raw.githubusercontent.com (raw.githubusercontent.com)|185.199.108.133|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 1671 (1.6K) [text/plain]
Saving to: ‘STDOUT’
- 0%[ ] 0 --.-KB/s
Installing NLU 3.0.0 with PySpark 3.0.2 and Spark NLP 3.0.1 for Google Colab ...
- 100%[===================>] 1.63K --.-KB/s in 0s
2021-05-05 05:38:30 (63.2 MB/s) - written to stdout [1671/1671]
[K |████████████████████████████████| 204.8MB 67kB/s
[K |████████████████████████████████| 153kB 73.9MB/s
[K |████████████████████████████████| 204kB 23.9MB/s
[K |████████████████████████████████| 204kB 65.5MB/s
[?25h Building wheel for pyspark (setup.py) ... [?25l[?25hdone
###Markdown
2. Download IMDB datasethttps://www.kaggle.com/lakshmi25npathi/imdb-dataset-of-50k-movie-reviewsIMDB dataset having 50K movie reviews for natural language processing or Text analytics.This is a dataset for binary sentiment classification containing substantially more data than previous benchmark datasets. We provide a set of 25,000 highly polar movie reviews for training and 25,000 for testing. So, predict the number of positive and negative reviews using either classification or deep learning algorithms.For more dataset information, please go through the following link,http://ai.stanford.edu/~amaas/data/sentiment/
###Code
! wget http://ckl-it.de/wp-content/uploads/2021/01/IMDB-Dataset.csv
import pandas as pd
train_path = '/content/IMDB-Dataset.csv'
train_df = pd.read_csv(train_path)
# the text data to use for classification should be in a column named 'text'
# the label column must have name 'y' name be of type str
columns=['text','y']
train_df = train_df[columns]
from sklearn.model_selection import train_test_split
train_df, test_df = train_test_split(train_df, test_size=0.2)
train_df
###Output
_____no_output_____
###Markdown
3. Train Deep Learning Classifier using nlu.load('train.sentiment')You dataset label column should be named 'y' and the feature column with text data should be named 'text'
###Code
import nlu
from sklearn.metrics import classification_report
# load a trainable pipeline by specifying the train. prefix and fit it on a datset with label and text columns
# by default the Universal Sentence Encoder (USE) Sentence embeddings are used for generation
trainable_pipe = nlu.load('train.sentiment')
fitted_pipe = trainable_pipe.fit(train_df.iloc[:50])
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df.iloc[:50],output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['trained_sentiment']))
preds
###Output
tfhub_use download started this may take some time.
Approximate size to download 923.7 MB
[OK!]
sentence_detector_dl download started this may take some time.
Approximate size to download 354.6 KB
[OK!]
precision recall f1-score support
negative 0.82 0.88 0.85 26
neutral 0.00 0.00 0.00 0
positive 0.85 0.71 0.77 24
accuracy 0.80 50
macro avg 0.56 0.53 0.54 50
weighted avg 0.84 0.80 0.81 50
###Markdown
4. Test the fitted pipe on new example
###Code
fitted_pipe.predict('It was one of the best films i have ever watched in my entire life !!')
###Output
_____no_output_____
###Markdown
5. Configure pipe training parameters
###Code
trainable_pipe.print_info()
###Output
The following parameters are configurable for this NLU pipeline (You can copy paste the examples) :
>>> pipe['sentiment_dl'] has settable params:
pipe['sentiment_dl'].setMaxEpochs(1) | Info: Maximum number of epochs to train | Currently set to : 1
pipe['sentiment_dl'].setLr(0.005) | Info: Learning Rate | Currently set to : 0.005
pipe['sentiment_dl'].setBatchSize(64) | Info: Batch size | Currently set to : 64
pipe['sentiment_dl'].setDropout(0.5) | Info: Dropout coefficient | Currently set to : 0.5
pipe['sentiment_dl'].setEnableOutputLogs(True) | Info: Whether to use stdout in addition to Spark logs. | Currently set to : True
pipe['sentiment_dl'].setThreshold(0.6) | Info: The minimum threshold for the final result otheriwse it will be neutral | Currently set to : 0.6
pipe['sentiment_dl'].setThresholdLabel('neutral') | Info: In case the score is less than threshold, what should be the label. Default is neutral. | Currently set to : neutral
>>> pipe['use@tfhub_use'] has settable params:
pipe['use@tfhub_use'].setDimension(512) | Info: Number of embedding dimensions | Currently set to : 512
pipe['use@tfhub_use'].setLoadSP(False) | Info: Whether to load SentencePiece ops file which is required only by multi-lingual models. This is not changeable after it's set with a pretrained model nor it is compatible with Windows. | Currently set to : False
pipe['use@tfhub_use'].setStorageRef('tfhub_use') | Info: unique reference name for identification | Currently set to : tfhub_use
>>> pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'] has settable params:
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setExplodeSentences(False) | Info: whether to explode each sentence into a different row, for better parallelization. Defaults to false. | Currently set to : False
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setStorageRef('SentenceDetectorDLModel_c83c27f46b97') | Info: storage unique identifier | Currently set to : SentenceDetectorDLModel_c83c27f46b97
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setEncoder(com.johnsnowlabs.nlp.annotators.sentence_detector_dl.SentenceDetectorDLEncoder@260a728d) | Info: Data encoder | Currently set to : com.johnsnowlabs.nlp.annotators.sentence_detector_dl.SentenceDetectorDLEncoder@260a728d
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setImpossiblePenultimates(['Bros', 'No', 'al', 'vs', 'etc', 'Fig', 'Dr', 'Prof', 'PhD', 'MD', 'Co', 'Corp', 'Inc', 'bros', 'VS', 'Vs', 'ETC', 'fig', 'dr', 'prof', 'PHD', 'phd', 'md', 'co', 'corp', 'inc', 'Jan', 'Feb', 'Mar', 'Apr', 'Jul', 'Aug', 'Sep', 'Sept', 'Oct', 'Nov', 'Dec', 'St', 'st', 'AM', 'PM', 'am', 'pm', 'e.g', 'f.e', 'i.e']) | Info: Impossible penultimates | Currently set to : ['Bros', 'No', 'al', 'vs', 'etc', 'Fig', 'Dr', 'Prof', 'PhD', 'MD', 'Co', 'Corp', 'Inc', 'bros', 'VS', 'Vs', 'ETC', 'fig', 'dr', 'prof', 'PHD', 'phd', 'md', 'co', 'corp', 'inc', 'Jan', 'Feb', 'Mar', 'Apr', 'Jul', 'Aug', 'Sep', 'Sept', 'Oct', 'Nov', 'Dec', 'St', 'st', 'AM', 'PM', 'am', 'pm', 'e.g', 'f.e', 'i.e']
pipe['deep_sentence_detector@SentenceDetectorDLModel_c83c27f46b97'].setModelArchitecture('cnn') | Info: Model architecture (CNN) | Currently set to : cnn
>>> pipe['document_assembler'] has settable params:
pipe['document_assembler'].setCleanupMode('shrink') | Info: possible values: disabled, inplace, inplace_full, shrink, shrink_full, each, each_full, delete_full | Currently set to : shrink
###Markdown
6. Retrain with new parameters
###Code
# Train longer!
trainable_pipe['sentiment_dl'].setMaxEpochs(5)
fitted_pipe = trainable_pipe.fit(train_df.iloc[:50])
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df.iloc[:50],output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['trained_sentiment']))
preds
###Output
precision recall f1-score support
negative 0.92 0.92 0.92 26
neutral 0.00 0.00 0.00 0
positive 1.00 0.75 0.86 24
accuracy 0.84 50
macro avg 0.64 0.56 0.59 50
weighted avg 0.96 0.84 0.89 50
###Markdown
7. Try training with different Embeddings
###Code
# We can use nlu.print_components(action='embed_sentence') to see every possibler sentence embedding we could use. Lets use bert!
nlu.print_components(action='embed_sentence')
trainable_pipe = nlu.load('en.embed_sentence.small_bert_L12_768 train.sentiment')
# We need to train longer and user smaller LR for NON-USE based sentence embeddings usually
# We could tune the hyperparameters further with hyperparameter tuning methods like gridsearch
# Also longer training gives more accuracy
trainable_pipe['sentiment_dl'].setMaxEpochs(120)
trainable_pipe['sentiment_dl'].setLr(0.0005)
fitted_pipe = trainable_pipe.fit(train_df)
# predict with the trainable pipeline on dataset and get predictions
preds = fitted_pipe.predict(train_df,output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['trained_sentiment']))
#preds
###Output
sent_small_bert_L12_768 download started this may take some time.
Approximate size to download 392.9 MB
[OK!]
sentence_detector_dl download started this may take some time.
Approximate size to download 354.6 KB
[OK!]
precision recall f1-score support
negative 0.87 0.77 0.82 988
neutral 0.00 0.00 0.00 0
positive 0.85 0.83 0.84 1012
accuracy 0.80 2000
macro avg 0.57 0.53 0.55 2000
weighted avg 0.86 0.80 0.83 2000
###Markdown
7.1 evaluate on Test Data
###Code
preds = fitted_pipe.predict(test_df,output_level='document')
#sentence detector that is part of the pipe generates sone NaNs. lets drop them first
preds.dropna(inplace=True)
print(classification_report(preds['y'], preds['trained_sentiment']))
###Output
precision recall f1-score support
negative 0.85 0.75 0.80 246
neutral 0.00 0.00 0.00 0
positive 0.84 0.81 0.83 254
accuracy 0.78 500
macro avg 0.56 0.52 0.54 500
weighted avg 0.85 0.78 0.81 500
###Markdown
8. Lets save the model
###Code
stored_model_path = './models/classifier_dl_trained'
fitted_pipe.save(stored_model_path)
###Output
Stored model in ./models/classifier_dl_trained
###Markdown
9. Lets load the model from HDD.This makes Offlien NLU usage possible! You need to call nlu.load(path=path_to_the_pipe) to load a model/pipeline from disk.
###Code
hdd_pipe = nlu.load(path=stored_model_path)
preds = hdd_pipe.predict('It was one of the best films i have ever watched in my entire life !!')
preds
hdd_pipe.print_info()
###Output
_____no_output_____ |
ontology/ontol_sim_nn.ipynb | ###Markdown
Load brain and text data
###Code
act_bin = utilities.load_coordinates().astype(float)
print("Document N={}, Structure N={}".format(act_bin.shape[0], act_bin.shape[1]))
version = 190325
dtm_bin = utilities.load_doc_term_matrix(version=version, binarize=True)
print("Document N={}, Term N={}".format(dtm_bin.shape[0], dtm_bin.shape[1]))
frameworks = ["data-driven", "rdoc", "dsm"]
list_suffixes = ["", "_opsim", "_opsim"]
circuit_suffixes = ["_nn", "", ""]
lists, circuits = {}, {}
for fw, list_suffix, circuit_suffix in zip(frameworks, list_suffixes, circuit_suffixes):
lists[fw], circuits[fw] = utilities.load_framework(fw, suffix=list_suffix, clf=circuit_suffix)
scores = {fw: utilities.score_lists(lists[fw], dtm_bin, label_var="DOMAIN") for fw in frameworks}
words = []
for fw in frameworks:
words += list(lists[fw]["TOKEN"])
words = sorted(list(set(words)))
structures = list(act_bin.columns)
print("Term N={}, Structure N={}".format(len(words), len(structures)))
domains = {fw: list(OrderedDict.fromkeys(lists[fw]["DOMAIN"])) for fw in frameworks}
pmids = act_bin.index.intersection(scores["rdoc"].index).intersection(scores["dsm"].index)
len(pmids)
for fw in frameworks:
scores[fw] = scores[fw].loc[pmids]
dtm_bin = dtm_bin.loc[pmids, words]
act_bin = act_bin.loc[pmids, structures]
###Output
_____no_output_____
###Markdown
Load frameworks
###Code
systems = {}
for fw in frameworks:
fw_df = pd.DataFrame(0.0, index=words+structures, columns=domains[fw])
for dom in domains[fw]:
for word in lists[fw].loc[lists[fw]["DOMAIN"] == dom, "TOKEN"]:
fw_df.loc[word, dom] = 1.0
for struct in structures:
fw_df.loc[struct, dom] = circuits[fw].loc[struct, dom]
fw_df[fw_df > 0.0] = 1.0
systems[fw] = fw_df
###Output
_____no_output_____
###Markdown
Similarity of RDoC and data-driven systems Observed values
###Code
from scipy.spatial.distance import dice, cdist
def compute_sim_obs(fw):
sims = pd.DataFrame(index=domains["data-driven"], columns=domains[fw])
for k in domains["data-driven"]:
for r in domains[fw]:
sims.loc[k,r] = 1.0 - dice(systems["data-driven"][k], systems[fw][r])
return sims
sims = compute_sim_obs("rdoc")
sims
###Output
_____no_output_____
###Markdown
Null distribution
###Code
def compute_sim_null(fw, n_iter=1000):
sims_null = np.empty((len(domains["data-driven"]), len(domains[fw]), n_iter))
for n in range(n_iter):
null = np.random.choice(words+structures,
size=len(words+structures), replace=False)
sims_null[:,:,n] = 1.0 - cdist(systems["data-driven"].loc[null].values.T,
systems[fw].values.T, metric="dice")
if n % (float(n_iter) / 10.0) == 0:
print("Iteration {}".format(n))
return sims_null
sims_null = compute_sim_null("rdoc")
###Output
Iteration 0
Iteration 100
Iteration 200
Iteration 300
Iteration 400
Iteration 500
Iteration 600
Iteration 700
Iteration 800
Iteration 900
###Markdown
False discovery rate
###Code
from statsmodels.stats.multitest import multipletests
def compute_sim_fdr(fw, sims, sims_null):
n_iter = sims_null.shape[2]
pvals = pd.DataFrame(index=domains["data-driven"], columns=domains[fw])
for i, k in enumerate(domains["data-driven"]):
for j, r in enumerate(domains[fw]):
pvals.loc[k,r] = np.sum(sims_null[i,j,:] > sims.loc[k,r]) / float(n_iter)
fdrs = multipletests(pvals.values.ravel(), method="fdr_bh")[1]
fdrs = np.reshape(fdrs, pvals.shape)
fdrs = pd.DataFrame(fdrs, index=domains["data-driven"], columns=domains[fw])
return fdrs
fdrs = compute_sim_fdr("rdoc", sims, sims_null)
fdrs
def compute_sim_star(fw, fdrs):
stars = pd.DataFrame("", index=domains["data-driven"], columns=domains[fw])
for k in domains["data-driven"]:
for r in domains[fw]:
fdr = fdrs.loc[k,r]
if fdr < 0.05:
stars.loc[k,r] = "*"
if fdr < 0.01:
stars.loc[k,r] = "**"
if fdr < 0.001:
stars.loc[k,r] = "***"
return stars
stars = compute_sim_star("rdoc", fdrs)
stars
###Output
_____no_output_____
###Markdown
Weights for figure
###Code
sims[fdrs < 0.05] * 40
###Output
_____no_output_____
###Markdown
Similarity of DSM and data-driven systems Observed values
###Code
sims = compute_sim_obs("dsm")
sims
###Output
_____no_output_____
###Markdown
Null distribution
###Code
sims_null = compute_sim_null("dsm")
###Output
Iteration 0
Iteration 100
Iteration 200
Iteration 300
Iteration 400
Iteration 500
Iteration 600
Iteration 700
Iteration 800
Iteration 900
###Markdown
False discovery rate
###Code
fdrs = compute_sim_fdr("dsm", sims, sims_null)
fdrs
stars = compute_sim_star("dsm", fdrs)
stars
###Output
_____no_output_____
###Markdown
Weights for figure
###Code
sims[fdrs < 0.05] * 40
###Output
_____no_output_____ |
eda_seattle_airbnb_data.ipynb | ###Markdown
Exploratory Data Analysis on Seattle Airbnb Data Table of Contents Introduction Data Wrangling Exploratory Data Analysis Conclusion IntroductionIn this project, exploratory data analysis was performed on Seattle Airbnb data with the aim of answering some questions related to the price of accomodations on Airbnb. Specifically, the data was used to answer the following questions.- How does price vary with different types of properties?- How do review scores affect the price?- How does price fluctuate over different months?The data can be found at [here](https://www.kaggle.com/airbnb/seattle/data).
###Code
# Import libraries
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
# Load data from CSV files
df_calendar = pd.read_csv('calendar.csv')
df_listings = pd.read_csv('listings.csv')
df_calendar.head()
df_listings.head()
###Output
_____no_output_____
###Markdown
Data WranglingData wrangling is performed on the dataset to clean the data, as well as to understand the data better. There are two steps to be carried out for data wrangling, which are data assessing and data cleaning. To answer the questions stated in the introduction, only certain columns of listings.csv and calendar.csv are needed, and therefore cleaned. Data AssessingData is assessed using programmatic assessment to look for data quality and tidiness issues
###Code
# Let check the data types of variables that will be used in subsequent analyses
df_listings.info()
df_calendar.info()
# Check if there is any duplicate data
print('Number of duplicates in listing.csv: {}'.format(df_listings.duplicated().sum()))
print('Number of duplicates in calendar.csv: {}'.format(df_calendar.duplicated().sum()))
# Check if there is any abnormal values in 'property_type' column of listing.csv
df_listings['property_type'].value_counts()
# Check if there is any abnormal values in 'review_scores_rating' column of listing.csv
df_listings[['review_scores_rating']].describe()
# Get columns of listing.csv with more than 25 % of their values missing
df_listings.columns[df_listings.isnull().mean() > 0.25 ]
# Check if there is any abnormal values in 'date' column of calendar.csv
df_calendar['date'].unique()
# Check if there is any abnormal values in 'available' column of calendar.csv
df_calendar['available'].unique()
###Output
_____no_output_____
###Markdown
Assessment Summary- There is no duplicate data.- There are missing values in 'review_scores_rating' and 'property_type' (they are among the columns of interest for subsequent analyses), but the percentage of missing values in these columns is not more than 25%. Since these missing values will not affect our subsequent analyses, they will be ignored.- The data type of 'price' column in listings.csv and calendar.csv should be float instead of string.- The data type of 'date' column in calendar.csv should datetime instead of string.- Day in the dates of 'date' column should be removed to reduce the number of unique dates for easier analyses and vizualization. For example, 2016-12-01 should become 2016-12.- 'property_type' column from listings.csv should be added to calendar.csv for subsequent analysis. Data CleaningData is cleaned based on the issues that were detected during data quality and tidiness assessment, as summarized in assessment summary.
###Code
# Make a copy of the dataframe
df_listings_clean = df_listings.copy()
df_calendar_clean = df_calendar.copy()
###Output
_____no_output_____
###Markdown
Step 1: Remove '$' and ',' from 'price' column in listings.csv and calendar.csv and convert it to float
###Code
# Remove '$' and ',' in 'price' column of listings.csv and calendar.csv
df_listings_clean['price'] = df_listings_clean['price'].str.replace('$', '').str.replace(',', '')
df_calendar_clean['price'] = df_calendar_clean['price'].str.replace('$', '').str.replace(',', '')
# Convert data type of 'price' column to float
df_listings_clean['price'] = df_listings_clean['price'].astype(float)
df_calendar_clean['price'] = df_calendar_clean['price'].astype(float)
# Verify that Step 1 is carried out correctly
df_listings_clean[['price']].info()
df_calendar_clean[['price']].info()
df_listings_clean['price'].describe()
###Output
_____no_output_____
###Markdown
Step 2: Convert data type of 'date' column in calendar.csv to 'datetime' and remove day from dates
###Code
# Convert data type of 'date' column to datetime
df_calendar_clean['date'] = pd.to_datetime(df_calendar_clean['date'],
format='%Y-%m-%d').apply(lambda x: x.strftime('%Y-%m'))
# Verify that Step 2 is carried out correctly
df_calendar_clean['date'].unique()
###Output
_____no_output_____
###Markdown
Step 3: Join 'property_type' column from listings.csv with calendar.csv
###Code
# Join 'property_type' column from listings.csv with calendar.csv
df_calendar_clean = df_calendar_clean.merge(df_listings_clean[['id', 'property_type']],
how='left', left_on='listing_id', right_on='id')
# Remove 'id' column
df_calendar_clean = df_calendar_clean.drop(['id'], axis=1)
# Verify that Step 3 is carried out correctly
df_calendar_clean.head(10)
###Output
_____no_output_____
###Markdown
Exploratory Data Analysis Question 1: How does price vary with different types of properties?
###Code
# Define the order of property types in box plot based on their median price.
# Median is chosen over mean is because the price of certain properties is not normailly distributed
boxplot_order = df_listings_clean.groupby('property_type')['price'].median().sort_values(ascending=False).index
# Use box plot to study the relationship between 'property_type' and 'price'
plt.figure(figsize=[10,10])
sns.boxplot(data=df_listings_clean, x='property_type', y='price', order=boxplot_order);
plt.title('Distribution of price for each type of property')
plt.xlabel('property_type');
plt.xticks(rotation=90);
plt.ylabel('price');
###Output
_____no_output_____
###Markdown
As there are many different types of properties shown on the box plot, it is difficult to digest the myriad of information that is delivered by the box plot. Thus, let's try to reduce the number of property types by considering some property types as others, so that there are fewer different property types on the box plot. Based on the results obtained during data wrangling, the number of each property type in the dataset is as follows:- House: 1733- Apartment: 1708- Townhouse: 118- Condominium: 91- Loft: 40- Bed & Breakfast: 37- Other: 22- Cabin: 21- Bungalow: 13- Camper/RV: 13- Boat: 8- Tent: 5- Treehouse: 3- Chalet: 2- Dorm: 2- Yurt: 1'Tent', 'Treehouse' and 'Dorm' each have less than 10 instances in the dataset. Furthermore, they have similar price as 'Other', as shown on the rightmost region of the box plot above. Thus, let's consider all of them as Other
###Code
# Replace 'Boat', 'Tent', 'Treehouse', 'Chalet', 'Dorm' and 'Yurt' with 'Other'
replace_func = lambda x: 'Other' if x in ['Tent', 'Treehouse', 'Dorm'] else x
df_listings_clean['property_type'] = df_listings_clean['property_type'].apply(replace_func)
# Define the order of property types in box plot based on their median price
boxplot_order = df_listings_clean.groupby('property_type')['price'].mean().sort_values(ascending=False).index
# Use box plot to study the relationship between 'property_type' and 'price'
plt.figure(figsize=[10,10])
sns.boxplot(data=df_listings_clean, x='property_type', y='price', order=boxplot_order);
plt.title('Distribution of price for each type of property')
plt.xlabel('property_type');
plt.xticks(rotation=90);
plt.ylabel('price');
###Output
_____no_output_____
###Markdown
>Finding(s): 'Boat' has the highest the median price and 'Other' has the lowest median price among all the different types of properties. As indicated by the box plot, there is a substantial amount of outliers in the price for 'House' and 'Apartment', as compared to other types of properties. This suggests that the variation in the price of 'House' and 'Apartment' is huge. Median is chosen over mean because the price of certain types of properties is not normally distributed. Question 2: How do review scores affect the price?
###Code
# Use scatter plot to study the relationship between 'review_scores_rating' and 'price'
plt.figure(figsize=[10,10])
sns.scatterplot(data=df_listings_clean, x='review_scores_rating', y='price');
plt.title('Relationship between review_scores_rating and price');
plt.xlabel('review_scores_rating');
plt.ylabel('price');
###Output
_____no_output_____
###Markdown
>Finding(s): Accommodations with higher price tend to have higher review_scores_rating. However, higher review_scores_rating does not always lead to higher price, as shown by the variation in price (0 - 1000) for accommodations with review_scores_rating above 90. In addition to that, lower review_scores_rating does not always to lead to lower price, as the price of accommodations with review_scores_rating lower than 50 is higher than the price of a handful of accommodations with much higher review_scores_rating. It suggests that review_scores_rating will affect the price, but the influence is minimal.Let's delve deeper into the relationship between review_scores_rating and price by generating the same scatter plot for each type of the property separately.
###Code
# Use scatter plot to study the relationship between 'review_scores_rating' and 'price' for each type of property
g = sns.FacetGrid(df_listings_clean, col='property_type', col_wrap=3, height=4, aspect=1);
g.fig.suptitle('Relationship between review_scores_rating and price', y=1);
g.map(sns.scatterplot, 'review_scores_rating', 'price');
###Output
_____no_output_____
###Markdown
>Finding(s): The scatter plots for Apartment and House support the two findings above. For other types of property, it was shown that most of them are priced around or below 200, and they have review_scores_rating that are higher than 80. However, the amount of data for these types of properties are substantially less than 'Apartment' and 'House'. Thus, the findings for these types of properties might not be accurate. Question 3: How does price fluctuate over different months?
###Code
# Calculate average accommodation price for each month
monthly_price = df_calendar_clean.groupby(['date'], as_index=False)['price'].mean()
# Use line plot to study the trend of average accommodation price over different months
plt.figure(figsize=[8,6])
sns.lineplot(data=monthly_price , x='date', y='price');
plt.title('Average accommodation price');
plt.xlabel('date');
plt.xticks(rotation=90)
plt.ylabel('average price');
###Output
_____no_output_____
###Markdown
>Finding(s): The line plot above shows that average accommodation price was at its lowest in January 2016. From then on, average accommodation price rose steadily until July 2016 to reach its peak. After the peak, average accommodation price started declining. The line plot shows that the average price of accommodations in Seattle is the highest between July 2016 and August 2016.Let's delve deeper into how average accommodation price fluctuates by generating the same line plot for each type of the property separately.
###Code
# Calculate average accommodation price of each type of property for each month
monthly_price_property = df_calendar_clean.groupby(['property_type', 'date'], as_index=False)['price'].mean()
# Use line plot to study the trend of average accommodation price over different months for each type of property
g = sns.FacetGrid(monthly_price_property , col='property_type', col_wrap=3, height=4, aspect=1.5);
g.map(sns.lineplot, 'date', 'price');
g.fig.suptitle('Average accommodation price', y=1);
g.set_xticklabels(rotation=90);
###Output
_____no_output_____
###Markdown
>Finding(s): In general, the average price of all the property types is slightly higher between June 2016 and August 2016, which is during summer in Seattle. This shows that accommodations in Seattle are more expensive during summer. The increase in price during summer is likely caused by the surging demand of accommodations during summer vacation.Let's plot the monthly occupancy of accommodations in Seattle to check if there is a surging demand of accommodations during summer.
###Code
# Calculate monthly occupancy
occupancy = df_calendar_clean.groupby(['available', 'date'], as_index=False)['listing_id'].count()
# Use line plot to study the fluctuation in occupancy
plt.figure(figsize=[8,6])
sns.lineplot(data=occupancy , x='date', y='listing_id', hue='available');
plt.title('Accommodation occupancy');
plt.xlabel('date');
plt.xticks(rotation=90);
plt.ylabel('count');
plt.legend(['Unavailable', 'Available']);
###Output
_____no_output_____ |
Reducer/convert_vector_to_raster.ipynb | ###Markdown
View source on GitHub Notebook Viewer Run in binder Run in Google Colab Install Earth Engine APIInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geehydro](https://github.com/giswqs/geehydro). The **geehydro** Python package builds on the [folium](https://github.com/python-visualization/folium) package and implements several methods for displaying Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, `Map.centerObject()`, and `Map.setOptions()`.The magic command `%%capture` can be used to hide output from a specific cell.
###Code
# %%capture
# !pip install earthengine-api
# !pip install geehydro
###Output
_____no_output_____
###Markdown
Import libraries
###Code
import ee
import folium
import geehydro
###Output
_____no_output_____
###Markdown
Authenticate and initialize Earth Engine API. You only need to authenticate the Earth Engine API once. Uncomment the line `ee.Authenticate()` if you are running this notebook for this first time or if you are getting an authentication error.
###Code
# ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map This step creates an interactive map using [folium](https://github.com/python-visualization/folium). The default basemap is the OpenStreetMap. Additional basemaps can be added using the `Map.setOptions()` function. The optional basemaps can be `ROADMAP`, `SATELLITE`, `HYBRID`, `TERRAIN`, or `ESRI`.
###Code
Map = folium.Map(location=[40, -100], zoom_start=4)
Map.setOptions('HYBRID')
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.setControlVisibility(layerControl=True, fullscreenControl=True, latLngPopup=True)
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in Google Colab Install Earth Engine API and geemapInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geemap](https://geemap.org). The **geemap** Python package is built upon the [ipyleaflet](https://github.com/jupyter-widgets/ipyleaflet) and [folium](https://github.com/python-visualization/folium) packages and implements several methods for interacting with Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, and `Map.centerObject()`.The following script checks if the geemap package has been installed. If not, it will install geemap, which automatically installs its [dependencies](https://github.com/giswqs/geemapdependencies), including earthengine-api, folium, and ipyleaflet.
###Code
# Installs geemap package
import subprocess
try:
import geemap
except ImportError:
print('Installing geemap ...')
subprocess.check_call(["python", '-m', 'pip', 'install', 'geemap'])
import ee
import geemap
###Output
_____no_output_____
###Markdown
Create an interactive map The default basemap is `Google Maps`. [Additional basemaps](https://github.com/giswqs/geemap/blob/master/geemap/basemaps.py) can be added using the `Map.add_basemap()` function.
###Code
Map = geemap.Map(center=[40,-100], zoom=4)
Map
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Add Earth Engine dataset
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.addLayerControl() # This line is not needed for ipyleaflet-based Map.
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in binder Run in Google Colab Install Earth Engine API and geemapInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geemap](https://github.com/giswqs/geemap). The **geemap** Python package is built upon the [ipyleaflet](https://github.com/jupyter-widgets/ipyleaflet) and [folium](https://github.com/python-visualization/folium) packages and implements several methods for interacting with Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, and `Map.centerObject()`.The following script checks if the geemap package has been installed. If not, it will install geemap, which automatically installs its [dependencies](https://github.com/giswqs/geemapdependencies), including earthengine-api, folium, and ipyleaflet.**Important note**: A key difference between folium and ipyleaflet is that ipyleaflet is built upon ipywidgets and allows bidirectional communication between the front-end and the backend enabling the use of the map to capture user input, while folium is meant for displaying static data only ([source](https://blog.jupyter.org/interactive-gis-in-jupyter-with-ipyleaflet-52f9657fa7a)). Note that [Google Colab](https://colab.research.google.com/) currently does not support ipyleaflet ([source](https://github.com/googlecolab/colabtools/issues/60issuecomment-596225619)). Therefore, if you are using geemap with Google Colab, you should use [`import geemap.eefolium`](https://github.com/giswqs/geemap/blob/master/geemap/eefolium.py). If you are using geemap with [binder](https://mybinder.org/) or a local Jupyter notebook server, you can use [`import geemap`](https://github.com/giswqs/geemap/blob/master/geemap/geemap.py), which provides more functionalities for capturing user input (e.g., mouse-clicking and moving).
###Code
# Installs geemap package
import subprocess
try:
import geemap
except ImportError:
print('geemap package not installed. Installing ...')
subprocess.check_call(["python", '-m', 'pip', 'install', 'geemap'])
# Checks whether this notebook is running on Google Colab
try:
import google.colab
import geemap.eefolium as emap
except:
import geemap as emap
# Authenticates and initializes Earth Engine
import ee
try:
ee.Initialize()
except Exception as e:
ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map The default basemap is `Google Satellite`. [Additional basemaps](https://github.com/giswqs/geemap/blob/master/geemap/geemap.pyL13) can be added using the `Map.add_basemap()` function.
###Code
Map = emap.Map(center=[40,-100], zoom=4)
Map.add_basemap('ROADMAP') # Add Google Map
Map
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Add Earth Engine dataset
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.addLayerControl() # This line is not needed for ipyleaflet-based Map.
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in Google Colab Install Earth Engine API and geemapInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geemap](https://github.com/giswqs/geemap). The **geemap** Python package is built upon the [ipyleaflet](https://github.com/jupyter-widgets/ipyleaflet) and [folium](https://github.com/python-visualization/folium) packages and implements several methods for interacting with Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, and `Map.centerObject()`.The following script checks if the geemap package has been installed. If not, it will install geemap, which automatically installs its [dependencies](https://github.com/giswqs/geemapdependencies), including earthengine-api, folium, and ipyleaflet.**Important note**: A key difference between folium and ipyleaflet is that ipyleaflet is built upon ipywidgets and allows bidirectional communication between the front-end and the backend enabling the use of the map to capture user input, while folium is meant for displaying static data only ([source](https://blog.jupyter.org/interactive-gis-in-jupyter-with-ipyleaflet-52f9657fa7a)). Note that [Google Colab](https://colab.research.google.com/) currently does not support ipyleaflet ([source](https://github.com/googlecolab/colabtools/issues/60issuecomment-596225619)). Therefore, if you are using geemap with Google Colab, you should use [`import geemap.eefolium`](https://github.com/giswqs/geemap/blob/master/geemap/eefolium.py). If you are using geemap with [binder](https://mybinder.org/) or a local Jupyter notebook server, you can use [`import geemap`](https://github.com/giswqs/geemap/blob/master/geemap/geemap.py), which provides more functionalities for capturing user input (e.g., mouse-clicking and moving).
###Code
# Installs geemap package
import subprocess
try:
import geemap
except ImportError:
print('geemap package not installed. Installing ...')
subprocess.check_call(["python", '-m', 'pip', 'install', 'geemap'])
# Checks whether this notebook is running on Google Colab
try:
import google.colab
import geemap.eefolium as emap
except:
import geemap as emap
# Authenticates and initializes Earth Engine
import ee
try:
ee.Initialize()
except Exception as e:
ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map The default basemap is `Google Satellite`. [Additional basemaps](https://github.com/giswqs/geemap/blob/master/geemap/geemap.pyL13) can be added using the `Map.add_basemap()` function.
###Code
Map = emap.Map(center=[40,-100], zoom=4)
Map.add_basemap('ROADMAP') # Add Google Map
Map
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Add Earth Engine dataset
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.addLayerControl() # This line is not needed for ipyleaflet-based Map.
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in binder Run in Google Colab Install Earth Engine APIInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geehydro](https://github.com/giswqs/geehydro). The **geehydro** Python package builds on the [folium](https://github.com/python-visualization/folium) package and implements several methods for displaying Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, `Map.centerObject()`, and `Map.setOptions()`.The magic command `%%capture` can be used to hide output from a specific cell. Uncomment these lines if you are running this notebook for the first time.
###Code
# %%capture
# !pip install earthengine-api
# !pip install geehydro
###Output
_____no_output_____
###Markdown
Import libraries
###Code
import ee
import folium
import geehydro
###Output
_____no_output_____
###Markdown
Authenticate and initialize Earth Engine API. You only need to authenticate the Earth Engine API once. Uncomment the line `ee.Authenticate()` if you are running this notebook for the first time or if you are getting an authentication error.
###Code
# ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map This step creates an interactive map using [folium](https://github.com/python-visualization/folium). The default basemap is the OpenStreetMap. Additional basemaps can be added using the `Map.setOptions()` function. The optional basemaps can be `ROADMAP`, `SATELLITE`, `HYBRID`, `TERRAIN`, or `ESRI`.
###Code
Map = folium.Map(location=[40, -100], zoom_start=4)
Map.setOptions('HYBRID')
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.setControlVisibility(layerControl=True, fullscreenControl=True, latLngPopup=True)
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in Google Colab Install Earth Engine API and geemapInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geemap](https://github.com/giswqs/geemap). The **geemap** Python package is built upon the [ipyleaflet](https://github.com/jupyter-widgets/ipyleaflet) and [folium](https://github.com/python-visualization/folium) packages and implements several methods for interacting with Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, and `Map.centerObject()`.The following script checks if the geemap package has been installed. If not, it will install geemap, which automatically installs its [dependencies](https://github.com/giswqs/geemapdependencies), including earthengine-api, folium, and ipyleaflet.**Important note**: A key difference between folium and ipyleaflet is that ipyleaflet is built upon ipywidgets and allows bidirectional communication between the front-end and the backend enabling the use of the map to capture user input, while folium is meant for displaying static data only ([source](https://blog.jupyter.org/interactive-gis-in-jupyter-with-ipyleaflet-52f9657fa7a)). Note that [Google Colab](https://colab.research.google.com/) currently does not support ipyleaflet ([source](https://github.com/googlecolab/colabtools/issues/60issuecomment-596225619)). Therefore, if you are using geemap with Google Colab, you should use [`import geemap.eefolium`](https://github.com/giswqs/geemap/blob/master/geemap/eefolium.py). If you are using geemap with [binder](https://mybinder.org/) or a local Jupyter notebook server, you can use [`import geemap`](https://github.com/giswqs/geemap/blob/master/geemap/geemap.py), which provides more functionalities for capturing user input (e.g., mouse-clicking and moving).
###Code
# Installs geemap package
import subprocess
try:
import geemap
except ImportError:
print('geemap package not installed. Installing ...')
subprocess.check_call(["python", '-m', 'pip', 'install', 'geemap'])
# Checks whether this notebook is running on Google Colab
try:
import google.colab
import geemap.eefolium as geemap
except:
import geemap
# Authenticates and initializes Earth Engine
import ee
try:
ee.Initialize()
except Exception as e:
ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map The default basemap is `Google Maps`. [Additional basemaps](https://github.com/giswqs/geemap/blob/master/geemap/basemaps.py) can be added using the `Map.add_basemap()` function.
###Code
Map = geemap.Map(center=[40,-100], zoom=4)
Map
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Add Earth Engine dataset
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.addLayerControl() # This line is not needed for ipyleaflet-based Map.
Map
###Output
_____no_output_____
###Markdown
View source on GitHub Notebook Viewer Run in binder Run in Google Colab Install Earth Engine APIInstall the [Earth Engine Python API](https://developers.google.com/earth-engine/python_install) and [geehydro](https://github.com/giswqs/geehydro). The **geehydro** Python package builds on the [folium](https://github.com/python-visualization/folium) package and implements several methods for displaying Earth Engine data layers, such as `Map.addLayer()`, `Map.setCenter()`, `Map.centerObject()`, and `Map.setOptions()`.The following script checks if the geehydro package has been installed. If not, it will install geehydro, which automatically install its dependencies, including earthengine-api and folium.
###Code
import subprocess
try:
import geehydro
except ImportError:
print('geehydro package not installed. Installing ...')
subprocess.check_call(["python", '-m', 'pip', 'install', 'geehydro'])
###Output
_____no_output_____
###Markdown
Import libraries
###Code
import ee
import folium
import geehydro
###Output
_____no_output_____
###Markdown
Authenticate and initialize Earth Engine API. You only need to authenticate the Earth Engine API once.
###Code
try:
ee.Initialize()
except Exception as e:
ee.Authenticate()
ee.Initialize()
###Output
_____no_output_____
###Markdown
Create an interactive map This step creates an interactive map using [folium](https://github.com/python-visualization/folium). The default basemap is the OpenStreetMap. Additional basemaps can be added using the `Map.setOptions()` function. The optional basemaps can be `ROADMAP`, `SATELLITE`, `HYBRID`, `TERRAIN`, or `ESRI`.
###Code
Map = folium.Map(location=[40, -100], zoom_start=4)
Map.setOptions('HYBRID')
###Output
_____no_output_____
###Markdown
Add Earth Engine Python script
###Code
# Load a collection of US counties.
counties = ee.FeatureCollection('TIGER/2018/Counties')
# Make an image out of the land area attribute.
landAreaImg = counties \
.filter(ee.Filter.notNull(['ALAND'])) \
.reduceToImage(**{
'properties': ['ALAND'],
'reducer': ee.Reducer.first()
})
# Display the county land area image.
Map.setCenter(-99.976, 40.38, 5)
Map.addLayer(landAreaImg, {
'min': 3e8,
'max': 1.5e10,
'palette': ['FCFDBF', 'FDAE78', 'EE605E', 'B63679', '711F81', '2C105C']
}, 'Land Area')
###Output
_____no_output_____
###Markdown
Display Earth Engine data layers
###Code
Map.setControlVisibility(layerControl=True, fullscreenControl=True, latLngPopup=True)
Map
###Output
_____no_output_____ |
001-rollercoaster-dataset/coaster-data-pull.ipynb | ###Markdown
Roller Coaster Data Pull- Twitch Stream Project
###Code
%load_ext autoreload
%autoreload 2
import pandas as pd
import wikipediaapi
from tqdm import tqdm
# Our code import
from coaster import parse_coaster_infobox
###Output
_____no_output_____
###Markdown
Pull Data for Operating Coasters
###Code
# wiki = wikipediaapi.Wikipedia('en')
# cat = wiki.page('Category:Operating roller coasters')
# len(cat.categorymembers)
# DEBUG = True
# ds = []
# i = 0
# for coaster, page in tqdm(cat.categorymembers.items()):
# url = page.fullurl
# ds.append(parse_coaster_infobox(url, filter_cols=False))
# i += 1
# if i == 30 and DEBUG:
# break
# if not DEBUG:
# coaster_db = pd.concat(ds)
# coaster_db.to_csv('dbv1.csv')
###Output
_____no_output_____
###Markdown
Data for All Coasters
###Code
DEBUG = False
wiki = wikipediaapi.Wikipedia('en')
cat = wiki.page('Category:Roller_coaster_introductions_by_year')
i = 0
ds = []
for year in tqdm(cat.categorymembers):
cat_year = wiki.page(year)
cat_year.categorymembers
for coaster, page in cat_year.categorymembers.items():
url = page.fullurl
parsed_df = parse_coaster_infobox(url, coaster, filter_cols=True)
parsed_df['year_introduced'] = int(year[-4:])
ds.append(parsed_df)
i += 1
if i == 20 and DEBUG:
break
if not DEBUG:
coaster_db = pd.concat(ds)
# (~coaster_db.isna()).sum().to_frame().rename(columns={0:'count'}) \
# .query('count > 20') \
# ['count'].sort_values(ascending=True) \
# .plot(kind='barh',
# figsize=(15, 20)
# )
MAIN_KEYS = ['coaster_name', 'Length', 'Speed', 'Location', 'Coordinates',
'Status', 'Opening date',
'General statistics', 'Type', 'Manufacturer',
'Height restriction',
'Model', 'Height', 'Inversions', 'Lift/launch system',
'Cost', 'Trains',
'Park section', 'Duration', 'Capacity',
'G-force', 'Designer',
'Max vertical angle', 'Drop', 'Soft opening date',
'Fast Lane available', 'Replaced', 'Track layout',
'Fastrack available','Soft opening date',
'Closing date', 'Opened', 'Replaced by', 'General Statistics',
'Website', 'Flash Pass Available', 'Must transfer from wheelchair',
'Theme', 'Single rider line available',
'Restraint Style',
'Flash Pass available', 'Acceleration',
'Restraints', 'Name','year_introduced']
coaster_db[MAIN_KEYS].to_csv('coaster_db_v2.csv')
###Output
_____no_output_____ |
ipython3/07_Input_Output.ipynb | ###Markdown
Python for Finance **Analyze Big Financial Data**O'Reilly (2014)Yves Hilpisch **Buy the book ** |O'Reilly |Amazon**All book codes & IPYNBs** |http://oreilly.quant-platform.com**The Python Quants GmbH** | http://tpq.io**Contact us** | [email protected] Input-Output Operations
###Code
import seaborn as sns; sns.set()
import matplotlib as mpl
mpl.rcParams['font.family'] = 'serif'
###Output
_____no_output_____
###Markdown
Basic I/O with Python Writing Objects to Disk
###Code
path = './data/'
import numpy as np
from random import gauss
a = [gauss(1.5, 2) for i in range(1000000)]
# generation of normally distributed randoms
import pickle
pkl_file = open(path + 'data.pkl', 'wb')
# open file for writing
# Note: existing file might be overwritten
%time pickle.dump(a, pkl_file)
pkl_file
pkl_file.close()
ll $path*
pkl_file = open(path + 'data.pkl', 'rb') # open file for reading
%time b = pickle.load(pkl_file)
b[:5]
a[:5]
np.allclose(np.array(a), np.array(b))
np.sum(np.array(a) - np.array(b))
pkl_file = open(path + 'data.pkl', 'wb') # open file for writing
%time pickle.dump(np.array(a), pkl_file)
%time pickle.dump(np.array(a) ** 2, pkl_file)
pkl_file.close()
ll $path*
pkl_file = open(path + 'data.pkl', 'rb') # open file for reading
x = pickle.load(pkl_file)
x
y = pickle.load(pkl_file)
y
pkl_file.close()
pkl_file = open(path + 'data.pkl', 'wb') # open file for writing
pickle.dump({'x' : x, 'y' : y}, pkl_file)
pkl_file.close()
pkl_file = open(path + 'data.pkl', 'rb') # open file for writing
data = pickle.load(pkl_file)
pkl_file.close()
for key in data.keys():
print(key, data[key][:4])
!rm -f $path*
###Output
_____no_output_____
###Markdown
Reading and Writing Text Files
###Code
rows = 5000
a = np.random.standard_normal((rows, 5)) # dummy data
a.round(4)
import pandas as pd
t = pd.date_range(start='2014/1/1', periods=rows, freq='H')
# set of hourly datetime objects
t
csv_file = open(path + 'data.csv', 'w') # open file for writing
header = 'date,no1,no2,no3,no4,no5\n'
csv_file.write(header)
for t_, (no1, no2, no3, no4, no5) in zip(t, a):
s = '%s,%f,%f,%f,%f,%f\n' % (t_, no1, no2, no3, no4, no5)
csv_file.write(s)
csv_file.close()
ll $path*
csv_file = open(path + 'data.csv', 'r') # open file for reading
for i in range(5):
print(csv_file.readline(), end='')
csv_file = open(path + 'data.csv', 'r')
content = csv_file.readlines()
for line in content[:5]:
print(line, end='')
csv_file.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
SQL Databases
###Code
import sqlite3 as sq3
query = 'CREATE TABLE numbs (Date date, No1 real, No2 real)'
con = sq3.connect(path + 'numbs.db')
con.execute(query)
con.commit()
import datetime as dt
con.execute('INSERT INTO numbs VALUES(?, ?, ?)',
(dt.datetime.now(), 0.12, 7.3))
data = np.random.standard_normal((10000, 2)).round(5)
for row in data:
con.execute('INSERT INTO numbs VALUES(?, ?, ?)',
(dt.datetime.now(), row[0], row[1]))
con.commit()
con.execute('SELECT * FROM numbs').fetchmany(10)
pointer = con.execute('SELECT * FROM numbs')
for i in range(3):
print(pointer.fetchone())
con.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
Writing and Reading Numpy Arrays
###Code
import numpy as np
dtimes = np.arange('2015-01-01 10:00:00', '2021-12-31 22:00:00',
dtype='datetime64[m]') # minute intervals
len(dtimes)
dty = np.dtype([('Date', 'datetime64[m]'), ('No1', 'f'), ('No2', 'f')])
data = np.zeros(len(dtimes), dtype=dty)
data['Date'] = dtimes
a = np.random.standard_normal((len(dtimes), 2)).round(5)
data['No1'] = a[:, 0]
data['No2'] = a[:, 1]
%time np.save(path + 'array', data) # suffix .npy is added
ll $path*
%time np.load(path + 'array.npy')
data = np.random.standard_normal((10000, 6000))
%time np.save(path + 'array', data)
ll $path*
%time np.load(path + 'array.npy')
data = 0.0
!rm -f $path*
###Output
_____no_output_____
###Markdown
I/O with pandas
###Code
import numpy as np
import pandas as pd
data = np.random.standard_normal((1000000, 5)).round(5)
# sample data set
filename = path + 'numbs'
###Output
_____no_output_____
###Markdown
SQL Database
###Code
import sqlite3 as sq3
query = 'CREATE TABLE numbers (No1 real, No2 real,\
No3 real, No4 real, No5 real)'
con = sq3.Connection(filename + '.db')
con.execute(query)
%%time
con.executemany('INSERT INTO numbers VALUES (?, ?, ?, ?, ?)', data)
con.commit()
ll $path*
%%time
temp = con.execute('SELECT * FROM numbers').fetchall()
print(temp[:2])
temp = 0.0
%%time
query = 'SELECT * FROM numbers WHERE No1 > 0 AND No2 < 0'
res = np.array(con.execute(query).fetchall()).round(3)
res = res[::100] # every 100th result
import matplotlib.pyplot as plt
%matplotlib inline
plt.plot(res[:, 0], res[:, 1], 'ro')
plt.grid(True); plt.xlim(-0.5, 4.5); plt.ylim(-4.5, 0.5)
# tag: scatter_query
# title: Plot of the query result
# size: 60
###Output
_____no_output_____
###Markdown
From SQL to pandas
###Code
import pandas.io.sql as pds
%time data = pds.read_sql('SELECT * FROM numbers', con)
data.head()
%time data[(data['No1'] > 0) & (data['No2'] < 0)].head()
%%time
res = data[['No1', 'No2']][((data['No1'] > 0.5) | (data['No1'] < -0.5))
& ((data['No2'] < -1) | (data['No2'] > 1))]
plt.plot(res.No1, res.No2, 'ro')
plt.grid(True); plt.axis('tight')
# tag: data_scatter_1
# title: Scatter plot of complex query results
# size: 55
h5s = pd.HDFStore(filename + '.h5s', 'w')
%time h5s['data'] = data
h5s
h5s.close()
%%time
h5s = pd.HDFStore(filename + '.h5s', 'r')
temp = h5s['data']
h5s.close()
np.allclose(np.array(temp), np.array(data))
temp = 0.0
ll $path*
###Output
-rw-r--r--@ 1 yves staff 52633600 Jan 4 12:57 ./data/numbs.db
-rw-r--r--@ 1 yves staff 48007192 Jan 4 12:57 ./data/numbs.h5s
###Markdown
Data as CSV File
###Code
%time data.to_csv(filename + '.csv')
ls data/
%%time
pd.read_csv(filename + '.csv')[['No1', 'No2',
'No3', 'No4']].hist(bins=20)
# tag: data_hist_3
# title: Histogram of 4 data sets
# size: 60
###Output
CPU times: user 1.29 s, sys: 139 ms, total: 1.43 s
Wall time: 1.43 s
###Markdown
Data as Excel File
###Code
%time data[:100000].to_excel(filename + '.xlsx')
%time pd.read_excel(filename + '.xlsx', 'Sheet1').cumsum().plot()
# tag: data_paths
# title: Paths of random data from Excel file
# size: 60
ll $path*
rm -f $path*
###Output
_____no_output_____
###Markdown
Fast I/O with PyTables
###Code
import numpy as np
import tables as tb
import datetime as dt
import matplotlib.pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
Working with Tables
###Code
filename = path + 'tab.h5'
h5 = tb.open_file(filename, 'w')
rows = 2000000
row_des = {
'Date': tb.StringCol(26, pos=1),
'No1': tb.IntCol(pos=2),
'No2': tb.IntCol(pos=3),
'No3': tb.Float64Col(pos=4),
'No4': tb.Float64Col(pos=5)
}
filters = tb.Filters(complevel=0) # no compression
tab = h5.create_table('/', 'ints_floats', row_des,
title='Integers and Floats',
expectedrows=rows, filters=filters)
tab
pointer = tab.row
ran_int = np.random.randint(0, 10000, size=(rows, 2))
ran_flo = np.random.standard_normal((rows, 2)).round(5)
%%time
for i in range(rows):
pointer['Date'] = dt.datetime.now()
pointer['No1'] = ran_int[i, 0]
pointer['No2'] = ran_int[i, 1]
pointer['No3'] = ran_flo[i, 0]
pointer['No4'] = ran_flo[i, 1]
pointer.append()
# this appends the data and
# moves the pointer one row forward
tab.flush()
tab
ll $path*
dty = np.dtype([('Date', 'S26'), ('No1', '<i4'), ('No2', '<i4'),
('No3', '<f8'), ('No4', '<f8')])
sarray = np.zeros(len(ran_int), dtype=dty)
sarray
%%time
sarray['Date'] = dt.datetime.now()
sarray['No1'] = ran_int[:, 0]
sarray['No2'] = ran_int[:, 1]
sarray['No3'] = ran_flo[:, 0]
sarray['No4'] = ran_flo[:, 1]
%%time
h5.create_table('/', 'ints_floats_from_array', sarray,
title='Integers and Floats',
expectedrows=rows, filters=filters)
h5
h5.remove_node('/', 'ints_floats_from_array')
tab[:3]
tab[:4]['No4']
%time np.sum(tab[:]['No3'])
%time np.sum(np.sqrt(tab[:]['No1']))
%%time
plt.hist(tab[:]['No3'], bins=30)
plt.grid(True)
print(len(tab[:]['No3']))
# tag: data_hist
# title: Histogram of data
# size: 60
%%time
res = np.array([(row['No3'], row['No4']) for row in
tab.where('((No3 < -0.5) | (No3 > 0.5)) \
& ((No4 < -1) | (No4 > 1))')])[::100]
plt.plot(res.T[0], res.T[1], 'ro')
plt.grid(True)
# tag: scatter_data
# title: Scatter plot of query result
# size: 70
%%time
values = tab.cols.No3[:]
print("Max %18.3f" % values.max())
print("Ave %18.3f" % values.mean())
print("Min %18.3f" % values.min())
print("Std %18.3f" % values.std())
%%time
results = [(row['No1'], row['No2']) for row in
tab.where('((No1 > 9800) | (No1 < 200)) \
& ((No2 > 4500) & (No2 < 5500))')]
for res in results[:4]:
print(res)
%%time
results = [(row['No1'], row['No2']) for row in
tab.where('(No1 == 1234) & (No2 > 9776)')]
for res in results:
print(res)
###Output
(1234, 9927)
(1234, 9899)
(1234, 9973)
(1234, 9803)
(1234, 9834)
(1234, 9785)
CPU times: user 27.7 ms, sys: 45.7 ms, total: 73.4 ms
Wall time: 47.3 ms
###Markdown
Working with Compressed Tables
###Code
filename = path + 'tab.h5c'
h5c = tb.open_file(filename, 'w')
filters = tb.Filters(complevel=4, complib='blosc')
tabc = h5c.create_table('/', 'ints_floats', sarray,
title='Integers and Floats',
expectedrows=rows, filters=filters)
%%time
res = np.array([(row['No3'], row['No4']) for row in
tabc.where('((No3 < -0.5) | (No3 > 0.5)) \
& ((No4 < -1) | (No4 > 1))')])[::100]
%time arr_non = tab.read()
%time arr_com = tabc.read()
ll $path*
h5c.close()
###Output
_____no_output_____
###Markdown
Working with Arrays
###Code
%%time
arr_int = h5.create_array('/', 'integers', ran_int)
arr_flo = h5.create_array('/', 'floats', ran_flo)
h5
ll $path*
h5.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
Out-of-Memory Computations
###Code
filename = path + 'array.h5'
h5 = tb.open_file(filename, 'w')
n = 100
ear = h5.create_earray(h5.root, 'ear',
atom=tb.Float64Atom(),
shape=(0, n))
%%time
rand = np.random.standard_normal((n, n))
for i in range(750):
ear.append(rand)
ear.flush()
ear
ear.size_on_disk
out = h5.create_earray(h5.root, 'out',
atom=tb.Float64Atom(),
shape=(0, n))
expr = tb.Expr('3 * sin(ear) + sqrt(abs(ear))')
# the numerical expression as a string object
expr.set_output(out, append_mode=True)
# target to store results is disk-based array
%time expr.eval()
# evaluation of the numerical expression
# and storage of results in disk-based array
out[0, :10]
%time imarray = ear.read()
# read whole array into memory
import numexpr as ne
expr = '3 * sin(imarray) + sqrt(abs(imarray))'
ne.set_num_threads(16)
%time ne.evaluate(expr)[0, :10]
h5.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
Python for Finance **Analyze Big Financial Data**O'Reilly (2014)Yves Hilpisch **Buy the book ** |O'Reilly |Amazon**All book codes & IPYNBs** |http://oreilly.quant-platform.com**The Python Quants GmbH** | http://tpq.io**Contact us** | [email protected] Input-Output Operations
###Code
from pylab import plt
plt.style.use('ggplot')
import matplotlib as mpl
mpl.rcParams['font.family'] = 'serif'
###Output
_____no_output_____
###Markdown
Basic I/O with Python Writing Objects to Disk
###Code
path = './data/'
import numpy as np
from random import gauss
a = [gauss(1.5, 2) for i in range(1000000)]
# generation of normally distributed randoms
import pickle
pkl_file = open(path + 'data.pkl', 'wb')
# open file for writing
# Note: existing file might be overwritten
%time pickle.dump(a, pkl_file)
pkl_file
pkl_file.close()
ll $path*
pkl_file = open(path + 'data.pkl', 'rb') # open file for reading
%time b = pickle.load(pkl_file)
b[:5]
a[:5]
np.allclose(np.array(a), np.array(b))
np.sum(np.array(a) - np.array(b))
pkl_file = open(path + 'data.pkl', 'wb') # open file for writing
%time pickle.dump(np.array(a), pkl_file)
%time pickle.dump(np.array(a) ** 2, pkl_file)
pkl_file.close()
ll $path*
pkl_file = open(path + 'data.pkl', 'rb') # open file for reading
x = pickle.load(pkl_file)
x
y = pickle.load(pkl_file)
y
pkl_file.close()
pkl_file = open(path + 'data.pkl', 'wb') # open file for writing
pickle.dump({'x' : x, 'y' : y}, pkl_file)
pkl_file.close()
pkl_file = open(path + 'data.pkl', 'rb') # open file for writing
data = pickle.load(pkl_file)
pkl_file.close()
for key in data.keys():
print(key, data[key][:4])
!rm -f $path*
###Output
_____no_output_____
###Markdown
Reading and Writing Text Files
###Code
rows = 5000
a = np.random.standard_normal((rows, 5)) # dummy data
a.round(4)
import pandas as pd
t = pd.date_range(start='2014/1/1', periods=rows, freq='H')
# set of hourly datetime objects
t
csv_file = open(path + 'data.csv', 'w') # open file for writing
header = 'date,no1,no2,no3,no4,no5\n'
csv_file.write(header)
for t_, (no1, no2, no3, no4, no5) in zip(t, a):
s = '%s,%f,%f,%f,%f,%f\n' % (t_, no1, no2, no3, no4, no5)
csv_file.write(s)
csv_file.close()
ll $path*
csv_file = open(path + 'data.csv', 'r') # open file for reading
for i in range(5):
print(csv_file.readline(), end='')
csv_file = open(path + 'data.csv', 'r')
content = csv_file.readlines()
for line in content[:5]:
print(line, end='')
csv_file.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
SQL Databases
###Code
import sqlite3 as sq3
query = 'CREATE TABLE numbs (Date date, No1 real, No2 real)'
con = sq3.connect(path + 'numbs.db')
con.execute(query)
con.commit()
import datetime as dt
con.execute('INSERT INTO numbs VALUES(?, ?, ?)',
(dt.datetime.now(), 0.12, 7.3))
data = np.random.standard_normal((10000, 2)).round(5)
for row in data:
con.execute('INSERT INTO numbs VALUES(?, ?, ?)',
(dt.datetime.now(), row[0], row[1]))
con.commit()
con.execute('SELECT * FROM numbs').fetchmany(10)
pointer = con.execute('SELECT * FROM numbs')
for i in range(3):
print(pointer.fetchone())
con.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
Writing and Reading Numpy Arrays
###Code
import numpy as np
dtimes = np.arange('2015-01-01 10:00:00', '2021-12-31 22:00:00',
dtype='datetime64[m]') # minute intervals
len(dtimes)
dty = np.dtype([('Date', 'datetime64[m]'), ('No1', 'f'), ('No2', 'f')])
data = np.zeros(len(dtimes), dtype=dty)
data['Date'] = dtimes
a = np.random.standard_normal((len(dtimes), 2)).round(5)
data['No1'] = a[:, 0]
data['No2'] = a[:, 1]
%time np.save(path + 'array', data) # suffix .npy is added
ll $path*
%time np.load(path + 'array.npy')
data = np.random.standard_normal((10000, 6000))
%time np.save(path + 'array', data)
ll $path*
%time np.load(path + 'array.npy')
data = 0.0
!rm -f $path*
###Output
_____no_output_____
###Markdown
I/O with pandas
###Code
import numpy as np
import pandas as pd
data = np.random.standard_normal((1000000, 5)).round(5)
# sample data set
filename = path + 'numbs'
###Output
_____no_output_____
###Markdown
SQL Database
###Code
import sqlite3 as sq3
query = 'CREATE TABLE numbers (No1 real, No2 real,\
No3 real, No4 real, No5 real)'
con = sq3.Connection(filename + '.db')
con.execute(query)
%%time
con.executemany('INSERT INTO numbers VALUES (?, ?, ?, ?, ?)', data)
con.commit()
ll $path*
%%time
temp = con.execute('SELECT * FROM numbers').fetchall()
print(temp[:2])
temp = 0.0
%%time
query = 'SELECT * FROM numbers WHERE No1 > 0 AND No2 < 0'
res = np.array(con.execute(query).fetchall()).round(3)
res = res[::100] # every 100th result
import matplotlib.pyplot as plt
%matplotlib inline
plt.plot(res[:, 0], res[:, 1], 'ro')
plt.grid(True); plt.xlim(-0.5, 4.5); plt.ylim(-4.5, 0.5)
# tag: scatter_query
# title: Plot of the query result
# size: 60
###Output
_____no_output_____
###Markdown
From SQL to pandas
###Code
import pandas.io.sql as pds
%time data = pds.read_sql('SELECT * FROM numbers', con)
data.head()
%time data[(data['No1'] > 0) & (data['No2'] < 0)].head()
%%time
res = data[['No1', 'No2']][((data['No1'] > 0.5) | (data['No1'] < -0.5))
& ((data['No2'] < -1) | (data['No2'] > 1))]
plt.plot(res.No1, res.No2, 'ro')
plt.grid(True); plt.axis('tight')
# tag: data_scatter_1
# title: Scatter plot of complex query results
# size: 55
h5s = pd.HDFStore(filename + '.h5s', 'w')
%time h5s['data'] = data
h5s
h5s.close()
%%time
h5s = pd.HDFStore(filename + '.h5s', 'r')
temp = h5s['data']
h5s.close()
np.allclose(np.array(temp), np.array(data))
temp = 0.0
ll $path*
###Output
-rw-r--r--@ 1 yves staff 52633600 Jul 11 19:24 ./data/numbs.db
-rw-r--r--@ 1 yves staff 48007192 Jul 11 19:24 ./data/numbs.h5s
###Markdown
Data as CSV File
###Code
%time data.to_csv(filename + '.csv')
ls data/
%%time
pd.read_csv(filename + '.csv')[['No1', 'No2',
'No3', 'No4']].hist(bins=20)
# tag: data_hist_3
# title: Histogram of 4 data sets
# size: 60
###Output
CPU times: user 1.25 s, sys: 151 ms, total: 1.41 s
Wall time: 1.41 s
###Markdown
Data as Excel File
###Code
%time data[:100000].to_excel(filename + '.xlsx')
%time pd.read_excel(filename + '.xlsx', 'Sheet1').cumsum().plot()
# tag: data_paths
# title: Paths of random data from Excel file
# size: 60
ll $path*
rm -f $path*
###Output
_____no_output_____
###Markdown
Fast I/O with PyTables
###Code
import numpy as np
import tables as tb
import datetime as dt
import matplotlib.pyplot as plt
%matplotlib inline
###Output
_____no_output_____
###Markdown
Working with Tables
###Code
filename = path + 'tab.h5'
h5 = tb.open_file(filename, 'w')
rows = 2000000
row_des = {
'Date': tb.StringCol(26, pos=1),
'No1': tb.IntCol(pos=2),
'No2': tb.IntCol(pos=3),
'No3': tb.Float64Col(pos=4),
'No4': tb.Float64Col(pos=5)
}
filters = tb.Filters(complevel=0) # no compression
tab = h5.create_table('/', 'ints_floats', row_des,
title='Integers and Floats',
expectedrows=rows, filters=filters)
tab
pointer = tab.row
ran_int = np.random.randint(0, 10000, size=(rows, 2))
ran_flo = np.random.standard_normal((rows, 2)).round(5)
%%time
for i in range(rows):
pointer['Date'] = dt.datetime.now()
pointer['No1'] = ran_int[i, 0]
pointer['No2'] = ran_int[i, 1]
pointer['No3'] = ran_flo[i, 0]
pointer['No4'] = ran_flo[i, 1]
pointer.append()
# this appends the data and
# moves the pointer one row forward
tab.flush()
tab
ll $path*
dty = np.dtype([('Date', 'S26'), ('No1', '<i4'), ('No2', '<i4'),
('No3', '<f8'), ('No4', '<f8')])
sarray = np.zeros(len(ran_int), dtype=dty)
sarray
%%time
sarray['Date'] = dt.datetime.now()
sarray['No1'] = ran_int[:, 0]
sarray['No2'] = ran_int[:, 1]
sarray['No3'] = ran_flo[:, 0]
sarray['No4'] = ran_flo[:, 1]
%%time
h5.create_table('/', 'ints_floats_from_array', sarray,
title='Integers and Floats',
expectedrows=rows, filters=filters)
h5
h5.remove_node('/', 'ints_floats_from_array')
tab[:3]
tab[:4]['No4']
%time np.sum(tab[:]['No3'])
%time np.sum(np.sqrt(tab[:]['No1']))
%%time
plt.hist(tab[:]['No3'], bins=30)
plt.grid(True)
print(len(tab[:]['No3']))
# tag: data_hist
# title: Histogram of data
# size: 60
%%time
res = np.array([(row['No3'], row['No4']) for row in
tab.where('((No3 < -0.5) | (No3 > 0.5)) \
& ((No4 < -1) | (No4 > 1))')])[::100]
plt.plot(res.T[0], res.T[1], 'ro')
plt.grid(True)
# tag: scatter_data
# title: Scatter plot of query result
# size: 70
%%time
values = tab.cols.No3[:]
print("Max %18.3f" % values.max())
print("Ave %18.3f" % values.mean())
print("Min %18.3f" % values.min())
print("Std %18.3f" % values.std())
%%time
results = [(row['No1'], row['No2']) for row in
tab.where('((No1 > 9800) | (No1 < 200)) \
& ((No2 > 4500) & (No2 < 5500))')]
for res in results[:4]:
print(res)
%%time
results = [(row['No1'], row['No2']) for row in
tab.where('(No1 == 1234) & (No2 > 9776)')]
for res in results:
print(res)
###Output
(1234, 9994)
(1234, 9997)
(1234, 9921)
(1234, 9902)
(1234, 9975)
(1234, 9970)
CPU times: user 28 ms, sys: 44.7 ms, total: 72.6 ms
Wall time: 51.7 ms
###Markdown
Working with Compressed Tables
###Code
filename = path + 'tab.h5c'
h5c = tb.open_file(filename, 'w')
filters = tb.Filters(complevel=4, complib='blosc')
tabc = h5c.create_table('/', 'ints_floats', sarray,
title='Integers and Floats',
expectedrows=rows, filters=filters)
%%time
res = np.array([(row['No3'], row['No4']) for row in
tabc.where('((No3 < -0.5) | (No3 > 0.5)) \
& ((No4 < -1) | (No4 > 1))')])[::100]
%time arr_non = tab.read()
%time arr_com = tabc.read()
ll $path*
h5c.close()
###Output
_____no_output_____
###Markdown
Working with Arrays
###Code
%%time
arr_int = h5.create_array('/', 'integers', ran_int)
arr_flo = h5.create_array('/', 'floats', ran_flo)
h5
ll $path*
h5.close()
!rm -f $path*
###Output
_____no_output_____
###Markdown
Out-of-Memory Computations
###Code
filename = path + 'array.h5'
h5 = tb.open_file(filename, 'w')
n = 100
ear = h5.create_earray(h5.root, 'ear',
atom=tb.Float64Atom(),
shape=(0, n))
%%time
rand = np.random.standard_normal((n, n))
for i in range(750):
ear.append(rand)
ear.flush()
ear
ear.size_on_disk
out = h5.create_earray(h5.root, 'out',
atom=tb.Float64Atom(),
shape=(0, n))
expr = tb.Expr('3 * sin(ear) + sqrt(abs(ear))')
# the numerical expression as a string object
expr.set_output(out, append_mode=True)
# target to store results is disk-based array
%time expr.eval()
# evaluation of the numerical expression
# and storage of results in disk-based array
out[0, :10]
%time imarray = ear.read()
# read whole array into memory
import numexpr as ne
expr = '3 * sin(imarray) + sqrt(abs(imarray))'
ne.set_num_threads(16)
%time ne.evaluate(expr)[0, :10]
h5.close()
!rm -f $path*
###Output
_____no_output_____ |
smartcab.ipynb | ###Markdown
**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
**Answer:While running the default agent code, a few observations i have taken into consideration would be that... 0. The agent state does not get updated. 1. The agent has not been given the parameters to learn. 2. The agent properly idled at a red lights many times with positive gain in reward (rewarded 1.25),(rewarded 1.77). 3. The agent idled at a green light with no oncoming traffic causing a negative loss in reward (rewarded -4.46). 4. Simulation ended 5. Simulated new trial, took different starting actions. 6. The agent takes 24 steps each trial for 10 test and 20 trials** **Answer:Agent: In the agent.py file, three flags that can be set to change simulation would be the... 1. To set a learning agent so that the agent knows that it is expected to learn given the environment so that the trials and test can update and have the agent "explore" its 'Q,s,a'. 2. The (simulator= n_test =nt...) changes the simulation with the number of trials increasing or decreasing given which decay function is used 3. Set the alpha/epsilon learning rate, to check through all actions, choosing random action which will explore the whole space and learn the True Q.. assuming the epsilon is small.. ( a drawback is that in some cases it doesn't use what it has learned where epsilon never decayse and does not explore). epsilon> "Initialize Q-learning so that the state gets updated givent the agents actions each(state, action) timestamp, that Q-hat says to take ."Environment: 1. The Environment class function called when agent performs an action is def_act(self, agent, action).To check if the agents current state, then determines the most legal action to take and supplying an accuarate reward based on the action. Updating with each state to action simulator: 1. render_text = This is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt. --Contains the simulation whether the agent properly idles at red lights, drives through green lights properly, avoids oncoming traffic... 2.render() = This is the GUI render display of the simulation. --Displays the visualisation of self driving car with pyGame planner: 1. In the planner.py file , the next_waypoint function would consider the East-West directions first if dx,dy-=0.**
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
**Answer:THE VISUALISATION ABOVE HAS THREE DIFFERENT GRAPHS DISPLAYING THE RELATIVE FREQUENCY OF BAD ACTIONS, ROLLING AVERAGE REWARDS PERACTION, AND RELIABILITY.THE GRAPH DISPLAYING RELATIVE FREQUENCY OF BAD ACTIONS, SHOWS MINOR-MAJOR ACCIDENTS BASED ON THE BAD ACTIONS, WITH EACH TRIAL NUMBER.THE AGENT IS MAKING MANY BAD DECISIONS BUT AROUND THE MIDDLE OF THE TRAINING THE AGENT FLUCTUATES BETWEEN THE GOOD-BAD DECSIONS. WHILE NEAR THE END OF THE TRIALS THE AGENT STARTED MAKING MORE STEADY DECISIONS.. THE NUMBER OF BAD ACTIONS TO DECREASE IS RELEVANT AND THIS STILL MAKES THE SMARTCAB UNSAFE.THE RATE OF RELIABILITY DOES MAKE SENSE GIVEN THAT THE AGENT IS DRIVING RANDOMLY. IT IS IN A RANDOM STATE AND HAS A STEADY RELIABLITY OF ABOUT %15,REACHES 20% RELIABILTY ANDSTARTS TO REDUCE BACK TO 15%.BECAUSE THE AGENT IS NOT LEARNING , THE REWARDS ARE RANGING FROM HIGH TO LOW. CONSIDERING THE RATE OF BAD ACTIONS IS RATHER HIGH IT IS SAFE TO ASSUME THAT THIS IS THE REASON THE SMARTCAB GET HEAVILY PENALIZED.AS THE NUMBER OF TRIALS INCREASE, GIVEN THE PARAMETERS, IT DOES NOT MATTER THE TRIAL NUMBER. IN THE MIDDLE OF THE TRIALS THE AGENT STARTED TO FLUCTUATE BETWEEN BAD-GOOD ACTIONS. NEAR THE END OF THE SIMULATION, THE AGENT STARTED MAKING STEADY DECISIONS BUT STILL NOT DECREASING IN BAD ACTIONS.WITH THE DATA GIVEN FROM THE TRIALS . THIS SMARTCAB IS NOT CONSIDERED SAFE AT THE MOMENT. NO MATTER THE TRIAL THE AGENT NEVER REACHES 0% FOR RELATIVE BAD ACTIONS. THE RATE OF RELIABILITY IS UNDER 40% MORE THAN HALF THE TIME SO THE SMARTCAB COMMITS MANY VIOLATIONS. THEREFORE 'GAINING' NEGATIVE REWARD AND CAUSING THE SMARTCAB TO FAIL. INCREASING NUMBER OF TRIALS CAN BE BENEFICIAL POSSIBLY??** ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. **Answer:THE MOST RELEVANT FEATURES FOR SAFETY AND EEFICIENCY WOULD BE THE {LEFT,RIGHT,ONCOMING,LIGHT} INPUT FEATURES FOR THE BUILD STATE. THESE FEATURES HAVE RELEVANCE BECAUSE IT WILL HELP THE AGENT DETERMINE THAT GIVEN ITS CURRENT STATE IF IT CAN MAKE THE APPRORIATE DECISION WHILE NOT CAUSING ANY ACCIDENTS OR VIOLATIONS WHILE STILL MEETING THE REQUIRED DEADLINE AND NOT HAVE TO ADD THE DEADLINE INPUT IN. IN CERTAIN AREAS WE CAN DISREGARD RIGHT BECAUSE IT IS SAFE TO TURN RIGHT ON RED IN MOST CASES. DEADLINE IS ALSO NOT RELEVANT BECAUSE THE AGENT WILL LEARN FROM ITS LOW REWARD AND BUILDS ON IT AS THE TRIALS INCREASE AND THE TRAINING OPTIMIZES, IMPROVING ITS RELIABILITY.** Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! **Answer:GIVEN THAT FOUR FEATURES HAVE BEEN USED, LIGHT(2INPUT), WAYPOINT(3INPUTS) AND FEATURES (WAYPOINT,LEFT,ONCOMING) MAKING A STATE SPACE OF 96 COMBINATIONS -> 2 X 3 X 4 X 4. NOT MAKING A POLICY TO COMPLICATED FOR AN AGENT TO LEARN WITH THE GIVEN TRIALS.** Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
**Answer:COMPARED TO THE OBSERVATIONS WITH THE BASIC DRIVING AGENT AND THE DEFAULT LEARNING AGENT, SUCH AS HIGH RATES OF MAJOR VIOLATIONS AND THE GRADES.THE AGENT REQUIRED 20 TRIALS BEFORE TESTING ,the epsilon was decreasing by 0.05 (0.05 X 20 = 1) WITH EACH TRIAL.WHEN EPSILON REACHED 0 REASONABLY,IT WILL BEGIN TESTING.THE DECAY FUNCTION self.trial = 0 -.05 HAS BEEN PROPERLY IMPLENTED IN THE PARAMTERS PANEL .IT STEADILY DECREASED ACROSS ALL 20 TRIALS AS THE NUMBER OF TRAINING TRIALS INCREASED , THE NUMBER OF BAD ACTIONS INCREASED TO AROUND >38% AND <45%, AND THE SCORE OF REWARDS WERE INCREASING COMPARED TO THE NON-LEARNERTHE AGENT HAS NOT IMPROVED MUCH .** ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$Use a decaying function for $\alpha$ (the learning rate). If so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** The smartcab does not move at all from the light. When the light is red, it is rewarded with a positive number. When it is green and the smartcab does not move, it is penalized with a negative number. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- num_dummies sets the number of dummy agents in the simulation- grid_size sets the number of intersections in the simulation- alpha sets the learning rate of the algorithm- Environment.act()- render_text() displays the simulation trial data on the terminal, while render() renders the image on the GUI.- East/West direction is checked first ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- The driving agent is making bad decisions about half the time. Accidents occur about 15% of the time.- Because the agent is driving randomly, there is no guarantee that it will move in any desired direction. Thus, the score of 'F' makes sense.- The agent gets positive rewards for good actions, and negative rewards for bad decisions. The 10-trial rolling average starts negative and stays negative at fairly large magnitudes. Since the agent is moving randomly, it can be expected that the average number of positve rewards should be roughly equal to the number of negative rewards. But because the average reward ammount is consistantly negative, the agent is being penalized heavily compared to the positive rewards.- The results do not change very much as the trials progress. The Reliability rate stays at 0%, and the relative frequency of bad actions remains in the neighborhood of 40%.- This smartcab would not be considered reliable for its passengers, given the score of 'F' for both safety and reliability. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**For safety, waypoint, light, and oncoming are the most important features, as they indicate whether or not the smartcab would be in any danger if it moves in a given direction. Oncoming will tell the smartcab if an oncoming car is moving in a direction that will intersect the smartcabs intended direction of travel. Right and Left vehicles are not as important because, assuming the cars observe the rules of the road, cars to the left will be at a red light while the agent is at a green, and thus cannot intersect the agent. Cars to the right at a red will have to yield to the agent for making right-on-red turns. And when the agent is at a red light, it technically can turn right on red, but for the sake of minimizing the state, it may be best to discourage the agent from turing righ ton red by not giving it Left information. Light tells the smartcab if it needs to stop at the intersection or not. If the light is red, and the smartcab does not stop, it will be at risk for colliding with a car traveling perpendicular to its heading. Waypoint tells the smart cab which directions are important to consider, because that/those directions will be the directions it needs to move.For efficiency, deadline is the most important. In order to be efficient, the smartcab must arrive before the deadline. However, since only reliability and safety are of concern in this project, deadline will not be included in the state. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**The state vector would be (waypoint, input['light'], input['oncoming']). Waypoint has 3 possible states: forward, right, left. input['light'] has two possible states: red and green. input['oncomming'] has four possible states: forward, right, left, and none. Thus the total number of combinations for the state vector is 3x2x4, or 24. This is a reasonable number of combinations for learning. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- No. The basic driving agent had no learning involved. Thus all metrics stayed relatively constant throughout the simluations. The default Q-learning agent made significant progress in learning driving. The rate of bad actions decreased from 30% to about 10%, the rate of reliability increased dramatically (from 50% to 80%), and the rolling average of reward per actions increased.- The agent was trained for 20 trials, which seams resonable for a 0.05 tolerance for epsilon.- The linearly decaying epsilon is accurately represented in the parameter panel- The relative frequency of bad actions decreased as the number of training trials increased. Also, as expected, the average reward per action increased with training, but never became positive.- The reliability of the default learner is much better than the basic driving agent in terms of reliability, scoring and A+. However, the safety rating remained at an F. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**- The Smartcab doesn't move at all.- Our Smartcab receives rewards as a numeric value during each iteration of simulation. This reward value can be negative or positive.- For the traffic light our Smartcab is facing, if it's red light (and our cab keeps still), we get a positive reward value, since we should wait at the crossroad. If it's green light (and our cab keeps still), we get a negative reward value, since we don't move when we should do so. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- `agent.py`: - learning: If set to be True, then the agent will use Q-Learning. - alpha: The learning rate for the agent, can be set bewteen 0 and 1. - enforce_deadline: If set to be True, then the deadline_metric is enforece for the agent. - `environment.py`: The class function `'act()'` will be called when agent performs an action, and perform the action if it is legal. Moreover, agent will receive a reward based on the traffic law.- `simulator.py`: The `'render_text()'` function is the non-GUI render display of simulation, i.e, it displays texts to your terminal to show how the simulation goes. The `'render()'` function is the GUI render display, i.e, it displays graphics to show how the simulation goes.- `planner.py`: The `'next_waypoint()'` function considers the East-West direction first, then the `'North-South'` direction. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- The drivining agent seems to make bad decisions in 40% of all the time. About 14% - 25% bad decisions cause accidents.- The agent is driving purely randomly, and each time, it just takes a random action from `'None'`, `'Left'`, `'Right'` and `'Forward'`. It knows nothing about the `waypoint`, and therefore doesn't know if an action will make it closer or further to its destination. Each time the probability our cab makes a **correct action** is about 25%. Therefore, reliability rate is slightly less than this value, which is not completely no-sence.- There is no doubt that our randomly driving agent is doing really badly, so that it continuesly receives heavy (negative) rewards all the time.- As the number of trials increases, it seems that, unfortunately, our agent is doing worse and worse. The average reward per action it receives goes from -5 to -7, meaning it is behaving more and more dangerously. On the other hand, the reliability also decreases from 10% to almost 0%, meaning it is almost very impossible for it to reach destination in time.To summarize, it is obvious that this `Smartcab` cannot be considered safe and reliable and it is no wonder that it receives two **F** scores for its safety and reliability. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**- Learning **Safety**: I choose to use the following features - - `light`: This is the most important feature about safety. Almost in any situations, traffic violations or accidents happen if the cab choose to move against a red light. The only exception, is that when the cab turns right on a red light, and there is no other car on the left side trying to move forward, which is completely safe. - `inputs[oncoming]`: This is also important. Suppose our agent is moving forward on a green light, then there is an oncoming car that is going to turn left. This will cause a serious accident. - `inputs[left]`: As mentioned to justify '`light`', the agent had better know cars on the left side. Because, if our cab tries to turn right on a red light, and suddenly a car on the left side tries to move forward, then cursh can still occur.- Learning **Efficiency**: I choose to the use following feature - - `waypoint`: It is clear that the `waypoint` attribute always tell the the next best action, for the cab to reach the destination as soon as possible. Therefore, in order to be efficient and not to hit the deadline, the cab should always consider the `waypoint` it is sugggested.**Therefore, the features I don't use are -**- `dead_line`: Normally, the `dead_line` will directly reflect the remaining time for a cab to reach its destination, and a cab should always avoiding using up its remaining time. But in my opinion, it doesn't make much sense to learn from this `numeric` value. For example, let's say, if the remaining time is 10 steps or 5 steps, what is the most efficient approch for the cab to reach its destination? Well, even if there are only 2 remaining steps, we are still not allowed to jump from our current position to our destination via a straight line in Euclidean space, instead, we still need to follow this Grid Network System. And `Waypoint` is always the best suggestion we can get in that situation, regardless of the remaining time.- inputs[right]: According to the US traffic rule, our cab actually needs not care about cars on the right side. If it's red light, and our agent of course cannot move forward or turn left, and turning right means our cab will never crush with a car on the right side (no matter whether that car is moving forward, turning left or right). On the other hand, if it's green light, our cab is of course safe to move in any direction. Therefore, ignoring traffic on the right side is completely safe.Also by not including these two features, we can keep our state space as small as possible. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**Following question 4, I use 4 features: `light`, `waypoint`, `input[oncoming]`, `input[left]` to construct the state space.- Possible values of `light`: **red**, **green**.- Possible values of `waypoint`: **forward**, **left**, **right**.- Possible values of `input[oncoming]`: **None**, **forward**, **left**, **right**.- Possible values of `input[left]`: **None**, **forward**, **left**, **right**.Therefore there are 2 x 3 x 4 x 4 = 96 possible states.Suppose all the states are independent with each other, then Monte Carlo simulation tells that when training for 750 steps, we have about 95.9% probability to visit all the 96 possible states. 750 steps doesn't seem too horrible, and also it is easily achievable when we use the epsilon-decaying function. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- The most obvious observation is that, the deafult Q-Learning agent **seems** to perform as bad as the basic driving agent (still receives **F** score for safety and **F** score for reliability).- We are using a linear decaying *epsilon*, and at each trial the *epsilon* will decrease for 0.05. If the starting *epsilon* is 1 then after 20 trials it will decay to 0, which is consistent with the trial number (20) we got, for the training process to finish.- According to the parameter panel, the decaying function works as planned, so that it makes the *epsilon* linearly decreased from 1 to 0, when the trial number goes from 0 to 20.- A good news for our Q-Learning algorithm is that, when the number of trials increased, frequency of total bad actions drops from about 27% to 17%, which is nicer than the basic agent. Moreover, any sub-type of bad actions also becomes less frequent: Major Violation drops from 13% to 10%; Minor Violation drops from 8% to 3%; Major Accident drops from 6% to 3%; Minor Accident stays at about 2%. On the other hand, average reward per action increased from -3.5 to about -1.2.- As I said, unfortunately, the **safety** and **reliability** scores haven't improved even when we have started using Q-learning. However, our Q-learning agent is already start learning! The frequency of bad actions continuesly drop as the training goes on. Also, the realibity rate as well as the average reward per action continuesly increase when we train our agent more and more. **Simply, it is just because our agent is not trained enough, so that its improvement in its behaviour is not enough.** In here, we have 20 training trials, and suppose each trail contains about 20 steps, we can only have 400 training steps. But as mentioned ealier, 750 steps are necessary if we want the agent to almost (95.9% chance) visit every possible state. Clearly, 400 steps is far from enough.To summerize, our Q-learning agent is starting to learn how to drive safely and efficently, but it is **not trained enough yet**. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** - The Smartcab does not move during the simulation. By default, the Smartcab (labeled with the Udacity logo) remains stationary at its initial intersection.- Random seeding is applied to the problem, i.e., the Smartcab is placed in a different starting position with each simulation, likewise, starting positions and travel directions by other cars are randomly seeded as well.- Visual information is quite coarse, e.g., turn-signals are not apparent on other cars (difficult to tell if on-coming traffic are making left turns, in which case, a Smartcab left turn would be allowable as well). Also relative velocities are hard to discern, e.g., if stopped at a red light, wishing to make a legal right turn, it's unclear how much time the Smartcar has before right-of-way traffic headed towards the intersection actually passes through it.- Floating point rewards (> 0) and penalities (0 <) are being assigned to the Smartcab after each time-step based on its previous action.- Penalities (negative rewards) are expected, e.g., idling at a green light with no oncoming traffic, as well are rewards, e.g., idling at red light.- Assigned rewards are not consistently valued, e.g., the following sequential rewards were observed: idling at a green light with no oncoming traffic was penalized (-5.83), but continuing to idle at the same green light lessened slightly (-5.59), and then worsened (-5.95). Idling at a green light with oncoming traffic was provided marginal reward (0.0 ~ 2.0). Idling at a red light provided a larger reward (1 ~ 3). Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**In `agent.py` several exist, e.g., several flags set for the driving environment:- `verbose`: _(boolean)_ setting to True displays additional output from the simulation- `num_dummies`: _(integer)_ number of dummy agents in the environment- `grid_size`: _(integer)_ number of intersections (columns, rows)In `environment.py`, the `act` function considers the action taken by the Smartcab and performs it if legal. In addition, it also assigns a reward according to the based on local traffic laws.In `simulator.py`, `render_text()` writes simulation updates into the console, while `render()` updates the GUI traffic map.In `planner.py`, the `next_waypoint()` function considers the East-West direction of travel, before North-South. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'Left'` (turn left), `'Right'` (turn right), or `'Forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliabilty make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:*** *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?* * On average, the agent is making bad decisions ~40% of the time. * On average, ~25% of these bad decisions are causing accidents, i.e., ~10% of all decisions lead to accidents.* *Given that the agent is driving randomly, does the rate of reliabilty make sense?* * Somewhat; it can certainly be justified. Given the actions (4 choices: None, L, R, F) and environment (traffic lights and other traffic), it seems like bad behavior is a bit of a coin flip. Let's further rationalize this: * Assume that agent is equally likely (p = 0.5) of encountering red or green light * For green lights, R, F, are always valid actions. Additionally, when there is no oncoming traffic (assume 80% of the time), L is also valid. * For red lights, None is always a valid action, as is R with no oncoming traffic (assume 80% of the time). * Computing the probabilities of success, if we assume R, F, L, None are all equally likely of occurring, for green lights, we're reliable 65% of the time (unreliable 35%); for red lights, a reliablilty of 45% is obtained (55% unreliable). * Averaging the unreliable probabilities for both light situations (since either is equally likely), the unreliable rate is 45%. Quite close to the ~40% observed!* *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?* * On average, the rewards are negative, and close to -5, which suggests that it has been heavily penalized.* *As the number of trials increases, does the outcome of results change significantly?* * Several observations can be made: * _Frequency of bad actions_, _exploration factor_, and _learning factor_ remain consistent over all trials. * Although there is some scatter with _average reward per action_ and _rate of reliability_, rerunning this simulation multiple times demonstrated no correlation to age of trial.* *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* * In no universe would this Smartcab be considered safe or reliable -- unless it's competing in a destruction derby, then yes.. Actions have a 40% chance of being violations or accidents - incredibly dangerous! Worse yet, the agent never achieves a reliability score above 20% -- abysmal. You'd likely accomplish similar performance while driving drunk, blindfolded, with earplugs, covered in ants. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate?* **Answer:**Tricky question - it asks, _which features_ (implying multiple features) _are the most relevant_ (implying a single prominent feature).First, let's begin with a discussion of features (assumed grouping of `'waypoint'`, `'inputs'`, and `'deadline'`):* `'input'` is the only one that prescribes what manuevers can safely be performed.* `'waypoint'` is the only feature that can direct the smartcar along the correct direction of travel. Not knowning the final destination turns the policy into a random walk, where we hope to (randomly) arrive at the destination.* `'deadline'` can be used to adjust policy, i.e., if time is not a consideration, the car can select safer actions, but if the deadline is of concern, it can be much more aggressive.Now, the features most relevant to safety and efficiency appear to be **`'input'`**, for safey, and **`'waypoint'`**, for efficiency. Next, a detailed discussion follows that Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the evironment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:** If `'inputs'` and `'waypoint'` are used to direct the smartcab, there are following states:* (2 states) `'input'` `'light'`: red (stop), green (go)* (0 states) `'input'` `'right'`:* (2 states) `'input'` `'left'`: forward (True/False)* (3 states) `'input'` `'oncoming'`: None, forward, right* (3 states) `'waypoint'`: forward, left, rightTalking through our minimum state set:* Light states (green/red) are mandatory. Additionally, based upon their state, we can apply common right-of-way rules to reduce the number of other states.* Vehicles travelling from the right are helpful but not necessary. As the smartcab moves through an intersection, regardless of its direction (forward, left, right), it does not need to know what vehicles are travelling from East to West, i.e., `'right'` vehicles.* Vehicles travelling from the left are helpful, but it is only necessary to know if they are going forward. Those that are turning right will never collide (when obeying traffic rules). Those that are turning left can only do so when they have a green light, which implies our smartcab has a red light.* Oncoming vehicles provide more information than right or left vehicles, but not each state is necessary. The smartcab requires knowledge of its action, except for left turns, since in undertaking this action, it would be foreced to yeild to the smartcab.* Waypoints are mandatory. Without them, it'd be a random walk to get to the destination.The product sum of all states (2, 2, 3, 3) is equal to the total number of combinations, which is 36. Had the number of states not been paired back (2, 4, 4, 4, 3) we would have had nearly a order of magnitude more (384)! Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the interative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.01). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning_saved.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:*** *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?* * Overall, although improvements are visible for the improved Q-Learning agent, the safety and reliability of the basic driving agent (no learning) and default Q-Learning agent are commensurate; both learners received failing grades for safety and reliability. * Rolling rate of reliability looks similar between agents. * Rolling (average) reward per action are _roughly_ similar between agents. There is a slight improvement towards 19th and 20th trials, but it may be the result of a stochastic process, rather than a true learning improvement. * Learning factors are constant (0.5) for both agents.* *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?* * Twenty training trials occured, which given the epsilon parameters (initial of 1.0, step sizes of -0.05) is expected, since 1.0/0.05 = 20. * *Is the decaying function you implemented for ϵϵ (the exploration factor) accurately represented in the parameters panel?* * Yes, a simple linear function, with a slope of -0.05 is shown.* *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?* * In a favorable manner, the number of bad actions decreased with the number of training trials, by roughly 30%. * Toward the end of training, the average rolling reward increased by a small margin. * *How does the safety and reliability rating compare to the initial driving agent?* * As mentioned earlier, unfortunately, the Q-Learning agent scored as poorly as the basic agent. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the impliciations it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_saved.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**- *Does the Smartcab move at all during the simulation?* **No**- *What kind of rewards is the driving agent receiving?* **"No action taken"**- *How does the light changing color affect the rewards?* **When the light is red, it receives a positive reward, when it is green a negative reward.** Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.* - learning - When set True the agent will learn using the Q-learning algorithm. - epsilon - It's possible to choose the Exploration factor. - apha - It's possible to choose the Learning rate. - *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?* - "class Agent(object)" - *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?* - The 'render_text()' will displays in terminal/command prompt, and will display the simulator screen.- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* - First consider East-West direction ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- *How frequently is the driving agent making bad decisions?* **About 45% of the time.**- *How many of those bad decisions cause accidents?* **About 10% of the time (minnor and major accidents). **- *Given that the agent is driving randomly, does the rate of reliability make sense?* **Yes, always there is a small possibility of getting the goal randomly.**- *What kind of rewards is the agent receiving for its actions?* **It gets positive rewards for good actions and negative rewards (penalties) for bad actions.**- *Do the rewards suggest it has been penalized heavily?* **Accidents generate heavier penalties**- *As the number of trials increases, does the outcome of results change significantly?* **No**- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **No, because of 10% of the time there was an accident.** ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**For **safety** the features should be the 'inputs' ('light', 'left', 'right', 'oncoming'), this features informs if is allowed or not to go there. For **efficiency** the feature used should be 'inputs' ('waypoint'), it informs the way to go. The feature 'deadline' isn't necessary. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**The state space:- light = 'green', 'red'- car_left = None, 'left', 'forward', 'right'- car_oncoming = None, 'left', 'forward', 'right'- car_right = None, 'left', 'forward', 'right'- waypoint = 'left', 'forward', 'right'So, the size of state space is 384. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?* **All the observations are similar, the differences look like random..**- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?* **About 20 training trials. The decay distribution of epsilon goes to zero after 20 interactions, then the number of training trials make sense.**- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?* **Yes**- *As the number of training trials increased, did the number of bad actions decrease?* **There is a small decrease.** - *Did the average reward increase?* **No**- *How does the safety and reliability rating compare to the initial driving agent?* **The same ratings** ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
#%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**During the entire simulation the driving agent stays idle while traffic inside the enviroment continues normaly. After waiting for a number of training trials I observed that staying idle yileds certain results as far as rewarding is concerned. Firstly, the agent is not enforced to meet a deadline, while it is rewarded for choosing an action when the traffic lights state changes. For example, the driving agent stays in the same place when the traffic ligh turns red and it is positevely rewarded for that decision,however, the agent still remains in the same place when the traffic light turns green again. For that decision is negatively rewarded. The repetition continues for as many time as it is the default configuration. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**1. agent.py: a) enforce_deadline: Setting this flag to True, the driving agent is enforced to meet a certain deadline and if it is due, probably it is going to receive a negative reward. b) learning: Learning falg is important since it enables the driving agent whether to learn or not by employing Bellman's equation (Q-Learning). c) Testing: Testing is set to False, however, when the value is changed ino True the testing trials begin.2. simulator.py: It seems that both function execue the same task but in two different visual ways. render_text() function displays the current state at each setp in cmd enviroment. On the other hand, render() function displays the results of the driving agent's current state in GUI. 3. entviroment.py: Given the input parameters of the act function, I believe that is the one called when an agent performs an action.4. planner.py: It appears that the second elif statement considers East-West first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**1. The driving agent took at least 45% of the time bad descisions which led to 5% major accidents until 14th iteration. Then the percentage of major accidents caused by bad decisions increased at least 2% after iteration 18th.2. The rate of reliability peaks at iteration 23 which is a little bit over 25%. Then it drops gradualy to 20%. It makes sense since the driving agent does not learn at all.3. The rewards start less than -4 where gradualy are reduced even more. I do not beleive that the agent is penalized heavilty since it constantly does not meet the deadline and takes bad decisions which results in major accidents.4. As the number of the trials increases the results are worse and that is reasonable since the actions taken by the agent are random. If another run the experiment one more time, probably the resutls will be better, however, we can not conclude that the agent improved it self.5. The analogy of actions taken and reliabiltiy indicate that the driving agent cannot be considered safe at all. If this experiment was conducted under real circumstances the agent would be a really dangerous vehicle. In my opinion the reason is that it takes naive decisions. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**From a first point of view all the features are considered relevant, however I will exclude two since I beleive that they do not favor safety and efficiency.Firstly, I will exclude deadline feature. I will assume that it is a regular driving agent and not an emergency driving agent for example. Thus, making the agent assign max Q-values in that feature it will force the agent to drive less carefully and take risky decision in order to be in time. Also, in case that deadline is a high value feature, I would consider other ways to make the driving agent more time efficient e.g. find the shortest route using heuristic algorithms.Secondly, I will exclude right feature. The specific feature indicates if there is car present on the right, however it does not gives the agent any important information since the other car in not in the same lane that the driving agent would consider take a right turn probably. In my opinion the rest of the features should be left because they provide valuable information for the agent's safety and the ability to learn efficiently. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**In order to calculate all the possible combinations of state space, first we need to check how many states each input has. Below are described the number of possible states for each feature selected:1. Left: None, Forward, right, left (4).2. Oncoming: None, Forward, right, left (4).3. Waypoint: Right,left,forward (3)4. Light: Red, green (2).Thus, the state space that the driving agent should learn includes 4x4x3x2 = 96 possible combinations. In my opinion is not a big state space, hence the agent will not have problem to learn them efficiently. Despite the fact that learning flag will be set to true, I do not beleive that 20 trials are not enough in order for the agent to be considered reliable. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**1. I would say that there reliability rate is similar between the naive driving agent and the default Q-learning agent. We observe an imorovement since the agent now has the ability to act based on past interactions with it's enviroment. Whereas the firt one was taking decisions at random.2. According to the visualization above the trials run before testing was 20 (epsillon = 1.0 x 0.05).3. With a decreasing rae at -0.05, the exploration factor seems to be accurately represented in the parameters panel during the trials.4. Visualizations indicate that both bad decisions decreased and average reward increased accordingly. More specificaly, at around 14th trial the bad decisions percentage fall from 34.42% to 22.95% after that increased for a while but during the last trails the percentage rate fall below 15%. At the same time, average reward was reducing until 14th trial which makes sense since the bad decision rate increased during that time. After 15th trial the average reward increased. 5. Reliability remained the same as before, however the safety rating got much better. A possible explanation is the enforce_dealine parameter is set to true and the agent fails to meet the deadlines. Despite that I will set that parameter to false as stated in question 4. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**In the initial simulation, without changing parameters, the agent does not move in the given environment.The agent recieves both negative and positive rewards.The agent is always idle in the environment. Thus, the rewards of the agent change dependent on the traffic signal at the intersection and the incoming traffic. Logically, staying idle during the red signal is suitable, but at green signals we want our car to move to the destination.In our simulation, the reward value confirms our logic. When our car is idle at the red signal, we get a positive reward typically in the range of 1 to 3. When the signal is green and the car is idle with no incoming traffic, our car is expected to move. As our car is always stationary, in such case it recieves a high negative reward of value above 3. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**Flags in agent.py:agent.learning = This flag shows whether our agent is testing or training. If we are training, we will update our Q-Tables, if we are not learning, we will drive through the environment without storing our experiences.agent.epsilon = This is the exploration factor. In an environment, we cannot only make ddecisions based on the best values in our Q-Table. We also need to explore actions, which seem inappropriate, or have lesser Q-Table values. This will help our agent's response when in unknown states be better, and our learning will be more complete. The rate of exploration is stored in epsilon.tolerance = If epsilon reaches this threshold value, we will pause our training and start testing our model. agent.alpha = This is our learning rate. This is the rate, at which we discard the previously learned information and accept the new information learned at each state by the model.n_test = The number of testing states for our model.When an agent makes an action, in environment.py we call the act() method. In this method, we pass our intended action and our agent object. The function, first checks the validity of our state, and the action. It will only let us execute an action in a state, if it exists amongst the list of predefined valid states and actions. Post that, the act checks the signal light, the surrounding dummies, and calculates the violation which will take place if the agent takes the particular action. It will then let the agent take that action in the environment, and then update the agent and environment metrics based on this action.render() is a function which updates and writes state data on our GUI in PyGame. render_text() is a function which write state data for display on our terminal window. We can edit information, and add additional stats, or metrics we would wish to see in these functons.In the function planner.py(), we first consider if the agent is east or west of our given destination and then check whether we are north of south of our destination. In both these condition we first check whether our destination is South_East and we are moving West or North, and act acordingly. We then check whether out destination is North_West and we are heading East or South and act accordingly. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?*
###Code
import pandas as pd
no_learning_data = pd.read_csv('raw_data/training_data_no_learning.csv')
for i in range(1,20):
#Finding out number of bad decisions, the average penalty and the number of accidents due bad decisions.
a = no_learning_data[no_learning_data['Trial']==i][no_learning_data['reward'] < 0].count()['reward']
inter = no_learning_data[no_learning_data['Trial']==i][no_learning_data['reward'] < 0]
b = inter.mean()['reward']
c= inter[inter['violation'] > 2].count()['reward']
print '\n\n Trial {}:'.format(i)
print 'Number of bad decisions taken are {}, number of accidents caused by them are {} and the average penalty for each bad decision is {}'.format(a,c,b)
#Mean reward per decision
d = no_learning_data[no_learning_data['Trial']==i].mean()['reward']
e = (no_learning_data[no_learning_data['Trial']==i].count()['reward']-a)/(a*1.0)
print 'The average reward per action is {}'.format(d)
print 'The ratio between valid to invalid actions is {}'.format(e)
###Output
/Users/akhilsethia/miniconda3/envs/ml2.7/lib/python2.7/site-packages/ipykernel_launcher.py:7: UserWarning: Boolean Series key will be reindexed to match DataFrame index.
import sys
/Users/akhilsethia/miniconda3/envs/ml2.7/lib/python2.7/site-packages/ipykernel_launcher.py:8: UserWarning: Boolean Series key will be reindexed to match DataFrame index.
###Markdown
**Answer:**There is no observable trend of improving results with each recurring trial. The results are random, and the ratio of valid to invalid action is variable. This is consitent with the fact that the agent does not learn from its past experiences and act differently each time.The rate of reliability is extremely low, this agrees with the fact that there is no logic while decision making for the car. The cab is not safe for driving, this is because there is no underlying method by which the cab makes it decisions. It is made by pure chance, and we cannot leave safety of the passengers to chance. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**I believe features most relevant for learning safety and efficiency are only few of the sensory input features that are available to the driver. RELEVANT STATE FEATURES:Input-Light: This feature is relevant to the cab, as the cab will decide to move or remain stationary in its current state dependent on the traffic light. To ensure maximum efficieny it is crucial for the cab to move towards its destination as soon as possible. This is only possible, if the can does not halt until it is absolutely necessary. The green light defines all possible instances, in which the smartcab can move towards its goal. Responsiveness to the green light is importat for efficiency. Similarly, the red light signifies times when other vehicales are moving across intersections and it is unsafe for the car to move as it might undergo an accident. Hence, halting at red light is important. Thus this input is needed in the state information.Input-Left: When the red light signal is indicated, all neighbouring cars will stop, so having information about the direction of the car on the left is redundant. When the signal is green, the car on the left can navigate 'None, Left, Right, Forward'. If this car moves 'Forward' and our cab decided to move 'Left', there can be a possibble accident. When the car on the left, steers to the 'Right' and we decide to move in the 'Forward' direction, there may be another accident. TO avoid this, we must have information about the car on our Left. This will ensure maximum safety for our smartcab.Input-Oncoming: This is an essential parameter in determining when the car is bound to move left. Oncoming traffic, cannot be forward or righ when we want to move left, as this will cause an majot violation, as seen in environment.py code. Hence, we would include this in our state.WayPoint: This is an important feature as it will keep informing the cab, the best possible action to take in the given deadline for reaching the deadline. This is also reflected in the Q-Table, but values in the Q-Table incorporate both security of the driver and the speed of fulfilling the task. When the driver is very close to the deadline, he may want to take steps which may compromise a bit of safety but maximise its reachine time. Hence, this is an important state feature to consider.IRRELEVANT STATE FEATURES:Deadline: The infotmation, about the deadline, would be considered, while calculating, the Q-values in the table. By choosing an action with maximum Q-value, we will choose an action which is most likely to get us to our destination as soon as possible. Hence, this data is incorporated in the Q-table and we need not have this as a state feature.Input-Right: This feature is not relevant to us. When we have a green signal, the right car will always have a red light, and will not move forward. When we wish to move right, when we have a red light, we would enter a different lane, than the reported car. Hence, this feature is not relevant to us. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**The size of the state space is 96. We have 3 features in our state, of which the light can take a binary value. The attribute waypoint can take 3 values and the attribute input features 'Oncoming' and 'Left' will take 4 values each. By computing all combinations we find out the total possible states, we get the total number of states to be 96. This state space is small and the driver can learn a policy for each intersection. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?*
###Code
q_learning_data = pd.read_csv('raw_data/training_data_default_learning.csv')
for i in range(1,20):
#Finding out number of bad decisions, the average penalty and the number of accidents due bad decisions.
q_a = q_learning_data[q_learning_data['Trial']==i][q_learning_data['reward'] < 0].count()['reward']
interim = q_learning_data[q_learning_data['Trial']==i][q_learning_data['reward'] < 0]
q_b = interim.mean()['reward']
q_c= interim[interim['violation'] > 2].count()['reward']
print '\n\n Trial {}:'.format(i)
print 'Number of bad decisions taken are {}, number of accidents caused by bad decsisons are {} and the average penalty for each bad decision is {} lesser than agent which has no learning'.format(-q_a+a,-q_c+c,-q_b+b)
#Mean reward per decision
q_d = no_learning_data[no_learning_data['Trial']==i].mean()['reward']
q_e = (no_learning_data[no_learning_data['Trial']==i].count()['reward']-q_a)/(q_a*1.0)
print 'The average reward per action is {} greater than that with no learning'.format(q_d-d)
print 'The ratio between valid to invalid actions is {} greater than that with no learning '.format(q_e-e)
###Output
/Users/akhilsethia/miniconda3/envs/ml2.7/lib/python2.7/site-packages/ipykernel_launcher.py:5: UserWarning: Boolean Series key will be reindexed to match DataFrame index.
"""
/Users/akhilsethia/miniconda3/envs/ml2.7/lib/python2.7/site-packages/ipykernel_launcher.py:6: UserWarning: Boolean Series key will be reindexed to match DataFrame index.
###Markdown
**Answer:**The performance parameters and metrics have been outlined above, and statistical comparision between no learning and Q learning has been made. Almost all trials show better performance with Q-Learning. The ratio between valid to invalid actions greatly increases as we go forward in the trial, thus when compared with the no learning model, the ratio is high. Observably, results get better as in the later trials. The agent required 20 trials before testing. This makes sense, because it will take 20 steps, before the epsilon value drops below our tolerance value.The decaying function is represented correctly in the parameters panel.Compared to the intitial driving agent, the reliability rating has spiked. The safety rating has not impoved, but the safety parameters have converged with each trial, showing that the trial is learning and getting better. The major violation curve, and the total bad actions cruve, are steeply reducing. This shows that our Q-learning agent has seen a big improvement, but yet cannot be trusted with handling passengers. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded! Model 1: Epsilon-decay = e^(-0.1*trial_number)alpha = 0.8tolerance = 0.00001Number of training trials = 150
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Model 2: Epsilon-decay = 0.3^(trial_number)alpha = 0.7tolerance = 0.00001Number of training trials = 20
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Model 4: Epsilon-decay = 1 / (trial_number^2 - 0.5125*trial_number)alpha = 0.7tolerance = 0.0002Number of training trials = 70
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Model 5: Epsilon-decay = 1 / (trial_number^2 + 0.5*trial_number)alpha = 0.5tolerance = 0.00001Number of training trials = 320
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Model 6: Epsilon-decay = 0.9 ^ (trial_number*0.8)alpha = 0.95tolerance = 0.00015Number of training trials = 120
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**Smartcab do not move at all during the simulation.Driving agent is receiving positive and negative rewards.If the lighting is Red color then Agent is assigned Positive rewards because the Agent was at Idle Position .If the lighting is Green color then Agent is assigned Negetive rewards because the Agent was at Idle Position .It was actually supposed to move and drive the car. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:****agent.py** I choose the flags "learning ""epsilon" "alpha". ** learning** This flag when enabled allows the model to learn from the training and the Q-table is updated. ** epsilon** This factor explains if the emphasis should be made on the exploration and exploitation . Ideally epsilon should be choosen in such a way that the exploitation increase onver the time and exploration derceases. **alpha**(Learning Rate) : The learning rate or step size determines to what extent the newly acquired information will override the old information. A factor of 0 will make the agent not learn anything, while a factor of 1 would make the agent consider only the most recent information. In fully deterministic environments, a learning rate of alpha =1 is optimal. When the problem is stochastic, the algorithm still converges under some technical conditions on the learning rate that require it to decrease to zero. In practice, often a constant learning rate is used,Ref: https://en.wikipedia.org/wiki/Q-learning **environment.py**:The act function is called when the action need to be performeddef act(self, agent, action):**simulator.py**:*render_text()* : This is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt.render():This is the GUI render display of the simulation.Supplementary trial data can be found from render_text.** planner.py**: * Next check if destination is cardinally East or West of location elif dx != 0:*next_waypoint() considers the East or West location first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**42% of the the decision made by the driving agent are bad decisions.6% of those are major accidents and 4 % of those are minor accidents.Reliability Rating is 'F' .As we can see that Reliabity is 10 % maximum as the agent does not improve the reliability even after many trails.10% reliablity is achieved beacause the agent takes action at randomAgent is receiving consistently Negetive reward of "-7" .yes,the model is being penalized heavily because the agent is not improving the actions taken .Even as the number of trail increase the outcome of the result does not change .Agent stops learning ,Rather it behaves even worse .For example look at the minor accidents graph ,it is increasing .This smartcab is not consider safe and Reliable for passenger . Both safety and Reliabilty Ratings as "F". ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**Safety and efficieny can be acheive by understanding the environment well ,Varibles like inputs['light'],inputs['left'],inputs['oncoming'] are very much importent .By understanding the inputs['light'] state whether it is in " RED" or "GREEN" colour will help the car to make decision to not cross the road when RED color is activated and Safety is ensured.Also by knowing the information like inputs['oncoming'] car can take decision to stop if there i oncoming traffic and avoid collision.inputs['Right'] does not contribute to the learning of the car in this environment .As the vehicle on the right will never interfere with cabs driving path.Deadline can be usefull but our main focus is the safety and reliability,By following the road direction and by avoiding collision ,cab can reach the destinatio in an optimum time. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**| Features | Combinations| total ||------|------|------|| Left | None, left, right, forward|4 || Oncoming | None, left, right, forward|4|| Waypoint | left, right, forward|3|| Light | red, green|2| Total number of possible combinations form the features selected would be 2x3x4x4 =96 For every state a car can take 4 possible action i.e., None ,Left,Right,Forward. Total number of state-action combination is 96x4 = 384 If the number of training trials to 100, and each trial had 20 time steps, we'd cover 2000 state-actions, which might be enough to cover all 384 state-actions. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation!
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning_update_state.csv')
###Output
_____no_output_____
###Markdown
----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**I observe that the Major accidents are decreasing as the trails are increment similar to basic driving agent.yes ,the decaying function is accurately represented ,the exploration factor is ramping down slowly for every training trial.yes , as the number of training trail increases the no of bad action are decreasing,they have almost decreas by 20% Relaibility is drastically improved from F to C ,however the cab still need to learn the aspects of the safety and does not show any improvement compared to that of the basic agent. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_epsilon_005.csv')
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_epsilon_alphaT.csv')
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_epsilon_alphaTT.csv')
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_epsilon_expMinusalphaT.csv')
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning_CosAlphaT.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to Drive> This is prepared for Udacity Machine Learning Engineer Nanodegree online class Author: jtmoogle @github.com All Rights Reserved Date: Aug-Sept 30, 2017 Welcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis. >The following steps were performed1. Understand the World2. Understand the Code3. Implement a Basic Driving Agent - Basic Agent Simulation Results4. nform the Driving Agent - Define a State Space - Update the Driving Agent State5. Implement a Q-Learning Driving Agent - Q-Learning Simulation Results - Define an Optimal Policy - Optional: Future Rewards - Discount Factor, 'gamma'
###Code
# Import the visualization code
import sys
from platform import python_version
import visuals as vs
from IPython.display import display # Allows the use of display() for DataFrames
from platform import python_version
print( '--> sys version= {} '.format(sys.version ))
print( '--> python version= {}'.format(python_version()))
# Pretty display for notebooks
%matplotlib inline
verbos=False
###Output
--> sys version= 2.7.13 |Anaconda 4.4.0 (64-bit)| (default, May 11 2017, 13:17:26) [MSC v.1500 64 bit (AMD64)]
--> python version= 2.7.13
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:1. *Does the Smartcab move at all during the simulation?*2. *What kind of rewards is the driving agent receiving?*3. *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'or'runsmart -c 1 > logs\logs-c1.txt` 1 : if running "no learning" (for question 2)'runsmart -c 2 > logs\logs-c2.txt` 2 : if running "default learning" (for question 5)'runsmart -c 3 > logs\logs-c3.txt` 3 : if running "improved learning" (for question 6)'runsmart -c 9 > logs\logs-c9.txt` 9 : running 1 -> 2 -> 3 in sequencial order``` >**Answer:** The first simulation was used the DEFAULT values describing below:>|learning|enforce_deadline|epsilon|alpha|n_dummies| grid_size|tolerance|testing trial simulated|update delay|optimized|| :--: |:--:|:--:|:--:|:--:| :--:|:--:|:--:|:--:||false|false| 1.0|0.5|100|(8, 6)|0.05|10|0.01|false|>1. I observed that the driving smartcab moved few blocked and stopped during the simulation. The stopped could be idle at red light, or idled at a green light with no oncoming traffic, or bad actions cause accidents2. + The agent received (negative) decreased rewards when having bad actions or violation such as - drove forward through a red light - caused major or minor accident - being idle at a green light with no oncoming traffic + The agent got (positive) increased rewards when having good actions such as - turned right at a red light when no traffic at right/left/oncoming traffic - headed forward at a green light - being idle at a red light - turned left at a green light when no traffic at right, left or oncoming path3. My observation was that the rewards did not have significant changes affected by the light changing color. But, if adding 'violation count' along with light changing color, I saw rewards changes as follows: - if changing green light to red light - If no violation, got increased (positive) rewards - If violation count > 0, got decreased (negative) rewards. More change to see violation count > 0 - If major accident, large negative rewards - if changing red light to green light - if no violation, got increased rewards - if violation count > 0, got decreased (negative) rewards>| changing light color | Violation Count | Rewards | Note || --------- | :---: | :----| :---- || Red -> Green Green -> Red | > zero | Get decreased (negative) rewards | The more violation count The less rewards received || Red -> Green Green -> Red | = zero | Get increased (positive) rewards | The zero violation The more rewards received | Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 21. In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*2. *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*3. *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*4. *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* >**Answer:** 1. In agent.py, I could configured the driving agent with associated parameters - Toggle 'learning' value: TURE/FALSE indicating if the driving agent was in the learning or no-learning mode. Default to FALSE. - if TRUE, the agent would use Q-learning technique to find an optimal action-selection policy for any given (finite) Markov decision process (MDP). - if FALSE, the agent would use random action-selection - Set 'epsilon' value: a float indicating random exploration factor, default to 1.0. - if value was between previous learning and risk-taking, the better - Note: - if too low, the agent would not learn new behavior because consistently in the 'SAFE' condition or using previous learnings - if too higher, the agent would keep learning new behavior, and taking risks consistently. The higher randomness the higher exploration - if zero, the agent would explore all the actions in as few steps as possible, being a pure exploiter - Set 'alpha' value: a float showing learning factor, how much the agent is going to learn, default to 0.5 - if value between 0 and 1.0, the better to enhance new learning on top of previous learning - Generally, 0 < alpha < 1.0 - Example: 0.5 constant learning rate: alpha(state, action) = 0.5 for all t(rial) - Note: if zero, no new learning, reuse previous learning, behave as usual if one, no inheritance algorithm from previous learning, consistent new learning 2. In environment.py, the 'act' function of environment class was called to perform the action, and would receive a reward for good action based on traffic law.3. In simulator.py, - 'render_text' function was called to render the simulated trial data output in non-GUI display such as terminal/command prompt. - 'render' function was called to render and display simulated trial data output in GUI display/interface.4. In planner.py, the next_waypoint function was called and conducted the following checks - (1) if the destination location was at this location - (2) if the agent's heading direction to the destination was East or West direction, - (3) if the agent's heading direction was North or South to destination ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of - 'None' (do nothing) - 'left' (turn left), - 'right' (turn right), - 'forward' (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:1. *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*2. *Given that the agent is driving randomly, does the rate of reliability make sense?*3. *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*4. *As the number of trials increases, does the outcome of results change significantly?*5. *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* >**Answer:**The initial simulation with no learning and simple random action had 'F' safety and Reliability ratings. From visualization, my observation described in the below matrix and sorted by relative frequencies percentage>|Topic Focused| Basic Driving Agent relative frequencies || :---------| :------------ || Total bad actions | 33% increased to 43%|| Major violation | 14% increased to 18% || Minor violation| 9% slightly increased to 13% || Major accidents | 5% decreased to 4% || Minor accidents | ~4% | |>1. The driving agent made bad decision started high frequency 33%, went up to 43% at the end trial 18. Major accident had approx. 4-5%2. The driving agent drove randomly, no learning, the reliability rating illustrated 20%, slightly went up to 30% at trail 17-18. It made sense to rank F because the driving agent DID 80% (100-20%) fail to reach destination on time.3. The driving agent got negative rewards starting negative 5% because over 40% bad decision causing accidents and violations. The rewards slight went down closing to negative 5.5% at trail number 15 to 17 as bad action increased to 42%. - Major accidents (violation:4) penalized/decreased rewards -40 attempted driving forward through a red light with traffic and cause a major accident - Minor accidents (violation:3) penalized/decreased rewards -20 attempted driving right through traffic and cause a minor accident - Major violation (violation:2) penalized/decreased rewards -10 attempted driving left through a red light; attempted driving forward through a red light - Minor violation (violation:1) penalized/decreased rewards -5 idled at a green light with no oncoming traffic4. As number of trials increased, outcome of result had no significantly change5. I would consider this smart cab not reliable neither safe, nor no learning at this moment. It caused many major accident, such as driving through a red light, and 80% (100-20%) failed to reach the destination on time. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 41. Which features available to the agent are most relevant for learning both **safety** and **efficiency**? 2. Why are these features appropriate for modeling the *Smartcab* in the environment? 3. If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.NOTE: You are not allowed to engineer new features for the smartcab. >**Answer:**1.The following table listed input features which were relevant to safety & efficiency consideration>| Evaluation for | More RelevantInput Features | Less Relevant Features ||:----: | :------------------------------ | :-- || Safety | (1) traffic `'Light'` representing state of traffic (2) `'Left'` traffic direction (3) `'Oncoming'` traffic direction | (1) `'Right'` traffic direction | | Efficiency | (1) `'Next waypoint'` indicating the direction of waypoint toward destination | (1) Count of remaining `'deadline'` action to destination | >2.I would choose above more relevant features to model the smartcab in the environment. They were appropriately used to determine intersection state and traffic action. - Safety: features of light state, left traffic direction, oncoming traffic direction would avoid/reduce traffic accidents or/and violations- Efficiency: feature of next waypoint could help driving agent to avoid/reduce mistakes or longer distances to reach destination >See examples of use cases>| |Use cases for Safety | logics translation | | -- | :------------------- | :-------------------------------- | | UC_1.0 |If green light, the driving agent should be **SAFE** | if light=green | | UC_1.1 |(1) go forward | action=forward | | UC_1.2 |(2) turn right | action=right | | UC_1.3 |(3) Yield (wait) for any oncoming traffic which would go straight or turn right,| and if oncoming=forward or right, action=Wait| | UC_1.4| (4) before turn left | and if oncoming=left, action=left | | UC_2.0 | If red light, the agent should be **SAFE** | if light=red | | UC_2.1 | (1) Yield(wait) for any oncoming traffic which would go straight, | and if oncoming=forward, action=Wait | | UC_2.2 | (2) before turn right | and if oncoming=right or left, action=right |>3.I did not choose the less relevant features - `'Right'` traffic direction would not provide significant help on the red or green light state. It is ok not including this feature, it wouldn't affect the agent driving experience. - If red light, the agent should stop, not action taken. - If green, the driving agent would still go forward because the right traffic should stop at their end. - Count of remaining `'deadline'` actions to destination would NOT significant impacting the driving agent action decision and shorten the agent movement. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, 1. what would be the size of the state space? 2. Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! >**Answer:**1.The matrix showed my chosen features, possible values and number of valid values. >| My Chosen Features | Possible values | Count of possible values || :---------- | :----------- | :-----: || Traffic light | true/ false (interpret as green/red) |2 valid states || Oncoming traffic | forward/ left/ right/ None |4 valid actions| | Left traffic | forward/ left/ right/ None |4 valid actions | | Next waypoint | forward/ left/ right/ None note 1 | 4 valid actions | >[note1]: The next_waypoint function in planner.py, would return states ('forward', 'left', 'right', 'None') 'None' indicated if the agent arrived the destination. I'd like to note that I haven't found 'None' for next_waypoint in actual log files yet. >My calculation for size of state space, pair (state, action) would be `'2 x 4 x 4 x 4 = 128'` >2.The environment was configured with associated parameters>|learning|enforce_deadline|epsilon|alpha|n_dummies| grid_size|tolerance|testing trial simulated|update delay|optimized|| :--: |:--:|:--:|:--:|:--:| :--:|:--:|:--:|:--:||false|false| 1.0|0.5|100|(8, 6)|0.05|10|0.01|false|> When simulation was started, the following executed in the sequence orders- Initiation - constructed class with parameter values 1. Created an environment with default values: 100 dummy drivers, (8,6) grid_size for road network 2. Created a driving agent no using Q-learning, epsilon-exploration factor to 1, alpha-learning rate to 0.5 3. followed the driving agent, not enforce a deadline metric 4. Created a simulator setting continuous time in 0.01 seconds, enabling the PyGame GUI, no log trial and simulation result to log file, no optimized. Simulation- Ran a simulation of the environment - The primary agent performed 10 testing trials simulated, 0.05 for epsilon tolerance before beginning testing - If no learning, it simulated 20 training trials, and followed 10 testing trials - If learning was enabled, the simulator would explore the action (1) build current state value (2) create 'state' in a Q-table (3) choose a random action or get max Q-value of all actions (4) receive a current reward (5) update learning rate Number of trial would be 128+, possible values of the features. >- As iterating each training or testing trail 1. The simulated environment would be reset at the beginning 2. Executed time step of simulation. - Primary agent and other agents updated involved as follows + Building the agent state + Choosing an action: either getting random action with epsilon probability, or choosing maximum Q-value of all actions based on 'state' the agent was in + Receiving a reward + Conducting learning Q-value= current Q-value * (1-alpha) + alpha * (current reward + discount factor * expected future reward) + Checking condition if hit hard time limit, or ran out of time. If yes, aborted trial. - update states of intersection lights and traffic - Since action was taken, reduced deadline count 3. Calculated timing, and collected metric from trial > The simulator would learn a policy by building up to 128 combined states in Q-table, recording rewards, final deadline, action taken, and success indicator to reach destination. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: - For every state the agent visits, create an entry in the Q-table for all state-action pairs available. - Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. - Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: 1. *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*2. *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*3. *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*4. *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*5. *How does the safety and reliability rating compare to the initial driving agent?* >**Answer:**The environment was configured with associated parameters for Q-learning agent >|learning|enforce_deadline|epsilon|alpha|n_dummies| grid_size|tolerance|testing trial simulated|update delay|optimized|| :--: |:--:|:--:|:--:|:--:| :--:|:--:|:--:|:--:||True|True| 1.0|0.5|100|(8, 6)|0.05|10|0.01|false|> Extended question 3 result with Q-learning agent relative frequencies>|Topic Focused| Basic Driving Agent frequencies | Default Q-learning Agent frequencies| | :---------| :---------- | :----------------- || Total bad actions | 33% increased to 43%| 31% went down to 12% || Major violation | 14% went down 19% at trial 18 | 14% went down to 2%|| Minor violation| 9% slightly increased to 13% | 8% went down below 5%|| Major accidents | 5% decreased to 4% | 5% slightly decreased to 3% || Minor accidents | ~4% | ~3% went down to 2% |>| Question | Basic Driving Agent | Default Q-learning Agent|| :------- | :------------------| :--------------- ||Q1. Observation for similarity | (1) sequence order of bad actions rewards % per action | (1) sequence order of bad actions first 20 trials reward % per action ||Q2.1 training trials before testing | 20 training trials 10 testing | 20 training 10 testing | |Q2.2 make sense given epsilon-tolerance | no learning, drove randomly| make sense given epsilon-tolerance $\epsilon$(t+1) = $\epsilon$(t) - 0.05, default was 1, decay subtraction from 0.05, after 20 trial, epsilon was close to 0 | |Q3. Decaying function $\epsilon$ | learning was disabled, couldn't compare | yes, The parameter panel graph matched the exploration factor starting at 1.0 decaying/subtracting 0.05, it was close to zero at trial 20. ||Q4. As number of trial increase, bad action? | Increased 10% range | significantly decreased 20% range | |Q5. Safety and reliability rating comparison| FF| CA | ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Question 7Using the visualization above that was produced from your improved Q-Learning simulation, provide a final analysis and make observations about the improved driving agent like in **Question 6**. Questions you should answer: 1. *What decaying function was used for epsilon (the exploration factor)?*2. *Approximately how many training trials were needed for your agent before begining testing?*3. *What epsilon-tolerance and alpha (learning rate) did you use? Why did you use them?*4. *How much improvement was made with this Q-Learner when compared to the default Q-Learner from the previous section?*5. *Would you say that the Q-Learner results show that your driving agent successfully learned an appropriate policy?*6. *Are you satisfied with the safety and reliability ratings of the *Smartcab*?* >**Answer:**>1. I have various attempts trying different decay functions. epsilon = 0.4 alpha=0.4>| Consider | Decay function | Optimized-learning || :-: | :--------- | :--: ||| self.epsilon -= 1.0 / 20000 * self.ntrial | FB ||| self.epsilon = math.exp( -1.0 * self.alpha * self.ntrial )| FF ||| self.epsilon = math.exp( -0.0125 * self.alpha * self.ntrial ) | A+A+ ||| self.epsilon = math.exp( -0.5 * self.alpha * self.ntrial ) | FF |||self.epsilon = math.exp( -0.1 * self.alpha * self.ntrial ) |FF|||self.epsilon = math.exp( -0.05 * self.alpha * self.ntrial ) |FA||[x]|self.epsilon = math.exp( -0.025 * self.alpha * self.ntrial ) |A+A||[x]| self.epsilon = math.exp( -0.0125 * self.alpha * self.ntrial )|A+A ||| self.epsilon = math.fabs(math.cos( self.alpha * self.ntrial)) |FA+||| self.epsilon = math.cos(self.alpha * self.ntrial) | FF||[x]|**self.epsilon = math.fabs( math.cos(0.0125 * self.alpha * self.ntrial))** |** A+A+ **|||self.epsilon = math.fabs( math.cos(0.02 * self.alpha * self.ntrial)) |FA||| self.epsilon = math.fabs( math.cos(0.125 * self.alpha * self.ntrial)) | FF|||self.epsilon = math.fabs( math.cos(0.05 * self.alpha * self.ntrial)) |FA|||self.epsilon = math.fabs( math.cos(0.025 * self.alpha * self.ntrial)) | CA||[x]| self.epsilon = math.fabs( math.cos(0.01 * self.alpha * self.ntrial)) |A+A+||| self.epsilon = math.pow(self.alpha, self.ntrial) | FF|||self.epsilon = math.pow( 0.025 * self.alpha, self.ntrial) | FF||| self.epsilon = math.pow(0.00125 * self.alpha, self.ntrial) | FF||| self.epsilon = math.pow (-0.00125 * self.alpha, self.ntrial) | FF|||self.epsilon = 1.025 * math.pow(self.alpha, self.ntrial) | FF||| self.epsilon = 0.00125 * math.pow(self.alpha, self.ntrial) | FF||| self.epsilon = 0.000125 * math.pow(self.alpha, self.ntrial) | FF||| self.epsilon = 0.0125 * 1.0/math.pow(self.ntrial, 2) | FF ||| self.epsilon = 1.0 / math.pow(self.ntrial, 2) | FC||| self.epsilon = 125 * 1.0/math.pow(self.ntrial, 2) | FD||| self.epsilon = 1.0 /math.pow(self.ntrial, 2) | FF|| self.epsilon = 1.0 /math.pow(default_seed * self.ntrial, 2) | FF||| self.epsilon = 1.0 /math.pow(self.ntrial, 2) | FF|>I chose the best decay function from above was - self.epsilon = math.fabs( math.cos(0.0125 * self.alpha * self.ntrial)) for 0 < alpha < 1 $$\epsilon = \cos(0.125*at), \textrm{for } 0 < a < 1$$ >2.Approx. 297 training trials were needed before beginning testing 3.I used 0.0875 for tolerance, 0.04 for epsilon, 0.4 for alpha (learning rate) to learn, and got 297 trials sufficient enough to train the model. 4.Significant improvement for safety and reliability>| Question | Basic Driving Agent | Default Q-learning Agent| Optimized Q-learning Agent || :------- | :------:| :-----: | :-------: ||Safety rating comparison| F | C | A+ ||Reliability rating comparison| F | A | A+ |>5.The driving agent became successfully Q-learner, learned an appropriate policy, and demonstrate as a safe and reliable driving agent in the simulated environment6.I am satified and happy my driving smartcab having the A+ rating for safety, as well as reliability Define an Optimal PolicySometimes, the answer to the important question *"what am I trying to get my agent to learn?"* only has a theoretical answer and cannot be concretely described. Here, however, you can concretely define what it is the agent is trying to learn, and that is the U.S. right-of-way traffic laws. - Since these laws are known information, you can further define, for each state the *Smartcab* is occupying, the optimal action for the driving agent based on these laws. - In that case, we call the set of optimal state-action pairs an **optimal policy**. Hence, unlike some theoretical answers, it is clear whether the agent is acting "incorrectly" not only by the reward (penalty) it receives, but also by pure observation. - If the agent drives through a red light, we both see it receive a negative reward but also know that it is not the correct behavior. - This can be used to your advantage for verifying whether the **policy** your driving agent has learned is the correct one, or if it is a **suboptimal policy**. Question 81. Please summarize what the optimal policy is for the smartcab in the given environment. What would be the best set of instructions possible given what we know about the environment? _You can explain with words or a table, but you should thoroughly discuss the optimal policy._2. Next, investigate the `'sim_improved-learning.txt'` text file to see the results of your improved Q-Learning algorithm. _For each state that has been recorded from the simulation, is the **policy** (the action with the highest value) correct for the given state? Are there any states where the policy is different than what would be expected from an optimal policy?_ 3. Provide a few examples from your recorded Q-table which demonstrate that your smartcab learned the optimal policy. Explain why these entries demonstrate the optimal policy.4. Try to find at least one entry where the smartcab did _not_ learn the optimal policy. Discuss why your cab may have not learned the correct policy for the given state.Be sure to document your `state` dictionary below, it should be easy for the reader to understand what each state represents. >**Answer:** The environment was configured with associated parameters>|learning|enforce_deadline|epsilon|alpha|n_dummies| grid_size|tolerance|testing trial simulated|update delay|optimized|| :--: |:--:|:--:|:--:|:--:| :--:|:--:|:--:|:--:||true|true| 0.4|0.4|100|(8, 6)|0.0875|25|0.01|true|>1.The optimal policy determined which action to take, based on current state which the agent was in. In order to ensure the agent to be safe and reliable, the agent should obey all traffic rules, have no/minimum bad behaviors, took minimum time reaching to destination. >>The definition of optimal policy: - If green light, the agent travelled across the intersection in the simulated environment, - If no oncoming traffic, the agent could go forward or turn right toward next way point - If any oncoming traffic would go straight or turn right, the agent should wait/yield - If any oncoming traffic would turn left, the agent could wait/yield and turn left >>- If red light, - For any oncoming traffic would go straight, the agent should wait/yield - If oncoming traffic turn right or left, the agent would wait/yield and turn right toward next way point - If next way point would turn left/forward (not right), the agent would wait/yield>During simulation, the learned policy action would be recorded: Q(s, a) which is Q-value of the (state, action) pair as well as R(s) reward.- format like ("light-state", "left-traffic", "oncoming-traffic", "next-waypoint") - State 1 = current light state. Possible value: green/red - State 2 = left traffic possible action: left/right/forward/none=no action - State 3 = oncoming traffic possible action: left/right/forward/none=no action - State 4 = next way point possible action: left/right/forward/none=no action>- R(s) the immediate/current reward to enter the State’s’. Increased/positive reward if the agent had good action; decreased/negative reward if bad actions (accidents or violations) - Updated Q-value = current Q-value * (1-alpha) + alpha * (current reward + discount factor * expected future reward)- Each step the agent passed info about its current state to the policy - The policy would be used to decide what action to perform next step - The positive/increased reward, would be a list of actions to consider, the highest reward would be best action for agent to take - The negative/decrease reward, would NOT recommend action could be accident or violation occurred in the history of actions.>Example 1: For the given environment, if (1) is-green-light (2) no left traffic (3) no oncoming traffic, the agent would ask Q-table for best next step for the current state it was in. From sim_improved-learning.txt, we could see the following visualization illustrate the optimal policy: comparison reward by action or/and next way point>| state4Next way point | Action | policy evaluation | Qvalue-Reward | | :------- | :---- | :---- | :--: | | if forward | forward | optimal | 1.92 | | if forward | right | suboptimal | 0.42 | | if forward | no action/yield/wait | incorrect | -5.21 | | if forward | left | suboptimal | 0.41 | >2.My observation from above result, This is correct policy, if next way point headed 'forward' direction, as expected - The best action was "forward" and had the highest Qvalue-reward. - The worst action was "no action/yield" at green light and had the lowest Qvalue-reward.
###Code
# example 1
import os
import pandas as pd
import matplotlib.pyplot as plt
import matplotlib
import seaborn as sns
# sim_improved-learning.txt
plt.close('defaultplot')
mycsv='sim_improved-qlearntbl.csv'
data1 = pd.read_csv(os.path.join("logs", mycsv))
mycsv='sim_improved-qlearntbl.2.csv'
data2 = pd.read_csv(os.path.join("logs", mycsv))
# The optimal policy: state1="light-state" 2="left-traffic" 3="oncoming-traffic" 4="next-waypoint"
filterstr='state1 == "green" & state2 == " notraffic" & state3 == " notraffic" '
sdata = data1.query( filterstr )
print( '-- In sim_improved-learning.txt, the optimal policy: Q-table filter criteria ->\n {}'.format(filterstr ))
print( '-- filtered result -->')
display( sdata )
sdata2= data2.query( filterstr ) # data format for graph
print('--> The Optimal Policy: Visual comparing reward by action, next way point' )
print('The given environment if is-green-light, no left traffic, no oncoming traffic')
fig, (ax1, ax2) = plt.subplots(1, 2,figsize=( 12, 6), sharex=False, sharey=True)
ax1.get_xaxis().set_visible(True)
ax1.set_title( 'Reward by action' )
sdata2.boxplot( ax=ax1, column='reward', by='action' )
ax2.get_xaxis().set_visible(False)
ax2.set_title( 'Avg reward by next way pt, action' )
(sdata2.groupby(['state4', 'action']).mean().unstack().plot.bar( ax=ax2 , table=True))
plt.show()
plt.close()
###Output
-- In sim_improved-learning.txt, the optimal policy: Q-table filter criteria ->
state1 == "green" & state2 == " notraffic" & state3 == " notraffic"
-- filtered result -->
###Markdown
>2.(continue...) >Example 2: If (1) is-green-light (2) left traffic - turn left (3) no oncoming traffic, Summary from the visualization for the optimal policy>|state4Next way point | Action| policy evaluation | Qvalue-Reward || :--- | :--- | :---- | :---- ||if forward | forward | suboptimal | 0.6 ||if forward | right | suboptimal | 0.58 ||if forward | no action/yield/wait | Incorrect | -5.41 ||if forward | left | optimal? not as expected | 2.13 ||if left | forward | suboptimal | 0.91 ||if left | right | optimal? not as expected| 1.58 ||if left | no action/yield/wait | Incorrect | -4.86 ||if left | left | optimal | 1.14 ||if right | forward | optimal? not as expected | 1.92 ||if right | right | suboptimal | 0.42 ||if right | no action/yield/wait | Incorrect | -5.21 ||if right | left | suboptimal | 0.41 |>My observation from above result, This policy was not I expected, if next way point headed 'forward' direction, I would expect 'forward' as the optimal policy- The best action was "left" which had the highest Qvalue-reward. - The worst action was "no action/yield" at green light and had the lowest Qvalue-reward.>This policy was not I expected, if next way point turned 'left' direction, I would expect 'left' as the optimal policy - The best action was "right" and had the highest Qvalue-reward. - The worst action was "no action/yield" at green light and had the lowest Qvalue-reward. >This policy was not I expected, if next way point turned 'right' direction, I would expect 'right' as the optimal policy - The best action was "left" and had the highest Qvalue-reward. - The worst action was "no action/yield" at green light and had the lowest Qvalue-reward.
###Code
# example 2
plt.close('defaultplot')
# The optimal policy: state1="light-state" 2="left-traffic" 3="oncoming-traffic" 4="next-waypoint"
filterstr='state1 == "green" & state2 == " notraffic" & state3 == " left" '
sdata = data1.query( filterstr )
print( '-- The optimal policy: Q-table filter criteria ->\n {}'.format(filterstr ))
print( '-- filtered result -->')
display( sdata )
sdata2= data2.query( filterstr ) # data format for graph
print('--> The Optimal Policy: Visual comparing reward by action, next way point' )
print('The given environment if is-green-light, left traffic-notraffic, oncoming traffic-turn left')
fig, (ax1, ax2) = plt.subplots(1, 2,figsize=( 12, 6), sharex=False, sharey=True)
ax1.get_xaxis().set_visible(True)
ax1.set_title( 'Reward by action' )
sdata2.boxplot( ax=ax1, column='reward', by='action' )
ax2.get_xaxis().set_visible(False)
ax2.set_title( 'Avg reward by next way pt, action' )
(sdata2.groupby(['state4', 'action']).mean().unstack().plot.bar( ax=ax2 , table=True))
plt.show()
plt.close()
###Output
-- The optimal policy: Q-table filter criteria ->
state1 == "green" & state2 == " notraffic" & state3 == " left"
-- filtered result -->
###Markdown
>Example 3: If (1) is-red-light (2) next way point is not right Summary from the visualization for the optimal policy >| left traffic | oncoming traffic | Action | policy evaluation | Qvalue-Reward | | :---- | :---- | :-------- | ---- | | all direction |all direction | left | incorrect | negative reward | | all direction |all direction |forward | incorrect |negative reward | | all direction |all direction |None | optimal | positive reward | | all direction |all direction |right | suboptimal | zero to negative reward |>My observation from above result, This is correct policy, if next way point would turn left/forward (not right), the agent would wait/yield>3.When simulation trial just started, I observed 3 / 6 (50%), which had violation count > 0 and negative rewards. - As trial runs increased, the observation decreased 4/15 (26%) which had negative rewards. References and Additional Resources: >4.Try to find at least one entry where the smartcab did not learn the optimal policy. Discuss why your cab may have not learned the correct policy for the given state.Conclusion:>Overall, the learned policy provided better decision to the agent to make better action in later trials>| Trial | Accident & Violation Count | Reward | Reliability | Exploration/Learning Factor | | --- | :------ | :--- | :------ | --- | | 150 | decreased to 25% | -4 | 75/40 % || 230 | decrease to 15% | -2 | 40/40 % || 250 | decreased to 2-3% | increased to above 0 | range of 80 | 30/40 % |
###Code
# example 3
plt.close('defaultplot')
# The optimal policy: state1="light-state" 2="left-traffic" 3="oncoming-traffic" 4="next-waypoint"
filterstr='state1 == "red" & state4 != " right"'
sdata = data1.query( filterstr )
print( '-- The optimal policy: Q-table filter criteria ->\n {}'.format(filterstr ))
if verbos:
print( '-- filtered result -->')
display( sdata )
sdata2= data2.query( filterstr ) # data format for graph
print('--> The Optimal Policy: Visual comparing reward by action, next way point' )
print('The given environment if is-red-light, next way point-not right')
fig, (ax1, ax2) = plt.subplots(1, 2,figsize=( 12, 6), sharex=False, sharey=True)
ax1.get_xaxis().set_visible(True)
ax1.set_title( 'Reward by action' )
sdata2.boxplot( ax=ax1, column='reward', by='action' )
ax2.get_xaxis().set_visible(False)
ax2.set_title( 'Avg reward by next way pt, action' )
(sdata2.groupby(['state4', 'action']).mean().unstack().plot.bar( ax=ax2 , table=True))
plt.show()
plt.close()
###Output
-- The optimal policy: Q-table filter criteria ->
state1 == "red" & state4 != " right"
--> The Optimal Policy: Visual comparing reward by action, next way point
The given environment if is-red-light, next way point-not right
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**Some things that I observed while running the simulation straight out of the box:- The Smartcab does not move at all.- The starting position of the Smartcab and the end position (the Udacity logo) are randomized for each trial run.- When the Smartcab is at a redlight, then the reward is positive for idling.- When the Smartcab is at a greenlight, then the reward is negitive for idling. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**`agent.py` flags: - `num_dummies`: This flag sets the number of vehicles other than the Smartcab driving around in the simulation. The default value is 100. The idea is that an increase in vehicles would make it more difficult for the Smartcab to complete its goal. But at the same time, an increase in vehicles may provide a higher probability for the Smartcab to encounter more states. - `grid_size`: This flag sets the physical column x row grid dimensions of the streets. The default value is (8, 6). By adjusting the grid size, we can see if changes in grid size change the length of time needed for the Smartcab to reach its goal. Also, the change in grid size may impact how much more or less the Smartcar may encounter states. This may especially be true for a tiny grid size (think 2 x 2) in which the Smartcar may randomly make it to the goal without needing to learn anything. - `learning`: This flag sets whether or not to force the driving agent to use the Q-learning technique to learn the optimal policy action-selection given a finite MDP [Wikipedia/Q-learning](https://en.wikipedia.org/wiki/Q-learning). Another way to look at this flag is if it is set to `False`, then the Smartcab will *only* use random choices to make decisions with no calculations, weights, etc. With the flag set to `True`, then the Smartcab will employ the Q-learning algorithm.`environment.py`: The class `act()` is called when the agent performs an action.`simulator.py`: The difference between 'render_text()' and 'render()' is that the former is a non-gui display of the output data to the terminal; Whereas the latter outputs the simulation to a fully realized graphical display along with data output.`planner.py`: The 'next_waypoint() function considers the East-West direction first before considering the North-South direction. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**Let's take a closer look at the invidual plots in the visualization:- **Relative Frequency of Bad Actions** According to the plot, it appears the agent is making roughly 35% to 40% total bad actions per trial. Out of all the bad actions the Smartcab is making, about a quarter (or 10% of all actions) caused both minor and major actions.- **Rate of Reliability** The rate of reliability shows the rate at which the Smartcab makes it to the goal. According to the plot, the Smartcab starts around 10% and steadily increases toward 50%. It appears it may be learning even though the flag settings for this specific trial run has learning turned off. This is merely coincidence because every action the Smartcab performed was completely random, thus this plot has no relevance.- **Average Reward per Action** It appears the average reward per action throughout the entire trial run is between -4 to -5. In other words, there are no penalization mechanics in place to help the Smartcab adjust its decisions. All the decisions made in the trials were random, thus no changes to penalization.- **Number of Trial Runs** As the number of trials increase, there doesn't appear to be a significant change in the all the outcomes. They're all pretty much pointing at a terrible performance. - **Safety/Reliability Rating** According to the built-in assessment tools, both safety and reliability are graded at "F". This is expected given our intial settings and flags. As the Smartcab stands, it is not safe nor reliable for passengers. We can also point to the other plots as evidence. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**I'll be honest, I am responding to this question "well-after-the-fact" of examining the various python files and running some of the steps further along in the project. That being said, my human-side says the features that are most relevant are "all of them."However, in context of how the dummy agents are driven and how the violation/reward rules are setup, the real answer to what features are appropriate for modeling the Smartcab are: `waypoint` and `inputs[light]`. I chose them because the way the violation detection rules are setup in the `environment.py` file only considers these two features.That means the features I'm ignoring are: `inputs[left]`, `inputs[right]`, `inputs[oncoming]`, and `deadline`. These features are not appropriate because their data inputs make no difference to the reward or violation detection scheme. Even for the edge case of making a right turn on a red light can be delegated to simply wait on red regardless of `inputs[left]` status and only go on green.**EDIT**After considerable feedback from mentors, the proper selection here is to incorporate as many features as possible. So I have edited the `agent.py` script to reflect this change. The selected features are now: `waypoint`, `inputs[light]`, `inputs[left]`, `inputs[right]`, and `inputs[oncoming]`. `deadline` is excluded because having the Smartcab learn to take actions based on how much time is left does not seem intuitive considering we want **safety** and efficiency. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**The state space based on my response in **Question 4** would be calculated from the number of `waypoints` times number of `inputs[light]`: `3 x 2 = 6`. Because there are only 6 states to learn and given the default environment the driving agent is learning in, I am confident it will learn the optimal policy within a reasonable number of training trials.**EDIT**The new state space calculation is $3 x 2 x 4 x 4 x 4 = 384$. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- There are no observable trends between this new visualization compared to the basic driving agent other than number of trials and tests. The number of trials and tests remain the same at 20 and 10 respectively.- The number of trials at 20 does make sense considering that our epsilon decay function decays at a linear rate of 0.05 from 1.0 down to the tolerance of 0.05.- As previously stated, the epsilon decay function is linear. So in the above visualization, it is accurately represented by the diagonal blue line.- As the the number of training trials increased, the number of bad actions decreased. The average reward also increased at the same time.- The new safety and reliability rating increased from F/F to A+/A+!.**EDIT**The safety and reliability ratings have decreased due to the increase number of features used for the reinforcement learnign model. However, the perception that the driving agent is still learning *something* still holds true due to the trends in the plots. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** During the simulation, the smartcab didnt move at all. The agent is receiving both positive and negative rewards randomly. When the light is red, the agent gets a positive reward. When the light is green with no oncoming traffic, the agent receives a negative reward. When the light is green with oncoming traffic, the agent receives a positive reward. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:** - In agent.py, - "verbose" flag can be set to True to display extra and detail information about the simulation and it will useful during debugging. - "display" flag can be set True to enable GUI simulation and False to disable GUI simulation. - "n_test" flag can used to set the number testing trial to perform during a simulation.- In environment.py, - The "act" funtion in "Environment" class is called when an agent performs an action.- In simulator.py, - The difference between 'render_text()' and 'render()' is that,'render_text()' is used to display the whole simulation data in text format in terminal or command prompt and 'render()' funtion is used to display the simulation in the GUI pygame window.- In planner.py, - The 'next_waypoint()' function considers East-West direction first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'Left'` (turn left), `'Right'` (turn right), or `'Forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliabilty make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:** For example in case of trial number 10, the driving agent is making bad decision at frequency of approximately 0.4(i.e. the sum of minor volations,major volations, minor accidents and major accidents). So 40% of actions out of all actions taken were bad. Around 30%(approx minor accident - 0.12, major accidents - 0.18, total = 0.30) of the total actions caused accidents. The rate of relability is very low(10% average) as the driving agent is only exploring by taking random action and not learning anything, signifying that the agent is not learning and not reliable. The driving agent is recieving an average of negative rewards throughout all the trials. The agent was forced to take the right decision by giving a negative reward but since the agent was randomly choosing the actions, it mostly got negative rewards, leading to an average negative reward(<-4). Increase in the number of trials doesn't seem change the results significantly except for little fluctuations in the output. The smartcab would not be considered as safe for passengers as the relabilty of the agent is < 20% and approx 30% action taken in a single drive can lead to accidents which can risk the passenger's and other's life. Also, knowing the fact that driving randomly will surely end up in bad situation, its not safe for passengers. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate?* **Answer:** The features that are most relevant to both learning and efficency are - 'waypoint' since, it helps in directing towards the goal and will help us reach the destination safely without taking wrong and random turns.- 'lights' since, traffic lights ensures safety by controlling the traffic. If violated, can lead to accidents.- 'oncoming' since, it is needed to check and follow the U.S. Right-of-Way rules. - 'left' since, it needed to confirm that no traffic in the left is moving forward when taking a right in red light as per the U.S Right-of-Way rules.The features that may not to be relevant to both learning and efficency are - 'deadline' since, there is rewards for following the traffic rules and no rewards for reaching early, adding it would only increase the size of the state significantly. - 'right' since it is not needed to follow the U.S. Right-of-Way rules and basic traffic rules. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the evironment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:** Considering the features 'waypoints' which can have 3 possible values(Forward, Left, Right), 'lights' which can 2 possible values(red, green),'left' which can 4 possible values(None,forward,left,right) and 'oncoming' which can 4 possible values(None,forward,left,right), the size of the state space would be 96. Yes, i think the driving could learn a policy for each possible state within a reasonable number of training trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the interative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.01). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:** There seems to be no similarity between the basic driving agent and this default Q-learning agent. It takes approximately 20 trials to train the model before testing as the epsilon value is decreased by 0.05 from 1 for each trial. It can be understood by looking at the Exploration(epsilon) - Learning(alpha) factor graph. The epsilon value is linearly decaying by value of 0.05 for each trial until it reaches the tolerance or threshold value of 0.01. As you can see the epsilon value reaches the tolerance 0.01 at around 20 trials where the training is stopped. The parameters panel in GUI and values printed in command line accurately represents the decaying function as the value of epsilon starts from 1 and decreases by 0.05 for each training trail. As the number of training trails increases, the number of bad actions decreases and in overall average reward increases. The agent got a F safety rating which is the worst rating as same as the initial driving agent. The agent got a C relability rating which is a average rating whereas the initial driving agent got the worst reliability rating of F. ----- Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** The Smartcab does not seem to move at all during the simulation as the agent state is never updated and is always idled at the light. During simulation at each step, the driving agent seems to be receiving different rewards based on how long it has been idling and what the color of the light is at the time. The Smartcab gets both positive and negative rewards for actions taken. As the light turns green it gets a positive reward for staying idle when there is incoming traffic, a negative reward for idling with a green light and no incoming traffic, and a positive reward for staying idle at a red light. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:** agent.py Flags are learning, epsilon, and alpha(the learning rate). As you switch learning to True it changes the simulation so that the agent learns and improves (hopefully), epsilon is a factor for making random moves, and the alpha flag sets the factor for which the agent learns.environment.py When an agent performs an action, the act function is called to assess the implications of the action that was taken, including the rewards, whether or not the agent can move based on the action chosen, etc. simulator.py The 'render()' function is the GUI render display of the simulation and the 'render_text()' function is the non-GUI render display of the simulation, or the command prompt or terminal interface. planner.py The 'next_waypoint()' function considers the East-West direction first so long as the current location is not the final destination. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'Left'` (turn left), `'Right'` (turn right), or `'Forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliabilty make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:** The driving agent is settling on a bad decision about 42% of the time because of which the driving agent is bringing on minor accidents approximately 5% of the time, and it is creating major accidents roughly 10% of the time. These bad decisions are additionally bringing about minor violations approximately 10% of the time, and major violations around 22% of the time.The agent is consistently receiving an average reward per action that is negative. This is indicative that it is being heavily penalized.As the number of trials increases though the results of the agent hasn't been changing significantly because it isn't learning from the rewards yet. I would not consider this a safe or reliable car for passengers yet as it currently takes bad and dangerous actions an unacceptable amount of the time shown in the first graphic. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate?* **Answer:** For safety 'light', 'left', and 'oncoming' are all relevant features, Trafic light is an important feature for safety as all the surrounding traffic is following Trafic light and rules (ie: Red means stop, Green means go) thus reduce a chance of violation and accident. Similarly 'left' and 'oncoming' are important as they are the only two side from which we have to deal with traffic , I excluded 'right' since simulation is following US traffic laws there will be no traffic from 'right' side assuming no violation of traffic law by surrounding traffic. I also excluded 'deadline' because deadline shouldn't improve safety and efficiency either because we are already traveling in the most efficient path according to our 'waypoint' feature as well as 'Deadline' could also encourage breaking traffic laws and decreasing safety as time runs out. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the evironment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:** The size of the state space using the features defined in Question 4: 2(light: green,red) x 3(Waypoint: forward, right,left) x 4(left: left, right,forward,none) x 4(oncoming: right, left, forward, none) which will give us 96 state space, I think this as a reasonable state space to learn a policy for each possible state in the reasonable amount of time. State space is neither small nor too big for the learning agent. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the interative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.01). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:** Default learning agent enhances with time while the basic driving agent does not demonstrate any change with time, thus we can say that it will improve if we train it more. Be that as it may, the consequence of Safety and Reliability for both the basic driving agent and the default Q-Learning agent score is F for Safety and Reliability, therefore, unsafe. There were 20 training trials which make sense mathematically as epsilon decays to 0 in exactly 20 trials with its current decay function. It is accurately represented in the panel as it linearly decays to 0 in 20 trials. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the impliciations it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Reinforcement Learning Project: Train a Smartcab to Drive ----- Getting StartedIn this project, presented work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, we need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**1. The smartcab doesn't move.2. The smartcab receives follow rewards: - Agent properly idled at a **red light** (approximately, +2) - Agent idled at a **green light** with no oncoming traffic (approximately, -5) - It is not clear what the reward for accident/collision while the smartcab doesn't move. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:****`agent.py`** fileFlags:- `num_dummies` - discrete number of dummy agents (other cars) in the environment, default is 100- `alpha` - continuous value for the learning rate, default is 0.5- `update_delay` - continuous time (in seconds) between actions, default is 2.0 seconds (simulation update rate)**`environment.py`** file- `act()` function is called when an agent performs an action.Consider an action and perform the action if it is legal.Receive a reward for the agent based on traffic laws.**`simulator.py`** file- `render_text()` - the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt.- `render()` - the GUI render display of the simulation.**`planner.py`** file- `next_waypoint()` function consider East-West direction first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:*** The driving agent making bad decisions from **37% to 43%** of the time. Around **8%** of those bad decisions cause major accidents and around **4%** cause minor accidents.* The rate of reliability is low (agent arrives to the destination correctly on random mode around 18% of the time) and it makes sense given that agent is not attempting to drive to the destination.* The average reward for the agent is around -5.5. That means it's getting heavily penalized: high rate of major violations and major accidents are the most probable causes.* Agent doesn't any learning at this point, so the more trials don't have a significant effect on the outcomes.* The smartcab is definitely not safe/reliable for its passengers. The rating for safety has been defined to have no major accidents and very low number of minor violations (8% of major violations for now). The reliability rating has been defined as whether the smartcab reaches its destination > 90 % of the time (around 18% for now). ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**Important for **Safety**:- `'light'` - is a critical and strong feature for safety. Agent should drive while light is green and stop while light is red.- `'left'` - is important because affecting on 2 cases: turning left and rule where is allowed to take a left turn during a red light.- `'oncoming'` - is important because the oncoming traffic should not intersect with the trajectory of the agent.Important for **Efficiency**:- `'waypoint'` - is important because if agent ignores this feature it will randomly drive around the grid.Features that **not appropriate** and can be ignored:- `'right'` - affects only for a scenario where the agent moves right. Ignoring this rule not much affects the safety. And agent still available to make U-turns.- `'deadline'` - there is some probability that agent will start to learn how to meet the deadline and will start to ignore critical for safety rules. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**- `'light'`: 2 possible values (1 - red, 2 - green)- `'left'`: 4 possible values (1 - None, 2 - left, 3 - right, 4 - forward)- `'oncoming'`: 4 possible values (1 - None, 2 - left, 3 - right, 4 - forward)- `'waypoint'`: 3 possible values (1 - left, 2 - right, 3 - forward)Number of states = 2 x 4 x 4 x 3 = **96**For given 20 training trials it would be difficult for an agent to 100% reliably learn all possible states.In case if we will apply more training trials ( a reasonable number), the quality significantly be increased. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- The safety score is still low (F value like in the basic driving agent case). But there are some improved results: the total number of bad actions decreased, the number of major violations decreased as well.- The agent required 20 training trials before testing. It makes sense because the epsilon decreasing by 0.05. When epsilon became zero, testing began.- Implemented Decaying function for the exploration facto is represented in the parameters panel.- As the number of trials increased, the number of bad actions decreased (from 33% to 15%). The Average reward increased from nearly -4.2 to around -1.2.- Reliability rating is 'A' now (F for the initial driving agent), but Safety rating still needs improvement because 15% of the time it makes a bad actions. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** - The Smartcab doesn't move at all. - Idle at green light with no oncoming traffic: large negative reward.- Idle at green light with oncoming traffic: small positive reward.- Idle at red light: small positive reward. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- *display: set to False to disable the GUI if PyGame is enabled; log_metrics: set to True to log trial and simulation results to logs; optimized: set to True to change the default log file name*- *act(self, agent, action)*- *`'render_text()'`*: This is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt. *`'render()'`*: This is the GUI render display of the simulation. Supplementary trial data can be found from render_text.- *East-West direction first* ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'Left'` (turn left), `'Right'` (turn right), or `'Forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliabilty make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- On average, the agent made bad decisions over 40% of the time. Among those bad decision, ~1/5 caused accidents.- Yes, since the agent didn't plan the route according to the destination (or waypoints), the reliabilty should be poor.- The average rewards were <-5 on average. This suggests the agent has been penalized heavily.- No, because the agent was set to *learning = false*- In this case, it's not safe nor reliable. It's not safe because it caused accidents and didn't obey traffic rules. It's not reliable because the agent didn't plan the route according to the destination. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate?* **Answer:**- `'waypoint'`, `'light'`, `'left'` and `'oncoming'` are most relevant for both safety and efficiency. To drive safely, the agent must consider some of the sensor data to obey traffic rules and avoid accidents. To drive efficiently, the agent must know the next waypoint.- `'right'` is not needed here because it won't affect the agent's decisions. (The agent should obey the traffic light anyway.)- `'deadline'` is less critical but it's also important. The agent must be aware of the remaining deadline so that it could arrive the destination in time. However, including the deadline feature means increasing our state space because deadline could be any number between 0 to the start time. As a result, it's not appropriate to include this featrue. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the evironment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**- 3(waypoint) x 2(light) x 4(left) x 4(oncoming) = 96- The driving agent may be able to learn a policy for each possible state with a resonable number of training trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the interative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.01). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- The two cases both start with high frequency of bad actions and low average rolling rewards.- 20 training trials. Yes, it took 20 rounds for the epsilon to reach the tolarance.- Yes, the plot shows a linear decaying function for $\epsilon$. - Yes for both of the two questions.- There's a significant improvement on the reliability rating while no changes on the safety rating. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the impliciations it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**1. The Smartcab idles all the time no matter the light is red or green.1. According to red and green, the driving agent receives positive and negative rewards respectly * green light with other traffic: small positive rewards * green light without other traffic: big negative rewards * red light: positive rewards Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**1. * learning: Whether the agent is expected to learn * destination: Select the destination as the new location to route to * testing: 'testing' is set to True if testing trials are being used once training trials have completed.1. act1. * render_text(): This is the non-GUI render display of the simulation.Simulated trial data will be rendered in the terminal/command prompt. * render(): This is the GUI render display of the simulation. Supplementary trial data can be found from render_text.1. East-West direction first ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'Left'` (turn left), `'Right'` (turn right), or `'Forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliabilty make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**1. * From 10-Trial Rolling Rate of Reliability, we can conclude that mean of total bad action is about 0.39 * Adding the mean of minor and major accident, we get about 0.04 + 0.07 = 0.111. Yes, we set the destination properly. If we get higher rate of reliabilty, we need to consider to set a new destination1. Mean of reward per action is about -5. The rewards suggest it has been penalized heavily.1. From the image of reliability and reward, the outcome of rate of reliability won't change while reward per action will increase as the number of trials increases1. NO. From the three images, almost 0 rate of reliability, negative rewards per action and double F of 10 test trials, we can conclude that this Smartcab can not be considered safe and reliable ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate?* **Answer:*** waypoint: * relevant for efficiency not safety* inputs: * relevant for safety not efficiency* deadline * relevant for efficiency not safety* If I have three opitions, I choose waypoint and inputs, for both safety and efficiency. If I have two options, I will not choose deadline, because I only need to know the right direction. * `state = (inputs['oncoming'], inputs['light'], waypoint)` * I choose these 3 features and omit `inputs['right'],inputs['left']` because 3 features have less states than 5 features which needs less training trials and these 3 features are the most relevant to both safety and efficiency Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the evironment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:*** I choose waypoint, inputs[light] and inputs[oncoming].* waypoint is [forward,left,right], inputs is [[red, green],[left,right,none,forward]], 24 = 3\*2\*4.* No.Number of training trials is 20, while the number of state is 24. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the interative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.01). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**1. Their total bad actions are decreasing. Both reward per action increase1. 20 training trials. It make sense,we keep training until epsilon less than tolerance.1. Yes,from 0.95 to 01. * yes, from the image * yes, from the image1. It does better. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the impliciations it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:*** No, the Smartcab currently does not move or make any actions within the simulation.* The agent is given positive rewards when the light is Red, for following the traffic rules by not moving or driving through a red light. The agent is given negative rewards when the light is Green and there is no on-coming traffic, for not attempting the reach the destination goal on time. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:*** For the agent.py file, the 3 flags are: - learning: this flag can be set to True to force the driving agent to use Q-learning and set to False when the driving agent is trained and using the developed model to act within the simulation. While the flag is set to True, the Reinforcement Model is still being trained so the processing of what action to take can change during the learning process. While when it is False, the driving agent will perform the same actions given the same environment states. - epsilon: allows for the Reinforcement Learning Model to make random actions based on the probability value, epsilon. This can be helpfuil by allowing the model to explore new actions and discover new reasons based on taking these randomized actions. While epsilon can have a value of 0 to 1, it would be in the best intrest of the model for the epsilon value to not be 1, i.e. completely random. - alpha: this flag represents the learning rate of the Reinforcement Learning Model. Alpha can have a value from 0 to 1, the higher the alpha value, the faster the model will converge but if the alpha is too high, then the model might miss the optium learning function.* For the environment.py file, the Environment class function called when an agent performs an action is environment.act() . * For the simulator.py file, the difference between 'render_text()' and 'render()' functions is that the 'render_text()' function outputs information concerning the simulation to the terminal. While 'render()' outputs information concerning the simulation to the GUI with a pygame backend.* For the planner.py file, the 'next_waypoint' function will check the East-West directions before it checks the North-South directions. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:*** The agent is making bad decisions ~45% of the time, or almost half of the time the agent makes a bad decision. Out of the agent's decisions, ~10% are Major Accidents and Minor Accidents. Therefore, ~22% of the bad decisions cause an accident.* Yes, the reliability rate is ~15%, which is slightly worse than random guessing which is 20%. If the simulation were run for more trials, the reliability rate should converge to 20%.* The average reward per action is ~-6, which suggests that the agent is being heavily penalized for making suboptimal actions.* Yes, as the trails increase, the results converge to the random guessing values based on the agent having access to 5 different actions, and 1 of the actions being optimal. * No, the Smartcab would not be considered safe and/or reliable currently, with a Safety and Reliability Rating of F. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**All of these features are important for an optimal path for the agent.* 'waypoint' tells the agent the optimal path, which will increase efficiency during the agent's decision making process.* 'inputs' tells the agent where other car agents are relative to the agent's current position, which will increase safety by helping the agent avoid accidents. While the color of the light will help the agent determine when not to make decisions that would cause traffic violations, increasing safety.* 'deadline' tells the agent the number of time steps left before the travel time runs out, increasing the agent's efficiency. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:*** 'waypoint': 3 possible states* 'light': 2 possible states* 'left': 4 possible states* 'right': 2 possible states* 'oncoming': 4 possible states* 'deadline': 5 x intersections of destination possible statesTotal Possible States: 3x2x2x2x2x(5x8) = **3840**The 'waypoint', 'light', 'oncoming' and 'left' will be used to decrease the state space size. Resulting in a state space of, **96**.Omitting 'right' is sensible for American traffic laws due to 'right' oncoming traffic to be required to yield to perpendicular traffic that has a green light, or Right of Way.Omitting 'deadline' is extremely helpful due to greatly reducing the state space size. Also, regardless of how many steps are remaining for a successfully on-time trip, the act of the agent should remain the same to find the optimal path while obeying traffic laws. If the 'deadline' is different but all other state information remains the same, the agent should make the same action regardless of the 'deadline' value. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:*** Both the basic driving agent and the default Q-Learning agent have Safety Ratings of F.* The agent required 20 trails. This makes sense given that the epsilon decay function is 1 - 0.5xt, with t being the number of trails, at t = 20, epsilon = 0.* Yes, it is actually represented in the Trial Number vs Parameter Value graph, shown with a linear decay.* The number of bad actions increased as the trials increased, until ~17 trials, where the states are being revisited and are no longer new for the agent.* The Safety Ratings are the same for the initial and current agents. While the Reliability Rating for the current agent is A+ compared to the F rating of the initial agent. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**- The Smartcab does ***not* move** at all during the simulation- **Idling** at a **red** light receives **positive** rewards- **Idling** at a **green** light receives **negative** rewards Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- `agent.py` * **`display`** - if `True`: enable the GUI if `PyGame` is enabled * **`log_metrics`** - if `True`: log trial and simulation results to `/logs` * **`optimized`** - if `True`: change the default log file name- `environment.py` * **`act()`** function is called when an agent performs an action- `simulator.py` * **`render_text()`** - **non-GUI**/pure-**text** (in the terminal/command prompt) render * **`render()`** - **GUI** render- `planner.py` * **`next_waypoint()`** function considers the direction **`East-West` first**, then the `North-South` ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- *Relative Frequency* of the **Bad Actions** $\approx$ 45~50%- *Relative Frequency* of the Minor/Major **Accidents** $\approx$ 5~10%- The **Reliability** of *Random Actions* could be a **naive baseline** to show the **trip difficulty**- The agent always receives **negative rewards**. Do not have a baseline to compare heavy or light.- The *outcome* does ***not* change** significantly as the number of trials increases- This cab is ***not* considered safe/reliable**/smart because the relative **fewer good actions** are **hardly chosen by random** ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**- **`'waypoint'`** is required for the **destination** and **Reliability/efficiency**- ~~`'deadline'`~~ is *not* considered when the **policy will *not* change over time** during testing- `'inputs'` ~ based on the **inspecting of the `environment.act()`** - **`'light'`** is required to avoid **all** the `violation`s - **`'left'`** is required to avoid the `violation 3` (`Accidents`) of the **`'right'`**-`action` when **`'red'`**-`light` - ~~`'right'`~~ can avoid *only the partial* `violation 4` (`Accidents`) of the **`'forward'`**/**`'left'`**-`action` when **`'red'`**-`light` - **`'oncoming'`** is required to avoid the `violation 3/4` (`Accidents`) of the **`'left'`**-`action` Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states!
###Code
# Answer:
valid_actions = [None, 'forward', 'left', 'right']
waypoint = valid_actions[1:]
inputs = {
'oncoming': valid_actions ,
'left' : valid_actions ,
'light' : ('green', 'red'),
}
from operator import mul
print 'The size of the state space is {} that could be learned within a reasonable number of training trials'.format(
reduce(mul, map(len, [waypoint] + inputs.values()), 1))
###Output
The size of the state space is 96 that could be learned within a reasonable number of training trials
###Markdown
Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- The *similar observation* between the basic driving agent and the default Q-Learning agent is the **same Safety Rating F**.The significant improvement is still not enough.- **20 training trials** = $$\frac{\epsilon_{Default} - tolerance_{Default}}{\epsilon_{Step}} + 1 = \frac{1 - 0.05}{0.05} + 1$$- The **decaying function** (exploration factor: $\epsilon -= 0.05$) is accurately represented in the parameters panel- The number of **bad actions decrease** and the average **reward increase** as the number of training **trials increased**- The **same Safety Rating F**, but the **Reliability Rating** is improved from **F** to **B** ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Question 7Using the visualization above that was produced from your improved Q-Learning simulation, provide a final analysis and make observations about the improved driving agent like in **Question 6**. Questions you should answer: - *What decaying function was used for epsilon (the exploration factor)?*- *Approximately how many training trials were needed for your agent before begining testing?*- *What epsilon-tolerance and alpha (learning rate) did you use? Why did you use them?*- *How much improvement was made with this Q-Learner when compared to the default Q-Learner from the previous section?*- *Would you say that the Q-Learner results show that your driving agent successfully learned an appropriate policy?*- *Are you satisfied with the safety and reliability ratings of the *Smartcab*?* **Answer:**- The **decaying function** (exploration factor) is $$\epsilon -= 0.003$$- **317 training trials** = $$\lceil\frac{\epsilon_{Default} - tolerance_{Default}}{\epsilon_{Step}}\rceil = \lceil\frac{1 - 0.05}{0.003}\rceil$$- The initial $\epsilon$, $tolerance$ and $\alpha$ (learning rate) are kept default 1, 0.05 and 0.5 in the first step because trying a simple *decaying function* to increase the **training trials** without any noise should be the first priority- The **Safety Rating** is improved from **F** to **A+** while the **Reliability Rating** is improved from **B** to **A+**- The Q-Learner results show that the driving agent **successfully learned** an appropriate policy- The **Safety Rating A+** & **Reliability Rating A+** are satisfying although 10 testing trials might be few Define an Optimal PolicySometimes, the answer to the important question *"what am I trying to get my agent to learn?"* only has a theoretical answer and cannot be concretely described. Here, however, you can concretely define what it is the agent is trying to learn, and that is the U.S. right-of-way traffic laws. Since these laws are known information, you can further define, for each state the *Smartcab* is occupying, the optimal action for the driving agent based on these laws. In that case, we call the set of optimal state-action pairs an **optimal policy**. Hence, unlike some theoretical answers, it is clear whether the agent is acting "incorrectly" not only by the reward (penalty) it receives, but also by pure observation. If the agent drives through a red light, we both see it receive a negative reward but also know that it is not the correct behavior. This can be used to your advantage for verifying whether the **policy** your driving agent has learned is the correct one, or if it is a **suboptimal policy**. Question 81. Please summarize what the optimal policy is for the smartcab in the given environment. What would be the best set of instructions possible given what we know about the environment? _You can explain with words or a table, but you should thoroughly discuss the optimal policy._2. Next, investigate the `'sim_improved-learning.txt'` text file to see the results of your improved Q-Learning algorithm. _For each state that has been recorded from the simulation, is the **policy** (the action with the highest value) correct for the given state? Are there any states where the policy is different than what would be expected from an optimal policy?_ 3. Provide a few examples from your recorded Q-table which demonstrate that your smartcab learned the optimal policy. Explain why these entries demonstrate the optimal policy.4. Try to find at least one entry where the smartcab did _not_ learn the optimal policy. Discuss why your cab may have not learned the correct policy for the given state.Be sure to document your `state` dictionary below, it should be easy for the reader to understand what each state represents. **Answer:*** **Optimal Policy**|waypoint|light |left |oncoming |Optimal actions|:-------|:-------------------------------------|:----------------|:-------------|:--------|forward |green|All |All |forward|forward |red |All |All |None|right |green|All |All |right|right |red |right, left, None|All |right|right |red |forward |All |None|left |green|All |left, None |left|left |green|All |forward, right|forward~~, right (opposite)~~|left |red |All |All |None* **Learned Policy** * All the `action`s with the highest Q are selected and compared with the **Optimal Policy** below. * The Policy **correctly avoids all `violation`s**, but based on the **few experiences**, only 44.79% `action`s have **Optimal `reward`**s. * More `trial`s should help to learn more Optimal `reward`s.
###Code
from numpy import sum
from pandas import DataFrame, concat
from IPython.display import display # Allows the use of display() for DataFrames
waypoints = [ 'forward', 'right', 'left']
state = ['waypoint', 'light', 'left', 'oncoming']
lights = [ 'green', 'red' ]
actions = waypoints + ['None' ]
def Policy (rule):
policy= DataFrame(rule, index = ['action']).T
policy.index.names = state
return policy
fold = lambda policy: concat([policy.loc[act] for act in waypoints], axis = 1, keys = [a + '-waypoint' for a in waypoints])
policy = open('logs/sim_improved-learning.txt').readlines()[4:]
policy = Policy({tuple(line[0][1:-2].translate(None, "',").split()):
sorted(map(lambda l: l.split(), line[1:]), key = lambda l: float(l[3]))[-1][1]
for line in [policy[i: i + 5] for i in range(0, len(policy), 6)]})
Optimal = Policy({(w, li, l, o): (w if li != 'red' else None)
for w in waypoints for li in lights for l in actions for o in actions})
Optimal.loc['right', 'red' , ['None' , 'right', 'left'], :] = 'right'
Optimal.loc['left' , 'green', :, ['forward', 'right' ] ] = 'forward'
print 'The Learned Policy is {:.2f}% optimized for the highest rewards'.format(float(sum(policy == Optimal))/len(Optimal)*100)
display(concat([fold(policy), fold(Optimal)], axis = 1, keys = ['Learned on the Q-Table', 'Optimal']))
###Output
The Learned Policy is 44.79% optimized for the highest rewards
###Markdown
机器学习工程师纳米学位 强化学习 项目 4: 训练智能出租车学会驾驶欢迎来到机器学习工程师纳米学位的第四个项目!在这个notebook文件中,模板代码已经提供给你,有助于你对*智能出租车*的分析和实现学习算法。你无须改动已包含的代码,除非另有要求。 你需要回答notebook文件中给出的与项目或可视化相关的问题。每一个你要回答的问题前都会冠以**'问题 X'**。仔细阅读每个问题,并在后面**'回答'**文本框内给出完整的回答。你提交的项目会根据你对于每个问题的回答以及提交的`agent.py`的实现来进行评分。 >**提示:** Code 和 Markdown 单元格可通过 **Shift + Enter** 快捷键来执行。此外,Markdown可以通过双击进入编辑模式。 ----- 开始在这个项目中,你将构建一个优化的Q-Learning驾驶代理程序,它会操纵*智能出租车* 通过它的周边环境到达目的地。因为人们期望*智能出租车*要将乘客从一个地方载到另一个地方,驾驶代理程序会以两个非常重要的指标来评价:**安全性**和**可靠性**。驾驶代理程序在红灯亮时仍然让*智能出租车*行驶往目的地或者勉强避开事故会被认为是**不安全**的。类似的,驾驶代理程序频繁地不能适时地到达目的地会被认为**不可靠**。最大化驾驶代理程序的**安全性**和**可靠性**保证了*智能出租车*会在交通行业获得长期的地位。**安全性**和**可靠性**用字母等级来评估,如下:| 等级 | 安全性 | 可靠性 ||:-----: |:------: |:-----------: || A+ | 代理程序没有任何妨害交通的行为,并且总是能选择正确的行动。| 代理程序在合理时间内到达目的地的次数占行驶次数的100%。 || A | 代理程序有很少的轻微妨害交通的行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的90%。 || B | 代理程序频繁地有轻微妨害交通行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的80%。 || C | 代理程序有至少一次重大的妨害交通行为,如闯红灯。| 代理程序在合理时间内到达目的地的次数占行驶次数的70%。 || D | 代理程序造成了至少一次轻微事故,如绿灯时在对面有车辆情况下左转。 | 代理程序在合理时间内到达目的地的次数占行驶次数的60%。 || F | 代理程序造成了至少一次重大事故,如有交叉车流时闯红灯。 | 代理程序在合理时间内到达目的地的次数未能达到行驶次数的60%。 |为了协助评估这些重要的指标,你会需要加载可视化模块的代码,会在之后的项目中用到。运行下面的代码格来导入这个代码,你的分析中会需要它。
###Code
# 检查你的Python版本
from sys import version_info
if version_info.major != 2 and version_info.minor != 7:
raise Exception('请使用Python 2.7来完成此项目')
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
了解世界在开始实现你的驾驶代理程序前,首先需要了解*智能出租车*和驾驶代理程序运行的这个世界(环境)。构建自我学习的代理程序重要的组成部分之一就是了解代理程序的特征,包括代理程序如何运作。原样直接运行`agent.py`代理程序的代码,不需要做任何额外的修改。让结果模拟运行一段时间,以观察各个不同的工作模块。注意在可视化模拟程序(如果启用了),**白色车辆**就是*智能出租车*。 问题 1用几句话,描述在运行默认的`agent.py`代理程序中,你在模拟程序里观察到了什么。一些你可以考虑的情况:- *在模拟过程中,智能出租车究竟移动了吗?*- *驾驶代理程序获得了什么样的奖励?*- *交通灯的颜色改变是如何影响奖励的?***提示:** 从顶层的`/smartcab/`目录(这个notebook所在的地方),运行命令```bash'python smartcab/agent.py'``` **回答:**默认状态下,白色小车一直未移动。当红灯亮起,因为小车处于未移动的状态,所以获得了较大正值的奖励(>1);当绿灯亮起但前方有即将到来的车辆时,因为小车依然未移动,奖励为小额正值奖励(0~1);当绿灯亮起而前方没有即将到来的车辆,因为小车未移动,得到的奖励为较大的负值(<-4)。到达某个阈值以后,小车因为一直未移动,模拟结束,失败。 理解代码除了要了解世界之外,还需要理解掌管世界、模拟程序等等如何运作的代码本身。如果一点也不去探索一下*“隐藏”*的器件,就试着去创建一个驾驶代理程序会很难。在顶层的`/smartcab/`的目录下,有两个文件夹:`/logs/` (之后会用到)和`/smartcab/`。打开`/smartcab/`文件夹,探索每个下面的Python文件,然后回答下面的问题。 问题 2- *在*`agent.py`* Python文件里,选择 3 个可以设定的 flag,并描述他们如何改变模拟程序的。*- *在*`environment.py`* Python文件里,当代理程序执行一个行动时,调用哪个Environment类的函数?*- *在*`simulator.py`* Python 文件里,*`'render_text()'`*函数和*`'render()'`*函数之间的区别是什么?*- *在*`planner.py`* Python文件里,*`'next_waypoint()`* 函数会先考虑南北方向还是东西方向?* **回答:**learning选择True或者False代表小车是否使用Q-learning策略,epsilon代表每次训练探索开始的状态,tolerance代表训练探索停止的状态,epsilon和tolerance加上探索衰减函数共同决定了训练的次数,alpha代表小车进行Q-learning运算时候进行新的学习的概率;当agent调用choose_action,environment会调用act()函数;render_text()函数把结果输出在command/terminal界面,render()函数会把结果展示在图形界面;next_waypoint()会优先考虑东西方向 ----- 实现一个基本的驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第一步,是让代理程序确实地执行有效的行动。在这个情况下,一个有效的行动是`None`(不做任何行动)、`'Left'`(左转)、`'Right'`(右转)或者`'Forward'`(前进)。作为你的第一个实现,到`'choose_action()'`代理程序函数,使驾驶代理程序随机选择其中的一个动作。注意你会访问到几个类的成员变量,它们有助于你编写这个功能,比如`'self.learning'`和`'self.valid_actions'`。实现后,运行几次代理程序文件和模拟程序来确认你的驾驶代理程序每步都执行随机的动作。 基本代理程序模拟结果要从最初的模拟程序获得结果,你需要调整下面的标志:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。可选的,你还可以通过将`'display'`标志设定为`False`来禁用可视化模拟(可以使得试验跑得更快)。调试时,设定的标志会返回到他们的默认设定。重要的是要理解每个标志以及它们如何影响到模拟。你成功完成了最初的模拟后(有20个训练试验和10个测试试验),运行下面的代码单元格来使结果可视化。注意运行同样的模拟时,日志文件会被覆写,所以留意被载入的日志文件!在 projects/smartcab 下运行 agent.py 文件。
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
问题 3利用上面的从你初始模拟中得到的可视化结果,给出关于驾驶代理程序的分析和若干观察。确保对于可视化结果上的每个面板你至少给出一条观察结果。你可以考虑的一些情况:- *驾驶代理程序多频繁地做出不良决策?有多少不良决策造成了事故?*- *假定代理程序是随机驾驶,那么可靠率是否合理?*- *代理程序对于它的行动会获得什么样的奖励?奖励是否表明了它收到严重的惩罚?*- *随着试验数增加,结果输出是否有重大变化?*- *这个智能出租车对于乘客来说,会被人为是安全的且/或可靠的吗?为什么或者为什么不?* **答案:**对于随机代理程序,35%-40%的决策属于不良决策,其中10%决策造成了至少微小以上的事故,可以理解为25%的不良决策会转化为事故,10%左右的可靠率基本和事故率差不多,属于合理的数值,从奖励的表格来看,随机驾驶受到了严重的惩罚,得分常年在-4以下,整个模型随实验次数增加未有重大变化,猜测是随机事件,趋势来说是平稳的。从最终的双F评分来看,此车极为不安全和不可靠。 ----- 通知驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第二步,是定义一系列代理程序会在环境中发生的状态。根据输入、感知数据和驾驶代理程序可用的变量,可以为代理程序定义一系列状态,使它最终可以*学习*在一个状态下它需要执行哪个动作。对于每个状态的`'如果这个处于这个状态就那个行动'`的状况称为**策略**,就是最终驾驶代理程序要学习的。没有定义状态,驾驶代理程序就不会明白哪个动作是最优的——或者甚至不会明白它要关注哪个环境变量和条件! 识别状态查看`'build_state()'`代理程序函数,它显示驾驶代理函数可以从环境中获得下列数据:- `'waypoint'`,*智能出租车*去向目的地应该行驶的方向,它是*智能出租车*车头方向的相对值。- `'inputs'`,*智能出租车*的感知器数据。它包括 - `'light'`,交通灯颜色。 - `'left'`,*智能出租车*左侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'right'`,*智能出租车*右侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'oncoming'`,*智能出租车*交叉方向车辆的目的方向。如果没有车辆,则返回`None`。- `'deadline'`,*智能出租车*在时间之内到达目的地还所需的剩余动作数目。 问题 4*代理程序的哪些可用特征与学习**安全性**和**效率**相关性最高?你为什么认为这些特征适合在环境中对**智能出租车**建模?如果你没有选择某些特征,放弃他们的原因是什么?* **回答:**waypoint影响车行驶的路线,进而影响效率性,input包含路口交通灯状态,左边右边和对面车辆的行进状态,进而会影响系统安全性,deadline特征虽然和效率性有相关,但是本身deadline数值是5*distance,而distance本身又不能小于4,所以加上deadline会让状态空间扩大至少20倍,distance本身又处于一个随机选择的状态(distance可能较大,上百),所以引入deadline的话会到导致状态空间太过膨胀,几百次的训练可能很难覆盖全,导致系统过于复杂。 定义状态空间当定义一系列代理程序会处于的状态,必需考虑状态空间的*大小*。就是说,如果你期望驾驶代理程序针对每个状态都学习一个**策略**,你会需要对于每一个代理状态都有一个最优的动作。如果所有可能状态的数量非常大,最后会变成这样的状况,驾驶代理程序对于某些状态学不到如何行动,会导致未学习过的决策。例如,考虑用下面的特征定义*智能出租车*的状态的情况:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.发生如`(False, True, True, True, False, False, '3AM')`的状态的频次如何?没有近乎无限数量的训练,很怀疑代理程序会学到一个合适的动作! 问题 5*如果用你在**问题4**中选择的特征来定义一个状态,状态空间的大小是多少?假定你了解环境以及它是如何模拟的,你觉得经过合理数量的训练之后,代理驾驶能学到一个较好的策略吗?(遇见绝大部分状态都能作出正确决策。)***提示:** 考虑特征*组合*来计算状态的总数! **回答:**从environment.py代码可知,waypoints包含right,left,forward,3个选择(planner.py中return none是指到达目的地的返回值,所以这里不做考虑),lights包含true,false,2个选择,oncoming,left,right都包含none,forward,left,right,4个选择,所以总的状态空间是3X2x4x4x4 = 384.鉴于总的状态空间并不是特别大,我觉得经过合理数量训练,代理是能找到一个较好的策略的。 更新驾驶代理程序的状态要完成你的第二个实现,去到`'build_state()'`代理程序函数。根据你在**问题4**给出的判断,你现在要将`'state'`变量设定为包含所有Q-Learning所需特征的元组。确认你的驾驶代理程序通过运行代理程序文件和模拟会更新它的状态,注意状态是否显示了。如果用了可视化模拟,确认更新的状态和在模拟程序里看到的一致。**注意:** 观察时记住重置模拟程序的标志到默认设定! ----- 实现Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是开始实现Q-Learning自身的功能。Q-Learning的概念相当直接:每个访问的状态,为所有可用的状态-行动配对在Q-table里创建一条记录。然后,当代理程序遇到一个状态并执行了一个动作,基于获得的奖励和设定的相互的更新规则,来更新关联的状态-动作配对的Q-value。当然,Q-Learning还带来其他的收益,如此我们可以让代理程序根据每个可能的状态-动作配对的Q-values,来为每个状态选择*最佳*动作。在这个项目里,你会实现一个*衰减* $\epsilon$ *-贪心* 的Q-learning算法,不含折扣因子。遵从每个代理程序函数的**TODO**下的实现指导。注意代理程序的属性`self.Q`是一个字典:这就是Q-table的构成。每个状态是`self.Q`字典的键,每个值是另一个字典,包含了*action*和*Q-value*。这里是个样例:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```此外,注意你要求利用一个*衰减*$\epsilon$*(探索)因子*。因此,随着试验的增加,$\epsilon$会向0减小。这是因为,代理程序会从它的行为中学习,然后根据习得的行为行动。而且当$\epsilon$达到特定阈值后(默认阈值为0.01),代理程序被以它所学到的东西来作检测。作为初始的Q-Learning实现,你将实现一个线性衰减$\epsilon$的函数。 Q-Learning模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。此外,使用下面的$\epsilon$衰减函数:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
问题 6利用上面的从你默认的Q-Learning模拟中得到的可视化结果,像在**问题3**那样,给出关于驾驶代理程序的分析和若干观察。注意模拟程序应该也产生了Q-table存在一个文本文件中,可以帮到你观察代理程序的算法。你可以考虑的一些情况:- *有没有观察到基本驾驶代理程序和默认的Q-Learning代理程序的相似之处?*- *在测试之前驾驶代理大约需要做多少训练试验?在给定的$\epsilon$ 容忍度下,这个数字是否合理?*- *你实现的$\epsilon$(探索因子)衰减函数是否准确地在参数面板中显示?*- *随着试验数增加,不良动作的数目是否减少?平均奖励是否增加?*- *与初始的驾驶代理程序相比,安全性和可靠性评分怎样?* **回答:**基本的驾驶代理程序和默认的Q-learning在rewards评分上都很低,评级都是F。在测试之前,采用epsilon初值为1,衰减速录为0.05,tolerance为0.05,根据之前的衰减公式可得,驾驶代理在做20次训练后停止开始测试阶段,从右侧下边的图标中,能明显看出衰减函数的下降曲线(蓝色曲线),并且衰减幅度符合0.05*次数的预期。从左侧上图能看出,随着实验的增加,违章率和不良策略呈下降趋势,事故率在前期有所下降后期又上升到同一水平,导致平均奖励虽然趋势变好,但评分依然偏低。此代理与初始代理相比,可靠性上有所增加,但是安全性能依然不行 ----- 改进Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是执行优化!现在Q-Learning算法已经实现并且驾驶代理程序已经成功学习了,需要调整设定、调节参数让驾驶代理程序学习**安全性**和**效率**。通常这一步需要很多试验和错误,因为某些设定必定会造成更糟糕的学习。要记住的一件事是学习的行为本身和需要的时间:理论上,我们可以允许代理程序用非常非常长的时间来学习;然而,Q-Learning另一个目的是*将没有习得行为的试验试验变为有习得行为的行动*。例如,训练中总让代理程序执行随机动作(如果$\epsilon = 1$并且永不衰减)当然可以使它*学习*,但是不会让它*行动*。当改进你的Q-Learning实现时,要考虑做一个特定的调整的意义,以及它是否逻辑上是否合理。 改进Q-Learning的模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。- `'optimized'` - 将此标志设定为`'True'`来告诉驾驶代理你在执行一个优化版本的Q-Learning实现。优化Q-Learning代理程序可以调整的额外的标志:- `'n_test'` - 将此标志设定为某个正数(之前是10)来执行那么多次测试试验。- `'alpha'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的学习率。- `'epsilon'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的起始探索因子。- `'tolerance'` - 将此标志设定为某个较小的大于0的值(默认是0.05)来设定测试的epsilon阈值。此外,使用一个你选择的$\epsilon$ (探索因子)衰减函数。注意无论你用哪个函数,**一定要以合理的速率衰减**到`'tolerance'`。Q-Learning代理程序到此才可以开始测试。某个衰减函数的例子($t$是试验的数目):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$如果你想的话,你也可以使用$\alpha$ (学习率) 的衰减函数,当然这通常比较少见。如果你这么做了,确保它满足不等式$0 \leq \alpha \leq 1$。如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化,请注意为了达到项目要求你需要在安全性和可靠性上获得至少都为A的评分。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
C:\Users\Eswar\Anaconda3\lib\site-packages\pandas\core\computation\__init__.py:18: UserWarning: The installed version of numexpr 2.3.1 is not supported in pandas and will be not be used
The minimum supported version is 2.4.6
ver=ver, min_ver=_MIN_NUMEXPR_VERSION), UserWarning)
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**When running the default agent.py agent code, I observed that the agent idled at a green light with no oncoming traffic. Actually the smartcab does not move at all.The rewarded is -4.02, which is negative.The diving agent receives a positive reward when it idles on a red light, and a negative reward when it idles on green. It also receives a positive reward when it idles when it is not allowed to turn left or right. The cab is basically receiving awards based on its following of the traffic laws, but it is not moving at all, so it is not receiving a reward for when the light is green. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**In the agent.py Python file, there are total 5 sets of flags.num_dummies - discrete number of dummy agents in the environment: The number of dummy agents will affect the performance of our agent. Since we have to wait for other cars who has higher priority, more dummy agents will delay our agent in the intersection. Also we may have higher chance to have accident in the intersection, then the safety will be affected.alpha - continuous calue for the learning rate, default is 0.5: The learning rate determines to what extent the newly acquired information will override the old information. A factor of 0 will make the agent not learn anything, while a factor of 1 would make the agent consider only the most recent information.display - set to False to disable the GUI if PyGame is enabled. This may save time when we are running large numbers of Monte Carlo runs and trying to find the optimal parameters such as epsilon and alphs.In the environment.py Python file, the class function 'act' is called when an agent performs an action.In the simulator.py Python file. The 'render_text()' is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt. The 'render()' function is the GUI render display of the simulation.In the planner.pyPython file, 'next_waypoint() function considers the East-West direction first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**The driving agent is making bad decisions somewhere between about 36% and 41% of the time. These bad decisions lead to a decent amount of accidents, with minor accidents occurring around 4.5% of the time, and major accidents between roughly 4.5% and 9% of the time. The rate of reliability is extremely low (looks like it did manage to randomly arrive on time during one or two of the trials), which makes sense given that it is not actually attempting to drive to the destination. The rewards received on average are between -4 and -6, showing it is getting heavily penalized (the relatively high rate of major violations and major accidents are probably driving this). Since we are not actually learning at this point, it makes sense that more trials does not have a significant effect on the outcomes. This Smartcab is both terribly unsafe and unreliable (getting F's in both), as it is substantially more likely that a bad outcome will occur than it actually arriving on time. To be safe, we would want very few accidents or violations, and to be reliable it would need to arrive on time much closer to 100% of the time. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**I would consider three of the features most relevant for learning both safety and efficiency:waypoint - the Smartcab heading must be known by the agent so it may head in the correct direction to reach its intented destination.inputs: light - the Smartcab must know the color of the lights so as to learn it will be penalized for being in idle state when the light is green, or going through intersections with red traffic lights which will cause traffic violations and even accidents.inputs: left - As I understand it now, this parameter is to indicate if there is a vehicle present on the left and its intented action/direction: None, forward, left or right. If there is a vehicle present and the inputs: light is red, then making a right turn maybe allow if there is no vehicle present or its intention is not to go forward. If there is a vehicle present and the inputs: light is red, then taking a right action will result in a major accident if there is also a vehicle on the left with the intent to go forward.inputs: oncoming - the Smartcab must know if there is a vehicle coming in the opposite direction when it is trying to make a left turn, so as to not go into a road with oncoming traffic when deciding to turn left. If the agent decides to drive into oncoming traffic, then it will cause either a minor traffic violation or, if there is a vehicle present, a major accident.I would consider the following features not as relivent for the following reasons:inputs: right - As I understand it now, this parameter is to indicate if there are vehicle present on the right and its intented action/direction: None, forward, left or right. If there is a vehicle present and the inputs: light is red, the Smartcab agent should not go forward or left in any case, so knowing if there is a car on the right does not provide any additional information. Similarly, making a right turn on red, does not require any information on if there is a vehicle on the right, since the lane that the right vehicle is on is separate for the direction of travel. As for the inputs: light is green cases, this is only valid for the case of the smartcab going forward, but this means the Smartcab agent has the right-of-way, so any accident would be the fault of the vehicle on the right making a decision that is not in-line with the US triffic laws.deadline not as relevent because I do not believe the Smartcab agent needs to know the deadline, but can learn about it in the reward it receives as it builds its Q table. Setting it this way may help improve on its reliability by causing minor safety violations to major accidents. This is something to avoid, but I think we want the agent to learn it on its own. Plus, doing it this way significantly reduces the Q table size and makes the learning algorthm more efficient. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**With the 4 inputs and the action to learn:**Features Number of states States**A: waypoint 3 forward, left, rightB: inputs: light 2 red, greenC: inputs: left 4 None, forward, left, rightD: inputs: oncoming 4 None, forward, left, rightE: possible actions 4 None, forward, left, rightThe possible combinations are N, based on A x B x C x D, or 3x2x4x4 or 96 total number of states for just the features, but if you also want to add the possible actions, then it is A x B x C x D x E, or 3x2x4x4x4 or 384 possible combinations.**N State combinations next action**1 {'forward', 'red', None, None} None2 {'forward', 'red', None, None} forward3 {'forward', 'red', None, None} left4 {'forward', 'red', None, None} right5 {'left', 'red', None, None} None6 {'left', 'red', None, None} forward... ... ...384 {'right', 'green', 'right', 'right'} rightThis is not a large number, so I believe the driving agent could learn a policy for each possible state within a reasonable number of training trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**The agent's safety score is still fairly poor, getting an F like the basic driving agent. However, this hides what actually occurred if you only look at the safety score. It has certainly improved its total number of bad actions, as the frequency of bad actions dropped below .2 by the end of trials. Other than beginning around the same values, this agent clearly got better as it went across all the measures above.The agent required 20 trials before testing, which makes sense given that the epsilon was decreasing by 0.05 (0.05 X 20 = 1). When epsilon hit zero, it began testing.My decaying function is accurately represented in the parameters panel, as it steadily decreased each trial across the 20 trials.As the number of trials increased, the rate of bad actions dropped from ~37% to under 20%, showing a clear improvement. Similarly, the average reward increased substantially from nearly -4.5 to around -1.5.As I mentioned above, the safety rating still needs improvement (20% of the time it still makes a bad action). However, reliability is now an A+, a complete flip from the F for the initial driving agent. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** After running the code for Smartcab agent, I was able to notice the following things regarding it:1) The agent doesn't appear to be moving at all. It is either idling at a red light or idling at a green light.2) The agent receives a negative reward if it idles at a green light. It receives a positive reward if it idles at a red light as it should be doing.3) When the light is green, it receives a negative reward for idling. When the light is red, it receives a positive reward for idling in the same location. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:** For the file agent.py, the following flags can be set that would impact the simulation: flags that create the driving agent and allow it to use Q-learning, flags to follow the driving agent, and flags for running the simulator. The first allows you to enable/disable Q-learning used by the agent along with setting the epsilon and alpha parameters (the exploration factor and learning rate respectively). Next, the "follow the driving agent" has an enforce_deadline flag that would make it enforce a given deadline for the agent to satisfy in order to be considered a pass or a fail. Finally, the flags for running the simulator include values for tolerance and n_test which set up the epsilon tolerance before beginning the test along with the number of trials to run for the model to learn with.For the file environment.py, the class function in Environment that is being called is the Environment constructor class. It is here that the agents are utilized and acted on with the other functions, including using the TrafficLight constructor to make the agent interact with the traffic light. In the Environment class, it contains the function "act" that takes as arguments the agent and the selected action. It is here that the agent will perform the given action in its environment and record any violations that the action may have resulted.For the file simulator.py, the difference between the render_text() and the render() function is that the render_text() function will render the results of the simulation as display text output for the user to digest and analyze, much as we saw the results being outputted while render() will display the results in a GUI format that can allow the person to see the simulation as an actual taxicab navigating the traffic lights.For the file planner.py, the function next_waypoint(), it will consider the East-West direction first before considering the North-South direction. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**Based on the simulation run and the display results from the log of the simulation, the following inferences can be made:1) Based on the graph above, the driver is making bad decisions approximately 40% of the time (oscillating between 38% and 43%). Minor accidents are about 5% of the time and major accidents start off at 2% and increase to about 7.5%.2) Based on the fact that the agent is driving randomly, it makes sense that the rate of reliability is exactly like this as given it is likely to make few correct decisions and mostly incorrect ones, the reliability would be considered extremely poor, especially in an environment where few mistakes can be tolerated.3) On average, the agent is receiving negative rewards overall. On closer inspection, it is receiving small rewards for positive actions and heavy negative rewards for wrong actions. It looks to be based on this that it is being penalized excessively for wrong actions. It would of course make sense that it is being penalized heavily due to the proportion of wrong decisions being made. Also with the not so insignificant amount of accidents, it makes sense that a lot of heavy penalties are being imposed.4) As the number of trials increased, the number of major accidents began to increase in proportion. Also, the reward per action began to decrease significantly and the rate of reliability began to increase somewhat before decreasing again. 5) I would not consider the Smartcab at this point as it causes a large proportion of bad decisions, along with a not insignificant amount of accidents. There is potential however as the reliability began to increase somewhat as the number of trials progressed. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**The features that we plan to use for both safety and efficiency are the inputs['light'], inputs['oncoming'], inputs['left'] and waypoint features. These features are appropriate for modeling the Smartcab in the environment due to the following reasons:1) Inputs['light'] is needed for safety and efficiency as the car would be more likely to get into an accident if it disregarded anything with the light.2) Inputs['oncoming'] is needed as it is very likely to get into a collision if it doesn't know whether a car is coming in the othe direction. It should be used so as to alert the user whether it is safe to turn or keep going.3) Inputs['left'] is needed as its important for right-on-red turns. Given that the agent can turn right on red turns, it needs to yield to the left traffic in order to avoid a collision.4) Waypoint I felt was needed as it needs to keep in mind the general direction it has to reach its destination. If it doesn't, then it might make too many short term turns to avoid traffic that eventually would cause it to fail in the long term of reaching it's destination.I've disregarded the rest of the following features: inputs['left'], inputs['right'] and deadline for the following reasons:1) Inputs['right'] is not as essential for safety and reliability and would add more dimensions that would make training trials cumbersome. While it might be somewhat important to have them, the system can learn overall with just traffic lights and oncoming traffic, with the car learning to wait for the left and right cars to pass by at a traffic light before making a turn. For driving straight, the information is irrelevant. 2) Deadline is also not that important as the car will learn to optimize rewards for getting to the destination based on previously failed trial runs. The information here is really more redundant and may even cause accidents as cars almost near the end of their run may be tempted more to get into accidents. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**Given that I am using inputs['oncoming'], inputs['light'], inputs['left'] and waypoint, they each have the following number of states: oncoming -> (left, right, forward, None), light -> (red, green), left -> (left, right, forward, None), waypoint -> (left, right, forward).Thus the number of states are: 4 X 2 X 4 X 3 = 96.I believe it could learn the correct number of sample space trials since we just have to set the number of trials to at least the sample space. Since there are 96 overall states, we can set the number of training trials to say 200. If there are redundant states, we can set it to 2 or 3 times the number of trials to make sure that all unique states are covered. It should train in a reasonable amount of time given the small number of states that we have. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**To answer the given parts of the given question here:1) What has been observed to be similar between the simple driving agent is that the reliability rating is F while the safety rating is also F.2) The simulation required 20 training trials before crossing the epsilon threshold for testing. This makes sense as given the decay rate, it would come out to 20 training trials.3) From the decay panel, I could see that the epsilon decay rate was represented accurately as each test showed a decay of 0.05.4) The number of bad actions decreased for all types of accidents, both major and minor, though at a gradual rate. The number of overall bad actions decreased from 37.77% at the beginning to 20.98% at the end of the testing trials. Also, the average reward increased from about -5 to about -2 during the run of the testing trials.5) The safety and reliability is still the same as compared to the initial driving agent. However, with the improvements in accidents and rewards, it has the potential to get a better rating if we tune the reinforcement learning algorithm. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
机器学习工程师纳米学位 强化学习 项目 4: 训练智能出租车学会驾驶欢迎来到机器学习工程师纳米学位的第四个项目!在这个notebook文件中,模板代码已经提供给你,有助于你对*智能出租车*的分析和实现学习算法。你无须改动已包含的代码,除非另有要求。 你需要回答notebook文件中给出的与项目或可视化相关的问题。每一个你要回答的问题前都会冠以**'问题 X'**。仔细阅读每个问题,并在后面**'回答'**文本框内给出完整的回答。你提交的项目会根据你对于每个问题的回答以及提交的`agent.py`的实现来进行评分。 >**提示:** Code 和 Markdown 单元格可通过 **Shift + Enter** 快捷键来执行。此外,Markdown可以通过双击进入编辑模式。 ----- 开始在这个项目中,你将构建一个优化的Q-Learning驾驶代理程序,它会操纵*智能出租车* 通过它的周边环境到达目的地。因为人们期望*智能出租车*要将乘客从一个地方载到另一个地方,驾驶代理程序会以两个非常重要的指标来评价:**安全性**和**可靠性**。驾驶代理程序在红灯亮时仍然让*智能出租车*行驶往目的地或者勉强避开事故会被认为是**不安全**的。类似的,驾驶代理程序频繁地不能适时地到达目的地会被认为**不可靠**。最大化驾驶代理程序的**安全性**和**可靠性**保证了*智能出租车*会在交通行业获得长期的地位。**安全性**和**可靠性**用字母等级来评估,如下:| 等级 | 安全性 | 可靠性 ||:-----: |:------: |:-----------: || A+ | 代理程序没有任何妨害交通的行为,并且总是能选择正确的行动。| 代理程序在合理时间内到达目的地的次数占行驶次数的100%。 || A | 代理程序有很少的轻微妨害交通的行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的90%。 || B | 代理程序频繁地有轻微妨害交通行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的80%。 || C | 代理程序有至少一次重大的妨害交通行为,如闯红灯。| 代理程序在合理时间内到达目的地的次数占行驶次数的70%。 || D | 代理程序造成了至少一次轻微事故,如绿灯时在对面有车辆情况下左转。 | 代理程序在合理时间内到达目的地的次数占行驶次数的60%。 || F | 代理程序造成了至少一次重大事故,如有交叉车流时闯红灯。 | 代理程序在合理时间内到达目的地的次数未能达到行驶次数的60%。 |为了协助评估这些重要的指标,你会需要加载可视化模块的代码,会在之后的项目中用到。运行下面的代码格来导入这个代码,你的分析中会需要它。
###Code
# 检查你的Python版本
from sys import version_info
if version_info.major != 2 and version_info.minor != 7:
raise Exception('请使用Python 2.7来完成此项目')
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
了解世界在开始实现你的驾驶代理程序前,首先需要了解*智能出租车*和驾驶代理程序运行的这个世界(环境)。构建自我学习的代理程序重要的组成部分之一就是了解代理程序的特征,包括代理程序如何运作。原样直接运行`agent.py`代理程序的代码,不需要做任何额外的修改。让结果模拟运行一段时间,以观察各个不同的工作模块。注意在可视化模拟程序(如果启用了),**白色车辆**就是*智能出租车*。 问题 1用几句话,描述在运行默认的`agent.py`代理程序中,你在模拟程序里观察到了什么。一些你可以考虑的情况:- *在模拟过程中,智能出租车究竟移动了吗?*- *驾驶代理程序获得了什么样的奖励?*- *交通灯的颜色改变是如何影响奖励的?***提示:** 从顶层的`/smartcab/`目录(这个notebook所在的地方),运行命令```bash'python smartcab/agent.py'``` **回答:**1. 在模拟过程中,智能出租车没有移动2. 驾驶代理程序以分数作为奖励,分数有正负之分,正的分数奖励正确的行为,负的分数奖励错误的行为,可以认为是惩罚3. 每个action的reward初始值是一个[-1, 1]的分数: -智能车直行,红灯,左右没有直行的车辆,2级违规,`reward += -10`,左右只要有一边有直行车辆,4级违规`reward += -40`,其他情况reward不变. -智能车左拐,红灯,左边或右边有直行车辆,4级违规`reward += -40`,如果交叉方向有车辆右拐,4级违规`reward += -40`,红灯的其他情况,2级违规`reward += -10`;绿灯,交叉方向有直行或右拐车辆,3级违规`reward += -30`,绿灯的其他情况reward不变. -智能车右拐,红灯,且左边有直行车辆,3级违规`reward += -30`,其他情况reward不变。 -智能车不动,绿灯,且交叉方向没有车左拐,1级违规`reward += -5`,其他情况,reward不变。 -最后,针对每个action,在没有违规的情况下,action的方向和next_waypoint(目的地方向)一致,`reward += 2 - penalty`;如果智能车红灯停了,也是`reward += 2 - penalty`,其他情况,`reward += 1 - penalty`。 penalty是智能车`enforce_deadline=True`情况下设置的一个惩罚值,是(0,1)的小数,智能车训练剩余时间越少,惩罚值penalty越大。 理解代码除了要了解世界之外,还需要理解掌管世界、模拟程序等等如何运作的代码本身。如果一点也不去探索一下*“隐藏”*的器件,就试着去创建一个驾驶代理程序会很难。在顶层的`/smartcab/`的目录下,有两个文件夹:`/logs/` (之后会用到)和`/smartcab/`。打开`/smartcab/`文件夹,探索每个下面的Python文件,然后回答下面的问题。 问题 2- *在*`agent.py`* Python文件里,选择 3 个可以设定的 flag,并描述他们如何改变模拟程序的。*- *在*`environment.py`* Python文件里,当代理程序执行一个行动时,调用哪个Environment类的函数?*- *在*`simulator.py`* Python 文件里,*`'render_text()'`*函数和*`'render()'`*函数之间的区别是什么?*- *在*`planner.py`* Python文件里,*`'next_waypoint()`* 函数会先考虑南北方向还是东西方向?* **回答:**1. 在agent.py Python文件里,新建环境的时候,Environment可以输入3个入参,verbose=True可以让程序运行时,打印更多的调试日志;num_dummies可以指定环境中小车的数量。比如`num_dummies=50`,环境里就会生成50个小车;grid_size可以设定有多少个交叉口或者说横竖有多少道路。比如,`grid_size = (10, 7)`指定环境里面7条横的道路,10条竖的道路,相互交叉,可以形成10*7=70个交叉口;2. 在environment.py Python文件里,当代理程序执行一个行动时,调用哪个Environment类的act函数.3. 在simulator.py Python 文件里,'render_text()'函数和'render()'函数之间的区别: render_text()是模拟数据的非图形化显示,模拟数据会在终端以文本的形式打印出来.render()是模拟数据的图形化显示,模拟数据以图形的形式在pygame窗口显示.4. 看代码,'next_waypoint() 函数会先考虑东西方向,然后再考虑南北方向. ----- 实现一个基本的驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第一步,是让代理程序确实地执行有效的行动。在这个情况下,一个有效的行动是`None`(不做任何行动)、`'Left'`(左转)、`'Right'`(右转)或者`'Forward'`(前进)。作为你的第一个实现,到`'choose_action()'`代理程序函数,使驾驶代理程序随机选择其中的一个动作。注意你会访问到几个类的成员变量,它们有助于你编写这个功能,比如`'self.learning'`和`'self.valid_actions'`。实现后,运行几次代理程序文件和模拟程序来确认你的驾驶代理程序每步都执行随机的动作。 基本代理程序模拟结果要从最初的模拟程序获得结果,你需要调整下面的标志:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。可选的,你还可以通过将`'display'`标志设定为`False`来禁用可视化模拟(可以使得试验跑得更快)。调试时,设定的标志会返回到他们的默认设定。重要的是要理解每个标志以及它们如何影响到模拟。你成功完成了最初的模拟后(有20个训练试验和10个测试试验),运行下面的代码单元格来使结果可视化。注意运行同样的模拟时,日志文件会被覆写,所以留意被载入的日志文件!在 projects/smartcab 下运行 agent.py 文件。
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
问题 3利用上面的从你初始模拟中得到的可视化结果,给出关于驾驶代理程序的分析和若干观察。确保对于可视化结果上的每个面板你至少给出一条观察结果。你可以考虑的一些情况:- *驾驶代理程序多频繁地做出不良决策?有多少不良决策造成了事故?*- *假定代理程序是随机驾驶,那么可靠率是否合理?*- *代理程序对于它的行动会获得什么样的奖励?奖励是否表明了它收到严重的惩罚?*- *随着试验数增加,结果输出是否有重大变化?*- *这个智能出租车对于乘客来说,会被人为是安全的且/或可靠的吗?为什么或者为什么不?* **答案:**1. 驾驶程序30%到45%的决策都是不良决策,大概25%的不良决策造成事故。2. 假定代理程序是随机驾驶,那么可靠率F(不可靠)是合理的,因为随机驾驶显然不可靠,智能驾驶才可靠。3. 代理程序对它的行动会或得一个数值奖励,可正可负。奖励表明它收到了严重的惩罚。4. 随着试验数增加,结果输出变化不大。5. 这个智能出租车对于乘客来说,是不安全的,因为safety rating F 代表:代理程序造成了至少一次重大事故,如有交叉车流时闯红灯。这个智能出租车是不可靠的,reliability rating显示F代表:代理程序在合理时间内到达目的地的次数未能达到行驶次数的60%。 ----- 通知驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第二步,是定义一系列代理程序会在环境中发生的状态。根据输入、感知数据和驾驶代理程序可用的变量,可以为代理程序定义一系列状态,使它最终可以*学习*在一个状态下它需要执行哪个动作。对于每个状态的`'如果这个处于这个状态就那个行动'`的状况称为**策略**,就是最终驾驶代理程序要学习的。没有定义状态,驾驶代理程序就不会明白哪个动作是最优的——或者甚至不会明白它要关注哪个环境变量和条件! 识别状态查看`'build_state()'`代理程序函数,它显示驾驶代理函数可以从环境中获得下列数据:- `'waypoint'`,*智能出租车*去向目的地应该行驶的方向,它是*智能出租车*车头方向的相对值。- `'inputs'`,*智能出租车*的感知器数据。它包括 - `'light'`,交通灯颜色。 - `'left'`,*智能出租车*左侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'right'`,*智能出租车*右侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'oncoming'`,*智能出租车*交叉方向车辆的目的方向。如果没有车辆,则返回`None`。- `'deadline'`,*智能出租车*在时间之内到达目的地还所需的剩余动作数目。 问题 4*代理程序的哪些可用特征与学习**安全性**和**效率**相关性最高?你为什么认为这些特征适合在环境中对**智能出租车**建模?如果你没有选择某些特征,放弃他们的原因是什么?* **回答:** 1. 代理程序的waypoint,inputs['light'],inputs['oncoming'],inputs['left'],inputs['right']等5个可用特征也学习安全性和效率相关性最高。waypoint代表智能出租车去向目的地应该行驶的方向,能保证效率。我们平时开车取某个地方,保证方向正确的情况下,有时候绕点路可能更快到达目的地,因为最近的那条路可能堵车。 至于交通灯颜色,以及智能车左右和交叉方向是否有车最为考虑因素,是因为只有考虑到这些,才能保证安全,否则不管红绿灯和各个方向是否有车,是很容易出交通事故的。 2. deadline是智能出租车在时间之内到达目的地还所需的剩余动作数目,是起点和终点之间的交叉路个数的5倍,系统生成的起点终点计算出来的deadline一般是20,也就是如果选deadline作为考虑的状态的话,状态样本空间会增加20倍,样本空间太大,不利于智能车学习,因为这样就要求训练次数更多。另外,本项目起点和终点随机生成的,不停变化,每一次训练起点和终点不一样,不能根据训练用的deadline来判断那一次训练好些,那一次训练结果差些,起点终点不一样导致用的deadline在不同训练的比较没有意义。3. mentor优化分析 -单纯来看,deadline 确实体现了“告诉合理时间内还剩下多少动作数目”,那么看起来体现了时效性地信息。 -但是首先这个时效性地信息是没有意义的,因为在每次训练过程中,起点、终点在随机变动,所以用它训练出来的 Q表在不同的环境下不具备泛化性,使用它反而会模糊小车的行动。(deadline在每次训练时候,值的分布都不同,那么一定程度上会干扰训练成果) -其次,它的值是5倍的小车与终点的曼哈顿距离,大概会将状态空间增加14倍(因为默认的地图尺寸是 6*8,1~6+8,共有14个取值),大幅增加状态空间的大小,导致小车很难训练。 -最后,这个deadline 还有某种 “急于求成” 的意思,即便有意义,也会过分追求时间效率——而这一特征和安全性在某种程度上存在对立关系,也会引入一定的问题。 定义状态空间当定义一系列代理程序会处于的状态,必需考虑状态空间的*大小*。就是说,如果你期望驾驶代理程序针对每个状态都学习一个**策略**,你会需要对于每一个代理状态都有一个最优的动作。如果所有可能状态的数量非常大,最后会变成这样的状况,驾驶代理程序对于某些状态学不到如何行动,会导致未学习过的决策。例如,考虑用下面的特征定义*智能出租车*的状态的情况:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.发生如`(False, True, True, True, False, False, '3AM')`的状态的频次如何?没有近乎无限数量的训练,很怀疑代理程序会学到一个合适的动作! 问题 5*如果用你在**问题4**中选择的特征来定义一个状态,状态空间的大小是多少?假定你了解环境以及它是如何模拟的,你觉得经过合理数量的训练之后,代理驾驶能学到一个较好的策略吗?(遇见绝大部分状态都能作出正确决策。)***提示:** 考虑特征*组合*来计算状态的总数! **回答:** 1. 在问题4选择了5个状态,分别是 waypoint,inputs['light'],inputs['oncoming'],inputs['left'],inputs['right']。2. waypoint 可能有forward,left,right,None 4种情况,但是查看代码,None代表到达目的地了,不需要考虑到状态空间里面了,已经到达目的地了,就不要再判断红绿灯和周围车辆请况了,inputs['light']有红绿灯2种情况,inputs['oncoming'],inputs['left'],inputs['right']分别有forward,left,right,none等4中情况,考虑这些特征的组合,共有`3*2*4*4*4=384`种组合。 状态最多有384中,每个状态有4种action,所以差不多`384*4=1536`次训练后,代理程序就能学到一个较好的策略了。 思考 -你对训练轮数进行了初步的分析,但这个分析是还可以更完全。更完整的分析可以包括三个部分: -遍历性问题,多少次遍历才能保证一定百分率的 state 被遍历? -微观训练(收敛)充分性问题,一个 state 遍历多少次才能被充分收敛? -宏观训练(收敛)充分性问题,整体来说,多少个 state 被充分训练了,才能保证小车基本上能够实现安全性、可靠性地驾驶(观察来看,并不一定要遍历所有 state 才能保证小车实现上述指标)? -为了进行分析,你应该对环境问题进行一定地简化与建模,使用到的数学工具主要来自概率论中的 独立事件模型、马尔科夫模型,以及 你需要对 bellman 公式有一定的认识与了解。 问:为什么我们这么强调对相同最大值的 action 的选择要随机呢? 答:在状态添加到Q-table里面的时候,该状态每个action的初始值都是0.0,那么初始状态的相同最大值的action就有4个None, 'forward', 'left', 'right',不随机选,每次都选第一个就失去了代理程序的探索能力,会降低程序的学习效率,也会到导致学习的结果不理想。 下图是取相同最大值的action中的第一个action的可视化结果:可见安全性和可靠性都得不到保证。 这边有两个考虑要素: 一个是起初的时候都为0的状态。 一个是有多个 action 经过较少次迭代,有相同的值,说明他们都不错,但是不知道哪个更好,所以要能够随机选取(避免总是选第一个)(配合 epsilon-random 让各种状态以较为平均的速度收敛)。 不随机选择 action 的后果往往是小车会转圈圈,所以你展示的图像中会出现可靠率为0的情况。
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
# action = max_value_actions[0] 取相同最大值的action中的第一个action
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
更新驾驶代理程序的状态要完成你的第二个实现,去到`'build_state()'`代理程序函数。根据你在**问题4**给出的判断,你现在要将`'state'`变量设定为包含所有Q-Learning所需特征的元组。确认你的驾驶代理程序通过运行代理程序文件和模拟会更新它的状态,注意状态是否显示了。如果用了可视化模拟,确认更新的状态和在模拟程序里看到的一致。**注意:** 观察时记住重置模拟程序的标志到默认设定! ----- 实现Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是开始实现Q-Learning自身的功能。Q-Learning的概念相当直接:每个访问的状态,为所有可用的状态-行动配对在Q-table里创建一条记录。然后,当代理程序遇到一个状态并执行了一个动作,基于获得的奖励和设定的相互的更新规则,来更新关联的状态-动作配对的Q-value。当然,Q-Learning还带来其他的收益,如此我们可以让代理程序根据每个可能的状态-动作配对的Q-values,来为每个状态选择*最佳*动作。在这个项目里,你会实现一个*衰减* $\epsilon$ *-贪心* 的Q-learning算法,不含折扣因子。遵从每个代理程序函数的**TODO**下的实现指导。注意代理程序的属性`self.Q`是一个字典:这就是Q-table的构成。每个状态是`self.Q`字典的键,每个值是另一个字典,包含了*action*和*Q-value*。这里是个样例:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```此外,注意你要求利用一个*衰减*$\epsilon$*(探索)因子*。因此,随着试验的增加,$\epsilon$会向0减小。这是因为,代理程序会从它的行为中学习,然后根据习得的行为行动。而且当$\epsilon$达到特定阈值后(默认阈值为0.01),代理程序被以它所学到的东西来作检测。作为初始的Q-Learning实现,你将实现一个线性衰减$\epsilon$的函数。 Q-Learning模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。此外,使用下面的$\epsilon$衰减函数:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件! Q: 如何理解 greed-epsilon 方法/如何设置 epsilon/如何理解 exploration & exploitation 权衡?A: (1) 我们的小车一开始接触到的 state 很少,并且如果小车按照已经学到的 qtable 执行,那么小车很有可能出错或者绕圈圈。同时我们希望小车一开始能随机的走一走,接触到更多的 state。(2) 基于上述原因,我们希望小车在一开始的时候不完全按照 Q learning 的结果运行,即以一定的概率 epsilon,随机选择 action,而不是根据 maxQ 来选择 action。然后随着不断的学习,那么我会降低这个随机的概率,使用一个衰减函数来降低 epsilon。(3) 这个就解决了所谓的 exploration and exploitation 的问题,在“探索”和“执行”之间寻找一个权衡。 [出自帖子](https://discussions.youdaxue.com/t/topic/33333)
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
问题 6利用上面的从你默认的Q-Learning模拟中得到的可视化结果,像在**问题3**那样,给出关于驾驶代理程序的分析和若干观察。注意模拟程序应该也产生了Q-table存在一个文本文件中,可以帮到你观察代理程序的算法。你可以考虑的一些情况:- *有没有观察到基本驾驶代理程序和默认的Q-Learning代理程序的相似之处?*- *在测试之前驾驶代理大约需要做多少训练试验?在给定的$\epsilon$ 容忍度下,这个数字是否合理?*- *你实现的$\epsilon$(探索因子)衰减函数是否准确地在参数面板中显示?*- *随着试验数增加,不良动作的数目是否减少?平均奖励是否增加?*- *与初始的驾驶代理程序相比,安全性和可靠性评分怎样?* **回答:**1. 基本驾驶代理程序和默认的Q-Learning代理程序训练次数都一样,都是20次。2. 在测试之前驾驶代理大约需要做多20次试验,在给定的容忍度tolerance=0.01下,衰减函数为:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$epsilon=1初始值=1,衰减20次后epsilon=0,小于tolerance,停止训练。 所以训练20次是对的。3. 衰减函数是准确地在参数面板中显示了,衰减因子以每次0.05的速率线性递减,直到衰减因子为0<tolerance=0.01,停止训练。4. 随着试验数增加,不良动作的数目逐渐减少。平均奖励逐渐增加。5. 与初始的驾驶代理程序相比,安全性没有提高F,可靠评分B,略有提高。 ----- 改进Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是执行优化!现在Q-Learning算法已经实现并且驾驶代理程序已经成功学习了,需要调整设定、调节参数让驾驶代理程序学习**安全性**和**效率**。通常这一步需要很多试验和错误,因为某些设定必定会造成更糟糕的学习。要记住的一件事是学习的行为本身和需要的时间:理论上,我们可以允许代理程序用非常非常长的时间来学习;然而,Q-Learning另一个目的是*将没有习得行为的试验试验变为有习得行为的行动*。例如,训练中总让代理程序执行随机动作(如果$\epsilon = 1$并且永不衰减)当然可以使它*学习*,但是不会让它*行动*。当改进你的Q-Learning实现时,要考虑做一个特定的调整的意义,以及它是否逻辑上是否合理。 改进Q-Learning的模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。- `'optimized'` - 将此标志设定为`'True'`来告诉驾驶代理你在执行一个优化版本的Q-Learning实现。优化Q-Learning代理程序可以调整的额外的标志:- `'n_test'` - 将此标志设定为某个正数(之前是10)来执行那么多次测试试验。- `'alpha'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的学习率。- `'epsilon'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的起始探索因子。- `'tolerance'` - 将此标志设定为某个较小的大于0的值(默认是0.05)来设定测试的epsilon阈值。此外,使用一个你选择的$\epsilon$ (探索因子)衰减函数。注意无论你用哪个函数,**一定要以合理的速率衰减**到`'tolerance'`。Q-Learning代理程序到此才可以开始测试。某个衰减函数的例子($t$是试验的数目):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$如果你想的话,你也可以使用$\alpha$ (学习率) 的衰减函数,当然这通常比较少见。如果你这么做了,确保它满足不等式$0 \leq \alpha \leq 1$。如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化,请注意为了达到项目要求你需要在安全性和可靠性上获得至少都为A的评分。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**- When running the default agent.py agent code, I observed that the agent idled at a green light with no oncoming traffic. Actually the smartcab does not move at all.- The agent receives positive rewards when the Smartcab is stopped at the red light. Later however, the majority of the rewards are negative and varies from -5.4 to -4 when the smartcab remains still at the current intersection. The reward becomes positive and range from 0.1 to 1.3 when the red light turns red again and the Smartcab is idle.- The Cab generally remains still during the whole simulation. Although the Smartcab is not moving at all when the traffic light is red and receives rewards which by time increases for this particular action. when the light turns green, the Smartcab does not move at all thus it does not receive rewards when the light changes from red to green and as long as the cab remains idle when the light is green the rewards decreases. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**-In agent.py I choose to describe the following flags: 1. learning: which shows whether the smartcab is in the learning mode or not. If it's in the learning mode, its actions will influence its decision making proccess in the next iterations. 2. display: indicates whether the GUI for the smartcab will be displayed on screen. In the environment.py Python file, the class function 'act' is called when an agent performs an action. 3. alpha: this flag indicates the numerical factor by which the smartcab will learn from its actions. In environment.py, the function which is named as *act* that is called when an agent performs an action. This functions enables the agent to act in the environment and receive the reward of his action.In simulator.py, render_text will give the user output in the command line, while render will give the user output in a GUI window.In planner.py, the East-West orientation is checked before the North-South ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- The agent is making bad decisions at a rate of about 44-39% of the time, approximately 7-10% of those mistakes lead to major accidents.- Due to the fact that the Smartcab agent drives randomly, the rate of reliabiliy around 10%-15% makes sense.- The rewards that the agent it seems to alter over time, it depends on the accident and the severity of the mistakes the agent makes.- As the the number of trials increases, the outcome of results barely changes. Relative Frequency of bad actions stays about the same, and the average reward per action is always negative without any significant improvement.- This Smartcab is totally and definately not safe at all because it is destined to make a lot of bad decisions. It has high accident rate. Furthermore, it never gets to the destination in time. The Safety Rating and Reliability Rating are both F. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**The inputs is definately the most vital information for the safety of the smartcab. Without this information it has no way of knowing the traffic conditions and will lead to accidents. It can do without the right information due to the fact that in Greece right of way traffic rules, in which cars on the right are not relevant for making any decisions. The waypoint information would be the most relevant information for making sure the agent actually goes in the correct direction to reach its destination. Deadline would be the least relevant information for making decisions as to which direction to move in, and would also increase the state space unnecesarially. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**The four features that were selected have the following possible values.- 'waypoints': None, 'forward', 'left', 'right', total 4 cases- 'light': green and red, total 2 cases- 'left': 'None', 'left, 'right', 'forward', total 4 cases- 'oncoming': 'None', 'left, 'right', 'forward', total 4 cases- Size of the states space: 3 x 2 x 4 x 4 = 96.I believe that the size of the state space is reasonable. We may need numerous training trails to pass over all the possible states and learn the optimal action for any state. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- In fact, they are very different, but the have something in common, both of them have very low safety rating.- My initial $\epsilon$ was 1, it decreases by 0.05 each time and my tolerance was 0.05. So, the number of training trials simply becomes by solving the following simple arithmetic operation: (1-0.05)/0.05, which is 19. Plus the first trial at $\epsilon=1$, there are total 20 trials.- Yes. the parameter panel represent both $\epsilon$ and $\alpha$, where $\epsilon$ is a straight line with a negative slope of 0.05. The $\alpha$ is constant at 0.5 as the graph shows.- As the number of training trials increased, the number of bad actions does decrease and the average reward does increase. If we have more learning trails, they may be even better.- Both the non-learning and the learning agent have safety rating "F". For the default-learning driving agent, the reliability rating now is "A" which is much better than the old one. However, the Rating is still "F", which means that our Smartcab did at least one major accident. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**Description : When running the default agent.py agent code we observe that the world is a 8 by 6 grid. Each column and each row has a road resulting in 48 intersections. The agent is a white car starting at a random location in the environment and a Udacity logo shows the destination it needs to reach. The simulation also involves 100 other cars moving in the world. Traffic lights are present at every intersections. Indications are written on top of the GUI to show informations about the simulation such as the number of trial, if the agent succeeded in the previous trial, the action chose by the agent and the associated reward for that action, the time remaining to reach destination...In the current state, the Smartcab is not moving at all during the simulation.The driving agent is receiving positive and negative rewards. After few minutes of observation, I see that the reward is slightly positive when "the agent is idled at red light" and strongly negative if not moving when "there was a green light with no oncoming traffic". According to the weight of each reward we can say that the agent is more penalized for doing nothing when it was supposed to move (unreliable) than rewarded for doing nothing when it was supposed to do nothing (safety). Therefore being unreliable is more penalizing here than it is rewarded to be safe. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- *In the agent.py Python file, choose three flags that can be set and explain how they change the simulation.*In the agent.py, I count 13 different flags. I choose to explain the first three in the class Environment.The first is the boolean verbose. When set to True, additional output from the simulation are displayed such as the time step or if the agent has reached destination. Those additional information can really useful while developing the simulation.There is also num_dummies which is a discrete number of dummy agents in the environment. It is set to 100 by default. This variable can impact the reliability of the agent as it might delay the agent's progression towards the destination. It can also impact the safety as it will increase the probability of the agent to be involved in an accident.Finally the grid_size is a discrete number that defines the number of intersections. By default columns = 8 and rows = 6. Changing the size of the world can have an impact on the time it will take for the agent to reach the destination and also impact the number of interactions tha agent will have with the environment.- *In the environment.py Python file, what Environment class function is called when an agent performs an action?*The function 'act()' is called when an agent performs an action. It is defined by the arguments agent and action and make the agent perform the action if it is legal.- *In the simulator.py Python file, what is the difference between the 'render_text()' function and the 'render()' function?*The 'render_text ()' function, as it is defined, is the non-GUI render display of the simulation and the simulated trial data are rendered in text format in the terminal/command prompt. Whereas the 'render()' function is the GUI render display of the simulation and allows to visualize the simulation.- *In the planner.py Python file, will the 'next_waypoint() function consider the North-South or East-West direction first?*The 'next_waypoint() function will first consider the East-West direction. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- In the '10-Trial Rolling Relative Frequency of Bad Actions' we can see that the relative frequency of bad actions over 10 trials is comprised between 0.4302 and 0.4840. The relative frequency of major accident is approximately 0.055 and for minor accidents approximately 0.025 (in average). We can then consider that around 18% of the bad decisions cause accidents. - In the '10-Trial Rolling Rate Reliability' we see that the rate of reliability is comprised between 0 and 20% which is very low. Considering that all actions are chosen randomly, this rate of reliability makes sense.- In the '10-Trial Rolling Average Reward Per Action' we can see that the average reward per action are mostly negative around -5. As we can see in environment.py def act() function, the rewards are -5 for minor violations, -10 for major violations, -20 for minor accidents, -40 for major accidents. This shows that in average, the agent made a minor violation per action and suggest that it has been heavily penalized for moving randomly.- In the '10-Trial Rolling Rate Reliability' we can see the the outcome does not change significantly as the number of trials increases and stays between 0 and 20%. Also, the '10-Trial Rolling Average Reward Per Action' shows a very consistent -5 rewards per action showing no improvement whatsoever. This observation makes sense considering that all decision are made randomly.- This Smartcab is far from being safe and/or reliable as we can see by the notations, Safety rating : F and Reliability rating : F. The F notation for the reliability rating means that the success ratio < 0.6 (as coded in visual.py 'calculate_reliability()' function). The F notation for the safety rating means that the agent has been involved in at least one major accident (as coded in the visual.py 'calculate_safety()' function). Therefore the smartcab cannot be considered safe because it's prone to accidents nor reliable because it gets to its destination on time less than 60 % of the time. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**The next waypoint, the intersection inputs, and the deadline are all features available to the agent. For learning both safety and efficiency, the most relevant features are:- 'waypoint' because it shows the direction to the agent which is primordial for efficiency- 'light', 'left', 'oncoming' because it let's the agent know is there are vehicules around before going a certain direction and traffic lights so it's important for safety. I don't have a driving license and we don't have this rule in France so I might be wrong on this one but I didn't include the 'right' because as far as I understand the U.S. Right-of-Way rules, the agent will not need to yield to traffic from the right side of the intersection when it is making any move. This is assuming that the dummy agents all follow traffic laws and right traffic will not run through a red light. Also in this environment, the agent can turn right at a red light as long as it avoids collision with left traffic moving forward.Also I didn't include 'deadline' because giving too much important on the time left to reach a destination might lead the agent to make violations or worse get into an accident. Since 'waypoint' is already supposed to give the best way, then it should be the shortest way and what we care about is to get there as soon as possible given that we are following the rules. It's good to get somewhere on time, it's always better alive. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**I chose the features 'waypoint', 'light', 'left' and 'oncoming'. For each features we have :- 'waypoint' 3 possible outcome : 'forward', 'right', 'left'- 'light' 2 possible outcome : 'green', 'red'- 'left' 4 possible outcome : 'None', 'forward', 'right', 'left'- 'oncoming' 4 possible outcome : 'None', 'forward', 'right', 'left'Therefore size of state space $= 3 \times 2 \times 4 \times 4 = 96$A size of state space of 96 is still fairly reasonable considering all the other features we could take into account. The environment has been extensively simplified and I think that the driving agent will be able to learn a policy for each possible state within a reasonable number of training trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- Looking at the notation we can see that the safety rating is the same between the basic driving agent and the default Q-Learning agent. Besides this part the basic driving agents and the default Q-Learning agent do not share more similarities.- As we can see in the 'sim_default-learning.csv', it took 20 training trials before testing. As asked, I used the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05 \hspace{10px}\textrm{for trial number } t$$And the $\epsilon_{tolerance}$ was 0.05. As the initial $\epsilon$ value was 1, the number of trials was then $\frac{\epsilon_{t}-\epsilon_{tolerance}}{0.05} = \frac{1-0.05}{0.05} = 19$. We can see in the 'sim_default-learning.csv' file that at trial number 19, $\epsilon = 0.049999999999999684$ and it took one more trial before starting testing maybe because of value approximation.- We see that the $\epsilon$ is accurately represented as a line with a slope of -0.05 as implemented above.- In the '10-Trial Rolling Relative Frequency of Bad Actions' we can see a significant decrease of the number of total bad actions as the number of training trails increases. When looking at the '10-Trial Rolling Average Reward per Action', we can see that the reward per action increases with the number of training trails. All those observations show that the agent is learning.- As mentionned above, the safety rating for both driving agent are equivalent with an F. This means that in both cases the agent has been involved in at least one major accident. However we observe that the reliability rating has significantly improve from F to A. This means that the success ratio is $\ge$ 0.90. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
机器学习工程师纳米学位 强化学习 项目 4: 训练智能出租车学会驾驶欢迎来到机器学习工程师纳米学位的第四个项目!在这个notebook文件中,模板代码已经提供给你,有助于你对*智能出租车*的分析和实现学习算法。你无须改动已包含的代码,除非另有要求。 你需要回答notebook文件中给出的与项目或可视化相关的问题。每一个你要回答的问题前都会冠以**'问题 X'**。仔细阅读每个问题,并在后面**'回答'**文本框内给出完整的回答。你提交的项目会根据你对于每个问题的回答以及提交的`agent.py`的实现来进行评分。 >**提示:** Code 和 Markdown 单元格可通过 **Shift + Enter** 快捷键来执行。此外,Markdown可以通过双击进入编辑模式。 ----- 开始在这个项目中,你将构建一个优化的Q-Learning驾驶代理程序,它会操纵*智能出租车* 通过它的周边环境到达目的地。因为人们期望*智能出租车*要将乘客从一个地方载到另一个地方,驾驶代理程序会以两个非常重要的指标来评价:**安全性**和**可靠性**。驾驶代理程序在红灯亮时仍然让*智能出租车*行驶往目的地或者勉强避开事故会被认为是**不安全**的。类似的,驾驶代理程序频繁地不能适时地到达目的地会被认为**不可靠**。最大化驾驶代理程序的**安全性**和**可靠性**保证了*智能出租车*会在交通行业获得长期的地位。**安全性**和**可靠性**用字母等级来评估,如下:| 等级 | 安全性 | 可靠性 ||:-----: |:------: |:-----------: || A+ | 代理程序没有任何妨害交通的行为,并且总是能选择正确的行动。| 代理程序在合理时间内到达目的地的次数占行驶次数的100%。 || A | 代理程序有很少的轻微妨害交通的行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的90%。 || B | 代理程序频繁地有轻微妨害交通行为,如绿灯时未能移动。| 代理程序在合理时间内到达目的地的次数占行驶次数的80%。 || C | 代理程序有至少一次重大的妨害交通行为,如闯红灯。| 代理程序在合理时间内到达目的地的次数占行驶次数的70%。 || D | 代理程序造成了至少一次轻微事故,如绿灯时在对面有车辆情况下左转。 | 代理程序在合理时间内到达目的地的次数占行驶次数的60%。 || F | 代理程序造成了至少一次重大事故,如有交叉车流时闯红灯。 | 代理程序在合理时间内到达目的地的次数未能达到行驶次数的60%。 |为了协助评估这些重要的指标,你会需要加载可视化模块的代码,会在之后的项目中用到。运行下面的代码格来导入这个代码,你的分析中会需要它。
###Code
# 检查你的Python版本
from sys import version_info
if version_info.major != 2 and version_info.minor != 7:
raise Exception('请使用Python 2.7来完成此项目')
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
了解世界在开始实现你的驾驶代理程序前,首先需要了解*智能出租车*和驾驶代理程序运行的这个世界(环境)。构建自我学习的代理程序重要的组成部分之一就是了解代理程序的特征,包括代理程序如何运作。原样直接运行`agent.py`代理程序的代码,不需要做任何额外的修改。让结果模拟运行一段时间,以观察各个不同的工作模块。注意在可视化模拟程序(如果启用了),**白色车辆**就是*智能出租车*。 问题 1用几句话,描述在运行默认的`agent.py`代理程序中,你在模拟程序里观察到了什么。一些你可以考虑的情况:- *在模拟过程中,智能出租车究竟移动了吗?*- *驾驶代理程序获得了什么样的奖励?*- *交通灯的颜色改变是如何影响奖励的?***提示:** 从顶层的`/smartcab/`目录(这个notebook所在的地方),运行命令```bash'python smartcab/agent.py'``` **回答:**- 没有- 有正数奖励,也有负数奖励- 小车前面为红灯,奖励为随机奖励+2;小车前面为绿灯,对面的车向它的左边行驶,奖励为随机奖励+1;小车前面为绿灯且对面的车不向它的左边行驶,奖励为随机奖励-5。 理解代码除了要了解世界之外,还需要理解掌管世界、模拟程序等等如何运作的代码本身。如果一点也不去探索一下*“隐藏”*的器件,就试着去创建一个驾驶代理程序会很难。在顶层的`/smartcab/`的目录下,有两个文件夹:`/logs/` (之后会用到)和`/smartcab/`。打开`/smartcab/`文件夹,探索每个下面的Python文件,然后回答下面的问题。 问题 2- *在*`agent.py`* Python文件里,选择 3 个可以设定的 flag,并描述他们如何改变模拟程序的。*- *在*`environment.py`* Python文件里,当代理程序执行一个行动时,调用哪个Environment类的函数?*- *在*`simulator.py`* Python 文件里,*`'render_text()'`*函数和*`'render()'`*函数之间的区别是什么?*- *在*`planner.py`* Python文件里,*`'next_waypoint()`* 函数会先考虑南北方向还是东西方向?* **回答:**- verbose用于调试输出额外信息。如果为True则输出额外信息,否则不输出额外信息;num_dummies创建dummy agents的最大个数;grid_size,横列十字路口个数- act函数- 'render_text()'函数在终端给出了小车的前一个状态,是否按规划方向走,是否按照交通规则行驶,有没有发生事故,对个状态的奖励和惩罚,有没有按时到达目的地,如果是学习小车则输出epsilon和alpha的值;'render()'函数画出矩形边界、路、中心线,十字路口,交通灯,动态显示学习小车和其它模拟小车,在图中描述学习小车前一个状态信息,行驶是否合法以及相应的奖励的相关信息,行驶是否违反交通规则,没有违反交通规则是否按规划方向行走,违反交通规则相应具体事故,是否按时到达目的地,当次训练是成功还是失败。- 'next_waypoint() 函数会先考虑东西方向。 ----- 实现一个基本的驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第一步,是让代理程序确实地执行有效的行动。在这个情况下,一个有效的行动是`None`(不做任何行动)、`'Left'`(左转)、`'Right'`(右转)或者`'Forward'`(前进)。作为你的第一个实现,到`'choose_action()'`代理程序函数,使驾驶代理程序随机选择其中的一个动作。注意你会访问到几个类的成员变量,它们有助于你编写这个功能,比如`'self.learning'`和`'self.valid_actions'`。实现后,运行几次代理程序文件和模拟程序来确认你的驾驶代理程序每步都执行随机的动作。 基本代理程序模拟结果要从最初的模拟程序获得结果,你需要调整下面的标志:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。可选的,你还可以通过将`'display'`标志设定为`False`来禁用可视化模拟(可以使得试验跑得更快)。调试时,设定的标志会返回到他们的默认设定。重要的是要理解每个标志以及它们如何影响到模拟。你成功完成了最初的模拟后(有20个训练试验和10个测试试验),运行下面的代码单元格来使结果可视化。注意运行同样的模拟时,日志文件会被覆写,所以留意被载入的日志文件!在 projects/smartcab 下运行 agent.py 文件。
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
问题 3利用上面的从你初始模拟中得到的可视化结果,给出关于驾驶代理程序的分析和若干观察。确保对于可视化结果上的每个面板你至少给出一条观察结果。你可以考虑的一些情况:- *驾驶代理程序多频繁地做出不良决策?有多少不良决策造成了事故?*- *假定代理程序是随机驾驶,那么可靠率是否合理?*- *代理程序对于它的行动会获得什么样的奖励?奖励是否表明了它收到严重的惩罚?*- *随着试验数增加,结果输出是否有重大变化?*- *这个智能出租车对于乘客来说,会被人为是安全的且/或可靠的吗?为什么或者为什么不?* **答案:**- 驾驶代理程序平均有0.4的概率做出不良决策,有平均0.17的概率不良决策造成了事故- 不合理- 负的奖励,是的- 没有- 不会,因为它的可靠率很低 ----- 通知驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第二步,是定义一系列代理程序会在环境中发生的状态。根据输入、感知数据和驾驶代理程序可用的变量,可以为代理程序定义一系列状态,使它最终可以*学习*在一个状态下它需要执行哪个动作。对于每个状态的`'如果这个处于这个状态就那个行动'`的状况称为**策略**,就是最终驾驶代理程序要学习的。没有定义状态,驾驶代理程序就不会明白哪个动作是最优的——或者甚至不会明白它要关注哪个环境变量和条件! 识别状态查看`'build_state()'`代理程序函数,它显示驾驶代理函数可以从环境中获得下列数据:- `'waypoint'`,*智能出租车*去向目的地应该行驶的方向,它是*智能出租车*车头方向的相对值。- `'inputs'`,*智能出租车*的感知器数据。它包括 - `'light'`,交通灯颜色。 - `'left'`,*智能出租车*左侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'right'`,*智能出租车*右侧车辆的目的方向。如果没有车辆,则返回`None`。 - `'oncoming'`,*智能出租车*交叉方向车辆的目的方向。如果没有车辆,则返回`None`。- `'deadline'`,*智能出租车*在时间之内到达目的地还所需的剩余动作数目。 问题 4*代理程序的哪些可用特征与学习**安全性**和**效率**相关性最高?你为什么认为这些特征适合在环境中对**智能出租车**建模?如果你没有选择某些特征,放弃他们的原因是什么?* **回答:**代理程序的input与安全性相关性最高,waypoint与效率相关性最高。智能出租车行驶方向是很重要的,所以选择waypoint,其次行驶中安全很重要,要遵守交通规则,还要注意前面右边左边的车辆状态,所以选择input。没有选择deadline,起始位置和终点位置不固定,deadline也不固定,同时deadline数值较大也会使状态空间过大。 定义状态空间当定义一系列代理程序会处于的状态,必需考虑状态空间的*大小*。就是说,如果你期望驾驶代理程序针对每个状态都学习一个**策略**,你会需要对于每一个代理状态都有一个最优的动作。如果所有可能状态的数量非常大,最后会变成这样的状况,驾驶代理程序对于某些状态学不到如何行动,会导致未学习过的决策。例如,考虑用下面的特征定义*智能出租车*的状态的情况:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.发生如`(False, True, True, True, False, False, '3AM')`的状态的频次如何?没有近乎无限数量的训练,很怀疑代理程序会学到一个合适的动作! 问题 5*如果用你在**问题4**中选择的特征来定义一个状态,状态空间的大小是多少?假定你了解环境以及它是如何模拟的,你觉得经过合理数量的训练之后,代理驾驶能学到一个较好的策略吗?(遇见绝大部分状态都能作出正确决策。)***提示:** 考虑特征*组合*来计算状态的总数! **回答:**- 384。waypoint有'forward'、'left'、'right'3种可能,'inputs'的'light'有绿灯、红灯2种可能,'inputs'的'left'、'right'和'oncoming'分别有'forward'、'left'、'right'和none4种可能。3x2x4x4x4=384- 能。如果每次训练经过十字路口个数为5个,且每一步state都不一样,则至少要384/5=77次训练才能遍历所有state;如果每次训练经过十字路口个数为7个,且每一步state都不一样,则至少要384/7=55次训练才能遍历所有state。一个状态要遍历4次。 更新驾驶代理程序的状态要完成你的第二个实现,去到`'build_state()'`代理程序函数。根据你在**问题4**给出的判断,你现在要将`'state'`变量设定为包含所有Q-Learning所需特征的元组。确认你的驾驶代理程序通过运行代理程序文件和模拟会更新它的状态,注意状态是否显示了。如果用了可视化模拟,确认更新的状态和在模拟程序里看到的一致。**注意:** 观察时记住重置模拟程序的标志到默认设定! ----- 实现Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是开始实现Q-Learning自身的功能。Q-Learning的概念相当直接:每个访问的状态,为所有可用的状态-行动配对在Q-table里创建一条记录。然后,当代理程序遇到一个状态并执行了一个动作,基于获得的奖励和设定的相互的更新规则,来更新关联的状态-动作配对的Q-value。当然,Q-Learning还带来其他的收益,如此我们可以让代理程序根据每个可能的状态-动作配对的Q-values,来为每个状态选择*最佳*动作。在这个项目里,你会实现一个*衰减* $\epsilon$ *-贪心* 的Q-learning算法,不含折扣因子。遵从每个代理程序函数的**TODO**下的实现指导。注意代理程序的属性`self.Q`是一个字典:这就是Q-table的构成。每个状态是`self.Q`字典的键,每个值是另一个字典,包含了*action*和*Q-value*。这里是个样例:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```此外,注意你要求利用一个*衰减*$\epsilon$*(探索)因子*。因此,随着试验的增加,$\epsilon$会向0减小。这是因为,代理程序会从它的行为中学习,然后根据习得的行为行动。而且当$\epsilon$达到特定阈值后(默认阈值为0.01),代理程序被以它所学到的东西来作检测。作为初始的Q-Learning实现,你将实现一个线性衰减$\epsilon$的函数。 Q-Learning模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'n_test'` - 将此标志设定为`'10'`则执行10次测试试验。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。此外,使用下面的$\epsilon$衰减函数:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
问题 6利用上面的从你默认的Q-Learning模拟中得到的可视化结果,像在**问题3**那样,给出关于驾驶代理程序的分析和若干观察。注意模拟程序应该也产生了Q-table存在一个文本文件中,可以帮到你观察代理程序的算法。你可以考虑的一些情况:- *有没有观察到基本驾驶代理程序和默认的Q-Learning代理程序的相似之处?*- *在测试之前驾驶代理大约需要做多少训练试验?在给定的$\epsilon$ 容忍度下,这个数字是否合理?*- *你实现的$\epsilon$(探索因子)衰减函数是否准确地在参数面板中显示?*- *随着试验数增加,不良动作的数目是否减少?平均奖励是否增加?*- *与初始的驾驶代理程序相比,安全性和可靠性评分怎样?* **回答:**- 安全率都很低- 20次,不合理,训练次数太低,不能遍历所有state。- 是的- 是的,是的。随着试验数增加,小车能将学习到的状态对应最佳action应用实际状态中,当小车按最佳action,奖励也会提高。- 安全性没有变化,可靠性提高了。 ----- 改进Q-Learning驾驶代理程序创建一个优化Q-Learning的驾驶代理程序的第三步,是执行优化!现在Q-Learning算法已经实现并且驾驶代理程序已经成功学习了,需要调整设定、调节参数让驾驶代理程序学习**安全性**和**效率**。通常这一步需要很多试验和错误,因为某些设定必定会造成更糟糕的学习。要记住的一件事是学习的行为本身和需要的时间:理论上,我们可以允许代理程序用非常非常长的时间来学习;然而,Q-Learning另一个目的是*将没有习得行为的试验试验变为有习得行为的行动*。例如,训练中总让代理程序执行随机动作(如果$\epsilon = 1$并且永不衰减)当然可以使它*学习*,但是不会让它*行动*。当改进你的Q-Learning实现时,要考虑做一个特定的调整的意义,以及它是否逻辑上是否合理。 改进Q-Learning的模拟结果要从最初的Q-learning程序获得结果,你需要调整下面的标志和设置:- `'enforce_deadline'` - 将此标志设定为`True`来强制驾驶代理程序捕获它是否在合理时间内到达目的地。- `'update_delay'` - 将此标志设定为较小数值(比如`0.01`)来减少每次试验中每步之间的时间。- `'log_metrics'` - 将此标志设定为`True`将模拟结果记录为在`/logs/`目录下的`.csv`文件,Q-table存为`.txt`文件。- `'learning'` - 将此标志设定为`'True'`来告诉驾驶代理使用你的Q-Learning实现。- `'optimized'` - 将此标志设定为`'True'`来告诉驾驶代理你在执行一个优化版本的Q-Learning实现。优化Q-Learning代理程序可以调整的额外的标志:- `'n_test'` - 将此标志设定为某个正数(之前是10)来执行那么多次测试试验。- `'alpha'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的学习率。- `'epsilon'` - 将此标志设定为0 - 1之间的实数来调整Q-Learning算法的起始探索因子。- `'tolerance'` - 将此标志设定为某个较小的大于0的值(默认是0.05)来设定测试的epsilon阈值。此外,使用一个你选择的$\epsilon$ (探索因子)衰减函数。注意无论你用哪个函数,**一定要以合理的速率衰减**到`'tolerance'`。Q-Learning代理程序到此才可以开始测试。某个衰减函数的例子($t$是试验的数目):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$如果你想的话,你也可以使用$\alpha$ (学习率) 的衰减函数,当然这通常比较少见。如果你这么做了,确保它满足不等式$0 \leq \alpha \leq 1$。如果你在实施时遇到困难,尝试把`'verbose'`标志设为`True`来调试。调试时,在这里设定的标志会返回到它们的默认设定。重要的是你要理解每个标志做什么并且解释它们怎么影响模拟!当你成功完成初始的Q-Learning模拟程序后,运行下面代码单元格来使结果可视化,请注意为了达到项目要求你需要在安全性和可靠性上获得至少都为A的评分。注意当相同的模拟运行时,log文件会被覆写,所以要留意载入的log文件!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:** In the default setting, the Smartcab does not move at all during the simulation, i.e. it is always idle. The driving agent receives positive or negative reward depending upon the environment state. For example, if the agent is idled at a green light then it receives a negative reward. On the other hand, if the agent is properly idled at a red light, then it gets a positive reward. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:** In the agent.py file, the three flags are: The boolean variable 'learning', 'epsilon' and 'alpha'. The 'learning' flag if True, then the agent is expected to learn. The 'epsilon' variable is the random exploration factor. If the value of 'epsilon' is more, then the agent has a higher probability to take a random action. 'alpha' is the learning factor, where a higher value suggests that the Q value convergence will be at a higher rate.When an agent performs an action, the 'act()' function is called.The 'render_text()' method is the non GUI version of the simulation display, i.e. the output is displayed on the terminal. The 'render()' method shows the simulation throught the PyGame GUI.The 'next_waypoint()' considers the East-West direction first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:** The agent is making a bad decision overall with a relative frequency of approximately 0.397, i.e. almost 40% of the time. Fatal accidents has occured with a relative frequency of about 0.05 or 5% of the time. Minor accidents, interestingly occur slightly less frequently than major accidents.Since at this point, the agent is not learning anything but only taking random actions, the low rate of reliability makes sense. The next time, this experiment runs the rate may go up or down as the actions are random. The average reward per action for the agent is negative and is around the -5 mark. These rewards do suggest that the agent is penalized mostly, because of choosing incorrect actions.No, as the number of trials is increased the outcome of the results do not change significantly. This makes sense, since the agent is not learning anything and is choosing every action in a random fashion. So even if the rate of reliability plot suggests that the reliability rate is increasing with increase in number of trials, it doesn't suggest anything in general.This version of smartcab will neither be safe nor reliable by it's passengers, due to it's low rate of reliability and it's high relative frequency of choosing bad actions. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:** In my opinion, the waypoint is a very critical feature for the agent, since it acts as a heuristic towards the destination. Reliability is greatly affected by this feature.Inputs from the Smartcab sensors are crucial for a safe ride. The 'inputs' basically provides the agent all the necessary information to choose which actions to take given a specific way point direction. All the data included inside the 'inputs' feature are essential. 'light' is required to avoid violations, while the other 3 are needed to avoid accidents. However, if we dive deeper, for our use case the inputs['right'] feature is of not much use. This is because our environment only consists of twin lane roads (one up and one down). As a result, the car coming from the right of the smartcab will not affect it's decisions or actions. 'Deadline' could be an essential feature for the agent and it's learning model. This is because depending on this value, the agent may be forced to take some actions over others. But for our purpose, keeping the deadline in the state space would be an overkill. Firstly, it would make the state-space really huge and thus would make it really difficult for the agent to learn the optimal policy in a reasonable amount of time. Secondly, 'Deadline' is actually not needed in our case as the 'waypoint' attribute gives the necessary information about the heading direction. Thirdly, because of the nature of the environment, the agent does not have control of how fast or slow to move. As a result, keeping 'Deadline' as a feature is not much useful.For my project, I shall be using a ('**waypoint**', '**light**', '**oncoming**', '**left**') as the set of features. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:** The size of the state space would be all combinations of the tuple ('waypoint', 'light', 'oncoming', 'left'). Light has 2 possible states (Red/Green). 'waypoint' has 3 possible states (forward/left/right), while 'left', and 'oncoming' has 4 possible states (None/forward/left/right). Therefore, the size of the state space would be : 3 x 2 x 4 x 4 = 96 states Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:** One similarity between the basic driving agent and the default Q learning agent is the fact that reliability has not improved much. Although the graph for the default agent does indicate the fact that the rolling rate of reliability is increasing as the trials keep increasing.For this analysis, the epsilon tolerance value was kept default (0.05). Also, the epsilon value was decreased by a 0.05 each time. As a result, the number of training trials that the driving agent required should be 19, since the first trial starts with epsilon value 0.95 in the given setting. But due to the minimum training constraint of 20 trials, the number of training trials is 20.Yes, the decaying function is accurately represented in the parameters panel. The learning factor (alpha) is obviously kept as a constant for now.As evident from the graph, the number of bad actions decreases as the training trails are increased. Major and minor accidents are low from the beginning anyway. Major and Minor violations show a decreasing trend with increasing training trials. Average rewards in general show a very positive trend. The average reward per action is increasing at a sharp rate.A safety rating of C here definitely suggests that the accidents and violations have decreased. But a reliability rating of F still indicates that the agent is not able to complete test trials on time. A deeper look into the .csv files show that the agent was able to complete the trip on time for 4 out of 10 testing trials. On the other hand, none of the trips were completed on time for the default agent. So, there is an improvement, but not enough to alter the grade in reliability. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**The smart cab does not move at all during the simulation and is stationary.The driving agent received numerical reqards, the values have a range of -6 to 0 - all negativeI observed the following : Agent idle at a red light - positive reward Agent idle at a green light - negative reward Agent idles with oncoming traffice - positive reward Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**qgent.py : i) learning: indicates if cab is in the learning mode or not. If the cab is in learning mode the cab learns from the environment and make decisions accordingly. ii) epsilon: It is the Random exploration factor. iii) alpha: Its the learning rate with a default value of 0.5, its an exploitation factor. The learning rate or step size determines to what extent the newly acquired information will override the old information. A factor of 0 will make the agent not learn anything, while a factor of 1 would make the agent consider only the most recent information.environment.pyThe class function act() is called when an agent performs an action.simulator.pyrender_text() : This is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt. Supplementary trial data can be found from render_text.render() : This is the GUI render display of the simulation. planner.py East-West orientation is considered before the North-South by next_waypoint() ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**i) According to "10-trial rolling relative frequency of bad actions" visualization, the agent has made bad decisions in approximately 43% percents of cases. Also, the frequence of accidents is approximately 1/7ii) The agent is driving randomly, the rate of reliability should be low and as per the visualization its around 15%. This should be fine.iii) As per "10-trial rolling average rewarding action" plot the reward per action is around -6, this shows high rate of penalization.iv) "10-trial rolling rate of Reliability" - As the the number of trials increases, the outcome of results didnt change much and has only decreased.v) The reliability and Safety ratings for the Smartcab are 'F' and 'F' respectively, hence its not safe and/or reliable for the passengers due to its doesn't reach destination, lot of accidents. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. The following features are most relevant for learning both safety and efficiency as they provide good information to the agent about the environment. i) waypoint : this feature guides the agent towards the destination thus help in efficiency. ii) inputs : features such as light and directions (left,right), oncoming help the agent in avoiding accidents thus keeping the vehicle safe. Not much Relevant deadline : this feature helps the car to guage the time towards the destination thus also helping in efficiency however in the context of the project we are more interested in making the car learn from the environment without accidents rather than rush it towards destination. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:** waypoint : 3 States : 'Left' , 'Right' , 'Forward' inputs : lights : 2 : 'Green' , 'Red' right : 4 : 'Left' , 'Right' , 'Forward' or 'None' left : 4 : 'Left' , 'Right' , 'Forward' or 'None' oncoming : 4 : 'Left' , 'Right' , 'Forward' or 'None'size of the states space: 3 x 2 x 4 x 4 x 4 = 384To make the size of state space optimal and reduce amount of training time. I will remove the input - right (we are dealing with single laned environment in the project)size of the states space: 3 x 2 x 4 x 4 = 96 Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**i) The safety rating and reliability rating for the basic driving agent are 'F' and 'F' respectively; however the same for the Q-Learning agent are 'F' and 'C' respectively. We can observe that there is a huge improvement in the Reliability rating. ii) The driving agent ran 20 training trials beacuse The default exploration factor is 0.5 and is reduced by 0.05 in every trial. iii) The decaying function is represented in the Trial Number vs Parameter value graph, where the exploration factor is the straight line which is decreasing at a constant rate.iv) We can observe from the Trial number vs Relative frequency graph that as the training trials increased the total bad actions have come down significantly. Also from Trial number vs Reward per action graph we can observe that the average award has increased but was still negative at the end of the testing trials.v) Safety Rating has been the same ('F') but the Reliability rating has significantly improved from 'F' to 'C'. However there has significant drop in the number of total bad actions. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**1- The Smartcab stay idle and does not move during the simulation. Since the Agent does not update its status there is no changes on displacement.2- The Agent receives positive rewards when it idles on red lights and on green lights during oncomming traffic. When the light changes from red to green the Agent receives highly amount of negative rewards since it is still idle.3- Once the Agent is static the light turns red again and then it receives another positive reward. Then, this process repeats consecutively. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**1- A) learning: This flag is set if the agent is expected to learn using Q-learning. This determines whether the agent will perform any Q-Learning (True), or instead choose wrong actions (False). B) epsilon: This flag is set to know the agent will make random decisions. C) enforce_deadline: This flag is set to see if the agent is qualified to a deadline goal. Based on its current statute finishing the goal is inconceivable due to the fact that flag has been set negatively (False). 2- environment.py: 'act()' function is the Environment class function that is called when an agent performs an action.3- The 'render_text()' function is for command-line based applications to output the result into the command line window. The 'render()' function is for to exibit the current state in a Graphical User Interface (GUI) using the PyGame module.4- 'next_waypoint()' function considers the East-West direction firstly, and then North-South direction. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**1- The driving agent was making bad decisions close 50% of the time at the beginning and ,almost 20% of them resulting major accidents. The percentage of making bad decisions has been dropped to around 40% and the resulting major accident rate flactuates between 4% to 6%. 2- The rate of reliability is like as I expected. It is extremely low which makes sense given that it is not actually attempting to drive to the destination. It started slightly over 10% and reaches to around 20% and then by the end of the trials falls down to 10%s again.3- Thte agent received negative rewards over the time. Some of them close to -9 and some others close to -4 due to the violation the traffic rules while received small amount of positive rewards since it is idled during the red lights on. The net_rewards that I hve seen from the "sim_no-learning" file are extremely negative rewards. I would say these are heavy penalties for the agent since it did not take any action in a random reward system. Dogru harekette positive yanlisda negatif hareketin siddetine gore4- As the number of trials increases, the Trial Rolling Rate of Reliablility has not changed significantly and stayed at a rate around slightly above 10%. In the mean time there are total bad actions that caused both major and minor accidents. The rate of minor accidents is falling to less than 5% and then start to increase above 5% whereas the rate of the major accidents was above 5% and tend to decrease below 5%. The minor thraffic violations has decreased from 15% to around 10% while major traffic violations has stayed between 18-20% over the time. Since there is not signicant enough positive rewards to receive the level of Reliability Rating stays as F grade.5- Currently, based on Safety Rate as F, I would say it is not safe since the Tatal Bad Action, Major-Minor Violation percentage is significantly higher than a safe agent. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:**APPROPRIATE FEATURES for SAFETY and EFFICIENCY:waypoint: Gives to smartcab directions about the next move to reach its final destination.inputs-light: The smartcab MUST know the color of the lights. When the color of the lights is green it must be aware of there is a penalty if the agent is idle or when the color is red moving is the penalty reason due to avoid possible traffic violations.inputs-left: This input informs the agent from the moves around it. For example, if the agent intent to take an action (left, right, forward, None) the "left" input let the agent wheter there is another agent on the left. Assuming that the smartcab wants to turn left and the light is red, then making a right tur is permissible unless another agent is present on the right direction that our agent wants to move. If so, then the agent must proceed forward when the light turns to green. inputs-oncoming: The smartcab MUST definitely know about oncaming other agent(s) from opposite direction. To avoid possible car crash/accident(s), our agent should intend to turn left/right for safe drive.Not APPROPRIATE FEATURES for SAFETY and EFFICIENCY:deadline: Although knowing the deadline is a nice feature, the smartcab may cause minor/major violations to acomplish his goal. The best way to set the deadline is allowing to our agent to lern itself by creating its own Q-table. I believe this method should decrease the size of the Q-table.inputs-right: Whenever I include to the "right" input tthe performance are getting lower. So I decied to exclude it from appropriate features. Thus, it is a not appropriate feature for safety and efficiency. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**light: 2onocomming: 4left: 4right: 4waypoint: 3: left, right, forwardwaypoint - [left, right, forward] : 3inputs-light - [red, green] : 2inputs-left - [left, right, forward, None] : 4inputs-oncoming - [left, right, forward, None] : 43x2x4x4=96. 96 is th number of states from the features. 96 is a practical number of dimension of state space matrix to handle for a Q learning algorithm. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**1- The observations are not similar between the basic driving agent and the default Q-Learning agent. The rate of all bad actions, violations, accidents went down dramatically conversely, rate of reliability and average reward per action went up regularly. I would be surprised after I check the graphs and "sim_default-learning" file after I compiled the file becaouse all the negative actions are converging to 0% and positive rates are approaching to 100%. Also, the safety rate is A+ and reliability is B. 2- As we can see from 'sim_improved-learning.csv' file after around 157 trials the driving agent is ready to test. Given the epsilon-tolerance:= 0.05, we obtain that 1-(157 x 0.05) = -6.85 < 0.05. So it does make sense.3- The graph of the decay function looks like $y =\mid cos(\alpha t)\mid$, where $\alpha$ is a constant. So, yes, the variable of ϵ accurately represented in the parameters panel. ϵ-tolerance means, if ϵ is less than the tolerace, the model hass trained enough. 4- Yes, as the number of training trials increased, the number of bad actions decreased from 40%s to 4%s. Also the average reward increased from -5 to 1.12 over the 157 trials.5- The safety rating is A+ and it was F before. The reliability is B at this time and it was F before at the initial driving agent. It seems the new Q-learning agent performance increased compare to no-learning case. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**- The Smartcab is not moving. Staying idle at all times. - Negative and positive rewards for not taking any action at every situation. - It gets a negative reward when the light is "green with no upcoming traffic" andit gets a positive reward when the light is red. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**- In agent.py:we can change the enviroment flags of verbose, num_dummies, and grid_size like env = Environment(verbose=True, num_dummies=50, grid_size = (5,5))Verbose will display more outputs like: Environment.step() and Environment.act().num_dummies will change the number of agents from the defualt 100 to the desired number. grid_size controls the number of intersections (columns, rows) with the default values of (8, 6)- In environment.py: when an agent performs an action, the act(self, agent, action) function is called to 'Consider an action and perform the action if it is legal.'- In simulator.py: The main b/t render_text() render() functions render_text() is 'the non-GUI render display of the simulation, Simulated trial data will be rendered in the terminal/command prompt.'render () is 'the GUI render display of the simulation. Supplementary trial data can be found from render_text.' - In planner.py: the 'next_waypoint()' function will conider the direction East-West first. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:**- 40% of the time the driving agent is making bad decisions. with 5% of those bad decisions causing accidents. - Since the agent is driving randomely, the rate of reliability is also random that it stays constant, decreases, stays constant then decreases and stays constant.- on average the driving agent is scoring -3.5 rewards. - No, the results correlated with the random state of the actions. - this smartcab would not be considered neither safe nor reliable. Because of the low rate of reliability, high rate of bad actions and lack of average positive rewards. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:** - For learning both safety and efficiency, we need 'waypoint' and some of the 'inputs' data, perhaps the intersection light and traffic. 'waypoint' addresses the efficiency need of knowing in which direction the cab should drive (hence, the shortest distance). the 'light' and 'oncoming' help the safety. The 'right' and 'left' are not neccessary, because agents' light == green means the left and right dummy agents have the red light and their action_okay = False (environment.py lines437-467), hence the the input['light'] is already providing us the same information we need for safety. The only case use for input['left'] would be when the agent's light == red and we wanna make right turn (a legal move in US) however I think the extra 4 states that the input['left'] would add does not justify its use. - 'deadline' feature can be eliminated, the cab is asked to reach the destination based on the shortest route and safest actions. The 'deadline' feature doesnt address the safety, the cab may not follow the traffic rules if it is trained to reach the destination in the deadline time which most likely will result in high bad actions. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:**Given the features selected in Q4: - Waypoint with 3 states (left, right, forward)- input['light'] with 2 states (red, green) - input['oncoming'] with 4 states (right, left, None, forward) the size of the state space will be 3X2X4 = 24! I think the driving agent can learn the policy for each possible state within a reasonable number of training trials of 24log24 = 76 trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:**- The default Q-Learning agent is not very similar to the initial driving agent(Q3), The only similarity is the decrease of minor violation . - there are ~20 training trials required before testing. Yes based on exploration factor of epsilon-0.05, the number of trials = 20 makes perfect sense.- The decaying exploration factor looks to be implemented correctly since the curve in the parameters panel is decreasing as the number of trials increases. The exploration is going down with each trial, hence the agent is learning. - As the number of tirals increases, the number of bad actions is decreasing, way better decrease than the initial agent in Q3. More trials is needed to know how the trials affect the bad actions. - the safety score has improved significantly to 'A+' . But the reliability score is the same 'F' as the initial agent in Q3. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____
###Markdown
Machine Learning Engineer Nanodegree Reinforcement Learning Project: Train a Smartcab to DriveWelcome to the fourth project of the Machine Learning Engineer Nanodegree! In this notebook, template code has already been provided for you to aid in your analysis of the *Smartcab* and your implemented learning algorithm. You will not need to modify the included code beyond what is requested. There will be questions that you must answer which relate to the project and the visualizations provided in the notebook. Each section where you will answer a question is preceded by a **'Question X'** header. Carefully read each question and provide thorough answers in the following text boxes that begin with **'Answer:'**. Your project submission will be evaluated based on your answers to each of the questions and the implementation you provide in `agent.py`. >**Note:** Code and Markdown cells can be executed using the **Shift + Enter** keyboard shortcut. In addition, Markdown cells can be edited by typically double-clicking the cell to enter edit mode. ----- Getting StartedIn this project, you will work towards constructing an optimized Q-Learning driving agent that will navigate a *Smartcab* through its environment towards a goal. Since the *Smartcab* is expected to drive passengers from one location to another, the driving agent will be evaluated on two very important metrics: **Safety** and **Reliability**. A driving agent that gets the *Smartcab* to its destination while running red lights or narrowly avoiding accidents would be considered **unsafe**. Similarly, a driving agent that frequently fails to reach the destination in time would be considered **unreliable**. Maximizing the driving agent's **safety** and **reliability** would ensure that *Smartcabs* have a permanent place in the transportation industry.**Safety** and **Reliability** are measured using a letter-grade system as follows:| Grade | Safety | Reliability ||:-----: |:------: |:-----------: || A+ | Agent commits no traffic violations,and always chooses the correct action. | Agent reaches the destination in timefor 100% of trips. || A | Agent commits few minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 90% of trips. || B | Agent commits frequent minor traffic violations,such as failing to move on a green light. | Agent reaches the destination on timefor at least 80% of trips. || C | Agent commits at least one major traffic violation, such as driving through a red light. | Agent reaches the destination on timefor at least 70% of trips. || D | Agent causes at least one minor accident, such as turning left on green with oncoming traffic. | Agent reaches the destination on timefor at least 60% of trips. || F | Agent causes at least one major accident,such as driving through a red light with cross-traffic. | Agent fails to reach the destination on timefor at least 60% of trips. |To assist evaluating these important metrics, you will need to load visualization code that will be used later on in the project. Run the code cell below to import this code which is required for your analysis.
###Code
# Import the visualization code
import visuals as vs
# Pretty display for notebooks
%matplotlib inline
###Output
_____no_output_____
###Markdown
Understand the WorldBefore starting to work on implementing your driving agent, it's necessary to first understand the world (environment) which the *Smartcab* and driving agent work in. One of the major components to building a self-learning agent is understanding the characteristics about the agent, which includes how the agent operates. To begin, simply run the `agent.py` agent code exactly how it is -- no need to make any additions whatsoever. Let the resulting simulation run for some time to see the various working components. Note that in the visual simulation (if enabled), the **white vehicle** is the *Smartcab*. Question 1In a few sentences, describe what you observe during the simulation when running the default `agent.py` agent code. Some things you could consider:- *Does the Smartcab move at all during the simulation?*- *What kind of rewards is the driving agent receiving?*- *How does the light changing color affect the rewards?* **Hint:** From the `/smartcab/` top-level directory (where this notebook is located), run the command ```bash'python smartcab/agent.py'``` **Answer:**The Agent state is not being updated so the Smartcab does not move during the simulation. The Agent receives positive reward when it idles at a red light and negative reward when it idles at a greenlight with no oncoming traffic. The light affects what kind of behavior gets rewarded. Understand the CodeIn addition to understanding the world, it is also necessary to understand the code itself that governs how the world, simulation, and so on operate. Attempting to create a driving agent would be difficult without having at least explored the *"hidden"* devices that make everything work. In the `/smartcab/` top-level directory, there are two folders: `/logs/` (which will be used later) and `/smartcab/`. Open the `/smartcab/` folder and explore each Python file included, then answer the following question. Question 2- *In the *`agent.py`* Python file, choose three flags that can be set and explain how they change the simulation.*- *In the *`environment.py`* Python file, what Environment class function is called when an agent performs an action?*- *In the *`simulator.py`* Python file, what is the difference between the *`'render_text()'`* function and the *`'render()'`* function?*- *In the *`planner.py`* Python file, will the *`'next_waypoint()`* function consider the North-South or East-West direction first?* **Answer:**agent.py - * n_test: discrete number of testing trials to perform, default is 0* display - set to False to disable the GUI if PyGame is enabled* enforce_deadline - set to True to enforce a deadline metricenvironment.py - act() is called to process an agent's actionsimulator.py - * render_text() is the non-GUI render display of the simulation. Simulated trial data will be rendered in the terminal/command prompt* render() is the GUI render display of the simulation. Supplementary trial data will be rendered in the terminal/command prompt as wellplanner.py - next_waypoint() function considers East-West direct before North-South. ----- Implement a Basic Driving AgentThe first step to creating an optimized Q-Learning driving agent is getting the agent to actually take valid actions. In this case, a valid action is one of `None`, (do nothing) `'left'` (turn left), `right'` (turn right), or `'forward'` (go forward). For your first implementation, navigate to the `'choose_action()'` agent function and make the driving agent randomly choose one of these actions. Note that you have access to several class variables that will help you write this functionality, such as `'self.learning'` and `'self.valid_actions'`. Once implemented, run the agent file and simulation briefly to confirm that your driving agent is taking a random action each time step. Basic Agent Simulation ResultsTo obtain results from the initial simulation, you will need to adjust following flags:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.Optionally, you may disable to the visual simulation (which can make the trials go faster) by setting the `'display'` flag to `False`. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation!Once you have successfully completed the initial simulation (there should have been 20 training trials and 10 testing trials), run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!Run the agent.py file after setting the flags from projects/smartcab folder instead of projects/smartcab/smartcab.
###Code
# Load the 'sim_no-learning' log file from the initial simulation results
vs.plot_trials('sim_no-learning.csv')
###Output
_____no_output_____
###Markdown
Question 3Using the visualization above that was produced from your initial simulation, provide an analysis and make several observations about the driving agent. Be sure that you are making at least one observation about each panel present in the visualization. Some things you could consider:- *How frequently is the driving agent making bad decisions? How many of those bad decisions cause accidents?*- *Given that the agent is driving randomly, does the rate of reliability make sense?*- *What kind of rewards is the agent receiving for its actions? Do the rewards suggest it has been penalized heavily?*- *As the number of trials increases, does the outcome of results change significantly?*- *Would this Smartcab be considered safe and/or reliable for its passengers? Why or why not?* **Answer:** The agent is making around .3513 to .4517 bad decisions. The agent's random decisions cause around 0.06 to 0.07 accidents. The Rate of Reliability seems to go up a little then hover little below 20%. Considering the agent is choosing actions randomly any trends in the data is likely a coincidence. The agent is clearly receiving negative rewards for its actions. Since, the agent is not learning there is no significant improvements in rewards. The agent only gets into a major accident trip around 7% of the time this would be considered unsafe. On average, a person takes 1500 trips per year (Bureau of Transportation Statistics - https://www.bts.gov/statistical-products/surveys/national-household-travel-survey-daily-travel-quick-facts) and files an insurance claim every 17.9 for an accident (Forbes - https://www.forbes.com/sites/moneybuilder/2011/07/27/how-many-times-will-you-crash-your-car/7a97d624e621) so this means that an average chance of accident is around 1 out of 26850 trips. By this comparison, the agent is considerably more unsafe. ----- Inform the Driving AgentThe second step to creating an optimized Q-learning driving agent is defining a set of states that the agent can occupy in the environment. Depending on the input, sensory data, and additional variables available to the driving agent, a set of states can be defined for the agent so that it can eventually *learn* what action it should take when occupying a state. The condition of `'if state then action'` for each state is called a **policy**, and is ultimately what the driving agent is expected to learn. Without defining states, the driving agent would never understand which action is most optimal -- or even what environmental variables and conditions it cares about! Identify StatesInspecting the `'build_state()'` agent function shows that the driving agent is given the following data from the environment:- `'waypoint'`, which is the direction the *Smartcab* should drive leading to the destination, relative to the *Smartcab*'s heading.- `'inputs'`, which is the sensor data from the *Smartcab*. It includes - `'light'`, the color of the light. - `'left'`, the intended direction of travel for a vehicle to the *Smartcab*'s left. Returns `None` if no vehicle is present. - `'right'`, the intended direction of travel for a vehicle to the *Smartcab*'s right. Returns `None` if no vehicle is present. - `'oncoming'`, the intended direction of travel for a vehicle across the intersection from the *Smartcab*. Returns `None` if no vehicle is present.- `'deadline'`, which is the number of actions remaining for the *Smartcab* to reach the destination before running out of time. Question 4*Which features available to the agent are most relevant for learning both **safety** and **efficiency**? Why are these features appropriate for modeling the *Smartcab* in the environment? If you did not choose some features, why are those features* not *appropriate? Please note that whatever features you eventually choose for your agent's state, must be argued for here. That is: your code in agent.py should reflect the features chosen in this answer.*NOTE: You are not allowed to engineer new features for the smartcab. **Answer:** **Waypoint:** Waypoint informs the Smartcab to be effecient in reaching destination. Also, it turns out that the most efficient path is also the safetest path as it minimizes the turns. Turning at the wrong time is the most dangerous thing that the SmartCab can do.**Inputs['light']: ** Accidents seem to happen most at crossroads. Learning the appropriate actions at the light will ensure SmartCab makes safe decisions.**Inputs['oncoming']: ** Originally, I was able to achieve A+ Safety and Reliability Ratings with **Waypoint** and **Inputs['light']** alone. This works because inputs learned from these two sensors can account for majority of the situation that the agent will encounter. However, there is a rare case where the agent will get into an accident when there is an oncoming traffic during left turn. Since the agent was able to achieve A+ Safety and Reliability rating within 20 iterations adding this sensor will not be too detrimental in terms of training time.**Inputs['left']" ** This sensor was added for the agent to learn the safest way to navigate intersections with cross traffic.I chose not to use **inputs['left']** and **inputs['right']** to limit the search space for SmartCab. As it turns out these inputs yield very little information in both safety and efficiency of the SmartCab.**Deadline** was dropped as it seems it will increase the search space for SmartCab unnecessarily. This feature will make the SmartCab try to learn appropriate actions depending on two time points even though the environmental conditions are the same and the action can be generalized. If the environmental condition is the same then actions at deadline 100 and 50 should be the same. However, this feature will make the SmartCab difficult to generalize that fact. Define a State SpaceWhen defining a set of states that the agent can occupy, it is necessary to consider the *size* of the state space. That is to say, if you expect the driving agent to learn a **policy** for each state, you would need to have an optimal action for *every* state the agent can occupy. If the number of all possible states is very large, it might be the case that the driving agent never learns what to do in some states, which can lead to uninformed decisions. For example, consider a case where the following features are used to define the state of the *Smartcab*:`('is_raining', 'is_foggy', 'is_red_light', 'turn_left', 'no_traffic', 'previous_turn_left', 'time_of_day')`.How frequently would the agent occupy a state like `(False, True, True, True, False, False, '3AM')`? Without a near-infinite amount of time for training, it's doubtful the agent would ever learn the proper action! Question 5*If a state is defined using the features you've selected from **Question 4**, what would be the size of the state space? Given what you know about the environment and how it is simulated, do you think the driving agent could learn a policy for each possible state within a reasonable number of training trials?* **Hint:** Consider the *combinations* of features to calculate the total number of states! **Answer:****Waypoint** has three possible states - forward, right, left - **inputs['light']** has two states - red, green. **inputs['oncoming']** has four states - none, left, right, forward, and **inputs['left']** has four - none, left, right, forward. The combination of two states are as follows:3 x 2 x 4 x 4 = 96The search space is 96 with the four chosen features. 96 is a reasonable search space so I remain optimistic that the driving agent will be able to learn the appropriate actions in a reasonable training trials. Update the Driving Agent StateFor your second implementation, navigate to the `'build_state()'` agent function. With the justification you've provided in **Question 4**, you will now set the `'state'` variable to a tuple of all the features necessary for Q-Learning. Confirm your driving agent is updating its state by running the agent file and simulation briefly and note whether the state is displaying. If the visual simulation is used, confirm that the updated state corresponds with what is seen in the simulation.**Note:** Remember to reset simulation flags to their default setting when making this observation! ----- Implement a Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to begin implementing the functionality of Q-Learning itself. The concept of Q-Learning is fairly straightforward: For every state the agent visits, create an entry in the Q-table for all state-action pairs available. Then, when the agent encounters a state and performs an action, update the Q-value associated with that state-action pair based on the reward received and the iterative update rule implemented. Of course, additional benefits come from Q-Learning, such that we can have the agent choose the *best* action for each state based on the Q-values of each state-action pair possible. For this project, you will be implementing a *decaying,* $\epsilon$*-greedy* Q-learning algorithm with *no* discount factor. Follow the implementation instructions under each **TODO** in the agent functions.Note that the agent attribute `self.Q` is a dictionary: This is how the Q-table will be formed. Each state will be a key of the `self.Q` dictionary, and each value will then be another dictionary that holds the *action* and *Q-value*. Here is an example:```{ 'state-1': { 'action-1' : Qvalue-1, 'action-2' : Qvalue-2, ... }, 'state-2': { 'action-1' : Qvalue-1, ... }, ...}```Furthermore, note that you are expected to use a *decaying* $\epsilon$ *(exploration) factor*. Hence, as the number of trials increases, $\epsilon$ should decrease towards 0. This is because the agent is expected to learn from its behavior and begin acting on its learned behavior. Additionally, The agent will be tested on what it has learned after $\epsilon$ has passed a certain threshold (the default threshold is 0.05). For the initial Q-Learning implementation, you will be implementing a linear decaying function for $\epsilon$. Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'n_test'` - Set this to `'10'` to perform 10 testing trials.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.In addition, use the following decay function for $\epsilon$:$$ \epsilon_{t+1} = \epsilon_{t} - 0.05, \hspace{10px}\textrm{for trial number } t$$If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the initial Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_default-learning' file from the default Q-Learning simulation
vs.plot_trials('sim_default-learning.csv')
###Output
_____no_output_____
###Markdown
Question 6Using the visualization above that was produced from your default Q-Learning simulation, provide an analysis and make observations about the driving agent like in **Question 3**. Note that the simulation should have also produced the Q-table in a text file which can help you make observations about the agent's learning. Some additional things you could consider: - *Are there any observations that are similar between the basic driving agent and the default Q-Learning agent?*- *Approximately how many training trials did the driving agent require before testing? Does that number make sense given the epsilon-tolerance?*- *Is the decaying function you implemented for $\epsilon$ (the exploration factor) accurately represented in the parameters panel?*- *As the number of training trials increased, did the number of bad actions decrease? Did the average reward increase?*- *How does the safety and reliability rating compare to the initial driving agent?* **Answer:** After about twenty trials, the agent was tested. This actually makes sense as Total Bad Actions decreased from .2938 to approximately 0.097. The decay function was a linear one so the decay function is accurately represented in the parameter panel. There is a clear evidence that the driving agent is learning as Violation and Accident Rates decreased to single digit percentage points. The average reward increased significantly starting at -3.5 per action to 0.5 per action. The dirving agent was able to achieve a Safety Rating of A+ while achieving a Reliability Rating of D. This is an improvement from initial driving agent which achieved a Safety and Reliability Rating of F. With few more training trials I believe the driving agent can achieve a greater safety rating. ----- Improve the Q-Learning Driving AgentThe third step to creating an optimized Q-Learning agent is to perform the optimization! Now that the Q-Learning algorithm is implemented and the driving agent is successfully learning, it's necessary to tune settings and adjust learning paramaters so the driving agent learns both **safety** and **efficiency**. Typically this step will require a lot of trial and error, as some settings will invariably make the learning worse. One thing to keep in mind is the act of learning itself and the time that this takes: In theory, we could allow the agent to learn for an incredibly long amount of time; however, another goal of Q-Learning is to *transition from experimenting with unlearned behavior to acting on learned behavior*. For example, always allowing the agent to perform a random action during training (if $\epsilon = 1$ and never decays) will certainly make it *learn*, but never let it *act*. When improving on your Q-Learning implementation, consider the implications it creates and whether it is logistically sensible to make a particular adjustment. Improved Q-Learning Simulation ResultsTo obtain results from the initial Q-Learning implementation, you will need to adjust the following flags and setup:- `'enforce_deadline'` - Set this to `True` to force the driving agent to capture whether it reaches the destination in time.- `'update_delay'` - Set this to a small value (such as `0.01`) to reduce the time between steps in each trial.- `'log_metrics'` - Set this to `True` to log the simluation results as a `.csv` file and the Q-table as a `.txt` file in `/logs/`.- `'learning'` - Set this to `'True'` to tell the driving agent to use your Q-Learning implementation.- `'optimized'` - Set this to `'True'` to tell the driving agent you are performing an optimized version of the Q-Learning implementation.Additional flags that can be adjusted as part of optimizing the Q-Learning agent:- `'n_test'` - Set this to some positive number (previously 10) to perform that many testing trials.- `'alpha'` - Set this to a real number between 0 - 1 to adjust the learning rate of the Q-Learning algorithm.- `'epsilon'` - Set this to a real number between 0 - 1 to adjust the starting exploration factor of the Q-Learning algorithm.- `'tolerance'` - set this to some small value larger than 0 (default was 0.05) to set the epsilon threshold for testing.Furthermore, use a decaying function of your choice for $\epsilon$ (the exploration factor). Note that whichever function you use, it **must decay to **`'tolerance'`** at a reasonable rate**. The Q-Learning agent will not begin testing until this occurs. Some example decaying functions (for $t$, the number of trials):$$ \epsilon = a^t, \textrm{for } 0 < a < 1 \hspace{50px}\epsilon = \frac{1}{t^2}\hspace{50px}\epsilon = e^{-at}, \textrm{for } 0 < a < 1 \hspace{50px} \epsilon = \cos(at), \textrm{for } 0 < a < 1$$You may also use a decaying function for $\alpha$ (the learning rate) if you so choose, however this is typically less common. If you do so, be sure that it adheres to the inequality $0 \leq \alpha \leq 1$.If you have difficulty getting your implementation to work, try setting the `'verbose'` flag to `True` to help debug. Flags that have been set here should be returned to their default setting when debugging. It is important that you understand what each flag does and how it affects the simulation! Once you have successfully completed the improved Q-Learning simulation, run the code cell below to visualize the results. Note that log files are overwritten when identical simulations are run, so be careful with what log file is being loaded!
###Code
# Load the 'sim_improved-learning' file from the improved Q-Learning simulation
vs.plot_trials('sim_improved-learning.csv')
###Output
_____no_output_____ |
pandas tutorial/Untitled.ipynb | ###Markdown
DataFrame with row labelsIf you supply an array to the index property of ```pd.DataFrame```, you can have custom row names
###Code
#example that shows how to add custom row names
indices = ['June', 'Robert', 'Lily', 'David']
purchases = pd.DataFrame(data, index=indices)
purchases
purchases.loc['June']
purchases.loc['June']['apples']
purchases.loc['June']['apples'] = 10
purchases
df = pd.read_csv('purchases.csv', index_col=0)
df
movies_df = pd.read_csv("IMDB-Movie-Data.csv", index_col="Title")
movies_df
movies_df.info()
movies_df.rename(columns={
'Runtime (Minutes)': 'Runtime',
'Revenue (Millions)': 'Revenue_millions'
}, inplace=True)
#movies_df.loc["Guardians of the Galaxy"]["Runtime (Minutes)"]
movies_df.loc["Guardians of the Galaxy"]["Runtime"]
movies_df.isnull()
movies_df.isnull().sum()
movies_no_nulls = movies_df.dropna()
movies_no_nulls.shape
#find average revenue
print(movies_no_nulls["Revenue_millions"].mean())
print(movies_df["Revenue_millions"].mean())
#describe revenue
movies_no_nulls["Revenue_millions"].describe()
movies_no_nulls["Revenue_millions"].median()
movies_no_nulls.corr()
#find movies by director
director_movies = movies_df[(movies_df['Director'] == "Ridley Scott")]
director_movies
#example of interpreted columns
def rating_function(x):
if x >= 8.0:
return "good"
else:
return "bad"
movies_df["rating_category"] = movies_df["Rating"].apply(rating_function)
movies_df.head(2)
movies_df.plot(kind='scatter', x='Rating', y='Revenue_millions', title='Revenue (millions) vs Rating')
movies_df.plot(kind='scatter', x='Votes', y='Revenue_millions', title='Revenue (millions) vs Votes')
movies_df['Rating'].plot(kind='hist', title='Rating')
movies_df['Rating'].plot(kind="box")
###Output
_____no_output_____ |
8-9 Machine Learning with Python/Final Project/The best classifier.ipynb | ###Markdown
Classification with Python In this notebook we try to practice all the classification algorithms that we learned in this course.We load a dataset using Pandas library, and apply the following algorithms, and find the best one for this specific dataset by accuracy evaluation methods.Lets first load required libraries:
###Code
import itertools
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.ticker import NullFormatter
import pandas as pd
import numpy as np
import matplotlib.ticker as ticker
from sklearn import preprocessing
%matplotlib inline
###Output
_____no_output_____
###Markdown
About dataset This dataset is about past loans. The __Loan_train.csv__ data set includes details of 346 customers whose loan are already paid off or defaulted. It includes following fields:| Field | Description ||----------------|---------------------------------------------------------------------------------------|| Loan_status | Whether a loan is paid off on in collection || Principal | Basic principal loan amount at the || Terms | Origination terms which can be weekly (7 days), biweekly, and monthly payoff schedule || Effective_date | When the loan got originated and took effects || Due_date | Since it’s one-time payoff schedule, each loan has one single due date || Age | Age of applicant || Education | Education of applicant || Gender | The gender of applicant | Lets download the dataset
###Code
!wget -O loan_train.csv https://s3-api.us-geo.objectstorage.softlayer.net/cf-courses-data/CognitiveClass/ML0101ENv3/labs/loan_train.csv
###Output
--2019-10-25 21:39:20-- https://s3-api.us-geo.objectstorage.softlayer.net/cf-courses-data/CognitiveClass/ML0101ENv3/labs/loan_train.csv
Resolving s3-api.us-geo.objectstorage.softlayer.net (s3-api.us-geo.objectstorage.softlayer.net)... 67.228.254.193
Connecting to s3-api.us-geo.objectstorage.softlayer.net (s3-api.us-geo.objectstorage.softlayer.net)|67.228.254.193|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 23101 (23K) [text/csv]
Saving to: ‘loan_train.csv’
100%[======================================>] 23,101 --.-K/s in 0.07s
2019-10-25 21:39:21 (303 KB/s) - ‘loan_train.csv’ saved [23101/23101]
###Markdown
Load Data From CSV File
###Code
df = pd.read_csv('loan_train.csv')
df.head()
df.shape
###Output
_____no_output_____
###Markdown
Convert to date time object
###Code
df['due_date'] = pd.to_datetime(df['due_date'])
df['effective_date'] = pd.to_datetime(df['effective_date'])
df.head()
###Output
_____no_output_____
###Markdown
Data visualization and pre-processing Let’s see how many of each class is in our data set
###Code
df['loan_status'].value_counts()
###Output
_____no_output_____
###Markdown
260 people have paid off the loan on time while 86 have gone into collection Lets plot some columns to underestand data better:
###Code
# notice: installing seaborn might takes a few minutes
!conda install -c anaconda seaborn -y
import seaborn as sns
bins = np.linspace(df.Principal.min(), df.Principal.max(), 10)
g = sns.FacetGrid(df, col="Gender", hue="loan_status", palette="Set1", col_wrap=2)
g.map(plt.hist, 'Principal', bins=bins, ec="k")
g.axes[-1].legend()
plt.show()
bins = np.linspace(df.age.min(), df.age.max(), 10)
g = sns.FacetGrid(df, col="Gender", hue="loan_status", palette="Set1", col_wrap=2)
g.map(plt.hist, 'age', bins=bins, ec="k")
g.axes[-1].legend()
plt.show()
###Output
_____no_output_____
###Markdown
Pre-processing: Feature selection/extraction Lets look at the day of the week people get the loan
###Code
df['dayofweek'] = df['effective_date'].dt.dayofweek
bins = np.linspace(df.dayofweek.min(), df.dayofweek.max(), 10)
g = sns.FacetGrid(df, col="Gender", hue="loan_status", palette="Set1", col_wrap=2)
g.map(plt.hist, 'dayofweek', bins=bins, ec="k")
g.axes[-1].legend()
plt.show()
###Output
_____no_output_____
###Markdown
We see that people who get the loan at the end of the week dont pay it off, so lets use Feature binarization to set a threshold values less then day 4
###Code
df['weekend'] = df['dayofweek'].apply(lambda x: 1 if (x>3) else 0)
df.head()
###Output
_____no_output_____
###Markdown
Convert Categorical features to numerical values Lets look at gender:
###Code
df.groupby(['Gender'])['loan_status'].value_counts(normalize=True)
###Output
_____no_output_____
###Markdown
86 % of female pay there loans while only 73 % of males pay there loan Lets convert male to 0 and female to 1:
###Code
df['Gender'].replace(to_replace=['male','female'], value=[0,1],inplace=True)
df.head()
###Output
_____no_output_____
###Markdown
One Hot Encoding How about education?
###Code
df.groupby(['education'])['loan_status'].value_counts(normalize=True)
###Output
_____no_output_____
###Markdown
Feature befor One Hot Encoding
###Code
df[['Principal','terms','age','Gender','education']].head()
###Output
_____no_output_____
###Markdown
Use one hot encoding technique to conver categorical varables to binary variables and append them to the feature Data Frame
###Code
Feature = df[['Principal','terms','age','Gender','weekend']]
Feature = pd.concat([Feature,pd.get_dummies(df['education'])], axis=1)
Feature.drop(['Master or Above'], axis = 1,inplace=True)
Feature.head()
###Output
_____no_output_____
###Markdown
Feature selection Lets defind feature sets, X:
###Code
X = Feature
X[0:5]
###Output
_____no_output_____
###Markdown
What are our lables?
###Code
y = df['loan_status'].values
y[0:5]
###Output
_____no_output_____
###Markdown
Normalize Data Data Standardization give data zero mean and unit variance (technically should be done after train test split )
###Code
X= preprocessing.StandardScaler().fit(X).transform(X)
X[0:5]
###Output
/opt/conda/envs/Python36/lib/python3.6/site-packages/sklearn/preprocessing/data.py:645: DataConversionWarning: Data with input dtype uint8, int64 were all converted to float64 by StandardScaler.
return self.partial_fit(X, y)
/opt/conda/envs/Python36/lib/python3.6/site-packages/ipykernel/__main__.py:1: DataConversionWarning: Data with input dtype uint8, int64 were all converted to float64 by StandardScaler.
if __name__ == '__main__':
###Markdown
Classification Now, it is your turn, use the training set to build an accurate model. Then use the test set to report the accuracy of the modelYou should use the following algorithm:- K Nearest Neighbor(KNN)- Decision Tree- Support Vector Machine- Logistic Regression__ Notice:__ - You can go above and change the pre-processing, feature selection, feature-extraction, and so on, to make a better model.- You should use either scikit-learn, Scipy or Numpy libraries for developing the classification algorithms.- You should include the code of the algorithm in the following cells. K Nearest Neighbor(KNN)Notice: You should find the best k to build the model with the best accuracy. **warning:** You should not use the __loan_test.csv__ for finding the best k, however, you can split your train_loan.csv into train and test to find the best __k__.
###Code
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
print ('Train set:', X_train.shape, y_train.shape)
print ('Test set:', X_test.shape, y_test.shape)
from sklearn.neighbors import KNeighborsClassifier
from sklearn import metrics
Ks = 10
mean_acc = np.zeros((Ks-1))
std_acc = np.zeros((Ks-1))
ConfustionMx = [];
for n in range(1,Ks):
#Train Model and Predict
neigh = KNeighborsClassifier(n_neighbors = n).fit(X_train,y_train)
yhat=neigh.predict(X_test)
mean_acc[n-1] = metrics.accuracy_score(y_test, yhat)
std_acc[n-1]=np.std(yhat==y_test)/np.sqrt(yhat.shape[0])
mean_acc
plt.plot(range(1,Ks),mean_acc,'g')
plt.fill_between(range(1,Ks),mean_acc - 1 * std_acc,mean_acc + 1 * std_acc, alpha=0.10)
plt.legend(('Accuracy ', '+/- 3xstd'))
plt.ylabel('Accuracy ')
plt.xlabel('Number of Nabors (K)')
plt.tight_layout()
plt.show()
print( "The best accuracy was with", mean_acc.max(), "with k =", mean_acc.argmax()+1)
# That's why for KNN model we should use k = 7:
kNN_model = KNeighborsClassifier(n_neighbors=7).fit(X_train,y_train)
kNN_model
###Output
_____no_output_____
###Markdown
Decision Tree
###Code
from sklearn.tree import DecisionTreeClassifier
dTree = DecisionTreeClassifier(criterion="entropy", max_depth = 4)
dTree.fit(X_train,y_train)
yhat = dTree.predict(X_test)
print("DecisionTrees's Accuracy: ", metrics.accuracy_score(y_test, yhat))
# So, Decision Tree model is:
DT_model = dTree
DT_model
###Output
_____no_output_____
###Markdown
Support Vector Machine
###Code
from sklearn import svm
clf = svm.SVC(kernel='rbf')
clf.fit(X_train, y_train)
yhat = clf.predict(X_test)
print("Support Vector Machine's Accuracy: ", metrics.accuracy_score(y_test, yhat))
# So, SVM model is:
SVM_model = clf
###Output
_____no_output_____
###Markdown
Logistic Regression
###Code
from sklearn.linear_model import LogisticRegression
LR = LogisticRegression(C=0.01, solver='liblinear').fit(X_train,y_train)
LR
yhat = LR.predict(X_test)
# yhat_prob = LR.predict_proba(X_test) # for LogLoss Evaluation
print("Logistic Regression's Accuracy: ", metrics.accuracy_score(y_test, yhat))
# So, Logistic Regression model is:
LR_model = LR
###Output
_____no_output_____
###Markdown
Model Evaluation using Test set
###Code
from sklearn.metrics import jaccard_similarity_score
from sklearn.metrics import f1_score
from sklearn.metrics import log_loss
###Output
_____no_output_____
###Markdown
First, download and load the test set:
###Code
!wget -O loan_test.csv https://s3-api.us-geo.objectstorage.softlayer.net/cf-courses-data/CognitiveClass/ML0101ENv3/labs/loan_test.csv
###Output
--2019-10-25 21:42:41-- https://s3-api.us-geo.objectstorage.softlayer.net/cf-courses-data/CognitiveClass/ML0101ENv3/labs/loan_test.csv
Resolving s3-api.us-geo.objectstorage.softlayer.net (s3-api.us-geo.objectstorage.softlayer.net)... 67.228.254.193
Connecting to s3-api.us-geo.objectstorage.softlayer.net (s3-api.us-geo.objectstorage.softlayer.net)|67.228.254.193|:443... connected.
HTTP request sent, awaiting response... 200 OK
Length: 3642 (3.6K) [text/csv]
Saving to: ‘loan_test.csv’
100%[======================================>] 3,642 --.-K/s in 0s
2019-10-25 21:42:41 (319 MB/s) - ‘loan_test.csv’ saved [3642/3642]
###Markdown
Load Test set for evaluation
###Code
test_df = pd.read_csv('loan_test.csv')
test_df.head()
## Preprocessing
test_df['due_date'] = pd.to_datetime(test_df['due_date'])
test_df['effective_date'] = pd.to_datetime(test_df['effective_date'])
test_df['dayofweek'] = test_df['effective_date'].dt.dayofweek
test_df['weekend'] = test_df['dayofweek'].apply(lambda x: 1 if (x>3) else 0)
test_df['Gender'].replace(to_replace=['male','female'], value=[0,1],inplace=True)
test_Feature = test_df[['Principal','terms','age','Gender','weekend']]
test_Feature = pd.concat([test_Feature,pd.get_dummies(test_df['education'])], axis=1)
test_Feature.drop(['Master or Above'], axis = 1,inplace=True)
test_X = preprocessing.StandardScaler().fit(test_Feature).transform(test_Feature)
test_y = test_df['loan_status'].values
knn_yhat = kNN_model.predict(test_X)
print("KNN Jaccard index: %.2f" % jaccard_similarity_score(test_y, knn_yhat))
print("KNN F1-score: %.2f" % f1_score(test_y, knn_yhat, average='weighted') )
DT_yhat = DT_model.predict(test_X)
print("DT Jaccard index: %.2f" % jaccard_similarity_score(test_y, DT_yhat))
print("DT F1-score: %.2f" % f1_score(test_y, DT_yhat, average='weighted') )
SVM_yhat = SVM_model.predict(test_X)
print("SVM Jaccard index: %.2f" % jaccard_similarity_score(test_y, SVM_yhat))
print("SVM F1-score: %.2f" % f1_score(test_y, SVM_yhat, average='weighted') )
LR_yhat = LR_model.predict(test_X)
LR_yhat_prob = LR_model.predict_proba(test_X)
print("LR Jaccard index: %.2f" % jaccard_similarity_score(test_y, LR_yhat))
print("LR F1-score: %.2f" % f1_score(test_y, LR_yhat, average='weighted') )
print("LR LogLoss: %.2f" % log_loss(test_y, LR_yhat_prob))
###Output
LR Jaccard index: 0.74
LR F1-score: 0.63
LR LogLoss: 0.58
|
datathon2019-target-5.ipynb | ###Markdown
Datathon 2019
###Code
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
path = './data/Dataset'
df_scores = pd.read_csv(path + '/Scores.csv', index_col = 0)
df_scores.head()
df_pre_contest = pd.read_csv(path + '/Pre_Contest.csv', index_col = 0)
df_pre_contest.head()
df_post_contest = pd.read_csv(path + '/Post_Contest.csv', index_col = 0)
df_post_contest.head()
df_pre_contest.describe()
df_post_contest.describe()
df_scores_pre_contest = df_scores.merge(df_pre_contest, left_on = 'Prutor ID', right_on='Prutor ID')
df_scores_pre_contest = df_scores_pre_contest[['Prutor ID','TOTAL', 'Rate your problem solving ability on the scale of 1 to 5', 'How comfortable are you coding in C?']]
df_scores_pre_contest.head()
df_scores_pre_contest.columns = ['Prutor ID', 'TOTAL', 'PreTestRate', 'PreTestCRate']
df_scores_pre_contest.head()
df_scores_post_contest = df_scores.merge(df_post_contest, left_on = 'Prutor ID', right_on='Prutor ID')
df_scores_post_contest = df_scores_post_contest[['Prutor ID','TOTAL', 'How would you rate your Problem Solving abilities after this contest?', 'How would you rate your proficiency in coding in C after this contest?']]
df_scores_post_contest.head()
df_scores_post_contest.columns = ['Prutor ID', 'TOTAL', 'PostTestRate', 'PostTestCRate']
df_scores_post_contest.head()
df_scores_pre_contest = df_scores.merge(df_pre_contest, left_on = 'Prutor ID', right_on='Prutor ID')
df_scores_pre_contest.groupby(['Department', 'Years of coding experience']).mean()
df_scores_pre_contest.groupby(['Department', 'Years of coding experience']).count()
###Output
_____no_output_____ |
SIFTt.ipynb | ###Markdown
SIFT[](https://colab.research.google.com/github/YoniChechik/AI_is_Math/blob/master/c_08_features/sift.ipynb)
###Code
# to run in google colab
import sys
if 'google.colab' in sys.modules:
import subprocess
subprocess.call("pip install -U opencv-python".split())
from google.colab import drive
drive.mount('/content/gdrive')
import os
path = '/content/gdrive/MyDrive/flowers/'
files = os.listdir(path)
print("Flowers dataset:")
for i in files:
print(f"- {i} : {len(os.listdir(path + i))}")
###Output
Flowers dataset:
- daisy : 764
- dandelion : 1052
- rose : 784
- sunflower : 733
- tulip : 984
- lotusflower : 604
- hydrangea : 669
- Gerbera : 600
- Lily : 600
###Markdown
SIFT code is adapted from:https://towardsdatascience.com/image-stitching-using-opencv-817779c86a83
###Code
from random import randrange
import matplotlib.pyplot as plt
import numpy as np
import cv2
figsize = (10, 10)
import os
path = '/content/gdrive/MyDrive/flowers/'
def readData(path):
X = [] #chứa image
y = [] #chứa label
label = 0
classes = os.listdir(path)
for folder in classes:
files = sorted(os.listdir(path + folder))
for i in files[:300]:
img = cv2.imread(path + folder + '/' + i)
gray= cv2.cvtColor(img,cv2.COLOR_BGR2GRAY)
# sift = cv2.SIFT_create()
# kp, des = sift.detectAndCompute(gray,None)
X.append(img)
y.append(label)
return X, y
label += 1
return X, y
import cv2
import os
import glob
def label_image(path_to_data):
list_label = os.listdir(path_to_data)
label2id = {}
for id_, value in enumerate(list_label):
label2id[value] = id_
data_train = []
data_label = []
for label in list_label:
print(f"Processing data {label} . . .")
count = 0
for img in glob.glob(path_to_data+"/" + label + '/*'):
if count == 300: break
image = cv2.imread(img)
label_image = label2id[label]
data_train.append(image)
data_label.append(label_image)
count += 1
print("Done!")
return data_train, data_label, label2id
import cv2
from sklearn.cluster import KMeans
import pickle
from scipy.spatial.distance import cdist
import os
import numpy as np
def extract_sift_features(list_image):
image_descriptors = []
sift = cv2.SIFT_create()#cv2.xfeatures2d.SIFT_create()
for image in list_image:
_, descriptor = sift.detectAndCompute(image, None)
image_descriptors.append(descriptor)
return image_descriptors
def kmean_bow(all_descriptors, num_cluster):
bow_dict = []
kmeans = KMeans(n_clusters = num_cluster)
kmeans.fit(all_descriptors)
bow_dict = kmeans.cluster_centers_
if not os.path.isfile('bow_dictionary.pkl'):
pickle.dump(bow_dict, open('bow_dictionary.pkl', 'wb'))
return bow_dict
def create_feature_bow(image_descriptors, BoW, num_cluster):
X_features = []
for i in range(len(image_descriptors)):
features = np.array([0] * num_cluster)
if image_descriptors[i] is not None:
distance = cdist(image_descriptors[i], BoW)
argmin = np.argmin(distance, axis = 1)
for j in argmin:
features[j] += 1
X_features.append(features)
return X_features
from sklearn.model_selection import train_test_split, GridSearchCV
import sklearn
import pickle
import argparse
import os
# arg = argparse.ArgumentParser()
# arg.add_argument("-dt", "--inputdata", required = True, help = "path to data training")
# args = vars(arg.parse_args())
path = '/content/gdrive/MyDrive/flowers'
print(path)
data_train, label, label2id = label_image(path)
image_desctiptors = extract_sift_features(data_train)
all_descriptors = []
for descriptor in image_desctiptors:
if descriptor is not None:
for des in descriptor:
all_descriptors.append(des)
num_cluster = 10
BoW = kmean_bow(all_descriptors, num_cluster)
X_features = create_feature_bow(image_desctiptors, BoW, num_cluster)
###Output
/content/gdrive/MyDrive/flowers
Processing data daisy . . .
Done!
Processing data dandelion . . .
Done!
Processing data rose . . .
Done!
Processing data sunflower . . .
Done!
Processing data tulip . . .
Done!
Processing data lotusflower . . .
Done!
Processing data hydrangea . . .
Done!
Processing data Gerbera . . .
Done!
Processing data Lily . . .
Done!
###Markdown
Test
###Code
import matplotlib.pyplot as plt
import itertools
def plot_confusion_matrix(cm, classes,
normalize=False,
title='Confusion matrix',
cmap=plt.cm.Blues):
"""
This function prints and plots the confusion matrix.
Normalization can be applied by setting normalize=True.
"""
if normalize:
cm = cm.astype('float') / cm.sum(axis=1, keepdims = True)
plt.imshow(cm, interpolation='nearest', cmap=cmap)
plt.title(title)
plt.colorbar()
tick_marks = np.arange(len(classes))
plt.xticks(tick_marks, classes, rotation=45)
plt.yticks(tick_marks, classes)
fmt = '.2f' if normalize else 'd'
thresh = cm.max() / 2.
for i, j in itertools.product(range(cm.shape[0]), range(cm.shape[1])):
plt.text(j, i, format(cm[i, j], fmt),
horizontalalignment="center",
color="white" if cm[i, j] > thresh else "black")
plt.tight_layout()
plt.ylabel('True label')
plt.xlabel('Predicted label')
import pandas as pd
import numpy as np
from sklearn.preprocessing import StandardScaler
from sklearn.model_selection import KFold
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC
from sklearn.naive_bayes import GaussianNB
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier
from sklearn.linear_model import SGDClassifier
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
def Experiment(X, y, model_used, classes):
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.33, random_state=42)
clf = model_used
clf.fit(X_train, y_train)
y_predict = clf.predict(X_test)
from sklearn.metrics import classification_report, confusion_matrix
print(classification_report(y_test, y_predict, target_names=classes))
print( confusion_matrix(y_test, y_predict))
from sklearn.metrics import accuracy_score
print(accuracy_score(y_test,y_predict))
# Plot non-normalized confusion matrix
plt.figure()
plot_confusion_matrix(confusion_matrix(y_test, y_predict), classes=classes,
title='Confusion matrix, without normalization')
# Plot normalized confusion matrix
plt.figure()
plot_confusion_matrix(confusion_matrix(y_test, y_predict), classes=classes, normalize=True,
title='Normalized confusion matrix')
plt.show()
###Output
_____no_output_____
###Markdown
Running
###Code
classes = list(label2id)
#Logistic Regression
Experiment(X_features, label, LogisticRegression(), classes)
#SVM
Experiment(X_features, label, SVC(C = 30), classes)
#Naive Bayes
Experiment(X_features, label, GaussianNB(), classes)
#Decision Tree
Experiment(X_features, label, DecisionTreeClassifier(), classes)
#Random Forest
Experiment(X_features, label, RandomForestClassifier(), classes)
#KNeighborsClassifier
Experiment(X_features, label, KNeighborsClassifier(), classes)
#SGDClassifier
Experiment(X_features, label, SGDClassifier(), classes)
###Output
precision recall f1-score support
daisy 0.29 0.44 0.35 91
dandelion 0.87 0.29 0.44 112
rose 0.26 0.77 0.38 91
sunflower 0.50 0.56 0.53 93
tulip 0.26 0.04 0.07 115
lotusflower 0.57 0.14 0.23 92
accuracy 0.36 594
macro avg 0.46 0.37 0.33 594
weighted avg 0.46 0.36 0.33 594
[[40 3 30 13 2 3]
[28 33 36 10 5 0]
[ 8 1 70 7 2 3]
[12 0 25 52 3 1]
[23 1 70 13 5 3]
[25 0 43 9 2 13]]
0.35858585858585856
|
patrones/08-bridge.ipynb | ###Markdown
Each Concrete Implementation corresponds to a specific platform and implements the Implementation interface using that platform's API.
###Code
class ConcreteImplementationA(Implementation):
def operation_implementation(self) -> str:
return "ConcreteImplementationA: Here's the result on the platform A."
class ConcreteImplementationB(Implementation):
def operation_implementation(self) -> str:
return "ConcreteImplementationB: Here's the result on the platform B."
def client_code(abstraction: Abstraction) -> None:
"""
Except for the initialization phase, where an Abstraction object gets linked
with a specific Implementation object, the client code should only depend on
the Abstraction class. This way the client code can support any abstraction-
implementation combination.
"""
# ...
print(abstraction.operation(), end="")
if __name__ == "__main__":
"""
The client code should be able to work with any pre-configured abstraction-
implementation combination.
"""
implementation = ConcreteImplementationA()
abstraction = Abstraction(implementation)
client_code(abstraction)
print("\n")
implementation = ConcreteImplementationB()
abstraction = ExtendedAbstraction(implementation)
client_code(abstraction)
print("\n")
###Output
Abstraction: Base operation with:
ConcreteImplementationA: Here's the result on the platform A.
ExtendedAbstraction: Extended operation with:
ConcreteImplementationB: Here's the result on the platform B.
###Markdown
Bridge Bridge es un patrón de diseño estructural que te permite dividir una clase grande, o un grupo de clases estrechamente relacionadas, en dos jerarquías separadas (abstracción e implementación) que pueden desarrollarse independientemente la una de la otra. 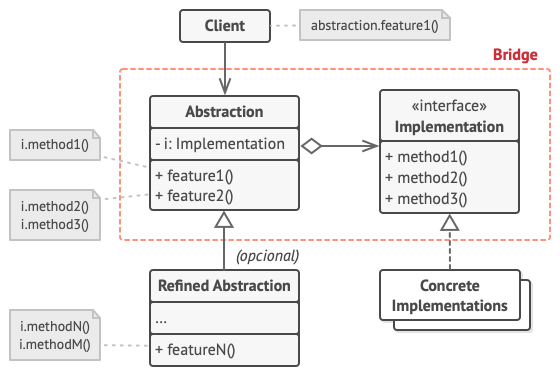 Cuando usarlo?* Utiliza el patrón Bridge cuando quieras dividir y organizar una clase monolítica que tenga muchas variantes de una sola funcionalidad (por ejemplo, si la clase puede trabajar con diversos servidores de bases de datos).* Utiliza el patrón cuando necesites extender una clase en varias dimensiones ortogonales (independientes).* Utiliza el patrón Bridge cuando necesites poder cambiar implementaciones durante el tiempo de ejecución. Pros y contras? Pros* Puedes crear clases y aplicaciones independientes de plataforma.* El código cliente funciona con abstracciones de alto nivel. No está expuesto a los detalles de la plataforma.* Principio de abierto/cerrado. Puedes introducir nuevas abstracciones e implementaciones independientes entre sí.* Principio de responsabilidad única. Puedes centrarte en la lógica de alto nivel en la abstracción y en detalles de la plataforma en la implementación. Contras* Puede ser que el código se complique si aplicas el patrón a una clase muy cohesionada. Codigo
###Code
from __future__ import annotations
from abc import ABC, abstractmethod
class Abstraction:
"""
The Abstraction defines the interface for the "control" part of the two
class hierarchies. It maintains a reference to an object of the
Implementation hierarchy and delegates all of the real work to this object.
"""
def __init__(self, implementation: Implementation) -> None:
self.implementation = implementation
def operation(self) -> str:
return (f"Abstraction: Base operation with:\n"
f"{self.implementation.operation_implementation()}")
class ExtendedAbstraction(Abstraction):
"""
You can extend the Abstraction without changing the Implementation classes.
"""
def operation(self) -> str:
return (f"ExtendedAbstraction: Extended operation with:\n"
f"{self.implementation.operation_implementation()}")
class Implementation(ABC):
"""
The Implementation defines the interface for all implementation classes. It
doesn't have to match the Abstraction's interface. In fact, the two
interfaces can be entirely different. Typically the Implementation interface
provides only primitive operations, while the Abstraction defines higher-
level operations based on those primitives.
"""
@abstractmethod
def operation_implementation(self) -> str:
pass
###Output
_____no_output_____ |
Neural_tdc.ipynb | ###Markdown
TIme depeNdent ConduiT flow neUral netwoRk Emulator (TINCTURE)A collaboration between Ying Qi Wong, PhD and Ben Mullet
###Code
import hashlib
import matplotlib.pyplot as plt
import numpy as np
import os
import pathlib
import pickle
import tqdm
import json
import uuid
import tensorflow as tf
from tensorflow import keras
from tensorflow.keras.layers import Dense
import matlab.engine
import domegoveqn
import constitutive
import utils
%load_ext autoreload
%autoreload 2
###Output
_____no_output_____
###Markdown
Gather training data(This section is adapted from YQ's notebook "RunModel")
###Code
eng = matlab.engine.start_matlab()
path_to_domeconduit_code = "/home/bmullet/Research/software/domeconduit/Code/" # on Ben's machine
path_to_domeconduit_code = "../domeconduit/Code/" # on YQ's machine
eng.addpath (eng.genpath(path_to_domeconduit_code), nargout= 0 )
###Output
_____no_output_____
###Markdown
Run time-dependent conduit model by calling matlab functions First start matlab and add paths for model functions Run the full time-dependent model using default parameters
###Code
def n_by_1(array):
"""Transform array to Nx1"""
array = np.array(array)
assert len(array.shape) <= 2, "Array dim should be <= 2"
if len(array.shape) < 2:
array = array[:,np.newaxis]
L, W = array.shape
if L < W:
array = array.T
return array
#TODO: Collect relevant pieces into a class that keeps list of inputs/outputs neat and clean
# Global constants
CONDUIT_VAR_NAMES = ["p", "v", "phi_g", "mh"] # TODO: change to mh
#ACTIVATIONS = [tf.keras.backend.abs, tf.keras.backend.abs, tf.nn.sigmoid, tf.nn.sigmoid] # should match the order of CONDUIT_VAR_NAMES
ACTIVATIONS = [tf.nn.sigmoid, tf.nn.sigmoid, tf.nn.sigmoid, tf.nn.sigmoid] # should match the order of CONDUIT_VAR_NAMES
DEFAULT_PARAMS = eng.tdcFV('setdef', nargout=1)
DEFAULT_PARAMS['phi_gc'] = 0
MODEL_PARAM_VARIABLES = DEFAULT_PARAMS.keys()
DATA_CACHE_DIR = "./data_cache"
# Make sure the cache directory exists
pathlib.Path(DATA_CACHE_DIR).mkdir(parents=False, exist_ok=True)
def check_model_params(params):
"""
Makes sure an object is a valid params dict
"""
assert DEFAULT_PARAMS.keys() == params.keys(), "model params dict is not valid"
class matlab_conduit_data_loader():
# Default parameter
Nz = 401
def __init__(self,
model_params=DEFAULT_PARAMS,
use_cache=True
):
check_model_params(model_params)
self.model_params = model_params
# Make a file name from hasing the input dictionary
hash_code = hashlib.sha1(json.dumps(self.model_params, sort_keys=True).encode()).hexdigest()
self.file_name = os.path.join(DATA_CACHE_DIR, hash_code)
# Load data
loaded_data = False
if pathlib.Path(self.file_name).is_file() and use_cache:
# We've already computed this and cached the values, so load the cached data
try:
with open(self.file_name, "rb") as f:
saved_data = pickle.load(f)
self.ss = saved_data['ss']
self.opts = saved_data['opts']
self.ssflag = saved_data['ss_flag']
loaded_data = True
except Exception as e:
print("Caught Exception:", e)
print("Cannot load data. Will generate it from scratch.")
if not loaded_data:
# Compute data
# adjust some parameters
opts = eng.tdcFV('ss_init',model_params, nargout=1)
#opts['plug_gas_loss'] = 0
ss, opts, ssflag = eng.tdcFV('run_ssc_opts', opts, nargout=3)
#ss, opts, ssflag = eng.tdcFV('run_ssc', model_params, nargout=3)
self.ss = ss
self.opts = constitutive.reformat_params(ss['m'])
self.ssflag = ssflag
# Cache data
if use_cache:
with open(self.file_name, "wb") as f:
data_to_save = {"ss" : self.ss, "opts" : self.opts,
"ss_flag": self.ssflag}
pickle.dump(data_to_save, f)
def get_transformed_data(self):
return conduit_data_transformer(self)
class conduit_data_transformer():
# Transform matlab conduit data into python and TF objects
def __init__(self, ml_conduit_data):
# Extract the variables and gradients
self.vars, self.grads = utils.get_steady_state_vars_and_grads(ml_conduit_data.ss)
self.opts = ml_conduit_data.opts
def create_tf_dataset(self):
# create a dict to store the data
tf_dict = {}
# adds z, p, phi_g, v, m_w
tf_dict.update({key : np.squeeze(var).astype(np.float32) for key, var in self.vars.items()})
# adds gradients as nested dictionaries
tf_dict["gradients"] = {key : np.squeeze(grad).astype(np.float32) for key, grad in self.grads.items()}
# adds model parameters.
uid = str(uuid.uuid4())
tf_dict["uuid"] = uid
opts = { uid : self.opts }
# Get boundary conditions
bottom_idx = np.argmin(tf_dict["z"])
top_idx = np.argmax(tf_dict["z"])
# Conduit bottom boundary conditions are set on p, phi_g, and m_h (not v)
bottom_bcs = ["p", "phi_g", "mh"]
# Conduit top boundary conditions are only set on p
top_bcs = ["p"]
# Creates a mask for those entries which are also boundary conditions
conduit_bcs = {}
for var in CONDUIT_VAR_NAMES:
conduit_bcs[var] = np.zeros_like(tf_dict["z"]).astype(np.float32)
if var in bottom_bcs:
conduit_bcs[var][bottom_idx] = 1.0
if var in top_bcs:
conduit_bcs[var][top_idx] = 1.0
tf_dict.update({"boundary_conditions" : conduit_bcs})
# Create tf dataset
dataset = tf.data.Dataset.from_tensors(tf_dict)
return dataset, opts
def data_assembler_template(opts, input_vars=["z"], input_model_params=[], output_vars=CONDUIT_VAR_NAMES):
"""
Creates a convenience function for splitting the dataset
Inputs:
opts: dictionary of opts from different models
input_vars: list of primary vars (z, phi_g, mh, p, v) to include in input to model
input_model_params: list of model params to include in input to model
output_vars: list of primary vars (z, phi_g, mh, p, v) to predict with model
Output:
data_assembler function
"""
assert input_vars[0] == "z", "First input variable needs to be z"
def data_assembler(dataset):
"""Convenience function for splitting up a tf.dataset
Inputs:
dataset: tf.dataset of the form created by conduit_data_transformer
Outputs:
model_input: tensor that matches input_vars
model_output: tensor that matches output_vars
model_output_gradients: d()/dz gradients for output_vars
model_params: dict of model_params tensors
boundary_conditions: boundary conditions tensor mask
"""
assert len(batch["uuid"]) == 1, "Data assembler is only set up for batch size of 1"
uid = batch["uuid"].numpy()[0].decode('utf-8')
# Load the opts associated with this batch
batch_opts = opts[uid]
# Create the input tensor
model_input = tf.stack([tf.squeeze(dataset[var]) for var in input_vars], axis=1)
# Add model parameters if we want to use those to train the model
if len(input_model_params) > 0:
dummy_tensor = tf.ones([model_input.shape[0]], dtype=tf.float32)
model_input_model_params = tf.stack([dummy_tensor*batch_opts[var] for var in input_model_params], axis=1)
model_input = tf.concat([model_input, model_input_model_params], axis=1)
# Create the output tensor
model_output = tf.stack([tf.squeeze(dataset[var]) for var in output_vars], axis=1)
# Gradients. Again we need to squeeze and reshape to make sure we have Nx1 vectors
model_output_gradients = {key : tf.squeeze(grad)[:,None] for key, grad in dataset["gradients"].items()}
# Boundary conditions
boundary_conditions = tf.stack([tf.squeeze(dataset["boundary_conditions"][var]) for var in output_vars], axis=1)
return model_input, model_output, model_output_gradients, boundary_conditions, batch_opts
return data_assembler
def plot_batch(model_input, model_output_true, model_output_pred=None, label=""):
"""Plot some output, given in_array and out_array"""
# Cast to numpy
if isinstance(model_input, tf.Tensor):
model_input = model_input.numpy()
if isinstance(model_output_true, tf.Tensor):
model_output_true = model_output_true.numpy()
if isinstance(model_output_pred, tf.Tensor):
model_output_pred = model_output_pred.numpy()
z = model_input[:,0]
plt.figure(figsize=(15, 3))
nsub = len(CONDUIT_VAR_NAMES)
for i, var_name in enumerate(CONDUIT_VAR_NAMES):
arrayi = model_output_true[:,i]
plt.subplot(1, nsub, i+1)
lines = plt.plot(arrayi, z)
if model_output_pred is not None:
arrayi2 = model_output_pred[:,i]
plt.plot(arrayi2, z, "--r")
plt.xlabel(label + var_name)
plt.ylabel("z")
plt.tight_layout()
plt.show()
class data_transformer():
def __init__(self, variable_bounds):
"""
Create functions to normalize (and undimensionalize) and unnormalize our data
Input:
variable_bounds: dict of maxes and mins for normalizing
Output:
to_nondim: function for nondimensionalizing data
to_dim: function for dimensionalizing data
"""
mins = tf.constant([variable_bounds[var]["min"] for var in CONDUIT_VAR_NAMES], dtype=tf.float32)
maxes = tf.constant([variable_bounds[var]["max"] for var in CONDUIT_VAR_NAMES], dtype=tf.float32)
def to_nondim(dimensional_tensor):
return (dimensional_tensor - mins)/(maxes - mins)
def to_dim(nondimensional_tensor):
return (nondimensional_tensor*(maxes-mins) + mins)
def to_nondim_z(x):
# Assumes that z is the first column of x
dimensional_z = x[:,0][:, None]
nondimensional_z = (dimensional_z - variable_bounds["z"]["min"])/(variable_bounds["z"]["max"] - variable_bounds["z"]["min"])
return tf.concat([nondimensional_z, x[:,1:]], axis=1)
def to_dim_z(x):
# Assumes that z is the first column of x
nondimensional_z = x[:,0][:, None]
dimensional_z = (nondimensional_z*(variable_bounds["z"]["max"] - variable_bounds["z"]["min"]) + variable_bounds["z"]["min"])
return tf.concat([dimensional_z, x[:,1:]], axis=1)
def unnormalize_z_gradient(nondimensional_gradient):
# Unnormalizes the z part of the gradient
if isinstance(nondimensional_gradient, list):
return [unnormalize_z_gradient(grad) for grad in nondimensional_gradient]
else:
return nondimensional_gradient/(variable_bounds["z"]["max"] - variable_bounds["z"]["min"])
self.to_nondim = to_nondim
self.to_dim = to_dim
self.to_nondim_z = to_nondim_z
self.to_dim_z = to_dim_z
self.unnormalize_z_gradient = unnormalize_z_gradient
f = tf.constant([[-4000,1,2,3],[0,4,5,6]], dtype = tf.float32)
print(tf.concat([f[:,0][:,None], f[:,1:]], axis=1))
dt = data_transformer(mins_and_maxes)
print(dt.to_nondim_z(f))
#mins_and_maxes["z"]
print(CONDUIT_VAR_NAMES)
c = matlab_conduit_data_loader(use_cache=False)
d = c.get_transformed_data()
print(c.opts.keys())
c.opts['vvfrac_thr']=0.
mins_and_maxes = {}
for var in CONDUIT_VAR_NAMES + ["z"]:
mins_and_maxes[var] = {
"min" : np.min(d.vars[var]),
"max" : np.max(d.vars[var])}
normalizer = data_transformer(mins_and_maxes)
dataset, opts = d.create_tf_dataset()
# Shows what one element of the dataset looks like
print(dataset.element_spec.keys())
print(dataset.element_spec["uuid"])
print(opts.keys())
print(list(opts.values())[0].keys())
assembler = data_assembler_template(opts=opts, input_vars=["z"], input_model_params=[], output_vars=CONDUIT_VAR_NAMES)
batcher = dataset.batch(1)
for batch in batcher:
model_input, model_output, model_output_gradients, boundary_conditions, batch_opts = assembler(batch)
plot_batch(model_input, model_output, model_output)
grads = np.concatenate([model_output_gradients[i] for i in model_output_gradients.keys()], axis=1)
plot_batch(model_input,grads,grads, label="grad_")
break
print(model_input[0:2])
print(model_input[1]-model_input[0])
print(model_output.shape)
print([f"{name} : {grad.shape}" for name, grad in model_output_gradients.items()])
print(boundary_conditions.shape)
print(model_output_gradients.keys())
print(np.concatenate([model_output_gradients[i] for i in model_output_gradients.keys()], axis=1))
#print(model_input)
print(batch_opts['fr']['A'])
###Output
tf.Tensor(
[[-4000. ]
[-3993.3333]], shape=(2, 1), dtype=float32)
tf.Tensor([6.666748], shape=(1,), dtype=float32)
(601, 4)
['p : (601, 1)', 'v : (601, 1)', 'phi_g : (601, 1)', 'mh : (601, 1)']
(601, 4)
dict_keys(['p', 'v', 'phi_g', 'mh'])
[[-2.1777895e+04 3.7924895e-08 4.6732886e-05 3.2517317e-05]
[-2.1771875e+04 3.8046732e-08 4.6823377e-05 3.2313947e-05]
[-2.1765828e+04 3.8168864e-08 4.6913992e-05 3.2112021e-05]
...
[-2.4179660e+04 5.6890511e-17 -1.1857537e-14 -1.1368684e-16]
[-2.3523125e+04 1.5909254e-17 -5.7576057e-15 -1.1101536e-16]
[-2.2866621e+04 -3.6337014e-18 1.7014556e-15 7.4477924e-16]]
9.079985952496972e-10
###Markdown
Set up model
###Code
# Neural network model
class PDENeuralNet(tf.keras.Model):
def __init__(self, n_inputs, n_outputs, activations=None):
assert (isinstance(activations, list)) or (activations is None), "Please provide a list of activations, if desired"
if activations is not None:
assert len(activations) == n_outputs, "Please provide an activation layer for each output"
super().__init__()
self.n_inputs = n_inputs
self.n_outputs = n_outputs
# Define model
inputs = keras.Input(shape=(self.n_inputs,))
x = Dense(100, activation=tf.nn.elu)(inputs)
x = Dense(100, activation=tf.nn.elu)(x)
x = Dense(40, activation=tf.nn.elu)(x)
x = Dense(30, activation=tf.nn.elu)(x)
x = Dense(10, activation=tf.nn.elu)(x)
x = Dense(self.n_outputs, activation=None)(x)
output = []
if activations is not None:
for xi, activation in zip(tf.unstack(x,axis=-1), activations):
output.append(activation(xi))
x = tf.stack(output, axis=1)
self.nn_model = keras.Model(inputs=inputs, outputs=x, name="PDEModel")
self(tf.zeros([1, self.n_inputs])) # dummy call to build the model
@tf.function
def call(self, x):
z = self.nn_model(x)
return z
# Neural network parameters
N_INPUTS = 1
N_OUTPUTS = 4
testmodel = PDENeuralNet(N_INPUTS, N_OUTPUTS, activations=ACTIVATIONS)
OUTPUT_SCALING = tf.constant([1e7, 1e-3, 1/3, 0.95], dtype=tf.float32) # A dumb normalization for scaling the loss
def get_optimzer(type="Adam", lr=2e-2):
"""Sets up NN model optimizer"""
if type=="Adam":
trainer = tf.keras.optimizers.Adam(learning_rate = lr)
else:
trainer = tf.keras.optimizers.SGD(learning_rate = lr)
return trainer
trainer = get_optimzer()
def get_conduit_vals(p, u, A):
raise NotImplementedError
return
def get_physics(x, y, dydx, batch_opts):
"""Get the the governing equations.
Inputs:
x: (N x K) tensor of (z, [input_variables])
y: (N x 4) tensor of (p,v,phi_g,m_g) primary conduit variables
dydx: 4 element list of gradients
batch_opts: dictionary of model parameters
Returns:
F: (N x M) tensor for the M governing equations for each of the N samples.
Each entry should equal zero if the physics are satisfied.
See the note at the bottom of this notebook for an explanation.
"""
z = x[:,0][:,None] # Enforce Nx1
conduit_values = [val for val in split_tensor(y)]
conduit_variables = {var : conduit_values[i] for i, var in enumerate(CONDUIT_VAR_NAMES)}
conduit_gradients = {var : {"z" : dydx[i][:,0][:,None]}
for i, var in enumerate(CONDUIT_VAR_NAMES)}
# Variables are accessible in the conduit_variables dictionary by key equal to their variable name
# e.g. conduit_variables["p"] is (Nx1) tensor for pressure
# Gradients are accessible in the "conduit_gradients" two-level dictionary. First key is variable name;
# second is the variable with respect to which to take the derivative.
# e.g. conduit_gradients["p"]["t"] is (Nx1) tensor for dpdt
# Feel free to rename these variables/use as desired!
####### YING QI'S CODE HERE #############
F = domegoveqn.ssc(z, conduit_variables, conduit_gradients, batch_opts)
#########################################
#raise NotImplementedError
return F
def split_tensor(tensor):
"""Splits NxM tensor into M Nx1 dimensional tensors"""
vectors = tf.unstack(tensor,axis=1)
for v in vectors:
yield v[:,None]
def conduit_loss(x, y, dydx, y_true, model_output_gradients, bc_mask, batch_opts, iteration):
"""Calculate the loss function"""
loss_multipliers = tf.constant([1, 1e-5, 1, 1], dtype=tf.float32)
# Start training by just trying to match the matlab output
if iteration < N_DATA_EPOCHS:
F = np.zeros((1,4))
loss = tf.reduce_mean(tf.square((normalizer.to_nondim(y) - normalizer.to_nondim(y_true))))
# After a certain number of steps, switch to a "physics-informed" loss
else:
# We can multiply each of the equations by a constant to make sure they are
# weighted equally (i.e. do a simple normalization)
loss_multipliers = tf.constant([1, 1e-5, 1, 1], dtype=tf.float32)
# F should be (Nx4), for the 4 governing equations
F = get_physics(x, y, dydx, batch_opts)
#import pdb; pdb.set_trace()
# loss_multipliers will broadcast to multiply F
physics_loss = tf.reduce_mean(tf.square(F*loss_multipliers)* (1-bc_mask))
# Boundary condition loss. We use a binary mask to mask out all contributions
# except for the values that represent the boundary conditions. (Note this
# assumes that the matlab solution [in y_truey] satisfied the BCs.)
bc_loss = tf.reduce_mean(tf.square((y - y_true)/y_true) * bc_mask)
loss = physics_loss + bc_loss
return loss, F*loss_multipliers
def train(model, dataset):
trainer = get_optimzer()
assembler = data_assembler_template(opts, input_vars=["z"], input_model_params=[], output_vars=CONDUIT_VAR_NAMES)
loss_best = 1e6 # arbitrary high number
lr_counter = 0
plot_every = 1000
# Iterate over epochs
for i in tqdm.trange(N_EPOCHS, desc="Training"):
batches = dataset.batch(601)
# Iterate over training set
for batch in batches:
x, y_true, gradients_true, bc_mask, batch_opts = assembler(batch)
x = normalizer.to_nondim_z(x)
with tf.GradientTape(persistent=True) as t:
t.watch(x)
# forward pass of the model
y = model(x)
y = normalizer.to_dim(y) # output of the nn is non-dimensional
outs = [tensor for tensor in split_tensor(y)]
# get gradients with respect to z
dydx = []
for j in range(len(outs)):
dydx.append(t.gradient(outs[j], x))
dydx = normalizer.unnormalize_z_gradient(dydx)
#Un-normalize the depths
x = normalizer.to_dim_z(x)
# Calculate loss
loss, F = conduit_loss(x, y, dydx, y_true, gradients_true, bc_mask, batch_opts, i)
# Reduce the learning rate if we hit a plateau
if loss < 0.9*loss_best:
lr_counter = 0
loss_best = loss
else:
lr_counter += 1
if lr_counter > 1000:
print("Reducing learning rate")
lr_counter = 0
learning_rate = trainer.lr.numpy()*0.5
trainer.lr.assign(learning_rate)
# Get gradients for model
gradients = t.gradient(loss, model.weights)
# Switch to new optimzer when we switch loss functions (see loss definition)
if i == N_DATA_EPOCHS:
trainer = get_optimzer("SGD", lr=1e-10)
# Apply gradients to model
trainer.apply_gradients(zip(gradients, model.weights))
# Plot every so often
if i % plot_every == 0:
print(f"Step {i} loss is {loss}")
print(f"Step {i} F is { tf.reduce_mean(tf.square(F), axis=0)}")
plot_batch(x, y, y_true)
plot_batch(x,tf.stack([np.squeeze(grad) for grad in dydx], axis=1), tf.stack([np.squeeze(gradients_true[i]) for i in gradients_true.keys()], axis=1),label="grad_")
N_EPOCHS=100000
N_DATA_EPOCHS=10000
conduit_nn = PDENeuralNet(N_INPUTS,N_OUTPUTS, activations= ACTIVATIONS) # should match the order of CONDUIT_VAR_NAMES) # First output is pressure, second is u
train(conduit_nn, dataset)
###Output
Training: 0%| | 0/100000 [00:00<?, ?it/s]
###Markdown
How to add the physics As a reminder, the neural network is set up to mimic the function $f(z,t) = [p, v, \phi_g, m_h](z,t)$, which is a map $\mathbb{R}^2 \to \mathbb{R}^4$.In practice, we train the neural network with a "batch" of $(z,t)$ samples. This adds an extra dimension to the tensors being passed in and out of the model, where different elements of a batch are stacked along the first axis. Therefore, during training we expect inputs of size $(N,2)$ (2 because of z and t) and outputs of size $(N,4)$ (4 because of the conduit model outputs), where $N$ is the batch size. Steady-State Governing EquationsWe can write the governing equations for the steady-state conduit model in the form (YQ is working on this)$$F(z, [\nu, \partial_z \nu]) = 0$$where $F$ is a matrix of size $(M,1)$ governing equations and $[\nu, \partial_z \nu]$ is a 8-element vector containing the primary conduit variables and their gradients with respect to $z$, e.g. a vector $[p, v, \phi_g, m_h, \partial_z p, \partial_z v, \partial_z \phi_g, \partial_z m_h]$. If we want to write this as a matrix-vector equation, we can express it as $$ F(z, [\nu, \partial_z \nu]) = J(z, \nu) \frac{\partial \nu}{\partial z} + Q(z, \nu, \partial_z p) = 0, $$where $ J(z, \nu)$ is a matrix of size $(M,4)$ containing the coefficients for $\partial_z \nu$ in the $M$ governing equations. However, one issue is that in the momentum balance equation, $\partial_z p$ appears in the sinh function for the frictional velocity and is therefore not linear in $\partial_z p$, thus $Q_{mbe}$ does contain the $\partial_z p$ term. **Q for Ben**: can we just rewrite the equations in the form $F(z, \nu) = 0$, instead of $J(z, \nu) \frac{\partial \nu}{\partial z} + Q(\nu) = 0$? Since $Q(\nu)$ is so weird and contains $\partial_z p$. Seems like the loss only calculates $F$ anyway. Maybe it would help if you just write down what the loss calculation would be?Also, how to implement boundary conditions?**A for YQ**: yes! We can definitely do that. No tensorflow reason to do one or the other -- I just figured you would have written the equations in the $J$, etc. way in your matlab code. I've re-written the loss function to show how that's done.The tensorflow code will produce conduit variables and their associated derivatives of size (N x 1) (where $N$ is the batch size). From these variables it is easy to assemble the vector $\nu$.**What we still need:** a function that takes in these variables and gradients, and assembles the matrix $J$. Note that because of the batch dimension, $J$ will no longer be of size $(M,12)$, but should be of size $(N,M,12)$. We can then take this tensor and multiply it with $\nu$ to get the governing equations. Time-Dependent Governing EquationsThe time-dependent governing equations can be rewritten as $$H(t, z, [\nu, \partial_z \nu, \partial_t \nu]) = 0, $$where $H$ is a matrix of size $(M,1)$ governing equations and $[\nu, \partial_z \nu, \partial_t \nu]$ is now a 12-element vector containing the primary conduit variables and their gradients with respect to $z$, e.g. a vector $[p, v, \phi_g, m_h, \partial_z p, \partial_z v, \partial_z \phi_g, \partial_z m_h, \partial_t p, \partial_t v, \partial_t \phi_g, \partial_t m_h]$. We can actually reduce this by one because $ \partial_t v$ does not appear in the governing equations due to low Reynolds number. But wait. Will this result in velocities being constant over time? The assumption here is that $v$ depends on the phase fractions and other properties, not on the inertial term.If we want to write this as a matrix-vector equation, we can express it as $$ H(t, z, [\nu, \partial_z \nu, \partial_t \nu]) = M(t, z, \nu) \frac{\partial \nu}{\partial t} - J(t, z, \nu) \frac{\partial \nu}{\partial z} - Q(t, z, \nu, \partial_z p) = 0, $$ Some numpy manipulations to show how to do the math once we have $J$ and $\nu$
###Code
J = np.arange(5*3*4).reshape(5,3,4)
nu = np.arange(5*4).reshape(5,4)
result = np.zeros((5,3))
for i in range(5):
result[i,:] = J[i,:,:].dot(nu[i,:])
print(result)
# Faster (?) alternative
result2 = np.einsum('ijk,ik->ij',J,nu)
print(result2)
result == result2
# A trainable variable
x0 = tf.Variable(3.0, name='x0')
# Not trainable
x1 = tf.Variable(3.0, name='x1', trainable=False)
# Not a Variable: A variable + tensor returns a tensor.
x2 = tf.Variable(2.0, name='x2') + 1.0
# Not a variable
x3 = tf.constant(3.0, name='x3')
with tf.GradientTape() as tape:
tape.watch(x1)
x4 = 2*x1
y = (x0**2) + (x1**2) + (x2**2)
grad = tape.gradient(y, [x0, x1, x2, x3, x4])
for g in grad:
print(g)
###Output
tf.Tensor(6.0, shape=(), dtype=float32)
tf.Tensor(6.0, shape=(), dtype=float32)
None
None
None
|
Lab 8/Fashion.ipynb | ###Markdown
Image Classification by MLP - Fashion MNIST In this exercise, we will try to use a neural network on a simple classification task: classifying images of clothes into 10 classes. We will first download the data:
###Code
from tensorflow.keras.datasets import fashion_mnist
#TODO: load dataset
(X_train, y_train), (X_test, y_test) = fashion_mnist.load_data()
# #TODO: Resample the dataset if needed
# X_train = ...
# y_train = ...
# X_test = ...
# y_test = ...
X_train.shape
###Output
_____no_output_____
###Markdown
This dataset contains 10 classes:* 0: T-shirt/top* 1: Trouser* 2: Pullover* 3: Dress* 4: Coat* 5: Sandal* 6: Shirt* 7: Sneaker* 8: Bag* 9: Ankle boot Now begin by exploring the data. Try to display some images with the associated label.
###Code
# TODO: Explore the data, display some input images
%matplotlib inline
import matplotlib.pyplot as plt
import numpy as np
label_class = ['top', 'trouser', 'pullover', 'dress', 'coat', 'sandal', 'shirt', 'sneaker', 'bag', 'ankle boot']
#np.random.seed(0)
idx = np.random.randint(X_train.shape[0])
plt.imshow(X_train[idx], cmap="gray_r")
plt.title(label_class[y_train[idx]])
plt.show()
###Output
_____no_output_____
###Markdown
**Before going further**: what methods could you use to perform such a classification task? --- The first method you will try is using neural networks. First step is the data preparation: data rescaling, label preparation.Hint: you can use the Keras function `to_categorical`
###Code
# TODO: Make the data preparation
from tensorflow.keras.utils import to_categorical
y_train_cat = to_categorical(y_train)
y_test_cat = to_categorical(y_test)
X_train_norm = X_train/255
X_test_norm = X_test/255
# TODO: reshape the image data (2D array) into input 1D array for a neural network
X_train_norm = X_train_norm.reshape(X_train_norm.shape[0], np.prod(X_train_norm.shape[1:]))
X_test_norm = X_test_norm.reshape(X_test_norm.shape[0], np.prod(X_test_norm.shape[1:]))
print(X_test_norm[:5])
###Output
[[0. 0. 0. ... 0. 0. 0.]
[0. 0. 0. ... 0. 0. 0.]
[0. 0. 0. ... 0. 0. 0.]
[0. 0. 0. ... 0. 0. 0.]
[0. 0. 0. ... 0. 0. 0.]]
###Markdown
Next step: model building with Keras. Build your neural network architecture. At first, I would recommend a light architecture: no more than 2 hidden layers, with about 10 units per layer. Put that model into a function, so that you can reuse it later.
###Code
# TODO: Build your model
from tensorflow.keras import Input
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
def my_model(input_dim):
# Create the Sequential object
model = Sequential()
# Add 2 dense layers with 10 neurons each using sigmoid or relu activation
model.add(Input(shape=(784,)))
model.add(Dense(10, activation="sigmoid"))
model.add(Dense(10, activation="relu"))
# Add the output layer with one unit: the predicted result
model.add(Dense(10, activation='softmax'))
return model
###Output
_____no_output_____
###Markdown
Now compile and fit your model on your training data. Since this is a multiclass classification, the loss is not `binary_crossentropy` anymore, but `categorical_crossentropy`.
###Code
import os
#https://stackoverflow.com/questions/53014306/error-15-initializing-libiomp5-dylib-but-found-libiomp5-dylib-already-initial
# os.environ['KMP_DUPLICATE_LIB_OK']='True'
# TODO: Compile and fit your model
model = my_model(X_train_norm.shape[1])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
model.fit(X_train_norm, y_train_cat, epochs=100, batch_size=128)
###Output
Epoch 1/100
469/469 [==============================] - 3s 3ms/step - loss: 1.7149 - accuracy: 0.4454
Epoch 2/100
469/469 [==============================] - 1s 3ms/step - loss: 0.7380 - accuracy: 0.7617
Epoch 3/100
469/469 [==============================] - 1s 3ms/step - loss: 0.5531 - accuracy: 0.8099: 0s - loss: 0.5594 -
Epoch 4/100
469/469 [==============================] - 2s 3ms/step - loss: 0.4873 - accuracy: 0.8309
Epoch 5/100
469/469 [==============================] - 1s 3ms/step - loss: 0.4597 - accuracy: 0.8388
Epoch 6/100
469/469 [==============================] - 1s 3ms/step - loss: 0.4388 - accuracy: 0.8461
Epoch 7/100
469/469 [==============================] - 1s 3ms/step - loss: 0.4256 - accuracy: 0.8509
Epoch 8/100
469/469 [==============================] - 1s 3ms/step - loss: 0.4074 - accuracy: 0.8558
Epoch 9/100
469/469 [==============================] - 1s 3ms/step - loss: 0.4003 - accuracy: 0.8602
Epoch 10/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3901 - accuracy: 0.8620
Epoch 11/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3814 - accuracy: 0.8646
Epoch 12/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3787 - accuracy: 0.8666
Epoch 13/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3738 - accuracy: 0.8664
Epoch 14/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3740 - accuracy: 0.8663
Epoch 15/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3697 - accuracy: 0.8701
Epoch 16/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3651 - accuracy: 0.8699
Epoch 17/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3650 - accuracy: 0.8705
Epoch 18/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3600 - accuracy: 0.8721
Epoch 19/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3567 - accuracy: 0.8742
Epoch 20/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3551 - accuracy: 0.8742
Epoch 21/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3503 - accuracy: 0.8754
Epoch 22/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3478 - accuracy: 0.8757
Epoch 23/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3440 - accuracy: 0.8778
Epoch 24/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3457 - accuracy: 0.8757
Epoch 25/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3431 - accuracy: 0.8777
Epoch 26/100
469/469 [==============================] - 2s 4ms/step - loss: 0.3398 - accuracy: 0.8786
Epoch 27/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3425 - accuracy: 0.8773
Epoch 28/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3380 - accuracy: 0.8792
Epoch 29/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3374 - accuracy: 0.8796
Epoch 30/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3403 - accuracy: 0.8789
Epoch 31/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3309 - accuracy: 0.8815
Epoch 32/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3387 - accuracy: 0.8781
Epoch 33/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3334 - accuracy: 0.8814
Epoch 34/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3306 - accuracy: 0.8830
Epoch 35/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3262 - accuracy: 0.8834
Epoch 36/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3307 - accuracy: 0.8817
Epoch 37/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3311 - accuracy: 0.8826
Epoch 38/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3264 - accuracy: 0.8821
Epoch 39/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3332 - accuracy: 0.8794
Epoch 40/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3270 - accuracy: 0.8830
Epoch 41/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3284 - accuracy: 0.8829
Epoch 42/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3234 - accuracy: 0.8841
Epoch 43/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3241 - accuracy: 0.8836
Epoch 44/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3234 - accuracy: 0.8832
Epoch 45/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3302 - accuracy: 0.8826
Epoch 46/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3188 - accuracy: 0.8861
Epoch 47/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3234 - accuracy: 0.8842
Epoch 48/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3194 - accuracy: 0.8858
Epoch 49/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3173 - accuracy: 0.8857
Epoch 50/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3177 - accuracy: 0.8861
Epoch 51/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3105 - accuracy: 0.8876
Epoch 52/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3225 - accuracy: 0.8856
Epoch 53/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3208 - accuracy: 0.8848
Epoch 54/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3098 - accuracy: 0.8882
Epoch 55/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3108 - accuracy: 0.8886
Epoch 56/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3143 - accuracy: 0.8862
Epoch 57/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3113 - accuracy: 0.8898
Epoch 58/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3113 - accuracy: 0.8882
Epoch 59/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3130 - accuracy: 0.8861
Epoch 60/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3100 - accuracy: 0.8875
Epoch 61/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3102 - accuracy: 0.8896
Epoch 62/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3177 - accuracy: 0.8863
Epoch 63/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3105 - accuracy: 0.8882
Epoch 64/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3098 - accuracy: 0.8871
Epoch 65/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3026 - accuracy: 0.8917
Epoch 66/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3081 - accuracy: 0.8884
Epoch 67/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3069 - accuracy: 0.8902
Epoch 68/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3063 - accuracy: 0.8892: 0s - loss: 0.3063 - accuracy: 0.88
Epoch 69/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3071 - accuracy: 0.8886
Epoch 70/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3103 - accuracy: 0.8890
Epoch 71/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3063 - accuracy: 0.8918
Epoch 72/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3034 - accuracy: 0.8906
Epoch 73/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3028 - accuracy: 0.8897
Epoch 74/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3030 - accuracy: 0.8903
Epoch 75/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3042 - accuracy: 0.8902
Epoch 76/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3058 - accuracy: 0.8912
Epoch 77/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3026 - accuracy: 0.8919
Epoch 78/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3038 - accuracy: 0.8932
Epoch 79/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2983 - accuracy: 0.8931
Epoch 80/100
469/469 [==============================] - 2s 3ms/step - loss: 0.3049 - accuracy: 0.8899
Epoch 81/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2982 - accuracy: 0.8917
Epoch 82/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3008 - accuracy: 0.8934
Epoch 83/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2997 - accuracy: 0.8926
Epoch 84/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2977 - accuracy: 0.8921
Epoch 85/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3011 - accuracy: 0.8937
Epoch 86/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2950 - accuracy: 0.8953
Epoch 87/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2982 - accuracy: 0.8922
Epoch 88/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2983 - accuracy: 0.8921
Epoch 89/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3003 - accuracy: 0.8919
Epoch 90/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2934 - accuracy: 0.8933
Epoch 91/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2952 - accuracy: 0.8939
Epoch 92/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2960 - accuracy: 0.8940
Epoch 93/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2954 - accuracy: 0.8949
Epoch 94/100
469/469 [==============================] - 1s 3ms/step - loss: 0.3020 - accuracy: 0.8923
Epoch 95/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2926 - accuracy: 0.8949
Epoch 96/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2964 - accuracy: 0.8931
Epoch 97/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2968 - accuracy: 0.8937
Epoch 98/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2936 - accuracy: 0.8946
Epoch 99/100
469/469 [==============================] - 2s 3ms/step - loss: 0.2940 - accuracy: 0.8929
Epoch 100/100
469/469 [==============================] - 1s 3ms/step - loss: 0.2942 - accuracy: 0.8946
###Markdown
Once your model has been trained, compute the accuracy (and other metrics if you want) on the train and test dataset.Be careful, Keras returns softmax output (so an array of 10 values between 0 and 1, for which the sum is equal to 1). To compute correctly the accuracy, you have to convert that array into a categorical array with zeros and a 1.
###Code
# TODO: Compute the accuracy of your model
print('accuracy on train with NN:', model.evaluate(X_train_norm, y_train_cat, verbose=0)[1])
print('accuracy on test with NN:', model.evaluate(X_test_norm, y_test_cat, verbose=0)[1])
###Output
accuracy on train with NN: 0.8936333060264587
accuracy on test with NN: 0.8531000018119812
###Markdown
What do you think of those results? Can you improve it by changing the number of layers? Of units per layer? The number of epochs? The activation functions?You should try! --- In order to compare your results with more traditional machine learning methods, you will do this work with another method: a PCA followed by a classification model (of your choice). Of course, you can perform hyperparameter optimization using a gridsearch on that model!Fit your model and display the performances.
###Code
# TODO: Redo the classification with PCA and classification model
from sklearn.decomposition import PCA
pca = PCA(n_components=100)
pca.fit(X_train_norm)
X_train_pca = pca.transform(X_train_norm)
X_test_pca = pca.transform(X_test_norm)
# TODO: use any classifier you want
from sklearn.ensemble import RandomForestClassifier
rf = RandomForestClassifier()
rf.fit(X_train_pca, y_train)
print('score with RF on train', rf.score(X_train_pca, y_train))
print('score with RF on train', rf.score(X_test_pca, y_test))
###Output
score with RF on train 1.0
score with RF on train 0.8612
|
workshop_1/02-Chromosome_k-mers.ipynb | ###Markdown
Session 02 - Chromosome $k$-mers Learning Outcomes* Read and manipulate prokaryotic genome sequences using [Biopython](https://www.biopython.org).* Extract bulk genome properties from a genome sequence* Visualisation of bulk genome properties using Python Introduction $k$-mersEmpirical frequencies of DNA $k$-mers in whole genome sequences provide an interesting perspective on genomic complexity, and the availability of large segments of genomic sequence from many organisms means that analysis of $k$-mers with non-trivial lengths is now possible, as can be seen in [Chor *et al.* (2009) *Genome Biol.* **10**:R108](http://dx.doi.org/10.1186/gb-2009-10-10-r108).You will visualise the distribution of $k$-mer counts as spectra, as in the image above, using Python. Python codeWe will use the [`Biopython`](http://www.biopython.org) libraries to interact with and manipulate sequence data, and the [`Pandas`](http://pandas.pydata.org/) data analysis libraries to manipulate numerical data.Some code is imported from the local `bs32010` module in this directory, to avoid clutter in this notebook. You can inspect this module if you are interested.
###Code
%matplotlib inline
from Bio import SeqIO # For working with sequence data files
from Bio.Seq import Seq # Seq object, needed for the last activity
from Bio.Alphabet import generic_dna # sequence alphabet, for the last activity
from bs32010 import ex02 # Local functions and data
###Output
_____no_output_____
###Markdown
Sequence dataLike Session 01, we will be dealing with sequence data directly, but there are again helper functions for this exercise, in the module `ex02`.There is a dictionary stored in the variable `ex02.bact_files`. This provides a tuple of sequence file names, for any organism name in the list stored in `ex02.bacteria`.You can see the contents of this list and dictionary with```pythonprint(list(ex02.bacteria))print(ex02.bact_files)```
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
To choose a particular organism, you can use the square bracket notation for dictionaries:```pythonprint(ex02.bact_files['Mycobacterium tuberculosis'])```
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
1. Counting $k$-mersA function is provided in the `ex02` module to help you:* `count_seq_kmers(inseq, k)`: this counts all subsequences of size $k$ in the sequence `inseq`Test the function using the code below, which conducts the analysis for a *Pectobacterium* chromosome:```pythoninseq = SeqIO.read('genome_data/Pectobacterium/GCA_000769535.1.fasta', 'fasta')kmer_count = ex02.count_seq_kmers(inseq, 6)kmer_count.head()```The `Pandas` dataframe that is returned lets us use the `.head()` method to view the first few rows of the dataframe. This shows a column of six-character strings (the $k$-mers), with a second column showing the number of times that $k$-mer occurs in the genome.
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
We can also inspect the `.shape` attribute to find out how large the returned results are, as this returns a `(rows, columns)` tuple, using the code below:```pythonkmer_count.shape```This tells us that there are 4096 distinct 6-mers in the sequence.
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
2. Plotting $k$-mer spectraYou can use the built-in `.hist()` method of `Pandas` dataframes, that will plot a histogram directly in this notebook. By default, this has quite a wide bin width, but this can be overridden with the `bins=n` argument, as with:```pythonkmer_count.hist(column='frequency', bins=100)```By default, the `.hist()` method will display the full range of data, but by specifying maximum and minimum values with the `range=(min, max)` argument, the extent of data displayed can be controlled.Use the code below to visualise the 6-mer spectrum```pythonkmer_count.hist(column='frequency', bins=100, range=(0, 1000))```
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
*** Exercise 1 (10min): Recreate the plot in the upper left corner of the figure in the introduction, for one of the *E. coli* genomes. **** **HINT:** Use `print(ex02.bact_files['Escherichia coli'])` to get a list of *E.coli* chromosome files.
###Code
# Enter code here
###Output
_____no_output_____
###Markdown
*** Exercise 2 (5min): The *E.coli* spectrum is unimodal, but how many modes does the Platypus chromosome 01 have? **** The platypus chromosome 01 file can be found in the file `genome_data/Platypus/oan_ref_Ornithorhynchus_anatinus_5.0.1_chr1.fa`
###Code
# Enter code here
###Output
_____no_output_____ |
data_processing/wind_power.ipynb | ###Markdown
Generate Synthetic Time Histories for Wind Power Generation Background: The University of Illinois has a power purchase agreement with Rail Splitter Wind Farm in Lincoln, IL. * The wind farm has a capacity of 100.5 MW from 67 GE 1.5 MW wind turbines.* UIUC buys 8.6% of power generated at 4 cents/kWh or $\$40$/MWh* The agreement is for 10 years (2016-2026). * Info about the specific wind turbines is available here: https://en.wind-turbine-models.com/turbines/655-general-electric-ge-1.5sle About the dataThe data used in this analysis was obtained from a subdivision of the National Oceanic and Atmospheric Administration (NOAA), National Centers for Environmental Information (NECI). The data was collected by a land-based weather station located at the Lincoln Illinois Airport for the years of 2010-2019. https://www.ncdc.noaa.gov/data-accessThe data is stored on Box rather than locally.
###Code
# Import necessary libraries
import pandas as pd
import matplotlib.pyplot as plt
import numpy as np
from data_funcs import to_float
import platform
###Output
_____no_output_____
###Markdown
Set up the Box API to access dataThese cells with Box API commands have been converted to raw text so they won't accidentally be run. To convert back to runnable cells: ``enter`` (enters command mode)``y`` (converts selected cell to code)
###Code
%%capture
!pip install boxsdk
# %%capture captures the output from pip install... comment out if there are installation issues.
from boxsdk import Client, OAuth2
# Defines client ID, secrete, and developer token
CLIENT_ID = 'ey8yzp3yxanb3jjujb2s95c1bnouz2o8'
CLIENT_SECRET = 'VGGJjHGWfBm0MHq1xIqncxZDolelVIDP'
ACCESS_TOKEN = 'RDQLBOw7M8wah5JxZk8Yxm6VLmsjyNM9' # only valid for 60 minutes...?
# Creates the client instance
oauth2 = OAuth2(CLIENT_ID, CLIENT_SECRET, access_token=ACCESS_TOKEN)
client = Client(oauth2)
# Folder IDs
gridload_id = 92229909420
solarfarm_id = 92974470362
solarmodel_id = 92182116657
steam_id = 92620146668
weather_id = 90175362988
weather_folder = client.folder(folder_id=weather_id).get_items()
for data in weather_folder:
print('{0} {1} is named "{2}"'.format(data.type.capitalize(), data.id, data.name))
###Output
_____no_output_____
###Markdown
A better way to obtain the data...? Since there is a specific file in Box that I am manipulating here, why not try using ``$curl`` with a shared link?First attempt: nothing downloaded.Solution: add ``-L`` flag to force the download by following redirects.This downloads the links... not the data. Not sure how to resolve at this point. It takes about 30 seconds to obtain a new developer key from box. But I want to be able to run this notebook without that (open source, right?) and I'm not sure how at this point (12/18/2019).
###Code
!rm -rf lincoln_weather.csv
# where the data will be downloaded
path = './lincoln_weather.csv'
# downloads the data
!curl -X GET https://uofi.box.com/s/3b4498ua7fziof4ex3fu75zfcvio0h1l --output lincoln_weather.csv \
-H "Authorization: BoxAuth api_key=API_KEY&auth_token=AUTH_TOKEN"
# read data as pandas dataframe
import pandas as pd
lincoln_wind = pd.read_csv(path, usecols=['HourlyWindSpeed', 'HourlyDryBulbTemperature',
'HourlyStationPressure','HourlyRelativeHumidity',
'DATE'])
# Removes the file from the local directory
!rm -rf lincoln_weather.csv
# convert to runnable cell by going to command mode --> y
# Gets the file with the data
lincoln_file = client.file(file_id=578216725033)
# Import to a pandas dataframe
import pandas as pd
path = './lincoln_weather.csv'
with open(path, 'wb') as file:
lincoln_file.download_to(file)
lincoln_wind = pd.read_csv(path, usecols=['HourlyWindSpeed', 'HourlyDryBulbTemperature',
'HourlyStationPressure','HourlyRelativeHumidity',
'DATE'])
# Removes the file from the local directory
!rm -rf lincoln_weather.csv
# when I'm using a windows computer
# !del lincoln_weather.csv
###Output
_____no_output_____
###Markdown
Simplest way to obtain data... download to local computer.
###Code
path = '../../data/weather_data/'
lincoln_wind = pd.read_csv(path+'lincoln_weather.csv', usecols=['HourlyWindSpeed', 'HourlyDryBulbTemperature',
'HourlyStationPressure','HourlyRelativeHumidity',
'DATE'])
# convert to datetime
lincoln_wind.DATE = pd.to_datetime(lincoln_wind.DATE)
# rename columns
lincoln_wind.rename(columns={'DATE':'time',
'HourlyDryBulbTemperature': 'temp',
'HourlyRelativeHumidity':'RH',
'HourlyStationPressure':'pressure',
'HourlyWindSpeed': 'u'}, inplace=True)
# Verify that the dataframe has float values
lincoln_wind = to_float(lincoln_wind, 'temp')
lincoln_wind = to_float(lincoln_wind, 'u')
lincoln_wind = to_float(lincoln_wind, 'RH')
lincoln_wind = to_float(lincoln_wind, 'pressure')
lincoln_wind.index = pd.to_datetime(lincoln_wind.time)
# check if there are NaN values
print("The data frame is missing values:", lincoln_wind.isnull().values.any())
# interpolate
lincoln_wind = lincoln_wind.interpolate(method='linear')
# check if there are NaN values
print("The data frame is missing values:", lincoln_wind.isnull().values.any())
# resample by hour to get single measurements for each hour
lincoln_wind = lincoln_wind.resample('H').mean()
###Output
_____no_output_____
###Markdown
Download the cleaned data only
###Code
lincoln_wind.to_csv(path+"lincoln_weather_clean.csv")
###Output
_____no_output_____
###Markdown
Calculate the Power to CampusThis analysis will use the following model from >H. E. Garcia et al., “Nuclear Hybrid Energy Systems Regional Studies: West Texas & Northeastern Arizona,” Idaho National Lab. (INL), Idaho Falls, ID (United States), INL/EXT-15-34503, Apr. 2015. Operating Regimes* Above the cut-out and below the cut-in speeds, the turbines produce no power. * Between the rated speed and the cut-out speeds the turbines produce a constant rated power.* Between the cut-in speed and the rated speeds the turbines produce power given by $P = 0.5\eta\rho U_h^3\frac{\pi d^2}{4} [\text{W}_e]$_(in Baker et. al they claim this formula gives the value in MW, this appears to be untrue)_where * $\eta$ is the conversion efficiency (not given for these turbines, so we assume 35%)* $\rho$ is the density of air at the location (based on temperature)* $d$ is the diameter of the turbine (2 x blade length). The density of air, $\rho$, can be calculated with the following: $\rho = \frac{P_{dry}}{R_{dry}T} + \frac{P_{vapor}}{R_{vapor}T}$The vapor pressure $P_{vapor}$ can be calculated using the Clausius-Clapeyron Equation which gives the saturation vapor pressure over water.$P_{vapor} = e_s * RH$$e_s = 0.6112e^{\frac{17.67T}{T+243.5}} [\text{kPa}]$>Iribarne, J. V., and W. L. Godson 1981. Atmospheric Thermodynamics. D. Reidel, . p. 65.* $T$ : Temperature in $^\circ C$* $R_{dry}$ : 287.058 J/(kg·K)* $R_{vapor}$ : 461.495 J/(kg·K)* $RH$ : Relative Humidity Solving the Units ProblemThe model given by Baker et. al is supposedly in MWe. However the results from this do not produce values similar to the values stated by Rail Splitter. Rail Splitter's Claim:>In November 2016, the campus received nearly 2400 MWh based on actual wind farm speeds.Let's nail this down by isolating the wind speed data from November 2016The model proposed by Garcia et. al corresponds the kinetic energy of wind to power produced. Start with 1. $P = \frac{1}{2}\dot{m}U^2$ with units of $[W] = \frac{[J]}{[s]} = \frac{[N][m]}{[s]} = \frac{[kg][m]^2}{[s^3]}$The mass flux through the wind turbines is given by: 2. $\dot{m} = \rho A_f U$ with units of $\frac{[kg]}{[m^3]}[m^2]\frac{[m]}{[s]} = \frac{[kg]}{[s]}$Plug (2) into (1) 3. $P = \frac{1}{2} (\rho A_f U)U^2$In order to obtain the model from Garcia et. al we must multiply by a conversion factor, $\eta$ because we cannot use 100% of the energy stored in wind. We can also replace the flow area, $A_f$, by $\frac{\pi d^2}{4}$ because that is the cross section area of the turbine. Finally, 4. $P = \frac{1}{2}\eta\rho \frac{\pi d^2}{4}U^3$ Solving the mismatch between Rail Splitter's claim and the data from Lincoln AirportAt the moment (12/22/19) the largest value for MW from the real data I curated was ~660 MWe. This is in stark contrast to Rail Splitter Wind Farm's stated 2400 MWe in November 2016. It is highly unlikely that there is a criminal conspiracy here so there must be something wrong with either: 1. How I'm calculating power2. The wind speeds I am usingInitially, I thought that the error must lie with how I was calculating power. After going through the quick derivation above, I am confident my calculations are correct. Thus there must be something wrong with the data. After some thought, there is actually nothing _wrong_ with the data, but it is likely that the wind speed measurements were taken somewhere around 10m above the ground, while the hub height of the wind turbines is around 60m. Wind speeds can be significantly greater at higher altitudes. After much digging, I found a way to extrapolate the wind speed to higher altitudes using a ''log law'' from J. F. Manwell, J. G. McGowan, and A. L. Rogers, Wind Energy Explained: Theory, Design and Application, 2nd ed. Wiley, 2010.and criteria for roughness lengths from the European Wind Atlas, I. Troen and E. L. Petersen, European Wind Atlas. Commission of the European Communities Directorate-General for Science, 1989.The formula is$v \approx v_{ref}\frac{\ln\left(\frac{z}{z_0}\right)}{\ln\left(\frac{z_{ref}}{z_0}\right)}$where* $z_{ref}$ is the reference height, in our case we are _assuming_ 10m.* $v_{ref}$ is the wind speed at the reference height.* $z_0$ is the roughness length for a certain terrain type as outlined in the Europen Wind Atlas (EWA).* $z$ is the height of interest. In this case we are interested in 50-60m. Because the wind speed measurements were taken at an airport, we will start by using $z_0 = 0.0024$ from EWA.
###Code
# convert windspeed to m/s from mph
to_ms = lambda u: u/2.237
lincoln_wind['u'] = lincoln_wind['u'].apply(to_ms)
# extrapolate windspeeds to a height of 50m
# z0 = 0.0024 # [m]
z0 = 0.165 # changing the roughness class to see if it improves the data
z = 80 # [m] increased the height from 50 --> 80, 50 gave 365 MW
zref = 1 # [m] lowered the ref height from 10 --> 5 to improve the difference...
speed_extrap = lambda v: v*(np.log(z/z0)/np.log(zref/z0))
lincoln_wind['u_ex'] = lincoln_wind['u'].apply(speed_extrap)
lincoln_wind.head(5)
# lincoln_wind[lincoln_wind['u'] >= 11.5]
# convert the temperature to Celsius
to_celsius = lambda T : (T-32)*5/9
lincoln_wind['temp'] = lincoln_wind['temp'].apply(to_celsius)
# lincoln_wind
# convert pressure to Pascals
to_pa = lambda P: P*3386.38867
lincoln_wind['pressure'] = lincoln_wind['pressure'].apply(to_pa)
# lincoln_wind
# calculate air density in g/m^3 --> wrong units.
# 12/20/19: Divide by 1000 to get kg/m^3
# 12/20/19: Edit 2, the density was already in kg/m^3.
# define constants
Rv = 461.495
Rd = 287.058
# define functions
es = lambda T: 611.2*np.exp(17.67*T/(T+243.5)) # saturation vapor pressure over water
rho = lambda T, P, Rh, Pv: (es(T)*(Rh/100))/(Rv*(T+273.15))+(P-(es(T)*(Rh/100)))/(Rd*(T+273.15)) # density of air
density = {'rho':[]}
for i in range(len(lincoln_wind)):
T = lincoln_wind.iloc[i]['temp']
P = lincoln_wind.iloc[i]['pressure']
Rh = lincoln_wind.iloc[i]['RH']
Pv = es(T)
density['rho'].append(rho(T, P, Rh, Pv))
lincoln_wind['rho'] = density['rho']
# lincoln_wind
# calculate the power generated
# define constants
N = 67 # the number of wind turbines
d = 77 # [m]
r = d/2 # [m]
u_in = 3.5 # [m/s]
u_out = 25.0 # [m/s]
u_ideal = 12 # [m/s]
eta = 0.31 # efficiency
ppa = 8.6/100 # the percentage of purchased power.
pmax = 1.5 # [MW], the rated power for a single turbine
P = lambda U, rho: N*ppa*(0.5*eta*rho*(U**3)*(np.pi*r**2))/1e6 # this is in Watts, dividing by 1e6 gives MW
# calculate all values of power
power = []
for i in range(len(lincoln_wind)):
U = lincoln_wind.iloc[i]['u_ex']
rho = lincoln_wind.iloc[i]['rho']
power.append(P(U, rho))
lincoln_wind['power'] = power
# fix based on cut in and cut out speeds
zero = (lincoln_wind.u_ex <= u_in) | (lincoln_wind.u_ex >= u_out)
peak = (lincoln_wind.u_ex > u_ideal) & (lincoln_wind.u_ex < u_out)
lincoln_wind['power'].mask(zero, 0, inplace=True)
lincoln_wind['power'].mask(peak, N*ppa*pmax, inplace=True)
fig = plt.figure(figsize=(12,9), edgecolor='k', facecolor='w')
plt.scatter(lincoln_wind['u_ex'], lincoln_wind['power'], lw=0.25, label='calculated power')
plt.axvline(x=12,color='r', linestyle='--', label='ideal speed : 12 m/s')
plt.xlabel('wind speed (u) [m/s]')
plt.ylabel('power [MW]')
plt.legend()
plt.title("Power generated as a function of extrapolated windspeed")
plt.show()
over_max = lincoln_wind['power'] > N*ppa*pmax
lincoln_wind['power'].mask(over_max, N*ppa*pmax, inplace=True)
fig = plt.figure(figsize=(12,9), edgecolor='k', facecolor='w')
plt.scatter(lincoln_wind['u_ex'], lincoln_wind['power'], lw=0.25, label='calculated power')
plt.axvline(x=12,color='r', linestyle='--', label='ideal speed : 12 m/s')
plt.xlabel('wind speed (u) [m/s]')
plt.ylabel('power [MW]')
plt.legend()
plt.title("Power generated as a function of extrapolated windspeed")
plt.show()
years = lincoln_wind.groupby(lincoln_wind.index.year)
data2016 = years.get_group(2016)
months = data2016.groupby(data2016.index.month)
november16 = months.get_group(11)
# november16
november16['power'].sum()
november16.plot(kind='scatter', x='u', y='power', lw=0.25)
plt.show()
###Output
_____no_output_____
###Markdown
The ''solution'' to the wind speed problemI found a helpful method to extrapolate wind speed to different heights based on a measured velocity and a terrain roughness measure (roughness length). I found that a* $Z_0 = 0.165$ meters* $Z_{ref} = 1$ meter* $Z = 80$ metersgives a reasonable sounding value for the power produced in November 2016 (~2400 MWe). Comparable to the number given by data from Rail Splitter (I am not sure who made the calculation, if it was Rail Splitter or F&S). This ''solution'' has obvious problems. 1. It's an approximation2. The roughness length of the measurement site and the wind farm are unknown (and thus a ''best guess''). 3. A reference height of one meter is almost totally unrealistic, but that quantity has the largest impact on the speed calculation thus it can't be increased much. It is necessary to strike a balance between the reference height and the roughness length. Step 2: Group by year
###Code
# check if there are NaN values
print("The data frame is missing values:", lincoln_wind.isnull().values.any())
# interpolate
lincoln_wind = lincoln_wind.interpolate(method='linear')
# check if there are NaN values
print("The data frame is missing values:", lincoln_wind.isnull().values.any())
# lincoln_w['time'] = lincoln_wind.index
# leap years drop the last day. Each year needs to be the same length.
grouped = lincoln_wind.groupby(lincoln_wind.index.year)
df11 = grouped.get_group(2011)
df12 = grouped.get_group(2012)[:8760]
df13 = grouped.get_group(2013)
df14 = grouped.get_group(2014)
df15 = grouped.get_group(2015)
df16 = grouped.get_group(2016)[:8760]
df17 = grouped.get_group(2017)
df18 = grouped.get_group(2018)
# create a column of hours
df11 = df11.reset_index(drop=True)
df12 = df12.reset_index(drop=True)
df13 = df13.reset_index(drop=True)
df14 = df14.reset_index(drop=True)
df15 = df15.reset_index(drop=True)
df16 = df16.reset_index(drop=True)
df17 = df17.reset_index(drop=True)
df18 = df18.reset_index(drop=True)
df11['time'] = df11.index
df12['time'] = df12.index
df13['time'] = df13.index
df14['time'] = df14.index
df15['time'] = df15.index
df16['time'] = df16.index
df17['time'] = df17.index
df18['time'] = df18.index
df11 = df11[['time','power','u_ex']]
df12 = df12[['time','power','u_ex']]
df13 = df13[['time','power','u_ex']]
df14 = df14[['time','power','u_ex']]
df15 = df15[['time','power','u_ex']]
df16 = df16[['time','power','u_ex']]
df17 = df17[['time','power','u_ex']]
df18 = df18[['time','power','u_ex']]
# converts from MW to kW
df11['power']=df11['power']*1000
df12['power']=df12['power']*1000
df13['power']=df13['power']*1000
df14['power']=df14['power']*1000
df15['power']=df15['power']*1000
df16['power']=df16['power']*1000
df17['power']=df17['power']*1000
df18['power']=df18['power']*1000
# =============================================
# writes the header file for RAVEN
fname = 'annual_wind201'
wind_keys = [fname + str(i) + '.csv' for i in range(1,9,1)]
header_file = pd.DataFrame({'scaling':np.zeros(len(wind_keys), dtype=np.int8), 'filename':wind_keys})
header_file.to_csv(path+'annual_wind_H.csv')
# header_file
# save the data frames as files that can be passed to RAVEN.
df11.to_csv(path+'annual_wind2011.csv')
df12.to_csv(path+'annual_wind2012.csv')
df13.to_csv(path+'annual_wind2013.csv')
df14.to_csv(path+'annual_wind2014.csv')
df15.to_csv(path+'annual_wind2015.csv')
df16.to_csv(path+'annual_wind2016.csv')
df17.to_csv(path+'annual_wind2017.csv')
df18.to_csv(path+'annual_wind2018.csv')
###Output
_____no_output_____
###Markdown
Pass the data to RAVENRAVEN will return a typical year of wind power production.
###Code
if platform.system() == 'Windows':
!git-bash %userprofile%/research/raven/raven_framework %userprofile%/research/pride/inputfiles/typical_wind.xml
else:
!~/research/raven/raven_framework ~/research/pride/inputfiles/typical_wind.xml
#upload the typical annual wind generation
path="../../data/"
typical_wind = pd.read_csv(path+"TypicalWind_0.csv")
typical_wind['date'] = pd.date_range(start='1/1/2018', end='1/1/2019', freq='H')[:8760]
import matplotlib.dates as mdates
# Set the locator
locator = mdates.MonthLocator() # every month
# Specify the format - %b gives us Jan, Feb...
fmt = mdates.DateFormatter('%b')
fig = plt.figure(figsize=(12,9), facecolor='w', edgecolor='k')
plt.plot(typical_wind['date'], typical_wind['power'])
plt.xlabel('Date',fontsize=24)
plt.ylabel('Power [kW]',fontsize=24)
plt.title('Typical Wind Power Received by UIUC',fontsize=32)
plt.ylim(top=12000)
X = plt.gca().xaxis
X.set_major_locator(locator)
# Specify formatter
X.set_major_formatter(fmt)
plt.show()
# let's calculate the annual power generated
power_gen = typical_wind['power'].sum()
power_gen
###Output
_____no_output_____
###Markdown
We will get to the bottom of this, let's look at the values predicted by Rail Splitter in the PPA
###Code
# from Rail Splitter Wind Farm
max_power = 100.5 #[MW]
#if 100% production
max_produced = max_power*8760
print(max_produced)
#UIUC gets 8.6% of produced power
uiuc_received = max_produced*0.086
print(uiuc_received)
###Output
880380.0
75712.68
###Markdown
When the analysis is finished we can safely delete the built files from the local directory
###Code
lincoln_wind
import datetime
fig,ax = plt.subplots(1,1,figsize=(12,9), edgecolor='k', facecolor='w')
ax.plot(lincoln_wind.index, lincoln_wind['u_ex'], lw=0.25, label='extrapolated windspeed')
ax.set_xlabel('Date')
ax.set_ylabel('wind speed [m/s]')
ax.legend()
ax.set_title("Wind Speed at 80m for 2011-2018")
ax.set_ylim(0,60)
ax.set_xlim(datetime.date(2011,1,1), datetime.date(2019,12,1))
plt.show()
lincoln_wind['u_ex'].mean()
###Output
_____no_output_____
###Markdown
The wind speed and power is much improved, but it's still not quite rightAccording to this grouphttps://web.stanford.edu/group/efmh/winds/global_winds.htmlCentral Illinois had an average wind speed at 80m of _at most_ 7.5 m/s (class 3). This calculation puts Illinois firmly 3 classes higher (it's windy but not that windy). I am proceeding with these calculations because they are the best I have with the available data.
###Code
typical_wind.power.mean()
if platform.system() == 'Windows':
!git-bash %userprofile%/research/raven/raven_framework %userprofile%/research/pride/inputfiles/wind_arma.xml
else:
!~/research/raven/raven_framework ~/research/pride/inputfiles/wind_arma.xml
synthetic_history = pd.read_csv(path+"windHistories_2.csv")
synthetic_history.keys()
# enforce max power
over_max = synthetic_history.power > N*ppa*pmax*1000
# lincoln_wind['power'].mask(over_max, N*ppa*pmax, inplace=True)
# peak = (synthetic_history.u_ex > u_ideal) & (synthetic_history.u_ex < u_out)
synthetic_history.power.mask(over_max, N*ppa*pmax, inplace=True)
N*ppa*pmax*1000
# plot synthetic history
fig,ax = plt.subplots(1,1,figsize=(12,9), edgecolor='k', facecolor='w')
ax.plot(synthetic_history.time, synthetic_history.power,
color='tab:red',lw = 0.5, label='Synthetic Wind')
ax.plot(typical_wind['time'], typical_wind['power'],
color='tab:blue', lw=0.5, label='Typical Wind')
ax.set_ylabel("Wind Power [kWh]", fontsize=18)
ax.set_xlabel("Time (hours)", fontsize=18)
ax.set_ylim(0,10000)
ax.set_xlim(0,8760)
ax.legend(fontsize=14, fancybox=True, shadow=True)
ax.set_title("Synthetic and Typical Wind Power Histories", fontsize=24)
synthetic_history.power.mean()
###Output
_____no_output_____ |
examples/money_creation/Ex4_Crises.ipynb | ###Markdown
[Money Creation Examples](http://www.siebenbrunner.com/moneycreation/) > **Example 4**: Banking crisesIn this example we revisit a situation from [Example 3](http://www.siebenbrunner.com/moneycreation/Ex3_Refinancing.html) where a a bank was holding a loan to an insolvent borrower. We now investigate what happens when the bank recognizes this loss.We start by importing required utilities:
###Code
import os
import sys
base_path = os.path.realpath(os.getcwd()+"/../..")
sys.path.append(base_path)
from abcFinance import Ledger, Account, AccountSide
###Output
_____no_output_____
###Markdown
Declaration of agentsThe set of agents is similar to the set in [Example 3](http://www.siebenbrunner.com/moneycreation/Ex3_Refinancing.html). We omit the central bank because it will not make any booking statements in this example.
###Code
bank1 = Ledger(residual_account_name="Equity")
bank2 = Ledger(residual_account_name="Equity")
bank3 = Ledger(residual_account_name="Equity")
private_agentA = Ledger(residual_account_name="Equity")
private_agentB = Ledger(residual_account_name="Equity")
bank1.make_asset_accounts(['Loans'])
bank1.make_liability_accounts(['Interbank Deposits'])
bank1.make_flow_accounts(['Impairments'])
bank2.make_asset_accounts(['Reserves','Interbank Loans'])
bank2.make_liability_accounts(['Deposits'])
bank2.make_flow_accounts(['Impairments','Expenses'])
bank3.make_asset_accounts(['Reserves'])
bank3.make_flow_accounts(['Income'])
private_agentA.make_asset_accounts(['Deposits'])
private_agentA.make_liability_accounts(['Loans'])
private_agentB.make_asset_accounts(['Deposits'])
private_agentB.make_liability_accounts(['Loans'])
private_agentB.make_flow_accounts(['Impairments'])
###Output
_____no_output_____
###Markdown
We further define a function that computes the money stocks according to our defined taxonomy:
###Code
from IPython.core.display import SVG
from IPython.display import display_svg
def print_money_stocks():
# Bank money: bank liabilities that are money
bank_money = private_agentB.get_balance('Deposits')[1]
print("Total (Bank) Money:",bank_money)
def print_balance_sheets_and_money_stocks(*args):
bank1.book_end_of_period()
bank2.book_end_of_period()
bank3.book_end_of_period()
if len(args)==0:
args = ("b1","b2","b3","pA","pB")
if "b1" in args and bank1.get_total_assets() > 0: display_svg(SVG(bank1.draw_balance_sheet("Bank 1 Balance Sheet", width=400)))
if "b2" in args and bank2.get_total_assets() > 0: display_svg(SVG(bank2.draw_balance_sheet("Bank 2 Balance Sheet", width=400)))
if "b3" in args and bank3.get_total_assets() > 0: display_svg(SVG(bank3.draw_balance_sheet("Bank 3 Balance Sheet", width=400)))
if "pA" in args and private_agentA.get_total_assets() > 0: display_svg(SVG(private_agentA.draw_balance_sheet("Private Agent A Balance Sheet", width=400)))
if "pB" in args and private_agentB.get_total_assets() > 0: display_svg(SVG(private_agentB.draw_balance_sheet("Private Agent B Balance Sheet", width=400)))
print_money_stocks()
###Output
_____no_output_____
###Markdown
InitializationWe start by recreating exactly the situation that occured at the end of the "Refinancing deposit outflows on the interbank market" section of [Example 3](http://www.siebenbrunner.com/moneycreation/Ex3_Refinancing.html):
###Code
bank1.book(debit=[('Loans',100)],credit=[('Equity',50),('Interbank Deposits',50)])
private_agentA.book(debit=[('Equity',100)],credit=[('Loans',100)])
bank2.book(debit=[('Reserves',200),('Interbank Loans',50)],credit=[('Equity',50),('Deposits',200)])
private_agentB.book(debit=[('Deposits',200)],credit=[('Equity',200)])
print_balance_sheets_and_money_stocks()
###Output
_____no_output_____
###Markdown
Bank defaultsAs already noted in [Example 3](http://www.siebenbrunner.com/moneycreation/Ex3_Refinancing.html), Bank 1 has a problem in this situation: it has a loan to a borrower who is completely insolvent. While we noted there that it usually takes some time before such losses are recognized and proceeded with the example, in this example we will consider the situation that arises when Bank 1 does indeed recognize this loss before the next transaction occurs. Note that for simplicity in this step we skip the step of creating a provision on the balance sheet and directly write off the loan on the balance sheet. This simplifies the presentation and has no impact on the results.
###Code
bank1.book(debit=[('Impairments',100)],credit=[('Loans',100)])
print_balance_sheets_and_money_stocks("b1")
###Output
_____no_output_____
###Markdown
Bank 1 is now insolvent and unable to repay its loan to Bank 2. Bank 2 makes an impairment in the same fashion:
###Code
bank2.book(debit=[('Impairments',50)],credit=[('Interbank Loans',50)])
print_balance_sheets_and_money_stocks("b2")
###Output
_____no_output_____
###Markdown
Bank 2 is now at the brink of insolvency. For the sake of the example we assume that it now faces a further loss, in the form of a payment that it has to make to Bank 3:
###Code
bank2.book(debit=[('Expenses',200)],credit=[('Reserves',200)])
bank3.book(debit=[('Reserves',200)],credit=[('Income',200)])
print_balance_sheets_and_money_stocks("b2","b3")
###Output
_____no_output_____
###Markdown
Now private agent B is faced with the situation of holding a deposit at a bank that has no more assets. In reality, other mechanisms like a deposit insurance, a government bail-out or bank resolution tools would step in at this point. We only note that in the absence of such mechanisms, the depositor would have to write down its savings, wiping out its entire balance sheet in this case, and destroying the money stock.
###Code
private_agentB.book(debit=[('Impairments',200)],credit=[('Deposits',200)])
print_balance_sheets_and_money_stocks("pB")
###Output
Total (Bank) Money: 0
|
demo-clase-2.ipynb | ###Markdown
Gráficos básicosEn esta clase vamos a aprender a hacer unos gráficos básicos con Altair. En el proceso vamos a aprender un poco mas sobre los componentes de un gráfico y como podríamos extender estos gráficos básicos a algo un tanto más complejo. **Información importante*** Documentación oficial de Altair: https://altair-viz.github.io/index.html* Espacio de trabajo de Notion de la clase: https://bit.ly/tacosdedatos-notion* Donde encontrar este _binder_: https://bit.ly.com/dataviz-demo Por explorar hoy* La manera de codificar (_encoding_) datos en Altair* Objetos en Altair (ejes, escalas, etc)* Configuraciones
###Code
import altair as alt
import pandas as pd
from vega_datasets import data as vega_data
data = vega_data.gapminder()
###Output
_____no_output_____ |
Mikala/ML_FINAL2.ipynb | ###Markdown
Feature Engineering
###Code
# Converting Date type from object to datetime
df['Date'] = pd.to_datetime(df['Date'], format='%d-%b-%y')
# creating variables from month data
df["Month"]=df["Date"].map(lambda x: x.month)
df["Months_high"]=df["Month"].map(lambda x: 1 if x>7 & x<12 else 0)
# 0 - Returner/ 1 - New_Access/ 2 - Other
dict_visitor = {'Returner': 0, 'New_Access': 1, 'Other': 0}
df['Type_of_Visitor_new'] = df['Type_of_Visitor'].map(dict_visitor)
dict_visitor = {'Returner': 1, 'New_Access': 0, 'Other': 0}
df['Type_of_Visitor_return'] = df['Type_of_Visitor'].map(dict_visitor)
###Output
_____no_output_____
###Markdown
Train Test Split
###Code
# .69 score on kaggle
# df_log3 = df[["Months_high","Type_of_Visitor_new",'GoogleAnalytics_ExitRate', 'GoogleAnalytics_PageValue',"Buy"]]
# undersampling of length 4.
# exit rate and page value both power transformed
# with outlier threshold of 350 only for page value
df_model = df[["Months_high","Type_of_Visitor_new",'GoogleAnalytics_ExitRate', 'GoogleAnalytics_PageValue',"Buy"]]
X = df_model.drop('Buy', axis=1)
y = df_model['Buy']
# split the dataset
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.25, random_state=5, stratify=y)
###Output
_____no_output_____
###Markdown
Balance the training set - undersampling
###Code
# Before undersampling
y_train.value_counts()
#joining y_train and x_train
under_df = pd.concat([X_train,y_train],axis=1)
#we randomly undersample our negative data until negatives our buy rate is 20%
negative = under_df.loc[under_df.Buy==0]
positive = under_df.loc[under_df.Buy==1]
# shuffling the negative data
negative = negative.sample(frac=1, random_state=0)
# selecting 4 times the length of positive data
negative_under = negative[:int(4*len(positive))]
# concatinating dataset
df_under = pd.concat([positive,negative_under],axis=0)
#shuffling
df_under = df_under.sample(frac=1, random_state=0)
# After undersampling
X_train_under = df_under.drop('Buy', axis=1)
y_train_under = df_under['Buy']
y_train_under.value_counts()
###Output
_____no_output_____
###Markdown
Variable power transformations after train_test_split
###Code
# Define metric and non-metric features
metric_features = ['GoogleAnalytics_ExitRate', 'GoogleAnalytics_PageValue']
non_metric_features = X.columns.drop(metric_features).to_list()
#separate numeric and non-numeric
X_train_num = X_train_under[metric_features]
X_train_cat = X_train_under[non_metric_features]
# DO IT for validation
X_val_num = X_val[metric_features]
X_val_cat = X_val[non_metric_features]
#use train to power transform train
power = PowerTransformer().fit(X_train_num)
X_train_num_power = power.transform(X_train_num)
X_train_num_power = pd.DataFrame(X_train_num_power, columns = X_train_num.columns).set_index(X_train_num.index)
#and for validation (using train data)
X_val_num_power = power.transform(X_val_num)
# Convert the array to a pandas dataframe
X_val_num_power = pd.DataFrame(X_val_num_power, columns = X_val_num.columns).set_index(X_val_num.index)
X_val_num_power.head(3)
X_train_power = pd.concat([X_train_num_power, X_train_cat], axis=1)
X_val_power = pd.concat([X_val_num_power, X_val_cat], axis=1)
###Output
_____no_output_____
###Markdown
final model
###Code
# model with 25% test set, undersampling of length 4, and 4 variables
model_log = LogisticRegression(class_weight='balanced',dual=False,
fit_intercept=True,penalty='l2',solver='sag',tol=.01).fit(X_train_power, y_train_under)
y_pred_train = model_log.predict(X_train_power)
y_pred_val = model_log.predict(X_val_power)
model_log.coef_
#low precision can be improved by increasing your threshold, making your model less sensitive
y_pred_val_new_threshold = (model_log.predict_proba(X_val_power)[:,1]>=0.7).astype(int)
print('f1_train:', f1_score(y_train_under, y_pred_train))
print(confusion_matrix(y_val, y_pred_val_new_threshold))
print('precision:', precision_score(y_val, y_pred_val_new_threshold))
print('f1_val:', f1_score(y_val, y_pred_val_new_threshold))
###Output
f1_train: 0.7101174687381584
[[1901 211]
[ 91 297]]
precision: 0.5846456692913385
f1_val: 0.6629464285714286
###Markdown
Dataset for test
###Code
test_set = pd.read_csv('test.csv')
# select the columns for the model
# 0 - Returner/ 1 - New_Access/ 2 - Other
dict_visitor = {'Returner': 0, 'New_Access': 1, 'Other': 0}
test_set['Type_of_Visitor_new'] = test_set['Type_of_Visitor'].map(dict_visitor)
#months variable
test_set['Date'] = pd.to_datetime(test_set['Date'], format='%d-%b-%y')
test_set["Month"]=test_set["Date"].map(lambda x: x.month)
test_set["Months_high"]=test_set["Month"].map(lambda x: 1 if x>7 & x<12 else 0)
test = test_set[["Months_high","Type_of_Visitor_new",
'GoogleAnalytics_ExitRate', 'GoogleAnalytics_PageValue']]
#define numeric features
metric_features = ['GoogleAnalytics_ExitRate','GoogleAnalytics_PageValue']
non_metric_features = X.columns.drop(metric_features).to_list()
test_num = test[metric_features]
test_cat = test[non_metric_features]
#power transform - still using training dataset
test_num_power = power.transform(test_num)
test_num_power = pd.DataFrame(test_num_power, columns = test_num.columns).set_index(test_num.index)
test_power = pd.concat([test_num_power, test_cat], axis=1)
test_power.columns
y_pred_new_threshold = (model_log.predict_proba(test_power)[:,1]>=0.7).astype(int)
df_submission = pd.concat([test_set['Access_ID'], pd.DataFrame(columns=['Buy'], data=y_pred_new_threshold)], axis=1)
df_submission['Buy'].value_counts()
#logistic_under with length 4_power_lessfeatures_newfeats_newthresh
df_submission.to_csv('Group17_Version32.csv', index=False)
###Output
_____no_output_____ |
014_nlp_part_2_sol.ipynb | ###Markdown
More NLP Truncated Singular Value Decomposition and Dimensionality ReductionWhen processing text we end up with feature sets that are large! There is up to one feature per different word in our text sample, far larger than a typical feature set that we're used to. One thing we can do when vectorizing is just to cap the number of features we end up with, but that doesn't seem to be the most sophisticated approach. TSVD is one thing that we can do to chop down the feature set - or reduce the dimensions - with a little more thought. Dimensionality ReductionDimensionality reduction is a common technique in machine learning, it does its name - reduces the dimensions in our feature data. Load Dataset from Last Time
###Code
#Load Data
df = pd.read_csv("data/spam.csv", encoding="ISO-8859-1")
df.drop(columns={"Unnamed: 2", "Unnamed: 3", "Unnamed: 4"}, inplace=True)
df.rename(columns={"v1":"target", "v2":"text"}, inplace=True)
#TF-IDF
vec_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
tmp = vec_tf.fit_transform(df["text"])
tok_cols = vec_tf.get_feature_names()
tok_df = pd.DataFrame(tmp.toarray(), columns=tok_cols)
print("original:", df["text"].shape)
print("vectorized:", tmp.shape)
y = df["target"]
X = df["text"]
tok_df.sample(5)
###Output
original: (5572,)
vectorized: (5572, 89635)
###Markdown
LSA - Latent Semantic AnalysisThe TSVD performs somehting called latent semantic analysis. The process of LSA and the math behind it are not something we need to explore in detail. (LSA is often called LSI - Latent Semantic Indexing)The idea of LSA is that it can generate "concepts" in the text. These concepts are found by looking at which terms occur in which documents - documents that have the same terms repeated are likely related to the same concept; other documents that share other words with those documents are likely on the same concept as well. An important part is the word "Latent" - i.e. the patterns detected are hidden, not explicit in the data. Implement SVD to Trim DatasetWe are starting with LOTS of feature inputs. Below we can loop through several models of different number of remaining components to see the accuracy depending on the number of features we keep in the feature set. The truncated part of truncated SVD trims the featureset down to the most significant features. We started with a lot of features - we can make predictions that are close to as accurate with far fewer, hopefully!
###Code
# get a list of models to evaluate
def get_models():
models = dict()
for i in range(99,100):
n = i*5
steps = [('svd', TruncatedSVD(n_components=n)), ('m', LogisticRegression())]
models[str(n)] = Pipeline(steps=steps)
return models
# evaluate a give model using cross-validation
def evaluate_model(model, X, y):
#Splits cut for speed
cv = RepeatedStratifiedKFold(n_splits=2, n_repeats=1)
scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1, error_score='raise')
return scores
models = get_models()
# evaluate the models and store results
results, names = list(), list()
for name, model in models.items():
scores = evaluate_model(model, tok_df, y)
results.append(scores)
names.append(name)
# plot model performance for comparison
plt.boxplot(results, labels=names, showmeans=True)
plt.xticks(rotation=45)
plt.show()
###Output
_____no_output_____
###Markdown
Exercise - Truncated SVDTry to use the same text for predictions from the newsgroups last time. Try to use the TSVD with a limited number of components and see if the accuracy can stay similar to what we got last time.
###Code
from sklearn.datasets import fetch_20newsgroups
remove = ("headers", "footers", "quotes")
categories = ["alt.atheism", "talk.religion.misc"]
data_train = fetch_20newsgroups(
subset="train", categories=categories, shuffle=True, random_state=42, remove=remove)
data_test = fetch_20newsgroups(
subset="test", categories=categories, shuffle=True, random_state=42, remove=remove)
# Vectorize and prep datasets
news_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
X_train = news_tf.fit_transform(data_train.data)
y_train = data_train.target
X_test = news_tf.transform(data_test.data)
y_test = data_test.target
print("Train (x,y):", X_train.shape, " Test (x,y):", X_test.shape)
# Create Models
tsvd = TruncatedSVD(n_components=50)
news_steps = [('svd', tsvd), ('m', LogisticRegression())]
news_model = Pipeline(steps=news_steps)
news_model.fit(X_train, y_train)
news_model.score(X_test, y_test)
terms = news_tf.get_feature_names()
for index, component in enumerate(tsvd.components_):
zipped = zip(terms, component)
top_terms_key=sorted(zipped, key = lambda t: t[1], reverse=True)[:5]
top_terms_list=list(dict(top_terms_key).keys())
print("Topic "+str(index)+": ",top_terms_list)
###Output
Topic 0: ['ico', 'ico tek', 'ico tek com', 'vice ico', 'vice ico tek']
Topic 1: ['just', 'god', 'people', 'don', 'think']
Topic 2: ['cobb', '3rd debate', '3rd debate cobb', '3rd debate cobb alexia', 'champaign urbana']
Topic 3: ['mom', 'men', 'isc', 'isc rit', 'isc rit edu']
Topic 4: ['freewill', 'angels freewill', 'angels freewill god', 'angels freewill god tells', 'freewill god']
Topic 5: ['deletion', 'argument', 'exist', 'alt atheism', 'statements']
Topic 6: ['deletion', 'ra', 'mcconkie', 'lds', 'mormon']
Topic 7: ['start', 'hand', 'account smile', 'account smile lot', 'account smile lot flunkies']
Topic 8: ['context', 'quote context', 'quotes context', 'jim', 'quote']
Topic 9: ['messenger', 'koresh', 'carried says', 'carried says character', 'carried says character messenger']
Topic 10: ['washed', 'washed blood', 'bull', 'blood', 'lamb']
Topic 11: ['rb', 'young', 'preying', 'preying young', 'altar boy']
Topic 12: ['objective', 'said surrender', 'morality', 'surrender', 'didn']
Topic 13: ['objective', 'just', 'petition', 'public schools', 'morality']
Topic 14: ['kent', 'cheers kent', 'cheers', 'said surrender', 'surrender']
Topic 15: ['just', 'broken', 'promises broken', 'promises', 'dean']
Topic 16: ['just', 'post', 'finished writing', 'finished writing sequel', 'finished writing sequel bible']
Topic 17: ['finished writing', 'finished writing sequel', 'finished writing sequel bible', 'koresh finished', 'koresh finished writing']
Topic 18: ['objective', 'god', 'morality', 'dean kaflowitz', 'dean']
Topic 19: ['broken', 'promises broken', 'promises', 'cheat hillary', 'hillary']
Topic 20: ['just', 'cheers kent', 'cheers', 'kent', 'ignorance strength']
Topic 21: ['believing god prepared', 'believing god prepared eternal', 'blashephemers', 'blashephemers hell', 'blashephemers hell believing']
Topic 22: ['cheat hillary', 'hillary', 'cheat', 'broken', 'promises broken']
Topic 23: ['just', 'objective', 'believing god prepared', 'believing god prepared eternal', 'blashephemers']
Topic 24: ['just', 'atheism', 'atheists', 'value', 'jose']
Topic 25: ['just', 'rosicrucian', 'order', 'animals', 'don']
Topic 26: ['cheat hillary', 'hillary', 'cheat', 'mary', 'think']
Topic 27: ['explain', 'explain pertains', 'explain pertains position', 'explain pertains position statement', 'pertains position']
Topic 28: ['moral', 'value', 'event', 'god', 'human']
Topic 29: ['did claim', 'objective', 'claim', 'god', 'claim objective']
Topic 30: ['science', 'values', 'value', 'cruel', 'sign']
Topic 31: ['theory', 'think', 'thing', 'paradise', 've']
Topic 32: ['theory', 'good', 'imaginative', 'imaginative theory', 'list']
Topic 33: ['sex', 'depression', 'marital', 'marital sex', 'extra marital']
Topic 34: ['liar', 'lunatic', 'moral', 'use', 'liar lunatic']
Topic 35: ['ignorance strength', 'ignorance', 'strength', 'just', 'religion']
Topic 36: ['lunacy', 'rosicrucian', 'world', 'morality', 'promises broken']
Topic 37: ['theory', 'christians', 'jesus', 'teachings jesus', 'teachings']
Topic 38: ['don', 'paradise', 'salvation', 'care', 'compuserve']
Topic 39: ['people', 'kill', 'explain pertains', 'explain pertains position', 'explain pertains position statement']
Topic 40: ['ignorance strength', 'strength', 'ignorance', 'claim', 'contradiction']
Topic 41: ['ignorance strength', 'strength', 'ignorance', 'theory', 'atheism']
Topic 42: ['sure wrong', 'sure', 'motto', 'compuserve', 'com']
Topic 43: ['did claim', 'claim', 'absolute', 'critus', 'evidence']
Topic 44: ['example', 'paradise', 'anti', 'jews', 'believe']
Topic 45: ['question', 'punishment', 'exist', 'life', 'good']
Topic 46: ['imaginative', 'imaginative theory', 'theory', 'didn', 'know']
Topic 47: ['time', 'theory', 'bible', 'words', 'deleted']
Topic 48: ['freedom', 'muslims', 'human', 'wouldn', 'laws']
Topic 49: ['freedom', 'sure', 'word', 'death', 'mormons']
###Markdown
Word2Vec and ClassificationIn addition to calculating things solely directly from our data, we can also use some external tools that can help create embeddings that are a little better (hopefully). This is also a neural network running behind the scenes to help us out. Word2Vec is an algorithm made by Google that can help process text and produce embeddings. Word2Vec looks for associations of words that occur with each other. Word2Vec in ProcessWord2Vec generates its embeddings by looking at words in a sentence, and the surrounding words in that same sentence. GensimGensim is a package that we can install that has an implementation of Word2Vec that we can use pretty easily.
###Code
from gensim.models import Word2Vec
import nltk
for package in ['stopwords','punkt','wordnet']:
nltk.download(package)
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
stop_words = set(stopwords.words('english'))
###Output
[nltk_data] Downloading package stopwords to
[nltk_data] /Users/akeems/nltk_data...
[nltk_data] Package stopwords is already up-to-date!
[nltk_data] Downloading package punkt to /Users/akeems/nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package wordnet to /Users/akeems/nltk_data...
[nltk_data] Package wordnet is already up-to-date!
###Markdown
TokenizerSince we are not using the vecorizer from sklearn, we need to provide our own tokenization. We can use the nltk based one from last time. We can also do any other types of processing here that we may want - stemming, customized stop words, etc... For this one I chopped out any 1 character tokens and added a regex filter to get rid of punctuation.
###Code
class lemmaTokenizer(object):
def __init__(self, stop_words):
self.stop_words = stop_words
from nltk.stem import WordNetLemmatizer
self.lemmatizer = WordNetLemmatizer()
def __call__(self, doc):
tokens = word_tokenize(doc)
filtered_tok = []
for tok in tokens:
if tok not in stop_words:
tok = re.sub('\W+','', tok) #Punctuation strip
tmp = self.lemmatizer.lemmatize(tok)
if len(tmp) >= 2:
filtered_tok.append(tmp)
return filtered_tok
###Output
_____no_output_____
###Markdown
Create Clan Text - Tokenize and LemmatizePrep some data. The "second half" of the dataframe is what we can use with the Word2Vec prediction models - we have cleaned up lists of tokens as well as translating the targets to 1 and 0.
###Code
tok = lemmaTokenizer(stop_words)
df["clean_text"] = df["text"].apply(lambda x: tok(x))
df["target2"] = pd.get_dummies(df["target"], drop_first=True)
df.head()
###Output
_____no_output_____
###Markdown
Create Word2VecWe can train our Word2Vec model with our cleaned up data. This will have Word2Vec do its magic behind the scenes and perform the training. W2V works in one of two ways, which are roughly opposites of each other, when doing this training: Continuous Bag of Words: looks at a window around each target word to try to predict surrounding words. Skip-Gram: looks at words surrounding target to try to predict it. We'll revisit the details of this stuff later on when we look at neural networks, since W2V is a neural network algorithm, it will make more sense in context. Note: this training is not making a model that we are using to make predictions. This is training inside the W2V algorithm to generate representations of our tokens.
###Code
# create Word2vec model
model = Word2Vec(df['clean_text'],min_count=1, vector_size=200, sg=1)
#min_count=1 means word should be present at least across all documents,
#if min_count=2 means if the word is present less than 2 times across all the documents then we shouldn't consider it
#combination of word and its vector
w2v = dict(zip(model.wv.index_to_key, model.wv.vectors))
#for converting sentence to vectors/numbers from word vectors result by Word2Vec
class MeanEmbeddingVectorizer(object):
def __init__(self, word2vec):
self.word2vec = word2vec
# if a text is empty we should return a vector of zeros
# with the same dimensionality as all the other vectors
self.dim = len(next(iter(word2vec.values())))
def fit(self, X, y):
return self
def transform(self, X):
return np.array([
np.mean([self.word2vec[w] for w in words if w in self.word2vec]
or [np.zeros(self.dim)], axis=0)
for words in X
])
###Output
_____no_output_____
###Markdown
Word2Vec ModelEach word in the vocabulary now has a vector representing it - of size 200. We can make a dataframe and see each token in our text and its vector representation. This vector is the internal representation of each token that is generated by Word2Vec. This is how the algorithm calculates things like similarity...
###Code
tmp = pd.DataFrame(w2v)
vectors = model.wv
tmp.head()
###Output
_____no_output_____
###Markdown
SimilarityOne of the things that Word2Vec allows us to do is to look at the similarity of words. This similarity is calculated via the cosine distance of the vectors. Cosine similarity is a technique to calculate the distance between two vectors - smaller distance, more similar. Once the vectors are derived by in the training process, these similarity calculations are pretty easy and quick. Note: the similarites here are calculated by the values derived from our trained model. So they are based on the relationships in our text. Word2Vec and other NLP packages also commonly have pretrained models that can be downloaded that are based on large amounts of text. Words may be represented very differently in those vs whatever we train here - the more data we have, the more consistent they'll be; the more "unique" our text is, the more different it will be.
###Code
#Find the most similar word to anything in our vocabulary
vectors.most_similar("think")[0:3]
# We can also see how similar different words are.
vectors.similarity("think", "determine")
###Output
_____no_output_____
###Markdown
Make PredictionsWe can take our actual data now and transform it through the Word2Vec model that we've made. This will generate our smaller feature set that we can build our models from.One of the things that the MeanEmbeddingVectorizer does is to collapse the data down to those 200 dimensions in the vector.
###Code
#SPLITTING THE TRAINING DATASET INTO TRAINING AND VALIDATION
# Split data - using the new dataframe parts that we cleaned up.
X_train, X_test, y_train, y_test = train_test_split(df["clean_text"],df["target2"])
#Word2vec
# Fit and transform
modelw = MeanEmbeddingVectorizer(w2v)
X_train_vectors_w2v = modelw.transform(X_train)
X_test_vectors_w2v = modelw.transform(X_test)
X_train_vectors_w2v.shape
###Output
_____no_output_____
###Markdown
Build ModelWe can now use the new data to make predictions.
###Code
# Make predictions
lr_w2v = RandomForestClassifier()
lr_w2v.fit(X_train_vectors_w2v, y_train) #model
#Predict y value for test dataset
y_predict = lr_w2v.predict(X_test_vectors_w2v)
y_prob = lr_w2v.predict_proba(X_test_vectors_w2v)[:,1]
print(classification_report(y_test,y_predict))
print('Confusion Matrix:',confusion_matrix(y_test, y_predict))
fpr, tpr, thresholds = roc_curve(y_test, y_prob)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.98 0.98 0.98 1212
1 0.88 0.84 0.86 181
accuracy 0.96 1393
macro avg 0.93 0.91 0.92 1393
weighted avg 0.96 0.96 0.96 1393
Confusion Matrix: [[1192 20]
[ 29 152]]
AUC: 0.9792407417537334
###Markdown
Exercise - Word2VecUse the newsgroup data and Word2Vec to make predictions.
###Code
# Prepare datsets and Tokenize
tok = lemmaTokenizer(stop_words)
X_w2v_news_train = [tok(x) for x in data_train.data]
X_w2v_news_test = [tok(x) for x in data_test.data]
y_train_news = data_train.target
y_test_news = data_test.target
# create Word2vec model
model_news = Word2Vec(X_w2v_news_train, min_count=1, vector_size=200)
w2v_news = dict(zip(model_news.wv.index_to_key, model_news.wv.vectors))
#Word2vec
# Fit and transform
model_news_w = MeanEmbeddingVectorizer(w2v_news)
X_train_vectors_w2v_news = modelw.transform(X_w2v_news_train)
X_val_vectors_w2v_news = modelw.transform(X_w2v_news_test)
X_train_vectors_w2v.shape
# Make predictions
news_clf = RandomForestClassifier()
news_clf.fit(X_train_vectors_w2v_news, y_train_news) #model
#Predict y value for test dataset
y_predict_news = news_clf.predict(X_val_vectors_w2v_news)
y_prob_news = news_clf.predict_proba(X_val_vectors_w2v_news)[:,1]
print(classification_report(y_test_news,y_predict_news))
print('Confusion Matrix:',confusion_matrix(y_test_news, y_predict_news))
fpr, tpr, thresholds = roc_curve(y_test_news, y_prob_news)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.56 0.65 0.60 319
1 0.43 0.34 0.38 251
accuracy 0.51 570
macro avg 0.49 0.50 0.49 570
weighted avg 0.50 0.51 0.50 570
Confusion Matrix: [[208 111]
[166 85]]
AUC: 0.5131324232849167
###Markdown
More NLP Truncated Singular Value Decomposition and Dimensionality ReductionWhen processing text we end up with feature sets that are large! There is up to one feature per different word in our text sample, far larger than a typical feature set that we're used to. One thing we can do when vectorizing is just to cap the number of features we end up with, but that doesn't seem to be the most sophisticated approach. TSVD is one thing that we can do to chop down the feature set - or reduce the dimensions - with a little more thought. Dimensionality ReductionDimensionality reduction is a common technique in machine learning, it does its name - reduces the dimensions in our feature data. Load Dataset from Last Time
###Code
#Load Data
df = pd.read_csv("data/spam.csv", encoding="ISO-8859-1")
df.drop(columns={"Unnamed: 2", "Unnamed: 3", "Unnamed: 4"}, inplace=True)
df.rename(columns={"v1":"target", "v2":"text"}, inplace=True)
df.head()
#TF-IDF
vec_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
tmp = vec_tf.fit_transform(df["text"])
tok_cols = vec_tf.get_feature_names()
tok_df = pd.DataFrame(tmp.toarray(), columns=tok_cols)
print("original:", df["text"].shape)
print("vectorized:", tmp.shape)
y = df["target"]
X = df["text"]
tok_df.sample(5)
###Output
original: (5572,)
vectorized: (5572, 89635)
###Markdown
LSA - Latent Semantic AnalysisThe TSVD performs somehting called latent semantic analysis. The process of LSA and the math behind it are not something we need to explore in detail. (LSA is often called LSI - Latent Semantic Indexing)The idea of LSA is that it can generate "concepts" in the text. These concepts are found by looking at which terms occur in which documents - documents that have the same terms repeated are likely related to the same concept; other documents that share other words with those documents are likely on the same concept as well. An important part is the word "Latent" - i.e. the patterns detected are hidden, not explicit in the data. Implement SVD to Trim DatasetWe are starting with LOTS of feature inputs. Below we can loop through several models of different number of remaining components to see the accuracy depending on the number of features we keep in the feature set. The truncated part of truncated SVD trims the featureset down to the most significant features. We started with a lot of features - we can make predictions that are close to as accurate with far fewer, hopefully!
###Code
# get a list of models to evaluate
def get_models():
models = dict()
for i in range(1,10):
n = i*50
steps = [('svd', TruncatedSVD(n_components=n)), ('m', LogisticRegression())]
models[str(n)] = Pipeline(steps=steps)
return models
# evaluate a give model using cross-validation
def evaluate_model(model, X, y):
#Splits cut for speed
cv = RepeatedStratifiedKFold(n_splits=2, n_repeats=1)
scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1, error_score='raise')
return scores
models = get_models()
# evaluate the models and store results
results, names = list(), list()
for name, model in models.items():
scores = evaluate_model(model, tok_df, y)
results.append(scores)
names.append(name)
# plot model performance for comparison
plt.boxplot(results, labels=names, showmeans=True)
plt.xticks(rotation=45)
plt.show()
###Output
_____no_output_____
###Markdown
Exercise - Truncated SVDTry to use the same text for predictions from the newsgroups last time. Try to use the TSVD with a limited number of components and see if the accuracy can stay similar to what we got last time.
###Code
from sklearn.datasets import fetch_20newsgroups
remove = ("headers", "footers", "quotes")
categories = ["alt.atheism", "talk.religion.misc"]
data_train = fetch_20newsgroups(
subset="train", categories=categories, shuffle=True, random_state=42, remove=remove)
data_test = fetch_20newsgroups(
subset="test", categories=categories, shuffle=True, random_state=42, remove=remove)
# Vectorize and prep datasets
news_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
X_train = news_tf.fit_transform(data_train.data)
y_train = data_train.target
X_test = news_tf.transform(data_test.data)
y_test = data_test.target
print("Train (x,y):", X_train.shape, " Test (x,y):", X_test.shape)
# Create Models
tsvd = TruncatedSVD(n_components=50)
news_steps = [('svd', tsvd), ('m', LogisticRegression())]
news_model = Pipeline(steps=news_steps)
news_model.fit(X_train, y_train)
news_model.score(X_test, y_test)
terms = news_tf.get_feature_names()
for index, component in enumerate(tsvd.components_):
zipped = zip(terms, component)
top_terms_key=sorted(zipped, key = lambda t: t[1], reverse=True)[:5]
top_terms_list=list(dict(top_terms_key).keys())
print("Topic "+str(index)+": ",top_terms_list)
###Output
Topic 0: ['ico', 'ico tek', 'ico tek com', 'vice ico', 'vice ico tek']
Topic 1: ['just', 'god', 'people', 'don', 'think']
Topic 2: ['cobb', '3rd debate', '3rd debate cobb', '3rd debate cobb alexia', 'champaign urbana']
Topic 3: ['mom', 'men', 'isc', 'isc rit', 'isc rit edu']
Topic 4: ['freewill', 'angels freewill', 'angels freewill god', 'angels freewill god tells', 'freewill god']
Topic 5: ['deletion', 'atheism', 'exist', 'definition', 'alt atheism']
Topic 6: ['deletion', 'ra', 'mcconkie', 'mormon', 'lds']
Topic 7: ['start', 'just', 'deletion', 'hand', 'account smile']
Topic 8: ['context', 'quote context', 'quotes context', 'jim', 'quote']
Topic 9: ['messenger', 'koresh', 'carried says', 'carried says character', 'carried says character messenger']
Topic 10: ['washed', 'washed blood', 'bull', 'blood', 'lamb']
Topic 11: ['rb', 'young', 'preying', 'preying young', 'altar boy']
Topic 12: ['said surrender', 'surrender', 'didn', 'broadcast message', 'broadcast message didn']
Topic 13: ['objective', 'morality', 'said surrender', 'surrender', 'did claim']
Topic 14: ['petition', 'public schools', 'dm', 'schools', 'reading']
Topic 15: ['kent', 'cheers kent', 'cheers', 'jose', 'san jose']
Topic 16: ['dean kaflowitz', 'dean', 'kaflowitz', 'post', 'thread']
Topic 17: ['just', 'finished writing', 'finished writing sequel', 'finished writing sequel bible', 'koresh finished']
Topic 18: ['just', 'objective', 'dean kaflowitz', 'dean', 'kaflowitz']
Topic 19: ['finished writing', 'finished writing sequel', 'finished writing sequel bible', 'koresh finished', 'koresh finished writing']
Topic 20: ['broken', 'promises broken', 'promises', 'god', 'atheism']
Topic 21: ['cheat hillary', 'hillary', 'cheat', 'just', 'broken']
Topic 22: ['just', 'broken', 'promises broken', 'promises', 'cheers kent']
Topic 23: ['rosicrucian', 'order', 'stephen', 'islam', 'took quote']
Topic 24: ['don', 'broken', 'think', 'promises broken', 'promises']
Topic 25: ['believing god prepared', 'believing god prepared eternal', 'blashephemers', 'blashephemers hell', 'blashephemers hell believing']
Topic 26: ['promises broken', 'broken', 'promises', 'did claim', 'objective']
Topic 27: ['just', 'atheism', 'god', 'atheist', 'question']
Topic 28: ['promises broken', 'broken', 'argument', 'promises', 'smoke']
Topic 29: ['did', 'statement', 'explain pertains', 'explain pertains position', 'explain pertains position statement']
Topic 30: ['animals', 'sex', 'think', 'depression', 'humans']
Topic 31: ['world', 'sex', 'objective', 'science', 'good']
Topic 32: ['question', 'word', 'god', 'children', 'jews']
Topic 33: ['just', 'ye', 'humans', 'human', 'promises broken']
Topic 34: ['cockroaches', 'explain pertains', 'explain pertains position', 'explain pertains position statement', 'pertains position']
Topic 35: ['sure wrong', 'wrong', 'sure', 'jews', 'koresh']
Topic 36: ['motto', 'think', 'does', 'christians', 'word']
Topic 37: ['did claim', 'claim objective', 'did claim objective', 'claim', 'did']
Topic 38: ['ignorance strength', 'ignorance', 'strength', 'values', 'like']
Topic 39: ['wrong', 'cruel', 'paradise', 'salvation', 'sure wrong']
Topic 40: ['theory', 'ignorance strength', 'imaginative', 'imaginative theory', 'strength']
Topic 41: ['ignorance strength', 'strength', 'just', 'ignorance', 'people']
Topic 42: ['think', 'ignorance strength', 'strength', 'question', 'quite']
Topic 43: ['paradise', 'salvation', 'know', 'explain pertains', 'explain pertains position']
Topic 44: ['ignorance strength', 'strength', 'ignorance', 'lunacy', 'car']
Topic 45: ['wrong', 'sure wrong', 'sure', 'know', 'material']
Topic 46: ['lunacy', 'wrong', 'motto', 'ignorance strength', 'theory']
Topic 47: ['laws', 'heard', 'religion', 'sure wrong', 'mormons']
Topic 48: ['cockroaches', 'theory', 'cruel', 'state', 'benefits']
Topic 49: ['lunacy', 'ignorance strength', 'years ago', 'ago', 'ignorance']
###Markdown
Word2Vec and ClassificationIn addition to calculating things solely directly from our data, we can also use some external tools that can help create embeddings that are a little better (hopefully). This is also a neural network running behind the scenes to help us out. Word2Vec is an algorithm made by Google that can help process text and produce embeddings. Word2Vec looks for associations of words that occur with each other. Word2Vec in ProcessWord2Vec generates its embeddings by looking at words in a sentence, and the surrounding words in that same sentence. GensimGensim is a package that we can install that has an implementation of Word2Vec that we can use pretty easily.
###Code
from gensim.models import Word2Vec
import nltk
for package in ['stopwords','punkt','wordnet']:
nltk.download(package)
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
stop_words = set(stopwords.words('english'))
###Output
[nltk_data] Downloading package stopwords to
[nltk_data] C:\Users\allyr\AppData\Roaming\nltk_data...
[nltk_data] Package stopwords is already up-to-date!
[nltk_data] Downloading package punkt to
[nltk_data] C:\Users\allyr\AppData\Roaming\nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package wordnet to
[nltk_data] C:\Users\allyr\AppData\Roaming\nltk_data...
[nltk_data] Package wordnet is already up-to-date!
###Markdown
TokenizerSince we are not using the vecorizer from sklearn, we need to provide our own tokenization. We can use the nltk based one from last time. We can also do any other types of processing here that we may want - stemming, customized stop words, etc... For this one I chopped out any 1 character tokens and added a regex filter to get rid of punctuation.
###Code
class lemmaTokenizer(object):
def __init__(self, stop_words):
self.stop_words = stop_words
from nltk.stem import WordNetLemmatizer
self.lemmatizer = WordNetLemmatizer()
def __call__(self, doc):
tokens = word_tokenize(doc)
filtered_tok = []
for tok in tokens:
if tok not in stop_words:
tok = re.sub('\W+','', tok) #Punctuation strip
tmp = self.lemmatizer.lemmatize(tok)
if len(tmp) >= 2:
filtered_tok.append(tmp)
return filtered_tok
###Output
_____no_output_____
###Markdown
Create Clan Text - Tokenize and LemmatizePrep some data. The "second half" of the dataframe is what we can use with the Word2Vec prediction models - we have cleaned up lists of tokens as well as translating the targets to 1 and 0.
###Code
tok = lemmaTokenizer(stop_words)
df["clean_text"] = df["text"].apply(lambda x: tok(x))
df["target2"] = pd.get_dummies(df["target"], drop_first=True)
df.head()
###Output
_____no_output_____
###Markdown
Create Word2VecWe can train our Word2Vec model with our cleaned up data. This will have Word2Vec do its magic behind the scenes and perform the training. W2V works in one of two ways, which are roughly opposites of each other, when doing this training: Continuous Bag of Words: looks at a window around each target word to try to predict surrounding words. Skip-Gram: looks at words surrounding target to try to predict it. We'll revisit the details of this stuff later on when we look at neural networks, since W2V is a neural network algorithm, it will make more sense in context. Note: this training is not making a model that we are using to make predictions. This is training inside the W2V algorithm to generate representations of our tokens.
###Code
# create Word2vec model
model = Word2Vec(df['clean_text'],min_count=1, vector_size=200, sg=1)
#min_count=1 means word should be present at least across all documents,
#if min_count=2 means if the word is present less than 2 times across all the documents then we shouldn't consider it
#combination of word and its vector
w2v = dict(zip(model.wv.index_to_key, model.wv.vectors))
#for converting sentence to vectors/numbers from word vectors result by Word2Vec
class MeanEmbeddingVectorizer(object):
def __init__(self, word2vec):
self.word2vec = word2vec
# if a text is empty we should return a vector of zeros
# with the same dimensionality as all the other vectors
self.dim = len(next(iter(word2vec.values())))
def fit(self, X, y):
return self
def transform(self, X):
return np.array([
np.mean([self.word2vec[w] for w in words if w in self.word2vec]
or [np.zeros(self.dim)], axis=0)
for words in X
])
###Output
_____no_output_____
###Markdown
Word2Vec ModelEach word in the vocabulary now has a vector representing it - of size 200. We can make a dataframe and see each token in our text and its vector representation. This vector is the internal representation of each token that is generated by Word2Vec. This is how the algorithm calculates things like similarity...
###Code
tmp = pd.DataFrame(w2v)
vectors = model.wv
tmp.head()
###Output
_____no_output_____
###Markdown
SimilarityOne of the things that Word2Vec allows us to do is to look at the similarity of words. This similarity is calculated via the cosine distance of the vectors. Cosine similarity is a technique to calculate the distance between two vectors - smaller distance, more similar. Once the vectors are derived by in the training process, these similarity calculations are pretty easy and quick. Note: the similarites here are calculated by the values derived from our trained model. So they are based on the relationships in our text. Word2Vec and other NLP packages also commonly have pretrained models that can be downloaded that are based on large amounts of text. Words may be represented very differently in those vs whatever we train here - the more data we have, the more consistent they'll be; the more "unique" our text is, the more different it will be.
###Code
#Find the most similar word to anything in our vocabulary
vectors.most_similar("think")[0:3]
# We can also see how similar different words are.
vectors.similarity("think", "determine")
###Output
_____no_output_____
###Markdown
Make PredictionsWe can take our actual data now and transform it through the Word2Vec model that we've made. This will generate our smaller feature set that we can build our models from.One of the things that the MeanEmbeddingVectorizer does is to collapse the data down to those 200 dimensions in the vector.
###Code
#SPLITTING THE TRAINING DATASET INTO TRAINING AND VALIDATION
# Split data - using the new dataframe parts that we cleaned up.
X_train, X_test, y_train, y_test = train_test_split(df["clean_text"],df["target2"])
#Word2vec
# Fit and transform
modelw = MeanEmbeddingVectorizer(w2v)
X_train_vectors_w2v = modelw.transform(X_train)
X_test_vectors_w2v = modelw.transform(X_test)
X_train_vectors_w2v.shape
###Output
_____no_output_____
###Markdown
Build ModelWe can now use the new data to make predictions.
###Code
# Make predictions
lr_w2v = RandomForestClassifier()
lr_w2v.fit(X_train_vectors_w2v, y_train) #model
#Predict y value for test dataset
y_predict = lr_w2v.predict(X_test_vectors_w2v)
y_prob = lr_w2v.predict_proba(X_test_vectors_w2v)[:,1]
print(classification_report(y_test,y_predict))
print('Confusion Matrix:',confusion_matrix(y_test, y_predict))
fpr, tpr, thresholds = roc_curve(y_test, y_prob)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.97 0.99 0.98 1205
1 0.93 0.83 0.88 188
accuracy 0.97 1393
macro avg 0.95 0.91 0.93 1393
weighted avg 0.97 0.97 0.97 1393
Confusion Matrix: [[1193 12]
[ 32 156]]
AUC: 0.9765471881345458
###Markdown
Exercise - Word2VecUse the newsgroup data and Word2Vec to make predictions.
###Code
# Prepare datsets and Tokenize
tok = lemmaTokenizer(stop_words)
X_w2v_news_train = [tok(x) for x in data_train.data]
X_w2v_news_test = [tok(x) for x in data_test.data]
y_train_news = data_train.target
y_test_news = data_test.target
# create Word2vec model
model_news = Word2Vec(X_w2v_news_train, min_count=1, vector_size=200)
w2v_news = dict(zip(model_news.wv.index_to_key, model_news.wv.vectors))
#Word2vec
# Fit and transform
model_news_w = MeanEmbeddingVectorizer(w2v_news)
X_train_vectors_w2v_news = modelw.transform(X_w2v_news_train)
X_val_vectors_w2v_news = modelw.transform(X_w2v_news_test)
X_train_vectors_w2v.shape
# Make predictions
news_clf = RandomForestClassifier()
news_clf.fit(X_train_vectors_w2v_news, y_train_news) #model
#Predict y value for test dataset
y_predict_news = news_clf.predict(X_val_vectors_w2v_news)
y_prob_news = news_clf.predict_proba(X_val_vectors_w2v_news)[:,1]
print(classification_report(y_test_news,y_predict_news))
print('Confusion Matrix:',confusion_matrix(y_test_news, y_predict_news))
fpr, tpr, thresholds = roc_curve(y_test_news, y_prob_news)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.55 0.64 0.59 319
1 0.42 0.34 0.38 251
accuracy 0.51 570
macro avg 0.49 0.49 0.48 570
weighted avg 0.50 0.51 0.50 570
Confusion Matrix: [[204 115]
[166 85]]
AUC: 0.48400129887971627
###Markdown
More NLP Truncated Singular Value Decomposition and Dimensionality ReductionWhen processing text we end up with feature sets that are large! There is up to one feature per different word in our text sample, far larger than a typical feature set that we're used to. One thing we can do when vectorizing is just to cap the number of features we end up with, but that doesn't seem to be the most sophisticated approach. TSVD is one thing that we can do to chop down the feature set - or reduce the dimensions - with a little more thought. Dimensionality ReductionDimensionality reduction is a common technique in machine learning, it does its name - reduces the dimensions in our feature data. Load Dataset from Last Time
###Code
#Load Data
df = pd.read_csv("data/spam.csv", encoding="ISO-8859-1")
df.drop(columns={"Unnamed: 2", "Unnamed: 3", "Unnamed: 4"}, inplace=True)
df.rename(columns={"v1":"target", "v2":"text"}, inplace=True)
#TF-IDF
vec_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
tmp = vec_tf.fit_transform(df["text"])
tok_cols = vec_tf.get_feature_names()
tok_df = pd.DataFrame(tmp.toarray(), columns=tok_cols)
print("original:", df["text"].shape)
print("vectorized:", tmp.shape)
y = df["target"]
X = df["text"]
tok_df.sample(5)
df.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 5572 entries, 0 to 5571
Data columns (total 4 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 target 5572 non-null object
1 text 5572 non-null object
2 clean_text 5572 non-null object
3 target2 5572 non-null uint8
dtypes: object(3), uint8(1)
memory usage: 136.2+ KB
###Markdown
LSA - Latent Semantic AnalysisThe TSVD performs somehting called latent semantic analysis. The process of LSA and the math behind it are not something we need to explore in detail. (LSA is often called LSI - Latent Semantic Indexing)The idea of LSA is that it can generate "concepts" in the text. These concepts are found by looking at which terms occur in which documents - documents that have the same terms repeated are likely related to the same concept; other documents that share other words with those documents are likely on the same concept as well. An important part is the word "Latent" - i.e. the patterns detected are hidden, not explicit in the data. Implement SVD to Trim DatasetWe are starting with LOTS of feature inputs. Below we can loop through several models of different number of remaining components to see the accuracy depending on the number of features we keep in the feature set. The truncated part of truncated SVD trims the featureset down to the most significant features. We started with a lot of features - we can make predictions that are close to as accurate with far fewer, hopefully!
###Code
# get a list of models to evaluate
def get_models():
models = dict()
for i in range(1,10):
n = i*50
steps = [('svd', TruncatedSVD(n_components=n)), ('m', LogisticRegression())]
models[str(n)] = Pipeline(steps=steps)
return models
# evaluate a give model using cross-validation
def evaluate_model(model, X, y):
#Splits cut for speed
cv = RepeatedStratifiedKFold(n_splits=2, n_repeats=1)
scores = cross_val_score(model, X, y, scoring='accuracy', cv=cv, n_jobs=-1, error_score='raise')
return scores
models = get_models()
# evaluate the models and store results
results, names = list(), list()
for name, model in models.items():
scores = evaluate_model(model, tok_df, y)
results.append(scores)
names.append(name)
# plot model performance for comparison
plt.boxplot(results, labels=names, showmeans=True)
plt.xticks(rotation=45)
plt.show()
tf_idf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,3), stop_words="english", strip_accents="unicode")
tmp_vec = tf_idf.fit_transform(df["text"])
tok_cols2 = tf_idf.get_feature_names()
tmp_df = pd.DataFrame(tmp_vec.toarray(), columns=tok_cols2)
tmp_df.head()
print(df["text"].sample(10))
X_tr, X_te, y_tr, y_te = train_test_split(tmp_df, y)
svd_tmp = TruncatedSVD(n_components=25)
pipe_steps = [("svd", svd_tmp), ("model", RandomForestClassifier())]
pipe_test = Pipeline(steps=pipe_steps)
pipe_test.fit(X_tr, y_tr)
pipe_test.score(X_te, y_te)
for index, component in enumerate(svd_tmp.components_):
zipped = zip(tok_cols2, component)
top_terms_key = sorted(zipped, key = lambda t : t[1], reverse=True)[1:6]
top_terms_list = list(dict(top_terms_key).keys())
print("Topic: " + str(index) + " ", top_terms_list)
###Output
Topic: 0 ['sorry ll later', 'sorry ll', 'later', 'sorry', 'll']
Topic: 1 ['lor', 'ok lor', 'ok prob', 'ok thanx', 'prob']
Topic: 2 ['gt', 'lt gt', 'come', 'home', 'like']
Topic: 3 ['come', 'lor', 'pick', 'send', 'way']
Topic: 4 ['pls', 'pick', 'pls send', 'pls send message', 'phone right']
Topic: 5 ['ok lor', 'wat', 'doing', 'ard', 'wat doing']
Topic: 6 ['way', 'coming home', 'lt gt', 'pick', 'gt']
Topic: 7 ['lor', 'pick', 'phone right', 'phone right pls', 'pick phone right']
Topic: 8 ['prize', 'claim', 'guaranteed', 'lor', 'urgent']
Topic: 9 ['wat', 'wat doing', 'prize', 'claim', 'guaranteed']
Topic: 10 ['text', 'll', 'txt', 'ur', 'nokia']
Topic: 11 ['looking', 'ur', 'reveal', 'admirer', 'reveal thinks']
Topic: 12 ['sent', 'know', 'll', 'way', 'yeah']
Topic: 13 ['da', 'way', 'work', 'meeting', 'got']
Topic: 14 ['da', 'sent', 'meeting', 'sir', 'waiting']
Topic: 15 ['dear', 'text', 'text way', 'okie', 'ur']
Topic: 16 ['work', 'like', 'princess', 'good', 'sent']
Topic: 17 ['okie', 'send', 'know', 'good', 'sir']
Topic: 18 ['ll', 'okie', 'yeah', 'waiting', 'good']
Topic: 19 ['dear', 'way', 'meeting', 'free', 'like']
Topic: 20 ['ll', 'meeting', 'like', 'text', 'da']
Topic: 21 ['know', 'need', 'draw', 'special', 'txt']
Topic: 22 ['dear', 'need', 'got', 'did', 'tell']
Topic: 23 ['meeting', 'sent', 'yes', 'savamob', 'today']
Topic: 24 ['time', 'okie', 'later', 'meeting later', 'free']
###Markdown
Exercise - Truncated SVDTry to use the same text for predictions from the newsgroups last time. Try to use the TSVD with a limited number of components and see if the accuracy can stay similar to what we got last time.
###Code
from sklearn.datasets import fetch_20newsgroups
remove = ("headers", "footers", "quotes")
categories = ["alt.atheism", "talk.religion.misc"]
data_train = fetch_20newsgroups(
subset="train", categories=categories, shuffle=True, random_state=42, remove=remove)
data_test = fetch_20newsgroups(
subset="test", categories=categories, shuffle=True, random_state=42, remove=remove)
# Vectorize and prep datasets
news_tf = TfidfVectorizer(sublinear_tf=True, ngram_range=(1,4), stop_words="english", strip_accents="unicode")
X_train = news_tf.fit_transform(data_train.data)
y_train = data_train.target
X_test = news_tf.transform(data_test.data)
y_test = data_test.target
print("Train (x,y):", X_train.shape, " Test (x,y):", X_test.shape)
# Create Models
tsvd = TruncatedSVD(n_components=50)
news_steps = [('svd', tsvd), ('m', LogisticRegression())]
news_model = Pipeline(steps=news_steps)
news_model.fit(X_train, y_train)
news_model.score(X_test, y_test)
terms = news_tf.get_feature_names()
for index, component in enumerate(tsvd.components_):
zipped = zip(terms, component)
top_terms_key=sorted(zipped, key = lambda t: t[1], reverse=True)[:5]
top_terms_list=list(dict(top_terms_key).keys())
print("Topic "+str(index)+": ",top_terms_list)
###Output
Topic 0: ['ico', 'ico tek', 'ico tek com', 'vice ico', 'vice ico tek']
Topic 1: ['just', 'god', 'people', 'don', 'think']
Topic 2: ['cobb', '3rd debate', '3rd debate cobb', '3rd debate cobb alexia', 'champaign urbana']
Topic 3: ['mom', 'men', 'isc', 'isc rit', 'isc rit edu']
Topic 4: ['freewill', 'angels freewill', 'angels freewill god', 'angels freewill god tells', 'freewill god']
Topic 5: ['deletion', 'argument', 'definition', 'exist', 'religion']
Topic 6: ['deletion', 'ra', 'mcconkie', 'lds', 'mormon']
Topic 7: ['start', 'hand', 'just', 'account smile', 'account smile lot']
Topic 8: ['context', 'quote context', 'quotes context', 'jim', 'quote']
Topic 9: ['messenger', 'koresh', 'carried says', 'carried says character', 'carried says character messenger']
Topic 10: ['washed', 'washed blood', 'bull', 'blood', 'lamb']
Topic 11: ['rb', 'young', 'preying', 'preying young', 'altar boy']
Topic 12: ['said surrender', 'surrender', 'didn', 'broadcast message', 'broadcast message didn']
Topic 13: ['kent', 'cheers kent', 'cheers', 'jesus', 'god']
Topic 14: ['petition', 'public schools', 'dm', 'schools', 'public']
Topic 15: ['just', 'god', 'petition', 'public schools', 'believe']
Topic 16: ['post', 'dean', 'dean kaflowitz', 'kaflowitz', 'thread']
Topic 17: ['just', 'just bet', 'oh just bet', 'oh just', 'bet']
Topic 18: ['broken', 'promises broken', 'promises', 'just', 'finished writing']
Topic 19: ['just', 'cheat hillary', 'hillary', 'cheat', 'objective']
Topic 20: ['broken', 'promises broken', 'promises', 'cheat hillary', 'hillary']
Topic 21: ['believing god prepared', 'believing god prepared eternal', 'blashephemers', 'blashephemers hell', 'blashephemers hell believing']
Topic 22: ['cheat hillary', 'hillary', 'cheat', 'objective', 'death cheat']
Topic 23: ['jose', 'san jose', 'san', 'objective', 'did claim']
Topic 24: ['said', 'explain', 'jose', 'san jose', 'stephen']
Topic 25: ['objective', 'atheism', 'god', 'cheers kent', 'com']
Topic 26: ['just', 'jesus', 'bible', 'objective', 'man']
Topic 27: ['atheists', 'atheism', 'rosicrucian', 'atheist', 'order']
Topic 28: ['jesus', 'god', 'christian', 'mary', 'christians']
Topic 29: ['islamic', 'mary', 'islam', 'does', 'just']
Topic 30: ['moral', 'animals', 'wrong', 'think', 'humans']
Topic 31: ['make', 'islamic', 'did', 'koresh', 'cruel']
Topic 32: ['religion', 'sex', 'objective', 'depression', 'people']
Topic 33: ['did claim', 'did', 'claim objective', 'did claim objective', 'claim']
Topic 34: ['theory', 'just', 'ignorance strength', 'critus', 'imaginative']
Topic 35: ['islam', 'muhammad', 'qur', 'muslims', 'ocean']
Topic 36: ['cruel', 'things', 'paradise', 'sure wrong', 'motto']
Topic 37: ['teachings', 'lord', 'sold sign', 'jesus', 'sold']
Topic 38: ['cruel', 'critus', 'muslims', 'islam', 'muslim']
Topic 39: ['christians', 'islamic', 'critus', 'practice', 'typing']
Topic 40: ['ignorance strength', 'strength', 'ignorance', 'theory', 'does']
Topic 41: ['cruel', 'punishment', 'benefits', 'qur', 'long']
Topic 42: ['million', 'sure wrong', 'talking', 'imaginative', 'imaginative theory']
Topic 43: ['wrong', 'sure wrong', 'sure', 'heard', 'ye']
Topic 44: ['ignorance strength', 'strength', 'ignorance', 'ye', 'bible']
Topic 45: ['sorry', 'hear', 'quote', 'bit argument', 'just bit argument']
Topic 46: ['read', 'origins', 'commandment', 'talk origins', 'critus']
Topic 47: ['religion', 'wrong', 'don', 'sure wrong', 'homosexuality']
Topic 48: ['sure wrong', 'wrong', 'quote', 'bit argument', 'just bit argument']
Topic 49: ['nanci', 'maybe', 'like', 'answer key', 'answer key nanci']
###Markdown
Word2Vec and ClassificationIn addition to calculating things solely directly from our data, we can also use some external tools that can help create embeddings that are a little better (hopefully). This is also a neural network running behind the scenes to help us out. Word2Vec is an algorithm made by Google that can help process text and produce embeddings. Word2Vec looks for associations of words that occur with each other. Word2Vec in ProcessWord2Vec generates its embeddings by looking at words in a sentence, and the surrounding words in that same sentence. GensimGensim is a package that we can install that has an implementation of Word2Vec that we can use pretty easily.
###Code
from gensim.models import Word2Vec
import nltk
for package in ['stopwords','punkt','wordnet']:
nltk.download(package)
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
stop_words = set(stopwords.words('english'))
###Output
[nltk_data] Downloading package stopwords to
[nltk_data] C:\Users\soniy\AppData\Roaming\nltk_data...
[nltk_data] Package stopwords is already up-to-date!
[nltk_data] Downloading package punkt to
[nltk_data] C:\Users\soniy\AppData\Roaming\nltk_data...
[nltk_data] Package punkt is already up-to-date!
[nltk_data] Downloading package wordnet to
[nltk_data] C:\Users\soniy\AppData\Roaming\nltk_data...
[nltk_data] Package wordnet is already up-to-date!
###Markdown
TokenizerSince we are not using the vecorizer from sklearn, we need to provide our own tokenization. We can use the nltk based one from last time. We can also do any other types of processing here that we may want - stemming, customized stop words, etc... For this one I chopped out any 1 character tokens and added a regex filter to get rid of punctuation.
###Code
class lemmaTokenizer(object):
def __init__(self, stop_words):
self.stop_words = stop_words
from nltk.stem import WordNetLemmatizer
self.lemmatizer = WordNetLemmatizer()
def __call__(self, doc):
tokens = word_tokenize(doc)
filtered_tok = []
for tok in tokens:
if tok not in stop_words:
tok = re.sub('\W+','', tok) #Punctuation strip
tmp = self.lemmatizer.lemmatize(tok)
if len(tmp) >= 2:
filtered_tok.append(tmp)
return filtered_tok
###Output
_____no_output_____
###Markdown
Create Clan Text - Tokenize and LemmatizePrep some data. The "second half" of the dataframe is what we can use with the Word2Vec prediction models - we have cleaned up lists of tokens as well as translating the targets to 1 and 0.
###Code
tok = lemmaTokenizer(stop_words)
df["clean_text"] = df["text"].apply(lambda x: tok(x))
df["target2"] = pd.get_dummies(df["target"], drop_first=True)
df.head()
###Output
_____no_output_____
###Markdown
Create Word2VecWe can train our Word2Vec model with our cleaned up data. This will have Word2Vec do its magic behind the scenes and perform the training. W2V works in one of two ways, which are roughly opposites of each other, when doing this training: Continuous Bag of Words: looks at a window around each target word to try to predict surrounding words. Skip-Gram: looks at words surrounding target to try to predict it. We'll revisit the details of this stuff later on when we look at neural networks, since W2V is a neural network algorithm, it will make more sense in context. Note: this training is not making a model that we are using to make predictions. This is training inside the W2V algorithm to generate representations of our tokens.
###Code
# create Word2vec model
model = Word2Vec(df['clean_text'],min_count=1, vector_size=200, sg=1)
#min_count=1 means word should be present at least across all documents,
#if min_count=2 means if the word is present less than 2 times across all the documents then we shouldn't consider it
#combination of word and its vector
w2v = dict(zip(model.wv.index_to_key, model.wv.vectors))
#for converting sentence to vectors/numbers from word vectors result by Word2Vec
class MeanEmbeddingVectorizer(object):
def __init__(self, word2vec):
self.word2vec = word2vec
# if a text is empty we should return a vector of zeros
# with the same dimensionality as all the other vectors
self.dim = len(next(iter(word2vec.values())))
def fit(self, X, y):
return self
def transform(self, X):
return np.array([
np.mean([self.word2vec[w] for w in words if w in self.word2vec]
or [np.zeros(self.dim)], axis=0)
for words in X
])
###Output
_____no_output_____
###Markdown
Word2Vec ModelEach word in the vocabulary now has a vector representing it - of size 200. We can make a dataframe and see each token in our text and its vector representation. This vector is the internal representation of each token that is generated by Word2Vec. This is how the algorithm calculates things like similarity...
###Code
tmp = pd.DataFrame(w2v)
vectors = model.wv
tmp.head()
###Output
_____no_output_____
###Markdown
SimilarityOne of the things that Word2Vec allows us to do is to look at the similarity of words. This similarity is calculated via the cosine distance of the vectors. Cosine similarity is a technique to calculate the distance between two vectors - smaller distance, more similar. Once the vectors are derived by in the training process, these similarity calculations are pretty easy and quick. Note: the similarites here are calculated by the values derived from our trained model. So they are based on the relationships in our text. Word2Vec and other NLP packages also commonly have pretrained models that can be downloaded that are based on large amounts of text. Words may be represented very differently in those vs whatever we train here - the more data we have, the more consistent they'll be; the more "unique" our text is, the more different it will be.
###Code
#Find the most similar word to anything in our vocabulary
vectors.most_similar("think")[0:3]
# We can also see how similar different words are.
vectors.similarity("think", "determine")
###Output
_____no_output_____
###Markdown
Make PredictionsWe can take our actual data now and transform it through the Word2Vec model that we've made. This will generate our smaller feature set that we can build our models from.One of the things that the MeanEmbeddingVectorizer does is to collapse the data down to those 200 dimensions in the vector.
###Code
#SPLITTING THE TRAINING DATASET INTO TRAINING AND VALIDATION
# Split data - using the new dataframe parts that we cleaned up.
X_train, X_test, y_train, y_test = train_test_split(df["clean_text"],df["target2"])
#Word2vec
# Fit and transform
modelw = MeanEmbeddingVectorizer(w2v)
X_train_vectors_w2v = modelw.transform(X_train)
X_test_vectors_w2v = modelw.transform(X_test)
X_train_vectors_w2v.shape
###Output
_____no_output_____
###Markdown
Build ModelWe can now use the new data to make predictions.
###Code
# Make predictions
lr_w2v = RandomForestClassifier()
lr_w2v.fit(X_train_vectors_w2v, y_train) #model
#Predict y value for test dataset
y_predict = lr_w2v.predict(X_test_vectors_w2v)
y_prob = lr_w2v.predict_proba(X_test_vectors_w2v)[:,1]
print(classification_report(y_test,y_predict))
print('Confusion Matrix:',confusion_matrix(y_test, y_predict))
fpr, tpr, thresholds = roc_curve(y_test, y_prob)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.97 0.99 0.98 1211
1 0.94 0.80 0.86 182
accuracy 0.97 1393
macro avg 0.96 0.89 0.92 1393
weighted avg 0.97 0.97 0.97 1393
Confusion Matrix: [[1202 9]
[ 37 145]]
AUC: 0.9668855092059057
###Markdown
Exercise - Word2VecUse the newsgroup data and Word2Vec to make predictions.
###Code
# Prepare datsets and Tokenize
tok = lemmaTokenizer(stop_words)
X_w2v_news_train = [tok(x) for x in data_train.data]
X_w2v_news_test = [tok(x) for x in data_test.data]
y_train_news = data_train.target
y_test_news = data_test.target
# create Word2vec model
model_news = Word2Vec(X_w2v_news_train, min_count=1, vector_size=200)
w2v_news = dict(zip(model_news.wv.index_to_key, model_news.wv.vectors))
#Word2vec
# Fit and transform
model_news_w = MeanEmbeddingVectorizer(w2v_news)
X_train_vectors_w2v_news = modelw.transform(X_w2v_news_train)
X_val_vectors_w2v_news = modelw.transform(X_w2v_news_test)
X_train_vectors_w2v.shape
# Make predictions
news_clf = RandomForestClassifier()
news_clf.fit(X_train_vectors_w2v_news, y_train_news) #model
#Predict y value for test dataset
y_predict_news = news_clf.predict(X_val_vectors_w2v_news)
y_prob_news = news_clf.predict_proba(X_val_vectors_w2v_news)[:,1]
print(classification_report(y_test_news,y_predict_news))
print('Confusion Matrix:',confusion_matrix(y_test_news, y_predict_news))
fpr, tpr, thresholds = roc_curve(y_test_news, y_prob_news)
roc_auc = auc(fpr, tpr)
print('AUC:', roc_auc)
###Output
precision recall f1-score support
0 0.55 0.63 0.59 319
1 0.43 0.35 0.39 251
accuracy 0.51 570
macro avg 0.49 0.49 0.49 570
weighted avg 0.50 0.51 0.50 570
Confusion Matrix: [[202 117]
[163 88]]
AUC: 0.5174162285029162
|
Predictive_model.ipynb | ###Markdown
Data Science Challenge - Model CreationBy Shyam Balagurumurthy Viswanathan Q4 - Build a predictive model for tip as a percentage of the total fareAs part of the challenge, we will use data collected by the New York City Taxi and Limousine commission about "Green" Taxis. We are using NYC Taxi and Limousine trip record data: (http://www.nyc.gov/html/tlc/html/about/trip_record_data.shtml). We will build a model to preWe will build a model to predict the 'Tip_percentage' provided on each trip.
###Code
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split, GridSearchCV
from sklearn.ensemble import RandomForestRegressor
from sklearn.preprocessing import Imputer, StandardScaler
from sklearn.pipeline import Pipeline
import dill
import os
import geopy.distance as geo
from sklearn.metrics import mean_squared_error, r2_score
import warnings
from sklearn.base import BaseEstimator, TransformerMixin
from sklearn.linear_model import ElasticNet
import json
import ast
def calculate_distance(x):
"""Return great circle distance of pickup and dropoff point"""
return(geo.great_circle((x.Pickup_latitude,x.Pickup_longitude),(x.Dropoff_latitude,x.Dropoff_longitude)).miles)
class DataPrep(BaseEstimator, TransformerMixin):
def fit(self, X, y =None):
return self
def transform(self, X):
"""Perform Feature Engineering and handle NAN"""
# Drop the column as it is NA
X = pd.DataFrame(X.drop('Ehail_fee',axis=1))
X['dates'] = pd.to_datetime(X.lpep_pickup_datetime)
# Day of ride
X['day'] = X['dates'].dt.day
# Hour of ride
X['hour'] = X['dates'].dt.hour
# Day of week ride
X['dayofweek'] = X['dates'].dt.dayofweek
# Convet to categorical variables and convert missing values to NA
X.VendorID = X.VendorID.astype('category',categories=[1, 2],ordered=False)
X.RateCodeID = X.RateCodeID.astype('category',categories=[1, 2, 3, 4, 5, 6],ordered=False)
X.Store_and_fwd_flag = X.Store_and_fwd_flag.astype('category',categories=['Y','N'],ordered=False)
X.Payment_type = X.Payment_type.astype('category',categories=[1, 2, 3, 4, 5, 6],ordered=False)
X.Trip_type = X.Trip_type.astype('category',categories=[1, 2],ordered=False)
# Drop uncessary columns
X.drop(configstore['drop_cols'],axis=1,inplace=True)
#print(X.info())
print("Data preparation completed successfully")
return X
class DataFrameImputer(TransformerMixin):
def __init__(self):
"""Perform Imputation for categorical variables"""
self.model = Imputer(strategy='median')
def fit(self, X, y=None):
#Fit the model on categorical missing cols
self.model.fit(X[configstore['categorical_cols']])
return self
def transform(self, X, y=None):
#Transform the model and convert it to dummies
X[configstore['categorical_cols']]= self.model.transform(X[configstore['categorical_cols']])
X_new = pd.get_dummies(X,drop_first=True,prefix_sep='_',columns=['VendorID','Store_and_fwd_flag','RateCodeID','Payment_type','Trip_type'])
#print(X_new.info())
print("Data Imputation on categorical variables completed successfully")
return X_new
# Main function which loads the datasets and calls other functions
if __name__=="__main__":
warnings.filterwarnings('ignore')
#Get year, month
year = str(input("Enter the year(4 digits): ")).zfill(4)
month = str(input("Enter the month number: ")).zfill(2)
url = 'https://s3.amazonaws.com/nyc-tlc/trip+data/green_tripdata_'+year+'-'+month+'.csv'
try:
df = pd.read_csv(url,sep=',')
except:
print("Error in inputs. Downloading default dataset of year 2015 and September month.")
df = pd.read_csv('https://s3.amazonaws.com/nyc-tlc/trip+data/green_tripdata_2015-09.csv',sep=',')
with open('./config/config.json','r') as f:
configstore = json.load(f)
print("Dataset and Config loaded successfully")
try:
df.columns = configstore['all_cols']
tip =((df['Tip_amount']/df['Total_amount']).round(2))*100
tip[tip.isna()]=0
df['Tip_percent']= tip
# Split the data between Train and test dataset. Have given a test size of 20%. Random split, or datewise split
if ast.literal_eval(configstore['normal_split']):
X_train, X_test, y_train, y_test = train_test_split(df.drop(['Tip_percent'], axis=1), df['Tip_percent'],test_size=configstore['split_size'], random_state=40)
else:
# Split around 20% of data
n = df.shape[0]
train_size= configstore['split_size']
X_train, X_test, y_train, y_test = df.drop(['Tip_percent'], axis=1).iloc[:int(n*train_size)] , df.drop(['Tip_percent'], axis=1).iloc[int(n*train_size):], \
df['Tip_percent'][:int(n*train_size)], df['Tip_percent'][int(n*train_size):]
print("Train/Test split performed successfully")
X_train['Tip_percent'] = y_train
print("Tip percentage calculated")
if ast.literal_eval(configstore['less_totals']):
#Totals less than 0 are removed. Tip % amount cant be calculated
X_train = X_train[X_train.Total_amount >0]
if ast.literal_eval(configstore['less_distance']):
#Distance less than or equal to 0 are removed. Need valid distance for a trip
X_train = X_train[X_train.Trip_distance > 0]
if ast.literal_eval(configstore['outliers']):
# Distance calculated from latitude/long for trip distance equal to 0
new_trip_distance = pd.Series(round(X_train[np.logical_and(X_train.Trip_distance == 0,~ np.logical_or(X_train.Pickup_longitude == 0,X_train.Dropoff_longitude == 0))]\
.apply(calculate_distance,axis=1),2),name='Trip_distance')
X_train.update(new_trip_distance)
if ast.literal_eval(configstore['correct_distance']):
#Removing the outliers which are wrong data for this dataset
try:
X_train.drop([X_train[X_train['Trip_distance'] >= mean + 100*sd].index.values[0]],inplace=True)
print('Records more than thresold miles are dropped')
except:
print('No records more than threshold miles')
# Perfrom split after cleaning the Training dataset
y_train = X_train['Tip_percent']
X_train.drop(['Tip_percent'],axis=1,inplace=True)
# Steps to be performed for pipeline
steps = [('clean_dataset',DataPrep()),('imputing',DataFrameImputer()),\
('scaler',StandardScaler()),#()
('regr',RandomForestRegressor())]
# Grid search parameters
parameters = [{'regr':[RandomForestRegressor()], 'regr__n_estimators':[30],'regr__random_state':[40]}]
# Sklearn pipeline
pl = Pipeline(steps)
# Gridsearch CV on the dataset not used due to memory limitations
#model = GridSearchCV(pl,parameters,cv=configstore['CV'],n_jobs=-1)
#model.fit(X_train,y_train)
# Fitting the model using pipeline
pl.fit(X_train,y_train)
# Performing prediction using the pipeline
y_pred = pl.predict(X_test)
#Store the model for future usage
if not os.path.exists('./generated_files'):
os.makedirs('./generated_files')
with open("./generated_files/model.pkl",'wb') as model_file:
dill.dump(pl,model_file)
print("Model output file stored as Pickle!")
print("Mean Squared Error: {}".format(mean_squared_error(y_test,y_pred)))
print("R-squared: {}".format(r2_score(y_test,y_pred)))
except:
print("Sorry, error while execution.")
###Output
Enter the year(4 digits): 2015
Enter the month number: 9
Dataset and Config loaded successfully
Train/Test split performed successfully
Tip percentage calculated
No records more than threshold miles
Data preparation completed successfully
Data Imputation on categorical variables completed successfully
Data preparation completed successfully
Data Imputation on categorical variables completed successfully
Model output file stored as Pickle!
Mean Squared Error: 0.01212023305439051
R-squared: 0.9998478634304127
|
Predictive Modeling Approach for Employee Salaries Estimations Based on Linear Regression Analysis.ipynb | ###Markdown
Predictive Modeling Approach for Employee Salaries Estimations Based on Linear Regression Analysis 1. IntroductionIn this notebook we will explore the linear regression model for learning predictive modeling. Linear Regression is a very simple machine learning algorithm for finding the relationship between two continuous variables.In this study, we have determined the linear relationship between the numbers of years experience of the employee in the company and the salary of an employee in the current time. 2. Import the Libraries 2.1. NumPy Numpy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
###Code
import numpy as np
###Output
_____no_output_____
###Markdown
2.2. PandasPandas is a software library written for the Python programming language for data processing, data manipulation and data analysis. In particular, it offers data structures and operations for manipulating numerical tables and time series.
###Code
import pandas as pd
###Output
_____no_output_____
###Markdown
2.3. MatplotlibMatplotlib is a Python 2D plotting library which produces publication quality figures in a variety of hardcopy formats and interactive environments across platforms.
###Code
import matplotlib.pyplot as plt
###Output
_____no_output_____
###Markdown
3. Import the datasetSalary_Data.csv file is obtained from the Kaggle.com athttps://www.kaggle.com/karthickveerakumar/salary-data-simple-linear-regression/version/1
###Code
dataset = pd.read_csv('Salary_Data.csv')
###Output
_____no_output_____
###Markdown
3.1. Loading the dataset in numpy matrix
###Code
X = dataset.iloc[:,:-1].values
y = dataset.iloc[:,-1].values
###Output
_____no_output_____
###Markdown
3.2. Dataset columnsSalary_Data.csv file consists of 2 columns, first one is "YearsExperience" and is used as independent variable "X" and second one is "Salary" used as dependent variable "y".
###Code
print(dataset)
###Output
YearsExperience Salary
0 1.1 39343.0
1 1.3 46205.0
2 1.5 37731.0
3 2.0 43525.0
4 2.2 39891.0
5 2.9 56642.0
6 3.0 60150.0
7 3.2 54445.0
8 3.2 64445.0
9 3.7 57189.0
10 3.9 63218.0
11 4.0 55794.0
12 4.0 56957.0
13 4.1 57081.0
14 4.5 61111.0
15 4.9 67938.0
16 5.1 66029.0
17 5.3 83088.0
18 5.9 81363.0
19 6.0 93940.0
20 6.8 91738.0
21 7.1 98273.0
22 7.9 101302.0
23 8.2 113812.0
24 8.7 109431.0
25 9.0 105582.0
26 9.5 116969.0
27 9.6 112635.0
28 10.3 122391.0
29 10.5 121872.0
###Markdown
4. Split the dataset into training and testing set When you’re working on a model and want to train it, you obviously have a dataset. But after training, we have to test the model on some test dataset. For this, we need a dataset which is different from the training set you used earlier. But it might not always be possible to have so much data during the development phase.In such cases, the obviously solution is to split the dataset you have into two sets, one for training and the other for testing; and you do this before you start training your model.
###Code
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
print(X_train)
print(y_train)
print(X_test)
print(y_test)
###Output
[ 37731. 122391. 57081. 63218. 116969. 109431.]
###Markdown
5. Linear Regression Model we import our linear regression model from sklearn library. Sklearn is a free software machine learning library for the Python programming language. It features various classification, regression and clustering algorithms.
###Code
from sklearn.linear_model import LinearRegression
###Output
_____no_output_____
###Markdown
5.1. Initialisation of linear regressionCreate an object called linear_model of Linear Regression class and fit the training data into it.
###Code
linear_model = LinearRegression()
###Output
_____no_output_____
###Markdown
5.2. Inserting the train data in model for training purpose
###Code
linear_model.fit(X_train,y_train)
###Output
_____no_output_____
###Markdown
5.3. Prediction ResultsSince the model has been trained with the training data now we will do predictions with the testing data. Y_pred will give the predicted values of test dataset.
###Code
y_pred = linear_model.predict(X_test)
print(y_pred)
###Output
[ 40748.96184072 122699.62295594 64961.65717022 63099.14214487
115249.56285456 107799.50275317]
###Markdown
5.4. Variance regression score function
###Code
from sklearn.metrics import explained_variance_score
explained_variance_score(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.5. Mean absolute error regression loss
###Code
from sklearn.metrics import mean_absolute_error
mean_absolute_error(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.6. Mean squared error regression loss
###Code
from sklearn.metrics import mean_squared_error
mean_squared_error(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.7. Mean squared logarithmic error regression loss
###Code
from sklearn.metrics import mean_squared_log_error
mean_squared_log_error(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.8. Median absolute error regression loss
###Code
from sklearn.metrics import median_absolute_error
median_absolute_error(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.9. R2 (coefficient of determination) regression score function
###Code
from sklearn.metrics import r2_score
r2_score(y_test, y_pred)
###Output
_____no_output_____
###Markdown
5.10. Plotting the graph for training data
###Code
plt.scatter(X_train,y_train, color= 'red')
plt.plot(X_train, linear_model.predict(X_train), color= 'blue' )
plt.title('Salary Prediction')
plt.xlabel('Years Experience')
plt.ylabel('Salary')
plt.show()
###Output
_____no_output_____
###Markdown
5.11. Plotting the graph for testing data
###Code
plt.scatter(X_test, y_test, color='red')
plt.plot(X_test, y_pred, color='blue')
plt.title("Salary Prediction")
plt.xlabel('Years Experience')
plt.ylabel('Salary')
plt.show()
###Output
_____no_output_____ |
experiment_CCstep.ipynb | ###Markdown
Response to hyperpolarising square current injection
###Code
import numpy as np
from matplotlib import pyplot as plt
%matplotlib inline
plt.rcParams['figure.figsize'] = [10, 5]
from neuron import h, gui
import Model
model = Model.without_ih()
# Some special place
middle_Bouton = model.boutons[model.axNum//2]
# Checking topology
s = h.Shape()
s.show(False)
s.color(2, sec=middle_Bouton) # color middle_Bouton red
# h.topology()
pre_interval = 1000.
sim_interval = 1000.
stim = h.IClamp(middle_Bouton(0.5))
stim.delay=pre_interval + 95 # stimulus delay in ms
stim.dur=200 # stimulus duration in ms
stim.amp=-0.1 # stimulus amplitude in nA
# Record voltage and time
v = h.Vector() # Membrane potential vector [mV]
t = h.Vector() # Time stamp vector [ms]
v.record(middle_Bouton(0.5)._ref_v)
t.record(h._ref_t)
h.tstop = pre_interval + sim_interval
h.run()
t.add(-pre_interval) # Discard the first 1000ms to equilibrate the membrane potential
fig = plt.figure()
plt.xlabel('time (ms)')
plt.ylabel('mV')
plt.plot(t, v, color='black')
plt.xlim((0,sim_interval))
plt.show()
###Output
_____no_output_____ |
2_automl/ml_data_prep/clusters_regions_monthly_data_prep.ipynb | ###Markdown
with mask
###Code
ds = xr.merge(dd[k][feature_ml[k]] for k in dd)\
.groupby('time.month').mean(dim=["time"]).rename({"month":"time"}).where(mask["mask"])
loc_name = list(r_mask)
df_train_ls = []
df_test_ls = []
for loc in loc_name:
# df_train
train_tmp = ds.sel(time=ds.time.isin([1,2,3,5,6,7,9,10,11,12]))\
.where(r_mask[loc]).to_dataframe().reset_index().dropna()
train_tmp["region"] = loc
df_train_ls.append(train_tmp.copy())
# df_test
test_tmp = ds.sel(time=ds.time.isin([4,8]))\
.where(r_mask[loc]).to_dataframe().reset_index().dropna()
test_tmp["region"] = loc
df_test_ls.append(test_tmp.copy())
train = pd.concat(df_train_ls)
test = pd.concat(df_test_ls)
del df_train_ls, df_test_ls
gc.collect()
print("shape of train:", train.shape)
print("shape of test:", test.shape)
###Output
shape of train: (39230, 42)
shape of test: (7846, 42)
###Markdown
save and load
###Code
# save
train.to_parquet('../data/c_r_monthly_train.gzip', compression='gzip')
test.to_parquet('../data/c_r_monthly_test.gzip', compression='gzip')
print("shape of train:", train.shape)
print("shape of test:", test.shape)
# load
train_l = pd.read_parquet('../data/c_r_monthly_train.gzip')
test_l = pd.read_parquet('../data/c_r_monthly_test.gzip')
print("shape of train_l:", train_l.shape)
print("shape of test_l:", test_l.shape)
# test
np.testing.assert_array_equal(train[feature_ls].values, train_l[feature_ls].values)
np.testing.assert_array_equal(test[feature_ls].values, test_l[feature_ls].values)
print("passed the test!")
###Output
shape of train: (39230, 42)
shape of test: (7846, 42)
shape of train_l: (39230, 42)
shape of test_l: (7846, 42)
passed the test!
|
examples/performance/00_overview.ipynb | ###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[y][z]/h_y - 6.66666667e-1F*r0[y + 1][z]/h_y + 6.66666667e-1F*r0[y + 3][z]/h_y - 8.33333333e-2F*r0[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r0)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
float (*r1)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r1[x + 2][y][z]/h_y - 6.66666667e-1F*r1[x + 2][y + 1][z]/h_y + 6.66666667e-1F*r1[x + 2][y + 3][z]/h_y - 8.33333333e-2F*r1[x + 2][y + 4][z]/h_y + 8.33333333e-2F*r0[x][y + 2][z]/h_x - 6.66666667e-1F*r0[x + 1][y + 2][z]/h_x + 6.66666667e-1F*r0[x + 3][y + 2][z]/h_x - 8.33333333e-2F*r0[x + 4][y + 2][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
free(r1);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 2 + 2][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r1[y][z]/h_y - 6.66666667e-1F*r1[y + 1][z]/h_y + 6.66666667e-1F*r1[y + 3][z]/h_y - 8.33333333e-2F*r1[y + 4][z]/h_y + 8.33333333e-2F*r0[x][y][z]/h_x - 6.66666667e-1F*r0[x + 1][y][z]/h_x + 6.66666667e-1F*r0[x + 3][y][z]/h_x - 8.33333333e-2F*r0[x + 4][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` whose divisor is a constant expression has a cost of 1.* A `/` whose divisor is not a constant expression has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-min-cost=31` makes the tensor temporary produced by `op7_omp` disappear. 30 is indeed the minimum cost such that the targeted sub-expression becomes an optimization candidate.```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* The cost of integer arithmetic for array indexing is always zero => 0.* Three `+` (or `-`) => 3* Four `/` by `h_y`, a constant symbol => 4* Four two-way `*` with operands `(1/h_y, u)` => 8So in total we have 15 operations. For a sub-expression to be optimizable away by CIRE, the resulting saving in operation count must be at least twice the `cire-mincost-sops` value OR there must be no increase in working set size. Here there is an increase in working set size -- the new tensor temporary -- and at the same time the threshold is set to 31, so the compiler decides not to optimize away the sub-expression. In short, the rational is that the saving in operation count does not justify the introduction of a new tensor temporary.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 30}))
print_kernel(op8_b1_omp)
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[y][z]/h_y - 6.66666667e-1F*r0[y + 1][z]/h_y + 6.66666667e-1F*r0[y + 3][z]/h_y - 8.33333333e-2F*r0[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
The `cire-mincost-inv` optionAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r0)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size + 2 + 2][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[x][y][z]/h_y - 6.66666667e-1F*r0[x][y + 1][z]/h_y + 6.66666667e-1F*r0[x][y + 3][z]/h_y - 8.33333333e-2F*r0[x][y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y0_blk0_size + 2 + 2][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr1);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= y0_blk0 + y0_blk0_size + 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[ys][z] - r1[ys + 4][z]) + 6.66666667e-1F*(-r1[ys + 1][z] + r1[ys + 3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r1` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r1` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[ysi][z] = (8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]))/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[yr0][z] - r1[yr2][z]) + 6.66666667e-1F*(r1[yr1][z] - r1[yr3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r1` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr1[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr0_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr0 = (float**) pr0_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r1 = 1.0F/h_y;
u[t1][x + 4][y + 4][z + 4] = r1*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r0[ys + 1][z]) + (-2*r1*r0[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r0` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr0_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr0 = (float**) pr0_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = 2*(-r0[ys][z] + r0[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)pr1);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[y][z] - r1[y + 4][z]) + 6.66666667e-1F*(-r1[y + 1][z] + r1[y + 3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r0_vec, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float (*r1)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
float (*r2)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r2, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
free(r1);
free(r2);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r1)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r1_vec;
float (*restrict r2)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r2_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]))/h_x;
r2[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r0_vec, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r1)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r1_vec;
float (*restrict r2)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r2_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((8.33333333e-2F*(r2[x + 2][y][z] - r2[x + 2][y + 4][z]) + 6.66666667e-1F*(-r2[x + 2][y + 1][z] + r2[x + 2][y + 3][z]))/h_y + (8.33333333e-2F*(r1[x][y + 2][z] - r1[x + 4][y + 2][z]) + 6.66666667e-1F*(-r1[x + 1][y + 2][z] + r1[x + 3][y + 2][z]))/h_x)*r0[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is two-fold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= MIN(x0_blk1 + x0_blk1_size - 1, x_M); x += 1)
{
for (int y = y0_blk1; y <= MIN(y0_blk1 + y0_blk1_size - 1, y_M); y += 1)
{
for (int z = z0_blk1; z <= MIN(z0_blk1 + z0_blk1_size - 1, z_M); z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2_vec;
posix_memalign((void**)&pr2_vec, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[y0_blk0_size + 4][z_size]));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size + 1, y_M + 2); y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(r0_vec);
free(pr2_vec);
return 0;
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body.allocs[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2_vec;
posix_memalign((void**)&pr2_vec, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[y_size + 4][z_size]));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(r0_vec);
free(pr2_vec);
return 0;
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float *r3_vec;
posix_memalign((void**)&r3_vec, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float *r4_vec;
posix_memalign((void**)&r4_vec, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m - 2; x0_blk0 <= x_M + 2; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m - 2; y0_blk0 <= y_M + 2; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M + 2); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M + 2); y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= MIN(x1_blk0 + x1_blk0_size - 1, x_M); x += 1)
{
for (int y = y1_blk0; y <= MIN(y1_blk0 + y1_blk0_size - 1, y_M); y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0_vec);
free(r3_vec);
free(r4_vec);
return 0;
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance.utils import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Always use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=2)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -40,7 +40,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications. In larger, more complex examples, the impact of factorization can be quite significant.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, the co-existance with other optimizations (e.g., blocking, vectorization), and much more. All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r3`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[y][z]/h_y + r5[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r7);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r5` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r7)[y_size + 1][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r8)[y_size + 1][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[x + 1][y + 1][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
r8[x + 1][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r8[x + 1][y][z]/h_y + r8[x + 1][y + 1][z]/h_y - r7[x][y + 1][z]/h_x + r7[x + 1][y + 1][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r7);
free(r8);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r8`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r8`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r8)[y_size][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size][z_size]));
float **r10;
posix_memalign((void**)&r10, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r10[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r8[x + 1][y][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r10[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r7[y][z]/h_y + r7[y + 1][z]/h_y - r8[x][y][z]/h_x + r8[x + 1][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r10[tid]);
}
free(r8);
free(r10);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that option, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 50.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 107}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
We observe how setting `cire-min-cost=107` makes the tensor temporary produced by `op7_omp` disappear. 106 is indeed the cost of the targeted sub-expression```-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y```* One `+` => 1* Two `/` by `h_y` => 50* One two-way `*` with operands `(-1/h_y, u)` => 1* One two-way `*` with operands `(1/h_y, u)` => 1* The sub-expression appears `n=2` times with shifted indices (precisely what CIRE looks for), so total cost to be multiplied by `n`.Note: the cost of integer arithmetic for array indexing is deliberately ignored.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 11}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
Hang on! This doesn't make any sense, does it? We just decreased `cire-mincost-sops` to `11` and we still get the same code as `op8_omp`. Well, yeah... we lied about one tiny yet important detail for ease of explanation. It's true the cost of division is generally 25, but there's one special case: when the divisor is made up of invariant values (i.e., read-only constants) the cost of division becomes 1. In this case we divide by `h_y`, that is the grid spacing along the `y` dimension, which the compiler knows to be read-only, so the actual cost of the expression is 10. This tweak reflects the fact that invariant sub-expressions are ultimately lifted out of inner loops. The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **r5;
posix_memalign((void**)&r5, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r5[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r5[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r5[tid]);
}
free(r5);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=7` or lower. `depth=7` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 9}))
print_kernel(op10_omp)
###Output
float **r4;
posix_memalign((void**)&r4, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r4[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y)/h_y + (-(-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 6][z + 2]/h_y + u[t0][x + 2][y + 7][z + 2]/h_y)/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r4[tid]);
}
free(r4);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to the `r1` temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r5)[y_size + 1][z_size];
posix_memalign((void**)&r5, 64, sizeof(float[x_size][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[x][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[x][y][z]/h_y + r5[x][y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r5);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y0_blk0_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[ys][z] + r7[ys + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r7` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 1`, while the second one at `y0_blk0`). Further, the size of `r7` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = -1; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = 0)
{
for (int yy = yii, ysi = (yy + ys + 1)%(2); yy <= 0; yy += 1, ysi = (yy + ys + 1)%(2))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ysi][z] = (-u[t0][x + 2][yy + y + 3][z + 2] + u[t0][x + 2][yy + y + 4][z + 2])/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r7[yr0][z] - r7[yr1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r7` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `r9[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[2][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`ys0`, `ys1`) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r4_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r4 = (float**) r4_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r3 = 1.0/h_y;
u[t1][x + 2][y + 2][z + 2] = r3*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r2[ys + 1][z]) + (-2*r3*r2[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r2` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r3_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r3 = (float**) r3_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r3[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = 2*(-r2[ys][z] + r2[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(1) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[y][z] + r7[y + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r10)[y_size + 1][z_size];
posix_memalign((void**)&r10, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r9)[y_size + 1][z_size];
posix_memalign((void**)&r9, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
free(r10);
free(r9);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[x + 1][y + 1][z] = (-u[t0][x + 3][y + 2][z + 2] + u[t0][x + 4][y + 2][z + 2])/h_x;
r10[x + 1][y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((-r10[x + 1][y][z] + r10[x + 1][y + 1][z])/h_y + (-r9[x][y + 1][z] + r9[x + 1][y + 1][z])/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r1)[y_size + 4][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
free(r1);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, float r1, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[y0_blk0_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,r1,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr2);
bf0(f_vec,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,r1,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr2);
bf0(f_vec,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,r1,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr2);
bf0(f_vec,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,r1,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr2);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(r0);
free(pr2);
return 0;
}
void bf0(struct dataobj *restrict f_vec, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, float r1, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr2 = (float**) pr2_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= y0_blk0 + y0_blk0_size + 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, float r1, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr2 = (float**) pr2_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, float r1, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[y_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,(float *)r0,u_vec,x_size,y_size,z_size,r1,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)pr2);
bf0(f_vec,(float *)r0,u_vec,x_size,y_size,z_size,r1,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)pr2);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(r0);
free(pr2);
return 0;
}
void bf0(struct dataobj *restrict f_vec, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, float r1, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr2 = (float**) pr2_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(float *restrict r3_vec, float *restrict r4_vec, struct dataobj *restrict u_vec, float r1, float r2, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, float *restrict r0_vec, float *restrict r3_vec, float *restrict r4_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, float r1, float r2, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float (*r3)[y_size + 4][z_size];
posix_memalign((void**)&r3, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r4)[y_size + 4][z_size];
posix_memalign((void**)&r4, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0((float *)r3,(float *)r4,u_vec,r1,r2,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0((float *)r3,(float *)r4,u_vec,r1,r2,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf0((float *)r3,(float *)r4,u_vec,r1,r2,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0((float *)r3,(float *)r4,u_vec,r1,r2,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,(float *)r0,(float *)r3,(float *)r4,u_vec,x_size,y_size,z_size,r1,r2,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,(float *)r0,(float *)r3,(float *)r4,u_vec,x_size,y_size,z_size,r1,r2,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,(float *)r0,(float *)r3,(float *)r4,u_vec,x_size,y_size,z_size,r1,r2,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,(float *)r0,(float *)r3,(float *)r4,u_vec,x_size,y_size,z_size,r1,r2,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
free(r3);
free(r4);
return 0;
}
void bf0(float *restrict r3_vec, float *restrict r4_vec, struct dataobj *restrict u_vec, float r1, float r2, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, float *restrict r0_vec, float *restrict r3_vec, float *restrict r4_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, float r1, float r2, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[y][z]/h_y - 6.66666667e-1F*r0[y + 1][z]/h_y + 6.66666667e-1F*r0[y + 3][z]/h_y - 8.33333333e-2F*r0[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r1)[y_size + 4][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r1[x + 2][y][z]/h_y - 6.66666667e-1F*r1[x + 2][y + 1][z]/h_y + 6.66666667e-1F*r1[x + 2][y + 3][z]/h_y - 8.33333333e-2F*r1[x + 2][y + 4][z]/h_y + 8.33333333e-2F*r0[x][y + 2][z]/h_x - 6.66666667e-1F*r0[x + 1][y + 2][z]/h_x + 6.66666667e-1F*r0[x + 3][y + 2][z]/h_x - 8.33333333e-2F*r0[x + 4][y + 2][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
free(r1);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r1[y][z]/h_y - 6.66666667e-1F*r1[y + 1][z]/h_y + 6.66666667e-1F*r1[y + 3][z]/h_y - 8.33333333e-2F*r1[y + 4][z]/h_y + 8.33333333e-2F*r0[x][y][z]/h_x - 6.66666667e-1F*r0[x + 1][y][z]/h_x + 6.66666667e-1F*r0[x + 3][y][z]/h_x - 8.33333333e-2F*r0[x + 4][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` whose divisor is a constant expression has a cost of 1.* A `/` whose divisor is not a constant expression has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-min-cost=31` makes the tensor temporary produced by `op7_omp` disappear. 30 is indeed the minimum cost such that the targeted sub-expression becomes an optimization candidate.```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* The cost of integer arithmetic for array indexing is always zero => 0.* Three `+` (or `-`) => 3* Four `/` by `h_y`, a constant symbol => 4* Four two-way `*` with operands `(1/h_y, u)` => 8So in total we have 15 operations. For a sub-expression to be optimizable away by CIRE, the resulting saving in operation count must be at least twice the `cire-mincost-sops` value OR there must be no increase in working set size. Here there is an increase in working set size -- the new tensor temporary -- and at the same time the threshold is set to 31, so the compiler decides not to optimize away the sub-expression. In short, the rational is that the saving in operation count does not justify the introduction of a new tensor temporary.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 30}))
print_kernel(op8_b1_omp)
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[y][z]/h_y - 6.66666667e-1F*r0[y + 1][z]/h_y + 6.66666667e-1F*r0[y + 3][z]/h_y - 8.33333333e-2F*r0[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
The `cire-mincost-inv` optionAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0[x][y][z]/h_y - 6.66666667e-1F*r0[x][y + 1][z]/h_y + 6.66666667e-1F*r0[x][y + 3][z]/h_y - 8.33333333e-2F*r0[x][y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y0_blk0_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr1);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= y0_blk0 + y0_blk0_size + 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[ys][z] - r1[ys + 4][z]) + 6.66666667e-1F*(-r1[ys + 1][z] + r1[ys + 3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r1` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r1` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[ysi][z] = (8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]))/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[yr0][z] - r1[yr2][z]) + 6.66666667e-1F*(r1[yr1][z] - r1[yr3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r1` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr1[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr0_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr0 = (float**) pr0_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r1 = 1.0F/h_y;
u[t1][x + 4][y + 4][z + 4] = r1*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r0[ys + 1][z]) + (-2*r1*r0[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r0` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr0_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr0 = (float**) pr0_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = 2*(-r0[ys][z] + r0[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)pr1);
bf0(f_vec,h_y,(float *)r0,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)pr1);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r0_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr1_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr1 = (float**) pr1_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r1[y][z] - r1[y + 4][z]) + 6.66666667e-1F*(-r1[y + 1][z] + r1[y + 3][z]))*r0[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r0_vec, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float (*r1)[y_size + 4][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r2)[y_size + 4][z_size];
posix_memalign((void**)&r2, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r1,(float *)r2,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r0,(float *)r1,(float *)r2,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
free(r1);
free(r2);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r1)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r1_vec;
float (*restrict r2)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r2_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]))/h_x;
r2[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r0_vec, float *restrict r1_vec, float *restrict r2_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r1)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r1_vec;
float (*restrict r2)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r2_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((8.33333333e-2F*(r2[x + 2][y][z] - r2[x + 2][y + 4][z]) + 6.66666667e-1F*(-r2[x + 2][y + 1][z] + r2[x + 2][y + 3][z]))/h_y + (8.33333333e-2F*(r1[x][y + 2][z] - r1[x + 4][y + 2][z]) + 6.66666667e-1F*(-r1[x + 1][y + 2][z] + r1[x + 3][y + 2][z]))/h_x)*r0[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance.utils import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Always use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=2)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications. In larger, more complex examples, the impact of factorization can be quite significant.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, the co-existance with other optimizations (e.g., blocking, vectorization), and much more. All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r3`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[y][z]/h_y + r5[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r7);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r5` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r7)[y_size + 1][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r8)[y_size + 1][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[x + 1][y + 1][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
r8[x + 1][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r8[x + 1][y][z]/h_y + r8[x + 1][y + 1][z]/h_y - r7[x][y + 1][z]/h_x + r7[x + 1][y + 1][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r7);
free(r8);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r8`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r8`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r8)[y_size][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size][z_size]));
float **r10;
posix_memalign((void**)&r10, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r10[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r8[x + 1][y][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r10[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r7[y][z]/h_y + r7[y + 1][z]/h_y - r8[x][y][z]/h_x + r8[x + 1][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r10[tid]);
}
free(r8);
free(r10);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that option, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 50.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 107}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-min-cost=107` makes the tensor temporary produced by `op7_omp` disappear. 106 is indeed the cost of the targeted sub-expression```-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y```* One `+` => 1* Two `/` by `h_y` => 50* One two-way `*` with operands `(-1/h_y, u)` => 1* One two-way `*` with operands `(1/h_y, u)` => 1* The sub-expression appears `n=2` times with shifted indices (precisely what CIRE looks for), so total cost to be multiplied by `n`.Note: the cost of integer arithmetic for array indexing is deliberately ignored.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 11}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Hang on! This doesn't make any sense, does it? We just decreased `cire-mincost-sops` to `11` and we still get the same code as `op8_omp`. Well, yeah... we lied about one tiny yet important detail for ease of explanation. It's true the cost of division is generally 25, but there's one special case: when the divisor is made up of invariant values (i.e., read-only constants) the cost of division becomes 1. In this case we divide by `h_y`, that is the grid spacing along the `y` dimension, which the compiler knows to be read-only, so the actual cost of the expression is 10. This tweak reflects the fact that invariant sub-expressions are ultimately lifted out of inner loops. The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **r5;
posix_memalign((void**)&r5, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r5[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r5[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r5[tid]);
}
free(r5);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=7` or lower. `depth=7` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 9}))
print_kernel(op10_omp)
###Output
float **r4;
posix_memalign((void**)&r4, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r4[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y)/h_y + (-(-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 6][z + 2]/h_y + u[t0][x + 2][y + 7][z + 2]/h_y)/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r4[tid]);
}
free(r4);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to the `r1` temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r5)[y_size + 1][z_size];
posix_memalign((void**)&r5, 64, sizeof(float[x_size][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[x][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[x][y][z]/h_y + r5[x][y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r5);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y0_blk0_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[ys][z] + r7[ys + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r7` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 1`, while the second one at `y0_blk0`). Further, the size of `r7` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = -1; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = 0)
{
for (int yy = yii, ysi = (yy + ys + 1)%(2); yy <= 0; yy += 1, ysi = (yy + ys + 1)%(2))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ysi][z] = (-u[t0][x + 2][yy + y + 3][z + 2] + u[t0][x + 2][yy + y + 4][z + 2])/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r7[yr0][z] - r7[yr1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r7` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `r9[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[2][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`ys0`, `ys1`) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r4_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r4 = (float**) r4_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r3 = 1.0/h_y;
u[t1][x + 2][y + 2][z + 2] = r3*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r2[ys + 1][z]) + (-2*r3*r2[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r2` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r3_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r3 = (float**) r3_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r3[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = 2*(-r2[ys][z] + r2[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(1) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[y][z] + r7[y + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r10)[y_size + 1][z_size];
posix_memalign((void**)&r10, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r9)[y_size + 1][z_size];
posix_memalign((void**)&r9, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
free(r10);
free(r9);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[x + 1][y + 1][z] = (-u[t0][x + 3][y + 2][z + 2] + u[t0][x + 4][y + 2][z + 2])/h_x;
r10[x + 1][y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((-r10[x + 1][y][z] + r10[x + 1][y + 1][z])/h_y + (-r9[x][y + 1][z] + r9[x + 1][y + 1][z])/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is two-fold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= MIN(x0_blk1 + x0_blk1_size - 1, x_M); x += 1)
{
for (int y = y0_blk1; y <= MIN(y0_blk1 + y0_blk1_size - 1, y_M); y += 1)
{
for (int z = z0_blk1; z <= MIN(z0_blk1 + z0_blk1_size - 1, z_M); z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*r0[x][y][z]*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
unsigned long * size;
unsigned long * npsize;
unsigned long * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, struct dataobj *restrict u_vec, const float h_y, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads, struct profiler * timers)
{
float **pr2_vec;
posix_memalign((void**)(&pr2_vec),64,nthreads*sizeof(float*));
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,z_size*(y0_blk0_size + 4)*sizeof(float));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size + 1, y_M + 2); y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(pr2_vec);
free(r0_vec);
return 0;
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + yy + 2][z + 4] - u[t0][x + 4][y + yy + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + yy + 3][z + 4] + u[t0][x + 4][y + yy + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body.allocs[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,5*z_size*sizeof(float));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
unsigned long * size;
unsigned long * npsize;
unsigned long * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, struct dataobj *restrict u_vec, const float h_y, const int time_M, const int time_m, const int x_M, const int x_m, const int x_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads, struct profiler * timers)
{
float **pr2_vec;
posix_memalign((void**)(&pr2_vec),64,nthreads*sizeof(float*));
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,z_size*(y_size + 4)*sizeof(float));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(pr2_vec);
free(r0_vec);
return 0;
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
unsigned long * size;
unsigned long * npsize;
unsigned long * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, struct dataobj *restrict u_vec, const float h_x, const float h_y, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
float *r3_vec;
posix_memalign((void**)(&r3_vec),64,z_size*(x_size + 4)*(y_size + 4)*sizeof(float));
float *r4_vec;
posix_memalign((void**)(&r4_vec),64,z_size*(x_size + 4)*(y_size + 4)*sizeof(float));
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m - 2; x0_blk0 <= x_M + 2; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m - 2; y0_blk0 <= y_M + 2; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M + 2); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M + 2); y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= MIN(x1_blk0 + x1_blk0_size - 1, x_M); x += 1)
{
for (int y = y1_blk0; y <= MIN(y1_blk0 + y1_blk0_size - 1, y_M); y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0_vec);
free(r3_vec);
free(r4_vec);
return 0;
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance.utils import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Always use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=2)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications. In larger, more complex examples, the impact of factorization can be quite significant.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, the co-existance with other optimizations (e.g., blocking, vectorization), and much more. All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r3`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[y][z]/h_y + r5[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r7);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r5` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r7)[y_size + 1][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r8)[y_size + 1][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[x + 1][y + 1][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
r8[x + 1][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r8[x + 1][y][z]/h_y + r8[x + 1][y + 1][z]/h_y - r7[x][y + 1][z]/h_x + r7[x + 1][y + 1][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r7);
free(r8);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r8`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r8`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r8)[y_size][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size][z_size]));
float **r10;
posix_memalign((void**)&r10, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r10[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r8[x + 1][y][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r10[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r7[y][z]/h_y + r7[y + 1][z]/h_y - r8[x][y][z]/h_x + r8[x + 1][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r10[tid]);
}
free(r8);
free(r10);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that option, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 50.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 107}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-min-cost=107` makes the tensor temporary produced by `op7_omp` disappear. 106 is indeed the cost of the targeted sub-expression```-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y```* One `+` => 1* Two `/` by `h_y` => 50* One two-way `*` with operands `(-1/h_y, u)` => 1* One two-way `*` with operands `(1/h_y, u)` => 1* The sub-expression appears `n=2` times with shifted indices (precisely what CIRE looks for), so total cost to be multiplied by `n`.Note: the cost of integer arithmetic for array indexing is deliberately ignored.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 11}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Hang on! This doesn't make any sense, does it? We just decreased `cire-mincost-sops` to `11` and we still get the same code as `op8_omp`. Well, yeah... we lied about one tiny yet important detail for ease of explanation. It's true the cost of division is generally 25, but there's one special case: when the divisor is made up of invariant values (i.e., read-only constants) the cost of division becomes 1. In this case we divide by `h_y`, that is the grid spacing along the `y` dimension, which the compiler knows to be read-only, so the actual cost of the expression is 10. This tweak reflects the fact that invariant sub-expressions are ultimately lifted out of inner loops. The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **r5;
posix_memalign((void**)&r5, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r5[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r5[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r5[tid]);
}
free(r5);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=7` or lower. `depth=7` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 9}))
print_kernel(op10_omp)
###Output
float **r4;
posix_memalign((void**)&r4, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r4[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y)/h_y + (-(-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 6][z + 2]/h_y + u[t0][x + 2][y + 7][z + 2]/h_y)/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r4[tid]);
}
free(r4);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to the `r1` temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r5)[y_size + 1][z_size];
posix_memalign((void**)&r5, 64, sizeof(float[x_size][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[x][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[x][y][z]/h_y + r5[x][y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r5);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y0_blk0_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[ys][z] + r7[ys + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r7` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 1`, while the second one at `y0_blk0`). Further, the size of `r7` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = -1; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = 0)
{
for (int yy = yii, ysi = (yy + ys + 1)%(2); yy <= 0; yy += 1, ysi = (yy + ys + 1)%(2))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[ysi][z] = (-u[t0][x + 2][yy + y + 3][z + 2] + u[t0][x + 2][yy + y + 4][z + 2])/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r7[yr0][z] - r7[yr1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r7` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `r9[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[2][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`ys0`, `ys1`) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r4_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r4 = (float**) r4_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r3 = 1.0/h_y;
u[t1][x + 2][y + 2][z + 2] = r3*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r2[ys + 1][z]) + (-2*r3*r2[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r2` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r3_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r3 = (float**) r3_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r3[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = 2*(-r2[ys][z] + r2[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r9;
posix_memalign((void**)&r9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r9[tid], 64, sizeof(float[y_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)r9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r9[tid]);
}
free(r1);
free(r9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r9 = (float**) r9_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r9[tid];
#pragma omp for collapse(1) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r7[y][z] + r7[y + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r10)[y_size + 1][z_size];
posix_memalign((void**)&r10, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r9)[y_size + 1][z_size];
posix_memalign((void**)&r9, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r10,(float *)r9,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r10,(float *)r9,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
free(r10);
free(r9);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[x + 1][y + 1][z] = (-u[t0][x + 3][y + 2][z + 2] + u[t0][x + 4][y + 2][z + 2])/h_x;
r10[x + 1][y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r10_vec, float *restrict r9_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r10)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r10_vec;
float (*restrict r9)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r9_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((-r10[x + 1][y][z] + r10[x + 1][y + 1][z])/h_y + (-r9[x][y + 1][z] + r9[x + 1][y + 1][z])/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) - 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + 6.66666667e-1F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]) - 8.33333333e-2F*r0*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r7`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **pr7;
posix_memalign((void**)&pr7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr7[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr7[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r7[y][z]/h_y - 6.66666667e-1F*r7[y + 1][z]/h_y + 6.66666667e-1F*r7[y + 3][z]/h_y - 8.33333333e-2F*r7[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr7[tid]);
}
free(pr7);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r7` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r11)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r11, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
float (*r12)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r12, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r11[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r12[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r12[x + 2][y][z]/h_y - 6.66666667e-1F*r12[x + 2][y + 1][z]/h_y + 6.66666667e-1F*r12[x + 2][y + 3][z]/h_y - 8.33333333e-2F*r12[x + 2][y + 4][z]/h_y + 8.33333333e-2F*r11[x][y + 2][z]/h_x - 6.66666667e-1F*r11[x + 1][y + 2][z]/h_x + 6.66666667e-1F*r11[x + 3][y + 2][z]/h_x - 8.33333333e-2F*r11[x + 4][y + 2][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r11);
free(r12);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r12`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r12`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r12)[y_size][z_size];
posix_memalign((void**)&r12, 64, sizeof(float[x_size + 2 + 2][y_size][z_size]));
float **pr11;
posix_memalign((void**)&pr11, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr11[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r12[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r11)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr11[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r11[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r11[y][z]/h_y - 6.66666667e-1F*r11[y + 1][z]/h_y + 6.66666667e-1F*r11[y + 3][z]/h_y - 8.33333333e-2F*r11[y + 4][z]/h_y + 8.33333333e-2F*r12[x][y][z]/h_x - 6.66666667e-1F*r12[x + 1][y][z]/h_x + 6.66666667e-1F*r12[x + 3][y][z]/h_x - 8.33333333e-2F*r12[x + 4][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr11[tid]);
}
free(r12);
free(pr11);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` whose divisor is a constant expression has a cost of 1.* A `/` whose divisor is not a constant expression has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-min-cost=31` makes the tensor temporary produced by `op7_omp` disappear. 30 is indeed the minimum cost such that the targeted sub-expression becomes an optimization candidate.```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* The cost of integer arithmetic for array indexing is always zero => 0.* Three `+` (or `-`) => 3* Four `/` by `h_y`, a constant symbol => 4* Four two-way `*` with operands `(1/h_y, u)` => 8So in total we have 15 operations. For a sub-expression to be optimizable away by CIRE, the resulting saving in operation count must be at least twice the `cire-mincost-sops` value OR there must be no increase in working set size. Here there is an increase in working set size -- the new tensor temporary -- and at the same time the threshold is set to 31, so the compiler decides not to optimize away the sub-expression. In short, the rational is that the saving in operation count does not justify the introduction of a new tensor temporary.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 30}))
print_kernel(op8_b1_omp)
###Output
float **pr7;
posix_memalign((void**)&pr7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr7[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r7)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr7[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r7[y][z]/h_y - 6.66666667e-1F*r7[y + 1][z]/h_y + 6.66666667e-1F*r7[y + 3][z]/h_y - 8.33333333e-2F*r7[y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr7[tid]);
}
free(pr7);
###Markdown
The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
u1 = TimeFunction(name='u', grid=grid, space_order=2) # Using lower space order for smaller expressions
eq = Eq(u1.forward, f**2*sin(f)*u1.dy.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **pr3;
posix_memalign((void**)&pr3, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr3[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr3[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr3[tid]);
}
free(pr3);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=7` or lower. `depth=7` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 9}))
print_kernel(op10_omp)
###Output
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y)/h_y + (-(-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 6][z + 2]/h_y + u[t0][x + 2][y + 7][z + 2]/h_y)/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(pr2);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y)/h_y - 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y)/h_y + 6.66666667e-1F*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y)/h_y - 8.33333333e-2F*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r7)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size][y_size + 2 + 2][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r7[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (8.33333333e-2F*r7[x][y][z]/h_y - 6.66666667e-1F*r7[x][y + 1][z]/h_y + 6.66666667e-1F*r7[x][y + 3][z]/h_y - 8.33333333e-2F*r7[x][y + 4][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r7);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **pr9;
posix_memalign((void**)&pr9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr9[tid], 64, sizeof(float[y0_blk0_size + 2 + 2][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)pr9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)pr9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr9[tid]);
}
free(r1);
free(pr9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr9 = (float**) pr9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r9)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr9[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= y0_blk0 + y0_blk0_size + 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r9[ys][z] - r9[ys + 4][z]) + 6.66666667e-1F*(-r9[ys + 1][z] + r9[ys + 3][z]))*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r9` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r9` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr9 = (float**) pr9_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r9)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr9[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[ysi][z] = (8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]))/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r9[yr0][z] - r9[yr2][z]) + 6.66666667e-1F*(r9[yr1][z] - r9[yr3][z]))*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r9` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `r11[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr9[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `cire-maxalias` optionLet's consider the following variation of our running example, in which the outer `y` derivative now comprises several terms, other than just `u.dy`.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy)
op16_omp = Operator(eq, opt=('advanced', {'openmp': True}))
print_kernel(op16_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr2 = (float**) pr2_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
float r3 = 1.0/h_y;
u[t1][x + 4][y + 4][z + 4] = r3*(2*(f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*r2[ys + 1][z]) + (-2*r3*r2[ys][z])*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1]);
}
}
}
}
}
}
}
###Markdown
By paying close attention to the generated code, we see that the `r2` temporary only stores the `u.dy` component, while the `2*f*f` term is left intact in the loop nest computing `u`. This is due to a heuristic applied by the Devito compiler: in a derivative, only the sub-expressions representing additions -- and therefore inner derivatives -- are used as CIRE candidates. The logic behind this heuristic is that of minimizing the number of required temporaries at the price of potentially leaving some redundancies on the table. One can disable this heuristic via the `cire-maxalias` option.
###Code
op16_b0_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
print_kernel(op16_b0_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int y0_blk0_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr2_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr2 = (float**) pr2_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = (f[x + 1][y + 2][z + 1]*f[x + 1][y + 2][z + 1])*(8.33333333e-2F*(u[t0][x + 4][y + 3][z + 4] - u[t0][x + 4][y + 7][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 4][z + 4] + u[t0][x + 4][y + 6][z + 4]))/((h_y*h_y));
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = 2*(-r2[ys][z] + r2[ys + 1][z]);
}
}
}
}
}
}
}
###Markdown
Now we have a "fatter" temporary, and that's good -- but if, instead, we had had an `Operator` such as the one below, the gain from a reduced operation count might be outweighed by the presence of more temporaries, which means larger working set and increased memory traffic.
###Code
eq = Eq(u.forward, (2*f*f*u.dy).dy + (3*f*u.dy).dy)
op16_b1_omp = Operator(eq, opt=('advanced', {'openmp': True}))
op16_b2_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-maxalias': True}))
# print_kernel(op16_b1_omp) # Uncomment to see generated code with one temporary but more flops
# print_kernel(op16_b2_omp) # Uncomment to see generated code with two temporaries but fewer flops
###Output
_____no_output_____
###Markdown
The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr9_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **pr9;
posix_memalign((void**)&pr9, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr9[tid], 64, sizeof(float[y_size + 2 + 2][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)pr9);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)pr9);
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr9[tid]);
}
free(r1);
free(pr9);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict pr9_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **pr9 = (float**) pr9_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r9)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr9[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r9[y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r9[y][z] - r9[y + 4][z]) + 6.66666667e-1F*(-r9[y + 1][z] + r9[y + 3][z]))*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r13_vec, float *restrict r14_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r13_vec, float *restrict r14_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r13)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r13, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
float (*r14)[y_size + 2 + 2][z_size];
posix_memalign((void**)&r14, 64, sizeof(float[x_size + 2 + 2][y_size + 2 + 2][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
bf0(h_x,h_y,(float *)r13,(float *)r14,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r13,(float *)r14,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 2,x_m - 2,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r13,(float *)r14,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,y0_blk0_size,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 2,y_m - 2,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r13,(float *)r14,u_vec,t0,(x_M - x_m + 5)%(x0_blk0_size),x_M + 2,x_M - (x_M - x_m + 5)%(x0_blk0_size) + 3,x_size,(y_M - y_m + 5)%(y0_blk0_size),y_M + 2,y_M - (y_M - y_m + 5)%(y0_blk0_size) + 3,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r13,(float *)r14,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r13,(float *)r14,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r13,(float *)r14,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r13,(float *)r14,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r1);
free(r13);
free(r14);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r13_vec, float *restrict r14_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r13)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r13_vec;
float (*restrict r14)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r14_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r13[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]))/h_x;
r14[x + 2][y + 2][z] = (8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]))/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r13_vec, float *restrict r14_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r13)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r13_vec;
float (*restrict r14)[y_size + 2 + 2][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 2 + 2][z_size]) r14_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((8.33333333e-2F*(r14[x + 2][y][z] - r14[x + 2][y + 4][z]) + 6.66666667e-1F*(-r14[x + 2][y + 1][z] + r14[x + 2][y + 3][z]))/h_y + (8.33333333e-2F*(r13[x][y + 2][z] - r13[x + 4][y + 2][z]) + 6.66666667e-1F*(-r13[x + 1][y + 2][z] + r13[x + 3][y + 2][z]))/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is two-fold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= MIN(x0_blk1 + x0_blk1_size - 1, x_M); x += 1)
{
for (int y = y0_blk1; y <= MIN(y0_blk1 + y0_blk1_size - 1, y_M); y += 1)
{
for (int z = z0_blk1; z <= MIN(z0_blk1 + z0_blk1_size - 1, z_M); z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*r0[x][y][z]*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', 'simd', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float **pr2_vec;
posix_memalign((void**)(&pr2_vec),64,nthreads*sizeof(float*));
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,z_size*(y0_blk0_size + 4)*sizeof(float));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size + 1, y_M + 2); y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(pr2_vec);
free(r0_vec);
return 0;
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + yy + 2][z + 4] - u[t0][x + 4][y + yy + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + yy + 3][z + 4] + u[t0][x + 4][y + yy + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body.allocs[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,5*z_size*sizeof(float));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float **pr2_vec;
posix_memalign((void**)(&pr2_vec),64,nthreads*sizeof(float*));
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)(&(pr2_vec[tid])),64,z_size*(y_size + 4)*sizeof(float));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(pr2_vec);
free(r0_vec);
return 0;
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)(&r0_vec),64,x_size*y_size*z_size*sizeof(float));
float *r3_vec;
posix_memalign((void**)(&r3_vec),64,z_size*(x_size + 4)*(y_size + 4)*sizeof(float));
float *r4_vec;
posix_memalign((void**)(&r4_vec),64,z_size*(x_size + 4)*(y_size + 4)*sizeof(float));
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m - 2; x0_blk0 <= x_M + 2; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m - 2; y0_blk0 <= y_M + 2; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M + 2); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M + 2); y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= MIN(x1_blk0 + x1_blk0_size - 1, x_M); x += 1)
{
for (int y = y1_blk0; y <= MIN(y1_blk0 + y1_blk0_size - 1, y_M); y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0_vec);
free(r3_vec);
free(r4_vec);
return 0;
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance.utils import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Always use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=2)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -40,7 +40,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications. In larger, more complex examples, the impact of factorization can be quite significant.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, the co-existance with other optimizations (e.g., blocking, vectorization), and much more. All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r3`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **r5;
posix_memalign((void**)&r5, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r5[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r5[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r3[y][z]/h_y + r3[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r5[tid]);
}
free(r5);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r3` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary is avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r6)[y_size + 1][z_size];
posix_memalign((void**)&r6, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r5)[y_size + 1][z_size];
posix_memalign((void**)&r5, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r6[x + 1][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
r5[x + 1][y + 1][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r6[x + 1][y][z]/h_y + r6[x + 1][y + 1][z]/h_y - r5[x][y + 1][z]/h_x + r5[x + 1][y + 1][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r6);
free(r5);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r6`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r6`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r6)[y_size][z_size];
posix_memalign((void**)&r6, 64, sizeof(float[x_size + 1][y_size][z_size]));
float **r8;
posix_memalign((void**)&r8, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r8[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r6[x + 1][y][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r8[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[y][z]/h_y + r5[y + 1][z]/h_y - r6[x][y][z]/h_x + r6[x + 1][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r8[tid]);
}
free(r6);
free(r8);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that option, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 50.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 107}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
We observe how setting `cire-min-cost=107` makes the tensor temporary produced by `op7_omp` disappear. 106 is indeed the cost of the targeted sub-expression```-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y```* One `+` => 1* Two `/` by `h_y` => 50* One two-way `*` with operands `(-1/h_y, u)` => 1* One two-way `*` with operands `(1/h_y, u)` => 1* The sub-expression appears `n=2` times with shifted indices (precisely what CIRE looks for), so total cost to be multiplied by `n`.Note: the cost of integer arithmetic for array indexing is deliberately ignored.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 11}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
Hang on! This doesn't make any sense, does it? We just decreased `cire-mincost-sops` to `11` and we still get the same code as `op8_omp`. Well, yeah... we lied about one tiny yet important detail for ease of explanation. It's true the cost of division is generally 25, but there's one special case: when the divisor is made up of invariant values (i.e., read-only constants) the cost of division becomes 1. In this case we divide by `h_y`, that is the grid spacing along the `y` dimension, which the compiler knows to be read-only, so the actual cost of the expression is 10. This tweak reflects the fact that invariant sub-expressions are ultimately lifted out of inner loops. The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **r6;
posix_memalign((void**)&r6, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r6[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r6[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r6[tid]);
}
free(r6);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=5` or lower. `depth=5` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 7}))
print_kernel(op10_omp)
###Output
float **r4;
posix_memalign((void**)&r4, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r4[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r4[tid]);
}
free(r4);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to the `r1` temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r3)[y_size + 1][z_size];
posix_memalign((void**)&r3, 64, sizeof(float[x_size][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[x][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r3[x][y][z]/h_y + r3[x][y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r3);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. All optimizations togetherThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y0_blk0_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r7);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r1);
free(r7);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r7 = (float**) r7_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0, xs = 0; x <= x0_blk0 + x0_blk0_size - 1; x += 1, xs += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r5[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r5[ys][z] + r5[ys + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r5` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 1`, while the second one at `y0_blk0`). Further, the size of `r5` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `advanced-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
op15_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op15_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)r7);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r1);
free(r7);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r7 = (float**) r7_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(1) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r5[y][z] + r5[y + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op15_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op15_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r7)[y_size + 1][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r8)[y_size + 1][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
free(r7);
free(r8);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r7)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r7_vec;
float (*restrict r8)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r8_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r8[x + 1][y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
r7[x + 1][y + 1][z] = (-u[t0][x + 3][y + 2][z + 2] + u[t0][x + 4][y + 2][z + 2])/h_x;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r7)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r7_vec;
float (*restrict r8)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r8_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((-r8[x + 1][y][z] + r8[x + 1][y + 1][z])/h_y + (-r7[x][y + 1][z] + r7[x + 1][y + 1][z])/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is twofold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance. Others, whose impact varies across different `Operator`'s, are instead to be enabled through specific flags.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`'s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance.utils import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Always use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from sympy import sin
from devito import Eq, Grid, Operator, Function, TimeFunction
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=2)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int t0, const int t1, const int x0_blk0_size, const int x0_blk1_size, const int x_M, const int x_m, const int y0_blk0_size, const int y0_blk1_size, const int y_M, const int y_m, const int z0_blk0_size, const int z0_blk1_size, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0 || z0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= x0_blk0 + x0_blk0_size - 1; x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= y0_blk0 + y0_blk0_size - 1; y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= z0_blk0 + z0_blk0_size - 1; z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= x0_blk1 + x0_blk1_size - 1; x += 1)
{
for (int y = y0_blk1; y <= y0_blk1 + y0_blk1_size - 1; y += 1)
{
for (int z = z0_blk1; z <= z0_blk1 + z0_blk1_size - 1; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r1[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*pow(f[x + 1][y + 1][z + 1], 2)*r1[x][y][z];
}
}
}
}
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -40,7 +40,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0/h_y;
+ u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications. In larger, more complex examples, the impact of factorization can be quite significant.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (-r0*(-r0*u[t0][x + 2][y + 2][z + 2] + r0*u[t0][x + 2][y + 3][z + 2]) + r0*(-r0*u[t0][x + 2][y + 3][z + 2] + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -41,7 +41,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0/h_y;
- u[t1][x + 2][y + 2][z + 2] = (r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r0*(-r0*(-u[t0][x + 2][y + 2][z + 2]) - r0*u[t0][x + 2][y + 3][z + 2]) + r0*(r0*(-u[t0][x + 2][y + 3][z + 2]) + r0*u[t0][x + 2][y + 4][z + 2]))*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, the co-existance with other optimizations (e.g., blocking, vectorization), and much more. All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r3`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what taking a derivative via finite differences produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **r5;
posix_memalign((void**)&r5, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r5[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r5[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r3[y][z]/h_y + r3[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r5[tid]);
}
free(r5);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r3` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary is avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r5)[y_size + 1][z_size];
posix_memalign((void**)&r5, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r6)[y_size + 1][z_size];
posix_memalign((void**)&r6, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[x + 1][y + 1][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
r6[x + 1][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r6[x + 1][y][z]/h_y + r6[x + 1][y + 1][z]/h_y - r5[x][y + 1][z]/h_x + r5[x + 1][y + 1][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r5);
free(r6);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r6`, now be a three-dimensional temporary when we know already it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r6`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r6)[y_size][z_size];
posix_memalign((void**)&r6, 64, sizeof(float[x_size + 1][y_size][z_size]));
float **r8;
posix_memalign((void**)&r8, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r8[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m - 1; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r6[x + 1][y][z] = -u[t0][x + 3][y + 2][z + 2]/h_x + u[t0][x + 4][y + 2][z + 2]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r8[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r5[y][z]/h_y + r5[y + 1][z]/h_y - r6[x][y][z]/h_x + r6[x + 1][y][z]/h_x)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r8[tid]);
}
free(r6);
free(r8);
###Markdown
The `cire-mincost-sops` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that option, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, we can tell Devito what the minimum cost of a sub-expression should be in order to be a CIRE candidate. The _cost_ is an integer number based on the operation count and the type of operations:* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 25.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 50.The `cire-mincost-sops` option can be used to control the minimum cost of CIRE candidates.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 107}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
We observe how setting `cire-min-cost=107` makes the tensor temporary produced by `op7_omp` disappear. 106 is indeed the cost of the targeted sub-expression```-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y```* One `+` => 1* Two `/` by `h_y` => 50* One two-way `*` with operands `(-1/h_y, u)` => 1* One two-way `*` with operands `(1/h_y, u)` => 1* The sub-expression appears `n=2` times with shifted indices (precisely what CIRE looks for), so total cost to be multiplied by `n`.Note: the cost of integer arithmetic for array indexing is deliberately ignored.Next, we try again with a smaller `cire-mincost-sops`.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mincost-sops': 11}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
Hang on! This doesn't make any sense, does it? We just decreased `cire-mincost-sops` to `11` and we still get the same code as `op8_omp`. Well, yeah... we lied about one tiny yet important detail for ease of explanation. It's true the cost of division is generally 25, but there's one special case: when the divisor is made up of invariant values (i.e., read-only constants) the cost of division becomes 1. In this case we divide by `h_y`, that is the grid spacing along the `y` dimension, which the compiler knows to be read-only, so the actual cost of the expression is 10. This tweak reflects the fact that invariant sub-expressions are ultimately lifted out of inner loops. The `cire-repeats-sops` optionHigher order derivatives -- typically higher than order two -- may lead to proportionately larger sum-of-products. Consider the following variant of the running example
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy.dy.dy)
op9_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op9_omp)
###Output
float **r6;
posix_memalign((void**)&r6, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r6[tid], 64, sizeof(float[y_size + 1 + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r3)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r6[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M + 1; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[y + 1][z] = -(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-r3[y][z]/h_y + r3[y + 1][z]/h_y)/h_y + (-r3[y + 1][z]/h_y + r3[y + 2][z]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r6[tid]);
}
free(r6);
###Markdown
At first glance, it's the same story as before, with a two-dimensional tensor temporary extracted and computed in a separate loop nest. By paying closer attention, however, we may note that there are now four occurrences of `r3` in the expression computing `u`, while before, in `op7_omp` for instance, we had only two. Devito seeks for a compromise between compilation speed and run-time performance, so it runs by default searching for redundancies across sum-of-products of `depth=5` or lower. `depth=5` sum-of-products are typically the by-product of second-order derivatives, which is what we find in many PDEs. There are other reasons behind such default value, which for brevity aren't discussed here. To search for deeper sum-of-products (and, therefore, for redundancies induced by higher order derivatives), one shall use the `cire-repeats-sops` option.
###Code
op10_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-repeats-sops': 7}))
print_kernel(op10_omp)
###Output
float **r4;
posix_memalign((void**)&r4, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r4[tid], 64, sizeof(float[y_size + 1][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r4[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 1][z] = -(-(-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y)/h_y + (-(-u[t0][x + 2][y + 4][z + 2]/h_y + u[t0][x + 2][y + 5][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 5][z + 2]/h_y + u[t0][x + 2][y + 6][z + 2]/h_y)/h_y)/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r2[y][z]/h_y + r2[y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r4[tid]);
}
free(r4);
###Markdown
The `cire-mincost-inv` and `cire-repeats-inv` optionsAkin to sum-of-products, cross-iteration redundancies may be searched across dimension-invariant sub-expressions, typically time-invariants. So, analogously to what we've seen before:
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
For convenience, the `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to the `r1` temporary. By setting a larger value for `cire-mincost-inv`, we avoid a grid-size temporary to be allocated, in exchange for a trascendental function, `sin`, to be computed at each iteration
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mincost-inv': 51}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-(-u[t0][x + 2][y + 2][z + 2]/h_y + u[t0][x + 2][y + 3][z + 2]/h_y)/h_y + (-u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y)/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r3)[y_size + 1][z_size];
posix_memalign((void**)&r3, 64, sizeof(float[x_size][y_size + 1][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r3[x][y + 1][z] = -u[t0][x + 2][y + 3][z + 2]/h_y + u[t0][x + 2][y + 4][z + 2]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (-r3[x][y][z]/h_y + r3[x][y + 1][z]/h_y)*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
}
free(r3);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g., `min-cost`, `cire-mincost-sops`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y0_blk0_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y0_blk0_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M - (y_M - y_m + 1)%(y0_blk0_size),y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,(y_M - y_m + 1)%(y0_blk0_size),y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_M - (y_M - y_m + 1)%(y0_blk0_size) + 1,z_M,z_m,nthreads,(float * *)r7);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r1);
free(r7);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r7 = (float**) r7_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0 - 1, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r5[ys][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y0_blk0, ys = 0; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r5[ys][z] + r5[ys + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r5` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 1`, while the second one at `y0_blk0`). Further, the size of `r5` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions are a function the loop blocking shape.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r7 = (float**) r7_vec;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = -1; y <= y0_blk0 + y0_blk0_size - 1; y += 1, ys += 1, yr0 = (ys + 1)%(2), yr1 = (ys)%(2), yii = 0)
{
for (int yy = yii, ysi = (yy + ys + 1)%(2); yy <= 0; yy += 1, ysi = (yy + ys + 1)%(2))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r5[ysi][z] = (-u[t0][x + 2][yy + y + 3][z + 2] + u[t0][x + 2][yy + y + 4][z + 2])/h_y;
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r5[yr0][z] - r5[yr1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
}
###Markdown
There are two key things to notice here:* The `r5` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `r7[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[2][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`ys0`, `ys1`) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at a each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
op16_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op16_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec);
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float **r7;
posix_memalign((void**)&r7, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&r7[tid], 64, sizeof(float[y_size + 1][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,x0_blk0_size,x_M - (x_M - x_m + 1)%(x0_blk0_size),x_m,y_M,y_m,z_M,z_m,nthreads,(float * *)r7);
bf0(f_vec,h_y,(float *)r1,u_vec,x_size,y_size,z_size,t0,t1,(x_M - x_m + 1)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x0_blk0_size) + 1,y_M,y_m,z_M,z_m,nthreads,(float * *)r7);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(r7[tid]);
}
free(r1);
free(r7);
return 0;
}
void bf0(struct dataobj *restrict f_vec, const float h_y, float *restrict r1_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t0, const int t1, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, float * *restrict r7_vec)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float **r7 = (float**) r7_vec;
if (x0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r5)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) r7[tid];
#pragma omp for collapse(1) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y_m - 1; y <= y_M; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r5[y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(-r5[y][z] + r5[y + 1][z])*r1[x][y][z]/h_y;
}
}
}
}
}
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op16_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op16_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
void bf0(const float h_x, const float h_y, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads);
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads);
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, struct profiler * timers, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*r1)[y_size][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size][y_size][z_size]));
float (*r7)[y_size + 1][z_size];
posix_memalign((void**)&r7, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
float (*r8)[y_size + 1][z_size];
posix_memalign((void**)&r8, 64, sizeof(float[x_size + 1][y_size + 1][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
struct timeval start_section0, end_section0;
gettimeofday(&start_section0, NULL);
/* Begin section0 */
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r1[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
/* End section0 */
gettimeofday(&end_section0, NULL);
timers->section0 += (double)(end_section0.tv_sec-start_section0.tv_sec)+(double)(end_section0.tv_usec-start_section0.tv_usec)/1000000;
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
struct timeval start_section1, end_section1;
gettimeofday(&start_section1, NULL);
/* Begin section1 */
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,x0_blk0_size,x_M - (x_M - x_m + 2)%(x0_blk0_size),x_m - 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,y0_blk0_size,y_M - (y_M - y_m + 2)%(y0_blk0_size),y_m - 1,y_size,z_M,z_m,z_size,nthreads);
bf0(h_x,h_y,(float *)r7,(float *)r8,u_vec,t0,(x_M - x_m + 2)%(x0_blk0_size),x_M,x_M - (x_M - x_m + 2)%(x0_blk0_size) + 1,x_size,(y_M - y_m + 2)%(y0_blk0_size),y_M,y_M - (y_M - y_m + 2)%(y0_blk0_size) + 1,y_size,z_M,z_m,z_size,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,x1_blk0_size,x_M - (x_M - x_m + 1)%(x1_blk0_size),x_m,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,y1_blk0_size,y_M - (y_M - y_m + 1)%(y1_blk0_size),y_m,z_M,z_m,nthreads);
bf1(f_vec,h_x,h_y,(float *)r1,(float *)r7,(float *)r8,u_vec,x_size,y_size,z_size,t1,(x_M - x_m + 1)%(x1_blk0_size),x_M,x_M - (x_M - x_m + 1)%(x1_blk0_size) + 1,(y_M - y_m + 1)%(y1_blk0_size),y_M,y_M - (y_M - y_m + 1)%(y1_blk0_size) + 1,z_M,z_m,nthreads);
/* End section1 */
gettimeofday(&end_section1, NULL);
timers->section1 += (double)(end_section1.tv_sec-start_section1.tv_sec)+(double)(end_section1.tv_usec-start_section1.tv_usec)/1000000;
}
free(r1);
free(r7);
free(r8);
return 0;
}
void bf0(const float h_x, const float h_y, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int t0, const int x0_blk0_size, const int x_M, const int x_m, const int x_size, const int y0_blk0_size, const int y_M, const int y_m, const int y_size, const int z_M, const int z_m, const int z_size, const int nthreads)
{
float (*restrict r7)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r7_vec;
float (*restrict r8)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r8_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x0_blk0_size == 0 || y0_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= x0_blk0 + x0_blk0_size - 1; x += 1)
{
for (int y = y0_blk0; y <= y0_blk0 + y0_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r7[x + 1][y + 1][z] = (-u[t0][x + 3][y + 2][z + 2] + u[t0][x + 4][y + 2][z + 2])/h_x;
r8[x + 1][y + 1][z] = (-u[t0][x + 2][y + 3][z + 2] + u[t0][x + 2][y + 4][z + 2])/h_y;
}
}
}
}
}
}
}
void bf1(struct dataobj *restrict f_vec, const float h_x, const float h_y, float *restrict r1_vec, float *restrict r7_vec, float *restrict r8_vec, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int t1, const int x1_blk0_size, const int x_M, const int x_m, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r1)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r1_vec;
float (*restrict r7)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r7_vec;
float (*restrict r8)[y_size + 1][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 1][z_size]) r8_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
if (x1_blk0_size == 0 || y1_blk0_size == 0)
{
return;
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= x1_blk0 + x1_blk0_size - 1; x += 1)
{
for (int y = y1_blk0; y <= y1_blk0 + y1_blk0_size - 1; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 2][y + 2][z + 2] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*((-r8[x + 1][y][z] + r8[x + 1][y + 1][z])/h_y + (-r7[x][y + 1][z] + r7[x + 1][y + 1][z])/h_x)*r1[x][y][z];
}
}
}
}
}
}
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is two-fold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= MIN(x0_blk1 + x0_blk1_size - 1, x_M); x += 1)
{
for (int y = y0_blk1; y <= MIN(y0_blk1 + y0_blk1_size - 1, y_M); y += 1)
{
for (int z = z0_blk1; z <= MIN(z0_blk1 + z0_blk1_size - 1, z_M); z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2_vec;
posix_memalign((void**)&pr2_vec, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[y0_blk0_size + 4][z_size]));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size + 1, y_M + 2); y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(r0_vec);
free(pr2_vec);
return 0;
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body.allocs[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2_vec;
posix_memalign((void**)&pr2_vec, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2_vec[tid], 64, sizeof(float[y_size + 4][z_size]));
}
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float **pr2 = (float**) pr2_vec;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2_vec[tid]);
}
free(r0_vec);
free(pr2_vec);
return 0;
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float *r0_vec;
posix_memalign((void**)&r0_vec, 64, sizeof(float[x_size][y_size][z_size]));
float *r3_vec;
posix_memalign((void**)&r3_vec, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float *r4_vec;
posix_memalign((void**)&r4_vec, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict r0)[y_size][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size][z_size]) r0_vec;
float (*restrict r3)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r3_vec;
float (*restrict r4)[y_size + 4][z_size] __attribute__ ((aligned (64))) = (float (*)[y_size + 4][z_size]) r4_vec;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m - 2; x0_blk0 <= x_M + 2; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m - 2; y0_blk0 <= y_M + 2; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M + 2); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M + 2); y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= MIN(x1_blk0 + x1_blk0_size - 1, x_M); x += 1)
{
for (int y = y1_blk0; y <= MIN(y1_blk0 + y1_blk0_size - 1, y_M); y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0_vec);
free(r3_vec);
free(r4_vec);
return 0;
}
###Markdown
Performance optimization overviewThe purpose of this tutorial is two-fold* Illustrate the performance optimizations applied to the code generated by an `Operator`.* Describe the options Devito provides to users to steer the optimization process.As we shall see, most optimizations are automatically applied as they're known to systematically improve performance.An Operator has several preset **optimization levels**; the fundamental ones are `noop` and `advanced`. With `noop`, no performance optimizations are introduced. With `advanced`, several flop-reducing and data locality optimization passes are applied. Examples of flop-reducing optimization passes are common sub-expressions elimination and factorization, while examples of data locality optimization passes are loop fusion and cache blocking. Optimization levels in Devito are conceptually akin to the `-O2, -O3, ...` flags in classic C/C++/Fortran compilers.An optimization pass may provide knobs, or **options**, for fine-grained tuning. As explained in the next sections, some of these options are given at compile-time, others at run-time.**\*\* Remark \*\***Parallelism -- both shared-memory (e.g., OpenMP) and distributed-memory (MPI) -- is _by default disabled_ and is _not_ controlled via the optimization level. In this tutorial we will also show how to enable OpenMP parallelism (you'll see it's trivial!). Another mini-guide about parallelism in Devito and related aspects is available [here](https://github.com/devitocodes/devito/tree/master/benchmarks/usera-step-back-configuring-your-machine-for-reliable-benchmarking). **\*\*\*\*** Outline* [API](API)* [Default values](Default-values)* [Running example](Running-example)* [OpenMP parallelism](OpenMP-parallelism)* [The `advanced` mode](The-advanced-mode)* [The `advanced-fsg` mode](The-advanced-fsg-mode) APIThe optimization level may be changed in various ways:* globally, through the `DEVITO_OPT` environment variable. For example, to disable all optimizations on all `Operator`'s, one could run with```DEVITO_OPT=noop python ...```* programmatically, adding the following lines to a program```from devito import configurationconfiguration['opt'] = 'noop'```* locally, as an `Operator` argument```Operator(..., opt='noop')```Local takes precedence over programmatic, and programmatic takes precedence over global.The optimization options, instead, may only be changed locally. The syntax to specify an option is```Operator(..., opt=('advanced', {})```A concrete example (you can ignore the meaning for now) is```Operator(..., opt=('advanced', {'blocklevels': 2})```That is, options are to be specified _together with_ the optimization level (`advanced`). Default valuesBy default, all `Operator`s are run with the optimization level set to `advanced`. So this```Operator(Eq(...))```is equivalent to```Operator(Eq(...), opt='advanced')```and obviously also to```Operator(Eq(...), opt=('advanced', {}))```In virtually all scenarios, regardless of application and underlying architecture, this ensures very good performance -- but not necessarily the very best. MiscThe following functions will be used throughout the notebook for printing generated code.
###Code
from examples.performance import unidiff_output, print_kernel
###Output
_____no_output_____
###Markdown
The following cell is only needed for Continuous Integration. But actually it's also an example of how "programmatic takes precedence over global" (see [API](API) section).
###Code
from devito import configuration
configuration['language'] = 'C'
configuration['platform'] = 'bdw' # Optimize for an Intel Broadwell
configuration['opt-options']['par-collapse-ncores'] = 1 # Maximize use loop collapsing
###Output
_____no_output_____
###Markdown
Running exampleThroughout the notebook we will generate `Operator`'s for the following time-marching `Eq`.
###Code
from devito import Eq, Grid, Operator, Function, TimeFunction, sin
grid = Grid(shape=(80, 80, 80))
f = Function(name='f', grid=grid)
u = TimeFunction(name='u', grid=grid, space_order=4)
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy)
###Output
_____no_output_____
###Markdown
Despite its simplicity, this `Eq` is all we need to showcase the key components of the Devito optimization engine. OpenMP parallelismThere are several ways to enable OpenMP parallelism. The one we use here consists of supplying an _option_ to an `Operator`. The next cell illustrates the difference between two `Operator`'s generated with the `noop` optimization level, but with OpenMP enabled on the latter one.
###Code
op0 = Operator(eq, opt=('noop'))
op0_omp = Operator(eq, opt=('noop', {'openmp': True}))
# print(op0)
# print(unidiff_output(str(op0), str(op0_omp))) # Uncomment to print out the diff only
print_kernel(op0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The OpenMP-ized `op0_omp` `Operator` includes: - the header file `"omp.h"` - a `pragma omp parallel num_threads(nthreads)` directive - a `pragma omp for collapse(...) schedule(dynamic,1)` directive More complex `Operator`'s will have more directives, more types of directives, different iteration scheduling strategies based on heuristics and empirical tuning (e.g., `static` instead of `dynamic`), etc.The reason for `collapse(1)`, rather than `collapse(3)`, boils down to using `opt=('noop', ...)`; if the default `advanced` mode had been used instead, we would see the latter clause.We note how the OpenMP pass introduces a new symbol, `nthreads`. This allows users to explicitly control the number of threads with which an `Operator` is run.```op0_omp.apply(time_M=0) Picks up `nthreads` from the standard environment variable OMP_NUM_THREADSop0_omp.apply(time_M=0, nthreads=2) Runs with 2 threads per parallel loop``` A few optimization options are available for this pass (but not on all platforms, see [here](https://github.com/devitocodes/devito/blob/master/examples/performance/README.md)), though in our experience the default values do a fine job:* `par-collapse-ncores`: use a collapse clause only if the number of available physical cores is greater than this value (default=4).* `par-collapse-work`: use a collapse clause only if the trip count of the collapsable loops is statically known to exceed this value (default=100).* `par-chunk-nonaffine`: a coefficient to adjust the chunk size in non-affine parallel loops. The larger the coefficient, the smaller the chunk size (default=3).* `par-dynamic-work`: use dynamic scheduling if the operation count per iteration exceeds this value. Otherwise, use static scheduling (default=10).* `par-nested`: use nested parallelism if the number of hyperthreads per core is greater than this value (default=2).So, for instance, we could switch to static scheduling by constructing the following `Operator`
###Code
op0_b0_omp = Operator(eq, opt=('noop', {'openmp': True, 'par-dynamic-work': 100}))
print_kernel(op0_b0_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `advanced` modeThe default optimization level in Devito is `advanced`. This mode performs several compilation passes to optimize the `Operator` for computation (number of flops), working set size, and data locality. In the next paragraphs we'll dissect the `advanced` mode to analyze, one by one, some of its key passes. Loop blockingThe next cell creates a new `Operator` that adds loop blocking to what we had in `op0_omp`.
###Code
op1_omp = Operator(eq, opt=('blocking', {'openmp': True}))
# print(op1_omp) # Uncomment to see the *whole* generated code
print_kernel(op1_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
**\*\* Remark \*\***`'blocking'` is **not** an optimization level -- it rather identifies a specific compilation pass. In other words, the `advanced` mode defines an ordered sequence of passes, and `blocking` is one such pass.**\*\*\*\*** The `blocking` pass creates additional loops over blocks. In this simple `Operator` there's just one loop nest, so only a pair of additional loops are created. In more complex `Operator`'s, several loop nests may individually be blocked, whereas others may be left unblocked -- this is decided by the Devito compiler according to certain heuristics. The size of a block is represented by the symbols `x0_blk0_size` and `y0_blk0_size`, which are runtime parameters akin to `nthreads`. By default, Devito applies 2D blocking and sets the default block shape to 8x8. There are two ways to set a different block shape:* passing an explicit value. For instance, below we run with a 24x8 block shape```op1_omp.apply(..., x0_blk0_size=24)```* letting the autotuner pick up a better block shape for us. There are several autotuning modes. A short summary is available [here](https://github.com/devitocodes/devito/wiki/FAQdevito_autotuning)```op1_omp.apply(..., autotune='aggressive')```Loop blocking also provides two optimization options:* `blockinner={False, True}` -- to enable 3D (or any nD, n>2) blocking* `blocklevels={int}` -- to enable hierarchical blocking, to exploit multiple levels of the cache hierarchy In the example below, we construct an `Operator` with six-dimensional loop blocking: the first three loops represent outer blocks, whereas the second three loops represent inner blocks within an outer block.
###Code
op1_omp_6D = Operator(eq, opt=('blocking', {'blockinner': True, 'blocklevels': 2, 'openmp': True}))
# print(op1_omp_6D) # Uncomment to see the *whole* generated code
print_kernel(op1_omp_6D)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int z0_blk0 = z_m; z0_blk0 <= z_M; z0_blk0 += z0_blk0_size)
{
for (int x0_blk1 = x0_blk0; x0_blk1 <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x0_blk1 += x0_blk1_size)
{
for (int y0_blk1 = y0_blk0; y0_blk1 <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y0_blk1 += y0_blk1_size)
{
for (int z0_blk1 = z0_blk0; z0_blk1 <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z0_blk1 += z0_blk1_size)
{
for (int x = x0_blk1; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk1; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1)
{
for (int z = z0_blk1; z <= MIN(z0_blk0 + z0_blk0_size - 1, z_M); z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
}
}
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
SIMD vectorizationDevito enforces SIMD vectorization through OpenMP pragmas.
###Code
op2_omp = Operator(eq, opt=('blocking', 'simd', {'openmp': True}))
# print(op2_omp) # Uncomment to see the generated code
# print(unidiff_output(str(op1_omp), str(op2_omp))) # Uncomment to print out the diff only
###Output
_____no_output_____
###Markdown
Code motionThe `advanced` mode has a code motion pass. In explicit PDE solvers, this is most commonly used to lift expensive time-invariant sub-expressions out of the inner loops. The pass is however quite general in that it is not restricted to the concept of time-invariance -- any sub-expression invariant with respect to a subset of `Dimension`s is a code motion candidate. In our running example, `sin(f)` gets hoisted out of the inner loops since it is determined to be an expensive invariant sub-expression. In other words, the compiler trades the redundant computation of `sin(f)` for additional storage (the `r0[...]` array).
###Code
op3_omp = Operator(eq, opt=('lift', {'openmp': True}))
print_kernel(op3_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r1)*(8.33333333e-2F*r1*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r1*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r1*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r1*u[t0][x + 4][y + 7][z + 4]))*pow(f[x + 1][y + 1][z + 1], 2)*r0[x][y][z];
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
###Markdown
Basic flop-reducing transformationsAmong the simpler flop-reducing transformations applied by the `advanced` mode we find:* "classic" common sub-expressions elimination (CSE),* factorization,* optimization of powersThe cell below shows how the computation of `u` changes by incrementally applying these three passes. First of all, we observe how the reciprocal of the symbolic spacing `h_y` gets assigned to a temporary, `r0`, as it appears in several sub-expressions. This is the effect of CSE.
###Code
op4_omp = Operator(eq, opt=('cse', {'openmp': True}))
print(unidiff_output(str(op0_omp), str(op4_omp)))
###Output
---
+++
@@ -42,7 +42,8 @@
{
for (int z = z_m; z <= z_M; z += 1)
{
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ float r0 = 1.0F/h_y;
+ u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
The factorization pass makes sure `r0` is collected to reduce the number of multiplications.
###Code
op5_omp = Operator(eq, opt=('cse', 'factorize', {'openmp': True}))
print(unidiff_output(str(op4_omp), str(op5_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 1][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 2][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 5][z + 4]) + (-8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 5][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 7][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 8][z + 4]) + (8.33333333e-2F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 1][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 3][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 4][z + 4]) + (6.66666667e-1F*r0)*(8.33333333e-2F*r0*u[t0][x + 4][y + 3][z + 4] - 6.66666667e-1F*r0*u[t0][x + 4][y + 4][z + 4] + 6.66666667e-1F*r0*u[t0][x + 4][y + 6][z + 4] - 8.33333333e-2F*r0*u[t0][x + 4][y + 7][z + 4]))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
###Markdown
Finally, `opt-pows` turns costly `pow` calls into multiplications.
###Code
op6_omp = Operator(eq, opt=('cse', 'factorize', 'opt-pows', {'openmp': True}))
print(unidiff_output(str(op5_omp), str(op6_omp)))
###Output
---
+++
@@ -43,7 +43,7 @@
for (int z = z_m; z <= z_M; z += 1)
{
float r0 = 1.0F/h_y;
- u[t1][x + 4][y + 4][z + 4] = pow(r0, 2)*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
+ u[t1][x + 4][y + 4][z + 4] = (r0*r0)*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(6.94444444e-3F*(u[t0][x + 4][y][z + 4] + u[t0][x + 4][y + 8][z + 4]) + 4.44444444e-1F*(u[t0][x + 4][y + 2][z + 4] + u[t0][x + 4][y + 6][z + 4]) + 1.11111111e-1F*(-u[t0][x + 4][y + 1][z + 4] + u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4] - u[t0][x + 4][y + 7][z + 4]) - 9.02777778e-1F*u[t0][x + 4][y + 4][z + 4])*sin(f[x + 1][y + 1][z + 1]);
}
}
}
###Markdown
Cross-iteration redundancy elimination (CIRE)This is perhaps the most advanced among the optimization passes in Devito. CIRE [1] searches for redundancies across consecutive loop iterations. These are often induced by a mixture of nested, high-order, partial derivatives. The underlying idea is very simple. Consider:```r0 = a[i-1] + a[i] + a[i+1]```at `i=1`, we have```r0 = a[0] + a[1] + a[2]```at `i=2`, we have```r0 = a[1] + a[2] + a[3]```So the sub-expression `a[1] + a[2]` is computed twice, by two consecutive iterations.What makes CIRE complicated is the generalization to arbitrary expressions, the presence of multiple dimensions, the scheduling strategy due to the trade-off between redundant compute and working set, and the co-existance with other optimizations (e.g., blocking, vectorization). All these aspects won't be treated here. What instead we will show is the effect of CIRE in our running example and the optimization options at our disposal to drive the detection and scheduling of the captured redundancies.In our running example, some cross-iteration redundancies are induced by the nested first-order derivatives along `y`. As we see below, these redundancies are captured and assigned to the two-dimensional temporary `r0`.Note: the name `cire-sops` means "Apply CIRE to sum-of-product expressions". A sum-of-product is what a finite difference derivative produces.
###Code
op7_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_omp)
# print(unidiff_output(str(op7_omp), str(op0_omp))) # Uncomment to print out the diff only
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
We note that since there are no redundancies along `x`, the compiler is smart to figure out that `r0` and `u` can safely be computed within the same `x` loop. This is nice -- not only is the reuse distance decreased, but also a grid-sized temporary avoided. The `min-storage` optionLet's now consider a variant of our running example
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op7_b0_omp = Operator(eq, opt=('cire-sops', {'openmp': True}))
print_kernel(op7_b0_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r1)[y_size + 4][z_size];
posix_memalign((void**)&r1, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
r1[x + 2][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y + 2][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y + 2][z] + (8.33333333e-2F/h_x)*r0[x][y + 2][z] + (6.66666667e-1F/h_x)*r0[x + 3][y + 2][z] + (-6.66666667e-1F/h_y)*r1[x + 2][y + 1][z] + (-8.33333333e-2F/h_y)*r1[x + 2][y + 4][z] + (8.33333333e-2F/h_y)*r1[x + 2][y][z] + (6.66666667e-1F/h_y)*r1[x + 2][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
free(r1);
###Markdown
As expected, there are now two temporaries, one stemming from `u.dy.dy` and the other from `u.dx.dx`. A key difference with respect to `op7_omp` here is that both are grid-size temporaries. This might seem odd at first -- why should the `u.dy.dy` temporary, that is `r1`, now be a three-dimensional temporary when we know already, from what seen in the previous cell, it could be a two-dimensional temporary? This is merely a compiler heuristic: by adding an extra dimension to `r1`, both temporaries can be scheduled within the same loop nest, thus augmenting data reuse and potentially enabling further cross-expression optimizations. We can disable this heuristic through the `min-storage` option.
###Code
op7_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'min-storage': True}))
print_kernel(op7_b1_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size + 4][y_size][z_size]));
float **pr1;
posix_memalign((void**)&pr1, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr1[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m - 2; x <= x_M + 2; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x + 2][y][z] = 8.33333333e-2F*u[t0][x + 2][y + 4][z + 4]/h_x - 6.66666667e-1F*u[t0][x + 3][y + 4][z + 4]/h_x + 6.66666667e-1F*u[t0][x + 5][y + 4][z + 4]/h_x - 8.33333333e-2F*u[t0][x + 6][y + 4][z + 4]/h_x;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r1)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr1[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r1[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_x)*r0[x + 1][y][z] + (-8.33333333e-2F/h_x)*r0[x + 4][y][z] + (8.33333333e-2F/h_x)*r0[x][y][z] + (6.66666667e-1F/h_x)*r0[x + 3][y][z] + (-6.66666667e-1F/h_y)*r1[y + 1][z] + (-8.33333333e-2F/h_y)*r1[y + 4][z] + (8.33333333e-2F/h_y)*r1[y][z] + (6.66666667e-1F/h_y)*r1[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr1[tid]);
}
free(r0);
free(pr1);
###Markdown
The `cire-mingain` optionSo far so good -- we've seen that Devito can capture and schedule cross-iteration redundancies. But what if, for example, we actually do _not_ want certain redundancies to be captured? There are a few reasons we may like that way, for example we're allocating too much extra memory for the tensor temporaries, and we rather prefer to avoid that. For this, the user can tell Devito what the *minimum gain*, or simply `mingain`, which stems from optimizing a CIRE candidate must be in order to trigger the transformation. Such `mingain` is an integer number reflecting the number and type of arithmetic operations in a CIRE candidate. The Devito compiler computes the `gain` of a CIRE candidate, compares it to the `mingain`, and acts as follows:* if `gain < mingain`, then the CIRE candidate is discarded;* if `mingain <= gain <= mingain*3`, then the CIRE candidate is optimized if and only if it doesn't lead to an increase in working set size;* if `gain > mingain*3`, then the CIRE candidate is optimized.The default `mingain` is set to 10, a value that was determined empirically. The coefficient `3` in the relationals above was also determined empirically. The user can change the `mingain`'s default value via the `cire-mingain` optimization option.To compute the `gain` of a CIRE candidate, Devito applies the following rules:* Any integer arithmetic operation (e.g. for array indexing) has a cost of 0.* A basic arithmetic operation such as `+` and `*` has a cost of 1.* A `/` has a cost of 5.* A power with non-integer exponent has a cost of 50.* A power with non-negative integer exponent `n` has a cost of `n-1` (i.e., the number of `*` it will be converted into).* A trascendental function (`sin`, `cos`, etc.) has a cost of 100.Further, if a CIRE candidate is invariant with respect to one or more dimensions, then the `gain` is proportionately scaled up.Let's now take a look at `cire-mingain` in action.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op8_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 31}))
print_kernel(op8_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
We observe how setting `cire-mingain=31` makes the tensor temporary produced by `op7_omp` disappear. Any `cire-mingain` greater than 31 will have the same effect. Let's see why. The CIRE candidate in question is `u.dy`, which expands to:```8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y```* Three `+` (or `-`) => 3*1 = 3* Four `/` by `h_y` => 4*5 = 20* Four two-way `*` with operands `(1/h_y, u)` => 8So we have a cost of 31. Since the CIRE candidate stems from `.dy`, and since `u`'s space order is 4, the CIRE candidates appears 4 times in total, with shifted indices along `y`. It follows that the gain due to optimizing it away through a tensor temporary would be 31\*(4 - 1) = 93. However, `mingain*3 = 31*3 = 93`, so `mingain < gain <= mingain*3`, and since there would be an increase in working set size (the new tensor temporary to be allocated), then the CIRE candidate is discarded.As shown below, any `cire-mingain` smaller than 31 will lead to capturing the tensor temporary.
###Code
op8_b1_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-mingain': 30}))
print_kernel(op8_b1_omp)
###Output
float **pr0;
posix_memalign((void**)&pr0, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr0[tid], 64, sizeof(float[y_size + 4][z_size]));
}
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r0)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr0[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[y + 1][z] + (-8.33333333e-2F/h_y)*r0[y + 4][z] + (8.33333333e-2F/h_y)*r0[y][z] + (6.66666667e-1F/h_y)*r0[y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr0[tid]);
}
free(pr0);
###Markdown
Dimension-invariant sub-expressionsCross-iteration redundancies may also be searched across dimension-invariant sub-expressions, typically time-invariants.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op11_omp = Operator(eq, opt=('lift', {'openmp': True}))
# print_kernel(op11_omp) # Uncomment to see the generated code
###Output
_____no_output_____
###Markdown
The `lift` pass triggers CIRE for dimension-invariant sub-expressions. As seen before, this leads to producing one tensor temporary. By setting `cire-mingain` to a larger value, we can avoid a grid-size temporary to be allocated, in exchange for a trascendental function (`sin(...)`) to be computed at each iteration.
###Code
op12_omp = Operator(eq, opt=('lift', {'openmp': True, 'cire-mingain': 34}))
print_kernel(op12_omp)
###Output
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 1][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 2][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 5][z + 4]/h_y) + (-8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 7][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 8][z + 4]/h_y) + (8.33333333e-2F/h_y)*(8.33333333e-2F*u[t0][x + 4][y][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 1][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 4][z + 4]/h_y) + (6.66666667e-1F/h_y)*(8.33333333e-2F*u[t0][x + 4][y + 3][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 4][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 6][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 7][z + 4]/h_y))*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
###Markdown
The `cire-maxpar` optionSometimes it's possible to trade storage for parallelism (i.e., for more parallel dimensions). For this, Devito provides the `cire-maxpar` option which is by default set to:* False on CPU backends* True on GPU backendsLet's see what happens when we switch it on
###Code
op13_omp = Operator(eq, opt=('cire-sops', {'openmp': True, 'cire-maxpar': True}))
print_kernel(op13_omp)
###Output
float (*r0)[y_size + 4][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size + 4][z_size]));
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y + 2][z] = 8.33333333e-2F*u[t0][x + 4][y + 2][z + 4]/h_y - 6.66666667e-1F*u[t0][x + 4][y + 3][z + 4]/h_y + 6.66666667e-1F*u[t0][x + 4][y + 5][z + 4]/h_y - 8.33333333e-2F*u[t0][x + 4][y + 6][z + 4]/h_y;
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(3) schedule(dynamic,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = ((-6.66666667e-1F/h_y)*r0[x][y + 1][z] + (-8.33333333e-2F/h_y)*r0[x][y + 4][z] + (8.33333333e-2F/h_y)*r0[x][y][z] + (6.66666667e-1F/h_y)*r0[x][y + 3][z])*sin(f[x + 1][y + 1][z + 1])*pow(f[x + 1][y + 1][z + 1], 2);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
}
free(r0);
###Markdown
The generated code uses a three-dimensional temporary that gets written and subsequently read in two separate `x-y-z` loop nests. Now, both loops can safely be openmp-collapsed, which is vital when running on GPUs. Impact of CIRE in the `advanced` modeThe `advanced` mode triggers all of the passes we've seen so far... and in fact, many more! Some of them, however, aren't visible in our running example (e.g., all of the MPI-related optimizations). These will be treated in a future notebook.Obviously, all of the optimization options (e.g. `cire-mingain`, `blocklevels`) are applicable and composable in any arbitrary way.
###Code
op14_omp = Operator(eq, openmp=True)
print(op14_omp)
# op14_b0_omp = Operator(eq, opt=('advanced', {'min-storage': True}))
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y0_blk0_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[y0_blk0_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0 - 2, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size + 1, y_M + 2); y += 1, ys += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ys][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y0_blk0, ys = 0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[ys][z] - r2[ys + 4][z]) + 6.66666667e-1F*(-r2[ys + 1][z] + r2[ys + 3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(r0);
free(pr2);
return 0;
}
###Markdown
A crucial observation here is that CIRE is applied on top of loop blocking -- the `r2` temporary is computed within the same block as `u`, which in turn requires an additional iteration at the block edge along `y` to be performed (the first `y` loop starts at `y0_blk0 - 2`, while the second one at `y0_blk0`). Further, the size of `r2` is now a function of the block shape. Altogether, this implements what in the literature is often referred to as "overlapped tiling" (or "overlapped blocking"): data reuse across consecutive loop nests is obtained by cross-loop blocking, which in turn requires a certain degree of redundant computation at the block edge. Clearly, there's a tension between the block shape and the amount of redundant computation. For example, a small block shape guarantees a small(er) working set, and thus better data reuse, but also requires more redundant computation. The `cire-rotate` optionSo far we've seen two ways to compute the tensor temporaries:* The temporary dimensions span the whole grid;* The temporary dimensions span a block created by the loop blocking pass.There are a few other ways, and in particular there's a third way supported in Devito, enabled through the `cire-rotate` option:* The temporary outermost-dimension is a function of the stencil radius; all other temporary dimensions are a function of the loop blocking shape.Let's jump straight into an example
###Code
op15_omp = Operator(eq, opt=('advanced', {'openmp': True, 'cire-rotate': True}))
print_kernel(op15_omp)
###Output
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[5][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m; y0_blk0 <= y_M; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y0_blk0, ys = 0, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = -2; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M); y += 1, ys += 1, yr0 = (ys)%(5), yr1 = (ys + 3)%(5), yr2 = (ys + 4)%(5), yr3 = (ys + 1)%(5), yii = 2)
{
for (int yy = yii, ysi = (yy + ys + 2)%(5); yy <= 2; yy += 1, ysi = (yy + ys + 2)%(5))
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[ysi][z] = r1*(8.33333333e-2F*(u[t0][x + 4][yy + y + 2][z + 4] - u[t0][x + 4][yy + y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][yy + y + 3][z + 4] + u[t0][x + 4][yy + y + 5][z + 4]));
}
}
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[yr0][z] - r2[yr2][z]) + 6.66666667e-1F*(r2[yr1][z] - r2[yr3][z]))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(r0);
free(pr2);
###Markdown
There are two key things to notice here:* The `r2` temporary is a pointer to a two-dimensional array of size `[2][z_size]`. It's obtained via casting of `pr2[tid]`, which in turn is defined as
###Code
print(op15_omp.body[1].header[4])
###Output
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[5][z_size]));
}
###Markdown
* Within the `y` loop there are several iteration variables, some of which (`yr0`, `yr1`, ...) employ modulo increment to cyclically produce the indices 0 and 1.In essence, with `cire-rotate`, instead of computing an entire slice of `y` values, at each `y` iteration we only keep track of the values that are strictly necessary to evaluate `u` at `y` -- only two values in this case. This results in a working set reduction, at the price of turning one parallel loop (`y`) into a sequential one. The `advanced-fsg` modeThe alternative `advanced-fsg` optimization level applies the same passes as `advanced`, but in a different order. The key difference is that `-fsg` does not generate overlapped blocking code across CIRE-generated loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*u.dy.dy) # Back to original running example
op17_omp = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_omp)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x_M, const int x_m, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float **pr2;
posix_memalign((void**)&pr2, 64, sizeof(float*)*nthreads);
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
posix_memalign((void**)&pr2[tid], 64, sizeof(float[y_size + 4][z_size]));
}
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
float (*restrict r2)[z_size] __attribute__ ((aligned (64))) = (float (*)[z_size]) pr2[tid];
#pragma omp for collapse(1) schedule(dynamic,1)
for (int x0_blk0 = x_m; x0_blk0 <= x_M; x0_blk0 += x0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M); x += 1)
{
for (int y = y_m - 2; y <= y_M + 2; y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r2[y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = r1*(f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(8.33333333e-2F*(r2[y][z] - r2[y + 4][z]) + 6.66666667e-1F*(-r2[y + 1][z] + r2[y + 3][z]))*r0[x][y][z];
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
#pragma omp parallel num_threads(nthreads)
{
const int tid = omp_get_thread_num();
free(pr2[tid]);
}
free(r0);
free(pr2);
return 0;
}
###Markdown
The `x` loop here is still shared by the two loop nests, but the `y` one isn't. Analogously, if we consider the alternative `eq` already used in `op7_b0_omp`, we get two completely separate, and therefore individually blocked, loop nests.
###Code
eq = Eq(u.forward, f**2*sin(f)*(u.dy.dy + u.dx.dx))
op17_b0 = Operator(eq, opt=('advanced-fsg', {'openmp': True}))
print(op17_b0)
###Output
#define _POSIX_C_SOURCE 200809L
#define MIN(a,b) (((a) < (b)) ? (a) : (b))
#define MAX(a,b) (((a) > (b)) ? (a) : (b))
#define START_TIMER(S) struct timeval start_ ## S , end_ ## S ; gettimeofday(&start_ ## S , NULL);
#define STOP_TIMER(S,T) gettimeofday(&end_ ## S, NULL); T->S += (double)(end_ ## S .tv_sec-start_ ## S.tv_sec)+(double)(end_ ## S .tv_usec-start_ ## S .tv_usec)/1000000;
#include "stdlib.h"
#include "math.h"
#include "sys/time.h"
#include "xmmintrin.h"
#include "pmmintrin.h"
#include "omp.h"
struct dataobj
{
void *restrict data;
int * size;
int * npsize;
int * dsize;
int * hsize;
int * hofs;
int * oofs;
} ;
struct profiler
{
double section0;
double section1;
} ;
int Kernel(struct dataobj *restrict f_vec, const float h_x, const float h_y, struct dataobj *restrict u_vec, const int x_size, const int y_size, const int z_size, const int time_M, const int time_m, const int x0_blk0_size, const int x1_blk0_size, const int x_M, const int x_m, const int y0_blk0_size, const int y1_blk0_size, const int y_M, const int y_m, const int z_M, const int z_m, const int nthreads, struct profiler * timers)
{
float (*restrict f)[f_vec->size[1]][f_vec->size[2]] __attribute__ ((aligned (64))) = (float (*)[f_vec->size[1]][f_vec->size[2]]) f_vec->data;
float (*restrict u)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]] __attribute__ ((aligned (64))) = (float (*)[u_vec->size[1]][u_vec->size[2]][u_vec->size[3]]) u_vec->data;
float (*r0)[y_size][z_size];
posix_memalign((void**)&r0, 64, sizeof(float[x_size][y_size][z_size]));
float (*r3)[y_size + 4][z_size];
posix_memalign((void**)&r3, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
float (*r4)[y_size + 4][z_size];
posix_memalign((void**)&r4, 64, sizeof(float[x_size + 4][y_size + 4][z_size]));
/* Flush denormal numbers to zero in hardware */
_MM_SET_DENORMALS_ZERO_MODE(_MM_DENORMALS_ZERO_ON);
_MM_SET_FLUSH_ZERO_MODE(_MM_FLUSH_ZERO_ON);
float r1 = 1.0F/h_x;
float r2 = 1.0F/h_y;
/* Begin section0 */
START_TIMER(section0)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(static,1)
for (int x = x_m; x <= x_M; x += 1)
{
for (int y = y_m; y <= y_M; y += 1)
{
#pragma omp simd aligned(f:32)
for (int z = z_m; z <= z_M; z += 1)
{
r0[x][y][z] = sin(f[x + 1][y + 1][z + 1]);
}
}
}
}
STOP_TIMER(section0,timers)
/* End section0 */
for (int time = time_m, t0 = (time)%(2), t1 = (time + 1)%(2); time <= time_M; time += 1, t0 = (time)%(2), t1 = (time + 1)%(2))
{
/* Begin section1 */
START_TIMER(section1)
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x0_blk0 = x_m - 2; x0_blk0 <= x_M + 2; x0_blk0 += x0_blk0_size)
{
for (int y0_blk0 = y_m - 2; y0_blk0 <= y_M + 2; y0_blk0 += y0_blk0_size)
{
for (int x = x0_blk0; x <= MIN(x0_blk0 + x0_blk0_size - 1, x_M + 2); x += 1)
{
for (int y = y0_blk0; y <= MIN(y0_blk0 + y0_blk0_size - 1, y_M + 2); y += 1)
{
#pragma omp simd aligned(u:32)
for (int z = z_m; z <= z_M; z += 1)
{
r3[x + 2][y + 2][z] = r1*(8.33333333e-2F*(u[t0][x + 2][y + 4][z + 4] - u[t0][x + 6][y + 4][z + 4]) + 6.66666667e-1F*(-u[t0][x + 3][y + 4][z + 4] + u[t0][x + 5][y + 4][z + 4]));
r4[x + 2][y + 2][z] = r2*(8.33333333e-2F*(u[t0][x + 4][y + 2][z + 4] - u[t0][x + 4][y + 6][z + 4]) + 6.66666667e-1F*(-u[t0][x + 4][y + 3][z + 4] + u[t0][x + 4][y + 5][z + 4]));
}
}
}
}
}
}
#pragma omp parallel num_threads(nthreads)
{
#pragma omp for collapse(2) schedule(dynamic,1)
for (int x1_blk0 = x_m; x1_blk0 <= x_M; x1_blk0 += x1_blk0_size)
{
for (int y1_blk0 = y_m; y1_blk0 <= y_M; y1_blk0 += y1_blk0_size)
{
for (int x = x1_blk0; x <= MIN(x1_blk0 + x1_blk0_size - 1, x_M); x += 1)
{
for (int y = y1_blk0; y <= MIN(y1_blk0 + y1_blk0_size - 1, y_M); y += 1)
{
#pragma omp simd aligned(f,u:32)
for (int z = z_m; z <= z_M; z += 1)
{
u[t1][x + 4][y + 4][z + 4] = (f[x + 1][y + 1][z + 1]*f[x + 1][y + 1][z + 1])*(r1*(8.33333333e-2F*(r3[x][y + 2][z] - r3[x + 4][y + 2][z]) + 6.66666667e-1F*(-r3[x + 1][y + 2][z] + r3[x + 3][y + 2][z])) + r2*(8.33333333e-2F*(r4[x + 2][y][z] - r4[x + 2][y + 4][z]) + 6.66666667e-1F*(-r4[x + 2][y + 1][z] + r4[x + 2][y + 3][z])))*r0[x][y][z];
}
}
}
}
}
}
STOP_TIMER(section1,timers)
/* End section1 */
}
free(r0);
free(r3);
free(r4);
return 0;
}
|
demo_pipeline/Pipeline-Inference.ipynb | ###Markdown
Inference Pipeline Imports
###Code
import numpy as np
import h5py
import sys
import logging
sys.path.append('..')
# Neural network stuff
from fielutil import load_verbatimnet
from featextractor import extract_imfeats
# Logging
# logging.getLogger('featextractor').setLevel(logging.DEBUG)
###Output
Using Theano backend.
Using gpu device 0: GeForce GTX 980M (CNMeM is disabled)
###Markdown
Parameters
###Code
# Do you want to load the features in? Or save them to a file?
load_features = True
# All the images that you require extraction should be in this HDF5 file
# hdf5images='icdar13data/benchmarking-processed/icdar_be.hdf5'
# hdf5images = 'icdar13data/experimental-processed/icdar13_ex.hdf5'
# hdf5images='nmecdata/nmec_scaled_flat.hdf5'
hdf5images='nmecdata/flat_nmec_bin_uint8.hdf5'
# This is the file that you will load the features from or save the features to
# featurefile = 'icdar13data/benchmarking-processed/icdar13be_fiel657.npy'
# featurefile = 'icdar13data/experimental-processed/icdar13ex_fiel657.npy'
# featurefile = 'nmecdata/globalfeatures/nmec_bw_fiel657_features_steps5_thresh.005.npy'
# featurefile = 'nmecdata/nmec_bw_fiel657_features_steps5_mean500.npy'
# featurefile = 'nmecdata/globalfeatures/nmec_cropped_fiel657_features_steps5_thresh.005.npy'
featurefile = 'nmecdata/globalfeatures/nmec_bw.fiel657.steps5_var005.npy'
# This is the neural networks and parameters you are deciding to use
paramsfile = '/fileserver/iam/iam-processed/models/fiel_657.hdf5'
###Output
_____no_output_____
###Markdown
Full image HDF5 fileEach entry in the HDF5 file is a full image/form/document
###Code
labels = h5py.File(hdf5images).keys()
###Output
_____no_output_____
###Markdown
Load feature extractor neural network
###Code
vnet = load_verbatimnet( 'fc7', paramsfile=paramsfile )
vnet.compile(loss='mse', optimizer='sgd')
###Output
Establishing Fiel's verbatim network
Loaded neural network up to fc7 layer
###Markdown
Image featuresCurrently taken as averages of all shard features in the image. You can either load them or extract everything manually, depending on if you have the .npy array.
###Code
if load_features:
print "Loading features in from "+featurefile
imfeats = np.load(featurefile)
print "Loaded features"
else:
print "Begin extracting features from "+hdf5images
imfeats = extract_imfeats( hdf5images, vnet, steps=(5,5), compthresh=500 )
print h5py.File(hdf5images).keys()
np.save( featurefile, imfeats )
###Output
Loading features in from nmecdata/globalfeatures/nmec_bw.fiel657.steps5_var005.npy
Loaded features
###Markdown
Build classifier
###Code
imfeats = ( imfeats.T / np.linalg.norm( imfeats, axis=1 ) ).T
F = imfeats.dot(imfeats.T)
np.fill_diagonal( F , -1 )
###Output
_____no_output_____
###Markdown
Evaluate classifier on HDF5 file You'll need to set the number of examples per image as follows:1. IAM: Not Implemented2. ICDAR 2013: g=43. NMEC: g=8Two criteria matched are:1. Precision (hard)2. Recall (soft)
###Code
# Top k (soft criteria)
k = 10
# Max top (hard criteria)
maxtop = 3
# Number of examples per image
g = 8
# Run through the adjacency matrix
softcorrect = 0
hardcorrect = 0
totalnum = 0
for j, i in enumerate(F):
topk = i.argsort()[-k:]
# Soft criteria
if j/g in topk/g:
softcorrect += 1
totalnum +=1
# Hard criteria
hardsample = sum([1 for jj in (j/g == topk[-maxtop:]/g) if jj])
if hardsample==maxtop:
hardcorrect += 1
# Print out results
print "Top %d (soft criteria) = %f" %( k, (softcorrect+0.0) / totalnum )
print "Top %d (hard criteria) = %f" %( k, (hardcorrect+0.0) / totalnum )
import matplotlib.pylab as plt
%matplotlib inline
plt.plot(range(16),np.log(F[2][0:16]))
###Output
_____no_output_____ |
tutorial2_zfit/custom_models.ipynb | ###Markdown
Custom modelsAll elements of zfit are built to be easily customized. Especially models offer many possibilities to be implemented by the user; in the end, regardless of how many models are provided by a library and of how many things are though, there is always a use-case that was not thought of. High flexibility is therefore a crucial aspect.This has disadvantages: the more freedom a model takes for itself, the less optimizations are potentially available. But this is usually not noticeable. Creating a modelFollowing the philosophy of zfit, there are different levels of customization. For the most simple use-case, all we need to do is to provide a function describing the shape and the name of the parameters. This can be done by overriding `_unnormalized_pdf`.To implement a mathematical function in zfit, TensorFlow or z should be used. The latter is a subset of TensorFlow and improves it in some aspects, such as automatic dtype casting, and therefore preferred to use.(_There are other ways to use arbitrary Python functions, they will be discussed later on_).
###Code
import zfit
from zfit import z
import numpy as np
import matplotlib.pyplot as plt
import tensorflow as tf
###Output
_____no_output_____
###Markdown
We can start with a simple model and implement a custom second order polynomial. Therefore we need to inherit from the right base class, the simpler one is `ZPDF`.For a minimal example, we need to override only `_unnormalized_pdf` and specify a list of parameters.`_unnormalized_pdf` gets (currently) one argument, x. This is a zfit `Data` object and should first be unstacked. If it is one dimensional - such as here - it will return a single Tensor, otherwise a list of Tensors that can directly be unpacked.
###Code
class SecondOrderPoly(zfit.pdf.ZPDF):
"""Second order polynomial `a + b * x + c * x^2`"""
_PARAMS = ['b', 'c'] # specify which parameters to take
def _unnormalized_pdf(self, x): # implement function, unnormalized
data = z.unstack_x(x)
b = self.params['b']
c = self.params['c']
return 1 + b * data + c * data ** 2
###Output
_____no_output_____
###Markdown
Note that we omitted _consciously_ any attempt to normalize the function, as this is usually done over a specific range. Also, no analytic sampling or integration has to be provided. The model handles all of this internally automatically and we have the full functionality available.First, we can instantiate the model:
###Code
obs = zfit.Space("obs1", limits=(-4, 4))
b = zfit.Parameter('b', 0.2, 0.1, 10)
custom_poly = SecondOrderPoly(obs=obs, b=b, c=1.4)
###Output
_____no_output_____
###Markdown
which lets us now fully access all the main methods of a model:
###Code
integral = custom_poly.integrate(limits=(-1, 2))
sample = custom_poly.sample(n=1000)
prob = custom_poly.pdf(sample)
print(f"integral={integral}, sample={sample}, prob={prob[:10]}")
###Output
integral=[0.11071254], sample=<zfit.Data: Data obs=('obs1',)>, prob=[0.1259812 0.18098075 0.29449112 0.18754387 0.25252336 0.30450077
0.30918563 0.29605061 0.32218707 0.33165225]
###Markdown
What happened?The model tries to use analytical functions for integration and sampling _if available_, otherwise (as happened above), it falls back to the numerical methods. To improve our model, we can add an analytic integral, a common use case. This has to be the _integral over the `_unnormalized_pdf`_.
###Code
# define the integral function
def cdf_poly(limit, b, c):
return limit + 0.5 * b * limit ** 2 + 1 / 3 * c * limit ** 3
def integral_func(limits, norm_range, params, model):
b = params['b']
c = params['c']
lower, upper = limits.limit1d
lower = z.convert_to_tensor(lower) # the limits are now 1-D, for axis 1
upper = z.convert_to_tensor(upper)
# calculate the integral
integral = cdf_poly(upper, b, c) - cdf_poly(lower, b, c)
print("Integral called")
return integral
# define the space over which it is defined. Here, we use the axes
integral_limits = zfit.Space(axes=(0,), limits=(zfit.Space.ANY, zfit.Space.ANY))
SecondOrderPoly.register_analytic_integral(func=integral_func, limits=integral_limits)
poly2 = SecondOrderPoly(obs=obs, b=b, c=1.2)
integral_analytic = custom_poly.integrate(limits=(-1, 2))
sample = custom_poly.sample(n=1000)
prob_analytic = custom_poly.pdf(sample)
print(f"integral={integral}, sample={sample}, prob={prob[:10]}")
###Output
<ipython-input-5-bbf4d8d50683>:10: UserWarning: The function <function Space.limit1d at 0x7f04fe8c1040> may does not return the actual area/limits but rather the rectangular limits. <zfit Space obs=('obs1',), axes=(0,), limits=(array([[-1.]]), array([[2.]]))> can also have functional limits that are arbitrarily defined and lay inside the rect_limits. To test if a value is inside, use `inside` or `filter`.
lower, upper = limits.limit1d
###Markdown
Multiple dimensions and parameters with angular observablesSo far, we used rather simple examples and many basic shapes, such as polynomials, already have an efficient implementation within zfit. Therefore, we will now create a three dimensional PDF measuring the angular observables of a $B^+ \rightarrow K^* l l$ decay.The implementation is not "special" or complicated at all, it rather shows how to deal with multiple dimensions and how to manage several parameters. It was created using the equation of the angular observables (taken from a paper)._Many thanks to Rafael Silva Coutinho for the implementation!_
###Code
class AngularPDF(zfit.pdf.ZPDF):
"""Full d4Gamma/dq2dOmega for Bd -> Kst ll (l=e,mu)
Angular distribution obtained in the total PDF (using LHCb convention JHEP 02 (2016) 104)
i.e. the valid of the angles is given for
- phi: [-pi, pi]
- theta_K: [0, pi]
- theta_l: [0, pi]
The function is normalized over a finite range and therefore a PDF.
Args:
FL (`zfit.Parameter`): Fraction of longitudinal polarisation of the Kst
S3 (`zfit.Parameter`): A_perp^2 - A_para^2 / A_zero^2 + A_para^2 + A_perp^2 (L, R)
S4 (`zfit.Parameter`): RE(A_zero*^2 * A_para^2) / A_zero^2 + A_para^2 + A_perp^2 (L, R)
S5 (`zfit.Parameter`): RE(A_zero*^2 * A_perp^2) / A_zero^2 + A_para^2 + A_perp^2 (L, R)
AFB (`zfit.Parameter`): Forward-backward asymmetry of the di-lepton system (also i.e. 3/4 * S6s)
S7 (`zfit.Parameter`): IM(A_zero*^2 * A_para^2) / A_zero^2 + A_para^2 + A_perp^2 (L, R)
S8 (`zfit.Parameter`): IM(A_zero*^2 * A_perp^2) / A_zero^2 + A_para^2 + A_perp^2 (L, R)
S9 (`zfit.Parameter`): IM(A_perp*^2 * A_para^2) / A_zero^2 + A_para^2 + A_perp^2 (L, R)
obs (`zfit.Space`):
name (str):
dtype (tf.DType):
"""
_PARAMS = ['FL', 'S3', 'S4', 'S5', 'AFB', 'S7', 'S8', 'S9']
_N_OBS = 3
def _unnormalized_pdf(self, x):
FL = self.params['FL']
S3 = self.params['S3']
S4 = self.params['S4']
S5 = self.params['S5']
AFB = self.params['AFB']
S7 = self.params['S7']
S8 = self.params['S8']
S9 = self.params['S9']
costheta_l, costheta_k, phi = z.unstack_x(x)
sintheta_k = tf.sqrt(1.0 - costheta_k * costheta_k)
sintheta_l = tf.sqrt(1.0 - costheta_l * costheta_l)
sintheta_2k = (1.0 - costheta_k * costheta_k)
sintheta_2l = (1.0 - costheta_l * costheta_l)
sin2theta_k = (2.0 * sintheta_k * costheta_k)
cos2theta_l = (2.0 * costheta_l * costheta_l - 1.0)
sin2theta_l = (2.0 * sintheta_l * costheta_l)
pdf = ((3.0 / 4.0) * (1.0 - FL) * sintheta_2k +
FL * costheta_k * costheta_k +
(1.0 / 4.0) * (1.0 - FL) * sintheta_2k * cos2theta_l +
-1.0 * FL * costheta_k * costheta_k * cos2theta_l +
S3 * sintheta_2k * sintheta_2l * tf.cos(2.0 * phi) +
S4 * sin2theta_k * sin2theta_l * tf.cos(phi) +
S5 * sin2theta_k * sintheta_l * tf.cos(phi) +
(4.0 / 3.0) * AFB * sintheta_2k * costheta_l +
S7 * sin2theta_k * sintheta_l * tf.sin(phi) +
S8 * sin2theta_k * sin2theta_l * tf.sin(phi) +
S9 * sintheta_2k * sintheta_2l * tf.sin(2.0 * phi))
return pdf
###Output
_____no_output_____
###Markdown
Multidimensional SpacesThis PDF now expects multidimensional data. Therefore, we need to provide a Space in multiple dimensions. The preferred way is to use the product operations to build this space from one dimensional `Space`s
###Code
costhetha_k = zfit.Space('costheta_k', (-1, 1))
costhetha_l = zfit.Space('costheta_l', (-1, 1))
phi = zfit.Space('phi', (-np.pi, np.pi))
angular_obs = costhetha_k * costhetha_l * phi
###Output
_____no_output_____
###Markdown
Managing parametersLuckily, we're in Python, which provides many tools out-of-the-box. Handling parameters in a `dict` can make things very easy, even for several parameters as here.
###Code
params_init = {'FL': 0.43, 'S3': -0.1, 'S4': -0.2, 'S5': -0.4, 'AFB': 0.343, 'S7': 0.001, 'S8': 0.003, 'S9': 0.002}
params = {name: zfit.Parameter(name, val, -1, 1) for name, val in params_init.items()}
angular_pdf = AngularPDF(obs=angular_obs, **params)
integral_analytic = angular_pdf.integrate(limits=angular_obs) # this should be one
sample = angular_pdf.sample(n=1000)
prob_analytic = angular_pdf.pdf(sample)
print(f"integral={integral}, sample={sample}, prob={prob[:10]}")
###Output
integral=[0.11071254], sample=<zfit.Data: Data obs=('costheta_k', 'costheta_l', 'phi')>, prob=[0.1259812 0.18098075 0.29449112 0.18754387 0.25252336 0.30450077
0.30918563 0.29605061 0.32218707 0.33165225]
###Markdown
Including another observableWe built our angular PDF successfully and can use this 3 dimensional PDF now. If we want, we could also include another observable. For example, the polynomial that we created above and make it 4 dimensional. Because it's so simple, let's do that!
###Code
full_pdf = angular_pdf * poly2
# equivalently
# full_pdf = zfit.pdf.ProductPDF([angular_pdf, poly2])
###Output
_____no_output_____
###Markdown
Done! This PDF is now 4 dimensional, which _had to be_, given that the observable of `poly2` is different from the observable of `angular_pdf`. If they would coincide, e.g. if `poly2` had the observable `phi`, this would now be a 3 dimensional PDF.
###Code
print(f"obs angular: {angular_pdf.obs} obs poly:{poly2.obs} obs product: {full_pdf.obs})")
###Output
obs angular: ('costheta_k', 'costheta_l', 'phi') obs poly:('obs1',) obs product: ('costheta_k', 'costheta_l', 'phi', 'obs1'))
###Markdown
What happened _exactly_ ?The model tries to be as smart as possible and calls the most explicit function. Then it starts falling back to alternatives and uses, whenever possible, the analytic version (if available), otherwise a numerical.The rule simplified: public (sanitizes input and) calls [...] private. So e.g. `pdf` calls `_pdf` and if this is not provided, it uses the fallback that may not be optimized, but general enough to work.The rule extended (in its current implementation): public calls a series of well defined methods and hooks before it calls the private method. These intermediate _can_ be used, they mostly automatically catch certain cases and handle them for us.**To remember**: in order to have full control over a public function such as `integrate`, `pdf`, `sample` or `normalization`, the private method, e.g. `_integrate` can be overriden and is _guaranteed_ to be called before other possibilities.In the case above, `pdf` called first `_pdf` (which is not implemented), so it calls `_unnormalized_pdf` and divides this by the `normalization`. The latter also does not have an explicit implementation (`_implementation`), so it uses the fallback and calls `integrate` over the `norm_range`. Since `_integrate` is not provided, the fallback tries to perform an analytic integral, which is not available. Therefore, it integrates the `_unnormalized_prob` numerically. In all of this calls, we can hook in by overriding the mentioned, specified methods.What we did not mention: `ZPDF` is just a wrapper around the actual `BasePDF` that should be preferred in general; it simply provides a convenient `__init__`. For the next example, we will implement a multidimensional PDF and use the custom `__init__` Overriding `pdf`Before, we used `_unnormalized_pdf`, which is the common use-case. Even if we want to add an analytic integral, we can register it. Or do more fancy stuff like overriding the `_normalization`. We can however also get the full control of what our model output by directly overriding `_pdf`. The signature does not contain only `x` but additionally `norm_range`. This can have no limits (`norm_range.has_limits` is False), in which case the "unnormalized pdf" is requested. Otherwise, `norm_range` can have different limits and we have to take care of the proper normalization.This is usually not needed and inside zfit, all PDFs are implemented using the `_unnormalized_pdf`.Therefore, it provides mostly a possibility to implement _whatever_ is wanted, any unforeseen use-case, any kind of hack to "just try out something".
###Code
class CustomPDF(zfit.pdf.BasePDF):
"""My custom pdf with three parameters.
"""
def __init__(self, param1, param2, param3, obs, name="CustomPDF", ):
# we can now do complicated stuff here if needed
# only thing: we have to specify explicitly here what is which parameter
params = {'super_param': param1, # we can change/compose etc parameters
'param2': param2, 'param3': param3}
super().__init__(obs, params, name=name)
def _pdf(self, x, norm_range):
data = z.unstack_x(x)
param1 = self.params['super_param']
param2 = self.params['param2']
param3 = self.params['param3']
# just a fantasy function
probs = 42 * param1 + (data * param3) ** param2
return probs
###Output
_____no_output_____
###Markdown
In a similar manner, other methods can be overriden as well. We won't go into further details here, as this provides a quite advanced task. Furthermore, if stability is a large concern or such special cases need to be implemented, it is recommended to get in contact with the developers and share the idea. Composed PDFsSo far, we only looked at creating a model that depends on parameters and data but did not include other models. This is crucial to create for example sums or products of PDFs. Instead of inheriting from `BasePDF`, we can use the `BaseFunctor` that contains a mixin which handles daughter PDFs correctly.The main difference is that we can now provide a list of PDFs that our model depends on. There can still be parameters (as for example the `fracs` for the sum) that describe the behavior of the models but they can also be omitted (e.g. for the product). _Sidenote: technically, a normal `BasePDF` can of course also have no parameters, however, since this is a constant function without dependencies, this will rarely be used in practice.
###Code
class SquarePDF(zfit.pdf.BaseFunctor):
"""Example of a functor pdf that takes the log of a single PDF.
DEMONSTRATION PURPOSE ONLY, DO **NOT** USE IN REAL CASE.
"""
def __init__(self, pdf1, name="SumOf3"):
pdfs = [pdf1] # we could have more of course, e.g. for sums
# no need for parameters here, so we can omit it
obs = pdf1.space
super().__init__(pdfs=pdfs, obs=obs, name=name)
def _unnormalized_pdf(self, x):
# we do not need to unstack x here as we want to feed it directly to the pdf1
pdf1 = self.pdfs[0]
return pdf1.pdf(x) ** 2
squarepdf = SquarePDF(pdf1=poly2)
squarepdf.integrate(limits=(-2, 3.2))
sample_square = squarepdf.sample(n=1000)
sample_square
squarepdf.pdf(sample_square)[:10]
###Output
Integral called
Integral called
###Markdown
...and now?We've implemented a custom PDF. Maybe spent quite some time fine tuning it, debugging it. Adding an integral. And now? Time to make it available to others: [zfit-physics](https://github.com/zfit/zfit-physics). This repository is meant for community contributions. It has less requirements to contribute than to zfit core and has a low threshold. Core devs can provide you with help and you can provide the community with a PDF.Make an issue or a PR, everything is welcome! Mixing with pure PythonWhenever possible, it is preferrable to write anything in TensorFlow. But there is the possibility to mix with pure Python, however losing many of the benefits that TensorFlow provides. To do so:- try to use `z.py_function` or `tf.py_function` to wrap pure Python code- if you write something and want to make sure it is run in eager mode, use `zfit.run.assert_executing_eagerly()`. This way, your function won't be compiled and an error would be raised.- set the graph mode and numerical gradient accordingly
###Code
x_tf = z.constant(42.)
def sqrt(x):
return np.sqrt(x)
y = z.py_function(func=sqrt, inp=[x_tf], Tout=tf.float64)
###Output
/home/jonas/Documents/physics/software/zfit_project/zfit_repo/zfit/z/zextension.py:250: AdvancedFeatureWarning: Either you're using an advanced feature OR causing unwanted behavior. To turn this warning off, use `zfit.settings.advanced_warnings['py_func_autograd']` = False` or 'all' (use with care) with `zfit.settings.advanced_warnings['all'] = False
Using py_function without numerical gradients. If the Python code does not contain any parametrization by `zfit.Parameter` or similar, this can work out. Otherwise, in case it depends on those, you may want to set `zfit.run.set_autograd_mode(=False)`.
warn_advanced_feature("Using py_function without numerical gradients. If the Python code does not contain any"
###Markdown
This raises a warning: since we do not use pure TensorFlow anymore, it means that the automatic gradient (potentially) fails, as it cannot be traced through Python operations. Depending on the use-case, this is not a problem. That's why the warning is an `AdvancedFeatureWarning`: it doesn't say what we're doing is wrong, it simply warns that we should know what we're doing; it can also be switched off as explained in the warning.It is technically not always required: if we e.g. use the internal, numerical gradient of a minimizer such as Minuit, the global setting does not really matter anyway.This follows strongly the zfit philosophy that there _must_ not be any bounds in terms of flexibility and even hackability of the framework, this should be an inherent part of it. However, the user should be made aware when leaving "the safe path".To do what the above warning told us to do, we can use `zfit.run.set_autograd_mode(False)`.This is needed whenever we want to use non-traceable Python calls in the dynamic calculations, be it by using `py_function` or be it by switching off the gradient mode as shown below. Sidestep: What is 'z'?This is a subset of TensorFlow, wrapped to improve dtype handling and sometimes even provide additional functionality, such as `z.function` decorator. Full Python compatibilityTo operate in a full Python compatible, yet (way) less efficient mode, we can switch off the automatic gradient, as discussed before, and the graph compilation, leaving us with a Numpy-like TensorFlow
###Code
zfit.run.set_graph_mode(False)
zfit.run.set_autograd_mode(False)
###Output
_____no_output_____
###Markdown
We can now build a Gaussian purely based on Numpy. As we have seen when building graphs with TensorFlow: anything Python-like will be converted to a static value in the graph. So we have to make sure that our code is never run in graph mode but only executed eagerly.This can be done by calling `zfit.run.assert_executing_eagerly()`, which raises an error if this code is run in graph mode.Note that omitting the graph mode means to loose many optimizations: Not only do we loose the whole TensorFlow speedup from the graph, we also perform redundant tasks that are not cached, since zfit itself is optimized to be run in the graph mode.However, practially, this mode should anyway be used rather rarely and compares still in the same order of magnitude as alternatives.
###Code
class NumpyGauss(zfit.pdf.ZPDF):
_PARAMS = ['mu', 'sigma']
def _unnormalized_pdf(self, x):
zfit.run.assert_executing_eagerly() # make sure we're eager
data = z.unstack_x(x)
mu = self.params['mu']
sigma = self.params['sigma']
return z.convert_to_tensor(np.exp( - 0.5 * (data - mu) ** 2 / sigma ** 2))
###Output
_____no_output_____
###Markdown
Make sure to return a Tensor again, otherwise there will be an error.
###Code
obs = zfit.Space('obs1', (-3, 3))
mu = zfit.Parameter('mu', 0., -1, 1)
sigma = zfit.Parameter('sigma', 1., 0.1, 10)
gauss_np = NumpyGauss(obs=obs, mu=mu, sigma=sigma)
gauss = zfit.pdf.Gauss(obs=obs, mu=mu, sigma=sigma)
integral_np = gauss_np.integrate((-1, 0))
integral = gauss.integrate((-1, 0))
print(integral_np, integral)
###Output
tf.Tensor([0.3422554], shape=(1,), dtype=float64) tf.Tensor([0.3422688], shape=(1,), dtype=float64)
|
onnx/onnx-inference-emotion-recognition.ipynb | ###Markdown
Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License. Facial Expression Recognition using ONNX Runtime on AzureMLThis example shows how to deploy an image classification neural network using the Facial Expression Recognition ([FER](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data)) dataset and Open Neural Network eXchange format ([ONNX](http://aka.ms/onnxdocarticle)) on the Azure Machine Learning platform. This tutorial will show you how to deploy a FER+ model from the [ONNX model zoo](https://github.com/onnx/models), use it to make predictions using ONNX Runtime Inference, and deploy it as a web service in Azure.Throughout this tutorial, we will be referring to ONNX, a neural network exchange format used to represent deep learning models. With ONNX, AI developers can more easily move models between state-of-the-art tools (CNTK, PyTorch, Caffe, MXNet, TensorFlow) and choose the combination that is best for them. ONNX is developed and supported by a community of partners including Microsoft AI, Facebook, and Amazon. For more information, explore the [ONNX website](http://onnx.ai) and [open source files](https://github.com/onnx).[ONNX Runtime](https://aka.ms/onnxruntime-python) is the runtime engine that enables evaluation of trained machine learning (Traditional ML and Deep Learning) models with high performance and low resource utilization. We use the CPU version of ONNX Runtime in this tutorial, but will soon be releasing an additional tutorial for deploying this model using ONNX Runtime GPU. Tutorial Objectives:1. Describe the FER+ dataset and pretrained Convolutional Neural Net ONNX model for Emotion Recognition, stored in the ONNX model zoo.2. Deploy and run the pretrained FER+ ONNX model on an Azure Machine Learning instance3. Predict labels for test set data points in the cloud using ONNX Runtime and Azure ML Prerequisites 1. Install Azure ML SDK and create a new workspacePlease follow [00.configuration.ipynb](https://github.com/Azure/MachineLearningNotebooks/blob/master/00.configuration.ipynb) notebook. 2. Install additional packages needed for this NotebookYou need to install the popular plotting library `matplotlib`, the image manipulation library `PIL`, and the `onnx` library in the conda environment where Azure Maching Learning SDK is installed.```sh(myenv) $ pip install matplotlib onnx Pillow``` 3. Download sample data and pre-trained ONNX model from ONNX Model Zoo.[Download the ONNX Emotion FER+ model and corresponding test data](https://www.cntk.ai/OnnxModels/emotion_ferplus/opset_7/emotion_ferplus.tar.gz) and place them in the same folder as this tutorial notebook. You can unzip the file through the following line of code.```sh(myenv) $ tar xvzf emotion_ferplus.tar.gz```More information can be found about the ONNX FER+ model on [github](https://github.com/onnx/models/tree/master/emotion_ferplus). For more information about the FER+ dataset, please visit Microsoft Researcher Emad Barsoum's [FER+ source data repository](https://github.com/ebarsoum/FERPlus). Load Azure ML workspaceWe begin by instantiating a workspace object from the existing workspace created earlier in the configuration notebook.
###Code
# Check core SDK version number
import azureml.core
print("SDK version:", azureml.core.VERSION)
from azureml.core import Workspace
ws = Workspace.from_config()
print(ws.name, ws.location, ws.resource_group, sep = '\n')
###Output
_____no_output_____
###Markdown
Registering your model with Azure ML
###Code
model_dir = "emotion_ferplus" # replace this with the location of your model files
# leave as is if it's in the same folder as this notebook
from azureml.core.model import Model
model = Model.register(model_path = model_dir + "/" + "model.onnx",
model_name = "onnx_emotion",
tags = {"onnx": "demo"},
description = "FER+ emotion recognition CNN from ONNX Model Zoo",
workspace = ws)
###Output
_____no_output_____
###Markdown
Optional: Displaying your registered modelsThis step is not required, so feel free to skip it.
###Code
models = ws.models()
for m in models:
print("Name:", m.name,"\tVersion:", m.version, "\tDescription:", m.description, m.tags)
###Output
_____no_output_____
###Markdown
ONNX FER+ Model MethodologyThe image classification model we are using is pre-trained using Microsoft's deep learning cognitive toolkit, [CNTK](https://github.com/Microsoft/CNTK), from the [ONNX model zoo](http://github.com/onnx/models). The model zoo has many other models that can be deployed on cloud providers like AzureML without any additional training. To ensure that our cloud deployed model works, we use testing data from the famous FER+ data set, provided as part of the [trained Emotion Recognition model](https://github.com/onnx/models/tree/master/emotion_ferplus) in the ONNX model zoo.The original Facial Emotion Recognition (FER) Dataset was released in 2013, but some of the labels are not entirely appropriate for the expression. In the FER+ Dataset, each photo was evaluated by at least 10 croud sourced reviewers, creating a better basis for ground truth. You can see the difference of label quality in the sample model input below. The FER labels are the first word below each image, and the FER+ labels are the second word below each image.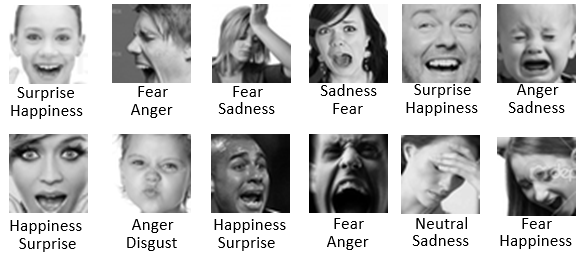***Input: Photos of cropped faces from FER+ Dataset******Task: Classify each facial image into its appropriate emotions in the emotion table***``` emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7} ```***Output: Emotion prediction for input image***Remember, once the application is deployed in Azure ML, you can use your own images as input for the model to classify.
###Code
# for images and plots in this notebook
import matplotlib.pyplot as plt
from IPython.display import Image
# display images inline
%matplotlib inline
###Output
_____no_output_____
###Markdown
Model DescriptionThe FER+ model from the ONNX Model Zoo is summarized by the graphic below. You can see the entire workflow of our pre-trained model in the following image from Barsoum et. al's paper ["Training Deep Networks for Facial Expression Recognitionwith Crowd-Sourced Label Distribution"](https://arxiv.org/pdf/1608.01041.pdf), with our (64 x 64) input images and our output probabilities for each of the labels.  Deploy our model on Azure ML We are now going to deploy our ONNX Model on AML with inference in ONNX Runtime. We begin by writing a score.py file, which will help us run the model in our Azure ML virtual machine (VM), and then specify our environment by writing a yml file.You will also notice that we import the onnxruntime library to do runtime inference on our ONNX models (passing in input and evaluating out model's predicted output). More information on the API and commands can be found in the [ONNX Runtime documentation](https://aka.ms/onnxruntime). Write Score FileA score file is what tells our Azure cloud service what to do. After initializing our model using azureml.core.model, we start an ONNX Runtime GPU inference session to evaluate the data passed in on our function calls.
###Code
%%writefile score.py
import json
import numpy as np
import onnxruntime
import sys
import os
from azureml.core.model import Model
import time
def init():
global session, input_name, output_name
model = Model.get_model_path(model_name = 'onnx_emotion')
session = onnxruntime.InferenceSession(model, None)
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
def run(input_data):
'''Purpose: evaluate test input in Azure Cloud using onnxruntime.
We will call the run function later from our Jupyter Notebook
so our azure service can evaluate our model input in the cloud. '''
try:
# load in our data, convert to readable format
data = np.array(json.loads(input_data)['data']).astype('float32')
start = time.time()
r = session.run([output_name], {input_name : data})
end = time.time()
result = emotion_map(postprocess(r[0]))
result_dict = {"result": result,
"time_in_sec": [end - start]}
except Exception as e:
result_dict = {"error": str(e)}
return json.dumps(result_dict)
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and returns the class IDs in decreasing
order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Write Environment File
###Code
from azureml.core.conda_dependencies import CondaDependencies
myenv = CondaDependencies()
myenv.add_pip_package("numpy")
myenv.add_pip_package("azureml-core")
myenv.add_pip_package("onnxruntime")
with open("myenv.yml","w") as f:
f.write(myenv.serialize_to_string())
###Output
_____no_output_____
###Markdown
Create the Container ImageThis step will likely take a few minutes.
###Code
from azureml.core.image import ContainerImage
image_config = ContainerImage.image_configuration(execution_script = "score.py",
runtime = "python",
conda_file = "myenv.yml",
description = "test",
tags = {"demo": "onnx"})
image = ContainerImage.create(name = "onnxtest",
# this is the model object
models = [model],
image_config = image_config,
workspace = ws)
image.wait_for_creation(show_output = True)
###Output
_____no_output_____
###Markdown
DebuggingIn case you need to debug your code, the next line of code accesses the log file.
###Code
print(image.image_build_log_uri)
###Output
_____no_output_____
###Markdown
We're all set! Let's get our model chugging. Deploy the container image
###Code
from azureml.core.webservice import AciWebservice
aciconfig = AciWebservice.deploy_configuration(cpu_cores = 1,
memory_gb = 1,
tags = {'demo': 'onnx'},
description = 'ONNX for emotion recognition model')
from azureml.core.webservice import Webservice
aci_service_name = 'onnx-demo-emotion'
print("Service", aci_service_name)
aci_service = Webservice.deploy_from_image(deployment_config = aciconfig,
image = image,
name = aci_service_name,
workspace = ws)
aci_service.wait_for_deployment(True)
print(aci_service.state)
###Output
_____no_output_____
###Markdown
The following cell will likely take a few minutes to run as well.
###Code
if aci_service.state != 'Healthy':
# run this command for debugging.
print(aci_service.get_logs())
# If your deployment fails, make sure to delete your aci_service before trying again!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Success!If you've made it this far, you've deployed a working VM with a facial emotion recognition model running in the cloud using Azure ML. Congratulations!Let's see how well our model deals with our test images. Testing and Evaluation Useful Helper FunctionsWe preprocess and postprocess our data (see score.py file) using the helper functions specified in the [ONNX FER+ Model page in the Model Zoo repository](https://github.com/onnx/models/tree/master/emotion_ferplus). Load Test Data
###Code
# to manipulate our arrays
import numpy as np
# read in test data protobuf files included with the model
import onnx
from onnx import numpy_helper
# to use parsers to read in our model/data
import json
import os
from score import emotion_map, softmax, postprocess
test_inputs = []
test_outputs = []
# read in 3 testing images from .pb files
test_data_size = 3
for i in np.arange(test_data_size):
input_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'input_0.pb')
output_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'output_0.pb')
# convert protobuf tensors to np arrays using the TensorProto reader from ONNX
tensor = onnx.TensorProto()
with open(input_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
input_data = numpy_helper.to_array(tensor)
test_inputs.append(input_data)
with open(output_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
output_data = numpy_helper.to_array(tensor)
output_processed = emotion_map(postprocess(output_data))[0]
test_outputs.append(output_processed)
###Output
_____no_output_____
###Markdown
Show some sample imagesWe use `matplotlib` to plot 3 test images from the model zoo with their labels over them.
###Code
plt.figure(figsize = (20, 20))
for test_image in np.arange(3):
test_inputs[test_image].reshape(1, 64, 64)
plt.subplot(1, 8, test_image+1)
plt.axhline('')
plt.axvline('')
plt.text(x = 10, y = -10, s = test_outputs[test_image], fontsize = 18)
plt.imshow(test_inputs[test_image].reshape(64, 64), cmap = plt.cm.Greys)
plt.show()
###Output
_____no_output_____
###Markdown
Run evaluation / prediction
###Code
plt.figure(figsize = (16, 6), frameon=False)
plt.subplot(1, 8, 1)
plt.text(x = 0, y = -30, s = "True Label: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -20, s = "Result: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -10, s = "Inference Time: ", fontsize = 13, color = 'black')
plt.text(x = 3, y = 14, s = "Model Input", fontsize = 12, color = 'black')
plt.text(x = 6, y = 18, s = "(64 x 64)", fontsize = 12, color = 'black')
plt.imshow(np.ones((28,28)), cmap=plt.cm.Greys)
for i in np.arange(test_data_size):
input_data = json.dumps({'data': test_inputs[i].tolist()})
# predict using the deployed model
r = json.loads(aci_service.run(input_data))
if "error" in r:
print(r['error'])
break
result = r['result'][0][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
ground_truth = test_outputs[i]
# compare actual value vs. the predicted values:
plt.subplot(1, 8, i+2)
plt.axhline('')
plt.axvline('')
# use different color for misclassified sample
font_color = 'red' if ground_truth != result else 'black'
clr_map = plt.cm.gray if ground_truth != result else plt.cm.Greys
# ground truth labels are in blue
plt.text(x = 10, y = -70, s = ground_truth, fontsize = 18, color = 'blue')
# predictions are in black if correct, red if incorrect
plt.text(x = 10, y = -45, s = result, fontsize = 18, color = font_color)
plt.text(x = 5, y = -22, s = str(time_ms) + ' ms', fontsize = 14, color = font_color)
plt.imshow(test_inputs[i].reshape(64, 64), cmap = clr_map)
plt.show()
###Output
_____no_output_____
###Markdown
Try classifying your own images!
###Code
from PIL import Image
def preprocess(image_path):
input_shape = (1, 1, 64, 64)
img = Image.open(image_path)
img = img.resize((64, 64), Image.ANTIALIAS)
img_data = np.array(img)
img_data = np.resize(img_data, input_shape)
return img_data
# Replace the following string with your own path/test image
# Make sure your image is square and the dimensions are equal (i.e. 100 * 100 pixels or 28 * 28 pixels)
# Any PNG or JPG image file should work
# Make sure to include the entire path with // instead of /
# e.g. your_test_image = "C://Users//vinitra.swamy//Pictures//emotion_test_images//img_1.png"
your_test_image = "<path to file>"
if your_test_image != "<path to file>":
img = preprocess(your_test_image)
plt.subplot(1,3,1)
plt.imshow(img.reshape((64,64)), cmap = plt.cm.gray)
else:
img = None
if img is None:
print("Add the path for your image data.")
else:
input_data = json.dumps({'data': img.tolist()})
try:
r = json.loads(aci_service.run(input_data))
result = r['result'][0][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
except Exception as e:
print(str(e))
plt.figure(figsize = (16, 6))
plt.subplot(1,8,1)
plt.axhline('')
plt.axvline('')
plt.text(x = -10, y = -35, s = "Model prediction: ", fontsize = 14)
plt.text(x = -10, y = -20, s = "Inference time: ", fontsize = 14)
plt.text(x = 100, y = -35, s = str(result), fontsize = 14)
plt.text(x = 100, y = -20, s = str(time_ms) + " ms", fontsize = 14)
plt.text(x = -10, y = -8, s = "Input image: ", fontsize = 14)
plt.imshow(img.reshape(64, 64), cmap = plt.cm.gray)
# remember to delete your service after you are done using it!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License. Facial Expression Recognition (Emotion FER+) using ONNX Runtime on Azure MLThis example shows how to deploy an image classification neural network using the Facial Expression Recognition ([FER](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data)) dataset and Open Neural Network eXchange format ([ONNX](http://aka.ms/onnxdocarticle)) on the Azure Machine Learning platform. This tutorial will show you how to deploy a FER+ model from the [ONNX model zoo](https://github.com/onnx/models), use it to make predictions using ONNX Runtime Inference, and deploy it as a web service in Azure.Throughout this tutorial, we will be referring to ONNX, a neural network exchange format used to represent deep learning models. With ONNX, AI developers can more easily move models between state-of-the-art tools (CNTK, PyTorch, Caffe, MXNet, TensorFlow) and choose the combination that is best for them. ONNX is developed and supported by a community of partners including Microsoft AI, Facebook, and Amazon. For more information, explore the [ONNX website](http://onnx.ai) and [open source files](https://github.com/onnx).[ONNX Runtime](https://aka.ms/onnxruntime-python) is the runtime engine that enables evaluation of trained machine learning (Traditional ML and Deep Learning) models with high performance and low resource utilization. We use the CPU version of ONNX Runtime in this tutorial, but will soon be releasing an additional tutorial for deploying this model using ONNX Runtime GPU. Tutorial Objectives:1. Describe the FER+ dataset and pretrained Convolutional Neural Net ONNX model for Emotion Recognition, stored in the ONNX model zoo.2. Deploy and run the pretrained FER+ ONNX model on an Azure Machine Learning instance3. Predict labels for test set data points in the cloud using ONNX Runtime and Azure ML Prerequisites 1. Install Azure ML SDK and create a new workspacePlease follow [Azure ML configuration notebook](https://github.com/Azure/MachineLearningNotebooks/blob/master/00.configuration.ipynb) to set up your environment. 2. Install additional packages needed for this NotebookYou need to install the popular plotting library `matplotlib`, the image manipulation library `opencv`, and the `onnx` library in the conda environment where Azure Maching Learning SDK is installed.```sh(myenv) $ pip install matplotlib onnx opencv-python```**Debugging tip**: Make sure that to activate your virtual environment (myenv) before you re-launch this notebook using the `jupyter notebook` comand. Choose the respective Python kernel for your new virtual environment using the `Kernel > Change Kernel` menu above. If you have completed the steps correctly, the upper right corner of your screen should state `Python [conda env:myenv]` instead of `Python [default]`. 3. Download sample data and pre-trained ONNX model from ONNX Model Zoo.In the following lines of code, we download [the trained ONNX Emotion FER+ model and corresponding test data](https://github.com/onnx/models/tree/master/emotion_ferplus) and place them in the same folder as this tutorial notebook. For more information about the FER+ dataset, please visit Microsoft Researcher Emad Barsoum's [FER+ source data repository](https://github.com/ebarsoum/FERPlus).
###Code
# urllib is a built-in Python library to download files from URLs
# Objective: retrieve the latest version of the ONNX Emotion FER+ model files from the
# ONNX Model Zoo and save it in the same folder as this tutorial
import urllib.request
onnx_model_url = "https://www.cntk.ai/OnnxModels/emotion_ferplus/opset_7/emotion_ferplus.tar.gz"
urllib.request.urlretrieve(onnx_model_url, filename="emotion_ferplus.tar.gz")
# the ! magic command tells our jupyter notebook kernel to run the following line of
# code from the command line instead of the notebook kernel
# We use tar and xvcf to unzip the files we just retrieved from the ONNX model zoo
!tar xvzf emotion_ferplus.tar.gz
###Output
_____no_output_____
###Markdown
Deploy a VM with your ONNX model in the Cloud Load Azure ML workspaceWe begin by instantiating a workspace object from the existing workspace created earlier in the configuration notebook.
###Code
# Check core SDK version number
import azureml.core
print("SDK version:", azureml.core.VERSION)
from azureml.core import Workspace
ws = Workspace.from_config()
print(ws.name, ws.location, ws.resource_group, sep = '\n')
###Output
_____no_output_____
###Markdown
Registering your model with Azure ML
###Code
model_dir = "emotion_ferplus" # replace this with the location of your model files
# leave as is if it's in the same folder as this notebook
from azureml.core.model import Model
model = Model.register(model_path = model_dir + "/" + "model.onnx",
model_name = "onnx_emotion",
tags = {"onnx": "demo"},
description = "FER+ emotion recognition CNN from ONNX Model Zoo",
workspace = ws)
###Output
_____no_output_____
###Markdown
Optional: Displaying your registered modelsThis step is not required, so feel free to skip it.
###Code
models = ws.models()
for name, m in models.items():
print("Name:", name,"\tVersion:", m.version, "\tDescription:", m.description, m.tags)
###Output
_____no_output_____
###Markdown
ONNX FER+ Model MethodologyThe image classification model we are using is pre-trained using Microsoft's deep learning cognitive toolkit, [CNTK](https://github.com/Microsoft/CNTK), from the [ONNX model zoo](http://github.com/onnx/models). The model zoo has many other models that can be deployed on cloud providers like AzureML without any additional training. To ensure that our cloud deployed model works, we use testing data from the well-known FER+ data set, provided as part of the [trained Emotion Recognition model](https://github.com/onnx/models/tree/master/emotion_ferplus) in the ONNX model zoo.The original Facial Emotion Recognition (FER) Dataset was released in 2013 by Pierre-Luc Carrier and Aaron Courville as part of a [Kaggle Competition](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data), but some of the labels are not entirely appropriate for the expression. In the FER+ Dataset, each photo was evaluated by at least 10 croud sourced reviewers, creating a more accurate basis for ground truth. You can see the difference of label quality in the sample model input below. The FER labels are the first word below each image, and the FER+ labels are the second word below each image.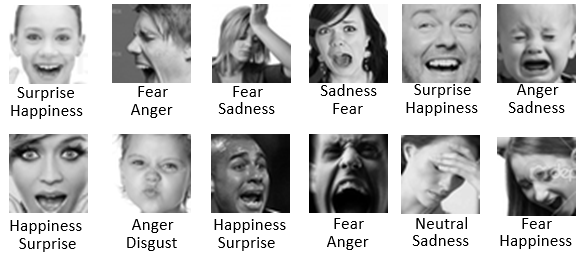***Input: Photos of cropped faces from FER+ Dataset******Task: Classify each facial image into its appropriate emotions in the emotion table***``` emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7} ```***Output: Emotion prediction for input image***Remember, once the application is deployed in Azure ML, you can use your own images as input for the model to classify.
###Code
# for images and plots in this notebook
import matplotlib.pyplot as plt
from IPython.display import Image
# display images inline
%matplotlib inline
###Output
_____no_output_____
###Markdown
Model DescriptionThe FER+ model from the ONNX Model Zoo is summarized by the graphic below. You can see the entire workflow of our pre-trained model in the following image from Barsoum et. al's paper ["Training Deep Networks for Facial Expression Recognitionwith Crowd-Sourced Label Distribution"](https://arxiv.org/pdf/1608.01041.pdf), with our (64 x 64) input images and our output probabilities for each of the labels.  Specify our Score and Environment Files We are now going to deploy our ONNX Model on AML with inference in ONNX Runtime. We begin by writing a score.py file, which will help us run the model in our Azure ML virtual machine (VM), and then specify our environment by writing a yml file. You will also notice that we import the onnxruntime library to do runtime inference on our ONNX models (passing in input and evaluating out model's predicted output). More information on the API and commands can be found in the [ONNX Runtime documentation](https://aka.ms/onnxruntime). Write Score FileA score file is what tells our Azure cloud service what to do. After initializing our model using azureml.core.model, we start an ONNX Runtime inference session to evaluate the data passed in on our function calls.
###Code
%%writefile score.py
import json
import numpy as np
import onnxruntime
import sys
import os
from azureml.core.model import Model
import time
def init():
global session, input_name, output_name
model = Model.get_model_path(model_name = 'onnx_emotion')
session = onnxruntime.InferenceSession(model, None)
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
def run(input_data):
'''Purpose: evaluate test input in Azure Cloud using onnxruntime.
We will call the run function later from our Jupyter Notebook
so our azure service can evaluate our model input in the cloud. '''
try:
# load in our data, convert to readable format
data = np.array(json.loads(input_data)['data']).astype('float32')
start = time.time()
r = session.run([output_name], {input_name : data})
end = time.time()
result = emotion_map(postprocess(r[0]))
result_dict = {"result": result,
"time_in_sec": [end - start]}
except Exception as e:
result_dict = {"error": str(e)}
return json.dumps(result_dict)
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the
top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3,
'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and
returns the class IDs in decreasing order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Write Environment File
###Code
from azureml.core.conda_dependencies import CondaDependencies
myenv = CondaDependencies()
myenv.add_pip_package("numpy")
myenv.add_pip_package("azureml-core")
myenv.add_pip_package("onnxruntime")
with open("myenv.yml","w") as f:
f.write(myenv.serialize_to_string())
###Output
_____no_output_____
###Markdown
Create the Container ImageThis step will likely take a few minutes.
###Code
from azureml.core.image import ContainerImage
image_config = ContainerImage.image_configuration(execution_script = "score.py",
runtime = "python",
conda_file = "myenv.yml",
description = "Emotion ONNX Runtime container",
tags = {"demo": "onnx"})
image = ContainerImage.create(name = "onnxtest",
# this is the model object
models = [model],
image_config = image_config,
workspace = ws)
image.wait_for_creation(show_output = True)
###Output
_____no_output_____
###Markdown
In case you need to debug your code, the next line of code accesses the log file.
###Code
print(image.image_build_log_uri)
###Output
_____no_output_____
###Markdown
We're all done specifying what we want our virtual machine to do. Let's configure and deploy our container image. Deploy the container image
###Code
from azureml.core.webservice import AciWebservice
aciconfig = AciWebservice.deploy_configuration(cpu_cores = 1,
memory_gb = 1,
tags = {'demo': 'onnx'},
description = 'ONNX for emotion recognition model')
from azureml.core.webservice import Webservice
aci_service_name = 'onnx-demo-emotion'
print("Service", aci_service_name)
aci_service = Webservice.deploy_from_image(deployment_config = aciconfig,
image = image,
name = aci_service_name,
workspace = ws)
aci_service.wait_for_deployment(True)
print(aci_service.state)
###Output
_____no_output_____
###Markdown
The following cell will likely take a few minutes to run as well.
###Code
if aci_service.state != 'Healthy':
# run this command for debugging.
print(aci_service.get_logs())
# If your deployment fails, make sure to delete your aci_service before trying again!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Success!If you've made it this far, you've deployed a working VM with a facial emotion recognition model running in the cloud using Azure ML. Congratulations!Let's see how well our model deals with our test images. Testing and Evaluation Useful Helper FunctionsWe preprocess and postprocess our data (see score.py file) using the helper functions specified in the [ONNX FER+ Model page in the Model Zoo repository](https://github.com/onnx/models/tree/master/emotion_ferplus).
###Code
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the
top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3,
'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and
returns the class IDs in decreasing order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Load Test DataThese are already in your directory from your ONNX model download (from the model zoo).Notice that our Model Zoo files have a .pb extension. This is because they are [protobuf files (Protocol Buffers)](https://developers.google.com/protocol-buffers/docs/pythontutorial), so we need to read in our data through our ONNX TensorProto reader into a format we can work with, like numerical arrays.
###Code
# to manipulate our arrays
import numpy as np
# read in test data protobuf files included with the model
import onnx
from onnx import numpy_helper
# to use parsers to read in our model/data
import json
import os
test_inputs = []
test_outputs = []
# read in 3 testing images from .pb files
test_data_size = 3
for i in np.arange(test_data_size):
input_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'input_0.pb')
output_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'output_0.pb')
# convert protobuf tensors to np arrays using the TensorProto reader from ONNX
tensor = onnx.TensorProto()
with open(input_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
input_data = numpy_helper.to_array(tensor)
test_inputs.append(input_data)
with open(output_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
output_data = numpy_helper.to_array(tensor)
output_processed = emotion_map(postprocess(output_data))[0]
test_outputs.append(output_processed)
###Output
_____no_output_____
###Markdown
Show some sample imagesWe use `matplotlib` to plot 3 test images from the dataset.
###Code
plt.figure(figsize = (20, 20))
for test_image in np.arange(3):
test_inputs[test_image].reshape(1, 64, 64)
plt.subplot(1, 8, test_image+1)
plt.axhline('')
plt.axvline('')
plt.text(x = 10, y = -10, s = test_outputs[test_image], fontsize = 18)
plt.imshow(test_inputs[test_image].reshape(64, 64), cmap = plt.cm.gray)
plt.show()
###Output
_____no_output_____
###Markdown
Run evaluation / prediction
###Code
plt.figure(figsize = (16, 6), frameon=False)
plt.subplot(1, 8, 1)
plt.text(x = 0, y = -30, s = "True Label: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -20, s = "Result: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -10, s = "Inference Time: ", fontsize = 13, color = 'black')
plt.text(x = 3, y = 14, s = "Model Input", fontsize = 12, color = 'black')
plt.text(x = 6, y = 18, s = "(64 x 64)", fontsize = 12, color = 'black')
plt.imshow(np.ones((28,28)), cmap=plt.cm.Greys)
for i in np.arange(test_data_size):
input_data = json.dumps({'data': test_inputs[i].tolist()})
# predict using the deployed model
r = json.loads(aci_service.run(input_data))
if "error" in r:
print(r['error'])
break
result = r['result'][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
ground_truth = test_outputs[i]
# compare actual value vs. the predicted values:
plt.subplot(1, 8, i+2)
plt.axhline('')
plt.axvline('')
# use different color for misclassified sample
font_color = 'red' if ground_truth != result else 'black'
clr_map = plt.cm.Greys if ground_truth != result else plt.cm.gray
# ground truth labels are in blue
plt.text(x = 10, y = -70, s = ground_truth, fontsize = 18, color = 'blue')
# predictions are in black if correct, red if incorrect
plt.text(x = 10, y = -45, s = result, fontsize = 18, color = font_color)
plt.text(x = 5, y = -22, s = str(time_ms) + ' ms', fontsize = 14, color = font_color)
plt.imshow(test_inputs[i].reshape(64, 64), cmap = clr_map)
plt.show()
###Output
_____no_output_____
###Markdown
Try classifying your own images!
###Code
# Preprocessing functions take your image and format it so it can be passed
# as input into our ONNX model
import cv2
def rgb2gray(rgb):
"""Convert the input image into grayscale"""
return np.dot(rgb[...,:3], [0.299, 0.587, 0.114])
def resize_img(img):
"""Resize image to MNIST model input dimensions"""
img = cv2.resize(img, dsize=(64, 64), interpolation=cv2.INTER_AREA)
img.resize((1, 1, 64, 64))
return img
def preprocess(img):
"""Resize input images and convert them to grayscale."""
if img.shape == (64, 64):
img.resize((1, 1, 64, 64))
return img
grayscale = rgb2gray(img)
processed_img = resize_img(grayscale)
return processed_img
# Replace the following string with your own path/test image
# Make sure your image is square and the dimensions are equal (i.e. 100 * 100 pixels or 28 * 28 pixels)
# Any PNG or JPG image file should work
# Make sure to include the entire path with // instead of /
# e.g. your_test_image = "C://Users//vinitra.swamy//Pictures//emotion_test_images//img_1.png"
import matplotlib.image as mpimg
if your_test_image != "<path to file>":
img = mpimg.imread(your_test_image)
plt.subplot(1,3,1)
plt.imshow(img, cmap = plt.cm.Greys)
print("Old Dimensions: ", img.shape)
img = preprocess(img)
print("New Dimensions: ", img.shape)
else:
img = None
if img is None:
print("Add the path for your image data.")
else:
input_data = json.dumps({'data': img.tolist()})
try:
r = json.loads(aci_service.run(input_data))
result = r['result'][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
except Exception as e:
print(str(e))
plt.figure(figsize = (16, 6))
plt.subplot(1,8,1)
plt.axhline('')
plt.axvline('')
plt.text(x = -10, y = -40, s = "Model prediction: ", fontsize = 14)
plt.text(x = -10, y = -25, s = "Inference time: ", fontsize = 14)
plt.text(x = 100, y = -40, s = str(result), fontsize = 14)
plt.text(x = 100, y = -25, s = str(time_ms) + " ms", fontsize = 14)
plt.text(x = -10, y = -10, s = "Model Input image: ", fontsize = 14)
plt.imshow(img.reshape((64, 64)), cmap = plt.cm.gray)
# remember to delete your service after you are done using it!
aci_service.delete()
###Output
_____no_output_____
###Markdown
Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License. Facial Expression Recognition using ONNX Runtime on AzureMLThis example shows how to deploy an image classification neural network using the Facial Expression Recognition ([FER](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data)) dataset and Open Neural Network eXchange format ([ONNX](http://aka.ms/onnxdocarticle)) on the Azure Machine Learning platform. This tutorial will show you how to deploy a FER+ model from the [ONNX model zoo](https://github.com/onnx/models), use it to make predictions using ONNX Runtime Inference, and deploy it as a web service in Azure.Throughout this tutorial, we will be referring to ONNX, a neural network exchange format used to represent deep learning models. With ONNX, AI developers can more easily move models between state-of-the-art tools (CNTK, PyTorch, Caffe, MXNet, TensorFlow) and choose the combination that is best for them. ONNX is developed and supported by a community of partners including Microsoft AI, Facebook, and Amazon. For more information, explore the [ONNX website](http://onnx.ai) and [open source files](https://github.com/onnx).[ONNX Runtime](https://aka.ms/onnxruntime-python) is the runtime engine that enables evaluation of trained machine learning (Traditional ML and Deep Learning) models with high performance and low resource utilization. We use the CPU version of ONNX Runtime in this tutorial, but will soon be releasing an additional tutorial for deploying this model using ONNX Runtime GPU. Tutorial Objectives:1. Describe the FER+ dataset and pretrained Convolutional Neural Net ONNX model for Emotion Recognition, stored in the ONNX model zoo.2. Deploy and run the pretrained FER+ ONNX model on an Azure Machine Learning instance3. Predict labels for test set data points in the cloud using ONNX Runtime and Azure ML Prerequisites 1. Install Azure ML SDK and create a new workspacePlease follow [00.configuration.ipynb](https://github.com/Azure/MachineLearningNotebooks/blob/master/00.configuration.ipynb) notebook. 2. Install additional packages needed for this NotebookYou need to install the popular plotting library `matplotlib`, the image manipulation library `PIL`, and the `onnx` library in the conda environment where Azure Maching Learning SDK is installed.```sh(myenv) $ pip install matplotlib onnx Pillow``` 3. Download sample data and pre-trained ONNX model from ONNX Model Zoo.[Download the ONNX Emotion FER+ model and corresponding test data](https://www.cntk.ai/OnnxModels/emotion_ferplus/opset_7/emotion_ferplus.tar.gz) and place them in the same folder as this tutorial notebook. You can unzip the file through the following line of code.```sh(myenv) $ tar xvzf emotion_ferplus.tar.gz```More information can be found about the ONNX FER+ model on [github](https://github.com/onnx/models/tree/master/emotion_ferplus). For more information about the FER+ dataset, please visit Microsoft Researcher Emad Barsoum's [FER+ source data repository](https://github.com/ebarsoum/FERPlus). Load Azure ML workspaceWe begin by instantiating a workspace object from the existing workspace created earlier in the configuration notebook.
###Code
# Check core SDK version number
import azureml.core
print("SDK version:", azureml.core.VERSION)
from azureml.core import Workspace
ws = Workspace.from_config()
print(ws.name, ws.location, ws.resource_group, sep = '\n')
###Output
_____no_output_____
###Markdown
Registering your model with Azure ML
###Code
model_dir = "emotion_ferplus" # replace this with the location of your model files
# leave as is if it's in the same folder as this notebook
from azureml.core.model import Model
model = Model.register(model_path = model_dir + "/" + "model.onnx",
model_name = "onnx_emotion",
tags = {"onnx": "demo"},
description = "FER+ emotion recognition CNN from ONNX Model Zoo",
workspace = ws)
###Output
_____no_output_____
###Markdown
Optional: Displaying your registered modelsThis step is not required, so feel free to skip it.
###Code
models = ws.models()
for m in models:
print("Name:", m.name,"\tVersion:", m.version, "\tDescription:", m.description, m.tags)
###Output
_____no_output_____
###Markdown
ONNX FER+ Model MethodologyThe image classification model we are using is pre-trained using Microsoft's deep learning cognitive toolkit, [CNTK](https://github.com/Microsoft/CNTK), from the [ONNX model zoo](http://github.com/onnx/models). The model zoo has many other models that can be deployed on cloud providers like AzureML without any additional training. To ensure that our cloud deployed model works, we use testing data from the famous FER+ data set, provided as part of the [trained Emotion Recognition model](https://github.com/onnx/models/tree/master/emotion_ferplus) in the ONNX model zoo.The original Facial Emotion Recognition (FER) Dataset was released in 2013, but some of the labels are not entirely appropriate for the expression. In the FER+ Dataset, each photo was evaluated by at least 10 croud sourced reviewers, creating a better basis for ground truth. You can see the difference of label quality in the sample model input below. The FER labels are the first word below each image, and the FER+ labels are the second word below each image.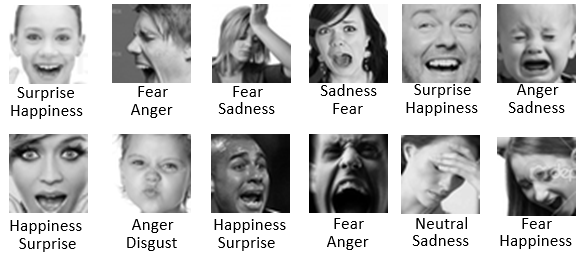***Input: Photos of cropped faces from FER+ Dataset******Task: Classify each facial image into its appropriate emotions in the emotion table***``` emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7} ```***Output: Emotion prediction for input image***Remember, once the application is deployed in Azure ML, you can use your own images as input for the model to classify.
###Code
# for images and plots in this notebook
import matplotlib.pyplot as plt
from IPython.display import Image
# display images inline
%matplotlib inline
###Output
_____no_output_____
###Markdown
Model DescriptionThe FER+ model from the ONNX Model Zoo is summarized by the graphic below. You can see the entire workflow of our pre-trained model in the following image from Barsoum et. al's paper ["Training Deep Networks for Facial Expression Recognitionwith Crowd-Sourced Label Distribution"](https://arxiv.org/pdf/1608.01041.pdf), with our (64 x 64) input images and our output probabilities for each of the labels.  Deploy our model on Azure ML We are now going to deploy our ONNX Model on AML with inference in ONNX Runtime. We begin by writing a score.py file, which will help us run the model in our Azure ML virtual machine (VM), and then specify our environment by writing a yml file.You will also notice that we import the onnxruntime library to do runtime inference on our ONNX models (passing in input and evaluating out model's predicted output). More information on the API and commands can be found in the [ONNX Runtime documentation](https://aka.ms/onnxruntime). Write Score FileA score file is what tells our Azure cloud service what to do. After initializing our model using azureml.core.model, we start an ONNX Runtime GPU inference session to evaluate the data passed in on our function calls.
###Code
%%writefile score.py
import json
import numpy as np
import onnxruntime
import sys
import os
from azureml.core.model import Model
import time
def init():
global session, input_name, output_name
model = Model.get_model_path(model_name = 'onnx_emotion')
session = onnxruntime.InferenceSession(model, None)
input_name = session.get_inputs()[0].name
output_name = session.get_outputs()[0].name
def run(input_data):
'''Purpose: evaluate test input in Azure Cloud using onnxruntime.
We will call the run function later from our Jupyter Notebook
so our azure service can evaluate our model input in the cloud. '''
try:
# load in our data, convert to readable format
data = np.array(json.loads(input_data)['data']).astype('float32')
start = time.time()
r = session.run([output_name], {input_name : data})
end = time.time()
result = emotion_map(postprocess(r[0]))
result_dict = {"result": result,
"time_in_sec": [end - start]}
except Exception as e:
result_dict = {"error": str(e)}
return json.dumps(result_dict)
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and returns the class IDs in decreasing
order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Write Environment File
###Code
from azureml.core.conda_dependencies import CondaDependencies
myenv = CondaDependencies()
myenv.add_pip_package("numpy")
myenv.add_pip_package("azureml-core")
myenv.add_pip_package("onnxruntime")
with open("myenv.yml","w") as f:
f.write(myenv.serialize_to_string())
###Output
_____no_output_____
###Markdown
Create the Container ImageThis step will likely take a few minutes.
###Code
from azureml.core.image import ContainerImage
image_config = ContainerImage.image_configuration(execution_script = "score.py",
runtime = "python",
conda_file = "myenv.yml",
description = "test",
tags = {"demo": "onnx"})
image = ContainerImage.create(name = "onnxtest",
# this is the model object
models = [model],
image_config = image_config,
workspace = ws)
image.wait_for_creation(show_output = True)
###Output
_____no_output_____
###Markdown
DebuggingIn case you need to debug your code, the next line of code accesses the log file.
###Code
print(image.image_build_log_uri)
###Output
_____no_output_____
###Markdown
We're all set! Let's get our model chugging. Deploy the container image
###Code
from azureml.core.webservice import AciWebservice
aciconfig = AciWebservice.deploy_configuration(cpu_cores = 1,
memory_gb = 1,
tags = {'demo': 'onnx'},
description = 'ONNX for emotion recognition model')
from azureml.core.webservice import Webservice
aci_service_name = 'onnx-demo-emotion'
print("Service", aci_service_name)
aci_service = Webservice.deploy_from_image(deployment_config = aciconfig,
image = image,
name = aci_service_name,
workspace = ws)
aci_service.wait_for_deployment(True)
print(aci_service.state)
###Output
_____no_output_____
###Markdown
The following cell will likely take a few minutes to run as well.
###Code
if aci_service.state != 'Healthy':
# run this command for debugging.
print(aci_service.get_logs())
# If your deployment fails, make sure to delete your aci_service before trying again!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Success!If you've made it this far, you've deployed a working VM with a facial emotion recognition model running in the cloud using Azure ML. Congratulations!Let's see how well our model deals with our test images. Testing and Evaluation Useful Helper FunctionsWe preprocess and postprocess our data (see score.py file) using the helper functions specified in the [ONNX FER+ Model page in the Model Zoo repository](https://github.com/onnx/models/tree/master/emotion_ferplus). Load Test Data
###Code
# to manipulate our arrays
import numpy as np
# read in test data protobuf files included with the model
import onnx
from onnx import numpy_helper
# to use parsers to read in our model/data
import json
import os
from score import emotion_map, softmax, postprocess
test_inputs = []
test_outputs = []
# read in 3 testing images from .pb files
test_data_size = 3
for i in np.arange(test_data_size):
input_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'input_0.pb')
output_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'output_0.pb')
# convert protobuf tensors to np arrays using the TensorProto reader from ONNX
tensor = onnx.TensorProto()
with open(input_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
input_data = numpy_helper.to_array(tensor)
test_inputs.append(input_data)
with open(output_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
output_data = numpy_helper.to_array(tensor)
output_processed = emotion_map(postprocess(output_data))[0]
test_outputs.append(output_processed)
###Output
_____no_output_____
###Markdown
Show some sample imagesWe use `matplotlib` to plot 3 test images from the model zoo with their labels over them.
###Code
plt.figure(figsize = (20, 20))
for test_image in np.arange(3):
test_inputs[test_image].reshape(1, 64, 64)
plt.subplot(1, 8, test_image+1)
plt.axhline('')
plt.axvline('')
plt.text(x = 10, y = -10, s = test_outputs[test_image], fontsize = 18)
plt.imshow(test_inputs[test_image].reshape(64, 64), cmap = plt.cm.Greys)
plt.show()
###Output
_____no_output_____
###Markdown
Run evaluation / prediction
###Code
plt.figure(figsize = (16, 6), frameon=False)
plt.subplot(1, 8, 1)
plt.text(x = 0, y = -30, s = "True Label: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -20, s = "Result: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -10, s = "Inference Time: ", fontsize = 13, color = 'black')
plt.text(x = 3, y = 14, s = "Model Input", fontsize = 12, color = 'black')
plt.text(x = 6, y = 18, s = "(64 x 64)", fontsize = 12, color = 'black')
plt.imshow(np.ones((28,28)), cmap=plt.cm.Greys)
for i in np.arange(test_data_size):
input_data = json.dumps({'data': test_inputs[i].tolist()})
# predict using the deployed model
r = json.loads(aci_service.run(input_data))
if "error" in r:
print(r['error'])
break
result = r['result'][0][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
ground_truth = test_outputs[i]
# compare actual value vs. the predicted values:
plt.subplot(1, 8, i+2)
plt.axhline('')
plt.axvline('')
# use different color for misclassified sample
font_color = 'red' if ground_truth != result else 'black'
clr_map = plt.cm.gray if ground_truth != result else plt.cm.Greys
# ground truth labels are in blue
plt.text(x = 10, y = -70, s = ground_truth, fontsize = 18, color = 'blue')
# predictions are in black if correct, red if incorrect
plt.text(x = 10, y = -45, s = result, fontsize = 18, color = font_color)
plt.text(x = 5, y = -22, s = str(time_ms) + ' ms', fontsize = 14, color = font_color)
plt.imshow(test_inputs[i].reshape(64, 64), cmap = clr_map)
plt.show()
###Output
_____no_output_____
###Markdown
Try classifying your own images!
###Code
from PIL import Image
def preprocess(image_path):
input_shape = (1, 1, 64, 64)
img = Image.open(image_path)
img = img.resize((64, 64), Image.ANTIALIAS)
img_data = np.array(img)
img_data = np.resize(img_data, input_shape)
return img_data
# Replace the following string with your own path/test image
# Make sure your image is square and the dimensions are equal (i.e. 100 * 100 pixels or 28 * 28 pixels)
# Any PNG or JPG image file should work
# Make sure to include the entire path with // instead of /
# e.g. your_test_image = "C://Users//vinitra.swamy//Pictures//emotion_test_images//img_1.png"
your_test_image = "<path to file>"
if your_test_image != "<path to file>":
img = preprocess(your_test_image)
plt.subplot(1,3,1)
plt.imshow(img.reshape((64,64)), cmap = plt.cm.gray)
else:
img = None
if img is None:
print("Add the path for your image data.")
else:
input_data = json.dumps({'data': img.tolist()})
try:
r = json.loads(aci_service.run(input_data))
result = r['result'][0][0]
time_ms = np.round(r['time_in_sec'][0] * 1000, 2)
except Exception as e:
print(str(e))
plt.figure(figsize = (16, 6))
plt.subplot(1,8,1)
plt.axhline('')
plt.axvline('')
plt.text(x = -10, y = -35, s = "Model prediction: ", fontsize = 14)
plt.text(x = -10, y = -20, s = "Inference time: ", fontsize = 14)
plt.text(x = 100, y = -35, s = str(result), fontsize = 14)
plt.text(x = 100, y = -20, s = str(time_ms) + " ms", fontsize = 14)
plt.text(x = -10, y = -8, s = "Input image: ", fontsize = 14)
plt.imshow(img.reshape(64, 64), cmap = plt.cm.gray)
# remember to delete your service after you are done using it!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License. 02. Facial Expression Recognition using ONNX Runtime GPU on AzureMLThis example shows how to deploy an image classification neural network using the Facial Expression Recognition ([FER](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data)) dataset and Open Neural Network eXchange format ([ONNX](http://aka.ms/onnxdocarticle)) on the Azure Machine Learning platform. This tutorial will show you how to deploy a FER+ model from the [ONNX model zoo](https://github.com/onnx/models), use it to make predictions using ONNX Runtime Inference, and deploy it as a web service in Azure.Throughout this tutorial, we will be referring to ONNX, a neural network exchange format used to represent deep learning models. With ONNX, AI developers can more easily move models between state-of-the-art tools (CNTK, PyTorch, Caffe, MXNet, TensorFlow) and choose the combination that is best for them. ONNX is developed and supported by a community of partners including Microsoft AI, Facebook, and Amazon. For more information, explore the [ONNX website](http://onnx.ai) and [open source files](https://github.com/onnx).[ONNX Runtime](https://aka.ms/onnxruntime) is the runtime engine that enables evaluation of trained machine learning (Traditional ML and Deep Learning) models with high performance and low resource utilization. Tutorial Objectives:1. Describe the FER+ dataset and pretrained Convolutional Neural Net ONNX model for Emotion Recognition, stored in the ONNX model zoo.2. Deploy and run the pretrained FER+ ONNX model on an Azure Machine Learning instance3. Predict labels for test set data points in the cloud using ONNX Runtime and Azure ML Prerequisites 1. Install Azure ML SDK and create a new workspacePlease follow [00.configuration.ipynb](https://github.com/Azure/MachineLearningNotebooks/blob/master/00.configuration.ipynb) notebook. 2. Install additional packages needed for this NotebookYou need to install the popular plotting library `matplotlib` and the `onnx` library in the conda environment where Azure Maching Learning SDK is installed.```sh(myenv) $ pip install matplotlib onnx``` 3. Download sample data and pre-trained ONNX model from ONNX Model Zoo.[Download the ONNX Emotion FER+ model and corresponding test data](https://www.cntk.ai/OnnxModels/emotion_ferplus/opset_7/emotion_ferplus.tar.gz) and place them in the same folder as this tutorial notebook. You can unzip the file through the following line of code.```sh(myenv) $ tar xvzf emotion_ferplus.tar.gz```More information can be found about the ONNX FER+ model on [github](https://github.com/onnx/models/tree/master/emotion_ferplus). For more information about the FER+ dataset, please visit Microsoft Researcher Emad Barsoum's [FER+ source data repository](https://github.com/ebarsoum/FERPlus). Load Azure ML workspaceWe begin by instantiating a workspace object from the existing workspace created earlier in the configuration notebook.
###Code
# Check core SDK version number
import azureml.core
print("SDK version:", azureml.core.VERSION)
from azureml.core import Workspace
ws = Workspace.from_config()
print(ws.name, ws.location, ws.resource_group, ws.location, sep = '\n')
###Output
_____no_output_____
###Markdown
Registering your model with Azure ML
###Code
model_dir = "emotion_ferplus" # replace this with the location of your model files
# leave as is if it's in the same folder as this notebook
from azureml.core.model import Model
model = Model.register(model_path = model_dir + "/" + "model.onnx",
model_name = "onnx_emotion",
tags = {"onnx": "demo"},
description = "FER+ emotion recognition CNN from ONNX Model Zoo",
workspace = ws)
###Output
_____no_output_____
###Markdown
Optional: Displaying your registered modelsThis step is not required, so feel free to skip it.
###Code
models = ws.models()
for m in models:
print("Name:", m.name,"\tVersion:", m.version, "\tDescription:", m.description, m.tags)
###Output
_____no_output_____
###Markdown
ONNX FER+ Model MethodologyThe image classification model we are using is pre-trained using Microsoft's deep learning cognitive toolkit, [CNTK](https://github.com/Microsoft/CNTK), from the [ONNX model zoo](http://github.com/onnx/models). The model zoo has many other models that can be deployed on cloud providers like AzureML without any additional training. To ensure that our cloud deployed model works, we use testing data from the famous FER+ data set, provided as part of the [trained Emotion Recognition model](https://github.com/onnx/models/tree/master/emotion_ferplus) in the ONNX model zoo.The original Facial Emotion Recognition (FER) Dataset was released in 2013, but some of the labels are not entirely appropriate for the expression. In the FER+ Dataset, each photo was evaluated by at least 10 croud sourced reviewers, creating a better basis for ground truth. You can see the difference of label quality in the sample model input below. The FER labels are the first word below each image, and the FER+ labels are the second word below each image.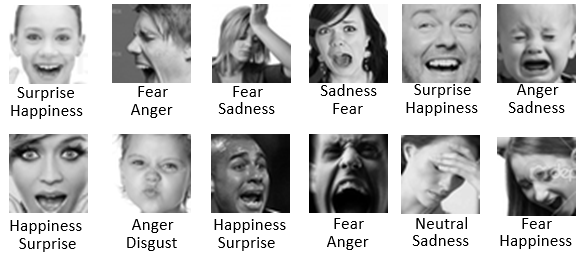***Input: Photos of cropped faces from FER+ Dataset******Task: Classify each facial image into its appropriate emotions in the emotion table***``` emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7} ```***Output: Emotion prediction for input image***Remember, once the application is deployed in Azure ML, you can use your own images as input for the model to classify.
###Code
# for images and plots in this notebook
import matplotlib.pyplot as plt
from IPython.display import Image
# display images inline
%matplotlib inline
###Output
_____no_output_____
###Markdown
Model DescriptionThe FER+ model from the ONNX Model Zoo is summarized by the graphic below. You can see the entire workflow of our pre-trained model in the following image from Barsoum et. al's paper ["Training Deep Networks for Facial Expression Recognitionwith Crowd-Sourced Label Distribution"](https://arxiv.org/pdf/1608.01041.pdf), with our (64,64) input images and our output probabilities for each of the labels.  Deploy our model on Azure ML We are now going to deploy our ONNX Model on AML with inference in ONNX Runtime. We begin by writing a score.py file, which will help us run the model in our Azure ML virtual machine (VM), and then specify our environment by writing a yml file.You will also notice that we import the onnxruntime library to do runtime inference on our ONNX models (passing in input and evaluating out model's predicted output). More information on the API and commands can be found in the [ONNX Runtime documentation](https://aka.ms/onnxruntime). Write Score FileA score file is what tells our Azure cloud service what to do. After initializing our model using azureml.core.model, we start an ONNX Runtime GPU inference session to evaluate the data passed in on our function calls.
###Code
%%writefile score.py
import json
import numpy as np
import onnxruntime
import sys
import os
from azureml.core.model import Model
import time
def init():
global session
model = Model.get_model_path(model_name = 'onnx_emotion')
session = onnxruntime.InferenceSession(model, None)
def run(input_data):
'''Purpose: evaluate test input in Azure Cloud using onnxruntime.
We will call the run function later from our Jupyter Notebook
so our azure service can evaluate our model input in the cloud. '''
try:
# load in our data, convert to readable format
start = time.time()
data = np.array(json.loads(input_data)['data']).astype('float32')
r = session.run(["Plus214_Output_0"], {"Input3": data})[0]
result = emotion_map(postprocess(r[0]))
end = time.time()
result_dict = {"result": np.array(result).tolist(),
"time": np.array(end - start).tolist()}
except Exception as e:
result_dict = {"error": str(e)}
return json.dumps(result_dict)
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and returns the class IDs in decreasing
order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Write Environment File
###Code
from azureml.core.conda_dependencies import CondaDependencies
myenv = CondaDependencies()
myenv.add_pip_package("numpy")
myenv.add_pip_package("azureml-core")
myenv.add_pip_package("onnxruntime-gpu")
with open("myenv.yml","w") as f:
f.write(myenv.serialize_to_string())
###Output
_____no_output_____
###Markdown
Create the Container ImageThis step will likely take a few minutes.
###Code
from azureml.core.image import ContainerImage
# enable_gpu = True to install CUDA 9.1 and cuDNN 7.0
image_config = ContainerImage.image_configuration(execution_script = "score.py",
runtime = "python",
conda_file = "myenv.yml",
description = "test",
tags = {"demo": "onnx"},
enable_gpu = True
)
image = ContainerImage.create(name = "onnxtest",
# this is the model object
models = [model],
image_config = image_config,
workspace = ws)
image.wait_for_creation(show_output = True)
###Output
_____no_output_____
###Markdown
DebuggingIn case you need to debug your code, the next line of code accesses the log file.
###Code
print(image.image_build_log_uri)
###Output
_____no_output_____
###Markdown
We're all set! Let's get our model chugging. Deploy the container image
###Code
from azureml.core.webservice import AciWebservice
aciconfig = AciWebservice.deploy_configuration(cpu_cores = 1,
memory_gb = 1,
tags = {'demo': 'onnx'},
description = 'ONNX for facial emotion recognition model')
###Output
_____no_output_____
###Markdown
The following cell will likely take a few minutes to run as well.
###Code
from azureml.core.webservice import Webservice
aci_service_name = 'onnx-emotion-demo'
print("Service", aci_service_name)
aci_service = Webservice.deploy_from_image(deployment_config = aciconfig,
image = image,
name = aci_service_name,
workspace = ws)
aci_service.wait_for_deployment(True)
print(aci_service.state)
if aci_service.state != 'Healthy':
# run this command for debugging.
print(aci_service.get_logs())
# If your deployment fails, make sure to delete your aci_service before trying again!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Success!If you've made it this far, you've deployed a working VM with a facial emotion recognition model running in the cloud using Azure ML. Congratulations!Let's see how well our model deals with our test images. Testing and Evaluation Useful Helper FunctionsWe preprocess and postprocess our data (see score.py file) using the helper functions specified in the [ONNX FER+ Model page in the Model Zoo repository](https://github.com/onnx/models/tree/master/emotion_ferplus).
###Code
def preprocess(img):
"""Convert image to the write format to be passed into the model"""
input_shape = (1, 64, 64)
img = np.reshape(img, input_shape)
img = np.expand_dims(img, axis=0)
return img
# to manipulate our arrays
import numpy as np
# read in test data protobuf files included with the model
import onnx
from onnx import numpy_helper
# to use parsers to read in our model/data
import json
import os
test_inputs = []
test_outputs = []
# read in 3 testing images from .pb files
test_data_size = 3
for i in np.arange(test_data_size):
input_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'input_0.pb')
output_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'output_0.pb')
# convert protobuf tensors to np arrays using the TensorProto reader from ONNX
tensor = onnx.TensorProto()
with open(input_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
input_data = preprocess(numpy_helper.to_array(tensor))
test_inputs.append(input_data)
with open(output_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
output_data = numpy_helper.to_array(tensor)
test_outputs.append(output_data)
###Output
_____no_output_____
###Markdown
Show some sample imagesWe use `matplotlib` to plot 3 images from the dataset with their labels over them.
###Code
plt.figure(figsize = (20, 20))
for test_image in np.arange(3):
test_inputs[test_image].reshape(1, 64, 64)
plt.subplot(1, 8, test_image+1)
plt.axhline('')
plt.axvline('')
plt.text(x = 10, y = -10, s = test_outputs[test_image][0], fontsize = 18)
plt.imshow(test_inputs[test_image].reshape(64, 64))
plt.show()
###Output
_____no_output_____
###Markdown
Run evaluation / prediction
###Code
plt.figure(figsize = (16, 6), frameon=False)
plt.subplot(1, 8, 1)
plt.text(x = 0, y = -30, s = "True Label: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -20, s = "Result: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -10, s = "Inference Time: ", fontsize = 13, color = 'black')
plt.text(x = 3, y = 14, s = "Model Input", fontsize = 12, color = 'black')
plt.text(x = 6, y = 18, s = "(64 x 64)", fontsize = 12, color = 'black')
plt.imshow(np.ones((28,28)), cmap=plt.cm.Greys)
for i in np.arange(test_data_size):
input_data = json.dumps({'data': test_inputs[i].tolist()})
# predict using the deployed model
r = json.loads(aci_service.run(input_data))
if len(r) == 1:
print(r['error'])
break
result = r['result']
time_ms = np.round(r['time'] * 1000, 2)
ground_truth = int(np.argmax(test_outputs[i]))
# compare actual value vs. the predicted values:
plt.subplot(1, 8, i+2)
plt.axhline('')
plt.axvline('')
# use different color for misclassified sample
font_color = 'red' if ground_truth != result else 'black'
clr_map = plt.cm.gray if ground_truth != result else plt.cm.Greys
# ground truth labels are in blue
plt.text(x = 10, y = -30, s = ground_truth, fontsize = 18, color = 'blue')
# predictions are in black if correct, red if incorrect
plt.text(x = 10, y = -20, s = result, fontsize = 18, color = font_color)
plt.text(x = 5, y = -10, s = str(time_ms) + ' ms', fontsize = 14, color = font_color)
plt.imshow(test_inputs[i].reshape(64, 64), cmap = clr_map)
plt.show()
###Output
_____no_output_____
###Markdown
Try classifying your own images!
###Code
# Replace the following string with your own path/test image
# Make sure the dimensions are 28 * 28 pixels
# Any PNG or JPG image file should work
# Make sure to include the entire path with // instead of /
# e.g. your_test_image = "C://Users//vinitra.swamy//Pictures//emotion_test_images//img_1.jpg"
your_test_image = "<path to file>"
import matplotlib.image as mpimg
if your_test_image != "<path to file>":
img = mpimg.imread(your_test_image)
plt.subplot(1,3,1)
plt.imshow(img, cmap = plt.cm.Greys)
img = img.reshape(1, 1, 64, 64)
else:
img = None
if img is None:
print("Add the path for your image data.")
else:
input_data = json.dumps({'data': img.tolist()})
try:
r = json.loads(aci_service.run(input_data))
result = r['result']
time_ms = np.round(r['time'] * 1000, 2)
except Exception as e:
print(json.loads(r)['error'])
plt.figure(figsize = (16, 6))
plt.subplot(1, 15,1)
plt.axhline('')
plt.axvline('')
plt.text(x = -100, y = -20, s = "Model prediction: ", fontsize = 14)
plt.text(x = -100, y = -10, s = "Inference time: ", fontsize = 14)
plt.text(x = 0, y = -20, s = str(result), fontsize = 14)
plt.text(x = 0, y = -10, s = str(time_ms) + " ms", fontsize = 14)
plt.text(x = -100, y = 14, s = "Input image: ", fontsize = 14)
plt.imshow(img.reshape(28, 28), cmap = plt.cm.Greys)
# remember to delete your service after you are done using it!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Copyright (c) Microsoft Corporation. All rights reserved. Licensed under the MIT License. 02. Facial Expression Recognition using ONNX Runtime GPU on AzureMLThis example shows how to deploy an image classification neural network using the Facial Expression Recognition ([FER](https://www.kaggle.com/c/challenges-in-representation-learning-facial-expression-recognition-challenge/data)) dataset and Open Neural Network eXchange format ([ONNX](http://aka.ms/onnxdocarticle)) on the Azure Machine Learning platform. This tutorial will show you how to deploy a FER+ model from the [ONNX model zoo](https://github.com/onnx/models), use it to make predictions using ONNX Runtime Inference, and deploy it as a web service in Azure.Throughout this tutorial, we will be referring to ONNX, a neural network exchange format used to represent deep learning models. With ONNX, AI developers can more easily move models between state-of-the-art tools (CNTK, PyTorch, Caffe, MXNet, TensorFlow) and choose the combination that is best for them. ONNX is developed and supported by a community of partners including Microsoft AI, Facebook, and Amazon. For more information, explore the [ONNX website](http://onnx.ai) and [open source files](https://github.com/onnx).[ONNX Runtime](https://aka.ms/onnxruntime) is the runtime engine that enables evaluation of trained machine learning (Traditional ML and Deep Learning) models with high performance and low resource utilization. Tutorial Objectives:1. Describe the FER+ dataset and pretrained Convolutional Neural Net ONNX model for Emotion Recognition, stored in the ONNX model zoo.2. Deploy and run the pretrained FER+ ONNX model on an Azure Machine Learning instance3. Predict labels for test set data points in the cloud using ONNX Runtime and Azure ML Prerequisites 1. Install Azure ML SDK and create a new workspacePlease follow [00.configuration.ipynb](https://github.com/Azure/MachineLearningNotebooks/blob/master/00.configuration.ipynb) notebook. 2. Install additional packages needed for this NotebookYou need to install the popular plotting library `matplotlib` and the `onnx` library in the conda environment where Azure Maching Learning SDK is installed.```sh(myenv) $ pip install matplotlib onnx``` 3. Download sample data and pre-trained ONNX model from ONNX Model Zoo.[Download the ONNX Emotion FER+ model and corresponding test data](https://www.cntk.ai/OnnxModels/emotion_ferplus/opset_7/emotion_ferplus.tar.gz) and place them in the same folder as this tutorial notebook. You can unzip the file through the following line of code.```sh(myenv) $ tar xvzf emotion_ferplus.tar.gz```More information can be found about the ONNX FER+ model on [github](https://github.com/onnx/models/tree/master/emotion_ferplus). For more information about the FER+ dataset, please visit Microsoft Researcher Emad Barsoum's [FER+ source data repository](https://github.com/ebarsoum/FERPlus). Load Azure ML workspaceWe begin by instantiating a workspace object from the existing workspace created earlier in the configuration notebook.
###Code
# Check core SDK version number
import azureml.core
print("SDK version:", azureml.core.VERSION)
from azureml.core import Workspace
ws = Workspace.from_config()
print(ws.name, ws.location, ws.resource_group, ws.location, sep = '\n')
###Output
_____no_output_____
###Markdown
Registering your model with Azure ML
###Code
model_dir = "emotion_ferplus" # replace this with the location of your model files
# leave as is if it's in the same folder as this notebook
from azureml.core.model import Model
model = Model.register(model_path = model_dir + "/" + "model.onnx",
model_name = "onnx_emotion",
tags = {"onnx": "demo"},
description = "FER+ emotion recognition CNN from ONNX Model Zoo",
workspace = ws)
###Output
_____no_output_____
###Markdown
Optional: Displaying your registered modelsThis step is not required, so feel free to skip it.
###Code
models = ws.models()
for m in models:
print("Name:", m.name,"\tVersion:", m.version, "\tDescription:", m.description, m.tags)
###Output
_____no_output_____
###Markdown
ONNX FER+ Model MethodologyThe image classification model we are using is pre-trained using Microsoft's deep learning cognitive toolkit, [CNTK](https://github.com/Microsoft/CNTK), from the [ONNX model zoo](http://github.com/onnx/models). The model zoo has many other models that can be deployed on cloud providers like AzureML without any additional training. To ensure that our cloud deployed model works, we use testing data from the famous FER+ data set, provided as part of the [trained Emotion Recognition model](https://github.com/onnx/models/tree/master/emotion_ferplus) in the ONNX model zoo.The original Facial Emotion Recognition (FER) Dataset was released in 2013, but some of the labels are not entirely appropriate for the expression. In the FER+ Dataset, each photo was evaluated by at least 10 croud sourced reviewers, creating a better basis for ground truth. You can see the difference of label quality in the sample model input below. The FER labels are the first word below each image, and the FER+ labels are the second word below each image.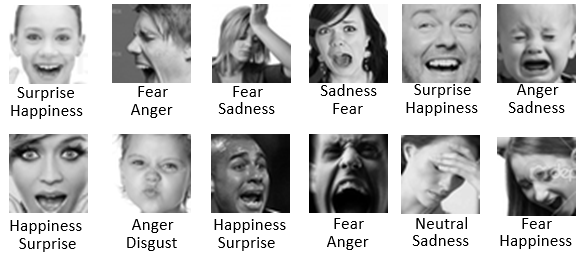***Input: Photos of cropped faces from FER+ Dataset******Task: Classify each facial image into its appropriate emotions in the emotion table***``` emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7} ```***Output: Emotion prediction for input image***Remember, once the application is deployed in Azure ML, you can use your own images as input for the model to classify.
###Code
# for images and plots in this notebook
import matplotlib.pyplot as plt
from IPython.display import Image
# display images inline
%matplotlib inline
###Output
_____no_output_____
###Markdown
Model DescriptionThe FER+ model from the ONNX Model Zoo is summarized by the graphic below. You can see the entire workflow of our pre-trained model in the following image from Barsoum et. al's paper ["Training Deep Networks for Facial Expression Recognitionwith Crowd-Sourced Label Distribution"](https://arxiv.org/pdf/1608.01041.pdf), with our (64,64) input images and our output probabilities for each of the labels.  Deploy our model on Azure ML We are now going to deploy our ONNX Model on AML with inference in ONNX Runtime. We begin by writing a score.py file, which will help us run the model in our Azure ML virtual machine (VM), and then specify our environment by writing a yml file.You will also notice that we import the onnxruntime library to do runtime inference on our ONNX models (passing in input and evaluating out model's predicted output). More information on the API and commands can be found in the [ONNX Runtime documentation](https://aka.ms/onnxruntime). Write Score FileA score file is what tells our Azure cloud service what to do. After initializing our model using azureml.core.model, we start an ONNX Runtime GPU inference session to evaluate the data passed in on our function calls.
###Code
%%writefile score.py
import json
import numpy as np
import onnxruntime
import sys
import os
from azureml.core.model import Model
import time
def init():
global session
model = Model.get_model_path(model_name = 'onnx_emotion')
session = onnxruntime.InferenceSession(model, None)
def run(input_data):
'''Purpose: evaluate test input in Azure Cloud using onnxruntime.
We will call the run function later from our Jupyter Notebook
so our azure service can evaluate our model input in the cloud. '''
try:
# load in our data, convert to readable format
start = time.time()
data = np.array(json.loads(input_data)['data']).astype('float32')
r = session.run(["Plus214_Output_0"], {"Input3": data})[0]
result = emotion_map(postprocess(r[0]))
end = time.time()
result_dict = {"result": np.array(result).tolist(),
"time": np.array(end - start).tolist()}
except Exception as e:
result_dict = {"error": str(e)}
return json.dumps(result_dict)
def emotion_map(classes, N=1):
"""Take the most probable labels (output of postprocess) and returns the top N emotional labels that fit the picture."""
emotion_table = {'neutral':0, 'happiness':1, 'surprise':2, 'sadness':3, 'anger':4, 'disgust':5, 'fear':6, 'contempt':7}
emotion_keys = list(emotion_table.keys())
emotions = []
for i in range(N):
emotions.append(emotion_keys[classes[i]])
return emotions
def softmax(x):
"""Compute softmax values (probabilities from 0 to 1) for each possible label."""
x = x.reshape(-1)
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum(axis=0)
def postprocess(scores):
"""This function takes the scores generated by the network and returns the class IDs in decreasing
order of probability."""
prob = softmax(scores)
prob = np.squeeze(prob)
classes = np.argsort(prob)[::-1]
return classes
###Output
_____no_output_____
###Markdown
Write Environment File
###Code
from azureml.core.conda_dependencies import CondaDependencies
myenv = CondaDependencies()
myenv.add_pip_package("numpy")
myenv.add_pip_package("azureml-core")
myenv.add_pip_package("onnxruntime-gpu")
with open("myenv.yml","w") as f:
f.write(myenv.serialize_to_string())
###Output
_____no_output_____
###Markdown
Create the Container ImageThis step will likely take a few minutes.
###Code
from azureml.core.image import ContainerImage
# enable_gpu = True to install CUDA 9.1 and cuDNN 7.0
image_config = ContainerImage.image_configuration(execution_script = "score.py",
runtime = "python",
conda_file = "myenv.yml",
description = "test",
tags = {"demo": "onnx"},
enable_gpu = True
)
image = ContainerImage.create(name = "onnxtest",
# this is the model object
models = [model],
image_config = image_config,
workspace = ws)
image.wait_for_creation(show_output = True)
###Output
_____no_output_____
###Markdown
DebuggingIn case you need to debug your code, the next line of code accesses the log file.
###Code
print(image.image_build_log_uri)
###Output
_____no_output_____
###Markdown
We're all set! Let's get our model chugging. Deploy the container image
###Code
from azureml.core.webservice import AciWebservice
aciconfig = AciWebservice.deploy_configuration(cpu_cores = 1,
memory_gb = 1,
tags = {'demo': 'onnx'},
description = 'ONNX for facial emotion recognition model')
###Output
_____no_output_____
###Markdown
The following cell will likely take a few minutes to run as well.
###Code
from azureml.core.webservice import Webservice
aci_service_name = 'onnx-emotion-demo'
print("Service", aci_service_name)
aci_service = Webservice.deploy_from_image(deployment_config = aciconfig,
image = image,
name = aci_service_name,
workspace = ws)
aci_service.wait_for_deployment(True)
print(aci_service.state)
if aci_service.state != 'Healthy':
# run this command for debugging.
print(aci_service.get_logs())
# If your deployment fails, make sure to delete your aci_service before trying again!
# aci_service.delete()
###Output
_____no_output_____
###Markdown
Success!If you've made it this far, you've deployed a working VM with a facial emotion recognition model running in the cloud using Azure ML. Congratulations!Let's see how well our model deals with our test images. Testing and Evaluation Useful Helper FunctionsWe preprocess and postprocess our data (see score.py file) using the helper functions specified in the [ONNX FER+ Model page in the Model Zoo repository](https://github.com/onnx/models/tree/master/emotion_ferplus).
###Code
def preprocess(img):
"""Convert image to the write format to be passed into the model"""
input_shape = (1, 64, 64)
img = np.reshape(img, input_shape)
img = np.expand_dims(img, axis=0)
return img
# to manipulate our arrays
import numpy as np
# read in test data protobuf files included with the model
import onnx
from onnx import numpy_helper
# to use parsers to read in our model/data
import json
import os
test_inputs = []
test_outputs = []
# read in 3 testing images from .pb files
test_data_size = 3
for i in np.arange(test_data_size):
input_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'input_0.pb')
output_test_data = os.path.join(model_dir, 'test_data_set_{0}'.format(i), 'output_0.pb')
# convert protobuf tensors to np arrays using the TensorProto reader from ONNX
tensor = onnx.TensorProto()
with open(input_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
input_data = preprocess(numpy_helper.to_array(tensor))
test_inputs.append(input_data)
with open(output_test_data, 'rb') as f:
tensor.ParseFromString(f.read())
output_data = numpy_helper.to_array(tensor)
test_outputs.append(output_data)
###Output
_____no_output_____
###Markdown
Show some sample imagesWe use `matplotlib` to plot 3 images from the dataset with their labels over them.
###Code
plt.figure(figsize = (20, 20))
for test_image in np.arange(3):
test_inputs[test_image].reshape(1, 64, 64)
plt.subplot(1, 8, test_image+1)
plt.axhline('')
plt.axvline('')
plt.text(x = 10, y = -10, s = test_outputs[test_image][0], fontsize = 18)
plt.imshow(test_inputs[test_image].reshape(64, 64))
plt.show()
###Output
_____no_output_____
###Markdown
Run evaluation / prediction
###Code
plt.figure(figsize = (16, 6), frameon=False)
plt.subplot(1, 8, 1)
plt.text(x = 0, y = -30, s = "True Label: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -20, s = "Result: ", fontsize = 13, color = 'black')
plt.text(x = 0, y = -10, s = "Inference Time: ", fontsize = 13, color = 'black')
plt.text(x = 3, y = 14, s = "Model Input", fontsize = 12, color = 'black')
plt.text(x = 6, y = 18, s = "(64 x 64)", fontsize = 12, color = 'black')
plt.imshow(np.ones((28,28)), cmap=plt.cm.Greys)
for i in np.arange(test_data_size):
input_data = json.dumps({'data': test_inputs[i].tolist()})
# predict using the deployed model
r = json.loads(aci_service.run(input_data))
if len(r) == 1:
print(r['error'])
break
result = r['result']
time_ms = np.round(r['time'] * 1000, 2)
ground_truth = int(np.argmax(test_outputs[i]))
# compare actual value vs. the predicted values:
plt.subplot(1, 8, i+2)
plt.axhline('')
plt.axvline('')
# use different color for misclassified sample
font_color = 'red' if ground_truth != result else 'black'
clr_map = plt.cm.gray if ground_truth != result else plt.cm.Greys
# ground truth labels are in blue
plt.text(x = 10, y = -30, s = ground_truth, fontsize = 18, color = 'blue')
# predictions are in black if correct, red if incorrect
plt.text(x = 10, y = -20, s = result, fontsize = 18, color = font_color)
plt.text(x = 5, y = -10, s = str(time_ms) + ' ms', fontsize = 14, color = font_color)
plt.imshow(test_inputs[i].reshape(64, 64), cmap = clr_map)
plt.show()
###Output
_____no_output_____
###Markdown
Try classifying your own images!
###Code
# Replace the following string with your own path/test image
# Make sure the dimensions are 28 * 28 pixels
# Any PNG or JPG image file should work
# Make sure to include the entire path with // instead of /
# e.g. your_test_image = "C://Users//vinitra.swamy//Pictures//emotion_test_images//img_1.jpg"
your_test_image = "<path to file>"
import matplotlib.image as mpimg
if your_test_image != "<path to file>":
img = mpimg.imread(your_test_image)
plt.subplot(1,3,1)
plt.imshow(img, cmap = plt.cm.Greys)
img = img.reshape(1, 1, 64, 64)
else:
img = None
if img is None:
print("Add the path for your image data.")
else:
input_data = json.dumps({'data': img.tolist()})
try:
r = json.loads(aci_service.run(input_data))
result = r['result']
time_ms = np.round(r['time'] * 1000, 2)
except Exception as e:
print(json.loads(r)['error'])
plt.figure(figsize = (16, 6))
plt.subplot(1, 15,1)
plt.axhline('')
plt.axvline('')
plt.text(x = -100, y = -20, s = "Model prediction: ", fontsize = 14)
plt.text(x = -100, y = -10, s = "Inference time: ", fontsize = 14)
plt.text(x = 0, y = -20, s = str(result), fontsize = 14)
plt.text(x = 0, y = -10, s = str(time_ms) + " ms", fontsize = 14)
plt.text(x = -100, y = 14, s = "Input image: ", fontsize = 14)
plt.imshow(img.reshape(28, 28), cmap = plt.cm.Greys)
# remember to delete your service after you are done using it!
# aci_service.delete()
###Output
_____no_output_____ |
notebooks/MakeFlagregionsCoast.ipynb | ###Markdown
Make Flagregions along the CoastThis example shows how to make a Ruled Rectangle for AMR flagging along the coast, based on first selecting points within some region where the water depth satisfies a costraint.
###Code
%matplotlib inline
from pylab import *
import os,sys
from imp import reload
from clawpack.geoclaw import topotools, dtopotools, kmltools
from clawpack.visclaw import colormaps
from IPython.display import Image
sys.path.insert(0,'../new_python')
import fgmax_tools
import region_tools, marching_front
zmin = -60.
zmax = 40.
land_cmap = colormaps.make_colormap({ 0.0:[0.1,0.4,0.0],
0.25:[0.0,1.0,0.0],
0.5:[0.8,1.0,0.5],
1.0:[0.8,0.5,0.2]})
sea_cmap = colormaps.make_colormap({ 0.0:[0,0,1], 1.:[.8,.8,1]})
cmap, norm = colormaps.add_colormaps((land_cmap, sea_cmap),
data_limits=(zmin,zmax),
data_break=0.)
sea_cmap_dry = colormaps.make_colormap({ 0.0:[1.0,0.8,0.8], 1.:[1.0,0.8,0.8]})
cmap_dry, norm_dry = colormaps.add_colormaps((land_cmap, sea_cmap_dry),
data_limits=(zmin,zmax),
data_break=0.)
###Output
_____no_output_____
###Markdown
Download etopo1 data coarsened to 4 minute resolutionEven if the computational grid will be finer than 4 arcminutes, this is sufficient resolution for creating a ruled rectangle to use as a flagregion.
###Code
extent = [-132, -122, 44, 52]
topo = topotools.read_netcdf('etopo1', extent=extent, coarsen=4)
topo.plot()
###Output
_____no_output_____
###Markdown
Restrict region of interest:We first define a simple ruled region with the region of interest. In this cae we are interested in making a flagregion that goes along the continental shelf from the Columbia River at about 46N to the northern tip of Vancouver Island at about 51N, and extending only slightly into the Strait of Juan de Fuca (since we used other flagregions in the Strait and Puget Sound).
###Code
# Specify a RuledRectangle the our flagregion should lie in:
rrect = region_tools.RuledRectangle()
rrect.ixy = 'y' # s = latitude
rrect.s = array([46,48,48.8,50,51.])
rrect.lower = -131*ones(rrect.s.shape)
rrect.upper = array([-123.,-124,-124,-126,-128])
rrect.method = 1
xr,yr = rrect.vertices()
figure(figsize=(10,10))
ax = axes()
topo.plot(axes=ax)
plot(xr,yr,'r')
###Output
_____no_output_____
###Markdown
We could use the red ruled rectangle plotted above directly as our flagregion, but instead we will trim this down to only cover the continental shelf a shore region:
###Code
# Start with a mask defined by the ruled rectangle `rrect` defined above:
mask_out = rrect.mask_outside(topo.X, topo.Y)
# select onshore points within 2 grip points of shore:
pts_chosen_Zabove0 = marching_front.select_by_flooding(topo.Z, mask=mask_out,
prev_pts_chosen=None,
Z1=0, Z2=1e6, max_iters=2)
# select offshore points down to 1000 m depth:
pts_chosen_Zbelow0 = marching_front.select_by_flooding(topo.Z, mask=None,
prev_pts_chosen=None,
Z1=0, Z2=-1000., max_iters=None)
# buffer offshore points with another 10 grid cells:
pts_chosen_Zbelow0 = marching_front.select_by_flooding(topo.Z, mask=None,
prev_pts_chosen=pts_chosen_Zbelow0,
Z1=0, Z2=-5000., max_iters=10)
# Take the intersection of the two sets of points selected above:
nearshore_pts = where(pts_chosen_Zabove0+pts_chosen_Zbelow0 == 2, 1, 0)
print('Number of nearshore points: %i' % nearshore_pts.sum())
###Output
_____no_output_____
###Markdown
Now create the minimal ruled rectangle `rr2` that covers the grid cells selected above:
###Code
rr2 = region_tools.ruledrectangle_covering_selected_points(topo.X, topo.Y,
nearshore_pts, ixy='y', method=0,
verbose=True)
###Output
_____no_output_____
###Markdown
Here's the topography, masked to only show the points selected in `nearshore_pts`, along with the minimal ruled rectangle that covers these points.
###Code
marching_front.plot_masked_Z(topo, nearshore_pts, zmin=-3000, zmax=1000)
x2,y2 = rr2.vertices()
plot(x2,y2,'r')
ylim(45,52);
###Output
_____no_output_____
###Markdown
And the ruled rectangle in the bigger context:
###Code
figure(figsize=(10,10))
ax = axes()
topo.plot(axes=ax)
x2,y2 = rr2.vertices()
plot(x2,y2,'r')
ylim(45,52);
###Output
_____no_output_____
###Markdown
From this we can make a `.data` file that can be used as a flagregion `spatial_extent_file`, see [FlagRegions.ipynb](FlagRegions.ipynb) for discussion.
###Code
rr_name = 'RuledRectangle_Coast_46_51'
rr2.write(rr_name + '.data')
rr2.make_kml(fname=rr_name+'.kml', name=rr_name)
###Output
_____no_output_____
###Markdown
The above cell also makes a kml file `RuledRectangle_Coast_46_51.kml` that can be opened on Google Earth to show this region. Here's a screenshot:
###Code
Image('figs/RuledRectangle_Coast_46_51_GE.png', width=500)
###Output
_____no_output_____ |
examples/notebooks/5_groundwater_surfacewater.ipynb | ###Markdown
Adding river levels*Developed by R.A. Collenteur & D. Brakenhoff*In this example it is shown how to create a Pastas model that not only includes precipitation and evaporation, but also observed river levels. We will consider observed heads that are strongly influenced by river level, based on a visual interpretation of the raw data.
###Code
import pandas as pd
import pastas as ps
import matplotlib.pyplot as plt
%matplotlib notebook
ps.show_versions()
ps.set_log_level("ERROR")
###Output
Python version: 3.7.6 | packaged by conda-forge | (default, Mar 23 2020, 22:45:16)
[Clang 9.0.1 ]
Numpy version: 1.17.5
Scipy version: 1.4.1
Pandas version: 1.0.3
Pastas version: 0.14.0b
###Markdown
1. import and plot dataBefore a model is created, it is generally a good idea to try and visually interpret the raw data and think about possible relationship between the time series and hydrological variables. Below the different time series are plotted.The top plot shows the observed heads, with different observation frequencies and some gaps in the data. Below that the observed river levels, precipitation and evaporation are shown. Especially the river level show a clear relationship with the observed heads. Note however how the range in the river levels is about twice the range in the heads. Based on these observations, we would expect the the final step response of the head to the river level to be around 0.5 [m/m].
###Code
oseries = pd.read_csv("../data/nb5_head.csv", parse_dates=True,
squeeze=True, index_col=0)
rain = pd.read_csv("../data/nb5_prec.csv", parse_dates=True, squeeze=True,
index_col=0)
evap = pd.read_csv("../data/nb5_evap.csv", parse_dates=True, squeeze=True,
index_col=0)
waterlevel = pd.read_csv("../data/nb5_riv.csv", parse_dates=True,
squeeze=True, index_col=0)
fig, axes = plt.subplots(4,1, figsize=(10, 5), sharex=True)
oseries.plot(ax=axes[0], x_compat=True, legend=True, marker=".", linestyle=" ")
waterlevel.plot(ax=axes[1], x_compat=True, legend=True)
rain.plot(ax=axes[2], x_compat=True, legend=True)
evap.plot(ax=axes[3], x_compat=True, legend=True)
plt.xlim("2000", "2020");
###Output
_____no_output_____
###Markdown
2. Create a timeseries modelFirst we create a model with precipitation and evaporation as explanatory time series. The results show that precipitation and evaporation can explain part of the fluctuations in the observed heads, but not all of them.
###Code
ml = ps.Model(oseries, name="River Model")
sm = ps.RechargeModel(rain, evap, rfunc=ps.Exponential, name="recharge")
ml.add_stressmodel(sm)
ml.solve(tmin="2000")
ml.plots.results(figsize=(12, 8));
###Output
Model Results River Model Fit Statistics
==================================================
nfev 21 EVP 38.52
nobs 6050 R2 0.38
noise True RMSE 0.46
tmin 2000-01-27 00:00:00 AIC 2.71
tmax 2020-01-21 00:00:00 BIC 36.25
freq D ___
warmup 3650 days 00:00:00 ___
solver LeastSquares ___
Parameters (5 were optimized)
==================================================
optimal stderr initial vary
recharge_A 545.641513 ±15.99% 183.785267 True
recharge_a 224.662550 ±15.71% 10.000000 True
recharge_f -1.532329 ±8.45% -1.000000 True
constant_d 8.480126 ±1.42% 8.530099 True
noise_alpha 64.922650 ±5.86% 1.000000 True
Parameter correlations |rho| > 0.5
==================================================
recharge_A recharge_a 0.97
recharge_f constant_d -0.97
###Markdown
3. Adding river water levelsBased on the analysis of the raw data, we expect that the river levels can help to explain the fluctuations in the observed heads. Here, we add a stress model (`ps.StressModel`) to add the rivers level as an explanatory time series to the model. The model fit is greatly improved, showing that the rivers help in explaining the observed fluctuations in the observed heads. It can also be observed how the response of the head to the river levels is a lot faster than the response to precipitation and evaporation.
###Code
w = ps.StressModel(waterlevel, rfunc=ps.Exponential, name="waterlevel",
settings="waterlevel")
ml.add_stressmodel(w)
ml.solve(tmin="2000")
axes = ml.plots.results(figsize=(12, 8));
axes[-1].set_xlim(0,10); # By default, the axes between responses are shared.
###Output
Model Results River Model Fit Statistics
===================================================
nfev 23 EVP 95.43
nobs 6050 R2 0.95
noise True RMSE 0.13
tmin 2000-01-27 00:00:00 AIC 11.39
tmax 2020-01-21 00:00:00 BIC 58.34
freq D ___
warmup 3650 days 00:00:00 ___
solver LeastSquares ___
Parameters (7 were optimized)
===================================================
optimal stderr initial vary
recharge_A 167.107980 ±12.59% 183.785267 True
recharge_a 191.849969 ±12.32% 10.000000 True
recharge_f -1.392901 ±7.56% -1.000000 True
waterlevel_A 0.476146 ±0.18% 1.000000 True
waterlevel_a 0.020297 ±0.00% 10.000000 True
constant_d 8.532987 ±0.38% 8.530099 True
noise_alpha 54.291165 ±5.52% 1.000000 True
Parameter correlations |rho| > 0.5
===================================================
recharge_A recharge_a 0.97
constant_d -0.59
recharge_f constant_d -0.95
waterlevel_a noise_alpha 0.99
###Markdown
Simulating groundwater levels with a surface water level*Developed by Raoul Collenteur* In this example it is shown how to create a time series model with not only evaporation and precipitation, but also including surface water levels. The following data is used:- groundwater.csv: groundwaterlevels- rain.csv: Precipitation- evap.csv: Potential evaporation- waterlevel.csv: Surface waterlevel
###Code
import pandas as pd
import pastas as ps
import matplotlib.pyplot as plt
%matplotlib notebook
###Output
_____no_output_____
###Markdown
1. import and plot the data
###Code
oseries = pd.read_csv("data_notebook_5/groundwater.csv", parse_dates=True, squeeze=True, index_col=0)
rain = pd.read_csv("data_notebook_5/rain.csv", parse_dates=True, squeeze=True, index_col=0)
evap = pd.read_csv("data_notebook_5/evap.csv", parse_dates=True, squeeze=True, index_col=0)
waterlevel = pd.read_csv("data_notebook_5/waterlevel.csv", parse_dates=True, squeeze=True, index_col=0)
fig, axes = plt.subplots(4,1, figsize=(12, 5), sharex=True)
oseries.plot(ax=axes[0], x_compat=True, legend=True)
rain.plot(ax=axes[1], x_compat=True, legend=True)
evap.plot(ax=axes[2], x_compat=True, legend=True)
waterlevel.plot(ax=axes[3], x_compat=True, legend=True)
###Output
_____no_output_____
###Markdown
2. Create a timeseries model
###Code
ml = ps.Model(oseries)
sm = ps.StressModel2([rain, evap], rfunc=ps.Gamma, name="recharge")
ml.add_stressmodel(sm)
ml.solve(tmin="2000")
ml.plots.results(tmin="2012", figsize=(16, 8))
print("The explained variance percentage over the period 2012-2017 is: %s" % ml.stats.evp(tmin="2012"))
###Output
INFO: Cannot determine frequency of series Groundwater
INFO: Time Series Groundwater: 5 nan-value(s) was/were found and filled with: drop
WARNING: User-provided name ' Groundwater' contains illegal character
INFO: Inferred frequency from time series Precipitation: freq=D
INFO: Inferred frequency from time series Evaporation: freq=D
###Markdown
3. Adding surface water level
###Code
w = ps.StressModel(waterlevel, rfunc=ps.Exponential, name="waterlevel", settings="waterlevel")
# Normalize the stress by the mean such that only the variation in the waterlevel matters
w.update_stress(norm="mean")
ml.add_stressmodel(w)
ml.solve(tmin="2012")
ml.plots.results(figsize=(16, 8))
###Output
_____no_output_____ |
samples/balloon/balloon_inspect-indo.ipynb | ###Markdown
1. Pertama kita mengambil dataset, dalam contoh kali ini kita menggunakan data berupa gambar balon2. Setelah itu gambar kita annotasikan di menggunakan `VIA (VGG Image Annotator) [VGG](https://https://www.robots.ox.ac.uk/~vgg/software/via/via_demo.html)`
###Code
import os
import sys
import itertools
import math
import logging
import json
import re
import random
from collections import OrderedDict
import numpy as np
import matplotlib
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.lines as lines
from matplotlib.patches import Polygon
# Root directory of the project
ROOT_DIR = os.path.abspath("../../")
# Import Mask RCNN
sys.path.append(ROOT_DIR) # To find local version of the library
from mrcnn import utils
from mrcnn import visualize
from mrcnn.visualize import display_images
import mrcnn.model as modellib
from mrcnn.model import log
from samples.balloon import balloon
%matplotlib inline
###Output
_____no_output_____ |
dmu26/dmu26_XID+PACS_ELAIS-N1/XID+PACS_prior_SWIRE.ipynb | ###Markdown
This notebook uses all the raw data from the XID+MIPS catalogue, maps, PSF and relevant MOCs to create XID+ prior object and relevant tiling scheme Read in MOCsThe selection functions required are the main MOC associated with the masterlist. As the prior for XID+ is based on IRAC detected sources coming from two different surveys at different depths (SERVS and SWIRE) I will split the XID+ run into two different runs. Here we use the SERVS depth.
###Code
Sel_func=pymoc.MOC()
Sel_func.read('../data/ELAIS_N1/MOCs/holes_ELAIS-N1_irac1_O16_MOC.fits')
SERVS_MOC=pymoc.MOC()
SERVS_MOC.read('../data/ELAIS_N1/MOCs/DF-SERVS_ELAIS-N1_MOC.fits')
SWIRE_MOC=pymoc.MOC()
SWIRE_MOC.read('../data/ELAIS_N1/MOCs/DF-SWIRE_ELAIS-N1_MOC.fits')
Final=Sel_func.intersection(SERVS_MOC)
Final=Sel_func.intersection(SWIRE_MOC)
Final=Final-SERVS_MOC
###Output
_____no_output_____
###Markdown
Read in XID+MIPS catalogue
###Code
XID_MIPS=Table.read('../data/ELAIS_N1/MIPS/dmu26_XID+MIPS_ELAIS-N1_SWIRE_cat_20170725.fits')
XID_MIPS[0:10]
skew=(XID_MIPS['FErr_MIPS_24_u']-XID_MIPS['F_MIPS_24'])/(XID_MIPS['F_MIPS_24']-XID_MIPS['FErr_MIPS_24_l'])
skew.name='(84th-50th)/(50th-16th) percentile'
g=sns.jointplot(x=np.log10(XID_MIPS['F_MIPS_24']),y=skew, kind='hex')
###Output
_____no_output_____
###Markdown
The uncertianties become Gaussian by $\sim 20 \mathrm{\mu Jy}$
###Code
good=XID_MIPS['F_MIPS_24']>20
good.sum()
###Output
_____no_output_____
###Markdown
Read in Maps
###Code
im100fits='../data/ELAIS_N1/PACS/ELAIS-N1-100um-img_wgls.fits'#PACS 100 map
nim100fits='../data/ELAIS_N1/PACS/ELAIS-N1-100um-img_noise.fits'#PACS 100 noise map
im160fits='../data/ELAIS_N1/PACS/ELAIS-N1-160um-img_wgls.fits'#PACS 160 map
nim160fits='../data/ELAIS_N1/PACS/ELAIS-N1-160um-img_noise.fits'#PACS 100 noise map
#output folder
output_folder='./'
from astropy.io import fits
from astropy import wcs
#-----100-------------
hdulist = fits.open(im100fits)
im100phdu=hdulist[0].header
im100hdu=hdulist[0].header
im100=hdulist[0].data
w_100 = wcs.WCS(hdulist[0].header)
pixsize100=3600.0*np.abs(hdulist[0].header['CDELT1']) #pixel size (in arcseconds)
hdulist.close()
hdulist = fits.open(nim100fits)
nim100=hdulist[0].data
hdulist.close()
#-----160-------------
hdulist = fits.open(im160fits)
im160phdu=hdulist[0].header
im160hdu=hdulist[0].header
im160=hdulist[0].data #convert to mJy
w_160 = wcs.WCS(hdulist[0].header)
pixsize160=3600.0*np.abs(hdulist[0].header['CDELT1']) #pixel size (in arcseconds)
hdulist.close()
hdulist = fits.open(nim160fits)
nim160=hdulist[0].data
hdulist.close()
###Output
_____no_output_____
###Markdown
Read in PSF
###Code
pacs100_psf=fits.open('../../dmu18/dmu18_ELAIS-N1/dmu18_PACS_100_PSF_ELAIS-N1_20170720.fits')
pacs160_psf=fits.open('../../dmu18/dmu18_ELAIS-N1/dmu18_PACS_160_PSF_ELAIS-N1_20170720.fits')
centre100=np.long((pacs100_psf[1].header['NAXIS1']-1)/2)
radius100=15
centre160=np.long((pacs160_psf[1].header['NAXIS1']-1)/2)
radius160=25
pind100=np.arange(0,radius100+1+radius100,1)*3600*np.abs(pacs100_psf[1].header['CDELT1'])/pixsize100 #get 100 scale in terms of pixel scale of map
pind160=np.arange(0,radius160+1+radius160,1)*3600*np.abs(pacs160_psf[1].header['CDELT1'])/pixsize160 #get 160 scale in terms of pixel scale of map
print(pind100)
import pylab as plt
plt.figure(figsize=(20,10))
plt.subplot(1,2,1)
plt.imshow(pacs100_psf[1].data[centre100-radius100:centre100+radius100+1,centre100-radius100:centre100+radius100+1])
plt.colorbar()
plt.subplot(1,2,2)
plt.imshow(pacs160_psf[1].data[centre160-radius160:centre160+radius160+1,centre160-radius160:centre160+radius160+1])
plt.colorbar()
###Output
_____no_output_____
###Markdown
Set XID+ prior class
###Code
#---prior100--------
prior100=xidplus.prior(im100,nim100,im100phdu,im100hdu, moc=Final)#Initialise with map, uncertianty map, wcs info and primary header
prior100.prior_cat(XID_MIPS['RA'][good],XID_MIPS['Dec'][good],'dmu26_XID+MIPS_ELAIS-N1-SERVS.fits',ID=XID_MIPS['help_id'][good])#Set input catalogue
prior100.prior_bkg(0.0,5)#Set prior on background (assumes Gaussian pdf with mu and sigma)
#---prior160--------
prior160=xidplus.prior(im160,nim160,im160phdu,im160hdu, moc=Final)
prior160.prior_cat(XID_MIPS['RA'][good],XID_MIPS['Dec'][good],'dmu26_XID+MIPS_ELAIS-N1-SERVS.fits',ID=XID_MIPS['help_id'][good])
prior160.prior_bkg(0.0,5)
# Divide by 1000 so that units are mJy
prior100.set_prf(pacs100_psf[1].data[centre100-radius100:centre100+radius100+1,centre100-radius100:centre100+radius100+1]/1000.0,
pind100,pind100)
prior160.set_prf(pacs160_psf[1].data[centre160-radius160:centre160+radius160+1,centre160-radius160:centre160+radius160+1]/1000.0,
pind160,pind160)
import pickle
#from moc, get healpix pixels at a given order
from xidplus import moc_routines
order=11
tiles=moc_routines.get_HEALPix_pixels(order,prior100.sra,prior100.sdec,unique=True)
order_large=6
tiles_large=moc_routines.get_HEALPix_pixels(order_large,prior100.sra,prior100.sdec,unique=True)
print('----- There are '+str(len(tiles))+' tiles required for input catalogue and '+str(len(tiles_large))+' large tiles')
output_folder='./'
outfile=output_folder+'Master_prior_SWIRE.pkl'
with open(outfile, 'wb') as f:
pickle.dump({'priors':[prior100,prior160],'tiles':tiles,'order':order,'version':xidplus.io.git_version()},f)
outfile=output_folder+'Tiles_SWIRE.pkl'
with open(outfile, 'wb') as f:
pickle.dump({'tiles':tiles,'order':order,'tiles_large':tiles_large,'order_large':order_large,'version':xidplus.io.git_version()},f)
raise SystemExit()
###Output
----- There are 8480 tiles required for input catalogue and 20 large tiles
|
labs/lab04.ipynb | ###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(
hp_prom = ("hp","mean"),
atk_min = ("sp_atk","min"),
atk_max = ("sp_atk","max"),
def_min = ("sp_def","min"),
def_max = ("sp_def","max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean()+0.4*(df["attack"].mean()+df["sp_atk"].mean())**2 +0.3*(df["defense"].mean()+df["sp_def"].mean())**(1.5) + 0.1*df["speed"].mean()
return oakgueda_ind
pkm_12 = pkm.groupby(["type1","type2"]).apply(lambda df: oakgueda_indicator(df) )
pkm_12.loc[lambda x: x == pkm12.max()]
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max()-s.min())
pkm.groupby("legendary").transform(lambda s: minmax_scale(s) ).drop("generation",axis=1)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg(
hp_mean=('hp','mean'), sp_atk_max=('sp_atk','max'), sp_atk_min=('sp_atk','min'), sp_def_max=('sp_def','max'), sp_def_min=('sp_def','min')
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df['hp'] + 0.4*(df['attack'] + df['sp_atk'])**2 + 0.3*(df['defense'] + df['sp_def'])**1.5 + 0.1*df['speed']
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(lambda df: oakgueda_indicator(df).mean()).sort_values(ascending = False) # Ordenamos de mayor a menor para encontrar el mejor #
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min())
pkm.groupby('legendary').transform(lambda s: minmax_scale(s))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1", "type2"]).apply(lambda df: oakgueda_indicator(df).mean()).loc[lambda df: df > 40000]
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(
hp_mean=("hp","mean")
)
).reset_index()
(
pkm.groupby(["generation","legendary"])
.agg(
sp_atk_max=("sp_atk", "max"),
sp_atk_min=("sp_atk", "min"),
sp_def_max=("sp_def", "max"),
sp_def_min=("sp_def", "min"),
)
).reset_index()
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
# Sacar un indicador, aplicarlo a todos los pokemones, agrupar por el tipo 1 y tipo 2
#sacar un valor de esto una agregacion, ordenarlos y decir cual es el mejor grupo de tipo 1 y tipo 2
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean()+0.4*(df["attack"]+df["sp_atk"]).mean()**2+0.3*(df["defense"]+df["sp_def"]).mean()**1.5+0.1*df["speed"].mean()# FIX ME PLEASE #
return oakgueda_ind
indicador=pkm.groupby(["type1","type2"]).apply(oakgueda_indicator)
indicador
indicador[indicador == indicador.max()]
print(f"RESPUESTA: En promedio los pokemones tipo Ground Fire tiene el mayor indicador con {indicador.max()} puntos.")
###Output
RESPUESTA: En promedio los pokemones tipo Ground Fire tiene el mayor indicador con 44774.85412256314 puntos.
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
#def minmax_scale(s):
#s_scaled=(s-s.min())/(s.max()-s.min())
# return s_scaled
(
pkm.groupby("legendary") #agrupa por generacion
.transform(lambda s: (s - s.min()) / (s.max()-s.min()) ) #aplica el transform, defino funcion anonima, toma la serie de n de largo
)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm_filtered = pkm.groupby(["type1","type2"]).filter(lambda df: 0.2*df["hp"].mean()+0.4*(df["attack"]+df["sp_atk"]).mean()**2+0.3*(df["defense"]+df["sp_def"]).mean()**1.5+0.1*df["speed"].mean()>40000)
pkm_filtered
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(
hp = ("hp","mean"),
sp_atk_min = ("sp_atk","min"),
sp_atk_max = ("sp_atk","max"),
sp_def_min = ("sp_def","min"),
sp_def_max = ("sp_def","max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean() + 0.4*(df["attack"].mean()+df["sp_atk"].mean())**2+0.3*(df["defense"].mean()+df["sp_def"].mean())**(1.5)+0.1*df["speed"].mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max()- s.min())
pkm.groupby(["legendary"]).transform(lambda s: minmax_scale(s) )
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).loc[lambda x: x>40000]
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg(
hp_mean = ('hp','mean'),
sp_atk_max = ('sp_atk','max'),
sp_atk_min = ('sp_atk','min'),
sp_def_max = ('sp_def','max'),
sp_def_min = ('sp_def','min')
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df['hp'].mean() + 0.4*((df['attack'].mean() + df['sp_atk'].mean())**2) + 0.3*((df['defense'].mean() + df['sp_def'].mean())**1.5) + 0.1*df['speed'].mean()
return oakgueda_ind
pkm.groupby(['type1','type2']).apply(oakgueda_indicator).sort_values().index[-1]
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min())
pkm.groupby('legendary').transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(['type1','type2']).apply(oakgueda_indicator).loc[lambda x: x >= 40000]
print(pkm.loc[lambda x: (x['type1']=='Ground')&(x['type2']=='Fire')].shape)
pkm.loc[lambda x: (x['type1']=='Psychic')&(x['type2']=='Dark')].shape
###Output
(1, 11)
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation", "legendary"])
.agg({"hp": "mean",
"sp_atk": ["min", "max"], "sp_def": ["min", "max"]}
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean()+0.4*(df["attack"].mean()+df["sp_atk"].mean())**2+0.3*(df["defense"].mean()+df["sp_def"].mean())**(1.5)+0.1*df["speed"].mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return ((s-s.min())/(s.max()-s.min()))
pkm.groupby("legendary").transform(lambda x: minmax_scale(x))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1", "type2"]).filter(lambda df: oakgueda_indicator(df) >= 40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg(
{"hp":"mean",'sp_atk':['min','max'],'sp_def':['min','max']}
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df['hp']+0.4*(df['attack']+df['sp_atk'])**2+0.3*(df['defense']+df['sp_def'])**(1.5)+0.1*df['speed']
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(lambda df: oakgueda_indicator(df).mean()).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max()-s.min())
pkm.groupby('legendary').transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(['type1','type2']).filter(lambda df: oakgueda_indicator(df).mean() >40000 ).shape[0]
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation", "legendary"]) # agrupa por generación y si son legendarios #
.agg(
hp_mean = ("hp", "mean"),
sp_atk_min = ("sp_atk", "min"),
sp_atk_max = ("sp_atk", "max"),
sp_def_min = ("sp_def", "min"),
sp_def_max = ("sp_def", "max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2 * df["hp"].mean() + 0.4 * ((df["attack"].mean() + df["sp_atk"].mean()) ** 2)
+ 0.3 * ((df["defense"].mean() + df["sp_def"].mean()) ** 1.5) + 0.1 * df["speed"].mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).idxmax() # idxmax para obtener el máximo #
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min()) # definir función #
pkm.groupby("legendary").transform(minmax_scale) # usar transform con la función definida #
# también se puede hacer sin definir la función usando lambda #
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1", "type2"]).filter(lambda df: (0.2 * df["hp"].mean() + 0.4 * ((df["attack"].mean() + df["sp_atk"].mean()) ** 2)
+ 0.3 * ((df["defense"].mean() + df["sp_def"].mean()) ** 1.5) + 0.1 * df["speed"].mean()) > 40000 )
# no supe cómo hacerlo con la función que definí en el ejercicio 2 #
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(
hp_mean = ("hp","mean"),
atk_max = ("sp_atk","max"),
atk_min = ("sp_atk","min"),
def_max = ("sp_def","max"),
def_min = ("sp_def","min")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean() + 0.4*(df["attack"].mean()+df["sp_atk"].mean())**2 + 0.3*(df["defense"].mean()+df["sp_def"].mean())**(1.5)+0.1*df["speed"].mean()
return oakgueda_ind
pkm12 = pkm.groupby(["type1","type2"]).apply(lambda df: oakgueda_indicator(df))
pkm12.loc[lambda x: x == pkm12.max()]
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max() - s.min())
pkm.groupby("legendary").transform(lambda s: if : minmax_scale(s))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: oakgueda_indicator(df)>40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg( {"hp": "mean","sp_atk":["max","min"], "sp_def":["max","min"]}
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = ((0.2*df["hp"])+ (0.4*((df["attack"]+df["sp_atk"])**2))+ (0.3*((df["defense"]+df["sp_def"])**(1.5)))+(0.1*df["speed"] )).mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s- s.min())/(s.max()-s.min())
pkm.groupby("legendary").transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: oakgueda_indicator(df)>40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
pkm.groupby("generation").agg({'hp':'mean', 'sp_atk':['min','max']}), pkm.groupby("legendary").agg({'hp':'mean', 'sp_atk':['min','max']})
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df['hp']+0.4*pow((df['attack']+df['sp_atk']),2)+0.3*pow((df['defense']+df['sp_def']),1.5)+0.1*df['speed']
return oakgueda_ind.mean()
indicador_por_tipo=pkm.groupby(['type1','type2']).apply(oakgueda_indicator)
print(f"En promedio, los pokemones {indicador_por_tipo.idxmax()} son mejores según el indicador del profesor oakgueda")
###Output
En promedio, los pokemones ('Ground', 'Fire') son mejores según el indicador del profesor oakgueda
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
s_scaled=(s-min(s))/(max(s)-min(s))
return s_scaled
legendary=pkm.loc[:,"legendary"]
pkm_scaled=pkm.groupby("legendary").transform(minmax_scale)
pkm_scaled.drop('generation', axis=1)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
filtrado=pkm.groupby(["type1","type2"]).filter(lambda x: oakgueda_indicator(x) > 40000)
filtrado.loc[:,"name"].count()
###Output
_____no_output_____
###Markdown
__Respuesta:__ Hay solo dos pokemones que cumplen esta condición. Bonus TrackHonestamente, ¿te causó risa el chiste del profesor Oakgueda? Responde del 1 al 5, donde 1 es equivalente a _"Me dio vergüenza ajena"_ y 5 a _"Me alegró el día y mi existencia en la UTFSM"_.
###Code
print("Mmm sí jaja. Le doy un 5")
###Output
Mmm sí jaja. Le doy un 5
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation", "legendary"])
.agg(
promediohp=("hp", "mean"),
min_sp_atk=("sp_atk", "min"),
Max_sp_atk=("sp_atk", "max"),
min_sp_def=("sp_def", "min"),
Max_sp_def=("sp_def", "min")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = (0.2*df["hp"].mean()+ 0.4*(df["attack"].mean() + df["sp_atk"].mean())**2+0.3*(df["defense"].mean()+df["sp_def"].mean())**(1.5)+0.1*df["speed"].mean())
return oakgueda_ind
pkm_indicador=oakgueda_indicator(pkm)
pkm_indicador.loc[lambda s: s== pkm_indicador.max()]
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min()) / (s.max()-s.min())
pkm.groupby("legendary").transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: oakgueda_indicator(df)>40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg(hp_mean = ('hp','mean'),
sp_atk_min=('sp_atk','min'), sp_atk_max=('sp_atk','max'),
sp_def_min=('sp_def','min'), sp_def_max=('sp_def','max')
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
oakgueda_ind = 0.2*pkm.loc[:,'hp'] + 0.4*(pkm.loc[:,'attack'] + pkm.loc[:,'sp_atk'])**2 + 0.3*(pkm.loc[:,'defense'] + pkm.loc[:,'sp_def'])**1.5 + 0.1*pkm.loc[:,'speed']
pkm_auxiliar = pkm.assign(indice= oakgueda_ind).groupby(['type1','type2']).agg(indice_mean=('indice','mean'))
pkm_auxiliar.head()
mejor_pkm = pkm_auxiliar[pkm_auxiliar == pkm_auxiliar.max()]
mejor_pkm = mejor_pkm.loc[lambda x: x.notnull().all(axis=1)].head()
for idx, row in mejor_pkm.iterrows():
print(f"En promedio, los pokemones {idx[0]}-{idx[1]} son mejores según el indicador del profesor Oakgueda.")
###Output
En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda.
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max()-s.min())
pkm.assign(
hp=lambda df: df.groupby("legendary")["hp"].transform(minmax_scale),
attack=lambda df: df.groupby("legendary")["attack"].transform(minmax_scale),
defense=lambda df: df.groupby("legendary")["defense"].transform(minmax_scale),
sp_atk=lambda df: df.groupby("legendary")["sp_atk"].transform(minmax_scale),
sp_def=lambda df: df.groupby("legendary")["sp_def"].transform(minmax_scale),
speed=lambda df: df.groupby("legendary")["speed"].transform(minmax_scale),
generation=lambda df: df.groupby("legendary")["generation"].transform(minmax_scale)
)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
contador= pkm_auxiliar[lambda x: x['indice_mean']>40000].count()[0]
print (f'Hay solo {contador} pokemones que cumplen esta condición.')
###Output
Hay solo 2 pokemones que cumplen esta condición.
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(
mean_hp = ("hp", "mean"), sp_atk_max = ("sp_atk", "max"), sp_atk_min = ("sp_atk","min"), sp_def_min = ("sp_def","min"), sp_def_max = ("sp_def","max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"] + 0.4*(df["attack"] + df["sp_atk"])**2 + 0.3*(df["defense"] + df["sp_def"])**1.5 + 0.1*df["speed"]
return df.assign(oakgueda_ind = oakgueda_ind)
pkm = oakgueda_indicator(pkm)
pkm.head(2)
u = pkm.groupby(["type1","type2"]).agg(mean_ind = ("oakgueda_ind", "mean"))
u.idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max() - s.min())
pkm.groupby("legendary").transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
n = (pkm.groupby(["type1","type2"]).agg(mean_ind = ("oakgueda_ind", "mean"))["mean_ind"]>40000).sum()
print(f"hay {n} pokemones que cumplen esta condicion")
###Output
hay 2 pokemones que cumplen esta condicion
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
pkm.groupby(["type1", "type2"]).agg(hp_mean=("hp", "mean"),sp_atk_min=("sp_atk", "min"),sp_atk_max=("sp_atk", "max"),sp_def_max=("sp_def", "max"),sp_def_min=("sp_def", "min"))
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df['hp'].mean()+0.4*(df['attack'].mean()+df['sp_atk'].mean())**2+0.3*(df['defense'].mean()+df['sp_def'].mean())**1.5+0.1*df['speed'].mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max()-s.min())
pkm.groupby("legendary").transform(lambda s: minmax_scale(s))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator).loc[lambda s: s >= 40000].shape
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
pkm.groupby(["generation", "legendary"]).agg(hp_mean=("hp","mean"), sp_atk_min = ("sp_atk","min"), sp_atk_max=("sp_atk","max"), sp_def_min=("sp_def","min"),sp_def_max=("sp_def","max"))
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = df.loc[:,"hp"]*0.2+0.4*(df.loc[:,"attack"]+df.loc[:,"sp_atk"])**2+0.3*(df.loc[:,"defense"]+df.loc[:,"sp_def"])**1.5+0.1*df.loc[:,"speed"]
return oakgueda_ind
max_ = pkm.assign( oak_ind = lambda df: oakgueda_indicator(df)).groupby(["type1","type2"])["oak_ind"].mean().loc[:,lambda df: df == df.max()]
print(f"En promedio, los pokemones {max_.keys()[0][0]}-{max_.keys()[0][1]} son los mejores según el indicador del profesor Oakgueda, con {round(max_.iloc[0],2)} puntos.")
###Output
En promedio, los pokemones Ground-Fire son los mejores según el indicador del profesor Oakgueda, con 44774.85 puntos.
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s-s.min())/(s.max()-s.min())
aux = pkm.copy()
aux[list(["hp","attack","defense","sp_atk","sp_def","speed"])] = pkm.groupby(["legendary"])[list(["hp","attack","defense","sp_atk","sp_def","speed"])].transform(minmax_scale)
display(aux)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
filtrados = pkm.groupby(["type1","type2"]).filter(lambda df: oakgueda_indicator(df).mean()>40000)
print(f"La cantidad de pokemones cuyo indicador promedio por grupo (type1 , type2) sea superior a 40000 es: {len(filtrados)}")
pkm_filtered = "Los pokemones son :"
for pkm_ in filtrados["name"]:
pkm_filtered += f" {pkm_},"
print(pkm_filtered.strip(","))
###Output
La cantidad de pokemones cuyo indicador promedio por grupo (type1 , type2) sea superior a 40000 es: 2
Los pokemones son : Primal Groudon, Hoopa Unbound
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation", "legendary"])
.agg(promedio_hp=("hp", "mean"),
min_sp_atk=("sp_atk","min"),
max_sp_atk=("sp_atk","max"),
min_sp_def=("sp_def","min"),
max_sp_def=("sp_def","max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2* df["hp"] + 0.4*(df["attack"]+df["sp_atk"])**2 + 0.3*(df["defense"]+df["sp_def"])**1.5 + 0.1*df["speed"]
return oakgueda_ind
oakgueda_indicator(pkm.groupby(["type1", "type2"]).mean()).sort_values().index[-1]
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min())
pkm.groupby("legendary").transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.assign(oak=lambda x : oakgueda_indicator(x)).groupby(["type1", "type2"]).filter(lambda df: df["oak"].mean() >40000).shape[0]
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg( hp_mean=("hp", "mean"),
sp_atk_max=("sp_atk","max"),
sp_atk_min=("sp_atk","min"),
sp_def_max=("sp_def","max"),
sp_def_min=("sp_def","min")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"].mean() + 0.4*(df["attack"].mean()+df["sp_atk"].mean())**2+0.3*(df["defense"].mean()+df["sp_def"].mean())**1.5+0.1*df["speed"].mean()
return oakgueda_ind
pkm.groupby(["type1", "type2"]).apply(oakgueda_indicator)
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return pkm.drop(['name','type1','type2','generation','legendary'],axis=1).transform(lambda s: (s - s.min()) / (s.max()-s.min()))
pkm.groupby("legendary").apply(minmax_scale) # FIX ME PLEASE #
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: df.groupby(["type1","type2"]).apply(oakgueda_indicator) > 40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve() / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation", "legendary"])
.agg(
hp_mean=("hp", "mean"),
sp_atk_min=("sp_atk", "min"),
sp_atk_max=("sp_atk", "max"),
sp_def_min=("sp_atk", "min"),
sp_def_max=("sp_atk", "max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_def})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = (0.2*df["hp"] + 0.4*(df["attack"] + df["sp_atk"])**2 + 0.3*(df["defense"] + df["sp_def"])**(1.5) + 0.1*df["speed"]).mean()
return oakgueda_ind
pkm.groupby(["type1","type2"]).apply(oakgueda_indicator).idxmax()
type1=pkm.groupby(['type1','type2']).apply(oakgueda_indicator).idxmax()[0]
type2=pkm.groupby(['type1','type2']).apply(oakgueda_indicator).idxmax()[1]
print(f"En promedio, los pokemones {type1}-{type2} son los mejores según el indicador del profesor Oakgueda.")
###Output
En promedio, los pokemones Ground-Fire son los mejores según el indicador del profesor Oakgueda.
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min())
pkm.groupby("legendary").transform(lambda s: minmax_scale(s))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: oakgueda_indicator(df) > 40000).shape[0]
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["legendary","generation"])
.agg(
hp_max=("hp", "mean"),
min_atk=("sp_atk","min"),
max_atk=("sp_atk","max"),
min_def=("sp_atk","min"),
max_def=("sp_atk","max")
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
pkm=pkm.assign(
indic=0.2*pkm.loc(1)["hp"]
+0.4*(pkm.loc(1)["attack"]+pkm.loc(1)["sp_atk"])**2
+0.3*(pkm.loc(1)["defense"]+pkm.loc(1)["sp_def"])**1.5
+0.1*pkm.loc(1)["speed"]
)
pkm.head(10)
pkm_filtered =(
pkm.groupby(["type1","type2"])
.agg(
indic_mean=("indic", "mean")
)
)
pkm_filtered.head()
pkm_filtered2=pkm_filtered[pkm_filtered == pkm_filtered.max()]
pkm_filtered2.loc[lambda x: x.notnull().all(axis=1)].head()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min()) / (s.max()-s.min())
pkm.assign(
attack=lambda df: df.groupby("legendary")["attack"].apply(minmax_scale),
defense=lambda df: df.groupby("legendary")["defense"].apply(minmax_scale),
hp=lambda df: df.groupby("legendary")["hp"].apply(minmax_scale),
sp_atk=lambda df: df.groupby("legendary")["sp_atk"].apply(minmax_scale),
sp_def=lambda df: df.groupby("legendary")["sp_def"].apply(minmax_scale),
speed=lambda df: df.groupby("legendary")["speed"].apply(minmax_scale),
indic=lambda df: df.groupby("legendary")["speed"].apply(minmax_scale)
)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda df: df["indic"].mean() > 40000)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(# FIX ME PLEASE #)
.agg(
# FIX ME PLEASE #
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = # FIX ME PLEASE #
return # FIX ME PLEASE #
pkm.# FIX ME PLEASE #
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return # FIX ME PLEASE #
pkm.# FIX ME PLEASE #
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.# FIX ME PLEASE #
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(['generation','legendary'])
.agg(
{'hp': 'mean',
'sp_atk': ['min', 'max'],
'sp_def': ['min', 'max']}
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = (0.2*df["attack"].mean() + 0.4*(df["attack"].mean() + df["sp_atk"].mean())**2 +
0.3*(df["defense"].mean() + df["sp_def"].mean())**1.5 + 0.1*df['speed'].mean())
return oakgueda_ind
pkm.groupby(['type1','type2']).apply(oakgueda_indicator).reset_index().sort_values(0, ascending=False).head(5)
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min()) / (s.max() - s.min())
pkm_transform = pkm.groupby('legendary').transform(lambda s: minmax_scale(s))
pkm_transform = pd.merge(pkm['name'], pkm_transform, left_index=True, right_index=True)
pkm_transform
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm_filtered = pkm.groupby(['type1','type2']).filter(lambda df: oakgueda_indicator(df) > 40000)
pkm_filtered.count()['name']
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
(
pkm.groupby(["generation","legendary"])
.agg(atk_min=("sp_atk","min"),
atk_max=("sp_atk","max"),
def_min=("sp_def","min"),
def_max=("sp_def","max"),
promedio_hp=("hp","mean"),
)
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
oakgueda_ind = 0.2*df["hp"] + 0.4*( df["attack"] + df["sp_atk"] )**2 + 0.3*(df["defense"] + df["sp_def"])**(1.5) + 0.1*df["speed"]
return oakgueda_ind.mean()
pkm.groupby(["type1","type2"]).apply(oakgueda_indicator).idxmax()
###Output
_____no_output_____
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return (s - s.min())/(s.max() - s.min())
pkm.groupby("legendary")[["hp","attack","defense","sp_atk","sp_def","speed"]].transform(minmax_scale)
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
pkm.groupby(["type1","type2"]).filter(lambda s: oakgueda_indicator(s) > 40000.00)
###Output
_____no_output_____
###Markdown
Laboratorio 4
###Code
import pandas as pd
from pathlib import Path
###Output
_____no_output_____
###Markdown
Utilizarás el conjunto de datos de pokemon.
###Code
pkm = (
pd.read_csv(Path().resolve().parent / "data" / "pokemon.csv", index_col="#")
.rename(columns=lambda x: x.replace(" ", "").replace(".", "_").lower())
)
pkm.head()
###Output
_____no_output_____
###Markdown
Ejercicio 1(1 pto)Agrupar por `generation` y `legendary` y obtener por grupo:* Promedio de `hp`* Mínimo y máximo de `sp_atk` y `sp_def`
###Code
pkm.groupby(["generation","legendary"]).agg(
mean_hp=("hp","mean"),
min_sp_atk=("sp_atk","min"),
max_sp_atk=("sp_atk","max"),
min_sp_def=("sp_def","min"),
max_sp_def=("sp_def","max")
)
###Output
_____no_output_____
###Markdown
Ejercicio 2(1 pto)El profesor Oakgueda determinó que una buen indicador de pokemones es: $$ 0.2 \, \textrm{hp} + 0.4 \,(\textrm{attack} + \textrm{sp_atk})^2 + 0.3 \,( \textrm{defense} + \textrm{sp_deff})^{1.5} + 0.1 \, \textrm{speed}$$Según este indicador, ¿Qué grupo de pokemones (`type1`, `type2`) es en promedio mejor que el resto?
###Code
def oakgueda_indicator(df):
#display(df)
temp_serie=df.apply(lambda row: 0.2*row["hp"]+0.4*(row["attack"]+row["sp_atk"])**2+0.3*(row["defense"]+row["sp_def"])**(1.5)+0.1*row["speed"],axis=1)
#display(temp_serie)
return temp_serie.mean()
type1,type2=pkm.groupby(["type1","type2"]).apply(oakgueda_indicator).idxmax()
print(f"En promedio, los pokemones {type1}-{type2} son mejores según el indicador del profesor Oakgueda")
###Output
En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda
###Markdown
__Respuesta__: En promedio, los pokemones Ground-Fire son mejores según el indicador del profesor Oakgueda. Ejercicio 3(1 pto)Define una función que escale los datos tal que, si $s$ es una columna: $$s\_scaled = \frac{s - \min(s)}{\max(s) - \min(s)}$$Y luego transforma cada columna numérica agrupando por si el pokemon es legendario o no.
###Code
def minmax_scale(s):
return s-s.min()/s.max()-s.min()
pkm.groupby("legendary")[["hp","attack","defense","sp_atk","sp_def","speed"]].transform(lambda s: minmax_scale(s))
###Output
_____no_output_____
###Markdown
Ejercicio 4(1 pto)El profesor Oakgueda necesita saber cuántos pokemones hay luego de filtrar el dataset tal que el grupo de (`type1`, `type2`) tenga en promedio un indicador (el del ejercicio 2) mayor a 40000.
###Code
filtered_table=pkm.groupby(["type1","type2"]).filter(lambda row: oakgueda_indicator(row)>=40000)
display(filtered_table)
print(f"Hay {filtered_table.shape[0]} pokemon(es) que cumplen esta condición, los cuales son:")
temp=filtered_table.loc[:,"name"]
for i in temp.values:
print(f"{i}")
###Output
Hay 2 pokemon(es) que cumplen esta condición, los cuales son:
Primal Groudon
Hoopa Unbound
|
Vital/ImpliedVol_v01.ipynb | ###Markdown
BSM formula Abstract- create GBM class- define a method for BSM formula for a given option type AnalysisBS model assumes the distribution of stock as lognormal. In particular, it writes $$\ln \frac{S(T)}{S(0)} \sim \mathcal N((r - \frac 1 2 \sigma^2) T, \sigma^2 T)$$with respect to risk neutral measure. In the above, the parameters stand for* $S(0)$: The initial stock price* $S(T)$: The stock price at $T$* $r$: interest rate* $\sigma$: volatility The call and put price with maturity $T$ and $K$ will be known as $C_0$ and $P_0$ given as below:$$C_0 = \mathbb E [e^{-rT} (S(T) - K)^+] = S_0 \Phi(d_1) - K e^{-rT} \Phi(d_2),$$and $$P_0 = \mathbb E [e^{-rT} (S(T) - K)^-] = K e^{-rT} \Phi(- d_2) - S_0 \Phi(- d_1),$$where $d_i$ are given as$$d_1 = \frac{1}{\sigma\sqrt{\left( T - t \right)}} \left[ \ln\frac{S_{0}}{K} + \left( r + \frac{\sigma^2}{2} \right) \left( T-t \right) \right],$$and$$d_2 = \frac{1}{\sigma\sqrt{\left( T - t \right)}} \left[ \ln\frac{S_{0}}{K} + \left( r - \frac{\sigma^2}{2} \right) \left( T-t \right) \right] = d_{1}-\sigma\sqrt{\left( T - t \right)}$$From $\textit{Stochastic Calculus for Finance II Continuous Time Models}$ by ShrevePut-call parity will be useful: $$C_0 - P_0 = S(0) - e^{-rT} K.$$ Code
###Code
import numpy as np
import scipy.stats as ss
from matplotlib import pyplot as plt
###Output
_____no_output_____
###Markdown
We reload the european option class created before.
###Code
'''=========
option class init
=========='''
class VanillaOption:
def __init__(
self,
otype = 1, # 1: 'call'
# -1: 'put'
strike = 110.,
maturity = 1.,
market_price = 10.):
self.otype = otype
self.strike = strike
self.maturity = maturity
self.market_price = market_price #this will be used for calibration
def payoff(self, s): #s: excercise price
otype = self.otype
k = self.strike
maturity = self.maturity
return np.max([0, (s - k)*otype])
###Output
_____no_output_____
###Markdown
Next, we create the gbm class, which is determined by three parameters. We shall initialize itas it is created.
###Code
'''============
Gbm class inherited from sde_1d
============='''
class Gbm:
def __init__(self,
init_state = 100.,
drift_ratio = .0475,
vol_ratio = .2
):
self.init_state = init_state
self.drift_ratio = drift_ratio
self.vol_ratio = vol_ratio
###Output
_____no_output_____
###Markdown
BSM formula is given by a method of Gbm class with an input of an option.
###Code
'''========
Black-Scholes-Merton formula.
=========='''
def bsm_price(self, vanilla_option):
s0 = self.init_state
sigma = self.vol_ratio
r = self.drift_ratio
otype = vanilla_option.otype
k = vanilla_option.strike
maturity = vanilla_option.maturity
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity)) # Need to be working with maturity minus current time t
d2 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r - np.power(sigma,2)/2)*(maturity)) # But how do we get the current time t
return (otype * s0 * ss.norm.cdf(otype * d1) #line break needs parenthesis
- otype * np.exp(-r * maturity) * k * ss.norm.cdf(otype * d2))
Gbm.bsm_price = bsm_price
'''===============
Test bsm_price
================='''
gbm1 = Gbm()
option1 = VanillaOption()
print('>>>>>>>>>>call value is ' + str(gbm1.bsm_price(option1)))
option2 = VanillaOption(otype=-1)
print('>>>>>>>>>>put value is ' + str(gbm1.bsm_price(option2)))
###############
# Arbitrage-Free Model Object-Oriented
#############
class ArbitrageFree:
def pc_parity(self, vanilla_option1, vanilla_option2, gbm):
call_price = gbm.bsm_price(vanilla_option1)
put_price = gbm.bsm_price(vanilla_option2)
k = vanilla_option1.strike #Note: Put and Call with same strike k
r = gbm.drift_ratio #and interest r
maturity = vanilla_option1.maturity #and maturity
s0 = gbm.init_state
#give some space for machine precision error
if call_price - put_price + np.exp(-(r*maturity)) - s0 <= 10^(-10):
return ">>>>>>>>>Option is arbitrage-free"
else:
return ">>>>>>>>>Option is not arbitrage-free"
###Output
_____no_output_____
###Markdown
###Code
'''===============
Test Arbitrage Free Model
================='''
arb_free = ArbitrageFree()
arb_free.pc_parity(option1, option2, gbm1)
'''===============
Define Greeks
================='''
class Greek:
#First time the current price of stock will affect equation
#Choose arbitrary price of 112
def __init__(self, s = 112.):
self.s = s
def delta(self,vanilla_option, gbm):
otype = vanilla_option.otype
maturity = vanilla_option.maturity
k = vanilla_option.strike
s0 = gbm.init_state
sigma = gbm.vol_ratio
r = gbm.drift_ratio
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity))
if otype == 1:
return ss.norm.cdf(d1)
else:
return ss.norm.cdf(d1) - 1
def gamma(self,vanilla_option, gbm):
otype = vanilla_option.otype
maturity = vanilla_option.maturity
k = vanilla_option.strike
s0 = gbm.init_state
sigma = gbm.vol_ratio
r = gbm.drift_ratio
s = self.s
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity))
return ss.norm.pdf(d1) / (s * sigma * np.sqrt(maturity))
def vega(self,vanilla_option, gbm):
otype = vanilla_option.otype
maturity = vanilla_option.maturity
k = vanilla_option.strike
s0 = gbm.init_state
sigma = gbm.vol_ratio
r = gbm.drift_ratio
s = self.s
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity))
return ss.norm.pdf(d1) * s * np.sqrt(maturity)
def theta(self,vanilla_option, gbm):
otype = vanilla_option.otype
maturity = vanilla_option.maturity
k = vanilla_option.strike
s0 = gbm.init_state
sigma = gbm.vol_ratio
r = gbm.drift_ratio
s = self.s
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity))
d2 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r - np.power(sigma,2)/2)*(maturity))
if otype == 1:
return (-s * ss.norm.pdf(d1) * sigma / (2 * np.sqrt(maturity))) - (r * k * np.exp(-r * maturity) * ss.norm.cdf(d2))
else:
return (-s * ss.norm.pdf(d1) * sigma / (2 * np.sqrt(maturity))) + (r * k * np.exp(-r * maturity) * ss.norm.cdf(-d2))
def rho(self,vanilla_option, gbm):
otype = vanilla_option.otype
maturity = vanilla_option.maturity
k = vanilla_option.strike
s0 = gbm.init_state
sigma = gbm.vol_ratio
r = gbm.drift_ratio
s = self.s
d2 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r - np.power(sigma,2)/2)*(maturity))
if otype == 1:
return k * ss.norm.cdf(d2) * maturity * np.exp(-r * maturity)
else:
return -k * ss.norm.cdf(-d2) * maturity * np.exp(-r * maturity)
'''===============
Test Arbitrage Free Model
================='''
greek = Greek()
#Delta
call_delta = greek.delta(option1, gbm1)
put_delta = greek.delta(option2, gbm1)
print(">>>>>>The Call Delta is " + str(call_delta))
print(">>>>>>The Put Delta is " + str(put_delta))
#Gamma
option_gamma = greek.gamma(option1,gbm1)
print(">>>>>>The Option's Gamma is " + str(option_gamma))
#Vega
option_vega = greek.vega(option1, gbm1)
print(">>>>>>The Option's Vega is " + str(option_vega))
#Theta
call_theta = greek.theta(option1, gbm1)
put_theta = greek.theta(option2, gbm1)
print(">>>>>>The Call Theta is " + str(call_theta))
print(">>>>>>The Put Theta is " + str(put_theta))
#Rho
call_rho = greek.rho(option1, gbm1)
put_rho = greek.rho(option2, gbm1)
print(">>>>>>The Call Rho is " + str(call_rho))
print(">>>>>>The Put Rho is " + str(put_rho))
'''===============
Implied Volatility Calculation through Newton Method divident paying asset
================='''
class ImpliedVolatility:
def __init__(self, q=0):
self.q = q
def newtonImpliedVolCalc(self, vanillaoption, gbm, greek, marketprice):
otype = vanillaoption.otype
maturity = vanillaoption.maturity
k=vanillaoption.strike
r = gbm.drift_ratio
sigma = gbm.vol_ratio
s0 = gbm.init_state
d1 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r + np.power(sigma,2)/2)*(maturity))
d2 = 1/(sigma*np.sqrt(maturity))*(np.log(s0/k) + (r - np.power(sigma,2)/2)*(maturity))
vega = greek.vega(vanillaoption, gbm)
optionprice = gbm.bsm_price(vanillaoption)
tolerance = 0.000001
x0 = sigma
xnew = x0
xold = x0 - 1
while abs(xnew - xold) > tolerance:
xold = xnew
xnew = (xnew - ((optionprice - marketprice) / vega))
return abs(xnew)
'''===============
Test Arbitrage Free Model
================='''
impliedVol = ImpliedVolatility()
impliedVolCall = impliedVol.newtonImpliedVolCalc(option1, gbm1, greek, 5.94)
impliedVolPut = impliedVol.newtonImpliedVolCalc(option2, gbm1, greek, 10.84)
print('>>>>>>>>> The Implied Volatility of the Call Option is ' + str(impliedVolCall))
print('>>>>>>>>> The Implied Volatility of the Put Option is ' + str(impliedVolPut))
'''===============
Plot Volatility Smile
================='''
marketpriceCall = 5.94
marketpricePut = 10.84
impliedVolCallArray = []
for i in [j for j in range(int(100*(marketpriceCall-3.0)), int(100*(marketpriceCall+3.0)), 1)]:
k = i * (0.01)
impliedVolCallArray.append(impliedVol.newtonImpliedVolCalc(option1, gbm1, greek, k))
impliedVolPutArray = []
for i in [j for j in range(int(100*(marketpricePut-3.0)), int(100*(marketpricePut+3.0)), 1)]:
k = float(i) * (0.01)
impliedVolPutArray.append(impliedVol.newtonImpliedVolCalc(option2, gbm1, greek, k))
callpriceRange = []
for i in [j for j in range(int(100*(marketpriceCall-3.0)), int(100*(marketpriceCall+3.0)), 1)]:
k = float(i) * (0.01)
callpriceRange.append(k)
putpriceRange = []
for i in [j for j in range(int(100*(marketpricePut-3.0)), int(100*(marketpricePut+3.0)), 1)]:
k = float(i) * (0.01)
putpriceRange.append(k)
print(">>>>>>>>>>", impliedVolCallArray)
print(">>>>>>>>>>", impliedVolPutArray)
print(">>>>>>>>>>", callpriceRange)
plt.plot(callpriceRange, impliedVolCallArray)
plt.show()
plt.plot(putpriceRange, impliedVolPutArray)
plt.show()
###Output
_____no_output_____ |
openmdao/docs/openmdao_book/features/building_blocks/components/cross_product_comp.ipynb | ###Markdown
CrossProductComp`CrossProductComp` performs a cross product between two 3-vector inputs. It may be vectorized to provide the result at one or more points simultaneously.$$ c_i = \bar{a}_i \times \bar{b}_i$$The first dimension of the inputs holds the vectorized dimension.The default `vec_size` is 1, providing the cross product of $a$ and $b$ at a singlepoint. The lengths of $a$ and $b$ at each point must be 3.The shape of $a$ and $b$ will always be `(vec_size, 3)`, but the connection rulesof OpenMDAO allow the incoming connection to have shape `(3,)` when `vec_size` is 1, sincethe storage order of the underlying data is the same. The output vector `c` ofCrossProductComp will always have shape `(vec_size, 3)`. CrossProductComp OptionsOptions for CrossProductComp allow the user to rename the input variables $a$ and $b$ and the output $c$, as well as specifying their units.
###Code
om.show_options_table("openmdao.components.cross_product_comp.CrossProductComp")
###Output
_____no_output_____
###Markdown
CrossProductComp ConstructorThe call signature for the `CrossProductComp` constructor is:```{eval-rst} .. automethod:: openmdao.components.cross_product_comp.CrossProductComp.__init__ :noindex:``` CrossProductComp UsageThere are often situations when numerous products need to be computed, essentially in parallel.You can reduce the number of components required by having one `CrossProductComp` perform multiple operations.This is also convenient when the different operations have common inputs.The `add_product` method is used to create additional products after instantiation.```{eval-rst} .. automethod:: openmdao.components.cross_product_comp.CrossProductComp.add_product :noindex:``` CrossProductComp ExampleIn the following example CrossProductComp is used to compute torque as thecross product of force ($F$) and radius ($r$) at 100 points simultaneously.Note the use of `a_name`, `b_name`, and `c_name` to assign names to the inputs and outputs.Units are assigned using `a_units`, `b_units`, and `c_units`.Note that no internal checks are performed to ensure that `c_units` are consistentwith `a_units` and `b_units`.
###Code
import numpy as np
n = 24
p = om.Problem()
p.model.add_subsystem(name='cross_prod_comp',
subsys=om.CrossProductComp(vec_size=n,
a_name='r', b_name='F', c_name='torque',
a_units='m', b_units='N', c_units='N*m'),
promotes_inputs=['r', 'F'])
p.setup()
p.set_val('r', np.random.rand(n, 3))
p.set_val('F', np.random.rand(n, 3))
p.run_model()
# Check the output in units of ft*lbf to ensure that our units work as expected.
expected = []
for i in range(n):
a_i = p.get_val('r')[i, :]
b_i = p.get_val('F')[i, :]
expected.append(np.cross(a_i, b_i) * 0.73756215)
actual_i = p.get_val('cross_prod_comp.torque', units='ft*lbf')[i]
rel_error = np.abs(expected[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
print(p.get_val('cross_prod_comp.torque', units='ft*lbf'))
from openmdao.utils.assert_utils import assert_near_equal
assert_near_equal(p.get_val('cross_prod_comp.torque', units='ft*lbf'), np.array(expected), tolerance=1e-8)
###Output
_____no_output_____
###Markdown
DotProductComp Example with Multiple ProductsWhen defining multiple products:- An input name in one call to `add_product` may not be an output name in another call, and vice-versa.- The units and shape of variables used across multiple products must be the same in each one.
###Code
n = 24
p = om.Problem()
cpc = om.CrossProductComp(vec_size=n,
a_name='r', b_name='F', c_name='torque',
a_units='m', b_units='N', c_units='N*m')
cpc.add_product(vec_size=n,
a_name='r', b_name='p', c_name='L',
a_units='m', b_units='kg*m/s', c_units='kg*m**2/s')
p.model.add_subsystem(name='cross_prod_comp', subsys=cpc,
promotes_inputs=['r', 'F', 'p'])
p.setup()
p.set_val('r', np.random.rand(n, 3))
p.set_val('F', np.random.rand(n, 3))
p.set_val('p', np.random.rand(n, 3))
p.run_model()
# Check the output.
expected_T = []
expected_L = []
for i in range(n):
a_i = p.get_val('r')[i, :]
b_i = p.get_val('F')[i, :]
expected_T.append(np.cross(a_i, b_i))
actual_i = p.get_val('cross_prod_comp.torque')[i]
rel_error = np.abs(expected_T[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
b_i = p.get_val('p')[i, :]
expected_L.append(np.cross(a_i, b_i))
actual_i = p.get_val('cross_prod_comp.L')[i]
rel_error = np.abs(expected_L[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
print(p.get_val('cross_prod_comp.torque'))
print(p.get_val('cross_prod_comp.L'))
assert_near_equal(p.get_val('cross_prod_comp.torque'), np.array(expected_T), tolerance=1e-8)
assert_near_equal(p.get_val('cross_prod_comp.L'), np.array(expected_L), tolerance=1e-8)
###Output
_____no_output_____
###Markdown
CrossProductComp`CrossProductComp` performs a cross product between two 3-vector inputs. It may be vectorized to provide the result at one or more points simultaneously.$$ c_i = \bar{a}_i \times \bar{b}_i$$The first dimension of the inputs holds the vectorized dimension.The default `vec_size` is 1, providing the cross product of $a$ and $b$ at a singlepoint. The lengths of $a$ and $b$ at each point must be 3.The shape of $a$ and $b$ will always be `(vec_size, 3)`, but the connection rulesof OpenMDAO allow the incoming connection to have shape `(3,)` when `vec_size` is 1, sincethe storage order of the underlying data is the same. The output vector `c` ofCrossProductComp will always have shape `(vec_size, 3)`. CrossProductComp OptionsOptions for CrossProductComp allow the user to rename the input variables $a$ and $b$ and the output $c$, as well as specifying their units.
###Code
import openmdao.api as om
om.show_options_table("openmdao.components.cross_product_comp.CrossProductComp")
###Output
_____no_output_____
###Markdown
CrossProductComp ConstructorThe call signature for the `CrossProductComp` constructor is:```{eval-rst} .. automethod:: openmdao.components.cross_product_comp.CrossProductComp.__init__ :noindex:``` CrossProductComp UsageThere are often situations when numerous products need to be computed, essentially in parallel.You can reduce the number of components required by having one `CrossProductComp` perform multiple operations.This is also convenient when the different operations have common inputs.The `add_product` method is used to create additional products after instantiation.```{eval-rst} .. automethod:: openmdao.components.cross_product_comp.CrossProductComp.add_product :noindex:``` CrossProductComp ExampleIn the following example CrossProductComp is used to compute torque as thecross product of force ($F$) and radius ($r$) at 100 points simultaneously.Note the use of `a_name`, `b_name`, and `c_name` to assign names to the inputs and outputs.Units are assigned using `a_units`, `b_units`, and `c_units`.Note that no internal checks are performed to ensure that `c_units` are consistentwith `a_units` and `b_units`.
###Code
import numpy as np
import openmdao.api as om
n = 24
p = om.Problem()
p.model.add_subsystem(name='cross_prod_comp',
subsys=om.CrossProductComp(vec_size=n,
a_name='r', b_name='F', c_name='torque',
a_units='m', b_units='N', c_units='N*m'),
promotes_inputs=['r', 'F'])
p.setup()
p.set_val('r', np.random.rand(n, 3))
p.set_val('F', np.random.rand(n, 3))
p.run_model()
# Check the output in units of ft*lbf to ensure that our units work as expected.
expected = []
for i in range(n):
a_i = p.get_val('r')[i, :]
b_i = p.get_val('F')[i, :]
expected.append(np.cross(a_i, b_i) * 0.73756215)
actual_i = p.get_val('cross_prod_comp.torque', units='ft*lbf')[i]
rel_error = np.abs(expected[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
print(p.get_val('cross_prod_comp.torque', units='ft*lbf'))
from openmdao.utils.assert_utils import assert_near_equal
assert_near_equal(p.get_val('cross_prod_comp.torque', units='ft*lbf'), np.array(expected), tolerance=1e-8)
###Output
_____no_output_____
###Markdown
DotProductComp Example with Multiple ProductsWhen defining multiple products:- An input name in one call to `add_product` may not be an output name in another call, and vice-versa.- The units and shape of variables used across multiple products must be the same in each one.
###Code
n = 24
p = om.Problem()
cpc = om.CrossProductComp(vec_size=n,
a_name='r', b_name='F', c_name='torque',
a_units='m', b_units='N', c_units='N*m')
cpc.add_product(vec_size=n,
a_name='r', b_name='p', c_name='L',
a_units='m', b_units='kg*m/s', c_units='kg*m**2/s')
p.model.add_subsystem(name='cross_prod_comp', subsys=cpc,
promotes_inputs=['r', 'F', 'p'])
p.setup()
p.set_val('r', np.random.rand(n, 3))
p.set_val('F', np.random.rand(n, 3))
p.set_val('p', np.random.rand(n, 3))
p.run_model()
# Check the output.
expected_T = []
expected_L = []
for i in range(n):
a_i = p.get_val('r')[i, :]
b_i = p.get_val('F')[i, :]
expected_T.append(np.cross(a_i, b_i))
actual_i = p.get_val('cross_prod_comp.torque')[i]
rel_error = np.abs(expected_T[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
b_i = p.get_val('p')[i, :]
expected_L.append(np.cross(a_i, b_i))
actual_i = p.get_val('cross_prod_comp.L')[i]
rel_error = np.abs(expected_L[i] - actual_i)/actual_i
assert np.all(rel_error < 1e-8), f"Relative error: {rel_error}"
print(p.get_val('cross_prod_comp.torque'))
print(p.get_val('cross_prod_comp.L'))
assert_near_equal(p.get_val('cross_prod_comp.torque'), np.array(expected_T), tolerance=1e-8)
assert_near_equal(p.get_val('cross_prod_comp.L'), np.array(expected_L), tolerance=1e-8)
###Output
_____no_output_____ |
notebooks/Computing_Wasserstein_distances_for_shock_locationOutput.ipynb | ###Markdown
Computing Wasserstein distances for the uncertain shock locationAt $T=0.2$, the exact solution is given as $$u(y;x,T)=\begin{cases}1&x\leq \frac{1}{2}(1+T)+y\\0&\text{otherwise}\end{cases}\qquad x\in[0,1], y\in[-0.1,0.1].$$Let $\mu_T$ be the law of $u(\cdot;\cdot,T)$ (ie. the statistical solution). Our approximate solution is an esemble of Dirac deltas centered at FVM solutions$$\mu^{\Delta x,M}_T=\frac{1}{M}\sum_{k=1}^M \delta_{u^{\Delta x}_k(\cdot,t)}$$After some disintegration and support considerations, we arrive at the final formula for the Wasserstein distance should be$$W_1(\mu_T,\mu^{\Delta x,M}_T)=\int_{-0.1}^{0.1} \|u_{\bar{k}(y)}^{\Delta x}-u(y,\cdot,T)\|_{L^1([0,1])}\frac{1}{0.2}\; dy$$where$$\bar{k}(y) = \lfloor \frac{M(y+0.1)}{0.2}\rfloor$$where the samples are sorted according to $$ u^{\Delta x}_k\leq u^{\Delta x}_l\Leftrightarrow u^{\Delta x}_k(x_i)\leq u^{\Delta x}_l(x_i)\qquad \text{for all }i=1,\ldots,N_{\Delta x}.$$That is, we divide the interval $[-0.1,0.1]$ into $M$ pieces, and assign $u_1$ the first interval, $u_2$ the second interval, and so on.**Claim** (Not requiring Miracle): The measure defined by$$f\mapsto \int_{-0.1}^{0.1}f(u(y,\cdot,T),u^{\Delta x}_{\bar{k}(y)})\frac{1}{0.2}\;dy$$is in $\Pi(\mu_t,\mu_t^{\Delta x,M})$.**Claim** (Miracle): The above defined measure is optimal. The proof goes through disintegrating the measure. Numerical algorithm1. Sort the samples according to (see next sections for when this is well-defined)$$ u^{\Delta x}_k\leq u^{\Delta x}_l\Leftrightarrow u^{\Delta x}_k(x_i)\leq u^{\Delta x}_l(x_i)\qquad \text{for all }i=1,\ldots,N_{\Delta x}$$2. Discretize the parameter space:$$Y=[-0.1, 0.1] =\bigcup_{y=1}^{N_y} [y_i,y_{i+1}]\qquad \text{where }|y_i-y_{i+1}|=\Delta y\approx \Delta x/8$$5. For $y = y_1,\ldots,y_{N_y}$: a) Compute exact solution $u(y,x_j,T)$ for $j=1,\ldots,N_{\Delta x}$ b) Compute current distance: $$d^{k}_y=\sum_i |u(y,x_i,T)-u^{\Delta x}_{\bar{k}(y)}(y,x_i,T)|\Delta y$$ e) Add contribution to the distance $$ distance \leftarrow distance + d^k(y)\Delta y$$
###Code
import numpy as np
import plot_info
import matplotlib
matplotlib.rcParams['errorbar.capsize'] = 3
%matplotlib inline
import matplotlib.pyplot as plt
import netCDF4
import functools
import matplotlib2tikz
import alsvinn
%%writefile shock_location.py
# Initial data file
Y = 0.2*X - 0.1
if x <= 0.5 + Y:
u = 1.0
else:
u = 0.0
%%writefile shock_location.xml
<config>
<fvm>
<name>
shocklocation
</name>
<platform>cpu</platform>
<grid>
<lowerCorner>0 0 0</lowerCorner>
<upperCorner>1 0 0</upperCorner>
<dimension>32 1 1</dimension>
</grid>
<boundary>neumann</boundary>
<flux>godunov</flux>
<endTime>.2</endTime>
<equation>burgers</equation>
<reconstruction>weno2</reconstruction>
<cfl>auto</cfl>
<integrator>auto</integrator>
<initialData>
<python>shock_location.py</python>
<parameters>
<parameter>
<name>X</name>
<length>1</length>
<value>0</value>
</parameter>
</parameters>
</initialData>
<writer>
<basename>shock_location</basename>
<type>netcdf</type>
<numberOfSaves>1</numberOfSaves>
</writer>
</fvm>
<uq>
<samples>1</samples>
<generator>auto</generator>
<parameters>
<parameter>
<name>X</name>
<length>1</length>
<type>uniform</type>
</parameter>
</parameters>
<stats>
</stats>
</uq>
</config>
def compare(u1, u2):
"""
Compares two numerical solutions against eachother.
We say that
u1 <= u2
if
u1[j] <= u2[j] for all j in range(len(u1))
"""
if not (u1<=u2).all() and not (u2<=u1).all():
raise Exception("Not well ordered!")
if (u1 <= u2).all():
return -1
else:
return 1
###Output
_____no_output_____
###Markdown
Some ordering testsThe main point below is that if the use WENO2 (meaning third order reconstruction), the results are not well-ordered according to our ordering definition above.
###Code
def test(reconstruction):
resolution = 2**14
number_of_samples=128
r = alsvinn.run(base_xml='shock_location.xml',
reconstruction=reconstruction,
dimension=[resolution, 1, 1],
samples=number_of_samples,
uq=True)
data = []
for k in range(number_of_samples):
plt.plot(r.get_data('u', timestep=0, sample=k))
data.append(r.get_data('u', timestep=0, sample=k))
plt.title(f"Some samples for reconstruction: {reconstruction}")
plt.show()
for d in range(0,len(data),16):
plt.plot(data[d],label='%d' % d)
plt.legend()
plt.title('Before sorting')
plt.show()
try:
data = sorted(data,key=functools.cmp_to_key(compare))
except:
print(f"Failed sorting samples with reconstruction: {reconstruction}")
return
for d in range(0,len(data),16):
plt.plot(data[d],label='%d' % d)
plt.legend()
plt.title(f"After sorting, reconstruction: {reconstruction}")
plt.show()
print("Everything went well")
# First we try with the low order:
test("none")
# Then with the high order:
test("weno2")
def runAndSort(resolution, samples, experiment=0, base_xml = 'shock_location.xml'):
sample_start = experiment * samples
r = alsvinn.run(base_xml = base_xml,
samples=samples,
sampleStart=sample_start,
reconstruction='none',
uq=True,
dimension=[int(resolution), 1, 1]
)
data = []
for k in range(sample_start, sample_start+samples):
d = r.get_data('u', sample=k, timestep=0)
data.append(d)
data = sorted(data,key=functools.cmp_to_key(compare))
return data
def upscale(d,N):
while d.shape[0] < N:
d = repeat(d,2,0)
return d
def l1distance(dataSample, shockLocation):
x = np.linspace(0,1,dataSample.shape[0])
uExact = x <= shockLocation
return np.sum(abs(dataSample - uExact))/dataSample.shape[0]
def l2distance(dataSample, shockLocation):
x = np.linspace(0,1,dataSample.shape[0])
uExact = x <= shockLocation
return np.sqrt(np.sum(abs(dataSample - uExact)**2)/dataSample.shape[0])
def wassersteinDistance(data, T):
shockLocation = lambda y: 0.5 + 0.5*T + y
M = len(data)
Y = np.linspace(-1/10.,1/10., 128*data[0].shape[0])
dy = Y[1]-Y[0]
distance = 0
for (ny, y) in enumerate(Y):
s = shockLocation(y)
currentDistance = l1distance(data[min(int((y+0.1)/0.2*M),M-1)], s)
distance += currentDistance * dy
return distance
import sys
def estimateConvergence(resolutions, errors, color='green'):
poly = np.polyfit(np.log(resolutions), np.log(errors), 1)
plt.loglog(resolutions, np.exp(poly[1])*resolutions**(poly[0]),
'--',label='$\\mathcal{O}(N^{%.2f})$' % poly[0],
color=color)
def runExperiments(resolution):
distances = []
for experiment in range(0,(4*4096)//resolution):
sys.stdout.write("#")
sys.stdout.flush()
data = runAndSort(resolution, resolution, experiment)
distance = wassersteinDistance(data,0.2)
distances.append(distance)
errorAvg = np.mean(distances)
errorStd = np.std(distances)
return errorAvg, errorStd
resolutions = [128,256,512,1024,2048,4096,2*4096]
errors = []
mean_errors = []
std_errors = []
for resolution in resolutions:
data = runAndSort(resolution, resolution)
distance = wassersteinDistance(data,0.2)
errors.append(distance)
avgError, stdError = runExperiments(resolution)
mean_errors.append(avgError)
std_errors.append(stdError)
plt.loglog(resolutions, errors,'-o',label='$W_1(\\mu_{0.2},\\mu_{0.2}^{\\Delta x,M})$')
line_ref = plt.loglog(resolutions, mean_errors,'-o',label='$\\mathbb{E}(W_1(\\mu_{0.2},\\mu_{0.2}^{\Delta x,M}))$')
plt.errorbar(resolutions, mean_errors,
yerr=std_errors,
fmt='o',label='$\\mathrm{std}(W_1(\\mu_{0.2},\\mu_{0.2}^{\\Delta x,M}))$')
estimateConvergence(resolutions, mean_errors, color=line_ref[0].get_color())
plt.grid('on')
plt.gca().set_xscale('log', basex=2)
plt.gca().set_yscale('log', basey=2)
plt.xlabel("$N$")
plt.ylabel("$W_1$")
plt.legend()
plot_info.showAndSave('wasserstein_shock')
###Output
##############################################################################################################################################################################################################################################################
###Markdown
Midpoint rule Configuration files
###Code
%%writefile shock_location_midpoint.xml
<config>
<fvm>
<name>
shock_location_midpoint
</name>
<platform>cpu</platform>
<grid>
<lowerCorner>0 0 0</lowerCorner>
<upperCorner>1 0 0</upperCorner>
<dimension>32 1 1</dimension>
</grid>
<boundary>neumann</boundary>
<flux>godunov</flux>
<endTime>.2</endTime>
<equation>burgers</equation>
<reconstruction>weno2</reconstruction>
<cfl>auto</cfl>
<integrator>auto</integrator>
<initialData>
<python>shock_location.py</python>
<parameters>
<parameter>
<name>X</name>
<length>1</length>
<value>0</value>
</parameter>
</parameters>
</initialData>
<writer>
<basename>shock_location</basename>
<type>netcdf</type>
<numberOfSaves>1</numberOfSaves>
</writer>
</fvm>
<uq>
<samples>1</samples>
<generator>auto</generator>
<parameters>
<parameter>
<name>X</name>
<length>1</length>
<type>uniform1d</type>
</parameter>
</parameters>
<stats>
</stats>
</uq>
</config>
resolutions = [128,256,512,1024,2048,4096,2*4096]
errors = []
for resolution in resolutions:
data = runAndSort(resolution, resolution, base_xml = 'shock_location_midpoint.xml')
distance = wassersteinDistance(data, 0.2)
errors.append(distance)
line_ref = plt.loglog(resolutions, errors,'-o',label='$W_1(\\mu_{0.2},\\mu_{0.2}^{\\Delta x,M})$')
estimateConvergence(resolutions, errors, color=line_ref[0].get_color())
plt.grid('on')
plt.gca().set_xscale('log', basex=2)
plt.gca().set_yscale('log', basey=2)
plt.xlabel("$N$")
plt.ylabel("$W_1$")
plt.legend()
plot_info.showAndSave('wasserstein_shock_uniform')
###Output
|
single_layered.ipynb | ###Markdown
Perceptron with decision boundary
###Code
import numpy as np
import pandas as pd
import math
import matplotlib
import matplotlib.pyplot as plt
data = pd.read_csv('hw3data', sep="\t", header=None)
x = np.hstack((np.ones((data.shape[0], 1)), data.drop(data.columns[2], axis=1)))
y = data[2]
y.head()
# Convert Class-1 and Class-2 into 0's and 1's
for i, val in enumerate(y):
if val == "Class-1":
y[i] = 0
else:
y[i] = 1
# Method to split data into 70% Train and 30% Test data
def split_data(df, X, Y):
arr_rand = np.random.rand(df.shape[0])
split = arr_rand < np.percentile(arr_rand, 70)
X_train = X[split]
Y_train = Y[split]
X_test = X[~split]
Y_test = Y[~split]
return X_train, Y_train, X_test, Y_test
# Splitting the data
X_train, Y_train, X_test, Y_test = split_data(data, x, y)
# Sigmoid activation function
def activation_func(value):
return (1 / (1 + np.exp(-value)))
# Function to return mea squared error
def mse(y_predicted, Y_test):
return np.sum((y_predicted - Y_test) ** 2) / len(y_predicted)
# Derivative of sigmoid function
def sigmoid_derivative(val):
return activation_func(val) * (1 - activation_func(val))
# Custom evaluate function to get the predicted value of Y using the sigmoid activation
def evaluate(theta, X_test, Y_test):
y_predicted = activation_func(np.dot(X_test, theta))
y_predicted = y_predicted.reshape(y_predicted.shape[0])
# Calculating the test error
test_error = mse(y_predicted, Y_test)
# Updating y values with 0's and 1's
for i, val in enumerate(y_predicted):
if val >= 0.5:
y_predicted[i] = 1
else:
y_predicted[i] = 0
ctr = 0
Y_test = np.array(Y_test)
# Counting the matched value count
for i, val in enumerate(y_predicted):
if y_predicted[i] == Y_test[i]:
ctr += 1
# Number of values matched
print('CORRECT', ctr)
accuracy = (ctr / len(Y_test)) * 100
return y_predicted, accuracy, test_error
# Model to train the perceptron using delta rule
def perceptron_train(x, y, alpha, iterations):
# converting into numpy
X = np.array(x)
# dtype is a numpy.object, converting the array into astype(float)
# otherwise it will show a message saying numpy.float64 has no attribute log10
X = X.astype(float)
n = X.shape[0]
Y = np.array(y).reshape(n, 1)
Y = Y.astype(float)
# Randomly initialising random weights
# weights = [L-1, L] where L-1 => no of neurons in prev layer, L => neurons in current neurons
weights = np.random.random((X.shape[1], 1))
weights = weights.reshape(3, 1)
# Final arr to include all errors
loss_arr = []
# Looping over through for N iterations
for i in range(iterations):
# Calcuating the predicted values of Y
z = np.dot(X, weights)
y_predicted = activation_func(z)
# Calucating mean squared loss
loss_j = mse(y_predicted, Y)
# Delta rule implementation
weights = weights - alpha * np.dot(np.transpose(X), (sigmoid_derivative(y_predicted) * (y_predicted - Y)))
# Append the loss error to the final array
loss_arr.append(loss_j)
return (weights, loss_arr)
weights, loss_arr = perceptron_train(X_train, Y_train, 0.001, 10000)
# Plotting the graph for the loss J decreases over time
x_arr = np.arange(0,10000,1)
loss_arr = np.array(loss_arr)
plt.plot(x_arr, loss_arr)
# Training error
print('Training error: ', loss_arr.mean() * 100, "%")
# Calculating the accuracy, test error
y_predicted, accuracy, test_error = evaluate(weights, X_test, Y_test)
print('Accuracy: ', accuracy)
print('Test Error: ', test_error * 100, "%" )
# Method to plot the decision boundary
# Get the transpose of the weights
weights = np.transpose(weights)
# Update the X, Y values
X = np.array(x)
X = X.astype(float)
n = X.shape[0]
Y = np.array(y).reshape(n, 1)
Y = Y.astype(float)
# Linear decision boundary in the input space
a = np.linspace(np.amin(X[:, 1]), np.amax(X[:, 1]), num=200)
b = np.array([])
for i in range(a.shape[0]):
c = -(weights[0, 1] / weights[0, 2]) * a[i] - weights[0, 0] / weights[0, 2]
b = np.append(b, np.array([c]))
# Plot the scatter points and decision boundary
colors=['#1B9CFC', '#FC427B']
plt.scatter(X[:, 1], X[:, 2], c=Y[:,0], cmap=matplotlib.colors.ListedColormap(colors))
plt.plot(a, b, 'black')
plt.grid(True)
plt.colorbar()
plt.show()
###Output
_____no_output_____ |
docs/source/notebooks/censored_data.ipynb | ###Markdown
Censored Data Models
###Code
import arviz as az
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import pymc3 as pm
import seaborn as sns
import theano.tensor as tt
print('Running on PyMC3 v{}'.format(pm.__version__))
%config InlineBackend.figure_format = 'retina'
az.style.use('arviz-darkgrid')
###Output
_____no_output_____
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lccdf, normal_lcdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [sigma, mu]
###Markdown
DiscussionAs we can see, both censored models appear to capture the mean and variance of the underlying distribution as well as the uncensored model! In addition, the imputed censored model is capable of generating data sets of censored values (sample from the posteriors of `left_censored` and `right_censored` to generate them), while the unimputed censored model scales much better with more censored data, and converges faster. Authors- Originally authored by [Luis Mario Domenzain](https://github.com/domenzain) on Mar 7, 2017.- Updated by [George Ho](https://github.com/eigenfoo) on Jul 14, 2018.
###Code
%load_ext watermark
%watermark -n -u -v -iv -w
###Output
numpy 1.18.5
pymc3 3.9.0
seaborn 0.10.1
pandas 1.0.4
arviz 0.8.3
last updated: Mon Jun 15 2020
CPython 3.7.7
IPython 7.15.0
watermark 2.0.2
###Markdown
Censored Data Models
###Code
%matplotlib inline
import pymc3 as pm
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import theano.tensor as tt
plt.style.use('seaborn-darkgrid')
print('Running on PyMC3 v{}'.format(pm.__version__))
###Output
Running on PyMC3 v3.6
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lcdf, normal_lccdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [sigma, mu]
Sampling 4 chains: 100%|██████████| 6000/6000 [00:01<00:00, 5044.47draws/s]
/home/canyon/repos/pymc3/pymc3/plots/__init__.py:40: UserWarning: Keyword argument `varnames` renamed to `var_names`, and will be removed in pymc3 3.8
warnings.warn('Keyword argument `{old}` renamed to `{new}`, and will be removed in pymc3 3.8'.format(old=old, new=new))
/home/canyon/miniconda3/envs/pymc/lib/python3.7/site-packages/arviz/data/io_pymc3.py:56: FutureWarning: arrays to stack must be passed as a "sequence" type such as list or tuple. Support for non-sequence iterables such as generators is deprecated as of NumPy 1.16 and will raise an error in the future.
chain_likelihoods.append(np.stack(log_like))
###Markdown
Censored Data Models
###Code
%matplotlib inline
import pymc3 as pm
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import theano.tensor as tt
plt.style.use('seaborn-darkgrid')
print('Running on PyMC3 v{}'.format(pm.__version__))
###Output
Running on PyMC3 v3.4.1
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sd=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sd=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sd=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sd=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sd=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sd=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sd=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sd=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lcdf, normal_lccdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sd=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sd=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sd=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (2 chains in 2 jobs)
NUTS: [sigma, mu]
Sampling 2 chains: 100%|██████████| 3000/3000 [00:01<00:00, 1960.47draws/s]
###Markdown
Censored Data Models
###Code
%matplotlib inline
import pymc3 as pm
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
import theano.tensor as tt
plt.style.use('seaborn-darkgrid')
print('Running on PyMC3 v{}'.format(pm.__version__))
###Output
Running on PyMC3 v3.4.1
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lcdf, normal_lccdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (2 chains in 2 jobs)
NUTS: [sigma, mu]
Sampling 2 chains: 100%|██████████| 3000/3000 [00:01<00:00, 1960.47draws/s]
###Markdown
Censored Data Models
###Code
import arviz as az
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import pymc3 as pm
import seaborn as sns
import theano.tensor as tt
print('Running on PyMC3 v{}'.format(pm.__version__))
%config InlineBackend.figure_format = 'retina'
az.style.use('arviz-darkgrid')
###Output
_____no_output_____
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lcdf, normal_lccdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [sigma, mu]
###Markdown
DiscussionAs we can see, both censored models appear to capture the mean and variance of the underlying distribution as well as the uncensored model! In addition, the imputed censored model is capable of generating data sets of censored values (sample from the posteriors of `left_censored` and `right_censored` to generate them), while the unimputed censored model scales much better with more censored data, and converges faster. Authors- Originally authored by [Luis Mario Domenzain](https://github.com/domenzain) on Mar 7, 2017.- Updated by [George Ho](https://github.com/eigenfoo) on Jul 14, 2018.
###Code
%load_ext watermark
%watermark -n -u -v -iv -w
###Output
numpy 1.18.5
pymc3 3.9.0
seaborn 0.10.1
pandas 1.0.4
arviz 0.8.3
last updated: Mon Jun 15 2020
CPython 3.7.7
IPython 7.15.0
watermark 2.0.2
###Markdown
Censored Data Models
###Code
import arviz as az
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import pymc3 as pm
import seaborn as sns
import theano.tensor as tt
print(f'Running on PyMC3 v{pm.__version__}')
%config InlineBackend.figure_format = 'retina'
az.style.use('arviz-darkgrid')
###Output
_____no_output_____
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.
sigma = 5.
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.
low = -1.
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title('Uncensored')
axarr[1].set_title('Censored')
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal('observed', mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
right_censored = pm.Bound(pm.Normal, lower=high)(
'right_censored', mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
'left_censored', mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
shape=n_observed
)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lccdf, normal_lcdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
''' Likelihood of left-censored data. '''
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
''' Likelihood of right-censored data. '''
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal('mu', mu=0., sigma=(high - low) / 2.)
sigma = pm.HalfNormal('sigma', sigma=(high - low) / 2.)
observed = pm.Normal(
'observed',
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
'left_censored',
left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
'right_censored',
right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=['mu', 'sigma']);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [sigma, mu]
###Markdown
DiscussionAs we can see, both censored models appear to capture the mean and variance of the underlying distribution as well as the uncensored model! In addition, the imputed censored model is capable of generating data sets of censored values (sample from the posteriors of `left_censored` and `right_censored` to generate them), while the unimputed censored model scales much better with more censored data, and converges faster. Authors- Originally authored by [Luis Mario Domenzain](https://github.com/domenzain) on Mar 7, 2017.- Updated by [George Ho](https://github.com/eigenfoo) on Jul 14, 2018.
###Code
%load_ext watermark
%watermark -n -u -v -iv -w
###Output
numpy 1.18.5
pymc3 3.9.0
seaborn 0.10.1
pandas 1.0.4
arviz 0.8.3
last updated: Mon Jun 15 2020
CPython 3.7.7
IPython 7.15.0
watermark 2.0.2
###Markdown
Censored Data Models
###Code
import arviz as az
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
import pymc3 as pm
import seaborn as sns
import theano.tensor as tt
print(f"Running on PyMC3 v{pm.__version__}")
%config InlineBackend.figure_format = 'retina'
az.style.use("arviz-darkgrid")
###Output
_____no_output_____
###Markdown
[This example notebook on Bayesian survivalanalysis](http://docs.pymc.io/notebooks/survival_analysis.html) touches on thepoint of censored data. _Censoring_ is a form of missing-data problem, in whichobservations greater than a certain threshold are clipped down to thatthreshold, or observations less than a certain threshold are clipped up to thatthreshold, or both. These are called right, left and interval censoring,respectively. In this example notebook we consider interval censoring.Censored data arises in many modelling problems. Two common examples are:1. _Survival analysis:_ when studying the effect of a certain medical treatment on survival times, it is impossible to prolong the study until all subjects have died. At the end of the study, the only data collected for many patients is that they were still alive for a time period $T$ after the treatment was administered: in reality, their true survival times are greater than $T$.2. _Sensor saturation:_ a sensor might have a limited range and the upper and lower limits would simply be the highest and lowest values a sensor can report. For instance, many mercury thermometers only report a very narrow range of temperatures.This example notebook presents two different ways of dealing with censored datain PyMC3:1. An imputed censored model, which represents censored data as parameters and makes up plausible values for all censored values. As a result of this imputation, this model is capable of generating plausible sets of made-up values that would have been censored. Each censored element introduces a random variable.2. An unimputed censored model, where the censored data are integrated out and accounted for only through the log-likelihood. This method deals more adequately with large amounts of censored data and converges more quickly.To establish a baseline we compare to an uncensored model of the uncensoreddata.
###Code
# Produce normally distributed samples
np.random.seed(1618)
size = 500
mu = 13.0
sigma = 5.0
samples = np.random.normal(mu, sigma, size)
# Set censoring limits
high = 16.0
low = -1.0
# Censor samples
censored = samples[(samples > low) & (samples < high)]
# Visualize uncensored and censored data
_, axarr = plt.subplots(ncols=2, figsize=[16, 4], sharex=True, sharey=True)
for i, data in enumerate([samples, censored]):
sns.distplot(data, ax=axarr[i])
axarr[0].set_title("Uncensored")
axarr[1].set_title("Censored")
plt.show()
###Output
_____no_output_____
###Markdown
Baseline - Uncensored Model of Uncensored Data
###Code
# Uncensored model
with pm.Model() as uncensored_model:
mu = pm.Normal("mu", mu=0.0, sigma=(high - low) / 2.0)
sigma = pm.HalfNormal("sigma", sigma=(high - low) / 2.0)
observed = pm.Normal("observed", mu=mu, sigma=sigma, observed=samples)
###Output
_____no_output_____
###Markdown
Model 1 - Imputed Censored Model of Censored DataIn this model, we impute the censored values from the same distribution as the uncensored data. Sampling from the posterior generates possible uncensored data sets.This model makes use of [PyMC3's bounded variables](https://docs.pymc.io/api/bounds.html).
###Code
# Imputed censored model
n_right_censored = len(samples[samples >= high])
n_left_censored = len(samples[samples <= low])
n_observed = len(samples) - n_right_censored - n_left_censored
with pm.Model() as imputed_censored_model:
mu = pm.Normal("mu", mu=0.0, sigma=(high - low) / 2.0)
sigma = pm.HalfNormal("sigma", sigma=(high - low) / 2.0)
right_censored = pm.Bound(pm.Normal, lower=high)(
"right_censored", mu=mu, sigma=sigma, shape=n_right_censored
)
left_censored = pm.Bound(pm.Normal, upper=low)(
"left_censored", mu=mu, sigma=sigma, shape=n_left_censored
)
observed = pm.Normal("observed", mu=mu, sigma=sigma, observed=censored, shape=n_observed)
###Output
_____no_output_____
###Markdown
Model 2 - Unimputed Censored Model of Censored DataIn this model, we do not impute censored data, but instead integrate them out through the likelihood.The implementations of the likelihoods are non-trivial. See the [Stan manual](https://github.com/stan-dev/stan/releases/download/v2.14.0/stan-reference-2.14.0.pdf) (section 11.3 on censored data) and the [original PyMC3 issue on GitHub](https://github.com/pymc-devs/pymc3/issues/1833) for more information.This model makes use of [PyMC3's `Potential`](https://docs.pymc.io/api/model.htmlpymc3.model.Potential).
###Code
# Import the log cdf and log complementary cdf of the normal Distribution from PyMC3
from pymc3.distributions.dist_math import normal_lccdf, normal_lcdf
# Helper functions for unimputed censored model
def left_censored_likelihood(mu, sigma, n_left_censored, lower_bound):
""" Likelihood of left-censored data. """
return n_left_censored * normal_lcdf(mu, sigma, lower_bound)
def right_censored_likelihood(mu, sigma, n_right_censored, upper_bound):
""" Likelihood of right-censored data. """
return n_right_censored * normal_lccdf(mu, sigma, upper_bound)
# Unimputed censored model
with pm.Model() as unimputed_censored_model:
mu = pm.Normal("mu", mu=0.0, sigma=(high - low) / 2.0)
sigma = pm.HalfNormal("sigma", sigma=(high - low) / 2.0)
observed = pm.Normal(
"observed",
mu=mu,
sigma=sigma,
observed=censored,
)
left_censored = pm.Potential(
"left_censored", left_censored_likelihood(mu, sigma, n_left_censored, low)
)
right_censored = pm.Potential(
"right_censored", right_censored_likelihood(mu, sigma, n_right_censored, high)
)
###Output
_____no_output_____
###Markdown
Sampling
###Code
# Uncensored model
with uncensored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace);
# Imputed censored model
with imputed_censored_model:
trace = pm.sample()
pm.plot_posterior(trace, varnames=["mu", "sigma"]);
# Unimputed censored model
with unimputed_censored_model:
trace = pm.sample(tune=1000) # Increase `tune` to avoid divergences
pm.plot_posterior(trace, varnames=["mu", "sigma"]);
###Output
Auto-assigning NUTS sampler...
Initializing NUTS using jitter+adapt_diag...
Multiprocess sampling (4 chains in 4 jobs)
NUTS: [sigma, mu]
###Markdown
DiscussionAs we can see, both censored models appear to capture the mean and variance of the underlying distribution as well as the uncensored model! In addition, the imputed censored model is capable of generating data sets of censored values (sample from the posteriors of `left_censored` and `right_censored` to generate them), while the unimputed censored model scales much better with more censored data, and converges faster. Authors- Originally authored by [Luis Mario Domenzain](https://github.com/domenzain) on Mar 7, 2017.- Updated by [George Ho](https://github.com/eigenfoo) on Jul 14, 2018.
###Code
%load_ext watermark
%watermark -n -u -v -iv -w
###Output
numpy 1.18.5
pymc3 3.9.0
seaborn 0.10.1
pandas 1.0.4
arviz 0.8.3
last updated: Mon Jun 15 2020
CPython 3.7.7
IPython 7.15.0
watermark 2.0.2
|
notebooks/welter_issue009-01_generate_synthetic_two_T_fake_data.ipynb | ###Markdown
Welter issue 9 Generate synthetic, noised-up two-temperature model spectra, then naively fit a single temperature model to it. Part 1- Can you dig it?Michael Gully-Santiago Wednesday, January 6, 2015 CAANNN YOUUUU DIGGG ITTTT
###Code
import warnings
warnings.filterwarnings("ignore")
import numpy as np
from astropy.io import fits
import matplotlib.pyplot as plt
% matplotlib inline
% config InlineBackend.figure_format = 'retina'
import seaborn as sns
sns.set_context('notebook')
###Output
_____no_output_____
###Markdown
Facts So, we are going to add two different temperature spectra together to create a *mixture model*:$$ \mathsf{M}_{mix} = c \cdot \mathsf{M}_A(\Theta_A, \phi_{\mathsf{P}}) + (1-c) \cdot \mathsf{M}_B(\Theta_B, \phi_{\mathsf{P}}) $$The $\phi_{\mathsf{P}}$ and $\theta_{ext}$ parameters will be the identical. The $\Theta$ parameters will be identical except for different effective temperatures:$$ \Theta_A = [\theta_{A,\ast}, \theta_{ext}] $$with: $$\theta_{A,\ast} = \{T_{\textrm{eff}, A}, \log g, [{\rm Fe}/{\rm H}] \}$$ $$\theta_{B,\ast} = \{T_{\textrm{eff}, B}, \log g, [{\rm Fe}/{\rm H}] \}$$But then we are going to *save* the noised up model as if it is *data*:$$ \mathsf{D}_{syn} = \mathsf{M}_{mix} + \mathsf{R}$$Where $\mathsf{R}$ is a single draw from the noise model, but I'm not quite sure how to illustrate that here. Getting the fluxes right. It will be easy to save fake data. We have already fit models to the data, and have noise estimates and calibration parameters. The one tricky thing is that Starfish automatically normalizes the stellar fluxes from the model grids. This makes sense for most cases, but in this case it is the wrong thing to do, since we care about the relative fluxes of the stars. To fix this problem we will un-normalize the spectra by calculating their bolometric flux ratio, $r$, before normalization: $$r = F_{B,bol}/F_{A,bol}$$ with: $$F_{B,bol} = \int{f_{B, \lambda}}{d\lambda}$$ $$F_{A,bol} = \int{f_{A, \lambda}}{d\lambda}$$So: $$f_{B, \lambda} = r \cdot \hat f_{A, \lambda}$$ where $\hat f$ denotes the normalized version of $f$. The $\mathsf{M}$ terms above assume the un-normalized $f$. (*n.b* Technically what we are doing is leaving $f_{A, \lambda}$ as normalized, and scaling $f_{B, \lambda}$ to ensure that their relative flux ratio is correct. This choice makes it so that we don't have to change too much else in the fitting procedure.) How to actually implement this. We will temporarily modify the `splot.py --noise` scripts. These dump out models, with the correlated residuals.Here are the specific bits of code from `splot.py` **line 66**. ```python Get many random draws from data_mat draws = random_draws(data_mat, num=9) min_spec, max_spec = std_envelope(draws)```The relevant vectors are conspicuous a few lines down.```python ax[0].plot(wl, data, "b", label="data") ax[0].plot(wl, model, "r", label="model")```What we will do is:0. Change all the $\log{g}$'s (and extrinsic parameters?) to the same value.1. Generate a model with high temperature, $T_{\textrm{eff}, A} = 4100$.2. Save a json file **model_A_spec.json** with `wl`, `model`, `noise_draw` for the warmer temperature.3. Generate a model with low temperature, $T_{\textrm{eff}, B} = 3300$.4. Save a json file **model_B_spec.json** with `wl`, `model`, `noise_draw` for the cooler temperature.5. Write a small script which does the following: - Scales the flux (and noise spectrum) by the cofactor $r$ as discussed above: $f_{B, \lambda} = r \cdot \hat f_{A, \lambda}$ - Scales the models (and noise spectrum) by the filling factor $c$, and $(1-c)$ - Adds the scaled models together - Adds the scaled noise spectrum - Save to an *HDF5* file that looks just like real data.The value of $r$ is: $r = 2.39894^{-1}$. See [this notebook](experiment_to_add_starpot_models-02.ipynb).One issue that comes to mind is scaling the noise to the "right level". If we linearly scale the data but not the noise, it will change the signal-to-noise ratio. So in the scheme described above, the uncertainty contained in the existing **data** *HDF5* file, the `['sigmas']` dataset, will altered. This is OK, since the code automatically estimates corrections in the `sigAmp` parameter. The error spectrum (correlated errors) will be change accross the spectrum as the warm and cool components change relative to each other. I'm not sure what all of this will mean... I know the code will pick up some of the slack in fitting a the `logAmp` parameter, but it could get weird. The other thing to keep in mind is that we have to *iterate* this for all the orders on all the instruments. Whew.Let's just try one by hand.
###Code
import os
import json
os.chdir('../sf/eo005/output/LkCa4_eo005/run01/')
try:
with open('model_A_spec.json') as f:
ma_raw = json.load(f)
with open('model_B_spec.json') as f:
mb_raw = json.load(f)
except FileNotFoundError:
print("You need to have the model_A and model_B spectral json files in place first.")
r_factor = 1.0/2.39894
wl = np.array(ma_raw['wl'])
fl_ma = np.array(ma_raw['model'])
nd_ma = np.array(ma_raw['noise_draw'])
fl_mb_raw = np.array(mb_raw['model'])
nd_mb_raw = np.array(mb_raw['noise_draw'])
#Scale the flux (and noise spectrum) by the cofactor $r$ as discussed above.
fl_mb = fl_mb_raw * r_factor
nd_mb = nd_mb_raw * r_factor
# Mixture model
c = 0.70
mmix = c * fl_ma + (1 - c) * fl_mb
ndmix = 0.5 * (c * nd_ma + (1 - c) * nd_mb) # Remove the factor of 2.
mmix_noised = mmix + ndmix
sns.set_context('paper', font_scale=1.2)
sns.set_style('ticks')
plt.figure(figsize=(18, 4))
plt.subplot('141')
plt.title('Normalized model spectra')
plt.plot(wl, fl_ma, label='$\hat f_{A}, T_{eff} = 4100$')
plt.plot(wl, fl_mb_raw, label='$\hat f_{B}, T_{eff} = 3300$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('normalized $f_\lambda$')
plt.subplot('142')
plt.title('Flux-scaled model spectra')
plt.plot(wl, fl_ma, label='$f_{A}, T_{eff} = 4100$')
plt.plot(wl, fl_mb, label='$f_{B}, T_{eff} = 3300$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('flux-scaled $f_\lambda$')
plt.subplot('143')
plt.title('Fill Factor c = {:.2f}'.format(c))
plt.plot(wl, fl_ma * c, label='$c \cdot f_{A}$')
plt.plot(wl, fl_mb * (1-c), label='$(1-c) \cdot f_{B}$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('flux-scaled $f_\lambda$')
plt.subplot('144')
plt.title('Mixture model')
plt.plot(wl, mmix, 'k', label='$f_{mix}$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('flux-scaled $f_\lambda$')
plt.figure(figsize=(14, 6))
plt.subplot('121')
plt.title('Mixture model')
plt.plot(wl, mmix, 'k', label='$f_{mix}$')
plt.plot(wl, fl_ma*0.83, 'b', alpha=0.5, label='$f_{A} \cdot 0.83$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('flux-scaled $f_\lambda$')
plt.subplot('122')
plt.title('Noised-up Mixture model')
plt.plot(wl, mmix, 'k', label='$f_{mix}$')
plt.plot(wl, mmix_noised, 'r', alpha=0.5, label='$f_{mix} + \mathsf{R}$')
plt.ylim(0,1.0)
plt.legend(loc='best')
plt.xlabel('$\lambda \,(\AA)$')
plt.ylabel('flux-scaled $f_\lambda$')
###Output
_____no_output_____
###Markdown
Prepare for automation We will save the default config and phi values to a DataFrame.This will allow us to compare what we get from the fits to what we input.
###Code
import pandas as pd
import yaml
def fill_DataFrame(config, phi):
dict_out = {
"logg":config['Theta']['grid'][1],
"Fe_H":config['Theta']['grid'][2],
"logOmega":config['Theta']['logOmega'],
"vsini":config['Theta']['vsini'],
"vz":config['Theta']['vz'],
"Av":config['Theta']['Av'],
"logAmp":phi['logAmp'],
"sigAmp":phi['sigAmp'],
"ll":phi['l'],
"cheb1":phi['cheb'][0],
"cheb2":phi['cheb'][1],
"cheb3":phi['cheb'][2]
}
return dict_out
os.chdir('/Users/gully/GitHub/welter/notebooks/')
df = pd.DataFrame()
for i in range(0, 34+1):
os.chdir('../sf/eo{:03d}/'.format(i))
if os.path.exists('config.yaml') & os.path.exists('s0_o0phi.json'):
#Let's just record the starting values of the config.yaml files in a DataFrame.
with open('config.yaml', 'r') as f:
config = yaml.load(f)
with open('s0_o0phi.json', 'r') as f:
phi = json.load(f)
this_df = pd.DataFrame(fill_DataFrame(config, phi), index=[i])
df = df.append(this_df)
os.chdir('/Users/gully/GitHub/welter/notebooks/')
df.head()
###Output
_____no_output_____
###Markdown
TODO Save to a file:
###Code
df.to_csv('../data/analysis/ESPaDOnS_mixture_params.csv')
###Output
_____no_output_____
###Markdown
Read in all the data.No spot-checking necessary, since we already did that in the previous notebook.
###Code
sf_dat = pd.read_csv('../data/analysis/IGRINS_ESPaDOnS_run01_last10kMCMC.csv')
sf_dat.rename(columns={"m_val_x":"m_val"}, inplace=True)
del sf_dat['m_val_y']
sf_dat.head(6)
###Output
_____no_output_____
###Markdown
Comparison: What is in the config files, versus what we learned from 20,000 MCMC samples.
###Code
plt.plot(df.index.values, df.logOmega, 'ro', label = 'In config files')
plt.plot(df.index.values, sf_dat.logO_50p, 's', label = '20,000 MCMC Samples')
plt.legend(loc='best');
###Output
_____no_output_____ |
simulate_singlecoil_from_multicoil.ipynb | ###Markdown
Following the approch by Mark Tygert and Jure Zbontar: Simulating single-coil MRIfrom the responses of multiple coils, Communications in Applied Mathematics and Computational Science, 2020.
###Code
# for optimization
import scipy as sp
from scipy import linalg
import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
# fastmri
import fastmri
from fastmri.data import subsample
from fastmri.data import transforms, mri_data
# other
import h5py
from tqdm.notebook import tqdm
import matplotlib.pyplot as plt
import os
os.environ["CUDA_VISIBLE_DEVICES"] = "0"
device = 'cuda'
# Get filenames from folder
mc_path = "/media/hdd1/fastMRIdata/brain/multicoil_train/" # multicoil data (from)
sc_path = "/root/workspace/singlecoil_train/" # singlecoil data path (save to)
filenames = []
for file in os.listdir(mc_path):
if file.endswith(".h5"):
filenames.append(file)
# Emulate single coil data
loss_curves = []
for i in tqdm(range(0, len(filenames))):
######################
##--Get file
#####################
fname = filenames[i]
path = os.path.join(mc_path, fname)
hf = h5py.File(path, mode='r')
#####################
##--Prepare data
#####################
volume_kspace = hf['kspace'][()]
volume_kspace = transforms.to_tensor(volume_kspace)
rss = hf['reconstruction_rss'][()]
coils = volume_kspace.shape[1]
slices, height, width = rss.shape
coil_images_full = fastmri.ifft2c(volume_kspace)
coil_images = transforms.complex_center_crop(coil_images_full, (height, width)).permute(1,0,2,3,4)
coil_images = transforms.tensor_to_complex_np(coil_images)
##########################################
##--Linear least-square initialization
##########################################
A = coil_images.reshape(coils, slices*height*width).T
b = rss.reshape(slices*height*width)
init = linalg.lstsq(A, b)
##########################################
##--Non-linear Least-square solution
##########################################
A = torch.tensor(A).to(device)
b = torch.tensor(b).to(device)
x = torch.tensor(init[0], requires_grad = True, device=device) # coil-weights
def obj_fun(x):
return torch.sum( ( (A@x).abs().sqrt()-b.abs().sqrt() )**2 )
# optimizer = optim.AdamW([x], lr=0.0, weight_decay=0.04)
optimizer = optim.SGD([x], lr=0.0, momentum=0.9)
scheduler = optim.lr_scheduler.OneCycleLR(optimizer, 0.05, total_steps=3000)
loss_hist = []
for _ in range(3000):
optimizer.zero_grad()
loss = obj_fun(x)
loss.backward()
nn.utils.clip_grad_norm_(x, max_norm=1, norm_type=2.)
optimizer.step()
scheduler.step()
loss_hist.append(loss.item())
loss_curves.append(loss_hist)
# print(loss.item())
# plt.plot(loss_hist)
# plt.show()
##########################################
##--Create emulated single coil data
##########################################
with torch.no_grad():
# Linear combination of coil images
xhat = x.view(1, coils, 1, 1).cpu().numpy()
coil_images_full = transforms.tensor_to_complex_np(coil_images_full)
single_coil_images = np.sum(coil_images_full * xhat, axis=1)
single_coil_images = transforms.to_tensor(single_coil_images)
# Volume single coil k-space
single_coil_kspace = fastmri.fft2c(single_coil_images)
single_coil_kspace = transforms.tensor_to_complex_np(single_coil_kspace)
# Volume single coil reconstruction
esc = transforms.complex_center_crop(single_coil_images, (height, width))
esc = np.abs(transforms.tensor_to_complex_np(esc))
# esc = (A@x).reshape(slices,height, width).abs().cpu().numpy() # Also valid
maxval = np.max(esc).astype(np.float64)
normval = linalg.norm(esc).astype(np.float64)
##########################################
##--Create and save as .h5 file
##########################################
with h5py.File(os.path.join(sc_path, fname), "w") as f:
f.create_dataset('ismrmrd_header', data=hf['ismrmrd_header'][()])
f.create_dataset('kspace', data=single_coil_kspace)
f.create_dataset('reconstruction_esc', data=esc)
f.create_dataset('reconstruction_rss', data=hf['reconstruction_rss'][()])
f.attrs.__setitem__('acquisition', hf.attrs.__getitem__('acquisition'))
f.attrs.__setitem__('max', maxval)
f.attrs.__setitem__('norm', normval)
f.attrs.__setitem__('patient_id', hf.attrs.__getitem__('patient_id'))
f.close()
torch.save(loss_curves, './loss_curves.pt')
# Check for one sample
file_name = '/root/workspace/singlecoil_train/file_brain_AXFLAIR_200_6002549.h5'
hf = h5py.File(file_name, mode='r')
print('Keys:', list(hf.keys()))
print('Attrs:', dict(hf.attrs))
esc = hf['reconstruction_esc'][()]
rss = hf['reconstruction_rss'][()]
idx = 8
fig= plt.figure(figsize=(15,10))
plt.subplot(1,3,1)
plt.imshow(esc[idx], cmap='gray')
plt.title('esc')
plt.subplot(1,3,2)
plt.imshow(rss[idx], cmap='gray')
plt.title('rss')
plt.subplot(1,3,3)
# plt.imshow(esc[idx]-rss[idx], cmap='bwr')
plt.imshow(esc[idx]-rss[idx], cmap='bwr')
plt.title('esc-rss')
plt.show()
###Output
Keys: ['ismrmrd_header', 'kspace', 'reconstruction_esc', 'reconstruction_rss']
Attrs: {'acquisition': 'AXFLAIR', 'max': 0.0002675850410014391, 'norm': 0.09161251783370972, 'patient_id': 'ce961206b6f8f8ccee820a43bc407f742be2c538cfaa7d33abbb5d7e3d0c015d'}
|
get_tarball.ipynb | ###Markdown
Retrieve the tarball
###Code
path = 'https://datasets.imdbws.com/title.basics.tsv.gz'
buf = urlopen(path)
buf.status
contents = buf.read()
b = io.BytesIO(contents)
with gzip.open(b) as f:
df = pd.read_csv(f, sep="\t")
df.head()
# filter adult
df = df[(df["runtimeMinutes"] >= 50) & (df["isAdult"] == 0)]
import numpy as np
import re
df["runtimeMinutes"].apply(lambda x: int(x) if re.match("\d+", x) else 0)
!cd web_scraping
from dotenv import load_dotenv
load_dotenv()
import os
import psycopg2
conn = psycopg2.connect(
database=os.getenv("DB_NAME"),
user=os.getenv("DB_USER"),
password=os.getenv("DB_PASSWORD"),
host=os.getenv("HOST"),
port=os.getenv("PORT"),
)
curs = conn.cursor()
curs.execute("""
SELECT *
FROM movie_reviews
WHERE source='letterboxd'
""")
res = curs.fetchall()
###Output
_____no_output_____ |
homework/Bonus1/Bonus1.ipynb | ###Markdown
Bonus 1: Numerical Optimization for Logistic Regression. Name: [Your-Name?] 0. You will do the following:1. Read the lecture note: [click here](https://github.com/wangshusen/DeepLearning/blob/master/LectureNotes/Logistic/paper/logistic.pdf)2. Read, complete, and run my code.3. **Implement mini-batch SGD** and evaluate the performance. (1 bonus point.)4. Convert the .IPYNB file to .HTML file. * The HTML file must contain **the code** and **the output after execution**. 5. Upload this .HTML file to your Google Drive, Dropbox, or your Github repo.6. Submit the link to this .HTML file to Canvas. * Example: https://github.com/wangshusen/CS583-2019F/blob/master/homework/Bonus1/Bonus1.html Grading criteria:1. When computing the ```gradient``` and ```objective function value``` using a batch of samples, use **matrix-vector multiplication** rather than a FOR LOOP of **vector-vector multiplications**.2. Plot ```objective function value``` against ```epochs```. In the plot, compare GD, SGD, and MB-SGD (with $b=8$ and $b=64$). The plot must look reasonable. 1. Data processing- Download the Diabete dataset from https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary/diabetes- Load the data using sklearn.- Preprocess the data. 1.1. Load the data
###Code
from sklearn import datasets
import numpy
x_sparse, y = datasets.load_svmlight_file('diabetes')
x = x_sparse.todense()
print('Shape of x: ' + str(x.shape))
print('Shape of y: ' + str(y.shape))
###Output
_____no_output_____
###Markdown
1.2. Partition to training and test sets
###Code
# partition the data to training and test sets
n = x.shape[0]
n_train = int(numpy.ceil(n * 0.8))
n_test = n - n_train
rand_indices = numpy.random.permutation(n)
train_indices = rand_indices[0:n_train]
test_indices = rand_indices[n_train:n]
x_train = x[train_indices, :]
x_test = x[test_indices, :]
y_train = y[train_indices].reshape(n_train, 1)
y_test = y[test_indices].reshape(n_test, 1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
print('Shape of y_train: ' + str(y_train.shape))
print('Shape of y_test: ' + str(y_test.shape))
###Output
_____no_output_____
###Markdown
1.3. Feature scaling Use the standardization to trainsform both training and test features
###Code
# Standardization
import numpy
# calculate mu and sig using the training set
d = x_train.shape[1]
mu = numpy.mean(x_train, axis=0).reshape(1, d)
sig = numpy.std(x_train, axis=0).reshape(1, d)
# transform the training features
x_train = (x_train - mu) / (sig + 1E-6)
# transform the test features
x_test = (x_test - mu) / (sig + 1E-6)
print('test mean = ')
print(numpy.mean(x_test, axis=0))
print('test std = ')
print(numpy.std(x_test, axis=0))
###Output
_____no_output_____
###Markdown
1.4. Add a dimension of all ones
###Code
n_train, d = x_train.shape
x_train = numpy.concatenate((x_train, numpy.ones((n_train, 1))), axis=1)
n_test, d = x_test.shape
x_test = numpy.concatenate((x_test, numpy.ones((n_test, 1))), axis=1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
###Output
_____no_output_____
###Markdown
2. Logistic regression modelThe objective function is $Q (w; X, y) = \frac{1}{n} \sum_{i=1}^n \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $.
###Code
# Calculate the objective function value
# Inputs:
# w: d-by-1 matrix
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# Return:
# objective function value (scalar)
def objective(w, x, y, lam):
n, d = x.shape
yx = numpy.multiply(y, x) # n-by-d matrix
yxw = numpy.dot(yx, w) # n-by-1 matrix
vec1 = numpy.exp(-yxw) # n-by-1 matrix
vec2 = numpy.log(1 + vec1) # n-by-1 matrix
loss = numpy.mean(vec2) # scalar
reg = lam / 2 * numpy.sum(w * w) # scalar
return loss + reg
# initialize w
d = x_train.shape[1]
w = numpy.zeros((d, 1))
# evaluate the objective function value at w
lam = 1E-6
objval0 = objective(w, x_train, y_train, lam)
print('Initial objective function value = ' + str(objval0))
###Output
_____no_output_____
###Markdown
3. Numerical optimization 3.1. Gradient descent The gradient at $w$ is $g = - \frac{1}{n} \sum_{i=1}^n \frac{y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$
###Code
# Calculate the gradient
# Inputs:
# w: d-by-1 matrix
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# Return:
# g: g: d-by-1 matrix, full gradient
def gradient(w, x, y, lam):
n, d = x.shape
yx = numpy.multiply(y, x) # n-by-d matrix
yxw = numpy.dot(yx, w) # n-by-1 matrix
vec1 = numpy.exp(yxw) # n-by-1 matrix
vec2 = numpy.divide(yx, 1+vec1) # n-by-d matrix
vec3 = -numpy.mean(vec2, axis=0).reshape(d, 1) # d-by-1 matrix
g = vec3 + lam * w
return g
# Gradient descent for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# stepsize: scalar
# max_iter: integer, the maximal iterations
# w: d-by-1 matrix, initialization of w
# Return:
# w: d-by-1 matrix, the solution
# objvals: a record of each iteration's objective value
def grad_descent(x, y, lam, stepsize, max_iter=100, w=None):
n, d = x.shape
objvals = numpy.zeros(max_iter) # store the objective values
if w is None:
w = numpy.zeros((d, 1)) # zero initialization
for t in range(max_iter):
objval = objective(w, x, y, lam)
objvals[t] = objval
print('Objective value at t=' + str(t) + ' is ' + str(objval))
g = gradient(w, x, y, lam)
w -= stepsize * g
return w, objvals
###Output
_____no_output_____
###Markdown
Run gradient descent.
###Code
lam = 1E-6
stepsize = 1.0
w, objvals_gd = grad_descent(x_train, y_train, lam, stepsize)
###Output
_____no_output_____
###Markdown
3.2. Stochastic gradient descent (SGD)Define $Q_i (w) = \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $.The stochastic gradient at $w$ is $g_i = \frac{\partial Q_i }{ \partial w} = -\frac{y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$.
###Code
# Calculate the objective Q_i and the gradient of Q_i
# Inputs:
# w: d-by-1 matrix
# xi: 1-by-d matrix
# yi: scalar
# lam: scalar, the regularization parameter
# Return:
# obj: scalar, the objective Q_i
# g: d-by-1 matrix, gradient of Q_i
def stochastic_objective_gradient(w, xi, yi, lam):
d = xi.shape[0]
yx = yi * xi # 1-by-d matrix
yxw = float(numpy.dot(yx, w)) # scalar
# calculate objective function Q_i
loss = numpy.log(1 + numpy.exp(-yxw)) # scalar
reg = lam / 2 * numpy.sum(w * w) # scalar
obj = loss + reg
# calculate stochastic gradient
g_loss = -yx.T / (1 + numpy.exp(yxw)) # d-by-1 matrix
g = g_loss + lam * w # d-by-1 matrix
return obj, g
# SGD for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# stepsize: scalar
# max_epoch: integer, the maximal epochs
# w: d-by-1 matrix, initialization of w
# Return:
# w: the solution
# objvals: record of each iteration's objective value
def sgd(x, y, lam, stepsize, max_epoch=100, w=None):
n, d = x.shape
objvals = numpy.zeros(max_epoch) # store the objective values
if w is None:
w = numpy.zeros((d, 1)) # zero initialization
for t in range(max_epoch):
# randomly shuffle the samples
rand_indices = numpy.random.permutation(n)
x_rand = x[rand_indices, :]
y_rand = y[rand_indices, :]
objval = 0 # accumulate the objective values
for i in range(n):
xi = x_rand[i, :] # 1-by-d matrix
yi = float(y_rand[i, :]) # scalar
obj, g = stochastic_objective_gradient(w, xi, yi, lam)
objval += obj
w -= stepsize * g
stepsize *= 0.9 # decrease step size
objval /= n
objvals[t] = objval
print('Objective value at epoch t=' + str(t) + ' is ' + str(objval))
return w, objvals
###Output
_____no_output_____
###Markdown
Run SGD.
###Code
lam = 1E-6
stepsize = 0.1
w, objvals_sgd = sgd(x_train, y_train, lam, stepsize)
###Output
_____no_output_____
###Markdown
4. Compare GD with SGDPlot objective function values against epochs.
###Code
import matplotlib.pyplot as plt
%matplotlib inline
fig = plt.figure(figsize=(6, 4))
epochs_gd = range(len(objvals_gd))
epochs_sgd = range(len(objvals_sgd))
line0, = plt.plot(epochs_gd, objvals_gd, '--b', LineWidth=4)
line1, = plt.plot(epochs_sgd, objvals_sgd, '-r', LineWidth=2)
plt.xlabel('Epochs', FontSize=20)
plt.ylabel('Objective Value', FontSize=20)
plt.xticks(FontSize=16)
plt.yticks(FontSize=16)
plt.legend([line0, line1], ['GD', 'SGD'], fontsize=20)
plt.tight_layout()
plt.show()
fig.savefig('compare_gd_sgd.pdf', format='pdf', dpi=1200)
###Output
_____no_output_____
###Markdown
5. Prediction
###Code
# Predict class label
# Inputs:
# w: d-by-1 matrix
# X: m-by-d matrix
# Return:
# f: m-by-1 matrix, the predictions
def predict(w, X):
xw = numpy.dot(X, w)
f = numpy.sign(xw)
return f
# evaluate training error
f_train = predict(w, x_train)
diff = numpy.abs(f_train - y_train) / 2
error_train = numpy.mean(diff)
print('Training classification error is ' + str(error_train))
# evaluate test error
f_test = predict(w, x_test)
diff = numpy.abs(f_test - y_test) / 2
error_test = numpy.mean(diff)
print('Test classification error is ' + str(error_test))
###Output
_____no_output_____
###Markdown
6. Mini-batch SGD (fill the code) 6.1. Compute the objective $Q_I$ and its gradient using a batch of samplesDefine $Q_I (w) = \frac{1}{b} \sum_{i \in I} \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $, where $I$ is a set containing $b$ indices randomly drawn from $\{ 1, \cdots , n \}$ without replacement.The stochastic gradient at $w$ is $g_I = \frac{\partial Q_I }{ \partial w} = \frac{1}{b} \sum_{i \in I} \frac{- y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$.
###Code
# Calculate the objective Q_I and the gradient of Q_I
# Inputs:
# w: d-by-1 matrix
# xi: b-by-d matrix
# yi: b-by-1 matrix
# lam: scalar, the regularization parameter
# b: integer, the batch size
# Return:
# obj: scalar, the objective Q_i
# g: d-by-1 matrix, gradient of Q_i
def mb_stochastic_objective_gradient(w, xi, yi, lam, b):
# Fill the function
# Follow the implementation of stochastic_objective_gradient
# Use matrix-vector multiplication; do not use FOR LOOP of vector-vector multiplications
...
return obj, g
###Output
_____no_output_____
###Markdown
6.2. Implement mini-batch SGDHints:1. In every epoch, randomly permute the $n$ samples (just like SGD).2. Each epoch has $\frac{n}{b}$ iterations. In every iteration, use $b$ samples, and compute the gradient and objective using the ``mb_stochastic_objective_gradient`` function. In the next iteration, use the next $b$ samples, and so on.3. Note that ``n mod b`` may not be zero. For example, if $n=1095$ and $b=100$, then there will be $95$ samples left. If the $95$ samples are not used, then the computed objective function will be incorrect. You need to take this effect into account in your implementation of the ``mb_sgd`` function.
###Code
# Mini-Batch SGD for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# b: integer, the batch size
# stepsize: scalar
# max_epoch: integer, the maximal epochs
# w: d-by-1 matrix, initialization of w
# Return:
# w: the solution
# objvals: record of each iteration's objective value
def mb_sgd(x, y, lam, b, stepsize, max_epoch=100, w=None):
# Fill the function
# Follow the implementation of sgd
# Record one objective value per epoch (not per iteration!)
...
return w, objvals
###Output
_____no_output_____
###Markdown
6.3. Run MB-SGD
###Code
# MB-SGD with batch size b=8
lam = 1E-6 # do not change
b = 8 # do not change
stepsize = 0.1 # you must tune this parameter
w, objvals_mbsgd8 = mb_sgd(x_train, y_train, lam, b, stepsize)
# MB-SGD with batch size b=64
lam = 1E-6 # do not change
b = 64 # do not change
stepsize = 0.1 # you must tune this parameter
w, objvals_mbsgd64 = mb_sgd(x_train, y_train, lam, b, stepsize)
###Output
_____no_output_____
###Markdown
7. Plot and compare GD, SGD, and MB-SGD You are required to compare the following algorithms:- Gradient descent (GD)- SGD- MB-SGD with b=8- MB-SGD with b=64Follow the code in Section 4 to plot ```objective function value``` against ```epochs```. There should be four curves in the plot; each curve corresponds to one algorithm. Hint: Logistic regression with $\ell_2$-norm regularization is a strongly convex optimization problem. All the algorithms will converge to the same solution. In the end, the ``objective function value`` of the 4 algorithms will be the same. If not the same, your implementation must be wrong.
###Code
# plot the 4 curves:
###Output
_____no_output_____
###Markdown
Bonus1: Parallel Algorithms Name: Ayman Elkfrawy 0. You will do the following:1. Read the lecture note: [click here](https://github.com/wangshusen/DeepLearning/blob/master/LectureNotes/Parallel/Parallel.pdf)2. Implement federated averaging or decentralized optimization.3. Plot the convergence curve. (The x-axis can be ```number of epochs``` or ```number of communication```. You must make sure the label is correct.)4. Convert the .IPYNB file to .HTML file. * The HTML file must contain **the code** and **the output after execution**. 5. Upload this .HTML file to your Google Drive, Dropbox, or your Github repo. (If it is submitted to Google Drive or Dropbox, you must make the file open-access.)6. Submit the link to this .HTML file to Canvas. * Example: https://github.com/wangshusen/CS583-2020S/blob/master/homework/Bonus1/Bonus1.html 1. Data processing- Download the Diabete dataset from https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary/diabetes- Load the data using sklearn.- Preprocess the data. 1.1. Load the data
###Code
from sklearn import datasets
import numpy
x_sparse, y = datasets.load_svmlight_file('diabetes')
x = x_sparse.todense()
print('Shape of x: ' + str(x.shape))
print('Shape of y: ' + str(y.shape))
###Output
Shape of x: (768, 8)
Shape of y: (768,)
###Markdown
1.2. Partition to training and test sets
###Code
from sklearn.model_selection import train_test_split
split_random_state = 376
y = y.reshape(len(y), 1)
x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.16)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
print('Shape of y_train: ' + str(y_train.shape))
print('Shape of y_test: ' + str(y_test.shape))
###Output
Shape of x_train: (645, 8)
Shape of x_test: (123, 8)
Shape of y_train: (645, 1)
Shape of y_test: (123, 1)
###Markdown
1.3. Feature scaling Use the standardization to trainsform both training and test features
###Code
# Standardization
import numpy
# calculate mu and sig using the training set
d = x_train.shape[1]
mu = numpy.mean(x_train, axis=0).reshape(1, d)
sig = numpy.std(x_train, axis=0).reshape(1, d)
# transform the training features
x_train = (x_train - mu) / (sig + 1E-6)
# transform the test features
x_test = (x_test - mu) / (sig + 1E-6)
print('test mean = ')
print(numpy.mean(x_test, axis=0))
print('test std = ')
print(numpy.std(x_test, axis=0))
###Output
test mean =
[[-0.27864308 0.03758729 -0.02998267 0.10722333 0.106165 0.01002021
-0.00890055 -0.11505426]]
test std =
[[0.82651628 1.14970787 0.99312144 1.01496174 1.11771912 1.17845981
0.81919962 0.99679393]]
###Markdown
1.4. Add a dimension of all ones
###Code
n_train, d = x_train.shape
x_train = numpy.concatenate((x_train, numpy.ones((n_train, 1))), axis=1)
n_test, d = x_test.shape
x_test = numpy.concatenate((x_test, numpy.ones((n_test, 1))), axis=1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
import math
VERBOSE = False
class Worker:
def __init__(self, worker_id, x, y):
self.x = x
self.y = y
self.n_samples = x.shape[0]
self.d_features = x.shape[1]
self.w = numpy.zeros((d, 1))
self.worker_id = worker_id
self.log('Created')
self.last_index = 0
self.epoch_objs = []
self.sgd_obj_acc = 0 # accumulated SGD objective value
def log(self, message):
if VERBOSE:
print(f'Worker ({self.worker_id}): {message}')
def set_params(self, w):
self.log('Received Updated Params')
self.w = w
def sgd_grad_objective(self, lam):
self.log('Calculating SGD grad/loss')
if self.last_index >= self.n_samples: # one epoch is done
# Shuffle the samples
rand_indices = numpy.random.permutation(self.n_samples)
self.x = self.x[rand_indices, :]
self.y = self.y[rand_indices, :]
self.epoch_objs.append(self.sgd_obj_acc / self.n_samples) # add per epoch obj value
self.sgd_obj_acc = 0
self.last_index = 0
xi = self.x[self.last_index, :]
yi = float(self.y[self.last_index, :])
self.last_index += 1
yx = yi * xi
yxw = float(numpy.dot(yx, self.w))
loss = numpy.log(1 + numpy.exp(-yxw))
obj = loss + lam / 2 * numpy.sum(self.w * self.w)
self.sgd_obj_acc += obj
g = -yx.T / (1 + numpy.exp(yxw)) + lam * self.w
return g, obj
def gd_grad_objective(self, lam):
self.log('Calculating GD grad/loss')
yx = numpy.multiply(self.y, self.x)
yxw = numpy.dot(yx, self.w)
loss = numpy.mean(numpy.log(1 + numpy.exp(-yxw)))
obj = loss + lam / 2 * numpy.sum(self.w * self.w)
self.epoch_objs.append(obj) # add per epoch obj value
g_vec = numpy.divide(yx, 1 + numpy.exp(yxw))
grad = -numpy.mean(g_vec, axis=0).reshape(self.d_features, 1) + lam * self.w
return grad, obj
def fed_avg(self, n_local_grads, algorithm, learn_rate, lam):
self.log('Performing FedAvg')
objval = 0
for iteration in range(n_local_grads):
if algorithm == 'sgd':
g, obj = self.sgd_grad_objective(lam)
else:
g, obj = self.gd_grad_objective(lam)
self.w -= learn_rate * g
objval += obj
objval /= n_local_grads
return self.w, objval
class Server:
def __init__(self, m, n, d):
self.m_workers = m
self.n_samples = n
self.d_features = d
self.w = numpy.zeros((d, 1))
self.obj = 0
self.log('Created')
def log(self, message):
if VERBOSE:
print(f'Server: {message}')
def broadcast(self):
self.log('Broadcasting')
return self.w
def aggregate(self, params, objvals):
self.log('Aggregating')
self.w = numpy.zeros((self.d_features, 1))
self.obj = 0
for worker in range(self.m_workers):
self.w += params[worker]
self.obj += objvals[worker]
# Average results
self.w /= self.m_workers
self.obj /= self.m_workers
def create_server_workers(m_workers, x, y):
n_samples, d_features = x.shape
s = math.floor(n_samples / m_workers)
server = Server(m_workers, n_samples, d_features)
workers = []
for i in range(m_workers):
indices = list(range(i*s, (i+1) * s))
worker = Worker('worker'+str(i), x[indices, :], y[indices, :])
workers.append(worker)
return server, workers
def run_fed_avg(x_train, y_train, q=8, algorithm='gd', m_workers=4, lam=1e-6, alpha=1e-1, max_epoch=50):
server, workers = create_server_workers(m_workers, x_train, y_train)
iterations_per_epoch = 1
if algorithm == 'sgd': # Iterate more for SGD to achieve target epochs
iterations_per_epoch = math.ceil(x_train.shape[0] / (q * m_workers))
adjusted_epoch = max_epoch
if algorithm == 'gd': # Adjust max_epochs in case GD with q > 1
adjusted_epoch = math.ceil(max_epoch / q)
for epoch in range(adjusted_epoch):
objval = 0
for iteration in range(iterations_per_epoch):
w = server.broadcast()
for i in range(m_workers):
workers[i].set_params(w)
params = []
objs = []
for worker in workers:
w_i, obj_i = worker.fed_avg(q, algorithm, alpha, lam)
params.append(w_i)
objs.append(obj_i)
server.aggregate(params, objs)
objval += server.obj
if algorithm == 'sgd':
alpha *= 0.9
objval = objval / iterations_per_epoch
print('Objective function value = ' + str(objval))
objvals = numpy.zeros(max_epoch)
for worker in workers:
objvals += worker.epoch_objs[: max_epoch]
objvals /= m_workers
return server.w, objvals
_, gd_1q_objvals = run_fed_avg(x_train, y_train, q=1, algorithm='gd', m_workers=4, lam=1e-6, alpha=1e-1, max_epoch=50)
print('-------------------------')
_, gd_8q_objvals = run_fed_avg(x_train, y_train, q=8, algorithm='gd', m_workers=4, lam=1e-6, alpha=1e-1, max_epoch=50)
print('-------------------------')
_, sgd_1q_objvals = run_fed_avg(x_train, y_train, q=1, algorithm='sgd', m_workers=4, lam=1e-6, alpha=1e-1, max_epoch=50)
print('-------------------------')
w_sgd8, sgd_8q_objvals = run_fed_avg(x_train, y_train, q=8, algorithm='sgd', m_workers=4, lam=1e-6, alpha=1e-1, max_epoch=50)
import matplotlib.pyplot as plt
%matplotlib inline
fig = plt.figure(figsize=(6, 4))
epochs = range(len(gd_1q_objvals))
line0, = plt.plot(epochs, gd_1q_objvals, '--b', linewidth=4)
line1, = plt.plot(epochs, gd_8q_objvals, '-r', linewidth=2)
line2, = plt.plot(epochs, sgd_1q_objvals, '--g', linewidth=1)
line3, = plt.plot(epochs, sgd_8q_objvals, '-m', linewidth=2)
plt.xlabel('Epochs', fontsize=20)
plt.ylabel('Objective Value', fontsize=20)
plt.xticks(fontsize=16)
plt.yticks(fontsize=16)
plt.legend([line0, line1, line2, line3], ['GD Q=1', 'GD Q=8', 'SGD Q=1', 'SGD Q=8'], fontsize=20)
plt.tight_layout()
plt.show()
def predict(w, X):
xw = numpy.dot(X, w)
f = numpy.sign(xw)
return f
# evaluate training error using SGD Q=8 params
w = w_sgd8
f_train = predict(w, x_train)
diff = numpy.abs(f_train - y_train) / 2
error_train = numpy.mean(diff)
print('Training classification error is ' + str(error_train))
# evaluate test error
f_test = predict(w, x_test)
diff = numpy.abs(f_test - y_test) / 2
error_test = numpy.mean(diff)
print('Test classification error is ' + str(error_test))
###Output
Test classification error is 0.21138211382113822
###Markdown
Bonus1: Parallel Algorithms Name: [Your-Name?] 0. You will do the following:1. Read the lecture note: [click here](https://github.com/wangshusen/DeepLearning/blob/master/LectureNotes/Parallel/Parallel.pdf)2. Implement federated averaging or decentralized optimization.3. Plot the convergence curve. (The x-axis can be ```number of epochs``` or ```number of communication```. You must make sure the label is correct.)4. Convert the .IPYNB file to .HTML file. * The HTML file must contain **the code** and **the output after execution**. 5. Upload this .HTML file to your Google Drive, Dropbox, or your Github repo. (If it is submitted to Google Drive or Dropbox, you must make the file open-access.)6. Submit the link to this .HTML file to Canvas. * Example: https://github.com/wangshusen/CS583-2020S/blob/master/homework/Bonus1/Bonus1.html 1. Data processing- Download the Diabete dataset from https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary/diabetes- Load the data using sklearn.- Preprocess the data. 1.1. Load the data
###Code
from sklearn import datasets
import numpy
x_sparse, y = datasets.load_svmlight_file('diabetes')
x = x_sparse.todense()
print('Shape of x: ' + str(x.shape))
print('Shape of y: ' + str(y.shape))
###Output
_____no_output_____
###Markdown
1.2. Partition to training and test sets
###Code
# partition the data to training and test sets
n = x.shape[0]
n_train = 640
n_test = n - n_train
rand_indices = numpy.random.permutation(n)
train_indices = rand_indices[0:n_train]
test_indices = rand_indices[n_train:n]
x_train = x[train_indices, :]
x_test = x[test_indices, :]
y_train = y[train_indices].reshape(n_train, 1)
y_test = y[test_indices].reshape(n_test, 1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
print('Shape of y_train: ' + str(y_train.shape))
print('Shape of y_test: ' + str(y_test.shape))
###Output
_____no_output_____
###Markdown
1.3. Feature scaling Use the standardization to trainsform both training and test features
###Code
# Standardization
import numpy
# calculate mu and sig using the training set
d = x_train.shape[1]
mu = numpy.mean(x_train, axis=0).reshape(1, d)
sig = numpy.std(x_train, axis=0).reshape(1, d)
# transform the training features
x_train = (x_train - mu) / (sig + 1E-6)
# transform the test features
x_test = (x_test - mu) / (sig + 1E-6)
print('test mean = ')
print(numpy.mean(x_test, axis=0))
print('test std = ')
print(numpy.std(x_test, axis=0))
###Output
_____no_output_____
###Markdown
1.4. Add a dimension of all ones
###Code
n_train, d = x_train.shape
x_train = numpy.concatenate((x_train, numpy.ones((n_train, 1))), axis=1)
n_test, d = x_test.shape
x_test = numpy.concatenate((x_test, numpy.ones((n_test, 1))), axis=1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
###Output
_____no_output_____
###Markdown
Bonus 1: Numerical Optimization for Logistic Regression. Name: [Your-Name?] 0. You will do the following:1. Read the lecture note: [click here](https://github.com/wangshusen/DeepLearning/blob/master/LectureNotes/Logistic/paper/logistic.pdf)2. Read, complete, and run my code.3. **Implement mini-batch SGD** and evaluate the performance. (1 bonus point.)4. Convert the .IPYNB file to .HTML file. * The HTML file must contain **the code** and **the output after execution**. 5. Upload this .HTML file to your Github repo.6. Submit the link to this .HTML file to Canvas. * Example: https://github.com/wangshusen/CS583-2019F/blob/master/homework/Bonus1/Bonus1.html Grading criteria:1. When computing the ```gradient``` and ```objective function value``` using a batch of samples, use **matrix-vector multiplication** rather than a FOR LOOP of **vector-vector multiplications**.2. Plot ```objective function value``` against ```epochs```. In the plot, compare GD, SGD, and MB-SGD (with $b=8$ and $b=64$). The plot must look reasonable. 1. Data processing- Download the Diabete dataset from https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary/diabetes- Load the data using sklearn.- Preprocess the data. 1.1. Load the data
###Code
from sklearn import datasets
import numpy
x_sparse, y = datasets.load_svmlight_file('diabetes')
x = x_sparse.todense()
print('Shape of x: ' + str(x.shape))
print('Shape of y: ' + str(y.shape))
###Output
_____no_output_____
###Markdown
1.2. Partition to training and test sets
###Code
# partition the data to training and test sets
n = x.shape[0]
n_train = int(numpy.ceil(n * 0.8))
n_test = n - n_train
rand_indices = numpy.random.permutation(n)
train_indices = rand_indices[0:n_train]
test_indices = rand_indices[n_train:n]
x_train = x[train_indices, :]
x_test = x[test_indices, :]
y_train = y[train_indices].reshape(n_train, 1)
y_test = y[test_indices].reshape(n_test, 1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
print('Shape of y_train: ' + str(y_train.shape))
print('Shape of y_test: ' + str(y_test.shape))
###Output
_____no_output_____
###Markdown
1.3. Feature scaling Use the standardization to trainsform both training and test features
###Code
# Standardization
import numpy
# calculate mu and sig using the training set
d = x_train.shape[1]
mu = numpy.mean(x_train, axis=0).reshape(1, d)
sig = numpy.std(x_train, axis=0).reshape(1, d)
# transform the training features
x_train = (x_train - mu) / (sig + 1E-6)
# transform the test features
x_test = (x_test - mu) / (sig + 1E-6)
print('test mean = ')
print(numpy.mean(x_test, axis=0))
print('test std = ')
print(numpy.std(x_test, axis=0))
###Output
_____no_output_____
###Markdown
1.4. Add a dimension of all ones
###Code
n_train, d = x_train.shape
x_train = numpy.concatenate((x_train, numpy.ones((n_train, 1))), axis=1)
n_test, d = x_test.shape
x_test = numpy.concatenate((x_test, numpy.ones((n_test, 1))), axis=1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
###Output
_____no_output_____
###Markdown
2. Logistic regression modelThe objective function is $Q (w; X, y) = \frac{1}{n} \sum_{i=1}^n \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $.
###Code
# Calculate the objective function value
# Inputs:
# w: d-by-1 matrix
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# Return:
# objective function value (scalar)
def objective(w, x, y, lam):
n, d = x.shape
yx = numpy.multiply(y, x) # n-by-d matrix
yxw = numpy.dot(yx, w) # n-by-1 matrix
vec1 = numpy.exp(-yxw) # n-by-1 matrix
vec2 = numpy.log(1 + vec1) # n-by-1 matrix
loss = numpy.mean(vec2) # scalar
reg = lam / 2 * numpy.sum(w * w) # scalar
return loss + reg
# initialize w
d = x_train.shape[1]
w = numpy.zeros((d, 1))
# evaluate the objective function value at w
lam = 1E-6
objval0 = objective(w, x_train, y_train, lam)
print('Initial objective function value = ' + str(objval0))
###Output
_____no_output_____
###Markdown
3. Numerical optimization 3.1. Gradient descent The gradient at $w$ is $g = - \frac{1}{n} \sum_{i=1}^n \frac{y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$
###Code
# Calculate the gradient
# Inputs:
# w: d-by-1 matrix
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# Return:
# g: g: d-by-1 matrix, full gradient
def gradient(w, x, y, lam):
n, d = x.shape
yx = numpy.multiply(y, x) # n-by-d matrix
yxw = numpy.dot(yx, w) # n-by-1 matrix
vec1 = numpy.exp(yxw) # n-by-1 matrix
vec2 = numpy.divide(yx, 1+vec1) # n-by-d matrix
vec3 = -numpy.mean(vec2, axis=0).reshape(d, 1) # d-by-1 matrix
g = vec3 + lam * w
return g
# Gradient descent for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# stepsize: scalar
# max_iter: integer, the maximal iterations
# w: d-by-1 matrix, initialization of w
# Return:
# w: d-by-1 matrix, the solution
# objvals: a record of each iteration's objective value
def grad_descent(x, y, lam, stepsize, max_iter=100, w=None):
n, d = x.shape
objvals = numpy.zeros(max_iter) # store the objective values
if w is None:
w = numpy.zeros((d, 1)) # zero initialization
for t in range(max_iter):
objval = objective(w, x, y, lam)
objvals[t] = objval
print('Objective value at t=' + str(t) + ' is ' + str(objval))
g = gradient(w, x, y, lam)
w -= stepsize * g
return w, objvals
###Output
_____no_output_____
###Markdown
Run gradient descent.
###Code
lam = 1E-6
stepsize = 1.0
w, objvals_gd = grad_descent(x_train, y_train, lam, stepsize)
###Output
_____no_output_____
###Markdown
3.2. Stochastic gradient descent (SGD)Define $Q_i (w) = \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $.The stochastic gradient at $w$ is $g_i = \frac{\partial Q_i }{ \partial w} = -\frac{y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$.
###Code
# Calculate the objective Q_i and the gradient of Q_i
# Inputs:
# w: d-by-1 matrix
# xi: 1-by-d matrix
# yi: scalar
# lam: scalar, the regularization parameter
# Return:
# obj: scalar, the objective Q_i
# g: d-by-1 matrix, gradient of Q_i
def stochastic_objective_gradient(w, xi, yi, lam):
d = xi.shape[0]
yx = yi * xi # 1-by-d matrix
yxw = float(numpy.dot(yx, w)) # scalar
# calculate objective function Q_i
loss = numpy.log(1 + numpy.exp(-yxw)) # scalar
reg = lam / 2 * numpy.sum(w * w) # scalar
obj = loss + reg
# calculate stochastic gradient
g_loss = -yx.T / (1 + numpy.exp(yxw)) # d-by-1 matrix
g = g_loss + lam * w # d-by-1 matrix
return obj, g
# SGD for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# stepsize: scalar
# max_epoch: integer, the maximal epochs
# w: d-by-1 matrix, initialization of w
# Return:
# w: the solution
# objvals: record of each iteration's objective value
def sgd(x, y, lam, stepsize, max_epoch=100, w=None):
n, d = x.shape
objvals = numpy.zeros(max_epoch) # store the objective values
if w is None:
w = numpy.zeros((d, 1)) # zero initialization
for t in range(max_epoch):
# randomly shuffle the samples
rand_indices = numpy.random.permutation(n)
x_rand = x[rand_indices, :]
y_rand = y[rand_indices, :]
objval = 0 # accumulate the objective values
for i in range(n):
xi = x_rand[i, :] # 1-by-d matrix
yi = float(y_rand[i, :]) # scalar
obj, g = stochastic_objective_gradient(w, xi, yi, lam)
objval += obj
w -= stepsize * g
stepsize *= 0.9 # decrease step size
objval /= n
objvals[t] = objval
print('Objective value at epoch t=' + str(t) + ' is ' + str(objval))
return w, objvals
###Output
_____no_output_____
###Markdown
Run SGD.
###Code
lam = 1E-6
stepsize = 0.1
w, objvals_sgd = sgd(x_train, y_train, lam, stepsize)
###Output
_____no_output_____
###Markdown
4. Compare GD with SGDPlot objective function values against epochs.
###Code
import matplotlib.pyplot as plt
%matplotlib inline
fig = plt.figure(figsize=(6, 4))
epochs_gd = range(len(objvals_gd))
epochs_sgd = range(len(objvals_sgd))
line0, = plt.plot(epochs_gd, objvals_gd, '--b', LineWidth=4)
line1, = plt.plot(epochs_sgd, objvals_sgd, '-r', LineWidth=2)
plt.xlabel('Epochs', FontSize=20)
plt.ylabel('Objective Value', FontSize=20)
plt.xticks(FontSize=16)
plt.yticks(FontSize=16)
plt.legend([line0, line1], ['GD', 'SGD'], fontsize=20)
plt.tight_layout()
plt.show()
fig.savefig('compare_gd_sgd.pdf', format='pdf', dpi=1200)
###Output
_____no_output_____
###Markdown
5. Prediction
###Code
# Predict class label
# Inputs:
# w: d-by-1 matrix
# X: m-by-d matrix
# Return:
# f: m-by-1 matrix, the predictions
def predict(w, X):
xw = numpy.dot(X, w)
f = numpy.sign(xw)
return f
# evaluate training error
f_train = predict(w, x_train)
diff = numpy.abs(f_train - y_train) / 2
error_train = numpy.mean(diff)
print('Training classification error is ' + str(error_train))
# evaluate test error
f_test = predict(w, x_test)
diff = numpy.abs(f_test - y_test) / 2
error_test = numpy.mean(diff)
print('Test classification error is ' + str(error_test))
###Output
_____no_output_____
###Markdown
6. Mini-batch SGD (fill the code)Define $Q_I (w) = \frac{1}{b} \sum_{i \in I} \log \Big( 1 + \exp \big( - y_i x_i^T w \big) \Big) + \frac{\lambda}{2} \| w \|_2^2 $, where $I$ is a set containing $b$ indices randomly drawn from $\{ 1, \cdots , n \}$ without replacement.The stochastic gradient at $w$ is $g_I = \frac{\partial Q_I }{ \partial w} = \frac{1}{b} \sum_{i \in I} \frac{- y_i x_i }{1 + \exp ( y_i x_i^T w)} + \lambda w$. 6.1. Compute the objective $Q_I$ and its gradient using a batch of samples
###Code
# Calculate the objective Q_I and the gradient of Q_I
# Inputs:
# w: d-by-1 matrix
# xi: 1-by-d matrix
# yi: scalar
# lam: scalar, the regularization parameter
# b: integer, the batch size
# Return:
# obj: scalar, the objective Q_i
# g: d-by-1 matrix, gradient of Q_i
def mb_stochastic_objective_gradient(w, xi, yi, lam, b):
# Fill the function
# Follow the implementation of stochastic_objective_gradient
# Use matrix-vector multiplication; do not use FOR LOOP of vector-vector multiplications
...
return obj, g
###Output
_____no_output_____
###Markdown
6.2. Implement mini-batch SGD
###Code
# Mini-Batch SGD for solving logistic regression
# Inputs:
# x: n-by-d matrix
# y: n-by-1 matrix
# lam: scalar, the regularization parameter
# b: integer, the batch size
# stepsize: scalar
# max_epoch: integer, the maximal epochs
# w: d-by-1 matrix, initialization of w
# Return:
# w: the solution
# objvals: record of each iteration's objective value
def mb_sgd(x, y, lam, b, stepsize, max_epoch=100, w=None):
# Fill the function
# Follow the implementation of sgd
# Record one objective value per epoch (not per iteration!)
...
return w, objvals
###Output
_____no_output_____
###Markdown
6.3. Run MB-SGD
###Code
# MB-SGD with batch size b=8
lam = 1E-6 # do not change
b = 8 # do not change
stepsize = 0.1 # you must tune this parameter
w, objvals_mbsgd8 = mb_sgd(x_train, y_train, lam, b, stepsize)
# MB-SGD with batch size b=64
lam = 1E-6 # do not change
b = 64 # do not change
stepsize = 0.1 # you must tune this parameter
w, objvals_mbsgd64 = mb_sgd(x_train, y_train, lam, b, stepsize)
###Output
_____no_output_____
###Markdown
7. Plot and compare GD, SGD, and MB-SGD You are required to compare the following algorithms:- Gradient descent (GD)- SGD- MB-SGD with b=8- MB-SGD with b=64Follow the code in Section 4 to plot ```objective function value``` against ```epochs```. There should be four curves in the plot; each curve corresponds to one algorithm.
###Code
# plot the 4 curves:
###Output
_____no_output_____
###Markdown
Bonus1: Parallel Algorithms Name: Ian Gomez 0. You will do the following:1. Read the lecture note: [click here](https://github.com/wangshusen/DeepLearning/blob/master/LectureNotes/Parallel/Parallel.pdf)2. Implement federated averaging or decentralized optimization.3. Plot the convergence curve. (The x-axis can be ```number of epochs``` or ```number of communication```. You must make sure the label is correct.)4. Convert the .IPYNB file to .HTML file. * The HTML file must contain **the code** and **the output after execution**. 5. Upload this .HTML file to your Google Drive, Dropbox, or your Github repo. (If it is submitted to Google Drive or Dropbox, you must make the file open-access.)6. Submit the link to this .HTML file to Canvas. * Example: https://github.com/wangshusen/CS583-2020S/blob/master/homework/Bonus1/Bonus1.html 1. Data processing- Download the Diabete dataset from https://www.csie.ntu.edu.tw/~cjlin/libsvmtools/datasets/binary/diabetes- Load the data using sklearn.- Preprocess the data. 1.1. Load the data
###Code
from sklearn import datasets
import numpy
x_sparse, y = datasets.load_svmlight_file('diabetes')
x = x_sparse.todense()
print('Shape of x: ' + str(x.shape))
print('Shape of y: ' + str(y.shape))
###Output
Shape of x: (768, 8)
Shape of y: (768,)
###Markdown
1.2. Partition to training and test sets
###Code
# partition the data to training and test sets
n = x.shape[0]
n_train = 640
n_test = n - n_train
rand_indices = numpy.random.permutation(n)
train_indices = rand_indices[0:n_train]
test_indices = rand_indices[n_train:n]
x_train = x[train_indices, :]
x_test = x[test_indices, :]
y_train = y[train_indices].reshape(n_train, 1)
y_test = y[test_indices].reshape(n_test, 1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
print('Shape of y_train: ' + str(y_train.shape))
print('Shape of y_test: ' + str(y_test.shape))
###Output
Shape of x_train: (640, 8)
Shape of x_test: (128, 8)
Shape of y_train: (640, 1)
Shape of y_test: (128, 1)
###Markdown
1.3. Feature scaling Use the standardization to trainsform both training and test features
###Code
# Standardization
import numpy
# calculate mu and sig using the training set
d = x_train.shape[1]
mu = numpy.mean(x_train, axis=0).reshape(1, d)
sig = numpy.std(x_train, axis=0).reshape(1, d)
# transform the training features
x_train = (x_train - mu) / (sig + 1E-6)
# transform the test features
x_test = (x_test - mu) / (sig + 1E-6)
print('test mean = ')
print(numpy.mean(x_test, axis=0))
print('test std = ')
print(numpy.std(x_test, axis=0))
###Output
test mean =
[[-0.13621832 0.05616356 -0.22971796 0.04707923 0.18478962 -0.07070564
0.00147609 -0.11279333]]
test std =
[[0.83179847 0.93211432 1.27557892 0.90068698 1.17443828 1.19893934
0.91767902 0.97604973]]
###Markdown
1.4. Add a dimension of all ones
###Code
n_train, d = x_train.shape
x_train = numpy.concatenate((x_train, numpy.ones((n_train, 1))), axis=1)
n_test, d = x_test.shape
x_test = numpy.concatenate((x_test, numpy.ones((n_test, 1))), axis=1)
print('Shape of x_train: ' + str(x_train.shape))
print('Shape of x_test: ' + str(x_test.shape))
import numpy as np
class Worker:
def __init__(self, x, y, lr, edges=None):
self.x = np.array(x)
self.y = np.array(y)
self.s = self.x.shape[0]
self.d = self.x.shape[1]
self.w = np.zeros((self.d, 1))
self.g = np.zeros((self.d, 1))
self.lr = lr
self.n_weights = []
self.edges = edges
def set_param(self, w):
self.w = w
def loss(self):
y, x, w = self.y, self.x, self.w
yx = y*x
yxw = yx@w
vec1 = np.exp(-yxw)
vec2 = np.log(1+vec1)
return 1/self.s * np.sum(vec2)
def step(self):
y, x, w = self.y, self.x, self.w
yx = y*x
yxw = yx@w
vec1 = np.exp(yxw)
vec2 = yx/(1+vec1)
g = -np.sum(vec2, axis=0).reshape(self.d, 1)
self.g = g
self.w -= self.lr * g
return g
def query(self):
edges = self.edges
#Currently just computing a non-weighted average
self.n_weights = np.array([neighbor.broadcast() for neighbor in edges]).reshape(-1, len(edges))
def update(self, weights=None):
if weights:
w1, w2 = weights
else:
w1, w2 = (1, 1)
self.w = w1 * self.w + w2 * np.sum(self.n_weights, axis=1).reshape(-1,1)
self.w /= len(edges)+1
return self.w
def broadcast(self):
return self.w
edges = [[1,2],[0,2,3],[0,1,4],[1,4,5,6],[2,3],[3,6],[3,5]]
def setup_graph(m, X, y, lr, edges):
s = X.shape[0]//m
samples = [(X[i*s:i*s+s], y[i*s:i*s+s]) for i in range(m)]
graph = [Worker(*sample, lr) for sample in samples]
edges = [list(map(lambda i: graph[i], edge)) for edge in edges]
for worker, edge in zip(graph, edges):
worker.edges = edge
return graph
def run_optimization(graph, epochs, weights=None):
losses = []
for _ in range(epochs):
L = 0
for worker in graph:
L += worker.loss()
for worker in graph:
worker.query()
for worker in graph:
worker.update(weights=weights)
for i, worker in enumerate(graph):
worker.step()
print(L/len(graph))
losses.append(L/len(graph))
return losses
def print_weights(graph):
print(*list(map(lambda x: x.w, graph)))
graph = setup_graph(7, x_train, y_train, 1e-2, edges)
graph2 = setup_graph(7, x_train, y_train, 1e-2, edges)
losses = run_optimization(graph, 20)
losses2 = run_optimization(graph2, 20, [0.25, 0.25])
print_weights(graph)
import matplotlib.pyplot as plt
plt.plot(range(len(losses)),losses, label="Unweighted")
plt.plot(range(len(losses2)),losses2, label="Weighted")
plt.legend()
plt.show()
def predict(graph, x):
w = np.mean(np.array([x.w for x in graph]), axis=0).reshape(-1,1)
return np.sign(x@w)
predictions = predict(graph, x_test)
predictions2 = predict(graph2, x_test)
print("Unweighted Test Accuracy:", np.sum(predictions == y_test)/len(y_test))
print("Weighted Test Accuracy:", np.sum(predictions2 == y_test)/len(y_test))
###Output
Unweighted Test Accuracy: 0.7578125
Weighted Test Accuracy: 0.7578125
|
ML English dataset.ipynb | ###Markdown
tokenizer
###Code
#lemmatizer and tokenization (good for noun)
import nltk
nltk.download('wordnet')
from nltk.stem import WordNetLemmatizer
Punctuations= "!'$%&()*+,-./:;”<=>?[\\]^_`{|}~\t\n"
wordnet_lemmatizer=WordNetLemmatizer() #lemmatizer object
tok =nltk.tokenize.WhitespaceTokenizer() #Tokenizer object
def lemma(sentence):
token_words=tok.tokenize(sentence) #Tokenization
token_words
for word in token_words:
if word in Punctuations:
token_words.remove(word)
stem_sentence=[]
for word in token_words:
stem_sentence.append(wordnet_lemmatizer.lemmatize(word)) #Stemming
stem_sentence.append(" ") #Join words
return "".join(stem_sentence)
Data['Lemma']=Data['text'].apply(lambda x:lemma(x))
Data[['Lemma']].head()
import sklearn
from sklearn.feature_extraction.text import TfidfVectorizer
tfidf1 = TfidfVectorizer(ngram_range=(1,1),min_df =2,max_df =0.5) #tfidf object for 1 gramm
features1 = tfidf1.fit_transform(Data['Lemma'])
vector1=features1.toarray()
vector1.shape
tfidf2 = TfidfVectorizer(ngram_range=(2,2),min_df =2,max_df =0.5) #tfidf object for 2 gramm
features2 = tfidf2.fit_transform(Data['Lemma'])
vector2=features2.toarray()
vector2.shape
tfidf12 = TfidfVectorizer(ngram_range=(1,2),min_df =2,max_df =0.5) #tfidf object for 1,2 gramm
features12 = tfidf12.fit_transform(Data['Lemma'])
vector12=features12.toarray()
vector12[1]
tfidf123 = TfidfVectorizer(ngram_range=(1,3),min_df =2,max_df =0.5) #tfidf object for 123gramm
features123 = tfidf123.fit_transform(Data['Lemma'])
vector123=features123.toarray()
vector123.shape
###Output
_____no_output_____
###Markdown
spliting into training and validation
###Code
from sklearn.model_selection import train_test_split
#train_1,val_1,train_2,val_2,train_12,val_12,train_123,val_123,train,test =train_test_split(vector1,vector2,vector12,vector123,label,test_size=0.30,random_state=24)
#print(train_1.shape)
#print(train_2.shape)
#print(train_12.shape)
#print(train_123.shape)
#print(train.shape)
#print(val_1.shape)
#print(val_2.shape)
#print(val_12.shape)
#print(val_123.shape)
#print(test.shape)
###Output
_____no_output_____
###Markdown
testing DATA
###Code
tDATA=pd.read_csv('/content/drive/MyDrive/Ankit/dec 2020 codalab/Hope speech/malyalam/malayalam_hope_dev.csv',names=["text","label","NaN"], sep='\t')
tDATA.pop('NaN')
#tDATA['text']=tDATA['tweet'].apply(lambda x:remove_sw(x))
tDATA['text']=tDATA['text'].apply(lambda x: deEmojify(x))
tDATA['text']=tDATA['text'].str.replace(r'\d+','')
#tDATA.head()
tDATA['Lemma']=tDATA['text'].apply(lambda x:lemma(x))
t_features1 = tfidf1.transform(tDATA['Lemma'])
t_vector1=t_features1.toarray()
t_vector1.shape
t_features2 = tfidf2.transform(tDATA['Lemma'])
t_vector2=t_features2.toarray()
t_vector2.shape
t_features12 = tfidf12.transform(tDATA['Lemma'])
t_vector12=t_features12.toarray()
t_vector12.shape
t_features123 = tfidf123.transform(tDATA['Lemma'])
t_vector123=t_features123.toarray()
t_vector123.shape
t_label=labelEncode.transform(tDATA['label'])
t_label
###Output
_____no_output_____
###Markdown
SVM for 1 gram
###Code
from sklearn.metrics import accuracy_score
from sklearn import metrics
from sklearn.svm import SVC
model_SVM = SVC()
model_SVM.fit(vector1,label)
pred_SVM = model_SVM.predict(t_vector1)
print("SVM")
print("Accuracy score =", accuracy_score(t_label, pred_SVM))
print(metrics.classification_report(t_label,pred_SVM))
###Output
SVM
Accuracy score = 0.8102803738317756
precision recall f1-score support
0 0.75 0.33 0.45 190
1 0.82 0.96 0.89 784
2 0.73 0.51 0.60 96
accuracy 0.81 1070
macro avg 0.77 0.60 0.65 1070
weighted avg 0.80 0.81 0.78 1070
###Markdown
for 2 gram
###Code
model_SVM2 = SVC()
model_SVM2.fit(vector2, label)
pred_SVM = model_SVM2.predict(t_vector2)
print("SVM")
print("Accuracy score =", accuracy_score(t_label, pred_SVM))
print(metrics.classification_report(t_label,pred_SVM))
###Output
SVM
Accuracy score = 0.7728971962616823
precision recall f1-score support
0 0.69 0.20 0.31 190
1 0.78 0.98 0.87 784
2 0.66 0.24 0.35 96
accuracy 0.77 1070
macro avg 0.71 0.47 0.51 1070
weighted avg 0.75 0.77 0.72 1070
###Markdown
for 12 gram
###Code
model_SVM12 = SVC()
model_SVM12.fit(vector12,label)
pred_SVM = model_SVM12.predict(t_vector12)
print("SVM")
print("Accuracy score =", accuracy_score(t_label, pred_SVM))
print(metrics.classification_report(t_label,pred_SVM))
###Output
SVM
Accuracy score = 0.8102803738317756
precision recall f1-score support
0 0.76 0.33 0.46 190
1 0.82 0.97 0.89 784
2 0.74 0.47 0.57 96
accuracy 0.81 1070
macro avg 0.77 0.59 0.64 1070
weighted avg 0.80 0.81 0.78 1070
###Markdown
for 123 gram
###Code
model_SVM123 = SVC()
model_SVM123.fit(vector123,label)
pred_SVM = model_SVM123.predict(t_vector123)
print("SVM")
print("Accuracy score =", accuracy_score(t_label, pred_SVM))
print(metrics.classification_report(t_label,pred_SVM))
###Output
SVM
Accuracy score = 0.811214953271028
precision recall f1-score support
0 0.77 0.33 0.46 190
1 0.82 0.97 0.89 784
2 0.74 0.47 0.57 96
accuracy 0.81 1070
macro avg 0.78 0.59 0.64 1070
weighted avg 0.80 0.81 0.78 1070
###Markdown
RANDOM FOREST
###Code
#for 1 gramm|
from sklearn.ensemble import RandomForestClassifier
rf = RandomForestClassifier(n_estimators=100,max_depth=None,min_samples_split=2, random_state=0)
rf.fit(vector1,label)
pred_rf = rf.predict(t_vector1)
print("random")
print("Accuracy score =", accuracy_score(t_label, pred_rf))
print(metrics.classification_report(t_label, pred_rf))
# for 2 gram
rf.fit(vector2,label)
pred_rf = rf.predict(t_vector2)
print("random")
print("Accuracy score =", accuracy_score(t_label, pred_rf))
print(metrics.classification_report(t_label, pred_rf))
# for 12 gram
rf.fit(vector12,label)
pred_rf12 = rf.predict(t_vector12)
print("random")
print("Accuracy score =", accuracy_score(t_label, pred_rf12))
print(metrics.classification_report(t_label, pred_rf12))
#for 123 gram
rf.fit(vector123,label)
pred_rf123 = rf.predict(t_vector123)
print("random")
print("Accuracy score =", accuracy_score(t_label, pred_rf123))
print(metrics.classification_report(t_label, pred_rf123))
###Output
random
Accuracy score = 0.8084112149532711
precision recall f1-score support
0 0.74 0.31 0.44 190
1 0.83 0.95 0.89 784
2 0.67 0.60 0.64 96
accuracy 0.81 1070
macro avg 0.75 0.62 0.65 1070
weighted avg 0.80 0.81 0.78 1070
###Markdown
LOGESTIC REGRESSION
###Code
# FOR 1 gram
from sklearn.linear_model import LogisticRegression
LR = LogisticRegression()
LR.fit(vector1,label)
pred_LR = LR.predict(t_vector1)
print("Logistic Regression")
print("Accuracy score =", accuracy_score(t_label, pred_LR))
print(metrics.classification_report(t_label, pred_LR ))
# for 2 gram
LR.fit(vector2,label)
pred_LR = LR.predict(t_vector2)
print("Logistic Regression")
print("Accuracy score =", accuracy_score(t_label, pred_LR))
print(metrics.classification_report(t_label, pred_LR ))
#for 12 gram
LR.fit(vector12,label)
pred_LR12 = LR.predict(t_vector12)
print("Logistic Regression")
print("Accuracy score =", accuracy_score(t_label, pred_LR12))
print(metrics.classification_report(t_label, pred_LR12 ))
# for 123 gram
LR.fit(vector123,label)
pred_LR123 = LR.predict(t_vector123)
print("Logistic Regression")
print("Accuracy score =", accuracy_score(t_label, pred_LR123))
print(metrics.classification_report(t_label, pred_LR123 ))
###Output
Logistic Regression
Accuracy score = 0.8158878504672897
precision recall f1-score support
0 0.75 0.40 0.52 190
1 0.83 0.96 0.89 784
2 0.74 0.45 0.56 96
accuracy 0.82 1070
macro avg 0.77 0.60 0.66 1070
weighted avg 0.81 0.82 0.79 1070
###Markdown
KNeighbors is bad
###Code
#for 1 gram
from sklearn.neighbors import KNeighborsClassifier
neigh = KNeighborsClassifier(n_neighbors = 5)
neigh.fit(vector1,label)
pred_KNN = neigh.predict(t_vector1)
print("KNN")
print("Accuracy score =", accuracy_score(t_label, pred_KNN))
print(metrics.classification_report(t_label,pred_KNN ))
# for 2 gram
neigh.fit(vector2,label)
pred_KNN = neigh.predict(t_vector2)
print("KNN")
print("Accuracy score =", accuracy_score(t_label, pred_KNN))
print(metrics.classification_report(t_label,pred_KNN ))
# for 12 gram
neigh.fit(vector12,label)
pred_KNN12 = neigh.predict(t_vector12)
print("KNN")
print("Accuracy score =", accuracy_score(t_label, pred_KNN12))
print(metrics.classification_report(t_label,pred_KNN12 ))
# for 123 gram
neigh.fit(vector123,label)
pred_KNN123 = neigh.predict(t_vector123)
print("KNN")
print("Accuracy score =", accuracy_score(t_label, pred_KNN123))
print(metrics.classification_report(t_label,pred_KNN123 ))
###Output
KNN
Accuracy score = 0.7635514018691589
precision recall f1-score support
0 0.62 0.11 0.19 190
1 0.78 0.97 0.86 784
2 0.57 0.40 0.47 96
accuracy 0.76 1070
macro avg 0.66 0.49 0.51 1070
weighted avg 0.73 0.76 0.71 1070
###Markdown
NAIVE BAYES
###Code
# for 1 gram
from sklearn.naive_bayes import GaussianNB
naive = GaussianNB()
naive.fit(vector1,label)
pred_naive = naive.predict(t_vector1)
print("Naive Bayes")
print("Accuracy score =", accuracy_score(t_label, pred_naive))
print(metrics.classification_report(t_label, pred_naive ))
# for 2 gram
naive.fit(vector2,label)
pred_naive = naive.predict(t_vector2)
print("Naive Bayes")
print("Accuracy score =", accuracy_score(t_label, pred_naive))
print(metrics.classification_report(t_label, pred_naive ))
# for 12 gram
naive.fit(vector12,label)
pred_naive = naive.predict(t_vector12)
print("Naive Bayes")
print("Accuracy score =", accuracy_score(t_label, pred_naive))
print(metrics.classification_report(t_label, pred_naive ))
# for 123 gram
naive.fit(vector123,label)
pred_naive = naive.predict(t_vector123)
print("Naive Bayes")
print("Accuracy score =", accuracy_score(t_label, pred_naive))
print(metrics.classification_report(t_label, pred_naive ))
###Output
Naive Bayes
Accuracy score = 0.6056074766355141
precision recall f1-score support
0 0.29 0.58 0.39 190
1 0.85 0.60 0.70 784
2 0.50 0.72 0.59 96
accuracy 0.61 1070
macro avg 0.55 0.63 0.56 1070
weighted avg 0.72 0.61 0.64 1070
###Markdown
GradientBoosting
###Code
# for 1 gram
from sklearn.ensemble import GradientBoostingClassifier
gradient = GradientBoostingClassifier(n_estimators=100,max_depth=None,min_samples_split=2, random_state=0)
gradient.fit(vector1,label)
pred_gradient = gradient.predict(t_vector1)
print("Gradient Boosting")
print("Accuracy score =", accuracy_score(t_label,pred_gradient))
print(metrics.classification_report(t_label, pred_gradient ))
# for 2 gram
gradient.fit(vector2,label)
pred_gradient = gradient.predict(t_vector2)
print("Gradient Boosting")
print("Accuracy score =", accuracy_score(t_label,pred_gradient))
print(metrics.classification_report(t_label, pred_gradient ))
# for 12 gram
gradient.fit(vector12,label)
pred_gradient12 = gradient.predict(t_vector12)
print("Gradient Boosting")
print("Accuracy score =", accuracy_score(t_label,pred_gradient12))
print(metrics.classification_report(t_label, pred_gradient12 ))
# for 123 gram
gradient.fit(vector123,label)
pred_gradient123 = gradient.predict(t_vector123)
print("Gradient Boosting")
print("Accuracy score =", accuracy_score(t_label,pred_gradient123))
print(metrics.classification_report(t_label, pred_gradient123 ))
###Output
_____no_output_____
###Markdown
DECISION TREE
###Code
# for 1 gram
from sklearn.tree import DecisionTreeClassifier
decision = DecisionTreeClassifier()
decision.fit(vector1,label)
pred_decision = decision.predict(t_vector1)
print("Decision Tree")
print("Accuracy score =", accuracy_score(t_label,pred_decision))
print(metrics.classification_report(t_label,pred_decision ))
# for 2 gram
decision.fit(vector2,label)
pred_decision2 = decision.predict(t_vector2)
print("Decision Tree")
print("Accuracy score =", accuracy_score(test,pred_decision2))
print(metrics.classification_report(test,pred_decision2 ))
# for 12 gram
decision.fit(vector12,label)
pred_decision12 = decision.predict(t_vector12)
print("Decision Tree")
print("Accuracy score =", accuracy_score(t_label,pred_decision12))
print(metrics.classification_report(t_label,pred_decision12 ))
# for 123 gram
decision.fit(vector123,label)
pred_decision123 = decision.predict(t_vector123)
print("Decision Tree")
print("Accuracy score =", accuracy_score(t_label,pred_decision123))
print(metrics.classification_report(t_label,pred_decision123 ))
###Output
_____no_output_____ |
caffe2/python/tutorials/MNIST_Dataset_and_Databases.ipynb | ###Markdown
MNIST Dataset & DatabaseIn the [MNIST tutorial](https://github.com/caffe2/caffe2/python/tutorials/MNIST.ipynb) we use a lmdb database. You can also use leveldb or even minidb by changing the type reference when you get ready to read from the db's. Dataset:You can download the raw [MNIST dataset](https://download.caffe2.ai/datasets/mnist/mnist.zip), g/unzip the dataset and labels, and make the database yourself. Databases:We provide a few database formats for you to try with the MNIST tutorial. The default is lmdb. * [MNIST-nchw-lmdb](https://download.caffe2.ai/databases/mnist-lmdb.zip) - contains both the train and test lmdb MNIST databases in NCHW format* [MNIST-nchw-leveldb](https://download.caffe2.ai/databases/mnist-leveldb.zip) - contains both the train and test leveldb MNIST databases in NCHW format* [MNIST-nchw-minidb](https://download.caffe2.ai/databases/mnist-minidb.zip) - contains both the train and test minidb MNIST databases in NCHW format Tools: make_mnist_dbIf you like LevelDB you can use Caffe2's `make_mnist_db` binary to generate leveldb databases. This binary is found in `/caffe2/build/caffe2/binaries/` or depending on your OS and installation, in `/usr/local/bin/`.Here is an example call to `make_mnist_db`:```./make_mnist_db --channel_first --db leveldb --image_file ~/Downloads/train-images-idx3-ubyte --label_file ~/Downloads/train-labels-idx1-ubyte --output_file ~/caffe2/caffe2/python/tutorials/tutorial_data/mnist/mnist-train-nchw-leveldb./make_mnist_db --channel_first --db leveldb --image_file ~/Downloads/t10k-images-idx3-ubyte --label_file ~/Downloads/t10k-labels-idx1-ubyte --output_file ~/caffe2/caffe2/python/tutorials/tutorial_data/mnist/mnist-test-nchw-leveldb```Note leveldb can get deadlocked if more than one user attempts to open the leveldb at the same time. This is why there is logic in the Python below to delete LOCK files if they're found.TODO: it would be great to extend this binary to create other database formats Python scriptYou can use the Python in the code block below to download the dataset with `DownloadResource`, call the `make_mnist_db` binary, and generate your database with `GenerateDB`. The `DownloadResource` function can also download and extract a database for you.**Downloads and extracts the MNIST dataset**The sample function below will download and extract the dataset for you.```pythonDownloadResource("http://download.caffe2.ai/datasets/mnist/mnist.zip", data_folder)```**Downloads and extracts the lmdb databases of MNIST images - both test and train**```pythonDownloadResource("http://download.caffe2.ai/databases/mnist-lmdb.zip", data_folder)```**(Re)generate the leveldb database (it can get locked with multi-user setups or abandoned threads)**Requires the download of the dataset (mnist.zip) - see above.```pythonGenerateDB(image_file_train, label_file_train, "mnist-train-nchw-leveldb")GenerateDB(image_file_test, label_file_test, "mnist-test-nchw-leveldb")```
###Code
import os
def DownloadResource(url, path):
'''Downloads resources from s3 by url and unzips them to the provided path'''
import requests, zipfile, StringIO
print("Downloading... {} to {}".format(url, path))
r = requests.get(url, stream=True)
z = zipfile.ZipFile(StringIO.StringIO(r.content))
z.extractall(path)
print("Completed download and extraction.")
def GenerateDB(image, label, name):
'''Calls the make_mnist_db binary to generate a leveldb from a mnist dataset'''
name = os.path.join(data_folder, name)
print 'DB: ', name
if not os.path.exists(name):
syscall = "/usr/local/bin/make_mnist_db --channel_first --db leveldb --image_file " + image + " --label_file " + label + " --output_file " + name
# print "Creating database with: ", syscall
os.system(syscall)
else:
print "Database exists already. Delete the folder if you have issues/corrupted DB, then rerun this."
if os.path.exists(os.path.join(name, "LOCK")):
# print "Deleting the pre-existing lock file"
os.remove(os.path.join(name, "LOCK"))
current_folder = os.path.join(os.path.expanduser('~'), 'caffe2_notebooks')
data_folder = os.path.join(current_folder, 'tutorial_data', 'mnist')
# Downloads and extracts the lmdb databases of MNIST images - both test and train
if not os.path.exists(os.path.join(data_folder,"mnist-train-nchw-lmdb")):
DownloadResource("http://download.caffe2.ai/databases/mnist-lmdb.zip", data_folder)
# Downloads and extracts the MNIST data set
if not os.path.exists(os.path.join(data_folder, "train-images-idx3-ubyte")):
DownloadResource("http://download.caffe2.ai/datasets/mnist/mnist.zip", data_folder)
# (Re)generate the leveldb database (it can get locked with multi-user setups or abandoned threads)
# Requires the download of the dataset (mnist.zip) - see DownloadResource above.
# You also need to change references in the MNIST tutorial code where you train or test from lmdb to leveldb
image_file_train = os.path.join(data_folder, "train-images-idx3-ubyte")
label_file_train = os.path.join(data_folder, "train-labels-idx1-ubyte")
image_file_test = os.path.join(data_folder, "t10k-images-idx3-ubyte")
label_file_test = os.path.join(data_folder, "t10k-labels-idx1-ubyte")
GenerateDB(image_file_train, label_file_train, "mnist-train-nchw-leveldb")
GenerateDB(image_file_test, label_file_test, "mnist-test-nchw-leveldb")
###Output
_____no_output_____
###Markdown
MNIST Dataset & DatabaseIn the [MNIST tutorial](https://github.com/caffe2/caffe2/blob/master/caffe2/python/tutorials/MNIST.ipynb) we use a lmdb database. You can also use leveldb or even minidb by changing the type reference when you get ready to read from the db's. Dataset:You can download the raw [MNIST dataset](https://download.caffe2.ai/datasets/mnist/mnist.zip), g/unzip the dataset and labels, and make the database yourself. Databases:We provide a few database formats for you to try with the MNIST tutorial. The default is lmdb. * [MNIST-nchw-lmdb](https://download.caffe2.ai/databases/mnist-lmdb.zip) - contains both the train and test lmdb MNIST databases in NCHW format* [MNIST-nchw-leveldb](https://download.caffe2.ai/databases/mnist-leveldb.zip) - contains both the train and test leveldb MNIST databases in NCHW format* [MNIST-nchw-minidb](https://download.caffe2.ai/databases/mnist-minidb.zip) - contains both the train and test minidb MNIST databases in NCHW format Tools: make_mnist_dbIf you like LevelDB you can use Caffe2's `make_mnist_db` binary to generate leveldb databases. This binary is found in `/caffe2/build/caffe2/binaries/` or depending on your OS and installation, in `/usr/local/bin/`.Here is an example call to `make_mnist_db`:```./make_mnist_db --channel_first --db leveldb --image_file ~/Downloads/train-images-idx3-ubyte --label_file ~/Downloads/train-labels-idx1-ubyte --output_file ~/caffe2/caffe2/python/tutorials/tutorial_data/mnist/mnist-train-nchw-leveldb./make_mnist_db --channel_first --db leveldb --image_file ~/Downloads/t10k-images-idx3-ubyte --label_file ~/Downloads/t10k-labels-idx1-ubyte --output_file ~/caffe2/caffe2/python/tutorials/tutorial_data/mnist/mnist-test-nchw-leveldb```Note leveldb can get deadlocked if more than one user attempts to open the leveldb at the same time. This is why there is logic in the Python below to delete LOCK files if they're found.TODO: it would be great to extend this binary to create other database formats Python scriptYou can use the Python in the code block below to download the dataset with `DownloadResource`, call the `make_mnist_db` binary, and generate your database with `GenerateDB`. The `DownloadResource` function can also download and extract a database for you.**Downloads and extracts the MNIST dataset**The sample function below will download and extract the dataset for you.```pythonDownloadResource("http://download.caffe2.ai/datasets/mnist/mnist.zip", data_folder)```**Downloads and extracts the lmdb databases of MNIST images - both test and train**```pythonDownloadResource("http://download.caffe2.ai/databases/mnist-lmdb.zip", data_folder)```**(Re)generate the leveldb database (it can get locked with multi-user setups or abandoned threads)**Requires the download of the dataset (mnist.zip) - see above.```pythonGenerateDB(image_file_train, label_file_train, "mnist-train-nchw-leveldb")GenerateDB(image_file_test, label_file_test, "mnist-test-nchw-leveldb")```
###Code
import os
def DownloadResource(url, path):
'''Downloads resources from s3 by url and unzips them to the provided path'''
import requests, zipfile, StringIO
print("Downloading... {} to {}".format(url, path))
r = requests.get(url, stream=True)
z = zipfile.ZipFile(StringIO.StringIO(r.content))
z.extractall(path)
print("Completed download and extraction.")
def GenerateDB(image, label, name):
'''Calls the make_mnist_db binary to generate a leveldb from a mnist dataset'''
name = os.path.join(data_folder, name)
print 'DB: ', name
if not os.path.exists(name):
syscall = "/usr/local/bin/make_mnist_db --channel_first --db leveldb --image_file " + image + " --label_file " + label + " --output_file " + name
# print "Creating database with: ", syscall
os.system(syscall)
else:
print "Database exists already. Delete the folder if you have issues/corrupted DB, then rerun this."
if os.path.exists(os.path.join(name, "LOCK")):
# print "Deleting the pre-existing lock file"
os.remove(os.path.join(name, "LOCK"))
current_folder = os.path.join(os.path.expanduser('~'), 'caffe2_notebooks')
data_folder = os.path.join(current_folder, 'tutorial_data', 'mnist')
# Downloads and extracts the lmdb databases of MNIST images - both test and train
if not os.path.exists(os.path.join(data_folder,"mnist-train-nchw-lmdb")):
DownloadResource("http://download.caffe2.ai/databases/mnist-lmdb.zip", data_folder)
# Downloads and extracts the MNIST data set
if not os.path.exists(os.path.join(data_folder, "train-images-idx3-ubyte")):
DownloadResource("http://download.caffe2.ai/datasets/mnist/mnist.zip", data_folder)
# (Re)generate the leveldb database (it can get locked with multi-user setups or abandoned threads)
# Requires the download of the dataset (mnist.zip) - see DownloadResource above.
# You also need to change references in the MNIST tutorial code where you train or test from lmdb to leveldb
image_file_train = os.path.join(data_folder, "train-images-idx3-ubyte")
label_file_train = os.path.join(data_folder, "train-labels-idx1-ubyte")
image_file_test = os.path.join(data_folder, "t10k-images-idx3-ubyte")
label_file_test = os.path.join(data_folder, "t10k-labels-idx1-ubyte")
GenerateDB(image_file_train, label_file_train, "mnist-train-nchw-leveldb")
GenerateDB(image_file_test, label_file_test, "mnist-test-nchw-leveldb")
###Output
_____no_output_____ |
notebooks/map_algebra.ipynb | ###Markdown
Geospatial Analysis I: global, zonal and focal operations, map algebraResources:* [GRASS GIS overview and manual](http://grass.osgeo.org/grass72/manuals/index.html)* [Recommendations](data_acquisition.htmlcommands)and [tutorial](http://www4.ncsu.edu/~akratoc/GRASS_intro/)how to use GUI from the first assignmentTo run _r.mapcalc_ expressions, you can eitherrun the entire command in the GUI _Console_, or in case of anyproblems, type or copy the expression (without the _r.mapcalc_) in the GRASS GIS Raster Map Calculatorwhich can be launched from _Layer Manager_ toolbar.Text files with color rules:* [srtmneddiff_color.txt](data/srtmneddiff_color.txt) Start GRASS GISIn startup pannel set GIS Data Directory to path to datasets,for example on MS Windows, `C:\Users\myname\grassdata`.For Project location select nc_spm_08_grass7 (North Carolina, State Plane, meters) andfor Accessible mapset create a new mapset (called e.g. HW_map_algebra) andclick Start GRASS.
###Code
# using Python to initialize GRASS GIS
# This is a quick introduction into Jupyter Notebook.
# Python code can be excecuted like this:
a = 6
b = 7
c = a * b
print "Answer is", c
# Python code can be mixed with command line code (Bash).
# It is enough just to prefix the command line with an exclamation mark:
!echo "Answer is $c"
# Use Shift+Enter to execute this cell. The result is below.
# using Python to initialize GRASS GIS
import os
import sys
import subprocess
from IPython.display import Image
# create GRASS GIS runtime environment
gisbase = subprocess.check_output(["grass", "--config", "path"]).strip()
os.environ['GISBASE'] = gisbase
sys.path.append(os.path.join(gisbase, "etc", "python"))
# do GRASS GIS imports
import grass.script as gs
import grass.script.setup as gsetup
# set GRASS GIS session data
rcfile = gsetup.init(gisbase, "/home/jovyan/grassdata", "nc_spm_08_grass7", "user1")
# using Python to initialize GRASS GIS
# default font displays
os.environ['GRASS_FONT'] = 'sans'
# overwrite existing maps
os.environ['GRASS_OVERWRITE'] = '1'
gs.set_raise_on_error(True)
gs.set_capture_stderr(True)
# using Python to initialize GRASS GIS
# set display modules to render into a file (named map.png by default)
os.environ['GRASS_RENDER_IMMEDIATE'] = 'cairo'
os.environ['GRASS_RENDER_FILE_READ'] = 'TRUE'
os.environ['GRASS_LEGEND_FILE'] = 'legend.txt'
###Output
_____no_output_____
###Markdown
Change working directory:_Settings_ > _GRASS working environment_ > _Change working directory_ > select/create any directoryor type `cd` (stands for change directory) into the GUI_Console_ and hit Enter:
###Code
# a proper directory is already set, download files
import urllib
urllib.urlretrieve("http://ncsu-geoforall-lab.github.io/geospatial-modeling-course/grass/data/srtmneddiff_color.txt", "srtmneddiff_color.txt")
urllib.urlretrieve("http://ncsu-geoforall-lab.github.io/geospatial-modeling-course/grass/data/srtmneddiff_color.txt", "srtmneddiff_color.txt")
###Output
_____no_output_____
###Markdown
Download all text files with color rules (see above)to the selected directory. Now you can use the commands from the assignment requiring the text filewithout the need to specify the full path to the file. Compute summariesCompute areas for each category at two different resolutions.Are results equal? Explain (see also Lecture 1).Copy and paste the report from the output window orsave the report in a text file: _Output window_ > _Save_.Use fixed width font (e.g., Courier, Andale Mono in your report to preserve formatting).
###Code
!g.region raster=landuse96_28m res=12 -ap
!r.report landuse96_28m unit=c,h,p
!g.region raster=landuse96_28m res=30 -ap
!r.report landuse96_28m unit=c,h,p
###Output
_____no_output_____
###Markdown
Compute areas for each category of land use for each zipcode.Compare 27601 Raleigh with 27511 Cary.Include only the relevant part of the table in your report.
###Code
!r.report zipcodes,landuse96_28m unit=h,p
###Output
_____no_output_____
###Markdown
Compute zonal statistics maps representingaverage slope for each basin.Add legends using _Add map elements_ in Map Display toolbar.Reminder: _d.out.file_ means Save to graphics file for your report.
###Code
!g.region raster=slope -p
!r.stats.zonal base=basin_50K cover=slope method=average output=slope_avgbasin
!r.colors slope_avgbasin color=gyr
!d.rast slope_avgbasin
!d.legend slope_avgbasin at=90,50,5,8
!d.vect streams color=15:25:110
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Compute zonal statistics maps representing most common land use for each basin.To get the best result, make sure you use all the flags from the examplebelow.
###Code
!g.region raster=landuse96_28m -p
!r.mode base=basin_50K cover=landuse96_28m output=landuse96_modebasin
!d.rast landuse96_modebasin
!d.vect streams
!d.legend landuse96_modebasin at=40,20,2,5 -n -f -c
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Apply neighborhood operatorsUse neighborhood operator to compute land use diversity map.How diverse is land use in NCSU area?Before you start to display the new results,remove all previously added map layers from the _Layer Manager_.
###Code
!g.region raster=landuse96_28m -p
!r.info -g landuse96_28m
!r.neighbors landuse96_28m output=lu_divers method=diversity size=7
!d.erase
!d.rast lu_divers
!d.legend lu_divers at=70,15,5,10 -v
!d.vect streets_wake
!r.report lu_divers unit=p
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Use neighborhood operator to smooth the SRTM elevation map and compare the global statistical measures for the original and smoothed DEM.How would size of the neighborhood influence the result?
###Code
!g.region raster=elev_srtm_30m -p
!r.neighbors elev_srtm_30m output=elev_srtm30m_sm5 method=average size=5
!d.rast elev_srtm_30m
!d.rast elev_srtm30m_sm5
!r.univar elev_srtm_30m
!r.univar elev_srtm30m_sm5
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Patch multiple raster layers into a single rasterPatch raster tiles for lidar based, 6m res. DEM for Centennial Campus.Before displaying new data, remove older map layers from _Layer Manager_.
###Code
!g.region raster=el_D793_6m,el_D783_6m,el_D782_6m,el_D792_6m -p
!r.patch input=el_D793_6m,el_D783_6m,el_D782_6m,el_D792_6m output=elevlidD_6m
!r.colors elevlidD_6m raster=elevation
!d.erase
!d.rast elevlidD_6m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Map AlgebraSee _r.mapcalc_ manual page for syntax and functions.If you are getting en error when running_r.mapcalc_ in GUI _Console_ or the systemcommand line, launch the GUI version from _Layer Manager_toolbar. NDVICompute Normalized Difference Vegetation Index (NDVI).Explain the difference between floating point and integerhandling in ndvi1, ndvi2 and ndvi3 result.
###Code
!g.region raster=lsat7_2002_40 -p
!r.mapcalc "ndvi1 = (lsat7_2002_40 - lsat7_2002_30) / (lsat7_2002_40 + lsat7_2002_30)"
!r.mapcalc "ndvi2 = 1.0 * (lsat7_2002_40 - lsat7_2002_30) / (lsat7_2002_40 + lsat7_2002_30)"
!r.mapcalc "ndvi3 = float(lsat7_2002_40 - lsat7_2002_30) / float(lsat7_2002_40 + lsat7_2002_30)"
!r.info -r ndvi1
!r.info -r ndvi2
!r.info -r ndvi3
!r.colors ndvi3 color=ndvi
!d.rast ndvi3
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Difference between DEM and DSMExplore the difference between the SRTM DSM and lidar-based NED DEM.Compute the map of elevation differences.
###Code
!g.region raster=elev_ned_30m -p
!r.mapcalc "srtm_ned30_dif = elev_srtm_30m - elev_ned_30m"
###Output
_____no_output_____
###Markdown
Create a custom color table to distinguish the negative and positive values:
###Code
!r.info -r srtm_ned30_dif
###Output
_____no_output_____
###Markdown
fp: Data range is -142.24... to 86.19...Assign custom color table [srtmneddiff_color.txt](data/srtmneddiff_color.txt).GUI: Right click on layer > _Properties_ > _Set color table_ > _Colors_ > _Path to rules file_.
###Code
!r.colors srtm_ned30_dif rules=srtmneddiff_color.txt
###Output
_____no_output_____
###Markdown
Zoom to computational region and switch off previous map layers. Display the difference map layer:
###Code
!d.rast srtm_ned30_dif
!d.legend srtm_ned30_dif at=70,15,5,10
!d.vect streets_wake
Image(filename="map.png")
!r.univar elev_srtm_30m
!r.univar elev_ned_30m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Is the srtm mostly higher or lower than elev_ned?Which result will you use to answer the above question -the srtm_ned30_dif map or numbers provided by _r.univar_? Working with if statementsCreate map of urban areas (high density and low density class)with 0 class elsewhere.
###Code
!g.region raster=landuse96_28m -p
!r.mapcalc "urban1_30m = if(landuse96_28m == 1,1,0) + if(landuse96_28m == 2,2,0)"
!d.rast urban1_30m
!d.legend urban1_30m at=10,30,5,8
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Alternatively with logical 'or' operator and null elsewhere:
###Code
!r.mapcalc "urban2_30m = if(landuse96_28m == 1 || landuse96_28m == 2,landuse96_28m,null())"
!d.rast urban2_30m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Handling null valuesCreate mask for low lying developed areas (e.g. vulnerable to flooding)with elevation between 60 and 100m and land use 1 or 2.Set the region, display the input maps and create a MASK.Before you start new computations, remove or switch off previous map layersin the _Layer Manger_.You may also zoom to computational region in _Map Display_once you set a new one.
###Code
!g.region raster=elevation -p
!d.erase
!d.rast elevation
!d.rast landuse96_28m
!r.mapcalc "low_elev_developed = if((elevation < 100 && elevation > 60) && (landuse96_28m == 1 || landuse96_28m == 2),1,null())"
!r.mask raster=low_elev_developed
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Command _r.mask_ creates a raster map "MASK" in your mapset.Remove "low_elev_developed" layer if it was added.Display watersheds to see the mask effect:
###Code
!d.rast basin_50K
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Disable mask, and display basin_50K againto show that the mask was removed.
###Code
!r.mask -r
!d.rast basin_50K
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Using coordinates of moving window in map algebraReplace section of SRTM DSM with NED DEM elevation.Try to explain how this _r.mapcalc_ expression works.
###Code
!r.mapcalc "elev_combined = if(y() < 224274. && x() > 637455., elevation, elev_srtm_30m)"
###Output
_____no_output_____
###Markdown
Optional - various additional useful tasks Tilted planeCreate a raster map representing tilted plane (e.g., geologic fault):
###Code
!g.region rural_1m -p
!r.mapcalc "tiltplane = 0.2*(0.1*row()+col())+50"
!r.mapcalc "tiltpl_under = if(tiltplane < elev_lid792_1m + 2,tiltplane,null())"
###Output
_____no_output_____
###Markdown
View the elevation surface and subsurface plane in 3D._Switch off all layers in the layer manager except for elev_lid792_1m and tiltpl_under_.Change display to 3D view, adjust viewing position to a view from South.Save an image for your report. Map subsetsUse map algebra to create map subsets.Change region to the airphoto tile 792 and resolution 10m.Since we will work in different are, it is a good idea to removeall previously used map layers from the _Layer Manager_.
###Code
!g.region raster=ortho_2001_t792_1m res=10 -ap
!d.erase
!d.rast ortho_2001_t792_1m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Create a subset of the map elevation for this subregion.
###Code
!r.mapcalc "elevation_792_10m = elevation"
!d.rast elevation_792_10m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Zoom out to see that it is a subset. Work with NULL and MASKSet the mask and check its effect.
###Code
!d.rast elevation
!d.vect streets_wake
!r.mask raster=urban maskcats=55
!d.rast elevation
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Remove mask:
###Code
!r.mask -r
###Output
_____no_output_____
###Markdown
Zonal statisticsCompute % area for each category in each zipcode.Which zipcode has the most high density development?
###Code
!r.stats -pl zipcodes,landuse96_28m
###Output
_____no_output_____
###Markdown
Working with relative coordinatesEnter the expression on a single line without \Again, it is a good idea to remove the previously used map layersbefore we start to work on a new task.
###Code
!g.region raster=elev_srtm_30m -p
!d.erase
!r.mapcalc "elev_srtm30m_smooth = (elev_srtm_30m[-1,-1] \
+ elev_srtm_30m[-1,0] + elev_srtm_30m[-1,1] \
+ elev_srtm_30m[0,-1] + elev_srtm_30m[0,0] \
+ elev_srtm_30m[0,1] + elev_srtm_30m[1,-1] \
+ elev_srtm_30m[1,0] + elev_srtm_30m[1,1])/9."
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Assign the resulting map the same color table as the original.Compare global statistics
###Code
!r.colors elev_srtm30m_smooth raster=elev_srtm_30m
!r.univar elev_srtm_30m
!r.univar elev_srtm30m_smooth
!d.rast elev_srtm_30m
!d.rast elev_srtm30m_smooth
Image(filename="map.png")
# end the GRASS session
os.remove(rcfile)
###Output
_____no_output_____
###Markdown
Geospatial Analysis I: global, zonal and focal operations, map algebraResources:* [GRASS GIS overview and manual](https://grass.osgeo.org/grass76/manuals/index.html)* [Recommendations](data_acquisition.htmlcommands)and [tutorial](./grass_intro.html)how to use GUI from the first assignmentTo run _r.mapcalc_ expressions, you can eitherrun the entire command in the GUI _Console_, or in case of anyproblems, type or copy the expression (without the _r.mapcalc_) in the GRASS GIS Raster Map Calculatorwhich can be launched from _Layer Manager_ toolbar.Text files with color rules:* [srtmneddiff_color.txt](data/srtmneddiff_color.txt) Start GRASS GISIn startup pannel set GRASS GIS Database Directory to path to datasets,for example on MS Windows, `C:\Users\myname\grassdata`.For GRASS Location select nc_spm_08_grass7 (North Carolina, State Plane, meters) andfor GRASS Mapset create a new mapset (called e.g. HW_map_algebra) andclick Start GRASS session.
###Code
# using Python to initialize GRASS GIS
# This is a quick introduction into Jupyter Notebook.
# Python code can be executed like this:
a = 6
b = 7
c = a * b
print("Answer is", c)
# Python code can be mixed with command line code (Bash).
# It is enough just to prefix the command line with an exclamation mark:
!echo "Answer is $c"
# Use Shift+Enter to execute this cell. The result is below.
# using Python to initialize GRASS GIS
import os
import sys
import subprocess
from IPython.display import Image
# create GRASS GIS runtime environment
gisbase = subprocess.check_output(["grass", "--config", "path"], text=True).strip()
os.environ['GISBASE'] = gisbase
sys.path.append(os.path.join(gisbase, "etc", "python"))
# do GRASS GIS imports
import grass.script as gs
import grass.script.setup as gsetup
# set GRASS GIS session data
rcfile = gsetup.init(gisbase, "/home/jovyan/grassdata", "nc_spm_08_grass7", "user1")
# using Python to initialize GRASS GIS
# default font displays
os.environ['GRASS_FONT'] = 'sans'
# overwrite existing maps
os.environ['GRASS_OVERWRITE'] = '1'
gs.set_raise_on_error(True)
gs.set_capture_stderr(True)
# using Python to initialize GRASS GIS
# set display modules to render into a file (named map.png by default)
os.environ['GRASS_RENDER_IMMEDIATE'] = 'cairo'
os.environ['GRASS_RENDER_FILE_READ'] = 'TRUE'
os.environ['GRASS_LEGEND_FILE'] = 'legend.txt'
###Output
_____no_output_____
###Markdown
Change working directory:_Settings_ > _GRASS working environment_ > _Change working directory_ > select/create any directoryor type `cd` (stands for change directory) into the GUI_Console_ and hit Enter:
###Code
# a proper directory is already set, download files
import urllib.request
urllib.request.urlretrieve("http://ncsu-geoforall-lab.github.io/geospatial-modeling-course/grass/data/srtmneddiff_color.txt", "srtmneddiff_color.txt")
urllib.request.urlretrieve("http://ncsu-geoforall-lab.github.io/geospatial-modeling-course/grass/data/srtmneddiff_color.txt", "srtmneddiff_color.txt")
###Output
_____no_output_____
###Markdown
Download all text files with color rules (see above)to the selected directory. Now you can use the commands from the assignment requiring the text filewithout the need to specify the full path to the file. Compute summariesCompute areas for each category at two different resolutions.Are results equal? Explain in detail why (see also Lecture 1).Copy and paste the report from the output window orsave the report in a text file: _Output window_ > _Save_.Use fixed width font (e.g., Courier, Andale Mono in your report to preserve formatting).
###Code
!g.region raster=landuse96_28m res=12 -ap
!r.report landuse96_28m unit=c,h,p
!g.region raster=landuse96_28m res=30 -ap
!r.report landuse96_28m unit=c,h,p
###Output
_____no_output_____
###Markdown
Compute areas for each category of land use for each zipcode.Compare 27601 Raleigh with 27511 Cary.Include only the relevant part of the table in your report.
###Code
!r.report zipcodes,landuse96_28m unit=h,p
###Output
_____no_output_____
###Markdown
Compute zonal statistics maps representingaverage slope for each basin.Add legends using _Add map elements_ in Map Display toolbar.Reminder: _d.out.file_ means Save to graphics file for your report.
###Code
!g.region raster=slope -p
!r.stats.zonal base=basin_50K cover=slope method=average output=slope_avgbasin
!r.colors slope_avgbasin color=gyr
!d.rast slope_avgbasin
!d.legend slope_avgbasin at=90,50,5,8
!d.vect streams color=15:25:110
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Compute zonal statistics maps representing most common land use for each basin.To get the best result, make sure you use all the flags from the examplebelow.
###Code
!g.region raster=landuse96_28m -p
!r.mode base=basin_50K cover=landuse96_28m output=landuse96_modebasin
!d.rast landuse96_modebasin
!d.vect streams
!d.legend landuse96_modebasin at=40,20,2,5 -n -f -c
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Apply neighborhood operatorsUse neighborhood operator to compute land use diversity map.How diverse is land use in NCSU area based on the following calculation?Explore how different sizes of the neighborhood used alter the results and why?Before you start to display the new results,remove all previously added map layers from the _Layer Manager_.
###Code
!g.region raster=landuse96_28m -p
!r.info -g landuse96_28m
!r.neighbors landuse96_28m output=lu_divers method=diversity size=7
!d.erase
!d.rast lu_divers
!d.legend lu_divers at=70,15,5,10 -v
!d.vect streets_wake
!r.report lu_divers unit=p
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Use neighborhood operator to smooth the SRTM elevation map and compare the global statistical measures for the original and smoothed DEM.How does the size of the neighborhood influence the result?(You can test different sizes yourself.)
###Code
!g.region raster=elev_srtm_30m -p
!r.neighbors elev_srtm_30m output=elev_srtm30m_sm5 method=average size=5
!d.rast elev_srtm_30m
!d.rast elev_srtm30m_sm5
!r.univar elev_srtm_30m
!r.univar elev_srtm30m_sm5
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Patch multiple raster layers into a single rasterPatch raster tiles for lidar based, 6m res. DEM for Centennial Campus.Before displaying new data, remove older map layers from _Layer Manager_.
###Code
!g.region raster=el_D793_6m,el_D783_6m,el_D782_6m,el_D792_6m -p
!r.patch input=el_D793_6m,el_D783_6m,el_D782_6m,el_D792_6m output=elevlidD_6m
!r.colors elevlidD_6m raster=elevation
!d.erase
!d.rast elevlidD_6m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Map AlgebraSee _r.mapcalc_ manual page for syntax and functions.If you are getting en error when running_r.mapcalc_ in GUI _Console_ or the systemcommand line, launch the GUI version from _Layer Manager_toolbar. NDVICompute Normalized Difference Vegetation Index (NDVI).Explain the difference between floating point and integerhandling in ndvi1, ndvi2 and ndvi3 result.
###Code
!g.region raster=lsat7_2002_40 -p
!r.mapcalc "ndvi1 = (lsat7_2002_40 - lsat7_2002_30) / (lsat7_2002_40 + lsat7_2002_30)"
!r.mapcalc "ndvi2 = 1.0 * (lsat7_2002_40 - lsat7_2002_30) / (lsat7_2002_40 + lsat7_2002_30)"
!r.mapcalc "ndvi3 = float(lsat7_2002_40 - lsat7_2002_30) / float(lsat7_2002_40 + lsat7_2002_30)"
!r.info -r ndvi1
!r.info -r ndvi2
!r.info -r ndvi3
!r.colors ndvi3 color=ndvi
!d.rast ndvi3
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Note that this is an example, for computing various vegetation indicesin GRASS GIS, we would use the _i.vi_ moduleafter performing atmospheric corrections. Difference between DEM and DSMExplore the difference between the SRTM DSM and lidar-based NED DEM.Compute the map of elevation differences.
###Code
!g.region raster=elev_ned_30m -p
!r.mapcalc "srtm_ned30_dif = elev_srtm_30m - elev_ned_30m"
###Output
_____no_output_____
###Markdown
Create a custom color table to distinguish the negative and positive values:
###Code
!r.info -r srtm_ned30_dif
###Output
_____no_output_____
###Markdown
fp: Data range is -142.24... to 86.19...Assign custom color table [srtmneddiff_color.txt](data/srtmneddiff_color.txt).GUI: Right click on layer > _Properties_ > _Set color table_ > _Colors_ > _Path to rules file_.
###Code
!r.colors srtm_ned30_dif rules=srtmneddiff_color.txt
###Output
_____no_output_____
###Markdown
Zoom to computational region and switch off previous map layers. Display the difference map layer:
###Code
!d.rast srtm_ned30_dif
!d.legend srtm_ned30_dif at=70,15,5,10
!d.vect streets_wake
Image(filename="map.png")
!r.univar elev_srtm_30m
!r.univar elev_ned_30m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Is the srtm mostly higher or lower than elev_ned?Which result will you use to answer the above question -the srtm_ned30_dif map or numbers provided by _r.univar_? Working with if statementsCreate map of urban areas (high density and low density class)with 0 class elsewhere.
###Code
!g.region raster=landuse96_28m -p
!r.mapcalc "urban1_30m = if(landuse96_28m == 1,1,0) + if(landuse96_28m == 2,2,0)"
!d.rast urban1_30m
!d.legend urban1_30m at=10,30,5,8
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Alternatively with logical 'or' operator and null elsewhere:
###Code
!r.mapcalc "urban2_30m = if(landuse96_28m == 1 || landuse96_28m == 2,landuse96_28m,null())"
!d.rast urban2_30m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Handling null valuesCreate mask for low lying developed areas (e.g. vulnerable to flooding)with elevation between 60 and 100m and land use 1 or 2.Set the region, display the input maps and create a MASK.Before you start new computations, remove or switch off previous map layersin the _Layer Manger_.You may also zoom to computational region in _Map Display_once you set a new one.
###Code
!g.region raster=elevation -p
!d.erase
!d.rast elevation
!d.rast landuse96_28m
!r.mapcalc "low_elev_developed = if((elevation < 100 && elevation > 60) && (landuse96_28m == 1 || landuse96_28m == 2),1,null())"
!r.mask raster=low_elev_developed
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Command _r.mask_ creates a raster map "MASK" in your mapset.Remove "low_elev_developed" layer if it was added.Display watersheds to see the mask effect:
###Code
!d.rast basin_50K
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Disable mask, and display basin_50K againto show that the mask was removed.
###Code
!r.mask -r
!d.rast basin_50K
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Using coordinates of moving window in map algebraReplace section of SRTM DSM with NED DEM elevation.Try to explain how this _r.mapcalc_ expression works.
###Code
!r.mapcalc "elev_combined = if(y() < 224274. && x() > 637455., elevation, elev_srtm_30m)"
###Output
_____no_output_____
###Markdown
Optional - various additional useful tasks Tilted planeCreate a raster map representing tilted plane (e.g., geologic fault):
###Code
!g.region rural_1m -p
!r.mapcalc "tiltplane = 0.2*(0.1*row()+col())+50"
!r.mapcalc "tiltpl_under = if(tiltplane < elev_lid792_1m + 2,tiltplane,null())"
###Output
_____no_output_____
###Markdown
View the elevation surface and subsurface plane in 3D._Switch off all layers in the layer manager except for elev_lid792_1m and tiltpl_under_.Change display to 3D view, adjust viewing position to a view from South.Save an image for your report. Map subsetsUse map algebra to create map subsets.Change region to the airphoto tile 792 and resolution 10m.Since we will work in different area, it is a good idea to removeall previously used map layers from _Layers_ inthe _Layer Manager_.
###Code
!g.region raster=ortho_2001_t792_1m res=10 -ap
!d.erase
!d.rast ortho_2001_t792_1m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Create a subset of the map elevation for this subregion.
###Code
!r.mapcalc "elevation_792_10m = elevation"
!d.rast elevation_792_10m
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Zoom out to see that it is a subset. Work with NULL and MASKSet the mask and check its effect.
###Code
!d.rast elevation
!d.vect streets_wake
!r.mask raster=urban maskcats=55
!d.rast elevation
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Remove mask:
###Code
!r.mask -r
###Output
_____no_output_____
###Markdown
Zonal statisticsFirst, set the computational region:
###Code
!g.region raster=urban2_30m
###Output
_____no_output_____
###Markdown
Then, compute % area for each category in each zipcode.Which zipcode has the most high density development?
###Code
!r.stats -pl zipcodes,urban2_30m
###Output
_____no_output_____
###Markdown
Working with relative coordinatesEnter the expression on a single line without \Again, it is a good idea to remove the previously used map layersbefore we start to work on a new task.
###Code
!g.region raster=elev_srtm_30m -p
!d.erase
!r.mapcalc "elev_srtm30m_smooth = (elev_srtm_30m[-1,-1] \
+ elev_srtm_30m[-1,0] + elev_srtm_30m[-1,1] \
+ elev_srtm_30m[0,-1] + elev_srtm_30m[0,0] \
+ elev_srtm_30m[0,1] + elev_srtm_30m[1,-1] \
+ elev_srtm_30m[1,0] + elev_srtm_30m[1,1])/9."
Image(filename="map.png")
###Output
_____no_output_____
###Markdown
Assign the resulting map the same color table as the original.Compare global statistics
###Code
!r.colors elev_srtm30m_smooth raster=elev_srtm_30m
!r.univar elev_srtm_30m
!r.univar elev_srtm30m_smooth
!d.rast elev_srtm_30m
!d.rast elev_srtm30m_smooth
Image(filename="map.png")
# end the GRASS session
os.remove(rcfile)
###Output
_____no_output_____ |
lotto6_45/collection.ipynb | ###Markdown
Collection- Determines what the most recent lottery draw was- Determines what the most recent lottery draw stored in the database is- Collects the winning numbers, of winners, total prize money for the recent draws not stored in the database- Collects the stores' info where the 1st & 2nd place winners bought their tickets- Collected data get stored into 'lottery.db' database.The notebook is programmed to collect and store only the data that are currently not stored in the database but are present to be collected.For example, if the lottery's most recent draw was 4149th and we have stored until 4146th in the database, then this notebook collects & saves 4147th to 4149th draws into the database by running it. Setup
###Code
import sys
sys.path.append('..')
import pandas as pd
import requests
import re
from bs4 import BeautifulSoup
from lib.progress_bar import progress_bar
import sqlite3
lotto_result_url = "https://www.dhlottery.co.kr/gameResult.do?method=byWin"
lotto_result_draw = lotto_result_url + "&drwNo="
store_url = "https://www.dhlottery.co.kr/store.do?method=topStore&pageGubun=L645&drwNo="
###Output
_____no_output_____
###Markdown
Most Recent DrawLet us determine what the most recent draw was in the real world.
###Code
def recentDraw():
request = requests.get(lotto_result_url)
soup = BeautifulSoup(request.text, "lxml")
content = soup.find("meta", {"id" : "desc", "name" : "description"})['content']
drawCount = re.compile(r'\d+회')
mo = drawCount.search(content)
return int(mo.group()[:-1])
mostRecent = recentDraw()
print(f"Most recent draw happend in the real world : {mostRecent}")
###Output
Most recent draw happend in the real world : 976
###Markdown
Most Recent Draw in our DatabaseLet us now determine what the most recent draw stored in our database is.
###Code
db_path = "lottery.db"
sql_draw_create = """CREATE TABLE IF NOT EXISTS
`DRAWS`(turn int, num_1 int, num_2 int, num_3 int, num_4 int, num_5 int, num_6 int, num_bonus int, UNIQUE(turn))"""
def recentDrawInDB(db_path):
con = sqlite3.connect(db_path)
cur = con.cursor()
cur.execute(sql_draw_create)
cur.execute("SELECT MAX(turn) FROM DRAWS")
info = cur.fetchall()
con.commit()
con.close()
return int(info[0][0]) if info[0][0] else 0
dbMostRecent = recentDrawInDB(db_path)
print(f"Most recent draw stored in our database : {dbMostRecent}")
###Output
Most recent draw stored in our database : 0
###Markdown
Draws Data CollectionThe function below crawls over the Korean 6/45 lottery web html files and collects:- Winning Numbers (1st, 2nd, ... 6th) + Bonus - \ of Winners (1st Place, ... 5th Place)- Total Prize Money (1st Place, ... 5th Place)
###Code
def collectDraws(lst, start=dbMostRecent + 1, end=mostRecent):
if start > end:
print(f"No new draw to be added.")
return
print(f"Collecting Draws from Draw #{start} to #{end}")
for idx in range(start, end + 1):
progress_bar(idx - start, end - start + 1)
draw = {'draw': idx}
req = requests.get(lotto_result_draw + str(idx))
soup = BeautifulSoup(req.text, 'lxml')
meta = soup.find("meta", {"id" : "desc", "name" : "description"})['content']
first, last = re.compile(r'(\d+,){4}\d+'), re.compile('\d+\+\d+')
first_mo, last_mo = first.search(meta), last.search(meta)
draw['nums'] = list(map(int, first_mo.group().split(',') + last_mo.group().split('+')))
date = meta = soup.find("p", {"class" : "desc"})
dateReg = re.compile(r'(\d){4}년 (\d){2}월 (\d){2}')
mo = dateReg.search(str(date))
draw['date'] = [int(mo.group()[:4]), int(mo.group()[6:8]), int(mo.group()[-2:])]
total, winners = [], [0] * 5
table = soup.find("table").find_all("td", {"class":"tar"})
regex = re.compile(r'>\d+.*원')
for i, c in enumerate(table):
mo = regex.search(str(c))
num = mo.group()[1:-1]
num = int(num.replace(",", ""))
if i % 2 == 0: total.append(num)
elif num > 0: winners[i // 2] = total[-1] // num
draw['winners'] = winners
draw['prize'] = total
lst.append(draw)
progress_bar(end, end)
print("")
print("Done Collecting Draws!")
draws = []
collectDraws(lst=draws)
if len(draws) > 0:
print(f"We have collected {len(draws)} draws")
print(f"First draw : {draws[0]}")
print(f"Last draw : {draws[-1]}")
else:
print("No additional draw collected.")
###Output
We have collected 976 draws
First draw : {'draw': 1, 'nums': [10, 23, 29, 33, 37, 40, 16], 'date': [2002, 12, 7], 'winners': [0, 1, 28, 2537, 40155], 'prize': [0, 143934100, 143934000, 287695800, 401550000]}
Last draw : {'draw': 976, 'nums': [4, 12, 14, 25, 35, 37, 2], 'date': [2021, 8, 14], 'winners': [7, 69, 3085, 142375, 2270249], 'prize': [22707070505, 3784511793, 3784514495, 7118750000, 11351245000]}
###Markdown
Stores Data CollectionThe functions below crawl over the Korean 6/45 lottery web html files and collect:- Stores' names (1st & 2nd Place Winners)- Stores' addresses (1st & 2nd Place Winners)- Whether the winning were chosen automatic or manual (1st Place Winners Only)
###Code
def parseStores(content, win = 1):
stores = []
rows = content.find_all("tr")
reg, regGen = re.compile(r'>.*<'), re.compile(r'[가-힣]+')
for r in rows:
tds = r.find_all('td')[1:4]
if win == 2: tds = tds[:-1]
store = []
for i, td in enumerate(tds):
if win == 1 and i == 1: td = str(regGen.search(str(td)).group())
else: td = str(reg.search(str(td)).group()[1:-1])
td = td.strip()
store.append(td)
stores.append(store)
return stores
sql_store_create = """CREATE TABLE IF NOT EXISTS
`STORES`(idx int, turn int, name varchar(255), auto BOOLEAN, firstPrize BOOLEAN, address varchar(255), UNIQUE(idx))"""
def dbStoresMostRecent(db_path):
con = sqlite3.connect(db_path)
cur = con.cursor()
cur.execute(sql_store_create)
cur.execute("SELECT MAX(turn) FROM STORES")
info = cur.fetchall()
con.commit()
con.close()
return int(info[0][0]) if info[0][0] else 923
dbStoresMostRecent = dbStoresMostRecent(db_path)
##### 924회부터 제공
def collectLocations(lst, start=dbStoresMostRecent + 1, end=mostRecent):
if start > end:
print(f"No new draws to be added.")
return
if start < 924:
print("Stores information is available since 924th draw.")
return
print(f"Collecting Locations from Draw #{start} to #{end}")
table_class = "tbl_data tbl_data_col"
for idx in range(start, end + 1):
progress_bar(idx - start, end - start + 1)
locations = {'draw': idx}
idx_url = store_url + str(idx)
soup = BeautifulSoup(requests.get(idx_url).text, 'lxml')
tables = soup.find_all("table", {"class":table_class})
tables = [x.find('tbody') for x in tables]
locations['first'] = parseStores(content = tables[0])
secondLocations = parseStores(content = tables[1], win = 2)
maxPage = len(soup.find('div', {"id": "page_box"}).find_all('a'))
for p in range(2, maxPage + 1):
soup = BeautifulSoup(requests.get(idx_url + "&nowPage=" + str(p)).text, 'lxml')
tables = soup.find_all("table", {"class":table_class})
tables = [x.find('tbody') if i == 1 else None for i, x in enumerate(tables)]
secondLocations.extend(parseStores(content = tables[1], win = 2))
locations['second'] = secondLocations
lst.append(locations)
progress_bar(end, end)
print("")
print("Done Collecting Locations!")
stores = []
collectLocations(lst=stores)
if len(stores) > 0:
print(f"We have collected {len(stores)} draws")
print(f"First Set : {stores[0]}")
print(f"Last Set : {stores[-1]}")
else:
print("No additional stores collected.")
###Output
We have collected 53 draws
First Set : {'draw': 924, 'first': [['복권백화점', '자동', '서울 마포구 월드컵로 157'], ['CU(초읍대공원점)', '자동', '부산 부산진구 성지로 160 목화빌딩'], ['이마트24 광안리굿-7', '수동', '부산 수영구 광안로 49'], ['우리들공업탑점', '자동', '울산 남구 수암로 11'], ['지화자', '자동', '경기 성남시 수정구 위례광장로 328 1층 116호(창곡동, 우성위례타워)'], ['복권백화점', '자동', '경기 파주시 평화로 70'], ['행운복권방', '자동', '경기 포천시 솔모루로 86-1'], ['로또복권방', '자동', '충남 당진시 반촌로 199'], ['로또명당인주점', '자동', '충남 아산시 서해로 519-2']], 'second': [['씨유역삼에클라트로또판매점', '서울 강남구 테헤란로20길 19 엘지에클라트 1층 씨유역삼에클라트점'], ['로또카페', '서울 강서구 강서로74길 3 가양빌딩 1층 6호'], ['로또복권', '서울 노원구 상계동 649-7번지 다모아빌딩103'], ['스파', '서울 노원구 동일로 1493 상계주공아파트(10단지) 주공10단지종합상가111'], ['웨이스탑(전농점)', '서울 동대문구 전농로 129,(전농동)'], ['연초2호 쇼케이스7호', '서울 서초구 신반포로 194 강남고속버스터미널 쇼케이스7호'], ['인터넷 복권판매사이트', '동행복권(dhlottery.co.kr)'], ['인터넷 복권판매사이트', '동행복권(dhlottery.co.kr)'], ['인터넷 복권판매사이트', '동행복권(dhlottery.co.kr)'], ['인터넷 복권판매사이트', '동행복권(dhlottery.co.kr)'], ['버스카드충전소', '서울 서초구 신반포로 205 반포쇼핑타운 6동쇼핑앞'], ['훼미리24', '서울 성동구 아차산로11길 26'], ['잠실매점', '서울 송파구 올림픽로 269 잠실역 8번출구 앞 가판'], ['미래상사', '서울 송파구 오금로 534 104호'], ['가판점(신문)', '서울 영등포구 양평로 48'], ['신암편의점', '부산 금정구 중앙대로 2002 복권판매점'], ['로또복권', '부산 남구 신선로 317-1'], ['로또판매점', '부산 남구 석포로 41 동아일보'], ['장림나눔로또복권', '부산 사하구 장림로 228 1층'], ['대박골드', '부산 중구 흑교로 51 1층 101호'], ['일등복권편의점', '대구 달서구 대명천로 220 1층'], ['희망복권방', '인천 계양구 효서로 293'], ['행운복권', '인천 미추홀구 길파로35번길 2 종합전기전자 맞은편'], ['우리동네마트(무한유통)', '광주 광산구 장신로 68 101호'], ['로또타운점', '대전 대덕구 우암로 466'], ['진플러스안경', '대전 중구 대종로 301-1'], ['노다지복권방', '울산 울주군 온양읍 태화8길 57,(운화리)'], ['오덕복권나라', '경기 광주시 회덕동 274-1'], ['드림복권방', '경기 남양주시 다산중앙로123번길 21-3 104호'], ['금곡가판', '경기 남양주시 금곡로 72 센타프라자'], ['역곡중앙슈퍼', '경기 부천시 부일로 724'], ['복권나라', '경기 수원시 장안구 장안로 288 수단란주점 1층'], ['종합복권슈퍼', '경기 시흥시 마유로 336 정일빌딩104'], ['아름드리(쉬어가기)', '경기 시흥시 목감중앙로 20 시흥목감엘에이치7단지'], ['오이도직판장마트', '경기 시흥시 오이도로 167 오이도이주단지B'], ['송가상사', '경기 안산시 단원구 중앙대로 462 안산역사쇼핑몰1층131-3호'], ['명당복권방', '경기 용인시 처인구 백암로201번길 15'], ['터미널유통마트', '경기 의정부시 신곡로 47 우신프라자1층101호'], ['행운로또복권방', '경기 파주시 광탄면 신산1리 215-20번지'], ['복권백화점', '경기 파주시 평화로 70'], ['잘찍어로또방', '경기 평택시 안현로서5길 62'], ['행복드림', '경기 화성시 메타폴리스로 42 디앤씨빌딩 104호'], ['로또판매점', '강원 삼척시 삼척로 425 1층 CU삼척호산점'], ['국가대표복권방', '강원 영월군 영월읍 중앙로 68 1층'], ['창원편의점', '강원 원주시 모란1길 69 1층'], ['행운복권방', '강원 인제군 북면 금강로 23 1층'], ['백억만로또', '강원 춘천시 퇴계로 15 7B-3L'], ['드림오피스문구', '강원 춘천시 퇴계로 213'], ['행복한로또', '강원 평창군 경강로 1652 1층'], ['로또나라', '충남 보령시 구시4길 40 세븐일레븐 보령구시점'], ['잉크와복권방', '충남 천안시 동남구 차돌로 84'], ['대박찬스', '전북 군산시 칠성로 164'], ['현텔레콤', '전북 전주시 덕진구 아중로 142'], ['현텔레콤', '전북 전주시 덕진구 아중로 142'], ['행운복권나라', '전남 광양시 대림오성로 146-1'], ['행복나눔', '전남 담양군 무정로 42'], ['신안비치아파트3차슈퍼', '전남 목포시 고하대로 641-9,(산정동, 신안비치3차아파트)'], ['GS25(경산사동점)', '경북 경산시 삼풍로2길 1'], ['부흥청과식품점', '경북 경산시 하양읍 하양로 35 우방3차상가'], ['역전로또', '경북 경주시 원화로 260 1층 짐케어내'], ['세븐일레븐영주가흥로또점', '경북 영주시 대학로 144,(가흥동)'], ['치산로또명당', '경북 영천시 충효로 118,(야사동)'], ['GS25(칠곡북삼점)', '경북 칠곡군 북삼읍 금오대로2길 59,(인평리)'], ['CU(두호점)', '경북 포항시 북구 삼호로 247,(두호동)'], ['복권판매점', '경남 거제시 거제대로 3441 지하1층'], ['진영로또판매점', '경남 김해시 진영읍 진영로 189-2'], ['GS25(김해가야점)', '경남 김해시 김해대로2355번길 31,(부원동)'], ['양산황금나눔', '경남 양산시 황산로 395 복권판매점'], ['삼양로또방 삼양점방', '제주 제주시 일주동로 382-1']]}
Last Set : {'draw': 976, 'first': [['제이복권방', '수동', '서울 종로구 종로 225-1 평창빌딩 1층 103호'], ['청솔서점', '자동', '부산 사하구 하신번영로 195,(신평동)'], ['천하명당복권', '자동', '대구 북구 동북로 152,(산격동)'], ['차밍플라워 로또', '자동', '광주 광산구 풍영로 41 동구빌딩1층'], ['바로전산', '자동', '경기 광명시 오리로 998-1 101호'], ['왕대박복권', '수동', '강원 속초시 번영로 142 1층, 102호'], ['애월한담일등로또복권판매점', '수동', '제주 제주시 애월로 66']], 'second': [['복드림복권방', '서울 강동구 상암로 99'], ['일등복권', '서울 강서구 초원로 83 101호'], ['복권나라', '서울 관악구 남부순환로 1739-9'], ['토큰판매소', '서울 광진구 구의강변로 42'], ['시흥식품', '서울 금천구 시흥대로63길 13 1층'], ['스파', '서울 노원구 동일로 1493 상계주공아파트(10단지) 주공10단지종합상가111'], ['그랜드마트앞가판점', '서울 마포구 신촌로 94'], ['대박복권', '서울 성북구 장월로 103 1층'], ['교통카드판매대', '서울 송파구 송파대로28길 27 송파성원쌍떼빌 공영주차장앞가판'], ['치즈마트', '서울 송파구 양재대로 932 가락동농수산물도매시장 가락몰 2관 1층 2-1호'], ['복권백화점', '서울 은평구 연서로29길 7-2 1층'], ['로또', '서울 중구 퇴계로86길 42,(신당동)'], ['로또복권방', '서울 중구 마른내로 21,(저동2가)'], ['승보슈퍼', '서울 중구 마장로9길 49-19'], ['씨스페이스(범어사역점)', '부산 금정구 중앙대로 2097,(남산동)'], ['천하명당초량점', '부산 동구 중앙대로221번길 3'], ['사이버정보통신', '부산 부산진구 동평로 409 백조상가동103-1호'], ['성광복권명당', '부산 연제구 과정로 335'], ['씨스페이스(영도대평점)', '부산 영도구 태종로 64-1 대교동 사거리'], ['GS로또(남대구IC점)', '대구 달서구 성서공단로 346 대구물류터미널 상가동 108-110호 1층 GS25편의점'], ['세진전자통신', '대구 서구 서대구로 156,(평리동)'], ['로또명당', '인천 남동구 호구포로 874'], ['풍인식품', '인천 남동구 남동대로934번길 13 2호'], ['노다지복권방진월점', '광주 남구 서문대로 649 백두장식 1층2호'], ['샹제르망베어커리', '광주 북구 북문대로159번길 25 샹제르망베이커리내'], ['새상무복권', '광주 서구 치평로 30 수암빌딩 1층 102호'], ['로또명당 황금돼지', '대전 중구 계백로 1691-10'], ['보람복권방', '울산 남구 화합로 194 18-1'], ['로또복권', '울산 남구 꽃대나리로 71'], ['황제복권', '울산 남구 수암로 42'], ['원흥나눔로또', '경기 고양시 덕양구 도래울3로 4 몽블랑타워 101호'], ['로또세계주류', '경기 광명시 오리로 899-1'], ['GS25(하안연서점)', '경기 광명시 안현로 52 중앙상가'], ['환영로또복권', '경기 군포시 금산로72번길 9 1층(산본동)'], ['100억로또점', '경기 김포시 양촌읍 김포대로 1610 나동 6호'], ['로또도곡점', '경기 남양주시 덕소로 277 1층 복권판매점'], ['세희사랑방', '경기 남양주시 경춘로1350번길 35 리치플러스빌딩 110'], ['로또암반수베팅샵', '경기 남양주시 와부읍 수레로 32,(덕소리)'], ['탑드림', '경기 성남시 분당구 새마을로1번길 2 1층 커피숍앞쪽가게'], ['노다지복권방', '경기 성남시 수정구 위례광장로 34 위례역푸르지오5단지 근린생활시설 제3동 1층 제5비 119호'], ['대왕판교로점', '경기 성남시 수정구 대왕판교로 812 B104, B105'], ['부현제일명당', '경기 시흥시 정왕시장길 26-1 102호'], ['로또휴게실', '경기 용인시 기흥구 용구대로 1885'], ['대박나라', '경기 용인시 처인구 포곡로234번길 2-14'], ['씨유 용인포곡둔전점', '경기 용인시 처인구 포곡로118번길 26-1 유성시티빌 103호'], ['행운복권방', '경기 의정부시 충의로 55'], ['일등복권방', '경기 의정부시 용민로 9'], ['대박복권방', '경기 파주시 문산읍 문향로 39'], ['복권백화점', '경기 파주시 평화로 70'], ['나눔로또', '경기 화성시 상신하길로274번길 21 서봉마을사랑으로부영7단지아파트 상가1동 104,105,106호'], ['행운의시작복권전문점', '강원 삼척시 사대안길 5 8통1반'], ['복덩어리', '충북 청주시 서원구 호국로25번길 1'], ['스타복권방', '충남 논산시 안심로 72'], ['장미슈퍼', '충남 부여군 부여읍 계백로 265 부여군청 로터리 부근'], ['로또명당', '충남 천안시 동남구 목천읍 천안대로 103 5호'], ['로또복권두정점', '충남 천안시 서북구 두정로 251 대원빌딩104호'], ['황금열쇠금고', '전남 목포시 영산로 135'], ['CU(화순중앙점)', '전남 화순군 화순읍 중앙로 92,(만연리)'], ['부엉이로또명당', '경북 구미시 인동36길 15 구평메디칼센터 104호'], ['에버빌마트', '경북 안동시 강남5길 92,(정하동, 현진에버빌1차)'], ['천하명당복권방', '경남 거제시 옥포성안로 60'], ['복슈퍼', '경남 김해시 가야로 19 분성마을5단지푸르지오아파트 푸르지오상가 111호 복권판매점'], ['천하명당', '경남 양산시 연호로 46 복권판매점'], ['제일복권', '경남 양산시 양산역2길 7 대상골드타워 103호 복권판매점'], ['새롬(GS25마산새롬점)', '경남 창원시 마산합포구 수산1길 181 새롬미리내아파트 새롬미리내상가113호'], ['블루25복권명당대원점', '경남 창원시 성산구 두대로 57'], ['행복복권방', '경남 창원시 의창구 팔용로 399,(팔용동)'], ['행운마트', '경남 함안군 칠원읍 오곡로 92'], ['마법사복권방', '제주 서귀포시 동홍중앙로52번길 13 하이PC방']]}
###Markdown
Raw Data to the DatabaseInsert each draws' data and stores' data to 'DRAWS' & 'STORES' tables in lottery.db'.
###Code
sql_draw = """INSERT INTO `DRAWS`(`turn`, `num_1`, `num_2`, `num_3`, `num_4`, `num_5`, `num_6`, `num_bonus`)
VALUES(?, ?, ?, ?, ?, ?, ?, ?)"""
sql_winner_create = """CREATE TABLE IF NOT EXISTS
`WINNERS`(turn int, winner_1 int, winner_2 int, winner_3 int, winner_4 int, winner_5 int, UNIQUE(turn))"""
sql_winner = """INSERT INTO `WINNERS`(`turn`, `winner_1`, `winner_2`, `winner_3`, `winner_4`, `winner_5`)
VALUES(?, ?, ?, ?, ?, ?)"""
sql_prize_create = """CREATE TABLE IF NOT EXISTS
`PRIZES`(turn int, prize_1 int, prize_2 int, prize_3 int, prize_4 int, prize_5 int, UNIQUE(turn))"""
sql_prize = """INSERT INTO `PRIZES`(`turn`, `prize_1`, `prize_2`, `prize_3`, `prize_4`, `prize_5`)
VALUES(?, ?, ?, ?, ?, ?)"""
sql_date_create = """CREATE TABLE IF NOT EXISTS `DATES`(turn int, year int, month int, day int, UNIQUE(turn))"""
sql_date = """INSERT INTO `DATES`(`turn`, `year`, `month`, `day`) VALUES(?, ?, ?, ?)"""
sql_store = """INSERT INTO `STORES`(`idx`, `turn`, `name`, `auto`, `firstPrize`, `address`)
VALUES(?, ?, ?, ?, ?, ?)"""
def rawToDB(draws, stores, db_path):
con = sqlite3.connect(db_path)
cur = con.cursor()
if len(draws) < 1 or len(stores) < 1:
print("No additional data to be added to the database.")
return
cur.execute(sql_draw_create)
cur.execute(sql_winner_create)
cur.execute(sql_prize_create)
cur.execute(sql_date_create)
cur.execute(sql_store_create)
for draw_info in draws:
draw = draw_info["draw"]
nums = draw_info["nums"]
winners = draw_info["winners"]
prizes = draw_info["prize"]
dates = draw_info["date"]
data = tuple([draw] + [int(n) for n in nums])
cur.execute(sql_draw, data)
data = tuple([draw] + [int(n) for n in winners])
cur.execute(sql_winner, data)
data = tuple([draw] + [int(n) for n in prizes])
cur.execute(sql_prize, data)
data = tuple([draw] + [int(n) for n in dates])
cur.execute(sql_date, data)
row_idx = 0
for stores_info in stores:
draw = stores_info["draw"]
for i in stores_info["first"]:
data = tuple([row_idx, draw, str(i[0]), \
1 if str(i[1]).strip() == "자동" else 0
, 1, str(i[-1])])
cur.execute(sql_store, data)
row_idx += 1
for i in stores_info["second"]:
data = tuple([row_idx, draw, str(i[0]), None, 0, str(i[-1])])
cur.execute(sql_store, data)
row_idx += 1
con.commit()
con.close()
rawToDB(draws, stores, db_path)
###Output
_____no_output_____ |
matplotlib/gallery_jupyter/statistics/errorbar_limits.ipynb | ###Markdown
Including upper and lower limits in error barsIn matplotlib, errors bars can have "limits". Applying limits to theerror bars essentially makes the error unidirectional. Because of that,upper and lower limits can be applied in both the y- and x-directionsvia the ``uplims``, ``lolims``, ``xuplims``, and ``xlolims`` parameters,respectively. These parameters can be scalar or boolean arrays.For example, if ``xlolims`` is ``True``, the x-error bars will onlyextend from the data towards increasing values. If ``uplims`` is anarray filled with ``False`` except for the 4th and 7th values, all of they-error bars will be bidirectional, except the 4th and 7th bars, whichwill extend from the data towards decreasing y-values.
###Code
import numpy as np
import matplotlib.pyplot as plt
# example data
x = np.array([0.5, 1.0, 1.5, 2.0, 2.5, 3.0, 3.5, 4.0, 4.5, 5.0])
y = np.exp(-x)
xerr = 0.1
yerr = 0.2
# lower & upper limits of the error
lolims = np.array([0, 0, 1, 0, 1, 0, 0, 0, 1, 0], dtype=bool)
uplims = np.array([0, 1, 0, 0, 0, 1, 0, 0, 0, 1], dtype=bool)
ls = 'dotted'
fig, ax = plt.subplots(figsize=(7, 4))
# standard error bars
ax.errorbar(x, y, xerr=xerr, yerr=yerr, linestyle=ls)
# including upper limits
ax.errorbar(x, y + 0.5, xerr=xerr, yerr=yerr, uplims=uplims,
linestyle=ls)
# including lower limits
ax.errorbar(x, y + 1.0, xerr=xerr, yerr=yerr, lolims=lolims,
linestyle=ls)
# including upper and lower limits
ax.errorbar(x, y + 1.5, xerr=xerr, yerr=yerr,
lolims=lolims, uplims=uplims,
marker='o', markersize=8,
linestyle=ls)
# Plot a series with lower and upper limits in both x & y
# constant x-error with varying y-error
xerr = 0.2
yerr = np.full_like(x, 0.2)
yerr[[3, 6]] = 0.3
# mock up some limits by modifying previous data
xlolims = lolims
xuplims = uplims
lolims = np.zeros(x.shape)
uplims = np.zeros(x.shape)
lolims[[6]] = True # only limited at this index
uplims[[3]] = True # only limited at this index
# do the plotting
ax.errorbar(x, y + 2.1, xerr=xerr, yerr=yerr,
xlolims=xlolims, xuplims=xuplims,
uplims=uplims, lolims=lolims,
marker='o', markersize=8,
linestyle='none')
# tidy up the figure
ax.set_xlim((0, 5.5))
ax.set_title('Errorbar upper and lower limits')
plt.show()
###Output
_____no_output_____ |
Conv1d_For_HEALPix(PyTorch).ipynb | ###Markdown
CNN for HEALPix data structureThis notebook served the same purpose as a PyTorch version of HEALPix CNN. We should keep in mind that this implementation of CNN on spheres is relatively naive, and there are some other sophisticated models in the field of geometric deep learning. However, what I would like to show is a quick and dirty way for astronomers to try CNN on spheres with their own hand and laptop. The other point is that the hierarchical pixelization scheme might be helpful for future CNN on spheres. HEALPix by its nature is not fully hierarchically arranged because of the 12 base pixels. Download Your Data
###Code
transet = MNIST(root='./mnist', train=True, download=True)
###Output
_____no_output_____
###Markdown
Data TransformerDataloader in PyTorch allows us to avoid loading everything into memory during the training process. We just designed a custom transformation class to allow us to load rectangle PIL images into HEALPix data structure.
###Code
class HEALPixTransform(object):
'''convert a square PIL img to a HEALPix array in numpy'''
def __init__(self, nside):
self.nside = nside
def __call__(self, img):
if isinstance(img, np.ndarray):
return self.img2healpix(img, self.nside)
else:
return self.img2healpix(np.array(img), self.nside)
def cart_healpix(self, cartview, nside):
'''read in an matrix and return a healpix pixelization map'''
# Generate a flat Healpix map and angular to pixels
healpix = np.zeros(hp.nside2npix(nside), dtype=np.double)
hptheta = np.linspace(0, np.pi, num=cartview.shape[0])[:, None]
hpphi = np.linspace(-np.pi, np.pi, num=cartview.shape[1])
pix = hp.ang2pix(nside, hptheta, hpphi)
# re-pixelize
healpix[pix] = np.fliplr(cartview)
return healpix
def ring2nest(self, healpix):
nest = np.zeros(healpix.shape)
ipix = hp.ring2nest(nside=hp.npix2nside(nest.shape[-1]),
ipix=np.arange(nest.shape[-1]))
nest[ipix] = healpix
return nest
def img2healpix(self, digit, nside):
'''
padding squre digits to 1*2 rectangles and
convert them to healpix with a given nside
'''
h, w = digit.shape
img = np.zeros((h, 2 * h))
img[:, h - w // 2 : h - w // 2 + w] = digit
return self.ring2nest(self.cart_healpix(img, nside))
class ToTensor(object):
'''convert ndarrays to Tensors'''
def __call__(self, healpix):
return torch.from_numpy(healpix).double()
class Normalize(object):
def __init__(self, mean, std):
self.mean = mean
self.std = std
def __call__(self, tensor):
tensor.div_(255)#.sub_(self.mean
# ).div_(self.std).clamp_(min=0., max=1.
# )
return tensor.unsqueeze(0)
###Output
_____no_output_____
###Markdown
You can test the functionality of HEALPix transformation class using you own image.
###Code
img = Image.open("hymenoptera_data/train/ants/0013035.jpg").convert('L') # please load your own image or skip this cell
hT = HEALPixTransform(nside=64)
hp.mollview(hT(img), nest=1)
###Output
_____no_output_____
###Markdown
1-D Conv NetSince HEALPix data structure grouped with each 4 elements, 1D Conv with kernel_size = 4 and stride = 4 would be elgible to apply on HEALPix.
###Code
class HEALPixNet(nn.Module):
def __init__(self):
super(HEALPixNet, self).__init__()
# nside = 8 -> nside = 4
self.conv1 = nn.Conv1d(1, 32, 4, stride=4)
# nside = 4 -> nside = 2
self.conv2 = nn.Conv1d(32, 64, 4, stride=4)
# nside**2 * 12 = 48
self.fc1 = nn.Linear(48 * 64, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = F.relu(self.conv1(x))
x = F.relu(self.conv2(x))
x = x.view(-1, 48 * 64)
x = F.relu(self.fc1(x))
x = F.dropout(x, training=self.training)
x = F.softmax(self.fc2(x), dim=1)
return x
net = HEALPixNet()
net = net.double()
net
###Output
_____no_output_____
###Markdown
Data Pre-Processing
###Code
batch_size = 32
data_transforms = {
'train' : transforms.Compose([
# transforms.RandomHorizontalFlip(),
HEALPixTransform(nside=8),
ToTensor(),
Normalize(0.083, 0.254) # mnist setting
]),
'val' : transforms.Compose([
HEALPixTransform(nside=8),
ToTensor(),
Normalize(0.083, 0.254) # mnist setting
]),
}
data_loaders = {
'train' : torch.utils.data.DataLoader(
MNIST(root='./mnist', train=True, download=True,
transform=data_transforms['train']),
batch_size=batch_size, shuffle=True,
),
'val' : torch.utils.data.DataLoader(
MNIST(root='./mnist', train=False,
transform=data_transforms['val']),
batch_size=batch_size, shuffle=True,
),
}
###Output
_____no_output_____
###Markdown
Visualize Some Samples
###Code
inputs, classes = next(iter(data_loaders['train']))
for i in range(5):
hp.orthview(inputs[i].numpy()[0, :], nest=True, sub=(1, 5, i + 1), half_sky=1, title='')
###Output
_____no_output_____
###Markdown
Optimizer
###Code
from torch.optim import lr_scheduler
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(net.parameters(), lr=0.001, betas=(0.9, 0.999), eps=1e-08)
scheduler = lr_scheduler.StepLR(optimizer, step_size=10, gamma=0.1)
###Output
_____no_output_____
###Markdown
Training Process
###Code
import copy
import time
t = time.time()
best_wts = copy.deepcopy(net.state_dict())
best_acc = 0.0
num_epochs = 100
use_gpu = False
history = {'train_loss' : [],
'val_loss' : [],
'train_acc' : [],
'val_acc' : []}
for epoch in range(num_epochs):
print('Epoch {}/{}'.format(epoch, num_epochs - 1))
print('=' * 10)
for phase in ['train', 'val']:
running_loss = 0.
running_corrects = 0
if phase == 'train':
scheduler.step()
net.train(True)
else:
net.train(False)
for data in data_loaders[phase]:
inputs, labels = data
if use_gpu:
inputs = Variable(inputs.cuda())
labels = Variable(labels.cuda())
else:
inputs = Variable(inputs)
labels = Variable(labels)
optimizer.zero_grad()
outputs = net(inputs)
_, preds = torch.max(outputs.data, 1)
loss = criterion(outputs, labels)
if phase == 'train':
loss.backward()
optimizer.step()
running_loss += loss.data.item() * inputs.size(0)
running_corrects += torch.sum(preds == labels.data)
epoch_loss = running_loss / len(data_loaders[phase].dataset)
epoch_acc = running_corrects.float() / len(data_loaders[phase].dataset)
print('{} Loss: {:.4f} Acc: {:.4f}'.format(phase, epoch_loss, epoch_acc))
if phase == 'val' and epoch_acc > best_acc:
best_acc = epoch_acc
best_wts = copy.deepcopy(net.state_dict())
# saving history
history['{}_loss'.format(phase)].append(epoch_loss)
history['{}_acc'.format(phase)].append(epoch_acc)
print()
time_elapsed = time.time() - t
print('Complete in {:.0f}min {:.0f}s'.format(
time_elapsed // 60, time_elapsed % 60))
print('Top Accuracy: {:4f}'.format(best_acc))
net.load_state_dict(best_wts)
###Output
Epoch 0/99
==========
train Loss: 1.6607 Acc: 0.8063
val Loss: 1.5394 Acc: 0.9233
Epoch 1/99
==========
train Loss: 1.5765 Acc: 0.8863
val Loss: 1.5228 Acc: 0.9385
Epoch 2/99
==========
train Loss: 1.5603 Acc: 0.9016
val Loss: 1.5150 Acc: 0.9462
Epoch 3/99
==========
train Loss: 1.5490 Acc: 0.9126
val Loss: 1.5086 Acc: 0.9527
Epoch 4/99
==========
train Loss: 1.5429 Acc: 0.9191
val Loss: 1.5055 Acc: 0.9556
Epoch 5/99
==========
train Loss: 1.5347 Acc: 0.9265
val Loss: 1.5019 Acc: 0.9595
Epoch 6/99
==========
train Loss: 1.5304 Acc: 0.9311
val Loss: 1.4999 Acc: 0.9610
Epoch 7/99
==========
train Loss: 1.5270 Acc: 0.9346
val Loss: 1.4965 Acc: 0.9650
Epoch 8/99
==========
train Loss: 1.5217 Acc: 0.9402
val Loss: 1.4968 Acc: 0.9645
Epoch 9/99
==========
train Loss: 1.5208 Acc: 0.9404
val Loss: 1.4949 Acc: 0.9657
Epoch 10/99
==========
train Loss: 1.5125 Acc: 0.9492
val Loss: 1.4908 Acc: 0.9700
Epoch 11/99
==========
train Loss: 1.5093 Acc: 0.9519
val Loss: 1.4903 Acc: 0.9706
Epoch 12/99
==========
train Loss: 1.5072 Acc: 0.9541
val Loss: 1.4898 Acc: 0.9715
Epoch 13/99
==========
train Loss: 1.5069 Acc: 0.9546
val Loss: 1.4900 Acc: 0.9714
Epoch 14/99
==========
train Loss: 1.5056 Acc: 0.9560
val Loss: 1.4895 Acc: 0.9718
Epoch 15/99
==========
train Loss: 1.5047 Acc: 0.9568
val Loss: 1.4890 Acc: 0.9721
Epoch 16/99
==========
train Loss: 1.5042 Acc: 0.9578
val Loss: 1.4885 Acc: 0.9729
Epoch 17/99
==========
train Loss: 1.5042 Acc: 0.9573
val Loss: 1.4886 Acc: 0.9731
Epoch 18/99
==========
train Loss: 1.5030 Acc: 0.9589
val Loss: 1.4883 Acc: 0.9729
Epoch 19/99
==========
train Loss: 1.5025 Acc: 0.9594
val Loss: 1.4883 Acc: 0.9728
Epoch 20/99
==========
train Loss: 1.5021 Acc: 0.9594
val Loss: 1.4882 Acc: 0.9731
Epoch 21/99
==========
train Loss: 1.5020 Acc: 0.9593
val Loss: 1.4881 Acc: 0.9730
Epoch 22/99
==========
train Loss: 1.5018 Acc: 0.9597
val Loss: 1.4880 Acc: 0.9730
Epoch 23/99
==========
train Loss: 1.5024 Acc: 0.9590
val Loss: 1.4879 Acc: 0.9732
Epoch 24/99
==========
train Loss: 1.5018 Acc: 0.9600
val Loss: 1.4880 Acc: 0.9729
Epoch 25/99
==========
train Loss: 1.5012 Acc: 0.9606
val Loss: 1.4880 Acc: 0.9728
Epoch 26/99
==========
train Loss: 1.5012 Acc: 0.9606
val Loss: 1.4880 Acc: 0.9730
Epoch 27/99
==========
train Loss: 1.5009 Acc: 0.9613
val Loss: 1.4880 Acc: 0.9731
Epoch 28/99
==========
train Loss: 1.5012 Acc: 0.9605
val Loss: 1.4879 Acc: 0.9731
Epoch 29/99
==========
train Loss: 1.5004 Acc: 0.9614
val Loss: 1.4879 Acc: 0.9732
Epoch 30/99
==========
train Loss: 1.5002 Acc: 0.9614
val Loss: 1.4879 Acc: 0.9733
Epoch 31/99
==========
train Loss: 1.5004 Acc: 0.9610
val Loss: 1.4879 Acc: 0.9733
Epoch 32/99
==========
train Loss: 1.5011 Acc: 0.9608
val Loss: 1.4879 Acc: 0.9731
Epoch 33/99
==========
train Loss: 1.5008 Acc: 0.9612
val Loss: 1.4879 Acc: 0.9731
Epoch 34/99
==========
train Loss: 1.5007 Acc: 0.9611
val Loss: 1.4879 Acc: 0.9732
Epoch 35/99
==========
train Loss: 1.5004 Acc: 0.9615
val Loss: 1.4879 Acc: 0.9732
Epoch 36/99
==========
train Loss: 1.5009 Acc: 0.9607
val Loss: 1.4879 Acc: 0.9731
Epoch 37/99
==========
train Loss: 1.5007 Acc: 0.9608
val Loss: 1.4879 Acc: 0.9730
Epoch 38/99
==========
train Loss: 1.5013 Acc: 0.9606
val Loss: 1.4879 Acc: 0.9733
Epoch 39/99
==========
train Loss: 1.5008 Acc: 0.9609
val Loss: 1.4878 Acc: 0.9732
Epoch 40/99
==========
train Loss: 1.5006 Acc: 0.9614
val Loss: 1.4878 Acc: 0.9732
Epoch 41/99
==========
train Loss: 1.5008 Acc: 0.9610
val Loss: 1.4878 Acc: 0.9733
Epoch 42/99
==========
train Loss: 1.5014 Acc: 0.9607
val Loss: 1.4878 Acc: 0.9733
Epoch 43/99
==========
train Loss: 1.5005 Acc: 0.9614
val Loss: 1.4878 Acc: 0.9733
Epoch 44/99
==========
train Loss: 1.5007 Acc: 0.9609
val Loss: 1.4878 Acc: 0.9733
Epoch 45/99
==========
train Loss: 1.5007 Acc: 0.9610
val Loss: 1.4878 Acc: 0.9733
Epoch 46/99
==========
train Loss: 1.5017 Acc: 0.9599
val Loss: 1.4878 Acc: 0.9733
Epoch 47/99
==========
train Loss: 1.5004 Acc: 0.9616
val Loss: 1.4878 Acc: 0.9733
Epoch 48/99
==========
train Loss: 1.5019 Acc: 0.9598
val Loss: 1.4878 Acc: 0.9733
Epoch 49/99
==========
train Loss: 1.5014 Acc: 0.9604
val Loss: 1.4878 Acc: 0.9733
Epoch 50/99
==========
train Loss: 1.5008 Acc: 0.9607
val Loss: 1.4878 Acc: 0.9733
Epoch 51/99
==========
train Loss: 1.5007 Acc: 0.9611
val Loss: 1.4878 Acc: 0.9733
Epoch 52/99
==========
train Loss: 1.5012 Acc: 0.9606
val Loss: 1.4878 Acc: 0.9733
Epoch 53/99
==========
train Loss: 1.5009 Acc: 0.9611
val Loss: 1.4878 Acc: 0.9733
Epoch 54/99
==========
train Loss: 1.5003 Acc: 0.9617
val Loss: 1.4878 Acc: 0.9733
Epoch 55/99
==========
train Loss: 1.5006 Acc: 0.9618
val Loss: 1.4878 Acc: 0.9733
Epoch 56/99
==========
train Loss: 1.5015 Acc: 0.9600
val Loss: 1.4878 Acc: 0.9733
Epoch 57/99
==========
train Loss: 1.5003 Acc: 0.9617
val Loss: 1.4878 Acc: 0.9733
Epoch 58/99
==========
train Loss: 1.5004 Acc: 0.9613
val Loss: 1.4878 Acc: 0.9733
Epoch 59/99
==========
train Loss: 1.5004 Acc: 0.9615
val Loss: 1.4878 Acc: 0.9733
Epoch 60/99
==========
train Loss: 1.5005 Acc: 0.9615
val Loss: 1.4878 Acc: 0.9733
Epoch 61/99
==========
train Loss: 1.5006 Acc: 0.9613
val Loss: 1.4878 Acc: 0.9733
Epoch 62/99
==========
train Loss: 1.5004 Acc: 0.9618
val Loss: 1.4878 Acc: 0.9733
Epoch 63/99
==========
train Loss: 1.5015 Acc: 0.9602
val Loss: 1.4878 Acc: 0.9733
Epoch 64/99
==========
train Loss: 1.5010 Acc: 0.9606
val Loss: 1.4878 Acc: 0.9733
Epoch 65/99
==========
train Loss: 1.5015 Acc: 0.9603
val Loss: 1.4878 Acc: 0.9733
Epoch 66/99
==========
train Loss: 1.5006 Acc: 0.9614
val Loss: 1.4878 Acc: 0.9733
Epoch 67/99
==========
train Loss: 1.5017 Acc: 0.9599
val Loss: 1.4878 Acc: 0.9733
Epoch 68/99
==========
train Loss: 1.5013 Acc: 0.9604
val Loss: 1.4878 Acc: 0.9733
Epoch 69/99
==========
train Loss: 1.5012 Acc: 0.9609
val Loss: 1.4878 Acc: 0.9733
Epoch 70/99
==========
train Loss: 1.5006 Acc: 0.9611
val Loss: 1.4878 Acc: 0.9733
Epoch 71/99
==========
train Loss: 1.5008 Acc: 0.9607
val Loss: 1.4878 Acc: 0.9733
Epoch 72/99
==========
train Loss: 1.5012 Acc: 0.9604
val Loss: 1.4878 Acc: 0.9733
Epoch 73/99
==========
train Loss: 1.5011 Acc: 0.9609
val Loss: 1.4878 Acc: 0.9733
Epoch 74/99
==========
train Loss: 1.5006 Acc: 0.9610
val Loss: 1.4878 Acc: 0.9733
Epoch 75/99
==========
train Loss: 1.5012 Acc: 0.9606
val Loss: 1.4878 Acc: 0.9733
Epoch 76/99
==========
train Loss: 1.5008 Acc: 0.9612
val Loss: 1.4878 Acc: 0.9733
Epoch 77/99
==========
train Loss: 1.5010 Acc: 0.9606
val Loss: 1.4878 Acc: 0.9733
Epoch 78/99
==========
train Loss: 1.5013 Acc: 0.9605
val Loss: 1.4878 Acc: 0.9733
Epoch 79/99
==========
train Loss: 1.5020 Acc: 0.9600
val Loss: 1.4878 Acc: 0.9733
Epoch 80/99
==========
train Loss: 1.5001 Acc: 0.9619
val Loss: 1.4878 Acc: 0.9733
Epoch 81/99
==========
train Loss: 1.5016 Acc: 0.9602
val Loss: 1.4878 Acc: 0.9733
Epoch 82/99
==========
train Loss: 1.5010 Acc: 0.9605
val Loss: 1.4878 Acc: 0.9733
Epoch 83/99
==========
train Loss: 1.5011 Acc: 0.9604
val Loss: 1.4878 Acc: 0.9733
Epoch 84/99
==========
train Loss: 1.5004 Acc: 0.9616
val Loss: 1.4878 Acc: 0.9733
Epoch 85/99
==========
train Loss: 1.5011 Acc: 0.9607
val Loss: 1.4878 Acc: 0.9733
Epoch 86/99
==========
train Loss: 1.5012 Acc: 0.9607
val Loss: 1.4878 Acc: 0.9733
Epoch 87/99
==========
train Loss: 1.5006 Acc: 0.9611
val Loss: 1.4878 Acc: 0.9733
Epoch 88/99
==========
train Loss: 1.5014 Acc: 0.9602
val Loss: 1.4878 Acc: 0.9733
Epoch 89/99
==========
train Loss: 1.5017 Acc: 0.9601
val Loss: 1.4878 Acc: 0.9733
Epoch 90/99
==========
train Loss: 1.5011 Acc: 0.9606
val Loss: 1.4878 Acc: 0.9733
Epoch 91/99
==========
train Loss: 1.5015 Acc: 0.9603
val Loss: 1.4878 Acc: 0.9733
Epoch 92/99
==========
train Loss: 1.5006 Acc: 0.9613
val Loss: 1.4878 Acc: 0.9733
Epoch 93/99
==========
train Loss: 1.5004 Acc: 0.9615
val Loss: 1.4878 Acc: 0.9733
Epoch 94/99
==========
train Loss: 1.5017 Acc: 0.9600
val Loss: 1.4878 Acc: 0.9733
Epoch 95/99
==========
train Loss: 1.4998 Acc: 0.9622
val Loss: 1.4878 Acc: 0.9733
Epoch 96/99
==========
train Loss: 1.4999 Acc: 0.9622
val Loss: 1.4878 Acc: 0.9733
Epoch 97/99
==========
train Loss: 1.5002 Acc: 0.9617
val Loss: 1.4878 Acc: 0.9733
Epoch 98/99
==========
###Markdown
Since I put a dropout layer during training, the training loss was higher than validation loss.
###Code
fig, ax = plt.subplots(1, 2, figsize=(14, 6))
ax[0].plot(history['train_loss'], label='train loss')
ax[0].plot(history['val_loss'], label='val loss')
ax[0].set_xlabel('epochs')
ax[1].plot(history['train_acc'], label='train acc')
ax[1].plot(history['val_acc'], label='val acc')
ax[1].set_xlabel('epochs')
ax[0].legend()
ax[1].legend();
###Output
_____no_output_____ |
ml_feature/03_Explore_Data/03_03/Begin/03_03.ipynb | ###Markdown
Explore The Data: Plot Continuous FeaturesUsing the Titanic dataset from [this](https://www.kaggle.com/c/titanic/overview) Kaggle competition.This dataset contains information about 891 people who were on board the ship when departed on April 15th, 1912. As noted in the description on Kaggle's website, some people aboard the ship were more likely to survive the wreck than others. There were not enough lifeboats for everybody so women, children, and the upper-class were prioritized. Using the information about these 891 passengers, the challenge is to build a model to predict which people would survive based on the following fields:- **Name** (str) - Name of the passenger- **Pclass** (int) - Ticket class (1st, 2nd, or 3rd)- **Sex** (str) - Gender of the passenger- **Age** (float) - Age in years- **SibSp** (int) - Number of siblings and spouses aboard- **Parch** (int) - Number of parents and children aboard- **Ticket** (str) - Ticket number- **Fare** (float) - Passenger fare- **Cabin** (str) - Cabin number- **Embarked** (str) - Port of embarkation (C = Cherbourg, Q = Queenstown, S = Southampton)**This section focuses on exploring the `Pclass`, `Age`, `SibSp`, `Parch`, and `Fare` features.** Read In Data
###Code
# Read in our data
import matplotlib.pyplot as plt
import seaborn as sns
%matplotlib inline
import numpy as np
import pandas as pd
titanic = pd.read_csv('../../../data/titanic.csv',
usecols=['Survived', 'Pclass', 'Age', 'SibSp', 'Parch', 'Fare'])
titanic.head()
###Output
_____no_output_____
###Markdown
Plot Continuous Features
###Code
# Plot overlaid histograms for continuous features
for i in ['Age', 'Fare']:
died = list(titanic[titanic['Survived'] == 0][i].dropna())
survived = list(titanic[titanic['Survived'] == 1][i].dropna())
xmin = min(min(died), min(survived))
xmax = max(max(died), max(survived))
width = (xmax - xmin) / 40
sns.distplot(died, color='r', kde=False, bins=np.arange(xmin, xmax, width))
sns.distplot(survived, color='g', kde=False, bins=np.arange(xmin, xmax, width))
plt.legend(['Did not survive', 'Survived'])
plt.title('Overlaid histogram for {}'.format(i))
plt.show()
# Generate categorical plots for ordinal features
for col in ['Pclass', 'SibSp', 'Parch']:
sns.catplot(x=col, y='Survived', data=titanic, kind='point', aspect=2, )
plt.ylim(0, 1)
# Create a new family count feature
###Output
_____no_output_____ |
file .ipynb/Titanic _ Visualization & Prediction.ipynb | ###Markdown
Titanic : Visualization & PredictionPredict survival on the Titanic and get familiar with ML basics. (⭐️ Upvote my notebook — it helps! )Getting started with competitive data science can be quite intimidating. So I build this notebook for quick overview on `Titanic: Machine Learning from Disaster` competition. If there is interest, I’m happy to do deep dives into the intuition behind the feature engineering and models used in this kernel.I encourage you to fork this kernel, play with the code and enter the competition. Good luck! Competition DescriptionIn this challenge, it ask you to build a predictive model that predicts which passengers survived the Titanic shipwreck and answers the question: “what sorts of people were more likely to survive?” using passenger data (ie name, age, gender, socio-economic class, etc). Executive SummaryI started this competition by just focusing on getting a good understanding of the dataset. The EDA & Visualizations are included to allow developers to dive into analysis of dataset. Key features of the model training process in this kernelK Fold Cross Validation: Using 5-fold cross-validation.First Level Learning Model: On each run of cross-validation tried fitting following models :-1. Random Forest classifier2. Extra Trees classifier3. AdaBoost classifer4. Gradient Boosting classifer5. Support Vector MachineSecond Level Learning Model : Trained a XGBClassifier using xgboost
###Code
# Load in our libraries
import random
import pandas as pd
import numpy as np
import re
import sklearn
from xgboost import XGBClassifier
import seaborn as sns
import matplotlib.pyplot as plt
%matplotlib inline
import plotly.offline as py
py.init_notebook_mode(connected=True)
import plotly.graph_objs as go
import plotly.tools as tls
import warnings
warnings.filterwarnings('ignore')
# Going to use these 5 base models for the stacking
from sklearn.ensemble import (RandomForestClassifier, AdaBoostClassifier,
GradientBoostingClassifier, ExtraTreesClassifier)
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
from sklearn.model_selection import KFold, cross_val_score
train = pd.read_csv('../input/titanic/train.csv')
test = pd.read_csv('../input/titanic/test.csv')
print("Train set size:", train.shape)
print("Test set size:", test.shape)
###Output
_____no_output_____
###Markdown
Data Pre-processing
###Code
# Store our passenger ID for easy access
PassengerId = test['PassengerId']
train.head(5)
train.info()
print('_'*40)
test.info()
###Output
_____no_output_____
###Markdown
What is the distribution of numerical feature values across the samples?This helps us determine, among other early insights, how representative is the training dataset of the actual problem domain.* Total samples are 891 or 40% of the actual number of passengers on board the Titanic (2,224).* Survived is a categorical feature with 0 or 1 values.* Around 38% samples survived representative of the actual survival rate at 32%.* Most passengers (> 75%) did not travel with parents or children.* Nearly 30% of the passengers had siblings and/or spouse aboard.* Fares varied significantly with few passengers (<1%) paying as high as $512.* Few elderly passengers (< 1%) within age range 65-80.
###Code
train.describe()
# Review survived rate using `percentiles=[.61, .62]` knowing our problem description mentions 38% survival rate.
# Review Parch distribution using `percentiles=[.75, .8]`
# SibSp distribution `[.68, .69]`
# Age and Fare `[.1, .2, .3, .4, .5, .6, .7, .8, .9, .99]`
###Output
_____no_output_____
###Markdown
What is the distribution of categorical features?* Names are unique across the dataset (count=unique=891).* Sex variable as two possible values with 65% male (top=male, freq=577/count=891).* Cabin values have several dupicates across samples. Alternatively several passengers shared a cabin.* Embarked takes three possible values. S port used by most passengers (top=S).* Ticket feature has high ratio (22%) of duplicate values (unique=681).
###Code
train.describe(include=['O'])
###Output
_____no_output_____
###Markdown
Visualisations 1. Pearson Correlation Heatmap
###Code
corr_matrix = train.corr()
plt.figure(figsize=(8, 7))
sns.heatmap(data = corr_matrix,cmap='BrBG', annot=True, linewidths=0.2)
###Output
_____no_output_____
###Markdown
There are no very highly correlated columns 2. Number of missing values
###Code
train.isnull().sum()
###Output
_____no_output_____
###Markdown
The columns 'Age' and 'Cabin' contains more null values. 3. Pclass wise - Survival probability
###Code
plt = train[['Pclass', 'Survived']].groupby('Pclass').mean().Survived.plot('bar')
plt.set_xlabel('Pclass')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
1st class has high chance of surviving than the other two classes. 4. Sex wise - Survival probability
###Code
plt = train[['Sex', 'Survived']].groupby('Sex').mean().Survived.plot('bar')
plt.set_xlabel('Sex')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
The survival probaility for Female is more. They might have given more priority to female than male. 5. Embarked wise - Survival probability
###Code
plt = train[['Embarked', 'Survived']].groupby('Embarked').mean().Survived.plot('bar')
plt.set_xlabel('Embarked')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
Survival probability: C > Q > S 6. SibSp - Siblings/Spouse wise - Survival probability
###Code
plt = train[['SibSp', 'Survived']].groupby('SibSp').mean().Survived.plot('bar')
plt.set_xlabel('SibSp')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
The passengers having one sibling/spouse has more survival probability.'1' > '2' > '0' > '3' > '4' 7. Parch - Children/Parents wise - Survival probability
###Code
plt = train[['Parch', 'Survived']].groupby('Parch').mean().Survived.plot('bar')
plt.set_xlabel('Parch')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
The passengers having three children/parents has more survival probability.'3' > '1' > '2' > '0' > '5' 8. Age wise - Survival probability
###Code
from matplotlib import pyplot as plt
fig = plt.figure(figsize=(20, 7))
sns.violinplot(x='Sex', y='Age',
hue='Survived', data=train,
split=True,
palette={0: "blue", 1: "yellow"}
);
###Output
_____no_output_____
###Markdown
Younger male tend to survive.Infants (Age <=4) had high survival rate.Oldest passengers (Age = 80) survived.A large number of passengers between 20 and 40 die.The age doesn't seem to have a direct impact on the female survival Feature Engineering
###Code
y = train.shape[0]
print(y)
y2 = test.shape[0]
features = pd.concat([train, test]).reset_index(drop=True)
print(features.shape)
features['Name_length'] = features['Name'].str.len()
features['Has_Cabin'] = features["Cabin"].apply(lambda x: 0 if type(x) == float else 1)
features['FamilySize'] = features['SibSp'] + features['Parch'] + 1
features['IsAlone'] = 0
features.loc[features['FamilySize'] == 1, 'IsAlone'] = 1
features['Embarked'] = features['Embarked'].fillna('S')
features['Fare'] = features['Fare'].fillna(train['Fare'].median())
# Mapping Fare
features.loc[ features['Fare'] <= 7.91, 'Fare'] = 0
features.loc[(features['Fare'] > 7.91) & (features['Fare'] <= 14.454), 'Fare'] = 1
features.loc[(features['Fare'] > 14.454) & (features['Fare'] <= 31), 'Fare'] = 2
features.loc[ features['Fare'] > 31, 'Fare'] = 3
features['Fare'] = features['Fare'].astype(int)
features.head(3)
features['Age'] = features['Age'].apply(lambda x: np.random.choice(features['Age'].dropna().values) if np.isnan(x) else x)
print(features['Age'].head(20))
features['Age'] = features['Age'].astype(int)
# Mapping Age
features.loc[ features['Age'] <= 16, 'Age'] = 0
features.loc[(features['Age'] > 16) & (features['Age'] <= 32), 'Age'] = 1
features.loc[(features['Age'] > 32) & (features['Age'] <= 48), 'Age'] = 2
features.loc[(features['Age'] > 48) & (features['Age'] <= 64), 'Age'] = 3
features.loc[ features['Age'] > 64, 'Age'] = 4 ;
features['Title'] = features['Name'].str.extract(pat = ' ([A-Za-z]+)\.' )
features['Title'] = features['Title'].replace('Mlle', 'Miss')
features['Title'] = features['Title'].replace('Ms', 'Miss')
features['Title'] = features['Title'].replace('Mme', 'Mrs')
features['Title'] = features['Title'].replace(['Lady', 'Countess','Capt', 'Col','Don', 'Dr', 'Major', 'Rev', 'Sir', 'Jonkheer', 'Dona'], 'Rare')
# Mapping titles
title_mapping = {"Mr": 1, "Miss": 2, "Mrs": 3, "Master": 4, "Rare": 5}
features['Title'] = features['Title'].map(title_mapping)
features['Title'] = features['Title'].fillna(0)
features.tail(3)
# Feature selection
drop_elements = ['PassengerId', 'Name', 'Ticket', 'Cabin', 'SibSp', 'Name_length']
features = features.drop(drop_elements, axis = 1)
# Mapping Categorical data
features['Embarked'] = features['Embarked'].map( {'S': 0, 'C': 1, 'Q': 2} ).astype(int)
features['Sex'] = features['Sex'].map( {'female': 0, 'male': 1} ).astype(int)
#features['Age*Class'] = features['Age']*features['Pclass']
#features = pd.get_dummies(features, prefix=['Embarked', 'Sex'], columns=['Embarked', 'Sex'])
# One hot encoding is not helping in this case
train = features.iloc[:y]
test = features.iloc[-y2:]
train.shape
test.shape
test = test.drop('Survived', axis = 1)
test.head(3)
###Output
_____no_output_____
###Markdown
All right so now having cleaned the features and extracted relevant information and dropped the redundant columns, converted categorical columns to numerical ones, a format suitable to feed into our Machine Learning models. 8. Title wise - Survival probability
###Code
plt = train[['Title', 'Survived']].groupby('Title').mean().Survived.plot('bar')
plt.set_xlabel('Title')
plt.set_ylabel('Survival Probability')
###Output
_____no_output_____
###Markdown
The survival probability for 'Mrs' and 'Miss' is high comapred to other classes. 9. Correlation between columns
###Code
import matplotlib.pyplot as plt
colormap = plt.cm.RdBu
plt.figure(figsize=(14,12))
plt.title('Pearson Correlation of Features', y=1.05, size=15)
sns.heatmap(train.astype(float).corr(),linewidths=0.1,vmax=1.0,
square=True, cmap=colormap, linecolor='white', annot=True)
###Output
_____no_output_____
###Markdown
Model Trying Different Model, predict and solve without Any Cross Validation We must understand the type of problem and solution requirement to narrow down to a select few models which we can evaluate. Our problem is a classification and regression problem. We want to identify relationship between output (Survived or not) with other variables or features (Gender, Age, Port...). We are also perfoming a category of machine learning which is called supervised learning as we are training our model with a given dataset. With these two criteria - Supervised Learning plus Classification and Regression, we can narrow down our choice of models to a few. These include:* Logistic Regression* KNN or k-Nearest Neighbors* Support Vector Machines* Naive Bayes classifier* Decision Tree* Random Forrest* Perceptron* Artificial neural network* RVM or Relevance Vector Machine
###Code
X_train = train.drop("Survived", axis=1)
Y_train = train["Survived"]
X_test = test.copy()
X_train.shape, Y_train.shape, X_test.shape
# machine learning
from sklearn.linear_model import LogisticRegression
from sklearn.svm import SVC, LinearSVC
from sklearn.ensemble import RandomForestClassifier
from sklearn.neighbors import KNeighborsClassifier
from sklearn.naive_bayes import GaussianNB
from sklearn.linear_model import Perceptron
from sklearn.linear_model import SGDClassifier
from sklearn.tree import DecisionTreeClassifier
# Logistic Regression
logreg = LogisticRegression()
logreg.fit(X_train, Y_train)
Y_pred = logreg.predict(X_test).astype(int)
acc_log = round(logreg.score(X_train, Y_train) * 100, 2)
acc_log
coeff_df = pd.DataFrame(train.columns.delete(0))
coeff_df.columns = ['Feature']
coeff_df["Correlation"] = pd.Series(logreg.coef_[0])
coeff_df.sort_values(by='Correlation', ascending=False)
# Support Vector Machines
svc = SVC()
svc.fit(X_train, Y_train)
Y_pred = svc.predict(X_test).astype(int)
acc_svc = round(svc.score(X_train, Y_train) * 100, 2)
acc_svc
knn = KNeighborsClassifier(n_neighbors = 3)
knn.fit(X_train, Y_train)
knn.predict(X_test).astype(int)
acc_knn = round(knn.score(X_train, Y_train) * 100, 2)
acc_knn
# Decision Tree
decision_tree = DecisionTreeClassifier()
decision_tree.fit(X_train, Y_train)
decision_tree.predict(X_test).astype(int)
acc_decision_tree = round(decision_tree.score(X_train, Y_train) * 100, 2)
acc_decision_tree
# Random Forest
random_forest = RandomForestClassifier(n_estimators=100)
random_forest.fit(X_train, Y_train)
random_forest.predict(X_test).astype(int)
random_forest.score(X_train, Y_train)
acc_random_forest = round(random_forest.score(X_train, Y_train) * 100, 2)
acc_random_forest
xgboost = XGBClassifier(
learning_rate = 0.95,
n_estimators= 5000,
max_depth= 4,
min_child_weight= 2,
gamma=1,
subsample=0.8,
colsample_bytree=0.8,
objective= 'binary:logistic',
nthread= -1,
scale_pos_weight=1)
xgboost.fit(X_train, Y_train)
xgboost.predict(X_test).astype(int)
xgboost.score(X_train, Y_train)
acc_xgboost = round(xgboost.score(X_train, Y_train) * 100, 2)
acc_xgboost
###Output
_____no_output_____
###Markdown
Plotting above XGBoost Decision Tree ( Without Cross Validation )
###Code
from xgboost import plot_tree
from matplotlib.pylab import rcParams
##set up the parameters
rcParams['figure.figsize'] = 80,50
# plot single tree
plot_tree(xgboost, rankdir='LR')
###Output
_____no_output_____
###Markdown
Model Comparison
###Code
models = pd.DataFrame({
'Model': ['Support Vector Machines', 'KNN', 'Logistic Regression',
'Random Forest', 'Decision Tree', 'Xgboost'],
'Confidence Score': [acc_svc, acc_knn, acc_log, acc_random_forest, acc_decision_tree,acc_xgboost],
'Real Score': [0.78947,0.74641,0.77511,0.76076,0.76076,0.74162]})
models.sort_values(by='Real Score', ascending=False)
submission = pd.DataFrame({
"PassengerId": PassengerId,
"Survived": Y_pred
})
submission.to_csv("Submission2new.csv", index=False)
###Output
_____no_output_____
###Markdown
Setup cross validation method "kf” means K-Folds cross-validator, so "kf = KFold(n_splits = NFOLDS, random_state = SEED)" will generates a K-Folds cross-validator which provides train/test indices to split data in train/test sets.
###Code
# Helpers via Python Classes
ntrain = train.shape[0]
ntest = test.shape[0]
# Choose a random seed
SEED = 0
NFOLDS = 5 # set folds for out-of-fold prediction
n_splits = 5 #needed later
kf = KFold(n_splits = NFOLDS, random_state = SEED)
# Class to extend the Sklearn classifier
# Standard code taken from http://blog.keyrus.co.uk/ensembling_ml_models.html.
class SklearnHelper(object):
def __init__(self, clf, seed=0, params=None):
"""Get the name of the model for labelling purposes and set a random seed."""
self.name = re.search("('.+')>$", str(clf)).group(1)
params['random_state'] = seed
self.clf = clf(**params)
def train(self, x_train, y_train):
"""Fit with training data."""
self.clf.fit(x_train, y_train)
def predict(self, x):
"""Make a prediction."""
return self.clf.predict(x)
def fit(self,x,y):
return self.clf.fit(x,y)
def feature_importances(self,x,y):
"""Refit and get the feature importances."""
print(self.clf.fit(x,y).feature_importances_)
return(self.clf.fit(x,y).feature_importances_)
# Class to extend XGboost classifer
###Output
_____no_output_____
###Markdown
Explaination for above & below code :-In each split of K-Folds, the original train data will be splited to new train data and new test data, which will be uesd to fit a model later. kf generates an index array based on current split, so the location selected as train data is marked as "trainindex", and the location selected as test data is marked as "testindex"."ooftrain[testindex] = clf.predict(xte)" can only provide prediction values on the “testindex” location, so other values on "trainindex" location of "ooftrain" will hold zero.In the next split, the values of "ooftrain" will hold the values which are provided in the last split, and only the values on the new "testindex" location in current split will be provided by current prediction.The location of "testindex" in each split is different. So "the last iteration will overwrite the previous oftrain[test_index] result" will not happen.After n splits, all of the values of "oof_train" will be given by n predictions. Out-of-Fold Predictions
###Code
def get_oof(clf, x_train, y_train, x_test):
"""Get out-of-fold predictions for a given classifier."""
# Initialise the correct sized dfs that we will need to store our results.
oof_train = np.zeros((ntrain,))
oof_test = np.zeros((ntest,))
oof_test_skf = np.empty((n_splits, ntest))
# Loop through our kfold object
for i, (train_index, test_index) in enumerate(kf.split(x_train)):
# Use kfold object indexes to select the fold for train/test split
x_tr = x_train[train_index]
y_tr = y_train[train_index]
x_te = x_train[test_index]
# Train the model
clf.train(x_tr, y_tr)
# Predict on the in-fold training set
oof_train[test_index] = clf.predict(x_te)
# Predict on the out-of-fold testing set
oof_test_skf[i, :] = clf.predict(x_test)
# Take the mean of the 5 folds predictions
oof_test[:] = oof_test_skf.mean(axis=0)
# Returns both the training and testing predictions
return oof_train.reshape(-1, 1), oof_test.reshape(-1, 1)
###Output
_____no_output_____
###Markdown
Our Base First-Level Models So now let us prepare five learning models as our first level classification. These models can all be conveniently invoked via the Sklearn library and are listed as follows:* Random Forest classifier* Extra Trees classifier* AdaBoost classifer* Gradient Boosting classifer* Support Vector Machine ParametersJust a quick summary of the parameters that we will be listing here for completeness,**n_jobs :** Number of cores used for the training process. If set to -1, all cores are used.**n_estimators :** Number of classification trees in your learning model ( set to 10 per default)**max_depth :** Maximum depth of tree, or how much a node should be expanded. Beware if set to too high a number would run the risk of overfitting as one would be growing the tree too deep**verbose :** Controls whether you want to output any text during the learning process. A value of 0 suppresses all text while a value of 3 outputs the tree learning process at every iteration.
###Code
# Random Forest parameters
rf_params = {
'n_jobs': -1,
'n_estimators': 575,
'warm_start': True,
#'max_features': 0.2,
'max_depth': 5,
'min_samples_leaf': 2,
'max_features' : 'sqrt',
'verbose': 3
}
# Extra Trees Parameters
et_params = {
'n_jobs': -1,
'n_estimators':575,
#'max_features': 0.5,
'max_depth': 8,
'min_samples_leaf': 3,
'verbose': 3
}
# AdaBoost parameters
ada_params = {
'n_estimators': 500,
'learning_rate' : 0.95
}
# Gradient Boosting parameters
gb_params = {
'n_estimators': 575,
#'max_features': 0.2,
'max_depth': 5,
'min_samples_leaf': 3,
'verbose': 3
}
# Support Vector Classifier parameters
svc_params = {
'kernel' : 'linear',
'C' : 0.025
}
###Output
_____no_output_____
###Markdown
Now, we can use our helper functions to initialise our level 1 classifiers and then return our results as a dataframe. We have already successfully imported all of your classifiers (from sklearn.ensemble import RandomForestClassifier) and created dictionaries of their respective hyper-parameters (rf_params).
###Code
# Create 5 objects that represent our 5 models
# # Initialize classifier objects
rf = SklearnHelper(clf=RandomForestClassifier, seed=SEED, params=rf_params)
et = SklearnHelper(clf=ExtraTreesClassifier, seed=SEED, params=et_params)
ada = SklearnHelper(clf=AdaBoostClassifier, seed=SEED, params=ada_params)
gb = SklearnHelper(clf=GradientBoostingClassifier, seed=SEED, params=gb_params)
svc = SklearnHelper(clf=SVC, seed=SEED, params=svc_params)
# Create Numpy arrays of train, test and target ( Survived) dataframes to feed into our models
y_train = train['Survived'].ravel()
train = train.drop(['Survived'], axis=1)
x_train = train.values # Creates an array of the train data
x_test = test.values # Creats an array of the test data
###Output
_____no_output_____
###Markdown
Output of the First level PredictionsWe now feed the training and test data into our 5 base classifiers and use the Out-of-Fold prediction function we defined earlier to generate our first level predictions.
###Code
# Create our out-of-fold train and test predictions. These base results will be used as new features.
et_oof_train, et_oof_test = get_oof(et, x_train, y_train, x_test) # Extra Trees
rf_oof_train, rf_oof_test = get_oof(rf,x_train, y_train, x_test) # Random Forest
ada_oof_train, ada_oof_test = get_oof(ada, x_train, y_train, x_test) # AdaBoost
gb_oof_train, gb_oof_test = get_oof(gb,x_train, y_train, x_test) # Gradient Boost
svc_oof_train, svc_oof_test = get_oof(svc,x_train, y_train, x_test) # Support Vector Classifier
print("Training is complete")
###Output
_____no_output_____
###Markdown
Feature importances generated from the different classifiersWe can utilise a very nifty feature of the Sklearn models and that is to output the importances of the various features in the training and test sets with one very simple line of code.
###Code
rf_feature = rf.feature_importances(x_train,y_train)
et_feature = et.feature_importances(x_train, y_train)
ada_feature = ada.feature_importances(x_train, y_train)
gb_feature = gb.feature_importances(x_train,y_train)
rf_features = list(rf_feature)
et_features = list(et_feature)
ada_features = list(ada_feature)
gb_features = list(gb_feature)
cols = train.columns.values
# Create a dataframe with features
feature_dataframe = pd.DataFrame( {'features': cols,
'Random Forest feature importances': rf_features,
'Extra Trees feature importances': et_features,
'AdaBoost feature importances': ada_features,
'Gradient Boost feature importances': gb_features
})
###Output
_____no_output_____
###Markdown
Interactive feature importances via Plotly scatterplots
###Code
# Scatter plot
trace = go.Scatter(
y = feature_dataframe['Random Forest feature importances'].values,
x = feature_dataframe['features'].values,
mode='markers',
marker=dict(
sizemode = 'diameter',
sizeref = 1,
size = 25,
# size= feature_dataframe['AdaBoost feature importances'].values,
#color = np.random.randn(500), #set color equal to a variable
color = feature_dataframe['Random Forest feature importances'].values,
colorscale='Portland',
showscale=True
),
text = feature_dataframe['features'].values
)
data = [trace]
layout= go.Layout(
autosize= True,
title= 'Random Forest Feature Importance',
hovermode= 'closest',
# xaxis= dict(
# title= 'Pop',
# ticklen= 5,
# zeroline= False,
# gridwidth= 2,
# ),
yaxis=dict(
title= 'Feature Importance',
ticklen= 5,
gridwidth= 2
),
showlegend= False
)
fig = go.Figure(data=data, layout=layout)
py.iplot(fig,filename='scatter2010')
# Scatter plot
trace = go.Scatter(
y = feature_dataframe['Extra Trees feature importances'].values,
x = feature_dataframe['features'].values,
mode='markers',
marker=dict(
sizemode = 'diameter',
sizeref = 1,
size = 25,
# size= feature_dataframe['AdaBoost feature importances'].values,
#color = np.random.randn(500), #set color equal to a variable
color = feature_dataframe['Extra Trees feature importances'].values,
colorscale='Portland',
showscale=True
),
text = feature_dataframe['features'].values
)
data = [trace]
layout= go.Layout(
autosize= True,
title= 'Extra Trees Feature Importance',
hovermode= 'closest',
# xaxis= dict(
# title= 'Pop',
# ticklen= 5,
# zeroline= False,
# gridwidth= 2,
# ),
yaxis=dict(
title= 'Feature Importance',
ticklen= 5,
gridwidth= 2
),
showlegend= False
)
fig = go.Figure(data=data, layout=layout)
py.iplot(fig,filename='scatter2010')
# Scatter plot
trace = go.Scatter(
y = feature_dataframe['AdaBoost feature importances'].values,
x = feature_dataframe['features'].values,
mode='markers',
marker=dict(
sizemode = 'diameter',
sizeref = 1,
size = 25,
# size= feature_dataframe['AdaBoost feature importances'].values,
#color = np.random.randn(500), #set color equal to a variable
color = feature_dataframe['AdaBoost feature importances'].values,
colorscale='Portland',
showscale=True
),
text = feature_dataframe['features'].values
)
data = [trace]
layout= go.Layout(
autosize= True,
title= 'AdaBoost Feature Importance',
hovermode= 'closest',
# xaxis= dict(
# title= 'Pop',
# ticklen= 5,
# zeroline= False,
# gridwidth= 2,
# ),
yaxis=dict(
title= 'Feature Importance',
ticklen= 5,
gridwidth= 2
),
showlegend= False
)
fig = go.Figure(data=data, layout=layout)
py.iplot(fig,filename='scatter2010')
# Scatter plot
trace = go.Scatter(
y = feature_dataframe['Gradient Boost feature importances'].values,
x = feature_dataframe['features'].values,
mode='markers',
marker=dict(
sizemode = 'diameter',
sizeref = 1,
size = 25,
# size= feature_dataframe['AdaBoost feature importances'].values,
#color = np.random.randn(500), #set color equal to a variable
color = feature_dataframe['Gradient Boost feature importances'].values,
colorscale='Portland',
showscale=True
),
text = feature_dataframe['features'].values
)
data = [trace]
layout= go.Layout(
autosize= True,
title= 'Gradient Boosting Feature Importance',
hovermode= 'closest',
# xaxis= dict(
# title= 'Pop',
# ticklen= 5,
# zeroline= False,
# gridwidth= 2,
# ),
yaxis=dict(
title= 'Feature Importance',
ticklen= 5,
gridwidth= 2
),
showlegend= False
)
fig = go.Figure(data=data, layout=layout)
py.iplot(fig,filename='scatter2010')
###Output
_____no_output_____
###Markdown
Now let us calculate the mean of all the feature importances and store it as a new column in the feature importance dataframe.
###Code
# Create the new column containing the average of values
feature_dataframe['mean'] = feature_dataframe.mean(axis= 1) # axis = 1 computes the mean row-wise
feature_dataframe.head(10)
###Output
_____no_output_____
###Markdown
Second-Level Predictions from the First-level Output We are therefore having as our new columns the first-level predictions from our earlier classifiers and we train the next classifier on this.
###Code
base_predictions_train = pd.DataFrame( {'RandomForest': rf_oof_train.ravel(),
'ExtraTrees': et_oof_train.ravel(),
'AdaBoost': ada_oof_train.ravel(),
'GradientBoost': gb_oof_train.ravel()
})
base_predictions_train.head()
###Output
_____no_output_____
###Markdown
Correlation Heatmap of the Second Level Training set
###Code
data = [
go.Heatmap(
z= base_predictions_train.astype(float).corr().values ,
x=base_predictions_train.columns.values,
y= base_predictions_train.columns.values,
colorscale='Viridis',
showscale=True,
reversescale = True
)
]
py.iplot(data, filename='labelled-heatmap')
# Concatenate the training and test sets
x_train = np.concatenate(( et_oof_train, rf_oof_train, ada_oof_train, gb_oof_train, svc_oof_train), axis=1)
x_test = np.concatenate(( et_oof_test, rf_oof_test, ada_oof_test, gb_oof_test, svc_oof_test), axis=1)
###Output
_____no_output_____
###Markdown
Now, we can use the results from our level 1 classifier as inputs for our level 2 classifier. For our level 2 learner, we are going to use an XGBoost model. Second level learning model via XGBoostHere we choose the eXtremely famous library for boosted tree learning model, XGBoost. It was built to optimize large-scale boosted tree algorithms.We call an XGBClassifier and fit it to the first-level train and target data and use the learned model to predict the test data.Just a quick run down of the XGBoost parameters used in the model:max_depth : How deep you want to grow your tree. Beware if set to too high a number might run the risk of overfitting.gamma : minimum loss reduction required to make a further partition on a leaf node of the tree. The larger, the more conservative the algorithm will be.
###Code
xgboost = XGBClassifier(
learning_rate = 0.95,
n_estimators= 5000,
max_depth= 4,
min_child_weight= 2,
gamma=1,
subsample=0.8,
colsample_bytree=0.8,
objective= 'binary:logistic',
nthread= -1,
scale_pos_weight=1)
xgb_model_full_data = xgboost.fit(x_train, y_train)
predictions = xgb_model_full_data.predict(x_test).astype(int)
###Output
_____no_output_____
###Markdown
Plot final XGBoost Decision Tree
###Code
from xgboost import plot_tree
from matplotlib.pylab import rcParams
##set up the parameters
rcParams['figure.figsize'] = 80,50
# plot single tree
plot_tree(xgb_model_full_data, rankdir='LR')
###Output
_____no_output_____
###Markdown
Producing the Submission fileFinally having trained and fit all our first-level and second-level models, we can now output the predictions into the proper format for submission to the Titanic competition as follows:
###Code
# Generate Submission File
StackingSubmission = pd.DataFrame({ 'PassengerId': PassengerId,'Survived': predictions })
StackingSubmission.to_csv("Submission.csv", index=False)
###Output
_____no_output_____ |
apache_beam_06_CompTrf.ipynb | ###Markdown
Composite TransformsTransforms can have a nested structure, where a complex transform performs multiple simpler transforms (such as more than one ParDo, Combine, GroupByKey, or even other composite transforms). These transforms are called composite transforms. Nesting multiple transforms inside a single composite transform can make your code more modular and easier to understand.
###Code
import apache_beam as beam
class MyTransform(beam.PTransform):
""" Transform with common tasks """
def expand(self, input_coll):
a = (
input_coll
| 'Group and sum' >> beam.CombinePerKey(sum)
| 'count filter' >> beam.Filter(filter_on_count)
| 'Regular employee' >> beam.Map(format_output)
)
return a
def SplitRow(element):
return element.split(',')
def filter_on_count(element):
name, count = element
if count > 30:
return element
def format_output(element):
name, count = element
# return ', '.join((name.encode('ascii'),str(count),'Regular employee'))
return (name, str(count), 'Regular employee')
with beam.Pipeline() as pipe:
input_collection = (
pipe
| "Read file" >> beam.io.ReadFromText('data/dept_data.txt')
| "Split rows" >> beam.Map(SplitRow)
)
accounts_count = (
input_collection
| 'Get all Accounts dept persons' >> beam.Filter(lambda record: record[3] == 'Accounts')
| 'Pair each accounts employee with 1' >> beam.Map(lambda record: ("Accounts, " +record[1], 1))
| 'composite accounts' >> MyTransform()
| 'Write results for account' >> beam.io.WriteToText('data/Account')
)
hr_count = (
input_collection
| 'Get all HR dept persons' >> beam.Filter(lambda record: record[3] == 'HR')
| 'Pair each hr employee with 1' >> beam.Map(lambda record: ("HR, " +record[1], 1))
| 'composite HR' >> MyTransform()
| 'Write results for hr' >> beam.io.WriteToText('data/HR')
)
# visualize output
!{('head -n 20 data/Account-00000-of-00001')}
!{('head -n 20 data/HR-00000-of-00001')}
###Output
WARNING:apache_beam.io.filebasedsink:Deleting 1 existing files in target path matching: -*-of-%(num_shards)05d
WARNING:apache_beam.io.filebasedsink:Deleting 1 existing files in target path matching: -*-of-%(num_shards)05d
|
1S2020/EA979A_Ex01_AndreMedeiros.ipynb | ###Markdown
Ex01 - Aprendendo Numpy1. Exercite o uso do Numpy, através de pequenos exemplos utilizando principalmente a criação de arrays. Testes conceitos vistos nos tutoriais, como: usando os índices de um array, índices negativos, índices bidimensionais, slicing, reshape, transposição. Aproveite para explorar os recursos de documentação do jupyter e explique a medida que for realizando os diversos testes. Exemplo de uma sequência para o exercício: - crie um array - acesse elementos, linhas e colunas específicas do array, modificando seus valores - altere o tamanho do array - teste operações matriciais com arrays Visualizando arrays em forma de imagem
###Code
import numpy as np
f = np.ones((100,120))
print("Imagem constante:\n",f)
%matplotlib inline
import matplotlib.pyplot as plt
plt.title('Constant image')
plt.imshow(f,cmap='gray')
plt.colorbar()
###Output
_____no_output_____
###Markdown
Parte 1 - aprendendo numpy Frequency Shift Keying Aqui temos um código que implementa modulação FSK. Desenvolvido como projeto final da disciplina EE881, vários conceitos da biblioteca numpy e de arrays são implementados (como por exemplo appending, convolving e slicing).OBS: para testar o código, entre com "2", seguido de "20" (como descrito nos comentários do código). Caso queira testar o 4-FSK, deve-se substituir "test_2fsk.wav" por "test_4fsk.wav". Estes arquivos tem de ser baixados (no repositório: https://github.com/andre91998/EE881/tree/master/Pythone) e inseridos na pasta em que está este notebook.OBSII: Como se entende pelos comentários no próprio código, o ruído de gravação é simulado (assumindo ruído gaussiano). Caso queira que a decodificação tenha 100% de acurácia, deve-se comentar as linhas que implementam o ruído.
###Code
import numpy as np
import matplotlib.pyplot as plt
def bintoimg(bin_string):
while not len(bin_string)%8:
bin_string=np.append(bin_string,'0')
byte_string=[]
for i in range(int(len(bin_string)/8)):
byte='0b'
for j in range(8):
byte=byte+bin_string[8*i+j]
byte=int(byte,2)
byte_string=np.append(byte_string,byte)
img_shape=byte_string[0:2]
img=np.array(byte_string[2:])
img=np.reshape(img,img_shape.astype(int))
return img
def bintotext(bin_string):
while not len(bin_string)%8:
bin_string=np.append(bin_string,'0')
byte_string=[]
for i in range(int(len(bin_string)/8)):
byte='0b'
for j in range(8):
byte=byte+bin_string[8*i+j]
byte_string=np.append(byte_string,byte)
string=''
for i in byte_string:
string=string+chr(int(i,2))
return string
def app_decoder(bit_stream):
bittype_code=bit_stream[0:15]
type_code=[]
for i in range(3):
bits=bittype_code[5*i:5*i+5]
if(sum(bits)>2):
type_code.append(1)
else:
type_code.append(0)
size=bit_stream[15:31]
size_byte='0b'
for j in size:
size_byte=size_byte+str(j)
length=int(size_byte,2)
try: recebido=bit_stream[31:31+length]
except: recebido=bit_stream[31:]
#Teste 1
if(type_code==[0,0,0]):
objetivo=np.array([0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0])
while len(recebido)<len(objetivo):
recebido=np.append(recebido,0)
recebido=recebido[0:len(objetivo)]
acerto=sum(recebido==objetivo)/len(objetivo)
print('Taxa de acerto: '+str(acerto))
try:
out=bintotext(recebido)
print(out)
except:
print('Não consigo exibir o dado recebido')
#Imagem
elif(type_code==[0,0,1]):
objetivo=np.array([0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 1, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 0, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 0, 1, 1, 1, 1, 1, 0, 0, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1])
while len(recebido)<len(objetivo):
recebido=np.append(recebido,0)
recebido=recebido[0:len(objetivo)]
acerto=sum(recebido==objetivo)/len(objetivo)
print('Taxa de acerto: '+str(acerto))
try:
out=bintoimg(recebido)
plt.imshow(out,cmap='gray')
plt.show()
except:
print('Não consigo exibir o dado recebido')
#Hello world
elif(type_code==[0,1,0]):
objetivo=np.array([0, 1, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0])
while len(recebido)<len(objetivo):
recebido=np.append(recebido,0)
recebido=recebido[0:len(objetivo)]
acerto=sum(recebido==objetivo)/len(objetivo)
print('Taxa de acerto: '+str(acerto))
try:
out=bintotext(recebido)
print(out)
except:
print('Não consigo exibir o dado recebido')
#Texto_feec
elif(type_code==[0,1,1]):
objetivo=np.array([0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 1, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 1, 0])
while len(recebido)<len(objetivo):
recebido=np.append(recebido,0)
recebido=recebido[0:len(objetivo)]
acerto=sum(recebido==objetivo)/len(objetivo)
print('Taxa de acerto: '+str(acerto))
try:
out=bintotext(recebido)
print(out)
except:
print('Não consigo exibir o dado recebido')
#Genérico
else:
print('Codigo não reconhecido')
try:
out=bintotext(recebido)
print(out)
except:
print('Não consigo exibir o dado recebido')
return
app_decoder([0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0])
#Algorithm:
#1. Choose 2/4 FSK and Baud Rate
#2. Record transimission
#3. Apply matched filters for the 2/4 frequencies (filters with sin/cossin decomposition)
#4. From the outputs of the matched filters, obtain bit sequence which was transmitted
#5. Sweep using Min. dist.Varredura or correlate to identify end of header and start of message
#6. Remove header to obtain binary message
#7. Decode message with app_decoder
import numpy as np
import matplotlib.pyplot as plt
import soundfile as sf
from scipy.io.wavfile import write
#import app_decoder
#Header to be removed from aquired signal (from Header.txt, see repository)
header = [1, 1, 1, 1, 0, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1]
#choose 2-FSK or 4-FSK
mode = int(input("For 2-FSK type '2' or for 4-FSK type '4': "))
if mode == 2:
print("2-FSK selected")
if mode == 4:
print("4-FSK selected")
#choose baud rate (bit/sec) - Always choose 20
baud_rate = int(input("Choose Baud Rate: "))
print("Baud Rate = "+str(baud_rate)+" bit/sec")
#record the transmission
"""
fs = int(input("Recording Sample Rate: ")) # Sample rate, usually 24000
seconds = 65 # Duration of recording
myrecording = sd.rec(int(seconds * fs), samplerate=fs, channels=1)
sd.wait() # Wait until recording is finished
write('output.wav', fs, myrecording) # Save as WAV file
"""
#recording simulation
data, Fs = sf.read('test_2fsk.wav') #comment above to use test files
data = np.concatenate((np.zeros(100000), data), axis=None) #adding noise to simulate recording
noise = np.random.normal(0,6,len(data)) #adding noise to simulate recording
data = np.add(data,noise) #adding noise to simulate recording
#Process the recorded transmission (with noise)
print("Signal Received")
if mode == 2: #2-FSK
F1=800 #0
F2=1200 #1
t_wave=np.arange(0,1/baud_rate,1/Fs)
wave1=np.cos(2*np.pi*F1*t_wave)
wave2=np.cos(2*np.pi*F2*t_wave)
wave1_sin=np.sin(2*np.pi*F1*t_wave+np.pi/2)
wave1_cos=np.cos(2*np.pi*F1*t_wave+np.pi/2)
wave2_sin=np.sin(2*np.pi*F2*t_wave+np.pi/2)
wave2_cos=np.cos(2*np.pi*F2*t_wave+np.pi/2)
#Apply matched filters for the 2 frequencies (filters with sin/cossin decomposition)
casado_1=np.power(np.convolve(data,np.flip(wave1_sin)),2)+np.power(np.convolve(data,np.flip(wave1_cos)),2)
casado_2=np.power(np.convolve(data,np.flip(wave2_sin)),2)+np.power(np.convolve(data,np.flip(wave2_cos)),2)
"""
#Apply matched filters fo the 2 frequencies (moving mean)
casado_1=np.convolve(data,np.flip(wave1))
casado_1=np.convolve(np.abs(casado_1),np.ones((int(len(t_wave)/2))))
casado_2=np.convolve(data,np.flip(wave2))
casado_2=np.convolve(np.abs(casado_2),np.ones((int(len(t_wave)/2))))
"""
step=int(Fs/baud_rate)
t=np.arange(0,len(data)/Fs,1/Fs)
amostra_casado1=casado_1[step::step]
amostra_casado2=casado_2[step::step]
t_amostra=np.arange(step/Fs,t[-1]+step/Fs,step/Fs)
#example plot to verify above code
fig, axs = plt.subplots(2)
fig.suptitle('Saída dos filtros casados + decomposição em seno e cosseno- Erro de 90°')
axs[0].plot(t,casado_1[0:len(t)])
axs[0].plot(t_amostra,amostra_casado1[0:len(t_amostra)],'or')
axs[1].plot(t,casado_2[0:len(t)])
axs[1].plot(t_amostra,amostra_casado2[0:len(t_amostra)],'or')
plt.show()
#From the outputs of the matched filters, obtain bit sequence which was transmitted
transmission_float1 = amostra_casado1[0:len(t_amostra)]
transmission_float2 = amostra_casado2[0:len(t_amostra)] #from step above
max_value=max(transmission_float2)
min_value=min(transmission_float2)
mean_value= (max_value-min_value)/2
transmission = np.zeros((len(t_amostra),), dtype=int)
for i in range(0,len(t_amostra),1): #transform transmission vector to pure binary
if transmission_float2[i]>transmission_float1[i]:
transmission[i]=1
elif transmission_float2[i]<transmission_float1[i]:
transmission[i]=0
#------------- End 2-FSK "if" section -----------------#
if mode == 4: #4-FSK
F1=600 #00
F2=800 #01
F3=1000 #11
F4=1200 #10
t_wave=np.arange(0,1/baud_rate,1/Fs)
wave1=np.cos(2*np.pi*F1*t_wave)
wave2=np.cos(2*np.pi*F2*t_wave)
wave3=np.cos(2*np.pi*F3*t_wave)
wave4=np.cos(2*np.pi*F4*t_wave)
wave1_sin=np.sin(2*np.pi*F1*t_wave+np.pi/2)
wave1_cos=np.cos(2*np.pi*F1*t_wave+np.pi/2)
wave2_sin=np.sin(2*np.pi*F2*t_wave+np.pi/2)
wave2_cos=np.cos(2*np.pi*F2*t_wave+np.pi/2)
wave3_sin=np.sin(2*np.pi*F3*t_wave+np.pi/2)
wave3_cos=np.cos(2*np.pi*F3*t_wave+np.pi/2)
wave4_sin=np.sin(2*np.pi*F4*t_wave+np.pi/2)
wave4_cos=np.cos(2*np.pi*F4*t_wave+np.pi/2)
# Apply matched filters for the 4 frequencies (filters with sin/cossin decomposition)
casado_1=np.power(np.convolve(data,np.flip(wave1_sin)),2)+np.power(np.convolve(data,np.flip(wave1_cos)),2)
casado_2=np.power(np.convolve(data,np.flip(wave2_sin)),2)+np.power(np.convolve(data,np.flip(wave2_cos)),2)
casado_3=np.power(np.convolve(data,np.flip(wave3_sin)),2)+np.power(np.convolve(data,np.flip(wave3_cos)),2)
casado_4=np.power(np.convolve(data,np.flip(wave4_sin)),2)+np.power(np.convolve(data,np.flip(wave4_cos)),2)
step=int(Fs/baud_rate)
t=np.arange(0,len(data)/Fs,1/Fs)
amostra_casado1=casado_1[step::step]
amostra_casado2=casado_2[step::step]
amostra_casado3=casado_3[step::step]
amostra_casado4=casado_4[step::step]
t_amostra=np.arange(step/Fs,t[-1]+step/Fs,step/Fs)
#example plot to verify above code
fig, axs = plt.subplots(4)
fig.suptitle('Saída dos filtros casados + decomposição em seno e cosseno- Erro de 90°')
axs[0].plot(t,casado_1[0:len(t)])
axs[0].plot(t_amostra,amostra_casado1[0:len(t_amostra)],'or')
axs[1].plot(t,casado_2[0:len(t)])
axs[1].plot(t_amostra,amostra_casado2[0:len(t_amostra)],'or')
axs[2].plot(t,casado_3[0:len(t)])
axs[2].plot(t_amostra,amostra_casado3[0:len(t_amostra)],'or')
axs[3].plot(t,casado_4[0:len(t)])
axs[3].plot(t_amostra,amostra_casado4[0:len(t_amostra)],'or')
plt.show()
#From the outputs of the matched filters, obtain bit sequence which was transmitted
transmission_float1 = amostra_casado1[0:len(t_amostra)] #from step above
transmission_float2 = amostra_casado2[0:len(t_amostra)]
transmission_float3 = amostra_casado3[0:len(t_amostra)]
transmission_float4 = amostra_casado4[0:len(t_amostra)]
#check something
check_vector=np.zeros(len(amostra_casado1))
for i in range(0,len(amostra_casado1),1):
check_vector[i]=transmission_float1[i]+transmission_float2[i]+transmission_float3[i]+transmission_float4[i]
max_value = max(transmission_float1)
min_value = min(transmission_float1)
mean_value = (max_value + min_value)/2
transmission = np.zeros((2*len(t_amostra)), dtype=int)
#Method 1
for i in range(0,2*len(t_amostra),2): #transform transmission vector to pure binary
if transmission_float1[int(i/2)] > transmission_float2[int(i/2)] and transmission_float1[int(i/2)] > transmission_float3[int(i/2)] and transmission_float1[int(i/2)] > transmission_float4[int(i/2)]:
transmission[i]=0
transmission[i+1]=0
elif transmission_float2[int(i/2)] > transmission_float1[int(i/2)] and transmission_float2[int(i/2)] > transmission_float3[int(i/2)] and transmission_float2[int(i/2)] > transmission_float4[int(i/2)]:
transmission[i]=0
transmission[i+1]=1
elif transmission_float4[int(i/2)] > transmission_float1[int(i/2)] and transmission_float4[int(i/2)] > transmission_float2[int(i/2)] and transmission_float4[int(i/2)] > transmission_float3[int(i/2)]:
transmission[i]=1
transmission[i+1]=0
elif transmission_float3[int(i/2)] > transmission_float1[int(i/2)] and transmission_float3[int(i/2)] > transmission_float2[int(i/2)] and transmission_float3[int(i/2)] > transmission_float4[int(i/2)]:
transmission[i]=1
transmission[i+1]=1
#------------- End 4-FSK "if" section -----------------#
#sweep signal with header array to find position that starts the transmission
header_size = len(header)
print("Header size: " + str(header_size))
transmission_size = len(transmission)
print("Signal size: " + str(transmission_size))
check=0
start_pos=0
best_check=1000000
for i in range(0, (transmission_size - (header_size-1)), 1): #MaxI is the number of times the header fits in the signal in one sweep
for j in range(i, i+header_size, 1): #Store in Var check the "distance" between the header and current position in Var signal. Will slice at MinDist position
#print(j)
check = check + (abs(transmission[j]-header[j-i])^2)
#print(check)
if check < best_check:
#print("New best starting position found")
best_check = check
start_pos = i+header_size #starting position for array slice (removing header)
#("New Min Dist: " + str(check))
print("New Optimal Starting Position: " + str(start_pos))
check=0
print("Start Position Found: " + str(start_pos))
print("Slicing Received Message")
message = transmission[(start_pos):] #Here we slice the received signal and remove the header and everything before the header
print("Message Array Sliced")
print("Message Received: " + str(message))
#print(message==[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 1, 0, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 1, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 1, 0, 0, 1, 0, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 1, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0, 0, 1, 1, 0, 1, 1, 1, 0, 1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 1, 0, 1, 1, 1, 0, 1, 0, 1, 0, 0, 1, 0, 1, 1, 1, 0])
app_decoder(message) #decode message received
###Output
For 2-FSK type '2' or for 4-FSK type '4': 2
2-FSK selected
Choose Baud Rate: 20
Baud Rate = 20 bit/sec
###Markdown
Parte 2 - Entendendo resolução espacial e radiométrica
###Code
#import all necessary libraries
import numpy as np
from numpy.lib.stride_tricks import as_strided #for strided_rescale Function
import matplotlib.pyplot as plt
from IPython.display import display
from PIL import Image
#Display Original, un-altered, camerman image
img = Image.open('../data/cameraman.tif')
display(img)
###Output
_____no_output_____
###Markdown
1. **Entendendo a resolução espacial:** Reproduza as figuras dos slides 19 e 20 (Cap1.pdf). Use, no lugar da imagem da rosa, a imagem do cameraman.2. **Entendendo a resolução radiométrica:** Visualize a imagem do cameraman em níveis de cinza e altere progressivamente a quantização (slide 26). Important Functions
###Code
# Function with sole objective of making following code cleaner and easier to read
def show_image(input):
if input=='true':
plt.axis('off')
plt.colorbar()
plt.show()
if input=='false':
plt.axis('off')
plt.show
# Function for rescaling bit representation of an image (Binning)
def strided_rescale(g, bin_fac): #g == image array & bin_fac == binning factor
strided = as_strided(g,
shape=(g.shape[0]//bin_fac, g.shape[1]//bin_fac, bin_fac, bin_fac),
strides=((g.strides[0]*bin_fac, g.strides[1]*bin_fac)+g.strides)) #strides are the number of bytes from one row to the next
return strided.mean(axis=-1).mean(axis=-1)
###Output
_____no_output_____
###Markdown
Entendendo a Resolução Espacial (i)
###Code
numpy_img = np.array(img) #transform image into array
shape = numpy_img.shape #get image dimensions
#show image in original dimensions
print("Original Image Dimensions: "+str(shape)+'\n')
plt.imshow(numpy_img, cmap = 'gray')
show_image('false')
#Reduce Spacial Resolution to (128,128)
new_img = strided_rescale(numpy_img,2)
shape = new_img.shape
print("New Image Dimensions: " + str(shape) + "\n")
plt.imshow(new_img, cmap = 'gray')
show_image('false')
#Reduce Spacial Resolution to (64,64)
new_img2 = strided_rescale(new_img,2)
shape = new_img2.shape
print("New Image Dimensions: " + str(shape) + "\n")
plt.imshow(new_img2, cmap = 'gray')
show_image('false')
#Reduce Spacial Resolution to (32,32)
new_img3 = strided_rescale(new_img2,2)
shape = new_img3.shape
print("New Image Dimensions: " + str(shape) + "\n")
plt.imshow(new_img3, cmap = 'gray')
show_image('false')
#Reduce Spacial Resolution to (16,16)
new_img4 = strided_rescale(new_img3,2)
shape = new_img4.shape
print("New Image Dimensions: " + str(shape) + "\n")
plt.imshow(new_img4, cmap = 'gray')
show_image('false')
#Reduce Spacial Resolution to (8,8)
new_img5 = strided_rescale(new_img4,2)
shape = new_img5.shape
print("New Image Dimensions: " + str(shape) + "\n")
plt.imshow(new_img5, cmap = 'gray')
show_image('false')
###Output
_____no_output_____
###Markdown
Entendendo a Resolução Espacial (ii)
###Code
# generate new, smaller images
new_image = img.resize((128,128))
new_image2 = img.resize((64,64))
new_image3 = img.resize((32,32))
# display original and new images with appropriate titles
print("Original Image: (256,256)")
display(img)
print("\nSecond Image: (128,128)")
display(new_image)
print("\nThird Image: (64,64)")
display(new_image2)
print("\nFourth Image; (32,32)")
display(new_image3)
# Second Method:
g = np.array(img)
g.shape
# generate image with half the columns and lines as the original
g1a = g[::2,::2]
# generate image with half the columns and lines as the previous image
g2a = g1a[::2, ::2]
# generate image with half the columns and lines as the previous image
g3a = g2a[::2, ::2]
# generate image with half the columns and lines as the previous image
g4a = g3a[::2, ::2]
# generate image with half the columns and lines as the previous image
g5a = g4a[::2, ::2]
# generate image with half the columns and lines as the previous image
g6a = g5a[::2, ::2]
display(Image.fromarray(g))
display(Image.fromarray(g1a))
display(Image.fromarray(g2a))
display(Image.fromarray(g3a))
display(Image.fromarray(g4a))
display(Image.fromarray(g5a))
display(Image.fromarray(g6a))
###Output
_____no_output_____
###Markdown
Entendendo a Resolução Radiométrica
###Code
#show image in original color map
print("\nOriginal Image: 256 Colors")
plt.imshow(numpy_img, cmap = 'gray')
show_image('true')
#show image in "half colormap"
print("\nNew Image: 128 Colors")
plt.imshow(numpy_img, cmap='gray', vmin=65, vmax=192)
show_image('true')
#show image in "quarter colormap"
print("\nNew Image: 64 Colors")
plt.imshow(numpy_img, cmap='gray', vmin=97, vmax=160)
show_image('true')
#show image in "one 8th colormap"
print("\nNew Image: 32 Colors")
plt.imshow(numpy_img, cmap='gray', vmin=113, vmax=144)
show_image('true')
#show image in "one 16th colormap"
print("\nNew Image: 16 Colors")
plt.imshow(numpy_img, cmap='gray', vmin=121, vmax=136)
show_image('true')
#show image in "one 32ndth colormap"
print("\nNew Image: 8 Colors")
plt.imshow(numpy_img, cmap='gray', vmin=125, vmax=132)
show_image('true')
#show image in "one 64th colormap"
print("\nNew Image: 4 colors")
plt.imshow(numpy_img, cmap='gray', vmin=127, vmax=130)
show_image('true')
#show image in "one 128th colormap"
print("\nFinal Image: binary colormap")
plt.imshow(numpy_img, cmap='gray', vmin=128, vmax=129)
show_image('true')
###Output
_____no_output_____ |
001-Pre-processamento/.ipynb_checkpoints/Untitled-checkpoint.ipynb | ###Markdown
Pré-processamento da base de dados census.csv**Para essa base de dados utilizaremos técnicas de pré-processamento um pouco diferentes das aplicadas na base de dados credit_data.csv, pois nessa base do censo já foram verificados que não existem valores inconsistentes, e por ela possuir atributos com variáveis categóricas, devemos convertê-las para variáveis numéricas discretas.**Bom, o primeiro passo será importarmos a biblioteca pandas, para a criação e manipulação do *dataframe* que armazenará a base de dados census.csv. Também criaremos dois novos arrays, onde chamaremos o primeiro de "previsores", pois nele serão armazenados os atributos que irão relizar a previsão dos resultados. Já o segundo será chamado de "classe", pois para ele será passado o atributo que classifica cada um dos respectivos previsores.
###Code
import pandas as pd
base = pd.read_csv('census.csv')
previsores = base.iloc[:, 0:14].values
classe = base.iloc[:, 14].values
###Output
_____no_output_____ |
Chapter 1/Section 1.1.ipynb | ###Markdown
[1.1 引言](http://www-inst.eecs.berkeley.edu/~cs61a/sp12/book/functions.htmlintroduction) 计算机科学是一个极其宽泛的学科。全球的分布式系统、人工智能、机器人、图形、安全、科学计算,计算机体系结构和许多新兴的二级领域,每年都会由于新技术和新发现而扩展。计算机科学的快速发展广泛影响了人类生活。商业、通信、科学、艺术、休闲和政治都被计算机领域彻底改造。计算机科学的巨大生产力可能只是因为它构建在一系列优雅且强大的基础概念上。所有计算都以表达信息、指定处理它所需的逻辑、以及设计管理逻辑复杂性的抽象作为开始。对这些基础的掌握需要我们精确理解计算机如何解释程序以及执行计算过程。这些基础概念在伯克利长期教授,使用由Harold Abelson、Gerald Jay Sussman和Julie Sussman创作的经典教科书*《计算机科学的构造与解释》*(SICP)。这个讲义大量借鉴了这本书,原作者慷慨地使它可用于改编和复用。我们的智力之旅一旦出发就不能回头了,我们也永远都不应该对此有所期待。> 我们将要学习计算过程的概念。计算过程是计算机中的抽象事物。在演化中,过程操纵着叫做数据的其它事物。过程的演化由叫做程序的一系列规则主导。人们创造程序来主导过程。实际上,我们使用我们的咒语来凭空创造出计算机的灵魂。>> 我们用于创造过程的程序就像巫师的魔法。它们由一些古怪且深奥的编程语言中的符号表达式所组成,这些语言指定了我们想让过程执行的任务。>> 在一台工作正确的计算机上,计算过程准确且严谨地执行程序。所以,就像巫师的学徒那样,程序员新手必须学会理解和预测他们的魔法产生的结果。>> --Abelson & Sussman, SICP (1993) 1.1.1 在Python中编程 > 语言并不是你学到的东西,而是你参与的东西。>> --[Arika Okrent](http://arikaokrent.com/)为了定义计算过程,我们需要一种编程语言,最好是一种许多人和大量计算机都能懂的语言。这门课中,我们将会使用[Python](http://docs.python.org/py3k/)语言。Python是一种广泛使用的编程语言,并且在许多职业中都有它的爱好者:Web程序员、游戏工程师、科学家、学者,甚至新编程语言的设计师。当你学习Python时,你就加入到了一个数百万人的开发者社群。开发者社群是一个极其重要的组织:成员可以互相帮助来解决问题,分享他们的代码和经验,以及一起开发软件和工具。投入的成员经常由于他们的贡献而出名,并且收到广泛的尊重。也许有一天你会被提名为Python开发者精英。Python语言自身就是一个[大型志愿者社群](http://www.python.org/psf/members/)的产物,并且为其贡献者的[多元化](http://python.org/community/diversity/)而自豪。这种语言在20世纪80年代末由[Guido van Rossum](http://en.wikipedia.org/wiki/Guido_van_Rossum)设计并首次实现。他的[Python3教程](http://docs.python.org/py3k/tutorial/appetite.html)的第一章解释了为什么Python在当今众多语言之中如此流行。Python适用于作为教学语言,因为纵观它的历史,Python的开发者强调了Python代码对人类的解释性,并在[Python之禅](http://www.python.org/dev/peps/pep-0020/)中美观、简约和可读的原则下进一步加强。Python尤其适用于课堂,因为它宽泛的特性支持大量的不同编程风格,我们将要探索它们。在Python中编程没有单一的解法,但是有一些习俗在开发者社群之间流传,它们可以使现有程序的阅读、理解,以及扩展变得容易。所以,Python的灵活性和易学性的组合可以让学生们探索许多编程范式,之后将它们新学到的知识用于数千个[正在开发的项目](http://pypi.python.org/pypi)中。这些讲义通过使用抽象设计的技巧和严谨的计算模型,来快速介绍Python的特性。此外,这些讲义提供了Python编程的实践简介,包含一些高级语言特性和展示示例。通过这门课,学习Python将会变成自然而然的事情。然而,Python是一门生态丰富的语言,带有大量特性和用法。我们讲到基本的计算机科学概念时,会刻意慢慢地介绍他们。对于有经验的学生,他们打算一口气学完语言的所有细节,我们推荐他们阅读Mark Pilgrim的书[深入Python 3](http://www.ttlsa.com/docs/dive-into-python3/),它在网上可以免费阅读。这本书的主题跟这门课极其不同,但是这本书包含了许多关于使用Python的宝贵的实用信息。事先告知:不像这些讲义,Dive Into Python 3需要一些编程经验。开始在Python中编程的最佳方法就是直接和解释器交互。这一章会描述如何安装Python3,使用解释器开始交互式会话,以及开始编程。 1.1.2 安装Python3 就像所有伟大的软件一样,Python具有许多版本。这门课会使用**Python3**最新的稳定版本(本notebook编写时是3.7)。许多计算机都已经安装了Python的旧版本,但是它们可能不满足这门课。你应该可以在这门课上使用任何能安装Python3的计算机。不要担心,Python是免费的。一个比较方便的选择是安装[Anaconda Distribution](https://www.anaconda.com/distribution/),该发行版包含了大部分需要用到的库,并且支持Win/Mac/Linux。 1.1.3 Jupyter Notebook `Jupyter Notebook`是以网页的形式打开,可以在网页页面中直接编写代码和运行代码,代码的运行结果也会直接在代码块下显示的程序。如在编程过程中需要编写说明文档,可在同一个页面中直接编写,便于作及时的说明和解释。如果你使用Anaconda发行版,那么你已经安装了`Jupyter Notebook`。打开`Jupyter Notebook`后,点击后缀名为`.ipynb`的文件就可以运行一个已经创建的notebook。或者你也可以如下图所示,新建一个notebook。 notebook中的每一个可输入空间被称为一个`cell`,主要有`markdown`和`code`两种。例如当前我们输入文字的位置就是一个`markdown cell`,它支持将以[Markdown](http://younghz.github.io/Markdown/)格式书写的文字渲染成网页。下面是一个`code cell`的例子,在里面输入表达式并键入`-`,Jupyter后端就会运行该段代码,并将结果显示在该`cell`下方。
###Code
2 + 2
###Output
_____no_output_____
###Markdown
进入notebook后,可以在上方菜单栏最右边的`帮助`中查看关于Jupyter使用的更多信息和快捷键。先去看看吧! 1.1.4 第一个例子> 想像会把不知名的事物用一种形式呈现出来,诗人的笔再使它们具有如实的形象,空虚的无物也会有了居处和名字。>> --威廉·莎士比亚,《仲夏夜之梦》为了介绍Python,我们会从一个使用多个语言特性的例子开始。下一节中,我们会从零开始,一步一步构建整个语言。你可以将这章视为即将到来的特性的预览。Python拥有常见编程功能的内建支持,例如文本操作、显示图形以及互联网通信。导入语句
###Code
from urllib.request import urlopen
###Output
_____no_output_____
###Markdown
为访问互联网上的数据加载功能。特别是,它提供了叫做`urlopen`的函数,可以访问到统一资源定位器(URL)处的内容,它是互联网上的某个位置。 语句和表达式:Python代码包含**语句**和**表达式**。广泛地说,计算机程序包含的语句1. 计算某个值2. 或执行某个操作语句通常用于描述操作。当Python解释器执行语句时,它执行相应操作。另一方面,表达式通常描述产生值的运算。当Python求解表达式时,就会计算出它的值。这一章介绍了几种表达式和语句。 赋值语句
###Code
shakespeare = urlopen('http://inst.eecs.berkeley.edu/~cs61a/fa11/shakespeare.txt')
###Output
_____no_output_____
###Markdown
将名称`shakespeare`和后面的表达式的值关联起来。这个表达式在URL上调用`urlopen`函数,URL包含了莎士比亚的37个剧本的完整文本,在单个文本文件中。 函数函数封装了操作数据的逻辑。Web地址是一块数据,莎士比亚的剧本文本是另一块数据。前者产生后者的过程可能有些复杂,但是我们可以只通过一个表达式来调用它们,因为复杂性都塞进函数里了。函数是这一章的主要话题。另一个赋值语句
###Code
words = set(shakespeare.read().decode().split())
###Output
_____no_output_____
###Markdown
将名称`words`关联到出现在莎士比亚剧本中的所有去重词汇的集合,总计33,721个。这个命令链调用了`read`、`decode`和`split`,每个都操作衔接的计算实体:从URL读取的数据、解码为文本的数据、以及分割为单词的文本。所有这些单词都放在`set`中。 对象集合是一种对象,它支持取交和测试成员的操作。对象整合了数据和操作数据的逻辑,并以一种隐藏其复杂性的方式。对象是第二章的主要话题。表达式
###Code
{w for w in words if len(w) >= 5 and w[::-1] in words}
###Output
_____no_output_____ |
Chapter01/Gradient_Boosting_in_Machine_Learning.ipynb | ###Markdown
Machine Learning - Classification
###Code
# Upload Census dataset (adult) from UCI Machine Learning Repository
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data')
# Display first 5 rows
df_census.head()
# Upload Census dataset with no header
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data', header=None)
# Display first 5 rows
df_census.head()
# Define df_census columns
df_census.columns = ['age', 'workclass', 'fnlwgt', 'education', 'education-num', 'marital-status', 'occupation',
'relationship', 'race', 'sex', 'capital-gain', 'capital-loss', 'hours-per-week', 'native-country',
'income']
# Display first 5 rows
df_census.head()
# Display df_census info
df_census.info()
# Drop education column
df_census = df_census.drop(['education'], axis=1)
# Convert non-numeric columns using get_dummies
df_census = pd.get_dummies(df_census)
# Display first 5 rows
df_census.head()
# Drop column 'income_ <=50K'
df_census = df_census.drop('income_ <=50K', axis=1)
# Split data into X and y
X = df_census.iloc[:,:-1]
y = df_census.iloc[:,-1]
# Import Logistic Regression
from sklearn.linear_model import LogisticRegression
# Import cross_val_score
from sklearn.model_selection import cross_val_score
# Define cross_val function with classifer and num_splits as input
def cross_val(classifier, num_splits=10):
# Initialize classifier
model = classifier
# Obtain scores of cross-validation
scores = cross_val_score(model, X, y, cv=num_splits)
# Display accuracy
print('Accuracy:', np.round(scores, 2))
# Display mean accuracy
print('Accuracy mean: %0.2f' % (scores.mean()))
# Use cross_val function to score LogisticRegression
cross_val(LogisticRegression())
# Import XGBoost Classifier
from xgboost import XGBClassifier
# Use cross_val function to score XGBoost
cross_val(XGBClassifier(n_estimators=5))
###Output
Accuracy: [0.85 0.86 0.87 0.85 0.86 0.86 0.86 0.87 0.86 0.86]
Accuracy mean: 0.86
###Markdown
1장. 머신러닝 개요 *아래 링크를 통해 이 노트북을 주피터 노트북 뷰어(nbviewer.org)로 보거나 구글 코랩(colab.research.google.com)에서 실행할 수 있습니다.* 주피터 노트북 뷰어로 보기 구글 코랩(Colab)에서 실행하기
###Code
# 노트북이 코랩에서 실행 중인지 체크합니다.
import sys
if 'google.colab' in sys.modules:
!pip install -q --upgrade xgboost
!wget -q https://raw.githubusercontent.com/rickiepark/handson-gb/main/Chapter01/bike_rentals.csv
###Output
_____no_output_____
###Markdown
데이터 랭글링 데이터셋 1 - 자전거 대여
###Code
# 판다스를 임포트합니다.
import pandas as pd
# 'bike_rentals.csv'를 데이터프레임으로 읽습니다.
df_bikes = pd.read_csv('bike_rentals.csv')
# 처음 다섯 개 행을 출력합니다.
df_bikes.head()
###Output
_____no_output_____
###Markdown
데이터 이해하기 describe()
###Code
# df_bikes의 통계를 출력합니다.
df_bikes.describe()
###Output
_____no_output_____
###Markdown
info()
###Code
# df_bikes 정보를 출력합니다.
df_bikes.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 731 entries, 0 to 730
Data columns (total 16 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 instant 731 non-null int64
1 dteday 731 non-null object
2 season 731 non-null float64
3 yr 730 non-null float64
4 mnth 730 non-null float64
5 holiday 731 non-null float64
6 weekday 731 non-null float64
7 workingday 731 non-null float64
8 weathersit 731 non-null int64
9 temp 730 non-null float64
10 atemp 730 non-null float64
11 hum 728 non-null float64
12 windspeed 726 non-null float64
13 casual 731 non-null int64
14 registered 731 non-null int64
15 cnt 731 non-null int64
dtypes: float64(10), int64(5), object(1)
memory usage: 91.5+ KB
###Markdown
누락된 값 처리하기 누락된 값의 개수 계산하기
###Code
# 누락된 값의 개수 더하기
df_bikes.isna().sum().sum()
###Output
_____no_output_____
###Markdown
누락된 값 출력하기
###Code
# df_bikes에 있는 누락된 값을 출력합니다.
df_bikes[df_bikes.isna().any(axis=1)]
###Output
_____no_output_____
###Markdown
누락된 값 고치기 중간값이나 평균으로 바꾸기
###Code
# windspeed의 누락된 값을 중간값으로 채웁니다.
df_bikes['windspeed'].fillna((df_bikes['windspeed'].median()), inplace=True)
# 인덱스 56과 81의 행을 출력합니다.
df_bikes.iloc[[56, 81]]
###Output
_____no_output_____
###Markdown
중간값이나 평균으로 그룹바이 하기
###Code
# season으로 groupby한 중간값을 얻습니다.
df_bikes.groupby(['season']).median()
# 'hum' 열의 누락된 값을 season의 중간값으로 바꿉니다.
df_bikes['hum'] = df_bikes['hum'].fillna(df_bikes.groupby('season')['hum'].transform('median'))
###Output
_____no_output_____
###Markdown
특정 행에서 중간값이나 평균을 구하기
###Code
# 'temp' 열의 누락된 값을 확인합니다.
df_bikes[df_bikes['temp'].isna()]
# temp와 atemp의 평균을 계산합니다.
mean_temp = (df_bikes.iloc[700]['temp'] + df_bikes.iloc[702]['temp'])/2
mean_atemp = (df_bikes.iloc[700]['atemp'] + df_bikes.iloc[702]['atemp'])/2
# 누락된 값을 평균 온도로 대체합니다.
df_bikes['temp'].fillna((mean_temp), inplace=True)
df_bikes['atemp'].fillna((mean_atemp), inplace=True)
###Output
_____no_output_____
###Markdown
날짜 추정하기
###Code
# 'dteday' 열을 datetime 객체로 바꿉니다.
df_bikes['dteday'] = pd.to_datetime(df_bikes['dteday'])
df_bikes['dteday'].apply(pd.to_datetime, infer_datetime_format=True, errors='coerce')
# datetime을 임포트합니다.
import datetime as dt
df_bikes['mnth'] = df_bikes['dteday'].dt.month
# 마지막 다섯 개 행을 출력합니다.
df_bikes.tail()
# 인덱스 730 행의 'yr' 열을 1.0으로 바꿉니다.
df_bikes.loc[730, 'yr'] = 1.0
# 마지막 다섯 개 행을 출력합니다.
df_bikes.tail()
###Output
_____no_output_____
###Markdown
수치형이 아닌 열 삭제하기
###Code
# 'dteday' 열을 삭제합니다.
df_bikes = df_bikes.drop('dteday', axis=1)
###Output
_____no_output_____
###Markdown
회귀 모델 만들기 자전거 대여 예측하기
###Code
# 'casual', 'registered' 열을 삭제합니다.
df_bikes = df_bikes.drop(['casual', 'registered'], axis=1)
###Output
_____no_output_____
###Markdown
나중을 위해서 데이터 저장하기
###Code
# 'bike_rentals_cleaned.csv' 파일로 저장합니다.
df_bikes.to_csv('bike_rentals_cleaned.csv', index=False)
###Output
_____no_output_____
###Markdown
특성과 타깃 준비하기
###Code
# X와 y로 데이터를 나눕니다.
X = df_bikes.iloc[:,:-1]
y = df_bikes.iloc[:,-1]
###Output
_____no_output_____
###Markdown
사이킷런 사용하기
###Code
# train_test_split 함수를 임포트합니다.
from sklearn.model_selection import train_test_split
# LinearRegression 클래스를 임포트합니다.
from sklearn.linear_model import LinearRegression
# 데이터를 훈련 세트와 테스트 세트로 나눕니다.
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=2)
###Output
_____no_output_____
###Markdown
경고 끄기
###Code
# 경고를 표시하지 않습니다.
import warnings
warnings.filterwarnings('ignore')
import xgboost as xgb
xgb.set_config(verbosity=0)
###Output
_____no_output_____
###Markdown
선형 회귀 모델 만들기
###Code
# LinearRegression 모델 객체를 만듭니다.
lin_reg = LinearRegression()
# 훈련 데이터로 lin_reg 객체를 훈련합니다.
lin_reg.fit(X_train, y_train)
# lin_reg 객체를 사용해 X_test에 대한 예측값을 만듭니다.
y_pred = lin_reg.predict(X_test)
# mean_squared_error 함수를 임포트합니다.
from sklearn.metrics import mean_squared_error
# numpy를 임포트합니다.
import numpy as np
# mean_squared_error 함수로 평균 제곱 오차를 계산합니다.
mse = mean_squared_error(y_test, y_pred)
# 평균 제곱근 오차를 계산합니다.
rmse = np.sqrt(mse)
# 평균 제곱근 오차를 출력합니다.
print("RMSE: %0.2f" % (rmse))
mean_squared_error(y_test, y_pred, squared=False)
# 'cnt' 열의 통계를 출력합니다.
df_bikes['cnt'].describe()
###Output
_____no_output_____
###Markdown
XGBRegressor
###Code
# XGBRegressor를 임포트합니다.
from xgboost import XGBRegressor
# XGBRegressor의 객체 xg_reg를 만듭니다.
xg_reg = XGBRegressor()
# 훈련 데이터로 xg_reg 객체를 훈련합니다.
xg_reg.fit(X_train, y_train)
# 테스트 세트의 레이블을 예측합니다.
y_pred = xg_reg.predict(X_test)
# 평균 제곱 오차를 계산합니다.
mse = mean_squared_error(y_test, y_pred)
# 평균 제곱근 오차를 계산합니다.
rmse = np.sqrt(mse)
# 평균 제곱근 오차를 출력합니다.
print("RMSE: %0.2f" % (rmse))
###Output
RMSE: 705.11
###Markdown
교차 검증 선형 회귀 교차 검증
###Code
# cross_val_score 함수를 임포트합니다.
from sklearn.model_selection import cross_val_score
# LinearRegression 클래스 객체를 만듭니다.
model = LinearRegression()
# 10-폴드 교차 검증으로 평균 제곱 오차를 구합니다.
scores = cross_val_score(model, X, y, scoring='neg_mean_squared_error', cv=10)
# 이 점수의 제곱근을 계산합니다.
rmse = np.sqrt(-scores)
# 평균 제곱근 오차를 출력합니다.
print('회귀 rmse:', np.round(rmse, 2))
# 평균 점수를 출력합니다.
print('RMSE 평균: %0.2f' % (rmse.mean()))
-np.mean(cross_val_score(model, X, y, scoring='neg_root_mean_squared_error', cv=10))
from sklearn.model_selection import cross_validate
cv_results = cross_validate(model, X, y, scoring='neg_root_mean_squared_error', cv=10)
-np.mean(cv_results['test_score'])
###Output
_____no_output_____
###Markdown
XGBoost 교차 검증
###Code
# XGBRegressor 객체를 만듭니다.
model = XGBRegressor(objective="reg:squarederror")
# 10-폴드 교차 검증으로 평균 제곱 오차를 구합니다.
scores = cross_val_score(model, X, y, scoring='neg_mean_squared_error', cv=10)
# 이 점수의 제곱근을 계산합니다.
rmse = np.sqrt(-scores)
# 평균 제곱근 오차를 출력합니다.
print('회귀 rmse:', np.round(rmse, 2))
# 평균 점수를 출력합니다.
print('RMSE 평균: %0.2f' % (rmse.mean()))
###Output
회귀 rmse: [ 717.65 692.8 520.7 737.68 835.96 1006.24 991.34 747.61 891.99
1731.13]
RMSE 평균: 887.31
###Markdown
분류 모델 만들기 데이터 랭글링 데이터 적재
###Code
# UCI 머신러닝 저장소에서 인구 조사 데이터셋(adult)을 로드합니다.
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data')
# 처음 다섯 개 행을 출력합니다.
df_census.head()
# 헤더가 없는 데이터셋을 로드합니다.
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data', header=None)
# 처음 다섯 개 행을 출력합니다.
df_census.head()
# df_census 열 이름을 정의합니다.
df_census.columns = ['age', 'workclass', 'fnlwgt', 'education', 'education-num', 'marital-status', 'occupation',
'relationship', 'race', 'sex', 'capital-gain', 'capital-loss', 'hours-per-week', 'native-country',
'income']
# 처음 다섯 개 행을 출력합니다.
df_census.head()
###Output
_____no_output_____
###Markdown
누락된 값
###Code
# df_census 정보를 출력합니다.
df_census.info()
###Output
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 32561 entries, 0 to 32560
Data columns (total 15 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 age 32561 non-null int64
1 workclass 32561 non-null object
2 fnlwgt 32561 non-null int64
3 education 32561 non-null object
4 education-num 32561 non-null int64
5 marital-status 32561 non-null object
6 occupation 32561 non-null object
7 relationship 32561 non-null object
8 race 32561 non-null object
9 sex 32561 non-null object
10 capital-gain 32561 non-null int64
11 capital-loss 32561 non-null int64
12 hours-per-week 32561 non-null int64
13 native-country 32561 non-null object
14 income 32561 non-null object
dtypes: int64(6), object(9)
memory usage: 3.7+ MB
###Markdown
수치형이 아닌 열
###Code
# education 열을 삭제합니다.
df_census = df_census.drop(['education'], axis=1)
# get_dummies를 사용해 숫자가 아닌 열을 바꿉니다.
df_census = pd.get_dummies(df_census)
# 처음 다섯 개 행을 출력합니다.
df_census.head()
###Output
_____no_output_____
###Markdown
특성과 타깃 데이터
###Code
# 'income_ <=50K' 열을 삭제합니다.
df_census = df_census.drop('income_ <=50K', axis=1)
# 데이터를 X와 y로 나눕니다.
X = df_census.iloc[:,:-1]
y = df_census.iloc[:,-1]
###Output
_____no_output_____
###Markdown
로지스틱 회귀
###Code
# LogisticRegression을 임포트합니다.
from sklearn.linear_model import LogisticRegression
###Output
_____no_output_____
###Markdown
교차 검증 함수
###Code
# classifier와 num_splits 매개변수를 가진 cross_val 함수를 정의합니다.
def cross_val(classifier, num_splits=10):
# 분류 모델 생성
model = classifier
# 교차 검증 점수 얻기
scores = cross_val_score(model, X, y, cv=num_splits)
# 정확도 출력
print('정확도:', np.round(scores, 2))
# 평균 정확도 출력
print('평균 정확도: %0.2f' % (scores.mean()))
# LogisticRegression으로 cross_val 함수를 호출합니다.
cross_val(LogisticRegression())
###Output
정확도: [0.8 0.8 0.79 0.8 0.79 0.81 0.79 0.79 0.8 0.8 ]
평균 정확도: 0.80
###Markdown
XGBClassifier
###Code
# XGBClassifier를 임포트합니다.
from xgboost import XGBClassifier
# XGBClassifier로 cross_val 함수를 호출합니다.
cross_val(XGBClassifier(n_estimators=5))
###Output
정확도: [0.85 0.86 0.87 0.85 0.86 0.86 0.86 0.87 0.86 0.86]
평균 정확도: 0.86
###Markdown
Machine Learning - Classification
###Code
# Upload Census dataset (adult) from UCI Machine Learning Repository
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data')
# Display first 5 rows
df_census.head()
# Upload Census dataset with no header
df_census = pd.read_csv('https://archive.ics.uci.edu/ml/machine-learning-databases/adult/adult.data', header=None)
# Display first 5 rows
df_census.head()
# Define df_census columns
df_census.columns = ['age', 'workclass', 'fnlwgt', 'education', 'education-num', 'marital-status', 'occupation',
'relationship', 'race', 'sex', 'capital-gain', 'capital-loss', 'hours-per-week', 'native-country',
'income']
# Display first 5 rows
df_census.head()
# Display df_census info
df_census.info()
# Drop education column
df_census = df_census.drop(['education'], axis=1)
# Convert non-numeric columns using get_dummies
df_census = pd.get_dummies(df_census)
# Display first 5 rows
df_census.head()
# Drop column 'income_ <=50K'
df_census = df_census.drop('income_ <=50K', axis=1)
# Split data into X and y
X = df_census.iloc[:,:-1]
y = df_census.iloc[:,-1]
# Import Logistic Regression
from sklearn.linear_model import LogisticRegression
# Import cross_val_score
from sklearn.model_selection import cross_val_score
# Define cross_val function with classifer and num_splits as input
def cross_val(classifier, num_splits=10):
# Initialize classifier
model = classifier
# Obtain scores of cross-validation
scores = cross_val_score(model, X, y, cv=num_splits)
# Display accuracy
print('Accuracy:', np.round(scores, 2))
# Display mean accuracy
print('Accuracy mean: %0.2f' % (scores.mean()))
# Use cross_val function to score LogisticRegression
cross_val(LogisticRegression())
# Import XGBoost Classifier
from xgboost import XGBClassifier
# Use cross_val function to score XGBoost
cross_val(XGBClassifier(n_estimators=5))
###Output
_____no_output_____ |
utf-8''C4_W3_Assignment.ipynb | ###Markdown
Assignment 3: Question AnsweringWelcome to this week's assignment of course 4. In this you will explore question answering. You will implement the "Text to Text Transfer from Transformers" (better known as T5). Since you implemented transformers from scratch last week you will now be able to use them. Outline- [Overview](0)- [Part 0: Importing the Packages](0)- [Part 1: C4 Dataset](1) - [1.1 Pre-Training Objective](1.1) - [1.2 Process C4](1.2) - [1.2.1 Decode to natural language](1.2.1) - [1.3 Tokenizing and Masking](1.3) - [Exercise 01](ex01) - [1.4 Creating the Pairs](1.4)- [Part 2: Transfomer](2) - [2.1 Transformer Encoder](2.1) - [2.1.1 The Feedforward Block](2.1.1) - [Exercise 02](ex02) - [2.1.2 The Encoder Block](2.1.2) - [Exercise 03](ex03) - [2.1.3 The Transformer Encoder](2.1.3) - [Exercise 04](ex04) OverviewThis assignment will be different from the two previous ones. Due to memory and time constraints of this environment you will not be able to train a model and use it for inference. Instead you will create the necessary building blocks for the transformer encoder model and will use a pretrained version of the same model in two ungraded labs after this assignment.After completing these 3 (1 graded and 2 ungraded) labs you will:* Implement the code neccesary for Bidirectional Encoder Representation from Transformer (BERT).* Understand how the C4 dataset is structured.* Use a pretrained model for inference.* Understand how the "Text to Text Transfer from Transformers" or T5 model works. Part 0: Importing the Packages
###Code
import ast
import string
import textwrap
import itertools
import numpy as np
import trax
from trax import layers as tl
from trax.supervised import decoding
# Will come handy later.
wrapper = textwrap.TextWrapper(width=70)
# Set random seed
np.random.seed(42)
###Output
INFO:tensorflow:tokens_length=568 inputs_length=512 targets_length=114 noise_density=0.15 mean_noise_span_length=3.0
###Markdown
Part 1: C4 DatasetThe [C4](https://www.tensorflow.org/datasets/catalog/c4) is a huge data set. For the purpose of this assignment you will use a few examples out of it which are present in `data.txt`. C4 is based on the [common crawl](https://commoncrawl.org/) project. Feel free to read more on their website. Run the cell below to see how the examples look like.
###Code
# load example jsons
example_jsons = list(map(ast.literal_eval, open('data.txt')))
# Printing the examples to see how the data looks like
for i in range(5):
print(f'example number {i+1}: \n\n{example_jsons[i]} \n')
###Output
example number 1:
{'content-length': b'1970', 'content-type': b'text/plain', 'text': b'Beginners BBQ Class Taking Place in Missoula!\nDo you want to get better at making delicious BBQ? You will have the opportunity, put this on your calendar now. Thursday, September 22nd join World Class BBQ Champion, Tony Balay from Lonestar Smoke Rangers. He will be teaching a beginner level class for everyone who wants to get better with their culinary skills.\nHe will teach you everything you need to know to compete in a KCBS BBQ competition, including techniques, recipes, timelines, meat selection and trimming, plus smoker and fire information.\nThe cost to be in the class is $35 per person, and for spectators it is free. Included in the cost will be either a t-shirt or apron and you will be tasting samples of each meat that is prepared.', 'timestamp': b'2019-04-25T12:57:54Z', 'url': b'https://klyq.com/beginners-bbq-class-taking-place-in-missoula/'}
example number 2:
{'content-length': b'12064', 'content-type': b'text/plain', 'text': b'Discussion in \'Mac OS X Lion (10.7)\' started by axboi87, Jan 20, 2012.\nI\'ve got a 500gb internal drive and a 240gb SSD.\nWhen trying to restore using disk utility i\'m given the error "Not enough space on disk ____ to restore"\nBut I shouldn\'t have to do that!!!\nAny ideas or workarounds before resorting to the above?\nUse Carbon Copy Cloner to copy one drive to the other. I\'ve done this several times going from larger HDD to smaller SSD and I wound up with a bootable SSD drive. One step you have to remember not to skip is to use Disk Utility to partition the SSD as GUID partition scheme HFS+ before doing the clone. If it came Apple Partition Scheme, even if you let CCC do the clone, the resulting drive won\'t be bootable. CCC usually works in "file mode" and it can easily copy a larger drive (that\'s mostly empty) onto a smaller drive. If you tell CCC to clone a drive you did NOT boot from, it can work in block copy mode where the destination drive must be the same size or larger than the drive you are cloning from (if I recall).\nI\'ve actually done this somehow on Disk Utility several times (booting from a different drive (or even the dvd) so not running disk utility from the drive your cloning) and had it work just fine from larger to smaller bootable clone. Definitely format the drive cloning to first, as bootable Apple etc..\nThanks for pointing this out. My only experience using DU to go larger to smaller was when I was trying to make a Lion install stick and I was unable to restore InstallESD.dmg to a 4 GB USB stick but of course the reason that wouldn\'t fit is there was slightly more than 4 GB of data.', 'timestamp': b'2019-04-21T10:07:13Z', 'url': b'https://forums.macrumors.com/threads/restore-from-larger-disk-to-smaller-disk.1311329/'}
example number 3:
{'content-length': b'5235', 'content-type': b'text/plain', 'text': b'Foil plaid lycra and spandex shortall with metallic slinky insets. Attached metallic elastic belt with O-ring. Headband included. Great hip hop or jazz dance costume. Made in the USA.', 'timestamp': b'2019-04-25T10:40:23Z', 'url': b'https://awishcometrue.com/Catalogs/Clearance/Tweens/V1960-Find-A-Way'}
example number 4:
{'content-length': b'4967', 'content-type': b'text/plain', 'text': b"How many backlinks per day for new site?\nDiscussion in 'Black Hat SEO' started by Omoplata, Dec 3, 2010.\n1) for a newly created site, what's the max # backlinks per day I should do to be safe?\n2) how long do I have to let my site age before I can start making more blinks?\nI did about 6000 forum profiles every 24 hours for 10 days for one of my sites which had a brand new domain.\nThere is three backlinks for every of these forum profile so thats 18 000 backlinks every 24 hours and nothing happened in terms of being penalized or sandboxed. This is now maybe 3 months ago and the site is ranking on first page for a lot of my targeted keywords.\nbuild more you can in starting but do manual submission and not spammy type means manual + relevant to the post.. then after 1 month you can make a big blast..\nWow, dude, you built 18k backlinks a day on a brand new site? How quickly did you rank up? What kind of competition/searches did those keywords have?", 'timestamp': b'2019-04-21T12:46:19Z', 'url': b'https://www.blackhatworld.com/seo/how-many-backlinks-per-day-for-new-site.258615/'}
example number 5:
{'content-length': b'4499', 'content-type': b'text/plain', 'text': b'The Denver Board of Education opened the 2017-18 school year with an update on projects that include new construction, upgrades, heat mitigation and quality learning environments.\nWe are excited that Denver students will be the beneficiaries of a four year, $572 million General Obligation Bond. Since the passage of the bond, our construction team has worked to schedule the projects over the four-year term of the bond.\nDenver voters on Tuesday approved bond and mill funding measures for students in Denver Public Schools, agreeing to invest $572 million in bond funding to build and improve schools and $56.6 million in operating dollars to support proven initiatives, such as early literacy.\nDenver voters say yes to bond and mill levy funding support for DPS students and schools. Click to learn more about the details of the voter-approved bond measure.\nDenver voters on Nov. 8 approved bond and mill funding measures for DPS students and schools. Learn more about what\xe2\x80\x99s included in the mill levy measure.', 'timestamp': b'2019-04-20T14:33:21Z', 'url': b'http://bond.dpsk12.org/category/news/'}
###Markdown
Notice the `b` before each string? This means that this data comes as bytes rather than strings. Strings are actually lists of bytes so for the rest of the assignments the name `strings` will be used to describe the data. To check this run the following cell:
###Code
type(example_jsons[0].get('text'))
###Output
_____no_output_____
###Markdown
1.1 Pre-Training Objective**Note:** The word "mask" will be used throughout this assignment in context of hiding/removing word(s)You will be implementing the BERT loss as shown in the following image. Assume you have the following text: **Thank you for inviting me to your party last week** Now as input you will mask the words in red in the text: **Input:** Thank you **X** me to your party **Y** week.**Output:** The model should predict the words(s) for **X** and **Y**. **Z** is used to represent the end. 1.2 Process C4C4 only has the plain string `text` field, so you will tokenize and have `inputs` and `targets` out of it for supervised learning. Given your inputs, the goal is to predict the targets during training. You will now take the `text` and convert it to `inputs` and `targets`.
###Code
# Grab text field from dictionary
natural_language_texts = [example_json['text'] for example_json in example_jsons]
# First text example
natural_language_texts[4]
###Output
_____no_output_____
###Markdown
1.2.1 Decode to natural languageThe following functions will help you `detokenize` and`tokenize` the text data. The `sentencepiece` vocabulary was used to convert from text to ids. This vocabulary file is loaded and used in this helper functions.`natural_language_texts` has the text from the examples we gave you. Run the cells below to see what is going on.
###Code
# Special tokens
PAD, EOS, UNK = 0, 1, 2
def detokenize(np_array):
return trax.data.detokenize(
np_array,
vocab_type='sentencepiece',
vocab_file='sentencepiece.model',
vocab_dir='.')
def tokenize(s):
# The trax.data.tokenize function operates on streams,
# that's why we have to create 1-element stream with iter
# and later retrieve the result with next.
return next(trax.data.tokenize(
iter([s]),
vocab_type='sentencepiece',
vocab_file='sentencepiece.model',
vocab_dir='.'))
# printing the encoding of each word to see how subwords are tokenized
tokenized_text = [(tokenize(word).tolist(), word) for word in natural_language_texts[0].split()]
print(tokenized_text, '\n')
# We can see that detokenize successfully undoes the tokenization
print(f"tokenized: {tokenize('Beginners')}\ndetokenized: {detokenize(tokenize('Beginners'))}")
###Output
tokenized: [12847 277]
detokenized: Beginners
###Markdown
As you can see above, you were able to take a piece of string and tokenize it. Now you will create `input` and `target` pairs that will allow you to train your model. T5 uses the ids at the end of the vocab file as sentinels. For example, it will replace: - `vocab_size - 1` by `` - `vocab_size - 2` by `` - and so forth. It assigns every word a `chr`.The `pretty_decode` function below, which you will use in a bit, helps in handling the type when decoding. Take a look and try to understand what the function is doing.Notice that:```pythonstring.ascii_letters = 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'```**NOTE:** Targets may have more than the 52 sentinels we replace, but this is just to give you an idea of things.
###Code
vocab_size = trax.data.vocab_size(
vocab_type='sentencepiece',
vocab_file='sentencepiece.model',
vocab_dir='.')
def get_sentinels(vocab_size=vocab_size, display=False):
sentinels = {}
for i, char in enumerate(reversed(string.ascii_letters), 1):
decoded_text = detokenize([vocab_size - i])
# Sentinels, ex: <Z> - <a>
sentinels[decoded_text] = f'<{char}>'
if display:
print(f'The sentinel is <{char}> and the decoded token is:', decoded_text)
return sentinels
sentinels = get_sentinels(vocab_size, display=True)
def pretty_decode(encoded_str_list, sentinels=sentinels):
# If already a string, just do the replacements.
if isinstance(encoded_str_list, (str, bytes)):
for token, char in sentinels.items():
encoded_str_list = encoded_str_list.replace(token, char)
return encoded_str_list
# We need to decode and then prettyfy it.
return pretty_decode(detokenize(encoded_str_list))
pretty_decode("I want to dress up as an Intellectual this halloween.")
###Output
_____no_output_____
###Markdown
The functions above make your `inputs` and `targets` more readable. For example, you might see something like this once you implement the masking function below. - Input sentence: Younes and Lukasz were working together in the lab yesterday after lunch. - Input: Younes and Lukasz **Z** together in the **Y** yesterday after lunch.- Target: **Z** were working **Y** lab. 1.3 Tokenizing and MaskingYou will now implement the `tokenize_and_mask` function. This function will allow you to tokenize and mask input words with a noise probability. We usually mask 15% of the words. Exercise 01
###Code
# UNQ_C1
# GRADED FUNCTION: tokenize_and_mask
def tokenize_and_mask(text, vocab_size=vocab_size, noise=0.15,
randomizer=np.random.uniform, tokenize=tokenize):
"""Tokenizes and masks a given input.
Args:
text (str or bytes): Text input.
vocab_size (int, optional): Size of the vocabulary. Defaults to vocab_size.
noise (float, optional): Probability of masking a token. Defaults to 0.15.
randomizer (function, optional): Function that generates random values. Defaults to np.random.uniform.
tokenize (function, optional): Tokenizer function. Defaults to tokenize.
Returns:
tuple: Tuple of lists of integers associated to inputs and targets.
"""
# current sentinel number (starts at 0)
cur_sentinel_num = 0
# inputs
inps = []
# targets
targs = []
### START CODE HERE (REPLACE INSTANCES OF 'None' WITH YOUR CODE) ###
# prev_no_mask is True if the previous token was NOT masked, False otherwise
# set prev_no_mask to True
prev_no_mask = True
# loop through tokenized `text`
for token in tokenize(text):
# check if the `noise` is greater than a random value (weighted coin flip)
if randomizer() < noise:
# check to see if the previous token was not masked
if prev_no_mask==True: # add new masked token at end_id
# number of masked tokens increases by 1
cur_sentinel_num += 1
# compute `end_id` by subtracting current sentinel value out of the total vocabulary size
end_id = vocab_size - cur_sentinel_num
# append `end_id` at the end of the targets
targs.append(end_id)
# append `end_id` at the end of the inputs
inps.append(end_id)
# append `token` at the end of the targets
targs.append(token)
# set prev_no_mask accordingly
prev_no_mask = False
else: # don't have two masked tokens in a row
# append `token ` at the end of the inputs
inps.append(token)
# set prev_no_mask accordingly
prev_no_mask = True
### END CODE HERE ###
return inps, targs
# Some logic to mock a np.random value generator
# Needs to be in the same cell for it to always generate same output
def testing_rnd():
def dummy_generator():
vals = np.linspace(0, 1, 10)
cyclic_vals = itertools.cycle(vals)
for _ in range(100):
yield next(cyclic_vals)
dumr = itertools.cycle(dummy_generator())
def dummy_randomizer():
return next(dumr)
return dummy_randomizer
input_str = natural_language_texts[0]
print(f"input string:\n\n{input_str}\n")
inps, targs = tokenize_and_mask(input_str, randomizer=testing_rnd())
print(f"tokenized inputs:\n\n{inps}\n")
print(f"targets:\n\n{targs}")
###Output
input string:
b'Beginners BBQ Class Taking Place in Missoula!\nDo you want to get better at making delicious BBQ? You will have the opportunity, put this on your calendar now. Thursday, September 22nd join World Class BBQ Champion, Tony Balay from Lonestar Smoke Rangers. He will be teaching a beginner level class for everyone who wants to get better with their culinary skills.\nHe will teach you everything you need to know to compete in a KCBS BBQ competition, including techniques, recipes, timelines, meat selection and trimming, plus smoker and fire information.\nThe cost to be in the class is $35 per person, and for spectators it is free. Included in the cost will be either a t-shirt or apron and you will be tasting samples of each meat that is prepared.'
tokenized inputs:
[31999, 15068, 4501, 3, 12297, 3399, 16, 5964, 7115, 31998, 531, 25, 241, 12, 129, 394, 44, 492, 31997, 58, 148, 56, 43, 8, 1004, 6, 474, 31996, 39, 4793, 230, 5, 2721, 6, 1600, 1630, 31995, 1150, 4501, 15068, 16127, 6, 9137, 2659, 5595, 31994, 782, 3624, 14627, 15, 12612, 277, 5, 216, 31993, 2119, 3, 9, 19529, 593, 853, 21, 921, 31992, 12, 129, 394, 28, 70, 17712, 1098, 5, 31991, 3884, 25, 762, 25, 174, 12, 214, 12, 31990, 3, 9, 3, 23405, 4547, 15068, 2259, 6, 31989, 6, 5459, 6, 13618, 7, 6, 3604, 1801, 31988, 6, 303, 24190, 11, 1472, 251, 5, 37, 31987, 36, 16, 8, 853, 19, 25264, 399, 568, 31986, 21, 21380, 7, 34, 19, 339, 5, 15746, 31985, 8, 583, 56, 36, 893, 3, 9, 3, 31984, 9486, 42, 3, 9, 1409, 29, 11, 25, 31983, 12246, 5977, 13, 284, 3604, 24, 19, 2657, 31982]
targets:
[31999, 12847, 277, 31998, 9, 55, 31997, 3326, 15068, 31996, 48, 30, 31995, 727, 1715, 31994, 45, 301, 31993, 56, 36, 31992, 113, 2746, 31991, 216, 56, 31990, 5978, 16, 31989, 379, 2097, 31988, 11, 27856, 31987, 583, 12, 31986, 6, 11, 31985, 26, 16, 31984, 17, 18, 31983, 56, 36, 31982, 5]
###Markdown
**Expected Output:**```CPPb'Beginners BBQ Class Taking Place in Missoula!\nDo you want to get better at making delicious BBQ? You will have the opportunity, put this on your calendar now. Thursday, September 22nd join World Class BBQ Champion, Tony Balay from Lonestar Smoke Rangers. He will be teaching a beginner level class for everyone who wants to get better with their culinary skills.\nHe will teach you everything you need to know to compete in a KCBS BBQ competition, including techniques, recipes, timelines, meat selection and trimming, plus smoker and fire information.\nThe cost to be in the class is $35 per person, and for spectators it is free. Included in the cost will be either a t-shirt or apron and you will be tasting samples of each meat that is prepared.'tokenized inputs:[31999, 15068, 4501, 3, 12297, 3399, 16, 5964, 7115, 31998, 531, 25, 241, 12, 129, 394, 44, 492, 31997, 58, 148, 56, 43, 8, 1004, 6, 474, 31996, 39, 4793, 230, 5, 2721, 6, 1600, 1630, 31995, 1150, 4501, 15068, 16127, 6, 9137, 2659, 5595, 31994, 782, 3624, 14627, 15, 12612, 277, 5, 216, 31993, 2119, 3, 9, 19529, 593, 853, 21, 921, 31992, 12, 129, 394, 28, 70, 17712, 1098, 5, 31991, 3884, 25, 762, 25, 174, 12, 214, 12, 31990, 3, 9, 3, 23405, 4547, 15068, 2259, 6, 31989, 6, 5459, 6, 13618, 7, 6, 3604, 1801, 31988, 6, 303, 24190, 11, 1472, 251, 5, 37, 31987, 36, 16, 8, 853, 19, 25264, 399, 568, 31986, 21, 21380, 7, 34, 19, 339, 5, 15746, 31985, 8, 583, 56, 36, 893, 3, 9, 3, 31984, 9486, 42, 3, 9, 1409, 29, 11, 25, 31983, 12246, 5977, 13, 284, 3604, 24, 19, 2657, 31982]targets:[31999, 12847, 277, 31998, 9, 55, 31997, 3326, 15068, 31996, 48, 30, 31995, 727, 1715, 31994, 45, 301, 31993, 56, 36, 31992, 113, 2746, 31991, 216, 56, 31990, 5978, 16, 31989, 379, 2097, 31988, 11, 27856, 31987, 583, 12, 31986, 6, 11, 31985, 26, 16, 31984, 17, 18, 31983, 56, 36, 31982, 5]``` You will now use the inputs and the targets from the `tokenize_and_mask` function you implemented above. Take a look at the masked sentence using your `inps` and `targs` from the sentence above.
###Code
print('Inputs: \n\n', pretty_decode(inps))
print('\nTargets: \n\n', pretty_decode(targs))
###Output
Inputs:
<Z> BBQ Class Taking Place in Missoul <Y> Do you want to get better at making <X>? You will have the opportunity, put <W> your calendar now. Thursday, September 22 <V> World Class BBQ Champion, Tony Balay <U>onestar Smoke Rangers. He <T> teaching a beginner level class for everyone<S> to get better with their culinary skills.<R> teach you everything you need to know to <Q> a KCBS BBQ competition,<P>, recipes, timelines, meat selection <O>, plus smoker and fire information. The<N> be in the class is $35 per person <M> for spectators it is free. Include <L> the cost will be either a <K>shirt or apron and you <J> tasting samples of each meat that is prepared <I>
Targets:
<Z> Beginners <Y>a! <X> delicious BBQ <W> this on <V>nd join <U> from L <T> will be<S> who wants<R> He will <Q> compete in<P> including techniques <O> and trimming<N> cost to <M>, and <L>d in <K>t- <J> will be <I>.
###Markdown
1.4 Creating the PairsYou will now create pairs using your dataset. You will iterate over your data and create (inp, targ) pairs using the functions that we have given you.
###Code
# Apply tokenize_and_mask
inputs_targets_pairs = [tokenize_and_mask(text) for text in natural_language_texts]
def display_input_target_pairs(inputs_targets_pairs):
for i, inp_tgt_pair in enumerate(inputs_targets_pairs, 1):
inps, tgts = inp_tgt_pair
inps, tgts = pretty_decode(inps), pretty_decode(tgts)
print(f'[{i}]\n\n'
f'inputs:\n{wrapper.fill(text=inps)}\n\n'
f'targets:\n{wrapper.fill(text=tgts)}\n\n\n\n')
display_input_target_pairs(inputs_targets_pairs)
###Output
[1]
inputs:
Beginners BBQ Class Taking <Z> in Missoul <Y>! Do you want to get
better at making delicious <X>? You will have the opportunity, <W>
this on <V> calendar now. Thursday <U> September 22 <T> join<S> Class
BBQ Champion, Tony Balay from Lonestar Smoke<R>ers <Q> He will be
teaching a beginner<P> class <O> everyone who wants<N> get better with
their <M> skills <L> He will teach <K> everything you need to know to
<J> in a KCBS BBQ <I> techniques, recipes, timelines, meat<H> and
trimming, plus smoker and fire information. The cost to be<G> the
class is $35 <F> person, and<E> spectators it is free. Included in the
cost will<D> either <C> t- <B> or apron and you will be tasting
samples <A> each meat that <z> prepared.
targets:
<Z> Place <Y>a <X> BBQ <W> put <V> your <U>, <T>nd<S> World<R> Rang
<Q>.<P> level <O> for<N> to <M> culinary <L>. <K> you <J> compete <I>
competition, including<H> selection<G> in <F> per<E> for<D> be<C>a
<B>shirt <A> of <z> is
[2]
inputs:
<Z> in 'Mac OS X <Y> (10 <X>7)' started by axb <W>i87, Jan 20, 2012.
I've got <V>a 500g <U> drive <T> a 240gb SSD. When trying to restore
using<S> utility i'm given the error "Not enough space on disk<R>____
to restore <Q> But I shouldn't have to do that!!! Any ideas or
work<P>s before <O>ing to the above? Use Carbon Copy Cloner to copy
one drive to the other. I'<N> done <M> several times going from <L>D
to <K> SSD and I wound <J> a bootable SSD drive. One step you <I>
remember not to skip is to use Disk Utility to partition the SSD as
GUID partition scheme<H> doing the <G>ne. If it came Apple <F>ition
Scheme, even if<E> let<D>CC do the clone, the resulting drive<C> boot
<B>. C <A> usually works <z> "file mode" and it can easily copy a
larger drive (that's mostly empty <y> onto a smaller drive.<x> you<w>
CCC to clone a drive you did<v> boot<u>, it can work <t> copy mode <s>
destination<r> must be<q> size or larger than the drive you
are<p>cloning from <o>if <n> recall <m>ve actually done this somehow
on Disk Utility <l> times<k>booting from <j>a different drive (or even
the dvd)<i> not running disk utility from the drive your clo<h>ing)
and had it work just fine from larger to smaller bootable clo<g>.
Definitely format the drive cloning to first <f> as bootable Apple
etc.. Thanks for <e> this out. My only experience <d> DU to go larger
to smaller was when <c> trying to make <b> install stick and I was
unable to restore InstallESD <a>dmg to a 4 GB Théâtre ofKeep the
reason that wouldn't fit isdürftig was slightly moreutti GB of data.
targets:
<Z> Discussion <Y> Lion <X>. <W>o <V> <U>b internal <T> and<S>
disk<R> <Q>"<P>around <O> resort<N>ve <M> this <L> larger HD <K>
smaller <J> up with <I> have to<H> HFS+ before<G>clo <F> Part<E>
you<D> C<C> won't be <B>able <A>CC <z> in <y>)<x> If<w> tell<v> NOT<u>
from <t> in block <s> where the<r> drive<q> the same<p> <o> ( <n> I
<m>). I' <l> several<k> ( <j> <i> so<h>n<g>ne <f>,<e>pointing <d>
using <c> I was <b>a Lion <a>. Théâtre USB stick butKeep coursedürftig
thereutti than 4
[3]
inputs:
<Z>il plaid <Y>lycra <X> spandex shortall with metallic slinky
<W>sets. Attache <V> metallic elastic belt with O <U>ring. Head <T>
included. Great hip hop<S> jazz dance costume.<R> in the USA.
targets:
<Z> Fo <Y> <X> and <W> in <V>d <U>- <T>band<S> or<R> Made
[4]
inputs:
How many backlink <Z> per day for new site? Discussion <Y> 'Black <X>
SEO' started by Omoplata, Dec 3, 2010. 1) for a <W> created site,
what's <V> max <U>links per day I should do to be safe? 2) how <T> do
I have<S> let my site<R> before I can start making more blinks? I did
about 6000 forum profiles every 24 hours for 10 days for <Q> of my
sites<P> had a brand new domain. There is <O> backlinks for every<N>
these <M> profile so <L>s 18 000 backlinks every 24 hours and nothing
happened in terms of being penalized <K> sandboxed. This is now maybe
3 months ago <J> the site <I> ranking on first page for<H>a lot<G> my
targeted keywords. build more you can in starting <F> do manual
submission and not spammy<E> means manual +<D> to<C> post.. <B> after
1 month you can <A> a <z> blast.. Wow, dude, you built 18k backlink
<y> a day<x> a brand<w>? How quickly did<v> rank up? What kind of
competition/search<u> did <t> keywords have?
targets:
<Z>s <Y> in <X> Hat <W> newly <V> the <U> # back <T> long<S> to<R> age
<Q> one<P> which <O> three<N> of <M> forum <L> that <K> or <J> and <I>
is<H> <G> of <F> but<E> type<D> relevant<C> the <B> then <A> make <z>
big <y>s<x> on<w> new site<v> you<u>es <t> those
[5]
inputs:
The Denver Board of Education opened the 2017-18 school year with an
update <Z> projects that include new construction <Y> upgrades, heat
mitigation <X> quality learning environments. We <W> excited <V>
Denver students will be the beneficiaries <U>a four year, $572 million
General Oblig <T> Bond.<S> the passage of the bond, our construction
team has worked to schedule<R> projects over <Q> four-year term<P>
bond. Denver voters on Tuesday approved bond and mill funding <O>
for<N> in Denver Public Schools, agreeing to invest $572 million in
bond funding <M> build and improve schools and <L>6.6 million in
operating dollars to support proven initiatives, <K> as early <J>
Denver voters say <I> to bond and mill levy funding<H> for<G>PS
students and schools. Click to learn more about the details of the
voter-approved <F> measure. Denver voters<E>. 8 approved bond and mill
funding<D> for DPS students and schools. Learn more about what’s
included in the mill <C>y measure.
targets:
<Z> on <Y>, <X> and <W> are <V> that <U> of <T>ation<S> Since<R> the
<Q> the<P> of the <O> measures<N> students <M> to <L> $5 <K> such <J>
literacy. <I> yes<H> support<G> D <F> bond<E> on Nov<D> measures<C>lev
###Markdown
Part 2: TransfomerWe now load a Transformer model checkpoint that has been pre-trained using the above C4 dataset and decode from it. This will save you a lot of time rather than have to train your model yourself. Later in this notebook, we will show you how to fine-tune your model.Start by loading in the model. We copy the checkpoint to local dir for speed, otherwise initialization takes a very long time. Last week you implemented the decoder part for the transformer. Now you will implement the encoder part. Concretely you will implement the following. 2.1 Transformer EncoderYou will now implement the transformer encoder. Concretely you will implement two functions. The first function is `FeedForwardBlock`. 2.1.1 The Feedforward BlockThe `FeedForwardBlock` function is an important one so you will start by implementing it. To do so, you need to return a list of the following: - [`tl.LayerNorm()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.normalization.LayerNorm) = layer normalization.- [`tl.Dense(d_ff)`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.core.Dense) = fully connected layer.- [`activation`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.activation_fns.Relu) = activation relu, tanh, sigmoid etc. - `dropout_middle` = we gave you this function (don't worry about its implementation).- [`tl.Dense(d_model)`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.core.Dense) = fully connected layer with same dimension as the model.- `dropout_final` = we gave you this function (don't worry about its implementation).You can always take a look at [trax documentation](https://trax-ml.readthedocs.io/en/latest/) if needed.**Instructions**: Implement the feedforward part of the transformer. You will be returning a list. Exercise 02
###Code
# UNQ_C2
# GRADED FUNCTION: FeedForwardBlock
def FeedForwardBlock(d_model, d_ff, dropout, dropout_shared_axes, mode, activation):
"""Returns a list of layers implementing a feed-forward block.
Args:
d_model: int: depth of embedding
d_ff: int: depth of feed-forward layer
dropout: float: dropout rate (how much to drop out)
dropout_shared_axes: list of integers, axes to share dropout mask
mode: str: 'train' or 'eval'
activation: the non-linearity in feed-forward layer
Returns:
A list of layers which maps vectors to vectors.
"""
dropout_middle = tl.Dropout(rate=dropout,
shared_axes=dropout_shared_axes,
mode=mode)
dropout_final = tl.Dropout(rate=dropout,
shared_axes=dropout_shared_axes,
mode=mode)
### START CODE HERE (REPLACE INSTANCES OF 'None' WITH YOUR CODE) ###
ff_block = [
# trax Layer normalization
tl.LayerNorm(),
# trax Dense layer using `d_ff`
tl.Dense(d_ff),
# activation() layer - you need to call (use parentheses) this func!
activation(),
# dropout middle layer
dropout_middle,
# trax Dense layer using `d_model`
tl.Dense(d_model),
# dropout final layer
dropout_final,
]
### END CODE HERE ###
return ff_block
# Print the block layout
feed_forward_example = FeedForwardBlock(d_model=512, d_ff=2048, dropout=0.8, dropout_shared_axes=0, mode = 'train', activation = tl.Relu)
print(feed_forward_example)
###Output
[LayerNorm, Dense_2048, Relu, Dropout, Dense_512, Dropout]
###Markdown
**Expected Output:**```CPP [LayerNorm, Dense_2048, Relu, Dropout, Dense_512, Dropout]``` 2.1.2 The Encoder BlockThe encoder block will use the `FeedForwardBlock`. You will have to build two residual connections. Inside the first residual connection you will have the `tl.layerNorm()`, `attention`, and `dropout_` layers. The second residual connection will have the `feed_forward`. You will also need to implement `feed_forward`, `attention` and `dropout_` blocks. So far you haven't seen the [`tl.Attention()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.attention.Attention) and [`tl.Residual()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.combinators.Residual) layers so you can check the docs by clicking on them. Exercise 03
###Code
# UNQ_C3
# GRADED FUNCTION: EncoderBlock
def EncoderBlock(d_model, d_ff, n_heads, dropout, dropout_shared_axes,
mode, ff_activation, FeedForwardBlock=FeedForwardBlock):
"""
Returns a list of layers that implements a Transformer encoder block.
The input to the layer is a pair, (activations, mask), where the mask was
created from the original source tokens to prevent attending to the padding
part of the input.
Args:
d_model (int): depth of embedding.
d_ff (int): depth of feed-forward layer.
n_heads (int): number of attention heads.
dropout (float): dropout rate (how much to drop out).
dropout_shared_axes (int): axes on which to share dropout mask.
mode (str): 'train' or 'eval'.
ff_activation (function): the non-linearity in feed-forward layer.
FeedForwardBlock (function): A function that returns the feed forward block.
Returns:
list: A list of layers that maps (activations, mask) to (activations, mask).
"""
### START CODE HERE (REPLACE INSTANCES OF 'None' WITH YOUR CODE) ###
# Attention block
attention = tl.Attention(
# Use dimension of the model
d_feature=d_model,
# Set it equal to number of attention heads
n_heads=n_heads,
# Set it equal `dropout`
dropout=dropout,
# Set it equal `mode`
mode=mode
)
# Call the function `FeedForwardBlock` (implemented before) and pass in the parameters
feed_forward = FeedForwardBlock(
d_model,
d_ff,
dropout,
dropout_shared_axes,
mode,
ff_activation
)
# Dropout block
dropout_ = tl.Dropout(
# set it equal to `dropout`
rate=dropout,
# set it equal to the axes on which to share dropout mask
shared_axes=dropout_shared_axes,
# set it equal to `mode`
mode=mode
)
encoder_block = [
# add `Residual` layer
tl.Residual(
# add norm layer
tl.LayerNorm(),
# add attention
attention,
# add dropout
dropout_,
),
# add another `Residual` layer
tl.Residual(
# add feed forward
feed_forward,
),
]
### END CODE HERE ###
return encoder_block
# Print the block layout
encoder_example = EncoderBlock(d_model=512, d_ff=2048, n_heads=6, dropout=0.8, dropout_shared_axes=0, mode = 'train', ff_activation=tl.Relu)
print(encoder_example)
###Output
[Serial_in2_out2[
Branch_in2_out3[
None
Serial_in2_out2[
LayerNorm
Serial_in2_out2[
Dup_out2
Dup_out2
Serial_in4_out2[
Parallel_in3_out3[
Dense_512
Dense_512
Dense_512
]
PureAttention_in4_out2
Dense_512
]
]
Dropout
]
]
Add_in2
], Serial[
Branch_out2[
None
Serial[
LayerNorm
Dense_2048
Relu
Dropout
Dense_512
Dropout
]
]
Add_in2
]]
###Markdown
**Expected Output:**```CPP[Serial_in2_out2[ Branch_in2_out3[ None Serial_in2_out2[ LayerNorm Serial_in2_out2[ Dup_out2 Dup_out2 Serial_in4_out2[ Parallel_in3_out3[ Dense_512 Dense_512 Dense_512 ] PureAttention_in4_out2 Dense_512 ] ] Dropout ] ] Add_in2], Serial[ Branch_out2[ None Serial[ LayerNorm Dense_2048 Relu Dropout Dense_512 Dropout ] ] Add_in2]]``` 2.1.3 The Transformer EncoderNow that you have implemented the `EncoderBlock`, it is time to build the full encoder. BERT, or Bidirectional Encoder Representations from Transformers is one such encoder. You will implement its core code in the function below by using the functions you have coded so far. The model takes in many hyperparameters, such as the `vocab_size`, the number of classes, the dimension of your model, etc. You want to build a generic function that will take in many parameters, so you can use it later. At the end of the day, anyone can just load in an API and call transformer, but we think it is important to make sure you understand how it is built. Let's get started. **Instructions:** For this encoder you will need a `positional_encoder` first (which is already provided) followed by `n_layers` encoder blocks, which are the same encoder blocks you previously built. Once you store the `n_layers` `EncoderBlock` in a list, you are going to encode a `Serial` layer with the following sublayers: - [`tl.Branch`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.combinators.Branch): helps with the branching and has the following sublayers: - `positional_encoder`. - [`tl.PaddingMask()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.attention.PaddingMask): layer that maps integer sequences to padding masks.- Your list of `EncoderBlock`s- [`tl.Select([0], n_in=2)`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.combinators.Select): Copies, reorders, or deletes stack elements according to indices.- [`tl.LayerNorm()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.normalization.LayerNorm).- [`tl.Mean()`](https://trax-ml.readthedocs.io/en/latest/trax.layers.htmltrax.layers.core.Mean): Mean along the first axis.- `tl.Dense()` with n_units set to n_classes. - `tl.LogSoftmax()` Please refer to the [trax documentation](https://trax-ml.readthedocs.io/en/latest/) for further information. Exercise 04
###Code
# UNQ_C4
# GRADED FUNCTION: TransformerEncoder
def TransformerEncoder(vocab_size=vocab_size,
n_classes=10,
d_model=512,
d_ff=2048,
n_layers=6,
n_heads=8,
dropout=0.1,
dropout_shared_axes=None,
max_len=2048,
mode='train',
ff_activation=tl.Relu,
EncoderBlock=EncoderBlock):
"""
Returns a Transformer encoder model.
The input to the model is a tensor of tokens.
Args:
vocab_size (int): vocab size. Defaults to vocab_size.
n_classes (int): how many classes on output. Defaults to 10.
d_model (int): depth of embedding. Defaults to 512.
d_ff (int): depth of feed-forward layer. Defaults to 2048.
n_layers (int): number of encoder/decoder layers. Defaults to 6.
n_heads (int): number of attention heads. Defaults to 8.
dropout (float): dropout rate (how much to drop out). Defaults to 0.1.
dropout_shared_axes (int): axes on which to share dropout mask. Defaults to None.
max_len (int): maximum symbol length for positional encoding. Defaults to 2048.
mode (str): 'train' or 'eval'. Defaults to 'train'.
ff_activation (function): the non-linearity in feed-forward layer. Defaults to tl.Relu.
EncoderBlock (function): Returns the encoder block. Defaults to EncoderBlock.
Returns:
trax.layers.combinators.Serial: A Transformer model as a layer that maps
from a tensor of tokens to activations over a set of output classes.
"""
positional_encoder = [
tl.Embedding(vocab_size, d_model),
tl.Dropout(rate=dropout, shared_axes=dropout_shared_axes, mode=mode),
tl.PositionalEncoding(max_len=max_len)
]
### START CODE HERE (REPLACE INSTANCES OF 'None' WITH YOUR CODE) ###
# Use the function `EncoderBlock` (implemented above) and pass in the parameters over `n_layers`
encoder_blocks = [EncoderBlock(d_model, d_ff, n_heads, dropout, dropout_shared_axes,
mode, ff_activation) for _ in range(n_layers)]
# Assemble and return the model.
return tl.Serial(
# Encode
tl.Branch(
# Use `positional_encoder`
positional_encoder,
# Use trax padding mask
tl.PaddingMask(),
),
# Use `encoder_blocks`
encoder_blocks,
# Use select layer
tl.Select([0],n_in=2),
# Use trax layer normalization
tl.LayerNorm(),
# Map to output categories.
# Use trax mean. set axis to 1
tl.Mean(axis=1),
# Use trax Dense using `n_classes`
tl.Dense(n_classes),
# Use trax log softmax
tl.LogSoftmax(),
)
### END CODE HERE ###
# Run this cell to see the structure of your model
# Only 1 layer is used to keep the output readable
TransformerEncoder(n_layers=1)
###Output
_____no_output_____ |
Utkarsh_NLP_Word2Vec.ipynb | ###Markdown
Implementing Word2Vec Model using Skip-Gram Importing the Necesssary Stuff
###Code
import numpy as np
import string
from nltk.corpus import stopwords
import re
###Output
_____no_output_____
###Markdown
Let's define some variables :V Number of unique words in our corpus of text ( Vocabulary )x Input layer (One hot encoding of our input word ). N Number of neurons in the hidden layer of neural networkW Weights between input layer and hidden layerW' Weights between hidden layer and output layery A softmax output layer having probabilities of every word in our vocabulary Softmax Function
###Code
def softmax(x):
"""Compute softmax values for each sets of scores in x."""
e_x = np.exp(x - np.max(x))
return e_x / e_x.sum()
###Output
_____no_output_____
###Markdown
Word2Vec Class Contains Functions for Forward Propogation, Backward Propogation, Training and Predicting the words. In Forward Propogation, We multiply one hot encoding of centre word (denoted by x) with the first weight matrix W to get hidden layer matrix h (of size N x 1). We then multiply the hidden layer vector h with second weight matrix W’ to get a new matrix u. We Then obtain our loss function, Which comes out to be E being our Loss Function.In Backward Propogation, We find the partial derivatives of our loss function with respect to W and W’ to apply gradient descent algorithm.
###Code
class word2vec(object):
def __init__(self):
self.N = 10
self.X_train = []
self.y_train = []
self.window_size = 2
self.alpha = 0.001
self.words = []
self.word_index = {}
def initialize(self,V,data):
self.V = V
self.W = np.random.uniform(-0.8, 0.8, (self.V, self.N))
self.W1 = np.random.uniform(-0.8, 0.8, (self.N, self.V))
self.words = data
for i in range(len(data)):
self.word_index[data[i]] = i
def feed_forward(self,X):
self.h = np.dot(self.W.T,X).reshape(self.N,1)
self.u = np.dot(self.W1.T,self.h)
#print(self.u)
self.y = softmax(self.u)
return self.y
def backpropagate(self,x,t):
e = self.y - np.asarray(t).reshape(self.V,1)
# e.shape is V x 1
dLdW1 = np.dot(self.h,e.T)
X = np.array(x).reshape(self.V,1)
dLdW = np.dot(X, np.dot(self.W1,e).T)
self.W1 = self.W1 - self.alpha*dLdW1
self.W = self.W - self.alpha*dLdW
def train(self,epochs):
for x in range(1,epochs):
self.loss = 0
for j in range(len(self.X_train)):
self.feed_forward(self.X_train[j])
self.backpropagate(self.X_train[j],self.y_train[j])
C = 0
for m in range(self.V):
if(self.y_train[j][m]):
self.loss += -1*self.u[m][0]
C += 1
self.loss += C*np.log(np.sum(np.exp(self.u)))
print("epoch ",x, " loss = ",self.loss)
self.alpha *= 1/( (1+self.alpha*x) )
def predict(self,word,number_of_predictions):
if word in self.words:
index = self.word_index[word]
X = [0 for i in range(self.V)]
X[index] = 1
prediction = self.feed_forward(X)
output = {}
for i in range(self.V):
output[prediction[i][0]] = i
top_context_words = []
for k in sorted(output,reverse=True):
top_context_words.append(self.words[output[k]])
if(len(top_context_words)>=number_of_predictions):
break
return top_context_words
else:
print("Word not found in dicitonary")
###Output
_____no_output_____
###Markdown
Functions for Preparing and Preprocessing Data
###Code
def preprocessing(corpus):
stop_words = set(stopwords.words('english'))
training_data = []
sentences = corpus.split(".")
for i in range(len(sentences)):
sentences[i] = sentences[i].strip()
sentence = sentences[i].split()
x = [word.strip(string.punctuation) for word in sentence
if word not in stop_words]
x = [word.lower() for word in x]
training_data.append(x)
return training_data
def prepare_data_for_training(sentences,w2v):
data = {}
for sentence in sentences:
for word in sentence:
if word not in data:
data[word] = 1
else:
data[word] += 1
V = len(data)
data = sorted(list(data.keys()))
vocab = {}
for i in range(len(data)):
vocab[data[i]] = i
#for i in range(len(words)):
for sentence in sentences:
for i in range(len(sentence)):
center_word = [0 for x in range(V)]
center_word[vocab[sentence[i]]] = 1
context = [0 for x in range(V)]
for j in range(i-w2v.window_size,i+w2v.window_size):
if i!=j and j>=0 and j<len(sentence):
context[vocab[sentence[j]]] += 1
w2v.X_train.append(center_word)
w2v.y_train.append(context)
w2v.initialize(V,data)
return w2v.X_train,w2v.y_train
###Output
_____no_output_____
###Markdown
Source Code
###Code
corpus = ""
corpus += "anarchism originated as a term of abuse first used against early working class radicals including the diggers of the english revolution and the sans culottes of the french revolution"
epochs = 1000
training_data = preprocessing(corpus)
w2v = word2vec()
prepare_data_for_training(training_data,w2v)
w2v.train(epochs)
print(w2v.predict("term",3))
###Output
1.29210593184752
epoch 484 loss = 141.29056401378705
epoch 485 loss = 141.28902844762388
epoch 486 loss = 141.2874991942975
epoch 487 loss = 141.2859762150668
epoch 488 loss = 141.2844594715055
epoch 489 loss = 141.28294892550002
epoch 490 loss = 141.28144453924565
epoch 491 loss = 141.2799462752441
epoch 492 loss = 141.2784540962994
epoch 493 loss = 141.2769679655161
epoch 494 loss = 141.2754878462951
epoch 495 loss = 141.2740137023315
epoch 496 loss = 141.2725454976114
epoch 497 loss = 141.2710831964087
epoch 498 loss = 141.2696267632827
epoch 499 loss = 141.26817616307508
epoch 500 loss = 141.26673136090713
epoch 501 loss = 141.26529232217675
epoch 502 loss = 141.26385901255594
epoch 503 loss = 141.26243139798834
epoch 504 loss = 141.2610094446858
epoch 505 loss = 141.25959311912683
epoch 506 loss = 141.25818238805297
epoch 507 loss = 141.2567772184668
epoch 508 loss = 141.255377577629
epoch 509 loss = 141.2539834330565
epoch 510 loss = 141.2525947525191
epoch 511 loss = 141.25121150403788
epoch 512 loss = 141.24983365588196
epoch 513 loss = 141.2484611765669
epoch 514 loss = 141.24709403485153
epoch 515 loss = 141.24573219973627
epoch 516 loss = 141.24437564046045
epoch 517 loss = 141.2430243265001
epoch 518 loss = 141.24167822756576
epoch 519 loss = 141.2403373135999
epoch 520 loss = 141.23900155477537
epoch 521 loss = 141.23767092149242
epoch 522 loss = 141.23634538437733
epoch 523 loss = 141.23502491427953
epoch 524 loss = 141.23370948226994
epoch 525 loss = 141.23239905963877
epoch 526 loss = 141.23109361789332
epoch 527 loss = 141.2297931287563
epoch 528 loss = 141.22849756416343
epoch 529 loss = 141.2272068962614
epoch 530 loss = 141.22592109740626
epoch 531 loss = 141.2246401401613
epoch 532 loss = 141.22336399729494
epoch 533 loss = 141.2220926417791
epoch 534 loss = 141.220826046787
epoch 535 loss = 141.2195641856916
epoch 536 loss = 141.2183070320636
epoch 537 loss = 141.21705455966963
epoch 538 loss = 141.21580674247045
epoch 539 loss = 141.21456355461896
epoch 540 loss = 141.21332497045887
epoch 541 loss = 141.21209096452262
epoch 542 loss = 141.21086151152966
epoch 543 loss = 141.20963658638496
epoch 544 loss = 141.208416164177
epoch 545 loss = 141.20720022017645
epoch 546 loss = 141.20598872983408
epoch 547 loss = 141.20478166877956
epoch 548 loss = 141.20357901281972
epoch 549 loss = 141.2023807379367
epoch 550 loss = 141.2011868202866
epoch 551 loss = 141.19999723619793
epoch 552 loss = 141.19881196216988
epoch 553 loss = 141.197630974871
epoch 554 loss = 141.1964542511375
epoch 555 loss = 141.19528176797178
epoch 556 loss = 141.19411350254111
epoch 557 loss = 141.1929494321762
epoch 558 loss = 141.19178953436904
epoch 559 loss = 141.1906337867725
epoch 560 loss = 141.18948216719826
epoch 561 loss = 141.18833465361547
epoch 562 loss = 141.18719122414956
epoch 563 loss = 141.1860518570808
epoch 564 loss = 141.18491653084263
epoch 565 loss = 141.18378522402088
epoch 566 loss = 141.18265791535185
epoch 567 loss = 141.18153458372137
epoch 568 loss = 141.1804152081634
epoch 569 loss = 141.17929976785862
epoch 570 loss = 141.17818824213344
epoch 571 loss = 141.17708061045823
epoch 572 loss = 141.1759768524467
epoch 573 loss = 141.17487694785405
epoch 574 loss = 141.17378087657636
epoch 575 loss = 141.17268861864864
epoch 576 loss = 141.1716001542442
epoch 577 loss = 141.1705154636734
epoch 578 loss = 141.16943452738224
epoch 579 loss = 141.16835732595135
epoch 580 loss = 141.1672838400947
epoch 581 loss = 141.16621405065877
epoch 582 loss = 141.16514793862103
epoch 583 loss = 141.16408548508903
epoch 584 loss = 141.16302667129935
epoch 585 loss = 141.16197147861624
epoch 586 loss = 141.16091988853097
epoch 587 loss = 141.15987188266016
epoch 588 loss = 141.15882744274532
epoch 589 loss = 141.15778655065154
epoch 590 loss = 141.1567491883662
epoch 591 loss = 141.15571533799834
epoch 592 loss = 141.15468498177754
epoch 593 loss = 141.1536581020528
epoch 594 loss = 141.15263468129152
epoch 595 loss = 141.15161470207846
epoch 596 loss = 141.1505981471153
epoch 597 loss = 141.14958499921877
epoch 598 loss = 141.14857524132043
epoch 599 loss = 141.14756885646543
epoch 600 loss = 141.14656582781146
epoch 601 loss = 141.14556613862817
epoch 602 loss = 141.1445697722958
epoch 603 loss = 141.14357671230462
epoch 604 loss = 141.14258694225387
epoch 605 loss = 141.14160044585077
epoch 606 loss = 141.14061720690998
epoch 607 loss = 141.1396372093524
epoch 608 loss = 141.1386604372042
epoch 609 loss = 141.13768687459654
epoch 610 loss = 141.1367165057639
epoch 611 loss = 141.13574931504397
epoch 612 loss = 141.13478528687637
epoch 613 loss = 141.13382440580193
epoch 614 loss = 141.1328666564621
epoch 615 loss = 141.1319120235977
epoch 616 loss = 141.1309604920484
epoch 617 loss = 141.13001204675197
epoch 618 loss = 141.12906667274325
epoch 619 loss = 141.12812435515366
epoch 620 loss = 141.12718507921025
epoch 621 loss = 141.12624883023483
epoch 622 loss = 141.1253155936434
epoch 623 loss = 141.12438535494556
epoch 624 loss = 141.12345809974312
epoch 625 loss = 141.12253381373023
epoch 626 loss = 141.12161248269172
epoch 627 loss = 141.1206940925034
epoch 628 loss = 141.11977862913042
epoch 629 loss = 141.11886607862715
epoch 630 loss = 141.117956427136
epoch 631 loss = 141.11704966088743
epoch 632 loss = 141.11614576619834
epoch 633 loss = 141.11524472947232
epoch 634 loss = 141.11434653719814
epoch 635 loss = 141.11345117594968
epoch 636 loss = 141.112558632385
epoch 637 loss = 141.1116688932458
epoch 638 loss = 141.1107819453565
epoch 639 loss = 141.10989777562392
epoch 640 loss = 141.10901637103666
epoch 641 loss = 141.108137718664
epoch 642 loss = 141.10726180565584
epoch 643 loss = 141.1063886192417
epoch 644 loss = 141.1055181467303
epoch 645 loss = 141.10465037550875
epoch 646 loss = 141.1037852930423
epoch 647 loss = 141.10292288687327
epoch 648 loss = 141.10206314462087
epoch 649 loss = 141.10120605398026
epoch 650 loss = 141.10035160272244
epoch 651 loss = 141.09949977869314
epoch 652 loss = 141.09865056981263
epoch 653 loss = 141.09780396407467
epoch 654 loss = 141.09695994954683
epoch 655 loss = 141.09611851436898
epoch 656 loss = 141.0952796467532
epoch 657 loss = 141.09444333498337
epoch 658 loss = 141.09360956741438
epoch 659 loss = 141.0927783324714
epoch 660 loss = 141.09194961864986
epoch 661 loss = 141.09112341451464
epoch 662 loss = 141.09029970869955
epoch 663 loss = 141.08947848990672
epoch 664 loss = 141.0886597469063
epoch 665 loss = 141.0878434685358
epoch 666 loss = 141.08702964369962
epoch 667 loss = 141.08621826136863
epoch 668 loss = 141.0854093105798
epoch 669 loss = 141.08460278043492
epoch 670 loss = 141.08379866010134
epoch 671 loss = 141.08299693881045
epoch 672 loss = 141.08219760585786
epoch 673 loss = 141.08140065060257
epoch 674 loss = 141.0806060624666
epoch 675 loss = 141.0798138309346
epoch 676 loss = 141.07902394555316
epoch 677 loss = 141.07823639593076
epoch 678 loss = 141.07745117173693
epoch 679 loss = 141.07666826270187
epoch 680 loss = 141.0758876586162
epoch 681 loss = 141.07510934933035
epoch 682 loss = 141.07433332475424
epoch 683 loss = 141.0735595748565
epoch 684 loss = 141.07278808966478
epoch 685 loss = 141.0720188592645
epoch 686 loss = 141.0712518737988
epoch 687 loss = 141.07048712346835
epoch 688 loss = 141.0697245985304
epoch 689 loss = 141.0689642892989
epoch 690 loss = 141.06820618614364
epoch 691 loss = 141.0674502794904
epoch 692 loss = 141.06669655981972
epoch 693 loss = 141.0659450176674
epoch 694 loss = 141.06519564362347
epoch 695 loss = 141.06444842833227
epoch 696 loss = 141.06370336249148
epoch 697 loss = 141.06296043685234
epoch 698 loss = 141.06221964221882
epoch 699 loss = 141.06148096944764
epoch 700 loss = 141.06074440944747
epoch 701 loss = 141.060009953179
epoch 702 loss = 141.05927759165417
epoch 703 loss = 141.0585473159359
epoch 704 loss = 141.057819117138
epoch 705 loss = 141.0570929864247
epoch 706 loss = 141.05636891500984
epoch 707 loss = 141.0556468941572
epoch 708 loss = 141.05492691517983
epoch 709 loss = 141.05420896943957
epoch 710 loss = 141.05349304834695
epoch 711 loss = 141.05277914336074
epoch 712 loss = 141.05206724598764
epoch 713 loss = 141.05135734778176
epoch 714 loss = 141.05064944034478
epoch 715 loss = 141.04994351532503
epoch 716 loss = 141.04923956441755
epoch 717 loss = 141.0485375793635
epoch 718 loss = 141.04783755195024
epoch 719 loss = 141.04713947401046
epoch 720 loss = 141.04644333742246
epoch 721 loss = 141.04574913410931
epoch 722 loss = 141.04505685603874
epoch 723 loss = 141.0443664952232
epoch 724 loss = 141.04367804371878
epoch 725 loss = 141.04299149362558
epoch 726 loss = 141.04230683708715
epoch 727 loss = 141.04162406629013
epoch 728 loss = 141.04094317346411
epoch 729 loss = 141.0402641508812
epoch 730 loss = 141.03958699085595
epoch 731 loss = 141.03891168574452
epoch 732 loss = 141.03823822794536
epoch 733 loss = 141.0375666098979
epoch 734 loss = 141.03689682408287
epoch 735 loss = 141.03622886302188
epoch 736 loss = 141.0355627192773
epoch 737 loss = 141.03489838545127
epoch 738 loss = 141.03423585418668
epoch 739 loss = 141.03357511816574
epoch 740 loss = 141.03291617011024
epoch 741 loss = 141.03225900278136
epoch 742 loss = 141.0316036089791
epoch 743 loss = 141.03094998154225
epoch 744 loss = 141.03029811334812
epoch 745 loss = 141.029647997312
epoch 746 loss = 141.02899962638745
epoch 747 loss = 141.02835299356542
epoch 748 loss = 141.02770809187442
epoch 749 loss = 141.02706491438013
epoch 750 loss = 141.02642345418533
epoch 751 loss = 141.02578370442916
epoch 752 loss = 141.02514565828767
epoch 753 loss = 141.02450930897254
epoch 754 loss = 141.02387464973202
epoch 755 loss = 141.02324167384967
epoch 756 loss = 141.02261037464476
epoch 757 loss = 141.0219807454718
epoch 758 loss = 141.0213527797203
epoch 759 loss = 141.0207264708144
epoch 760 loss = 141.02010181221317
epoch 761 loss = 141.01947879740976
epoch 762 loss = 141.01885741993166
epoch 763 loss = 141.01823767333985
epoch 764 loss = 141.0176195512295
epoch 765 loss = 141.017003047229
epoch 766 loss = 141.01638815499985
epoch 767 loss = 141.01577486823678
epoch 768 loss = 141.01516318066723
epoch 769 loss = 141.0145530860513
epoch 770 loss = 141.0139445781814
epoch 771 loss = 141.01333765088225
epoch 772 loss = 141.01273229801026
epoch 773 loss = 141.0121285134539
epoch 774 loss = 141.01152629113312
epoch 775 loss = 141.0109256249991
epoch 776 loss = 141.01032650903437
epoch 777 loss = 141.00972893725225
epoch 778 loss = 141.00913290369667
epoch 779 loss = 141.00853840244253
epoch 780 loss = 141.00794542759485
epoch 781 loss = 141.00735397328876
epoch 782 loss = 141.00676403368948
epoch 783 loss = 141.00617560299202
epoch 784 loss = 141.00558867542088
epoch 785 loss = 141.00500324522997
epoch 786 loss = 141.00441930670257
epoch 787 loss = 141.0038368541509
epoch 788 loss = 141.00325588191592
epoch 789 loss = 141.0026763843674
epoch 790 loss = 141.0020983559037
epoch 791 loss = 141.0015217909512
epoch 792 loss = 141.00094668396457
epoch 793 loss = 141.0003730294264
epoch 794 loss = 140.9998008218472
epoch 795 loss = 140.99923005576483
epoch 796 loss = 140.99866072574466
epoch 797 loss = 140.99809282637938
epoch 798 loss = 140.99752635228884
epoch 799 loss = 140.99696129811957
epoch 800 loss = 140.99639765854513
epoch 801 loss = 140.99583542826542
epoch 802 loss = 140.9952746020069
epoch 803 loss = 140.99471517452218
epoch 804 loss = 140.99415714059006
epoch 805 loss = 140.99360049501524
epoch 806 loss = 140.99304523262805
epoch 807 loss = 140.9924913482847
epoch 808 loss = 140.99193883686664
epoch 809 loss = 140.9913876932806
epoch 810 loss = 140.99083791245857
epoch 811 loss = 140.99028948935745
epoch 812 loss = 140.9897424189591
epoch 813 loss = 140.98919669626974
epoch 814 loss = 140.98865231632044
epoch 815 loss = 140.98810927416642
epoch 816 loss = 140.98756756488737
epoch 817 loss = 140.9870271835868
epoch 818 loss = 140.98648812539224
epoch 819 loss = 140.98595038545508
epoch 820 loss = 140.98541395895032
epoch 821 loss = 140.98487884107632
epoch 822 loss = 140.98434502705496
epoch 823 loss = 140.98381251213135
epoch 824 loss = 140.9832812915734
epoch 825 loss = 140.98275136067235
epoch 826 loss = 140.98222271474194
epoch 827 loss = 140.98169534911852
epoch 828 loss = 140.98116925916128
epoch 829 loss = 140.98064444025158
epoch 830 loss = 140.98012088779296
epoch 831 loss = 140.97959859721124
epoch 832 loss = 140.979077563954
epoch 833 loss = 140.97855778349088
epoch 834 loss = 140.97803925131333
epoch 835 loss = 140.9775219629341
epoch 836 loss = 140.9770059138875
epoch 837 loss = 140.97649109972917
epoch 838 loss = 140.9759775160361
epoch 839 loss = 140.9754651584063
epoch 840 loss = 140.9749540224585
epoch 841 loss = 140.97444410383255
epoch 842 loss = 140.97393539818881
epoch 843 loss = 140.97342790120848
epoch 844 loss = 140.97292160859274
epoch 845 loss = 140.97241651606376
epoch 846 loss = 140.97191261936342
epoch 847 loss = 140.97140991425385
epoch 848 loss = 140.97090839651725
epoch 849 loss = 140.9704080619556
epoch 850 loss = 140.9699089063906
epoch 851 loss = 140.96941092566368
epoch 852 loss = 140.9689141156357
epoch 853 loss = 140.96841847218695
epoch 854 loss = 140.96792399121708
epoch 855 loss = 140.96743066864482
epoch 856 loss = 140.966938500408
epoch 857 loss = 140.9664474824634
epoch 858 loss = 140.96595761078663
epoch 859 loss = 140.96546888137206
epoch 860 loss = 140.9649812902327
epoch 861 loss = 140.96449483340007
epoch 862 loss = 140.96400950692404
epoch 863 loss = 140.9635253068728
epoch 864 loss = 140.9630422293329
epoch 865 loss = 140.96256027040872
epoch 866 loss = 140.96207942622272
epoch 867 loss = 140.96159969291543
epoch 868 loss = 140.96112106664492
epoch 869 loss = 140.960643543587
epoch 870 loss = 140.96016711993522
epoch 871 loss = 140.9596917919003
epoch 872 loss = 140.95921755571072
epoch 873 loss = 140.95874440761193
epoch 874 loss = 140.9582723438665
epoch 875 loss = 140.9578013607545
epoch 876 loss = 140.95733145457254
epoch 877 loss = 140.9568626216345
epoch 878 loss = 140.95639485827078
epoch 879 loss = 140.95592816082834
epoch 880 loss = 140.95546252567124
epoch 881 loss = 140.95499794917959
epoch 882 loss = 140.95453442775016
epoch 883 loss = 140.95407195779586
epoch 884 loss = 140.95361053574604
epoch 885 loss = 140.95315015804601
epoch 886 loss = 140.9526908211572
epoch 887 loss = 140.95223252155688
epoch 888 loss = 140.9517752557385
epoch 889 loss = 140.95131902021095
epoch 890 loss = 140.95086381149895
epoch 891 loss = 140.9504096261428
epoch 892 loss = 140.94995646069833
epoch 893 loss = 140.94950431173675
epoch 894 loss = 140.94905317584463
epoch 895 loss = 140.9486030496239
epoch 896 loss = 140.94815392969144
epoch 897 loss = 140.94770581267943
epoch 898 loss = 140.94725869523484
epoch 899 loss = 140.9468125740198
epoch 900 loss = 140.9463674457111
epoch 901 loss = 140.94592330700033
epoch 902 loss = 140.9454801545937
epoch 903 loss = 140.9450379852121
epoch 904 loss = 140.94459679559085
epoch 905 loss = 140.94415658247988
epoch 906 loss = 140.94371734264317
epoch 907 loss = 140.94327907285918
epoch 908 loss = 140.94284176992053
epoch 909 loss = 140.94240543063376
epoch 910 loss = 140.94197005181988
epoch 911 loss = 140.94153563031347
epoch 912 loss = 140.941102162963
epoch 913 loss = 140.94066964663105
epoch 914 loss = 140.9402380781936
epoch 915 loss = 140.9398074545405
epoch 916 loss = 140.9393777725751
epoch 917 loss = 140.93894902921429
epoch 918 loss = 140.93852122138833
epoch 919 loss = 140.93809434604094
epoch 920 loss = 140.93766840012898
epoch 921 loss = 140.93724338062262
epoch 922 loss = 140.9368192845053
epoch 923 loss = 140.93639610877327
epoch 924 loss = 140.935973850436
epoch 925 loss = 140.93555250651573
epoch 926 loss = 140.93513207404766
epoch 927 loss = 140.9347125500799
epoch 928 loss = 140.93429393167298
epoch 929 loss = 140.93387621590034
epoch 930 loss = 140.93345939984786
epoch 931 loss = 140.93304348061415
epoch 932 loss = 140.93262845530998
epoch 933 loss = 140.93221432105867
epoch 934 loss = 140.93180107499592
epoch 935 loss = 140.93138871426962
epoch 936 loss = 140.93097723603978
epoch 937 loss = 140.93056663747856
epoch 938 loss = 140.93015691577034
epoch 939 loss = 140.92974806811125
epoch 940 loss = 140.9293400917095
epoch 941 loss = 140.9289329837852
epoch 942 loss = 140.92852674157012
epoch 943 loss = 140.92812136230796
epoch 944 loss = 140.9277168432539
epoch 945 loss = 140.9273131816749
epoch 946 loss = 140.92691037484937
epoch 947 loss = 140.92650842006734
epoch 948 loss = 140.92610731463012
epoch 949 loss = 140.9257070558505
epoch 950 loss = 140.92530764105254
epoch 951 loss = 140.92490906757158
epoch 952 loss = 140.92451133275424
epoch 953 loss = 140.92411443395812
epoch 954 loss = 140.92371836855202
epoch 955 loss = 140.92332313391555
epoch 956 loss = 140.9229287274395
epoch 957 loss = 140.92253514652583
epoch 958 loss = 140.9221423885867
epoch 959 loss = 140.9217504510455
epoch 960 loss = 140.92135933133622
epoch 961 loss = 140.92096902690363
epoch 962 loss = 140.92057953520307
epoch 963 loss = 140.92019085370052
epoch 964 loss = 140.91980297987223
epoch 965 loss = 140.91941591120516
epoch 966 loss = 140.91902964519667
epoch 967 loss = 140.91864417935435
epoch 968 loss = 140.9182595111962
epoch 969 loss = 140.91787563825034
epoch 970 loss = 140.91749255805524
epoch 971 loss = 140.91711026815946
epoch 972 loss = 140.9167287661215
epoch 973 loss = 140.9163480495102
epoch 974 loss = 140.9159681159041
epoch 975 loss = 140.91558896289175
epoch 976 loss = 140.91521058807177
epoch 977 loss = 140.9148329890523
epoch 978 loss = 140.91445616345155
epoch 979 loss = 140.9140801088973
epoch 980 loss = 140.91370482302705
epoch 981 loss = 140.913330303488
epoch 982 loss = 140.91295654793686
epoch 983 loss = 140.9125835540398
epoch 984 loss = 140.91221131947276
epoch 985 loss = 140.91183984192082
epoch 986 loss = 140.91146911907856
epoch 987 loss = 140.91109914864998
epoch 988 loss = 140.9107299283482
epoch 989 loss = 140.91036145589592
epoch 990 loss = 140.90999372902465
epoch 991 loss = 140.90962674547532
epoch 992 loss = 140.90926050299788
epoch 993 loss = 140.90889499935128
epoch 994 loss = 140.90853023230358
epoch 995 loss = 140.90816619963203
epoch 996 loss = 140.9078028991222
epoch 997 loss = 140.90744032856918
epoch 998 loss = 140.9070784857766
epoch 999 loss = 140.9067173685569
['originated', 'anarchism', 'class']
|
Neural network in FPGA Editted STEP = 10 (820k~939k).ipynb | ###Markdown
Рекуррентные нейронных сетей
###Code
import tensorflow as tf
import matplotlib as mpl
import matplotlib.pyplot as plt
import numpy as np
import os
import pandas as pd
mpl.rcParams['figure.figsize'] = (8, 6)
mpl.rcParams['axes.grid'] = False
df = pd.read_csv("C:/Users/Zhastay/Downloads/jena_climate_2009_2016/realistic2.csv")
df.head()
def univariate_data(dataset, start_index, end_index, history_size, target_size):
data = []
labels = []
start_index = start_index + history_size
if end_index is None:
end_index = len(dataset) - target_size
for i in range(start_index, end_index):
indices = range(i-history_size, i)
# Reshape data from (history_size,) to (history_size, 1)
data.append(np.reshape(dataset[indices], (history_size, 1)))
labels.append(dataset[i+target_size])
return np.array(data), np.array(labels)
TRAIN_SPLIT = 8330
tf.random.set_seed(13)
uni_data = df['x']
uni_data.index = df['step']
uni_data.head()
uni_data.plot(subplots=True)
uni_data = uni_data.values
uni_train_mean = uni_data[:TRAIN_SPLIT].mean()
uni_train_std = uni_data[:TRAIN_SPLIT].std()
uni_data = (uni_data-uni_train_mean)/uni_train_std
uni_data = tf.keras.utils.normalize(uni_data)[0]
univariate_past_history = 1000
univariate_future_target = 0
x_train_uni, y_train_uni = univariate_data(uni_data, 0, TRAIN_SPLIT,
univariate_past_history,
univariate_future_target)
x_val_uni, y_val_uni = univariate_data(uni_data, TRAIN_SPLIT, None,
univariate_past_history,
univariate_future_target)
print ('Single window of past history')
print (x_train_uni[0])
print ('\n Target temperature to predict')
print (y_train_uni[0])
def create_time_steps(length):
return list(range(-length, 0))
def show_plot(plot_data, delta, title):
labels = ['History', 'True Future', 'Model Prediction']
marker = ['.-', 'rx', 'go']
time_steps = create_time_steps(plot_data[0].shape[0])
if delta:
future = delta
else:
future = 0
plt.title(title)
for i, x in enumerate(plot_data):
if i:
plt.plot(future, plot_data[i], marker[i], markersize=10,
label=labels[i])
else:
plt.plot(time_steps, plot_data[i].flatten(), marker[i], label=labels[i])
plt.legend()
plt.xlim([time_steps[0], (future+5)*2])
plt.xlabel('Time-Step')
return plt
show_plot([x_train_uni[0], y_train_uni[0]], 0, 'Sample Example')
def baseline(history):
return np.mean(history)
show_plot([x_train_uni[0], y_train_uni[0], baseline(x_train_uni[0])], 0,
'Baseline Prediction Example')
###Output
_____no_output_____
###Markdown
LSTM-модель для прогнозирование
###Code
BATCH_SIZE = 256
BUFFER_SIZE = 10000
train_univariate = tf.data.Dataset.from_tensor_slices((x_train_uni, y_train_uni))
train_univariate
train_univariate = train_univariate.cache().shuffle(BUFFER_SIZE).batch(BATCH_SIZE).repeat()
train_univariate
val_univariate = tf.data.Dataset.from_tensor_slices((x_val_uni, y_val_uni))
val_univariate = val_univariate.batch(BATCH_SIZE).repeat()
simple_lstm_model = tf.keras.models.Sequential([
tf.keras.layers.LSTM(8, input_shape=x_train_uni.shape[-2:]),
tf.keras.layers.Dense(1)
])
simple_lstm_model.compile(optimizer='adam', loss='mae')
for x, y in val_univariate.take(1):
print(simple_lstm_model.predict(x).shape)
print(x_train_uni.shape)
EVALUATION_INTERVAL = 200
EPOCHS = 10
single_step_history = simple_lstm_model.fit(train_univariate, epochs=EPOCHS,
steps_per_epoch=EVALUATION_INTERVAL,
validation_data=val_univariate, validation_steps=50)
for x, y in val_univariate.take(3):
plot = show_plot([x[0].numpy(), y[0].numpy(),
simple_lstm_model.predict(x)[0]], 0, 'Simple LSTM model')
plot.show()
def plot_train_history(history, title):
loss = history.history['loss']
val_loss = history.history['val_loss']
epochs = range(len(loss))
plt.figure()
plt.plot(epochs, loss, 'b', label='Training loss')
plt.plot(epochs, val_loss, 'r', label='Validation loss')
plt.title(title)
plt.legend()
plt.show()
plot_train_history(single_step_history,
'Single Step Training and validation loss')
###Output
_____no_output_____ |
01.air_quality_prediction/01.Solution_with_SVM.ipynb | ###Markdown
Import Libraries
###Code
import pandas as pd
import matplotlib.pyplot as plt
from sklearn import svm
from sklearn.preprocessing import LabelEncoder
import numpy as np
import sklearn
###Output
_____no_output_____
###Markdown
Explore the Dataset
###Code
df = pd.read_csv('data.csv')
df.head()
###Output
_____no_output_____
###Markdown
Preprosessing the Dataset
###Code
# drop date part because svm can not utilize time information
df.drop('year', axis=1, inplace=True)
df.head()
df.drop('month', axis=1, inplace=True)
df.drop('day', axis=1, inplace=True)
df.drop('hour', axis=1, inplace=True)
df.drop('No', axis=1, inplace=True)
df.head()
# manually specify column names
df.columns = ['pollution', 'dew', 'temp', 'press', 'wnd_dir', 'wnd_spd', 'snow', 'rain']
df.head()
df.index.name = 'date'
df.head()
# mark all NA values with 0
df['pollution'].fillna(0, inplace=True)
df.head()
# drop the first 24 hours as it has 0 value
df = df[24:]
df.head()
df.to_csv('pollution_svm_byBurak.csv')
df=pd.read_csv('pollution_svm_byBurak.csv',header=0, index_col=0)
df.head()
#plot various parameters of csv into graph
df[['dew', 'temp', 'press', 'wnd_spd', 'snow', 'rain']].plot()
plt.show()
#Store training dataset in X
X=df.values
print(X.shape)
#convert wind direction from string to numerical value
encoder = LabelEncoder()
X[:,4] = encoder.fit_transform(X[:,4])
print(X[:,4])
X = X.astype('float32')
print(X)
#remove polution colunm from data into label
#Split X
y=X[:,0]
X=X[:,1:]
#create SVM model and train it
clf = svm.SVC(kernel = 'linear', C = 1.0)
# ensure all data is float
y = (y > 60).astype(float)
clf.fit(X, y)
#predict values
y_pred=clf.predict(X)
print("Actual Y:")
print(y)
print("Predicted Y:")
print(y_pred)
a=sklearn.metrics.accuracy_score(y, y_pred)
print("accuracy",a)
###Output
accuracy 0.7277625570776256
|
notebook/L14_PCA_face_IRIS.ipynb | ###Markdown
NOW one basic example on using PCA on Tabular-IRIS data adapted from: https://scikit-learn.org/stable/auto_examples/decomposition/plot_pca_iris.html
###Code
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
from sklearn import decomposition
from sklearn import datasets
np.random.seed(5)
iris = datasets.load_iris()
target_names = iris.target_names
iris.data.shape
X = iris.data
X.shape
y_r = iris.target
pca = decomposition.PCA(n_components=2)
X_r = pca.fit(X).transform(X)
# Percentage of variance explained for each components
print('explained variance ratio (first two components): %s'
% str(pca.explained_variance_ratio_))
plt.figure()
colors = ['navy', 'turquoise', 'darkorange']
lw = 2
for color, i, target_name in zip(colors, [0, 1, 2], target_names):
plt.scatter(X_r[y_r == i, 0], X_r[y_r == i, 1], color=color, alpha=.8, lw=lw,
label=target_name)
plt.legend(loc='best', shadow=False, scatterpoints=1)
plt.title('PCA of IRIS dataset')
X = iris.data
pca = decomposition.PCA(n_components=3)
pca.fit(X)
X = pca.transform(X)
y = iris.target
# Percentage of variance explained for each components
print('explained variance ratio (first two components): %s'
% str(pca.explained_variance_ratio_))
centers = [[1, 1], [-1, -1], [1, -1]]
fig = plt.figure(1, figsize=(4, 3))
plt.clf()
ax = Axes3D(fig, rect=[0, 0, .95, 1], elev=48, azim=134)
plt.cla()
pca = decomposition.PCA(n_components=3)
pca.fit(X)
X = pca.transform(X)
for name, label in [('Setosa', 0), ('Versicolour', 1), ('Virginica', 2)]:
ax.text3D(X[y == label, 0].mean(),
X[y == label, 1].mean() + 1.5,
X[y == label, 2].mean(), name,
horizontalalignment='center',
bbox=dict(alpha=.5, edgecolor='w', facecolor='w'))
# Reorder the labels to have colors matching the cluster results
y = np.choose(y, [1, 2, 0]).astype(np.float)
ax.scatter(X[:, 0], X[:, 1], X[:, 2], c=y, cmap=plt.cm.nipy_spectral,
edgecolor='k')
ax.w_xaxis.set_ticklabels([])
ax.w_yaxis.set_ticklabels([])
ax.w_zaxis.set_ticklabels([])
ax.set_xlabel('PC1')
ax.set_ylabel('PC2')
ax.set_zlabel('PC3')
###Output
explained variance ratio (first two components): [0.92461872 0.05306648 0.01710261]
###Markdown
Faces recognition example using eigenfaces and SVMsThe dataset used in this example is a preprocessed of the"Labeled Faces in the Wild", aka LFW_: http://vis-www.cs.umass.edu/lfw/lfw-funneled.tgz (233MB) This is adpated from: * https://scikit-learn.org/stable/auto_examples/applications/plot_face_recognition.html
###Code
for name in dir():
if not name.startswith('_'):
del globals()[name]
from time import time
import logging
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.model_selection import GridSearchCV
from sklearn.metrics import classification_report
from sklearn.metrics import confusion_matrix
from sklearn.decomposition import PCA
from sklearn.svm import SVC
print(__doc__)
from sklearn.datasets import fetch_lfw_people
# Display progress logs on stdout
logging.basicConfig(level=logging.INFO, format='%(asctime)s %(message)s')
# #############################################################################
# Download the data, if not already on disk and load it as numpy arrays
lfw_people = fetch_lfw_people(min_faces_per_person=70, resize=0.4)
# introspect the images arrays to find the shapes (for plotting)
n_samples, h, w = lfw_people.images.shape
# for machine learning we use the 2 data directly (as relative pixel
# positions info is ignored by this model)
X = lfw_people.data
n_features = X.shape[1]
# the label to predict is the id of the person
y = lfw_people.target
target_names = lfw_people.target_names
n_classes = target_names.shape[0]
print("Total dataset size:")
print("n_samples: %d" % n_samples)
print("n_features: %d" % n_features)
print("n_classes: %d" % n_classes)
# #############################################################################
# Split into a training set and a test set using a stratified k fold
# split into a training and testing set
X_train, X_test, y_train, y_test = train_test_split(
X, y, test_size=0.25, random_state=42)
# #############################################################################
# Compute a PCA (eigenfaces) on the face dataset (treated as unlabeled
# dataset): unsupervised feature extraction / dimensionality reduction
n_components = 150
print("Extracting the top %d eigenfaces from %d faces"
% (n_components, X_train.shape[0]))
t0 = time()
pca = PCA(n_components=n_components, svd_solver='randomized',
whiten=True).fit(X_train)
print("done in %0.3fs" % (time() - t0))
eigenfaces = pca.components_.reshape((n_components, h, w))
print("Projecting the input data on the eigenfaces orthonormal basis")
t0 = time()
X_train_pca = pca.transform(X_train)
X_test_pca = pca.transform(X_test)
print("done in %0.3fs" % (time() - t0))
# Percentage of variance explained for each components
print('explained variance ratio (first 150 components): %s'
% str(pca.explained_variance_ratio_))
# #############################################################################
# Train a SVM classification model
print("Fitting the classifier to the training set")
t0 = time()
param_grid = {'C': [1e3, 5e3, 1e4, 5e4, 1e5],
'gamma': [0.0001, 0.0005, 0.001, 0.005, 0.01, 0.1], }
clf = GridSearchCV(
SVC(kernel='rbf', class_weight='balanced'), param_grid
)
clf = clf.fit(X_train_pca, y_train)
print("done in %0.3fs" % (time() - t0))
print("Best estimator found by grid search:")
print(clf.best_estimator_)
# #############################################################################
# Quantitative evaluation of the model quality on the test set
print("Predicting people's names on the test set")
t0 = time()
y_pred = clf.predict(X_test_pca)
print("done in %0.3fs" % (time() - t0))
print(classification_report(y_test, y_pred, target_names=target_names))
print(confusion_matrix(y_test, y_pred, labels=range(n_classes)))
# #############################################################################
# Qualitative evaluation of the predictions using matplotlib
def plot_gallery(images, titles, h, w, n_row=3, n_col=4):
"""Helper function to plot a gallery of portraits"""
plt.figure(figsize=(1.8 * n_col, 2.4 * n_row))
plt.subplots_adjust(bottom=0, left=.01, right=.99, top=.90, hspace=.35)
for i in range(n_row * n_col):
plt.subplot(n_row, n_col, i + 1)
plt.imshow(images[i].reshape((h, w)), cmap=plt.cm.gray)
plt.title(titles[i], size=12)
plt.xticks(())
plt.yticks(())
# plot the result of the prediction on a portion of the test set
def title(y_pred, y_test, target_names, i):
pred_name = target_names[y_pred[i]].rsplit(' ', 1)[-1]
true_name = target_names[y_test[i]].rsplit(' ', 1)[-1]
return 'predicted: %s\ntrue: %s' % (pred_name, true_name)
prediction_titles = [title(y_pred, y_test, target_names, i)
for i in range(y_pred.shape[0])]
plot_gallery(X_test, prediction_titles, h, w)
# plot the gallery of the most significative eigenfaces
eigenface_titles = ["eigenface %d" % i for i in range(eigenfaces.shape[0])]
plot_gallery(eigenfaces, eigenface_titles, h, w)
plt.show()
###Output
_____no_output_____ |
PDF2TXT_CellOCR.ipynb | ###Markdown
PDF Data Extraction Notebook -- Cell Based This notebook demonstrates how to extract text from a PDF when the PDF is a highly structured document.Many types of government forms (e.g. IRS' 1040) have been designed to guide the human eye when filling out the form. The page is highly structured, with tables, boxes, dividing lines, white space, etc. Such forms can confuse OCR processes. Vertical lines can be read as '1' or 'l', horizontal lines can make '.' and ',' invisible, and all the white space can confuse the system on when to insert line breaks.To overcome this issue, I've found it works well to take that complex initial document and split it into many simplier image. I do this by identifying long, straight lines on the page, and subdividing the image of the page into chunks based on those lines. The example in this notebook only looks for the longest lines in the form, but a full application would be an iterative process. Looking for long lines in each new subimage, which would in turn keep breaking the subimages into smaller and simplier pieces. Pipeline:1. Given pdf, convert into images with ghostscript2. Given an image, identify long horizontal and vertical lines.3. Split image into many subimages (cells) based on the found horizontal and vertical lines.4. OCR each cell.5. Combine results in csv. Tools:* ghostscript -- to convert pdfs to images so that they can be OCR'd.* tesseract -- to extract text from images* python -- glue to run ghostscript, tesseract, perform image manipulations, and compile results The main features of this notebook (converting PDFs to PNGs and OCRing PNGs to extract text) are not done by Python. They are being done by command line tools GhostScript and Tesseract. Those need to be installed before this notebook will do anything.Starter places for easy installs of those tools:1. Tesseract: https://github.com/UB-Mannheim/tesseract/wiki -- A full installer for Windows. But hosted outside the US. Domestic installers for older versions of Tesseract may be found here: https://github.com/tesseract-ocr/tesseract/wiki/4.0-with-LSTM400-alpha-for-windows2. Ghostscript: https://www.ghostscript.com/download/gsdnld.html Ghostscript and Tesseract where both installed on a Windows 10 laptop when creating this demo. The tools were installed in subfolders of this projects folder. When being called by the Python OS package in the code below, the relative filepath is being given to the executable application files. 0. Importing required Python packages.
###Code
import os #for navigating folder structures and running command line tools ghostscript and tesseract
import matplotlib.pyplot as plt #for visualizing steps in this notebook
from PIL import Image #for visualizing results and manipulating images
from matplotlib.patches import Rectangle #for visualizing image manipulation
import numpy as np #for maths and manipulating images
import pandas as pd#for storing results at end of pipeline
import tempfile #for creating temp folder to store images and text files
tempDir=tempfile.mkdtemp(dir=os.getcwd())
###Output
_____no_output_____
###Markdown
1. Convert a PDF into image(s)Tesseract works on images, so the first step needs to be converting the PDFs into images. If the PDF is multipage, we want to have access to each of those pages, so we'll save each page as its own file. When working with a collection of, say, 100+ page PDFs, this can quickly generate a huge amount of image data. For those production level processes, the images should be deleted after OCRing to reduce storage issues.GhostScript is the command line tool I will use to convert the PDF into PNGs. Ghostscript has additional options than those shown here (see https://www.ghostscript.com/doc/current/Use.htm ). The ones used here:* -sDEVICE -- Tell ghostscript what to convert the PDF into. In this case, PNGs.* -sOutputFile -- The filepath and name of image to save.* -dBATCH -- Quit ghostscript after completing the batch of 1+ PDF to PNG conversions.* -dNOPAUSE -- Skip any user prompts that Ghostscript would normally ask for when running as command line tool.* -rnumber -- Hor. and ver. resolution in pixels per inch. Higher number--> more detail and often better OCR, but Ghostscript takes longer.* The final option is the input PDF file to convert.Below, making use the of %s string variable to insert the filepaths for the location to save the image(s) and the location of the original PDF.
###Code
os.listdir('examplePDFs')
#target pdf. Currently being pulled from the examples included in the GitHub project.
targetPDF=os.path.join('examplePDFs','f1040.pdf')
#save location:
PDFImages=os.path.join(tempDir, 'PDFImages')
try:
os.mkdir(PDFImages)
except:
print('directory already exists. Cleaning out for next data run')
for file in os.listdir(PDFImages):
os.remove(os.path.join(PDFImages, file))
imageSave=os.path.join(PDFImages,
'.'.join(os.path.basename(targetPDF).split('.')[:-1])+'-%03d.png') #add page number for multipage PDFs when creating images.
p = os.popen("GhostScript\\gs9.27\\bin\\gswin64c.exe -sDEVICE=pngalpha -sOutputFile=%s -dBATCH -dNOPAUSE -r288 %s"%(imageSave, targetPDF))
print(p.read())
###Output
GPL Ghostscript 9.27 (2019-04-04)
Copyright (C) 2018 Artifex Software, Inc. All rights reserved.
This software is supplied under the GNU AGPLv3 and comes with NO WARRANTY:
see the file COPYING for details.
Processing pages 1 through 2.
Page 1
Page 2
###Markdown
1.5 Visualize the converted image:
###Code
targetImage='f1040-001.png'
targetImage=os.path.join(PDFImages, targetImage)
im = Image.open(targetImage)
plt.figure(figsize=(12,8))
plt.imshow(np.array(im))
###Output
_____no_output_____
###Markdown
3. Image manipulation Finding long horizontal and vertical lines in the form and using those to create many simplier subimages for OCRing.Because this can generate a large number of extra images and eventually text files, creating folder to save them in.
###Code
subimagepath=os.path.join(tempDir, 'subimages')
try:
os.mkdir(subimagepath)
except:
print('subimage folder already exists. Cleaning out for next run...')
for file in os.listdir(subimagepath):
os.remove(os.path.join(subimagepath, file))
im2=np.array(im)[:,:,1] #easier to work with 2D array, but am losing some info about the other color channels.
#Collapsing pixels down along vertical axis. This will cause long horizontal lines to show up as deep drops in the total:
dim=1 #setting to look at horizontal lines first:
pixelSum=im2.sum(axis=dim)
threshold=pixelSum.mean()*.35 #If value is lesss than this, consider it a long ddark line for splitting the image.
#option to plot:
# plt.plot(pixelSum)
# plt.axhline(y=threshold)
# plt.show()
#collecting locations of all horizontal lines
cuts0=[i for i, x in enumerate(pixelSum) if x<=threshold]
cuts0=[0]+cuts0+[len(im2)]
#Now looking at vertical lines in each of the strips found above:
for x in range(0,len(cuts0)-1):
a0=x#topcut
b0=x+1#bottomcut
imSub=im2[cuts0[a0]:cuts0[b0]]
if len(imSub)>5: #ignore close horizontal lines (prob. same multipixel think line)
#split along vertical lines:
dim=0
pixelSum2=imSub.sum(axis=dim)
threshold2=pixelSum2.mean()*.25
cuts1=[i for i, x in enumerate(pixelSum2) if x<=threshold2]
cuts1=[0]+cuts1+[len(imSub[0])]
for y in range(0, len(cuts1)-1):
a1=y
b1=y+1
imSub1=imSub[:,cuts1[a1]:cuts1[b1]] #found subbox.
if len(imSub1[0]>5):#ignore close vertical lines (prob. same multipixel think line)
name='.'.join(os.path.basename(targetImage).split('.')[:-1])\
+'_'+'_'.join([str(elm) for elm in [cuts0[a0],cuts0[b0],cuts1[a1],cuts1[b1]]])+'.png'
#plt.figure(figsize=(8, 24))
#plt.imshow(imSub1, cmap='gray')
#plt.show()
#below: dropping a few pixel rows at top and bottom to remove horizontal lines.\
# adding white space padding on top and bottom to improve OCRing
imSub1pad=np.concatenate([np.ones((50,len(imSub1[0])))*255,imSub1[4:-4],np.ones((50,len(imSub1[0])))*255])
imSub1pad=imSub1pad.astype('uint8')
subImage=Image.fromarray(imSub1pad)
subImage.save(os.path.join(subimagepath, name)) #saving all the subimages in the same location
#print(name)
#plt.imshow(imSub[:,cuts1[a1]:cuts1[b1]])
###Output
_____no_output_____
###Markdown
Tesseract to extract textAgain, because there could be so many files, I'm creating a subfolder to stash everything.
###Code
subimageTextPath=os.path.join(tempDir, 'subimageText')
try:
os.mkdir(subimageTextPath)
except:
print('subimage folder already exists. Cleaning out for next run...')
for file in os.listdir(subimageTextPath):
os.remove(os.path.join(subimageTextPath, file))
for subimage in os.listdir(subimagepath):
targetPath=os.path.join(subimagepath,subimage)
savePath=os.path.join(subimageTextPath,'.'.join(subimage.split('.')[:-1]))
p = os.popen('Tesseract\\tesseract.exe %s %s'%(targetPath, savePath))
###Output
_____no_output_____
###Markdown
Note: The notebook cell above could show as fully run, but tesseract could still be operating in the background. Give Tesseract a moment to finish up if no data is being seen in the extracted text folder. Compile data
###Code
df=pd.DataFrame(columns=['boxname','text'])
boxOCR=os.listdir(subimageTextPath)
for x in range (0, len(boxOCR)):
try:
df.loc[x,'boxname']=boxOCR[x]
df.loc[x,'text']=open(os.path.join(subimageTextPath,boxOCR[x])).read()
except:
pass
df['HT']=-1
df['HB']=-1
df['VL']=-1
df['VR']=-1
for x in range(0, len(df)):
df.loc[x, ['HT','HB','VL','VR']]=[int(elm.split('.')[0]) for elm in df.loc[x,'boxname'].split('_')[-4:]]
fig, ax = plt.subplots(1, figsize=(12,20))
ax.imshow(im2, cmap='gray')
for i in range(0, len(df)):
#i=0
xy=(df.loc[i,'VL'],df.loc[i,'HT']);
width=df.loc[i,'VR']-df.loc[i,'VL'];
height=df.loc[i,'HB']-df.loc[i,'HT'];
if width > 20 and height >20 and len(str(df.loc[i,'text']).strip())>0:
rect=Rectangle(xy, width, height,
edgecolor='r',facecolor='none')
ax.add_patch(rect)
ax.annotate(i, (int(xy[0]+width/2),int(xy[1]+height/1.2)), color='blue',fontsize=20)
n=0
target='.'.join(df.loc[n,'boxname'].split('.')[:-1])+'.png'
plt.figure(figsize=[8, 12])
plt.imshow(np.array(Image.open(os.path.join(subimagepath,target))), cmap='gray');
print(df.loc[n,'text'])
###Output
E 1 040 Department of the Treasury—Internal Revenue Service 2 O 4 8
& U.S. Individual Income Tax Return OMB No. 1545-0074 | IRS Use Only—Do not write or staple in this space.
###Markdown
Again, this example only looked for the longest horizontal and vertical lines to split the original image. So you may see some subimages above that could have been further split into smaller cells. To do that, change the code above to iterate over each subimage, performing the same test for lines to split the image on. 4. Clean up temp directoryOnce everything has been completed, the temp files that were generated should be deleted.
###Code
for folder in ['PDFImages','subimages','subimageText']:
try:
for file in os.listdir(os.path.join(tempDir, folder)):
os.remove(os.path.join(tempDir, folder,file))
os.rmdir(os.path.join(tempDir, folder))
except:
pass
os.rmdir(tempDir)
###Output
_____no_output_____ |
examples/e2e/mnist.ipynb | ###Markdown
Load Dataset Loading MNIST code taken from https://github.com/oreilly-japan/deep-learning-from-scratch/blob/master/dataset/mnist.py
###Code
import urllib.request
import os.path
import gzip
import pickle
import os
import numpy as np
url_base = 'http://yann.lecun.com/exdb/mnist/'
key_file = {
'train_img':'train-images-idx3-ubyte.gz',
'train_label':'train-labels-idx1-ubyte.gz',
'test_img':'t10k-images-idx3-ubyte.gz',
'test_label':'t10k-labels-idx1-ubyte.gz'
}
dataset_dir = "."
save_file = dataset_dir + "/mnist.pkl"
train_num = 60000
test_num = 10000
img_dim = (1, 28, 28)
img_size = 784
def _download(file_name):
file_path = dataset_dir + "/" + file_name
if os.path.exists(file_path):
return
headers = {"User-Agent": "Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:47.0) Gecko/20100101 Firefox/47.0"}
request = urllib.request.Request(url_base+file_name, headers=headers)
response = urllib.request.urlopen(request).read()
with open(file_path, mode='wb') as f:
f.write(response)
def download_mnist():
for v in key_file.values():
_download(v)
def _load_label(file_name):
file_path = dataset_dir + "/" + file_name
with gzip.open(file_path, 'rb') as f:
labels = np.frombuffer(f.read(), np.uint8, offset=8)
return labels
def _load_img(file_name):
file_path = dataset_dir + "/" + file_name
with gzip.open(file_path, 'rb') as f:
data = np.frombuffer(f.read(), np.uint8, offset=16)
data = data.reshape(-1, img_size)
return data
def _convert_numpy():
dataset = {}
dataset['train_img'] = _load_img(key_file['train_img'])
dataset['train_label'] = _load_label(key_file['train_label'])
dataset['test_img'] = _load_img(key_file['test_img'])
dataset['test_label'] = _load_label(key_file['test_label'])
return dataset
def init_mnist():
download_mnist()
dataset = _convert_numpy()
with open(save_file, 'wb') as f:
pickle.dump(dataset, f, -1)
def _change_one_hot_label(X):
T = np.zeros((X.size, 10))
for idx, row in enumerate(T):
row[X[idx]] = 1
return T
def load_mnist(normalize=True, flatten=True, one_hot_label=False):
"""MNISTデータセットの読み込み
Parameters
----------
normalize : 画像のピクセル値を0.0~1.0に正規化する
one_hot_label :
one_hot_labelがTrueの場合、ラベルはone-hot配列として返す
one-hot配列とは、たとえば[0,0,1,0,0,0,0,0,0,0]のような配列
flatten : 画像を一次元配列に平にするかどうか
Returns
-------
(訓練画像, 訓練ラベル), (テスト画像, テストラベル)
"""
if not os.path.exists(save_file):
init_mnist()
with open(save_file, 'rb') as f:
dataset = pickle.load(f)
if normalize:
for key in ('train_img', 'test_img'):
dataset[key] = dataset[key].astype(np.float32)
dataset[key] /= 255.0
if one_hot_label:
dataset['train_label'] = _change_one_hot_label(dataset['train_label'])
dataset['test_label'] = _change_one_hot_label(dataset['test_label'])
if not flatten:
for key in ('train_img', 'test_img'):
dataset[key] = dataset[key].reshape(-1, 1, 28, 28)
return dataset['train_img'], dataset['train_label'], dataset['test_img'], dataset['test_label']
X_train, y_train, X_test, y_test = load_mnist()
X_train.shape, y_train.shape
X_test.shape, y_test .shape
###Output
_____no_output_____
###Markdown
SimpleNN
###Code
!pip install -e ../../
from simplenn import Network
from simplenn.layer import Dense
from simplenn.activation import ReLu
from simplenn.activation import SoftMaxLoss
from simplenn.layer.dropout import Dropout
from simplenn.metrics.loss import CategoricalCrossEntropy
from simplenn.metrics import Accuracy
from simplenn.optimizers import Adam
class Model(Network):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
self.l1 = Dense(img_size, 64, W_l1=5e-4, b_l1=5e-4)
self.dropout1 = Dropout(rate=0.2)
self.activation1 = ReLu()
self.l2 = Dense(64, 64)
self.dropout2 = Dropout(rate=0.2)
self.activation2 = ReLu()
self.l3 = Dense(64, 10)
self.output = SoftMaxLoss(loss=CategoricalCrossEntropy())
def forward(self, x, targets):
# forward pass
x = self.l1(x)
x = self.dropout1(x)
x = self.activation1(x)
x = self.l2(x)
x = self.dropout2(x)
x = self.activation2(x)
x = self.l3(x)
return self.output(x, targets)
optimizer = Adam(lr=0.001, decay=5e-5, b1=0.9, b2=0.999)
acc = Accuracy()
model = Model(optimizer=optimizer)
model.fit(X_train, y_train, epochs=15, batch_size=64, metrics=[acc], X_val=X_test, y_val=y_test)
yprob_train = model.predict(X_train)
train_acc = acc(yprob_train, y_train)
print(f"Train Accuracy: {train_acc}")
yprob_test= model.predict(X_test)
test_acc = acc(yprob_test, y_test)
print(f"Test Accuracy: {test_acc}")
# model.save('mnist_model.pkl')
###Output
_____no_output_____ |
orttraining/tools/mnist_model_builder/mnist_fc_builder.ipynb | ###Markdown
Building a FC model for MNIST
###Code
import math
import numpy as np
import scipy.stats as stats
def truncated_normal(dims):
dim0, dim1 = dims
mu, stddev = 0, 1/math.sqrt(dim0)
lower, upper = -2 * stddev, 2 * stddev
X = stats.truncnorm( (lower - mu) / stddev, (upper - mu) / stddev, loc = mu, scale = stddev)
return X.rvs(dim0 * dim1).tolist()
def zeros(dim):
return [0] * dim[0]
W1_dims = [784, 128]
W2_dims = [128, 32]
W3_dims = [32, 10]
W1 = onnx.helper.make_tensor(name="W1", data_type=onnx.TensorProto.FLOAT, dims=W1_dims, vals=truncated_normal(W1_dims))
W2 = helper.make_tensor(name="W2", data_type=onnx.TensorProto.FLOAT, dims=W2_dims, vals=truncated_normal(W2_dims))
W3 = helper.make_tensor(name="W3", data_type=onnx.TensorProto.FLOAT, dims=W3_dims, vals=truncated_normal(W3_dims))
B1_dims = [128]
B2_dims = [32]
B3_dims = [10]
B1 = helper.make_tensor(name="B1", data_type=onnx.TensorProto.FLOAT, dims=B1_dims, vals=zeros(B1_dims))
B2 = helper.make_tensor(name="B2", data_type=onnx.TensorProto.FLOAT, dims=B2_dims, vals=zeros(B2_dims))
B3 = helper.make_tensor(name="B3", data_type=onnx.TensorProto.FLOAT, dims=B3_dims, vals=zeros(B3_dims))
node1 = helper.make_node('MatMul', inputs=['X', 'W1'], outputs=['T1'])
node2 = helper.make_node('Add', inputs=['T1', 'B1'], outputs=['T2'])
node3 = helper.make_node('Relu', inputs=['T2'], outputs=['T3'])
node4 = helper.make_node('MatMul', inputs=['T3', 'W2'], outputs=['T4'])
node5 = helper.make_node('Add', inputs=['T4', 'B2'], outputs=['T5'])
node6 = helper.make_node('Relu', inputs=['T5'], outputs=['T6'])
node7 = helper.make_node('MatMul', inputs=['T6', 'W3'], outputs=['T7'])
node8 = helper.make_node('Add', inputs=['T7', 'B3'], outputs=['predictions'])
graph = helper.make_graph(
[node1, node2, node3, node4, node5, node6, node7, node8],
'fully_connected_mnist',
[helper.make_tensor_value_info('X', TensorProto.FLOAT, ([-1, 784])),
helper.make_tensor_value_info('W1', TensorProto.FLOAT, W1_dims),
helper.make_tensor_value_info('W2', TensorProto.FLOAT, W2_dims),
helper.make_tensor_value_info('W3', TensorProto.FLOAT, W3_dims),
helper.make_tensor_value_info('B1', TensorProto.FLOAT, B1_dims),
helper.make_tensor_value_info('B2', TensorProto.FLOAT, B2_dims),
helper.make_tensor_value_info('B3', TensorProto.FLOAT, B3_dims),
],
[helper.make_tensor_value_info('predictions', TensorProto.FLOAT, ([-1, 10]))],
[W1, W2, W3, B1, B2, B3]
)
original_model = helper.make_model(graph, producer_name='onnx-examples')
onnx.checker.check_model(original_model)
inferred_model = shape_inference.infer_shapes(original_model)
onnx.save_model(inferred_model, "mnist_fc.onnx")
###Output
_____no_output_____
###Markdown
Inferencing session
###Code
import onnxruntime as lotus
import numpy as np
sess = lotus.InferenceSession('mnist_fc_model_with_cost.onnx', None)
X_dims = [1, 784]
data = np.random.uniform(size=X_dims).astype(np.float32)
labels = np.zeros(10).astype(np.float32)
labels[3] = 1
result = sess.run(['predictions', 'loss'], {'X': data, 'labels': labels})
print(result[0])
print(result[1])
###Output
_____no_output_____ |
Blackjack Reinforcement Learning.ipynb | ###Markdown
The Agent
###Code
# The agent is designed to be very easy
# to instantiate and begin learning. Hyperparameter
# selection and loading previous models are both
# available, but not required.
env = gym.make('blackjack-v0')
env.reset()
agent = Monte_Carlo_Search(env)
for i in range(1):
agent.one_game()
agent.value_fn.to_csv("trained_model.csv")
###Output
_____no_output_____
###Markdown
EvaluationBlackjack is a solved game with a mathematically-proven optimal strategy. We will evaluate the RL agent based on its convergence to this optimal strategy. To do this, we will create a DataFrame containing every possible state and the right correct play, then pass each state to the model to predict.
###Code
from itertools import product
states_list = list(product(range(12,22), range(2,11), range(2), [0]))
states_aces = list(product(range(12,22), [11], range(2), [1]))
states_list.extend(states_aces)
import pandas as pd
df = pd.DataFrame(data=states_list, columns=['Player Value', 'Dealer Upcard', 'Player Ace', 'Dealer Ace'])
df['State'] = df.apply(lambda x: tuple(x), axis=1)
df.head()
# Initialize the Correct Action column full of hits
df['Correct'] = 1
# Modify the Correct Action column to include when it
# is appropriate to stay (no double down or splits)
# Taken from:
# https://www.blackjackapprenticeship.com/blackjack-strategy-charts/
df.loc[(df['Player Value'] >= 17) & (df['Player Ace'] == 0), 'Correct'] = 0
df.loc[(df['Player Value'] >= 13) & (df['Player Ace'] == 0) &\
(df['Player Value'] <= 16) & (df['Dealer Upcard'] <=6), 'Correct'] = 0
df.loc[(df['Player Value'] == 12) & (df['Player Ace'] == 0) &\
(df['Dealer Upcard'] >= 4) & (df['Dealer Upcard'] <=6), 'Correct'] = 0
df.loc[(df['Player Value'] >= 19) & (df['Player Ace'] == 1), 'Correct'] = 0
df.loc[(df['Player Value'] == 18) & (df['Player Ace'] == 1) &\
(df['Dealer Upcard'] <= 8), 'Correct'] = 0
df.drop(['Player Value', 'Dealer Upcard', 'Player Ace', 'Dealer Ace'], axis=1, inplace=True)
df.head()
# Prepare the agent's action choices
agent = pd.read_csv("test_monte_carlo.csv")
agent['1_ratio'] = agent['1_win'] / agent['1_count']
agent['0_ratio'] = agent['0_win'] / agent['0_count']
agent['Agent'] = 0
agent.loc[agent['1_ratio'] > agent['0_ratio'], 'Agent'] = 1
agent.head()
# Compare the generated Agent with a mathematically perfect game
# Show all places where the Agent is wrong
df['Agent'] = agent['Agent']
df[df['Correct'] != df['Agent']]
###Output
_____no_output_____ |
Naive Bees_ Image Loading and Processing/notebook.ipynb | ###Markdown
1. Import Python librariesA honey bee.The question at hand is: can a machine identify a bee as a honey bee or a bumble bee? These bees have different behaviors and appearances, but given the variety of backgrounds, positions, and image resolutions it can be a challenge for machines to tell them apart.Being able to identify bee species from images is a task that ultimately would allow researchers to more quickly and effectively collect field data. Pollinating bees have critical roles in both ecology and agriculture, and diseases like colony collapse disorder threaten these species. Identifying different species of bees in the wild means that we can better understand the prevalence and growth of these important insects.A bumble bee.This notebook walks through loading and processing images. After loading and processing these images, they will be ready for building models that can automatically detect honeybees and bumblebees.
###Code
# Used to change filepaths
from pathlib import Path
# We set up matplotlib, pandas, and the display function
%matplotlib inline
import matplotlib.pyplot as plt
from IPython.display import display
import pandas as pd
# import numpy to use in this cell
import numpy as np
# import Image from PIL so we can use it later
from PIL import Image
# generate test_data
test_data = np.random.beta(1, 1, size=(100, 100, 3))
# display the test_data
plt.imshow(test_data)
###Output
_____no_output_____
###Markdown
2. Opening images with PILNow that we have all of our imports ready, it is time to work with some real images.Pillow is a very flexible image loading and manipulation library. It works with many different image formats, for example, .png, .jpg, .gif and more. For most image data, one can work with images using the Pillow library (which is imported as PIL).Now we want to load an image, display it in the notebook, and print out the dimensions of the image. By dimensions, we mean the width of the image and the height of the image. These are measured in pixels. The documentation for Image in Pillow gives a comprehensive view of what this object can do.
###Code
# open the image
img = Image.open('datasets/bee_1.jpg')
# Get the image size
img_size = img.size
print("The image size is: {}".format(img_size))
# Just having the image as the last line in the cell will display it in the notebook
img
###Output
The image size is: (100, 100)
###Markdown
3. Image manipulation with PILPillow has a number of common image manipulation tasks built into the library. For example, one may want to resize an image so that the file size is smaller. Or, perhaps, convert an image to black-and-white instead of color. Operations that Pillow provides include:resizingcroppingrotatingflippingconverting to greyscale (or other color modes)Often, these kinds of manipulations are part of the pipeline for turning a small number of images into more images to create training data for machine learning algorithms. This technique is called data augmentation, and it is a common technique for image classification.We'll try a couple of these operations and look at the results.
###Code
# Crop the image to 25, 25, 75, 75
img_cropped = img.crop([25,25,75,75])
display(img_cropped)
# rotate the image by 45 degrees
img_rotated = img.rotate(45, expand=25)
display(img_rotated)
# flip the image left to right
img_flipped = img.transpose(Image.FLIP_LEFT_RIGHT)
display(img_flipped)
###Output
_____no_output_____
###Markdown
4. Images as arrays of dataWhat is an image? So far, PIL has handled loading images and displaying them. However, if we're going to use images as data, we need to understand what that data looks like.Most image formats have three color "channels": red, green, and blue (some images also have a fourth channel called "alpha" that controls transparency). For each pixel in an image, there is a value for every channel.The way this is represented as data is as a three-dimensional matrix. The width of the matrix is the width of the image, the height of the matrix is the height of the image, and the depth of the matrix is the number of channels. So, as we saw, the height and width of our image are both 100 pixels. This means that the underlying data is a matrix with the dimensions 100x100x3.
###Code
# Turn our image object into a NumPy array
img_data = np.array(img)
# get the shape of the resulting array
img_data_shape = img_data.shape
print("Our NumPy array has the shape: {}".format(img_data_shape))
# plot the data with `imshow`
plt.show()
# plot the red channel
plt.imshow(img_data[:, :, 0], cmap=plt.cm.Reds_r)
plt.show()
# plot the green channel
plt.imshow(img_data[:,:,1], cmap=plt.cm.Greens_r)
plt.show()
# plot the blue channel
plt.imshow(img_data[:,:,2], cmap=plt.cm.Blues_r)
plt.show()
###Output
Our NumPy array has the shape: (100, 100, 3)
###Markdown
5. Explore the color channelsColor channels can help provide more information about an image. A picture of the ocean will be more blue, whereas a picture of a field will be more green. This kind of information can be useful when building models or examining the differences between images.We'll look at the kernel density estimate for each of the color channels on the same plot so that we can understand how they differ.When we make this plot, we'll see that a shape that appears further to the right means more of that color, whereas further to the left means less of that color.
###Code
def plot_kde(channel, color):
""" Plots a kernel density estimate for the given data.
`channel` must be a 2d array
`color` must be a color string, e.g. 'r', 'g', or 'b'
"""
data = channel.flatten()
return pd.Series(data).plot.density(c=color)
# create the list of channels
channels = ['r','g','b']
def plot_rgb(image_data):
# use enumerate to loop over colors and indexes
for ix, color in enumerate(channels):
plot_kde(img_data[:, :, ix], color)
plt.show()
plot_rgb(img_data)
###Output
_____no_output_____
###Markdown
6. Honey bees and bumble bees (i)Now we'll look at two different images and some of the differences between them. The first image is of a honey bee, and the second image is of a bumble bee.First, let's look at the honey bee.
###Code
# load bee_12.jpg as honey
honey = Image.open('datasets/bee_12.jpg')
# display the honey bee image
display(honey)
# NumPy array of the honey bee image data
honey_data = np.array(honey)
# plot the rgb densities for the honey bee image
plot_rgb(honey_data)
###Output
_____no_output_____
###Markdown
7. Honey bees and bumble bees (ii)Now let's look at the bumble bee.When one compares these images, it is clear how different the colors are. The honey bee image above, with a blue flower, has a strong peak on the right-hand side of the blue channel. The bumble bee image, which has a lot of yellow for the bee and the background, has almost perfect overlap between the red and green channels (which together make yellow).
###Code
# load bee_3.jpg as bumble
bumble = Image.open('datasets/bee_3.jpg')
# display the bumble bee image
display(bumble)
# NumPy array of the bumble bee image data
bumble_data = np.array(bumble)
# plot the rgb densities for the bumble bee image
plot_rgb(bumble_data)
###Output
_____no_output_____
###Markdown
8. Simplify, simplify, simplifyWhile sometimes color information is useful, other times it can be distracting. In this examples where we are looking at bees, the bees themselves are very similar colors. On the other hand, the bees are often on top of different color flowers. We know that the colors of the flowers may be distracting from separating honey bees from bumble bees, so let's convert these images to black-and-white, or "grayscale."Grayscale is just one of the modes that Pillow supports. Switching between modes is done with the .convert() method, which is passed a string for the new mode.Because we change the number of color "channels," the shape of our array changes with this change. It also will be interesting to look at how the KDE of the grayscale version compares to the RGB version above.
###Code
# convert honey to grayscale
honey_bw = honey.convert("L")
display(honey_bw)
# convert the image to a NumPy array
honey_bw_arr = np.array(honey_bw)
# get the shape of the resulting array
honey_bw_arr_shape = honey_bw_arr.shape
print("Our NumPy array has the shape: {}".format(honey_bw_arr_shape))
# plot the array using matplotlib
plt.imshow(honey_bw_arr, cmap=plt.cm.gray)
plt.show()
# plot the kde of the new black and white array
plot_kde(honey_bw_arr, 'k')
###Output
_____no_output_____
###Markdown
9. Save your work!We've been talking this whole time about making changes to images and the manipulations that might be useful as part of a machine learning pipeline. To use these images in the future, we'll have to save our work after we've made changes.Now, we'll make a couple changes to the Image object from Pillow and save that. We'll flip the image left-to-right, just as we did with the color version. Then, we'll change the NumPy version of the data by clipping it. Using the np.maximum function, we can take any number in the array smaller than 100 and replace it with 100. Because this reduces the range of values, it will increase the contrast of the image. We'll then convert that back to an Image and save the result.
###Code
# flip the image left-right with transpose
honey_bw_flip = honey_bw.transpose(Image.FLIP_LEFT_RIGHT)
# show the flipped image
display(honey_bw_flip)
# save the flipped image
honey_bw_flip.save("saved_images/bw_flipped.jpg")
# create higher contrast by reducing range
honey_hc_arr = np.maximum(honey_bw_arr,100)
# show the higher contrast version
plt.imshow(honey_hc_arr, cmap=plt.cm.gray)
# convert the NumPy array of high contrast to an Image
honey_bw_hc = Image.fromarray(honey_hc_arr)
# save the high contrast version
honey_bw_hc.save("saved_images/bw_hc.jpg")
###Output
_____no_output_____
###Markdown
10. Make a pipelineNow it's time to create an image processing pipeline. We have all the tools in our toolbox to load images, transform them, and save the results.In this pipeline we will do the following:Load the image with Image.open and create paths to save our images toConvert the image to grayscaleSave the grayscale imageRotate, crop, and zoom in on the image and save the new image
###Code
image_paths = ['datasets/bee_1.jpg', 'datasets/bee_12.jpg', 'datasets/bee_2.jpg', 'datasets/bee_3.jpg']
def process_image(path):
img = Image.open(path)
# create paths to save files to
bw_path = "saved_images/bw_{}.jpg".format(path.stem)
rcz_path = "saved_images/rcz_{}.jpg".format(path.stem)
print("Creating grayscale version of {} and saving to {}.".format(path, bw_path))
bw = img.convert("L")
bw.save(bw_path)
print("Creating rotated, cropped, and zoomed version of {} and saving to {}.".format(path, rcz_path))
rcz = ...
# ... YOUR CODE FOR TASK 10 ...
# for loop over image paths
for img_path in image_paths:
process_image(Path(img_path))
###Output
Creating grayscale version of datasets/bee_1.jpg and saving to saved_images/bw_bee_1.jpg.
Creating rotated, cropped, and zoomed version of datasets/bee_1.jpg and saving to saved_images/rcz_bee_1.jpg.
Creating grayscale version of datasets/bee_12.jpg and saving to saved_images/bw_bee_12.jpg.
Creating rotated, cropped, and zoomed version of datasets/bee_12.jpg and saving to saved_images/rcz_bee_12.jpg.
Creating grayscale version of datasets/bee_2.jpg and saving to saved_images/bw_bee_2.jpg.
Creating rotated, cropped, and zoomed version of datasets/bee_2.jpg and saving to saved_images/rcz_bee_2.jpg.
Creating grayscale version of datasets/bee_3.jpg and saving to saved_images/bw_bee_3.jpg.
Creating rotated, cropped, and zoomed version of datasets/bee_3.jpg and saving to saved_images/rcz_bee_3.jpg.
|
docs/source/examples/powerlaw.ipynb | ###Markdown
Powerlaw curves=========
###Code
import matplotlib.pyplot as plt
import numpy as np
import seaborn
%matplotlib inline
seaborn.set_context('poster')
seaborn.set_style("dark")
from simlightcurve import curves
hr = 60*60
decay_tau=1.*24*hr
rise_tau=decay_tau*0.3
t_min = 0.1
break_one_t_offset = 0.2*decay_tau
unbroken_pl = curves.Powerlaw(init_amp=1,
alpha_one=-0.5,
t_offset_min=t_min,
t0=None)
offset_pl = curves.Powerlaw(init_amp=1,
alpha_one=-0.5,
t_offset_min=t_min+1.0,
t0=-10
)
broken_pl = curves.SingleBreakPowerlaw(init_amp=.1,
alpha_one=-0.2,
break_one_t_offset=break_one_t_offset,
alpha_two=-0.8,
t_offset_min=t_min,
t0=None
)
tsteps = np.linspace(t_min, decay_tau, 1e5, dtype=np.float)
fig, axes = plt.subplots(2,1)
fig.suptitle('Powerlaws', fontsize=36)
ax=axes[0]
# ax.axvline(0, ls='--')
ax.axvline(break_one_t_offset, ls=':')
ax.set_xlabel('Time')
ax.set_ylabel('Flux')
ax.plot(tsteps, unbroken_pl(tsteps), label='Unbroken powerlaw')
ax.plot(tsteps, broken_pl(tsteps), label='Broken powerlaw')
ax.plot(tsteps, offset_pl(tsteps), label='Offset powerlaw')
ax.set_yscale('log')
ax.set_xscale('log')
# ax.set_ylim(0.001,.1)
# ax.set_xlim(t_min, 0.8*decay_tau)
ax.legend()
ax=axes[1]
# ax.axvline(0, ls='--')
ax.axvline(break_one_t_offset, ls=':')
ax.set_xlabel('Time')
ax.set_ylabel('Flux')
ax.plot(tsteps, unbroken_pl(tsteps), label='Unbroken powerlaw')
ax.plot(tsteps, broken_pl(tsteps), label='Broken powerlaw')
ax.plot(tsteps, offset_pl(tsteps), label='Offset powerlaw')
# ax.set_yscale('log')
# ax.set_xscale('log')
ax.set_ylim(0.001,.05)
ax.legend()
ax.set_xlim(t_min, 0.3*decay_tau)
# plt.gcf().tight_layout()
###Output
_____no_output_____ |
SimilarSkills.ipynb | ###Markdown
*Skills Recommendation*: For a set of skills provided by user, return n skills which are the most similar
###Code
# Reading in and setting up the adjacency matrix
Adj=pd.read_csv('https://datajam2018.blob.core.windows.net/eventopendata/ne_data/dwp_data/adzuna_adjacency_201709_201808.csv')
Adj=Adj.set_index('skills')
# Calculating correlation matrix
Diag=Adj.max()
B=Adj.divide(Diag)
Corr=B.multiply(B.transpose())
# Choosing n skills most correlated to user's skills (mySkills)
def SimilarSkills(mySkills, n) :
m=len(mySkills)
Similar=Corr.loc[mySkills].sum()
Similar=Similar.sort_values(ascending=False)[m:]
return Similar[:n]
# Test
#mySkills = ['.Net', 'Sales','Marketing','Business Development']
#SimilarSkills(mySkills, 10)
###Output
_____no_output_____
###Markdown
*Salary Information*: For each recommended skill return median salary from July 2018
###Code
# Get Salary information for skills, use latest reliable data
SkillSalary=pd.read_csv('https://datajam2018.blob.core.windows.net/eventopendata/ne_data/dwp_data/adzuna_skill_salary_median.csv', index_col=0)
Salary=SkillSalary.loc[201807]
# For each recommended skill return median salary
def SimilarSalary (mySkills, n) :
mySimilarSkills=SimilarSkills(mySkills, 10)
mySimilarSalary=Salary[mySimilarSkills.index.values]
return mySimilarSalary
# Test
#mySkills = ['Sales','Marketing','Business Development','Project Management']
#SimilarSalary(mySkills,10)
###Output
_____no_output_____ |
notebooks/Getting Started with Excalibur.ipynb | ###Markdown
Getting StartedThis notebook assumes you have followed the steps to download the Excalibur package onto your local machine. If you haven't, please visit this site: https://excalibur-alpha.readthedocs.io/en/latest/installation.html.The Run.py file is where the end-user will spend most of their time. If you want to run it, please make sure it is in the same directory as the Run.py folder in your Excalibur package. The user can use the `summon` function to download line lists, the `compute_cross_section` function to compute cross-sections, and the `plot_sigma_wl` function to plot the results of the output cross-sections.We will work through each of these in turn to demonstrate how to use the Run.py file. 1) The `summon` functionLet’s take a look at the `summon` function and the arguments it takes before seeing how to use it.We see that users can specify:- the database- species- isotope- VALD_data_dir (the relative path to the folder we have provided containing VALD line lists)- linelist (usually for ExoMol where there are multiple line lists for each molecule) - ionization_state (for VALD atomic line lists)You can use as many, or as few of these arguments as desired. The minimum required is the database and species. Example 1: Downloading the H20 line list from HITRAN
###Code
from excalibur.core import summon
species = 'H2O'
database = 'HITRAN'
# Download line list
summon(database=database, species = species)
###Output
Fetching data from HITRAN...
Molecule: H2O
Isotopologue H2(16O)
This .trans file took 2.4 seconds to reformat to HDF.
Line list ready.
###Markdown
Example 2: Downloading the BT2 line list for H2O from ExoMol The default line list for H2O is the Pokazatel line list, but say we want to download the BT2 line list for some reason.
###Code
from excalibur.core import summon
species = 'H2O'
database = 'ExoMol'
linelist = 'BT2'
# Download line list
summon(database=database, species = species, linelist = linelist)
###Output
_____no_output_____
###Markdown
Example 3: Downloading the line list of the second ionization state of oxygen from VALD
###Code
from excalibur.core import summon
species = 'O'
database = 'VALD'
ionization_state = 2
VALD_data_dir = './VALD Line Lists/'
# Download line list
summon(database=database, species = species, ionization_state = ionization_state, VALD_data_dir = VALD_data_dir)
###Output
_____no_output_____
###Markdown
Keep in mind that a path needs to be specified to read in the VALD line lists we have provided. Assuming that the user hasn’t moved this ‘VALD Line Lists’ folder anywhere, the path we use here will work as a default.The line lists will all be downloaded in an ‘input’ folder that is one directory above where the exclibur-alpha folder is stored on your local machine. 2) The `compute_cross_section` functionThis section describes how to compute the cross-section of a species at a given temperature and presssure. For info on computing cross sections for a grid of temperatures and pressures on a cluster, please see the ‘Cluster Run’ section.Let’s look at the `compute_cross_section` function.
###Code
def compute_cross_section(input_dir, database, species, log_pressure, temperature, isotope = 'default',
ionization_state = 1, linelist = 'default', cluster_run = False,
nu_out_min = 200, nu_out_max = 25000, dnu_out = 0.01, broad_type = 'default',
X_H2 = 0.85, X_He = 0.15, Voigt_cutoff = 500, Voigt_sub_spacing = (1.0/6.0),
N_alpha_samples = 500, S_cut = 1.0e-100, cut_max = 30.0, **kwargs):
###Output
_____no_output_____
###Markdown
Most of these arguments take on a default value. The ones the user has to worry about is:- input_dir (the prefix of the folder where the line list is stored, use the one we provided as a default)- database- species- log_pressure- temperatureSpecify the database, species, log_pressure, and temperature to compute a cross-section for a species with those specifications (assuming the line list has already been downloaded) Example 1: Compute the cross-section for H2O (HITRAN line list) at 1 bar and 1200 Kelvin
###Code
species = 'H2O'
database = 'HITRAN'
P = 1 # Pressure (bar)
T = 1200 # Temperature (K)
nu, sigma = compute_cross_section(input_dir = './input/', database = database,
species = species, log_pressure = np.log10(P),
temperature = T)
###Output
_____no_output_____
###Markdown
The results of this are stored in .txt files with wavenumber in the left column and sigma in the right column. Note that `nu` and `sigma` are returned by the `compute_cross_section` function. These are used, as shown in the next section, to plot cross-section. 3) The `plot_sigma_wl` functionThis section shows how to plot an outputted cross-section.Let’s look at the `plot_sigma_wl` function.
###Code
def plot_sigma_wl(species, temperature, log_pressure, nu_arr = [], sigma_arr = [],
file = '', database = '', plot_dir = './plots/', **kwargs):
###Output
_____no_output_____
###Markdown
Most of these arguments take on a default value. The ones the user has to worry about is:- species- temperature- log_pressure- nu_arr (nu array to plot)- sigma_arr (sigma array to plot)- plot_dir (where the plot will be stored, default is a folder called ‘plots’ one directory out)`species`, `temperature`, and `log_pressure` are used to name the outputted file appropriately. Currently, we do not support passing in files to be plotted by this function, but that is in the works.Here is an example of the `plot_sigma_wl` function and the plot it produces, using the nu and sigma arrays returned from Example 2.
###Code
species = 'H2O'
database = 'HITRAN'
P = 1 # Pressure (bar)
T = 1200 # Temperature (K)
# Plot cross section
plot_sigma_wl(nu_arr = nu, sigma_arr = sigma, species = species, temperature = T,
log_pressure = np.log10(P), database = database, plot_dir = './plots/')
###Output
_____no_output_____ |
site/en/r2/tutorials/load_data/csv.ipynb | ###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. The model will predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
try:
%tensorflow_version 2.x # Colab only.
except Exception:
pass
from __future__ import absolute_import, division, print_function, unicode_literals
import functools
import numpy as np
import tensorflow as tf
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataTo start, let's look at the top of the CSV file to see how it is formatted.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
You can [load this using pandas](pandas.ipynb), and pass the NumPy arrays to TensorFlow. If you need to scale up to a large set of files, or need a loader that integrates with [TensorFlow and tf.data](../../guide/data,ipynb) then use the `tf.data.experimental.make_csv_dataset` function: The only column you need to identify explicitly is the one with the value that the model is intended to predict.
###Code
LABEL_COLUMN = 'survived'
LABELS = [0, 1]
###Output
_____no_output_____
###Markdown
Now read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path, **kwargs):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=5, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True,
**kwargs)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
def show_batch(dataset):
for batch, label in dataset.take(1):
for key, value in batch.items():
print("{:20s}: {}".format(key,value.numpy()))
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
show_batch(raw_train_data)
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are named. The dataset constructor will pick these names up automatically. If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.
###Code
CSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']
temp_dataset = get_dataset(train_file_path, column_names=CSV_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'class', 'deck', 'alone']
temp_dataset = get_dataset(train_file_path, select_columns=SELECT_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
Data preprocessingA CSV file can contain a variety of data types. Typically you want to convert from those mixed types to a fixed length vector before feeding the data into your model.TensorFlow has a built-in system for describing common input conversions: `tf.feature_column`, see [this tutorial](../keras/feature_columns) for details.You can preprocess your data using any tool you like (like [nltk](https://www.nltk.org/) or [sklearn](https://scikit-learn.org/stable/)), and just pass the processed output to TensorFlow. The primary advantage of doing the preprocessing inside your model is that when you export the model it includes the preprocessing. This way you can pass the raw data directly to your model. Continuous data If your data is already in an apropriate numeric format, you can pack the data into a vector before passing it off to the model:
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'parch', 'fare']
DEFAULTS = [0, 0.0, 0.0, 0.0, 0.0]
temp_dataset = get_dataset(train_file_path,
select_columns=SELECT_COLUMNS,
column_defaults = DEFAULTS)
show_batch(temp_dataset)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
Here's a simple function that will pack together all the columns:
###Code
def pack(features, label):
return tf.stack(list(features.values()), axis=-1), label
###Output
_____no_output_____
###Markdown
Apply this to each element of the dataset:
###Code
packed_dataset = temp_dataset.map(pack)
for features, labels in packed_dataset.take(1):
print(features.numpy())
print()
print(labels.numpy())
###Output
_____no_output_____
###Markdown
If you have mixed datatypes you may want to separate out these simple-numeric fields. The `tf.feature_column` api can handle them, but this incurs some overhead and should be avoided unless really necessary. Switch back to the mixed dataset:
###Code
show_batch(raw_train_data)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
So define a more general preprocessor that selects a list of numeric features and packs them into a single column:
###Code
class PackNumericFeatures(object):
def __init__(self, names):
self.names = names
def __call__(self, features, labels):
numeric_freatures = [features.pop(name) for name in self.names]
numeric_features = [tf.cast(feat, tf.float32) for feat in numeric_freatures]
numeric_features = tf.stack(numeric_features, axis=-1)
features['numeric'] = numeric_features
return features, labels
NUMERIC_FEATURES = ['age','n_siblings_spouses','parch', 'fare']
packed_train_data = raw_train_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
packed_test_data = raw_test_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
show_batch(packed_train_data)
example_batch, labels_batch = next(iter(packed_train_data))
###Output
_____no_output_____
###Markdown
Data NormalizationContinuous data should always be normalized.
###Code
import pandas as pd
desc = pd.read_csv(train_file_path)[NUMERIC_FEATURES].describe()
desc
MEAN = np.array(desc.T['mean'])
STD = np.array(desc.T['std'])
def normalize_numeric_data(data, mean, std):
# Center the data
return (data-mean)/std
###Output
_____no_output_____
###Markdown
Now create a numeric column. The `tf.feature_columns.numeric_column` API accepts a `normalizer_fn` argument, which will be run on each batch.Bind the `MEAN` and `STD` to the normalizer fn using [`functools.partial`](https://docs.python.org/3/library/functools.htmlfunctools.partial).
###Code
# See what you just created.
normalizer = functools.partial(normalize_numeric_data, mean=MEAN, std=STD)
numeric_column = tf.feature_column.numeric_column('numeric', normalizer_fn=normalizer, shape=[len(NUMERIC_FEATURES)])
numeric_columns = [numeric_column]
numeric_column
###Output
_____no_output_____
###Markdown
When you train the model, include this feature column to select and center this block of numeric data:
###Code
example_batch['numeric']
numeric_layer = tf.keras.layers.DenseFeatures(numeric_columns)
numeric_layer(example_batch).numpy()
###Output
_____no_output_____
###Markdown
The mean based normalization used here requires knowing the means of each column ahead of time. Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.Use the `tf.feature_column` API to create a collection with a `tf.feature_column.indicator_column` for each categorical column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
categorical_columns = []
for feature, vocab in CATEGORIES.items():
cat_col = tf.feature_column.categorical_column_with_vocabulary_list(
key=feature, vocabulary_list=vocab)
categorical_columns.append(tf.feature_column.indicator_column(cat_col))
# See what you just created.
categorical_columns
categorical_layer = tf.keras.layers.DenseFeatures(categorical_columns)
print(categorical_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
This will be become part of a data processing input later when you build the model. Combined preprocessing layer Add the two feature column collections and pass them to a `tf.keras.layers.DenseFeatures` to create an input layer that will extract and preprocess both input types:
###Code
preprocessing_layer = tf.keras.layers.DenseFeatures(categorical_columns+numeric_columns)
print(preprocessing_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
Build the model Build a `tf.keras.Sequential`, starting with the `preprocessing_layer`.
###Code
model = tf.keras.Sequential([
preprocessing_layer,
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid'),
])
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
train_data = packed_train_data.shuffle(500)
test_data = packed_test_data
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, you can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta1
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/beta/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta0
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/beta/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-alpha0
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/alpha/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. The model will predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
try:
# %tensorflow_version only exists in Colab.
%tensorflow_version 2.x
except Exception:
pass
from __future__ import absolute_import, division, print_function, unicode_literals
import functools
import numpy as np
import tensorflow as tf
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataTo start, let's look at the top of the CSV file to see how it is formatted.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
You can [load this using pandas](pandas.ipynb), and pass the NumPy arrays to TensorFlow. If you need to scale up to a large set of files, or need a loader that integrates with [TensorFlow and tf.data](../../guide/data,ipynb) then use the `tf.data.experimental.make_csv_dataset` function: The only column you need to identify explicitly is the one with the value that the model is intended to predict.
###Code
LABEL_COLUMN = 'survived'
LABELS = [0, 1]
###Output
_____no_output_____
###Markdown
Now read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path, **kwargs):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=5, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True,
**kwargs)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
def show_batch(dataset):
for batch, label in dataset.take(1):
for key, value in batch.items():
print("{:20s}: {}".format(key,value.numpy()))
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
show_batch(raw_train_data)
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are named. The dataset constructor will pick these names up automatically. If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.
###Code
CSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']
temp_dataset = get_dataset(train_file_path, column_names=CSV_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'class', 'deck', 'alone']
temp_dataset = get_dataset(train_file_path, select_columns=SELECT_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
Data preprocessingA CSV file can contain a variety of data types. Typically you want to convert from those mixed types to a fixed length vector before feeding the data into your model.TensorFlow has a built-in system for describing common input conversions: `tf.feature_column`, see [this tutorial](../keras/feature_columns) for details.You can preprocess your data using any tool you like (like [nltk](https://www.nltk.org/) or [sklearn](https://scikit-learn.org/stable/)), and just pass the processed output to TensorFlow. The primary advantage of doing the preprocessing inside your model is that when you export the model it includes the preprocessing. This way you can pass the raw data directly to your model. Continuous data If your data is already in an apropriate numeric format, you can pack the data into a vector before passing it off to the model:
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'parch', 'fare']
DEFAULTS = [0, 0.0, 0.0, 0.0, 0.0]
temp_dataset = get_dataset(train_file_path,
select_columns=SELECT_COLUMNS,
column_defaults = DEFAULTS)
show_batch(temp_dataset)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
Here's a simple function that will pack together all the columns:
###Code
def pack(features, label):
return tf.stack(list(features.values()), axis=-1), label
###Output
_____no_output_____
###Markdown
Apply this to each element of the dataset:
###Code
packed_dataset = temp_dataset.map(pack)
for features, labels in packed_dataset.take(1):
print(features.numpy())
print()
print(labels.numpy())
###Output
_____no_output_____
###Markdown
If you have mixed datatypes you may want to separate out these simple-numeric fields. The `tf.feature_column` api can handle them, but this incurs some overhead and should be avoided unless really necessary. Switch back to the mixed dataset:
###Code
show_batch(raw_train_data)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
So define a more general preprocessor that selects a list of numeric features and packs them into a single column:
###Code
class PackNumericFeatures(object):
def __init__(self, names):
self.names = names
def __call__(self, features, labels):
numeric_freatures = [features.pop(name) for name in self.names]
numeric_features = [tf.cast(feat, tf.float32) for feat in numeric_freatures]
numeric_features = tf.stack(numeric_features, axis=-1)
features['numeric'] = numeric_features
return features, labels
NUMERIC_FEATURES = ['age','n_siblings_spouses','parch', 'fare']
packed_train_data = raw_train_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
packed_test_data = raw_test_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
show_batch(packed_train_data)
example_batch, labels_batch = next(iter(packed_train_data))
###Output
_____no_output_____
###Markdown
Data NormalizationContinuous data should always be normalized.
###Code
import pandas as pd
desc = pd.read_csv(train_file_path)[NUMERIC_FEATURES].describe()
desc
MEAN = np.array(desc.T['mean'])
STD = np.array(desc.T['std'])
def normalize_numeric_data(data, mean, std):
# Center the data
return (data-mean)/std
###Output
_____no_output_____
###Markdown
Now create a numeric column. The `tf.feature_columns.numeric_column` API accepts a `normalizer_fn` argument, which will be run on each batch.Bind the `MEAN` and `STD` to the normalizer fn using [`functools.partial`](https://docs.python.org/3/library/functools.htmlfunctools.partial).
###Code
# See what you just created.
normalizer = functools.partial(normalize_numeric_data, mean=MEAN, std=STD)
numeric_column = tf.feature_column.numeric_column('numeric', normalizer_fn=normalizer, shape=[len(NUMERIC_FEATURES)])
numeric_columns = [numeric_column]
numeric_column
###Output
_____no_output_____
###Markdown
When you train the model, include this feature column to select and center this block of numeric data:
###Code
example_batch['numeric']
numeric_layer = tf.keras.layers.DenseFeatures(numeric_columns)
numeric_layer(example_batch).numpy()
###Output
_____no_output_____
###Markdown
The mean based normalization used here requires knowing the means of each column ahead of time. Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.Use the `tf.feature_column` API to create a collection with a `tf.feature_column.indicator_column` for each categorical column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
categorical_columns = []
for feature, vocab in CATEGORIES.items():
cat_col = tf.feature_column.categorical_column_with_vocabulary_list(
key=feature, vocabulary_list=vocab)
categorical_columns.append(tf.feature_column.indicator_column(cat_col))
# See what you just created.
categorical_columns
categorical_layer = tf.keras.layers.DenseFeatures(categorical_columns)
print(categorical_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
This will be become part of a data processing input later when you build the model. Combined preprocessing layer Add the two feature column collections and pass them to a `tf.keras.layers.DenseFeatures` to create an input layer that will extract and preprocess both input types:
###Code
preprocessing_layer = tf.keras.layers.DenseFeatures(categorical_columns+numeric_columns)
print(preprocessing_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
Build the model Build a `tf.keras.Sequential`, starting with the `preprocessing_layer`.
###Code
model = tf.keras.Sequential([
preprocessing_layer,
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid'),
])
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
train_data = packed_train_data.shuffle(500)
test_data = packed_test_data
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, you can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. The model will predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta1
from __future__ import absolute_import, division, print_function, unicode_literals
import functools
import numpy as np
import tensorflow as tf
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataTo start, let's look at the top of the CSV file to see how it is formatted.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
You can [load this using pandas](pandas.ipynb), and pass the NumPy arrays to TensorFlow. If you need to scale up to a large set of files, or need a loader that integrates with [TensorFlow and tf.data](../../guide/data,ipynb) then use the `tf.data.experimental.make_csv_dataset` function: The only column you need to identify explicitly is the one with the value that the model is intended to predict.
###Code
LABEL_COLUMN = 'survived'
LABELS = [0, 1]
###Output
_____no_output_____
###Markdown
Now read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path, **kwargs):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=5, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True,
**kwargs)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
def show_batch(dataset):
for batch, label in dataset.take(1):
for key, value in batch.items():
print("{:20s}: {}".format(key,value.numpy()))
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
show_batch(raw_train_data)
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are named. The dataset constructor will pick these names up automatically. If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.
###Code
CSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']
temp_dataset = get_dataset(train_file_path, column_names=CSV_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'class', 'deck', 'alone']
temp_dataset = get_dataset(train_file_path, select_columns=SELECT_COLUMNS)
show_batch(temp_dataset)
###Output
_____no_output_____
###Markdown
Data preprocessingA CSV file can contain a variety of data types. Typically you want to convert from those mixed types to a fixed length vector before feeding the data into your model.TensorFlow has a built-in system for describing common input conversions: `tf.feature_column`, see [this tutorial](../keras/feature_columns) for details.You can preprocess your data using any tool you like (like [nltk](https://www.nltk.org/) or [sklearn](https://scikit-learn.org/stable/)), and just pass the processed output to TensorFlow. The primary advantage of doing the preprocessing inside your model is that when you export the model it includes the preprocessing. This way you can pass the raw data directly to your model. Continuous data If your data is already in an apropriate numeric format, you can pack the data into a vector before passing it off to the model:
###Code
SELECT_COLUMNS = ['survived', 'age', 'n_siblings_spouses', 'parch', 'fare']
DEFAULTS = [0, 0.0, 0.0, 0.0, 0.0]
temp_dataset = get_dataset(train_file_path,
select_columns=SELECT_COLUMNS,
column_defaults = DEFAULTS)
show_batch(temp_dataset)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
Here's a simple function that will pack together all the columns:
###Code
def pack(features, label):
return tf.stack(list(features.values()), axis=-1), label
###Output
_____no_output_____
###Markdown
Apply this to each element of the dataset:
###Code
packed_dataset = temp_dataset.map(pack)
for features, labels in packed_dataset.take(1):
print(features.numpy())
print()
print(labels.numpy())
###Output
_____no_output_____
###Markdown
If you have mixed datatypes you may want to separate out these simple-numeric fields. The `tf.feature_column` api can handle them, but this incurs some overhead and should be avoided unless really necessary. Switch back to the mixed dataset:
###Code
show_batch(raw_train_data)
example_batch, labels_batch = next(iter(temp_dataset))
###Output
_____no_output_____
###Markdown
So define a more general preprocessor that selects a list of numeric features and packs them into a single column:
###Code
class PackNumericFeatures(object):
def __init__(self, names):
self.names = names
def __call__(self, features, labels):
numeric_freatures = [features.pop(name) for name in self.names]
numeric_features = [tf.cast(feat, tf.float32) for feat in numeric_freatures]
numeric_features = tf.stack(numeric_features, axis=-1)
features['numeric'] = numeric_features
return features, labels
NUMERIC_FEATURES = ['age','n_siblings_spouses','parch', 'fare']
packed_train_data = raw_train_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
packed_test_data = raw_test_data.map(
PackNumericFeatures(NUMERIC_FEATURES))
show_batch(packed_train_data)
example_batch, labels_batch = next(iter(packed_train_data))
###Output
_____no_output_____
###Markdown
Data NormalizationContinuous data should always be normalized.
###Code
import pandas as pd
desc = pd.read_csv(train_file_path)[NUMERIC_FEATURES].describe()
desc
MEAN = np.array(desc.T['mean'])
STD = np.array(desc.T['std'])
def normalize_numeric_data(data, mean, std):
# Center the data
return (data-mean)/std
###Output
_____no_output_____
###Markdown
Now create a numeric column. The `tf.feature_columns.numeric_column` API accepts a `normalizer_fn` argument, which will be run on each batch.Bind the `MEAN` and `STD` to the normalizer fn using [`functools.partial`](https://docs.python.org/3/library/functools.htmlfunctools.partial).
###Code
# See what you just created.
normalizer = functools.partial(normalize_numeric_data, mean=MEAN, std=STD)
numeric_column = tf.feature_column.numeric_column('numeric', normalizer_fn=normalizer, shape=[len(NUMERIC_FEATURES)])
numeric_columns = [numeric_column]
numeric_column
###Output
_____no_output_____
###Markdown
When you train the model, include this feature column to select and center this block of numeric data:
###Code
example_batch['numeric']
numeric_layer = tf.keras.layers.DenseFeatures(numeric_columns)
numeric_layer(example_batch).numpy()
###Output
_____no_output_____
###Markdown
The mean based normalization used here requires knowing the means of each column ahead of time. Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.Use the `tf.feature_column` API to create a collection with a `tf.feature_column.indicator_column` for each categorical column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
categorical_columns = []
for feature, vocab in CATEGORIES.items():
cat_col = tf.feature_column.categorical_column_with_vocabulary_list(
key=feature, vocabulary_list=vocab)
categorical_columns.append(tf.feature_column.indicator_column(cat_col))
# See what you just created.
categorical_columns
categorical_layer = tf.keras.layers.DenseFeatures(categorical_columns)
print(categorical_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
This will be become part of a data processing input later when you build the model. Combined preprocessing layer Add the two feature column collections and pass them to a `tf.keras.layers.DenseFeatures` to create an input layer that will extract and preprocess both input types:
###Code
preprocessing_layer = tf.keras.layers.DenseFeatures(categorical_columns+numeric_columns)
print(preprocessing_layer(example_batch).numpy()[0])
###Output
_____no_output_____
###Markdown
Build the model Build a `tf.keras.Sequential`, starting with the `preprocessing_layer`.
###Code
model = tf.keras.Sequential([
preprocessing_layer,
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid'),
])
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
train_data = packed_train_data.shuffle(500)
test_data = packed_test_data
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, you can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. The model will predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta1
from __future__ import absolute_import, division, print_function, unicode_literals
import functools
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataTo start, let's look at the top of the CSV file to see how it is formatted.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are named. The dataset constructor will pick these names up automatically. If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function. ```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` The only column you need to identify explicitly is the one with the value that the model is intended to predict.
###Code
LABEL_COLUMN = 'survived'
LABELS = [0, 1]
###Output
_____no_output_____
###Markdown
Now read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.Use the `tf.feature_column` API to create a collection with a `tf.feature_column.indicator_column` for each categorical column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
categorical_columns = []
for feature, vocab in CATEGORIES.items():
cat_col = tf.feature_column.categorical_column_with_vocabulary_list(
key=feature, vocabulary_list=vocab)
categorical_columns.append(tf.feature_column.indicator_column(cat_col))
# See what you just created.
categorical_columns
###Output
_____no_output_____
###Markdown
This will be become part of a data processing input later when you build the model. Continuous data Continuous data needs to be normalized.Write a function that normalizes the values and reshapes them into two-dimensional tensors.
###Code
def process_continuous_data(mean, data):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
Now create a collection of numeric columns. The `tf.feature_columns.numeric_column` API takes a `normalizer_fn`. Pass in a [`functools.partial`](https://docs.python.org/3/library/functools.htmlfunctools.partial) made from the processing function, loaded with the mean of each column.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
numerical_columns = []
for feature in MEANS.keys():
num_col = tf.feature_column.numeric_column(feature, normalizer_fn=functools.partial(process_continuous_data, MEANS[feature]))
numerical_columns.append(num_col)
# See what you just created.
numerical_columns
###Output
_____no_output_____
###Markdown
The means based normalization used here requires knowing the means of each column ahead of time. To calculate normalized values in a continuous data stream, use [TensorFlow Transform](https://www.tensorflow.org/tfx/transform/get_started). Create a preprocessing layer Add the two feature column collections and pass them to `tf.keras.layers.DenseFeatures` to create an input layer that will handle your preprocessing.
###Code
preprocessing_layer = tf.keras.layers.DenseFeatures(categorical_columns+numerical_columns)
###Output
_____no_output_____
###Markdown
Build the model Build a `tf.keras.Sequential`, starting with the `preprocessing_layer`.
###Code
model = tf.keras.Sequential([
preprocessing_layer,
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid'),
])
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
train_data = raw_train_data.shuffle(500)
test_data = raw_test_data
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, you can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta1
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/beta/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta0
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/beta/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. We'll try to predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-alpha0
from __future__ import absolute_import, division, print_function, unicode_literals
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataSo we know what we're doing, lets look at the top of the CSV file we're working with.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are labeled. We need the list later on, so let's read it out of the file.
###Code
# CSV columns in the input file.
with open(train_file_path, 'r') as f:
names_row = f.readline()
CSV_COLUMNS = names_row.rstrip('\n').split(',')
print(CSV_COLUMNS)
###Output
_____no_output_____
###Markdown
The dataset constructor will pick these labels up automatically.If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function.```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondrop_columns = ['fare', 'embark_town']columns_to_use = [col for col in CSV_COLUMNS if col not in drop_columns]dataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` We also have to identify which column will serve as the labels for each example, and what those labels are.
###Code
LABELS = [0, 1]
LABEL_COLUMN = 'survived'
FEATURE_COLUMNS = [column for column in CSV_COLUMNS if column != LABEL_COLUMN]
###Output
_____no_output_____
###Markdown
Now that these constructor argument values are in place, read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.In the CSV, these options are represented as text. This text needs to be converted to numbers before the model can be trained. To facilitate that, we need to create a list of categorical columns, along with a list of the options available in each column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
###Output
_____no_output_____
###Markdown
Write a function that takes a tensor of categorical values, matches it to a list of value names, and then performs a one-hot encoding.
###Code
def process_categorical_data(data, categories):
"""Returns a one-hot encoded tensor representing categorical values."""
# Remove leading ' '.
data = tf.strings.regex_replace(data, '^ ', '')
# Remove trailing '.'.
data = tf.strings.regex_replace(data, r'\.$', '')
# ONE HOT ENCODE
# Reshape data from 1d (a list) to a 2d (a list of one-element lists)
data = tf.reshape(data, [-1, 1])
# For each element, create a new list of boolean values the length of categories,
# where the truth value is element == category label
data = tf.equal(categories, data)
# Cast booleans to floats.
data = tf.cast(data, tf.float32)
# The entire encoding can fit on one line:
# data = tf.cast(tf.equal(categories, tf.reshape(data, [-1, 1])), tf.float32)
return data
###Output
_____no_output_____
###Markdown
To help you visualize this, we'll take a single category-column tensor from the first batch, preprocess it, and show the before and after state.
###Code
class_tensor = examples['class']
class_tensor
class_categories = CATEGORIES['class']
class_categories
processed_class = process_categorical_data(class_tensor, class_categories)
processed_class
###Output
_____no_output_____
###Markdown
Notice the relationship between the lengths of the two inputs and the shape of the output.
###Code
print("Size of batch: ", len(class_tensor.numpy()))
print("Number of category labels: ", len(class_categories))
print("Shape of one-hot encoded tensor: ", processed_class.shape)
###Output
_____no_output_____
###Markdown
Continuous data Continuous data needs to be normalized, so that the values fall between 0 and 1. To do that, write a function that multiplies each value by 1 over twice the mean of the column values.The function should also reshape the data into a two dimensional tensor.
###Code
def process_continuous_data(data, mean):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
To do this calculation, you need the column means. You would obviously need to compute these in real life, but for this example we'll just provide them.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
###Output
_____no_output_____
###Markdown
Again, to see what this function is actually doing, we'll take a single tensor of continuous data and show it before and after processing.
###Code
age_tensor = examples['age']
age_tensor
process_continuous_data(age_tensor, MEANS['age'])
###Output
_____no_output_____
###Markdown
Preprocess the data Now assemble these preprocessing tasks into a single function that can be mapped to each batch in the dataset.
###Code
def preprocess(features, labels):
# Process categorial features.
for feature in CATEGORIES.keys():
features[feature] = process_categorical_data(features[feature],
CATEGORIES[feature])
# Process continuous features.
for feature in MEANS.keys():
features[feature] = process_continuous_data(features[feature],
MEANS[feature])
# Assemble features into a single tensor.
features = tf.concat([features[column] for column in FEATURE_COLUMNS], 1)
return features, labels
###Output
_____no_output_____
###Markdown
Now apply that function with `tf.Dataset.map`, and shuffle the dataset to avoid overfitting.
###Code
train_data = raw_train_data.map(preprocess).shuffle(500)
test_data = raw_test_data.map(preprocess)
###Output
_____no_output_____
###Markdown
And let's see what a single example looks like.
###Code
examples, labels = next(iter(train_data))
examples, labels
###Output
_____no_output_____
###Markdown
The examples are in a two dimensional arrays of 12 items each (the batch size). Each item represents a single row in the original CSV file. The labels are a 1d tensor of 12 values. Build the model This example uses the [Keras Functional API](https://www.tensorflow.org/alpha/guide/keras/functional) wrapped in a `get_model` constructor to build up a simple model.
###Code
def get_model(input_dim, hidden_units=[100]):
"""Create a Keras model with layers.
Args:
input_dim: (int) The shape of an item in a batch.
labels_dim: (int) The shape of a label.
hidden_units: [int] the layer sizes of the DNN (input layer first)
learning_rate: (float) the learning rate for the optimizer.
Returns:
A Keras model.
"""
inputs = tf.keras.Input(shape=(input_dim,))
x = inputs
for units in hidden_units:
x = tf.keras.layers.Dense(units, activation='relu')(x)
outputs = tf.keras.layers.Dense(1, activation='sigmoid')(x)
model = tf.keras.Model(inputs, outputs)
return model
###Output
_____no_output_____
###Markdown
The `get_model` constructor needs to know the input shape of your data (not including the batch size).
###Code
input_shape, output_shape = train_data.output_shapes
input_dimension = input_shape.dims[1] # [0] is the batch size
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
model = get_model(input_dimension)
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, we can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____
###Markdown
Copyright 2019 The TensorFlow Authors.
###Code
#@title Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# https://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
###Output
_____no_output_____
###Markdown
Load CSV with tf.data View on TensorFlow.org Run in Google Colab View source on GitHub Download notebook This tutorial provides an example of how to load CSV data from a file into a `tf.data.Dataset`.The data used in this tutorial are taken from the Titanic passenger list. The model will predict the likelihood a passenger survived based on characteristics like age, gender, ticket class, and whether the person was traveling alone. Setup
###Code
!pip install tensorflow==2.0.0-beta1
from __future__ import absolute_import, division, print_function, unicode_literals
import functools
import numpy as np
import tensorflow as tf
import tensorflow_datasets as tfds
TRAIN_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/train.csv"
TEST_DATA_URL = "https://storage.googleapis.com/tf-datasets/titanic/eval.csv"
train_file_path = tf.keras.utils.get_file("train.csv", TRAIN_DATA_URL)
test_file_path = tf.keras.utils.get_file("eval.csv", TEST_DATA_URL)
# Make numpy values easier to read.
np.set_printoptions(precision=3, suppress=True)
###Output
_____no_output_____
###Markdown
Load dataTo start, let's look at the top of the CSV file to see how it is formatted.
###Code
!head {train_file_path}
###Output
_____no_output_____
###Markdown
As you can see, the columns in the CSV are named. The dataset constructor will pick these names up automatically. If the file you are working with does not contain the column names in the first line, pass them in a list of strings to the `column_names` argument in the `make_csv_dataset` function. ```pythonCSV_COLUMNS = ['survived', 'sex', 'age', 'n_siblings_spouses', 'parch', 'fare', 'class', 'deck', 'embark_town', 'alone']dataset = tf.data.experimental.make_csv_dataset( ..., column_names=CSV_COLUMNS, ...) ``` This example is going to use all the available columns. If you need to omit some columns from the dataset, create a list of just the columns you plan to use, and pass it into the (optional) `select_columns` argument of the constructor.```pythondataset = tf.data.experimental.make_csv_dataset( ..., select_columns = columns_to_use, ...)``` The only column you need to identify explicitly is the one with the value that the model is intended to predict.
###Code
LABEL_COLUMN = 'survived'
LABELS = [0, 1]
###Output
_____no_output_____
###Markdown
Now read the CSV data from the file and create a dataset. (For the full documentation, see `tf.data.experimental.make_csv_dataset`)
###Code
def get_dataset(file_path):
dataset = tf.data.experimental.make_csv_dataset(
file_path,
batch_size=12, # Artificially small to make examples easier to show.
label_name=LABEL_COLUMN,
na_value="?",
num_epochs=1,
ignore_errors=True)
return dataset
raw_train_data = get_dataset(train_file_path)
raw_test_data = get_dataset(test_file_path)
###Output
_____no_output_____
###Markdown
Each item in the dataset is a batch, represented as a tuple of (*many examples*, *many labels*). The data from the examples is organized in column-based tensors (rather than row-based tensors), each with as many elements as the batch size (12 in this case).It might help to see this yourself.
###Code
examples, labels = next(iter(raw_train_data)) # Just the first batch.
print("EXAMPLES: \n", examples, "\n")
print("LABELS: \n", labels)
###Output
_____no_output_____
###Markdown
Data preprocessing Categorical dataSome of the columns in the CSV data are categorical columns. That is, the content should be one of a limited set of options.Use the `tf.feature_column` API to create a collection with a `tf.feature_column.indicator_column` for each categorical column.
###Code
CATEGORIES = {
'sex': ['male', 'female'],
'class' : ['First', 'Second', 'Third'],
'deck' : ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'],
'embark_town' : ['Cherbourg', 'Southhampton', 'Queenstown'],
'alone' : ['y', 'n']
}
categorical_columns = []
for feature, vocab in CATEGORIES.items():
cat_col = tf.feature_column.categorical_column_with_vocabulary_list(
key=feature, vocabulary_list=vocab)
categorical_columns.append(tf.feature_column.indicator_column(cat_col))
# See what you just created.
categorical_columns
###Output
_____no_output_____
###Markdown
This will be become part of a data processing input later when you build the model. Continuous data Continuous data needs to be normalized.Write a function that normalizes the values and reshapes them into two-dimensional tensors.
###Code
def process_continuous_data(mean, data):
# Normalize data
data = tf.cast(data, tf.float32) * 1/(2*mean)
return tf.reshape(data, [-1, 1])
###Output
_____no_output_____
###Markdown
Now create a collection of numeric columns. The `tf.feature_columns.numeric_column` API takes a `normalizer_fn`. Pass in a [`functools.partial`](https://docs.python.org/3/library/functools.htmlfunctools.partial) made from the processing function, loaded with the mean of each column.
###Code
MEANS = {
'age' : 29.631308,
'n_siblings_spouses' : 0.545455,
'parch' : 0.379585,
'fare' : 34.385399
}
numerical_columns = []
for feature in MEANS.keys():
num_col = tf.feature_column.numeric_column(feature, normalizer_fn=functools.partial(process_continuous_data, MEANS[feature]))
numerical_columns.append(num_col)
# See what you just created.
numerical_columns
###Output
_____no_output_____
###Markdown
The means based normalization used here requires knowing the means of each column ahead of time. To calculate normalized values in a continuous data stream, use [TensorFlow Transform](https://www.tensorflow.org/tfx/transform/get_started). Create a preprocessing layer Add the two feature column collections and pass them to `tf.keras.layers.DenseFeatures` to create an input layer that will handle your preprocessing.
###Code
preprocessing_layer = tf.keras.layers.DenseFeatures(categorical_columns+numerical_columns)
###Output
_____no_output_____
###Markdown
Build the model Build a `tf.keras.Sequential`, starting with the `preprocessing_layer`.
###Code
model = tf.keras.Sequential([
preprocessing_layer,
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid'),
])
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
###Output
_____no_output_____
###Markdown
Train, evaluate, and predict Now the model can be instantiated and trained.
###Code
train_data = raw_train_data.shuffle(500)
test_data = raw_test_data
model.fit(train_data, epochs=20)
###Output
_____no_output_____
###Markdown
Once the model is trained, you can check its accuracy on the `test_data` set.
###Code
test_loss, test_accuracy = model.evaluate(test_data)
print('\n\nTest Loss {}, Test Accuracy {}'.format(test_loss, test_accuracy))
###Output
_____no_output_____
###Markdown
Use `tf.keras.Model.predict` to infer labels on a batch or a dataset of batches.
###Code
predictions = model.predict(test_data)
# Show some results
for prediction, survived in zip(predictions[:10], list(test_data)[0][1][:10]):
print("Predicted survival: {:.2%}".format(prediction[0]),
" | Actual outcome: ",
("SURVIVED" if bool(survived) else "DIED"))
###Output
_____no_output_____ |
spark-training/spark-python/jupyter-introduction/PySpark Introduction.ipynb | ###Markdown
PySpark IntroductionThis introdactionary Jupyter notebook is intended to provide a self-learning basis for getting started with Apache Spark with Python. It contains all basic operations (transofmrations, filtering, joins, grouping and aggregation) and serves as a small reference for later training exercises. OrganisationThe Notebook contains multiple sections, each with a small introduction on the specific topic, some PySpark example code and some small exercises, where you can directly apply the newly learned material. PrerequisitesYou need a Jupyter Notebook environment with an embedded Spark context. You might need to ask your administrator to setup an appropiate environment. Moreover some small test data is also required to be at a location accessible from Spark.
###Code
spark.version
# Set the base directory according to your environment
basedir = "s3://dimajix-training/data/"
###Output
_____no_output_____
###Markdown
1. Reading DataFor working with data, we need to get some data first. Spark supports various file formats, we will use CSV in the following example.The entrypoint for creating Spark objects is an object called `spark` which is provided in the notebook and read to use. We will read a file containing some informations on a couple of persons, which will serve as the basis for the next examples.
###Code
persons = (
spark.read.option("inferSchema", True)
.option("header", True)
.csv(basedir + "persons_header.csv")
)
###Output
_____no_output_____
###Markdown
Inspecting DataThe read operation returns a so called Spark *DataFrame* object. This object is similar to a table, it contains rows of records, which all conform to a common schema with named columns and specific types. On the surface it heavily borrows concepts from Pandas DataFrames or R DataFrames, although the syntax and many operations are syntactically very different.As the first step, we want to see the contents of the DataFrame. This can be easily done by using the `show` method.
###Code
persons.show()
###Output
_____no_output_____
###Markdown
Inspecting the SchemaIt may also be interesting not to inspect the data directly, but to inspect the schema. The schema contains the meta information about which columns are present and which types are used in the columns. You can either directly work with the schema object by using the `schema` variable of a DataFrame, or you can print the schema with the `printSchema()` method as follows:
###Code
persons.printSchema()
###Output
_____no_output_____
###Markdown
Converting to PandasSpark also provides some convenience method for converting a Spark DataFrame into a Pandas DataFrame. This is not only useful for using Pandas algorithms, but this is particular handy in Jupyter notebooks which have built-in support for displaying Pandas DataFrames nicely. Therefore we will prefer to use the `toPandas()` method for displaying the contents of a DataFrame instead of the `show()` method above.
###Code
persons.toPandas()
###Output
_____no_output_____
###Markdown
Attention: Beware of huge DatFrames!Do not forget hat Apache Spark has been designed and built to handle really huge data sets, which do not need to fit into memory. Spark DataFrames con contain billions of rows and are stored in a distributed way on many nodes in a cluster. Actually the contents do not even need to be physically present at all, as long as the input data is accessible.But calling the `toPandas()` method will transfer all the records to a single machine (where the Jupyter Notebook runs on) - but maybe this computer does not have enough memory to hold all the data. In this case, you risk that the notebook process will crash with an Out-Of-Memory error (OOM). So you should only use `toPandas()` when you are really sure that the DataFrame contains a limited amount of records. Exercise1. Load in the file "persons.json". This file contains exactly the same data, but is stored as a JSON file instead of a CSV file. 2. Inspect the schema3. Show the contents of the file4. Convert the Spark DataFrame to a Pandas DataFrame 2. Simple Transformations ProjectionsThe simplest thing to do is to create a new DataFrame with a subset of the available columns
###Code
from pyspark.sql.functions import *
result = persons.select(persons.name, persons['sex'], col('age'))
result.toPandas()
###Output
_____no_output_____
###Markdown
One noteable concept of Spark is that every transformation will return a new DataFrame. The original DataFrame remains unchanged. This is a deep architectural decision of Spark which simplifies parallel processing under the hood. Addressing ColumnsSpark supports multiple different ways for *addressing* a columns. We just saw one way, but also the following methods are supported for specifying a column:```df.column_namedf['column_name']col('column_name')```All these methods return a `Column` object, which is an abstract representative of the data in the column. As we will see soon, transformations can be applied to `Column` in order to derive new values. ExerciseUse all three different methods for addressing a column, and select the following columns:* name* age* city TransformationsThe `select` method actually accepts any *column* object. A *column* object conceptually represents a column in a DataFrame. The column may either refer directly to an existing column of the input DataFrame, or it may represent the result of a calculation or transformation of one or multiple columns of the input DataFrame. For example if we simply want to transform the name into upper case, we can do so by using a function `upper` provided by PySpark.
###Code
from pyspark.sql.functions import *
result = persons.select(upper(persons.name))
result.toPandas()
###Output
_____no_output_____
###Markdown
Lets look at a differnt example where we want to create a new DataFrame with the appropriate salutation in front of the name. We achieve this by the following `select` statement:
###Code
from pyspark.sql.functions import *
result = persons.select(
concat(when(persons.sex == 'male', "Mr ").otherwise("Mrs "), persons.name)
)
result.toPandas()
###Output
_____no_output_____
###Markdown
Common FunctionsYou can find the full list of available functions at [PySpark SQL Module](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlmodule-pyspark.sql.functions). Commonly used functions for example are as follows:* [`concat(*cols)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.concat) - Concatenates multiple input columns together into a single column.* [`substring(col,start,len)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.substring) - Substring starts at pos and is of length len when str is String type or returns the slice of byte array that starts at pos in byte and is of length len when str is Binary type.* [`instr(col,substr)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.instr) - Locate the position of the first occurrence of substr column in the given string. Returns null if either of the arguments are null.* [`locate(col,substr, pos)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.locate) - Locate the position of the first occurrence of substr in a string column, after position pos.* [`length(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.length) - Computes the character length of string data or number of bytes of binary data. * [`upper(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.upper) - Converts a string column to upper case.* [`lower(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.lower) - Converts a string column to lower case.* [`coalesce(*cols)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.coalesce) - Returns the first column that is not null.* [`isnull(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.isnull) - An expression that returns true iff the column is null.* [`isnan(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.isnan) - An expression that returns true iff the column is NaN.* [`hash(cols*)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.hash) - Calculates the hash code of given columns.Spark also supports conditional expressions, like the SQL `CASE WHEN` construct* [`when(condition, value)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.when) - Evaluates a list of conditions and returns one of multiple possible result expressions.There are also some special functions often required* [`col(str)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.col) - Returns a Column based on the given column name.* [`lit(val)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.lit) - Creates a Column of literal value.* [`expr(str)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.expr) - Parses the expression string into the column that it represents User Defined FunctionsUnfortunately you cannot directly use normal Python functions for transforming DataFrame columns. Although PySpark already provides many useful functions, this might not always sufficient. But fortunately you can *convert* a standard Python function into a PySpark function, thereby defining a so called *user defined function* (UDF). Details will be explained in detail in the training. Defining new Column NamesThe resulting DataFrame again has a schema, but the column names to not look very nice. But by using the `alias` method of a `Column` object, you can immediately rename the newly created column like you are already used to in SQL with `SELECT complex_operation(...) AS nice_name FROM ...`. Technically specifying a new name for the resulting column is not required (as we already saw above), if the name is not specified, PySpark will generate a name from the expression. But since this generated name tends to be rather long and contains the logic instead of the intention, it is highly recommended to always explicitly specify the name of the resulting column using `as`.
###Code
result = persons.select(
concat(when(persons.sex == 'male', "Mr ").otherwise("Mrs "), persons.name).alias(
"qualified_name"
)
)
result.toPandas()
###Output
_____no_output_____
###Markdown
Adding ColumnsA special variant of a `select` statement is the `withColumn` method. While the `select` statement requires all resulting columns to be defined in as arguments, the `withColumn` method keeps all existing columns and adds a new one. This operation is quite useful since in many cases new columns are derived from the existing ones, while the old ones still should be contained in the result.Let us have a look at a simple example, which only adds the salutation as a new column:
###Code
result = persons.withColumn(
"salutation", when(persons.sex == 'male', "Mr ").otherwise("Mrs ")
)
result.toPandas()
###Output
_____no_output_____
###Markdown
As you can see from the example above, `withColumn` always takes two arguments: The first one is the name of the new column (and it has to be a string), and the second argument is the expression containing the logic for calculating the actual contents. Removing ColumnsPySpark also supports the opposite operation which simply removes some columns from a dataframe. This is useful if you need to remove some sensitive data before saving it to disk:
###Code
result = persons.drop("name")
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseUsing the `persons` DataFrame, perform the following operations:* Add a new column `status` which should be `child` if the person is younger than 18 and `adult` otherwise* Replace the column `name` by a new column `hashed_name` containing the hash value of the name* Drop the column `sex` 3. Filtering*Filtering* denotes the process of keeping only rows which meet a certain filter criteria. PySpark support two different approaches. The first approach specifies the filtering expression as a PySpark expression using columns:
###Code
result = persons.filter(persons.age > 20)
result.show()
###Output
_____no_output_____
###Markdown
The second approach simply uses a string containing an SQL expression:
###Code
result = persons.filter("age > 20")
result.show()
###Output
_____no_output_____
###Markdown
Of course you can also combine multiple conditions using `&` (and) and `|` (or) with the first approach or by using the SQL keywords `AND` and `OR` in the second approach. ExercisePerform different filter operations:* Select all women with a height of at least 160* Select all persons which are younger than 20 or older than 30 4. Grouping & AggregationAn important class of operation is grouping and aggregation, which is equivalnt to an SQL `SELECT aggregation GROUP BY grouping` statement. In PySpark, grouping and aggregation is always performed by first creating groups using `groupBy` immediately followed by aggregation expressions inside an `agg` method. (Actually there are also some predefined aggregations which can be used instead of `agg`, but they do not offer the flexiviliby which is required most of the time).Note that in the `agg` method you only need to specify the aggregation expression, the grouping columns are added automatically by PySpark to the resulting DataFrame.
###Code
result = persons.groupBy(persons.sex).agg(
avg(persons.age).alias('avg_age'),
min(persons.height).alias('min_height'),
max(persons.height).alias('max_height'),
)
result.toPandas()
###Output
_____no_output_____
###Markdown
Aggregation FunctionsPySpark supports many aggregation functions, they can be found in the documentation at [PySpark Function Documentation](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlmodule-pyspark.sql.functions). Aggregation functions are marked as such in the documentation, unfortunately there is no simple overview. Among common aggregation functions, there are for example:* count* sum* avg* corr* first* last ExerciseUsing the `persons` DataFrame, calculate the average height and the number of records per sex. 5. SortingPySpark also supports sorting data with the `orderBy` method. For example we can sort all persons by their name as follows:
###Code
result = persons.orderBy(persons.name)
result.toPandas()
###Output
_____no_output_____
###Markdown
If nothing else is specified, PySpark will sort the records in increasing order of the sort columns. If you require descending order, this can be specified by manipulating the sort column with the `desc()` method as follows:
###Code
result = persons.orderBy(persons.age.desc())
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseAs an exercise we want to sort all persons first by their sex and then by their descening age. Sorting by multiple columns can easily be achieved by specifying multiple columns as arguments in the `orderBy` method. 6. Joining DataEvery relation algebra also contains join operations which lets you combine multiple tables by a matching criterion. PySpark also supports joins of multiple DataFrames. In order to shed some light on that, we need a second DataFrame in addition to the `persons` DataFrame. Therefore we load some address data as follows:
###Code
addresses = spark.read.json(basedir + "addresses.json")
addresses.toPandas()
###Output
_____no_output_____
###Markdown
Now that we have the `addresses` DataFrame, we want to combine it with the `persons` DataFrame such that the city of every person is added as a new column. This is achieved by the `join` method which essentially takes two parameters: The first parameter specifies the second DataFrame to join with, and the second parameter specifies the join condition. In this case we want to join all records, where the `name` column matches.
###Code
result = persons.join(addresses, persons.name == addresses.name)
result.toPandas()
###Output
_____no_output_____
###Markdown
Let me make some relevant remarks:* The resulting DataFrame now contains two `name` columns - one comes from the `persons` DataFrame, the other from the `addresses` DataFrame. Since the join condition could have used some more complex expression, this behaviour is only logical since PySpark cannot assume that all joins simply use directly some column value. For example we could also have transformed the column on the fly by converting the name to upper case directly inside the join condition.* The result contains only persons where an address was found, although the original `persons` DataFrame contained more persons.* There are no records of addresses without any person, although the `addresses` DataFrame contains information about some persons not available in the `persons` DataFrame.So let us first address the first observation. We can easily get rid of the copied `name` column by either performing an explicit select of the desired columns, or by dropping the duplicate columns. Since PySpark records the lineage of every column, the duplicate `name` columns can be addressed by their original DataFrame even after the join operation:
###Code
result = persons.join(addresses, persons.name == addresses.name).select(
persons.name, persons.age, addresses.city
)
result.toPandas()
###Output
_____no_output_____
###Markdown
Now let us explain the last two observations. These are due to the used join type, which was a so called *inner* join. In this case, only records with information from both DataFrames are included in the result.In addition to the *inner* join, PySpark also supports some additional joins:* *outer join* will contain records for all elements from both DataFrames. If either the left or right DataFrames doesn't contain any information, the result will contain `None` values (= `NULL` values) for the corresponding columns.* In a *right join*, the second DataFrame (the right DataFrame) as specified as an argument is the leading element. The result will contain records for every record in that DataFrame.* In a *left join*, the first DataFrame (the left DataFrame) as specified as the object iteself is the leading element. The result will contain records for every record in that DataFrame.
###Code
result = persons.join(addresses, persons.name == addresses.name, how="outer")
result.toPandas()
result = persons.join(addresses, persons.name == addresses.name, how="right")
result.toPandas()
result = persons.join(addresses, persons.name == addresses.name, how="left")
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseAs an exercise, we use another DataFrame loaded from a file called `lastnames.json`, which can be joined to the `persons` DataFrame again:
###Code
lastnames = spark.read.json(basedir + "lastnames.json")
lastnames.toPandas()
###Output
_____no_output_____
###Markdown
PySpark IntroductionThis introdactionary Jupyter notebook is intended to provide a self-learning basis for getting started with Apache Spark with Python. It contains all basic operations (transofmrations, filtering, joins, grouping and aggregation) and serves as a small reference for later training exercises. OrganisationThe Notebook contains multiple sections, each with a small introduction on the specific topic, some PySpark example code and some small exercises, where you can directly apply the newly learned material. PrerequisitesYou need a Jupyter Notebook environment with an embedded Spark context. You might need to ask your administrator to setup an appropiate environment. Moreover some small test data is also required to be at a location accessible from Spark.
###Code
spark.version
# Set the base directory according to your environment
basedir = "s3://dimajix-training/data/"
###Output
_____no_output_____
###Markdown
1. Reading DataFor working with data, we need to get some data first. Spark supports various file formats, we will use CSV in the following example.The entrypoint for creating Spark objects is an object called `spark` which is provided in the notebook and read to use. We will read a file containing some informations on a couple of persons, which will serve as the basis for the next examples.
###Code
persons = spark.read \
.option("inferSchema", True) \
.option("header", True) \
.csv(basedir + "persons_header.csv")
###Output
_____no_output_____
###Markdown
Inspecting DataThe read operation returns a so called Spark *DataFrame* object. This object is similar to a table, it contains rows of records, which all conform to a common schema with named columns and specific types. On the surface it heavily borrows concepts from Pandas DataFrames or R DataFrames, although the syntax and many operations are syntactically very different.As the first step, we want to see the contents of the DataFrame. This can be easily done by using the `show` method.
###Code
persons.show()
###Output
_____no_output_____
###Markdown
Inspecting the SchemaIt may also be interesting not to inspect the data directly, but to inspect the schema. The schema contains the meta information about which columns are present and which types are used in the columns. You can either directly work with the schema object by using the `schema` variable of a DataFrame, or you can print the schema with the `printSchema()` method as follows:
###Code
persons.printSchema()
###Output
_____no_output_____
###Markdown
Converting to PandasSpark also provides some convenience method for converting a Spark DataFrame into a Pandas DataFrame. This is not only useful for using Pandas algorithms, but this is particular handy in Jupyter notebooks which have built-in support for displaying Pandas DataFrames nicely. Therefore we will prefer to use the `toPandas()` method for displaying the contents of a DataFrame instead of the `show()` method above.
###Code
persons.toPandas()
###Output
_____no_output_____
###Markdown
Attention: Beware of huge DatFrames!Do not forget hat Apache Spark has been designed and built to handle really huge data sets, which do not need to fit into memory. Spark DataFrames con contain billions of rows and are stored in a distributed way on many nodes in a cluster. Actually the contents do not even need to be physically present at all, as long as the input data is accessible.But calling the `toPandas()` method will transfer all the records to a single machine (where the Jupyter Notebook runs on) - but maybe this computer does not have enough memory to hold all the data. In this case, you risk that the notebook process will crash with an Out-Of-Memory error (OOM). So you should only use `toPandas()` when you are really sure that the DataFrame contains a limited amount of records. Exercise1. Load in the file "persons.json". This file contains exactly the same data, but is stored as a JSON file instead of a CSV file. 2. Inspect the schema3. Show the contents of the file4. Convert the Spark DataFrame to a Pandas DataFrame 2. Simple Transformations ProjectionsThe simplest thing to do is to create a new DataFrame with a subset of the available columns
###Code
from pyspark.sql.functions import *
result = persons.select(persons.name, persons['sex'], col('age'))
result.toPandas()
###Output
_____no_output_____
###Markdown
One noteable concept of Spark is that every transformation will return a new DataFrame. The original DataFrame remains unchanged. This is a deep architectural decision of Spark which simplifies parallel processing under the hood. Addressing ColumnsSpark supports multiple different ways for *addressing* a columns. We just saw one way, but also the following methods are supported for specifying a column:```df.column_namedf['column_name']col('column_name')```All these methods return a `Column` object, which is an abstract representative of the data in the column. As we will see soon, transformations can be applied to `Column` in order to derive new values. ExerciseUse all three different methods for addressing a column, and select the following columns:* name* age* city TransformationsThe `select` method actually accepts any *column* object. A *column* object conceptually represents a column in a DataFrame. The column may either refer directly to an existing column of the input DataFrame, or it may represent the result of a calculation or transformation of one or multiple columns of the input DataFrame. For example if we simply want to transform the name into upper case, we can do so by using a function `upper` provided by PySpark.
###Code
from pyspark.sql.functions import *
result = persons.select(
upper(persons.name)
)
result.toPandas()
###Output
_____no_output_____
###Markdown
Lets look at a differnt example where we want to create a new DataFrame with the appropriate salutation in front of the name. We achieve this by the following `select` statement:
###Code
from pyspark.sql.functions import *
result = persons.select(concat(when(persons.sex == 'male', "Mr ").otherwise("Mrs "), persons.name))
result.toPandas()
###Output
_____no_output_____
###Markdown
Common FunctionsYou can find the full list of available functions at [PySpark SQL Module](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlmodule-pyspark.sql.functions). Commonly used functions for example are as follows:* [`concat(*cols)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.concat) - Concatenates multiple input columns together into a single column.* [`substring(col,start,len)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.substring) - Substring starts at pos and is of length len when str is String type or returns the slice of byte array that starts at pos in byte and is of length len when str is Binary type.* [`instr(col,substr)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.instr) - Locate the position of the first occurrence of substr column in the given string. Returns null if either of the arguments are null.* [`locate(col,substr, pos)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.locate) - Locate the position of the first occurrence of substr in a string column, after position pos.* [`length(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.length) - Computes the character length of string data or number of bytes of binary data. * [`upper(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.upper) - Converts a string column to upper case.* [`lower(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.lower) - Converts a string column to lower case.* [`coalesce(*cols)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.coalesce) - Returns the first column that is not null.* [`isnull(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.isnull) - An expression that returns true iff the column is null.* [`isnan(col)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.isnan) - An expression that returns true iff the column is NaN.* [`hash(cols*)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.hash) - Calculates the hash code of given columns.Spark also supports conditional expressions, like the SQL `CASE WHEN` construct* [`when(condition, value)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.when) - Evaluates a list of conditions and returns one of multiple possible result expressions.There are also some special functions often required* [`col(str)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.col) - Returns a Column based on the given column name.* [`lit(val)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.lit) - Creates a Column of literal value.* [`expr(str)`](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlpyspark.sql.functions.expr) - Parses the expression string into the column that it represents User Defined FunctionsUnfortunately you cannot directly use normal Python functions for transforming DataFrame columns. Although PySpark already provides many useful functions, this might not always sufficient. But fortunately you can *convert* a standard Python function into a PySpark function, thereby defining a so called *user defined function* (UDF). Details will be explained in detail in the training. Defining new Column NamesThe resulting DataFrame again has a schema, but the column names to not look very nice. But by using the `alias` method of a `Column` object, you can immediately rename the newly created column like you are already used to in SQL with `SELECT complex_operation(...) AS nice_name FROM ...`. Technically specifying a new name for the resulting column is not required (as we already saw above), if the name is not specified, PySpark will generate a name from the expression. But since this generated name tends to be rather long and contains the logic instead of the intention, it is highly recommended to always explicitly specify the name of the resulting column using `as`.
###Code
result = persons.select(concat(when(persons.sex == 'male', "Mr ").otherwise("Mrs "), persons.name).alias("qualified_name"))
result.toPandas()
###Output
_____no_output_____
###Markdown
Adding ColumnsA special variant of a `select` statement is the `withColumn` method. While the `select` statement requires all resulting columns to be defined in as arguments, the `withColumn` method keeps all existing columns and adds a new one. This operation is quite useful since in many cases new columns are derived from the existing ones, while the old ones still should be contained in the result.Let us have a look at a simple example, which only adds the salutation as a new column:
###Code
result = persons.withColumn("salutation", when(persons.sex == 'male', "Mr ").otherwise("Mrs "))
result.toPandas()
###Output
_____no_output_____
###Markdown
As you can see from the example above, `withColumn` always takes two arguments: The first one is the name of the new column (and it has to be a string), and the second argument is the expression containing the logic for calculating the actual contents. Removing ColumnsPySpark also supports the opposite operation which simply removes some columns from a dataframe. This is useful if you need to remove some sensitive data before saving it to disk:
###Code
result = persons.drop("name")
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseUsing the `persons` DataFrame, perform the following operations:* Add a new column `status` which should be `child` if the person is younger than 18 and `adult` otherwise* Replace the column `name` by a new column `hashed_name` containing the hash value of the name* Drop the column `sex` 3. Filtering*Filtering* denotes the process of keeping only rows which meet a certain filter criteria. PySpark support two different approaches. The first approach specifies the filtering expression as a PySpark expression using columns:
###Code
result = persons.filter(persons.age > 20)
result.show()
###Output
_____no_output_____
###Markdown
The second approach simply uses a string containing an SQL expression:
###Code
result = persons.filter("age > 20")
result.show()
###Output
_____no_output_____
###Markdown
Of course you can also combine multiple conditions using `&` (and) and `|` (or) with the first approach or by using the SQL keywords `AND` and `OR` in the second approach. ExercisePerform different filter operations:* Select all women with a height of at least 160* Select all persons which are younger than 20 or older than 30 4. Grouping & AggregationAn important class of operation is grouping and aggregation, which is equivalnt to an SQL `SELECT aggregation GROUP BY grouping` statement. In PySpark, grouping and aggregation is always performed by first creating groups using `groupBy` immediately followed by aggregation expressions inside an `agg` method. (Actually there are also some predefined aggregations which can be used instead of `agg`, but they do not offer the flexiviliby which is required most of the time).Note that in the `agg` method you only need to specify the aggregation expression, the grouping columns are added automatically by PySpark to the resulting DataFrame.
###Code
result = persons.groupBy(persons.sex).agg(
avg(persons.age).alias('avg_age'),
min(persons.height).alias('min_height'),
max(persons.height).alias('max_height'))
result.toPandas()
###Output
_____no_output_____
###Markdown
Aggregation FunctionsPySpark supports many aggregation functions, they can be found in the documentation at [PySpark Function Documentation](http://spark.apache.org/docs/latest/api/python/pyspark.sql.htmlmodule-pyspark.sql.functions). Aggregation functions are marked as such in the documentation, unfortunately there is no simple overview. Among common aggregation functions, there are for example:* count* sum* avg* corr* first* last ExerciseUsing the `persons` DataFrame, calculate the average height and the number of records per sex. 5. SortingPySpark also supports sorting data with the `orderBy` method. For example we can sort all persons by their name as follows:
###Code
result = persons.orderBy(persons.name)
result.toPandas()
###Output
_____no_output_____
###Markdown
If nothing else is specified, PySpark will sort the records in increasing order of the sort columns. If you require descending order, this can be specified by manipulating the sort column with the `desc()` method as follows:
###Code
result = persons.orderBy(persons.age.desc())
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseAs an exercise we want to sort all persons first by their sex and then by their descening age. Sorting by multiple columns can easily be achieved by specifying multiple columns as arguments in the `orderBy` method. 6. Joining DataEvery relation algebra also contains join operations which lets you combine multiple tables by a matching criterion. PySpark also supports joins of multiple DataFrames. In order to shed some light on that, we need a second DataFrame in addition to the `persons` DataFrame. Therefore we load some address data as follows:
###Code
addresses = spark.read.json(basedir + "addresses.json")
addresses.toPandas()
###Output
_____no_output_____
###Markdown
Now that we have the `addresses` DataFrame, we want to combine it with the `persons` DataFrame such that the city of every person is added as a new column. This is achieved by the `join` method which essentially takes two parameters: The first parameter specifies the second DataFrame to join with, and the second parameter specifies the join condition. In this case we want to join all records, where the `name` column matches.
###Code
result = persons.join(addresses,persons.name == addresses.name)
result.toPandas()
###Output
_____no_output_____
###Markdown
Let me make some relevant remarks:* The resulting DataFrame now contains two `name` columns - one comes from the `persons` DataFrame, the other from the `addresses` DataFrame. Since the join condition could have used some more complex expression, this behaviour is only logical since PySpark cannot assume that all joins simply use directly some column value. For example we could also have transformed the column on the fly by converting the name to upper case directly inside the join condition.* The result contains only persons where an address was found, although the original `persons` DataFrame contained more persons.* There are no records of addresses without any person, although the `addresses` DataFrame contains information about some persons not available in the `persons` DataFrame.So let us first address the first observation. We can easily get rid of the copied `name` column by either performing an explicit select of the desired columns, or by dropping the duplicate columns. Since PySpark records the lineage of every column, the duplicate `name` columns can be addressed by their original DataFrame even after the join operation:
###Code
result = persons.join(addresses,persons.name == addresses.name).select(persons.name,persons.age,addresses.city)
result.toPandas()
###Output
_____no_output_____
###Markdown
Now let us explain the last two observations. These are due to the used join type, which was a so called *inner* join. In this case, only records with information from both DataFrames are included in the result.In addition to the *inner* join, PySpark also supports some additional joins:* *outer join* will contain records for all elements from both DataFrames. If either the left or right DataFrames doesn't contain any information, the result will contain `None` values (= `NULL` values) for the corresponding columns.* In a *right join*, the second DataFrame (the right DataFrame) as specified as an argument is the leading element. The result will contain records for every record in that DataFrame.* In a *left join*, the first DataFrame (the left DataFrame) as specified as the object iteself is the leading element. The result will contain records for every record in that DataFrame.
###Code
result = persons.join(addresses,persons.name == addresses.name, how="outer")
result.toPandas()
result = persons.join(addresses,persons.name == addresses.name, how="right")
result.toPandas()
result = persons.join(addresses,persons.name == addresses.name, how="left")
result.toPandas()
###Output
_____no_output_____
###Markdown
ExerciseAs an exercise, we use another DataFrame loaded from a file called `lastnames.json`, which can be joined to the `persons` DataFrame again:
###Code
lastnames = spark.read.json(basedir + "lastnames.json")
lastnames.toPandas()
###Output
_____no_output_____ |
PowerOutagePredictor/Tree/.ipynb_checkpoints/BuildingTheTree-AllData-checkpoint.ipynb | ###Markdown
import data
###Code
data = pd.read_csv('../../Data/WeatherOutagesAllJerry.csv')
data = data.dropna(how = 'all')
data.head(5)
np.shape(data)
#data = data.fillna(0)
#data = data.round(4)
train,test=train_test_split(data,test_size=0.1,random_state=567)
x_train = train.iloc[:,6:]
y_train = train.iloc[:,1]
x_test = test.iloc[:,6:]
y_test = test.iloc[:,1]
y_train.size
y_test.size
###Output
_____no_output_____
###Markdown
Decision Tree
###Code
predictor = DecisionTreeRegressor(criterion='mse',max_leaf_nodes=5)
clf = predictor.fit(x_train,y_train)
y_trainpred = predictor.predict(x_train)
y_pred = predictor.predict(x_test)
score = mean_squared_error(y_test, y_pred)
print("Train error",mean_squared_error(y_train,y_trainpred))
print("Test error",mean_squared_error(y_test,y_pred))
tree_dot = export_graphviz(clf)
graph = pydotplus.graphviz.graph_from_dot_file('tree.dot')
Image(graph.create_png())
y_test.size
plt.scatter(y_test,y_pred,alpha=0.2)
plt.xlabel('real test value')
plt.ylabel('predicted test value')
###Output
_____no_output_____
###Markdown
Random Forests
###Code
from sklearn.ensemble import RandomForestRegressor, ExtraTreesRegressor
from sklearn.cross_validation import ShuffleSplit
from sklearn.learning_curve import validation_curve
Param_name = 'max_features'
Param_range = range(1,x.shape[1]+1)
for Forest, color, lable in [(RandomForestRegressor,'g','RF'),
(ExtraTreesRegressor),'r','ETs']
_, test_scores = validation_curve(
Forest(n_estimators=100, n_jobs=-1),x,y,
cv=ShuttleSplit(n=len(x),n_iter=10, test_size=0.25),
scoring='mean_squared_error')
test_scores_mean = np.mean(-test_scores, axis=1)
plt.plot(param_range, test_scores_mean, label=label, color=color)
###Output
_____no_output_____ |
Neural Networks and Deep Learning/Week 2/Python Basics with Numpy/Python Basics With Numpy v3.ipynb | ###Markdown
Python Basics with Numpy (optional assignment)Welcome to your first assignment. This exercise gives you a brief introduction to Python. Even if you've used Python before, this will help familiarize you with functions we'll need. **Instructions:**- You will be using Python 3.- Avoid using for-loops and while-loops, unless you are explicitly told to do so.- Do not modify the ( GRADED FUNCTION [function name]) comment in some cells. Your work would not be graded if you change this. Each cell containing that comment should only contain one function.- After coding your function, run the cell right below it to check if your result is correct.**After this assignment you will:**- Be able to use iPython Notebooks- Be able to use numpy functions and numpy matrix/vector operations- Understand the concept of "broadcasting"- Be able to vectorize codeLet's get started! About iPython Notebooks iPython Notebooks are interactive coding environments embedded in a webpage. You will be using iPython notebooks in this class. You only need to write code between the START CODE HERE and END CODE HERE comments. After writing your code, you can run the cell by either pressing "SHIFT"+"ENTER" or by clicking on "Run Cell" (denoted by a play symbol) in the upper bar of the notebook. We will often specify "(≈ X lines of code)" in the comments to tell you about how much code you need to write. It is just a rough estimate, so don't feel bad if your code is longer or shorter.**Exercise**: Set test to `"Hello World"` in the cell below to print "Hello World" and run the two cells below.
###Code
### START CODE HERE ### (≈ 1 line of code)
test = "Hello World"
### END CODE HERE ###
print ("test: " + test)
###Output
test: Hello World
###Markdown
**Expected output**:test: Hello World **What you need to remember**:- Run your cells using SHIFT+ENTER (or "Run cell")- Write code in the designated areas using Python 3 only- Do not modify the code outside of the designated areas 1 - Building basic functions with numpy Numpy is the main package for scientific computing in Python. It is maintained by a large community (www.numpy.org). In this exercise you will learn several key numpy functions such as np.exp, np.log, and np.reshape. You will need to know how to use these functions for future assignments. 1.1 - sigmoid function, np.exp() Before using np.exp(), you will use math.exp() to implement the sigmoid function. You will then see why np.exp() is preferable to math.exp().**Exercise**: Build a function that returns the sigmoid of a real number x. Use math.exp(x) for the exponential function.**Reminder**:$sigmoid(x) = \frac{1}{1+e^{-x}}$ is sometimes also known as the logistic function. It is a non-linear function used not only in Machine Learning (Logistic Regression), but also in Deep Learning.To refer to a function belonging to a specific package you could call it using package_name.function(). Run the code below to see an example with math.exp().
###Code
# GRADED FUNCTION: basic_sigmoid
import math
def basic_sigmoid(x):
"""
Compute sigmoid of x.
Arguments:
x -- A scalar
Return:
s -- sigmoid(x)
"""
### START CODE HERE ### (≈ 1 line of code)
s = 1 / (1 + math.exp(-x))
### END CODE HERE ###
return s
basic_sigmoid(3)
###Output
_____no_output_____
###Markdown
**Expected Output**: ** basic_sigmoid(3) ** 0.9525741268224334 Actually, we rarely use the "math" library in deep learning because the inputs of the functions are real numbers. In deep learning we mostly use matrices and vectors. This is why numpy is more useful.
###Code
### One reason why we use "numpy" instead of "math" in Deep Learning ###
x = [1, 2, 3]
basic_sigmoid(x) # you will see this give an error when you run it, because x is a vector.
###Output
_____no_output_____
###Markdown
In fact, if $ x = (x_1, x_2, ..., x_n)$ is a row vector then $np.exp(x)$ will apply the exponential function to every element of x. The output will thus be: $np.exp(x) = (e^{x_1}, e^{x_2}, ..., e^{x_n})$
###Code
import numpy as np
# example of np.exp
x = np.array([1, 2, 3])
print(np.exp(x)) # result is (exp(1), exp(2), exp(3))
###Output
[ 2.71828183 7.3890561 20.08553692]
###Markdown
Furthermore, if x is a vector, then a Python operation such as $s = x + 3$ or $s = \frac{1}{x}$ will output s as a vector of the same size as x.
###Code
# example of vector operation
x = np.array([1, 2, 3])
print (x + 3)
###Output
[4 5 6]
###Markdown
Any time you need more info on a numpy function, we encourage you to look at [the official documentation](https://docs.scipy.org/doc/numpy-1.10.1/reference/generated/numpy.exp.html). You can also create a new cell in the notebook and write `np.exp?` (for example) to get quick access to the documentation.**Exercise**: Implement the sigmoid function using numpy. **Instructions**: x could now be either a real number, a vector, or a matrix. The data structures we use in numpy to represent these shapes (vectors, matrices...) are called numpy arrays. You don't need to know more for now.$$ \text{For } x \in \mathbb{R}^n \text{, } sigmoid(x) = sigmoid\begin{pmatrix} x_1 \\ x_2 \\ ... \\ x_n \\\end{pmatrix} = \begin{pmatrix} \frac{1}{1+e^{-x_1}} \\ \frac{1}{1+e^{-x_2}} \\ ... \\ \frac{1}{1+e^{-x_n}} \\\end{pmatrix}\tag{1} $$
###Code
# GRADED FUNCTION: sigmoid
import numpy as np # this means you can access numpy functions by writing np.function() instead of numpy.function()
def sigmoid(x):
"""
Compute the sigmoid of x
Arguments:
x -- A scalar or numpy array of any size
Return:
s -- sigmoid(x)
"""
### START CODE HERE ### (≈ 1 line of code)
s = 1 / (1 + np.exp(-x))
### END CODE HERE ###
return s
x = np.array([1, 2, 3])
print ("sigmoid(x) = " + str(sigmoid(x)))
###Output
sigmoid(x) = [0.73105858 0.88079708 0.95257413]
###Markdown
**Expected Output**: **sigmoid([1,2,3])** array([ 0.73105858, 0.88079708, 0.95257413]) 1.2 - Sigmoid gradientAs you've seen in lecture, you will need to compute gradients to optimize loss functions using backpropagation. Let's code your first gradient function.**Exercise**: Implement the function sigmoid_grad() to compute the gradient of the sigmoid function with respect to its input x. The formula is: $$sigmoid\_derivative(x) = \sigma'(x) = \sigma(x) (1 - \sigma(x))\tag{2}$$(This is similiar to da/dz = a*(1-a))You often code this function in two steps:1. Set s to be the sigmoid of x. You might find your sigmoid(x) function useful.2. Compute $\sigma'(x) = s(1-s)$
###Code
# GRADED FUNCTION: sigmoid_derivative
def sigmoid_derivative(x):
"""
Compute the gradient (also called the slope or derivative) of the sigmoid function with respect to its input x.
You can store the output of the sigmoid function into variables and then use it to calculate the gradient.
Arguments:
x -- A scalar or numpy array
Return:
ds -- Your computed gradient.
"""
### START CODE HERE ### (≈ 2 lines of code)
s = sigmoid(x)
ds = s*(1-s)
### END CODE HERE ###
return ds
x = np.array([1, 2, 3])
print ("sigmoid_derivative(x) = " + str(sigmoid_derivative(x)))
###Output
sigmoid_derivative(x) = [0.19661193 0.10499359 0.04517666]
###Markdown
**Expected Output**: **sigmoid_derivative([1,2,3])** [ 0.19661193 0.10499359 0.04517666] 1.3 - Reshaping arrays Two common numpy functions used in deep learning are [np.shape](https://docs.scipy.org/doc/numpy/reference/generated/numpy.ndarray.shape.html) and [np.reshape()](https://docs.scipy.org/doc/numpy/reference/generated/numpy.reshape.html). - X.shape is used to get the shape (dimension) of a matrix/vector X. - X.reshape(...) is used to reshape X into some other dimension. For example, in computer science, an image is represented by a 3D array of shape $(length, height, depth = 3)$. However, when you read an image as the input of an algorithm you convert it to a vector of shape $(length*height*3, 1)$. In other words, you "unroll", or reshape, the 3D array into a 1D vector.**Exercise**: Implement `image2vector()` that takes an input of shape (length, height, 3) and returns a vector of shape (length\*height\*3, 1). For example, if you would like to reshape an array v of shape (a, b, c) into a vector of shape (a*b,c) you would do:``` pythonv = v.reshape((v.shape[0]*v.shape[1], v.shape[2])) v.shape[0] = a ; v.shape[1] = b ; v.shape[2] = c```- Please don't hardcode the dimensions of image as a constant. Instead look up the quantities you need with `image.shape[0]`, etc.
###Code
# GRADED FUNCTION: image2vector
def image2vector(image):
"""
Argument:
image -- a numpy array of shape (length, height, depth)
Returns:
v -- a vector of shape (length*height*depth, 1)
"""
### START CODE HERE ### (≈ 1 line of code)
v = image.reshape((image.shape[0]*image.shape[1]*image.shape[2]), 1)
### END CODE HERE ###
return v
# This is a 3 by 3 by 2 array, typically images will be (num_px_x, num_px_y,3) where 3 represents the RGB values
image = np.array([[[ 0.67826139, 0.29380381],
[ 0.90714982, 0.52835647],
[ 0.4215251 , 0.45017551]],
[[ 0.92814219, 0.96677647],
[ 0.85304703, 0.52351845],
[ 0.19981397, 0.27417313]],
[[ 0.60659855, 0.00533165],
[ 0.10820313, 0.49978937],
[ 0.34144279, 0.94630077]]])
print ("image2vector(image) = " + str(image2vector(image)))
###Output
image2vector(image) = [[0.67826139]
[0.29380381]
[0.90714982]
[0.52835647]
[0.4215251 ]
[0.45017551]
[0.92814219]
[0.96677647]
[0.85304703]
[0.52351845]
[0.19981397]
[0.27417313]
[0.60659855]
[0.00533165]
[0.10820313]
[0.49978937]
[0.34144279]
[0.94630077]]
###Markdown
**Expected Output**: **image2vector(image)** [[ 0.67826139] [ 0.29380381] [ 0.90714982] [ 0.52835647] [ 0.4215251 ] [ 0.45017551] [ 0.92814219] [ 0.96677647] [ 0.85304703] [ 0.52351845] [ 0.19981397] [ 0.27417313] [ 0.60659855] [ 0.00533165] [ 0.10820313] [ 0.49978937] [ 0.34144279] [ 0.94630077]] 1.4 - Normalizing rowsAnother common technique we use in Machine Learning and Deep Learning is to normalize our data. It often leads to a better performance because gradient descent converges faster after normalization. Here, by normalization we mean changing x to $ \frac{x}{\| x\|} $ (dividing each row vector of x by its norm / absolute value / length).For example, if $$x = \begin{bmatrix} 0 & 3 & 4 \\ 2 & 6 & 4 \\\end{bmatrix}\tag{3}$$ then $$\| x\| = np.linalg.norm(x, axis = 1, keepdims = True) = \begin{bmatrix} 5 \\ \sqrt{56} \\\end{bmatrix}\tag{4} $$and $$ x\_normalized = \frac{x}{\| x\|} = \begin{bmatrix} 0 & \frac{3}{5} & \frac{4}{5} \\ \frac{2}{\sqrt{56}} & \frac{6}{\sqrt{56}} & \frac{4}{\sqrt{56}} \\\end{bmatrix}\tag{5}$$ Note that you can divide matrices of different sizes and it works fine: this is called broadcasting and you're going to learn about it in part 5.**Exercise**: Implement normalizeRows() to normalize the rows of a matrix. After applying this function to an input matrix x, each row of x should be a vector of unit length (meaning length 1).
###Code
# GRADED FUNCTION: normalizeRows
def normalizeRows(x):
"""
Implement a function that normalizes each row of the matrix x (to have unit length).
Argument:
x -- A numpy matrix of shape (n, m)
Returns:
x -- The normalized (by row) numpy matrix. You are allowed to modify x.
"""
### START CODE HERE ### (≈ 2 lines of code)
xNorm = np.linalg.norm(x, axis = 1, keepdims = True)
###divide each row by its norm
x = x/xNorm
### END CODE HERE ###
return x
x = np.array([
[0, 3, 4],
[1, 6, 4]])
print("normalizeRows(x) = " + str(normalizeRows(x)))
###Output
normalizeRows(x) = [[0. 0.6 0.8 ]
[0.13736056 0.82416338 0.54944226]]
###Markdown
**Expected Output**: **normalizeRows(x)** [[ 0. 0.6 0.8 ] [ 0.13736056 0.82416338 0.54944226]] **Note**:In normalizeRows(), you can try to print the shapes of x_norm and x, and then rerun the assessment. You'll find out that they have different shapes. This is normal given that x_norm takes the norm of each row of x. So x_norm has the same number of rows but only 1 column. So how did it work when you divided x by x_norm? This is called broadcasting and we'll talk about it now! 1.5 - Broadcasting and the softmax function A very important concept to understand in numpy is "broadcasting". It is very useful for performing mathematical operations between arrays of different shapes. For the full details on broadcasting, you can read the official [broadcasting documentation](http://docs.scipy.org/doc/numpy/user/basics.broadcasting.html). **Exercise**: Implement a softmax function using numpy. You can think of softmax as a normalizing function used when your algorithm needs to classify two or more classes. You will learn more about softmax in the second course of this specialization.**Instructions**:- $ \text{for } x \in \mathbb{R}^{1\times n} \text{, } softmax(x) = softmax(\begin{bmatrix} x_1 && x_2 && ... && x_n \end{bmatrix}) = \begin{bmatrix} \frac{e^{x_1}}{\sum_{j}e^{x_j}} && \frac{e^{x_2}}{\sum_{j}e^{x_j}} && ... && \frac{e^{x_n}}{\sum_{j}e^{x_j}} \end{bmatrix} $ - $\text{for a matrix } x \in \mathbb{R}^{m \times n} \text{, $x_{ij}$ maps to the element in the $i^{th}$ row and $j^{th}$ column of $x$, thus we have: }$ $$softmax(x) = softmax\begin{bmatrix} x_{11} & x_{12} & x_{13} & \dots & x_{1n} \\ x_{21} & x_{22} & x_{23} & \dots & x_{2n} \\ \vdots & \vdots & \vdots & \ddots & \vdots \\ x_{m1} & x_{m2} & x_{m3} & \dots & x_{mn}\end{bmatrix} = \begin{bmatrix} \frac{e^{x_{11}}}{\sum_{j}e^{x_{1j}}} & \frac{e^{x_{12}}}{\sum_{j}e^{x_{1j}}} & \frac{e^{x_{13}}}{\sum_{j}e^{x_{1j}}} & \dots & \frac{e^{x_{1n}}}{\sum_{j}e^{x_{1j}}} \\ \frac{e^{x_{21}}}{\sum_{j}e^{x_{2j}}} & \frac{e^{x_{22}}}{\sum_{j}e^{x_{2j}}} & \frac{e^{x_{23}}}{\sum_{j}e^{x_{2j}}} & \dots & \frac{e^{x_{2n}}}{\sum_{j}e^{x_{2j}}} \\ \vdots & \vdots & \vdots & \ddots & \vdots \\ \frac{e^{x_{m1}}}{\sum_{j}e^{x_{mj}}} & \frac{e^{x_{m2}}}{\sum_{j}e^{x_{mj}}} & \frac{e^{x_{m3}}}{\sum_{j}e^{x_{mj}}} & \dots & \frac{e^{x_{mn}}}{\sum_{j}e^{x_{mj}}}\end{bmatrix} = \begin{pmatrix} softmax\text{(first row of x)} \\ softmax\text{(second row of x)} \\ ... \\ softmax\text{(last row of x)} \\\end{pmatrix} $$
###Code
# GRADED FUNCTION: softmax
def softmax(x):
"""Calculates the softmax for each row of the input x.
Your code should work for a row vector and also for matrices of shape (n, m).
Argument:
x -- A numpy matrix of shape (n,m)
Returns:
s -- A numpy matrix equal to the softmax of x, of shape (n,m)
"""
### START CODE HERE ### (≈ 3 lines of code)
##sum only over each row (axis = 1), keep dims to get matrix (n,1) and not array
s = np.exp(x) / np.sum(np.exp(x), axis = 1, keepdims = True)
### END CODE HERE ###
return s
x = np.array([
[9, 2, 5, 0, 0],
[7, 5, 0, 0 ,0]])
print("softmax(x) = " + str(softmax(x)))
###Output
softmax(x) = [[9.80897665e-01 8.94462891e-04 1.79657674e-02 1.21052389e-04
1.21052389e-04]
[8.78679856e-01 1.18916387e-01 8.01252314e-04 8.01252314e-04
8.01252314e-04]]
###Markdown
**Expected Output**: **softmax(x)** [[ 9.80897665e-01 8.94462891e-04 1.79657674e-02 1.21052389e-04 1.21052389e-04] [ 8.78679856e-01 1.18916387e-01 8.01252314e-04 8.01252314e-04 8.01252314e-04]] **Note**:- If you print the shapes of x_exp, x_sum and s above and rerun the assessment cell, you will see that x_sum is of shape (2,1) while x_exp and s are of shape (2,5). **x_exp/x_sum** works due to python broadcasting.Congratulations! You now have a pretty good understanding of python numpy and have implemented a few useful functions that you will be using in deep learning. **What you need to remember:**- np.exp(x) works for any np.array x and applies the exponential function to every coordinate- the sigmoid function and its gradient- image2vector is commonly used in deep learning- np.reshape is widely used. In the future, you'll see that keeping your matrix/vector dimensions straight will go toward eliminating a lot of bugs. - numpy has efficient built-in functions- broadcasting is extremely useful 2) Vectorization In deep learning, you deal with very large datasets. Hence, a non-computationally-optimal function can become a huge bottleneck in your algorithm and can result in a model that takes ages to run. To make sure that your code is computationally efficient, you will use vectorization. For example, try to tell the difference between the following implementations of the dot/outer/elementwise product.
###Code
import time
x1 = [9, 2, 5, 0, 0, 7, 5, 0, 0, 0, 9, 2, 5, 0, 0]
x2 = [9, 2, 2, 9, 0, 9, 2, 5, 0, 0, 9, 2, 5, 0, 0]
### CLASSIC DOT PRODUCT OF VECTORS IMPLEMENTATION ###
tic = time.process_time()
dot = 0
for i in range(len(x1)):
dot+= x1[i]*x2[i]
toc = time.process_time()
print ("dot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC OUTER PRODUCT IMPLEMENTATION ###
tic = time.process_time()
outer = np.zeros((len(x1),len(x2))) # we create a len(x1)*len(x2) matrix with only zeros
for i in range(len(x1)):
for j in range(len(x2)):
outer[i,j] = x1[i]*x2[j]
toc = time.process_time()
print ("outer = " + str(outer) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC ELEMENTWISE IMPLEMENTATION ###
tic = time.process_time()
mul = np.zeros(len(x1))
for i in range(len(x1)):
mul[i] = x1[i]*x2[i]
toc = time.process_time()
print ("elementwise multiplication = " + str(mul) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC GENERAL DOT PRODUCT IMPLEMENTATION ###
W = np.random.rand(3,len(x1)) # Random 3*len(x1) numpy array
tic = time.process_time()
gdot = np.zeros(W.shape[0])
for i in range(W.shape[0]):
for j in range(len(x1)):
gdot[i] += W[i,j]*x1[j]
toc = time.process_time()
print ("gdot = " + str(gdot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
x1 = [9, 2, 5, 0, 0, 7, 5, 0, 0, 0, 9, 2, 5, 0, 0]
x2 = [9, 2, 2, 9, 0, 9, 2, 5, 0, 0, 9, 2, 5, 0, 0]
### VECTORIZED DOT PRODUCT OF VECTORS ###
tic = time.process_time()
dot = np.dot(x1,x2)
toc = time.process_time()
print ("dot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED OUTER PRODUCT ###
tic = time.process_time()
outer = np.outer(x1,x2)
toc = time.process_time()
print ("outer = " + str(outer) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED ELEMENTWISE MULTIPLICATION ###
tic = time.process_time()
mul = np.multiply(x1,x2)
toc = time.process_time()
print ("elementwise multiplication = " + str(mul) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED GENERAL DOT PRODUCT ###
tic = time.process_time()
dot = np.dot(W,x1)
toc = time.process_time()
print ("gdot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
###Output
dot = 278
----- Computation time = 0.10599999999971743ms
outer = [[81 18 18 81 0 81 18 45 0 0 81 18 45 0 0]
[18 4 4 18 0 18 4 10 0 0 18 4 10 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[63 14 14 63 0 63 14 35 0 0 63 14 35 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[81 18 18 81 0 81 18 45 0 0 81 18 45 0 0]
[18 4 4 18 0 18 4 10 0 0 18 4 10 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]]
----- Computation time = 0.1290000000002678ms
elementwise multiplication = [81 4 10 0 0 63 10 0 0 0 81 4 25 0 0]
----- Computation time = 0.08700000000017027ms
gdot = [20.36807117 22.18965098 16.67003702]
----- Computation time = 0.10599999999971743ms
###Markdown
As you may have noticed, the vectorized implementation is much cleaner and more efficient. For bigger vectors/matrices, the differences in running time become even bigger. **Note** that `np.dot()` performs a matrix-matrix or matrix-vector multiplication. This is different from `np.multiply()` and the `*` operator (which is equivalent to `.*` in Matlab/Octave), which performs an element-wise multiplication. 2.1 Implement the L1 and L2 loss functions**Exercise**: Implement the numpy vectorized version of the L1 loss. You may find the function abs(x) (absolute value of x) useful.**Reminder**:- The loss is used to evaluate the performance of your model. The bigger your loss is, the more different your predictions ($ \hat{y} $) are from the true values ($y$). In deep learning, you use optimization algorithms like Gradient Descent to train your model and to minimize the cost.- L1 loss is defined as:$$\begin{align*} & L_1(\hat{y}, y) = \sum_{i=0}^m|y^{(i)} - \hat{y}^{(i)}| \end{align*}\tag{6}$$
###Code
# GRADED FUNCTION: L1
def L1(yhat, y):
"""
Arguments:
yhat -- vector of size m (predicted labels)
y -- vector of size m (true labels)
Returns:
loss -- the value of the L1 loss function defined above
"""
### START CODE HERE ### (≈ 1 line of code)
# use abs value of y - yhat
loss = np.sum(abs(y - yhat))
### END CODE HERE ###
return loss
yhat = np.array([.9, 0.2, 0.1, .4, .9])
y = np.array([1, 0, 0, 1, 1])
print("L1 = " + str(L1(yhat,y)))
###Output
L1 = 1.1
###Markdown
**Expected Output**: **L1** 1.1 **Exercise**: Implement the numpy vectorized version of the L2 loss. There are several way of implementing the L2 loss but you may find the function np.dot() useful. As a reminder, if $x = [x_1, x_2, ..., x_n]$, then `np.dot(x,x)` = $\sum_{j=0}^n x_j^{2}$. - L2 loss is defined as $$\begin{align*} & L_2(\hat{y},y) = \sum_{i=0}^m(y^{(i)} - \hat{y}^{(i)})^2 \end{align*}\tag{7}$$
###Code
# GRADED FUNCTION: L2
def L2(yhat, y):
"""
Arguments:
yhat -- vector of size m (predicted labels)
y -- vector of size m (true labels)
Returns:
loss -- the value of the L2 loss function defined above
"""
### START CODE HERE ### (≈ 1 line of code)
loss = np.sum(np.dot(y - yhat, y - yhat))
### END CODE HERE ###
return loss
yhat = np.array([.9, 0.2, 0.1, .4, .9])
y = np.array([1, 0, 0, 1, 1])
print("L2 = " + str(L2(yhat,y)))
###Output
L2 = 0.43
###Markdown
Python Basics with Numpy (optional assignment)Welcome to your first assignment. This exercise gives you a brief introduction to Python. Even if you've used Python before, this will help familiarize you with functions we'll need. **Instructions:**- You will be using Python 3.- Avoid using for-loops and while-loops, unless you are explicitly told to do so.- Do not modify the ( GRADED FUNCTION [function name]) comment in some cells. Your work would not be graded if you change this. Each cell containing that comment should only contain one function.- After coding your function, run the cell right below it to check if your result is correct.**After this assignment you will:**- Be able to use iPython Notebooks- Be able to use numpy functions and numpy matrix/vector operations- Understand the concept of "broadcasting"- Be able to vectorize codeLet's get started! About iPython Notebooks iPython Notebooks are interactive coding environments embedded in a webpage. You will be using iPython notebooks in this class. You only need to write code between the START CODE HERE and END CODE HERE comments. After writing your code, you can run the cell by either pressing "SHIFT"+"ENTER" or by clicking on "Run Cell" (denoted by a play symbol) in the upper bar of the notebook. We will often specify "(≈ X lines of code)" in the comments to tell you about how much code you need to write. It is just a rough estimate, so don't feel bad if your code is longer or shorter.**Exercise**: Set test to `"Hello World"` in the cell below to print "Hello World" and run the two cells below.
###Code
### START CODE HERE ### (≈ 1 line of code)
test = "Hello World"
### END CODE HERE ###
print ("test: " + test)
###Output
test: Hello World
###Markdown
**Expected output**:test: Hello World **What you need to remember**:- Run your cells using SHIFT+ENTER (or "Run cell")- Write code in the designated areas using Python 3 only- Do not modify the code outside of the designated areas 1 - Building basic functions with numpy Numpy is the main package for scientific computing in Python. It is maintained by a large community (www.numpy.org). In this exercise you will learn several key numpy functions such as np.exp, np.log, and np.reshape. You will need to know how to use these functions for future assignments. 1.1 - sigmoid function, np.exp() Before using np.exp(), you will use math.exp() to implement the sigmoid function. You will then see why np.exp() is preferable to math.exp().**Exercise**: Build a function that returns the sigmoid of a real number x. Use math.exp(x) for the exponential function.**Reminder**:$sigmoid(x) = \frac{1}{1+e^{-x}}$ is sometimes also known as the logistic function. It is a non-linear function used not only in Machine Learning (Logistic Regression), but also in Deep Learning.To refer to a function belonging to a specific package you could call it using package_name.function(). Run the code below to see an example with math.exp().
###Code
# GRADED FUNCTION: basic_sigmoid
import math
def basic_sigmoid(x):
"""
Compute sigmoid of x.
Arguments:
x -- A scalar
Return:
s -- sigmoid(x)
"""
### START CODE HERE ### (≈ 1 line of code)
s = 1/(1+math.exp(-x))
### END CODE HERE ###
return s
basic_sigmoid(3)
###Output
_____no_output_____
###Markdown
**Expected Output**: ** basic_sigmoid(3) ** 0.9525741268224334 Actually, we rarely use the "math" library in deep learning because the inputs of the functions are real numbers. In deep learning we mostly use matrices and vectors. This is why numpy is more useful.
###Code
### One reason why we use "numpy" instead of "math" in Deep Learning ###
x = [1, 2, 3]
basic_sigmoid(x) # you will see this give an error when you run it, because x is a vector.
###Output
_____no_output_____
###Markdown
In fact, if $ x = (x_1, x_2, ..., x_n)$ is a row vector then $np.exp(x)$ will apply the exponential function to every element of x. The output will thus be: $np.exp(x) = (e^{x_1}, e^{x_2}, ..., e^{x_n})$
###Code
import numpy as np
# example of np.exp
x = np.array([1, 2, 3])
print(np.exp(x)) # result is (exp(1), exp(2), exp(3))
###Output
[ 2.71828183 7.3890561 20.08553692]
###Markdown
Furthermore, if x is a vector, then a Python operation such as $s = x + 3$ or $s = \frac{1}{x}$ will output s as a vector of the same size as x.
###Code
# example of vector operation
x = np.array([1, 2, 3])
print (x + 3)
###Output
[4 5 6]
###Markdown
Any time you need more info on a numpy function, we encourage you to look at [the official documentation](https://docs.scipy.org/doc/numpy-1.10.1/reference/generated/numpy.exp.html). You can also create a new cell in the notebook and write `np.exp?` (for example) to get quick access to the documentation.**Exercise**: Implement the sigmoid function using numpy. **Instructions**: x could now be either a real number, a vector, or a matrix. The data structures we use in numpy to represent these shapes (vectors, matrices...) are called numpy arrays. You don't need to know more for now.$$ \text{For } x \in \mathbb{R}^n \text{, } sigmoid(x) = sigmoid\begin{pmatrix} x_1 \\ x_2 \\ ... \\ x_n \\\end{pmatrix} = \begin{pmatrix} \frac{1}{1+e^{-x_1}} \\ \frac{1}{1+e^{-x_2}} \\ ... \\ \frac{1}{1+e^{-x_n}} \\\end{pmatrix}\tag{1} $$
###Code
# GRADED FUNCTION: sigmoid
import numpy as np # this means you can access numpy functions by writing np.function() instead of numpy.function()
def sigmoid(x):
"""
Compute the sigmoid of x
Arguments:
x -- A scalar or numpy array of any size
Return:
s -- sigmoid(x)
"""
### START CODE HERE ### (≈ 1 line of code)
s = 1/(1+np.exp(-x))
### END CODE HERE ###
return s
x = np.array([1, 2, 3])
sigmoid(x)
###Output
_____no_output_____
###Markdown
**Expected Output**: **sigmoid([1,2,3])** array([ 0.73105858, 0.88079708, 0.95257413]) 1.2 - Sigmoid gradientAs you've seen in lecture, you will need to compute gradients to optimize loss functions using backpropagation. Let's code your first gradient function.**Exercise**: Implement the function sigmoid_grad() to compute the gradient of the sigmoid function with respect to its input x. The formula is: $$sigmoid\_derivative(x) = \sigma'(x) = \sigma(x) (1 - \sigma(x))\tag{2}$$You often code this function in two steps:1. Set s to be the sigmoid of x. You might find your sigmoid(x) function useful.2. Compute $\sigma'(x) = s(1-s)$
###Code
# GRADED FUNCTION: sigmoid_derivative
def sigmoid_derivative(x):
"""
Compute the gradient (also called the slope or derivative) of the sigmoid function with respect to its input x.
You can store the output of the sigmoid function into variables and then use it to calculate the gradient.
Arguments:
x -- A scalar or numpy array
Return:
ds -- Your computed gradient.
"""
### START CODE HERE ### (≈ 2 lines of code)
s = sigmoid(x)
ds = s*(1-s)
### END CODE HERE ###
return ds
x = np.array([1, 2, 3])
print ("sigmoid_derivative(x) = " + str(sigmoid_derivative(x)))
###Output
sigmoid_derivative(x) = [ 0.19661193 0.10499359 0.04517666]
###Markdown
**Expected Output**: **sigmoid_derivative([1,2,3])** [ 0.19661193 0.10499359 0.04517666] 1.3 - Reshaping arrays Two common numpy functions used in deep learning are [np.shape](https://docs.scipy.org/doc/numpy/reference/generated/numpy.ndarray.shape.html) and [np.reshape()](https://docs.scipy.org/doc/numpy/reference/generated/numpy.reshape.html). - X.shape is used to get the shape (dimension) of a matrix/vector X. - X.reshape(...) is used to reshape X into some other dimension. For example, in computer science, an image is represented by a 3D array of shape $(length, height, depth = 3)$. However, when you read an image as the input of an algorithm you convert it to a vector of shape $(length*height*3, 1)$. In other words, you "unroll", or reshape, the 3D array into a 1D vector.**Exercise**: Implement `image2vector()` that takes an input of shape (length, height, 3) and returns a vector of shape (length\*height\*3, 1). For example, if you would like to reshape an array v of shape (a, b, c) into a vector of shape (a*b,c) you would do:``` pythonv = v.reshape((v.shape[0]*v.shape[1], v.shape[2])) v.shape[0] = a ; v.shape[1] = b ; v.shape[2] = c```- Please don't hardcode the dimensions of image as a constant. Instead look up the quantities you need with `image.shape[0]`, etc.
###Code
# GRADED FUNCTION: image2vector
def image2vector(image):
"""
Argument:
image -- a numpy array of shape (length, height, depth)
Returns:
v -- a vector of shape (length*height*depth, 1)
"""
### START CODE HERE ### (≈ 1 line of code)
v = image.reshape((image.shape[0]*image.shape[1]*image.shape[2], 1))
### END CODE HERE ###
return v
# This is a 3 by 3 by 2 array, typically images will be (num_px_x, num_px_y,3) where 3 represents the RGB values
image = np.array([[[ 0.67826139, 0.29380381],
[ 0.90714982, 0.52835647],
[ 0.4215251 , 0.45017551]],
[[ 0.92814219, 0.96677647],
[ 0.85304703, 0.52351845],
[ 0.19981397, 0.27417313]],
[[ 0.60659855, 0.00533165],
[ 0.10820313, 0.49978937],
[ 0.34144279, 0.94630077]]])
print ("image2vector(image) = " + str(image2vector(image)))
###Output
image2vector(image) = [[ 0.67826139]
[ 0.29380381]
[ 0.90714982]
[ 0.52835647]
[ 0.4215251 ]
[ 0.45017551]
[ 0.92814219]
[ 0.96677647]
[ 0.85304703]
[ 0.52351845]
[ 0.19981397]
[ 0.27417313]
[ 0.60659855]
[ 0.00533165]
[ 0.10820313]
[ 0.49978937]
[ 0.34144279]
[ 0.94630077]]
###Markdown
**Expected Output**: **image2vector(image)** [[ 0.67826139] [ 0.29380381] [ 0.90714982] [ 0.52835647] [ 0.4215251 ] [ 0.45017551] [ 0.92814219] [ 0.96677647] [ 0.85304703] [ 0.52351845] [ 0.19981397] [ 0.27417313] [ 0.60659855] [ 0.00533165] [ 0.10820313] [ 0.49978937] [ 0.34144279] [ 0.94630077]] 1.4 - Normalizing rowsAnother common technique we use in Machine Learning and Deep Learning is to normalize our data. It often leads to a better performance because gradient descent converges faster after normalization. Here, by normalization we mean changing x to $ \frac{x}{\| x\|} $ (dividing each row vector of x by its norm).For example, if $$x = \begin{bmatrix} 0 & 3 & 4 \\ 2 & 6 & 4 \\\end{bmatrix}\tag{3}$$ then $$\| x\| = np.linalg.norm(x, axis = 1, keepdims = True) = \begin{bmatrix} 5 \\ \sqrt{56} \\\end{bmatrix}\tag{4} $$and $$ x\_normalized = \frac{x}{\| x\|} = \begin{bmatrix} 0 & \frac{3}{5} & \frac{4}{5} \\ \frac{2}{\sqrt{56}} & \frac{6}{\sqrt{56}} & \frac{4}{\sqrt{56}} \\\end{bmatrix}\tag{5}$$ Note that you can divide matrices of different sizes and it works fine: this is called broadcasting and you're going to learn about it in part 5.**Exercise**: Implement normalizeRows() to normalize the rows of a matrix. After applying this function to an input matrix x, each row of x should be a vector of unit length (meaning length 1).
###Code
# GRADED FUNCTION: normalizeRows
def normalizeRows(x):
"""
Implement a function that normalizes each row of the matrix x (to have unit length).
Argument:
x -- A numpy matrix of shape (n, m)
Returns:
x -- The normalized (by row) numpy matrix. You are allowed to modify x.
"""
### START CODE HERE ### (≈ 2 lines of code)
# Compute x_norm as the norm 2 of x. Use np.linalg.norm(..., ord = 2, axis = ..., keepdims = True)
x_norm = np.linalg.norm(x,ord=2,axis=1,keepdims = True)
# Divide x by its norm.
x = x/x_norm
### END CODE HERE ###
return x
x = np.array([
[0, 3, 4],
[1, 6, 4]])
print("normalizeRows(x) = " + str(normalizeRows(x)))
###Output
normalizeRows(x) = [[ 0. 0.6 0.8 ]
[ 0.13736056 0.82416338 0.54944226]]
###Markdown
**Expected Output**: **normalizeRows(x)** [[ 0. 0.6 0.8 ] [ 0.13736056 0.82416338 0.54944226]] **Note**:In normalizeRows(), you can try to print the shapes of x_norm and x, and then rerun the assessment. You'll find out that they have different shapes. This is normal given that x_norm takes the norm of each row of x. So x_norm has the same number of rows but only 1 column. So how did it work when you divided x by x_norm? This is called broadcasting and we'll talk about it now! 1.5 - Broadcasting and the softmax function A very important concept to understand in numpy is "broadcasting". It is very useful for performing mathematical operations between arrays of different shapes. For the full details on broadcasting, you can read the official [broadcasting documentation](http://docs.scipy.org/doc/numpy/user/basics.broadcasting.html). **Exercise**: Implement a softmax function using numpy. You can think of softmax as a normalizing function used when your algorithm needs to classify two or more classes. You will learn more about softmax in the second course of this specialization.**Instructions**:- $ \text{for } x \in \mathbb{R}^{1\times n} \text{, } softmax(x) = softmax(\begin{bmatrix} x_1 && x_2 && ... && x_n \end{bmatrix}) = \begin{bmatrix} \frac{e^{x_1}}{\sum_{j}e^{x_j}} && \frac{e^{x_2}}{\sum_{j}e^{x_j}} && ... && \frac{e^{x_n}}{\sum_{j}e^{x_j}} \end{bmatrix} $ - $\text{for a matrix } x \in \mathbb{R}^{m \times n} \text{, $x_{ij}$ maps to the element in the $i^{th}$ row and $j^{th}$ column of $x$, thus we have: }$ $$softmax(x) = softmax\begin{bmatrix} x_{11} & x_{12} & x_{13} & \dots & x_{1n} \\ x_{21} & x_{22} & x_{23} & \dots & x_{2n} \\ \vdots & \vdots & \vdots & \ddots & \vdots \\ x_{m1} & x_{m2} & x_{m3} & \dots & x_{mn}\end{bmatrix} = \begin{bmatrix} \frac{e^{x_{11}}}{\sum_{j}e^{x_{1j}}} & \frac{e^{x_{12}}}{\sum_{j}e^{x_{1j}}} & \frac{e^{x_{13}}}{\sum_{j}e^{x_{1j}}} & \dots & \frac{e^{x_{1n}}}{\sum_{j}e^{x_{1j}}} \\ \frac{e^{x_{21}}}{\sum_{j}e^{x_{2j}}} & \frac{e^{x_{22}}}{\sum_{j}e^{x_{2j}}} & \frac{e^{x_{23}}}{\sum_{j}e^{x_{2j}}} & \dots & \frac{e^{x_{2n}}}{\sum_{j}e^{x_{2j}}} \\ \vdots & \vdots & \vdots & \ddots & \vdots \\ \frac{e^{x_{m1}}}{\sum_{j}e^{x_{mj}}} & \frac{e^{x_{m2}}}{\sum_{j}e^{x_{mj}}} & \frac{e^{x_{m3}}}{\sum_{j}e^{x_{mj}}} & \dots & \frac{e^{x_{mn}}}{\sum_{j}e^{x_{mj}}}\end{bmatrix} = \begin{pmatrix} softmax\text{(first row of x)} \\ softmax\text{(second row of x)} \\ ... \\ softmax\text{(last row of x)} \\\end{pmatrix} $$
###Code
# GRADED FUNCTION: softmax
def softmax(x):
"""Calculates the softmax for each row of the input x.
Your code should work for a row vector and also for matrices of shape (n, m).
Argument:
x -- A numpy matrix of shape (n,m)
Returns:
s -- A numpy matrix equal to the softmax of x, of shape (n,m)
"""
### START CODE HERE ### (≈ 3 lines of code)
# Apply exp() element-wise to x. Use np.exp(...).
x_exp = np.exp(x)
# Create a vector x_sum that sums each row of x_exp. Use np.sum(..., axis = 1, keepdims = True).
x_sum = np.sum(x,axis=1,keepdims=True)
# Compute softmax(x) by dividing x_exp by x_sum. It should automatically use numpy broadcasting.
s = np.divide(x_exp,x_sum)
print(x_exp.shape, x_sum.shape)
### END CODE HERE ###
return s
x = np.array([
[9, 2, 5, 0, 0],
[7, 5, 0, 0 ,0]])
print("softmax(x) = " + str(softmax(x)))
###Output
(2, 5) (2, 1)
softmax(x) = [[ 5.06442745e+02 4.61816006e-01 9.27582244e+00 6.25000000e-02
6.25000000e-02]
[ 9.13860965e+01 1.23677633e+01 8.33333333e-02 8.33333333e-02
8.33333333e-02]]
###Markdown
**Expected Output**: **softmax(x)** [[ 9.80897665e-01 8.94462891e-04 1.79657674e-02 1.21052389e-04 1.21052389e-04] [ 8.78679856e-01 1.18916387e-01 8.01252314e-04 8.01252314e-04 8.01252314e-04]] **Note**:- If you print the shapes of x_exp, x_sum and s above and rerun the assessment cell, you will see that x_sum is of shape (2,1) while x_exp and s are of shape (2,5). **x_exp/x_sum** works due to python broadcasting.Congratulations! You now have a pretty good understanding of python numpy and have implemented a few useful functions that you will be using in deep learning. **What you need to remember:**- np.exp(x) works for any np.array x and applies the exponential function to every coordinate- the sigmoid function and its gradient- image2vector is commonly used in deep learning- np.reshape is widely used. In the future, you'll see that keeping your matrix/vector dimensions straight will go toward eliminating a lot of bugs. - numpy has efficient built-in functions- broadcasting is extremely useful 2) Vectorization In deep learning, you deal with very large datasets. Hence, a non-computationally-optimal function can become a huge bottleneck in your algorithm and can result in a model that takes ages to run. To make sure that your code is computationally efficient, you will use vectorization. For example, try to tell the difference between the following implementations of the dot/outer/elementwise product.
###Code
import time
x1 = [9, 2, 5, 0, 0, 7, 5, 0, 0, 0, 9, 2, 5, 0, 0]
x2 = [9, 2, 2, 9, 0, 9, 2, 5, 0, 0, 9, 2, 5, 0, 0]
### CLASSIC DOT PRODUCT OF VECTORS IMPLEMENTATION ###
tic = time.process_time()
dot = 0
for i in range(len(x1)):
dot+= x1[i]*x2[i]
toc = time.process_time()
print ("dot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC OUTER PRODUCT IMPLEMENTATION ###
tic = time.process_time()
outer = np.zeros((len(x1),len(x2))) # we create a len(x1)*len(x2) matrix with only zeros
for i in range(len(x1)):
for j in range(len(x2)):
outer[i,j] = x1[i]*x2[j]
toc = time.process_time()
print ("outer = " + str(outer) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC ELEMENTWISE IMPLEMENTATION ###
tic = time.process_time()
mul = np.zeros(len(x1))
for i in range(len(x1)):
mul[i] = x1[i]*x2[i]
toc = time.process_time()
print ("elementwise multiplication = " + str(mul) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### CLASSIC GENERAL DOT PRODUCT IMPLEMENTATION ###
W = np.random.rand(3,len(x1)) # Random 3*len(x1) numpy array
tic = time.process_time()
gdot = np.zeros(W.shape[0])
for i in range(W.shape[0]):
for j in range(len(x1)):
gdot[i] += W[i,j]*x1[j]
toc = time.process_time()
print ("gdot = " + str(gdot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
x1 = [9, 2, 5, 0, 0, 7, 5, 0, 0, 0, 9, 2, 5, 0, 0]
x2 = [9, 2, 2, 9, 0, 9, 2, 5, 0, 0, 9, 2, 5, 0, 0]
### VECTORIZED DOT PRODUCT OF VECTORS ###
tic = time.process_time()
dot = np.dot(x1,x2)
toc = time.process_time()
print ("dot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED OUTER PRODUCT ###
tic = time.process_time()
outer = np.outer(x1,x2)
toc = time.process_time()
print ("outer = " + str(outer) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED ELEMENTWISE MULTIPLICATION ###
tic = time.process_time()
mul = np.multiply(x1,x2)
toc = time.process_time()
print ("elementwise multiplication = " + str(mul) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
### VECTORIZED GENERAL DOT PRODUCT ###
tic = time.process_time()
dot = np.dot(W,x1)
toc = time.process_time()
print ("gdot = " + str(dot) + "\n ----- Computation time = " + str(1000*(toc - tic)) + "ms")
###Output
dot = 278
----- Computation time = 0.16332400000007574ms
outer = [[81 18 18 81 0 81 18 45 0 0 81 18 45 0 0]
[18 4 4 18 0 18 4 10 0 0 18 4 10 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[63 14 14 63 0 63 14 35 0 0 63 14 35 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[81 18 18 81 0 81 18 45 0 0 81 18 45 0 0]
[18 4 4 18 0 18 4 10 0 0 18 4 10 0 0]
[45 10 10 45 0 45 10 25 0 0 45 10 25 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]
[ 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0]]
----- Computation time = 0.14671599999993568ms
elementwise multiplication = [81 4 10 0 0 63 10 0 0 0 81 4 25 0 0]
----- Computation time = 0.11316999999988475ms
gdot = [ 23.95551969 28.84522088 19.9943449 ]
----- Computation time = 0.15018200000005422ms
###Markdown
As you may have noticed, the vectorized implementation is much cleaner and more efficient. For bigger vectors/matrices, the differences in running time become even bigger. **Note** that `np.dot()` performs a matrix-matrix or matrix-vector multiplication. This is different from `np.multiply()` and the `*` operator (which is equivalent to `.*` in Matlab/Octave), which performs an element-wise multiplication. 2.1 Implement the L1 and L2 loss functions**Exercise**: Implement the numpy vectorized version of the L1 loss. You may find the function abs(x) (absolute value of x) useful.**Reminder**:- The loss is used to evaluate the performance of your model. The bigger your loss is, the more different your predictions ($ \hat{y} $) are from the true values ($y$). In deep learning, you use optimization algorithms like Gradient Descent to train your model and to minimize the cost.- L1 loss is defined as:$$\begin{align*} & L_1(\hat{y}, y) = \sum_{i=0}^m|y^{(i)} - \hat{y}^{(i)}| \end{align*}\tag{6}$$
###Code
# GRADED FUNCTION: L1
def L1(yhat, y):
"""
Arguments:
yhat -- vector of size m (predicted labels)
y -- vector of size m (true labels)
Returns:
loss -- the value of the L1 loss function defined above
"""
### START CODE HERE ### (≈ 1 line of code)
loss = np.sum(np.abs(y - yhat))
### END CODE HERE ###
return loss
yhat = np.array([.9, 0.2, 0.1, .4, .9])
y = np.array([1, 0, 0, 1, 1])
print("L1 = " + str(L1(yhat,y)))
###Output
L1 = 1.1
###Markdown
**Expected Output**: **L1** 1.1 **Exercise**: Implement the numpy vectorized version of the L2 loss. There are several way of implementing the L2 loss but you may find the function np.dot() useful. As a reminder, if $x = [x_1, x_2, ..., x_n]$, then `np.dot(x,x)` = $\sum_{j=0}^n x_j^{2}$. - L2 loss is defined as $$\begin{align*} & L_2(\hat{y},y) = \sum_{i=0}^m(y^{(i)} - \hat{y}^{(i)})^2 \end{align*}\tag{7}$$
###Code
# GRADED FUNCTION: L2
def L2(yhat, y):
"""
Arguments:
yhat -- vector of size m (predicted labels)
y -- vector of size m (true labels)
Returns:
loss -- the value of the L2 loss function defined above
"""
### START CODE HERE ### (≈ 1 line of code)
loss = np.sum(np.dot(y - yhat, y - yhat))
### END CODE HERE ###
return loss
yhat = np.array([.9, 0.2, 0.1, .4, .9])
y = np.array([1, 0, 0, 1, 1])
print("L2 = " + str(L2(yhat,y)))
###Output
L2 = 0.43
|
deep-learning/Tensorflow-2.x/Browser-Based-Models/Horse-or-Human-WithDropouts.ipynb | ###Markdown
Building a Small Model from ScratchBut before we continue, let's start defining the model:Step 1 will be to import tensorflow.
###Code
import tensorflow as tf
###Output
_____no_output_____
###Markdown
We then add convolutional layers as in the previous example, and flatten the final result to feed into the densely connected layers. Finally we add the densely connected layers. Note that because we are facing a two-class classification problem, i.e. a *binary classification problem*, we will end our network with a [*sigmoid* activation](https://wikipedia.org/wiki/Sigmoid_function), so that the output of our network will be a single scalar between 0 and 1, encoding the probability that the current image is class 1 (as opposed to class 0).
###Code
model = tf.keras.models.Sequential([
# Note the input shape is the desired size of the image 300x300 with 3 bytes color
# This is the first convolution
tf.keras.layers.Conv2D(16, (3,3), activation='relu', input_shape=(300, 300, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
# The second convolution
tf.keras.layers.Conv2D(32, (3,3), activation='relu'),
tf.keras.layers.Dropout(0.5),
tf.keras.layers.MaxPooling2D(2,2),
# The third convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.Dropout(0.5),
tf.keras.layers.MaxPooling2D(2,2),
# The fourth convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# The fifth convolution
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
# Flatten the results to feed into a DNN
tf.keras.layers.Flatten(),
tf.keras.layers.Dropout(0.5),
# 512 neuron hidden layer
tf.keras.layers.Dense(512, activation='relu'),
# Only 1 output neuron. It will contain a value from 0-1 where 0 for 1 class ('horses') and 1 for the other ('humans')
tf.keras.layers.Dense(1, activation='sigmoid')
])
from tensorflow.keras.optimizers import RMSprop
model.compile(loss='binary_crossentropy',
optimizer=RMSprop(lr=1e-4),
metrics=['acc'])
from tensorflow.keras.preprocessing.image import ImageDataGenerator
# All images will be rescaled by 1./255
train_datagen = ImageDataGenerator(
rescale=1./255,
rotation_range=40,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest')
validation_datagen = ImageDataGenerator(rescale=1/255)
# Flow training images in batches of 128 using train_datagen generator
train_generator = train_datagen.flow_from_directory(
'/tmp/horse-or-human/', # This is the source directory for training images
target_size=(300, 300), # All images will be resized to 150x150
batch_size=128,
# Since we use binary_crossentropy loss, we need binary labels
class_mode='binary')
# Flow training images in batches of 128 using train_datagen generator
validation_generator = validation_datagen.flow_from_directory(
'/tmp/validation-horse-or-human/', # This is the source directory for training images
target_size=(300, 300), # All images will be resized to 150x150
batch_size=32,
# Since we use binary_crossentropy loss, we need binary labels
class_mode='binary')
history = model.fit_generator(
train_generator,
steps_per_epoch=8,
epochs=100,
verbose=1,
validation_data = validation_generator,
validation_steps=8)
import matplotlib.pyplot as plt
acc = history.history['acc']
val_acc = history.history['val_acc']
loss = history.history['loss']
val_loss = history.history['val_loss']
epochs = range(len(acc))
plt.plot(epochs, acc, 'bo', label='Training accuracy')
plt.plot(epochs, val_acc, 'b', label='Validation accuracy')
plt.title('Training and validation accuracy')
plt.figure()
plt.plot(epochs, loss, 'bo', label='Training Loss')
plt.plot(epochs, val_loss, 'b', label='Validation Loss')
plt.title('Training and validation loss')
plt.legend()
plt.show()
###Output
_____no_output_____ |
Coursera Machine Learning Python Assignments/Exercise3/exercise3.ipynb | ###Markdown
Programming Exercise 3 Multi-class Classification and Neural Networks IntroductionIn this exercise, you will implement one-vs-all logistic regression and neural networks to recognize handwritten digits. Before starting the programming exercise, we strongly recommend watching the video lectures and completing the review questions for the associated topics. All the information you need for solving this assignment is in this notebook, and all the code you will be implementing will take place within this notebook. The assignment can be promptly submitted to the coursera grader directly from this notebook (code and instructions are included below).Before we begin with the exercises, we need to import all libraries required for this programming exercise. Throughout the course, we will be using [`numpy`](http://www.numpy.org/) for all arrays and matrix operations, [`matplotlib`](https://matplotlib.org/) for plotting, and [`scipy`](https://docs.scipy.org/doc/scipy/reference/) for scientific and numerical computation functions and tools. You can find instructions on how to install required libraries in the README file in the [github repository](https://github.com/dibgerge/ml-coursera-python-assignments).
###Code
# used for manipulating directory paths
import os
# Scientific and vector computation for python
import numpy as np
# Plotting library
from matplotlib import pyplot
# Optimization module in scipy
from scipy import optimize
# will be used to load MATLAB mat datafile format
from scipy.io import loadmat
# library written for this exercise providing additional functions for assignment submission, and others
import utils
# define the submission/grader object for this exercise
grader = utils.Grader()
# tells matplotlib to embed plots within the notebook
%matplotlib inline
###Output
_____no_output_____
###Markdown
Submission and GradingAfter completing each part of the assignment, be sure to submit your solutions to the grader. The following is a breakdown of how each part of this exercise is scored.| Section | Part | Submission function | Points | :- |:- | :- | :-: | 1 | [Regularized Logistic Regression](section1) | [`lrCostFunction`](lrCostFunction) | 30 | 2 | [One-vs-all classifier training](section2) | [`oneVsAll`](oneVsAll) | 20 | 3 | [One-vs-all classifier prediction](section3) | [`predictOneVsAll`](predictOneVsAll) | 20 | 4 | [Neural Network Prediction Function](section4) | [`predict`](predict) | 30| | Total Points | | 100 You are allowed to submit your solutions multiple times, and we will take only the highest score into consideration.At the end of each section in this notebook, we have a cell which contains code for submitting the solutions thus far to the grader. Execute the cell to see your score up to the current section. For all your work to be submitted properly, you must execute those cells at least once. They must also be re-executed everytime the submitted function is updated. 1 Multi-class ClassificationFor this exercise, you will use logistic regression and neural networks to recognize handwritten digits (from 0 to 9). Automated handwritten digit recognition is widely used today - from recognizing zip codes (postal codes)on mail envelopes to recognizing amounts written on bank checks. This exercise will show you how the methods you have learned can be used for this classification task.In the first part of the exercise, you will extend your previous implementation of logistic regression and apply it to one-vs-all classification. 1.1 DatasetYou are given a data set in `ex3data1.mat` that contains 5000 training examples of handwritten digits (This is a subset of the [MNIST](http://yann.lecun.com/exdb/mnist) handwritten digit dataset). The `.mat` format means that that the data has been saved in a native Octave/MATLAB matrix format, instead of a text (ASCII) format like a csv-file. We use the `.mat` format here because this is the dataset provided in the MATLAB version of this assignment. Fortunately, python provides mechanisms to load MATLAB native format using the `loadmat` function within the `scipy.io` module. This function returns a python dictionary with keys containing the variable names within the `.mat` file. There are 5000 training examples in `ex3data1.mat`, where each training example is a 20 pixel by 20 pixel grayscale image of the digit. Each pixel is represented by a floating point number indicating the grayscale intensity at that location. The 20 by 20 grid of pixels is “unrolled” into a 400-dimensional vector. Each of these training examples becomes a single row in our data matrix `X`. This gives us a 5000 by 400 matrix `X` where every row is a training example for a handwritten digit image.$$ X = \begin{bmatrix} - \: (x^{(1)})^T \: - \\ -\: (x^{(2)})^T \:- \\ \vdots \\ - \: (x^{(m)})^T \:- \end{bmatrix} $$The second part of the training set is a 5000-dimensional vector `y` that contains labels for the training set. We start the exercise by first loading the dataset. Execute the cell below, you do not need to write any code here.
###Code
# 20x20 Input Images of Digits
input_layer_size = 400
# 10 labels, from 1 to 10 (note that we have mapped "0" to label 10)
num_labels = 10
# training data stored in arrays X, y
data = loadmat(os.path.join('Data', 'ex3data1.mat'))
X, y = data['X'], data['y'].ravel()
# set the zero digit to 0, rather than its mapped 10 in this dataset
# This is an artifact due to the fact that this dataset was used in
# MATLAB where there is no index 0
y[y == 10] = 0
m = y.size
###Output
_____no_output_____
###Markdown
1.2 Visualizing the dataYou will begin by visualizing a subset of the training set. In the following cell, the code randomly selects selects 100 rows from `X` and passes those rows to the `displayData` function. This function maps each row to a 20 pixel by 20 pixel grayscale image and displays the images together. We have provided the `displayData` function in the file `utils.py`. You are encouraged to examine the code to see how it works. Run the following cell to visualize the data.
###Code
# Randomly select 100 data points to display
rand_indices = np.random.choice(m, 100, replace=False)
sel = X[rand_indices, :]
utils.displayData(sel)
###Output
_____no_output_____
###Markdown
1.3 Vectorizing Logistic RegressionYou will be using multiple one-vs-all logistic regression models to build a multi-class classifier. Since there are 10 classes, you will need to train 10 separate logistic regression classifiers. To make this training efficient, it is important to ensure that your code is well vectorized. In this section, you will implement a vectorized version of logistic regression that does not employ any `for` loops. You can use your code in the previous exercise as a starting point for this exercise. To test your vectorized logistic regression, we will use custom data as defined in the following cell.
###Code
# test values for the parameters theta
theta_t = np.array([-2, -1, 1, 2], dtype=float)
# test values for the inputs
X_t = np.concatenate([np.ones((5, 1)), np.arange(1, 16).reshape(5, 3, order='F')/10.0], axis=1)
# test values for the labels
y_t = np.array([1, 0, 1, 0, 1])
# test value for the regularization parameter
lambda_t = 3
###Output
_____no_output_____
###Markdown
1.3.1 Vectorizing the cost function We will begin by writing a vectorized version of the cost function. Recall that in (unregularized) logistic regression, the cost function is$$ J(\theta) = \frac{1}{m} \sum_{i=1}^m \left[ -y^{(i)} \log \left( h_\theta\left( x^{(i)} \right) \right) - \left(1 - y^{(i)} \right) \log \left(1 - h_\theta \left( x^{(i)} \right) \right) \right] $$To compute each element in the summation, we have to compute $h_\theta(x^{(i)})$ for every example $i$, where $h_\theta(x^{(i)}) = g(\theta^T x^{(i)})$ and $g(z) = \frac{1}{1+e^{-z}}$ is the sigmoid function. It turns out that we can compute this quickly for all our examples by using matrix multiplication. Let us define $X$ and $\theta$ as$$ X = \begin{bmatrix} - \left( x^{(1)} \right)^T - \\ - \left( x^{(2)} \right)^T - \\ \vdots \\ - \left( x^{(m)} \right)^T - \end{bmatrix} \qquad \text{and} \qquad \theta = \begin{bmatrix} \theta_0 \\ \theta_1 \\ \vdots \\ \theta_n \end{bmatrix} $$Then, by computing the matrix product $X\theta$, we have: $$ X\theta = \begin{bmatrix} - \left( x^{(1)} \right)^T\theta - \\ - \left( x^{(2)} \right)^T\theta - \\ \vdots \\ - \left( x^{(m)} \right)^T\theta - \end{bmatrix} = \begin{bmatrix} - \theta^T x^{(1)} - \\ - \theta^T x^{(2)} - \\ \vdots \\ - \theta^T x^{(m)} - \end{bmatrix} $$In the last equality, we used the fact that $a^Tb = b^Ta$ if $a$ and $b$ are vectors. This allows us to compute the products $\theta^T x^{(i)}$ for all our examples $i$ in one line of code. 1.3.2 Vectorizing the gradientRecall that the gradient of the (unregularized) logistic regression cost is a vector where the $j^{th}$ element is defined as$$ \frac{\partial J }{\partial \theta_j} = \frac{1}{m} \sum_{i=1}^m \left( \left( h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x_j^{(i)} \right) $$To vectorize this operation over the dataset, we start by writing out all the partial derivatives explicitly for all $\theta_j$,$$\begin{align*}\begin{bmatrix} \frac{\partial J}{\partial \theta_0} \\\frac{\partial J}{\partial \theta_1} \\\frac{\partial J}{\partial \theta_2} \\\vdots \\\frac{\partial J}{\partial \theta_n}\end{bmatrix} = &\frac{1}{m} \begin{bmatrix}\sum_{i=1}^m \left( \left(h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x_0^{(i)}\right) \\\sum_{i=1}^m \left( \left(h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x_1^{(i)}\right) \\\sum_{i=1}^m \left( \left(h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x_2^{(i)}\right) \\\vdots \\\sum_{i=1}^m \left( \left(h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x_n^{(i)}\right) \\\end{bmatrix} \\= & \frac{1}{m} \sum_{i=1}^m \left( \left(h_\theta\left(x^{(i)}\right) - y^{(i)} \right)x^{(i)}\right) \\= & \frac{1}{m} X^T \cdot \left( h_\theta(x) - y\right)\end{align*}$$where$$ h_\theta(x) - y = \begin{bmatrix}h_\theta\left(x^{(1)}\right) - y^{(1)} \\h_\theta\left(x^{(2)}\right) - y^{(2)} \\\vdots \\h_\theta\left(x^{(m)}\right) - y^{(m)} \end{bmatrix} $$Note that $x^{(i)}$ is a vector, while $h_\theta\left(x^{(i)}\right) - y^{(i)}$ is a scalar (single number).To understand the last step of the derivation, let $\beta_i = (h_\theta\left(x^{(m)}\right) - y^{(m)})$ andobserve that:$$ \sum_i \beta_ix^{(i)} = \begin{bmatrix} | & | & & | \\x^{(1)} & x^{(2)} & \cdots & x^{(m)} \\| & | & & | \end{bmatrix}\begin{bmatrix}\beta_1 \\\beta_2 \\\vdots \\\beta_m\end{bmatrix} = x^T \beta$$where the values $\beta_i = \left( h_\theta(x^{(i)} - y^{(i)} \right)$.The expression above allows us to compute all the partial derivativeswithout any loops. If you are comfortable with linear algebra, we encourage you to work through the matrix multiplications above to convince yourself that the vectorized version does the same computations. Your job is to write the unregularized cost function `lrCostFunction` which returns both the cost function $J(\theta)$ and its gradient $\frac{\partial J}{\partial \theta}$. Your implementation should use the strategy we presented above to calculate $\theta^T x^{(i)}$. You should also use a vectorized approach for the rest of the cost function. A fully vectorized version of `lrCostFunction` should not contain any loops.**Debugging Tip:** Vectorizing code can sometimes be tricky. One common strategy for debugging is to print out the sizes of the matrices you are working with using the `shape` property of `numpy` arrays. For example, given a data matrix $X$ of size $100 \times 20$ (100 examples, 20 features) and $\theta$, a vector with size $20$, you can observe that `np.dot(X, theta)` is a valid multiplication operation, while `np.dot(theta, X)` is not. Furthermore, if you have a non-vectorized version of your code, you can compare the output of your vectorized code and non-vectorized code to make sure that they produce the same outputs.
###Code
def lrCostFunction(theta, X, y, lambda_):
"""
Computes the cost of using theta as the parameter for regularized
logistic regression and the gradient of the cost w.r.t. to the parameters.
Parameters
----------
theta : array_like
Logistic regression parameters. A vector with shape (n, ). n is
the number of features including any intercept.
X : array_like
The data set with shape (m x n). m is the number of examples, and
n is the number of features (including intercept).
y : array_like
The data labels. A vector with shape (m, ).
lambda_ : float
The regularization parameter.
Returns
-------
J : float
The computed value for the regularized cost function.
grad : array_like
A vector of shape (n, ) which is the gradient of the cost
function with respect to theta, at the current values of theta.
Instructions
------------
Compute the cost of a particular choice of theta. You should set J to the cost.
Compute the partial derivatives and set grad to the partial
derivatives of the cost w.r.t. each parameter in theta
Hint 1
------
The computation of the cost function and gradients can be efficiently
vectorized. For example, consider the computation
sigmoid(X * theta)
Each row of the resulting matrix will contain the value of the prediction
for that example. You can make use of this to vectorize the cost function
and gradient computations.
Hint 2
------
When computing the gradient of the regularized cost function, there are
many possible vectorized solutions, but one solution looks like:
grad = (unregularized gradient for logistic regression)
temp = theta
temp[0] = 0 # because we don't add anything for j = 0
grad = grad + YOUR_CODE_HERE (using the temp variable)
Hint 3
------
We have provided the implementatation of the sigmoid function within
the file `utils.py`. At the start of the notebook, we imported this file
as a module. Thus to access the sigmoid function within that file, you can
do the following: `utils.sigmoid(z)`.
"""
#Initialize some useful values
m = y.size
# convert labels to ints if their type is bool
if y.dtype == bool:
y = y.astype(int)
# You need to return the following variables correctly
J = 0
grad = np.zeros(theta.shape)
# ====================== YOUR CODE HERE ======================
J = -1/m * np.sum(
(y*np.log(utils.sigmoid(np.dot(X,theta))))+((1-y)*np.log(1-utils.sigmoid(np.dot(X,theta))))
) + lambda_/(2*m)*np.sum(theta[1:]**2)
grad = 1/m* np.dot(X.T, utils.sigmoid(np.dot(X, theta))-y)
grad[1:] = grad[1:] + lambda_/m*theta[1:]
# =============================================================
return J, grad
###Output
_____no_output_____
###Markdown
1.3.3 Vectorizing regularized logistic regressionAfter you have implemented vectorization for logistic regression, you will nowadd regularization to the cost function. Recall that for regularized logisticregression, the cost function is defined as$$ J(\theta) = \frac{1}{m} \sum_{i=1}^m \left[ -y^{(i)} \log \left(h_\theta\left(x^{(i)} \right)\right) - \left( 1 - y^{(i)} \right) \log\left(1 - h_\theta \left(x^{(i)} \right) \right) \right] + \frac{\lambda}{2m} \sum_{j=1}^n \theta_j^2 $$Note that you should not be regularizing $\theta_0$ which is used for the bias term.Correspondingly, the partial derivative of regularized logistic regression cost for $\theta_j$ is defined as$$\begin{align*}& \frac{\partial J(\theta)}{\partial \theta_0} = \frac{1}{m} \sum_{i=1}^m \left( h_\theta\left( x^{(i)} \right) - y^{(i)} \right) x_j^{(i)} & \text{for } j = 0 \\& \frac{\partial J(\theta)}{\partial \theta_0} = \left( \frac{1}{m} \sum_{i=1}^m \left( h_\theta\left( x^{(i)} \right) - y^{(i)} \right) x_j^{(i)} \right) + \frac{\lambda}{m} \theta_j & \text{for } j \ge 1\end{align*}$$Now modify your code in lrCostFunction in the [**previous cell**](lrCostFunction) to account for regularization. Once again, you should not put any loops into your code.**python/numpy Tip:** When implementing the vectorization for regularized logistic regression, you might often want to only sum and update certain elements of $\theta$. In `numpy`, you can index into the matrices to access and update only certain elements. For example, A[:, 3:5]= B[:, 1:3] will replaces the columns with index 3 to 5 of A with the columns with index 1 to 3 from B. To select columns (or rows) until the end of the matrix, you can leave the right hand side of the colon blank. For example, A[:, 2:] will only return elements from the $3^{rd}$ to last columns of $A$. If you leave the left hand size of the colon blank, you will select elements from the beginning of the matrix. For example, A[:, :2] selects the first two columns, and is equivalent to A[:, 0:2]. In addition, you can use negative indices to index arrays from the end. Thus, A[:, :-1] selects all columns of A except the last column, and A[:, -5:] selects the $5^{th}$ column from the end to the last column. Thus, you could use this together with the sum and power ($^{**}$) operations to compute the sum of only the elements you are interested in (e.g., `np.sum(z[1:]**2)`). In the starter code, `lrCostFunction`, we have also provided hints on yet another possible method computing the regularized gradient.Once you finished your implementation, you can call the function `lrCostFunction` to test your solution using the following cell:
###Code
J, grad = lrCostFunction(theta_t, X_t, y_t, lambda_t)
print('Cost : {:.6f}'.format(J))
print('Expected cost: 2.534819')
print('-----------------------')
print('Gradients:')
print(' [{:.6f}, {:.6f}, {:.6f}, {:.6f}]'.format(*grad))
print('Expected gradients:')
print(' [0.146561, -0.548558, 0.724722, 1.398003]');
###Output
Cost : 2.534819
Expected cost: 2.534819
-----------------------
Gradients:
[0.146561, -0.548558, 0.724722, 1.398003]
Expected gradients:
[0.146561, -0.548558, 0.724722, 1.398003]
###Markdown
After completing a part of the exercise, you can submit your solutions for grading by first adding the function you modified to the submission object, and then sending your function to Coursera for grading. The submission script will prompt you for your login e-mail and submission token. You can obtain a submission token from the web page for the assignment. You are allowed to submit your solutions multiple times, and we will take only the highest score into consideration.*Execute the following cell to grade your solution to the first part of this exercise.*
###Code
# appends the implemented function in part 1 to the grader object
grader[1] = lrCostFunction
# send the added functions to coursera grader for getting a grade on this part
grader.grade()
###Output
Submitting Solutions | Programming Exercise multi-class-classification-and-neural-networks
Login (email address): [email protected]
Token: uU0rS1oTJwNSlwp5
Part Name | Score | Feedback
--------- | ----- | --------
Regularized Logistic Regression | 30 / 30 | Nice work!
One-vs-All Classifier Training | 0 / 20 |
One-vs-All Classifier Prediction | 0 / 20 |
Neural Network Prediction Function | 0 / 30 |
--------------------------------
| 30 / 100 |
###Markdown
1.4 One-vs-all ClassificationIn this part of the exercise, you will implement one-vs-all classification by training multiple regularized logistic regression classifiers, one for each of the $K$ classes in our dataset. In the handwritten digits dataset, $K = 10$, but your code should work for any value of $K$. You should now complete the code for the function `oneVsAll` below, to train one classifier for each class. In particular, your code should return all the classifier parameters in a matrix $\theta \in \mathbb{R}^{K \times (N +1)}$, where each row of $\theta$ corresponds to the learned logistic regression parameters for one class. You can do this with a “for”-loop from $0$ to $K-1$, training each classifier independently.Note that the `y` argument to this function is a vector of labels from 0 to 9. When training the classifier for class $k \in \{0, ..., K-1\}$, you will want a K-dimensional vector of labels $y$, where $y_j \in 0, 1$ indicates whether the $j^{th}$ training instance belongs to class $k$ $(y_j = 1)$, or if it belongs to a differentclass $(y_j = 0)$. You may find logical arrays helpful for this task. Furthermore, you will be using scipy's `optimize.minimize` for this exercise.
###Code
def oneVsAll(X, y, num_labels, lambda_):
"""
Trains num_labels logistic regression classifiers and returns
each of these classifiers in a matrix all_theta, where the i-th
row of all_theta corresponds to the classifier for label i.
Parameters
----------
X : array_like
The input dataset of shape (m x n). m is the number of
data points, and n is the number of features. Note that we
do not assume that the intercept term (or bias) is in X, however
we provide the code below to add the bias term to X.
y : array_like
The data labels. A vector of shape (m, ).
num_labels : int
Number of possible labels.
lambda_ : float
The logistic regularization parameter.
Returns
-------
all_theta : array_like
The trained parameters for logistic regression for each class.
This is a matrix of shape (K x n+1) where K is number of classes
(ie. `numlabels`) and n is number of features without the bias.
Instructions
------------
You should complete the following code to train `num_labels`
logistic regression classifiers with regularization parameter `lambda_`.
Hint
----
You can use y == c to obtain a vector of 1's and 0's that tell you
whether the ground truth is true/false for this class.
Note
----
For this assignment, we recommend using `scipy.optimize.minimize(method='CG')`
to optimize the cost function. It is okay to use a for-loop
(`for c in range(num_labels):`) to loop over the different classes.
Example Code
------------
# Set Initial theta
initial_theta = np.zeros(n + 1)
# Set options for minimize
options = {'maxiter': 50}
# Run minimize to obtain the optimal theta. This function will
# return a class object where theta is in `res.x` and cost in `res.fun`
res = optimize.minimize(lrCostFunction,
initial_theta,
(X, (y == c), lambda_),
jac=True,
method='TNC',
options=options)
"""
# Some useful variables
m, n = X.shape
# You need to return the following variables correctly
all_theta = np.zeros((num_labels, n + 1))
# Add ones to the X data matrix
X = np.concatenate([np.ones((m, 1)), X], axis=1)
# ====================== YOUR CODE HERE ======================
for c in range(num_labels):
# Set Initial theta
initial_theta = np.zeros(n + 1)
# Set options for minimize
options = {'maxiter': 50}
# Run minimize to obtain the optimal theta. This function will
# return a class object where theta is in `res.x` and cost in `res.fun`
res = optimize.minimize(lrCostFunction,
initial_theta,
(X, (y == c), lambda_),
jac=True,
method='TNC',
options=options)
all_theta[c, :] = res.x
# ============================================================
return all_theta
###Output
_____no_output_____
###Markdown
After you have completed the code for `oneVsAll`, the following cell will use your implementation to train a multi-class classifier.
###Code
lambda_ = 0.1
all_theta = oneVsAll(X, y, num_labels, lambda_)
###Output
_____no_output_____
###Markdown
*You should now submit your solutions.*
###Code
grader[2] = oneVsAll
grader.grade()
###Output
Submitting Solutions | Programming Exercise multi-class-classification-and-neural-networks
Use token from last successful submission ([email protected])? (Y/n): Y
Part Name | Score | Feedback
--------- | ----- | --------
Regularized Logistic Regression | 30 / 30 | Nice work!
One-vs-All Classifier Training | 20 / 20 | Nice work!
One-vs-All Classifier Prediction | 0 / 20 |
Neural Network Prediction Function | 0 / 30 |
--------------------------------
| 50 / 100 |
###Markdown
1.4.1 One-vs-all PredictionAfter training your one-vs-all classifier, you can now use it to predict the digit contained in a given image. For each input, you should compute the “probability” that it belongs to each class using the trained logistic regression classifiers. Your one-vs-all prediction function will pick the class for which the corresponding logistic regression classifier outputs the highest probability and return the class label (0, 1, ..., K-1) as the prediction for the input example. You should now complete the code in the function `predictOneVsAll` to use the one-vs-all classifier for making predictions.
###Code
def predictOneVsAll(all_theta, X):
"""
Return a vector of predictions for each example in the matrix X.
Note that X contains the examples in rows. all_theta is a matrix where
the i-th row is a trained logistic regression theta vector for the
i-th class. You should set p to a vector of values from 0..K-1
(e.g., p = [0, 2, 0, 1] predicts classes 0, 2, 0, 1 for 4 examples) .
Parameters
----------
all_theta : array_like
The trained parameters for logistic regression for each class.
This is a matrix of shape (K x n+1) where K is number of classes
and n is number of features without the bias.
X : array_like
Data points to predict their labels. This is a matrix of shape
(m x n) where m is number of data points to predict, and n is number
of features without the bias term. Note we add the bias term for X in
this function.
Returns
-------
p : array_like
The predictions for each data point in X. This is a vector of shape (m, ).
Instructions
------------
Complete the following code to make predictions using your learned logistic
regression parameters (one-vs-all). You should set p to a vector of predictions
(from 0 to num_labels-1).
Hint
----
This code can be done all vectorized using the numpy argmax function.
In particular, the argmax function returns the index of the max element,
for more information see '?np.argmax' or search online. If your examples
are in rows, then, you can use np.argmax(A, axis=1) to obtain the index
of the max for each row.
"""
m = X.shape[0];
num_labels = all_theta.shape[0]
# You need to return the following variables correctly
p = np.zeros(m)
# Add ones to the X data matrix
X = np.concatenate([np.ones((m, 1)), X], axis=1)
# ====================== YOUR CODE HERE ======================
p = np.argmax(np.dot(X, all_theta.T), axis = 1)
# ============================================================
return p
###Output
_____no_output_____
###Markdown
Once you are done, call your `predictOneVsAll` function using the learned value of $\theta$. You should see that the training set accuracy is about 95.1% (i.e., it classifies 95.1% of the examples in the training set correctly).
###Code
pred = predictOneVsAll(all_theta, X)
print('Training Set Accuracy: {:.2f}%'.format(np.mean(pred == y) * 100))
###Output
Training Set Accuracy: 95.36%
###Markdown
*You should now submit your solutions.*
###Code
grader[3] = predictOneVsAll
grader.grade()
###Output
Submitting Solutions | Programming Exercise multi-class-classification-and-neural-networks
Use token from last successful submission ([email protected])? (Y/n):
Part Name | Score | Feedback
--------- | ----- | --------
Regularized Logistic Regression | 30 / 30 | Nice work!
One-vs-All Classifier Training | 20 / 20 | Nice work!
One-vs-All Classifier Prediction | 20 / 20 | Nice work!
Neural Network Prediction Function | 0 / 30 |
--------------------------------
| 70 / 100 |
###Markdown
2 Neural NetworksIn the previous part of this exercise, you implemented multi-class logistic regression to recognize handwritten digits. However, logistic regression cannot form more complex hypotheses as it is only a linear classifier (You could add more features - such as polynomial features - to logistic regression, but that can be very expensive to train).In this part of the exercise, you will implement a neural network to recognize handwritten digits using the same training set as before. The neural network will be able to represent complex models that form non-linear hypotheses. For this week, you will be using parameters from a neural network that we have already trained. Your goal is to implement the feedforward propagation algorithm to use our weights for prediction. In next week’s exercise, you will write the backpropagation algorithm for learning the neural network parameters. We start by first reloading and visualizing the dataset which contains the MNIST handwritten digits (this is the same as we did in the first part of this exercise, we reload it here to ensure the variables have not been modified).
###Code
# training data stored in arrays X, y
data = loadmat(os.path.join('Data', 'ex3data1.mat'))
X, y = data['X'], data['y'].ravel()
# set the zero digit to 0, rather than its mapped 10 in this dataset
# This is an artifact due to the fact that this dataset was used in
# MATLAB where there is no index 0
y[y == 10] = 0
# get number of examples in dataset
m = y.size
# randomly permute examples, to be used for visualizing one
# picture at a time
indices = np.random.permutation(m)
# Randomly select 100 data points to display
rand_indices = np.random.choice(m, 100, replace=False)
sel = X[rand_indices, :]
utils.displayData(sel)
###Output
_____no_output_____
###Markdown
2.1 Model representation Our neural network is shown in the following figure.It has 3 layers: an input layer, a hidden layer and an output layer. Recall that our inputs are pixel values of digit images. Since the images are of size 20×20, this gives us 400 input layer units (excluding the extra bias unit which always outputs +1). As before, the training data will be loaded into the variables X and y. You have been provided with a set of network parameters ($\Theta^{(1)}$, $\Theta^{(2)}$) already trained by us. These are stored in `ex3weights.mat`. The following cell loads those parameters into `Theta1` and `Theta2`. The parameters have dimensions that are sized for a neural network with 25 units in the second layer and 10 output units (corresponding to the 10 digit classes).
###Code
# Setup the parameters you will use for this exercise
input_layer_size = 400 # 20x20 Input Images of Digits
hidden_layer_size = 25 # 25 hidden units
num_labels = 10 # 10 labels, from 0 to 9
# Load the .mat file, which returns a dictionary
weights = loadmat(os.path.join('Data', 'ex3weights.mat'))
# get the model weights from the dictionary
# Theta1 has size 25 x 401
# Theta2 has size 10 x 26
Theta1, Theta2 = weights['Theta1'], weights['Theta2']
# swap first and last columns of Theta2, due to legacy from MATLAB indexing,
# since the weight file ex3weights.mat was saved based on MATLAB indexing
Theta2 = np.roll(Theta2, 1, axis=0)
###Output
_____no_output_____
###Markdown
2.2 Feedforward Propagation and PredictionNow you will implement feedforward propagation for the neural network. You will need to complete the code in the function `predict` to return the neural network’s prediction. You should implement the feedforward computation that computes $h_\theta(x^{(i)})$ for every example $i$ and returns the associated predictions. Similar to the one-vs-all classification strategy, the prediction from the neural network will be the label that has the largest output $\left( h_\theta(x) \right)_k$.**Implementation Note:** The matrix $X$ contains the examples in rows. When you complete the code in the function `predict`, you will need to add the column of 1’s to the matrix. The matrices `Theta1` and `Theta2` contain the parameters for each unit in rows. Specifically, the first row of `Theta1` corresponds to the first hidden unit in the second layer. In `numpy`, when you compute $z^{(2)} = \theta^{(1)}a^{(1)}$, be sure that you index (and if necessary, transpose) $X$ correctly so that you get $a^{(l)}$ as a 1-D vector.
###Code
def predict(Theta1, Theta2, X):
"""
Predict the label of an input given a trained neural network.
Parameters
----------
Theta1 : array_like
Weights for the first layer in the neural network.
It has shape (2nd hidden layer size x input size)
Theta2: array_like
Weights for the second layer in the neural network.
It has shape (output layer size x 2nd hidden layer size)
X : array_like
The image inputs having shape (number of examples x image dimensions).
Return
------
p : array_like
Predictions vector containing the predicted label for each example.
It has a length equal to the number of examples.
Instructions
------------
Complete the following code to make predictions using your learned neural
network. You should set p to a vector containing labels
between 0 to (num_labels-1).
Hint
----
This code can be done all vectorized using the numpy argmax function.
In particular, the argmax function returns the index of the max element,
for more information see '?np.argmax' or search online. If your examples
are in rows, then, you can use np.argmax(A, axis=1) to obtain the index
of the max for each row.
Note
----
Remember, we have supplied the `sigmoid` function in the `utils.py` file.
You can use this function by calling `utils.sigmoid(z)`, where you can
replace `z` by the required input variable to sigmoid.
"""
# Make sure the input has two dimensions
if X.ndim == 1:
X = X[None] # promote to 2-dimensions
# useful variables
m = X.shape[0]
num_labels = Theta2.shape[0]
# You need to return the following variables correctly
p = np.zeros(X.shape[0])
# ====================== YOUR CODE HERE ======================
a1 = np.concatenate([np.ones((m,1)), X], axis = 1)
a2 = utils.sigmoid(np.dot(a1, Theta1.T))
a2 = np.concatenate([np.ones((a2.shape[0],1)),a2], axis = 1)
a3 = utils.sigmoid(np.dot(a2, Theta2.T))
p = np.argmax(a3, axis = 1)
# =============================================================
return p
###Output
_____no_output_____
###Markdown
Once you are done, call your predict function using the loaded set of parameters for `Theta1` and `Theta2`. You should see that the accuracy is about 97.5%.
###Code
pred = predict(Theta1, Theta2, X)
print('Training Set Accuracy: {:.1f}%'.format(np.mean(pred == y) * 100))
###Output
Training Set Accuracy: 97.5%
###Markdown
After that, we will display images from the training set one at a time, while at the same time printing out the predicted label for the displayed image. Run the following cell to display a single image the the neural network's prediction. You can run the cell multiple time to see predictions for different images.
###Code
if indices.size > 0:
i, indices = indices[0], indices[1:]
utils.displayData(X[i, :], figsize=(4, 4))
pred = predict(Theta1, Theta2, X[i, :])
print('Neural Network Prediction: {}'.format(*pred))
else:
print('No more images to display!')
###Output
Neural Network Prediction: 9
###Markdown
*You should now submit your solutions.*
###Code
grader[4] = predict
grader.grade()
###Output
Submitting Solutions | Programming Exercise multi-class-classification-and-neural-networks
Use token from last successful submission ([email protected])? (Y/n):
Part Name | Score | Feedback
--------- | ----- | --------
Regularized Logistic Regression | 30 / 30 | Nice work!
One-vs-All Classifier Training | 20 / 20 | Nice work!
One-vs-All Classifier Prediction | 20 / 20 | Nice work!
Neural Network Prediction Function | 30 / 30 | Nice work!
--------------------------------
| 100 / 100 |
|
scripts/125_omim_standard/00_ukb_biobank_to_efo.ipynb | ###Markdown
Load gene mappings
###Code
with open(os.path.join(conf.GENES_METADATA_DIR, 'genes_mapping_simplified-0.pkl'), 'rb') as f:
genes_mapping_0 = pickle.load(f)
with open(os.path.join(conf.GENES_METADATA_DIR, 'genes_mapping_simplified-1.pkl'), 'rb') as f:
genes_mapping_1 = pickle.load(f)
###Output
_____no_output_____
###Markdown
Load S-MultiXcan results
###Code
# s-predixcan
smultixcan_genes_associations_filename = os.path.join(conf.GENE_ASSOC_DIR, f'smultixcan-mashr-pvalues.pkl.xz')
display(smultixcan_genes_associations_filename)
smultixcan_genes_associations = pd.read_pickle(smultixcan_genes_associations_filename)
smultixcan_genes_associations.shape
smultixcan_genes_associations.head(5)
###Output
_____no_output_____
###Markdown
Create UKB data with traits
###Code
ukb_data = pd.DataFrame(index=smultixcan_genes_associations.columns).reset_index().rename(columns={'index': 'ukb_fullcode'})
ukb_data = ukb_data.assign(ukb_code=ukb_data['ukb_fullcode'].str.split('-').str[0])
ukb_data.head()
###Output
_____no_output_____
###Markdown
Take a look at the UKB-EFO mappings file **Note:** `UK_Biobank_master_file.tsv` was downloaded from https://github.com/EBISPOT/EFO-UKB-mappings
###Code
ukb_map = pd.read_csv(conf.OMIM_SILVER_STANDARD_UKB_EFO_MAP_FILE, sep='\t')
ukb_map = ukb_map.dropna(subset=['ICD10_CODE/SELF_REPORTED_TRAIT_FIELD_CODE'])
ukb_map.shape
ukb_map.head()
ukb_map['MAPPING_TYPE'].unique()
ukb_map[ukb_map['MAPPING_TYPE'] == 'Narrow'].shape
ukb_map[ukb_map['MAPPING_TYPE'] == 'Broad'].shape
ukb_map[ukb_map['MAPPING_TYPE'] == 'Exact'].shape
ukb_map[ukb_map['ICD10_CODE/SELF_REPORTED_TRAIT_FIELD_CODE'].str.contains('20001_1073')]
###Output
_____no_output_____
###Markdown
Group traits according to EFO terms
###Code
from efo import EFO
efo_file = os.path.join(conf.OMIM_SILVER_STANDARD_EFO_FILE)
display(efo_file)
efo = EFO(efo_file)
efo.efo_data_full.head(10)
#ukb_data_with_efo = efo.assign_efo_from_ukb(ukb_data, map_type='Exact')
ukb_data_with_efo = efo.assign_efo_from_ukb(ukb_data, map_types=('Exact', 'Broad', 'Narrow'))
ukb_data_with_efo = ukb_data_with_efo.dropna(subset=['efo_name'])
display(ukb_data_with_efo.shape)
display(ukb_data_with_efo.head())
# How does "asthma" get grouped?
ukb_data_with_efo[ukb_data_with_efo['efo_name'].str.lower().str.contains('asthma')]
# Save only ukb traits
ukb_data_with_efo.to_csv('ukbiobank_efo_mappings.tsv', index=False, sep='\t')
###Output
_____no_output_____
###Markdown
Add GTEX GWAS traits description
###Code
# import metadata
# metadata.GTEX_GWAS_PHENO_INFO.head()
# gtex_gwas_traits_info = metadata.GTEX_GWAS_PHENO_INFO[['EFO', 'HPO', 'Description', 'Phenotype']]
# gtex_gwas_traits_info = gtex_gwas_traits_info[gtex_gwas_traits_info.index.isin(smultixcan_genes_associations.columns)]
# gtex_gwas_traits_info.shape
# gtex_gwas_traits_info.head()
# gtex_gwas_data_with_efo = efo.assign_efo_label_from_efo_code(gtex_gwas_traits_info, efo_code_column='EFO')
# gtex_gwas_data_with_efo.head()
# gtex_gwas_data_with_efo = gtex_gwas_data_with_efo[['efo_name']]
# gtex_gwas_data_with_efo = gtex_gwas_data_with_efo.assign(ukb_code=gtex_gwas_data_with_efo.index)
# gtex_gwas_data_with_efo = gtex_gwas_data_with_efo.reset_index()[['Tag', 'ukb_code', 'efo_name']].rename(columns={'Tag': 'ukb_fullcode'})
# gtex_gwas_data_with_efo.head()
# df = pd.concat((ukb_data_with_efo, gtex_gwas_data_with_efo), ignore_index=True)
# df.shape
# df.head()
# df.to_csv('ukbiobank_efo_mappings.tsv', index=False, sep='\t')
###Output
_____no_output_____
###Markdown
Run map_trait_to_hpo_and_mim.R
###Code
omim_silver_standard_dir = conf.OMIM_SILVER_STANDARD_DATA_DIR
%%bash -s "$omim_silver_standard_dir"
Rscript map_trait_to_hpo_and_mim.R $1
###Output
Attaching package: ‘dplyr’
The following objects are masked from ‘package:stats’:
filter, lag
The following objects are masked from ‘package:base’:
intersect, setdiff, setequal, union
###Markdown
Run mim-to-gene.R (generates OMIM silver standard)
###Code
%%bash -s "$omim_silver_standard_dir"
Rscript mim-to-gene.R $1
###Output
Attaching package: ‘dplyr’
The following objects are masked from ‘package:data.table’:
between, first, last
The following objects are masked from ‘package:stats’:
filter, lag
The following objects are masked from ‘package:base’:
intersect, setdiff, setequal, union
Taking input= as a system command ('cat /home/miltondp/projects/labs/hakyimlab/phenomexcan/base/results/omim_silver_standard/data/mim2gene.txt | tail -n +5') and a variable has been used in the expression passed to `input=`. Please use fread(cmd=...). There is a security concern if you are creating an app, and the app could have a malicious user, and the app is not running in a secure envionment; e.g. the app is running as root. Please read item 5 in the NEWS file for v1.11.6 for more information and for the option to suppress this message.
Taking input= as a system command ('cat /home/miltondp/projects/labs/hakyimlab/phenomexcan/base/results/omim_silver_standard/data/genemap2.txt | head -n 16943 | tail -n +4') and a variable has been used in the expression passed to `input=`. Please use fread(cmd=...). There is a security concern if you are creating an app, and the app could have a malicious user, and the app is not running in a secure envionment; e.g. the app is running as root. Please read item 5 in the NEWS file for v1.11.6 for more information and for the option to suppress this message.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
361
362
363
364
365
366
367
368
369
370
371
372
373
374
375
376
377
378
379
380
381
382
383
384
385
386
387
388
389
390
391
392
393
394
395
396
397
398
399
400
401
402
403
404
405
406
407
408
409
410
411
412
413
414
415
416
417
418
419
420
421
422
423
424
425
426
427
428
429
430
431
432
433
434
435
436
437
438
439
440
441
442
443
444
445
446
447
448
449
450
451
452
453
454
455
456
457
458
459
460
461
462
463
464
465
466
467
468
469
470
471
472
473
474
475
476
477
478
479
480
481
482
483
484
485
486
487
488
489
490
491
492
493
494
495
496
497
498
499
500
501
502
503
504
505
506
507
508
509
510
511
512
513
514
515
516
517
518
519
520
521
522
523
524
525
526
527
528
529
530
531
532
533
534
535
536
537
538
539
540
541
542
543
544
545
546
547
548
549
550
551
552
553
554
555
556
557
558
559
560
561
562
563
564
565
566
567
568
569
570
571
572
573
574
575
576
577
578
579
580
581
582
583
584
585
586
587
588
589
590
591
592
593
594
595
596
597
598
599
600
601
602
603
604
605
606
607
608
609
610
611
612
613
614
615
616
617
618
619
620
621
622
623
624
625
626
627
628
629
630
631
632
633
634
635
636
637
638
639
640
641
642
643
644
645
646
647
648
649
650
651
652
653
654
655
656
657
658
659
660
661
662
663
664
665
666
667
668
669
670
671
672
673
674
675
676
677
678
679
680
681
682
683
684
685
686
687
688
689
690
691
692
693
694
695
696
697
698
699
700
701
702
703
704
705
706
707
708
709
710
711
712
713
714
715
716
717
718
719
720
721
722
723
724
725
726
727
728
729
730
731
732
733
734
735
736
737
738
739
740
741
742
743
744
745
746
747
748
749
750
751
752
753
754
755
756
757
758
759
760
761
762
763
764
765
766
767
768
769
770
771
772
773
774
775
776
777
778
779
780
781
782
783
784
785
786
787
788
789
790
791
792
793
794
795
796
797
798
799
800
801
802
803
804
805
806
807
808
809
810
811
812
813
814
815
816
817
818
819
820
821
822
823
824
825
826
827
828
829
830
831
832
833
834
835
836
837
838
839
840
841
842
843
844
845
846
847
848
849
850
851
852
853
854
855
856
857
858
859
860
861
862
863
864
865
866
867
868
869
870
871
872
873
874
875
876
877
878
879
880
881
882
883
884
885
886
887
888
889
890
891
892
893
894
895
896
897
898
899
900
901
902
903
904
905
906
907
908
909
910
911
912
913
914
915
916
917
918
919
920
921
922
923
924
925
926
927
928
929
930
931
932
933
934
935
936
937
938
939
940
941
942
943
944
945
946
947
948
949
950
951
952
953
954
955
956
957
958
959
960
961
962
963
964
965
966
967
968
969
970
971
972
973
974
975
976
977
978
979
980
981
982
983
984
985
986
987
988
989
990
991
992
993
994
995
996
997
998
999
1000
1001
1002
1003
1004
1005
1006
1007
###Markdown
Move OMIM silver standard file
###Code
new_omim_file_location = conf.OMIM_SILVER_STANDARD_FINAL_FILE
%%bash -s "$new_omim_file_location"
mv omim_silver_standard.tsv $1
###Output
_____no_output_____ |
LogisticClassifier.ipynb | ###Markdown
LOGISTIC CLASSIFIER FROM SCRATCH Log loss as loss function and Gradient descent as optimizer
###Code
import numpy as np
import math
import matplotlib.pyplot as plt
import sklearn.datasets as datasets
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
iris = datasets.load_iris()
x = iris.data[:, :2]
y = (iris.target != 0) * 1
# shuffling data
arr = np.concatenate((x,y.reshape(150, 1)), axis=1)
np.random.shuffle(arr)
x = arr[:, :-1]
y = arr[:, -1]
plt.figure(figsize=(10,6))
plt.scatter(x[y==0][:,0], x[y==0][:,1], c='r', label='0')
plt.scatter(x[y==1][:,0], x[y==1][:,1], c='b', label='1')
plt.legend()
plt.show()
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# Log_loss
def calc_loss(y_pred, y_true):
soma = np.sum((y_true * np.log(y_pred) + (1-y_true) *
np.log(1 - y_pred)))
return -soma/y_pred.size
# Gradient descent
def gradient(X, y_pred, y_true):
return np.dot(X.T, (y_pred-y_true))/y_pred.size
def predict(X,w):
return np.dot(X,w)
def calc_acc(y_hat, y):
return ((y_hat.round() == y).mean() * 100).round(2)
def fit(X,y, num_iter, lr, loss_min, verbose=50):
err = []
curr_epoch = 0
Xu = np.concatenate((np.ones((X.shape[0],1)),X), axis=1)
weights = np.zeros(Xu.shape[1])
while curr_epoch <= num_iter:
z = predict(Xu,weights)
h = sigmoid(z)
loss = calc_loss(h, y)
grad = gradient(Xu, h, y)
weights -= lr*grad
if curr_epoch%verbose == 0:
acc = calc_acc(h, y)
print(f'Epoch: {curr_epoch} - Loss: {loss} - Accuracy: {acc}\n')
err.append(loss)
if loss <= loss_min:
break
curr_epoch = curr_epoch + 1
return err, weights
err, weights = fit(x, y, 1500, 0.05, 0, verbose=300)
plt.plot(err, label='Loss')
plt.legend(loc='best')
###Output
_____no_output_____ |
docs/_utility/generate_images.ipynb | ###Markdown
Generate qlibrary component images By running this notebook the qlibrary component image files will be update. After the docs build is rerun the images in the docs will be updated automatically. Start gui
###Code
%load_ext autoreload
%autoreload 2
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict
design = designs.DesignPlanar()
gui = MetalGUI(design)
###Output
_____no_output_____
###Markdown
Qlibrary component imports and define worker function
###Code
from qiskit_metal.qlibrary.sample_shapes.circle_caterpillar import CircleCaterpillar
from qiskit_metal.qlibrary.sample_shapes.circle_raster import CircleRaster
from qiskit_metal.qlibrary.sample_shapes.n_gon import NGon
from qiskit_metal.qlibrary.sample_shapes.n_square_spiral import NSquareSpiral
from qiskit_metal.qlibrary.sample_shapes.rectangle import Rectangle
from qiskit_metal.qlibrary.sample_shapes.rectangle_hollow import RectangleHollow
from qiskit_metal.qlibrary.lumped.cap_3_interdigital import Cap3Interdigital
from qiskit_metal.qlibrary.lumped.cap_n_interdigital import CapNInterdigital
from qiskit_metal.qlibrary.lumped.resonator_coil_rect import ResonatorCoilRect
from qiskit_metal.qlibrary.couplers.coupled_line_tee import CoupledLineTee
from qiskit_metal.qlibrary.couplers.line_tee import LineTee
from qiskit_metal.qlibrary.couplers.cap_n_interdigital_tee import CapNInterdigitalTee
from qiskit_metal.qlibrary.couplers.tunable_coupler_01 import TunableCoupler01
from qiskit_metal.qlibrary.terminations.launchpad_wb import LaunchpadWirebond
from qiskit_metal.qlibrary.terminations.launchpad_wb_coupled import LaunchpadWirebondCoupled
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
from qiskit_metal.qlibrary.qubits.JJ_Dolan import jj_dolan
from qiskit_metal.qlibrary.qubits.JJ_Manhattan import jj_manhattan
from qiskit_metal.qlibrary.qubits.transmon_concentric import TransmonConcentric
from qiskit_metal.qlibrary.qubits.transmon_cross import TransmonCross
from qiskit_metal.qlibrary.qubits.transmon_cross_fl import TransmonCrossFL
from qiskit_metal.qlibrary.qubits.Transmon_Interdigitated import TransmonInterdigitated
from qiskit_metal.qlibrary.qubits.transmon_pocket import TransmonPocket
from qiskit_metal.qlibrary.qubits.transmon_pocket_cl import TransmonPocketCL
from qiskit_metal.qlibrary.qubits.transmon_pocket_6 import TransmonPocket6
from qiskit_metal.qlibrary.qubits.transmon_pocket_teeth import TransmonPocketTeeth
from qiskit_metal.qlibrary.qubits.SQUID_loop import SQUID_LOOP
from qiskit_metal.qlibrary.qubits.star_qubit import StarQubit
import shutil, os
def create_image(component, component_name, file_name):
obj = component(design, component_name)
gui.rebuild()
gui.autoscale()
gui.zoom_on_components([component_name])
gui.figure.savefig(file_name, dpi=30)
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image(file_name, **_disp_ops))
design.delete_component(component_name)
shutil.copy(file_name, '../apidocs/')
os.remove(file_name)
###Output
_____no_output_____
###Markdown
sample_shapes
###Code
create_image(CircleCaterpillar, 'circlecaterpillar', 'CircleCaterpillar.png')
create_image(CircleRaster, 'circleraster', 'CircleRaster.png')
create_image(NGon, 'ngon', 'NGon.png')
create_image(NSquareSpiral, 'nsquarespiral', 'NSquareSpiral.png')
create_image(Rectangle, 'rectangle', 'Rectangle.png')
create_image(RectangleHollow, 'rectanglehollow', 'RectangleHollow.png')
###Output
_____no_output_____
###Markdown
lumped
###Code
create_image(Cap3Interdigital, 'cap3interdigital', 'Cap3Interdigital.png')
create_image(CapNInterdigital, 'capninterdigital', 'CapNInterdigital.png')
create_image(ResonatorCoilRect, 'resonatorcoilrect', 'ResonatorCoilRect.png')
###Output
_____no_output_____
###Markdown
couplers
###Code
create_image(CoupledLineTee, 'coupledlintee', 'CoupledLineTee.png')
create_image(LineTee, 'linetee', 'LineTee.png')
create_image(CapNInterdigitalTee, 'capninterdigitaltee', 'CapNInterdigitalTee.png')
create_image(TunableCoupler01, 'tunablecoupler01', 'TunableCoupler01.png')
###Output
_____no_output_____
###Markdown
terminations
###Code
create_image(LaunchpadWirebond, 'launchpadwirebond', 'LaunchpadWirebond.png')
create_image(LaunchpadWirebondCoupled, 'launchpadwirebondcoupled', 'LaunchpadWirebondCoupled.png')
create_image(OpenToGround, 'opentoground', 'OpenToGround.png')
###Output
_____no_output_____
###Markdown
qubits
###Code
create_image(jj_dolan, 'jj_dolan', 'JJDolan.png')
create_image(jj_manhattan, 'jj_manhattan', 'JJManhattan.png')
create_image(TransmonConcentric, 'transmonconcentric', 'TransmonConcentric.png')
create_image(TransmonCross, 'transmoncross', 'TransmonCross.png')
create_image(TransmonCrossFL, 'transmoncrossfl', 'TransmonCrossFL.png')
create_image(TransmonInterdigitated, 'transmoninterdigitated', 'TransmonInterdigitated.png')
create_image(TransmonPocket, 'transmonpocket', 'TransmonPocket.png')
create_image(TransmonPocketCL, 'transmonpocketcl', 'TransmonPocketCL.png')
create_image(TransmonPocket6, 'transmonpocket6', 'TransmonPocket6.png')
create_image(TransmonPocketTeeth, 'transmonpocketteeth', 'Transmon_Pocket_Teeth.png')
create_image(SQUID_LOOP, 'squidloop', 'SQUID_LOOP.png')
create_image(StarQubit, 'starqubit', 'StarQubit.png')
###Output
_____no_output_____
###Markdown
Closing the Qiskit Metal GUI
###Code
gui.main_window.close()
###Output
_____no_output_____
###Markdown
Generate qlibrary component images By running this notebook the qlibrary component image files will be update. After the docs build is rerun the images in the docs will be updated automatically. Start gui
###Code
%load_ext autoreload
%autoreload 2
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict
design = designs.DesignPlanar()
gui = MetalGUI(design)
###Output
_____no_output_____
###Markdown
Qlibrary component imports and define worker function
###Code
from qiskit_metal.qlibrary.sample_shapes.circle_caterpillar import CircleCaterpillar
from qiskit_metal.qlibrary.sample_shapes.circle_raster import CircleRaster
from qiskit_metal.qlibrary.sample_shapes.n_gon import NGon
from qiskit_metal.qlibrary.sample_shapes.n_square_spiral import NSquareSpiral
from qiskit_metal.qlibrary.sample_shapes.rectangle import Rectangle
from qiskit_metal.qlibrary.sample_shapes.rectangle_hollow import RectangleHollow
from qiskit_metal.qlibrary.lumped.cap_3_interdigital import Cap3Interdigital
from qiskit_metal.qlibrary.lumped.cap_n_interdigital import CapNInterdigital
from qiskit_metal.qlibrary.lumped.resonator_coil_rect import ResonatorCoilRect
from qiskit_metal.qlibrary.couplers.coupled_line_tee import CoupledLineTee
from qiskit_metal.qlibrary.couplers.line_tee import LineTee
from qiskit_metal.qlibrary.couplers.cap_n_interdigital_tee import CapNInterdigitalTee
from qiskit_metal.qlibrary.couplers.tunable_coupler_01 import TunableCoupler01
from qiskit_metal.qlibrary.terminations.launchpad_wb import LaunchpadWirebond
from qiskit_metal.qlibrary.terminations.launchpad_wb_coupled import LaunchpadWirebondCoupled
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
from qiskit_metal.qlibrary.qubits.JJ_Dolan import jj_dolan
from qiskit_metal.qlibrary.qubits.JJ_Manhattan import jj_manhattan
from qiskit_metal.qlibrary.qubits.transmon_concentric import TransmonConcentric
from qiskit_metal.qlibrary.qubits.transmon_cross import TransmonCross
from qiskit_metal.qlibrary.qubits.transmon_cross_fl import TransmonCrossFL
from qiskit_metal.qlibrary.qubits.Transmon_Interdigitated import TransmonInterdigitated
from qiskit_metal.qlibrary.qubits.transmon_pocket import TransmonPocket
from qiskit_metal.qlibrary.qubits.transmon_pocket_cl import TransmonPocketCL
from qiskit_metal.qlibrary.qubits.transmon_pocket_6 import TransmonPocket6
from qiskit_metal.qlibrary.qubits.transmon_pocket_teeth import TransmonPocketTeeth
import shutil, os
def create_image(component, component_name, file_name):
obj = component(design, component_name)
gui.rebuild()
gui.autoscale()
gui.zoom_on_components([component_name])
gui.figure.savefig(file_name, dpi=30)
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image(file_name, **_disp_ops))
design.delete_component(component_name)
shutil.copy(file_name, '../apidocs/')
os.remove(file_name)
###Output
_____no_output_____
###Markdown
sample_shapes
###Code
create_image(CircleCaterpillar, 'circlecaterpillar', 'CircleCaterpillar.png')
create_image(CircleRaster, 'circleraster', 'CircleRaster.png')
create_image(NGon, 'ngon', 'NGon.png')
create_image(NSquareSpiral, 'nsquarespiral', 'NSquareSpiral.png')
create_image(Rectangle, 'rectangle', 'Rectangle.png')
create_image(RectangleHollow, 'rectanglehollow', 'RectangleHollow.png')
###Output
_____no_output_____
###Markdown
lumped
###Code
create_image(Cap3Interdigital, 'cap3interdigital', 'Cap3Interdigital.png')
create_image(CapNInterdigital, 'capninterdigital', 'CapNInterdigital.png')
create_image(ResonatorCoilRect, 'resonatorcoilrect', 'ResonatorCoilRect.png')
###Output
_____no_output_____
###Markdown
couplers
###Code
create_image(CoupledLineTee, 'coupledlintee', 'CoupledLineTee.png')
create_image(LineTee, 'linetee', 'LineTee.png')
create_image(CapNInterdigitalTee, 'capninterdigitaltee', 'CapNInterdigitalTee.png')
create_image(TunableCoupler01, 'tunablecoupler01', 'TunableCoupler01.png')
###Output
_____no_output_____
###Markdown
terminations
###Code
create_image(LaunchpadWirebond, 'launchpadwirebond', 'LaunchpadWirebond.png')
create_image(LaunchpadWirebondCoupled, 'launchpadwirebondcoupled', 'LaunchpadWirebondCoupled.png')
create_image(OpenToGround, 'opentoground', 'OpenToGround.png')
###Output
_____no_output_____
###Markdown
qubits
###Code
create_image(jj_dolan, 'jj_dolan', 'JJDolan.png')
create_image(jj_manhattan, 'jj_manhattan', 'JJManhattan.png')
create_image(TransmonConcentric, 'transmonconcentric', 'TransmonConcentric.png')
create_image(TransmonCross, 'transmoncross', 'TransmonCross.png')
create_image(TransmonCrossFL, 'transmoncrossfl', 'TransmonCrossFL.png')
create_image(TransmonInterdigitated, 'transmoninterdigitated', 'TransmonInterdigitated.png')
create_image(TransmonPocket, 'transmonpocket', 'TransmonPocket.png')
create_image(TransmonPocketCL, 'transmonpocketcl', 'TransmonPocketCL.png')
create_image(TransmonPocket6, 'transmonpocket6', 'TransmonPocket6.png')
create_image(TransmonPocketTeeth, 'transmonpocketteeth', 'Transmon_Pocket_Teeth.png')
###Output
_____no_output_____
###Markdown
Closing the Qiskit Metal GUI
###Code
gui.main_window.close()
###Output
_____no_output_____
###Markdown
Generate qlibrary component images By running this notebook the qlibrary component image files will be update. After the docs build is rerun the images in the docs will be updated automatically. Start gui
###Code
%load_ext autoreload
%autoreload 2
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict
design = designs.DesignPlanar()
gui = MetalGUI(design)
###Output
_____no_output_____
###Markdown
Qlibrary component imports and define worker function
###Code
from qiskit_metal.qlibrary.sample_shapes.circle_caterpillar import CircleCaterpillar
from qiskit_metal.qlibrary.sample_shapes.circle_raster import CircleRaster
from qiskit_metal.qlibrary.sample_shapes.n_gon import NGon
from qiskit_metal.qlibrary.sample_shapes.n_square_spiral import NSquareSpiral
from qiskit_metal.qlibrary.sample_shapes.rectangle import Rectangle
from qiskit_metal.qlibrary.sample_shapes.rectangle_hollow import RectangleHollow
from qiskit_metal.qlibrary.lumped.cap_3_interdigital import Cap3Interdigital
from qiskit_metal.qlibrary.lumped.cap_n_interdigital import CapNInterdigital
from qiskit_metal.qlibrary.lumped.resonator_coil_rect import ResonatorCoilRect
from qiskit_metal.qlibrary.couplers.coupled_line_tee import CoupledLineTee
from qiskit_metal.qlibrary.couplers.line_tee import LineTee
from qiskit_metal.qlibrary.couplers.cap_n_interdigital_tee import CapNInterdigitalTee
from qiskit_metal.qlibrary.couplers.tunable_coupler_01 import TunableCoupler01
from qiskit_metal.qlibrary.terminations.launchpad_wb import LaunchpadWirebond
from qiskit_metal.qlibrary.terminations.launchpad_wb_coupled import LaunchpadWirebondCoupled
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
from qiskit_metal.qlibrary.qubits.JJ_Dolan import jj_dolan
from qiskit_metal.qlibrary.qubits.JJ_Manhattan import jj_manhattan
from qiskit_metal.qlibrary.qubits.transmon_concentric import TransmonConcentric
from qiskit_metal.qlibrary.qubits.transmon_cross import TransmonCross
from qiskit_metal.qlibrary.qubits.transmon_cross_fl import TransmonCrossFL
from qiskit_metal.qlibrary.qubits.Transmon_Interdigitated import TransmonInterdigitated
from qiskit_metal.qlibrary.qubits.transmon_pocket import TransmonPocket
from qiskit_metal.qlibrary.qubits.transmon_pocket_cl import TransmonPocketCL
from qiskit_metal.qlibrary.qubits.transmon_pocket_6 import TransmonPocket6
from qiskit_metal.qlibrary.qubits.transmon_pocket_teeth import TransmonPocketTeeth
from qiskit_metal.qlibrary.qubits.SQUID_loop import SQUID_LOOP
import shutil, os
def create_image(component, component_name, file_name):
obj = component(design, component_name)
gui.rebuild()
gui.autoscale()
gui.zoom_on_components([component_name])
gui.figure.savefig(file_name, dpi=30)
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image(file_name, **_disp_ops))
design.delete_component(component_name)
shutil.copy(file_name, '../apidocs/')
os.remove(file_name)
###Output
_____no_output_____
###Markdown
sample_shapes
###Code
create_image(CircleCaterpillar, 'circlecaterpillar', 'CircleCaterpillar.png')
create_image(CircleRaster, 'circleraster', 'CircleRaster.png')
create_image(NGon, 'ngon', 'NGon.png')
create_image(NSquareSpiral, 'nsquarespiral', 'NSquareSpiral.png')
create_image(Rectangle, 'rectangle', 'Rectangle.png')
create_image(RectangleHollow, 'rectanglehollow', 'RectangleHollow.png')
###Output
_____no_output_____
###Markdown
lumped
###Code
create_image(Cap3Interdigital, 'cap3interdigital', 'Cap3Interdigital.png')
create_image(CapNInterdigital, 'capninterdigital', 'CapNInterdigital.png')
create_image(ResonatorCoilRect, 'resonatorcoilrect', 'ResonatorCoilRect.png')
###Output
_____no_output_____
###Markdown
couplers
###Code
create_image(CoupledLineTee, 'coupledlintee', 'CoupledLineTee.png')
create_image(LineTee, 'linetee', 'LineTee.png')
create_image(CapNInterdigitalTee, 'capninterdigitaltee', 'CapNInterdigitalTee.png')
create_image(TunableCoupler01, 'tunablecoupler01', 'TunableCoupler01.png')
###Output
_____no_output_____
###Markdown
terminations
###Code
create_image(LaunchpadWirebond, 'launchpadwirebond', 'LaunchpadWirebond.png')
create_image(LaunchpadWirebondCoupled, 'launchpadwirebondcoupled', 'LaunchpadWirebondCoupled.png')
create_image(OpenToGround, 'opentoground', 'OpenToGround.png')
###Output
_____no_output_____
###Markdown
qubits
###Code
create_image(jj_dolan, 'jj_dolan', 'JJDolan.png')
create_image(jj_manhattan, 'jj_manhattan', 'JJManhattan.png')
create_image(TransmonConcentric, 'transmonconcentric', 'TransmonConcentric.png')
create_image(TransmonCross, 'transmoncross', 'TransmonCross.png')
create_image(TransmonCrossFL, 'transmoncrossfl', 'TransmonCrossFL.png')
create_image(TransmonInterdigitated, 'transmoninterdigitated', 'TransmonInterdigitated.png')
create_image(TransmonPocket, 'transmonpocket', 'TransmonPocket.png')
create_image(TransmonPocketCL, 'transmonpocketcl', 'TransmonPocketCL.png')
create_image(TransmonPocket6, 'transmonpocket6', 'TransmonPocket6.png')
create_image(TransmonPocketTeeth, 'transmonpocketteeth', 'Transmon_Pocket_Teeth.png')
create_image(SQUID_LOOP, 'squidloop', 'SQUID_LOOP.png')
###Output
_____no_output_____
###Markdown
Closing the Qiskit Metal GUI
###Code
gui.main_window.close()
###Output
_____no_output_____
###Markdown
Generate qlibrary component images By running this notebook the qlibrary component image files will be update. After the docs build is rerun the images in the docs will be updated automatically. Start gui
###Code
%load_ext autoreload
%autoreload 2
import qiskit_metal as metal
from qiskit_metal import designs, draw
from qiskit_metal import MetalGUI, Dict
design = designs.DesignPlanar()
gui = MetalGUI(design)
###Output
_____no_output_____
###Markdown
Qlibrary component imports and define worker function
###Code
from qiskit_metal.qlibrary.sample_shapes.circle_caterpillar import CircleCaterpillar
from qiskit_metal.qlibrary.sample_shapes.circle_raster import CircleRaster
from qiskit_metal.qlibrary.sample_shapes.n_gon import NGon
from qiskit_metal.qlibrary.sample_shapes.n_square_spiral import NSquareSpiral
from qiskit_metal.qlibrary.sample_shapes.rectangle import Rectangle
from qiskit_metal.qlibrary.sample_shapes.rectangle_hollow import RectangleHollow
from qiskit_metal.qlibrary.lumped.cap_3_interdigital import Cap3Interdigital
from qiskit_metal.qlibrary.lumped.cap_n_interdigital import CapNInterdigital
from qiskit_metal.qlibrary.lumped.resonator_coil_rect import ResonatorCoilRect
from qiskit_metal.qlibrary.couplers.coupled_line_tee import CoupledLineTee
from qiskit_metal.qlibrary.couplers.line_tee import LineTee
from qiskit_metal.qlibrary.couplers.cap_n_interdigital_tee import CapNInterdigitalTee
from qiskit_metal.qlibrary.couplers.tunable_coupler_01 import TunableCoupler01
from qiskit_metal.qlibrary.terminations.launchpad_wb import LaunchpadWirebond
from qiskit_metal.qlibrary.terminations.launchpad_wb_coupled import LaunchpadWirebondCoupled
from qiskit_metal.qlibrary.terminations.open_to_ground import OpenToGround
from qiskit_metal.qlibrary.qubits.JJ_Dolan import jj_dolan
from qiskit_metal.qlibrary.qubits.JJ_Manhattan import jj_manhattan
from qiskit_metal.qlibrary.qubits.transmon_concentric import TransmonConcentric
from qiskit_metal.qlibrary.qubits.transmon_cross import TransmonCross
from qiskit_metal.qlibrary.qubits.transmon_cross_fl import TransmonCrossFL
from qiskit_metal.qlibrary.qubits.Transmon_Interdigitated import TransmonInterdigitated
from qiskit_metal.qlibrary.qubits.transmon_pocket import TransmonPocket
from qiskit_metal.qlibrary.qubits.transmon_pocket_cl import TransmonPocketCL
from qiskit_metal.qlibrary.qubits.transmon_pocket_6 import TransmonPocket6
import shutil, os
def create_image(component, component_name, file_name):
obj = component(design, component_name)
gui.rebuild()
gui.autoscale()
gui.zoom_on_components([component_name])
gui.figure.savefig(file_name, dpi=30)
from IPython.display import Image, display
_disp_ops = dict(width=500)
display(Image(file_name, **_disp_ops))
design.delete_component(component_name)
shutil.copy(file_name, '../apidocs/')
os.remove(file_name)
###Output
_____no_output_____
###Markdown
sample_shapes
###Code
create_image(CircleCaterpillar, 'circlecaterpillar', 'CircleCaterpillar.png')
create_image(CircleRaster, 'circleraster', 'CircleRaster.png')
create_image(NGon, 'ngon', 'NGon.png')
create_image(NSquareSpiral, 'nsquarespiral', 'NSquareSpiral.png')
create_image(Rectangle, 'rectangle', 'Rectangle.png')
create_image(RectangleHollow, 'rectanglehollow', 'RectangleHollow.png')
###Output
_____no_output_____
###Markdown
lumped
###Code
create_image(Cap3Interdigital, 'cap3interdigital', 'Cap3Interdigital.png')
create_image(CapNInterdigital, 'capninterdigital', 'CapNInterdigital.png')
create_image(ResonatorCoilRect, 'resonatorcoilrect', 'ResonatorCoilRect.png')
###Output
_____no_output_____
###Markdown
couplers
###Code
create_image(CoupledLineTee, 'coupledlintee', 'CoupledLineTee.png')
create_image(LineTee, 'linetee', 'LineTee.png')
create_image(CapNInterdigitalTee, 'capninterdigitaltee', 'CapNInterdigitalTee.png')
create_image(TunableCoupler01, 'tunablecoupler01', 'TunableCoupler01.png')
###Output
_____no_output_____
###Markdown
terminations
###Code
create_image(LaunchpadWirebond, 'launchpadwirebond', 'LaunchpadWirebond.png')
create_image(LaunchpadWirebondCoupled, 'launchpadwirebondcoupled', 'LaunchpadWirebondCoupled.png')
create_image(OpenToGround, 'opentoground', 'OpenToGround.png')
###Output
_____no_output_____
###Markdown
qubits
###Code
create_image(jj_dolan, 'jj_dolan', 'JJDolan.png')
create_image(jj_manhattan, 'jj_manhattan', 'JJManhattan.png')
create_image(TransmonConcentric, 'transmonconcentric', 'TransmonConcentric.png')
create_image(TransmonCross, 'transmoncross', 'TransmonCross.png')
create_image(TransmonCrossFL, 'transmoncrossfl', 'TransmonCrossFL.png')
create_image(TransmonInterdigitated, 'transmoninterdigitated', 'TransmonInterdigitated.png')
create_image(TransmonPocket, 'transmonpocket', 'TransmonPocket.png')
create_image(TransmonPocketCL, 'transmonpocketcl', 'TransmonPocketCL.png')
create_image(TransmonPocket6, 'transmonpocket6', 'TransmonPocket6.png')
###Output
_____no_output_____
###Markdown
Closing the Qiskit Metal GUI
###Code
gui.main_window.close()
###Output
_____no_output_____ |
module2-random-forests/Copy_of_LS_DS17_222_assignment.ipynb | ###Markdown
Lambda School Data Science*Unit 2, Sprint 2, Module 2*--- Random Forests Assignment- [ ] Read [“Adopting a Hypothesis-Driven Workflow”](http://archive.is/Nu3EI), a blog post by a Lambda DS student about the Tanzania Waterpumps challenge.- [ ] Continue to participate in our Kaggle challenge.- [ ] Define a function to wrangle train, validate, and test sets in the same way. Clean outliers and engineer features.- [ ] Try Ordinal Encoding.- [ ] Try a Random Forest Classifier.- [ ] Submit your predictions to our Kaggle competition. (Go to our Kaggle InClass competition webpage. Use the blue **Submit Predictions** button to upload your CSV file. Or you can use the Kaggle API to submit your predictions.)- [ ] Commit your notebook to your fork of the GitHub repo. Stretch Goals Doing- [ ] Add your own stretch goal(s) !- [ ] Do more exploratory data analysis, data cleaning, feature engineering, and feature selection.- [ ] Try other [categorical encodings](https://contrib.scikit-learn.org/category_encoders/).- [ ] Get and plot your feature importances.- [ ] Make visualizations and share on Slack. ReadingTop recommendations in _**bold italic:**_ Decision Trees- A Visual Introduction to Machine Learning, [Part 1: A Decision Tree](http://www.r2d3.us/visual-intro-to-machine-learning-part-1/), and _**[Part 2: Bias and Variance](http://www.r2d3.us/visual-intro-to-machine-learning-part-2/)**_- [Decision Trees: Advantages & Disadvantages](https://christophm.github.io/interpretable-ml-book/tree.htmladvantages-2)- [How a Russian mathematician constructed a decision tree — by hand — to solve a medical problem](http://fastml.com/how-a-russian-mathematician-constructed-a-decision-tree-by-hand-to-solve-a-medical-problem/)- [How decision trees work](https://brohrer.github.io/how_decision_trees_work.html)- [Let’s Write a Decision Tree Classifier from Scratch](https://www.youtube.com/watch?v=LDRbO9a6XPU) Random Forests- [_An Introduction to Statistical Learning_](http://www-bcf.usc.edu/~gareth/ISL/), Chapter 8: Tree-Based Methods- [Coloring with Random Forests](http://structuringtheunstructured.blogspot.com/2017/11/coloring-with-random-forests.html)- _**[Random Forests for Complete Beginners: The definitive guide to Random Forests and Decision Trees](https://victorzhou.com/blog/intro-to-random-forests/)**_ Categorical encoding for trees- [Are categorical variables getting lost in your random forests?](https://roamanalytics.com/2016/10/28/are-categorical-variables-getting-lost-in-your-random-forests/)- [Beyond One-Hot: An Exploration of Categorical Variables](http://www.willmcginnis.com/2015/11/29/beyond-one-hot-an-exploration-of-categorical-variables/)- _**[Categorical Features and Encoding in Decision Trees](https://medium.com/data-design/visiting-categorical-features-and-encoding-in-decision-trees-53400fa65931)**_- _**[Coursera — How to Win a Data Science Competition: Learn from Top Kagglers — Concept of mean encoding](https://www.coursera.org/lecture/competitive-data-science/concept-of-mean-encoding-b5Gxv)**_- [Mean (likelihood) encodings: a comprehensive study](https://www.kaggle.com/vprokopev/mean-likelihood-encodings-a-comprehensive-study)- [The Mechanics of Machine Learning, Chapter 6: Categorically Speaking](https://mlbook.explained.ai/catvars.html) Imposter Syndrome- [Effort Shock and Reward Shock (How The Karate Kid Ruined The Modern World)](http://www.tempobook.com/2014/07/09/effort-shock-and-reward-shock/)- [How to manage impostor syndrome in data science](https://towardsdatascience.com/how-to-manage-impostor-syndrome-in-data-science-ad814809f068)- ["I am not a real data scientist"](https://brohrer.github.io/imposter_syndrome.html)- _**[Imposter Syndrome in Data Science](https://caitlinhudon.com/2018/01/19/imposter-syndrome-in-data-science/)**_ More Categorical Encodings**1.** The article **[Categorical Features and Encoding in Decision Trees](https://medium.com/data-design/visiting-categorical-features-and-encoding-in-decision-trees-53400fa65931)** mentions 4 encodings:- **"Categorical Encoding":** This means using the raw categorical values as-is, not encoded. Scikit-learn doesn't support this, but some tree algorithm implementations do. For example, [Catboost](https://catboost.ai/), or R's [rpart](https://cran.r-project.org/web/packages/rpart/index.html) package.- **Numeric Encoding:** Synonymous with Label Encoding, or "Ordinal" Encoding with random order. We can use [category_encoders.OrdinalEncoder](https://contrib.scikit-learn.org/category_encoders/ordinal.html).- **One-Hot Encoding:** We can use [category_encoders.OneHotEncoder](https://contrib.scikit-learn.org/category_encoders/onehot.html).- **Binary Encoding:** We can use [category_encoders.BinaryEncoder](https://contrib.scikit-learn.org/category_encoders/binary.html).**2.** The short video **[Coursera — How to Win a Data Science Competition: Learn from Top Kagglers — Concept of mean encoding](https://www.coursera.org/lecture/competitive-data-science/concept-of-mean-encoding-b5Gxv)** introduces an interesting idea: use both X _and_ y to encode categoricals.Category Encoders has multiple implementations of this general concept:- [CatBoost Encoder](https://contrib.scikit-learn.org/category_encoders/catboost.html)- [Generalized Linear Mixed Model Encoder](https://contrib.scikit-learn.org/category_encoders/glmm.html)- [James-Stein Encoder](https://contrib.scikit-learn.org/category_encoders/jamesstein.html)- [Leave One Out](https://contrib.scikit-learn.org/category_encoders/leaveoneout.html)- [M-estimate](https://contrib.scikit-learn.org/category_encoders/mestimate.html)- [Target Encoder](https://contrib.scikit-learn.org/category_encoders/targetencoder.html)- [Weight of Evidence](https://contrib.scikit-learn.org/category_encoders/woe.html)Category Encoder's mean encoding implementations work for regression problems or binary classification problems. For multi-class classification problems, you will need to temporarily reformulate it as binary classification. For example:```pythonencoder = ce.TargetEncoder(min_samples_leaf=..., smoothing=...) Both parameters > 1 to avoid overfittingX_train_encoded = encoder.fit_transform(X_train, y_train=='functional')X_val_encoded = encoder.transform(X_train, y_val=='functional')```For this reason, mean encoding won't work well within pipelines for multi-class classification problems.**3.** The **[dirty_cat](https://dirty-cat.github.io/stable/)** library has a Target Encoder implementation that works with multi-class classification.```python dirty_cat.TargetEncoder(clf_type='multiclass-clf')```It also implements an interesting idea called ["Similarity Encoder" for dirty categories](https://www.slideshare.net/GaelVaroquaux/machine-learning-on-non-curated-data-154905090).However, it seems like dirty_cat doesn't handle missing values or unknown categories as well as category_encoders does. And you may need to use it with one column at a time, instead of with your whole dataframe.**4. [Embeddings](https://www.kaggle.com/colinmorris/embedding-layers)** can work well with sparse / high cardinality categoricals._**I hope it’s not too frustrating or confusing that there’s not one “canonical” way to encode categoricals. It’s an active area of research and experimentation — maybe you can make your own contributions!**_ SetupYou can work locally (follow the [local setup instructions](https://lambdaschool.github.io/ds/unit2/local/)) or on Colab (run the code cell below).
###Code
%%capture
import sys
# If you're on Colab:
if 'google.colab' in sys.modules:
DATA_PATH = 'https://raw.githubusercontent.com/LambdaSchool/DS-Unit-2-Kaggle-Challenge/master/data/'
!pip install category_encoders==2.*
# If you're working locally:
else:
DATA_PATH = '../data/'
import pandas as pd
from sklearn.model_selection import train_test_split
train = pd.merge(pd.read_csv(DATA_PATH+'waterpumps/train_features.csv'),
pd.read_csv(DATA_PATH+'waterpumps/train_labels.csv'))
test = pd.read_csv(DATA_PATH+'waterpumps/test_features.csv')
sample_submission = pd.read_csv(DATA_PATH+'waterpumps/sample_submission.csv')
train.shape, test.shape
# Split train into train & val (already have test)
train, val = train_test_split(train, train_size=0.80, test_size=0.20,
stratify=train['status_group'], random_state=42)
train.shape, val.shape, test.shape
# Defining a function to wrangle train, validate, and test sets
import numpy as np
def wrangle(X):
# Prevent SettingWithCopyWarning
X = X.copy()
# Setting latitude values near zero equal to zero
X['latitude'] = X['latitude'].replace(-2e-08, 0)
# replace zeros with nulls
cols_with_zeros = ['longitude', 'latitude','district_code','amount_tsh',
'population','construction_year','num_private']
for col in cols_with_zeros:
X[col] = X[col].replace(0, np.nan)
# Drop duplicate columns
duplicates = ['quantity_group', 'payment_type', 'waterpoint_type_group',
'extraction_type_group','extraction_type_class','source_type']
X = X.drop(columns=duplicates)
# Drop unusable columns
unusable_variance = ['recorded_by', 'id']
X = X.drop(columns=unusable_variance)
# Convert date_recorded to datetime
X['date_recorded'] = pd.to_datetime(X['date_recorded'], infer_datetime_format=True)
# Extract components from date_recorded, then drop the original column
# This will give us just the year for the next feature
X['year_recorded'] = X['date_recorded'].dt.year
X['month_recorded'] = X['date_recorded'].dt.month
X['day_recorded'] = X['date_recorded'].dt.day
X = X.drop(columns='date_recorded')
# creating columns 'years' and 'years_MISSING'
# to represent years from construction_year to date_recorded
X['years'] = X['year_recorded'] - X['construction_year']
X['years_MISSING'] = X['years'].isnull()
# return the wrangled dataframe
return X
train = wrangle(train)
val = wrangle(val)
test = wrangle(test)
# setting the target
target = 'status_group'
# dropping target
train_features = train.drop(columns=[target])
# list of the numeric features
numeric_features = train_features.select_dtypes(include='number').columns.tolist()
# series with the cardinality of the nonnumeric features
cardinality = train_features.select_dtypes(exclude='number').nunique()
# list of all categorical features with cardinality <= 50
categorical_features = cardinality[cardinality <= 50].index.tolist()
# Combine the lists
features = numeric_features + categorical_features
print(features)
###Output
['amount_tsh', 'gps_height', 'longitude', 'latitude', 'num_private', 'region_code', 'district_code', 'population', 'construction_year', 'year_recorded', 'month_recorded', 'day_recorded', 'years', 'basin', 'region', 'public_meeting', 'scheme_management', 'permit', 'extraction_type', 'management', 'management_group', 'payment', 'water_quality', 'quality_group', 'quantity', 'source', 'source_class', 'waterpoint_type', 'years_MISSING']
###Markdown
Try Ordinal Encoding & Random Forest Classifier.
###Code
# Creating X features matrix and y target for ordinal encoding
# - we will use ALL features, inc. high cardinality categoricals
X_train = train.drop(columns=target)
y_train = train[target]
X_val = val.drop(columns=target)
y_val = val[target]
X_test = test
X_train.shape, y_train.shape, X_val.shape, y_val.shape, X_test.shape
# Pipeline for ordinal encoding with a Random Forest Classifier
import category_encoders as ce
from sklearn.tree import DecisionTreeClassifier
from sklearn.ensemble import RandomForestClassifier
from sklearn.impute import SimpleImputer
from sklearn.pipeline import make_pipeline
pipeline = make_pipeline(
ce.OrdinalEncoder(),
SimpleImputer(strategy='median'),
RandomForestClassifier(random_state=0, n_jobs=-1)
)
# Fit on train, score on val
pipeline.fit(X_train, y_train)
print('Validation Accuracy', pipeline.score(X_val, y_val))
#predict on test
y_pred = pipeline.predict(X_test)
###Output
Validation Accuracy 0.8087542087542088
###Markdown
Submit your predictions to our Kaggle competition.
###Code
submission = sample_submission.copy()
submission['status_group'] = y_pred
submission.to_csv('EvanGrinalds222Prediction.csv', index=False)
###Output
_____no_output_____ |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.