licenses
listlengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 5023 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
import Base: setindex!, getindex
"""
HierarchicalImage{T<:Number} <: AbstractArray{T,2}
Image type which dynamically allocated memory for pixels when their value is set, the value of unset pixels is assumed to be zero.
This is used for the detector image of [`AbstractOpticalSystem`](@ref)s which can typically be very high resolution, but often have a large proportion of the image blank.
"""
struct HierarchicalImage{T<:Number} <: AbstractArray{T,2} #allow for complex values. May want to expand this even further to allow more complicated detector image types
toplevel::Array{Array{T,2},2}
secondlevelrows::Int64
secondlevelcols::Int64
apparentrows::Int64
apparentcols::Int64
function HierarchicalImage{T}(height, width) where {T<:Number}
heightfirst, heightsecond = nearestsqrts(height)
widthfirst, widthsecond = nearestsqrts(width)
return HierarchicalImage{T}(height, width, heightfirst, widthfirst, heightsecond, widthsecond)
end
HierarchicalImage{T}(apparentheight, apparentwidth, height, width, secondlevelheight, secondlevelwidth) where {T<:Number} = new{T}(Array{Array{T,2},2}(undef, height, width), secondlevelheight, secondlevelwidth, apparentheight, apparentwidth)
end
export HierarchicalImage
function nearestsqrts(num)
rt1 = Int64(ceil(sqrt(num)))
rt2 = Int64(floor(sqrt(num)))
if rt1 * rt2 >= num
return (rt1, rt2)
else
return (rt1, rt2 + 1)
end
end
toplevel(a::HierarchicalImage) = a.toplevel
secondlevelrows(a::HierarchicalImage) = a.secondlevelrows
secondlevelcols(a::HierarchicalImage) = a.secondlevelcols
apparentrows(a::HierarchicalImage) = a.apparentrows
apparentcols(a::HierarchicalImage) = a.apparentcols
topindex(a::HierarchicalImage, index1, index2)::Int = linearindex(size(toplevel(a), 1), 1 + (index1 - 1) ÷ secondlevelrows(a), 1 + (index2 - 1) ÷ secondlevelcols(a))
lowerindex(a::HierarchicalImage, index1, index2)::Int = linearindex(secondlevelrows(a), 1 + mod(index1 - 1, secondlevelrows(a)), 1 + mod(index2 - 1, secondlevelcols(a)))
linearindex(rows::Int, index1::Int, index2::Int)::Int = index1 + rows * (index2 - 1)
function outofbounds(a::HierarchicalImage, indices::Tuple{Int,Int})
if any(indices .> size(a))
throw(BoundsError(a, indices))
end
end
Base.eltype(::HierarchicalImage{T}) where {T<:Number} = T
Base.length(a::HierarchicalImage{T}) where {T<:Number} = reduce(*, size(a))
Base.size(a::HierarchicalImage{T}) where {T<:Number} = (apparentrows(a), apparentcols(a))
function Base.getindex(a::HierarchicalImage{T}, indices::Vararg{Int,2}) where {T<:Number}
outofbounds(a, indices)
top = topindex(a, indices[1], indices[2])
bott = lowerindex(a, indices[1], indices[2])
return getindexdirect(a, top, bott)
end
function getindexdirect(a::HierarchicalImage{T}, topidx::Int, botidx::Int) where {T<:Real}
if !isassigned(toplevel(a), topidx) #by default any part of the image that was not written to has the value zero
return zero(T)
else
return toplevel(a)[topidx][botidx]
end
end
function Base.setindex!(a::HierarchicalImage, v::T, indices::Vararg{Int,2}) where {T<:Number} #if use HierarchicalImage{T} get error "setindex! not defined". No idea why this happens
outofbounds(a, indices)
top = topindex(a, indices[1], indices[2])
bott = lowerindex(a, indices[1], indices[2])
setindexdirect!(a, v, top, bott)
end
function setindexdirect!(a::HierarchicalImage{T}, v::T, topidx::Int, botidx::Int) where {T<:Real}
if !isassigned(toplevel(a), LinearIndices(toplevel(a))[topidx]) #by default any part of the image that has not been not written to is set to zero
toplevel(a)[topidx] = fill(zero(T), secondlevelrows(a), secondlevelcols(a))
end
toplevel(a)[topidx][botidx] = v
end
"""
reset!(a::HierarchicalImage{T})
Resets the pixels in the image to `zero(T)`.
Do this rather than `image .= zero(T)` because that will cause every pixel to be accessed, and therefore allocated.
For large images this can cause huge memory traffic.
"""
function reset!(a::HierarchicalImage{T}) where {T}
for index in 1:length(toplevel(a))
if isassigned(toplevel(a), index)
temp = toplevel(a)[index]
for k in 1:length(temp)
temp[k] = zero(T)
end
end
end
end
"""
sum!(a::HierarchicalImage{T}, b::HierarchicalImage{T})
Add the contents of `b` to `a` in an efficient way.
"""
function sum!(a::HierarchicalImage{T}, b::HierarchicalImage{T}) where {T}
@assert size(a) == size(b)
for index in 1:length(toplevel(b))
if isassigned(toplevel(b), index)
temp = toplevel(b)[index]
for k in 1:length(temp)
setindexdirect!(a, getindexdirect(a, index, k) + temp[k], index, k)
end
end
end
end
export sum!, reset!
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 15783 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
LensAssembly{T<:Real}
Structure which contains the elements of the optical system, these can be [`CSGTree`](@ref) or [`Surface`](@ref) objects.
In order to prevent type ambiguities bespoke structs are created for each possible number of elements e.g. `LensAssembly3`.
These are parameterized by the types of the elements to prevent ambiguities.
Basic surface types such as [`Rectangle`](@ref) (which can occur in large numbers) are stored independently in `Vector`s, so type paramters are only needed for CSG objects.
Each struct looks like this:
```julia
struct LensAssemblyN{T,T1,T2,...,TN} <: LensAssembly{T}
axis::SVector{3,T}
rectangles::Vector{Rectangle{T}}
ellipses::Vector{Ellipse{T}}
hexagons::Vector{Hexagon{T}}
paraxials::Vector{ParaxialLens{T}}
E1::T1
E2::T2
...
EN::TN
end
```
Where `Ti <: Union{Surface{T},CSGTree{T}}`.
To create a LensAssembly object the following functions can be used:
```julia
LensAssembly(elements::Vararg{Union{Surface{T},CSGTree{T},LensAssembly{T}}}; axis = SVector(0.0, 0.0, 1.0)) where {T<:Real}
```
"""
abstract type LensAssembly{T<:Real} end
export LensAssembly
Base.show(io::IO, ::Type{<:LensAssembly}) = print(io, "LensAssembly")
Base.show(io::IO, a::LensAssembly{T}) where {T<:Real} = print(io, "LensAssembly{$T}($(length(a.rectangles) + length(a.ellipses) + length(a.hexagons) + length(typed_elements(a))) elements)")
# in order to prevent type ambiguities lens assembly must be paramaterised by its elements, but we want lens assembly to have an arbitrary number of elements
# as such we have to use macros to create lens assembly structs of a given size, and methods to handle them in a type unambiguous way
# we may often end up with lots of simple surfaces (Rectangle, Ellipse) in the assembly, and we can store these more efficiently in Vectors
macro lensassembly_constructor(N)
# generate the type definition for a lens assembly of size N
typesig = [:($(Symbol("T$(i)")) <: Union{Surface{T},CSGTree{T}}) for i in 1:N]
fields = [:($(Symbol("E$(i)"))::$(Symbol("T$(i)"))) for i in 1:N]
name = Symbol("LensAssembly$N")
return esc(quote
struct $(name){T,$(typesig...)} <: LensAssembly{T}
axis::SVector{3,T}
rectangles::Vector{Rectangle{T}}
ellipses::Vector{Ellipse{T}}
hexagons::Vector{Hexagon{T}}
paraxials::Vector{ParaxialLens{T}}
$(fields...)
end
end)
end
# This is the basic idea of what each closestintersection implementation does:
# function closestintersection(ass::LensAssembly{T}, r::AbstractRay{T,N})::Union{Nothing,Intersection{T,N}} where {T<:Real,N}
# αmin = typemax(T)
# closest = nothing
# for element in elements(ass)
# allintscts = surfaceintersection(element, r)
# if !(allintscts isa EmptyInterval)
# intsct = closestintersection(allintscts)
# if intsct !== nothing
# αcurr = α(intsct)
# if αcurr < αmin
# αmin = αcurr
# closest = intsct
# end
# end
# end
# end
# return closest
# end
macro lensassembly_intersection(N)
# generates the _closestintersection() function for a lens assembly of size N, this should never be called directly, instead call closestintersection() in all cases
type = :($(Symbol("LensAssembly$(N)")))
fieldnames = [Symbol("E$(i)") for i in 1:N]
checks = [quote
intvl = surfaceintersection(obj.$(fieldnames[i]), r)
if !(intvl isa EmptyInterval)
intsct = closestintersection(intvl::Union{Interval{T},DisjointUnion{T}})
if intsct !== nothing
αcurr = α(intsct)
if αcurr < αmin
αmin = αcurr
closest = intsct
end
end
end
end for i in 1:N]
return esc(quote
function _closestintersection(obj::$(type){T}, r::AbstractRay{T,N})::Union{Nothing,Intersection{T,N}} where {T<:Real,N}
αmin = typemax(T)
closest = nothing
for rect in obj.rectangles
intvl = surfaceintersection(rect, r)
if !(intvl isa EmptyInterval)
intsct = closestintersection(intvl::Union{Interval{T},DisjointUnion{T}})
if intsct !== nothing
αcurr = α(intsct)
if αcurr < αmin
αmin = αcurr
closest = intsct
end
end
end
end
for ell in obj.ellipses
intvl = surfaceintersection(ell, r)
if !(intvl isa EmptyInterval)
intsct = closestintersection(intvl::Union{Interval{T},DisjointUnion{T}})
if intsct !== nothing
αcurr = α(intsct)
if αcurr < αmin
αmin = αcurr
closest = intsct
end
end
end
end
for hex in obj.hexagons
intvl = surfaceintersection(hex, r)
if !(intvl isa EmptyInterval)
intsct = closestintersection(intvl::Union{Interval{T},DisjointUnion{T}})
if intsct !== nothing
αcurr = α(intsct)
if αcurr < αmin
αmin = αcurr
closest = intsct
end
end
end
end
for par in obj.paraxials
intvl = surfaceintersection(par, r)
if !(intvl isa EmptyInterval)
intsct = closestintersection(intvl::Union{Interval{T},DisjointUnion{T}})
if intsct !== nothing
αcurr = α(intsct)
if αcurr < αmin
αmin = αcurr
closest = intsct
end
end
end
end
$(checks...)
return closest
end
end)
end
macro lensassembly_elements(N)
# generates the _elements() function for a lens assembly of size N, this should never be called directly, instead call elements() in all cases
type = :($(Symbol("LensAssembly$(N)")))
fieldnames = [Symbol("E$(i)") for i in 1:N]
tuple = [:(obj.$(fieldnames[i])) for i in 1:N]
return esc(quote
function _typed_elements(obj::$(type){T}) where {T<:Real}
return tuple($(tuple...))
end
end)
end
# pregenerate a small number at compile time
for N in 1:PREGENERATED_LENS_ASSEMBLY_SIZE
type = :($(Symbol("LensAssembly$(N)")))
if !isdefined(OpticSim, type)
@eval @lensassembly_constructor($N)
@eval @lensassembly_intersection($N)
@eval @lensassembly_elements($N)
end
end
function LensAssembly(elements::Vararg{Union{Surface{T},CSGTree{T},LensAssembly{T}}}; axis::SVector{3,T} = SVector{3,T}(0.0, 0.0, 1.0)) where {T<:Real}
# make the actual object
actual_elements = []
rectangles = Vector{Rectangle{T}}(undef, 0)
ellipses = Vector{Ellipse{T}}(undef, 0)
hexagons = Vector{Hexagon{T}}(undef, 0)
paraxials = Vector{ParaxialLens{T}}(undef, 0)
for e in elements
if e isa Rectangle{T}
push!(rectangles, e)
elseif e isa Ellipse{T}
push!(ellipses, e)
elseif e isa Hexagon{T}
push!(hexagons, e)
elseif e isa ParaxialLens{T}
push!(paraxials, e)
elseif e isa Surface{T} || e isa CSGTree{T}
push!(actual_elements, e)
else
# unpack the lens assembly
rectangles = vcat(rectangles, e.rectangles)
ellipses = vcat(ellipses, e.ellipses)
hexagons = vcat(hexagons, e.hexagons)
for lae in OpticSim.typed_elements(e)
push!(actual_elements, lae)
end
end
end
N = length(actual_elements)
# make the methods for this N
type = :($(Symbol("LensAssembly$(N)")))
if !isdefined(OpticSim, type)
@eval @lensassembly_constructor($N)
@eval @lensassembly_intersection($N)
@eval @lensassembly_elements($N)
end
eltypes = typeof.(actual_elements)
naxis = normalize(axis)
return eval(:($(type){$T,$(eltypes...)}($naxis, $rectangles, $ellipses, $hexagons, $paraxials, $(actual_elements...))))
end
function typed_elements(ass::LensAssembly{T}) where {T<:Real}
# under certain circumstances we run into the world age problem (see https://discourse.julialang.org/t/how-to-bypass-the-world-age-problem/7012)
# to avoid this we need to use invokelatest(), but this is type ambiguous and ruins performance, and in most cases isn't necessary
# so we can use try/catch to only call it when we need (and warn appropriately), retaining performance in almost all cases
# NEVER call _typed_elements directly, always use this method
try
_typed_elements(ass)
catch MethodError
@warn "First time call to LensAssembly of previously unused size, performance will be worse than normal." maxlog = 1
Base.invokelatest(_typed_elements, ass)
end
end
elements(ass::LensAssembly{T}) where {T<:Real} = (ass.rectangles..., ass.ellipses..., ass.hexagons..., ass.paraxials..., typed_elements(ass)...)
export elements
function closestintersection(ass::LensAssembly{T}, r::AbstractRay{T,N})::Union{Nothing,Intersection{T,N}} where {T<:Real,N}
# under certain circumstances we run into the world age problem (see https://discourse.julialang.org/t/how-to-bypass-the-world-age-problem/7012)
# to avoid this we need to use invokelatest(), but this is type ambiguous and ruins performance, and in most cases isn't necessary
# so we can use try/catch to only call it when we need (and warn appropriately), retaining performance in almost all cases
# NEVER call _closestintersection directly, always use this method
try
_closestintersection(ass, r)
catch MethodError
@warn "First time call to LensAssembly of previously unused size, performance will be worse than normal." maxlog = 1
Base.invokelatest(_closestintersection, ass, r)
end
end
function BoundingBox(la::LensAssembly{T}) where {T<:Real}
es = elements(la)
bbox = BoundingBox(es[1])
for b in es[2:end]
if b isa ParametricSurface{T} || b isa CSGTree{T}
bbox = union(bbox, b)
end
end
return bbox
end
axis(a::LensAssembly{T}) where {T<:Real} = a.axis
export axis
#############################################################################
"""
LensTrace{T<:Real,N}
Contains an intersection point and the ray segment leading to it from within an optical trace.
The ray carries the path length, power, wavelength, number of intersections and source number, all of which are accessible directly on this class too.
Has the following accessor methods:
```julia
ray(a::LensTrace{T,N}) -> OpticalRay{T,N}
intersection(a::LensTrace{T,N}) -> Intersection{T,N}
power(a::LensTrace{T,N}) -> T
wavelength(a::LensTrace{T,N}) -> T
pathlength(a::LensTrace{T,N}) -> T
point(a::LensTrace{T,N}) -> SVector{N,T}
uv(a::LensTrace{T,N}) -> SVector{2,T}
sourcenum(a::LensTrace{T,N}) -> Int
nhits(a::LensTrace{T,N}) -> Int
```
"""
struct LensTrace{T<:Real,N}
ray::OpticalRay{T,N}
intersection::Intersection{T,N}
end
export LensTrace
ray(a::LensTrace{T,N}) where {T<:Real,N} = a.ray
intersection(a::LensTrace{T,N}) where {T<:Real,N} = a.intersection
power(a::LensTrace{T,N}) where {T<:Real,N} = power(ray(a))
wavelength(a::LensTrace{T,N}) where {T<:Real,N} = wavelength(ray(a))
pathlength(a::LensTrace{T,N}) where {T<:Real,N} = pathlength(ray(a))
point(a::LensTrace{T,N}) where {T<:Real,N} = point(intersection(a))
uv(a::LensTrace{T,N}) where {T<:Real,N} = uv(intersection(a))
sourcenum(a::LensTrace{T,N}) where {T<:Real,N} = sourcenum(ray(a))
nhits(a::LensTrace{T,N}) where {T<:Real,N} = nhits(ray(a))
export intersection
function Base.print(io::IO, a::LensTrace{T,N}) where {T,N}
println(io, "Ray\n$(ray(a))")
println(io, "Lensintersection\n$(intersection(a))")
end
struct NoPower end
const nopower = NoPower()
# isnopower(::NoPower) = true
# isnopower(::Any) = false
#############################################################################
"""
trace(assembly::LensAssembly{T}, r::OpticalRay{T}, temperature::T = 20.0, pressure::T = 1.0; trackrays = nothing, test = false)
Returns the ray as it exits the assembly in the form of a [`LensTrace`](@ref) object if it hits any element in the assembly, otherwise `nothing`.
Recursive rays are offset by a small amount (`RAY_OFFSET`) to prevent it from immediately reintersecting the same lens element.
`trackrays` can be passed an empty vector to accumulate the `LensTrace` objects at each intersection of `ray` with a surface in the assembly.
"""
function trace(assembly::LensAssembly{T}, r::OpticalRay{T,N}, temperature::T = T(OpticSim.GlassCat.TEMP_REF), pressure::T = T(OpticSim.GlassCat.PRESSURE_REF); trackrays::Union{Nothing,Vector{LensTrace{T,N}}} = nothing, test::Bool = false, recursion::Int = 0)::Union{Nothing,NoPower,LensTrace{T,N}} where {T<:Real,N}
if power(r) < POWER_THRESHOLD || recursion > TRACE_RECURSION_LIMIT
return nopower
end
intsct = closestintersection(assembly, r)
if intsct === nothing
return nothing
else
surfintsct = point(intsct)
nml = normal(intsct)
opticalinterface = interface(intsct)
λ = wavelength(r)
if VERSION < v"1.6.0-DEV"
# TODO REMOVE
temp = @unionsplit OpticalInterface T opticalinterface processintersection(opticalinterface, surfintsct, nml, r, temperature, pressure, test, recursion == 0)
else
temp = processintersection(opticalinterface, surfintsct, nml, r, temperature, pressure, test, recursion == 0)
end
if temp === nothing
return nopower
else
raydirection, raypower, raypathlength = temp
if trackrays !== nothing
# we want the power that is hitting the surface, i.e. power on incident ray, but the path length at this intersection
# i.e. with the path length for this intersection added and power modulated by absorption only
push!(trackrays, LensTrace(OpticalRay(ray(r), raypower, λ, opl = raypathlength, nhits = nhits(r), sourcenum = sourcenum(r), sourcepower = sourcepower(r)), intsct))
end
offsetray = OpticalRay(surfintsct + RAY_OFFSET * raydirection, raydirection, raypower, λ, opl = raypathlength, nhits = nhits(r) + 1, sourcenum = sourcenum(r), sourcepower = sourcepower(r))
res = trace(assembly, offsetray, temperature, pressure, trackrays = trackrays, test = test, recursion = recursion + 1)
if res === nothing
return LensTrace(OpticalRay(surfintsct, raydirection, raypower, λ, opl = raypathlength, nhits = nhits(r), sourcenum = sourcenum(r), sourcepower = sourcepower(r)), intsct)
else
return res
end
end
end
end
# """approximate focal length of simple double convex lens. For plano convex set one of the radii to Inf"""
focallength(index::Float64, rf::Float64, rb::Float64) = 1 / ((index - 1) * (1 / rf - 1 / rb))
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 15230 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
opticinterface(::Type{S}, insidematerial, outsidematerial, reflectance = zero(S), interfacemode = ReflectOrTransmit) where {S<:Real} = FresnelInterface{S}(insidematerial, outsidematerial, reflectance = reflectance, transmission = one(S) - reflectance, interfacemode = interfacemode)
"""
SphericalLens(insidematerial, frontvertex, frontradius, backradius, thickness, semidiameter; lastmaterial = OpticSim.GlassCat.Air, nextmaterial = OpticSim.GlassCat.Air, frontsurfacereflectance = 0.0, backsurfacereflectance = 0.0, frontdecenter = (0, 0), backdecenter = (0, 0), interfacemode = ReflectOrTransmit)
Constructs a simple cylindrical lens with spherical front and back surfaces. The side walls of the lens are absorbing.
"""
function SphericalLens(insidematerial::T, frontvertex::S, frontradius::S, backradius::S, thickness::S, semidiameter::S; lastmaterial::Q = OpticSim.GlassCat.Air, nextmaterial::R = OpticSim.GlassCat.Air, frontsurfacereflectance::S = zero(S), backsurfacereflectance::S = zero(S), frontdecenter::Tuple{S,S} = (zero(S), zero(S)), backdecenter::Tuple{S,S} = (zero(S), zero(S)), interfacemode = ReflectOrTransmit) where {R<:OpticSim.GlassCat.AbstractGlass,Q<:OpticSim.GlassCat.AbstractGlass,T<:OpticSim.GlassCat.AbstractGlass,S<:Real}
@assert !isnan(frontradius)
@assert !isnan(backradius)
return ConicLens(insidematerial, frontvertex, frontradius, zero(S), backradius, zero(S), thickness, semidiameter, lastmaterial = lastmaterial, nextmaterial = nextmaterial, frontsurfacereflectance = frontsurfacereflectance, backsurfacereflectance = backsurfacereflectance, frontdecenter = frontdecenter, backdecenter = backdecenter, interfacemode = interfacemode)
end
export SphericalLens
"""
ConicLens(insidematerial, frontvertex, frontradius, frontconic, backradius, backconic, thickness, semidiameter; lastmaterial = OpticSim.GlassCat.Air, nextmaterial = OpticSim.GlassCat.Air, frontsurfacereflectance = 0.0, backsurfacereflectance = 0.0, frontdecenter = (0, 0), backdecenter = (0, 0), interfacemode = ReflectOrTransmit)
Constructs a simple cylindrical lens with front and back surfaces with a radius and conic term. The side walls of the lens are absorbing.
"""
function ConicLens(insidematerial::T, frontvertex::S, frontradius::S, frontconic::S, backradius::S, backconic::S, thickness::S, semidiameter::S; lastmaterial::Q = OpticSim.GlassCat.Air, nextmaterial::R = OpticSim.GlassCat.Air, frontsurfacereflectance::S = zero(S), backsurfacereflectance::S = zero(S), nsamples::Int = 17, frontdecenter::Tuple{S,S} = (zero(S), zero(S)), backdecenter::Tuple{S,S} = (zero(S), zero(S)), interfacemode = ReflectOrTransmit) where {R<:OpticSim.GlassCat.AbstractGlass,Q<:OpticSim.GlassCat.AbstractGlass,T<:OpticSim.GlassCat.AbstractGlass,S<:Real}
@assert !isnan(frontradius)
@assert !isnan(frontconic)
return AsphericLens(insidematerial, frontvertex, frontradius, frontconic, nothing, backradius, backconic, nothing, thickness, semidiameter, lastmaterial = lastmaterial, nextmaterial = nextmaterial, frontsurfacereflectance = frontsurfacereflectance, backsurfacereflectance = backsurfacereflectance, nsamples = nsamples, frontdecenter = frontdecenter, backdecenter = backdecenter, interfacemode = interfacemode)
end
export ConicLens
"""
AsphericLens(insidematerial, frontvertex, frontradius, frontconic, frontaspherics, backradius, backconic, backaspherics, thickness, semidiameter; lastmaterial = OpticSim.GlassCat.Air, nextmaterial = OpticSim.GlassCat.Air, frontsurfacereflectance = 0.0, backsurfacereflectance = 0.0, frontdecenter = (0, 0), backdecenter = (0, 0), interfacemode = ReflectOrTransmit)
Cosntructs a simple cylindrical lens with front and back surfaces with a radius, conic and apsheric terms. The side walls of the lens are absorbing.
"""
function AsphericLens(insidematerial::T, frontvertex::S, frontradius::S, frontconic::S, frontaspherics::Union{Nothing,Vector{Pair{Int,S}}}, backradius::S, backconic::S, backaspherics::Union{Nothing,Vector{Pair{Int,S}}}, thickness::S, semidiameter::S; lastmaterial::Q = OpticSim.GlassCat.Air, nextmaterial::R = OpticSim.GlassCat.Air, frontsurfacereflectance::S = zero(S), backsurfacereflectance::S = zero(S), nsamples::Int = 17, frontdecenter::Tuple{S,S} = (zero(S), zero(S)), backdecenter::Tuple{S,S} = (zero(S), zero(S)), interfacemode = ReflectOrTransmit) where {R<:OpticSim.GlassCat.AbstractGlass,Q<:OpticSim.GlassCat.AbstractGlass,T<:OpticSim.GlassCat.AbstractGlass,S<:Real}
@assert semidiameter > zero(S)
@assert !isnan(frontradius)
frontdecenter_l = abs(frontdecenter[1]) > eps(S) && abs(frontdecenter[2]) > eps(S) ? sqrt(frontdecenter[1]^2 + frontdecenter[2]^2) : zero(S)
backdecenter_l = abs(backdecenter[1]) > eps(S) && abs(backdecenter[2]) > eps(S) ? sqrt(backdecenter[1]^2 + backdecenter[2]^2) : zero(S)
if isinf(frontradius) && (frontaspherics === nothing)
#planar
lens_front = leaf(Plane(SVector{3,S}(0, 0, 1), SVector{3,S}(0, 0, frontvertex), vishalfsizeu = semidiameter, vishalfsizev = semidiameter, interface = interface = opticinterface(S, insidematerial, lastmaterial, frontsurfacereflectance, interfacemode)))
elseif frontconic == zero(S) && (frontaspherics === nothing)
#spherical
if frontradius < zero(S)
ϕmax = NaNsafeasin(min(abs((min(semidiameter, abs(frontradius)) + frontdecenter_l) / frontradius), one(S))) + S(π / 50)
lens_front = leaf(SphericalCap(abs(frontradius), ϕmax, SVector{3,S}(0, 0, 1), SVector{3,S}(frontdecenter[1], frontdecenter[2], frontvertex), interface = opticinterface(S, insidematerial, lastmaterial, frontsurfacereflectance, interfacemode)))
else
offset = translation(S, frontdecenter[1], frontdecenter[2], frontvertex - frontradius)
surf = Sphere(abs(frontradius), interface = opticinterface(S, insidematerial, lastmaterial, frontsurfacereflectance, interfacemode))
lens_front = leaf(surf, offset)
end
if abs(frontradius) - frontdecenter_l <= semidiameter
# if optical surface is smaller than semidiameter then use a plane to fill the gap
plane = leaf(Plane(SVector{3,S}(0, 0, 1), SVector{3,S}(0, 0, frontvertex - frontradius), vishalfsizeu = semidiameter, vishalfsizev = semidiameter, interface = interface = opticinterface(S, insidematerial, lastmaterial, frontsurfacereflectance, interfacemode)))
if frontradius > zero(S)
lens_front = lens_front ∪ plane
else
lens_front = lens_front ∩ plane
end
end
else
#conic or aspheric
if frontaspherics !== nothing
frontaspherics = [(i, -k) for (i, k) in frontaspherics]
end
surf = AcceleratedParametricSurface(AsphericSurface(semidiameter + frontdecenter_l + S(0.01), radius = -frontradius, conic = frontconic, aspherics = frontaspherics), nsamples, interface = opticinterface(S, insidematerial, lastmaterial, frontsurfacereflectance, interfacemode))
lens_front = leaf(surf, translation(S, frontdecenter[1], frontdecenter[2], frontvertex))
end
if isinf(backradius) && (backaspherics === nothing)
#planar
lens_rear = leaf(Plane(SVector{3,S}(0, 0, -1), SVector{3,S}(0, 0, frontvertex - thickness), vishalfsizeu = semidiameter, vishalfsizev = semidiameter, interface = interface = opticinterface(S, insidematerial, nextmaterial, backsurfacereflectance, interfacemode)))
elseif backconic == zero(S) && (backaspherics === nothing)
#spherical
if backradius < zero(S)
offset = translation(S, backdecenter[1], backdecenter[2], (frontvertex - thickness) - backradius)
surf = Sphere(abs(backradius), interface = opticinterface(S, insidematerial, nextmaterial, backsurfacereflectance, interfacemode))
lens_rear = leaf(surf, offset)
if backradius == semidiameter
# in this case we need to clip the sphere
plane = leaf(Plane(SVector{3,S}(0, 0, 1), SVector{3,S}(0, 0, frontvertex - thickness + backradius)))
lens_rear = lens_rear ∩ plane
end
else
ϕmax = NaNsafeasin(min(abs((min(semidiameter, abs(backradius)) + backdecenter_l) / backradius), one(S))) + S(π / 50)
lens_rear = leaf(SphericalCap(abs(backradius), ϕmax, SVector{3,S}(0, 0, -1), SVector{3,S}(backdecenter[1], backdecenter[2], frontvertex - thickness), interface = opticinterface(S, insidematerial, nextmaterial, backsurfacereflectance, interfacemode)))
end
if abs(backradius) - backdecenter_l <= semidiameter
plane = leaf(Plane(SVector{3,S}(0, 0, -1), SVector{3,S}(0, 0, frontvertex - thickness - backradius), vishalfsizeu = semidiameter, vishalfsizev = semidiameter, interface = interface = opticinterface(S, insidematerial, lastmaterial, backsurfacereflectance, interfacemode)))
if backradius < zero(S)
lens_rear = lens_rear ∪ plane
else
lens_rear = lens_rear ∩ plane
end
end
else
#conic or aspheric
if backaspherics !== nothing
backaspherics = Tuple{Int,S}.(backaspherics)
end
surf = AcceleratedParametricSurface(AsphericSurface(semidiameter + backdecenter_l + S(0.01), radius = backradius, conic = backconic, aspherics = backaspherics), nsamples, interface = opticinterface(S, insidematerial, nextmaterial, backsurfacereflectance, interfacemode))
lens_rear = leaf(surf, Transform(zero(S), S(π), zero(S), backdecenter[1], backdecenter[2], frontvertex - thickness))
end
extra_front = frontradius >= zero(S) || isinf(frontradius) ? zero(S) : abs(frontradius) - sqrt(frontradius^2 - semidiameter^2)
extra_back = backradius >= zero(S) || isinf(backradius) ? zero(S) : abs(backradius) - sqrt(backradius^2 - semidiameter^2)
barrel_center = ((frontvertex + extra_front) + (frontvertex - thickness - extra_back)) / 2
lens_barrel = leaf(Cylinder(semidiameter, (thickness + extra_back + extra_front) * 2, interface = FresnelInterface{S}(insidematerial, OpticSim.GlassCat.Air, reflectance = zero(S), transmission = zero(S))), translation(S, zero(S), zero(S), barrel_center))
lens_csg = lens_front ∩ lens_rear ∩ lens_barrel
return lens_csg
end
export AsphericLens
"""
FresnelLens(insidematerial, frontvertex, radius, thickness, semidiameter, groovedepth; conic = 0.0, aspherics = nothing, outsidematerial = OpticSim.GlassCat.Air)
Create a Fresnel lens as a CSG object, can be concave or convex. Groove positions are found iteratively based on `groovedepth`. For negative radii the vertex on the central surface is at `frontvertex`, so the total thickness of the lens is `thickness` + `groovedepth`.
**Aspherics currently not supported**.
"""
function FresnelLens(insidematerial::G, frontvertex::T, radius::T, thickness::T, semidiameter::T, groovedepth::T; conic::T = 0.0, aspherics::Union{Nothing,Vector{Pair{Int,T}}} = nothing, outsidematerial::H = OpticSim.GlassCat.Air, reverse::Bool = false) where {T<:Real,G<:OpticSim.GlassCat.AbstractGlass,H<:OpticSim.GlassCat.AbstractGlass}
@assert abs(radius) > semidiameter
interface = FresnelInterface{T}(insidematerial, outsidematerial)
if aspherics !== nothing
aspherics = [(i, -k) for (i, k) in aspherics]
end
# make the fresnel
if conic == zero(T)
if radius == zero(T) || isinf(radius)
@error "Invalid radius"
end
# spherical lens which makes things much easier, we can just caluclate groove positions directly
n = 1
if radius > zero(T)
sphere = Sphere(radius, interface = interface)
fresnel = leaf(sphere, translation(T, zero(T), zero(T), frontvertex - radius))
else
ϕmax = NaNsafeasin(abs(semidiameter / radius)) + T(π / 50)
sphere = SphericalCap(abs(radius), ϕmax, interface = interface)
fresnel = leaf(sphere, translation(T, zero(T), zero(T), frontvertex))
end
while true
offset = n * groovedepth
cylrad = sqrt(offset * (2 * abs(radius) - offset))
if cylrad >= semidiameter
break
end
if radius > zero(T)
cylinder = leaf(Cylinder(cylrad, groovedepth * 1.5, interface = interface), translation(T, zero(T), zero(T), frontvertex - groovedepth / 2))
newsurf = leaf(sphere, translation(T, zero(T), zero(T), frontvertex - radius + offset)) - cylinder
fresnel = newsurf ∪ fresnel
else
cylinder = leaf(Cylinder(cylrad, groovedepth * 1.5, interface = interface), translation(T, zero(T), zero(T), frontvertex + groovedepth / 2))
newsurf = leaf(sphere, translation(T, zero(T), zero(T), frontvertex - offset)) ∪ cylinder
fresnel = newsurf ∩ fresnel
end
n += 1
end
elseif (aspherics === nothing)
if radius == zero(T) || isinf(radius)
@error "Invalid radius"
end
n = 1
surface = AcceleratedParametricSurface(ZernikeSurface(semidiameter + T(0.01), radius = -radius, conic = conic), interface = interface)
fresnel = leaf(surface, translation(T, zero(T), zero(T), frontvertex))
while true
offset = n * groovedepth
if radius < zero(T)
offset *= -1
end
cylrad = sqrt(2 * radius * offset - (conic + one(T)) * offset^2)
if cylrad >= semidiameter
break
end
if radius > zero(T)
cylinder = leaf(Cylinder(cylrad, groovedepth * 1.5, interface = interface), translation(T, zero(T), zero(T), frontvertex - groovedepth / 2))
newsurf = leaf(surface, translation(T, zero(T), zero(T), frontvertex + offset)) - cylinder
fresnel = newsurf ∪ fresnel
else
cylinder = leaf(Cylinder(cylrad, groovedepth * 1.5, interface = interface), translation(T, zero(T), zero(T), frontvertex + groovedepth / 2))
newsurf = leaf(surface, translation(T, zero(T), zero(T), frontvertex + offset)) ∪ cylinder
fresnel = newsurf ∩ fresnel
end
n += 1
end
else
# TODO - CSG gets more complicated as the surface can be both convex and concave and we need to find the groove positions iteratively
@error "Ashperics not supported for Fresnel lenses"
end
outer_barrel = leaf(Cylinder(semidiameter, thickness * 2, interface = interface), translation(T, zero(T), zero(T), frontvertex - thickness / 2))
fresnel = outer_barrel ∩ fresnel
backplane = leaf(Plane(zero(T), zero(T), -one(T), zero(T), zero(T), frontvertex - thickness, vishalfsizeu = semidiameter, vishalfsizev = semidiameter))
fresnel = backplane ∩ fresnel
if !reverse
fresnel = leaf(fresnel, rotationd(T, zero(T), T(180.0), zero(T)))
end
return fresnel
end
export FresnelLens
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 468 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# OpticalInterface.jl is included in Geometry.jl so is omitted here
include("OpticalRay.jl")
include("RayGenerator.jl")
include("Emitters/Emitters.jl")
include("Fresnel.jl")
include("Paraxial.jl")
include("Grating.jl")
include("LensAssembly.jl")
include("HierarchicalImage.jl")
include("OpticalSystem.jl")
include("Lenses.jl")
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 16767 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
OpticalInterface{T<:Real}
Any subclass of OpticalInterface **must** implement the following:
```julia
processintersection(opticalinterface::OpticalInterface{T}, point::SVector{N,T}, normal::SVector{N,T}, incidentray::OpticalRay{T,N}, temperature::T, pressure::T, ::Bool, firstray::Bool = false) -> Tuple{SVector{N,T}, T, T}
```
See documentation for [`processintersection`](@ref) for details.
These methods are also commonly implemented, but not essential:
```julia
insidematerialid(i::OpticalInterface{T}) -> OpticSim.GlassCat.AbstractGlass
outsidematerialid(i::OpticalInterface{T}) -> OpticSim.GlassCat.AbstractGlass
reflectance(i::OpticalInterface{T}) -> T
transmission(i::OpticalInterface{T}) -> T
```
"""
abstract type OpticalInterface{T<:Real} end
export OpticalInterface
"""
Valid modes for deterministic raytracing
"""
@enum InterfaceMode Reflect Transmit ReflectOrTransmit
export InterfaceMode, Reflect, Transmit, ReflectOrTransmit
######################################################################
"""
NullInterface{T} <: OpticalInterface{T}
Interface which will be ignored totally by any rays, used only in construction of CSG objects.
```julia
NullInterface(T = Float64)
NullInterface{T}()
```
"""
struct NullInterface{T} <: OpticalInterface{T}
NullInterface(::Type{T} = Float64) where {T<:Real} = new{T}()
NullInterface{T}() where {T<:Real} = new{T}()
end
insidematerialid(::NullInterface{T}) where {T<:Real} = glassid(OpticSim.GlassCat.Air)
outsidematerialid(::NullInterface{T}) where {T<:Real} = glassid(OpticSim.GlassCat.Air)
reflectance(::NullInterface{T}) where {T<:Real} = zero(T)
transmission(::NullInterface{T}) where {T<:Real} = one(T)
export NullInterface
######################################################################
"""
ParaxialInterface{T} <: OpticalInterface{T}
Interface describing an idealized planar lens, i.e. one that is thin and with no aberrations.
**In general this interface should not be constructed directly, the `ParaxialLensEllipse` and `ParaxialLensRect` functions should be used to create a [`ParaxialLens`](@ref) object directly.**
```julia
ParaxialInterface(focallength::T, centroid::SVector{3,T}, outsidematerial::Y)
```
"""
struct ParaxialInterface{T} <: OpticalInterface{T}
focallength::T
outsidematerial::GlassID
centroid::SVector{3,T}
function ParaxialInterface(focallength::T, centroid::SVector{3,T}, outsidematerial::Y) where {Y<:OpticSim.GlassCat.AbstractGlass,T<:Real}
return new{T}(focallength, glassid(outsidematerial), centroid)
end
end
export ParaxialInterface
function Base.show(io::IO, a::ParaxialInterface{R}) where {R<:Real}
print(io, "ParaxialInterface($(a.focallength), $(glassname(a.outsidematerial)))")
end
insidematerialid(a::ParaxialInterface{T}) where {T<:Real} = a.outsidematerial
outsidematerialid(a::ParaxialInterface{T}) where {T<:Real} = a.outsidematerial
reflectance(::ParaxialInterface{T}) where {T<:Real} = zero(T)
transmission(::ParaxialInterface{T}) where {T<:Real} = one(T)
opticalcenter(a::ParaxialInterface) = a.centroid
focallength(a::ParaxialInterface) = a.focallength
######################################################################
"""
FresnelInterface{T} <: OpticalInterface{T}
Interface between two materials with behavior defined according to the [Fresnel equations](https://en.wikipedia.org/wiki/Fresnel_equations), with a specified reflectance and transmission.
Assumes unpolarized light.
```julia
FresnelInterface{T}(insidematerial, outsidematerial; reflectance = 0, transmission = 1, interfacemode = ReflectOrTransmit)
```
The interfacemode can be used to trace rays deterministically. Valid values are defined in the InterfaceMode enum.
Reflect means that all values are reflected, Transmit means that all values are transmitted. ReflectOrTransmit will randomly
reflect and transmit rays with the distribution given by the reflection and transmission arguments. This is also the default.
In all cases the power recorded with the ray is correctly updated. This can be used to fake sequential raytracing. For
example a beamsplitter surface may be set to either Reflect or Transmit to switch between the two outgoing ray paths.
"""
struct FresnelInterface{T} <: OpticalInterface{T}
# storing glasses as IDs (integer) rather than the whole thing seems to improve performance significantly, even when the Glass type is a fixed size (i.e. the interface is pointer-free)
insidematerial::GlassID
outsidematerial::GlassID
reflectance::T
transmission::T
interfacemode::InterfaceMode
function FresnelInterface{T}(insidematerial::Z, outsidematerial::Y; reflectance::T = zero(T), transmission::T = one(T), interfacemode = ReflectOrTransmit) where {Z<:OpticSim.GlassCat.AbstractGlass,Y<:OpticSim.GlassCat.AbstractGlass,T<:Real}
return FresnelInterface{T}(glassid(insidematerial), glassid(outsidematerial), reflectance = reflectance, transmission = transmission, interfacemode = interfacemode)
end
function FresnelInterface{T}(insidematerialid::GlassID, outsidematerialid::GlassID; reflectance::T = zero(T), transmission::T = one(T), interfacemode = ReflectOrTransmit) where {T<:Real}
@assert zero(T) <= reflectance <= one(T)
@assert zero(T) <= transmission <= one(T)
@assert reflectance + transmission <= one(T)
return new{T}(insidematerialid, outsidematerialid, reflectance, transmission, interfacemode)
end
end
export FresnelInterface
function Base.show(io::IO, a::FresnelInterface{R}) where {R<:Real}
print(io, "FresnelInterface($(glassname(a.insidematerial)), $(glassname(a.outsidematerial)), $(a.reflectance), $(a.transmission), $(a.interfacemode))")
end
insidematerialid(a::FresnelInterface{T}) where {T<:Real} = a.insidematerial
outsidematerialid(a::FresnelInterface{T}) where {T<:Real} = a.outsidematerial
reflectance(a::FresnelInterface{T}) where {T<:Real} = a.reflectance
transmission(a::FresnelInterface{T}) where {T<:Real} = a.transmission
interfacemode(a::FresnelInterface{T}) where {T<:Real} = a.interfacemode
transmissiveinterface(::Type{T}, insidematerial::X, outsidematerial::Y) where {T<:Real,X<:OpticSim.GlassCat.AbstractGlass,Y<:OpticSim.GlassCat.AbstractGlass} = FresnelInterface{T}(insidematerial, outsidematerial, reflectance = zero(T), transmission = one(T))
reflectiveinterface(::Type{T}, insidematerial::X, outsidematerial::Y) where {T<:Real,X<:OpticSim.GlassCat.AbstractGlass,Y<:OpticSim.GlassCat.AbstractGlass} = FresnelInterface{T}(insidematerial, outsidematerial, reflectance = one(T), transmission = zero(T))
opaqueinterface(::Type{T} = Float64) where {T<:Real} = FresnelInterface{T}(OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, reflectance = zero(T), transmission = zero(T))
export opaqueinterface
######################################################################
"""
ThinGratingInterface{T} <: OpticalInterface{T}
Interface representing an idealized thin grating. `period` is in microns, `vector` should lie in the plane of the surface.
Transmission and reflectance can be specified for an arbitrary number of orders up to 10, selected using the `maxorder` and `minorder` parameters.
If `nothing` then `reflectance` is assumed to be **0** and `transmission` is assumed to be **1**.
```julia
ThinGratingInterface(vector, period, insidematerial, outsidematerial; maxorder = 1, minorder = -1, reflectance = nothing, transmission = nothing)
```
"""
struct ThinGratingInterface{T} <: OpticalInterface{T}
insidematerial::GlassID
outsidematerial::GlassID
vector::SVector{3,T}
period::T
maxorder::Int
minorder::Int
transmission::SVector{GRATING_MAX_ORDERS,T}
reflectance::SVector{GRATING_MAX_ORDERS,T}
function ThinGratingInterface(vector::SVector{3,T}, period::T, insidematerial::Z, outsidematerial::Y; maxorder::Int = 1, minorder::Int = -1, reflectance::Union{Nothing,AbstractVector{T}} = nothing, transmission::Union{Nothing,AbstractVector{T}} = nothing) where {T<:Real,Z<:OpticSim.GlassCat.AbstractGlass,Y<:OpticSim.GlassCat.AbstractGlass}
@assert maxorder >= minorder
norders = maxorder - minorder + 1
@assert norders <= GRATING_MAX_ORDERS "Thin grating is limited to $GRATING_MAX_ORDERS orders"
@assert ((reflectance === nothing) || length(reflectance) == norders) && ((transmission === nothing) || length(transmission) == norders)
if reflectance !== nothing
sreflectance = vcat(SVector{length(reflectance),T}(reflectance), zeros(SVector{GRATING_MAX_ORDERS - length(reflectance),T}))
else
sreflectance = zeros(SVector{GRATING_MAX_ORDERS,T})
end
if transmission !== nothing
stransmission = vcat(SVector{length(transmission),T}(transmission), zeros(SVector{GRATING_MAX_ORDERS - length(transmission),T}))
else
stransmission = ones(SVector{GRATING_MAX_ORDERS,T}) / norders
end
@assert zero(T) <= sum(reflectance) <= one(T)
@assert zero(T) <= sum(transmission) <= one(T)
@assert zero(T) <= sum(reflectance) + sum(transmission) <= one(T)
new{T}(glassid(insidematerial), glassid(outsidematerial), normalize(vector), period, maxorder, minorder, sreflectance, stransmission)
end
end
export ThinGratingInterface
insidematerialid(a::ThinGratingInterface{T}) where {T<:Real} = a.insidematerial
outsidematerialid(a::ThinGratingInterface{T}) where {T<:Real} = a.outsidematerial
reflectance(a::ThinGratingInterface{T}, order::Int) where {T<:Real} = a.reflectance[order - a.minorder + 1]
transmission(a::ThinGratingInterface{T}, order::Int) where {T<:Real} = a.transmission[order - a.minorder + 1]
function Base.show(io::IO, a::ThinGratingInterface{R}) where {R<:Real}
print(io, "ThinGratingInterface($(glassname(a.insidematerial)), $(glassname(a.outsidematerial)), $(a.vector), $(a.period), $(a.transmission), $(a.reflectance))")
end
######################################################################
"""
`ConvergingBeam`, `DivergingBeam` or `CollimatedBeam`, defines the behavior of a beam in a [`HologramInterface`](@ref).
"""
@enum BeamState ConvergingBeam DivergingBeam CollimatedBeam
export BeamState, ConvergingBeam, DivergingBeam, CollimatedBeam
"""
HologramInterface{T} <: OpticalInterface{T}
Interface representing a _thick_ hologram (though geometrically thin).
The efficiency, `η`, is calculated using Kogelnik's coupled wave theory so is only valid for the first order.
If the zero order is included then it has efficiency `1 - η`.
Also assumes that the HOE was recorded under similar conditions to the playback conditions, `thickness` is in microns.
`BeatState` arguments can be one of `ConvergingBeam`, `DivergingBeam` and `CollimatedBeam`. In the first two cases `signalpointordir` and `referencepointordir` are 3D point in global coordinate space. For `CollimatedBeam` they are normalized direction vectors.
For reference, see:
- _Coupled Wave Theory for Thick Hologram Gratings_ - H Kogelnik, 1995
- _Sequential and non-sequential simulation of volume holographic gratings_ - M Kick et al, 2018
```julia
HologramInterface(signalpointordir::SVector{3,T}, signalbeamstate::BeamState, referencepointordir::SVector{3,T}, referencebeamstate::BeamState, recordingλ::T, thickness::T, beforematerial, substratematerial, aftermaterial, signalrecordingmaterial, referencerecordingmaterial, RImodulation::T, include0order = false)
```
"""
struct HologramInterface{T} <: OpticalInterface{T}
beforematerial::GlassID
substratematerial::GlassID
aftermaterial::GlassID
signalpointordir::SVector{3,T}
signalbeamstate::BeamState
referencepointordir::SVector{3,T}
referencebeamstate::BeamState
recordingλ::T
signalrecordingmaterial::GlassID
referencerecordingmaterial::GlassID
thickness::T
RImodulation::T
include0order::Bool
function HologramInterface(signalpointordir::SVector{3,T}, signalbeamstate::BeamState, referencepointordir::SVector{3,T}, referencebeamstate::BeamState, recordingλ::T, thickness::T, beforematerial::Z, substratematerial::X, aftermaterial::Y, signalrecordingmaterial::P, referencerecordingmaterial::Q, RImodulation::T, include0order::Bool = false) where {T<:Real,X<:OpticSim.GlassCat.AbstractGlass,Z<:OpticSim.GlassCat.AbstractGlass,Y<:OpticSim.GlassCat.AbstractGlass,P<:OpticSim.GlassCat.AbstractGlass,Q<:OpticSim.GlassCat.AbstractGlass}
@assert substratematerial !== OpticSim.GlassCat.Air "Substrate can't be air"
if signalbeamstate === CollimatedBeam
signalpointordir = normalize(signalpointordir)
end
if referencebeamstate === CollimatedBeam
referencepointordir = normalize(referencepointordir)
end
new{T}(glassid(beforematerial), glassid(substratematerial), glassid(aftermaterial), signalpointordir, signalbeamstate, referencepointordir, referencebeamstate, recordingλ, glassid(signalrecordingmaterial), glassid(referencerecordingmaterial), thickness, RImodulation, include0order)
end
# 'zero' constructor to use for blank elements in SVector - not ideal...
HologramInterface{T}() where {T<:Real} = new{T}(NullInterface(T), 0, NullInterface(T), zeros(SVector{3,T}), CollimatedBeam, zeros(SVector{3,T}), CollimatedBeam, zero(T), 0, 0, zero(T), zero(T), false)
end
export HologramInterface
insidematerialid(a::HologramInterface{T}) where {T<:Real} = a.beforematerial
outsidematerialid(a::HologramInterface{T}) where {T<:Real} = a.aftermaterial
substratematerialid(a::HologramInterface{T}) where {T<:Real} = a.substratematerial
function Base.show(io::IO, a::HologramInterface{R}) where {R<:Real}
print(io, "HologramInterface($(glassname(a.beforematerial)), $(glassname(a.substratematerial)), $(glassname(a.aftermaterial)), $(a.signalpointordir), $(a.signalbeamstate), $(a.referencepointordir), $(a.referencebeamstate), $(a.recordingλ), $(a.thickness), $(a.RImodulation))")
end
"""
MultiHologramInterface{T} <: OpticalInterface{T}
Interface to represent multiple overlapped [`HologramInterface`](@ref)s on a single surface. Each ray randomly selects an interface to use.
```julia
MultiHologramInterface(interfaces::Vararg{HologramInterface{T}})
MultiHologramInterface(interfaces::Vector{HologramInterface{T}})
```
"""
struct MultiHologramInterface{T} <: OpticalInterface{T}
interfaces::Ptr{HologramInterface{T}} # pointer to the array of hologram interfaces TODO!! fix this to not be hacky...
numinterfaces::Int
MultiHologramInterface(interfaces::Vararg{HologramInterface{T},N}) where {T<:Real,N} = MultiHologramInterface(collect(interfaces))
function MultiHologramInterface(interfaces::Vector{HologramInterface{T}}) where {T<:Real}
N = length(interfaces)
@assert N > 1 "Don't need to use MultiHologramInterface if only 1 hologram"
p = pointer(interfaces)
push!(MultiHologramInterfaceRefCache, interfaces)
new{T}(p, N)
end
end
export MultiHologramInterface
interface(m::MultiHologramInterface{T}, i::Int) where {T<:Real} = (@assert i <= m.numinterfaces; return unsafe_load(m.interfaces, i))
# need this to keep julia references to the arrays used in MultiHologramInterface so they don't get garbage collected
const MultiHologramInterfaceRefCache = []
######################################################################
# a few things need a concrete type to prevent allocations resulting from the ambiguities introduce by an abstract type (i.e. OpticalInterface{T})
# if adding new subtypes of OpticalInterface they must be added to this definition as well (and only paramaterised by T so they are fully specified)
AllOpticalInterfaces{T} = Union{NullInterface{T},ParaxialInterface{T},FresnelInterface{T},HologramInterface{T},MultiHologramInterface{T},ThinGratingInterface{T}}
NullOrFresnel{T} = Union{NullInterface{T},FresnelInterface{T}}
# can't have more than 4 types here or we get allocations because inference on the union stops: https://github.com/JuliaLang/julia/blob/1e6e65691254a7fe81f5da8706bb30aa6cb3f8d2/base/compiler/types.jl#L113
# use the macro to get around this: @unionsplit OpticalInterface T x f(x)
import InteractiveUtils
# TODO REMOVE
macro unionsplit(type, paramtype, var, call)
st = InteractiveUtils.subtypes(@eval $type)
orig_expr = Expr(:if, :($var isa $(st[1]){$paramtype}), call)
expr = orig_expr
for t in st[2:end]
push!(expr.args, Expr(:elseif, :($var isa $t{$paramtype}), call))
expr = expr.args[end]
end
push!(expr.args, :(error("unhandled type")))
return :($(esc(orig_expr)))
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4305 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
OpticalRay{T,N} <: AbstractRay{T,N}
Ray with power, wavelength and optical path length.
**NOTE**: we use monte carlo integration to get accurate results on the detector, this means that all rays essentially hit the detector with power = 1 and some rays are thrown away at any interface to correctly match the reflection/transmission at that interface. For inspection purposes we also track the 'instantaneous' power of the ray in the `power` field of the `OpticalRay`.
```julia
OpticalRay(ray::Ray{T,N}, power::T, wavelength::T, opl=zero(T))
OpticalRay(origin::SVector{N,T}, direction::SVector{N,T}, power::T, wavelength::T, opl=zero(T))
```
Has the following accessor methods:
```julia
ray(r::OpticalRay{T,N}) -> Ray{T,N}
direction(r::OpticalRay{T,N}) -> SVector{N,T}
origin(r::OpticalRay{T,N}) -> SVector{N,T}
power(r::OpticalRay{T,N}) -> T
wavelength(r::OpticalRay{T,N}) -> T
pathlength(r::OpticalRay{T,N}) -> T
sourcepower(r::OpticalRay{T,N}) -> T
nhits(r::OpticalRay{T,N}) -> Int
sourcenum(r::OpticalRay{T,N}) -> Int
```
"""
struct OpticalRay{T,N} <: AbstractRay{T,N}
ray::Ray{T,N}
power::T
wavelength::T
opl::T
nhits::Int
sourcepower::T
sourcenum::Int
function OpticalRay(ray::Ray{T,N}, power::T, wavelength::T; opl::T = zero(T), nhits::Int = 0, sourcenum::Int = 0, sourcepower::T = power) where {T<:Real,N}
return new{T,N}(ray, power, wavelength, opl, nhits, sourcepower, sourcenum)
end
function OpticalRay(origin::SVector{N,T}, direction::SVector{N,T}, power::T, wavelength::T; opl::T = zero(T), nhits::Int = 0, sourcenum::Int = 0, sourcepower::T = power) where {T<:Real,N}
return new{T,N}(Ray(origin, normalize(direction)), power, wavelength, opl, nhits, sourcepower, sourcenum)
end
function OpticalRay(origin::AbstractArray{T,1}, direction::AbstractArray{T,1}, power::T, wavelength::T; opl::T = zero(T), nhits::Int = 0, sourcenum::Int = 0, sourcepower::T = power) where {T<:Real}
@assert length(origin) == length(direction) "origin (dimension $(length(origin))) and direction (dimension $(length(direction))) vectors do not have the same dimension"
N = length(origin)
return new{T,N}(Ray(SVector{N,T}(origin), normalize(SVector{N,T}(direction))), power, wavelength, opl, nhits, sourcepower, sourcenum)
end
# Convenience constructor. Not as much typing
OpticalRay(ox::T, oy::T, oz::T, dx::T, dy::T, dz::T; wavelength = 0.55) where {T<:Real} = OpticalRay(SVector{3,T}(ox, oy, oz), SVector{3,T}(dx, dy, dz), one(T), T(wavelength), one(T)) #doesn't have to be inside struct definition but if it is then VSCode displays hover information. If it's outside the struct definition it doesn't.
end
export OpticalRay
ray(r::OpticalRay{T,N}) where {T<:Real,N} = r.ray
direction(r::OpticalRay{T,N}) where {T<:Real,N} = direction(ray(r))
origin(r::OpticalRay{T,N}) where {T<:Real,N} = origin(ray(r))
power(r::OpticalRay{T,N}) where {T<:Real,N} = r.power
wavelength(r::OpticalRay{T,N}) where {T<:Real,N} = r.wavelength
pathlength(r::OpticalRay{T,N}) where {T<:Real,N} = r.opl
nhits(r::OpticalRay{T,N}) where {T<:Real,N} = r.nhits
sourcepower(r::OpticalRay{T,N}) where {T<:Real,N} = r.sourcepower
sourcenum(r::OpticalRay{T,N}) where {T<:Real,N} = r.sourcenum
export ray, power, wavelength, pathlength, nhits, sourcepower, sourcenum
function Base.print(io::IO, a::OpticalRay{T,N}) where {T,N}
println(io, "$(rpad("Origin:", 25)) $(origin(a))")
println(io, "$(rpad("Direction:", 25)) $(direction(a))")
println(io, "$(rpad("Power:", 25)) $(power(a))")
println(io, "$(rpad("Source Power:", 25)) $(sourcepower(a))")
println(io, "$(rpad("Wavelength (in air):", 25)) $(wavelength(a))")
println(io, "$(rpad("Optical Path Length:", 25)) $(pathlength(a))")
println(io, "$(rpad("Hits:", 25)) $(nhits(a))")
if sourcenum(a) != 0
println(io, "$(rpad("Source Number:", 25)) $(sourcenum(a))")
end
end
function Base.:*(a::Transform{T}, r::OpticalRay{T,N}) where {T,N}
return OpticalRay(a * ray(r), power(r), wavelength(r), opl = pathlength(r), nhits = nhits(r), sourcenum = sourcenum(r), sourcepower = sourcepower(r))
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 31629 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using DataFrames: nrow
using Unitful.DefaultSymbols
using .OpticSim.GlassCat: AbstractGlass, TEMP_REF, PRESSURE_REF, Glass, Air
export AbstractOpticalSystem
export CSGOpticalSystem, temperature, pressure, detectorimage, resetdetector!, assembly
export AxisymmetricOpticalSystem, semidiameter
export trace, traceMT, tracehits, tracehitsMT
"""
AbstractOpticalSystem{T<:Real}
Abstract type for any optical system, must parameterized by the datatype of entities within the system `T`.
"""
abstract type AbstractOpticalSystem{T<:Real} end
"""
CSGOpticalSystem{T,D<:Real,S<:Surface{T},L<:LensAssembly{T}} <: AbstractOpticalSystem{T}
An optical system containing a lens assembly with all optical elements and a detector surface with associated image. The
system can be at a specified temperature and pressure.
There are two number types in the type signature. The `T` type parameter is the numeric type for geometry in the optical
system, the `D` type parameter is the numeric type of the pixels in the detector image. This way you can have `Float64`
geometry, where high precision is essential, but the pixels in the detector can be `Float32` since precision is much
less critical for image data, or Complex if doing wave optic simulations.
The detector can be any [`Surface`](@ref) which implements [`uv`](@ref), [`uvtopix`](@ref) and [`onsurface`](@ref),
typically this is one of [`Rectangle`](@ref), [`Ellipse`](@ref) or [`SphericalCap`](@ref).
```julia
CSGOpticalSystem(
assembly::LensAssembly,
detector::Surface,
detectorpixelsx = 1000,
detectorpixelsy = 1000, ::Type{D} = Float32;
temperature = OpticSim.GlassCat.TEMP_REF,
pressure = OpticSim.GlassCat.PRESSURE_REF
)
```
"""
struct CSGOpticalSystem{T,D<:Number,S<:Surface{T},L<:LensAssembly{T}} <: AbstractOpticalSystem{T}
assembly::L
detector::S
detectorimage::HierarchicalImage{D}
temperature::T
pressure::T
function CSGOpticalSystem(
assembly::L,
detector::S,
detectorpixelsx::Int = 1000,
detectorpixelsy::Int = 1000,
::Type{D} = Float32;
temperature::Union{T,Unitful.Temperature} = convert(T, TEMP_REF),
pressure::T = convert(T, PRESSURE_REF)
) where {T<:Real,S<:Surface{T},L<:LensAssembly{T},D<:Number}
@assert hasmethod(uv, (S, SVector{3,T})) "Detector must implement uv()"
@assert hasmethod(uvtopix, (S, SVector{2,T}, Tuple{Int,Int})) "Detector must implement uvtopix()"
@assert hasmethod(onsurface, (S, SVector{3,T})) "Detector must implement onsurface()"
opticalinterface = interface(detector)
@assert insidematerialid(opticalinterface) == outsidematerialid(opticalinterface) "Detector must have same material either side"
@assert interface(detector) !== NullInterface(T) "Detector can't have null interface"
image = HierarchicalImage{D}(detectorpixelsy, detectorpixelsx)
if temperature isa Unitful.Temperature
temperature = Unitful.ustrip(T, °C, temperature)
end
return new{T,D,S,L}(assembly, detector, image, temperature, convert(T, pressure))
end
end
Base.copy(a::CSGOpticalSystem) = CSGOpticalSystem(
a.assembly,
a.detector,
size(a.detectorimage)...,
temperature = (a.temperature)°C,
pressure = a.pressure
)
# added this show method because the type of CSGOpticalSystem is gigantic and printing it in the REPL can crash the
# system
function Base.show(io::IO, a::CSGOpticalSystem{T}) where {T}
print(io, "CSGOpticalSystem{$T}($(temperature(a)), $(pressure(a)), $(assembly(a)), $(detector(a)))")
end
"""
assembly(system::AbstractOpticalSystem{T}) -> LensAssembly{T}
Get the [`LensAssembly`](@ref) of `system`.
"""
assembly(system::CSGOpticalSystem{T}) where {T<:Real} = system.assembly
detector(system::CSGOpticalSystem) = system.detector
"""
detectorimage(system::AbstractOpticalSystem{T}) -> HierarchicalImage{D}
Get the detector image of `system`.
`D` is the datatype of the detector image and is not necessarily the same as the datatype of the system `T`.
"""
detectorimage(system::CSGOpticalSystem) = system.detectorimage
detectorsize(system::CSGOpticalSystem) = size(system.detectorimage)
"""
temperature(system::AbstractOpticalSystem{T}) -> T
Get the temperature of `system` in °C.
"""
temperature(system::CSGOpticalSystem{T}) where {T<:Real} = system.temperature
"""
pressure(system::AbstractOpticalSystem{T}) -> T
Get the pressure of `system` in Atm.
"""
pressure(system::CSGOpticalSystem{T}) where {T<:Real} = system.pressure
"""
resetdetector!(system::AbstractOpticalSystem{T})
Reset the deterctor image of `system` to zero.
"""
resetdetector!(system::CSGOpticalSystem{T}) where {T<:Real} = reset!(system.detectorimage)
Base.Float32(a::T) where {T<:ForwardDiff.Dual} = Float32(ForwardDiff.value(a))
"""
trace(system::AbstractOpticalSystem{T}, ray::OpticalRay{T}; trackrays = nothing, test = false)
Traces `system` with `ray`, if `test` is enabled then fresnel reflections are disabled and the power distribution will
not be correct. Returns either a [`LensTrace`](@ref) if the ray hits the detector or `nothing` otherwise.
`trackrays` can be passed an empty vector to accumulate the `LensTrace` objects at each intersection of `ray` with a
surface in the system.
"""
function trace(
system::CSGOpticalSystem{T,D},
r::OpticalRay{T,N};
trackrays::Union{Nothing,Vector{LensTrace{T,N}}} = nothing,
test::Bool = false
) where {T<:Real,N,D<:Number}
if power(r) < POWER_THRESHOLD
return nothing
end
result = trace(system.assembly, r, temperature(system), pressure(system), trackrays = trackrays, test = test)
if result === nothing || result === nopower
emptyintervalpool!(T)
return nothing
else #ray intersected lens assembly so continue to see if ray intersects detector
intsct = surfaceintersection(detector(system), ray(result))
if intsct === nothing # no intersection of final ray with detector
emptyintervalpool!(T)
return nothing
end
detintsct = closestintersection(intsct)
if detintsct === nothing
emptyintervalpool!(T)
return nothing
else
# need to modify power and path length accordingly for the intersection with the detector
surfintsct = point(detintsct)
nml = normal(detintsct)
opticalinterface = interface(detintsct)::FresnelInterface{T}
λ = wavelength(r)
# Optical path length is measured in mm
# in this case the result ray is exact so no correction for RAY_OFFSET is needed
geometricpathlength = norm(surfintsct - origin(ray(result)))
opticalpathlength = geometricpathlength
pow = power(result)
m = outsidematerialid(opticalinterface)
# compute updated power based on absorption coefficient of material using Beer's law
# this will almost always not apply as the detector will be in air, but it's possible that the detector is
# not in air, in which case this is necessary
if !isair(m)
mat::Glass = glassforid(m)
nᵢ = index(mat, λ, temperature = temperature(system), pressure = pressure(system))::T
α = absorption(mat, λ, temperature = temperature(system), pressure = pressure(system))::T
if α > zero(T)
internal_trans = exp(-α * geometricpathlength)
if rand() >= internal_trans
return nothing
end
pow = pow * internal_trans
end
opticalpathlength = nᵢ * geometricpathlength
end
temp = LensTrace{T,N}(
OpticalRay(
ray(ray(result)),
pow,
wavelength(result),
opl = pathlength(result) + opticalpathlength,
nhits = nhits(result) + 1,
sourcenum = sourcenum(r),
sourcepower = sourcepower(r)),
detintsct
)
if trackrays !== nothing
push!(trackrays, temp)
end
# increment the detector image
pixu, pixv = uvtopix(detector(system), uv(detintsct), size(system.detectorimage))
system.detectorimage[pixv, pixu] += convert(D, sourcepower(r)) # TODO will need to handle different detector
# image types a bit better than this
# should be okay to assume intersection will not be a DisjointUnion for all the types of detectors we will
# be using
emptyintervalpool!(T)
return temp
end
end
end
######################################################################################################################
function validate_axisymmetricopticalsystem_dataframe(prescription::DataFrame)
# note: there's a slight difference between `col_types` and `surface_types` below: the former refers to the types of
# the prescription DataFrame columns; the former refers to the actual `surface_type` values, which are all strings
required_cols = ["SurfaceType", "Radius", "Thickness", "Material", "SemiDiameter"]
supported_col_types = Dict(
"SurfaceType" => AbstractString,
"Radius" => Real,
"Thickness" => Real,
"Material" => AbstractGlass,
"SemiDiameter" => Real,
"Conic" => Real,
"Reflectance" => Real,
"Parameters" => Vector{<:Pair{<:AbstractString,<:Real}},
)
cols = names(prescription)
supported_surface_types = ["Object", "Stop", "Image", "Standard", "Aspheric", "Zernike"]
surface_types = prescription[!, "SurfaceType"]
comma_join(l::Vector{<:AbstractString}) = join(l, ", ", " and ")
missing_cols = setdiff(required_cols, cols)
@assert isempty(missing_cols) "missing required columns: $(comma_join(missing_cols))"
unsupported_cols = setdiff(cols, keys(supported_col_types))
@assert isempty(unsupported_cols) "unsupported columns: $(comma_join(unsupported_cols))"
col_type_errors = ["$col: $T1 should be $T2" for (col, T1, T2) in
[(col, eltype(prescription[!, col]), supported_col_types[col]) for col in cols]
if !(T1 <: Union{Missing, T2})
]
@assert isempty(col_type_errors) "incorrect column types: $(comma_join(col_type_errors))"
unsupported_surface_types = setdiff(surface_types, supported_surface_types)
@assert isempty(unsupported_surface_types) "unsupported surface types: $(comma_join(unsupported_surface_types))"
@assert(
findall(s->s==="Object", surface_types) == [1],
"there should only be one Object surface and it should be the first row"
)
@assert(
findall(s->s==="Image", surface_types) == [nrow(prescription)],
"there should only be one Image surface and it should be the last row"
)
end
function get_front_back_property(prescription::DataFrame, rownum::Int, property::String, default=nothing)
properties = (
property ∈ names(prescription) ?
[prescription[rownum, property], prescription[rownum + 1, property]] : repeat([missing], 2)
)
return replace(properties, missing => default)
end
turnEmptyIntoNothing(list) = length(list)==0 ? nothing : list
"""
AxisymmetricOpticalSystem{T,C<:CSGOpticalSystem{T}} <: AbstractOpticalSystem{T}
Optical system which has lens elements and an image detector, created from a `DataFrame` containing prescription data.
These tags are supported for columns: `:Radius`, `:SemiDiameter`, `:SurfaceType`, `:Thickness`, `:Conic`, `:Parameters`,
`:Reflectance`, `:Material`.
These tags are supported for entries in a `SurfaceType` column: `Object`, `Image`, `Stop`. Assumes the `Image` row will
be the last row in the `DataFrame`.
In practice a [`CSGOpticalSystem`](@ref) is generated automatically and stored within this system.
```julia
AxisymmetricOpticalSystem{T}(
prescription::DataFrame,
detectorpixelsx = 1000,
detectorpixelsy:: = 1000,
::Type{D} = Float32;
temperature = OpticSim.GlassCat.TEMP_REF,
pressure = OpticSim.GlassCat.PRESSURE_REF
)
```
"""
struct AxisymmetricOpticalSystem{T,C<:CSGOpticalSystem{T}} <: AbstractOpticalSystem{T}
system::C # CSGOpticalSystem
prescription::DataFrame
semidiameter::T # semidiameter of first element (default = 0.0)
function AxisymmetricOpticalSystem{T}(
prescription::DataFrame,
detectorpixelsx::Int = 1000,
detectorpixelsy::Int = 1000,
::Type{D} = Float32;
temperature::Union{T,Unitful.Temperature} = convert(T, TEMP_REF),
pressure::T = convert(T, PRESSURE_REF)
) where {T<:Real,D<:Number}
validate_axisymmetricopticalsystem_dataframe(prescription)
elements::Vector{Union{Surface{T},CSGTree{T}}} = []
systemsemidiameter::T = zero(T)
firstelement::Bool = true
# track sequential movement along the z-axis
vertices::Vector{T} = -cumsum(replace(prescription[!, "Thickness"], Inf => 0, missing => 0))
# pre-construct list of rows which we will skip over (e.g. air gaps, but never Stop surfaces)
# later on, this may get more complicated as we add in compound surfaces
function skip_row(i::Int)
return (
prescription[i, "SurfaceType"] != "Stop" &&
(prescription[i, "Material"] === missing || prescription[i, "Material"] == Air)
)
end
skips::Vector{Bool} = skip_row.(1:nrow(prescription))
for i in 2:nrow(prescription)-1
if skips[i]
continue
end
surface_type::String = prescription[i, "SurfaceType"]
lastmaterial::AbstractGlass, material::AbstractGlass, nextmaterial::AbstractGlass = prescription[i-1:i+1, "Material"]
thickness::T = prescription[i, "Thickness"]
frontradius::T, backradius::T = get_front_back_property(prescription, i, "Radius")
frontsurfacereflectance::T, backsurfacereflectance::T = get_front_back_property(
prescription, i, "Reflectance", zero(T)
)
frontconic::T, backconic::T = get_front_back_property(prescription, i, "Conic", zero(T))
frontparams::Vector{Pair{String,T}}, backparams::Vector{Pair{String,T}} = get_front_back_property(
prescription, i, "Parameters", Vector{Pair{String,T}}()
)
semidiameter::T = max(get_front_back_property(prescription, i, "SemiDiameter", zero(T))...)
if surface_type == "Stop"
semidiameter = prescription[i, "SemiDiameter"]
newelement = CircularAperture(semidiameter, SVector{3,T}(0, 0, 1), SVector{3,T}(0, 0, vertices[i-1]))
elseif surface_type == "Standard"
if frontconic != zero(T) || backconic != zero(T)
newelement = ConicLens(
material, vertices[i-1], frontradius, frontconic, backradius, backconic, thickness,
semidiameter; lastmaterial, nextmaterial, frontsurfacereflectance, backsurfacereflectance
)()
else
newelement = SphericalLens(
material, vertices[i-1], frontradius, backradius, thickness, semidiameter;
lastmaterial, nextmaterial, frontsurfacereflectance, backsurfacereflectance
)()
end
elseif surface_type == "Aspheric"
frontaspherics::Vector{Pair{Int,T}}, backaspherics::Vector{Pair{Int,T}} = [
[parse(Int, k) => v for (k, v) in params] for params in [frontparams, backparams]
]
fa = turnEmptyIntoNothing(frontaspherics)
ba = turnEmptyIntoNothing(backaspherics)
if fa === nothing && ba === nothing #it should be a CONIC. Note: if aspheric terms are present but all zero then an AshpericLens will be created
newelement = ConicLens(
material, vertices[i-1], frontradius, frontconic, backradius, backconic, thickness,
semidiameter; lastmaterial, nextmaterial, frontsurfacereflectance, backsurfacereflectance
)()
else
newelement = AsphericLens(
material, vertices[i-1], frontradius, frontconic, fa, backradius, backconic,
ba, thickness, semidiameter; lastmaterial, nextmaterial, frontsurfacereflectance,
backsurfacereflectance
)()
end
else
error(
"Unsupported surface type \"$surface_type\". If you'd like to add support for this surface, ",
"please create an issue at https://github.com/microsoft/OpticSim.jl/issues/new."
)
end
if firstelement
systemsemidiameter = semidiameter
firstelement = false
end
push!(elements, newelement)
end
# make the detector (Image)
imagesize::T = prescription[end, "SemiDiameter"]
imagerad::T = prescription[end, "Radius"]
if imagerad != zero(T) && imagerad != typemax(T)
det = SphericalCap(
abs(imagerad),
NaNsafeasin(imagesize / abs(imagerad)),
imagerad < 0 ? SVector{3,T}(0, 0, 1) : SVector{3,T}(0, 0, -1),
SVector{3,T}(0, 0, vertices[end-1]),
interface = opaqueinterface(T)
)
else
det = Rectangle(
imagesize,
imagesize,
SVector{3,T}(0, 0, 1),
SVector{3,T}(0, 0, vertices[end-1]),
interface = opaqueinterface(T)
)
end
system = CSGOpticalSystem(
OpticSim.LensAssembly(elements...), det, detectorpixelsx, detectorpixelsy, D; temperature, pressure
)
return new{T,typeof(system)}(system, prescription, systemsemidiameter)
end
AxisymmetricOpticalSystem(prescription::DataFrame) = AxisymmetricOpticalSystem{Float64}(prescription)
end
Base.show(io::IO, a::AxisymmetricOpticalSystem) = print(io, a.prescription)
function Base.copy(a::AxisymmetricOpticalSystem)
temperature = temperature(a)
pressure = pressure(a)
AxisymmetricOpticalSystem(a.prescription, size(detectorimage(a))...; temperature, pressure)
end
function trace(
system::AxisymmetricOpticalSystem{T,C},
r::OpticalRay{T,N};
trackrays::Union{Nothing,Vector{LensTrace{T,N}}} = nothing,
test::Bool = false
) where {T<:Real,N,C<:CSGOpticalSystem{T}}
trace(system.system, r, trackrays = trackrays, test = test)
end
"""
semidiameter(system::AxisymmetricOpticalSystem{T}) -> T
Get the semidiameter of `system`, that is the semidiameter of the entrance pupil (i.e. first surface) of the system.
"""
semidiameter(a::AxisymmetricOpticalSystem) = a.semidiameter
assembly(system::AxisymmetricOpticalSystem) = assembly(system.system)
detector(system::AxisymmetricOpticalSystem) = detector(system.system)
detectorimage(system::AxisymmetricOpticalSystem) = detectorimage(system.system)
detectorsize(system::AxisymmetricOpticalSystem) = detectorsize(system.system)
resetdetector!(system::AxisymmetricOpticalSystem) = resetdetector!(system.system)
temperature(system::AxisymmetricOpticalSystem) = temperature(system.system)
pressure(system::AxisymmetricOpticalSystem) = pressure(system.system)
######################################################################################################################
function trace(
system::AxisymmetricOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false,
outpath::Union{Nothing,String} = nothing
) where {T<:Real}
trace(system.system, raygenerator; printprog, test, outpath)
end
"""
trace(system::AbstractOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; printprog = true, test = false)
Traces `system` with rays generated by `raygenerator` on a single thread.
Optionally the progress can be printed to the REPL.
If `test` is enabled then fresnel reflections are disabled and the power distribution will not be correct.
If `outpath` is specified then the result will be saved to this path.
Returns the detector image of the system.
"""
function trace(
system::CSGOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false,
outpath::Union{Nothing,String} = nothing
) where {T<:Real}
start_time = time()
update_timesteps = 1000
total_traced = 0
for (k, r) in enumerate(raygenerator)
if k % update_timesteps == 0
total_traced += update_timesteps
dif = round(time() - start_time, digits = 1)
left = round((time() - start_time) * (length(raygenerator) / total_traced - 1), digits = 1)
if printprog
print("\rTraced: ~ $k / $(length(raygenerator)) Elapsed: $(dif)s Left: $(left)s ")
end
end
trace(system, r, test = test)
end
numrays = length(raygenerator)
tracetime = round(time() - start_time)
if printprog
print("\rFinished tracing $numrays rays in $(tracetime)s")
if tracetime != 0.0
print(", $(Int32(round(numrays/tracetime))) rays per second")
end
println()
end
det = detectorimage(system)
if outpath !== nothing
save(outpath, colorview(Gray, det ./ maximum(det)))
end
return det
end
function traceMT(
system::AxisymmetricOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false,
outpath::Union{Nothing,String} = nothing
) where {T<:Real}
traceMT(system.system, raygenerator, printprog = printprog, test = test, outpath = outpath)
end
"""
traceMT(system::AbstractOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; printprog = true, test = false)
Traces `system` with rays generated by `raygenerator` using as many threads as possible.
Optionally the progress can be printed to the REPL.
If `test` is enabled then fresnel reflections are disabled and the power distribution will not be correct.
If `outpath` is specified then the result will be saved to this path.
Returns the accumulated detector image from all threads.
"""
function traceMT(
system::CSGOpticalSystem{T,S},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false,
outpath::Union{Nothing,String} = nothing
) where {T<:Real,S<:Number}
if printprog
println("Initialising...")
end
all_start_time = time()
N = Threads.nthreads()
copies = Vector{Tuple{CSGOpticalSystem{T},OpticalRayGenerator{T}}}(undef, N)
for i in eachindex(copies)
copies[i] = (copy(system), copy(raygenerator))
end
if printprog
println("Tracing...")
end
total_traced = Threads.Atomic{Int}(0)
update_timesteps = 1000
Threads.@threads for sys in copies
(system, sources) = sys
i = Threads.threadid()
len, rem = divrem(length(sources), N)
# not enough iterations for all the threads
if len == 0
if i > rem
return
end
len, rem = 1, 0
end
# compute this thread's iterations
f = firstindex(sources) + ((i - 1) * len)
l = f + len - 1
# distribute remaining iterations evenly
if rem > 0
if i <= rem
f = f + (i - 1)
l = l + i
else
f = f + rem
l = l + rem
end
end
if i == 1
start_time = time()
for k in f:l
if k % update_timesteps == 0
Threads.atomic_add!(total_traced, update_timesteps)
dif = round(time() - start_time, digits = 1)
t = total_traced[]
left = round((time() - start_time) * (length(sources) / t - 1), digits = 1)
if printprog
print("\rTraced: ~ $t / $(length(sources)) Elapsed: $(dif)s Left: $(left)s ")
end
end
trace(system, sources[k]; test)
end
else
for k in f:l
if k % update_timesteps == 0
Threads.atomic_add!(total_traced, update_timesteps)
end
trace(system, sources[k]; test)
end
end
end
if printprog
println("\rAccumulating images... ")
end
det = OpticSim.detectorimage(copies[1][1])
# sum all the copies
@inbounds for i in 2:N
OpticSim.sum!(det, OpticSim.detectorimage(copies[i][1]))
end
numrays = length(raygenerator)
tracetime = round(time() - all_start_time)
if printprog
print("\rFinished tracing $numrays rays in $(tracetime)s, ")
if tracetime != 0.0
print("$(Int32(round(numrays/tracetime))) rays per second")
end
println()
end
if outpath !== nothing
save(outpath, colorview(Gray, det ./ maximum(det)))
end
return det
end
function tracehitsMT(
system::AxisymmetricOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true, test::Bool = false
) where {T<:Real}
tracehitsMT(system.system, raygenerator, printprog = printprog, test = test)
end
"""
tracehitsMT(system::AbstractOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; printprog = true, test = false)
Traces `system` with rays generated by `raygenerator` using as many threads as possible.
Optionally the progress can be printed to the REPL.
If `test` is enabled then fresnel reflections are disabled and the power distribution will not be correct.
Returns a list of [`LensTrace`](@ref)s which hit the detector, accumulated from all threads.
"""
function tracehitsMT(
system::CSGOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false
) where {T<:Real}
if printprog
println("Initialising...")
end
all_start_time = time()
N = Threads.nthreads()
copies = Vector{Tuple{CSGOpticalSystem{T},OpticalRayGenerator{T}}}(undef, N)
results = Vector{Vector{LensTrace{T,3}}}(undef, N)
for i in eachindex(copies)
copies[i] = (copy(system), copy(raygenerator))
results[i] = Vector{LensTrace{T,3}}(undef, 0)
end
if printprog
println("Tracing...")
end
total_traced = Threads.Atomic{Int}(0)
update_timesteps = 1000
Threads.@threads for sys in copies
(system, sources) = sys
i = Threads.threadid()
len, rem = divrem(length(sources), N)
# not enough iterations for all the threads
if len == 0
if i > rem
return
end
len, rem = 1, 0
end
# compute this thread's iterations
f = firstindex(sources) + ((i - 1) * len)
l = f + len - 1
# distribute remaining iterations evenly
if rem > 0
if i <= rem
f = f + (i - 1)
l = l + i
else
f = f + rem
l = l + rem
end
end
if i == 1
start_time = time()
for k in f:l
if k % update_timesteps == 0
Threads.atomic_add!(total_traced, update_timesteps)
dif = round(time() - start_time, digits = 1)
t = total_traced[]
left = round((time() - start_time) * (length(sources) / t - 1), digits = 1)
if printprog
print("\rTraced: ~ $t / $(length(sources)) Elapsed: $(dif)s Left: $(left)s ")
end
end
lt = trace(system, sources[k]; test)
if lt !== nothing
push!(results[i], lt)
end
end
else
for k in f:l
if k % update_timesteps == 0
Threads.atomic_add!(total_traced, update_timesteps)
end
lt = trace(system, sources[k]; test)
if lt !== nothing
push!(results[i], lt)
end
end
end
end
if printprog
println("\rAccumulating results... ")
end
accumres = results[1]
# sum all the copies
@inbounds for i in 2:N
accumres = vcat(accumres, results[i])
end
numrays = length(raygenerator)
tracetime = round(time() - all_start_time)
if printprog
print("\rFinished tracing $numrays rays in $(tracetime)s")
if tracetime != 0.0
print(", $(Int32(round(numrays/tracetime))) rays per second")
end
println()
end
return accumres
end
function tracehits(
system::AxisymmetricOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false
) where {T<:Real}
tracehits(system.system, raygenerator; printprog, test)
end
"""
tracehits(system::AbstractOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; printprog = true, test = false)
Traces `system` with rays generated by `raygenerator` on a single thread.
Optionally the progress can be printed to the REPL.
If `test` is enabled then fresnel reflections are disabled and the power distribution will not be correct.
Returns a list of [`LensTrace`](@ref)s which hit the detector.
"""
function tracehits(
system::CSGOpticalSystem{T},
raygenerator::OpticalRayGenerator{T};
printprog::Bool = true,
test::Bool = false
) where {T<:Real}
start_time = time()
update_timesteps = 1000
total_traced = 0
res = Vector{LensTrace{T,3}}(undef, 0)
for (k, r) in enumerate(raygenerator)
if k % update_timesteps == 0
total_traced += update_timesteps
dif = round(time() - start_time, digits = 1)
left = round((time() - start_time) * (length(sources) / total_traced - 1), digits = 1)
if printprog
print("\rTraced: ~ $t / $(length(sources)) Elapsed: $(dif)s Left: $(left)s ")
end
end
lt = trace(system, r, test = test)
if lt !== nothing
push!(res, lt)
end
end
numrays = length(raygenerator)
tracetime = round(time() - all_start_time)
if printprog
print("\rFinished tracing $numrays rays in $(tracetime)s")
if tracetime != 0.0
print(", $(Int32(round(numrays/tracetime))) rays per second")
end
println()
end
return res
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 11451 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
ParaxialLens{T} <: Surface{T}
`surfacenormal` is the **output** direction of the lens.
Paraxial lens cannot act as the interface between two materials, hence only a single outside material is specified, by default Air.
Create with the following functions
```julia
ParaxialLensEllipse(focaldistance, halfsizeu, halfsizev, surfacenormal, centrepoint; rotationvec = [0.0, 1.0, 0.0], outsidematerial = OpticSim.GlassCat.Air, decenteruv = (0.0, 0.0))
ParaxialLensRect(focaldistance, halfsizeu, halfsizev, surfacenormal, centrepoint; rotationvec = [0.0, 1.0, 0.0], outsidematerial = OpticSim.GlassCat.Air, decenteruv = (0.0, 0.0))
ParaxialLensHex(focaldistance, side_length, surfacenormal, centrepoint; rotationvec = [0.0, 1.0, 0.0], outsidematerial = OpticSim.GlassCat.Air, decenteruv = (0.0, 0.0))
ParaxialLensConvexPoly(focaldistance, local_frame, local_polygon_points, local_center_point; outsidematerial = OpticSim.GlassCat.Air)
```
"""
struct ParaxialLens{T} <: Surface{T}
shape::Union{Rectangle{T},Ellipse{T},Hexagon{T},ConvexPolygon{N, T} where {N}}
interface::ParaxialInterface{T}
function ParaxialLens(shape::Union{Rectangle{T},Ellipse{T},Hexagon{T},ConvexPolygon{N, T} where {N}}, interface::ParaxialInterface{T}) where {T<:Real}
new{T}(shape, interface)
end
end
shape(a::ParaxialLens) = a.shape
export shape
opticalcenter(a::ParaxialLens) = opticalcenter(a.interface)
export opticalcenter
focallength(a::ParaxialLens) = focallength(a.interface)
export focallength
"""returns the 2 dimensional vertex points of the shape defining the lens aperture. These points lie in the plane of the shape"""
vertices(a::ParaxialLens) = vertices(a.shape)
"""All paraxial lens surfaces are planar"""
plane(a::ParaxialLens) = plane(a.shape)
struct VirtualPoint{T<:Real}
center::SVector{3,T}
direction::SVector{3,T}
distance::T
function VirtualPoint(center::AbstractVector{T},direction::AbstractVector{T},distance::T) where{T<:Real}
@assert distance ≥ 0 #direction is encoded in the direction vector, but distance might have a sign. Need to discard it.
new{T}(SVector{3,T}(center),SVector{3,T}(direction),distance)
end
end
"""This will return (Inf,Inf,Inf) if the point is at infinity. In this case you probably should be using the direction of the VirtualPoint rather than its position"""
point(a::VirtualPoint) = a.center + a.direction*a.distance
distancefromplane(lens::ParaxialLens,point::AbstractVector) = distancefromplane(lens.shape,SVector{3}(point))
export distancefromplane
"""returns the virtual distance of the point from the lens plane. When |distance| == focallength then virtualdistance = ∞"""
function virtualdistance(focallength::T,distance::T) where{T<:Real}
if abs(distance) == focallength
return sign(distance)*T(Inf)
else
return distance*focallength/(focallength-abs(distance))
end
end
"""computes the virtual point position corresponding to the input `point`, or returns nothing for points at infinity. `point` is specified in the lens coordinate frame"""
function virtualpoint(lens::ParaxialLens{T}, point::AbstractVector{T}) where{T}
oc = opticalcenter(lens)
point_oc = normalize(point - oc)
distance = distancefromplane(lens,point)
vdistance = virtualdistance(focallength(lens),distance)
return VirtualPoint(oc,point_oc,abs(vdistance))
end
export virtualpoint
function ParaxialLensRect(focaldistance::T, halfsizeu::T, halfsizev::T, surfacenormal::AbstractArray{T,1}, centrepoint::AbstractArray{T,1}; rotationvec::AbstractArray{T,1} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
@assert length(surfacenormal) == 3 && length(centrepoint) == 3
return ParaxialLensRect(focaldistance, halfsizeu, halfsizev, SVector{3,T}(surfacenormal), SVector{3,T}(centrepoint), rotationvec = SVector{3,T}(rotationvec), outsidematerial = outsidematerial, decenteruv = decenteruv)
end
function ParaxialLensRect(focaldistance::T, halfsizeu::T, halfsizev::T, surfacenormal::SVector{3,T}, centrepoint::SVector{3,T}; rotationvec::SVector{3,T} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
r = Rectangle(halfsizeu, halfsizev, surfacenormal, centrepoint)
centrepoint = centrepoint + decenteruv[1] * r.uvec + decenteruv[2] * r.vvec
return ParaxialLens(r, ParaxialInterface(focaldistance, centrepoint, outsidematerial))
end
function ParaxialLensHex(focaldistance::T, side_length::T, surfacenormal::AbstractArray{T,1}, centrepoint::AbstractArray{T,1}; rotationvec::AbstractArray{T,1} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
@assert length(surfacenormal) == 3 && length(centrepoint) == 3
return ParaxialLensHex(focaldistance, side_length, SVector{3,T}(surfacenormal), SVector{3,T}(centrepoint), rotationvec = SVector{3,T}(rotationvec), outsidematerial = outsidematerial, decenteruv = decenteruv)
end
function ParaxialLensHex(focaldistance::T, side_length::T, surfacenormal::SVector{3,T}, centrepoint::SVector{3,T}; rotationvec::SVector{3,T} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
h = Hexagon(side_length, surfacenormal, centrepoint)
centrepoint = centrepoint + decenteruv[1] * h.uvec + decenteruv[2] * h.vvec
return ParaxialLens(h, ParaxialInterface(focaldistance, centrepoint, outsidematerial))
end
function ParaxialLensConvexPoly(focallength::T,convpoly::ConvexPolygon{N,T},local_center_point::SVector{2,T}; outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air) where {N, T<:Real}
# the local frame information is stored in convpoly. The polygon points are 2D stored in the z = 0 local plane. The shape and vertices functions automatically apply local to world transformations so the points are represented in world space.
centrepoint = SVector{3, T}(local2world(localframe(convpoly)) * Vec3(local_center_point[1], local_center_point[2], zero(T)))
return ParaxialLens(convpoly, ParaxialInterface(focallength, centrepoint, outsidematerial))
end
function ParaxialLensConvexPoly(focaldistance::T, local_frame::Transform{T}, local_polygon_points::Vector{SVector{2, T}}, local_center_point::SVector{2, T}; outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air) where {N, T<:Real}
# the local frame information is stored in convpoly. The polygon points are 2D stored in the z = 0 local plane. The shape and vertices functions automatically apply local to world transformations so the points are represented in world space.
poly = ConvexPolygon(local_frame, local_polygon_points)
centrepoint = SVector{3, T}(local2world(local_frame) * Vec3(local_center_point[1], local_center_point[2], zero(T)))
return ParaxialLens(poly, ParaxialInterface(focaldistance, centrepoint, outsidematerial))
end
function ParaxialLensEllipse(focaldistance::T, halfsizeu::T, halfsizev::T, surfacenormal::AbstractArray{T,1}, centrepoint::AbstractArray{T,1}; rotationvec::AbstractArray{T,1} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
@assert length(surfacenormal) == 3 && length(centrepoint) == 3
return ParaxialLensEllipse(focaldistance, halfsizeu, halfsizev, SVector{3,T}(surfacenormal), SVector{3,T}(centrepoint), rotationvec = SVector{3,T}(rotationvec), outsidematerial = outsidematerial, decenteruv = decenteruv)
end
function ParaxialLensEllipse(focaldistance::T, halfsizeu::T, halfsizev::T, surfacenormal::SVector{3,T}, centrepoint::SVector{3,T}; rotationvec::SVector{3,T} = SVector{3,T}(0.0, 1.0, 0.0), outsidematerial::OpticSim.GlassCat.AbstractGlass = OpticSim.GlassCat.Air, decenteruv::Tuple{T,T} = (zero(T), zero(T))) where {T<:Real}
e = Ellipse(halfsizeu, halfsizev, surfacenormal, centrepoint)
centrepoint = centrepoint + decenteruv[1] * e.uvec + decenteruv[2] * e.vvec
return ParaxialLens(e, ParaxialInterface(focaldistance, centrepoint, outsidematerial))
end
export ParaxialLens, ParaxialLensRect, ParaxialLensEllipse, ParaxialLensHex, ParaxialLensConvexPoly
interface(r::ParaxialLens{T}) where {T<:Real} = r.interface
centroid(r::ParaxialLens{T}) where {T<:Real} = centroid(r.shape)
normal(r::ParaxialLens{T}) where {T<:Real} = normal(r.shape)
point(r::ParaxialLens{T}, u::T, v::T) where {T<:Real} = point(r.shape, u, v)
uv(r::ParaxialLens{T}, x::T, y::T, z::T) where {T<:Real} = uv(r, SVector{3,T}(x, y, z))
uv(r::ParaxialLens{T}, p::SVector{3,T}) where {T<:Real} = uv(r.shape, p)
function surfaceintersection(l::ParaxialLens{T}, r::AbstractRay{T,3}) where {T<:Real}
#this code seems unnecessary. If the ParaxialInterface is stored in the shape instead of the ParaxialLens then can do this:
#surfaceintersection(l::ParaxialLens....) = surfaceintersection(l.shape). Should be much faster than this code.
itvl = surfaceintersection(l.shape, r)
if itvl isa EmptyInterval{T}
return EmptyInterval(T)
else
intsct = halfspaceintersection(itvl)
u, v = uv(intsct)
intsct = Intersection(α(intsct), point(intsct), normal(intsct), u, v, interface(l), flippednormal = flippednormal(intsct))
return positivehalfspace(intsct)
end
end
makemesh(l::ParaxialLens{T}, ::Int = 0) where {T<:Real} = makemesh(l.shape)
function processintersection(opticalinterface::ParaxialInterface{T}, point::SVector{N,T}, normal::SVector{N,T}, incidentray::OpticalRay{T,N}, temperature::T, pressure::T, ::Bool, firstray::Bool = false) where {T<:Real,N}
raypower = power(incidentray)
m = outsidematerialid(opticalinterface) # necessarily both the same
n = one(T)
if !isair(m)
mat = glassforid(m)::OpticSim.GlassCat.Glass
n = index(mat, wavelength(incidentray), temperature = temperature, pressure = pressure)::T
end
geometricpathlength = norm(point - origin(incidentray)) + (firstray ? zero(T) : T(RAY_OFFSET))
thisraypathlength = n * geometricpathlength
raypathlength = pathlength(incidentray) + thisraypathlength
#TODO there is an error in this formula for refraction. If the ray and the normal have a negative dot product the refracted ray will be the negative of the correct direction. Fix this.
# refraction calculated directly for paraxial lens
# raydirection = normalize((opticalinterface.centroid - point) + direction(incidentray) * opticalinterface.focallength / dot(normal, direction(incidentray)))
#this works for both positive and negative focal lengths
raydirection = normalize(sign(opticalinterface.focallength)*(opticalinterface.centroid - point) + direction(incidentray) * abs(opticalinterface.focallength / dot(normal, direction(incidentray))))
# if opticalinterface.focallength < 0
# # flip for negative focal lengths
# raydirection = -raydirection
# end
return raydirection, raypower, raypathlength
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 735 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
abstract type AbstractRayGenerator{T<:Real} end
"""
GeometricRayGenerator{T,O<:RayOriginGenerator{T}} <: AbstractRayGenerator{T}
Generates geometric [`Ray`](@ref)s according to the specific implementation of the subclass.
"""
abstract type GeometricRayGenerator{T} <: AbstractRayGenerator{T} end
"""
OpticalRayGenerator{T} <: AbstractRayGenerator{T}
Generates [`OpticalRay`](@ref)s according to the specific implementation of the subclass.
"""
abstract type OpticalRayGenerator{T} <: AbstractRayGenerator{T} end
export AbstractRayGenerator, GeometricRayGenerator, OpticalRayGenerator
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1954 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module AngularPower
export Lambertian, Cosine, Gaussian
using ....OpticSim
using ...Emitters
using ...Geometry
using LinearAlgebra
abstract type AbstractAngularPowerDistribution{T<:Real} end
"""
Lambertian{T} <: AbstractAngularPowerDistribution{T}
Ray power is unaffected by angle.
"""
struct Lambertian{T} <: AbstractAngularPowerDistribution{T}
Lambertian(::Type{T} = Float64) where {T<:Real} = new{T}()
end
Emitters.apply(::Lambertian, ::Transform, power::Real, ::OpticSim.Ray{<:Real,3}) = power
"""
Cosine{T} <: AbstractAngularPowerDistribution{T}
Cosine power distribution. Ray power is calculated by:
`power = power * (cosine_angle ^ cosine_exp)`
"""
struct Cosine{T} <: AbstractAngularPowerDistribution{T}
cosine_exp::T
function Cosine(cosine_exp::T = one(Float64)) where {T<:Real}
new{T}(cosine_exp)
end
end
function Emitters.apply(d::Cosine, tr::Transform, power::Real, ray::OpticSim.Ray{<:Real,3})
cosanglebetween = dot(OpticSim.direction(ray), forward(tr))
return power * cosanglebetween ^ d.cosine_exp
end
"""
Gaussian{T} <: AbstractAngularPowerDistribution{T}
GGaussian power distribution. Ray power is calculated by:
`power = power * exp(-(gaussianu * l^2 + gaussianv * m^2))`
where l and m are the cos_angles between the two axes respectivly.
"""
struct Gaussian{T} <: AbstractAngularPowerDistribution{T}
gaussianu::T
gaussianv::T
function Gaussian(gaussianu::T, gaussianv::T) where {T<:Real}
new{T}(gaussianu, gaussianv)
end
end
function Emitters.apply(d::Gaussian, tr::Transform, power::Real, ray::OpticSim.Ray{<:Real,3})
l = dot(OpticSim.direction(ray), right(tr))
m = dot(OpticSim.direction(ray), up(tr))
return power * exp(-(d.gaussianu * l^2 + d.gaussianv * m^2))
end
end # module Angular Power
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6425 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Directions
export Constant, RectGrid, UniformCone, HexapolarCone
using ....OpticSim
using ...Emitters
using ...Geometry
using LinearAlgebra
using Random
abstract type AbstractDirectionDistribution{T<:Real} end
Base.iterate(a::AbstractDirectionDistribution, state = 1) = state > length(a) ? nothing : (generate(a, state - 1), state + 1)
Base.getindex(a::AbstractDirectionDistribution, index) = generate(a, index)
Base.firstindex(a::AbstractDirectionDistribution) = 0
Base.lastindex(a::AbstractDirectionDistribution) = length(a) - 1
Base.copy(a::AbstractDirectionDistribution) = a # most don't have any heap allocated stuff so don't really need copying
"""
Constant{T} <: AbstractDirectionDistribution{T}
Encapsulates a single ray direction, where the default direction is unitZ3 [0, 0, 1].
```julia
Constant(direction::Vec3{T}) where {T<:Real}
Constant(::Type{T} = Float64) where {T<:Real}
```
"""
struct Constant{T} <: AbstractDirectionDistribution{T}
direction::Vec3{T}
function Constant(dirx::T,diry::T,dirz::T) where{T<:Real}
return new{T}(Vec3(dirx,diry,dirz))
end
function Constant(direction::Vec3{T}) where {T<:Real}
return new{T}(direction)
end
function Constant(::Type{T} = Float64) where {T<:Real}
direction = unitZ3(T)
return new{T}(direction)
end
end
Base.length(d::Constant) = 1
Emitters.generate(d::Constant, ::Int64) = d.direction
"""
RectGrid{T} <: AbstractDirectionDistribution{T}
Encapsulates a single ray direction, where the default direction is unitZ3 [0, 0, 1].
```julia
Constant(direction::Vec3{T}) where {T<:Real}
Constant(::Type{T} = Float64) where {T<:Real}
```
"""
struct RectGrid{T} <: AbstractDirectionDistribution{T}
direction::Vec3{T}
halfangleu::T
halfanglev::T
nraysu::Int64
nraysv::Int64
uvec::Vec3{T}
vvec::Vec3{T}
function RectGrid(direction::Vec3{T}, halfangleu::T, halfanglev::T, numraysu::Int64, numraysv::Int64) where {T<:Real}
(uvec, vvec) = get_orthogonal_vectors(direction)
return new{T}(direction, halfangleu, halfanglev, numraysu, numraysv, uvec, vvec)
end
function RectGrid(halfangleu::T, halfanglev::T, numraysu::Int64, numraysv::Int64) where {T<:Real}
direction = unitZ3(T)
return RectGrid(direction, halfangleu, halfanglev, numraysu, numraysv)
end
end
Base.length(d::RectGrid) = d.nraysu * d.nraysv
function Emitters.generate(d::RectGrid{T}, n::Int64) where {T<:Real}
direction = d.direction
uvec = d.uvec
vvec = d.vvec
# distributing evenly across the area of the rectangle which subtends the given angle (*not* evenly across the angle range)
dindex = mod(n, d.nraysu * d.nraysv)
v = d.nraysv == 1 ? zero(T) : 2 * Int64(floor(dindex / d.nraysu)) / (d.nraysv - 1) - 1.0
u = d.nraysu == 1 ? zero(T) : 2 * mod(dindex, d.nraysu) / (d.nraysu - 1) - 1.0
θu = atan(u * tan(d.halfangleu) / 2) * d.halfangleu
θv = atan(v * tan(d.halfanglev) / 2) * d.halfanglev
dir = cos(θv) * (cos(θu) * direction + sin(θu) * uvec) + sin(θv) * vvec
return dir
end
"""
UniformCone{T} <: AbstractDirectionDistribution{T}
Encapsulates `numsamples` rays sampled uniformly from a cone with max angle θmax.
```julia
UniformCone(direction::Vec3{T}, θmax::T, numsamples::Int64) where {T<:Real}
UniformCone(θmax::T, numsamples::Int64) where {T<:Real}
```
"""
struct UniformCone{T} <: AbstractDirectionDistribution{T}
direction::Vec3{T}
θmax::T
numsamples::Int64
uvec::Vec3{T}
vvec::Vec3{T}
rng::Random.AbstractRNG
function UniformCone(direction::Vec3{T}, θmax::T, numsamples::Int64; rng=Random.GLOBAL_RNG) where {T<:Real}
(uvec, vvec) = get_orthogonal_vectors(direction)
return new{T}(direction, θmax, numsamples, uvec, vvec, rng)
end
function UniformCone(θmax::T, numsamples::Int64; rng=Random.GLOBAL_RNG) where {T<:Real}
direction = unitZ3(T)
return UniformCone(direction, θmax, numsamples, rng=rng)
end
end
Base.length(d::UniformCone) = d.numsamples
function Emitters.generate(d::UniformCone{T}, ::Int64) where {T<:Real}
direction = d.direction
θmax = d.θmax
uvec = d.uvec
vvec = d.vvec
ϕ = rand(d.rng, T) * 2π
θ = acos(clamp(one(T) + rand(d.rng, T) * (cos(θmax) - 1), -one(T), one(T)))
return normalize(sin(θ) * (cos(ϕ) * uvec + sin(ϕ) * vvec) + cos(θ) * direction)
end
"""
HexapolarCone{T} <: AbstractDirectionDistribution{T}
Rays are generated by sampling a cone with θmax angle in an hexapolar fashion. The number of rays depends on the requested rings and is computed using the following formula:
`1 + round(Int64, (nrings * (nrings + 1) / 2) * 6)`
```julia
HexapolarCone(direction::Vec3{T}, θmax::T, nrings::Int64) where {T<:Real}
HexapolarCone(θmax::T, nrings::Int64 = 3) where {T<:Real}
```
"""
struct HexapolarCone{T} <: AbstractDirectionDistribution{T}
direction::Vec3{T}
θmax::T
nrings::Int64
uvec::Vec3{T}
vvec::Vec3{T}
function HexapolarCone(direction::Vec3{T}, θmax::T, nrings::Int64) where {T<:Real}
(uvec, vvec) = get_orthogonal_vectors(direction)
return new{T}(direction, θmax, nrings, uvec, vvec)
end
# assume canonical directions
function HexapolarCone(θmax::T, nrings::Int64 = 3) where {T<:Real}
direction = unitZ3(T)
return HexapolarCone(direction, θmax, nrings)
end
end
Base.length(d::HexapolarCone) = 1 + round(Int64, (d.nrings * (d.nrings + 1) / 2) * 6)
function Emitters.generate(d::HexapolarCone{T}, n::Int64) where {T<:Real}
dir = d.direction
θmax = d.θmax
uvec = d.uvec
vvec = d.vvec
n = mod(n, length(d))
if n == 0
return normalize(dir)
else
t = 1
ringi = 1
for i in 1:(d.nrings)
t += 6 * i
if n < t
ringi = i
break
end
end
ρ = ringi / d.nrings
pind = n - (t - 6 * ringi)
ϕ = (pind / (6 * ringi)) * 2π
# elevation calculated as ring fraction multipled by max angle
θ = acos(clamp(one(T) + (cos(ρ * θmax) - 1), -one(T), one(T)))
return normalize(sin(θ) * (cos(ϕ) * uvec + sin(ϕ) * vvec) + cos(θ) * dir)
end
end
end # module Directions
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1055 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#=
Emitters are defined by Pixels and SpatialLayouts. An emitter has a spectrum, and an optical power distribution over the hemisphere.
These are intrinsic physical properties of the emitter.
=#
module Emitters
export generate, visual_size, apply
export right, up, forward
export Spectrum, Directions, Origins, AngularPower, Sources
export pointemitter, collimatedemitter
using ...OpticSim, ..Geometry
using LinearAlgebra
import Unitful.Length
using Unitful.DefaultSymbols
# defining name placeholders to override in nested modules
"""
generate(???)
[TODO]
"""
function generate end
"""
visual_size(???)
[TODO]
"""
function visual_size end
"""
apply(???)
[TODO] Returns ray power
"""
function apply end
include("Spectrum.jl")
include("Directions.jl")
include("Origins.jl")
include("AngularPower.jl")
include("Sources.jl")
include("StandardEmitters.jl")
end # module Emitters
export Emitters
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6308 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Origins
export Point, RectUniform, RectGrid, Hexapolar, RectJitterGrid
using ....OpticSim
using ...Emitters
using ...Geometry
using LinearAlgebra
using Distributions
using Random
abstract type AbstractOriginDistribution{T<:Real} end
Base.iterate(a::AbstractOriginDistribution, state = 1) = state > length(a) ? nothing : (generate(a, state - 1), state + 1)
Base.getindex(a::AbstractOriginDistribution, index) = generate(a, index)
Base.firstindex(a::AbstractOriginDistribution) = 0
Base.lastindex(a::AbstractOriginDistribution) = length(a) - 1
Base.copy(a::AbstractOriginDistribution) = a # most don't have any heap allocated stuff so don't really need copying
"""
Point{T} <: AbstractOriginDistribution{T}
Encapsulates a single point origin.
```julia
Point(position::Vec3{T}) where {T<:Real}
Point(x::T, y::T, z::T) where {T<:Real}
Point(::Type{T} = Float64) where {T<:Real}
```
"""
struct Point{T} <: AbstractOriginDistribution{T}
origin::Vec3{T}
function Point(position::Vec3{T}) where {T<:Real}
return new{T}(position)
end
function Point(x::T, y::T, z::T) where {T<:Real}
return new{T}(Vec3{T}(x, y, z))
end
function Point(::Type{T} = Float64) where {T<:Real}
return new{T}(zero(Vec3))
end
end
Base.length(::Point) = 1
Emitters.visual_size(::Point) = 1
Emitters.generate(o::Point, ::Int64) = o.origin
"""
RectUniform{T} <: AbstractOriginDistribution{T}
Encapsulates a uniformly sampled rectangle with user defined number of samples.
```julia
RectUniform(width::T, height::T, samples_count::Int64) where {T<:Real}
```
"""
struct RectUniform{T} <: AbstractOriginDistribution{T}
width::T
height::T
samples_count::Int64
rng::Random.AbstractRNG
function RectUniform(width::T, height::T, samples_count::Int64; rng=Random.GLOBAL_RNG) where {T<:Real}
return new{T}(width, height, samples_count, rng)
end
end
Base.length(o::RectUniform) = o.samples_count
Emitters.visual_size(o::RectUniform) = max(o.width, o.height)
# generate origin on the agrid
function Emitters.generate(o::RectUniform{T}, n::Int64) where {T<:Real}
n = mod(n, length(o))
u = rand(o.rng, Distributions.Uniform(-one(T), one(T)))
v = rand(o.rng, Distributions.Uniform(-one(T), one(T)))
return zero(Vec3{T}) + ((o.width / 2) * u * unitX3(T)) + ((o.height/2) * v * unitY3(T))
end
"""
RectGrid{T} <: AbstractOriginDistribution{T}
Encapsulates a rectangle sampled in a grid fashion.
```julia
RectGrid(width::T, height::T, usamples::Int64, vsamples::Int64) where {T<:Real}
```
"""
struct RectGrid{T} <: AbstractOriginDistribution{T}
width::T
height::T
usamples::Int64
vsamples::Int64
ustep::T
vstep::T
function RectGrid(width::T, height::T, usamples::Int64, vsamples::Int64) where {T<:Real}
return new{T}(width, height, usamples, vsamples, width / (usamples - 1), height / (vsamples - 1))
end
end
Base.length(o::RectGrid) = o.usamples * o.vsamples
Emitters.visual_size(o::RectGrid) = max(o.width, o.height)
# generate origin on the grid
function Emitters.generate(o::RectGrid{T}, n::Int64) where {T<:Real}
n = mod(n, length(o))
v = o.vsamples == 1 ? zero(T) : 2 * Int64(floor(n / o.usamples)) / (o.vsamples - 1) - 1.0
u = o.usamples == 1 ? zero(T) : 2 * mod(n, o.usamples) / (o.usamples - 1) - 1.0
return zeros(Vec3{T}) + ((o.width / 2) * u * unitX3(T)) + ((o.height/2) * v * unitY3(T))
end
"""
RectJitterGrid{T} <: AbstractOriginDistribution{T}
Encapsulates a rectangle sampled in a grid fashion with jitter.
```julia
RectGrid(width::T, height::T, ures::Int64, vres::Int64, samplesPerRegion::Int64) where {T<:Real}
```
"""
struct RectJitterGrid{T} <: AbstractOriginDistribution{T}
width::T
height::T
uResolution::Int64
vResolution::Int64
samplesPerRegion::Int64
ustep::T
vstep::T
rng::Random.AbstractRNG
function RectJitterGrid(width::T, height::T, ures::Int64, vres::Int64, samplesPerRegion::Int64; rng=Random.GLOBAL_RNG) where {T<:Real}
return new{T}(width, height, ures, vres, samplesPerRegion, width / ures, height / vres, rng)
end
end
Base.length(o::RectJitterGrid) = o.uResolution * o.vResolution * o.samplesPerRegion
Emitters.visual_size(o::RectJitterGrid) = max(o.width, o.height)
# generate origin on the grid
function Emitters.generate(o::RectJitterGrid{T}, n::Int64) where {T<:Real}
n = mod(n, length(o))
uu = rand(o.rng, Distributions.Uniform(zero(T), o.ustep))
vv = rand(o.rng, Distributions.Uniform(zero(T), o.vstep))
nn = Int64(floor(n / o.samplesPerRegion))
v = (o.vResolution == 1) ? zero(T) : Int64(floor(nn / o.uResolution))
u = (o.uResolution == 1) ? zero(T) : Int64(floor(mod(nn, o.uResolution)))
v = v * o.vstep + vv - (o.height / 2.0)
u = u * o.ustep + uu - (o.width / 2.0)
return zeros(Vec3{T}) + u * unitX3(T) + v * unitY3(T)
end
"""
Hexapolar{T} <: AbstractOriginDistribution{T}
Encapsulates an ellipse (or a circle where halfsizeu=halfsizev) sampled in an hexapolar fashion (rings).
```julia
Hexapolar(nrings::Int64, halfsizeu::T, halfsizev::T) where {T<:Real}
```
"""
struct Hexapolar{T} <: AbstractOriginDistribution{T}
halfsizeu::T
halfsizev::T
nrings::Int64
function Hexapolar(nrings::Int64, halfsizeu::T, halfsizev::T) where {T<:Real}
return new{T}(halfsizeu, halfsizev, nrings)
end
end
Base.length(o::Hexapolar) = 1 + round(Int64, (o.nrings * (o.nrings + 1) / 2) * 6)
Emitters.visual_size(o::Hexapolar) = max(o.halfsizeu*2, o.halfsizev*2)
function Emitters.generate(o::Hexapolar{T}, n::Int64) where {T<:Real}
n = mod(n, length(o))
if n == 0
return zeros(Vec3{T})
else
t = 1
ringi = 1
for i in 1:(o.nrings)
t += 6 * i
if n < t
ringi = i
break
end
end
ρ = ringi / o.nrings
pind = n - (t - 6 * ringi)
ϕ = (pind / (6 * ringi)) * 2π
u = cos(ϕ) * o.halfsizeu
v = sin(ϕ) * o.halfsizev
return zeros(Vec3{T}) + ρ * (u * unitX3(T) + v * unitY3(T))
end
end
end # module Origins
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 9926 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Sources
export Source, CompositeSource
using ....OpticSim
using ...Emitters
using ...Geometry
using ..Spectrum
using ..Directions
using ..Origins
using ..AngularPower
using LinearAlgebra
using Distributions
"""AbstractSource interface:
for your new type S{T}<:AbstractSource{T} you must define
Base.length(s::S) returns number of rays in the source s
Base.iterate(s::S) returns first ray and first state
Base.iterate(s::S, state) returns next ray and next state
origins(s::S) returns origins of the rays generated by the source s
transform(s::S) returns the transform associated with the source s
"""
abstract type AbstractSource{T} <: OpticSim.OpticalRayGenerator{T} end
Base.getindex(s::AbstractSource, index) = generate(s, index)
Base.firstindex(s::AbstractSource) = 0
Base.lastindex(s::AbstractSource) = length(s) - 1
Base.copy(a::AbstractSource) = a # most don't have any heap allocated stuff so don't really need copying
""" Simple ray generator that takes a vector of OpticalRay objects."""
struct RayListSource{T,N} <: AbstractSource{T}
rays::Vector{OpticalRay{T,N}}
end
Base.length(raylist::RayListSource) = length(raylist.rays)
Base.iterate(raylist::RayListSource) = length(raylist.rays) == 0 ? nothing : (raylist.rays[1],1)
Base.iterate(raylist::RayListSource, state) = state >= length(raylist.rays) ? nothing : (raylist.rays[state+1],state+1)
origins(raylist::RayListSource) = map(x->origin(x),raylist.rays)
transform(::RayListSource) = identitytransform()
"""
Source{T<:Real, Tr<:Transform{T}, S<:Spectrum.AbstractSpectrum{T}, O<:Origins.AbstractOriginDistribution{T}, D<:Directions.AbstractDirectionDistribution{T}, P<:AngularPower.AbstractAngularPowerDistribution{T}} <: AbstractSource{T}
This data-type represents the basic emitter (Source), which is a combination of a Spectrum, Angular Power Distribution, Origins and Directions distibution and a 3D Transform.
```julia
Source(::Type{T} = Float64;
transform::Tr = Transform(),
spectrum::S = Spectrum.Uniform(),
origins::O = Origins.Point(),
directions::D = Directions.Constant(),
power::P = AngularPower.Lambertian(),
sourcenum::Int64 = 0) where {
Tr<:Transform,
S<:Spectrum.AbstractSpectrum,
O<:Origins.AbstractOriginDistribution,
D<:Directions.AbstractDirectionDistribution,
P<:AngularPower.AbstractAngularPowerDistribution,
T<:Real}
Source(transform::Tr, spectrum::S, origins::O, directions::D, power::P, ::Type{T} = Float64; sourcenum::Int64 = 0) where {
Tr<:Transform,
S<:Spectrum.AbstractSpectrum,
O<:Origins.AbstractOriginDistribution,
D<:Directions.AbstractDirectionDistribution,
P<:AngularPower.AbstractAngularPowerDistribution,
T<:Real}
```
"""
struct Source{
T<:Real,
Tr<:Transform{T},
S<:Spectrum.AbstractSpectrum{T},
O<:Origins.AbstractOriginDistribution{T},
D<:Directions.AbstractDirectionDistribution{T},
P<:AngularPower.AbstractAngularPowerDistribution{T}
} <: AbstractSource{T}
transform::Tr
spectrum::S
origins::O
directions::D
power_distribution::P
sourcenum::Int64
function Source(
::Type{T} = Float64;
transform::Tr = Transform(T),
spectrum::S = Spectrum.Uniform(T),
origins::O = Origins.Point(T),
directions::D = Directions.Constant(T),
power::P = AngularPower.Lambertian(T),
sourcenum::Int64 = 0
) where {
Tr<:Transform,
S<:Spectrum.AbstractSpectrum,
O<:Origins.AbstractOriginDistribution,
D<:Directions.AbstractDirectionDistribution,
P<:AngularPower.AbstractAngularPowerDistribution,
T<:Real
}
new{T, Tr, S, O, D, P}(transform, spectrum, origins, directions, power, sourcenum)
end
function Source(
transform::Tr,
spectrum::S,
origins::O,
directions::D,
power::P,
::Type{T} = Float64;
sourcenum::Int64 = 0
) where {
Tr<:Transform,
S<:Spectrum.AbstractSpectrum,
O<:Origins.AbstractOriginDistribution,
D<:Directions.AbstractDirectionDistribution,
P<:AngularPower.AbstractAngularPowerDistribution,
T<:Real
}
new{T, Tr, S, O, D, P}(transform, spectrum, origins, directions, power, sourcenum)
end
end
origins(s::Source) = s.origins
transform(s::Source) = s.transform
# used to not generate new origin points if we can use last points - mainly to keep consistency of origin generation when randomness is involved
struct SourceGenerationState{T<:Real}
n::Int64
last_index_used::Int64
last_point_generated::Vec3{T}
end
Base.length(s::Source) = length(s.origins) * length(s.directions)
Base.iterate(s::Source{T}) where {T<:Real} = iterate(s, SourceGenerationState(1, -1, zeros(Vec3{T})))
Base.iterate(s::Source, state::SourceGenerationState) = state.n > length(s) ? nothing : generate(s, state)
function Emitters.generate(s::Source{T}, n::Int64) where {T<:Real}
return generate(s, SourceGenerationState(n+1, -1, zeros(Vec3{T})))[1]
end
function Emitters.generate(s::Source{T}, state::SourceGenerationState{T} = SourceGenerationState(-1, -1, zeros(Vec3{T}))) where {T<:Real}
# @info "New State Generation"
n::Int64 = state.n - 1
origin_index = floor(Int64, (n / length(s.directions)))
direction_index = mod(n, length(s.directions))
if (origin_index == state.last_index_used)
o = state.last_point_generated
else
o = generate(s.origins, origin_index)
o = s.transform * o
end
# o = generate(s.origins, origin_index)
d = generate(s.directions, direction_index)
# rotate the direction according to the orientation of the ray-originator
d = rotation(s.transform) * d
ray = OpticSim.Ray(o, d)
power, wavelength = generate(s.spectrum)
power = apply(s.power_distribution, s.transform, power, ray)
# return (OpticSim.Ray(o, d), SourceGenerationState(state.n+1, origin_index, o))
return (OpticSim.OpticalRay(ray, power, wavelength; sourcenum=s.sourcenum), SourceGenerationState(state.n+1, origin_index, o))
end
"""
CompositeSource{T} <: AbstractSource{T}
This data-type represents the composite emitter (Source) which is constructed with a list of basic or composite emitters and a 3D Transform.
```julia
CompositeSource(transform::Transform{T}, sources::Vector{<:AbstractSource}) where {T<:Real}
```
"""
struct CompositeSource{T} <: AbstractSource{T}
transform::Transform{T}
sources::Vector{<:AbstractSource}
uniform_length::Int
total_length::Int
start_indexes::Vector{Int}
function CompositeSource(transform::Transform{T}, sources::Vector{<:AbstractSource}) where {T<:Real}
lens = [length(src) for src in sources]
size1 = findmin(lens)[1]
size2 = findmax(lens)[1]
if (size1 == size2)
uniform_length = size1
start_indexes = Vector{Int}()
total_length = length(sources) * uniform_length
# @info "Uniform Length $size1 total=$total_length"
else
uniform_length = -1
start_indexes = Vector{Int}()
start = 0
for s in sources
push!(start_indexes, start)
start = start + length(s)
end
push!(start_indexes, start)
# @info start_indexes
total_length = start
end
new{T}(transform, sources, uniform_length, total_length, start_indexes)
end
end
transform(s::CompositeSource) = s.transform
Base.length(s::CompositeSource) = s.total_length
Base.iterate(s::CompositeSource{T}) where {T<:Real} = iterate(s, SourceGenerationState(1, -1, zeros(Vec3{T})))
Base.iterate(s::CompositeSource, state::SourceGenerationState) = state.n > length(s) ? nothing : generate(s, state)
function Emitters.generate(s::CompositeSource{T}, n::Int64) where {T<:Real}
return generate(s, SourceGenerationState(n+1, -1, zeros(Vec3{T})))[1]
end
function Emitters.generate(s::CompositeSource{T}, state::SourceGenerationState{T} = SourceGenerationState(-1, -1, zeros(Vec3{T}))) where {T<:Real}
# @info "New Composite State Generation"
n::Int64 = state.n - 1
if (s.uniform_length != -1)
source_index = floor(Int64, (n / s.uniform_length))
ray_index = mod(n, s.uniform_length)
# @info "New Composite State Generation: source=$source_index ray=$ray_index ($(length(s.sources)))"
else
n = mod(n, s.total_length)
## perform a binary search to find out which source can generate the spesific ray
low = 1
high = length(s.start_indexes) - 1 # last cell in this vector is a dummy cell containing the index after the last ray
source_index = -1
while (low <= high)
mid = floor(Int64, (low + high) / 2)
if (n < s.start_indexes[mid])
high = mid - 1
elseif (n >= s.start_indexes[mid+1])
low = mid + 1
else
source_index = mid
break;
end
end
@assert source_index != -1
# @info "Bin Search n=$n source_index=$source_index"
source_index = source_index - 1
ray_index = n - s.start_indexes[source_index+1]
end
ray, new_state = generate(s.sources[source_index+1], SourceGenerationState(ray_index+1, state.last_index_used, state.last_point_generated))
ray = s.transform * ray
return (ray, SourceGenerationState(state.n+1, new_state.last_index_used, new_state.last_point_generated))
end
end # module Sources
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4242 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Spectrum
export Uniform, DeltaFunction, Measured
using ....OpticSim
using ...Emitters
using DataFrames: DataFrame
using Distributions
import Unitful: Length, ustrip
using Unitful.DefaultSymbols
using Random
const UNIFORMSHORT = 0.450 #um
const UNIFORMLONG = 0.680 #um
abstract type AbstractSpectrum{T<:Real} end
"""
Uniform{T} <: AbstractSpectrum{T}
Encapsulates a flat spectrum range which is sampled uniformly. Unless stated diferrently, the range used will be 450nm to 680nm.
```julia
Uniform(low_end::T, high_end::T) where {T<:Real}
Uniform(::Type{T} = Float64) where {T<:Real}
```
"""
struct Uniform{T} <: AbstractSpectrum{T}
low_end::T
high_end::T
rng::Random.AbstractRNG
# user defined range of spectrum
function Uniform(low_end::T, high_end::T; rng=Random.GLOBAL_RNG) where {T<:Real}
return new{T}(low_end, high_end, rng)
end
# with no specific range we will use the constants' values
function Uniform(::Type{T} = Float64; rng=Random.GLOBAL_RNG) where {T<:Real}
return new{T}(UNIFORMSHORT, UNIFORMLONG, rng)
end
end
Emitters.generate(s::Uniform{T}) where {T<:Real} = (one(T), rand(s.rng, Distributions.Uniform(s.low_end, s.high_end)))
"""
DeltaFunction{T} <: AbstractSpectrum{T}
Encapsulates a constant spectrum.
```julia
DeltaFunction{T<:Real}
```
"""
struct DeltaFunction{T} <: AbstractSpectrum{T}
λ::T
end
DeltaFunction(λ::Length) = DeltaFunction{Float64}(ustrip(μm, λ))
Emitters.generate(s::DeltaFunction{T}) where {T<:Real} = (one(T), s.λ)
"""
Measured{T} <: AbstractSpectrum{T}
Encapsulates a measured spectrum to compute emitter power. Create spectrum by reading CSV files.
Evaluate spectrum at arbitrary wavelength with [`spectrumpower`](@ref) (**more technical details coming soon**)
```julia
Measured(samples::DataFrame)
```
"""
struct Measured{T} <: AbstractSpectrum{T}
low_wave_length::Int64
high_wave_length::Int64
wave_length_step::Int64
power_samples::Vector{T}
function Measured(samples::DataFrame)
colnames = names(samples)
@assert "Wavelength" in colnames
@assert "Power" in colnames
wavelengths = samples[!, :Wavelength]
@assert eltype(wavelengths) <: Int64
power::Vector{T} where {T<:Real} = samples[!, :Power] # no missing values allowed and must be real numbers
maxpower = maximum(power)
p = sortperm(wavelengths)
wavelengths = wavelengths[p]
power = power[p] ./ maxpower #make sure power values have the same sorted permutation as wavelengths normalized with maximum power equal to 1
λmin = wavelengths[p[1]]
λmax = wavelengths[p[end]]
step = wavelengths[2] - wavelengths[1]
for i in 3:length(wavelengths)
@assert wavelengths[i] - wavelengths[i - 1] == step # no missing values allowed and step size must be consistent
end
return new{T}(λmin, λmax, step, power)
end
end
function Emitters.generate(spectrum::Measured{T}) where {T<:Real}
λ = rand(Distributions.Uniform(convert(T, spectrum.low_wave_length), convert(T, spectrum.high_wave_length)))
power = spectrumpower(spectrum, λ)
if power === nothing #added this condition because the compiler was allocating if just returned values directly.
return (nothing, nothing)
else
return (power, λ / convert(T, 1000)) #convert from nm to um
end
end
"""expects wavelength in nm not um"""
function spectrumpower(spectrum::Measured{T}, λ::T) where {T<:Real}
if λ < spectrum.low_wave_length || λ > spectrum.high_wave_length
return nothing
end
lowindex = floor(Int64, (λ - spectrum.low_wave_length)) ÷ spectrum.wave_length_step + 1
if lowindex == length(spectrum.power_samples)
return convert(T, spectrum.power_samples[end])
else
highindex = lowindex + 1
α = mod(λ, spectrum.wave_length_step) / spectrum.wave_length_step
return convert(T, (1 - α) * spectrum.power_samples[lowindex] + α * spectrum.power_samples[highindex])
end
end
end # module Spectrum
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1958 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# These utility functions provide a simple interface to the Emitters package to create emitters that are commonly used in optical systems. Many optical systems by convention have their optical axis parallel to the z axis. These emitters direct the rays in the negative z direction, toward the entrance of the optical system.
"""
pointemitter(origin::AbstractVector{T}, coneangle; λ::Length = 500nm, numrays = 100) where {T<:Real}
Creates a point source with Lambertian emission power and cone distribution of rays, emitting in the -z direction. λ is
a unitful Length quantity, e.g., 550nm.
"""
function pointemitter(origin::AbstractVector{T}, coneangle; λ::Length = 500nm, numrays = 100) where {T<:Real}
@assert length(origin) == 3
return Sources.Source(
Geometry.identitytransform(T),
Spectrum.DeltaFunction(λ),
Origins.Point(Geometry.Vec3(origin)),
Directions.UniformCone(-Geometry.unitZ3(T), coneangle, numrays),
AngularPower.Lambertian()
)
end
"""
collimatedemitter(origin::AbstractVector{T}, halfsquaresize; λ::Length = 500nm, numrays = 100) where {T<:Real}
Creates a square collimated emitter, emitting rays in the -z direction. Rays are emitted on a square grid with
sqrt(numrays) on a side. λ can be a unitful quantity, e.g., 550nm, or a number. In the latter case the units are
implicitly microns.
"""
function collimatedemitter(origin::AbstractVector{T}, halfsquaresize; λ::Length = 500nm, numrays = 100) where {T<:Real}
samples = Int(round(sqrt(numrays)))
return Sources.Source(
Geometry.translation(origin...),
Spectrum.DeltaFunction(λ),
Origins.RectGrid(2 * halfsquaresize, 2 * halfsquaresize, samples, samples),
Directions.Constant(-Geometry.unitZ3(T)),
AngularPower.Lambertian()
)
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4732 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
Optimization interface consists of two functions `optimizationvariables` and `updateoptimizationvariables`.
`optimizationvariables` packs variables to be optimized into a vector.
`updateoptimizationvariables` receives a vector of variables and creates a new optical system with the variable values.
"""
module Optimization
using ..OpticSim
using ..OpticSim: detectorsize, temperature, pressure
"""
optimizationvariables(a::AxisymmetricOpticalSystem{T}) -> Vector{T}
Pack variables that have been marked to be optimized into a vector in a form suitable for the optimizer.
Variables are marked for optimization by having a true value in the `:OptimizeName` column, where `Name` can be `Radius`, `Thickness` or `Conic`.
"""
function optimizationvariables(a::AxisymmetricOpticalSystem{T}) where {T<:Real}
prescription = a.prescription
radiusvariables = in(:OptimizeRadius, propertynames(prescription)) ? prescription[prescription[!, :OptimizeRadius], :Radius] : []
radiuslower = [typemin(T) for _ in 1:length(radiusvariables)]
radiusupper = [typemax(T) for _ in 1:length(radiusvariables)]
thicknessvariables = in(:OptimizeThickness, propertynames(prescription)) ? prescription[prescription[!, :OptimizeThickness], :Thickness] : []
thicknesslower = [zero(T) for _ in 1:length(thicknessvariables)] # TODO
thicknessupper = [T(100) for _ in 1:length(thicknessvariables)] # TODO
conicvariables = in(:OptimizeConic, propertynames(prescription)) ? prescription[prescription[!, :OptimizeConic], :Conic] : []
coniclower = [typemin(T) for _ in 1:length(radiusvariables)]
conicupper = [typemax(T) for _ in 1:length(radiusvariables)]
#convert to Vector{T} because may have missing values and would otherwise get Array{Union{Missing,Float64}} which optimization routines won't like.
return Vector{T}(vcat(radiusvariables, thicknessvariables, conicvariables)), Vector{T}(vcat(radiuslower, thicknesslower, coniclower)), Vector{T}(vcat(radiusupper, thicknessupper, conicupper))
end
"""
updateoptimizationvariables(a::AxisymmetricOpticalSystem{T}, optimizationvariables::Vector{S}) -> AxisymmetricOpticalSystem{S}
Creates a new optical system with updated variables corresponding to the optimization variables.
"""
function updateoptimizationvariables(a::AxisymmetricOpticalSystem{T}, optimizationvariables::Vector{S}) where {T<:Real,S<:Real}
prescription = a.prescription
#assume these two types of prescription variables will always be present
prescradii = prescription[!, :Radius]
prescthicknesses = prescription[!, :Thickness]
@assert reduce(&, map((x) -> !isnan(x), optimizationvariables)) #make sure no optimization variables are NaN
conic = in(:Conic, propertynames(prescription)) ? prescription[!, :Conic] : nothing
radii = Vector{Union{Missing,S}}(undef, 0)
thicknesses = Vector{Union{Missing,S}}(undef, 0)
newconic = Vector{Union{Missing,S}}(undef, 0)
optindex = 1
if in(:OptimizeRadius, propertynames(prescription))
radiusflags = prescription[!, :OptimizeRadius]
for index in eachindex(radiusflags)
if radiusflags[index]
push!(radii, optimizationvariables[optindex])
optindex += 1
else
push!(radii, prescradii[index])
end
end
end
if in(:OptimizeThickness, propertynames(prescription))
thicknessflags = prescription[!, :OptimizeThickness]
for index in eachindex(thicknessflags)
if thicknessflags[index]
push!(thicknesses, optimizationvariables[optindex])
optindex += 1
else
push!(thicknesses, prescthicknesses[index])
end
end
end
if conic !== nothing
if in(:OptimizeConic, propertynames(prescription))
conicflags = prescription[!, :OptimizeConic]
for index in eachindex(conicflags)
if conicflags[index]
push!(newconic, optimizationvariables[optindex])
optindex += 1
else
push!(newconic, conic[index])
end
end
end
end
newprescription = copy(prescription)
newprescription.Radius = radii
newprescription.Thickness = thicknesses
if conic !== nothing
newprescription.Conic = newconic
end
return AxisymmetricOpticalSystem{S}(newprescription, detectorsize(a)..., S, temperature = S(temperature(a)), pressure = S(pressure(a)))
end
end #module
export Optimization
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1475 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
struct UnitCell
coordinateframe #this should be computed once when the UnitCell is created. It incorporates the parent coordinateframe transformation and the transformation from the parent to the unit cell.
emitter
# lens::LensAssembly
detectors #have multiple eye positions that get computed all at once. Maybe. Lots of space so might be inefficient.
end
""" coordinateframe is a coordinate frame defined at a point in the repeating structure which all other positions will be measured with respect to. Used to define a globally consistent coordinate frame that pixels in each unit call map to."""
struct RepeatingStructure{N}
coordinateframe #need to think about how to define this. Maybe using a Transform?
cells::SVector{N,UnitCell} #may want to access cells not just by vector index, i.e., x,y, or something more complicated for hexagonal systems.
end
""" Maps a pixel position from an emitter in a unit cell into a globally consistent angular coordinate frame with coordinates (θ,ϕ). This allows the contributions from multiple cells to be summed correctly."""
function globalcoordinates(px,py,cell::UnitCell,repeat::RepeatingStructure)::NamedTuple{(:θ,:ϕ),Tuple{Real,Real}}
#result will be like this (θ = val1, ϕ = val2)
end
function rectangulararray(nrows,ncols)
end
function hexagonalarray()
end | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 11232 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""A Cluster is a repeating pattern of indices defined in a lattice called the element lattice. The cluster of elements has its own lattice, which by definition is different from the element lattice unless each cluster consists of a single element. A LatticeCluster subtype must implement these methods:
clusterbasis(a::AbstractLatticeCluster) returns the AbstractBasis object for the cluster. This is not generally the same as the basis for the underlying lattice.
elementbasis(a::AbstractLatticeCluster) returns the AbstractBasis object the underlying lattice of the cluster.
clusterelements(a::AbstractLatticeCluster) returns the lattice indices, represented in the underlying lattice basis, for each of the elements in a unit cluster cell.
clustersize(a::AbstractLatticeCluster) returns the number of elements in a unit cluster cell.
For example this defines a Cluster of three hexagonal elements:
```
julia> function hex3cluster()
clusterelts = SVector((0,0),(-1,0),(-1,1))
eltlattice = HexBasis1()
clusterbasis = LatticeBasis(( -1,2),(2,-1))
return LatticeCluster(clusterbasis,eltlattice,clusterelts)
end
julia> lattice = hex3cluster()
LatticeCluster{3, 2, Float64, LatticeBasis{2, Int64}, HexBasis1{2, Float64}}(LatticeBasis{2, Int64}([-1 2; 2 -1]), HexBasis1{2, Float64}(), [(0, 0), (-1, 0), (-1, 1)])
julia> lattice[1,1] #returns the locations of the 3 elements in the cluster at the cluster offset of 1,1
2×3 SMatrix{2, 3, Float64, 6} with indices SOneTo(2)×SOneTo(3):
1.0 -0.5 1.0
1.0 0.133975 -0.732051
```
"""
abstract type AbstractLatticeCluster{N1,N} end
"""returns the euclidean distance between lattice clusters (norm of the longest lattice basis vector). The companion function `latticediameter` returns the distance in lattice space which does not take into account the size the element basis underlying the cluster."""
euclideandiameter(a::AbstractLatticeCluster) = euclideandiameter(elementbasis(a))*latticediameter(a)
export euclideandiameter
"""Basic lattic cluster type. N1 is the number of tiles in the cluster, N is the dimension."""
struct LatticeCluster{N1, N, T<:Real, B1<:AbstractBasis{N,Int},B2<:AbstractBasis{N,T}} <: AbstractLatticeCluster{N1,N}
clusterbasis::B1 #this basis defines the offsets of the clusters
elementbasis::B2 #this basis defines the underlying lattice
clusterelements::SVector{N1,NTuple{N,Int64}} #vector containing the lattice indices of the elements in the cluster. Each column is one lattice coordinate. These indices are assumed to be offsets from the origin of the lattice.
LatticeCluster(clusterbasis::B1,eltlattice::B2,clusterelements::SVector{N1,NTuple{N,Int64}}) where{N1,N,T<:Real,B1<:AbstractBasis{N,Int64},B2<:AbstractBasis{N,T}} = new{N1,N,T,B1,B2}(clusterbasis,eltlattice,clusterelements)
end
export LatticeCluster
LatticeCluster(clusterbasis::B1,eltlattice::B2,clusterelements::SMatrix{N,N1,Int64}) where{N1,N,T<:Real,B1<:AbstractBasis{N,Int64},B2<:AbstractBasis{N,T}} = LatticeCluster(clusterbasis,eltlattice,SVector{N1}(reinterpret(reshape,NTuple{N,Int64},clusterelements)))
clusterelements(a::LatticeCluster) = a.clusterelements
export clusterelements
elementbasis(a::LatticeCluster) = a.elementbasis
export elementbasis
clustersize(a::LatticeCluster) = length(a.clusterelements)
clusterbasis(a::LatticeCluster) = a.clusterbasis
export clusterbasis
""" Lattice clusters have integer basis vectors. These represent the lattice in unit steps of the underlying element basis, not the Euclidean distance between lattice cluster centers. For example, the lattice cluster basis vectors for a hex3 lattice are (-1,2),(2,-1), where the units of the basis vectors represent steps in the underlying element basis. To get a Euclidean distance measure between cluster centers you need to multiply by the size of the element basis diameter. In the case of lenslet layout you need to multiply the cluster diameter by the lenslet diameter."""
latticediameter(a::Repeat.AbstractLatticeCluster) = euclideandiameter(Repeat.basismatrix(Repeat.clusterbasis(a)))
export latticediameter
"""returns the positions of every element in a cluster given the cluster indices"""
function Base.getindex(A::LatticeCluster{N1,N,T,B1,B2}, indices::Vararg{Int, N}) where{N1,N,T,B1<:AbstractBasis{N,Int},B2<:AbstractBasis{N,T}}
clusteroffset = A.clusterbasis[indices...]
temp = MMatrix{N,N1,T}(undef)
for i in 1:N1
temp[:,i] = A.elementbasis[A.clusterelements[i]...] + clusteroffset
end
return SMatrix{N,N1,T}(temp) #positions of the cluster elements, not the lattice coordinates.
end
"""Can access any cluster in a cluster lattice so the range of indices is unlimited"""
function Base.size(::LatticeCluster{N1,N}) where{N1,N}
return ntuple((i)->Base.IsInfinite(),N)
end
"""returns the integer coordinates of the tile at tileindex in the cluster at for a cluster with location cᵢ,cⱼ"""
function tilecoordinates(cluster::LatticeCluster{N1,N},cᵢ,cⱼ,tileindex) where{N1,N}
@assert tileindex <= N1
clustercenter = basismatrix(clusterbasis(cluster))*SVector(cᵢ,cⱼ)
tileoffset = cluster.clusterelements[tileindex]
return clustercenter .+ tileoffset
end
export tilecoordinates
Base.setindex!(A::AbstractBasis{N}, v, I::Vararg{Int, N}) where{N} = nothing #can't set lattice points. Might want to throw an exception instead.
""" returns the lattice indices of the elements in the cluster. These are generally not the positions of the elements"""
function clustercoordinates(a::LatticeCluster{N1,N},indices::Vararg{Int,N}) where{N1,N}
clusteroffset = a.clusterbasis[indices...]
temp = MMatrix{N,N1,Int}(undef)
for i in 1:N1
temp[:,i] = SVector{N,Int}(a.clusterelements[i]) + clusteroffset
end
return SMatrix{N,N1,Int}(temp) #the lattice coordinates in the underlying element basis of the cluster elements
end
export clustercoordinates
"""Given the (i,j) coordinates of a tile defined in the the underlying lattice basis of elements of the cluster compute the coordinates (cᵢ,cⱼ) of the cluster containing the tile, and the tile number of the tile in that cluster"""
function cluster_coordinates_from_tile_coordinates(cluster::S,i::Int,j::Int) where{N1,N, S<:AbstractLatticeCluster{N1,N}}
found = false
bmatrix = Rational.(basismatrix(clusterbasis(cluster)))
local clustercoords::SVector{}
tileindex = 0 #initialize to illegal value
for coords in eachcol(clustercoordinates(cluster, 0,0)) #don't know which tile in the cluster the i,j coords come from so try all of them until find one that yields an integer value for the cluster coordinate.
tileindex += 1
possiblecenter = SVector{N,Rational}((i,j)) .- Rational.(coords)
clustercoords = bmatrix \ possiblecenter
if all(1 .== denominator.(clustercoords)) #If coordinates are integer this is the correct cluster value. If coordinates are not integer keep trying.
found = true #every tile position i,j corresponds to some cluster (cᵢ,cⱼ) so will always find an answer
break
end
end
@assert found == true #should always find a cluster corresponding to any i,j. If not then something is seriously wrong.
return Int64.(clustercoords),tileindex #return coordinates of the cluster and the index of the tile within that cluster
end
export cluster_coordinates_from_tile_coordinates
cluster_coordinates_from_tile_coordinates(cluster::S,coords::NTuple{2,Int64}) where{N1,N, S<:AbstractLatticeCluster{N1,N}} = cluster_coordinates_from_tile_coordinates(cluster,coords...)
abstract type ClusterColors end
abstract type MonochromeCluster <: ClusterColors end
abstract type RGBCluster <: ClusterColors end
"""
May want to have many properties associated with the elements in a cluster, which is why properties is represented as a DataFrame. The DataFrame in the properties field should have as many rows as there are elements in a cluster. At a minimum it must have a :Color and a :Name column.
Clusters can be type tagged as being either MonochromeCluster or RGBCluster. If you are using the lenslet assignment functions in Multilens
then you should explicitly include an RGB type if the cluster has RGB lenslets.
Example: a lattice cluster of three hexagonal elements, labeled R,G,B with colors red,green,blue.
```
function hex3RGB()
clusterelements = SVector((0,0),(-1,0),(-1,1)) #lattice indices of cluster elements represent in the element lattice basis
colors = [colorant"red",colorant"green",colorant"blue"]
names = ["R","G","B"]
eltlattice = HexBasis1()
clusterbasis = LatticeBasis(( -1,2),(2,-1)) #lattice indices, in the element lattice basis, representing the repeating pattern of the cluster
lattice = LatticeCluster(clusterbasis,eltlattice,clusterelements)
properties = DataFrame(Color = colors, Name = names)
return ClusterWithProperties{RGBCluster}(lattice,properties) #NOTE: this is an RGB cluster so it has been explicitly tagged with an RGBCluster type.
end
```
"""
struct ClusterWithProperties{N1,N,T,Q<:ClusterColors} <: AbstractLatticeCluster{N1,N}
cluster::LatticeCluster{N1,N,T}
properties::DataFrame
ClusterWithProperties(cluster::L,properties::D) where{L<:LatticeCluster,D<:DataFrame} = ClusterWithProperties{MonochromeCluster}(cluster,properties)
ClusterWithProperties{Q}(cluster::L,properties::D) where{N1,N,T,Q<:ClusterColors,L<:LatticeCluster{N1,N,T},D<:DataFrame} = new{N1,N,T,Q}(cluster,properties)
end
export ClusterWithProperties
Base.getindex(A::ClusterWithProperties{N1,N,T}, indices::Vararg{Int,N}) where{N1,N,T} = cluster(A)[indices...]
Base.setindex!(A::ClusterWithProperties, v, I::Vararg{Int, N}) where{N} = nothing #can't set lattice points. Might want to throw an exception instead.
properties(a::ClusterWithProperties) = a.properties
export properties
cluster(a::ClusterWithProperties) = a.cluster
export cluster
clustercoordinates(a::ClusterWithProperties,indices...) = clustercoordinates(cluster(a),indices...)
elementbasis(a::ClusterWithProperties) = elementbasis(cluster(a))
export elementbasis
clustersize(a::ClusterWithProperties) = clustersize(a.cluster)
export clustersize
clusterbasis(a::ClusterWithProperties) = clusterbasis(a.cluster)
export clusterbasis
"""return 3 Vectors, call them r,g,b. The r tuple contains the indices of all tiles colored red, g contains those colored green, etc. Not efficient but shouldn't be in a critical inner loop."""
function rgbtileindices(a::ClusterWithProperties{A,B,C,RGBCluster}) where{A,B,C}
red = Vector{Int64}(undef,0)
green = Vector{Int64}(undef,0)
blue = Vector{Int64}(undef,0)
colors = properties(a)[:,:Color]
for (i,color) in enumerate(colors)
if color == "red"
push!(red,i)
elseif color == "green"
push!(green,i)
elseif color == "blue"
push!(blue,i)
else
throw(Error("only handles the three colors red, green, and blue. Found another color."))
end
end
return red,green,blue
end
export rgbtileindices
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6049 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
const sin60 = .5*sqrt(3)
const cos60 = .5
"""coordinates of a unit hexagon tile polygon with unit length sides centered about the point (0,0)"""
const hexcoords = [
1 0;
cos60 -sin60;
-cos60 -sin60;
-1 0;
-cos60 sin60;
cos60 sin60
]
"""`scale` will scale the canonical basis vectors of this hexagonal tiling"""
struct HexBasis1{N,T} <: AbstractBasis{N,T}
scale::T
HexBasis1(scale::T = 1.0) where{T<:Real} = new{2,T}(scale)
end
export HexBasis1
scale(a::HexBasis1) = a.scale
basismatrix(a::HexBasis1{2,T}) where{T} = scale(a) * SMatrix{2,2,T}(T(1.5),T(.5)*sqrt(T(3)),T(1.5),T(-.5)*(sqrt(T(3))))
# SVector{2,SVector{2,T}}(hexe₁(T),hexe₂(T))
"""Returns the vertices of the unit tile polygon for the basis"""
function tilevertices(a::HexBasis1{2,T}) where{T}
sin60 = T(.5)*sqrt(T(3))
cos60 = T(.5)
return scale(a)*SMatrix{2,6}(
1, 0,
cos60, -sin60,
-cos60, -sin60,
-1, 0,
-cos60, sin60,
cos60, sin60)
end
export tilevertices
# coordinate offsets to move up, down, etc., numsteps hex cells in a hex lattice defined in HexBasis1
hexup(numsteps = 1) = numsteps .* (1,-1)
hexdown(numsteps = 1) = numsteps .* (-1,1)
hexupright(numsteps = 1) = numsteps .* (1,0)
hexdownleft(numsteps = 1) = numsteps .* (-1,0)
hexdownright(numsteps = 1) = numsteps .* (0,1)
hexupleft(numsteps = 1) = numsteps .* (0,-1)
""" (i,j) offsets of a ring represented in the HexBasis1 lattice basis. The hexagonal grid is assumed to start from the bottom center hex cell. To generate larger rings repeat this pattern
ring 1 = (1, 0) (1, -1) (0, -1) (-1, 0) (-1, 1) (0, 1)
ring 2 = (1, 0)(1, 0)(1, -1)(1, -1)(0, -1)(0, -1)(-1, 0)(-1, 0)(-1, 1)(-1, 1)(0, 1)(0, 1)
"""
const hexcycle = SVector{6,NTuple{2,Int64}}(hexupright(),hexup(),hexupleft(),hexdownleft(),hexdown(),hexdownright())
"""returns (i,j) offsets of ring n defined in the HexBasis1 lattice basis."""
ringoffsets(n::Int64) = ringoffsets(Val{n})
function ringoffsets(::Type{Val{N}}) where N
temp = MVector{N*6,NTuple{2,Int64}}(undef)
for i in 1:6
for j in 1:N
temp[(i-1)*N + j] = hexcycle[i]
end
end
return SVector{N*6,NTuple{2,Int64}}(temp)
end
export ringoffsets
"""Returns ```centerpoint``` plus all its neighbors in a ring of size n. This is a hexagonal region of the lattice n times as large as the unit hexagon cell.
Example:
```
Vis.@wrapluxor Vis.drawhexcells(50, hexregion((0,0),2))
```
"""
function region(::Type{HexBasis1},centerpoint::Tuple{Int64,Int64}, n::Int64)
f(i) = i==0 ? () : (neighbors(HexBasis1,centerpoint,i)...,f(i-1)...)
cells = (centerpoint...,f(n)...)
numcells = length(cells) ÷ 2
# convert to matrix form since that is what the drawing code expects
temp = MMatrix{2,numcells,Int64}(undef)
for i in 1:numcells
temp[1,i] = cells[2*i-1]
temp[2,i] = cells[2*i]
end
return SMatrix{2,numcells,Int64}(temp)
end
export region
"""Returns all hex cells contained in the ring of size n centered around centerpoint. Use region if you want all the cells contained in the ring of size n."""
function neighbors(::Type{HexBasis1}, centerpoint::Tuple{Int64,Int64},n::Int) where{T}
temp = MMatrix{2,n*6,Int64}(undef)
hoffsets = ringoffsets(Val{n})
latticepoint = centerpoint .+ n .* (hexdown())
#convert hexoffsets to absolute coordinate positions
for i in 1:size(temp)[2]
temp[1,i] = latticepoint[1]
temp[2,i] = latticepoint[2]
latticepoint = latticepoint .+ hoffsets[i] #last computed value of latticepoint won't be used
end
return SMatrix{2,n*6,Int64}(temp)
end
export neighbors
function xbounds(numi)
numevens = div(numi,2)
numodds = numevens + mod(numi,2)
basewidth = numodds + 2*numevens + 1
if iseven(numi)
maxx = basewidth
else
maxx = basewidth + sin(deg2rad(30))
end
minx = -maxx
return (minx,maxx)
end
function ybounds(numj)
maxy = 2*sin60*(numj + 1)
return (-maxy,maxy-sin60)
end
"""computes bounding box of hex tiles arranged in an approximately rectangular layout, with 2*numj+1 hexagons in a row, and 2*numi+1 hexagons in a column"""
function bbox(numi,numj)
return (xbounds(numi),ybounds(numj))
end
export bbox
"""computes the basis coordinates of hexagonal cells defined in the Hex1Basis in a rectangular pattern 2*numi+1 by 2*numj+1 wide and high respectively."""
function hexcellsinbox(numi,numj)
#i2 and j2 are coordinates in the basis (1.5,.5√3),(0,-√3). The conditional tests are simpler in this basis. Convert to Hex1Basis1 coordinates before returning.
hexbasis2to1(i,j) = [i+j,-j]
result = Matrix{Int}(undef,2,(2*numi+1)*(2*numj+1))
for i2 in -numi:numi
let offsetj
if i2 < 0
offsetj = -abs(div(i2,2))
else
offsetj = div(i2+1,2)
end
for j2 in -numj:numj
# conversion from Hex2Basis i2,j2 to Hex1Basis i,j is
# (i,j) = (i2,0) + j2*(1,-1)
jtemp = j2-offsetj
# i1 = i2 + jtemp
# j1 = -jtemp
result[:,(i2+numi)*(2*numj+1) + j2+numj+1] = hexbasis2to1(i2,jtemp)
end
end
end
return result
end
export Repeat
"""`scale` will scale the canonical basis vectors of this hexagonal tiling"""
struct HexBasis3{N,T}<:AbstractBasis{N,T}
scale::T
HexBasis3(scale::T = 1.0) where{T<:Real} = new{2,T}(scale)
end
export HexBasis3
scale(a::HexBasis3) = a.scale
function tilevertices(a::HexBasis3{2,T}) where{T}
sin60 = T(.5)*sqrt(T(3))
cos60 = T(.5)
return scale(a) * SMatrix{2,6,T}(
0, 1,
-sin60, cos60,
-sin60, -cos60,
0, -1,
sin60, -cos60,
sin60, cos60)
end
basismatrix(a::HexBasis3{2,T}) where{T} = scale(a)*SMatrix{2,2,T}(T(2*sin60),T(0),T(sin60),T(1.5))
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 8355 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
""" Base class for defining points on a lattice. Lattice points are defined by basis vectors eᵢ and lattice indices indexᵢ:
point = Σᵢ indexᵢ*eᵢ
Example: define a 2D basis.
```julia
julia> a = LatticeBasis([1,2],[3,4])
LatticeBasis{2, Int64}(SVector{2, Int64}[[1, 2], [3, 4]])
```
Array indexing is used to generate a lattice point: a[1,2] = 1*[1,2] + 2*[3,4]
```julia
julia> a[1,2]
2-element SVector{2, Int64} with indices SOneTo(2):
7
10
```
Subtypes supporting the AbstractBasis interface should implement these functions:
Returns the neighbors in ring n surrounding centerpoint, excluding centerpoint
```
neighbors(::Type{B},centerpoint::Tuple{T,T},neighborhoodsize::Int) where{T<:Real,B<:AbstractBasis}
```
Returns the lattice basis vectors that define the lattice
```
basis(a::S) where{S<:AbstractBasis}
```
Returns the vertices of the unit polygon that tiles the plane for the basis
```
tilevertices(a::S) where{S<:AbstractBasis}
```
"""
abstract type AbstractBasis{N,T <: Real} end
export AbstractBasis
function Base.getindex(A::B1, indices::Vararg{Int,N}) where {N,T,B1 <: AbstractBasis{N,T}}
return basismatrix(A) * SVector{N,Int}(indices)
end
# temp::SVector{N,T} = (basis(A)*SVector{N,Int}(indices))::SVector{N,T}
# return temp
# end
Base.setindex!(A::AbstractBasis{N,T}, v, I::Vararg{Int,N}) where {T,N} = nothing # can't set lattice points. Might want to throw an exception instead.
"""computes the tile vertices at the offset lattice coordinate."""
tilevertices(latticecoords::Union{AbstractVector,NTuple},lattice::AbstractBasis) = lattice[latticecoords...] .+ tilevertices(lattice)
struct LatticeBasis{N,T <: Real} <: AbstractBasis{N,T}
basisvectors::SMatrix{N,N,T}
""" Convenience constructor that lets you use tuple arguments to describe the basis instead of SVector
Example:
```
basis = LatticeBasis{2}((1.0,0.0),(0.0,1.0)) #basis for rectangular lattice
```
This allocates 48 bytes for a 2D basis in Julia 1.6.2. It shouldn't allocate anything but not performance critical.
"""
function LatticeBasis(vectors::Vararg{NTuple{N,T},N}) where {T,N}
temp = MMatrix{N,N,T}(undef)
for (j, val) in pairs(vectors)
for i in 1:N
temp[i,j] = val[i]
end
end
return new{N,T}(SMatrix{N,N,T}(temp))
end
LatticeBasis(vectors::SMatrix{N,N,T}) where {N,T <: Real} = new{N,T}(vectors)
end
export LatticeBasis
function basismatrix(a::LatticeBasis{N,T})::SMatrix{N,N,T,N * N} where {N,T}
return a.basisvectors
end
"""Can access any point in a lattice so the range of indices is unlimited"""
function Base.size(a::LatticeBasis{N,T}) where {N,T}
return ntuple((i) -> Base.IsInfinite(), N)
end
# These functions are used to find the lattice tiles that lie inside a polygonal shape
"""computes the matrix that transforms the basis set into the canonical basic vectors, eᵢ"""
basisnormalization(a::Repeat.AbstractBasis) = Matrix(inv(Repeat.basismatrix(a))) # unfortunately LazySets doesn't work with StaticArrays. If you return a static Array is messes with their functions.
"""warp the matrix into a coordinate frame where the basis vectors are canonical, eᵢ"""
function normalizedshape(a::Repeat.AbstractBasis, shape::LazySets.VPolygon)
normalizer = basisnormalization(a)
return normalizer * shape
end
export normalizedshape
""" compute a conservative range of lattice indices that might intersect containingshape"""
function latticebox(containingshape::LazySets.VPolygon, lattice::Repeat.AbstractBasis)
warpedshape = normalizedshape(lattice, containingshape)
box = LazySets.interval_hull(warpedshape)
return LazySets.Hyperrectangle(round.(box.center), (1, 1) .+ ceil.(box.radius)) # center the hyperrectangle on an integer point and enlarge the radius to take this into account
end
export latticebox
"""Returns the lattice coordinates of all tiles that lie at least partly inside containingshape.
Compute the lattice tiles with non-zero intersection with containingshape. First compute a transformation that maps the lattice basis vectors to canonical unit basis vectors eᵢ (this is the inverse of the lattice basis matrix). Then transform containingshape into this coordinate frame and compute a bounding box. Unit steps along the coordinate axes in this space represent unit *lattice* steps in the original space. This makes it simple to determine coordinate bounds in the original space. Then test for intersection in the original space."""
function tilesinside(containingshape::LazySets.VPolygon, lattice::Repeat.AbstractBasis{2,T}) where {T}
coordtype = Int64
box = latticebox(containingshape, lattice)
coords = coordtype.(box.radius)
tilevertices = LazySets.VPolygon(Matrix(Repeat.tilevertices(lattice))) # VPolygon will accept StaticArrays but other LazySets function will barf.
result = Matrix{coordtype}(undef, 2, 0)
for i in -coords[1]:coords[1]
for j in -coords[2]:coords[2]
offsetcoords = (Int64.(box.center) + [i,j])
center = Vector(lattice[offsetcoords...]) #[] indexer for lattices returns SVector which LazySets doesn't handle. Convert to conventional Vector.
offsethex = LazySets.translate(tilevertices, center)
if !isempty(offsethex ∩ containingshape)
result = hcat(result, offsetcoords)
end
end
end
return result
end
export tilesinside
"""The vertices of the containing shape are the columns of the matrix containingshape"""
tilesinside(containingshape::AbstractMatrix,lattice::Repeat.AbstractBasis) = tilesinside(LazySets.VPolygon(containingshape), lattice)
tilesinside(xmin::T,ymin::T,xmax::T,ymax::T,lattice::Repeat.AbstractBasis) where {T <: AbstractFloat} = tilesinside(SMatrix{2,4,T}(xmin, ymin, xmin, ymax, xmax, ymax, xmax, ymin), lattice)
using Plots
""" to see what the objects look like in the warped coordinate frame use inv(basismatrix(lattice)) as the transform"""
function plotall(containingshape, lattice, transform=[1.0 0.0;0.0 1.0])
temp = Vector{SMatrix}(undef, 0)
for coordinate in eachcol(tilesinside(containingshape, lattice))
println(typeof(coordinate))
push!(temp, tilevertices(coordinate, lattice))
end
tiles = LazySets.VPolygon.(temp)
for tile in tiles
plot!(transform * tile, aspectratio=1)
end
plot!(containingshape, aspectratio=1)
end
export plotall
function testtilesinside()
# poly = LazySets.VPolygon([-3.0 3.0 3.0; -3.0 3.0 -3.0])
hex = HexBasis1()
poly = LazySets.VPolygon(3 * tilevertices(hex))
tilecoords = tilesinside(poly, hex)
insidetiles = LazySets.VPolygon.([tilevertices(x,hex) for x in eachcol(tilecoords)])
# plotall(poly, hex)
for tile in insidetiles
plot!(tile)
end
end
export testtilesinside
"""This function returns the radius of the longest basis vector of the lattice cluster. Most lattices defined in this project have symmetric basis vectors so the radii of all basis vectors will be identical. This function is used in determing which clusters "fit" within the eye pupil."""
function euclideandiameter(basismatrix::SMatrix)
maximum(norm.([basismatrix[:,i] for i in 1:size(basismatrix)[2]]))
end
export euclideandiameter
""" Lattic bases can have real basis vectors. This returns the maximum Euclidean distance between lattice basis center points."""
euclideandiameter(a::Repeat.AbstractBasis) = euclideandiameter(Repeat.basismatrix(a))
"""Only will work properly if lattice basis matrix contains only integer or rational terms. Returns the integer lattice coords of point in the given basis if the point is in the span of latticebasis. Otherwise returns nothing"""
function latticepoint(lattice_basis_matrix::AbstractMatrix, origin, point)
Ainv = inv(Rational.(lattice_basis_matrix))
b = [(point .- origin)...]
x = Ainv * b
if reduce(&, (1, 1) .== denominator.(x))
return Integer.(x)
else
return nothing
end
end
export latticepoint
latticepoint(latticebasis::AbstractBasis,origin,point) = latticepoint(basismatrix(latticebasis),origin,point)
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6591 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""Encapsulates the lens assembly and the display for one lenslet."""
struct Display{T} <: OpticSim.Surface{T}
surface::OpticSim.Rectangle{T}
pixellattice::LatticeBasis{2,T}
pixelpitch::Tuple{T,T} # x,y pitch of pixels
xresolution::T
yresolution::T
transform::OpticSim.Geometry.Transform{T}
function Display(xres::Int64, yres::Int64, xpitch::Unitful.Length, ypitch::Unitful.Length, transform::OpticSim.Geometry.Transform{T}) where {T <: Real}
# convert xpitch,ypitch to unitless numbers representing μm
pitchx = Unitful.ustrip(Unitful.μm, xpitch)
pitchy = Unitful.ustrip(Unitful.μm, ypitch)
size = (xres * pitchx, yres * pitchy)
surface = OpticSim.Rectangle(size[1] / T(2), size[2] / T(2), SVector(T(0), T(0), T(1)), SVector(T(0), T(0), T(0))) # surface normal is +z axis
pixellattice = Repeat.rectangularlattice(size[2], size[1]) # lattice takes ypitch,xpitch in that order
return new{T}(surface, pixellattice, (pitchx, pitchy), xres, yres, transform)
end
end
surfaceintersection(a::Display) = surfaceintersection(a.surface)
normal(a::Display) = normal(a.surface)
interface(a::Display) = interface(a.surface)
makemesh(a::Display) = makemesh(a.surface)
uv(a::Display,p::SVector{3,T}) where {T <: Real} = uv(a.surface, p)
onsurface(a::Display, point::SVector{3,T}) where {T <: Real} = onsurface(a.surface, point)
pixelposition(xindex::Int,yindex::Int) = transform * pixellattice[yindex,xindex]
struct LensletAssembly{T}
lens::OpticSim.ParaxialLens{T}
transform::OpticSim.Geometry.Transform{T}
display::Display{T}
worldtodisplay::OpticSim.Geometry.Transform{T}
LensletAssembly(lens::OpticSim.ParaxialLens{T}, transform::OpticSim.Geometry.Transform{T}, display::Display{T}) where {T} = new{T}(lens, transform, display, display.transform * transform)
end
lens(a::LensletAssembly) = a.lens
display(a::LensletAssembly) = a.display
transform(a::LensletAssembly) = a.transform
worldtolens(a::LensletAssembly, pt::SVector{3}) = a.transform * pt
worldtolens(a::LensletAssembly,mat::SMatrix{3}) = a.transform * mat
lenstodisplay(a::LensletAssembly,pt::SVector{3,T}) where {T <: Real} = a.display.transform * pt
worldtodisplay(a::LensletAssembly,pt::SVector{3,T}) where {T <: Real} = a.worldtodisplay * pt
vertices3d(vertices::SMatrix{2,N,T}) where {N,T <: Real} = vcat(vertices, zero(SMatrix{1,N,T}))
export vertices3d
"""Projects vertexpoints in world space onto the lens plane and converts them to two dimensional points represented in the local x,y coordinates of the lens coordinate frame. Used for projecting eye pupil onto lens plane."""
function project(lenslet::LensletAssembly{T}, displaypoint::SVector{3,T}, vertexpoints::SMatrix{3,N,T}) where {T <: Real,N}
projectedpoints = MMatrix{2,N,T}(undef)
locvertices = worldtolens(lenslet, vertexpoints) # transform pupil vertices into local coordinate frame of lens
virtpoint = point(virtualpoint(lens(lenslet), displaypoint)) # compute virtual point corresponding to physical display point
for i in 1:N
ppoint = locvertices[:,i]
vec = ppoint - virtpoint
vecdist = ppoint[3]
virtdist = virtpoint[3]
scale = abs(virtdist / (vecdist - virtdist))
planepoint = scale * vec + virtpoint
projectedpoints[1:2,i] = SVector{2,T}(planepoint[1], planepoint[2]) # local lens coordinate frame has z axis aligned with the positive normal to the lens plane.
end
return SMatrix{2,N,T}(projectedpoints)
end
function convertlazysets(verts::LazySets.VectorIterator)
M = length(verts)
T = eltype(eltype(verts))
temp = MMatrix{3,M,T}(undef)
i = 1
for vertex in verts
temp[1:2,i] = vertex
temp[3,i] = 0
i += 1
end
return SMatrix{3,M,T}(temp)
end
"""Returns a number between 0 and 1 representing the ratio of the lens radiance to the pupil radiance. Assume lᵣ is the radiance transmitted through the lens from the display point. Some of this radiance, pᵣ, passes through the pupil. The beam energy is the ratio pᵣ/lᵣ. Returns beam energy and the centroid of the intersection polygon to simplify ray casting."""
function beamenergy(assy::LensletAssembly{T}, displaypoint::AbstractVector{T}, pupilpoints::SMatrix{3,N,T}) where {N,T <: Real}
llens::OpticSim.ParaxialLens{T} = lens(assy)
virtpoint = point(virtualpoint(llens, displaypoint))
projectedpoints = project(assy, displaypoint, pupilpoints)
lensverts = OpticSim.vertices(llens)
rows, cols = size(lensverts)
temp = SMatrix{1,cols,T}(reshape(fill(0, cols), 1, cols))
lensverts3D = vcat(lensverts, temp)
beamlens = SphericalPolygon(lensverts3D, virtpoint, T(1))
projpoly = LazySets.VPolygon(projectedpoints)
lenspoly = LazySets.VPolygon(lensverts)
intsct = projpoly ∩ lenspoly # this could be slow, especially multithreaded, because it will allocate. Lazysets.vertices returns Vector{SVector}, rather than SMatrix or SVector{SVector}.
if isempty(intsct)
return T(0)
else
verts = convertlazysets(LazySets.vertices(intsct))
centroid = sum(eachcol(verts)) ./ size(verts)[2]
beamintsct = SphericalPolygon(verts, virtpoint, T(1))
return OpticSim.area(beamintsct) / OpticSim.area(beamlens), centroid
end
end
"""Compute the bounding box in the display plane of the image of the worldpoly on the display plane. Pixels inside this area, conservatively, need to be turned on because some of their rays may pass through the worldpoly"""
function activebox(lenslet::LensletAssembly{T}, worldpoly::SVector{N,SVector{3,T}}) where {T <: Real,N}
maxx = typemin(T)
maxy = typemin(T)
minx = typemax(T)
miny = typemax(T)
lens = lens(lenslet)
display = display(lenslet)
for point in worldpoly
locpoint = transform(lenslet) * point
for lenspoint in OpticSim.vertices(lens)
ray = Ray(locpoint, lenspoint - locpoint)
refractedray, _, _ = processintersection(interface(lens), lenspoint, ray, T(OpticSim.GlassCat.TEMP_REF), T(OpticSim.GlassCat.PRESSURE_REF))
displaypoint = surfaceintersection(display, refractedray)
maxx = max(maxx, displaypoint[1])
minx = min(minx, displaypoint[1])
maxy = max(maxy, displaypoint[2])
miny = min(miny, displaypoint[2])
end
end
return minx, miny, maxx, maxy
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 774 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
struct RectangularBasis{N,T} <: AbstractBasis{N,T}
RectangularBasis(::Type{T} = Float64) where{T<:Real} = new{2,T}()
end
export RectangularBasis
basismatrix(::RectangularBasis{2,T}) where{T} = SMatrix{2,2,T}(T(1),T(0),T(0),T(1))
# SVector{2,SVector{2,T}}(hexe₁(T),hexe₂(T))
"""Returns the vertices of the unit tile polygon for the basis"""
function tilevertices(::RectangularBasis{2,T}) where{T}
return SMatrix{2,4,T}(
-.5, -.5,
-.5, .5,
.5, .5,
.5, -.5)
end
rectangularlattice(ipitch::T = 1.0,jpitch::T = 1.0) where{T<:Real} = LatticeBasis(SMatrix{2,2,T}(
ipitch, 0,
0, jpitch))
export rectangularlattice | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 855 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Repeat
export basismatrix
using Base: offset_if_vec
using StaticArrays:SVector,MVector,SMatrix,MMatrix
using DataFrames:DataFrame
using Colors
import LazySets
using LinearAlgebra:norm
import ..OpticSim #only LensletAssembly uses OpticSim. This doesn't seem like a great idea. Probably should move LensletAssembly somewhere else or at least remove the dependency.
import OpticSim: surfaceintersection
using OpticSim: virtualpoint,SphericalPolygon,processintersection,point
import Unitful
include("Lattice.jl")
include("HexagonalLattice.jl")
include("RectangularLattice.jl")
include("Array.jl")
include("Cluster.jl")
include("Multilens/Multilens.jl")
include("LensletAssembly.jl")
end #module
export Repeat | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 15754 |
# MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using Roots
import DataFrames
import OpticSim.Repeat
#Really should extend AbstractLattice to support cosets, then wouldn't need this ad hoc stuff. TODO
colorbasis(::Repeat.HexBasis1) = SMatrix{2,2}(2, -1, 1, 1)
colorbasis(::Repeat.HexBasis3) = SMatrix{2,2}(2, -1, 1, 1)
colororigins(::Repeat.HexBasis1) = ((0, 0), (-1, 0), (-1, 1))
colororigins(::Repeat.HexBasis3) = ((0, 0), (0, -1), (1, -1))
""" For lenslets arranged in a hexagonal pattern cross talk between lenslets can be reduced by arranging the color of the lenslets so that each lenslet is surrounded only by lenslets of a different color. This function computes the color to assign to any lattice point to ensure this property holds."""
function pointcolor(point, cluster::Repeat.AbstractLatticeCluster)
latticematrix = colorbasis(Repeat.elementbasis(cluster))
origins = colororigins(Repeat.elementbasis(cluster))
colors = zip(origins, ("red", "green", "blue"))
for (origin, color) in colors
if nothing !== Repeat.latticepoint(latticematrix, origin, point)
return color
end
end
end
export pointcolor
defaultclusterproperties() = (mtf = .2, minfnumber = 2.0, cyclesperdegree = 11, λ = 530nm, pixelpitch = .9μm)
export defaultclusterproperties
"""maximum allowable display for visibility reasons"""
maxlensletdisplaysize() = (250μm, 250μm)
const ρ_quartervalue = 2.21509 # value of ρ at which the airy disk function has magnitude .25
export ρ_quartervalue
const ρ_zerovalue = 3.832 # value of ρ at which the airy disk function has magnitude 0
"""compute focal length given maximum display size, fov, etc."""
function compute_focal_length(fov,lensletdiameter, minfnumber, maxdisplaysize)
maxangle = deg2rad(max(fov...)) #leaving angles as degrees caused trouble for not obvious reason
tempfl = uconvert(mm, maxdisplaysize/(2*tan(maxangle/2.0)))
fnum = tempfl/lensletdiameter
return tempfl*minfnumber/fnum
end
export compute_focal_length
"""compute focal length required for a given pixel pitch and angular size of the pixel"""
compute_focal_length(pixel_pitch::Unitful.Length,pixel_angle) = .5*pixel_pitch/tan(.5*pixel_angle)
"""minimum lenslet diameter given `ppd`, `fov`, and `pixel_pitch` assuming a Bayer pattern 2x2 pixel. `pixel_pitch` is the distance between individual pixels. The pitch for the Bayer pattern is 2*`pixel_pitch``."""
minimum_lenslet_diameter(ppd,fov::Tuple{T,T},pixel_pitch::Unitful.Length) where{T} = norm(ppd .* fov .* 2 .* pixel_pitch)
export minimum_lenslet_diameter
pixelsperdegree(focal_length,pixelpitch) = 1/(2.0*atand(uconvert(Unitful.NoUnits,pixelpitch/(2.0*focal_length))))
export pixelsperdegree
function lensletdisplaysize(fov,focal_length)
uconvert.(μm,2*focal_length .* tand.(fov./2))
end
export lenseletdisplaysize
"""diffraction limited response for a circular aperture, normalized by maximum cutoff frequency"""
function mtfcircular(freq, freqcutoff)
s = freq / freqcutoff
return 2 / π * (acos(s) - s * ((1 - s^2)^.5))
end
export mtfcircular
"""
# Returns the diffraction limit frequency in cycles/deg. At this frequeny the response of the system is zero.
## Derivation from the more common formula relating f number and diffraction limit.
focal length = 𝒇𝒍
diffraction cutoff frequency,fc, in cycles/mm = 1/λF# = diameter/λ*𝒇𝒍
cutoff wavelength, Wc, = 1/cutoff frequency = λ*𝒇𝒍/diameter
angular wavelength, θc, radians/cycle, corresponding to cutoff wavelength:
θ ≈ tanθ for small θ
θc corresponding to Wc: θc ≈ tanθ = Wc/𝒇𝒍
Wc = θc*𝒇𝒍
from equation for Wc:
λ*𝒇𝒍/diameter = Wc = θc*𝒇𝒍
θc = λ/diameter
cycles/rad = 1/θc = diameter/λ
"""
diffractionlimit(λ::Unitful.Length,diameter::Unitful.Length) = uconvert(Unitful.NoUnits, diameter / λ) / rad2deg(1)
export diffractionlimit
"""computes the diameter of a diffraction limited lens that has response mtf at frequency cyclesperdeg"""
function diameter_for_cycles_deg(mtf, cyclesperdeg, λ::Unitful.Length)
cyclesperrad = rad2deg(1) * cyclesperdeg
f(x) = mtfcircular(x, 1.0) - mtf
normalizedfrequency = find_zero(f, (0.0, 1.0))
fc = cyclesperrad / normalizedfrequency
return uconvert(mm, λ * fc)
end
export diameter_for_cycles_deg
airydisk(ρ) = (2 * SpecialFunctions.besselj1(ρ) / ρ)^2
"""The f# required for the first zero of the airy diffraction disk to be at the next sample point"""
diffractionfnumber(λ::Unitful.Length,pixelpitch::Unitful.Length,indexofrefraction) = uconvert(Unitful.NoUnits, pixelpitch / (2.44λ / indexofrefraction))
export diffractionfnumber
"""returns ρ, the normalized distance, at which the airy disk will have the value airyvalue"""
function ρatairyvalue(airyvalue)
ρ = 1e-5 # start at small angle
while airydisk(ρ) > airyvalue
ρ += 1e-5 # numerical precision not an issue here
end
return ρ
end
export ρatairyvalue
""" Spacing between lenslets which guarantess that for any pixel visible in all lenslets every point on the eyebox plane is covered. This is closest packing of circles."""
closestpackingdistance(pupildiameter::Unitful.Length) = pupildiameter * cosd(30)
export closestpackingdistance
"""Tries clusters of various sizes to choose the largest one which fits within the eye pupil. Larger clusters allow for greater reduction of the fov each lenslet must cover so it returns the largest feasible cluster"""
function choosecluster(pupildiameter::Unitful.Length, lensletdiameter::Unitful.Length, no_eyebox_subdivision::Bool = false)
#for now don't use cluster sizes that are not divisible by 3 since this causes problems with RGB subdivision of the lenslets. Maybe add back in later.
# clusters = (hex3RGB, hex4RGB, hex7RGB , hex9RGB, hex12RGB,hex19RGB) #, hex37RGB) # hex37RGB leave out for now. Leads to designs with thousands of small lenslets. May not be practical.
if no_eyebox_subdivision
clusters = (hex3RGB,)
else
clusters = (hex3RGB, hex9RGB, hex12RGB,hex18RGB) #, hex37RGB) # hex37RGB leave out for now. Leads to designs with thousands of small lenslets. May not be practical.
end
pupildiameter
ratio = 0.0
clusterindex = 0
scale = 0
for clusterfunc in clusters
cluster = clusterfunc() #create an instance of the cluster type with default unit scale
scale = ustrip(mm,lensletdiameter)/euclideandiameter(elementbasis(cluster)) #scale the element basis of the cluster to match lenslet diameter
scaledcluster = clusterfunc(scale) #make a new instance of the cluster type scaled so the element basis has diameter equal to lenslet diameter
temp = ustrip(mm,pupildiameter) / (euclideandiameter(scaledcluster))
if temp >= 1.0
ratio = temp
clusterindex += 1
else
break
end
end
maxcluster = clusters[clusterindex](ratio*scale)
@assert isapprox(Repeat.euclideandiameter(maxcluster), ustrip(mm,pupildiameter))
scaledlensletdiameter = lensletdiameter*ratio
return (cluster = maxcluster, lensletdiameter = scaledlensletdiameter, packingdistance = pupildiameter * ustrip(mm, lensletdiameter))
end
export choosecluster
"""Computes the largest feasible cluster size meeting constraints"""
function choosecluster(pupildiameter::Unitful.Length, λ::Unitful.Length, mtf, cyclesperdeg::T, no_eyebox_subdivisions::Bool = false) where {T <: Real}
diam = diameter_for_cycles_deg(mtf, cyclesperdeg, λ)
return choosecluster(pupildiameter, diam, no_eyebox_subdivisions) # use minimum diameter for now.
end
"""This computes the approximate size of the entire display, not the individual lenslet displays."""
sizeofdisplay(fov,eyerelief) = @. 2 * tand(fov / 2) * eyerelief
export sizeofdisplay
"""Computes the number of lenslets required to create a specified field of view at the given eyerelief"""
function numberoflenslets(fov, eyerelief::Unitful.Length, lensletdiameter::Unitful.Length)
lensletarea = π * (lensletdiameter / 2)^2
dispsize = sizeofdisplay(fov, eyerelief)
return dispsize[1] * dispsize[2] / lensletarea
end
export numberoflenslets
"""angular size of the eyebox when viewed from distance eyerelief"""
eyeboxangles(eyebox,eyerelief) = @. atand(uconvert(Unitful.NoUnits, eyebox / eyerelief))
export eyeboxangles
"""This code is tightly linked to the cluster sizes returned by choosecluster. This is not goood design to link these two functions. Think about how to decouple these two functions. computes how the fov can be subdivided among lenslets based on cluster size. Assumes the horizontal size of the eyebox is larger than the vertical so the larger number of subdivisions will always be the first number in the returns Tuple."""
function anglesubdivisions(cluster, pupildiameter::Unitful.Length, λ::Unitful.Length, mtf, cyclesperdegree;RGB=true)
numelements = Repeat.clustersize(cluster)
if numelements == 37
return RGB ? (4,3) : (6,6)
elseif numelements == 19
return RGB ? (3, 2) : (5, 3)
elseif numelements == 18
return RGB ? (3,2) : (6,3)
elseif numelements == 12
return RGB ? (2, 2) : (4, 3)
elseif numelements == 9
return RGB ? (3, 1) : (3, 3)
elseif numelements == 7
return RGB ? (3, 1) : (3, 2)
elseif numelements == 4 || numelements == 3
return RGB ? (1, 1) : (3, 1)
else throw(ErrorException("this should never happen"))
end
end
export anglesubdivisions
"""Multilens displays tradeoff pixel redundancy for a reduction in total track of the display, by using many short focal length lenses to cover the eyebox. This function computes the ratio of pixels in the multilens display vs. a conventional display of the same nominal resolution"""
function pixelredundancy(fov, eyerelief::Unitful.Length, eyebox::NTuple{2,Unitful.Length}, pupildiameter::Unitful.Length, lensletdiameter,angles; RGB=true)
nominalresolution = ustrip.(°, fov) #remove degree units so pixel redundancy doesn't have units of °^-2
numlenses = numberoflenslets(fov, eyerelief, lensletdiameter)
return (numlenses * angles[1] * angles[2]) / ( nominalresolution[1] * nominalresolution[2])
end
export pixelredundancy
label(color) = color ? "RGB" : "Monochrome"
"""generates a contour plot of lenslet display size as a function of ppd and pupil diameter"""
function displaysize_ppdvspupildiameter()
x = 20:2:45
y = 3.0:.05:4
RGB = true
Plots.plot(Plots.contour(x, y, (x, y) -> maximum(ustrip.(μm, lensletdisplaysize((50, 35), 18mm, (10mm, 6mm), y * mm, x, RGB=RGB))), fill=true, xlabel="pixels per degree", ylabel="pupil diameter", legendtitle="display size μm", title="$(label(RGB)) lenslets"))
end
export displaysize_ppdvspupildiameter
""" Compute high level properties of a lenslet display system. Uses basic geometric and optical constraints to compute approximate values which should be within 10% or so of a real physical system.
`eyerelief` is in mm: `18mm` not `18`.
`eyebox` is a 2 tuple of lengths, also in mm, that specifies the size of the rectangular eyebox, assumed to lie on vertex of the cornea when the eye is looking directly forward.
`fov` is the field of view of the display as seen by the eye, in degrees (no unit type necessary here).
`pupildiameter` is the diameter of the eye pupil in mm.
`mtf` is the MTF response of the system at the angular frequency `cyclesperdegree`.
`pixelsperdegree` is the number of pixels per degree on the display, as seen by the eye through the lenslets. This is not a specification of the optical resolution of the system (use `mtf` and `cyclesperdegree` for that). It is a physical characteristic of the display.
Example:
```
julia> system_properties(18mm,(10mm,9mm),(55°,45°),4.0mm,.2,11.0)
Dict{Symbol, Any} with 14 entries:
:lenslet_diameter => 0.742781 mm
:lenslet_display_size => (251.708 μm, 345.939 μm)
:fnumber => 2.0
:number_lenslets => 644.903
:total_silicon_area => 56.1555 mm^2
:subdivisions => (3, 2)
:pixel_redundancy => 33.5192
:diffraction_limit => 24.4603
:eyebox_angles => (29.0546, 26.5651)
:pixels_per_degree => 28.8088
:lenslet_fov => (9.68487, 13.2825)
:display_size => (18.7404 mm, 14.9117 mm)
:cluster_data => (cluster = ClusterWithProperties{19, 2, Float64}(Lat…
:focal_length => 1.48556 mm
```
"""
function system_properties(eyerelief::Unitful.Length, eyebox::NTuple{2,Unitful.Length}, fov, pupildiameter::Unitful.Length, mtf, cyclesperdegree; minfnumber=2.0,RGB=true,λ=530nm,pixelpitch=.9μm, maxdisplaysize = 250μm, no_eyebox_subdivision::Bool = false)::Dict{Symbol,Any}
diameter = diameter_for_cycles_deg(mtf, cyclesperdegree, λ)
clusterdata = choosecluster(pupildiameter, diameter,no_eyebox_subdivision)
difflimit = diffractionlimit(λ, clusterdata.lensletdiameter)
numlenses = numberoflenslets(fov, eyerelief, clusterdata.lensletdiameter)
subdivisions = anglesubdivisions(clusterdata.cluster, pupildiameter, λ, mtf, cyclesperdegree, RGB=RGB)
eyebox_angles = eyeboxangles(eyebox,eyerelief)
angles = eyebox_angles ./ subdivisions
redundancy = pixelredundancy(fov, eyerelief, eyebox, pupildiameter,clusterdata.lensletdiameter, angles, RGB=RGB)
focal_length = compute_focal_length(angles,clusterdata.lensletdiameter,minfnumber,maxdisplaysize)
dispsize = lensletdisplaysize(angles,focal_length)
fnumber = focal_length/clusterdata.lensletdiameter
siliconarea = uconvert(mm^2,numlenses * dispsize[1]*dispsize[2])
fulldisplaysize = sizeofdisplay(fov,eyerelief)
pixels_per_degree = pixelsperdegree(focal_length,pixelpitch)
return Dict(:cluster_data => clusterdata, :lenslet_diameter => clusterdata.lensletdiameter,:pixels_per_degree => pixels_per_degree, :diffraction_limit => difflimit, :fnumber => fnumber, :focal_length => focal_length, :display_size => fulldisplaysize, :lenslet_display_size => dispsize, :total_silicon_area => siliconarea, :number_lenslets => numlenses, :pixel_redundancy => redundancy, :eyebox_angles => eyebox_angles, :lenslet_fov => angles, :subdivisions => subdivisions)
end
export system_properties
"""prints system properties nicely"""
function printsystemproperties(eyerelief::Unitful.Length, eyebox::NTuple{2,Unitful.Length}, fov, pupildiameter::Unitful.Length, mtf, cyclesperdegree; minfnumber=2.0,RGB=true,λ=530nm,pixelpitch=.9μm,maxdisplaysize = 350μm)
println("Input parameters")
println()
println("pixel pitch $pixelpitch")
println("eye relief = $eyerelief")
println("eye box = $eyebox")
println("fov = $(fov)")
println("pupil diameter = $pupildiameter")
println("mtf = $mtf @ $cyclesperdegree cycles/°")
println()
printsystemproperties(system_properties(eyerelief, eyebox, fov, pupildiameter, mtf, cyclesperdegree, minfnumber = minfnumber,RGB=RGB,λ=λ,pixelpitch=pixelpitch,maxdisplaysize = maxdisplaysize))
end
export printsystemproperties
function printsystemproperties(props)
println("Output values")
println()
for (key,value) in pairs(props)
if key == :cluster_data
println("cluster = $(typeof(props[:cluster_data][:cluster]))")
elseif key == :diffraction_limit
println("$key = $value cycles/°")
else
println("$key = $value")
end
end
println("occlusion ratio $(π*uconvert(Unitful.NoUnits,*(props[:lenslet_display_size]...)/(props[:lenslet_diameter])^2))")
cluster = props[:cluster_data][:cluster]
clusterdiameter = Repeat.euclideandiameter(cluster)
println("cluster diameter (approx): $(clusterdiameter)")
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 9859 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#display lenslet systems: emitter, paraxial lenslet, paraxial eye lens, retina detector.
#start with eyebox plane and hexagonal tiling. Project onto display surface, generate lenslets automatically.
using OpticSim.Geometry:Transform,world2local
using OpticSim:plane_from_points,surfaceintersection,closestintersection,Ray,Plane,ConvexPolygon,Sphere
using OpticSim
using OpticSim.Repeat:tilevertices,HexBasis1,tilesinside
using Unitful:upreferred
using Unitful.DefaultSymbols:°
"""compute the mean of the columns of `a`. If `a` is an `SMatrix` this is very fast and will not allocate."""
columncentroid(a::AbstractMatrix) = sum(eachcol(a))/size(a)[2] #works except for the case of zero dimensional matrix.
export columncentroid
function project(point::AbstractVector{T},projectionvector::AbstractVector{T},surface::Union{OpticSim.LeafNode{T,N}, S}) where {T,N,S<:ParametricSurface{T,N}}
ray = Ray(point, projectionvector)
intsct = surfaceintersection(surface, ray)
pointintsct = closestintersection(intsct,false)
if pointintsct === nothing #point didn't project to a point on the surface
return nothing
else
return OpticSim.point(pointintsct)
end
end
export project
"""project the vertices of a polygon represented by `vertices` onto `surface` using the point as the origin and `projectionvector` as the projection direction. Return nothing if any of the projected points do not intersect the surface. The projected vertices are not guaranteed to be coplanar."""
function project(vertices::R, projectionvector::AbstractVector{T}, surface::Union{OpticSim.LeafNode{T,N}, S}) where {T,N,S<:ParametricSurface{T,N},R<:AbstractMatrix{T}}
result = similar(vertices)
for i in 1:size(vertices)[2]
origin = vertices[:, i]
pt = project(origin,projectionvector,surface)
if pt === nothing #one of the polygon points didn't project to a point on the surface so reject the polygon
return nothing
else
result[:, i] = pt
end
end
return result
end
"""Finds the best fit plane to `vertices` then projects `vertices` onto this plane by transforming from the global to the local coordinate frame. The projected points are represented in the local coordinate frame of the plane."""
function projectonbestfitplane(vertices::AbstractMatrix{T},positive_z_direction::AbstractVector) where{T}
@assert size(vertices)[1] == 3 "projection only works for 3D points"
center, _, localrotation = plane_from_points(vertices)
if localrotation[3,:] ⋅ positive_z_direction < 0 #want the local frame z axis to be aligned with positive_z_direction
lr = localrotation
localrotation = SMatrix{3,3,T}(lr[:,1]...,-lr[:,2]...,-lr[:,3]...) #flip zaxis to align with positive_z_direction. Also flip sign of one other column to maintain a +1 determinant
end
toworld = Transform(localrotation,center) #compute local to world transformation
tolocal = world2local(toworld)
numpts = size(vertices)[2]
result = tolocal * SMatrix{3,numpts}(vertices)
return result,toworld,tolocal #project onto best fit plane by setting z coordinate to zero.
end
export projectonbestfitplane
"""projects convex polygon, represented by `vertices`, onto `surface` along vector `normal`. Assumes original polygon is convex and that the projection will be convex. No guarantee that this will be true but for smoothly curved surfaces that are not varying too quickly relative to the size of the polygon it should be true."""
function planarpoly(projectedpoints::AbstractMatrix{T},desired_normal::AbstractVector) where{T}
planarpoints,toworld,_ = projectonbestfitplane(projectedpoints,desired_normal)
numpts = size(planarpoints)[2]
temp = SMatrix{2,numpts}(planarpoints[1:2,:])
vecofpts = collect(reinterpret(reshape,SVector{2,T},temp))
#this code is inefficient because of different data structures used by convex polygon and most other code for representing lists of vertices.
return ConvexPolygon(toworld,vecofpts)
end
spherepoint(radius,θ,ϕ) = radius .* SVector(cos(θ)sin(ϕ),sin(θ),cos(θ)cos(ϕ))
export spherepoint
"""Computes points on the edges of the spherical rectangle defined by the range of θ,ϕ. This is used to determine lattice boundaries on the eyebox surface."""
function spherepoints(radius, θmin,θmax,ϕmin,ϕmax)
@assert abs(θmax-θmin) > 0
@assert abs(ϕmax-ϕmin) > 0
θedges = [spherepoint(radius,ϕ,θ) for θ in θmin:.01:θmax, ϕ in (ϕmin,ϕmax)]
ϕedges = [spherepoint(radius,ϕ,θ) for ϕ in ϕmin:.01:ϕmax, θ in (θmin,θmax)]
@assert length(θedges) > 0
@assert length(ϕedges) > 0
allpoints = vcat(reshape(θedges,reduce(*,size(θedges))),reshape(ϕedges,reduce(*,size(ϕedges))))
pts = collect(reshape(reinterpret(Float64,allpoints),3,length(allpoints))) #return points as 3xn matrix with points as columns. Collect is not strictly necessary but it makes debugging easier
@assert length(pts) > 0
return pts
end
export spherepoints
"""given a total fov in θ and ϕ compute sample points on the edges of the spherical rectangle."""
function spherepoints(eyerelief,radius,θ,ϕ)
hθ = tan(θ/2)*eyerelief
nθ = atan(uconvert(Unitful.NoUnits,hθ/radius))
hϕ = tan(ϕ/2)*eyerelief
nϕ = atan(uconvert(Unitful.NoUnits,hϕ/radius))
spherepoints(radius,-nθ,nθ,-nϕ,nϕ)
end
function bounds(pts::AbstractMatrix{T}) where{T}
return [extrema(row) for row in eachrow(pts)]
end
export bounds
"""projects points on the sphere onto the eyebox along the -z axis direction."""
function eyeboxbounds(eyebox::OpticSim.Plane,eyerelief, dir::AbstractVector, radius,fovθ,fovϕ)
pts = spherepoints(eyerelief,radius,fovθ,fovϕ)
projectedpts = project(pts,dir,eyebox)
@assert projectedpts !== nothing && length(projectedpts) > 0
return bounds(projectedpts)
end
export eyeboxbounds
function boxtiles(bbox,lattice)
tiles = tilesinside(bbox[1][1],bbox[2][1],bbox[1][2],bbox[2][2],lattice)
end
export boxtiles
eyeboxtiles(eyebox,eyerelief,dir,radius,fovθ,fovϕ,lattice) = boxtiles(eyeboxbounds(eyebox,eyerelief,dir,radius,fovθ,fovϕ),lattice)
export eyeboxtiles
function spherepolygon(vertices,projectiondirection,sph::OpticSim.LeafNode)
@assert typeof(vertices) <: SMatrix
projectedpts = project(vertices,projectiondirection,sph)
return planarpoly(projectedpts,-projectiondirection) #polygon on best fit plane to projectedpts. Make normal of polygon be opposite projection, which points from eyebox plane to projection sphere
end
#TODO need to figure out what to use as the normal (eventually this will need to take into account the part of the eyebox the lenslet should cover)
#TODO write test for planarpoly and generation of Paraxial lens system using planar hexagon as lenslet.
"""
# Generate hexagonal polygons on a spherical display surface.
Each hexagonal polygon corresponds to one lenslet. A hexagonal lattice is first generated in the eyebox plane and then the vertices of these hexagonal tiles are projected onto the spherical display surface. The projected points are not necessarily planar so they are projected onto a best fit plane. For systems with fields of view less that 90° the error is small.
`dir` is the 3d direction vector to project points from the eyebox plane to the spherical display surface.
`radius` is the radius of the spherical display surface.
`fovθ,fovϕ` correspond to the field of view of the display as seen from the center of the eyebox plane.
`lattice` is the hexagonal lattice to tile the sphere with, HexBasis1 or HexBasis3, which are rotated versions of each other."""
function spherepolygons(eyebox::Plane{T,N},eyerelief,sphereradius,dir,fovθ,fovϕ,lattice)::Tuple{Vector{ConvexPolygon{6,T}},Vector{Tuple{Int64,Int64}}} where{T,N}
# if fovθ, fovϕ are in degrees convert to radians. If they are unitless then the assumption is that they represent radians
eyeboxz = eyebox.pointonplane[3]
sphereoriginoffset = eyeboxz + ustrip(mm,eyerelief - sphereradius) #we don't use mm when creating shapes because Transform doesn't work properly with unitful values. Add the units back on here.
sph = OpticSim.LeafNode(Sphere(ustrip(mm,sphereradius)),Geometry.translation(0.0,0.0,sphereoriginoffset)) #Sphere objects are always centered at the origin so have to make
θ = upreferred(fovθ) #converts to radians if in degrees
ϕ = upreferred(fovϕ) #converts to radians if in degrees
tiles = eyeboxtiles(eyebox,eyerelief,-dir,sphereradius,θ,ϕ,lattice)
shapes = Vector{ConvexPolygon{6,T}}(undef,0)
lattice_coordinates = Vector{Tuple{Int64,Int64}}(undef,0)
for coords in eachcol(tiles)
twodverts = tilevertices(coords,lattice)
numverts = size(twodverts)[2]
temp = MMatrix{1,numverts,T}(undef)
fill!(temp,eyeboxz)
zerorow = SMatrix{1,numverts,T}(temp)
vertices = SMatrix{N,numverts,T}(vcat(twodverts,zerorow))
@assert typeof(vertices) <: SMatrix
push!(shapes,spherepolygon(vertices,dir,sph))
push!(lattice_coordinates,Tuple{T,T}(coords))
end
shapes,lattice_coordinates
end
function spherelenslets(eyeboxplane::Plane{T,N},eyerelief,focallength,dir,sphereradius,fovθ,fovϕ,lattice)::Tuple{Vector{ParaxialLens{T}},Vector{Tuple{Int64,Int64}}} where{T,N}
lenspolys,tilecoords = spherepolygons(eyeboxplane,eyerelief, sphereradius, dir,fovθ,fovϕ,lattice)
result = Vector{ParaxialLens{T}}(undef,length(lenspolys))
empty!(result)
for poly in lenspolys
lenslet = ParaxialLensConvexPoly(focallength,poly,SVector{2,T}(T.((0,0))))
push!(result,lenslet)
end
return result,tilecoords
end
export spherelenslets | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 15447 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
""" Typical properties for near eye VR display """
nominal_system_inputs() = (eye_relief = 20mm, fov = (20°,20°),eyebox = (10mm,8mm),display_radius = 125.0mm, pupil_diameter = 3.5mm,pixel_pitch = .9μm, minfnumber = 2.0, mtf = .2, cycles_per_degree = 11, max_display_size = 250μm, )
export nominal_system_inputs
xcoords(a::SMatrix{3,4}) = a[1,:]
ycoords(a::SMatrix{3,4}) = a[2,:]
zcoords(a::SMatrix{3,4}) = a[3,:]
function matnormal(a::SMatrix{3,4})
side1 = a[:,4] - a[:,1]
side2 = a[:,2] - a[:,1]
return normalize(cross(side1,side2))
end
matcentroid(a::SMatrix{3,4}) = Statistics.mean(eachcol(a))
function matrix2rectangle(a::SMatrix{3,4,T}) where{T}
center = Statistics.mean(eachcol(a))
xmin,xmax = extrema(xcoords(a))
halfsizeu = (xmax-xmin)/2.0
ymin,ymax = extrema(ycoords(a))
halfsizev = (ymax-ymin)/2.0
Rectangle(halfsizeu,halfsizev,matnormal(a),center, interface = opaqueinterface())
end
function test_paraxial_lens()
lens = ParaxialLensRect(10.0,1.0,1.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
displaypoint = [0.0,0.0,-2.5]
direction = [0.0,0.0,1.0]
r1 = Ray(displaypoint,direction)
intsct1 = OpticSim.surfaceintersection(lens,r1)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac1,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct1),OpticSim.normal(lens),OpticalRay(r1,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
r2 = Ray(-displaypoint,-direction)
intsct2= OpticSim.surfaceintersection(lens,r2)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac2,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct2),OpticSim.normal(lens),OpticalRay(r2,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
# isapprox(-refrac2[1],refrac1[1]), refrac2[1], refrac1[1]
Vis.draw(lens)
Vis.draw!([Ray(OpticSim.point(intsct1)+ [.5,.5,0.0],refrac1),r1]) #offset first ray so it can be seen. The other ray will be written over it and obscure it.
Vis.draw!([Ray(OpticSim.point(intsct2),refrac2),r2], color = "green") #visualization should have two rays in opposite directions coming out of lens plane but only see one. Error in ParaxialLens.
end
export test_paraxial_lens
"""Example that shows how to call setup_system with typical values"""
function setup_nominal_system(;no_eyebox_subdivision::Bool = false)::LensletSystem
(;eye_relief,fov,eyebox,display_radius,pupil_diameter,minfnumber,pixel_pitch) = nominal_system_inputs()
setup_system(eyebox,fov,eye_relief,pupil_diameter,display_radius,minfnumber,pixel_pitch,no_eyebox_subdivision = no_eyebox_subdivision)
end
export setup_nominal_system
function compute_eyebox_rays(sys = setup_nominal_system())
(;lenses,projected_eyeboxes,eyebox_rectangle) = sys
centers = [x for x in centroid.(shape.(lenses))] #use the geometric center of the lenses not the optical center_point
display_centers = matcentroid.(projected_eyeboxes)
rays = Ray.(display_centers, centers.-display_centers)
ray_traces = Vector{LensTrace{Float64,3}}(undef,0)
tracked_rays = [trace(CSGOpticalSystem(LensAssembly(lens),eyebox_rectangle),OpticalRay(ray,1.0,.530),trackrays = ray_traces) for (lens,ray) in zip(lenses,rays)]
return [x for x in ray_traces]
end
export compute_eyebox_rays
"""Example call of system_properties function"""
function systemproperties_call()
(;eye_relief,fov,eyebox,pupil_diameter,minfnumber,pixel_pitch) = nominal_system_inputs()
system_properties(eye_relief,eyebox,fov,pupil_diameter,.2,20,minfnumber = minfnumber,pixelpitch = pixel_pitch)
end
export systemproperties_call
"""Computes the lenslets on the display sphere and draws them."""
function drawspherelenslets()
(;fov, eye_relief,eyebox,display_radius,pupil_diameter,pixel_pitch,minfnumber,mtf,cycles_per_degree) = nominal_system_inputs()
computedprops = system_properties(eye_relief,eyebox,fov,pupil_diameter,mtf,cycles_per_degree,minfnumber = minfnumber,maxdisplaysize = 250μm,pixelpitch = pixel_pitch)
focallength = ustrip(mm,computedprops[:focal_length])
lenses,_ = spherelenslets(Plane(0.0,0.0,1.0,0.0,0.0,18.0),eye_relief,focallength,[0.0,0.0,1.0],display_radius,fov[1],fov[2],HexBasis1())
Vis.draw() #clear screen
Vis.draw!.(lenses)
return nothing
end
export drawspherelenslets
"""returns the hex polygons on the sphere that will eventually be turned into hexagonal lenslets"""
function testspherepolygons()
eyebox = Plane(0.0,0.0,1.0,0.0,0.0,12.0)
dir = [0.0,0.0,1.0]
fovθ = 10.0°
fovϕ = 5.0°
lattice = HexBasis1()
displayradius = 125.0mm
eyerelief = 20mm
return spherepolygons(eyebox,eyerelief,displayradius,dir,fovθ,fovϕ,lattice)
end
export testspherepolygons
function testprojectonplane()
verts = tilevertices(HexBasis1())
verts = vcat(verts,[0 for _ in 1:6]')
projectonbestfitplane(SMatrix{size(verts)...}(verts),[0.0,0.0,1.0])
end
export testprojectonplane
function testproject()
norml = SVector(0.0, 0, 1)
hex = HexBasis1()
verts =vcat(tilevertices((0, 0), hex), [0.0 0 0 0 0 0])
surf = Plane(norml, SVector(0.0, 0, 10))
project(verts, norml, surf)
end
export testproject
function testprojection()
pts = spherepoints(1.0,-.2,-.2,1.0,1.1)
surf = Plane(0.0,0.0,-1.0,0.0,0.0,0.0)
dir = [0.0,0.0,-1.0]
project(pts,dir,surf)
end
export testprojection
"""returns hexagonal lenslets on the display sphere"""
function testspherelenslets()
(;fov, eye_relief,eyebox,display_radius,pupil_diameter,pixel_pitch,minfnumber,mtf,cycles_per_degree,max_display_size) = nominal_system_inputs()
fov = (10°,10°)
computedprops = system_properties(eye_relief,eyebox,fov,pupil_diameter,mtf,cycles_per_degree,minfnumber = minfnumber,maxdisplaysize = max_display_size,pixelpitch = pixel_pitch)
focallength = ustrip(mm,computedprops[:focal_length])
spherelenslets(Plane(0.0,0.0,1.0,0.0,0.0,18.0),eye_relief,focallength,[0.0,0.0,1.0],display_radius,fov[1],fov[2],HexBasis1())
end
export testspherelenslets
function draw_projected_corners(system = setup_nominal_system())
(;lenslet_eye_boxes, subdivisions_of_eyebox,lenses,displayplanes,projected_eyeboxes) = system
colors = distinguishable_colors(reduce(*,subdivisions_of_eyebox))
for (lenslet_eyebox,lens,display_plane,projected_eyebox) in zip(lenslet_eye_boxes,lenses,displayplanes,projected_eyeboxes)
center = opticalcenter(lens)
for (eyeboxpt,projected_point) in zip(eachcol(lenslet_eyebox),eachcol(projected_eyebox))
r = Ray(eyeboxpt,center-eyeboxpt)
intsct = surfaceintersection(display_plane,r)
closest = closestintersection(intsct)
display_intersection = point(closest)
@assert isapprox(display_intersection,projected_point) "error display_intersection $display_intersection projected_point $projected_point"
lenstrace = LensTrace(OpticalRay(r,1.0,.5),closest)
Vis.draw!(lenstrace)
end
end
end
export draw_projected_corners
"""assigns each lenslet/display subsystem a rectangular sub part of the eyebox"""
function draw_eyebox_assignment(system = setup_nominal_system(),clear_screen = true;draw_eyebox = true)
(;eyebox_rectangle,
lenses,
lenslet_colors,
lattice_coordinates,
subdivisions_of_eyebox,
projected_eyeboxes,
displayplanes,
lenslet_eyebox_numbers) = system
if clear_screen
Vis.draw() #clear screen
end
if draw_eyebox
Vis.draw!(eyebox_rectangle)
end
for (lens,lattice_coordinates,color) in zip(lenses,lattice_coordinates,lenslet_colors)
Vis.draw!(lens,color = color)
end
num_distinct_eyeboxes = reduce(*,subdivisions_of_eyebox)
colors = distinguishable_colors(num_distinct_eyeboxes)
#THIS is almost certainly wrong
# for (eyebox,plane,eyeboxnum,lens) in zip(projected_eyeboxes,displayplanes,lenslet_eyebox_numbers,lenses)
# localframe = OpticSim.translation(-OpticSim.normal(lens))*OpticSim.localframe(shape(lens)) #the local frame of the lens is, unfortunately, stored only in the ConvexPoly shape. No other lens type has a local frame which is terrible design. Should be fixed.
# transformedpoints = (inv(localframe)*eyebox)[1:2,:]
# pts = [SVector{2}(x...) for x in eachcol(transformedpoints)]
# # Vis.draw!(plane,color = colors[eyeboxnum])
# Vis.draw!(ConvexPolygon(localframe,pts),color = colors[eyeboxnum])
# end
end
export draw_eyebox_assignment
#compute the ray from each lenslet's eyebox center to the geometric center of the lenslet. Trace this ray through the lens and draw the refracted ray. The refracted ray should line up exactly with -normal(lenslet).
function draw_eyebox_rays(system = setup_nominal_system())
(;lenses, lenslet_eyebox_centers,) = system
for (lens,eyebox_center) in zip(lenses,lenslet_eyebox_centers)
r = Ray(centroid(lens),centroid(lens)-eyebox_center)
refrac_ray = ray(trace(LensAssembly(lens),OpticalRay(r,1.0,.5)))
Vis.draw!(refrac_ray,color = "yellow")
end
end
function draw_eyebox_polygons(system = setup_nominal_system())
(;projected_eyebox_polygons) = system
for poly in projected_eyebox_polygons
Vis.draw!(poly)
end
end
export draw_eyebox_polygons
function draw_system(system = setup_nominal_system())
draw_eyebox_assignment(system,draw_eyebox=false)
draw_subdivided_eyeboxes(system,false)
# Vis.draw!(compute_eyebox_rays(system))
draw_eyebox_rays(system)
draw_projected_corners(system)
draw_eyebox_polygons(system)
end
export draw_system
"""draw subdivided eyeboxes to make sure they are in the right place"""
function draw_subdivided_eyeboxes(system = setup_nominal_system(), clear_screen = true)
(;subdivided_eyebox_polys) = system
colors = distinguishable_colors(length(subdivided_eyebox_polys))
if clear_screen
Vis.draw()
end
for (eyebox,color) in zip(subdivided_eyebox_polys,colors)
center = matcentroid(eyebox)
Vis.draw!(matrix2rectangle(eyebox),color = color)
r = Ray(center,matnormal(eyebox))
Vis.draw!(r)
Vis.draw!(leaf(Sphere(.1),Geometry.translation(center)),color = "white")
end
end
export draw_subdivided_eyeboxes
function test_project_eyebox_to_display_plane()
boxpoly = SMatrix{3,4}(
-1.0,1,0.0,
1.0,1.0,0.0,
1.0,-1.0,0.0,
-1.0,-1.0,0.0
)
correct_answer = SMatrix{3,4}(
1.3,1.0, 11.5,
1.0,1.0, 11.5,
1.0,1.3, 11.5,
1.3,1.3, 11.5)
fl = 1.5
lenscenter = [1.0,1.0,10.0]
lens = ParaxialLensRect(fl,.5,.5,[0.0,0.0,1.0],lenscenter)
displayplane = Plane([0.0,0.0,1.0],[0.0,0.0,lenscenter[3]+fl])
answer,polygons = project_eyebox_to_display_plane(boxpoly,lens,displayplane)
@assert isapprox(answer,correct_answer) "computed points $answer"
@info "eyebox points $answer"
@info "eyebox polygons $polygons"
end
export test_project_eyebox_to_display_plane
"""For each display computes a ray from the display center to the geometric center of the lenslet. Then it computes the refracted ray and intersects it with the subdivided eyebox associated with the display. Draws all these rays. The rays from the displays should hit the center points of the subdivided eyeboxes"""
function test_ray_cast_from_display_center(clear_display = true)
system = setup_nominal_system()
(;eyebox_rectangle,lenses,projected_eyeboxes) = system
if clear_display
Vis.draw()
end
# draw_system(system)
draw_subdivided_eyeboxes(system,false)
for (lens,display_eyebox) in zip(lenses,projected_eyeboxes)
lens_geometric_center = centroid(lens)
display_center = matcentroid(display_eyebox)
diff = lens_geometric_center-display_center
r = OpticalRay(Ray(display_center,diff),1.0,.53)
# Vis.draw!(eyebox_rectangle)
system = CSGOpticalSystem(LensAssembly(lens),eyebox_rectangle)
Vis.draw!(r,color = "black")
tr = trace(system,r)
if tr !== nothing
Vis.draw!(tr,color = "black")
end
end
end
export test_ray_cast_from_display_center
"""refracts the ray from the center of the display passing through the geometric center of the lens and then computes the closes point on this ray to the eyeboxcentroid. If the lens optical center has been calculated correctly the ray should pass through the eyeboxcentroid"""
function test_replace_optical_center(eyeboxcentroid,lensgeometriccenter,displaycenter,lens)
rdir = lensgeometriccenter-displaycenter
r = Ray(displaycenter,rdir)
assy = LensAssembly(lens)
refracted_trace = trace(assy,OpticalRay(r,1.0,.530))
r2 = Ray(eyeboxcentroid,lensgeometriccenter-eyeboxcentroid)
#this doesn't work because of a bug in processintersection for paraxial interfaces. Ray points in the wrong direction.
reverse_trace = trace(assy,OpticalRay(r2,1.0,.530))
reverse_point = closestpointonray(ray(reverse_trace),displaycenter)
return closestpointonray(ray(refracted_trace),eyeboxcentroid)
end
export test_replace_optical_center
function center_data()
a = HexBasis1()
verts = 5*tilevertices(a)
vecofmat = collect(reinterpret(reshape,SVector{2,eltype(verts)},verts))
lens = ParaxialLensConvexPoly(
1.0,
Geometry.translation(1.0,0.0,1.0)*Geometry.rotation(0.0,Float64(π),0.0),
vecofmat,
SVector(0.0,0.0)
)
display_center = [1.0,0.0,2.0]
eyebox_center = [0.0,0.0,0.0]
return lens,eyebox_center,display_center
end
export center_data
"""verify that paraxial lens properly transorms rays into local coordinate frame to compute intersections with convex poly shape"""
function test_paraxial_convex_poly()
lens,eyebox_center,display_center = center_data()
@info "normal of lens $(OpticSim.normal(lens))"
@info "center of lens $(centroid(lens)) center of lens geometry $(centroid(shape(lens)))"
display_ray = OpticalRay(Ray([1.0,0.0,2.0],[0.0,0.0,-1.0]),1.0,.53)
ltrace = trace(LensAssembly(lens),display_ray)
@info "trace with optical center aligned with geometric center $ltrace"
new_center = compute_optical_center(eyebox_center,display_center,lens)
@info "offset center $new_center"
displaced_lens = replace_optical_center(eyebox_center,display_center,lens)
@info "optical center of displacedlens $(opticalcenter(displaced_lens)) center of geometry $(centroid(shape(displaced_lens)))"
dtrace = trace(LensAssembly(displaced_lens),display_ray)
@info "trace with optical center displaced from geometric center $dtrace"
refracted_ray = ray(dtrace)
intsct = surfaceintersection(Plane([0.0,0.0,1.0],eyebox_center),refracted_ray)
@info "intsct should be [0,0,0] $(point(OpticSim.lower(intsct)))" #ray was computed to refract from the geometric lens center to the point [0,0,0] on the eyebox plane
return intsct
end
export test_paraxial_convex_poly
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3057 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#Various sizes of hexagonal clusters that are useful in lenslet design. To see what they look like use the functions in HexTilings to make lattices with color properties and then draw them with Vis.draw
#example: Vis.draw(hex3RGB())
function hex3(scale::T = 1.0) where{T<:Real}
clusterelements = SVector((0, 0), (-1, 0), (-1, 1))
eltlattice = HexBasis1(scale)
clusterbasis = LatticeBasis((-1, 2), (2, -1))
return LatticeCluster(clusterbasis, eltlattice, clusterelements)
end
export hex3
function hex4(scale::T = 1.0) where{T<:Real}
clusterelements = SVector((-1, 0), (-1, 1), (0, 0), (0, 1))
eltlattice = HexBasis1(scale)
clusterbasis = LatticeBasis((2, 0), (0, 2))
return LatticeCluster(clusterbasis, eltlattice, clusterelements)
end
export hex4
hex7(scale::T = 1.0) where{T<:Real} = hexn(1,scale)
export hex7
function hex9(scale::T = 1.0) where{T<:Real}
clusterelements = SVector((-1, -1), (-1, 0), (-2, 1), (0, -1), (0, 0), (-1, 1), (1, -1), (1, 0), (0, 1))
eltlattice = HexBasis1(scale)
clusterbasis = LatticeBasis((3, 0), (0, 3))
return LatticeCluster(clusterbasis, eltlattice, clusterelements)
end
export hex9
function hex12(scale::T = 1.0) where{T<:Real}
clusterelements = SVector(
(-1, 1),(0, 1),
(-1, 0),(0, 0),(1, 0),
(-1, -1),(0, -1),(1, -1),(2, -1),
(0, -2),(1, -2),(2, -2)
)
eltlattice = HexBasis3(scale)
clusterbasis = LatticeBasis((2, 2), (-4, 2))
return LatticeCluster(clusterbasis, eltlattice, clusterelements)
end
export hex12
function hex18(scale::T = 1.0) where{T<:Real}
# throw(ErrorException("not yet working"))
clusterelements = SVector(
(-2,1),(-1, 1),(0, 1),(1,1),
(-2,0),(-1, 0),(0, 0),(1, 0),(2,0),
(-1, -1),(0, -1),(1, -1),(2, -1),
(-1,-2),(0, -2),(1, -2),(2, -2),(3,-2)
)
eltlattice = HexBasis3(scale)
clusterbasis = LatticeBasis((4, 1), (-2,4))
return LatticeCluster(clusterbasis, eltlattice, clusterelements)
end
export hex18
hex19(scale::T = 1.0) where{T<:Real} = hexn(2,scale)
export hex19
hex37(scale::T = 1.0) where{T<:Real} = hexn(3,scale)
export hex37
""" function for creating symmetric clusters consisting of all lattice cells within regionsize of the origin. This includes hex1 (use regionsize = 0),hex7 (regionsize = 1),hex19 (region size = 2),hex37 (regionsize = 3), etc."""
function hexn(regionsize::Int64,scale::T = 1.0) where{T<:Real}
eltlattice = HexBasis1(scale)
clusterbasis = LatticeBasis((2*regionsize+1, -regionsize), (regionsize, regionsize+1))
return LatticeCluster(clusterbasis, eltlattice, region(HexBasis1,(0,0),regionsize))
end
export hexn
function testhexclusters()
for clusterfunc in (hex3,hex4,hex9,hex12,hex19,hex37)
cluster = clusterfunc()
println("$clusterfunc euclidean diameter $(euclideandiameter(cluster))")
end
end
export testhexclusters | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3244 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# All of the objects can be displayed with Vis.draw. Example:
# Vis.draw(hex4RGB())
colornames(colors) = uppercase.(x[1]*string((i-1)÷3 + 1) for (i,x) in pairs(colors))
export colornames
function clustercolors(lattice)
elements = Repeat.clusterelements(lattice)
colors = Vector{String}(undef, length(elements))
for (i, element) in pairs(elements)
colors[i] = pointcolor(element, lattice)
end
return colors
end
export clustercolors
function hex3RGB(scale::T = 1.0) where{T<:Real}
lattice = hex3(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(hex3(scale), properties)
end
export hex3RGB
function hex4RGB(scale::T = 1.0) where{T<:Real}
lattice = hex4(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(hex4(scale), properties)
end
export hex4RGB
function hex7RGB(scale::T = 1.0) where{T<:Real}
lattice = hex7(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(hex7(scale), properties)
end
export hex7RGB
function hex9RGB(scale::T = 1.0) where{T<:Real}
lattice = hex9(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(hex9(scale), properties)
end
export hex9RGB
function hex12RGB(scale::T = 1.0) where{T<:Real}
lattice = hex12(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(lattice, properties)
end
export hex12RGB
function hex18RGB(scale::T = 1.0) where{T<:Real}
lattice = hex18(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
# properties = DataFrames.DataFrame(Color = colors)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(lattice, properties)
end
export hex18RGB
function hex19RGB(scale::T = 1.0) where{T<:Real}
lattice = hex19(scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
# properties = DataFrames.DataFrame(Color = colors)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(lattice, properties)
end
export hex19RGB
function hex37RGB(scale::T = 1.0) where{T<:Real}
lattice = hexn(3,scale)
colors = clustercolors(lattice)
names = colornames(colors)
properties = DataFrames.DataFrame(Color=colors, Name=names)
# properties = DataFrames.DataFrame(Color = colors)
return Repeat.ClusterWithProperties{Repeat.RGBCluster}(lattice, properties)
end | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 17058 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
import Statistics
"""
@unzip xs, ys, ... = us
will expand the assignment into the following code
xs, ys, ... = map(x -> x[1], us), map(x -> x[2], us), ...
"""
macro unzip(args)
args.head != :(=) && error("Expression needs to be of form `xs, ys, ... = us`")
lhs, rhs = args.args
items = isa(lhs, Symbol) ? [lhs] : lhs.args
rhs_items = [:(map(x -> x[$i], $rhs)) for i in 1:length(items)]
rhs_expand = Expr(:tuple, rhs_items...)
esc(Expr(:(=), lhs, rhs_expand))
end
export @unzip
"""Contains the information defining a lenslet array."""
struct LensletSystem{T<:Real}
eyebox_rectangle::Rectangle{T}
subdivisions_of_eyebox::Tuple{Int64,Int64}
lenses::Vector{ParaxialLens{T}}
lattice_coordinates::Vector{Tuple{Int64,Int64}}
lenslet_colors::Vector{String}
projected_eyeboxes::Vector{SMatrix{3,4,T}}
displayplanes::Vector{Plane{T,3}}
lenslet_eye_boxes::Vector{SMatrix{3, 4, T, 12}}
lenslet_eyebox_numbers::Vector{Int64}
lenslet_eyebox_centers::Vector{SVector{3,T}}
system_properties::Dict
subdivided_eyebox_polys::Vector{SMatrix{3,4,T}}
projected_eyebox_polygons::Vector{ConvexPolygon{N,T}} where{N}
end
# """ Computes the location of the optical center of the lens that will project the centroid of the display to the centroid of the eyebox. Normally the display centroid will be aligned with the geometric centroid of the lens, rather than the optical center of the lens."""
# function compute_optical_center(eyeboxcentroid,displaycenter,lensplane)
# v = displaycenter - eyeboxcentroid
# r = Ray(eyeboxcentroid,v)
# intsct = point(closestintersection(surfaceintersection(lensplane,r),false)) #this seems complicated when you don't need CSG
# @assert intsct !== nothing
# return intsct
# end
""" Computes the location of the optical center of the lens that will project the centroid of the display to the centroid of the eyebox. Normally the display centroid will be aligned with the geometric centroid of the lens, rather than the optical center of the lens."""
function compute_optical_center(eyeboxcentroid,display_center,lens)
lens_geometric_center = centroid(lens) #geometric and optical center coincide at this point
v = eyeboxcentroid - lens_geometric_center
r = Ray(display_center,v)
#optical center may not lie inside the shape of the lens. surfaceintersection(lens,r) will return nothing in this case which will cause the setup_system code to crash. Instead intersect with the plane of the shape of the lens, which has infinite extent.
surf = OpticSim.plane(lens)
intsct = point(closestintersection(surfaceintersection(surf,r),false)) #this seems complicated when you don't need CSG
@assert intsct !== nothing
return intsct
end
export compute_optical_center
function localframe(a::ParaxialLens)
if OpticSim.shape(a) isa ConvexPolygon
return OpticSim.localframe(OpticSim.shape(a))
else
return nothing
end
end
"""Paraxial lenses are created with a local transform of identity. This is confusing because the lens has a local transform and the shape in the lens has a local transform."""
function replace_optical_center(eyeboxcentroid,displaycenter,lens)
world_optic_center = compute_optical_center(eyeboxcentroid,displaycenter,lens)
world_to_local_lens = inv(localframe(lens))
local_optic_center = world_to_local_lens * world_optic_center
@assert isapprox(0.0, local_optic_center[3],atol = 1e-12) "z should be zero in the local frame but instead it is $(local_optic_center[3])"
local_optic_center = SVector{2}((local_optic_center)[1:2]) #this is defined in the 2D lens plane so z = 0
# need the untransformed convexpoly vertices
return ParaxialLensConvexPoly(focallength(lens),shape(lens),local_optic_center)
end
"""returns display plane represented in world coordinates, and the center point of the display"""
function display_plane(lens)
center_point = centroid(lens) + -OpticSim.normal(lens)* OpticSim.focallength(lens)
pln = Plane(OpticSim.normal(lens), center_point, vishalfsizeu = .5, vishalfsizev = .5,interface = opaqueinterface())
return pln,center_point
end
export display_plane
""" eyebox rectangle represented as a 3x4 SMatrix with x,y,z coordinates in rows 1,2,3 respectively. By default the eyebox z is 0.0"""
function eyeboxpolygon(xsize,ysize)::SMatrix{3,4}
xext,yext = (xsize,ysize) ./ 2.0 #divide by 2 to get min and max extensions
return SMatrix{3,4}([xext;yext;0.0 ;; xext;-yext;0.0 ;; -xext;-yext;0.0 ;; -xext;yext;0.0])
end
subpoints(pts::AbstractVector,subdivisions) = reshape(collect(range(extrema(pts)...,subdivisions)),1,subdivisions)
export subpoints
subdivide(poly::AbstractMatrix,xsubdivisions,ysubdivisions) = subdivide(SMatrix{3,4}(poly),xsubdivisions,ysubdivisions)
"""poly is a two dimensional rectangle represented as a 3x4 SMatrix with x values in row 1 and y values in row 2. Returns a vector of length xsubdivisions*ysubdivisions containing all the subdivided rectangles."""
function subdivide(poly::T, xsubdivisions, ysubdivisions)::Vector{T} where{T<:SMatrix{3,4}}
result = Vector{T}(undef,0) #efficiency not an issue here so don't need to preallocate vector
x = subpoints(poly[1,:],xsubdivisions+1) #range function makes one less subdivision that people will be expecting, e.g., collect(range(1,3,2)) returns [1,3]
y = subpoints(poly[2,:],ysubdivisions+1)
for i in 1:length(x) -1
for j in 1:length(y) -1
push!(result,T([x[i];y[j];0.0mm ;; x[i+1];y[j];0.0mm ;; x[i+1];y[j+1];0.0mm ;; x[i];y[j+1];0.0mm])) #this is klunky but the polygon vertices have units of mm so the 0.0 terms must as well.
end
end
return result
end
export subdivide
"""Assigns an eyebox to each lenslet in the cluster"""
function eyebox_number(tilecoords::NTuple{2,Int64},cluster::R,num_eyeboxes::Integer)::Int64 where {R<:AbstractLatticeCluster}
_, tile_index = cluster_coordinates_from_tile_coordinates(cluster,tilecoords)
eyeboxnum = mod(tile_index-1,num_eyeboxes) + 1
return eyeboxnum
end
"""For RGB clusters have to extract the indices of the color tiles and distribute the eyeboxes separately among RGB.
Example:
Assume there are 4 eyeboxes. The red,green, and blue tiles should each have the 4 eyebox numbers distributed evenly among them.
julia> hex12RGB()
ClusterWithProperties{12, 2, Float64, OpticSim.Repeat.RGBCluster}(LatticeCluster{12, 2, Float64,
LatticeBasis{2, Int64}, HexBasis3{2, Float64}}(LatticeBasis{2, Int64}([2 -3; 2 2]), HexBasis3{2,
Float64}(1.0), [(-1, 1), (0, 1), (-1, 0), (0, 0), (1, 0), (-1, -1), (0, -1), (1, -1), (2, -1), (0, -2), (1, -2), (2, -2)]), 12×2 DataFrame
Row │ Color Name
│ String String
─────┼────────────────
1 │ green G1
2 │ blue B1
3 │ blue B1
4 │ red R2
5 │ green G2
6 │ red R2
7 │ green G3
8 │ blue B3
9 │ red R3
10 │ blue B4
11 │ red R4
12 │ green G4)
julia> rgbtileindices(ans)
([4, 6, 9, 11], [1, 5, 7, 12], [2, 3, 8, 10])
Assume eyebox_number is called with tilecoords that correspond to tile_index 9. The red tiles have indices [4, 6, 9, 11]. 9 is the third element in this vector so it should get eyebox number 3. If tile_index is 2 then this is a blue tile. The index of the element 2 in the blue vector is 1 so this tile should get eyebox 1.
"""
function eyebox_number(tilecoords::NTuple{2,Int64},cluster::ClusterWithProperties{A,B,C,Repeat.RGBCluster},num_eyeboxes::Integer)::Int64 where{A,B,C}
_, tile_index = cluster_coordinates_from_tile_coordinates(cluster,tilecoords)
rindices,gindices,bindices = Repeat.rgbtileindices(cluster)
@assert length(rindices) == length(gindices)
@assert length(gindices) == length(bindices)
@assert mod(length(rindices), num_eyeboxes) == 0 #if num_eyeboxes doesn't evenly divide the number of available r,g,b, lenslets then the system won't work properly
allcolors = [rindices;gindices;bindices] #stack all the indices for the different colors into a single vector
matchingindex = findfirst(x->x==tile_index,allcolors) #
eyeboxnum = mod(matchingindex-1,num_eyeboxes) + 1
return eyeboxnum
end
function eyebox_assignment(tilecoords::NTuple{2,Int64},cluster::R,eyeboxes) where{R<:AbstractLatticeCluster}
#compute cluster
eyeboxnum = eyebox_number(tilecoords,cluster,length(eyeboxes))
return eyeboxes[eyeboxnum] #use linear index for Matrix.
end
"""given the eye box polygon and how it is to be subdivided computes the subdivided eyeboxes and centroids"""
function compute_lenslet_eyebox_data(eyeboxtransform,eyeboxpoly::SMatrix{3,4,T,12},subdivisions::Tuple{Int64,Int64})::Vector{SMatrix{3,4,Float64}} where{T} #can't figure out how to get the system to accept a type for an SMatrix of a Unitful quantity which is what eyeboxpoly is. Want to extract the number type from the Quantity type but this is not happening.
subdivided_eyeboxpolys = subdivide(eyeboxpoly,subdivisions...)
strippedpolys = map(x-> ustrip.(mm,x), subdivided_eyeboxpolys)
subdivided_eyeboxpolys =[eyeboxtransform * x for x in strippedpolys] #these have units of mm which don't interact well with Transform.
return subdivided_eyeboxpolys
end
function test_compute_lenslet_eyebox_data(eyeboxtransform,eyeboxpoly,subdivisions)
subpolys = compute_lenslet_eyebox_data(eyeboxtransform,eyeboxpoly,subdivisions)
end
function project_eyebox_to_display_plane(eyeboxpoly::AbstractMatrix{T},lens,displayplane) where{T<:Real}
rowdim,coldim = size(eyeboxpoly)
rays = [Ray(SVector{rowdim}(point),opticalcenter(lens)-SVector{rowdim}(point)) for point in eachcol(eyeboxpoly)]
points = collect([point(closestintersection(surfaceintersection(displayplane,ray),false)) for ray in rays])
eyebox = SMatrix{rowdim,coldim}(reinterpret(Float64,points)...)
threeDpts, toworld, _ = projectonbestfitplane(eyebox,[0.0,0.0,-1.0])
twoDpts = reshape(threeDpts[1:2,:],2*size(threeDpts)[2])
twoDpts = collect(reinterpret(SVector{2,T},twoDpts))
polygon = ConvexPolygon(toworld,twoDpts,opaqueinterface())
for (eyept,polypt) in zip(eachcol(eyebox),eachcol(vertices(polygon)))
@assert isapprox(eyept,polypt)
end
return eyebox,polygon
end
export project_eyebox_to_display_plane
"""System parameters for a typical HMD."""
function systemparameters()
return (eye_box = (10.0mm,9.0mm),fov = (90.0°,60.0°),eye_relief = 20.0mm, pupil_diameter = 4.0mm, display_sphere_radius = 40.0mm,min_fnumber = 1.6, pixel_pitch = .7μm) #these numbers give a reasonable system, albeit with 2000 lenslets. However displays are quite small (184x252)μm
end
"""System parameters which will yield fewer lenslets which will draw much faster"""
function smallsystemparameters()
return (eye_box = (10.0mm,9.0mm),fov = (20.0°,20.0°),eye_relief = 20.0mm, pupil_diameter = 2mm, display_sphere_radius = 40.0mm,min_fnumber = 1.6, pixel_pitch = .7μm) #these numbers give a reasonable system, albeit with 2000 lenslets. However displays are quite small (184x252)μm
end
export smallsystemparameters
"""Coordinate frames for the eye/display system. The origin of this frame is at the geometric center of the eyeball. Positive Z axis is assumed to be the forward direction, the default direction of the eye's optical axis when looking directly ahead."""
function setup_coordinate_frames()
eyeballframe = Transform()
corneavertex = OpticSim.Data.cornea_to_eyecenter()
eyeboxtransform = eyeballframe*OpticSim.translation(0.0,0.0,ustrip(mm,corneavertex)) #unfortunately can't use unitful values in transforms because the rotation and translation components would have different types which is not allowed in a Matrix.
return (eyeball_frame = eyeballframe,eye_box_frame = eyeboxtransform)
end
"""repeat vec1 enough times to fill a vector of length(vec2)."""
function extend(vec1,vec2)
numrepeats = Int64(ceil(length(vec1)/length(vec2)))
remaining = mod(length(vec1),length(vec2))
subdivs= similar(vec2,numrepeats*length(vec2)+remaining)
empty!(subdivs)
for i in 1:numrepeats-1
append!(subdivs,vec2)
end
append!(subdivs,vec2[1:remaining])
return subdivs
end
"""Create lenslet system that will cover the eyebox and fov. If the numbers in the fov tuple do not have units the system assumes they represent an angle in radians. Is is better to use unitful angles though, either rad or °:
```
setup_system(eye_box,(1rad,1.2rad),...)
setup_system(eye_box,(1°,1.2°),...)
```
If you do this
```
setup_system(eye_box,(1,1.2),...)
```
the system will assume the angle is in radians."""
function setup_system(eye_box,fov,eye_relief,pupil_diameter,display_sphere_radius,min_fnumber,pixel_pitch;no_eyebox_subdivision::Bool = false)
#All coordinates are ultimately transformed into the eyeball_frame coordinate systems
(eyeball_frame,eye_box_frame) = setup_coordinate_frames()
@info "Input parameters\n"
parameters = (eye_box = eye_box,fov = fov,eye_relief = eye_relief,pupil_diameter = pupil_diameter,display_sphere_radius = display_sphere_radius,min_fnumber = min_fnumber,pixel_pitch = pixel_pitch)
for key in keys(parameters) @info "$key = $(parameters[key])" end
println("\n\n")
eyeboxz = (eye_box_frame*SVector(0.0,0.0,0.0))[3]
eyebox_plane = Plane([0.0,0.0,1.0],[0.0,0.0,eyeboxz])
#get system properties
props = system_properties(eye_relief,eye_box,fov,pupil_diameter,.2,11,pixelpitch = pixel_pitch, minfnumber = min_fnumber,no_eyebox_subdivision = no_eyebox_subdivision)
subdivisions = props[:subdivisions] #tuple representing how the eyebox can be subdivided given the cluster used for the lenslets
clusterdata = props[:cluster_data]
cluster = clusterdata[:cluster] #cluster that is repeated across the display to ensure continuous coverage of the eyebox and fov.
focallength = ustrip(mm,props[:focal_length]) #strip units off because these don't work well with Transform
#compute lenslets based on system properties. lattice_coordinates are the (i,j) integer lattice coordinates of the hexagonal lattice making up the display. These coordinates are used to properly assign color and subdivided eyebox to the lenslets.
lenses,lattice_coordinates = spherelenslets(eyebox_plane,eye_relief,focallength,[0.0,0.0,1.0],display_sphere_radius,fov[1],fov[2],elementbasis(cluster))
@info "$(length(lenses)) lenses required for this design"
temp = display_plane.(lenses)
displayplanes = [x[1] for x in temp]
display_plane_centers = [x[2] for x in temp]
lensletcolors = pointcolor.(lattice_coordinates,Ref(cluster))
#compute subdivided eyebox polygons and assign to appropriate lenslets
eyeboxpoly::SMatrix{3,4} = mm * (eye_box_frame * eyeboxpolygon(ustrip.(mm,eye_box)...)) #four corners of the eyebox frame which is assumed centered around the positive Z axis. Transformed to the eyeballframe. Have to switch back and forth between Unitful and unitless quantities because Transform doesn't work with Unitful values.
@info "Subdividing eyebox polygon into $subdivisions sub boxes"
subdivided_eyeboxpolys::Vector{SMatrix{3,4}} = compute_lenslet_eyebox_data(eye_box_frame,eyeboxpoly,subdivisions)
test_compute_lenslet_eyebox_data(eye_box_frame,eyeboxpoly,subdivisions)
#assign each lenslet one of the subdivided eyebox polygons
lenslet_eye_boxes = eyebox_assignment.(lattice_coordinates,Ref(cluster),Ref(subdivided_eyeboxpolys))
#assign each lenslet the index into subdivided_eyeboxpolys that corresponds to the subdivided eyebox polygon the lenslet is supposed to cover.
lenslet_eyebox_numbers = eyebox_number.(lattice_coordinates,Ref(cluster),Ref(length(subdivided_eyeboxpolys)))
#compute centroids of the subdivided eyeboxes on the eyebox plane
lensleteyeboxcenters = [Statistics.mean(eachcol(x)) for x in lenslet_eye_boxes]
offset_lenses = replace_optical_center.(lensleteyeboxcenters,display_plane_centers,lenses) #make new lenses with optical centers of lenses to put display centers directly behind lenslets
projected = project_eyebox_to_display_plane.(lenslet_eye_boxes,offset_lenses,displayplanes) #repeate subdivided_eyeboxpolys enough times to cover all lenses
projected_eyeboxes = [x[1] for x in projected]
projected_polygons = [x[2] for x in projected]
@info "Lenslet diameter $(props[:lenslet_diameter])"
eyebox_rectangle = Rectangle(ustrip(mm,eye_box[1]/2),ustrip(mm,eye_box[2]/2),[0.0,0.0,1.0],[0.0,0.0,eyeboxz], interface = opaqueinterface())
return LensletSystem{Float64}(eyebox_rectangle,subdivisions,offset_lenses,lattice_coordinates,lensletcolors,projected_eyeboxes,displayplanes,lenslet_eye_boxes,lenslet_eyebox_numbers,lensleteyeboxcenters,props,subdivided_eyeboxpolys,projected_polygons)
end
export setup_system
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1090 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Multilens
using LinearAlgebra
import Unitful
using Unitful:uconvert,ustrip
using Unitful.DefaultSymbols:mm,μm,nm #Unitful.DefaultSymbols exports a variable T which can cause major confusion with type declarations since T is a common parametric type symbol. Import only what is needed.
using Colors
using StaticArrays
import DataFrames
import ..Repeat
import SpecialFunctions
import Plots
import ...OpticSim
using ...OpticSim:plane_from_points,centroid,pointonplane,focallength,ParaxialLens
import ...OpticSim.Geometry
using ...OpticSim.Repeat:region,HexBasis1,HexBasis3,LatticeBasis,LatticeCluster,clustersize,ClusterWithProperties,cluster_coordinates_from_tile_coordinates, AbstractLatticeCluster,elementbasis,euclideandiameter
using ...OpticSim.Data
include("HexClusters.jl")
include("HexTilings.jl")
include("Analysis.jl")
include("DisplayGeneration.jl")
include("LensletAssignment.jl")
include("Example.jl")
end # module
export Multilens | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6664 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using ..OpticSim, .Geometry
using LinearAlgebra
using Distributions
using StaticArrays
import Makie
using .Emitters
using .Emitters.Spectrum
using .Emitters.Directions
using .Emitters.Origins
using .Emitters.AngularPower
using .Emitters.Sources
const ARRROW_LENGTH = 0.5
const ARRROW_SIZE = 0.01
const MARKER_SIZE = 1
#-------------------------------------
# draw debug information - local axes and positions
#-------------------------------------
function maybe_draw_debug_info(scene::Makie.LScene, o::Origins.AbstractOriginDistribution; transform::Geometry.Transform = Transform(), debug::Bool=false, kwargs...) where {T<:Real}
dir = forward(transform)
uv = SVector{3}(right(transform))
vv = SVector{3}(up(transform))
pos = origin(transform)
if (debug)
# this is a stupid hack to force makie to render in 3d - for some scenes, makie decide with no apperent reason to show in 2d instead of 3d
Makie.scatter!(scene, [pos[1], pos[1]+0.1], [pos[2], pos[2]+0.1], [pos[3], pos[3]+0.1], color=:red, markersize=0)
# draw the origin and normal of the surface
Makie.scatter!(scene, pos, color=:blue, markersize = MARKER_SIZE * visual_size(o))
# normal
arrow_size = ARRROW_SIZE * visual_size(o)
arrow_start = pos
arrow_end = dir * ARRROW_LENGTH * visual_size(o)
Makie.arrows!(scene.scene, [Makie.Point3f(arrow_start)], [Makie.Point3f(arrow_end)], arrowsize=arrow_size, linewidth=arrow_size * 0.5, linecolor=:blue, arrowcolor=:blue)
arrow_end = uv * 0.5 * ARRROW_LENGTH * visual_size(o)
Makie.arrows!(scene.scene, [Makie.Point3f(arrow_start)], [Makie.Point3f(arrow_end)], arrowsize= 0.5 * arrow_size, linewidth=arrow_size * 0.5, linecolor=:red, arrowcolor=:red)
arrow_end = vv * 0.5 * ARRROW_LENGTH * visual_size(o)
Makie.arrows!(scene.scene, [Makie.Point3f(arrow_start)], [Makie.Point3f(arrow_end)], arrowsize= 0.5 * arrow_size, linewidth=arrow_size * 0.5, linecolor=:green, arrowcolor=:green)
# draw all the samples origins
positions = map(x -> transform*x, collect(o))
positions = collect(Makie.Point3f, positions)
Makie.scatter!(scene, positions, color=:green, markersize = MARKER_SIZE * visual_size(o))
# positions = collect(Makie.Point3f, o)
# Makie.scatter!(scene, positions, color=:green, markersize = MARKER_SIZE * visual_size(o))
end
end
#-------------------------------------
# draw point origin
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, o::Origins.Point; transform::Geometry.Transform = Transform(), kwargs...) where {T<:Real}
maybe_draw_debug_info(scene, o; transform=transform, kwargs...)
end
#-------------------------------------
# draw RectGrid and RectUniform origins
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, o::Union{Origins.RectGrid, Origins.RectUniform}; transform::Geometry.Transform = Transform(), kwargs...) where {T<:Real}
dir = forward(transform)
uv = SVector{3}(right(transform))
vv = SVector{3}(up(transform))
pos = origin(transform)
# @info "RECT: transform $(pos)"
plane = OpticSim.Plane(dir, pos)
rect = OpticSim.Rectangle(plane, o.width / 2, o.height / 2, uv, vv)
OpticSim.Vis.draw!(scene, rect; kwargs...)
maybe_draw_debug_info(scene, o; transform=transform, kwargs...)
end
#-------------------------------------
# draw hexapolar origin
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, o::Origins.Hexapolar; transform::Geometry.Transform = Transform(), kwargs...) where {T<:Real}
dir = forward(transform)
uv = SVector{3}(right(transform))
vv = SVector{3}(up(transform))
pos = origin(transform)
plane = OpticSim.Plane(dir, pos)
ellipse = OpticSim.Ellipse(plane, o.halfsizeu, o.halfsizev, uv, vv)
OpticSim.Vis.draw!(scene, ellipse; kwargs...)
maybe_draw_debug_info(scene, o; transform=transform, kwargs...)
end
#-------------------------------------
# draw source
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, s::S; parent_transform::Geometry.Transform = Transform(), debug::Bool=false, kwargs...) where {T<:Real,S<:Sources.AbstractSource{T}}
OpticSim.Vis.draw!(scene, Emitters.Sources.origins(s); transform=parent_transform * Emitters.Sources.transform(s), debug=debug, kwargs...)
if (debug)
m = zeros(T, length(s), 7)
for (index, optical_ray) in enumerate(s)
ray = OpticSim.ray(optical_ray)
ray = parent_transform * ray
m[index, 1:7] = [ray.origin... ray.direction... OpticSim.power(optical_ray)]
end
m[:, 4:6] .*= m[:, 7] * ARRROW_LENGTH * visual_size(Emitters.Sources.origins(s))
# Makie.arrows!(scene, [Makie.Point3f(origin(ray))], [Makie.Point3f(rayscale * direction(ray))]; kwargs..., arrowsize = min(0.05, rayscale * 0.05), arrowcolor = color, linecolor = color, linewidth = 2)
color = :yellow
arrow_size = ARRROW_SIZE * visual_size(Emitters.Sources.origins(s))
Makie.arrows!(scene, m[:,1], m[:,2], m[:,3], m[:,4], m[:,5], m[:,6]; kwargs..., arrowcolor=color, linecolor=color, arrowsize=arrow_size, linewidth=arrow_size*0.5)
end
# for ray in o
# OpticSim.Vis.draw!(scene, ray)
# end
end
#-------------------------------------
# draw optical rays
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, rays::AbstractVector{OpticSim.OpticalRay{T, 3}}; kwargs...) where {T<:Real}
m = zeros(T, length(rays)*2, 3)
for (index, optical_ray) in enumerate(rays)
ray = OpticSim.ray(optical_ray)
m[(index-1)*2+1, 1:3] = [origin(optical_ray)...]
m[(index-1)*2+2, 1:3] = [(OpticSim.origin(optical_ray) + OpticSim.direction(optical_ray) * 1 * OpticSim.power(optical_ray))... ]
end
color = :green
Makie.linesegments!(scene, m[:,1], m[:,2], m[:,3]; kwargs..., color = color, linewidth = 2, )
end
#-------------------------------------
# draw composite source
#-------------------------------------
function OpticSim.Vis.draw!(scene::Makie.LScene, s::Sources.CompositeSource{T}; parent_transform::Geometry.Transform = Transform(), kwargs...) where {T<:Real}
for source in s.sources
OpticSim.Vis.draw!(scene, source; parent_transform=parent_transform*Emitters.Sources.transform(s), kwargs...)
end
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 647 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Vis
using ..OpticSim
using ..OpticSim: euclideancontrolpoints, evalcsg, vertex, makiemesh, detector, centroid, lower, upper, intervals, α
using ..OpticSim.Geometry
using ..OpticSim.Repeat
using Unitful
using ImageView
using Images
using ColorTypes
using ColorSchemes
using StaticArrays
using LinearAlgebra
import Makie
import GeometryBasics
import Plots
import Luxor
using FileIO
include("Visualization.jl")
include("Emitters.jl")
include("VisRepeatingStructures.jl")
end # module Vis
export Vis
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3135 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#############################################################################
# lattice visualizations are drawn with Luxor because it is easier to do 2D drawings with Luxor than with Makie.
function draw(tilebasis::AbstractBasis, tilesize, i, j, color, name)
vertices = Repeat.tilevertices(tilebasis)
tile = tilesize * [Luxor.Point(vertices[:,i]...) for i in 1:size(vertices)[2]]
pt = tilesize * tilebasis[i,j]
offset = Luxor.Point(pt[1], -pt[2]) # flip y so indices show up correctly
Luxor.translate(offset)
Luxor.sethue(color)
Luxor.poly(tile, :fill, close=true)
Luxor.sethue("lightgrey")
Luxor.poly(tile, :stroke, close=true)
Luxor.sethue("black")
# scale and offset text so coordinates are readable
Luxor.fontsize(tilesize / 3)
Luxor.text("$i, $j", Luxor.Point(-tilesize / 3, tilesize / 2.5))
if name !== nothing
Luxor.text(name, Luxor.Point(-tilesize / 4, -tilesize / 4))
end
Luxor.translate(-offset)
end
function drawcells(clstr::Repeat.ClusterWithProperties,scale,points)
_,npts = size(points)
repeats = npts ÷ clustersize(clstr)
props = repeat(Repeat.properties(clstr), repeats)
drawcells(elementbasis(clstr), scale, points, color=props[:,:Color], name=props[:,:Name])
end
drawcells(clstr::Repeat.LatticeCluster,scale,points) = drawcells(elementbasis(clstr),scale,points)
"""Draws a list of hexagonal cells, represented by their lattice coordinates, which are represented as a 2D matrix, with each column being one lattice coordinate."""
function drawcells(tilebasis::AbstractBasis, tilesize, cells::AbstractMatrix; color::Union{AbstractArray,Nothing}=nothing, name::Union{AbstractArray{String},Nothing}=nothing, format=:png, resolution=(1000, 1000))
numcells = size(cells)[2]
Luxor.Drawing(resolution[1], resolution[2], format)
Luxor.origin()
Luxor.background(Colors.RGBA(1, 1, 1, 0.0))
if color === nothing
color = Colors.distinguishable_colors(numcells, lchoices=30:250) # type unstable but not performance critical code
end
for i in 1:numcells
cell = cells[:,i]
cellname = name === nothing ? nothing : name[i]
draw(tilebasis, tilesize, cell[1], cell[2], color[i], cellname)
end
Luxor.finish()
if (format == :svg)
res = Luxor.svgstring()
return res
end
if (format == :png)
Luxor.preview()
end
end
""" draw the ClusterWithProperties at coordinates specified by lattice_coordinate_offset """
function draw(clstr::Repeat.AbstractLatticeCluster, cluster_coordinate_offset::AbstractMatrix{T} = [0;0;;] , scale=50.0) where{T}
dims = size(cluster_coordinate_offset)
clstrsize = clustersize(clstr)
points = Matrix{Int64}(undef, dims[1], dims[2] * clstrsize)
for i in 1:dims[2]
points[:,(i - 1) * clstrsize + 1:i * clstrsize] = clustercoordinates(clstr, cluster_coordinate_offset[:,i]...)
end
drawcells(clstr,scale,points)
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 44725 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#############################################################################
function drawcurve!(canvassize::Int, curve::Spline{P,S,N,M}, numpoints::Int; linewidth = 0.5, curvecolor = RGB(1, 0, 1), controlpointcolor = RGB(0, 0, 0), controlpointsize = 5, controlpolygoncolor = RGB(0, 0, 0)) where {P,S,N,M}
step = 1.0 / numpoints
point1 = point(curve, 0.0) .* canvassize
# println("point1 $point1")
point1 = [point1[1], canvassize - point1[2]] # flip y because of the coordinate system SVG uses
Luxor.setline(linewidth)
Luxor.sethue(curvecolor)
for u in step:step:1.0
point2 = point(curve, u) .* canvassize
point2 = [point2[1], canvassize - point2[2]]
Luxor.line(Luxor.Point(point1...), Luxor.Point(point2...), :fillstroke)
point1 = copy(point2)
end
Luxor.sethue(controlpointcolor)
# draw control points
controlpoints = euclideancontrolpoints(curve)
for point in controlpoints
pt = point .* canvassize
pt = [pt[1], canvassize - pt[2]]
Luxor.circle(Luxor.Point(pt...), controlpointsize, :fill)
end
Luxor.sethue(controlpolygoncolor)
for i in 1:(size(controlpoints)[1] - 1)
pt1 = controlpoints[i] .* canvassize
pt1 = [pt1[1], canvassize - pt1[2]]
pt2 = controlpoints[i + 1] .* canvassize
pt2 = [pt2[1], canvassize - pt2[2]]
Luxor.line(Luxor.Point(pt1...), Luxor.Point(pt2...), :fillstroke)
end
end
function drawcurves(curves::Vararg{Spline{P,S,N,M}}; numpoints::Int = 200, canvassize::Int = 2000) where {P,S,N,M}
canvas = Luxor.Drawing(canvassize, canvassize)
Luxor.background("white")
drawcurve!(canvassize, curves[1], numpoints, linewidth = 5, curvecolor = RGB(0, 1, 1))
for curve in curves[2:end]
drawcurve!(canvassize, curve, numpoints, linewidth = 1, controlpointcolor = RGB(1, 0, 0), controlpointsize = 3, controlpolygoncolor = RGB(0, 1, 0))
end
Luxor.finish()
Luxor.preview()
end
#############################################################################
# all functions follow the pattern draw(obj) and draw!(scene, obj) where the first case draws the object in a blank scene and displays it, and
# the second case draws the object in an existing scene, draw!(obj) can also be used to draw the object in the current scene
global current_main_scene = nothing
global current_layout_scene = nothing
global current_3d_scene = nothing
global current_mode = nothing # modes: nothing, :default -> Original Vis beheviour
# :pluto -> support pluto notebooks
# :docs -> support documenter figures
# added the following 2 functions to allow us to hack the drawing mechanisim while in a pluto notebook
set_current_main_scene(scene) = (global current_main_scene = scene)
set_current_3d_scene(lscene) = (global current_3d_scene = lscene)
get_current_mode() = begin global current_mode; return current_mode end
set_current_mode(mode) = (global current_mode = mode)
show(image) = imshow(image)
display(scene = current_main_scene) = begin
global current_mode;
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return scene
end
Makie.display(scene)
end
"""
scene(resolution = (1000, 1000))
Create a new Makie scene with the given resolution including control buttons.
"""
function scene(resolution = (1000, 1000))
@assert resolution[1] > 0 && resolution[2] > 0
scene, layout = Makie.layoutscene(resolution = resolution)
global current_main_scene = scene
global current_layout_scene = layout
lscene = layout[1, 1] = Makie.LScene(scene, scenekw = (camera = Makie.cam3d_cad!, axis_type = Makie.axis3d!, raw = false))
global current_3d_scene = lscene
# in these modes we want to skip the creation of the utility buttons as these modes are not interactive
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return scene, lscene
end
threedbutton = Makie.Button(scene, label = "3D", buttoncolor = RGB(0.8, 0.8, 0.8), height = 40, width = 80)
twodxbutton = Makie.Button(scene, label = "2D-x", buttoncolor = RGB(0.8, 0.8, 0.8), height = 40, width = 80)
twodybutton = Makie.Button(scene, label = "2D-y", buttoncolor = RGB(0.8, 0.8, 0.8), height = 40, width = 80)
savebutton = Makie.Button(scene, label = "Screenshot", buttoncolor = RGB(0.8, 0.8, 0.8), height = 40, width = 160)
Makie.on(threedbutton.clicks) do nclicks
make3d(lscene)
yield()
end
Makie.on(twodybutton.clicks) do nclicks
make2dy(lscene)
yield()
end
Makie.on(twodxbutton.clicks) do nclicks
make2dx(lscene)
yield()
end
Makie.on(savebutton.clicks) do nclicks
Vis.save("screenshot.png")
yield()
end
layout[2, 1] = Makie.grid!(hcat(threedbutton, twodxbutton, twodybutton, savebutton), tellwidth = false, tellheight = true)
return scene, lscene
end
function make3d(scene::Makie.LScene = current_3d_scene)
s = scene.scene
# use 3d camera
Makie.cam3d_cad!(s)
# reset scene rotation
s.transformation.rotation[] = Makie.Quaternion(0.0, 0.0, 0.0, 1.0)
# show all the axis ticks
s[Makie.OldAxis].attributes.showticks[] = (true, true, true)
# reset tick and axis label rotation and position
rot = (Makie.Quaternion(0.0, 0.0, -0.7071067811865476, -0.7071067811865475), Makie.Quaternion(0.0, 0.0, 1.0, 0.0), Makie.Quaternion(0.0, 0.7071067811865475, 0.7071067811865476, 0.0))
s[Makie.OldAxis].attributes.ticks.rotation[] = rot
s[Makie.OldAxis].attributes.names.rotation[] = rot
s[Makie.OldAxis].attributes.ticks.align = ((:left, :center), (:right, :center), (:right, :center))
s[Makie.OldAxis].attributes.names.align = ((:left, :center), (:right, :center), (:right, :center))
# reset scene limits to automatic
s.limits[] = Makie.Automatic()
Makie.update!(s)
end
function make2dy(scene::Makie.LScene = current_3d_scene)
s = scene.scene
# use 2d camera
Makie.cam2d!(s)
scene_transform = Makie.qrotation(Makie.Vec3f(0, 1, 0), 0.5pi)
scene_transform_inv = Makie.qrotation(Makie.Vec3f(0, 1, 0), -0.5pi) # to use with the ticks and names
# set rotation to look onto yz plane
s.transformation.rotation[] = scene_transform
# hide x ticks
# there is a bug in Makie 0.14.2 which cause an exception setting the X showticks to false.
# we work wround it by making sure the labels we want to turn off are ortogonal to the view direction
# s[Makie.OldAxis].attributes.showticks[] = (false, true, true)
s[Makie.OldAxis].attributes.showticks[] = (true, true, true)
# set tick and axis label rotation and position
s[Makie.OldAxis].attributes.ticks.rotation[] = (0.0, scene_transform_inv, scene_transform_inv)
s[Makie.OldAxis].attributes.names.rotation[] = s[Makie.OldAxis].attributes.ticks.rotation[]
s[Makie.OldAxis].attributes.ticks.align = ((:right, :center), (:right, :center), (:center, :right))
s[Makie.OldAxis].attributes.names.align = ((:left, :center), (:left, :center), (:center, :left))
# update the scene limits automatically to get true reference values
s.limits[] = Makie.Automatic()
Makie.update_limits!(s)
# manually set the scene limits to draw the axes correctly
o, w = Makie.origin(s.data_limits[]), Makie.widths(s.data_limits[])
s.limits[] = Makie.FRect3D((1000.0f0, o[2], o[3]), (w[2], w[2], w[3]))
# set the eye (i.e. light) position to behind the camera
s.camera.eyeposition[] = (0, 0, -100)
Makie.update!(s)
end
function make2dx(scene::Makie.LScene = current_3d_scene)
s = scene.scene
# use 2d camera
Makie.cam2d!(s)
scene_transform= Makie.qrotation(Makie.Vec3f(0, 0, 1), 0.5pi) * Makie.qrotation(Makie.Vec3f(1, 0, 0), 0.5pi)
scene_transform_inv=Makie.qrotation(Makie.Vec3f(1, 0, 0), -0.5pi) * Makie.qrotation(Makie.Vec3f(0, 0, 1), -0.5pi)
# set rotation to look onto yz plane
s.transformation.rotation[] = scene_transform
# hide y ticks
# there is a bug in Makie 0.14.2 which cause an exception setting the X showticks to false.
# we work wround it by making sure the labels we want to turn off are ortogonal to the view direction
s[Makie.OldAxis].attributes.showticks[] = (true, false, true)
# set tick and axis label rotation and position
s[Makie.OldAxis].attributes.ticks.rotation[] = (scene_transform_inv, 0.0, scene_transform_inv)
s[Makie.OldAxis].attributes.names.rotation[] = s[Makie.OldAxis].attributes.ticks.rotation[]
s[Makie.OldAxis].attributes.ticks.align = ((:right, :center), (:right, :center), (:center, :center))
s[Makie.OldAxis].attributes.names.align = ((:left, :center), (:right, :center), (:center, :center))
# update the scene limits automatically to get true reference values
s.limits[] = Makie.Automatic()
Makie.update_limits!(s)
# manually set the scene limits to draw the axes correctly
o, w = Makie.origin(s.data_limits[]), Makie.widths(s.data_limits[])
s.limits[] = Makie.FRect3D((o[1], -1000.0f0, o[3]), (w[1], w[1], w[3]))
# set the eye (i.e. light) position to behind the camera
s.camera.eyeposition[] = (0, 0, -100)
Makie.update!(s)
end
"""
draw(ob; resolution = (1000, 1000), kwargs...)
Draw an object in a new scene.
`kwargs` depends on the object type.
"""
function draw(ob; resolution = (1000, 1000), kwargs...)
scene, lscene = Vis.scene(resolution)
draw!(lscene, ob; kwargs...)
display(scene)
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return scene
end
end
"""
draw!([scene = currentscene], ob; kwargs...)
Draw an object in an existing scene.
`kwargs` depends on the object type.
"""
function draw!(ob; kwargs...)
if current_3d_scene === nothing
scene, lscene = Vis.scene()
else
scene = current_main_scene
lscene = current_3d_scene
end
Makie.cameracontrols(lscene.scene).attributes.mouse_rotationspeed[] = .0001f0 #doesn't seem to do anything
draw!(lscene, ob; kwargs...)
display(scene)
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return scene
end
end
"""
save(path::String)
Save the current Makie scene to an image file.
"""
function save(path::String)
# save closes the window so just display it again as a work-around
# for some reason the size isn't maintained automatically so we just reset it manually
size = Makie.size(current_main_scene)
Makie.save(path, current_3d_scene.scene)
Makie.resize!(current_main_scene, size)
display(current_main_scene)
end
function save(::Nothing) end
#############################################################################
function λtoRGB(λ::T, gamma::T = 0.8) where {T<:Real}
wavelength = λ * 1000 # λ is in um, need in nm
if (wavelength >= 380 && wavelength <= 440)
attenuation = 0.3 + 0.7 * (wavelength - 380) / (440 - 380)
R = ((-(wavelength - 440) / (440 - 380)) * attenuation)^gamma
G = 0.0
B = (1.0 * attenuation)^gamma
elseif (wavelength >= 440 && wavelength <= 490)
R = 0.0
G = ((wavelength - 440) / (490 - 440))^gamma
B = 1.0
elseif (wavelength >= 490 && wavelength <= 510)
R = 0.0
G = 1.0
B = (-(wavelength - 510) / (510 - 490))^gamma
elseif (wavelength >= 510 && wavelength <= 580)
R = ((wavelength - 510) / (580 - 510))^gamma
G = 1.0
B = 0.0
elseif (wavelength >= 580 && wavelength <= 645)
R = 1.0
G = (-(wavelength - 645) / (645 - 580))^gamma
B = 0.0
elseif (wavelength >= 645 && wavelength <= 750)
attenuation = 0.3 + 0.7 * (750 - wavelength) / (750 - 645)
R = (1.0 * attenuation)^gamma
G = 0.0
B = 0.0
else
R = 0.0
G = 0.0
B = 0.0
end
return RGB(R, G, B)
end
indexedcolor(i::Int) = ColorSchemes.hsv[rem(i / (2.1 * π), 1.0)]
indexedcolor2(i::Int) = ColorSchemes.hsv[1.0 - rem(i / (2.1 * π), 1.0)] .* 0.5
#############################################################################
## displaying imported meshes
Base.:*(a::Transform, p::GeometryBasics.PointMeta) = a * p.main
Base.:*(a::Real, p::GeometryBasics.PointMeta{N,S}) where {S<:Real,N} = GeometryBasics.Point{N,S}((a * SVector{N,S}(p))...)
Base.:*(a::Transform, p::GeometryBasics.Point{N,S}) where {S<:Real,N} = GeometryBasics.Point{N,S}((a.rotation * SVector{N,S}(p) + a.translation)...)
function draw!(scene::Makie.LScene, ob::AbstractString; color = :gray, linewidth = 3, shaded::Bool = true, wireframe::Bool = false, transform::Transform{Float64} = identitytransform(Float64), scale::Float64 = 1.0, kwargs...)
if any(endswith(lowercase(ob), x) for x in [".obj", "ply", ".2dm", ".off", ".stl"])
meshdata = FileIO.load(ob)
if transform != identitytransform(Float64) || scale != 1.0
coords = [transform * (scale * p) for p in GeometryBasics.coordinates(meshdata)]
meshdata = GeometryBasics.Mesh(coords, GeometryBasics.faces(meshdata))
end
Makie.mesh!(GeometryBasics.normal_mesh(meshdata); kwargs..., color = color, shading = shaded, visible = shaded)
if wireframe
if shaded
Makie.wireframe!(scene[end][1], color = (:black, 0.1), linewidth = linewidth)
else
Makie.wireframe!(scene[end][1], color = color, linewidth = linewidth)
end
end
else
@error "Unsupported file type"
end
end
## GEOMETRY
"""
draw!(scene::Makie.LScene, surf::Surface{T}; numdivisions = 20, normals = false, normalcolor = :blue, kwargs...)
Transforms `surf` into a mesh using [`makemesh`](@ref) and draws the result.
`normals` of the surface can be drawn at evenly sampled points with provided `normalcolor`.
`numdivisions` determines the resolution with which the mesh is triangulated.
`kwargs` is passed on to the [`TriangleMesh`](@ref) drawing function.
"""
function draw!(scene::Makie.LScene, surf::Surface{T}; numdivisions::Int = 30, normals::Bool = false, normalcolor = :blue, kwargs...) where {T<:Real}
mesh = makemesh(surf, numdivisions)
if nothing === mesh
return
end
draw!(scene, mesh; kwargs..., normals = false)
if normals
ndirs = Makie.Point3f.(samplesurface(surf, normal, numdivisions ÷ 10))
norigins = Makie.Point3f.(samplesurface(surf, point, numdivisions ÷ 10))
Makie.arrows!(scene, norigins, ndirs, arrowsize = 0.2, arrowcolor = normalcolor, linecolor = normalcolor, linewidth = 2)
end
end
"""
draw!(scene::Makie.LScene, tmesh::TriangleMesh{T}; linewidth = 3, shaded = true, wireframe = false, color = :orange, normals = false, normalcolor = :blue, transparency = false, kwargs...)
Draw a [`TriangleMesh`](@ref), optionially with a visible `wireframe`. `kwargs` are passed on to [`Makie.mesh`](http://makie.juliaplots.org/stable/plotting_functions.html#mesh).
"""
function draw!(scene::Makie.LScene, tmesh::TriangleMesh{T}; linewidth = 3, shaded::Bool = true, wireframe::Bool = false, color = :orange, normals::Bool = false, normalcolor = :blue, transparency::Bool = false, kwargs...) where {T<:Real}
points, indices = makiemesh(tmesh)
if length(points) > 0 && length(indices) > 0
Makie.mesh!(scene, points, indices; kwargs..., color = color, shading = shaded, transparency = transparency, visible = shaded)
if wireframe
mesh = scene.scene[end][1]
if shaded
Makie.wireframe!(scene, mesh, color = (:black, 0.1), linewidth = linewidth)
else
Makie.wireframe!(scene, mesh, color = color, linewidth = linewidth)
end
end
end
if normals
@warn "Normals being drawn from triangulated mesh, precision may be low"
norigins = [Makie.Point3f(centroid(t)) for t in tmesh.triangles[1:10:end]]
ndirs = [Makie.Point3f(normal(t)) for t in tmesh.triangles[1:10:end]]
if length(norigins) > 0
Makie.arrows!(scene, norigins, ndirs, arrowsize = 0.2, arrowcolor = normalcolor, linecolor = normalcolor, linewidth = 2)
end
end
end
"""
draw!(scene::Makie.LScene, meshes::Vararg{S}; colors::Bool = false, kwargs...) where {T<:Real,S<:Union{TriangleMesh{T},Surface{T}}}
Draw a series of [`TriangleMesh`](@ref) or [`Surface`](@ref) objects, if `colors` is true then each mesh will be colored automatically with a diverse series of colors.
`kwargs` are is passed on to the drawing function for each element.
"""
function draw!(scene::Makie.LScene, meshes::Vararg{S}; colors::Bool = false, kwargs...) where {T<:Real,S<:Union{TriangleMesh{T},Surface{T}}}
for i in 1:length(meshes)
if colors
col = indexedcolor2(i)
else
col = :orange
end
draw!(scene, meshes[i]; kwargs..., color = col)
end
end
function draw(meshes::Vararg{S}; kwargs...) where {T<:Real,S<:Union{TriangleMesh{T},Surface{T}}}
scene, lscene = Vis.scene()
draw!(lscene, meshes...; kwargs...)
Makie.display(scene)
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return scene
end
end
"""
draw!(scene::Makie.LScene, csg::Union{CSGTree,CSGGenerator}; numdivisions::Int = 20, kwargs...)
Convert a CSG object ([`CSGTree`](@ref) or [`CSGGenerator`](@ref)) to a mesh using [`makemesh`](@ref) with resolution set by `numdivisions` and draw the resulting [`TriangleMesh`](@ref).
"""
draw!(scene::Makie.LScene, csg::CSGTree{T}; numdivisions::Int = 30, kwargs...) where {T<:Real} = draw!(scene, makemesh(csg, numdivisions); kwargs...)
draw!(scene::Makie.LScene, csg::CSGGenerator{T}; kwargs...) where {T<:Real} = draw!(scene, csg(); kwargs...)
"""
draw!(scene::Makie.LScene, bbox::BoundingBox{T}; kwargs...)
Draw a [`BoundingBox`](@ref) as a wireframe, ie series of lines.
"""
function draw!(scene::Makie.LScene, bbox::BoundingBox{T}; kwargs...) where {T<:Real}
p1 = SVector{3,T}(bbox.xmin, bbox.ymin, bbox.zmin)
p2 = SVector{3,T}(bbox.xmin, bbox.ymax, bbox.zmin)
p3 = SVector{3,T}(bbox.xmin, bbox.ymax, bbox.zmax)
p4 = SVector{3,T}(bbox.xmin, bbox.ymin, bbox.zmax)
p5 = SVector{3,T}(bbox.xmax, bbox.ymin, bbox.zmin)
p6 = SVector{3,T}(bbox.xmax, bbox.ymax, bbox.zmin)
p7 = SVector{3,T}(bbox.xmax, bbox.ymax, bbox.zmax)
p8 = SVector{3,T}(bbox.xmax, bbox.ymin, bbox.zmax)
Makie.linesegments!(scene, [p1, p2, p2, p3, p3, p4, p4, p1, p1, p5, p2, p6, p3, p7, p4, p8, p5, p6, p6, p7, p7, p8, p8, p5]; kwargs...)
end
## OPTICS
"""
draw!(scene::Makie.LScene, ass::LensAssembly; kwargs...)
Draw each element in a [`LensAssembly`](@ref), with each element automatically colored differently.
"""
function draw!(scene::Makie.LScene, ass::LensAssembly{T}; kwargs...) where {T<:Real}
for (i, e) in enumerate(elements(ass))
draw!(scene, e; kwargs..., color = indexedcolor2(i))
end
end
"""
draw!(scene::Makie.LScene, sys::AbstractOpticalSystem; kwargs...)
Draw each element in the lens assembly of an [`AbstractOpticalSystem`](@ref), with each element automatically colored differently, as well as the detector of the system.
"""
function draw!(scene::Makie.LScene, sys::CSGOpticalSystem{T}; kwargs...) where {T<:Real}
draw!(scene, sys.assembly; kwargs...)
draw!(scene, sys.detector; kwargs...)
end
draw!(scene::Makie.LScene, sys::AxisymmetricOpticalSystem{T}; kwargs...) where {T<:Real} = draw!(scene, sys.system; kwargs...)
onlydetectorrays(system::Q, tracevalue::LensTrace{T,3}) where {T<:Real,Q<:AbstractOpticalSystem{T}} = onsurface(detector(system), point(tracevalue))
## RAY GEN
"""
drawtracerays(system::Q; raygenerator::S = Source(transform = Transform.translation(0.0,0.0,10.0), origins = Origins.RectGrid(10.0,10.0,25,25),directions = Constant(0.0,0.0,-1.0)), test::Bool = false, trackallrays::Bool = false, colorbysourcenum::Bool = false, colorbynhits::Bool = false, rayfilter::Union{Nothing,Function} = onlydetectorrays, kwargs...)
Displays a model of the optical system.
`raygenerator` is an iterator that generates rays.
If `trackallrays` is true then ray paths from the emitter will be displayed otherwise just the final rays that intersect the image detector will be shown, not the entire ray path.
`colorbysourcenum` and `colorbynhits` will color rays accordingly, otherwise rays will be colored according to their wavelength.
By default only ray paths that eventually intersect the detector surface are displayed. If you want to display all ray paths set `rayfilter = nothing`.
Also `drawtracerays!` to add to an existing scene, with `drawsys` and `drawgen` to specify whether `system` and `raygenerator` should be drawn respectively.
"""
function drawtracerays(system::Q; raygenerator::S = Source(transform = translation(0.0,0.0,10.0), origins = Origins.RectGrid(10.0,10.0,25,25),directions = Constant(0.0,0.0,-1.0)), test::Bool = false, trackallrays::Bool = false, colorbysourcenum::Bool = false, colorbynhits::Bool = false, rayfilter::Union{Nothing,Function} = onlydetectorrays, verbose::Bool = false, resolution::Tuple{Int,Int} = (1000, 1000), kwargs...) where {T<:Real,Q<:AbstractOpticalSystem{T},S<:AbstractRayGenerator{T}}
verbose && println("Drawing System...")
s, ls = Vis.scene(resolution)
drawtracerays!(ls, system, raygenerator = raygenerator, test = test, colorbysourcenum = colorbysourcenum, colorbynhits = colorbynhits, rayfilter = rayfilter, trackallrays = trackallrays, verbose = verbose, drawsys = true, drawgen = true; kwargs...)
display(s)
if (get_current_mode() == :pluto || get_current_mode() == :docs)
return s
end
end
drawtracerays!(system::Q; kwargs...) where {T<:Real,Q<:AbstractOpticalSystem{T}} = drawtracerays!(current_3d_scene, system; kwargs...)
function drawtracerays!(scene::Makie.LScene, system::Q; raygenerator::S = Source(transform = translation(0.0,0.0,10.0), origins = Origins.RectGrid(10.0,10.0,25,25),directions = Constant(0.0,0.0,-1.0)), test::Bool = false, trackallrays::Bool = false, colorbysourcenum::Bool = false, colorbynhits::Bool = false, rayfilter::Union{Nothing,Function} = onlydetectorrays, verbose::Bool = false, drawsys::Bool = false, drawgen::Bool = false, kwargs...) where {T<:Real,Q<:AbstractOpticalSystem{T},S<:AbstractRayGenerator{T}}
raylines = Vector{LensTrace{T,3}}(undef, 0)
drawgen && draw!(scene, raygenerator, norays = true; kwargs...)
drawsys && draw!(scene, system; kwargs...)
verbose && println("Tracing...")
for (i, r) in enumerate(raygenerator)
if i % 1000 == 0 && verbose
print("\r $i / $(length(raygenerator))")
end
allrays = Vector{LensTrace{T,3}}(undef, 0)
if trackallrays
res = trace(system, r, trackrays = allrays, test = test)
else
res = trace(system, r, test = test)
end
if trackallrays && !isempty(allrays)
if rayfilter === nothing || rayfilter(system, allrays[end])
# filter on the trace that is hitting the detector
for r in allrays
push!(raylines, r)
end
end
elseif res !== nothing
if rayfilter === nothing || rayfilter(system, res)
push!(raylines, res)
end
end
end
verbose && print("\r")
verbose && println("Drawing Rays...")
draw!(scene, raylines, colorbysourcenum = colorbysourcenum, colorbynhits = colorbynhits; kwargs...)
end
"""
drawtraceimage(system::Q; raygenerator::S = Source(transform = translation(0.0,0.0,10.0), origins = Origins.RectGrid(10.0,10.0,25,25),directions = Constant(0.0,0.0,-1.0)), test::Bool = false)
Traces rays from `raygenerator` through `system` and shows and returns the detector image. `verbose` will print progress updates.
"""
function drawtraceimage(system::Q; raygenerator::S = Source(transform = translation(0.0,0.0,10.0), origins = Origins.RectGrid(10.0,10.0,25,25),directions = Constant(0.0,0.0,-1.0)), test::Bool = false, verbose::Bool = false) where {T<:Real,Q<:AbstractOpticalSystem{T},S<:AbstractRayGenerator{T}}
resetdetector!(system)
start_time = time()
for (i, r) in enumerate(raygenerator)
if i % 1000 == 0 && verbose
dif = round(time() - start_time, digits = 1)
left = round((time() - start_time) / i * (length(raygenerator) - i), digits = 1)
print("\rTraced: $i / $(length(raygenerator)) Elapsed: $(dif)s Left: $(left)s")
end
res = trace(system, r, test = test)
end
verbose && print("\r")
tot = round(time() - start_time, digits = 1)
verbose && println("Completed in $(tot)s")
show(detectorimage(system))
return detectorimage(system)
end
## RAYS, LINES AND POINTS
"""
draw!(scene::Makie.LScene, rays::AbstractVector{<:AbstractRay{T,N}}; kwargs...)
Draw a vector of [`Ray`](@ref) or [`OpticalRay`](@ref) objects.
"""
function draw!(scene::Makie.LScene, rays::AbstractVector{<:AbstractRay{T,N}}; kwargs...) where {T<:Real,N}
for r in rays
draw!(scene, r; kwargs...)
end
end
"""
draw!(scene::Makie.LScene, traces::AbstractVector{LensTrace{T,N}}; kwargs...)
Draw a vector of [`LensTrace`](@ref) objects.
"""
function draw!(scene::Makie.LScene, traces::AbstractVector{LensTrace{T,N}}; kwargs...) where {T<:Real,N}
for t in traces
draw!(scene, t; kwargs...)
end
end
"""
draw!(scene::Makie.LScene, trace::LensTrace{T,N}; colorbysourcenum::Bool = false, colorbynhits::Bool = false, kwargs...)
Draw a [`LensTrace`](@ref) as a line which can be colored automatically by its `sourcenum` or `nhits` attributes.
The alpha is determined by the `power` attribute of `trace`.
"""
function draw!(scene::Makie.LScene, trace::LensTrace{T,N}; colorbysourcenum::Bool = false, colorbynhits::Bool = false, kwargs...) where {T<:Real,N}
if colorbysourcenum
color = indexedcolor(sourcenum(trace))
elseif colorbynhits
color = indexedcolor(nhits(trace))
else
color = λtoRGB(wavelength(trace))
end
draw!(scene, (origin(ray(trace)), point(intersection(trace))); kwargs..., color = RGBA(color.r, color.g, color.b, sqrt(power(trace))), transparency = true)
end
"""
draw!(scene::Makie.LScene, ray::OpticalRay{T,N}; colorbysourcenum::Bool = false, colorbynhits::Bool = false, kwargs...)
Draw an [`OpticalRay`](@ref) which can be colored automatically by its `sourcenum` or `nhits` attributes.
The alpha of the ray is determined by the `power` attribute of `ray`.
`kwargs` are passed to `draw!(scene, ray::Ray)`.
"""
function draw!(scene::Makie.LScene, r::OpticalRay{T,N}; colorbysourcenum::Bool = false, colorbynhits::Bool = false, kwargs...) where {T<:Real,N}
if colorbysourcenum
color = indexedcolor(sourcenum(r))
elseif colorbynhits
color = indexedcolor(nhits(r))
else
color = λtoRGB(wavelength(r))
end
draw!(scene, ray(r); kwargs..., color = RGBA(color.r, color.g, color.b, sqrt(power(r))), transparency = true, rayscale = power(r))
end
"""
draw!(scene::Makie.LScene, ray::Ray{T,N}; color = :yellow, rayscale = 1.0, kwargs...)
Draw a [`Ray`](@ref) in a given `color` optionally scaling the size using `rayscale`.
`kwargs` are passed to [`Makie.arrows`](http://makie.juliaplots.org/stable/plotting_functions.html#arrows).
"""
function draw!(scene::Makie.LScene, ray::AbstractRay{T,N}; color = :yellow, rayscale = 1.0, kwargs...) where {T<:Real,N}
arrow_size = min(0.05, rayscale * 0.05)
Makie.arrows!(scene, [Makie.Point3f(origin(ray))], [Makie.Point3f(rayscale * direction(ray))]; kwargs..., arrowsize = arrow_size, arrowcolor = color, linecolor = color, linewidth=arrow_size * 0.5)
end
"""
draw!(scene::Makie.LScene, du::DisjointUnion{T}; kwargs...)
Draw each [`Interval`](@ref) in a [`DisjointUnion`](@ref).
"""
function draw!(scene::Makie.LScene, du::DisjointUnion{T}; kwargs...) where {T<:Real}
draw!(scene, intervals(du); kwargs...)
end
"""
draw!(scene::Makie.LScene, intervals::AbstractVector{Interval{T}}; kwargs...)
Draw a vector of [`Interval`](@ref)s.
"""
function draw!(scene::Makie.LScene, intervals::AbstractVector{Interval{T}}; kwargs...) where {T<:Real}
for i in intervals
draw!(scene, i; kwargs...)
end
end
"""
draw!(scene::Makie.LScene, interval::Interval{T}; kwargs...)
Draw an [`Interval`](@ref) as a line with circles at each [`Intersection`](@ref) point.
"""
function draw!(scene::Makie.LScene, interval::Interval{T}; kwargs...) where {T<:Real}
if !(interval isa EmptyInterval)
l = lower(interval)
u = upper(interval)
if !(l isa RayOrigin)
draw!(scene, l)
else
@warn "Negative half space, can't draw ray origin"
end
if !(u isa Infinity)
draw!(scene, u)
else
@warn "Positive half space, can't draw end point"
end
if !(l isa RayOrigin) && !(u isa Infinity)
draw!(scene, (point(l), point(u)); kwargs...)
end
end
end
"""
draw!(scene::Makie.LScene, intersection::Intersection; normal::Bool = false, kwargs...)
Draw an [`Intersection`](@ref) as a circle, optionally showing the surface normal at the point.
"""
function draw!(scene::Makie.LScene, intersection::Intersection; normal::Bool = false, kwargs...)
draw!(scene, point(intersection); kwargs...)
if normal
draw!(scene, Ray(point(intersection), normal(intersection)); kwargs...)
end
end
"""
draw!(scene::Makie.LScene, lines::AbstractVector{Tuple{AbstractVector{T},AbstractVector{T}}}; kwargs...)
Draw a vector of lines.
"""
function draw!(scene::Makie.LScene, lines::AbstractVector{Tuple{P,P}}; kwargs...) where {T<:Real,P<:AbstractVector{T}}
for l in lines
draw!(scene, l; kwargs...)
end
end
"""
draw!(scene::Makie.LScene, line::Tuple{AbstractVector{T},AbstractVector{T}}; color = :yellow, kwargs...)
Draw a line between two points, `kwargs` are passed to [`Makie.linesegments`](http://makie.juliaplots.org/stable/plotting_functions.html#linesegments).
"""
function draw!(scene::Makie.LScene, line::Tuple{P,P}; color = :yellow, kwargs...) where {T<:Real,P<:AbstractVector{T}}
Makie.linesegments!(scene, [line[1], line[2]]; kwargs..., color = color)
end
"""
draw!(s::Makie.LScene, point::AbstractVector{T}; kwargs...)
Draw a single point, `kwargs` are passed to `draw!(scene, points::AbstractVector{AbstractVector{T}})`.
"""
function draw!(s::Makie.LScene, point::AbstractVector{T}; kwargs...) where {T<:Real}
draw!(s, [point]; kwargs...)
end
"""
draw!(scene::Makie.LScene, points::AbstractVector{AbstractVector{T}}; markersize = 20, color = :black, kwargs...)
Draw a vector of points.
`kwargs` are passed to [`Makie.scatter`](http://makie.juliaplots.org/stable/plotting_functions.html#scatter).
"""
function draw!(scene::Makie.LScene, points::AbstractVector{P}; markersize = 20, color = :black, kwargs...) where {T<:Real,P<:AbstractVector{T}}
Makie.scatter!(scene, points, markersize = markersize, color = color, strokewidth = 0; kwargs...)
end
#######################################################
function plotOPD!(sys::AxisymmetricOpticalSystem{T}; label = nothing, color = nothing, collimated::Bool = true, axis::Int = 1, samples::Int = 5, wavelength::T = 0.55, sourcepos::SVector{3,T} = SVector{3,T}(0.0, 0.0, 10.0), kwargs...) where {T<:Real}
sysdiam = semidiameter(sys)
rays = Vector{OpticalRay{T,3}}(undef, 0)
positions = Vector{T}(undef, 0)
if axis == 2
if collimated
dir = -sourcepos
for v in 0:samples
s = (2 * (v / samples) - 1.0) * sysdiam
p = SVector{3,T}(0, s, 0)
push!(positions, s)
push!(rays, OpticalRay(p - dir, dir, 1.0, wavelength))
end
else
for v in 0:samples
s = (2 * (v / samples) - 1.0) * sysdiam
p = SVector{3,T}(0, s, 0)
dir = p - sourcepos
push!(positions, s)
push!(rays, OpticalRay(sourcepos, dir, 1.0, wavelength))
end
end
elseif axis == 1
if collimated
dir = -sourcepos
for v in 0:samples
s = (2 * (v / samples) - 1.0) * sysdiam
p = SVector{3,T}(s, 0, 0)
push!(positions, s)
push!(rays, OpticalRay(p - dir, dir, 1.0, wavelength))
end
else
for v in 0:samples
s = (2 * (v / samples) - 1.0) * sysdiam
p = SVector{3,T}(s, 0, 0)
dir = p - sourcepos
push!(positions, s)
push!(rays, OpticalRay(sourcepos, dir, 1.0, wavelength))
end
end
end
raygenerator = Emitters.Sources.RayListSource(rays)
chiefray = OpticalRay(sourcepos, -sourcepos, 1.0, wavelength)
chiefres = trace(sys, chiefray, test = true)
xs = Vector{T}()
opls = Vector{T}()
for (i, r) in enumerate(raygenerator)
lt = trace(sys, r, test = true)
if !(nothing === lt)
push!(xs, positions[i + 1])
push!(opls, (pathlength(lt) - pathlength(chiefres)) / (wavelength / 1000)) # wavelength is um while OPD is mm
end
end
p = Plots.plot!(xs, opls, color = nothing === color ? λtoRGB(wavelength) : color, label = label)
Plots.xlabel!("Relative position on entrance pupil")
Plots.ylabel!("OPD (waves)")
return p
end
"""
spotdiag(sys::CSGOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; size = (500, 500), kwargs...)
Plot a spot diagram for an arbitrary [`CSGOpticalSystem`](@ref) and [`OpticalRayGenerator`](@ref).
All rays from `raygenerator` will be traced through `sys` and their intersection location on the detector plotted.
Also `spotdiag!` of the same arguments to add to an existing plot.
"""
function spotdiag(sys::CSGOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; kwargs...) where {T<:Real}
Plots.plot()
return spotdiag!(sys, raygenerator; kwargs...)
end
function spotdiag!(sys::CSGOpticalSystem{T}, raygenerator::OpticalRayGenerator{T}; color = nothing, label = nothing, size = (500, 500), kwargs...) where {T<:Real}
xs = Vector{T}()
ys = Vector{T}()
det = detector(sys)
for (i, r) in enumerate(raygenerator)
lt = trace(sys, r, test = true)
if !(nothing === lt)
push!(xs, dot(point(lt) - centroid(det), det.uvec))
push!(ys, dot(point(lt) - centroid(det), det.vvec))
end
end
# relative to centroid
cen = sum(xs) / length(xs), sum(ys) / length(ys)
xs .-= cen[1]
ys .-= cen[2]
p = Plots.scatter!(xs, ys, color = (color === nothing) ? λtoRGB(wavelength(raygenerator[0])) : color, label = label, marker = :+, markerstrokewidth = 0, markerstrokealpha = 0, size = size, aspect_ratio = :equal)
Plots.xlabel!(p, "x (mm)")
Plots.ylabel!(p, "y (mm)")
return p
end
spotdiaggrid(sys::AxisymmetricOpticalSystem{T}, raygenerators::Vector{<:OpticalRayGenerator{T}}; kwargs...) where {T<:Real} = spotdiaggrid(sys.system, raygenerators; kwargs...)
function spotdiaggrid(sys::CSGOpticalSystem{T}, raygenerators::Vector{<:OpticalRayGenerator{T}}; colorbynum::Bool = false, kwargs...) where {T<:Real}
plots = []
x = Int(floor(sqrt(length(raygenerators))))
y = length(raygenerators) ÷ x
size = 2000 / max(x, y)
xmin = 0
xmax = 0
ymin = 0
ymax = 0
for (i, r) in enumerate(raygenerators)
if colorbynum
p = spotdiag(sys, r; color = indexedcolor2(i), kwargs..., size = (size, size))
else
p = spotdiag(sys, r; kwargs..., size = (size, size))
end
xmin = min(min(Plots.xlims(p)...), xmin)
xmax = max(max(Plots.xlims(p)...), xmax)
ymin = min(min(Plots.ylims(p)...), ymin)
ymax = max(max(Plots.ylims(p)...), ymax)
push!(plots, p)
end
# set all plots to the same range
for p in plots
Plots.xlims!(p, xmin, xmax)
Plots.ylims!(p, ymin, ymax)
end
return Plots.plot(plots..., layout = Plots.grid(x, y), size = (y * size, x * size))
end
"""
surfacesag(object::Union{CSGTree{T},Surface{T}}, resolution::Tuple{Int,Int}, halfsizes::Tuple{T,T}; offset::T = T(10), position::SVector{3,T} = SVector{3,T}(0.0, 0.0, 10.0), direction::SVector{3,T} = SVector{3,T}(0.0, 0.0, -1.0), rotationvec::SVector{3,T} = SVector{3,T}(0.0, 1.0, 0.0))
Calculates and displays the surface sag of an arbitrary [`Surface`](@ref) or [`CSGTree`](@ref).
Rays are shot in a grid of size defined by `resolution` across a arectangular area defined by `halfsizes`.
This rectangle is centred at `postion` with normal along `direction` and rotation defined by `rotationvec`.
`offset` is subtracted from the sag measurements to provide values relative to the appropriate zero level.
"""
function surfacesag(object::Union{CSGTree{T},Surface{T}}, resolution::Tuple{Int,Int}, halfsizes::Tuple{T,T}; offset::T = T(10), position::SVector{3,T} = SVector{3,T}(0.0, 0.0, 10.0), direction::SVector{3,T} = SVector{3,T}(0.0, 0.0, -1.0), rotationvec::SVector{3,T} = SVector{3,T}(0.0, 1.0, 0.0)) where {T<:Real}
direction = normalize(direction)
if abs(dot(rotationvec, direction)) == one(T)
rotationvec = SVector{3,T}(1.0, 0.0, 0.0)
end
uvec = normalize(cross(normalize(rotationvec), direction))
vvec = normalize(cross(direction, uvec))
image = Array{T,2}(undef, resolution)
image .= NaN
Threads.@threads for x in 0:(resolution[1] - 1)
for y in 0:(resolution[2] - 1)
u = 2 * (x / (resolution[1] - 1)) - 1.0
v = 2 * (y / (resolution[2] - 1)) - 1.0
pos = position + (halfsizes[1] * u * uvec) + (halfsizes[2] * v * vvec)
r = Ray(pos, direction)
int = closestintersection(surfaceintersection(object, r), false)
if int !== nothing
int = int::Intersection{T,3}
sag = α(int) - offset
image[y + 1, x + 1] = -sag
end
end
end
p = Plots.heatmap((-halfsizes[1]):(2 * halfsizes[1] / resolution[1]):halfsizes[1], (-halfsizes[2]):(2 * halfsizes[2] / resolution[2]):halfsizes[2], image, c = :jet1, aspect_ratio = :equal, size = (1000, 1000))
Plots.xlims!(p, -halfsizes[1], halfsizes[1])
Plots.ylims!(p, -halfsizes[2], halfsizes[2])
return p
end
"""
eyebox_eval_eye(assembly::LensAssembly{T}, raygen::OpticalRayGenerator{T}, eye_rotation_x::T, eye_rotation_y::T, sample_points_x::Int, sample_points_y::Int; pupil_radius::T = T(2.0), resolution::Int = 512, eye_transform::Transform{T} = identitytransform(T))
Visualise the images formed when tracing `assembly` with a human eye for an evenly sampled `sample_points_x × sample_points_y` grid in the angular range of eyeball rotations `-eye_rotation_x:eye_rotation_x` and `-eye_rotation_y:eye_rotation_y` in each dimension respectively.
`resolution` is the size of the detector image (necessarily square).
The eye must be positioned appropriately relative to the system using `eye_transform`, this should transform the eye to the correct position and orientation when at 0 rotation in both dimensions.
By default the eye is directed along the positive z-axis with the vertex of the cornea at the origin.
The result is displayed as a 4D image - the image seen by the eye is shown in 2D as normal with sliders to vary eye rotation in x and y.
The idea being that the whole image should be visible for all rotations in the range.
"""
function eyebox_eval_eye(assembly::LensAssembly{T}, raygen::OpticalRayGenerator{T}, eye_rotation_x::T, eye_rotation_y::T, sample_points_x::Int, sample_points_y::Int; pupil_radius::T = T(2.0), resolution::Int = 512, eye_transform::Transform{T} = identitytransform(T)) where {T<:Real}
out_image = zeros(Float32, resolution, resolution, sample_points_y, sample_points_x)
xrange = sample_points_x > 1 ? range(-eye_rotation_x, stop = eye_rotation_x, length = sample_points_x) : [0.0]
yrange = sample_points_y > 1 ? range(-eye_rotation_y, stop = eye_rotation_y, length = sample_points_y) : [0.0]
for (xi, erx) in enumerate(xrange)
for (yi, ery) in enumerate(yrange)
print("Position: ($xi, $yi) / ($sample_points_x, $sample_points_y)\r")
r = OpticSim.rotmatd(T, ery, erx, 0.0)
ov = OpticSim.rotate(eye_transform, SVector{3,T}(0.0, 0.0, 13.0))
t = r * ov - ov
sys = HumanEye.ModelEye(assembly, pupil_radius = pupil_radius, detpixels = resolution, transform = eye_transform * Transform(r, t))
# Vis.draw(sys)
# Vis.draw!((eye_transform.translation - ov - SVector(0.0, 20.0, 0.0), eye_transform.translation - ov + SVector(0.0, 20.0, 0.0)))
# Vis.draw!((eye_transform.translation - ov - SVector(20.0, 0.0, 0.0), eye_transform.translation - ov + SVector(20.0, 0.0, 0.0)))
# Vis.draw!(eye_transform.translation, markersize = 500.0)
out_image[:, :, yi, xi] = traceMT(sys, raygen, printprog = false)
end
end
# this is a 4D image - ImageView makes sliders to visualise the dimensions >2!
Vis.show(out_image)
end
"""
eyebox_eval_planar(assembly::LensAssembly{T}, raygen::OpticalRayGenerator{T}, eyebox::Rectangle{T}, sample_points_x::Int, sample_points_y::Int, vsize::T; pupil_radius::T = T(2.0), resolution::Int = 512)
Visualise the images formed when tracing `assembly` for multiple pupil positions within a planar `eyebox`.
Any angles which are present in a circle radius `pupil_radius` around each sampling point on an even `sample_points_x × sample_points_y` grid on the `eyebox` are added to the sub-image at that grid point.
A paraxial lens focal length 1mm is placed at the eyebox and a detector of size `vsize × vsize`mm placed 1mm behind it.
The normal of the detector rectangle should point towards the system (and away from the fake detector).
Any rays which miss the detector are ignored.
The pupil is always fully contained in the eyebox, i.e., the extreme sample position in u would be `eyebox.halfsizeu - pupil_radius`, for example.
The result is displayed as a 4D image - each sub-image is shown as normal with sliders to vary eye rotation in x and y.
The idea being that the whole FoV should be visible for all rotations in the range.
"""
function eyebox_eval_planar(assembly::LensAssembly{T}, raygen::OpticalRayGenerator{T}, eyebox::Rectangle{T}, sample_points_x::Int, sample_points_y::Int, vsize::T; pupil_radius::T = T(2.0), resolution::Int = 512) where {T<:Real}
sys = CSGOpticalSystem(assembly, eyebox, 1, 1)
hits = tracehitsMT(sys, raygen)
println("Calculating results...")
det = Rectangle(vsize, vsize, normal(eyebox), centroid(eyebox) - normal(eyebox))
if length(hits) > 0
out_image = zeros(Float32, resolution, resolution, sample_points_y, sample_points_x)
for res in hits
dir = direction(ray(res))
u, v = uv(res)
x = u * eyebox.halfsizeu
y = v * eyebox.halfsizev
raydirection = normalize((centroid(eyebox) - point(res)) + direction(ray(res)) / dot(-normal(eyebox), direction(ray(res))))
focal_plane_hit = surfaceintersection(det, Ray(point(res), raydirection))
if focal_plane_hit isa EmptyInterval
continue
end
px, py = OpticSim.uvtopix(det, uv(OpticSim.halfspaceintersection(focal_plane_hit)), (resolution, resolution))
hsu = eyebox.halfsizeu - pupil_radius
hsv = eyebox.halfsizev - pupil_radius
xrange = sample_points_x > 1 ? range(-hsu, stop = hsu, length = sample_points_x) : [0.0]
yrange = sample_points_y > 1 ? range(-hsv, stop = hsv, length = sample_points_y) : [0.0]
for (xi, posx) in enumerate(xrange)
for (yi, posy) in enumerate(yrange)
if (x - posx)^2 + (y - posy)^2 < pupil_radius^2
out_image[py, px, yi, xi] += sourcepower(ray(res))
end
end
end
end
# this is a 4D image - ImageView makes sliders to visualise the dimensions >2!
Vis.show(out_image)
end
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3533 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using Test
using FiniteDifferences
using StaticArrays
using LinearAlgebra
using Suppressor
using Random
using Unitful
using Plots
using DataFrames
using OpticSim
# Geometry Module
using OpticSim.Geometry
# curve/surface imports
using OpticSim: findspan, makemesh, knotstoinsert, coefficients, inside, quadraticroots, tobeziersegments, evalcsg, makiemesh
# interval imports
using OpticSim: α, halfspaceintersection, positivehalfspace, lower, upper, EmptyInterval, rayorigininterval, intervalcomplement, intervalintersection, intervalunion, RayOrigin, Infinity, Intersection
include("TestData/TestData.jl")
# bounding box imports
using OpticSim: doesintersect
# RBT imports
using OpticSim: rotmat, rotmatd
# lens imports
using OpticSim: reflectedray, snell, refractedray, trace, intersection, pathlength, power
#Bounding volume hierarchy imports
# using OpticSim: partition!
const COMP_TOLERANCE = 25 * eps(Float64)
const RTOLERANCE = 1e-10
const ATOLERANCE = 10 * eps(Float64)
const SEED = 12312487
const ALL_TESTS = isempty(ARGS) || "all" in ARGS || "All" in ARGS || "ALL" in ARGS
const TESTSET_DIR = "testsets"
"""Evalute all functions not requiring arguments in a given module and test they don't throw anything"""
macro test_all_no_arg_functions(m)
quote
for n in names($m, all = true)
# get all the valid functions
if occursin("#", string(n)) || string(n) == string(nameof($m))
continue
end
f = Core.eval($m, n)
# get the methods of this function
for meth in methods(f)
# if this method has no args then try and evaluate it
if meth.nargs == 1
# suppress STDOUT to prevent loads of stuff spamming the console
@test (@suppress_out begin
f()
end;
true)
end
end
end
end
end
"""
@wrappedallocs(expr)
https://github.com/JuliaAlgebra/TypedPolynomials.jl/blob/master/test/runtests.jl
Given an expression, this macro wraps that expression inside a new function
which will evaluate that expression and measure the amount of memory allocated
by the expression. Wrapping the expression in a new function allows for more
accurate memory allocation detection when using global variables (e.g. when
at the REPL).
For example, `@wrappedallocs(x + y)` produces:
```julia
function g(x1, x2)
@allocated x1 + x2
end
g(x, y)
```
You can use this macro in a unit test to verify that a function does not
allocate:
```
@test @wrappedallocs(x + y) == 0
```
"""
macro wrappedallocs(expr)
argnames = [gensym() for a in expr.args]
quote
function g($(argnames...))
@allocated $(Expr(expr.head, argnames...))
end
$(Expr(:call, :g, [esc(a) for a in expr.args]...))
end
end
include("Benchmarks/Benchmarks.jl")
alltestsets = [
"Repeat",
"Emitters",
"Lenses",
"Comparison",
"Paraxial",
"ParaxialAnalysis",
"Transform",
"JuliaLang",
# "BVH",
# "Examples", # slow
"General",
"SurfaceDefs",
"Intersection",
"OpticalSystem",
"Allocations",
"GlassCat",
"Visualization"
]
runtestsets = ALL_TESTS ? alltestsets : intersect(alltestsets, ARGS)
include.([joinpath(TESTSET_DIR, "$(testset).jl") for testset in runtestsets])
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6436 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
module Benchmarks
using BenchmarkTools
using Unitful
using StaticArrays
using OpticSim
using OpticSim: Sphere, Ray, replprint, trace, Cylinder, AcceleratedParametricSurface, newton, Infinity, RayOrigin, NullInterface, IntervalPoint, intervalintersection, LensTrace, FresnelInterface, wavelength, mᵢandmₜ, refractedray, direction, origin, pathlength, LensAssembly, NoPower, power, snell, fresnel, reflectedray, BoundingBox
using OpticSim.Examples
include("../TestData/TestData.jl")
rayz() = Ray([0.0, 0.0, 10.0], [0.0, 0.0, -1.0])
perturbrayz() = Ray([0.0, 0.0, 10.0], [0.001, 0.001, -1.0])
rayx() = Ray([10.0, 0.0, 0.0], [-1.0, 0.0, 0.0])
bezierray() = Ray([0.5, 0.5, 10.0], [0.0, 0.0, -1.0])
optray() = OpticalRay(origin(rayz()), direction(rayz()), 1.0, 0.55)
perturboptray() = OpticalRay(origin(perturbrayz()), direction(perturbrayz()), 1.0, 0.55)
#############################
### BENCHMARK DEFINITIONS ###
#############################
# benchmarks are listed in the form: benchmark() = function, (args...)
# e.g. surfaceintersection, (surface, ray)
double_convex() = trace, (TestData.doubleconvex(), optray())
double_concave() = trace, (TestData.doubleconcave(), optray())
zernike_lens() = trace, (TestData.zernikesystem(), perturboptray())
aspheric_lens() = trace, (TestData.asphericsystem(), perturboptray())
cooke_triplet() = trace, (TestData.cooketriplet(), optray())
chebyshev_lens() = trace, (TestData.chebyshevsystem(), perturboptray())
gridsag_lens() = trace, (TestData.gridsagsystem(), perturboptray())
multi_hoe() = trace, (TestData.multiHOE(), OpticalRay(SVector(0.0, 3.0, 3.0), SVector(0.0, -1.0, -1.0), 1.0, 0.55))
planar_shapes() = trace, (TestData.planarshapes(), optray())
system_benchmarks() = double_concave, double_convex, aspheric_lens, zernike_lens, chebyshev_lens, gridsag_lens, cooke_triplet, multi_hoe, planar_shapes
triangle() = surfaceintersection, (Triangle(SVector(-1.0, -1.0, 0.0), SVector(1.0, 0.0, 0.0), SVector(0.0, 1.0, 0.0)), rayz())
sphericalcap() = surfaceintersection, (SphericalCap(1.0, π / 3), rayz())
sphere_outside() = surfaceintersection, (Sphere(0.5), rayz())
sphere_inside() = surfaceintersection, (Sphere(0.5), Ray([0.0, 0.0, 0.0], [0.0, 0.0, -1.0]))
plane() = surfaceintersection, (Plane(0.0, 0.0, 1.0, 0.0, 0.0, 0.0), rayz())
rectangle() = surfaceintersection, (Rectangle(1.0, 1.0, SVector(0.0, 0.0, 1.0), SVector(0.0, 0.0, 0.0)), rayz())
ellipse() = surfaceintersection, (Ellipse(1.0, 1.0, SVector(0.0, 0.0, 1.0), SVector(0.0, 0.0, 0.0)), rayz())
convexPolygon() = surfaceintersection, (ConvexPolygon(Geometry.Transform(), [SVector(-1.0, -1.0), SVector(1.0, -1.0), SVector(1.0, 1.0), SVector(-1.0, 1.0)]), rayz())
cylinder_parallel() = surfaceintersection, (Cylinder(1.0), rayz())
cylinder_perp() = surfaceintersection, (Cylinder(1.0), rayx())
bbox_parallel() = surfaceintersection, (BoundingBox(-1.0, 1.0, -1.0, 1.0, -5.0, 5.0), rayz())
bbox_perp() = surfaceintersection, (BoundingBox(-1.0, 1.0, -1.0, 1.0, -5.0, 5.0), rayx())
infiniteStopConvexPolygon() = surfaceintersection, (InfiniteStopConvexPoly(ConvexPolygon(Geometry.Transform(), [SVector(-1.0, -1.0), SVector(1.0, -1.0), SVector(1.0, 1.0), SVector(-1.0, 1.0)])), rayz())
simple_surface_benchmarks() = plane, rectangle, ellipse, convexPolygon, infiniteStopConvexPolygon, triangle, sphere_outside, sphere_inside, sphericalcap, cylinder_parallel, cylinder_perp, bbox_parallel, bbox_perp
zernike_surface1() = surfaceintersection, (AcceleratedParametricSurface(TestData.zernikesurface1(), 30), perturbrayz())
zernike_surface2() = surfaceintersection, (AcceleratedParametricSurface(TestData.zernikesurface3(), 30), perturbrayz())
evenaspheric_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.evenasphericsurface(), 30), perturbrayz())
oddaspheric_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.oddasphericsurface(), 30), perturbrayz())
oddevenaspheric_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.oddevenasphericsurface(), 30), perturbrayz())
qtype_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.qtypesurface1(), 30), perturbrayz())
bezier_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.beziersurface(), 30), bezierray())
chebyshev_surface1() = surfaceintersection, (AcceleratedParametricSurface(TestData.chebyshevsurface1(), 30), perturbrayz())
chebyshev_surface2() = surfaceintersection, (AcceleratedParametricSurface(TestData.chebyshevsurface2(), 30), perturbrayz())
gridsag_surface() = surfaceintersection, (AcceleratedParametricSurface(TestData.gridsagsurfacebicubic(), 30), perturbrayz())
accel_surface_benchmarks() = zernike_surface1, zernike_surface2, evenaspheric_surface, oddaspheric_surface, oddevenaspheric_surface, qtype_surface, bezier_surface, chebyshev_surface1, chebyshev_surface2, gridsag_surface
all_benchmarks() = (simple_surface_benchmarks()..., accel_surface_benchmarks()..., system_benchmarks()...)
#############################
function runbenchmark(b; kwargs...)
f, args = b()
qkwargs = [:($(keys(kwargs)[i]) = $(values(kwargs)[i])) for i in 1:length(kwargs)]
if f === trace
return @eval (@benchmark($f(($args)..., test = true), $(qkwargs...)))
else
return @eval (@benchmark($f(($args)...), setup = (OpticSim.emptyintervalpool!()), $(qkwargs...)))
end
end
timings(f::Function) = timings(f()...)
function timings(functions::Vararg{Function})
for func in functions
println("timing $func")
replprint(runbenchmark(func))
println("\n\n***************\n")
end
end
function runforpipeline(ismaster::Bool)
io = open(ismaster ? "timings_master.csv" : "timings_pr.csv", "w")
write(io, "Function, Memory, Allocs, Min Time, Mean Time, Max Time\n")
for f in all_benchmarks()
b = runbenchmark(f)
join(io, [
"$f",
"$(BenchmarkTools.prettymemory(b.memory))",
"$(b.allocs)",
"$(BenchmarkTools.prettytime(minimum(b.times)))",
"$(BenchmarkTools.prettytime(BenchmarkTools.mean(b.times)))",
"$(BenchmarkTools.prettytime(maximum(b.times)))\n"
], ", ")
end
close(io)
end
########################################################
end #module
export Benchmarks
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 16287 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# Programs used to visualize output, profile code or perform debugging tasks, as opposed to unit testing
# WARNING: many of the functions in this module depend on old functions such as HexapolarField which no longer exist
module Diagnostics
using OpticSim
using OpticSim: replprint
using OpticSim.Vis
using OpticSim.Optimization
using LinearAlgebra
using StaticArrays
import Unitful
import Plots
include("../TestData/TestData.jl")
function testbigfloat()
λ = 550 * Unitful.u"nm"
temp = 20 * Unitful.u"°C"
TOLERANCE = 1e-8
function printintersections(a::Type{T}) where {T<:Real}
println("* Intersections for type $T *")
r1 = OpticalRay(Vector{T}([0.0, 0.0, 1.0]), Vector{T}([0.0, 0.0, -1.0]), one(T), T(0.55))
r2 = OpticalRay(Vector{T}([2.0, 2.0, 1.0]), Vector{T}([0.0, 0.0, -1.0]), one(T), T(0.55))
r3 = OpticalRay(Vector{T}([5.0, 5.0, 1.0]), Vector{T}([0.0, 0.0, -1.0]), one(T), T(0.55))
r4 = OpticalRay(Vector{T}([0.0, -5.0, 1.0]), Vector{T}([0.0, sind(5.0), -cosd(5.0)]), one(T), T(0.55))
r5 = OpticalRay(Vector{T}([-5.0, -5.0, 1.0]), Vector{T}([sind(5.0), sind(2.0), -cosd(2.0) * cosd(5.0)]), one(T), T(0.55))
a = TestData.doubleconvex(T, temperature = temp)
for r in (r1, r2, r3, r4, r5)
println(point(OpticSim.intersection(OpticSim.trace(a, r, test = true))))
end
println()
end
printintersections(Float64)
printintersections(BigFloat)
a = TestData.doubleconvex(BigFloat)
r2 = OpticalRay(Vector{BigFloat}([2.0, 2.0, 1.0]), Vector{BigFloat}([0.0, 0.0, -1.0]), one(BigFloat), BigFloat(0.55))
r3 = OpticalRay(Vector{BigFloat}([5.0, 5.0, 1.0]), Vector{BigFloat}([0.0, 0.0, -1.0]), one(BigFloat), BigFloat(0.55))
r4 = OpticalRay(Vector{BigFloat}([0.0, -5.0, 1.0]), Vector{BigFloat}([0.0, sind(5.0), -cosd(5.0)]), one(BigFloat), BigFloat(0.55))
r5 = OpticalRay(Vector{BigFloat}([-5.0, -5.0, 1.0]), Vector{BigFloat}([sind(5.0), sind(2.0), -cosd(2.0) * cosd(5.0)]), one(BigFloat), BigFloat(0.55))
@assert isapprox(point(OpticSim.intersection(OpticSim.trace(a, r2, test = true))), [-0.06191521590711035, -0.06191521590711035, -67.8], atol = TOLERANCE)
@assert isapprox(point(OpticSim.intersection(OpticSim.trace(a, r3, test = true))), [-0.2491105067897657, -0.2491105067897657, -67.8], atol = TOLERANCE)
@assert isapprox(point(OpticSim.intersection(OpticSim.trace(a, r4, test = true))), [0.0, 5.639876913179362, -67.8], atol = TOLERANCE)
@assert isapprox(point(OpticSim.intersection(OpticSim.trace(a, r5, test = true))), [5.75170097290395, 2.504152441922817, -67.8], atol = TOLERANCE)
end
function vistest(sys::AbstractOpticalSystem{Float64}; kwargs...)
λ = 0.550
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ)
raygen = Emitters.Sources.RayListSource([r1, r2, r3, r4, r5])
Vis.drawtracerays(sys, raygenerator = raygen, trackallrays = true, test = true; kwargs...)
for (i, r) in enumerate(raygen)
t = OpticSim.trace(sys, r, test = true)
if t !== nothing
println("=== RAY $i ===")
println(OpticSim.point(t))
println(OpticSim.pathlength(t))
println()
end
end
end
function visualizerefraction()
nₛ = SVector{3,Float64}([0.0, 0.0, 1.0])
c = 1 / sqrt(2.0)
r = Ray([0.0, c, c], [0.0, -c, -c])
rray = OpticSim.refractedray(1.0, 1.5, nₛ, direction(r))
Vis.draw(Ray([0.0, 0.0, 0.0], Array{Float64,1}(nₛ)), color = :black)
Vis.draw!(r, color = :green)
Vis.draw!(Ray([0.0, 0.0, 0.0], Array{Float64,1}(rray)), color = :red)
Vis.draw!(Ray([0.0, 0.0, 0.0], Array{Float64,1}(-nₛ)), color = :yellow)
temp = OpticSim.refractedray(1.0, 1.5, nₛ, direction(r))
temp = [0.0, temp[2], -temp[3]]
r = Ray([0.0, -temp[2], -temp[3]], temp)
rray = OpticSim.refractedray(1.5, 1.0, nₛ, direction(r))
Vis.draw!(Ray([0.0, 0.0, 0.0], Array{Float64,1}(nₛ)), color = :black)
Vis.draw!(r, color = :green)
Vis.draw!(Ray([0.0, 0.0, 0.0], Array{Float64,1}(rray)), color = :red)
Vis.draw!(Ray([0.0, 0.0, 0.0], Array{Float64,1}(-nₛ)), color = :yellow)
end
function plotreflectedvsrefractedpower()
lens = OpticSim.SphericalLens(OpticSim.Examples.Examples_BAK50, 0.0, Inf64, Inf64, 5.0, 10.0)
reflectpow = Array{Float64,1}(undef, 0)
refractpow = Array{Float64,1}(undef, 0)
green = 500 * Unitful.u"nm"
glass = OpticSim.GlassCat.SCHOTT.BAK50
incidentindex = OpticSim.GlassCat.index(glass, green)
transmittedindex = OpticSim.GlassCat.index(OpticSim.GlassCat.Air, green)
for θ in 0.0:0.01:(π / 2.0)
origin = [0.0, tan(θ), 1.0]
dir = -origin
r = Ray(origin, dir)
intsct = OpticSim.closestintersection(OpticSim.surfaceintersection(lens(), r))
nml = SVector{3,Float64}(0.0, 0.0, 1.0)
rdir = direction(r)
(nᵢ, nₜ) = OpticSim.mᵢandmₜ(incidentindex, transmittedindex, nml, r)
reflected = OpticSim.reflectedray(nml, rdir)
refracted = OpticSim.refractedray(nᵢ, nₜ, nml, rdir)
(sinθᵢ, sinθₜ) = OpticSim.snell(nml, direction(r), nᵢ, nₜ)
(powᵣ, powₜ) = OpticSim.fresnel(nᵢ, nₜ, sinθᵢ, sinθₜ)
push!(reflectpow, powᵣ)
push!(refractpow, powₜ)
end
Plots.plot((0.0:0.01:(π / 2.0)), [reflectpow, refractpow], label = ["reflected" "refracted"])
end
### OPTIMIZATION TESTING
using FiniteDifferences
using ForwardDiff
using DataFrames: DataFrame
using Optim
using JuMP
using Ipopt
using Zygote
using NLopt
doubleconvexprescription() = DataFrame(SurfaceType = ["Object", "Standard", "Standard", "Image"], Radius = [(Inf64), 60.0, -60.0, (Inf64)], Thickness = [(Inf64), (10.0), (77.8), missing], Material = [OpticSim.GlassCat.Air, OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, missing], SemiDiameter = [(Inf64), (9.0), (9.0), (15.0)])
function doubleconvex(a::AbstractVector{T}; detpix::Int = 100) where {T<:Real}
frontradius = a[1]
rearradius = a[2]
#! format: off
AxisymmetricOpticalSystem{T}(DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [T(Inf64), frontradius, rearradius, T(Inf64)],
Conic = [missing, -1.0, 1.0, missing],
Thickness = [T(Inf64), T(10.0), T(77.8), missing],
Material = [OpticSim.GlassCat.Air, OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, missing],
SemiDiameter = [T(Inf64), T(9.0), T(9.0), T(15.0)],
), detpix, detpix, T, temperature = OpticSim.GlassCat.TEMP_REF_UNITFUL, pressure = OpticSim.GlassCat.PRESSURE_REF)
#! format: on
end
function testfinitedifferences()
lens = Examples.doubleconvex(60.0, -60.0)
start = Optimization.optimizationvariables(lens)
u = 60.0
println("time to compute objective function $(@time RMS_spot_size([u,-60.0],lens))")
fdm = central_fdm(3, 1)
f1(u) = RMS_spot_size([u, -60.0], lens)
fu = 0.0
for i in 1:100
fu = fdm(f1, 60.0)
end
return fu
end
export testfinitedifferences
function testoptimization(; lens = Examples.cooketriplet(), constrained = false, algo = constrained ? IPNewton() : LBFGS(), chunk_size = 1, samples = 3)
@info "NaN safe: $(ForwardDiff.NANSAFE_MODE_ENABLED)"
start, lower, upper = Optimization.optimizationvariables(lens)
optimobjective = (arg) -> RMS_spot_size(arg, lens, samples)
if chunk_size == 0
gcfg = ForwardDiff.GradientConfig(optimobjective, start)
hcfg = ForwardDiff.HessianConfig(optimobjective, start)
else
gcfg = ForwardDiff.GradientConfig(optimobjective, start, ForwardDiff.Chunk{chunk_size}())
hcfg = ForwardDiff.HessianConfig(optimobjective, start, ForwardDiff.Chunk{chunk_size}())
@info "$(length(start)) optimization variables, chunk size = $chunk_size"
end
g! = (G, x) -> ForwardDiff.gradient!(G, optimobjective, x, gcfg)
h! = (H, x) -> ForwardDiff.hessian!(H, optimobjective, x, hcfg)
@info "Starting objective function $(optimobjective(start))"
if constrained && !all(isinf.(abs.(lower))) && !all(isinf.(upper))
if !(algo isa Optim.ConstrainedOptimizer)
@error "This algorithm can't handle constraints, use IPNewton"
return
end
@info "Constraints: $lower, $upper"
df = TwiceDifferentiable(optimobjective, g!, h!, start)
dfc = TwiceDifferentiableConstraints(lower, upper)
res = optimize(df, dfc, start, algo, Optim.Options(show_trace = true, iterations = 100, allow_f_increases = true))
else
if constrained
@warn "No constraints to apply, using unconstrained optimization"
end
res = optimize(optimobjective, g!, h!, start, algo, Optim.Options(show_trace = true, iterations = 100, allow_f_increases = true))
end
# println("== Computing gradient")
# println("First call:")
# @time g!(zeros(length(start)), start)
# println("Second call:")
# @time g!(zeros(length(start)), start)
# println("== Computing hessian")
# println("First call:")
# @time h!(zeros(length(start), length(start)), start)
# println("Second call:")
# @time h!(zeros(length(start), length(start)), start)
# println()
final = Optim.minimizer(res)
newlens = Optimization.updateoptimizationvariables(lens, final)
@info "Result: $final"
field = HexapolarField(newlens, collimated = true, samples = samples)
Vis.drawtraceimage(newlens, raygenerator = field)
Vis.drawtracerays(newlens, raygenerator = field, trackallrays = true, test = true)
end
function testnlopt()
lens = Examples.doubleconvex(60.0, -60.0)
# lens = Examples.doubleconvex()
# lens = Examples.telephoto(6,.5)
# Vis.drawtraceimage(lens)
start = OpticSim.optimizationvariables(lens)
optimobjective = (arg) -> RMS_spot_size(arg, lens)
println("starting objective function $(optimobjective(start))")
model = Model(NLopt.optimizer)
@variable(model, rad1, start = 60.0)
@variable(model, rad2, start = -60.0)
register(model, :f, 2, f, autodiff = true)
@NLconstraint(model, 30.0 <= rad1 <= 85.0)
@NLconstraint(model, -100.0 <= rad1 <= -55.0)
@NLobjective(model, Min, f(rad1, rad2))
optimize!(model)
println("rad1 $(value(rad1)) rad2 $(value(rad2))")
end
function testJuMP()
function innerfunc(radius1::T, radius2::T) where {T<:Real}
lens = Examples.doubleconvex(radius1, radius2)
field = HexapolarField(lens, collimated = true, samples = 10)
error = zero(T)
hits = 0
for r in field
traceres = OpticSim.trace(lens, r, test = true)
if !(nothing === traceres)
hitpoint = point(traceres)
if abs(hitpoint[1]) > eps(T) && abs(hitpoint[2]) > eps(T)
dist_to_axis = sqrt(hitpoint[1]^2 + hitpoint[2]^2)
error += dist_to_axis
end
hits += 1
end
end
# if isa(radius1,ForwardDiff.Dual)
# println("radius1 $(realpart(radius1)) radius2 $(realpart(radius2))")
# end
error /= hits
# println("error in my code $error")
# Vis.drawtracerays(doubleconvex(radiu1,radius2),trackallrays = true, test = true)
return error
end
function f(radius1::T, radius2::T) where {T<:Real}
innerfunc(radius1, radius2)
# try
# innerfunc(radius1, radius2)
# catch err
# return zero(T)
# end
end
# Vis.drawtracerays(doubleconvex(60.0,-60.0), trackallrays = true, test = true)
model = Model(Ipopt.Optimizer)
set_time_limit_sec(model, 10.0)
register(model, :f, 2, f, autodiff = true)
@variable(model, 10.0 <= rad1 <= 85.0, start = 60.0)
@variable(model, -150 <= rad2 <= 20.0, start = -10.0)
@NLobjective(model, Min, f(rad1, rad2))
optimize!(model)
println("rad1 $(value(rad1)) rad2 $(value(rad2))")
Vis.drawtracerays(Examples.doubleconvex(value(rad1), value(rad2)), trackallrays = true, test = true)
end
function simpletest()
lens = Examples.cooketriplet()
# lens = Examples.doubleconvex(60.0,-60.0)
testgenericoptimization(lens, RMS_spot_size)
end
function RMS_spot_size(lens, x::T...) where {T}
lens = Optimization.updateoptimizationvariables(lens, collect(x))
field = HexapolarField(lens, collimated = true, samples = 10)
error = zero(T)
hits = 0
for r in field
traceres = OpticSim.trace(lens, r, test = true)
if !(nothing === traceres)
hitpoint = point(traceres)
if abs(hitpoint[1]) > eps(T) && abs(hitpoint[2]) > eps(T)
dist_to_axis = sqrt(hitpoint[1]^2 + hitpoint[2]^2)
error += dist_to_axis
end
hits += 1
end
end
error /= hits
return error
end
function testZygoteGradient(lens::S, objective::Function) where {S<:AbstractOpticalSystem}
vars = Optimization.optimizationvariables(lens)
gradient = objective'(vars)
println(gradient)
end
function testgenericoptimization(lens::S, objective::Function) where {S<:AbstractOpticalSystem}
vars = Optimization.optimizationvariables(lens)
function newobjective(a)
objective(lens, a...)
end
numvars = length(vars)
model = Model(Ipopt.Optimizer)
set_time_limit_sec(model, 10.0)
register(model, :newobjective, numvars, newobjective, autodiff = true)
# @variable(model, bounds[i][1] <= x[i=1:numvars] <= bounds[i][2],start = vars)
@variable(model, x[i = 1:numvars], start = vars[i])
@NLobjective(model, Min, newobjective(x...))
# d = NLPEvaluator(model)
# MOI.initialize(d, [:Grad])
# println("objective $(MOI.eval_objective(d, vars))")
# ∇f = zeros(length(vars))
# println(vars)
# MOI.eval_objective_gradient(d, ∇f, vars)
# println("gradient $∇f")
# println("starting forward diff")
# time = @time ForwardDiff.gradient(newobjective,vars)
# println("forward diff time1 $time")
# time = @time ForwardDiff.gradient(newobjective,vars)
# println("forward diff time2 $time")
println("starting reverse diff")
time = @time ReverseDiff.gradient(newobjective, vars)
println("reverse diff time1 $time")
time = @time ReverseDiff.gradient(newobjective, vars)
println("reverse diff time2 $time")
println("ending forward diff")
# fdm = central_fdm(2,1)
# replprint( @benchmark(FiniteDifferences.grad($fdm,$newobjective,$vars...)))
# println("finite difference gradient $(FiniteDifferences.grad(fdm,newobjective,vars...))")
# optimize!(model)
lens = Optimization.updateoptimizationvariables(lens, value.(x))
Vis.drawtracerays(lens, trackallrays = true, test = true)
end
function testIpopt()
model = Model(Ipopt.Optimizer)
@variable(model, x1, start = 1)
@variable(model, x2, start = 5)
@variable(model, x3, start = 5)
@variable(model, x4, start = 1)
f(x1, x2, x3, x4) = x1 * x4 * (x1 + x2 + x3) + x3
register(model, :f, 4, f, autodiff = true)
@NLobjective(model, Min, f(x1, x2, x3, x4))
@NLconstraint(model, x1 * x2 * x3 * x4 >= 25)
@NLconstraint(model, x1^2 + x2^2 + x3^2 + x4^2 == 40)
optimize!(model)
println("x1 $(value(x1)) x2 $(value(x2)) x3 $(value(x3)) x4 $(value(x4))")
end
function testtypesignature()
a = OpticSim.Sphere(1.0)
b = (a ∪ a) ∩ (a ∪ a)
end
function testoptimizationvariables()
lens = Examples.cooketriplet()
vars = OpticSim.optimizationvariables(lens)
vars .= [Float64(i) for i in 1:length(vars)]
newlens = OpticSim.updateoptimizationvariables(lens, vars)
println("original lens")
show(lens)
println("updated lens")
show(newlens)
end
end #module
export Diagnostics
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4006 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
"""
Contains all complex data used for testing and benchmarking.
"""
module TestData
using OpticSim
using OpticSim: tobeziersegments # try to use only exported functions so this list should stay short
using OpticSim.Geometry
using OpticSim.Emitters
using OpticSim.GlassCat
using StaticArrays
using DataFrames: DataFrame
using Unitful
using Images
using Base: @.
using LinearAlgebra
#define glasses locally rather than relying on GlassCat. These tests used to rely on downloaded glasses which made the tests brittle.
#If a glass didn't download the test would fail.
const Examples_N_BK7 = GlassCat.Glass(GlassCat.GlassID(GlassCat.AGF, 885), 2, 1.03961212, 0.00600069867, 0.231792344, 0.0200179144, 1.01046945, 103.560653, 0.0, 0.0, NaN, NaN, 0.3, 2.5, 1.86e-6, 1.31e-8, -1.37e-11, 4.34e-7, 6.27e-10, 0.17, 20.0, -0.0009, 2.3, 1.0, 7.1, 1.0, 1, 1.0, [(0.3, 0.05, 25.0), (0.31, 0.25, 25.0), (0.32, 0.52, 25.0), (0.334, 0.78, 25.0), (0.35, 0.92, 25.0), (0.365, 0.971, 25.0), (0.37, 0.977, 25.0), (0.38, 0.983, 25.0), (0.39, 0.989, 25.0), (0.4, 0.992, 25.0), (0.405, 0.993, 25.0), (0.42, 0.993, 25.0), (0.436, 0.992, 25.0), (0.46, 0.993, 25.0), (0.5, 0.994, 25.0), (0.546, 0.996, 25.0), (0.58, 0.995, 25.0), (0.62, 0.994, 25.0), (0.66, 0.994, 25.0), (0.7, 0.996, 25.0), (1.06, 0.997, 25.0), (1.53, 0.98, 25.0), (1.97, 0.84, 25.0), (2.325, 0.56, 25.0), (2.5, 0.36, 25.0)], 1.5168, 2.3, 0.0, 0, 64.17, 0, 2.51, 0.0)
const Examples_N_SK16 =GlassCat.Glass(GlassCat.GlassID(GlassCat.AGF, 828), 2, 1.34317774, 0.00704687339, 0.241144399, 0.0229005, 0.994317969, 92.7508526, 0.0, 0.0, NaN, NaN, 0.31, 2.5, -2.37e-8, 1.32e-8, -1.29e-11, 4.09e-7, 5.17e-10, 0.17, 20.0, -0.0011, 3.2, 1.4, 6.3, 4.0, 1, 53.3, [(0.31, 0.02, 25.0), (0.32, 0.11, 25.0), (0.334, 0.4, 25.0), (0.35, 0.7, 25.0), (0.365, 0.86, 25.0), (0.37, 0.89, 25.0), (0.38, 0.93, 25.0), (0.39, 0.956, 25.0), (0.4, 0.97, 25.0), (0.405, 0.974, 25.0), (0.42, 0.979, 25.0), (0.436, 0.981, 25.0), (0.46, 0.984, 25.0), (0.5, 0.991, 25.0), (0.546, 0.994, 25.0), (0.58, 0.994, 25.0), (0.62, 0.993, 25.0), (0.66, 0.994, 25.0), (0.7, 0.996, 25.0), (1.06, 0.995, 25.0), (1.53, 0.973, 25.0), (1.97, 0.88, 25.0), (2.325, 0.54, 25.0), (2.5, 0.26, 25.0)], 1.62041, 3.3, 4.0, 0, 60.32, 0, 3.58, 0.0)
const Examples_N_SF2 = GlassCat.Glass(GlassCat.GlassID(GlassCat.AGF, 815), 2, 1.47343127, 0.0109019098, 0.163681849, 0.0585683687, 1.36920899, 127.404933, 0.0, 0.0, NaN, NaN, 0.365, 2.5, 3.1e-6, 1.75e-8, 6.62e-11, 7.51e-7, 8.99e-10, 0.277, 20.0, 0.0081, 1.0, 1.4, 6.68, 1.0, 1, 1.0, [(0.365, 0.007, 25.0), (0.37, 0.06, 25.0), (0.38, 0.4, 25.0), (0.39, 0.68, 25.0), (0.4, 0.83, 25.0), (0.405, 0.865, 25.0), (0.42, 0.926, 25.0), (0.436, 0.949, 25.0), (0.46, 0.961, 25.0), (0.5, 0.975, 25.0), (0.546, 0.986, 25.0), (0.58, 0.987, 25.0), (0.62, 0.984, 25.0), (0.66, 0.984, 25.0), (0.7, 0.987, 25.0), (1.06, 0.997, 25.0), (1.53, 0.984, 25.0), (1.97, 0.93, 25.0), (2.325, 0.76, 25.0), (2.5, 0.67, 25.0)], 1.64769, 1.2, 0.0, 0, 33.82, 0, 2.718, 0.0)
const Examples_N_SF14 = GlassCat.Glass(GlassCat.GlassID(GlassCat.AGF, 896), 2, 1.69022361, 0.0130512113, 0.288870052, 0.061369188, 1.7045187, 149.517689, 0.0, 0.0, NaN, NaN, 0.365, 2.5, -5.56e-6, 7.09e-9, -1.09e-11, 9.85e-7, 1.39e-9, 0.287, 20.0, 0.013, 1.0, 2.2, 9.41, 1.0, 1, 1.0, [(0.365, 0.004, 25.0), (0.37, 0.04, 25.0), (0.38, 0.33, 25.0), (0.39, 0.61, 25.0), (0.4, 0.75, 25.0), (0.405, 0.79, 25.0), (0.42, 0.87, 25.0), (0.436, 0.91, 25.0), (0.46, 0.938, 25.0), (0.5, 0.964, 25.0), (0.546, 0.983, 25.0), (0.58, 0.987, 25.0), (0.62, 0.987, 25.0), (0.66, 0.987, 25.0), (0.7, 0.985, 25.0), (1.06, 0.998, 25.0), (1.53, 0.98, 25.0), (1.97, 0.88, 25.0), (2.325, 0.64, 25.0), (2.5, 0.57, 25.0)], 1.76182, 1.0, 0.0, 0, 26.53, 0, 3.118, 0.0)
include("curves.jl")
include("surfaces.jl")
include("lenses.jl")
include("systems.jl")
include("other.jl")
end # module TestData
export TestData
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1248 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
function bsplinecurve()
knots = KnotVector{Float64}([0.0, 0.0, 0.0, 0.0, 0.3, 0.6, 1.0, 1.0, 1.0, 1.0])
points = Array{MVector{2,Float64},1}([(0.05, 0.05), (0.05, 0.8), (0.6, 0.0), (0.7, 0.5), (0.5, 0.95), (0.95, 0.5)])
curve = BSplineCurve{OpticSim.Euclidean,Float64,2,3}(knots, points)
return curve
end
function homogeneousbsplinecurve()
knots = KnotVector{Float64}([0.0, 0.0, 0.0, 0.0, 0.3, 0.6, 1.0, 1.0, 1.0, 1.0])
points = Array{MVector{3,Float64},1}([(0.05, 0.0, 1.0), (0.05, 0.8, 1.0), (0.6, 0.0, 1.0), (0.7, 0.5, 1.0), (0.5, 0.95, 1.0), (0.95, 0.5, 1.0)])
curve = BSplineCurve{OpticSim.Rational,Float64,3,3}(knots, points)
return curve
end
function beziercurve()
orig = onespanspline()
return BezierCurve{OpticSim.Euclidean,Float64,2,3}(tobeziersegments(orig)[1])
end
function onespanspline()
knots = KnotVector{Float64}([0.0, 0.0, 0.0, 0.0, 1.0, 1.0, 1.0, 1.0])
points = Array{MVector{2,Float64},1}([(0.05, 0.05), (0.05, 0.95), (0.5, 0.95), (0.95, 0.5)])
curve = BSplineCurve{OpticSim.Euclidean,Float64,2,3}(knots, points)
return curve
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 7204 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
function zernikelens()
topsurface = leaf(AcceleratedParametricSurface(ZernikeSurface(9.5, zcoeff = [(1, 0.0), (4, -1.0), (7, 0.5)]), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function evenasphericlens()
topsurface = leaf(AcceleratedParametricSurface(AsphericSurface(9.5, radius = -50.0,aspherics = [(2, 0.0), (4, -0.001)]), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), Geometry.translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function oddasphericlens()
topsurface = leaf(AcceleratedParametricSurface(AsphericSurface(9.5, radius = -50.0,aspherics = [(3, 0.001), (5, -0.0001)]), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), Geometry.translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function asphericlens()
topsurface = leaf(AcceleratedParametricSurface(AsphericSurface(9.5, aspherics = [(3, 0.0), (4, -0.001)]), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function coniclensA()
topsurface = leaf(AcceleratedParametricSurface(AsphericSurface(9.5, radius = -15.0, conic = -5.0), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function coniclensQ()
topsurface = leaf(AcceleratedParametricSurface(QTypeSurface(9.5, radius = -15.0, conic = -5.0), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function qtypelens()
topsurface = leaf(AcceleratedParametricSurface(QTypeSurface(9.0, radius = -25.0, conic = 0.3, αcoeffs = [(1, 0, 0.3), (1, 1, 1.0)], βcoeffs = [(1, 0, -0.1), (2, 0, 0.4), (3, 0, -0.6)], normradius = 9.5), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function chebyshevlens()
topsurface = leaf(AcceleratedParametricSurface(ChebyshevSurface(9.0, 9.0, [(1, 0, -0.081), (2, 0, -0.162), (0, 1, 0.243), (1, 1, 0.486), (2, 1, 0.081), (0, 2, -0.324), (1, 2, -0.405), (2, 2, -0.81)], radius = -25.0), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
function gridsaglens()
topsurface = leaf(AcceleratedParametricSurface(GridSagSurface{Float64}(joinpath(@__DIR__, "../../test/test.GRD"), radius = -25.0, conic = 0.4, interpolation = GridSagBicubic), interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)), translation(0.0, 0.0, 5.0))
botsurface = leaf(Plane(0.0, 0.0, -1.0, 0.0, 0.0, -5.0, vishalfsizeu = 9.5, vishalfsizev = 9.5, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air)))
barrel = leaf(Cylinder(9.0, 20.0, interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, reflectance = zero(Float64), transmission = zero(Float64))))
return (barrel ∩ topsurface ∩ botsurface)(Transform(0.0, Float64(π), 0.0, 0.0, 0.0, -5.0))
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1935 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
intersectionat(α::Float64) = Intersection(α, [0.7071067811865475, 0.7071067811865475, 0.0] * α, [0.0, 0.0, 0.0], 0.0, 0.0, NullInterface())
const greenwavelength = Unitful.u"550nm"
const redwavelength = Unitful.u"650nm"
const bluewavelength = Unitful.u"450nm"
const roomtemperature = 20 * Unitful.u"°C"
transmissivethingrating(period, orders) = ThinGratingInterface(SVector(0.0, 1.0, 0.0), period, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, minorder = -orders, maxorder = orders, reflectance = [0.0 for _ in (-orders):orders], transmission = [0.2 for _ in (-orders):orders])
reflectivethingrating(period, orders) = ThinGratingInterface(SVector(0.0, 1.0, 0.0), period, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, minorder = -orders, maxorder = orders, transmission = [0.0 for _ in (-orders):orders], reflectance = [0.2 for _ in (-orders):orders])
function multiHOE()
rect = Rectangle(5.0, 5.0, SVector(0.0, 0.0, 1.0), SVector(0.0, 0.0, 0.0))
inbeam = SVector(0.0, 0.0, -1.0), CollimatedBeam
int1 = HologramInterface(SVector(-5.0, 0.0, -20.0), ConvergingBeam, SVector(0.0, -1.0, -1.0), CollimatedBeam, 0.55, 100.0, OpticSim.GlassCat.Air, OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, 0.05, false)
int2 = HologramInterface(SVector(5.0, 0.0, -20.0), ConvergingBeam, SVector(0.0, 1.0, -1.0), CollimatedBeam, 0.55, 100.0, OpticSim.GlassCat.Air, OpticSim.Examples.Examples_N_BK7, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, OpticSim.GlassCat.Air, 0.05, false)
mint = MultiHologramInterface(int1, int2)
obj = MultiHologramSurface(rect, mint)
sys = CSGOpticalSystem(LensAssembly(obj), Rectangle(50.0, 50.0, SVector(0.0, 0.0, 1.0), SVector(0.0, 0.0, -20.0), interface = opaqueinterface()))
return sys
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 6084 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
function bsplinesurface()
vknots = KnotVector{Float64}([0.0, 0.0, 0.0, 0.0, 0.5, 1.0, 1.0, 1.0, 1.0])
uknots = KnotVector{Float64}([0.0, 0.0, 0.0, 0.0, 1.0, 1.0, 1.0, 1.0])
points = map(
x -> collect(x),
[
(0.0, 0.0, 0.0) (0.33, 0.0, 0.0) (0.66, 0.0, 0.0) (1.0, 0.0, 0.0) (1.33, 0.0, 0.0)
(0.0, 0.33, 0.0) (0.33, 0.33, 1.0) (0.66, 0.33, 1.0) (1.0, 0.33, 0.5) (1.33, 0.33, 0.0)
(0.0, 0.66, 0.0) (0.33, 0.66, 1.0) (0.66, 0.66, 1.0) (1.0, 0.66, 0.5) (1.33, 0.66, 0.0)
(0.0, 1.0, 0.0) (0.33, 1.0, 0.0) (0.66, 1.0, 0.0) (1.0, 1.0, 0.0) (1.33, 1.0, 0.0)
],
)
return BSplineSurface{OpticSim.Euclidean,Float64,3,3}(uknots, vknots, points)
end
function beziersurface()
# only simple way I could think of to write this array literal as 2D array of 1D arrays.
# Julia doesn't parse a 2D array of 1D arrays properly. Maybe should use tuples instead though.
points = map(
x -> collect(x),
[
(0.0, 0.0, 0.0) (0.0, 0.33, 0.0) (0.0, 0.66, 0.0) (0.0, 1.0, 0.0)
(0.33, 0.0, 0.0) (0.33, 0.33, 1.0) (0.33, 0.66, 1.0) (0.33, 1.0, 0.0)
(0.66, 0.0, 0.0) (0.66, 0.33, 1.0) (0.66, 0.66, 1.0) (0.66, 1.0, 0.0)
(1.0, 0.0, 0.0) (1.0, 0.33, 0.0) (1.0, 0.66, 0.0) (1.0, 1.0, 0.0)
],
)
return BezierSurface{OpticSim.Euclidean,Float64,3,3}(points)
end
function upsidedownbeziersurface()
points = map(
x -> collect(x),
[
(0.0, 0.0, 0.0) (0.33, 0.0, 0.0) (0.66, 0.0, 0.0) (1.0, 0.0, 0.0)
(0.0, 0.33, 0.0) (0.33, 0.33, -1.0) (0.66, 0.33, -1.0) (1.0, 0.33, -.5)
(0.0, 0.66, 0.0) (0.33, 0.66, -1.0) (0.66, 0.66, -1.0) (1.0, 0.66, -.5)
(0.0, 1.0, 0.0) (0.33, 1.0, 0.0) (0.66, 1.0, 0.0) (1.0, 1.0, 0.0)
],
)
return BezierSurface{OpticSim.Euclidean,Float64,3,3}(points)
end
function wavybeziersurface()
points = map(
x -> collect(x),
[
(0.0, 0.0, -0.3) (0.0, 0.33, 1.0) (0.0, 0.66, -1.0) (0.0, 1.0, 0.3)
(0.33, 0.0, -0.3) (0.33, 0.33, 1.0) (0.33, 0.66, -1.0) (0.33, 1.0, 0.3)
(0.66, 0.0, -0.3) (0.66, 0.33, 1.0) (0.66, 0.66, -1.0) (0.66, 1.0, 0.3)
(1.0, 0.0, -0.3) (1.0, 0.33, 1.0) (1.0, 0.66, -1.0) (1.0, 1.0, 0.3)
],
)
return BezierSurface{OpticSim.Euclidean,Float64,3,3}(points)
end
function verywavybeziersurface()
points = map(
x -> collect(x),
[
(0.0, 0.0, 0.5) (0.0, 0.2, 1.0) (0.0, 0.4, -3.0) (0.0, 0.6, 3.0) (0.0, 0.8, -1.0) (0.0, 1.0, 0.5)
(0.2, 0.0, 0.5) (0.2, 0.2, 1.0) (0.2, 0.4, -3.0) (0.2, 0.6, 3.0) (0.2, 0.8, -1.0) (0.2, 1.0, 0.5)
(0.4, 0.0, 0.0) (0.4, 0.2, 1.0) (0.4, 0.4, -3.0) (0.4, 0.6, 3.0) (0.4, 0.8, -1.0) (0.4, 1.0, 0.5)
(0.6, 0.0, 0.0) (0.6, 0.2, 1.0) (0.6, 0.4, -3.0) (0.6, 0.6, 3.0) (0.6, 0.8, -1.0) (0.6, 1.0, 0.5)
(0.8, 0.0, -0.5) (0.8, 0.2, 1.0) (0.8, 0.4, -3.0) (0.8, 0.6, 3.0) (0.8, 0.8, -1.0) (0.8, 1.0, 0.5)
(1.0, 0.0, -0.5) (1.0, 0.2, 1.0) (1.0, 0.4, -3.0) (1.0, 0.6, 3.0) (1.0, 0.8, -1.0) (1.0, 1.0, 0.5)
],
)
return BezierSurface{OpticSim.Euclidean,Float64,3,5}(points)
end
qtypesurface1() = QTypeSurface(1.5, radius = 5.0, conic = 1.0, αcoeffs = [(0, 1, -0.3), (5, 4, 0.1)], βcoeffs = [(3, 7, 0.1)], normradius = 1.6)
zernikesurface1() = ZernikeSurface(1.5, radius = 5.0, conic = 1.0, zcoeff = [(1, 0.0), (4, -0.1), (7, 0.05), (33, 0.03)], aspherics = [(2, 0.0), (10, 0.01)])
zernikesurface1a() = ZernikeSurface(1.5, radius = 5.0, conic = 1.0, zcoeff = [(1, 0.0), (4, -0.1), (7, 0.05), (33, 0.03)], aspherics = [(2, 0.0), (10, 0.01)], normradius = 1.6)
zernikesurface2() = ZernikeSurface(1.5, radius = 5.0, conic = 1.0, zcoeff = [(7, 0.2), (9, 0.2)], aspherics = [(10, -0.01)])
zernikesurface3() = ZernikeSurface(9.5, zcoeff = [(1, 0.0), (4, -1.0), (7, 0.5)])
conicsurface() = AcceleratedParametricSurface(ZernikeSurface(9.5, radius = -15.0, conic = -5.0)) #this also tests the CONIC parameter of AsphericSurface
oddasphericsurface() = OddAsphericSurface(9.5, 1.0/-50.0, 1.0, [0.0, 0.001, -0.0001])
evenasphericsurface() = EvenAsphericSurface(9.5, 1.0/-50.0, 1.0, [0.001, -0.0001])
oddevenasphericsurface() = OddEvenAsphericSurface(9.5, 1.0/-50.0, 1.0, [0.0, 0.001, -0.0001])
function simplesaggrid()
points = map(
x -> collect(x),
[
(0.1, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0) (0.0, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0)
(0.4, 0.0, 0.0, 0.0) (0.1, 0.0, 0.0, 0.0) (0.0, 0.0, 0.0, 0.0) (0.1, 0.0, 0.0, 0.0)
(-0.3, 0.0, 0.0, 0.0) (0.1, 0.0, 0.0, 0.0) (0.2, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0)
(0.2, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0) (-0.1, 0.0, 0.0, 0.0) (-0.1, 0.0, 0.0, 0.0)
(0.1, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0) (0.0, 0.0, 0.0, 0.0) (0.3, 0.0, 0.0, 0.0)
(0.4, 0.0, 0.0, 0.0) (0.1, 0.0, 0.0, 0.0) (0.0, 0.0, 0.0, 0.0) (0.1, 0.0, 0.0, 0.0)
],
)
return points
end
gridsagsurfacelinear() = GridSagSurface(ZernikeSurface(1.5, radius = 5.0, conic = 1.0, zcoeff = [(1, 0.0), (4, -0.1), (7, 0.05), (33, 0.03)], aspherics = [(2, 0.0), (10, 0.01)]), simplesaggrid(), interpolation = GridSagLinear)
gridsagsurfacebicubic() = GridSagSurface(ZernikeSurface(1.5, radius = 25.0, conic = 1.0, zcoeff = [(1, 0.0), (4, -0.1), (7, 0.05), (33, 0.03)], aspherics = [(2, 0.0), (10, 0.01)]), simplesaggrid(), interpolation = GridSagBicubic)
gridsagsurfacebicubiccheby() = GridSagSurface(ChebyshevSurface(1.5, 2.0, radius = 25.0, conic = 0.2, [(0, 1, 0.03), (2, 1, -0.01)]), simplesaggrid(), interpolation = GridSagBicubic)
chebyshevsurface1() = ChebyshevSurface(2.0, 2.0, [(1, 1, 0.1), (4, 5, -0.3), (2, 1, 0.0), (22, 23, 0.01)], radius = 25.0, conic = 0.2)
chebyshevsurface2() = ChebyshevSurface(2.0, 2.0, [(1, 1, 0.1), (0, 2, 0.3), (4, 2, -0.1)], radius = 10.0, conic = 0.2)
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 7314 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
#TODO this is redundant. Many of these systems are already defined in Examples.otherexamples.jl. We should only have one copy
function cooketriplet(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Standard", "Stop", "Standard", "Standard", "Image"],
Radius = [Inf, 26.777, 66.604, -35.571, 35.571, 35.571, -26.777, Inf],
Thickness = [Inf, 4.0, 2.0, 4.0, 2.0, 4.0, 44.748, missing],
Material = [Air, OpticSim.Examples.Examples_N_SK16, Air, OpticSim.Examples.Examples_N_SF2, Air, OpticSim.Examples.Examples_N_SK16, Air, missing],
SemiDiameter = [Inf, 8.580, 7.513, 7.054, 6.033, 7.003, 7.506, 15.0]
)
)
end
function cooketripletfirstelement(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf, -35.571, 35.571, Inf],
Thickness = [Inf, 4.0, 44.748, missing],
Material = [Air, OpticSim.Examples.Examples_N_SK16, Air, missing],
SemiDiameter = [Inf, 7.054, 6.033, 15.0]
)
)
end
function convexplano(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf, 60.0, Inf, Inf],
Thickness = [Inf, 10.0, 57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf, 9.0, 9.0, 15.0]
)
)
end
function doubleconvex(
frontradius::T, rearradius::T;
temperature::Unitful.Temperature = GlassCat.TEMP_REF_UNITFUL, pressure::T = T(PRESSURE_REF)
) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [T(Inf64), frontradius, rearradius, T(Inf64)],
Thickness = [T(Inf64), T(10.0), T(57.8), missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [T(Inf64), T(9.0), T(9.0), T(15.0)]
);
temperature,
pressure
)
end
function doubleconvex(
::Type{T} = Float64; temperature::Unitful.Temperature = GlassCat.TEMP_REF_UNITFUL, pressure::T = T(PRESSURE_REF)
) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(
SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf64, 60, -60, Inf64],
Thickness = [Inf64, 10.0, 57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf64, 9.0, 9.0, 15.0]
);
temperature,
pressure
)
end
function doubleconcave(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf64, -41.0, 41.0, Inf64],
Thickness = [Inf64, 10.0, 57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf64, 9.0, 9.0, 15.0]
)
)
end
function planoconcaverefl(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf64, Inf64, -41.0, Inf64],
Thickness = [Inf64, 10.0, -57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf64, 9.0, 9.0, 25.0],
Reflectance = [missing, missing, 1.0, missing]
)
)
end
function concaveplano(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf64, -41.0, Inf64, Inf64],
Thickness = [Inf64, 10.0, 57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf64, 9.0, 9.0, 15.0]
)
)
end
function planoplano(::Type{T} = Float64) where {T<:Real}
return AxisymmetricOpticalSystem{T}(
DataFrame(SurfaceType = ["Object", "Standard", "Standard", "Image"],
Radius = [Inf64, Inf64, Inf64, Inf64],
Thickness = [Inf64, 10.0, 57.8, missing],
Material = [Air, OpticSim.Examples.Examples_N_BK7, Air, missing],
SemiDiameter = [Inf64, 9.0, 9.0, 15.0]
)
)
end
"""
planarshapes()
Returns a CSGOpticalSystem containing a vertical stack (along the z-axis) of alternating planar shapes and a detector
placed at the end of the stack. This is for the purpose of benchmarking allocations when planar shapes are stored in a
shared Vector within LensAssembly.
"""
function planarshapes()
interface = FresnelInterface{Float64}(OpticSim.Examples.Examples_N_BK7, Air; interfacemode=Transmit)
s = 10.0
surfacenormal = SVector(0., 0., 1.)
elements = []
for i = 1:9
centrepoint = SVector(0., 0., -i)
if i % 3 == 1
push!(elements, Ellipse(s, s, surfacenormal, centrepoint; interface))
elseif i % 3 == 2
push!(elements, Hexagon(s, surfacenormal, centrepoint; interface))
elseif i % 3 == 0
push!(elements, Rectangle(s, s, surfacenormal, centrepoint; interface))
end
end
lensassembly = LensAssembly(elements...)
detector = Rectangle(s, s, surfacenormal, SVector(0., 0., -10.); interface = opaqueinterface())
return CSGOpticalSystem(lensassembly, detector)
end
zernikesystem() = CSGOpticalSystem(LensAssembly(zernikelens()), Rectangle(40.0, 40.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
asphericsystem() = CSGOpticalSystem(LensAssembly(asphericlens()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
evenasphericsystem()=CSGOpticalSystem(LensAssembly(evenasphericlens()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
oddasphericsystem()=CSGOpticalSystem(LensAssembly(oddasphericlens()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
conicsystemA() = CSGOpticalSystem(LensAssembly(coniclensA()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
conicsystemQ() = CSGOpticalSystem(LensAssembly(coniclensQ()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
qtypesystem() = CSGOpticalSystem(LensAssembly(qtypelens()), Rectangle(25.0, 25.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
chebyshevsystem() = CSGOpticalSystem(LensAssembly(chebyshevlens()), Rectangle(40.0, 40.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
gridsagsystem() = CSGOpticalSystem(LensAssembly(gridsaglens()), Rectangle(40.0, 40.0, [0.0, 0.0, 1.0], [0.0, 0.0, -67.8], interface = opaqueinterface()))
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 385 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Allocations" begin
# ensure that there are 0 allocations for all the benchmarks
for b in Benchmarks.all_benchmarks()
@test Benchmarks.runbenchmark(b, samples = 1, evals = 1).allocs == 0
end
end # testset Allocations
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 963 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "BVH" begin
@testset "partition!" begin
split = 0.5
for i in 1:100000
a = rand(5)
b = copy(a)
badresult::Bool = false
lower, upper = partition!(a, (x) -> x, split)
if lower !== nothing
for i in lower
if i >= split
badresult = true
break
end
end
end
if upper !== nothing
for i in upper
if i <= split
badresult = true
end
end
end
if badresult
throw(ErrorException("array didn't partition: $(b)"))
end
end
end
end # testset BVH
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 20894 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Comparison" begin
@testset "Refraction" begin
λ = 0.550
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ)
# Test a number of rays under standard environmental conditions
a = TestData.planoplano()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [2.0, 2.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [5.0, 5.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 0.7192321011619232, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.234903851062, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [0.7200535362928893, -6.141047252697188, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.2448952769065, rtol = COMP_TOLERANCE)
a = TestData.concaveplano()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [3.633723967570758, 3.633723967570758, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.0772722321026, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [9.153654757938119, 9.153654757938119, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.5695599263722, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, -3.401152468994745, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.1609946836496, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [-3.457199906282556, -10.32836827735406, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.5393056652617, rtol = COMP_TOLERANCE)
a = TestData.doubleconcave()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [5.235579681684886, 5.235579681684886, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.2624909340242, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [13.42351905137007, 13.42351905137007, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.8131310483928, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, -6.904467939352202, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.3286918571586, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [-7.089764102262856, -14.61837033417989, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.4286280772859, rtol = COMP_TOLERANCE)
a = TestData.convexplano()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [0.8867891519368289, 0.8867891519368289, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9698941263224, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [2.212067233969831, 2.212067233969831, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.8895474037682, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 3.489759589087602, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.4310036252394, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [3.491858246934857, -3.331802834115089, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.344370622227, rtol = COMP_TOLERANCE)
a = TestData.doubleconvex()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-0.06191521590711035, -0.06191521590711035, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.987421926598, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-0.2491105067897657, -0.2491105067897657, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.0110871422915, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 5.639876913179362, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.6758349889372, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [5.705452331562673, -0.7679713587894854, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.6175821043825, rtol = COMP_TOLERANCE)
end # testset Refraction
@testset "Temperature/Pressure" begin
λ = 0.550
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ)
# Test a component whose material does have a ΔT component
a = TestData.doubleconvex(temperature = 40 * u"°C")
@test isapprox(point(intersection(trace(a, r1, test = true))), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(point(intersection(trace(a, r2, test = true))), [-0.06214282132053373, -0.06214282132053373, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(point(intersection(trace(a, r3, test = true))), [-0.2497005807710933, -0.2497005807710933, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(point(intersection(trace(a, r4, test = true))), [0.0, 5.640416741927821, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(point(intersection(trace(a, r5, test = true))), [5.706006107804734, -0.7673622008537766, -67.8], rtol = COMP_TOLERANCE)
# note that this material doesn't have a ΔT component
λ2 = 0.533
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ2)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ2)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ2)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ2)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ2)
end # testset Temperature/Pressure
@testset "Reflection" begin
λ = 0.550
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ)
a = TestData.planoconcaverefl()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, 47.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 79.1704477524159, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-6.19723306038804, -6.19723306038804, 47.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 80.0979229417755, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-17.7546255175372, -17.7546255175372, 47.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 86.2063094599075, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 19.5349836031196, 47.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 83.5364646376395, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [21.2477706541112, 17.3463704045055, 47.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 87.4033932087711, rtol = COMP_TOLERANCE)
end # testset Reflection
@testset "Complex Lenses" begin
λ = 0.550
r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
r4 = OpticalRay([0.0, -5.0, 1.0], [0.0, 0.08715574274765818, -0.9961946980917454], 1.0, λ)
r5 = OpticalRay([-5.0, -5.0, 1.0], [0.08715574274765818, -0.01738599476176408, -0.9960429728140486], 1.0, λ)
a = TestData.conicsystemA()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-1.80279270185495, -1.80279270185495, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.1000975813922, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-2.8229241607807, -2.8229241607807, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.282094499601, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 9.01421545841289, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.2211078071893, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [8.13946939328266, 2.23006981338816, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.1025133542546, rtol = COMP_TOLERANCE)
a = TestData.asphericsystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [0.0735282671574837, 0.0735282671574837, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.0161411455851, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r3, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [-5.0, -5.0, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 46.5556716286238, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 11.9748998399885, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 76.0760286320348, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r5, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [5.49905367197174, 5.66882664623822, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 47.6825931025333, rtol = COMP_TOLERANCE)
a = TestData.evenasphericsystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-1.1537225076569997, -1.153722507656998, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.08191763367685, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r3, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [-4.684043128742365, -4.684043128742363, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 45.610874251552275, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r4, test = true, trackrays = track)
@test isapprox(point(res), [0.0, 15.887441944041871, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 77.14042689028618, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r5, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [5.095756154983988, 5.24166010736873, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 45.618062468023375, rtol = COMP_TOLERANCE)
a = TestData.oddasphericsystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [0.6256581890889898, 0.6256581890889894, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.97868203031574, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r3, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [-6.097868437446996, -6.097868437446997, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 48.34832912579036, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r4, test = true, trackrays = track)
@test isapprox(point(res), [0.0, 7.485281534548376, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.07125754375438, rtol = COMP_TOLERANCE)
track = Vector{OpticSim.LensTrace{Float64,3}}(undef, 0)
res = trace(a, r5, test = true, trackrays = track)
@test (res === nothing) # TIR
@test isapprox(point(track[end]), [6.197436823172213, 6.440993733499131, 0.0], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(track[end]), 48.292537161700146, rtol = COMP_TOLERANCE)
a = TestData.zernikesystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, -8.9787010034042, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.5977029819277, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-1.58696749235066, -9.71313213852721, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.7603266537023, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [0.790348081563859, -0.762155123619682, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.1933359669848, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true, trackrays = track)
@test isapprox(point(res), [0.0, 10.0037899692172, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 76.8041236301678, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [35.5152289731456, 28.3819941055557, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 94.3059947823954, rtol = COMP_TOLERANCE)
a = TestData.conicsystemQ()
res = trace(a, r1, test = true)
@test isapprox(point(res), [0.0, 0.0, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 73.9852238762079, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-1.80279270185495, -1.80279270185495, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.1000975813922, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-2.8229241607807, -2.8229241607807, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.282094499601, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [0.0, 9.01421545841289, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.2211078071893, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [8.13946939328266, 2.23006981338816, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.1025133542546, rtol = COMP_TOLERANCE)
# No NSC qtype so have less precise SC values and no angled rays
a = TestData.qtypesystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [-4.3551074306, -0.318112811010, -67.8], rtol = 1e-12)
# TODO these are the values that I get for comparison - I'm pretty certain that they are just wrong...
# res = trace(a, r2, test = true)
# @test isapprox(point(res), [-0.091773202667, 3.9362695851, -67.8], rtol = 1e-12)
# res = trace(a, r3, test = true)
# @test isapprox(point(res), [-7.0993098547, -2.6848726242, -67.8], rtol = 1e-12)
# No NSC chebyshev so have less precise SC values and no angled rays
a = TestData.chebyshevsystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [-1.07963031980, 0.53981515992, -67.8], rtol = 1e-12)
res = trace(a, r2, test = true)
@test isapprox(point(res), [0.00851939011, 1.25870229260, -67.8], rtol = 1e-12)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-1.20441411240, -0.07554605390, -67.8], rtol = 1e-12)
a = TestData.gridsagsystem()
res = trace(a, r1, test = true)
@test isapprox(point(res), [21.0407756733608, 21.724638830759, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 82.0777022031378, rtol = COMP_TOLERANCE)
res = trace(a, r2, test = true)
@test isapprox(point(res), [-0.489183765274452, 0.405160352533666, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 75.536902213118, rtol = COMP_TOLERANCE)
res = trace(a, r3, test = true)
@test isapprox(point(res), [-12.0770793886528, -8.7705340321259, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 77.5651917266687, rtol = COMP_TOLERANCE)
res = trace(a, r4, test = true)
@test isapprox(point(res), [-1.20535362019229, 6.07973526939659, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.7349148204077, rtol = COMP_TOLERANCE)
res = trace(a, r5, test = true)
@test isapprox(point(res), [6.5112407340601, -0.22055440245024, -67.8], rtol = COMP_TOLERANCE)
@test isapprox(pathlength(res), 74.6955888563913, rtol = COMP_TOLERANCE)
end #testset complex lenses
# @testset "Power" begin
# λ = 0.550
# r1 = OpticalRay([0.0, 0.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
# r2 = OpticalRay([2.0, 2.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
# r3 = OpticalRay([5.0, 5.0, 1.0], [0.0, 0.0, -1.0], 1.0, λ)
# a = TestData.doubleconvex()
# res = trace(a, r1, test = true)
# @test isapprox(power(res), 0.915508396)
# res = trace(a, r2, test = true)
# @test isapprox(power(res), 0.915526077)
# res = trace(a, r3, test = true)
# @test isapprox(power(res), 0.915577586)
# end
end # testset Comparison
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 14082 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using Test
using OpticSim
using OpticSim.Emitters
using OpticSim.Geometry
using Random
using StaticArrays
@testset "Emitters" begin
@testset "RayListSource" begin
rays = Vector{OpticalRay{Float64,3}}(undef, 0)
for i in 0:7
λ = ((i / 7) * 200 + 450) / 1000
r = OpticalRay(SVector(0.0, -3.0, 10.0), SVector(0.0, 0.5, -1.0), 1.0, λ)
push!(rays, r)
end
raygen = Emitters.Sources.RayListSource(rays)
for (i,ray) in enumerate(raygen)
@assert ray == rays[i]
end
end
@testset "AngularPower" begin
@testset "Lambertian" begin
@test typeof(AngularPower.Lambertian()) === AngularPower.Lambertian{Float64}
@test_throws MethodError AngularPower.Lambertian(String)
@test apply(AngularPower.Lambertian(), Transform(), 1, Ray(zeros(3), ones(3))) === 1
end
@testset "Cosine" begin
@test AngularPower.Cosine().cosine_exp === one(Float64)
@test_throws MethodError AngularPower.Cosine(String)
@test apply(AngularPower.Cosine(), Transform(), 1, Ray(zeros(3), ones(3))) === 0.5773502691896258
end
@testset "Gaussian" begin
@test AngularPower.Gaussian(0, 1).gaussianu === 0
@test AngularPower.Gaussian(0, 1).gaussianv === 1
@test apply(AngularPower.Gaussian(0, 1), Transform(), 1, Ray(zeros(3), ones(3))) === 0.7165313105737892
end
end
@testset "Directions" begin
@testset "Constant" begin
@test Directions.Constant(0, 0, 0).direction === Vec3(Int)
@test Directions.Constant(Vec3()).direction === Vec3()
@test Directions.Constant().direction === unitZ3()
@test Base.length(Directions.Constant()) === 1
@test Emitters.generate(Directions.Constant(), 0) === unitZ3()
@test Base.iterate(Directions.Constant()) === (unitZ3(), 2)
@test Base.iterate(Directions.Constant(), 2) === nothing
@test Base.getindex(Directions.Constant(), 1) === unitZ3()
@test Base.getindex(Directions.Constant(), 2) === unitZ3()
@test Base.firstindex(Directions.Constant()) === 0
@test Base.lastindex(Directions.Constant()) === 0
@test Base.copy(Directions.Constant()) === Directions.Constant()
end
@testset "RectGrid" begin
@test Directions.RectGrid(unitX3(), 0., 0., 0, 0).uvec === unitY3()
@test Directions.RectGrid(unitX3(), 0., 0., 0, 0).vvec === unitZ3()
@test Directions.RectGrid(0., 0., 0, 0).uvec === unitX3()
@test Directions.RectGrid(0., 0., 0, 0).vvec === unitY3()
@test Base.length(Directions.RectGrid(0., 0., 2, 3)) === 6
@test collect(Directions.RectGrid(ones(Vec3), π/4, π/4, 2, 3)) == [
[0.6014252485829169, 0.7571812496798511, 1.2608580392339483],
[1.1448844430815608, 0.4854516524305293, 0.9891284419846265],
[0.643629619770125, 1.0798265148205617, 1.0798265148205617],
[1.225225479837374, 0.7890285847869373, 0.7890285847869373],
[0.6014252485829169, 1.2608580392339483, 0.7571812496798511],
[1.1448844430815608, 0.9891284419846265, 0.4854516524305293],
]
end
@testset "UniformCone" begin
@test Directions.UniformCone(unitX3(), 0., 0).uvec === unitY3()
@test Directions.UniformCone(unitX3(), 0., 0).vvec === unitZ3()
@test Directions.UniformCone(0., 0).uvec === unitX3()
@test Directions.UniformCone(0., 0).vvec === unitY3()
@test Base.length(Directions.UniformCone(0., 1)) === 1
@test collect(Directions.UniformCone(π/4, 2, rng=Random.MersenneTwister(0))) == [
[0.30348115383395624, -0.6083405920618145, 0.7333627433388552],
[0.16266571675478964, 0.2733479444418462, 0.9480615833700192],
]
end
@testset "HexapolarCone" begin
@test Directions.HexapolarCone(unitX3(), 0., 0).uvec === unitY3()
@test Directions.HexapolarCone(unitX3(), 0., 0).vvec === unitZ3()
@test Directions.HexapolarCone(0., 0).uvec === unitX3()
@test Directions.HexapolarCone(0., 0).vvec === unitY3()
@test Base.length(Directions.HexapolarCone(0., 1)) === 7
@test Base.length(Directions.HexapolarCone(0., 2)) === 19
@test collect(Directions.HexapolarCone(π/4, 1)) == [
[0.0, 0.0, 1.0],
[0.7071067811865475, 0.0, 0.7071067811865476],
[0.3535533905932738, 0.6123724356957945, 0.7071067811865476],
[-0.3535533905932736, 0.6123724356957946, 0.7071067811865477],
[-0.7071067811865475, 8.659560562354932e-17, 0.7071067811865476],
[-0.35355339059327406, -0.6123724356957944, 0.7071067811865476],
[0.3535533905932738, -0.6123724356957945, 0.7071067811865476],
]
end
end
@testset "Origins" begin
@testset "Point" begin
@test Origins.Point(Vec3()).origin === Vec3()
@test Origins.Point(0, 0, 0).origin === Vec3(Int)
@test Origins.Point().origin === Vec3()
@test Base.length(Origins.Point()) === 1
@test Emitters.visual_size(Origins.Point()) === 1
@test Emitters.visual_size(Origins.Point()) === 1
@test Emitters.generate(Origins.Point(), 1) === Vec3()
@test Base.iterate(Origins.Point(), 1) === (Vec3(), 2)
@test Base.iterate(Origins.Point(), 2) === nothing
@test Base.getindex(Origins.Point(), 1) === Vec3()
@test Base.getindex(Origins.Point(), 2) === Vec3()
@test Base.firstindex(Origins.Point()) === 0
@test Base.lastindex(Origins.Point()) === 0
@test Base.copy(Origins.Point()) === Origins.Point()
end
@testset "RectUniform" begin
@test Origins.RectUniform(1, 2, 3).width === 1
@test Origins.RectUniform(1, 2, 3).height === 2
@test Origins.RectUniform(1, 2, 3).samples_count === 3
@test Base.length(Origins.RectUniform(1, 2, 3)) === 3
@test Emitters.visual_size(Origins.RectUniform(1, 2, 3)) === 2
@test collect(Origins.RectUniform(1, 2, 3, rng=Random.MersenneTwister(0))) == [
[0.3236475079774124, 0.8207130758528729, 0.0],
[-0.3354342018663148, -0.6453423070674709, 0.0],
[-0.221119890668799, -0.5930468839161547, 0.0],
]
end
@testset "RectGrid" begin
@test Origins.RectGrid(1., 2., 3, 4).ustep === .5
@test Origins.RectGrid(1., 2., 3, 4).vstep === 2/3
@test Base.length(Origins.RectGrid(1., 2., 3, 4)) === 12
@test Emitters.visual_size(Origins.RectGrid(1., 2., 3, 4)) === 2.
@test collect(Origins.RectGrid(1., 2., 3, 4)) == [
[-0.5, -1.0, 0.0],
[0.0, -1.0, 0.0],
[0.5, -1.0, 0.0],
[-0.5, -0.33333333333333337, 0.0],
[0.0, -0.33333333333333337, 0.0],
[0.5, -0.33333333333333337, 0.0],
[-0.5, 0.33333333333333326, 0.0],
[0.0, 0.33333333333333326, 0.0],
[0.5, 0.33333333333333326, 0.0],
[-0.5, 1.0, 0.0],
[0.0, 1.0, 0.0],
[0.5, 1.0, 0.0],
]
end
@testset "RectJitterGrid" begin
@test Origins.RectJitterGrid(1., 2., 3, 4, 1).width === 1.0
@test Origins.RectJitterGrid(1., 2., 3, 4, 1).height === 2.0
@test Origins.RectJitterGrid(1., 2., 2, 2, 3).ustep === 0.5
@test Origins.RectJitterGrid(1., 2., 2, 2, 3).vstep === 1.0
@test Base.length(Origins.RectJitterGrid(1., 2., 5, 6, 7)) === 210
@test Emitters.visual_size(Origins.RectJitterGrid(1., 2., 5, 6, 7)) === 2.0
@test collect(Origins.RectJitterGrid(1., 2., 2, 2, 2, rng=Random.MersenneTwister(0))) == [
[-0.08817624601129381, -0.08964346207356355, 0.0],
[-0.4177171009331574, -0.8226711535337354, 0.0],
[0.1394400546656005, -0.7965234419580773, 0.0],
[0.021150832966014832, -0.9317307444943552, 0.0],
[-0.3190858046118913, 0.9732164043865108, 0.0],
[-0.2070942241283379, 0.5392892841426182, 0.0],
[0.13001792513452393, 0.910046541351011, 0.0],
[0.08351809722107484, 0.6554484126999125, 0.0],
]
end
@testset "Hexapolar" begin
@test Origins.Hexapolar(1, 0, 0).nrings === 1
@test_throws MethodError Origins.Hexapolar(1., 0, 0)
@test Base.length(Origins.Hexapolar(1, 0, 0)) === 7
@test Base.length(Origins.Hexapolar(2, 0, 0)) === 19
@test Emitters.visual_size(Origins.Hexapolar(0, 1, 2)) === 4
@test collect(Origins.Hexapolar(1, π/4, π/4)) == [
[0.0, 0.0, 0.0],
[0.7853981633974483, 0.0, 0.0],
[0.39269908169872425, 0.6801747615878316, 0.0],
[-0.392699081698724, 0.6801747615878317, 0.0],
[-0.7853981633974483, 9.618353468608949e-17, 0.0],
[-0.3926990816987245, -0.6801747615878315, 0.0],
[0.39269908169872425, -0.6801747615878316, 0.0],
]
end
end
@testset "Spectrum" begin
@testset "Uniform" begin
@test Spectrum.UNIFORMSHORT === 0.450
@test Spectrum.UNIFORMLONG === 0.680
@test Spectrum.Uniform(0, 1).low_end === 0
@test Spectrum.Uniform(0, 1).high_end === 1
@test Spectrum.Uniform().low_end === 0.450
@test Spectrum.Uniform().high_end === 0.680
@test Emitters.generate(Spectrum.Uniform(rng=Random.MersenneTwister(0))) === (1.0, 0.6394389268348049)
end
@testset "DeltaFunction" begin
@test Emitters.generate(Spectrum.DeltaFunction(2)) === (1, 2)
@test Emitters.generate(Spectrum.DeltaFunction(2.)) === (1., 2.)
@test Emitters.generate(Spectrum.DeltaFunction(π)) === (true, π) # hopefully this is ok!
end
@testset "Measured" begin
# TODO
end
end
@testset "Sources" begin
expected_rays = [
OpticalRay([0., 0., 0.], [0., 0., 1.], 1., 0.6394389268348049),
OpticalRay([0., 0., 0.], [0., 0., 1.], 1., 0.6593820037230804),
OpticalRay([0., 0., 0.], [0., 0., 1.], 1., 0.48785013357074763),
]
@testset "Source" begin
@test Sources.Source().transform === Transform()
@test Sources.Source().spectrum === Spectrum.Uniform()
@test Sources.Source().origins === Origins.Point()
@test Sources.Source().directions === Directions.Constant()
@test Sources.Source().power_distribution === AngularPower.Lambertian()
@test Sources.Source().sourcenum === 0
@test Sources.Source() === Sources.Source(
Transform(),
Spectrum.Uniform(),
Origins.Point(),
Directions.Constant(),
AngularPower.Lambertian())
@test Base.length(Sources.Source()) === 1
@test Base.length(Sources.Source(; origins=Origins.Hexapolar(1, 0., 0.))) === 7
@test Base.length(Sources.Source(; directions=Directions.HexapolarCone(0., 1))) === 7
@test Base.length(Sources.Source(;
origins=Origins.Hexapolar(1, 0., 0.),
directions=Directions.HexapolarCone(0., 1)
)) === 49
@test Base.iterate(Sources.Source(spectrum=Spectrum.Uniform(rng=Random.MersenneTwister(0)))) === (expected_rays[1], Sources.SourceGenerationState(2, 0, Vec3()))
@test Base.getindex(Sources.Source(spectrum=Spectrum.Uniform(rng=Random.MersenneTwister(0))), 0) === expected_rays[1]
@test Emitters.generate(Sources.Source(spectrum=Spectrum.Uniform(rng=Random.MersenneTwister(0))), 0) === expected_rays[1]
@test Emitters.generate(Sources.Source(spectrum=Spectrum.Uniform(rng=Random.MersenneTwister(0)))) === (expected_rays[1], Sources.SourceGenerationState(0, -2, Vec3()))
@test Base.firstindex(Sources.Source()) === 0
@test Base.lastindex(Sources.Source()) === 0
@test Base.copy(Sources.Source()) === Sources.Source()
end
@testset "CompositeSource" begin
s() = Sources.Source(spectrum=Spectrum.Uniform(rng=Random.MersenneTwister(0)))
tr = Transform()
cs1 = Sources.CompositeSource(tr, [s()])
cs2 = Sources.CompositeSource(tr, [s(), s()])
cs3 = Sources.CompositeSource(tr, [s(), cs2])
@test cs1.transform === tr
@test cs1.uniform_length === 1
@test cs2.uniform_length === 1
@test cs3.uniform_length === -1
@test cs1.total_length === 1
@test cs2.total_length === 2
@test cs3.total_length === 3
@test cs1.start_indexes == []
@test cs2.start_indexes == []
@test cs3.start_indexes == [0, 1, 3]
@test Base.length(cs1) === 1
@test Base.length(cs2) === 2
@test Base.length(cs3) === 3
@test Base.iterate(cs1) === (expected_rays[1], Sources.SourceGenerationState(2, 0, Vec3()))
@test collect(cs2) == vcat(expected_rays[1:1], expected_rays[1:1])
@test collect(cs3) == vcat(expected_rays[1:1], expected_rays[2:2], expected_rays[2:2])
end
end
end # testset Emitters
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 285 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# TODO may want to test this but it's very slow on azure
@testset "Examples" begin
@test_all_no_arg_functions Examples
end # testset Examples
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 17071 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "General" begin
@testset "QuadraticRoots" begin
Random.seed!(SEED)
similarroots(r1, r2, x1, x2) = isapprox([r1, r2], [x1, x2], rtol = 1e-9) || isapprox([r2, r1], [x1, x2], rtol = 1e-9)
for i in 1:10000
r1, r2, scale = rand(3) .- 0.5
a, b, c = scale .* (1, r1 + r2, r1 * r2)
x1, x2 = quadraticroots(a, b, c)
@test similarroots(-r1, -r2, x1, x2)
end
a, b, c = 1, 0, -1
x1, x2 = quadraticroots(a, b, c)
@test similarroots(1, -1, x1, x2)
a, b, c = 1, 2, 1 # (x+1)(x+1)= x^2 + 2x + 1, double root at one
x1, x2 = quadraticroots(a, b, c)
@test similarroots(-1, -1, x1, x2)
end # testset QuadraticRoots
@testset "Transform" begin
Random.seed!(SEED)
@test isapprox(rotmatd(180, 0, 0), [1.0 0.0 0.0; 0.0 -1.0 0.0; 0.0 0.0 -1.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(rotmatd(0.0, 180.0, 0.0), [-1.0 0.0 0.0; 0.0 1.0 0.0; 0.0 0.0 -1.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(rotmatd(0, 0, 180), [-1.0 0.0 0.0; 0.0 -1.0 0.0; 0.0 0.0 1.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(rotmatd(0, 90, 0), [0.0 0.0 1.0; 0.0 1.0 0.0; -1.0 0.0 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(rotmatd(45, -45, 45), [0.5 -0.8535533905932737 0.1464466094067261; 0.5 0.14644660940672644 -0.8535533905932737; 0.7071067811865475 0.5 0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
x, y, z = rand(3)
@test isapprox(rotmatd(x * 180 / π, y * 180 / π, z * 180 / π), rotmat(x, y, z), rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(Transform(rotmatd(0, 90, 0), SVector(0.0, 0.0, 1.0)) * SVector(1.0, 0.0, 0.0), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
ta = Transform(rotmatd(0, 90, 0), SVector(0.0, 0.0, 1.0))
tb = Transform(rotmatd(90, 0, 0), SVector(1.0, 0.0, 0.0))
@test isapprox(collect(ta * tb), collect(Transform(rotmatd(90, 90, 0), SVector(0.0, 0.0, 0.0))), rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(collect(ta * inv(ta)), collect(identitytransform()), rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(collect(tb * inv(tb)), collect(identitytransform()), rtol = RTOLERANCE, atol = ATOLERANCE)
@test isapprox(collect(inv(ta)), collect(Transform(rotmatd(0, -90, 0), SVector(1.0, 0.0, 0.0))), rtol = RTOLERANCE, atol = ATOLERANCE)
#verify that matrix and vector{vector} forms of point representations are transformed the same way
pts = SVector(SVector(1.0,1.0,1.0),SVector(2.0,2.0,2.0),SVector(3.0,3.0,3.0))
ptsmat = SMatrix{3,3}(1.0,1.0,1.0,2.0,2.0,2.0,3.0,3.0,3.0)
rottrans = rotationd(20,30,40)
for i in 1:3
@test isapprox((rottrans*pts[i]),(rottrans*ptsmat)[:,i])
end
end # testset Transform
@testset "Interval" begin
pt1 = Intersection(0.3, [4.0, 5.0, 6.0], normalize(rand(3)), 0.4, 0.5, NullInterface())
pt2 = Intersection(0.5, [1.0, 2.0, 3.0], normalize(rand(3)), 0.2, 0.3, NullInterface())
@test pt1 < pt2 && pt1 <= pt2 && pt2 > pt1 && pt2 >= pt1 && pt1 != pt2 && pt1 <= pt1
intvl2 = positivehalfspace(pt1)
@test α(halfspaceintersection(intvl2)) == α(pt1)
### interval intersection
intersectionat = TestData.intersectionat
## interval/interval
# one in another
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.0), intersectionat(0.3))
@test intervalintersection(a, b) == intervalintersection(b, a) == a
# overlap
a = Interval(intersectionat(0.1), intersectionat(0.3))
b = Interval(intersectionat(0.2), intersectionat(0.4))
@test intervalintersection(a, b) == intervalintersection(b, a) == Interval(intersectionat(0.2), intersectionat(0.3))
# no overlap
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.3), intersectionat(0.4))
@test intervalintersection(a, b) == intervalintersection(b, a) isa EmptyInterval
# start == end
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.2), intersectionat(0.3))
@test intervalintersection(a, b) == intervalintersection(b, a) isa EmptyInterval
## interval/du
# encompass one
a = Interval(intersectionat(0.0), intersectionat(0.3))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalintersection(a, b) == intervalintersection(b, a) == b[1]
# encompass two
a = Interval(intersectionat(0.0), intersectionat(0.8))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalintersection(a, b) == intervalintersection(b, a) == b
# overlap one
a = Interval(intersectionat(0.0), intersectionat(0.2))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalintersection(a, b) == intervalintersection(b, a) == Interval(intersectionat(0.1), intersectionat(0.2))
# overlap two
a = Interval(intersectionat(0.2), intersectionat(0.5))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.6)))
res = intervalintersection(a, b)
@test res == intervalintersection(b, a) && res[1] == Interval(intersectionat(0.2), intersectionat(0.3)) && res[2] == Interval(intersectionat(0.4), intersectionat(0.5))
# no overlap
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = DisjointUnion(Interval(intersectionat(0.3), intersectionat(0.4)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalintersection(a, b) == intervalintersection(b, a) isa EmptyInterval
## du/du
# no overlap
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.1)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.2), intersectionat(0.3)), Interval(intersectionat(0.6), intersectionat(0.7)))
@test intervalintersection(a, b) == intervalintersection(b, a) isa EmptyInterval
# one in a overlaps one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.2)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.6), intersectionat(0.7)))
@test intervalintersection(a, b) == intervalintersection(b, a) == Interval(intersectionat(0.1), intersectionat(0.2))
# one in a encompasses one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.6), intersectionat(0.7)))
@test intervalintersection(a, b) == intervalintersection(b, a) == b[1]
# one in a overlaps two in b
a = DisjointUnion(Interval(intersectionat(0.2), intersectionat(0.5)), Interval(intersectionat(0.7), intersectionat(0.8)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.6)))
res = intervalintersection(a, b)
@test res == intervalintersection(b, a) && res[1] == Interval(intersectionat(0.2), intersectionat(0.3)) && res[2] == Interval(intersectionat(0.4), intersectionat(0.5))
# one in a encompasses two in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.5)), Interval(intersectionat(0.7), intersectionat(0.8)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.3), intersectionat(0.4)))
@test intervalintersection(a, b) == intervalintersection(b, a) == b
# each in a overlap one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.2)), Interval(intersectionat(0.4), intersectionat(0.6)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.5), intersectionat(0.7)))
res = intervalintersection(a, b)
@test res == intervalintersection(b, a) && res[1] == Interval(intersectionat(0.1), intersectionat(0.2)) && res[2] == Interval(intersectionat(0.5), intersectionat(0.6))
# each in a encompass one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.7)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalintersection(a, b) == intervalintersection(b, a) == b
## empties
intvl = positivehalfspace(pt1)
du = intervalcomplement(Interval(pt1, pt2))
@test intervalintersection(EmptyInterval(), EmptyInterval()) isa EmptyInterval
@test intervalintersection(EmptyInterval(), intvl) isa EmptyInterval
@test intervalintersection(intvl, EmptyInterval()) isa EmptyInterval
@test intervalintersection(EmptyInterval(), du) isa EmptyInterval
@test intervalintersection(du, EmptyInterval()) isa EmptyInterval
### interval union
## interval/interval
# one in another
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.0), intersectionat(0.3))
@test intervalunion(a, b) == intervalunion(b, a) == b
# overlap
a = Interval(intersectionat(0.1), intersectionat(0.3))
b = Interval(intersectionat(0.2), intersectionat(0.4))
@test intervalunion(a, b) == intervalunion(b, a) == Interval(intersectionat(0.1), intersectionat(0.4))
# no overlap
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.3), intersectionat(0.4))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == a && res[2] == b
# start == end
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = Interval(intersectionat(0.2), intersectionat(0.3))
@test intervalunion(a, b) == intervalunion(b, a) == Interval(intersectionat(0.1), intersectionat(0.3))
## interval/du
# encompass one
a = Interval(intersectionat(0.0), intersectionat(0.3))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == a && res[2] == b[2]
# encompass two
a = Interval(intersectionat(0.0), intersectionat(0.8))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalunion(a, b) == intervalunion(b, a) == a
# overlap one
a = Interval(intersectionat(0.0), intersectionat(0.2))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.5), intersectionat(0.6)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == Interval(intersectionat(0.0), intersectionat(0.3)) && res[2] == b[2]
# overlap two
a = Interval(intersectionat(0.2), intersectionat(0.5))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.6)))
@test intervalunion(a, b) == intervalunion(b, a) == Interval(intersectionat(0.1), intersectionat(0.6))
# no overlap
a = Interval(intersectionat(0.1), intersectionat(0.2))
b = DisjointUnion(Interval(intersectionat(0.3), intersectionat(0.4)), Interval(intersectionat(0.5), intersectionat(0.6)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == a && res[2] == b[1] && res[3] == b[2]
## du/du
# no overlap
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.1)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.2), intersectionat(0.3)), Interval(intersectionat(0.6), intersectionat(0.7)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == a[1] && res[2] == b[1] && res[3] == a[2] && res[4] == b[2]
# one in a overlaps one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.2)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.6), intersectionat(0.7)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == Interval(intersectionat(0.0), intersectionat(0.3)) && res[2] == a[2] && res[3] == b[2]
# one in a encompasses one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.5)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.6), intersectionat(0.7)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == a[1] && res[2] == a[2] && res[3] == b[2]
# one in a overlaps two in b
a = DisjointUnion(Interval(intersectionat(0.2), intersectionat(0.5)), Interval(intersectionat(0.7), intersectionat(0.8)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.6)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == Interval(intersectionat(0.1), intersectionat(0.6)) && res[2] == a[2]
# one in a encompasses two in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.5)), Interval(intersectionat(0.7), intersectionat(0.8)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.3), intersectionat(0.4)))
@test intervalunion(a, b) == intervalunion(b, a) == a
# each in a overlap one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.2)), Interval(intersectionat(0.4), intersectionat(0.6)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.5), intersectionat(0.7)))
res = intervalunion(a, b)
@test res == intervalunion(b, a) && res[1] == Interval(intersectionat(0.0), intersectionat(0.3)) && res[2] == Interval(intersectionat(0.4), intersectionat(0.7))
# each in a encompass one in b
a = DisjointUnion(Interval(intersectionat(0.0), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.7)))
b = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.2)), Interval(intersectionat(0.5), intersectionat(0.6)))
@test intervalunion(a, b) == intervalunion(b, a) == a
## empties
@test intervalunion(EmptyInterval(), EmptyInterval()) isa EmptyInterval
@test intervalunion(EmptyInterval(), intvl) == intvl
@test intervalunion(intvl, EmptyInterval()) == intvl
@test intervalunion(EmptyInterval(), du) == du
@test intervalunion(du, EmptyInterval()) == du
# interval complement
int = intervalcomplement(EmptyInterval())
@test lower(int) isa RayOrigin && upper(int) isa Infinity
int = intervalcomplement(rayorigininterval(Infinity()))
@test int isa EmptyInterval
int = intervalcomplement(rayorigininterval(pt1))
@test lower(int) == pt1 && upper(int) isa Infinity
int = intervalcomplement(positivehalfspace(pt1))
@test lower(int) isa RayOrigin && upper(int) == pt1
int = intervalcomplement(Interval(pt1, pt2))
@test lower(int[1]) isa RayOrigin && upper(int[1]) == pt1 && lower(int[2]) == pt2 && upper(int[2]) isa Infinity
a = DisjointUnion(Interval(intersectionat(0.1), intersectionat(0.3)), Interval(intersectionat(0.4), intersectionat(0.7)))
res = intervalcomplement(a)
@test res[1] == rayorigininterval(intersectionat(0.1)) && res[2] == Interval(intersectionat(0.3), intersectionat(0.4)) && res[3] == positivehalfspace(intersectionat(0.7))
a = DisjointUnion(rayorigininterval(intersectionat(0.2)), Interval(intersectionat(0.4), intersectionat(0.7)))
res = intervalcomplement(a)
@test res[1] == Interval(intersectionat(0.2), intersectionat(0.4)) && res[2] == positivehalfspace(intersectionat(0.7))
end # testset interval
end # testset General
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 16813 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using Test
using OpticSim.GlassCat
using Base.Filesystem
using DataFrames: DataFrame
using StaticArrays
using Unitful
using Unitful.DefaultSymbols
@testset "GlassCat" begin
@testset "Build Tests" begin
# check that all automatic downloads are working
# this shouldn't be a test because we cannot guarantee that all downloads will work. Random network issues, changes in webpages, etc. can temporarily prevent downloads.
# for catname in split("HOYA NIKON OHARA SCHOTT SUMITA")
# agffile = joinpath(GlassCat.AGF_DIR, catname * ".agf")
# @test isfile(agffile)
# end
# check that particularly problematic glasses are parsing correctly
@test !isnan(NIKON.LLF6.C10)
end
@testset "sources.jl" begin
@testset "add_agf" begin
tmpdir = mktempdir()
agfdir = mktempdir(tmpdir)
sourcefile, _ = mktemp(tmpdir)
@testset "bad implicit catalog names" begin
for agffile in split("a 1.agf a.Agf")
@test_logs (:error, "invalid implicit catalog name \"$agffile\". Should be purely alphabetical with a .agf/.AGF extension.") add_agf(agffile; agfdir, sourcefile)
end
end
@testset "file not found" begin
@test_logs(
(:info, "Downloading source file from nonexistentfile.agf"),
(:error, ArgumentError("missing or unsupported scheme in URL (expected http(s) or ws(s)): nonexistentfile.agf")),
(:error, "failed to download from nonexistentfile.agf"),
add_agf("nonexistentfile.agf"; agfdir, sourcefile)
)
end
@testset "add to source file" begin
for name in split("a b")
open(joinpath(tmpdir, "$name.agf"), "w") do io
write(io, "")
end
end
empty_sha = "e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855"
@test isempty(readlines(sourcefile))
add_agf(joinpath(tmpdir, "a.agf"); agfdir, sourcefile, rebuild=false)
@test length(readlines(sourcefile)) === 1
@test readlines(sourcefile)[1] === "A $empty_sha"
add_agf(joinpath(tmpdir, "b.agf"); agfdir, sourcefile, rebuild=false)
@test length(readlines(sourcefile)) === 2
@test readlines(sourcefile)[1] === "A $empty_sha"
@test readlines(sourcefile)[2] === "B $empty_sha"
@test_logs(
(:error, "adding the catalog name \"A\" would create a duplicate entry in source file $sourcefile"),
add_agf(joinpath(tmpdir, "a.agf"); agfdir, sourcefile)
)
end
# TODO rebuild=true
end
# integration test
@testset "verify_sources!" begin
tmpdir = mktempdir()
agfdir = mktempdir(tmpdir)
sources = split.(readlines(GlassCat.SOURCES_PATH))
GlassCat.verify_sources!(sources, agfdir)
# this doesn't work if any of the glass catalogs can't be downloaded. Need a different test
# @test first.(sources) == first.(split.(readlines(GlassCat.SOURCES_PATH)))
# TODO missing_sources
end
# TODO unit tests
end
@testset "generate.jl" begin
CATALOG_NAME = "TEST_CAT"
SOURCE_DIR = joinpath(@__DIR__, "..", "..", "test")
SOURCE_FILE = joinpath(SOURCE_DIR, "$(CATALOG_NAME).agf")
TMP_DIR = mktempdir()
MAIN_FILE = joinpath(TMP_DIR, "AGF_TEST_CAT.jl")
TEST_CAT_VALUES = DataFrame(
name = split("MG463_ MG523 _5MG448 MG452_STAR"),
raw_name = split("MG463& MG523 5MG448 MG452*"),
dispform = repeat([2], 4),
Nd = repeat([1.0], 4),
Vd = repeat([0.0], 4),
exclude_sub = [5, 0, 0, 0],
status = [6, 0, 0, 0],
meltfreq = repeat([-1], 4),
TCE = [19.8, 18.3, 30.2, 25.7],
p = [4.63, 5.23, 4.48, 4.52],
ΔPgF = repeat([0.0], 4),
ignore_thermal_exp = [3, 0, 0, 0],
C1 = [6.781409960E+00, 4.401393677E+00, 8.427948454E+00, 4.656568861E+00],
C2 = [9.868538020E-02, 2.234722960E-02, 6.325268390E-02, 1.170534894E-01],
C3 = [3.469529960E-03, 4.562935070E+00, -2.441576539E+00, 1.409322578E+00],
C4 = [2.277823700E+00, 3.239318260E-01, 1.997453950E-03, 2.445302672E-04],
C5 = [2.126055280E+00, 6.863473749E-01, 4.975938104E-01, 6.352738622E-01],
C6 = [3.227490100E+03, 1.625888344E+03, 1.443307391E+03, 1.397305161E+03],
C7 = repeat([0.0], 4),
C8 = repeat([0.0], 4),
C9 = repeat([0.0], 4),
C10 = repeat([0.0], 4),
D₀ = [2.226000000E-05, 1.122401430E-04, -1.718689957E-05, -2.545621409E-07],
D₁ = [5.150100000E-08, 2.868995096E-13, -6.499841076E-13, 1.258087492E-10],
D₂ = [1.309700000E-10, -4.157332734E-16, -4.153311088E-16, 1.119703940E-11],
E₀ = [4.382400000E-05, 9.532969159E-05, 8.220254442E-06, 2.184273853E-05],
E₁ = [4.966000000E-07, -1.045247704E-11, 6.086168578E-12, -2.788674395E-09],
λₜₖ = [3.728000000E-01, 6.338475915E-01, 3.610058073E-01, -1.948154058E+00],
temp = [2.002000000E+01, 2.000000000E+01, 2.000000000E+01, 2.000000000E+01],
relcost = [1.1, -1.0, -1.0, -1.0],
CR = [1.2, -1.0, -1.0, -1.0],
FR = [1.3, -1.0, -1.0, -1.0],
SR = [1.4, -1.0, -1.0, -1.0],
AR = [1.5, -1.0, -1.0, -1.0],
PR = [1.6, -1.0, -1.0, -1.0],
λmin = repeat([2.0], 4),
λmax = repeat([14.0], 4),
transmission = [
[[2.0, 1.0, 3.0], [14.0, 4.0, 5.0]],
[[2.0, 1.0, 1.0], [14.0, 1.0, 1.0]],
[[2.0, 1.0, 1.0], [14.0, 1.0, 1.0]],
[[2.0, 1.0, 1.0], [14.0, 1.0, 1.0]],
],
)
FIELDS = names(TEST_CAT_VALUES)[2:end]
@testset "Parsing Tests" begin
cat = GlassCat.agffile_to_catalog(SOURCE_FILE)
for glass in eachrow(TEST_CAT_VALUES)
name = glass["name"]
@test name ∈ keys(cat)
for field in FIELDS
@test glass[field] == cat[name][field]
end
end
end
@testset "Module Gen Tests" begin
OpticSim.GlassCat.generate_jls([CATALOG_NAME], MAIN_FILE, TMP_DIR, SOURCE_DIR, test=true)
include(MAIN_FILE)
for row in eachrow(TEST_CAT_VALUES)
name = row["name"]
@test "$CATALOG_NAME.$name" ∈ TEST_GLASS_NAMES
glass = getfield(getfield(Main, Symbol(CATALOG_NAME)), Symbol(name))
@test glass ∈ TEST_GLASSES
for field in FIELDS
if field === "raw_name"
elseif field === "transmission"
@test row[field] == getfield(glass, Symbol(field))[1:length(row[field])]
@test zero(SVector{100-length(row[field]),SVector{3,Float64}}) == getfield(glass, Symbol(field))[length(row[field])+1:end]
else
@test row[field] == getfield(glass, Symbol(field))
end
end
end
# these used to be in the "Glass Tests" testset, but they rely on the generated AGF_TEST_CAT.jl file
g = TEST_CAT.MG523
@test index(g, ((g.λmin + g.λmax) / 2)μm) ≈ 3.1560980389455593 atol=1e-14
@test_throws ErrorException index(g, (g.λmin - 1)μm)
@test_throws ErrorException index(g, (g.λmax + 1)μm)
end
end
@testset "Glass Tests" begin
@test absairindex(500nm) ≈ 1.0002741948670688 atol=1e-14
@test absairindex(600nm, temperature=35°C, pressure=2.0) ≈ 1.0005179096900811 atol=1e-14
@test index(Air, 500nm) == 1.0
@test index(Air, 600nm) == 1.0
# test against true values
g = OpticSim.Examples.Examples_N_BK7
@test index(g, 533nm) ≈ 1.519417351519283 atol=1e-14
@test index(g, 533nm, temperature=35°C) ≈ 1.519462486258311 atol=1e-14
@test index(g, 533nm, pressure=2.0) ≈ 1.518994119690216 atol=1e-14
@test index(g, 533nm, temperature=35°C, pressure=2.0) ≈ 1.519059871499476 atol=1e-14
# test transmission
@test absorption(Air, 500nm) == 0.0
@test absorption(Air, 600nm) == 0.0
# TODO these are currently taken from this package (i.e. regression tests), ideally we would get true values somehow
@test absorption(g, 500nm) == 0.0002407228930225164
@test absorption(g, 600nm) == 0.00022060722752440445
@test absorption(g, 3000nm) == 0.04086604990127926
@test absorption(g, 600nm, temperature=35°C, pressure=2.0) == 0.00022075540719494738
# test that everything is alloc-less
@test (@wrappedallocs absorption(g, 600nm)) == 0
@test (@wrappedallocs index(g, 533nm)) == 0
@test (@allocated OpticSim.Examples.Examples_N_BK7) == 0
@test glassforid(glassid(OpticSim.Examples.Examples_N_BK7)) == OpticSim.Examples.Examples_N_BK7
@test isair(Air) == true
@test isair(OpticSim.Examples.Examples_N_BK7) == false
# test MIL and model glasses
fit_acc = 0.001
bk7 = glassfromMIL(517642)
@test glassforid(glassid(bk7)) == bk7
@test index(bk7, 0.5875618) ≈ 1.517 atol=fit_acc
@test index(bk7, 0.533) ≈ 1.519417351519283 atol=fit_acc
@test index(bk7, 0.743) ≈ 1.511997032563557 atol=fit_acc
@test index(bk7, 533nm, temperature=35°C, pressure=2.0) ≈ 1.519059871499476 atol=fit_acc
t2 = glassfromMIL(1.135635)
@test index(t2, 0.5875618) ≈ 2.135 atol=fit_acc
fit_acc = 0.0001
bk7 = modelglass(1.5168, 64.167336, -0.0009)
@test glassforid(glassid(bk7)) == bk7
@test index(bk7, 0.5875618) ≈ 1.5168 atol=fit_acc
@test index(bk7, 0.533) ≈ 1.519417351519283 atol=fit_acc
@test index(bk7, 0.743) ≈ 1.511997032563557 atol=fit_acc
@test index(bk7, 533nm, temperature=35°C, pressure=2.0) ≈ 1.519059871499476 atol=fit_acc
# test other glass
@test index(CARGILLE.OG0608, 0.578) == 1.4596475735607324
# make sure that the other functions work
plot_indices(OpticSim.Examples.Examples_N_BK7; polyfit = true, fiterror = true)
end
@testset "Air.jl" begin
@test repr(Air) === "Air"
@test glassname(Air) === "GlassCat.Air"
info_lines = [
"GlassCat.Air",
"Material representing air, RI is always 1.0 at system temperature and pressure, absorption is always 0.0.",
""
]
io = IOBuffer()
info(io, Air)
@test String(take!(io)) === join(info_lines, '\n')
end
@testset "GlassTypes.jl" begin
@test repr(GlassID(GlassCat.MODEL, 1)) === "MODEL:1"
@test repr(GlassID(GlassCat.MIL, 1)) === "MIL:1"
@test repr(GlassID(GlassCat.AGF, 1)) === "AGF:1"
@test repr(GlassID(GlassCat.OTHER, 1)) === "OTHER:1"
@test repr(GlassID(GlassCat.AIR, 1)) === "AIR:1" # TODO should this fail?
empty_args = SVector{36,Union{Int,Nothing}}(repeat([0], 27)..., nothing, repeat([0], 8)...)
@test repr(GlassCat.Glass(GlassID(GlassCat.MODEL, 1), empty_args...)) === "GlassCat.ModelGlass.1"
@test repr(GlassCat.Glass(GlassID(GlassCat.MIL, 1), empty_args...)) === "GlassCat.GlassFromMIL.1"
@test repr(GlassCat.Glass(GlassID(GlassCat.AGF, 1), empty_args...)) === "NIKON.SF9" # fails if sources.txt is changed
@test repr(GlassCat.Glass(GlassID(GlassCat.OTHER, 1), empty_args...)) === "CARGILLE.OG0607"
@test repr(GlassCat.Glass(GlassID(GlassCat.AIR, 1), empty_args...)) === "GlassCat.Air" # repeated code
@test glassforid(GlassID(GlassCat.AIR, 1)) === Air
@test glassforid(GlassID(GlassCat.OTHER, 1)) === CARGILLE.OG0607
info_lines = [
"ID: OTHER:2",
"Dispersion formula: Cauchy (-2)",
"Dispersion formula coefficients:",
" A: 1.44503",
" B: 0.0044096",
" C: -2.85878e-5",
" D: 0.0",
" E: 0.0",
" F: 0.0",
"Valid wavelengths: 0.32μm to 1.55μm",
"Reference temperature: 25.0°C",
"Thermal ΔRI coefficients:",
" D₀: -0.0009083144750540808",
" D₁: 0.0",
" D₂: 0.0",
" E₀: 0.0",
" E₁: 0.0",
" λₜₖ: 0.0",
"TCE (÷1e-6): 700.0",
"Ignore thermal expansion: false",
"Density (p): 0.878g/m³",
"ΔPgF: 0.008",
"RI at sodium D-Line (587nm): 1.457587",
"Abbe Number: 57.19833",
"Cost relative to N_BK7: ?",
"Status: Standard (0)",
"Melt frequency: ?",
"Exclude substitution: false",
"Transmission data:",
" Wavelength Transmission Thickness",
" 0.32μm 0.15 10.0mm",
" 0.365μm 0.12 100.0mm",
" 0.4047μm 0.42 100.0mm",
" 0.48μm 0.78 100.0mm",
" 0.4861μm 0.79 100.0mm",
" 0.5461μm 0.86 100.0mm",
" 0.5893μm 0.9 100.0mm",
" 0.6328μm 0.92 100.0mm",
" 0.6439μm 0.9 100.0mm",
" 0.6563μm 0.92 100.0mm",
" 0.6943μm 0.98 100.0mm",
" 0.84μm 0.99 100.0mm",
" 0.10648μm 0.61 100.0mm",
" 0.13μm 0.39 100.0mm",
" 0.155μm 0.11 100.0mm",
""
]
io = IOBuffer()
info(io, CARGILLE.OG0607)
@test String(take!(io)) === join(info_lines, '\n')
# TODO the rest of the string tests
end
@testset "search.jl" begin
#this test set doesn't work as is. It assumes that all the glass catalogs have downloaded correctly which may not happen. Commenting them all out till we figure out a better set of tests.
# @test glasscatalogs() == [
# CARGILLE,
# HOYA,
# NIKON,
# OHARA,
# SCHOTT,
# SUMITA,
# ]
# @test glassnames(CARGILLE) == [
# :OG0607,
# :OG0608,
# :OG081160,
# ]
# @test first.(glassnames()) == [
# CARGILLE,
# HOYA,
# NIKON,
# OHARA,
# SCHOTT,
# SUMITA,
# ]
# @test length.(last.(glassnames())) == [
# 3,
# 210,
# 379,
# 160,
# 160,
# 178,
# ]
# @test findglass(x -> (x.Nd > 2.1 && x.λmin < 0.5 && x.λmax > 0.9)) == [
# HOYA.E_FDS3,
# SUMITA.K_PSFn214P,
# SUMITA.K_PSFn214P_M_,
# ]
# TODO _child_modules() unit test
end
end # testset GlassCat
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 67127 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Intersection" begin
function samplepoints(numsamples, lowu, highu, lowv, highv)
samples = Array{Tuple{Float64,Float64},1}(undef, 0)
for i in 0:numsamples
u = lowu * i / numsamples + (1 - i / numsamples) * highu
for j in 0:numsamples
v = lowv * j / numsamples + (1 - j / numsamples) * highv
push!(samples, (u, v))
end
end
return samples
end
@testset "Cylinder" begin
# Random.seed!(SEED)
# # random point intersections
# ur, vr = uvrange(Cylinder)
# for i in 1:10000
# cyl = Cylinder(rand() * 3.0, rand() * 50.0)
# u, v = rand() * (ur[2] - ur[1]) + ur[1], rand() * (vr[2] - vr[1]) + vr[1]
# pointon = point(cyl, u, v)
# origin = SVector(4.0, 4.0, 4.0)
# r = Ray(origin, pointon .- origin)
# halfspace = surfaceintersection(cyl, r)
# @test halfspace !== nothing && !((lower(halfspace) isa RayOrigin) || (upper(halfspace) isa Infinity))
# @test isapprox(pointon, point(lower(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE) || isapprox(pointon, point(upper(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE)
# end
# on xy
cyl = Cylinder(0.5)
r = Ray([1.0, 1.0, 0.0], [-1.0, -1.0, 0.0])
intscts = surfaceintersection(cyl, r)
xy = sqrt(2) / 4.0
pt1 = [xy, xy, 0.0]
pt2 = [-xy, -xy, 0.0]
cpt1 = point(lower(intscts))
cpt2 = point(upper(intscts))
@test isapprox(pt1, cpt1, rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(pt2, cpt2, rtol = RTOLERANCE, atol = ATOLERANCE)
# starting inside
r = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
intscts = surfaceintersection(cyl, r)
@test lower(intscts) isa RayOrigin && isapprox(pt1, point(upper(intscts)), rtol = RTOLERANCE, atol = ATOLERANCE)
# inside infinite
r = Ray([0.0, 0.0, 0.0], [0.0, 0.0, 1.0])
intscts = surfaceintersection(cyl, r)
@test lower(intscts) isa RayOrigin && upper(intscts) isa Infinity
# on surface
r = Ray([0.5, 0.0, 0.0], [0.0, 0.0, 1.0])
intscts = surfaceintersection(cyl, r)
@test lower(intscts) isa RayOrigin && upper(intscts) isa Infinity
# on diagonal
r = Ray([1.0, 1.0, 1.0], [-1.0, -1.0, -1.0])
intscts = surfaceintersection(cyl, r)
cpt1 = point(lower(intscts))
cpt2 = point(upper(intscts))
pt1 = [xy, xy, xy]
pt2 = [-xy, -xy, -xy]
@test isapprox(pt1, cpt1, rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(pt2, cpt2, rtol = RTOLERANCE, atol = ATOLERANCE)
# missing
r = Ray([5.0, 5.0, 5.0], [0.0, 0.0, -1.0])
intscts = surfaceintersection(cyl, r)
@test intscts isa EmptyInterval
end # testset cylinder
@testset "Sphere" begin
# Random.seed!(SEED)
# # random point intersections
# ur, vr = uvrange(Sphere)
# for i in 1:10000
# sph = Sphere(rand() * 3)
# u, v = rand() * (ur[2] - ur[1]) + ur[1], rand() * (vr[2] - vr[1]) + vr[1]
# pointon = point(sph, u, v)
# origin = SVector(4.0, 4.0, 4.0)
# r = Ray(origin, pointon .- origin)
# halfspace = surfaceintersection(sph, r)
# @test halfspace !== nothing && !((lower(halfspace) isa RayOrigin) || (upper(halfspace) isa Infinity))
# @test isapprox(pointon, point(lower(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE) || isapprox(pointon, point(upper(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE)
# end
# on xy plane
sph = Sphere(0.5)
r = Ray([1.0, 1.0, 0.0], [-1.0, -1.0, 0.0])
intscts = surfaceintersection(sph, r)
xy = sqrt(2) / 4.0
pt1 = [xy, xy, 0.0]
pt2 = [-xy, -xy, 0.0]
cpt1 = point(lower(intscts))
cpt2 = point(upper(intscts))
@test isapprox(pt1, cpt1, rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(pt2, cpt2, rtol = RTOLERANCE, atol = ATOLERANCE)
# starting inside
r = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
intscts = surfaceintersection(sph, r)
@test lower(intscts) isa RayOrigin && isapprox(pt1, point(upper(intscts)), rtol = RTOLERANCE, atol = ATOLERANCE)
# on diagonal
r = Ray([1.0, 1.0, 1.0], [-1.0, -1.0, -1.0])
intscts = surfaceintersection(sph, r)
cpt1 = point(lower(intscts))
cpt2 = point(upper(intscts))
xyz = sqrt(3) / 6.0
pt1 = [xyz, xyz, xyz]
pt2 = [-xyz, -xyz, -xyz]
@test isapprox(pt1, cpt1, rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(pt2, cpt2, rtol = RTOLERANCE, atol = ATOLERANCE)
# missing
r = Ray([5.0, 5.0, 5.0], [0.0, 0.0, -1.0])
intscts = surfaceintersection(sph, r)
@test intscts isa EmptyInterval
# tangent
r = Ray([0.5, 0.5, 0.0], [0.0, -1.0, 0.0])
intscts = surfaceintersection(sph, r)
@test intscts isa EmptyInterval
end # testset sphere
@testset "Spherical Cap" begin
@test_throws AssertionError SphericalCap(0.0, 1.0)
@test_throws AssertionError SphericalCap(1.0, 0.0)
sph = SphericalCap(0.5, π / 3, SVector(1.0, 1.0, 0.0), SVector(1.0, 1.0, 1.0))
r = Ray([10.0, 10.0, 1.0], [-1.0, -1.0, 0.0])
int = surfaceintersection(sph, r)
@test isapprox(point(lower(int)), [1.0, 1.0, 1.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(int) isa Infinity
r = Ray([0.8, 1.4, 1.2], [1.0, -1.0, 0.0])
int = surfaceintersection(sph, r)
@test isapprox(point(lower(int)), [0.898231126982741, 1.301768873017259, 1.2], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [1.301768873017259, 0.8982311269827411, 1.2], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([2.0, 2.0, 1.5], [-1.0, -1.0, 0.0])
@test surfaceintersection(sph, r) isa EmptyInterval
end # testset spherical cap
@testset "Triangle" begin
Random.seed!(SEED)
tri = Triangle(SVector{3}(2.0, 1.0, 1.0), SVector{3}(1.0, 2.0, 1.0), SVector{3}(1.0, 1.0, 2.0), SVector{2}(0.0, 0.0), SVector{2}(1.0, 0.0), SVector{2}(0.5, 0.5))
# random point intersections
for i in 1:1000
barycentriccoords = rand(3)
barycentriccoords /= sum(barycentriccoords)
pointontri = point(tri, barycentriccoords...)
origin = SVector(3.0, 3.0, 3.0)
r = Ray(origin, pointontri .- origin)
halfspace = surfaceintersection(tri, r)
@test !(halfspace isa EmptyInterval) && upper(halfspace) isa Infinity
t = α(lower(halfspace))
@test isapprox(point(r, t), point(lower(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(pointontri, point(lower(halfspace)), rtol = RTOLERANCE, atol = ATOLERANCE)
end
# front and back face intersections
tri = Triangle(SVector{3}(0.0, 0.0, 0.0), SVector{3}(1.0, 0.0, 0.0), SVector{3}(0.0, 1.0, 0.0), SVector{2}(0.0, 0.0), SVector{2}(1.0, 0.0), SVector{2}(0.0, 1.0))
r1 = Ray([0.1, 0.1, 1.0], [0.0, 0.0, -1.0])
r2 = Ray([0.1, 0.1, -1.0], [0.0, 0.0, 1.0])
intsct1 = halfspaceintersection(surfaceintersection(tri, r1))
intsct2 = halfspaceintersection(surfaceintersection(tri, r2))
@test isapprox(point(intsct1), point(intsct2), rtol = RTOLERANCE, atol = ATOLERANCE)
# ray should miss
r = Ray([1.0, 1.0, 1.0], [0.0, 0.0, -1.0])
intsct = surfaceintersection(tri, r)
@test intsct isa EmptyInterval
end # testset triangle
@testset "Plane" begin
rinplane = Ray([0.0, 0.0, 1.0], [1.0, 1.0, 0.0])
routside = Ray([0.0, 0.0, 2.0], [1.0, 1.0, 1.0])
rinside = Ray([0.0, 0.0, -1.0], [1.0, 1.0, -1.0])
routintersects = Ray([0.0, 0.0, 2.0], [0.0, 0.0, -1.0])
rinintersects = Ray([0.0, 0.0, -1.0], [0.0, 0.0, 1.0])
pln = Plane(0.0, 0.0, 1.0, 0.0, 0.0, 1.0)
# parallel to plane and outside
@test surfaceintersection(pln, routside) isa EmptyInterval
# NOTE for coplanar faces to work with visualization, rays in the plane must count as being 'inside'
# parallel and on plane
res = surfaceintersection(pln, rinplane)
@test lower(res) isa RayOrigin && upper(res) isa Infinity
# inside but not hitting
res = surfaceintersection(pln, rinside)
@test lower(res) isa RayOrigin && upper(res) isa Infinity
# starts outside and hits
res = surfaceintersection(pln, routintersects)
@test isapprox(point(lower(res)), [0.0, 0.0, 1.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits
res = surfaceintersection(pln, rinintersects)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.0, 0.0, 1.0], rtol = RTOLERANCE, atol = ATOLERANCE)
end # testset plane
@testset "Rectangle" begin
@test_throws AssertionError Rectangle(0.0, 1.0)
@test_throws AssertionError Rectangle(1.0, 0.0)
rinplane = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
routside = Ray([0.0, 0.0, 1.0], [1.0, 1.0, 1.0])
rinside = Ray([0.0, 0.0, -1.0], [1.0, 1.0, -1.0])
routintersects = Ray([0.0, 0.0, 1.0], [0.0, 0.0, -1.0])
rinintersects = Ray([0.0, 0.0, -1.0], [0.0, 0.0, 1.0])
routmiss = Ray([0.0, 2.0, 1.0], [0.0, 0.0, -1.0])
rinmiss = Ray([0.0, 2.0, -1.0], [0.0, 0.0, 1.0])
rinbounds = Ray([0.5, 0.5, -1.0], [0.0, 0.0, 1.0])
routbounds = Ray([0.5, 0.5, 1.0], [0.0, 0.0, -1.0])
rect = Rectangle(0.5, 0.5)
# parallel to plane and outside
@test surfaceintersection(rect, routside) isa EmptyInterval
# parallel and on plane
@test surfaceintersection(rect, rinplane) isa EmptyInterval
# inside but not hitting
@test surfaceintersection(rect, rinside) isa EmptyInterval
# starts outside and hits
res = surfaceintersection(rect, routintersects)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits
res = surfaceintersection(rect, rinintersects)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# starts inside and misses bounds
@test surfaceintersection(rect, rinmiss) isa EmptyInterval
# starts outside and misses bounds
@test surfaceintersection(rect, routmiss) isa EmptyInterval
# starts outside and hits bounds
res = surfaceintersection(rect, routbounds)
@test isapprox(point(lower(res)), [0.5, 0.5, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits bounds
res = surfaceintersection(rect, rinbounds)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.5, 0.5, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# test a rectangle with translation and rotation
rect2 = Rectangle(0.3, 0.5, SVector(3.0, 1.0, 4.0), SVector(0.2, 0.3, 0.4))
rayhit = Ray([0.6, 0.6, 0.6], [-0.3, -0.1, -0.3])
raymiss = Ray([0.7, 0.4, 0.4], [-0.3, -0.1, -0.3])
res = surfaceintersection(rect2, rayhit)
@test isapprox(point(lower(res)), [0.2863636363636363, 0.4954545454545454, 0.2863636363636363], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
@test surfaceintersection(rect2, raymiss) isa EmptyInterval
end # testset Rectangle
@testset "Ellipse" begin
@test_throws AssertionError Ellipse(0.0, 1.0)
@test_nowarn Circle(0.5)
rinplane = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
routside = Ray([0.0, 0.0, 1.0], [1.0, 1.0, 1.0])
rinside = Ray([0.0, 0.0, -1.0], [1.0, 1.0, -1.0])
routintersects = Ray([0.0, 0.0, 1.0], [0.0, 0.0, -1.0])
rinintersects = Ray([0.0, 0.0, -1.0], [0.0, 0.0, 1.0])
routmiss = Ray([0.0, 2.0, 1.0], [0.0, 0.0, -1.0])
rinmiss = Ray([0.0, 2.0, -1.0], [0.0, 0.0, 1.0])
rinbounds = Ray([0.5, 0.0, -1.0], [0.0, 0.0, 1.0])
routbounds = Ray([0.5, 0.0, 1.0], [0.0, 0.0, -1.0])
rasymhit = Ray([0.0, 0.9, 1.0], [0.0, 0.0, -1.0])
rasymmiss = Ray([0.9, 0.0, 1.0], [0.0, 0.0, -1.0])
ell = Ellipse(0.5, 1.0)
# parallel to plane and outside
@test surfaceintersection(ell, routside) isa EmptyInterval
# parallel and on plane
@test surfaceintersection(ell, rinplane) isa EmptyInterval
# inside but not hitting
@test surfaceintersection(ell, rinside) isa EmptyInterval
# starts outside and hits
res = surfaceintersection(ell, routintersects)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits
res = surfaceintersection(ell, rinintersects)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# starts inside and misses bounds
@test surfaceintersection(ell, rinmiss) isa EmptyInterval
# starts outside and misses bounds
@test surfaceintersection(ell, routmiss) isa EmptyInterval
# starts outside and hits bounds
res = surfaceintersection(ell, routbounds)
@test isapprox(point(lower(res)), [0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits bounds
res = surfaceintersection(ell, rinbounds)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# asymmetric hit
res = surfaceintersection(ell, rasymhit)
@test isapprox(point(lower(res)), [0.0, 0.9, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# asymmetric miss
@test surfaceintersection(ell, rasymmiss) isa EmptyInterval
# test an ellipse with translation and rotation
ell2 = Ellipse(0.3, 0.5, SVector(3.0, 1.0, 4.0), SVector(0.2, 0.3, 0.4))
rayhit = Ray([0.6, 0.6, 0.6], [-0.3, -0.1, -0.3])
raymiss = Ray([0.7, 0.4, 0.4], [-0.3, -0.1, -0.3])
res = surfaceintersection(ell2, rayhit)
@test isapprox(point(lower(res)), [0.2863636363636363, 0.4954545454545454, 0.2863636363636363], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
@test surfaceintersection(ell2, raymiss) isa EmptyInterval
end # testset Ellipse
@testset "Hexagon" begin
@test_nowarn Hexagon(0.5)
rinplane = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
routside = Ray([0.0, 0.0, 1.0], [1.0, 1.0, 1.0])
rinside = Ray([0.0, 0.0, -1.0], [1.0, 1.0, -1.0])
routintersects = Ray([0.0, 0.0, 1.0], [0.0, 0.0, -1.0])
rinintersects = Ray([0.0, 0.0, -1.0], [0.0, 0.0, 1.0])
routmiss = Ray([0.0, 2.0, 1.0], [0.0, 0.0, -1.0])
rinmiss = Ray([0.0, 2.0, -1.0], [0.0, 0.0, 1.0])
rinbounds = Ray([sqrt(3) / 2, 0.0, -1.0], [0.0, 0.0, 1.0])
routbounds = Ray([sqrt(3) / 2, 0.0, 1.0], [0.0, 0.0, -1.0])
rasymhit = Ray([0.0, 1.0, 1.0], [0.0, 0.0, -1.0])
rasymmiss = Ray([1.0, 0.0, 1.0], [0.0, 0.0, -1.0])
hex = Hexagon(1.0)
# parallel to plane and outside
@test surfaceintersection(hex, routside) isa EmptyInterval
# parallel and on plane
@test surfaceintersection(hex, rinplane) isa EmptyInterval
# inside but not hitting
@test surfaceintersection(hex, rinside) isa EmptyInterval
# starts outside and hits
res = surfaceintersection(hex, routintersects)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits
res = surfaceintersection(hex, rinintersects)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# starts inside and misses bounds
@test surfaceintersection(hex, rinmiss) isa EmptyInterval
# starts outside and misses bounds
@test surfaceintersection(hex, routmiss) isa EmptyInterval
# starts outside and hits bounds
res = surfaceintersection(hex, routbounds)
@test isapprox(point(lower(res)), [sqrt(3) / 2, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits bounds
res = surfaceintersection(hex, rinbounds)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [sqrt(3) / 2, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# asymmetric hit
res = surfaceintersection(hex, rasymhit)
@test isapprox(point(lower(res)), [0.0, 1.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# asymmetric miss
@test surfaceintersection(hex, rasymmiss) isa EmptyInterval
# test an ellipse with translation and rotation
hex2 = Hexagon(0.4, SVector(3.0, 1.0, 4.0), SVector(0.2, 0.3, 0.4))
rayhit = Ray([0.6, 0.6, 0.6], [-0.3, -0.1, -0.3])
raymiss = Ray([0.7, 0.4, 0.4], [-0.3, -0.1, -0.3])
res = surfaceintersection(hex2, rayhit)
@test isapprox(point(lower(res)), [0.2863636363636363, 0.4954545454545454, 0.2863636363636363], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
@test surfaceintersection(hex2, raymiss) isa EmptyInterval
end # testset Hexagon
@testset "Convex Polygon" begin
t = identitytransform()
local_points_2d = [
SVector(-1.0, -1.0),
SVector(1.0, -1.0),
SVector(2.0, 0.0),
SVector(1.0, 1.0),
SVector(-1.0, 1.0),
SVector(-2.0, 0.0)
]
@test_nowarn ConvexPolygon(t, local_points_2d)
rinplane = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
routside = Ray([0.0, 0.0, 1.0], [1.0, 1.0, 1.0])
rinside = Ray([0.0, 0.0, -1.0], [1.0, 1.0, -1.0])
routintersects = Ray([0.0, 0.0, 1.0], [0.0, 0.0, -1.0])
rinintersects = Ray([0.0, 0.0, -1.0], [0.0, 0.0, 1.0])
routmiss = Ray([0.0, 2.0, 1.0], [0.0, 0.0, -1.0])
rinmiss = Ray([0.0, 2.0, -1.0], [0.0, 0.0, 1.0])
rinbounds = Ray([sqrt(3) / 2, 0.0, -1.0], [0.0, 0.0, 1.0])
routbounds = Ray([sqrt(3) / 2, 0.0, 1.0], [0.0, 0.0, -1.0])
rasymhit = Ray([0.0, 0.9, 1.0], [0.0, 0.0, -1.0])
rasymmiss = Ray([3.0, 0.0, 1.0], [0.0, 0.0, -1.0])
poly = ConvexPolygon(t, local_points_2d)
# parallel to plane and outside
@test surfaceintersection(poly, routside) isa EmptyInterval
# parallel and on plane
@test surfaceintersection(poly, rinplane) isa EmptyInterval
# inside but not hitting
@test surfaceintersection(poly, rinside) isa EmptyInterval
# starts outside and hits
res = surfaceintersection(poly, routintersects)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && OpticSim.upper(res) isa Infinity
# starts inside and hits
res = surfaceintersection(poly, rinintersects)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# starts inside and misses bounds
@test surfaceintersection(poly, rinmiss) isa EmptyInterval
# starts outside and misses bounds
@test surfaceintersection(poly, routmiss) isa EmptyInterval
# starts outside and hits bounds
res = surfaceintersection(poly, routbounds)
@test isapprox(point(lower(res)), [sqrt(3) / 2, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# starts inside and hits bounds
res = surfaceintersection(poly, rinbounds)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [sqrt(3) / 2, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# asymmetric hit
res = surfaceintersection(poly, rasymhit)
@test isapprox(point(lower(res)), [0.0, 0.9, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# asymmetric miss
@test surfaceintersection(poly, rasymmiss) isa EmptyInterval
end # testset Convex Polygon
@testset "Stops" begin
infiniterect = RectangularAperture(0.4, 0.8, SVector(0.0, 1.0, 0.0), SVector(0.0, 0.0, 1.0))
finiterect = RectangularAperture(0.4, 0.8, 1.0, 2.0, SVector(0.0, 1.0, 0.0), SVector(0.0, 0.0, 1.0))
# through hole
r = Ray([0.0, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(infiniterect, r) isa EmptyInterval
@test surfaceintersection(finiterect, r) isa EmptyInterval
r = Ray([0.0, -1.0, 1.0], [0.0, 1.0, 0.0])
@test surfaceintersection(infiniterect, r) isa EmptyInterval
@test surfaceintersection(finiterect, r) isa EmptyInterval
# on edge
r = Ray([0.0, 1.0, 0.6], [0.0, -1.0, 0.0])
res = surfaceintersection(infiniterect, r)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.6], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
res = surfaceintersection(finiterect, r)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.6], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# through hole asym
r = Ray([0.7, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(infiniterect, r) isa EmptyInterval
@test surfaceintersection(finiterect, r) isa EmptyInterval
# on finite edge
r = Ray([1.0, -1.0, 2.0], [0.0, 1.0, 0.0])
res = surfaceintersection(infiniterect, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [1.0, 0.0, 2.0], rtol = RTOLERANCE, atol = ATOLERANCE)
res = surfaceintersection(finiterect, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [1.0, 0.0, 2.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# outside finite
r = Ray([2.0, 1.0, 3.0], [0.0, -1.0, 0.0])
@test isapprox(point(surfaceintersection(infiniterect, r)), [2.0, 0.0, 3.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test surfaceintersection(finiterect, r) isa EmptyInterval
infinitecirc = CircularAperture(0.4, SVector(0.0, 1.0, 0.0), SVector(0.0, 0.0, 1.0))
finitecirc = CircularAperture(0.4, 1.0, 2.0, SVector(0.0, 1.0, 0.0), SVector(0.0, 0.0, 1.0))
# through hole
r = Ray([0.0, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(infinitecirc, r) isa EmptyInterval
@test surfaceintersection(finitecirc, r) isa EmptyInterval
r = Ray([0.0, -1.0, 1.0], [0.0, 1.0, 0.0])
@test surfaceintersection(infinitecirc, r) isa EmptyInterval
@test surfaceintersection(finitecirc, r) isa EmptyInterval
# on edge
r = Ray([0.0, 1.0, 0.6], [0.0, -1.0, 0.0])
res = surfaceintersection(infinitecirc, r)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.6], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
res = surfaceintersection(finitecirc, r)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.6], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# through hole 2
r = Ray([0.3, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(infinitecirc, r) isa EmptyInterval
@test surfaceintersection(finitecirc, r) isa EmptyInterval
# on finite edge
r = Ray([1.0, -1.0, 2.0], [0.0, 1.0, 0.0])
res = surfaceintersection(infinitecirc, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [1.0, 0.0, 2.0], rtol = RTOLERANCE, atol = ATOLERANCE)
res = surfaceintersection(finitecirc, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [1.0, 0.0, 2.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# outside finite
r = Ray([2.0, 1.0, 3.0], [0.0, -1.0, 0.0])
@test isapprox(point(surfaceintersection(infinitecirc, r)), [2.0, 0.0, 3.0], rtol = RTOLERANCE, atol = ATOLERANCE)
@test surfaceintersection(finitecirc, r) isa EmptyInterval
annulus = Annulus(0.5, 1.0, SVector(0.0, 1.0, 0.0), SVector(0.0, 0.0, 1.0))
# through hole
r = Ray([0.0, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(annulus, r) isa EmptyInterval
r = Ray([0.0, -1.0, 1.0], [0.0, 1.0, 0.0])
@test surfaceintersection(annulus, r) isa EmptyInterval
# on edge
r = Ray([0.0, 1.0, 0.5], [0.0, -1.0, 0.0])
res = surfaceintersection(annulus, r)
@test isapprox(point(lower(res)), [0.0, 0.0, 0.5], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# through hole 2
r = Ray([0.3, 1.0, 1.0], [0.0, -1.0, 0.0])
@test surfaceintersection(infiniterect, r) isa EmptyInterval
@test surfaceintersection(annulus, r) isa EmptyInterval
# on finite edge
r = Ray([1.0, -1.0, 1.0], [0.0, 1.0, 0.0])
res = surfaceintersection(annulus, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [1.0, 0.0, 1.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# outside finite
r = Ray([0.9, 1.0, 1.9], [0.0, -1.0, 0.0])
@test surfaceintersection(annulus, r) isa EmptyInterval
# polygon stop intesection
polygon_stop_frame = Transform()
polygon_stop_poly = ConvexPolygon(polygon_stop_frame, [SVector(0.0, 0.0), SVector(1.0, 0.0), SVector(0.5, 0.5)], opaqueinterface(Float64))
polygon_stop = InfiniteStopConvexPoly(polygon_stop_poly)
# through polygon which should lead to non intersection
r = Ray([0.2, 0.1, 1.0], [0.0, 0.0, -1.0])
@test surfaceintersection(polygon_stop, r) isa EmptyInterval
# not through the polygon
r = Ray([-0.2, 0.2, 1.0], [0.0, 0.0, -1.0])
res = surfaceintersection(polygon_stop, r)
@test OpticSim.lower(surfaceintersection(polygon_stop, r)).point == SVector(-0.2, 0.2, 0.0)
# parallel to the polygon's plane
r = Ray([0.0, 0.0, 1.0], [1.0, 0.0, 0.0])
@test surfaceintersection(polygon_stop, r) isa EmptyInterval
end # testset Stops
@testset "Bezier" begin
surf = TestData.beziersurface()
accelsurf = AcceleratedParametricSurface(surf)
numsamples = 100
samples = samplepoints(numsamples, 0.05, 0.95, 0.05, 0.95)
missedintersections = 0
for uv in samples
pt = point(surf, uv[1], uv[2])
origin = [0.5, 0.5, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(accelsurf, r)
if allintersections isa EmptyInterval
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
@test isapprox(point(halfspaceintersection(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test upper(intsct) isa Infinity
end
end
end
if missedintersections > 0
@warn "$missedintersections out of total $(length(samples)) bezier suface intersections were missed"
end
# miss from outside
r = Ray([0.5, 0.5, 5.0], [0.0, 0.0, 1.0])
@test surfaceintersection(accelsurf, r) isa EmptyInterval
r = Ray([5.0, 0.5, -5.0], [0.0, 0.0, -1.0])
@test surfaceintersection(accelsurf, r) isa EmptyInterval
# miss from inside
r = Ray([0.5, 0.5, -5.0], [0.0, 0.0, -1.0])
res = surfaceintersection(accelsurf, r)
# TODO!! Fix bezier surface to create halfspace
# @test lower(res) isa RayOrigin && upper(res) isa Infinity
# hit from inside
r = Ray([0.5, 0.5, 0.2], [0.0, 1.0, 0.0])
res = surfaceintersection(accelsurf, r)
@test lower(res) isa RayOrigin && isapprox(point(upper(res)), [0.5, 0.8996900152986361, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit from outside
r = Ray([0.0, 0.5, 0.2], [1.0, 0.0, -1.0])
res = surfaceintersection(accelsurf, r)
@test isapprox(point(lower(res)), [0.06398711204047353, 0.5, 0.13601288795952649], rtol = RTOLERANCE, atol = ATOLERANCE) && upper(res) isa Infinity
# two hits from outside
r = Ray([0.0, 0.5, 0.25], [1.0, 0.0, 0.0])
res = surfaceintersection(accelsurf, r)
@test isapprox(point(lower(res)), [0.12607777053030267, 0.5, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res)), [0.8705887077060419, 0.5, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
surf = TestData.wavybeziersurface()
accelsurf = AcceleratedParametricSurface(surf)
# 3 hit starting outside
r = Ray([0.5, 0.0, 0.0], [0.0, 1.0, 0.0])
res = surfaceintersection(accelsurf, r)
@test isa(res, DisjointUnion) && length(res) == 2 && isapprox(point(lower(res[1])), [0.5, 0.10013694786182059, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[1])), [0.5, 0.49625, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(lower(res[2])), [0.5, 0.8971357794109067, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(res[2]) isa Infinity)
# 3 hit starting inside
r = Ray([0.5, 1.0, 0.0], [0.0, -1.0, 0.0])
res = surfaceintersection(accelsurf, r)
@test isa(res, DisjointUnion) && length(res) == 2 && (lower(res[1]) isa RayOrigin) && isapprox(point(upper(res[1])), [0.5, 0.8971357794109067, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(lower(res[2])), [0.5, 0.49625, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.5, 0.10013694786182059, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
surf = TestData.verywavybeziersurface()
accelsurf = AcceleratedParametricSurface(surf, 20)
# five hits starting outside
r = Ray([0.9, 0.0, -0.3], [0.0, 1.0, 0.7])
res = surfaceintersection(accelsurf, r)
a = isapprox(point(lower(res[1])), [0.9, 0.03172286522032046, -0.2777939943457758], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[1])), [0.9, 0.1733979947040411, -0.17862140370717122], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.9, 0.5335760974594397, 0.07350326822160776], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.9, 0.7767707607392784, 0.24373953251749486], rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(point(lower(res[3])), [0.9, 0.9830891958374246, 0.3881624370861975], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(res[3]) isa Infinity)
@test isa(res, DisjointUnion) && length(res) == 3 && a && b && c
# five hits starting inside
r = Ray([0.9, 1.0, 0.4], [0.0, -1.0, -0.7])
res = surfaceintersection(accelsurf, r)
a = (lower(res[1]) isa RayOrigin) && isapprox(point(upper(res[1])), [0.9, 0.9830891958374246, 0.3881624370861975], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.9, 0.7767707607392784, 0.24373953251749486], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.9, 0.5335760974594397, 0.07350326822160776], rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(point(lower(res[3])), [0.9, 0.17339799470404108, -0.1786214037071712], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[3])), [0.9, 0.03172286522032046, -0.27779399434577573], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(res, DisjointUnion) && length(res) == 3 && a && b && c
# 4 hits starting inside
r = Ray([0.1, 0.0, -0.3], [0.0, 1.0, 0.7])
res = surfaceintersection(accelsurf, r)
a = (lower(res[1]) isa RayOrigin) && isapprox(point(upper(res[1])), [0.1, 0.2851860296285551, -0.10036977926001144], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.1, 0.5166793625025807, 0.06167555375180668], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.1, 0.7770862508789854, 0.24396037561528983], rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(point(lower(res[3])), [0.1, 0.98308919558696, 0.3881624369108719], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(res[3]) isa Infinity)
@test isa(res, DisjointUnion) && length(res) == 3 && a && b && c
# 4 hits starting outside
r = Ray([0.9, 0.9, 0.4], [0.0, -0.9, -0.7])
res = surfaceintersection(accelsurf, r)
a = isapprox(point(lower(res[1])), [0.9, 0.736072142615238, 0.2725005553674076], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[1])), [0.9, 0.567439326091764, 0.141341698071372], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.9, 0.16601081959179267, -0.1708804736508277], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.9, 0.032434058775915924, -0.274773509840954], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(res, DisjointUnion) && length(res) == 2 && a && b
end # testset Bezier
@testset "Zernike" begin
@test_nowarn ZernikeSurface(1.5)
@test_throws AssertionError ZernikeSurface(0.0)
z1 = TestData.zernikesurface1()
az1 = AcceleratedParametricSurface(z1, 20)
numsamples = 100
# test that an on axis ray doesn't miss
@test !(surfaceintersection(AcceleratedParametricSurface(z1), Ray([0.0, 0.0, 10.0], [0.0, 0.0, -1.0])) isa EmptyInterval)
samples = samplepoints(numsamples, 0.01, 0.99, 0.01, 2π - 0.01)
missedintersections = 0
for uv in samples
pt = point(z1, uv[1], uv[2])
origin = [0.0, 0.0, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(az1, r)
if (allintersections isa EmptyInterval)
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
if lower(intsct) isa RayOrigin
missedintersections += 1
else
# closest point should be on the surface, furthest should be on bounding prism
@test isapprox(point(lower(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(upper(intsct), Intersection{Float64,3}) || (OpticSim.direction(r)[3] == -1 && isa(upper(intsct), Infinity{Float64}))
end
end
end
end
if missedintersections > 0
@warn "$missedintersections out of total $(length(samples)) zernike suface intersections were missed"
end
# hit from inside
r = Ray([0.0, 0.0, -0.5], [0.1, 0.2, 0.5])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.12363711269619936, 0.24727422539239863, 0.11818556348099664], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit from outside
r = Ray([0.0, 0.0, 0.5], [0.1, 0.2, -0.5])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.07118311042701463, 0.1423662208540292, 0.144084447864927], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.6708203932499369, 1.3416407864998738, -2.8541019662496843], rtol = RTOLERANCE, atol = ATOLERANCE)
# miss from inside
r = Ray([0.2, 0.2, -0.5], [0.0, 0.0, -1.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && (upper(intvl) isa Infinity)
# miss from outside
r = Ray([0.2, 2.0, -0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 0.5], [0.0, 0.0, -1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 0.2, 0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 2.0], [0.0, -1.0, 0.0])
@test surfaceintersection(az1, r) isa EmptyInterval
# two hits from outside
r = Ray([2.0, 0.0, 0.25], [-1.0, 0.0, 0.0])
hit = surfaceintersection(az1, r)
a = isapprox(point(lower(hit[1])), [1.5, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[1])), [1.3571758210851095, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(hit[2])), [-1.2665828947521165, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[2])), [-1.5, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(hit, DisjointUnion) && length(hit) == 2 && a && b
# hit cyl from outside
r = Ray([0.0, 2.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.0, 1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.0, -1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit cyl from inside
r = Ray([0.0, 0.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.0, -1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
z2 = TestData.zernikesurface2()
az2 = AcceleratedParametricSurface(z2, 20)
# two hits
r = Ray([2.0, -1.0, 0.2], [-1.0, 0.0, 0.0])
intvl = surfaceintersection(az2, r)
@test isapprox(point(lower(intvl)), [0.238496383738369, -1.0, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [-0.8790518227874484, -1.0, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE)
# three hits
r = Ray([-0.7, 1.0, 0.2], [0.0, -1.0, 0.0])
hit = surfaceintersection(az2, r)
a = (lower(hit[1]) isa RayOrigin) && isapprox(point(upper(hit[1])), [-0.7, 0.8732489598020176, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(hit[2])), [-0.7, -0.34532502965048606, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[2])), [-0.7, -1.1615928003236047, 0.2], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(hit, DisjointUnion) && length(hit) == 2 && a && b
# four hits
r = Ray([1.5, 0.1, 0.35], [-1.0, -0.5, 0.0])
hit = surfaceintersection(az2, r)
a = isapprox(point(lower(hit[1])), [1.4371467631969683, 0.06857338159848421, 0.35], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[1])), [1.1428338578611368, -0.07858307106943167, 0.35], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(hit[2])), [0.12916355683491865, -0.5854182215825409, 0.35], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[2])), [-0.7290713614371003, -1.0145356807185504, 0.35], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(hit, DisjointUnion) && length(hit) == 2 && a && b
# failure case, had a bug where rounding error would cause o2 to fail
o1 = leaf(AcceleratedParametricSurface(ZernikeSurface(1.4 * 1.15)), translation(0.0, 0.0, 3.0))()
o2 = leaf(AcceleratedParametricSurface(ZernikeSurface(1.4 * 1.15)), translation(2.0, 0.0, 3.0))()
r = Ray([-5.0, 0.0, 0.0], [1.0, 0.0, 0.0])
@test !(surfaceintersection(o1, r) isa EmptyInterval)
@test !(surfaceintersection(o2, r) isa EmptyInterval)
end # testset Zernike
@testset "QType" begin
@test_nowarn QTypeSurface(1.5)
@test_throws AssertionError QTypeSurface(0.0)
q1 = TestData.qtypesurface1()
aq1 = AcceleratedParametricSurface(q1, 20)
numsamples = 100
# test that an on axis ray doesn't miss
@test !(surfaceintersection(AcceleratedParametricSurface(q1), Ray([0.0, 0.0, 10.0], [0.0, 0.0, -1.0])) isa EmptyInterval)
samples = samplepoints(numsamples, 0.01, 0.99, 0.01, 2π - 0.01)
missedintersections = 0
for uv in samples
pt = point(q1, uv[1], uv[2])
origin = [0.0, 0.0, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(aq1, r)
if (allintersections isa EmptyInterval)
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
if lower(intsct) isa RayOrigin
missedintersections += 1
else
# closest point should be on the surface, furthest should be on bounding prism
@test isapprox(point(lower(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(upper(intsct), Intersection{Float64,3}) || (OpticSim.direction(r)[3] == -1 && isa(upper(intsct), Infinity{Float64}))
end
end
end
end
if missedintersections > 0
@warn "$missedintersections out of total $(length(samples)) qtype suface intersections were missed"
end
# hit from inside
r = Ray([0.0, 0.0, -0.5], [0.1, 0.2, 0.5])
intvl = surfaceintersection(aq1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.09758258750208074, 0.19516517500416142, -0.01208706248959643], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit from outside
r = Ray([0.0, 0.0, 0.5], [0.1, 0.2, -0.5])
intvl = surfaceintersection(aq1, r)
@test isapprox(point(lower(intvl)), [0.10265857811957124, 0.20531715623914257, -0.013292890597856405], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.6708203932499369, 1.3416407864998738, -2.8541019662496843], rtol = RTOLERANCE, atol = ATOLERANCE)
# miss from inside
r = Ray([0.2, 0.2, -0.5], [0.0, 0.0, -1.0])
intvl = surfaceintersection(aq1, r)
@test (lower(intvl) isa RayOrigin) && (upper(intvl) isa Infinity)
# miss from outside
r = Ray([0.2, 2.0, -0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(aq1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 0.5], [0.0, 0.0, -1.0])
@test surfaceintersection(aq1, r) isa EmptyInterval
r = Ray([0.2, 0.2, 0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(aq1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 2.0], [0.0, -1.0, 0.0])
@test surfaceintersection(aq1, r) isa EmptyInterval
end # testset QType
@testset "BoundingBox" begin
function boundingval(a::BoundingBox{T}, axis::Int, plane::Bool) where {T<:Real}
if axis === 1
return plane ? a.xmax : a.xmin
elseif axis === 2
return plane ? a.ymax : a.ymin
elseif axis == 3
return plane ? a.zmax : a.zmin
else
throw(ErrorException("Invalid axis: $axis"))
end
end
Random.seed!(SEED)
axis(x) = (x + 1) ÷ 2
face(x) = mod(x, 2)
facevalue(a::BoundingBox, x) = boundingval(a, axis(x), Bool(face(x)))
boundingindices = ((2, 3), (1, 3), (1, 2))
function facepoint(a::BoundingBox, facenumber)
axisindex = axis(facenumber)
indices = boundingindices[axisindex]
b1min, b1max = boundingval(a, indices[1], false), boundingval(a, indices[1], true)
b2min, b2max = boundingval(a, indices[2], false), boundingval(a, indices[2], true)
step = 0.00001
pt1val = rand((b1min + step):step:(b1max - step))
pt2val = rand((b2min + step):step:(b2max - step))
result = Array{Float64,1}(undef, 3)
result[indices[1]] = pt1val
result[indices[2]] = pt2val
result[axisindex] = facevalue(a, facenumber)
return result
end
bbox = BoundingBox(-1.0, 1.0, -1.0, 1.0, -1.0, 1.0)
for i in 1:10000
# randomly pick two planes
face1 = rand(1:6)
face2 = rand(1:6)
while face2 == face1
face2 = rand(1:6)
end
# pick a point on each face
pt1 = facepoint(bbox, face1)
pt2 = facepoint(bbox, face2)
direction = normalize(pt1 - pt2)
origin = pt2 - 3 * direction
r = Ray(origin, direction)
@test doesintersect(bbox, r)
end
# should miss
@test !doesintersect(bbox, Ray([-2.0, -2.0, 0.0], [1.0, 0.0, 0.0]))
@test !doesintersect(bbox, Ray([-2.0, -2.0, 0.0], [0.0, 1.0, 0.0]))
@test !doesintersect(bbox, Ray([-2.0, -2.0, 0.0], [0.0, 0.0, 1.0]))
@test !doesintersect(bbox, Ray([-2.0, 0.0, 0.0], [-1.0, 0.0, 0.0]))
@test !doesintersect(bbox, Ray([0.0, -2.0, 0.0], [0.0, -1.0, 0.0]))
@test !doesintersect(bbox, Ray([0.0, 0.0, -2.0], [0.0, 0.0, -1.0]))
bbox = BoundingBox(-0.5, 0.5, -0.75, 0.75, typemin(Float64), typemax(Float64))
# starting outside
r = Ray([-1.0, -1.1, 0.0], [1.0, 1.0, 0.0])
intscts = surfaceintersection(bbox, r)
@test isapprox(point(lower(intscts)), [-0.5, -0.6, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intscts)), [0.5, 0.4, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# starting inside
r = Ray([0.0, 0.0, 0.0], [1.0, 1.0, 0.0])
intscts = surfaceintersection(bbox, r)
@test lower(intscts) isa RayOrigin && isapprox(point(upper(intscts)), [0.5, 0.5, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# inside infinite
r = Ray([0.0, 0.0, 0.0], [0.0, 0.0, 1.0])
intscts = surfaceintersection(bbox, r)
@test lower(intscts) isa RayOrigin && upper(intscts) isa Infinity
# on surface
r = Ray([0.5, 0.0, 0.0], [0.0, 0.0, 1.0])
intscts = surfaceintersection(bbox, r)
@test lower(intscts) isa RayOrigin && upper(intscts) isa Infinity
# missing
r = Ray([5.0, 5.0, 5.0], [0.0, 0.0, -1.0])
intscts = surfaceintersection(bbox, r)
@test intscts isa EmptyInterval
r = Ray([5.0, 5.0, 5.0], [0.0, -1.0, 0.0])
intscts = surfaceintersection(bbox, r)
@test intscts isa EmptyInterval
end # testset BoundingBox
@testset "CSG" begin
pln1 = Plane([0.0, 0.0, -1.0], [0.0, 0.0, -1.0])
pln2 = Plane([0.0, 0.0, 1.0], [0.0, 0.0, 1.0])
r = Ray([0.0, 0, 2.0], [0.0, 0.0, -1.0])
gen1 = pln1 ∩ pln2
gen2 = pln1 ∪ pln2
csg = gen1(identitytransform())
intsct = evalcsg(csg, r)
intvlpt1 = lower(intsct)
intvlpt2 = upper(intsct)
@test isapprox(point(intvlpt1), SVector{3}(0.0, 0.0, 1.0)) && isapprox(point(intvlpt2), SVector{3}(0.0, 0.0, -1.0))
csg = gen2(identitytransform())
intsct = evalcsg(csg, r)
@test (lower(intsct) isa RayOrigin) && (upper(intsct) isa Infinity)
# test simple rays for intersection, union and difference operations
# INTERSECTION
intersection_obj = (leaf(Cylinder(0.5, 3.0), OpticSim.rotationd(90.0, 0.0, 0.0)) ∩ Sphere(1.0))()
r = Ray([0.7, 0.0, 0.0], [-1.0, 0.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test isapprox(point(lower(int)), [0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [-0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([5.0, 0.0, 0.0], [-1.0, 0.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test isapprox(point(lower(int)), [0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [-0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([0.7, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test (int isa EmptyInterval)
r = Ray([0.0, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([0.0, 0.0, 0.0], [0.0, 1.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [0.0, 1.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([0.0, 2.0, 0.0], [0.0, -1.0, 0.0])
int = OpticSim.evalcsg(intersection_obj, r)
@test isapprox(point(lower(int)), [0.0, 1.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [0.0, -1.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# UNION
union_obj = (Cylinder(0.5, 3.0) ∪ Sphere(1.0))(OpticSim.translation(1.0, 0.0, 0.0))
r = Ray([1.0, 0.0, 0.0], [0.0, 0.0, 1.0])
int = OpticSim.evalcsg(union_obj, r)
@test (lower(int) isa RayOrigin) && (upper(int) isa Infinity)
r = Ray([1.0, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(union_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [2.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([1.6, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(union_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [2.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([1.6, 0.0, 0.0], [-1.0, 0.0, 0.0])
int = OpticSim.evalcsg(union_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([-1.0, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(union_obj, r)
@test isapprox(point(lower(int)), [0.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [2.0, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([-1.0, 0.0, 4.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(union_obj, r)
@test isapprox(point(lower(int)), [0.5, 0.0, 4.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [1.5, 0.0, 4.0], rtol = RTOLERANCE, atol = ATOLERANCE)
# DIFFERENCE
difference_obj = (Cylinder(0.5, 3.0) - leaf(Sphere(1.0), OpticSim.translation(0.75, 0.0, 0.2)))()
r = Ray([0.25, 0.0, 0.0], [-1.0, 0.0, 0.0])
int = OpticSim.evalcsg(difference_obj, r)
@test isapprox(point(lower(int)), [-0.2297958971132712, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(int)), [-0.5, 0.0, 0.0], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([0.25, 0.0, 0.0], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(difference_obj, r)
@test int isa EmptyInterval
r = Ray([0.25, 0.0, 0.0], [0.0, 0.0, 1.0])
int = OpticSim.evalcsg(difference_obj, r)
@test isapprox(point(lower(int)), [0.25, 0.0, 1.0660254037844386], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(int) isa Infinity)
r = Ray([0.25, 0.0, 1.5], [0.0, 0.0, -1.0])
int = OpticSim.evalcsg(difference_obj, r)
@test (lower(int[1]) isa RayOrigin) && isapprox(point(upper(int[1])), [0.25, 0.0, 1.0660254037844386], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(lower(int[2])), [0.25, 0.0, -0.6660254037844386], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(int[2]) isa Infinity)
r = Ray([0.25, 0.0, 1.5], [1.0, 0.0, 0.0])
int = OpticSim.evalcsg(difference_obj, r)
@test (lower(int) isa RayOrigin) && isapprox(point(upper(int)), [0.5, 0.0, 1.5], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([0.25, 0.0, 1.5], [0.0, 0.0, 1.0])
int = OpticSim.evalcsg(difference_obj, r)
@test (lower(int) isa RayOrigin) && (upper(int) isa Infinity)
# DisjointUnion result on CSG
surf = TestData.verywavybeziersurface()
accelsurf = leaf(AcceleratedParametricSurface(surf, 20))()
# five hits starting outside
r = Ray([0.9, 0.0, -0.3], [0.0, 1.0, 0.7])
res = surfaceintersection(accelsurf, r)
a = isapprox(point(lower(res[1])), [0.9, 0.03172286522032046, -0.2777939943457758], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[1])), [0.9, 0.1733979947040411, -0.17862140370717122], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.9, 0.5335760974594397, 0.07350326822160776], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.9, 0.7767707607392784, 0.24373953251749486], rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(point(lower(res[3])), [0.9, 0.9830891958374246, 0.3881624370861975], rtol = RTOLERANCE, atol = ATOLERANCE) && (upper(res[3]) isa Infinity)
@test isa(res, DisjointUnion) && length(res) == 3 && a && b && c
# five hits starting inside
r = Ray([0.9, 1.0, 0.4], [0.0, -1.0, -0.7])
res = surfaceintersection(accelsurf, r)
a = (lower(res[1]) isa RayOrigin) && isapprox(point(upper(res[1])), [0.9, 0.9830891958374246, 0.3881624370861975], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(res[2])), [0.9, 0.7767707607392784, 0.24373953251749486], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[2])), [0.9, 0.5335760974594397, 0.07350326822160776], rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(point(lower(res[3])), [0.9, 0.17339799470404108, -0.1786214037071712], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(res[3])), [0.9, 0.03172286522032046, -0.27779399434577573], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(res, DisjointUnion) && length(res) == 3 && a && b && c
end # testset CSG
@testset "ThinGrating" begin
angle_from_ray(raydirection) = 90 + atand(raydirection[3], raydirection[2])
true_diff(order, λ, period, θi) = asind((order * λ / period + sind(θi)))
period = 3.0
int = TestData.transmissivethingrating(period, 2)
for k in 1:50
for θi in [-5.0, 0.0, 5.0, 10.0]
for λ in [0.35, 0.55, 1.0]
ray = OpticalRay(SVector(0.0, 0.0, 2.0), SVector(0.0, sind(θi), -cosd(θi)), 1.0, λ)
raydir, _, _ = OpticSim.processintersection(int, SVector(0.0, 0.0, 0.0), SVector(0.0, 0.0, 1.0), ray, 20.0, 1.0, true, true)
# order is random so just check that it is correct for one of them
@test any([isapprox(x, angle_from_ray(raydir), rtol = RTOLERANCE, atol = ATOLERANCE) for x in [true_diff(m, λ, period, θi) for m in -2:2]])
end
end
end
end # testset ThinGrating
# TODO Hologram intersection tests
@testset "Chebyshev" begin
@test_throws AssertionError ChebyshevSurface(0.0, 1.0, [(1, 2, 1.0)])
@test_throws AssertionError ChebyshevSurface(1.0, 0.0, [(1, 2, 1.0)])
z1 = TestData.chebyshevsurface2()
az1 = AcceleratedParametricSurface(z1, 20)
numsamples = 100
# test that an on axis ray doesn't miss
@test !(surfaceintersection(AcceleratedParametricSurface(z1), Ray([0.0, 0.0, 10.0], [0.0, 0.0, -1.0])) isa EmptyInterval)
samples = samplepoints(numsamples, -0.95, 0.95, -0.95, 0.95)
missedintersections = 0
for uv in samples
pt = point(z1, uv[1], uv[2])
origin = [0.0, 0.0, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(az1, r)
if (allintersections isa EmptyInterval)
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
if lower(intsct) isa RayOrigin
missedintersections += 1
else
# closest point should be on the surface, furthest should be on bounding prism
@test isapprox(point(lower(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(upper(intsct), Intersection{Float64,3}) || (OpticSim.direction(r)[3] == -1 && isa(upper(intsct), Infinity{Float64}))
end
end
end
end
if missedintersections > 0
@warn "$missedintersections out of total $(length(samples)) chebychev suface intersections were missed"
end
z1 = TestData.chebyshevsurface1()
az1 = AcceleratedParametricSurface(z1, 20)
# hit from inside
r = Ray([0.0, 0.0, -0.5], [0.1, 0.2, 0.5])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.07870079995991423, 0.15740159991982847, -0.10649600020042879], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit from outside
r = Ray([0.0, 0.0, 0.5], [0.1, 0.2, -0.5])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.13584702907541094, 0.2716940581508219, -0.1792351453770547], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [1.0, 2.0, -4.5], rtol = RTOLERANCE, atol = ATOLERANCE)
# miss from inside
r = Ray([0.2, 0.2, -0.5], [0.0, 0.0, -1.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && (upper(intvl) isa Infinity)
# miss from outside
r = Ray([0.2, 3.0, -0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 3.0, 0.5], [0.0, 0.0, -1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 0.2, 0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 3.0, 2.0], [0.0, -1.0, 0.0])
@test surfaceintersection(az1, r) isa EmptyInterval
# two hits from outside
r = Ray([0.0, -1.5, 0.25], [-1.0, 0.0, 0.0])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [-1.030882920068228, -1.5, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [-1.786071230727613, -1.5, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
r = Ray([1.5, -0.8, 0.25], [-1.0, 0.0, 0.0])
hit = surfaceintersection(az1, r)
a = isapprox(point(lower(hit[1])), [0.21526832427065165, -0.8, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[1])), [-0.25523748548950076, -0.8, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(hit[2])), [-1.9273057166986296, -0.8, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[2])), [-2.0, -0.8, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(hit, DisjointUnion) && length(hit) == 2 && a && b
# hit cyl from outside
r = Ray([0.0, 3.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.0, 2.0, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.0, -2.0, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit cyl from inside
r = Ray([0.0, 0.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.0, -2.0, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
end # testset Chebyshev
@testset "GridSag" begin
z1 = TestData.gridsagsurfacebicubic()
az1 = AcceleratedParametricSurface(z1, 20)
numsamples = 100
# test that an on axis ray doesn't miss
@test !(surfaceintersection(AcceleratedParametricSurface(z1), Ray([0.0, 0.0, 10.0], [0.0, 0.0, -1.0])) isa EmptyInterval)
samples1 = samplepoints(numsamples, 0.01, 0.99, 0.01, 2π - 0.01)
missedintersections = 0
for uv in samples1
pt = point(z1, uv[1], uv[2])
origin = [0.0, 0.0, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(az1, r)
if (allintersections isa EmptyInterval)
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
if lower(intsct) isa RayOrigin
missedintersections += 1
else
# closest point should be on the surface, furthest should be on bounding prism
@test isapprox(point(lower(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(upper(intsct), Intersection{Float64,3}) || (OpticSim.direction(r)[3] == -1 && isa(upper(intsct), Infinity{Float64}))
end
end
end
end
z2 = TestData.gridsagsurfacebicubiccheby()
az2 = AcceleratedParametricSurface(z2, 20)
samples2 = samplepoints(numsamples, -0.95, 0.95, -0.95, 0.95)
for uv in samples2
pt = point(z2, uv[1], uv[2])
origin = [0.0, 0.0, 5.0]
r = Ray(origin, pt .- origin)
allintersections = surfaceintersection(az2, r)
if (allintersections isa EmptyInterval)
# sampled triangle acceleration structure doesn't guarantee all intersecting rays will be detected.
# Have to use patch subdivision and convex hull polyhedron to do this.
missedintersections += 1
else
if isa(allintersections, Interval)
allintersections = (allintersections,)
end
for intsct in allintersections
if lower(intsct) isa RayOrigin
missedintersections += 1
else
# closest point should be on the surface, furthest should be on bounding prism
@test isapprox(point(lower(intsct)), pt, rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(upper(intsct), Intersection{Float64,3}) || (OpticSim.direction(r)[3] == -1 && isa(upper(intsct), Infinity{Float64}))
end
end
end
end
if missedintersections > 0
@warn "$missedintersections out of total $(length(samples1) + length(samples2)) gridsag suface intersections were missed"
end
# hit from inside
r = Ray([0.0, 0.0, -0.5], [0.1, 0.2, 0.5])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.13038780645120746, 0.26077561290241486, 0.15193903225603717], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit from outside
r = Ray([0.0, 0.0, 0.5], [0.1, 0.2, -0.5])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.046677740288643785, 0.0933554805772876, 0.26661129855678095], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.6708203932499369, 1.3416407864998738, -2.8541019662496843], rtol = RTOLERANCE, atol = ATOLERANCE)
# miss from inside
r = Ray([0.2, 0.2, -0.5], [0.0, 0.0, -1.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && (upper(intvl) isa Infinity)
# miss from outside
r = Ray([0.2, 2.0, -0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 0.5], [0.0, 0.0, -1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 0.2, 0.5], [0.0, 0.0, 1.0])
@test surfaceintersection(az1, r) isa EmptyInterval
r = Ray([0.2, 2.0, 2.0], [0.0, -1.0, 0.0])
@test surfaceintersection(az1, r) isa EmptyInterval
# two hits from outside
r = Ray([2.0, 0.0, 0.25], [-1.0, 0.0, 0.0])
hit = surfaceintersection(az1, r)
a = isapprox(point(lower(hit[1])), [1.5, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[1])), [1.394132887629247, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(point(lower(hit[2])), [0.30184444700168467, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(hit[2])), [-0.6437764440680096, 0.0, 0.25], rtol = RTOLERANCE, atol = ATOLERANCE)
@test isa(hit, DisjointUnion) && length(hit) == 2 && a && b
# hit cyl from outside
r = Ray([0.0, 2.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test isapprox(point(lower(intvl)), [0.0, 1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(point(upper(intvl)), [0.0, -1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
# hit cyl from inside
r = Ray([0.0, 0.0, -0.5], [0.0, -1.0, 0.0])
intvl = surfaceintersection(az1, r)
@test (lower(intvl) isa RayOrigin) && isapprox(point(upper(intvl)), [0.0, -1.5, -0.5], rtol = RTOLERANCE, atol = ATOLERANCE)
end # testset GridSag
end # testset intersection
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 2509 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
# FIXING ERRONEOUS UNBOUND TYPE ERRORS THAT OCCUR WITH VARARG
#=
The set will contain something like this:
MultiHologramInterface(interfaces::Vararg{HologramInterface{T}, N}) where {T<:Real, N}
To get the signature run:
methods(OpticSim.MultiHologramInterface).ms[I].sig where I is typically 1 or 2 depending on the number of methods
This gives:
Tuple{Type{MultiHologramInterface}, Vararg{HologramInterface{T}, N}} where N where T<:Real
We then have to use which to find the method:
which(OpticSim.MultiHologramInterface, Tuple{Vararg{HologramInterface{T}, N}} where N where T<:Real)
and pop it from the set
=#
@testset "JuliaLang" begin
# here we ignore the specific methods which we know are ok but are still failing
# for some weird reason the unbound args check seems to fail for some (seemingly random) methods with Vararg arguments
methods_to_ignore = Dict(
OpticSim => VERSION >= v"1.7" ? [
(OpticSim.LensAssembly, Tuple{Vararg{Union{CSGTree{T},LensAssembly{T},OpticSim.Surface{T}},N} where N} where {T<:Real}),
(OpticSim.MultiHologramInterface, Tuple{Vararg{HologramInterface{T},N}} where {N} where {T<:Real}),
] : [],
OpticSim.Zernike => [],
OpticSim.QType => [],
OpticSim.Vis => [
(OpticSim.Vis.drawcurves, Tuple{Vararg{Spline{P,S,N,M},N1} where N1} where {M} where {N} where {S} where {P}),
(OpticSim.Vis.draw, Tuple{Vararg{S,N} where N} where {S<:Union{OpticSim.Surface{T},OpticSim.TriangleMesh{T}}} where {T<:Real}),
(OpticSim.Vis.draw!, Tuple{OpticSim.Vis.Makie.LScene,Vararg{S,N} where N} where {S<:Union{OpticSim.Surface{T},OpticSim.TriangleMesh{T}}} where {T<:Real})
],
OpticSim.Examples => [],
OpticSim.Chebyshev => [],
OpticSim.GlassCat => [],
OpticSim.Optimization => [],
)
for (mod, ignore_list) in methods_to_ignore
unbound = setdiff(
detect_unbound_args(mod),
[which(method, signature) for (method, signature) in ignore_list]
)
# also ignore any generate methods created due to default or keyword arguments
filter!(m -> !occursin("#", string(m.name)), unbound)
@test isempty(unbound)
ambiguous = detect_ambiguities(mod)
@test isempty(ambiguous)
end
end # testset JuliaLang
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4052 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Lenses" begin
test_n = 5000
"""Creates a 3D vector uniformly distributed on the sphere by rejection sampling, i.e., discarding all points with norm > 1.0"""
function randunit()
let v = rand(3)
while (norm(v) > 1.0)
v = rand(3)
end
return normalize(v)
end
end
@testset "Refraction" begin
Random.seed!(SEED)
ηᵢ = 1.4
ηₜ = 1.2
for i in 1:test_n
nₛ = randunit()
rᵢ = randunit()
rₜ = refractedray(ηᵢ, ηₜ, nₛ, rᵢ)
if !(rₜ === nothing)
sinθₜ = norm(cross(-nₛ, rₜ))
sinθᵢ = norm(cross(nₛ, rᵢ))
a = isapprox(ηₜ * sinθₜ, ηᵢ * sinθᵢ, rtol = RTOLERANCE, atol = ATOLERANCE) #verify snell's law for the the transmitted and incident ray
b = isapprox(1.0, norm(rₜ), rtol = RTOLERANCE, atol = ATOLERANCE) #verify transmitted ray is unit
perp = normalize(cross(nₛ, rᵢ))
c = isapprox(0.0, rₜ ⋅ perp, rtol = RTOLERANCE, atol = ATOLERANCE) #verify incident and transmitted ray are in the same plane
@test a && b && c
end
end
end # testset refraction
# snell
@testset "Snell" begin
Random.seed!(SEED)
for i in 1:test_n
r = randunit()
nₛ = randunit()
n1 = 1.0
n2 = 1.4
sθ1, sθ2 = snell(nₛ, r, n1, n2)
sθ3, sθ4 = snell(nₛ, r, n2, n1)
@test isapprox(sθ1 * n1, sθ2 * n2, rtol = RTOLERANCE, atol = ATOLERANCE) && isapprox(sθ3 * n2, sθ4 * n1, rtol = RTOLERANCE, atol = ATOLERANCE)
end
end # testset snell
@testset "Reflection" begin
Random.seed!(SEED)
# reflection
for i in 1:test_n
r = randunit()
nₛ = randunit()
if abs(r ⋅ nₛ) < 0.9999 # if r and n are exactly aligned then their sum will be zero, not a vector pointing in the direction of the normal
reflected = reflectedray(nₛ, r)
#ensure reflected and refracted rays are in the same plane
a = isapprox(norm(reflected ⋅ cross(r, nₛ)), 0.0, rtol = RTOLERANCE, atol = ATOLERANCE)
b = isapprox(norm(reflected), 1.0, rtol = RTOLERANCE, atol = ATOLERANCE)
c = isapprox(0.0, reflected ⋅ nₛ + r ⋅ nₛ, rtol = RTOLERANCE, atol = ATOLERANCE)
#ensure sum of reflected and origin ray are in the direction of the normal
d = isapprox(0.0, norm(nₛ - sign(reflected ⋅ nₛ) * (normalize(reflected - r))), rtol = RTOLERANCE, atol = ATOLERANCE)
@test a & b & c & d
end
end
end # testset reflection
@testset "Paraxial" begin
# check that normally incident rays are focussed across the lens
l = ParaxialLensEllipse(100.0, 10.0, 10.0, [1.0, 1.0, 0.0], [3.0, 3.0, 3.0])
r = OpticalRay([2.0, 2.0, 3.0], [1.0, 1.0, 0.0], 1.0, 0.55)
intsct = halfspaceintersection(surfaceintersection(l, r))
ref, _, _ = OpticSim.processintersection(OpticSim.interface(intsct), OpticSim.point(intsct), OpticSim.normal(intsct), r, 20.0, 1.0, true, true)
@test isapprox(ref, normalize([1.0, 1.0, 0.0]), rtol = RTOLERANCE, atol = ATOLERANCE)
l = ParaxialLensRect(100.0, 10.0, 10.0, [1.0, 1.0, 0.0], [3.0, 3.0, 3.0])
r = OpticalRay([2.3, 2.4, 3.1], [1.0, 1.0, 0.0], 1.0, 0.55)
intsct = halfspaceintersection(surfaceintersection(l, r))
fp = [3.0, 3.0, 3.0] + 100 * normalize([1.0, 1.0, 0.0])
ref, _, _ = OpticSim.processintersection(OpticSim.interface(intsct), OpticSim.point(intsct), OpticSim.normal(intsct), r, 20.0, 1.0, true, true)
@test isapprox(ref, normalize(fp - OpticSim.point(intsct)), rtol = RTOLERANCE, atol = ATOLERANCE)
end # testset Paraxial
end # testset lenses
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 526 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "OpticalSystem" begin
@testset "Single threaded trace makes sure function executes properly" begin
conv = Examples.doubleconvex()
rays = Emitters.Sources.CompositeSource(translation(-unitZ3()), repeat([Emitters.Sources.Source()], 10000))
trace(conv, rays)
@test true #just want to verify that the trace function executed properly
end
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3792 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "ParaxialLens" begin
@testset "Virtual point" begin
lens = ParaxialLensRect(10.0,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
displaypoint = [0.0,0.0,-8.0]
r1 = Ray(displaypoint,[1.0,0.0,1.0])
intsct = OpticSim.surfaceintersection(lens,r1)
intsctpt = point(intsct)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct),OpticSim.normal(lens),OpticalRay(r1,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
# compute intersection of ray with optical axis. this should match the position of the virtual point
slope = refrac[1]/refrac[3]
virtptfromslope = [0.0,0.0,-intsctpt[1]/slope]
# insert test virtptfromslope == point(virtualpoint(lens,displaypoint))
@test isapprox(virtptfromslope,point(virtualpoint(lens,displaypoint)))
end
function rayintersection(lens,incomingray)
intsct = OpticSim.surfaceintersection(lens,incomingray)
refrac,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct),OpticSim.normal(lens),OpticalRay(incomingray,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
return refrac
end
@testset "Refracted rays" begin
#test all 4 combinations: n⋅r > 0, n⋅r < 0, focal length > 0, focal length < 0
#n⋅r > 0 focal length > 0
lens = ParaxialLensRect(1.0,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
r = Ray([1.0,0.0,-1.0],[0.0,0.0,1.0])
refrac = rayintersection(lens,r)
@test isapprox([-sqrt(2)/2,0.0,sqrt(2)/2],refrac)
#n⋅r < 0 focal length > 0
r = Ray([1.0,0.0,1.0],[0.0,0.0,-1.0])
refrac = rayintersection(lens,r)
@test isapprox([-sqrt(2)/2,0.0,-sqrt(2)/2],refrac)
#n⋅r > 0 focal length < 0
lens = ParaxialLensRect(-1.0,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
r = Ray([1.0,0.0,-1.0],[0.0,0.0,1.0])
refrac = rayintersection(lens,r)
@test isapprox([sqrt(2)/2,0.0,sqrt(2)/2],refrac)
#n⋅r < 0 focal length < 0
r = Ray([1.0,0.0,1.0],[0.0,0.0,-1.0])
refrac = rayintersection(lens,r)
@test isapprox([sqrt(2)/2,0.0,-sqrt(2)/2],refrac)
end
@testset "Reversibility of rays for paraxial lenses" begin
lens = ParaxialLensRect(10.0,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
displaypoint = [0.0,0.0,-8.0]
direction = [0.0,0.0,1.0]
r1 = Ray(displaypoint,direction)
intsct = OpticSim.surfaceintersection(lens,r1)
intsctpt = point(intsct)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac1,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct),OpticSim.normal(lens),OpticalRay(r1,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
r2 = Ray(-displaypoint,-direction)
intsct = OpticSim.surfaceintersection(lens,r2)
intsctpt = point(intsct)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac2,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct),OpticSim.normal(lens),OpticalRay(r2,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
@test isapprox(-refrac2,refrac1)
end
end | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 4604 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
using OpticSim:area,Rectangle,ParaxialLensRect,virtualpoint
using Unitful.DefaultSymbols
@testset "ParaxialAnalysis" begin
@testset "Lens" begin
lens = ParaxialLensRect(10.0,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
displaypoint = [0.0,0.0,-8.0]
r1 = Ray(displaypoint,[1.0,0.0,1.0])
intsct = OpticSim.surfaceintersection(lens,r1)
intsctpt = point(intsct)
# compute the refracted ray and project this backwards to its intersection with the optical axis. This should be the same position as returned by virtualpoint()
refrac,_,_ = OpticSim.processintersection(OpticSim.interface(lens),OpticSim.point(intsct),OpticSim.normal(lens),OpticalRay(r1,1.0,.55),OpticSim.TEMP_REF,OpticSim.PRESSURE_REF,false)
# compute intersection of ray with optical axis. this should match the position of the virtual point
slope = refrac[1]/refrac[3]
virtptfromslope = [0.0,0.0,-intsctpt[1]/slope]
@test isapprox(virtptfromslope, point(OpticSim.virtualpoint(lens,displaypoint)))
end
@testset "Projection" begin
focallength = 10.0
lens = ParaxialLensRect(focallength,100.0,100.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
display = OpticSim.Repeat.Display(1000,1000,1.0μm,1.0μm,translation(0.0,0.0,-focallength))
lenslet = OpticSim.Repeat.LensletAssembly(lens,identitytransform(),display)
displaypoint = SVector(0.0,0.0,-8.0)
pupilpoints = SMatrix{3,2}(10.0,10.0,10.0,-10.0,-10.0,20.0)
Repeat.project(lenslet,displaypoint,pupilpoints)
end
@testset "BeamEnergy" begin
focallength = 10.0
lens = ParaxialLensRect(focallength,1.0,1.0,[0.0,0.0,1.0],[0.0,0.0,0.0])
display = OpticSim.Repeat.Display(1000,1000,1.0μm,1.0μm,translation(0.0,0.0,-focallength))
lenslet = OpticSim.Repeat.LensletAssembly(lens,identitytransform(),display)
displaypoint = SVector(0.0,0.0,-8.0)
#pupil is placed so that only 1/4 of it (approximately) is illuminated by lens beam
pupil = Rectangle(1.0,1.0,SVector(0.0,0.0,-1.0),SVector(2.0,2.0,40.0))
energy,centroid = OpticSim.Repeat.beamenergy(lenslet,displaypoint,Geometry.vertices3d(pupil))
@test isapprox(1/16, energy,atol = 1e-4)
@test isapprox([.75,.75,0.0],centroid)
end
@testset "SphericalPolygon" begin
"""creates a circular polygon that subtends a half angle of θ"""
function sphericalcircle(θ, nsides = 10)
temp = MMatrix{3,nsides,Float64}(undef)
for i in 0:1:(nsides-1)
ϕ = i*2π/nsides
temp[1,i+1] = sin(θ)*cos(ϕ)
temp[2,i+1] = cos(θ)
temp[3,i+1] = sin(θ)*sin(ϕ)
end
return SphericalPolygon(SMatrix{3,nsides,Float64}(temp),SVector(0.0,0.0,0.0),1.0)
end
oneeigthsphere() = SphericalTriangle(SMatrix{3,3,Float64}(
0.0,1.0,0.0,
1.0,0.0,0.0,
0.0,0.0,1.0),
SVector(0.0,0.0,0.0),
1.0)
onesixteenthphere() = SphericalTriangle(SMatrix{3,3,Float64}(
0.0,1.0,0.0,
1.0,1.0,0.0,
0.0,0.0,1.0),
SVector(0.0,0.0,0.0),
1.0)
onesixteenthspherepoly() = SphericalPolygon(SMatrix{3,3,Float64}(
0.0,1.0,0.0,
1.0,1.0,0.0,
0.0,0.0,1.0),
SVector(0.0,0.0,0.0),
1.0)
threesidedpoly() = SphericalPolygon(SMatrix{3,3,Float64}(
0.0,1.0,0.0,
1.0,0.0,0.0,
0.0,0.0,1.0),
SVector(0.0,0.0,0.0),
1.0)
foursidedpoly() = SphericalPolygon(SMatrix{3,4,Float64}(
0.0,1.0,0.0,
1.0,1.0,-.9,
1.0,0.0,0.0,
0.0,0.0,1.0),
SVector(0.0,0.0,0.0),
1.0)
#test that spherical triangle and spherical polygon area algorithms return the same values for the same spherical areas
@test isapprox(area(oneeigthsphere()), area(threesidedpoly()))
@test isapprox(area(onesixteenthphere()),area(onesixteenthspherepoly()))
#halve the spherical area and make sure area function computes 1/2 the area
@test isapprox(area(onesixteenthphere()), area(oneeigthsphere())/2.0)
@test isapprox(area(sphericalcircle(π/8.0,1000))/4.0,area(sphericalcircle(π/16.0,1000)), atol = 1e-2)
end
end
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 3736 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Repeat" begin
function hex3RGB()
clusterelements = SVector((0,0),(-1,0),(-1,1))
colors = [colorant"red",colorant"green",colorant"blue"]
names = ["R","G","B"]
eltlattice = Repeat.HexBasis1()
clusterbasis = Repeat.LatticeBasis(( -1,2),(2,-1))
lattice = Repeat.LatticeCluster(clusterbasis,eltlattice,clusterelements)
properties = DataFrame(Color = colors, Name = names)
return Repeat.ClusterWithProperties(lattice,properties)
end
#spherepoint tests
@test isapprox(Repeat.Multilens.spherepoint(1,π/2,0.0), [0.0,1.0,0.0])
@test isapprox(Repeat.Multilens.spherepoint(1,0.0,π/2), [1.0,0.0,0.0])
@test isapprox(Repeat.Multilens.spherepoint(1,0,0.0), [0.0,0.0,1.0])
@test isapprox(Repeat.Multilens.spherepoint(1,0.0,π/4), [sqrt(2)/2,0.0,sqrt(2)/2])
""" Create a LatticeCluser with three elements at (0,0),(-1,0),(-1,1) coordinates in the HexBasis1 lattice"""
function hex3cluster()
clusterelts = SVector((0,0),(-1,0),(-1,1))
eltlattice = Repeat.HexBasis1()
clusterbasis = Repeat.LatticeBasis(( -1,2),(2,-1))
return Repeat.LatticeCluster(clusterbasis,eltlattice,clusterelts)
end
@test [-1 2;2 -1] == Repeat.basismatrix(Repeat.clusterbasis(hex3RGB()))
function basistest(a::Repeat.AbstractLatticeCluster)
return Repeat.clusterbasis(a)
end
@test basistest(hex3cluster()) == basistest(hex3RGB())
#LatticeCluster testset
cluster = Repeat.Multilens.hex9()
#generate many tile coordinates. Compute the cluster index and tile index in that cluster for each tile coordinate.
for iter in 1:100
(i,j) = rand.((1:1000,1:1000))
coords,tileindex = Repeat.cluster_coordinates_from_tile_coordinates(cluster,i,j)
reconstructed = Repeat.tilecoordinates(cluster,coords...,tileindex)
@test all((i,j) .== reconstructed)
end
function testassignment()
#test assignment of eyebox numbers to RGB clusters
rgb_cluster = Repeat.Multilens.hex12RGB()
cluster_coords = map(x->Tuple(x),eachcol(Repeat.clustercoordinates(rgb_cluster,0,0))) #create the cluster coordinates corresponding to each of the tiles in the cluster
eyeboxnumbers = (1,1,2,1,2,2,3,3,3,4,4,4) #correct eyebox number assignment for the tiles in the cluster
for (index,coord) in enumerate(cluster_coords)
boxnum = eyeboxnumbers[index]
num = Repeat.Multilens.eyebox_number(coord,rgb_cluster,4)
if num != boxnum
return false
end
end
return true
end
@test testassignment()
#verify that the 0,0 cluster is correct
for (index,element) in pairs(Repeat.clusterelements(cluster))
coords, tileindex = Repeat.cluster_coordinates_from_tile_coordinates(cluster, element...)
@test all(coords .== 0)
@test tileindex == index
end
#verify that choosecluster assertion doesn't fire incorrectly
using Unitful,Unitful.DefaultSymbols
function generate_clusters()
freq = Vector{Int64}(undef,0)
subdivs = Vector{Tuple{Int64,Int64}}(undef,0)
areas = Vector(undef,0)
try
for cycles in 15:30
OpticSim.Repeat.Multilens.system_properties(15mm,(10mm,9mm),(100°,70°),3.5mm,.1,cycles)
end
catch err #if any errors then failure
return false
end
return true
end
@test generate_clusters() #shouldn't get assertion failures for any of the frequencies between 15:30
end | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 11676 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "SurfaceDefs" begin
@testset "Knots" begin
# find span
knots = KnotVector{Int64}([0, 0, 0, 1, 2, 3, 4, 4, 5, 5, 5])
function apply(knots::KnotVector, curveorder, u, correctindex)
knotnum = findspan(knots, curveorder, u)
return knotnum == correctindex
end
@test apply(knots, 2, 2.5, 5) && apply(knots, 2, 0, 3) && apply(knots, 2, 4, 6) && apply(knots, 2, 5, 8)
# insert knots
knots = [0, 0, 0, 0, 1, 2, 3, 3, 4, 4, 5, 5, 6, 7, 7, 7, 7]
insertions = knotstoinsert(knots, 3)
@test insertions == [(5, 2), (6, 2), (7, 1), (9, 1), (11, 1), (13, 2)]
end
@testset "BSpline" begin
# bspline conversion
correctverts = [[0.0, 0.0, 0.0], [0.0, 0.49625, 0.0], [0.6625, 0.49625, 0.65625], [0.0, 0.0, 0.0], [0.6625, 0.49625, 0.65625], [0.6625, 0.0, 0.0], [0.6625, 0.0, 0.0], [0.6625, 0.49625, 0.65625], [1.33, 0.49625, 0.0], [0.6625, 0.0, 0.0], [1.33, 0.49625, 0.0], [1.33, 0.0, 0.0], [0.0, 0.49625, 0.0], [0.0, 1.0, 0.0], [0.6625, 1.0, 0.0], [0.0, 0.49625, 0.0], [0.6625, 1.0, 0.0], [0.6625, 0.49625, 0.65625], [0.6625, 0.49625, 0.65625], [0.6625, 1.0, 0.0], [1.33, 1.0, 0.0], [0.6625, 0.49625, 0.65625], [1.33, 1.0, 0.0], [1.33, 0.49625, 0.0]]
correcttris = [
0x00000001 0x00000002 0x00000003
0x00000004 0x00000005 0x00000006
0x00000007 0x00000008 0x00000009
0x0000000a 0x0000000b 0x0000000c
0x0000000d 0x0000000e 0x0000000f
0x00000010 0x00000011 0x00000012
0x00000013 0x00000014 0x00000015
0x00000016 0x00000017 0x00000018
]
surf = TestData.bsplinesurface()
points, triangles = makiemesh(makemesh(surf, 2))
segments = tobeziersegments(surf) # TODO just testing that this evaluates for now
@test triangles == correcttris
@test all(isapprox.(points, correctverts, rtol = RTOLERANCE, atol = ATOLERANCE))
end # testset BSpline
@testset "PowerBasis" begin
# polynomial is 3x^2 + 2x + 1
coeff = [1.0 2.0 3.0]
curve = PowerBasisCurve{OpticSim.Euclidean,Float64,1,2}(coeff)
@test !all(isapprox.(coeff, coefficients(curve, 1), rtol = RTOLERANCE, atol = ATOLERANCE)) # TODO not sure if this is correct/what this is testing?
correct = true
for x in -10.0:0.1:10
exact = 3 * x^2 + 2 * x + 1
calculated = point(curve, x)[1]
@test isapprox(exact, calculated, rtol = RTOLERANCE, atol = ATOLERANCE)
end
end # testset PowerBasis
@testset "BezierSurface" begin
surf = TestData.beziersurface()
fdm = central_fdm(10, 1)
for u in 0:0.1:1, v in 0:0.1:1
(du, dv) = partials(surf, u, v)
fu(u) = point(surf, u, v)
fv(v) = point(surf, u, v)
# compute accurate finite difference approximation to derivative
fdu, fdv = (fdm(fu, u), fdm(fv, v))
@test isapprox(du, fdu, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dv, fdv, rtol = 1e-12, atol = 2 * eps(Float64))
end
end # testset BezierSurface
@testset "ZernikeSurface" begin
surf = TestData.zernikesurface1a() # with normradius
fdm = central_fdm(10, 1)
for ρ in 0.05:0.1:0.95, ϕ in 0:(π / 10):(2π)
(dρ, dϕ) = partials(surf, ρ, ϕ)
fρ(ρ) = point(surf, ρ, ϕ)
fϕ(ϕ) = point(surf, ρ, ϕ)
# compute accurate finite difference approximation to derivative
fdρ, fdϕ = (fdm(fρ, ρ), fdm(fϕ, ϕ))
@test isapprox(dρ, fdρ, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dϕ, fdϕ, rtol = 1e-12, atol = 2 * eps(Float64))
end
@test !any(isnan.(normal(TestData.conicsurface(), 0.0, 0.0)))
end # testset ZernikeSurface
@testset "QTypeSurface" begin
# test predefined values against papers
# Forbes, G. W. "Robust, efficient computational methods for axially symmetric optical aspheres." OpticSim express 18.19 (2010): 19700-19712.
# Forbes, G. W. "Characterizing the shape of freeform optics." OpticSim express 20.3 (2012): 2483-2499.
f0_true = [2, sqrt(19 / 4), 4 * sqrt(10 / 19), 1 / 2 * sqrt(509 / 10), 6 * sqrt(259 / 509), 1 / 2 * sqrt(25607 / 259)]
for i in 1:length(f0_true)
@test isapprox(OpticSim.QType.f0(i - 1), f0_true[i], rtol = 2 * eps(Float64))
end
g0_true = [-1 / 2, -5 / (2 * sqrt(19)), -17 / (2 * sqrt(190)), -91 / (2 * sqrt(5090)), -473 / (2 * sqrt(131831))]
for i in 1:length(g0_true)
@test isapprox(OpticSim.QType.g0(i - 1), g0_true[i], rtol = 2 * eps(Float64))
end
h0_true = [-1 / 2, -6 / sqrt(19), -3 / 2 * sqrt(19 / 10), -20 * sqrt(10 / 509)]
for i in 1:length(h0_true)
@test isapprox(OpticSim.QType.h0(i - 1), h0_true[i], rtol = 2 * eps(Float64))
end
F_true = [1/4 1/2 27/32 5/4; 15/32 7/8 35/16 511/128; 17/72 35/36 35/16 23/6; 29/40 67/40 243/80 12287/2560]
N, M = size(F_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.F(m, n), F_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
G_true = [1/4 3/8 15/32 35/64; -1/24 -5/48 -7/32 -21/64; -7/40 -7/16 -117/160 -33/32]
N, M = size(G_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.G(m, n), G_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
f_true = [1/2 1/sqrt(2) 3 / 4*sqrt(3 / 2) sqrt(5)/2; 1 / 4*sqrt(7 / 2) 1 / 4*sqrt(19 / 2) 1 / 4*sqrt(185 / 6) 3 / 32*sqrt(427); 1 / 6*sqrt(115 / 14) 1 / 2*sqrt(145 / 38) 1 / 4*sqrt(12803 / 370) 1 / 2*sqrt(2785 / 183); 1 / 5*sqrt(3397 / 230) 1 / 4*sqrt(6841 / 290) 9 / 4*sqrt(14113 / 25606) 1 / 16*sqrt(1289057 / 1114)]
N, M = size(f_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.f(m, n), f_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
g_true = [1/2 3/(4 * sqrt(2)) 5/(4 * sqrt(6)) 7 * sqrt(5)/32; -1/(3 * sqrt(14)) -5/(6 * sqrt(38)) -7 / 4*sqrt(3 / 370) -1 / 2*sqrt(7 / 61); -21 / 10*sqrt(7 / 230) -7 / 4*sqrt(19 / 290) -117 / 4*sqrt(37 / 128030) -33 / 16*sqrt(183 / 2785)]
N, M = size(g_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.g(m, n), g_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
A_true = [2 3 5 7 9 11; -4/3 2/3 2 76/27 85/24 106/25; 9/5 26/15 2 117/50 203/75 108/35; 55/28 66/35 2 536/245 135/56 130/49; 161/81 122/63 2 515/243 143/63 161/66]
N, M = size(A_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.A(m, n), A_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
B_true = [-1 -2 -4 -6 -8 -10; -8/3 -4 -4 -40/9 -5 -28/5; -24/5 -4 -4 -21/5 -112/25 -24/5; -30/7 -4 -4 -144/35 -30/7 -220/49; -112/27 -4 -4 -110/27 -88/21 -13/3]
N, M = size(B_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.B(m, n), B_true[n + 1, m], rtol = 2 * eps(Float64))
end
end
C_true = [NaN NaN NaN NaN NaN NaN; -11/3 -3 -5/3 -35/27 -9/8 -77/75; 0 5/9 7/15 21/50 88/225 13/35; 27/28 21/25 27/35 891/1225 39/56 33/49; 80/81 45/49 55/63 1430/1701 40/49 1105/1386]
N, M = size(C_true)
for m in 1:M
for n in 0:(N - 1)
@test isapprox(OpticSim.QType.C(m, n), C_true[n + 1, m], rtol = 2 * eps(Float64)) || (isnan(OpticSim.QType.C(m, n)) && isnan(C_true[n + 1, m]))
end
end
# test deriv with finite differences
surf = TestData.qtypesurface1()
fdm = central_fdm(10, 1)
for ρ in 0.05:0.1:0.95, ϕ in 0:(π / 10):(2π)
(dρ, dϕ) = partials(surf, ρ, ϕ)
fρ(ρ) = point(surf, ρ, ϕ)
fϕ(ϕ) = point(surf, ρ, ϕ)
# compute accurate finite difference approximation to derivative
fdρ, fdϕ = (fdm(fρ, ρ), fdm(fϕ, ϕ))
@test isapprox(dρ, fdρ, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dϕ, fdϕ, rtol = 1e-12, atol = 2 * eps(Float64))
end
end # testset QTypeSurface
@testset "GridSag" begin
surf = TestData.gridsagsurfacelinear()
fdm = central_fdm(10, 1)
# doesn't enforce C1 across patch boundaries meaning that finite differences won't match at all
# just test within a patch to make sure partials() is working
for ρ in 0.02:0.01:0.18, ϕ in 0:(π / 30):(2π)
(dρ, dϕ) = partials(surf, ρ, ϕ)
fρ(ρ) = point(surf, ρ, ϕ)
fϕ(ϕ) = point(surf, ρ, ϕ)
# compute accurate finite difference approximation to derivative
fdρ, fdϕ = (fdm(fρ, ρ), fdm(fϕ, ϕ))
@test isapprox(dρ, fdρ, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dϕ, fdϕ, rtol = 1e-12, atol = 2 * eps(Float64))
end
surf = TestData.gridsagsurfacebicubic()
fdm = central_fdm(10, 1)
# doesn't enforce C2 across patch boundaries meaning that finite differences won't match exactly
# just test within a patch to make sure partials() is working
for ρ in 0.02:0.01:0.18, ϕ in 0:(π / 30):(2π)
(dρ, dϕ) = partials(surf, ρ, ϕ)
fρ(ρ) = point(surf, ρ, ϕ)
fϕ(ϕ) = point(surf, ρ, ϕ)
# compute accurate finite difference approximation to derivative
fdρ, fdϕ = (fdm(fρ, ρ), fdm(fϕ, ϕ))
@test isapprox(dρ, fdρ, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dϕ, fdϕ, rtol = 1e-12, atol = 2 * eps(Float64))
end
surf = TestData.gridsagsurfacebicubiccheby()
fdm = central_fdm(10, 1)
# not valid at the very boundary of the surface as stuff gets weird outside |u| < 1 and |v| < 1
for u in -0.3:0.01:0.3, v in -0.15:0.01:0.15
(du, dv) = partials(surf, u, v)
fu(u) = point(surf, u, v)
fv(v) = point(surf, u, v)
# compute accurate finite difference approximation to derivative
fdu, fdv = (fdm(fu, u), fdm(fv, v))
@test isapprox(du, fdu, rtol = 1e-12, atol = 2 * eps(Float64))
@test isapprox(dv, fdv, rtol = 1e-12, atol = 2 * eps(Float64))
end
end # testset GridSag
@testset "ChebyshevSurface" begin
surf = TestData.chebyshevsurface1() # with normradius
fdm = central_fdm(10, 1)
# not valid at the very boundary of the surface as stuff gets weird outside |u| < 1 and |v| < 1
for u in -0.99:0.1:0.99, v in -0.99:0.1:0.99
(du, dv) = partials(surf, u, v)
fu(u) = point(surf, u, v)
fv(v) = point(surf, u, v)
# compute accurate finite difference approximation to derivative
fdu, fdv = (fdm(fu, u), fdm(fv, v))
@test isapprox(du, fdu, rtol = 1e-11, atol = 2 * eps(Float64)) #changed rtol from 1e-12 to 1e-11. FiniteDifferences approximation to the derivative had larger than expected error.
@test isapprox(dv, fdv, rtol = 1e-11, atol = 2 * eps(Float64)) #changed rtol from 1e-12 to 1e-11. FiniteDifferences approximation to the derivative had larger than expected error.
end
end # testset ChebyshevSurface
end # testset SurfaceDefs
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 576 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Transform" begin
A = [
1 2 3 4;
4 5 6 7;
8 9 10 11;
12 13 14 15
]
x,y,z,w = Vec4.([A[:,i] for i in 1:4])
@test Geometry.matrix(Geometry.Transform(x,y,z,w)) == A
B = [
1 2 3 4;
4 5 6 7;
8 9 10 11;
0 0 0 1
]
x,y,z,w = Vec3.([B[1:3,i] for i in 1:4])
@test B == Geometry.matrix(Geometry.Transform(x,y,z,w))
end # testset Allocations
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | code | 1727 | # MIT license
# Copyright (c) Microsoft Corporation. All rights reserved.
# See LICENSE in the project root for full license information.
@testset "Visualization" begin
if get(ENV, "CI", nothing) == "true" && Sys.iswindows() return #OpenGL is unreliable on headless Windows VMs on github. This fails unpredictably and prevents pull requests from being approved. These tests are not essential, so turn them off when running on windows.
else
# empty Vis.draw() call to clear Makie @info message:
# "Info: Makie/Makie is caching fonts, this may take a while. Needed only on first run!"
Vis.draw()
# test that this all at least runs
surf1 = AcceleratedParametricSurface(TestData.beziersurface(), 15)
surf2 = AcceleratedParametricSurface(TestData.upsidedownbeziersurface(), 15)
m = (
leaf(surf1, translation(-0.5, -0.5, 0.0)) ∩
Cylinder(0.3, 5.0) ∩
leaf(surf1, Transform(0.0, Float64(π), 0.0, 0.5, -0.5, 0.0))
)()
Vis.draw(m)
@test_nowarn Vis.draw(m)
m = (
leaf(surf1, translation(-0.5, -0.5, 0.0)) ∩
Cylinder(0.3, 5.0) ∩
leaf(surf2, translation(-0.5, -0.5, 0.0))
)()
@test_nowarn Vis.draw(m)
m = (Cylinder(0.5, 3.0) ∪ Sphere(1.0))()
@test_nowarn Vis.draw(m)
m = (Cylinder(0.5, 3.0) ∩ Sphere(1.0))()
@test_nowarn Vis.draw(m)
m = (Cylinder(0.5, 3.0) - Sphere(1.0))()
@test_nowarn Vis.draw(m)
@test_nowarn Vis.draw(Repeat.Multilens.hex3RGB(),[0 1 0;0 0 1])
@test_nowarn Examples.drawhexrect()
@test_nowarn Examples.drawhexneighbors()
end
end # testset Visualization
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 444 | # Microsoft Open Source Code of Conduct
This project has adopted the [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/).
Resources:
- [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/)
- [Microsoft Code of Conduct FAQ](https://opensource.microsoft.com/codeofconduct/faq/)
- Contact [[email protected]](mailto:[email protected]) with questions or concerns
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 493 | ## Contributing to OpticSim.jl
👋 Hi, it's great to see you here!
Our aim is to be as collaborator-friendly as possible, but we're a small team so please bear with us while we get set up. This project started out as an in-house tool for specific optical simulations, but we'd like to see it growing into a modern replacement for general optical engineering tasks.
WIP:
- Bug reports
- Contributing guidelines
- Code style
- Branch naming conventions
- Forking workflow
- Development roadmap
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 3885 | <p align="center">
<a href="https://microsoft.github.io/OpticSim.jl/dev/">
<img src=docs/src/assets/logo.svg height=128px style="text-align:center">
</a>
</p>
# OpticSim.jl
<table>
<thead>
<tr>
<th>Documentation</th>
<th>Build Status</th>
</tr>
</thead>
<tbody>
<tr>
<td>
<a href="https://microsoft.github.io/OpticSim.jl/stable/">
<img src="https://img.shields.io/badge/docs-stable-blue.svg" alt="docs stable">
</a>
<a href="https://microsoft.github.io/OpticSim.jl/dev/">
<img src="https://img.shields.io/badge/docs-dev-blue.svg" alt="docs dev">
</a>
</td>
<td>
<a href="https://github.com/microsoft/OpticSim.jl/actions/workflows/CI.yml">
<img src="https://github.com/microsoft/OpticSim.jl/workflows/CI/badge.svg" alt="CI action">
</a>
<a href="https://codecov.io/gh/microsoft/OpticSim.jl">
<img src="https://codecov.io/gh/microsoft/OpticSim.jl/branch/main/graph/badge.svg?token=9QxvIHt5F5" alt="codecov">
</a>
</td>
</tr>
</tbody>
</table>
OpticSim.jl is a [Julia](https://julialang.org/) package for geometric optics (ray tracing) simulation and optimization of complex optical systems developed by the Microsoft Research Interactive Media Group and the Microsoft Hardware Architecture Incubation Team (HART).
It is designed to allow optical engineers to create optical systems procedurally and then to simulate and optimize them. Unlike Zemax, Code V, or other interactive optical design systems OpticSim.jl has limited support for interactivity, primarily in the tools for visualizing optical systems.
A large variety of surface types are supported, and these can be composed into complex 3D objects through the use of constructive solid geometry (CSG). A substantial catalog of optical materials is provided through the GlassCat submodule.
This software provides extensive control over the modelling, simulation, visualization and optimization of optical systems. It is especially suited for designs that have a procedural architecture.
# Installation
Before you can use the software you will need to download glass files. See the documentation for detailed information about how to do this.
## Contributing
[](https://github.com/SciML/ColPrac)
This project welcomes contributions and suggestions. Most contributions require you to agree to a
Contributor License Agreement (CLA) declaring that you have the right to, and actually do, grant us
the rights to use your contribution. For details, visit <https://cla.opensource.microsoft.com>.
When you submit a pull request, a CLA bot will automatically determine whether you need to provide
a CLA and decorate the PR appropriately (e.g., status check, comment). Simply follow the instructions
provided by the bot. You will only need to do this once across all repositories using our CLA.
This project has adopted the [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/).
For more information see the [Code of Conduct FAQ](https://opensource.microsoft.com/codeofconduct/faq/) or
contact [[email protected]](mailto:[email protected]) with any additional questions or comments.
## Trademarks
This project may contain trademarks or logos for projects, products, or services. Authorized use of Microsoft
trademarks or logos is subject to and must follow
[Microsoft's Trademark & Brand Guidelines](https://www.microsoft.com/en-us/legal/intellectualproperty/trademarks/usage/general).
Use of Microsoft trademarks or logos in modified versions of this project must not cause confusion or imply Microsoft sponsorship.
Any use of third-party trademarks or logos are subject to those third-party's policies.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 2780 | <!-- BEGIN MICROSOFT SECURITY.MD V0.0.5 BLOCK -->
## Security
Microsoft takes the security of our software products and services seriously, which includes all source code repositories managed through our GitHub organizations, which include [Microsoft](https://github.com/Microsoft), [Azure](https://github.com/Azure), [DotNet](https://github.com/dotnet), [AspNet](https://github.com/aspnet), [Xamarin](https://github.com/xamarin), and [our GitHub organizations](https://opensource.microsoft.com/).
If you believe you have found a security vulnerability in any Microsoft-owned repository that meets [Microsoft's definition of a security vulnerability](https://docs.microsoft.com/en-us/previous-versions/tn-archive/cc751383(v=technet.10)), please report it to us as described below.
## Reporting Security Issues
**Please do not report security vulnerabilities through public GitHub issues.**
Instead, please report them to the Microsoft Security Response Center (MSRC) at [https://msrc.microsoft.com/create-report](https://msrc.microsoft.com/create-report).
If you prefer to submit without logging in, send email to [[email protected]](mailto:[email protected]). If possible, encrypt your message with our PGP key; please download it from the [Microsoft Security Response Center PGP Key page](https://www.microsoft.com/en-us/msrc/pgp-key-msrc).
You should receive a response within 24 hours. If for some reason you do not, please follow up via email to ensure we received your original message. Additional information can be found at [microsoft.com/msrc](https://www.microsoft.com/msrc).
Please include the requested information listed below (as much as you can provide) to help us better understand the nature and scope of the possible issue:
* Type of issue (e.g. buffer overflow, SQL injection, cross-site scripting, etc.)
* Full paths of source file(s) related to the manifestation of the issue
* The location of the affected source code (tag/branch/commit or direct URL)
* Any special configuration required to reproduce the issue
* Step-by-step instructions to reproduce the issue
* Proof-of-concept or exploit code (if possible)
* Impact of the issue, including how an attacker might exploit the issue
This information will help us triage your report more quickly.
If you are reporting for a bug bounty, more complete reports can contribute to a higher bounty award. Please visit our [Microsoft Bug Bounty Program](https://microsoft.com/msrc/bounty) page for more details about our active programs.
## Preferred Languages
We prefer all communications to be in English.
## Policy
Microsoft follows the principle of [Coordinated Vulnerability Disclosure](https://www.microsoft.com/en-us/msrc/cvd).
<!-- END MICROSOFT SECURITY.MD BLOCK --> | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 1315 | # TODO: The maintainer of this repo has not yet edited this file
**REPO OWNER**: Do you want Customer Service & Support (CSS) support for this product/project?
- **No CSS support:** Fill out this template with information about how to file issues and get help.
- **Yes CSS support:** Fill out an intake form at [aka.ms/spot](https://aka.ms/spot). CSS will work with/help you to determine next steps. More details also available at [aka.ms/onboardsupport](https://aka.ms/onboardsupport).
- **Not sure?** Fill out a SPOT intake as though the answer were "Yes". CSS will help you decide.
*Then remove this first heading from this SUPPORT.MD file before publishing your repo.*
# Support
## How to file issues and get help
This project uses GitHub Issues to track bugs and feature requests. Please search the existing
issues before filing new issues to avoid duplicates. For new issues, file your bug or
feature request as a new Issue.
For help and questions about using this project, please **REPO MAINTAINER: INSERT INSTRUCTIONS HERE
FOR HOW TO ENGAGE REPO OWNERS OR COMMUNITY FOR HELP. COULD BE A STACK OVERFLOW TAG OR OTHER
CHANNEL. WHERE WILL YOU HELP PEOPLE?**.
## Microsoft Support Policy
Support for this **PROJECT or PRODUCT** is limited to the resources listed above.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 686 | ---
name: Bug report
about: Create a report to help us improve
title: ''
labels: ''
assignees: ''
---
**Describe the bug**
A clear and concise description of what the bug is.
**To Reproduce**
Steps to reproduce the behavior:
1. Go to '...'
2. Click on '....'
3. Scroll down to '....'
4. See error
**Expected behavior**
A clear and concise description of what you expected to happen.
**Screenshots**
If applicable, add screenshots to help explain your problem.
**Desktop (please complete the following information):**
- OS: [e.g. Ubuntu 20.04]
- Julia version [e.g. 1.5.2]
- OpticSim.jl version [e.g. 0.3.1]
**Additional context**
Add any other context about the problem here.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 595 | ---
name: Feature request
about: Suggest an idea for this project
title: ''
labels: ''
assignees: ''
---
**Is your feature request related to a problem? Please describe.**
A clear and concise description of what the problem is. Ex. I'm always frustrated when [...]
**Describe the solution you'd like**
A clear and concise description of what you want to happen.
**Describe alternatives you've considered**
A clear and concise description of any alternative solutions or features you've considered.
**Additional context**
Add any other context or screenshots about the feature request here.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 1426 | # Pull Request Template
## Description
Please include a summary of the change and which issue is fixed. Please also include relevant motivation and context. List any dependencies that are required for this change.
Fixes # (issue)
## Type of change
- [ ] Bug fix (non-breaking change which fixes an issue)
- [ ] New feature (non-breaking change which adds functionality)
- [ ] Breaking change (fix or feature that would cause existing functionality to not work as expected)
- [ ] This change requires a documentation update
## How Has This Been Tested?
Please describe the tests that you ran to verify your changes. Provide instructions so we can reproduce. Please also list any relevant details for your test configuration
- [ ] Test A
- [ ] Test B
**Test Configuration(s)**:
* Firmware version:
* Hardware:
* Toolchain:
* SDK:
## Checklist:
- [ ] My code follows the style guidelines of this project
- [ ] I have performed a self-review of my own code
- [ ] I have commented my code, particularly in hard-to-understand areas
- [ ] I have made corresponding changes to the documentation
- [ ] My changes generate no new warnings
- [ ] I have added tests that prove my fix is effective or that my feature works
- [ ] New and existing unit tests pass locally with my changes
- [ ] Any dependent changes have been merged and published in downstream modules
- [ ] I have checked my code and corrected any misspellings
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 1194 | # Basic Types
The following are basic geometric types and utilities, such as vectors and transforms, that are used all acoross the package. Using these types requires, in addition to the `using OpticSim` statement to also use the OpticSim.Geoemtry module like:
```@example
using OpticSim, OpticSim.Geometry
```
## Vec3
Representing a 3D vector.
```@docs
OpticSim.Geometry.Vec3
OpticSim.Geometry.unitX3
OpticSim.Geometry.unitY3
OpticSim.Geometry.unitZ3
```
## Vec4
Representing a 4D vector
```@docs
OpticSim.Geometry.Vec4
OpticSim.Geometry.unitX4
OpticSim.Geometry.unitY4
OpticSim.Geometry.unitZ4
OpticSim.Geometry.unitW4
```
## Transform
Representing a general 3D transform (4x4 matrix). Currently only used as a rigid-body transform.
Transforms are used to position surfaces within the CSG tree, position emitters in 3D, etc.
```@docs
OpticSim.Geometry.Transform
OpticSim.Geometry.identitytransform
OpticSim.Geometry.rotationX
OpticSim.Geometry.rotationY
OpticSim.Geometry.rotationZ
OpticSim.Geometry.rotation
OpticSim.Geometry.rotationd
OpticSim.Geometry.rotate
OpticSim.Geometry.translation
OpticSim.Geometry.rotmat
OpticSim.Geometry.rotmatd
OpticSim.Geometry.rotmatbetween
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 2073 | # Cloud Execution
## Azure
A key benefit and design motivation of OpticSim is being able to execute many simulations/optimizations at once.
This is best enabled through the use of cloud computing services such as Azure.
As part of the base package, we provide support for cloud execution using an [Azure Machine Learning](https://azure.microsoft.com/en-gb/free/machine-learning) workspace.
To use this functionally you'll first need to set up an AML workspace with a compute cluster. Then you'll need to provide a few bits of information to OpticSim:
- Subscription ID, of the form `XXXXXXXX-XXX-XXX-XXXX-XXXXXXXXXXXX`
- Resource group name
- Workspace name
- Compute cluster name
This information can be cached either to a specific file, or globally:
```@docs
OpticSim.Cloud.cache_run_config
OpticSim.Cloud.get_cached_run_config
```
You should also include an `.amlignore` file in the root of your project.
This is similar to a `.gitignore` file and should include any files which should not be uploaded to AML as part of your source snapshot, for examples `test/`.
!!! note
**`Manifest.toml` must be listed in your `.amlignore` file.**
If an `.amlignore` doesn't already exist then one will be created on the first submission of a run to AML.
Once everything is configured, you can submit a run:
```@docs
OpticSim.Cloud.submit_run_to_AML
```
To retrieve outputs from your run simply write files to the `outputs/` directory and the files will automatically appear as part of the AML run.
### Examples
```julia
using OpticSim.Cloud
cache_run_config([subscription_id], [resource_group_name], [workspace_name], [compute_name], [path_to_config])
submit_run_to_AML("example-run", [path_to_script], ["--arg1", "1", "--arg2", "2"], nothing, [path_to_config])
submit_run_to_AML("example-hyperdrive-run", [path_to_script], ["--arg1", "1"], Dict("--arg2" => ["1", "2", "3"]), [path_to_config])
```
## Other Cloud Services
Currently no other services are supported, though it should be reasonably straightforward to add similar functionality to that for AML.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 1952 | # CSG
## CSG Operations
There are three binary csg operations which can construct extremely complex objects from very simple primitives: union (``\cup``), intersection (``\cap``) and subtraction (i.e. difference).
This diagram shows the basic idea:
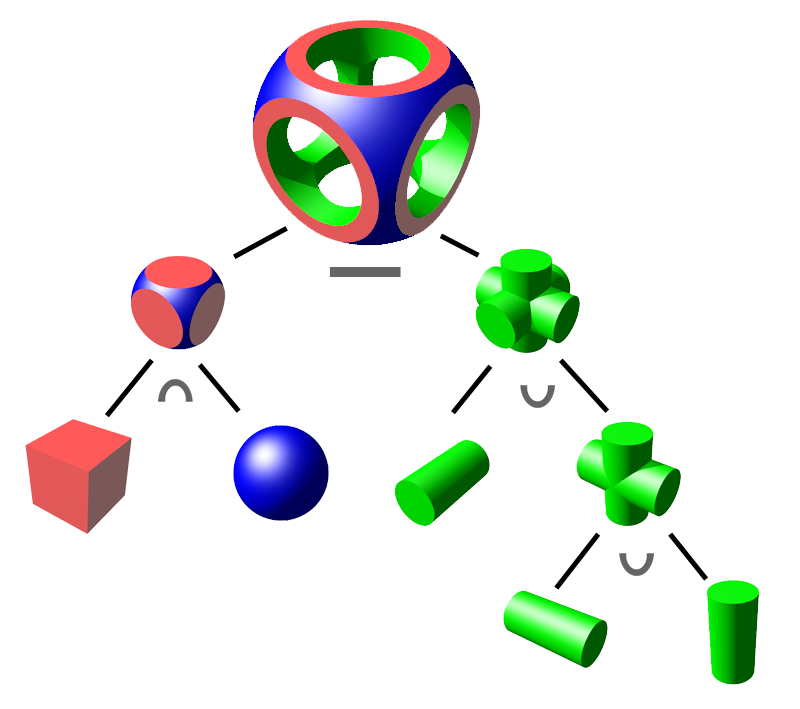
The code for this in our system would look this this:
```@example
using OpticSim # hide
cyl = Cylinder(0.7)
cyl_cross = cyl ∪ leaf(cyl, Geometry.rotationd(90, 0, 0)) ∪ leaf(cyl, Geometry.rotationd(0, 90, 0))
cube = Cuboid(1.0, 1.0, 1.0)
sph = Sphere(1.3)
rounded_cube = cube ∩ sph
result = rounded_cube - cyl_cross
Vis.draw(result, numdivisions=100)
Vis.save("assets/csg_ex.png"); nothing # hide
```

```@docs
leaf
∪
∩
-
```
## Pre-made CSG Shapes
There are also some shortcut methods available to create common CSG objects more easily:
```@docs
BoundedCylinder
Cuboid
HexagonalPrism
RectangularPrism
TriangularPrism
Spider
```
## CSG Types
These are the types of the primary CSG elements, i.e. the nodes in the CSG tree.
```@docs
OpticSim.CSGTree
OpticSim.CSGGenerator
OpticSim.ComplementNode
OpticSim.UnionNode
OpticSim.IntersectionNode
OpticSim.LeafNode
```
## Additional Functions and Types
These are the internal types and functions used for geometric/CSG operations.
### Functions
```@docs
surfaceintersection(::CSGTree{T}, ::AbstractRay{T,N}) where {T<:Real,N}
inside(a::CSGTree{T}, x::T, y::T, z::T) where {T<:Real}
onsurface(a::CSGTree{T}, x::T, y::T, z::T) where {T<:Real}
```
### Intervals
```@docs
Interval
EmptyInterval
DisjointUnion
OpticSim.isemptyinterval
OpticSim.ispositivehalfspace
OpticSim.israyorigininterval
OpticSim.halfspaceintersection
OpticSim.closestintersection
OpticSim.IntervalPool
```
### Intersections
```@docs
OpticSim.IntervalPoint
RayOrigin
Infinity
Intersection
OpticSim.isinfinity
OpticSim.israyorigin
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 8319 | # Emitters
!!! warning
**The old emitter implementation is deprecated as of v0.5!** See below for the new API.
Emitters create rays in a certain pattern, usually controlled by some parameters. Emitters are defined by Pixels and Spatial Layouts, and have a spectrum and an optical power distribution over the hemisphere. These are intrinsic physical properties of the emitter.
The **basic emitter** ([`Source`](@ref sources)) is constructed as a combination of 4 basic elements and a 3D [`Transform`](@ref). The basic elements include:
- [`Spectrum`](@ref spectrum)
- [`Angular Power Distribution`](@ref angular_power_distribution)
- [`Rays Origins Distribution`](@ref rays_origins_distribution)
- [`Rays Directions Distribution`](@ref rays_directions_distribution)
The [`OpticSim`](index.html) package comes with various implementations of each of these basic elements:
- Spectrum - the **generate** interface returns a tuple (power, wavelength)
* [`Emitters.Spectrum.Uniform`](@ref) - A flat spectrum bounded (default: from 450nm to 680nm). the range is sampled uniformly.
* [`Emitters.Spectrum.DeltaFunction`](@ref) - Constant wave length.
* [`Emitters.Spectrum.Measured`](@ref) - measured spectrum to compute emitter power and wavelength (created by reading CSV files – more details will follow).
- Angular Power Distribution - the interface **apply** returns an OpticalRay with modified power
* [`Emitters.AngularPower.Lambertian`](@ref)
* [`Emitters.AngularPower.Cosine`](@ref)
* [`Emitters.AngularPower.Gaussian`](@ref)
- Rays Origins Distribution - the interface **length** returns the number of samples, and **generate** returns the n'th sample.
* [`Emitters.Origins.Point`](@ref) - a single point
* [`Emitters.Origins.RectUniform`](@ref) - a uniformly sampled rectangle with user defined number of samples
* [`Emitters.Origins.RectGrid`](@ref) - a rectangle sampled in a grid fashion
* [`Emitters.Origins.Hexapolar`](@ref) - a circle (or an ellipse) sampled in an hexapolar fashion (rings)
- Rays Directions Distribution - the interface **length** returns the number of samples, and **generate** returns the n'th sample.
* [`Emitters.Directions.Constant`](@ref)
* [`Emitters.Directions.RectGrid`](@ref)
* [`Emitters.Directions.UniformCone`](@ref)
* [`Emitters.Directions.HexapolarCone`](@ref)
## [Examples of Basic Emitters](@id basic_emitters)
**Note**: All of the examples on this page assume that the following statement was executed:
```@example
dummy = "switching to WGLMakie in order to produce interactive figures with Makie" # hide
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
OpticSim.NotebooksUtils.SetDocsBackend("Web") # hide
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters
```
### Simple functions for creating commonly used emitters
Many optical systems by convention have their optical axis parallel to the z axis. These utility functions provide a simple interface to the Emitters package to create emitters that direct their rays in the negative z direction, toward the entrance of the optical system.
```@docs
Emitters.pointemitter
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
pt = Emitters.pointemitter([0.0,0.0,.5],.3)
Vis.draw(pt, debug=true, resolution = (800, 600))
```
```@docs
Emitters.collimatedemitter
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
pt = Emitters.collimatedemitter([0.0,0.0,1.0],.5)
Vis.draw(pt, debug=true, resolution = (800, 600))
```
### Point origin with various Direction distributions
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(origins=Origins.Point(), directions=Directions.RectGrid(π/4, π/4, 15, 15))
Vis.draw(src, debug=true, resolution = (800, 600))
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(origins=Origins.Point(), directions=Directions.UniformCone(π/6, 1000))
Vis.draw(src, debug=true, resolution = (800, 600))
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(origins=Origins.Point(), directions=Directions.HexapolarCone(π/6, 10))
Vis.draw(src, debug=true, resolution = (800, 600))
```
### Various origins distributions
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(origins=Origins.RectGrid(1.0, 1.0, 10, 10), directions=Directions.Constant())
Vis.draw(src, debug=true, resolution = (800, 600))
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(origins=Origins.Hexapolar(5, 1.0, 2.0), directions=Directions.Constant())
Vis.draw(src, debug=true, resolution = (800, 600))
```
### Examples of Angular Power Distribution
In these example, the arrow width is proportional to the ray power.
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(
origins=Origins.Hexapolar(1, 8.0, 8.0), # Hexapolar Origins
directions=Directions.RectGrid(π/6, π/6, 15, 15), # RectGrid Directions
power=AngularPower.Cosine(10.0) # Cosine Angular Power
)
Vis.draw(src, debug=true, resolution = (800, 600))
```
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
src = Sources.Source(
origins=Origins.RectGrid(1.0, 1.0, 3, 3), # RectGrid Origins
directions=Directions.HexapolarCone(π/6, 10), # HexapolarCone Directions
power=AngularPower.Gaussian(2.0, 2.0) # Gaussian Angular Power
)
Vis.draw(src, debug=true, resolution = (800, 600))
```
### Composite Sources - Display Example
```@example
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
# construct the emitter's basic components
S = Spectrum.Uniform()
P = AngularPower.Lambertian()
O = Origins.RectGrid(1.0, 1.0, 3, 3)
D = Directions.HexapolarCone(deg2rad(5.0), 3)
# construct the source. in this example a "pixel" source will contain only one source as we are simulating a "b/w" display.
# for RGB displays we can combine 3 sources to simulate "a pixel".
Tr = Transform(Vec3(0.5, 0.5, 0.0))
source1 = Sources.Source(Tr, S, O, D, P)
# create a list of pixels - each one is a composite source
pixels = Vector{Sources.CompositeSource{Float64}}(undef, 0)
for y in 1:5 # image_height
for x in 1:10 # image_width
# pixel position relative to the display's origin
local pixel_position = Vec3((x-1) * 1.1, (y-1) * 1.5, 0.0)
local Tr = Transform(pixel_position)
# constructing the "pixel"
pixel = Sources.CompositeSource(Tr, [source1])
push!(pixels, pixel)
end
end
Tr = Transform(Vec3(0.0, 0.0, 0.0))
my_display = Sources.CompositeSource(Tr, pixels)
Vis.draw(my_display) # render the display - nothing but the origins primitives
rays = AbstractArray{OpticalRay{Float64, 3}}(collect(my_display)) # collect the rays generated by the display
Vis.draw!(rays) # render the rays
```
```@example
dummy = "switching Back to the GLMakie to allow the rest of the pages to work with static figures" # hide
using OpticSim, OpticSim.Geometry, OpticSim.Emitters # hide
OpticSim.NotebooksUtils.SetDocsBackend("Static") # hide
```
## [Spectrum](@id spectrum)
```@docs
Emitters.Spectrum.Uniform
Emitters.Spectrum.DeltaFunction
Emitters.Spectrum.Measured
Emitters.Spectrum.spectrumpower
```
## [Angular Power Distribution](@id angular_power_distribution)
```@docs
Emitters.AngularPower.Lambertian
Emitters.AngularPower.Cosine
Emitters.AngularPower.Gaussian
```
## [Rays Origins Distribution](@id rays_origins_distribution)
```@docs
Emitters.Origins.Point
Emitters.Origins.RectUniform
Emitters.Origins.RectGrid
Emitters.Origins.Hexapolar
```
## [Rays Directions Distribution](@id rays_directions_distribution)
```@docs
Emitters.Directions.Constant
Emitters.Directions.RectGrid
Emitters.Directions.UniformCone
Emitters.Directions.HexapolarCone
```
## [Sources](@id sources)
```@docs
Emitters.Sources.Source
Emitters.Sources.CompositeSource
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 2926 | # Examples
```@setup highlight
using CodeTracking, Markdown, OpticSim.Examples
mdparse(s) = Markdown.parse("```julia\n$s\n```")
```
```@setup example
using OpticSim.Examples
```
## Pluto Notebooks
The [`OpticSim`](index.html) package comes with several [`Pluto`](https://github.com/fonsp/Pluto.jl) notebooks (code snippets are coming soon) that allow the user to change and run sample code and view the results in real-time. We highly recommend for you to try these out.
The notebooks are located in the **samples** folder, and you can try them by running:
```julia
import OpticSim.NotebooksUtils as NB # the **as** option was added in Julia v1.6
NB.run_sample("EmittersIntro.jl")
```
The **run_sample** method will **copy** the notebook to your current folder (if it does not exist) and launch Pluto to run the notebook in the browser.
## Cooke Triplet
```@example highlight
mdparse(@code_string draw_cooketriplet()) # hide
```
```@example example
sys = draw_cooketriplet("assets/cooke.png"); @show sys; nothing # hide
```

## Zoom Lens
```@example highlight
mdparse(@code_string draw_zoomlenses()) # hide
```
```@example example
syss = draw_zoomlenses(["assets/zoom$i.png" for i in 1:3]); @show syss[1]; nothing # hide
```



## Schmidt Cassegrain Telescope
```@example highlight
mdparse(@code_string draw_schmidtcassegraintelescope()) # hide
```
```@example example
draw_schmidtcassegraintelescope("assets/tele.png") # hide
```

## Lens Construction
```@example highlight
mdparse(@code_string draw_lensconstruction()) # hide
```
```@example example
draw_lensconstruction("assets/qtype.png") # hide
```

## HOEs
### Focusing
```@example highlight
mdparse(@code_string draw_HOEfocus()) # hide
```
```@example example
draw_HOEfocus("assets/hoe_f.png") # hide
```

### Collimating
```@example highlight
mdparse(@code_string draw_HOEcollimate()) # hide
```
```@example example
draw_HOEcollimate("assets/hoe_c.png") # hide
```

### Multi
```@example highlight
mdparse(@code_string draw_multiHOE()) # hide
```
```@example example
draw_multiHOE("assets/hoe_m.png") # hide
```

## Deterministic Raytracing
```@example highlight
mdparse(@code_string draw_stackedbeamsplitters()) # hide
```
```@example example
draw_stackedbeamsplitters(["assets/deterministic_trace_$i.png" for i in 1:3]) # hide
```


 | OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 4155 | # GlassCat
This submodule is used to download, parse, install and manage AGF glass specifications for use in OpticSim.
The central configuration file for GlassCat is located at `src/GlassCat/data/sources.txt`, which ships with the
following default entries.
```
NIKON a49714470fa875ad4fd8d11cbc0edbf1adfe877f42926d7612c1649dd9441e75 https://www.nikon.com/products/components/assets/pdf/nikon_zemax_data.zip
OHARA 0c9021bf11b8d4e660012191818685ad3110d4f9490699cabdc89aae1fd26d2e https://www.oharacorp.com/xls/OHARA_201130_CATALOG.zip
HOYA b02c203e5a5b7a8918cc786badf0a9db1fe2572372c1c163dc306b0a8a908854 http://www.hoya-opticalworld.com/common/agf/HOYA20210105.agf
SCHOTT e9aabbb8ebff116ba0c106a71afd86e72f2a397ac9bc447469129e325e795f4e https://www.schott.com/d/advanced_optics/6959f9a4-0e4f-4ef2-a302-2347468a82f5/1.31/schott-optical-glass-overview-zemax-format.zip
SUMITA c1093e42a1d08acbe30698aba730161e3b43f8f0d50533f65de8b6b11100fdc8 https://www.sumita-opt.co.jp/en/wp/wp-content/themes/sumita-opt-en/download-files.php files%5B%5D=new_sumita.agf&category=data
```
Each line corresponds to one AGF source, which is described by 2 to 4 space-delimited columns. The first column provides
the installed module name for the catalog, e.g. `GlassCat.NIKON`. The second column is the expected SHA256 checksum for
the AGF file.
The final two columns are optional, specifying download instructions for acquiring the zipped AGF files
automatically from the web. The fourth column allows us to use POST requests to acquire files from interactive sites.
When `] build OpticSim` is run, the sources are verified and parsed into corresponding Julia files. These are then
included in OpticSim via `AGFGlassCat.jl`. These steps are run automatically when the package is first installed using
`] add OpticSim`, creating a sufficient working environment for our examples and tests.
## Adding glass catalogs
`sources.txt` can be edited freely to add more glass catalogs. However, this is a somewhat tedious process, so we have a
convenience function for adding a locally downloaded AGF file to the source list.
```@docs
OpticSim.GlassCat.add_agf
```
## Using installed glasses
Glass types are accessed like so: `OpticSim.GlassCat.CATALOG_NAME.GLASS_NAME`, e.g.
```julia
OpticSim.GlassCat.SUMITA.LAK7
OpticSim.GlassCat.SCHOTT.PK3
```
All glasses and catalogs are exported in their respective modules, so it is possible to invoke `using` calls for convenience, e.g.
```julia
using OpticSim
GlassCat.SUMITA.LAK7
using OpticSim.GlassCat
SCHOTT.PK3
using OpticsSim.GlassCat.SCHOTT
N_BK7
```
Autocompletion can be used to see available catalogs and glasses. All catalog glasses are of type [`OpticSim.GlassCat.Glass`](@ref).
Note that special characters in glass/catalog names are replaced with `_`.
There is a special type and constant value for air: [`OpticSim.GlassCat.Air`](@ref).
[Unitful.jl](https://github.com/PainterQubits/Unitful.jl) is used to manage units, meaning any valid unit can be used for all arguments, e.g., wavelength can be passed in as μm or nm (or cm, mm, m, etc.).
Non-unitful options are also available, in which case units are assumed to be μm, °C and Atm for length, temperature and pressure respectively.
`TEMP_REF` and `PRESSURE_REF` are constants:
```julia
const TEMP_REF = 20.0 # °C
const PRESSURE_REF = 1.0 # Atm
```
## Types
```@docs
OpticSim.GlassCat.AbstractGlass
OpticSim.GlassCat.Glass
OpticSim.GlassCat.Air
OpticSim.GlassCat.GlassID
```
## Functions
```@docs
OpticSim.GlassCat.index
OpticSim.GlassCat.absairindex
OpticSim.GlassCat.absorption
```
---
```@docs
OpticSim.GlassCat.glassfromMIL
OpticSim.GlassCat.modelglass
```
---
```@docs
OpticSim.GlassCat.glasscatalogs
OpticSim.GlassCat.glassnames
OpticSim.GlassCat.info
OpticSim.GlassCat.findglass
OpticSim.GlassCat.isair
```
---
```@docs
OpticSim.GlassCat.glassname
OpticSim.GlassCat.glassid
OpticSim.GlassCat.glassforid
```
---
```@docs
OpticSim.GlassCat.polyfit_indices
OpticSim.GlassCat.plot_indices
OpticSim.GlassCat.drawglassmap
```
---
```@docs
OpticSim.GlassCat.verify_sources!
OpticSim.GlassCat.verify_source
OpticSim.GlassCat.download_source
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 3958 | # OpticSim.jl
OpticSim.jl is a [Julia](https://julialang.org/) package for geometric optics (ray tracing) simulation and optimization of complex optical systems developed by the Microsoft Research Interactive Media Group and the Microsoft Hardware Architecture Incubation Team (HART).
It is designed to allow optical engineers to create optical systems procedurally and then to simulate and optimize them. Unlike Zemax, Code V, or other interactive optical design systems OpticSim.jl has limited support for interactivity, primarily in the tools for visualizing optical systems.
A large variety of surface types are supported, and these can be composed into complex 3D objects through the use of constructive solid geometry (CSG). A complete catalog of optical materials is provided through the complementary GlassCat submodule.
This software provides extensive control over the modelling, simulation, visualization and optimization of optical systems. It is especially suited for designs that have a procedural architecture.
## Installation
Install the Julia programming language from the [official download page](https://julialang.org/downloads/).
OpticSim.jl is optimized for use with Julia 1.7.x; using other versions will probably not work.
The system will automatically download glass catalog (.agf) files from some manufacturers when the package is built for the first time. These files are in an industry standard format and can be downloaded from many optical glass manufacturers.
Here are links to several publicly available glass files:
* [NIKON](https://www.nikon.com/products/components/assets/pdf/nikon_zemax_data.zip) (automatically downloaded)
* [NHG](http://hbnhg.com/down/data/nhgagp.zip) (you have to manually download)
* [OHARA](https://www.oharacorp.com/xls/OHARA_201130_CATALOG.zip) (automatically downloaded)
* [HOYA](https://hoyaoptics.com/wp-content/uploads/2019/10/HOYA20170401.zip) (automatically downloaded)
* [SUMITA](https://www.sumita-opt.co.jp/en/download/) (automatically downloaded)
* [SCHOTT](https://www.schott.com/advanced_optics/english/download/index.html) (automatically downloaded)
OpticSim.jl will generate a glass database from the available files in `deps/downloads/glasscat/` and store it in the file `AGFClassCat.jl`. See [GlassCat](@ref) for a detailed description, including instructions on how to add more catalogs.
Run this example to check that everything installed properly:
```@example
using OpticSim
Vis.draw(SphericalLens(OpticSim.GlassCat.SCHOTT.N_BK7, 0.0, 10.0, 10.0, 5.0, 5.0))
Vis.save("assets/test_install.png") # hide
nothing # hide
```

## System Image
If you are using Julia 1.5, we recommend compiling a custom [Julia system image](https://julialang.github.io/PackageCompiler.jl/dev/sysimages) for the OpticSim.jl package to reduce startup time and improve first-time performance.
With VSCode, you can create a sysimage by opening the commant palette (CTRL-shift-P) and selecting `Tasks: Run Build Task, julia: Build custom sysimage for current environment`.
Alternatively, we provide a Julia script that will build the sysimage using a representative workload. To do this, activate a Julia environment which has OpticSim installed and run
```julia
include("deps/sysimage.jl")
compile()
```
By default, the sysimage is located in the current working directory. On Linux, it will be called `JuliaSysimage.so`; on Windows, the extension will be `.dll`. A custom path can be used instead which is passed as an argument to `compile()`.
To use the generated system image, run Julia with the `--sysimage` flag:
```bash
julia --project=[your_project] --sysimage=[path_to_sysimage]
```
If OpticSim.jl is installed in the base project, then there is no need for the `--project` flag in the above command. If your current working directory is OpticSim.jl, then you can use the `--project` flag without needing to specify an argument.
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 459 | # Optical Interfaces
Every [`Surface`](@ref) must have an `OpticalInterface` associated with it to defined the behavior of any ray when it intersects that surface.
```@docs
OpticSim.OpticalInterface
OpticSim.NullInterface
FresnelInterface
ParaxialInterface
ThinGratingInterface
HologramInterface
MultiHologramInterface
```
The critical behavior of each interface is defined in the `processintersection` function:
```@docs
OpticSim.processintersection
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 979 | # Lenses and Other Optical Components
## Lenses
A number of helper functions are provided to make constructing simple lenses easier.
Firstly ordinary thick lenses:
```@docs
SphericalLens
ConicLens
AsphericLens
FresnelLens
```
As well as idealized lenses:
```@docs
ParaxialLens
```
## Other Components
We also have some holographic elements implemented, note that these have not been extensively tested and should not be treated as wholely accurate at this stage.
It is relatively simple to extend the existing code to add these kinds of specialized surfaces providing a paired [`OpticalInterface`](@ref) subclass is also defined. In this case the `WrapperSurface` can often serve as a suitable base for extension.
```@docs
WrapperSurface
ThinGratingSurface
HologramSurface
MultiHologramSurface
```
## Eye Models
Eye models are often very useful in simulation of head mounted display systems. We have two models implemented currently.
```@docs
ModelEye
ArizonaEye
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 127 | # Notebook utilities
```@meta
CurrentModule = NotebooksUtils
```
[TODO]
```@docs
run_sample
SetBackend
run
InitNotebook
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 3177 | # Optimization
We are currently in the early stages of implementing optimization for lens surfaces.
We have had success using [Optim.jl](https://julianlsolvers.github.io/Optim.jl/stable/) and [ForwardDiff.jl](http://www.juliadiff.org/ForwardDiff.jl/stable/) among other packages, though ForwardDiff.jl gradient and hessian pre-compilation can be very slow for complex systems.
Other optimization packages _should_ integrate easily with the system too: [JuMP.jl](https://github.com/jump-dev/JuMP.jl), [Ipopt.jl](https://github.com/jump-dev/Ipopt.jl) and [NLOpt.jl](https://github.com/JuliaOpt/NLopt.jl) are some options.
Merit functions must currently be implemented by the user, this is quite straight-forward as [`trace`](@ref) returns the vast majority of information that could be needed in the form of a [`LensTrace`](@ref) which hits the detector (or `nothing` if the ray doesn't reach the detector).
There are some helper functions implemented for [`AxisymmetricOpticalSystem`](@ref)s which can make optimization of basic systems much easier:
```@docs
OpticSim.Optimization
OpticSim.Optimization.optimizationvariables
OpticSim.Optimization.updateoptimizationvariables
```
It is of course possible to write your own optimization loop for more complex (i.e. non-AxisymmetricOpticalSystem) systems and this _should_ work without issue.
## Example
```julia
using OpticSim
using ForwardDiff
using Optim
function objective(a::AbstractVector{T}, b::AxisymmetricOpticalSystem{T}, samples::Int = 3) where {T}
# RMSE spot size
system = Optimization.updateoptimizationvariables(b, a)
# distribute rays evenly across entrance pupil using HexapolarField. The latest version of OpticSim no longer supports HexapolarField.
field = HexapolarField(system, collimated = true, samples = samples)
error = zero(T)
hits = 0
for r in field
traceres = trace(system, r, test = true)
if traceres !== nothing # ignore rays which miss
hitpoint = point(traceres)
if abs(hitpoint[1]) > eps(T) && abs(hitpoint[2]) > eps(T)
dist_to_axis = hitpoint[1]^2 + hitpoint[2]^2
error += dist_to_axis
end
hits += 1
end
end
if hits > 0
error = sqrt(error / hits)
end
# if hits == 0 returns 0 - not ideal!
return error
end
start, lower, upper = Optimization.optimizationvariables(system)
optimobjective = arg -> objective(arg, system)
gcfg = ForwardDiff.GradientConfig(optimobjective, start, ForwardDiff.Chunk{1}()) # speed up ForwardDiff significantly
hcfg = ForwardDiff.HessianConfig(optimobjective, start, ForwardDiff.Chunk{1}())
g! = (G, x) -> ForwardDiff.gradient!(G, optimobjective, x, gcfg)
h! = (H, x) -> ForwardDiff.hessian!(H, optimobjective, x, hcfg)
df = TwiceDifferentiable(optimobjective, g!, h!, start)
dfc = TwiceDifferentiableConstraints(lower, upper) # constrain the optimization to avoid e.g. thickness < 0
res = optimize(df, dfc, start, algo, Optim.Options(show_trace = true, iterations = 100, allow_f_increases = true))
final = Optim.minimizer(res)
new_system = Optimization.updateoptimizationvariables(system, final)
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 2842 | # Primitives
All geometry is built up from a small(ish) number of primitives and a number of constructive solid geometry (CSG) operations (see [CSG](@ref)).
Primitives are split into two types, `Surface`s and `ParametricSurface`s, the latter being a subset of the former.
`Surface`s are standalone surfaces which cannot be used in CSG operations, e.g. an aperture or rectangle.
`ParametricSurface`s are valid csg objects and can be composed into very complex structures.
## Surfaces
A surface can be any surface in 3D space, it can be bounded and not create a half-space (i.e. not partition space into _inside_ and _outside_).
```@docs
Surface
```
### Basic Shapes
These are the simple shapes with are provided already, they act only as standalone objects and cannot be used in CSG objects.
Adding a new `Surface` is easy, the new structure must simply follow the interface defined above.
```@docs
Ellipse
Circle
Rectangle
Hexagon
Triangle
TriangleMesh
```
### Stops
A number of simple occlusive apertures are provided as constructing such objects using CSG can be inefficient and error-prone.
```@docs
InfiniteStop
FiniteStop
RectangularAperture
CircularAperture
Annulus
```
## Parametric Surfaces
A parametric surface must partition space into two valid half-spaces, i.e. _inside_ and _outside_.
The surface must also be parameterized by two variables, nominally `u` and `v`.
Typically these surfaces cannot be intersected with a ray analytically and so must be triangulated and an iterative solution found.
```@docs
ParametricSurface
AcceleratedParametricSurface
```
### Parametric Surface Types
These are the available types of parametric surfaces which are already implemented, all of which can be used in the creation of CSG objects.
New `ParametricSurface`s can be added with relative ease providing they follow the interface defined above.
```@docs
Cylinder
Plane
Sphere
SphericalCap
AsphericSurface
ZernikeSurface
BezierSurface
BSplineSurface
QTypeSurface
ChebyshevSurface
GridSagSurface
```
## Functions
These are some useful functions related to `Surface` objects.
```@docs
point(::ParametricSurface{T}, ::T, ::T) where {T<:Real}
normal
partials(::ParametricSurface{T}, ::T, ::T) where {T<:Real}
uvrange
uv
OpticSim.uvtopix
inside(s::ParametricSurface{T,3}, x::T, y::T, z::T) where {T<:Real}
onsurface(s::ParametricSurface{T,3}, x::T, y::T, z::T) where {T<:Real}
interface
surfaceintersection(surf::AcceleratedParametricSurface{S,N}, r::AbstractRay{S,N}) where {S,N}
samplesurface
triangulate
makemesh
```
## Bounding Boxes
Bounding boxes are mostly used internally for efficiency, but are also exposed to the user for visualization (and any other) purposes.
All bounding boxes are axis aligned.
```@docs
BoundingBox
doesintersect
surfaceintersection(::BoundingBox{T}, ::AbstractRay{T,3}) where {T<:Real}
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 529 | # Complete Reference
This page contains what should be a complete list of all docstrings in the OpticSim module, and its submodule.
## Index
```@index
Pages = ["ref.md"]
```
## OpticSim
```@autodocs
Modules = [OpticSim]
```
## Geometry
```@autodocs
Modules = [OpticSim.Geometry]
```
## Zernike
```@autodocs
Modules = [OpticSim.Zernike]
```
## QType
```@autodocs
Modules = [OpticSim.QType]
```
## Chebyshev
```@autodocs
Modules = [OpticSim.Chebyshev]
```
## Examples
```@autodocs
Modules = [OpticSim.Examples]
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 2347 | # Repeating Structures
The Repeat module contains functions for creating regular repeated patterns. This could be pixels in a display grid, or mirrors in an active optics telescope. Repeated patterns are defined by creating an object that inherits from the abstract type [`Basis`](@ref sources).
Subtypes supporting the Basis interface should implement these functions:
Returns the neighbors in ring n surrounding centerpoint, excluding centerpoint
```
neighbors(::Type{B},centerpoint::Tuple{T,T},neighborhoodsize::Int) where{T<:Real,B<:Basis}
```
Returns the lattice basis vectors that define the lattice
```
basis(a::S) where{S<:Basis}
```
Returns the vertices of the unit polygon for the basis that tiles the plane
```
tilevertices(a::S) where{S<:Basis}
```
A lattice is described by a set of lattice vectors eᵢ which are stored in a [`Basis`](@ref sources) object. You can create bases in any dimension. Points in the lattice are indexed by integer coordinates. These lattice coordinates can be converted to Cartesian coordinates by indexing the LatticeBasis object.
``` @example example
using OpticSim, OpticSim.Repeat
a = LatticeBasis((1.0,5.0),(0.0,1.0))
a[3,3]
```
The Lattice points are defined by a weighted sum of the basis vectors:
```
latticepoint = ∑αᵢ*eᵢ
```
where the αᵢ are integer weights.
The [`HexBasis1`](@ref sources) constructor defines a symmetric basis for hexagonal lattices
```@example
using OpticSim, OpticSim.Repeat
basis(HexBasis1())
```
The [`rectangularlattice`](@ref sources) function creates a rectangular lattice basis.
There are a few visualization functions for special 2D lattices. [`Vis.drawcells`](@ref sources) draws a set of hexagonal cells. Using [`Repeat.hexcellsinbox`](@ref sources) we can draw all the hexagonal cells that fit in a rectangular box:
```@example
using OpticSim
Vis.drawcells(Repeat.HexBasis1(),50,Repeat.hexcellsinbox(2,2))
```
There is also a function to compute the n rings of a cell x, i.e., the cells which can be reached by taking no more than n steps along the lattice from x:
```@example
using OpticSim
Vis.drawcells(Repeat.HexBasis1(),50,Repeat.neighbors(Repeat.HexBasis1,(0,0),2))
```
You can also draw all the cells contained within an n ring:
```@example
using OpticSim
Vis.drawcells(Repeat.HexBasis1(),50,Repeat.region(Repeat.HexBasis1,(0,0),2))
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 1177 | # Roadmap
OpticSim.jl is still under active development. Here are things we are considering:
## User Interface
- Improvements to visualization tools
- Better control of 3D/2D system views
- More drawing options (e.g. wireframe)
- More analysis tools e.g. grids of spot diagrams, OPD diagrams etc.
- Interactive editor, probably in a spreadsheet format or similar
- Easily usable optimization features
## Optimization
- Performance improvements
- More rigorous local optimization (better parameter limits)
- Global optimization/smart initialization
- Better interface (particularly for merit function design)
## Raytracing
- Improvements to ray energy accuracy
- Improve HOE implementations
- Finish implementation of Bezier and BSpline surfaces (mostly done)
## Other
- Properly support Julia 1.6 once released
- Add automatic 'run on azure' options
- Some long standing bug fixes/improvements to implementations
## Long-Term
- Simulation of gradient index (GRIN) materials
- Simulation of meta-materials
- CSG file import/export
- Simulate physical effects of thermal variation (physical expansion)
- Support polarization of rays and elements relying on this
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 952 | # Optical Systems
## Assemblies
All systems are made up of a `LensAssembly` which contains all the optical components in the system, excluding any sources (see [Emitters](@ref)) and the detector (see below).
```@docs
LensAssembly
```
## Images
The detector image is stored within the system as a `HierarchicalImage` for memory efficiency.
```@docs
HierarchicalImage
OpticSim.reset!
OpticSim.sum!
```
## Systems
There are two types of `AbstractOpticalSystem` which can be used depending on the requirements.
```@docs
AbstractOpticalSystem
CSGOpticalSystem
AxisymmetricOpticalSystem
temperature
pressure
detectorimage
resetdetector!
assembly
semidiameter
```
## Tracing
We can trace an individual [`OpticalRay`](@ref) through the system (or directly through a [`LensAssembly`](@ref)), or we can trace using an [`OpticalRayGenerator`](@ref) to create a large number of rays.
```@docs
trace
traceMT
tracehits
tracehitsMT
OpticSim.LensTrace
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 0.6.0 | 525b470e4e94930a59024823b40a24756c750a89 | docs | 3012 | # Visualization
There are a number of powerful visualization tools available, we primarily rely on 3D visualization of systems using [Makie](http://makie.juliaplots.org/stable/).
There are a number of helper methods, as well as the ability to draw objects, surfaces, points, rays and more individually. For example, looking at rays passing through a system in 3D and 2D:
```julia
Vis.drawtracerays(Examples.cooketriplet(), trackallrays=true, test=true, numdivisions=100)
```
```@setup base
using OpticSim
```
```@example base
Vis.drawtracerays(Examples.cooketriplet(), trackallrays=true, test=true, numdivisions=100)
Vis.save("assets/vis_ex_3d.png") # hide
Vis.drawtracerays(Examples.cooketriplet(), trackallrays=true, test=true, numdivisions=100, drawsys=true, resolution = (1000, 700))
Vis.make2dy()
Vis.save("assets/vis_ex_2d.png"); nothing #hide
```


And the image on the detector for a trace of a system:
```julia
Vis.drawtraceimage(Examples.cooketriplet(), test=true)
```
```@example base
using Images # hide
im = Vis.drawtraceimage(Examples.cooketriplet(Float64, 400), test=true)
save("assets/vis_ex_im.png", colorview(Gray, real.(im ./ maximum(im)))); nothing # hide
```

## Basic Drawing
These methods are all you need to build up a visualization piece by piece.
For example:
```@example base
obj = (Sphere(0.5) ∩ Plane(0.0, 1.0, 0.0, 0.0, 0.1, 0.0))()
ray1 = Ray([0.0, -0.1, 1.0], [0.0, 0.0, -1.0])
ray2 = Ray([0.8, 0.0, 0.0], [-1.0, 0.0, 0.0])
Vis.draw(obj)
Vis.draw!(ray1, rayscale=0.2)
Vis.draw!(ray2, rayscale=0.2, color=:blue)
Vis.draw!(surfaceintersection(obj, ray1), color=:red)
Vis.draw!(surfaceintersection(obj, ray2), color=:green)
Vis.save("assets/vis_ex_3d_parts.png"); nothing # hide
```

```@docs
OpticSim.Vis.scene
OpticSim.Vis.draw
OpticSim.Vis.draw!(::Any; kwargs...)
OpticSim.Vis.save
```
## Helper Methods
These are the helper methods to provide common visualizations more easily, as used above. Another example:
```julia
Vis.surfacesag(AcceleratedParametricSurface(TestData.zernikesurface2()), (256, 256), (1.55, 1.55))
```
```@example base
using Plots; include("../../test/TestData/TestData.jl") # hide
p = Vis.surfacesag(AcceleratedParametricSurface(TestData.zernikesurface2()), (256, 256), (1.55, 1.55))
Plots.savefig(p, "assets/surface_sag.svg"); nothing # hide
```

```@docs
OpticSim.Vis.drawtracerays
OpticSim.Vis.drawtraceimage
OpticSim.Vis.spotdiag
OpticSim.Vis.surfacesag
OpticSim.Vis.eyebox_eval_eye
OpticSim.Vis.eyebox_eval_planar
```
## Complete Drawing Functions
As mentioned above, `Vis.draw!` can be used to draw a large variety of objects, each with their own additional arguments.
Here is a full list of the available drawing function and their associated options.
```@docs
OpticSim.Vis.draw!
```
| OpticSim | https://github.com/brianguenter/OpticSim.jl.git |
|
[
"MIT"
]
| 1.1.0 | d36e5f4b91f8fd4a0aea50927cdb98492d3d318e | code | 25284 | module PerfectPacking
export Alg, rectanglePacking, backtracking, integerProgramming, dancingLinks
using ProgressMeter
using JuMP
using HiGHS
using DataStructures
@enum Alg begin
backtracking = 1
integerProgramming = 2
dancingLinks = 3
end
"""
rectanglePacking(h, w, rects, rot, alg)
Decides if perfect rectangle packing is possible and if so return it.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
- `rot`: If rectangles are allowed to be rotated by 90 degrees
- `alg`: Which exhaustive algorithm to use
"""
function rectanglePacking(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}}, rot::Bool, alg::Alg)
# verify that area is valid
totalArea = 0
for i in rects
totalArea += i[1] * i[2]
end
if totalArea != h * w
println("Total area of rectangles (" * string(totalArea) * ") is unequal to area of bounding rectangle (" * string(h * w) * ").")
return false
end
# verify that all rectangles fit
for i in rects
if !rot
if i[1] > h || i[2] > w
println("Not all rectangles fit in bounding rectangle.")
return false
end
else
if max(i[1], i[2]) > max(h, w) || min(i[1], i[2]) > min(h, w)
println("Not all rectangles fit in bounding rectangle.")
return false
end
end
end
result = false
output = nothing
if alg == backtracking
result, output = runBacktracking(h, w, rects, rot)
elseif alg == integerProgramming
result, output = runIntegerProgramming(h, w, rects, rot)
elseif alg == dancingLinks
result, output = runDancingLinks(h, w, rects, rot)
end
return result, output
end
"""
runBacktracking(h, w, rects, rot)
Perfect rectangle packing using backtracking with top-left heuristic. The rectangles are sorted
by width and in each step the frist free tile beginning on the top-left is covered. If rotation
is allowed, squares need to handeled seperatly.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
- `rot`: If rectangles are allowed to be rotated by 90 degrees
"""
function runBacktracking(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}}, rot::Bool)
if rot
# filter out squares, since they don't need to be rotated
squares = filter(x -> x[1] == x[2], rects)
filter!(x -> x[1] != x[2], rects)
sort!(rects, rev=true, by = x -> x[2]) # sort rectangles with descending width in preparation for top-left heuristic
switch = x->Pair(x[2], x[1]) # generate rotated version of rectangles
rectsRot = reverse(switch.(rects))
return solveBacktrackingRot(h, w, vcat(rects, squares, rectsRot), length(rects))
else
sort!(rects, rev=true, by = x -> x[2]) # sort rectangles with descending width in preparation for top-left heuristic
return solveBacktracking(h, w, rects)
end
end
"""
solveBacktracking(h, w, rects)
Perfect rectangle packing without rotations using backtracking with top-left heuristic. The
concept of using the height in each row to determine validity of a placement is an unpublished
idea of Prof. Dimitri Leemans at the Université libre de Bruxelles.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
"""
function solveBacktracking(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}})
s = length(rects)
prog = Progress(s-1, "Top nodes explored: ") # number of rectangles already tried as first rectangle
height = fill(0, h) # number of tiles covered in each row
used = fill(0, s) # rectangles used
coords = Vector{Pair{Int64, Int64}}() # remember coordinates
count = 0 # number of rectangles used
x = y = kStart = 1
while true
# Try to place a rectangle on (x, y)
done = false
k = kStart # only rectangles after kStart are allowed
while k <= s && !done
if used[k] == 0 && (x + rects[k][1] - 1 <= h && y + rects[k][2] - 1 <= w) # piece not used and fits
done = true
# check permiter of rectangle for collisions with other rectangles
for l = 1 : rects[k][1] - 1
if height[x+l] > height[x]
done = false
break
end
end
if !done
k += 1
end
else
k += 1 # try next piece
end
end
if done # rectangle k can be placed on (x, y)
push!(coords, Pair(x, y))
height[x : x + rects[k][1] - 1] .+= rects[k][2] # add rectangle
if count == 0 # choice for first rectangle placed is switched
ProgressMeter.update!(prog, k-1)
end
count += 1
used[k] = count
kStart = 1
else # no rectangle can be placed anymore, backtrack
k = argmax(used) # find which piece was last piece
if !isempty(coords)
last = pop!(coords) # find coordinates of last piece
height[last[1] : last[1] + rects[k][1] - 1] .-= rects[k][2] # remove rectangle
end
count -= 1
used[k] = 0
kStart = k + 1 # k does not work as next piece, do not include in next attempt
end
y = minimum(height) + 1
x = findfirst(height .== y-1) # can't use argmin, since x needs to be minimal such that height[x] = minimum(height)
if count == s # all s rectangles are placed
tiles = fill(0, h, w) # output square
for l in 1 : length(used)
if used[l] >= 1
x = coords[used[l]][1]
y = coords[used[l]][2]
tiles[x : x + rects[l][1] - 1, y : y + rects[l][2] - 1] = fill(used[l], rects[l][1], rects[l][2])
end
end
return true, tiles
elseif count == -1 # no rectangles are placed but kStart > s, so all combinations have been tried
return false, nothing
end
end
end
"""
solveBacktrackingRot(h, w, rects)
Perfect rectangle packing with rotations using backtracking with top-left heuristic. In rects
each rectangle is given twice, one for each possible rotation. If a rectangle that is not a
square is choosen, used[s - k + 1] prevents us from choosing the other orientation of that
rectangle. The concept of using the height in each row to determine validity of a placement
is an unpublished idea of Prof. Dimitri Leemans at the Université libre de Bruxelles.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
- `numRects`: Number of rectangles that are not a square
"""
function solveBacktrackingRot(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}}, numRects::Int64)
s = length(rects)
steps = h == w ? s-numRects-1 : s-1
prog = Progress(steps, "Top nodes explored: ") # number of rectangles already tried as first rectangle
height = fill(0, h) # number of tiles covered in each row
used = fill(0, s) # rectangles used
coords = Vector{Pair{Int64, Int64}}() # remember coordinates
count = 0 # number of rectangles used
x = y = kStart = 1
while true
# Try to place a rectangle on (x, y)
done = false
k = kStart # only rectangles after kStart are allowed
while k <= s && !done
if h == w && count == 0 && k > s-numRects # if bounding box is a square we can use symmetry and restrict ourself to not rotating the first rectangle
break
end
if used[k] == 0 && (x + rects[k][1] - 1 <= h && y + rects[k][2] - 1 <= w) # piece not used and fits
done = true
# check permiter of rectangle for collisions with other rectangles
for l = 1 : rects[k][1] - 1
if height[x+l] > height[x]
done = false
break
end
end
if !done
k += 1
end
else
k += 1 # try next piece
end
end
if done # rectangle k can be placed on (x, y)
push!(coords, Pair(x, y))
height[x : x + rects[k][1] - 1] .+= rects[k][2] # add rectangle
if count == 0 # choice for first rectangle placed is switched
ProgressMeter.update!(prog, k-1)
end
count += 1
if k <= numRects || k > s-numRects # check if true rectangle and not square
used[s - k + 1] = -1 # different rotation can't be used anymore
end
used[k] = count
kStart = 1
else # no rectangle can be placed anymore, backtrack
k = argmax(used) # find which piece was last piece
if !isempty(coords)
last = pop!(coords) # find coordinates of last piece
height[last[1] : last[1] + rects[k][1] - 1] .-= rects[k][2] # remove rectangle
end
count -= 1
used[k] = 0
if k <= numRects || k > s-numRects # check if true rectangle and not square
used[s - k + 1] = 0
end
kStart = k + 1 # k does not work as next piece, do not include in next attempt
end
y = minimum(height) + 1
x = findfirst(height .== y-1) # can't use argmin, since x needs to be minimal such that height[x] = minimum(height)
if count == s-numRects # all s rectangles are placed
tiles = fill(0, h, w) # output square
for l in 1 : length(used)
if used[l] >= 1
x = coords[used[l]][1]
y = coords[used[l]][2]
tiles[x : x + rects[l][1] - 1, y : y + rects[l][2] - 1] = fill(used[l], rects[l][1], rects[l][2])
end
end
return true, tiles
elseif count == -1 # no rectangles are placed but kStart > s-numRects, so all combinations have been tried
return false, nothing
end
end
end
"""
runIntegerProgramming(h, w, rects, rot)
Perfect rectangle packing using Integer Programming and the open-source solver HiGHS. The model
is taken from M. Berger, M. Schröder, K.-H. Küfer, "A constraint programming approach for the
two-dimensional rectangular packing problem with orthogonal orientations", Berichte des
Fraunhofer ITWM, Nr. 147 (2008).
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
- `rot`: If rectangles are allowed to be rotated by 90 degrees
"""
function runIntegerProgramming(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}}, rot::Bool)
if rot
return solveIntegerProgrammingRot(h, w, rects)
else
return solveIntegerProgramming(h, w, rects)
end
end
"""
solveIntegerProgramming(h, w, rects)
Perfect rectangle packing without rotations using Integer Programming and the open-source
solver HiGHS.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
"""
function solveIntegerProgramming(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}})
s = length(rects)
model = Model(HiGHS.Optimizer)
set_optimizer_attribute(model, "log_to_console", false)
@variable(model, px[1:s], Int) # x position
@variable(model, py[1:s], Int) # y position
@variable(model, overlap[1:s, 1:s, 1:4], Bin) # help variable to prevent overlap
for i in 1 : s
@constraint(model, px[i] >= 0) # position is non-negative
@constraint(model, py[i] >= 0)
@constraint(model, px[i] + rects[i][2] <= w) # rectangle is contained in square
@constraint(model, py[i] + rects[i][1] <= h)
end
for i in 1 : s
for j in i + 1 : s
@constraint(model, px[i] - px[j] + rects[i][2] <= w * (1 - overlap[i, j, 1])) # rectangle i is left of rectangle j
@constraint(model, px[j] - px[i] + rects[j][2] <= w * (1 - overlap[i, j, 2])) # rectangle i is right of rectangle j
@constraint(model, py[i] - py[j] + rects[i][1] <= h * (1 - overlap[i, j, 3])) # rectangle i is below rectangle j
@constraint(model, py[j] - py[i] + rects[j][1] <= h * (1 - overlap[i, j, 4])) # rectangle i is above rectangle j
@constraint(model, sum(overlap[i, j, :]) >= 1) # one of the cases must be true so that rectangles don't overlap
end
end
optimize!(model)
if (has_values(model)) # if solution was found
output = fill(0, h, w)
for i in 1 : s
iPX = Int(round(value(px[i])))
iPY = Int(round(value(py[i])))
for x in iPX + 1 : iPX + rects[i][2]
for y in iPY + 1 : iPY + rects[i][1]
output[y, x] = i
end
end
end
return true, output
end
return false, nothing
end
"""
solveIntegerProgrammingRot(h, w, rects)
Perfect rectangle packing with rotations using Integer Programming and the open-source
solver HiGHS.
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
"""
function solveIntegerProgrammingRot(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}})
s = length(rects)
model = Model(HiGHS.Optimizer)
set_optimizer_attribute(model, "log_to_console", false)
@variable(model, sx[1:s], Int) # width of rectangle i
@variable(model, sy[1:s], Int) # height of rectangle i
@variable(model, px[1:s], Int) # x position
@variable(model, py[1:s], Int) # y position
@variable(model, o[1:s], Bin) # orientation of rectangle
@variable(model, overlap[1:s, 1:s, 1:4], Bin) # help variable to prevent overlap
for i in 1 : s
@constraint(model, px[i] >= 0) # position is non-negative
@constraint(model, py[i] >= 0)
@constraint(model, px[i] + sx[i] <= w) # rectangle is contained in square
@constraint(model, py[i] + sy[i] <= h)
@constraint(model, (1 - o[i]) * rects[i][1] + o[i] * rects[i][2] == sx[i]) # determine size from orientation
@constraint(model, o[i] * rects[i][1] + (1 - o[i]) * rects[i][2] == sy[i])
end
for i in 1 : s
for j in i + 1 : s
@constraint(model, px[i] - px[j] + sx[i] <= w * (1 - overlap[i, j, 1])) # rectangle i is left of rectangle j
@constraint(model, px[j] - px[i] + sx[j] <= w * (1 - overlap[i, j, 2])) # rectangle i is right of rectangle j
@constraint(model, py[i] - py[j] + sy[i] <= h * (1 - overlap[i, j, 3])) # rectangle i is below rectangle j
@constraint(model, py[j] - py[i] + sy[j] <= h * (1 - overlap[i, j, 4])) # rectangle i is above rectangle j
@constraint(model, sum(overlap[i, j, :]) >= 1) # one of the cases must be true so that rectangles don't overlap
end
end
optimize!(model)
if (has_values(model)) # if solution was found
output = fill(0, h, w)
for i in 1 : s
iPX = Int(round(value(px[i])))
iPY = Int(round(value(py[i])))
iSX = Int(round(value(sx[i])))
iSY = Int(round(value(sy[i])))
for x in iPX + 1 : iPX + iSX
for y in iPY + 1 : iPY + iSY
output[y, x] = i
end
end
end
return true, output
end
return false, nothing
end
"""
runDancingLinks(h, w, rects, rot)
Perfect rectangle packing using Knuth's Dancing Links algorithm. The details are described
in his [paper](https://arxiv.org/abs/cs/0011047) and the implementation uses dictionaries
inspired from this [blog post](https://www.cs.mcgill.ca/~aassaf9/python/algorithm_x.html).
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
- `rot`: If rectangles are allowed to be rotated by 90 degrees
"""
function runDancingLinks(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}}, rot::Bool)
if rot
return solveDancingLinksRot(h, w, rects)
else
return solveDancingLinks(h, w, rects)
end
end
"""
solveDancingLinks(h, w, rects)
Perfect rectangle packing without rotations using Knuth's Dancing Links algorithm.
+---------------------------------------------+--------------------+--------------------+
| - | Tile covered (h*w) | Rectangle used (s) |
+---------------------------------------------+--------------------+--------------------+
| Rectangle 1 on (1, 1) | | |
| Rectangle 1 on (1, 2) | | |
| ... | | |
| Rectangle 1 on (h - h(rect1), w - w(rect1)) | | |
| ... | | |
| Rectangle s on (h - h(rect1), w - w(rect1)) | | |
+---------------------------------------------+--------------------+--------------------+
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
"""
function solveDancingLinks(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}})
s = length(rects)
row = 0
lookup = Dict{Int64, NTuple{3, Int64}}() # (row) -> (rect, px, py), allow reconstruction of rectangles from row number
dictX = Dict{Int64, Set{Int64}}()
dictY = Dict{Int64, Vector{Int64}}()
for i in 1 : h*w + s
dictX[i] = Set{Int64}()
end
# generate table
for i in 1 : s
for px in 0 : w - rects[i][2]
for py in 0 : h - rects[i][1]
row += 1
dictY[row] = Vector{Int64}()
# i-th rectangle is used
push!(dictY[row], h*w + i)
push!(dictX[h*w + i], row)
for x in px + 1 : px + rects[i][2]
for y in py + 1 : py + rects[i][1]
push!(dictY[row], x + (y - 1) * w)
push!(dictX[x + (y - 1) * w], row)
end
end
lookup[row] = (i, px, py)
end
end
end
solution = Stack{Int64}()
dancingLink!(dictX, dictY, solution)
if !(isempty(solution)) # if solution was found
output = fill(0, h, w)
for i in solution
rect, px, py = lookup[i]
for x in px + 1 : px + rects[rect][2]
for y in py + 1 : py + rects[rect][1]
output[y, x] = rect
end
end
end
return true, output
end
return false, nothing
end
"""
solveDancingLinksRot(h, w, rects)
Perfect rectangle packing with rotations using Knuth's Dancing Links algorithm.
+---------------------------------------------+--------------------+--------------------+
| - | Tile covered (h*w) | Rectangle used (s) |
+---------------------------------------------+--------------------+--------------------+
| Rectangle 1 on (1, 1) | | |
| Rectangle 1 on (1, 2) | | |
| ... | | |
| Rectangle 1 on (h - h(rect1), w - w(rect1)) | | |
| Rectangle 1 rotated on (1, 1) | | |
| ... | | |
| Rectangle s on (h - h(rect1), w - w(rect1)) | | |
+---------------------------------------------+--------------------+--------------------+
# Arguments
- `h`: Height of rectangle to fill
- `w`: Width of rectangle to fill
- `rects`: Vector of rectangles to use for the packing; Encoded as Pair(height, width)
"""
function solveDancingLinksRot(h::Int64, w::Int64, rects::Vector{Pair{Int64, Int64}})
s = length(rects)
row = 0
lookup = Dict{Int64, NTuple{5, Int64}}() # (row) -> (rect, px, py, sx, sy), allow reconstruction of rectangles from row number
dictX = Dict{Int64, Set{Int64}}()
dictY = Dict{Int64, Vector{Int64}}()
for i in 1 : h*w + s
dictX[i] = Set{Int64}()
end
# generate table
for i in 1 : s
for rot in [true, false]
sx = rot ? rects[i][1] : rects[i][2]
sy = rot ? rects[i][2] : rects[i][1]
for px in 0 : w - sx
for py in 0 : h - sy
row += 1
dictY[row] = Vector{Int64}()
# i-th rectangle is used
push!(dictY[row], h*w + i)
push!(dictX[h*w + i], row)
for x in px + 1 : px + sx
for y in py + 1 : py + sy
push!(dictY[row], x + (y - 1) * w)
push!(dictX[x + (y - 1) * w], row)
end
end
lookup[row] = (i, px, py, sx, sy)
end
end
end
end
solution = Stack{Int64}()
dancingLink!(dictX, dictY, solution)
if !(isempty(solution)) # if solution was found
output = fill(0, h, w)
for i in solution
rect, px, py, sx, sy = lookup[i]
for x in px + 1 : px + sx
for y in py + 1 : py + sy
output[y, x] = rect
end
end
end
return true, output
end
return false, nothing
end
"""
dancingLink!(dictX, dictY, solution)
Recursivly run Dancing Links algorithm.
# Arguments
- `dictX`: Dictionary to replace pointer arithmetic
- `dictY`: Dictionary to replace toroidal double-linked matrix
- `solution`: Stack for backtracking
"""
function dancingLink!(dictX::Dict{Int64, Set{Int64}}, dictY::Dict{Int64, Vector{Int64}}, solution::Stack{Int64})
if isempty(dictX) # no constraints left
return true
end
# Knuths MRV heuristic, which always chooses the column that can be covered by the least number
c = valMin = typemax(Int64)
for (key, value) in dictX
if length(value) < valMin
valMin = length(value)
c = key
end
end
# backtracking step
for i in dictX[c]
push!(solution, i)
cols = select!(dictX, dictY, i) # cover rows
if dancingLink!(dictX, dictY, solution)
return true
end
deselect!(dictX, dictY, i, cols) # uncover rows
pop!(solution)
end
return false
end
"""
select!(dictX, dictY, solution)
Cover operation of the Dancing Links algorithm.
# Arguments
- `dictX`: Dictionary to replace pointer arithmetic
- `dictY`: Dictionary to replace toroidal double-linked matrix
- `r`: Row to cover
"""
@inline function select!(dictX::Dict{Int64, Set{Int64}}, dictY::Dict{Int64, Vector{Int64}}, r::Int64)
cols = Stack{Set{Int64}}()
for j in dictY[r]
for i in dictX[j]
for k in dictY[i]
if k != j
delete!(dictX[k], i)
end
end
end
push!(cols, pop!(dictX, j)) # remember all rows removed while covering
end
return cols
end
"""
deselect!(dictX, dictY, solution)
Unover operation of the Dancing Links algorithm.
# Arguments
- `dictX`: Dictionary to replace pointer arithmetic
- `dictY`: Dictionary to replace toroidal double-linked matrix
- `r`: Row to uncover
- `cols`: Columns removed in cover operation on r
"""
@inline function deselect!(dictX::Dict{Int64, Set{Int64}}, dictY::Dict{Int64, Vector{Int64}}, r::Int64, cols::Stack{Set{Int64}})
for j in reverse(dictY[r])
dictX[j] = pop!(cols)
for i in dictX[j]
for k in dictY[i]
if k != j
push!(dictX[k], i)
end
end
end
end
end
end # module PerfectPacking
| PerfectPacking | https://github.com/PhoenixSmaug/PerfectPacking.jl.git |
|
[
"MIT"
]
| 1.1.0 | d36e5f4b91f8fd4a0aea50927cdb98492d3d318e | code | 941 | println("Testing...")
using Test, PerfectPacking
result, _ = rectanglePacking(6, 6, [(1 => 6), (1 => 3), (5 => 1), (2 => 2), (3 => 2), (4 => 2), (4 => 1)], false, backtracking)
@test result == true
result, _ = rectanglePacking(6, 6, [(5 => 1), (1 => 3), (5 => 1), (2 => 2), (3 => 2), (3 => 3), (4 => 1)], true, backtracking)
@test result == true
result, _ = rectanglePacking(6, 7, [(1 => 4), (6 => 1), (2 => 2), (4 => 2), (2 => 3), (5 => 1), (3 => 3)], false, integerProgramming)
@test result == true
result, _ = rectanglePacking(6, 7, [(1 => 4), (1 => 6), (2 => 2), (2 => 4), (3 => 2), (5 => 1), (3 => 3)], true, integerProgramming)
@test result == true
result, _ = rectanglePacking(10, 10, [(4 => 3), (1 => 7), (3 => 7), (6 => 2), (6 => 5), (6 => 3)], false, dancingLinks)
@test result == true
result, _ = rectanglePacking(10, 10, [(4 => 3), (7 => 1), (7 => 3), (6 => 2), (5 => 6), (6 => 3)], true, dancingLinks)
@test result == true | PerfectPacking | https://github.com/PhoenixSmaug/PerfectPacking.jl.git |
|
[
"MIT"
]
| 1.1.0 | d36e5f4b91f8fd4a0aea50927cdb98492d3d318e | docs | 1778 | # PerfectPacking.jl
This library solves the NP-complete perfect rectangle packing problem, where smaller rectangles must be placed in a bounding rectangle without overlapping or leaving tiles uncovered. It can also solve the perfect rectangle packing problem with orthogonal rotation, where the smaller rectangles are allowed to be rotated. The common solving algorithms from literature are implemented.
### Backtracking
```julia
feasibility, solution = rectanglePacking(height, width, rectangles, rotAllowed, backtracking)
```
The most popular and generally the fastest algorithm is backtracking with the top-left heuristic. In each step, an attempt is made to place a new rectangle in the first free tile, proceeding first by rows and then by columns. To further reduce the search space, the rectangles are sorted by descending width.
### Integer Programming
```julia
feasibility, solution = rectanglePacking(height, width, rectangles, rotAllowed, integerProgramming)
```
The perfect rectangle packing problem is translated into an Integer Programming feasibility problem using a model from [this article](https://link.springer.com/chapter/10.1007/978-3-642-00142-0_69). Then it is solved with the open source ILP solver HiGHS. In many cases, it provides the quickest way to prove non-feasibility of the packing problem.
### Dancing Links
```julia
feasibility, solution = rectanglePacking(height, width, rectangles, rotAllowed, dancingLinks)
```
Knuth's famous dancing links algorithm can be used to solve the perfect rectangle packing problem, since it can be reduced to an exact cover problem. When the problem is feasible, it often outperforms the Integer Programming model, but can rarely compete with the classical backtracking approach.
(c) Christoph Muessig
| PerfectPacking | https://github.com/PhoenixSmaug/PerfectPacking.jl.git |
|
[
"MIT"
]
| 0.1.5 | 0d7ee0a1acad90d544fa87cc3d6f463e99abb77a | code | 4744 | module BetterFileWatching
using Deno_jll
import JSON
abstract type FileEvent end
struct Modified <: FileEvent
paths::Vector{String}
end
struct Removed <: FileEvent
paths::Vector{String}
end
struct Created <: FileEvent
paths::Vector{String}
end
struct Accessed <: FileEvent
paths::Vector{String}
end
const mapFileEvent = Dict(
"modify" => Modified,
"create" => Created,
"remove" => Removed,
"access" => Accessed,
)
export watch_folder, watch_file
function _doc_examples(folder)
f = folder ? "folder" : "file"
args = folder ? "\".\"" : "\"file.txt\""
"""
# Example
```julia
watch_$(f)($(args)) do event
@info "Something changed!" event
end
```
You can watch a $(f) asynchronously, and interrupt the task later:
```julia
watch_task = @async watch_$(f)($(args)) do event
@info "Something changed!" event
end
sleep(5)
# stop watching the $(f)
schedule(watch_task, InterruptException(); error=true)
```
"""
end
"""
```julia
watch_folder(f::Function, dir=".")
```
Watch a folder recursively for any changes. Includes changes to file contents. A [`FileEvent`](@ref) is passed to the callback function `f`.
Use the single-argument `watch_folder(dir::AbstractString=".")` to create a **blocking call** until the folder changes (like the FileWatching standard library).
$(_doc_examples(true))
# Differences with the FileWatching stdlib
- `BetterFileWatching.watch_folder` works _recursively_, i.e. subfolders are also watched.
- `BetterFileWatching.watch_folder` also watching file _contents_ for changes.
- BetterFileWatching.jl is based on [Deno.watchFs](https://doc.deno.land/builtin/stable#Deno.watchFs), made available through the [Deno_jll](https://github.com/JuliaBinaryWrappers/Deno_jll.jl) package.
"""
function watch_folder(on_event::Function, dir::AbstractString="."; ignore_accessed::Bool=true, ignore_dotgit::Bool=true)
script = """
const watcher = Deno.watchFs($(JSON.json(dir)));
for await (const event of watcher) {
try {
await Deno.stdout.write(new TextEncoder().encode("\\n" + JSON.stringify(event) + "\\n"));
} catch(e) {
Deno.exit();
}
}
"""
outpipe = Pipe()
function on_stdout(str)
for s in split(str, "\n"; keepempty=false)
local event_raw = nothing
event = try
event_raw = JSON.parse(s)
T = mapFileEvent[event_raw["kind"]]
T(String.(event_raw["paths"]))
catch e
@warn "Unrecognized file watching event. Please report this to https://github.com/JuliaPluto/BetterFileWatching.jl" event_raw ex=(e,catch_backtrace())
end
if !(ignore_accessed && event isa Accessed)
if !(ignore_dotgit && event isa FileEvent && all(".git" ∈ splitpath(relpath(path, dir)) for path in event.paths))
on_event(event)
end
end
end
end
deno_task = @async run(pipeline(`$(deno()) eval $(script)`; stdout=outpipe))
watch_task = @async try
sleep(.1)
while true
on_stdout(String(readavailable(outpipe)))
end
catch e
if !istaskdone(deno_task)
schedule(deno_task, e; error=true)
end
if !(e isa InterruptException)
showerror(stderr, e, catch_backtrace())
end
end
try wait(watch_task) catch; end
end
function watch_folder(dir::AbstractString="."; kwargs...)::Union{Nothing,FileEvent}
event = Ref{Union{Nothing,FileEvent}}(nothing)
task = Ref{Task}()
task[] = @async watch_folder(dir; kwargs...) do e
event[] = e
try
schedule(task[], InterruptException(); error=true)
catch; end
end
wait(task[])
event[]
end
"""
```julia
watch_file(f::Function, filename::AbstractString)
```
Watch a file recursively for any changes. A [`FileEvent`](@ref) is passed to the callback function `f` when a change occurs.
Use the single-argument `watch_file(filename::AbstractString)` to create a **blocking call** until the file changes (like the FileWatching standard library).
$(_doc_examples(false))
# Differences with the FileWatching stdlib
- BetterFileWatching.jl is based on [Deno.watchFs](https://doc.deno.land/builtin/stable#Deno.watchFs), made available through the [Deno_jll](https://github.com/JuliaBinaryWrappers/Deno_jll.jl) package.
"""
watch_file(filename::AbstractString; kwargs...) = watch_folder(filename; kwargs...)
watch_file(f::Function, filename::AbstractString; kwargs...) = watch_folder(f, filename; kwargs...)
end
| BetterFileWatching | https://github.com/JuliaPluto/BetterFileWatching.jl.git |
|
[
"MIT"
]
| 0.1.5 | 0d7ee0a1acad90d544fa87cc3d6f463e99abb77a | code | 1943 | using BetterFileWatching
using Test
function poll(query::Function, timeout::Real=Inf64, interval::Real=1/20)
start = time()
while time() < start + timeout
if query()
return true
end
sleep(interval)
end
return false
end
"Like @async except it prints errors to the terminal. 👶"
macro asynclog(expr)
quote
@async begin
# because this is being run asynchronously, we need to catch exceptions manually
try
$(esc(expr))
catch ex
bt = stacktrace(catch_backtrace())
showerror(stderr, ex, bt)
rethrow(ex)
end
end
end
end
@testset "Basic - $(method)" for method in ["new", "legacy"]
test_dir = tempname(cleanup=false)
mkpath(test_dir)
j(args...) = joinpath(test_dir, args...)
write(j("script.jl"), "nice(123)")
mkdir(j("mapje"))
write(j("mapje", "cool.txt"), "hello")
events = []
last_length = Ref(0)
watch_task = if method == "new"
@asynclog watch_folder(test_dir) do e
@info "Event" e
push!(events, e)
end
else
@asynclog while true
e = watch_folder(test_dir)
@info "Event" e
push!(events, e)
end
end
sleep(2)
@test length(events) == 0
function somethingdetected()
result = poll(10, 1/100) do
length(events) > last_length[]
end
sleep(.5)
last_length[] = length(events)
result
end
write(j("mapje", "cool2.txt"), "hello")
@test somethingdetected()
write(j("mapje", "cool2.txt"), "hello again!")
@test somethingdetected()
write(j("asdf.txt"), "")
@test somethingdetected()
mv(j("mapje", "cool2.txt"), j("mapje", "cool3.txt"))
@test somethingdetected()
rm(j("mapje", "cool3.txt"))
@test somethingdetected()
sleep(2)
@test_nowarn schedule(watch_task, InterruptException(); error=true)
end
| BetterFileWatching | https://github.com/JuliaPluto/BetterFileWatching.jl.git |
|
[
"MIT"
]
| 0.1.5 | 0d7ee0a1acad90d544fa87cc3d6f463e99abb77a | docs | 1904 | # BetterFileWatching.jl
```julia
watch_folder(f::Function, dir=".")
```
Watch a folder recursively for any changes. Includes changes to file contents. A [`FileEvent`](@ref) is passed to the callback function `f`.
```julia
watch_file(f::Function, filename=".")
```
Watch a file for changes. A [`FileEvent`](@ref) is passed to the callback function `f`.
# Example
```julia
watch_folder(".") do event
@info "Something changed!" event
end
```
You can watch a folder asynchronously, and interrupt the task later:
```julia
watch_task = @async watch_folder(".") do event
@info "Something changed!" event
end
sleep(5)
# stop watching the folder
schedule(watch_task, InterruptException(); error=true)
```
# Differences with the FileWatching stdlib
`BetterFileWatching.watch_file` is an alternative to `FileWatching.watch_file`. The differences are:
- We offer an additional callback API (`watch_file(::Function, ::String)`, like the examples above), which means that *handling* events does not block *receiving new events*: we keep listening to changes asynchronously while your callback runs.
- BetterFileWatching.jl is just a small wrapper around [`Deno.watchFs`](https://doc.deno.land/builtin/stable#Deno.watchFs), made available through the [Deno_jll](https://github.com/JuliaBinaryWrappers/Deno_jll.jl) package. `Deno.watchFs` is well-tested and widely used.
`BetterFileWatching.watch_folder` is an alternative to `FileWatching.watch_folder`. The differences are, in addition to those mentioned above for `watch_file`:
- `BetterFileWatching.watch_folder` works _recursively_, i.e. subfolders are also watched.
- `BetterFileWatching.watch_folder` also watches for changes to the _contents_ of files contained in the folder.
---
In fact, `BetterFileWatching.watch_file` and `BetterFileWatching.watch_folder` are actually just the same function! It handles both files and folders. | BetterFileWatching | https://github.com/JuliaPluto/BetterFileWatching.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 1636 | module SegyIO
myRoot=dirname(dirname(pathof(SegyIO)))
CHUNKSIZE = 2048
TRACE_CHUNKSIZE = 512
MB2B = 1024^2
# what's being used
using Distributed
using Printf
#Types
include("types/IBMFloat32.jl")
include("types/BinaryFileHeader.jl")
include("types/BinaryTraceHeader.jl")
include("types/FileHeader.jl")
include("types/SeisBlock.jl")
include("types/BlockScan.jl")
include("types/SeisCon.jl")
#Reader
include("read/read_fileheader.jl")
include("read/read_traceheader.jl")
include("read/read_trace.jl")
include("read/read_file.jl")
include("read/segy_read.jl")
include("read/read_block.jl")
include("read/read_block_headers.jl")
include("read/read_con.jl")
include("read/read_con_headers.jl")
include("read/extract_con_headers.jl")
# Writer
include("write/write_fileheader.jl")
include("write/segy_write.jl")
include("write/segy_write_append.jl")
include("write/check_fileheader.jl")
include("write/write_trace.jl")
# Scanner
include("scan/scan_file.jl")
include("scan/segy_scan.jl")
include("scan/scan_chunk.jl")
include("scan/scan_block.jl")
include("scan/scan_shots.jl")
include("scan/delim_vector.jl")
include("scan/find_next_delim.jl")
# Workspace
th_b2s = SegyIO.th_byte2sample()
blank_th = BinaryTraceHeader()
# Methods
include("methods/ordered_pmap.jl")
include("methods/merge.jl")
include("methods/split.jl")
include("methods/set_header.jl")
include("methods/get_header.jl")
include("methods/get_sources.jl")
end # module
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 2580 | export get_header
"""
getheader(block, header)
Returns a vector of 'header' values from each trace in 'block'. Trace order is preserved.
# Example
sx = get_header(block, "SourceX")
sy = get_header(block, :SourceX)
"""
function get_header(block::SeisBlock, name_in::Union{Symbol, String}; scale::Bool = true)
name = Symbol(name_in)
ntraces = length(block)
ftype = fieldtype(BinaryTraceHeader, name)
out = zeros(ftype, ntraces)
for i in 1:ntraces
out[i] = getfield(block.traceheaders[i], name)
end
# Check, and apply scaling
is_scalable, scale_name = check_scale(name)
if scale && is_scalable
scaling_factor = get_header(block, scale_name)
out = Float64.(out)
for ii in 1:ntraces
fact = scaling_factor[ii]
fact > 0 ? out[ii] *= fact : out[ii] /= abs(fact)
end
end
return out
end
"""
is_scalable, scale_name = check_scale(sym)
Checks to see if the BinaryTraceHeader field `sym` requires scaling.
`is_scalable` returns true if the field can be scaled, and `scale_name` returns
the symbol of the appropriate scaling field.
"""
function check_scale(sym::Symbol)
recsrc = false
el = false
scale_name = :Null
recsrc_str = "SourceX
SourceY
GroupX
GroupY
CDPX
CDPY"
el_str = " RecGroupElevation
SourceSurfaceElevation
SourceDepth
RecDatumElevation
SourceDatumElevation
SourceWaterDepth
GroupWaterDepth"
if occursin(String(sym), recsrc_str)
recsrc = true
scale_name = :RecSourceScalar
elseif occursin(String(sym), el_str)
el = true
scale_name = :ElevationScalar
end
return (recsrc||el), scale_name
end
"""
get_header(con::SeisCon, name::String)
Gets the metadata summary, `name`, from every `BlockScan` in `con`.
Column 1 contains the minimum value of `name` within the block, and Column 2
contains the maximum value of `name` within the block. Src/Rec scaling is not applied.
To get source coordinate pairs as an array, see `get_sources`.
# Example
trace = get_header(s, "TraceNumber")
"""
function get_header(con::SeisCon,name::String)
minmax = [con.blocks[i].summary[name] for i in 1:length(con)]
I = length(minmax)
vals = Array{Int32,2}(undef, I, 2)
for i in 1:I
vals[i,1] = minmax[i][1]
vals[i,2] = minmax[i][2]
end
return vals
end
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 903 | export get_sources
"""
get_sources(con::SeisCon)
Returns an array of the source location coordinate pairs, NOT the minimum and maximum values.
Unlike `get_headers`, `get_sources` checks to make sure that SourceX and SourceY are consistant
throughout each block, that is `min == max`.
Column 1 of the returned array is SourceX, and Column 2 is SourceY.
# Example
sx = get_sources(s)
"""
function get_sources(con::SeisCon)
sx_v = get_header(con, "SourceX")
sy_v = get_header(con, "SourceY")
sx_const = all([sx_v[i,1] == sx_v[i,2] for i in 1:size(sx_v)[1]])
sy_const = all([sy_v[i,1] == sy_v[i,2] for i in 1:size(sy_v)[1]])
if sx_const && sy_const
sx = [sx_v[i,1] for i in 1:size(sx_v)[1]]
sy = [sy_v[i,1] for i in 1:size(sy_v)[1]]
else
@error "Source locations are not constistant for all blocks."
end
return hcat(sx,sy)
end
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 3489 | import Base.merge,Base.==
export merge, ==
"""
merge(cons::Array{SeisCon,1})
Merge the elements of `con`, a vector of SeisCon objects, into one SeisCon object.
"""
function merge(cons::Array{SeisCon,1})
# Check similar metadata
ns = get_confield(cons, :ns)
dsf = get_confield(cons, :dsf)
if all(ns.==ns[1]) && all(dsf.==dsf[1])
d = [cons[i].blocks for i in 1:length(cons)]
return SeisCon(ns[1], dsf[1], vcat(d...))
else
@error "Dissimilar metadata, cannot merge"
end
end
"""
merge(a::SeisCon, b::SeisCon)
Merge two SeisCon objects together
"""
merge(a::SeisCon, b::SeisCon) = merge([a; b])
"""
merge(a::SeisBlock, b::SeisBlock; kwargs...)
Merge two SeisBlocks into one.
"""
function merge(a::SeisBlock{DT}, b::SeisBlock{DT};
force::Bool = false, consume::Bool = false) where {DT<:Union{IBMFloat32, Float32}}
merge([a; b], force = force, consume = consume)
end
"""
merge(a::SeisBlock{DT}, b::SeisBlock{DT};
force::Bool = false, consume::Bool = false) where {DT<:Union{IBMFloat32, Float32}}
Merge `blocks`, a vector of `SeisBlock` objects into one `SeisBlock` object.
By default, `merge` checks to ensure that each `SeisBlock` has matching fileheaders. This ensures
that the returned `SeisBlock` is consistant with it's fileheader. This check can be turned off
using the `force` keyword. If set to false, `merge` will attempt to combine `blocks` without
checking fileheader metadata. The first fileheader in `blocks` will be used for the returned
`SeisBlock`'s fileheader.
By default, `merge` references traceheaders, but copies data from the elements of `blocks`.
If memory use is a concern, the `consume` keyword will clear the traceheader and data field of each
element of `block` after it has been copied. This will prevent duplicating the data in memory,
at the cost of clearing the elements of `blocks`.
# Examples
julia> s = segy_scan(joinpath(dirname(pathof(SegyIO)),"data/"), "overthrust", ["GroupX"; "GroupY"], verbosity = 0);
julia> a = s[1:4]; b = s[5:8];
julia> c = merge([a; b]);
julia> c.traceheaders[1] === a.traceheaders[1]
true
julia> c = merge([a; b], consume = true);
julia> a.traceheaders
0-element Array{SegyIO.BinaryTraceHeader,1}
"""
function merge(blocks::Vector{SeisBlock{DT}};
force::Bool = false, consume::Bool = false) where {DT<:Union{IBMFloat32, Float32}}
# Check common file header
n = length(blocks)
if !force && !all([blocks[i].fileheader == blocks[i+1].fileheader for i in 1:n-1])
@error "Fileheaders do not match for all blocks, use kw force=true to ignore"
end
fh = blocks[1].fileheader
bth = Vector{BinaryTraceHeader}()
data = Vector{DT}()
for ii in 1:n
if consume
append!(bth, blocks[ii].traceheaders[:])
append!(data, blocks[ii].data[:])
(blocks[ii].data = Array{DT}(undef,0,0))
(blocks[ii].traceheaders = Array{BinaryTraceHeader}(undef,0))
else
append!(bth, @view blocks[ii].traceheaders[:])
append!(data, blocks[ii].data[:])
end
end
out = SeisBlock(fh, bth, reshape(data, Int64(fh.bfh.ns), :))
end
function ==(a::FileHeader, b::FileHeader)
match_th = a.th == b.th
match_bfh = [getfield(a.bfh, field) == getfield(b.bfh,field) for field in fieldnames(BinaryFileHeader)]
return match_th && all(match_bfh)
end
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 975 | export ordered_pmap
function ordered_pmap(pool::WorkerPool, f, list)
pids = pool.workers
np = length(pids)
n = length(list)
results = Vector{Any}(undef,n)
i = 1
nextidx() = (idx=i; i+=1; idx)
@sync begin
for p in pids
if p != myid() || np == 1
@async begin
while true
idx = nextidx()
if idx > n
break
end
results[idx] = remotecall_fetch(f, p, list[idx])
end
end
end
end
end
return results
end
ordered_pmap(f, c1,) = ordered_pmap(default_worker_pool(), f, c1)
ordered_pmap(f, c1, c...) = ordered_pmap(default_worker_pool(), a->f(a...), zip(c1,c))
ordered_pmap(p::WorkerPool, f, c1, c...) = ordered_pmap(p, a->f(a...),zip(c1,c))
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 2129 | export set_header!, set_traceheader!, set_fileheader!
# Set traceheader for vector
function set_traceheader!(traceheaders::Array{BinaryTraceHeader,1},
name::Symbol, x::ET) where {ET<:Array{<:Integer,1}}
ftype = fieldtype(BinaryTraceHeader, name)
try
x_typed = convert.(ftype, x)
for t in 1:length(traceheaders)
setfield!(traceheaders[t], name, x_typed[t])
end
catch e
@warn "Unable to convert $x to $(ftype)"
throw(e)
end
end
# Set fileheader
function set_fileheader!(fileheader::BinaryFileHeader,
name::Symbol, x::ET) where {ET<:Integer}
ftype = fieldtype(BinaryFileHeader, name)
try
x_typed = convert.(ftype, x)
setfield!(fileheader, name, x_typed)
catch e
@warn "Unable to convert $x to $(ftype)"
throw(e)
end
end
"""
set_header!(block, header, value)
Set the 'header' field in 'block' to 'value', where 'header' is either a string or symbol of a valid field in BinaryTraceHeader.
If 'value' is an Int, it will be applied to each header.
If 'value' is a vector, the i-th 'value' will be set in the i-th traceheader.
# Example
set_header!(block, "SourceX", 100)
set_header!(block, :SourceY, Array(1:100))
"""
function set_header!(block::SeisBlock, name_in::Union{Symbol, String}, x::ET) where {ET<:Integer}
name = Symbol(name_in)
ntraces = size(block)[2]
x_vec = x.*ones(Int32, ntraces)
# Try setting trace headers
try
set_traceheader!(block.traceheaders, name, x_vec)
catch
end
# Try setting file header
try
set_fileheader!(block.fileheader.bfh, name, x)
catch
end
end
function set_header!(block::SeisBlock, name_in::Union{Symbol, String},
x::Array{ET,1}) where {ET<:Integer}
name = Symbol(name_in)
ntraces = size(block)[2]
# Try setting trace headers
try
set_traceheader!(block.traceheaders, name, x)
catch
end
# Try setting file header
try
set_fileheader!(block.fileheader.bfh, name, x)
catch
end
end
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 3013 | import Base.split
export split
"""
c = split(s::SeisCon, inds)
Creates a new SeisCon object `c` without copying by referencing `s.blocks[inds]`
`inds` can be an array of indicies, a range, or a scalar.
# Example
```julia-repl
julia> b = split(s, 1:10);
julia> c = split(b, [1; 7; 9]);
julia> d = split(c, 1);
```
And we can confirm that `d` and `s` reference the same place in memory.
```julia-repl
julia> s.blocks[1] === d.blocks[1]
true
```
"""
function split(s::SeisCon, inds::Union{Vector{Ti}, AbstractRange{Ti}}) where {Ti<:Integer}
c = SeisCon(s.ns, s.dsf, view(s.blocks, inds))
end
split(s::SeisCon, inds::Integer) = split(s, [inds])
"""
split(s::SeisBlock, inds::Union{Vector{Ti}, AbstractRange{Ti}};
consume::Bool = false) where {Ti<:Integer}
Split the 'ind' traces of `s` into a sepretate `SeisBlock` object.
The default behaviour does not change the input object `s`. The returned `SeisBlock` is
created by referencing the traceheaders of `s`, and copying the subset of the data.
If memory use is a concern, the `consume` keyword changes the behaviour of `merge`.
If `consume` is true, then the `ind` traces of `s` are REMOVED and placed into the returned
`SeisBlock` object. In this case, `merge` will operate in place on `s`, while still returning a
subset of `s`.
# Examples
julia> using SegyIO
julia> s = segy_scan(joinpath(dirname(pathof(SegyIO)),"data/"), "overthrust", ["GroupX"; "GroupY"], verbosity = 0);
julia> d = s[1:length(s)]; @time b = split(d, 1:10000);
0.005683 seconds (23 allocations: 28.649 MiB, 17.99% gc time)
julia> println("d: ", size(d)); println("b: ", size(b))
d: (751, 20013)
b: (751, 10000)
julia> data_in_memory = Base.summarysize(d.data) + Base.summarysize(b.data)
90159052
Note that the default behaviour of `merge` is to create `b` by copying `d`.
julia> d = s[1:length(s)]; @time b = split(d, 1:10000, consume=true);
0.062364 seconds (37 allocations: 116.537 MiB, 19.44% gc time)
julia> println("d: ", size(d)); println("b: ", size(b))
d: (751, 10013)
b: (751, 10000)
julia> data_in_memory = Base.summarysize(d.data) + Base.summarysize(b.data)
60119052
In this case, because `consume` was set to true, `merge` created `b` by removing traces
from `d`. `consume` prevents the duplication of data in memory at the cost of performance,
memory allocation, and risk.
"""
function split(s::SeisBlock, inds::Union{Vector{Ti}, AbstractRange{Ti}};
consume::Bool = false) where {Ti<:Integer}
if consume
c = SeisBlock(s.fileheader, s.traceheaders[inds], s.data[:, inds])
ii = BitArray(size(s.data))
ii[:, inds] = true
deleteat!(vec(s.data), vec(ii))
deleteat!(s.traceheaders, inds)
s.data = s.data[:, 1:end-length(inds)]
else
c = SeisBlock(s.fileheader, view(s.traceheaders, inds), s.data[:, inds])
end
return c
end
split(s::SeisBlock, inds::Integer) = split(s, [inds])
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
|
[
"MIT"
]
| 0.8.5 | 0fc24db28695a80aa59c179372b11165d371188a | code | 4949 | export bytes_to_samples
"""
Return bytes to samples dict
"""
function bytes_to_samples()
Dict{String, Int32}(
"TraceNumWithinLine" => 1 -1,
"TraceNumWithinFile" => 5 -1,
"FieldRecord" => 9 -1,
"TraceNumber" => 13 -1,
"EnergySourcePoint" => 17 -1,
"CDP" => 21 -1,
"CDPTrace" => 25 -1,
"TraceIDCode" => 29 -1,
"NSummedTraces" => 31 -1,
"NStackedTraces" => 33 -1,
"DataUse" => 35 -1,
"Offset" => 37 -1,
"RecGroupElevation" => 41 -1,
"SourceSurfaceElevation" => 45 -1,
"SourceDepth" => 49 -1,
"RecDatumElevation" => 53 -1,
"SourceDatumElevation" => 57 -1,
"SourceWaterDepth" => 61 -1,
"GroupWaterDepth" => 65 -1,
"ElevationScalar" => 69 -1,
"RecSourceScalar" => 71 -1,
"SourceX" => 73 -1,
"SourceY" => 77 -1,
"GroupX" => 81 -1,
"GroupY" => 85 -1,
"CoordUnits" => 89 -1,
"WeatheringVelocity" => 91 -1,
"SubWeatheringVelocity" => 93 -1,
"UpholeTimeSource" => 95 -1,
"UpholeTimeGroup" => 97 -1,
"StaticCorrectionSource" => 99 -1,
"StaticCorrectionGroup" => 101-1,
"TotalStaticApplied" => 103-1,
"LagTimeA" => 105-1,
"LagTimeB" => 107-1,
"DelayRecordingTime" => 109-1,
"MuteTimeStart" => 111-1,
"MuteTimeEnd" => 113-1,
"ns" => 115-1,
"dt" => 117-1,
"GainType" => 119-1,
"InstrumentGainConstant" => 121-1,
"InstrumntInitialGain" => 123-1,
"Correlated" => 125-1,
"SweepFrequencyStart" => 127-1,
"SweepFrequencyEnd" => 129-1,
"SweepLength" => 131-1,
"SweepType" => 133-1,
"SweepTraceTaperLengthStart" => 135-1,
"SweepTraceTaperLengthEnd" => 137-1,
"TaperType" => 139-1,
"AliasFilterFrequency" => 141-1,
"AliasFilterSlope" => 143-1,
"NotchFilterFrequency" => 145-1,
"NotchFilterSlope" => 147-1,
"LowCutFrequency" => 149-1,
"HighCutFrequency" => 151-1,
"LowCutSlope" => 153-1,
"HighCutSlope" => 155-1,
"Year" => 157-1,
"DayOfYear" => 159-1,
"HourOfDay" => 161-1,
"MinuteOfHour" => 163-1,
"SecondOfMinute" => 165-1,
"TimeCode" => 167-1,
"TraceWeightingFactor" => 169-1,
"GeophoneGroupNumberRoll" => 171-1,
"GeophoneGroupNumberTraceStart" => 173-1,
"GeophoneGroupNumberTraceEnd" => 175-1,
"GapSize" => 177-1,
"OverTravel" => 179-1,
"CDPX" => 181-1,
"CDPY" => 185-1,
"Inline3D" => 189-1,
"Crossline3D" => 193-1,
"ShotPoint" => 197-1,
"ShotPointScalar" => 201-1,
"TraceValueMeasurmentUnit" => 203-1,
"TransductionConstnatMantissa" => 205-1,
"TransductionConstantPower" => 209-1,
"TransductionUnit" => 211-1,
"TraceIdentifier" => 213-1,
"ScalarTraceHeader" => 215-1,
"SourceType" => 217-1,
"SourceEnergyDirectionMantissa" => 219-1,
"SourceEnergyDirectionExponent" => 223-1,
"SourceMeasurmentMantissa" => 225-1,
"SourceMeasurementExponent" => 229-1,
"SourceMeasurmentUnit" => 231-1,
"Unassigned1" => 233-1,
"Unassigned2" => 237-1)
end
| SegyIO | https://github.com/slimgroup/SegyIO.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.